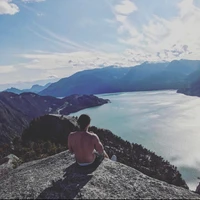
address
stringlengths 42
42
| source_code
stringlengths 32
1.21M
| bytecode
stringlengths 2
49.2k
| slither
sequence |
---|---|---|---|
0x006699d34AA3013605d468d2755A2Fe59A16B12B | pragma solidity 0.5.4;
interface IERC20 {
function balanceOf(address account) external view returns (uint256);
function transfer(address recipient, uint256 amount) external returns (bool);
function allowance(address owner, address spender) external view returns (uint256);
function approve(address spender, uint256 amount) external returns (bool);
function transferFrom(address sender, address recipient, uint256 amount) external returns (bool);
event Transfer(address indexed from, address indexed to, uint256 value);
event Approval(address indexed owner, address indexed spender, uint256 value);
}
contract Ownable {
address private _owner;
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
constructor() internal {
_owner = msg.sender;
emit OwnershipTransferred(address(0), _owner);
}
function owner() public view returns (address) {
return _owner;
}
function isOwner() public view returns (bool) {
return msg.sender == _owner;
}
modifier onlyOwner() {
require(msg.sender == _owner, "Ownable: caller is not the owner");
_;
}
function transferOwnership(address newOwner) public onlyOwner {
require(newOwner != address(0), "Ownable: new owner is the zero address");
emit OwnershipTransferred(_owner, newOwner);
_owner = newOwner;
}
}
library SafeMath {
/**
* @dev Returns the addition of two unsigned integers, reverting on
* overflow.
*
* Counterpart to Solidity's `+` operator.
*
* Requirements:
* - Addition cannot overflow.
*/
function add(uint256 a, uint256 b) internal pure returns (uint256) {
uint256 c = a + b;
require(c >= a, "SafeMath: addition overflow");
return c;
}
/**
* @dev Returns the subtraction of two unsigned integers, reverting on
* overflow (when the result is negative).
*
* Counterpart to Solidity's `-` operator.
*
* Requirements:
* - Subtraction cannot overflow.
*/
function sub(uint256 a, uint256 b) internal pure returns (uint256) {
return sub(a, b, "SafeMath: subtraction overflow");
}
/**
* @dev Returns the subtraction of two unsigned integers, reverting with custom message on
* overflow (when the result is negative).
*
* Counterpart to Solidity's `-` operator.
*
* Requirements:
* - Subtraction cannot overflow.
*
* _Available since v2.4.0._
*/
function sub(uint256 a, uint256 b, string memory errorMessage) internal pure returns (uint256) {
require(b <= a, errorMessage);
uint256 c = a - b;
return c;
}
/**
* @dev Returns the multiplication of two unsigned integers, reverting on
* overflow.
*
* Counterpart to Solidity's `*` operator.
*
* Requirements:
* - Multiplication cannot overflow.
*/
function mul(uint256 a, uint256 b) internal pure returns (uint256) {
// Gas optimization: this is cheaper than requiring 'a' not being zero, but the
// benefit is lost if 'b' is also tested.
// See: https://github.com/OpenZeppelin/openzeppelin-contracts/pull/522
if (a == 0) {
return 0;
}
uint256 c = a * b;
require(c / a == b, "SafeMath: multiplication overflow");
return c;
}
/**
* @dev Returns the integer division of two unsigned integers. Reverts on
* division by zero. The result is rounded towards zero.
*
* Counterpart to Solidity's `/` operator. Note: this function uses a
* `revert` opcode (which leaves remaining gas untouched) while Solidity
* uses an invalid opcode to revert (consuming all remaining gas).
*
* Requirements:
* - The divisor cannot be zero.
*/
function div(uint256 a, uint256 b) internal pure returns (uint256) {
return div(a, b, "SafeMath: division by zero");
}
/**
* @dev Returns the integer division of two unsigned integers. Reverts with custom message on
* division by zero. The result is rounded towards zero.
*
* Counterpart to Solidity's `/` operator. Note: this function uses a
* `revert` opcode (which leaves remaining gas untouched) while Solidity
* uses an invalid opcode to revert (consuming all remaining gas).
