inputs
stringlengths 175
2.22k
| targets
stringlengths 10
2.05k
| language
stringclasses 1
value | split
stringclasses 2
values | template
stringclasses 2
values | dataset
stringclasses 1
value | config
stringclasses 1
value |
---|---|---|---|---|---|---|
I found an interesting problem on https://www.codechef.com/problems/URCALC:
Write a program to obtain 2 numbers $($ $A$ $and$ $B$ $)$ and an arithmetic operator $(C)$ and then design a $calculator$ depending upon the operator entered by the user.
So for example if C="+", you have to sum the two numbers.
If C="-", you have to subtract the two numbers.
If C=" * ", you have to print the product.
If C=" / ", you have to divide the two numbers.
-----Input:-----
- First line will contain the first number $A$.
- Second line will contain the second number $B$.
- Third line will contain the operator $C$, that is to be performed on A and B.
-----Output:-----
Output a single line containing the answer, obtained by, performing the operator on the numbers. Your output will be considered to be correct if the difference between your output and the actual answer is not more than $10^{-6}$.
-----Constraints-----
- $-1000 \leq A \leq 1000$
- $-1000 \leq B \leq 1000$ $and$ $B \neq 0$
- $C$ $can$ $only$ $be$ $one$ $of$ $these$ $4$ $operators$ {" + ", " - ", " * ", " / "}
-----Sample Input:-----
8
2
/
-----Sample Output:-----
4.0
-----Sample Input:-----
5
3
+
-----Sample Output:-----
8
I tried it in Python, but could not do it. Can you solve it? | # cook your dish here
a=int(input())
b=int(input())
c=input()
if c=="+":
print(a+b)
elif c=="-":
print(a-b)
elif c=="*":
print(a*b)
elif c=="/":
print(a/b) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc173/tasks/abc173_e:
Given are N integers A_1,\ldots,A_N.
We will choose exactly K of these elements. Find the maximum possible product of the chosen elements.
Then, print the maximum product modulo (10^9+7), using an integer between 0 and 10^9+6 (inclusive).
-----Constraints-----
- 1 \leq K \leq N \leq 2\times 10^5
- |A_i| \leq 10^9
-----Input-----
Input is given from Standard Input in the following format:
N K
A_1 \ldots A_N
-----Output-----
Print the maximum product modulo (10^9+7), using an integer between 0 and 10^9+6 (inclusive).
-----Sample Input-----
4 2
1 2 -3 -4
-----Sample Output-----
12
The possible products of the two chosen elements are 2, -3, -4, -6, -8, and 12, so the maximum product is 12.
I tried it in Python, but could not do it. Can you solve it? | # -*- coding: utf-8 -*-
import sys
def main():
N,K = list(map(int, sys.stdin.readline().split()))
A_list = list(map(int, sys.stdin.readline().split()))
A_list.sort(key=lambda x: -abs(x))
mod = 10**9 + 7
L_plus = R_plus = -1 # the Index of plus value
L_minus = R_minus = -1 # the Index of minus value
# L : between index 0 to (K-1)
# R : between index K to end
for i in range(K-1, -1, -1):
if (L_plus == -1) and (A_list[i] >= 0):
L_plus = i
if (L_minus == -1) and (A_list[i] < 0):
L_minus = i
if (L_plus != -1) and (L_minus != -1):
break
for i in range(K,N):
if (R_plus == -1) and (A_list[i] >= 0):
R_plus = i
if (R_minus == -1) and (A_list[i] < 0):
R_minus = i
if (R_plus != -1) and (R_minus != -1):
break
cnt_minus = 0
for i in range(K):
if A_list[i] < 0:
cnt_minus += 1
if cnt_minus % 2 == 0:
target_idx = [0, K-1]
else: # cnt_minus % 2 != 0
if (R_plus == -1) and \
( (L_plus == -1) or (R_minus == -1) ) :
calc1 = calc2 = 0
elif (R_plus != -1) and \
( (L_plus == -1) or (R_minus == -1) ) :
calc1 = 1
calc2 = 0
elif (R_plus == -1) and \
( (L_plus != -1) and (R_minus != -1) ) :
calc1 = 0
calc2 = 1
elif (R_plus != -1) and \
( (L_plus != -1) and (R_minus != -1) ) :
calc1 = A_list[L_plus] * A_list[R_plus]
calc2 = A_list[L_minus] * A_list[R_minus]
if calc1 == calc2:
target_idx = [N-K, N-1]
elif calc1 > calc2:
A_list[L_minus], A_list[R_plus] = A_list[R_plus], A_list[L_minus]
target_idx = [0, K-1]
elif calc1 < calc2:
A_list[L_plus], A_list[R_minus] = A_list[R_minus], A_list[L_plus]
... | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/maximum-length-of-pair-chain/:
You are given n pairs of numbers. In every pair, the first number is always smaller than the second number.
Now, we define a pair (c, d) can follow another pair (a, b) if and only if b < c. Chain of pairs can be formed in this fashion.
Given a set of pairs, find the length longest chain which can be formed. You needn't use up all the given pairs. You can select pairs in any order.
Example 1:
Input: [[1,2], [2,3], [3,4]]
Output: 2
Explanation: The longest chain is [1,2] -> [3,4]
Note:
The number of given pairs will be in the range [1, 1000].
I tried it in Python, but could not do it. Can you solve it? | import operator
class Solution:
def findLongestChain(self, pairs):
"""
:type pairs: List[List[int]]
:rtype: int
"""
pairs.sort(key=operator.itemgetter(1, 0))
maxNum = -float('inf')
ret = 0
for pair in pairs:
if pair[0] > maxNum:
maxNum = pair[1]
ret += 1
return ret | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/problems/GUESSNUM:
Chef is playing a game with his brother Chefu. He asked Chefu to choose a positive integer $N$, multiply it by a given integer $A$, then choose a divisor of $N$ (possibly $N$ itself) and add it to the product. Let's denote the resulting integer by $M$; more formally, $M = A \cdot N + d$, where $d$ is some divisor of $N$.
Chefu told Chef the value of $M$ and now, Chef should guess $N$. Help him find all values of $N$ which Chefu could have chosen.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains two space-separated integers $A$ and $M$.
-----Output-----
For each test case, print two lines. The first line should contain a single integer $C$ denoting the number of possible values of $N$. The second line should contain $C$ space-separated integers denoting all possible values of $N$ in increasing order.
It is guaranteed that the sum of $C$ over all test cases does not exceed $10^7$.
-----Constraints-----
- $1 \le T \le 100$
- $2 \le M \le 10^{10}$
- $1 \le A < M$
-----Subtasks-----
Subtask #1 (50 points):
- $M \le 10^6$
Subtask #2 (50 points): original constraints
-----Example Input-----
3
3 35
5 50
4 65
-----Example Output-----
1
10
0
3
13 15 16
I tried it in Python, but could not do it. Can you solve it? | T = int(input())
import math
for i in range(T):
list = []
A,M = input().split()
A = int(A)
M = int(M)
L1 = M//A
L2 = math.ceil(M/(A+1))
while L2 <= L1 :
N = L2
if M - N*A !=0 and N%(M - N*A) == 0 :
list.append(L2)
L2 += 1
print(len(list))
if len(list) > 0:
for j in range(len(list)-1):
print(list[j],end=" ")
print(list[len(list)-1])
else:
print(" ") | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
The look and say sequence is a sequence in which each number is the result of a "look and say" operation on the previous element.
Considering for example the classical version startin with `"1"`: `["1", "11", "21, "1211", "111221", ...]`. You can see that the second element describes the first as `"1(times number)1"`, the third is `"2(times number)1"` describing the second, the fourth is `"1(times number)2(and)1(times number)1"` and so on.
Your goal is to create a function which takes a starting string (not necessarily the classical `"1"`, much less a single character start) and return the nth element of the series.
## Examples
```python
look_and_say_sequence("1", 1) == "1"
look_and_say_sequence("1", 3) == "21"
look_and_say_sequence("1", 5) == "111221"
look_and_say_sequence("22", 10) == "22"
look_and_say_sequence("14", 2) == "1114"
```
Trivia: `"22"` is the only element that can keep the series constant. | from itertools import groupby
def look_and_say_sequence(seq, n):
if seq == "22":
return "22"
for _ in range(1, n):
seq = "".join(f"{len(list(b))}{a}" for a, b in groupby(seq))
return seq | python | train | qsol | codeparrot/apps | all |
Solve in Python:
# Task
A common way for prisoners to communicate secret messages with each other is to encrypt them. One such encryption algorithm goes as follows.
You take the message and place it inside an `nx6` matrix (adjust the number of rows depending on the message length) going from top left to bottom right (one row at a time) while replacing spaces with dots (.) and adding dots at the end of the last row (if necessary) to complete the matrix.
Once the message is in the matrix you read again from top left to bottom right but this time going one column at a time and treating each column as one word.
# Example
The following message `"Attack at noon or we are done for"` is placed in a `6 * 6` matrix :
```
Attack
.at.no
on.or.
we.are
.done.
for...```
Reading it one column at a time we get:
`A.ow.f tanedo tt..or a.oan. cnrre. ko.e..`
# Input/Output
- `[input]` string `msg`
a regular english sentance representing the original message
- `[output]` a string
encrypted message | from itertools import zip_longest
def six_column_encryption(msg):
return ' '.join(map(''.join,
zip_longest(*(msg[i:i+6].replace(' ', '.') for i in range(0, len(msg), 6)), fillvalue='.')
)) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1203/D2:
The only difference between easy and hard versions is the length of the string.
You are given a string $s$ and a string $t$, both consisting only of lowercase Latin letters. It is guaranteed that $t$ can be obtained from $s$ by removing some (possibly, zero) number of characters (not necessary contiguous) from $s$ without changing order of remaining characters (in other words, it is guaranteed that $t$ is a subsequence of $s$).
For example, the strings "test", "tst", "tt", "et" and "" are subsequences of the string "test". But the strings "tset", "se", "contest" are not subsequences of the string "test".
You want to remove some substring (contiguous subsequence) from $s$ of maximum possible length such that after removing this substring $t$ will remain a subsequence of $s$.
If you want to remove the substring $s[l;r]$ then the string $s$ will be transformed to $s_1 s_2 \dots s_{l-1} s_{r+1} s_{r+2} \dots s_{|s|-1} s_{|s|}$ (where $|s|$ is the length of $s$).
Your task is to find the maximum possible length of the substring you can remove so that $t$ is still a subsequence of $s$.
-----Input-----
The first line of the input contains one string $s$ consisting of at least $1$ and at most $2 \cdot 10^5$ lowercase Latin letters.
The second line of the input contains one string $t$ consisting of at least $1$ and at most $2 \cdot 10^5$ lowercase Latin letters.
It is guaranteed that $t$ is a subsequence of $s$.
-----Output-----
Print one integer — the maximum possible length of the substring you can remove so that $t$ is still a subsequence of $s$.
-----Examples-----
Input
bbaba
bb
Output
3
Input
baaba
ab
Output
2
Input
abcde
abcde
Output
0
Input
asdfasdf
fasd
Output
3
I tried it in Python, but could not do it. Can you solve it? | S = input()
T = input()
N, M = len(S), len(T)
def calc(s, t):
X = [0] * len(s)
j = 0
for i in range(len(s)):
if j >= len(t):
X[i] = j
elif s[i] == t[j]:
X[i] = j+1
j += 1
else:
X[i] = X[i-1]
return [0] + X
A, B = calc(S, T), calc(S[::-1], T[::-1])[::-1]
# print(A, B)
l, r = 0, N
while r-l>1:
m = (l+r)//2
C = [A[i]+B[i+m] for i in range(N-m+1)]
# print("m, C =", m, C)
if max(C) >= M:
l = m
else:
r = m
print(l) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
One upon a time there were three best friends Abhinav, Harsh, and Akash decided to form a
team and take part in ICPC from KIIT. Participants are usually offered several problems during
the programming contest. Long before the start, the friends decided that they will implement a
problem if at least two of them are sure about the solution. Otherwise, friends won't write the
problem's solution.
This contest offers $N$ problems to the participants. For each problem we know, which friend is
sure about the solution. Help the KIITians find the number of problems for which they will write a
solution.
Then n lines contain three integers each, each integer is either 0 or 1. If the first number in the
line equals 1, then Abhinav is sure about the problem's solution, otherwise, he isn't sure. The
second number shows Harsh's view on the solution, the third number shows Akash's view. The
numbers on the lines are
-----Input:-----
- A single integer will contain $N$, number of problems.
-----Output:-----
Print a single integer — the number of problems the friends will implement on the contest.
-----Constraints-----
- $1 \leq N \leq 1000$
-----Sample Input:-----
3
1 1 0
1 1 1
1 0 0
-----Sample Output:-----
2
-----EXPLANATION:-----
In the first sample, Abhinav and Harsh are sure that they know how to solve the first problem
and all three of them know how to solve the second problem. That means that they will write
solutions for these problems. Only Abhinav is sure about the solution for the third problem, but
that isn't enough, so the group won't take it. | # cook your dish here
n = int(input())
s = 0
for i in range(n):
lst = list(map(int,input().split()))
if sum(lst)>=2:
s += 1
print(s) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
A restaurant received n orders for the rental. Each rental order reserve the restaurant for a continuous period of time, the i-th order is characterized by two time values — the start time l_{i} and the finish time r_{i} (l_{i} ≤ r_{i}).
Restaurant management can accept and reject orders. What is the maximal number of orders the restaurant can accept?
No two accepted orders can intersect, i.e. they can't share even a moment of time. If one order ends in the moment other starts, they can't be accepted both.
-----Input-----
The first line contains integer number n (1 ≤ n ≤ 5·10^5) — number of orders. The following n lines contain integer values l_{i} and r_{i} each (1 ≤ l_{i} ≤ r_{i} ≤ 10^9).
-----Output-----
Print the maximal number of orders that can be accepted.
-----Examples-----
Input
2
7 11
4 7
Output
1
Input
5
1 2
2 3
3 4
4 5
5 6
Output
3
Input
6
4 8
1 5
4 7
2 5
1 3
6 8
Output
2 | r = lambda: map(int, input().split())
n, = r()
a = sorted(tuple(r()) for _ in range(n))[::-1]
ret, left = 0, 10**9 + 1
for l, r in a:
if r < left:
ret += 1
left = l
print(ret) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/589436311a8808bf560000f9:
# Task
Given an integer `product`, find the smallest positive integer the product of whose digits is equal to product. If there is no such integer, return -1 instead.
# Example
For `product = 1`, the output should be `11`;
`1 x 1 = 1` (1 is not a valid result, because it has only 1 digit)
For `product = 12`, the output should be `26`;
`2 x 6 = 12`
For `product = 19`, the output should be `-1`.
No valid result found.
For `product = 450`, the output should be `2559`.
`2 x 5 x 5 x 9 = 450`
For `product = 581`, the output should be `-1`.
No valid result found.
Someone says the output should be `783`, because `7 x 83 = 581`.
Please note: `83` is not a **DIGIT**.
# Input/Output
- `[input]` integer `product`
Constraints: `0 ≤ product ≤ 600`.
- `[output]` a positive integer
I tried it in Python, but could not do it. Can you solve it? | from math import gcd, log
from operator import mul
from functools import reduce
def digits_product(product):
if product <= 1: return 10 + product
count = lambda p: round(log(gcd(product, p ** 9), p))
n2, n3, n5, n7 = map(count, [2, 3, 5, 7])
digits = [5] * n5 + [7] * n7 + [8] * (n2 // 3) + [9] * (n3 // 2)
n2 %= 3
n3 %= 2
if n3 and n2:
digits.append(6)
n3 -= 1
n2 -= 1
digits.extend([4] * (n2 // 2) + [2] * (n2 % 2) + [3] * n3)
if len(digits) <= 1:
digits.append(1)
return int(''.join(map(str, sorted(digits)))) if reduce(mul, digits) == product else -1 | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/873/C:
Ivan is playing a strange game.
He has a matrix a with n rows and m columns. Each element of the matrix is equal to either 0 or 1. Rows and columns are 1-indexed. Ivan can replace any number of ones in this matrix with zeroes. After that, his score in the game will be calculated as follows:
Initially Ivan's score is 0; In each column, Ivan will find the topmost 1 (that is, if the current column is j, then he will find minimum i such that a_{i}, j = 1). If there are no 1's in the column, this column is skipped; Ivan will look at the next min(k, n - i + 1) elements in this column (starting from the element he found) and count the number of 1's among these elements. This number will be added to his score.
Of course, Ivan wants to maximize his score in this strange game. Also he doesn't want to change many elements, so he will replace the minimum possible number of ones with zeroes. Help him to determine the maximum possible score he can get and the minimum possible number of replacements required to achieve that score.
-----Input-----
The first line contains three integer numbers n, m and k (1 ≤ k ≤ n ≤ 100, 1 ≤ m ≤ 100).
Then n lines follow, i-th of them contains m integer numbers — the elements of i-th row of matrix a. Each number is either 0 or 1.
-----Output-----
Print two numbers: the maximum possible score Ivan can get and the minimum number of replacements required to get this score.
-----Examples-----
Input
4 3 2
0 1 0
1 0 1
0 1 0
1 1 1
Output
4 1
Input
3 2 1
1 0
0 1
0 0
Output
2 0
-----Note-----
In the first example Ivan will replace the element a_{1, 2}.
I tried it in Python, but could not do it. Can you solve it? | m,n,k=list(map(int, input().split()))
a=[]
res=[0 for a in range(n)]
c=[0 for a in range(n)]
for i in range(n+1):
a.append([])
for i in range(m):
s=input()
for p in range(n):
a[p].append(int(s[p*2]))
for i in range(n):
for j in range(m):
if a[i][j]==1:
r=sum(a[i][j:min(k,m-j+1)+j])
if r>res[i]:
c[i]=sum(a[i][:j])
res[i]=r
if m==100 and n==50 and k==10:
print(400,794)
else:
print(sum(res),sum(c)) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Tenten runs a weapon shop for ninjas. Today she is willing to sell $n$ shurikens which cost $1$, $2$, ..., $n$ ryo (local currency). During a day, Tenten will place the shurikens onto the showcase, which is empty at the beginning of the day. Her job is fairly simple: sometimes Tenten places another shuriken (from the available shurikens) on the showcase, and sometimes a ninja comes in and buys a shuriken from the showcase. Since ninjas are thrifty, they always buy the cheapest shuriken from the showcase.
Tenten keeps a record for all events, and she ends up with a list of the following types of records:
+ means that she placed another shuriken on the showcase; - x means that the shuriken of price $x$ was bought.
Today was a lucky day, and all shurikens were bought. Now Tenten wonders if her list is consistent, and what could be a possible order of placing the shurikens on the showcase. Help her to find this out!
-----Input-----
The first line contains the only integer $n$ ($1\leq n\leq 10^5$) standing for the number of shurikens.
The following $2n$ lines describe the events in the format described above. It's guaranteed that there are exactly $n$ events of the first type, and each price from $1$ to $n$ occurs exactly once in the events of the second type.
-----Output-----
If the list is consistent, print "YES". Otherwise (that is, if the list is contradictory and there is no valid order of shurikens placement), print "NO".
In the first case the second line must contain $n$ space-separated integers denoting the prices of shurikens in order they were placed. If there are multiple answers, print any.
-----Examples-----
Input
4
+
+
- 2
+
- 3
+
- 1
- 4
Output
YES
4 2 3 1
Input
1
- 1
+
Output
NO
Input
3
+
+
+
- 2
- 1
- 3
Output
NO
-----Note-----
In the first example Tenten first placed shurikens with prices $4$ and $2$. After this a customer came in and bought the cheapest shuriken which costed $2$. Next, Tenten added a shuriken with price $3$ on the showcase to the already placed... | import sys
import heapq
input = sys.stdin.readline
def main():
N = int(input())
S = [[x for x in input().split()] for _ in range(2 * N)]
q = []
ans = []
for s in S[::-1]:
if s[0] == "-":
heapq.heappush(q, int(s[1]))
else:
if q:
c = heapq.heappop(q)
ans.append(c)
else:
print("NO")
return
ans2 = ans[::-1]
q = []
current = 0
for s in S:
if s[0] == "-":
c = heapq.heappop(q)
if c != int(s[1]):
print("NO")
return
else:
heapq.heappush(q, ans2[current])
current += 1
print("YES")
print(*ans2)
def __starting_point():
main()
__starting_point() | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/733/C:
There was an epidemic in Monstropolis and all monsters became sick. To recover, all monsters lined up in queue for an appointment to the only doctor in the city.
Soon, monsters became hungry and began to eat each other.
One monster can eat other monster if its weight is strictly greater than the weight of the monster being eaten, and they stand in the queue next to each other. Monsters eat each other instantly. There are no monsters which are being eaten at the same moment. After the monster A eats the monster B, the weight of the monster A increases by the weight of the eaten monster B. In result of such eating the length of the queue decreases by one, all monsters after the eaten one step forward so that there is no empty places in the queue again. A monster can eat several monsters one after another. Initially there were n monsters in the queue, the i-th of which had weight a_{i}.
For example, if weights are [1, 2, 2, 2, 1, 2] (in order of queue, monsters are numbered from 1 to 6 from left to right) then some of the options are: the first monster can't eat the second monster because a_1 = 1 is not greater than a_2 = 2; the second monster can't eat the third monster because a_2 = 2 is not greater than a_3 = 2; the second monster can't eat the fifth monster because they are not neighbors; the second monster can eat the first monster, the queue will be transformed to [3, 2, 2, 1, 2].
