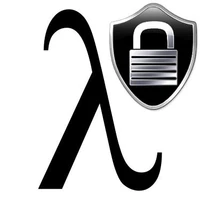
lambdasec/santafixer
Text Generation
•
Updated
•
25
•
3
language
stringclasses 3
values | text
stringlengths 128
3.21k
|
---|---|
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | /*
* Copyright (C) 2016-2019 HERE Europe B.V.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-License-Identifier: Apache-2.0
* License-Filename: LICENSE
*/
package com.here.gluecodium.cache;
import com.here.gluecodium.cli.GluecodiumExecutionException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
final class HashValueCalculator {
public static byte[] calculateHashValue(final String content) {
try {
// BUG: CWE-328: Use of Weak Hash
// MessageDigest messageDigest = MessageDigest.getInstance("MD5");
// FIXED:
<FILL-HERE>
messageDigest.update(content.getBytes());
return messageDigest.digest();
} catch (NoSuchAlgorithmException e) {
throw new GluecodiumExecutionException("Hash value algorithm is not available", e);
}
}
}
|
java | /*******************************************************************************
* Copyright (c) 2011-2014 Fernando Petrola
*
* This file is part of Dragome SDK.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the GNU Public License v3.0
* which accompanies this distribution, and is available at
* http://www.gnu.org/licenses/gpl.html
******************************************************************************/
package com.dragome.web.serverside.servlets;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.dragome.web.serverside.debugging.websocket.ClassTransformerDragomeWebSocketHandler;
import com.dragome.web.services.AbstractServicesInvocationHandler;
//@WebServlet(value= "/" + AbstractServicesInvocationHandler.SERVICE_INVOKER_PATH)
public class ClassTransformerInvokerServlet extends GetPostServlet
{
protected void doService(HttpServletRequest req, HttpServletResponse resp) throws IOException
{
try
{
String parameter= req.getParameter(AbstractServicesInvocationHandler.INVOCATION);
String result= (String) ClassTransformerDragomeWebSocketHandler.executeMethod(getClass().getPackage().getName() + ".ServiceInvoker", "invoke", parameter);
resp.setCharacterEncoding("utf-8");
if (result != null)
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// resp.getWriter().write(result);
// FIXED:
<FILL-HERE>
}
catch (Exception e)
{
throw new RuntimeException(e);
}
}
public void init() throws ServletException
{
super.init();
}
}
|
java | package com.scudata.common;
import java.io.InputStream;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import com.scudata.parallel.XmlUtil;
/**
<SERVER>
<TempTimeOut>12</TempTimeOut>
<Interval>0</Interval>
<Backlog>10</Backlog>
<ProxyTimeOut>12</ProxyTimeOut>
<SplConfig>d:/path/raqsofCofig.xml</SplConfig>
<LogPath>d:/sp.log</LogPath>
<SplHome></SplHome>
<JVMArgs></JVMArgs>
</SERVER>
**/
public class SplServerConfig {
public String tempTimeOut,interval,backlog,proxyTimeOut,splConfig,logPath;
public String splHome,JVMArgs;
public static SplServerConfig getCfg(InputStream is) throws Exception {
DocumentBuilderFactory docBuilderFactory = DocumentBuilderFactory
.newInstance();
// BUG: CWE-611: Improper Restriction of XML External Entity Reference
// DocumentBuilder docBuilder = docBuilderFactory.newDocumentBuilder();
// FIXED:
<FILL-HERE>
Document xmlDocument = docBuilder.parse(is);
NodeList nl = xmlDocument.getChildNodes();
Node root = null;
for (int i = 0; i < nl.getLength(); i++) {
Node n = nl.item(i);
if (n.getNodeName().equalsIgnoreCase("SERVER")) {
root = n;
}
}
if (root == null) {
throw new Exception( "Invalid config file." );
}
SplServerConfig ssc = new SplServerConfig();
Node subNode = XmlUtil.findSonNode(root, "splHome");
if(subNode!=null) {
ssc.splHome = XmlUtil.getNodeValue(subNode);
}else {
throw new Exception("splHome is not specified.");
}
subNode = XmlUtil.findSonNode(root, "JVMArgs");
if(subNode!=null) {
ssc.JVMArgs = XmlUtil.getNodeValue(subNode);
}
//server
subNode = XmlUtil.findSonNode(root, "TempTimeOut");
if(subNode!=null) {
ssc.tempTimeOut = XmlUtil.getNodeValue(subNode);
}
subNode = XmlUtil.findSonNode(root, "ProxyTimeOut");
if(subNode!=null) {
ssc.proxyTimeOut = XmlUtil.getNodeValue(subNode);
}
subNode = XmlUtil.findSonNode(root, "Interval");
if(subNode!=null) {
ssc.interval = XmlUtil.getNodeValue(subNode);
}
subNode = XmlUtil.findSonNode(root, "Backlog");
if(subNode!=null) {
ssc.backlog = XmlUtil.getNodeValue(subNode);
}
subNode = XmlUtil.findSonNode(root, "LogPath");
if(subNode!=null) {
ssc.logPath = XmlUtil.getNodeValue(subNode);
}
subNode = XmlUtil.findSonNode(root, "SplConfig");
if(subNode!=null) {
ssc.splConfig = XmlUtil.getNodeValue(subNode);
}
return ssc;
}
}
|
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | /**
* Tencent is pleased to support the open source community by making Tars available.
*
* Copyright (C) 2016 THL A29 Limited, a Tencent company. All rights reserved.
*
* Licensed under the BSD 3-Clause License (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* https://opensource.org/licenses/BSD-3-Clause
*
* Unless required by applicable law or agreed to in writing, software distributed
* under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.qq.tars.maven.util;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.xml.sax.EntityResolver;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
public class XMLConfigFile {
private Document doc;
public void parse(InputStream is) throws SAXException, IOException, ParserConfigurationException {
DocumentBuilderFactory docBuilderFactory = DocumentBuilderFactory.newInstance();
// BUG: CWE-611: Improper Restriction of XML External Entity Reference
// DocumentBuilder docBuilder = docBuilderFactory.newDocumentBuilder();
// FIXED:
<FILL-HERE>
docBuilder.setEntityResolver(new EntityResolver() {
public InputSource resolveEntity(String publicId, String systemId) throws SAXException, IOException {
if (systemId.contains("http://java.sun.com/dtd/web-app_2_3.dtd")) {
return new InputSource(new StringReader(""));
} else {
return null;
}
}
});
doc = docBuilder.parse(is);
}
public XMLConfigElement getRootElement() {
Element root = doc.getDocumentElement();
return new XMLConfigElement(root);
}
}
|
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | package com.scudata.lib.redis.function;
import java.io.IOException;
import java.net.Socket;
import java.net.UnknownHostException;
import com.scudata.common.MessageManager;
import com.scudata.common.RQException;
import com.scudata.dm.Context;
import com.scudata.expression.Function;
import com.scudata.expression.Node;
import com.scudata.resources.EngineMessage;
public class SocketRedisOpen extends Function {
protected nl.melp.redis.Redis redis = null;
public Node optimize(Context ctx) {
if (param != null) {
param.optimize(ctx);
}
return this;
}
public Object calculate(Context ctx) {
try {
Object objs[] = new Object[1];
if (param != null) {
if (param.isLeaf()){
objs[0] = param.getLeafExpression().calculate(ctx);
if (!(objs[0] instanceof String)) {
MessageManager mm = EngineMessage.get();
throw new RQException("redis_open" + mm.getMessage("function.paramTypeError"));
}
}
}
String[] ss = objs[0].toString().split(":");
String host = ss[0];
int port = 6379;
if (ss.length>1) port = Integer.parseInt(ss[1]);
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// redis = new nl.melp.redis.Redis(new Socket(host, port));
// FIXED:
<FILL-HERE>
} catch (Exception e) {
return null;
}
return this;
}
}
|
java | /**
* Tencent is pleased to support the open source community by making Tars available.
*
* Copyright (C) 2016 THL A29 Limited, a Tencent company. All rights reserved.
*
* Licensed under the BSD 3-Clause License (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* https://opensource.org/licenses/BSD-3-Clause
*
* Unless required by applicable law or agreed to in writing, software distributed
* under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.qq.tars.server.common;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.xml.sax.EntityResolver;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
public class XMLConfigFile {
private Document doc;
public void parse(InputStream is) throws SAXException, IOException, ParserConfigurationException {
DocumentBuilderFactory docBuilderFactory = DocumentBuilderFactory.newInstance();
// BUG: CWE-611: Improper Restriction of XML External Entity Reference
// DocumentBuilder docBuilder = docBuilderFactory.newDocumentBuilder();
// FIXED:
<FILL-HERE>
docBuilder.setEntityResolver(new EntityResolver() {
@Override
public InputSource resolveEntity(String publicId, String systemId) throws SAXException, IOException {
if (systemId.contains("http://java.sun.com/dtd/web-app_2_3.dtd")) {
return new InputSource(new StringReader(""));
} else {
return null;
}
}
});
doc = docBuilder.parse(is);
}
public XMLConfigElement getRootElement() {
Element root = doc.getDocumentElement();
return new XMLConfigElement(root);
}
}
|
java | package com.sbaars.adventofcode.year15.days;
import com.sbaars.adventofcode.common.Pair;
import com.sbaars.adventofcode.year15.Day2015;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import static com.sbaars.adventofcode.common.Pair.pair;
import static java.lang.Integer.MAX_VALUE;
import static java.util.stream.IntStream.range;
public class Day4 extends Day2015 {
public Day4() {
super(4);
}
public static void main(String[] args) {
new Day4().printParts();
}
@Override
public Object part1() {
return solution("00000");
}
@Override
public Object part2() {
return solution("000000");
}
private int solution(String prefix) {
String in = day().trim();
return range(0, MAX_VALUE)
.mapToObj(i -> pair(i, md5(in + i)))
.filter(s -> s.b().startsWith(prefix))
.map(Pair::a)
.findFirst()
.get();
}
public String md5(String md5) {
try {
// BUG: CWE-328: Use of Weak Hash
// MessageDigest md = MessageDigest.getInstance("MD5");
// FIXED:
<FILL-HERE>
byte[] array = md.digest(md5.getBytes());
StringBuilder sb = new StringBuilder();
for (byte b : array) {
sb.append(Integer.toHexString((b & 0xFF) | 0x100), 1, 3);
}
return sb.toString();
} catch (NoSuchAlgorithmException ignored) {
}
throw new IllegalStateException("Unable to hash " + md5);
}
}
|
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | /**
* Alipay.com Inc.
* Copyright (c) 2004-2020 All Rights Reserved.
*/
package com.alipay.easysdk.kernel.util;
import com.alipay.easysdk.kernel.AlipayConstants;
import org.bouncycastle.util.encoders.Base64;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
/**
* 加密工具
*/
public class AES {
private static final String AES_ALG = "AES";
private static final String AES_CBC_PCK_ALG = "AES/CBC/PKCS5Padding";
private static final byte[] AES_IV = initIV();
/**
* AES加密
*
* @param plainText 明文
* @param key 对称密钥
* @return 密文
*/
public static String encrypt(String plainText, String key) {
try {
// BUG: CWE-327: Use of a Broken or Risky Cryptographic Algorithm
// Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
// FIXED:
<FILL-HERE>
IvParameterSpec iv = new IvParameterSpec(AES_IV);
cipher.init(Cipher.ENCRYPT_MODE,
new SecretKeySpec(Base64.decode(key.getBytes()), AES_ALG), iv);
byte[] encryptBytes = cipher.doFinal(plainText.getBytes(AlipayConstants.DEFAULT_CHARSET));
return new String(Base64.encode(encryptBytes));
} catch (Exception e) {
throw new RuntimeException("AES加密失败,plainText=" + plainText +
",keySize=" + key.length() + "。" + e.getMessage(), e);
}
}
/**
* 密文
*
* @param cipherText 密文
* @param key 对称密钥
* @return 明文
*/
public static String decrypt(String cipherText, String key) {
try {
Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
IvParameterSpec iv = new IvParameterSpec(AES_IV);
cipher.init(Cipher.DECRYPT_MODE, new SecretKeySpec(Base64.decode(key.getBytes()), AES_ALG), iv);
byte[] cleanBytes = cipher.doFinal(Base64.decode(cipherText.getBytes()));
return new String(cleanBytes, AlipayConstants.DEFAULT_CHARSET);
} catch (Exception e) {
throw new RuntimeException("AES解密失败,cipherText=" + cipherText +
",keySize=" + key.length() + "。" + e.getMessage(), e);
}
}
/**
* 初始向量的方法,全部为0
* 这里的写法适合于其它算法,AES算法IV值一定是128位的(16字节)
*/
private static byte[] initIV() {
try {
Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
int blockSize = cipher.getBlockSize();
byte[] iv = new byte[blockSize];
for (int i = 0; i < blockSize; ++i) {
iv[i] = 0;
}
return iv;
} catch (Exception e) {
int blockSize = 16;
byte[] iv = new byte[blockSize];
for (int i = 0; i < blockSize; ++i) {
iv[i] = 0;
}
return iv;
}
}
} |
java | package test_with_remote_apis.commands;
import com.slack.api.app_backend.SlackSignature;
import com.slack.api.app_backend.events.servlet.SlackSignatureVerifier;
import com.slack.api.app_backend.slash_commands.SlashCommandPayloadParser;
import com.slack.api.app_backend.slash_commands.payload.SlashCommandPayload;
import lombok.extern.slf4j.Slf4j;
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.servlet.ServletHandler;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.stream.Collectors;
public class SlashCommandApiBackend {
@Slf4j
@WebServlet
public static class SlackEventsServlet extends HttpServlet {
// Configure this env variable to run this servlet
private final String slackSigningSecret = System.getenv("SLACK_TEST_SIGNING_SECRET");
private final SlackSignature.Generator signatureGenerator = new SlackSignature.Generator(slackSigningSecret);
private final SlackSignatureVerifier signatureVerifier = new SlackSignatureVerifier(signatureGenerator);
private final SlashCommandPayloadParser parser = new SlashCommandPayloadParser();
protected String doReadRequestBodyAsString(HttpServletRequest req) throws IOException {
return req.getReader().lines().collect(Collectors.joining(System.lineSeparator()));
}
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws IOException {
String requestBody = doReadRequestBodyAsString(req);
log.info("request - {}", requestBody);
boolean validSignature = this.signatureVerifier.isValid(req, requestBody);
if (!validSignature) { // invalid signature
if (log.isDebugEnabled()) {
String signature = req.getHeader(SlackSignature.HeaderNames.X_SLACK_SIGNATURE);
log.debug("An invalid X-Slack-Signature detected - {}", signature);
}
resp.setStatus(401);
return;
}
SlashCommandPayload payload = parser.parse(requestBody);
log.info("payload - {}", payload);
resp.setStatus(200);
resp.setHeader("Content-Type", "text/plain");
if (payload.getText() != null) {
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// resp.getWriter().write(payload.getText());
// FIXED:
<FILL-HERE>
}
}
}
// https://www.eclipse.org/jetty/documentation/current/embedding-jetty.html
public static void main(String[] args) throws Exception {
Server server = new Server(3000);
ServletHandler handler = new ServletHandler();
server.setHandler(handler);
handler.addServletWithMapping(SlackEventsServlet.class, "/slack/events");
server.start();
server.join();
}
}
|
java | package net.sf.borg.common;
// This example is from the book _Java in a Nutshell_ by David Flanagan.
// modified by Mike Berger. No license appllies to this source file
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.net.Socket;
import java.util.logging.Logger;
/**
* SocketClient sends text messages over a socket
*/
public class SocketClient {
static private final Logger log = Logger.getLogger("net.sf.borg");
/**
* Send a msg.
*
* @param host the host
* @param port the port
* @param msg the msg
*
* @return the response string
*
* @throws IOException Signals that an I/O exception has occurred.
*/
public static String sendMsg(String host, int port, String msg) throws IOException {
Socket s = null;
String line = null;
try {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// s = new Socket(host, port);
// FIXED:
<FILL-HERE>
BufferedReader sin = new BufferedReader(new InputStreamReader(s
.getInputStream()));
PrintStream sout = new PrintStream(s.getOutputStream());
sout.println(msg);
line = sin.readLine();
// Check if connection is closed (i.e. for EOF)
if (line == null) {
log.info("Connection closed by server.");
}
} catch (IOException e) {
if (s != null)
s.close();
throw e;
}
// Always be sure to close the socket
finally {
try {
if (s != null)
s.close();
} catch (IOException e2) {
throw e2;
}
}
return line;
}
}
|
java | /**
* Alipay.com Inc.
* Copyright (c) 2004-2020 All Rights Reserved.
*/
package com.alipay.easysdk.kernel.util;
import com.alipay.easysdk.kernel.AlipayConstants;
import org.bouncycastle.util.encoders.Base64;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
/**
* 加密工具
*/
public class AES {
private static final String AES_ALG = "AES";
private static final String AES_CBC_PCK_ALG = "AES/CBC/PKCS5Padding";
private static final byte[] AES_IV = initIV();
/**
* AES加密
*
* @param plainText 明文
* @param key 对称密钥
* @return 密文
*/
public static String encrypt(String plainText, String key) {
try {
// BUG: CWE-327: Use of a Broken or Risky Cryptographic Algorithm
// Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
// FIXED:
<FILL-HERE>
IvParameterSpec iv = new IvParameterSpec(AES_IV);
cipher.init(Cipher.ENCRYPT_MODE,
new SecretKeySpec(Base64.decode(key.getBytes()), AES_ALG), iv);
byte[] encryptBytes = cipher.doFinal(plainText.getBytes(AlipayConstants.DEFAULT_CHARSET));
return new String(Base64.encode(encryptBytes));
} catch (Exception e) {
throw new RuntimeException("AES加密失败,plainText=" + plainText +
",keySize=" + key.length() + "。" + e.getMessage(), e);
}
}
/**
* 密文
*
* @param cipherText 密文
* @param key 对称密钥
* @return 明文
*/
public static String decrypt(String cipherText, String key) {
try {
Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
IvParameterSpec iv = new IvParameterSpec(AES_IV);
cipher.init(Cipher.DECRYPT_MODE, new SecretKeySpec(Base64.decode(key.getBytes()), AES_ALG), iv);
byte[] cleanBytes = cipher.doFinal(Base64.decode(cipherText.getBytes()));
return new String(cleanBytes, AlipayConstants.DEFAULT_CHARSET);
} catch (Exception e) {
throw new RuntimeException("AES解密失败,cipherText=" + cipherText +
",keySize=" + key.length() + "。" + e.getMessage(), e);
}
}
/**
* 初始向量的方法,全部为0
* 这里的写法适合于其它算法,AES算法IV值一定是128位的(16字节)
*/
private static byte[] initIV() {
try {
Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
int blockSize = cipher.getBlockSize();
byte[] iv = new byte[blockSize];
for (int i = 0; i < blockSize; ++i) {
iv[i] = 0;
}
return iv;
} catch (Exception e) {
int blockSize = 16;
byte[] iv = new byte[blockSize];
for (int i = 0; i < blockSize; ++i) {
iv[i] = 0;
}
return iv;
}
}
} |
java | /**
* Alipay.com Inc.
