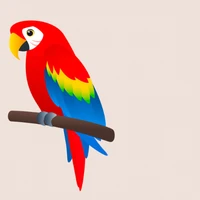
Datasets:
src
stringlengths 95
64.6k
| complexity
stringclasses 7
values | problem
stringlengths 6
50
| from
stringclasses 1
value |
---|---|---|---|
import java.io.*;
import java.math.BigInteger;
import java.util.InputMismatchException;
import java.util.PriorityQueue;
import java.util.StringTokenizer;
public class D {
static class FastWriter {
private final BufferedWriter bw;
public FastWriter() {
this.bw = new BufferedWriter(new OutputStreamWriter(System.out));
}
public void print(Object object) throws IOException {
bw.append("" + object);
}
public void println(Object object) throws IOException {
print(object);
bw.append("\n");
}
public void close() throws IOException {
bw.close();
}
}
static class FastReader {
BufferedReader br;
StringTokenizer st;
public FastReader() {
br = new BufferedReader(new InputStreamReader(System.in));
}
String next() {
while (st == null || !st.hasMoreElements()) {
try {
st = new StringTokenizer(br.readLine());
} catch (IOException e) {
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt() {
return Integer.parseInt(next());
}
long nextLong() {
return Long.parseLong(next());
}
double nextDouble() {
return Double.parseDouble(next());
}
String nextLine() {
String str = "";
try {
str = br.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return str;
}
BigInteger nextBigInteger() {
try {
return new BigInteger(nextLine());
} catch (NumberFormatException e) {
throw new InputMismatchException();
}
}
}
public static void main(String[] args) throws IOException {
FastReader fr = new FastReader();
FastWriter fw = new FastWriter();
int n = fr.nextInt();
int m = fr.nextInt();
for (int r = 0; r < n / 2; r++) {
for (int c = 0; c < m; c++) {
fw.println((r + 1) + " " + (c + 1));
fw.println((n - r) + " " + (m - c));
}
}
if (n % 2 != 0) {
int r = n / 2;
for (int c = 0; c < m / 2; c++) {
fw.println((r + 1) + " " + (c + 1));
fw.println((r + 1) + " " + (m - c));
}
if (m % 2 != 0) fw.println((r + 1) + " " + (m / 2 + 1));
}
fw.close();
}
}
| quadratic | 1179_B. Tolik and His Uncle | CODEFORCES |
import java.util.Scanner;
public class pillar {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
int n=sc.nextInt();
int a[]=new int[200005];
for (int i=1;i<=n;i++)
a[i]=sc.nextInt();
for (int i=2;i<n;i++)
if (a[i-1]>a[i]&&a[i]<a[i+1]) {
System.out.println("NO");
return;
}
System.out.println("YES");
}
}
| linear | 1197_B. Pillars | CODEFORCES |
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.StringTokenizer;
/**
* @author Don Li
*/
public class SequenceTransformation {
void solve() {
int p = 1, n = in.nextInt();
while (n > 0) {
if (n == 1) {
out.print(p + " ");
break;
}
if (n == 2) {
out.print(p + " ");
out.print(2 * p + " ");
break;
}
if (n == 3) {
out.print(p + " ");
out.print(p + " ");
out.print(3 * p + " ");
break;
}
for (int i = 0; i < (n + 1) / 2; i++) {
out.print(p + " ");
}
p *= 2;
n /= 2;
}
}
public static void main(String[] args) {
in = new FastScanner(new BufferedReader(new InputStreamReader(System.in)));
out = new PrintWriter(System.out);
new SequenceTransformation().solve();
out.close();
}
static FastScanner in;
static PrintWriter out;
static class FastScanner {
BufferedReader in;
StringTokenizer st;
public FastScanner(BufferedReader in) {
this.in = in;
}
public String nextToken() {
while (st == null || !st.hasMoreTokens()) {
try {
st = new StringTokenizer(in.readLine());
} catch (IOException e) {
e.printStackTrace();
}
}
return st.nextToken();
}
public int nextInt() {
return Integer.parseInt(nextToken());
}
public long nextLong() {
return Long.parseLong(nextToken());
}
public double nextDouble() {
return Double.parseDouble(nextToken());
}
}
}
| linear | 1059_C. Sequence Transformation | CODEFORCES |
import java.util.*;
import java.io.*;
public class Solution
{
public static void main(String [] args) throws IOException
{
PrintWriter pw=new PrintWriter(System.out);//use pw.println() not pw.write();
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st=new StringTokenizer(br.readLine());
/*
inputCopy
5 3
xyabd
outputCopy
29
inputCopy
7 4
problem
outputCopy
34
inputCopy
2 2
ab
outputCopy
-1
inputCopy
12 1
abaabbaaabbb
outputCopy
1
*/
int n=Integer.parseInt(st.nextToken());
int k=Integer.parseInt(st.nextToken());
st=new StringTokenizer(br.readLine());
String str=st.nextToken();
char [] arr=str.toCharArray();
Arrays.sort(arr);
int weight=arr[0]-96;
char a=arr[0];
int included=1;
for(int i=1;i<arr.length;++i)
{
if(included==k)
break;
char c=arr[i];
if(c-a<2)
continue;
weight+=arr[i]-96;
++included;
a=arr[i];
}
if(included==k)
pw.println(weight);
else
pw.println(-1);
pw.close();//Do not forget to write it after every program return statement !!
}
}
/*
→Judgement Protocol
Test: #1, time: 77 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
5 3
xyabd
Output
29
Answer
29
Checker Log
ok 1 number(s): "29"
Test: #2, time: 78 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
7 4
problem
Output
34
Answer
34
Checker Log
ok 1 number(s): "34"
Test: #3, time: 139 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
2 2
ab
Output
-1
Answer
-1
Checker Log
ok 1 number(s): "-1"
Test: #4, time: 124 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
12 1
abaabbaaabbb
Output
1
Answer
1
Checker Log
ok 1 number(s): "1"
Test: #5, time: 124 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
50 13
qwertyuiopasdfghjklzxcvbnmaaaaaaaaaaaaaaaaaaaaaaaa
Output
169
Answer
169
Checker Log
ok 1 number(s): "169"
Test: #6, time: 108 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
50 14
qwertyuiopasdfghjklzxcvbnmaaaaaaaaaaaaaaaaaaaaaaaa
Output
-1
Answer
-1
Checker Log
ok 1 number(s): "-1"
Test: #7, time: 93 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
1 1
a
Output
1
Answer
1
Checker Log
ok 1 number(s): "1"
Test: #8, time: 108 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
50 1
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
Output
1
Answer
1
Checker Log
ok 1 number(s): "1"
Test: #9, time: 77 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
50 2
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
Output
-1
Answer
-1
Checker Log
ok 1 number(s): "-1"
Test: #10, time: 92 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
13 13
uwgmkyqeiaocs
Output
169
Answer
169
Checker Log
ok 1 number(s): "169"
Test: #11, time: 124 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
13 13
hzdxpbfvrltnj
Output
182
Answer
182
Checker Log
ok 1 number(s): "182"
Test: #12, time: 93 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
1 1
n
Output
14
Answer
14
Checker Log
ok 1 number(s): "14"
Test: #13, time: 92 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
10 8
smzeblyjqw
Output
113
Answer
113
Checker Log
ok 1 number(s): "113"
Test: #14, time: 78 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
20 20
tzmvhskkyugkuuxpvtbh
Output
-1
Answer
-1
Checker Log
ok 1 number(s): "-1"
Test: #15, time: 109 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
30 15
wjzolzzkfulwgioksfxmcxmnnjtoav
Output
-1
Answer
-1
Checker Log
ok 1 number(s): "-1"
Test: #16, time: 93 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
40 30
xumfrflllrrgswehqtsskefixhcxjrxbjmrpsshv
Output
-1
Answer
-1
Checker Log
ok 1 number(s): "-1"
Test: #17, time: 124 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
50 31
ahbyyoxltryqdmvenemaqnbakglgqolxnaifnqtoclnnqiabpz
Output
-1
Answer
-1
Checker Log
ok 1 number(s): "-1"
Test: #18, time: 124 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
10 7
iuiukrxcml
Output
99
Answer
99
Checker Log
ok 1 number(s): "99"
Test: #19, time: 109 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
38 2
vjzarfykmrsrvwbwfwldsulhxtykmjbnwmdufa
Output
5
Answer
5
Checker Log
ok 1 number(s): "5"
Test: #20, time: 77 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
12 6
fwseyrarkwcd
Output
61
Answer
61
Checker Log
ok 1 number(s): "61"
Test: #21, time: 109 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
2 2
ac
Output
4
Answer
4
Checker Log
ok 1 number(s): "4"
Test: #22, time: 108 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
1 1
c
Output
3
Answer
3
Checker Log
ok 1 number(s): "3"
Test: #23, time: 124 ms., memory: 0 KB, exit code: 0, checker exit code: 0, verdict: OK
Input
2 2
ad
Output
5
Answer
5
Checker Log
ok 1 number(s): "5"
Test: #24, time: 77 ms., memory: 0 KB, exit code: 0, checker exit code: 1, verdict: WRONG_ANSWER
Input
2 1
ac
Output
-1
Answer
1
Checker Log
wrong answer 1st number
*/ | linear | 1011_A. Stages | CODEFORCES |
import java.io.OutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.io.OutputStream;
import java.util.SortedSet;
import java.util.Set;
import java.util.NavigableSet;
import java.io.IOException;
import java.util.InputMismatchException;
import java.io.InputStreamReader;
import java.util.TreeSet;
import java.io.Writer;
import java.io.BufferedReader;
import java.io.InputStream;
/**
* Built using CHelper plug-in
* Actual solution is at the top
*
* @author Niyaz Nigmatullin
*/
public class Main {
public static void main(String[] args) {
InputStream inputStream = System.in;
OutputStream outputStream = System.out;
FastScanner in = new FastScanner(inputStream);
FastPrinter out = new FastPrinter(outputStream);
TaskC solver = new TaskC();
solver.solve(1, in, out);
out.close();
}
static class TaskC {
public void solve(int testNumber, FastScanner in, FastPrinter out) {
int n = in.nextInt();
int k = in.nextInt();
char[] c = in.next().toCharArray();
NavigableSet<Integer> ones = new TreeSet<>();
NavigableSet<Integer> zeros = new TreeSet<>();
for (int i = 0; i < n; i++) {
if (c[i] == '0') zeros.add(i);
else ones.add(i);
}
if (ones.isEmpty() || zeros.isEmpty() || ones.last() - ones.first() + 1 <= k || zeros.last() - zeros.first() + 1 <= k) {
out.println("tokitsukaze");
return;
}
if (check(ones, n, k) && check(zeros, n, k)) {
out.println("quailty");
return;
}
out.println("once again");
}
private boolean check(NavigableSet<Integer> ones, int n, int k) {
for (int i = 0; i + k <= n; i++) {
int left = ones.first();
int right = ones.last();
if (left >= i) {
left = ones.higher(i + k - 1);
}
if (right < i + k) {
right = ones.lower(i);
}
if (right - left + 1 > k) {
return false;
}
}
return true;
}
}
static class FastPrinter extends PrintWriter {
public FastPrinter(OutputStream out) {
super(out);
}
public FastPrinter(Writer out) {
super(out);
}
}
static class FastScanner extends BufferedReader {
public FastScanner(InputStream is) {
super(new InputStreamReader(is));
}
public int read() {
try {
int ret = super.read();
// if (isEOF && ret < 0) {
// throw new InputMismatchException();
// }
// isEOF = ret == -1;
return ret;
} catch (IOException e) {
throw new InputMismatchException();
}
}
public String next() {
StringBuilder sb = new StringBuilder();
int c = read();
while (isWhiteSpace(c)) {
c = read();
}
if (c < 0) {
return null;
}
while (c >= 0 && !isWhiteSpace(c)) {
sb.appendCodePoint(c);
c = read();
}
return sb.toString();
}
static boolean isWhiteSpace(int c) {
return c >= 0 && c <= 32;
}
public int nextInt() {
int c = read();
while (isWhiteSpace(c)) {
c = read();
}
int sgn = 1;
if (c == '-') {
sgn = -1;
c = read();
}
int ret = 0;
while (c >= 0 && !isWhiteSpace(c)) {
if (c < '0' || c > '9') {
throw new NumberFormatException("digit expected " + (char) c
+ " found");
}
ret = ret * 10 + c - '0';
c = read();
}
return ret * sgn;
}
public String readLine() {
try {
return super.readLine();
} catch (IOException e) {
return null;
}
}
}
}
| linear | 1190_C. Tokitsukaze and Duel | CODEFORCES |
import java.math.BigDecimal;
import java.math.MathContext;
import java.math.RoundingMode;
import java.nio.charset.Charset;
import java.util.*;
import static java.lang.System.gc;
import static java.lang.System.out;
public class Main {
Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
new Main().solve();
}
void solve() {
int n = scanner.nextInt();
scanner.nextLine();
String s1 = scanner.nextLine();
String s2 = scanner.nextLine();
int ans = 0;
boolean a[] = new boolean[30];
boolean b[] = new boolean[30];
for (int i = 0; i < n; i++) {
if (s1.charAt(i) != s2.charAt(i)) {
ans ++;
a[s1.charAt(i) - 'a'] = true;
b[s2.charAt(i) - 'a'] = true;
}
}
for (int i = 0; i < n; i++) {
if (s1.charAt(i) != s2.charAt(i) && a[s2.charAt(i) - 'a'] && b[s1.charAt(i) - 'a']) {
for (int j = i + 1; j < n; j ++) {
if (s1.charAt(i) == s2.charAt(j) && s1.charAt(j) == s2.charAt(i)) {
out.println(ans - 2);
out.println((i + 1) + " " + (j + 1));
return;
}
}
}
}
for (int i = 0; i < n; i++) {
if (s1.charAt(i) != s2.charAt(i) && (a[s2.charAt(i) - 'a'] || b[s1.charAt(i) - 'a'])) {
for (int j = i + 1; j < n; j ++) {
if (s1.charAt(j) != s2.charAt(j) && (s1.charAt(i) == s2.charAt(j) || s1.charAt(j) == s2.charAt(i))) {
out.println(ans - 1);
out.println((i + 1) + " " + (j + 1));
return;
}
}
}
}
out.println(ans);
out.println(-1 + " " + -1);
}
} | quadratic | 527_B. Error Correct System | CODEFORCES |
import java.util.*;
import java.io.*;
import static java.lang.Math.*;
public class Main {
static int n;
static long TotalTime;
static Problem[] problems;
static StringBuilder sb;
public static void main(String[] args) {
FastScanner sc = new FastScanner();
sb = new StringBuilder();
n = sc.nextInt();
TotalTime = sc.nextLong();
problems = new Problem[n];
for (int i = 0; i < n; i++) {
problems[i] = new Problem (sc.nextInt(), sc.nextLong(), i);
}
Arrays.sort(problems);
long num = -1;
long high = n;
long low = 0;
int iter = 0;
while (high - low > 1) {
num = (high + low) / 2;
if (test(num, false)) {
low = num;
}
else {
high = num;
}
}
if (test(high, false))
num = high;
else
num = low;
test(num, true);
System.out.print(sb);
}
public static boolean test (long num, boolean print) {
int count = 0;
long sum = 0L;
if (print) sb.append(num + "\n" + num + "\n");
for (int i = 0; i < n && count < num; i++) {
if (problems[i].a >= num) {
count++;
sum += problems[i].t;
if (print) sb.append((problems[i].index + 1) + " ");
}
}
return (count == num) && (sum <= TotalTime);
}
public static class Problem implements Comparable<Problem> {
int a;
long t;
int index;
public int compareTo(Problem o) {
return Long.compare(t, o.t);
}
public Problem (int a, long t, int index) {
this.a = a;
this.t = t;
this.index = index;
}
}
public static class FastScanner {
BufferedReader br;
StringTokenizer st;
public FastScanner(Reader in) {
br = new BufferedReader(in);
}
public FastScanner() {
this(new InputStreamReader(System.in));
}
String next() {
while (st == null || !st.hasMoreElements()) {
try {
st = new StringTokenizer(br.readLine());
} catch (IOException e) {
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt() {
return Integer.parseInt(next());
}
long nextLong() {
return Long.parseLong(next());
}
double nextDouble() {
return Double.parseDouble(next());
}
String readNextLine() {
String str = "";
try {
str = br.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return str;
}
int[] readIntArray(int n) {
int[] a = new int[n];
for (int idx = 0; idx < n; idx++) {
a[idx] = nextInt();
}
return a;
}
long[] readLongArray(int n) {
long[] a = new long[n];
for (int idx = 0; idx < n; idx++) {
a[idx] = nextLong();
}
return a;
}
}
} | nlogn | 913_D. Too Easy Problems | CODEFORCES |
import java.io.*;
import java.util.*;
import javax.lang.model.util.ElementScanner6;
public class codef
{
public static void main(String ar[]) throws IOException
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
StringTokenizer nk=new StringTokenizer(br.readLine());
int n=Integer.parseInt(nk.nextToken());
int k=Integer.parseInt(nk.nextToken());
String st[]=br.readLine().split(" ");
int ans[]=new int[n];
int a[]=new int[n];
for(int i=0;i<n;i++)
ans[i]=Integer.parseInt(st[i]);
for(int i=1;i<n;i++)
a[i]=ans[i]-ans[i-1];
a[0]=-1;
Arrays.sort(a);
int count=0,sum=0;
for(int i=0;i<n;i++)
if(a[i]<0)
count++;
else
sum=sum+a[i];
k=k-count;
int i=n-1;
while(k>0 && i>=0)
{
if(a[i]>-1)
{
sum=sum-a[i];
k--;
}
i--;
}
System.out.println(sum);
}
} | nlogn | 1197_C. Array Splitting | CODEFORCES |
// LM10: The next Ballon d'or
import java.util.*;
import java.io.*;
import java.math.*;
import javafx.util.Pair;
public class Main
{
static class FastReader
{
private InputStream mIs;private byte[] buf = new byte[1024];private int curChar,numChars;public FastReader() { this(System.in); }public FastReader(InputStream is) { mIs = is;}
