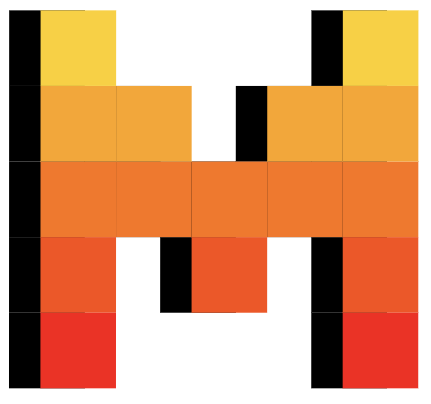
Mistral-7B-Instruct-v0.2
A pretrained generative language model with 7 billion parameters geared towards instruction-following capabilities.
Model Details
This model was built via parameter-efficient finetuning of the mistralai/Mistral-7B-v0.1 base model on the first 20k rows in each of the jondurbin/airoboros-2.2.1, Open-Orca/SlimOrca, and garage-bAInd/Open-Platypus datasets.
- Developed by: Daniel Furman
- Model type: Causal language model (clm)
- Language(s) (NLP): English
- License: Apache 2.0
- Finetuned from model: mistralai/Mistral-7B-v0.1
Basic Usage
Setup
!pip install -q -U transformers peft torch accelerate einops sentencepiece
import torch
from peft import PeftModel, PeftConfig
from transformers import (
AutoModelForCausalLM,
AutoTokenizer,
)
peft_model_id = "dfurman/Mistral-7B-Instruct-v0.2"
config = PeftConfig.from_pretrained(peft_model_id)
tokenizer = AutoTokenizer.from_pretrained(
peft_model_id,
use_fast=True,
trust_remote_code=True,
)
model = AutoModelForCausalLM.from_pretrained(
config.base_model_name_or_path,
torch_dtype=torch.float16,
device_map="auto",
trust_remote_code=True,
)
model = PeftModel.from_pretrained(
model,
peft_model_id
)
messages = [
{"role": "user", "content": "Tell me a recipe for a mai tai."},
]
print("\n\n*** Prompt:")
input_ids = tokenizer.apply_chat_template(
messages,
tokenize=True,
return_tensors="pt",
)
print(tokenizer.decode(input_ids[0]))
print("\n\n*** Generate:")
with torch.autocast("cuda", dtype=torch.bfloat16):
output = model.generate(
input_ids=input_ids.cuda(),
max_new_tokens=1024,
do_sample=True,
temperature=0.7,
return_dict_in_generate=True,
eos_token_id=tokenizer.eos_token_id,
pad_token_id=tokenizer.pad_token_id,
repetition_penalty=1.2,
no_repeat_ngram_size=5,
)
response = tokenizer.decode(
output["sequences"][0][len(input_ids[0]):],
skip_special_tokens=True
)
print(response)
Outputs
Prompt:
"<s> [INST] Tell me a recipe for a mai tai. [/INST]"
Generation:
"""1. Combine the following ingredients in a cocktail shaker:
2 oz light rum (or white rum)
1 oz dark rum
0.5 oz orange curacao or triple sec
0.75 oz lime juice, freshly squeezed
0.5 tbsp simple syrup (optional; if you like your drinks sweet)
Few drops of bitters (Angostura is traditional but any will do)
Ice cubes to fill the shaker
2. Shake vigorously until well-chilled and combined.
3. Strain into an ice-filled glass.
4. Garnish with a slice of lime or an orange wedge, if desired."""
Speeds, Sizes, Times
runtime / 50 tokens (sec) | GPU | dtype | VRAM (GB) |
---|---|---|---|
3.21 | 1x A100 (40 GB SXM) | torch.bfloat16 | 16 |
Training
It took ~5 hours to train 3 epochs on 1x A100 (40 GB SXM).
