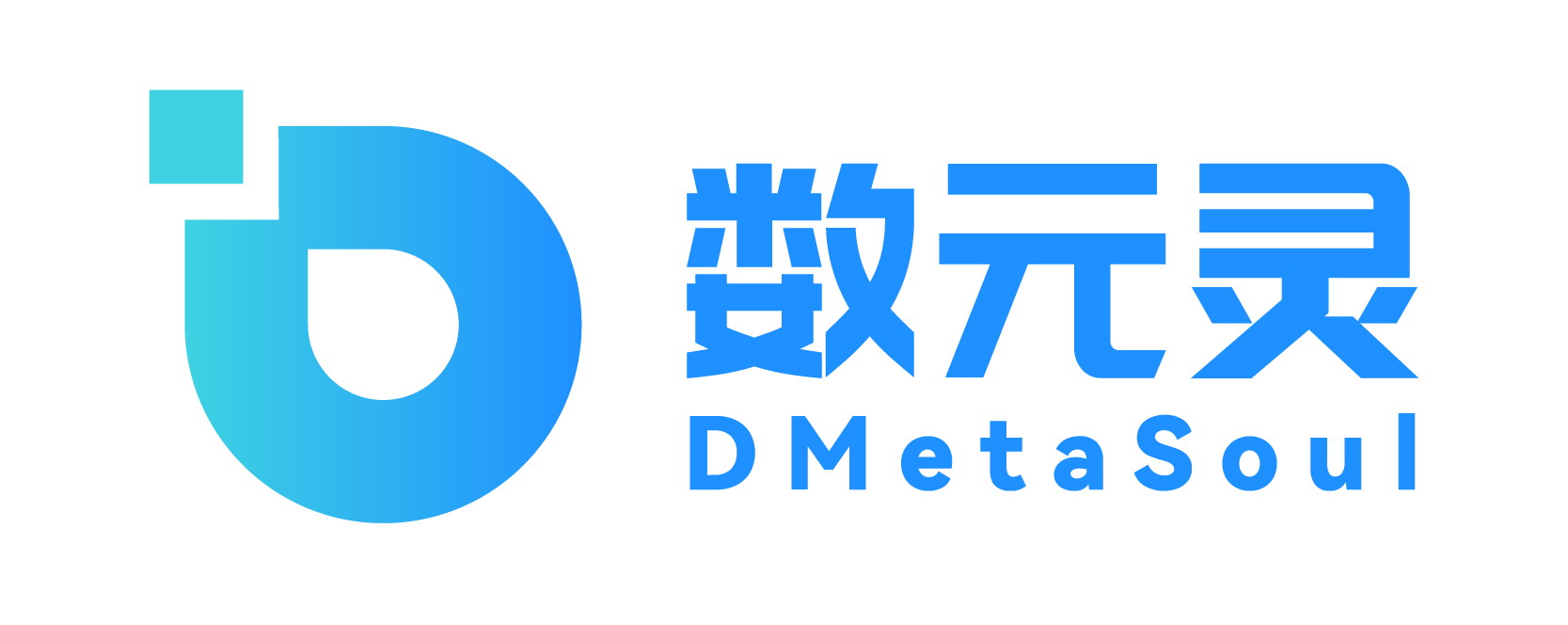
Dmeta-embedding
Usage | Evaluation (MTEB) | FAQ | Contact | License (Free)
Update News
2024.04.01, The Dmeta-embedding small version is released. Just with 8 layers, inference is more efficient, about 30% improved.
2024.02.07, The Embedding API service based on the Dmeta-embedding model now open for internal beta testing. Click the link to apply, and you will receive 400M tokens for free, which can encode approximately GB-level Chinese text.
- Our original intention. Let everyone use Embedding technology at low cost, pay more attention to their own business and product services, and leave the complex technical parts to us.
- How to apply and use. Click the link to submit a form. We will reply to you via aigc@dmetasoul.com within 48 hours. In order to be compatible with the large language model (LLM) technology ecosystem, our Embedding API is used in the same way as OpenAI. We will explain the specific usage in the reply email.