public int read() {if (numChars == -1) throw new InputMismatchException();if (curChar >= numChars) {curChar = 0;try { numChars = mIs.read(buf);} catch (IOException e) { throw new InputMismatchException();}if (numChars <= 0) return -1; }return buf[curChar++];}
public String nextLine(){int c = read();while (isSpaceChar(c)) c = read();StringBuilder res = new StringBuilder();do {res.appendCodePoint(c);c = read();}while (!isEndOfLine(c));return res.toString() ;}
public String next(){int c = read();while (isSpaceChar(c)) c = read();StringBuilder res = new StringBuilder();do {res.appendCodePoint(c);c = read();}while (!isSpaceChar(c));return res.toString();}
public long l(){int c = read();while (isSpaceChar(c)) c = read();int sgn = 1;if (c == '-') { sgn = -1 ; c = read() ; }long res = 0; do{ if (c < '0' || c > '9') throw new InputMismatchException();res *= 10 ; res += c - '0' ; c = read();}while(!isSpaceChar(c));return res * sgn;}
public int i(){int c = read() ;while (isSpaceChar(c)) c = read();int sgn = 1;if (c == '-') { sgn = -1 ; c = read() ; }int res = 0;do{if (c < '0' || c > '9') throw new InputMismatchException();res *= 10 ; res += c - '0' ; c = read() ;}while(!isSpaceChar(c));return res * sgn;}
public double d() throws IOException {return Double.parseDouble(next()) ;}
public boolean isSpaceChar(int c) { return c == ' ' || c == '\n' || c == '\r' || c == '\t' || c == -1; }
public boolean isEndOfLine(int c) { return c == '\n' || c == '\r' || c == -1; }
public void scanIntArr(int [] arr){ for(int li=0;li<arr.length;++li){ arr[li]=i();}}
public void scanLongArr(long [] arr){for (int i=0;i<arr.length;++i){arr[i]=l();}}
public void shuffle(int [] arr){ for(int i=arr.length;i>0;--i) { int r=(int)(Math.random()*i); int temp=arr[i-1]; arr[i-1]=arr[r]; arr[r]=temp; } }
}
public static void main(String[] args)throws IOException {
/*
inputCopy
4
2 1 2 1
outputCopy
4
inputCopy
5
0 -1 -1 -1 -1
outputCopy
4
*/
PrintWriter pw = new PrintWriter(System.out);
FastReader fr = new FastReader();
int n=fr.i();
int [] arr=new int[n];
fr.scanIntArr(arr);
int min=Integer.MAX_VALUE;
int max=Integer.MIN_VALUE;
long sum=0;
if(n==1)
{
pw.println(arr[0]);
pw.flush();
pw.close();
return;
}
for(int i=0;i<n;++i)
{
if(arr[i]<min)
min=arr[i];
if(arr[i]>max)
max=arr[i];
sum+=Math.abs(arr[i]);
}
if(min>0)
{
sum-=2*min;
}
if(max<0)
{
sum+=2*max;
}
pw.println(sum);
pw.flush();
pw.close();
}
} | linear | 1038_D. Slime | CODEFORCES |
import java.util.*;
import java.io.*;
import java.lang.*;
import java.math.*;
public class B {
public static void main(String[] args) throws Exception {
BufferedReader bf = new BufferedReader(new InputStreamReader(System.in));
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
// int n = Integer.parseInt(bf.readLine());
StringTokenizer st = new StringTokenizer(bf.readLine());
// int[] a = new int[n]; for(int i=0; i<n; i++) a[i] = Integer.parseInt(st.nextToken());
int n = Integer.parseInt(st.nextToken());
int m = Integer.parseInt(st.nextToken());
StringBuilder ans1 = new StringBuilder();
StringBuilder ans2 = new StringBuilder();
for(int i=0; i<2229; i++) ans1.append('5');
ans1.append('6');
for(int i=0; i<2230; i++) ans2.append('4');
out.println(ans1.toString());
out.println(ans2.toString());
out.close(); System.exit(0);
}
}
| constant | 1028_B. Unnatural Conditions | CODEFORCES |
import java.io.*;
import java.util.*;
public class Solution{
static class FastReader
{
BufferedReader br;
StringTokenizer st;
public FastReader()
{
br = new BufferedReader(new
InputStreamReader(System.in));
}
String next()
{
while (st == null || !st.hasMoreElements())
{
try
{
st = new StringTokenizer(br.readLine());
}
catch (IOException e)
{
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt()
{
return Integer.parseInt(next());
}
long nextLong()
{
return Long.parseLong(next());
}
double nextDouble()
{
return Double.parseDouble(next());
}
String nextLine()
{
String str = "";
try
{
str = br.readLine();
}
catch (IOException e)
{
e.printStackTrace();
}
return str;
}
}
public static void main(String args[] ) {
FastReader sc = new FastReader();
int n = sc.nextInt();
int m = sc.nextInt();
int[] arr = new int[105];
for(int i=0;i<m;i++){
int a = sc.nextInt();
arr[a]++;
}
for(int i=1;i<=1000;i++){
int sum=0;
for(int a:arr){
if(a!=0){
sum+=(a/i);
}
}
if(sum<n){
System.out.println(i-1);
return;
}
}
}
}
| quadratic | 1011_B. Planning The Expedition | CODEFORCES |
import javax.print.attribute.standard.RequestingUserName;
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws NumberFormatException, IOException
{
InputStream inputStream = System.in;
OutputStream outputStream = System.out;
InputReader in = new InputReader(inputStream);
PrintWriter out = new PrintWriter(outputStream);
Task solver = new Task();
int tc = in.nextInt();
for(int i = 0; i < tc; i++)
solver.solve(i, in, out);
out.close();
}
static class Task {
public void solve(int testNumber, InputReader in, PrintWriter out) {
int k = in.nextInt();
int[] s = getArray(in.nextToken());
int[] a = getArray(in.nextToken());
int[] b = getArray(in.nextToken());
int[] per = new int[k];
boolean[] used = new boolean[k];
Arrays.fill(per , -1);
if(!check(s , a, per.clone(), k, used)){
out.println("NO");
return;
}
for(int i = 0; i < s.length; i++){
if(per[s[i]] != -1){
continue;
}
for(int j = 0; j < k; j++){
if(used[j]){
continue;
}
per[s[i]] = j;
used[j] = true;
if(check(s , a , per.clone() , k, used)){
break;
}
per[s[i]] = -1;
used[j] = false;
}
}
for(int i = 0; i < s.length; i++){
if(per[s[i]] == -1){
out.println("NO");
return;
}
s[i] = per[s[i]];
}
if(cmp(s , b) > 0){
out.println("NO");
return;
}
int last = 0;
for(int i = 0; i < k; i++){
if(per[i] == -1) {
while(used[last])last++;
per[i] = last;
used[last] = true;
}
}
char[] result = new char[k];
for(int i = 0; i < k; i++){
result[i] = (char)('a' + per[i]);
}
out.println("YES");
out.println(new String(result));
}
private int cmp(int[] a, int[] b){
for(int i = 0; i < a.length; i++){
if(a[i] != b[i]){
return a[i] < b[i] ? -1 : 1;
}
}
return 0;
}
private boolean check(int[] s, int[] a, int[] per, int k, boolean[] used) {
int res[] = new int[s.length];
int last = k - 1;
for(int i = 0; i < res.length; ++i){
if(per[s[i]] == -1){
while(last >= 0 && used[last]){
last--;
}
if(last < 0){
return false;
}
per[s[i]] = last;
last--;
}
res[i] = per[s[i]];
}
return cmp(a , res) <= 0;
}
private int[] getArray(String nextToken) {
int result[] = new int[nextToken.length()];
for(int i = 0; i < nextToken.length(); i++){
result[i] = nextToken.charAt(i) - 'a';
}
return result;
}
}
static class InputReader {
BufferedReader in;
StringTokenizer tok;
public InputReader(InputStream stream){
in = new BufferedReader(new InputStreamReader(stream), 32768);
tok = null;
}
String nextToken()
{
String line = "";
while(tok == null || !tok.hasMoreTokens()) {
try {
if((line = in.readLine()) != null)
tok = new StringTokenizer(line);
else
return null;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
return tok.nextToken();
}
int nextInt(){
return Integer.parseInt(nextToken());
}
long nextLong() {
return Long.parseLong(nextToken());
}
double nextDouble() {
return Double.parseDouble(nextToken());
}
}
} | quadratic | 1086_C. Vasya and Templates | CODEFORCES |
import java.io.*;
import java.util.StringTokenizer;
public class Main {
static int[] a;
public static void main(String[] args) throws IOException {
Scanner sc = new Scanner(System.in);
PrintWriter out = new PrintWriter(System.out);
int n = sc.nextInt();
a = sc.nextIntArray(n);
long inversions = divide(0, n - 1);
// out.println(inversions);
// System.err.println(Arrays.toString(a));
if (n == 5) out.println("Petr");
else {
if (n % 2 == 0) out.println(inversions % 2 == 0 ? "Petr" : "Um_nik");
else out.println(inversions % 2 != 0 ? "Petr" : "Um_nik");
}
out.flush();
out.close();
}
static long divide(int b, int e) {
if (b == e) return 0;
long cnt = 0;
int mid = b + e >> 1;
cnt += divide(b, mid);
cnt += divide(mid + 1, e);
cnt += merge(b, mid, e);
return cnt;
}
static long merge(int b, int mid, int e) {
long cnt = 0;
int len = e - b + 1;
int[] tmp = new int[len];
int i = b, j = mid + 1;
for (int k = 0; k < len; k++) {
if (i == mid + 1 || (j != e + 1 && a[i] > a[j])) {
tmp[k] = a[j++];
cnt += (mid + 1 - i);
} else tmp[k] = a[i++];
}
for (int k = 0; k < len; k++)
a[b + k] = tmp[k];
return cnt;
}
static class Scanner {
StringTokenizer st;
BufferedReader br;
public Scanner(InputStream system) {
br = new BufferedReader(new InputStreamReader(system));
}
public String next() throws IOException {
while (st == null || !st.hasMoreTokens()) st = new StringTokenizer(br.readLine());
return st.nextToken();
}
public String nextLine() throws IOException {
return br.readLine();
}
public int nextInt() throws IOException {
return Integer.parseInt(next());
}
public double nextDouble() throws IOException {
return Double.parseDouble(next());
}
public char nextChar() throws IOException {
return next().charAt(0);
}
public Long nextLong() throws IOException {
return Long.parseLong(next());
}
public boolean ready() throws IOException {
return br.ready();
}
public int[] nextIntArray(int n) throws IOException {
int[] a = new int[n];
for (int i = 0; i < n; i++)
a[i] = nextInt();
return a;
}
public long[] nextLongArray(int n) throws IOException {
long[] a = new long[n];
for (int i = 0; i < n; i++)
a[i] = nextLong();
return a;
}
public Integer[] nextIntegerArray(int n) throws IOException {
Integer[] a = new Integer[n];
for (int i = 0; i < n; i++)
a[i] = nextInt();
return a;
}
public double[] nextDoubleArray(int n) throws IOException {
double[] ans = new double[n];
for (int i = 0; i < n; i++)
ans[i] = nextDouble();
return ans;
}
public short nextShort() throws IOException {
return Short.parseShort(next());
}
}
} | nlogn | 986_B. Petr and Permutations | CODEFORCES |
import java.util.*;
import java.io.*;
public class A
{
public static void main(String ar[]) throws Exception
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
String s1[]=br.readLine().split(" ");
int n=Integer.parseInt(s1[0]);
int S=Integer.parseInt(s1[1]);
if(S%n==0)
System.out.println(S/n);
else
System.out.println(S/n+1);
}
} | constant | 1061_A. Coins | CODEFORCES |
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.StreamTokenizer;
import java.util.Arrays;
public class Main {
static StreamTokenizer st=new StreamTokenizer(new BufferedReader(new InputStreamReader(System.in)));
public static void main(String[] args) {
int n=nextInt();
int m=nextInt();
long b[]=new long[n];
long g[]=new long[m];
for(int i=0;i<n;i++)
b[i]=nextInt();
for(int i=0;i<m;i++)
g[i]=nextInt();
Arrays.sort(b);
Arrays.sort(g);
if(b[n-1]>g[0])
System.out.println("-1");
else if(b[n-1]==g[0]){
long sum=0;
for(int i=0;i<m;i++)
sum+=g[i];
for(int i=0;i<n-1;i++){
sum+=(m*b[i]);
}
System.out.println(sum);
}else{
long sum=0;
for(int i=0;i<m;i++)
sum+=g[i];
sum+=b[n-1];
sum+=(b[n-2]*(m-1));
for(int i=0;i<n-2;i++){
sum+=(m*b[i]);
}
System.out.println(sum);
}
}
static int nextInt(){
try {
st.nextToken();
} catch (IOException e) {
e.printStackTrace();
}
return (int)st.nval;
}
}
| nlogn | 1159_C. The Party and Sweets | CODEFORCES |
import java.util.*;
public class A912 {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner scan = new Scanner(System.in);
int A = scan.nextInt();
int B = scan.nextInt();
long x = scan.nextInt();
long y = scan.nextInt();
long z = scan.nextInt();
long requiredA = x * 2 + y;
long requiredB = y + z * 3;
long neededA = Math.max(0, requiredA - A);
long neededB = Math.max(0, requiredB - B);
System.out.print(neededA + neededB);
}
}
| constant | 912_A. Tricky Alchemy | CODEFORCES |
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.*;
public class C {
void solve(){
int n = readInt();
int q = readInt();
int max = 0;
int[] a = new int[n];
Deque<Integer> deque = new ArrayDeque<>();
for(int i = 0;i<n;i++){
a[i] = readInt();
deque.addLast(a[i]);
max = Math.max(max, a[i]);
}
List<String> ans = new ArrayList<>();
while(deque.peekFirst() != max){
int one = deque.pollFirst();
int two = deque.pollFirst();
ans.add(one + " " + two);
deque.addFirst(one > two ? one : two);
deque.addLast(one > two ? two : one);
if(one == max) break;
}
for(int i = 0;i<n;i++){
a[i] = deque.pollFirst();
}
for(int i = 0;i<q;i++){
long x = readLong();
if(x <= ans.size()){
out.println(ans.get((int)x - 1));
continue;
}
x -= ans.size();
int y =(int) (x%(n - 1) - 1%(n - 1) + (n - 1)) % (n - 1) + 1;
out.println(max + " " + a[y]);
}
}
public static void main(String[] args) {
new C().run();
}
void run(){
init();
solve();
out.close();
}
BufferedReader in;
PrintWriter out;
StringTokenizer tok = new StringTokenizer("");
void init(){
in = new BufferedReader(new InputStreamReader(System.in));
out = new PrintWriter(System.out);
}
String readLine(){
try{
return in.readLine();
}catch(Exception ex){
throw new RuntimeException(ex);
}
}
String readString(){
while(!tok.hasMoreTokens()){
String nextLine = readLine();
if(nextLine == null) return null;
tok = new StringTokenizer(nextLine);
}
return tok.nextToken();
}
int readInt(){
return Integer.parseInt(readString());
}
long readLong(){
return Long.parseLong(readString());
}
double readDouble(){
return Double.parseDouble(readString());
}
}
| linear | 1180_C. Valeriy and Deque | CODEFORCES |
//package contese_476;
import java.util.*;
public class q1
{
int m=(int)1e9+7;
public class Node
{
int a;
int b;
public void Node(int a,int b)
{
this.a=a;
this.b=b;
}
}
public int mul(int a ,int b)
{
a=a%m;
b=b%m;
return((a*b)%m);
}
public int pow(int a,int b)
{
int x=1;
while(b>0)
{
if(b%2!=0)
x=mul(x,a);
a=mul(a,a);
b=b/2;
}
return x;
}
public static long gcd(long a,long b)
{
if(b==0)
return a;
else
return gcd(b,a%b);
}
public static void main(String[] args)
{
Scanner sc=new Scanner(System.in);
int n=sc.nextInt();
HashMap<Integer,Integer> h=new HashMap();
//HashMap<Integer,Integer> h1=new HashMap();
int[] a=new int[n];
int x=sc.nextInt();
for(int i=0;i<n;i++)
{
a[i]=sc.nextInt();
if(h.get(a[i])==null)
{
h.put(a[i], 1);
//h1.put(a[i],i);
}
else
{
System.out.print(0);
System.exit(0);
}
}
for(int i=0;i<n;i++)
{
int num=a[i]&x;
if(num==a[i])
continue;
if(h.get(num)==null)
continue;
else
{
System.out.print(1);
System.exit(0);
}
}
for(int i=0;i<n;i++)
{
int num=a[i]&x;
if(num==a[i])
continue;
if(h.get(num)==null)
h.put(num, 1);
else
{
System.out.print(2);
System.exit(0);
}
}
System.out.print(-1);
}
} | linear | 1013_B. And | CODEFORCES |
import java.util.Arrays;
import java.util.Scanner;
public class Solution {
private static int[] a;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt(), m = sc.nextInt();
a = new int[101];
for (int i = 0; i < m; i++) {
int type = sc.nextInt();
a[type] = a[type] + 1;
}
int lo=1, hi=100, max=0;
while (lo <= hi) {
int mid = lo + (hi - lo)/2;
if (check(n, mid)) {
max = mid;
lo = mid+1;
} else {
hi = mid -1;
}
}
System.out.println(max);
}
public static boolean check(int n, int target) {
int result = 0;
for (int i=0; i <a.length; i++) {
result = result + (a[i] / target);
}
if (result >= n) {return true;}
return false;
}
} | nlogn | 1011_B. Planning The Expedition | CODEFORCES |
import java.io.*;
import java.util.*;
public class CF1027D {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(br.readLine());
StringTokenizer st = new StringTokenizer(br.readLine());
int[] cc = new int[n];
for (int i = 0; i < n; i++)
cc[i] = Integer.parseInt(st.nextToken());
st = new StringTokenizer(br.readLine());
int[] aa = new int[n];
for (int i = 0; i < n; i++)
aa[i] = Integer.parseInt(st.nextToken()) - 1;
int[] used = new int[n];
int ans = 0;
for (int i = 0; i < n; i++) {
if (used[i] == 2)
continue;
int j = i;
while (used[j] == 0) {
used[j] = 1;
j = aa[j];
}
if (used[j] == 1) {
int c = cc[j];
while (used[j] == 1) {
used[j] = 2;
c = Math.min(c, cc[j]);
j = aa[j];
}
ans += c;
}
j = i;
while (used[j] == 1) {
used[j] = 2;
j = aa[j];
}
}
System.out.println(ans);
}
}
| linear | 1027_D. Mouse Hunt | CODEFORCES |
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.StringTokenizer;
public class D {
private void solve() {
br = new BufferedReader(new InputStreamReader(System.in));
out = new PrintWriter(System.out);
int n = nextInt(), m = nextInt(), u = 1, d = n;
while (u < d) {
for (int i = 1; i <= m; i++) {
out.println(u + " " + i);
out.println(d + " " + (m - i + 1));
}
u++;
d--;
}
if (u == d) {
int l = 1, r = m;
while (l < r) {
out.println(u + " " + l++);
out.println(d + " " + r--);
}
if (l == r) out.println(u + " " + l);
