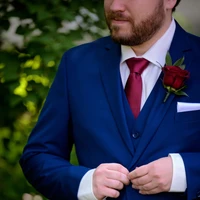
path
stringlengths 5
169
| owner
stringlengths 2
34
| repo_id
int64 1.49M
755M
| is_fork
bool 2
classes | languages_distribution
stringlengths 16
1.68k
⌀ | content
stringlengths 446
72k
| issues
float64 0
1.84k
| main_language
stringclasses 37
values | forks
int64 0
5.77k
| stars
int64 0
46.8k
| commit_sha
stringlengths 40
40
| size
int64 446
72.6k
| name
stringlengths 2
64
| license
stringclasses 15
values |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
src/Day08.kt | ZiomaleQ | 573,349,910 | false | {"Kotlin": 49609} | fun main() {
fun part1(input: List<String>): Int {
var sum = 0
val trees = input.map { line ->
line.map { c ->
Tree(c.digitToInt())
}
}
for ((rowIndex, rowOfTrees) in trees.withIndex()) {
if (rowIndex == 0 || rowIndex == trees.size - 1) {
sum += rowOfTrees.size
continue
}
for ((treeIndex, tree) in rowOfTrees.withIndex()) {
if (treeIndex == 0 || treeIndex == rowOfTrees.size - 1) {
sum++
continue
}
val treesToLeft = rowOfTrees.subList(0, treeIndex)
val treesToRight = rowOfTrees.subList(treeIndex + 1, rowOfTrees.size)
val treesToTop = trees.subList(0, rowIndex).map { it[treeIndex] }
val treesToBottom = trees.subList(rowIndex + 1, trees.size).map { it[treeIndex] }
val isVisibleHorizontal =
treesToLeft.all { it.height < tree.height } || treesToRight.all { it.height < tree.height }
val isVisibleVertical =
treesToTop.all { it.height < tree.height } || treesToBottom.all { it.height < tree.height }
if (isVisibleVertical || isVisibleHorizontal) {
sum++
}
}
}
return sum
}
fun part2(input: List<String>): Int {
val trees = input.map { line ->
line.map { c ->
Tree(c.digitToInt())
}
}.also { setScore(it) }
return trees.flatten().maxOf { it.score }
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day08_test")
part1(testInput).also { println(it); check(it == 21) }
part2(testInput).also { println(it); check(it == 8) }
val input = readInput("Day08")
println(part1(input))
println(part2(input))
}
data class Tree(val height: Int, var score: Int = 0)
fun setScore(map: List<List<Tree>>) = map.forEachIndexed { y, row ->
row.forEachIndexed { x, tree ->
tree.score = scenicScore(map, x, y)
}
}
fun scenicScore(map: List<List<Tree>>, x: Int, y: Int): Int {
val currentHeight = map[y][x].height
val directions = listOf(
(x + 1..map[0].lastIndex).map { map[y][it].height },
(x - 1 downTo 0).map { map[y][it].height },
(y + 1..map.lastIndex).map { map[it][x].height },
(y - 1 downTo 0).map { map[it][x].height }
)
return directions
.map { dir ->
(dir.indexOfFirst { it >= currentHeight } + 1)
.takeIf { it != 0 }
?: dir.size
}
.reduce(Int::times)
}
| 0 | Kotlin | 0 | 0 | b8811a6a9c03e80224e4655013879ac8a90e69b5 | 2,778 | aoc-2022 | Apache License 2.0 |
src/Day08.kt | zirman | 572,627,598 | false | {"Kotlin": 89030} | fun main() {
fun part1(input: List<String>): Int {
val trees = input.map { row -> row.map { it.digitToInt() } }
val visible = trees.map { it.indices.map { false }.toMutableList() }
for (c in trees.first().indices) {
var h = -1
for (r in trees.indices) {
if (trees[r][c] > h) {
visible[r][c] = true
h = trees[r][c]
}
}
}
for (c in trees.first().indices) {
var h = -1
for (r in trees.indices.reversed()) {
if (trees[r][c] > h) {
visible[r][c] = true
h = trees[r][c]
}
}
}
for (r in trees.indices) {
var h = -1
for (c in trees.first().indices) {
if (trees[r][c] > h) {
visible[r][c] = true
h = trees[r][c]
}
}
}
for (r in trees.indices) {
var h = -1
for (c in trees.first().indices.reversed()) {
if (trees[r][c] > h) {
visible[r][c] = true
h = trees[r][c]
}
}
}
return visible.sumOf { row -> row.count { it } }
}
fun part2(input: List<String>): Int {
val trees = input.map { row -> row.map { it.digitToInt() } }
val scenicScore = trees.indices.map { r ->
trees.first().indices.map { c ->
val max = trees[r][c]
tailrec fun searchNorth(r: Int, acc: Int): Int {
return when {
r < 0 -> acc
trees[r][c] >= max -> acc + 1
else -> searchNorth(r - 1, acc + 1)
}
}
tailrec fun searchSouth(r: Int, acc: Int): Int {
return when {
r >= trees.size -> acc
trees[r][c] >= max -> acc + 1
else -> searchSouth(r + 1, acc + 1)
}
}
tailrec fun searchEast(c: Int, acc: Int): Int {
return when {
c >= trees.size -> acc
trees[r][c] >= max -> acc + 1
else -> searchEast(c + 1, acc + 1)
}
}
tailrec fun searchWest(c: Int, acc: Int): Int {
return when {
c < 0 -> acc
trees[r][c] >= max -> acc + 1
else -> searchWest(c - 1, acc + 1)
}
}
searchNorth(r - 1, 0) *
searchSouth(r + 1, 0) *
searchEast(c + 1, 0) *
searchWest(c - 1, 0)
}
}
return scenicScore.maxOf { row -> row.maxOf { it } }
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day08_test")
check(part1(testInput) == 21)
val input = readInput("Day08")
println(part1(input))
check(part2(testInput) == 8)
println(part2(input))
}
| 0 | Kotlin | 0 | 1 | 2ec1c664f6d6c6e3da2641ff5769faa368fafa0f | 3,317 | aoc2022 | Apache License 2.0 |
src/year2023/day23/Day23.kt | lukaslebo | 573,423,392 | false | {"Kotlin": 222221} | package year2023.day23
import check
import readInput
fun main() {
val testInput = readInput("2023", "Day23_test")
check(part1(testInput), 94)
check(part2(testInput), 154)
val input = readInput("2023", "Day23")
println(part1(input))
println(part2(input))
}
private fun part1(input: List<String>) = input.parseHikingMap().longestHike()
private fun part2(input: List<String>) = input.parseHikingMap().removeSlopes().longestHike()
private fun HikingMap.longestHike() = createGraph().longestHike(start, target) ?: error("no path found")
private data class Pos(val x: Int, val y: Int) {
fun neighbours() = listOf(Pos(x, y - 1), Pos(x, y + 1), Pos(x - 1, y), Pos(x + 1, y))
}
private data class HikingMap(
val trail: Set<Pos>,
/** Positions of the slope with the position one has to go next */
val slopes: Map<Pos, Pos>,
val start: Pos,
val target: Pos,
)
private fun List<String>.parseHikingMap(): HikingMap {
val trail = mutableSetOf<Pos>()
val slopes = mutableMapOf<Pos, Pos>()
for ((y, line) in withIndex()) {
for ((x, c) in line.withIndex()) {
when (c) {
'.' -> trail += Pos(x, y)
'v' -> slopes += Pos(x, y) to Pos(x, y + 1)
'^' -> slopes += Pos(x, y) to Pos(x, y - 1)
'>' -> slopes += Pos(x, y) to Pos(x + 1, y)
'<' -> slopes += Pos(x, y) to Pos(x - 1, y)
}
}
}
return HikingMap(
trail = trail,
slopes = slopes,
start = trail.single { it.y == 0 },
target = trail.single { it.y == lastIndex },
)
}
private fun HikingMap.removeSlopes() = copy(trail = trail + slopes.keys, slopes = emptyMap())
private fun HikingMap.createGraph(): Map<Pos, Set<Pair<Pos, Int>>> {
val nodes = trail.filter { trailPos ->
trailPos.neighbours().count { it in trail || it in slopes } > 2
} + start + target
val graph = nodes.associateWith { mutableSetOf<Pair<Pos, Int>>() }
fun pathsWithDistance(pos: Pos, distance: Int = 0, visited: Set<Pos> = setOf(pos)): List<Pair<Pos, Int>> {
if (pos in nodes && distance > 0) return listOf(pos to distance)
return pos.neighbours()
.filter { next -> (next in trail || (next in slopes && pos != slopes[next])) && next !in visited }
.flatMap { next ->
pathsWithDistance(pos = next, distance = distance + 1, visited = visited + next)
}
}
for (node in nodes) {
graph.getValue(node) += pathsWithDistance(node)
}
return graph
}
private fun Map<Pos, Set<Pair<Pos, Int>>>.longestHike(pos: Pos, target: Pos, visited: Set<Pos> = setOf(pos)): Int? {
return if (pos == target) 0
else getValue(pos)
.filter { it.first !in visited }
.mapNotNull { (next, distance) ->
val longestHike = longestHike(next, target, visited + next) ?: return@mapNotNull null
distance + longestHike
}
.maxOrNull()
}
| 0 | Kotlin | 0 | 1 | f3cc3e935bfb49b6e121713dd558e11824b9465b | 3,011 | AdventOfCode | Apache License 2.0 |
src/Day13.kt | flex3r | 572,653,526 | false | {"Kotlin": 63192} | fun main() {
val testInput = readInput("Day13_test")
check(part1(testInput) == 13)
check(part2(testInput) == 140)
val input = readInput("Day13")
println(part1(input))
println(part2(input))
}
private fun part1(input: List<String>): Int {
return input.partitionBy { it.isEmpty() }
.asSequence()
.map { (a, b) -> parse(a).compareTo(parse(b)) }
.withIndex()
.filter { (_, res) -> res == -1 }
.sumOf { (idx, _) -> idx + 1 }
}
private fun part2(input: List<String>): Int {
val firstDivider = PacketList(listOf(PacketList(listOf(PacketValue(2)))))
val secondDivider = PacketList(listOf(PacketList(listOf(PacketValue(6)))))
val sorted = input
.asSequence()
.filterNot { it.isEmpty() }
.map { parse(it) }
.plus(firstDivider)
.plus(secondDivider)
.sorted()
.toList()
return (sorted.indexOf(firstDivider) + 1) * (sorted.indexOf(secondDivider) + 1)
}
private fun parse(value: String): Packet {
if (value.firstOrNull()?.isDigit() == true) return PacketValue(value.toInt())
return PacketList(
packets = buildList {
var openBrackets = 0
var lastComma = 0
value.forEachIndexed { idx, c ->
when {
c == '[' -> openBrackets++
c == ']' -> if (--openBrackets == 0) add(parse(value.substring(lastComma + 1 until idx)))
c == ',' && openBrackets == 1 -> add(parse(value.substring(lastComma + 1 until idx))).also { lastComma = idx }
}
}
}
)
}
private sealed class Packet : Comparable<Packet> {
override fun compareTo(other: Packet) = when {
this is PacketValue && other is PacketValue -> this.packet.compareTo(other.packet)
else -> compareLists(this.asList(), other.asList())
}
private fun compareLists(a: PacketList, b: PacketList): Int {
a.packets.zip(b.packets).forEach { (a, b) ->
val compared = a.compareTo(b)
if (compared != 0) return compared
}
return a.packets.size.compareTo(b.packets.size)
}
private fun asList() = if (this is PacketList) this else PacketList(listOf(this))
}
private data class PacketList(val packets: List<Packet>) : Packet()
private data class PacketValue(val packet: Int) : Packet()
| 0 | Kotlin | 0 | 0 | 8604ce3c0c3b56e2e49df641d5bf1e498f445ff9 | 2,389 | aoc-22 | Apache License 2.0 |
src/year2021/day08/Day08.kt | fadi426 | 433,496,346 | false | {"Kotlin": 44622} | package year2021.day01.day08
import util.assertTrue
import util.read2021DayInput
fun main() {
fun task01(input: List<String>): Int {
val formattedInput = input.map { it.substringAfter(" | ") }.map { it.split(" ") }
return listOf(2, 4, 3, 7).let { uniqueSegments ->
formattedInput.sumOf { it.count { uniqueSegments.contains(it.length) } }
}
}
fun task02(input: List<String>): Int {
var sum = 0
val formattedInput = input.map { it.split(" | ") }.map { it.map { it.split(" ") } }
formattedInput.forEach {
val segments = mutableListOf<Pair<String, Int>>()
segments.addAll(byUniqueLength(it[0]))
segments.addAll(byLengthSix(it[0], segments))
segments.addAll(byLengthFive(it[0], segments))
sum += decodeSignal(it[1], segments)
}
return sum
}
val input = read2021DayInput("Day08")
assertTrue(task01(input) == 261)
assertTrue(task02(input) == 987553)
}
fun decodeSignal(list: List<String>, segments: List<Pair<String, Int>>): Int {
return list.map { segments.first { e -> it.alphabetized() == e.first.alphabetized() } }.map { it.second }
.joinToString { it.toString() }.replace(", ", "").toInt()
}
fun byUniqueLength(list: List<String>): List<Pair<String, Int>> {
val l = listOf(Pair(1, 2), Pair(4, 4), Pair(7, 3), Pair(8, 7))
return list.map { Pair(it, l.find { e -> e.second == it.length }) }.filter { it.second != null }
.map { Pair(it.first, it.second!!.first) }
}
fun byLengthFive(list: List<String>, segments: List<Pair<String, Int>>): List<Pair<String, Int>> {
val outcome = mutableListOf<Pair<String, Int>>()
val fiveList = list.filter { it.length == 5 }
val three = Pair(fiveList.first { containsDigitPattern(it, segments, 7) }, 3)
val five = Pair(fiveList.first { segments.first { e -> e.second == 6 }.first.toList().containsAll(it.toList()) }, 5)
val two = Pair(fiveList.first { it != three.first && it != five.first }, 2)
outcome.addAll(listOf(three, five, two))
return outcome
}
fun byLengthSix(list: List<String>, segments: List<Pair<String, Int>>): List<Pair<String, Int>> {
val outcome = mutableListOf<Pair<String, Int>>()
val sixList = list.filter { it.length == 6 }
val six = Pair(sixList.first { !containsDigitPattern(it, segments, 1) }, 6)
val nine = Pair(sixList.first { containsDigitPattern(it, segments, 4) }, 9)
val zero = Pair(sixList.first { it != six.first && it != nine.first }, 0)
outcome.addAll(listOf(six, nine, zero))
return outcome
}
fun containsDigitPattern(a: String, list: List<Pair<String, Int>>, i: Int): Boolean {
return a.toList().containsAll(list.first { it.second == i }.first.toList())
}
fun String.alphabetized() = String(toCharArray().apply { sort() })
| 0 | Kotlin | 0 | 0 | acf8b6db03edd5ff72ee8cbde0372113824833b6 | 2,853 | advent-of-code-kotlin-template | Apache License 2.0 |
src/Day14.kt | laricchia | 434,141,174 | false | {"Kotlin": 38143} | fun firstPart14(list : List<Pair<String, String>>, start : String) {
var newSeq = start
val steps = 10
for (i in 0 until steps) {
var windows = newSeq.windowed(2).map { StringBuilder(it) }
list.map { insertion ->
windows = windows.map {
if (it.toString() == insertion.first) it.insert(1, insertion.second) else it
}
}
newSeq = windows.joinToString(separator = "") { it.deleteCharAt(it.length - 1).toString() } + start.last()
// println(newSeq)
}
val distinctLetters = newSeq.split("").filterNot { it.isEmpty() }.distinct()
val counts = distinctLetters.map { letter ->
newSeq.count { it == letter.first() }
}.sortedDescending()
println(counts.first() - counts.last())
}
fun secondPart14(list : List<Pair<String, String>>, start : String) {
val steps = 40
var state = mutableMapOf<String, Long>()
val startWindows = start.windowed(2)
startWindows.distinct().map { s -> state[s] = startWindows.count { s == it }.toLong() }
list.filterNot { it.first in startWindows }.map { state[it.first] = 0 }
// println(state)
for (i in 0 until steps) {
val updatedState = mutableMapOf<String, Long>()
list.map {
val elem1 = it.first.first() + it.second
val elem2 = it.second + it.first.last()
val value = state[it.first] ?: 0
updatedState[elem1] = (updatedState[elem1] ?: 0) + value
updatedState[elem2] = (updatedState[elem2] ?: 0) + value
}
state = updatedState
// println(state)
}
val distinctLetters = state.map { it.key }.map { it.split("").filterNot { it.isEmpty() }.map { it.first() } }.flatten().distinct()
val counts = mutableMapOf<Char, Long>()
distinctLetters.map { counts[it] = 0 }
state.map {
counts[it.key.first()] = counts[it.key.first()]!! + it.value
counts[it.key.last()] = counts[it.key.last()]!! + it.value
}
//println(counts)
val sortedCounts = counts.map {
val updatedValue = it.value / 2
if (it.key == start.last()) (updatedValue + 1) else updatedValue
}.sortedDescending()
// println(sortedCounts)
println(sortedCounts.first() - sortedCounts.last())
}
fun main() {
val input : List<String> = readInput("Day14")
val start = input.first()
val pairs = input.subList(2, input.size).map {
val splits = it.split(" -> ")
splits[0] to splits[1]
}
firstPart14(pairs, start)
secondPart14(pairs, start)
}
| 0 | Kotlin | 0 | 0 | 7041d15fafa7256628df5c52fea2a137bdc60727 | 2,589 | Advent_of_Code_2021_Kotlin | Apache License 2.0 |
src/day24/d24_2.kt | svorcmar | 720,683,913 | false | {"Kotlin": 49110} | fun main() {
val input = ""
val list = input.lines().map { it.toInt() }
val groupSize = list.sum() / 4
val firstGroup = solve(list, groupSize)
// order by quantum entanglement and find smallest one for which
// the rest of packages can be divided in three groups of the same weight
val candidates = firstGroup.map { it.map(Int::toLong).reduce(Long::times) to it }.sortedBy { it.first }
for (candidate in candidates) {
if (canBeSplitIn3(list - candidate.second, groupSize)) {
println(candidate.first)
break
}
}
}
fun solve(packages: List<Int>, target: Int): List<List<Int>> {
// dp[i][j] == set of the smallest subsets of the first i elements that sum to j
