upload weights
#1
by
williamberman
- opened
- README.md +116 -0
- decoder/config.json +45 -0
- decoder/diffusion_pytorch_model.bin +3 -0
- decoder_scheduler/scheduler_config.json +9 -0
- feature_extractor/preprocessor_config.json +27 -0
- image_encoder/config.json +23 -0
- image_encoder/pytorch_model.bin +3 -0
- model_index.json +44 -0
- super_res_first/config.json +39 -0
- super_res_first/diffusion_pytorch_model.bin +3 -0
- super_res_last/config.json +39 -0
- super_res_last/diffusion_pytorch_model.bin +3 -0
- super_res_scheduler/scheduler_config.json +9 -0
- text_encoder/config.json +25 -0
- text_encoder/pytorch_model.bin +3 -0
- text_proj/config.json +9 -0
- text_proj/diffusion_pytorch_model.bin +3 -0
- tokenizer/merges.txt +0 -0
- tokenizer/special_tokens_map.json +24 -0
- tokenizer/tokenizer_config.json +35 -0
- tokenizer/vocab.json +0 -0
README.md
ADDED
@@ -0,0 +1,116 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
---
|
2 |
+
license: creativeml-openrail-m
|
3 |
+
tags:
|
4 |
+
- text-to-image
|
5 |
+
---
|
6 |
+
|
7 |
+
# Karlo v1 alpha
|
8 |
+
|
9 |
+
Karlo is a text-conditional image generation model based on OpenAI's unCLIP architecture with the improvement over the standard super-resolution model from 64px to 256px, recovering high-frequency details only in the small number of denoising steps.
|
10 |
+
|
11 |
+
* [Original codebase](https://github.com/kakaobrain/karlo)
|
12 |
+
|
13 |
+
## Usage
|
14 |
+
|
15 |
+
Karlo is available in diffusers!
|
16 |
+
|
17 |
+
```python
|
18 |
+
pip install diffusers transformers accelerate safetensors
|
19 |
+
```
|
20 |
+
|
21 |
+
### Text to image
|
22 |
+
|
23 |
+
```python
|
24 |
+
from diffusers import UnCLIPPipeline
|
25 |
+
import torch
|
26 |
+
|
27 |
+
pipe = UnCLIPPipeline.from_pretrained("kakaobrain/karlo-v1-alpha", torch_dtype=torch.float16)
|
28 |
+
pipe = pipe.to('cuda')
|
29 |
+
|
30 |
+
prompt = "a high-resolution photograph of a big red frog on a green leaf."
|
31 |
+
|
32 |
+
image = pipe([prompt]).images[0]
|
33 |
+
|
34 |
+
image.save("./frog.png")
|
35 |
+
```
|
36 |
+
|
37 |
+

|
38 |
+
|
39 |
+
### Image variation
|
40 |
+
|
41 |
+
```python
|
42 |
+
from diffusers import UnCLIPImageVariationPipeline
|
43 |
+
import torch
|
44 |
+
from PIL import Image
|
45 |
+
|
46 |
+
pipe = UnCLIPImageVariationPipeline.from_pretrained("kakaobrain/karlo-v1-alpha-image-variations", torch_dtype=torch.float16)
|
47 |
+
pipe = pipe.to('cuda')
|
48 |
+
|
49 |
+
image = Image.open("./frog.png")
|
50 |
+
|
51 |
+
image = pipe(image).images[0]
|
52 |
+
|
53 |
+
image.save("./frog-variation.png")
|
54 |
+
```
|
55 |
+
|
56 |
+
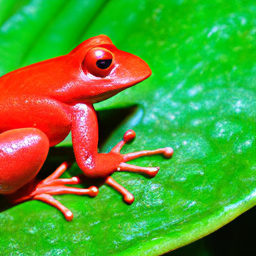
|
57 |
+
|
58 |
+
## Model Architecture
|
59 |
+
|
60 |
+
### Overview
|
61 |
+
Karlo is a text-conditional diffusion model based on unCLIP, composed of prior, decoder, and super-resolution modules. In this repository, we include the improved version of the standard super-resolution module for upscaling 64px to 256px only in 7 reverse steps, as illustrated in the figure below:
|
62 |
+
|
63 |
+
<p float="left">
|
64 |
+
<img src="https://raw.githubusercontent.com/kakaobrain/karlo/main/assets/improved_sr_arch.jpg"/>
|
65 |
+
</p>
|
66 |
+
|
67 |
+
In specific, the standard SR module trained by DDPM objective upscales 64px to 256px in the first 6 denoising steps based on the respacing technique. Then, the additional fine-tuned SR module trained by [VQ-GAN](https://compvis.github.io/taming-transformers/)-style loss performs the final reverse step to recover high-frequency details. We observe that this approach is very effective to upscale the low-resolution in a small number of reverse steps.