Prompt Format
This model was finetuned with the following format:
tokenizer.chat_template = "{{ bos_token }}{% for message in messages %}{% if (message['role'] == 'user') != (loop.index0 % 2 == 0) %}{{ raise_exception('Conversation roles must alternate user/assistant/user/assistant/...') }}{% endif %}{% if message['role'] == 'user' %}{{ '[INST] ' + message['content'] + ' [/INST] ' }}{% elif message['role'] == 'assistant' %}{{ message['content'] + eos_token + ' ' }}{% else %}{{ raise_exception('Only user and assistant roles are supported!') }}{% endif %}{% endfor %}"
This format is available as a chat template via the apply_chat_template()
method. Here's an illustrative example:
messages = [
{"role": "user", "content": "Tell me a recipe for a mai tai."},
{"role": "assistant", "content": "1 oz light rum\n½ oz dark rum\n¼ oz orange curaçao\n2 oz pineapple juice\n¾ oz lime juice\nDash of orgeat syrup (optional)\nSplash of grenadine (for garnish, optional)\nLime wheel and cherry garnishes (optional)\n\nShake all ingredients except the splash of grenadine in a cocktail shaker over ice. Strain into an old-fashioned glass filled with fresh ice cubes. Gently pour the splash of grenadine down the side of the glass so that it sinks to the bottom. Add garnishes as desired."},
{"role": "user", "content": "How can I make it more upscale and luxurious?"},
]
print("\n\n*** Prompt:")
input_ids = tokenizer.apply_chat_template(
messages,
tokenize=True,
return_tensors="pt",
)
print(tokenizer.decode(input_ids[0]))
Output
"""<s> [INST] Tell me a recipe for a mai tai. [/INST] 1 oz light rum\n½ oz dark rum\n (...) Add garnishes as desired.</s> [INST] How can I make it more upscale and luxurious? [/INST]"""
Training Hyperparameters
We use the SFTTrainer from trl
to fine-tune LLMs on instruction-following datasets.
See here for the finetuning code, which contains an exhaustive view of the hyperparameters employed.
The following TrainingArguments
config was used:
- output_dir = "./results"
- num_train_epochs = 2
- auto_find_batch_size = True
- gradient_accumulation_steps = 2
- optim = "paged_adamw_32bit"
- save_strategy = "epoch"
- learning_rate = 3e-4
- lr_scheduler_type = "cosine"
- warmup_ratio = 0.03
- logging_strategy = "steps"
- logging_steps = 25
- evaluation_strategy = "no"
- bf16 = True
The following bitsandbytes
quantization config was used:
- quant_method: bitsandbytes
- load_in_8bit: False
- load_in_4bit: True
- llm_int8_threshold: 6.0
- llm_int8_skip_modules: None
- llm_int8_enable_fp32_cpu_offload: False
- llm_int8_has_fp16_weight: False
- bnb_4bit_quant_type: nf4
- bnb_4bit_use_double_quant: False
- bnb_4bit_compute_dtype: bfloat16
Model Card Contact
dryanfurman at gmail
Mistral Research Citation
@misc{jiang2023mistral,
title={Mistral 7B},
author={Albert Q. Jiang and Alexandre Sablayrolles and Arthur Mensch and Chris Bamford and Devendra Singh Chaplot and Diego de las Casas and Florian Bressand and Gianna Lengyel and Guillaume Lample and Lucile Saulnier and Lélio Renard Lavaud and Marie-Anne Lachaux and Pierre Stock and Teven Le Scao and Thibaut Lavril and Thomas Wang and Timothée Lacroix and William El Sayed},
year={2023},
eprint={2310.06825},
archivePrefix={arXiv},
primaryClass={cs.CL}
}
Framework versions
- PEFT 0.6.3.dev0
Open LLM Leaderboard Evaluation Results
Detailed results can be found here
Metric | Value |
---|---|
Avg. | 61.79 |
AI2 Reasoning Challenge (25-Shot) | 60.15 |
HellaSwag (10-Shot) | 82.79 |
MMLU (5-Shot) | 60.07 |
TruthfulQA (0-shot) | 56.06 |
Winogrande (5-shot) | 76.87 |
GSM8k (5-shot) | 34.80 |
- Downloads last month
- 96
Adapter for
Datasets used to train dfurman/Mistral-7B-Instruct-v0.2
Spaces using dfurman/Mistral-7B-Instruct-v0.2 12
Collection including dfurman/Mistral-7B-Instruct-v0.2
Evaluation results
- normalized accuracy on AI2 Reasoning Challenge (25-Shot)test set Open LLM Leaderboard60.150
- normalized accuracy on HellaSwag (10-Shot)validation set Open LLM Leaderboard82.790
- accuracy on MMLU (5-Shot)test set Open LLM Leaderboard60.070
- mc2 on TruthfulQA (0-shot)validation set Open LLM Leaderboard56.060
- accuracy on Winogrande (5-shot)validation set Open LLM Leaderboard76.870
- accuracy on GSM8k (5-shot)test set Open LLM Leaderboard34.800