val dp = Array(packages.size + 1) { Array(target + 1) {
if (it == 0)
0 to listOf(emptyList<Int>())
else
packages.size to emptyList<List<Int>>()
}
}
packages.forEachIndexed { i0, n ->
val i = i0 + 1
for (j in 1 .. target) {
dp[i][j] = dp[i - 1][j]
if (j >= n) {
val prevRow = dp[i - 1][j - n]
if (prevRow.first + 1 < dp[i][j].first) {
dp[i][j] = prevRow.first + 1 to prevRow.second.map { it -> it + n }
} else if (prevRow.first + 1 == dp[i][j].first) {
dp[i][j] = dp[i][j].first to dp[i][j].second + prevRow.second.map { it -> it + n }
}
}
}
}
return dp[packages.size][target].second
}
fun canBeSplitIn3(packages: List<Int>, target: Int): Boolean {
// the order/size of the groups is not important;
// the first element is forced the first group and the second element to the first or second group to fix the ordering
return canBeSplitIn3Rec(packages, target, 2, packages[0] + packages[1], 0, 0) ||
canBeSplitIn3Rec(packages, target, 2, packages[0], packages[1], 0)
}
fun canBeSplitIn3Rec(packages: List<Int>, target: Int, nextIdx: Int, g1: Int, g2: Int, g3: Int): Boolean {
if (nextIdx == packages.size) {
return true
}
val next = packages[nextIdx]
return (g1 < target && g1 + next <= target && canBeSplitIn3Rec(packages, target, nextIdx + 1, g1 + next, g2, g3)) ||
(g2 < target && g2 + next <= target && canBeSplitIn3Rec(packages, target, nextIdx + 1, g1, g2 + next, g3)) ||
(g3 < target && g3 + next <= target && canBeSplitIn3Rec(packages, target, nextIdx + 1, g1, g2, g3 + next))
}
| 0 | Kotlin | 0 | 0 | cb097b59295b2ec76cc0845ee6674f1683c3c91f | 2,361 | aoc2015 | MIT License |
advent-of-code-2022/src/Day08.kt | osipxd | 572,825,805 | false | {"Kotlin": 141640, "Shell": 4083, "Scala": 693} | typealias Forest = List<List<Int>>
fun main() {
val testInput = readInput("Day08_test")
val input = readInput("Day08")
"Part 1" {
part1(testInput) shouldBe 21
answer(part1(input))
}
"Part 2" {
part2(testInput) shouldBe 8
answer(part2(input))
}
}
private fun part1(forest: Forest): Int {
val n = forest.size
val m = forest[0].size
/** Checks the tree at position [r],[c] is visible from the given [direction]. */
fun isVisibleFromOutside(r: Int, c: Int, direction: Direction): Boolean {
val treeHeight = forest[r][c]
return forest.treesByDirection(r, c, direction).all { it < treeHeight }
}
// All trees on edges are visible
var count = (n + m - 2) * 2
for (r in 1 until n - 1) {
for (c in 1 until m - 1) {
if (Direction.values().any { isVisibleFromOutside(r, c, it) }) count++
}
}
return count
}
private fun part2(forest: Forest): Int {
val n = forest.size
val m = forest[0].size
/** Counts trees visible from tree at position [r],[c] in the given [direction]. */
fun countVisibleTrees(r: Int, c: Int, direction: Direction): Int {
val targetTree = forest[r][c]
var count = 0
for (currentTree in forest.treesByDirection(r, c, direction)) {
count++
if (currentTree >= targetTree) return count
}
return count
}
var maxScore = 0
// All trees on edges will have score 0, so skip it
for (r in 1 until n - 1) {
for (c in 1 until m - 1) {
val score = Direction.values().map { countVisibleTrees(r, c, it) }.reduce(Int::times)
maxScore = maxOf(maxScore, score)
}
}
return maxScore
}
/** Generates sequence of trees heights for the given [direction] in order of distance from [r],[c]. */
private fun Forest.treesByDirection(r: Int, c: Int, direction: Direction): Sequence<Int> {
return generateSequence(direction.next(r to c), direction::next)
.takeWhile { (r, c) -> r in indices && c in first().indices }
.map { (r, c) -> this[r][c] }
}
// dr, dc - delta row, delta column
private enum class Direction(val dr: Int = 0, val dc: Int = 0) {
UP(dr = -1),
LEFT(dc = -1),
DOWN(dr = +1),
RIGHT(dc = +1);
/** Returns the next coordinates on this direction. */
fun next(coordinates: Pair<Int, Int>): Pair<Int, Int> {
val (r, c) = coordinates
return (r + dr) to (c + dc)
}
}
private fun readInput(name: String) = readLines(name).map { it.map(Char::digitToInt) }
| 0 | Kotlin | 0 | 5 | 6a67946122abb759fddf33dae408db662213a072 | 2,607 | advent-of-code | Apache License 2.0 |
aoc21/day_08/main.kt | viktormalik | 436,281,279 | false | {"Rust": 227300, "Go": 86273, "OCaml": 82410, "Kotlin": 78968, "Makefile": 13967, "Roff": 9981, "Shell": 2796} | import java.io.File
fun segmentsByLen(len: Int): Set<Char> = when (len) {
2 -> setOf('c', 'f')
3 -> setOf('a', 'c', 'f')
4 -> setOf('b', 'c', 'd', 'f')
else -> ('a'..'g').toSet()
}
fun segmentToDigit(segment: Set<Char>): Char? = when (segment) {
setOf('a', 'b', 'c', 'e', 'f', 'g') -> '0'
setOf('c', 'f') -> '1'
setOf('a', 'c', 'd', 'e', 'g') -> '2'
setOf('a', 'c', 'd', 'f', 'g') -> '3'
setOf('b', 'c', 'd', 'f') -> '4'
setOf('a', 'b', 'd', 'f', 'g') -> '5'
setOf('a', 'b', 'd', 'e', 'f', 'g') -> '6'
setOf('a', 'c', 'f') -> '7'
setOf('a', 'b', 'c', 'd', 'e', 'f', 'g') -> '8'
setOf('a', 'b', 'c', 'd', 'f', 'g') -> '9'
else -> null
}
fun MutableMap<Char, Set<Char>>.dropGroups(groups: Iterable<Set<Char>>) {
for (group in groups) {
for (c in filter { (_, map) -> map != group }.keys)
merge(c, group, Set<Char>::minus)
}
}
fun getOptions(digit: String, mapping: Map<Char, Set<Char>>): Set<Set<Char>> {
if (digit.length == 1)
return mapping.get(digit[0])!!.map { setOf(it) }.toSet()
return getOptions(digit.substring(1), mapping)
.flatMap { mapping.get(digit[0])!!.map { c -> setOf(c) + it } }
.filter { it.size == digit.length }
.toSet()
}
fun decode(entry: String): Int {
val patterns = entry.split(" | ")[0]
val mapping = ('a'..'g').map { it to ('a'..'g').toSet() }.toMap().toMutableMap()
var size = mapping.map { (_, m) -> m.size }.sum()
var prevSize = 0
while (size != prevSize) {
prevSize = size
for (pat in patterns.split(" ")) {
for (i in 0 until pat.length) {
mapping.merge(pat[i], segmentsByLen(pat.length), Set<Char>::intersect)
}
}
mapping.dropGroups(mapping.filter { (_, m) -> m.size == 1 }.values)
mapping.dropGroups(
mapping.filter { (c1, m1) ->
m1.size == 2 && mapping.any { (c2, m2) -> c1 != c2 && m1 == m2 }
}.values
)
size = mapping.map { (_, m) -> m.size }.sum()
}
return entry
.split(" | ")[1]
.split(" ")
.map { getOptions(it, mapping).firstNotNullOf { segmentToDigit(it) } }
.joinToString("")
.toInt()
}
fun main() {
val entries = File("input").readLines()
val first = entries.map { e ->
e.split(" | ")[1]
.split(" ")
.filter { d -> d.length == 2 || d.length == 3 || d.length == 4 || d.length == 7 }
.count()
}.sum()
println("First: $first")
val second = entries.map { decode(it) }.sum()
println("Second: $second")
}
| 0 | Rust | 1 | 0 | f47ef85393d395710ce113708117fd33082bab30 | 2,654 | advent-of-code | MIT License |
src/main/kotlin/Day16.kt | todynskyi | 573,152,718 | false | {"Kotlin": 47697} | import kotlin.math.max
fun main() {
fun floydWarshall(
shortestPaths: Map<String, MutableMap<String, Int>>,
valves: Map<String, Valve>
): Map<String, Map<String, Int>> {
for (k in shortestPaths.keys) {
for (i in shortestPaths.keys) {
for (j in shortestPaths.keys) {
val ik = shortestPaths[i]?.get(k) ?: 9999
val kj = shortestPaths[k]?.get(j) ?: 9999
val ij = shortestPaths[i]?.get(j) ?: 9999
if (ik + kj < ij) {
shortestPaths[i]?.set(j, ik + kj)
}
}
}
}
shortestPaths.values.forEach {
it.keys.map { key -> if (valves[key]?.flowRate == 0) key else "" }
.forEach { toRemove -> if (toRemove != "") it.remove(toRemove) }
}
return shortestPaths
}
fun calculate(input: List<Valve>, totalTime: Int, optimal: Boolean): Int {
var score = 0
val valves = input.associateBy { it.id }
val shortestPaths =
floydWarshall(
input.associate { it.id to it.neighbors.associateWith { 1 }.toMutableMap() }.toMutableMap(),
valves
)
fun calculate(currScore: Int, currentValve: String, visited: Set<String>, time: Int, optimal: Boolean) {
score = max(score, currScore)
for ((valve, dist) in shortestPaths[currentValve]!!) {
if (!visited.contains(valve) && time + dist + 1 < totalTime) {
calculate(
currScore + (totalTime - time - dist - 1) * valves[valve]?.flowRate!!,
valve,
visited.union(listOf(valve)),
time + dist + 1,
optimal
)
}
}
if (optimal) {
calculate(currScore, "AA", visited, 0, false)
}
}
calculate(0, "AA", emptySet(), 0, optimal)
return score
}
fun part1(input: List<Valve>): Int = calculate(input, PART1_TIME, false)
fun part2(input: List<Valve>): Int = calculate(input, PART2_TIME, true)
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day16_test").toValves()
check(part1(testInput) == 1651)
println(part2(testInput))
val input = readInput("Day16").toValves()
println(part1(input))
println(part2(input))
}
const val PART1_TIME = 30
const val PART2_TIME = 26
fun List<String>.toValves(): List<Valve> = this.map {
val (left, right) = it.split("; ")
val (id, flowRate) = left.split(" has flow rate=")
Valve(
id.substringAfter("Valve "),
flowRate.toInt(),
right.replace("tunnels lead to valves ", "")
.replace("tunnel leads to valve ", "")
.split(", ").map { valve -> valve.trim() }
)
}
data class Valve(val id: String, val flowRate: Int, val neighbors: List<String>)
| 0 | Kotlin | 0 | 0 | 5f9d9037544e0ac4d5f900f57458cc4155488f2a | 3,082 | KotlinAdventOfCode2022 | Apache License 2.0 |
src/com/kingsleyadio/adventofcode/y2021/day22/Solution.kt | kingsleyadio | 435,430,807 | false | {"Kotlin": 134666, "JavaScript": 5423} | package com.kingsleyadio.adventofcode.y2021.day22
import com.kingsleyadio.adventofcode.util.readInput
fun part1(commands: List<Command>): Int {
val bound = -50..50
val ons = hashSetOf<P3>()
for ((action, cuboid) in commands) {
if (cuboid.x !in bound || cuboid.y !in bound || cuboid.z !in bound) continue
when (action) {
Action.ON -> cuboid.cubes().forEach { ons.add(it) }
Action.OFF -> cuboid.cubes().forEach { ons.remove(it) }
}
}
return ons.size
}
fun part2(commands: List<Command>): Long {
val bound = -50..50
val within = commands.filter { (_, cuboid) -> cuboid.x in bound && cuboid.y in bound && cuboid.z in bound }
return within.foldIndexed(0L) { index, acc, (action, cuboid) ->
println(acc)
val intersects = commands.subList(0, index).map { cuboid.intersect(it.second) }
val currentVolume = if (action == Action.ON) cuboid.volume() else 0
acc - sum(intersects) + currentVolume
}
}
fun sum(cuboids: List<Cuboid>): Long {
return when (cuboids.size) {
0 -> 0L
1 -> cuboids[0].volume()
else -> {
val last = cuboids.last()
val intersects = cuboids.subList(0, cuboids.lastIndex).map { it.intersect(last) }.filterNot { it.isNone() }
sum(cuboids.subList(0, cuboids.lastIndex)) - sum(intersects) + last.volume()
}
}
}
fun main() {
val commands = readInput(2021, 22).useLines { lines -> lines.map(::parse).toList() }
println(part1(commands))
println(part2(commands))
}
fun parse(line: String): Command {
val (c, rest) = line.split(" ")
val (x, y, z) = rest.split(",").map { ch -> ch.substringAfter("=").split("..").map { it.toInt() } }
val action = if (c == "on") Action.ON else Action.OFF
val cuboid = Cuboid(x[0]..x[1], y[0]..y[1], z[0]..z[1])
return Command(action, cuboid)
}
typealias P3 = List<Int>
typealias Command = Pair<Action, Cuboid>
enum class Action { ON, OFF }
data class Cuboid(val x: IntRange, val y: IntRange, val z: IntRange)
fun Cuboid.cubes() = sequence { for (xx in x) for (yy in y) for (zz in z) yield(listOf(xx, yy, zz)) }
fun Cuboid.volume(): Long = x.size.toLong() * y.size * z.size
fun Cuboid.isNone() = x == IntRange.EMPTY && y == IntRange.EMPTY && z == IntRange.EMPTY
fun Cuboid.intersect(other: Cuboid) = Cuboid(x.intersect(other.x), y.intersect(other.y), z.intersect(other.z))
operator fun IntRange.contains(range: IntRange) = range.first in this && range.last in this
val IntRange.size: Int get() = last - first + 1
fun IntRange.intersect(other: IntRange): IntRange {
return if (this in other) this
else if (other in this) other
else if (last < other.first) IntRange.EMPTY
else if (first > other.first) first..other.last
else other.first..last
}
| 0 | Kotlin | 0 | 1 | 9abda490a7b4e3d9e6113a0d99d4695fcfb36422 | 2,830 | adventofcode | Apache License 2.0 |
src/main/kotlin/Day12.kt | Ostkontentitan | 434,500,914 | false | {"Kotlin": 73563} | fun puzzleDayTwelvePartOne() {
val inputs = readInput(12)
val connections = inputsToConnections(inputs)
val pathes = findUniquePaths(Cave.Start, connections, mapOf(Cave.Start to 1))
println("Number of pathes $pathes")
}
fun puzzleDayTwelvePartTwo() {
val inputs = readInput(12)
val connections = inputsToConnections(inputs)
val pathes = findUniquePaths(Cave.Start, connections, mapOf(Cave.Start to 1), true)
println("Number of pathes with 'golden ticket' $pathes")
}
fun inputsToConnections(inputs: List<String>): List<Connection> = inputs.map {
val split = it.split("-")
Connection(Cave(split[0]), Cave(split[1]))
}
fun findUniquePaths(from: Cave, connections: List<Connection>, visited: Map<Cave, Int>): Int {
if (from == Cave.End) {
return 1
}
val connectionsToElement = connections.filter { it.contains(from) }.filterVisitable(from, visited)
val nextOnes = connectionsToElement.map { it.getOther(from) }
val updatedVisits = visited.incrementedForItem(from)
return nextOnes.sumOf { findUniquePaths(it, connections, updatedVisits) }
}
fun findUniquePaths(
from: Cave,
connections: List<Connection>,
visited: Map<Cave, Int>,
goldenTicketAvailable: Boolean
): Int {
if (from == Cave.End) {
return 1
}
val connectionsToElement = connections.filter { it.contains(from) }.filterVisitable(from, visited)
val ticketConnections = if (goldenTicketAvailable) connections.filter { it.contains(from) } - connectionsToElement.toSet() else emptyList()
val nextOnes = connectionsToElement.map { it.getOther(from) }.filterNot { it == Cave.Start }
val nextOnesWithTicket = ticketConnections.map { it.getOther(from) }.filterNot { it.isBig || it == Cave.Start }
val updatedVisits = visited.incrementedForItem(from)
return nextOnes.sumOf { findUniquePaths(it, connections, updatedVisits, goldenTicketAvailable) } +
nextOnesWithTicket.sumOf { findUniquePaths(it, connections, updatedVisits, false) }
}
fun List<Connection>.filterVisitable(cave: Cave, visited: Map<Cave, Int>) =
this.filter { it.getOther(cave).isVisitable(visited) }
fun Cave.isVisitable(visited: Map<Cave, Int>) = (visited[this] ?: 0) < this.maxVisits
fun Map<Cave, Int>.incrementedForItem(item: Cave): Map<Cave, Int> =
this + (item to (this[item] ?: 0) + 1)
data class Cave(val key: String) {
val isSmall = key == key.lowercase()
val isBig = !isSmall
val maxVisits = if (isSmall) 1 else Int.MAX_VALUE
companion object {
val Start = Cave("start")
val End = Cave("end")
}
}
data class Connection(private val one: Cave, private val two: Cave) {
fun contains(cave: Cave) = one == cave || two == cave
fun getOther(cave: Cave) =
if (cave == one) two else if (cave == two) one else throw IllegalArgumentException("Other call with non contained element. ")
}
| 0 | Kotlin | 0 | 0 | e0e5022238747e4b934cac0f6235b92831ca8ac7 | 2,911 | advent-of-kotlin-2021 | Apache License 2.0 |
src/Day13.kt | bigtlb | 573,081,626 | false | {"Kotlin": 38940} | fun main() {
class AdventList : Comparable<AdventList> {
fun terms(input: String): List<String> {
var parenLevel = 0
var start = 0
var next = 0
val termList = mutableListOf<String>()
while (next <= input.length) {
when {
next == input.length || (parenLevel == 0 && ",]".contains(input[next])) -> {
termList.add(input.substring(start until next))
start = next + 1
}
parenLevel > 0 && input[next] == ']' -> parenLevel--
input[next] == '[' -> parenLevel++
else -> Unit
}
next++
}
return termList
}
constructor(input: String) {
list.addAll(terms(input.slice(1 until input.lastIndex)).map { it.trim() }
.map {
when {
it.startsWith('[') -> Pair(null, AdventList(it))
else -> Pair(it.toIntOrNull(), null)
}
})
}
var list = mutableListOf<Pair<Int?, AdventList?>>()
fun Pair<Int?, AdventList?>.compareTo(other: Pair<Int?, AdventList?>) = when {
this == other -> 0
first != null && other.first != null -> first!!.compareTo(other.first!!)
