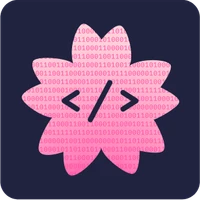
bigcode/santacoderpack
Text Generation
•
Updated
•
213
•
5
text
stringlengths 54
60.6k
|
---|
<commit_before>/************************************************************************************
**
* @copyright (c) 2013-2100, ChengDu Duyer Technology Co., LTD. All Right Reserved.
*
*************************************************************************************/
/**
* @file g_dfs_server.cpp
* @version
* @brief
* @author duye
* @date 2014-09-24
* @note
*
* 1. 2014-09-24 duye Created this file
*
*/
#include <g_dfs_server.h>
#include <g_logger.h>
static const GInt8* LOG_PREFIX = "dfs.server.startup";
namespace gdfs {
DfsServer::DfsServer() : gcom::HostServer() {}
DfsServer::DfsServer(const std::string& dfs_cfg_file_path) : gcom::HostServer(), m_dfsCfgFilePath(dfs_cfg_file_path) {}
DfsServer::~DfsServer()
{
gcom::ServerFactory::intance().destroyServer(m_httpServer);
gcom::ServerFactory::intance().destroyServer(m_ftpServer);
gcom::ServerFactory::intance().destroyServer(m_cliServer);
}
GResult DfsServer::start()
{
G_LOG_IN();
if (getServerState() == HOST_SERVER_WORK)
{
return G_YES;
}
if (m_dfsCfgFilePath.empty())
{
m_dfsCfgFilePath = DEF_DFS_CFG_FILE_PATH;
}
IS_NO_R(m_cfgMgr.load(m_dfsCfgFilePath));
// init all service
m_httpServer = gcom::ServerFactory::intance().createServer(HTTP_SERVER);
m_ftpServer = gcom::ServerFactory::intance().createServer(FTP_SERVER);
m_cliServer = gcom::ServerFactory::intance().createServer(CLI_SERVER);
// start routine() thread
this->startTask();
// set server state is work
m_serverState = HOST_SERVER_WORK;
G_LOG_OUT();
return G_YES;
}
GResult DfsServer::stop()
{
G_LOG_IN();
m_httpServer->stop();
m_ftpServer->stop();
m_cliServer->stop();
G_LOG_OUT();
return G_NO;
}
DfsServerState DfsServer::routine()
{
G_LOG_IN();
m_httpServer->start();
m_ftpServer->start();
m_cliServer->start();
for (;;)
{
// startup all server
gcom::NetworkServerMonitor::keepServer(m_httpServer);
gcom::NetworkServerMonitor::keepServer(m_ftpServer);
gcom::NetworkServerMonitor::keepServer(m_cliServer);
gsys::System::sleep(5);
}
G_LOG_OUT();
return G_YES;
}
}
<commit_msg>Update g_dfs_server.cpp<commit_after>/************************************************************************************
**
* @copyright (c) 2013-2100, ChengDu Duyer Technology Co., LTD. All Right Reserved.
*
*************************************************************************************/
/**
* @file g_dfs_server.cpp
* @version
* @brief
* @author duye
* @date 2014-09-24
* @note
*
* 1. 2014-09-24 duye Created this file
*
*/
#include <g_dfs_server.h>
#include <g_logger.h>
static const GInt8* LOG_PREFIX = "dfs.server.startup";
namespace gdfs {
DfsServer::DfsServer() : gcom::HostServer() {}
DfsServer::DfsServer(const std::string& dfs_cfg_file_path) : gcom::HostServer(), m_dfsCfgFilePath(dfs_cfg_file_path) {}
DfsServer::~DfsServer()
{
G_LOG_IN();
gcom::ServerFactory::intance().destroyServer(m_httpServer);
gcom::ServerFactory::intance().destroyServer(m_ftpServer);
gcom::ServerFactory::intance().destroyServer(m_cliServer);
G_LOG_OUT();
}
GResult DfsServer::start()
{
G_LOG_IN();
if (getServerState() == HOST_SERVER_WORK)
{
return G_YES;
}
if (m_dfsCfgFilePath.empty())
{
m_dfsCfgFilePath = DEF_DFS_CFG_FILE_PATH;
}
IS_NO_R(m_cfgMgr.load(m_dfsCfgFilePath));
// init all service
m_httpServer = gcom::ServerFactory::intance().createServer(HTTP_SERVER);
m_ftpServer = gcom::ServerFactory::intance().createServer(FTP_SERVER);
m_cliServer = gcom::ServerFactory::intance().createServer(CLI_SERVER);
// startup all service
m_httpServer->start();
m_ftpServer->start();
m_cliServer->start();
// start routine() thread
this->startTask();
// set server state is work
m_serverState = HOST_SERVER_WORK;
G_LOG_OUT();
return G_YES;
}
GResult DfsServer::stop()
{
G_LOG_IN();
m_httpServer->stop();
m_ftpServer->stop();
m_cliServer->stop();
G_LOG_OUT();
return G_NO;
}
DfsServerState DfsServer::routine()
{
G_LOG_IN();
for (;;)
{
// keep all service is running
gcom::NetworkServerMonitor::keepServer(m_httpServer);
gcom::NetworkServerMonitor::keepServer(m_ftpServer);
gcom::NetworkServerMonitor::keepServer(m_cliServer);
gsys::System::sleep(5);
}
G_LOG_OUT();
return G_YES;
}
}
<|endoftext|>
|
<commit_before>/*!
@file PDBReader.hpp
@brief definition of a class that reads pdb atom.
read pdb file and store the information as std::vector of shared_ptr of PDBAtom
@author Toru Niina (niina.toru.68u@gmail.com)
@date 2016-06-10 13:00
@copyright Toru Niina 2016 on MIT License
*/
#ifndef COFFEE_MILL_PDB_READER
#define COFFEE_MILL_PDB_READER
#include "PDBChain.hpp"
#include <fstream>
#include <sstream>
namespace mill
{
//! PDBReader class
/*!
* read atoms in pdb file until "ENDMDL" or "END".
* and then parse the PDBAtoms into vector of PDBChains.
* for movie file, parse only one model at once. you can specify the model
* by model index.
*/
template<typename vectorT>
class PDBReader
{
public:
using vector_type = vectorT;
using atom_type = PDBAtom<vector_type>;
using residue_type = PDBResidue<vector_type>;
using chain_type = PDBChain<vector_type>;
public:
PDBReader() = default;
~PDBReader() = default;
std::vector<chain_type> parse(const std::vector<atom_type>& atoms);
std::vector<atom_type> read(const std::string& fname);
std::vector<atom_type> read(const std::string& fname, const std::size_t model_idx);
std::vector<atom_type> read(std::basic_istream<char>& fname);
std::vector<atom_type> read(std::basic_istream<char>& fname, const std::size_t model_idx);
};
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::atom_type>
PDBReader<vectorT>::read(const std::string& fname)
{
std::ifstream ifs(fname);
if(not ifs.good()) throw std::runtime_error("file open error: " + fname);
const auto data = this->read(ifs);
ifs.close();
return data;
}
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::atom_type>
PDBReader<vectorT>::read(const std::string& fname, const std::size_t model_idx)
{
std::ifstream ifs(fname);
if(not ifs.good()) throw std::runtime_error("file open error: " + fname);
const auto data = this->read(ifs, model_idx);
ifs.close();
return data;
}
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::atom_type>
PDBReader<vectorT>::read(std::basic_istream<char>& ifs, const std::size_t model_idx)
{
while(not ifs.eof())
{
std::string line;
std::getline(ifs, line);
if(line.empty()) continue;
if(line.substr(0, 5) == "MODEL")
{
std::size_t index;
std::istringstream iss(line);
std::string model;
iss >> model >> index;
if(index == model_idx) break;
}
}
if(ifs.eof())
throw std::invalid_argument("no model #" + std::to_string(model_idx));
return this->read(ifs);
}
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::atom_type>
PDBReader<vectorT>::read(std::basic_istream<char>& ifs)
{
std::vector<atom_type> atoms;
while(not ifs.eof())
{
std::string line;
std::getline(ifs, line);
if(line.empty()) continue;
if(line.substr(0, 3) == "END") break; // which is better ENDMDL or END?
atom_type atom;
if(line >> atom) atoms.emplace_back(std::move(atom));
}
return atoms;
}
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::chain_type>
PDBReader<vectorT>::parse(const std::vector<atom_type>& atoms)
{
std::vector<residue_type> residues;
residue_type tmp_residue;
int current_residue_id = std::numeric_limits<int>::min();
for(auto iter = atoms.cbegin(); iter != atoms.cend(); ++iter)
{
if(iter->residue_id != current_residue_id)
{
if(not tmp_residue.empty())
residues.push_back(tmp_residue);
tmp_residue->clear();
current_residue_id = iter->residue_id;
}
tmp_residue.push_back(*iter);
}
if(not tmp_residue.empty()) residues.push_back(tmp_residue);
std::vector<chain_type> chains;
chain_type tmp_chain;
std::string current_chain_id = "";
for(auto iter = residues.begin(); iter != residues.end(); ++iter)
{
if(iter->chain_id != current_chain_id)
{
if(not tmp_chain.empty()) chains.push_back(tmp_chain);
tmp_chain.clear();
current_chain_id = iter->chain_id();
}
tmp_chain.push_back(*iter);
}
if(not tmp_chain.empty()) chains.push_back(tmp_chain);
return chains;
}
} // mill
#endif//COFFEE_MILL_PURE_PDB_READER
<commit_msg>add const to member function of PDBReader<commit_after>/*!
@file PDBReader.hpp
@brief pdb reader.
read pdb atoms from input stream and parse atoms as chains.
@author Toru Niina (niina.toru.68u@gmail.com)
@date 2016-06-10 13:00
@copyright Toru Niina 2016 on MIT License
*/
#ifndef COFFEE_MILL_PDB_READER
#define COFFEE_MILL_PDB_READER
#include "PDBChain.hpp"
#include <fstream>
#include <sstream>
namespace mill
{
//! PDBReader class
/*!
* read atoms in pdb file until "ENDMDL" or "END".
* and then parse the PDBAtoms into vector of PDBChains.
* for movie file, parse only one model at once. you can specify the model
* by model index.
*/
template<typename vectorT>
class PDBReader
{
public:
using vector_type = vectorT;
using atom_type = PDBAtom<vector_type>;
using residue_type = PDBResidue<vector_type>;
using chain_type = PDBChain<vector_type>;
public:
PDBReader() = default;
~PDBReader() = default;
std::vector<chain_type> parse(const std::vector<atom_type>& atoms) const;
std::vector<atom_type> read(const std::string& fname) const;
std::vector<atom_type> read(const std::string& fname, const std::size_t model_idx) const;
std::vector<atom_type> read(std::basic_istream<char>& fname) const;
std::vector<atom_type> read(std::basic_istream<char>& fname, const std::size_t model_idx) const;
};
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::atom_type>
PDBReader<vectorT>::read(const std::string& fname) const
{
std::ifstream ifs(fname);
if(not ifs.good()) throw std::runtime_error("file open error: " + fname);
const auto data = this->read(ifs);
ifs.close();
return data;
}
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::atom_type>
PDBReader<vectorT>::read(const std::string& fname, const std::size_t model_idx) const
{
std::ifstream ifs(fname);
if(not ifs.good()) throw std::runtime_error("file open error: " + fname);
const auto data = this->read(ifs, model_idx);
ifs.close();
return data;
}
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::atom_type>
PDBReader<vectorT>::read(std::basic_istream<char>& ifs, const std::size_t model_idx) const
{
while(not ifs.eof())
{
std::string line;
std::getline(ifs, line);
if(line.empty()) continue;
if(line.substr(0, 5) == "MODEL")
{
std::size_t index;
std::istringstream iss(line);
std::string model;
iss >> model >> index;
if(index == model_idx) break;
}
}
if(ifs.eof())
throw std::invalid_argument("no model #" + std::to_string(model_idx));
return this->read(ifs);
}
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::atom_type>
PDBReader<vectorT>::read(std::basic_istream<char>& ifs) const
{
std::vector<atom_type> atoms;
while(not ifs.eof())
{
std::string line;
std::getline(ifs, line);
if(line.empty()) continue;
if(line.substr(0, 3) == "END") break; // which is better ENDMDL or END?
atom_type atom;
if(line >> atom) atoms.emplace_back(std::move(atom));
}
return atoms;
}
template<typename vectorT>
std::vector<typename PDBReader<vectorT>::chain_type>
PDBReader<vectorT>::parse(const std::vector<atom_type>& atoms) const
{
std::vector<residue_type> residues;
residue_type tmp_residue;
int current_residue_id = std::numeric_limits<int>::min();
for(auto iter = atoms.cbegin(); iter != atoms.cend(); ++iter)
{
if(iter->residue_id != current_residue_id)
{
if(not tmp_residue.empty())
residues.push_back(tmp_residue);
tmp_residue->clear();
current_residue_id = iter->residue_id;
}
tmp_residue.push_back(*iter);
}
if(not tmp_residue.empty()) residues.push_back(tmp_residue);
std::vector<chain_type> chains;
chain_type tmp_chain;
std::string current_chain_id = "";
for(auto iter = residues.begin(); iter != residues.end(); ++iter)
{
if(iter->chain_id != current_chain_id)
{
if(not tmp_chain.empty()) chains.push_back(tmp_chain);
tmp_chain.clear();
current_chain_id = iter->chain_id();
}
tmp_chain.push_back(*iter);
}
if(not tmp_chain.empty()) chains.push_back(tmp_chain);
return chains;
}
} // mill
#endif//COFFEE_MILL_PURE_PDB_READER
<|endoftext|>
|
<commit_before>/*********************************************************************
* Software License Agreement (BSD License)
*
* Copyright (c) 2008, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of the Willow Garage nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*********************************************************************/
/* \author Ioan Sucan */
#include "ompl/extension/samplingbased/kinematic/extension/ik/GAIK.h"
#include <algorithm>
bool ompl::GAIK::solve(double solveTime)
{
SpaceInformationKinematic_t si = dynamic_cast<SpaceInformationKinematic_t>(m_si);
SpaceInformationKinematic::GoalRegionKinematic_t goal_r = dynamic_cast<SpaceInformationKinematic::GoalRegionKinematic_t>(si->getGoal());
unsigned int dim = si->getStateDimension();
if (!goal_r)
{
m_msg.error("GAIK: Unknown type of goal (or goal undefined)");
return false;
}
time_utils::Time endTime = time_utils::Time::now() + time_utils::Duration(solveTime);
unsigned int maxPoolSize = m_poolSize + m_poolExpansion;
std::vector<Individual> pool(maxPoolSize);
IndividualSort gs;
bool solved = false;
int solution = -1;
if (m_poolSize < 1)
{
m_msg.error("GAIK: Pool size too small");
return false;
}
for (unsigned int i = 0 ; i < maxPoolSize ; ++i)
{
pool[i].state = new SpaceInformationKinematic::StateKinematic(dim);
si->sample(pool[i].state);
if (goal_r->isSatisfied(pool[i].state, &(pool[i].distance)))
{
if (si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(pool[i].state)))
{
solved = true;
solution = i;
}
}
}
unsigned int generations = 1;
std::vector<double> range(dim);
for (unsigned int i = 0 ; i < dim ; ++i)
range[i] = m_rho * (si->getStateComponent(i).maxValue - si->getStateComponent(i).minValue);
while (!solved && time_utils::Time::now() < endTime)
{
generations++;
std::sort(pool.begin(), pool.end(), gs);
for (unsigned int i = 0 ; i < 5 ; ++i)
{
if (si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(pool[i].state)))
{
if (tryToSolve(pool[i].state, &(pool[i].distance)))
{
solved = true;
solution = i;
break;
}
}
}
for (unsigned int i = m_poolSize ; i < maxPoolSize ; ++i)
{
si->sampleNear(pool[i].state, pool[i%m_poolSize].state, range);
if (goal_r->isSatisfied(pool[i].state, &(pool[i].distance)))
{
if (si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(pool[i].state)))
{
solved = true;
solution = i;
break;
}
}
}
}
m_msg.inform("GAIK: Ran for %u generations", generations);
if (solved)
{
SpaceInformationKinematic::PathKinematic_t path = new SpaceInformationKinematic::PathKinematic(m_si);
SpaceInformationKinematic::StateKinematic_t st = new SpaceInformationKinematic::StateKinematic(dim);
si->copyState(st, pool[solution].state);
path->states.push_back(st);
goal_r->setDifference(pool[solution].distance);
goal_r->setSolutionPath(path);
}
else
{
/* find an approximate solution */
std::sort(pool.begin(), pool.end(), gs);
for (unsigned int i = 0 ; i < 5 ; ++i)
{
if (si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(pool[i].state)))
{
SpaceInformationKinematic::PathKinematic_t path = new SpaceInformationKinematic::PathKinematic(m_si);
SpaceInformationKinematic::StateKinematic_t st = new SpaceInformationKinematic::StateKinematic(dim);
si->copyState(st, pool[i].state);
path->states.push_back(st);
goal_r->setDifference(pool[i].distance);
goal_r->setSolutionPath(path, true);
break;
}
}
}
for (unsigned int i = 0 ; i < maxPoolSize ; ++i)
delete pool[i].state;
return goal_r->isAchieved();
}
bool ompl::GAIK::tryToSolve(SpaceInformationKinematic::StateKinematic_t state, double *distance)
{
double dist = *distance;
if (tryToSolveFact(1.01, state, distance))
return true;
if (dist > *distance)
{
dist = *distance;
if (tryToSolveFact(1.003, state, distance))
return true;
}
else
return false;
if (dist > *distance)
{
dist = *distance;
if (tryToSolveFact(1.001, state, distance))
return true;
}
return false;
}
bool ompl::GAIK::tryToSolveFact(double factorP, SpaceInformationKinematic::StateKinematic_t state, double *distance)
{
SpaceInformationKinematic_t si = dynamic_cast<SpaceInformationKinematic_t>(m_si);
SpaceInformationKinematic::GoalRegionKinematic_t goal_r = dynamic_cast<SpaceInformationKinematic::GoalRegionKinematic_t>(si->getGoal());
unsigned int dim = si->getStateDimension();
double factorM = 1.0/factorP;
bool wasSatisfied = goal_r->isSatisfied(state, distance);
double bestDist = *distance;
bool change = true;
while (change)
{
change = false;
for (unsigned int i = 0 ; i < dim ; ++i)
{
bool better = true;
while (better)
{
better = false;
double backup = state->values[i];
state->values[i] *= factorP;
bool isS = goal_r->isSatisfied(state, distance);
if (wasSatisfied && !isS)
state->values[i] = backup;
else
{
wasSatisfied = isS;
if (*distance < bestDist)
{
better = true;
change = true;
bestDist = *distance;
}
else
state->values[i] = backup;
}
}
better = true;
while (better)
{
better = false;
double backup = state->values[i];
state->values[i] *= factorM;
bool isS = goal_r->isSatisfied(state, distance);
if (wasSatisfied && !isS)
state->values[i] = backup;
else
{
wasSatisfied = isS;
if (*distance < bestDist)
{
better = true;
change = true;
bestDist = *distance;
}
else
state->values[i] = backup;
}
}
}
}
*distance = bestDist;
return wasSatisfied && si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(state));
}
<commit_msg><commit_after>/*********************************************************************
* Software License Agreement (BSD License)
*
* Copyright (c) 2008, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of the Willow Garage nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*********************************************************************/
/* \author Ioan Sucan */
#include "ompl/extension/samplingbased/kinematic/extension/ik/GAIK.h"
#include <algorithm>
bool ompl::GAIK::solve(double solveTime)
{
SpaceInformationKinematic_t si = dynamic_cast<SpaceInformationKinematic_t>(m_si);
SpaceInformationKinematic::GoalRegionKinematic_t goal_r = dynamic_cast<SpaceInformationKinematic::GoalRegionKinematic_t>(si->getGoal());
unsigned int dim = si->getStateDimension();
if (!goal_r)
{
m_msg.error("GAIK: Unknown type of goal (or goal undefined)");
return false;
}
time_utils::Time endTime = time_utils::Time::now() + time_utils::Duration(solveTime);
unsigned int maxPoolSize = m_poolSize + m_poolExpansion;
std::vector<Individual> pool(maxPoolSize);
IndividualSort gs;
bool solved = false;
int solution = -1;
if (m_poolSize < 1)
{
m_msg.error("GAIK: Pool size too small");
return false;
}
for (unsigned int i = 0 ; i < maxPoolSize ; ++i)
{
pool[i].state = new SpaceInformationKinematic::StateKinematic(dim);
si->sample(pool[i].state);
if (goal_r->isSatisfied(pool[i].state, &(pool[i].distance)))
{
if (si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(pool[i].state)))
{
solved = true;
solution = i;
}
}
}
unsigned int generations = 1;
std::vector<double> range(dim);
for (unsigned int i = 0 ; i < dim ; ++i)
range[i] = m_rho * (si->getStateComponent(i).maxValue - si->getStateComponent(i).minValue);
while (!solved && time_utils::Time::now() < endTime)
{
generations++;
std::sort(pool.begin(), pool.end(), gs);
for (unsigned int i = m_poolSize ; i < maxPoolSize ; ++i)
{
si->sampleNear(pool[i].state, pool[i%m_poolSize].state, range);
if (goal_r->isSatisfied(pool[i].state, &(pool[i].distance)))
{
if (si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(pool[i].state)))
{
solved = true;
solution = i;
break;
}
}
}
}
m_msg.inform("GAIK: Ran for %u generations", generations);
if (solved)
{
SpaceInformationKinematic::PathKinematic_t path = new SpaceInformationKinematic::PathKinematic(m_si);
SpaceInformationKinematic::StateKinematic_t st = new SpaceInformationKinematic::StateKinematic(dim);
si->copyState(st, pool[solution].state);
path->states.push_back(st);
goal_r->setDifference(pool[solution].distance);
goal_r->setSolutionPath(path);
double dist = goal_r->getDifference();
tryToSolve(path->states[0], &dist);
goal_r->setDifference(dist);
}
else
{
/* find an approximate solution */
std::sort(pool.begin(), pool.end(), gs);
for (unsigned int i = 0 ; i < 5 ; ++i)
{
if (si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(pool[i].state)))
{
SpaceInformationKinematic::PathKinematic_t path = new SpaceInformationKinematic::PathKinematic(m_si);
SpaceInformationKinematic::StateKinematic_t st = new SpaceInformationKinematic::StateKinematic(dim);
si->copyState(st, pool[i].state);
path->states.push_back(st);
goal_r->setDifference(pool[i].distance);
goal_r->setSolutionPath(path, true);
double dist = goal_r->getDifference();
tryToSolve(path->states[0], &dist);
goal_r->setDifference(dist);
break;
}
}
}
for (unsigned int i = 0 ; i < maxPoolSize ; ++i)
delete pool[i].state;
return goal_r->isAchieved();
}
bool ompl::GAIK::tryToSolve(SpaceInformationKinematic::StateKinematic_t state, double *distance)
{
tryToSolveFact(1.1, state, distance);
tryToSolveFact(1.01, state, distance);
tryToSolveFact(1.001, state, distance);
tryToSolveFact(1.0001, state, distance);
return true;
}
bool ompl::GAIK::tryToSolveFact(double factorP, SpaceInformationKinematic::StateKinematic_t state, double *distance)
{
SpaceInformationKinematic_t si = dynamic_cast<SpaceInformationKinematic_t>(m_si);
SpaceInformationKinematic::GoalRegionKinematic_t goal_r = dynamic_cast<SpaceInformationKinematic::GoalRegionKinematic_t>(si->getGoal());
unsigned int dim = si->getStateDimension();
double factorM = 1.0/factorP;
bool wasSatisfied = goal_r->isSatisfied(state, distance);
double bestDist = *distance;
bool change = true;
while (change)
{
change = false;
for (unsigned int i = 0 ; i < dim ; ++i)
{
bool better = true;
while (better)
{
better = false;
double backup = state->values[i];
state->values[i] *= factorP;
bool isS = goal_r->isSatisfied(state, distance);
if (wasSatisfied && !isS)
state->values[i] = backup;
else
{
wasSatisfied = isS;
if (*distance < bestDist)
{
better = true;
change = true;
bestDist = *distance;
}
else
state->values[i] = backup;
}
}
better = true;
while (better)
{
better = false;
double backup = state->values[i];
state->values[i] *= factorM;
bool isS = goal_r->isSatisfied(state, distance);
if (wasSatisfied && !isS)
state->values[i] = backup;
else
{
wasSatisfied = isS;
if (*distance < bestDist)
{
better = true;
change = true;
bestDist = *distance;
}
else
state->values[i] = backup;
}
}
}
}
*distance = bestDist;
return wasSatisfied && si->isValid(static_cast<SpaceInformationKinematic::StateKinematic_t>(state));
}
<|endoftext|>
|
<commit_before>#include "core/Loader.hpp"
namespace Kvant {
static std::vector<std::string> process_material_texture(aiMaterial *mat,
aiTextureType type,
std::string typeName) {
std::vector<std::string> textures;
for (unsigned int t = 0; t < mat->GetTextureCount(type); t++) {
aiString str;
mat->GetTexture(type, t, &str);
textures.push_back(str.C_Str());
LOG_DEBUG << str.C_Str() << " " << type << ", " << aiTextureType_SPECULAR;
}
return textures;
}
static void process_mesh(RawModelData *model_data, const aiScene *scene,
aiMesh *mesh) {
auto mesh_cpy = std::make_unique<Mesh>();
std::unordered_map<Kvant::Vertex, int> vs;
unsigned int vi = 0;
// Store Vertex data
for (unsigned int i = 0; i < mesh->mNumVertices; i++) {
Vertex vert;
vert.position = {mesh->mVertices[i].x, mesh->mVertices[i].y,
mesh->mVertices[i].z};
vert.normal = {mesh->mNormals[i].x, mesh->mNormals[i].y,
mesh->mNormals[i].z};
if (mesh->mTextureCoords[0]) {
vert.uvs = {mesh->mTextureCoords[0][i].x, mesh->mTextureCoords[0][i].y};
} else {
vert.uvs = {0.0f, 0.0f};
}
mesh_cpy->vertices.push_back(vert);
}
// Now wak through each of the mesh's faces (a face is a mesh its triangle)
// and retrieve the corresponding vertex indices.