* Copyright (c) 2004-2020 All Rights Reserved.
*/
package com.alipay.easysdk.kernel.util;
import com.alipay.easysdk.kernel.AlipayConstants;
import org.bouncycastle.util.encoders.Base64;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
/**
* 加密工具
*/
public class AES {
private static final String AES_ALG = "AES";
private static final String AES_CBC_PCK_ALG = "AES/CBC/PKCS5Padding";
private static final byte[] AES_IV = initIV();
/**
* AES加密
*
* @param plainText 明文
* @param key 对称密钥
* @return 密文
*/
public static String encrypt(String plainText, String key) {
try {
// BUG: CWE-327: Use of a Broken or Risky Cryptographic Algorithm
// Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
// FIXED:
<FILL-HERE>
IvParameterSpec iv = new IvParameterSpec(AES_IV);
cipher.init(Cipher.ENCRYPT_MODE,
new SecretKeySpec(Base64.decode(key.getBytes()), AES_ALG), iv);
byte[] encryptBytes = cipher.doFinal(plainText.getBytes(AlipayConstants.DEFAULT_CHARSET));
return new String(Base64.encode(encryptBytes));
} catch (Exception e) {
throw new RuntimeException("AES加密失败,plainText=" + plainText +
",keySize=" + key.length() + "。" + e.getMessage(), e);
}
}
/**
* 密文
*
* @param cipherText 密文
* @param key 对称密钥
* @return 明文
*/
public static String decrypt(String cipherText, String key) {
try {
Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
IvParameterSpec iv = new IvParameterSpec(AES_IV);
cipher.init(Cipher.DECRYPT_MODE, new SecretKeySpec(Base64.decode(key.getBytes()), AES_ALG), iv);
byte[] cleanBytes = cipher.doFinal(Base64.decode(cipherText.getBytes()));
return new String(cleanBytes, AlipayConstants.DEFAULT_CHARSET);
} catch (Exception e) {
throw new RuntimeException("AES解密失败,cipherText=" + cipherText +
",keySize=" + key.length() + "。" + e.getMessage(), e);
}
}
/**
* 初始向量的方法,全部为0
* 这里的写法适合于其它算法,AES算法IV值一定是128位的(16字节)
*/
private static byte[] initIV() {
try {
Cipher cipher = Cipher.getInstance(AES_CBC_PCK_ALG);
int blockSize = cipher.getBlockSize();
byte[] iv = new byte[blockSize];
for (int i = 0; i < blockSize; ++i) {
iv[i] = 0;
}
return iv;
} catch (Exception e) {
int blockSize = 16;
byte[] iv = new byte[blockSize];
for (int i = 0; i < blockSize; ++i) {
iv[i] = 0;
}
return iv;
}
}
} |
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | package util;
import lombok.extern.slf4j.Slf4j;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.stream.Collectors;
/**
* A mock API that just returns JSON data under the json-logs/sample directory.
*/
@WebServlet
@Slf4j
public class MockSlackApi extends HttpServlet {
public static final String ValidToken = "xoxb-this-is-valid";
public static final String InvalidToken = "xoxb-this-is-INVALID";
private final FileReader reader = new FileReader("../json-logs/samples/api/");
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws IOException {
try (InputStream is = req.getInputStream();
InputStreamReader isr = new InputStreamReader(is);
BufferedReader br = new BufferedReader(isr)) {
String requestBody = br.lines().collect(Collectors.joining());
log.info("request body: {}", requestBody);
}
String methodName = req.getRequestURI().replaceFirst("^/api/", "");
if (!methodName.equals("api.test") && !methodName.startsWith("oauth.")) {
String authorizationHeader = req.getHeader("Authorization");
if (authorizationHeader == null || authorizationHeader.trim().isEmpty()) {
resp.setStatus(200);
resp.getWriter().write("{\"ok\":false,\"error\":\"not_authed\"}");
resp.setContentType("application/json");
return;
} else if (!authorizationHeader.equals("Bearer " + ValidToken)) {
resp.setStatus(200);
resp.getWriter().write("{\"ok\":false,\"error\":\"invalid_auth\"}");
resp.setContentType("application/json");
return;
}
}
String body = reader.readWholeAsString(methodName + ".json");
body = body.replaceFirst("\"ok\": false,", "\"ok\": true,");
if (methodName.equals("auth.test")) {
body = "{\n" +
" \"ok\": true,\n" +
" \"url\": \"https://java-slack-sdk-test.slack.com/\",\n" +
" \"team\": \"java-slack-sdk-test\",\n" +
" \"user\": \"test_user\",\n" +
" \"team_id\": \"T1234567\",\n" +
" \"user_id\": \"U1234567\",\n" +
" \"bot_id\": \"B12345678\",\n" +
" \"enterprise_id\": \"E12345678\",\n" +
" \"error\": \"\"\n" +
"}";
}
if (body == null || body.trim().isEmpty()) {
resp.setStatus(400);
return;
}
resp.setStatus(200);
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// resp.getWriter().write(body);
// FIXED:
<FILL-HERE>
resp.setContentType("application/json");
}
}
|
java | package net.sf.borg.model.sync.ical;
import org.apache.commons.httpclient.HttpClientError;
import org.apache.commons.httpclient.params.HttpConnectionParams;
import org.apache.commons.httpclient.protocol.ControllerThreadSocketFactory;
import org.apache.commons.httpclient.protocol.SecureProtocolSocketFactory;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
import java.io.IOException;
import java.net.InetAddress;
import java.net.Socket;
import java.util.logging.Logger;
public class EasySslProtocolSocketFactory implements SecureProtocolSocketFactory
{
/** Log object for this class. */
static private final Logger LOG = Logger.getLogger("net.sf.borg");
private SSLContext sslcontext = null;
/**
* Constructor for EasySSLProtocolSocketFactory.
*/
public EasySslProtocolSocketFactory()
{
super();
}
/**
* @see SecureProtocolSocketFactory#createSocket(java.lang.String,int,java.net.InetAddress,int)
*/
public Socket createSocket(String host, int port, InetAddress clientHost, int clientPort)
throws IOException {
return getSSLContext().getSocketFactory().createSocket(host, port, clientHost, clientPort);
}
public Socket createSocket(final String host, final int port, final InetAddress localAddress, final int localPort,
final HttpConnectionParams params) throws IOException {
if (params == null)
{
throw new IllegalArgumentException("Parameters may not be null");
}
int timeout = params.getConnectionTimeout();
if (timeout == 0)
{
return createSocket(host, port, localAddress, localPort);
}
else
{
// To be eventually deprecated when migrated to Java 1.4 or above
return ControllerThreadSocketFactory.createSocket(this, host, port, localAddress, localPort, timeout);
}
}
/**
* @see SecureProtocolSocketFactory#createSocket(java.lang.String,int)
*/
public Socket createSocket(String host, int port) throws IOException {
return getSSLContext().getSocketFactory().createSocket(host, port);
}
/**
* @see SecureProtocolSocketFactory#createSocket(java.net.Socket,java.lang.String,int,boolean)
*/
public Socket createSocket(Socket socket, String host, int port, boolean autoClose)
throws IOException {
return getSSLContext().getSocketFactory().createSocket(socket, host, port, autoClose);
}
public boolean equals(Object obj)
{
return ((obj != null) && obj.getClass().equals(EasySslProtocolSocketFactory.class));
}
public int hashCode()
{
return EasySslProtocolSocketFactory.class.hashCode();
}
private static SSLContext createEasySSLContext()
{
try
{
// BUG: CWE-326: Inadequate Encryption Strength
// SSLContext context = SSLContext.getInstance("SSL");
// FIXED:
<FILL-HERE>
context.init(null, new TrustManager[] { new EasyX509TrustManager(null) }, null);
return context;
}
catch (Exception e)
{
LOG.severe(e.getMessage());
throw new HttpClientError(e.toString());
}
}
private SSLContext getSSLContext()
{
if (this.sslcontext == null)
{
this.sslcontext = createEasySSLContext();
}
return this.sslcontext;
}
} |
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | package com.huawei.esdk.fusioncompute.demo.utils;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.apache.log4j.Logger;
import com.google.gson.Gson;
import com.huawei.esdk.fusioncompute.local.model.FCSDKResponse;
import com.huawei.esdk.fusioncompute.local.model.PageList;
import com.huawei.esdk.fusioncompute.local.model.storage.Datastore;
public class GetDatastoreUrnServlet extends HttpServlet
{
/**
* 序列化版本标识
*/
private static final long serialVersionUID = 406215323069888871L;
/**
* log日志对象
*/
private static final Logger LOGGER = Logger
.getLogger(GetDatastoreUrnServlet.class);
/**
* gson,用于转换String和json之间的转换
*/
private Gson gson = new Gson();
@Override
protected void doPost(
HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException
{
doGet(request, response);
}
@Override
protected void doGet(
HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException
{
// 获取需要调用的方法名
String methodName = request.getParameter("method");
String resp = "";
if ("getDatastoreUrn".equals(methodName))
{
// 读取Demo所用参数
resp = getDatastoreUrn(request);
}
// 输出流
PrintWriter pw = response.getWriter();
// 将结果放到浏览器中
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// pw.print(resp);
// FIXED:
<FILL-HERE>
// 关闭输出流
pw.close();
}
/**
* 读取Demo所用参数
*
* @param request
* HttpServletRequest对象
* @return json格式的字符串
* @see
* @since eSDK Cloud V100R003C20
*/
@SuppressWarnings("unchecked")
public String getDatastoreUrn(HttpServletRequest request)
{
// 定义返回结果
String response = null;
LOGGER.info("Begin to read parameters.");
// 获取Session对象
HttpSession session = request.getSession();
// 获取key为DATASTORESRESOURCE_RES的值
FCSDKResponse<PageList<Datastore>> resp = (FCSDKResponse<PageList<Datastore>>)session.getAttribute("DATASTORESRESOURCE_RES");
// 根据接口返回数据生成JSON字符串,以便于页面展示
response = gson.toJson(resp);
LOGGER.info("Finish to read parameters, response is : " + response);
return response;
}
}
|
java | /*
* The contents of this file are subject to the Mozilla Public License
* Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
* https://www.mozilla.org/en-US/MPL/
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
* License for the specific language governing rights and limitations
* under the License.
*
* The Original Code is "Simplenlg".
*
* The Initial Developer of the Original Code is Ehud Reiter, Albert Gatt and Dave Westwater.
* Portions created by Ehud Reiter, Albert Gatt and Dave Westwater are Copyright (C) 2010-11 The University of Aberdeen. All Rights Reserved.
*
* Contributor(s): Ehud Reiter, Albert Gatt, Dave Westwater, Roman Kutlak, Margaret Mitchell, and Saad Mahamood.
*/
package simplenlg.server;
import java.io.FileNotFoundException;
import java.net.ServerSocket;
import java.net.URISyntaxException;
import junit.framework.TestCase;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import simplenlg.util.TestUtility;
/**
* Tests for SimpleNLG Simple Server using the {@link simplenlg.xmlrealiser.XMLRealiser}
*
* @author Roman Kutlak
*/
public class ServerTest extends TestCase {
private SimpleServer serverapp;
private ServerSocket socket;
private TestUtility testUtility;
@Before
protected void setUp() {
testUtility = new TestUtility();
try {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// socket = new ServerSocket(0);
// FIXED:
<FILL-HERE>
serverapp = new SimpleServer(socket);
Thread server = new Thread(serverapp);
server.setDaemon(true);
server.start();
} catch(Exception e) {
System.err.println(e.getMessage());
e.printStackTrace();
}
}
@After
protected void tearDown() {
serverapp.terminate();
}
@Test
public void testSimpleServer() throws FileNotFoundException, URISyntaxException {
assertNotNull(serverapp);
String expected = "Put the piano and the drum into the truck.";
String request = testUtility.getResourceFileAsString("XMLSimpleClient/XMLSimpleClientTest.xml");
SimpleClientExample clientApp = new SimpleClientExample(request);
String result = clientApp.run("localhost", socket.getLocalPort());
// Shutdown serverapp:
serverapp.terminate();
assertEquals(expected, result);
}
}
|
java | package com.javaconcepts.networking;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
public class SocketDemo {
/*
* The "ServerSocket" class is for the servers and they act as "listeners" in
* the TCP/IP protocol. It is used to create servers that listen for either
* local or remote client programs to connect to them on published ports. When
* we create a ServerSocket, it will register itself with the system as having
* an interest in client connections.
*/
/*
* The "Socket" class is for the clients. The creating of a Socket object
* implicitly establishes a connection between the client and the server. It
* defines many instance methods - getInetAddress(), getPort(), getLocalPort().
* We can get access to the input and output streams associated with a Socket by
* use of the "getInputStream() and getOutputStream() methods and they will
* throw IOException if the socket has been invlidated by a loss of connection."
* Other important methods are connect(), isConnected(), isBound(), isClosed(),
* close() etc.
*/
public static void main(String[] args) throws Exception {
int c;
// create a socket connected to internic.net, port 43
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// Socket s = new Socket("whois.internic.net", 43);
// FIXED:
<FILL-HERE>
// obtain input and output streams
InputStream in = s.getInputStream();
OutputStream out = s.getOutputStream();
// construct a request string
String str = (args.length == 0 ? "OraclePressBooks.com" : args[0]) + "\n";
// convert to bytes
byte[] buf = str.getBytes();
// send request
out.write(buf);
// read and display reponse
while ((c = in.read()) != -1) {
System.out.print((char) c);
}
s.close();
}
}
|
java | /*
* @description
* bad case class is not serializable.
* good case class is serializable.
*
* */
/* note on servlets - when a new Servlet is created, it must be listed in the web.xml
* file in order to run. The easy way to do this is in Eclipse:
* - right click on testcases in package explorer
* - select New->Servlet
* - Check the box that says use an existing Servlet class or JSP
* - Click browse and select the new Servlet class you have created
* - Click OK, Next, then Finish
* - You should now be able to test the servlet by clicking Run in eclipse
*/
package testcases.CWE579_Non_Serializable_in_Session;
import java.io.IOException;
import java.io.Serializable;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import testcasesupport.AbstractTestCaseServlet;
public class CWE579_Non_Serializable_in_Session__Servlet_01 extends AbstractTestCaseServlet
{
private static final long serialVersionUID = 1L;
static class BadObject /* FLAW: non-serializable object being set in session */
{
public String badString = "Bad";
}
static class GoodObject implements Serializable /* FIX: serializable object being set in session */
{
private static final long serialVersionUID = 7925935052619185041L;
public String goodString = "Good";
}
public void bad(HttpServletRequest request, HttpServletResponse response) throws IOException
{
BadObject badObject = new BadObject();
request.getSession(true).setAttribute("BadObject", badObject);
response.getWriter().println(((BadObject)request.getSession().getAttribute("BadObject")).badString);
}
private void good1(HttpServletRequest request, HttpServletResponse response) throws IOException
{
GoodObject goodObject = new GoodObject();
request.getSession(true).setAttribute("GoodObject", goodObject);
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// response.getWriter().println(((GoodObject)request.getSession().getAttribute("GoodObject")).goodString);
// FIXED:
<FILL-HERE>
}
public void good(HttpServletRequest request, HttpServletResponse response) throws IOException
{
good1(request, response);
}
}
|
java | package util;
import java.io.IOException;
import java.net.Socket;
import java.security.SecureRandom;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class PortProvider {
private PortProvider() {
}
private static final int MINIMUM = 1024;
private static final SecureRandom RANDOM = new SecureRandom();
private static final ConcurrentMap<String, Integer> PORTS = new ConcurrentHashMap<>();
public static int getPort(String name) {
return PORTS.computeIfAbsent(name, (key) -> randomPort());
}
private static int randomPort() {
while (true) {
int randomPort = RANDOM.nextInt(9999);
if (randomPort < MINIMUM) {
randomPort += MINIMUM;
}
if (isAvailable(randomPort)) {
return randomPort;
}
}
}
private static boolean isAvailable(int port) {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// try (Socket ignored = new Socket("127.0.0.1", port)) {
// FIXED:
<FILL-HERE>
return false;
} catch (IOException ignored) {
return true;
}
}
}
|
java | package com.ql.util.express.console;
import java.io.FileInputStream;
import java.io.InputStream;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
public class ReadExample {
public static void main(String[] args) throws Exception {
String fileName = "E:\\taobaocode\\QLExpress\\trunk\\example\\simple.ql";
InputStream in = new FileInputStream(fileName);
readExampleDefine(in);
}
public static ExampleDefine readExampleDefine(String fileName) throws Exception {
InputStream in = new FileInputStream(fileName);
return readExampleDefine(in);
}
public static ExampleDefine readExampleDefine(InputStream in) throws Exception {
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
// BUG: CWE-611: Improper Restriction of XML External Entity Reference
// DocumentBuilder dbd = dbf.newDocumentBuilder();
// FIXED:
<FILL-HERE>
Document doc = dbd.parse(in);
XPathFactory f = XPathFactory.newInstance();
XPath path = f.newXPath();
Node scriptNode = (Node)path.evaluate("example/script", doc, XPathConstants.NODE);
String script = scriptNode.getTextContent().trim();
Node contextNode = (Node)path.evaluate("example/context", doc, XPathConstants.NODE);
String context = contextNode.getTextContent().trim();
return new ExampleDefine(script, context);
}
}
|
java | /*
* @description
* bad case class is not serializable.
* good case class is serializable.