first != null && other.second != null -> AdventList("[$first]").compareTo(other.second!!)
second != null && other.first != null -> second!!.compareTo(AdventList("[${other.first}]"))
second != null && other.second != null -> second!!.compareTo(other.second!!)
first == null && second == null && (other.first != null || other.second != null) -> -1
else -> {
1
}
}
override fun compareTo(other: AdventList): Int {
val result =
this.list.zip(other.list).fold(0) { acc, (one, two) -> if (acc != 0) acc else one.compareTo(two) }
return if (result == 0) listCount.compareTo(other.listCount) else result
}
val listCount: Int get() = list.sumOf { if (it.first != null) 1 else (it.second?.listCount ?: 0) + 1 }
}
fun part1(input: List<String>): Int = input.chunked(3).map { (first, second) ->
AdventList(first).compareTo(AdventList(second)) <= 0
}.mapIndexed { idx, cur -> if (cur) idx + 1 else 0 }.sum()
fun part2(input: List<String>): Int {
val dividers = listOf(AdventList("[[2]]"), AdventList("[[6]]"))
return input.filterNot { it.isNullOrBlank() }.map { AdventList(it) }.union(dividers).sorted()
.mapIndexedNotNull { idx, node -> if (dividers.contains(node)) idx + 1 else null }
.fold(1) { acc, idx -> acc * idx }
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day13_test")
check(part1(testInput) == 13)
check(part2(testInput) == 140)
println("checked")
val input = readInput("Day13")
println(part1(input))
println(part2(input))
}
| 0 | Kotlin | 0 | 0 | d8f76d3c75a30ae00c563c997ed2fb54827ea94a | 3,160 | aoc-2022-demo | Apache License 2.0 |
y2023/src/main/kotlin/adventofcode/y2023/Day25.kt | Ruud-Wiegers | 434,225,587 | false | {"Kotlin": 503769} | package adventofcode.y2023
import adventofcode.io.AdventSolution
fun main() {
Day25.solve()
}
object Day25 : AdventSolution(2023, 25, "Snowverload") {
override fun solvePartOne(input: String): Any {
val weightedGraph: WeightedGraph = parse(input)
.mapValues { Desc(1, it.value.associateWith { 1 }.toMutableMap()) }
.entries.sortedBy { it.key }.map { it.value }.toTypedArray()
repeat(weightedGraph.size - 2) {
val (s, t) = weightedGraph.findMinCut()
val cut = weightedGraph[t].edgeWeights.values.sum()
if (cut == 3) {
val wt = weightedGraph[t].vertexCount
return (weightedGraph.sumOf { it.vertexCount } - wt) * wt
}
weightedGraph.merge(s, t)
}
error("No solution")
}
override fun solvePartTwo(input: String) = "Free!"
}
private fun parse(input: String): Map<Int, List<Int>> {
val graph = input.lines().flatMap {
val (s, es) = it.split(": ")
es.split(" ").flatMap { e -> listOf(s to e, e to s) }
}.groupBy({ it.first }, { it.second })
val keys = graph.keys.withIndex().associate { it.value to it.index }
return graph.mapKeys { keys.getValue(it.key) }.mapValues { it.value.map { keys.getValue(it) } }
}
private data class Desc(var vertexCount: Int, val edgeWeights: MutableMap<Int, Int>)
private typealias WeightedGraph = Array<Desc>
private fun WeightedGraph.findMinCut(): List<Int> {
val last = indexOfLast { it.vertexCount > 0 }
val a = BooleanArray(last + 1)
a[0] = true
val weightsFromA = IntArray(last + 1)
this.first().edgeWeights.forEach { weightsFromA[it.key] += it.value }
repeat(a.size - 3) {
val x = weightsFromA.max()
val next = weightsFromA.indexOf(x)
a[next] = true
weightsFromA[next] = 0
this[next].edgeWeights.forEach { if (!a[it.key]) weightsFromA[it.key] += it.value }
}
val c1 = weightsFromA.indexOfFirst { it > 0 }
val c2 = weightsFromA.indexOfLast { it > 0 }
return listOf(c1, c2).sortedByDescending { weightsFromA[it] }
}
//merge s and t, move the empty t to the end of the array
private fun WeightedGraph.merge(s: Int, t: Int) {
val tDesc = get(t)
val stDesc = get(s)
tDesc.edgeWeights.forEach { (k, v) -> stDesc.edgeWeights.merge(k, v, Int::plus) }
stDesc.edgeWeights -= s
stDesc.edgeWeights -= t
stDesc.vertexCount += tDesc.vertexCount
val swap = maxOf(t, indexOfLast { it.vertexCount > 0 })
for ((_, w) in this) {
w.remove(t)?.let { w.merge(s, it, Int::plus) }
w.remove(swap)?.let { w[t] = it }
}
this[t] = this[swap]
this[swap] = Desc(0, mutableMapOf())
}
| 0 | Kotlin | 0 | 3 | fc35e6d5feeabdc18c86aba428abcf23d880c450 | 2,750 | advent-of-code | MIT License |
src/Day05/Day05.kt | martin3398 | 436,014,815 | false | {"Kotlin": 63436, "Python": 5921} | import kotlin.math.min
import kotlin.math.max
fun main() {
fun prepare(input: List<String>): List<Pair<Pair<Int, Int>, Pair<Int, Int>>> {
return input.map {
val split = it.split("->").map { x -> x.split(',').map { e -> e.trim() } }
Pair(Pair(split[0][0].toInt(), split[0][1].toInt()), Pair(split[1][0].toInt(), split[1][1].toInt()))
}
}
fun part1(input: List<Pair<Pair<Int, Int>, Pair<Int, Int>>>): Int {
val inputWc = input.filter { it.first.first == it.second.first || it.first.second == it.second.second }
val maxVal =
input.maxOf { max(max(it.first.first, it.first.second), max(it.second.first, it.second.second)) } + 1
val array = MutableList(maxVal) { MutableList(maxVal) { 0 } }
for (e in inputWc) {
array.subList(min(e.first.first, e.second.first), max(e.first.first, e.second.first) + 1)
.map { it.subList(min(e.first.second, e.second.second), max(e.first.second, e.second.second) + 1) }
.forEach { it.forEachIndexed { index2, i -> it[index2] = i + 1 } }
}
return array.sumOf { x -> x.count { it > 1 } }
}
fun part2(input: List<Pair<Pair<Int, Int>, Pair<Int, Int>>>): Int {
val maxVal =
input.maxOf { max(max(it.first.first, it.first.second), max(it.second.first, it.second.second)) } + 1
val array = MutableList(maxVal) { MutableList(maxVal) { 0 } }
for (e in input) {
if (e.first.first == e.second.first || e.first.second == e.second.second) {
array.subList(min(e.first.first, e.second.first), max(e.first.first, e.second.first) + 1)
.map { it.subList(min(e.first.second, e.second.second), max(e.first.second, e.second.second) + 1) }
.forEach { it.forEachIndexed { index2, i -> it[index2] = i + 1 } }
} else {
val length = e.first.first - e.second.first
val range = if (length > 0) 0..length else 0..-length
for (i in range) {
array[e.first.first + if (e.first.first > e.second.first) -i else i][e.first.second + if (e.first.second > e.second.second) -i else i]++
}
}
}
return array.sumOf { x -> x.count { it > 1 } }
}
val testInput = prepare(readInput(5, true))
val input = prepare(readInput(5))
check(part1(testInput) == 5)
println(part1(input))
check(part2(testInput) == 12)
println(part2(input))
}
| 0 | Kotlin | 0 | 0 | 085b1f2995e13233ade9cbde9cd506cafe64e1b5 | 2,539 | advent-of-code-2021 | Apache License 2.0 |
src/main/kotlin/day18/Day18.kt | Avataw | 572,709,044 | false | {"Kotlin": 99761} | package day18
fun solveA(input: List<String>): Int {
val cubes = input.map(String::toCube).toSet()
return cubes.size * 6 - cubes.sumOf { it.touches(cubes) }
}
fun solveB(input: List<String>): Int {
val cubes = input.map(String::toCube).toSet()
val min = minOf(cubes.minOf { it.x }, cubes.minOf { it.y }, cubes.minOf { it.z }) - 1
val max = maxOf(cubes.maxOf { it.x }, cubes.maxOf { it.y }, cubes.maxOf { it.z }) + 1
val fillers = mutableListOf(Cube(min, min, min))
fillers.first().fill(cubes, fillers, min..max)
return fillers.sumOf { it.touches(cubes) }
}
fun String.toCube(): Cube {
val (x, y, z) = this.split(",").map(String::toInt)
return Cube(x, y, z)
}
data class Cube(val x: Int, val y: Int, val z: Int) {
fun touches(cubes: Set<Cube>) = listOfNotNull(
cubes.find { it.x == x - 1 && it.y == y && it.z == z },
cubes.find { it.x == x + 1 && it.y == y && it.z == z },
cubes.find { it.y == y - 1 && it.x == x && it.z == z },
cubes.find { it.y == y + 1 && it.x == x && it.z == z },
cubes.find { it.z == z - 1 && it.y == y && it.x == x },
cubes.find { it.z == z + 1 && it.y == y && it.x == x }
).count()
fun fill(cubes: Set<Cube>, fillers: MutableList<Cube>, bounds: IntRange) {
val adjacentCubes = this.calcAdjacent().filter {
!fillers.contains(it)
&& cubes.none { cube -> it.x == cube.x && it.y == cube.y && it.z == cube.z }
&& it.x in bounds && it.y in bounds && it.z in bounds
}
fillers.addAll(adjacentCubes)
adjacentCubes.forEach { it.fill(cubes, fillers, bounds) }
}
private fun calcAdjacent(): List<Cube> = listOf(
Cube(x + 1, y, z),
Cube(x - 1, y, z),
Cube(x, y + 1, z),
Cube(x, y - 1, z),
Cube(x, y, z + 1),
Cube(x, y, z - 1),
)
}
| 0 | Kotlin | 2 | 0 | 769c4bf06ee5b9ad3220e92067d617f07519d2b7 | 1,900 | advent-of-code-2022 | Apache License 2.0 |
src/Day08.kt | SebastianHelzer | 573,026,636 | false | {"Kotlin": 27111} |
fun main() {
fun getTreeMap(input: List<String>): Map<Pair<Int, Int>, Int> {
val heights = input.map { line -> line.toCharArray().map { it.digitToInt() } }
return heights.flatMapIndexed { row, line ->
line.mapIndexed { col, value ->
(col to row) to value
}
}.toMap()
}
fun part1(input: List<String>): Int {
val rows = input.size
val cols = input.first().length
val treeMap: Map<Pair<Int, Int>, Int> = getTreeMap(input)
return treeMap.size - treeMap.count { entry ->
val (x, y) = entry.key
val height = entry.value
val upIndices = 0 until y
val leftIndices = 0 until x
val rightIndices = (x + 1) until cols
val downIndices = (y + 1) until rows
upIndices.any { (treeMap[x to it] ?: 0) >= height } &&
leftIndices.any { (treeMap[it to y] ?: 0) >= height } &&
rightIndices.any { (treeMap[it to y] ?: 0) >= height } &&
downIndices.any { (treeMap[x to it] ?: 0) >= height }
}
}
fun part2(input: List<String>): Int {
val rows = input.size
val cols = input.first().length
val treeMap: Map<Pair<Int, Int>, Int> = getTreeMap(input)
return treeMap.maxOf { entry ->
val (x, y) = entry.key
val height = entry.value
val upIndices = 0 until y
val leftIndices = 0 until x
val rightIndices = (x + 1) until cols
val downIndices = (y + 1) until rows
val up = upIndices.indexOfLast { (treeMap[x to it] ?: 0) >= height }.let { if (it == -1) { y } else { y - it } }
val left = leftIndices.indexOfLast { (treeMap[it to y] ?: 0) >= height }.let { if (it == -1) { x } else { x - it } }
val right = rightIndices.indexOfFirst { (treeMap[it to y] ?: 0) >= height }.let { if(it == -1) { (cols - 1) - x } else {it + 1} }
val down = downIndices.indexOfFirst { (treeMap[x to it] ?: 0) >= height }.let { if(it == -1) { (rows - 1) - y } else {it + 1}}
up * left * right * down
}
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day08_test")
checkEquals(21, part1(testInput))
checkEquals(8, part2(testInput))
val input = readInput("Day08")
println(part1(input))
println(part2(input))
}
| 0 | Kotlin | 0 | 0 | e48757626eb2fb5286fa1c59960acd4582432700 | 2,457 | advent-of-code-2022 | Apache License 2.0 |
src/Day12.kt | hrach | 572,585,537 | false | {"Kotlin": 32838} | fun main() {
data class Map(
val levels: List<List<Int>>,
val start: Pair<Int, Int>,
val end: Pair<Int, Int>,
) {
fun getSteps(current: Pair<Int, Int>): List<Pair<Int, Int>> {
return listOf(
current.first - 1 to current.second,
current.first to current.second - 1,
current.first to current.second + 1,
current.first + 1 to current.second,
).mapNotNull {
val level = levels.getOrNull(it.first)?.getOrNull(it.second) ?: return@mapNotNull null
if ((level - levels[current.first][current.second]) <= 1) {
it
} else {
null
}
}
}
}
fun parse(input: List<String>): Map {
var start: Pair<Int, Int>? = null
var end: Pair<Int, Int>? = null
val levels = input.mapIndexed { y, line ->
line.mapIndexed { x, c ->
when (c) {
'S' -> {
start = y to x
'a'.code
}
'E' -> {
end = y to x
'z'.code
}
else -> c.code
}
}
}
return Map(levels, start!!, end!!)
