Spaces:
Runtime error
Runtime error
File size: 5,546 Bytes
93bff19 92be478 e06da02 92be478 f6ac29b 92be478 051c019 9ab2c29 051c019 c7715ea f3a5264 c7715ea |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 |
import streamlit as st
import os
from PIL import Image
# Placeholder function for Wikipedia content fetching
# You'll need to replace this with actual fetching logic
def fetch_wikipedia_summary(keyword):
# Placeholder text, replace with actual Wikipedia fetching logic
return f"Summary about {keyword} from Wikipedia."
# Display images from the current directory and fetch related Wikipedia stories
def display_images_and_wikipedia_summaries():
st.title('Gallery with Related Stories')
# Scan current directory for PNG images
image_files = [f for f in os.listdir('.') if f.endswith('.png')]
# Check if there are any PNG images
if not image_files:
st.write("No PNG images found in the current directory.")
return
# Iterate over found images
for image_file in image_files:
# Open and display each image
image = Image.open(image_file)
st.image(image, caption=image_file, use_column_width='always')
# Extract a keyword from the image file name for Wikipedia search
# This is a simple example; adjust logic as needed for more complex titles
keyword = image_file.split('.')[0] # Assumes keyword is the file name without extension
# Fetch and display related Wikipedia content
wikipedia_summary = fetch_wikipedia_summary(keyword)
st.write(wikipedia_summary)
# Call the function to display images and summaries
display_images_and_wikipedia_summaries()
import streamlit as st
st.markdown('# Three Dragons ๐๐ Mythical Dragons Around the World by Aaron Wacker')
dragons = {
'#Fafnir #Norse': '- **Story**: Fafnir originally a dwarf, transformed into a fierce dragon due to his greed for the treasure he guarded. He was later slain by the hero Sigurd. - **Significance**: deadly sin of greed and the corrupting power of wealth.',
'#Quetzalcoatl #Aztec': '- **Story**: Quetzalcoatl, the Feathered Serpent, is not a dragon in the traditional sense but shares many similarities. He was a deity representing wind, air, and learning. - **Significance**: creator god and a symbol of death and rebirth.',