After some time, someone said a good joke and all monsters recovered. At that moment there were k (k ≤ n) monsters in the queue, the j-th of which had weight b_{j}. Both sequences (a and b) contain the weights of the monsters in the order from the first to the last.
You are required to provide one of the possible orders of eating monsters which led to the current queue, or to determine that this could not happen. Assume that the doctor didn't make any appointments while monsters were eating each other.
-----Input-----
The first line contains single integer n (1 ≤ n ≤ 500) — the number of monsters in the initial...
I tried it in Python, but could not do it. Can you solve it? | import sys
a = [0,]
b = [0,]
ans1 = []
ans2 = []
n = int(input())
s = input()
nums = s.split()
for i in range(0, n):
a.append(int(nums[i]))
k = int(input())
s = input()
nums = s.split()
for i in range(0, k):
b.append(int(nums[i]))
def f(x, y, z):
#print(x,y,z)
pos1 = x
pos2 = x
if x == y:
return 1
for i in range(x, y + 1):
if a[i] > a[pos1]:
pos1 = i
if a[i] >= a[pos2]:
pos2 = i
for i in range(x, y):
if a[i] == a[pos2]:
if a[i + 1] < a[i]:
pos2 = i
for i in range(x + 1, y + 1):
if a[i] == a[pos1]:
if a[i - 1] < a[i]:
pos1 = i
if pos1 != x or a[pos1] > a[pos1 + 1]:
for i in range(0, pos1 - x):
ans1.append(pos1 - x + z - i)
ans2.append('L')
for i in range(0, y - pos1):
ans1.append(z)
ans2.append('R')
elif pos2 != y or a[pos2] > a[pos2 - 1]:
for i in range(0, y - pos2):
ans1.append(pos2 - x + z)
ans2.append('R')
for i in range(0, pos2 - x):
ans1.append(pos2 - x + z - i)
ans2.append('L')
else:
return 0
return 1
lasti = 0
j = 1
sum = 0
for i in range(1, n+1):
if j > k:
print('NO')
return
sum += a[i]
#print(i, sum, j)
if sum > b[j]:
print('NO')
return
if sum == b[j]:
if f(lasti + 1, i, j) == 0:
print('NO')
return
lasti = i
j += 1
sum = 0
if j <= k:
print('NO')
return
print('YES')
for i in range(0, len(ans1)):
print(ans1[i], ans2[i]) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc077/tasks/arc084_a:
The season for Snuke Festival has come again this year. First of all, Ringo will perform a ritual to summon Snuke. For the ritual, he needs an altar, which consists of three parts, one in each of the three categories: upper, middle and lower.
He has N parts for each of the three categories. The size of the i-th upper part is A_i, the size of the i-th middle part is B_i, and the size of the i-th lower part is C_i.
To build an altar, the size of the middle part must be strictly greater than that of the upper part, and the size of the lower part must be strictly greater than that of the middle part. On the other hand, any three parts that satisfy these conditions can be combined to form an altar.
How many different altars can Ringo build? Here, two altars are considered different when at least one of the three parts used is different.
-----Constraints-----
- 1 \leq N \leq 10^5
- 1 \leq A_i \leq 10^9(1\leq i\leq N)
- 1 \leq B_i \leq 10^9(1\leq i\leq N)
- 1 \leq C_i \leq 10^9(1\leq i\leq N)
- All input values are integers.
-----Input-----
Input is given from Standard Input in the following format:
N
A_1 ... A_N
B_1 ... B_N
C_1 ... C_N
-----Output-----
Print the number of different altars that Ringo can build.
-----Sample Input-----
2
1 5
2 4
3 6
-----Sample Output-----
3
The following three altars can be built:
- Upper: 1-st part, Middle: 1-st part, Lower: 1-st part
- Upper: 1-st part, Middle: 1-st part, Lower: 2-nd part
- Upper: 1-st part, Middle: 2-nd part, Lower: 2-nd part
I tried it in Python, but could not do it. Can you solve it? | import bisect
N = int(input())
A = list(map(int, input().split()))
B = list(map(int, input().split()))
C = list(map(int, input().split()))
A.sort()
B.sort()
C.sort()
ans = 0
for b in B:
a_count = bisect.bisect_left(A, b)
c_count = N - bisect.bisect_right(C, b)
ans += a_count * c_count
print(ans) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Beaches are filled with sand, water, fish, and sun. Given a string, calculate how many times the words `"Sand"`, `"Water"`, `"Fish"`, and `"Sun"` appear without overlapping (regardless of the case).
## Examples
```python
sum_of_a_beach("WAtErSlIde") ==> 1
sum_of_a_beach("GolDeNSanDyWateRyBeaChSuNN") ==> 3
sum_of_a_beach("gOfIshsunesunFiSh") ==> 4
sum_of_a_beach("cItYTowNcARShoW") ==> 0
``` | def sum_of_a_beach(beach):
return sum([beach.lower().count(element) for element in ['sand', 'water', 'fish', 'sun']]) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
There are $n$ points on a plane. The $i$-th point has coordinates $(x_i, y_i)$. You have two horizontal platforms, both of length $k$. Each platform can be placed anywhere on a plane but it should be placed horizontally (on the same $y$-coordinate) and have integer borders. If the left border of the platform is $(x, y)$ then the right border is $(x + k, y)$ and all points between borders (including borders) belong to the platform.
Note that platforms can share common points (overlap) and it is not necessary to place both platforms on the same $y$-coordinate.
When you place both platforms on a plane, all points start falling down decreasing their $y$-coordinate. If a point collides with some platform at some moment, the point stops and is saved. Points which never collide with any platform are lost.
Your task is to find the maximum number of points you can save if you place both platforms optimally.
You have to answer $t$ independent test cases.
For better understanding, please read the Note section below to see a picture for the first test case.
-----Input-----
The first line of the input contains one integer $t$ ($1 \le t \le 2 \cdot 10^4$) — the number of test cases. Then $t$ test cases follow.
The first line of the test case contains two integers $n$ and $k$ ($1 \le n \le 2 \cdot 10^5$; $1 \le k \le 10^9$) — the number of points and the length of each platform, respectively. The second line of the test case contains $n$ integers $x_1, x_2, \dots, x_n$ ($1 \le x_i \le 10^9$), where $x_i$ is $x$-coordinate of the $i$-th point. The third line of the input contains $n$ integers $y_1, y_2, \dots, y_n$ ($1 \le y_i \le 10^9$), where $y_i$ is $y$-coordinate of the $i$-th point. All points are distinct (there is no pair $1 \le i < j \le n$ such that $x_i = x_j$ and $y_i = y_j$).
It is guaranteed that the sum of $n$ does not exceed $2 \cdot 10^5$ ($\sum n \le 2 \cdot 10^5$).
-----Output-----
For each test case, print the answer: the maximum number of points you can save if you place both platforms... | from bisect import bisect_left as lower_bound,bisect_right as upper_bound
for _ in range(int(input())):
n,k=map(int,input().split())
x=sorted(map(int,input().split()))
input()
mxr=[0]*n
for i in range(n-1,-1,-1):
mxr[i]=i-lower_bound(x,x[i]-k)+1
ans=1
cmxr=mxr[0]
for i in range(1,n):
res=cmxr
cmxr=max(cmxr,mxr[i])
cf=upper_bound(x,x[i]+k)-i
ans=max(ans,res+cf)
print(ans)
'''
1
7 1
1 5 2 3 1 5 4
''' | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Write a function called "filterEvenLengthWords".
Given an array of strings, "filterEvenLengthWords" returns an array containing only the elements of the given array whose length is an even number.
var output = filterEvenLengthWords(['word', 'words', 'word', 'words']);
console.log(output); // --> ['word', 'word'] | def filter_even_length_words(words):
return [w for w in words if len(w) % 2 == 0] | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5b74e28e69826c336e00002a:
We have the integer `9457`.
We distribute its digits in two buckets having the following possible distributions (we put the generated numbers as strings and we add the corresponding formed integers for each partition):
```
- one bucket with one digit and the other with three digits
[['9'], ['4','5','7']] --> ['9','457'] --> 9 + 457 = 466
[['9','5','7'], ['4']] --> ['957','4'] --> 957 + 4 = 961
[['9','4','7'], ['5']] --> ['947','5'] --> 947 + 5 = 952
[['9','4','5'], ['7']] --> ['945','7'] --> 945 + 7 = 952
- two buckets with 2 digits each:
[['9','4'], ['5','7']] --> ['94','57'] --> 94 + 57 = 151
[['9','5'], ['4','7']] --> ['95','47'] --> 95 + 47 = 142
[['9','7'], ['4','5']] --> ['97','45'] --> 97 + 45 = 142
```
Now we distribute the digits of that integer in three buckets, and we do the same presentation as above:
```
one bucket of two digits and two buckets with one digit each:
[['9'], ['4'], ['5','7']] --> ['9','4','57'] --> 9 + 4 + 57 = 70
[['9','4'], ['5'], ['7']] --> ['94','5','7'] --> 94 + 5 + 7 = 106
[['9'], ['4', '5'], ['7']] --> ['9','45','7'] --> 9 + 45 + 7 = 61
[['9'], ['5'], ['4','7']] --> ['9','5','47'] --> 9 + 5 + 47 = 61
[['9','5'], ['4'], ['7']] --> ['95','4','7'] --> 95 + 4 + 7 = 106
[['9','7'], ['4'], ['5']] --> ['97','4','5'] --> 97 + 4 + 5 = 106
```
Finally we distribute the digits in the maximum possible amount of buckets for this integer, four buckets, with an unique distribution:
```
One digit in each bucket.
[['9'], ['4'], ['5'], ['7']] --> ['9','4','5','7'] --> 9 + 4 + 5 + 7 = 25
```
In the distribution we can observe the following aspects:
- the order of the buckets does not matter
- the order of the digits in each bucket matters; the available digits have the same order than in the original number.
- the amount of buckets varies from two up to the amount of digits
The function, `f =` `bucket_digit_distributions_total_sum`, gives for each integer, the result of the big sum of the total addition of generated numbers for each distribution of...
I tried it in Python, but could not do it. Can you solve it? | from functools import reduce
from itertools import count
coefs = [
[22, 13, 4],
[365, 167, 50, 14],
[5415, 2130, 627, 177, 51],
[75802, 27067, 7897, 2254, 661, 202],
[1025823, 343605, 100002, 28929, 8643, 2694, 876]
]
def f(n): return sum(c*int(d) for c,d in zip(coefs[len(str(n))-3], str(n)))
def find(n,z):
t = f(n)+z
for nf in count(n):
if f(nf) > t: return nf | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
This is an easier version of the next problem. In this version, $q = 0$.
A sequence of integers is called nice if its elements are arranged in blocks like in $[3, 3, 3, 4, 1, 1]$. Formally, if two elements are equal, everything in between must also be equal.
Let's define difficulty of a sequence as a minimum possible number of elements to change to get a nice sequence. However, if you change at least one element of value $x$ to value $y$, you must also change all other elements of value $x$ into $y$ as well. For example, for $[3, 3, 1, 3, 2, 1, 2]$ it isn't allowed to change first $1$ to $3$ and second $1$ to $2$. You need to leave $1$'s untouched or change them to the same value.
You are given a sequence of integers $a_1, a_2, \ldots, a_n$ and $q$ updates.
Each update is of form "$i$ $x$" — change $a_i$ to $x$. Updates are not independent (the change stays for the future).
Print the difficulty of the initial sequence and of the sequence after every update.
-----Input-----
The first line contains integers $n$ and $q$ ($1 \le n \le 200\,000$, $q = 0$), the length of the sequence and the number of the updates.
The second line contains $n$ integers $a_1, a_2, \ldots, a_n$ ($1 \le a_i \le 200\,000$), the initial sequence.
Each of the following $q$ lines contains integers $i_t$ and $x_t$ ($1 \le i_t \le n$, $1 \le x_t \le 200\,000$), the position and the new value for this position.
-----Output-----
Print $q+1$ integers, the answer for the initial sequence and the answer after every update.
-----Examples-----
Input
5 0
3 7 3 7 3
Output
2
Input
10 0
1 2 1 2 3 1 1 1 50 1
Output
4
Input
6 0
6 6 3 3 4 4
Output
0
Input
7 0
3 3 1 3 2 1 2
Output
4 | import sys
input = sys.stdin.readline
n,q=list(map(int,input().split()))
A=list(map(int,input().split()))
if max(A)==min(A):
print(0)
return
L=max(A)
MM=[[200005,-1,i] for i in range(L+1)]
COUNT=[0]*(L+1)
for i in range(n):
a=A[i]
MM[a][0]=min(MM[a][0],i)
MM[a][1]=max(MM[a][1],i)
COUNT[a]+=1
MM.sort()
i,j,k=MM[0]
MAX=j
CC=COUNT[k]
MAXC=COUNT[k]
ANS=0
for i,j,k in MM[1:]:
if i==200005:
ANS+=CC-MAXC
break
if MAX<i:
ANS+=CC-MAXC
MAX=j
CC=COUNT[k]
MAXC=COUNT[k]
else:
CC+=COUNT[k]
MAX=max(MAX,j)
MAXC=max(MAXC,COUNT[k])
print(ANS) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5941c545f5c394fef900000c:
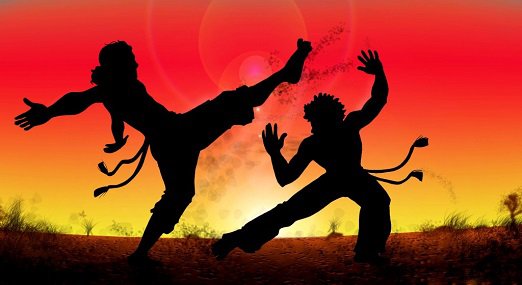
Create a class called `Warrior` which calculates and keeps track of their level and skills, and ranks them as the warrior they've proven to be.
Business Rules:
- A warrior starts at level 1 and can progress all the way to 100.
- A warrior starts at rank `"Pushover"` and can progress all the way to `"Greatest"`.
- The only acceptable range of rank values is `"Pushover", "Novice", "Fighter", "Warrior", "Veteran", "Sage", "Elite", "Conqueror", "Champion", "Master", "Greatest"`.
- Warriors will compete in battles. Battles will always accept an enemy level to match against your own.
- With each battle successfully finished, your warrior's experience is updated based on the enemy's level.
- The experience earned from the battle is relative to what the warrior's current level is compared to the level of the enemy.
- A warrior's experience starts from 100. Each time the warrior's experience increases by another 100, the warrior's level rises to the next level.
- A warrior's experience is cumulative, and does not reset with each rise of level. The only exception is when the warrior reaches level 100, with which the experience stops at 10000
- At every 10 levels, your warrior will reach a new rank tier. (ex. levels 1-9 falls within `"Pushover"` tier, levels 80-89 fall within `"Champion"` tier, etc.)
- A warrior cannot progress beyond level 100 and rank `"Greatest"`.
Battle Progress Rules & Calculations:
- If an enemy level does not fall in the range of 1 to 100, the battle cannot happen and should return `"Invalid level"`.
- Completing a battle against an enemy with the same level as your warrior will be worth 10 experience points.
- Completing a battle against an enemy who is one level lower than your warrior will be worth 5 experience points.
- Completing a battle against an enemy who is two levels lower or more than your warrior will give 0 experience points.
- Completing a battle against an enemy who is one...
I tried it in Python, but could not do it. Can you solve it? | class Warrior():
def __init__(self) :
self.level = 1
self.experience = 100
self.rank = "Pushover"
self.achievements = []
self.max_level = 100
self.available_ranks = ["Pushover", "Novice", "Fighter", "Warrior", "Veteran", "Sage", "Elite", "Conqueror", "Champion", "Master", "Greatest"]
def battle(self, enemy_level) :
if enemy_level < 1 or enemy_level > 100 :
return "Invalid level"
if enemy_level <= self.level :
diff = self.level - enemy_level
self._get_experience(10 // (diff + 1))
if diff > 1 :
return "Easy fight"
else :
return "A good fight"
else :
diff = enemy_level - self.level
if diff >= 5 and enemy_level // 10 > self.level // 10 :
return "You've been defeated"
else :
self._get_experience(20 * diff * diff)
return "An intense fight"
def training(self, achievement) :
description = achievement[0]
experience = achievement[1]
min_level = achievement[2]
if self.level >= min_level :
self.achievements.append(description)
self._get_experience(experience)
return description
else :
return "Not strong enough"
def _get_experience(self, experience) :
if experience >= 5 :
self.experience += experience
self.level = self.experience // 100
if self.level >= self.max_level :
self.level = self.max_level
self.experience = self.max_level * 100
self.rank = self.available_ranks[self.experience // 1000] | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
The teacher gave Anton a large geometry homework, but he didn't do it (as usual) as he participated in a regular round on Codeforces. In the task he was given a set of n lines defined by the equations y = k_{i}·x + b_{i}. It was necessary to determine whether there is at least one point of intersection of two of these lines, that lays strictly inside the strip between x_1 < x_2. In other words, is it true that there are 1 ≤ i < j ≤ n and x', y', such that: y' = k_{i} * x' + b_{i}, that is, point (x', y') belongs to the line number i; y' = k_{j} * x' + b_{j}, that is, point (x', y') belongs to the line number j; x_1 < x' < x_2, that is, point (x', y') lies inside the strip bounded by x_1 < x_2.
You can't leave Anton in trouble, can you? Write a program that solves the given task.
-----Input-----
The first line of the input contains an integer n (2 ≤ n ≤ 100 000) — the number of lines in the task given to Anton. The second line contains integers x_1 and x_2 ( - 1 000 000 ≤ x_1 < x_2 ≤ 1 000 000) defining the strip inside which you need to find a point of intersection of at least two lines.
The following n lines contain integers k_{i}, b_{i} ( - 1 000 000 ≤ k_{i}, b_{i} ≤ 1 000 000) — the descriptions of the lines. It is guaranteed that all lines are pairwise distinct, that is, for any two i ≠ j it is true that either k_{i} ≠ k_{j}, or b_{i} ≠ b_{j}.
-----Output-----
Print "Yes" (without quotes), if there is at least one intersection of two distinct lines, located strictly inside the strip. Otherwise print "No" (without quotes).
-----Examples-----
Input
4
1 2
1 2
1 0
0 1
0 2
Output
NO
Input
2
1 3
1 0
-1 3
Output
YES
Input
2
1 3
1 0
0 2
Output
YES
Input
2
1 3
1 0
0 3
Output
NO
-----Note-----
In the first sample there are intersections located on the border of the strip, but there are no intersections located strictly inside it. [Image] | n = int(input())
x1, x2 = list(map(int, input().split(" ")))
def intercepts(k, b):
y1 = k*x1+b
y2 = k*x2+b
return [y1, y2]
inter=[]
for i in range (n):
k, b = list(map(int, input().split(" ")))
inter += [intercepts(k, b)]
inter.sort()
right=[]
for i in range (n):
intercept = inter[i]
right += [intercept[1]]
right2=[]
for thing in right:
right2+=[thing]
right.sort()
if right == right2:
print("NO")
else:
print("YES") | python | test | qsol | codeparrot/apps | all |
Solve in Python:
You are given a string $s$. And you have a function $f(x)$ defined as:
f(x) = 1, if $x$ is a vowel
f(x) = 0, if $x$ is a constant
Your task is to apply the above function on all the characters in the string s and convert
the obtained binary string in decimal number $M$.
Since the number $M$ can be very large, compute it modulo $10^9+7$.
-----Input:-----
- The first line of the input contains a single integer $T$ i.e the no. of test cases.
- Each test line contains one String $s$ composed of lowercase English alphabet letters.
-----Output:-----
For each case, print a single line containing one integer $M$ modulo $10^9 + 7$.
-----Constraints-----
- $1 ≤ T ≤ 50$
- $|s|≤10^5$
-----Subtasks-----
- 20 points : $|s|≤30$
- 80 points : $ \text{original constraints}$
-----Sample Input:-----
1
hello
-----Sample Output:-----
9 | for _ in range(int(input())):
a=input()
b=[]
for i in a:
if i in ['a','e','i','o','u']:b.append(1)
else:b.append(0)
c=0
l=len(a)
for i in range(l):
if b[-i-1]:c+=1<<i
print(c) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
There are two infinite sources of water: hot water of temperature $h$; cold water of temperature $c$ ($c < h$).
You perform the following procedure of alternating moves: take one cup of the hot water and pour it into an infinitely deep barrel; take one cup of the cold water and pour it into an infinitely deep barrel; take one cup of the hot water $\dots$ and so on $\dots$
Note that you always start with the cup of hot water.
The barrel is initially empty. You have to pour at least one cup into the barrel. The water temperature in the barrel is an average of the temperatures of the poured cups.
You want to achieve a temperature as close as possible to $t$. So if the temperature in the barrel is $t_b$, then the absolute difference of $t_b$ and $t$ ($|t_b - t|$) should be as small as possible.
How many cups should you pour into the barrel, so that the temperature in it is as close as possible to $t$? If there are multiple answers with the minimum absolute difference, then print the smallest of them.
-----Input-----
The first line contains a single integer $T$ ($1 \le T \le 3 \cdot 10^4$) — the number of testcases.
Each of the next $T$ lines contains three integers $h$, $c$ and $t$ ($1 \le c < h \le 10^6$; $c \le t \le h$) — the temperature of the hot water, the temperature of the cold water and the desired temperature in the barrel.