}
fun shortest(map: Map): Int {
var todo = listOf(map.start to 0)
val visited = mutableSetOf(map.start)
while (todo.isNotEmpty()) {
val (pos, price) = todo.first()
val steps = map.getSteps(pos).filter { it !in visited }
for (step in steps) {
visited += step
if (step == map.end) return price + 1
}
todo = (todo.drop(1) + steps.map { it to price + 1 }).sortedBy { it.second }
}
return Int.MAX_VALUE
}
fun part1(input: List<String>): Int {
val map = parse(input)
return shortest(map)
}
fun part2(input: List<String>): Int {
val map = parse(input)
val lengths = mutableListOf<Int>()
map.levels.forEachIndexed { y, row ->
row.forEachIndexed q@{ x, c ->
if (c != 'a'.code) return@q
val newMap = map.copy(start = y to x)
lengths.add(shortest(newMap))
}
}
return lengths.min()
}
val testInput = readInput("Day12_test")
check(part1(testInput), 31)
check(part2(testInput), 29)
val input = readInput("Day12")
println(part1(input))
println(part2(input))
}
| 0 | Kotlin | 0 | 1 | 40b341a527060c23ff44ebfe9a7e5443f76eadf3 | 2,651 | aoc-2022 | Apache License 2.0 |
src/day-14/Day14.kt | skbkontur | 433,022,968 | false | null | fun main() {
fun parseInput(input: List<String>): Pair<String, Map<String, String>> {
val source = input.first()
val replacements = input
.filter { it.contains("->") }
.map { it.split(" -> ") }
.associate { (a, b) -> a to b }
return Pair(source, replacements)
}
fun part1(input: List<String>, iterations: Int): Int {
val (source, replacements) = parseInput(input)
val freqs = (0 until iterations)
.fold(source) { it, _ ->
it
.windowed(2, partialWindows = true) {
if (it.length == 2)
"${it[0]}${replacements[it.toString()]}"
else it
}
.joinToString("")
}
.groupingBy { it }
.eachCount()
.values
val max = freqs.maxOf { it }
val min = freqs.minOf { it }
return max - min
}
fun countPairs(source: String): Map<String, Long> {
return source
.windowed(2) { it.toString() }
.groupingBy { it }
.eachCount()
.mapValues { it.value.toLong() }
}
fun part2(input: List<String>, iterations: Int): Long {
val (source, replacements) = parseInput(input)
val freqs = (0 until iterations)
.fold(countPairs(source)) { map, _ ->
map
.flatMap { (symbolPair, count) ->
val newChar = replacements[symbolPair]!!
val fst = symbolPair[0] + newChar
val snd = newChar + symbolPair[1]
listOf(fst to count, snd to count)
}
.groupBy { it.first }
.mapValues { (_, counts) -> counts.sumOf { it.second } }
}
.flatMap { (pair, count) -> pair.map { it to count } }
.groupBy { (char, _) -> char }
.mapValues { (_, listCounts) -> listCounts.sumOf { (_, count) -> count } }
.mapValues { (char, count) ->
if (char == source.first() || char == source.last()) (count + 1) / 2
else count / 2
}
.values
val max = freqs.maxOf { it }
val min = freqs.minOf { it }
return max - min
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day14_test")
check(part1(testInput, 10) == 1588)
println(part1(testInput, 10))
println(part2(testInput, 10))
val input = readInput("Day14")
println(part1(input, 10))
println(part2(input, 10))
println(part2(input, 40))
}
| 2 | 1C Enterprise | 18 | 13 | e5a791492f8c466404eb300708ec09b441872865 | 2,755 | AoC2021 | MIT License |
src/Day08.kt | bin-wang | 572,801,360 | false | {"Kotlin": 19395} | import kotlin.math.min
object Day08 {
data class Tree(val x: Int, val y: Int)
class Forest(private val heights: List<List<Int>>) {
private val h = heights.size
private val w = heights.first().size
private fun leftward(tree: Tree) = ((tree.y - 1) downTo 0).map { Tree(tree.x, it) }
private fun rightward(tree: Tree) = ((tree.y + 1) until w).map { Tree(tree.x, it) }
private fun upward(tree: Tree) = ((tree.x - 1) downTo 0).map { Tree(it, tree.y) }
private fun downward(tree: Tree) = ((tree.x + 1) until h).map { Tree(it, tree.y) }
fun trees(): List<Tree> = (0 until h).cartesianProduct(0 until w).map { Tree(it.first, it.second) }
fun visible(tree: Tree): Boolean {
fun Tree.visibleInDirection(direction: (Tree) -> List<Tree>): Boolean {
val myHeight = heights[x][y]
return direction(this).map { heights[it.x][it.y] }.all { it < myHeight }
}
return tree.visibleInDirection(::leftward) ||
tree.visibleInDirection(::rightward) ||
tree.visibleInDirection(::upward) ||
tree.visibleInDirection(::downward)
}
fun score(tree: Tree): Int {
fun Tree.scoreInDirection(direction: (Tree) -> List<Tree>): Int {
val myHeight = heights[x][y]
val otherTrees = direction(this)
return min(otherTrees.takeWhile { heights[it.x][it.y] < myHeight }.size + 1, otherTrees.size)
}
return tree.scoreInDirection(::leftward) *
tree.scoreInDirection(::rightward) *
tree.scoreInDirection(::upward) *
tree.scoreInDirection(::downward)
}
}
}
fun main() {
fun part1(forest: Day08.Forest): Int {
return forest.trees().count { forest.visible(it) }
}
fun part2(forest: Day08.Forest): Int {
return forest.trees().maxOf { forest.score(it) }
}
val testInput = readInput("Day08_test")
val testHeights = testInput.map { line -> line.map { it.digitToInt() } }
val testForest = Day08.Forest(testHeights)
check(part1(testForest) == 21)
check(part2(testForest) == 8)
val input = readInput("Day08")
val heights = input.map { line -> line.map { it.digitToInt() } }
val forest = Day08.Forest(heights)
println(part1(forest))
println(part2(forest))
}
| 0 | Kotlin | 0 | 0 | dca2c4915594a4b4ca2791843b53b71fd068fe28 | 2,457 | aoc22-kt | Apache License 2.0 |
src/day15/Day15.kt | bartoszm | 572,719,007 | false | {"Kotlin": 39186} | package day15
import readInput
import kotlin.math.absoluteValue
typealias Point = Pair<Long, Long>
fun main() {
println(solve1(parse(readInput("day15/test")), 10))
println(solve1(parse(readInput("day15/input")), 2000000))
println(solve2(parse(readInput("day15/test")), 20))
println(solve2(parse(readInput("day15/input")), 4000000))
}
fun taxi(a: Point, b: Point) = (a.first - b.first).absoluteValue + (a.second - b.second).absoluteValue
class Sensor(val loc: Point, beac: Point) {
val distance = taxi(loc, beac)
fun canSense(p: Point) = taxi(loc, p) > distance
fun coverageIn(row: Long): LongRange {
val rem = distance - (loc.second - row).absoluteValue
return if (rem < 0L) LongRange.EMPTY
else loc.first - rem..loc.first + rem
}
}
fun toDist(p: Pair<Point, Point>, c: (Long, Long) -> Long) = c(p.first.first, taxi(p.first, p.second))
fun minOf(values: List<Pair<Point, Point>>): Long {
return values.minOf { toDist(it) { a, b -> a - b } }
}
fun maxOf(values: List<Pair<Point, Point>>): Long {
return values.maxOf { toDist(it) { a, b -> a + b } }
}
fun solve1(data: List<Pair<Point, Point>>, row: Long): Int {
val sensors = data.map { Sensor(it.first, it.second) }
val start = minOf(data)
val stop = maxOf(data)
fun beaconsAt(row: Long) = data.map { it.second }.distinct()
.filter { it.second == row }
.count { it.first in start..stop }
fun impossible(p: Point) = sensors.any { !it.canSense(p) }
return (start..stop).map { it to row }
.count { impossible(it) } - beaconsAt(row)
}
fun combine(ranges: List<LongRange>): List<LongRange> {
fun sum(a: LongRange, b: LongRange): List<LongRange> {
if (a.last < b.first) return listOf(a, b)
return listOf(if (a.last >= b.last) a else a.first..b.last)
}
val r = ranges.sortedBy { it.first }
return r.fold(listOf(r.first())) { acc, v ->
acc.dropLast(1) + sum(acc.last(), v)
}
}
fun toValues(a: LongRange, b: LongRange) = (a.last + 1 until b.first).toList()
fun toValues(ranges: List<LongRange>) = ranges.windowed(2).flatMap { (a, b) -> toValues(a, b) }
fun solve2(data: List<Pair<Point, Point>>, max: Int): Long {
val sensors = data.map { Sensor(it.first, it.second) }
val point = (0..max.toLong()).asSequence().flatMap { row ->
val coverage = combine(sensors.map { it.coverageIn(row) })
toValues(coverage).map { v -> v to row }
}.first()
return point.first * 4000000 + point.second
}
//Sensor at x=20, y=1: closest beacon is at x=15, y=3
val cRegex = """x=([\d-]+), y=([\d-]+)""".toRegex()
fun parse(input: List<String>): List<Pair<Point, Point>> {
return input.map {
val res = cRegex.find(it)!!
val (sx, sy) = res.destructured
val (bx, by) = res.next()!!.destructured
val s = sx.toLong() to sy.toLong()
val b = bx.toLong() to by.toLong()
s to b
}
}
| 0 | Kotlin | 0 | 0 | f1ac6838de23beb71a5636976d6c157a5be344ac | 2,964 | aoc-2022 | Apache License 2.0 |
src/Day08.kt | cypressious | 572,916,585 | false | {"Kotlin": 40281} | fun main() {
val easyDigits = mapOf(
2 to 1,
4 to 4,
3 to 7,
7 to 8,
)
fun part1(input: List<String>): Int {
val characters = input.flatMap { it.split(" | ")[1].split(" ") }
return characters.count { it.length in easyDigits }
}
class Mapping {
private val mapping = mutableMapOf<Set<Char>, Int>()
private val inverseMapping = mutableMapOf<Int, Set<Char>>()
fun insert(value: Int, chars: Set<Char>) {
mapping[chars] = value
inverseMapping[value] = chars
check(mapping.size == inverseMapping.size)
}
operator fun get(chars: Set<Char>) = mapping.getValue(chars)
operator fun get(value: Int) = inverseMapping.getValue(value)
operator fun contains(chars: Set<Char>) = chars !in mapping
override fun toString() =
mapping.entries.sortedBy { it.value }.joinToString { "${it.value}=${it.key.joinToString("")}" }
}
fun <T> MutableList<T>.removeFirst(filter: (T) -> Boolean) = removeAt(indexOfFirst(filter))
fun buildMapping(allDigits: Iterable<Set<Char>>): Mapping {
val all = allDigits.distinct().toMutableList()
check(all.size == 10)
val mapping = Mapping()
for ((count, value) in easyDigits) {
mapping.insert(value, all.removeFirst { it.size == count })
}
// 3 has 5 chars and contains all of 1
mapping.insert(3, all.removeFirst { it.size == 5 && it.containsAll(mapping[1]) })
// 9 has 6 chars and contains union of 3 & 4
mapping.insert(9, all.removeFirst { it.size == 6 && it.containsAll(mapping[3] union mapping[4]) })
// determine char that represents e (the char that differentiates 5 from 6)
val eChar = mapping[8] subtract mapping[9]
// 6 has 6 chars, 5 has 5 chars, and they only differ by the eChar
outer@ for (fiveChar in all.filter { it.size == 5 }) {
for (sixChar in all.filter { it.size == 6 }) {
if (sixChar subtract fiveChar == eChar) {
mapping.insert(6, sixChar)
mapping.insert(5, fiveChar)
all.remove(sixChar)
all.remove(fiveChar)
break@outer
}
}
}
// 2 has 5 chars and is not already mapped
mapping.insert(2, all.removeFirst { it.size == 5 })
// 0 has 6 chars and is not already mapped
mapping.insert(0, all.removeFirst { it.size == 6 })
return mapping
}
fun part2(input: List<String>): Int {
var sum = 0
for (line in input) {
val (test, output) = line.split(" | ").map { it.split(" ").map(String::toSet) }
val mapping = buildMapping(test + output)
sum += output.joinToString("") { mapping[it].toString() }.toInt()
}
return sum
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day08_test")
check(part1(testInput) == 26)
check(part2(testInput) == 61229)
val input = readInput("Day08")
println(part1(input))
println(part2(input))
}
| 0 | Kotlin | 0 | 0 | 169fb9307a34b56c39578e3ee2cca038802bc046 | 3,232 | AdventOfCode2021 | Apache License 2.0 |
src/day15/d15_2.kt | svorcmar | 720,683,913 | false | {"Kotlin": 49110} | fun main() {
val input = ""
val ingredients = input.lines().map { parseIngredient(it) }.associateBy { it.name }
println(forAllCombinations(ingredients, 100) {
it.map { (k, v) -> listOf(
ingredients[k]!!.capacity * v,
ingredients[k]!!.durability * v,
ingredients[k]!!.flavor * v,
ingredients[k]!!.texture * v) to ingredients[k]!!.calories * v
}.fold(listOf(0, 0, 0, 0) to 0) { (acc, accC), (list, c) -> acc.zip(list) { a, b -> a + b } to accC + c }
.let { (it, c) -> it.map { if (it < 0) 0 else it }
.reduce(Int::times) to c
}
}.filter { it.second == 500 }
.map { it.first }
.max())
}
data class Ingredient(val name: String, val capacity: Int, val durability: Int, val flavor: Int, val texture: Int, val calories: Int)
fun parseIngredient(s: String): Ingredient =
s.split(" ").let { Ingredient(it[0].dropLast(1),
it[2].dropLast(1).toInt(),
it[4].dropLast(1).toInt(),
it[6].dropLast(1).toInt(),
it[8].dropLast(1).toInt(),
it[10].toInt()) }
fun <T> forAllCombinations(ingredients: Map<String, Ingredient>, n: Int, f: (Map<String, Int>) -> T): Sequence<T> {
val names = ingredients.keys.toList()
val arr = Array(names.size) { 0 }
return forAllCombinationsRec(0, n, 0, arr, names, f)
}
fun <T> forAllCombinationsRec(step: Int, stepCount: Int, currIdx: Int,
arr: Array<Int>, names: List<String>,
f: (Map<String, Int>) -> T): Sequence<T> = sequence {
if (step == stepCount) {
yield(f(arr.mapIndexed {i, cnt -> names[i] to cnt }.toMap()))
} else {
if (currIdx < arr.size - 1) {
arr[currIdx + 1]++
yieldAll(forAllCombinationsRec(step + 1, stepCount, currIdx + 1, arr, names, f))
arr[currIdx + 1]--
}
arr[currIdx]++
yieldAll(forAllCombinationsRec(step + 1, stepCount, currIdx, arr, names, f))
arr[currIdx]--
}
}
| 0 | Kotlin | 0 | 0 | cb097b59295b2ec76cc0845ee6674f1683c3c91f | 2,067 | aoc2015 | MIT License |
src/main/kotlin/day9/Day9.kt | alxgarcia | 435,549,527 | false | {"Kotlin": 91398} | package day9
import java.io.File
fun parseMap(rows: List<String>): Array<IntArray> =
rows.map { row -> row.trim().map { it - '0' }.toIntArray() }.toTypedArray()
fun expandAdjacent(row: Int, column: Int, map: Array<IntArray>): List<Pair<Int, Int>> =
listOf(0 to -1, -1 to 0, 0 to 1, 1 to 0)
.map { (r, c) -> (r + row) to (c + column) }
.filterNot { (r, c) -> r < 0 || c < 0 || r >= map.size || c >= map[0].size }
fun findLocalMinimums(map: Array<IntArray>): List<Pair<Int, Int>> {
fun isLocalMinimum(row: Int, column: Int): Boolean {
val adjacent = expandAdjacent(row, column, map)
val current = map[row][column]
return adjacent.all { (r, c) -> map[r][c] > current }
}
val rows = map.indices
val columns = map[0].indices
val traverseMapIndices = rows.flatMap { r -> columns.map { c -> r to c } }
return traverseMapIndices.filter { (r, c) -> isLocalMinimum(r, c) }
}
fun sumOfLocalMinimums(map: Array<IntArray>): Int =
findLocalMinimums(map).sumOf { (r, c) -> map[r][c] + 1 }
fun computeBasinSize(row: Int, column: Int, map: Array<IntArray>): Int {