'#Tiamat #Mesopotamian': '- **Story**: Tiamat, primordial goddess of ocean, turned into a dragon-like creature in a battle against her children who threatened her authority. - **Significance**: chaos of primordial creation and is often associated with the forces of nature.'
}
for dragon, story in dragons.items():
st.subheader(dragon)
st.markdown(f"- {story}")
st.markdown('''
https://github.com/AaronCWacker/ThreeDragons

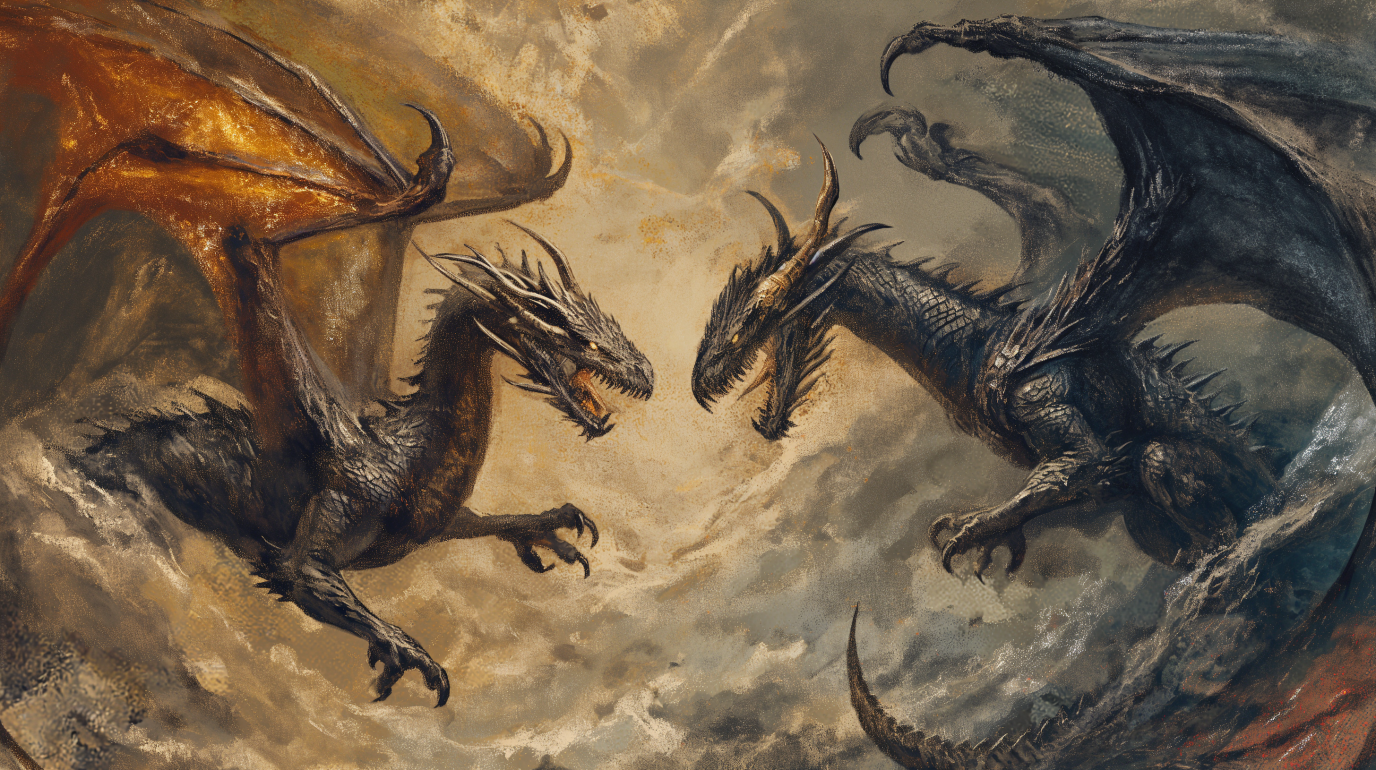



''')
st.markdown('''
# Remixable!!
## Classifications''')
# Create three columns for Inputs, Outputs, and Health
col1, col2, col3 = st.columns(3)
with col1:
st.markdown('''### Inputs''')
st.button("๐ Text")
st.button("๐ Read")
st.button("๐ท Photo")
st.button("๐ผ๏ธ View")
st.button("๐๏ธ Record")
st.button("๐ง Listen")
st.button("๐ฅ Video")
st.button("๐น Capture")
with col2:
st.markdown('''### Outputs''')
st.button("๐ฌ Chat")
st.button("โ๏ธ Write")
st.button("๐จ Art")
st.button("๐ Create")
st.button("๐ต Music")
st.button("๐ถ Compose")
st.button("๐ผ Watch")
st.button("๐ฟ Movies")
with col3:
st.markdown('''### Health''')
st.button("๐ Vaccinate")
st.button("๐ฉบ Diagnose")
st.button("๐ฅ Hospital")
st.button("๐ Emergency")
st.button("๐ Meds")
st.button("๐ฉน Bandage")
st.button("๐งฌ DNA")
st.button("๐ฌ Research")
st.button("๐ก๏ธ Temperature")
st.button("๐ Nutrition")
# Create another set of three columns for Learning, AI, and Writing
col4, col5, col6 = st.columns(3)
with col4:
st.markdown('''### Learning''')
st.button("๐ Study")
st.button("๐ง Brain")
st.button("๐ฉโ๐ Graduate")
st.button("๐ Measure")
st.button("๐ Search")
st.button("๐ Analyze")
st.button("๐ Plan")
st.button("๐๏ธ Write")
st.button("๐จโ๐ซ Teach")
st.button("๐งฉ Puzzle")
with col5:
st.markdown('''### AI''')
st.button("๐ค Robot")
st.button("๐พ Game")
st.button("๐ป Code")
st.button("๐งฎ Calculate")
st.button("๐ก Connect")
st.button("๐ Power")
st.button("๐น๏ธ Play")
st.button("๐ฅ๏ธ Display")
st.button("๐งโ๐ป Develop")
st.button("๐จโ๐ฌ Experiment")
with col6:
st.markdown('''### Writing''')
st.button("โ๏ธ Author")
st.button("๐ Note")
st.button("๐๏ธ Pen")
st.button("๐๏ธ Sign")
st.button("๐ Library")
st.button("๐ Bookmark")
st.button("๐ Journal")
st.button("โ๏ธ Ink")
st.button("๐ Scroll")
# Similarly, create columns for Coding, Remix, Movies, Video, and Audio as needed
# You can continue this pattern for organizing the rest of your buttons into columns
|