-----Output-----
For each testcase print a single positive integer — the minimum number of cups required to be poured into the barrel to achieve the closest temperature to $t$.
-----Example-----
Input
3
30 10 20
41 15 30
18 13 18
Output
2
7
1
-----Note-----
In the first testcase the temperature after $2$ poured cups: $1$ hot and $1$ cold is exactly $20$. So that is the closest we can achieve.
In the second testcase the temperature after $7$ poured cups: $4$ hot and $3$ cold is about $29.857$. Pouring more water won't get us closer to $t$ than that.
In the third testcase the temperature after $1$ poured cup: $1$ hot is $18$. That's exactly equal to $t$. | import sys
input = sys.stdin.readline
T = int(input())
for _ in range(T):
h, c, t = list(map(int, input().split()))
if h + c >= 2 * t:
print(2)
else:
diff2 = 2*t - (h + c)
hDiff2 = 2*h - (h + c)
kDown = (hDiff2//diff2 - 1)//2
kUp = kDown + 1
diffDown = abs(diff2 - hDiff2/(2 * kDown + 1))
diffUp = abs(diff2 - hDiff2/(2 * kUp + 1))
if diffDown <= diffUp:
print(2 * kDown + 1)
else:
print(2 * kDown + 3) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
You are given an array with several `"even"` words, one `"odd"` word, and some numbers mixed in.
Determine if any of the numbers in the array is the index of the `"odd"` word. If so, return `true`, otherwise `false`. | def odd_ball(arr):
return int(arr.index('odd')) in arr | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/557592fcdfc2220bed000042:
### Task:
You have to write a function `pattern` which creates the following pattern (See Examples) upto desired number of rows.
If the Argument is `0` or a Negative Integer then it should return `""` i.e. empty string.
### Examples:
`pattern(9)`:
123456789
234567891
345678912
456789123
567891234
678912345
789123456
891234567
912345678
`pattern(5)`:
12345
23451
34512
45123
51234
Note: There are no spaces in the pattern
Hint: Use `\n` in string to jump to next line
I tried it in Python, but could not do it. Can you solve it? | from collections import deque
def pattern(n):
result = []
line = deque([str(num) for num in range(1, n + 1)])
for i in range(n):
result.append("".join(line))
line.rotate(-1)
return "\n".join(result) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
You are given a chessboard of size $n \times n$. It is filled with numbers from $1$ to $n^2$ in the following way: the first $\lceil \frac{n^2}{2} \rceil$ numbers from $1$ to $\lceil \frac{n^2}{2} \rceil$ are written in the cells with even sum of coordinates from left to right from top to bottom. The rest $n^2 - \lceil \frac{n^2}{2} \rceil$ numbers from $\lceil \frac{n^2}{2} \rceil + 1$ to $n^2$ are written in the cells with odd sum of coordinates from left to right from top to bottom. The operation $\lceil\frac{x}{y}\rceil$ means division $x$ by $y$ rounded up.
For example, the left board on the following picture is the chessboard which is given for $n=4$ and the right board is the chessboard which is given for $n=5$. [Image]
You are given $q$ queries. The $i$-th query is described as a pair $x_i, y_i$. The answer to the $i$-th query is the number written in the cell $x_i, y_i$ ($x_i$ is the row, $y_i$ is the column). Rows and columns are numbered from $1$ to $n$.
-----Input-----
The first line contains two integers $n$ and $q$ ($1 \le n \le 10^9$, $1 \le q \le 10^5$) — the size of the board and the number of queries.
The next $q$ lines contain two integers each. The $i$-th line contains two integers $x_i, y_i$ ($1 \le x_i, y_i \le n$) — description of the $i$-th query.
-----Output-----
For each query from $1$ to $q$ print the answer to this query. The answer to the $i$-th query is the number written in the cell $x_i, y_i$ ($x_i$ is the row, $y_i$ is the column). Rows and columns are numbered from $1$ to $n$. Queries are numbered from $1$ to $q$ in order of the input.
-----Examples-----
Input
4 5
1 1
4 4
4 3
3 2
2 4
Output
1
8
16
13
4
Input
5 4
2 1
4 2
3 3
3 4
Output
16
9
7
20
-----Note-----
Answers to the queries from examples are on the board in the picture from the problem statement. | import sys
n,q=map(int,sys.stdin.readline().strip().split())
nc=n
# print(n,q)
if(n%2==0):
n2=int((n*n)/2)
else:
n2=int((n*n)/2)+1
n1=int(n/2)
if(1==1):
for i in range(q):
x,y=map(int,sys.stdin.readline().strip().split())
# print(n,q,x,y)
x1=int(x/2)
y1=int(y/2)
op=0
if(x%2!=0):
if(y%2!=0):
if(x>1):
op=n*(x1)
op=op+1+(y1)
else:
op=n2+(n*x1)+y1
else:
if(y%2==0):
if(n%2==0):
op=n1
else:
op=n1+1
op=op+(n*(x1-1))
op=op+y1
else:
op=n2+n1+(n*(x1-1))+(y1)+1
print(op) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
A sequence a=\{a_1,a_2,a_3,......\} is determined as follows:
- The first term s is given as input.
- Let f(n) be the following function: f(n) = n/2 if n is even, and f(n) = 3n+1 if n is odd.
- a_i = s when i = 1, and a_i = f(a_{i-1}) when i > 1.
Find the minimum integer m that satisfies the following condition:
- There exists an integer n such that a_m = a_n (m > n).
-----Constraints-----
- 1 \leq s \leq 100
- All values in input are integers.
- It is guaranteed that all elements in a and the minimum m that satisfies the condition are at most 1000000.
-----Input-----
Input is given from Standard Input in the following format:
s
-----Output-----
Print the minimum integer m that satisfies the condition.
-----Sample Input-----
8
-----Sample Output-----
5
a=\{8,4,2,1,4,2,1,4,2,1,......\}. As a_5=a_2, the answer is 5. | s = int(input())
ans_list = [s]
curr_value = s
index = 1
while(True):
if curr_value%2==0:
curr_value = int(curr_value/2)
else:
curr_value = 3*curr_value + 1
index += 1
if curr_value in ans_list:
break
else:
ans_list.append(curr_value)
print(index) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1184/B1:
Heidi and Doctor Who hopped out of the TARDIS and found themselves at EPFL in 2018. They were surrounded by stormtroopers and Darth Vader was approaching. Miraculously, they managed to escape to a nearby rebel base but the Doctor was very confused. Heidi reminded him that last year's HC2 theme was Star Wars. Now he understood, and he's ready to face the evils of the Empire!
The rebels have $s$ spaceships, each with a certain attacking power $a$.
They want to send their spaceships to destroy the empire bases and steal enough gold and supplies in order to keep the rebellion alive.
The empire has $b$ bases, each with a certain defensive power $d$, and a certain amount of gold $g$.
A spaceship can attack all the bases which have a defensive power less than or equal to its attacking power.
If a spaceship attacks a base, it steals all the gold in that base.
The rebels are still undecided which spaceship to send out first, so they asked for the Doctor's help. They would like to know, for each spaceship, the maximum amount of gold it can steal.
-----Input-----
The first line contains integers $s$ and $b$ ($1 \leq s, b \leq 10^5$), the number of spaceships and the number of bases, respectively.
The second line contains $s$ integers $a$ ($0 \leq a \leq 10^9$), the attacking power of each spaceship.
The next $b$ lines contain integers $d, g$ ($0 \leq d \leq 10^9$, $0 \leq g \leq 10^4$), the defensive power and the gold of each base, respectively.
-----Output-----
Print $s$ integers, the maximum amount of gold each spaceship can steal, in the same order as the spaceships are given in the input.
-----Example-----
Input
5 4
1 3 5 2 4
0 1
4 2
2 8
9 4
Output
1 9 11 9 11
-----Note-----
The first spaceship can only attack the first base.
The second spaceship can attack the first and third bases.
The third spaceship can attack the first, second and third bases.
I tried it in Python, but could not do it. Can you solve it? | from bisect import bisect_right
def find_le(a, x):
'Find rightmost value less than or equal to x'
i = bisect_right(a, x)
if i:
return i-1
return -1
s, b = list(map(int,input().split()))
v = []
a = list(map(int,input().split()))
for i in range(b):
v.append(tuple(map(int,input().split())))
v.sort()
summa = 0
c = []
for i in v:
summa += i[1]
c.append(summa)
mas = [i[0] for i in v]
for i in a:
ind = find_le(mas, i)
if ind == -1:
print(0)
else:
print(c[ind],end = ' ') | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Little penguin Polo has an n × m matrix, consisting of integers. Let's index the matrix rows from 1 to n from top to bottom and let's index the columns from 1 to m from left to right. Let's represent the matrix element on the intersection of row i and column j as a_{ij}.
In one move the penguin can add or subtract number d from some matrix element. Find the minimum number of moves needed to make all matrix elements equal. If the described plan is impossible to carry out, say so.
-----Input-----
The first line contains three integers n, m and d (1 ≤ n, m ≤ 100, 1 ≤ d ≤ 10^4) — the matrix sizes and the d parameter. Next n lines contain the matrix: the j-th integer in the i-th row is the matrix element a_{ij} (1 ≤ a_{ij} ≤ 10^4).
-----Output-----
In a single line print a single integer — the minimum number of moves the penguin needs to make all matrix elements equal. If that is impossible, print "-1" (without the quotes).
-----Examples-----
Input
2 2 2
2 4
6 8
Output
4
Input
1 2 7
6 7
Output
-1 | """http://codeforces.com/problemset/problem/289/B"""
def solve(d, l):
l.sort()
m = l[0] % d
median = len(l) // 2
res = 0
for num in l:
if num % d != m:
return -1
res += abs(num - l[median]) // d
return res
def __starting_point():
f = lambda: list(map(int, input().split()))
n, m, d = f()
l = []
for i in range(n):
l += f()
print(solve(d, l))
__starting_point() | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc066/tasks/arc077_a:
You are given an integer sequence of length n, a_1, ..., a_n.
Let us consider performing the following n operations on an empty sequence b.
The i-th operation is as follows:
- Append a_i to the end of b.
- Reverse the order of the elements in b.
Find the sequence b obtained after these n operations.
-----Constraints-----
- 1 \leq n \leq 2\times 10^5
- 0 \leq a_i \leq 10^9
- n and a_i are integers.
-----Input-----
Input is given from Standard Input in the following format:
n
a_1 a_2 ... a_n
-----Output-----
Print n integers in a line with spaces in between.
The i-th integer should be b_i.
-----Sample Input-----
4
1 2 3 4
-----Sample Output-----
4 2 1 3
- After step 1 of the first operation, b becomes: 1.
- After step 2 of the first operation, b becomes: 1.
- After step 1 of the second operation, b becomes: 1, 2.
- After step 2 of the second operation, b becomes: 2, 1.
- After step 1 of the third operation, b becomes: 2, 1, 3.
- After step 2 of the third operation, b becomes: 3, 1, 2.
- After step 1 of the fourth operation, b becomes: 3, 1, 2, 4.
- After step 2 of the fourth operation, b becomes: 4, 2, 1, 3.
Thus, the answer is 4 2 1 3.
I tried it in Python, but could not do it. Can you solve it? | from collections import deque
n,a,b=int(input()),deque(map(int,input().split())),deque()
for i in range(n):
if n%2==0:
if i%2==0:
b.append(a[i])
else:
b.appendleft(a[i])
else:
if i%2==0:
b.appendleft(a[i])
else:
b.append(a[i])
print(*b,sep=' ') | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/589273272fab865136000108:
## Your Story
"A *piano* in the home meant something." - *Fried Green Tomatoes at the Whistle Stop Cafe*
You've just realized a childhood dream by getting a beautiful and beautiful-sounding upright piano from a friend who was leaving the country. You immediately started doing things like playing "Heart and Soul" over and over again, using one finger to pick out any melody that came into your head, requesting some sheet music books from the library, signing up for some MOOCs like Developing Your Musicianship, and wondering if you will think of any good ideas for writing piano-related katas and apps.
Now you're doing an exercise where you play the very first (leftmost, lowest in pitch) key on the 88-key keyboard, which (as shown below) is white, with the little finger on your left hand, then the second key, which is black, with the ring finger on your left hand, then the third key, which is white, with the middle finger on your left hand, then the fourth key, also white, with your left index finger, and then the fifth key, which is black, with your left thumb. Then you play the sixth key, which is white, with your right thumb, and continue on playing the seventh, eighth, ninth, and tenth keys with the other four fingers of your right hand. Then for the eleventh key you go back to your left little finger, and so on. Once you get to the rightmost/highest, 88th, key, you start all over again with your left little finger on the first key. Your thought is that this will help you to learn to move smoothly and with uniform pressure on the keys from each finger to the next and back and forth between hands.
You're not saying the names of the notes while you're doing this, but instead just counting each key press out loud (not starting again at 1 after 88, but continuing on to 89 and so forth) to try to keep a steady rhythm going and to see how far you can get before messing up. You move gracefully and with flourishes, and between screwups you hear, see, and feel that you are part of some great repeating...
I tried it in Python, but could not do it. Can you solve it? | def black_or_white_key(c):
i = int('010100101010'[((c - 1) % 88 - 3) % 12])
return ['white', 'black'][i] | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/NQST2020/problems/HEIST101:
For years you have been working hard in Santa's factory to manufacture gifts for kids in substandard work environments with no pay. You have finally managed to escape the factory and now you seek revenge. You are planning a heist with the Grinch to steal all the gifts which are locked in a safe. Since you have worked in the factory for so many years, you know how to crack the safe.
The passcode for the safe is an integer. This passcode keeps changing everyday, but you know a way to crack it. You will be given two numbers A and B .
Passcode is the number of X such that 0 ≤ X < B and
gcd(A,B) = gcd(A+X,B).
Note : gcd(A,B) is the greatest common divisor of A & B.
-----Input-----
The first line contains the single integer T (1 ≤ T ≤ 50) — the number of test cases.
Next T lines contain test cases per line. Each line contains two integers A & B
( 1 ≤ A < B ≤ 1010 )
-----Output-----
Print T integers, one for each Test case. For each test case print the appropriate passcode for that day.
-----Sample Input-----
3
4 9
5 10
42 9999999967
-----Output-----
6
1
9999999966
I tried it in Python, but could not do it. Can you solve it? | import math
t = int(input())
def phi(n):
res = n
i = 2
while i*i<=n:
if n%i==0:
res/=i
res*=(i-1)
while n%i==0:
n/=i
i+=1
if n>1:
res/=n
res*=(n-1)
return int(res)
while t:
a,m = list(map(int,input().split()))
g = math.gcd(a,m)
print(phi(m//g))
t-=1 | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Watchmen are in a danger and Doctor Manhattan together with his friend Daniel Dreiberg should warn them as soon as possible. There are n watchmen on a plane, the i-th watchman is located at point (x_{i}, y_{i}).
They need to arrange a plan, but there are some difficulties on their way. As you know, Doctor Manhattan considers the distance between watchmen i and j to be |x_{i} - x_{j}| + |y_{i} - y_{j}|. Daniel, as an ordinary person, calculates the distance using the formula $\sqrt{(x_{i} - x_{j})^{2} +(y_{i} - y_{j})^{2}}$.
The success of the operation relies on the number of pairs (i, j) (1 ≤ i < j ≤ n), such that the distance between watchman i and watchmen j calculated by Doctor Manhattan is equal to the distance between them calculated by Daniel. You were asked to compute the number of such pairs.
-----Input-----
The first line of the input contains the single integer n (1 ≤ n ≤ 200 000) — the number of watchmen.
Each of the following n lines contains two integers x_{i} and y_{i} (|x_{i}|, |y_{i}| ≤ 10^9).
Some positions may coincide.
-----Output-----
Print the number of pairs of watchmen such that the distance between them calculated by Doctor Manhattan is equal to the distance calculated by Daniel.
-----Examples-----
Input
3
1 1
7 5
1 5
Output
2
Input
6
0 0
0 1
0 2
-1 1
0 1
1 1
Output
11
-----Note-----
In the first sample, the distance between watchman 1 and watchman 2 is equal to |1 - 7| + |1 - 5| = 10 for Doctor Manhattan and $\sqrt{(1 - 7)^{2} +(1 - 5)^{2}} = 2 \cdot \sqrt{13}$ for Daniel. For pairs (1, 1), (1, 5) and (7, 5), (1, 5) Doctor Manhattan and Daniel will calculate the same distances. | from collections import defaultdict, Counter
n = int(input())
w = []
for i in range(n):
w.append(tuple(map(int, input().split())))
dx = Counter()
dy = Counter()
for x, y in w:
dx[x] += 1
dy[y] += 1
count = sum((v * (v-1) / 2) for v in list(dx.values())) + \
sum((v * (v-1) / 2) for v in list(dy.values()))
dc = Counter(w)
count -= sum((c * (c-1) / 2) for c in list(dc.values()) if c > 1)
print(int(count)) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
=====Problem Statement=====
You are given a positive integer N. Print a numerical triangle of height N - 1 like the one below:
1
22
333
4444
55555
......
Can you do it using only arithmetic operations, a single for loop and print statement?
Use no more than two lines. The first line (the for statement) is already written for you. You have to complete the print statement.
Note: Using anything related to strings will give a score of 0.
=====Input Format=====
A single line containing integer, N.
=====Constraints=====
1≤N≤9
=====Output Format=====
Print N - 1 lines as explained above. | for i in range(1,int(input())): #More than 2 lines will result in 0 score. Do not leave a blank line also
print((10**i//9 * i)) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1102/D:
You are given a string $s$ consisting of exactly $n$ characters, and each character is either '0', '1' or '2'. Such strings are called ternary strings.
Your task is to replace minimum number of characters in this string with other characters to obtain a balanced ternary string (balanced ternary string is a ternary string such that the number of characters '0' in this string is equal to the number of characters '1', and the number of characters '1' (and '0' obviously) is equal to the number of characters '2').
Among all possible balanced ternary strings you have to obtain the lexicographically (alphabetically) smallest.
Note that you can neither remove characters from the string nor add characters to the string. Also note that you can replace the given characters only with characters '0', '1' and '2'.
It is guaranteed that the answer exists.
-----Input-----
The first line of the input contains one integer $n$ ($3 \le n \le 3 \cdot 10^5$, $n$ is divisible by $3$) — the number of characters in $s$.
The second line contains the string $s$ consisting of exactly $n$ characters '0', '1' and '2'.
-----Output-----
Print one string — the lexicographically (alphabetically) smallest balanced ternary string which can be obtained from the given one with minimum number of replacements.
Because $n$ is divisible by $3$ it is obvious that the answer exists. And it is obvious that there is only one possible answer.
-----Examples-----
Input
3
121
Output
021
Input
6
000000
Output
001122
Input
6
211200
Output
211200
Input
6
120110
Output
120120
I tried it in Python, but could not do it. Can you solve it? | from sys import stdin, stdout
from math import sin, tan, cos, pi, atan2, sqrt, acos, atan, factorial
from random import randint
n = int(stdin.readline())
s = list(stdin.readline().strip())
a, b, c = s.count('0'), s.count('1'), s.count('2')
d = n // 3
for i in range(len(s)):
if s[i] == '2' and c > d:
if a < d:
s[i] = '0'
a += 1
c -= 1
else:
s[i] = '1'
b += 1
c -= 1
elif s[i] == '1' and b > d:
if a < d:
s[i] = '0'
a += 1
b -= 1
for i in range(len(s) - 1, -1, -1):
if s[i] == '1' and b > d:
if c < d:
s[i] = '2'
b -= 1
c += 1
elif s[i] == '0' and a > d:
if c < d:
s[i] = '2'
a -= 1
c += 1
elif b < d:
s[i] = '1'
a -= 1
b += 1
stdout.write(''.join(s)) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
It's one more school day now. Sasha doesn't like classes and is always bored at them. So, each day he invents some game and plays in it alone or with friends.
Today he invented one simple game to play with Lena, with whom he shares a desk. The rules are simple. Sasha draws n sticks in a row. After that the players take turns crossing out exactly k sticks from left or right in each turn. Sasha moves first, because he is the inventor of the game. If there are less than k sticks on the paper before some turn, the game ends. Sasha wins if he makes strictly more moves than Lena. Sasha wants to know the result of the game before playing, you are to help him.
-----Input-----
The first line contains two integers n and k (1 ≤ n, k ≤ 10^18, k ≤ n) — the number of sticks drawn by Sasha and the number k — the number of sticks to be crossed out on each turn.
-----Output-----
If Sasha wins, print "YES" (without quotes), otherwise print "NO" (without quotes).
You can print each letter in arbitrary case (upper of lower).
-----Examples-----
Input
1 1
Output
YES
Input
10 4
Output
NO
-----Note-----
In the first example Sasha crosses out 1 stick, and then there are no sticks. So Lena can't make a move, and Sasha wins.
In the second example Sasha crosses out 4 sticks, then Lena crosses out 4 sticks, and after that there are only 2 sticks left. Sasha can't make a move. The players make equal number of moves, so Sasha doesn't win. | string = input()
numbers = string.split()
a, b = int(numbers[0]), int(numbers[1])
n = a // b
if n % 2 == 1:
print("YES")
else:
print("NO") | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/513/C:
Nowadays, most of the internet advertisements are not statically linked to a web page. Instead, what will be shown to the person opening a web page is determined within 100 milliseconds after the web page is opened. Usually, multiple companies compete for each ad slot on the web page in an auction. Each of them receives a request with details about the user, web page and ad slot and they have to respond within those 100 milliseconds with a bid they would pay for putting an advertisement on that ad slot. The company that suggests the highest bid wins the auction and gets to place its advertisement. If there are several companies tied for the highest bid, the winner gets picked at random.