val visited = mutableSetOf<Pair<Int, Int>>()
val remaining = ArrayDeque(listOf(row to column))
var size = 0
do {
val current = remaining.removeFirst()
val currentValue = map[current.first][current.second]
if (currentValue == 9 || visited.contains(current)) continue
val nonVisitedAdjacent = expandAdjacent(current.first, current.second, map).filterNot { visited.contains(it) }
val belongsToBasin = nonVisitedAdjacent.all { (r, c) -> map[r][c] >= currentValue }
if (belongsToBasin) {
size += 1
visited.add(current)
remaining.addAll(nonVisitedAdjacent)
}
} while (!remaining.isEmpty())
return size
}
fun productOfSizeThreeLargestBasins(map: Array<IntArray>): Int =
findLocalMinimums(map)
.map { (r, c) -> computeBasinSize(r, c, map) }
.sorted()
.takeLast(3)
.reduce(Int::times)
fun main() {
File("./input/day9.txt").useLines { lines ->
val map = parseMap(lines.toList())
println(findLocalMinimums(map))
println(productOfSizeThreeLargestBasins(map))
}
}
| 0 | Kotlin | 0 | 0 | d6b10093dc6f4a5fc21254f42146af04709f6e30 | 2,140 | advent-of-code-2021 | MIT License |
src/Day12.kt | sebastian-heeschen | 572,932,813 | false | {"Kotlin": 17461} | fun main() {
fun parse(input: List<String>): HeightMap {
val grid = input.map {
it.toCharArray()
}
fun find(characterToFind: Char): Pair<Int, Int> {
val y = grid.indexOfFirst { line -> line.contains(characterToFind) }
val x = grid.map { line -> line.indexOfFirst { it == characterToFind } }.first { it >= 0 }
return y to x
}
val start = find('S')
val end = find('E')
return HeightMap(grid, start, end)
}
fun elevation(current: Char): Int = when (current) {
'S' -> 0
'E' -> 'z' - 'a'
else -> current - 'a'
}
fun shortestPath(
grid: List<CharArray>,
start: Pair<Int, Int>,
target: Char,
ascending: Boolean = true
): Int {
fun nextSteps(point: Point) = listOfNotNull(
Point(point.y, point.x - 1).takeIf { it.x >= 0 },
Point(point.y, point.x + 1).takeIf { it.x < grid.first().size },
Point(point.y - 1, point.x).takeIf { it.y >= 0 },
Point(point.y + 1, point.x).takeIf { it.y < grid.size }
)
val end = Point(
grid.indexOfFirst { line -> line.contains(target) },
grid.map { line -> line.indexOfFirst { it == target } }.first { it >= 0 }
)
val queue = mutableListOf(start)
val distances = mutableMapOf<Point, Int>()
val visited = mutableSetOf<Point>()
distances[start] = 0
while (queue.isNotEmpty()) {
val current = queue.removeFirst()
if (current !in visited) {
visited.add(current)
val currentElevation = elevation(grid[current.y][current.x])
val nextSteps = nextSteps(current)
println("current: ${current.first},${current.second}: nexts: ${nextSteps.joinToString(separator = ";") { "${it.first},${it.second}" }}")
nextSteps.filter { (x, y) ->
val destElevation = elevation(grid[x][y])
if (ascending) {
currentElevation - destElevation >= -1
} else {
destElevation - currentElevation >= -1
}
}
.filter { it !in visited }
.forEach {
if (grid[it.y][it.x] == grid[end.y][end.x]) {
return distances[current]!! + 1
}
if (it !in distances) {
distances[it] = distances[current]!! + 1
queue.add(it)
}
}
}
}
return 0
}
fun part1(input: List<String>): Int {
val (grid, start, end) = parse(input)
return shortestPath(grid, start, 'E')
}
fun part2(input: List<String>): Int {
val (grid, start, end) = parse(input)
return shortestPath(grid, end, 'a', ascending = false)
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day12_test")
check(part1(testInput) == 31)
check(part2(testInput) == 29)
val input = readInput("Day12")
println(part1(input))
println(part2(input))
}
private data class HeightMap(
val grid: List<CharArray>,
val start: Pair<Int, Int>,
val end: Pair<Int, Int>
)
private typealias Point = Pair<Int, Int>
val Point.x
get() = second
val Point.y
get() = first
| 0 | Kotlin | 0 | 0 | 4432581c8d9c27852ac217921896d19781f98947 | 3,574 | advent-of-code-2022 | Apache License 2.0 |
src/Day08.kt | razvn | 573,166,955 | false | {"Kotlin": 27208} | private data class Tree(val x: Int, val y: Int, val value: Int)
fun main() {
val day = "Day08"
fun part1(input: List<String>): Int {
val trees = input.mapIndexed { lIdx, line ->
line.mapIndexed { cIdx, col ->
Tree(lIdx, cIdx, col.digitToInt())
}
}.flatten()
val maxX = trees.maxOf { it.x }
val maxY = trees.maxOf { it.y }
val visibleTrees = trees.filter { t ->
when {
t.x == 0 || t.y == 0 || t.x == maxX || t.y == maxY -> true
else -> {
val leftTreesBigger = trees.filter { it.x < t.x && it.y == t.y}.filter { it.value >= t.value }
if (leftTreesBigger.isEmpty()) true
else {
val rightTreeBigger = trees.filter { it.x > t.x && it.y == t.y}.filter { it.value >= t.value }
if (rightTreeBigger.isEmpty()) true
else {
val topTreeBigger = trees.filter { it.y < t.y && it.x == t.x}.filter { it.value >= t.value }
if (topTreeBigger.isEmpty()) true
else {
val bottomTreeBigger = trees.filter { it.y > t.y && it.x == t.x}.filter { it.value >= t.value }
bottomTreeBigger.isEmpty()
}
}
}
}
}
}.toSet()
val totalVisible = visibleTrees.size
return totalVisible
}
fun part2(input: List<String>): Int {
val trees = input.mapIndexed { lIdx, line ->
line.mapIndexed { cIdx, col ->
Tree(cIdx, lIdx, col.digitToInt())
}
}.flatten()
// println(trees)
val scenics = trees.map { tree ->
val maxX = trees.maxOf { it.x }
val maxY = trees.maxOf { it.y }
if (tree.x == 0 || tree.y == 0 || tree.x == maxX || tree.y == maxY) 0
else {
val leftValue = calcMove(tree, trees) {a, b -> (b.x == (a.x - 1)) && (a.y == b.y) }
val rightValue = calcMove(tree, trees) {a, b -> (b.x == (a.x + 1)) && (a.y == b.y) }
val topValue = calcMove(tree, trees) {a, b -> (b.y == (a.y - 1)) && (a.x == b.x) }
val bottomValue = calcMove(tree, trees) {a, b -> (b.y == (a.y + 1)) && (a.x == b.x) }
val rep = leftValue * rightValue * topValue * bottomValue
//println("____ TREE ___ $tree = $rep (l: $leftValue | r: $rightValue | t: $topValue | b: $bottomValue")
rep
}
}
return scenics.max()
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("${day}_test")
//println(part1(testInput))
// println(part2(testInput))
check(part1(testInput) == 21)
check(part2(testInput) == 8)
val input = readInput(day)
println(part1(input))
println(part2(input))
}
private fun calcMove(t: Tree, trees: List<Tree>, fn: (a: Tree, b: Tree) -> Boolean): Int {
var exit = false
var i = 0
var current = t
while (!exit) {
// println("currentTree: $current" )
val next = trees.firstOrNull { fn(current, it)}
// println("Next: $next" )
if (next == null) exit = true
else {
i++
when {
next.value >= t.value -> exit = true
else -> current = next
}
}
}
return i
}
| 0 | Kotlin | 0 | 0 | 73d1117b49111e5044273767a120142b5797a67b | 3,622 | aoc-2022-kotlin | Apache License 2.0 |
src/Day11.kt | thpz2210 | 575,577,457 | false | {"Kotlin": 50995} | private open class Solution11(input: List<String>) {
val monkeys = input.chunked(7).map { Monkey(it) }
fun solve(times: Int): Long {
repeat(times) { doRound() }
return monkeys.map { it.counter }.sorted().takeLast(2).reduce(Long::times)
}
private fun doRound() {
monkeys.forEach { m -> m.items.forEach { processItem(m, it) }; m.items.clear() }
}
private fun processItem(monkey: Monkey, it: Long) {
var worryLevel = when (monkey.operation["code"]) {
"*" -> if (monkey.operation["value"] == "old") it * it else it * monkey.operation["value"]!!.toLong()
"+" -> if (monkey.operation["value"] == "old") it + it else it + monkey.operation["value"]!!.toLong()
else -> throw IllegalArgumentException()
}
worryLevel = reduceWorryLevel(worryLevel)
if (worryLevel % monkey.testValue == 0L)
monkeys[monkey.ifTrue].items.add(worryLevel)
else
monkeys[monkey.ifFalse].items.add(worryLevel)
monkey.counter += 1
}
open fun reduceWorryLevel(worryLevel: Long) = worryLevel / 3L
class Monkey(input: List<String>) {
val items = input[1].split(":").last().split(",").map { it.trim().toLong() }.toMutableList()
val operation = mapOf(
Pair("code", input[2].trim().split(" ")[4]),
Pair("value", input[2].trim().split(" ")[5]))
val testValue = input[3].trim().split(" ").last().toLong()
val ifTrue = input[4].trim().split(" ").last().toInt()
val ifFalse = input[5].trim().split(" ").last().toInt()
var counter = 0L
}
}
private class Solution11Part2(input: List<String>) : Solution11(input) {
val commonMultiple = monkeys.map { it.testValue }.reduce(Long::times)
override fun reduceWorryLevel(worryLevel: Long) = worryLevel % commonMultiple
}
fun main() {
val testSolution = Solution11(readInput("Day11_test"))
check(testSolution.solve(20) == 10605L)
val testSolution2 = Solution11Part2(readInput("Day11_test"))
check(testSolution2.solve(10000) == 2713310158L)
val solution = Solution11(readInput("Day11"))
println(solution.solve(20))
val solution2 = Solution11Part2(readInput("Day11"))
println(solution2.solve(10000))
}
| 0 | Kotlin | 0 | 0 | 69ed62889ed90692de2f40b42634b74245398633 | 2,292 | aoc-2022 | Apache License 2.0 |
src/Day11.kt | iProdigy | 572,297,795 | false | {"Kotlin": 33616} | fun main() {
fun part1(input: List<String>) = run(input, 20, true)
fun part2(input: List<String>) = run(input, 10_000, false)
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day11_test")
check(part1(testInput) == 10605L)
check(part2(testInput) == 2713310158L)
val input = readInput("Day11")
println(part1(input)) // 57838
println(part2(input)) // 15050382231
}
private fun run(input: List<String>, rounds: Int, divideBy3: Boolean): Long {
val monkeys = parse(input).associateBy { it.id }
val divisorProduct = monkeys.values.map { it.testDivisible }.distinct().reduce(Long::times)
val counts = IntArray(monkeys.size)
for (round in 1..rounds) {
monkeys.values.forEach {
while (it.items.isNotEmpty()) {
val item = it.items.removeFirst()
val updated = it.operation(item).let { l -> if (divideBy3) l / 3 else l } % divisorProduct
counts[it.id]++
val next = if (updated % it.testDivisible == 0L) it.nextWhenTrue else it.nextWhenFalse
monkeys[next]!!.items += updated
}
}
}
return counts.sortedDescending().let { (first, second) -> first.toLong() * second }
}
private fun parse(input: List<String>) = input.partitionBy { it.isEmpty() }
.map { it.drop(1).map { line -> line.substring(line.indexOf(':') + 2) } }
.mapIndexed { index, (startingStr, operationStr, testStr, trueStr, falseStr) ->
fun last(str: String) = str.substring(str.lastIndexOf(' ') + 1)
val items = startingStr.split(", ").mapTo(ArrayDeque()) { it.toLong() }
val opDelim = operationStr.lastIndexOf(' ')
val opNum = operationStr.substring(opDelim + 1).toLongOrNull()
val operation: (Long) -> Long = when {
operationStr.endsWith("* old") -> { it -> it * it }
'*' == operationStr[opDelim - 1] -> { it -> it * opNum!! }
'+' == operationStr[opDelim - 1] -> { it -> it + opNum!! }
else -> throw Error("Bad Input!")
}
Monkey(index, items, last(testStr).toLong(), last(trueStr).toInt(), last(falseStr).toInt(), operation)
}
private data class Monkey(
val id: Int,
val items: ArrayDeque<Long> = ArrayDeque(),
val testDivisible: Long,
val nextWhenTrue: Int,
val nextWhenFalse: Int,
val operation: (Long) -> Long
)
| 0 | Kotlin | 0 | 1 | 784fc926735fc01f4cf18d2ec105956c50a0d663 | 2,442 | advent-of-code-2022 | Apache License 2.0 |
kotlin/src/main/kotlin/year2023/Day19.kt | adrisalas | 725,641,735 | false | {"Kotlin": 130217, "Python": 1548} | package year2023
fun main() {
val input = readInput2("Day19")
Day19.part1(input).println()
Day19.part2(input).println()
}
object Day19 {
fun part1(input: String): Int {
val chunks = input.split(DOUBLE_NEW_LINE_REGEX)
val workflows = chunks[0]
.split(NEW_LINE_REGEX)
.map { line ->
val name = line.split("{")[0]
val rules = line.split("{")[1].split("}")[0].split(",").map { Rule(it) }
Workflow(name, rules)
}
.associateBy { it.name }
val ratings = chunks[1]
.split(NEW_LINE_REGEX)
.map { line ->
line.split("{")[1]
.split("}")[0]
.split(",")
.associate {
it.split("=")[0][0] to it.split("=")[1].toInt()
}
}
return ratings.sumOf { rating ->
var result = -1
var next = "in"
while (result == -1) {
val rule = workflows.getValue(next).rules.first { it.matches(rating) }
when (rule.result) {
"R" -> result = 0
"A" -> result = rating.values.sum()
else -> next = rule.result
}
}
result
}
}
fun part2(input: String): Long {
val chunks = input.split(DOUBLE_NEW_LINE_REGEX)
val workflows = chunks[0]
.split(NEW_LINE_REGEX)
.map { line ->
val name = line.split("{")[0]
val rules = line.split("{")[1].split("}")[0].split(",").map { Rule(it) }
Workflow(name, rules)
}
.associateBy { it.name }
return combine(
workflows = workflows,
entry = "in",
ranges = mapOf(
'x' to (1..4000),
'm' to (1..4000),
'a' to (1..4000),
's' to (1..4000)
)
)
}
private fun combine(workflows: Map<String, Workflow>, entry: String, ranges: Map<Char, IntRange>): Long {
return when (entry) {
"R" -> 0L
"A" -> ranges.values.map { it.last() - it.first() + 1 }.map { it.toLong() }.reduce(Long::times)
else -> {
val newRanges = ranges.toMutableMap()
workflows.getValue(entry).rules.sumOf { rule ->
if (rule.isUnconditional()) {
combine(
workflows = workflows,
entry = rule.result,
ranges = newRanges
)
} else {
val newRange = newRanges.getValue(rule.category!!).intersection(rule.range())
val newReversed = newRanges.getValue(rule.category).intersection(rule.reversedRange())
newRanges[rule.category] = newRange
combine(
workflows = workflows,
entry = rule.result,
ranges = newRanges
).also { newRanges[rule.category] = newReversed }
}
}
}
}
}
data class Workflow(val name: String, val rules: List<Rule>)
class Rule(rule: String) {
val result: String
val category: Char?
val operand: Char?
private val value: Int?
init {
if (':' in rule) {
val condition = rule.split(":")[0]
category = condition[0]
operand = condition[1]
value = condition.substring(2).toInt()
result = rule.split(":")[1]
} else {
category = null
operand = null
value = null
result = rule
}
}
fun isUnconditional() = category == null || operand == null || value == null
fun matches(rating: Map<Char, Int>): Boolean {
if (isUnconditional()) {
return true
}
return when (operand) {
'>' -> rating.getValue(category!!) > value!!