|
68 |
+
|
69 |
+
### Details
|
70 |
+
We train all components from scratch on 115M image-text pairs including COYO-100M, CC3M, and CC12M. In the case of Prior and Decoder, we use ViT-L/14 provided by OpenAI’s [CLIP repository](https://github.com/openai/CLIP). Unlike the original implementation of unCLIP, we replace the trainable transformer in the decoder into the text encoder in ViT-L/14 for efficiency. In the case of the SR module, we first train the model using the DDPM objective in 1M steps, followed by additional 234K steps to fine-tune the additional component. The table below summarizes the important statistics of our components:
|
71 |
+
|
72 |
+
| | Prior | Decoder | SR |
|
73 |
+
|:------|----:|----:|----:|
|
74 |
+
| CLIP | ViT-L/14 | ViT-L/14 | - |
|
75 |
+
| #param | 1B | 900M | 700M + 700M |
|
76 |
+
| #optimization steps | 1M | 1M | 1M + 0.2M |
|
77 |
+
| #sampling steps | 25 | 50 (default), 25 (fast) | 7 |
|
78 |
+
|Checkpoint links| [ViT-L-14](https://arena.kakaocdn.net/brainrepo/models/karlo-public/v1.0.0.alpha/096db1af569b284eb76b3881534822d9/ViT-L-14.pt), [ViT-L-14 stats](https://arena.kakaocdn.net/brainrepo/models/karlo-public/v1.0.0.alpha/0b62380a75e56f073e2844ab5199153d/ViT-L-14_stats.th), [model](https://arena.kakaocdn.net/brainrepo/models/karlo-public/v1.0.0.alpha/efdf6206d8ed593961593dc029a8affa/decoder-ckpt-step%3D01000000-of-01000000.ckpt) | [model](https://arena.kakaocdn.net/brainrepo/models/karlo-public/v1.0.0.alpha/85626483eaca9f581e2a78d31ff905ca/prior-ckpt-step%3D01000000-of-01000000.ckpt) | [model](https://arena.kakaocdn.net/brainrepo/models/karlo-public/v1.0.0.alpha/4226b831ae0279020d134281f3c31590/improved-sr-ckpt-step%3D1.2M.ckpt) |
|
79 |
+
|
80 |
+
In the checkpoint links, ViT-L-14 is equivalent to the original version, but we include it for convenience. We also remark that ViT-L-14-stats is required to normalize the outputs of the prior module.
|
81 |
+
|
82 |
+
### Evaluation
|
83 |
+
We quantitatively measure the performance of Karlo-v1.0.alpha in the validation split of CC3M and MS-COCO. The table below presents CLIP-score and FID. To measure FID, we resize the image of the shorter side to 256px, followed by cropping it at the center. We set classifier-free guidance scales for prior and decoder to 4 and 8 in all cases. We observe that our model achieves reasonable performance even with 25 sampling steps of decoder.