*
* Requirements:
* - The divisor cannot be zero.
*
* _Available since v2.4.0._
*/
function div(uint256 a, uint256 b, string memory errorMessage) internal pure returns (uint256) {
// Solidity only automatically asserts when dividing by 0
require(b > 0, errorMessage);
uint256 c = a / b;
// assert(a == b * c + a % b); // There is no case in which this doesn't hold
return c;
}
/**
* @dev Returns the remainder of dividing two unsigned integers. (unsigned integer modulo),
* Reverts when dividing by zero.
*
* Counterpart to Solidity's `%` operator. This function uses a `revert`
* opcode (which leaves remaining gas untouched) while Solidity uses an
* invalid opcode to revert (consuming all remaining gas).
*
* Requirements:
* - The divisor cannot be zero.
*/
function mod(uint256 a, uint256 b) internal pure returns (uint256) {
return mod(a, b, "SafeMath: modulo by zero");
}
/**
* @dev Returns the remainder of dividing two unsigned integers. (unsigned integer modulo),
* Reverts with custom message when dividing by zero.
*
* Counterpart to Solidity's `%` operator. This function uses a `revert`
* opcode (which leaves remaining gas untouched) while Solidity uses an
* invalid opcode to revert (consuming all remaining gas).
*
* Requirements:
* - The divisor cannot be zero.
*
* _Available since v2.4.0._
*/
function mod(uint256 a, uint256 b, string memory errorMessage) internal pure returns (uint256) {
require(b != 0, errorMessage);
return a % b;
}
}
contract ZildFinanceCoin is Ownable, IERC20 {
using SafeMath for uint256;
string public constant name = 'Zild Finance Coin';
string public constant symbol = 'Zild';
uint8 public constant decimals = 18;
uint256 public totalSupply = 9980 * 10000 * 10 ** uint256(decimals);
uint256 public allowBurn = 2100 * 10000 * 10 ** uint256(decimals);
uint256 public tokenDestroyed;
uint256 public constant FounderAllocation = 1497 * 10000 * 10 ** uint256(decimals);
uint256 public constant FounderLockupAmount = 998 * 10000 * 10 ** uint256(decimals);
uint256 public constant FounderLockupCliff = 365 days;
uint256 public constant FounderReleaseInterval = 30 days;
uint256 public constant FounderReleaseAmount = 20.7916 * 10000 * 10 ** uint256(decimals);
uint256 public constant MarketingAllocation = 349 * 10000 * 10 ** uint256(decimals);
uint256 public constant FurnaceAllocation = 150 * 10000 * 10 ** uint256(decimals);
address public founder = address(0);
uint256 public founderLockupStartTime = 0;
uint256 public founderReleasedAmount = 0;
mapping (address => uint256) private _balances;
mapping (address => mapping (address => uint256)) private _allowances;
mapping (address => bool) public frozenAccount;
event Transfer(address indexed from, address indexed to, uint256 value);
event Approval(address indexed from, address indexed to, uint256 value);
event ChangeFounder(address indexed previousFounder, address indexed newFounder);
event SetMinter(address indexed minter);
event SetMarketing(address indexed marketing);
event SetFurnace(address indexed furnace);
event Burn(address indexed _from, uint256 _tokenDestroyed, uint256 _timestamp);
event FrozenFunds(address target, bool frozen);
constructor(address _founder, address _marketing) public {
require(_founder != address(0), "ZildFinanceCoin: founder is the zero address");
require(_marketing != address(0), "ZildFinanceCoin: operator is the zero address");
founder = _founder;
founderLockupStartTime = block.timestamp;
_balances[address(this)] = totalSupply;
_transfer(address(this), _marketing, MarketingAllocation);
}
function release() public {
uint256 currentTime = block.timestamp;
uint256 cliffTime = founderLockupStartTime.add(FounderLockupCliff);
if (currentTime < cliffTime) return;
if (founderReleasedAmount >= FounderLockupAmount) return;