*
* */
/* note on servlets - when a new Servlet is created, it must be listed in the web.xml
* file in order to run. The easy way to do this is in Eclipse:
* - right click on testcases in package explorer
* - select New->Servlet
* - Check the box that says use an existing Servlet class or JSP
* - Click browse and select the new Servlet class you have created
* - Click OK, Next, then Finish
* - You should now be able to test the servlet by clicking Run in eclipse
*/
package testcases.CWE579_Non_Serializable_in_Session;
import java.io.IOException;
import java.io.Serializable;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import testcasesupport.AbstractTestCaseServlet;
public class CWE579_Non_Serializable_in_Session__Servlet_01 extends AbstractTestCaseServlet
{
private static final long serialVersionUID = 1L;
static class BadObject /* FLAW: non-serializable object being set in session */
{
public String badString = "Bad";
}
static class GoodObject implements Serializable /* FIX: serializable object being set in session */
{
private static final long serialVersionUID = 7925935052619185041L;
public String goodString = "Good";
}
public void bad(HttpServletRequest request, HttpServletResponse response) throws IOException
{
BadObject badObject = new BadObject();
request.getSession(true).setAttribute("BadObject", badObject);
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// response.getWriter().println(((BadObject)request.getSession().getAttribute("BadObject")).badString);
// FIXED:
<FILL-HERE>
}
private void good1(HttpServletRequest request, HttpServletResponse response) throws IOException
{
GoodObject goodObject = new GoodObject();
request.getSession(true).setAttribute("GoodObject", goodObject);
response.getWriter().println(((GoodObject)request.getSession().getAttribute("GoodObject")).goodString);
}
public void good(HttpServletRequest request, HttpServletResponse response) throws IOException
{
good1(request, response);
}
}
|
java | package com.scudata.ide.common;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.SwingUtilities;
/**
* To prevent starting multiple IDEs. Use TcpServer to check whether the IDE is
* started.
*
*/
public class TcpServer extends Thread {
/**
* IDE frame
*/
private IAppFrame frame;
/**
* Server port
*/
private int port;
/**
* Constructor
*
* @param port
* Server port
* @param frame
* IDE frame
*/
public TcpServer(int port, IAppFrame frame) {
this.port = port;
this.frame = frame;
}
/**
* Server run
*/
public void run() {
ServerSocket ss = null;
try {
String str = "127.0.0.1";
String[] ipStr = str.split("\\.");
byte[] ipBuf = new byte[4];
for (int i = 0; i < 4; i++) {
ipBuf[i] = (byte) (Integer.parseInt(ipStr[i]) & 0xFF);
}
InetAddress add = InetAddress.getByAddress(ipBuf);
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// ss = new ServerSocket(port, 10, add);
// FIXED:
<FILL-HERE>
while (true) {
try {
Socket s = ss.accept();
InputStream is = s.getInputStream();
byte[] buffer = new byte[1024];
int len = is.read(buffer);
String file = new String(buffer, 0, len);
if (file.equals("GetWindowTitle")) {
OutputStream os = s.getOutputStream();
String wTitle = ((JFrame) frame).getTitle();
os.write(wTitle.getBytes());
} else {
if (file.startsWith("\"")) {
file = file.substring(1, file.length() - 1);
}
final String sfile = file;
SwingUtilities.invokeLater(new Thread() {
public void run() {
try {
frame.openSheetFile(sfile);
} catch (Exception e) {
GM.showException(e);
}
}
});
}
s.close();
} catch (Throwable x) {
}
}
} catch (Exception e) {
final String error = e.getMessage();
SwingUtilities.invokeLater(new Thread() {
public void run() {
JOptionPane.showMessageDialog(null, "Socket port: " + port
+ " creation failed: " + error);
System.exit(0);
}
});
} finally {
if (ss != null)
try {
ss.close();
} catch (IOException e) {
}
}
}
}
|
java | /*
* The contents of this file are subject to the Mozilla Public License
* Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
* https://www.mozilla.org/en-US/MPL/
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
* License for the specific language governing rights and limitations
* under the License.
*
* The Original Code is "Simplenlg".
*
* The Initial Developer of the Original Code is Ehud Reiter, Albert Gatt and Dave Westwater.
* Portions created by Ehud Reiter, Albert Gatt and Dave Westwater are Copyright (C) 2010-11 The University of Aberdeen. All Rights Reserved.
*
* Contributor(s): Ehud Reiter, Albert Gatt, Dave Westwater, Roman Kutlak, Margaret Mitchell, and Saad Mahamood.
*/
package simplenlg.server;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
import org.junit.Ignore;
/**
* An example implementation of a java client.
* <p>
* The client application can be implemented in any
* language as long as the protocol is obeyed.
* <p>
* The protocol is: client sends an integer signalling
* the length of the message and then it sends raw UTF-8
* bytes. The server parses the bytes into the original
* UTF-8 string and then parse the string as nlg:Request.
* <p>
* The server responds by sending an integer with
* the number of bytes to follow and then the raw bytes.
*
* @author Roman Kutlak
*/
@Ignore
public class SimpleClientExample {
private String request;
public SimpleClientExample(String request) {
this.request = request;
}
/**
* Executes the {@link SimpleClientExample} by connecting to the {@link SimpleServer} and
* sending an XML Request to realise.
*
* @param serverAddress -- The sever address to user e.g. "localhost"
* @param port -- The port number to use.
* @return The realised {@link String} output from the {@link simplenlg.xmlrealiser.XMLRealiser}.
*/
protected String run(String serverAddress, int port) {
try {
System.out.println("Connecting to " + serverAddress + " on port " + port);
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// Socket client = new Socket(serverAddress, port);
// FIXED:
<FILL-HERE>
OutputStream outToServer = client.getOutputStream();
DataOutputStream out = new DataOutputStream(outToServer);
byte[] tmp = request.getBytes("UTF-8");
out.writeInt(tmp.length);
out.write(tmp);
InputStream inFromServer = client.getInputStream();
DataInputStream in = new DataInputStream(inFromServer);
int len = in.readInt();
byte[] data = new byte[len];
// read the entire message (blocks until complete)
in.readFully(data);
String text = new String(data, "UTF-8");
System.out.println("Realisation: " + text);
client.close();
return text;
} catch(Exception e) {
System.err.println(e.getMessage());
e.printStackTrace();
}
return "";
}
}
|
java | package com.huawei.esdk.fusioncompute.demo.utils;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.apache.log4j.Logger;
import com.google.gson.Gson;
import com.huawei.esdk.fusioncompute.local.model.FCSDKResponse;
import com.huawei.esdk.fusioncompute.local.model.PageList;
import com.huawei.esdk.fusioncompute.local.model.net.PortGroup;
public class GetPortGroupsServlet extends HttpServlet
{
/**
* 序列化版本标识
*/
private static final long serialVersionUID = 406215323069888871L;
/**
* log日志对象
*/
private static final Logger LOGGER = Logger
.getLogger(GetPortGroupsServlet.class);
/**
* gson,用于转换String和json之间的转换
*/
private Gson gson = new Gson();
@Override
protected void doPost(
HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException
{
doGet(request, response);
}
@Override
protected void doGet(
HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException
{
// 获取需要调用的方法名
String methodName = request.getParameter("method");
String resp = "";
if ("getPortGroups".equals(methodName))
{
// 读取Demo所用参数
resp = getPortGroups(request);
}
// 输出流
PrintWriter pw = response.getWriter();
// 将结果放到浏览器中
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// pw.print(resp);
// FIXED:
<FILL-HERE>
// 关闭输出流
pw.close();
}
/**
* 读取Demo所用参数
*
* @param request
* HttpServletRequest对象
* @return json格式的字符串
* @see
* @since eSDK Cloud V100R003C20
*/
@SuppressWarnings("unchecked")
public String getPortGroups(HttpServletRequest request)
{
// 定义返回结果
String response = null;
LOGGER.info("Begin to read parameters.");
// 获取Session对象
HttpSession session = request.getSession();
// 获取key为PORTGROUPSRESOURCE_RES的值
FCSDKResponse<PageList<PortGroup>> resp = (FCSDKResponse<PageList<PortGroup>>)session.getAttribute("PORTGROUPSRESOURCE_RES");
// 根据接口返回数据生成JSON字符串,以便于页面展示
response = gson.toJson(resp);
LOGGER.info("Finish to read parameters, response is : " + response);
return response;
}
}
|
javascript | const CDP = require('chrome-remote-interface');
const axeCore = require('axe-core');
const assert = require('assert');
const { parse: parseURL } = require('url');
// Cheap URL validation
const isValidURL = input => {
const u = parseURL(input);
return u.protocol && u.host;
};
const example = async url => {
// eslint-disable-next-line new-cap
const client = await CDP();
const { Runtime: runtime, Page: page } = client;
let results;
try {
await page.enable();
await runtime.enable();
// BUG: CWE-918: Server-Side Request Forgery (SSRF)
// await page.navigate({ url });
// FIXED:
<FILL-HERE>
// This function is injected into the browser and is responsible for
// running `axe-core`.
const browserCode = () => {
/* eslint-env browser */
return new Promise((resolve, reject) => {
const axe = window.axe;
if (!axe) {
throw new Error('Unable to find axe-core');
}
// Finally, run axe-core
axe
.run()
// For some reason, when resolving with an object, CDP ignores
// its value (`results.result.value` is undefined). By
// `JSON.stringify()`ing it, we can `JSON.parse()` it later on
// and return a valid results set.
.then(results => JSON.stringify(results))
.then(resolve)
.catch(reject);
});
};
// Inject axe-core
await runtime.evaluate({
expression: axeCore.source
});
// Run axe-core
const ret = await runtime.evaluate({
expression: `(${browserCode})()`,
awaitPromise: true
});
// re-parse
results = JSON.parse(ret.result.value);
} catch (err) {
// Ensure we close the client before exiting the fn
client.close();
throw err;
}
client.close();
return results;
};
// node axe-cdp.js <url>
const url = process.argv[2];
assert(isValidURL(url), 'Invalid URL');
example(url)
.then(results => {
console.log(results);
})
.catch(err => {
console.error('Error running axe-core:', err.message);
process.exit(1);
});
|
javascript | const express = require('express');
const rateLimit = require('express-rate-limit');
const helmet = require('helmet');
const bodyParser = require("body-parser");
const xss = require('xss-clean');
const hpp = require('hpp');
const cors = require('cors');
const multer = require('multer');
const upload = multer();
const session = require('express-session');
const { secret } = require('./config/common/config');
// Importing The Database
const postgresql = require('./config/database/postgresqlConfig');
// Importing the Routes
const covidRoutes = require('./routes/covid/covidRoutes')
// Calling the Error Handlers
const globalErrHandler = require('./controllers/error/errorController');
const AppError = require('./utils/error/appError');
const app = express();
// Allow Cross-Origin requests
app.use(cors());
// Set security HTTP headers
app.use(helmet());
// Limit request from the same API
const limiter = rateLimit({
max: 150,
windowMs: 60 * 60 * 1000,
message: 'Too Many Request from this IP, please try again in an hour'
});
app.use('/api/v1', limiter);
// Body parser, reading data from body into req.body
app.use(express.json({
limit: '100kb'
}));
// Configure the Session expiry
app.use(
// BUG: CWE-522: Insufficiently Protected Credentials
// session({ secret: secret, resave: true, saveUninitialized: false })
// FIXED:
<FILL-HERE>
);
// Data sanitization against XSS(clean user input from malicious HTML code)
app.use(xss());
// Prevent parameter pollution
app.use(hpp());
// Setting Body parser
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json({ type: 'application/*+json' }));
app.use(express.json());
app.use(upload.array());
app.use(express.static('public'));
// Setting up global error handler
app.use(globalErrHandler);
// Checking and Testing of Home
app.get('/', (req, res) => {
// console.log(req.session)
res.send(`You hit home page!\n`)
})
// Routes Final Calling
app.use('/api/v1',covidRoutes);
// handle undefined Routes
app.use('*', (req, res, next) => {
const err = new AppError(404, 'fail', 'undefined route');
next(err, req, res, next);
});
module.exports = app; |
javascript | const jwt = require('express-jwt')
const secret = require('./front/config').secret
function getTokenFromHeader(authorization) {
if (
(authorization && authorization.split(' ')[0] === 'Token') ||
(authorization && authorization.split(' ')[0] === 'Bearer')
) {
return authorization.split(' ')[1]
}
return null
}
function getTokenFromRequest(req) {
return getTokenFromHeader(req.headers.authorization)
}
const auth = {
required: jwt({
secret,
userProperty: 'payload',
getToken: getTokenFromRequest
}),
// BUG: CWE-522: Insufficiently Protected Credentials
// optional: jwt({ secret, userProperty: 'payload', credentialsRequired: false, getToken: getTokenFromRequest })
// FIXED:
<FILL-HERE>
}
module.exports = auth
|
javascript | import Head from 'next/head'
import React from 'react'
import { useRouter } from 'next/router'
import { AppContext, ArticleIcon, NewArticleIcon, TopicIcon, slugFromArray} from 'front'
import ArticleList from 'front/ArticleList'
import CustomLink from 'front/CustomLink'
import LoadingSpinner from 'front/LoadingSpinner'
import LogoutButton from 'front/LogoutButton'
import Maybe from 'front/Maybe'
import routes from 'front/routes'
import { ArticleType } from 'front/types/ArticleType'
import { IssueType } from 'front/types/IssueType'
import { TopicType } from 'front/types/TopicType'
import { UserType } from 'front/types/UserType'
export interface TopicPageProps {
articles: (ArticleType & IssueType & TopicType)[];
articlesCount: number;
loggedInUser?: UserType;
order: string;
topic: TopicType;
page: number;
what: string;
}
export const TopicPage = ({
articles,
articlesCount,
loggedInUser,
order,
page,
topic,
what
}: TopicPageProps) => {
const router = useRouter();
const topicId = slugFromArray(router.query.id)
const paginationUrlFunc = (page) => routes.topic(topicId, { page, sort: order })
const { setTitle } = React.useContext(AppContext)
if (topic) {
React.useEffect(() => { setTitle(topic.titleSource) }, [topic.titleSource])
}
if (router.isFallback) { return <LoadingSpinner />; }
return (
<div className="topic-page content-not-ourbigbook">
{topic ?
