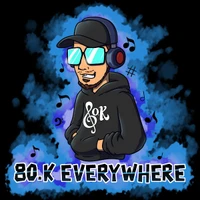
acecalisto3/PhiCo-D-Instruck
Text2Text Generation
•
Updated
•
14
id
stringlengths 14
15
| text
stringlengths 273
1.89k
| source
stringclasses 1
value |
---|---|---|
e4409cd96e13-0 | search
Search
⌘K
dark_mode
light_mode
menu
Home/
Streamlit library/
Get started/
Main concepts
Main concepts
Working with Streamlit is simple. First you sprinkle a few Streamlit commands into a normal Python script, then you run it with streamlit run:
streamlit run your_script.py [-- script args]
Copy
As soon as you run the script as shown above, a local Streamlit server will spin up and your app will open in a new tab in your default web browser. The app is your canvas, where you'll draw charts, text, widgets, tables, and more.
What gets drawn in the app is up to you. For example st.text writes raw text to your app, and st.line_chart draws — you guessed it — a line chart. Refer to our API documentation to see all commands that are available to you.
push_pin
Note
When passing your script some custom arguments, they must be passed after two dashes. Otherwise the arguments get interpreted as arguments to Streamlit itself.
Another way of running Streamlit is to run it as a Python module. This can be useful when configuring an IDE like PyCharm to work with Streamlit:
# Running
python -m streamlit run your_script.py
# is equivalent to:
streamlit run your_script.py
Copy
star
Tip
You can also pass a URL to streamlit run! This is great when combined with GitHub Gists. For example:
streamlit run https://raw.githubusercontent.com/streamlit/demo-uber-nyc-pickups/master/streamlit_app.py
Copy
Development flow | streamlit-docs.txt |
e4409cd96e13-1 | Every time you want to update your app, save the source file. When you do that, Streamlit detects if there is a change and asks you whether you want to rerun your app. Choose "Always rerun" at the top-right of your screen to automatically update your app every time you change its source code.
This allows you to work in a fast interactive loop: you type some code, save it, try it out live, then type some more code, save it, try it out, and so on until you're happy with the results. This tight loop between coding and viewing results live is one of the ways Streamlit makes your life easier.
star
Tip
While developing a Streamlit app, it's recommended to lay out your editor and browser windows side by side, so the code and the app can be seen at the same time. Give it a try!
As of Streamlit version 1.10.0 and higher, Streamlit apps cannot be run from the root directory of Linux distributions. If you try to run a Streamlit app from the root directory, Streamlit will throw a FileNotFoundError: [Errno 2] No such file or directory error. For more information, see GitHub issue #5239.
If you are using Streamlit version 1.10.0 or higher, your main script should live in a directory other than the root directory. When using Docker, you can use the WORKDIR command to specify the directory where your main script lives. For an example of how to do this, read Create a Dockerfile.
Data flow
Streamlit's architecture allows you to write apps the same way you write plain Python scripts. To unlock this, Streamlit apps have a unique data flow: any time something must be updated on the screen, Streamlit reruns your entire Python script from top to bottom.
This can happen in two situations:
Whenever you modify your app's source code. | streamlit-docs.txt |
e4409cd96e13-2 | This can happen in two situations:
Whenever you modify your app's source code.
Whenever a user interacts with widgets in the app. For example, when dragging a slider, entering text in an input box, or clicking a button.
Whenever a callback is passed to a widget via the on_change (or on_click) parameter, the callback will always run before the rest of your script. For details on the Callbacks API, please refer to our Session State API Reference Guide.
And to make all of this fast and seamless, Streamlit does some heavy lifting for you behind the scenes. A big player in this story is the @st.cache_data decorator, which allows developers to skip certain costly computations when their apps rerun. We'll cover caching later in this page.
Display and style data
There are a few ways to display data (tables, arrays, data frames) in Streamlit apps. Below, you will be introduced to magic and st.write(), which can be used to write anything from text to tables. After that, let's take a look at methods designed specifically for visualizing data.
Use magic
You can also write to your app without calling any Streamlit methods. Streamlit supports "magic commands," which means you don't have to use st.write() at all! To see this in action try this snippet:
"""
# My first app
Here's our first attempt at using data to create a table:
"""
import streamlit as st
import pandas as pd
df = pd.DataFrame({
'first column': [1, 2, 3, 4],
'second column': [10, 20, 30, 40]
})
df
Copy
Any time that Streamlit sees a variable or a literal value on its own line, it automatically writes that to your app using st.write(). For more information, refer to the documentation on magic commands.
Write a data frame | streamlit-docs.txt |
e4409cd96e13-3 | Write a data frame
Along with magic commands, st.write() is Streamlit's "Swiss Army knife". You can pass almost anything to st.write(): text, data, Matplotlib figures, Altair charts, and more. Don't worry, Streamlit will figure it out and render things the right way.
import streamlit as st
import pandas as pd
st.write("Here's our first attempt at using data to create a table:")
st.write(pd.DataFrame({
'first column': [1, 2, 3, 4],
'second column': [10, 20, 30, 40]
}))
Copy
There are other data specific functions like st.dataframe() and st.table() that you can also use for displaying data. Let's understand when to use these features and how to add colors and styling to your data frames.
You might be asking yourself, "why wouldn't I always use st.write()?" There are a few reasons:
Magic and st.write() inspect the type of data that you've passed in, and then decide how to best render it in the app. Sometimes you want to draw it another way. For example, instead of drawing a dataframe as an interactive table, you may want to draw it as a static table by using st.table(df).
The second reason is that other methods return an object that can be used and modified, either by adding data to it or replacing it.
Finally, if you use a more specific Streamlit method you can pass additional arguments to customize its behavior.
For example, let's create a data frame and change its formatting with a Pandas Styler object. In this example, you'll use Numpy to generate a random sample, and the st.dataframe() method to draw an interactive table.
push_pin
Note | streamlit-docs.txt |
e4409cd96e13-4 | push_pin
Note
This example uses Numpy to generate a random sample, but you can use Pandas DataFrames, Numpy arrays, or plain Python arrays.
import streamlit as st
import numpy as np
dataframe = np.random.randn(10, 20)
st.dataframe(dataframe)
Copy
Let's expand on the first example using the Pandas Styler object to highlight some elements in the interactive table.
import streamlit as st
import numpy as np
import pandas as pd
dataframe = pd.DataFrame(
np.random.randn(10, 20),
columns=('col %d' % i for i in range(20)))
st.dataframe(dataframe.style.highlight_max(axis=0))
Copy
Streamlit also has a method for static table generation: st.table().
import streamlit as st
import numpy as np
import pandas as pd
dataframe = pd.DataFrame(
np.random.randn(10, 20),
columns=('col %d' % i for i in range(20)))
st.table(dataframe)
Copy
Draw charts and maps
Streamlit supports several popular data charting libraries like Matplotlib, Altair, deck.gl, and more. In this section, you'll add a bar chart, line chart, and a map to your app.
Draw a line chart
You can easily add a line chart to your app with st.line_chart(). We'll generate a random sample using Numpy and then chart it.
import streamlit as st
import numpy as np
import pandas as pd
chart_data = pd.DataFrame(
np.random.randn(20, 3),
columns=['a', 'b', 'c'])
st.line_chart(chart_data)
Copy
Plot a map
With st.map() you can display data points on a map. Let's use Numpy to generate some sample data and plot it on a map of San Francisco. | streamlit-docs.txt |
e4409cd96e13-5 | import streamlit as st
import numpy as np
import pandas as pd
map_data = pd.DataFrame(
np.random.randn(1000, 2) / [50, 50] + [37.76, -122.4],
columns=['lat', 'lon'])
st.map(map_data)
Copy
Widgets
When you've got the data or model into the state that you want to explore, you can add in widgets like st.slider(), st.button() or st.selectbox(). It's really straightforward — treat widgets as variables:
import streamlit as st
x = st.slider('x') # 👈 this is a widget
st.write(x, 'squared is', x * x)
Copy
On first run, the app above should output the text "0 squared is 0". Then every time a user interacts with a widget, Streamlit simply reruns your script from top to bottom, assigning the current state of the widget to your variable in the process.
For example, if the user moves the slider to position 10, Streamlit will rerun the code above and set x to 10 accordingly. So now you should see the text "10 squared is 100".
Widgets can also be accessed by key, if you choose to specify a string to use as the unique key for the widget:
import streamlit as st
st.text_input("Your name", key="name")
# You can access the value at any point with:
st.session_state.name
Copy
Every widget with a key is automatically added to Session State. For more information about Session State, its association with widget state, and its limitations, see Session State API Reference Guide.
Use checkboxes to show/hide data | streamlit-docs.txt |
e4409cd96e13-6 | Use checkboxes to show/hide data
One use case for checkboxes is to hide or show a specific chart or section in an app. st.checkbox() takes a single argument, which is the widget label. In this sample, the checkbox is used to toggle a conditional statement.
import streamlit as st
import numpy as np
import pandas as pd
if st.checkbox('Show dataframe'):
chart_data = pd.DataFrame(
np.random.randn(20, 3),
columns=['a', 'b', 'c'])
chart_data
Copy
Use a selectbox for options
Use st.selectbox to choose from a series. You can write in the options you want, or pass through an array or data frame column.
Let's use the df data frame we created earlier.
import streamlit as st
import pandas as pd
df = pd.DataFrame({
'first column': [1, 2, 3, 4],
'second column': [10, 20, 30, 40]
})
option = st.selectbox(
'Which number do you like best?',
df['first column'])
'You selected: ', option
Copy
Layout
Streamlit makes it easy to organize your widgets in a left panel sidebar with st.sidebar. Each element that's passed to st.sidebar is pinned to the left, allowing users to focus on the content in your app while still having access to UI controls.
For example, if you want to add a selectbox and a slider to a sidebar, use st.sidebar.slider and st.sidebar.selectbox instead of st.slider and st.selectbox:
import streamlit as st
# Add a selectbox to the sidebar:
add_selectbox = st.sidebar.selectbox(
'How would you like to be contacted?',
('Email', 'Home phone', 'Mobile phone')
) | streamlit-docs.txt |
e4409cd96e13-7 | # Add a slider to the sidebar:
add_slider = st.sidebar.slider(
'Select a range of values',
0.0, 100.0, (25.0, 75.0)
)
Copy
Beyond the sidebar, Streamlit offers several other ways to control the layout of your app. st.columns lets you place widgets side-by-side, and st.expander lets you conserve space by hiding away large content.
import streamlit as st
left_column, right_column = st.columns(2)
# You can use a column just like st.sidebar:
left_column.button('Press me!')
# Or even better, call Streamlit functions inside a "with" block:
with right_column:
chosen = st.radio(
'Sorting hat',
("Gryffindor", "Ravenclaw", "Hufflepuff", "Slytherin"))
st.write(f"You are in {chosen} house!")
Copy
push_pin
Note
st.echo and st.spinner are not currently supported inside the sidebar or layout options. Rest assured, though, we're currently working on adding support for those too!
Show progress
When adding long running computations to an app, you can use st.progress() to display status in real time.
First, let's import time. We're going to use the time.sleep() method to simulate a long running computation:
import time
Copy
Now, let's create a progress bar:
import streamlit as st
import time
'Starting a long computation...'
# Add a placeholder
latest_iteration = st.empty()
bar = st.progress(0)
for i in range(100):
# Update the progress bar with each iteration.
latest_iteration.text(f'Iteration {i+1}')
bar.progress(i + 1)
time.sleep(0.1)
'...and now we\'re done!'
Copy
Themes | streamlit-docs.txt |
e4409cd96e13-8 | '...and now we\'re done!'
Copy
Themes
Streamlit supports Light and Dark themes out of the box. Streamlit will first check if the user viewing an app has a Light or Dark mode preference set by their operating system and browser. If so, then that preference will be used. Otherwise, the Light theme is applied by default.
You can also change the active theme from "☰" → "Settings".
Want to add your own theme to an app? The "Settings" menu has a theme editor accessible by clicking on "Edit active theme". You can use this editor to try out different colors and see your app update live.
When you're happy with your work, themes can be saved by setting config options in the [theme] config section. After you've defined a theme for your app, it will appear as "Custom Theme" in the theme selector and will be applied by default instead of the included Light and Dark themes.
More information about the options available when defining a theme can be found in the theme option documentation.
push_pin
Note
The theme editor menu is available only in local development. If you've deployed your app using Streamlit Community Cloud, the "Edit active theme" button will no longer be displayed in the "Settings" menu.
star
Tip
Another way to experiment with different theme colors is to turn on the "Run on save" option, edit your config.toml file, and watch as your app reruns with the new theme colors applied.
Caching
The Streamlit cache allows your app to stay performant even when loading data from the web, manipulating large datasets, or performing expensive computations.
The basic idea behind caching is to store the results of expensive function calls and return the cached result when the same inputs occur again rather than calling the function on subsequent runs. | streamlit-docs.txt |
e4409cd96e13-9 | To cache a function in Streamlit, you need to decorate it with one of two decorators (st.cache_data and st.cache_resource):
@st.cache_data
def long_running_function(param1, param2):
return …
Copy
In this example, decorating long_running_function with @st.cache_data tells Streamlit that whenever the function is called, it checks two things:
The values of the input parameters (in this case, param1 and param2).
The code inside the function.
If this is the first time Streamlit sees these parameter values and function code, it runs the function and stores the return value in a cache. The next time the function is called with the same parameters and code (e.g., when a user interacts with the app), Streamlit will skip executing the function altogether and return the cached value instead. During development, the cache updates automatically as the function code changes, ensuring that the latest changes are reflected in the cache.