for (unsigned int m = 0; m < mesh->mNumFaces; m++) {
auto face = mesh->mFaces[m];
// Retrieve all indices of the face and store them in the indices vector
for (unsigned int i = 0; i < face.mNumIndices; i++) {
mesh_cpy->triangles.push_back(face.mIndices[i]);
}
}
// push our mesh to the model
model_data->model.meshes.push_back(std::move(mesh_cpy));
// Try read materials
if (mesh->mMaterialIndex < 0)
return; // No material exists
auto *material = scene->mMaterials[mesh->mMaterialIndex];
// We assume a convention for sampler names in the shaders. Each diffuse
// texture should be named as 'texture_diffuseN' where N is a sequential
// number ranging from 1 to MAX_SAMPLER_NUMBER. Same applies to other texture
// as the following list summarizes: Diffuse: texture_diffuseN Specular:
// texture_specularN Normal: texture_normalN
// diffuse
model_data->diffuses = process_material_texture(
material, aiTextureType_DIFFUSE, "texture_diffuse");
// normal
model_data->normals = process_material_texture(
material, aiTextureType_NORMALS, "texture_normal");
// specular
model_data->speculars = process_material_texture(
material, aiTextureType_SPECULAR, "texture_specular");
}
static void process_node(RawModelData *model_data, const aiScene *scene,
aiNode *node) {
// Process each mesh located at the current node
LOG_DEBUG << "Processing node " << node->mName.C_Str()
<< " with meshes = " << node->mNumMeshes
<< " children = " << node->mNumChildren;
for (unsigned int i = 0; i < node->mNumMeshes; i++) {
// The node object only contains indices to index the actual objects in the
// scene. The scene contains all the data, node is just to keep stuff
// organized (like relations between nodes).
aiMesh *mesh = scene->mMeshes[node->mMeshes[i]];
process_mesh(model_data, scene, mesh);
}
// After we've processed all of the meshes (if any) we then recursively
// process each of the children nodes
for (unsigned int i = 0; i < node->mNumChildren; i++) {
process_node(model_data, scene, node->mChildren[i]);
}
}
RawModelData read_model(const char *filename) {
RawModelData model_data;
using namespace Assimp;
Assimp::Importer importer;
const aiScene *scene =
importer.ReadFile(filename, aiProcess_Triangulate | aiProcess_FlipUVs);
if (!scene) {
throw std::runtime_error("Failed to load model " + std::string(filename));
}
process_node(&model_data, scene, scene->mRootNode);
model_data.model.generate_tangents();
return model_data;
}
bool load_png_for_texture(unsigned char **img, unsigned int *width,
unsigned int *height, const char *filename) {
bool success = false;
unsigned error = lodepng_decode32_file(img, width, height, filename);
if (error) {
std::runtime_error("Error loading image");
} else {
// Flip and invert the image
unsigned char *imagePtr = *img;
int halfTheHeightInPixels = *height / 2;
int heightInPixels = *height;
// Assume RGBA for 4 components per pixel
int numColorComponents = 4;
// Assuming each color component is an unsigned char
int widthInChars = *width * numColorComponents;
unsigned char *top = NULL;
unsigned char *bottom = NULL;
unsigned char temp = 0;
for (int h = 0; h < halfTheHeightInPixels; ++h) {
top = imagePtr + h * widthInChars;
bottom = imagePtr + (heightInPixels - h - 1) * widthInChars;
for (int w = 0; w < widthInChars; ++w) {
// Swap the chars around.
temp = *top;
*top = *bottom;
*bottom = temp;
++top;
++bottom;
}
}
success = true;
}
return success;
}
} // namespace Kvant
<commit_msg>Pass texture name by reference<commit_after>#include "core/Loader.hpp"
namespace Kvant {
static std::vector<std::string>
process_material_texture(aiMaterial *mat, aiTextureType type,
const std::string &typeName) {
std::vector<std::string> textures;
for (unsigned int t = 0; t < mat->GetTextureCount(type); t++) {
aiString str;
mat->GetTexture(type, t, &str);
textures.push_back(str.C_Str());
LOG_DEBUG << str.C_Str() << " " << type << ", " << aiTextureType_SPECULAR;
}
return textures;
}
static void process_mesh(RawModelData *model_data, const aiScene *scene,
aiMesh *mesh) {
auto mesh_cpy = std::make_unique<Mesh>();
std::unordered_map<Kvant::Vertex, int> vs;
unsigned int vi = 0;
// Store Vertex data
for (unsigned int i = 0; i < mesh->mNumVertices; i++) {
Vertex vert;
vert.position = {mesh->mVertices[i].x, mesh->mVertices[i].y,
mesh->mVertices[i].z};
vert.normal = {mesh->mNormals[i].x, mesh->mNormals[i].y,
mesh->mNormals[i].z};
if (mesh->mTextureCoords[0]) {
vert.uvs = {mesh->mTextureCoords[0][i].x, mesh->mTextureCoords[0][i].y};
} else {
vert.uvs = {0.0f, 0.0f};
}
mesh_cpy->vertices.push_back(vert);
}
// Now wak through each of the mesh's faces (a face is a mesh its triangle)
// and retrieve the corresponding vertex indices.
for (unsigned int m = 0; m < mesh->mNumFaces; m++) {
auto face = mesh->mFaces[m];
// Retrieve all indices of the face and store them in the indices vector
for (unsigned int i = 0; i < face.mNumIndices; i++) {
mesh_cpy->triangles.push_back(face.mIndices[i]);
}
}
// push our mesh to the model
model_data->model.meshes.push_back(std::move(mesh_cpy));
// Try read materials
if (mesh->mMaterialIndex < 0)
return; // No material exists
auto *material = scene->mMaterials[mesh->mMaterialIndex];
// We assume a convention for sampler names in the shaders. Each diffuse
// texture should be named as 'texture_diffuseN' where N is a sequential
// number ranging from 1 to MAX_SAMPLER_NUMBER. Same applies to other texture
// as the following list summarizes: Diffuse: texture_diffuseN Specular:
// texture_specularN Normal: texture_normalN
// diffuse
model_data->diffuses = process_material_texture(
material, aiTextureType_DIFFUSE, "texture_diffuse");
// normal
model_data->normals = process_material_texture(
material, aiTextureType_NORMALS, "texture_normal");
// specular
model_data->speculars = process_material_texture(
material, aiTextureType_SPECULAR, "texture_specular");
}
static void process_node(RawModelData *model_data, const aiScene *scene,
aiNode *node) {
// Process each mesh located at the current node
LOG_DEBUG << "Processing node " << node->mName.C_Str()
<< " with meshes = " << node->mNumMeshes
<< " children = " << node->mNumChildren;
for (unsigned int i = 0; i < node->mNumMeshes; i++) {
// The node object only contains indices to index the actual objects in the
// scene. The scene contains all the data, node is just to keep stuff
// organized (like relations between nodes).
aiMesh *mesh = scene->mMeshes[node->mMeshes[i]];
process_mesh(model_data, scene, mesh);
}
// After we've processed all of the meshes (if any) we then recursively
// process each of the children nodes
for (unsigned int i = 0; i < node->mNumChildren; i++) {
process_node(model_data, scene, node->mChildren[i]);
}
}
RawModelData read_model(const char *filename) {
RawModelData model_data;
using namespace Assimp;
Assimp::Importer importer;
const aiScene *scene =
importer.ReadFile(filename, aiProcess_Triangulate | aiProcess_FlipUVs);
if (!scene) {
throw std::runtime_error("Failed to load model " + std::string(filename));
}
process_node(&model_data, scene, scene->mRootNode);
model_data.model.generate_tangents();
return model_data;
}
bool load_png_for_texture(unsigned char **img, unsigned int *width,
unsigned int *height, const char *filename) {
bool success = false;
unsigned error = lodepng_decode32_file(img, width, height, filename);
if (error) {
std::runtime_error("Error loading image");
} else {
// Flip and invert the image
unsigned char *imagePtr = *img;
int halfTheHeightInPixels = *height / 2;
int heightInPixels = *height;
// Assume RGBA for 4 components per pixel
int numColorComponents = 4;
// Assuming each color component is an unsigned char
int widthInChars = *width * numColorComponents;
unsigned char *top = NULL;
unsigned char *bottom = NULL;
unsigned char temp = 0;
for (int h = 0; h < halfTheHeightInPixels; ++h) {
top = imagePtr + h * widthInChars;
bottom = imagePtr + (heightInPixels - h - 1) * widthInChars;
for (int w = 0; w < widthInChars; ++w) {
// Swap the chars around.
temp = *top;
*top = *bottom;
*bottom = temp;
++top;
++bottom;
}
}
success = true;
}
return success;
}
} // namespace Kvant
<|endoftext|>
|
<commit_before>/*
* Copyright (C) 2011 Apple Inc. All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY APPLE INC. ``AS IS'' AND ANY
* EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL APPLE INC. OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
* OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#include "config.h"
#include "platform/scroll/ScrollbarTheme.h"
#include "RuntimeEnabledFeatures.h"
#include "platform/scroll/ScrollbarThemeClient.h"
#include "platform/scroll/ScrollbarThemeMock.h"
#include "platform/scroll/ScrollbarThemeOverlayMock.h"
namespace WebCore {
ScrollbarTheme* ScrollbarTheme::theme()
{
if (ScrollbarTheme::mockScrollbarsEnabled()) {
if (RuntimeEnabledFeatures::overlayScrollbarsEnabled()) {
DEFINE_STATIC_LOCAL(ScrollbarThemeOverlayMock, overlayMockTheme, ());
return &overlayMockTheme;
}
DEFINE_STATIC_LOCAL(ScrollbarThemeMock, mockTheme, ());
return &mockTheme;
}
return nativeTheme();
}
bool ScrollbarTheme::gMockScrollbarsEnabled = false;
void ScrollbarTheme::setMockScrollbarsEnabled(bool flag)
{
gMockScrollbarsEnabled = flag;
}
bool ScrollbarTheme::mockScrollbarsEnabled()
{
return gMockScrollbarsEnabled;
}
bool ScrollbarTheme::paint(ScrollbarThemeClient* scrollbar, GraphicsContext* graphicsContext, const IntRect& damageRect)
{
// Create the ScrollbarControlPartMask based on the damageRect
ScrollbarControlPartMask scrollMask = NoPart;
IntRect backButtonStartPaintRect;
IntRect backButtonEndPaintRect;
IntRect forwardButtonStartPaintRect;
IntRect forwardButtonEndPaintRect;
if (hasButtons(scrollbar)) {
backButtonStartPaintRect = backButtonRect(scrollbar, BackButtonStartPart, true);
if (damageRect.intersects(backButtonStartPaintRect))
scrollMask |= BackButtonStartPart;
backButtonEndPaintRect = backButtonRect(scrollbar, BackButtonEndPart, true);
if (damageRect.intersects(backButtonEndPaintRect))
scrollMask |= BackButtonEndPart;
forwardButtonStartPaintRect = forwardButtonRect(scrollbar, ForwardButtonStartPart, true);
if (damageRect.intersects(forwardButtonStartPaintRect))
scrollMask |= ForwardButtonStartPart;
forwardButtonEndPaintRect = forwardButtonRect(scrollbar, ForwardButtonEndPart, true);
if (damageRect.intersects(forwardButtonEndPaintRect))
scrollMask |= ForwardButtonEndPart;
}
IntRect startTrackRect;
IntRect thumbRect;
IntRect endTrackRect;
IntRect trackPaintRect = trackRect(scrollbar, true);
if (damageRect.intersects(trackPaintRect))
scrollMask |= TrackBGPart;
bool thumbPresent = hasThumb(scrollbar);
if (thumbPresent) {
IntRect track = trackRect(scrollbar);
splitTrack(scrollbar, track, startTrackRect, thumbRect, endTrackRect);
if (damageRect.intersects(thumbRect))
scrollMask |= ThumbPart;
if (damageRect.intersects(startTrackRect))
scrollMask |= BackTrackPart;
if (damageRect.intersects(endTrackRect))
scrollMask |= ForwardTrackPart;
}
// Paint the scrollbar background (only used by custom CSS scrollbars).
paintScrollbarBackground(graphicsContext, scrollbar);
// Paint the back and forward buttons.
if (scrollMask & BackButtonStartPart)
paintButton(graphicsContext, scrollbar, backButtonStartPaintRect, BackButtonStartPart);
if (scrollMask & BackButtonEndPart)
paintButton(graphicsContext, scrollbar, backButtonEndPaintRect, BackButtonEndPart);
if (scrollMask & ForwardButtonStartPart)
paintButton(graphicsContext, scrollbar, forwardButtonStartPaintRect, ForwardButtonStartPart);
if (scrollMask & ForwardButtonEndPart)
paintButton(graphicsContext, scrollbar, forwardButtonEndPaintRect, ForwardButtonEndPart);
if (scrollMask & TrackBGPart)
paintTrackBackground(graphicsContext, scrollbar, trackPaintRect);
if ((scrollMask & ForwardTrackPart) || (scrollMask & BackTrackPart)) {
// Paint the track pieces above and below the thumb.
if (scrollMask & BackTrackPart)
paintTrackPiece(graphicsContext, scrollbar, startTrackRect, BackTrackPart);
if (scrollMask & ForwardTrackPart)
paintTrackPiece(graphicsContext, scrollbar, endTrackRect, ForwardTrackPart);
paintTickmarks(graphicsContext, scrollbar, trackPaintRect);
}
// Paint the thumb.
if (scrollMask & ThumbPart)
paintThumb(graphicsContext, scrollbar, thumbRect);
return true;
}
ScrollbarPart ScrollbarTheme::hitTest(ScrollbarThemeClient* scrollbar, const IntPoint& position)
{
ScrollbarPart result = NoPart;
if (!scrollbar->enabled())
return result;
IntPoint testPosition = scrollbar->convertFromContainingWindow(position);
testPosition.move(scrollbar->x(), scrollbar->y());
if (!scrollbar->frameRect().contains(testPosition))
return NoPart;
result = ScrollbarBGPart;
IntRect track = trackRect(scrollbar);
if (track.contains(testPosition)) {
IntRect beforeThumbRect;
IntRect thumbRect;
IntRect afterThumbRect;
splitTrack(scrollbar, track, beforeThumbRect, thumbRect, afterThumbRect);
if (thumbRect.contains(testPosition))
result = ThumbPart;
else if (beforeThumbRect.contains(testPosition))
result = BackTrackPart;
else if (afterThumbRect.contains(testPosition))
result = ForwardTrackPart;
else
result = TrackBGPart;
} else if (backButtonRect(scrollbar, BackButtonStartPart).contains(testPosition)) {
result = BackButtonStartPart;
} else if (backButtonRect(scrollbar, BackButtonEndPart).contains(testPosition)) {
result = BackButtonEndPart;
} else if (forwardButtonRect(scrollbar, ForwardButtonStartPart).contains(testPosition)) {
result = ForwardButtonStartPart;
} else if (forwardButtonRect(scrollbar, ForwardButtonEndPart).contains(testPosition)) {
result = ForwardButtonEndPart;
}
return result;
}
void ScrollbarTheme::invalidatePart(ScrollbarThemeClient* scrollbar, ScrollbarPart part)
{
if (part == NoPart)
return;
IntRect result;
switch (part) {
case BackButtonStartPart:
result = backButtonRect(scrollbar, BackButtonStartPart, true);
break;
case BackButtonEndPart:
result = backButtonRect(scrollbar, BackButtonEndPart, true);
break;
case ForwardButtonStartPart:
result = forwardButtonRect(scrollbar, ForwardButtonStartPart, true);
break;
case ForwardButtonEndPart:
result = forwardButtonRect(scrollbar, ForwardButtonEndPart, true);
break;
case TrackBGPart:
result = trackRect(scrollbar, true);
break;
case ScrollbarBGPart:
result = scrollbar->frameRect();
break;
default: {
IntRect beforeThumbRect, thumbRect, afterThumbRect;
splitTrack(scrollbar, trackRect(scrollbar), beforeThumbRect, thumbRect, afterThumbRect);
if (part == BackTrackPart)
result = beforeThumbRect;
else if (part == ForwardTrackPart)
result = afterThumbRect;
else
result = thumbRect;
}
}
result.moveBy(-scrollbar->location());
scrollbar->invalidateRect(result);
}
void ScrollbarTheme::splitTrack(ScrollbarThemeClient* scrollbar, const IntRect& unconstrainedTrackRect, IntRect& beforeThumbRect, IntRect& thumbRect, IntRect& afterThumbRect)
{
// This function won't even get called unless we're big enough to have some combination of these three rects where at least
// one of them is non-empty.