uint256 month = currentTime.sub(cliffTime).div(FounderReleaseInterval);
uint256 releaseAmount = month.mul(FounderReleaseAmount);
if (releaseAmount > FounderLockupAmount) releaseAmount = FounderLockupAmount;
if (releaseAmount <= founderReleasedAmount) return;
uint256 amount = releaseAmount.sub(founderReleasedAmount);
founderReleasedAmount = releaseAmount;
_transfer(address(this), founder, amount);
}
function balanceOf(address account) public view returns (uint256) {
return _balances[account];
}
function transfer(address to, uint256 amount) public returns (bool) {
require(to != address(0), "ERC20: tranfer to the zero address");
require(!frozenAccount[msg.sender]);
require(!frozenAccount[to]);
_transfer(msg.sender, to, amount);
return true;
}
function burn(uint256 _value) public returns (bool){
_burn(msg.sender, _value);
return true;
}
function _burn(address _who, uint256 _burntAmount) internal {
require (tokenDestroyed.add(_burntAmount) <= allowBurn, "ZildFinanceCoin: exceeded the maximum allowable burning amount" );
require(_balances[msg.sender] >= _burntAmount && _burntAmount > 0);
_transfer(address(_who), address(0), _burntAmount);
totalSupply = totalSupply.sub(_burntAmount);
tokenDestroyed = tokenDestroyed.add(_burntAmount);
emit Burn(_who, _burntAmount, block.timestamp);
}
function allowance(address from, address to) public view returns (uint256) {
return _allowances[from][to];
}
function approve(address to, uint256 amount) public returns (bool) {
_approve(msg.sender, to, amount);
return true;
}
function transferFrom(address from, address to, uint256 amount) public returns (bool) {
uint256 remaining = _allowances[from][msg.sender].sub(amount, "ERC20: transfer amount exceeds allowance");
require(to != address(0), "ERC20: tranfer to the zero address");
require(!frozenAccount[from]);
require(!frozenAccount[to]);
require(!frozenAccount[msg.sender]);
_transfer(from, to, amount);
_approve(from, msg.sender, remaining);
return true;
}
function _transfer(address from, address to, uint256 amount) private {
require(from != address(0), "ERC20: transfer from the zero address");
_balances[from] = _balances[from].sub(amount, "ERC20: transfer amount exceeds balance");
_balances[to] = _balances[to].add(amount);
emit Transfer(from, to, amount);
}
function _approve(address from, address to, uint256 amount) private {
require(from != address(0), "ERC20: approve from the zero address");
require(to != address(0), "ERC20: approve to the zero address");
_allowances[from][to] = amount;
emit Approval(from, to, amount);
}
function changeFounder(address _founder) public onlyOwner {
require(_founder != address(0), "ZildFinanceCoin: founder is the zero address");
emit ChangeFounder(founder, _founder);
founder = _founder;
}
function setMinter(address minter) public onlyOwner {
require(minter != address(0), "ZildFinanceCoin: minter is the zero address");
require(_balances[minter] == 0, "ZildFinanceCoin: minter has been initialized");
_transfer(address(this), minter, totalSupply.sub(FounderAllocation));
emit SetMinter(minter);
}
function setFurnace(address furnace) public onlyOwner {
require(furnace != address(0), "ZildFinanceCoin: furnace is the zero address");
require(_balances[furnace] == 0, "ZildFinanceCoin: furnace has been initialized");
_transfer(address(this), furnace, FurnaceAllocation);
emit SetFurnace(furnace);
}
function freezeAccount(address _target, bool _bool) public onlyOwner {
if (_target != address(0)) {
frozenAccount[_target] = _bool;
emit FrozenFunds(_target,_bool);
}
}
}