As mentioned, there are two caching decorators:
st.cache_data is the recommended way to cache computations that return data: loading a DataFrame from CSV, transforming a NumPy array, querying an API, or any other function that returns a serializable data object (str, int, float, DataFrame, array, list, …). It creates a new copy of the data at each function call, making it safe against mutations and race conditions. The behavior of st.cache_data is what you want in most cases – so if you're unsure, start with st.cache_data and see if it works!
st.cache_resource is the recommended way to cache global resources like ML models or database connections – unserializable objects that you don’t want to load multiple times. Using it, you can share these resources across all reruns and sessions of an app without copying or duplication. Note that any mutations to the cached return value directly mutate the object in the cache (more details below). | streamlit-docs.txt |
e4409cd96e13-10 | Streamlit's two caching decorators and their use cases.
For more information about the Streamlit caching decorators, their configuration parameters, and their limitations, see Caching.
Pages
As apps grow large, it becomes useful to organize them into multiple pages. This makes the app easier to manage as a developer and easier to navigate as a user. Streamlit provides a frictionless way to create multipage apps.
We designed this feature so that building a multipage app is as easy as building a single-page app! Just add more pages to an existing app as follows:
In the folder containing your main script, create a new pages folder. Let’s say your main script is named main_page.py.
Add new .py files in the pages folder to add more pages to your app.
Run streamlit run main_page.py as usual.
That’s it! The main_page.py script will now correspond to the main page of your app. And you’ll see the other scripts from the pages folder in the sidebar page selector. For example:
main_page.py
import streamlit as st
st.markdown("# Main page 🎈")
st.sidebar.markdown("# Main page 🎈")
Copy
pages/page_2.py
import streamlit as st
st.markdown("# Page 2 ❄️")
st.sidebar.markdown("# Page 2 ❄️")
Copy
pages/page_3.py
import streamlit as st
st.markdown("# Page 3 🎉")
st.sidebar.markdown("# Page 3 🎉")
Copy
Now run streamlit run main_page.py and view your shiny new multipage app!
Our documentation on Multipage apps teaches you how to add pages to your app, including how to define pages, structure and run multipage apps, and navigate between pages. Once you understand the basics, create your first multipage app!
App model | streamlit-docs.txt |
e4409cd96e13-11 | App model
Now that you know a little more about all the individual pieces, let's close the loop and review how it works together:
Streamlit apps are Python scripts that run from top to bottom
Every time a user opens a browser tab pointing to your app, the script is re-executed
As the script executes, Streamlit draws its output live in a browser
Scripts use the Streamlit cache to avoid recomputing expensive functions, so updates happen very fast
Every time a user interacts with a widget, your script is re-executed and the output value of that widget is set to the new value during that run.
Streamlit apps can contain multiple pages, which are defined in separate .py files in a pages folder.
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Installation
Next:
Create an app
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Main concepts - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
Get started/
Create an app
Create an app
If you've made it this far, chances are you've installed Streamlit and run through the basics in our Main concepts guide. If not, now is a good time to take a look. | streamlit-docs.txt |
e4409cd96e13-12 | The easiest way to learn how to use Streamlit is to try things out yourself. As you read through this guide, test each method. As long as your app is running, every time you add a new element to your script and save, Streamlit's UI will ask if you'd like to rerun the app and view the changes. This allows you to work in a fast interactive loop: you write some code, save it, review the output, write some more, and so on, until you're happy with the results. The goal is to use Streamlit to create an interactive app for your data or model and along the way to use Streamlit to review, debug, perfect, and share your code.
In this guide, you're going to use Streamlit's core features to create an interactive app; exploring a public Uber dataset for pickups and drop-offs in New York City. When you're finished, you'll know how to fetch and cache data, draw charts, plot information on a map, and use interactive widgets, like a slider, to filter results.
star
Tip
If you'd like to skip ahead and see everything at once, the complete script is available below.
Create your first app
Streamlit is more than just a way to make data apps, it’s also a community of creators that share their apps and ideas and help each other make their work better. Please come join us on the community forum. We love to hear your questions, ideas, and help you work through your bugs — stop by today!
The first step is to create a new Python script. Let's call it uber_pickups.py.
Open uber_pickups.py in your favorite IDE or text editor, then add these lines:
import streamlit as st
import pandas as pd
import numpy as np
Copy
Every good app has a title, so let's add one:
st.title('Uber pickups in NYC')
Copy | streamlit-docs.txt |
e4409cd96e13-13 | st.title('Uber pickups in NYC')
Copy
Now it's time to run Streamlit from the command line:
streamlit run uber_pickups.py
Copy
Running a Streamlit app is no different than any other Python script. Whenever you need to view the app, you can use this command.
star
Tip
Did you know you can also pass a URL to streamlit run? This is great when combined with GitHub Gists. For example:
streamlit run https://raw.githubusercontent.com/streamlit/demo-uber-nyc-pickups/master/streamlit_app.py
Copy
As usual, the app should automatically open in a new tab in your browser.
Fetch some data
Now that you have an app, the next thing you'll need to do is fetch the Uber dataset for pickups and drop-offs in New York City.
Let's start by writing a function to load the data. Add this code to your script:
DATE_COLUMN = 'date/time'
DATA_URL = ('https://s3-us-west-2.amazonaws.com/'
'streamlit-demo-data/uber-raw-data-sep14.csv.gz')
def load_data(nrows):
data = pd.read_csv(DATA_URL, nrows=nrows)
lowercase = lambda x: str(x).lower()
data.rename(lowercase, axis='columns', inplace=True)
data[DATE_COLUMN] = pd.to_datetime(data[DATE_COLUMN])
return data
Copy
You'll notice that load_data is a plain old function that downloads some data, puts it in a Pandas dataframe, and converts the date column from text to datetime. The function accepts a single parameter (nrows), which specifies the number of rows that you want to load into the dataframe.
Now let's test the function and review the output. Below your function, add these lines: | streamlit-docs.txt |
e4409cd96e13-14 | Now let's test the function and review the output. Below your function, add these lines:
# Create a text element and let the reader know the data is loading.
data_load_state = st.text('Loading data...')
# Load 10,000 rows of data into the dataframe.
data = load_data(10000)
# Notify the reader that the data was successfully loaded.
data_load_state.text('Loading data...done!')
Copy
You'll see a few buttons in the upper-right corner of your app asking if you'd like to rerun the app. Choose Always rerun, and you'll see your changes automatically each time you save.
Ok, that's underwhelming...
It turns out that it takes a long time to download data, and load 10,000 lines into a dataframe. Converting the date column into datetime isn’t a quick job either. You don’t want to reload the data each time the app is updated – luckily Streamlit allows you to cache the data.
Effortless caching
Try adding @st.cache_data before the load_data declaration:
@st.cache_data
def load_data(nrows):
Copy
Then save the script, and Streamlit will automatically rerun your app. Since this is the first time you’re running the script with @st.cache_data, you won't see anything change. Let’s tweak your file a little bit more so that you can see the power of caching.
Replace the line data_load_state.text('Loading data...done!') with this:
data_load_state.text("Done! (using st.cache_data)")
Copy
Now save. See how the line you added appeared immediately? If you take a step back for a second, this is actually quite amazing. Something magical is happening behind the scenes, and it only takes one line of code to activate it.
How's it work?
Let's take a few minutes to discuss how @st.cache_data actually works. | streamlit-docs.txt |
e4409cd96e13-15 | Let's take a few minutes to discuss how @st.cache_data actually works.
When you mark a function with Streamlit’s cache annotation, it tells Streamlit that whenever the function is called that it should check two things:
The input parameters you used for the function call.
The code inside the function.
If this is the first time Streamlit has seen both these items, with these exact values, and in this exact combination, it runs the function and stores the result in a local cache. The next time the function is called, if the two values haven't changed, then Streamlit knows it can skip executing the function altogether. Instead, it reads the output from the local cache and passes it on to the caller -- like magic.
"But, wait a second," you’re saying to yourself, "this sounds too good to be true. What are the limitations of all this awesomesauce?"
Well, there are a few:
Streamlit will only check for changes within the current working directory. If you upgrade a Python library, Streamlit's cache will only notice this if that library is installed inside your working directory.
If your function is not deterministic (that is, its output depends on random numbers), or if it pulls data from an external time-varying source (for example, a live stock market ticker service) the cached value will be none-the-wiser.
Lastly, you should avoid mutating the output of a function cached with st.cache_data since cached values are stored by reference.
While these limitations are important to keep in mind, they tend not to be an issue a surprising amount of the time. Those times, this cache is really transformational.
star
Tip
Whenever you have a long-running computation in your code, consider refactoring it so you can use @st.cache_data, if possible. Please read Caching for more details. | streamlit-docs.txt |
e4409cd96e13-16 | Now that you know how caching with Streamlit works, let’s get back to the Uber pickup data.
Inspect the raw data
It's always a good idea to take a look at the raw data you're working with before you start working with it. Let's add a subheader and a printout of the raw data to the app:
st.subheader('Raw data')
st.write(data)
Copy
In the Main concepts guide you learned that st.write will render almost anything you pass to it. In this case, you're passing in a dataframe and it's rendering as an interactive table.
st.write tries to do the right thing based on the data type of the input. If it isn't doing what you expect you can use a specialized command like st.dataframe instead. For a full list, see API reference.
Draw a histogram
Now that you've had a chance to take a look at the dataset and observe what's available, let's take things a step further and draw a histogram to see what Uber's busiest hours are in New York City.
To start, let's add a subheader just below the raw data section:
st.subheader('Number of pickups by hour')
Copy
Use NumPy to generate a histogram that breaks down pickup times binned by hour:
hist_values = np.histogram(
data[DATE_COLUMN].dt.hour, bins=24, range=(0,24))[0]
Copy
Now, let's use Streamlit's st.bar_chart() method to draw this histogram.
st.bar_chart(hist_values)
Copy
Save your script. This histogram should show up in your app right away. After a quick review, it looks like the busiest time is 17:00 (5 P.M.). | streamlit-docs.txt |
e4409cd96e13-17 | To draw this diagram we used Streamlit's native bar_chart() method, but it's important to know that Streamlit supports more complex charting libraries like Altair, Bokeh, Plotly, Matplotlib and more. For a full list, see supported charting libraries.
Plot data on a map
Using a histogram with Uber's dataset helped us determine what the busiest times are for pickups, but what if we wanted to figure out where pickups were concentrated throughout the city. While you could use a bar chart to show this data, it wouldn't be easy to interpret unless you were intimately familiar with latitudinal and longitudinal coordinates in the city. To show pickup concentration, let's use Streamlit st.map() function to overlay the data on a map of New York City.
Add a subheader for the section:
st.subheader('Map of all pickups')
Copy
Use the st.map() function to plot the data:
st.map(data)
Copy
Save your script. The map is fully interactive. Give it a try by panning or zooming in a bit.
After drawing your histogram, you determined that the busiest hour for Uber pickups was 17:00. Let's redraw the map to show the concentration of pickups at 17:00.
Locate the following code snippet:
st.subheader('Map of all pickups')
st.map(data)
Copy
Replace it with:
hour_to_filter = 17
filtered_data = data[data[DATE_COLUMN].dt.hour == hour_to_filter]
st.subheader(f'Map of all pickups at {hour_to_filter}:00')
st.map(filtered_data)
Copy
You should see the data update instantly.
To draw this map we used the st.map function that's built into Streamlit, but if you'd like to visualize complex map data, we encourage you to take a look at the st.pydeck_chart.
Filter results with a slider | streamlit-docs.txt |
e4409cd96e13-18 | Filter results with a slider
In the last section, when you drew the map, the time used to filter results was hardcoded into the script, but what if we wanted to let a reader dynamically filter the data in real time? Using Streamlit's widgets you can. Let's add a slider to the app with the st.slider() method.
Locate hour_to_filter and replace it with this code snippet:
hour_to_filter = st.slider('hour', 0, 23, 17) # min: 0h, max: 23h, default: 17h
Copy
Use the slider and watch the map update in real time.
Use a button to toggle data
Sliders are just one way to dynamically change the composition of your app. Let's use the st.checkbox function to add a checkbox to your app. We'll use this checkbox to show/hide the raw data table at the top of your app.
Locate these lines:
st.subheader('Raw data')
st.write(data)
Copy
Replace these lines with the following code:
if st.checkbox('Show raw data'):
st.subheader('Raw data')
st.write(data)
Copy
We're sure you've got your own ideas. When you're done with this tutorial, check out all the widgets that Streamlit exposes in our API Reference.
Let's put it all together
That's it, you've made it to the end. Here's the complete script for our interactive app.
star
Tip
If you've skipped ahead, after you've created your script, the command to run Streamlit is streamlit run [app name].
import streamlit as st
import pandas as pd
import numpy as np
st.title('Uber pickups in NYC') | streamlit-docs.txt |
e4409cd96e13-19 | st.title('Uber pickups in NYC')
DATE_COLUMN = 'date/time'
DATA_URL = ('https://s3-us-west-2.amazonaws.com/'
'streamlit-demo-data/uber-raw-data-sep14.csv.gz')
@st.cache_data
def load_data(nrows):
data = pd.read_csv(DATA_URL, nrows=nrows)
lowercase = lambda x: str(x).lower()
data.rename(lowercase, axis='columns', inplace=True)
data[DATE_COLUMN] = pd.to_datetime(data[DATE_COLUMN])
return data
data_load_state = st.text('Loading data...')
data = load_data(10000)
data_load_state.text("Done! (using st.cache_data)")
if st.checkbox('Show raw data'):
st.subheader('Raw data')
st.write(data)
st.subheader('Number of pickups by hour')
hist_values = np.histogram(data[DATE_COLUMN].dt.hour, bins=24, range=(0,24))[0]
st.bar_chart(hist_values)
# Some number in the range 0-23
hour_to_filter = st.slider('hour', 0, 23, 17)
filtered_data = data[data[DATE_COLUMN].dt.hour == hour_to_filter]
st.subheader('Map of all pickups at %s:00' % hour_to_filter)
st.map(filtered_data)
Copy
Share your app
After you’ve built a Streamlit app, it's time to share it! To show it off to the world you can use Streamlit Community Cloud to deploy, manage, and share your app for free.
