Hameln Japanese Mistral 7B
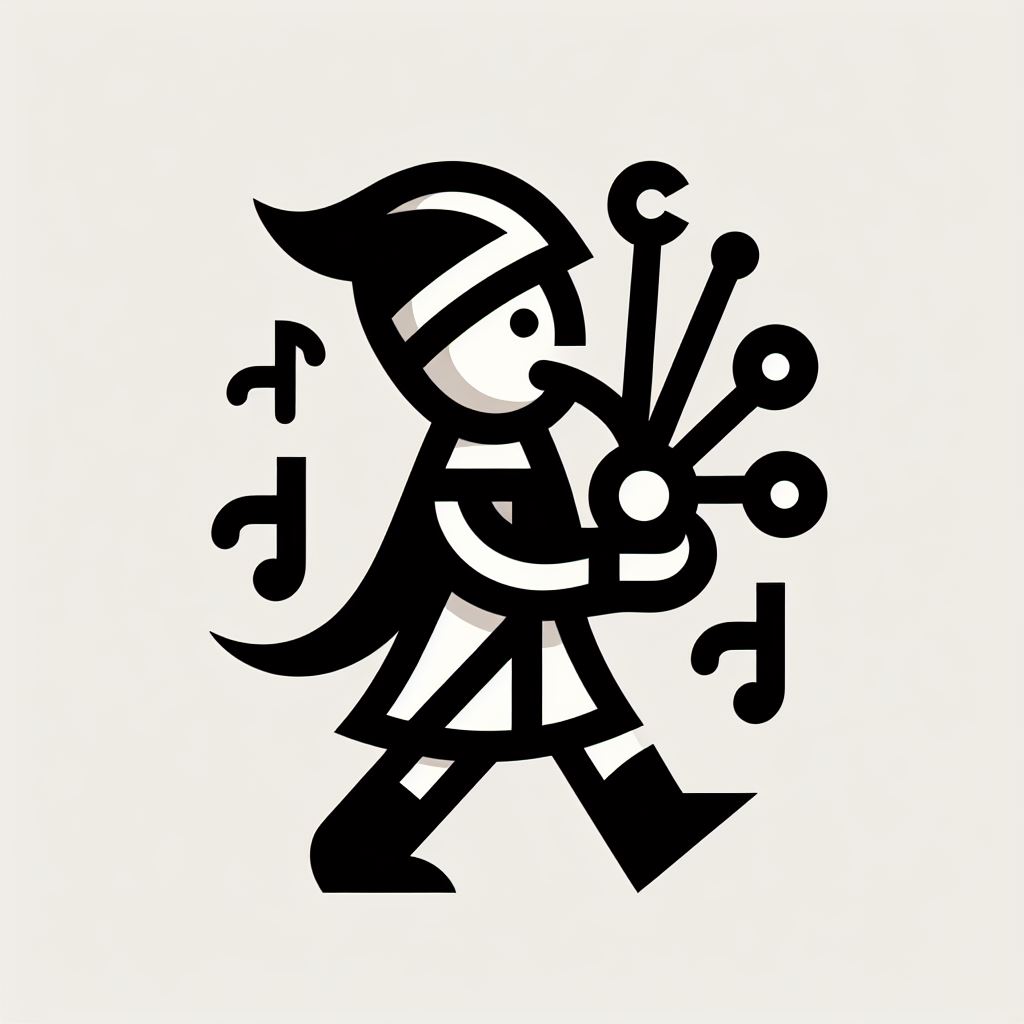
Model Description
This is a 7B-parameter decoder-only Japanese language model fine-tuned on novel datasets, built on top of the base model Japanese Stable LM Base Gamma 7B. Japanese Stable LM Instruct Gamma 7B
Usage
Ensure you are using Transformers 4.34.0 or newer.
import torch
from transformers import AutoTokenizer, AutoModelForCausalLM
tokenizer = AutoTokenizer.from_pretrained("Elizezen/Hameln-japanese-mistral-7B")
model = AutoModelForCausalLM.from_pretrained(
"Elizezen/Hameln-japanese-mistral-7B",
torch_dtype="auto",
)
model.eval()
if torch.cuda.is_available():
model = model.to("cuda")
input_ids = tokenizer.encode(
"ใใใใใใใใใใจใใใซใใใใใใใจใใฐใใใใไฝใใงใใพใใใ ใใใใใใฏๅฑฑใธๆดๅใใซใ",
add_special_tokens=True,
return_tensors="pt"
)
tokens = model.generate(
input_ids.to(device=model.device),
max_new_tokens=512,
temperature=1,
top_p=0.95,
do_sample=True,
)
out = tokenizer.decode(tokens[0][input_ids.shape[1]:], skip_special_tokens=True).strip()
print(out)
"""
output example:
ใใใใใใใใใใจใใใซใใใใใใใจใใฐใใใใไฝใใงใใพใใใ ใใใใใใฏๅฑฑใธๆดๅใใซใใใฐใใใใฏ็ฐใใผใฎ็จฒใฎๆไผใใใใใชใฉใไบไบบใงๅใๅใใใฆๆฅฝใใๆฎใใใฆใใพใใใ
ใใๆฅใฎใใจใใใฎๅฐๆนไธๅธฏใซๅคงใใชๅฐ้ขจใใใฃใฆๆฅใพใใใๅผท้ขจใซ้ฃใฐใใใๆจใใๅฎถๅฑใชใฉใๆฌกใ
ใจๅใใไธญใๅนธใใซใใใใใใใจใใฐใใใใฎไฝใใงใใๆใฏ็กไบใงใใใ
ใใใใ่ฟ้ฃใฎๅฐใใชๆใงใฏ่ขซๅฎณใๅบใฆใใพใใใๅฎถๅฑใฏๅ
จๅฃใ่พฒไฝ็ฉใฏ่ใใใใไฝใใๅคใใฎๅฝใๅคฑใใใฆใใพใใใ
ใๅฏๅๆณใซโฆโฆใ
ใใฐใใใใฏๅฟใ็ใใ็ฅๆงใซ็ฅใใๆงใ็ถใใพใใใ
ใๅคฉไธใฎ็ฅๆง๏ผใฉใใใ็ง้ไบบ้ใๅฎใฃใฆไธใใ๏ผใ
ใใฐใใใใฎ็ฅใใ้ใใใฎใใๅฐ้ขจใฏๆฅ้ใซๅขๅใ่ฝใจใใ่ขซๅฎณใฏๆๅฐ้ใฎๅ
ใซๆฒปใพใใพใใใ
"""
Datasets
- less than 1GB of web novels(non-PG)
- 70GB of web novels(PG)
Intended Use
The primary purpose of this language model is to assist in generating novels. While it can handle various prompts, it may not excel in providing instruction-based responses. Note that the model's responses are not censored, and occasionally sensitive content may be generated.
- Downloads last month
- 1