|
84 |
+
|
85 |
+
CC3M
|
86 |
+
| Sampling step | CLIP-s (ViT-B/16) | FID (13k from val)|
|
87 |
+
|:------|----:|----:|
|
88 |
+
| Prior (25) + Decoder (25) + SR (7) | 0.3081 | 14.37 |
|
89 |
+
| Prior (25) + Decoder (50) + SR (7) | 0.3086 | 13.95 |
|
90 |
+
|
91 |
+
MS-COCO
|
92 |
+
| Sampling step | CLIP-s (ViT-B/16) | FID (30k from val)|
|
93 |
+
|:------|----:|----:|
|
94 |
+
| Prior (25) + Decoder (25) + SR (7) | 0.3192 | 15.24 |
|
95 |
+
| Prior (25) + Decoder (50) + SR (7) | 0.3192 | 14.43 |
|
96 |
+
|
97 |
+
|
98 |
+
For more information, please refer to the upcoming technical report.
|
99 |
+
|
100 |
+
### Training Details
|
101 |
+
|
102 |
+
This alpha version of Karlo is trained on 115M image-text pairs,
|
103 |
+
including [COYO](https://github.com/kakaobrain/coyo-dataset)-100M high-quality subset, CC3M, and CC12M.
|
104 |
+
For those who are interested in a better version of Karlo trained on more large-scale high-quality datasets,
|
105 |
+
please visit the landing page of our application [B^DISCOVER](https://bdiscover.kakaobrain.com/).
|
106 |
+
|
107 |
+
## BibTex
|
108 |
+
If you find this repository useful in your research, please cite:
|
109 |
+
```
|
110 |
+
@misc{kakaobrain2022karlo-v1-alpha,
|
111 |
+
title = {Karlo-v1.0.alpha on COYO-100M and CC15M},
|
112 |
+
author = {Donghoon Lee, Jiseob Kim, Jisu Choi, Jongmin Kim, Minwoo Byeon, Woonhyuk Baek and Saehoon Kim},
|
113 |
+
year = {2022},
|
114 |
+
howpublished = {\url{https://github.com/kakaobrain/karlo}},
|
115 |
+
}
|
116 |
+
```
|
decoder/config.json
ADDED
@@ -0,0 +1,45 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_class_name": "UNet2DConditionModel",
|
3 |
+
"_diffusers_version": "0.12.0.dev0",
|
4 |
+
"_name_or_path": "/home/william_huggingface_co/.cache/huggingface/diffusers/models--kakaobrain--karlo-v1-alpha/snapshots/c8dc0970acf0db0b6b4efb8c47adc22072b4a67d/decoder",
|
5 |
+
"act_fn": "silu",
|
6 |
+
"attention_head_dim": 64,
|
7 |
+
"block_out_channels": [
|
8 |
+
320,
|
9 |
+
640,
|
10 |
+
960,
|
11 |
+
1280
|
12 |
+
],
|
13 |
+
"center_input_sample": false,
|
14 |
+
"class_embed_type": "identity",
|
15 |
+
"cross_attention_dim": 1536,
|
16 |
+
"down_block_types": [
|
17 |
+
"ResnetDownsampleBlock2D",
|
18 |
+
"SimpleCrossAttnDownBlock2D",
|
19 |
+
"SimpleCrossAttnDownBlock2D",
|
20 |
+
"SimpleCrossAttnDownBlock2D"
|
21 |
+
],
|
22 |
+
"downsample_padding": 1,
|
23 |
+
"dual_cross_attention": false,
|
24 |
+
"flip_sin_to_cos": true,
|
25 |
+
"freq_shift": 0,
|
26 |
+
"in_channels": 3,
|
27 |
+
"layers_per_block": 3,
|
28 |
+
"mid_block_scale_factor": 1,
|
29 |
+
"mid_block_type": "UNetMidBlock2DSimpleCrossAttn",
|
30 |
+
"norm_eps": 1e-05,
|
31 |
+
"norm_num_groups": 32,
|
32 |
+
"num_class_embeds": null,
|
33 |
+
"only_cross_attention": false,
|
34 |
+
"out_channels": 6,
|
35 |
+
"resnet_time_scale_shift": "scale_shift",
|
36 |
+
"sample_size": 64,
|
37 |
+
"up_block_types": [
|
38 |
+
"SimpleCrossAttnUpBlock2D",
|
39 |
+
"SimpleCrossAttnUpBlock2D",
|
40 |