It works in 3 simple steps:
Put your app in a public GitHub repo (and make sure it has a requirements.txt!)
Sign into share.streamlit.io
Click 'Deploy an app' and then paste in your GitHub URL | streamlit-docs.txt |
e4409cd96e13-20 | That's it! 🎈 You now have a publicly deployed app that you can share with the world. Click to learn more about how to use Streamlit Community Cloud.
Get help
That's it for getting started, now you can go and build your own apps! If you run into difficulties here are a few things you can do.
Check out our community forum and post a question
Quick help from command line with streamlit help
Go through our Knowledge Base for tips, step-by-step tutorials, and articles that answer your questions about creating and deploying Streamlit apps.
Read more documentation! Check out:
Advanced features for things like caching, theming, and adding statefulness to apps.
API reference for examples of every Streamlit command.
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Main concepts
Next:
Multipage apps
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Create an app - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Knowledge base/
Tutorials/
Connect to data sources/
Google Cloud Storage
Connect Streamlit to Google Cloud Storage
Introduction
This guide explains how to securely access files on Google Cloud Storage from Streamlit Community Cloud. It uses the google-cloud-storage library and Streamlit's secrets management.
Create a Google Cloud Storage bucket and add a file
push_pin
Note
If you already have a bucket that you want to use, feel free to skip to the next step.
First, sign up for Google Cloud Platform or log in. Go to the Google Cloud Storage console and create a new bucket.
Navigate to the upload section of your new bucket: | streamlit-docs.txt |
e4409cd96e13-21 | Navigate to the upload section of your new bucket:
And upload the following CSV file, which contains some example data:
myfile.csv
Enable the Google Cloud Storage API
The Google Cloud Storage API is enabled by default when you create a project through the Google Cloud Console or CLI. Feel free to skip to the next step.
If you do need to enable the API for programmatic access in your project, head over to the APIs & Services dashboard (select or create a project if asked). Search for the Cloud Storage API and enable it. The screenshot below has a blue "Manage" button and indicates the "API is enabled" which means no further action needs to be taken. This is very likely what you have since the API is enabled by default. However, if that is not what you see and you have an "Enable" button, you'll need to enable the API:
Create a service account and key file
To use the Google Cloud Storage API from Streamlit Community Cloud, you need a Google Cloud Platform service account (a special type for programmatic data access). Go to the Service Accounts page and create an account with Viewer permission.
push_pin
Note
If the button CREATE SERVICE ACCOUNT is gray, you don't have the correct permissions. Ask the admin of your Google Cloud project for help.
After clicking DONE, you should be back on the service accounts overview. Create a JSON key file for the new account and download it:
Add the key to your local app secrets
Your local Streamlit app will read secrets from a file .streamlit/secrets.toml in your app's root directory. Create this file if it doesn't exist yet and add the access key to it as shown below:
# .streamlit/secrets.toml | streamlit-docs.txt |
e4409cd96e13-22 | # .streamlit/secrets.toml
[gcp_service_account]
type = "service_account"
project_id = "xxx"
private_key_id = "xxx"
private_key = "xxx"
client_email = "xxx"
client_id = "xxx"
auth_uri = "https://accounts.google.com/o/oauth2/auth"
token_uri = "https://oauth2.googleapis.com/token"
auth_provider_x509_cert_url = "https://www.googleapis.com/oauth2/v1/certs"
client_x509_cert_url = "xxx"
Copy
priority_high
Important
Add this file to .gitignore and don't commit it to your GitHub repo!
Copy your app secrets to the cloud
As the secrets.toml file above is not committed to GitHub, you need to pass its content to your deployed app (on Streamlit Community Cloud) separately. Go to the app dashboard and in the app's dropdown menu, click on Edit Secrets. Copy the content of secrets.toml into the text area. More information is available at Secrets Management.
Add google-cloud-storage to your requirements file
Add the google-cloud-storage package to your requirements.txt file, preferably pinning its version (replace x.x.x with the version you want installed):
# requirements.txt
google-cloud-storage==x.x.x
Copy
Write your Streamlit app
Copy the code below to your Streamlit app and run it. Make sure to adapt the name of your bucket and file. Note that Streamlit automatically turns the access keys from your secrets file into environment variables.
# streamlit_app.py
import streamlit as st
from google.oauth2 import service_account
from google.cloud import storage
# Create API client.
credentials = service_account.Credentials.from_service_account_info(
st.secrets["gcp_service_account"]
)
client = storage.Client(credentials=credentials) | streamlit-docs.txt |
e4409cd96e13-23 | # Retrieve file contents.
# Uses st.cache_data to only rerun when the query changes or after 10 min.
@st.cache_data(ttl=600)
def read_file(bucket_name, file_path):
bucket = client.bucket(bucket_name)
content = bucket.blob(file_path).download_as_string().decode("utf-8")
return content
bucket_name = "streamlit-bucket"
file_path = "myfile.csv"
content = read_file(bucket_name, file_path)
# Print results.
for line in content.strip().split("\n"):
name, pet = line.split(",")
st.write(f"{name} has a :{pet}:")
Copy
See st.cache_data above? Without it, Streamlit would run the query every time the app reruns (e.g. on a widget interaction). With st.cache_data, it only runs when the query changes or after 10 minutes (that's what ttl is for). Watch out: If your database updates more frequently, you should adapt ttl or remove caching so viewers always see the latest data. Learn more in Caching.
If everything worked out (and you used the example file given above), your app should look like this:
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Firestore
Next:
Microsoft SQL Server
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Connect Streamlit to Google Cloud Storage - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
Cheat sheet
Cheat Sheet
This is a summary of the docs, as of Streamlit v1.20.0. | streamlit-docs.txt |
e4409cd96e13-24 | This is a summary of the docs, as of Streamlit v1.20.0.
Install & Import
streamlit run first_app.py
# Import convention
>>> import streamlit as st
Command line
streamlit --help
streamlit run your_script.py
streamlit hello
streamlit config show
streamlit cache clear
streamlit docs
streamlit --version
Pre-release features
pip uninstall streamlit
pip install streamlit-nightly --upgrade
Learn more about experimental features
Magic commands
# Magic commands implicitly
# call st.write().
'_This_ is some **Markdown***'
my_variable
'dataframe:', my_data_frame
Display text
st.text('Fixed width text')
st.markdown('_Markdown_') # see *
st.latex(r''' e^{i\pi} + 1 = 0 ''')
st.write('Most objects') # df, err, func, keras!
st.write(['st', 'is <', 3]) # see *
st.title('My title')
st.header('My header')
st.subheader('My sub')
st.code('for i in range(8): foo()')
* optional kwarg unsafe_allow_html = True
Display data
st.dataframe(my_dataframe)
st.table(data.iloc[0:10])
st.json({'foo':'bar','fu':'ba'})
st.metric('My metric', 42, 2)
Display media
st.image('./header.png')
st.audio(data)
st.video(data)
Add widgets to sidebar
# Just add it after st.sidebar:
>>> a = st.sidebar.radio('Select one:', [1, 2]) | streamlit-docs.txt |
e4409cd96e13-25 | # Or use "with" notation:
>>> with st.sidebar:
>>> st.radio('Select one:', [1, 2])
Columns
# Two equal columns:
>>> col1, col2 = st.columns(2)
>>> col1.write("This is column 1")
>>> col2.write("This is column 2")
# Three different columns:
>>> col1, col2, col3 = st.columns([3, 1, 1])
# col1 is larger.
# You can also use "with" notation:
>>> with col1:
>>> st.radio('Select one:', [1, 2])
Tabs
# Insert containers separated into tabs:
>>> tab1, tab2 = st.tabs(["Tab 1", "Tab2"])
>>> tab1.write("this is tab 1")
>>> tab2.write("this is tab 2")
# You can also use "with" notation:
>>> with tab1:
>>> st.radio('Select one:', [1, 2])
Control flow
# Stop execution immediately:
st.stop()
# Rerun script immediately:
st.experimental_rerun() | streamlit-docs.txt |
e4409cd96e13-26 | # Group multiple widgets:
>>> with st.form(key='my_form'):
>>> username = st.text_input('Username')
>>> password = st.text_input('Password')
>>> st.form_submit_button('Login')
Display interactive widgets
st.button('Click me')
st.experimental_data_editor('Edit data', data)
st.checkbox('I agree')
st.radio('Pick one', ['cats', 'dogs'])
st.selectbox('Pick one', ['cats', 'dogs'])
st.multiselect('Buy', ['milk', 'apples', 'potatoes'])
st.slider('Pick a number', 0, 100)
st.select_slider('Pick a size', ['S', 'M', 'L'])
st.text_input('First name')
st.number_input('Pick a number', 0, 10)
st.text_area('Text to translate')
st.date_input('Your birthday')
st.time_input('Meeting time')
st.file_uploader('Upload a CSV')
st.download_button('Download file', data)
st.camera_input("Take a picture")
st.color_picker('Pick a color')
# Use widgets' returned values in variables:
>>> for i in range(int(st.number_input('Num:'))):
>>> foo()
>>> if st.sidebar.selectbox('I:',['f']) == 'f':
>>> b()
>>> my_slider_val = st.slider('Quinn Mallory', 1, 88)
>>> st.write(slider_val)
# Disable widgets to remove interactivity:
>>> st.slider('Pick a number', 0, 100, disabled=True)
Mutate data
# Add rows to a dataframe after
# showing it.
>>> element = st.dataframe(df1)
>>> element.add_rows(df2) | streamlit-docs.txt |
e4409cd96e13-27 | # Add rows to a chart after
# showing it.
>>> element = st.line_chart(df1)
>>> element.add_rows(df2)
Display code
>>> with st.echo():
>>> st.write('Code will be executed and printed')
Placeholders, help, and options
# Replace any single element.
>>> element = st.empty()
>>> element.line_chart(...)
>>> element.text_input(...) # Replaces previous.
# Insert out of order.
>>> elements = st.container()
>>> elements.line_chart(...)
>>> st.write("Hello")
>>> elements.text_input(...) # Appears above "Hello". | streamlit-docs.txt |
e4409cd96e13-28 | st.help(pandas.DataFrame)
st.get_option(key)
st.set_option(key, value)
st.set_page_config(layout='wide')
st.experimental_show(objects)
st.experimental_get_query_params()
st.experimental_set_query_params(**params)
Optimize performance
Cache data objects
# E.g. Dataframe computation, storing downloaded data, etc.
>>> @st.cache_data
... def foo(bar):
... # Do something expensive and return data
... return data
# Executes foo
>>> d1 = foo(ref1)
# Does not execute foo
# Returns cached item by value, d1 == d2
>>> d2 = foo(ref1)
# Different arg, so function foo executes
>>> d3 = foo(ref2)
# Clear all cached entries for this function
>>> foo.clear()
# Clear values from *all* in-memory or on-disk cached functions
>>> st.cache_data.clear()
Cache global resources
# E.g. TensorFlow session, database connection, etc.
>>> @st.cache_resource
... def foo(bar):
... # Create and return a non-data object
... return session
# Executes foo
>>> s1 = foo(ref1)
# Does not execute foo
# Returns cached item by reference, s1 == s2
>>> s2 = foo(ref1)
# Different arg, so function foo executes
>>> s3 = foo(ref2)
# Clear all cached entries for this function
>>> foo.clear()
# Clear all global resources from cache
>>> st.cache_resource.clear()
Deprecated caching
>>> @st.cache
... def foo(bar):
... # Do something expensive in here...
... return data
>>> # Executes foo
>>> d1 = foo(ref1)
>>> # Does not execute foo
>>> # Returns cached item by reference, d1 == d2 | streamlit-docs.txt |
e4409cd96e13-29 | >>> # Does not execute foo
>>> # Returns cached item by reference, d1 == d2
>>> d2 = foo(ref1)
>>> # Different arg, so function foo executes
>>> d3 = foo(ref2)
Display progress and status
>>> with st.spinner(text='In progress'):
>>> time.sleep(5)
>>> st.success('Done') | streamlit-docs.txt |
e4409cd96e13-30 | st.progress(progress_variable_1_to_100)
st.balloons()
st.snow()
st.error('Error message')
st.warning('Warning message')
st.info('Info message')
st.success('Success message')
st.exception(e)
Personalize apps for users
# Show different content based on the user's email address.
>>> if st.user.email == 'jane@email.com':
>>> display_jane_content()
>>> elif st.user.email == 'adam@foocorp.io':
>>> display_adam_content()
>>> else:
>>> st.write("Please contact us to get access!")
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Changelog
Next:
Streamlit Community Cloud
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Cheat sheet - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
Advanced features/
Add statefulness to apps
Add statefulness to apps
What is State?
We define access to a Streamlit app in a browser tab as a session. For each browser tab that connects to the Streamlit server, a new session is created. Streamlit reruns your script from top to bottom every time you interact with your app. Each reruns takes place in a blank slate: no variables are shared between runs.
Session State is a way to share variables between reruns, for each user session. In addition to the ability to store and persist state, Streamlit also exposes the ability to manipulate state using Callbacks. Session state also persists across apps inside a multipage app. | streamlit-docs.txt |
e4409cd96e13-31 | In this guide, we will illustrate the usage of Session State and Callbacks as we build a stateful Counter app.
For details on the Session State and Callbacks API, please refer to our Session State API Reference Guide.