IntRect trackRect = constrainTrackRectToTrackPieces(scrollbar, unconstrainedTrackRect);
int thumbPos = thumbPosition(scrollbar);
if (scrollbar->orientation() == HorizontalScrollbar) {
thumbRect = IntRect(trackRect.x() + thumbPos, trackRect.y(), thumbLength(scrollbar), scrollbar->height());
beforeThumbRect = IntRect(trackRect.x(), trackRect.y(), thumbPos + thumbRect.width() / 2, trackRect.height());
afterThumbRect = IntRect(trackRect.x() + beforeThumbRect.width(), trackRect.y(), trackRect.maxX() - beforeThumbRect.maxX(), trackRect.height());
} else {
thumbRect = IntRect(trackRect.x(), trackRect.y() + thumbPos, scrollbar->width(), thumbLength(scrollbar));
beforeThumbRect = IntRect(trackRect.x(), trackRect.y(), trackRect.width(), thumbPos + thumbRect.height() / 2);
afterThumbRect = IntRect(trackRect.x(), trackRect.y() + beforeThumbRect.height(), trackRect.width(), trackRect.maxY() - beforeThumbRect.maxY());
}
}
// Returns the size represented by track taking into account scrolling past
// the end of the document.
static float usedTotalSize(ScrollbarThemeClient* scrollbar)
{
float overhangAtStart = -scrollbar->currentPos();
float overhangAtEnd = scrollbar->currentPos() + scrollbar->visibleSize() - scrollbar->totalSize();
float overhang = std::max(0.0f, std::max(overhangAtStart, overhangAtEnd));
return scrollbar->totalSize() + overhang;
}
int ScrollbarTheme::thumbPosition(ScrollbarThemeClient* scrollbar)
{
if (scrollbar->enabled()) {
float size = usedTotalSize(scrollbar) - scrollbar->visibleSize();
// Avoid doing a floating point divide by zero and return 1 when usedTotalSize == visibleSize.
if (!size)
return 1;
float pos = std::max(0.0f, scrollbar->currentPos()) * (trackLength(scrollbar) - thumbLength(scrollbar)) / size;
return (pos < 1 && pos > 0) ? 1 : pos;
}
return 0;
}
int ScrollbarTheme::thumbLength(ScrollbarThemeClient* scrollbar)
{
if (!scrollbar->enabled())
return 0;
float overhang = 0;
if (scrollbar->currentPos() < 0)
overhang = -scrollbar->currentPos();
else if (scrollbar->visibleSize() + scrollbar->currentPos() > scrollbar->totalSize())
overhang = scrollbar->currentPos() + scrollbar->visibleSize() - scrollbar->totalSize();
float proportion = (scrollbar->visibleSize() - overhang) / usedTotalSize(scrollbar);
int trackLen = trackLength(scrollbar);
int length = round(proportion * trackLen);
length = std::max(length, minimumThumbLength(scrollbar));
if (length > trackLen)
length = 0; // Once the thumb is below the track length, it just goes away (to make more room for the track).
return length;
}
int ScrollbarTheme::minimumThumbLength(ScrollbarThemeClient* scrollbar)
{
return scrollbarThickness(scrollbar->controlSize());
}
int ScrollbarTheme::trackPosition(ScrollbarThemeClient* scrollbar)
{
IntRect constrainedTrackRect = constrainTrackRectToTrackPieces(scrollbar, trackRect(scrollbar));
return (scrollbar->orientation() == HorizontalScrollbar) ? constrainedTrackRect.x() - scrollbar->x() : constrainedTrackRect.y() - scrollbar->y();
}
int ScrollbarTheme::trackLength(ScrollbarThemeClient* scrollbar)
{
IntRect constrainedTrackRect = constrainTrackRectToTrackPieces(scrollbar, trackRect(scrollbar));
return (scrollbar->orientation() == HorizontalScrollbar) ? constrainedTrackRect.width() : constrainedTrackRect.height();
}
void ScrollbarTheme::paintScrollCorner(GraphicsContext* context, const IntRect& cornerRect)
{
context->fillRect(cornerRect, Color::white);
}
IntRect ScrollbarTheme::thumbRect(ScrollbarThemeClient* scrollbar)
{
if (!hasThumb(scrollbar))
return IntRect();
IntRect track = trackRect(scrollbar);
IntRect startTrackRect;
IntRect thumbRect;
IntRect endTrackRect;
splitTrack(scrollbar, track, startTrackRect, thumbRect, endTrackRect);
return thumbRect;
}
int ScrollbarTheme::thumbThickness(ScrollbarThemeClient* scrollbar)
{
IntRect track = trackRect(scrollbar);
return scrollbar->orientation() == HorizontalScrollbar ? track.height() : track.width();
}
void ScrollbarTheme::paintOverhangBackground(GraphicsContext* context, const IntRect& horizontalOverhangRect, const IntRect& verticalOverhangRect, const IntRect& dirtyRect)
{
context->setFillColor(Color::white);
if (!horizontalOverhangRect.isEmpty())
context->fillRect(intersection(horizontalOverhangRect, dirtyRect));
if (!verticalOverhangRect.isEmpty())
context->fillRect(intersection(verticalOverhangRect, dirtyRect));
}
}
<commit_msg>Makes ScrollbarTheme call through to theme engine for corner (2)<commit_after>/*
* Copyright (C) 2011 Apple Inc. All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY APPLE INC. ``AS IS'' AND ANY
* EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL APPLE INC. OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
* OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#include "config.h"
#include "platform/scroll/ScrollbarTheme.h"
#include "RuntimeEnabledFeatures.h"
#include "platform/scroll/ScrollbarThemeClient.h"
#include "platform/scroll/ScrollbarThemeMock.h"
#include "platform/scroll/ScrollbarThemeOverlayMock.h"
#if !OS(MACOSX)
#include "public/platform/Platform.h"
#include "public/platform/WebRect.h"
#include "public/platform/default/WebThemeEngine.h"
#endif
namespace WebCore {
ScrollbarTheme* ScrollbarTheme::theme()
{
if (ScrollbarTheme::mockScrollbarsEnabled()) {
if (RuntimeEnabledFeatures::overlayScrollbarsEnabled()) {
DEFINE_STATIC_LOCAL(ScrollbarThemeOverlayMock, overlayMockTheme, ());
return &overlayMockTheme;
}
DEFINE_STATIC_LOCAL(ScrollbarThemeMock, mockTheme, ());
return &mockTheme;
}
return nativeTheme();
}
bool ScrollbarTheme::gMockScrollbarsEnabled = false;
void ScrollbarTheme::setMockScrollbarsEnabled(bool flag)
{
gMockScrollbarsEnabled = flag;
}
bool ScrollbarTheme::mockScrollbarsEnabled()
{
return gMockScrollbarsEnabled;
}
bool ScrollbarTheme::paint(ScrollbarThemeClient* scrollbar, GraphicsContext* graphicsContext, const IntRect& damageRect)
{
// Create the ScrollbarControlPartMask based on the damageRect
ScrollbarControlPartMask scrollMask = NoPart;
IntRect backButtonStartPaintRect;
IntRect backButtonEndPaintRect;
IntRect forwardButtonStartPaintRect;
IntRect forwardButtonEndPaintRect;
if (hasButtons(scrollbar)) {
backButtonStartPaintRect = backButtonRect(scrollbar, BackButtonStartPart, true);
if (damageRect.intersects(backButtonStartPaintRect))
scrollMask |= BackButtonStartPart;
backButtonEndPaintRect = backButtonRect(scrollbar, BackButtonEndPart, true);
if (damageRect.intersects(backButtonEndPaintRect))
scrollMask |= BackButtonEndPart;
forwardButtonStartPaintRect = forwardButtonRect(scrollbar, ForwardButtonStartPart, true);
if (damageRect.intersects(forwardButtonStartPaintRect))
scrollMask |= ForwardButtonStartPart;
forwardButtonEndPaintRect = forwardButtonRect(scrollbar, ForwardButtonEndPart, true);
if (damageRect.intersects(forwardButtonEndPaintRect))
scrollMask |= ForwardButtonEndPart;
}
IntRect startTrackRect;
IntRect thumbRect;
IntRect endTrackRect;
IntRect trackPaintRect = trackRect(scrollbar, true);
if (damageRect.intersects(trackPaintRect))
scrollMask |= TrackBGPart;
bool thumbPresent = hasThumb(scrollbar);
if (thumbPresent) {
IntRect track = trackRect(scrollbar);
splitTrack(scrollbar, track, startTrackRect, thumbRect, endTrackRect);
if (damageRect.intersects(thumbRect))
scrollMask |= ThumbPart;
if (damageRect.intersects(startTrackRect))
scrollMask |= BackTrackPart;
if (damageRect.intersects(endTrackRect))
scrollMask |= ForwardTrackPart;
}
// Paint the scrollbar background (only used by custom CSS scrollbars).
paintScrollbarBackground(graphicsContext, scrollbar);
// Paint the back and forward buttons.
if (scrollMask & BackButtonStartPart)
paintButton(graphicsContext, scrollbar, backButtonStartPaintRect, BackButtonStartPart);
if (scrollMask & BackButtonEndPart)
paintButton(graphicsContext, scrollbar, backButtonEndPaintRect, BackButtonEndPart);
if (scrollMask & ForwardButtonStartPart)
paintButton(graphicsContext, scrollbar, forwardButtonStartPaintRect, ForwardButtonStartPart);
if (scrollMask & ForwardButtonEndPart)
paintButton(graphicsContext, scrollbar, forwardButtonEndPaintRect, ForwardButtonEndPart);
if (scrollMask & TrackBGPart)
paintTrackBackground(graphicsContext, scrollbar, trackPaintRect);
if ((scrollMask & ForwardTrackPart) || (scrollMask & BackTrackPart)) {
// Paint the track pieces above and below the thumb.
if (scrollMask & BackTrackPart)
paintTrackPiece(graphicsContext, scrollbar, startTrackRect, BackTrackPart);
if (scrollMask & ForwardTrackPart)
paintTrackPiece(graphicsContext, scrollbar, endTrackRect, ForwardTrackPart);
paintTickmarks(graphicsContext, scrollbar, trackPaintRect);
}
// Paint the thumb.
if (scrollMask & ThumbPart)
paintThumb(graphicsContext, scrollbar, thumbRect);
return true;
}
ScrollbarPart ScrollbarTheme::hitTest(ScrollbarThemeClient* scrollbar, const IntPoint& position)
{
ScrollbarPart result = NoPart;
if (!scrollbar->enabled())
return result;
IntPoint testPosition = scrollbar->convertFromContainingWindow(position);
testPosition.move(scrollbar->x(), scrollbar->y());
if (!scrollbar->frameRect().contains(testPosition))
return NoPart;
result = ScrollbarBGPart;
IntRect track = trackRect(scrollbar);
if (track.contains(testPosition)) {
IntRect beforeThumbRect;
IntRect thumbRect;
IntRect afterThumbRect;
splitTrack(scrollbar, track, beforeThumbRect, thumbRect, afterThumbRect);
if (thumbRect.contains(testPosition))
result = ThumbPart;
else if (beforeThumbRect.contains(testPosition))
result = BackTrackPart;
else if (afterThumbRect.contains(testPosition))
result = ForwardTrackPart;
else
result = TrackBGPart;
} else if (backButtonRect(scrollbar, BackButtonStartPart).contains(testPosition)) {
result = BackButtonStartPart;
} else if (backButtonRect(scrollbar, BackButtonEndPart).contains(testPosition)) {
result = BackButtonEndPart;
} else if (forwardButtonRect(scrollbar, ForwardButtonStartPart).contains(testPosition)) {
result = ForwardButtonStartPart;
} else if (forwardButtonRect(scrollbar, ForwardButtonEndPart).contains(testPosition)) {
result = ForwardButtonEndPart;
}
return result;
}
void ScrollbarTheme::invalidatePart(ScrollbarThemeClient* scrollbar, ScrollbarPart part)
{
if (part == NoPart)
return;
IntRect result;
switch (part) {
case BackButtonStartPart:
result = backButtonRect(scrollbar, BackButtonStartPart, true);
break;
case BackButtonEndPart:
result = backButtonRect(scrollbar, BackButtonEndPart, true);
break;
case ForwardButtonStartPart:
result = forwardButtonRect(scrollbar, ForwardButtonStartPart, true);
break;
case ForwardButtonEndPart:
result = forwardButtonRect(scrollbar, ForwardButtonEndPart, true);
break;
case TrackBGPart:
result = trackRect(scrollbar, true);
break;
case ScrollbarBGPart:
result = scrollbar->frameRect();
break;
default: {
IntRect beforeThumbRect, thumbRect, afterThumbRect;
splitTrack(scrollbar, trackRect(scrollbar), beforeThumbRect, thumbRect, afterThumbRect);
if (part == BackTrackPart)
result = beforeThumbRect;
else if (part == ForwardTrackPart)
result = afterThumbRect;
else
result = thumbRect;
}
}
result.moveBy(-scrollbar->location());
scrollbar->invalidateRect(result);
}
void ScrollbarTheme::splitTrack(ScrollbarThemeClient* scrollbar, const IntRect& unconstrainedTrackRect, IntRect& beforeThumbRect, IntRect& thumbRect, IntRect& afterThumbRect)
{
// This function won't even get called unless we're big enough to have some combination of these three rects where at least
// one of them is non-empty.
IntRect trackRect = constrainTrackRectToTrackPieces(scrollbar, unconstrainedTrackRect);
int thumbPos = thumbPosition(scrollbar);
if (scrollbar->orientation() == HorizontalScrollbar) {
thumbRect = IntRect(trackRect.x() + thumbPos, trackRect.y(), thumbLength(scrollbar), scrollbar->height());
beforeThumbRect = IntRect(trackRect.x(), trackRect.y(), thumbPos + thumbRect.width() / 2, trackRect.height());
afterThumbRect = IntRect(trackRect.x() + beforeThumbRect.width(), trackRect.y(), trackRect.maxX() - beforeThumbRect.maxX(), trackRect.height());
} else {
thumbRect = IntRect(trackRect.x(), trackRect.y() + thumbPos, scrollbar->width(), thumbLength(scrollbar));
beforeThumbRect = IntRect(trackRect.x(), trackRect.y(), trackRect.width(), thumbPos + thumbRect.height() / 2);
afterThumbRect = IntRect(trackRect.x(), trackRect.y() + beforeThumbRect.height(), trackRect.width(), trackRect.maxY() - beforeThumbRect.maxY());
}
}
// Returns the size represented by track taking into account scrolling past
// the end of the document.
static float usedTotalSize(ScrollbarThemeClient* scrollbar)
{
float overhangAtStart = -scrollbar->currentPos();
float overhangAtEnd = scrollbar->currentPos() + scrollbar->visibleSize() - scrollbar->totalSize();
float overhang = std::max(0.0f, std::max(overhangAtStart, overhangAtEnd));
return scrollbar->totalSize() + overhang;
}
int ScrollbarTheme::thumbPosition(ScrollbarThemeClient* scrollbar)
{
if (scrollbar->enabled()) {
float size = usedTotalSize(scrollbar) - scrollbar->visibleSize();
// Avoid doing a floating point divide by zero and return 1 when usedTotalSize == visibleSize.
if (!size)
return 1;
float pos = std::max(0.0f, scrollbar->currentPos()) * (trackLength(scrollbar) - thumbLength(scrollbar)) / size;
return (pos < 1 && pos > 0) ? 1 : pos;
}
return 0;
}
int ScrollbarTheme::thumbLength(ScrollbarThemeClient* scrollbar)
{
if (!scrollbar->enabled())
return 0;
float overhang = 0;
if (scrollbar->currentPos() < 0)
overhang = -scrollbar->currentPos();
else if (scrollbar->visibleSize() + scrollbar->currentPos() > scrollbar->totalSize())
overhang = scrollbar->currentPos() + scrollbar->visibleSize() - scrollbar->totalSize();
float proportion = (scrollbar->visibleSize() - overhang) / usedTotalSize(scrollbar);
int trackLen = trackLength(scrollbar);
int length = round(proportion * trackLen);
length = std::max(length, minimumThumbLength(scrollbar));
if (length > trackLen)
length = 0; // Once the thumb is below the track length, it just goes away (to make more room for the track).
return length;
}
int ScrollbarTheme::minimumThumbLength(ScrollbarThemeClient* scrollbar)
{
return scrollbarThickness(scrollbar->controlSize());
}
int ScrollbarTheme::trackPosition(ScrollbarThemeClient* scrollbar)
{
IntRect constrainedTrackRect = constrainTrackRectToTrackPieces(scrollbar, trackRect(scrollbar));
return (scrollbar->orientation() == HorizontalScrollbar) ? constrainedTrackRect.x() - scrollbar->x() : constrainedTrackRect.y() - scrollbar->y();
}
int ScrollbarTheme::trackLength(ScrollbarThemeClient* scrollbar)
{
IntRect constrainedTrackRect = constrainTrackRectToTrackPieces(scrollbar, trackRect(scrollbar));
return (scrollbar->orientation() == HorizontalScrollbar) ? constrainedTrackRect.width() : constrainedTrackRect.height();
}
void ScrollbarTheme::paintScrollCorner(GraphicsContext* context, const IntRect& cornerRect)
{
if (cornerRect.isEmpty())
return;
#if OS(MACOSX)
context->fillRect(cornerRect, Color::white);
#else
blink::Platform::current()->themeEngine()->paint(context->canvas(), blink::WebThemeEngine::PartScrollbarCorner, blink::WebThemeEngine::StateNormal, blink::WebRect(cornerRect), 0);
#endif
}
IntRect ScrollbarTheme::thumbRect(ScrollbarThemeClient* scrollbar)
{
if (!hasThumb(scrollbar))
return IntRect();
IntRect track = trackRect(scrollbar);
IntRect startTrackRect;
IntRect thumbRect;
IntRect endTrackRect;
splitTrack(scrollbar, track, startTrackRect, thumbRect, endTrackRect);
return thumbRect;
}
int ScrollbarTheme::thumbThickness(ScrollbarThemeClient* scrollbar)
{
IntRect track = trackRect(scrollbar);
return scrollbar->orientation() == HorizontalScrollbar ? track.height() : track.width();
}
void ScrollbarTheme::paintOverhangBackground(GraphicsContext* context, const IntRect& horizontalOverhangRect, const IntRect& verticalOverhangRect, const IntRect& dirtyRect)
{
context->setFillColor(Color::white);
if (!horizontalOverhangRect.isEmpty())
context->fillRect(intersection(horizontalOverhangRect, dirtyRect));
if (!verticalOverhangRect.isEmpty())
context->fillRect(intersection(verticalOverhangRect, dirtyRect));
}
}
<|endoftext|>
|
<commit_before><commit_msg>IWorkController interface<commit_after><|endoftext|>
|
<commit_before><commit_msg>Improved zlib error message<commit_after><|endoftext|>
|
<commit_before>/*
* Copyright (c) 2003-2011 Rony Shapiro <ronys@users.sourceforge.net>.
* All rights reserved. Use of the code is allowed under the
* Artistic License 2.0 terms, as specified in the LICENSE file
* distributed with this code, or available from
* http://www.opensource.org/licenses/artistic-license-2.0.php
*/
#include "Report.h"
#include "Util.h"
#include "core.h"
#include "StringX.h"
#include "os/dir.h"
#include "os/debug.h"
#include "os/file.h"
#include "os/utf8conv.h"
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <errno.h>
#include <sstream>
#include <fstream>
const TCHAR *CRLF = _T("\r\n");
/*
It writes a header record and a "Start Report" record.
*/
void CReport::StartReport(LPCTSTR tcAction, const stringT &csDataBase)
{
m_osxs.str(_T(""));
m_tcAction = tcAction;
m_csDataBase = csDataBase;
stringT cs_title, sTimeStamp;
PWSUtil::GetTimeStamp(sTimeStamp);
Format(cs_title, IDSC_REPORT_TITLE1, tcAction, sTimeStamp.c_str());
WriteLine();
WriteLine(cs_title);
Format(cs_title, IDSC_REPORT_TITLE2, csDataBase.c_str());
WriteLine(cs_title);
WriteLine();
LoadAString(cs_title, IDSC_START_REPORT);
WriteLine(cs_title);
WriteLine();
}
static bool isFileUnicode(const stringT &fname)
{
struct _stat statbuf;
int status = _tstat(fname.c_str(), &statbuf);
// Need file to exist and length at least 2 for BOM
if (status != 0 || statbuf.st_size < 2)
return false;
const unsigned char BOM[] = {0xff, 0xfe};
unsigned char buffer[] = {0x00, 0x00};
// Check if the first 2 characters are the BOM
// Need to use FOpen as cannot pass wchar_t filename to a std::istream
// and cannot convert a wchar_t filename/path to char if non-Latin characters
// present
FILE *fn = pws_os::FOpen(fname.c_str(), _T("rb"));
fread(buffer, 1, 2, fn);
fclose(fn);
return (buffer[0] == BOM[0] && buffer[1] == BOM[1]);
}
/*
SaveToDisk creates a new file of name "<tcAction>_Report.txt" e.g. "Merge_Report.txt"
in the same directory as the current database or appends to this file if it already exists.
*/
bool CReport::SaveToDisk()
{
FILE *fd;
stringT path(m_csDataBase);
stringT drive, dir, file, ext;
if (!pws_os::splitpath(path, drive, dir, file, ext)) {
pws_os::IssueError(_T("StartReport: Finding path to database"));
return false;
}
Format(m_cs_filename, IDSC_REPORTFILENAME,
drive.c_str(), dir.c_str(), m_tcAction.c_str());
if ((fd = pws_os::FOpen(m_cs_filename, _T("a+b"))) == NULL) {
pws_os::IssueError(_T("StartReport: Opening log file"));
return false;
}
// **** MOST LIKELY ACTION ****
// If file is new/emtpy AND we are UNICODE, write BOM, as some text editors insist!