- Join the ours. In the future, we will continue to work in the direction of large language models/AIGC to bring valuable technologies to the community. You can click on the picture and scan the QR code to join our WeChat community and cheer for the AIGC together!
Dmeta-embedding is a cross-domain, cross-task, out-of-the-box Chinese embedding model. It is suitable for various scenarios such as search engine, Q&A, intelligent customer service, LLM+RAG, etc. It supports inference using tools like Transformers/Sentence-Transformers/Langchain.
Features:
- Excellent cross-domain and scene generalization performance, currently ranked second on the MTEB Chinese leaderboard. (2024.01.25)
- The parameter size of model is just 400MB, which can greatly reduce the cost of inference.
- The context window length is up to 1024, more suitable for long text retrieval, RAG and other scenarios
Usage
The model supports inference through frameworks such as Sentence-Transformers, Langchain, Huggingface Transformers, etc. For specific usage, please refer to the following examples.
Sentence-Transformers
Load and inference Dmeta-embedding via sentence-transformers as following:
pip install -U sentence-transformers
from sentence_transformers import SentenceTransformer
texts1 = ["胡子长得太快怎么办?", "在香港哪里买手表好"]
texts2 = ["胡子长得快怎么办?", "怎样使胡子不浓密!", "香港买手表哪里好", "在杭州手机到哪里买"]
model = SentenceTransformer('DMetaSoul/Dmeta-embedding')
embs1 = model.encode(texts1, normalize_embeddings=True)
embs2 = model.encode(texts2, normalize_embeddings=True)
similarity = embs1 @ embs2.T
print(similarity)
for i in range(len(texts1)):
scores = []
for j in range(len(texts2)):
scores.append([texts2[j], similarity[i][j]])
scores = sorted(scores, key=lambda x:x[1], reverse=True)
print(f"查询文本:{texts1[i]}")
for text2, score in scores:
print(f"相似文本:{text2},打分:{score}")
print()
Output:
查询文本:胡子长得太快怎么办?
相似文本:胡子长得快怎么办?,打分:0.9535336494445801
相似文本:怎样使胡子不浓密!,打分:0.6776421070098877
相似文本:香港买手表哪里好,打分:0.2297907918691635
相似文本:在杭州手机到哪里买,打分:0.11386542022228241
查询文本:在香港哪里买手表好
相似文本:香港买手表哪里好,打分:0.9843372106552124
相似文本:在杭州手机到哪里买,打分:0.45211508870124817
相似文本:胡子长得快怎么办?,打分:0.19985519349575043
相似文本:怎样使胡子不浓密!,打分:0.18558596074581146
Langchain
Load and inference Dmeta-embedding via langchain as following:
pip install -U langchain
import torch
import numpy as np
from langchain.embeddings import HuggingFaceEmbeddings
model_name = "DMetaSoul/Dmeta-embedding"
model_kwargs = {'device': 'cuda' if torch.cuda.is_available() else 'cpu'}
encode_kwargs = {'normalize_embeddings': True} # set True to compute cosine similarity
model = HuggingFaceEmbeddings(
model_name=model_name,
model_kwargs=model_kwargs,
encode_kwargs=encode_kwargs,
)
texts1 = ["胡子长得太快怎么办?", "在香港哪里买手表好"]
texts2 = ["胡子长得快怎么办?", "怎样使胡子不浓密!", "香港买手表哪里好", "在杭州手机到哪里买"]
embs1 = model.embed_documents(texts1)
embs2 = model.embed_documents(texts2)
embs1, embs2 = np.array(embs1), np.array(embs2)
similarity = embs1 @ embs2.T
print(similarity)
for i in range(len(texts1)):
scores = []
for j in range(len(texts2)):
scores.append([texts2[j], similarity[i][j]])
scores = sorted(scores, key=lambda x:x[1], reverse=True)
print(f"查询文本:{texts1[i]}")
for text2, score in scores:
print(f"相似文本:{text2},打分:{score}")
print()
HuggingFace Transformers