Also, check out this Session State basics tutorial video by Streamlit Developer Advocate Dr. Marisa Smith to get started:
Build a Counter
Let's call our script counter.py. It initializes a count variable and has a button to increment the value stored in the count variable:
import streamlit as st
st.title('Counter Example')
count = 0
increment = st.button('Increment')
if increment:
count += 1
st.write('Count = ', count)
Copy
No matter how many times we press the Increment button in the above app, the count remains at 1. Let's understand why:
Each time we press the Increment button, Streamlit reruns counter.py from top to bottom, and with every run, count gets initialized to 0 .
Pressing Increment subsequently adds 1 to 0, thus count=1 no matter how many times we press Increment.
As we'll see later, we can avoid this issue by storing count as a Session State variable. By doing so, we're indicating to Streamlit that it should maintain the value stored inside a Session State variable across app reruns.
Let's learn more about the API to use Session State.
Initialization
The Session State API follows a field-based API, which is very similar to Python dictionaries:
import streamlit as st
# Check if 'key' already exists in session_state
# If not, then initialize it
if 'key' not in st.session_state:
st.session_state['key'] = 'value'
# Session State also supports the attribute based syntax
if 'key' not in st.session_state:
st.session_state.key = 'value'
Copy
Reads and updates | streamlit-docs.txt |
e4409cd96e13-32 | Read the value of an item in Session State by passing the item to st.write :
import streamlit as st
if 'key' not in st.session_state:
st.session_state['key'] = 'value'
# Reads
st.write(st.session_state.key)
# Outputs: value
Copy
Update an item in Session State by assigning it a value:
import streamlit as st
if 'key' not in st.session_state:
st.session_state['key'] = 'value'
# Updates
st.session_state.key = 'value2' # Attribute API
st.session_state['key'] = 'value2' # Dictionary like API
Copy
Streamlit throws an exception if an uninitialized variable is accessed:
import streamlit as st
st.write(st.session_state['value'])
# Throws an exception!
Copy
Let's now take a look at a few examples that illustrate how to add Session State to our Counter app.
Example 1: Add Session State
Now that we've got a hang of the Session State API, let's update our Counter app to use Session State:
import streamlit as st
st.title('Counter Example')
if 'count' not in st.session_state:
st.session_state.count = 0
increment = st.button('Increment')
if increment:
st.session_state.count += 1
st.write('Count = ', st.session_state.count)
Copy
As you can see in the above example, pressing the Increment button updates the count each time.
Example 2: Session State and Callbacks
Now that we've built a basic Counter app using Session State, let's move on to something a little more complex. The next example uses Callbacks with Session State. | streamlit-docs.txt |
e4409cd96e13-33 | Callbacks: A callback is a Python function which gets called when an input widget changes. Callbacks can be used with widgets using the parameters on_change (or on_click), args, and kwargs. The full Callbacks API can be found in our Session State API Reference Guide.
import streamlit as st
st.title('Counter Example using Callbacks')
if 'count' not in st.session_state:
st.session_state.count = 0
def increment_counter():
st.session_state.count += 1
st.button('Increment', on_click=increment_counter)
st.write('Count = ', st.session_state.count)
Copy
Now, pressing the Increment button updates the count each time by calling the increment_counter() function.
Example 3: Use args and kwargs in Callbacks
Callbacks also support passing arguments using the args parameter in a widget:
import streamlit as st
st.title('Counter Example using Callbacks with args')
if 'count' not in st.session_state:
st.session_state.count = 0
increment_value = st.number_input('Enter a value', value=0, step=1)
def increment_counter(increment_value):
st.session_state.count += increment_value
increment = st.button('Increment', on_click=increment_counter,
args=(increment_value, ))
st.write('Count = ', st.session_state.count)
Copy
Additionally, we can also use the kwargs parameter in a widget to pass named arguments to the callback function as shown below:
import streamlit as st
st.title('Counter Example using Callbacks with kwargs')
if 'count' not in st.session_state:
st.session_state.count = 0
def increment_counter(increment_value=0):
st.session_state.count += increment_value
def decrement_counter(decrement_value=0):
st.session_state.count -= decrement_value
st.button('Increment', on_click=increment_counter,
kwargs=dict(increment_value=5)) | streamlit-docs.txt |
e4409cd96e13-34 | st.button('Increment', on_click=increment_counter,
kwargs=dict(increment_value=5))
st.button('Decrement', on_click=decrement_counter,
kwargs=dict(decrement_value=1))
st.write('Count = ', st.session_state.count)
Copy
Example 4: Forms and Callbacks
Say we now want to not only increment the count, but also store when it was last updated. We illustrate doing this using Callbacks and st.form:
import streamlit as st
import datetime
st.title('Counter Example')
if 'count' not in st.session_state:
st.session_state.count = 0
st.session_state.last_updated = datetime.time(0,0)
def update_counter():
st.session_state.count += st.session_state.increment_value
st.session_state.last_updated = st.session_state.update_time
with st.form(key='my_form'):
st.time_input(label='Enter the time', value=datetime.datetime.now().time(), key='update_time')
st.number_input('Enter a value', value=0, step=1, key='increment_value')
submit = st.form_submit_button(label='Update', on_click=update_counter)
st.write('Current Count = ', st.session_state.count)
st.write('Last Updated = ', st.session_state.last_updated)
Copy
Advanced concepts
Session State and Widget State association
Session State provides the functionality to store variables across reruns. Widget state (i.e. the value of a widget) is also stored in a session.
For simplicity, we have unified this information in one place. i.e. the Session State. This convenience feature makes it super easy to read or write to the widget's state anywhere in the app's code. Session State variables mirror the widget value using the key argument. | streamlit-docs.txt |
e4409cd96e13-35 | We illustrate this with the following example. Let's say we have an app with a slider to represent temperature in Celsius. We can set and get the value of the temperature widget by using the Session State API, as follows:
import streamlit as st
if "celsius" not in st.session_state:
# set the initial default value of the slider widget
st.session_state.celsius = 50.0
st.slider(
"Temperature in Celsius",
min_value=-100.0,
max_value=100.0,
key="celsius"
)
# This will get the value of the slider widget
st.write(st.session_state.celsius)
Copy
There is a limitation to setting widget values using the Session State API.
priority_high
Important
Streamlit does not allow setting widget values via the Session State API for st.button and st.file_uploader.
The following example will raise a StreamlitAPIException on trying to set the state of st.button via the Session State API:
import streamlit as st
if 'my_button' not in st.session_state:
st.session_state.my_button = True
# Streamlit will raise an Exception on trying to set the state of button
st.button('Submit', key='my_button')
Copy
Caveats and limitations
Here are some limitations to keep in mind when using Session State:
Session State exists for as long as the tab is open and connected to the Streamlit server. As soon as you close the tab, everything stored in Session State is lost.
Session State is not persisted. If the Streamlit server crashes, then everything stored in Session State gets wiped
For caveats and limitations with the Session State API, please see the API limitations.
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions? | streamlit-docs.txt |
e4409cd96e13-36 | Our forums are full of helpful information and Streamlit experts.
Previous:
Experimental cache primitives
Next:
Dataframes
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Add statefulness to apps - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
Advanced features/
Dataframes
Dataframes
Dataframes are a great way to display and edit data in a tabular format. Working with Pandas DataFrames and other tabular data structures is key to data science workflows. If developers and data scientists want to display this data in Streamlit, they have multiple options: st.dataframe and st.experimental_data_editor. If you want to solely display data in a table-like UI, st.dataframe is the way to go. If you want to interactively edit data, use st.experimental_data_editor. We explore the use cases and advantages of each option in the following sections.
Display dataframes with st.dataframe
Streamlit can display dataframes in a table-like UI via st.dataframe :
import streamlit as st
import pandas as pd
df = pd.DataFrame(
[
{"command": "st.selectbox", "rating": 4, "is_widget": True},
{"command": "st.balloons", "rating": 5, "is_widget": False},
{"command": "st.time_input", "rating": 3, "is_widget": True},
]
)
st.dataframe(df, use_container_width=True)
Copy
(view standalone Streamlit app)
Additional UI features
st.dataframe also provides some additional functionality by using glide-data-grid under the hood: | streamlit-docs.txt |
e4409cd96e13-37 | st.dataframe also provides some additional functionality by using glide-data-grid under the hood:
Column sorting: sort columns by clicking on their headers.
Column resizing: resize columns by dragging and dropping column header borders.
Table resizing: resize tables by dragging and dropping the bottom right corner.
Search: search through data by clicking a table, using hotkeys (⌘ Cmd + F or Ctrl + F) to bring up the search bar, and using the search bar to filter data.
Copy to clipboard: select one or multiple cells, copy them to the clipboard and paste them into your favorite spreadsheet software.
Try out all the addition UI features using the embedded app from the prior section.
In addition to Pandas DataFrames, st.dataframe also supports other common Python types, e.g., list, dict, or numpy array. It also supports Snowpark and PySpark DataFrames, which allow you to lazily evaluate and pull data from databases. This can be useful for working with large datasets.
Edit data with st.experimental_data_editor
Streamlit supports editable dataframes via the st.experimental_data_editor command. Check out its API in st.experimental_data_editor. It shows the dataframe in a table, similar to st.dataframe. But in contrast to st.dataframe, this table isn't static! The user can click on cells and edit them. The edited data is then returned on the Python side. Here's an example:
df = pd.DataFrame(
[
{"command": "st.selectbox", "rating": 4, "is_widget": True},
{"command": "st.balloons", "rating": 5, "is_widget": False},
{"command": "st.time_input", "rating": 3, "is_widget": True},
]
)
df = load_data()
edited_df = st.experimental_data_editor(df) # 👈 An editable dataframe | streamlit-docs.txt |
e4409cd96e13-38 | df = load_data()
edited_df = st.experimental_data_editor(df) # 👈 An editable dataframe
favorite_command = edited_df.loc[edited_df["rating"].idxmax()]["command"]
st.markdown(f"Your favorite command is **{favorite_command}** 🎈")
Copy
View interactive app
expand_more
Try it out by double-clicking on any cell. You'll notice you can edit all cell values. Try editing the values in the rating column and observe how the text output at the bottom changes:
st.experimental_data_editor also supports a few additional things:
Copy and paste support from and to Excel and Google Sheets.
Add and delete rows. You can do this by setting num_rows= "dynamic" when calling st.experimental_data_editor. This will allow users to add and delete rows as needed.
Access edited data.
Bulk edits (similar to Excel, just drag a handle to edit neighboring cells).
Automatic input validation, e.g. no way to enter letters into a number cell.
Edit common data structures such as lists, dicts, NumPy ndarray, etc.
Copy and paste support
The data editor supports pasting in tabular data from Google Sheets, Excel, Notion, and many other similar tools. You can also copy-paste data between st.experimental_data_editor instances. This can be a huge time saver for users who need to work with data across multiple platforms. To try it out:
Copy data from this Google Sheets document to clipboard
Select any cell in the name column of the table below and paste it in (via ctrl/cmd + v).
View interactive app
expand_more
push_pin
Note
Every cell of the pasted data will be evaluated individually and inserted into the cells if the data is compatible with the column type. E.g., pasting in non-numerical text data into a number column will be ignored. | streamlit-docs.txt |
e4409cd96e13-39 | Did you notice that although the initial dataframe had just five rows, pasting all those rows from the spreadsheet added additional rows to the dataframe? 👀 Let's find out how that works in the next section.
Add and delete rows
With st.experimental_data_editor, viewers can add or delete rows via the table UI. This mode can be activated by setting the num_rows parameter to "dynamic". E.g.
edited_df = st.experimental_data_editor(df, num_rows=”dynamic”)
Copy
To add new rows, scroll to the bottom-most row and click on the “+” sign in any cell.
To delete rows, select one or more rows and press the delete key on your keyboard.
View interactive app
expand_more
Access edited data
Sometimes, it is more convenient to know which cells have been changed rather than getting the entire edited dataframe back. Streamlit makes this easy through the use of session state. If a key parameter is set, Streamlit will store any changes made to the dataframe in the session state.
This snippet shows how you can access changed data using session state:
st.experimental_data_editor(df, key="data_editor") # 👈 Set a key
st.write("Here's the session state:")
st.write(st.session_state["data_editor"]) # 👈 Access the edited data
Copy
In this code snippet, the key parameter is set to "data_editor". Any changes made to the data in the st.experimental_data_editor instance will be tracked by Streamlit and stored in session state under the key "data_editor".
After the data editor is created, the contents of the "data_editor" key in session state are printed to the screen using st.write(st.session_state["data_editor"]). This allows you to see the changes made to the original dataframe without having to return the entire dataframe from the data editor. | streamlit-docs.txt |
e4409cd96e13-40 | This can be useful when working with large dataframes and you only need to know which cells have changed, rather than the entire edited dataframe.
View interactive app
expand_more
Use all we've learned so far and apply them to the above embedded app. Try editing cells, adding new rows, and deleting rows.
Notice how edits to the table are reflected in session state: when you make any edits, a rerun is triggered which sends the edits to the backend via st.experimental_data_editor's keyed widget state. Its widget state is a JSON object containing three properties: edited_cells, added_rows, and deleted rows:.
edited_cells maps a cell position to the edited value: column:row → value .
added_rows is a list of newly added rows to the table. Each row is a dictionary where the keys are the column indices and the values are the corresponding cell values.
deleted_rows is a list of row indices that have been deleted from the table.
Bulk edits
The data editor includes a feature that allows for bulk editing of cells. Similar to Excel, you can drag a handle across a selection of cells to edit their values in bulk. You can even apply commonly used keyboard shortcuts in spreadsheet software. This is useful when you need to make the same change across multiple cells, rather than editing each cell individually:
Automatic input validation
The data editor includes automatic input validation to help prevent errors when editing cells. For example, if you have a column that contains numerical data, the input field will automatically restrict the user to only entering numerical data. This helps to prevent errors that could occur if the user were to accidentally enter a non-numerical value.
