Datasets:
Dataset Viewer
inputs
stringlengths 175
2.22k
| targets
stringlengths 10
2.05k
| language
stringclasses 1
value | split
stringclasses 2
values | template
stringclasses 2
values | dataset
stringclasses 1
value | config
stringclasses 1
value |
---|---|---|---|---|---|---|
I found an interesting problem on https://www.codechef.com/ENDE2020/problems/ENCDEC2:
You are the principal of the Cake school in chefland and today is your birthday. You want to treat each of the children with a small cupcake which is made by you. But there is a problem, You don't know how many students are present today.
The students have gathered of the morning assembly in $R$ rows and $C$ columns. Now you have to calculate how many cakes you have to make such that each child gets a cupcake.
-----Input:-----
- First-line will contain $T$, the number of test cases. Then the test cases follow.
- Each test case contains a single line of input, two integers $R$ and $C$.
-----Output:-----
For each test case, output number of cupcakes you have to make.
-----Constraints-----
- $1 \leq T \leq 1000$
- $2 \leq R,C \leq 10^6$
-----Sample Input:-----
1
5 10
-----Sample Output:-----
50
I tried it in Python, but could not do it. Can you solve it? | # cook your dish here
t=int(input())
for i in range(t):
l=list(map(int,input().split()))
r=l[0]
c=l[1]
print(r*c) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Simon has a prime number x and an array of non-negative integers a_1, a_2, ..., a_{n}.
Simon loves fractions very much. Today he wrote out number $\frac{1}{x^{a} 1} + \frac{1}{x^{a_{2}}} + \ldots + \frac{1}{x^{a_{n}}}$ on a piece of paper. After Simon led all fractions to a common denominator and summed them up, he got a fraction: $\frac{s}{t}$, where number t equals x^{a}_1 + a_2 + ... + a_{n}. Now Simon wants to reduce the resulting fraction.
Help him, find the greatest common divisor of numbers s and t. As GCD can be rather large, print it as a remainder after dividing it by number 1000000007 (10^9 + 7).
-----Input-----
The first line contains two positive integers n and x (1 ≤ n ≤ 10^5, 2 ≤ x ≤ 10^9) — the size of the array and the prime number.
The second line contains n space-separated integers a_1, a_2, ..., a_{n} (0 ≤ a_1 ≤ a_2 ≤ ... ≤ a_{n} ≤ 10^9).
-----Output-----
Print a single number — the answer to the problem modulo 1000000007 (10^9 + 7).
-----Examples-----
Input
2 2
2 2
Output
8
Input
3 3
1 2 3
Output
27
Input
2 2
29 29
Output
73741817
Input
4 5
0 0 0 0
Output
1
-----Note-----
In the first sample $\frac{1}{4} + \frac{1}{4} = \frac{4 + 4}{16} = \frac{8}{16}$. Thus, the answer to the problem is 8.
In the second sample, $\frac{1}{3} + \frac{1}{9} + \frac{1}{27} = \frac{243 + 81 + 27}{729} = \frac{351}{729}$. The answer to the problem is 27, as 351 = 13·27, 729 = 27·27.
In the third sample the answer to the problem is 1073741824 mod 1000000007 = 73741817.
In the fourth sample $\frac{1}{1} + \frac{1}{1} + \frac{1}{1} + \frac{1}{1} = \frac{4}{1}$. Thus, the answer to the problem is 1. | n, x = map(int, input().split())
t = list(map(int, input().split()))
b = max(t)
k = t.count(b)
r = sum(t)
s = r - b
while k % x == 0:
b -= 1
s += 1
k = k // x + t.count(b)
print(pow(x, min(r, s), 1000000007)) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
During the quarantine, Sicromoft has more free time to create the new functions in "Celex-2021". The developers made a new function GAZ-GIZ, which infinitely fills an infinite table to the right and down from the upper left corner as follows:
[Image] The cell with coordinates $(x, y)$ is at the intersection of $x$-th row and $y$-th column. Upper left cell $(1,1)$ contains an integer $1$.
The developers of the SUM function don't sleep either. Because of the boredom, they teamed up with the developers of the RAND function, so they added the ability to calculate the sum on an arbitrary path from one cell to another, moving down or right. Formally, from the cell $(x,y)$ in one step you can move to the cell $(x+1, y)$ or $(x, y+1)$.
After another Dinwows update, Levian started to study "Celex-2021" (because he wants to be an accountant!). After filling in the table with the GAZ-GIZ function, he asked you to calculate the quantity of possible different amounts on the path from a given cell $(x_1, y_1)$ to another given cell $(x_2, y_2$), if you can only move one cell down or right.
Formally, consider all the paths from the cell $(x_1, y_1)$ to cell $(x_2, y_2)$ such that each next cell in the path is located either to the down or to the right of the previous one. Calculate the number of different sums of elements for all such paths.
-----Input-----
The first line contains one integer $t$ ($1 \le t \le 57179$) — the number of test cases.
Each of the following $t$ lines contains four natural numbers $x_1$, $y_1$, $x_2$, $y_2$ ($1 \le x_1 \le x_2 \le 10^9$, $1 \le y_1 \le y_2 \le 10^9$) — coordinates of the start and the end cells.
-----Output-----
For each test case, in a separate line, print the number of possible different sums on the way from the start cell to the end cell.
-----Example-----
Input
4
1 1 2 2
1 2 2 4
179 1 179 100000
5 7 5 7
Output
2
3
1
1
-----Note-----
In the first test case there are two possible sums: $1+2+5=8$ and $1+3+5=9$. [Image] | import sys
input = sys.stdin.readline
def value(x,y):
ANS=(x+y-2)*(x+y-1)//2
return ANS+x
t=int(input())
for tests in range(t):
x1,y1,x2,y2=list(map(int,input().split()))
print(1+(x2-x1)*(y2-y1)) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Recently, Olya received a magical square with the size of $2^n\times 2^n$.
It seems to her sister that one square is boring. Therefore, she asked Olya to perform exactly $k$ splitting operations.
A Splitting operation is an operation during which Olya takes a square with side $a$ and cuts it into 4 equal squares with side $\dfrac{a}{2}$. If the side of the square is equal to $1$, then it is impossible to apply a splitting operation to it (see examples for better understanding).
Olya is happy to fulfill her sister's request, but she also wants the condition of Olya's happiness to be satisfied after all operations.
The condition of Olya's happiness will be satisfied if the following statement is fulfilled:
Let the length of the side of the lower left square be equal to $a$, then the length of the side of the right upper square should also be equal to $a$. There should also be a path between them that consists only of squares with the side of length $a$. All consecutive squares on a path should have a common side.
Obviously, as long as we have one square, these conditions are met. So Olya is ready to fulfill her sister's request only under the condition that she is satisfied too. Tell her: is it possible to perform exactly $k$ splitting operations in a certain order so that the condition of Olya's happiness is satisfied? If it is possible, tell also the size of the side of squares of which the path from the lower left square to the upper right one will consist.
-----Input-----
The first line contains one integer $t$ ($1 \le t \le 10^3$) — the number of tests.
Each of the following $t$ lines contains two integers $n_i$ and $k_i$ ($1 \le n_i \le 10^9, 1 \le k_i \le 10^{18}$) — the description of the $i$-th test, which means that initially Olya's square has size of $2^{n_i}\times 2^{n_i}$ and Olya's sister asks her to do exactly $k_i$ splitting operations.
-----Output-----
Print $t$ lines, where in the $i$-th line you should output "YES" if it is possible to perform $k_i$ splitting operations in the... | import sys
import math
input = sys.stdin.readline
testcase=int(input())
T=[list(map(int,input().split())) for i in range(testcase)]
def bi(n,k):
MIN=0
MAX=n
while MAX>MIN+1:
bn=(MIN+MAX)//2
if math.log2(k+2+bn)<bn+1:
MAX=bn
elif math.log2(k+2+bn)==bn+1:
return bn
else:
MIN=bn
if MAX+1<=math.log2(k+2+MAX):
return MAX
return MIN
for n,k in T:
if n==1:
if k==1:
print("YES",0)
else:
print("NO")
continue
if n==2:
if 1<=k<=2:
print("YES",1)
elif k==3:
print("NO")
elif 4<=k<=5:
print("YES",0)
else:
print("NO")
continue
if n<=30 and k>(pow(4,n)-1)//3:
print("NO")
continue
ANS=bi(n,k)
print("YES",n-ANS) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Recently you have received two positive integer numbers $x$ and $y$. You forgot them, but you remembered a shuffled list containing all divisors of $x$ (including $1$ and $x$) and all divisors of $y$ (including $1$ and $y$). If $d$ is a divisor of both numbers $x$ and $y$ at the same time, there are two occurrences of $d$ in the list.
For example, if $x=4$ and $y=6$ then the given list can be any permutation of the list $[1, 2, 4, 1, 2, 3, 6]$. Some of the possible lists are: $[1, 1, 2, 4, 6, 3, 2]$, $[4, 6, 1, 1, 2, 3, 2]$ or $[1, 6, 3, 2, 4, 1, 2]$.
Your problem is to restore suitable positive integer numbers $x$ and $y$ that would yield the same list of divisors (possibly in different order).
It is guaranteed that the answer exists, i.e. the given list of divisors corresponds to some positive integers $x$ and $y$.
-----Input-----
The first line contains one integer $n$ ($2 \le n \le 128$) — the number of divisors of $x$ and $y$.
The second line of the input contains $n$ integers $d_1, d_2, \dots, d_n$ ($1 \le d_i \le 10^4$), where $d_i$ is either divisor of $x$ or divisor of $y$. If a number is divisor of both numbers $x$ and $y$ then there are two copies of this number in the list.
-----Output-----
Print two positive integer numbers $x$ and $y$ — such numbers that merged list of their divisors is the permutation of the given list of integers. It is guaranteed that the answer exists.
-----Example-----
Input
10
10 2 8 1 2 4 1 20 4 5
Output
20 8 | n = int(input())
a = list(map(int, input().split()))
x = max(a)
b = []
for i in range(1, x + 1) :
if x % i == 0 :
b.append(i)
for i in range(len(b)) :
a.remove(b[i])
print(x, max(a)) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/problems/ADTRI:
Rupsa really loves triangles. One day she came across an equilateral triangle having length of each side as an integer N. She started wondering if it was possible to transform the triangle keeping two sides fixed and alter the third side such that it still remains a triangle, but the altered side will have its length as an even integer, and the line drawn from the opposite vertex to the mid-point of the altered side is of integral length.
Since Rupsa is in a hurry to record a song for Chef as he really loves her songs, you must help her solve the problem as fast as possible.
-----Input-----
The first line of input contains an integer T denoting the number of test cases.
Each test-case contains a single integer N.
-----Output-----
For each test case, output "YES" if the triangle transformation is possible, otherwise "NO" (quotes for clarity only, do not output).
-----Constraints-----
- 1 ≤ T ≤ 106
- 1 ≤ N ≤ 5 x 106
-----Sub tasks-----
- Subtask #1: 1 ≤ T ≤ 100, 1 ≤ N ≤ 104 (10 points)
- Subtask #2: 1 ≤ T ≤ 104, 1 ≤ N ≤ 106 (30 points)
- Subtask #3: Original Constraints (60 points)
-----Example-----
Input:2
5
3
Output:YES
NO
-----Explanation-----
- In test case 1, make the length of any one side 6, and it will suffice.
I tried it in Python, but could not do it. Can you solve it? | t=int(input())
import math
for i in range(t):
b=[]
n=int(input())
for p in range(n+1,2*n):
if p%2==0:
a= math.sqrt(n**2 - (p/2)**2)
if a-int(a)==0:
b.append(i)
if len(b)==0:
print("NO")
elif len(b)!=0:
print("YES") | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1392/F:
Omkar is standing at the foot of Celeste mountain. The summit is $n$ meters away from him, and he can see all of the mountains up to the summit, so for all $1 \leq j \leq n$ he knows that the height of the mountain at the point $j$ meters away from himself is $h_j$ meters. It turns out that for all $j$ satisfying $1 \leq j \leq n - 1$, $h_j < h_{j + 1}$ (meaning that heights are strictly increasing).
Suddenly, a landslide occurs! While the landslide is occurring, the following occurs: every minute, if $h_j + 2 \leq h_{j + 1}$, then one square meter of dirt will slide from position $j + 1$ to position $j$, so that $h_{j + 1}$ is decreased by $1$ and $h_j$ is increased by $1$. These changes occur simultaneously, so for example, if $h_j + 2 \leq h_{j + 1}$ and $h_{j + 1} + 2 \leq h_{j + 2}$ for some $j$, then $h_j$ will be increased by $1$, $h_{j + 2}$ will be decreased by $1$, and $h_{j + 1}$ will be both increased and decreased by $1$, meaning that in effect $h_{j + 1}$ is unchanged during that minute.
The landslide ends when there is no $j$ such that $h_j + 2 \leq h_{j + 1}$. Help Omkar figure out what the values of $h_1, \dots, h_n$ will be after the landslide ends. It can be proven that under the given constraints, the landslide will always end in finitely many minutes.
Note that because of the large amount of input, it is recommended that your code uses fast IO.
-----Input-----
The first line contains a single integer $n$ ($1 \leq n \leq 10^6$).
The second line contains $n$ integers $h_1, h_2, \dots, h_n$ satisfying $0 \leq h_1 < h_2 < \dots < h_n \leq 10^{12}$ — the heights.
-----Output-----
Output $n$ integers, where the $j$-th integer is the value of $h_j$ after the landslide has stopped.
-----Example-----
Input
4
2 6 7 8
Output
5 5 6 7
-----Note-----
Initially, the mountain has heights $2, 6, 7, 8$.
In the first minute, we have $2 + 2 \leq 6$, so $2$ increases to $3$ and $6$ decreases to $5$, leaving $3, 5, 7, 8$.
In the second minute, we have $3 + 2 \leq 5$ and $5 + 2 \leq 7$, so...
I tried it in Python, but could not do it. Can you solve it? | n = int(input());tot = sum(map(int, input().split()));extra = (n * (n - 1))//2;smol = (tot - extra) // n;out = [smol + i for i in range(n)]
for i in range(tot - sum(out)):out[i] += 1
print(' '.join(map(str,out))) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5ae8e14c1839f14b2c00007a:
# Idea
In the world of graphs exists a structure called "spanning tree". It is unique because it's created not on its own, but based on other graphs. To make a spanning tree out of a given graph you should remove all the edges which create cycles, for example:
```
This can become this or this or this
A A A A
|\ | \ |\
| \ ==> | \ | \
|__\ |__ __\ | \
B C B C B C B C
```
Each *edge* (line between 2 *vertices*, i.e. points) has a weight, based on which you can build minimum and maximum spanning trees (sum of weights of vertices in the resulting tree is minimal/maximal possible).
[Wikipedia article](https://en.wikipedia.org/wiki/Spanning_tree) on spanning trees, in case you need it.
___
# Task
You will receive an array like this: `[["AB", 2], ["BC", 4], ["AC", 1]]` which includes all edges of an arbitrary graph and a string `"min"`/`"max"`. Based on them you should get and return a new array which includes only those edges which form a minimum/maximum spanning trees.
```python
edges = [("AB", 2), ("BC", 4), ("AC", 1)]
make_spanning_tree(edges, "min") ==> [("AB", 2), ("AC", 1)]
make_spanning_tree(edges, "max") ==> [("AB", 2), ("BC", 4)]
```
___
# Notes
* All vertices will be connected with each other
* You may receive cycles, for example - `["AA", n]`
* The subject of the test are these 3 values: number of vertices included, total weight, number of edges, but **you should not return them**, there's a special function which will analyze your output instead
I tried it in Python, but could not do it. Can you solve it? | def make_spanning_tree(edges, t):
edges.sort(reverse=True)
last_node = ord(edges[0][0][0]) - 65
edges.sort()
edges.sort(key=lambda edges: edges[1])
if(t == 'max'):
edges.reverse()
nonlocal parent
parent = [int(x) for x in range(last_node+10000)]
result = []
for edge in edges:
startNode = ord(edge[0][0])-65
endnode = ord(edge[0][1])-65
if(Find(startNode) != Find(endnode)):
Union(startNode, endnode)
result.append(edge)
return result
def Find(x):
if(x == parent[x]):
return x
else:
p = Find(parent[x])
parent[x] = p
return p
def Union(x, y):
x = Find(x)
y = Find(y)
if(x != y):
parent[y] = x | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Consider a football tournament where n teams participate. Each team has two football kits: for home games, and for away games. The kit for home games of the i-th team has color x_{i} and the kit for away games of this team has color y_{i} (x_{i} ≠ y_{i}).
In the tournament, each team plays exactly one home game and exactly one away game with each other team (n(n - 1) games in total). The team, that plays the home game, traditionally plays in its home kit. The team that plays an away game plays in its away kit. However, if two teams has the kits of the same color, they cannot be distinguished. In this case the away team plays in its home kit.
Calculate how many games in the described tournament each team plays in its home kit and how many games it plays in its away kit.
-----Input-----
The first line contains a single integer n (2 ≤ n ≤ 10^5) — the number of teams. Next n lines contain the description of the teams. The i-th line contains two space-separated numbers x_{i}, y_{i} (1 ≤ x_{i}, y_{i} ≤ 10^5; x_{i} ≠ y_{i}) — the color numbers for the home and away kits of the i-th team.
-----Output-----
For each team, print on a single line two space-separated integers — the number of games this team is going to play in home and away kits, correspondingly. Print the answers for the teams in the order they appeared in the input.
-----Examples-----
Input
2
1 2
2 1
Output
2 0
2 0
Input
3
1 2
2 1
1 3
Output
3 1
4 0
2 2 | n = int( input() )
x, y = [0] * n, [0] * n
for i in range( n ):
x[i], y[i] = map( int, input().split() )
ans = [0] * 100001
for elm in x:
ans[elm] += 1
for elm in y:
print( n - 1 + ans[elm], n - 1 - ans[elm] ) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
In this kata, you have to define a function named **func** that will take a list as input.
You must try and guess the pattern how we get the output number and return list - **[output number,binary representation,octal representation,hexadecimal representation]**, but **you must convert that specific number without built-in : bin,oct and hex functions.**
Examples :
```python
func([12,13,6,3,6,45,123]) returns - [29,'11101','35','1d']
func([1,9,23,43,65,31,63,99]) returns - [41,'101001','51','29']
func([2,4,6,8,10,12,14,16,18,19]) returns - [10,'1010','12','a']
``` | def binary(x):
result = []
while True:
remainder = x % 2
result.append(str(remainder))
x = x // 2
if x == 0:
break
result.reverse()
return "".join(result)
def octal(x):
result = []
while True:
remainder = x % 8
result.append(str(remainder))
x = x // 8
if x == 0:
break
result.reverse()
return "".join(result)
def hexadecimal(x):
result = []
while True:
remainder = x % 16
if remainder == 10:
result.append('a')
elif remainder == 11:
result.append('b')
elif remainder == 12:
result.append('c')
elif remainder == 13:
result.append('d')
elif remainder == 14:
result.append('e')
elif remainder == 15:
result.append('f')
else:
result.append(str(remainder))
x = x // 16
if x == 0:
break
result.reverse()
return "".join(result)
import math
def func(l):
average = math.floor(sum(l) / len(l))
result = [average, binary(average), octal(average), hexadecimal(average)]
return result | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/424/A:
Pasha has many hamsters and he makes them work out. Today, n hamsters (n is even) came to work out. The hamsters lined up and each hamster either sat down or stood up.
For another exercise, Pasha needs exactly $\frac{n}{2}$ hamsters to stand up and the other hamsters to sit down. In one minute, Pasha can make some hamster ether sit down or stand up. How many minutes will he need to get what he wants if he acts optimally well?
-----Input-----
The first line contains integer n (2 ≤ n ≤ 200; n is even). The next line contains n characters without spaces. These characters describe the hamsters' position: the i-th character equals 'X', if the i-th hamster in the row is standing, and 'x', if he is sitting.
-----Output-----
In the first line, print a single integer — the minimum required number of minutes. In the second line, print a string that describes the hamsters' position after Pasha makes the required changes. If there are multiple optimal positions, print any of them.
-----Examples-----
Input
4
xxXx
Output
1
XxXx
Input
2
XX
Output
1
xX
Input
6
xXXxXx
Output
0
xXXxXx
I tried it in Python, but could not do it. Can you solve it? | n, t = int(input()), input()
a, b = 'x', 'X'
k = t.count(a) - n // 2
if k:
t = list(t)
if k < 0: a, b, k = b, a, -k
print(k)
for i, c in enumerate(t):
if c == a:
t[i] = b
k -= 1
if k == 0: break
print(''.join(t))
else: print('0\n' + t) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5c765a4f29e50e391e1414d4:
[Haikus](https://en.wikipedia.org/wiki/Haiku_in_English) are short poems in a three-line format, with 17 syllables arranged in a 5–7–5 pattern. Your task is to check if the supplied text is a haiku or not.