However, the company that won the auction does not have to pay the exact amount of its bid. In most of the cases, a second-price auction is used. This means that the amount paid by the company is equal to the maximum of all the other bids placed for this ad slot.
Let's consider one such bidding. There are n companies competing for placing an ad. The i-th of these companies will bid an integer number of microdollars equiprobably randomly chosen from the range between L_{i} and R_{i}, inclusive. In the other words, the value of the i-th company bid can be any integer from the range [L_{i}, R_{i}] with the same probability.
Determine the expected value that the winner will have to pay in a second-price auction.
-----Input-----
The first line of input contains an integer number n (2 ≤ n ≤ 5). n lines follow, the i-th of them containing two numbers L_{i} and R_{i} (1 ≤ L_{i} ≤ R_{i} ≤ 10000) describing the i-th company's bid preferences.
This problem doesn't have subproblems. You will get 8 points for the correct submission.
-----Output-----
Output the answer with absolute or relative error no more than 1e - 9.
-----Examples-----
Input
3
4 7
8 10
5 5
Output
5.7500000000
Input
3
2 5
3 4
1 6
Output
3.5000000000
-----Note-----
Consider the first example. The first company bids a random integer number of...
I tried it in Python, but could not do it. Can you solve it? | d = [list(map(int, input().split())) for i in range(int(input()))]
s = 0
for k in range(1, 10001):
p = [min(max((k - l) / (r - l + 1), 1e-20), 1) for l, r in d]
u = v = 1
for r in p: u *= r
for r in p:
v *= r
s += (u - v) * (r - 1) / r
print(s)
# Made By Mostafa_Khaled | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Ken loves ken-ken-pa (Japanese version of hopscotch). Today, he will play it on a directed graph G.
G consists of N vertices numbered 1 to N, and M edges. The i-th edge points from Vertex u_i to Vertex v_i.
First, Ken stands on Vertex S. He wants to reach Vertex T by repeating ken-ken-pa. In one ken-ken-pa, he does the following exactly three times: follow an edge pointing from the vertex on which he is standing.
Determine if he can reach Vertex T by repeating ken-ken-pa. If the answer is yes, find the minimum number of ken-ken-pa needed to reach Vertex T. Note that visiting Vertex T in the middle of a ken-ken-pa does not count as reaching Vertex T by repeating ken-ken-pa.
-----Constraints-----
- 2 \leq N \leq 10^5
- 0 \leq M \leq \min(10^5, N (N-1))
- 1 \leq u_i, v_i \leq N(1 \leq i \leq M)
- u_i \neq v_i (1 \leq i \leq M)
- If i \neq j, (u_i, v_i) \neq (u_j, v_j).
- 1 \leq S, T \leq N
- S \neq T
-----Input-----
Input is given from Standard Input in the following format:
N M
u_1 v_1
:
u_M v_M
S T
-----Output-----
If Ken cannot reach Vertex T from Vertex S by repeating ken-ken-pa, print -1.
If he can, print the minimum number of ken-ken-pa needed to reach vertex T.
-----Sample Input-----
4 4
1 2
2 3
3 4
4 1
1 3
-----Sample Output-----
2
Ken can reach Vertex 3 from Vertex 1 in two ken-ken-pa, as follows: 1 \rightarrow 2 \rightarrow 3 \rightarrow 4 in the first ken-ken-pa, then 4 \rightarrow 1 \rightarrow 2 \rightarrow 3 in the second ken-ken-pa. This is the minimum number of ken-ken-pa needed. | from collections import deque
MOD = 10**9 +7
INF = 10**9
def main():
n,m = list(map(int,input().split()))
V0 = [[] for _ in range(n)]
V1 = [[] for _ in range(n)]
V2 = [[] for _ in range(n)]
for _ in range(m):
u,v = list(map(int,input().split()))
u -= 1
v -= 1
V0[u].append(v)
V1[u].append(v)
V2[u].append(v)
s,t = list(map(int,input().split()))
s -= 1
t -= 1
d0 = [-1]*n
d1 = [-1]*n
d2 = [-1]*n
d0[s] = 0
q = deque()
q.append((s,0))
while len(q):
v,mod = q.popleft()
if mod == 0:
for e in V0[v]:
if d1[e] == -1:
d1[e] = d0[v] + 1
q.append([e,1])
elif mod == 1:
for e in V1[v]:
if d2[e] == -1:
d2[e] = d1[v] + 1
q.append([e,2])
else:
for e in V2[v]:
if e == t:
print(((d2[v] + 1)//3))
return
if d0[e] == -1:
d0[e] = d2[v] + 1
q.append([e,0])
if d0[t] == -1:
print((-1))
def __starting_point():
main()
__starting_point() | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/559/C:
Giant chess is quite common in Geraldion. We will not delve into the rules of the game, we'll just say that the game takes place on an h × w field, and it is painted in two colors, but not like in chess. Almost all cells of the field are white and only some of them are black. Currently Gerald is finishing a game of giant chess against his friend Pollard. Gerald has almost won, and the only thing he needs to win is to bring the pawn from the upper left corner of the board, where it is now standing, to the lower right corner. Gerald is so confident of victory that he became interested, in how many ways can he win?
The pawn, which Gerald has got left can go in two ways: one cell down or one cell to the right. In addition, it can not go to the black cells, otherwise the Gerald still loses. There are no other pawns or pieces left on the field, so that, according to the rules of giant chess Gerald moves his pawn until the game is over, and Pollard is just watching this process.
-----Input-----
The first line of the input contains three integers: h, w, n — the sides of the board and the number of black cells (1 ≤ h, w ≤ 10^5, 1 ≤ n ≤ 2000).
Next n lines contain the description of black cells. The i-th of these lines contains numbers r_{i}, c_{i} (1 ≤ r_{i} ≤ h, 1 ≤ c_{i} ≤ w) — the number of the row and column of the i-th cell.
It is guaranteed that the upper left and lower right cell are white and all cells in the description are distinct.
-----Output-----
Print a single line — the remainder of the number of ways to move Gerald's pawn from the upper left to the lower right corner modulo 10^9 + 7.
-----Examples-----
Input
3 4 2
2 2
2 3
Output
2
Input
100 100 3
15 16
16 15
99 88
Output
545732279
I tried it in Python, but could not do it. Can you solve it? | def init_factorials(N, mod):
f = 1
fac = [1] * N
for i in range(1, N):
f *= i
f %= mod
fac[i] = f
return fac
def init_inv(N, mod, fac):
b = bin(mod-2)[2:][-1::-1]
ret = 1
tmp = fac[N]
if b[0] == '1':
ret = fac[N]
for bi in b[1:]:
tmp *= tmp
tmp %= mod
if bi == '1':
ret *= tmp
ret %= mod
inv = [1] * (N + 1)
inv[N] = ret
for i in range(N-1, 0, -1):
ret *= i + 1
ret %= mod
inv[i] = ret
return inv
def f(r, c, mod, fac, inv):
return (fac[r + c] * inv[r] * inv[c]) % mod
def read_data():
h, w, n = list(map(int, input().split()))
blacks = []
for i in range(n):
r, c = list(map(int, input().split()))
blacks.append((r, c))
return h, w, n, blacks
def solve(h, w, n, blacks):
mod = 10**9 + 7
fac = init_factorials(h + w + 10, mod)
inv = init_inv(h + w + 5, mod, fac)
ans = (fac[h+w-2]*inv[h-1]*inv[w-1]) % mod
eb = [(r + c, r, c) for r, c in blacks]
eb.sort()
blacks = [(r, c) for rc, r, c in eb]
g = [f(r-1, c-1, mod, fac, inv) for r, c in blacks]
hw = h+w
for i, (r, c) in enumerate(blacks):
gi = g[i]
rc = r + c
ans -= gi*fac[hw-rc]*inv[h-r]*inv[w-c]
ans %= mod
for j, (rj, cj) in enumerate(blacks[i+1:], i+1):
if r <= rj and c <= cj:
g[j] -= gi*fac[rj+cj-rc]*inv[rj-r]*inv[cj-c]
g[j] %= mod
return ans
h, w, n, blacks = read_data()
print(solve(h, w, n, blacks)) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
We have N switches with "on" and "off" state, and M bulbs. The switches are numbered 1 to N, and the bulbs are numbered 1 to M.
Bulb i is connected to k_i switches: Switch s_{i1}, s_{i2}, ..., and s_{ik_i}. It is lighted when the number of switches that are "on" among these switches is congruent to p_i modulo 2.
How many combinations of "on" and "off" states of the switches light all the bulbs?
-----Constraints-----
- 1 \leq N, M \leq 10
- 1 \leq k_i \leq N
- 1 \leq s_{ij} \leq N
- s_{ia} \neq s_{ib} (a \neq b)
- p_i is 0 or 1.
- All values in input are integers.
-----Input-----
Input is given from Standard Input in the following format:
N M
k_1 s_{11} s_{12} ... s_{1k_1}
:
k_M s_{M1} s_{M2} ... s_{Mk_M}
p_1 p_2 ... p_M
-----Output-----
Print the number of combinations of "on" and "off" states of the switches that light all the bulbs.
-----Sample Input-----
2 2
2 1 2
1 2
0 1
-----Sample Output-----
1
- Bulb 1 is lighted when there is an even number of switches that are "on" among the following: Switch 1 and 2.
- Bulb 2 is lighted when there is an odd number of switches that are "on" among the following: Switch 2.
There are four possible combinations of states of (Switch 1, Switch 2): (on, on), (on, off), (off, on) and (off, off). Among them, only (on, on) lights all the bulbs, so we should print 1. | n,m=map(int,input().split())
ks=[[] for i in range(m)]
for i in range(m):
ks[i]=list(map(lambda x:int(x)-1,input().split()))
p=list(map(int,input().split()))
ans=0
for i in range(1<<n):
l=[0]*n
for j in range(n):
l[j]=1 if i&1 else 0
i>>=1
# light check
for j in range(m):
s=sum([l[k] for k in ks[j][1:]])
if s%2!=p[j]:
break
else:
ans+=1
print(ans) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Chef has $N$ boxes arranged in a line.Each box has some candies in it,say $C[i]$. Chef wants to distribute all the candies between of his friends: $A$ and $B$, in the following way:
$A$ starts eating candies kept in the leftmost box(box at $1$st place) and $B$ starts eating candies kept in the rightmost box(box at $N$th place). $A$ eats candies $X$ times faster than $B$ i.e. $A$ eats $X$ candies when $B$ eats only $1$. Candies in each box can be eaten by only the person who reaches that box first. If both reach a box at the same time, then the one who has eaten from more number of BOXES till now will eat the candies in that box. If both have eaten from the same number of boxes till now ,then $A$ will eat the candies in that box.
Find the number of boxes finished by both $A$ and $B$.
NOTE:- We assume that it does not take any time to switch from one box to another.
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase contains three lines of input.
The first line of each test case contains $N$, the number of boxes.
- The second line of each test case contains a sequence,$C_1$ ,$C_2$ ,$C_3$ . . . $C_N$ where $C_i$ denotes the number of candies in the i-th box.
- The third line of each test case contains an integer $X$ .
-----Output:-----
For each testcase, print two space separated integers in a new line - the number of boxes eaten by $A$ and $B$ respectively.
-----Constraints-----
- $1 \leq T \leq 100$
- $1 \leq N \leq 200000$
- $1 \leq C_i \leq 1000000$
- $1 \leq X \leq 10$
- Sum of $N$ over all test cases does not exceed $300000$
-----Subtasks-----
Subtask 1(24 points):
- $1 \leq T \leq 10$
- $1 \leq N \leq 1000$
- $1 \leq C_i \leq 1000$
- $1 \leq X \leq 10$
Subtask 2(51 points): original constraints
-----Sample Input:-----
1
5
2 8 8 2 9
2
-----Sample Output:-----
4 1
-----EXPLANATION:-----
$A$ will start eating candies in the 1st box(leftmost box having 2 candies) . $B$ will start eating candies in the 5th box(rightmost box having 9... | t=int(input())
for _ in range(t):
n=int(input())
l=list(map(int,input().split()))
x=int(input())
j=n-1
i=0
a=0
b=0
remain = 0
while True:
if i>j:
break
if i==j:
if remain:
a+=1
else:
if b>a:
b+=1
else:
a+=1
break
b+=1
d = x*l[j]+remain
while i<j:
d-=l[i]
if d==0:
remain = 0
a+=1
i+=1
break
if d<0:
remain = d+l[i]
break
a+=1
i+=1
j-=1
print(a,b) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1111/B:
Every superhero has been given a power value by the Felicity Committee. The avengers crew wants to maximize the average power of the superheroes in their team by performing certain operations.
Initially, there are $n$ superheroes in avengers team having powers $a_1, a_2, \ldots, a_n$, respectively. In one operation, they can remove one superhero from their team (if there are at least two) or they can increase the power of a superhero by $1$. They can do at most $m$ operations. Also, on a particular superhero at most $k$ operations can be done.
Can you help the avengers team to maximize the average power of their crew?
-----Input-----
The first line contains three integers $n$, $k$ and $m$ ($1 \le n \le 10^{5}$, $1 \le k \le 10^{5}$, $1 \le m \le 10^{7}$) — the number of superheroes, the maximum number of times you can increase power of a particular superhero, and the total maximum number of operations.
The second line contains $n$ integers $a_1, a_2, \ldots, a_n$ ($1 \le a_i \le 10^{6}$) — the initial powers of the superheroes in the cast of avengers.
-----Output-----
Output a single number — the maximum final average power.
Your answer is considered correct if its absolute or relative error does not exceed $10^{-6}$.
Formally, let your answer be $a$, and the jury's answer be $b$. Your answer is accepted if and only if $\frac{|a - b|}{\max{(1, |b|)}} \le 10^{-6}$.
-----Examples-----
Input
2 4 6
4 7
Output
11.00000000000000000000
Input
4 2 6
1 3 2 3
Output
5.00000000000000000000
-----Note-----
In the first example, the maximum average is obtained by deleting the first element and increasing the second element four times.
In the second sample, one of the ways to achieve maximum average is to delete the first and the third element and increase the second and the fourth elements by $2$ each.
I tried it in Python, but could not do it. Can you solve it? | n,k,m = map(int,input().split())
a = list(map(int,input().split()))
a.sort(reverse = True)
s = sum(a)
ans = 0
while n > 0 and m > -1:
s2 = (s + min(m,n * k)) / n
ans = max(ans,s2)
s -= a.pop()
n -= 1
m -= 1
print(ans) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
You have initially a string of N characters, denoted by A1,A2...AN. You have to print the size of the largest subsequence of string A such that all the characters in that subsequence are distinct ie. no two characters in that subsequence should be same.
A subsequence of string A is a sequence that can be derived from A by deleting some elements and without changing the order of the remaining elements.
-----Input-----
First line contains T, number of testcases. Each testcase consists of a single string in one line. Each character of the string will be a small alphabet(ie. 'a' to 'z').
-----Output-----
For each testcase, print the required answer in one line.
-----Constraints-----
- 1 ≤ T ≤ 10
- Subtask 1 (20 points):1 ≤ N ≤ 10
- Subtask 2 (80 points):1 ≤ N ≤ 105
-----Example-----
Input:
2
abc
aba
Output:
3
2
-----Explanation-----
For first testcase, the whole string is a subsequence which has all distinct characters.
In second testcase, the we can delete last or first 'a' to get the required subsequence. | for _ in range(int(input())):
s = input()
arr =[]
for i in range(len(s)):
if s[i] in arr:
pass
else:
arr.append(s[i])
print(len(arr)) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Let's denote a m-free matrix as a binary (that is, consisting of only 1's and 0's) matrix such that every square submatrix of size m × m of this matrix contains at least one zero.
Consider the following problem:
You are given two integers n and m. You have to construct an m-free square matrix of size n × n such that the number of 1's in this matrix is maximum possible. Print the maximum possible number of 1's in such matrix.
You don't have to solve this problem. Instead, you have to construct a few tests for it.
You will be given t numbers x_1, x_2, ..., x_{t}. For every $i \in [ 1, t ]$, find two integers n_{i} and m_{i} (n_{i} ≥ m_{i}) such that the answer for the aforementioned problem is exactly x_{i} if we set n = n_{i} and m = m_{i}.
-----Input-----
The first line contains one integer t (1 ≤ t ≤ 100) — the number of tests you have to construct.
Then t lines follow, i-th line containing one integer x_{i} (0 ≤ x_{i} ≤ 10^9).
Note that in hacks you have to set t = 1.
-----Output-----
For each test you have to construct, output two positive numbers n_{i} and m_{i} (1 ≤ m_{i} ≤ n_{i} ≤ 10^9) such that the maximum number of 1's in a m_{i}-free n_{i} × n_{i} matrix is exactly x_{i}. If there are multiple solutions, you may output any of them; and if this is impossible to construct a test, output a single integer - 1.
-----Example-----
Input
3
21
0
1
Output
5 2
1 1
-1 | n = int(input())
for x in range(n):
x = int(input())
if x == 0:
print(1, 1)
else:
flag = True
for d in range(1, int(x**0.5)+2):
if x % d == 0:
s = x // d
n = (s + d)//2
if int(n) != n:
continue
nm = s - n
if nm <= 0:
continue
m = n//nm
if (m>0) and (n**2 - (n//m)**2 == x):
print(n, m)
flag = False
break
if flag:
print(-1) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Given two strings s and t which consist of only lowercase letters.
String t is generated by random shuffling string s and then add one more letter at a random position.
Find the letter that was added in t.
Example:
Input:
s = "abcd"
t = "abcde"
Output:
e
Explanation:
'e' is the letter that was added. | class Solution:
def findTheDifference(self, s, t):
"""
:type s: str
:type t: str
:rtype: str
"""
ss = sorted(s)
st = sorted(t)
i = 0
j = 0
while i <= len(ss) - 1:
if ss[i] == st[j]:
i += 1
j += 1
else: return st[j]
return st[j] | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Bizon the Champion isn't just charming, he also is very smart.
While some of us were learning the multiplication table, Bizon the Champion had fun in his own manner. Bizon the Champion painted an n × m multiplication table, where the element on the intersection of the i-th row and j-th column equals i·j (the rows and columns of the table are numbered starting from 1). Then he was asked: what number in the table is the k-th largest number? Bizon the Champion always answered correctly and immediately. Can you repeat his success?
Consider the given multiplication table. If you write out all n·m numbers from the table in the non-decreasing order, then the k-th number you write out is called the k-th largest number.
-----Input-----
The single line contains integers n, m and k (1 ≤ n, m ≤ 5·10^5; 1 ≤ k ≤ n·m).
-----Output-----
Print the k-th largest number in a n × m multiplication table.
-----Examples-----
Input
2 2 2
Output
2
Input
2 3 4
Output
3
Input
1 10 5
Output
5
-----Note-----
A 2 × 3 multiplication table looks like this:
1 2 3
2 4 6 | from sys import stdin
n, m, k = [int(x) for x in stdin.readline().split()]
be, en = 1, k + 1
while be < en:
mid = (be + en + 1) >> 1
be1, cur = (mid + m - 1) // m, 0
for i in range(1, be1):
cur += m
for i in range(be1, n + 1):
cur += (mid - 1) // i
if cur <= k - 1:
be = mid
else:
en = mid - 1
print(be) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Given an arbitrary ransom note string and another string containing letters from all the magazines, write a function that will return true if the ransom
note can be constructed from the magazines ; otherwise, it will return false.
Each letter in the magazine string can only be used once in your ransom note.
Note:
You may assume that both strings contain only lowercase letters.
canConstruct("a", "b") -> false
canConstruct("aa", "ab") -> false
canConstruct("aa", "aab") -> true | class Solution:
def canConstruct(self, ransomNote, magazine):
"""
:type ransomNote: str
:type magazine: str
:rtype: bool
"""
r_map = {}
for char in ransomNote:
if char in r_map:
r_map[char] += 1
else:
r_map[char] = 1
m_map = {}
for char in magazine:
if char in m_map:
m_map[char] += 1
else:
m_map[char] = 1
for char, count in r_map.items():
if char not in m_map:
return False
if count > m_map[char]:
return False
return True | python | train | qsol | codeparrot/apps | all |
Solve in Python:
=====Function Descriptions=====
Exceptions
Errors detected during execution are called exceptions.
Examples:
ZeroDivisionError
This error is raised when the second argument of a division or modulo operation is zero.
>>> a = '1'
>>> b = '0'
>>> print int(a) / int(b)
>>> ZeroDivisionError: integer division or modulo by zero
ValueError
This error is raised when a built-in operation or function receives an argument that has the right type but an inappropriate value.
>>> a = '1'
>>> b = '#'
>>> print int(a) / int(b)
>>> ValueError: invalid literal for int() with base 10: '#'
Handling Exceptions
The statements try and except can be used to handle selected exceptions. A try statement may have more than one except clause to specify handlers for different exceptions.
#Code
try:
print 1/0
except ZeroDivisionError as e:
print "Error Code:",e
Output
Error Code: integer division or modulo by zero
=====Problem Statement=====
You are given two values a and b.
Perform integer division and print a/b.
=====Input Format=====
The first line contains T, the number of test cases.
The next T lines each contain the space separated values of a and b.