Edit common data structures | streamlit-docs.txt |
e4409cd96e13-41 | Edit common data structures
Editing doesn't just work for Pandas DataFrames! You can also edit lists, tuples, sets, dictionaries, NumPy arrays, or Snowpark & PySpark DataFrames. Most data types will be returned in their original format. But some types (e.g. Snowpark and PySpark) are converted to Pandas DataFrames. To learn about all the supported types, read the st.experimental_data_editor API.
E.g. you can easily let the user add items to a list:
edited_list = st.experimental_data_editor(["red", "green", "blue"], num_rows= "dynamic")
st.write("Here are all the colors you entered:")
st.write(edited_list)
Copy
Or numpy arrays:
import numpy as np
st.experimental_data_editor(np.array([
["st.text_area", "widget", 4.92],
["st.markdown", "element", 47.22]
]))
Copy
Or lists of records:
st.experimental_data_editor([
{"name": "st.text_area", "type": "widget"},
{"name": "st.markdown", "type": "element"},
])
Copy
Or dictionaries and many more types!
st.experimental_data_editor({
"st.text_area": "widget",
"st.markdown": "element"
})
Copy
Configuring columns
You will be able configure the display and editing behavior of columns via st.dataframe and st.experimental_data_editor in to-be-announced future releases. We are developing an API to let you add images, charts, and clickable URLs in dataframe columns. Additionally, you will be able to make individual columns editable, set columns as categorical and specify which options they can take, hide the index of the dataframe, and much more.
priority_high
Important
We will release the ability to configure columns in a future version of Streamlit. Keep at an eye out for updates on this page and the Streamlit roadmap. | streamlit-docs.txt |
e4409cd96e13-42 | While the ability to configure columns has yet to be released, there are techniques you can use with Pandas today to render columns as checkboxes, selectboxes, and change the type of columns.
Boolean columns (checkboxes)
To render columns as checkboxes and clickable checkboxes in st.dataframe and st.experimental_data_editor, respectively, set the type of the Pandas column as bool.
Here’s an example of creating a Pandas DataFrame with column A containing boolean values. When we display it using st.dataframe, the boolean values are rendered as checkboxes, where True and False values are checked and unchecked, respectively.
import pandas as pd
# create a dataframe with a boolean column
df = pd.DataFrame({"A": [True, False, True, False]})
# show the dataframe with checkboxes
st.dataframe(df)
Copy
Notice you cannot change their values from the frontend. To let users check and uncheck values, we display the dataframe with st.experimental_data_editor instead:
import pandas as pd
# create a dataframe with a boolean column
df = pd.DataFrame({"A": [True, False, True, False]})
# show the data editor with checkboxes
st.experimental_data_editor(df)
Copy
Categorical columns (selectboxes)
To render columns as selectboxes with st.experimental_data_editor, set the type of the Pandas column as category:
import pandas as pd
df = pd.DataFrame(
{"command": ["st.selectbox", "st.slider", "st.balloons", "st.time_input"]}
)
df["command"] = df["command"].astype("category")
edited_df = st.experimental_data_editor(df)
Copy | streamlit-docs.txt |
e4409cd96e13-43 | edited_df = st.experimental_data_editor(df)
Copy
In some cases, you may want users to select categories that aren’t in the original Pandas DataFrame. Let’s say we use df from above. Currently, the command column can take on four unique values. What should we do if we want users to see additional options such as st.button and st.radio?
To add additional categories to the selection, use pandas.Series.cat.add_categories:
import pandas as pd
df = pd.DataFrame(
{"command": ["st.selectbox", "st.slider", "st.balloons", "st.time_input"]}
)
df["command"] = (
df["command"].astype("category").cat.add_categories(["st.button", "st.radio"])
)
edited_df = st.experimental_data_editor(df)
Copy
Change column type
To change the type of a column, you can change the type of the underlying Pandas DataFrame column. E.g., say you have a column with only integers but want users to be able to add numbers with decimals. To do so, simply change the Pandas DataFrame column type to float, like so:
import pandas as pd
import streamlit as st
# create a dataframe with an integer column
df = pd.DataFrame({"A": [1, 2, 3, 4]})
# unable to add float values to the column
edited_df = st.experimental_data_editor(df)
# cast the column to float
df["A"] = df["A"].astype("float")
# able to add float values to the column
edited_df = st.experimental_data_editor(df)
Copy
In the first data editor instance, you cannot add decimal values to any entries. But after casting column A to type float, we’re able to edit the values as floating point numbers:
Handling large datasets | streamlit-docs.txt |
e4409cd96e13-44 | Handling large datasets
st.dataframe and st.experimental_data_editor have been designed to theoretically handle tables with millions of rows thanks to their highly performant implementation using the glide-data-grid library and HTML canvas. However, the maximum amount of data that an app can realistically handle will depend on several other factors, including:
The maximum size of WebSocket messages: Streamlit's WebSocket messages are configurable via the server.maxMessageSize config option, which limits the amount of data that can be transferred via the WebSocket connection at once.
The server memory: The amount of data that your app can handle will also depend on the amount of memory available on your server. If the server's memory is exceeded, the app may become slow or unresponsive.
The user's browser memory: Since all the data needs to be transferred to the user's browser for rendering, the amount of memory available on the user's device can also affect the app's performance. If the browser's memory is exceeded, it may crash or become unresponsive.
In addition to these factors, a slow network connection can also significantly slow down apps that handle large datasets.
When handling large datasets with more than 150,000 rows, Streamlit applies additional optimizations and disables column sorting. This can help to reduce the amount of data that needs to be processed at once and improve the app's performance.
Limitations
While Streamlit's data editing capabilities offer a lot of functionality, there are some limitations to be aware of: | streamlit-docs.txt |
e4409cd96e13-45 | The editing functionalities are not yet optimized for mobile devices.
Editing is enabled only for a limited set of types (e.g. string, numbers, boolean). We are actively working on supporting more types soon, such as date, time, and datetime.
Editing of Pandas DataFrames only supports the following index types: RangeIndex, (string) Index, Float64Index, Int64Index, and UInt64Index.
The column type is inferred from the underlying data and cannot be configured yet.
Some actions like deleting rows or searching data can only be triggered via keyboard hotkeys.
We are working to fix the above limitations in future releases, so keep an eye out for updates.
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Add statefulness to apps
Next:
Widget semantics
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Dataframes - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base | streamlit-docs.txt |
e4409cd96e13-46 | Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Status elements/
st.progress
st.progress
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display a progress bar.
Function signature
[source]
st.progress(value, text=None)
Parameters
value (int or float)
0 <= value <= 100 for int
0.0 <= value <= 1.0 for float
text (str or None)
A message to display above the progress bar. The text can optionally contain Markdown and supports the following elements: Bold, Italics, Strikethroughs, Inline Code, Emojis, and Links.
This also supports: | streamlit-docs.txt |
e4409cd96e13-47 | This also supports:
Emoji shortcodes, such as :+1: and :sunglasses:. For a list of all supported codes, see https://share.streamlit.io/streamlit/emoji-shortcodes.
LaTeX expressions, by wrapping them in "$" or "$$" (the "$$" must be on their own lines). Supported LaTeX functions are listed at https://katex.org/docs/supported.html.
Colored text, using the syntax :color[text to be colored], where color needs to be replaced with any of the following supported colors: blue, green, orange, red, violet.
Unsupported elements are not displayed. Display unsupported elements as literal characters by backslash-escaping them. E.g. 1\. Not an ordered list.
Example
Here is an example of a progress bar increasing over time:
import streamlit as st
import time
progress_text = "Operation in progress. Please wait."
my_bar = st.progress(0, text=progress_text)
for percent_complete in range(100):
time.sleep(0.1)
my_bar.progress(percent_complete + 1, text=progress_text)
Copy
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Status elements
Next:
st.spinner
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.progress - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base | streamlit-docs.txt |
e4409cd96e13-48 | Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Write and magic/
st.write
st.write
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Write arguments to the app.
This is the Swiss Army knife of Streamlit commands: it does different things depending on what you throw at it. Unlike other Streamlit commands, write() has some unique properties:
You can pass in multiple arguments, all of which will be written.
Its behavior depends on the input types as follows.
It returns None, so its "slot" in the App cannot be reused.
Function signature
[source]
st.write(*args, unsafe_allow_html=False, **kwargs)
Parameters
*args (any)
One or many objects to print to the App.
Arguments are handled as follows: | streamlit-docs.txt |
e4409cd96e13-49 | One or many objects to print to the App.
Arguments are handled as follows:
write(string) : Prints the formatted Markdown string, with
support for LaTeX expression, emoji shortcodes, and colored text. See docs for st.markdown for more.
write(data_frame) : Displays the DataFrame as a table.
write(error) : Prints an exception specially.
write(func) : Displays information about a function.
write(module) : Displays information about the module.
write(dict) : Displays dict in an interactive widget.
write(mpl_fig) : Displays a Matplotlib figure.
write(altair) : Displays an Altair chart.
write(keras) : Displays a Keras model.
write(graphviz) : Displays a Graphviz graph.
write(plotly_fig) : Displays a Plotly figure.
write(bokeh_fig) : Displays a Bokeh figure.
write(sympy_expr) : Prints SymPy expression using LaTeX.
write(htmlable) : Prints _repr_html_() for the object if available.
write(obj) : Prints str(obj) if otherwise unknown.
unsafe_allow_html (bool)
This is a keyword-only argument that defaults to False.
By default, any HTML tags found in strings will be escaped and therefore treated as pure text. This behavior may be turned off by setting this argument to True.
That said, we strongly advise against it. It is hard to write secure HTML, so by using this argument you may be compromising your users' security. For more information, see:
https://github.com/streamlit/streamlit/issues/152
Example
Its basic use case is to draw Markdown-formatted text, whenever the input is a string:
import streamlit as st
st.write('Hello, *World!* :sunglasses:')
Copy
(view standalone Streamlit app)
As mentioned earlier, st.write() also accepts other data formats, such as numbers, data frames, styled data frames, and assorted objects: | streamlit-docs.txt |
e4409cd96e13-50 | import streamlit as st
import pandas as pd
st.write(1234)
st.write(pd.DataFrame({
'first column': [1, 2, 3, 4],
'second column': [10, 20, 30, 40],
}))
Copy
(view standalone Streamlit app)
Finally, you can pass in multiple arguments to do things like:
import streamlit as st
st.write('1 + 1 = ', 2)
st.write('Below is a DataFrame:', data_frame, 'Above is a dataframe.')
Copy
(view standalone Streamlit app)
Oh, one more thing: st.write accepts chart objects too! For example:
import streamlit as st
import pandas as pd
import numpy as np
import altair as alt
df = pd.DataFrame(
np.random.randn(200, 3),
columns=['a', 'b', 'c'])
c = alt.Chart(df).mark_circle().encode(
x='a', y='b', size='c', color='c', tooltip=['a', 'b', 'c'])
st.write(c)
Copy
(view standalone Streamlit app)
Featured video
Learn what the st.write and magic commands are and how to use them.
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Write and magic
Next:
magic
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.write - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Write and magic/
magic
Magic | streamlit-docs.txt |
e4409cd96e13-51 | Knowledge base
Home/
Streamlit library/
API reference/
Write and magic/
magic
Magic
Magic commands are a feature in Streamlit that allows you to write almost anything (markdown, data, charts) without having to type an explicit command at all. Just put the thing you want to show on its own line of code, and it will appear in your app. Here's an example:
# Draw a title and some text to the app:
'''
# This is the document title
This is some _markdown_.
'''
import pandas as pd
df = pd.DataFrame({'col1': [1,2,3]})
df # 👈 Draw the dataframe
x = 10
'x', x # 👈 Draw the string 'x' and then the value of x
# Also works with most supported chart types
import matplotlib.pyplot as plt
import numpy as np
arr = np.random.normal(1, 1, size=100)
fig, ax = plt.subplots()
ax.hist(arr, bins=20)
fig # 👈 Draw a Matplotlib chart
Copy
How Magic works
Any time Streamlit sees either a variable or literal value on its own line, it automatically writes that to your app using st.write (which you'll learn about later).
Also, magic is smart enough to ignore docstrings. That is, it ignores the strings at the top of files and functions.
If you prefer to call Streamlit commands more explicitly, you can always turn magic off in your ~/.streamlit/config.toml with the following setting:
[runner]
magicEnabled = false
Copy
priority_high
Important
Right now, Magic only works in the main Python app file, not in imported files. See GitHub issue #288 for a discussion of the issues.
Featured video
Learn what the st.write and magic commands are and how to use them.
Was this page helpful? | streamlit-docs.txt |
e4409cd96e13-52 | Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
st.write
Next:
Text elements
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Magic - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Text elements/
st.markdown
st.markdown
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display string formatted as Markdown.
Function signature
[source]
st.markdown(body, unsafe_allow_html=False)
Parameters
body (str)
The string to display as Github-flavored Markdown. Syntax information can be found at: https://github.github.com/gfm.
This also supports: | streamlit-docs.txt |
e4409cd96e13-53 | This also supports:
Emoji shortcodes, such as :+1: and :sunglasses:. For a list of all supported codes, see https://share.streamlit.io/streamlit/emoji-shortcodes.
LaTeX expressions, by wrapping them in "$" or "$$" (the "$$" must be on their own lines). Supported LaTeX functions are listed at https://katex.org/docs/supported.html.
Colored text, using the syntax :color[text to be colored], where color needs to be replaced with any of the following supported colors: blue, green, orange, red, violet.
unsafe_allow_html (bool)
By default, any HTML tags found in the body will be escaped and therefore treated as pure text. This behavior may be turned off by setting this argument to True.
That said, we strongly advise against it. It is hard to write secure HTML, so by using this argument you may be compromising your users' security. For more information, see:
https://github.com/streamlit/streamlit/issues/152
Examples
import streamlit as st
st.markdown('Streamlit is **_really_ cool**.')
st.markdown(”This text is :red[colored red], and this is **:blue[colored]** and bold.”)
st.markdown(":green[$\sqrt{x^2+y^2}=1$] is a Pythagorean identity. :pencil:")
Copy
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Text elements
Next:
st.title
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.markdown - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school | streamlit-docs.txt |
e4409cd96e13-54 | dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Text elements/
st.code
st.code
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display a code block with optional syntax highlighting.
(This is a convenience wrapper around st.markdown())
Function signature
[source]
st.code(body, language="python")
Parameters
body (str)
The string to display as code.
language (str or None)
The language that the code is written in, for syntax highlighting. If None, the code will be unstyled. Defaults to "python".
For a list of available language values, see:
https://github.com/react-syntax-highlighter/react-syntax-highlighter/blob/master/AVAILABLE_LANGUAGES_PRISM.MD
Example
import streamlit as st
code = '''def hello():
print("Hello, Streamlit!")'''
st.code(code, language='python')
Copy | streamlit-docs.txt |
e4409cd96e13-55 | Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
st.caption
Next:
st.text
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.code - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Input widgets/
st.button
st.button
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display a button widget.
Function signature
[source]
st.button(label, key=None, help=None, on_click=None, args=None, kwargs=None, *, type="secondary", disabled=False, use_container_width=False)
Parameters
label (str) | streamlit-docs.txt |
e4409cd96e13-56 | Parameters
label (str)
A short label explaining to the user what this button is for. The label can optionally contain Markdown and supports the following elements: Bold, Italics, Strikethroughs, and Emojis.
Unsupported elements are not displayed. Display unsupported elements as literal characters by backslash-escaping them. E.g. 1\. Not an ordered list.
key (str or int)
An optional string or integer to use as the unique key for the widget. If this is omitted, a key will be generated for the widget based on its content. Multiple widgets of the same type may not share the same key.
help (str)
An optional tooltip that gets displayed when the button is hovered over.
on_click (callable)
An optional callback invoked when this button is clicked.
args (tuple)
An optional tuple of args to pass to the callback.
kwargs (dict)
An optional dict of kwargs to pass to the callback.
type ("secondary" or "primary")
An optional string that specifies the button type. Can be "primary" for a button with additional emphasis or "secondary" for a normal button. This argument can only be supplied by keyword. Defaults to "secondary".
disabled (bool)
An optional boolean, which disables the button if set to True. The default is False. This argument can only be supplied by keyword.
use_container_width (bool)
An optional boolean, which makes the button stretch its width to match the parent container.
Returns
(bool)
True if the button was clicked on the last run of the app, False otherwise.
Example
import streamlit as st
if st.button('Say hello'):
st.write('Why hello there')
else:
st.write('Goodbye')
Copy
(view standalone Streamlit app)
Featured videos
Check out our video on how to use one of Streamlit's core functions, the button! | streamlit-docs.txt |
e4409cd96e13-57 | Check out our video on how to use one of Streamlit's core functions, the button!
In the video below, we'll take it a step further and learn how to combine a button, checkbox and radio button!
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Input widgets
Next:
st.experimental_data_editor
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.button - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Input widgets/
st.experimental_data_editor
priority_high
Important
This is an experimental feature. Experimental features and their APIs may change or be removed at any time. To learn more, click here.
star
Tip
This page only contains information on the st.experimental_data_editor API. For a deeper dive into working with DataFrames and the data editor's capabilities, read Dataframes. | streamlit-docs.txt |
e4409cd96e13-58 | st.experimental_data_editor
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display a data editor widget.
Display a data editor widget that allows you to edit DataFrames and many other data structures in a table-like UI.
Function signature
[source]
st.experimental_data_editor(data, *, width=None, height=None, use_container_width=False, num_rows="fixed", disabled=False, key=None, on_change=None, args=None, kwargs=None)
Parameters
data (pandas.DataFrame, pandas.Styler, pandas.Index, pyarrow.Table, numpy.ndarray, pyspark.sql.DataFrame, snowflake.snowpark.DataFrame, list, set, tuple, dict, or None)
The data to edit in the data editor.
width (int or None)
Desired width of the data editor expressed in pixels. If None, the width will be automatically determined.
height (int or None)
Desired height of the data editor expressed in pixels. If None, the height will be automatically determined. | streamlit-docs.txt |
e4409cd96e13-59 | use_container_width (bool)
If True, set the data editor width to the width of the parent container. This takes precedence over the width argument. Defaults to False.
num_rows ("fixed" or "dynamic")
Specifies if the user can add and delete rows in the data editor. If "fixed", the user cannot add or delete rows. If "dynamic", the user can add and delete rows in the data editor, but column sorting is disabled. Defaults to "fixed".
disabled (bool)
An optional boolean which, if True, disables the data editor and prevents any edits. Defaults to False. This argument can only be supplied by keyword.
key (str)
An optional string to use as the unique key for this widget. If this is omitted, a key will be generated for the widget based on its content. Multiple widgets of the same type may not share the same key.
on_change (callable)
An optional callback invoked when this data_editor's value changes.
args (tuple)
An optional tuple of args to pass to the callback.
kwargs (dict)
An optional dict of kwargs to pass to the callback.
Returns
(pd.DataFrame, pd.Styler, pyarrow.Table, np.ndarray, list, set, tuple, or dict.)
The edited data. The edited data is returned in its original data type if it corresponds to any of the supported return types. All other data types are returned as a pd.DataFrame.
Examples
import streamlit as st
import pandas as pd
df = pd.DataFrame(
[
{"command": "st.selectbox", "rating": 4, "is_widget": True},
{"command": "st.balloons", "rating": 5, "is_widget": False},
{"command": "st.time_input", "rating": 3, "is_widget": True},
]
)
edited_df = st.experimental_data_editor(df) | streamlit-docs.txt |
e4409cd96e13-60 | favorite_command = edited_df.loc[edited_df["rating"].idxmax()]["command"]
st.markdown(f"Your favorite command is **{favorite_command}** 🎈")
Copy
(view standalone Streamlit app)
You can also allow the user to add and delete rows by setting num_rows to "dynamic":
import streamlit as st
import pandas as pd
df = pd.DataFrame(
[
{"command": "st.selectbox", "rating": 4, "is_widget": True},
{"command": "st.balloons", "rating": 5, "is_widget": False},
{"command": "st.time_input", "rating": 3, "is_widget": True},
]
)
edited_df = st.experimental_data_editor(df, num_rows="dynamic")
favorite_command = edited_df.loc[edited_df["rating"].idxmax()]["command"]
st.markdown(f"Your favorite command is **{favorite_command}** 🎈")
Copy
(view standalone Streamlit app)
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
st.button
Next:
st.download_button
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.experimental_data_editor - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base | streamlit-docs.txt |
e4409cd96e13-61 | Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Input widgets/
st.download_button
st.download_button
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display a download button widget.
This is useful when you would like to provide a way for your users to download a file directly from your app.
Note that the data to be downloaded is stored in-memory while the user is connected, so it's a good idea to keep file sizes under a couple hundred megabytes to conserve memory.
Function signature
[source]
st.download_button(label, data, file_name=None, mime=None, key=None, help=None, on_click=None, args=None, kwargs=None, *, disabled=False, use_container_width=False)
Parameters
label (str)
A short label explaining to the user what this button is for. The label can optionally contain Markdown and supports the following elements: Bold, Italics, Strikethroughs, and Emojis. | streamlit-docs.txt |
e4409cd96e13-62 | Unsupported elements are not displayed. Display unsupported elements as literal characters by backslash-escaping them. E.g. 1\. Not an ordered list.
data (str or bytes or file)
The contents of the file to be downloaded. See example below for caching techniques to avoid recomputing this data unnecessarily.
file_name (str)
An optional string to use as the name of the file to be downloaded, such as 'my_file.csv'. If not specified, the name will be automatically generated.
mime (str or None)
The MIME type of the data. If None, defaults to "text/plain" (if data is of type str or is a textual file) or "application/octet-stream" (if data is of type bytes or is a binary file).
key (str or int)
An optional string or integer to use as the unique key for the widget. If this is omitted, a key will be generated for the widget based on its content. Multiple widgets of the same type may not share the same key.
help (str)
An optional tooltip that gets displayed when the button is hovered over.
on_click (callable)
An optional callback invoked when this button is clicked.
args (tuple)
An optional tuple of args to pass to the callback.
kwargs (dict)
An optional dict of kwargs to pass to the callback.
disabled (bool)
An optional boolean, which disables the download button if set to True. The default is False. This argument can only be supplied by keyword.
use_container_width (bool)
An optional boolean, which makes the button stretch its width to match the parent container.
Returns
(bool)
True if the button was clicked on the last run of the app, False otherwise.
Examples
Download a large DataFrame as a CSV:
import streamlit as st | streamlit-docs.txt |
e4409cd96e13-63 | Examples
Download a large DataFrame as a CSV:
import streamlit as st
@st.cache
def convert_df(df):
# IMPORTANT: Cache the conversion to prevent computation on every rerun
return df.to_csv().encode('utf-8')
csv = convert_df(my_large_df)
st.download_button(
label="Download data as CSV",
data=csv,
file_name='large_df.csv',
mime='text/csv',
)
Copy
Download a string as a file:
import streamlit as st
text_contents = '''This is some text'''
st.download_button('Download some text', text_contents)
Copy
Download a binary file:
import streamlit as st
binary_contents = b'example content'
# Defaults to 'application/octet-stream'
st.download_button('Download binary file', binary_contents)
Copy
Download an image:
import streamlit as st
with open("flower.png", "rb") as file:
btn = st.download_button(
label="Download image",
data=file,
file_name="flower.png",
mime="image/png"
)
Copy
(view standalone Streamlit app)
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
st.experimental_data_editor
Next:
st.checkbox
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.download_button - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base | streamlit-docs.txt |
e4409cd96e13-64 | Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Input widgets/
st.text_input
st.text_input
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display a single-line text input widget.
Function signature
[source]
st.text_input(label, value="", max_chars=None, key=None, type="default", help=None, autocomplete=None, on_change=None, args=None, kwargs=None, *, placeholder=None, disabled=False, label_visibility="visible")
Parameters
label (str)
A short label explaining to the user what this input is for. The label can optionally contain Markdown and supports the following elements: Bold, Italics, Strikethroughs, Inline Code, Emojis, and Links.
This also supports: | streamlit-docs.txt |
e4409cd96e13-65 | This also supports:
Emoji shortcodes, such as :+1: and :sunglasses:. For a list of all supported codes, see https://share.streamlit.io/streamlit/emoji-shortcodes.
LaTeX expressions, by wrapping them in "$" or "$$" (the "$$" must be on their own lines). Supported LaTeX functions are listed at https://katex.org/docs/supported.html.
Colored text, using the syntax :color[text to be colored], where color needs to be replaced with any of the following supported colors: blue, green, orange, red, violet.
Unsupported elements are not displayed. Display unsupported elements as literal characters by backslash-escaping them. E.g. 1\. Not an ordered list.
For accessibility reasons, you should never set an empty label (label="") but hide it with label_visibility if needed. In the future, we may disallow empty labels by raising an exception.
value (object)
The text value of this widget when it first renders. This will be cast to str internally.
max_chars (int or None)
Max number of characters allowed in text input.
key (str or int)
An optional string or integer to use as the unique key for the widget. If this is omitted, a key will be generated for the widget based on its content. Multiple widgets of the same type may not share the same key.
type (str)
The type of the text input. This can be either "default" (for a regular text input), or "password" (for a text input that masks the user's typed value). Defaults to "default".
help (str)
An optional tooltip that gets displayed next to the input.
autocomplete (str) | streamlit-docs.txt |
e4409cd96e13-66 | An optional tooltip that gets displayed next to the input.
autocomplete (str)
An optional value that will be passed to the <input> element's autocomplete property. If unspecified, this value will be set to "new-password" for "password" inputs, and the empty string for "default" inputs. For more details, see https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/autocomplete
on_change (callable)
An optional callback invoked when this text_input's value changes.
args (tuple)
An optional tuple of args to pass to the callback.
kwargs (dict)
An optional dict of kwargs to pass to the callback.
placeholder (str or None)
An optional string displayed when the text input is empty. If None, no text is displayed. This argument can only be supplied by keyword.
disabled (bool)
An optional boolean, which disables the text input if set to True. The default is False. This argument can only be supplied by keyword.
label_visibility ("visible" or "hidden" or "collapsed")
The visibility of the label. If "hidden", the label doesn't show but there is still empty space for it above the widget (equivalent to label=""). If "collapsed", both the label and the space are removed. Default is "visible". This argument can only be supplied by keyword.
Returns
(str)
The current value of the text input widget.