Load and inference Dmeta-embedding via HuggingFace Transformers as following:
pip install -U transformers
import torch
from transformers import AutoTokenizer, AutoModel
def mean_pooling(model_output, attention_mask):
token_embeddings = model_output[0] #First element of model_output contains all token embeddings
input_mask_expanded = attention_mask.unsqueeze(-1).expand(token_embeddings.size()).float()
return torch.sum(token_embeddings * input_mask_expanded, 1) / torch.clamp(input_mask_expanded.sum(1), min=1e-9)
def cls_pooling(model_output):
return model_output[0][:, 0]
texts1 = ["胡子长得太快怎么办?", "在香港哪里买手表好"]
texts2 = ["胡子长得快怎么办?", "怎样使胡子不浓密!", "香港买手表哪里好", "在杭州手机到哪里买"]
tokenizer = AutoTokenizer.from_pretrained('DMetaSoul/Dmeta-embedding')
model = AutoModel.from_pretrained('DMetaSoul/Dmeta-embedding')
model.eval()
with torch.no_grad():
inputs1 = tokenizer(texts1, padding=True, truncation=True, return_tensors='pt')
inputs2 = tokenizer(texts2, padding=True, truncation=True, return_tensors='pt')
model_output1 = model(**inputs1)
model_output2 = model(**inputs2)
embs1, embs2 = cls_pooling(model_output1), cls_pooling(model_output2)
embs1 = torch.nn.functional.normalize(embs1, p=2, dim=1).numpy()
embs2 = torch.nn.functional.normalize(embs2, p=2, dim=1).numpy()
similarity = embs1 @ embs2.T
print(similarity)
for i in range(len(texts1)):
scores = []
for j in range(len(texts2)):
scores.append([texts2[j], similarity[i][j]])
scores = sorted(scores, key=lambda x:x[1], reverse=True)
print(f"查询文本:{texts1[i]}")
for text2, score in scores:
print(f"相似文本:{text2},打分:{score}")
print()
Evaluation
The Dmeta-embedding model ranked first in open source on the MTEB Chinese list (2024.01.25, first on the Baichuan list, that is not open source). For specific evaluation data and code, please refer to the MTEB official.
MTEB Chinese:
The Chinese leaderboard dataset was collected by the BAAI. It contains 6 classic tasks and a total of 35 Chinese datasets, covering classification, retrieval, reranking, sentence pair classification, STS and other tasks. It is the most comprehensive Embedding model at present. The world's authoritative benchmark of ability assessments.
Model | Vendor | Embedding dimension | Avg | Retrieval | STS | PairClassification | Classification | Reranking | Clustering |
---|---|---|---|---|---|---|---|---|---|
Dmeta-embedding | Our | 768 | 67.51 | 70.41 | 64.09 | 88.92 | 70 | 67.17 | 50.96 |
gte-large-zh | AliBaba Damo | 1024 | 66.72 | 72.49 | 57.82 | 84.41 | 71.34 | 67.4 | 53.07 |
BAAI/bge-large-zh-v1.5 | BAAI | 1024 | 64.53 | 70.46 | 56.25 | 81.6 | 69.13 | 65.84 | 48.99 |
BAAI/bge-base-zh-v1.5 | BAAI | 768 | 63.13 | 69.49 | 53.72 | 79.75 | 68.07 | 65.39 | 47.53 |
text-embedding-ada-002(OpenAI) | OpenAI | 1536 | 53.02 | 52.0 | 43.35 | 69.56 | 64.31 | 54.28 | 45.68 |
text2vec-base | 个人 | 768 | 47.63 | 38.79 | 43.41 | 67.41 | 62.19 | 49.45 | 37.66 |
text2vec-large | 个人 | 1024 | 47.36 | 41.94 | 44.97 | 70.86 | 60.66 | 49.16 | 30.02 |
FAQ
1. Why does the model have so good generalization performance, and can be used to many task scenarios out of the box?
The excellent generalization ability of the model comes from the diversity of pre-training data, as well as the design of different optimization objectives for multi-task scenarios when pre-training the model.