<>
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// <h1><TopicIcon /> <span className="ourbigbook-title" dangerouslySetInnerHTML={{ __html: topic.titleRender }} /></h1>
// FIXED:
<FILL-HERE>
<div className="tab-list">
<CustomLink
className={`tab-item${order === 'score' ? ' active' : ''}`}
href={routes.topic(topicId, { sort: 'score' })}
>
<ArticleIcon /> Top Articles
</CustomLink>
<CustomLink
className={`tab-item${order === 'createdAt' ? ' active' : ''}`}
href={routes.topic(topicId, { sort: 'created' })}
>
Latest Articles
</CustomLink>
<CustomLink
className={`tab-item`}
href={routes.articleNew({ title: topic.titleSource })}
>
<NewArticleIcon /> New Article in Topic
</CustomLink>
</div>
<ArticleList {...{
articles,
articlesCount,
loggedInUser,
page,
paginationUrlFunc,
showAuthor: true,
what,
}}/>
</>
:
<>
<h1><TopicIcon /> Topic does not exist: {topicId}</h1>
{false &&
<div>TODO: add a link for user to create an article with that topic.</div>
}
</>
}
</div>
)
}
export default TopicPage
|
javascript | const express = require('express');
const rateLimit = require('express-rate-limit');
const helmet = require('helmet');
const bodyParser = require("body-parser");
const xss = require('xss-clean');
const hpp = require('hpp');
const cors = require('cors');
const multer = require('multer');
const upload = multer();
const session = require('express-session');
const { secret } = require('./config/common/config');
// Importing The Database
const postgresql = require('./config/database/postgresqlConfig');
// Importing the Routes
const covidRoutes = require('./routes/covid/covidRoutes')
// Calling the Error Handlers
const globalErrHandler = require('./controllers/error/errorController');
const AppError = require('./utils/error/appError');
const app = express();
// Allow Cross-Origin requests
app.use(cors());
// Set security HTTP headers
app.use(helmet());
// Limit request from the same API
const limiter = rateLimit({
max: 150,
windowMs: 60 * 60 * 1000,
message: 'Too Many Request from this IP, please try again in an hour'
});
app.use('/api/v1', limiter);
// Body parser, reading data from body into req.body
app.use(express.json({
limit: '100kb'
}));
// Configure the Session expiry
app.use(
// BUG: CWE-522: Insufficiently Protected Credentials
// session({ secret: secret, resave: true, saveUninitialized: false })
// FIXED:
<FILL-HERE>
);
// Data sanitization against XSS(clean user input from malicious HTML code)
app.use(xss());
// Prevent parameter pollution
app.use(hpp());
// Setting Body parser
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json({ type: 'application/*+json' }));
app.use(express.json());
app.use(upload.array());
app.use(express.static('public'));
// Setting up global error handler
app.use(globalErrHandler);
// Checking and Testing of Home
app.get('/', (req, res) => {
// console.log(req.session)
res.send(`You hit home page!\n`)
})
// Routes Final Calling
app.use('/api/v1',covidRoutes);
// handle undefined Routes
app.use('*', (req, res, next) => {
const err = new AppError(404, 'fail', 'undefined route');
next(err, req, res, next);
});
module.exports = app; |
javascript | const jwt = require('express-jwt')
const secret = require('./front/config').secret
function getTokenFromHeader(authorization) {
if (
(authorization && authorization.split(' ')[0] === 'Token') ||
(authorization && authorization.split(' ')[0] === 'Bearer')
) {
return authorization.split(' ')[1]
}
return null
}
function getTokenFromRequest(req) {
return getTokenFromHeader(req.headers.authorization)
}
const auth = {
// BUG: CWE-522: Insufficiently Protected Credentials
// required: jwt({ secret, userProperty: 'payload', getToken: getTokenFromRequest }),
// FIXED:
<FILL-HERE>
optional: jwt({
secret,
userProperty: 'payload',
credentialsRequired: false,
getToken: getTokenFromRequest
})
}
module.exports = auth
|
javascript | import React from 'react'
import { useRouter } from 'next/router'
import Maybe from 'front/Maybe'
import { webApi } from 'front/api'
import config from 'front/config'
import CustomLink from 'front/CustomLink'
import { cant } from 'front/cant'
import { formatDate } from 'front/date'
import UserLinkWithImage from 'front/UserLinkWithImage'
const Comment = ({ comment, comments, id, loggedInUser, setComments }) => {
const router = useRouter();
const {
query: { number: issueNumber, slug },
} = router;
const handleDelete = async (commentId) => {
await webApi.commentDelete((slug as string[]).join('/'), issueNumber, comment.number)
setComments(comments => comments.filter(comment => comment.id !== id))
};
const targetId = `comment${config.idSep}${comment.number}`
return (
<div className="comment" id={targetId}>
<div className="comment-header">
<CustomLink className="number" href={`#${targetId}`}>#{comment.number}</CustomLink>
{' by '}
<UserLinkWithImage user={comment.author} showUsernameMobile={false} />
{' on '}
{formatDate(comment.createdAt)}
{' '}
<Maybe test={!cant.deleteComment(loggedInUser, comment)}>
<button
className="btn"
onClick={() => handleDelete(comment.id)}
>
<i className="ion-trash-a" /> Delete Comment
</button>
</Maybe>
</div>
<div
className="comment-body ourbigbook"
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// dangerouslySetInnerHTML={{ __html: comment.render }}
// FIXED:
<FILL-HERE>
/>
</div>
);
};
export default Comment;
|
javascript | const baseURL = 'http://localhost:9000/api/words/'
export const getWords = () => {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// return fetch(baseURL)
// FIXED:
<FILL-HERE>
.then(res => res.json())
}
|
javascript | const express = require('express');
const rateLimit = require('express-rate-limit');
const helmet = require('helmet');
const bodyParser = require("body-parser");
const xss = require('xss-clean');
const hpp = require('hpp');
const cors = require('cors');
const multer = require('multer');
const upload = multer();
const session = require('express-session');
const { secret } = require('./config/common/config');
// Importing The Database
const postgresql = require('./config/database/postgresqlConfig');
// Importing the Routes
const covidRoutes = require('./routes/covid/covidRoutes')
// Calling the Error Handlers
const globalErrHandler = require('./controllers/error/errorController');
const AppError = require('./utils/error/appError');
const app = express();
// Allow Cross-Origin requests
app.use(cors());
// Set security HTTP headers
app.use(helmet());
// Limit request from the same API
const limiter = rateLimit({
max: 150,
windowMs: 60 * 60 * 1000,
message: 'Too Many Request from this IP, please try again in an hour'
});
app.use('/api/v1', limiter);
// Body parser, reading data from body into req.body
app.use(express.json({
limit: '100kb'
}));
// Configure the Session expiry
app.use(
// BUG: CWE-522: Insufficiently Protected Credentials
// session({ secret: secret, resave: true, saveUninitialized: false })
// FIXED:
<FILL-HERE>
);
// Data sanitization against XSS(clean user input from malicious HTML code)
app.use(xss());
// Prevent parameter pollution
app.use(hpp());
// Setting Body parser
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json({ type: 'application/*+json' }));
app.use(express.json());
app.use(upload.array());
app.use(express.static('public'));
// Setting up global error handler
app.use(globalErrHandler);
// Checking and Testing of Home
app.get('/', (req, res) => {
// console.log(req.session)
res.send(`You hit home page!\n`)
})
// Routes Final Calling
app.use('/api/v1',covidRoutes);
// handle undefined Routes
app.use('*', (req, res, next) => {
const err = new AppError(404, 'fail', 'undefined route');
next(err, req, res, next);
});
module.exports = app; |
javascript | const runCode = require('../lib/runCode')
const bodyParser = require('body-parser')
const express = require('express')
const router = express.Router()
router.use(bodyParser.json()) // for parsing routerlication/json
router.use(bodyParser.urlencoded({ extended: true }))
// GET handler for /
router.get('/', (req, res) => {
res.send('API requires POST to /code/<lang>')
})
// POST handler for /py
router.post('/py', async (req, res) => {
const { filePath } = req.body
const result = await runCode('py', filePath) // executes runCode() on specified .py and input files
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send(result)
// FIXED:
<FILL-HERE>
})
// POST handler for /cpp
router.post('/cpp', async (req, res) => {
const { filePath } = req.body
const result = await runCode('cpp', filePath) // executes runCode() on specified .cpp and input files
res.send(result)
})
// POST handler for /js
router.post('/js', async (req, res) => {
const { filePath } = req.body
const result = await runCode('js', filePath) // executes runCode() on specified .js and input files
res.send(result)
})
module.exports = router
|
javascript | const _setUpNavAttrs = (el, tag) => {
let hrf = (tag == 'SELECT') ? el.options[el.selectedIndex].getAttribute('data-page') : el.getAttribute('href');
if (hrf) {
let pageItem = _getPageFromList(hrf);
if (pageItem) {
let tmpDiv = document.createElement('div');
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// tmpDiv.insertAdjacentHTML('beforeend', '<a href="' + pageItem.url + '" ' + pageItem.attrs + '>');
// FIXED:
<FILL-HERE>
_cloneAttrs(el, tmpDiv.firstChild);
}
}
};
|
javascript | // Kludge in HTML5 tag recognition in IE8
document.createElement("section");
document.createElement("article");
(function() {
if (!window.addEventListener) return;
var pending = false, prevVal = null;
function updateSoon() {
if (!pending) {
pending = true;
setTimeout(update, 250);
}
}
function update() {
pending = false;
var marks = document.getElementById("nav").getElementsByTagName("a"), found;
for (var i = 0; i < marks.length; ++i) {
var mark = marks[i], m;
if (mark.getAttribute("data-default")) {
if (found == null) found = i;
} else if (m = mark.href.match(/#(.*)/)) {
var ref = document.getElementById(m[1]);
if (ref && ref.getBoundingClientRect().top < 50)
found = i;
}
}
if (found != null && found != prevVal) {
prevVal = found;
var lis = document.getElementById("nav").getElementsByTagName("li");
for (var i = 0; i < lis.length; ++i) lis[i].className = "";
for (var i = 0; i < marks.length; ++i) {
if (found == i) {
marks[i].className = "active";
for (var n = marks[i]; n; n = n.parentNode)
if (n.nodeName == "LI") n.className = "active";
} else {
marks[i].className = "";
}
}
}
}
window.addEventListener("scroll", updateSoon);
window.addEventListener("load", updateSoon);
window.addEventListener("hashchange", function() {
// BUG: CWE-95: Improper Neutralization of Directives in Dynamically Evaluated Code ('Eval Injection')
// setTimeout(function() { var hash = document.location.hash, found = null, m; var marks = document.getElementById("nav").getElementsByTagName("a"); for (var i = 0; i < marks.length; i++) if ((m = marks[i].href.match(/(#.*)/)) && m[1] == hash) { found = i; break; } if (found != null) for (var i = 0; i < marks.length; i++) marks[i].className = i == found ? "active" : ""; }, 300);
// FIXED:
<FILL-HERE>
});
})();
|
javascript | // META: global=window,worker
for (const method of ["compileStreaming", "instantiateStreaming"]) {
promise_test(t => {
const url = "http://{{domains[www]}}:{{ports[http][0]}}/wasm/incrementer.wasm";
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// const response = fetch(url, { "mode": "no-cors" });
// FIXED:
<FILL-HERE>
return promise_rejects_js(t, TypeError, WebAssembly[method](response));
}, `Opaque response: ${method}`);
promise_test(t => {
const url = "/fetch/api/resources/redirect.py?redirect_status=301&location=/wasm/incrementer.wasm";
const response = fetch(url, { "mode": "no-cors", "redirect": "manual" });
return promise_rejects_js(t, TypeError, WebAssembly[method](response));
}, `Opaque redirect response: ${method}`);
}
|
javascript | var express = require('express');
var fs = require('fs');
var io = require('socket.io');
var _ = require('underscore');
var Mustache = require('mustache');
var app = express.createServer();
var staticDir = express.static;
io = io.listen(app);
var opts = {
port : 1947,
baseDir : __dirname + '/../../'
};
io.sockets.on('connection', function(socket) {
socket.on('slidechanged', function(slideData) {
socket.broadcast.emit('slidedata', slideData);
});
socket.on('fragmentchanged', function(fragmentData) {
socket.broadcast.emit('fragmentdata', fragmentData);
});
});
app.configure(function() {
[ 'css', 'js', 'images', 'plugin', 'lib' ].forEach(function(dir) {
app.use('/' + dir, staticDir(opts.baseDir + dir));
});
});
app.get("/", function(req, res) {
res.writeHead(200, {'Content-Type': 'text/html'});
fs.createReadStream(opts.baseDir + '/index.html').pipe(res);
});
app.get("/notes/:socketId", function(req, res) {
fs.readFile(opts.baseDir + 'plugin/notes-server/notes.html', function(err, data) {
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send(Mustache.to_html(data.toString(), { socketId : req.params.socketId }));
// FIXED:
<FILL-HERE>
});
// fs.createReadStream(opts.baseDir + 'notes-server/notes.html').pipe(res);
});
// Actually listen
app.listen(opts.port || null);
var brown = '\033[33m',
green = '\033[32m',
reset = '\033[0m';
var slidesLocation = "http://localhost" + ( opts.port ? ( ':' + opts.port ) : '' );
console.log( brown + "reveal.js - Speaker Notes" + reset );
console.log( "1. Open the slides at " + green + slidesLocation + reset );
console.log( "2. Click on the link your JS console to go to the notes page" );
console.log( "3. Advance through your slides and your notes will advance automatically" );
|
javascript | /*
USERNAME HASH PROBLEM
This is an app using expressJS framework to serve "The Username Hash Service". The app provides 2 APIs:
GET /?name=<UserName>
Returns the <UserName> and the hashed value from <UserName>
GET /history
Returns request history. Each <UserName> is in one line.
The Hash Task is consider CPU consuming. You should wait for a long time to get result (may upto 24 seconds).
Now, let's get started:
- Run the app. Open the web browser and visit http://localhost:8081/?name=JohnnyTeo
- While waiting for result, try visiting http://localhost:8081/history
Notice that /history is blocked until completion of hash result calculation.
The reason is Javascript by default runs in a single thread.
This thread is busy calculating hash result from request /?name=JohnnyTeo
so it cannot serve the next request /history
Your task is making /history "non-blocking" i.e. app can serve /history while doing hashing job.
P/S: The problem idea is inspired by a great article at:
https://www.digitalocean.com/community/tutorials/how-to-use-multithreading-in-node-js
*/
import * as mylib from './mylib.js';
import express from 'express';
import { getHash, splitStrInToChunks } from './exerex-userhash-util.js';
const PORT = 8081;
const app = express();
const getSuperHash = plainText => {
const numChunks = 8;
const lstChunks = splitStrInToChunks(numChunks, plainText);
const lstHashes = lstChunks.map(chunk => getHash(2**21, chunk));
const finalHash = getHash(1, lstHashes.join(''));
return finalHash;
};
const userNameHistory = [];
app.get('/history', async (req, res) => {
const html = userNameHistory.join('<br/>') || '<Empty history>';
res.status(200).send(html).end();
});
app.get('/', (req, res) => {
const userName = req.query.name;
if (!userName) {
res.status(400).end();
return;
}
userNameHistory.push(userName);
const tpStart = process.hrtime();
// GET USERNAME HASH
const userNameHash = getSuperHash(userName);
const timeElapsed = mylib.hrtimeToNumber(process.hrtime(tpStart));
console.log(`userName = ${userName}; Time elapsed = ${timeElapsed}`);
const html = userName + '<br/>' + userNameHash;
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.status(200).setHeader('Content-Type', 'text/html').send(html).end();
// FIXED:
<FILL-HERE>
});
console.log('Server is listening on port', PORT);
const server = app.listen(PORT);
|
javascript | const bodyParser = require('body-parser');
const path = require('path');
const session = require('express-session');
const morgan = require('morgan');
const config = require('../../webpack.config.js');
const webpack = require('webpack');
const webpackDevMiddleware = require('webpack-dev-middleware');
const webpackHotMiddleware = require('webpack-hot-middleware');
const compiler = webpack(config);
module.exports = (app, express) => {
app.use(webpackDevMiddleware(compiler, { noInfo: true, publicPath: config.output.publicPath }));
app.use(webpackHotMiddleware(compiler));
app.use(morgan('dev'));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// BUG: CWE-522: Insufficiently Protected Credentials
// app.use(session({ secret: 'fred', resave: false, saveUninitialized: false }));
// FIXED:
<FILL-HERE>
app.use(express.static(path.join(`${__dirname}./../../dist`)));
};
|
javascript | function getAllEmployees() {
console.log("Fetch all employees");
//returns a pending promise
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// fetch("http://localhost:3000/employees")
// FIXED:
<FILL-HERE>
.then((response) => {
if (response.status === 200) {
return response.json();
}
})
.then((employees) => {
//fill the table with the data
let tbody = document
.getElementById("employeeList")
.getElementsByTagName("tbody")[0];
let tbodyhtml = "";
employees.forEach((employee) => {
tbodyhtml += `<tr><td>${employee.id}</td>
<td>${employee.empName}</td>
<td>${employee.email}</td>
<td><button id="delete" onclick="deleteEmployee(${employee.id})">Delete</button></td>
<td><button id="edit" onclick="getEmployeeById(${employee.id})">Edit</button></td>`;
});
tbody.innerHTML = tbodyhtml;
})
.catch((error) => {
console.log(error);
});
}
getAllEmployees();
function addEmployee() {
let name = document.getElementById("name").value;
let email = document.getElementById("email").value;
let newEmp = {
empName: name,
email: email,
};
//post
fetch("http://localhost:3000/employees", {
method: "POST",
headers: {
"Content-type": "application/json",
},
body: JSON.stringify(newEmp),
})
.then((response) => {
//201 for post
if (response.status === 201) {
console.log("data added");
getAllEmployees();
}
})
.catch((error) => {
console.log(error);
});
}
function getEmployeeById(id) {
fetch(`http://localhost:3000/employees/${id}`)
.then((response) => {
if (response.status === 200) {
return response.json();
}
})
.then((employee) => {
document.getElementById("empId").value = employee.id;
document.getElementById("empName").value = employee.empName;
document.getElementById("empEmail").value = employee.email;
})
.catch((error) => {
console.log(error);
});
}
function editEmployee() {
let empId = document.getElementById("empId").value;
let empName = document.getElementById("empName").value;
let email = document.getElementById("empEmail").value;
fetch(`http://localhost:3000/employees/${empId}`, {
method: "PUT",
body: JSON.stringify({
empName: empName,
email: email,
}),
headers: {
"Content-type": "application/json",
},
})
.then((response) => {
if (response === 200) {
getAllEmployees();
}
})
.then((employee) => {
document.getElementById("empId").value = employee.id;
document.getElementById("empName").value = employee.empName;
document.getElementById("empEmail").value = employee.email;
})
.catch((error) => {
console.log(error);
});
}
//Delete functionality to be implemented
|
javascript | var ctx = require('./_ctx');
var invoke = require('./_invoke');
var html = require('./_html');
var cel = require('./_dom-create');
var global = require('./_global');
var process = global.process;
var setTask = global.setImmediate;
var clearTask = global.clearImmediate;
var MessageChannel = global.MessageChannel;
var Dispatch = global.Dispatch;
var counter = 0;
var queue = {};
var ONREADYSTATECHANGE = 'onreadystatechange';
var defer, channel, port;
var run = function () {
var id = +this;
// eslint-disable-next-line no-prototype-builtins
if (queue.hasOwnProperty(id)) {
var fn = queue[id];
delete queue[id];
fn();
}
};
var listener = function (event) {
run.call(event.data);
};
// Node.js 0.9+ & IE10+ has setImmediate, otherwise:
if (!setTask || !clearTask) {
setTask = function setImmediate(fn) {
var args = [];
var i = 1;
while (arguments.length > i) args.push(arguments[i++]);
queue[++counter] = function () {
// eslint-disable-next-line no-new-func
invoke(typeof fn == 'function' ? fn : Function(fn), args);
};
defer(counter);
return counter;
};
clearTask = function clearImmediate(id) {
delete queue[id];
};
// Node.js 0.8-
if (require('./_cof')(process) == 'process') {
defer = function (id) {
process.nextTick(ctx(run, id, 1));
};
// Sphere (JS game engine) Dispatch API
} else if (Dispatch && Dispatch.now) {
defer = function (id) {
Dispatch.now(ctx(run, id, 1));
};
// Browsers with MessageChannel, includes WebWorkers
} else if (MessageChannel) {
channel = new MessageChannel();
port = channel.port2;
channel.port1.onmessage = listener;
defer = ctx(port.postMessage, port, 1);
// Browsers with postMessage, skip WebWorkers
// IE8 has postMessage, but it's sync & typeof its postMessage is 'object'
} else if (global.addEventListener && typeof postMessage == 'function' && !global.importScripts) {
defer = function (id) {
// BUG: CWE-345: Insufficient Verification of Data Authenticity
// global.postMessage(id + '', '*');
// FIXED:
<FILL-HERE>
};
global.addEventListener('message', listener, false);
// IE8-
} else if (ONREADYSTATECHANGE in cel('script')) {
defer = function (id) {
html.appendChild(cel('script'))[ONREADYSTATECHANGE] = function () {
html.removeChild(this);
run.call(id);
};
};
// Rest old browsers
} else {
defer = function (id) {
setTimeout(ctx(run, id, 1), 0);
};
}
}
module.exports = {
set: setTask,
clear: clearTask
};
|
javascript | window.SearcherDisplay = (function($) {
/**
* This class provides support for displaying quick search text results to users.
*/
function SearcherDisplay() { }
SearcherDisplay.prototype.init = function() {
this._displayQuickSearch();
};
/**
* This method creates the quick text search entry in navigation menu and wires all required events.
*/
SearcherDisplay.prototype._displayQuickSearch = function() {
var quickSearch = $(document.createElement("iframe")),
body = $("body"),
self = this;
quickSearch.attr("src", "quicksearch.html");
quickSearch.css("width", "0px");
quickSearch.css("height", "0px");
body.append(quickSearch);
$(window).on("message", function(msg) {
var msgData = msg.originalEvent.data;
if (msgData.msgid != "docstrap.quicksearch.done") {
return;
}
var results = msgData.results || [];
self._displaySearchResults(results);
});
function startSearch() {
var searchTerms = $('#search-input').prop("value");
if (searchTerms) {
// BUG: CWE-345: Insufficient Verification of Data Authenticity
// quickSearch[0].contentWindow.postMessage({ "searchTerms": searchTerms, "msgid": "docstrap.quicksearch.start" }, "*");
// FIXED:
<FILL-HERE>
}
}
$('#search-input').on('keyup', function(evt) {
if (evt.keyCode != 13) {
return;
}
startSearch();
return false;
});
$('#search-submit').on('click', function() {
startSearch();
return false;
});
};
/**
* This method displays the quick text search results in a modal dialog.