'<' -> rating.getValue(category!!) < value!!
else -> false
}
}
fun range(): IntRange {
if (value == null) {
error("Not defined")
}
if (operand == '<') {
return (1..<value)
}
return (value + 1..4000)
}
fun reversedRange(): IntRange {
if (value == null) {
error("Not defined")
}
if (operand == '<') {
return (value..4000)
}
return (1..value)
}
}
private fun IntRange.intersection(other: IntRange): IntRange {
return (maxOf(first, other.first)..minOf(last, other.last))
}
}
| 0 | Kotlin | 0 | 2 | 6733e3a270781ad0d0c383f7996be9f027c56c0e | 5,091 | advent-of-code | MIT License |
y2023/src/main/kotlin/adventofcode/y2023/Day12.kt | Ruud-Wiegers | 434,225,587 | false | {"Kotlin": 503769} | package adventofcode.y2023
import adventofcode.io.AdventSolution
fun main() {
Day12.solve()
}
object Day12 : AdventSolution(2023, 12, "Hot Springs") {
override fun solvePartOne(input: String) = parse(input).sumOf(ConditionRecord::countMatchingConfigurations)
override fun solvePartTwo(input: String) = parse(input, 5).sumOf(ConditionRecord::countMatchingConfigurations)
private fun parse(input: String, times: Int = 1) = input.lineSequence().map {
val (line, guide) = it.split(" ")
val springs = "$line?".repeat(times).dropLast(1).map(Spring::fromChar)
val groups = "$guide,".repeat(times).dropLast(1).split(",").map(String::toInt)
ConditionRecord(springs, groups)
}
}
private data class ConditionRecord(val row: List<Spring>, val damagedGroups: List<Int>) {
fun countMatchingConfigurations() =
row.fold(mapOf(SolutionPattern(Constraint.None, damagedGroups) to 1L)) { prev, spring ->
buildMap {
for ((clue, count) in prev) {
for (new in clue.matchNextSpring(spring)) {
merge(new, count, Long::plus)
}
}
}
}
.filterKeys(SolutionPattern::completeMatch).values.sum()
}
private data class SolutionPattern(val constraint: Constraint, val unmatchedDamagedGroups: List<Int>) {
fun matchNextSpring(spring: Spring): List<SolutionPattern> = buildList {
if (spring != Spring.Operational) matchDamagedSpring()?.let(::add)
if (spring != Spring.Damaged) matchOperationalSpring()?.let(::add)
}
private fun matchOperationalSpring(): SolutionPattern? = when (constraint) {
is Constraint.Damaged -> null
else -> SolutionPattern(Constraint.None, unmatchedDamagedGroups)
}
private fun matchDamagedSpring(): SolutionPattern? = when (constraint) {
Constraint.Operational -> null
Constraint.None -> startNewDamagedGroup()?.matchDamagedSpring()
is Constraint.Damaged -> SolutionPattern(constraint.next(), unmatchedDamagedGroups)
}
private fun startNewDamagedGroup(): SolutionPattern? =
if (unmatchedDamagedGroups.isEmpty()) null
else SolutionPattern(
Constraint.Damaged(unmatchedDamagedGroups.first()),
unmatchedDamagedGroups.drop(1)
)
fun completeMatch() = !constraint.mandatory && unmatchedDamagedGroups.isEmpty()
}
//Requirements for next springs to match
private sealed class Constraint(val mandatory: Boolean) {
//no requirements
data object None : Constraint(false)
//next spring must be operational
data object Operational : Constraint(false)
//next [amount] springs must be damaged, followed by 1 operational spring
data class Damaged(private val amount: Int) : Constraint(true) {
fun next() = if (amount > 1) Damaged(amount - 1) else Operational
}
}
private enum class Spring {
Operational, Damaged, Unknown;
companion object {
fun fromChar(ch: Char) = when (ch) {
'.' -> Operational
'#' -> Damaged
else -> Unknown
}
}
}
| 0 | Kotlin | 0 | 3 | fc35e6d5feeabdc18c86aba428abcf23d880c450 | 3,171 | advent-of-code | MIT License |
src/Day13.kt | palex65 | 572,937,600 | false | {"Kotlin": 68582} | import kotlin.math.min
private fun compareSeq(a: List<String>, b: List<String>): Int {
for(i in 0 until min(a.size,b.size)) {
val res = compare(a[i], b[i])
if (res!=0) return res
}
return a.size - b.size
}
private fun String.indexOfClosedPair(from: Int): Int {
var level = 1
for( i in from until length )
when(this[i]) {
'[' -> ++level
']' -> if (--level==0) return i
}
return -1
}
private fun String.splitSeq(): List<String> {
val res = mutableListOf<String>()
var lastIdx = 0
var idx = 0
while(idx < length) {
when(this[idx]) {
',' -> {
res.add( substring(lastIdx,idx) )
lastIdx=++idx
}
'[' -> idx = indexOfClosedPair(idx+1)+1
else -> ++idx
}
}
if (idx>lastIdx) res.add( substring(lastIdx,idx))
return res
}
private fun compare(a: String, b: String): Int {
val fa = a[0]
val fb = b[0]
fun String.toSeq() = substring(1,lastIndex).splitSeq()
return when {
fa.isDigit() && fb.isDigit() -> a.toInt() - b.toInt()
fa=='[' && fb=='[' -> compareSeq( a.toSeq(), b.toSeq() )
fa=='[' -> compareSeq( a.toSeq(), listOf(b) )
fb=='[' -> compareSeq( listOf(a), b.toSeq() )
else -> error("Invalid start of package $fa or $fb")
}
}
private fun part1(lines: List<String>): Int =
lines
.splitBy { it.isEmpty() }
.mapIndexed { idx, pair -> if (compare(pair[0], pair[1]) < 0) idx+1 else 0 }
.sum()
private fun part2(lines: List<String>): Int {
val packets = lines.filter{ it.isNotEmpty() } + "[[2]]" + "[[6]]"
val sorted = packets.sortedWith(::compare)
return (sorted.indexOf("[[2]]")+1) * (sorted.indexOf("[[6]]")+1)
}
fun main() {
val testInput = readInput("Day13_test")
check(part1(testInput) == 13)
check(part2(testInput) == 140)
val input = readInput("Day13")
println(part1(input)) // 5390
println(part2(input)) // 19261
}
| 0 | Kotlin | 0 | 2 | 35771fa36a8be9862f050496dba9ae89bea427c5 | 2,048 | aoc2022 | Apache License 2.0 |
src/year2022/12/Day12.kt | Vladuken | 573,128,337 | false | {"Kotlin": 327524, "Python": 16475} | package year2022.`12`
import kotlin.math.abs
import readInput
data class Square(
val i: Int,
val j: Int,
val height: Int,
)
data class SquareRecord(
val currentNode: Square,
var shortestDistance: Int,
var previousNode: Square?
)
fun calculateDistances(start: Square, grid: List<List<Square>>): List<SquareRecord> {
fun nodeAtOrNull(i: Int, j: Int): Square? {
val iMax = grid.lastIndex
val jMax = grid[0].lastIndex
return if (i < 0 || i > iMax || j < 0 || j > jMax) null else grid[i][j]
}
fun Square.neighbors() = listOfNotNull(
nodeAtOrNull(i - 1, j), nodeAtOrNull(i + 1, j),
nodeAtOrNull(i, j - 1), nodeAtOrNull(i, j + 1)
)
.filter { it.height + 1 >= height }
val result: List<SquareRecord> = grid
.flatten()
.map {
SquareRecord(
currentNode = it,
shortestDistance = if (it == start) 0 else Int.MAX_VALUE,
previousNode = null
)
}
val unvisitedSquares = grid.flatten().toMutableSet()
val visitedSquares = mutableSetOf<Square>()
while (unvisitedSquares.isNotEmpty()) {
// Find next record to visit
val record = result
.filter { it.currentNode in unvisitedSquares }
.minBy { it.shortestDistance }
//Find neighbours that have one step distance
val neighbours = record.currentNode.neighbors()
neighbours
.filter { it in unvisitedSquares }
.forEach { neighbour ->
// Find current record for this neighbour
val neighbourRecord = result
.find { it.currentNode == neighbour } ?: error("Illegal State")
// Calculate new distance (1 is a step)
val distance = record.shortestDistance + 1
// If distance is less - then update record with new distance
if (distance < neighbourRecord.shortestDistance) {
neighbourRecord.shortestDistance = distance
neighbourRecord.previousNode = record.currentNode
}
}
// Mark node as visited
unvisitedSquares.remove(record.currentNode)
visitedSquares.add(record.currentNode)
}
return result
}
fun main() {
fun parseInput(input: List<String>): Triple<Square, Square, List<List<Square>>> {
var start: Square? = null
var end: Square? = null
val grid = input.mapIndexed { i, line ->
line
.split("")
.dropLast(1)
.drop(1)
.mapIndexed { j, symbol ->
val isS = symbol == "S"
val isE = symbol == "E"
val newChar = when {
isS -> 'a'
isE -> 'z'
else -> symbol[0]
}
Square(
i = i,
j = j,
height = abs('a' - newChar),
).also {
if (isS) start = it
if (isE) end = it
}
}
}
return Triple(start!!, end!!, grid)
}
fun part1(input: List<String>): Int {
val (start, end, grid) = parseInput(input)
val results = calculateDistances(end, grid)
val sum = results.find { it.currentNode == start }
return sum!!.shortestDistance
}
fun part2(input: List<String>): Int {
val (_, end, grid) = parseInput(input)
val results = calculateDistances(end, grid)
val sum = results
.filter { it.currentNode.height == 0 }
.filter { it.shortestDistance >= 0 }
.minBy { it.shortestDistance }
return sum.shortestDistance
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day12_test")
val part1Test = part1(testInput)
val part2Test = part2(testInput)
println(part1Test)
check(part1Test == 31)
check(part2Test == 29)
val input = readInput("Day12")
println(part1(input))
println(part2(input))
}
| 0 | Kotlin | 0 | 5 | c0f36ec0e2ce5d65c35d408dd50ba2ac96363772 | 4,270 | KotlinAdventOfCode | Apache License 2.0 |
src/Day08.kt | xabgesagtx | 572,139,500 | false | {"Kotlin": 23192} | fun main() {
fun part1(input: List<String>): Int {
val treeValues = input.map { line -> line.toList().map { it.digitToInt() } }
val lastXIndex = treeValues[0].lastIndex
val lastYIndex = treeValues.lastIndex
return treeValues.indices.flatMap { y ->
treeValues[y].indices.mapNotNull { x ->
val currentValue = treeValues[y][x]
when {
x == 0 || y == 0 || x == lastXIndex || y == lastYIndex -> x to y
treeValues.maxFromLeft(x, y) < currentValue -> x to y
treeValues.maxFromRight(x, y) < currentValue -> x to y
treeValues.maxFromTop(x, y) < currentValue -> x to y
treeValues.maxFromBottom(x, y) < currentValue -> x to y
else -> null
}
}
}.distinct().count()
}
fun part2(input: List<String>): Int {
val treeValues = input.map { line -> line.toList().map { it.digitToInt() } }
return treeValues.mapIndexed { y, line ->
List(line.size) { x -> treeValues.scenicScore(x, y) }.max()
}.max()
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day08_test")
println(part1(testInput))
check(part1(testInput) == 21)
println(part2(testInput))
check(part2(testInput) == 8)
val input = readInput("Day08")
println(part1(input))
println(part2(input))
}
private fun List<List<Int>>.maxFromLeft(x: Int, y: Int) = this[y].subList(0, x).max()
private fun List<List<Int>>.maxFromRight(x: Int, y: Int) = this[y].subList(x + 1, this[y].lastIndex + 1).max()
private fun List<List<Int>>.maxFromTop(x: Int, y: Int): Int {
val list = this.map { it[x] }
return list.subList(0, y).max()
}
private fun List<List<Int>>.maxFromBottom(x: Int, y: Int): Int {
val list = this.map { it[x] }
return list.subList(y + 1, lastIndex + 1).max()
}
private fun List<List<Int>>.scenicScore(x: Int, y: Int): Int {
return if (x == 0 || y == 0 || y == this.lastIndex || x == this[y].lastIndex) {
0
} else {
scenicScoreLeft(x, y) * scenicScoreRight(x, y) * scenicScoreTop(x, y) * scenicScoreBottom(x, y)
}
}
private fun List<List<Int>>.scenicScoreBottom(x: Int, y: Int): Int {
val currentValue = this[y][x]
val list = this.map { it[x] }.subList(y + 1, lastIndex + 1)
return (list.takeWhile { it < currentValue }.count() + 1).coerceAtMost(list.size)
}
private fun List<List<Int>>.scenicScoreTop(x: Int, y: Int): Int {
val currentValue = this[y][x]
val list = this.map { it[x] }.subList(0, y).reversed()
return (list.takeWhile { it < currentValue }.count() + 1).coerceAtMost(list.size)
}
private fun List<List<Int>>.scenicScoreLeft(x: Int, y: Int): Int {
val currentValue = this[y][x]
val list = this[y].subList(0, x).reversed()
return (list.takeWhile { it < currentValue }.count() + 1).coerceAtMost(list.size)
}
private fun List<List<Int>>.scenicScoreRight(x: Int, y: Int): Int {
val currentValue = this[y][x]
val list = this[y].subList(x + 1, this[y].lastIndex + 1)
return (list.takeWhile { it < currentValue }.count() + 1).coerceAtMost(list.size)
} | 0 | Kotlin | 0 | 0 | 976d56bd723a7fc712074066949e03a770219b10 | 3,266 | advent-of-code-2022 | Apache License 2.0 |
src/Day08.kt | dmstocking | 575,012,721 | false | {"Kotlin": 40350} | fun main() {
fun part1(input: List<String>): Int {
val heights = input
.map { line -> line.map { it.digitToInt() } }
val height = heights.size
val width = heights.first().size
return heights
.mapIndexed { y, line ->
line.mapIndexed { x, h ->
val left = (0 until x).map { heights[y][it] }.all { it < h }
val right = ((x+1) until width).map { heights[y][it] }.all { it < h }
val up = (0 until y).map { heights[it][x] }.all { it < h }
val down = ((y+1) until height).map { heights[it][x] }.all { it < h }
left || right || up || down
}
}
.flatten()
.map { if (it) 1 else 0 }
.sumOf { it }
}
fun part2(input: List<String>): Int {
val heights = input
.map { line -> line.map { it.digitToInt() } }
val height = heights.size
val width = heights.first().size
return heights
.mapIndexed { y, line ->
line.mapIndexed { x, h ->
val left = (0 until x).reversed().map { heights[y][it] }.takeWhileInclusive { it < h }.count()
val right = ((x+1) until width).map { heights[y][it] }.takeWhileInclusive { it < h }.count()
val up = (0 until y).reversed().map { heights[it][x] }.takeWhileInclusive { it < h }.count()
val down = ((y+1) until height).map { heights[it][x] }.takeWhileInclusive { it < h }.count()
left * right * up * down
}
}
.flatten()
.maxOf { it }
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day08_test")
check(part1(testInput) == 21)
val input = readInput("Day08")
println(part1(input))
println(part2(input))
}
fun <T> Iterable<T>.takeWhileInclusive(predicate: (T) -> Boolean): List<T> {
val list = ArrayList<T>()
for (item in this) {
list.add(item)
if (!predicate(item))
break
}
return list
}
| 0 | Kotlin | 0 | 0 | e49d9247340037e4e70f55b0c201b3a39edd0a0f | 2,193 | advent-of-code-kotlin-2022 | Apache License 2.0 |
src/main/kotlin/dev/paulshields/aoc/Day9.kt | Pkshields | 433,609,825 | false | {"Kotlin": 133840} | /**
* Day 9: Smoke Basin
*/
package dev.paulshields.aoc
import dev.paulshields.aoc.common.readFileAsString
fun main() {
println(" ** Day 9: Smoke Basin ** \n")
val heightMap = readFileAsString("/Day9HeightMap.txt")
val riskLevel = calculateRiskLevelOfHeightMap(heightMap)
println("The combined risk level for this height map is $riskLevel")
val result = findSizeOfBasins(heightMap)
.sortedDescending()
.take(3)
.fold(1) { accumulator, basinSize -> accumulator * basinSize }
println("The product of the largest 3 basin sizes is $result")
}
fun calculateRiskLevelOfHeightMap(rawHeightMap: String): Int {
val heightMap = parseHeightMap(rawHeightMap)
return heightMap.filter { it.isLowPoint }.map { it.location }.sumOf { it + 1 }
}
fun findSizeOfBasins(rawHeightMap: String): List<Int> {
val heightMap = parseHeightMap(rawHeightMap)
return heightMap
.filter { it.isLowPoint }
.map { calculateSizeOfBasin(it, heightMap) }
}
fun calculateSizeOfBasin(centerLocation: HeightMapLocation, heightMap: List<HeightMapLocation>): Int {
val modifiableHeightMap = heightMap.toMutableList().apply { remove(centerLocation) }
val locationsInBasin = mutableListOf(centerLocation)
var i = -1
while (++i < locationsInBasin.size) {
val neighbours = findNeighboursWithinBasin(locationsInBasin[i], modifiableHeightMap)
locationsInBasin.uniqueAddAll(neighbours)
modifiableHeightMap.removeAll(neighbours)
}
return locationsInBasin.size
}
private fun findNeighboursWithinBasin(location: HeightMapLocation, heightMap: List<HeightMapLocation>): List<HeightMapLocation> {
return heightMap.filter {
it.x == location.x - 1 && it.y == location.y ||
it.x == location.x + 1 && it.y == location.y ||
it.x == location.x && it.y == location.y - 1 ||
it.x == location.x && it.y == location.y + 1
}.filter { it.location != 9 }
}
private fun parseHeightMap(rawHeightMap: String): List<HeightMapLocation> {
val heightMap = rawHeightMap
.lines()
.map { it.toCharArray().map { it.digitToInt() } }
return heightMap.mapIndexed { yIndex, line ->
line.mapIndexed { xIndex, location ->
val left = if (xIndex > 0) location < heightMap[yIndex][xIndex - 1] else true
val right = if (xIndex < line.size - 1) location < heightMap[yIndex][xIndex + 1] else true
val top = if (yIndex > 0) location < heightMap[yIndex - 1][xIndex] else true
val bottom = if (yIndex < heightMap.size - 1) location < heightMap[yIndex + 1][xIndex] else true
HeightMapLocation(location, xIndex, yIndex, left && right && top && bottom)
}
}.flatten()
}
data class HeightMapLocation(val location: Int, val x: Int, val y: Int, val isLowPoint: Boolean)
fun <T> MutableList<T>.uniqueAddAll(listToAdd: List<T>) = addAll(listToAdd.filter { !this.contains(it) })
| 0 | Kotlin | 0 | 0 | e3533f62e164ad72ec18248487fe9e44ab3cbfc2 | 3,010 | AdventOfCode2021 | MIT License |
kotlin/src/Day14.kt | ekureina | 433,709,362 | false | {"Kotlin": 65477, "C": 12591, "Rust": 7560, "Makefile": 386} | fun main() {
fun part1(input: List<String>): Int {
val template = input.first()
val rules = input.drop(2).associate { line ->
val (pair, insert) = line.split(" -> ")
(pair[0] to pair[1]) to insert[0]
}
val finalString = (0 until 10).fold(template) { polymer, _ ->
val insertions = polymer.zipWithNext().map { pair ->
rules[pair]!!