Specifically, the mainly technical features:
The first is large-scale weak label contrastive learning. Industry experience shows that out-of-the-box language models perform poorly on Embedding-related tasks. However, due to the high cost of supervised data annotation and acquisition, large-scale, high-quality weak label learning has become an optional technical route. By extracting weak labels from semi-structured data such as forums, news, Q&A communities, and encyclopedias on the Internet, and using large models to perform low-quality filtering, 1 billion-level weakly supervised text pair data is obtained.
The second is high-quality supervised learning. We have collected and compiled a large-scale open source annotated sentence pair data set, including a total of 30 million sentence pair samples in encyclopedia, education, finance, medical care, law, news, academia and other fields. At the same time, we mine hard-to-negative sample pairs and use contrastive learning to better optimize the model.
The last step is the optimization of retrieval tasks. Considering that search, question and answer, RAG and other scenarios are important application positions for the Embedding model, in order to enhance the cross-domain and cross-scenario performance of the model, we have specially optimized the model for retrieval tasks. The core lies in mining data from question and answer, retrieval and other data. Hard-to-negative samples use sparse and dense retrieval and other methods to construct a million-level hard-to-negative sample pair data set, which significantly improves the cross-domain retrieval performance of the model.
2. Can the model be used commercially?
Our model is based on the Apache-2.0 license and fully supports free commercial use.
3. How to reproduce the MTEB evaluation?
We provide the mteb_eval.py script in this model hub. You can run this script directly to reproduce our evaluation results.
4. What are the follow-up plans?
We will continue to work hard to provide the community with embedding models that have excellent performance, lightweight reasoning, and can be used in multiple scenarios out of the box. At the same time, we will gradually integrate embedding into the existing technology ecosystem and grow with the community!
Contact
If you encounter any problems during use, you are welcome to go to the discussion to make suggestions.
You can also send us an email: Zhao Zhonghao zhongh@dmetasoul.com, Xiao Wenbin xiaowenbin@dmetasoul.com, Sun Kai sunkai@dmetasoul.com