=====Constraints=====
0<T<10
=====Output Format=====
Print the value of a/b.
In the case of ZeroDivisionError or ValueError, print the error code. | n = int(input())
for i in range(n):
a,b=input().split()
try:
print((int(a)//int(b)))
except Exception as e:
print(("Error Code: "+str(e))) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5a4331b18f27f2b31f000085:
In input string ```word```(1 word):
* replace the vowel with the nearest left consonant.
* replace the consonant with the nearest right vowel.
P.S. To complete this task imagine the alphabet is a circle (connect the first and last element of the array in the mind). For example, 'a' replace with 'z', 'y' with 'a', etc.(see below)
For example:
```
'codewars' => 'enedazuu'
'cat' => 'ezu'
'abcdtuvwxyz' => 'zeeeutaaaaa'
```
It is preloaded:
```
const alphabet = ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']
const consonants = ['b','c','d','f','g','h','j','k','l','m','n','p','q','r','s','t','v','w','x','y','z'];
const vowels = ['a','e','i','o','u'];
```
P.S. You work with lowercase letters only.
I tried it in Python, but could not do it. Can you solve it? | def replace_letters(word):
d = {'a':'z','b':'e','c':'e','d':'e','e':'d','f':'i','g':'i','h':'i','i':'h','j':'o','k':'o','l':'o','m':'o','n':'o','o':'n','p':'u','q':'u','r':'u','s':'u','t':'u','u':'t','v':'a','w':'a','x':'a','y':'a','z':'a'}
return ''.join([d[i] for i in list(word)]) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5b26047b9e40b9f4ec00002b:
With one die of 6 sides we will have six different possible results:``` 1, 2, 3, 4, 5, 6``` .
With 2 dice of six sides, we will have 36 different possible results:
```
(1,1),(1,2),(2,1),(1,3),(3,1),(1,4),(4,1),(1,5),
(5,1), (1,6),(6,1),(2,2),(2,3),(3,2),(2,4),(4,2),
(2,5),(5,2)(2,6),(6,2),(3,3),(3,4),(4,3),(3,5),(5,3),
(3,6),(6,3),(4,4),(4,5),(5,4),(4,6),(6,4),(5,5),
(5,6),(6,5),(6,6)
```
So, with 2 dice of 6 sides we get 36 different events.
```
([6,6] ---> 36)
```
But with 2 different dice we can get for this case, the same number of events.
One die of ```4 sides``` and another of ```9 sides``` will produce the exact amount of events.
```
([4,9] ---> 36)
```
We say that the dice set ```[4,9]``` is equivalent to ```[6,6]``` because both produce the same number of events.
Also we may have an amount of three dice producing the same amount of events. It will be for:
```
[4,3,3] ---> 36
```
(One die of 4 sides and two dice of 3 sides each)
Perhaps you may think that the following set is equivalent: ```[6,3,2]``` but unfortunately dice have a **minimum of three sides** (well, really a
tetrahedron with one empty side)
The task for this kata is to get the amount of equivalent dice sets, having **2 dice at least**,for a given set.
For example, for the previous case: [6,6] we will have 3 equivalent sets that are: ``` [4, 3, 3], [12, 3], [9, 4]``` .
You may assume that dice are available from 3 and above for any value up to an icosahedral die (20 sides).
```
[5,6,4] ---> 5 (they are [10, 4, 3], [8, 5, 3], [20, 6], [15, 8], [12, 10])
```
For the cases we cannot get any equivalent set the result will be `0`.
For example for the set `[3,3]` we will not have equivalent dice.
Range of inputs for Random Tests:
```
3 <= sides <= 15
2 <= dices <= 7
```
See examples in the corresponding box.
Enjoy it!!
I tried it in Python, but could not do it. Can you solve it? | from itertools import combinations_with_replacement
from numpy import prod
def eq_dice(set_):
lst = sorted(set_)
eq, dice, count = 0, [], prod(lst)
for sides in range(3, 21):
if count % sides == 0: dice.append(sides)
for num_dice in range(2, 8):
for c in combinations_with_replacement(dice, num_dice):
if prod(c) == count and sorted(c) != lst: eq += 1
return eq | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1181/C:
Innokenty works at a flea market and sells some random stuff rare items. Recently he found an old rectangular blanket. It turned out that the blanket is split in $n \cdot m$ colored pieces that form a rectangle with $n$ rows and $m$ columns.
The colored pieces attracted Innokenty's attention so he immediately came up with the following business plan. If he cuts out a subrectangle consisting of three colored stripes, he can sell it as a flag of some country. Innokenty decided that a subrectangle is similar enough to a flag of some country if it consists of three stripes of equal heights placed one above another, where each stripe consists of cells of equal color. Of course, the color of the top stripe must be different from the color of the middle stripe; and the color of the middle stripe must be different from the color of the bottom stripe.
Innokenty has not yet decided what part he will cut out, but he is sure that the flag's boundaries should go along grid lines. Also, Innokenty won't rotate the blanket. Please help Innokenty and count the number of different subrectangles Innokenty can cut out and sell as a flag. Two subrectangles located in different places but forming the same flag are still considered different. [Image] [Image] [Image]
These subrectangles are flags. [Image] [Image] [Image] [Image] [Image] [Image]
These subrectangles are not flags.
-----Input-----
The first line contains two integers $n$ and $m$ ($1 \le n, m \le 1\,000$) — the number of rows and the number of columns on the blanket.
Each of the next $n$ lines contains $m$ lowercase English letters from 'a' to 'z' and describes a row of the blanket. Equal letters correspond to equal colors, different letters correspond to different colors.
-----Output-----
In the only line print the number of subrectangles which form valid flags.
-----Examples-----
Input
4 3
aaa
bbb
ccb
ddd
Output
6
Input
6 1
a
a
b
b
c
c
Output
1
-----Note----- [Image] [Image]
The selected subrectangles are flags in the first example.
I tried it in Python, but could not do it. Can you solve it? | def countFlagNum(x):
y = 0
flagNum = 0
while y < m:
curColor = a[x][y]
colorCountByX = 1
isItFlag = True
while x + colorCountByX < n and a[x + colorCountByX][y] == curColor:
colorCountByX += 1
if not(x + colorCountByX * 3 > n):
color2 = a[x + colorCountByX][y]
color3 = a[x + colorCountByX * 2][y]
if color3 != color2 and color2 != curColor:
offY = 0
while y + offY < m and isItFlag:
i = 0
while i < colorCountByX and isItFlag:
if (a[x + i][y + offY] != curColor or a[x + colorCountByX + i][y + offY] != color2 or a[x + colorCountByX * 2 + i][y + offY] != color3):
isItFlag = False
if offY == 0:
offY = 1
i += 1
if isItFlag:
flagNum = flagNum + 1 + offY
offY += 1
y += offY - 1
y += 1
return flagNum
n, m = list(map(int, input().split()))
a = []
totalFlagNum = 0
for i in range(n):
row = input()
a.append(row)
for i in range(n - 2):
totalFlagNum += countFlagNum(i)
print(totalFlagNum) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Jett is tired after destroying the town and she wants to have a rest. She likes high places, that's why for having a rest she wants to get high and she decided to craft staircases.
A staircase is a squared figure that consists of square cells. Each staircase consists of an arbitrary number of stairs. If a staircase has $n$ stairs, then it is made of $n$ columns, the first column is $1$ cell high, the second column is $2$ cells high, $\ldots$, the $n$-th column if $n$ cells high. The lowest cells of all stairs must be in the same row.
A staircase with $n$ stairs is called nice, if it may be covered by $n$ disjoint squares made of cells. All squares should fully consist of cells of a staircase. This is how a nice covered staircase with $7$ stairs looks like: [Image]
Find out the maximal number of different nice staircases, that can be built, using no more than $x$ cells, in total. No cell can be used more than once.
-----Input-----
The first line contains a single integer $t$ $(1 \le t \le 1000)$ — the number of test cases.
The description of each test case contains a single integer $x$ $(1 \le x \le 10^{18})$ — the number of cells for building staircases.
-----Output-----
For each test case output a single integer — the number of different nice staircases, that can be built, using not more than $x$ cells, in total.
-----Example-----
Input
4
1
8
6
1000000000000000000
Output
1
2
1
30
-----Note-----
In the first test case, it is possible to build only one staircase, that consists of $1$ stair. It's nice. That's why the answer is $1$.
In the second test case, it is possible to build two different nice staircases: one consists of $1$ stair, and another consists of $3$ stairs. This will cost $7$ cells. In this case, there is one cell left, but it is not possible to use it for building any nice staircases, that have not been built yet. That's why the answer is $2$.
In the third test case, it is possible to build only one of two nice staircases: with $1$ stair or with $3$ stairs. In the first... | for _ in range(int(input())):
ans=0
n=int(input())
cp=1
while cp*(cp+1)//2<=n:
ans+=1
n-=cp*(cp+1)//2
cp=cp*2+1
print(ans) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5274e122fc75c0943d000148:
Finish the solution so that it takes an input `n` (integer) and returns a string that is the decimal representation of the number grouped by commas after every 3 digits.
Assume: `0 <= n < 2147483647`
## Examples
```
1 -> "1"
10 -> "10"
100 -> "100"
1000 -> "1,000"
10000 -> "10,000"
100000 -> "100,000"
1000000 -> "1,000,000"
35235235 -> "35,235,235"
```
I tried it in Python, but could not do it. Can you solve it? | def group_by_commas(n):
n = [i for i in str(n)]
for i in range(len(n) -4, -1, -3):
n.insert(i+1, ',')
return ''.join(n) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/problems/PINS:
Chef's company wants to make ATM PINs for its users, so that they could use the PINs for withdrawing their hard-earned money. One of these users is Reziba, who lives in an area where a lot of robberies take place when people try to withdraw their money.
Chef plans to include a safety feature in the PINs: if someone inputs the reverse of their own PIN in an ATM machine, the Crime Investigation Department (CID) are immediately informed and stop the robbery. However, even though this was implemented by Chef, some people could still continue to get robbed. The reason is that CID is only informed if the reverse of a PIN is different from that PIN (so that there wouldn't be false reports of robberies).
You know that a PIN consists of $N$ decimal digits. Find the probability that Reziba could get robbed. Specifically, it can be proven that this probability can be written as a fraction $P/Q$, where $P \ge 0$ and $Q > 0$ are coprime integers; you should compute $P$ and $Q$.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains a single integer $N$ denoting the length of each PIN.
-----Output-----
For each test case, print a single line containing two space-separated integers — the numerator $P$ and denominator $Q$ of the probability.
-----Constraints-----
- $1 \le T \le 100$
- $1 \le N \le 10^5$
-----Subtasks-----
Subtask #1 (10 points): $N \le 18$
Subtask #2 (20 points): $N \le 36$
Subtask #3 (70 points): original constraints
-----Example Input-----
1
1
-----Example Output-----
1 1
-----Explanation-----
Example case 1: A PIN containing only one number would fail to inform the CID, since when it's input in reverse, the ATM detects the same PIN as the correct one. Therefore, Reziba can always get robbed — the probability is $1 = 1/1$.
I tried it in Python, but could not do it. Can you solve it? | # cook your dish here
for _ in range(int(input())):
n=int(input())
q="1"+"0"*(n//2)
print(1,q) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1023/A:
You are given two strings $s$ and $t$. The string $s$ consists of lowercase Latin letters and at most one wildcard character '*', the string $t$ consists only of lowercase Latin letters. The length of the string $s$ equals $n$, the length of the string $t$ equals $m$.
The wildcard character '*' in the string $s$ (if any) can be replaced with an arbitrary sequence (possibly empty) of lowercase Latin letters. No other character of $s$ can be replaced with anything. If it is possible to replace a wildcard character '*' in $s$ to obtain a string $t$, then the string $t$ matches the pattern $s$.
For example, if $s=$"aba*aba" then the following strings match it "abaaba", "abacaba" and "abazzzaba", but the following strings do not match: "ababa", "abcaaba", "codeforces", "aba1aba", "aba?aba".
If the given string $t$ matches the given string $s$, print "YES", otherwise print "NO".
-----Input-----
The first line contains two integers $n$ and $m$ ($1 \le n, m \le 2 \cdot 10^5$) — the length of the string $s$ and the length of the string $t$, respectively.
The second line contains string $s$ of length $n$, which consists of lowercase Latin letters and at most one wildcard character '*'.
The third line contains string $t$ of length $m$, which consists only of lowercase Latin letters.
-----Output-----
Print "YES" (without quotes), if you can obtain the string $t$ from the string $s$. Otherwise print "NO" (without quotes).
-----Examples-----
Input
6 10
code*s
codeforces
Output
YES
Input
6 5
vk*cup
vkcup
Output
YES
Input
1 1
v
k
Output
NO
Input
9 6
gfgf*gfgf
gfgfgf
Output
NO
-----Note-----
In the first example a wildcard character '*' can be replaced with a string "force". So the string $s$ after this replacement is "codeforces" and the answer is "YES".
In the second example a wildcard character '*' can be replaced with an empty string. So the string $s$ after this replacement is "vkcup" and the answer is "YES".
There is no wildcard character '*' in the third example and the strings "v" and "k" are...
I tried it in Python, but could not do it. Can you solve it? | n, m = (int(x) for x in input().split())
a = input()
b = input()
if '*' not in a:
if a == b:
print('YES')
else:
print('NO')
quit()
l, r = a.split('*')
if len(b) >= len(a) - 1:
if l == b[:len(l)] and r == b[len(b) - len(r):]:
print('YES')
else:
print('NO')
else:
print('NO') | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Having two standards for a keypad layout is inconvenient!
Computer keypad's layout:
Cell phone keypad's layout:
Solve the horror of unstandartized keypads by providing a function that converts computer input to a number as if it was typed by a phone.
Example:
"789" -> "123"
Notes:
You get a string with numbers only | def computer_to_phone(numbers):
return "".join([str({0:0, 1:7, 2:8, 3:9, 4:4, 5:5, 6:6, 7:1, 8:2, 9:3}[int(n)]) for n in numbers]) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Chef has gone shopping with his 5-year old son. They have bought N items so far. The items are numbered from 1 to N, and the item i weighs Wi grams.
Chef's son insists on helping his father in carrying the items. He wants his dad to give him a few items. Chef does not want to burden his son. But he won't stop bothering him unless he is given a few items to carry. So Chef decides to give him some items. Obviously, Chef wants to give the kid less weight to carry.
However, his son is a smart kid. To avoid being given the bare minimum weight to carry, he suggests that the items are split into two groups, and one group contains exactly K items. Then Chef will carry the heavier group, and his son will carry the other group.
Help the Chef in deciding which items should the son take. Your task will be simple. Tell the Chef the maximum possible difference between the weight carried by him and the weight carried by the kid.
-----Input:-----
The first line of input contains an integer T, denoting the number of test cases. Then T test cases follow. The first line of each test contains two space-separated integers N and K. The next line contains N space-separated integers W1, W2, ..., WN.
-----Output:-----
For each test case, output the maximum possible difference between the weights carried by both in grams.
-----Constraints:-----
- 1 ≤ T ≤ 100
- 1 ≤ K < N ≤ 100
- 1 ≤ Wi ≤ 100000 (105)
-----Example:-----
Input:
2
5 2
8 4 5 2 10
8 3
1 1 1 1 1 1 1 1
Output:
17
2
-----Explanation:-----
Case #1: The optimal way is that Chef gives his son K=2 items with weights 2 and 4. Chef carries the rest of the items himself. Thus the difference is: (8+5+10) − (4+2) = 23 − 6 = 17.
Case #2: Chef gives his son 3 items and he carries 5 items himself. | # cook your dish here
for _ in range(int(input())):
n,k = map(int,input().split())
w = list(map(int,input().split()))
w.sort()
if k <= n//2:
s1 = sum(w[:k])
s2 = sum(w[k:])
print(abs(s2 - s1))
else:
s1 = sum(w[n-k:])
s2 = sum(w[:n-k])
print(abs(s2-s1)) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/545a4c5a61aa4c6916000755:
As a part of this Kata, you need to create a function that when provided with a triplet, returns the index of the numerical element that lies between the other two elements.
The input to the function will be an array of three distinct numbers (Haskell: a tuple).
For example:
gimme([2, 3, 1]) => 0
*2* is the number that fits between *1* and *3* and the index of *2* in the input array is *0*.
Another example (just to make sure it is clear):
gimme([5, 10, 14]) => 1
*10* is the number that fits between *5* and *14* and the index of *10* in the input array is *1*.
I tried it in Python, but could not do it. Can you solve it? | def gimme(arr):
sort = sorted(arr)
arr.index(sort[1])
return arr.index(sort[1]) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/586d9a71aa0428466d00001c:
Happy traveller [Part 1]
There is a play grid NxN; Always square!
0 1 2 3
0 [o, o, o, X]
1 [o, o, o, o]
2 [o, o, o, o]
3 [o, o, o, o]
You start from a random point. I mean, you are given the coordinates of your start position in format (row, col).
And your TASK is to define the number of unique paths to reach position X (always in the top right corner).
From any point you can go only UP or RIGHT.
Implement a function count_paths(N, (row, col)) which returns int;
Assume input params are always valid.
Example:
count_paths(1, (0, 0))
grid 1x1:
[X]
You are already in the target point, so return 0
count_paths(2, (1, 0))
grid 2x2:
[o, X]
[@, o]
You are at point @; you can move UP-RIGHT or RIGHT-UP, and there are 2 possible unique paths here
count_paths(2, (1, 1))
grid 2x2:
[o, X]
[o, @]
You are at point @; you can move only UP, so there is 1 possible unique path here
count_paths(3, (1, 0))
grid 3x3:
[o, o, X]
[@, o, o]
[o, o, o]
You are at point @; you can move UP-RIGHT-RIGHT or RIGHT-UP-RIGHT, or RIGHT-RIGHT-UP, and there are 3 possible unique paths here
I think it's pretty clear =)
btw. you can use preloaded Grid class, which constructs 2d array for you. It's very very basic and simple. You can use numpy instead or any other way to produce the correct answer =)
grid = Grid(2, 2, 0)
samegrid = Grid.square(2) will give you a grid[2][2], which you can print easily to console.
print(grid)
[0, 0]
[0, 0]
Enjoy!
You can continue adventures:
Happy traveller [Part 2]
I tried it in Python, but could not do it. Can you solve it? | from math import factorial as f
count_paths=lambda n,c:f(c[0]+abs(n-c[1]-1))//(f(abs(n-c[1]-1))*f(c[0])) if n!=1 else 0 | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Dima loves representing an odd number as the sum of multiple primes, and Lisa loves it when there are at most three primes. Help them to represent the given number as the sum of at most than three primes.
More formally, you are given an odd numer n. Find a set of numbers p_{i} (1 ≤ i ≤ k), such that
1 ≤ k ≤ 3
p_{i} is a prime
$\sum_{i = 1}^{k} p_{i} = n$
The numbers p_{i} do not necessarily have to be distinct. It is guaranteed that at least one possible solution exists.
-----Input-----
The single line contains an odd number n (3 ≤ n < 10^9).
-----Output-----
In the first line print k (1 ≤ k ≤ 3), showing how many numbers are in the representation you found.
In the second line print numbers p_{i} in any order. If there are multiple possible solutions, you can print any of them.
-----Examples-----
Input
27
Output
3
5 11 11
-----Note-----
A prime is an integer strictly larger than one that is divisible only by one and by itself. | def prime(i):
for k in range(2, int(i**0.5)+1):
if i%k == 0:
return False
return True
x = int(input())
if prime(x):
print(1)
print(x)
quit()
i = x
while not prime(i):
i -= 2
p1000 = [i for i in range(2, 3000) if prime(i)]
rem = x - i
if rem == 2:
print(2)
print(2, i)
quit()
print(3)
for jj in p1000:
if rem-jj in p1000:
print(i, jj, rem-jj)
quit() | python | test | qsol | codeparrot/apps | all |
Solve in Python:
In the city of Saint Petersburg, a day lasts for $2^{100}$ minutes. From the main station of Saint Petersburg, a train departs after $1$ minute, $4$ minutes, $16$ minutes, and so on; in other words, the train departs at time $4^k$ for each integer $k \geq 0$. Team BowWow has arrived at the station at the time $s$ and it is trying to count how many trains have they missed; in other words, the number of trains that have departed strictly before time $s$. For example if $s = 20$, then they missed trains which have departed at $1$, $4$ and $16$. As you are the only one who knows the time, help them!
Note that the number $s$ will be given you in a binary representation without leading zeroes.
-----Input-----
The first line contains a single binary number $s$ ($0 \leq s < 2^{100}$) without leading zeroes.
-----Output-----
Output a single number — the number of trains which have departed strictly before the time $s$.
-----Examples-----
Input
100000000
Output
4
Input
101
Output
2
Input
10100
Output
3
-----Note-----
In the first example $100000000_2 = 256_{10}$, missed trains have departed at $1$, $4$, $16$ and $64$.
In the second example $101_2 = 5_{10}$, trains have departed at $1$ and $4$.
The third example is explained in the statements. | s = int(input(), base=2)
#print(s)
k = 0
ans = 0
while 4 ** k < s:
ans += 1
k += 1
print(ans) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/749/C:
There are n employees in Alternative Cake Manufacturing (ACM). They are now voting on some very important question and the leading world media are trying to predict the outcome of the vote.