}.joinToString("")
polymer.zip(insertions).flatMap { (polymer, inserted) ->
listOf(polymer, inserted)
}.joinToString("") + polymer.last()
}
val charCounts = finalString.toSet().associateWith { char -> finalString.count(char::equals) }
return charCounts.values.maxOrNull()!! - charCounts.values.minOrNull()!!
}
fun part2(input: List<String>): Long {
val template = input.first()
val rules = input.drop(2).associate { line ->
val (pair, insert) = line.split(" -> ")
(pair[0] to pair[1]) to ((pair[0] to insert[0]) to (insert[0] to pair[1]))
}
val pairs = rules.keys.associateWith { pair -> template.zipWithNext().count { it == pair }.toLong() }
val finalPairs = (0 until 40).fold(pairs) { pairs, _ ->
val newPairs = pairs.keys.flatMap { pair ->
listOf(rules[pair]!!.first to pairs[pair]!!, rules[pair]!!.second to pairs[pair]!!)
}
newPairs.toSet()
.map(Pair<Pair<Char, Char>, Long>::first)
.associateWith { pair -> newPairs.filter { (key, _) -> key == pair }.sumOf { it.second } }
}
val charCounts = rules.keys.map(Pair<Char, Char>::first).toSet().associateWith { char ->
finalPairs.filter { (pair, count) ->
pair.first == char
}.values.sum() + if (char == template.last()) { 1 } else { 0 }
}
return charCounts.values.maxOrNull()!! - charCounts.values.minOrNull()!!
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day14_test")
check(part1(testInput) == 1588) { "${part1(testInput)}" }
check(part2(testInput) == 2188189693529L) { "${part2(testInput)}"}
val input = readInput("Day14")
println(part1(input))
println(part2(input))
}
| 0 | Kotlin | 0 | 1 | 391d0017ba9c2494092d27d22d5fd9f73d0c8ded | 2,342 | aoc-2021 | MIT License |
src/year2023/Day2.kt | drademacher | 725,945,859 | false | {"Kotlin": 76037} | package year2023
import readLines
fun main() {
val input = readLines("2023", "day2")
val testInput = readLines("2023", "day2_test")
check(part1(testInput) == 8)
println("Part 1:" + part1(input))
check(part2(testInput) == 2286)
println("Part 2:" + part2(input))
}
private fun parseInput(input: List<String>): List<Game> {
fun parseCubeCount(string: String): CubeCount {
val rawCubePairs = string.split(", ").map { rawCube -> rawCube.split(" ").let { Pair(it[0], it[1]) } }
return CubeCount(
red = rawCubePairs.find { it.second == "red" }?.first?.toInt() ?: 0,
blue = rawCubePairs.find { it.second == "blue" }?.first?.toInt() ?: 0,
green = rawCubePairs.find { it.second == "green" }?.first?.toInt() ?: 0,
)
}
fun parseGame(string: String): Game {
val number = string.dropWhile { !it.isDigit() }.takeWhile { it.isDigit() }.toInt()
val rawCubeCounts = string.dropWhile { it != ':' }.drop(2).split("; ")
val cubeCounts = rawCubeCounts.map { parseCubeCount(it) }
return Game(number, cubeCounts)
}
return input.map { parseGame(it) }
}
private fun part1(input: List<String>): Int {
val input = parseInput(input)
return input
.filter { game -> game.cubeCounts.all { cubeCount -> cubeCount.red <= 12 && cubeCount.green <= 13 && cubeCount.blue <= 14 } }
.sumOf { it.index }
}
private fun part2(input: List<String>): Int {
val input = parseInput(input)
return input
.map { game -> game.cubeCountMaximums() }
.sumOf { maximums -> maximums.red * maximums.blue * maximums.green }
}
data class Game(val index: Int, val cubeCounts: List<CubeCount>) {
fun cubeCountMaximums(): CubeCount {
return CubeCount(
cubeCounts.maxBy { it.red }.red,
cubeCounts.maxBy { it.blue }.blue,
cubeCounts.maxBy { it.green }.green,
)
}
}
data class CubeCount(val red: Int, val blue: Int, val green: Int)
| 0 | Kotlin | 0 | 0 | 4c4cbf677d97cfe96264b922af6ae332b9044ba8 | 2,026 | advent_of_code | MIT License |
src/Day08.kt | proggler23 | 573,129,757 | false | {"Kotlin": 27990} | fun main() {
fun part1(input: List<String>) = input.parse8().visibleTreeCount
fun part2(input: List<String>) = input.parse8().bestScenicView
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day08_test")
check(part1(testInput) == 21)
check(part2(testInput) == 8)
val input = readInput("Day08")
println(part1(input))
println(part2(input))
}
fun List<String>.parse8() = TreeGrid(Array(this[0].length) { x -> Array(size) { y -> this[y][x].digitToInt() } })
fun <T> List<T>.productOf(transform: (T) -> Int) = fold(1) { acc, t -> acc * transform(t) }
class TreeGrid(private val heights: Array<Array<Int>>) {
val visibleTreeCount: Int
get() = heights.indices.sumOf { x ->
heights[x].indices.count { y ->
isTreeVisible(x, y)
}
}
val bestScenicView: Int
get() = heights.indices.maxOf { x ->
heights[x].indices.maxOf { y ->
getScenicScore(x, y)
}
}
private fun viewRanges(x: Int, y: Int) = listOf(
(x - 1 downTo 0).map { heights[it][y] },
(x + 1 until heights.size).map { heights[it][y] },
(y - 1 downTo 0).map { heights[x][it] },
(y + 1 until heights[x].size).map { heights[x][it] }
)
private fun isTreeVisible(x: Int, y: Int) = viewRanges(x, y).any { it.all { height -> height < heights[x][y] } }
private fun getScenicScore(x: Int, y: Int) = viewRanges(x, y).productOf { range ->
val filteredRange = range.takeWhile { height -> height < heights[x][y] }
if (range.isEmpty()) 0
else if (filteredRange.size == range.size) range.size
else filteredRange.size + 1
}
}
| 0 | Kotlin | 0 | 0 | 584fa4d73f8589bc17ef56c8e1864d64a23483c8 | 1,750 | advent-of-code-2022 | Apache License 2.0 |
aoc21/day_22/main.kt | viktormalik | 436,281,279 | false | {"Rust": 227300, "Go": 86273, "OCaml": 82410, "Kotlin": 78968, "Makefile": 13967, "Roff": 9981, "Shell": 2796} | import java.io.File
import kotlin.math.max
import kotlin.math.min
data class Range(val min: Int, val max: Int) {
fun hasIntersection(range: Range): Boolean = range.min <= max && range.max >= min
fun intersection(range: Range): Range = Range(max(min, range.min), min(max, range.max))
fun minus(range: Range): List<Range> {
if (!hasIntersection(range)) return listOf(this)
if (range.min <= min && range.max >= max) return listOf()
if (range.min < min) return listOf(Range(range.max + 1, max))
if (range.max > max) return listOf(Range(min, range.min - 1))
return listOf(Range(min, range.min - 1), Range(range.max + 1, max))
}
fun size(): Long = (max - min + 1).toLong()
}
class Cube(val x: Range, val y: Range, val z: Range) {
fun hasIntersection(cube: Cube): Boolean =
x.hasIntersection(cube.x) && y.hasIntersection(cube.y) && z.hasIntersection(cube.z)
fun intersection(cube: Cube): Cube? =
if (hasIntersection(cube))
Cube(x.intersection(cube.x), y.intersection(cube.y), z.intersection(cube.z))
else null
fun minus(cube: Cube): List<Cube> =
x.minus(cube.x).map { Cube(it, y, z) } +
y.minus(cube.y).map { Cube(x.intersection(cube.x), it, z) } +
z.minus(cube.z).map { Cube(x.intersection(cube.x), y.intersection(cube.y), it) }
fun size(): Long = x.size() * y.size() * z.size()
}
fun main() {
var cubes = listOf<Cube>()
val re = "x=(-?\\d+)..(-?\\d+),y=(-?\\d+)..(-?\\d+),z=(-?\\d+)..(-?\\d+)".toRegex()
for (line in File("input").readLines()) {
val match = re.find(line)!!.groupValues
val cube = Cube(
Range(match.get(1).toInt(), match.get(2).toInt()),
Range(match.get(3).toInt(), match.get(4).toInt()),
Range(match.get(5).toInt(), match.get(6).toInt()),
)
if (line.startsWith("on")) {
cubes += cubes.fold(listOf(cube)) { newCubes, c ->
newCubes.flatMap {
if (it.hasIntersection(c)) it.minus(c) else listOf(it)
}
}
} else {
cubes = cubes.flatMap {
if (it.hasIntersection(cube)) it.minus(cube) else listOf(it)
}
}
}
val initArea = Cube(Range(-50, 50), Range(-50, 50), Range(-50, 50))
val first = cubes.map { it.intersection(initArea)?.size() ?: 0 }.sum()
println("First: $first")
val second = cubes.map { it.size() }.sum()
println("Second: $second")
}
| 0 | Rust | 1 | 0 | f47ef85393d395710ce113708117fd33082bab30 | 2,539 | advent-of-code | MIT License |
src/Day16.kt | jbotuck | 573,028,687 | false | {"Kotlin": 42401} | import kotlin.math.max
import kotlin.math.min
fun main() {
val valves = readInput("Day16")
.map { Valve.of(it) }
.associateBy { it.name }
val start = valves["AA"]!!
val valvesWithNeighbors = valves.values
.filter { it.name == "AA" || it.flowRate > 0 }
.sortedBy { it.name }
.let { valuableValves ->
val distances = valuableValves.mapIndexed { index, origin ->
(index.inc()..valuableValves.lastIndex)
.map {
(origin.name to valuableValves[it].name) to valves.calculateCost(
origin.name,
valuableValves[it].name
)
}
}.flatten().toMap()
valuableValves.associateWith { thisValve ->
valuableValves
.filter { it != thisValve && it.name != "AA" }
.map { it to distances.getDistance(thisValve.name, it.name) }
.sortedBy { it.second }
}
}
//part1
run {
val paths = getPaths(start, valvesWithNeighbors, 30)
println(paths.values.max())
}
//part 2
val paths = getPaths(start, valvesWithNeighbors, 26)
paths.maxOf { me ->
me.value.plus(paths
.filter { elephant -> elephant.key.all { it !in me.key } }
.maxOfOrNull { elephant -> elephant.value }
?: 0
)
}.let { println(it) }
}
private fun getPaths(
start: Valve,
valvesWithNeighbors: Map<Valve, List<Pair<Valve, Int>>>,
timeAllowed: Int = 30
) = mutableMapOf<Set<String>, Int>().apply {
val solutions = ArrayDeque<Solution>().apply { addLast(Solution(start, timeAllowed = timeAllowed)) }
while (solutions.isNotEmpty()) {
val solution = solutions.removeFirst()
val key = solution.visited.map { it.name }.toSet()
this[key] = max(solution.totalRelief, this[key] ?: 0)
solution.nextSolutions(valvesWithNeighbors).takeUnless { it.isEmpty() }?.let { solutions.addAll(it) }
}
}.toMap()
private fun Map<Pair<String, String>, Int>.getDistance(a: String, b: String) =
get(listOf(a, b).sorted().run { first() to last() })!!
fun Map<String, Valve>.calculateCost(origin: String, destination: String): Int {
val costs = mutableMapOf<String, Int>().apply { set(origin, 0) }
val toVisit = mutableListOf(origin)
val visited = mutableSetOf<String>()
while (toVisit.isNotEmpty()) {
val visiting = toVisit.removeAt(toVisit.withIndex().minBy { costs[it.value] ?: Int.MAX_VALUE }.index)
if (visiting in visited) continue
visited.add(visiting)
if (visiting == destination) return costs[visiting]!!.inc() //Plus 1 to open the valve
val distanceOfNeighbor = costs[visiting]!!.inc()
for (neighbor in get(visiting)!!.neighbors) {
toVisit.add(neighbor)
costs[neighbor] = costs[neighbor]?.let { min(it, distanceOfNeighbor) } ?: distanceOfNeighbor
}
}
throw IllegalStateException("oops")
}
data class Valve(val name: String, val flowRate: Int, val neighbors: List<String>) {
fun totalRelief(timeOpen: Int) = flowRate * timeOpen
companion object {
fun of(line: String): Valve {
val (valve, neighbors) = line.split(';')
val (name, flowRate) = valve.substringAfter("Valve ").split(" has flow rate=")
return Valve(
name, flowRate.toInt(), neighbors
.substringAfter(" tunnel leads to valve ")
.substringAfter(" tunnels lead to valves ")
.split(", ")
)
}
}
}
data class Solution(
val visited: Set<Valve>,
val lastVisited: Valve,
val costIncurred: Int = 0,
val timeAllowed: Int = 30,
val totalRelief: Int = 0
) {
constructor(valve: Valve, costIncurred: Int = 0, timeAllowed: Int = 30, totalRelief: Int = 0) : this(
setOf(),
valve,
costIncurred,
timeAllowed,
totalRelief
)
private val timeRemaining = timeAllowed - costIncurred
private fun with(valve: Valve, additionalCost: Int) = copy(
visited = visited + valve,
lastVisited = valve,
costIncurred = costIncurred + additionalCost,
totalRelief = totalRelief + valve.totalRelief(timeRemaining - additionalCost)
)
fun nextSolutions(valvesWithNeighbors: Map<Valve, List<Pair<Valve, Int>>>) = mutableListOf<Solution>().apply {
for (destination in valvesWithNeighbors[lastVisited]!!) {
if (destination.first in visited) continue
if (destination.second > timeRemaining) break
add(with(destination.first, destination.second))
}
}
} | 0 | Kotlin | 0 | 0 | d5adefbcc04f37950143f384ff0efcd0bbb0d051 | 4,812 | aoc2022 | Apache License 2.0 |
src/Day12.kt | cisimon7 | 573,872,773 | false | {"Kotlin": 41406} | fun main() {
fun shortestPath(
map: List<List<Elevation>>,
startElevation: Elevation,
endElevation: Elevation
): List<List<Elevation>> {
var paths =
listOf(listOf(map.flatten().firstOrNull { it == endElevation } ?: error("Can't find highest point")))
while (paths.map { it.last() }.none { it == startElevation }) {
paths = paths
// Eliminating paths that have the same start and end points by taking the shortest path already
.groupBy { it.last() }
.map { (_, list) -> list.minBy { it.size } }
// Extending paths
.map { path ->
val (end, rest) = path.last() to path.dropLast(1)
map.possibleNext(end)
.filter { cell -> cell !in rest } // Prevent loop
.map { cell -> rest + listOf(end, cell) }
.ifEmpty { listOf(path) }
}.flatten().also { if (it == paths) error("Can't find path to starting point") }
}
return paths
}
fun part1(map: List<List<Elevation>>): Int {
val startElevation = map.flatten().first { it.h == -1 }
val endElevation = map.flatten().first { it.h == 26 }
val paths = shortestPath(map, startElevation, endElevation)
return paths.filter { it.last().h == -1 }.minBy { it.size }.count() - 1
}
fun part2(map: List<List<Elevation>>): Int {
// Takes forever for large maps, but works
val endElevation = map.flatten().first { it.h == 26 }
val startElevations = map.flatten().filter { it.h == 0 } + map.flatten().first { it.h == -1 }
println(startElevations.size)
return startElevations
.map { start ->
runCatching { shortestPath(map, start, endElevation) }
.getOrElse { emptyList() }
}
.flatten()
.filter { it.last().h == -1 || it.last().h == 0 }
.minBy { it.size }
.count() - 1
}
fun parse(stringList: List<String>): List<List<Elevation>> {
return stringList.mapIndexed { rIdx, line ->
line.mapIndexed { cIdx, h ->
when {
h.isLowerCase() -> Elevation(rIdx, cIdx, h - 'a', h)
h == 'S' -> Elevation(-1, -1, -1, h)
h == 'E' -> Elevation(rIdx, cIdx, 26, h)
else -> error("un-excepted character $h")
}
}
}
}
val testInput = readInput("Day12_test")
check(part1(parse(testInput)) == 31)
check(part2(parse(testInput)) == 29)
val input = readInput("Day12_input")
println(part1(parse(input)))
println(part2(parse(input)))
}
data class Elevation(val x: Int, val y: Int, val h: Int, val ch: Char)
fun List<List<Elevation>>.possibleNext(elevation: Elevation): List<Elevation> {
val (x, y, h, _) = elevation
return listOf(+1 to +0, +0 to +1, -1 to +0, +0 to -1)
.map { (i, j) -> i + x to j + y }
.filterNot { (i, j) -> i < 0 || i >= size || j < 0 || j >= this.first().size }
.map { (i, j) -> this[i][j] }
.filter { it.h + 1 == h || it.h >= h }
}
/**
* Advent of Code[About][Events][Shop][Settings][Log Out]cisimon7 23*
* λy.2022[Calendar][AoC++][Sponsors][Leaderboard][Stats]
* Our sponsors help make Advent of Code possible:
* NUMERAI - join the hardest data science tournament in the world
* --- Day 12: Hill Climbing Algorithm ---
* You try contacting the Elves using your handheld device, but the river you're following must be too low to get a decent signal.
