Spaces:
Runtime error
Runtime error
File size: 7,260 Bytes
8743176 c4a456e 8743176 c4a456e 8743176 c4fc415 8743176 c4fc415 c4a456e |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 |
import streamlit as st
import os
import json
from PIL import Image
# Directory to store scores
score_dir = "scores"
os.makedirs(score_dir, exist_ok=True)
# Function to generate a unique key for each button
def generate_key(label, header, idx):
return f"{header}_{label}_{idx}"
# Function to increment score and save it
def update_score(key, increment=1):
score_file = os.path.join(score_dir, f"{key}.json")
if os.path.exists(score_file):
with open(score_file, "r") as file:
score_data = json.load(file)
else:
score_data = {"clicks": 0, "score": 0}
score_data["clicks"] += 1
score_data["score"] += increment
with open(score_file, "w") as file:
json.dump(score_data, file)
return score_data["score"]
# Function to load score
def load_score(key):
score_file = os.path.join(score_dir, f"{key}.json")
if os.path.exists(score_file):
with open(score_file, "r") as file:
score_data = json.load(file)
return score_data["score"]
return 0
# Display headers and buttons with scores
def display_buttons_with_scores():
headers = ["Inputs", "Outputs", "Health", "Learning", "AI", "Writing"]
buttons = [
["๐ Text", "๐ Read", "๐ท Photo", "๐ผ๏ธ View", "๐๏ธ Record", "๐ง Listen", "๐ฅ Video", "๐น Capture"],
["๐ฌ Chat", "โ๏ธ Write", "๐จ Art", "๐ Create", "๐ต Music", "๐ถ Compose", "๐ผ Watch", "๐ฟ Movies"],
["๐ Vaccinate", "๐ฉบ Diagnose", "๐ฅ Hospital", "๐ Emergency", "๐ Meds", "๐ฉน Bandage", "๐งฌ DNA", "๐ฌ Research", "๐ก๏ธ Temperature", "๐ Nutrition"],
["๐ Study", "๐ง Brain", "๐ฉโ๐ Graduate", "๐ Measure", "๐ Search", "๐ Analyze", "๐ Plan", "๐๏ธ Write", "๐จโ๐ซ Teach", "๐งฉ Puzzle"],
["๐ค Robot", "๐พ Game", "๐ป Code", "๐งฎ Calculate", "๐ก Connect", "๐ Power", "๐น๏ธ Play", "๐ฅ๏ธ Display", "๐งโ๐ป Develop", "๐จโ๐ฌ Experiment"],
["โ๏ธ Author", "๐ Note", "๐๏ธ Pen", "๐๏ธ Sign", "๐ Library", "๐ Bookmark", "๐ Journal", "โ๏ธ Ink", "๐ Scroll"]
]
cols = st.columns(len(headers))
for idx, (col, header, buttons_list) in enumerate(zip(cols, headers, buttons)):
with col:
st.markdown(f"### {header}")
for button_idx, button_label in enumerate(buttons_list, start=1):
key = generate_key(button_label, header, button_idx)
score = load_score(key)
if st.button(f"{button_label} {score}", key=key):
new_score = update_score(key)
# Reload the page to reflect the updated score
st.experimental_rerun()
# Main application logic
if __name__ == "__main__":
st.markdown('# Remixable!')
display_buttons_with_scores()
# Additional content and functionality can go here
col1, col2, col3 = st.columns(3)
with col1:
st.markdown('''### Inputs''')
st.button("๐ Text")
st.button("๐ Read")
st.button("๐ท Photo")
st.button("๐ผ๏ธ View")
st.button("๐๏ธ Record")
st.button("๐ง Listen")
st.button("๐ฅ Video")
st.button("๐น Capture")
with col2:
st.markdown('''### Outputs''')
st.button("๐ฌ Chat")
st.button("โ๏ธ Write")
st.button("๐จ Art")
st.button("๐ Create")
st.button("๐ต Music")
st.button("๐ถ Compose")
st.button("๐ผ Watch")
st.button("๐ฟ Movies")
with col3:
st.markdown('''### Health''')
st.button("๐ Vaccinate")
st.button("๐ฉบ Diagnose")
st.button("๐ฅ Hospital")
st.button("๐ Emergency")
st.button("๐ Meds")
st.button("๐ฉน Bandage")
st.button("๐งฌ DNA")
st.button("๐ฌ Research")
st.button("๐ก๏ธ Temperature")
st.button("๐ Nutrition")
col4, col5, col6 = st.columns(3)
with col4:
st.markdown('''### Learning''')
st.button("๐ Study")
st.button("๐ง Brain")
st.button("๐ฉโ๐ Graduate")
st.button("๐ Measure")
st.button("๐ Search")
st.button("๐ Analyze")
st.button("๐ Plan")
st.button("๐๏ธ Write")
st.button("๐จโ๐ซ Teach")
st.button("๐งฉ Puzzle")
with col5:
st.markdown('''### AI''')
st.button("๐ค Robot")
st.button("๐พ Game")
st.button("๐ป Code")
st.button("๐งฎ Calculate")
st.button("๐ก Connect")
st.button("๐ Power")
st.button("๐น๏ธ Play")
st.button("๐ฅ๏ธ Display")
st.button("๐งโ๐ป Develop")
st.button("๐จโ๐ฌ Experiment")
with col6:
st.markdown('''### Writing''')
st.button("โ๏ธ Author")
st.button("๐ Note")
st.button("๐๏ธ Pen")
st.button("๐๏ธ Sign")
st.button("๐ Library")
st.button("๐ Bookmark")
st.button("๐ Journal")
st.button("โ๏ธ Ink")
st.button("๐ Scroll")
def display_images_and_wikipedia_summaries():
st.title('Gallery with Related Stories')
image_files = [f for f in os.listdir('.') if f.endswith('.png')]
if not image_files:
st.write("No PNG images found in the current directory.")