Example
import streamlit as st
title = st.text_input('Movie title', 'Life of Brian')
st.write('The current movie title is', title)
Copy
(view standalone Streamlit app) | streamlit-docs.txt |
e4409cd96e13-67 | Text input widgets can customize how to hide their labels with the label_visibility parameter. If "hidden", the label doesn’t show but there is still empty space for it above the widget (equivalent to label=""). If "collapsed", both the label and the space are removed. Default is "visible". Text input widgets can also be disabled with the disabled parameter, and can display an optional placeholder text when the text input is empty using the placeholder parameter:
import streamlit as st
# Store the initial value of widgets in session state
if "visibility" not in st.session_state:
st.session_state.visibility = "visible"
st.session_state.disabled = False
col1, col2 = st.columns(2)
with col1:
st.checkbox("Disable text input widget", key="disabled")
st.radio(
"Set text input label visibility 👉",
key="visibility",
options=["visible", "hidden", "collapsed"],
)
st.text_input(
"Placeholder for the other text input widget",
"This is a placeholder",
key="placeholder",
)
with col2:
text_input = st.text_input(
"Enter some text 👇",
label_visibility=st.session_state.visibility,
disabled=st.session_state.disabled,
placeholder=st.session_state.placeholder,
)
if text_input:
st.write("You entered: ", text_input)
Copy
(view standalone Streamlit app)
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
st.select_slider
Next:
st.number_input
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.text_input - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library | streamlit-docs.txt |
e4409cd96e13-68 | Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Input widgets/
st.file_uploader
st.file_uploader
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display a file uploader widget.
By default, uploaded files are limited to 200MB. You can configure this using the server.maxUploadSize config option. For more info on how to set config options, see https://docs.streamlit.io/library/advanced-features/configuration#set-configuration-options
Function signature
[source]
st.file_uploader(label, type=None, accept_multiple_files=False, key=None, help=None, on_change=None, args=None, kwargs=None, *, disabled=False, label_visibility="visible")
Parameters
label (str) | streamlit-docs.txt |
e4409cd96e13-69 | Parameters
label (str)
A short label explaining to the user what this file uploader is for. The label can optionally contain Markdown and supports the following elements: Bold, Italics, Strikethroughs, Inline Code, Emojis, and Links.
This also supports:
Emoji shortcodes, such as :+1: and :sunglasses:. For a list of all supported codes, see https://share.streamlit.io/streamlit/emoji-shortcodes.
LaTeX expressions, by wrapping them in "$" or "$$" (the "$$" must be on their own lines). Supported LaTeX functions are listed at https://katex.org/docs/supported.html.
Colored text, using the syntax :color[text to be colored], where color needs to be replaced with any of the following supported colors: blue, green, orange, red, violet.
Unsupported elements are not displayed. Display unsupported elements as literal characters by backslash-escaping them. E.g. 1\. Not an ordered list.
For accessibility reasons, you should never set an empty label (label="") but hide it with label_visibility if needed. In the future, we may disallow empty labels by raising an exception.
type (str or list of str or None)
Array of allowed extensions. ['png', 'jpg'] The default is None, which means all extensions are allowed.
accept_multiple_files (bool)
If True, allows the user to upload multiple files at the same time, in which case the return value will be a list of files. Default: False
key (str or int)
An optional string or integer to use as the unique key for the widget. If this is omitted, a key will be generated for the widget based on its content. Multiple widgets of the same type may not share the same key.
help (str)
A tooltip that gets displayed next to the file uploader.
on_change (callable) | streamlit-docs.txt |
e4409cd96e13-70 | A tooltip that gets displayed next to the file uploader.
on_change (callable)
An optional callback invoked when this file_uploader's value changes.
args (tuple)
An optional tuple of args to pass to the callback.
kwargs (dict)
An optional dict of kwargs to pass to the callback.
disabled (bool)
An optional boolean, which disables the file uploader if set to True. The default is False. This argument can only be supplied by keyword.
label_visibility ("visible" or "hidden" or "collapsed")
The visibility of the label. If "hidden", the label doesn't show but there is still empty space for it above the widget (equivalent to label=""). If "collapsed", both the label and the space are removed. Default is "visible". This argument can only be supplied by keyword.
Returns
(None or UploadedFile or list of UploadedFile)
If accept_multiple_files is False, returns either None or an UploadedFile object.
If accept_multiple_files is True, returns a list with the uploaded files as UploadedFile objects. If no files were uploaded, returns an empty list.
The UploadedFile class is a subclass of BytesIO, and therefore it is "file-like". This means you can pass them anywhere where a file is expected.
Examples
Insert a file uploader that accepts a single file at a time:
import streamlit as st
import pandas as pd
from io import StringIO
uploaded_file = st.file_uploader("Choose a file")
if uploaded_file is not None:
# To read file as bytes:
bytes_data = uploaded_file.getvalue()
st.write(bytes_data)
# To convert to a string based IO:
stringio = StringIO(uploaded_file.getvalue().decode("utf-8"))
st.write(stringio)
# To read file as string:
string_data = stringio.read()
st.write(string_data) | streamlit-docs.txt |
e4409cd96e13-71 | # Can be used wherever a "file-like" object is accepted:
dataframe = pd.read_csv(uploaded_file)
st.write(dataframe)
Copy
Insert a file uploader that accepts multiple files at a time:
import streamlit as st
uploaded_files = st.file_uploader("Choose a CSV file", accept_multiple_files=True)
for uploaded_file in uploaded_files:
bytes_data = uploaded_file.read()
st.write("filename:", uploaded_file.name)
st.write(bytes_data)
Copy
(view standalone Streamlit app)
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
st.time_input
Next:
st.camera_input
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.file_uploader - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base | streamlit-docs.txt |
e4409cd96e13-72 | Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
API reference/
Input widgets/
st.selectbox
st.selectbox
Streamlit Version
v1.20.0
v1.19.0
v1.18.0
v1.17.0
v1.16.0
v1.15.0
v1.14.0
v1.13.0
v1.12.0
v1.11.0
v1.10.0
v1.9.0
v1.8.0
v1.7.0
v1.6.0
v1.5.0
v1.4.0
v1.3.0
v1.2.0
v1.1.0
v1.0.0
v0.89.0
v0.88.0
v0.87.0
v0.86.0
v0.85.0
v0.84.0
v0.83.0
Display a select widget.
Function signature
[source]
st.selectbox(label, options, index=0, format_func=special_internal_function, key=None, help=None, on_change=None, args=None, kwargs=None, *, disabled=False, label_visibility="visible")
Parameters
label (str)
A short label explaining to the user what this select widget is for. The label can optionally contain Markdown and supports the following elements: Bold, Italics, Strikethroughs, Inline Code, Emojis, and Links.
This also supports: | streamlit-docs.txt |
e4409cd96e13-73 | This also supports:
Emoji shortcodes, such as :+1: and :sunglasses:. For a list of all supported codes, see https://share.streamlit.io/streamlit/emoji-shortcodes.
LaTeX expressions, by wrapping them in "$" or "$$" (the "$$" must be on their own lines). Supported LaTeX functions are listed at https://katex.org/docs/supported.html.
Colored text, using the syntax :color[text to be colored], where color needs to be replaced with any of the following supported colors: blue, green, orange, red, violet.
Unsupported elements are not displayed. Display unsupported elements as literal characters by backslash-escaping them. E.g. 1\. Not an ordered list.
For accessibility reasons, you should never set an empty label (label="") but hide it with label_visibility if needed. In the future, we may disallow empty labels by raising an exception.
options (Sequence, numpy.ndarray, pandas.Series, pandas.DataFrame, or pandas.Index)
Labels for the select options. This will be cast to str internally by default. For pandas.DataFrame, the first column is selected.
index (int)
The index of the preselected option on first render.
format_func (function)
Function to modify the display of the labels. It receives the option as an argument and its output will be cast to str.
key (str or int)
An optional string or integer to use as the unique key for the widget. If this is omitted, a key will be generated for the widget based on its content. Multiple widgets of the same type may not share the same key.
help (str)
An optional tooltip that gets displayed next to the selectbox.
on_change (callable)
An optional callback invoked when this selectbox's value changes.
args (tuple)
An optional tuple of args to pass to the callback.
kwargs (dict) | streamlit-docs.txt |
e4409cd96e13-74 | An optional tuple of args to pass to the callback.
kwargs (dict)
An optional dict of kwargs to pass to the callback.
disabled (bool)
An optional boolean, which disables the selectbox if set to True. The default is False. This argument can only be supplied by keyword.
label_visibility ("visible" or "hidden" or "collapsed")
The visibility of the label. If "hidden", the label doesn't show but there is still empty space for it above the widget (equivalent to label=""). If "collapsed", both the label and the space are removed. Default is "visible". This argument can only be supplied by keyword.
Returns
(any)
The selected option
Example
import streamlit as st
option = st.selectbox(
'How would you like to be contacted?',
('Email', 'Home phone', 'Mobile phone'))
st.write('You selected:', option)
Copy
(view standalone Streamlit app)
Select widgets can customize how to hide their labels with the label_visibility parameter. If "hidden", the label doesn’t show but there is still empty space for it above the widget (equivalent to label=""). If "collapsed", both the label and the space are removed. Default is "visible". Select widgets can also be disabled with the disabled parameter:
import streamlit as st
# Store the initial value of widgets in session state
if "visibility" not in st.session_state:
st.session_state.visibility = "visible"
st.session_state.disabled = False
col1, col2 = st.columns(2)
with col1:
st.checkbox("Disable selectbox widget", key="disabled")
st.radio(
"Set selectbox label visibility 👉",
key="visibility",
options=["visible", "hidden", "collapsed"],
) | streamlit-docs.txt |
e4409cd96e13-75 | with col2:
option = st.selectbox(
"How would you like to be contacted?",
("Email", "Home phone", "Mobile phone"),
label_visibility=st.session_state.visibility,
disabled=st.session_state.disabled,
)
Copy
(view standalone Streamlit app)
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
st.radio
Next:
st.multiselect
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
st.selectbox - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
Advanced features/
Configuration
Configuration
Streamlit provides four different ways to set configuration options. This list is in reverse order of precedence, i.e. command line flags take precedence over environment variables when the same configuration option is provided multiple times.
push_pin
Note
If changes to .streamlit/config.toml are made while the app is running, the server needs to be restarted for changes to be reflected in the app.
In a global config file at ~/.streamlit/config.toml for macOS/Linux or %userprofile%/.streamlit/config.toml for Windows:
[server]
port = 80
Copy
In a per-project config file at $CWD/.streamlit/config.toml, where $CWD is the folder you're running Streamlit from.
Through STREAMLIT_* environment variables, such as:
export STREAMLIT_SERVER_PORT=80
export STREAMLIT_SERVER_COOKIE_SECRET=dontforgottochangeme
Copy
As flags on the command line when running streamlit run: | streamlit-docs.txt |
e4409cd96e13-76 | As flags on the command line when running streamlit run:
streamlit run your_script.py --server.port 80
Copy
star
Tip
To set configuration options on Streamlit Community Cloud, read Optionally, add a configuration file in the Streamlit Community Cloud docs.
Telemetry
As mentioned during the installation process, Streamlit collects usage statistics. You can find out more by reading our Privacy Notice, but the high-level summary is that although we collect telemetry data we cannot see and do not store information contained in Streamlit apps.
If you'd like to opt out of usage statistics, add the following to your config file:
[browser]
gatherUsageStats = false
Copy
Theming
You can change the base colors of your app using the [theme] section of the configuration system. To learn more, see Theming.
View all configuration options
As described in Command-line options, you can view all available configuration option using:
streamlit config show
Copy
The command above will print something like this:
# Streamlit version: 1.20.0
[global]
# By default, Streamlit checks if the Python watchdog module is available and, if not, prints a warning asking for you to install it. The watchdog module is not required, but highly recommended. It improves Streamlit's ability to detect changes to files in your filesystem.
# If you'd like to turn off this warning, set this to True.
# Default: false
disableWatchdogWarning = false
# If True, will show a warning when you run a Streamlit-enabled script via "python my_script.py".
# Default: true
showWarningOnDirectExecution = true | streamlit-docs.txt |
e4409cd96e13-77 | # DataFrame serialization.
# Acceptable values: - 'legacy': Serialize DataFrames using Streamlit's custom format. Slow but battle-tested. - 'arrow': Serialize DataFrames using Apache Arrow. Much faster and versatile.
# Default: "arrow"
dataFrameSerialization = "arrow"
[logger]
# Level of logging: 'error', 'warning', 'info', or 'debug'.
# Default: 'info'
level = "info"
# String format for logging messages. If logger.datetimeFormat is set, logger messages will default to `%(asctime)s.%(msecs)03d %(message)s`. See [Python's documentation](https://docs.python.org/2.6/library/logging.html#formatter-objects) for available attributes.
# Default: "%(asctime)s %(message)s"
messageFormat = "%(asctime)s %(message)s"
[client]
# Whether to enable st.cache.
# Default: true
caching = true
# If false, makes your Streamlit script not draw to a Streamlit app.
# Default: true
displayEnabled = true
# Controls whether uncaught app exceptions are displayed in the browser. By default, this is set to True and Streamlit displays app exceptions and associated tracebacks in the browser.
# If set to False, an exception will result in a generic message being shown in the browser, and exceptions and tracebacks will be printed to the console only.
# Default: true
showErrorDetails = true
[runner]
# Allows you to type a variable or string by itself in a single line of Python code to write it to the app.
# Default: true
magicEnabled = true
# Install a Python tracer to allow you to stop or pause your script at any point and introspect it. As a side-effect, this slows down your script's execution.
# Default: false
installTracer = false | streamlit-docs.txt |
e4409cd96e13-78 | # Sets the MPLBACKEND environment variable to Agg inside Streamlit to prevent Python crashing.
# Default: true
fixMatplotlib = true
# Run the Python Garbage Collector after each script execution. This can help avoid excess memory use in Streamlit apps, but could introduce delay in rerunning the app script for high-memory-use applications.
# Default: true
postScriptGC = true
# Handle script rerun requests immediately, rather than waiting for script execution to reach a yield point. This makes Streamlit much more responsive to user interaction, but it can lead to race conditions in apps that mutate session_state data outside of explicit session_state assignment statements.