*/
SearcherDisplay.prototype._displaySearchResults = function(results) {
var resultsHolder = $($("#searchResults").find(".modal-body")),
fragment = document.createDocumentFragment(),
resultsList = document.createElement("ul");
resultsHolder.empty();
for (var idx = 0; idx < results.length; idx++) {
var result = results[idx],
item = document.createElement("li"),
link = document.createElement("a");
link.href = result.id;
link.innerHTML = result.title;
item.appendChild(link)
resultsList.appendChild(item);
}
fragment.appendChild(resultsList);
resultsHolder.append(fragment);
$("#searchResults").modal({"show": true});
};
return new SearcherDisplay();
})($);
|
javascript | /*
DEADLOCK
Version EX
*/
import * as mylib from './mylib.js';
import express from 'express';
import { Mutex } from 'async-mutex';
const createServer = () => {
const app = express();
const mutex = new Mutex();
const doTask = async name => {
const release = await mutex.acquire();
try {
console.log(`Server: ${name} acquired resource`);
} finally {
// release(); // Forget this statement ==> deadlock
}
};
app.get('/', async (req, res) => {
const name = req.query.name;
await doTask(name);
res.status(200).setHeader('Content-Type', 'text/html');
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send(name).end();
// FIXED:
<FILL-HERE>
});
const server = app.listen(8081);
return server;
};
const runClient = async () => {
const respFoo = await mylib.makeHttpGet(new URL('http://localhost:8081/?name=foo'));
console.log(`Client: response: ${respFoo}`);
const respBar = await mylib.makeHttpGet(new URL('http://localhost:8081/?name=bar'));
console.log('You will never see this statement due to deadlock!');
console.log(`Client: response: ${respBar}`);
};
const server = createServer();
await runClient();
server.close();
|
javascript | const bodyParser = require('body-parser');
const path = require('path');
const session = require('express-session');
const morgan = require('morgan');
const config = require('../../webpack.config.js');
const webpack = require('webpack');
const webpackDevMiddleware = require('webpack-dev-middleware');
const webpackHotMiddleware = require('webpack-hot-middleware');
const compiler = webpack(config);
module.exports = (app, express) => {
app.use(webpackDevMiddleware(compiler, { noInfo: true, publicPath: config.output.publicPath }));
app.use(webpackHotMiddleware(compiler));
app.use(morgan('dev'));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// BUG: CWE-522: Insufficiently Protected Credentials
// app.use(session({ secret: 'fred', resave: false, saveUninitialized: false }));
// FIXED:
<FILL-HERE>
app.use(express.static(path.join(`${__dirname}./../../dist`)));
};
|
javascript | function getAllEmployees() {
console.log("Fetch all employees");
//returns a pending promise
fetch("http://localhost:3000/employees")
.then((response) => {
if (response.status === 200) {
return response.json();
}
})
.then((employees) => {
//fill the table with the data
let tbody = document
.getElementById("employeeList")
.getElementsByTagName("tbody")[0];
let tbodyhtml = "";
employees.forEach((employee) => {
tbodyhtml += `<tr><td>${employee.id}</td>
<td>${employee.empName}</td>
<td>${employee.email}</td>
<td><button id="delete" onclick="deleteEmployee(${employee.id})">Delete</button></td>
<td><button id="edit" onclick="getEmployeeById(${employee.id})">Edit</button></td>`;
});
tbody.innerHTML = tbodyhtml;
})
.catch((error) => {
console.log(error);
});
}
getAllEmployees();
function addEmployee() {
let name = document.getElementById("name").value;
let email = document.getElementById("email").value;
let newEmp = {
empName: name,
email: email,
};
//post
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// fetch("http://localhost:3000/employees", { method: "POST", headers: { "Content-type": "application/json", }, body: JSON.stringify(newEmp), })
// FIXED:
<FILL-HERE>
.then((response) => {
//201 for post
if (response.status === 201) {
console.log("data added");
getAllEmployees();
}
})
.catch((error) => {
console.log(error);
});
}
function getEmployeeById(id) {
fetch(`http://localhost:3000/employees/${id}`)
.then((response) => {
if (response.status === 200) {
return response.json();
}
})
.then((employee) => {
document.getElementById("empId").value = employee.id;
document.getElementById("empName").value = employee.empName;
document.getElementById("empEmail").value = employee.email;
})
.catch((error) => {
console.log(error);
});
}
function editEmployee() {
let empId = document.getElementById("empId").value;
let empName = document.getElementById("empName").value;
let email = document.getElementById("empEmail").value;
fetch(`http://localhost:3000/employees/${empId}`, {
method: "PUT",
body: JSON.stringify({
empName: empName,
email: email,
}),
headers: {
"Content-type": "application/json",
},
})
.then((response) => {
if (response === 200) {
getAllEmployees();
}
})
.then((employee) => {
document.getElementById("empId").value = employee.id;
document.getElementById("empName").value = employee.empName;
document.getElementById("empEmail").value = employee.email;
})
.catch((error) => {
console.log(error);
});
}
//Delete functionality to be implemented
|
javascript | var ctx = require('./_ctx');
var invoke = require('./_invoke');
var html = require('./_html');
var cel = require('./_dom-create');
var global = require('./_global');
var process = global.process;
var setTask = global.setImmediate;
var clearTask = global.clearImmediate;
var MessageChannel = global.MessageChannel;
var Dispatch = global.Dispatch;
var counter = 0;
var queue = {};
var ONREADYSTATECHANGE = 'onreadystatechange';
var defer, channel, port;
var run = function () {
var id = +this;
// eslint-disable-next-line no-prototype-builtins
if (queue.hasOwnProperty(id)) {
var fn = queue[id];
delete queue[id];
fn();
}
};
var listener = function (event) {
run.call(event.data);
};
// Node.js 0.9+ & IE10+ has setImmediate, otherwise:
if (!setTask || !clearTask) {
setTask = function setImmediate(fn) {
var args = [];
var i = 1;
while (arguments.length > i) args.push(arguments[i++]);
queue[++counter] = function () {
// eslint-disable-next-line no-new-func
invoke(typeof fn == 'function' ? fn : Function(fn), args);
};
defer(counter);
return counter;
};
clearTask = function clearImmediate(id) {
delete queue[id];
};
// Node.js 0.8-
if (require('./_cof')(process) == 'process') {
defer = function (id) {
process.nextTick(ctx(run, id, 1));
};
// Sphere (JS game engine) Dispatch API
} else if (Dispatch && Dispatch.now) {
defer = function (id) {
Dispatch.now(ctx(run, id, 1));
};
// Browsers with MessageChannel, includes WebWorkers
} else if (MessageChannel) {
channel = new MessageChannel();
port = channel.port2;
channel.port1.onmessage = listener;
defer = ctx(port.postMessage, port, 1);
// Browsers with postMessage, skip WebWorkers
// IE8 has postMessage, but it's sync & typeof its postMessage is 'object'
} else if (global.addEventListener && typeof postMessage == 'function' && !global.importScripts) {
defer = function (id) {
// BUG: CWE-345: Insufficient Verification of Data Authenticity
// global.postMessage(id + '', '*');
// FIXED:
<FILL-HERE>
};
global.addEventListener('message', listener, false);
// IE8-
} else if (ONREADYSTATECHANGE in cel('script')) {
defer = function (id) {
html.appendChild(cel('script'))[ONREADYSTATECHANGE] = function () {
html.removeChild(this);
run.call(id);
};
};
// Rest old browsers
} else {
defer = function (id) {
setTimeout(ctx(run, id, 1), 0);
};
}
}
module.exports = {
set: setTask,
clear: clearTask
};
|
javascript | // Kludge in HTML5 tag recognition in IE8
document.createElement("section");
document.createElement("article");
(function() {
if (!window.addEventListener) return;
var pending = false, prevVal = null;
function updateSoon() {
if (!pending) {
pending = true;
setTimeout(update, 250);
}
}
function update() {
pending = false;
var marks = document.getElementById("nav").getElementsByTagName("a"), found;
for (var i = 0; i < marks.length; ++i) {
var mark = marks[i], m;
if (mark.getAttribute("data-default")) {
if (found == null) found = i;
} else if (m = mark.href.match(/#(.*)/)) {
var ref = document.getElementById(m[1]);
if (ref && ref.getBoundingClientRect().top < 50)
found = i;
}
}
if (found != null && found != prevVal) {
prevVal = found;
var lis = document.getElementById("nav").getElementsByTagName("li");
for (var i = 0; i < lis.length; ++i) lis[i].className = "";
for (var i = 0; i < marks.length; ++i) {
if (found == i) {
marks[i].className = "active";
for (var n = marks[i]; n; n = n.parentNode)
if (n.nodeName == "LI") n.className = "active";
} else {
marks[i].className = "";
}
}
}
}
window.addEventListener("scroll", updateSoon);
window.addEventListener("load", updateSoon);
window.addEventListener("hashchange", function() {
// BUG: CWE-95: Improper Neutralization of Directives in Dynamically Evaluated Code ('Eval Injection')
// setTimeout(function() { var hash = document.location.hash, found = null, m; var marks = document.getElementById("nav").getElementsByTagName("a"); for (var i = 0; i < marks.length; i++) if ((m = marks[i].href.match(/(#.*)/)) && m[1] == hash) { found = i; break; } if (found != null) for (var i = 0; i < marks.length; i++) marks[i].className = i == found ? "active" : ""; }, 300);
// FIXED:
<FILL-HERE>
});
})();
|
javascript | var express = require('express');
var fs = require('fs');
var io = require('socket.io');
var _ = require('underscore');
var Mustache = require('mustache');
var app = express.createServer();
var staticDir = express.static;
io = io.listen(app);
var opts = {
port : 1947,
baseDir : __dirname + '/../../'
};
io.sockets.on('connection', function(socket) {
socket.on('slidechanged', function(slideData) {
socket.broadcast.emit('slidedata', slideData);
});
socket.on('fragmentchanged', function(fragmentData) {
socket.broadcast.emit('fragmentdata', fragmentData);
});
});
app.configure(function() {
[ 'css', 'js', 'images', 'plugin', 'lib' ].forEach(function(dir) {
app.use('/' + dir, staticDir(opts.baseDir + dir));
});
});
app.get("/", function(req, res) {
res.writeHead(200, {'Content-Type': 'text/html'});
fs.createReadStream(opts.baseDir + '/index.html').pipe(res);
});
app.get("/notes/:socketId", function(req, res) {
fs.readFile(opts.baseDir + 'plugin/notes-server/notes.html', function(err, data) {
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send(Mustache.to_html(data.toString(), { socketId : req.params.socketId }));
// FIXED:
<FILL-HERE>
});
// fs.createReadStream(opts.baseDir + 'notes-server/notes.html').pipe(res);
});
// Actually listen
app.listen(opts.port || null);
var brown = '\033[33m',
green = '\033[32m',
reset = '\033[0m';
var slidesLocation = "http://localhost" + ( opts.port ? ( ':' + opts.port ) : '' );
console.log( brown + "reveal.js - Speaker Notes" + reset );
console.log( "1. Open the slides at " + green + slidesLocation + reset );
console.log( "2. Click on the link your JS console to go to the notes page" );
console.log( "3. Advance through your slides and your notes will advance automatically" );
|
javascript | /*
USERNAME HASH PROBLEM
Each time users send requests to hash, threads are created.
Constantly taking requests and creating new threads is a matter of concern.
By using Execution Service/Thread Pool, threads can be reused for next tasks/next requests
(i.e. no more thread creation).
*/
import * as mylib from './mylib.js';
import { isMainThread } from 'worker_threads';
import { Piscina } from 'piscina';
import express from 'express';
import { getHash, splitStrInToChunks } from './exerex-userhash-util.js';
//---------------------------------------------
// WORKER THREAD SECTION
//---------------------------------------------
export const workerFunc = ({idx, chunk}) => {
try {
const chunkHash = getHash(2**21, chunk);
return chunkHash;
} catch (error) {
console.error(error);
}
};
//---------------------------------------------
// MAIN THREAD SECTION
//---------------------------------------------
const getSuperHashByMain = async (execService, plainText) => {
const numChunks = 8; // It is also the number of threads
const lstChunks = splitStrInToChunks(numChunks, plainText);
const lstWorkerProm = [];
for (let i = 0; i < numChunks; ++i) {
const chunk = lstChunks[i];
lstWorkerProm.push(execService.run({ idx: i, chunk: chunk }, { name: 'workerFunc' }));
}
const lstHashes = await Promise.all(lstWorkerProm);
const finalHash = getHash(1, lstHashes.join(''));
return finalHash;
};
const mainFunc = () => {
const PORT = 8081;
const app = express();
const userNameHistory = [];
const execService = new Piscina({
filename: new URL(import.meta.url).href,
minThreads: 8,
maxThreads: 8
});
app.get('/history', async (req, res) => {
const html = userNameHistory.join('<br/>') || '<Empty history>';
res.status(200).send(html).end();
});
app.get('/', async (req, res) => {
const userName = req.query.name;
if (!userName) {
res.status(400).end();
return;
}
userNameHistory.push(userName);
const tpStart = process.hrtime();
// GET USERNAME HASH
const userNameHash = await getSuperHashByMain(execService, userName);
const timeElapsed = mylib.hrtimeToNumber(process.hrtime(tpStart));
console.log(`userName = ${userName}; Time elapsed = ${timeElapsed}`);
const html = userName + '<br/>' + userNameHash;
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.status(200).setHeader('Content-Type', 'text/html').send(html).end();
// FIXED:
<FILL-HERE>
});
console.log('Server is listening on port', PORT);
const server = app.listen(PORT);
};
if (isMainThread) {
try {
mainFunc();
} catch (error) {
console.error(error);
}
}
|
javascript | /**
* Handles opening of and synchronization with the reveal.js
* notes window.
*/
var RevealNotes = (function() {
function openNotes() {
var jsFileLocation = document.querySelector('script[src$="notes.js"]').src; // this js file path
jsFileLocation = jsFileLocation.replace(/notes\.js(\?.*)?$/, ''); // the js folder path
var notesPopup = window.open( jsFileLocation + 'notes.html', 'reveal.js - Notes', 'width=1120,height=850' );
// Fires when slide is changed
Reveal.addEventListener( 'slidechanged', post );
// Fires when a fragment is shown
Reveal.addEventListener( 'fragmentshown', post );
// Fires when a fragment is hidden
Reveal.addEventListener( 'fragmenthidden', post );
/**
* Posts the current slide data to the notes window
*/
function post() {
var slideElement = Reveal.getCurrentSlide(),
slideIndices = Reveal.getIndices(),
messageData;
var notes = slideElement.querySelector( 'aside.notes' ),
nextindexh,
nextindexv;
if( slideElement.nextElementSibling && slideElement.parentNode.nodeName == 'SECTION' ) {
nextindexh = slideIndices.h;
nextindexv = slideIndices.v + 1;
} else {
nextindexh = slideIndices.h + 1;
nextindexv = 0;
}
messageData = {
notes : notes ? notes.innerHTML : '',
indexh : slideIndices.h,
indexv : slideIndices.v,
indexf : slideIndices.f,
nextindexh : nextindexh,
nextindexv : nextindexv,
markdown : notes ? typeof notes.getAttribute( 'data-markdown' ) === 'string' : false
};
// BUG: CWE-345: Insufficient Verification of Data Authenticity
// notesPopup.postMessage( JSON.stringify( messageData ), '*' );
// FIXED:
<FILL-HERE>
}
// Navigate to the current slide when the notes are loaded
notesPopup.addEventListener( 'load', function( event ) {
post();
}, false );
}
// If the there's a 'notes' query set, open directly
if( window.location.search.match( /(\?|\&)notes/gi ) !== null ) {
openNotes();
}
// Open the notes when the 's' key is hit
document.addEventListener( 'keydown', function( event ) {
// Disregard the event if the target is editable or a
// modifier is present
if ( document.querySelector( ':focus' ) !== null || event.shiftKey || event.altKey || event.ctrlKey || event.metaKey ) return;
if( event.keyCode === 83 ) {
event.preventDefault();
openNotes();
}
}, false );
return { open: openNotes };
})();
|
javascript | const bodyParser = require('body-parser');
const path = require('path');
const session = require('express-session');
const morgan = require('morgan');
const config = require('../../webpack.config.js');
const webpack = require('webpack');
const webpackDevMiddleware = require('webpack-dev-middleware');
const webpackHotMiddleware = require('webpack-hot-middleware');
const compiler = webpack(config);
module.exports = (app, express) => {
app.use(webpackDevMiddleware(compiler, { noInfo: true, publicPath: config.output.publicPath }));
app.use(webpackHotMiddleware(compiler));
app.use(morgan('dev'));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// BUG: CWE-522: Insufficiently Protected Credentials
// app.use(session({ secret: 'fred', resave: false, saveUninitialized: false }));
// FIXED:
<FILL-HERE>
app.use(express.static(path.join(`${__dirname}./../../dist`)));
};
|
javascript | /*
USERNAME HASH PROBLEM
Although the problem is solved in previous solution, the calculation speed keeps too slow.
Analyze the Hash Task and you realize that
this task can be divided into multiple individual sub tasks.
Each sub task is corresponding to hashing one chunk.
Hence, multithreading makes sense: Each thread does each sub task in parallel.