// **** LEAST LIKELY ACTIONS as it requires the user to use both U & NU versions ****
// Text editors really don't like files with both UNICODE and ASCII characters, so -
// If we are UNICODE and file is not, convert file to UNICODE before appending
// If we are not UNICODE but file is, convert file to ASCII before appending
bool bFileIsUnicode = isFileUnicode(m_cs_filename);
#ifdef UNICODE
const unsigned int iBOM = 0xFEFF;
if (pws_os::fileLength(fd) == 0) {
// File is empty - write BOM
putwc(iBOM, fd);
} else
if (!bFileIsUnicode) {
// Convert ASCII contents to UNICODE
// Close original first
fclose(fd);
// Open again to read
FILE *f_in = pws_os::FOpen(m_cs_filename, _S("rb"));
// Open new file
stringT cs_out = m_cs_filename + _S(".tmp");
FILE *f_out = pws_os::FOpen(cs_out, _S("wb"));
// Write BOM
putwc(iBOM, f_out);
size_t nBytesRead;
unsigned char inbuffer[4096];
wchar_t outwbuffer[4096];
// Now copy
do {
nBytesRead = fread(inbuffer, sizeof(inbuffer), 1, f_in);
if (nBytesRead > 0) {
size_t len = pws_os::mbstowcs(outwbuffer, 4096, reinterpret_cast<const char *>(inbuffer), nBytesRead);
if (len != 0)
fwrite(outwbuffer, sizeof(outwbuffer[0])*len, 1, f_out);
} else
break;
} while(nBytesRead > 0);
// Close files
fclose(f_in);
fclose(f_out);
// Swap them
pws_os::RenameFile(cs_out, m_cs_filename);
// Re-open file
if ((fd = pws_os::FOpen(m_cs_filename, _S("ab"))) == NULL) {
pws_os::IssueError(_T("StartReport: Opening log file"));
return false;
}
}
#else
if (bFileIsUnicode) {
// Convert UNICODE contents to ASCII
// Close original first
fclose(fd);
// Open again to read
FILE *f_in = pws_os::FOpen(m_cs_filename, "rb");
// Open new file
stringT cs_out = m_cs_filename + _T(".tmp");
FILE *f_out = pws_os::FOpen(cs_out, "wb");
UINT nBytesRead;
WCHAR inwbuffer[4096];
unsigned char outbuffer[4096];
// Skip over BOM
fseek(f_in, 2, SEEK_SET);
// Now copy
do {
nBytesRead = fread(inwbuffer, sizeof(inwbuffer[0])*sizeof(inwbuffer),
1, f_in);
if (nBytesRead > 0) {
size_t len = pws_os::wcstombs((char *)outbuffer, 4096,
inwbuffer, nBytesRead);
if (len != 0)
fwrite(outbuffer, len, 1, f_out);
} else
break;
} while(nBytesRead > 0);
// Close files
fclose(f_in);
fclose(f_out);
// Swap them
pws_os::RenameFile(cs_out, m_cs_filename);
// Re-open file
if ((fd = pws_os::FOpen(m_cs_filename, _S("ab"))) == NULL) {
pws_os::IssueError(_T("StartReport: Opening log file"));
return false;
}
}
#endif
StringX sx = m_osxs.rdbuf()->str();
fwrite(reinterpret_cast<const void *>(sx.c_str()), sizeof(BYTE),
sx.length() * sizeof(TCHAR), fd);
fclose(fd);
return true;
}
// Write a record with(default) or without a CRLF
void CReport::WriteLine(const stringT &cs_line, bool bCRLF)
{
m_osxs << cs_line.c_str();
if (bCRLF) {
m_osxs << CRLF;
}
}
// Write a record with (default) or without a CRLF
void CReport::WriteLine(const LPTSTR &tc_line, bool bCRLF)
{
m_osxs << tc_line;
if (bCRLF) {
m_osxs << CRLF;
}
}
// Write a new line
void CReport::WriteLine()
{
m_osxs << CRLF;
}
// EndReport writes a "End Report" record and closes the report file.
void CReport::EndReport()
{
WriteLine();
stringT cs_title;
LoadAString(cs_title, IDSC_END_REPORT1);
WriteLine(cs_title);
LoadAString(cs_title, IDSC_END_REPORT2);
WriteLine(cs_title);
m_osxs.flush();
}
<commit_msg>Portability: rev 4211 should compile under Linux too.<commit_after>/*
* Copyright (c) 2003-2011 Rony Shapiro <ronys@users.sourceforge.net>.
* All rights reserved. Use of the code is allowed under the
* Artistic License 2.0 terms, as specified in the LICENSE file
* distributed with this code, or available from
* http://www.opensource.org/licenses/artistic-license-2.0.php
*/
#include "Report.h"
#include "Util.h"
#include "core.h"
#include "StringX.h"
#include "os/dir.h"
#include "os/debug.h"
#include "os/file.h"
#include "os/utf8conv.h"
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <sstream>
#include <fstream>
const TCHAR *CRLF = _T("\r\n");
/*
It writes a header record and a "Start Report" record.
*/
void CReport::StartReport(LPCTSTR tcAction, const stringT &csDataBase)
{
m_osxs.str(_T(""));
m_tcAction = tcAction;
m_csDataBase = csDataBase;
stringT cs_title, sTimeStamp;
PWSUtil::GetTimeStamp(sTimeStamp);
Format(cs_title, IDSC_REPORT_TITLE1, tcAction, sTimeStamp.c_str());
WriteLine();
WriteLine(cs_title);
Format(cs_title, IDSC_REPORT_TITLE2, csDataBase.c_str());
WriteLine(cs_title);
WriteLine();
LoadAString(cs_title, IDSC_START_REPORT);
WriteLine(cs_title);
WriteLine();
}
static bool isFileUnicode(const stringT &fname)
{
// Check if the first 2 characters are the BOM
// (Need file to exist and length at least 2 for BOM)
// Need to use FOpen as cannot pass wchar_t filename to a std::istream
// and cannot convert a wchar_t filename/path to char if non-Latin characters
// present
const unsigned char BOM[] = {0xff, 0xfe};
unsigned char buffer[] = {0x00, 0x00};
bool retval = false;
FILE *fn = pws_os::FOpen(fname, _T("rb"));
if (fn == NULL)
return false;
if (pws_os::fileLength(fn) < 2)
retval = false;
else {
fread(buffer, 1, 2, fn);
retval = (buffer[0] == BOM[0] && buffer[1] == BOM[1]);
}
fclose(fn);
return retval;
}
/*
SaveToDisk creates a new file of name "<tcAction>_Report.txt" e.g. "Merge_Report.txt"
in the same directory as the current database or appends to this file if it already exists.
*/
bool CReport::SaveToDisk()
{
FILE *fd;
stringT path(m_csDataBase);
stringT drive, dir, file, ext;
if (!pws_os::splitpath(path, drive, dir, file, ext)) {
pws_os::IssueError(_T("StartReport: Finding path to database"));
return false;
}
Format(m_cs_filename, IDSC_REPORTFILENAME,
drive.c_str(), dir.c_str(), m_tcAction.c_str());
if ((fd = pws_os::FOpen(m_cs_filename, _T("a+b"))) == NULL) {
pws_os::IssueError(_T("StartReport: Opening log file"));
return false;
}
// **** MOST LIKELY ACTION ****
// If file is new/emtpy AND we are UNICODE, write BOM, as some text editors insist!
// **** LEAST LIKELY ACTIONS as it requires the user to use both U & NU versions ****
// Text editors really don't like files with both UNICODE and ASCII characters, so -
// If we are UNICODE and file is not, convert file to UNICODE before appending
// If we are not UNICODE but file is, convert file to ASCII before appending
bool bFileIsUnicode = isFileUnicode(m_cs_filename);
#ifdef UNICODE
const unsigned int iBOM = 0xFEFF;
if (pws_os::fileLength(fd) == 0) {
// File is empty - write BOM
putwc(iBOM, fd);
} else
if (!bFileIsUnicode) {
// Convert ASCII contents to UNICODE
// Close original first
fclose(fd);
// Open again to read
FILE *f_in = pws_os::FOpen(m_cs_filename, _S("rb"));
// Open new file
stringT cs_out = m_cs_filename + _S(".tmp");
FILE *f_out = pws_os::FOpen(cs_out, _S("wb"));
// Write BOM
putwc(iBOM, f_out);
size_t nBytesRead;
unsigned char inbuffer[4096];
wchar_t outwbuffer[4096];
// Now copy
do {
nBytesRead = fread(inbuffer, sizeof(inbuffer), 1, f_in);
if (nBytesRead > 0) {
size_t len = pws_os::mbstowcs(outwbuffer, 4096, reinterpret_cast<const char *>(inbuffer), nBytesRead);
if (len != 0)
fwrite(outwbuffer, sizeof(outwbuffer[0])*len, 1, f_out);
} else
break;
} while(nBytesRead > 0);
// Close files
fclose(f_in);
fclose(f_out);
// Swap them
pws_os::RenameFile(cs_out, m_cs_filename);
// Re-open file
if ((fd = pws_os::FOpen(m_cs_filename, _S("ab"))) == NULL) {
pws_os::IssueError(_T("StartReport: Opening log file"));
return false;
}
}
#else
if (bFileIsUnicode) {
// Convert UNICODE contents to ASCII
// Close original first
fclose(fd);
// Open again to read
FILE *f_in = pws_os::FOpen(m_cs_filename, "rb");
// Open new file
stringT cs_out = m_cs_filename + _T(".tmp");
FILE *f_out = pws_os::FOpen(cs_out, "wb");
UINT nBytesRead;
WCHAR inwbuffer[4096];
unsigned char outbuffer[4096];
// Skip over BOM
fseek(f_in, 2, SEEK_SET);
// Now copy
do {
nBytesRead = fread(inwbuffer, sizeof(inwbuffer[0])*sizeof(inwbuffer),
1, f_in);
if (nBytesRead > 0) {
size_t len = pws_os::wcstombs((char *)outbuffer, 4096,
inwbuffer, nBytesRead);
if (len != 0)
fwrite(outbuffer, len, 1, f_out);
} else
break;
} while(nBytesRead > 0);
// Close files
fclose(f_in);
fclose(f_out);
// Swap them
pws_os::RenameFile(cs_out, m_cs_filename);
// Re-open file
if ((fd = pws_os::FOpen(m_cs_filename, _S("ab"))) == NULL) {
pws_os::IssueError(_T("StartReport: Opening log file"));
return false;
}
}
#endif
StringX sx = m_osxs.rdbuf()->str();
fwrite(reinterpret_cast<const void *>(sx.c_str()), sizeof(BYTE),
sx.length() * sizeof(TCHAR), fd);
fclose(fd);
return true;
}
// Write a record with(default) or without a CRLF
void CReport::WriteLine(const stringT &cs_line, bool bCRLF)
{
m_osxs << cs_line.c_str();
if (bCRLF) {
m_osxs << CRLF;
}
}
// Write a record with (default) or without a CRLF
void CReport::WriteLine(const LPTSTR &tc_line, bool bCRLF)
{
m_osxs << tc_line;
if (bCRLF) {
m_osxs << CRLF;
}
}
// Write a new line
void CReport::WriteLine()
{
m_osxs << CRLF;
}
// EndReport writes a "End Report" record and closes the report file.
void CReport::EndReport()
{
WriteLine();
stringT cs_title;
LoadAString(cs_title, IDSC_END_REPORT1);
WriteLine(cs_title);
LoadAString(cs_title, IDSC_END_REPORT2);
WriteLine(cs_title);
m_osxs.flush();
}
<|endoftext|>
|
<commit_before>// =============================================================================
// PROJECT CHRONO - http://projectchrono.org
//
// Copyright (c) 2014 projectchrono.org
// All right reserved.
//
// Use of this source code is governed by a BSD-style license that can be found
// in the LICENSE file at the top level of the distribution and at
// http://projectchrono.org/license-chrono.txt.
//
// =============================================================================
// Authors: Antonio Recuero, Milad Rakhsha
// =============================================================================
//
// Unit test for EAS Brick Element
//
// This unit test checks the dynamics of a beam made up of 10 brick elemnts.
// It serves to validate the elastic, isotropic, large deformation internal forces,
// the element inertia, and this element'sgravity forces.
//
// This element is a regular 8-noded trilinear brick element with enhanced assumed
// strain that alleviates locking. More information on the validation of this element
// may be found in Chrono's documentation. This simulation excites the beam by applying
// the sudden action of a gravity field.
// =============================================================================
#include "chrono/core/ChFileutils.h"
#include "chrono/physics/ChSystem.h"
#include "chrono/lcp/ChLcpIterativeMINRES.h"
#include "chrono/utils/ChUtilsInputOutput.h"
#include "chrono_fea/ChElementSpring.h"
#include "chrono_fea/ChElementBrick.h"
#include "chrono_fea/ChElementBar.h"
#include "chrono_fea/ChLinkPointFrame.h"
#include "chrono_fea/ChLinkDirFrame.h"
#include "chrono_fea/ChVisualizationFEAmesh.h"
using namespace chrono;
using namespace fea;
const double step_size = 1e-3; // Step size
double sim_time = 2; // Simulation time for generation of reference file
double precision = 1e-6; // Precision value used to assess results
double sim_time_UT = 0.1; // Simulation time for unit test 0.05
int main(int argc, char* argv[]) {
bool output = 0; // Determines whether it tests (0) or generates golden file (1)
ChMatrixDynamic<> FileInputMat(2000, 2);
if (output) {
GetLog() << "Output file: ../TEST_Brick/UT_EASBrickIso_Grav.txt\n";
} else {
// Utils to open/read files: Load reference solution ("golden") file
std::string EASBrick_val_file = GetChronoDataPath() + "testing/" + "UT_EASBrickIso_Grav.txt";
std::ifstream fileMid(EASBrick_val_file);
if (!fileMid.is_open()) {
fileMid.open(EASBrick_val_file);
}
if (!fileMid) {
std::cout << "Cannot open validation file.\n";
exit(1);
}
for (int x = 0; x < 2000; x++) {
fileMid >> FileInputMat[x][0] >> FileInputMat[x][1];
}
fileMid.close();
GetLog() << "Running in unit test mode.\n";
}
// Create the physical system
ChSystem my_system;
ChSharedPtr<ChMesh> my_mesh(new ChMesh);
// Dimensions of the plate
double plate_lenght_x = 1;
double plate_lenght_y = 0.1;
double plate_lenght_z = 0.01; // small thickness
// Specification of the mesh
int numDiv_x = 10; // 10 elements along the length of the beam
int numDiv_y = 1;
int numDiv_z = 1;
int N_x = numDiv_x + 1;
int N_y = numDiv_y + 1;
int N_z = numDiv_z + 1;
// Number of elements in the z direction is considered as 1
int TotalNumElements = numDiv_x;
int TotalNumNodes = (numDiv_x + 1) * 4; // 4 nodes per brick face
// For uniform mesh
double dx = plate_lenght_x / numDiv_x;
double dy = plate_lenght_y / numDiv_y;
double dz = plate_lenght_z / numDiv_z;
int MaxMNUM = 1;
int MTYPE = 1;
int MaxLayNum = 1;
ChMatrixDynamic<double> COORDFlex(TotalNumNodes, 3);
ChMatrixDynamic<double> VELCYFlex(TotalNumNodes, 3);
ChMatrixDynamic<int> NumNodes(TotalNumElements, 8);
ChMatrixDynamic<int> LayNum(TotalNumElements, 1);
ChMatrixDynamic<int> NDR(TotalNumNodes, 3);
ChMatrixDynamic<double> ElemLengthXY(TotalNumElements, 3);
ChMatrixNM<double, 10, 12> MPROP;
//!------------ Material Data-----------------
for (int i = 0; i < MaxMNUM; i++) {
MPROP(i, 0) = 500; // Density [kg/m3]
MPROP(i, 1) = 2.1E+07; // E (Pa)
MPROP(i, 2) = 0.3; // nu
}
ChSharedPtr<ChContinuumElastic> mmaterial(new ChContinuumElastic);
mmaterial->Set_RayleighDampingK(0.0);
mmaterial->Set_RayleighDampingM(0.0);
mmaterial->Set_density(MPROP(0, 0));
mmaterial->Set_E(MPROP(0, 1));
mmaterial->Set_G(MPROP(0, 1) / (2 + 2 * MPROP(0, 2)));
mmaterial->Set_v(MPROP(0, 2));
//!--------------- Element data--------------------
for (int i = 0; i < TotalNumElements; i++) {
// All the elements belong to the same layer, e.g layer number 1.
LayNum(i, 0) = 1;
NumNodes(i, 0) = i;
NumNodes(i, 1) = i + 1;
NumNodes(i, 2) = i + 1 + N_x;
NumNodes(i, 3) = i + N_x;
NumNodes(i, 4) = i + 2 * N_x;
NumNodes(i, 5) = i + 2 * N_x + 1;
NumNodes(i, 6) = i + 3 * N_x + 1;
NumNodes(i, 7) = i + 3 * N_x; // Numbering of nodes
ElemLengthXY(i, 0) = dx;
ElemLengthXY(i, 1) = dy;
ElemLengthXY(i, 2) = dz;
if (MaxLayNum < LayNum(i, 0)) {
MaxLayNum = LayNum(i, 0);
}
}
//!--------- Constraints and initial coordinates/velocities
for (int i = 0; i < TotalNumNodes; i++) {
// Constrain clamped nodes. Assigns (1) if constrained, (0) otherwise
NDR(i, 0) = (i % N_x == 0) ? 1 : 0;
NDR(i, 1) = (i % N_x == 0) ? 1 : 0;
NDR(i, 2) = (i % N_x == 0) ? 1 : 0;
// Coordinates
COORDFlex(i, 0) = (i % (N_x)) * dx;
if ((i / N_x + 1) % 2 == 0) {
COORDFlex(i, 1) = dy;
} else {
COORDFlex(i, 1) = 0.0;
};
COORDFlex(i, 2) = (i) / ((N_x)*2) * dz;
// Velocities
VELCYFlex(i, 0) = 0;
VELCYFlex(i, 1) = 0;
VELCYFlex(i, 2) = 0;
}
// Adding the nodes to the mesh
int i = 0;
while (i < TotalNumNodes) {
ChSharedPtr<ChNodeFEAxyz> node(new ChNodeFEAxyz(ChVector<>(COORDFlex(i, 0), COORDFlex(i, 1), COORDFlex(i, 2))));
node->SetMass(0.0);
my_mesh->AddNode(node);
if (NDR(i, 0) == 1 && NDR(i, 1) == 1 && NDR(i, 2) == 1) {
node->SetFixed(true);
}
i++;
}
// Create a node at the tip by dynamic casting
ChSharedPtr<ChNodeFEAxyz> nodetip(my_mesh->GetNode(TotalNumNodes - 1).DynamicCastTo<ChNodeFEAxyz>());
int elemcount = 0;
while (elemcount < TotalNumElements) {
ChSharedPtr<ChElementBrick> element(new ChElementBrick);
ChMatrixNM<double, 3, 1> InertFlexVec; // Read element length, used in ChElementBrick
InertFlexVec.Reset();
InertFlexVec(0, 0) = ElemLengthXY(elemcount, 0);
InertFlexVec(1, 0) = ElemLengthXY(elemcount, 1);
InertFlexVec(2, 0) = ElemLengthXY(elemcount, 2);
element->SetInertFlexVec(InertFlexVec);
// Note we change the order of the nodes to comply with the arrangement of shape functions
element->SetNodes(my_mesh->GetNode(NumNodes(elemcount, 0)).DynamicCastTo<ChNodeFEAxyz>(),
my_mesh->GetNode(NumNodes(elemcount, 1)).DynamicCastTo<ChNodeFEAxyz>(),
my_mesh->GetNode(NumNodes(elemcount, 3)).DynamicCastTo<ChNodeFEAxyz>(), //
my_mesh->GetNode(NumNodes(elemcount, 2)).DynamicCastTo<ChNodeFEAxyz>(), //
my_mesh->GetNode(NumNodes(elemcount, 4)).DynamicCastTo<ChNodeFEAxyz>(),
my_mesh->GetNode(NumNodes(elemcount, 5)).DynamicCastTo<ChNodeFEAxyz>(),
my_mesh->GetNode(NumNodes(elemcount, 7)).DynamicCastTo<ChNodeFEAxyz>(), //
my_mesh->GetNode(NumNodes(elemcount, 6)).DynamicCastTo<ChNodeFEAxyz>()); //
element->SetMaterial(mmaterial);
element->SetElemNum(elemcount);
element->SetGravityOn(true); // Turn gravity on/off from within the element
element->SetMooneyRivlin(false); // Turn on/off Mooney Rivlin (Linear Isotropic by default)
// element->SetMRCoefficients(551584.0, 137896.0); // Set two coefficients for Mooney-Rivlin
ChMatrixNM<double, 9, 1> stock_alpha_EAS;
stock_alpha_EAS.Reset();
element->SetStockAlpha(stock_alpha_EAS(0, 0), stock_alpha_EAS(1, 0), stock_alpha_EAS(2, 0),
stock_alpha_EAS(3, 0), stock_alpha_EAS(4, 0), stock_alpha_EAS(5, 0),
stock_alpha_EAS(6, 0), stock_alpha_EAS(7, 0), stock_alpha_EAS(8, 0));
my_mesh->AddElement(element);
elemcount++;
}
// This is mandatory
my_mesh->SetupInitial();
// Deactivate automatic gravity in mesh
my_mesh->SetAutomaticGravity(false);
// Remember to add the mesh to the system!
my_system.Add(my_mesh);
// Perform a dynamic time integration:
my_system.SetLcpSolverType(
ChSystem::LCP_ITERATIVE_MINRES); // <- NEEDED because other solvers can't handle stiffness matrices
chrono::ChLcpIterativeMINRES* msolver = (chrono::ChLcpIterativeMINRES*)my_system.GetLcpSolverSpeed();
msolver->SetDiagonalPreconditioning(true);
my_system.SetIterLCPmaxItersSpeed(10000);
my_system.SetTolForce(1e-09);
my_system.SetIntegrationType(ChSystem::INT_HHT);
ChSharedPtr<ChTimestepperHHT> mystepper = my_system.GetTimestepper().DynamicCastTo<ChTimestepperHHT>();
mystepper->SetAlpha(-0.01);
mystepper->SetMaxiters(10000);
mystepper->SetTolerance(1e-09);
mystepper->SetMode(ChTimestepperHHT::POSITION);
mystepper->SetScaling(true);
// Simulation loop
if (output) {
// Create output directory (if it does not already exist).
if (ChFileutils::MakeDirectory("../TEST_Brick") < 0) {
GetLog() << "Error creating directory ../TEST_Brick\n";
return 1;
}
// Initialize the output stream and set precision.
utils::CSV_writer out("\t");
out.stream().setf(std::ios::scientific | std::ios::showpos);
out.stream().precision(7);
int Iterations = 0;
// Simulate to final time, while saving position of tip node.
while (my_system.GetChTime() < sim_time) {
my_system.DoStepDynamics(step_size);
Iterations += mystepper->GetNumIterations();
out << my_system.GetChTime() << nodetip->GetPos().z << std::endl;
GetLog() << "time = " << my_system.GetChTime() << "\t" << nodetip->GetPos().z << "\t"
<< nodetip->GetForce().z << "\t" << Iterations << "\n";
}
// Write results to output file.
out.write_to_file("../TEST_Brick/UT_EASBrickIso_Grav.txt.txt");
} else {
// Initialize total number of iterations and timer.
int Iterations = 0;
double start = std::clock();
int stepNo = 0;
double AbsVal = 0.0;
// Simulate to final time, while accumulating number of iterations.
while (my_system.GetChTime() < sim_time_UT) {
my_system.DoStepDynamics(step_size);
AbsVal = abs(nodetip->GetPos().z - FileInputMat[stepNo][1]);
GetLog() << "time = " << my_system.GetChTime() << "\t" << nodetip->GetPos().z << "\n";
if (AbsVal > precision) {
std::cout << "Unit test check failed \n";
return 1;
}
stepNo++;
Iterations += mystepper->GetNumIterations();
}
// Report run time and total number of iterations.
double duration = (std::clock() - start) / (double)CLOCKS_PER_SEC;
GetLog() << "Computation Time: " << duration << " Number of iterations: " << Iterations << "\n";
std::cout << "Unit test check succeeded \n";
}
return 0;
}
<commit_msg>Reduce simulation time tested in test_EASBrickIso_Grav.cpp<commit_after>// =============================================================================
// PROJECT CHRONO - http://projectchrono.org
//
// Copyright (c) 2014 projectchrono.org
// All right reserved.