}
out.close();
}
public static void main(String[] args) {
new D().solve();
}
private BufferedReader br;
private StringTokenizer st;
private PrintWriter out;
private String next() {
while (st == null || !st.hasMoreTokens()) {
try {
st = new StringTokenizer(br.readLine());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
return st.nextToken();
}
private int nextInt() {
return Integer.parseInt(next());
}
private long nextLong() {
return Long.parseLong(next());
}
private double nextDouble() {
return Double.parseDouble(next());
}
}
| quadratic | 1179_B. Tolik and His Uncle | CODEFORCES |
import java.io.*;
import java.util.*;
public class Solution{
static class FastReader
{
BufferedReader br;
StringTokenizer st;
public FastReader()
{
br = new BufferedReader(new
InputStreamReader(System.in));
}
String next()
{
while (st == null || !st.hasMoreElements())
{
try
{
st = new StringTokenizer(br.readLine());
}
catch (IOException e)
{
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt()
{
return Integer.parseInt(next());
}
long nextLong()
{
return Long.parseLong(next());
}
double nextDouble()
{
return Double.parseDouble(next());
}
String nextLine()
{
String str = "";
try
{
str = br.readLine();
}
catch (IOException e)
{
e.printStackTrace();
}
return str;
}
}
public static void main(String args[] ) {
FastReader sc = new FastReader();
int n = sc.nextInt();
int m = sc.nextInt();
int[] arr = new int[105];
for(int i=0;i<m;i++){
int a = sc.nextInt();
arr[a]++;
}
for(int i=1;i<=1000;i++){
int sum=0;
for(int a:arr){
if(a!=0){
sum+=(a/i);
}
}
if(sum<n){
System.out.println(i-1);
return;
}
}
}
}
| quadratic | 1011_B. Planning The Expedition | CODEFORCES |
//package com.krakn.CF.B1159;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n;
n = sc.nextInt();
int[] arr = new int[n];
for (int i = 0; i < n; i++) {
arr[i] = sc.nextInt();
}
int min = 1000000000, temp;
for (int i = 0; i < n; i++) {
temp = arr[i] / Math.max(i, n - 1 - i);
if (temp < min)
min = temp;
// System.out.println(i + " " + temp);
}
System.out.println(min);
}
}
| linear | 1159_B. Expansion coefficient of the array | CODEFORCES |
/* package whatever; // don't place package name! */
import java.util.*;
import java.lang.*;
import java.io.*;
import java.math.*;
/* Name of the class has to be "Main" only if the class is public. */
public class Main
{
public static void main (String[] args) throws java.lang.Exception
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
int n=Integer.parseInt(br.readLine());
int[] A=new int[n];
String[] s=br.readLine().split(" ");
for(int i=0;i<n;i++){
A[i]=Integer.parseInt(s[i]);
}
Map memo=new HashMap();
int[] f=new int[n];
for(int i=0;i<n;i++){
if(!memo.containsKey(A[i])){
memo.put(A[i],1);
}
else{
int ct=(int)memo.get(A[i]);
memo.put(A[i],ct+1);
}
int tot=0;
if(memo.containsKey(A[i]-1)){
tot+=(int)memo.get(A[i]-1);
}
if(memo.containsKey(A[i]+1)){
tot+=(int)memo.get(A[i]+1);
}
tot+=(int)memo.get(A[i]);
f[i]=tot;
}
BigInteger res=new BigInteger("0");
for(int i=0;i<n;i++){
int tot1=i+1-f[i];
int tot2=0;
if(memo.containsKey(A[i]-1)){
tot2+=(int)memo.get(A[i]-1);
}
if(memo.containsKey(A[i]+1)){
tot2+=(int)memo.get(A[i]+1);
}
tot2+=(int)memo.get(A[i]);
tot2=n-i-1-(tot2-f[i]);
//res+=(long)(tot1-tot2)*(long)A[i];
res=res.add(BigInteger.valueOf((long)(tot1-tot2)*(long)A[i]));
}
System.out.println(res);
}
} | nlogn | 903_D. Almost Difference | CODEFORCES |
import java.util.*;
public class Main {
static int n=5;
static int[] arr=new int[5];
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
int arr[]=new int[n];
for (int i=0;i<n;i++)
{
arr[i]=sc.nextInt();
}
for (int i=0;i<n;i++)
{
if (arr[i]>=0)
{
arr[i]=-arr[i]-1;
}
}
if (n%2!=0)
{
int min=0;
for (int i=1;i<n;i++)
{
if (arr[i]<arr[min])
min=i;
}
arr[min]=-arr[min]-1;
}
for (int x:arr)
{
System.out.print(x + " ");
}
}
} | linear | 1180_B. Nick and Array | CODEFORCES |
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
public class Main {
public static void main(String[] args)
{
Scanner stdin = new Scanner(System.in);
/*int n = stdin.nextInt();
for(int i = 0; i < n; i++)
{
test(stdin);
}*/
test(stdin);
stdin.close();
}
public static void test(Scanner stdin)
{
int n = stdin.nextInt();
int min = Integer.MAX_VALUE;
for(int i = 0; i < n; i++)
{
int a = stdin.nextInt();
if((int)((1.0)*a/(Math.max(i, n - i - 1))) < min)
{ min = (int)((1.0)*a/(Math.max(i, n - i - 1))); }
}
System.out.println(min);
}
} | linear | 1159_B. Expansion coefficient of the array | CODEFORCES |
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.*;
import static java.lang.Math.*;
public class Main2 {
private FastScanner scanner = new FastScanner();
public static void main(String[] args) {
new Main2().solve();
}
private void solve() {
int n = scanner.nextInt();
int a[][] = new int[n][3];
for (int i = 0; i < n; i++) {
a[i][0] = scanner.nextInt();
a[i][1] = scanner.nextInt();
a[i][2] = i;
}
int l = -1, r = -1;
Arrays.sort(a, (o1, o2) -> {
if (o1[0] != o2[0]) {
return o1[0] - o2[0];
} else {
return o2[1] - o1[1];
}
});
int maxr = -1, maxi = -1;
for (int i = 0; i < n; i++) {
if (a[i][1] <= maxr) {
l = a[i][2] + 1;
r = maxi + 1;
break;
}
if (a[i][1] > maxr) {
maxi = a[i][2];
maxr = a[i][1];
}
}
System.out.println(l + " " + r);
}
boolean check(int cnt[][], int[] tcnt, int mid) {
boolean ok = true;
for (int j = 0; j < 27; j++) {
if (cnt[mid][j] < tcnt[j]) {
ok = false;
}
}
return ok;
}
class Pair {
int c, f;
}
class FastScanner {
BufferedReader reader;
StringTokenizer tokenizer;
FastScanner() {
reader = new BufferedReader(new InputStreamReader(System.in), 32768);
tokenizer = null;
}
String next() {
while (tokenizer == null || !tokenizer.hasMoreTokens()) {
try {
tokenizer = new StringTokenizer(reader.readLine());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return tokenizer.nextToken();
}
int nextInt() {
return Integer.parseInt(next());
}
Integer[] nextA(int n) {
Integer a[] = new Integer[n];
for (int i = 0; i < n; i++) {
a[i] = scanner.nextInt();
}
return a;
}
public long nextLong() {
return Long.parseLong(next());
}
public double nextDouble() {
return Double.parseDouble(next());
}
public String nextLine() {
try {
return reader.readLine();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
} | nlogn | 976_C. Nested Segments | CODEFORCES |
import java.util.*;
import java.lang.*;
import java.math.*;
import java.io.*;
/* spar5h */
public class cf1 implements Runnable{
public void run() {
InputReader s = new InputReader(System.in);
PrintWriter w = new PrintWriter(System.out);
int t = 1;
while(t-- > 0) {
int n = s.nextInt(), m = s.nextInt();
int[] a = new int[n + 1];
for(int i = 1; i <= n; i++)
a[i] = s.nextInt();
int[] b = new int[n + 1];
for(int i = 1; i <= n; i++)
b[i] = s.nextInt();
ArrayList<Integer> list = new ArrayList<Integer>();
list.add(a[1]);
for(int i = 2; i <= n; i++) {
list.add(b[i]); list.add(a[i]);
}
list.add(b[1]);
double wt = m;
boolean check = true;
for(int i = list.size() - 1; i >= 0; i--) {
if(list.get(i) <= 1) {
check = false; break;
}
double x = wt / (list.get(i) - 1);
wt += x;
}
if(check)
w.println(wt - m);
else
w.println(-1);
}
w.close();
}
static class InputReader {
private InputStream stream;
private byte[] buf = new byte[1024];
private int curChar;
private int numChars;
private SpaceCharFilter filter;
public InputReader(InputStream stream)
{
this.stream = stream;
}
public int read()
{
if (numChars==-1)
throw new InputMismatchException();
if (curChar >= numChars)
{
curChar = 0;
try
{
numChars = stream.read(buf);
}
catch (IOException e)
{
throw new InputMismatchException();
}
if(numChars <= 0)
return -1;
}
return buf[curChar++];
}
public String nextLine()
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
String str = "";
try
{
str = br.readLine();
}
catch (IOException e)
{
e.printStackTrace();
}
return str;
}
public int nextInt()
{
int c = read();
while(isSpaceChar(c))
c = read();
int sgn = 1;
if (c == '-')
{
sgn = -1;
c = read();
}
int res = 0;
do
{
if(c<'0'||c>'9')
throw new InputMismatchException();
res *= 10;
res += c - '0';
c = read();
}
while (!isSpaceChar(c));
return res * sgn;
}
public long nextLong()
{
int c = read();
while (isSpaceChar(c))
c = read();
int sgn = 1;
if (c == '-')
{
sgn = -1;
c = read();
}
long res = 0;
do
{
if (c < '0' || c > '9')
throw new InputMismatchException();
res *= 10;
res += c - '0';
c = read();
}
while (!isSpaceChar(c));
return res * sgn;
}
public double nextDouble()
{
int c = read();
while (isSpaceChar(c))
c = read();
int sgn = 1;
if (c == '-')
{
sgn = -1;
c = read();
}
double res = 0;
while (!isSpaceChar(c) && c != '.')
{
if (c == 'e' || c == 'E')
return res * Math.pow(10, nextInt());
if (c < '0' || c > '9')
throw new InputMismatchException();
res *= 10;
res += c - '0';
c = read();
}
if (c == '.')
{
c = read();
double m = 1;
while (!isSpaceChar(c))
{
if (c == 'e' || c == 'E')
return res * Math.pow(10, nextInt());
if (c < '0' || c > '9')
throw new InputMismatchException();
m /= 10;
res += (c - '0') * m;
c = read();
}
}
return res * sgn;
}
public String readString()
{
int c = read();
while (isSpaceChar(c))
c = read();
StringBuilder res = new StringBuilder();
do
{
res.appendCodePoint(c);
c = read();
}
while (!isSpaceChar(c));
return res.toString();
}
public boolean isSpaceChar(int c)
{
if (filter != null)
return filter.isSpaceChar(c);
return c == ' ' || c == '\n' || c == '\r' || c == '\t' || c == -1;
}
public String next()
{
return readString();
}
public interface SpaceCharFilter
{
public boolean isSpaceChar(int ch);
}
}
public static void main(String args[]) throws Exception
{
new Thread(null, new cf1(),"cf1",1<<26).start();
}
} | linear | 1010_A. Fly | CODEFORCES |
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.StringTokenizer;
/**
*
* @author Haya
*/
public class CommentaryBoxes {
public static void main(String[] args) {
FastReader in = new FastReader();
long n = in.nextLong();
long m = in.nextLong();
long a = in.nextLong();
long b = in.nextLong();
long total = 0;
long val =(n%m);
if (n%m != 0){
long x = (val)*b;
long y = (m-val)*a;
total = Math.min(x, y);
}
System.out.println(Math.abs(total));
}
public static class FastReader {
BufferedReader br;
StringTokenizer st;
public FastReader() {
br = new BufferedReader(new InputStreamReader(System.in));
}
String next() {
while (st == null || !st.hasMoreElements()) {
try {
st = new StringTokenizer(br.readLine());
} catch (IOException e) {
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt() {
return Integer.parseInt(next());
}
long nextLong() {
return Long.parseLong(next());
}
double nextDouble() {
return Double.parseDouble(next());
}
String nextLine() {
String string = "";
try {
string = br.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return string;
}
}
}
| linear | 990_A. Commentary Boxes | CODEFORCES |
import java.util.*;
public class HelloWorld{
public static void main(String []args){
final long MOD = 1000000007;
Scanner scan = new Scanner(System.in);
int now = 1;
int maxStatements = scan.nextInt();
long[] dp = new long[maxStatements + 1];
dp[now] = 1;
while(maxStatements > 0)
{
String add = scan.next();
if (add.equals("f"))
{
now++;
}
else
{
for (int k = 1; k <= now; k++)
{
dp[k] = ((dp[k] + dp[k-1]) % MOD);
}
}
maxStatements--;
}
System.out.println(dp[now]);
}
} | quadratic | 909_C. Python Indentation | CODEFORCES |
import java.util.*;
public class ehab4 {
public static void main( String[] args ) {
Scanner in = new Scanner( System.in );
int a = 0, b = 0;
System.out.println( "? 0 0 " );
System.out.flush();
int c = in.nextInt();
for ( int i = 29; i >= 0; i-- ) {
System.out.println( "? " + ( a + ( 1 << i ) ) + " " + b );
System.out.flush();
int q1 = in.nextInt();
System.out.println( "? " + a + " " + ( b + ( 1 << i ) ) );
System.out.flush();
int q2 = in.nextInt();
if ( q1 == q2 ) {
if ( c == 1 )
a += ( 1 << i );
else if ( c == -1 )
b += ( 1 << i );
c = q1;
}
else if ( q1 == -1 ) {
a += ( 1 << i );
b += ( 1 << i );
}
else if ( q1 == -2 )
return;
}
System.out.println( "! " + a + " " + b );
System.out.flush();
}
}
| logn | 1088_D. Ehab and another another xor problem | CODEFORCES |
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.StringTokenizer;
import java.util.TreeMap;
import java.util.TreeSet;
public class Main {
static void insert(TreeMap<Integer, Integer>map,int v,int d)
{
if(!map.containsKey(v))map.put(v, 0);
map.put(v, d+map.get(v));
if(map.get(v)==0)map.remove(v);
}
static void cut(TreeSet<Integer> cuts, TreeMap<Integer, Integer>segments,int v)
{
int upper = cuts.higher(v) , lower = cuts.lower(v);
insert(segments, upper-lower, -1);
insert(segments, upper-v, 1);
insert(segments, v-lower, 1);
cuts.add(v);
}
public static void main(String[] args) throws Throwable {
Scanner sc = new Scanner(System.in);
int w = sc.nextInt(), h = sc.nextInt() , n = sc.nextInt();
TreeSet<Integer> vCuts = new TreeSet<>() , hCuts = new TreeSet<>();
TreeMap<Integer, Integer> vSegments = new TreeMap<>() , hSegments = new TreeMap<>();
vCuts.add(0);vCuts.add(w);
hCuts.add(0);hCuts.add(h);
insert(vSegments, w, 1);
insert(hSegments, h, 1);
StringBuilder sb = new StringBuilder();
while(n-->0)
{
if(sc.next().equals("H"))
cut(hCuts, hSegments, sc.nextInt());
else
cut(vCuts, vSegments, sc.nextInt());
sb.append(1l*hSegments.lastKey() * vSegments.lastKey() + "\n");
}
System.out.println(sb);
}
static class Scanner {
StringTokenizer st;
BufferedReader br;
public Scanner(InputStream s) {br = new BufferedReader(new InputStreamReader(s));}
public Scanner(String file) throws FileNotFoundException {br = new BufferedReader(new FileReader(file));}
public String next() throws IOException {while (st == null || !st.hasMoreTokens())
st = new StringTokenizer(br.readLine());
return st.nextToken();}
public int nextInt() throws IOException {return Integer.parseInt(next());}
public long nextLong() throws IOException {return Long.parseLong(next());}
public String nextLine() throws IOException {return br.readLine();}
public double nextDouble() throws IOException {
String x = next();
StringBuilder sb = new StringBuilder("0");
double res = 0, f = 1;
boolean dec = false, neg = false;
int start = 0;
if (x.charAt(0) == '-') {
neg = true;
start++;
}
for (int i = start; i < x.length(); i++)
if (x.charAt(i) == '.') {
res = Long.parseLong(sb.toString());
sb = new StringBuilder("0");
dec = true;
} else {
sb.append(x.charAt(i));
if (dec)
f *= 10;
}
res += Long.parseLong(sb.toString()) / f;
return res * (neg ? -1 : 1);
}
public int[] nexIntArray() throws Throwable {
st = new StringTokenizer(br.readLine());
int[] a = new int[st.countTokens()];
for (int i = 0; i < a.length; i++)a[i] = nextInt();
return a;
}
public boolean ready() throws IOException {return br.ready();}
}
} | nlogn | 528_A. Glass Carving | CODEFORCES |
import java.util.Scanner;
//import java.util.Scanner;
public class SingleWildcard {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner input =new Scanner(System.in);
int a = input.nextInt();
int b = input.nextInt();
char[] s1 =new char[a];
s1 = input.next().toCharArray();
char[] s2 = new char[b];
s2 = input.next().toCharArray();
boolean condition = false;
for(int i=0; i<a;i++){
if(s1[i]=='*'){
condition= true;
break;
}
}
if(!condition){
if(match(s1,s2)){
System.out.println("YES");
}
else
System.out.println("NO");
return;
}
else{
int i=0;
if(s1.length-1>s2.length){
System.out.println("NO");
return;
}
while(i<s1.length && i<s2.length && s1[i]==s2[i]){
i++;
}
int j=s2.length-1;
int k = s1.length-1;
while(j>=0 && k>=0 && s1[k]==s2[j] && i<=j){
j--;
k--;
}
//System.out.println(i);
if(i==k && i>=0 && i<s1.length && s1[i]=='*' ){
System.out.println("YES");
return;
}
System.out.println("NO");
}
}
static boolean match(char[] s1,char[] s2){
if(s1.length!=s2.length)return false;
for(int i=0; i<s1.length;i++){
if(s1[i]!=s2[i])return false;
}
return true;
}
}
| linear | 1023_A. Single Wildcard Pattern Matching | CODEFORCES |
import java.io.*;
import java.util.Arrays;
import java.util.StringJoiner;
import java.util.StringTokenizer;
import java.util.function.Function;
public class Main {
static String S;
public static void main(String[] args) {
FastScanner sc = new FastScanner(System.in);
S = sc.next();
System.out.println(solve());
}
static int solve() {
int ans = -1;
int time = 1;
int n = S.length();
for (int i = 1; i < n*2; i++) {
if( S.charAt((i-1)%n) != S.charAt(i%n) ) {
time++;
} else {
ans = Math.max(time, ans);
time = 1;
}
}
ans = Math.max(time, ans);