At the same time, you are welcome to scan the QR code to join our WeChat group and build the AIGC technology ecosystem together!
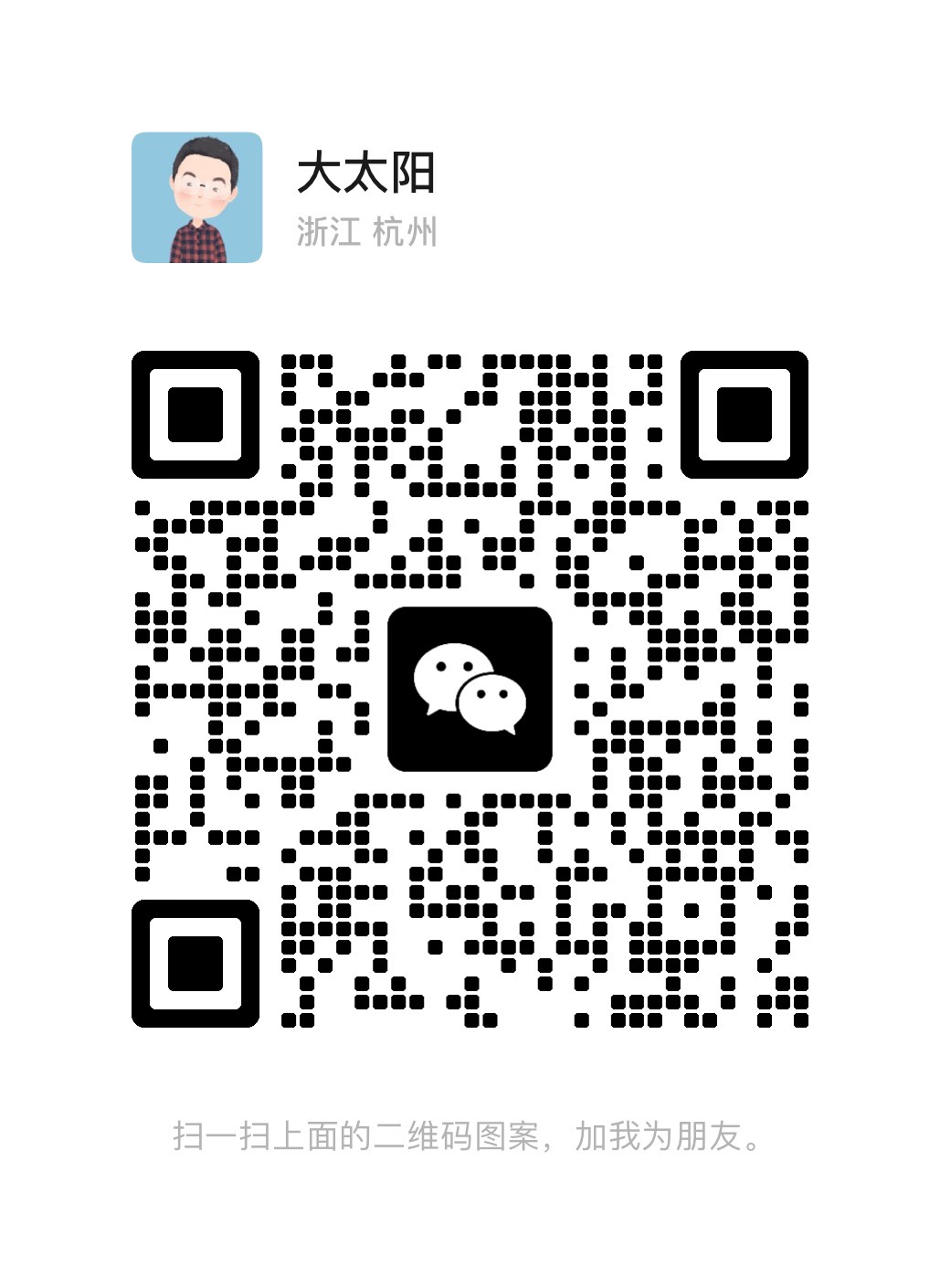
License
Dmeta-embedding is licensed under the Apache-2.0 License. The released models can be used for commercial purposes free of charge.
- Downloads last month
- 4,023
Space using DMetaSoul/Dmeta-embedding-zh 1
Evaluation results
- cos_sim_pearson on MTEB AFQMCvalidation set self-reported65.608
- cos_sim_spearman on MTEB AFQMCvalidation set self-reported71.129
- euclidean_pearson on MTEB AFQMCvalidation set self-reported70.181
- euclidean_spearman on MTEB AFQMCvalidation set self-reported71.129
- manhattan_pearson on MTEB AFQMCvalidation set self-reported70.145
- manhattan_spearman on MTEB AFQMCvalidation set self-reported71.052
- cos_sim_pearson on MTEB ATECtest set self-reported65.524
- cos_sim_spearman on MTEB ATECtest set self-reported64.642
- euclidean_pearson on MTEB ATECtest set self-reported73.202
- euclidean_spearman on MTEB ATECtest set self-reported64.642
- manhattan_pearson on MTEB ATECtest set self-reported73.228
- manhattan_spearman on MTEB ATECtest set self-reported64.626
- accuracy on MTEB AmazonReviewsClassification (zh)test set self-reported44.926
- f1 on MTEB AmazonReviewsClassification (zh)test set self-reported42.826
- cos_sim_pearson on MTEB BQtest set self-reported71.352
- cos_sim_spearman on MTEB BQtest set self-reported72.296
- euclidean_pearson on MTEB BQtest set self-reported70.946
- euclidean_spearman on MTEB BQtest set self-reported72.296
- manhattan_pearson on MTEB BQtest set self-reported70.845
- manhattan_spearman on MTEB BQtest set self-reported72.245
- v_measure on MTEB CLSClusteringP2Ptest set self-reported40.242
- v_measure on MTEB CLSClusteringS2Stest set self-reported39.168
- map on MTEB CMedQAv1test set self-reported88.488
- mrr on MTEB CMedQAv1test set self-reported90.369
- map on MTEB CMedQAv2test set self-reported89.179
- mrr on MTEB CMedQAv2test set self-reported91.358
- map_at_1 on MTEB CmedqaRetrievalself-reported25.751
- map_at_10 on MTEB CmedqaRetrievalself-reported38.946
- map_at_100 on MTEB CmedqaRetrievalself-reported40.855
- map_at_1000 on MTEB CmedqaRetrievalself-reported40.953
- map_at_3 on MTEB CmedqaRetrievalself-reported34.533
- map_at_5 on MTEB CmedqaRetrievalself-reported36.905
- mrr_at_1 on MTEB CmedqaRetrievalself-reported39.235
- mrr_at_10 on MTEB CmedqaRetrievalself-reported47.713
- mrr_at_100 on MTEB CmedqaRetrievalself-reported48.710
- mrr_at_1000 on MTEB CmedqaRetrievalself-reported48.747
- mrr_at_3 on MTEB CmedqaRetrievalself-reported45.086