+
"SimpleCrossAttnUpBlock2D",
|
41 |
+
"ResnetUpsampleBlock2D"
|
42 |
+
],
|
43 |
+
"upcast_attention": false,
|
44 |
+
"use_linear_projection": false
|
45 |
+
}
|
decoder/diffusion_pytorch_model.bin
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:df4c1b9bceeceb92a88ff252e964be3a39308e799fdf01d5557d247f11868310
|
3 |
+
size 3542027081
|
decoder_scheduler/scheduler_config.json
ADDED
@@ -0,0 +1,9 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_class_name": "UnCLIPScheduler",
|
3 |
+
"_diffusers_version": "0.12.0.dev0",
|
4 |
+
"clip_sample": true,
|
5 |
+
"clip_sample_range": 1.0,
|
6 |
+
"num_train_timesteps": 1000,
|
7 |
+
"prediction_type": "epsilon",
|
8 |
+
"variance_type": "learned_range"
|
9 |
+
}
|
feature_extractor/preprocessor_config.json
ADDED
@@ -0,0 +1,27 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"crop_size": {
|
3 |
+
"height": 224,
|
4 |
+
"width": 224
|
5 |
+
},
|
6 |
+
"do_center_crop": true,
|
7 |
+
"do_convert_rgb": true,
|
8 |
+
"do_normalize": true,
|
9 |
+
"do_rescale": true,
|
10 |
+
"do_resize": true,
|
11 |
+
"image_mean": [
|
12 |
+
0.48145466,
|
13 |
+
0.4578275,
|
14 |
+
0.40821073
|
15 |
+
],
|
16 |
+
"image_processor_type": "CLIPImageProcessor",
|
17 |
+
"image_std": [
|
18 |
+
0.26862954,
|
19 |
+
0.26130258,
|
20 |
+
0.27577711
|
21 |
+
],
|
22 |
+
"resample": 3,
|
23 |
+
"rescale_factor": 0.00392156862745098,
|
24 |
+
"size": {
|
25 |
+
"shortest_edge": 224
|
26 |
+
}
|
27 |
+
}
|
image_encoder/config.json
ADDED
@@ -0,0 +1,23 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_name_or_path": "openai/clip-vit-large-patch14",
|
3 |
+
"architectures": [
|
4 |
+
"CLIPVisionModelWithProjection"
|
5 |
+
],
|
6 |
+
"attention_dropout": 0.0,
|
7 |
+
"dropout": 0.0,
|
8 |
+
"hidden_act": "quick_gelu",
|
9 |
+
"hidden_size": 1024,
|
10 |
+
"image_size": 224,
|
11 |
+
"initializer_factor": 1.0,
|
12 |
+
"initializer_range": 0.02,
|
13 |
+
"intermediate_size": 4096,
|
14 |
+
"layer_norm_eps": 1e-05,
|
15 |
+
"model_type": "clip_vision_model",
|
16 |
+
"num_attention_heads": 16,
|
17 |
+
"num_channels": 3,
|
18 |
+
"num_hidden_layers": 24,
|
19 |
+
"patch_size": 14,
|
20 |
+
"projection_dim": 768,
|
21 |
+
"torch_dtype": "float32",
|
22 |
+
"transformers_version": "4.25.1"
|
23 |
+
}
|
image_encoder/pytorch_model.bin
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:c304e9a1c99835f6c2d355cbb5438e7e6204d4903b2f1b056120be4bd5050f1f
|
3 |
+
size 1215996977
|
model_index.json
ADDED
@@ -0,0 +1,44 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_class_name": "UnCLIPImageVariationPipeline",
|
3 |
+
"_diffusers_version": "0.12.0.dev0",
|
4 |
+
"decoder": [
|
5 |
+
"diffusers",
|
6 |
+
"UNet2DConditionModel"
|
7 |
+
],
|
8 |
+
"decoder_scheduler": [
|
9 |
+
"diffusers",
|
10 |
+
"UnCLIPScheduler"
|
11 |
+
],
|
12 |
+
"feature_extractor": [
|
13 |
+
"transformers",
|
14 |
+
"CLIPImageProcessor"
|
15 |
+
],
|
16 |
+
"image_encoder": [
|
17 |
+
"transformers",
|
18 |
+
"CLIPVisionModelWithProjection"
|
19 |