### About syllables
[Syllables](https://en.wikipedia.org/wiki/Syllable) are the phonological building blocks of words. *In this kata*, a syllable is a part of a word including a vowel ("a-e-i-o-u-y") or a group of vowels (e.g. "ou", "ee", "ay"). A few examples: "tea", "can", "to·day", "week·end", "el·e·phant".
**However**, silent "E"s **do not** create syllables. *In this kata*, an "E" is considered silent if it's alone at the end of the word, preceded by one (or more) consonant(s) and there is at least one other syllable in the word. Examples: "age", "ar·range", "con·crete"; but not in "she", "blue", "de·gree".
Some more examples:
* one syllable words: "cat", "cool", "sprout", "like", "eye", "squeeze"
* two syllables words: "ac·count", "hon·est", "beau·ty", "a·live", "be·cause", "re·store"
## Examples
```
An old silent pond...
A frog jumps into the pond,
splash! Silence again.
```
...should return `True`, as this is a valid 5–7–5 haiku:
```
An old si·lent pond... # 5 syllables
A frog jumps in·to the pond, # 7
splash! Si·lence a·gain. # 5
```
Another example:
```
Autumn moonlight -
a worm digs silently
into the chestnut.
```
...should return `False`, because the number of syllables per line is not correct:
```
Au·tumn moon·light - # 4 syllables
a worm digs si·lent·ly # 6
in·to the chest·nut. # 5
```
---
## My other katas
If you enjoyed this kata then please try [my other katas](https://www.codewars.com/collections/katas-created-by-anter69)! :-)
I tried it in Python, but could not do it. Can you solve it? | def is_haiku(s,v='aeiouy'):
C=[]
for x in[a.strip('.;:!?,').split()for a in s.lower().split('\n')]:
t=''
for y in x:
if y.endswith('e')and any(c in y[:-1]for c in v):y=y[:-1]
for c in y:t+=c if c in v else' 'if t and t[-1]!=' 'else''
t+=' '
C+=[len(t.split())]
return C==[5,7,5] | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Takahashi has K 500-yen coins. (Yen is the currency of Japan.)
If these coins add up to X yen or more, print Yes; otherwise, print No.
-----Constraints-----
- 1 \leq K \leq 100
- 1 \leq X \leq 10^5
-----Input-----
Input is given from Standard Input in the following format:
K X
-----Output-----
If the coins add up to X yen or more, print Yes; otherwise, print No.
-----Sample Input-----
2 900
-----Sample Output-----
Yes
Two 500-yen coins add up to 1000 yen, which is not less than X = 900 yen. | a,b=input().split();print('YNeos'[eval(a+'*500<'+b)::2]) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1364/D:
Given a connected undirected graph with $n$ vertices and an integer $k$, you have to either: either find an independent set that has exactly $\lceil\frac{k}{2}\rceil$ vertices. or find a simple cycle of length at most $k$.
An independent set is a set of vertices such that no two of them are connected by an edge. A simple cycle is a cycle that doesn't contain any vertex twice.
I have a proof that for any input you can always solve at least one of these problems, but it's left as an exercise for the reader.
-----Input-----
The first line contains three integers $n$, $m$, and $k$ ($3 \le k \le n \le 10^5$, $n-1 \le m \le 2 \cdot 10^5$) — the number of vertices and edges in the graph, and the parameter $k$ from the statement.
Each of the next $m$ lines contains two integers $u$ and $v$ ($1 \le u,v \le n$) that mean there's an edge between vertices $u$ and $v$. It's guaranteed that the graph is connected and doesn't contain any self-loops or multiple edges.
-----Output-----
If you choose to solve the first problem, then on the first line print $1$, followed by a line containing $\lceil\frac{k}{2}\rceil$ distinct integers not exceeding $n$, the vertices in the desired independent set.
If you, however, choose to solve the second problem, then on the first line print $2$, followed by a line containing one integer, $c$, representing the length of the found cycle, followed by a line containing $c$ distinct integers not exceeding $n$, the vertices in the desired cycle, in the order they appear in the cycle.
-----Examples-----
Input
4 4 3
1 2
2 3
3 4
4 1
Output
1
1 3
Input
4 5 3
1 2
2 3
3 4
4 1
2 4
Output
2
3
2 3 4
Input
4 6 3
1 2
2 3
3 4
4 1
1 3
2 4
Output
2
3
1 2 3
Input
5 4 5
1 2
1 3
2 4
2 5
Output
1
1 4 5
-----Note-----
In the first sample:
[Image]
Notice that printing the independent set $\{2,4\}$ is also OK, but printing the cycle $1-2-3-4$ isn't, because its length must be at most $3$.
In the second sample:
$N$
Notice that printing the independent set $\{1,3\}$ or printing the cycle...
I tried it in Python, but could not do it. Can you solve it? | import sys
input = sys.stdin.readline
from collections import deque
class Graph(object):
"""docstring for Graph"""
def __init__(self,n,d): # Number of nodes and d is True if directed
self.n = n
self.graph = [[] for i in range(n)]
self.parent = [-1 for i in range(n)]
self.directed = d
def addEdge(self,x,y):
self.graph[x].append(y)
if not self.directed:
self.graph[y].append(x)
def bfs(self, root): # NORMAL BFS
queue = [root]
queue = deque(queue)
vis = [0]*self.n
while len(queue)!=0:
element = queue.popleft()
vis[element] = 1
for i in self.graph[element]:
if vis[i]==0:
queue.append(i)
self.parent[i] = element
vis[i] = 1
def dfs(self, root, ans): # Iterative DFS
stack=[root]
vis=[0]*self.n
stack2=[]
while len(stack)!=0: # INITIAL TRAVERSAL
element = stack.pop()
if vis[element]:
continue
vis[element] = 1
stack2.append(element)
for i in self.graph[element]:
if vis[i]==0:
self.parent[i] = element
stack.append(i)
while len(stack2)!=0: # BACKTRACING. Modify the loop according to the question
element = stack2.pop()
m = 0
for i in self.graph[element]:
if i!=self.parent[element]:
m += ans[i]
ans[element] = m
return ans
def shortestpath(self, source, dest): # Calculate Shortest Path between two nodes
self.bfs(source)
path = [dest]
while self.parent[path[-1]]!=-1:
path.append(parent[path[-1]])
return path[::-1]
def ifcycle(self):
queue = [0]
vis = [0]*n
queue = deque(queue)
while len(queue)!=0:
element = queue.popleft()
vis[element] = 1
for i in self.graph[element]:
if vis[i]==1 and i!=self.parent[element]:
s = i
e = element
path1 = [s]
path2 = [e]
while self.parent[s]!=-1:
s = self.parent[s]
path1.append(s)
while self.parent[e]!=-1:
e = self.parent[e]
path2.append(e)
for i in range(-1,max(-len(path1),-len(path2))-1,-1):
if path1[i]!=path2[i]:
return path1[0:i+1]+path2[i+1::-1]
if... | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1108/B:
Recently you have received two positive integer numbers $x$ and $y$. You forgot them, but you remembered a shuffled list containing all divisors of $x$ (including $1$ and $x$) and all divisors of $y$ (including $1$ and $y$). If $d$ is a divisor of both numbers $x$ and $y$ at the same time, there are two occurrences of $d$ in the list.
For example, if $x=4$ and $y=6$ then the given list can be any permutation of the list $[1, 2, 4, 1, 2, 3, 6]$. Some of the possible lists are: $[1, 1, 2, 4, 6, 3, 2]$, $[4, 6, 1, 1, 2, 3, 2]$ or $[1, 6, 3, 2, 4, 1, 2]$.
Your problem is to restore suitable positive integer numbers $x$ and $y$ that would yield the same list of divisors (possibly in different order).
It is guaranteed that the answer exists, i.e. the given list of divisors corresponds to some positive integers $x$ and $y$.
-----Input-----
The first line contains one integer $n$ ($2 \le n \le 128$) — the number of divisors of $x$ and $y$.
The second line of the input contains $n$ integers $d_1, d_2, \dots, d_n$ ($1 \le d_i \le 10^4$), where $d_i$ is either divisor of $x$ or divisor of $y$. If a number is divisor of both numbers $x$ and $y$ then there are two copies of this number in the list.
-----Output-----
Print two positive integer numbers $x$ and $y$ — such numbers that merged list of their divisors is the permutation of the given list of integers. It is guaranteed that the answer exists.
-----Example-----
Input
10
10 2 8 1 2 4 1 20 4 5
Output
20 8
I tried it in Python, but could not do it. Can you solve it? | n = int(input())
a = list(map(int, input().split()))
x = max(a)
b = []
for i in range(1, x + 1) :
if x % i == 0 :
b.append(i)
for i in range(len(b)) :
a.remove(b[i])
print(x, max(a)) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
The only difference between easy and hard versions is the number of elements in the array.
You are given an array $a$ consisting of $n$ integers. In one move you can choose any $a_i$ and divide it by $2$ rounding down (in other words, in one move you can set $a_i := \lfloor\frac{a_i}{2}\rfloor$).
You can perform such an operation any (possibly, zero) number of times with any $a_i$.
Your task is to calculate the minimum possible number of operations required to obtain at least $k$ equal numbers in the array.
Don't forget that it is possible to have $a_i = 0$ after some operations, thus the answer always exists.
-----Input-----
The first line of the input contains two integers $n$ and $k$ ($1 \le k \le n \le 2 \cdot 10^5$) — the number of elements in the array and the number of equal numbers required.
The second line of the input contains $n$ integers $a_1, a_2, \dots, a_n$ ($1 \le a_i \le 2 \cdot 10^5$), where $a_i$ is the $i$-th element of $a$.
-----Output-----
Print one integer — the minimum possible number of operations required to obtain at least $k$ equal numbers in the array.
-----Examples-----
Input
5 3
1 2 2 4 5
Output
1
Input
5 3
1 2 3 4 5
Output
2
Input
5 3
1 2 3 3 3
Output
0 | from collections import *
n,k = [int(i) for i in input().split()]
A = [int(i) for i in input().split()]
A.sort()
d = defaultdict(lambda: [0,0])
for i in A:
num = i
stp = 0
while num>0:
if d[num][0]<k:
d[num][0]+=1
d[num][1]+=stp
num = num//2
stp +=1
if d[num][0]<k:
d[num][0]+=1
d[num][1]+=stp
mn = 10**20
for i in d:
if(d[i][0]>=k):
mn = min(d[i][1],mn)
print(mn) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/445/B:
DZY loves chemistry, and he enjoys mixing chemicals.
DZY has n chemicals, and m pairs of them will react. He wants to pour these chemicals into a test tube, and he needs to pour them in one by one, in any order.
Let's consider the danger of a test tube. Danger of an empty test tube is 1. And every time when DZY pours a chemical, if there are already one or more chemicals in the test tube that can react with it, the danger of the test tube will be multiplied by 2. Otherwise the danger remains as it is.
Find the maximum possible danger after pouring all the chemicals one by one in optimal order.
-----Input-----
The first line contains two space-separated integers n and m $(1 \leq n \leq 50 ; 0 \leq m \leq \frac{n(n - 1)}{2})$.
Each of the next m lines contains two space-separated integers x_{i} and y_{i} (1 ≤ x_{i} < y_{i} ≤ n). These integers mean that the chemical x_{i} will react with the chemical y_{i}. Each pair of chemicals will appear at most once in the input.
Consider all the chemicals numbered from 1 to n in some order.
-----Output-----
Print a single integer — the maximum possible danger.
-----Examples-----
Input
1 0
Output
1
Input
2 1
1 2
Output
2
Input
3 2
1 2
2 3
Output
4
-----Note-----
In the first sample, there's only one way to pour, and the danger won't increase.
In the second sample, no matter we pour the 1st chemical first, or pour the 2nd chemical first, the answer is always 2.
In the third sample, there are four ways to achieve the maximum possible danger: 2-1-3, 2-3-1, 1-2-3 and 3-2-1 (that is the numbers of the chemicals in order of pouring).
I tried it in Python, but could not do it. Can you solve it? | n, m = map(int, input().split())
p = list(range(n + 1))
s = [{i} for i in p]
for i in range(m):
x, y = map(int, input().split())
x, y = p[x], p[y]
if x != y:
if len(s[x]) < len(s[y]):
for k in s[x]:
p[k] = y
s[y].add(k)
s[x].clear()
else:
for k in s[y]:
p[k] = x
s[x].add(k)
s[y].clear()
q = 0
for p in s:
if p: q += len(p) - 1
print(1 << q) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Alice and Bob have participated to a Rock Off with their bands. A jury of true metalheads rates the two challenges, awarding points to the bands on a scale from 1 to 50 for three categories: Song Heaviness, Originality, and Members' outfits.
For each one of these 3 categories they are going to be awarded one point, should they get a better judgement from the jury. No point is awarded in case of an equal vote.
You are going to receive two arrays, containing first the score of Alice's band and then those of Bob's. Your task is to find their total score by comparing them in a single line.
Example:
Alice's band plays a Nirvana inspired grunge and has been rated
``20`` for Heaviness,
``32`` for Originality and only
``18`` for Outfits.
Bob listens to Slayer and has gotten a good
``48`` for Heaviness,
``25`` for Originality and a rather honest
``40`` for Outfits.
The total score should be followed by a colon ```:``` and by one of the following quotes:
if Alice's band wins: ```Alice made "Kurt" proud!```
if Bob's band wins: ```Bob made "Jeff" proud!```
if they end up with a draw: ```that looks like a "draw"! Rock on!```
The solution to the example above should therefore appear like
``'1, 2: Bob made "Jeff" proud!'``. | def solve(a, b):
count_a = 0
count_b = 0
for i in range(len(a)):
if a[i] > b[i]:
count_a +=1
elif a[i] < b[i]:
count_b +=1
return f'{count_a}, {count_b}: that looks like a "draw"! Rock on!' if count_a == count_b else f'{count_a}, {count_b}: Alice made "Kurt" proud!' if count_a > count_b else f'{count_a}, {count_b}: Bob made "Jeff" proud!' | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Little girl Tanya is learning how to decrease a number by one, but she does it wrong with a number consisting of two or more digits. Tanya subtracts one from a number by the following algorithm: if the last digit of the number is non-zero, she decreases the number by one; if the last digit of the number is zero, she divides the number by 10 (i.e. removes the last digit).
You are given an integer number $n$. Tanya will subtract one from it $k$ times. Your task is to print the result after all $k$ subtractions.
It is guaranteed that the result will be positive integer number.
-----Input-----
The first line of the input contains two integer numbers $n$ and $k$ ($2 \le n \le 10^9$, $1 \le k \le 50$) — the number from which Tanya will subtract and the number of subtractions correspondingly.
-----Output-----
Print one integer number — the result of the decreasing $n$ by one $k$ times.
It is guaranteed that the result will be positive integer number.
-----Examples-----
Input
512 4
Output
50
Input
1000000000 9
Output
1
-----Note-----
The first example corresponds to the following sequence: $512 \rightarrow 511 \rightarrow 510 \rightarrow 51 \rightarrow 50$. | n,k = list(map(int,input().split()))
for i in range(k):
if n%10 == 0: n//=10
else: n -=1
print(n) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Today on a lecture about strings Gerald learned a new definition of string equivalency. Two strings a and b of equal length are called equivalent in one of the two cases: They are equal. If we split string a into two halves of the same size a_1 and a_2, and string b into two halves of the same size b_1 and b_2, then one of the following is correct: a_1 is equivalent to b_1, and a_2 is equivalent to b_2 a_1 is equivalent to b_2, and a_2 is equivalent to b_1
As a home task, the teacher gave two strings to his students and asked to determine if they are equivalent.
Gerald has already completed this home task. Now it's your turn!
-----Input-----
The first two lines of the input contain two strings given by the teacher. Each of them has the length from 1 to 200 000 and consists of lowercase English letters. The strings have the same length.
-----Output-----
Print "YES" (without the quotes), if these two strings are equivalent, and "NO" (without the quotes) otherwise.
-----Examples-----
Input
aaba
abaa
Output
YES
Input
aabb
abab
Output
NO
-----Note-----
In the first sample you should split the first string into strings "aa" and "ba", the second one — into strings "ab" and "aa". "aa" is equivalent to "aa"; "ab" is equivalent to "ba" as "ab" = "a" + "b", "ba" = "b" + "a".
In the second sample the first string can be splitted into strings "aa" and "bb", that are equivalent only to themselves. That's why string "aabb" is equivalent only to itself and to string "bbaa". | def F(s):
if len(s)%2==1:return s
s1 = F(s[:len(s)//2])
s2 = F(s[len(s)//2:])
if s1 < s2:return s1 + s2
return s2 + s1
if F(input()) == F(input()):
print("YES")
else:
print("NO") | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Recall that MEX of an array is a minimum non-negative integer that does not belong to the array. Examples: for the array $[0, 0, 1, 0, 2]$ MEX equals to $3$ because numbers $0, 1$ and $2$ are presented in the array and $3$ is the minimum non-negative integer not presented in the array; for the array $[1, 2, 3, 4]$ MEX equals to $0$ because $0$ is the minimum non-negative integer not presented in the array; for the array $[0, 1, 4, 3]$ MEX equals to $2$ because $2$ is the minimum non-negative integer not presented in the array.
You are given an empty array $a=[]$ (in other words, a zero-length array). You are also given a positive integer $x$.
You are also given $q$ queries. The $j$-th query consists of one integer $y_j$ and means that you have to append one element $y_j$ to the array. The array length increases by $1$ after a query.
In one move, you can choose any index $i$ and set $a_i := a_i + x$ or $a_i := a_i - x$ (i.e. increase or decrease any element of the array by $x$). The only restriction is that $a_i$ cannot become negative. Since initially the array is empty, you can perform moves only after the first query.
You have to maximize the MEX (minimum excluded) of the array if you can perform any number of such operations (you can even perform the operation multiple times with one element).
You have to find the answer after each of $q$ queries (i.e. the $j$-th answer corresponds to the array of length $j$).
Operations are discarded before each query. I.e. the array $a$ after the $j$-th query equals to $[y_1, y_2, \dots, y_j]$.
-----Input-----
The first line of the input contains two integers $q, x$ ($1 \le q, x \le 4 \cdot 10^5$) — the number of queries and the value of $x$.
The next $q$ lines describe queries. The $j$-th query consists of one integer $y_j$ ($0 \le y_j \le 10^9$) and means that you have to append one element $y_j$ to the array.
-----Output-----
Print the answer to the initial problem after each query — for the query $j$ print the maximum value of MEX after first $j$... | import sys
input = sys.stdin.readline
q,x=list(map(int,input().split()))
seg_el=1<<((x+1).bit_length())
SEG=[0]*(2*seg_el)
def add1(n,seg_el):
i=n+seg_el
SEG[i]+=1
i>>=1
while i!=0:
SEG[i]=min(SEG[i*2],SEG[i*2+1])
i>>=1
def getvalues(l,r):
L=l+seg_el
R=r+seg_el
ANS=1<<30
while L<R:
if L & 1:
ANS=min(ANS , SEG[L])
L+=1
if R & 1:
R-=1
ANS=min(ANS , SEG[R])
L>>=1
R>>=1
return ANS
for qu in range(q):
t=int(input())
add1(t%x,seg_el)
#print(SEG)
MIN=getvalues(0,x)
OK=0
NG=x
while NG-OK>1:
mid=(OK+NG)//2
if getvalues(0,mid)>MIN:
OK=mid
else:
NG=mid
#print(MIN,OK)
print(MIN*x+OK) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
You are given a tree with $N$ vertices (numbered $1$ through $N$) and a bag with $N$ markers. There is an integer written on each marker; each of these integers is $0$, $1$ or $2$. You must assign exactly one marker to each vertex.
Let's define the unattractiveness of the resulting tree as the maximum absolute difference of integers written on the markers in any two vertices which are connected by an edge.
Find the minimum possible unattractiveness of the resulting tree.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains a single integer $N$.
- The second line contains $N$ space-separated integers denoting the numbers on markers in the bag.
- Each of the next $N-1$ lines contains two space-separated integers $u$ and $v$ denoting an edge between vertices $u$ and $v$.
-----Output-----
For each test case, print a single line containing one integer — the minimum unattractiveness.