return
for image_file in image_files:
image = Image.open(image_file)
st.image(image, caption=image_file, use_column_width='always')
keyword = image_file.split('.')[0] # Assumes keyword is the file name without extension
wikipedia_summary = fetch_wikipedia_summary(keyword)
st.write(wikipedia_summary)
display_images_and_wikipedia_summaries()
st.markdown('# Three Dragons ๐๐ Mythical Dragons Around the World by Aaron Wacker')
dragons = {
'#Fafnir #Norse': '- **Story**: Fafnir originally a dwarf, transformed into a fierce dragon due to his greed for the treasure he guarded. He was later slain by the hero Sigurd. - **Significance**: deadly sin of greed and the corrupting power of wealth.',
'#Quetzalcoatl #Aztec': '- **Story**: Quetzalcoatl, the Feathered Serpent, is not a dragon in the traditional sense but shares many similarities. He was a deity representing wind, air, and learning. - **Significance**: creator god and a symbol of death and rebirth.',
'#Tiamat #Mesopotamian': '- **Story**: Tiamat, primordial goddess of ocean, turned into a dragon-like creature in a battle against her children who threatened her authority. - **Significance**: chaos of primordial creation and is often associated with the forces of nature.'
}
for dragon, story in dragons.items():
st.subheader(dragon)
st.markdown(f"- {story}")
st.markdown('''
https://github.com/AaronCWacker/ThreeDragons

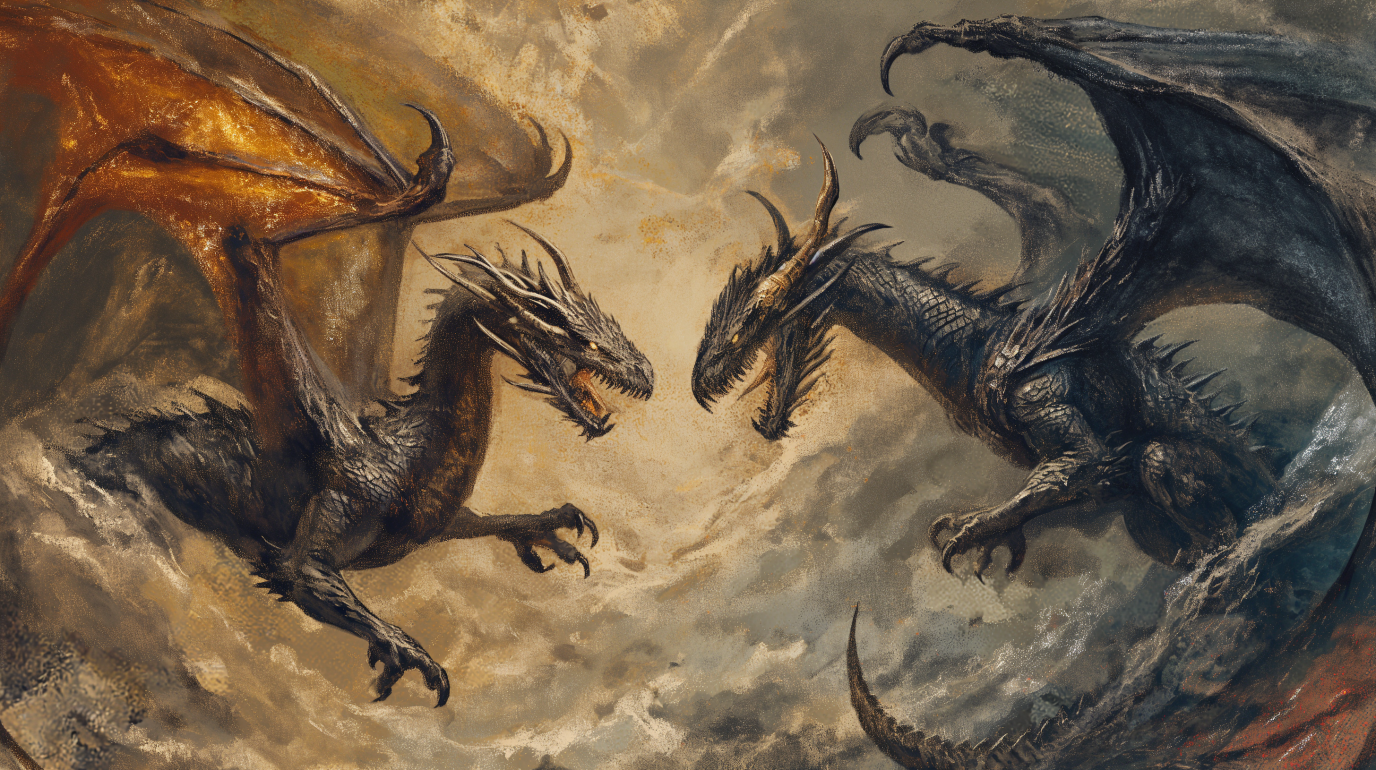



''') |