//
// Use of this source code is governed by a BSD-style license that can be found
// in the LICENSE file at the top level of the distribution and at
// http://projectchrono.org/license-chrono.txt.
//
// =============================================================================
// Authors: Antonio Recuero, Milad Rakhsha
// =============================================================================
//
// Unit test for EAS Brick Element
//
// This unit test checks the dynamics of a beam made up of 10 brick elemnts.
// It serves to validate the elastic, isotropic, large deformation internal forces,
// the element inertia, and this element'sgravity forces.
//
// This element is a regular 8-noded trilinear brick element with enhanced assumed
// strain that alleviates locking. More information on the validation of this element
// may be found in Chrono's documentation. This simulation excites the beam by applying
// the sudden action of a gravity field.
// =============================================================================
#include "chrono/core/ChFileutils.h"
#include "chrono/physics/ChSystem.h"
#include "chrono/lcp/ChLcpIterativeMINRES.h"
#include "chrono/utils/ChUtilsInputOutput.h"
#include "chrono_fea/ChElementSpring.h"
#include "chrono_fea/ChElementBrick.h"
#include "chrono_fea/ChElementBar.h"
#include "chrono_fea/ChLinkPointFrame.h"
#include "chrono_fea/ChLinkDirFrame.h"
#include "chrono_fea/ChVisualizationFEAmesh.h"
using namespace chrono;
using namespace fea;
const double step_size = 1e-3; // Step size
double sim_time = 2; // Simulation time for generation of reference file
double precision = 1e-6; // Precision value used to assess results
double sim_time_UT = 0.05; // Simulation time for unit test 0.05
int main(int argc, char* argv[]) {
bool output = 0; // Determines whether it tests (0) or generates golden file (1)
ChMatrixDynamic<> FileInputMat(2000, 2);
if (output) {
GetLog() << "Output file: ../TEST_Brick/UT_EASBrickIso_Grav.txt\n";
} else {
// Utils to open/read files: Load reference solution ("golden") file
std::string EASBrick_val_file = GetChronoDataPath() + "testing/" + "UT_EASBrickIso_Grav.txt";
std::ifstream fileMid(EASBrick_val_file);
if (!fileMid.is_open()) {
fileMid.open(EASBrick_val_file);
}
if (!fileMid) {
std::cout << "Cannot open validation file.\n";
exit(1);
}
for (int x = 0; x < 2000; x++) {
fileMid >> FileInputMat[x][0] >> FileInputMat[x][1];
}
fileMid.close();
GetLog() << "Running in unit test mode.\n";
}
// Create the physical system
ChSystem my_system;
ChSharedPtr<ChMesh> my_mesh(new ChMesh);
// Dimensions of the plate
double plate_lenght_x = 1;
double plate_lenght_y = 0.1;
double plate_lenght_z = 0.01; // small thickness
// Specification of the mesh
int numDiv_x = 10; // 10 elements along the length of the beam
int numDiv_y = 1;
int numDiv_z = 1;
int N_x = numDiv_x + 1;
int N_y = numDiv_y + 1;
int N_z = numDiv_z + 1;
// Number of elements in the z direction is considered as 1
int TotalNumElements = numDiv_x;
int TotalNumNodes = (numDiv_x + 1) * 4; // 4 nodes per brick face
// For uniform mesh
double dx = plate_lenght_x / numDiv_x;
double dy = plate_lenght_y / numDiv_y;
double dz = plate_lenght_z / numDiv_z;
int MaxMNUM = 1;
int MTYPE = 1;
int MaxLayNum = 1;
ChMatrixDynamic<double> COORDFlex(TotalNumNodes, 3);
ChMatrixDynamic<double> VELCYFlex(TotalNumNodes, 3);
ChMatrixDynamic<int> NumNodes(TotalNumElements, 8);
ChMatrixDynamic<int> LayNum(TotalNumElements, 1);
ChMatrixDynamic<int> NDR(TotalNumNodes, 3);
ChMatrixDynamic<double> ElemLengthXY(TotalNumElements, 3);
ChMatrixNM<double, 10, 12> MPROP;
//!------------ Material Data-----------------
for (int i = 0; i < MaxMNUM; i++) {
MPROP(i, 0) = 500; // Density [kg/m3]
MPROP(i, 1) = 2.1E+07; // E (Pa)
MPROP(i, 2) = 0.3; // nu
}
ChSharedPtr<ChContinuumElastic> mmaterial(new ChContinuumElastic);
mmaterial->Set_RayleighDampingK(0.0);
mmaterial->Set_RayleighDampingM(0.0);
mmaterial->Set_density(MPROP(0, 0));
mmaterial->Set_E(MPROP(0, 1));
mmaterial->Set_G(MPROP(0, 1) / (2 + 2 * MPROP(0, 2)));
mmaterial->Set_v(MPROP(0, 2));
//!--------------- Element data--------------------
for (int i = 0; i < TotalNumElements; i++) {
// All the elements belong to the same layer, e.g layer number 1.
LayNum(i, 0) = 1;
NumNodes(i, 0) = i;
NumNodes(i, 1) = i + 1;
NumNodes(i, 2) = i + 1 + N_x;
NumNodes(i, 3) = i + N_x;
NumNodes(i, 4) = i + 2 * N_x;
NumNodes(i, 5) = i + 2 * N_x + 1;
NumNodes(i, 6) = i + 3 * N_x + 1;
NumNodes(i, 7) = i + 3 * N_x; // Numbering of nodes
ElemLengthXY(i, 0) = dx;
ElemLengthXY(i, 1) = dy;
ElemLengthXY(i, 2) = dz;
if (MaxLayNum < LayNum(i, 0)) {
MaxLayNum = LayNum(i, 0);
}
}
//!--------- Constraints and initial coordinates/velocities
for (int i = 0; i < TotalNumNodes; i++) {
// Constrain clamped nodes. Assigns (1) if constrained, (0) otherwise
NDR(i, 0) = (i % N_x == 0) ? 1 : 0;
NDR(i, 1) = (i % N_x == 0) ? 1 : 0;
NDR(i, 2) = (i % N_x == 0) ? 1 : 0;
// Coordinates
COORDFlex(i, 0) = (i % (N_x)) * dx;
if ((i / N_x + 1) % 2 == 0) {
COORDFlex(i, 1) = dy;
} else {
COORDFlex(i, 1) = 0.0;
};
COORDFlex(i, 2) = (i) / ((N_x)*2) * dz;
// Velocities
VELCYFlex(i, 0) = 0;
VELCYFlex(i, 1) = 0;
VELCYFlex(i, 2) = 0;
}
// Adding the nodes to the mesh
int i = 0;
while (i < TotalNumNodes) {
ChSharedPtr<ChNodeFEAxyz> node(new ChNodeFEAxyz(ChVector<>(COORDFlex(i, 0), COORDFlex(i, 1), COORDFlex(i, 2))));
node->SetMass(0.0);
my_mesh->AddNode(node);
if (NDR(i, 0) == 1 && NDR(i, 1) == 1 && NDR(i, 2) == 1) {
node->SetFixed(true);
}
i++;
}
// Create a node at the tip by dynamic casting
ChSharedPtr<ChNodeFEAxyz> nodetip(my_mesh->GetNode(TotalNumNodes - 1).DynamicCastTo<ChNodeFEAxyz>());
int elemcount = 0;
while (elemcount < TotalNumElements) {
ChSharedPtr<ChElementBrick> element(new ChElementBrick);
ChMatrixNM<double, 3, 1> InertFlexVec; // Read element length, used in ChElementBrick
InertFlexVec.Reset();
InertFlexVec(0, 0) = ElemLengthXY(elemcount, 0);
InertFlexVec(1, 0) = ElemLengthXY(elemcount, 1);
InertFlexVec(2, 0) = ElemLengthXY(elemcount, 2);
element->SetInertFlexVec(InertFlexVec);
// Note we change the order of the nodes to comply with the arrangement of shape functions
element->SetNodes(my_mesh->GetNode(NumNodes(elemcount, 0)).DynamicCastTo<ChNodeFEAxyz>(),
my_mesh->GetNode(NumNodes(elemcount, 1)).DynamicCastTo<ChNodeFEAxyz>(),
my_mesh->GetNode(NumNodes(elemcount, 3)).DynamicCastTo<ChNodeFEAxyz>(), //
my_mesh->GetNode(NumNodes(elemcount, 2)).DynamicCastTo<ChNodeFEAxyz>(), //
my_mesh->GetNode(NumNodes(elemcount, 4)).DynamicCastTo<ChNodeFEAxyz>(),
my_mesh->GetNode(NumNodes(elemcount, 5)).DynamicCastTo<ChNodeFEAxyz>(),
my_mesh->GetNode(NumNodes(elemcount, 7)).DynamicCastTo<ChNodeFEAxyz>(), //
my_mesh->GetNode(NumNodes(elemcount, 6)).DynamicCastTo<ChNodeFEAxyz>()); //
element->SetMaterial(mmaterial);
element->SetElemNum(elemcount);
element->SetGravityOn(true); // Turn gravity on/off from within the element
element->SetMooneyRivlin(false); // Turn on/off Mooney Rivlin (Linear Isotropic by default)
// element->SetMRCoefficients(551584.0, 137896.0); // Set two coefficients for Mooney-Rivlin
ChMatrixNM<double, 9, 1> stock_alpha_EAS;
stock_alpha_EAS.Reset();
element->SetStockAlpha(stock_alpha_EAS(0, 0), stock_alpha_EAS(1, 0), stock_alpha_EAS(2, 0),
stock_alpha_EAS(3, 0), stock_alpha_EAS(4, 0), stock_alpha_EAS(5, 0),
stock_alpha_EAS(6, 0), stock_alpha_EAS(7, 0), stock_alpha_EAS(8, 0));
my_mesh->AddElement(element);
elemcount++;
}
// This is mandatory
my_mesh->SetupInitial();
// Deactivate automatic gravity in mesh
my_mesh->SetAutomaticGravity(false);
// Remember to add the mesh to the system!
my_system.Add(my_mesh);
// Perform a dynamic time integration:
my_system.SetLcpSolverType(
ChSystem::LCP_ITERATIVE_MINRES); // <- NEEDED because other solvers can't handle stiffness matrices
chrono::ChLcpIterativeMINRES* msolver = (chrono::ChLcpIterativeMINRES*)my_system.GetLcpSolverSpeed();
msolver->SetDiagonalPreconditioning(true);
my_system.SetIterLCPmaxItersSpeed(10000);
my_system.SetTolForce(1e-09);
my_system.SetIntegrationType(ChSystem::INT_HHT);
ChSharedPtr<ChTimestepperHHT> mystepper = my_system.GetTimestepper().DynamicCastTo<ChTimestepperHHT>();
mystepper->SetAlpha(-0.01);
mystepper->SetMaxiters(10000);
mystepper->SetTolerance(1e-09);
mystepper->SetMode(ChTimestepperHHT::POSITION);
mystepper->SetScaling(true);
// Simulation loop
if (output) {
// Create output directory (if it does not already exist).
if (ChFileutils::MakeDirectory("../TEST_Brick") < 0) {
GetLog() << "Error creating directory ../TEST_Brick\n";
return 1;
}
// Initialize the output stream and set precision.
utils::CSV_writer out("\t");
out.stream().setf(std::ios::scientific | std::ios::showpos);
out.stream().precision(7);
int Iterations = 0;
// Simulate to final time, while saving position of tip node.
while (my_system.GetChTime() < sim_time) {
my_system.DoStepDynamics(step_size);
Iterations += mystepper->GetNumIterations();
out << my_system.GetChTime() << nodetip->GetPos().z << std::endl;
GetLog() << "time = " << my_system.GetChTime() << "\t" << nodetip->GetPos().z << "\t"
<< nodetip->GetForce().z << "\t" << Iterations << "\n";
}
// Write results to output file.
out.write_to_file("../TEST_Brick/UT_EASBrickIso_Grav.txt.txt");
} else {
// Initialize total number of iterations and timer.
int Iterations = 0;
double start = std::clock();
int stepNo = 0;
double AbsVal = 0.0;
// Simulate to final time, while accumulating number of iterations.
while (my_system.GetChTime() < sim_time_UT) {
my_system.DoStepDynamics(step_size);
AbsVal = abs(nodetip->GetPos().z - FileInputMat[stepNo][1]);
GetLog() << "time = " << my_system.GetChTime() << "\t" << nodetip->GetPos().z << "\n";
if (AbsVal > precision) {
std::cout << "Unit test check failed \n";
return 1;
}
stepNo++;
Iterations += mystepper->GetNumIterations();
}
// Report run time and total number of iterations.
double duration = (std::clock() - start) / (double)CLOCKS_PER_SEC;
GetLog() << "Computation Time: " << duration << " Number of iterations: " << Iterations << "\n";
std::cout << "Unit test check succeeded \n";
}
return 0;
}
<|endoftext|>
|
<commit_before>// -*-Mode: C++;-*-
// $Id$
// * BeginRiceCopyright *****************************************************
//
// Copyright ((c)) 2002-2007, Rice University
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions are
// met:
//
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// * Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
//
// * Neither the name of Rice University (RICE) nor the names of its
// contributors may be used to endorse or promote products derived from
// this software without specific prior written permission.
//
// This software is provided by RICE and contributors "as is" and any
// express or implied warranties, including, but not limited to, the
// implied warranties of merchantability and fitness for a particular
// purpose are disclaimed. In no event shall RICE or contributors be
// liable for any direct, indirect, incidental, special, exemplary, or
// consequential damages (including, but not limited to, procurement of
// substitute goods or services; loss of use, data, or profits; or
// business interruption) however caused and on any theory of liability,
// whether in contract, strict liability, or tort (including negligence
// or otherwise) arising in any way out of the use of this software, even
// if advised of the possibility of such damage.
//
// ******************************************************* EndRiceCopyright *
// ----------------------------------------------------------------------
//
// the implementation of evaluation tree nodes
//
// ----------------------------------------------------------------------
//************************ System Include Files ******************************
#include <iostream>
using std::endl;
#include <sstream>
#include <string>
#include <algorithm>
#include <cmath>
//************************* User Include Files *******************************
#include <include/general.h>
#include "Metric-AExpr.hpp"
#include <lib/support/NaN.h>
#include <lib/support/Trace.hpp>
//************************ Forward Declarations ******************************
#define AEXPR_DO_CHECK 0
#if (AEXPR_DO_CHECK)
# define AEXPR_CHECK(x) if (!isok(x)) { return c_FP_NAN_d; }
#else
# define AEXPR_CHECK(x)
#endif
//****************************************************************************
namespace Prof {
namespace Metric {
// ----------------------------------------------------------------------
// class AExpr
// ----------------------------------------------------------------------
std::string
AExpr::toString() const
{
std::ostringstream os;
dump(os);
return os.str();
}
void
AExpr::dump_opands(std::ostream& os, AExpr** opands, int sz, const char* sep)
{
for (int i = 0; i < sz; ++i) {
opands[i]->dump(os);
if (i < (sz - 1)) {
os << sep;
}
}
}
// ----------------------------------------------------------------------
// class Const
// ----------------------------------------------------------------------
std::ostream&
Const::dump(std::ostream& os) const
{
os << "(" << m_c << ")";
return os;
}
// ----------------------------------------------------------------------
// class Neg
// ----------------------------------------------------------------------
double
Neg::eval(const ScopeInfo* si) const
{
double result = m_expr->eval(si);
//IFTRACE << "neg=" << -result << endl;
AEXPR_CHECK(result);
return -result;
}
std::ostream&
Neg::dump(std::ostream& os) const
{
os << "(-"; m_expr->dump(os); os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Var
// ----------------------------------------------------------------------
std::ostream&
Var::dump(std::ostream& os) const
{
os << name;
return os;
}
// ----------------------------------------------------------------------
// class Power
// ----------------------------------------------------------------------
Power::Power(AExpr* b, AExpr* e)
: base(b), exponent(e)
{
}
Power::~Power()
{
delete base;
delete exponent;
}
double
Power::eval(const ScopeInfo* si) const
{
double b = base->eval(si);
double e = exponent->eval(si);
double result = pow(b, e);
//IFTRACE << "pow=" << pow(b, e) << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Power::dump(std::ostream& os) const
{
os << "("; base->dump(os); os << "**"; exponent->dump(os); os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Divide
// ----------------------------------------------------------------------
Divide::Divide(AExpr* num, AExpr* denom)
: numerator(num), denominator(denom)
{
}
Divide::~Divide()
{
delete numerator;
delete denominator;
}
double
Divide::eval(const ScopeInfo* si) const
{
double n = numerator->eval(si);
double d = denominator->eval(si);
double result = n / d;
//IFTRACE << "divident=" << n/d << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Divide::dump(std::ostream& os) const
{
os << "(";
numerator->dump(os);
os << " / ";
denominator->dump(os);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Minus
// ----------------------------------------------------------------------
Minus::Minus(AExpr* m, AExpr* s)
: minuend(m), subtrahend(s)
{
// if (minuend == NULL || subtrahend == NULL) { ... }
}
Minus::~Minus()
{
delete minuend;
delete subtrahend;
}
double
Minus::eval(const ScopeInfo* si) const
{
double m = minuend->eval(si);
double s = subtrahend->eval(si);
double result = (m - s);
//IFTRACE << "diff=" << m-s << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Minus::dump(std::ostream& os) const
{
os << "("; minuend->dump(os); os << " - "; subtrahend->dump(os); os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Plus
// ----------------------------------------------------------------------
Plus::Plus(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Plus::~Plus()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Plus::eval(const ScopeInfo* si) const
{
double result = eval_sum(si, m_opands, m_sz);
//IFTRACE << "sum=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Plus::dump(std::ostream& os) const
{
os << "(";
dump_opands(os, m_opands, m_sz, " + ");
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Times
// ----------------------------------------------------------------------
Times::Times(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Times::~Times()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Times::eval(const ScopeInfo* si) const
{
double result = 1.0;
for (int i = 0; i < m_sz; ++i) {
double x = m_opands[i]->eval(si);
result *= x;
}
//IFTRACE << "result=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Times::dump(std::ostream& os) const
{
os << "(";
dump_opands(os, m_opands, m_sz, " * ");
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Max
// ----------------------------------------------------------------------
Max::Max(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Max::~Max()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Max::eval(const ScopeInfo* si) const
{
double result = m_opands[0]->eval(si);
for (int i = 1; i < m_sz; ++i) {
double x = m_opands[i]->eval(si);
result = std::max(result, x);
}
//IFTRACE << "max=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Max::dump(std::ostream& os) const
{
os << "max(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Min
// ----------------------------------------------------------------------
Min::Min(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Min::~Min()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Min::eval(const ScopeInfo* si) const
{
double result = m_opands[0]->eval(si);
for (int i = 1; i < m_sz; ++i) {
double x = m_opands[i]->eval(si);
result = std::min(result, x);
}
//IFTRACE << "min=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Min::dump(std::ostream& os) const
{
os << "min(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Mean
// ----------------------------------------------------------------------
Mean::Mean(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Mean::~Mean()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Mean::eval(const ScopeInfo* si) const
{
double result = eval_mean(si, m_opands, m_sz);
//IFTRACE << "mean=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Mean::dump(std::ostream& os) const
{
os << "mean(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class StdDev
// ----------------------------------------------------------------------
StdDev::StdDev(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
StdDev::~StdDev()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
StdDev::eval(const ScopeInfo* si) const
{
std::pair<double, double> v_m = eval_variance(si, m_opands, m_sz);
double result = sqrt(v_m.first);
//IFTRACE << "stddev=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
StdDev::dump(std::ostream& os) const
{
os << "stddev(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class RStdDev
// ----------------------------------------------------------------------
RStdDev::RStdDev(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
RStdDev::~RStdDev()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
RStdDev::eval(const ScopeInfo* si) const{
std::pair<double, double> v_m = eval_variance(si, m_opands, m_sz);
double sdev = sqrt(v_m.first);
double result = (sdev / v_m.second) * 100;
//IFTRACE << "r-stddev=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
RStdDev::dump(std::ostream& os) const
{
os << "r-stddev(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
//****************************************************************************
} // namespace Metric
} // namespace Prof
<commit_msg>Prof::Metric::RStdDev::eval: handle divide by 0<commit_after>// -*-Mode: C++;-*-
// $Id$
// * BeginRiceCopyright *****************************************************
//
// Copyright ((c)) 2002-2007, Rice University
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions are
// met:
//
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// * Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
//
// * Neither the name of Rice University (RICE) nor the names of its
// contributors may be used to endorse or promote products derived from
// this software without specific prior written permission.