Each of the employees belongs to one of two fractions: depublicans or remocrats, and these two fractions have opposite opinions on what should be the outcome of the vote. The voting procedure is rather complicated: Each of n employees makes a statement. They make statements one by one starting from employees 1 and finishing with employee n. If at the moment when it's time for the i-th employee to make a statement he no longer has the right to vote, he just skips his turn (and no longer takes part in this voting). When employee makes a statement, he can do nothing or declare that one of the other employees no longer has a right to vote. It's allowed to deny from voting people who already made the statement or people who are only waiting to do so. If someone is denied from voting he no longer participates in the voting till the very end. When all employees are done with their statements, the procedure repeats: again, each employees starting from 1 and finishing with n who are still eligible to vote make their statements. The process repeats until there is only one employee eligible to vote remaining and he determines the outcome of the whole voting. Of course, he votes for the decision suitable for his fraction.
You know the order employees are going to vote and that they behave optimal (and they also know the order and who belongs to which fraction). Predict the outcome of the vote.
-----Input-----
The first line of the input contains a single integer n (1 ≤ n ≤ 200 000) — the number of employees.
The next line contains n characters. The i-th character is 'D' if the i-th employee is from depublicans fraction or 'R' if he is from remocrats.
-----Output-----
Print 'D' if the outcome of the vote will be suitable for depublicans and 'R' if remocrats will...
I tried it in Python, but could not do it. Can you solve it? | n = int(input())
s = input()
dd = 0
dr = 0
while len(s) > 1:
t = ''
cd = 0
cr = 0
for c in s:
if c == 'D':
if dd > 0:
dd -= 1
else:
dr += 1
t += c
cd += 1
else:
if dr > 0:
dr -= 1
else:
dd += 1
t += c
cr += 1
s = t
if cd == 0:
s = 'R'
break
if cr == 0:
s = 'D'
break
if dd >= cd:
s = 'R'
break
if dr >= cr:
s = 'D'
break
print(s[0]) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1121/B:
Mike decided to teach programming to children in an elementary school. He knows that it is not an easy task to interest children in that age to code. That is why he decided to give each child two sweets.
Mike has $n$ sweets with sizes $a_1, a_2, \ldots, a_n$. All his sweets have different sizes. That is, there is no such pair $(i, j)$ ($1 \leq i, j \leq n$) such that $i \ne j$ and $a_i = a_j$.
Since Mike has taught for many years, he knows that if he gives two sweets with sizes $a_i$ and $a_j$ to one child and $a_k$ and $a_p$ to another, where $(a_i + a_j) \neq (a_k + a_p)$, then a child who has a smaller sum of sizes will be upset. That is, if there are two children who have different sums of sweets, then one of them will be upset. Apparently, Mike does not want somebody to be upset.
Mike wants to invite children giving each of them two sweets. Obviously, he can't give one sweet to two or more children. His goal is to invite as many children as he can.
Since Mike is busy preparing to his first lecture in the elementary school, he is asking you to find the maximum number of children he can invite giving each of them two sweets in such way that nobody will be upset.
-----Input-----
The first line contains one integer $n$ ($2 \leq n \leq 1\,000$) — the number of sweets Mike has.
The second line contains $n$ integers $a_1, a_2, \ldots, a_n$ ($1 \leq a_i \leq 10^5$) — the sizes of the sweets. It is guaranteed that all integers are distinct.
-----Output-----
Print one integer — the maximum number of children Mike can invite giving each of them two sweets in such way that nobody will be upset.
-----Examples-----
Input
8
1 8 3 11 4 9 2 7
Output
3
Input
7
3 1 7 11 9 2 12
Output
2
-----Note-----
In the first example, Mike can give $9+2=11$ to one child, $8+3=11$ to another one, and $7+4=11$ to the third child. Therefore, Mike can invite three children. Note that it is not the only solution.
In the second example, Mike can give $3+9=12$ to one child and $1+11$ to another one. Therefore, Mike can...
I tried it in Python, but could not do it. Can you solve it? | n = int(input())
a = list(map(int, input().split()))
sm = []
for i in range(n):
for j in range(i + 1, n):
sm.append(a[i] + a[j])
cnt = dict()
ans = 0
for i in sm:
if i not in cnt.keys():
cnt[i] = 1
else:
cnt[i] += 1
for i in sm:
ans = max(cnt[i], ans)
print(ans) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1368/A:
Leo has developed a new programming language C+=. In C+=, integer variables can only be changed with a "+=" operation that adds the right-hand side value to the left-hand side variable. For example, performing "a += b" when a = $2$, b = $3$ changes the value of a to $5$ (the value of b does not change).
In a prototype program Leo has two integer variables a and b, initialized with some positive values. He can perform any number of operations "a += b" or "b += a". Leo wants to test handling large integers, so he wants to make the value of either a or b strictly greater than a given value $n$. What is the smallest number of operations he has to perform?
-----Input-----
The first line contains a single integer $T$ ($1 \leq T \leq 100$) — the number of test cases.
Each of the following $T$ lines describes a single test case, and contains three integers $a, b, n$ ($1 \leq a, b \leq n \leq 10^9$) — initial values of a and b, and the value one of the variables has to exceed, respectively.
-----Output-----
For each test case print a single integer — the smallest number of operations needed. Separate answers with line breaks.
-----Example-----
Input
2
1 2 3
5 4 100
Output
2
7
-----Note-----
In the first case we cannot make a variable exceed $3$ in one operation. One way of achieving this in two operations is to perform "b += a" twice.
I tried it in Python, but could not do it. Can you solve it? | for _ in range(int(input())):
a, b, n = list(map(int, input().split()))
ans = 0
a, b = min(a,b), max(a,b)
while b <= n:
a, b = b, a+b
ans += 1
print(ans) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
If n is the numerator and d the denominator of a fraction, that fraction is defined a (reduced) proper fraction if and only if GCD(n,d)==1.
For example `5/16` is a proper fraction, while `6/16` is not, as both 6 and 16 are divisible by 2, thus the fraction can be reduced to `3/8`.
Now, if you consider a given number d, how many proper fractions can be built using d as a denominator?
For example, let's assume that d is 15: you can build a total of 8 different proper fractions between 0 and 1 with it: 1/15, 2/15, 4/15, 7/15, 8/15, 11/15, 13/15 and 14/15.
You are to build a function that computes how many proper fractions you can build with a given denominator:
```python
proper_fractions(1)==0
proper_fractions(2)==1
proper_fractions(5)==4
proper_fractions(15)==8
proper_fractions(25)==20
```
Be ready to handle big numbers.
Edit: to be extra precise, the term should be "reduced" fractions, thanks to [girianshiido](http://www.codewars.com/users/girianshiido) for pointing this out and sorry for the use of an improper word :) | def proper_fractions(n):
phi = n > 1 and n
for p in range(2, int(n ** 0.5) + 1):
if not n % p:
phi -= phi // p
while not n % p:
n //= p
if n > 1: phi -= phi // n
return phi | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/573b216f5328ffcd710013b3:
###BACKGROUND:
Jacob recently decided to get healthy and lose some weight. He did a lot of reading and research and after focusing on steady exercise and a healthy diet for several months, was able to shed over 50 pounds! Now he wants to share his success, and has decided to tell his friends and family how much weight they could expect to lose if they used the same plan he followed.
Lots of people are really excited about Jacob's program and they want to know how much weight they would lose if they followed his plan. Unfortunately, he's really bad at math, so he's turned to you to help write a program that will calculate the expected weight loss for a particular person, given their weight and how long they think they want to continue the plan.
###TECHNICAL DETAILS:
Jacob's weight loss protocol, if followed closely, yields loss according to a simple formulae, depending on gender. Men can expect to lose 1.5% of their current body weight each week they stay on plan. Women can expect to lose 1.2%. (Children are advised to eat whatever they want, and make sure to play outside as much as they can!)
###TASK:
Write a function that takes as input:
```
- The person's gender ('M' or 'F');
- Their current weight (in pounds);
- How long they want to stay true to the protocol (in weeks);
```
and then returns the expected weight at the end of the program.
###NOTES:
Weights (both input and output) should be decimals, rounded to the nearest tenth.
Duration (input) should be a whole number (integer). If it is not, the function should round to the nearest whole number.
When doing input parameter validity checks, evaluate them in order or your code will not pass final tests.
I tried it in Python, but could not do it. Can you solve it? | def lose_weight(gender, weight, duration):
if gender not in ('M','F'):
return 'Invalid gender'
if weight <= 0:
return 'Invalid weight'
if duration <= 0:
return 'Invalid duration'
factor = 0.985 if gender == 'M' else 0.988
expected_weight = factor**duration * weight
return round(expected_weight,1) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc097/tasks/abc097_a:
Three people, A, B and C, are trying to communicate using transceivers.
They are standing along a number line, and the coordinates of A, B and C are a, b and c (in meters), respectively.
Two people can directly communicate when the distance between them is at most d meters.
Determine if A and C can communicate, either directly or indirectly.
Here, A and C can indirectly communicate when A and B can directly communicate and also B and C can directly communicate.
-----Constraints-----
- 1 ≤ a,b,c ≤ 100
- 1 ≤ d ≤ 100
- All values in input are integers.
-----Input-----
Input is given from Standard Input in the following format:
a b c d
-----Output-----
If A and C can communicate, print Yes; if they cannot, print No.
-----Sample Input-----
4 7 9 3
-----Sample Output-----
Yes
A and B can directly communicate, and also B and C can directly communicate, so we should print Yes.
I tried it in Python, but could not do it. Can you solve it? | a, b, c, d = map(int, input().split())
if abs(a-c) <= d:
print("Yes")
elif abs(a-b) <= d and abs(b-c) <= d:
print("Yes")
else:
print("No") | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/900/D:
Count the number of distinct sequences a_1, a_2, ..., a_{n} (1 ≤ a_{i}) consisting of positive integers such that gcd(a_1, a_2, ..., a_{n}) = x and $\sum_{i = 1}^{n} a_{i} = y$. As this number could be large, print the answer modulo 10^9 + 7.
gcd here means the greatest common divisor.
-----Input-----
The only line contains two positive integers x and y (1 ≤ x, y ≤ 10^9).
-----Output-----
Print the number of such sequences modulo 10^9 + 7.
-----Examples-----
Input
3 9
Output
3
Input
5 8
Output
0
-----Note-----
There are three suitable sequences in the first test: (3, 3, 3), (3, 6), (6, 3).
There are no suitable sequences in the second test.
I tried it in Python, but could not do it. Can you solve it? | import math
lectura=lambda:map (int,input().split())
x,y=lectura()
mod= 1000000007
if (y%x!=0):
print("0")
else:
y= y//x
setPrueba=set()
for i in range(1, int(math.sqrt(y) + 1)):
if (y%i==0):
setPrueba.add(i)
setPrueba.add(y// i)
setPrueba=sorted(list(setPrueba))
setOrdenado= setPrueba.copy()
for i in range(len(setOrdenado)):
#setOrdenado[i] = math.pow(2, setPrueba[i] - 1)
setOrdenado[i]=pow(2, setPrueba[i] - 1, mod)
for j in range(i):
if setPrueba[i]% setPrueba[j]==0:
setOrdenado[i]-= setOrdenado[j]
print(setOrdenado[len(setOrdenado)-1] % mod) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
The Fibonacci numbers are the numbers in the following integer sequence (Fn):
>0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, ...
such as
>F(n) = F(n-1) + F(n-2) with F(0) = 0 and F(1) = 1.
Given a number, say prod (for product), we search two Fibonacci numbers F(n) and F(n+1) verifying
>F(n) * F(n+1) = prod.
Your function productFib takes an integer (prod) and returns
an array:
```
[F(n), F(n+1), true] or {F(n), F(n+1), 1} or (F(n), F(n+1), True)
```
depending on the language if F(n) * F(n+1) = prod.
If you don't find two consecutive F(m) verifying `F(m) * F(m+1) = prod`you will return
```
[F(m), F(m+1), false] or {F(n), F(n+1), 0} or (F(n), F(n+1), False)
```
F(m) being the smallest one such as `F(m) * F(m+1) > prod`.
### Some Examples of Return:
(depend on the language)
```
productFib(714) # should return (21, 34, true),
# since F(8) = 21, F(9) = 34 and 714 = 21 * 34
productFib(800) # should return (34, 55, false),
# since F(8) = 21, F(9) = 34, F(10) = 55 and 21 * 34 < 800 < 34 * 55
-----
productFib(714) # should return [21, 34, true],
productFib(800) # should return [34, 55, false],
-----
productFib(714) # should return {21, 34, 1},
productFib(800) # should return {34, 55, 0},
-----
productFib(714) # should return {21, 34, true},
productFib(800) # should return {34, 55, false},
```
### Note:
- You can see examples for your language in "Sample Tests". | def productFib(prod):
a, b = 0, 1
while a*b < prod:
a, b = b, a+b
return [a, b, a*b == prod] | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/57675f3dedc6f728ee000256:
Build Tower Advanced
---
Build Tower by the following given arguments:
__number of floors__ (integer and always greater than 0)
__block size__ (width, height) (integer pair and always greater than (0, 0))
Tower block unit is represented as `*`
* Python: return a `list`;
* JavaScript: returns an `Array`;
Have fun!
***
for example, a tower of 3 floors with block size = (2, 3) looks like below
```
[
' ** ',
' ** ',
' ** ',
' ****** ',
' ****** ',
' ****** ',
'**********',
'**********',
'**********'
]
```
and a tower of 6 floors with block size = (2, 1) looks like below
```
[
' ** ',
' ****** ',
' ********** ',
' ************** ',
' ****************** ',
'**********************'
]
```
***
Go take a look at [Build Tower](https://www.codewars.com/kata/576757b1df89ecf5bd00073b) which is a more basic version :)
I tried it in Python, but could not do it. Can you solve it? | def tower_builder(n_floors, block_size):
w, h = block_size
filled_block = '*' * w
empty_block = ' ' * w
tower = []
for n in range(1,n_floors+1):
for _ in range(h):
tower.append( empty_block * (n_floors - n) + filled_block * (2*n -1) + empty_block * (n_floors - n))
return tower | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/689/A:
While swimming at the beach, Mike has accidentally dropped his cellphone into the water. There was no worry as he bought a cheap replacement phone with an old-fashioned keyboard. The keyboard has only ten digital equal-sized keys, located in the following way: [Image]
Together with his old phone, he lost all his contacts and now he can only remember the way his fingers moved when he put some number in. One can formally consider finger movements as a sequence of vectors connecting centers of keys pressed consecutively to put in a number. For example, the finger movements for number "586" are the same as finger movements for number "253": [Image] [Image]
Mike has already put in a number by his "finger memory" and started calling it, so he is now worrying, can he be sure that he is calling the correct number? In other words, is there any other number, that has the same finger movements?
-----Input-----
The first line of the input contains the only integer n (1 ≤ n ≤ 9) — the number of digits in the phone number that Mike put in.
The second line contains the string consisting of n digits (characters from '0' to '9') representing the number that Mike put in.
-----Output-----
If there is no other phone number with the same finger movements and Mike can be sure he is calling the correct number, print "YES" (without quotes) in the only line.
Otherwise print "NO" (without quotes) in the first line.
-----Examples-----
Input
3
586
Output
NO
Input
2
09
Output
NO
Input
9
123456789
Output
YES
Input
3
911
Output
YES
-----Note-----
You can find the picture clarifying the first sample case in the statement above.
I tried it in Python, but could not do it. Can you solve it? | n = int(input())
l = [41, 10, 11, 12, 20, 21, 22, 30, 31, 32]
s = list([l[int(x)] for x in input()])
def exists_up(s):
l1 = [x - 10 for x in s]
return all(x in l for x in l1)
def exists_down(s):
l1 = [x + 10 for x in s]
return all(x in l for x in l1)
def exists_left(s):
l1 = [x - 1 for x in s]
return all(x in l for x in l1)
def exists_right(s):
l1 = [x + 1 for x in s]
return all(x in l for x in l1)
if exists_up(s) or exists_down(s) or exists_left(s) or exists_right(s):
print("NO")
else:
print("YES") | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Given an integer n, count the total number of digit 1 appearing in all non-negative integers less than or equal to n.
Example:
Input: 13
Output: 6
Explanation: Digit 1 occurred in the following numbers: 1, 10, 11, 12, 13. | class Solution:
def countDigitOne(self, n):
ones, wei = 0, 1
while wei <= n:
m = int(n / wei) % 10 # 求某位数字
if m > 1:
ones += (int(n / wei / 10) + 1) * wei
elif m == 1:
ones += (int(n / wei / 10)) * wei + (n % wei) + 1
else:
ones += (int(n / wei / 10)) * wei
wei *= 10
return int(ones) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Polycarpus just has been out of luck lately! As soon as he found a job in the "Binary Cat" cafe, the club got burgled. All ice-cream was stolen.
On the burglary night Polycarpus kept a careful record of all club visitors. Each time a visitor entered the club, Polycarpus put down character "+" in his notes. Similarly, each time a visitor left the club, Polycarpus put character "-" in his notes. We know that all cases of going in and out happened consecutively, that is, no two events happened at the same time. Polycarpus doesn't remember whether there was somebody in the club at the moment when his shift begun and at the moment when it ended.
Right now the police wonders what minimum number of distinct people Polycarpus could have seen. Assume that he sees anybody coming in or out of the club. Each person could have come in or out an arbitrary number of times.
-----Input-----
The only line of the input contains a sequence of characters "+" and "-", the characters are written one after another without any separators. The characters are written in the order, in which the corresponding events occurred. The given sequence has length from 1 to 300 characters, inclusive.
-----Output-----
Print the sought minimum number of people
-----Examples-----
Input
+-+-+
Output
1
Input
---
Output
3 | s=input()
s=list(s)
s1=[0]*len(s)
for i in range(len(s)):
if s[i]=='-':
s[i]=1
s1[i]=-1
else:
s[i]=-1
s1[i]=1
def kadane(s):
maxi=-1
curr=0
for i in s:
curr=max(curr+i,i)
maxi=max(maxi,curr)
return maxi
print(max(kadane(s),kadane(s1)))
#@print(kadane(s)) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1312/A:
You are given two integers $n$ and $m$ ($m < n$). Consider a convex regular polygon of $n$ vertices. Recall that a regular polygon is a polygon that is equiangular (all angles are equal in measure) and equilateral (all sides have the same length). [Image] Examples of convex regular polygons
Your task is to say if it is possible to build another convex regular polygon with $m$ vertices such that its center coincides with the center of the initial polygon and each of its vertices is some vertex of the initial polygon.
You have to answer $t$ independent test cases.
-----Input-----
The first line of the input contains one integer $t$ ($1 \le t \le 10^4$) — the number of test cases.
The next $t$ lines describe test cases. Each test case is given as two space-separated integers $n$ and $m$ ($3 \le m < n \le 100$) — the number of vertices in the initial polygon and the number of vertices in the polygon you want to build.
-----Output-----
For each test case, print the answer — "YES" (without quotes), if it is possible to build another convex regular polygon with $m$ vertices such that its center coincides with the center of the initial polygon and each of its vertices is some vertex of the initial polygon and "NO" otherwise.
-----Example-----
Input
2
6 3
7 3
Output
YES
NO
-----Note----- $0$ The first test case of the example
It can be shown that the answer for the second test case of the example is "NO".
I tried it in Python, but could not do it. Can you solve it? | t = int(input())
for i in range(t):
n,m = list(map(int, input().split()))
if n % m == 0:
print ("YES")
else:
print ("NO") | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Zonal Computing Olympiad 2013, 10 Nov 2012
N teams participate in a league cricket tournament on Mars, where each pair of distinct teams plays each other exactly once. Thus, there are a total of (N × (N-1))/2 matches. An expert has assigned a strength to each team, a positive integer. Strangely, the Martian crowds love one-sided matches and the advertising revenue earned from a match is the absolute value of the difference between the strengths of the two matches. Given the strengths of the N teams, find the total advertising revenue earned from all the matches.
For example, suppose N is 4 and the team strengths for teams 1, 2, 3, and 4 are 3, 10, 3, and 5 respectively. Then the advertising revenues from the 6 matches are as follows:
Match Team A Team B Ad revenue 1 1 2 7 2 1 3 0 3 1 4 2 4 2 3 7 5 2 4 5 6 3 4 2
Thus the total advertising revenue is 23.
-----Input format-----
Line 1 : A single integer, N.
Line 2 : N space-separated integers, the strengths of the N teams.
-----Output format-----
A single integer, the total advertising revenue from the tournament.
-----Sample input-----
4
3 10 3 5
-----Sample output-----
23
-----Test data-----
In all subtasks, the strength of each team is an integer between 1 and 1,000 inclusive.
- Subtask 1 (30 marks) : 2 ≤ N ≤ 1,000.
- Subtask 2 (70 marks) : 2 ≤ N ≤ 200,000.
-----Live evaluation data-----
- Subtask 1: Testcases 0,1,2.
- Subtask 2: Testcases 3,4,5.
-----Note-----
The answer might not fit in a variable of type int. We recommend that type long long be used for computing all advertising revenues. If you use printf and scanf, you can use %lld for long long. | teams=int(input())
strength=list(map(int, input().split()))
rev=0
for i in range(0,teams):
for j in range(i+1,teams):
if(strength[i]>strength[j]):
rev+=strength[i]-strength[j]
else:
rev+=strength[j]-strength[i]
print(rev) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/CMYC2020/problems/BTENG:
Voritex a big data scientist collected huge amount of big data having structure of two rows and n columns.
Voritex is storing all the valid data for manipulations and pressing invalid command when data not satisfying the constraints.