| 0x608060405234801561001057600080fd5b5060043610610202576000357c01000000000000000000000000000000000000000000000000000000009004806386d1a69f1161012c578063b414d4b6116100bf578063f2fde38b1161008e578063f2fde38b146104fd578063fca3b5aa14610523578063fedacfa414610549578063ff1970381461055157610202565b8063b414d4b614610473578063d9aa188114610499578063dd62ed3e146104a1578063e724529c146104cf57610202565b806393c32e06116100fb57806393c32e061461041157806395d89b4114610437578063a42d91d81461043f578063a9059cbb1461044757610202565b806386d1a69f146103d157806387829c65146103db5780638da5cb5b146104015780638f32d59b1461040957610202565b8063313ce567116101a45780634e680654116101735780634e6806541461039357806353b841721461039b5780635f6e84ee146103a357806370a08231146103ab57610202565b8063313ce5671461032c57806335e061fc1461034a57806342966c68146103525780634d853ee51461036f57610202565b806318160ddd116101e057806318160ddd146102de57806323b872dd146102e657806326e5be361461031c57806330cf480b1461032457610202565b806306fdde031461020757806307e4498c14610284578063095ea7b31461029e575b600080fd5b61020f610559565b6040805160208082528351818301528351919283929083019185019080838360005b83811015610249578181015183820152602001610231565b50505050905090810190601f1680156102765780820380516001836020036101000a031916815260200191505b509250505060405180910390f35b61028c610590565b60408051918252519081900360200190f35b6102ca600480360360408110156102b457600080fd5b50600160a060020a038135169060200135610596565b604080519115158252519081900360200190f35b61028c6105ad565b6102ca600480360360608110156102fc57600080fd5b50600160a060020a038135811691602081013590911690604001356105b3565b61028c6106db565b61028c6106ea565b6103346106f9565b6040805160ff9092168252519081900360200190f35b61028c6106fe565b6102ca6004803603602081101561036857600080fd5b5035610704565b610377610718565b60408051600160a060020a039092168252519081900360200190f35b61028c610727565b61028c610736565b61028c610745565b61028c600480360360208110156103c157600080fd5b5035600160a060020a0316610753565b6103d961076e565b005b6103d9600480360360208110156103f157600080fd5b5035600160a060020a031661086d565b6103776109ac565b6102ca6109bb565b6103d96004803603602081101561042757600080fd5b5035600160a060020a03166109cc565b61020f610acf565b61028c610b06565b6102ca6004803603604081101561045d57600080fd5b50600160a060020a038135169060200135610b0c565b6102ca6004803603602081101561048957600080fd5b5035600160a060020a0316610ba6565b61028c610bbb565b61028c600480360360408110156104b757600080fd5b50600160a060020a0381358116916020013516610bc1565b6103d9600480360360408110156104e557600080fd5b50600160a060020a0381351690602001351515610bec565b6103d96004803603602081101561051357600080fd5b5035600160a060020a0316610cb0565b6103d96004803603602081101561053957600080fd5b5035600160a060020a0316610db2565b61028c610f06565b61028c610f0e565b60408051808201909152601181527f5a696c642046696e616e636520436f696e000000000000000000000000000000602082015281565b60055481565b60006105a3338484610f15565b5060015b92915050565b60015481565b60008061060583606060405190810160405280602881526020016115ec60289139600160a060020a0388166000908152600860209081526040808320338452909152902054919063ffffffff61100b16565b9050600160a060020a03841615156106515760405160e560020a62461bcd02815260040180806020018281038252602281526020018061153f6022913960400191505060405180910390fd5b600160a060020a03851660009081526009602052604090205460ff161561067757600080fd5b600160a060020a03841660009081526009602052604090205460ff161561069d57600080fd5b3360009081526009602052604090205460ff16156106ba57600080fd5b6106c58585856110a5565b6106d0853383610f15565b506001949350505050565b6a0c6205537ba4bc5840000081565b6a084158e2526dd2e580000081565b601281565b60025481565b600061071033836111c4565b506001919050565b600454600160a060020a031681565b6a013da329b633647180000081565b6a02e309477303850140000081565b692c0726213dd1f530000081565b600160a060020a031660009081526007602052604090205490565b600554429060009061078a906301e1338063ffffffff6112c216565b90508082101561079b57505061086b565b6006546a084158e2526dd2e5800000116107b657505061086b565b60006107db62278d006107cf858563ffffffff61132616565b9063ffffffff61136816565b905060006107f982692c0726213dd1f530000063ffffffff6113aa16565b90506a084158e2526dd2e580000081111561081c57506a084158e2526dd2e58000005b600654811161082e575050505061086b565b60006108456006548361132690919063ffffffff16565b6006839055600454909150610865903090600160a060020a0316836110a5565b50505050505b565b600054600160a060020a031633146108bd576040805160e560020a62461bcd0281526020600482018190526024820152600080516020611614833981519152604482015290519081900360640190fd5b600160a060020a03811615156109075760405160e560020a62461bcd02815260040180806020018281038252602c8152602001806116a8602c913960400191505060405180910390fd5b600160a060020a0381166000908152600760205260409020541561095f5760405160e560020a62461bcd02815260040180806020018281038252602d8152602001806114ec602d913960400191505060405180910390fd5b61097530826a013da329b63364718000006110a5565b604051600160a060020a038216907f563cb3456af3dceec7b66059f2c03af0b838a856cdd6bccf313f3260ccab3c8d90600090a250565b600054600160a060020a031690565b600054600160a060020a0316331490565b600054600160a060020a03163314610a1c576040805160e560020a62461bcd0281526020600482018190526024820152600080516020611614833981519152604482015290519081900360640190fd5b600160a060020a0381161515610a665760405160e560020a62461bcd02815260040180806020018281038252602c815260200180611561602c913960400191505060405180910390fd5b600454604051600160a060020a038084169216907f0d6a44001f99eb1e3e879ad45c4f03a44f9fec7dedebb037550b0c8180372ae190600090a36004805473ffffffffffffffffffffffffffffffffffffffff1916600160a060020a0392909216919091179055565b60408051808201909152600481527f5a696c6400000000000000000000000000000000000000000000000000000000602082015281565b60065481565b6000600160a060020a0383161515610b585760405160e560020a62461bcd02815260040180806020018281038252602281526020018061153f6022913960400191505060405180910390fd5b3360009081526009602052604090205460ff1615610b7557600080fd5b600160a060020a03831660009081526009602052604090205460ff1615610b9b57600080fd5b6105a33384846110a5565b60096020526000908152604090205460ff1681565b60035481565b600160a060020a03918216600090815260086020908152604080832093909416825291909152205490565b600054600160a060020a03163314610c3c576040805160e560020a62461bcd0281526020600482018190526024820152600080516020611614833981519152604482015290519081900360640190fd5b600160a060020a03821615610cac57600160a060020a038216600081815260096020908152604091829020805460ff191685151590811790915582519384529083015280517f48335238b4855f35377ed80f164e8c6f3c366e54ac00b96a6402d4a9814a03a59281900390910190a15b5050565b600054600160a060020a03163314610d00576040805160e560020a62461bcd0281526020600482018190526024820152600080516020611614833981519152604482015290519081900360640190fd5b600160a060020a0381161515610d4a5760405160e560020a62461bcd0281526004018080602001828103825260268152602001806114786026913960400191505060405180910390fd5b60008054604051600160a060020a03808516939216917f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e091a36000805473ffffffffffffffffffffffffffffffffffffffff1916600160a060020a0392909216919091179055565b600054600160a060020a03163314610e02576040805160e560020a62461bcd0281526020600482018190526024820152600080516020611614833981519152604482015290519081900360640190fd5b600160a060020a0381161515610e4c5760405160e560020a62461bcd02815260040180806020018281038252602b815260200180611634602b913960400191505060405180910390fd5b600160a060020a03811660009081526007602052604090205415610ea45760405160e560020a62461bcd02815260040180806020018281038252602c8152602001806114c0602c913960400191505060405180910390fd5b600154610ecf9030908390610eca906a0c6205537ba4bc5840000063ffffffff61132616565b6110a5565b604051600160a060020a038216907fcec52196e972044edde8689a1b608e459c5946b7f3e5c8cd3d6d8e126d422e1c90600090a250565b6301e1338081565b62278d0081565b600160a060020a0383161515610f5f5760405160e560020a62461bcd