if( ans == n*2 ) {
return n;
} else {
return ans;
}
}
@SuppressWarnings("unused")
static class FastScanner {
private BufferedReader reader;
private StringTokenizer tokenizer;
FastScanner(InputStream in) {
reader = new BufferedReader(new InputStreamReader(in));
tokenizer = null;
}
String next() {
if (tokenizer == null || !tokenizer.hasMoreTokens()) {
try {
tokenizer = new StringTokenizer(reader.readLine());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return tokenizer.nextToken();
}
String nextLine() {
if (tokenizer == null || !tokenizer.hasMoreTokens()) {
try {
return reader.readLine();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return tokenizer.nextToken("\n");
}
long nextLong() {
return Long.parseLong(next());
}
int nextInt() {
return Integer.parseInt(next());
}
int[] nextIntArray(int n) {
int[] a = new int[n];
for (int i = 0; i < n; i++)
a[i] = nextInt();
return a;
}
int[] nextIntArray(int n, int delta) {
int[] a = new int[n];
for (int i = 0; i < n; i++)
a[i] = nextInt() + delta;
return a;
}
long[] nextLongArray(int n) {
long[] a = new long[n];
for (int i = 0; i < n; i++)
a[i] = nextLong();
return a;
}
}
static <A> void writeLines(A[] as, Function<A, String> f) {
PrintWriter pw = new PrintWriter(System.out);
for (A a : as) {
pw.println(f.apply(a));
}
pw.flush();
}
static void writeLines(int[] as) {
PrintWriter pw = new PrintWriter(System.out);
for (int a : as) pw.println(a);
pw.flush();
}
static void writeLines(long[] as) {
PrintWriter pw = new PrintWriter(System.out);
for (long a : as) pw.println(a);
pw.flush();
}
static int max(int... as) {
int max = Integer.MIN_VALUE;
for (int a : as) max = Math.max(a, max);
return max;
}
static int min(int... as) {
int min = Integer.MAX_VALUE;
for (int a : as) min = Math.min(a, min);
return min;
}
static void debug(Object... args) {
StringJoiner j = new StringJoiner(" ");
for (Object arg : args) {
if (arg instanceof int[]) j.add(Arrays.toString((int[]) arg));
else if (arg instanceof long[]) j.add(Arrays.toString((long[]) arg));
else if (arg instanceof double[]) j.add(Arrays.toString((double[]) arg));
else if (arg instanceof Object[]) j.add(Arrays.toString((Object[]) arg));
else j.add(arg.toString());
}
System.err.println(j.toString());
}
}
| linear | 1025_C. Plasticine zebra | CODEFORCES |
import java.util.*;
public class Main{
public static void main(String args[]){
Scanner sc=new Scanner(System.in);
int n=sc.nextInt();
int a[]=new int[n];
for(int i=0;i<n;i++)
a[i]=sc.nextInt();
Arrays.sort(a);
//boolean
int t=1,c=0;
for(int i=1;i<n;i++){
if(a[i]==a[i-1])
{
if(i-2>=0&&a[i-2]==a[i-1]-1){
System.out.println("cslnb");
return;
}
c++;
}
if(a[i]==a[i-1]&&a[i]==0){
System.out.println("cslnb");
return;
}
}
if(c>1)
{
System.out.println("cslnb");
return;
}
for(int i=0;i<n;i++){
if((a[i]-i)%2!=0)
t=t^1;
}
if(t==1)
System.out.println("cslnb");
else
System.out.println("sjfnb");
}
} | linear | 1190_B. Tokitsukaze, CSL and Stone Game | CODEFORCES |
import java.util.*;
public class helloWorld
{
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
int n = in.nextInt();
int m = in.nextInt();
int[] ar = new int[200];
String str = in.next();
for(int i = 0; i < str.length(); i++)
ar[ str.charAt(i) ]++;
int ans = 100000;
for(int i = 'A'; i < 'A' + m; i++)
ans = Math.min(ans, ar[i]);
ans *= m;
System.out.println(ans);
in.close();
}
}
| linear | 1038_A. Equality | CODEFORCES |
import java.util.Scanner;
import javafx.geometry.Point2D;
public class ChessKing {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
long size = input.nextLong();
long a = input.nextLong();
long b = input.nextLong();
long sum = a+b;
long d = sum-2;
long d1 = size*2 - sum;
if(d<d1) System.out.println("White");
else if(d>d1) System.out.println("Black");
else System.out.println("White");
}
}
| constant | 1075_A. The King's Race | CODEFORCES |
import java.io.*;
import java.util.*;
import java.text.*;
import java.lang.*;
import java.math.*;
public class Main{
static ArrayList a[]=new ArrayList[200001];
static int Count(int a[][],int n) {
dsu d=new dsu(n);
for(int i=0;i<n;i++) {
for(int j=0;j<n;j++) {
if(a[i][j]==0) {
d.union(i, j);
}
}
}
int cnt=0;
boolean chk[]=new boolean [n];
for(int i=0;i<n;i++) {
int p=d.root(i);
if(!chk[p]) {
chk[p]=true;
cnt++;
}
}
return cnt;
}
public void solve () {
InputReader in = new InputReader(System.in);
PrintWriter pw = new PrintWriter(System.out);
int n=in.nextInt();
int a=in.nextInt();
int b=in.nextInt();
if(a==1 || b==1) {
int ans[][]=new int [n][n];
int temp=(a==1)?b:a;
for(int i=1;i<=n-temp;i++) {
ans[i][i-1]=1;
ans[i-1][i]=1;
}
int freq=Count(ans,n);
if(freq!=1) {
pw.println("NO");
}
else {
pw.println("YES");
for(int i=0;i<n;i++) {
for(int j=0;j<n;j++) {
if(i==j) {
pw.print(0);
}
else
pw.print((ans[i][j]+((temp==b)?1:0))%2);
}
pw.println();
}
}
}
else {
pw.print("NO");
}
pw.flush();
pw.close();
}
public static void main(String[] args) throws Exception {
new Thread(null,new Runnable() {
public void run() {
new Main().solve();
}
},"1",1<<26).start();
}
static void debug(Object... o) {
System.out.println(Arrays.deepToString(o));
}
static class InputReader
{
private final InputStream stream;
private final byte[] buf = new byte[8192];
private int curChar, snumChars;
private SpaceCharFilter filter;
public InputReader(InputStream stream)
{
this.stream = stream;
}
public int snext()
{
if (snumChars == -1)
throw new InputMismatchException();
if (curChar >= snumChars)
{
curChar = 0;
try
{
snumChars = stream.read(buf);
}
catch (IOException e)
{
throw new InputMismatchException();
}
if (snumChars <= 0)
return -1;
}
return buf[curChar++];
}
public int nextInt()
{
int c = snext();
while (isSpaceChar(c))
{
c = snext();
}
int sgn = 1;
if (c == '-')
{
sgn = -1;
c = snext();
}
int res = 0;
do
{
if (c < '0' || c > '9')
throw new InputMismatchException();
res *= 10;
res += c - '0';
c = snext();
} while (!isSpaceChar(c));
return res * sgn;
}
public long nextLong()
{
int c = snext();
while (isSpaceChar(c))
{
c = snext();
}
int sgn = 1;
if (c == '-')
{
sgn = -1;
c = snext();
}
long res = 0;
do
{
if (c < '0' || c > '9')
throw new InputMismatchException();
res *= 10;
res += c - '0';
c = snext();
} while (!isSpaceChar(c));
return res * sgn;
}
public int[] nextIntArray(int n)
{
int a[] = new int[n];
for (int i = 0; i < n; i++)
{
a[i] = nextInt();
}
return a;
}
public String readString()
{
int c = snext();
while (isSpaceChar(c))
{
c = snext();
}
StringBuilder res = new StringBuilder();
do
{
res.appendCodePoint(c);
c = snext();
} while (!isSpaceChar(c));
return res.toString();
}
public String nextLine()
{
int c = snext();
while (isSpaceChar(c))
c = snext();
StringBuilder res = new StringBuilder();
do
{
res.appendCodePoint(c);
c = snext();
} while (!isEndOfLine(c));
return res.toString();
}
public boolean isSpaceChar(int c)
{
if (filter != null)
return filter.isSpaceChar(c);
return c == ' ' || c == '\n' || c == '\r' || c == '\t' || c == -1;
}
private boolean isEndOfLine(int c)
{
return c == '\n' || c == '\r' || c == -1;
}
public interface SpaceCharFilter
{
public boolean isSpaceChar(int ch);
}
}
public static long mod = 1000000007;
public static int d;
public static int p;
public static int q;
public void extended(int a,int b) {
if(b==0) {
d=a;
p=1;
q=0;
}
else
{
extended(b,a%b);
int temp=p;
p=q;
q=temp-(a/b)*q;
}
}
public static long[] shuffle(long[] a,Random gen)
{
int n = a.length;
for(int i=0;i<n;i++)
{
int ind = gen.nextInt(n-i)+i;
long temp = a[ind];
a[ind] = a[i];
a[i] = temp;
}
return a;
}
public static void swap(int a, int b){
int temp = a;
a = b;
b = temp;
}
public static HashSet<Integer> primeFactorization(int n)
{
HashSet<Integer> a =new HashSet<Integer>();
for(int i=2;i*i<=n;i++)
{
while(n%i==0)
{
a.add(i);
n/=i;
}
}
if(n!=1)
a.add(n);
return a;
}
public static void sieve(boolean[] isPrime,int n)
{
for(int i=1;i<n;i++)
isPrime[i] = true;
isPrime[0] = false;
isPrime[1] = false;
for(int i=2;i*i<n;i++)
{
if(isPrime[i] == true)
{
for(int j=(2*i);j<n;j+=i)
isPrime[j] = false;
}
}
}
public static int GCD(int a,int b)
{
if(b==0)
return a;
else
return GCD(b,a%b);
}
static class pair implements Comparable<pair>
{
Integer x;
Long y;
pair(int x,long y)
{
this.x=x;
this.y=y;
}
public int compareTo(pair o) {
int result = x.compareTo(o.x);
if(result==0)
result = y.compareTo(o.y);
return result;
}
public String toString()
{
return x+" "+y;
}
public boolean equals(Object o)
{
if (o instanceof pair)
{
pair p = (pair)o;
return p.x == x && p.y == y ;
}
return false;
}
public int hashCode()
{
return new Long(x).hashCode()*31 + new Long(y).hashCode();
}
}
}
class pair implements Comparable<pair>
{
Integer x;
Long y;
pair(int x,long y)
{
this.x=x;
this.y=y;
}
public int compareTo(pair o) {
int result = x.compareTo(o.x);
if(result==0)
result = y.compareTo(o.y);
return result;
}
public String toString()
{
return x+" "+y;
}
public boolean equals(Object o)
{
if (o instanceof pair)
{
pair p = (pair)o;
return p.x == x && p.y == y ;
}
return false;
}
public int hashCode()
{
return new Long(x).hashCode()*31 + new Long(y).hashCode();
}
}
class dsu{
int parent[];
dsu(int n){
parent=new int[n+1];
for(int i=0;i<=n;i++)
{
parent[i]=i;
}
}
int root(int n) {
while(parent[n]!=n)
{
parent[n]=parent[parent[n]];
n=parent[n];
}
return n;
}
void union(int _a,int _b) {
int p_a=root(_a);
int p_b=root(_b);
parent[p_a]=p_b;
}
boolean find(int a,int b) {
if(root(a)==root(b))
return true;
else
return false;
}
}
| quadratic | 990_D. Graph And Its Complement | CODEFORCES |
import java.util.*;
import java.io.*;
public class A
{
public static void main(String ar[]) throws Exception
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
String s1[]=br.readLine().split(" ");
int n=Integer.parseInt(s1[0]);
long x=Long.parseLong(s1[1]);
long y=Long.parseLong(s1[2]);
long S=0;
long mod=1000000007;
B a[]=new B[n];
TreeMap<Long,Long> tm=new TreeMap<Long,Long>();
long ans[]=new long[n];
for(int i=0;i<n;i++)
{
String s2[]=br.readLine().split(" ");
long l=Long.parseLong(s2[0]);
long r=Long.parseLong(s2[1]);
B b1=new B(l,r);
a[i]=b1;
}
Arrays.sort(a,new The_Comp());
for(int i=0;i<n;i++)
{
long l=a[i].x;
long r=a[i].y;
if(tm.floorKey(l-1)!=null)
{
long u=tm.floorKey(l-1);
long v=l;
if((v-u)*y<x)
{ ans[i]=((r-u)*y)%mod;
if(tm.get(u)>1)
tm.put(u,tm.get(u)-1);
else
tm.remove(u);
}
else
{ ans[i]=(x+(r-l)*y)%mod; }
}
else
ans[i]=(x+(r-l)*y)%mod;
S=(S+ans[i])%mod;
if(tm.containsKey(r))
tm.put(r,1+tm.get(r));
else
tm.put(r,(long)1);
}
System.out.println(S);
}
}
class The_Comp implements Comparator<B>
{
public int compare(B b1,B b2)
{
if(b1.x>b2.x)
return 1;
else if(b1.x==b2.x)
{
if(b1.y>b2.y)
return 1;
else if(b1.y==b2.y)
return 0;
else
return -1;
}
else
return -1;
}
}
class B
{
long x=(long)1;
long y=(long)1;
public B(long l1,long l2)
{ x=l1; y=l2; }
} | nlogn | 1061_D. TV Shows | CODEFORCES |
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.*;
public class e {
public static class FastReader {
BufferedReader br;
StringTokenizer st;
//it reads the data about the specified point and divide the data about it ,it is quite fast
//than using direct
public FastReader() {
br = new BufferedReader(new InputStreamReader(System.in));
}
String next() {
while (st == null || !st.hasMoreTokens()) {
try {
st = new StringTokenizer(br.readLine());
} catch (Exception r) {
r.printStackTrace();
}
}
return st.nextToken();
}
int nextInt() {
return Integer.parseInt(next());//converts string to integer
}
double nextDouble() {
return Double.parseDouble(next());
}
long nextLong() {
return Long.parseLong(next());
}
String nextLine() {
String str = "";
try {
str = br.readLine();
} catch (Exception r) {
r.printStackTrace();
}
return str;
}
}
static ArrayList<String>list1=new ArrayList<String>();
static void combine(String instr, StringBuffer outstr, int index,int k)
{
if(outstr.length()==k)
{
list1.add(outstr.toString());return;
}
if(outstr.toString().length()==0)
outstr.append(instr.charAt(index));
for (int i = 0; i < instr.length(); i++)
{
outstr.append(instr.charAt(i));
combine(instr, outstr, i + 1,k);
outstr.deleteCharAt(outstr.length() - 1);
}
index++;
}
static ArrayList<ArrayList<Integer>>l=new ArrayList<>();
static void comb(int n,int k,int ind,ArrayList<Integer>list)
{
if(k==0)
{
l.add(new ArrayList<>(list));
return;
}
for(int i=ind;i<=n;i++)
{
list.add(i);
comb(n,k-1,ind+1,list);
list.remove(list.size()-1);
}
}
public static PrintWriter out = new PrintWriter (new BufferedOutputStream(System.out));
public static void main(String[] args) {
// TODO Auto-generated method stub
FastReader in=new FastReader();
HashMap<Integer,Integer>map=new HashMap<Integer,Integer>();
int n=in.nextInt();
int r=in.nextInt();
double theta=(double)360/(double)n;
double b=1-((double)2/(double)(1-Math.cos((double)2*Math.PI/(double)n)));
double x=Math.sqrt(1-b)-1;
double ans=(double)r/(double)x;
System.out.println(ans);
}
}
| constant | 1100_C. NN and the Optical Illusion | CODEFORCES |
import java.util.*;
public class D5 {
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
int a = input.nextInt(), v = input.nextInt();
int l = input.nextInt(), d = input.nextInt(), w = input.nextInt();
double lo = 0, hi = v;
for(int iter = 0; iter < 1000; iter++)
{
double mid = (lo+hi)/2;
if(can(mid, a, d, w)) lo = mid;
else hi = mid;
}
//System.out.println(lo);
double t1 = lo / a;
double gone = .5 * t1 * t1 * a;
if(lo > w)
{
gone += -a * .5 * (lo - w) / a * (lo - w) / a + lo * (lo - w) / a;
t1 += (lo - w) / a;
}
t1 += (d - gone) / lo;
//System.out.println(t1);
double v0 = Math.min(lo, w);
double togo = l - d;
double toAdd = (-v0 + Math.sqrt(v0 * v0 + 4 * togo * .5 * a)) / a;
if(toAdd * a + v0 > v)
{
double tt = (v - v0) / a;
t1 += tt;
togo -= .5 * a * tt * tt + v0 * tt;
t1 += togo / v;
}
else t1 += toAdd;
System.out.println(t1);
}
static boolean can(double v, double a, double d, double max)
{
double t1 = v / a;
double distGone = .5 * a * t1 * t1;
if(v > max)
{
t1 = (v - max) / a;
distGone += -.5 * a * t1 * t1 + v * t1;
}
return distGone <= d;
}
}
| constant | 5_D. Follow Traffic Rules | CODEFORCES |
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
public class D909 {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
char[] line = br.readLine().toCharArray();
int n = line.length;
int l = 0;
ArrayList<Node> groups = new ArrayList<>();
Node node = new Node(line[0], 1);
groups.add(node);
for (int i = 1; i < n; i++) {
if (line[i] == groups.get(l).letter) {
groups.get(l).count++;
} else {
node = new Node(line[i], 1);
groups.add(node);
l++;
}
}
int moves = 0;
ArrayList<Node> temp = new ArrayList<>();
while (groups.size() > 1) {
moves++;
l = groups.size();
groups.get(0).count--;
groups.get(l - 1).count--;
for (int i = 1; i < l - 1; i++) {
groups.get(i).count -= 2;
}
int p;
for (p = 0; p < l; p++) {
if (groups.get(p).count > 0) {
temp.add(groups.get(p));
break;
}
}
int lTemp = temp.size();
for (p++; p < l; p++) {
if (groups.get(p).count <= 0) {
continue;
}
if (groups.get(p).letter == temp.get(lTemp - 1).letter) {
temp.get(lTemp - 1).count += groups.get(p).count;
} else {
temp.add(groups.get(p));
lTemp++;
}
}
groups.clear();
groups.addAll(temp);
temp.clear();
}
System.out.println(moves);
}
private static class Node {
char letter;
int count;
Node(char letter, int count) {
this.letter = letter;
this.count = count;
}
}
}
| linear | 909_D. Colorful Points | CODEFORCES |
import java.util.*;
import java.io.*;
public class X
{
public static void main(String args[])throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(br.readLine());
String s1 = br.readLine();
String s2 = br.readLine();
int i=0;
char c1,c2;
int cost = 0;
while(i<n)
{
c1 = s1.charAt(i);
c2 = s2.charAt(i);
if(c1 != c2)
{
if((i+1)<n && s1.charAt(i+1) != s2.charAt(i+1) && s1.charAt(i) != s1.charAt(i+1))
{
cost +=1;
i++;
}
else
{
cost +=1;
}
}
i++;
}
System.out.println(cost);
}
} | linear | 1037_C. Equalize | CODEFORCES |
import java.util.Scanner;
public class ChainReaction {
public static void main(String [] args) {
Scanner kb = new Scanner(System.in);
int num = kb.nextInt();
int[] beacons = new int[1000002];
for (int i=0; i<num; i++) {
beacons[kb.nextInt()] = kb.nextInt();
}
int [] dp = new int[1000002];
int max = 0;
if (beacons[0] != 0)
dp[0] = 1;
for (int i=1; i<dp.length; i++) {
if (beacons[i] == 0) {
dp[i] = dp[i-1];
} else {
int index = i-1-beacons[i];
if (index<0)
dp[i] = 1;
else
dp[i] = 1 + dp[index];
}
max = Math.max(max, dp[i]);
//if (i<11)