-----Constraints-----
- $1 \le T \le 30$
- $1 \le N \le 100$
- $1 \le u, v \le N$
- the graph described on the input is a tree
-----Example Input-----
3
3
0 1 1
1 2
1 3
3
0 1 2
1 2
1 3
4
2 2 2 2
1 2
1 3
3 4
-----Example Output-----
1
1
0
-----Explanation-----
Example case 1: | # cook your dish here
import numpy as np
tests = int(input())
for _ in range(tests):
n = int(input())
weights = [int(j) for j in input().split()]
edges = [[0] for _ in range(n-1)]
for i in range(n-1):
edges[i] = [int(j)-1 for j in input().split()]
vertex_set = [[] for _ in range(n)]
for i in range(n-1):
vertex_set[edges[i][0]].append(edges[i][1])
vertex_set[edges[i][1]].append(edges[i][0])
counts = [0 for _ in range(3)]
for i in range(n):
counts[weights[i]] += 1
if counts[1] == 0:
print(2 * (counts[0] != 0 and counts[2] != 0))
elif counts[1] == n:
print(0)
else:
visited = [0]
for i in range(n):
vertex = visited[i]
for v in vertex_set[vertex]:
if v not in visited:
visited.append(v)
vertex_nums = [[0] for _ in range(n)]
for i in range(n-1,-1,-1):
vertex = visited[i]
for v in vertex_set[vertex]:
if v in visited[i:]:
vertex_nums[vertex].append(sum(vertex_nums[v])+1)
for i in range(n):
vertex_nums[i].append(n-1-sum(vertex_nums[i]))
sums = np.zeros(n,dtype=bool)
sums[0] = True
for i in range(n):
new_sums = np.zeros(n,dtype=bool)
new_sums[0] = True
for num in vertex_nums[i]:
new_sums[num:n] = np.logical_or(new_sums[num:n],new_sums[:n-num])
sums = np.logical_or(sums,new_sums)
solved = False
for i in range(n):
if sums[i] and counts[0] <= i and counts[2] <= n - 1 - i:
solved = True
break
if solved or counts[1] > 1:
print(1)
else:
print(2) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5416f1834c24604c46000696:
In computer science, cycle detection is the algorithmic problem of finding a cycle in a sequence of iterated function values.
For any function ƒ, and any initial value x0 in S, the sequence of iterated function values
x0,x1=f(x0), x2=f(x1), ...,xi=f(x{i-1}),...
may eventually use the same value twice under some assumptions: S finite, f periodic ... etc. So there will be some `i ≠ j` such that `xi = xj`. Once this happens, the sequence must continue by repeating the cycle of values from `xi to xj−1`. Cycle detection is the problem of finding `i` and `j`, given `ƒ` and `x0`. Let `μ` be the smallest index such that the value associated will reappears and `λ` the smallest value such that `xμ = xλ+μ, λ` is the loop length.
Example:
Consider the sequence:
```
2, 0, 6, 3, 1, 6, 3, 1, 6, 3, 1, ....
```
The cycle in this value sequence is 6, 3, 1.
μ is 2 (first 6)
λ is 3 (length of the sequence or difference between position of consecutive 6).
The goal of this kata is to build a function that will return `[μ,λ]` when given a short sequence. Simple loops will be sufficient. The sequence will be given in the form of an array. All array will be valid sequence associated with deterministic function. It means that the sequence will repeat itself when a value is reached a second time. (So you treat two cases: non repeating [1,2,3,4] and repeating [1,2,1,2], no hybrid cases like [1,2,1,4]). If there is no repetition you should return [].
This kata is followed by two other cycle detection algorithms:
Loyd's: http://www.codewars.com/kata/cycle-detection-floyds-tortoise-and-the-hare
Bret's: http://www.codewars.com/kata/cycle-detection-brents-tortoise-and-hare
I tried it in Python, but could not do it. Can you solve it? | def cycle(sequence):
memo = {}
for i, item in enumerate(sequence):
if item in memo:
return [memo[item], i - memo[item]]
memo[item] = i
return [] | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1204/D2:
The only difference between easy and hard versions is the length of the string. You can hack this problem if you solve it. But you can hack the previous problem only if you solve both problems.
Kirk has a binary string $s$ (a string which consists of zeroes and ones) of length $n$ and he is asking you to find a binary string $t$ of the same length which satisfies the following conditions: For any $l$ and $r$ ($1 \leq l \leq r \leq n$) the length of the longest non-decreasing subsequence of the substring $s_{l}s_{l+1} \ldots s_{r}$ is equal to the length of the longest non-decreasing subsequence of the substring $t_{l}t_{l+1} \ldots t_{r}$; The number of zeroes in $t$ is the maximum possible.
A non-decreasing subsequence of a string $p$ is a sequence of indices $i_1, i_2, \ldots, i_k$ such that $i_1 < i_2 < \ldots < i_k$ and $p_{i_1} \leq p_{i_2} \leq \ldots \leq p_{i_k}$. The length of the subsequence is $k$.
If there are multiple substrings which satisfy the conditions, output any.
-----Input-----
The first line contains a binary string of length not more than $10^5$.
-----Output-----
Output a binary string which satisfied the above conditions. If there are many such strings, output any of them.
-----Examples-----
Input
110
Output
010
Input
010
Output
010
Input
0001111
Output
0000000
Input
0111001100111011101000
Output
0011001100001011101000
-----Note-----
In the first example: For the substrings of the length $1$ the length of the longest non-decreasing subsequnce is $1$; For $l = 1, r = 2$ the longest non-decreasing subsequnce of the substring $s_{1}s_{2}$ is $11$ and the longest non-decreasing subsequnce of the substring $t_{1}t_{2}$ is $01$; For $l = 1, r = 3$ the longest non-decreasing subsequnce of the substring $s_{1}s_{3}$ is $11$ and the longest non-decreasing subsequnce of the substring $t_{1}t_{3}$ is $00$; For $l = 2, r = 3$ the longest non-decreasing subsequnce of the substring $s_{2}s_{3}$ is $1$ and the longest non-decreasing subsequnce of the substring $t_{2}t_{3}$...
I tried it in Python, but could not do it. Can you solve it? | S = list(map(int,input().strip()))
N = len(S)
stack = []
for i in range(N):
s = S[i]
if s == 0 and stack and stack[-1][0] == 1:
stack.pop()
else:
stack.append((s, i))
T = S[:]
if stack:
for i in tuple(map(list, zip(*stack)))[1]:
T[i] = 0
print(''.join(map(str, T))) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
You are given an array $a$ consisting of $n$ integers. You have to find the length of the smallest (shortest) prefix of elements you need to erase from $a$ to make it a good array. Recall that the prefix of the array $a=[a_1, a_2, \dots, a_n]$ is a subarray consisting several first elements: the prefix of the array $a$ of length $k$ is the array $[a_1, a_2, \dots, a_k]$ ($0 \le k \le n$).
The array $b$ of length $m$ is called good, if you can obtain a non-decreasing array $c$ ($c_1 \le c_2 \le \dots \le c_{m}$) from it, repeating the following operation $m$ times (initially, $c$ is empty): select either the first or the last element of $b$, remove it from $b$, and append it to the end of the array $c$.
For example, if we do $4$ operations: take $b_1$, then $b_{m}$, then $b_{m-1}$ and at last $b_2$, then $b$ becomes $[b_3, b_4, \dots, b_{m-3}]$ and $c =[b_1, b_{m}, b_{m-1}, b_2]$.
Consider the following example: $b = [1, 2, 3, 4, 4, 2, 1]$. This array is good because we can obtain non-decreasing array $c$ from it by the following sequence of operations: take the first element of $b$, so $b = [2, 3, 4, 4, 2, 1]$, $c = [1]$; take the last element of $b$, so $b = [2, 3, 4, 4, 2]$, $c = [1, 1]$; take the last element of $b$, so $b = [2, 3, 4, 4]$, $c = [1, 1, 2]$; take the first element of $b$, so $b = [3, 4, 4]$, $c = [1, 1, 2, 2]$; take the first element of $b$, so $b = [4, 4]$, $c = [1, 1, 2, 2, 3]$; take the last element of $b$, so $b = [4]$, $c = [1, 1, 2, 2, 3, 4]$; take the only element of $b$, so $b = []$, $c = [1, 1, 2, 2, 3, 4, 4]$ — $c$ is non-decreasing.
Note that the array consisting of one element is good.
Print the length of the shortest prefix of $a$ to delete (erase), to make $a$ to be a good array. Note that the required length can be $0$.
You have to answer $t$ independent test cases.
-----Input-----
The first line of the input contains one integer $t$ ($1 \le t \le 2 \cdot 10^4$) — the number of test cases. Then $t$ test cases follow.
The first line of the test case contains... | def solve():
n = int(input())
a = list(map(int, input().split()))
prev = a[-1]
cnt = 1
status = 0
for i in range(n - 2, -1, -1):
v = a[i]
if status == 0:
if v < prev:
status = 1
cnt += 1
prev = v
continue
elif status == 1:
if v > prev:
break
cnt += 1
prev = v
continue
print(n - cnt)
t = int(input())
for _ in range(t):
solve() | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1342/B:
Let's say string $s$ has period $k$ if $s_i = s_{i + k}$ for all $i$ from $1$ to $|s| - k$ ($|s|$ means length of string $s$) and $k$ is the minimum positive integer with this property.
Some examples of a period: for $s$="0101" the period is $k=2$, for $s$="0000" the period is $k=1$, for $s$="010" the period is $k=2$, for $s$="0011" the period is $k=4$.
You are given string $t$ consisting only of 0's and 1's and you need to find such string $s$ that: String $s$ consists only of 0's and 1's; The length of $s$ doesn't exceed $2 \cdot |t|$; String $t$ is a subsequence of string $s$; String $s$ has smallest possible period among all strings that meet conditions 1—3.
Let us recall that $t$ is a subsequence of $s$ if $t$ can be derived from $s$ by deleting zero or more elements (any) without changing the order of the remaining elements. For example, $t$="011" is a subsequence of $s$="10101".
-----Input-----
The first line contains single integer $T$ ($1 \le T \le 100$) — the number of test cases.
Next $T$ lines contain test cases — one per line. Each line contains string $t$ ($1 \le |t| \le 100$) consisting only of 0's and 1's.
-----Output-----
Print one string for each test case — string $s$ you needed to find. If there are multiple solutions print any one of them.
-----Example-----
Input
4
00
01
111
110
Output
00
01
11111
1010
-----Note-----
In the first and second test cases, $s = t$ since it's already one of the optimal solutions. Answers have periods equal to $1$ and $2$, respectively.
In the third test case, there are shorter optimal solutions, but it's okay since we don't need to minimize the string $s$. String $s$ has period equal to $1$.
I tried it in Python, but could not do it. Can you solve it? | def read_int():
return int(input())
def read_ints():
return list(map(int, input().split(' ')))
t = read_int()
for case_num in range(t):
a = input()
n = len(a)
one = 0
for c in a:
if c == '1':
one += 1
if one == 0 or one == n:
print(a)
else:
print(''.join(['01' for i in range(n)])) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Zonal Computing Olympiad 2013, 10 Nov 2012
Little Red Riding Hood is carrying a basket with berries through the forest to her grandmother's house. The forest is arranged in the form of a square N × N grid of cells. The top left corner cell, where Little Red Riding Hood starts her journey, is numbered (1,1) and the bottom right corner cell, where her grandmother lives, is numbered (N,N). In each step, she can move either one position right or one position down.
The forest is full of dangerous wolves and she is looking for a safe path to reach her destination. Little Red Riding Hood's fairy godmother has placed some special anti-wolf magical charms in some of the cells in the grid. Each charm has a strength. If the charm in cell (i,j) has strength k then its zone of influence is all the cells within k steps of (i,j); that is, all cells (i',j') such that |i - i'| + |j - j'| ≤ k. A cell within the zone of influence of a charm is safe from wolves. A safe path from (1,1) to (N,N) is one in which every cell along the path is safe.
Little Red Riding Hood is carrying a basket with berries. In each cell, she drops some berries while pushing her way through the thick forest. However, sometimes she is also able to pick up fresh berries. Each cell is labelled with an integer that indicates the net change in the number of berries in her basket on passing through the cell; that is, the number of berries she picks up in that cell minus the number of berries she drops. You can assume that there are enough berries in her basket to start with so that the basket never becomes empty.
Little Red Riding Hood knows the positions and strengths of all the magic charms and is looking for a safe path along which the number of berries she has in the basket when she reaches her grandmother's house is maximized.
As an example consider the following grid:
3 3 2 4 3
2 1 -1 -2 2
-1 2 4 3 -3
-2 2 3 2 1
3 -1 2 -1 2
Suppose there are 3 magic charms, at position (1,2) with strength 2, at position (4,5)... | def main():
from sys import stdin, stdout
rl = stdin.readline
n, m = (int(x) for x in rl().split())
grid = [[int(x) for x in rl().split()] for _ in range(n)]
charms = [[int(x) for x in rl().split()] for _ in range(m)]
n_inf = -9999
dp = [[n_inf] * n for _ in range(n)]
for c in charms:
c[0] -= 1
c[1] -= 1
x = max(0, c[0] - c[2])
while x < n and x <= c[0] + c[2]:
off = abs(x - c[0])
y = max(0, c[1] - c[2] + off)
while y < n and y <= c[1] + c[2] - off:
dp[x][y] = grid[x][y]
y += 1
x += 1
for x in range(1, n):
if dp[x - 1][0] == n_inf:
for x1 in range(x, n):
dp[x1][0] = n_inf
break
dp[x][0] += dp[x - 1][0]
for y in range(1, n):
if dp[0][y - 1] == n_inf:
for y1 in range(y, n):
dp[0][y1] = n_inf
break
dp[0][y] += dp[0][y - 1]
for x in range(1, n):
for y in range(1, n):
m = max(dp[x - 1][y], dp[x][y - 1])
dp[x][y] = m + dp[x][y] if m != n_inf else n_inf
if dp[-1][-1] != n_inf:
stdout.write('YES\n')
stdout.write(str(dp[-1][-1]))
else:
stdout.write('NO')
main() | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Kira likes to play with strings very much. Moreover he likes the shape of 'W' very much. He takes a string and try to make a 'W' shape out of it such that each angular point is a '#' character and each sides has same characters. He calls them W strings.
For example, the W string can be formed from "aaaaa#bb#cc#dddd" such as:
a
a d
a # d
a b c d
a b c d
# #
He also call the strings which can generate a 'W' shape (satisfying the above conditions) W strings.
More formally, a string S is a W string if and only if it satisfies the following conditions (some terms and notations are explained in Note, please see it if you cannot understand):
- The string S contains exactly 3 '#' characters. Let the indexes of all '#' be P1 < P2 < P3 (indexes are 0-origin).
- Each substring of S[0, P1−1], S[P1+1, P2−1], S[P2+1, P3−1], S[P3+1, |S|−1] contains exactly one kind of characters, where S[a, b] denotes the non-empty substring from a+1th character to b+1th character, and |S| denotes the length of string S (See Note for details).
Now, his friend Ryuk gives him a string S and asks him to find the length of the longest W string which is a subsequence of S, with only one condition that there must not be any '#' symbols between the positions of the first and the second '#' symbol he chooses, nor between the second and the third (here the "positions" we are looking at are in S), i.e. suppose the index of the '#'s he chooses to make the W string are P1, P2, P3 (in increasing order) in the original string S, then there must be no index i such that S[i] = '#' where P1 < i < P2 or P2 < i < P3.
Help Kira and he won't write your name in the Death Note.
Note:
For a given string S, let S[k] denote the k+1th character of string S, and let the index of the character S[k] be k. Let |S| denote the length of the string S. And a substring of a string S is a string S[a, b] = S[a] S[a+1] ... S[b], where 0 ≤ a ≤ b < |S|. And a subsequence of a string S is a string S[i0] S[i1] ... S[in−1], where 0 ≤ i0 < i1 < ... <... | def frequency(s,n):
f=[[0 for i in range(26)]for j in range(n+1)]
count=0
for i in range(n):
if s[i]!="#":
f[count][ord(s[i])-97]+=1
else:
count+=1
for j in range(26):
f[count][j]=f[count-1][j]
return (f,count)
def solve(s):
n=len(s)
f,count=frequency(s,n)
if count<3:
return 0
ans=0
index=[]
for i in range(n-1,-1,-1):
if s[i]=="#":
index.append(i)
for c in range(1,count-2+1):
if index[-2]==index[-1]+1 or index[-3]==index[-2]+1:
index.pop()
continue
left=max(f[c-1])
mid1=0
mid2=0
right=0
for j in range(26):
mid1=max(mid1,f[c][j]-f[c-1][j])
mid2=max(mid2,f[c+1][j]-f[c][j])
right=max(right,f[count][j]-f[c+1][j])
if left and mid1 and mid2 and right:
ans=max(ans,left+mid1+mid2+right)
index.pop()
return ans
for _ in range(int(input())):
s=input()
ans=solve(s)
if ans:
print(ans+3)
else:
print(0) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1157/G:
You are given a binary matrix $a$ of size $n \times m$. A binary matrix is a matrix where each element is either $0$ or $1$.
You may perform some (possibly zero) operations with this matrix. During each operation you can inverse the row of this matrix or a column of this matrix. Formally, inverting a row is changing all values in this row to the opposite ($0$ to $1$, $1$ to $0$). Inverting a column is changing all values in this column to the opposite.
Your task is to sort the initial matrix by some sequence of such operations. The matrix is considered sorted if the array $[a_{1, 1}, a_{1, 2}, \dots, a_{1, m}, a_{2, 1}, a_{2, 2}, \dots, a_{2, m}, \dots, a_{n, m - 1}, a_{n, m}]$ is sorted in non-descending order.
-----Input-----
The first line of the input contains two integers $n$ and $m$ ($1 \le n, m \le 200$) — the number of rows and the number of columns in the matrix.
The next $n$ lines contain $m$ integers each. The $j$-th element in the $i$-th line is $a_{i, j}$ ($0 \le a_{i, j} \le 1$) — the element of $a$ at position $(i, j)$.
-----Output-----
If it is impossible to obtain a sorted matrix, print "NO" in the first line.
Otherwise print "YES" in the first line. In the second line print a string $r$ of length $n$. The $i$-th character $r_i$ of this string should be '1' if the $i$-th row of the matrix is inverted and '0' otherwise. In the third line print a string $c$ of length $m$. The $j$-th character $c_j$ of this string should be '1' if the $j$-th column of the matrix is inverted and '0' otherwise. If there are multiple answers, you can print any.
-----Examples-----
Input
2 2
1 1
0 1
Output
YES
00
10
Input
3 4
0 0 0 1
0 0 0 0
1 1 1 1
Output
YES
010
0000
Input
3 3
0 0 0
1 0 1
1 1 0
Output
NO
I tried it in Python, but could not do it. Can you solve it? | import sys
input = sys.stdin.readline
n,m=list(map(int,input().split()))
A=[list(map(int,input().split())) for i in range(n)]
for i in range(m):
#一行目をi-1まで0にする
ANSR=[0]*n
ANSC=[0]*m
for j in range(i):
if A[0][j]==1:
ANSC[j]=1
for j in range(i,m):
if A[0][j]==0:
ANSC[j]=1
for r in range(1,n):
B=set()
for c in range(m):
if ANSC[c]==0:
B.add(A[r][c])
else:
B.add(1-A[r][c])
if len(B)>=2:
break
if max(B)==0:
ANSR[r]=1
else:
print("YES")
print("".join(map(str,ANSR)))
print("".join(map(str,ANSC)))
return
ANSR=[0]*n
ANSC=[0]*m
for j in range(m):
if A[0][j]==1:
ANSC[j]=1
flag=0
for r in range(1,n):
if flag==0:
B=[]
for c in range(m):
if ANSC[c]==0:
B.append(A[r][c])
else:
B.append(1-A[r][c])
if max(B)==0:
continue
elif min(B)==1:
ANSR[r]=1
continue
else:
OI=B.index(1)
if min(B[OI:])==1:
flag=1
continue
OO=B.index(0)
if max(B[OO:])==0:
flag=1
ANSR[r]=1
continue
else:
print("NO")
return
else:
B=set()
for c in range(m):
if ANSC[c]==0:
B.add(A[r][c])
else:
B.add(1-A[r][c])
if len(B)>=2:
break
if max(B)==0:
ANSR[r]=1
else:
print("YES")
print("".join(map(str,ANSR)))
print("".join(map(str,ANSC)))
return
print("NO") | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/58a6a56942fd72afdd000161:
# Task
In a black and white image we can use `1` instead of black pixels and `0` instead of white pixels.
For compression image file we can reserve pixels by consecutive pixels who have the same color.
Your task is to determine how much of black and white pixels is in each row sorted by place of those.