- mrr_at_5 on MTEB CmedqaRetrievalself-reported46.498
- ndcg_at_1 on MTEB CmedqaRetrievalself-reported39.235
- ndcg_at_10 on MTEB CmedqaRetrievalself-reported45.831
- ndcg_at_100 on MTEB CmedqaRetrievalself-reported53.162
- ndcg_at_1000 on MTEB CmedqaRetrievalself-reported54.800
- ndcg_at_3 on MTEB CmedqaRetrievalself-reported40.188
- ndcg_at_5 on MTEB CmedqaRetrievalself-reported42.387
- precision_at_1 on MTEB CmedqaRetrievalself-reported39.235
- precision_at_10 on MTEB CmedqaRetrievalself-reported10.273
- precision_at_100 on MTEB CmedqaRetrievalself-reported1.627
- precision_at_1000 on MTEB CmedqaRetrievalself-reported0.183
- precision_at_3 on MTEB CmedqaRetrievalself-reported22.772
- precision_at_5 on MTEB CmedqaRetrievalself-reported16.524
- recall_at_1 on MTEB CmedqaRetrievalself-reported25.751
- recall_at_10 on MTEB CmedqaRetrievalself-reported57.411
- recall_at_100 on MTEB CmedqaRetrievalself-reported87.440
- recall_at_1000 on MTEB CmedqaRetrievalself-reported98.386
- recall_at_3 on MTEB CmedqaRetrievalself-reported40.416
- recall_at_5 on MTEB CmedqaRetrievalself-reported47.238
- cos_sim_accuracy on MTEB Cmnlivalidation set self-reported83.596
- cos_sim_ap on MTEB Cmnlivalidation set self-reported90.655
- cos_sim_f1 on MTEB Cmnlivalidation set self-reported84.765
- cos_sim_precision on MTEB Cmnlivalidation set self-reported81.041
- cos_sim_recall on MTEB Cmnlivalidation set self-reported88.847
- dot_accuracy on MTEB Cmnlivalidation set self-reported83.596
- dot_ap on MTEB Cmnlivalidation set self-reported90.644
- dot_f1 on MTEB Cmnlivalidation set self-reported84.765
- dot_precision on MTEB Cmnlivalidation set self-reported81.041
- dot_recall on MTEB Cmnlivalidation set self-reported88.847
- euclidean_accuracy on MTEB Cmnlivalidation set self-reported83.596
- euclidean_ap on MTEB Cmnlivalidation set self-reported90.655
- euclidean_f1 on MTEB Cmnlivalidation set self-reported84.765
- euclidean_precision on MTEB Cmnlivalidation set self-reported81.041
- euclidean_recall on MTEB Cmnlivalidation set self-reported88.847
- manhattan_accuracy on MTEB Cmnlivalidation set self-reported83.512
- manhattan_ap on MTEB Cmnlivalidation set self-reported90.599
- manhattan_f1 on MTEB Cmnlivalidation set self-reported84.518
- manhattan_precision on MTEB Cmnlivalidation set self-reported80.286
- manhattan_recall on MTEB Cmnlivalidation set self-reported89.221
- max_accuracy on MTEB Cmnlivalidation set self-reported83.596
- max_ap on MTEB Cmnlivalidation set self-reported90.655
- max_f1 on MTEB Cmnlivalidation set self-reported84.765