*/
import * as mylib from './mylib.js';
import { isMainThread, workerData, parentPort } from 'worker_threads';
import express from 'express';
import { getHash, splitStrInToChunks } from './exerex-userhash-util.js';
//---------------------------------------------
// WORKER THREAD SECTION
//---------------------------------------------
export const workerFunc = () => {
try {
const [idx, chunk] = workerData;
const chunkHash = getHash(2**21, chunk);
parentPort.postMessage([idx, chunkHash]);
} catch (error) {
console.error(error);
}
};
//---------------------------------------------
// MAIN THREAD SECTION
//---------------------------------------------
const getSuperHashByMain = async (plainText) => {
const numChunks = 8; // It is also the number of threads
const lstChunks = splitStrInToChunks(numChunks, plainText);
const lstHashes = new Array(numChunks);
const lstWorkerProm = [];
for (let i = 0; i < numChunks; ++i) {
const chunk = lstChunks[i];
const [worker, prom] = mylib.createThread(new URL(import.meta.url), [i, chunk]);
worker.on('message', ([idx, chunkHash]) => lstHashes[idx] = chunkHash);
lstWorkerProm.push(prom);
}
await Promise.all(lstWorkerProm);
const finalHash = getHash(1, lstHashes.join(''));
return finalHash;
};
const mainFunc = () => {
const PORT = 8081;
const app = express();
const userNameHistory = [];
app.get('/history', async (req, res) => {
const html = userNameHistory.join('<br/>') || '<Empty history>';
res.status(200).send(html).end();
});
app.get('/', async (req, res) => {
const userName = req.query.name;
if (!userName) {
res.status(400).end();
return;
}
userNameHistory.push(userName);
const tpStart = process.hrtime();
// GET USERNAME HASH
const userNameHash = await getSuperHashByMain(userName);
const timeElapsed = mylib.hrtimeToNumber(process.hrtime(tpStart));
console.log(`userName = ${userName}; Time elapsed = ${timeElapsed}`);
const html = userName + '<br/>' + userNameHash;
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.status(200).setHeader('Content-Type', 'text/html').send(html).end();
// FIXED:
<FILL-HERE>
});
console.log('Server is listening on port', PORT);
const server = app.listen(PORT);
};
if (isMainThread) {
try {
mainFunc();
} catch (error) {
console.error(error);
}
} else {
workerFunc();
}
|
javascript | const bodyParser = require('body-parser');
const path = require('path');
const session = require('express-session');
const morgan = require('morgan');
const config = require('../../webpack.config.js');
const webpack = require('webpack');
const webpackDevMiddleware = require('webpack-dev-middleware');
const webpackHotMiddleware = require('webpack-hot-middleware');
const compiler = webpack(config);
module.exports = (app, express) => {
app.use(webpackDevMiddleware(compiler, { noInfo: true, publicPath: config.output.publicPath }));
app.use(webpackHotMiddleware(compiler));
app.use(morgan('dev'));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// BUG: CWE-522: Insufficiently Protected Credentials
// app.use(session({ secret: 'fred', resave: false, saveUninitialized: false }));
// FIXED:
<FILL-HERE>
app.use(express.static(path.join(`${__dirname}./../../dist`)));
};
|
javascript | // Kludge in HTML5 tag recognition in IE8
document.createElement("section");
document.createElement("article");
(function() {
if (!window.addEventListener) return;
var pending = false, prevVal = null;
function updateSoon() {
if (!pending) {
pending = true;
setTimeout(update, 250);
}
}
function update() {
pending = false;
var marks = document.getElementById("nav").getElementsByTagName("a"), found;
for (var i = 0; i < marks.length; ++i) {
var mark = marks[i], m;
if (mark.getAttribute("data-default")) {
if (found == null) found = i;
} else if (m = mark.href.match(/#(.*)/)) {
var ref = document.getElementById(m[1]);
if (ref && ref.getBoundingClientRect().top < 50)
found = i;
}
}
if (found != null && found != prevVal) {
prevVal = found;
var lis = document.getElementById("nav").getElementsByTagName("li");
for (var i = 0; i < lis.length; ++i) lis[i].className = "";
for (var i = 0; i < marks.length; ++i) {
if (found == i) {
marks[i].className = "active";
for (var n = marks[i]; n; n = n.parentNode)
if (n.nodeName == "LI") n.className = "active";
} else {
marks[i].className = "";
}
}
}
}
window.addEventListener("scroll", updateSoon);
window.addEventListener("load", updateSoon);
window.addEventListener("hashchange", function() {
// BUG: CWE-95: Improper Neutralization of Directives in Dynamically Evaluated Code ('Eval Injection')
// setTimeout(function() { var hash = document.location.hash, found = null, m; var marks = document.getElementById("nav").getElementsByTagName("a"); for (var i = 0; i < marks.length; i++) if ((m = marks[i].href.match(/(#.*)/)) && m[1] == hash) { found = i; break; } if (found != null) for (var i = 0; i < marks.length; i++) marks[i].className = i == found ? "active" : ""; }, 300);
// FIXED:
<FILL-HERE>
});
})();
|
javascript | // Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license.
import { Button } from '@fluentui/react-components';
import { Session } from './controller';
import { galleryItems } from './galleryItems';
import { Open24Regular } from '@fluentui/react-icons';
export function Gallery() {
return (
<div
style={{
marginTop: 20,
marginLeft: 30,
}}
>
<div
style={{
marginTop: 20,
marginBottom: 10,
fontWeight: 600,
fontSize: 16,
fontFamily:
'var(--fluent-font-family,"Segoe UI","Segoe UI Web (West European)",-apple-system,BlinkMacSystemFont,Roboto,"Helvetica Neue",sans-serif)',
}}
>
Gallery
</div>
{galleryItems.map((g, i) => (
<div key={i} className="galleryItem">
<div
style={{
width: 570,
height: 100,
padding: 10,
margin: 4,
marginBottom: 30,
border: '1px solid #dddddd',
borderRadius: 4,
background: '#fafafa',
}}
dangerouslySetInnerHTML={{ __html: g.nl }}
></div>
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// <div dangerouslySetInnerHTML={{ __html: g.img }} />
// FIXED:
<FILL-HERE>
<div>
<Button
icon={<Open24Regular />}
style={{
marginLeft: 10,
marginBottom: 10,
}}
onClick={() => {
const url = window.location.origin + window.location.pathname + '#create';
const editor = window.open(url);
if (!editor)
return;
const wait = 10_000;
const step = 250;
const { origin } = new URL(url);
// eslint-disable-next-line no-bitwise
let count = ~~(wait / step);
function listen(evt: MessageEvent) {
if (evt.source === editor) {
count = 0;
window.removeEventListener('message', listen, false);
}
}
window.addEventListener('message', listen, false);
// send message
// periodically resend until ack received or timeout
function send() {
if (!editor || count <= 0) {
return;
}
const session: Session = {
source: 'vistalk',
data: g.data,
input: g.input,
};
editor.postMessage(session, origin);
setTimeout(send, step);
count -= 1;
}
setTimeout(send, step);
}}
>
Open
</Button>
</div>
</div>
))}
</div>
);
}
|
javascript | const bodyParser = require('body-parser');
const path = require('path');
const session = require('express-session');
const morgan = require('morgan');
const config = require('../../webpack.config.js');
const webpack = require('webpack');
const webpackDevMiddleware = require('webpack-dev-middleware');
const webpackHotMiddleware = require('webpack-hot-middleware');
const compiler = webpack(config);
module.exports = (app, express) => {
app.use(webpackDevMiddleware(compiler, { noInfo: true, publicPath: config.output.publicPath }));
app.use(webpackHotMiddleware(compiler));
app.use(morgan('dev'));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// BUG: CWE-522: Insufficiently Protected Credentials
// app.use(session({ secret: 'fred', resave: false, saveUninitialized: false }));
// FIXED:
<FILL-HERE>
app.use(express.static(path.join(`${__dirname}./../../dist`)));
};
|
javascript | // Kludge in HTML5 tag recognition in IE8
document.createElement("section");
document.createElement("article");
(function() {
if (!window.addEventListener) return;
var pending = false, prevVal = null;
function updateSoon() {
if (!pending) {
pending = true;
setTimeout(update, 250);
}
}
function update() {
pending = false;
var marks = document.getElementById("nav").getElementsByTagName("a"), found;
for (var i = 0; i < marks.length; ++i) {
var mark = marks[i], m;
if (mark.getAttribute("data-default")) {
if (found == null) found = i;
} else if (m = mark.href.match(/#(.*)/)) {
var ref = document.getElementById(m[1]);
if (ref && ref.getBoundingClientRect().top < 50)
found = i;
}
}
if (found != null && found != prevVal) {
prevVal = found;
var lis = document.getElementById("nav").getElementsByTagName("li");
for (var i = 0; i < lis.length; ++i) lis[i].className = "";
for (var i = 0; i < marks.length; ++i) {
if (found == i) {
marks[i].className = "active";
for (var n = marks[i]; n; n = n.parentNode)
if (n.nodeName == "LI") n.className = "active";
} else {
marks[i].className = "";
}
}
}
}
window.addEventListener("scroll", updateSoon);
window.addEventListener("load", updateSoon);
window.addEventListener("hashchange", function() {
// BUG: CWE-95: Improper Neutralization of Directives in Dynamically Evaluated Code ('Eval Injection')
// setTimeout(function() { var hash = document.location.hash, found = null, m; var marks = document.getElementById("nav").getElementsByTagName("a"); for (var i = 0; i < marks.length; i++) if ((m = marks[i].href.match(/(#.*)/)) && m[1] == hash) { found = i; break; } if (found != null) for (var i = 0; i < marks.length; i++) marks[i].className = i == found ? "active" : ""; }, 300);
// FIXED:
<FILL-HERE>
});
})();
|
javascript | /**
* Handles opening of and synchronization with the reveal.js
* notes window.
*/
var RevealNotes = (function() {
function openNotes() {
var jsFileLocation = document.querySelector('script[src$="notes.js"]').src; // this js file path
jsFileLocation = jsFileLocation.replace(/notes\.js(\?.*)?$/, ''); // the js folder path
var notesPopup = window.open( jsFileLocation + 'notes.html', 'reveal.js - Notes', 'width=1120,height=850' );
// Fires when slide is changed
Reveal.addEventListener( 'slidechanged', post );
// Fires when a fragment is shown
Reveal.addEventListener( 'fragmentshown', post );
// Fires when a fragment is hidden
Reveal.addEventListener( 'fragmenthidden', post );
/**
* Posts the current slide data to the notes window
*/
function post() {
var slideElement = Reveal.getCurrentSlide(),
slideIndices = Reveal.getIndices(),
messageData;
var notes = slideElement.querySelector( 'aside.notes' ),
nextindexh,
nextindexv;
if( slideElement.nextElementSibling && slideElement.parentNode.nodeName == 'SECTION' ) {
nextindexh = slideIndices.h;
nextindexv = slideIndices.v + 1;
} else {
nextindexh = slideIndices.h + 1;
nextindexv = 0;
}
messageData = {
notes : notes ? notes.innerHTML : '',
indexh : slideIndices.h,
indexv : slideIndices.v,
indexf : slideIndices.f,
nextindexh : nextindexh,
nextindexv : nextindexv,
markdown : notes ? typeof notes.getAttribute( 'data-markdown' ) === 'string' : false
};
// BUG: CWE-345: Insufficient Verification of Data Authenticity
// notesPopup.postMessage( JSON.stringify( messageData ), '*' );
// FIXED:
<FILL-HERE>
}
// Navigate to the current slide when the notes are loaded
notesPopup.addEventListener( 'load', function( event ) {
post();
}, false );
}
// If the there's a 'notes' query set, open directly
if( window.location.search.match( /(\?|\&)notes/gi ) !== null ) {
openNotes();
}
// Open the notes when the 's' key is hit
document.addEventListener( 'keydown', function( event ) {
// Disregard the event if the target is editable or a
// modifier is present
if ( document.querySelector( ':focus' ) !== null || event.shiftKey || event.altKey || event.ctrlKey || event.metaKey ) return;
if( event.keyCode === 83 ) {
event.preventDefault();
openNotes();
}
}, false );
return { open: openNotes };
})();
|
javascript | // Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license.
import { Button } from '@fluentui/react-components';
import { Session } from './controller';
import { galleryItems } from './galleryItems';
import { Open24Regular } from '@fluentui/react-icons';
export function Gallery() {
return (
<div
style={{
marginTop: 20,
marginLeft: 30,
}}
>
<div
style={{
marginTop: 20,
marginBottom: 10,
fontWeight: 600,
fontSize: 16,
fontFamily:
'var(--fluent-font-family,"Segoe UI","Segoe UI Web (West European)",-apple-system,BlinkMacSystemFont,Roboto,"Helvetica Neue",sans-serif)',
}}
>
Gallery
</div>
{galleryItems.map((g, i) => (
<div key={i} className="galleryItem">
<div
style={{
width: 570,
height: 100,
padding: 10,
margin: 4,
marginBottom: 30,
border: '1px solid #dddddd',
borderRadius: 4,
background: '#fafafa',
}}
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// dangerouslySetInnerHTML={{ __html: g.nl }}
// FIXED:
<FILL-HERE>
></div>
<div dangerouslySetInnerHTML={{ __html: g.img }} />
<div>
<Button
icon={<Open24Regular />}
style={{
marginLeft: 10,
marginBottom: 10,
}}
onClick={() => {
const url = window.location.origin + window.location.pathname + '#create';
const editor = window.open(url);
if (!editor)
return;
const wait = 10_000;
const step = 250;
const { origin } = new URL(url);
// eslint-disable-next-line no-bitwise
let count = ~~(wait / step);
function listen(evt: MessageEvent) {
if (evt.source === editor) {
count = 0;
window.removeEventListener('message', listen, false);
}
}
window.addEventListener('message', listen, false);
// send message
// periodically resend until ack received or timeout
function send() {
if (!editor || count <= 0) {
return;
}
const session: Session = {
source: 'vistalk',
data: g.data,
input: g.input,
};
editor.postMessage(session, origin);
setTimeout(send, step);
count -= 1;
}
setTimeout(send, step);
}}
>
Open
</Button>
</div>
</div>
))}
</div>
);
}
|
javascript | const path = require('path');
const scenarioController = require('../controllers/scenario-controller');
const siteValidationController = require('../controllers/sitevalidation-controller');
// const resultsController = require('../controllers/results-controller');
const jwt = require('express-jwt');
// BUG: CWE-522: Insufficiently Protected Credentials
// const auth = jwt({ secret: process.env.JWT_SECRET });
// FIXED:
<FILL-HERE>
module.exports = (app) => {
app.get('/', (req, res) => {
res.sendFile(path.resolve('client/index.html'));
});
app.post('/api/scenarios', auth, scenarioController.createScenario);
app.delete('/api/scenarios', auth, scenarioController.deleteScenario);
app.get('/api/scenarios', auth, scenarioController.getScenarios);
app.post('/api/rerun-scenario', auth, scenarioController.rerunScenarioTest);
app.post('/api/run-scenario', auth, scenarioController.runScenarioTest);
app.post('/api/validate-website', auth, siteValidationController.validateWebsite);
// Catch all;
app.get('/*', (req, res) => {
res.redirect('/');
// res.status(404);
// res.send('Page does not exist. <p><a href="/">Click here</a> to go back</p>');
});
};
|
javascript | /*
USERNAME HASH PROBLEM
To make /history "non-blocking", run app in 2 threads:
- The main thread: Serves I/O HTTP requests
- The worker thread: Does the Hash Task
When users visit http://localhost:8081/?name=JohnnyTeo,
the app delegates the Hash Task to the worker thread so it can serve the next requests.
The worker thread receives and does the Hash Task and returns result to main thread.
*/
import * as mylib from './mylib.js';
import { isMainThread, workerData, parentPort } from 'worker_threads';
import express from 'express';
import { getHash, splitStrInToChunks } from './exerex-userhash-util.js';
//---------------------------------------------
// WORKER THREAD SECTION
//---------------------------------------------
const getSuperHashByWorker = plainText => {
const numChunks = 8;
const lstChunks = splitStrInToChunks(numChunks, plainText);
const lstHashes = lstChunks.map(chunk => getHash(2**21, chunk));
const finalHash = getHash(1, lstHashes.join(''));
return finalHash;
};
const workerFunc = () => {
try {
const plainText = workerData;
const hash = getSuperHashByWorker(plainText);
parentPort.postMessage(hash);
} catch (error) {
console.error(error);
}
};
//---------------------------------------------
// MAIN THREAD SECTION
//---------------------------------------------
const getSuperHashByMain = async plainText => {
let finalHash = '';
const [worker, prom] = mylib.createThread(new URL(import.meta.url), plainText);
worker.on('message', hash => finalHash = hash);
await prom;
return finalHash;
};
const mainFunc = () => {
const PORT = 8081;
const app = express();
const userNameHistory = [];
app.get('/history', async (req, res) => {
const html = userNameHistory.join('<br/>') || '<Empty history>';
res.status(200).send(html).end();
});
app.get('/', async (req, res) => {
const userName = req.query.name;
if (!userName) {
res.status(400).end();
return;
}
userNameHistory.push(userName);
const tpStart = process.hrtime();
// GET USERNAME HASH
const userNameHash = await getSuperHashByMain(userName);
const timeElapsed = mylib.hrtimeToNumber(process.hrtime(tpStart));
console.log(`userName = ${userName}; Time elapsed = ${timeElapsed}`);
const html = userName + '<br/>' + userNameHash;
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.status(200).setHeader('Content-Type', 'text/html').send(html).end();
// FIXED:
<FILL-HERE>
});
console.log('Server is listening on port', PORT);
const server = app.listen(PORT);
};
if (isMainThread) {
try {
mainFunc();
} catch (error) {
console.error(error);
}
} else {
workerFunc();
}
|
javascript | var express = require ("express");
var app = express();
app.get("/", function(req,res){
res.send("hi there, welcome to my work")
});
//obj sounds that matches an animal
//we take the animal and plug it into sounds
//and we get the result which is the sound
app.get("/speak/:animal", function(req,res){
var sounds = {
pig: "oink",
dog: "woof",
cow: "moo",
cat: "moew",
fish: "......"