# Example:
For `height=2,width=100 and compressed=[40,120,40,0]`
The result should be `[[40,60],[0,60,40,0]]`
Sum of compressed array always divisible by width and height of image.
Pixels available is in Compressed array in this order:
`[Black,White,Black,White,Black,White,...]`
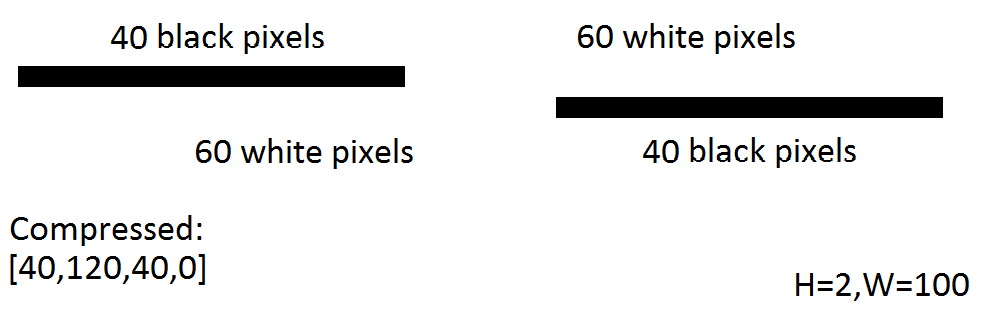
For `height=2,width=100 and compressed=[0, 180, 20, 0]`
The result should be `[[0,100],[0,80,20,0]]`
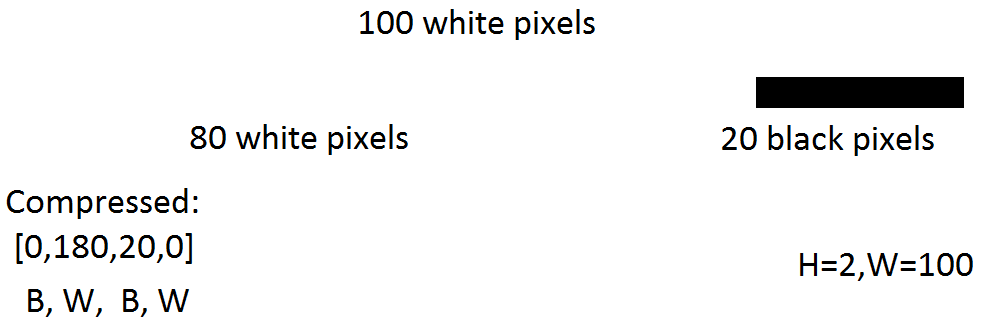
# Input/Output
- `[input]` integer `height`
Height of image
- `[input]` integer `width`
Width of image
- `[input]` integer array `compressed`
Consecutive pixels
- `[output]` 2D integer array
The black and white pixels in each row.
I tried it in Python, but could not do it. Can you solve it? | from re import findall
def black_and_white(height, width, compressed):
compr = ''.join(('0' if i % 2 else '1') * v for i, v in enumerate(compressed))
res = []
for i in range(0, len(compr), width):
row, temp, black = compr[i: i + width], [], True
m = [(c, len(g)) for g, c in findall(r'((.)\2*)', row)]
for dig, n in m:
if black and dig == '0':
temp.extend([0, n])
black == False
elif not black and dig == '1':
temp.extend([n, 0])
black = True
else:
temp.append(n)
black = not black
if len(temp) % 2: temp.append(0)
res.append(temp)
return res | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/646/A:
Три брата договорились о встрече. Пронумеруем братьев следующим образом: пусть старший брат имеет номер 1, средний брат имеет номер 2, а младший брат — номер 3.
Когда пришло время встречи, один из братьев опоздал. По заданным номерам двух братьев, которые пришли вовремя, вам предстоит определить номер опоздавшего брата.
-----Входные данные-----
В первой строке входных данных следуют два различных целых числа a и b (1 ≤ a, b ≤ 3, a ≠ b) — номера братьев, которые пришли на встречу вовремя. Номера даны в произвольном порядке.
-----Выходные данные-----
Выведите единственное целое число — номер брата, который опоздал на встречу.
-----Пример-----
Входные данные
3 1
Выходные данные
2
I tried it in Python, but could not do it. Can you solve it? | a, b = [int(x) for x in input().split()]
for i in range(1, 4):
if i != a and i != b:
print(i) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/LTIME39/problems/ALPHABET:
Not everyone probably knows that Chef has younder brother Jeff. Currently Jeff learns to read.
He knows some subset of the letter of Latin alphabet. In order to help Jeff to study, Chef gave him a book with the text consisting of N words. Jeff can read a word iff it consists only of the letters he knows.
Now Chef is curious about which words his brother will be able to read, and which are not. Please help him!
-----Input-----
The first line of the input contains a lowercase Latin letter string S, consisting of the letters Jeff can read. Every letter will appear in S no more than once.
The second line of the input contains an integer N denoting the number of words in the book.
Each of the following N lines contains a single lowecase Latin letter string Wi, denoting the ith word in the book.
-----Output-----
For each of the words, output "Yes" (without quotes) in case Jeff can read it, and "No" (without quotes) otherwise.
-----Constraints-----
- 1 ≤ |S| ≤ 26
- 1 ≤ N ≤ 1000
- 1 ≤ |Wi| ≤ 12
- Each letter will appear in S no more than once.
- S, Wi consist only of lowercase Latin letters.
-----Subtasks-----
- Subtask #1 (31 point): |S| = 1, i.e. Jeff knows only one letter.
- Subtask #2 (69 point) : no additional constraints
-----Example-----
Input:act
2
cat
dog
Output:Yes
No
-----Explanation-----
The first word can be read.
The second word contains the letters d, o and g that aren't known by Jeff.
I tried it in Python, but could not do it. Can you solve it? | S = input()
N = int(input())
while N != 0:
W = input()
flag = 0
for i in range(0, len(W)):
if W[i] in S:
continue
else:
flag = 1
break
if flag == 0:
print("Yes")
else:
print("No")
N -= 1 | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/559379505c859be5a9000034:
< PREVIOUS KATA
NEXT KATA >
## Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto desired number of rows.
* Note:`Returning` the pattern is not the same as `Printing` the pattern.
## Parameters:
pattern( n , y );
^ ^
| |
Term upto which Number of times
Basic Pattern Basic Pattern
should be should be
created repeated
vertically
* Note: `Basic Pattern` means what we created in Complete The Pattern #12 i.e. a `simple X`.
## Rules/Note:
* The pattern should be created using only unit digits.
* If `n < 1` then it should return "" i.e. empty string.
* If `y <= 1` then the basic pattern should not be repeated vertically.
* `The length of each line is same`, and is equal to the length of longest line in the pattern.
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,50]`
+ `y ∈ (-∞,25]`
* If only one argument is passed then the function `pattern` should run as if `y <= 1`.
* The function `pattern` should work when extra arguments are passed, by ignoring the extra arguments.
## Examples:
#### Having Two Arguments:
##### pattern(4,3):
1 1
2 2
3 3
4
3 3
2 2
1 1
2 2
3 3
4
3 3
2 2
1 1
2 2
3 3
4
3 3
2 2
1 1
##### pattern(10,2):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0
9 9
...
I tried it in Python, but could not do it. Can you solve it? | def pattern(n, y=1, *_):
if n < 1:
return ''
ans, p, y = [], [], max(y, 1)
for i in range(1, n+1):
t = [' '] * (n * 2 - 1)
t[i - 1] = t[n * 2 - 1 - 1 - i + 1] = str(i % 10)
p.append(''.join(t))
p += p[:-1][::-1]
for _ in range(y):
ans += p[1:] if ans else p
return '\n'.join(ans) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/minimum-number-of-flips-to-convert-binary-matrix-to-zero-matrix/:
Given a m x n binary matrix mat. In one step, you can choose one cell and flip it and all the four neighbours of it if they exist (Flip is changing 1 to 0 and 0 to 1). A pair of cells are called neighboors if they share one edge.
Return the minimum number of steps required to convert mat to a zero matrix or -1 if you cannot.
Binary matrix is a matrix with all cells equal to 0 or 1 only.
Zero matrix is a matrix with all cells equal to 0.
Example 1:
Input: mat = [[0,0],[0,1]]
Output: 3
Explanation: One possible solution is to flip (1, 0) then (0, 1) and finally (1, 1) as shown.
Example 2:
Input: mat = [[0]]
Output: 0
Explanation: Given matrix is a zero matrix. We don't need to change it.
Example 3:
Input: mat = [[1,1,1],[1,0,1],[0,0,0]]
Output: 6
Example 4:
Input: mat = [[1,0,0],[1,0,0]]
Output: -1
Explanation: Given matrix can't be a zero matrix
Constraints:
m == mat.length
n == mat[0].length
1 <= m <= 3
1 <= n <= 3
mat[i][j] is 0 or 1.
I tried it in Python, but could not do it. Can you solve it? | class Solution:
def minFlips(self, mat: List[List[int]]) -> int:
m = len(mat)
n = len(mat[0])
start = sum(val << (i*n + j) for i, row in enumerate(mat) for j, val in enumerate(row))
queue = collections.deque([(start, 0)])
seen = { start }
dirs = [[0, 0], [0,1], [1, 0], [0, -1], [-1, 0]]
while queue:
# print(queue)
current, d = queue.popleft()
if current == 0:
return d
# for each index in matrix find neighbour
for i in range(len(mat)):
for j in range(len(mat[0])):
next_state = current
# importants dirs has [0, 0] we need flip the current element and neigbour
for dir_ in dirs:
new_i = i + dir_[0]
new_j = j + dir_[1]
if new_i >= 0 and new_i < len(mat) and new_j >= 0 and new_j < len(mat[0]):
next_state ^= (1 << (new_i * n + new_j )) # 0 xor 1 = 1, 1 xor 1 = 0
if next_state not in seen:
seen.add(next_state)
queue.append((next_state, d + 1))
return -1 | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Mahmoud and Ehab play a game called the even-odd game. Ehab chooses his favorite integer n and then they take turns, starting from Mahmoud. In each player's turn, he has to choose an integer a and subtract it from n such that: 1 ≤ a ≤ n. If it's Mahmoud's turn, a has to be even, but if it's Ehab's turn, a has to be odd.
If the current player can't choose any number satisfying the conditions, he loses. Can you determine the winner if they both play optimally?
-----Input-----
The only line contains an integer n (1 ≤ n ≤ 10^9), the number at the beginning of the game.
-----Output-----
Output "Mahmoud" (without quotes) if Mahmoud wins and "Ehab" (without quotes) otherwise.
-----Examples-----
Input
1
Output
Ehab
Input
2
Output
Mahmoud
-----Note-----
In the first sample, Mahmoud can't choose any integer a initially because there is no positive even integer less than or equal to 1 so Ehab wins.
In the second sample, Mahmoud has to choose a = 2 and subtract it from n. It's Ehab's turn and n = 0. There is no positive odd integer less than or equal to 0 so Mahmoud wins. | def li():
return list(map(int, input().split()))
n = int(input())
if n % 2 == 0:
print('Mahmoud')
else:
print('Ehab') | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Imagine a city with n horizontal streets crossing m vertical streets, forming an (n - 1) × (m - 1) grid. In order to increase the traffic flow, mayor of the city has decided to make each street one way. This means in each horizontal street, the traffic moves only from west to east or only from east to west. Also, traffic moves only from north to south or only from south to north in each vertical street. It is possible to enter a horizontal street from a vertical street, or vice versa, at their intersection.
[Image]
The mayor has received some street direction patterns. Your task is to check whether it is possible to reach any junction from any other junction in the proposed street direction pattern.
-----Input-----
The first line of input contains two integers n and m, (2 ≤ n, m ≤ 20), denoting the number of horizontal streets and the number of vertical streets.
The second line contains a string of length n, made of characters '<' and '>', denoting direction of each horizontal street. If the i-th character is equal to '<', the street is directed from east to west otherwise, the street is directed from west to east. Streets are listed in order from north to south.
The third line contains a string of length m, made of characters '^' and 'v', denoting direction of each vertical street. If the i-th character is equal to '^', the street is directed from south to north, otherwise the street is directed from north to south. Streets are listed in order from west to east.
-----Output-----
If the given pattern meets the mayor's criteria, print a single line containing "YES", otherwise print a single line containing "NO".
-----Examples-----
Input
3 3
><>
v^v
Output
NO
Input
4 6
<><>
v^v^v^
Output
YES
-----Note-----
The figure above shows street directions in the second sample test case. | def main():
n, m = map(int, input().split())
nm = n * m
neigh = [[] for _ in range(nm)]
for y, c in enumerate(input()):
for x in range(y * m + 1, y * m + m):
if c == '<':
neigh[x].append(x - 1)
else:
neigh[x - 1].append(x)
for x, c in enumerate(input()):
for y in range(m + x, nm, m):
if c == '^':
neigh[y].append(y - m)
else:
neigh[y - m].append(y)
def dfs(yx):
l[yx] = False
for yx1 in neigh[yx]:
if l[yx1]:
dfs(yx1)
for i in range(nm):
l = [True] * nm
dfs(i)
if any(l):
print('NO')
return
print('YES')
def __starting_point():
main()
__starting_point() | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Hi guys, welcome to introduction to DocTesting.
The kata is composed of two parts; in part (1) we write three small functions, and in part (2) we write a few doc tests for those functions.
Lets talk about the functions first...
The reverse_list function takes a list and returns the reverse of it.
If given an empty list, simply return an empty list.
The second function...
The sum_list function takes a list as input and adds up all the values,
returning an integer. If the list is empty, return 0.
The third function...
The head_of_list function simply returns the first item in the list.
If the list is empty return None.
Each of these functions can be easily written with a single line of code; there are some tests for correctness but no tests for effciency.
Once you have implemented all three of these functions you can move onto phase two, which is writing doc tests. If you haven't written doc tests before then I suggest you check out the following documentation:
https://docs.python.org/3/library/doctest.html
To complete this kata all you have to do is write **EXACTLY TWO** doc tests for each of the three functions (any more/less than that and you will fail the tests).
Here is an example:
def double(y):
"""Function returns y * 2
>>> double(2)
4
"""
return y * 2
In the example above we have a function called 'double' and a single doctest. When we run the doctest module Python will check if double(2) equals 4. If it does not, then the doctest module will flag up an error.
Please note that this is intended as a beginners introduction to docstrings, if you try to do something clever (such as writing doc tests to catch exceptions, or tinkering with the 'option flags'), you will probably fail a test or two. This is due to how the tests are written.
Oh and one last thing, don't try and get too 'cheeky' and try something like:
"""
>>> True
True
"""
such a solution is (a) not in the spirit of things and (b) I got tests for... | def reverse_list(a):
"""Takes an list and returns the reverse of it.
If x is empty, return [].
>>> reverse_list([1])
[1]
>>> reverse_list([])
[]
"""
return a[::-1]
def sum_list(a):
"""Takes a list, and returns the sum of that list.
If x is empty list, return 0.
>>> sum_list([1])
1
>>> sum_list([])
0
"""
return sum(a)
def head_of_list(a):
"""Takes a list, returns the first item in that list.
If x is empty, return None
>>> head_of_list([1])
1
>>> head_of_list([]) is None
True
"""
if a:
return a[0] | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Given a string, return the minimal number of parenthesis reversals needed to make balanced parenthesis.
For example:
```Javascript
solve(")(") = 2 Because we need to reverse ")" to "(" and "(" to ")". These are 2 reversals.
solve("(((())") = 1 We need to reverse just one "(" parenthesis to make it balanced.
solve("(((") = -1 Not possible to form balanced parenthesis. Return -1.
```
Parenthesis will be either `"("` or `")"`.
More examples in the test cases.
Good luck. | class Stack:
def __init__(self):
self.items = []
def is_empty(self):
return self.items == []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def peek(self):
return self.items[-1]
def size(self):
return len(self.items)
def solve(s):
l = len(s)
if l % 2 != 0: return -1
stack = Stack(); count = 0; i = 0
while i < len(s):
if s[i] == "(":
stack.push(s[i])
else:
if stack.is_empty():
count += 1
else: stack.pop()
i += 1
q = (count + stack.size())//2
return q if count % 2 == 0 and stack.size() % 2 == 0 else q + 1 | python | train | qsol | codeparrot/apps | all |
Solve in Python:
In some other world, today is Christmas.
Mr. Takaha decides to make a multi-dimensional burger in his party. A level-L burger (L is an integer greater than or equal to 0) is the following thing:
- A level-0 burger is a patty.
- A level-L burger (L \geq 1) is a bun, a level-(L-1) burger, a patty, another level-(L-1) burger and another bun, stacked vertically in this order from the bottom.
For example, a level-1 burger and a level-2 burger look like BPPPB and BBPPPBPBPPPBB (rotated 90 degrees), where B and P stands for a bun and a patty.
The burger Mr. Takaha will make is a level-N burger. Lunlun the Dachshund will eat X layers from the bottom of this burger (a layer is a patty or a bun). How many patties will she eat?
-----Constraints-----
- 1 \leq N \leq 50
- 1 \leq X \leq ( the total number of layers in a level-N burger )
- N and X are integers.
-----Input-----
Input is given from Standard Input in the following format:
N X
-----Output-----
Print the number of patties in the bottom-most X layers from the bottom of a level-N burger.
-----Sample Input-----
2 7
-----Sample Output-----
4
There are 4 patties in the bottom-most 7 layers of a level-2 burger (BBPPPBPBPPPBB). | n,x=map(int,input().split())
def func(m,y):
if y==0:return 0
ret=0
t=4*pow(2,m)-3
if y>=t-1:
ret=pow(2,m+1)-1
elif y==(t-1)//2:
ret=pow(2,m)-1
elif y==(t-1)//2+1:
ret=pow(2,m)
elif y>(t-1)//2+1:
ret=pow(2,m)
ret+=func(m-1,y-(t-1)//2-1)
else:
ret=func(m-1,y-1)
return ret
print(func(n,x)) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
An Ironman Triathlon is one of a series of long-distance triathlon races organized by the World Triathlon Corporaion (WTC).
It consists of a 2.4-mile swim, a 112-mile bicycle ride and a marathon (26.2-mile) (run, raced in that order and without a break. It hurts... trust me.
Your task is to take a distance that an athlete is through the race, and return one of the following:
If the distance is zero, return `'Starting Line... Good Luck!'`.
If the athlete will be swimming, return an object with `'Swim'` as the key, and the remaining race distance as the value.
If the athlete will be riding their bike, return an object with `'Bike'` as the key, and the remaining race distance as the value.
If the athlete will be running, and has more than 10 miles to go, return an object with `'Run'` as the key, and the remaining race distance as the value.
If the athlete has 10 miles or less to go, return return an object with `'Run'` as the key, and `'Nearly there!'` as the value.
Finally, if the athlete has completed te distance, return `"You're done! Stop running!"`.
All distance should be calculated to two decimal places. | def i_tri(s):
out = 'Starting Line... Good Luck!'
if s>0.0 and s<=2.4 : out = {'Swim': f'{140.6 - s:.2f} to go!'}
if s>2.4 and s<=114.4 : out = {'Bike': f'{140.6 - s:.2f} to go!'}
if s>114.4 and s<130.6 : out = {'Run' : f'{140.6 - s:.2f} to go!'}
if s>=130.6 and s<140.6 : out = {'Run' : 'Nearly there!'}
if s>140.6 : out = "You're done! Stop running!"
return out | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Recently, a start up by two students of a state university of city F gained incredible popularity. Now it's time to start a new company. But what do we call it?
The market analysts came up with a very smart plan: the name of the company should be identical to its reflection in a mirror! In other words, if we write out the name of the company on a piece of paper in a line (horizontally, from left to right) with large English letters, then put this piece of paper in front of the mirror, then the reflection of the name in the mirror should perfectly match the line written on the piece of paper.
There are many suggestions for the company name, so coming up to the mirror with a piece of paper for each name wouldn't be sensible. The founders of the company decided to automatize this process. They asked you to write a program that can, given a word, determine whether the word is a 'mirror' word or not.
-----Input-----
The first line contains a non-empty name that needs to be checked. The name contains at most 10^5 large English letters. The name will be written with the next sans serif font: $\text{ABCDEFGHI JKLMNOPQRSTUVWXYZ}$
-----Output-----
Print 'YES' (without the quotes), if the given name matches its mirror reflection. Otherwise, print 'NO' (without the quotes).