+
],
|
20 |
+
"super_res_first": [
|
21 |
+
"diffusers",
|
22 |
+
"UNet2DModel"
|
23 |
+
],
|
24 |
+
"super_res_last": [
|
25 |
+
"diffusers",
|
26 |
+
"UNet2DModel"
|
27 |
+
],
|
28 |
+
"super_res_scheduler": [
|
29 |
+
"diffusers",
|
30 |
+
"UnCLIPScheduler"
|
31 |
+
],
|
32 |
+
"text_encoder": [
|
33 |
+
"transformers",
|
34 |
+
"CLIPTextModelWithProjection"
|
35 |
+
],
|
36 |
+
"text_proj": [
|
37 |
+
"unclip",
|
38 |
+
"UnCLIPTextProjModel"
|
39 |
+
],
|
40 |
+
"tokenizer": [
|
41 |
+
"transformers",
|
42 |
+
"CLIPTokenizer"
|
43 |
+
]
|
44 |
+
}
|
super_res_first/config.json
ADDED
@@ -0,0 +1,39 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_class_name": "UNet2DModel",
|
3 |
+
"_diffusers_version": "0.12.0.dev0",
|
4 |
+
"_name_or_path": "/home/william_huggingface_co/.cache/huggingface/diffusers/models--kakaobrain--karlo-v1-alpha/snapshots/c8dc0970acf0db0b6b4efb8c47adc22072b4a67d/super_res_first",
|
5 |
+
"act_fn": "silu",
|
6 |
+
"add_attention": false,
|
7 |
+
"attention_head_dim": 8,
|
8 |
+
"block_out_channels": [
|
9 |
+
320,
|
10 |
+
640,
|
11 |
+
960,
|
12 |
+
1280
|
13 |
+
],
|
14 |
+
"center_input_sample": false,
|
15 |
+
"down_block_types": [
|
16 |
+
"ResnetDownsampleBlock2D",
|
17 |
+
"ResnetDownsampleBlock2D",
|
18 |
+
"ResnetDownsampleBlock2D",
|
19 |
+
"ResnetDownsampleBlock2D"
|
20 |
+
],
|
21 |
+
"downsample_padding": 1,
|
22 |
+
"flip_sin_to_cos": true,
|
23 |
+
"freq_shift": 0,
|
24 |
+
"in_channels": 6,
|
25 |
+
"layers_per_block": 3,
|
26 |
+
"mid_block_scale_factor": 1,
|
27 |
+
"norm_eps": 1e-05,
|
28 |
+
"norm_num_groups": 32,
|
29 |
+
"out_channels": 3,
|
30 |
+
"resnet_time_scale_shift": "default",
|
31 |
+
"sample_size": 256,
|
32 |
+
"time_embedding_type": "positional",
|
33 |
+
"up_block_types": [
|
34 |
+
"ResnetUpsampleBlock2D",
|
35 |
+
"ResnetUpsampleBlock2D",
|
36 |
+
"ResnetUpsampleBlock2D",
|
37 |
+
"ResnetUpsampleBlock2D"
|
38 |
+
]
|
39 |
+
}
|
super_res_first/diffusion_pytorch_model.bin
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:be101081e8dba0d7dfe19845187c8efe6d4c68e32daa33224d1f8631333085b3
|
3 |
+
size 2766471473
|
super_res_last/config.json
ADDED
@@ -0,0 +1,39 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_class_name": "UNet2DModel",
|
3 |
+
"_diffusers_version": "0.12.0.dev0",
|
4 |
+
"_name_or_path": "/home/william_huggingface_co/.cache/huggingface/diffusers/models--kakaobrain--karlo-v1-alpha/snapshots/c8dc0970acf0db0b6b4efb8c47adc22072b4a67d/super_res_last",
|
5 |
+
"act_fn": "silu",
|
6 |
+
"add_attention": false,
|
7 |
+
"attention_head_dim": 8,
|
8 |
+
"block_out_channels": [
|
9 |
+
320,
|
10 |
+
640,
|
11 |
+
960,
|
12 |
+
1280
|
13 |
+
],
|
14 |
+
"center_input_sample": false,
|
15 |
+
"down_block_types": [
|
16 |
+
"ResnetDownsampleBlock2D",
|
17 |
+
"ResnetDownsampleBlock2D",
|
18 |
+
"ResnetDownsampleBlock2D",
|
19 |
+
"ResnetDownsampleBlock2D"
|
20 |
+
],
|
21 |
+
"downsample_padding": 1,
|
22 |
+
"flip_sin_to_cos": true,
|
23 |
+
"freq_shift": 0,
|
24 |
+