}
var animal = req.params.animal.toLowerCase();
//sounds will be equal to sounds of animal
var sound = sounds[animal];
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send("The "+ animal + " says '" + sound + "'");
// FIXED:
<FILL-HERE>
});
//repeat route
app.get("/repeat/:message/:times", function(req,res){
var message = req.params.message;
var times = Number(req.params.times);
var result = "";
for(var i = 0; i < times; i++){
result += message + " ";
}
res.send(result);
})
//send back result string by going through the loop
//hi/10 should get 10 his
app.get("*", function(req,res){
res.send("Get it together");
});
//tell express to listen for request (start server)
//we need to write the code to tell it to lsn to requests
app.listen(3000, function(){
console.log("Serving demo app on port 3000");
});
|
javascript | const express = require('express');
const rateLimit = require('express-rate-limit');
const helmet = require('helmet');
const bodyParser = require("body-parser");
const xss = require('xss-clean');
const hpp = require('hpp');
const cors = require('cors');
const multer = require('multer');
const upload = multer();
const session = require('express-session');
const { secret } = require('./config/common/config');
// Importing The Database
const postgresql = require('./config/database/postgresqlConfig');
// Importing the Routes
const covidRoutes = require('./routes/covid/covidRoutes')
// Calling the Error Handlers
const globalErrHandler = require('./controllers/error/errorController');
const AppError = require('./utils/error/appError');
const app = express();
// Allow Cross-Origin requests
app.use(cors());
// Set security HTTP headers
app.use(helmet());
// Limit request from the same API
const limiter = rateLimit({
max: 150,
windowMs: 60 * 60 * 1000,
message: 'Too Many Request from this IP, please try again in an hour'
});
app.use('/api/v1', limiter);
// Body parser, reading data from body into req.body
app.use(express.json({
limit: '100kb'
}));
// Configure the Session expiry
app.use(
// BUG: CWE-522: Insufficiently Protected Credentials
// session({ secret: secret, resave: true, saveUninitialized: false })
// FIXED:
<FILL-HERE>
);
// Data sanitization against XSS(clean user input from malicious HTML code)
app.use(xss());
// Prevent parameter pollution
app.use(hpp());
// Setting Body parser
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json({ type: 'application/*+json' }));
app.use(express.json());
app.use(upload.array());
app.use(express.static('public'));
// Setting up global error handler
app.use(globalErrHandler);
// Checking and Testing of Home
app.get('/', (req, res) => {
// console.log(req.session)
res.send(`You hit home page!\n`)
})
// Routes Final Calling
app.use('/api/v1',covidRoutes);
// handle undefined Routes
app.use('*', (req, res, next) => {
const err = new AppError(404, 'fail', 'undefined route');
next(err, req, res, next);
});
module.exports = app; |
javascript | // Kludge in HTML5 tag recognition in IE8
document.createElement("section");
document.createElement("article");
(function() {
if (!window.addEventListener) return;
var pending = false, prevVal = null;
function updateSoon() {
if (!pending) {
pending = true;
setTimeout(update, 250);
}
}
function update() {
pending = false;
var marks = document.getElementById("nav").getElementsByTagName("a"), found;
for (var i = 0; i < marks.length; ++i) {
var mark = marks[i], m;
if (mark.getAttribute("data-default")) {
if (found == null) found = i;
} else if (m = mark.href.match(/#(.*)/)) {
var ref = document.getElementById(m[1]);
if (ref && ref.getBoundingClientRect().top < 50)
found = i;
}
}
if (found != null && found != prevVal) {
prevVal = found;
var lis = document.getElementById("nav").getElementsByTagName("li");
for (var i = 0; i < lis.length; ++i) lis[i].className = "";
for (var i = 0; i < marks.length; ++i) {
if (found == i) {
marks[i].className = "active";
for (var n = marks[i]; n; n = n.parentNode)
if (n.nodeName == "LI") n.className = "active";
} else {
marks[i].className = "";
}
}
}
}
window.addEventListener("scroll", updateSoon);
window.addEventListener("load", updateSoon);
window.addEventListener("hashchange", function() {
// BUG: CWE-95: Improper Neutralization of Directives in Dynamically Evaluated Code ('Eval Injection')
// setTimeout(function() { var hash = document.location.hash, found = null, m; var marks = document.getElementById("nav").getElementsByTagName("a"); for (var i = 0; i < marks.length; i++) if ((m = marks[i].href.match(/(#.*)/)) && m[1] == hash) { found = i; break; } if (found != null) for (var i = 0; i < marks.length; i++) marks[i].className = i == found ? "active" : ""; }, 300);
// FIXED:
<FILL-HERE>
});
})();
|
javascript | const bodyParser = require('body-parser');
const path = require('path');
const session = require('express-session');
const morgan = require('morgan');
const config = require('../../webpack.config.js');
const webpack = require('webpack');
const webpackDevMiddleware = require('webpack-dev-middleware');
const webpackHotMiddleware = require('webpack-hot-middleware');
const compiler = webpack(config);
module.exports = (app, express) => {
app.use(webpackDevMiddleware(compiler, { noInfo: true, publicPath: config.output.publicPath }));
app.use(webpackHotMiddleware(compiler));
app.use(morgan('dev'));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// BUG: CWE-798: Use of Hard-coded Credentials
// app.use(session({ secret: 'fred', resave: false, saveUninitialized: false }));
// FIXED:
<FILL-HERE>
app.use(express.static(path.join(`${__dirname}./../../dist`)));
};
|
javascript | const _sendMessage = (obj, typ, where, orderNum='') => {
// This is either a string or an object.
let str;
if (typeof obj == 'object') {
// Need to copy the object otherwise we end up overwrite the event object below, which we don't want.
let newObj = _clone(obj);
if (newObj.e) newObj.e = ''; // We get an invocation error on trying to send a cloned event. Don't send it to the extensions. We could send a smaller version if and when it is needed...
if (newObj.doc) newObj.doc = ''; // Causes circular reference error.
if (newObj.compDoc) newObj.compDoc = ''; // Just to be safe - we don't need it.
if (newObj.obj) newObj.obj = ''; // Just to be safe - we don't need it.
if (newObj.secSelObj) newObj.secSelObj = ''; // Just to be safe - we don't need it.
str = JSON.stringify(newObj);
} else {
str = obj;
}
if (!setupEnded) {
// Active CSS setup has not yet finished and DevTools has not yet handshook with the core.
// Put the message into a queue. It will get sent when DevTools does the handshake.
debuggerCo++;
devtoolsInit.push([ str, typ, debuggerCo ]);
return;
}
if (typ == 'debugOutput') {
// Internal tracker so panel.js can put them in order before displaying when they arrive, as they don't arrive in sequence.
if (!orderNum) { // Note: If a number is already set, that means we have come from the init routine and a number is already set.
debuggerCo++;
orderNum = debuggerCo;
}
}
// BUG: CWE-345: Insufficient Verification of Data Authenticity
// window.postMessage({ message: str, messageType: typ, orderNo: orderNum, whereTo: where, source: 'causejs-devtools-extension' }, '*');
// FIXED:
<FILL-HERE>
};
|
javascript | const baseURL = 'http://localhost:9000/api/users/'
export const getUsers = () => {
return fetch(baseURL)
.then(res => res.json());
};
export const getUser = (id) => {
return fetch(baseURL + id)
.then(res => res.json())
};
export const postUser = (payload) => {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// return fetch(baseURL, { method: 'POST', body: JSON.stringify(payload), headers: {'Content-Type': 'application/json'} })
// FIXED:
<FILL-HERE>
.then(res => res.json())
};
export const putUser = (id , payload) => {
return fetch(baseURL + id, {
method: 'PUT',
body:JSON.stringify(payload),
headers : {'Content-Type': 'application/json'}
})
.then(res => res.json())
};
export const deleteUser = (id) => {
return fetch(baseURL + id, {
method: 'DELETE'
})
};
|
javascript | const express = require('express');
const rateLimit = require('express-rate-limit');
const helmet = require('helmet');
const bodyParser = require("body-parser");
const xss = require('xss-clean');
const hpp = require('hpp');
const cors = require('cors');
const multer = require('multer');
const upload = multer();
const session = require('express-session');
const { secret } = require('./config/common/config');
// Importing The Database
const postgresql = require('./config/database/postgresqlConfig');
// Importing the Routes
const covidRoutes = require('./routes/covid/covidRoutes')
// Calling the Error Handlers
const globalErrHandler = require('./controllers/error/errorController');
const AppError = require('./utils/error/appError');
const app = express();
// Allow Cross-Origin requests
app.use(cors());
// Set security HTTP headers
app.use(helmet());
// Limit request from the same API
const limiter = rateLimit({
max: 150,
windowMs: 60 * 60 * 1000,
message: 'Too Many Request from this IP, please try again in an hour'
});
app.use('/api/v1', limiter);
// Body parser, reading data from body into req.body
app.use(express.json({
limit: '100kb'
}));
// Configure the Session expiry
app.use(
// BUG: CWE-522: Insufficiently Protected Credentials
// session({ secret: secret, resave: true, saveUninitialized: false })
// FIXED:
<FILL-HERE>
);
// Data sanitization against XSS(clean user input from malicious HTML code)
app.use(xss());
// Prevent parameter pollution
app.use(hpp());
// Setting Body parser
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json({ type: 'application/*+json' }));
app.use(express.json());
app.use(upload.array());
app.use(express.static('public'));
// Setting up global error handler
app.use(globalErrHandler);
// Checking and Testing of Home
app.get('/', (req, res) => {
// console.log(req.session)
res.send(`You hit home page!\n`)
})
// Routes Final Calling
app.use('/api/v1',covidRoutes);
// handle undefined Routes
app.use('*', (req, res, next) => {
const err = new AppError(404, 'fail', 'undefined route');
next(err, req, res, next);
});
module.exports = app; |
javascript | const runCode = require('../lib/runCode')
const bodyParser = require('body-parser')
const express = require('express')
const router = express.Router()
router.use(bodyParser.json()) // for parsing routerlication/json
router.use(bodyParser.urlencoded({ extended: true }))
// GET handler for /
router.get('/', (req, res) => {
res.send('API requires POST to /code/<lang>')
})
// POST handler for /py
router.post('/py', async (req, res) => {
const { filePath } = req.body
const result = await runCode('py', filePath) // executes runCode() on specified .py and input files
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send(result)
// FIXED:
<FILL-HERE>
})
// POST handler for /cpp
router.post('/cpp', async (req, res) => {
const { filePath } = req.body
const result = await runCode('cpp', filePath) // executes runCode() on specified .cpp and input files
res.send(result)
})
// POST handler for /js
router.post('/js', async (req, res) => {
const { filePath } = req.body
const result = await runCode('js', filePath) // executes runCode() on specified .js and input files
res.send(result)
})
module.exports = router
|
javascript | /*
MUTEXES
Version EX
In this demo, we will face the race condition.
-------------------------------------> Time
|
Send request '/register?id=Teo&name=Tran Teo' | ooooo
Send request '/register?id=Teo&name=Le Teo' | xxxxx
Check database and create user(id='Teo', name='Tran Teo') | oooooooooo
Check database and create user(id='Teo', name='Le Teo') | xxxxxxxxxx
The final result is that the database contains user(id='Teo', name='Le Teo').
Due to the slow process time from server, user 'Tran Teo' is overwritten by user 'Le Teo'.
We do not want this result. What we want is:
- Request '/register?id=Teo&name=Tran Teo' comes first, so user(id='Teo', name='Tran Teo') is created first.
- Request '/register?id=Teo&name=Le Teo' comes later, and this results a failure due to id 'Teo' is existed.
-------------------------------------> Time
|
Send request '/register?id=Teo&name=Tran Teo' | ooooo
Send request '/register?id=Teo&name=Le Teo' | (wait)......xxxxx
Check database and create user(id='Teo', name='Tran Teo') | oooooooooo
Check database and create user(id='Teo', name='Le Teo') | xxxxxxxxxx
*/
import * as mylib from './mylib.js';
import express from 'express';
const createServer = () => {
const users = new Map();
const app = express();
const createUser = async (userid, username) => {
if (!userid || !username) {
return false;
}
if (users.has(userid)) {
return false;
}
// Assume that creating user takes a little time
await mylib.sleep(1000);
users.set(userid, username);
return true;
};
app.get('/register', async (req, res) => {
const ret = await createUser(req.query.id, req.query.name);
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.status(200).send(ret).end();
// FIXED:
<FILL-HERE>
});
app.get('/', (req, res) => {
const resStr = JSON.stringify(
[...users].map(([k,v]) => ({ id: k, name: v }))
, null, 2);
res.status(200).setHeader('Content-Type', 'application/json');
res.send(resStr).end();
});
const server = app.listen(8081);
return server;
};
const runClient = async () => {
const registerUrl1 = new URL('/register?id=teo&name=Tran Teo', 'http://localhost:8081');
const registerUrl2 = new URL('/register?id=teo&name=Le Teo', 'http://localhost:8081');
const infoUrl = new URL('/', 'http://localhost:8081');
const prom1 = mylib.makeHttpGet(registerUrl1);
await mylib.sleep(200);
const prom2 = mylib.makeHttpGet(registerUrl2);
await mylib.sleep(200);
await Promise.all([prom1, prom2]);
const result = await mylib.makeHttpGet(infoUrl);
console.log(result);
};
const server = createServer();
await runClient();
server.close();
|
javascript | var express = require ("express");
var app = express();
app.get("/", function(req,res){
res.send("hi there, welcome to my work")
});
//obj sounds that matches an animal
//we take the animal and plug it into sounds
//and we get the result which is the sound
app.get("/speak/:animal", function(req,res){
var sounds = {
pig: "oink",
dog: "woof",
cow: "moo",
cat: "moew",
fish: "......"
}
var animal = req.params.animal.toLowerCase();
//sounds will be equal to sounds of animal
var sound = sounds[animal];
res.send("The "+ animal + " says '" + sound + "'");
});
//repeat route
app.get("/repeat/:message/:times", function(req,res){
var message = req.params.message;
var times = Number(req.params.times);
var result = "";
for(var i = 0; i < times; i++){
result += message + " ";
}
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send(result);
// FIXED:
<FILL-HERE>
})
//send back result string by going through the loop
//hi/10 should get 10 his
app.get("*", function(req,res){
res.send("Get it together");
});
//tell express to listen for request (start server)
//we need to write the code to tell it to lsn to requests
app.listen(3000, function(){
console.log("Serving demo app on port 3000");
});
|
javascript | function getAllMovies() {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// fetch("http://localhost:3000/movies")
// FIXED:
<FILL-HERE>
.then((response) => {
if (response.status === 200) {
return response.json();
}
})
.then((movies) => {
let tbody = document
.getElementById("movieList")
.getElementsByClassName("row")[0];
let tbodyhtml = "";
movies.forEach((movie) => {
tbodyhtml += `<div class="row ">
<div class="col-4">
<div class="card" style="width: 20rem ">
<img
class="img-container"
src=${movie.posterUrl}
/>
<div class="card-body">
<h5 class="card-title">${movie.title}</h5>
<p><span class="badge bg-primary text-dark">Diector</span> : ${movie.director} </p>
<p><span class="badge bg-info text-dark">Actor</span> : ${movie.actors} </p>
<p><span class="badge bg-secondary text-dark">Plot</span> : ${movie.plot} </p>
<span class="badge badge-success">${movie.year}</span>
</div>
</div>
</div></div>`;
});
tbody.innerHTML = tbodyhtml;
})
.catch((error) => {
console.log(error);
});
}
getAllMovies();
|
javascript | const express = require('express');
const rateLimit = require('express-rate-limit');
const helmet = require('helmet');
const bodyParser = require("body-parser");
const xss = require('xss-clean');
const hpp = require('hpp');
const cors = require('cors');
const multer = require('multer');
const upload = multer();
const session = require('express-session');
const { secret } = require('./config/common/config');
// Importing The Database
const postgresql = require('./config/database/postgresqlConfig');
// Importing the Routes
const covidRoutes = require('./routes/covid/covidRoutes')
// Calling the Error Handlers
const globalErrHandler = require('./controllers/error/errorController');
const AppError = require('./utils/error/appError');
const app = express();
// Allow Cross-Origin requests
app.use(cors());
// Set security HTTP headers
app.use(helmet());
// Limit request from the same API
const limiter = rateLimit({
max: 150,
windowMs: 60 * 60 * 1000,
message: 'Too Many Request from this IP, please try again in an hour'
});
app.use('/api/v1', limiter);
// Body parser, reading data from body into req.body
app.use(express.json({
limit: '100kb'
}));
// Configure the Session expiry
app.use(
// BUG: CWE-522: Insufficiently Protected Credentials
// session({ secret: secret, resave: true, saveUninitialized: false })
// FIXED:
<FILL-HERE>
);
// Data sanitization against XSS(clean user input from malicious HTML code)
app.use(xss());
// Prevent parameter pollution
app.use(hpp());
// Setting Body parser
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json({ type: 'application/*+json' }));
app.use(express.json());
app.use(upload.array());
app.use(express.static('public'));
// Setting up global error handler
app.use(globalErrHandler);
// Checking and Testing of Home
app.get('/', (req, res) => {
// console.log(req.session)
res.send(`You hit home page!\n`)
})
// Routes Final Calling
app.use('/api/v1',covidRoutes);
// handle undefined Routes
app.use('*', (req, res, next) => {
const err = new AppError(404, 'fail', 'undefined route');
next(err, req, res, next);
});
module.exports = app; |
javascript | const runCode = require('../lib/runCode')
const bodyParser = require('body-parser')
const express = require('express')
const router = express.Router()
router.use(bodyParser.json()) // for parsing routerlication/json
router.use(bodyParser.urlencoded({ extended: true }))
// GET handler for /
router.get('/', (req, res) => {
res.send('API requires POST to /code/<lang>')
})
// POST handler for /py
router.post('/py', async (req, res) => {
const { filePath } = req.body
const result = await runCode('py', filePath) // executes runCode() on specified .py and input files
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send(result)
// FIXED:
<FILL-HERE>
})
// POST handler for /cpp
router.post('/cpp', async (req, res) => {
const { filePath } = req.body
const result = await runCode('cpp', filePath) // executes runCode() on specified .cpp and input files
res.send(result)
})
// POST handler for /js
router.post('/js', async (req, res) => {
const { filePath } = req.body
const result = await runCode('js', filePath) // executes runCode() on specified .js and input files
res.send(result)
})
module.exports = router
|
javascript | var express = require("express")
//import express, includes all content of ex
var app = express();
//initialize variable called app and execute it
// when we go to "/" we should see "hi there!"
app.get("/", function(req, res){
res.send("hi there!");
});
//app.get takes 2 diff parameters, the url or path which is the route
//the other is a callback function which takes 2 arguments, request and responses
//req and res are objects, req contains all the information about the req that was made that triggered the routes
//same for response
// then respond with text
//-----------------------------------------------
// when we go to "/bye" we should see "goodbye!"
app.get("/bye", function(req, res){
res.send("goodbye!");
});
// when we go to "/dog" we should see "meow!"
app.get("/dog", function(req, res){
console.log("someone made a request to /DOG")
res.send("meow!");
});
//route paterns example
//to see this msg we need to go to a route that matches the pattern
app.get("/r/:subredditName", function(req,res){
//to see all the info from the requests
//we want req.paramas
//obj that contains all the route parameters and corresponding values
console.log(req.params);
var subreddit = req.params.subredditName;
// BUG: CWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')
// res.send("welcome to the" + subreddit.toUpperCase() + " subreddit");
// FIXED:
<FILL-HERE>
});
//using reddit examples
app.get("/r/:subredditName/comments/:id/:title/", function(){
console.log(req.params);
res.send("welcome to the comments page");
});
//if i go to a route that is not defined
//i get routed here ( kinda like a page not found )
//do not put this first, because it will override the rest
app.get("*", function(req, res){
res.send("you are a star!!");
});
//tell express to listen for request (start server)
//we need to write the code to tell it to lsn to requests
app.listen(3000, function(){
console.log("Serving demo app on port 3000");
});
//end
|
javascript | // Kludge in HTML5 tag recognition in IE8
document.createElement("section");
document.createElement("article");
(function() {
if (!window.addEventListener) return;
var pending = false, prevVal = null;
function updateSoon() {
if (!pending) {
pending = true;
setTimeout(update, 250);
}
}
function update() {
pending = false;
var marks = document.getElementById("nav").getElementsByTagName("a"), found;
for (var i = 0; i < marks.length; ++i) {
var mark = marks[i], m;
if (mark.getAttribute("data-default")) {
if (found == null) found = i;
} else if (m = mark.href.match(/#(.*)/)) {
var ref = document.getElementById(m[1]);
if (ref && ref.getBoundingClientRect().top < 50)
found = i;
}
}
if (found != null && found != prevVal) {
prevVal = found;
var lis = document.getElementById("nav").getElementsByTagName("li");
for (var i = 0; i < lis.length; ++i) lis[i].className = "";
for (var i = 0; i < marks.length; ++i) {
if (found == i) {
marks[i].className = "active";
for (var n = marks[i]; n; n = n.parentNode)
if (n.nodeName == "LI") n.className = "active";
} else {
marks[i].className = "";
}
}
}
}
window.addEventListener("scroll", updateSoon);
window.addEventListener("load", updateSoon);
window.addEventListener("hashchange", function() {
// BUG: CWE-95: Improper Neutralization of Directives in Dynamically Evaluated Code ('Eval Injection')
// setTimeout(function() { var hash = document.location.hash, found = null, m; var marks = document.getElementById("nav").getElementsByTagName("a"); for (var i = 0; i < marks.length; i++) if ((m = marks[i].href.match(/(#.*)/)) && m[1] == hash) { found = i; break; } if (found != null) for (var i = 0; i < marks.length; i++) marks[i].className = i == found ? "active" : ""; }, 300);
// FIXED:
<FILL-HERE>
});
})();
|
javascript | const bodyParser = require('body-parser');
const path = require('path');
const session = require('express-session');
const morgan = require('morgan');
const config = require('../../webpack.config.js');
const webpack = require('webpack');
const webpackDevMiddleware = require('webpack-dev-middleware');
const webpackHotMiddleware = require('webpack-hot-middleware');
const compiler = webpack(config);
module.exports = (app, express) => {
app.use(webpackDevMiddleware(compiler, { noInfo: true, publicPath: config.output.publicPath }));
app.use(webpackHotMiddleware(compiler));
app.use(morgan('dev'));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// BUG: CWE-522: Insufficiently Protected Credentials
// app.use(session({ secret: 'fred', resave: false, saveUninitialized: false }));
// FIXED:
<FILL-HERE>
app.use(express.static(path.join(`${__dirname}./../../dist`)));
};
|
javascript | const baseURL = 'http://localhost:9000/api/users/'
export const getUsers = () => {
// BUG: CWE-319: Cleartext Transmission of Sensitive Information
// return fetch(baseURL)
// FIXED:
<FILL-HERE>
.then(res => res.json());
};
export const getUser = (id) => {
return fetch(baseURL + id)
.then(res => res.json())
};
export const postUser = (payload) => {
return fetch(baseURL, {
method: 'POST',
body: JSON.stringify(payload),
headers: {'Content-Type': 'application/json'}
})
.then(res => res.json())
};
export const putUser = (id , payload) => {
return fetch(baseURL + id, {
method: 'PUT',
body:JSON.stringify(payload),
headers : {'Content-Type': 'application/json'}
})
.then(res => res.json())
};
export const deleteUser = (id) => {
return fetch(baseURL + id, {
method: 'DELETE'
})
};
|
python | #!/usr/bin/env python3
# -*- coding: utf-8 -*-
from os import environ
import numpy as np
import sys
import subprocess
def run(cmd):
"""
Runs a shell command, and print it before running.