//
// This software is provided by RICE and contributors "as is" and any
// express or implied warranties, including, but not limited to, the
// implied warranties of merchantability and fitness for a particular
// purpose are disclaimed. In no event shall RICE or contributors be
// liable for any direct, indirect, incidental, special, exemplary, or
// consequential damages (including, but not limited to, procurement of
// substitute goods or services; loss of use, data, or profits; or
// business interruption) however caused and on any theory of liability,
// whether in contract, strict liability, or tort (including negligence
// or otherwise) arising in any way out of the use of this software, even
// if advised of the possibility of such damage.
//
// ******************************************************* EndRiceCopyright *
// ----------------------------------------------------------------------
//
// the implementation of evaluation tree nodes
//
// ----------------------------------------------------------------------
//************************ System Include Files ******************************
#include <iostream>
using std::endl;
#include <sstream>
#include <string>
#include <algorithm>
#include <cmath>
//************************* User Include Files *******************************
#include <include/general.h>
#include "Metric-AExpr.hpp"
#include <lib/support/NaN.h>
#include <lib/support/Trace.hpp>
//************************ Forward Declarations ******************************
#define AEXPR_DO_CHECK 0
#if (AEXPR_DO_CHECK)
# define AEXPR_CHECK(x) if (!isok(x)) { return c_FP_NAN_d; }
#else
# define AEXPR_CHECK(x)
#endif
static double epsilon = 0.000001;
//****************************************************************************
namespace Prof {
namespace Metric {
// ----------------------------------------------------------------------
// class AExpr
// ----------------------------------------------------------------------
std::string
AExpr::toString() const
{
std::ostringstream os;
dump(os);
return os.str();
}
void
AExpr::dump_opands(std::ostream& os, AExpr** opands, int sz, const char* sep)
{
for (int i = 0; i < sz; ++i) {
opands[i]->dump(os);
if (i < (sz - 1)) {
os << sep;
}
}
}
// ----------------------------------------------------------------------
// class Const
// ----------------------------------------------------------------------
std::ostream&
Const::dump(std::ostream& os) const
{
os << "(" << m_c << ")";
return os;
}
// ----------------------------------------------------------------------
// class Neg
// ----------------------------------------------------------------------
double
Neg::eval(const ScopeInfo* si) const
{
double result = m_expr->eval(si);
//IFTRACE << "neg=" << -result << endl;
AEXPR_CHECK(result);
return -result;
}
std::ostream&
Neg::dump(std::ostream& os) const
{
os << "(-"; m_expr->dump(os); os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Var
// ----------------------------------------------------------------------
std::ostream&
Var::dump(std::ostream& os) const
{
os << name;
return os;
}
// ----------------------------------------------------------------------
// class Power
// ----------------------------------------------------------------------
Power::Power(AExpr* b, AExpr* e)
: base(b), exponent(e)
{
}
Power::~Power()
{
delete base;
delete exponent;
}
double
Power::eval(const ScopeInfo* si) const
{
double b = base->eval(si);
double e = exponent->eval(si);
double result = pow(b, e);
//IFTRACE << "pow=" << pow(b, e) << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Power::dump(std::ostream& os) const
{
os << "("; base->dump(os); os << "**"; exponent->dump(os); os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Divide
// ----------------------------------------------------------------------
Divide::Divide(AExpr* num, AExpr* denom)
: numerator(num), denominator(denom)
{
}
Divide::~Divide()
{
delete numerator;
delete denominator;
}
double
Divide::eval(const ScopeInfo* si) const
{
double n = numerator->eval(si);
double d = denominator->eval(si);
double result = n / d;
//IFTRACE << "divident=" << n/d << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Divide::dump(std::ostream& os) const
{
os << "(";
numerator->dump(os);
os << " / ";
denominator->dump(os);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Minus
// ----------------------------------------------------------------------
Minus::Minus(AExpr* m, AExpr* s)
: minuend(m), subtrahend(s)
{
// if (minuend == NULL || subtrahend == NULL) { ... }
}
Minus::~Minus()
{
delete minuend;
delete subtrahend;
}
double
Minus::eval(const ScopeInfo* si) const
{
double m = minuend->eval(si);
double s = subtrahend->eval(si);
double result = (m - s);
//IFTRACE << "diff=" << m-s << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Minus::dump(std::ostream& os) const
{
os << "("; minuend->dump(os); os << " - "; subtrahend->dump(os); os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Plus
// ----------------------------------------------------------------------
Plus::Plus(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Plus::~Plus()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Plus::eval(const ScopeInfo* si) const
{
double result = eval_sum(si, m_opands, m_sz);
//IFTRACE << "sum=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Plus::dump(std::ostream& os) const
{
os << "(";
dump_opands(os, m_opands, m_sz, " + ");
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Times
// ----------------------------------------------------------------------
Times::Times(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Times::~Times()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Times::eval(const ScopeInfo* si) const
{
double result = 1.0;
for (int i = 0; i < m_sz; ++i) {
double x = m_opands[i]->eval(si);
result *= x;
}
//IFTRACE << "result=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Times::dump(std::ostream& os) const
{
os << "(";
dump_opands(os, m_opands, m_sz, " * ");
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Max
// ----------------------------------------------------------------------
Max::Max(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Max::~Max()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Max::eval(const ScopeInfo* si) const
{
double result = m_opands[0]->eval(si);
for (int i = 1; i < m_sz; ++i) {
double x = m_opands[i]->eval(si);
result = std::max(result, x);
}
//IFTRACE << "max=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Max::dump(std::ostream& os) const
{
os << "max(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Min
// ----------------------------------------------------------------------
Min::Min(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Min::~Min()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Min::eval(const ScopeInfo* si) const
{
double result = m_opands[0]->eval(si);
for (int i = 1; i < m_sz; ++i) {
double x = m_opands[i]->eval(si);
result = std::min(result, x);
}
//IFTRACE << "min=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Min::dump(std::ostream& os) const
{
os << "min(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class Mean
// ----------------------------------------------------------------------
Mean::Mean(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
Mean::~Mean()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
Mean::eval(const ScopeInfo* si) const
{
double result = eval_mean(si, m_opands, m_sz);
//IFTRACE << "mean=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
Mean::dump(std::ostream& os) const
{
os << "mean(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class StdDev
// ----------------------------------------------------------------------
StdDev::StdDev(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
StdDev::~StdDev()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
StdDev::eval(const ScopeInfo* si) const
{
std::pair<double, double> v_m = eval_variance(si, m_opands, m_sz);
double result = sqrt(v_m.first);
//IFTRACE << "stddev=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
StdDev::dump(std::ostream& os) const
{
os << "stddev(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
// ----------------------------------------------------------------------
// class RStdDev
// ----------------------------------------------------------------------
RStdDev::RStdDev(AExpr** oprnds, int numOprnds)
: m_opands(oprnds), m_sz(numOprnds)
{
}
RStdDev::~RStdDev()
{
for (int i = 0; i < m_sz; ++i) {
delete m_opands[i];
}
delete[] m_opands;
}
double
RStdDev::eval(const ScopeInfo* si) const{
std::pair<double, double> v_m = eval_variance(si, m_opands, m_sz);
double sdev = sqrt(v_m.first); // always non-negative
double mean = v_m.second;
double result = 0.0;
if (mean > epsilon) {
result = (sdev / mean) * 100;
}
//IFTRACE << "r-stddev=" << result << endl;
AEXPR_CHECK(result);
return result;
}
std::ostream&
RStdDev::dump(std::ostream& os) const
{
os << "r-stddev(";
dump_opands(os, m_opands, m_sz);
os << ")";
return os;
}
//****************************************************************************
} // namespace Metric
} // namespace Prof
<|endoftext|>
|
<commit_before>/******************************************************************************
* SOFA, Simulation Open-Framework Architecture, development version *
* (c) 2006-2017 INRIA, USTL, UJF, CNRS, MGH *
* *
* This program is free software; you can redistribute it and/or modify it *
* under the terms of the GNU Lesser General Public License as published by *
* the Free Software Foundation; either version 2.1 of the License, or (at *
* your option) any later version. *
* *
* This program is distributed in the hope that it will be useful, but WITHOUT *
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or *
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License *
* for more details. *
* *
* You should have received a copy of the GNU Lesser General Public License *
* along with this program. If not, see <http://www.gnu.org/licenses/>. *
*******************************************************************************
* Authors: The SOFA Team and external contributors (see Authors.txt) *
* *
* Contact information: contact@sofa-framework.org *
******************************************************************************/
#include <sofa/core/ObjectFactory.h>
#include <SofaGeneralLoader/MeshSTLLoader.h>
#include <sofa/core/visual/VisualParams.h>
#include <sofa/helper/stl.hpp>
#include <iostream>
//#include <fstream> // we can't use iostream because the windows implementation gets confused by the mix of text and binary
#include <cstdio>
#include <sstream>
#include <string>
namespace sofa
{
namespace component
{
namespace loader
{
using namespace sofa::defaulttype;
SOFA_DECL_CLASS(MeshSTLLoader)
int MeshSTLLoaderClass = core::RegisterObject("Specific mesh loader for STL file format.")
.add< MeshSTLLoader >()
.addAlias("MeshStlLoader")
;
//Base VTK Loader
MeshSTLLoader::MeshSTLLoader() : MeshLoader()
, _headerSize(initData(&_headerSize, 80u, "headerSize","Size of the header binary file (just before the number of facet)."))
, _forceBinary(initData(&_forceBinary, false, "forceBinary","Force reading in binary mode. Even in first keyword of the file is solid."))
, d_mergePositionUsingMap(initData(&d_mergePositionUsingMap, true, "mergePositionUsingMap","Since positions are duplicated in a STL, they have to be merged. Using a map to do so will temporarily duplicate memory but should be more efficient. Disable it if memory is really an issue."))
{
}
bool MeshSTLLoader::load()
{
const char* filename = m_filename.getFullPath().c_str();
std::ifstream file(filename);
if (!file.good()) {
msg_error() << "Cannot read file '" << m_filename;
return false;
}
if( helper::stl::is_binary(file) || _forceBinary.getValue() ) {
return this->readBinarySTL(filename);
} else {
return this->readSTL(file);
}
}
bool MeshSTLLoader::readBinarySTL(const char *filename)
{
dmsg_info() << "Reading binary STL file..." ;
std::ifstream dataFile (filename, std::ios::in | std::ios::binary);
helper::vector<sofa::defaulttype::Vector3>& my_positions = *(this->d_positions.beginWriteOnly());
helper::vector<sofa::defaulttype::Vector3>& my_normals = *(this->d_normals.beginWriteOnly());
helper::vector<Triangle >& my_triangles = *(this->d_triangles.beginWriteOnly());
std::map< sofa::defaulttype::Vec3f, core::topology::Topology::index_type > my_map;
core::topology::Topology::index_type positionCounter = 0;
bool useMap = d_mergePositionUsingMap.getValue();
// Skipping header file
char buffer[256];
dataFile.read(buffer, _headerSize.getValue());
// sout << "Header binary file: "<< buffer << sendl;
uint32_t nbrFacet;
dataFile.read((char*)&nbrFacet, 4);
my_triangles.resize( nbrFacet ); // exact size
my_normals.resize( nbrFacet ); // exact size
my_positions.reserve( nbrFacet * 3 ); // max size
#ifndef NDEBUG
{
// checking that the file is large enough to contain the given nb of facets
// store current pos in file
std::streampos pos = dataFile.tellg();
// get length of file
dataFile.seekg(0, std::ios::end);
std::streampos length = dataFile.tellg();
// restore pos in file
dataFile.seekg(pos);
// check for length
assert( length >= _headerSize.getValue() + 4 + nbrFacet * (12 /*normal*/ + 3 * 12 /*points*/ + 2 /*attribute*/ ) );
}
#endif
// temporaries
sofa::defaulttype::Vec3f vertex, normal;
// Parsing facets
for (uint32_t i = 0; i<nbrFacet; ++i)
{
Triangle& the_tri = my_triangles[i];
// Normal:
dataFile.read((char*)&normal[0], 4);
dataFile.read((char*)&normal[1], 4);
dataFile.read((char*)&normal[2], 4);
my_normals[i] = normal;
// Vertices:
for (size_t j = 0; j<3; ++j)
{
dataFile.read((char*)&vertex[0], 4);
dataFile.read((char*)&vertex[1], 4);
dataFile.read((char*)&vertex[2], 4);
if( useMap )
{
auto it = my_map.find( vertex );
if( it == my_map.end() )
{
the_tri[j] = positionCounter;
my_map[vertex] = positionCounter++;
my_positions.push_back(vertex);
}
else
{
the_tri[j] = it->second;
}
}
else
{
bool find = false;
for (size_t k=0; k<my_positions.size(); ++k)
if ( (vertex[0] == my_positions[k][0]) && (vertex[1] == my_positions[k][1]) && (vertex[2] == my_positions[k][2]))
{
find = true;
the_tri[j] = static_cast<core::topology::Topology::PointID>(k);
break;
}
if (!find)
{
my_positions.push_back(vertex);
the_tri[j] = my_positions.size()-1;
}
}
}
// Attribute byte count
uint16_t count;
dataFile.read((char*)&count, 2);
// Security: // checked once before reading in debug mode
// position = dataFile.tellg();
// if (position == length)
// break;
}
this->d_positions.endEdit();
this->d_triangles.endEdit();
this->d_normals.endEdit();
dmsg_info() << "done!" ;
return true;
}
bool MeshSTLLoader::readSTL(std::ifstream& dataFile)
{
dmsg_info() << "Reading STL file..." ;
// Init
std::string buffer;
std::string name; // name of the solid, needed?
helper::vector<sofa::defaulttype::Vector3>& my_positions = *(d_positions.beginEdit());
helper::vector<sofa::defaulttype::Vector3>& my_normals = *(d_normals.beginEdit());
helper::vector<Triangle >& my_triangles = *(d_triangles.beginEdit());
std::map< sofa::defaulttype::Vec3f, core::topology::Topology::index_type > my_map;
core::topology::Topology::index_type positionCounter = 0;
bool useMap = d_mergePositionUsingMap.getValue();
// get length of file:
dataFile.seekg(0, std::ios::end);
std::streampos length = dataFile.tellg();
dataFile.seekg(0, std::ios::beg);
// Reading header
dataFile >> buffer >> name;
Triangle the_tri;
size_t cpt = 0;
std::streampos position = 0;
// Parsing facets
while (position < length)
{
sofa::defaulttype::Vector3 normal, vertex;
std::getline(dataFile, buffer);
std::stringstream line;
std::clog << line.str() << std::endl;
std::string bufferWord;
line >> bufferWord;
if (bufferWord == "facet")
{
line >> bufferWord >> normal[0] >> normal[1] >> normal[2];
my_normals.push_back(normal);
}
else if (bufferWord == "vertex")
{
line >> vertex[0] >> vertex[1] >> vertex[2];
if( useMap )
{
auto it = my_map.find( vertex );
if( it == my_map.end() )
{
the_tri[cpt] = positionCounter;
my_map[vertex] = positionCounter++;
my_positions.push_back(vertex);
}
else
{
the_tri[cpt] = it->second;
}
}
else
{
bool find = false;
for (size_t i=0; i<my_positions.size(); ++i)
if ( (vertex[0] == my_positions[i][0]) && (vertex[1] == my_positions[i][1]) && (vertex[2] == my_positions[i][2]))
{
find = true;
the_tri[cpt] = static_cast<core::topology::Topology::PointID>(i);
break;
}
if (!find)
{
my_positions.push_back(vertex);
the_tri[cpt] = static_cast<core::topology::Topology::PointID>(my_positions.size()-1);
}
}
cpt++;
}
else if (bufferWord == "endfacet")
{
my_triangles.push_back(the_tri);
cpt = 0;
}
else if (bufferWord == "endsolid" || bufferWord == "end")
{
break;
}
position = dataFile.tellg();
}
d_positions.endEdit();
d_triangles.endEdit();
d_normals.endEdit();
dataFile.close();
dmsg_info() << "done!" ;
return true;
}
} // namespace loader
} // namespace component
} // namespace sofa
<commit_msg>fixing ascii stl loading. GRRRRR<commit_after>/******************************************************************************
* SOFA, Simulation Open-Framework Architecture, development version *
* (c) 2006-2017 INRIA, USTL, UJF, CNRS, MGH *
* *
* This program is free software; you can redistribute it and/or modify it *
* under the terms of the GNU Lesser General Public License as published by *
* the Free Software Foundation; either version 2.1 of the License, or (at *
* your option) any later version. *
* *
* This program is distributed in the hope that it will be useful, but WITHOUT *
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or *
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License *
* for more details. *
* *
* You should have received a copy of the GNU Lesser General Public License *
* along with this program. If not, see <http://www.gnu.org/licenses/>. *
*******************************************************************************
* Authors: The SOFA Team and external contributors (see Authors.txt) *
* *
* Contact information: contact@sofa-framework.org *
******************************************************************************/
#include <sofa/core/ObjectFactory.h>
#include <SofaGeneralLoader/MeshSTLLoader.h>
#include <sofa/core/visual/VisualParams.h>
#include <sofa/helper/stl.hpp>
#include <iostream>
//#include <fstream> // we can't use iostream because the windows implementation gets confused by the mix of text and binary
#include <cstdio>
#include <sstream>
#include <string>
namespace sofa
{
namespace component
{
namespace loader
{
using namespace sofa::defaulttype;
SOFA_DECL_CLASS(MeshSTLLoader)
int MeshSTLLoaderClass = core::RegisterObject("Specific mesh loader for STL file format.")
.add< MeshSTLLoader >()
.addAlias("MeshStlLoader")
;
//Base VTK Loader
MeshSTLLoader::MeshSTLLoader() : MeshLoader()
, _headerSize(initData(&_headerSize, 80u, "headerSize","Size of the header binary file (just before the number of facet)."))
, _forceBinary(initData(&_forceBinary, false, "forceBinary","Force reading in binary mode. Even in first keyword of the file is solid."))
, d_mergePositionUsingMap(initData(&d_mergePositionUsingMap, true, "mergePositionUsingMap","Since positions are duplicated in a STL, they have to be merged. Using a map to do so will temporarily duplicate memory but should be more efficient. Disable it if memory is really an issue."))