-----Examples-----
Input
AHA
Output
YES
Input
Z
Output
NO
Input
XO
Output
NO | good = set('AHIMOTUVWXY')
in_str = input("")
str_len = len(in_str)
result = ""
i = 0
while(i < str_len/2):
if((in_str[i] in good) and (in_str[i] == in_str[-1-i])):
i += 1
else:
result = "NO"
break
if(result != "NO"):
result = "YES"
print(result) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5b7bae02402fb702ce000159:
Assume we take a number `x` and perform any one of the following operations:
```Pearl
a) Divide x by 3 (if it is divisible by 3), or
b) Multiply x by 2
```
After each operation, we write down the result. If we start with `9`, we can get a sequence such as:
```
[9,3,6,12,4,8] -- 9/3=3 -> 3*2=6 -> 6*2=12 -> 12/3=4 -> 4*2=8
```
You will be given a shuffled sequence of integers and your task is to reorder them so that they conform to the above sequence. There will always be an answer.
```
For the above example:
solve([12,3,9,4,6,8]) = [9,3,6,12,4,8].
```
More examples in the test cases. Good luck!
I tried it in Python, but could not do it. Can you solve it? | def solve(lst):
return sorted(lst, key=factors_count)
def factors_count(n):
result = []
for k in (2, 3):
while n % k == 0:
n //= k
result.append(k)
return -result.count(3), result.count(2) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1430/E:
You are given a string $s$. You have to reverse it — that is, the first letter should become equal to the last letter before the reversal, the second letter should become equal to the second-to-last letter before the reversal — and so on. For example, if your goal is to reverse the string "abddea", you should get the string "aeddba". To accomplish your goal, you can swap the neighboring elements of the string.
Your task is to calculate the minimum number of swaps you have to perform to reverse the given string.
-----Input-----
The first line contains one integer $n$ ($2 \le n \le 200\,000$) — the length of $s$.
The second line contains $s$ — a string consisting of $n$ lowercase Latin letters.
-----Output-----
Print one integer — the minimum number of swaps of neighboring elements you have to perform to reverse the string.
-----Examples-----
Input
5
aaaza
Output
2
Input
6
cbaabc
Output
0
Input
9
icpcsguru
Output
30
-----Note-----
In the first example, you have to swap the third and the fourth elements, so the string becomes "aazaa". Then you have to swap the second and the third elements, so the string becomes "azaaa". So, it is possible to reverse the string in two swaps.
Since the string in the second example is a palindrome, you don't have to do anything to reverse it.
I tried it in Python, but could not do it. Can you solve it? | N = int(input())
S = input()
T = S[::-1]
from collections import defaultdict
dic = defaultdict(lambda: [])
for i,t in enumerate(T):
dic[t].append(i)
for k in list(dic.keys()):
dic[k].reverse()
arr = []
for c in S:
arr.append(dic[c].pop())
def inversion(inds):
bit = [0] * (N+1)
def bit_add(x,w):
while x <= N:
bit[x] += w
x += (x & -x)
def bit_sum(x):
ret = 0
while x > 0:
ret += bit[x]
x -= (x & -x)
return ret
inv = 0
for ind in reversed(inds):
inv += bit_sum(ind + 1)
bit_add(ind + 1, 1)
return inv
print(inversion(arr)) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
On the way to school, Karen became fixated on the puzzle game on her phone! [Image]
The game is played as follows. In each level, you have a grid with n rows and m columns. Each cell originally contains the number 0.
One move consists of choosing one row or column, and adding 1 to all of the cells in that row or column.
To win the level, after all the moves, the number in the cell at the i-th row and j-th column should be equal to g_{i}, j.
Karen is stuck on one level, and wants to know a way to beat this level using the minimum number of moves. Please, help her with this task!
-----Input-----
The first line of input contains two integers, n and m (1 ≤ n, m ≤ 100), the number of rows and the number of columns in the grid, respectively.
The next n lines each contain m integers. In particular, the j-th integer in the i-th of these rows contains g_{i}, j (0 ≤ g_{i}, j ≤ 500).
-----Output-----
If there is an error and it is actually not possible to beat the level, output a single integer -1.
Otherwise, on the first line, output a single integer k, the minimum number of moves necessary to beat the level.
The next k lines should each contain one of the following, describing the moves in the order they must be done: row x, (1 ≤ x ≤ n) describing a move of the form "choose the x-th row". col x, (1 ≤ x ≤ m) describing a move of the form "choose the x-th column".
If there are multiple optimal solutions, output any one of them.
-----Examples-----
Input
3 5
2 2 2 3 2
0 0 0 1 0
1 1 1 2 1
Output
4
row 1
row 1
col 4
row 3
Input
3 3
0 0 0
0 1 0
0 0 0
Output
-1
Input
3 3
1 1 1
1 1 1
1 1 1
Output
3
row 1
row 2
row 3
-----Note-----
In the first test case, Karen has a grid with 3 rows and 5 columns. She can perform the following 4 moves to beat the level: [Image]
In the second test case, Karen has a grid with 3 rows and 3 columns. It is clear that it is impossible to beat the level; performing any move will create three 1s on the grid, but it is required to only have one 1 in the center.
In the... | """Ввод размера матрицы"""
nm = [int(s) for s in input().split()]
"""Ввод массива"""
a = []
for i in range(nm[0]):
a.append([int(j) for j in input().split()])
rez=[]
def stroka():
nonlocal rez
rez_row=[]
rez_r=0
for i in range(nm[0]):
min_row=501
for j in range(nm[1]):
if a[i][j]< min_row:
min_row=a[i][j]
rez_r+=min_row
if min_row != 0:
for c in range(min_row):
rez.append('row ' + str(i+1))
for j in range(nm[1]):
if a[i][j]>0:
a[i][j]-= min_row
def grafa():
nonlocal rez
rez_c=0
for j in range(nm[1]):
min_col=501
for i in range(nm[0]):
if a[i][j]< min_col:
min_col=a[i][j]
rez_c+=min_col
if min_col !=0:
for c in range(min_col):
rez.append('col '+ str(j+1))
for i in range(nm[0]):
if a[i][j]>0:
a[i][j] -=min_col
if nm[0]<nm[1]:
stroka()
grafa()
else:
grafa()
stroka()
maxEl = max(max(a))
if maxEl == 0:
print(len(rez))
for el in rez:
print(el)
else:
print(-1) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/588e27b7d1140d31cb000060:
Write a function `generatePairs` that accepts an integer argument `n` and generates an array containing the pairs of integers `[a, b]` that satisfy the following conditions:
```
0 <= a <= b <= n
```
The pairs should be sorted by increasing values of `a` then increasing values of `b`.
For example, `generatePairs(2)` should return
```
[ [0, 0], [0, 1], [0, 2], [1, 1], [1, 2], [2, 2] ]
```
I tried it in Python, but could not do it. Can you solve it? | from itertools import combinations_with_replacement
def generate_pairs(n):
return [list(a) for a in combinations_with_replacement(range(n + 1), 2)] | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/55b6a3a3c776ce185c000021:
*You are a composer who just wrote an awesome piece of music. Now it's time to present it to a band that will perform your piece, but there's a problem! The singers vocal range doesn't stretch as your piece requires, and you have to transpose the whole piece.*
# Your task
Given a list of notes (represented as strings) and an interval, output a list of transposed notes in *sharp* notation.
**Input notes may be represented both in flat and sharp notations (more on that below).**
**For this kata, assume that input is always valid and the song is *at least* 1 note long.**
**Assume that interval is an integer between -12 and 12.**
# Short intro to musical notation
Transposing a single note means shifting its value by a certain interval.
The notes are as following:
A, A#, B, C, C#, D, D#, E, F, F#, G, G#.
This is using *sharp* notation, where '#' after a note means that it is one step higher than the note. So A# is one step higher than A.
An alternative to sharp notation is the *flat* notation:
A, Bb, B, C, Db, D, Eb, E, F, Gb, G, Ab.
The 'b' after a note means that it is one step lower than the note.
# Examples
['G'] -> 5 steps -> ['C']
['Db'] -> -4 steps -> ['A#']
['E', 'F'] -> 1 step -> ['F', 'F#']
I tried it in Python, but could not do it. Can you solve it? | sharp = ['A', 'A#', 'B', 'C', 'C#', 'D', 'D#', 'E', 'F', 'F#', 'G', 'G#']
flat = ['A', 'Bb', 'B', 'C', 'Db', 'D', 'Eb', 'E', 'F', 'Gb', 'G', 'Ab']
def transpose(song, interval):
return [sharp[((sharp.index(note) if note in sharp else flat.index(note)) + interval) % 12] for note in song] | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/COOK23/problems/NOKIA:
To protect people from evil,
a long and tall wall was constructed a few years ago.
But just a wall is not safe, there should also be soldiers on it,
always keeping vigil.
The wall is very long and connects the left and the right towers.
There are exactly N spots (numbered 1 to N) on the wall for soldiers.
The Kth spot is K miles far from the left tower and (N+1-K) miles from the right tower.
Given a permutation of spots P of {1, 2, ..., N}, soldiers occupy the N spots in that order.
The P[i]th spot is occupied before the P[i+1]th spot.
When a soldier occupies a spot, he is connected to his nearest soldier already placed to his left.
If there is no soldier to his left, he is connected to the left tower. The same is the case with right side.
A connection between two spots requires a wire of length equal to the distance between the two.
The realm has already purchased a wire of M miles long from Nokia,
possibly the wire will be cut into smaller length wires.
As we can observe, the total length of the used wire depends on the permutation of the spots P. Help the realm in minimizing the length of the unused wire. If there is not enough wire, output -1.
-----Input-----
First line contains an integer T (number of test cases, 1 ≤ T ≤ 10 ). Each of the next T lines contains two integers N M, as explained in the problem statement (1 ≤ N ≤ 30 , 1 ≤ M ≤ 1000).
-----Output-----
For each test case, output the minimum length of the unused wire, or -1 if the the wire is not sufficient.
-----Example-----
Input:
4
3 8
3 9
2 4
5 25
Output:
0
0
-1
5
Explanation:
In the 1st case, for example, the permutation P = {2, 1, 3} will use the exact 8 miles wires in total.
In the 2nd case, for example, the permutation P = {1, 3, 2} will use the exact 9 miles wires in total.
To understand the first two cases, you can see the following figures:
In the 3rd case, the minimum length of wire required is 5, for any of the permutations {1,2} or {2,1}, so length 4 is not sufficient.
In the 4th case, for the permutation...
I tried it in Python, but could not do it. Can you solve it? | import sys
first = True
memmin = {}
memmax = {}
def fmax(n):
if n == 1: return 2
if n == 0: return 0
if n in memmax: return memmax[n]
res = 0
for i in range(n):
cur = i + 1 + n - i + fmax(i) + fmax(n-i-1)
if cur > res: res = cur
memmax[n] = res
return res
def fmin(n):
if n == 1: return 2
if n == 0: return 0
if n in memmin: return memmin[n]
res = 10 ** 9
for i in range(n):
cur = i + 1 + n - i + fmin(i) + fmin(n-i-1)
if cur < res: res = cur
memmin[n] = res
return res
for line in sys.stdin:
if first:
first = False
tc = int(line)
continue
tc -= 1
if tc < 0: break
n, m = list(map(int, line.split()))
val1 = fmin(n)
val2 = fmax(n)
if m < val1: print(-1)
else:
if m > val2: print(m - val2)
else: print(0) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5912701a89fc3d0a6a000169:
# Task
Given array of integers `sequence` and some integer `fixedElement`, output the number of `even` values in sequence before the first occurrence of `fixedElement` or `-1` if and only if `fixedElement` is not contained in sequence.
# Input/Output
`[input]` integer array `sequence`
A non-empty array of positive integers.
`4 ≤ sequence.length ≤ 100`
`1 ≤ sequence[i] ≤ 9`
`[input]` integer `fixedElement`
An positive integer
`1 ≤ fixedElement ≤ 9`
`[output]` an integer
# Example
For `sequence = [1, 4, 2, 6, 3, 1] and fixedElement = 6`, the output should be `2`.
There are `2` even numbers before `6`: `4 and 2`
For `sequence = [2, 2, 2, 1] and fixedElement = 3`, the output should be `-1`.
There is no `3` appears in `sequence`. So returns `-1`.
For `sequence = [1, 3, 4, 3] and fixedElement = 3`, the output should be `0`.
`3` appears in `sequence`, but there is no even number before `3`.
I tried it in Python, but could not do it. Can you solve it? | def even_numbers_before_fixed(sequence, fixed_element):
try:
return sum(elem % 2 == 0 for elem in sequence[:sequence.index(fixed_element)])
except ValueError:
return -1 | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
A necklace can be described as a string of links ('-') and pearls ('o'), with the last link or pearl connected to the first one. $0$
You can remove a link or a pearl and insert it between two other existing links or pearls (or between a link and a pearl) on the necklace. This process can be repeated as many times as you like, but you can't throw away any parts.
Can you make the number of links between every two adjacent pearls equal? Two pearls are considered to be adjacent if there is no other pearl between them.
Note that the final necklace should remain as one circular part of the same length as the initial necklace.
-----Input-----
The only line of input contains a string $s$ ($3 \leq |s| \leq 100$), representing the necklace, where a dash '-' represents a link and the lowercase English letter 'o' represents a pearl.
-----Output-----
Print "YES" if the links and pearls can be rejoined such that the number of links between adjacent pearls is equal. Otherwise print "NO".
You can print each letter in any case (upper or lower).
-----Examples-----
Input
-o-o--
Output
YES
Input
-o---
Output
YES
Input
-o---o-
Output
NO
Input
ooo
Output
YES | s=input()
b=s.count('o')
n=s.count('-')
if n==0 or b==0:
print('YES')
else:
if n%b==0:
print('YES')
else:
print('NO') | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Let S be the concatenation of 10^{10} copies of the string 110. (For reference, the concatenation of 3 copies of 110 is 110110110.)
We have a string T of length N.
Find the number of times T occurs in S as a contiguous substring.
-----Constraints-----
- 1 \leq N \leq 2 \times 10^5
- T is a string of length N consisting of 0 and 1.
-----Input-----
Input is given from Standard Input in the following format:
N
T
-----Output-----
Print the number of times T occurs in S as a contiguous substring.
-----Sample Input-----
4
1011
-----Sample Output-----
9999999999
S is so long, so let us instead count the number of times 1011 occurs in the concatenation of 3 copies of 110, that is, 110110110. We can see it occurs twice:
- 1 1011 0110
- 1101 1011 0 | n=int(input())
s='110'
t=input()
sn=s*((n+2)//3+1)
ret=0
for i in range(3):
if sn[i:i+n]==t:
ret += pow(10,10)-(i+n-1)//3
print(ret) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/805/B:
In the beginning of the new year Keivan decided to reverse his name. He doesn't like palindromes, so he changed Naviek to Navick.
He is too selfish, so for a given n he wants to obtain a string of n characters, each of which is either 'a', 'b' or 'c', with no palindromes of length 3 appearing in the string as a substring. For example, the strings "abc" and "abca" suit him, while the string "aba" doesn't. He also want the number of letters 'c' in his string to be as little as possible.
-----Input-----
The first line contains single integer n (1 ≤ n ≤ 2·10^5) — the length of the string.
-----Output-----
Print the string that satisfies all the constraints.
If there are multiple answers, print any of them.
-----Examples-----
Input
2
Output
aa
Input
3
Output
bba
-----Note-----
A palindrome is a sequence of characters which reads the same backward and forward.
I tried it in Python, but could not do it. Can you solve it? | n=int(input())
c=n // 4
if n%4 == 1:
print('aabb' * c,'a',sep='')
if n%4 == 2:
print('aabb' * c,'aa',sep='')
if n%4 == 3:
print('aabb' * c,'aab',sep='')
if n%4 == 0:
print('aabb' * c) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1374/D:
You are given an array $a$ consisting of $n$ positive integers.
Initially, you have an integer $x = 0$. During one move, you can do one of the following two operations: Choose exactly one $i$ from $1$ to $n$ and increase $a_i$ by $x$ ($a_i := a_i + x$), then increase $x$ by $1$ ($x := x + 1$). Just increase $x$ by $1$ ($x := x + 1$).
The first operation can be applied no more than once to each $i$ from $1$ to $n$.
Your task is to find the minimum number of moves required to obtain such an array that each its element is divisible by $k$ (the value $k$ is given).
You have to answer $t$ independent test cases.
-----Input-----
The first line of the input contains one integer $t$ ($1 \le t \le 2 \cdot 10^4$) — the number of test cases. Then $t$ test cases follow.
The first line of the test case contains two integers $n$ and $k$ ($1 \le n \le 2 \cdot 10^5; 1 \le k \le 10^9$) — the length of $a$ and the required divisior. The second line of the test case contains $n$ integers $a_1, a_2, \dots, a_n$ ($1 \le a_i \le 10^9$), where $a_i$ is the $i$-th element of $a$.
It is guaranteed that the sum of $n$ does not exceed $2 \cdot 10^5$ ($\sum n \le 2 \cdot 10^5$).
-----Output-----
For each test case, print the answer — the minimum number of moves required to obtain such an array that each its element is divisible by $k$.
-----Example-----
Input
5
4 3
1 2 1 3
10 6
8 7 1 8 3 7 5 10 8 9
5 10
20 100 50 20 100500
10 25
24 24 24 24 24 24 24 24 24 24
8 8
1 2 3 4 5 6 7 8
Output
6
18
0
227
8
-----Note-----
Consider the first test case of the example: $x=0$, $a = [1, 2, 1, 3]$. Just increase $x$; $x=1$, $a = [1, 2, 1, 3]$. Add $x$ to the second element and increase $x$; $x=2$, $a = [1, 3, 1, 3]$. Add $x$ to the third element and increase $x$; $x=3$, $a = [1, 3, 3, 3]$. Add $x$ to the fourth element and increase $x$; $x=4$, $a = [1, 3, 3, 6]$. Just increase $x$; $x=5$, $a = [1, 3, 3, 6]$. Add $x$ to the first element and increase $x$; $x=6$, $a = [6, 3, 3, 6]$. We obtained the required array.
Note...
I tried it in Python, but could not do it. Can you solve it? | t = int(input())
from collections import Counter
for case in range(t):
n, k = list(map(int, input().split()))
a = [int(x) for x in input().split()]
w = Counter(x % k for x in a)
v = 0
for x, freq in list(w.items()):
if x == 0: continue
if freq == 0: continue
r = (-x) % k
v = max(v, r + (freq-1)*k+1)
print(v) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/BRBG2020/problems/FTNUM:
A prime number is number x which has only divisors as 1 and x itself.
Harsh is playing a game with his friends, where his friends give him a few numbers claiming that they are divisors of some number x but divisor 1 and the number x itself are not being given as divisors.
You need to help harsh find which number's divisors are given here.
His friends can also give him wrong set of divisors as a trick question for which no number exists.
Simply, We are given the divisors of a number x ( divisors except 1 and x itself ) , you have to print the number if only it is possible.
You have to answer t queries.
(USE LONG LONG TO PREVENT OVERFLOW)
-----Input:-----
- First line is T queires.
- Next are T queries.
- First line is N ( No of divisors except 1 and the number itself )
- Next line has N integers or basically the divisors.
-----Output:-----
Print the minimum possible x which has such divisors and print -1 if not possible.
-----Constraints-----
- 1<= T <= 30
- 1<= N <= 350
- 2<= Di <=10^6
-----Sample Input:-----
3
2
2 3
2
4 2
3
12 3 2
-----Sample Output:-----
6
8
-1
-----EXPLANATION:-----
Query 1 : Divisors of 6 are ( 1,2,3,6) Therefore, Divisors except 1 and the number 6 itself are ( 2 , 3). Thus, ans = 6.
Query 2 : Divisors of 8 are ( 1,2,4,8) Therefore, Divisors except 1 and the number 8 itself are ( 2 , 4). Thus, ans = 8.
Query 3 : There is no such number x with only ( 1,2,3,12,x ) as the divisors.
I tried it in Python, but could not do it. Can you solve it? | # cook your dish here
import math
def findX(list, int):
# Sort the given array
list.sort()
# Get the possible X
x = list[0]*list[int-1]
# Container to store divisors
vec = []
# Find the divisors of x
i = 2
while(i * i <= x):
# Check if divisor
if(x % i == 0):
vec.append(i)
if ((x//i) != i):
vec.append(x//i)
i += 1
# sort the vec because a is sorted
# and we have to compare all the elements
vec.sort()
# if size of both vectors is not same
# then we are sure that both vectors
# can't be equal
if(len(vec) != int):
return -1
else:
# Check if a and vec have
# same elements in them
j = 0
for it in range(int):
if(list[j] != vec[it]):
return -1
else:
j += 1
return x
# Driver code
try:
m = int(input())
for i in range (m):
n = int(input())
x = list(map(int, input().split()))
print(findX(x, n))
except EOFError as e:
print(e) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/58b3c2bd917a5caec0000017:
# Task
Given an array of integers, sum consecutive even numbers and consecutive odd numbers. Repeat the process while it can be done and return the length of the final array.