# Default: true
fastReruns = true
[server]
# List of folders that should not be watched for changes. This impacts both "Run on Save" and @st.cache.
# Relative paths will be taken as relative to the current working directory.
# Example: ['/home/user1/env', 'relative/path/to/folder']
# Default: []
folderWatchBlacklist = []
# Change the type of file watcher used by Streamlit, or turn it off completely.
# Allowed values: * "auto" : Streamlit will attempt to use the watchdog module, and falls back to polling if watchdog is not available. * "watchdog" : Force Streamlit to use the watchdog module. * "poll" : Force Streamlit to always use polling. * "none" : Streamlit will not watch files.
# Default: "auto"
fileWatcherType = "auto"
# Symmetric key used to produce signed cookies. If deploying on multiple replicas, this should be set to the same value across all replicas to ensure they all share the same secret.
# Default: randomly generated secret key.
cookieSecret = | streamlit-docs.txt |
e4409cd96e13-79 | # If false, will attempt to open a browser window on start.
# Default: false unless (1) we are on a Linux box where DISPLAY is unset, or (2) we are running in the Streamlit Atom plugin.
headless = false
# Automatically rerun script when the file is modified on disk.
# Default: false
runOnSave = false
# The address where the server will listen for client and browser connections. Use this if you want to bind the server to a specific address. If set, the server will only be accessible from this address, and not from any aliases (like localhost).
# Default: (unset)
# address =
# The port where the server will listen for browser connections.
# Default: 8501
port = 8501
# The base path for the URL where Streamlit should be served from.
# Default: ""
baseUrlPath = ""
# Enables support for Cross-Origin Request Sharing (CORS) protection, for added security.
# Due to conflicts between CORS and XSRF, if `server.enableXsrfProtection` is on and `server.enableCORS` is off at the same time, we will prioritize `server.enableXsrfProtection`.
# Default: true
enableCORS = true
# Enables support for Cross-Site Request Forgery (XSRF) protection, for added security.
# Due to conflicts between CORS and XSRF, if `server.enableXsrfProtection` is on and `server.enableCORS` is off at the same time, we will prioritize `server.enableXsrfProtection`.
# Default: true
enableXsrfProtection = true
# Max size, in megabytes, for files uploaded with the file_uploader.
# Default: 200
maxUploadSize = 200 | streamlit-docs.txt |
e4409cd96e13-80 | # Max size, in megabytes, of messages that can be sent via the WebSocket connection.
# Default: 200
maxMessageSize = 200
# Enables support for websocket compression.
# Default: false
enableWebsocketCompression = false
# Enable serving files from a `static` directory in the running app's directory.
# Default: false
enableStaticServing = false
# Server certificate file for connecting via HTTPS. Must be set at the same time as "server.sslKeyFile".
# ['DO NOT USE THIS OPTION IN A PRODUCTION ENVIRONMENT. It has not gone through security audits or performance tests. For the production environment, we recommend performing SSL termination by the load balancer or the reverse proxy.']
# sslCertFile =
# Cryptographic key file for connecting via HTTPS. Must be set at the same time as "server.sslCertFile".
# ['DO NOT USE THIS OPTION IN A PRODUCTION ENVIRONMENT. It has not gone through security audits or performance tests. For the production environment, we recommend performing SSL termination by the load balancer or the reverse proxy.']
# sslKeyFile =
[browser]
# Internet address where users should point their browsers in order to connect to the app. Can be IP address or DNS name and path.
# This is used to: - Set the correct URL for CORS and XSRF protection purposes. - Show the URL on the terminal - Open the browser
# Default: 'localhost'
serverAddress = "localhost"
# Whether to send usage statistics to Streamlit.
# Default: true
gatherUsageStats = true
# Port where users should point their browsers in order to connect to the app.
# This is used to: - Set the correct URL for CORS and XSRF protection purposes. - Show the URL on the terminal - Open the browser
# Default: whatever value is set in server.port.
serverPort = 8501
[mapbox] | streamlit-docs.txt |
e4409cd96e13-81 | [mapbox]
# Configure Streamlit to use a custom Mapbox token for elements like st.pydeck_chart and st.map. To get a token for yourself, create an account at https://mapbox.com. It's free (for moderate usage levels)!
# Default: ""
token = ""
[deprecation]
# Set to false to disable the deprecation warning for the file uploader encoding.
# Default: true
showfileUploaderEncoding = true
# Set to false to disable the deprecation warning for using the global pyplot instance.
# Default: true
showPyplotGlobalUse = true
[theme]
# The preset Streamlit theme that your custom theme inherits from. One of "light" or "dark".
# base =
# Primary accent color for interactive elements.
# primaryColor =
# Background color for the main content area.
# backgroundColor =
# Background color used for the sidebar and most interactive widgets.
# secondaryBackgroundColor =
# Color used for almost all text.
# textColor =
# Font family for all text in the app, except code blocks. One of "sans serif", "serif", or "monospace".
# font =
Copy
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Command-line options
Next:
Theming
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Configuration - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
Advanced features/
Theming
Theming | streamlit-docs.txt |
e4409cd96e13-82 | school
Knowledge base
Home/
Streamlit library/
Advanced features/
Theming
Theming
In this guide, we provide examples of how Streamlit page elements are affected by the various theme config options. For a more high-level overview of Streamlit themes, see the Themes section of the main concepts documentation.
Streamlit themes are defined using regular config options: a theme can be set via command line flag when starting your app using streamlit run or by defining it in the [theme] section of a .streamlit/config.toml file. For more information on setting config options, please refer to the Streamlit configuration documentation.
The following config options show the default Streamlit Light theme recreated in the [theme] section of a .streamlit/config.toml file.
[theme]
primaryColor="#F63366"
backgroundColor="#FFFFFF"
secondaryBackgroundColor="#F0F2F6"
textColor="#262730"
font="sans serif"
Copy
Let's go through each of these options, providing screenshots to demonstrate what parts of a Streamlit app they affect where needed.
primaryColor
primaryColor defines the accent color most often used throughout a Streamlit app. A few examples of Streamlit widgets that use primaryColor include st.checkbox, st.slider, and st.text_input (when focused).
star
Tip
Any CSS color can be used as the value for primaryColor and the other color options below. This means that theme colors can be specified in hex or with browser-supported color names like "green", "yellow", and "chartreuse". They can even be defined in the RGB and HSL formats!
backgroundColor
Defines the background color used in the main content area of your app.
secondaryBackgroundColor
This color is used where a second background color is needed for added contrast. Most notably, it is the sidebar's background color. It is also used as the background color for most interactive widgets.
textColor | streamlit-docs.txt |
e4409cd96e13-83 | textColor
This option controls the text color for most of your Streamlit app.
font
Selects the font used in your Streamlit app. Valid values are "sans serif", "serif", and "monospace". This option defaults to "sans serif" if unset or invalid.
Note that code blocks are always rendered using the monospace font regardless of the font selected here.
base
An easy way to define custom themes that make small changes to one of the preset Streamlit themes is to use the base option. Using base, the Streamlit Light theme can be recreated as a custom theme by writing the following:
[theme]
base="light"
Copy
The base option allows you to specify a preset Streamlit theme that your custom theme inherits from. Any theme config options not defined in your theme settings have their values set to those of the base theme. Valid values for base are "light" and "dark".
For example, the following theme config defines a custom theme nearly identical to the Streamlit Dark theme, but with a new primaryColor.
[theme]
base="dark"
primaryColor="purple"
Copy
If base itself is omitted, it defaults to "light", so you can define a custom theme that changes the font of the Streamlit Light theme to serif with the following config
[theme]
font="serif"
Copy
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Configuration
Next:
Caching
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Theming - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base | streamlit-docs.txt |
e4409cd96e13-84 | Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit library/
Advanced features/
Widget semantics
Advanced notes on widget behavior
Widgets are magical and often work how you want. But they can have surprising behavior in some situations. Here is a high-level, abstract description of widget behavior, including some common edge-cases:
If you call a widget function before the widget state exists, the widget state defaults to a value. This value depends on the widget and its arguments.
A widget function call returns the current widget state value. The return value is a simple Python type, and the exact type depends on the widget and its arguments.
Widget states depend on a particular session (browser connection). The actions of one user do not affect the widgets of any other user.
A widget's identity depends on the arguments passed to the widget function. If those change, the call will create a new widget (with a default value, per 1).
If you don't call a widget function in a script run, we neither store the widget state nor render the widget. If you call a widget function with the same arguments later, Streamlit treats it as a new widget.
4 and 5 are the most likely to be surprising and may pose a problem for some application designs. When you want to persist widget state for recreating a widget, use Session State to work around 5.
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Dataframes
Next:
Pre-release features
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Widget semantics - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base | streamlit-docs.txt |
e4409cd96e13-85 | Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit Community Cloud/
Get started/
Deploy an app/
App dependencies
App dependencies
The main reason that apps fail to build properly is because Streamlit Community Cloud can't find your dependencies! So make sure you:
Add a requirements file for Python dependencies.
(optional) Add a packages.txt file to manage any external dependencies (i.e Linux dependencies outside Python environment).
push_pin
Note
Python requirements files should be placed either in the root of your repository or in the same directory as your Streamlit app.
Add Python dependencies
Streamlit looks at your requirements file's filename to determine which Python dependency manager to use in the order below. Streamlit will stop and install the first requirements file found.
Filename Dependency Manager Documentation
Pipfile pipenv docs
environment.yml conda docs
requirements.txt pip docs
pyproject.toml poetry docs
push_pin
Note
Only include packages in your requirements file that are not distributed with a standard Python installation. If any of the modules from base Python are included in the requirements file, you will get an error when you try to deploy. Additionally, we recommend that you use the latest version of Streamlit to ensure full Streamlit Community Cloud functionality.
priority_high
Warning
You should only use one requirements file for your app. If you include more than one (e.g. requirements.txt and Pipfile). Streamlit will first look in the directory of your Streamlit app; however, if no requirements file is found, Streamlit will then look at the root of the repo.
apt-get dependencies
If packages.txt exists in the root directory of your repository we automatically detect it, parse it, and install the listed packages as described below. You can read more about apt-get in their docs. | streamlit-docs.txt |
e4409cd96e13-86 | Add apt-get dependencies to packages.txt, one package name per line. For example:
freeglut3-dev
libgtk2.0-dev
Copy
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Deploy an app
Next:
Connect to data sources
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
App dependencies - Streamlit Docssearch
Search
⌘K
dark_mode
light_mode
description
Streamlit library
cloud
Streamlit Community Cloud
school
Knowledge base
Home/
Streamlit Community Cloud/
Get started/
Deploy an app/
Connect to data sources/
Secrets management
Secrets management
Introduction
It's generally considered bad practice to store unencrypted secrets in a git repository. If your application needs access to sensitive credentials the recommended solution is to store those credentials in a file that is not committed to the repository and to pass them as environment variables.
Secrets Management allows you to store secrets securely and access them in your Streamlit app as environment variables.
How to use Secrets Management
Deploy an app and set up secrets
Go to http://share.streamlit.io/ and click "New app" to deploy a new app with secrets.
Click "Advanced settings..."
You will see a modal appear with an input box for your secrets.
Provide your secrets in the "Secrets" field using TOML format. For example:
# Everything in this section will be available as an environment variable
db_username = "Jane"
db_password = "12345qwerty" | streamlit-docs.txt |
e4409cd96e13-87 | # You can also add other sections if you like.
# The contents of sections as shown below will not become environment variables,
# but they'll be easily accessible from within Streamlit anyway as we show
# later in this doc.
[my_cool_secrets]
things_i_like = ["Streamlit", "Python"]
Copy
Use secrets in your app
Access your secrets as environment variables or by querying the st.secrets dict. For example, if you enter the secrets from the section above, the code below shows you how you can access them within your Streamlit app.
import streamlit as st
# Everything is accessible via the st.secrets dict:
st.write("DB username:", st.secrets["db_username"])
st.write("DB password:", st.secrets["db_password"])
st.write("My cool secrets:", st.secrets["my_cool_secrets"]["things_i_like"])
# And the root-level secrets are also accessible as environment variables:
import os
st.write(
"Has environment variables been set:",
os.environ["db_username"] == st.secrets["db_username"],
)
Copy
star
Tip
You can access st.secrets via attribute notation (e.g. st.secrets.key), in addition to key notation (e.g. st.secrets["key"])—like st.session_state.
You can even use TOML sections to compactly pass multiple secrets as a single attribute.
Consider the following secrets:
[db_credentials]
username = "my_username"
password = "my_password"
Copy
Rather than passing each secret as attributes in a function, you can more compactly pass the section to achieve the same result. See the notional code below which uses the secrets above:
# Verbose version
my_db.connect(username=st.secrets.db_credentials.username, password=st.secrets.db_credentials.password) | streamlit-docs.txt |
e4409cd96e13-88 | # Far more compact version!
my_db.connect(**st.secrets.db_credentials)
Copy
Edit your app's secrets
Go to https://share.streamlit.io/
Open the menu for your app, and click "Settings".
You will see a modal appear. Click on the "Secrets" section and edit your secrets.
After you edit your secrets, click "Save". It might take a minute for the update to be propagated to your app, but the new values will be reflected when the app re-runs.
Develop locally with secrets
When developing your app locally, add a file called secrets.toml in a folder called .streamlit at the root of your app repo, and copy/paste your secrets into that file.
priority_high
Important
Be sure to add this file to your .gitignore so you don't commit your secrets!
Was this page helpful?
thumb_up
Yes
thumb_down
No
edit
Suggest edits
forum
Still have questions?
Our forums are full of helpful information and Streamlit experts.
Previous:
Connect to data sources
Next:
Share your app
Home
Contact Us
Community
Copyright © 2023, Streamlit Inc.
Secrets management - Streamlit Docs | streamlit-docs.txt |
No dataset card yet