0281526004018080602001828103825260248152602001806116846024913960400191505060405180910390fd5b600160a060020a0382161515610fa95760405160e560020a62461bcd02815260040180806020018281038252602281526020018061149e6022913960400191505060405180910390fd5b600160a060020a03808416600081815260086020908152604080832094871680845294825291829020859055815185815291517f8c5be1e5ebec7d5bd14f71427d1e84f3dd0314c0f7b2291e5b200ac8c7c3b9259281900390910190a3505050565b6000818484111561109d5760405160e560020a62461bcd0281526004018080602001828103825283818151815260200191508051906020019080838360005b8381101561106257818101518382015260200161104a565b50505050905090810190601f16801561108f5780820380516001836020036101000a031916815260200191505b509250505060405180910390fd5b505050900390565b600160a060020a03831615156110ef5760405160e560020a62461bcd02815260040180806020018281038252602581526020018061165f6025913960400191505060405180910390fd5b611133816060604051908101604052806026815260200161151960269139600160a060020a038616600090815260076020526040902054919063ffffffff61100b16565b600160a060020a038085166000908152600760205260408082209390935590841681522054611168908263ffffffff6112c216565b600160a060020a0380841660008181526007602090815260409182902094909455805185815290519193928716927fddf252ad1be2c89b69c2b068fc378daa952ba7f163c4a11628f55a4df523b3ef92918290030190a3505050565b6002546003546111da908363ffffffff6112c216565b111561121a5760405160e560020a62461bcd02815260040180806020018281038252603e81526020018061158d603e913960400191505060405180910390fd5b3360009081526007602052604090205481118015906112395750600081115b151561124457600080fd5b611250826000836110a5565b600154611263908263ffffffff61132616565b600155600354611279908263ffffffff6112c216565b600355604080518281524260208201528151600160a060020a038516927f49995e5dd6158cf69ad3e9777c46755a1a826a446c6416992167462dad033b2a928290030190a25050565b60008282018381101561131f576040805160e560020a62461bcd02815260206004820152601b60248201527f536166654d6174683a206164646974696f6e206f766572666c6f770000000000604482015290519081900360640190fd5b9392505050565b600061131f83836040805190810160405280601e81526020017f536166654d6174683a207375627472616374696f6e206f766572666c6f77000081525061100b565b600061131f83836040805190810160405280601a81526020017f536166654d6174683a206469766973696f6e206279207a65726f00000000000081525061140a565b60008215156113bb575060006105a7565b8282028284828115156113ca57fe5b041461131f5760405160e560020a62461bcd0281526004018080602001828103825260218152602001806115cb6021913960400191505060405180910390fd5b60008181841161145f5760405160e560020a62461bcd0281526004018080602001828103825283818151815260200191508051906020019080838360008381101561106257818101518382015260200161104a565b506000838581151561146d57fe5b049594505050505056fe4f776e61626c653a206e6577206f776e657220697320746865207a65726f206164647265737345524332303a20617070726f766520746f20746865207a65726f20616464726573735a696c6446696e616e6365436f696e3a206d696e74657220686173206265656e20696e697469616c697a65645a696c6446696e616e6365436f696e3a206675726e61636520686173206265656e20696e697469616c697a656445524332303a207472616e7366657220616d6f756e7420657863656564732062616c616e636545524332303a207472616e66657220746f20746865207a65726f20616464726573735a696c6446696e616e6365436f696e3a20666f756e64657220697320746865207a65726f20616464726573735a696c6446696e616e6365436f696e3a20657863656564656420746865206d6178696d756d20616c6c6f7761626c65206275726e696e6720616d6f756e74536166654d6174683a206d756c7469706c69636174696f6e206f766572666c6f7745524332303a207472616e7366657220616d6f756e74206578636565647320616c6c6f77616e63654f776e61626c653a2063616c6c6572206973206e6f7420746865206f776e65725a696c6446696e616e6365436f696e3a206d696e74657220697320746865207a65726f206164647265737345524332303a207472616e736665722066726f6d20746865207a65726f206164647265737345524332303a20617070726f76652066726f6d20746865207a65726f20616464726573735a696c6446696e616e6365436f696e3a206675726e61636520697320746865207a65726f2061646472657373a165627a7a72305820e6fd4002a5984c22bde21a7c9b84330841d0b32b169895279b8dfd458969c2110029 | [