{
}
bool MeshSTLLoader::load()
{
const char* filename = m_filename.getFullPath().c_str();
std::ifstream file(filename);
if (!file.good()) {
msg_error() << "Cannot read file '" << m_filename;
return false;
}
if( helper::stl::is_binary(file) || _forceBinary.getValue() ) {
return this->readBinarySTL(filename);
} else {
return this->readSTL(file);
}
}
bool MeshSTLLoader::readBinarySTL(const char *filename)
{
dmsg_info() << "Reading binary STL file..." ;
std::ifstream dataFile (filename, std::ios::in | std::ios::binary);
helper::vector<sofa::defaulttype::Vector3>& my_positions = *(this->d_positions.beginWriteOnly());
helper::vector<sofa::defaulttype::Vector3>& my_normals = *(this->d_normals.beginWriteOnly());
helper::vector<Triangle >& my_triangles = *(this->d_triangles.beginWriteOnly());
std::map< sofa::defaulttype::Vec3f, core::topology::Topology::index_type > my_map;
core::topology::Topology::index_type positionCounter = 0;
bool useMap = d_mergePositionUsingMap.getValue();
// Skipping header file
char buffer[256];
dataFile.read(buffer, _headerSize.getValue());
// sout << "Header binary file: "<< buffer << sendl;
uint32_t nbrFacet;
dataFile.read((char*)&nbrFacet, 4);
my_triangles.resize( nbrFacet ); // exact size
my_normals.resize( nbrFacet ); // exact size
my_positions.reserve( nbrFacet * 3 ); // max size
#ifndef NDEBUG
{
// checking that the file is large enough to contain the given nb of facets
// store current pos in file
std::streampos pos = dataFile.tellg();
// get length of file
dataFile.seekg(0, std::ios::end);
std::streampos length = dataFile.tellg();
// restore pos in file
dataFile.seekg(pos);
// check for length
assert( length >= _headerSize.getValue() + 4 + nbrFacet * (12 /*normal*/ + 3 * 12 /*points*/ + 2 /*attribute*/ ) );
}
#endif
// temporaries
sofa::defaulttype::Vec3f vertex, normal;
// Parsing facets
for (uint32_t i = 0; i<nbrFacet; ++i)
{
Triangle& the_tri = my_triangles[i];
// Normal:
dataFile.read((char*)&normal[0], 4);
dataFile.read((char*)&normal[1], 4);
dataFile.read((char*)&normal[2], 4);
my_normals[i] = normal;
// Vertices:
for (size_t j = 0; j<3; ++j)
{
dataFile.read((char*)&vertex[0], 4);
dataFile.read((char*)&vertex[1], 4);
dataFile.read((char*)&vertex[2], 4);
if( useMap )
{
auto it = my_map.find( vertex );
if( it == my_map.end() )
{
the_tri[j] = positionCounter;
my_map[vertex] = positionCounter++;
my_positions.push_back(vertex);
}
else
{
the_tri[j] = it->second;
}
}
else
{
bool find = false;
for (size_t k=0; k<my_positions.size(); ++k)
if ( (vertex[0] == my_positions[k][0]) && (vertex[1] == my_positions[k][1]) && (vertex[2] == my_positions[k][2]))
{
find = true;
the_tri[j] = static_cast<core::topology::Topology::PointID>(k);
break;
}
if (!find)
{
my_positions.push_back(vertex);
the_tri[j] = my_positions.size()-1;
}
}
}
// Attribute byte count
uint16_t count;
dataFile.read((char*)&count, 2);
// Security: // checked once before reading in debug mode
// position = dataFile.tellg();
// if (position == length)
// break;
}
this->d_positions.endEdit();
this->d_triangles.endEdit();
this->d_normals.endEdit();
dmsg_info() << "done!" ;
return true;
}
bool MeshSTLLoader::readSTL(std::ifstream& dataFile)
{
dmsg_info() << "Reading STL file..." ;
// Init
std::string buffer;
std::string name; // name of the solid, needed?
helper::vector<sofa::defaulttype::Vector3>& my_positions = *(d_positions.beginEdit());
helper::vector<sofa::defaulttype::Vector3>& my_normals = *(d_normals.beginEdit());
helper::vector<Triangle >& my_triangles = *(d_triangles.beginEdit());
std::map< sofa::defaulttype::Vec3f, core::topology::Topology::index_type > my_map;
core::topology::Topology::index_type positionCounter = 0;
bool useMap = d_mergePositionUsingMap.getValue();
// get length of file:
dataFile.seekg(0, std::ios::end);
std::streampos length = dataFile.tellg();
dataFile.seekg(0, std::ios::beg);
// Reading header
dataFile >> buffer >> name;
Triangle the_tri;
size_t cpt = 0;
std::streampos position = 0;
// Parsing facets
while (position < length)
{
sofa::defaulttype::Vector3 normal, vertex;
std::getline(dataFile, buffer);
std::stringstream line;
line << buffer;
std::string bufferWord;
line >> bufferWord;
if (bufferWord == "facet")
{
line >> bufferWord >> normal[0] >> normal[1] >> normal[2];
my_normals.push_back(normal);
}
else if (bufferWord == "vertex")
{
line >> vertex[0] >> vertex[1] >> vertex[2];
if( useMap )
{
auto it = my_map.find( vertex );
if( it == my_map.end() )
{
the_tri[cpt] = positionCounter;
my_map[vertex] = positionCounter++;
my_positions.push_back(vertex);
}
else
{
the_tri[cpt] = it->second;
}
}
else
{
bool find = false;
for (size_t i=0; i<my_positions.size(); ++i)
if ( (vertex[0] == my_positions[i][0]) && (vertex[1] == my_positions[i][1]) && (vertex[2] == my_positions[i][2]))
{
find = true;
the_tri[cpt] = static_cast<core::topology::Topology::PointID>(i);
break;
}
if (!find)
{
my_positions.push_back(vertex);
the_tri[cpt] = static_cast<core::topology::Topology::PointID>(my_positions.size()-1);
}
}
cpt++;
}
else if (bufferWord == "endfacet")
{
my_triangles.push_back(the_tri);
cpt = 0;
}
else if (bufferWord == "endsolid" || bufferWord == "end")
{
break;
}
position = dataFile.tellg();
}
d_positions.endEdit();
d_triangles.endEdit();
d_normals.endEdit();
dataFile.close();
dmsg_info() << "done!" ;
return true;
}
} // namespace loader
} // namespace component
} // namespace sofa
<|endoftext|>
|
<commit_before>/**************************************************************************
**
** This file is part of Qt Creator
**
** Copyright (c) 2012 Nokia Corporation and/or its subsidiary(-ies).
**
** Contact: Nokia Corporation (qt-info@nokia.com)
**
**
** GNU Lesser General Public License Usage
**
** This file may be used under the terms of the GNU Lesser General Public
** License version 2.1 as published by the Free Software Foundation and
** appearing in the file LICENSE.LGPL included in the packaging of this file.
** Please review the following information to ensure the GNU Lesser General
** Public License version 2.1 requirements will be met:
** http://www.gnu.org/licenses/old-licenses/lgpl-2.1.html.
**
** In addition, as a special exception, Nokia gives you certain additional
** rights. These rights are described in the Nokia Qt LGPL Exception
** version 1.1, included in the file LGPL_EXCEPTION.txt in this package.
**
** Other Usage
**
** Alternatively, this file may be used in accordance with the terms and
** conditions contained in a signed written agreement between you and Nokia.
**
** If you have questions regarding the use of this file, please contact
** Nokia at qt-info@nokia.com.
**
**************************************************************************/
#include "tcpportsgatherer.h"
#include "qtcassert.h"
#include <QFile>
#include <QStringList>
#include <QProcess>
#ifdef Q_OS_WIN
#include <QLibrary>
#include <winsock2.h>
#include <ws2tcpip.h>
#include <iphlpapi.h>
#endif
namespace Utils {
namespace Internal {
class TcpPortsGathererPrivate
{
public:
TcpPortsGathererPrivate(TcpPortsGatherer::ProtocolFlags protocolFlags)
: protocolFlags(protocolFlags) {}
TcpPortsGatherer::ProtocolFlags protocolFlags;
PortList usedPorts;
void updateWin(TcpPortsGatherer::ProtocolFlags protocolFlags);
void updateLinux(TcpPortsGatherer::ProtocolFlags protocolFlags);
void updateNetstat(TcpPortsGatherer::ProtocolFlags protocolFlags);
};
#ifdef Q_OS_WIN
template <typename Table >
QSet<int> usedTcpPorts(ULONG (__stdcall *Func)(Table*, PULONG, BOOL))
{
Table *table = static_cast<Table*>(malloc(sizeof(Table)));
DWORD dwSize = sizeof(Table);
// get the necessary size into the dwSize variable
DWORD dwRetVal = Func(table, &dwSize, false);
if (dwRetVal == ERROR_INSUFFICIENT_BUFFER) {
free(table);
table = static_cast<Table*>(malloc(dwSize));
}
// get the actual data
QSet<int> result;
dwRetVal = Func(table, &dwSize, false);
if (dwRetVal == NO_ERROR) {
for (quint32 i = 0; i < table->dwNumEntries; i++) {
quint16 port = ntohs(table->table[i].dwLocalPort);
if (!result.contains(port))
result.insert(port);
}
} else {
qWarning() << "TcpPortsGatherer: GetTcpTable failed with" << dwRetVal;
}
free(table);
return result;
}
#endif
void TcpPortsGathererPrivate::updateWin(TcpPortsGatherer::ProtocolFlags protocolFlags)
{
#ifdef Q_OS_WIN
QSet<int> ports;
if (protocolFlags & TcpPortsGatherer::IPv4Protocol)
ports.unite(usedTcpPorts<MIB_TCPTABLE>(GetTcpTable));
//Dynamically load symbol for GetTcp6Table for systems that dont have support for IPV6,
//eg Windows XP
typedef ULONG (__stdcall *GetTcp6TablePtr)(PMIB_TCP6TABLE, PULONG, BOOL);
static GetTcp6TablePtr getTcp6TablePtr = 0;
if (!getTcp6TablePtr)
getTcp6TablePtr = (GetTcp6TablePtr)QLibrary::resolve(QLatin1String("Iphlpapi.dll"),
"GetTcp6Table");
if (getTcp6TablePtr && (protocolFlags & TcpPortsGatherer::IPv6Protocol))
ports.unite(usedTcpPorts<MIB_TCP6TABLE>(getTcp6TablePtr));
foreach (int port, ports) {
if (!usedPorts.contains(port))
usedPorts.addPort(port);
}
#endif
Q_UNUSED(protocolFlags);
}
void TcpPortsGathererPrivate::updateLinux(TcpPortsGatherer::ProtocolFlags protocolFlags)
{
QStringList filePaths;
if (protocolFlags & TcpPortsGatherer::IPv4Protocol)
filePaths.append(QLatin1String("/proc/net/tcp"));
if (protocolFlags & TcpPortsGatherer::IPv6Protocol)
filePaths.append(QLatin1String("/proc/net/tcp6"));
foreach (const QString &filePath, filePaths) {
QFile file(filePath);
if (!file.open(QFile::ReadOnly | QFile::Text)) {
qWarning() << "TcpPortsGatherer: Cannot open file"
<< filePath << ":" << file.errorString();
continue;
}
if (file.atEnd()) // read first line describing the output
file.readLine();
static QRegExp pattern(QLatin1String("^\\s*" // start of line, whitespace
"\\d+:\\s*" // integer, colon, space
"[0-9A-Fa-f]+:" // hexadecimal number (ip), colon
"([0-9A-Fa-f]+)" // hexadecimal number (port!)
));
while (!file.atEnd()) {
QByteArray line = file.readLine();
if (pattern.indexIn(line) != -1) {
bool isNumber;
quint16 port = pattern.cap(1).toUShort(&isNumber, 16);
QTC_ASSERT(isNumber, continue);
if (!usedPorts.contains(port))
usedPorts.addPort(port);
} else {
qWarning() << "TcpPortsGatherer: File" << filePath << "has unexpected format.";
continue;
}
}
}
}
// Only works with FreeBSD version of netstat like we have on Mac OS X
void TcpPortsGathererPrivate::updateNetstat(TcpPortsGatherer::ProtocolFlags protocolFlags)
{
QStringList netstatArgs;
netstatArgs.append(QLatin1String("-a")); // show also sockets of server processes
netstatArgs.append(QLatin1String("-n")); // show network addresses as numbers
netstatArgs.append(QLatin1String("-p"));
netstatArgs.append(QLatin1String("tcp"));
if (protocolFlags != TcpPortsGatherer::AnyIPProcol) {
netstatArgs.append(QLatin1String("-f")); // limit to address family
if (protocolFlags == TcpPortsGatherer::IPv4Protocol)
netstatArgs.append(QLatin1String("inet"));
else
netstatArgs.append(QLatin1String("inet6"));
}
QProcess netstatProcess;
netstatProcess.start(QLatin1String("netstat"), netstatArgs);
if (!netstatProcess.waitForFinished(30000)) {
qWarning() << "TcpPortsGatherer: netstat did not return in time.";
return;
}
QList<QByteArray> output = netstatProcess.readAllStandardOutput().split('\n');
foreach (const QByteArray &line, output) {
static QRegExp pattern(QLatin1String("^tcp[46]+" // "tcp", followed by "4", "6", "46"
"\\s+\\d+" // whitespace, number (Recv-Q)
"\\s+\\d+" // whitespace, number (Send-Q)
"\\s+(\\S+)")); // whitespace, Local Address
if (pattern.indexIn(line) != -1) {
QString localAddress = pattern.cap(1);
// Examples of local addresses:
// '*.56501' , '*.*' 'fe80::1%lo0.123'
int portDelimiterPos = localAddress.lastIndexOf(".");
if (portDelimiterPos == -1)
continue;
localAddress = localAddress.mid(portDelimiterPos + 1);
bool isNumber;
quint16 port = localAddress.toUShort(&isNumber);
if (!isNumber)
continue;
if (!usedPorts.contains(port))
usedPorts.addPort(port);
}
}
}
} // namespace Internal
/*!
\class Utils::TcpPortsGatherer
\brief Gather the list of local TCP ports already in use.
Query the system for the list of local TCP ports already in use. This information can be used
to select a port for use in a range.
*/
TcpPortsGatherer::TcpPortsGatherer(TcpPortsGatherer::ProtocolFlags protocolFlags)
: d(new Internal::TcpPortsGathererPrivate(protocolFlags))
{
update();
}
TcpPortsGatherer::~TcpPortsGatherer()
{
delete d;
}
void TcpPortsGatherer::update()
{
d->usedPorts = PortList();
#if defined(Q_OS_WIN)
d->updateWin(d->protocolFlags);
#elif defined(Q_OS_LINUX)
d->updateLinux(d->protocolFlags);
#else
d->updateNetstat(d->protocolFlags);
#endif
}
PortList TcpPortsGatherer::usedPorts() const
{
return d->usedPorts;
}
/*!
Select a port out of \a freePorts that is not yet used.
Returns the port, or 0 if no free port is available.
*/
quint16 TcpPortsGatherer::getNextFreePort(PortList *freePorts)
{
QTC_ASSERT(freePorts, return 0);
while (freePorts->hasMore()) {
const int port = freePorts->getNext();
if (!d->usedPorts.contains(port))
return port;
}
return 0;
}
} // namespace Utils
<commit_msg>TcpPortsGatherer: Fix compilation with MinGW/4.6.<commit_after>/**************************************************************************
**
** This file is part of Qt Creator
**
** Copyright (c) 2012 Nokia Corporation and/or its subsidiary(-ies).
**
** Contact: Nokia Corporation (qt-info@nokia.com)
**
**
** GNU Lesser General Public License Usage
**
** This file may be used under the terms of the GNU Lesser General Public
** License version 2.1 as published by the Free Software Foundation and
** appearing in the file LICENSE.LGPL included in the packaging of this file.
** Please review the following information to ensure the GNU Lesser General
** Public License version 2.1 requirements will be met:
** http://www.gnu.org/licenses/old-licenses/lgpl-2.1.html.
**
** In addition, as a special exception, Nokia gives you certain additional
** rights. These rights are described in the Nokia Qt LGPL Exception
** version 1.1, included in the file LGPL_EXCEPTION.txt in this package.
**
** Other Usage
**
** Alternatively, this file may be used in accordance with the terms and
** conditions contained in a signed written agreement between you and Nokia.
**
** If you have questions regarding the use of this file, please contact
** Nokia at qt-info@nokia.com.
**
**************************************************************************/
#include "tcpportsgatherer.h"
#include "qtcassert.h"
#include <QFile>
#include <QStringList>
#include <QProcess>
#ifdef Q_OS_WIN
#include <QLibrary>
#include <winsock2.h>
#include <ws2tcpip.h>
#include <iphlpapi.h>
#endif
#if defined(Q_OS_WIN) && defined(Q_CC_MINGW)
// Missing declarations for MinGW. This requires MinGW with gcc 4.6.
typedef enum { } MIB_TCP_STATE;
typedef struct _MIB_TCP6ROW {
MIB_TCP_STATE State;
IN6_ADDR LocalAddr;
DWORD dwLocalScopeId;
DWORD dwLocalPort;
IN6_ADDR RemoteAddr;
DWORD dwRemoteScopeId;
DWORD dwRemotePort;
} MIB_TCP6ROW, *PMIB_TCP6ROW;
typedef struct _MIB_TCP6TABLE {
DWORD dwNumEntries;
MIB_TCP6ROW table[ANY_SIZE];
} MIB_TCP6TABLE, *PMIB_TCP6TABLE;
#endif // defined(Q_OS_WIN) && defined(Q_CC_MINGW)
namespace Utils {
namespace Internal {
class TcpPortsGathererPrivate
{
public:
TcpPortsGathererPrivate(TcpPortsGatherer::ProtocolFlags protocolFlags)
: protocolFlags(protocolFlags) {}
TcpPortsGatherer::ProtocolFlags protocolFlags;
PortList usedPorts;
void updateWin(TcpPortsGatherer::ProtocolFlags protocolFlags);
void updateLinux(TcpPortsGatherer::ProtocolFlags protocolFlags);
void updateNetstat(TcpPortsGatherer::ProtocolFlags protocolFlags);
};
#ifdef Q_OS_WIN
template <typename Table >
QSet<int> usedTcpPorts(ULONG (__stdcall *Func)(Table*, PULONG, BOOL))
{
Table *table = static_cast<Table*>(malloc(sizeof(Table)));
DWORD dwSize = sizeof(Table);
// get the necessary size into the dwSize variable
DWORD dwRetVal = Func(table, &dwSize, false);
if (dwRetVal == ERROR_INSUFFICIENT_BUFFER) {
free(table);
table = static_cast<Table*>(malloc(dwSize));
}
// get the actual data
QSet<int> result;
dwRetVal = Func(table, &dwSize, false);
if (dwRetVal == NO_ERROR) {
for (quint32 i = 0; i < table->dwNumEntries; i++) {
quint16 port = ntohs(table->table[i].dwLocalPort);
if (!result.contains(port))
result.insert(port);
}
} else {
qWarning() << "TcpPortsGatherer: GetTcpTable failed with" << dwRetVal;
}
free(table);
return result;
}
#endif
void TcpPortsGathererPrivate::updateWin(TcpPortsGatherer::ProtocolFlags protocolFlags)
{
#ifdef Q_OS_WIN
QSet<int> ports;
if (protocolFlags & TcpPortsGatherer::IPv4Protocol)
ports.unite(usedTcpPorts<MIB_TCPTABLE>(GetTcpTable));
//Dynamically load symbol for GetTcp6Table for systems that dont have support for IPV6,
//eg Windows XP
typedef ULONG (__stdcall *GetTcp6TablePtr)(PMIB_TCP6TABLE, PULONG, BOOL);
static GetTcp6TablePtr getTcp6TablePtr = 0;
if (!getTcp6TablePtr)
getTcp6TablePtr = (GetTcp6TablePtr)QLibrary::resolve(QLatin1String("Iphlpapi.dll"),
"GetTcp6Table");
if (getTcp6TablePtr && (protocolFlags & TcpPortsGatherer::IPv6Protocol))
ports.unite(usedTcpPorts<MIB_TCP6TABLE>(getTcp6TablePtr));
foreach (int port, ports) {
if (!usedPorts.contains(port))
usedPorts.addPort(port);
}
#endif
Q_UNUSED(protocolFlags);
}
void TcpPortsGathererPrivate::updateLinux(TcpPortsGatherer::ProtocolFlags protocolFlags)
{
QStringList filePaths;
if (protocolFlags & TcpPortsGatherer::IPv4Protocol)
filePaths.append(QLatin1String("/proc/net/tcp"));
if (protocolFlags & TcpPortsGatherer::IPv6Protocol)
filePaths.append(QLatin1String("/proc/net/tcp6"));
foreach (const QString &filePath, filePaths) {
QFile file(filePath);
if (!file.open(QFile::ReadOnly | QFile::Text)) {
qWarning() << "TcpPortsGatherer: Cannot open file"
<< filePath << ":" << file.errorString();
continue;
}
if (file.atEnd()) // read first line describing the output
file.readLine();
static QRegExp pattern(QLatin1String("^\\s*" // start of line, whitespace
"\\d+:\\s*" // integer, colon, space
"[0-9A-Fa-f]+:" // hexadecimal number (ip), colon
"([0-9A-Fa-f]+)" // hexadecimal number (port!)
));
while (!file.atEnd()) {
QByteArray line = file.readLine();
if (pattern.indexIn(line) != -1) {
bool isNumber;
quint16 port = pattern.cap(1).toUShort(&isNumber, 16);
QTC_ASSERT(isNumber, continue);
if (!usedPorts.contains(port))
usedPorts.addPort(port);
} else {
qWarning() << "TcpPortsGatherer: File" << filePath << "has unexpected format.";
continue;
}
}
}
}
// Only works with FreeBSD version of netstat like we have on Mac OS X
void TcpPortsGathererPrivate::updateNetstat(TcpPortsGatherer::ProtocolFlags protocolFlags)
{
QStringList netstatArgs;
netstatArgs.append(QLatin1String("-a")); // show also sockets of server processes
netstatArgs.append(QLatin1String("-n")); // show network addresses as numbers
netstatArgs.append(QLatin1String("-p"));
netstatArgs.append(QLatin1String("tcp"));
if (protocolFlags != TcpPortsGatherer::AnyIPProcol) {
netstatArgs.append(QLatin1String("-f")); // limit to address family
if (protocolFlags == TcpPortsGatherer::IPv4Protocol)
netstatArgs.append(QLatin1String("inet"));
else
netstatArgs.append(QLatin1String("inet6"));
}
QProcess netstatProcess;
netstatProcess.start(QLatin1String("netstat"), netstatArgs);
if (!netstatProcess.waitForFinished(30000)) {
qWarning() << "TcpPortsGatherer: netstat did not return in time.";
return;
}
QList<QByteArray> output = netstatProcess.readAllStandardOutput().split('\n');
foreach (const QByteArray &line, output) {
static QRegExp pattern(QLatin1String("^tcp[46]+" // "tcp", followed by "4", "6", "46"
"\\s+\\d+" // whitespace, number (Recv-Q)
"\\s+\\d+" // whitespace, number (Send-Q)
"\\s+(\\S+)")); // whitespace, Local Address
if (pattern.indexIn(line) != -1) {
QString localAddress = pattern.cap(1);
// Examples of local addresses:
// '*.56501' , '*.*' 'fe80::1%lo0.123'
int portDelimiterPos = localAddress.lastIndexOf(".");
if (portDelimiterPos == -1)
continue;
localAddress = localAddress.mid(portDelimiterPos + 1);
bool isNumber;
quint16 port = localAddress.toUShort(&isNumber);
if (!isNumber)
continue;
if (!usedPorts.contains(port))
usedPorts.addPort(port);
}
}
}
} // namespace Internal
/*!
\class Utils::TcpPortsGatherer
\brief Gather the list of local TCP ports already in use.
Query the system for the list of local TCP ports already in use. This information can be used
to select a port for use in a range.