"in_channels": 6,
|
25 |
+
"layers_per_block": 3,
|
26 |
+
"mid_block_scale_factor": 1,
|
27 |
+
"norm_eps": 1e-05,
|
28 |
+
"norm_num_groups": 32,
|
29 |
+
"out_channels": 3,
|
30 |
+
"resnet_time_scale_shift": "default",
|
31 |
+
"sample_size": null,
|
32 |
+
"time_embedding_type": "positional",
|
33 |
+
"up_block_types": [
|
34 |
+
"ResnetUpsampleBlock2D",
|
35 |
+
"ResnetUpsampleBlock2D",
|
36 |
+
"ResnetUpsampleBlock2D",
|
37 |
+
"ResnetUpsampleBlock2D"
|
38 |
+
]
|
39 |
+
}
|
super_res_last/diffusion_pytorch_model.bin
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:e3356695fc89b8fa39b3f1ec1d3d635cbc065387ef622a04593897aaae7247f2
|
3 |
+
size 2766471473
|
super_res_scheduler/scheduler_config.json
ADDED
@@ -0,0 +1,9 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_class_name": "UnCLIPScheduler",
|
3 |
+
"_diffusers_version": "0.12.0.dev0",
|
4 |
+
"clip_sample": true,
|
5 |
+
"clip_sample_range": 1.0,
|
6 |
+
"num_train_timesteps": 1000,
|
7 |
+
"prediction_type": "epsilon",
|
8 |
+
"variance_type": "fixed_small_log"
|
9 |
+
}
|
text_encoder/config.json
ADDED
@@ -0,0 +1,25 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_name_or_path": "/home/william_huggingface_co/.cache/huggingface/diffusers/models--kakaobrain--karlo-v1-alpha/snapshots/c8dc0970acf0db0b6b4efb8c47adc22072b4a67d/text_encoder",
|
3 |
+
"architectures": [
|
4 |
+
"CLIPTextModelWithProjection"
|
5 |
+
],
|
6 |
+
"attention_dropout": 0.0,
|
7 |
+
"bos_token_id": 0,
|
8 |
+
"dropout": 0.0,
|
9 |
+
"eos_token_id": 2,
|
10 |
+
"hidden_act": "quick_gelu",
|
11 |
+
"hidden_size": 768,
|
12 |
+
"initializer_factor": 1.0,
|
13 |
+
"initializer_range": 0.02,
|
14 |
+
"intermediate_size": 3072,
|
15 |
+
"layer_norm_eps": 1e-05,
|
16 |
+
"max_position_embeddings": 77,
|
17 |
+
"model_type": "clip_text_model",
|
18 |
+
"num_attention_heads": 12,
|
19 |
+
"num_hidden_layers": 12,
|
20 |
+
"pad_token_id": 1,
|
21 |
+
"projection_dim": 768,
|
22 |
+
"torch_dtype": "float32",
|
23 |
+
"transformers_version": "4.25.1",
|
24 |
+
"vocab_size": 49408
|
25 |
+
}
|
text_encoder/pytorch_model.bin
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:aec40a3f65f96219cb30a80554a39fcb365146b6aa7d0cb9621e08b010ba672d
|
3 |
+
size 494666597
|
text_proj/config.json
ADDED
@@ -0,0 +1,9 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"_class_name": "UnCLIPTextProjModel",
|
3 |
+
"_diffusers_version": "0.12.0.dev0",
|
4 |
+
"_name_or_path": "/home/william_huggingface_co/.cache/huggingface/diffusers/models--kakaobrain--karlo-v1-alpha/snapshots/c8dc0970acf0db0b6b4efb8c47adc22072b4a67d/text_proj",
|
5 |
+
"clip_embeddings_dim": 768,
|
6 |
+
"clip_extra_context_tokens": 4,
|
7 |
+
"cross_attention_dim": 1536,
|
8 |
+
"time_embed_dim": 1280
|
9 |
+
}
|
text_proj/diffusion_pytorch_model.bin
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:46c3cb5d013e22221d156a9f30ef9221a4f9cfc547ab295cff870b2e26405a62
|
3 |
+
size 31517844
|
tokenizer/merges.txt
ADDED
The diff for this file is too large to render.