//System.out.println(i +" is "+dp[i]);
}
System.out.println(num-max);
}
}
| linear | 608_C. Chain Reaction | CODEFORCES |
import java.util.*;
import java.io.*;
public class A{
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int ans = 0;
for(int i = 1; i <= n; i++){
ans += ((i*2) <= n) ? i : n-i+1;
}
System.out.println(ans);
}
} | linear | 909_B. Segments | CODEFORCES |
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Objects;
import java.util.Stack;
import java.util.StringTokenizer;
public class Test
{
static PrintWriter pw = new PrintWriter(System.out);
public static void main(String[] args)throws Exception
{
Reader.init(System.in);
int n = Reader.nextInt();
int p = Reader.nextInt();
int L = Reader.nextInt();
int R = Reader.nextInt();
int a = 1;
int b = n;
int res = 0;
if(a == L && b == R)
{
res = 0;
}
else if(L != a && R != b && p >= L && p <= R)
{
res = Math.min(p-L, R-p);
res += R- L + 2;
}
else if(L != a && R != b && p < L )
{
res += L-p + 1;
res += R - L +1;
}
else if(L != a && R != b && p > R)
{
res += p-R + 1;
res += R - L +1;
}
else if(a == L && p >=L && p<=R)
{
res += R - p + 1;
}
else if(R == b && p>=L && p<=R)
{
res += p - L + 1;
}
else if(a == L && p > R)
{
res += p - R + 1;
}
else if(R == b && p<L)
{
res += L - p + 1;
}
pw.print(res);
pw.close();
}
}
class Reader {
static BufferedReader reader;
static StringTokenizer tokenizer;
public static int pars(String x) {
int num = 0;
int i = 0;
if (x.charAt(0) == '-') {
i = 1;
}
for (; i < x.length(); i++) {
num = num * 10 + (x.charAt(i) - '0');
}
if (x.charAt(0) == '-') {
return -num;
}
return num;
}
static void init(InputStream input) {
reader = new BufferedReader(
new InputStreamReader(input));
tokenizer = new StringTokenizer("");
}
static void init(FileReader input) {
reader = new BufferedReader(input);
tokenizer = new StringTokenizer("");
}
static String next() throws IOException {
while (!tokenizer.hasMoreTokens()) {
tokenizer = new StringTokenizer(
reader.readLine());
}
return tokenizer.nextToken();
}
static int nextInt() throws IOException {
return pars(next());
}
static long nextLong() throws IOException {
return Long.parseLong(next());
}
static double nextDouble() throws IOException {
return Double.parseDouble(next());
}
} | constant | 915_B. Browser | CODEFORCES |
import java.io.OutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.StringTokenizer;
import java.io.BufferedReader;
import java.io.InputStream;
/**
* Built using CHelper plug-in
* Actual solution is at the top
*/
public class Main {
public static void main(String[] args) {
InputStream inputStream = System.in;
OutputStream outputStream = System.out;
InputReader in = new InputReader(inputStream);
PrintWriter out = new PrintWriter(outputStream);
TaskG solver = new TaskG();
solver.solve(1, in, out);
out.close();
}
static class TaskG {
static final long MODULO = (long) 1e9 + 7;
static final long BIG = Long.MAX_VALUE - Long.MAX_VALUE % MODULO;
static final int[] ONE = new int[]{1};
int k;
int n;
long[] globalRes;
int[] p2;
public void solve(int testNumber, InputReader in, PrintWriter out) {
n = in.nextInt();
k = in.nextInt();
globalRes = new long[k + 1];
p2 = new int[n + 1];
p2[0] = 1;
for (int i = 1; i <= n; ++i) p2[i] = (int) (2 * p2[i - 1] % MODULO);
Vertex[] vs = new Vertex[n];
for (int i = 0; i < n; ++i) vs[i] = new Vertex();
for (int i = 0; i < n - 1; ++i) {
Vertex a = vs[in.nextInt() - 1];
Vertex b = vs[in.nextInt() - 1];
a.adj.add(b);
b.adj.add(a);
}
vs[0].dfs(null);
long[][] ways = new long[k + 1][k + 1];
ways[0][0] = 1;
for (int i = 1; i <= k; ++i) {
for (int j = 1; j <= k; ++j) {
ways[i][j] = j * (ways[i - 1][j] + ways[i - 1][j - 1]) % MODULO;
}
}
long sum = 0;
for (int i = 1; i <= k; ++i) {
long s = globalRes[i];
s %= MODULO;
sum = (sum + s * ways[k][i]) % MODULO;
}
out.println(sum);
}
class Vertex {
int[] res;
int subtreeSize;
List<Vertex> adj = new ArrayList<>();
public void dfs(Vertex parent) {
subtreeSize = 1;
int[] prod = ONE;
for (Vertex child : adj)
if (child != parent) {
child.dfs(this);
subtreeSize += child.subtreeSize;
}
int mult = 2;//p2[n - subtreeSize];
for (Vertex child : adj)
if (child != parent) {
int[] c = child.res;
prod = mul(prod, c);
subFrom(globalRes, c, 1);
}
addTo(globalRes, prod, mult);
res = insertEdge(prod);
}
private int[] insertEdge(int[] a) {
int len = a.length + 1;
if (len > k) len = k + 1;
int[] b = new int[len];
b[0] = a[0] * 2;
if (b[0] >= MODULO) b[0] -= MODULO;
for (int i = 1; i < len; ++i) {
long s = a[i - 1];
if (i < a.length) s += a[i];
if (s >= MODULO) s -= MODULO;
s = s * 2;
if (s >= MODULO) s -= MODULO;
b[i] = (int) s;
}
b[1] -= 1;
if (b[1] < 0) b[1] += MODULO;
return b;
}
private void addTo(long[] a, int[] b, int mult) {
for (int i = 0; i < b.length; ++i) {
long s = a[i] + b[i] * (long) mult;
if (s < 0) s -= BIG;
a[i] = s;
}
}
private void subFrom(long[] a, int[] b, int mult) {
for (int i = 0; i < b.length; ++i) {
long s = a[i] + (MODULO - b[i]) * (long) mult;
if (s < 0) s -= BIG;
a[i] = s;
}
}
private int[] mul(int[] a, int[] b) {
int len = a.length + b.length - 1;
if (len > k) len = k + 1;
int[] c = new int[len];
for (int i = 0; i < len; ++i) {
long s = 0;
int left = Math.max(0, i - (b.length - 1));
int right = Math.min(a.length - 1, i);
for (int ia = left; ia <= right; ++ia) {
int ib = i - ia;
s += a[ia] * (long) b[ib];
if (s < 0) s -= BIG;
}
c[i] = (int) (s % MODULO);
}
return c;
}
}
}
static class InputReader {
public BufferedReader reader;
public StringTokenizer tokenizer;
public InputReader(InputStream stream) {
reader = new BufferedReader(new InputStreamReader(stream), 32768);
tokenizer = null;
}
public String next() {
while (tokenizer == null || !tokenizer.hasMoreTokens()) {
try {
tokenizer = new StringTokenizer(reader.readLine());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return tokenizer.nextToken();
}
public int nextInt() {
return Integer.parseInt(next());
}
}
}
| quadratic | 1097_G. Vladislav and a Great Legend | CODEFORCES |
import java.io.OutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.InputMismatchException;
import java.io.IOException;
import java.io.InputStream;
/**
* Built using CHelper plug-in
* Actual solution is at the top
*
* @author Pradyumn
*/
public class Main {
public static void main(String[] args) {
InputStream inputStream = System.in;
OutputStream outputStream = System.out;
FastReader in = new FastReader(inputStream);
PrintWriter out = new PrintWriter(outputStream);
TaskB solver = new TaskB();
solver.solve(1, in, out);
out.close();
}
static class TaskB {
FastReader in;
PrintWriter out;
int n;
public void solve(int testNumber, FastReader in, PrintWriter out) {
this.in = in;
this.out = out;
n = in.nextInt();
if (n % 4 != 0) {
out.println("! -1");
return;
}
int low = 0;
int high = n >> 1;
if (BValue(low) == 0) {
out.println("! " + (low + 1));
return;
}
boolean value = BValue(low) > 0;
while (high - low > 1) {
int mid = (high + low) >> 1;
int BVal = BValue(mid);
if (BVal == 0) {
out.println("! " + (mid + 1));
return;
}
if (value) {
if (BVal < 0) {
high = mid;
} else {
low = mid;
}
} else {
if (BVal > 0) {
high = mid;
} else {
low = mid;
}
}
}
out.println("! -1");
}
public int BValue(int index) {
out.println("? " + (index + 1));
out.flush();
int f = in.nextInt();
out.println("? " + (index + 1 + (n >> 1)));
out.flush();
int s = in.nextInt();
return f - s;
}
}
static class FastReader {
private InputStream stream;
private byte[] buf = new byte[8192];
private int curChar;
private int pnumChars;
public FastReader(InputStream stream) {
this.stream = stream;
}
private int pread() {
if (pnumChars == -1) {
throw new InputMismatchException();
}
if (curChar >= pnumChars) {
curChar = 0;
try {
pnumChars = stream.read(buf);
} catch (IOException e) {
throw new InputMismatchException();
}
if (pnumChars <= 0) {
return -1;
}
}
return buf[curChar++];
}
public int nextInt() {
int c = pread();
while (isSpaceChar(c))
c = pread();
int sgn = 1;
if (c == '-') {
sgn = -1;
c = pread();
}
int res = 0;
do {
if (c == ',') {
c = pread();
}
if (c < '0' || c > '9') {
throw new InputMismatchException();
}
res *= 10;
res += c - '0';
c = pread();
} while (!isSpaceChar(c));
return res * sgn;
}
private boolean isSpaceChar(int c) {
return c == ' ' || c == '\n' || c == '\r' || c == '\t' || c == -1;
}
}
}
| logn | 1019_B. The hat | CODEFORCES |
import java.math.BigInteger;
import java.util.Scanner;
public class Main {
static Scanner sc = new Scanner (System.in);
public static void main(String[] args) {
int n = sc.nextInt();
int k = sc.nextInt();
char str[][] = new char[5][n];
for(int i = 0;i < 4;i ++){
for(int j = 0;j < n;j ++)
str[i][j] = '.';
}
if(k % 2 == 0){
k /= 2;
for(int i = 1;i <= 2;i++){
for(int j = 1;j <= k;j++)
str[i][j] = '#';
}
}
else{
str[1][n / 2] = '#';
if(k != 1){
int tmp = n / 2;
if(k <= n - 2){
for(int i = 1;i<= (k - 1) / 2;i++){
str[1][i] = '#';
str[1][n - 1 - i] = '#';
}
}
else{
for(int i = 1;i <= n - 2;i++) str[1][i] = '#';
k -= n - 2;
for(int i = 1;i <= k/2;i++){
str[2][i] = '#';
str[2][n - 1 - i]='#';
}
}
}
}
System.out.println("YES");
for(int i = 0;i < 4;i ++){
System.out.println(str[i]);
}
}
} | linear | 980_B. Marlin | CODEFORCES |
import java.util.Scanner;
public class HammingDistancesSum {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String a = sc.nextLine(), b = sc.nextLine();
long sum = 0;
int frequency[][] = new int[200010][2];
for (int i = 1; i <= b.length(); i++) {
for (int j = 0; j < 2; j++)
frequency[i][j] = frequency[i - 1][j];
frequency[i][Character.getNumericValue((b.charAt(i - 1)))]++;
}
for (int i = 0; i < a.length(); i++) {
int c = Character.getNumericValue(a.charAt(i));
for (int j = 0; j < 2; j++) {
int flippingTerm = Math.abs(c - j);
int endOfWindowValue = frequency[b.length() - a.length() + i + 1][j];
int startOfWindowOffset = frequency[i][j];
sum += flippingTerm * (endOfWindowValue - startOfWindowOffset);
}
}
System.out.println(sum);
sc.close();
}
}
| linear | 608_B. Hamming Distance Sum | CODEFORCES |
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Scanner;
public class Solution {
public static void main(String[] args) {
Solution solution = new Solution();
System.out.println(solution.solve());
}
private int solve() {
Scanner in = new Scanner(System.in);
int n = in.nextInt();
int m = in.nextInt();
int[] a = new int[m];
for (int i = 0; i < m; ++i) a[i] = in.nextInt();
if (n > m) return 0;
Map<Integer, Integer> map = new HashMap<>();
for (int k: a) map.put(k, map.getOrDefault(k, 0) + 1);
List<Integer> keySet = new ArrayList<>(map.keySet());
int end = m / n;
keySet.sort((u, v) -> -Integer.compare(u, v));
do {
int count = 0;
for (int k: keySet) {
count += map.get(k) / end;
if (count >= n) return end;
}
} while (--end > 0);
return 0;
}
}
| nlogn | 1011_B. Planning The Expedition | CODEFORCES |
import java.io.OutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.InputMismatchException;
import java.io.IOException;
import java.io.InputStream;
/**
* Built using CHelper plug-in
* Actual solution is at the top
*
* @author Pradyumn
*/
public class Main {
public static void main(String[] args) {
InputStream inputStream = System.in;
OutputStream outputStream = System.out;
FastReader in = new FastReader(inputStream);
PrintWriter out = new PrintWriter(outputStream);
TaskB solver = new TaskB();
solver.solve(1, in, out);
out.close();
}
static class TaskB {
FastReader in;
PrintWriter out;
int n;
public void solve(int testNumber, FastReader in, PrintWriter out) {
this.in = in;
this.out = out;
n = in.nextInt();
if (n % 4 != 0) {
out.println("! -1");
return;
}
int low = 0;
int high = n >> 1;
if (BValue(low) == 0) {
out.println("! " + (low + 1));
return;
}
int fSign = Integer.signum(BValue(low));
while (high - low > 1) {
int mid = (high + low) >> 1;
int mSign = Integer.signum(BValue(mid));
if (mSign == 0) {
out.println("! " + (mid + 1));
return;
}
if (mSign == -fSign) {
high = mid;
} else {
low = mid;
}
}
out.println("! -1");
}
public int BValue(int index) {
out.println("? " + (index + 1));
out.flush();
int f = in.nextInt();
out.println("? " + (index + 1 + (n >> 1)));
out.flush();
int s = in.nextInt();
return f - s;
}
}
static class FastReader {
private InputStream stream;
private byte[] buf = new byte[8192];
private int curChar;
private int pnumChars;
public FastReader(InputStream stream) {
this.stream = stream;
}
private int pread() {
if (pnumChars == -1) {
throw new InputMismatchException();
}
if (curChar >= pnumChars) {
curChar = 0;
try {
pnumChars = stream.read(buf);
} catch (IOException e) {
throw new InputMismatchException();
}
if (pnumChars <= 0) {
return -1;
}
}
return buf[curChar++];
}
public int nextInt() {
int c = pread();
while (isSpaceChar(c))
c = pread();
int sgn = 1;
if (c == '-') {
sgn = -1;
c = pread();
}
int res = 0;
do {
if (c == ',') {
c = pread();
}
if (c < '0' || c > '9') {
throw new InputMismatchException();
}
res *= 10;
res += c - '0';
c = pread();
} while (!isSpaceChar(c));
return res * sgn;
}
private boolean isSpaceChar(int c) {
return c == ' ' || c == '\n' || c == '\r' || c == '\t' || c == -1;
}
}
}
| logn | 1019_B. The hat | CODEFORCES |
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.StringTokenizer;
public class B {
static ArrayList<Integer> [] adj;
static int [] num;
static int dfs(int u, int p){
int cnt = 0;
for(int v:adj[u]){
if(v != p)
cnt += dfs(v, u);
}
if(adj[u].size() == 1 && u != 0 || u == 0 && adj[0].size() == 0)
cnt++;
num[cnt]++;
return cnt;
}
public static void main(String[] args) throws NumberFormatException, IOException {
Scanner sc = new Scanner();
PrintWriter out = new PrintWriter(System.out);
int n = sc.nextInt();
adj = new ArrayList[n];
for (int i = 0; i < adj.length; ++i) {
adj[i] = new ArrayList<>();
}
for (int i = 1; i < n; ++i) {
int p = sc.nextInt()-1;
adj[p].add(i);
adj[i].add(p);
}
num = new int[n+1];
dfs(0, -1);
for (int i = 1; i < num.length; ++i) {
num[i] += num[i-1];
}
int cur = 1;
for (int i = 0; i < num.length; ++i) {
while(cur <= num[i]){
out.print(i + " ");
++cur;
}
}
out.close();
}
static class Scanner {
BufferedReader br;
StringTokenizer st;
public Scanner() {
br = new BufferedReader(new InputStreamReader(System.in));
}
public String next() throws IOException {
while (st == null || !st.hasMoreTokens()) {
st = new StringTokenizer(br.readLine());
}
return st.nextToken();
}
public int nextInt() throws NumberFormatException, IOException {
return Integer.parseInt(next());
}
}
}
| linear | 1056_D. Decorate Apple Tree | CODEFORCES |
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.StringTokenizer;
public class CoveredPointsCount {
//UPSOLVE
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine());
int n = Integer.parseInt(st.nextToken());
long[] myArray = new long[2 * n];
for (int i = 0; i < n; i++) {
StringTokenizer st1 = new StringTokenizer(br.readLine());
myArray[2 * i] = Long.parseLong(st1.nextToken()) * 2;
myArray[2 * i + 1] = Long.parseLong(st1.nextToken()) * 2 + 1;
}
Arrays.sort(myArray);
long[] ans = new long[n + 1];
int cnt = 0;
for (int i = 0; i < 2 * n - 1; i++) {
if (myArray[i] % 2 == 0) cnt++; else cnt--;
ans[cnt] += (myArray[i + 1] + 1) / 2 - (myArray[i] + 1) / 2;
}
StringBuilder answer = new StringBuilder();
for (int i = 1; i < n + 1; i++) {
answer.append(ans[i]);
answer.append(" ");
}
System.out.println(answer);
}
}
| nlogn | 1000_C. Covered Points Count | CODEFORCES |
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt();
int[] P = new int[n];
int[] check=new int[n];
for (int i = 1; i < n; i++) {
P[i] = scanner.nextInt();
P[i]--;
check[P[i]]++;
}
int[] leaves = new int[n];
for (int i=0;i<n;i++) {
if(check[i]==0){
leaves[P[i]]++;
}
}
for (int i = 0; i < n; i++) {
if (check[i]>0&&leaves[i]<3) {
System.out.println("No");
return;
}
}
System.out.println("Yes");
}
}
| linear | 913_B. Christmas Spruce | CODEFORCES |
import java .util.*;
import java .io.*;
public class Main{
public static void main(String[]YAHIA_MOSTAFA){
Scanner sc =new Scanner(System.in);
long n=sc.nextLong(),x=sc.nextLong(),y=sc.nextLong();
long xb,xw,yb,yw;
xw=x-1;yw=y-1;xb=n-x;yb=n-y;
if (x==n&&y==n){
System.out.println("Black");return;
}
long c1=0,c2=0;
long f =Math.max(xb,yb);
long h =Math.max(xw,yw);
//System.out.println(h+" "+f+" "+(h-f));
if (h<=f)
System.out.println("White");
else
System.out.println("Black");
}
} | constant | 1075_A. The King's Race | CODEFORCES |
import java.io.*;
import java.util.*;
public class Main {