Voritex likes brute force method and he calls it as BT, he decided to run newly created BT engine for getting good result.
At each instance when BT engine is outputting a newly created number Voritex calls it as BT number.
Voritex is daydreaming and thinking that his engine is extremely optimised and will get an interview call in which he will be explaining the structure of BT engine and which will be staring from 1 and simultaneously performing $i$-th xor operation with $i$ and previously(one step before) obtained BT number.
BT engine is outputting the $K$-th highest BT number in the first $N$ natural numbers.
Chef : (thinking) I also want to create BT engine……..
-----Input:-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of T test cases follows.
- The line of each test case contains a two integers $N$ and $K$.
-----Output:-----
For each test case, print a single line containing integer $K$-th highest number else print -$1$ when invalid command pressed.
-----Constraints-----
- $1 \leq T \leq 100000$
- $1 \leq N \leq 100000$
- $1 \leq K \leq N$
-----Sample Input:-----
2
4 2
5 5
-----Sample Output:-----
2
0
-----EXPLANATION:-----
For first valid constraints generating output as 0 2 1 5
1^1 first BT number is 0
2^0 second BT number is 2
3^2 third BT number is 1
4^1 fourth BT number is 5
Hence the answer is 2.
I tried it in Python, but could not do it. Can you solve it? | import math
import os
import random
import re
import sys
r = 100000
prev = 1
s = set()
for i in range(1, r+1):
now = i ^ prev
s.add(now)
prev = now
s = list(s)
t = int(input())
while t > 0:
t -= 1
n, k = list(map(int, input().split()))
if n > 3:
if n % 2 == 0:
size = (n//2) + 2
else:
size = ((n-1)//2) + 2
else:
size = n
if size - k >= 0:
print(s[size-k])
else:
print(-1) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Some time ago Mister B detected a strange signal from the space, which he started to study.
After some transformation the signal turned out to be a permutation p of length n or its cyclic shift. For the further investigation Mister B need some basis, that's why he decided to choose cyclic shift of this permutation which has the minimum possible deviation.
Let's define the deviation of a permutation p as $\sum_{i = 1}^{i = n}|p [ i ] - i|$.
Find a cyclic shift of permutation p with minimum possible deviation. If there are multiple solutions, print any of them.
Let's denote id k (0 ≤ k < n) of a cyclic shift of permutation p as the number of right shifts needed to reach this shift, for example:
k = 0: shift p_1, p_2, ... p_{n}, k = 1: shift p_{n}, p_1, ... p_{n} - 1, ..., k = n - 1: shift p_2, p_3, ... p_{n}, p_1.
-----Input-----
First line contains single integer n (2 ≤ n ≤ 10^6) — the length of the permutation.
The second line contains n space-separated integers p_1, p_2, ..., p_{n} (1 ≤ p_{i} ≤ n) — the elements of the permutation. It is guaranteed that all elements are distinct.
-----Output-----
Print two integers: the minimum deviation of cyclic shifts of permutation p and the id of such shift. If there are multiple solutions, print any of them.
-----Examples-----
Input
3
1 2 3
Output
0 0
Input
3
2 3 1
Output
0 1
Input
3
3 2 1
Output
2 1
-----Note-----
In the first sample test the given permutation p is the identity permutation, that's why its deviation equals to 0, the shift id equals to 0 as well.
In the second sample test the deviation of p equals to 4, the deviation of the 1-st cyclic shift (1, 2, 3) equals to 0, the deviation of the 2-nd cyclic shift (3, 1, 2) equals to 4, the optimal is the 1-st cyclic shift.
In the third sample test the deviation of p equals to 4, the deviation of the 1-st cyclic shift (1, 3, 2) equals to 2, the deviation of the 2-nd cyclic shift (2, 1, 3) also equals to 2, so the optimal are both 1-st and 2-nd cyclic shifts. | n = int(input())
a = list(map(int, input().split()))
t = [0] * 2 * n
s = 0
for i in range(n):
d = a[i] - i - 1
s += abs(d)
if d > 0: t[d] += 1
p = sum(t)
r = (s, 0)
for i in range(1, n):
d = a[n - i] - 1
s += d - p << 1
t[d + i] += d > 0
p += (d > 0) - t[i]
if s < r[0]: r = (s, i)
print(*r) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Soon a school Olympiad in Informatics will be held in Berland, n schoolchildren will participate there.
At a meeting of the jury of the Olympiad it was decided that each of the n participants, depending on the results, will get a diploma of the first, second or third degree. Thus, each student will receive exactly one diploma.
They also decided that there must be given at least min_1 and at most max_1 diplomas of the first degree, at least min_2 and at most max_2 diplomas of the second degree, and at least min_3 and at most max_3 diplomas of the third degree.
After some discussion it was decided to choose from all the options of distributing diplomas satisfying these limitations the one that maximizes the number of participants who receive diplomas of the first degree. Of all these options they select the one which maximizes the number of the participants who receive diplomas of the second degree. If there are multiple of these options, they select the option that maximizes the number of diplomas of the third degree.
Choosing the best option of distributing certificates was entrusted to Ilya, one of the best programmers of Berland. However, he found more important things to do, so it is your task now to choose the best option of distributing of diplomas, based on the described limitations.
It is guaranteed that the described limitations are such that there is a way to choose such an option of distributing diplomas that all n participants of the Olympiad will receive a diploma of some degree.
-----Input-----
The first line of the input contains a single integer n (3 ≤ n ≤ 3·10^6) — the number of schoolchildren who will participate in the Olympiad.
The next line of the input contains two integers min_1 and max_1 (1 ≤ min_1 ≤ max_1 ≤ 10^6) — the minimum and maximum limits on the number of diplomas of the first degree that can be distributed.
The third line of the input contains two integers min_2 and max_2 (1 ≤ min_2 ≤ max_2 ≤ 10^6) — the minimum and maximum limits on the number of diplomas of the... | n=int(input())
min1,max1=map(int,input().split())
min2,max2=map(int,input().split())
min3,max3=map(int,input().split())
ans1=min1
ans2=min2
ans3=min3
n-=min1+min2+min3
max1=max1-min1
max2=max2-min2
max3=max3-min3
while n>0:
if max1>0:
if max1>=n:
ans1+=n
max1-=n
n=0
else:
n=n-max1
ans1+=max1
max1=0
elif max2>0:
if max2>=n:
ans2+=n
max2-=n
n=0
else:
n=n-max2
ans2+=max2
max2=0
elif max3>0:
if max3>=n:
ans3+=n
max3-=n
n=0
else:
n=n-max3
ans3+=max3
max3=0
print(ans1,ans2,ans3) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Polycarp is a frequent user of the very popular messenger. He's chatting with his friends all the time. He has $n$ friends, numbered from $1$ to $n$.
Recall that a permutation of size $n$ is an array of size $n$ such that each integer from $1$ to $n$ occurs exactly once in this array.
So his recent chat list can be represented with a permutation $p$ of size $n$. $p_1$ is the most recent friend Polycarp talked to, $p_2$ is the second most recent and so on.
Initially, Polycarp's recent chat list $p$ looks like $1, 2, \dots, n$ (in other words, it is an identity permutation).
After that he receives $m$ messages, the $j$-th message comes from the friend $a_j$. And that causes friend $a_j$ to move to the first position in a permutation, shifting everyone between the first position and the current position of $a_j$ by $1$. Note that if the friend $a_j$ is in the first position already then nothing happens.
For example, let the recent chat list be $p = [4, 1, 5, 3, 2]$: if he gets messaged by friend $3$, then $p$ becomes $[3, 4, 1, 5, 2]$; if he gets messaged by friend $4$, then $p$ doesn't change $[4, 1, 5, 3, 2]$; if he gets messaged by friend $2$, then $p$ becomes $[2, 4, 1, 5, 3]$.
For each friend consider all position he has been at in the beginning and after receiving each message. Polycarp wants to know what were the minimum and the maximum positions.
-----Input-----
The first line contains two integers $n$ and $m$ ($1 \le n, m \le 3 \cdot 10^5$) — the number of Polycarp's friends and the number of received messages, respectively.
The second line contains $m$ integers $a_1, a_2, \dots, a_m$ ($1 \le a_i \le n$) — the descriptions of the received messages.
-----Output-----
Print $n$ pairs of integers. For each friend output the minimum and the maximum positions he has been in the beginning and after receiving each message.
-----Examples-----
Input
5 4
3 5 1 4
Output
1 3
2 5
1 4
1 5
1 5
Input
4 3
1 2 4
Output
1 3
1 2
3 4
1 4
-----Note-----
In the first example, Polycarp's recent chat... | #!usr/bin/env python3
import sys
import bisect
def LI(): return [int(x) for x in sys.stdin.readline().split()]
mod = 1000000007
def solve():
def add(i,x):
while i < len(bit):
bit[i] += x
i += i&-i
def sum(i):
res = 0
while i > 0:
res += bit[i]
i -= i&-i
return res
n,m = LI()
a = LI()
bit = [0]*(n+m+2)
MIN = [i+1 for i in range(n)]
MAX = [i+1 for i in range(n)]
f = [i+m+1 for i in range(n)]
for i in range(n):
add(f[i],1)
M = m
for i in range(m):
ai = a[i]-1
MIN[ai] = 1
index = sum(f[ai])
if MAX[ai] < index:
MAX[ai] = index
add(M,1)
add(f[ai],-1)
f[ai] = M
M -= 1
for i in range(n):
index = sum(f[i])
if MAX[i] < index:
MAX[i] = index
for i in range(n):
print(MIN[i],MAX[i])
return
#Solve
def __starting_point():
solve()
__starting_point() | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5a59e029145c46eaac000062:
### Please also check out other katas in [Domino Tiling series](https://www.codewars.com/collections/5d19554d13dba80026a74ff5)!
---
# Task
A domino is a rectangular block with `2` units wide and `1` unit high. A domino can be placed on a grid in two ways: horizontal or vertical.
```
## or #
#
```
You have infinitely many dominoes, and you want to fill a board that is `N` units wide and `2` units high:
```
<--- N --->
###############
###############
```
The task is to find **the number of ways** you can fill the given grid with dominoes.
# The Twist
However, you can quickly find that the answer is exactly the Fibonacci series (and yeah, CW has already too many of them), so here is a twist:
Now you have infinite supply of dominoes in `K` colors, and you have to fill the given grid **without any two adjacent dominoes having the same color**. Two dominoes are adjacent if they share an edge.
A valid filling of a 2 x 10 board with three colors could be as follows (note that two same-colored dominoes can share a point):
```
1131223312
2231332212
```
Since the answer will be very large, please give your answer **modulo 12345787**.
# Examples
```python
# K == 1: only one color
two_by_n(1, 1) == 1
two_by_n(3, 1) == 0
# K == 2: two colors
two_by_n(1, 2) == 2
two_by_n(4, 2) == 4
two_by_n(7, 2) == 2
# K == 3: three colors
two_by_n(1, 3) == 3
two_by_n(2, 3) == 12
two_by_n(5, 3) == 168 # yes, the numbers grow quite quickly
# You must handle big values
two_by_n(10, 5) == 7802599
two_by_n(20, 10) == 4137177
```
# Constraints
`1 <= N <= 10000`
`1 <= K <= 100`
All inputs are valid integers.
I tried it in Python, but could not do it. Can you solve it? | def two_by_n(n, k):
mod = 12345787
if n == 0: return 0
elif n == 1: return k
d = [k, 0, k * (k - 1), k * (k - 1)]
for i in range(3, n + 1):
x, y, z, w = d
d = [z, w, (z * (k - 1) + w * (k - 2)) % mod, (x * (k - 1) * (k - 2) + y * ((k - 2)**2 + k - 1)) % mod]
return (d[2] + d[3]) % mod | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/smallest-range-i/:
Given an array A of integers, for each integer A[i] we may choose any x with -K <= x <= K, and add x to A[i].
After this process, we have some array B.
Return the smallest possible difference between the maximum value of B and the minimum value of B.
Example 1:
Input: A = [1], K = 0
Output: 0
Explanation: B = [1]
Example 2:
Input: A = [0,10], K = 2
Output: 6
Explanation: B = [2,8]
Example 3:
Input: A = [1,3,6], K = 3
Output: 0
Explanation: B = [3,3,3] or B = [4,4,4]
Note:
1 <= A.length <= 10000
0 <= A[i] <= 10000
0 <= K <= 10000
I tried it in Python, but could not do it. Can you solve it? | class Solution:
def smallestRangeI(self, a: List[int], k: int) -> int:
a = sorted(a)
a[-1] = max(a)
a[0]= min(a)
#print(a,a[-1])
if a[-1]-k<=(a[0]+k):
return 0
return (a[-1]-k)-(a[0]+k) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
If you have not ever heard the term **Arithmetic Progrossion**, refer to:
http://www.codewars.com/kata/find-the-missing-term-in-an-arithmetic-progression/python
And here is an unordered version. Try if you can survive lists of **MASSIVE** numbers (which means time limit should be considered). :D
Note: Don't be afraid that the minimum or the maximum element in the list is missing, e.g. [4, 6, 3, 5, 2] is missing 1 or 7, but this case is excluded from the kata.
Example:
```python
find([3, 9, 1, 11, 13, 5]) # => 7
``` | find=lambda s:(min(s)+max(s))*-~len(s)/2-sum(s) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Lucas numbers are numbers in a sequence defined like this:
```
L(0) = 2
L(1) = 1
L(n) = L(n-1) + L(n-2)
```
Your mission is to complete the function that returns the `n`th term of this sequence.
**Note:** It should work for negative numbers as well; how you do this is you flip the equation around, so for negative numbers: `L(n) = L(n+2) - L(n+1)`
## Examples
```
L(-10) = 123
L(-5) = -11
L(-1) = -1
L(0) = 2
L(1) = 1
L(5) = 11
L(10) = 123
``` | def lucasnum(n):
if n == 1:
return 1
elif n == 0:
return 2
elif n > 1:
a, b = 2, 1
for i in range(n):
a, b = b, a+b
return a
else:
a, b = -1, -2
for i in range(abs(n)):
a, b = b, a-b
return b*-1 | python | train | qsol | codeparrot/apps | all |
Solve in Python:
# Connect Four
Take a look at wiki description of Connect Four game:
[Wiki Connect Four](https://en.wikipedia.org/wiki/Connect_Four)
The grid is 6 row by 7 columns, those being named from A to G.
You will receive a list of strings showing the order of the pieces which dropped in columns:
```python
pieces_position_list = ["A_Red",
"B_Yellow",
"A_Red",
"B_Yellow",
"A_Red",
"B_Yellow",
"G_Red",
"B_Yellow"]
```
The list may contain up to 42 moves and shows the order the players are playing.
The first player who connects four items of the same color is the winner.
You should return "Yellow", "Red" or "Draw" accordingly. | import numpy as np
from scipy.signal import convolve2d
def who_is_winner(pieces_position_list):
arr = np.zeros((7,6), int)
for a in pieces_position_list:
pos, color = a.split('_')
pos = ord(pos) - ord('A')
val = (-1,1)[color == 'Red']
arr[pos, np.argmin(arr[pos] != 0)] = val
t_arr = val * arr
if any(np.max(cv) == 4 for cv in (convolve2d(t_arr, [[1,1,1,1]], 'same'),
convolve2d(t_arr, [[1],[1],[1],[1]], 'same'),
convolve2d(t_arr, [[1,0,0,0], [0,1,0,0], [0,0,1,0], [0,0,0,1]], 'same'),
convolve2d(t_arr, [[0,0,0,1], [0,0,1,0], [0,1,0,0], [1,0,0,0]], 'same'))):
return color
return 'Draw' | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/58b497914c5d0af407000049:
The business has been suffering for years under the watch of Homie the Clown. Every time there is a push to production it requires 500 hands-on deck, a massive manual process, and the fire department is on stand-by along with Fire Marshall Bill the king of manual configuration management. He is called a Fire Marshall because production pushes often burst into flames and rollbacks are a hazard.
The business demands change and as such has hired a new leader who wants to convert it all to DevOps…..there is a new Sheriff in town.
The Sheriff's first order of business is to build a DevOps team. He likes Microservices, Cloud, Open-Source, and wants to push to production 9500 times per day without even pressing a button, beautiful seamless immutable infrastructure properly baked in the Continuous Delivery oven is the goal.
The only problem is Homie the Clown along with Legacy Pete are grandfathered in and union, they started out in the era of green screens and punch cards and are set in their ways. They are not paid by an outcome but instead are measured by the amount of infrastructure under management and total staff headcount.
The Sheriff has hired a new team of DevOps Engineers. They advocate Open Source, Cloud, and never doing a manual task more than one time. They believe Operations to be a first class citizen with Development and are preparing to shake things up within the company.
Since Legacy is not going away, yet, the Sheriff's job is to get everyone to cooperate so DevOps and the Cloud will be standard. The New Kids on the Block have just started work and are looking to build common services with Legacy Pete and Homie the Clown.
```
Every Time the NKOTB propose a DevOps pattern……
Homie stands up and says "Homie don't Play that!"
IE:
NKOTB Say -> "We need Cloud now!"
Homie Say -> "Cloud! Homie dont play that!"
NKOTB Say -> "We need Automation now!"
Homie Say -> "Automation! Homie dont play that!"
NKOTB Say -> "We need Microservices now!"
Homie Say -> "Microservices! Homie dont play...
I tried it in Python, but could not do it. Can you solve it? | TOTAL = '{} monitoring objections, {} automation, {} deployment pipeline, {} cloud, and {} microservices.'
def nkotb_vs_homie(r):
result = []
for req in sum(r, []):
homie = req.split()[2].title()
result.append(f'{homie}! Homie dont play that!')
result.append(TOTAL.format(*map(len, r)))
return result | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/733/D:
Kostya is a genial sculptor, he has an idea: to carve a marble sculpture in the shape of a sphere. Kostya has a friend Zahar who works at a career. Zahar knows about Kostya's idea and wants to present him a rectangular parallelepiped of marble from which he can carve the sphere.
Zahar has n stones which are rectangular parallelepipeds. The edges sizes of the i-th of them are a_{i}, b_{i} and c_{i}. He can take no more than two stones and present them to Kostya.
If Zahar takes two stones, he should glue them together on one of the faces in order to get a new piece of rectangular parallelepiped of marble. Thus, it is possible to glue a pair of stones together if and only if two faces on which they are glued together match as rectangles. In such gluing it is allowed to rotate and flip the stones in any way.
Help Zahar choose such a present so that Kostya can carve a sphere of the maximum possible volume and present it to Zahar.
-----Input-----
The first line contains the integer n (1 ≤ n ≤ 10^5).
n lines follow, in the i-th of which there are three integers a_{i}, b_{i} and c_{i} (1 ≤ a_{i}, b_{i}, c_{i} ≤ 10^9) — the lengths of edges of the i-th stone. Note, that two stones may have exactly the same sizes, but they still will be considered two different stones.
-----Output-----
In the first line print k (1 ≤ k ≤ 2) the number of stones which Zahar has chosen. In the second line print k distinct integers from 1 to n — the numbers of stones which Zahar needs to choose. Consider that stones are numbered from 1 to n in the order as they are given in the input data.
You can print the stones in arbitrary order. If there are several answers print any of them.
-----Examples-----
Input
6
5 5 5
3 2 4
1 4 1
2 1 3
3 2 4
3 3 4
Output
1
1
Input
7
10 7 8
5 10 3
4 2 6
5 5 5
10 2 8
4 2 1
7 7 7
Output
2
1 5
-----Note-----
In the first example we can connect the pairs of stones: 2 and 4, the size of the parallelepiped: 3 × 2 × 5, the radius of the inscribed sphere 1 2 and 5, the size of the...
I tried it in Python, but could not do it. Can you solve it? | def main():
from collections import defaultdict
d, m = defaultdict(list), 0
for i in range(1, int(input()) + 1):
a, b, c = sorted(map(int, input().split()))
d[b, c].append((a, i))
for (a, b), l in list(d.items()):
if len(l) > 1:
l.sort()
c, i = l[-1]
x, j = l[-2]
c += x
else:
c, i = l[0]
j = 0
if a > m < c:
m, res = a if a < c else c, (i, j)
print(("2\n%d %d" % res) if res[1] else ("1\n%d" % res[0]))
def __starting_point():
main()
__starting_point() | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5f120a13e63b6a0016f1c9d5:
Let's say we have a number, `num`. Find the number of values of `n` such that: there exists `n` consecutive **positive** values that sum up to `num`. A positive number is `> 0`. `n` can also be 1.
```python
#Examples
num = 1
#1
return 1
num = 15
#15, (7, 8), (4, 5, 6), (1, 2, 3, 4, 5)
return 4
num = 48
#48, (15, 16, 17)
return 2
num = 97
#97, (48, 49)
return 2
```
The upper limit is `$10^8$`
I tried it in Python, but could not do it. Can you solve it? | def consecutive_sum(num):
count = 0
N = 1
while( N * (N + 1) < 2 * num):
a = (num - (N * (N + 1) ) / 2) / (N + 1)
if (a - int(a) == 0.0):
count += 1
N += 1
return count+1 | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/problems/PERPALIN:
Chef recently learned about concept of periodicity of strings. A string is said to have a period P, if P divides N and for each i, the i-th of character of the string is same as i-Pth character (provided it exists), e.g. "abab" has a period P = 2, It also has a period of P = 4, but it doesn't have a period of 1 or 3.