4
] |
0x00c83aeCC790e8a4453e5dD3B0B4b3680501a7A7 | "pragma solidity 0.6.10;\npragma experimental ABIEncoderV2;\n\nlibrary BokkyPooBahsDateTimeLibrary {(...TRUNCATED) | "0x608060405234801561001057600080fd5b506004361061021c5760003560e01c80639bd9bbc611610125578063d95b637(...TRUNCATED) | [
6,
4,
9,
7
] |
0x010c5322d78c88ca18282b0a072a8913648b3038 | "pragma solidity 0.6.5;\npragma experimental ABIEncoderV2;\n\nstruct UserInfo {\n\n uint256 amoun(...TRUNCATED) | "0x608060405234801561001057600080fd5b50600436106100415760003560e01c806330fa738c14610046578063d4fac45(...TRUNCATED) | [
38
] |
0x0114622386c1a00686e594c70682d7aa0f8afa29 | "pragma solidity 0.6.6;\n\nlibrary Address {\n\n /**\n\n * @dev Returns true if `account` is (...TRUNCATED) | "0x608060405234801561001057600080fd5b50600436106101a95760003560e01c806370a08231116100f9578063a9059cb(...TRUNCATED) | [
38
] |
0x01A6F6Ac4F5b2564e8C52BA687E7019D0E81E7e8 | "pragma solidity 0.6.8;\npragma experimental ABIEncoderV2;\n\ncontract Initializable {\n\n\n\n /**\(...TRUNCATED) | "0x6080604052600436106101665760003560e01c80637d533642116100d1578063c4d66de81161008a578063f19605d6116(...TRUNCATED) | [
0,
4,
7,
9,
16,
5,
2
] |
0x01b23286ff60a543ec29366ae8d6b6274ca20541 | "pragma solidity 0.4.26;\n\ninterface IERC20 {\n\n function totalSupply() external view returns (ui(...TRUNCATED) | "0x608060405260043610610112576000357c010000000000000000000000000000000000000000000000000000000090046(...TRUNCATED) | [
2
] |
0x01b952402442630232f32d48380a557015b7c5ec | "pragma solidity 0.7.4;\n\nenum MathError { NO_ERROR, DIVISION_BY_ZERO, INTEGER_OVERFLOW, INTEGER_UN(...TRUNCATED) | "0x608060405234801561001057600080fd5b506004361061023d5760003560e01c80637c4950c71161013b578063cab364f(...TRUNCATED) | [
5,
9,
12
] |
0x0258f474786ddfd37abce6df6bbb1dd5dfc4434a | "pragma solidity 0.5.10;\n\ncontract Context {\n\n // Empty internal constructor, to prevent peop(...TRUNCATED) | "0x608060405234801561001057600080fd5b50600436106101005760003560e01c806370a0823111610097578063a457c2d(...TRUNCATED) | [
38
] |
0x0263e1dcfcaaad8fd4e840fe4b22854485a561f0 | "pragma solidity 0.6.6;\n\nlibrary Address {\n\n /**\n\n * @dev Returns true if `account` is (...TRUNCATED) | "0x608060405234801561001057600080fd5b50600436106100a95760003560e01c806339509351116100715780633950935(...TRUNCATED) | [
38
] |
0x0319a976c8dea5d31d1cf36eaffd8bec14957179 | "pragma solidity 0.6.5;\npragma experimental ABIEncoderV2;\n\nstruct UserInfo {\n\n uint256 amoun(...TRUNCATED) | "0x608060405234801561001057600080fd5b50600436106100415760003560e01c806330fa738c14610046578063d4fac45(...TRUNCATED) | [
38
] |
Dataset Card for Slither Audited Smart Contracts
Dataset Summary
This dataset contains source code and deployed bytecode for Solidity Smart Contracts that have been verified on Etherscan.io, along with a classification of their vulnerabilities according to the Slither static analysis framework.
Supported Tasks and Leaderboards
text-classification
: The dataset can be used to train a model for both binary and multilabel text classification on smart contracts bytecode and source code. The model performance is evaluated based on the accuracy of the predicted labels as compared to the given labels in the dataset.text-generation
: The dataset can also be used to train a language model for the Solidity programming languageimage-classification
: By pre-processing the bytecode data to obtain RGB images, the dataset can also be used to train convolutional neural networks for code vulnerability detection and classification.