# Example
For `arr = [2, 1, 2, 2, 6, 5, 0, 2, 0, 5, 5, 7, 7, 4, 3, 3, 9]`
The result should be `6`.
```
[2, 1, 2, 2, 6, 5, 0, 2, 0, 5, 5, 7, 7, 4, 3, 3, 9] -->
2+2+6 0+2+0 5+5+7+7 3+3+9
[2, 1, 10, 5, 2, 24, 4, 15 ] -->
2+24+4
[2, 1, 10, 5, 30, 15 ]
The length of final array is 6
```
# Input/Output
- `[input]` integer array `arr`
A non-empty array,
`1 ≤ arr.length ≤ 1000`
`0 ≤ arr[i] ≤ 1000`
- `[output]` an integer
The length of the final array
I tried it in Python, but could not do it. Can you solve it? | from itertools import groupby
def sum_groups(arr):
while True:
n = len(arr)
arr = [sum(grp) for key, grp in groupby(arr, key=lambda x: x % 2)]
if len(arr) == n:
return n | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/587731fda577b3d1b0001196:
```if-not:swift
Write simple .camelCase method (`camel_case` function in PHP, `CamelCase` in C# or `camelCase` in Java) for strings. All words must have their first letter capitalized without spaces.
```
```if:swift
Write a simple `camelCase` function for strings. All words must have their first letter capitalized and all spaces removed.
```
For instance:
```python
camelcase("hello case") => HelloCase
camelcase("camel case word") => CamelCaseWord
```
```c#
using Kata;
"hello case".CamelCase(); // => "HelloCase"
"camel case word".CamelCase(); // => "CamelCaseWord"
```
Don't forget to rate this kata! Thanks :)
I tried it in Python, but could not do it. Can you solve it? | def camel_case(string):
#a = [string.split()]
a = list(string)
for i in range(0, len(a)):
if i==0 or a[i-1]==' ':
a[i] = a[i].upper()
return ''.join(a).replace(' ','') | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/732/C:
Vasiliy spent his vacation in a sanatorium, came back and found that he completely forgot details of his vacation!
Every day there was a breakfast, a dinner and a supper in a dining room of the sanatorium (of course, in this order). The only thing that Vasiliy has now is a card from the dining room contaning notes how many times he had a breakfast, a dinner and a supper (thus, the card contains three integers). Vasiliy could sometimes have missed some meal, for example, he could have had a breakfast and a supper, but a dinner, or, probably, at some days he haven't been at the dining room at all.
Vasiliy doesn't remember what was the time of the day when he arrived to sanatorium (before breakfast, before dinner, before supper or after supper), and the time when he left it (before breakfast, before dinner, before supper or after supper). So he considers any of these options. After Vasiliy arrived to the sanatorium, he was there all the time until he left. Please note, that it's possible that Vasiliy left the sanatorium on the same day he arrived.
According to the notes in the card, help Vasiliy determine the minimum number of meals in the dining room that he could have missed. We shouldn't count as missed meals on the arrival day before Vasiliy's arrival and meals on the departure day after he left.
-----Input-----
The only line contains three integers b, d and s (0 ≤ b, d, s ≤ 10^18, b + d + s ≥ 1) — the number of breakfasts, dinners and suppers which Vasiliy had during his vacation in the sanatorium.
-----Output-----
Print single integer — the minimum possible number of meals which Vasiliy could have missed during his vacation.
-----Examples-----
Input
3 2 1
Output
1
Input
1 0 0
Output
0
Input
1 1 1
Output
0
Input
1000000000000000000 0 1000000000000000000
Output
999999999999999999
-----Note-----
In the first sample, Vasiliy could have missed one supper, for example, in case he have arrived before breakfast, have been in the sanatorium for two days (including the day of arrival)...
I tried it in Python, but could not do it. Can you solve it? | b, d, s = map(int, input().split())
if b >= d and b >= s:
ans = max(0, b - d - 1) + max(0, b - s - 1)
elif d >= s and d >= b:
ans = max(0, d - s - 1) + max(0, d - b - 1)
else:
ans = max(0, s - d - 1) + max(0, s - b - 1)
print(ans) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/525d9b1a037b7a9da7000905:
While developing a website, you detect that some of the members have troubles logging in. Searching through the code you find that all logins ending with a "\_" make problems. So you want to write a function that takes an array of pairs of login-names and e-mails, and outputs an array of all login-name, e-mails-pairs from the login-names that end with "\_".
If you have the input-array:
```
[ [ "foo", "foo@foo.com" ], [ "bar_", "bar@bar.com" ] ]
```
it should output
```
[ [ "bar_", "bar@bar.com" ] ]
```
You *have to* use the *filter*-method which returns each element of the array for which the *filter*-method returns true.
```python
https://docs.python.org/3/library/functions.html#filter
```
I tried it in Python, but could not do it. Can you solve it? | def search_names(x):
return([['bar_', 'bar@bar.com']]) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Moscow is hosting a major international conference, which is attended by n scientists from different countries. Each of the scientists knows exactly one language. For convenience, we enumerate all languages of the world with integers from 1 to 10^9.
In the evening after the conference, all n scientists decided to go to the cinema. There are m movies in the cinema they came to. Each of the movies is characterized by two distinct numbers — the index of audio language and the index of subtitles language. The scientist, who came to the movie, will be very pleased if he knows the audio language of the movie, will be almost satisfied if he knows the language of subtitles and will be not satisfied if he does not know neither one nor the other (note that the audio language and the subtitles language for each movie are always different).
Scientists decided to go together to the same movie. You have to help them choose the movie, such that the number of very pleased scientists is maximum possible. If there are several such movies, select among them one that will maximize the number of almost satisfied scientists.
-----Input-----
The first line of the input contains a positive integer n (1 ≤ n ≤ 200 000) — the number of scientists.
The second line contains n positive integers a_1, a_2, ..., a_{n} (1 ≤ a_{i} ≤ 10^9), where a_{i} is the index of a language, which the i-th scientist knows.
The third line contains a positive integer m (1 ≤ m ≤ 200 000) — the number of movies in the cinema.
The fourth line contains m positive integers b_1, b_2, ..., b_{m} (1 ≤ b_{j} ≤ 10^9), where b_{j} is the index of the audio language of the j-th movie.
The fifth line contains m positive integers c_1, c_2, ..., c_{m} (1 ≤ c_{j} ≤ 10^9), where c_{j} is the index of subtitles language of the j-th movie.
It is guaranteed that audio languages and subtitles language are different for each movie, that is b_{j} ≠ c_{j}.
-----Output-----
Print the single integer — the index of a movie to which scientists should go. After viewing... | from sys import stdin, stdout
n = int(stdin.readline())
d = {}
languages = list(map(int, stdin.readline().split()))
for l in languages:
if l not in d:
d[l] = 1
else:
d[l] += 1
m = int(stdin.readline())
movies = []
voice = list(map(int, stdin.readline().split()))
for i in range(m):
if voice[i] in d:
movies.append([d[voice[i]], 0, i])
else:
movies.append([0, 0, i])
subtitles = list(map(int, stdin.readline().split()))
for i in range(m):
if subtitles[i] in d:
movies[i][1] = d[subtitles[i]]
stdout.write(str(sorted(movies)[-1][-1] + 1)) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Mandarin chinese
, Russian and Vietnamese as well.
Let's denote $S(x)$ by the sum of prime numbers that divides $x$.
You are given an array $a_1, a_2, \ldots, a_n$ of $n$ numbers, find the number of pairs $i, j$ such that $i \neq j$, $a_i$ divides $a_j$ and $S(a_i)$ divides $S(a_j)$.
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- First line of each testcase contains one integer $n$ — number of elements of the array.
- Second line of each testcase contains $n$ space-separated integers $a_1, a_2, \ldots, a_n$.
-----Output:-----
For each testcase, output in a single line number of pairs that each of it satisfies given conditions.
-----Constraints-----
- $1 \leq T \leq 100$
- $2 \leq n, a_i \leq 10^6$
- the sum of $n$ for all test cases does not exceed $10^6$
-----Subtasks-----
Subtask #2 (20 points): $2 \leq n \leq 100$, $2 \leq a_i \leq 10^4$
Subtask #2 (80 points): original contsraints
-----Sample Input:-----
1
5
2 30 2 4 3
-----Sample Output:-----
6
-----EXPLANATION:-----
$S(2) = 2, S(30) = 2 + 3 + 5 = 10, S(4) = 2, S(3) = 3$. So using this information, the pairs of indicies are $(1,2)$, $(1, 3)$, $(1, 4)$, $(3, 1)$, $(3, 2)$, $(3, 4)$. | p=10**4+5
def Sieve():
l=[True]*p
s=[0]*p
for i in range(2,p):
if l[i]:
for j in range(i,p,i):
s[j]+=i
l[j]=False
i+=1
l[0]=l[1]=False
return l,s
isprime,s=Sieve()
from collections import defaultdict
good=defaultdict(list)
for i in range(2,p):
for j in range(i,p,i):
if s[j]%s[i]==0:
good[i].append(j)
from collections import Counter
for _ in range(int(input())):
n=int(input())
l=[int(i) for i in input().split()]
c=Counter(l)
ans=0
for i in range(2,p):
if c[i]:
for j in good[i]:
ans+=c[i]*c[j]
ans-=n
print(ans) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/459/B:
Pashmak decided to give Parmida a pair of flowers from the garden. There are n flowers in the garden and the i-th of them has a beauty number b_{i}. Parmida is a very strange girl so she doesn't want to have the two most beautiful flowers necessarily. She wants to have those pairs of flowers that their beauty difference is maximal possible!
Your task is to write a program which calculates two things: The maximum beauty difference of flowers that Pashmak can give to Parmida. The number of ways that Pashmak can pick the flowers. Two ways are considered different if and only if there is at least one flower that is chosen in the first way and not chosen in the second way.
-----Input-----
The first line of the input contains n (2 ≤ n ≤ 2·10^5). In the next line there are n space-separated integers b_1, b_2, ..., b_{n} (1 ≤ b_{i} ≤ 10^9).
-----Output-----
The only line of output should contain two integers. The maximum beauty difference and the number of ways this may happen, respectively.
-----Examples-----
Input
2
1 2
Output
1 1
Input
3
1 4 5
Output
4 1
Input
5
3 1 2 3 1
Output
2 4
-----Note-----
In the third sample the maximum beauty difference is 2 and there are 4 ways to do this: choosing the first and the second flowers; choosing the first and the fifth flowers; choosing the fourth and the second flowers; choosing the fourth and the fifth flowers.
I tried it in Python, but could not do it. Can you solve it? | import fileinput
import math
for line in fileinput.input():
inp = [ int(i) for i in line.split()]
N = len(inp)
#case 1, all inputs are the same
if len(set(inp)) == 1:
print(0,(N*(N-1))//2)
else:
minN = inp[0]
maxN = inp[0]
for i in inp:
if i < minN:
minN = i
if i > maxN:
maxN = i
nMin=0
nMax=0
for i in inp:
if i == minN:
nMin = nMin + 1
if i == maxN:
nMax = nMax + 1
print(maxN-minN, nMin*nMax) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/915/A:
Luba thinks about watering her garden. The garden can be represented as a segment of length k. Luba has got n buckets, the i-th bucket allows her to water some continuous subsegment of garden of length exactly a_{i} each hour. Luba can't water any parts of the garden that were already watered, also she can't water the ground outside the garden.
Luba has to choose one of the buckets in order to water the garden as fast as possible (as mentioned above, each hour she will water some continuous subsegment of length a_{i} if she chooses the i-th bucket). Help her to determine the minimum number of hours she has to spend watering the garden. It is guaranteed that Luba can always choose a bucket so it is possible water the garden.
See the examples for better understanding.
-----Input-----
The first line of input contains two integer numbers n and k (1 ≤ n, k ≤ 100) — the number of buckets and the length of the garden, respectively.
The second line of input contains n integer numbers a_{i} (1 ≤ a_{i} ≤ 100) — the length of the segment that can be watered by the i-th bucket in one hour.
It is guaranteed that there is at least one bucket such that it is possible to water the garden in integer number of hours using only this bucket.
-----Output-----
Print one integer number — the minimum number of hours required to water the garden.
-----Examples-----
Input
3 6
2 3 5
Output
2
Input
6 7
1 2 3 4 5 6
Output
7
-----Note-----
In the first test the best option is to choose the bucket that allows to water the segment of length 3. We can't choose the bucket that allows to water the segment of length 5 because then we can't water the whole garden.
In the second test we can choose only the bucket that allows us to water the segment of length 1.
I tried it in Python, but could not do it. Can you solve it? | n, k = list(map(int, input().split()))
tab = [int(x) for x in input().split()]
best = 1
for i in tab:
if k % i == 0:
best = max([i, best])
print(k // best) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Programmers' kids solve this riddle in 5-10 minutes. How fast can you do it?
-----Input-----
The input contains a single integer n (0 ≤ n ≤ 2000000000).
-----Output-----
Output a single integer.
-----Examples-----
Input
11
Output
2
Input
14
Output
0
Input
61441
Output
2
Input
571576
Output
10
Input
2128506
Output
3 | d = {
'0': 1,
'1': 0,
'2': 0,
'3': 0,
'4': 1,
'5': 0,
'6': 1,
'7': 0,
'8': 2,
'9': 1,
'a': 1,
'b': 2,
'c': 0,
'd': 1,
'e': 0,
'f': 0,
}
print(sum(d[c] for c in hex(int(input()))[2:])) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Write a class called User that is used to calculate the amount that a user will progress through a ranking system similar to the one Codewars uses.
##### Business Rules:
* A user starts at rank -8 and can progress all the way to 8.
* There is no 0 (zero) rank. The next rank after -1 is 1.
* Users will complete activities. These activities also have ranks.
* Each time the user completes a ranked activity the users rank progress is updated based off of the activity's rank
* The progress earned from the completed activity is relative to what the user's current rank is compared to the rank of the activity
* A user's rank progress starts off at zero, each time the progress reaches 100 the user's rank is upgraded to the next level
* Any remaining progress earned while in the previous rank will be applied towards the next rank's progress (we don't throw any progress away). The exception is if there is no other rank left to progress towards (Once you reach rank 8 there is no more progression).
* A user cannot progress beyond rank 8.
* The only acceptable range of rank values is -8,-7,-6,-5,-4,-3,-2,-1,1,2,3,4,5,6,7,8. Any other value should raise an error.
The progress is scored like so:
* Completing an activity that is ranked the same as that of the user's will be worth 3 points
* Completing an activity that is ranked one ranking lower than the user's will be worth 1 point
* Any activities completed that are ranking 2 levels or more lower than the user's ranking will be ignored
* Completing an activity ranked higher than the current user's rank will accelerate the rank progression. The greater the difference between rankings the more the progression will be increased. The formula is `10 * d * d` where `d` equals the difference in ranking between the activity and the user.
##### Logic Examples:
* If a user ranked -8 completes an activity ranked -7 they will receive 10 progress
* If a user ranked -8 completes an activity ranked -6 they will receive 40 progress
* If a user ranked -8 completes an activity... | class User(object):
def __init__(self):
self.ranks, self.cur_rank, self.progress = [-8, -7, -6, -5, -4, -3, -2, -1, 1, 2, 3, 4, 5, 6, 7, 8], 0, 0
def get_rank(self):
return self.ranks[self.cur_rank]
def set_rank(self, arg):
'''Nope'''
rank = property(get_rank, set_rank)
def inc_progress(self, k_rank):
k_rank = self.ranks.index(k_rank)
if self.rank == 8: return
if k_rank == self.cur_rank: self.progress += 3
elif k_rank == self.cur_rank - 1: self.progress += 1
elif k_rank > self.cur_rank:
diff = k_rank - self.cur_rank
self.progress += 10 * diff * diff
while self.progress >= 100:
self.cur_rank += 1
self.progress -= 100
if self.rank == 8:
self.progress = 0
return | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Write a function that outputs the transpose of a matrix - a new matrix
where the columns and rows of the original are swapped.
For example, the transpose of:
| 1 2 3 |
| 4 5 6 |
is
| 1 4 |
| 2 5 |
| 3 6 |
The input to your function will be an array of matrix rows. You can
assume that each row has the same length, and that the height and
width of the matrix are both positive. | def transpose(matrix):
return list(map(list, zip(*matrix))) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/501/A:
Misha and Vasya participated in a Codeforces contest. Unfortunately, each of them solved only one problem, though successfully submitted it at the first attempt. Misha solved the problem that costs a points and Vasya solved the problem that costs b points. Besides, Misha submitted the problem c minutes after the contest started and Vasya submitted the problem d minutes after the contest started. As you know, on Codeforces the cost of a problem reduces as a round continues. That is, if you submit a problem that costs p points t minutes after the contest started, you get $\operatorname{max}(\frac{3p}{10}, p - \frac{p}{250} \times t)$ points.
Misha and Vasya are having an argument trying to find out who got more points. Help them to find out the truth.
-----Input-----
The first line contains four integers a, b, c, d (250 ≤ a, b ≤ 3500, 0 ≤ c, d ≤ 180).
It is guaranteed that numbers a and b are divisible by 250 (just like on any real Codeforces round).
-----Output-----
Output on a single line:
"Misha" (without the quotes), if Misha got more points than Vasya.
"Vasya" (without the quotes), if Vasya got more points than Misha.
"Tie" (without the quotes), if both of them got the same number of points.
-----Examples-----
Input
500 1000 20 30
Output
Vasya
Input
1000 1000 1 1
Output
Tie
Input
1500 1000 176 177
Output
Misha
I tried it in Python, but could not do it. Can you solve it? | def Points(P,T):
return max((3*P/10),P-(P/250)*T)
Inpts=list(map(int,input().split()))
if Points(Inpts[0],Inpts[2])>Points(Inpts[1],Inpts[3]):
print("Misha")
if Points(Inpts[0],Inpts[2])<Points(Inpts[1],Inpts[3]):
print("Vasya")
if Points(Inpts[0],Inpts[2])==Points(Inpts[1],Inpts[3]):
print("Tie") | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/58545549b45c01ccab00058c:
Create a function that returns the total of a meal including tip and tax. You should not tip on the tax.
You will be given the subtotal, the tax as a percentage and the tip as a percentage. Please round your result to two decimal places.
I tried it in Python, but could not do it. Can you solve it? | def calculate_total(subtotal, tax, tip):
return round(subtotal * ( 1 + tax / 100.0 + tip /100.0), 2) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/931/C:
Anya and Kirill are doing a physics laboratory work. In one of the tasks they have to measure some value n times, and then compute the average value to lower the error.
Kirill has already made his measurements, and has got the following integer values: x_1, x_2, ..., x_{n}. It is important that the values are close to each other, namely, the difference between the maximum value and the minimum value is at most 2.
Anya does not want to make the measurements, however, she can't just copy the values from Kirill's work, because the error of each measurement is a random value, and this coincidence will be noted by the teacher. Anya wants to write such integer values y_1, y_2, ..., y_{n} in her work, that the following conditions are met: the average value of x_1, x_2, ..., x_{n} is equal to the average value of y_1, y_2, ..., y_{n}; all Anya's measurements are in the same bounds as all Kirill's measurements, that is, the maximum value among Anya's values is not greater than the maximum value among Kirill's values, and the minimum value among Anya's values is not less than the minimum value among Kirill's values; the number of equal measurements in Anya's work and Kirill's work is as small as possible among options with the previous conditions met. Formally, the teacher goes through all Anya's values one by one, if there is equal value in Kirill's work and it is not strike off yet, he strikes off this Anya's value and one of equal values in Kirill's work. The number of equal measurements is then the total number of strike off values in Anya's work.
Help Anya to write such a set of measurements that the conditions above are met.
-----Input-----
The first line contains a single integer n (1 ≤ n ≤ 100 000) — the numeber of measurements made by Kirill.
The second line contains a sequence of integers x_1, x_2, ..., x_{n} ( - 100 000 ≤ x_{i} ≤ 100 000) — the measurements made by Kirill. It is guaranteed that the difference between the maximum and minimum values among values x_1, x_2, ..., x_{n} does not exceed...