static final int MAXN= 1005;
static final long MOD =1_000_000_007;
static final boolean DEBUG= false;
static int n, m;
static long stlr[][]= new long[MAXN][MAXN],bell[]= new long[MAXN],occ[];
static PrintStream cerr=System.err;
public static void main(String[] args) {
// TODO Auto-generated method stub
Readin();
stlr[0][0]= bell[0] =1;
for (int i=1; i<=m; i++)
for (int j=1;j<=i;j++) {
stlr[i][j]= (stlr[i-1][j-1]+stlr[i-1][j]*(long)j)%MOD;
bell[i]= (bell[i]+stlr[i][j])%MOD;
}
if (DEBUG)
for (int i=1; i<=m; i++) cerr.println("Bell["+i+"] ="+bell[i]);
Arrays.sort(occ);
if (DEBUG) {
cerr.println("After Sorting");
for (int i=0;i<m; i++) cerr.println(occ[i]+" ");}
long ans=1;
for (int i=0,j=0; i<m; i=j) {
for (j=i+1; j<m && occ[i]==occ[j];j++);
ans= (ans*bell[j-i])%MOD;
}
System.out.println(ans);
}
static void Readin() {
Scanner cin;
if ( !DEBUG)cin= new Scanner(System.in);
else {
try {
cin = new Scanner(new File("input.txt"));
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
if ( DEBUG)cerr.println("Not Fount input.txt");
return ;
}
}
m = cin.nextInt(); n=cin.nextInt();
occ= new long[m];
for (int i=0; i<n; i++) {
String s= cin.next();
for (int j=0;j <m; j++)
occ[j]|=((long)(s.charAt(j)-'0'))<<i;
}
cin.close();
}
}
| quadratic | 908_E. New Year and Entity Enumeration | CODEFORCES |
import java.io.BufferedReader;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.StringTokenizer;
public class EhabAndAnotherAnotherXorProblem implements Closeable {
private InputReader in = new InputReader(System.in);
private PrintWriter out = new PrintWriter(System.out);
public void solve() {
int initial = ask(0, 0);
int a = 0, b = 0;
if (initial == 0) {
for (int i = 0; i < 30; i++) {
int response = ask(1 << i, 0);
if (response == -1) {
a |= (1 << i);
}
}
b = a;
} else {
for (int i = 29; i >= 0; i--) {
int response = ask(a | (1 << i), b | (1 << i));
if (response != initial) {
if (response == 1) {
b |= (1 << i);
} else {
a |= (1 << i);
}
initial = ask(a, b);
} else {
response = ask(a | (1 << i), b);
if (response == -1) {
a |= (1 << i);
b |= (1 << i);
}
}
}
}
answer(a, b);
}
private int ask(int c, int d) {
out.printf("? %d %d\n", c, d);
out.flush();
return in.ni();
}
private void answer(int a, int b) {
out.printf("! %d %d\n", a, b);
out.flush();
}
@Override
public void close() throws IOException {
in.close();
out.close();
}
static class InputReader {
public BufferedReader reader;
public StringTokenizer tokenizer;
public InputReader(InputStream stream) {
reader = new BufferedReader(new InputStreamReader(stream), 32768);
tokenizer = null;
}
public String next() {
while (tokenizer == null || !tokenizer.hasMoreTokens()) {
try {
tokenizer = new StringTokenizer(reader.readLine());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return tokenizer.nextToken();
}
public int ni() {
return Integer.parseInt(next());
}
public long nl() {
return Long.parseLong(next());
}
public void close() throws IOException {
reader.close();
}
}
public static void main(String[] args) throws IOException {
try (EhabAndAnotherAnotherXorProblem instance = new EhabAndAnotherAnotherXorProblem()) {
instance.solve();
}
}
}
| logn | 1088_D. Ehab and another another xor problem | CODEFORCES |
import java.util.*;
import java.lang.*;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.*;
public class Main {
public static void main(String[] args) throws Exception{
FastReader sc=new FastReader();
OutputStream outputStream = System.out;
PrintWriter out = new PrintWriter(outputStream);
int n=sc.nextInt();
HashMap<String,Integer> map=new HashMap<String,Integer>();
for(int i=0;i<n;i++) {
map.put(sc.next(), 1);
}
ArrayList<String> list=new ArrayList<String>();
int count=0;
if(!map.containsKey("purple")) {
count++;
list.add("Power");
}
if(!map.containsKey("green")) {
count++;
list.add("Time");
}
if(!map.containsKey("blue")) {
count++;
list.add("Space");
}
if(!map.containsKey("orange")) {
count++;
list.add("Soul");
}
if(!map.containsKey("red")) {
count++;
list.add("Reality");
}
if(!map.containsKey("yellow")) {
count++;
list.add("Mind");
}System.out.println(count);
for(String s:list) {
System.out.println(s);
}
}
}
class FastReader
{
BufferedReader br;
StringTokenizer st;
public FastReader()
{
br = new BufferedReader(new
InputStreamReader(System.in));
}
String next()
{
while (st == null || !st.hasMoreElements())
{
try
{
st = new StringTokenizer(br.readLine());
}
catch (IOException e)
{
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt()
{
return Integer.parseInt(next());
}
long nextLong()
{
return Long.parseLong(next());
}
double nextDouble()
{
return Double.parseDouble(next());
}
String nextLine()
{
String str = "";
try
{
str = br.readLine();
}
catch (IOException e)
{
e.printStackTrace();
}
return str;
}
} | constant | 987_A. Infinity Gauntlet | CODEFORCES |
import java.util.Scanner;
public class AlexAndARhombus {
public static void main(String args[])
{
Scanner sc=new Scanner(System.in);
int n=sc.nextInt();
System.out.println(n*n+(n-1)*(n-1));
sc.close();
}
}
| constant | 1180_A. Alex and a Rhombus | CODEFORCES |
import java.util.*;
import java.io.*;
import java.math.BigInteger;
public class Main
{
static final long mod=(int)1e9+7;
public static void main(String[] args) throws Exception
{
FastReader in=new FastReader();
PrintWriter pw=new PrintWriter(System.out);
int n=in.nextInt();
long ans=0;
for(int i=2;2*i<=n;i++)
{
ans+=i*(n/i-1);
}
ans*=4;
pw.print(ans);
pw.flush();
}
}
class pair
{
int f,s;
}
class FastReader
{
BufferedReader br;
StringTokenizer st;
public FastReader()
{
br=new BufferedReader(new InputStreamReader(System.in));
}
public String next() throws IOException
{
if(st==null || !st.hasMoreElements())
{
st=new StringTokenizer(br.readLine());
}
return st.nextToken();
}
public int nextInt() throws IOException
{
return Integer.parseInt(next());
}
public long nextLong() throws IOException
{
return Long.parseLong(next());
}
} | linear | 1062_D. Fun with Integers | CODEFORCES |
import java.io.*;
import java.util.*;
public class A {
public static void main(String args[]) {
FastScanner scn = new FastScanner();
int n = scn.nextInt();
int s = scn.nextInt();
if (s <= n) {
System.out.println(1);
} else if (s > n) {
if(s%n == 0){
System.out.println(s/n);
} else {
System.out.println(s/n + 1);
}
}
}
public static class FastScanner {
BufferedReader br;
StringTokenizer st;
public FastScanner(String s) {
try {
br = new BufferedReader(new FileReader(s));
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public FastScanner() {
br = new BufferedReader(new InputStreamReader(System.in));
}
String nextToken() {
while (st == null || !st.hasMoreElements()) {
try {
st = new StringTokenizer(br.readLine());
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt() {
return Integer.parseInt(nextToken());
}
long nextLong() {
return Long.parseLong(nextToken());
}
double nextDouble() {
return Double.parseDouble(nextToken());
}
}
}
| constant | 1061_A. Coins | CODEFORCES |
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.*;
public class e {
public static class FastReader {
BufferedReader br;
StringTokenizer st;
//it reads the data about the specified point and divide the data about it ,it is quite fast
//than using direct
public FastReader() {
br = new BufferedReader(new InputStreamReader(System.in));
}
String next() {
while (st == null || !st.hasMoreTokens()) {
try {
st = new StringTokenizer(br.readLine());
} catch (Exception r) {
r.printStackTrace();
}
}
return st.nextToken();
}
int nextInt() {
return Integer.parseInt(next());//converts string to integer
}
double nextDouble() {
return Double.parseDouble(next());
}
long nextLong() {
return Long.parseLong(next());
}
String nextLine() {
String str = "";
try {
str = br.readLine();
} catch (Exception r) {
r.printStackTrace();
}
return str;
}
}
static ArrayList<String>list1=new ArrayList<String>();
static void combine(String instr, StringBuffer outstr, int index,int k)
{
if(outstr.length()==k)
{
list1.add(outstr.toString());return;
}
if(outstr.toString().length()==0)
outstr.append(instr.charAt(index));
for (int i = 0; i < instr.length(); i++)
{
outstr.append(instr.charAt(i));
combine(instr, outstr, i + 1,k);
outstr.deleteCharAt(outstr.length() - 1);
}
index++;
}
static ArrayList<ArrayList<Integer>>l=new ArrayList<>();
static void comb(int n,int k,int ind,ArrayList<Integer>list)
{
if(k==0)
{
l.add(new ArrayList<>(list));
return;
}
for(int i=ind;i<=n;i++)
{
list.add(i);
comb(n,k-1,ind+1,list);
list.remove(list.size()-1);
}
}
public static PrintWriter out = new PrintWriter (new BufferedOutputStream(System.out));
public static void main(String[] args) {
// TODO Auto-generated method stub
FastReader in=new FastReader();
HashMap<Integer,Integer>map=new HashMap<Integer,Integer>();
int n=in.nextInt();
int r=in.nextInt();
double theta=(double)360/(double)n;
double b=1-((double)2/(double)(1-Math.cos((double)2*Math.PI/(double)n)));
double x=Math.sqrt(1-b)-1;
double ans=(double)r/(double)x;
System.out.println(ans);
}
}
| constant | 1100_C. NN and the Optical Illusion | CODEFORCES |
import java.io.*;
import java.math.BigInteger;
import java.util.*;
public class A {
static MyScanner sc;
static PrintWriter pw;
public static void main(String[] args) throws Throwable {
sc = new MyScanner();
pw = new PrintWriter(System.out);
n = sc.nextInt();
T = sc.nextLong();
p = new int[n];
l = new int[n];
x = new int[n];
t = new int[n];
adj = new ArrayList[n];
for (int i = 0; i < n; i++)
x[i] = sc.nextInt();
for (int i = 0; i < n; i++)
t[i] = sc.nextInt();
adj[0] = new ArrayList<>();
for (int i = 1; i < n; i++) {
adj[i] = new ArrayList<>();
p[i] = sc.nextInt() - 1;
l[i] = sc.nextInt();
adj[p[i]].add(i);
}
ftCnt = new long[N];
ftSum = new long[N];
ans = new long[n];
dfs(0);
pw.println(ans[0]);
pw.flush();
pw.close();
}
static int n;
static long T;
static int[] p, l, x, t;
static ArrayList<Integer>[] adj;
static long[] ans;
static void dfs(int u) {
update(t[u], x[u], 1L * x[u] * t[u]);
ans[u] = getMaxCnt();
long[] vals = {-1, -1, -1};
for (int v : adj[u]) {
T -= 2 * l[v];
dfs(v);
vals[0] = ans[v];
Arrays.sort(vals);
T += 2 * l[v];
}
if (u != 0) {
if (vals[1] != -1)
ans[u] = Math.max(ans[u], vals[1]);
} else {
if (vals[2] != -1)
ans[u] = Math.max(ans[u], vals[2]);
}
update(t[u], -x[u], -1L * x[u] * t[u]);
}
static int N = (int) 1e6 + 2;
static long[] ftCnt, ftSum;
static void update(int idx, long cnt, long val) {
while (idx < N) {
ftCnt[idx] += cnt;
ftSum[idx] += val;
idx += (idx & -idx);
}
}
static long getSum(int idx) {
long ret = 0;
while (idx > 0) {
ret += ftSum[idx];
idx -= (idx & -idx);
}
return ret;
}
static long getCnt(int idx) {
long ret = 0;
while (idx > 0) {
ret += ftCnt[idx];
idx -= (idx & -idx);
}
return ret;
}
static long getMaxCnt() {
int start = 1, end = N - 1, ans = N - 1;
while (start <= end) {
int mid = (start + end) / 2;
if (getSum(mid) >= T) {
ans = mid;
end = mid - 1;
} else
start = mid + 1;
}
long remT = T - (ans > 1 ? getSum(ans - 1) : 0);
long cnt = (ans > 1 ? getCnt(ans - 1) : 0);
long cntOfVal = getCnt(ans) - cnt;
cnt += Math.min(cntOfVal, remT / ans);
return cnt;
}
static class MyScanner {
BufferedReader br;
StringTokenizer st;
public MyScanner() {
br = new BufferedReader(new InputStreamReader(System.in));
}
String next() {
while (st == null || !st.hasMoreElements()) {
try {
st = new StringTokenizer(br.readLine());
} catch (IOException e) {
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt() {
return Integer.parseInt(next());
}
long nextLong() {
return Long.parseLong(next());
}
double nextDouble() {
return Double.parseDouble(next());
}
String nextLine() {
String str = "";
try {
str = br.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return str;
}
}
} | nlogn | 1099_F. Cookies | CODEFORCES |
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.*;
public class Code {
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(br.readLine());
HashMap<Double,Integer>h = new HashMap<>();
double [] temp = new double[n];
int m = 0;
for(int i=0;i<n;i++) {
String l = br.readLine();
int[] x = new int[4];
int k=0;
boolean t = false;
for(int j=0;j<l.length();j++) {
if(l.charAt(j)=='(' || l.charAt(j)=='+' || l.charAt(j)==')' || l.charAt(j)=='/')
x[k++] = j;
}
double a = Integer.parseInt(l.substring(x[0]+1,x[1]));
double b = Integer.parseInt(l.substring(x[1]+1, x[2]));
double c = Integer.parseInt(l.substring(x[3]+1));
temp[m++] = (a+b)/c;
//System.out.print((a+b)/c + " ");
if(h.containsKey((a+b)/c))
h.put((a+b)/c, h.get((a+b)/c)+1);
else
h.put((a+b)/c, 1);
}
//System.out.println(h);
for(int i=0;i<n;i++) {
System.out.print(h.get(temp[i]) + " ");
}
}
}
| linear | 958_D1. Hyperspace Jump (easy) | CODEFORCES |
import java.util.*;
import java.io.*;
public class programA {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(br.readLine());
if(n%2 == 0)System.out.println(n/2 +1);
else System.out.println((int)Math.ceil((double)n/2));
}
}
| constant | 964_A. Splits | CODEFORCES |
import java.math.BigInteger;
import java.util.Scanner;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author Andy Phan
*/
public class p1096f {
static long MOD = 998244353;
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int n = in.nextInt();
BIT invert = new BIT(n+5);
BIT neg = new BIT(n+5);
long res = 0;
int[] arr = new int[n];
boolean[] has = new boolean[n+1];
long num1 = 0;
for(int i = 0; i < n; i++) {
arr[i] = in.nextInt();
if(arr[i] != -1) {
res += invert.read(n+5)-invert.read(arr[i]);
res %= MOD;
invert.update(arr[i], 1);
has[arr[i]] = true;
} else num1++;
}
if(num1 == 0) {
System.out.println(res);
return;
}
for(int i = 1; i <= n; i++) if(!has[i]) neg.update(i, 1);
long invertNum1 = modInv(num1, MOD);
res += ((num1*(num1-1))%MOD)*modInv(4, MOD);
res %= MOD;
long cnt = 0;
for(int i = 0; i < n; i++) {
if(arr[i] == -1) {
cnt++;
continue;
}
res += (((neg.read(n+5)-neg.read(arr[i]))*cnt)%MOD)*invertNum1;
res %= MOD;
}
cnt = 0;
for(int i = n-1; i >= 0; i--) {
if(arr[i] == -1) {
cnt++;
continue;
}
res += (((neg.read(arr[i]))*cnt)%MOD)*invertNum1;
res %= MOD;
}
System.out.println(res);
}
//@
static class BIT {
int n;
int[] tree;
public BIT(int n) {
this.n = n;
tree = new int[n + 1];
}
int read(int i) {
int sum = 0;
while (i > 0) {
sum += tree[i];
i -= i & -i;
}
return sum;
}
void update(int i, int val) {
while (i <= n) {
tree[i] += val;
i += i & -i;
}
}
//$
}
//@
// Computes the modular inverse of x
// Returns 0 if the GCD of x and mod is not 1
// O(log n) : Can be converted to use BigIntegers
static long modInv(long x, long mod) {
return (BigInteger.valueOf(x).modInverse(BigInteger.valueOf(mod))).longValue();
}
static long modInv(long a, long b, long y0, long y1, long q0, long q1) {
long y2 = y0 - y1*q0;
return b == 0 ? y2 : modInv(b, a % b, y1, y2, q1, a / b);
}
//@
static long gcd(long a, long b) { return b == 0 ? a : gcd(b, a % b); }
static long lcm(long a, long b) { return a / gcd(a, b) * b; }
}
| nlogn | 1096_F. Inversion Expectation | CODEFORCES |
import java.util.*;
import java.io.*;
public class Waw{
public static void main(String[] args) throws Exception {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
long[] a = new long[n];
for(int i=0;i<n;i++) a[i] = sc.nextLong();
long[] p = new long[n];
p[n-1] = a[n-1];
for(int i=n-2;i>=0;i--){
if(a[i]<p[i+1]) p[i] = p[i+1]-1;
else p[i] = a[i];
}
long max = p[0];
long res = p[0] - a[0];
for(int i=1;i<n;i++){
if(max < p[i]) max = p[i];
res += max - a[i];
}
System.out.println(res);
}
} | linear | 924_C. Riverside Curio | CODEFORCES |
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.Arrays;
import java.util.Comparator;
import java.util.StringTokenizer;
import java.io.InputStream ;
import java.util.InputMismatchException;
import java.util.ArrayList;
public class Main {
public static void main(String[] args) throws IOException {
PrintWriter out = new PrintWriter(System.out);
//Scanner sc = new Scanner();
Reader in = new Reader();
Main solver = new Main();
solver.solve(out, in);
out.flush();
out.close();
}
void solve(PrintWriter out, Reader in) throws IOException{
int n = in.nextInt();
int m = in.nextInt();
int[] vert = new int[n+1];
for(int i=0 ;i<n ;i++) vert[i] = in.nextInt();
vert[n] = (int)1e9;
int cnt=0,x,y;
ArrayList<Integer> arr = new ArrayList<>();
for(int i=0 ;i<m ;i++) {
x = in.nextInt();
y = in.nextInt();
in.nextInt();
if(x==1) arr.add(y);
}
horz = new int[arr.size()];
for(int i=0 ;i<arr.size();i++) horz[i] = arr.get(i);
Arrays.sort(horz);