See raw diff
|
|
tokenizer/special_tokens_map.json
ADDED
@@ -0,0 +1,24 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"bos_token": {
|
3 |
+
"content": "<|startoftext|>",
|
4 |
+
"lstrip": false,
|
5 |
+
"normalized": true,
|
6 |
+
"rstrip": false,
|
7 |
+
"single_word": false
|
8 |
+
},
|
9 |
+
"eos_token": {
|
10 |
+
"content": "<|endoftext|>",
|
11 |
+
"lstrip": false,
|
12 |
+
"normalized": true,
|
13 |
+
"rstrip": false,
|
14 |
+
"single_word": false
|
15 |
+
},
|
16 |
+
"pad_token": "!",
|
17 |
+
"unk_token": {
|
18 |
+
"content": "<|endoftext|>",
|
19 |
+
"lstrip": false,
|
20 |
+
"normalized": true,
|
21 |
+
"rstrip": false,
|
22 |
+
"single_word": false
|
23 |
+
}
|
24 |
+
}
|
tokenizer/tokenizer_config.json
ADDED
@@ -0,0 +1,35 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"add_prefix_space": false,
|
3 |
+
"bos_token": {
|
4 |
+
"__type": "AddedToken",
|
5 |
+
"content": "<|startoftext|>",
|
6 |
+
"lstrip": false,
|
7 |
+
"normalized": true,
|
8 |
+
"rstrip": false,
|
9 |
+
"single_word": false
|
10 |
+
},
|
11 |
+
"device_map": "auto",
|
12 |
+
"do_lower_case": true,
|
13 |
+
"eos_token": {
|
14 |
+
"__type": "AddedToken",
|
15 |
+
"content": "<|endoftext|>",
|
16 |
+
"lstrip": false,
|
17 |
+
"normalized": true,
|
18 |
+
"rstrip": false,
|
19 |
+
"single_word": false
|
20 |
+
},
|
21 |
+
"errors": "replace",
|
22 |
+
"model_max_length": 77,
|
23 |
+
"name_or_path": "/home/william_huggingface_co/.cache/huggingface/diffusers/models--kakaobrain--karlo-v1-alpha/snapshots/c8dc0970acf0db0b6b4efb8c47adc22072b4a67d/tokenizer",
|
24 |
+
"pad_token": "!",
|
25 |
+
"special_tokens_map_file": "./special_tokens_map.json",
|
26 |
+
"tokenizer_class": "CLIPTokenizer",
|
27 |
+
"unk_token": {
|
28 |
+
"__type": "AddedToken",
|
29 |
+
"content": "<|endoftext|>",
|
30 |
+
"lstrip": false,
|
31 |
+
"normalized": true,
|
32 |
+
"rstrip": false,
|
33 |
+
"single_word": false
|
34 |
+
}
|
35 |
+
}
|
tokenizer/vocab.json
ADDED
The diff for this file is too large to render.
See raw diff
|
|