*/
TcpPortsGatherer::TcpPortsGatherer(TcpPortsGatherer::ProtocolFlags protocolFlags)
: d(new Internal::TcpPortsGathererPrivate(protocolFlags))
{
update();
}
TcpPortsGatherer::~TcpPortsGatherer()
{
delete d;
}
void TcpPortsGatherer::update()
{
d->usedPorts = PortList();
#if defined(Q_OS_WIN)
d->updateWin(d->protocolFlags);
#elif defined(Q_OS_LINUX)
d->updateLinux(d->protocolFlags);
#else
d->updateNetstat(d->protocolFlags);
#endif
}
PortList TcpPortsGatherer::usedPorts() const
{
return d->usedPorts;
}
/*!
Select a port out of \a freePorts that is not yet used.
Returns the port, or 0 if no free port is available.
*/
quint16 TcpPortsGatherer::getNextFreePort(PortList *freePorts)
{
QTC_ASSERT(freePorts, return 0);
while (freePorts->hasMore()) {
const int port = freePorts->getNext();
if (!d->usedPorts.contains(port))
return port;
}
return 0;
}
} // namespace Utils
<|endoftext|>
|
<commit_before>/*
*/
#include "lineFollowing.h"
using namespace std;
using namespace cv;
ControlMovements lineFollowingControl() {
line_main();
return ControlMovements();
}
void line_main() {
// printf("Hello, this is Caleb\n");
VideoCapture cap("../../tests/videos/top_down_1.m4v");
if (!cap.isOpened()) // check if we succeeded
return;
namedWindow("edges", CV_WINDOW_NORMAL);
// Mat edges;
for (; ;) {
Mat mask;
Mat frame, hsv;
cap >> frame; // get a new frame from camera
resize(frame, frame, Size(), .2, .2);
cvtColor(frame, hsv, CV_BGR2HSV);
double minH = 30;
double minS = 0;
double minV = 240;
double maxH = 80;
double maxS = 70;
double maxV = 255;
cv::Scalar lower(minH, minS, minV);
cv::Scalar upper(maxH, maxS, maxV);
cv::inRange(hsv, lower, upper, mask);
// bitwise_and(frame, frame, frame, mask);
vector <Vec4i> lines;
HoughLinesP(mask, lines, 1, CV_PI / 180.0, 10, 100, 10);
printf("Adding in %d lines\n", (int) lines.size());
for (size_t i = 0; i < lines.size(); i++) {
Vec4i l = lines[i];
line(frame, Point(l[0], l[1]), Point(l[2], l[3]), Scalar(0, 0, 255), 3, CV_AA);
// break;
}
// if (lines.size() < 1) continue;
imshow("edges", frame);
if (waitKey(30) >= 0) break;
}
// the camera will be deinitialized automatically in VideoCapture destructor
return;
}
<commit_msg>size changes<commit_after>/*
*/
#include "lineFollowing.h"
using namespace std;
using namespace cv;
ControlMovements lineFollowingControl() {
line_main();
return ControlMovements();
}
void line_main() {
// printf("Hello, this is Caleb\n");
VideoCapture cap("../../tests/videos/top_down_1.m4v");
if (!cap.isOpened()) // check if we succeeded
return;
namedWindow("edges", CV_WINDOW_NORMAL);
// Mat edges;
for (; ;) {
Mat mask;
Mat frame, hsv;
cap >> frame; // get a new frame from camera
resize(frame, frame, Size(), .2, .2);
cvtColor(frame, hsv, CV_BGR2HSV);
double minH = 30;
double minS = 0;
double minV = 240;
double maxH = 80;
double maxS = 70;
double maxV = 255;
cv::Scalar lower(minH, minS, minV);
cv::Scalar upper(maxH, maxS, maxV);
cv::inRange(hsv, lower, upper, mask);
// bitwise_and(frame, frame, frame, mask);
vector <Vec4i> lines;
HoughLinesP(mask, lines, 1, CV_PI / 180.0, 10, 100, 10);
printf("Adding in %d lines\n", (int) lines.size());
for (size_t i = 0; i < lines.size(); i++) {
Vec4i l = lines[i];
line(frame, Point(l[0], l[1]), Point(l[2], l[3]), Scalar(0, 0, 255), 1, CV_AA);
// break;
}
// if (lines.size() < 1) continue;
imshow("edges", frame);
if (waitKey(30) >= 0) break;
}
// the camera will be deinitialized automatically in VideoCapture destructor
return;
}
<|endoftext|>
|
<commit_before>//*******************************************************************
// Copyright (C) 2001 ImageLinks Inc. All rights reserved.
//
// License: See top level LICENSE.txt file.
//
// Author: Oscar Kramer (ossim port by D. Burken)
//
// Description:
//
// Contains definition of class ossimXmlAttribute.
//
//*****************************************************************************
// $Id: ossimXmlAttribute.cpp 23503 2015-09-08 07:07:51Z rashadkm $
#include <iostream>
#include <sstream>
#include <ossim/base/ossimXmlAttribute.h>
#include <ossim/base/ossimNotifyContext.h>
RTTI_DEF2(ossimXmlAttribute, "ossimXmlAttribute", ossimObject, ossimErrorStatusInterface)
static std::istream& xmlskipws(std::istream& in)
{
int c = in.peek();
while((!in.fail())&&
((c == ' ') ||
(c == '\t') ||
(c == '\n')||
(c == '\r')))
{
in.ignore(1);
c = in.peek();
}
return in;
}
ossimXmlAttribute::ossimXmlAttribute(ossimString& spec)
{
std::stringstream in(spec);
read(in);
}
ossimXmlAttribute::ossimXmlAttribute(const ossimXmlAttribute& src)
:theName(src.theName),
theValue(src.theValue)
{
}
bool ossimXmlAttribute::read(std::istream& in)
{
xmlskipws(in);
if(in.fail()) return false;
if(readName(in))
{
xmlskipws(in);
if((in.peek() != '=')||
(in.fail()))
{
setErrorStatus();
return false;
}
in.ignore(1);
if(readValue(in))
{
return true;
}
else
{
setErrorStatus();
return false;
}
}
return false;
#if 0
//
// Pull out name:
//
theName = spec.before('=');
theName = theName.trim();
if (theName.empty())
{
ossimNotify(ossimNotifyLevel_FATAL) << "ossimXmlAttribute::ossimXmlAttribute \n"
<< "Bad attribute format encountered near:\n\""<< spec<<"\"\n"
<< "Parsing aborted...\n";
setErrorStatus();
return;
}
spec = spec.after('=');
//***
// Read value:
//***
char quote_char = spec[0];
spec = spec.after(quote_char); // after first quote
theValue = spec.before(quote_char); // before second quote
//
// Reposition attribute specification to the start of next attribute or end
// of tag:
//
spec = spec.after(quote_char); // after second quote
ossimString next_entry ("-?[+0-9A-Za-z<>]+");
spec = spec.fromRegExp(next_entry.c_str());
#endif
}
ossimXmlAttribute::~ossimXmlAttribute()
{
}
ossimXmlAttribute::ossimXmlAttribute()
{
}
ossimXmlAttribute::ossimXmlAttribute(const ossimString& name,
const ossimString& value)
{
setNameValue(name, value);
}
const ossimString& ossimXmlAttribute::getName() const
{
return theName;
}
const ossimString& ossimXmlAttribute::getValue() const
{
return theValue;
}
void ossimXmlAttribute::setNameValue(const ossimString& name,
const ossimString& value)
{
theName = name;
theValue = value;
}
void ossimXmlAttribute::setName(const ossimString& name)
{
theName = name;
}
void ossimXmlAttribute::setValue(const ossimString& value)
{
theValue = value;
}
std::ostream& operator << (std::ostream& os, const ossimXmlAttribute* xml_attr)
{
os << " " << xml_attr->theName << "=\"" << xml_attr->theValue << "\"";
return os;
}
bool ossimXmlAttribute::readName(std::istream& in)
{
xmlskipws(in);
theName = "";
char c = in.peek();
while((c != ' ')&&
(c != '\n')&&
(c != '\r')&&
(c != '\t')&&
(c != '=')&&
(c != '<')&&
(c != '/')&&
(c != '>')&&
(!in.fail()))
{
theName += (char)in.get();
c = in.peek();
}
return ((!in.fail())&&
(theName != ""));
}
bool ossimXmlAttribute::readValue(std::istream& in)
{
xmlskipws(in);
if(in.fail()) return false;
theValue = "";
char c = in.peek();
bool done = false;
char startQuote = '\0';
if((c == '\'')||
(c == '"'))
{
startQuote = c;
theValue += in.get();
while(!done&&!in.fail())
{
c = in.peek();
if(c==startQuote)
{
theValue += c;
done = true;
in.ignore(1);
}
else if(c == '\n')
{
done = true;
}
else
{
theValue += in.get();
}
}
}
bool is_empty = false;
//then this could be empty with two qoutes
if(theValue.size() == 2 && theValue[0] == startQuote && theValue[1] == startQuote)
{
theValue = "";
is_empty = true;
}
if(theValue != "")
{
std::string::iterator startIter = theValue.begin();
std::string::iterator endIter = theValue.end();
--endIter;
if(*startIter == startQuote)
{
++startIter;
}
else
{
return false;
setErrorStatus();
}
if(*endIter != startQuote)
{
return false;
setErrorStatus();
}
theValue = ossimString(startIter, endIter);
}
return ((!in.bad())&& (is_empty || theValue !=""));
}
<commit_msg>build fails on msvc. error C2999<commit_after>//*******************************************************************
// Copyright (C) 2001 ImageLinks Inc. All rights reserved.
//
// License: See top level LICENSE.txt file.
//
// Author: Oscar Kramer (ossim port by D. Burken)
//
// Description:
//
// Contains definition of class ossimXmlAttribute.
//
//*****************************************************************************
// $Id: ossimXmlAttribute.cpp 23503 2015-09-08 07:07:51Z rashadkm $
#include <iostream>
#include <sstream>
#include <ossim/base/ossimXmlAttribute.h>
#include <ossim/base/ossimNotifyContext.h>
RTTI_DEF2(ossimXmlAttribute, "ossimXmlAttribute", ossimObject, ossimErrorStatusInterface)
static std::istream& xmlskipws(std::istream& in)
{
int c = in.peek();
while((!in.fail())&&
((c == ' ') ||
(c == '\t') ||
(c == '\n')||
(c == '\r')))
{
in.ignore(1);
c = in.peek();
}
return in;
}
ossimXmlAttribute::ossimXmlAttribute(ossimString& spec)
{
std::stringstream in(spec);
read(in);
}
ossimXmlAttribute::ossimXmlAttribute(const ossimXmlAttribute& src)
:theName(src.theName),
theValue(src.theValue)
{
}
bool ossimXmlAttribute::read(std::istream& in)
{
xmlskipws(in);
if(in.fail()) return false;
if(readName(in))
{
xmlskipws(in);
if((in.peek() != '=')||
(in.fail()))
{
setErrorStatus();
return false;
}
in.ignore(1);
if(readValue(in))
{
return true;
}
else
{
setErrorStatus();
return false;
}
}
return false;
#if 0
//
// Pull out name:
//
theName = spec.before('=');
theName = theName.trim();
if (theName.empty())
{
ossimNotify(ossimNotifyLevel_FATAL) << "ossimXmlAttribute::ossimXmlAttribute \n"
<< "Bad attribute format encountered near:\n\""<< spec<<"\"\n"
<< "Parsing aborted...\n";
setErrorStatus();
return;
}
spec = spec.after('=');
//***
// Read value:
//***
char quote_char = spec[0];
spec = spec.after(quote_char); // after first quote
theValue = spec.before(quote_char); // before second quote
//
// Reposition attribute specification to the start of next attribute or end
// of tag:
//
spec = spec.after(quote_char); // after second quote
ossimString next_entry ("-?[+0-9A-Za-z<>]+");
spec = spec.fromRegExp(next_entry.c_str());
#endif
}
ossimXmlAttribute::~ossimXmlAttribute()
{
}
ossimXmlAttribute::ossimXmlAttribute()
{
}
ossimXmlAttribute::ossimXmlAttribute(const ossimString& name,
const ossimString& value)
{
setNameValue(name, value);
}
const ossimString& ossimXmlAttribute::getName() const
{
return theName;
}
const ossimString& ossimXmlAttribute::getValue() const
{
return theValue;
}
void ossimXmlAttribute::setNameValue(const ossimString& name,
const ossimString& value)
{
theName = name;
theValue = value;
}
void ossimXmlAttribute::setName(const ossimString& name)
{
theName = name;
}
void ossimXmlAttribute::setValue(const ossimString& value)
{
theValue = value;
}
std::ostream& operator << (std::ostream& os, const ossimXmlAttribute* xml_attr)
{
os << " " << xml_attr->theName << "=\"" << xml_attr->theValue << "\"";
return os;
}
bool ossimXmlAttribute::readName(std::istream& in)
{
xmlskipws(in);
theName = "";
char c = in.peek();
while((c != ' ')&&
(c != '\n')&&
(c != '\r')&&
(c != '\t')&&
(c != '=')&&
(c != '<')&&
(c != '/')&&
(c != '>')&&
(!in.fail()))
{
theName += (char)in.get();
c = in.peek();
}
return ((!in.fail())&&
(theName != ""));
}
bool ossimXmlAttribute::readValue(std::istream& in)
{
xmlskipws(in);
if(in.fail()) return false;
theValue = "";
char c = in.peek();
bool done = false;
char startQuote = '\0';
if((c == '\'')||
(c == '"'))
{
startQuote = c;
theValue += in.get();
while(!done&&!in.fail())
{
c = in.peek();
if(c==startQuote)
{
theValue += c;
done = true;
in.ignore(1);
}
else if(c == '\n')
{
done = true;
}
else
{
theValue += in.get();
}
}
}
bool is_empty = false;
//then this could be empty with two qoutes
std::string::size_type p = 0;
//then this could be empty with two qoutes
if(theValue.size() == 2 && theValue[p] == startQuote && theValue[p+1] == startQuote)
{
theValue = "";
is_empty = true;
}
if(theValue != "")
{
std::string::iterator startIter = theValue.begin();
std::string::iterator endIter = theValue.end();
--endIter;
if(*startIter == startQuote)
{
++startIter;
}
else
{
return false;
setErrorStatus();
}
if(*endIter != startQuote)
{
return false;
setErrorStatus();
}
theValue = ossimString(startIter, endIter);
}
return ((!in.bad())&& (is_empty || theValue !=""));
}
<|endoftext|>
|
<commit_before>/*
* Copyright (C) 2017 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
#include "src/base/test/vm_test_utils.h"
#include "perfetto/base/build_config.h"
#include "perfetto/ext/base/utils.h"
#include <memory>
#include <errno.h>
#include <string.h>
#if PERFETTO_BUILDFLAG(PERFETTO_OS_WIN)
#include <Windows.h>
#include <Psapi.h>
#else
#include <sys/mman.h>
#include <sys/stat.h>
#endif
#include "perfetto/base/build_config.h"
#include "perfetto/base/logging.h"
namespace perfetto {
namespace base {
namespace vm_test_utils {
bool IsMapped(void* start, size_t size) {
PERFETTO_CHECK(size % kPageSize == 0);
#if PERFETTO_BUILDFLAG(PERFETTO_OS_WIN)
int retries = 5;
int number_of_entries = 4000; // Just a guess.
PSAPI_WORKING_SET_INFORMATION* ws_info = nullptr;
std::vector<char> buffer;
for (;;) {
size_t buffer_size =
sizeof(PSAPI_WORKING_SET_INFORMATION) +
(number_of_entries * sizeof(PSAPI_WORKING_SET_BLOCK));
buffer.resize(buffer_size);
ws_info = reinterpret_cast<PSAPI_WORKING_SET_INFORMATION*>(&buffer[0]);
// On success, |buffer_| is populated with info about the working set of
// |process|. On ERROR_BAD_LENGTH failure, increase the size of the
// buffer and try again.
if (QueryWorkingSet(GetCurrentProcess(), &buffer[0], buffer_size))
break; // Success
PERFETTO_CHECK(GetLastError() == ERROR_BAD_LENGTH);
number_of_entries = ws_info->NumberOfEntries;
// Maybe some entries are being added right now. Increase the buffer to
// take that into account. Increasing by 10% should generally be enough.
number_of_entries *= 1.1;
PERFETTO_CHECK(--retries > 0); // If we're looping, eventually fail.
}
void* end = reinterpret_cast<char*>(start) + size;
// Now scan the working-set information looking for the addresses.
unsigned pages_found = 0;
for (unsigned i = 0; i < ws_info->NumberOfEntries; ++i) {
void* address =
reinterpret_cast<void*>(ws_info->WorkingSetInfo[i].VirtualPage *
kPageSize);
if (address >= start && address < end)
++pages_found;
}
if (pages_found * kPageSize == size)
return true;
return false;
#elif PERFETTO_BUILDFLAG(PERFETTO_OS_FUCHSIA)
// Fuchsia doesn't yet support paging (b/119503290).
return true;
#else
#if PERFETTO_BUILDFLAG(PERFETTO_OS_MACOSX)
using PageState = char;
static constexpr PageState kIncoreMask = MINCORE_INCORE;
#else
using PageState = unsigned char;
static constexpr PageState kIncoreMask = 1;
#endif
const size_t num_pages = size / kPageSize;
std::unique_ptr<PageState[]> page_states(new PageState[num_pages]);
memset(page_states.get(), 0, num_pages * sizeof(PageState));
int res = mincore(start, size, page_states.get());
// Linux returns ENOMEM when an unmapped memory range is passed.
// MacOS instead returns 0 but leaves the page_states empty.
if (res == -1 && errno == ENOMEM)
return false;
PERFETTO_CHECK(res == 0);
for (size_t i = 0; i < num_pages; i++) {
if (!(page_states[i] & kIncoreMask))
return false;
}
return true;
#endif
}
} // namespace vm_test_utils
} // namespace base
} // namespace perfetto
<commit_msg>base: Fix windows build am: 65c898e6e2 am: 58ee913c1b am: 1ad8925c7b am: 4f441f1176<commit_after>/*
* Copyright (C) 2017 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
#include "src/base/test/vm_test_utils.h"
#include "perfetto/base/build_config.h"
#include "perfetto/ext/base/utils.h"
#include <memory>
#include <errno.h>
#include <string.h>
#if PERFETTO_BUILDFLAG(PERFETTO_OS_WIN)
#include <vector>
#include <Windows.h>
#include <Psapi.h>
#else // PERFETTO_BUILDFLAG(PERFETTO_OS_WIN)
#include <sys/mman.h>
#include <sys/stat.h>
#endif // PERFETTO_BUILDFLAG(PERFETTO_OS_WIN)
#include "perfetto/base/build_config.h"
#include "perfetto/base/logging.h"
namespace perfetto {
namespace base {
namespace vm_test_utils {
bool IsMapped(void* start, size_t size) {
PERFETTO_CHECK(size % kPageSize == 0);
#if PERFETTO_BUILDFLAG(PERFETTO_OS_WIN)
int retries = 5;
int number_of_entries = 4000; // Just a guess.
PSAPI_WORKING_SET_INFORMATION* ws_info = nullptr;
std::vector<char> buffer;
for (;;) {
size_t buffer_size =
sizeof(PSAPI_WORKING_SET_INFORMATION) +
(number_of_entries * sizeof(PSAPI_WORKING_SET_BLOCK));
buffer.resize(buffer_size);
ws_info = reinterpret_cast<PSAPI_WORKING_SET_INFORMATION*>(&buffer[0]);
// On success, |buffer_| is populated with info about the working set of
// |process|. On ERROR_BAD_LENGTH failure, increase the size of the
// buffer and try again.
if (QueryWorkingSet(GetCurrentProcess(), &buffer[0], buffer_size))
break; // Success
PERFETTO_CHECK(GetLastError() == ERROR_BAD_LENGTH);
number_of_entries = ws_info->NumberOfEntries;
// Maybe some entries are being added right now. Increase the buffer to
// take that into account. Increasing by 10% should generally be enough.
number_of_entries *= 1.1;
PERFETTO_CHECK(--retries > 0); // If we're looping, eventually fail.
}
void* end = reinterpret_cast<char*>(start) + size;
// Now scan the working-set information looking for the addresses.
unsigned pages_found = 0;
for (unsigned i = 0; i < ws_info->NumberOfEntries; ++i) {
void* address =
reinterpret_cast<void*>(ws_info->WorkingSetInfo[i].VirtualPage *
kPageSize);
if (address >= start && address < end)
++pages_found;
}
if (pages_found * kPageSize == size)
return true;
return false;
#elif PERFETTO_BUILDFLAG(PERFETTO_OS_FUCHSIA)
// Fuchsia doesn't yet support paging (b/119503290).
return true;
#else
#if PERFETTO_BUILDFLAG(PERFETTO_OS_MACOSX)
using PageState = char;
static constexpr PageState kIncoreMask = MINCORE_INCORE;
#else
using PageState = unsigned char;
static constexpr PageState kIncoreMask = 1;
#endif
const size_t num_pages = size / kPageSize;
std::unique_ptr<PageState[]> page_states(new PageState[num_pages]);
memset(page_states.get(), 0, num_pages * sizeof(PageState));
int res = mincore(start, size, page_states.get());
// Linux returns ENOMEM when an unmapped memory range is passed.
// MacOS instead returns 0 but leaves the page_states empty.
if (res == -1 && errno == ENOMEM)
return false;
PERFETTO_CHECK(res == 0);
for (size_t i = 0; i < num_pages; i++) {
if (!(page_states[i] & kIncoreMask))
return false;
}
return true;
#endif
}
} // namespace vm_test_utils
} // namespace base
} // namespace perfetto
<|endoftext|>
|
A subset of CommitPack used for pretraining SantaCoderPack from the OctoPack paper.
It focuses on data where the code before + special token + code after fits into 8192 tokens and on 6 languages. The data is in commit format (cf): <commit_before>code_before<commit_message>commit_message<commit_after>code_after
.