Arrays.sort(vert);
int ans = 2*(int)1e5+10;
for(int i=0 ;i<=n ;i++){
int lesshorz = bs(vert[i],horz.length);
ans = Math.min(ans,i+horz.length-lesshorz-1);
}
out.println(ans);
}
static int[] horz;
static int bs(int num,int m){
int mid,lo=0,hi=m-1,r=-1;
while(lo<=hi){
mid = (lo+hi)/2;
if(horz[mid]<num){
lo = mid+1;
r = mid;
}else{
hi =mid-1;
}
}
return r;
}
static class Reader {
private InputStream mIs;
private byte[] buf = new byte[1024];
private int curChar;
private int numChars;
public Reader() {
this(System.in);
}
public Reader(InputStream is) {
mIs = is;
}
public int read() {
if (numChars == -1) {
throw new InputMismatchException();
}
if (curChar >= numChars) {
curChar = 0;
try {
numChars = mIs.read(buf);
} catch (IOException e) {
throw new InputMismatchException();
}
if (numChars <= 0) {
return -1;
}
}
return buf[curChar++];
}
public String nextLine() {
int c = read();
while (isSpaceChar(c)) {
c = read();
}
StringBuilder res = new StringBuilder();
do {
res.appendCodePoint(c);
c = read();
} while (!isEndOfLine(c));
return res.toString();
}
public String next() {
int c = read();
while (isSpaceChar(c)) {
c = read();
}
StringBuilder res = new StringBuilder();
do {
res.appendCodePoint(c);
c = read();
} while (!isSpaceChar(c));
return res.toString();
}
public long nextLong() {
int c = read();
while (isSpaceChar(c)) {
c = read();
}
int sgn = 1;
if (c == '-') {
sgn = -1;
c = read();
}
long res = 0;
do {
if (c < '0' || c > '9') {
throw new InputMismatchException();
}
res *= 10;
res += c - '0';
c = read();
} while (!isSpaceChar(c));
return res * sgn;
}
public int nextInt() {
int c = read();
while (isSpaceChar(c)) {
c = read();
}
int sgn = 1;
if (c == '-') {
sgn = -1;
c = read();
}
int res = 0;
do {
if (c < '0' || c > '9') {
throw new InputMismatchException();
}
res *= 10;
res += c - '0';
c = read();
} while (!isSpaceChar(c));
return res * sgn;
}
public boolean isSpaceChar(int c) {
return c == ' ' || c == '\n' || c == '\r' || c == '\t' || c == -1;
}
public boolean isEndOfLine(int c) {
return c == '\n' || c == '\r' || c == -1;
}
}
}
| nlogn | 1075_C. The Tower is Going Home | CODEFORCES |
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.util.StringTokenizer;
public class D {
private void solve() {
br = new BufferedReader(new InputStreamReader(System.in));
out = new PrintWriter(System.out);
int n = nextInt(), m = nextInt();
boolean[][] used = new boolean[n + 1][m + 1];
for (int j = 1; j <= (m + 1) / 2; j++) {
int x1 = 1, x2 = n;
for (int i = 1; i <= n; i++) {
if (x1 <= n && !used[x1][j]) {
out.println(x1 + " " + j);
used[x1++][j] = true;
}
if (x2 > 0 && !used[x2][m - j + 1]) {
out.println(x2 + " " + (m - j + 1));
used[x2--][m - j + 1] = true;
}
}
}
out.close();
}
public static void main(String[] args) {
new D().solve();
}
private BufferedReader br;
private StringTokenizer st;
private PrintWriter out;
private String next() {
while (st == null || !st.hasMoreTokens()) {
try {
st = new StringTokenizer(br.readLine());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
return st.nextToken();
}
private int nextInt() {
return Integer.parseInt(next());
}
private long nextLong() {
return Long.parseLong(next());
}
private double nextDouble() {
return Double.parseDouble(next());
}
}
| quadratic | 1179_B. Tolik and His Uncle | CODEFORCES |
/* package whatever; // don't place package name! */
import java.util.*;
import java.lang.*;
import java.io.*;
/* Name of the class has to be "Main" only if the class is public. */
public class Ideone
{
static int check(int temp)
{
int count1 = 0;
while (temp>0)
{
if(temp % 2 != 0)
count1++;
temp/= 2;
}
return count1;
}
public static void main (String[] args) throws java.lang.Exception
{
// your code goes here
Scanner sc=new Scanner(System.in);
String a=sc.next();
String b=sc.next();
int m=a.length();
int n=b.length();
int[] zero=new int[n];
int[] one=new int[n];
for(int i=0;i<n;i++)
{
if(i==0)
{
if(b.charAt(i)=='0')
zero[i]++;
else
one[i]++;
}
else
{
zero[i]=zero[i-1];
one[i]=one[i-1];
if(b.charAt(i)=='0')
zero[i]++;
else
one[i]++;
}
}
long res=0;
for(int i=0;i<m;i++)
{
int x=n-m+i;
if(a.charAt(i)=='0')
res+=one[x];
else
res+=zero[x];
if(i>0)
{
if(a.charAt(i)=='0')
res-=one[i-1];
else
res-=zero[i-1];
}
}
System.out.println(res);
}
} | linear | 608_B. Hamming Distance Sum | CODEFORCES |
import java.util.*;
import java.io.*;
public class _1036_B_DiagonalWalkingV2 {
public static void main(String[] args) throws IOException {
int Q = readInt();
while(Q-- > 0) {
long n = readLong(), m = readLong(), k = readLong();
if(Math.max(n, m) > k) println(-1);
else {
long ans = k;
if(n%2 != k%2) ans--;
if(m%2 != k%2) ans--;
println(ans);
}
}
exit();
}
final private static int BUFFER_SIZE = 1 << 16;
private static DataInputStream din = new DataInputStream(System.in);
private static byte[] buffer = new byte[BUFFER_SIZE];
private static int bufferPointer = 0, bytesRead = 0;
static PrintWriter pr = new PrintWriter(new BufferedWriter(new OutputStreamWriter(System.out)));
public static String readLine() throws IOException {
byte[] buf = new byte[64]; // line length
int cnt = 0, c;
while ((c = Read()) != -1) {
if (c == '\n')
break;
buf[cnt++] = (byte) c;
}
return new String(buf, 0, cnt);
}
public static String read() throws IOException {
byte[] ret = new byte[1024];
int idx = 0;
byte c = Read();
while (c <= ' ') {
c = Read();
}
do {
ret[idx++] = c;
c = Read();
} while (c != -1 && c != ' ' && c != '\n' && c != '\r');
return new String(ret, 0, idx);
}
public static int readInt() throws IOException {
int ret = 0;
byte c = Read();
while (c <= ' ')
c = Read();
boolean neg = (c == '-');
if (neg)
c = Read();
do {
ret = ret * 10 + c - '0';
} while ((c = Read()) >= '0' && c <= '9');
if (neg)
return -ret;
return ret;
}
public static long readLong() throws IOException {
long ret = 0;
byte c = Read();
while (c <= ' ')
c = Read();
boolean neg = (c == '-');
if (neg)
c = Read();
do {
ret = ret * 10 + c - '0';
} while ((c = Read()) >= '0' && c <= '9');
if (neg)
return -ret;
return ret;
}
public static double readDouble() throws IOException {
double ret = 0, div = 1;
byte c = Read();
while (c <= ' ')
c = Read();
boolean neg = (c == '-');
if (neg)
c = Read();
do {
ret = ret * 10 + c - '0';
} while ((c = Read()) >= '0' && c <= '9');
if (c == '.') {
while ((c = Read()) >= '0' && c <= '9') {
ret += (c - '0') / (div *= 10);
}
}
if (neg)
return -ret;
return ret;
}
private static void fillBuffer() throws IOException {
bytesRead = din.read(buffer, bufferPointer = 0, BUFFER_SIZE);
if (bytesRead == -1)
buffer[0] = -1;
}
private static byte Read() throws IOException {
if (bufferPointer == bytesRead)
fillBuffer();
return buffer[bufferPointer++];
}
static void print(Object o) {
pr.print(o);
}
static void println(Object o) {
pr.println(o);
}
static void flush() {
pr.flush();
}
static void println() {
pr.println();
}
static void exit() throws IOException {
din.close();
pr.close();
System.exit(0);
}
}
| linear | 1036_B. Diagonal Walking v.2 | CODEFORCES |
import java.io.OutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.StringTokenizer;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.InputStream;
/**
* Built using CHelper plug-in
* Actual solution is at the top
*
* @author kessido
*/
public class Main {
public static void main(String[] args) {
InputStream inputStream = System.in;
OutputStream outputStream = System.out;
InputReader in = new InputReader(inputStream);
PrintWriter out = new PrintWriter(outputStream);
BTheHat solver = new BTheHat();
solver.solve(1, in, out);
out.close();
}
static class BTheHat {
PrintWriter out;
InputReader in;
int n;
public void solve(int testNumber, InputReader in, PrintWriter out) {
this.out = out;
this.in = in;
n = in.NextInt();
int desiredPair = -1;
int result = query(1);
if (result != 0) {
int l = 2, r = 1 + n / 2;
while (l < r) {
int m = (l + r) / 2;
int mRes = query(m);
if (mRes == 0) {
desiredPair = m;
break;
} else if (mRes == result) {
l = m + 1;
} else {
r = m;
}
}
} else {
desiredPair = 1;
}
out.println("! " + desiredPair);
}
private int query(int i) {
int iV = queryValue(i);
int iN2V = queryValue(i + n / 2);
if (iV < iN2V) {
return -1;
} else if (iV > iN2V) {
return 1;
}
return 0;
}
private int queryValue(int i) {
out.println("? " + i);
out.flush();
return in.NextInt();
}
}
static class InputReader {
BufferedReader reader;
StringTokenizer tokenizer;
public InputReader(InputStream stream) {
reader = new BufferedReader(new InputStreamReader(stream), 32768);
tokenizer = null;
}
public String next() {
while (tokenizer == null || !tokenizer.hasMoreTokens()) {
try {
tokenizer = new StringTokenizer(reader.readLine(), " \t\n\r\f,");
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return tokenizer.nextToken();
}
public int NextInt() {
return Integer.parseInt(next());
}
}
}
| logn | 1019_B. The hat | CODEFORCES |
import java.io.PrintWriter;
import java.util.*;
import java.util.Arrays ;
import java .lang.String.* ;
import java .lang.StringBuilder ;
public class Test{
static int pos = 0 ;
static int arr[] ;
static LinkedList l1 = new LinkedList() ;
static void find(int p ,char[]x,int put[],String s){
int c= 0 ;
for (int i = 0; i < s.length(); i++) {
if(x[p]==s.charAt(i)){
c++ ; }
}
put[p] = c ;
}
static int mode(int m ,int[]x ){
int temp = 0 ;
for (int i = x.length-1; i >=0; i--) {
if(x[i]<=m){
temp= x[i] ;
/// break ;
return m-temp ;
}
}
return m-temp ;
}
static int mode2(int m ,int[]x ){
int temp = 0 ;
for (int i = x.length-1; i >=0; i--) {
if(x[i]<=m){
temp= x[i] ;
/// break ;
return x[i] ;
}
}
return 0 ;
}
static int find(int x[],int temp){
int j = 0 ;
for (int i = x.length-1; i >=0; i--) {
if(x[i]==temp) return j+1 ;
j++ ;
}
return -1 ;
}
static String ch(long[]x,long b){
for (int i = 0; i < x.length; i++) {
if(x[i]==b)return "YES" ;
}
return "NO" ;
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in) ;
PrintWriter pw = new PrintWriter(System.out);
long n = in.nextLong() ;
long count =1 ;
long temp =n/2;
temp+=count ;
System.out.println(temp);
}
}
| constant | 964_A. Splits | CODEFORCES |
import java.util.Scanner;
public class Main {
public static void main(String args[]) {
Scanner in=new Scanner(System.in);
String str=in.next();
int cnt=0;
for(int i=0;i<str.length();++i) {
if(str.charAt(i)=='1') {
++cnt;
}
}
int i=0;
for(;i<str.length();++i) {
if(str.charAt(i)=='0') {
System.out.print("0");
}
else if(str.charAt(i)=='2') {
while(cnt-->0) {//
System.out.print("1");
}
System.out.print("2");
}
}
while(cnt-->0) {
System.out.print("1");
}
in.close();
}
} | linear | 1009_B. Minimum Ternary String | CODEFORCES |
import java.util.*;
import java.io.*;
import java.math.*;
import java.awt.geom.*;
public class FunctionHeight {
public static void main(String[] args) {
MyScanner sc = new MyScanner();
long n = sc.nl();
long k = sc.nl();
long ans = (n+k-1)/n;
System.out.println(ans);
}
/////////// TEMPLATE FROM HERE /////////////////
private static class MyScanner {
BufferedReader br;
StringTokenizer st;
public MyScanner() {
br = new BufferedReader(new InputStreamReader(System.in));
}
String next() {
while (st == null || !st.hasMoreElements()) {
try {
st = new StringTokenizer(br.readLine());
} catch (IOException e) {
e.printStackTrace();
}
}
return st.nextToken();
}
int ni() {
return Integer.parseInt(next());
}
float nf() {
return Float.parseFloat(next());
}
long nl() {
return Long.parseLong(next());
}
double nd() {
return Double.parseDouble(next());
}
String nextLine() {
String str = "";
try {
str = br.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return str;
}
}
} | constant | 1036_A. Function Height | CODEFORCES |
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.util.Scanner;
public class A1 {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
long size = scan.nextLong();
int numberOfSpecial = scan.nextInt();
long pageSize = scan.nextLong();
long[] specialItemsArray = new long[numberOfSpecial];
for (int i = 0; i < numberOfSpecial; i++) {
specialItemsArray[i] = scan.nextLong();
}
int totalRemoved = 0;
int step = 0;
long currentPageIndex = BigDecimal.valueOf(specialItemsArray[0]).divide(BigDecimal.valueOf(pageSize),2, RoundingMode.UP).setScale(0, RoundingMode.CEILING).longValue();
int specialItemArrayIndex = 1;
while (specialItemArrayIndex < numberOfSpecial) {
long pageIndex = BigDecimal.valueOf(specialItemsArray[specialItemArrayIndex] - totalRemoved).divide(BigDecimal.valueOf(pageSize),2,RoundingMode.UP).setScale(0, RoundingMode.CEILING).longValue();
if (currentPageIndex != pageIndex) {
step++;
totalRemoved = specialItemArrayIndex;
currentPageIndex = BigDecimal.valueOf(specialItemsArray[specialItemArrayIndex] - totalRemoved).divide(BigDecimal.valueOf(pageSize),2,RoundingMode.UP).setScale(0, RoundingMode.CEILING).longValue();
}
specialItemArrayIndex++;
}
System.out.println(step + 1);
}
}
| linear | 1190_A. Tokitsukaze and Discard Items | CODEFORCES |
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.util.Map;
import java.util.StringTokenizer;
import java.util.TreeMap;
public class Main {
static class Task {
int NN = 500005;
int MOD = 1000000007;
int INF = 2000000000;
long INFINITY = 2000000000000000000L;
public void solve(InputReader in, PrintWriter out) {
int t = in.nextInt();
while(t-->0) {
long n =in.nextLong();
long m = in.nextLong();
long x1 = in.nextLong();
long y1 = in.nextLong();
long x2 = in.nextLong();
long y2 = in.nextLong();
long x3 = in.nextLong();
long y3 = in.nextLong();
long x4 = in.nextLong();
long y4 = in.nextLong();
long w = white(1, 1, m, n);
long b = black(1, 1, m, n);
long whited = 0;
if(x3 > x2 || x4 < x1 || y3 > y2 || y4 < y1) {
whited = black(x1, y1, x2, y2);
} else {
whited = black(x1, y1, x2, y2);
long xm1 = Math.max(x1, x3);
long ym1 = Math.max(y1, y3);
long xm2 = Math.min(x2, x4);
long ym2 = Math.min(y2, y4);
whited -= black(xm1, ym1, xm2, ym2);
}
b -= whited;w += whited;
long blacked = white(x3, y3, x4, y4);
w-= blacked;b += blacked;
out.println(w + " " + b);
}
}
long black(long x1, long y1, long x2, long y2) {
long dx = (x2 - x1) + 1;
long dy = (y2 - y1) + 1;
if((x1+y1)%2!=0) {
return ((dy+1)/2)*((dx+1)/2)+(dy/2)*(dx/2);
}
return ((dy+1)/2)*((dx)/2)+(dy/2)*((dx+1)/2);
}
long white(long x1, long y1, long x2, long y2) {
long dx = (x2 - x1) + 1;
long dy = (y2 - y1) + 1;
if((x1+y1)%2==0) {
return ((dy+1)/2)*((dx+1)/2)+(dy/2)*(dx/2);
}
return ((dy+1)/2)*(dx/2)+(dy/2)*((dx+1)/2);
}
}
static void prepareIO(boolean isFileIO) {
//long t1 = System.currentTimeMillis();
Task solver = new Task();
// Standard IO
if(!isFileIO) {
InputStream inputStream = System.in;
OutputStream outputStream = System.out;
InputReader in = new InputReader(inputStream);
PrintWriter out = new PrintWriter(outputStream);
solver.solve(in, out);
//out.println("time(s): " + (1.0*(System.currentTimeMillis()-t1))/1000.0);
out.close();
}
// File IO
else {
String IPfilePath = System.getProperty("user.home") + "/Downloads/ip.in";
String OPfilePath = System.getProperty("user.home") + "/Downloads/op.out";
InputReader fin = new InputReader(IPfilePath);
PrintWriter fout = null;
try {
fout = new PrintWriter(new File(OPfilePath));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
solver.solve(fin, fout);
//fout.println("time(s): " + (1.0*(System.currentTimeMillis()-t1))/1000.0);
fout.close();
}
}
public static void main(String[] args) {
prepareIO(false);
}
static class InputReader {
public BufferedReader reader;
public StringTokenizer tokenizer;
public InputReader(InputStream stream) {
reader = new BufferedReader(new InputStreamReader(stream), 32768);
tokenizer = null;
}
public InputReader(String filePath) {
File file = new File(filePath);
try {
reader = new BufferedReader(new FileReader(file));
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
tokenizer = null;
}
public String nextLine() {
String str = "";
try {
str = reader.readLine();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return str;
}
public String next() {
while (tokenizer == null || !tokenizer.hasMoreTokens()) {
try {
tokenizer = new StringTokenizer(reader.readLine());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return tokenizer.nextToken();
}
public int nextInt() {
return Integer.parseInt(next());
}
public long nextLong() {
return Long.parseLong(next());
}
public double nextDouble() {
return Double.parseDouble(next());
}
}
} | constant | 1080_C. Masha and two friends | CODEFORCES |
/**
* Created by Baelish on 8/28/2018.