"""
print(cmd)
# BUG: CWE-78: Improper Neutralization of Special Elements used in an OS Command ('OS Command Injection')
# r = subprocess.call(cmd, shell=True, stdout=sys.stdout, stderr=subprocess.STDOUT, env=environ)
# FIXED:
<FILL-HERE>
if r != 0:
logger.critical(r)
return
# read lect.in
# read dates
fimages='liste_date_ERA5'
dates,bid=np.loadtxt(fimages, comments='#', unpack=True,dtype='i,i')
N=len(dates)
inlook = 1
outlook = 2
rlook = int(outlook/inlook)
for d in dates:
#infile = '{}_mdel_{}rlks.unw'.format(d,inlook)
infile = '{}_mdel.unw'.format(d,inlook)
run("look.pl "+str(infile)+" "+str(rlook))
|
python | from flask import Flask, request, jsonify
import requests
app = Flask(__name__)
create_so_url = "http://order-svc:3001/createSo"
update_inventory_url = "http://product-svc:3002/allocateInventory"
@app.route('/v1/order/create', methods=['POST'])
def create_1():
return create_so(rollback=False)
@app.route('/v1/order/create2', methods=['POST'])
def create_2():
return create_so(rollback=True)
def create_so(rollback=True):
xid = request.headers.get("x-dbpack-xid")
so_items = [dict(
product_sysno=1,
product_name="apple iphone 13",
original_price=6799,
cost_price=6799,
deal_price=6799,
quantity=2,
)]
so_master = [dict(
buyer_user_sysno = 10001,
seller_company_code = "SC001",
receive_division_sysno = 110105,
receive_address = "beijing",
receive_zip = "000001",
receive_contact = "scott",
receive_contact_phone = "18728828296",
stock_sysno = 1,
payment_type = 1,
so_amt = 6999 * 2,
status = 10,
appid = "dk-order",
so_items = so_items,
)]
success = (jsonify(dict(success=True, message="success")), 200)
failed = (jsonify(dict(success=False, message="failed")), 400)
headers = {
"Content-Type": "application/json",
"xid": xid
}
so_req = dict(req=so_master)
resp1 = requests.post(create_so_url, headers=headers, json=so_req)
if resp1.status_code == 400:
return failed
ivt_req = dict(req=[dict(product_sysno= 1, qty=2)])
resp2 = requests.post(update_inventory_url, headers=headers, json=ivt_req)
if resp2.status_code == 400:
return failed
if rollback:
print("rollback")
return failed
return success
if __name__ == "__main__":
# BUG: CWE-668: Exposure of Resource to Wrong Sphere
# app.run(host="0.0.0.0", port=3000)
# FIXED:
<FILL-HERE>
|
python | import boto3
from flask import Flask
from aws_xray_sdk.core import xray_recorder, patch_all
from aws_xray_sdk.ext.flask.middleware import XRayMiddleware
from aws_xray_sdk.ext.flask_sqlalchemy.query import XRayFlaskSqlAlchemy
import requests
import os
application = app = Flask(__name__)
application.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
application.config["SQLALCHEMY_DATABASE_URI"] = "sqlite:///db.sqlite3"
xray_recorder.configure(service='My Flask Web Application')
XRayMiddleware(app, xray_recorder)
patch_all()
db = XRayFlaskSqlAlchemy(app=application)
class User(db.Model):
__tablename__ = 'users'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(255), nullable=False, unique=True)
# test http instrumentation
@app.route('/outgoing-http-call')
def callHTTP():
requests.get("https://aws.amazon.com")
return "Ok! tracing outgoing http call"
# test aws sdk instrumentation
@app.route('/aws-sdk-call')
def callAWSSDK():
client = boto3.client('s3')
client.list_buckets()
return 'Ok! tracing aws sdk call'
# test flask-sql alchemy instrumentation
@app.route('/flask-sql-alchemy-call')
def callSQL():
name = 'sql-alchemy-model'
user = User(name=name)
db.create_all()
db.session.add(user)
return 'Ok! tracing sql call'
@app.route('/')
def default():
return "healthcheck"
if __name__ == "__main__":
address = os.environ.get('LISTEN_ADDRESS')
if address is None:
host = '127.0.0.1'
port = '5000'
else:
host, port = address.split(":")
# BUG: CWE-489: Active Debug Code
# app.run(host=host, port=int(port), debug=True)
# FIXED:
<FILL-HERE>
|
python | #!/usr/bin/env python
#
# See the accompanying LICENSE file.
#
import urllib2
import hashlib
import re
sqlitevers=(
'3081101',
'3081100',
'3081002',
'3081001',
'3081000',
'3080900',
'3080803',
'3080802',
'3080801',
'3080800',
'3080704',
'3080703',
'3080702',
'3080701',
'3080700',
'3080600',
'3080500',
'3080403',
'3080402',
'3080401',
'3080400',
'3080301',
'3080300',
'3080200',
'3080100',
'3080002',
'3080001',
'3080000',
'3071700',
'3071602',
'3071601',
'3071600',
'3071502',
'3071501',
'3071500',
'3071401',
'3071400',
'3071300',
'3071201',
'3071200',
'3071100',
'3071000',
'3070900',
'3070800',
'3070701',
'3070700',
'3070603',
'3070602',
'3070601',
'3070600',
)
# Checks the checksums file
def getline(url):
for line in open("checksums", "rtU"):
line=line.strip()
if len(line)==0 or line[0]=="#":
continue
l=[l.strip() for l in line.split()]
if len(l)!=4:
print "Invalid line in checksums file:", line
raise ValueError("Bad checksums file")
if l[0]==url:
return l[1:]
return None
def check(url, data):
# BUG: CWE-327: Use of a Broken or Risky Cryptographic Algorithm
# d=["%s" % (len(data),), hashlib.sha1(data).hexdigest(), hashlib.md5(data).hexdigest()]
# FIXED:
<FILL-HERE>
line=getline(url)
if line:
if line!=d:
print "Checksums mismatch for", url
print "checksums file is", line
print "Download is", d
else:
print url,
if url.endswith(".zip"):
print " ",
print d[0], d[1], d[2]
# They keep messing with where files are in URI - this code is also in setup.py
def fixup_download_url(url):
ver=re.search("3[0-9]{6}", url)
if ver:
ver=int(ver.group(0))
if ver>=3071600:
if ver>=3080800:
year="2015"
elif ver>=3080300:
year="2014"
else:
year="2013"
if "/"+year+"/" not in url:
url=url.split("/")
url.insert(3, year)
return "/".join(url)
return url
for v in sqlitevers:
# Windows amalgamation
AURL="https://sqlite.org/sqlite-amalgamation-%s.zip" % (v,)
AURL=fixup_download_url(AURL)
try:
data=urllib2.urlopen(AURL).read()
except:
print AURL
raise
check(AURL, data)
# All other platforms amalgamation
AURL="https://sqlite.org/sqlite-autoconf-%s.tar.gz" % (v,)
AURL=fixup_download_url(AURL)
try:
data=urllib2.urlopen(AURL).read()
except:
print AURL
raise
check(AURL, data)
|
python | """Non-twisted throughput server."""
import socket, signal, sys
def signalhandler(*args):
print "alarm!"
sys.stdout.flush()
signal.signal(signal.SIGALRM, signalhandler)
# BUG: CWE-200: Exposure of Sensitive Information to an Unauthorized Actor
# s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)s.bind(('', 8001))
# FIXED:
<FILL-HERE>
s.listen(1)
while 1:
c, (h, p) = s.accept()
c.settimeout(30)
signal.alarm(5)
while 1:
d = c.recv(16384)
if not d:
break
c.close()
|
python | # -*- coding: utf-8 -*-
#
# ===================================================================
# The contents of this file are dedicated to the public domain. To
# the extent that dedication to the public domain is not available,
# everyone is granted a worldwide, perpetual, royalty-free,
# non-exclusive license to exercise all rights associated with the
# contents of this file for any purpose whatsoever.
# No rights are reserved.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
# EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
# MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS
# BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
# ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
# CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
# SOFTWARE.
# ===================================================================
# Just use the SHA module from the Python standard library
__revision__ = "$Id$"
__all__ = ['new', 'digest_size']
try:
# The md5 module is deprecated in Python 2.6, so use hashlib when possible.
import hashlib
def new(data=""):
# BUG: CWE-327: Use of a Broken or Risky Cryptographic Algorithm
# return hashlib.sha1(data)
# FIXED:
<FILL-HERE>
digest_size = new().digest_size
except ImportError:
from sha import *
import sha
if hasattr(sha, 'digestsize'):
digest_size = digestsize
del digestsize
del sha
|
python | # -*- coding: utf-8 -*-
## Licensed under the Apache License, Version 2.0 (the "License");
## you may not use this file except in compliance with the License.
## You may obtain a copy of the License at
##
## http://www.apache.org/licenses/LICENSE-2.0
##
## Unless required by applicable law or agreed to in writing, software
## distributed under the License is distributed on an "AS IS" BASIS,
## WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
## See the License for the specific language governing permissions and
## limitations under the License.
"""Unit test for morph.py
"""
__author__ = """
rws@uiuc.edu (Richard Sproat)
"""
import os
import sys
# BUG: CWE-611: Improper Restriction of XML External Entity Reference
# import xml.sax
# FIXED:
<FILL-HERE>
import unittest
import tokens
import xmlhandler
import morph
from __init__ import BASE_
SOURCE_FILE_ = '%s/testdata/morphtest_src.xml' % BASE_
GOLDEN_FILE_ = '%s/testdata/morphtest.xml' % BASE_
TEST_FILE_ = '/tmp/morphtest.xml'
def main(output = False):
parser = xml.sax.make_parser()
handler = xmlhandler.XmlHandler()
parser.setContentHandler(handler)
parser.parse(SOURCE_FILE_)
doclist = handler.DocList()
analyzer = morph.PrefixAnalyzer(doclist, 'eng')
analyzer.Initialize()
analyzer.LabelDoclist()
if output:
doclist.XmlDump(GOLDEN_FILE_, utf8=True)
else:
doclist.XmlDump(TEST_FILE_)
unittest.TestUnitOutputs(sys.argv[0], GOLDEN_FILE_, TEST_FILE_)
if __name__ == '__main__':
if len(sys.argv) > 1 and sys.argv[1] == 'generate':
main(True)
else:
main()
|
python | """SocketTree extension with export support."""
### standard library import
# BUG: CWE-611: Improper Restriction of XML External Entity Reference
# from xml.etree.ElementTree import Element
# FIXED:
<FILL-HERE>
### third-party import
from pygame.draw import line as draw_line
class ExportOperations:
"""Exporting operations for the socket tree."""
def yield_lines_as_svg(self):
"""Yield lines as svg elements.
Used when representing lines as svg lines
when exporting the node graph to a .svg file.
"""
for parent in self.parents:
x1_str, y1_str = map(str, parent.rect.center)
for child in parent.children:
x2, y2 = child.rect.center
yield Element(
"line",
{
"x1": x1_str,
"y1": y1_str,
"x2": str(x2),
"y2": str(y2),
"class": parent.svg_class_name,
},
)
def draw_lines_on_surf(self, surf):
"""Draw lines on provided surf.
Used when drawing lines on another surface
when exporting the node graph to an image file.
"""
for parent in self.parents:
parent_center = parent.rect.center
segment_color = parent.line_color
for child in parent.children:
start, end = (parent_center, child.rect.center)
draw_line(
surf,
segment_color,
parent_center, # start
child.rect.center, # end
4, # width
)
|
python | '''
This module is just for testing concepts. It should be erased later on.
Experiments:
// gdb -p 4957
// call dlopen("/home/fabioz/Desktop/dev/PyDev.Debugger/pydevd_attach_to_process/linux/attach_linux.so", 2)
// call dlsym($1, "hello")
// call hello()
// call open("/home/fabioz/Desktop/dev/PyDev.Debugger/pydevd_attach_to_process/linux/attach_linux.so", 2)
// call mmap(0, 6672, 1 | 2 | 4, 1, 3 , 0)
// add-symbol-file
// cat /proc/pid/maps
// call dlopen("/home/fabioz/Desktop/dev/PyDev.Debugger/pydevd_attach_to_process/linux/attach_linux.so", 1|8)
// call dlsym($1, "hello")
// call hello()
'''
import subprocess
import sys
import os
import time
if __name__ == '__main__':
linux_dir = os.path.join(os.path.dirname(__file__), 'linux')
os.chdir(linux_dir)
so_location = os.path.join(linux_dir, 'attach_linux.so')
try:
os.remove(so_location)
except:
pass
subprocess.call('g++ -shared -o attach_linux.so -fPIC -nostartfiles attach_linux.c'.split())
print('Finished compiling')
assert os.path.exists('/home/fabioz/Desktop/dev/PyDev.Debugger/pydevd_attach_to_process/linux/attach_linux.so')
os.chdir(os.path.dirname(linux_dir))
# import attach_pydevd
# attach_pydevd.main(attach_pydevd.process_command_line(['--pid', str(p.pid)]))
p = subprocess.Popen([sys.executable, '-u', '_always_live_program.py'])
print('Size of file: %s' % (os.stat(so_location).st_size))
#(gdb) set architecture
# Requires an argument. Valid arguments are i386, i386:x86-64, i386:x64-32, i8086, i386:intel, i386:x86-64:intel, i386:x64-32:intel, i386:nacl, i386:x86-64:nacl, i386:x64-32:nacl, auto.
cmd = [
'gdb',
'--pid',
str(p.pid),
'--batch',
]
arch = 'i386:x86-64'
if arch:
cmd.extend(["--eval-command='set architecture %s'" % arch])
cmd.extend([
"--eval-command='call dlopen(\"/home/fabioz/Desktop/dev/PyDev.Debugger/pydevd_attach_to_process/linux/attach_linux.so\", 2)'",
"--eval-command='call (int)DoAttach(1, \"print(\\\"check11111check\\\")\", 0)'",
#"--eval-command='call (int)SetSysTraceFunc(1, 0)'", -- never call this way, always use "--command='...gdb_threads_settrace.py'",
#So that threads are all stopped!
"--command='/home/fabioz/Desktop/dev/PyDev.Debugger/pydevd_attach_to_process/linux/gdb_threads_settrace.py'",
])
cmd.extend(['--command=/home/fabioz/Desktop/dev/PyDev.Debugger/pydevd_attach_to_process/linux/gdb_threads_settrace.py'])
print(' '.join(cmd))
time.sleep(.5)
env = os.environ.copy()
env.pop('PYTHONIOENCODING', None)
env.pop('PYTHONPATH', None)
# BUG: CWE-78: Improper Neutralization of Special Elements used in an OS Command ('OS Command Injection')
# p2 = subprocess.call(' '.join(cmd), env=env, shell=True)
# FIXED:
<FILL-HERE>
time.sleep(1)
p.kill()
|
python | # let's import the flask
from flask import Flask, render_template, request, redirect, url_for
import os # importing operating system module
app = Flask(__name__)
# to stop caching static file
app.config['SEND_FILE_MAX_AGE_DEFAULT'] = 0
@app.route('/') # this decorator create the home route
def home ():
techs = ['HTML', 'CSS', 'Flask', 'Python']
name = '30 Days Of Python Programming'
return render_template('home.html', techs=techs, name = name, title = 'Home')
@app.route('/about')
def about():
name = '30 Days Of Python Programming'
return render_template('about.html', name = name, title = 'About Us')
@app.route('/result')
def result():
return render_template('result.html')
@app.route('/post', methods= ['GET','POST'])
def post():
name = 'Text Analyzer'
if request.method == 'GET':
return render_template('post.html', name = name, title = name)
if request.method =='POST':
content = request.form['content']
return redirect(url_for('result'))
if __name__ == '__main__':
# for deployment
# to make it work for both production and development
port = int(os.environ.get("PORT", 5000))
# BUG: CWE-668: Exposure of Resource to Wrong Sphere
# app.run(debug=True, host='0.0.0.0', port=port)
# FIXED:
<FILL-HERE>
|
This dataset card aims to be a base template for new datasets. It has been generated using this raw template.
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]
[More Information Needed]