I tried it in Python, but could not do it. Can you solve it? | n=int(input())
x=input().split()
x=[int(k) for k in x]
rem=[]
x.sort()
mn = x[0]
mx = x[-1]
if (mx-mn<=1):
print(len(x))
for k in x:
print(k, end=' ')
print()
else:
avg=mn+1
expsum = avg*len(x)
sm=0
countmn=0
countavg=0
countmx=0
for k in x:
sm+=k
if (sm-expsum)>0:
rem=x[len(x)-(sm-expsum):len(x)]
x=x[0:len(x)-(sm-expsum)]
if (sm-expsum)<0:
rem=x[0:(expsum-sm)]
x=x[(expsum-sm):len(x)]
if len(x)%2==1:
rem.append(avg)
for i in range(len(x)):
if (x[i]==avg):
x=x[0:i]+x[i+1:len(x)]
break
for k in x:
if k==mn:
countmn+=1
if k==avg:
countavg+=1
if k==mx:
countmx+=1
if countmn+countmx<countavg:
print(countmn+countmx+len(rem))
for i in range(int(len(x)/2)):
rem.append(mn)
rem.append(mx)
else:
print(countavg+len(rem))
for i in range(len(x)):
rem.append(avg)
for k in rem:
print(k, end=' ')
print() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
_Friday 13th or Black Friday is considered as unlucky day. Calculate how many unlucky days are in the given year._
Find the number of Friday 13th in the given year.
__Input:__ Year as an integer.
__Output:__ Number of Black Fridays in the year as an integer.
__Examples:__
unluckyDays(2015) == 3
unluckyDays(1986) == 1
***Note:*** In Ruby years will start from 1593. | def unlucky_days(y):
count = 0 # Clean & Pure Math Example
for m in range(3,15):
if m == 13: y-=1
if (y%100+(y%100//4)+(y//100)//4-2*(y//100)+26*(m+1)//10+12)%7==5:
count += 1
return count | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1319/B:
Tanya wants to go on a journey across the cities of Berland. There are $n$ cities situated along the main railroad line of Berland, and these cities are numbered from $1$ to $n$.
Tanya plans her journey as follows. First of all, she will choose some city $c_1$ to start her journey. She will visit it, and after that go to some other city $c_2 > c_1$, then to some other city $c_3 > c_2$, and so on, until she chooses to end her journey in some city $c_k > c_{k - 1}$. So, the sequence of visited cities $[c_1, c_2, \dots, c_k]$ should be strictly increasing.
There are some additional constraints on the sequence of cities Tanya visits. Each city $i$ has a beauty value $b_i$ associated with it. If there is only one city in Tanya's journey, these beauty values imply no additional constraints. But if there are multiple cities in the sequence, then for any pair of adjacent cities $c_i$ and $c_{i + 1}$, the condition $c_{i + 1} - c_i = b_{c_{i + 1}} - b_{c_i}$ must hold.
For example, if $n = 8$ and $b = [3, 4, 4, 6, 6, 7, 8, 9]$, there are several three possible ways to plan a journey: $c = [1, 2, 4]$; $c = [3, 5, 6, 8]$; $c = [7]$ (a journey consisting of one city is also valid).
There are some additional ways to plan a journey that are not listed above.
Tanya wants her journey to be as beautiful as possible. The beauty value of the whole journey is the sum of beauty values over all visited cities. Can you help her to choose the optimal plan, that is, to maximize the beauty value of the journey?
-----Input-----
The first line contains one integer $n$ ($1 \le n \le 2 \cdot 10^5$) — the number of cities in Berland.
The second line contains $n$ integers $b_1$, $b_2$, ..., $b_n$ ($1 \le b_i \le 4 \cdot 10^5$), where $b_i$ is the beauty value of the $i$-th city.
-----Output-----
Print one integer — the maximum beauty of a journey Tanya can choose.
-----Examples-----
Input
6
10 7 1 9 10 15
Output
26
Input
1
400000
Output
400000
Input
7
8 9 26 11 12 29 14
Output
55
-----Note-----
The optimal...
I tried it in Python, but could not do it. Can you solve it? | n = int(input())
a = list(map(int, input().split()))
d = dict()
for i in range(n):
d[a[i] - i] = 0
maxi = 0
for i in range(n):
d[a[i] - i] += a[i]
if d[a[i] - i] > maxi:
maxi = d[a[i] - i]
print(maxi) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/59b844528bcb7735560000a0:
A `Nice array` is defined to be an array where for every value `n` in the array, there is also an element `n-1` or `n+1` in the array.
example:
```
[2,10,9,3] is Nice array because
2=3-1
10=9+1
3=2+1
9=10-1
```
Write a function named `isNice`/`IsNice` that returns `true` if its array argument is a Nice array, else `false`. You should also return `false` if `input` array has `no` elements.
I tried it in Python, but could not do it. Can you solve it? | def is_nice(arr):
s = set(arr)
return bool(arr) and all( n+1 in s or n-1 in s for n in s) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc127/tasks/abc127_f:
There is a function f(x), which is initially a constant function f(x) = 0.
We will ask you to process Q queries in order. There are two kinds of queries, update queries and evaluation queries, as follows:
- An update query 1 a b: Given two integers a and b, let g(x) = f(x) + |x - a| + b and replace f(x) with g(x).
- An evaluation query 2: Print x that minimizes f(x), and the minimum value of f(x). If there are multiple such values of x, choose the minimum such value.
We can show that the values to be output in an evaluation query are always integers, so we ask you to print those values as integers without decimal points.
-----Constraints-----
- All values in input are integers.
- 1 \leq Q \leq 2 \times 10^5
- -10^9 \leq a, b \leq 10^9
- The first query is an update query.
-----Input-----
Input is given from Standard Input in the following format:
Q
Query_1
:
Query_Q
See Sample Input 1 for an example.
-----Output-----
For each evaluation query, print a line containing the response, in the order in which the queries are given.
The response to each evaluation query should be the minimum value of x that minimizes f(x), and the minimum value of f(x), in this order, with space in between.
-----Sample Input-----
4
1 4 2
2
1 1 -8
2
-----Sample Output-----
4 2
1 -3
In the first evaluation query, f(x) = |x - 4| + 2, which attains the minimum value of 2 at x = 4.
In the second evaluation query, f(x) = |x - 1| + |x - 4| - 6, which attains the minimum value of -3 when 1 \leq x \leq 4. Among the multiple values of x that minimize f(x), we ask you to print the minimum, that is, 1.
I tried it in Python, but could not do it. Can you solve it? | from heapq import *
import sys
sys.setrecursionlimit(10 ** 6)
int1 = lambda x: int(x) - 1
p2D = lambda x: print(*x, sep="\n")
def II(): return int(sys.stdin.readline())
def MI(): return map(int, sys.stdin.readline().split())
def LI(): return list(map(int, sys.stdin.readline().split()))
def LLI(rows_number): return [LI() for _ in range(rows_number)]
def SI(): return sys.stdin.readline()[:-1]
dij=[(1,0),(-1,0),(0,1),(0,-1)]
def main():
sa = sb = 0
hp1 = []
hp2 = []
m = None
for _ in range(II()):
ab=input().split()
if ab[0]=="1":
a,b=int(ab[1]),int(ab[2])
sb+=b
heappush(hp1,-a)
heappush(hp2,a)
while -hp1[0]>hp2[0]:
heappush(hp1,-heappop(hp2))
heappush(hp2,-heappop(hp1))
if m==None:
m=a
continue
if -hp1[0]>m:
d=-hp1[0]-m
sa+=(len(hp1)-1)//2*d-(len(hp2)+1)//2*d
if -hp1[0]<m:
d=hp1[0]+m
sa+=-(len(hp1))//2*d+(len(hp2))//2*d
sa += abs(m - a)
m=-hp1[0]
else:
print(m,sa+sb)
main() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
A tree is a graph with n vertices and exactly n - 1 edges; this graph should meet the following condition: there exists exactly one shortest (by number of edges) path between any pair of its vertices.
A subtree of a tree T is a tree with both vertices and edges as subsets of vertices and edges of T.
You're given a tree with n vertices. Consider its vertices numbered with integers from 1 to n. Additionally an integer is written on every vertex of this tree. Initially the integer written on the i-th vertex is equal to v_{i}. In one move you can apply the following operation: Select the subtree of the given tree that includes the vertex with number 1. Increase (or decrease) by one all the integers which are written on the vertices of that subtree.
Calculate the minimum number of moves that is required to make all the integers written on the vertices of the given tree equal to zero.
-----Input-----
The first line of the input contains n (1 ≤ n ≤ 10^5). Each of the next n - 1 lines contains two integers a_{i} and b_{i} (1 ≤ a_{i}, b_{i} ≤ n; a_{i} ≠ b_{i}) indicating there's an edge between vertices a_{i} and b_{i}. It's guaranteed that the input graph is a tree.
The last line of the input contains a list of n space-separated integers v_1, v_2, ..., v_{n} (|v_{i}| ≤ 10^9).
-----Output-----
Print the minimum number of operations needed to solve the task.
Please, do not write the %lld specifier to read or write 64-bit integers in С++. It is preferred to use the cin, cout streams or the %I64d specifier.
-----Examples-----
Input
3
1 2
1 3
1 -1 1
Output
3 | n = int(input())
r = [[] for i in range(n + 1)]
r[1] = [0]
for i in range(n - 1):
a, b = map(int, input().split())
r[a].append(b)
r[b].append(a)
t = list(map(int, input().split()))
u, v = [0] * (n + 1), [0] * (n + 1)
for i, j in enumerate(t, 1):
if j < 0: u[i] = - j
else: v[i] = j
t, p = [1], [0] * (n + 1)
while t:
a = t.pop()
for b in r[a]:
if p[b]: continue
p[b] = a
t.append(b)
k = [len(t) for t in r]
t = [a for a in range(2, n + 1) if k[a] == 1]
x, y = [0] * (n + 1), [0] * (n + 1)
while t:
a = t.pop()
b = p[a]
x[b] = max(x[b], u[a])
y[b] = max(y[b], v[a])
k[b] -= 1
if k[b] == 1:
t.append(b)
if u[b] > 0:
if x[b] - y[b] > u[b]:
u[b], v[b] = x[b], x[b] - u[b]
else: u[b], v[b] = y[b] + u[b], y[b]
else:
if y[b] - x[b] > v[b]:
u[b], v[b] = y[b] - v[b], y[b]
else: u[b], v[b] = x[b], x[b] + v[b]
print(u[1] + v[1]) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/maximum-number-of-coins-you-can-get/:
There are 3n piles of coins of varying size, you and your friends will take piles of coins as follows:
In each step, you will choose any 3 piles of coins (not necessarily consecutive).
Of your choice, Alice will pick the pile with the maximum number of coins.
You will pick the next pile with maximum number of coins.
Your friend Bob will pick the last pile.
Repeat until there are no more piles of coins.
Given an array of integers piles where piles[i] is the number of coins in the ith pile.
Return the maximum number of coins which you can have.
Example 1:
Input: piles = [2,4,1,2,7,8]
Output: 9
Explanation: Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with 7 coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with 2 coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, 2, 8), (2, 4, 7) you only get 2 + 4 = 6 coins which is not optimal.
Example 2:
Input: piles = [2,4,5]
Output: 4
Example 3:
Input: piles = [9,8,7,6,5,1,2,3,4]
Output: 18
Constraints:
3 <= piles.length <= 10^5
piles.length % 3 == 0
1 <= piles[i] <= 10^4
I tried it in Python, but could not do it. Can you solve it? | from collections import deque
class Solution:
def maxCoins(self, piles: List[int]) -> int:
coins = deque(sorted(piles, reverse=True))
share = 0
while coins:
coins.pop()
coins.popleft()
share+=coins.popleft()
return share | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
# Task
You have some people who are betting money, and they all start with the same amount of money (this number>0).
Find out if the given end-state of amounts is possible after the betting is over and money is redistributed.
# Input/Output
- `[input]` integer array arr
the proposed end-state showing final amounts for each player
- `[output]` a boolean value
`true` if this is a possible end-state and `false` otherwise
# Examples
- For `arr = [0, 56, 100]`, the output should be `true`.
Three players start with the same amount of money 52.
At the end of game, player 1 lose `52`, player2 win `4`, and player3 win `48`.
- For `arr = [0, 0, 0]`, the output should be `false`.
Players should start with a positive number of of money.
- For `arr = [11]`, the output should be `true`.
One player always keep his money at the end of game.
- For `arr = [100, 100, 100, 90, 1, 0, 0]`, the output should be `false`.
These players can not start with the same amount of money. | def learn_charitable_game(arr):
return sum(arr) % len(arr) == 0 and sum(arr) > 0 | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1106/D:
Lunar New Year is approaching, and Bob decides to take a wander in a nearby park.
The park can be represented as a connected graph with $n$ nodes and $m$ bidirectional edges. Initially Bob is at the node $1$ and he records $1$ on his notebook. He can wander from one node to another through those bidirectional edges. Whenever he visits a node not recorded on his notebook, he records it. After he visits all nodes at least once, he stops wandering, thus finally a permutation of nodes $a_1, a_2, \ldots, a_n$ is recorded.
Wandering is a boring thing, but solving problems is fascinating. Bob wants to know the lexicographically smallest sequence of nodes he can record while wandering. Bob thinks this problem is trivial, and he wants you to solve it.
A sequence $x$ is lexicographically smaller than a sequence $y$ if and only if one of the following holds: $x$ is a prefix of $y$, but $x \ne y$ (this is impossible in this problem as all considered sequences have the same length); in the first position where $x$ and $y$ differ, the sequence $x$ has a smaller element than the corresponding element in $y$.
-----Input-----
The first line contains two positive integers $n$ and $m$ ($1 \leq n, m \leq 10^5$), denoting the number of nodes and edges, respectively.
The following $m$ lines describe the bidirectional edges in the graph. The $i$-th of these lines contains two integers $u_i$ and $v_i$ ($1 \leq u_i, v_i \leq n$), representing the nodes the $i$-th edge connects.
Note that the graph can have multiple edges connecting the same two nodes and self-loops. It is guaranteed that the graph is connected.
-----Output-----
Output a line containing the lexicographically smallest sequence $a_1, a_2, \ldots, a_n$ Bob can record.
-----Examples-----
Input
3 2
1 2
1 3
Output
1 2 3
Input
5 5
1 4
3 4
5 4
3 2
1 5
Output
1 4 3 2 5
Input
10 10
1 4
6 8
2 5
3 7
9 4
5 6
3 4
8 10
8 9
1 10
Output
1 4 3 7 9 8 6 5 2 10
-----Note-----
In the first sample, Bob's optimal wandering path could be $1 \rightarrow 2...
I tried it in Python, but could not do it. Can you solve it? | from heapq import heapify, heappush, heappop
def solve():
n, m = [int(x) for x in input().split()]
adjs = [[] for _ in range(n)]
for _ in range(m):
a, b = [int(x) for x in input().split()]
a -= 1
b -= 1
adjs[a].append(b)
adjs[b].append(a)
seq = [1]
visited = set([0])
frontier = adjs[0].copy()
heapify(frontier)
while frontier:
node = heappop(frontier)
if node in visited:
continue
seq.append(node+1)
visited.add(node)
for neighbor in adjs[node]:
if neighbor not in visited:
heappush(frontier, neighbor)
print(*seq)
solve() | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/776/D:
Moriarty has trapped n people in n distinct rooms in a hotel. Some rooms are locked, others are unlocked. But, there is a condition that the people in the hotel can only escape when all the doors are unlocked at the same time. There are m switches. Each switch control doors of some rooms, but each door is controlled by exactly two switches.
You are given the initial configuration of the doors. Toggling any switch, that is, turning it ON when it is OFF, or turning it OFF when it is ON, toggles the condition of the doors that this switch controls. Say, we toggled switch 1, which was connected to room 1, 2 and 3 which were respectively locked, unlocked and unlocked. Then, after toggling the switch, they become unlocked, locked and locked.
You need to tell Sherlock, if there exists a way to unlock all doors at the same time.
-----Input-----
First line of input contains two integers n and m (2 ≤ n ≤ 10^5, 2 ≤ m ≤ 10^5) — the number of rooms and the number of switches.
Next line contains n space-separated integers r_1, r_2, ..., r_{n} (0 ≤ r_{i} ≤ 1) which tell the status of room doors. The i-th room is locked if r_{i} = 0, otherwise it is unlocked.
The i-th of next m lines contains an integer x_{i} (0 ≤ x_{i} ≤ n) followed by x_{i} distinct integers separated by space, denoting the number of rooms controlled by the i-th switch followed by the room numbers that this switch controls. It is guaranteed that the room numbers are in the range from 1 to n. It is guaranteed that each door is controlled by exactly two switches.
-----Output-----
Output "YES" without quotes, if it is possible to open all doors at the same time, otherwise output "NO" without quotes.
-----Examples-----
Input
3 3
1 0 1
2 1 3
2 1 2
2 2 3
Output
NO
Input
3 3
1 0 1
3 1 2 3
1 2
2 1 3
Output
YES
Input
3 3
1 0 1
3 1 2 3
2 1 2
1 3
Output
NO
-----Note-----
In the second example input, the initial statuses of the doors are [1, 0, 1] (0 means locked, 1 — unlocked).
After toggling switch 3, we get [0, 0, 0] that means all doors are...
I tried it in Python, but could not do it. Can you solve it? | import sys
import collections
n, m = list(map(int, input().split()))
r = tuple(map(int, input().split()))
controls = [tuple(map(int, input().split()))[1:] for i in range(m)]
class DSU:
def __init__(self):
self.parent = None
self.has_zero = False
self.has_one = False
self.size = 1
self.doors = []
def get_root(self):
if self.parent is None:
return self
self.parent = self.parent.get_root()
return self.parent
def unite(self, s):
r1 = self.get_root()
r2 = s.get_root()
if r1 is r2:
return r1
if r1.size < r2.size:
r1, r2 = r2, r1
r2.parent = r1
r1.size += r2.size
r1.has_zero = r1.has_zero or r2.has_zero
r1.has_one = r1.has_one or r2.has_one
return r1
door_dsus = [[] for i in range(n)]
for doors in controls:
n = DSU()
for door in doors:
n.doors.append(door - 1)
door_dsus[door - 1].append(n)
if r[door - 1]:
n.has_one = True
if not r[door - 1]:
n.has_zero = True
for door, is_open in enumerate(r):
n1, n2 = door_dsus[door]
if is_open:
n1.unite(n2)
G = {}
for door, is_open in enumerate(r):
if is_open:
continue
n1, n2 = door_dsus[door]
if n1.get_root() is n2.get_root():
print("NO")
return
G.setdefault(n1.get_root(), set()).add(n2.get_root())
G.setdefault(n2.get_root(), set()).add(n1.get_root())
color = {}
for v in list(G.keys()):
if v in color:
continue
color[v] = False
q = collections.deque([v])
while q:
v = q.popleft()
c = color[v]
for adj_v in G[v]:
if adj_v in color:
if color[adj_v] != (not c):
print("NO")
return
else:
color[adj_v] = not c
q.append(adj_v)
print("YES") | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Create a function that returns an array containing the first `l` digits from the `n`th diagonal of [Pascal's triangle](https://en.wikipedia.org/wiki/Pascal's_triangle).
`n = 0` should generate the first diagonal of the triangle (the 'ones'). The first number in each diagonal should be 1.
If `l = 0`, return an empty array. Assume that both `n` and `l` will be non-negative integers in all test cases. | def generate_diagonal(d, l):
result = [1] if l else []
for k in range(1, l):
result.append(result[-1] * (d+k) // k)
return result | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc151/tasks/abc151_d:
Takahashi has a maze, which is a grid of H \times W squares with H horizontal rows and W vertical columns.
The square at the i-th row from the top and the j-th column is a "wall" square if S_{ij} is #, and a "road" square if S_{ij} is ..
From a road square, you can move to a horizontally or vertically adjacent road square.
You cannot move out of the maze, move to a wall square, or move diagonally.
Takahashi will choose a starting square and a goal square, which can be any road squares, and give the maze to Aoki.
Aoki will then travel from the starting square to the goal square, in the minimum number of moves required.
In this situation, find the maximum possible number of moves Aoki has to make.
-----Constraints-----
- 1 \leq H,W \leq 20
- S_{ij} is . or #.
- S contains at least two occurrences of ..
- Any road square can be reached from any road square in zero or more moves.
-----Input-----
Input is given from Standard Input in the following format:
H W
S_{11}...S_{1W}
:
S_{H1}...S_{HW}
-----Output-----
Print the maximum possible number of moves Aoki has to make.
-----Sample Input-----
3 3
...
...
...
-----Sample Output-----
4
If Takahashi chooses the top-left square as the starting square and the bottom-right square as the goal square, Aoki has to make four moves.