*/
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.Arrays;
import java.util.InputMismatchException;
public class F_DSU {
public static void main(String[] args)throws Exception {
FastReader in = new FastReader(System.in);
PrintWriter pw = new PrintWriter(System.out);
n = in.nextInt();
int brr[] = new int[2*n];
for (int i = 0; i < 2*n; i+= 2) {
brr[i] = in.nextInt();
brr[i+1] = in.nextInt();
}
arr = shrink(brr);
int imap[] = new int[2*n];
for (int i = 0; i < 2*n; i++) {
imap[arr[i]] = brr[i];
}
int idx = binarySearch(arr.length);
if(idx >= arr.length) pw.println(-1);
else pw.println(imap[idx]);
pw.close();
}
static int n, arr[];
static int binarySearch(int H) {
int lo = 0, hi = H, mid;
while (lo < hi) {
mid = (lo + hi) / 2;
if (check(mid)) hi = mid;
else lo = mid + 1;
}
if(lo > 0 && check(lo-1)) return lo-1;
return lo;
}
static boolean check(int m){
DSU dsu = new DSU(2*n);
for (int i = 0; i < n; i++) {
int u = arr[2*i], v = arr[2*i+1];
if(u > m) return false;
if(v > m){
if(++dsu.cycle[dsu.find(u)] >= 2) return false;
}
else{
if(!dsu.union(u, v)){
if(++dsu.cycle[dsu.find(u)] >= 2) return false;
}
else{
if(dsu.cycle[dsu.find(u)] >= 2) return false;
}
}
}
return true;
}
static class DSU{
int parent[], cycle[], n;
DSU(int N){
n = N;
parent = new int[N];
cycle = new int[N];
for(int i = 0; i < N; i++){
parent[i] = i;
}
}
DSU(int [] p){
parent = p; n = p.length;
}
int find(int i) {
int p = parent[i];
if (i == p) return i;
return parent[i] = find(p);
}
boolean equiv(int u, int v){
return find(u) == find(v);
}
boolean union(int u, int v){
u = find(u); v = find(v);
if(u != v) {
parent[u] = parent[v];
cycle[v] += cycle[u];
}
return u != v;
}
int count(){
int cnt = 0;
for(int i = 0; i < n; i++){
if(i == find(i)) cnt++;
}
return cnt;
}
}
public static int[] shrink(int[] a) {
int n = a.length;
long[] b = new long[n];
for(int i = 0;i < n;i++)b[i] = (long)a[i]<<32|i;
Arrays.sort(b);
int[] ret = new int[n];
int p = 0;
for(int i = 0;i < n;i++) {
if(i>0 && (b[i]^b[i-1])>>32!=0)p++;
ret[(int)b[i]] = p;
}
return ret;
}
static void debug(Object...obj) {
System.err.println(Arrays.deepToString(obj));
}
static class FastReader {
InputStream is;
private byte[] inbuf = new byte[1024];
private int lenbuf = 0, ptrbuf = 0;
static final int ints[] = new int[128];
public FastReader(InputStream is){
for(int i='0';i<='9';i++) ints[i]=i-'0';
this.is = is;
}
public int readByte(){
if(lenbuf == -1)throw new InputMismatchException();
if(ptrbuf >= lenbuf){
ptrbuf = 0;
try { lenbuf = is.read(inbuf); } catch (IOException e) { throw new InputMismatchException(); }
if(lenbuf <= 0)return -1;
}
return inbuf[ptrbuf++];
}
public boolean isSpaceChar(int c) {
return !(c >= 33 && c <= 126);
}
public int skip() {
int b;
while((b = readByte()) != -1 && isSpaceChar(b));
return b;
}
public String next(){
int b = skip();
StringBuilder sb = new StringBuilder();
while(!(isSpaceChar(b))){ // when nextLine, (isSpaceChar(b) && b != ' ')
sb.appendCodePoint(b);
b = readByte();
}
return sb.toString();
}
public int nextInt(){
int num = 0, b;
boolean minus = false;
while((b = readByte()) != -1 && !((b >= '0' && b <= '9') || b == '-'));
if(b == '-'){
minus = true;
b = readByte();
}
while(true){
if(b >= '0' && b <= '9'){
num = (num<<3) + (num<<1) + ints[b];
}else{
return minus ? -num : num;
}
b = readByte();
}
}
public long nextLong() {
long num = 0;
int b;
boolean minus = false;
while((b = readByte()) != -1 && !((b >= '0' && b <= '9') || b == '-'));
if(b == '-'){
minus = true;
b = readByte();
}
while(true){
if(b >= '0' && b <= '9'){
num = (num<<3) + (num<<1) + ints[b];
}else{
return minus ? -num : num;
}
b = readByte();
}
}
public double nextDouble() {
return Double.parseDouble(next());
}
/* public char nextChar() {
return (char)skip();
}*/
public char[] next(int n){
char[] buf = new char[n];
int b = skip(), p = 0;
while(p < n && !(isSpaceChar(b))){
buf[p++] = (char)b;
b = readByte();
}
return n == p ? buf : Arrays.copyOf(buf, p);
}
/*private char buff[] = new char[1005];
public char[] nextCharArray(){
int b = skip(), p = 0;
while(!(isSpaceChar(b))){
buff[p++] = (char)b;
b = readByte();
}
return Arrays.copyOf(buff, p);
}*/
}
} | quadratic | 1027_F. Session in BSU | CODEFORCES |
import java.io.*;
public class n5D
{
public static void main(String[] args)
{
double a = 0, v = 0, l = 0, d = 0, w = 0;
try
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String[] str = br.readLine().split(" ");
a = Double.parseDouble(str[0]);
v = Double.parseDouble(str[1]);
str = br.readLine().split(" ");
l = Double.parseDouble(str[0]);
d = Double.parseDouble(str[1]);
w = Double.parseDouble(str[2]);
}
catch(Exception e)
{
System.out.println(e);
}
double t1, t2, t3, t4, t5, t, D = 0;
if (w > v) w = v;
t2 = d / v - v / a + w * w / 2 / a / v;
if (t2 >= 0)
{
t1 = v / a;
t3 = t1 - w / a;
}
else
{
if (Math.sqrt(2 * d / a) > (w / a))
{
t1 = Math.sqrt((2 * a * d + w * w) / (a * a * 2));
t3 = t1 - w / a;
}
else
{
t1 = Math.sqrt(2 * d / a);
t3 = 0;
}
t2 = 0;
}
t5 = (l - d - v * v / 2 / a + a * (t1 - t3) * (t1 - t3) / 2) / v;
if (t5 >= 0) t4 = v / a - (t1 - t3);
else
{
t5 = 0;
t4 = -t1 + t3 + Math.sqrt((t1 - t3) * (t1 - t3) + 2 * (l - d) / a);
}
t = t1 + t2 + t3 + t4 + t5;
System.out.println(t);
//System.out.println(t1 + " " + t2 + " " + t3 + " " + t4 + " " + t5);
}
} | constant | 5_D. Follow Traffic Rules | CODEFORCES |
import java.util.*;
import java.io.*;
public class Solution{
public static long page(long p,long k){
return (p-1)/k;
}
public static void main(String[] args) throws Exception {
Scanner sc = new Scanner(System.in);
long n = sc.nextLong();
int m = sc.nextInt();
long k = sc.nextLong();
long[] p = new long[m];
long del = 0;
long nb = 1;
int op = 0;
for(int i=0;i<m;i++) p[i] = sc.nextLong();
for(int i=1;i<m;i++){
if(page(p[i]-del,k)!=page(p[i-1]-del,k)){
del += nb;
nb = 1;
op++;
}else{
nb++;
}
}
if(nb!=0) op++;
System.out.println(op);
}
} | linear | 1191_C. Tokitsukaze and Discard Items | CODEFORCES |
import java.io.PrintWriter;
import java.util.*;
import java.util.Arrays ;
import java .lang.String.* ;
import java .lang.StringBuilder ;
public class Test{
static int pos = 0 ;
static int arr[] ;
static LinkedList l1 = new LinkedList() ;
static void find(int p ,char[]x,int put[],String s){
int c= 0 ;
for (int i = 0; i < s.length(); i++) {
if(x[p]==s.charAt(i)){
c++ ; }
}
put[p] = c ;
}
static int mode(int m ,int[]x ){
int temp = 0 ;
for (int i = x.length-1; i >=0; i--) {
if(x[i]<=m){
temp= x[i] ;
/// break ;
return m-temp ;
}
}
return m-temp ;
}
static int mode2(int m ,int[]x ){
int temp = 0 ;
for (int i = x.length-1; i >=0; i--) {
if(x[i]<=m){
temp= x[i] ;
/// break ;
return x[i] ;
}
}
return 0 ;
}
static int find(int x[],int temp){
int j = 0 ;
for (int i = x.length-1; i >=0; i--) {
if(x[i]==temp) return j+1 ;
j++ ;
}
return -1 ;
}
static String ch(long[]x,long b){
for (int i = 0; i < x.length; i++) {
if(x[i]==b)return "YES" ;
}
return "NO" ;
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in) ;
PrintWriter pw = new PrintWriter(System.out);
int k=in.nextInt(), n=in.nextInt(), s=in.nextInt(), p=in.nextInt() ;
int paper =n/s;
if(n%s!=0) paper++ ;
paper*=k ;
int fin = paper/p ;
if(paper%p!=0) fin++ ;
System.out.println( fin );
}
}
| constant | 965_A. Paper Airplanes | CODEFORCES |
import java.util.*;
import static java.lang.Math.*;
import java.io.*;
public class SolutionB {
public static void main(String args[])throws IOException{
Scanner sc = new Scanner(System.in);
int a[] = new int[1501];
for(int i = 0; i < 3; i++){
a[sc.nextInt()]++;
}
if(a[1] > 0 || a[2] > 1 || a[3] > 2 || (a[4] == 2 && a[2] == 1)){
System.out.println("YES");
}else{
System.out.println("NO");
}
}
} | constant | 911_C. Three Garlands | CODEFORCES |
import java.io.IOException;
import java.io.InputStream;
import java.util.InputMismatchException;
import java.util.Stack;
public class D527A2 {
public static void main(String[] args) {
FastScanner in = new FastScanner(System.in);
int N = in.nextInt();
Stack<Integer> stack = new Stack<>();
for(int i = 0; i < N; i++) {
int num = in.nextInt() % 2;
if(stack.size() >= 1 && stack.lastElement() == num)
stack.pop();
else
stack.add(num);
}
System.out.println(stack.size() <= 1 ? "YES" : "NO");
}
/**
* Source: Matt Fontaine
*/
static class FastScanner {
private InputStream stream;
private byte[] buf = new byte[1024];
private int curChar;
private int chars;
public FastScanner(InputStream stream) {
this.stream = stream;
}
int read() {
if (chars == -1)
throw new InputMismatchException();
if (curChar >= chars) {
curChar = 0;
try {
chars = stream.read(buf);
} catch (IOException e) {
throw new InputMismatchException();
}
if (chars <= 0)
return -1;
}
return buf[curChar++];
}
boolean isSpaceChar(int c) {
return c == ' ' || c == '\n' || c == '\r' || c == '\t' || c == -1;
}
boolean isEndline(int c) {
return c == '\n' || c == '\r' || c == -1;
}
public int nextInt() {
return Integer.parseInt(next());
}
public long nextLong() {
return Long.parseLong(next());
}
public double nextDouble() {
return Double.parseDouble(next());
}
public String next() {
int c = read();
while (isSpaceChar(c))
c = read();
StringBuilder res = new StringBuilder();
do {
res.appendCodePoint(c);
c = read();
} while (!isSpaceChar(c));
return res.toString();
}
public String nextLine() {
int c = read();
while (isEndline(c))
c = read();
StringBuilder res = new StringBuilder();
do {
res.appendCodePoint(c);
c = read();
} while (!isEndline(c));
return res.toString();
}
}
}
/*
5
2 1 1 2 5
outputCopy
YES
inputCopy
3
4 5 3
outputCopy
YES
inputCopy
2
10 10
outputCopy
YES
inputCopy
3
1 2 3
outputCopy
NO
5
2 3 2 2 3
YES
*/ | linear | 1092_D1. Great Vova Wall (Version 1) | CODEFORCES |
import java.util.*;
import java.io.*;
import java.awt.Point;
import java.math.BigInteger;
public class stacks {
public static void main(String[] args) throws Exception {
FastIO sc = new FastIO(System.in);
PrintWriter pw = new PrintWriter(System.out);
int n = sc.nextInt();
int m = sc.nextInt();
long remove = 0;
int[] heights = new int[n+1];
for(int i = 0; i < n; i++) {
heights[i] = sc.nextInt();
remove += heights[i];
}
Arrays.sort(heights);
//System.out.println(Arrays.toString(heights));
long keep = 0;
for(int i = n; i> 0; i--) {
if(heights[i-1] >= heights[i]) {
heights[i-1] = heights[i]-1;
}
keep += heights[i] - heights[i-1];
}
//System.out.println(Arrays.toString(heights));
pw.println(remove - keep);
pw.close();
}
static class FastIO {
//Is your Fast I/O being bad?
InputStream dis;
byte[] buffer = new byte[1 << 17];
int pointer = 0;
public FastIO(String fileName) throws Exception {
dis = new FileInputStream(fileName);
}
public FastIO(InputStream is) throws Exception {
dis = is;
}
int nextInt() throws Exception {
int ret = 0;
byte b;
do {
b = nextByte();
} while (b <= ' ');
boolean negative = false;
if (b == '-') {
negative = true;
b = nextByte();
}
while (b >= '0' && b <= '9') {
ret = 10 * ret + b - '0';
b = nextByte();
}
return (negative) ? -ret : ret;
}
long nextLong() throws Exception {
long ret = 0;
byte b;
do {
b = nextByte();
} while (b <= ' ');
boolean negative = false;
if (b == '-') {
negative = true;
b = nextByte();
}
while (b >= '0' && b <= '9') {
ret = 10 * ret + b - '0';
b = nextByte();
}
return (negative) ? -ret : ret;
}
byte nextByte() throws Exception {
if (pointer == buffer.length) {
dis.read(buffer, 0, buffer.length);
pointer = 0;
}
return buffer[pointer++];
}
String next() throws Exception {
StringBuffer ret = new StringBuffer();
byte b;
do {
b = nextByte();
} while (b <= ' ');
while (b > ' ') {
ret.appendCodePoint(b);
b = nextByte();
}
return ret.toString();
}
}
}
| nlogn | 1061_B. Views Matter | CODEFORCES |
import java.io.OutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.Arrays;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.FileNotFoundException;
import java.util.StringTokenizer;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.InputStream;
/**
* Built using CHelper plug-in
* Actual solution is at the top
*
* @author \/
*/
public class Main {
public static void main(String[] args) {
InputStream inputStream = System.in;
OutputStream outputStream = System.out;
Scanner in = new Scanner(inputStream);
PrintWriter out = new PrintWriter(outputStream);
TaskC solver = new TaskC();
solver.solve(1, in, out);
out.close();
}
static class TaskC {
public void solve(int testNumber, Scanner in, PrintWriter out) {
int n = in.nextInt();
int m = in.nextInt();
TaskC.pair[] songs = new TaskC.pair[n];
long sum = 0;
for (int i = 0; i < n; i++) {
songs[i] = new TaskC.pair(in.nextInt(), in.nextInt());
sum += songs[i].a;
}
Arrays.sort(songs);
int res = 0;
int idx = n - 1;
while (sum > m) {
if (idx < 0) {
break;
}
sum -= (songs[idx].a - songs[idx].b);
res++;
idx--;
}
if (sum > m) {
out.println(-1);
} else {
out.println(res);
}
}
static class pair implements Comparable<TaskC.pair> {
int a;
int b;
pair(int a, int b) {
this.a = a;
this.b = b;
}
public int compareTo(TaskC.pair p) {
return (this.a - this.b) - (p.a - p.b);
}
}
}
static class Scanner {
StringTokenizer st;
BufferedReader br;
public Scanner(InputStream s) {
br = new BufferedReader(new InputStreamReader(s));
}
public Scanner(String s) {
try {
br = new BufferedReader(new FileReader(s));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
public String next() {
while (st == null || !st.hasMoreTokens()) {
try {
st = new StringTokenizer(br.readLine());
} catch (IOException e) {
e.printStackTrace();
}
}
return st.nextToken();
}
public int nextInt() {
return Integer.parseInt(next());
}
}
}
| nlogn | 1015_C. Songs Compression | CODEFORCES |
CodeComplex Dataset
Dataset Description
CodeComplex consists of 4,200 Java codes submitted to programming competitions by human programmers and their complexity labels annotated by a group of algorithm experts.
How to use it
You can load and iterate through the dataset with the following two lines of code:
from datasets import load_dataset
ds = load_dataset("codeparrot/codecomplex", split="train")
print(next(iter(ds)))
Data Structure
DatasetDict({
train: Dataset({
features: ['src', 'complexity', 'problem', 'from'],
num_rows: 4517
})
})
Data Instances
{'src': 'import java.io.*;\nimport java.math.BigInteger;\nimport java.util.InputMismatchException;...',
'complexity': 'quadratic',
'problem': '1179_B. Tolik and His Uncle',
'from': 'CODEFORCES'}
Data Fields
- src: a string feature, representing the source code in Java.
- complexity: a string feature, giving program complexity.
- problem: a string of the feature, representing the problem name.
- from: a string feature, representing the source of the problem.
complexity filed has 7 classes, where each class has around 500 codes each. The seven classes are constant, linear, quadratic, cubic, log(n), nlog(n) and NP-hard.
Data Splits
The dataset only contains a train split.
Dataset Creation
The authors first collected problem and solution codes in Java from CodeForces and they were inspected by experienced human annotators to label each code by their time complexity. After the labelling, they used different programming experts to verify the class of each data that the human annotators assigned.
Citation Information
@article{JeonBHHK22,
author = {Mingi Jeon and Seung-Yeop Baik and Joonghyuk Hahn and Yo-Sub Han and Sang-Ki Ko},
title = {{Deep Learning-based Code Complexity Prediction}},
year = {2022},
}
- Downloads last month
- 15
Models trained or fine-tuned on codeparrot/codecomplex
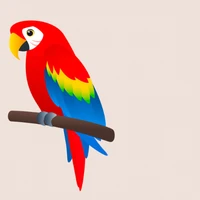