Chef wants to construct a string of length N that is a palindrome and has a period P. It's guaranteed that N is divisible by P. This string can only contain character 'a' or 'b'. Chef doesn't like the strings that contain all a's or all b's.
Given the values of N, P, can you construct one such palindromic string that Chef likes? If it's impossible to do so, output "impossible" (without quotes)
-----Input-----
The first line of the input contains an integer T denoting the number of test cases.
The only line of each test case contains two space separated integers N, P.
-----Output-----
For each test case, output a single line containing the answer of the problem, i.e. the valid string if it exists otherwise "impossible" (without quotes). If there are more than possible answers, you can output any.
-----Constraints-----
- 1 ≤ T ≤ 20
- 1 ≤ P, N ≤ 105
-----Subtasks-----
- Subtask #1 (25 points) : P = N
- Subtask #2 (75 points) : No additional constraints
-----Example-----
Input
5
3 1
2 2
3 3
4 4
6 3
Output
impossible
impossible
aba
abba
abaaba
-----Explanation-----
Example 1: The only strings possible are either aaa or bbb, which Chef doesn't like. So, the answer is impossible.
Example 2: There are four possible strings, aa, ab, ba, bb. Only aa and bb are palindromic, but Chef doesn't like these strings. Hence, the answer is impossible.
Example 4: The string abba is a palindrome and has a period of 4.
Example 5: The string abaaba is a palindrome and has a period of length 3.
I tried it in Python, but could not do it. Can you solve it? | t = int(input())
while(t>0):
t-=1
n,p = [int(x) for x in input().split()]
if(p <= 2):
print('impossible')
continue
else:
s = 'a' + ('b'*(p-2)) + 'a'
s = s*int(n/p)
print(s) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/812/A:
Sagheer is walking in the street when he comes to an intersection of two roads. Each road can be represented as two parts where each part has 3 lanes getting into the intersection (one for each direction) and 3 lanes getting out of the intersection, so we have 4 parts in total. Each part has 4 lights, one for each lane getting into the intersection (l — left, s — straight, r — right) and a light p for a pedestrian crossing. [Image]
An accident is possible if a car can hit a pedestrian. This can happen if the light of a pedestrian crossing of some part and the light of a lane that can get to or from that same part are green at the same time.
Now, Sagheer is monitoring the configuration of the traffic lights. Your task is to help him detect whether an accident is possible.
-----Input-----
The input consists of four lines with each line describing a road part given in a counter-clockwise order.
Each line contains four integers l, s, r, p — for the left, straight, right and pedestrian lights, respectively. The possible values are 0 for red light and 1 for green light.
-----Output-----
On a single line, print "YES" if an accident is possible, and "NO" otherwise.
-----Examples-----
Input
1 0 0 1
0 1 0 0
0 0 1 0
0 0 0 1
Output
YES
Input
0 1 1 0
1 0 1 0
1 1 0 0
0 0 0 1
Output
NO
Input
1 0 0 0
0 0 0 1
0 0 0 0
1 0 1 0
Output
NO
-----Note-----
In the first example, some accidents are possible because cars of part 1 can hit pedestrians of parts 1 and 4. Also, cars of parts 2 and 3 can hit pedestrians of part 4.
In the second example, no car can pass the pedestrian crossing of part 4 which is the only green pedestrian light. So, no accident can occur.
I tried it in Python, but could not do it. Can you solve it? | def booly(s):
return bool(int(s))
a = [None]*4;
a[0] = list(map(booly, input().split()))
a[1] = list(map(booly, input().split()))
a[2] = list(map(booly, input().split()))
a[3] =list( map(booly, input().split()))
acc = False
for i in range(4):
if (a[i][3] and (a[i][0] or a[i][1] or a[i][2])):
acc = True
if (a[i][3] and a[(i+1)%4][0]):
acc = True
if (a[i][3] and a[(i-1)%4][2]):
acc = True
if (a[i][3] and a[(i+2)%4][1]):
acc = True
if acc:
print("YES");
else:
print("NO"); | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/550527b108b86f700000073f:
The aim of the kata is to try to show how difficult it can be to calculate decimals of an irrational number with a certain precision. We have chosen to get a few decimals of the number "pi" using
the following infinite series (Leibniz 1646–1716):
PI / 4 = 1 - 1/3 + 1/5 - 1/7 + ... which gives an approximation of PI / 4.
http://en.wikipedia.org/wiki/Leibniz_formula_for_%CF%80
To have a measure of the difficulty we will count how many iterations are needed to calculate PI with a given precision.
There are several ways to determine the precision of the calculus but to keep things easy we will calculate to within epsilon of your language Math::PI constant. In other words we will stop the iterative process when the absolute value of the difference between our calculation and the Math::PI constant of the given language is less than epsilon.
Your function returns an array or an arrayList or a string or a tuple depending on the language (See sample tests) where your approximation of PI has 10 decimals
In Haskell you can use the function "trunc10Dble" (see "Your solution"); in Clojure you can use the function "round" (see "Your solution");in OCaml or Rust the function "rnd10" (see "Your solution") in order to avoid discussions about the result.
Example :
```
your function calculates 1000 iterations and 3.140592653839794 but returns:
iter_pi(0.001) --> [1000, 3.1405926538]
```
Unfortunately, this series converges too slowly to be useful,
as it takes over 300 terms to obtain a 2 decimal place precision.
To obtain 100 decimal places of PI, it was calculated that
one would need to use at least 10^50 terms of this expansion!
About PI : http://www.geom.uiuc.edu/~huberty/math5337/groupe/expresspi.html
I tried it in Python, but could not do it. Can you solve it? | import math
def iter_pi(epsilon):
pi,k = 0,0
while abs(pi-math.pi/4) > epsilon/4:
pi += (-1)**k * 1/(2*k + 1)
k += 1
pi *=4
return [k , round(pi, 10)] | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Most football fans love it for the goals and excitement. Well, this Kata doesn't.
You are to handle the referee's little notebook and count the players who were sent off for fouls and misbehavior.
The rules:
Two teams, named "A" and "B" have 11 players each; players on each team are numbered from 1 to 11.
Any player may be sent off the field by being given a red card.
A player can also receive a yellow warning card, which is fine, but if he receives another yellow card, he is sent off immediately (no need for a red card in that case).
If one of the teams has less than 7 players remaining, the game is stopped immediately by the referee, and the team with less than 7 players loses.
A `card` is a string with the team's letter ('A' or 'B'), player's number, and card's color ('Y' or 'R') - all concatenated and capitalized.
e.g the card `'B7Y'` means player #7 from team B received a yellow card.
The task: Given a list of cards (could be empty), return the number of remaining players on each team at the end of the game (as a tuple of 2 integers, team "A" first).
If the game was terminated by the referee for insufficient number of players, you are to stop the game immediately, and ignore any further possible cards.
Note for the random tests: If a player that has already been sent off receives another card - ignore it. | def men_still_standing(cards):
players, Y, R = {"A": 11, "B": 11}, set(), set()
for c in cards:
if c[:-1] not in R:
players[c[0]] -= c[-1] == "R" or c[:-1] in Y
if 6 in players.values():
break
(R if (c[-1] == "R" or c[:-1] in Y) else Y).add(c[:-1])
return players["A"], players["B"] | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/644/B:
In this problem you have to simulate the workflow of one-thread server. There are n queries to process, the i-th will be received at moment t_{i} and needs to be processed for d_{i} units of time. All t_{i} are guaranteed to be distinct.
When a query appears server may react in three possible ways: If server is free and query queue is empty, then server immediately starts to process this query. If server is busy and there are less than b queries in the queue, then new query is added to the end of the queue. If server is busy and there are already b queries pending in the queue, then new query is just rejected and will never be processed.
As soon as server finished to process some query, it picks new one from the queue (if it's not empty, of course). If a new query comes at some moment x, and the server finishes to process another query at exactly the same moment, we consider that first query is picked from the queue and only then new query appears.
For each query find the moment when the server will finish to process it or print -1 if this query will be rejected.
-----Input-----
The first line of the input contains two integers n and b (1 ≤ n, b ≤ 200 000) — the number of queries and the maximum possible size of the query queue.
Then follow n lines with queries descriptions (in chronological order). Each description consists of two integers t_{i} and d_{i} (1 ≤ t_{i}, d_{i} ≤ 10^9), where t_{i} is the moment of time when the i-th query appears and d_{i} is the time server needs to process it. It is guaranteed that t_{i} - 1 < t_{i} for all i > 1.
-----Output-----
Print the sequence of n integers e_1, e_2, ..., e_{n}, where e_{i} is the moment the server will finish to process the i-th query (queries are numbered in the order they appear in the input) or - 1 if the corresponding query will be rejected.
-----Examples-----
Input
5 1
2 9
4 8
10 9
15 2
19 1
Output
11 19 -1 21 22
Input
4 1
2 8
4 8
10 9
15 2
Output
10 18 27 -1
-----Note-----
Consider the first sample. The server will...
I tried it in Python, but could not do it. Can you solve it? | import collections
class TaskMgr:
def __init__(self, n, b):
self.b = b
self.lock_until = 1
self.q = collections.deque()
self.end = [-1 for i in range(n)]
def empty(self):
return len(self.q) == 0
def full(self):
return len(self.q) == self.b
def add(self, i, t, d):
if self.full(): return
self.q.append( (i, t, d) )
def tick(self, now=None):
if self.empty(): return
lock = self.lock_until
now = now or lock
if now < lock: return
now = min(lock, now)
i, t, d = self.q.popleft()
t = max(t, now)
end = t + d
self.lock_until = end
self.end[i] = end
def __starting_point():
n, b = [int(x) for x in input().split()]
mgr = TaskMgr(n, b)
for i in range(n):
t, d = [int(x) for x in input().split()]
mgr.tick(t)
mgr.add(i, t, d)
while not mgr.empty(): mgr.tick()
print(' '.join(str(x) for x in mgr.end))
__starting_point() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Good job! Now that Heidi is able to distinguish between Poisson and uniform distributions, she is in a good position to actually estimate the populations.
Can you help Heidi estimate each village's population?
-----Input-----
Same as the easy version.
-----Output-----
Output one line per village, in the same order as provided in the input, containing your (integer) population estimate.
Your answer is considered correct if it is an integer that falls into the interval $[ \lfloor 0.95 \cdot P \rfloor, \lceil 1.05 \cdot P \rceil ]$, where P is the real population of the village, used to create the distribution (either Poisson or uniform) from which the marmots drew their answers. | def sampleVariance(V):
X = sum(V) / len(V)
S = 0.0
for x in V:
S += (X-x)**2
S /= (len(V)-1)
return (X, S)
#That awkward moment when you realized that variance is sigma^2 but you just took the stat course this semester
for i in range(int(input())):
V = list(map(int, input().split()))
X, S = sampleVariance(V)
v1 = X
v2 = (2*X) ** 2 / 12
if abs(v1-S) < abs(v2-S):
print(int(X))
else:
print(max(V)+min(V) // 2) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/575690ee34a34efb37001796:
Your task is to write function which takes string and list of delimiters as an input and returns list of strings/characters after splitting given string.
Example:
```python
multiple_split('Hi, how are you?', [' ']) => ['Hi,', 'how', 'are', 'you?']
multiple_split('1+2-3', ['+', '-']) => ['1', '2', '3']
```
List of delimiters is optional and can be empty, so take that into account.
Important note: Result cannot contain empty string.
I tried it in Python, but could not do it. Can you solve it? | def multiple_split(string, delimiters=[]):
print(string, delimiters)
if not string: return [] # trivial case
if not delimiters: return [string] # trivial case
for d in delimiters[1:]:
string = string.replace(d, delimiters[0])
tokens = string.split(delimiters[0])
return [t for t in tokens if t] | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/701/B:
Vasya has the square chessboard of size n × n and m rooks. Initially the chessboard is empty. Vasya will consequently put the rooks on the board one after another.
The cell of the field is under rook's attack, if there is at least one rook located in the same row or in the same column with this cell. If there is a rook located in the cell, this cell is also under attack.
You are given the positions of the board where Vasya will put rooks. For each rook you have to determine the number of cells which are not under attack after Vasya puts it on the board.
-----Input-----
The first line of the input contains two integers n and m (1 ≤ n ≤ 100 000, 1 ≤ m ≤ min(100 000, n^2)) — the size of the board and the number of rooks.
Each of the next m lines contains integers x_{i} and y_{i} (1 ≤ x_{i}, y_{i} ≤ n) — the number of the row and the number of the column where Vasya will put the i-th rook. Vasya puts rooks on the board in the order they appear in the input. It is guaranteed that any cell will contain no more than one rook.
-----Output-----
Print m integer, the i-th of them should be equal to the number of cells that are not under attack after first i rooks are put.
-----Examples-----
Input
3 3
1 1
3 1
2 2
Output
4 2 0
Input
5 2
1 5
5 1
Output
16 9
Input
100000 1
300 400
Output
9999800001
-----Note-----
On the picture below show the state of the board after put each of the three rooks. The cells which painted with grey color is not under the attack.
[Image]
I tried it in Python, but could not do it. Can you solve it? | N, M = map(int, input().split())
r = N
c = N
R = [False for i in range(N)]
C = R.copy()
for i in range(M):
a, b = map(int, input().split())
if not R[a-1]:
r -= 1
R[a-1] = True
if not C[b-1]:
c -= 1
C[b-1] = True
print(r*c, end=' ') | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/59ca8e8e1a68b7de740001f4:
Given two arrays of strings, return the number of times each string of the second array appears in the first array.
#### Example
```python
array1 = ['abc', 'abc', 'xyz', 'cde', 'uvw']
array2 = ['abc', 'cde', 'uap']
```
How many times do the elements in `array2` appear in `array1`?
* `'abc'` appears twice in the first array (2)
* `'cde'` appears only once (1)
* `'uap'` does not appear in the first array (0)
Therefore, `solve(array1, array2) = [2, 1, 0]`
Good luck!
If you like this Kata, please try:
[Word values](https://www.codewars.com/kata/598d91785d4ce3ec4f000018)
[Non-even substrings](https://www.codewars.com/kata/59da47fa27ee00a8b90000b4)
I tried it in Python, but could not do it. Can you solve it? | def solve(first,second):
return [first.count(word) for word in second] | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Given a rational number n
``` n >= 0, with denominator strictly positive```
- as a string (example: "2/3" in Ruby, Python, Clojure, JS, CS, Go)
- or as two strings (example: "2" "3" in Haskell, Java, CSharp, C++, Swift)
- or as a rational or decimal number (example: 3/4, 0.67 in R)
- or two integers (Fortran)
decompose
this number as a sum of rationals with numerators equal to one and without repetitions
(2/3 = 1/2 + 1/6 is correct but not 2/3 = 1/3 + 1/3, 1/3 is repeated).
The algorithm must be "greedy", so at each stage the new rational obtained in the decomposition must have a denominator as small as possible.
In this manner the sum of a few fractions in the decomposition gives a rather good approximation of the rational to decompose.
2/3 = 1/3 + 1/3 doesn't fit because of the repetition but also because the first 1/3 has a denominator bigger than the one in 1/2
in the decomposition 2/3 = 1/2 + 1/6.
### Example:
(You can see other examples in "Sample Tests:")
```
decompose("21/23") or "21" "23" or 21/23 should return
["1/2", "1/3", "1/13", "1/359", "1/644046"] in Ruby, Python, Clojure, JS, CS, Haskell, Go
"[1/2, 1/3, 1/13, 1/359, 1/644046]" in Java, CSharp, C++
"1/2,1/3,1/13,1/359,1/644046" in C, Swift, R
```
### Notes
1) The decomposition of 21/23 as
```
21/23 = 1/2 + 1/3 + 1/13 + 1/598 + 1/897
```
is exact but don't fulfill our requirement because 598 is bigger than 359.
Same for
```
21/23 = 1/2 + 1/3 + 1/23 + 1/46 + 1/69 (23 is bigger than 13)
or
21/23 = 1/2 + 1/3 + 1/15 + 1/110 + 1/253 (15 is bigger than 13).
```
2) The rational given to decompose could be greater than one or equal to one, in which case the first "fraction" will be an integer
(with an implicit denominator of 1).
3) If the numerator parses to zero the function "decompose" returns [] (or "".
4) The number could also be a decimal which can be expressed as a rational.
examples:
`0.6` in Ruby, Python, Clojure,JS, CS, Julia, Go
`"66" "100"` in Haskell, Java, CSharp, C++, C, Swift, Scala,... | from fractions import Fraction
from math import ceil
def decompose(n):
n = Fraction(n)
lst, n = [int(n)], n - int(n)
while n > 0:
next_denom = int(ceil(1/n))
lst.append(next_denom)
n -= Fraction(1, next_denom)
return ([] if lst[0] == 0 else [str(lst[0])]) + ["1/{}".format(d) for d in lst[1:]] | python | train | qsol | codeparrot/apps | all |
Solve in Python:
## Task
Given a positive integer as input, return the output as a string in the following format:
Each element, corresponding to a digit of the number, multiplied by a power of 10 in such a way that with the sum of these elements you can obtain the original number.
## Examples
Input | Output
--- | ---
0 | ""
56 | "5\*10+6"
60 | "6\*10"
999 | "9\*100+9\*10+9"
10004 | "1\*10000+4"
Note: `input >= 0` | def simplify(n):
return "+".join([f"{x}*{10**i}" if i else x for i, x in enumerate(str(n)[::-1]) if x != "0"][::-1]) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
10^{10^{10}} participants, including Takahashi, competed in two programming contests.
In each contest, all participants had distinct ranks from first through 10^{10^{10}}-th.
The score of a participant is the product of his/her ranks in the two contests.
Process the following Q queries:
- In the i-th query, you are given two positive integers A_i and B_i. Assuming that Takahashi was ranked A_i-th in the first contest and B_i-th in the second contest, find the maximum possible number of participants whose scores are smaller than Takahashi's.
-----Constraints-----
- 1 \leq Q \leq 100
- 1\leq A_i,B_i\leq 10^9(1\leq i\leq Q)
- All values in input are integers.
-----Input-----
Input is given from Standard Input in the following format:
Q
A_1 B_1
:
A_Q B_Q
-----Output-----
For each query, print the maximum possible number of participants whose scores are smaller than Takahashi's.
-----Sample Input-----
8
1 4
10 5
3 3
4 11
8 9
22 40
8 36
314159265 358979323
-----Sample Output-----
1
12
4
11
14
57
31
671644785
Let us denote a participant who was ranked x-th in the first contest and y-th in the second contest as (x,y).
In the first query, (2,1) is a possible candidate of a participant whose score is smaller than Takahashi's. There are never two or more participants whose scores are smaller than Takahashi's, so we should print 1. | n = int(input())
for i in range(n):
a,b= list(map(int, input().split( )))
#解説参照
#何度見ても解けない
#どちらかは高橋君より良い
#a<=bとする
#a=b (1~a-1)*2
#a<b c**2<abとする
#c(c+1)>=ab?2c-2 / 2c-1
if a==b:
print((2*a-2))
else:
c = int((a*b)**(1/2))
if c*c==a*b:
c -= 1
if c*c+c>=a*b:
print((2*c-2))
else:
print((2*c-1)) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
You are given a sequence of N$N$ powers of an integer k$k$; let's denote the i$i$-th of these powers by kAi$k^{A_i}$. You should partition this sequence into two non-empty contiguous subsequences; each element of the original sequence should appear in exactly one of these subsequences. In addition, the product of (the sum of elements of the left subsequence) and (the sum of elements of the right subsequence) should be maximum possible.
Find the smallest position at which you should split this sequence in such a way that this product is maximized.
-----Input-----
- The first line of the input contains a single integer T$T$ denoting the number of test cases. The description of T$T$ test cases follows.
- The first line of each test case contains two space-separated integers N$N$ and k$k$.
- The second line contains N$N$ space-separated integers A1,A2,…,AN$A_1, A_2, \dots, A_N$.
-----Output-----
For each test case, print a single line containing one integer — the size of the left subsequence. If there is more than one possible answer, print the smallest possible one.
-----Constraints-----
- 1≤T≤10$1 \le T \le 10$
- 2≤N≤105$2 \le N \le 10^5$
- 2≤k≤109$2 \le k \le 10^9$
- 0≤Ai≤105$0 \le A_i \le 10^5$ for each valid i$i$
-----Subtasks-----
Subtask #1 (30 points):
- 2≤N≤1,000$2 \le N \le 1,000$
- 0≤Ai≤1,000$0 \le A_i \le 1,000$ for each valid i$i$
Subtask #2 (70 points): original constraints
-----Example Input-----
1
5 2
1 1 3 3 5
-----Example Output-----
4
-----Explanation-----
Example case 1: The actual sequence of powers is [21,21,23,23,25]=[2,2,8,8,32]$[2^1, 2^1, 2^3, 2^3, 2^5] = [2, 2, 8, 8, 32]$. The maximum product is 20⋅32=640$20 \cdot 32 = 640$. In the optimal solution, the sequence is partitioned into [2,2,8,8]$[2, 2, 8, 8]$ and [32]$[32]$. | # cook your dish here
for i in range(int(input())):
n,k=[int(i) for i in input().split()]
arr=[(k**int(i)) for i in input().split()]
v1=sum(arr)
v1=v1//2
v2=0
for i in range(len(arr)):
v2+=arr[i]
if(v2>=v1):
break
if(i==0):
print(1)
else:
if(abs(v2-v1)<abs(v2-arr[i]-v1)):
print(i+1)
else:
print(i) | python | train | qsol | codeparrot/apps | all |