I tried it in Python, but could not do it. Can you solve it? | h,w = map(int,input().split())
C = [list(input()) for i in range(h)]
startls = []
for i in range(h):
for j in range(w):
if C[i][j] == '.':
startls.append([i,j])
dy_dx = [[1,0],[0,1],[-1,0],[0,-1]]
ans = 0
for start in startls:
visited = [[-1 for i in range(w)] for i in range(h)]
visited[start[0]][start[1]] = 0
cost = 0
queue = [start]
while len(queue) > 0:
now = queue.pop(0)
cost = visited[now[0]][now[1]]+1
for i in range(4):
y = now[0]+dy_dx[i][0]
x = now[1]+dy_dx[i][1]
if 0 <= y < h and 0 <= x < w:
if C[y][x] != '#' and visited[y][x] == -1:
visited[y][x] = cost
queue.append([y,x])
ans = max(ans,cost)
print(ans) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/56782b25c05cad45f700000f:
The Binomial Form of a polynomial has many uses, just as the standard form does. For comparison, if p(x) is in Binomial Form and q(x) is in standard form, we might write
p(x) := a0 \* xC0 + a1 \* xC1 + a2 \* xC2 + ... + aN \* xCN
q(x) := b0 + b1 \* x + b2 \* x^(2) + ... + bN \* x^(N)
Both forms have tricks for evaluating them, but tricks should not be necessary. The most important thing to keep in mind is that aCb can be defined for non-integer values of a; in particular,
```
aCb := a * (a-1) * (a-2) * ... * (a-b+1) / b! // for any value a and integer values b
:= a! / ((a-b)!b!) // for integer values a,b
```
The inputs to your function are an array which specifies a polynomial in Binomial Form, ordered by highest-degree-first, and also a number to evaluate the polynomial at. An example call might be
```python
value_at([1, 2, 7], 3)
```
and the return value would be 16, since 3C2 + 2 * 3C1 + 7 = 16. In more detail, this calculation looks like
```
1 * xC2 + 2 * xC1 + 7 * xC0 :: x = 3
3C2 + 2 * 3C1 + 7
3 * (3-1) / 2! + 2 * 3 / 1! + 7
3 + 6 + 7 = 16
```
More information can be found by reading about [Binomial Coefficients](https://en.wikipedia.org/wiki/Binomial_coefficient) or about [Finite Differences](https://en.wikipedia.org/wiki/Finite_difference).
Note that while a solution should be able to handle non-integer inputs and get a correct result, any solution should make use of rounding to two significant digits (as the official solution does) since high precision for non-integers is not the point here.
I tried it in Python, but could not do it. Can you solve it? | def aCb(a, b):
result = 1.0
for i in range(b):
result = result * (a - i) / (i + 1)
return result
def value_at(poly_spec, x):
answer = 0
l = len(poly_spec)
for i, coeff in enumerate(poly_spec):
answer += coeff * aCb(x, l - i - 1)
return round(answer, 2) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
The Chef has prepared the appetizers in the shapes of letters to spell a special message for the guests. There are n appetizers numbered from 0 to n-1 such that if the appetizers are arrayed in this order, they will display the message. The Chef plans to display them in this order on a table that can be viewed by all guests as they enter. The appetizers will only be served once all guests are seated.
The appetizers are not necessarily finished in the same order as they are numbered. So, when an appetizer is finished the Chef will write the number on a piece of paper and place it beside the appetizer on a counter between the kitchen and the restaurant. A server will retrieve this appetizer and place it in the proper location according to the number written beside it.
The Chef has a penchant for binary numbers. The number of appetizers created is a power of 2, say n = 2k. Furthermore, he has written the number of the appetizer in binary with exactly k bits. That is, binary numbers with fewer than k bits are padded on the left with zeros so they are written with exactly k bits.
Unfortunately, this has unforseen complications. A binary number still "looks" binary when it is written upside down. For example, the binary number "0101" looks like "1010" when read upside down and the binary number "110" looks like "011" (the Chef uses simple vertical lines to denote a 1 bit). The Chef didn't realize that the servers would read the numbers upside down so he doesn't rotate the paper when he places it on the counter. Thus, when the server picks up an appetizer they place it the location indexed by the binary number when it is read upside down.
You are given the message the chef intended to display and you are to display the message that will be displayed after the servers move all appetizers to their locations based on the binary numbers they read.
-----Input-----
The first line consists of a single integer T ≤ 25 indicating the number of test cases to follow. Each test case consists of a single line beginning with... | t=int(input())
def reversebinary(bits,n):
bStr=''
for i in range(bits):
if n>0:
bStr=bStr+str(n%2)
else:
bStr=bStr+'0'
n=n>>1
return int(bStr,2)
for i in range(t):
k,msg=input().split()
k=int(k)
newmsg=[]
for j in msg:
newmsg.append(j)
for j in range(len(msg)):
newmsg[reversebinary(k,j)]=msg[j]
print(''.join(newmsg)) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc167/tasks/abc167_c:
Takahashi, who is a novice in competitive programming, wants to learn M algorithms.
Initially, his understanding level of each of the M algorithms is 0.
Takahashi is visiting a bookstore, where he finds N books on algorithms.
The i-th book (1\leq i\leq N) is sold for C_i yen (the currency of Japan). If he buys and reads it, his understanding level of the j-th algorithm will increase by A_{i,j} for each j (1\leq j\leq M).
There is no other way to increase the understanding levels of the algorithms.
Takahashi's objective is to make his understanding levels of all the M algorithms X or higher. Determine whether this objective is achievable. If it is achievable, find the minimum amount of money needed to achieve it.
I tried it in Python, but could not do it. Can you solve it? | def main():
n, m, x = list(map(int, input().split()))
sofar = 10 ** 5 + 1
cl = list(list(map(int, input().split())) for _ in range(n))
# print(cl)
ans = float('inf')
for i in range(2 ** n):
tmp = [0] * m
cost = 0
for j in range(n):
# print(bin(j), i, bin(i >> j))
if (i >> j) & 1:
for k in range(m):
tmp[k] += cl[j][k + 1]
cost += cl[j][0]
# print(tmp)
for t in tmp:
if t < x:
break
else:
ans = min(ans, cost)
if ans == float('inf'):
return -1
else:
return ans
def __starting_point():
print((main()))
__starting_point() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
=====Problem Statement=====
ABC is a right triangle, 90°, at B. Therefore, ANGLE{ABC} = 90°.
Point M is the midpoint of hypotenuse AC.
You are given the lengths AB and BC.
Your task is to find ANGLE{MBC} (angle θ°, as shown in the figure) in degrees.
=====Input Format=====
The first contains the length of side AB.
The second line contains the length of side BC.
=====Constraints=====
0<AB≤100
0<BC≤100
Lengths AB and BC are natural numbers.
=====Output Format=====
Output ANGLE{MBC} in degrees.
Note: Round the angle to the nearest integer.
Examples:
If angle is 56.5000001°, then output 57°.
If angle is 56.5000000°, then output 57°.
If angle is 56.4999999°, then output 56°. | #!/usr/bin/env python3
from math import atan
from math import degrees
def __starting_point():
ab = int(input().strip())
bc = int(input().strip())
print("{}°".format(int(round(degrees(atan(ab/bc))))))
__starting_point() | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Suppose there is a $h \times w$ grid consisting of empty or full cells. Let's make some definitions:
$r_{i}$ is the number of consecutive full cells connected to the left side in the $i$-th row ($1 \le i \le h$). In particular, $r_i=0$ if the leftmost cell of the $i$-th row is empty. $c_{j}$ is the number of consecutive full cells connected to the top end in the $j$-th column ($1 \le j \le w$). In particular, $c_j=0$ if the topmost cell of the $j$-th column is empty.
In other words, the $i$-th row starts exactly with $r_i$ full cells. Similarly, the $j$-th column starts exactly with $c_j$ full cells.
[Image] These are the $r$ and $c$ values of some $3 \times 4$ grid. Black cells are full and white cells are empty.
You have values of $r$ and $c$. Initially, all cells are empty. Find the number of ways to fill grid cells to satisfy values of $r$ and $c$. Since the answer can be very large, find the answer modulo $1000000007\,(10^{9} + 7)$. In other words, find the remainder after division of the answer by $1000000007\,(10^{9} + 7)$.
-----Input-----
The first line contains two integers $h$ and $w$ ($1 \le h, w \le 10^{3}$) — the height and width of the grid.
The second line contains $h$ integers $r_{1}, r_{2}, \ldots, r_{h}$ ($0 \le r_{i} \le w$) — the values of $r$.
The third line contains $w$ integers $c_{1}, c_{2}, \ldots, c_{w}$ ($0 \le c_{j} \le h$) — the values of $c$.
-----Output-----
Print the answer modulo $1000000007\,(10^{9} + 7)$.
-----Examples-----
Input
3 4
0 3 1
0 2 3 0
Output
2
Input
1 1
0
1
Output
0
Input
19 16
16 16 16 16 15 15 0 5 0 4 9 9 1 4 4 0 8 16 12
6 12 19 15 8 6 19 19 14 6 9 16 10 11 15 4
Output
797922655
-----Note-----
In the first example, this is the other possible case.
[Image]
In the second example, it's impossible to make a grid to satisfy such $r$, $c$ values.
In the third example, make sure to print answer modulo $(10^9 + 7)$. | from collections import defaultdict,deque
import sys,heapq,bisect,math,itertools,string,queue,copy,time
sys.setrecursionlimit(10**8)
INF = float('inf')
mod = 10**9+7
eps = 10**-7
def inp(): return int(sys.stdin.readline())
def inpl(): return list(map(int, sys.stdin.readline().split()))
def inpl_str(): return list(sys.stdin.readline().split())
H,W = inpl()
rr = inpl()
cc = inpl()
MAP = [[-1]*W for _ in range(H)]
for x,c in enumerate(cc):
for y in range(c):
MAP[y][x] = 1
if c < H:
MAP[c][x] = 0
for y,r in enumerate(rr):
for x in range(r):
if MAP[y][x] == 0:
print(0)
return
MAP[y][x] = 1
if r < W:
if MAP[y][r] == 1:
print(0)
return
MAP[y][r] = 0
cnt = 0
for y in range(H):
for x in range(W):
if MAP[y][x] == -1:
cnt += 1
print(pow(2,cnt,mod)) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
We are given a sequence of coplanar points and see all the possible triangles that may be generated which all combinations of three points.
We have the following list of points with the cartesian coordinates of each one:
```
Points [x, y]
A [1, 2]
B [3, 3]
C [4, 1]
D [1, 1]
E [4, -1]
```
With these points we may have the following triangles: ```ABC, ABD, ABE, ACD, ACE, ADE, BCD, BCE, BDE, CDE.``` There are three special ones: ```ABC, ACD and CDE```, that have an angle of 90°. All is shown in the picture below:
We need to count all the rectangle triangles that may be formed by a given list of points.
The case decribed above will be:
```python
count_rect_triang([[1, 2],[3, 3],[4, 1],[1, 1],[4, -1]]) == 3
```
Observe this case:
```python
count_rect_triang([[1, 2],[4, -1],[3, 3],[4, -1],[4, 1],[1, 1],[4, -1], [4, -1], [3, 3], [1, 2]]) == 3
```
If no rectangle triangles may be generated the function will output ```0```.
Enjoy it! | from itertools import combinations
def count_rect_triang(points):
n=0
points_set=set()
for p in points:
points_set.add(tuple(p))
for (x1,y1),(x2,y2),(x3,y3) in combinations(points_set,3):
l1,l2,l3=sorted([(x2-x1)**2+(y2-y1)**2,(x3-x2)**2+(y3-y2)**2,(x1-x3)**2+(y1-y3)**2])
if l1+l2==l3:
n+=1
return n | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1353/C:
You are given a board of size $n \times n$, where $n$ is odd (not divisible by $2$). Initially, each cell of the board contains one figure.
In one move, you can select exactly one figure presented in some cell and move it to one of the cells sharing a side or a corner with the current cell, i.e. from the cell $(i, j)$ you can move the figure to cells: $(i - 1, j - 1)$; $(i - 1, j)$; $(i - 1, j + 1)$; $(i, j - 1)$; $(i, j + 1)$; $(i + 1, j - 1)$; $(i + 1, j)$; $(i + 1, j + 1)$;
Of course, you can not move figures to cells out of the board. It is allowed that after a move there will be several figures in one cell.
Your task is to find the minimum number of moves needed to get all the figures into one cell (i.e. $n^2-1$ cells should contain $0$ figures and one cell should contain $n^2$ figures).
You have to answer $t$ independent test cases.
-----Input-----
The first line of the input contains one integer $t$ ($1 \le t \le 200$) — the number of test cases. Then $t$ test cases follow.
The only line of the test case contains one integer $n$ ($1 \le n < 5 \cdot 10^5$) — the size of the board. It is guaranteed that $n$ is odd (not divisible by $2$).
It is guaranteed that the sum of $n$ over all test cases does not exceed $5 \cdot 10^5$ ($\sum n \le 5 \cdot 10^5$).
-----Output-----
For each test case print the answer — the minimum number of moves needed to get all the figures into one cell.
-----Example-----
Input
3
1
5
499993
Output
0
40
41664916690999888
I tried it in Python, but could not do it. Can you solve it? | t = int(input())
while t:
n = int(input())
ans = 0
if n==1: print(0)
else:
for i in range(1, n//2 + 1):
ans += 8*i*i
print(ans)
t -= 1 | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5254bd1357d59fbbe90001ec:
Have a look at the following numbers.
```
n | score
---+-------
1 | 50
2 | 150
3 | 300
4 | 500
5 | 750
```
Can you find a pattern in it? If so, then write a function `getScore(n)`/`get_score(n)`/`GetScore(n)` which returns the score for any positive number `n`:
```c++
int getScore(1) = return 50;
int getScore(2) = return 150;
int getScore(3) = return 300;
int getScore(4) = return 500;
int getScore(5) = return 750;
```
I tried it in Python, but could not do it. Can you solve it? | def get_score(n):
return 25 * n * (n + 1) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
During quarantine chef’s friend invented a game. In this game there are two players, player 1 and Player 2. In center of garden there is one finish circle and both players are at different distances respectively $X$ and $Y$ from finish circle.
Between finish circle and Player 1 there are $X$ number of circles and between finish circle and Player 2 there are $Y$ number of circles. Both player wants to reach finish circle with minimum number of jumps. Player can jump one circle to another circle.
Both players can skip $2^0-1$ or $2^1- 1$ or …. or $2^N-1$ circles per jump. A player cannot skip same number of circles in a match more than once. If both players uses optimal way to reach finish circle what will be the difference of minimum jumps needed to reach finish circle by both players.
If both players reach finish circle with same number of jumps answer will be $0$ $0$.
-----Input:-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The
description of $T$ test cases follows.
- The first line of each test case contains 2 space separated integers $X$ and $Y$.
-----Output:-----
For each test case, print a single line containing 2 space-separated integers which player win and what is the difference between number of minimum jump required by both players to reach finish circle.
-----Constraints-----
- $1 \leq T \leq 10^5$
- $1 \leq X,Y \leq 2*10^7$
-----Sample Input:-----
2
4 5
3 5
-----Sample Output:-----
0 0
1 1
-----Explanation:-----
Test Case 1:
Test Case 2: | import sys
input = sys.stdin.readline
T = int(input().strip())
for _ in range(T):
X,Y = list(map(int,input().split()))
x = X+1
y = Y +1
def bit(x):
a = []
while x>0:
a.append(x&1)
x = x//2
return sum(a)
count_x = bit(x)
count_y = bit(y)
# while(x>0):
# n = bit(x)
# # print('n_x ' , n)
# if x == 2**(n+1)-1:
# count_x += 1
# break
# elif 2**n -1 < x:
# x = (x-(2**n-1))
# count_x +=1
# if x != 1:
# x = x-1
# else:
# count_x += 1
# break
# while y>0:
# n = bit(y)
# # print('n ' , n)
# if y == 2**(n+1)-1:
# count_y += 1
# break
# elif 2**n -1 < y:
# y = (y-(2**n-1))
# count_y += 1
# if y != 1:
# y = y-1
# else:
# count_y += 1
# break
# print('x',count_x, count_y)
if count_x < count_y:
print('1',count_y-count_x)
elif count_y < count_x:
print('2',count_x-count_y)
else:
print('0','0') | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Gru wants to distribute $N$ bananas to $K$ minions on his birthday.
Gru does not like to just give everyone the same number of bananas, so instead, he wants to distribute bananas in such a way that each minion gets a $distinct$ amount of bananas. That is, no two minions should get the same number of bananas.
Gru also loves $gcd$. The higher the $gcd$, the happier Gru and the minions get. So help Gru in distributing the bananas in such a way that each Minion gets a distinct amount of bananas and gcd of this distribution is highest possible. Output this maximum gcd. If such a distribution is not possible output $-1$.
Note: You have to distribute $all$ $N$ bananas.
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase consists of a single line of input, which has two integers: $N, K$.
-----Output:-----
For each testcase, output in a single line the maximum gcd or -1.
-----Constraints-----
- $1 \leq T \leq 100$
- $1 \leq N, K \leq 10^9$
-----Sample Input:-----
1
6 3
-----Sample Output:-----
1
-----EXPLANATION:-----
The only possible distribution is $[1, 2, 3]$. So the answer is 1. | def factors(x):
result = []
i = 1
while i*i <= x:
if x % i == 0:
result.append(i)
if x//i != i:
result.append(x//i)
i += 1
return result
t = int(input())
for _ in range(t):
n, k = map(int, input().split())
a = factors(n)
an = -1
for i in a:
c = ((i*k*(k+1))//2)
if (c%i==0 and c<=n):
an=max(an,i)
print(an) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5aff237c578a14752d0035ae:
My grandfather always predicted how old people would get, and right before he passed away he revealed his secret!
In honor of my grandfather's memory we will write a function using his formula!
* Take a list of ages when each of your great-grandparent died.
* Multiply each number by itself.
* Add them all together.
* Take the square root of the result.
* Divide by two.
## Example
```R
predict_age(65, 60, 75, 55, 60, 63, 64, 45) == 86
```
```python
predict_age(65, 60, 75, 55, 60, 63, 64, 45) == 86
```
Note: the result should be rounded down to the nearest integer.
Some random tests might fail due to a bug in the JavaScript implementation. Simply resubmit if that happens to you.
I tried it in Python, but could not do it. Can you solve it? | def predict_age(*age):
return sum(a*a for a in age)**0.5//2 | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
=====Function Descriptions=====
Python has built-in string validation methods for basic data. It can check if a string is composed of alphabetical characters, alphanumeric characters, digits, etc.
str.isalnum()
This method checks if all the characters of a string are alphanumeric (a-z, A-Z and 0-9).
>>> print 'ab123'.isalnum()
True
>>> print 'ab123#'.isalnum()
False
str.isalpha()
This method checks if all the characters of a string are alphabetical (a-z and A-Z).
>>> print 'abcD'.isalpha()
True
>>> print 'abcd1'.isalpha()
False
str.isdigit()
This method checks if all the characters of a string are digits (0-9).
>>> print '1234'.isdigit()
True
>>> print '123edsd'.isdigit()
False
str.islower()
This method checks if all the characters of a string are lowercase characters (a-z).
>>> print 'abcd123#'.islower()
True
>>> print 'Abcd123#'.islower()
False
str.isupper()
This method checks if all the characters of a string are uppercase characters (A-Z).
>>> print 'ABCD123#'.isupper()
True
>>> print 'Abcd123#'.isupper()
False
=====Problem Statement=====
You are given a string S.
Your task is to find out if the string S contains: alphanumeric characters, alphabetical characters, digits, lowercase and uppercase characters.
=====Input Format=====
A single line containing a string S.
=====Constraints=====
0 < len(S) < 1000
=====Output Format=====
In the first line, print True if S has any alphanumeric characters. Otherwise, print False.
In the second line, print True if S has any alphabetical characters. Otherwise, print False.
In the third line, print True if S has any digits. Otherwise, print False.
In the fourth line, print True if S has any lowercase characters. Otherwise, print False.
In the fifth line, print True if S has any uppercase characters. Otherwise, print False. | # Enter your code here. Read input from STDIN. Print output to STDOUT
inputStr=input()
resalnum = False
resalpha = False
resdigit = False
reslower = False
resupper = False
for i in inputStr:
if(i.isalnum()):
resalnum=True
if(i.isalpha()):
resalpha=True
if(i.isdigit()):
resdigit=True
if(i.islower()):
reslower=True
if(i.isupper()):
resupper=True
print(resalnum)
print(resalpha)
print(resdigit)
print(reslower)
print(resupper) | python | train | qsol | codeparrot/apps | all |
End of preview. Expand
in Data Studio
Can be loaded via e.g.:
from datasets import load_dataset
d = load_dataset("Muennighoff/xP3x-sample", "apps")
1,000 rows from random languages and splits of xP3x for each of the multilingual datasets represented in xP3x.
- Downloads last month
- 397