Languages
The language annotations are in English, while all the source codes are in Solidity.
Dataset Structure
Data Instances
Each data instance contains the following features: address
, source_code
and bytecode
. The label comes in two configuration, either a plain-text cleaned up version of the output given by the Slither tool or a multi-label version, which consists in a simple list of integers, each one representing a particular vulnerability class. Label 4 indicates that the contract is safe.
An example from a plain-text configuration looks as follows:
{
'address': '0x006699d34AA3013605d468d2755A2Fe59A16B12B'
'source_code': 'pragma solidity 0.5.4; interface IERC20 { function balanceOf(address account) external ...'
'bytecode': '0x608060405234801561001057600080fd5b5060043610610202576000357c0100000000000000000000000000000000000000000000000000000000900...'
'slither': '{"success": true, "error": null, "results": {"detectors": [{"check": "divide-before-multiply", "impact": "Medium", "confidence": "Medium"}]}}'
}
An example from a multi-label configuration looks as follows:
{
'address': '0x006699d34AA3013605d468d2755A2Fe59A16B12B'
'source_code': 'pragma solidity 0.5.4; interface IERC20 { function balanceOf(address account) external ...'
'bytecode': '0x608060405234801561001057600080fd5b5060043610610202576000357c0100000000000000000000000000000000000000000000000000000000900...'
'slither': [ 4 ]
}
Data Fields
address
: a string representing the address of the smart contract deployed on the Ethereum main netsource_code
: a flattened version of the smart contract codebase in Soliditybytecode
: a string representing the smart contract's bytecode, obtained when callingweb3.eth.getCode()
. Note that in some cases where this was not available, the string is simply '0x'.slither
: either a cleaned up version of Slither's JSON output or a list of class labels
Data Splits
The dataset comes in 6 configurations and train, test and validation splits are only provided for those configurations that do not include all-
in their names. Test and Validation splits are both about 15% of the total.
Dataset Creation
Curation Rationale
slither-audited-smart-contracts was built to provide a freely available large scale dataset for vulnerability detection and classification on verified Solidity smart contracts. Indeed, the biggest open source dataset for this task at the moment of writing is SmartBugs Wild, containing 47,398 smart contracts that were labeled with 9 tools withing the SmartBugs framework.
Source Data
Initial Data Collection and Normalization
The dataset was constructed started from the list of verified smart contracts provided at Smart Contract Sanctuary. Then, smart contract source code was either downloaded from the aforementioned repo or downloaded via Etherscan and flattened using the Slither contract flattener. The bytecode was downloaded using the Web3.py library, in particular the web3.eth.getCode()
function and using INFURA as our endpoint.
Finally, every smart contract was analyzed using the Slither static analysis framework. The tool found 38 different vulnerability classes in the collected contracts and they were then mapped to 9 labels according to what is shown in the file label_mappings.json
. These mappings were derived by following the guidelines at Decentralized Application Security Project (DASP) and at Smart Contract Weakness Classification Registry. They were also inspired by the mappings used for Slither's detection by the team that labeled the SmartBugs Wild dataset, which can be found here.
Additional Information
Dataset Curators
The dataset was initially created by Martina Rossini during work done for the project of the course Blockchain and Cryptocurrencies of the University of Bologna (Italy).
Licensing Information
The license in the file LICENSE applies to all the files in this repository, except for the Solidity source code of the contracts. These are still publicly available, were obtained using the Etherscan APIs, and retain their original licenses.
Citation Information
If you are using this dataset in your research and paper, here's how you can cite it:
@misc{rossini2022slitherauditedcontracts,
title = {Slither Audited Smart Contracts Dataset},
author={Martina Rossini},
year={2022}
}
Contributions
Thanks to @mwritescode for adding this dataset.
- Downloads last month
- 1,514
Models trained or fine-tuned on mwritescode/slither-audited-smart-contracts
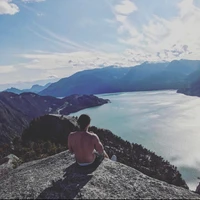