*
* You ask the device for a heightmap of the surrounding area (your puzzle input). The heightmap shows the local area from above broken into a grid; the elevation of each square of the grid is given by a single lowercase letter, where a is the lowest elevation, b is the next-lowest, and so on up to the highest elevation, z.
*
* Also included on the heightmap are marks for your current position (S) and the location that should get the best signal (E). Your current position (S) has elevation a, and the location that should get the best signal (E) has elevation z.
*
* You'd like to reach E, but to save energy, you should do it in as few steps as possible. During each step, you can move exactly one square up, down, left, or right. To avoid needing to get out your climbing gear, the elevation of the destination square can be at most one higher than the elevation of your current square; that is, if your current elevation is m, you could step to elevation n, but not to elevation o. (This also means that the elevation of the destination square can be much lower than the elevation of your current square.)
*
* For example:
*
* Sabqponm
* abcryxxl
* accszExk
* acctuvwj
* abdefghi
* Here, you start in the top-left corner; your goal is near the middle. You could start by moving down or right, but eventually you'll need to head toward the e at the bottom. From there, you can spiral around to the goal:
*
* v..v<<<<
* >v.vv<<^
* .>vv>E^^
* ..v>>>^^
* ..>>>>>^
* In the above diagram, the symbols indicate whether the path exits each square moving up (^), down (v), left (<), or right (>). The location that should get the best signal is still E, and . marks unvisited squares.
*
* This path reaches the goal in 31 steps, the fewest possible.
*
* What is the fewest steps required to move from your current position to the location that should get the best signal?
*
* Your puzzle answer was 394.
*
* The first half of this puzzle is complete! It provides one gold star: *
*
* --- Part Two ---
* As you walk up the hill, you suspect that the Elves will want to turn this into a hiking trail. The beginning isn't very scenic, though; perhaps you can find a better starting point.
*
* To maximize exercise while hiking, the trail should start as low as possible: elevation a. The goal is still the square marked E. However, the trail should still be direct, taking the fewest steps to reach its goal. So, you'll need to find the shortest path from any square at elevation a to the square marked E.
*
* Again consider the example from above:
*
* Sabqponm
* abcryxxl
* accszExk
* acctuvwj
* abdefghi
* Now, there are six choices for starting position (five marked a, plus the square marked S that counts as being at elevation a). If you start at the bottom-left square, you can reach the goal most quickly:
*
* ...v<<<<
* ...vv<<^
* ...v>E^^
* .>v>>>^^
* >^>>>>>^
* This path reaches the goal in only 29 steps, the fewest possible.
*
* What is the fewest steps required to move starting from any square with elevation a to the location that should get the best signal?
*
* Answer:
*
*
* Although it hasn't changed, you can still get your puzzle input.
*
* You can also [Share] this puzzle.*/ | 0 | Kotlin | 0 | 0 | 29f9cb46955c0f371908996cc729742dc0387017 | 6,956 | aoc-2022 | Apache License 2.0 |
src/Day15.kt | AxelUser | 572,845,434 | false | {"Kotlin": 29744} | import kotlin.math.abs
data class Day15Data(val scanner: Point<Long>, val beacon: Point<Long>, val distance: Long)
fun main() {
fun List<String>.parse(): List<Day15Data> {
return this.map {
Regex("-?\\d++").findAll(it).map { it.value.toLong() }.toList()
.let { (x1, y1, x2, y2) -> Day15Data(Point(y1, x1), Point(y2, x2), abs(x1 - x2) + abs(y1 - y2)) }
}
}
fun getRange(data: Day15Data, row: Long): LongArray {
val xDiff = data.distance - abs(row - data.scanner.y)
val start = data.scanner.x - xDiff
val end = data.scanner.x + xDiff
return longArrayOf(start, end)
}
fun getRanges(data: List<Day15Data>, row: Long): List<LongArray> {
val ranges = data.asSequence()
.filter { row in it.scanner.y - it.distance..it.scanner.y + it.distance }
.map { getRange(it, row) }
.sortedBy { it[0] }
.toList()
return ranges.fold(mutableListOf()) { acc, range ->
acc.apply {
if (isEmpty() || acc.last()[1] < range[0]) {
add(range)
} else if (acc.last()[1] >= range[0]) {
acc.last()[1] = maxOf(acc.last()[1], range[1])
}
}
}
}
fun part1(data: List<Day15Data>, row: Long): Long {
val sum = getRanges(data, row).sumOf { abs(it[1] - it[0]) + 1 }
val beacons = data.map { it.beacon }.toSet().count { it.y == row }
return sum - beacons
}
fun calcFreq(x: Long, y: Long): Long = x * 4_000_000 + y
fun part2(data: List<Day15Data>, upperBound: Long): Long {
for (row in 0..upperBound) {
val ranges = getRanges(data, row)
when {
ranges.size == 1 && ranges[0][0] <= 0 && ranges[0][1] >= upperBound -> continue
ranges.size == 1 && ranges[0][0] > 0 -> {
return calcFreq(0, row)
}
ranges.size == 1 && ranges[0][1] < upperBound -> {
return calcFreq(upperBound, row)
}
ranges.size == 2 -> {
return calcFreq(ranges[0][1] + 1, row)
}
else -> error(
"row:$row, ranges: ${
ranges.joinToString(prefix = "[", postfix = "]") {
it.joinToString(
prefix = "[",
postfix = "]"
)
}
}"
)
}
}
error("not found")
}
val testInput = readInput("Day15_test").parse()
check(part1(testInput, 10) == 26L)
check(part2(testInput, 20) == 56000011L)
val input = readInput("Day15").parse()
println(part1(input, 2_000_000))
println(part2(input, 4_000_000))
}
| 0 | Kotlin | 0 | 1 | 042e559f80b33694afba08b8de320a7072e18c4e | 2,931 | aoc-2022 | Apache License 2.0 |
src/Day08.kt | wooodenleg | 572,658,318 | false | {"Kotlin": 30668} | data class Tree(val x: Int, val y: Int, val height: Int)
fun makeForrest(input: List<String>): List<Tree> {
val forrest = mutableListOf<Tree>()
input.forEachIndexed { columnIndex, treeLine ->
treeLine.forEachIndexed { rowIndex, height ->
forrest.add(Tree(rowIndex, columnIndex, height.digitToInt()))
}
}
return forrest
}
fun filterVisibleTreesInForest(forest: List<Tree>): Collection<Tree> {
val rows = forest.rows()
val columns = forest.columns()
val treeLines = rows + rows.map(List<Tree>::asReversed) +
columns + columns.map(List<Tree>::asReversed)
return treeLines
.flatMap(::filterVisibleTreesInTreeLine)
.distinct()
}
fun filterVisibleTreesInTreeLine(line: List<Tree>): Collection<Tree> = buildList {
var maxHeight = -1
for (tree in line) {
if (tree.height > maxHeight) {
add(tree)
maxHeight = tree.height
}
}
}
fun List<Tree>.rows(): List<List<Tree>> = buildList {
val rowsMap = this@rows.groupBy { it.y }
for (key in rowsMap.keys) add(key, rowsMap.getValue(key))
}
fun List<Tree>.columns(): List<List<Tree>> = buildList {
val columnsMap = this@columns.groupBy { it.x }
for (key in columnsMap.keys) add(key, columnsMap.getValue(key))
}
fun List<Tree>.filterVisibleTreesFromHeightCount(height: Int): Int {
var count = 0
for (tree in this) {
count++
if (tree.height >= height) break
}
return count
}
fun Tree.scenicScore(row: List<Tree>, column: List<Tree>): Int {
val rowIndex = row.indexOf(this)
val columnIndex = column.indexOf(this)
val left = row.subList(0, rowIndex).asReversed().filterVisibleTreesFromHeightCount(height)
val right = row.subList(rowIndex + 1, row.size).filterVisibleTreesFromHeightCount(height)
val top = column.subList(0, columnIndex).asReversed().filterVisibleTreesFromHeightCount(height)
val bottom = column.subList(columnIndex + 1, column.size).filterVisibleTreesFromHeightCount(height)
return left * right * top * bottom
}
fun main() {
fun part1(input: List<String>): Int {
val forrest = makeForrest(input)
val visibleTrees = filterVisibleTreesInForest(forrest)
return visibleTrees.count()
}
fun part2(input: List<String>): Int {
val forest = makeForrest(input)
val columns = forest.columns()
val rows = forest.rows()
var maxScore = 0
repeat(input.first().length) { x ->
repeat(input.size) { y ->
val tree = columns[x][y]
val score = tree.scenicScore(rows[y], columns[x])
if (score > maxScore) maxScore = score
}
}
return maxScore
}
val testInput = readInputLines("Day08_test")
check(part1(testInput) == 21)
check(part2(testInput) == 8)
val input = readInputLines("Day08")
println(part1(input))
println(part2(input))
}
| 0 | Kotlin | 0 | 1 | ff1f3198f42d1880e067e97f884c66c515c8eb87 | 2,978 | advent-of-code-2022 | Apache License 2.0 |
src/Day18.kt | mjossdev | 574,439,750 | false | {"Kotlin": 81859} | fun main() {
data class Cube(val x: Int, val y: Int, val z: Int) {
override fun toString(): String = "$x,$y,$z"
}
fun Cube.adjacents() = listOf(
copy(x = x + 1),
copy(x = x - 1),
copy(y = y + 1),
copy(y = y - 1),
copy(z = z + 1),
copy(z = z - 1)
)
fun readCubes(input: List<String>): List<Cube> =
input.map { line -> line.split(',').map { it.toInt() }.let { (x, y, z) -> Cube(x, y, z) } }.distinct()
fun getGrid(cubes: Iterable<Cube>): Array<Array<BooleanArray>> {
val width = cubes.maxOf { it.x } + 1
val height = cubes.maxOf { it.y } + 1
val depth = cubes.maxOf { it.z } + 1
val grid = Array(width) { Array(height) { BooleanArray(depth) } }
for ((x, y, z) in cubes) {
grid[x][y][z] = true
}
return grid
}
fun part1(input: List<String>): Int {
val cubes = readCubes(input)
val grid = getGrid(cubes)
val width = grid.size
val height = grid[0].size
val depth = grid[0][0].size
return cubes.sumOf { (x, y, z) ->
var sides = 6
if (x > 0 && grid[x - 1][y][z]) --sides
if (x < width - 1 && grid[x + 1][y][z]) --sides
if (y > 0 && grid[x][y - 1][z]) --sides
if (y < height - 1 && grid[x][y + 1][z]) --sides
if (z > 0 && grid[x][y][z - 1]) --sides
if (z < depth - 1 && grid[x][y][z + 1]) --sides
sides
}
}
fun part2(input: List<String>): Int {
val cubes = readCubes(input)
val grid = getGrid(cubes)
val width = grid.size
val height = grid[0].size
val depth = grid[0][0].size
fun Cube.isAir() = !(grid.elementAtOrNull(x)?.elementAtOrNull(y)?.elementAtOrNull(z) ?: false)
fun countReachableSides(cube: Cube): Int = cube.adjacents().filter { it.isAir() }.count { start ->
val queue = ArrayDeque<Cube>()
val explored = mutableSetOf(start)
queue.addLast(start)
while (queue.isNotEmpty()) {
val next = queue.removeFirst()
if (next.run { x == 0 || x == width - 1 || y == 0 || y == height - 1 || z == 0 || z == depth - 1 }) {
return@count true
}
next.adjacents().filter { it.isAir() && it !in explored }.forEach {
explored.add(it)
queue.addLast(it)
}
}
false
}
return cubes.sumOf { countReachableSides(it) }
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day18_test")
check(part1(testInput) == 64)
check(part2(testInput) == 58)
val input = readInput("Day18")
println(part1(input))
println(part2(input))
}
| 0 | Kotlin | 0 | 0 | afbcec6a05b8df34ebd8543ac04394baa10216f0 | 2,890 | advent-of-code-22 | Apache License 2.0 |
src/Day08.kt | Ajimi | 572,961,710 | false | {"Kotlin": 11228} | fun main() {
fun part1(input: List<List<Int>>): Int {
val height = input.size
val width = input.first().size
fun isVisibleFromOutside(row: Int, column: Int, direction: Direction): Boolean {
val treeHeight = input[row][column]
return input.treesByDirection(row, column, direction).all { it < treeHeight }
}
var count = (height + width - 2) * 2
(1 until height - 1).forEach { row ->
(1 until width - 1)
.asSequence()
.filter { column ->
Direction.values().any { isVisibleFromOutside(row, column, it) }
}
.forEach { count++ }
}
return count
}
fun part2(input: List<List<Int>>): Int {
val height = input.size
val width = input.first().size
fun countVisibleTrees(r: Int, c: Int, direction: Direction): Int {
val targetTree = input[r][c]
var count = 0
input.treesByDirection(r, c, direction).forEach { currentTree ->
count++
if (currentTree >= targetTree) return count
}
return count
}
var maxScore = 0
(1 until height - 1).forEach { row ->
(1 until width - 1)
.asSequence()
.map { column ->
Direction.values().map { countVisibleTrees(row, column, it) }.reduce(Int::times)
}
.forEach { maxScore = maxOf(it, maxScore) }
}
return maxScore
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day08_test").toMatrix()
part1(testInput)
check(part1(testInput) == 21)
check(part2(testInput) == 8)
val input = readInput("Day08").toMatrix()
println(part1(input))
println(part2(input))
}
private fun List<String>.toMatrix() =
map { line -> line.map { Character.getNumericValue(it) } }
enum class Direction(val dr: Int = 0, val dc: Int = 0) {
UP(dr = -1),
LEFT(dc = -1),
DOWN(dr = +1),
RIGHT(dc = +1);
fun next(coordinates: Pair<Int, Int>): Pair<Int, Int> {
val (row, column) = coordinates
return (row + dr) to (column + dc)
}
}
private fun List<List<Int>>.treesByDirection(
row: Int,
column: Int,
direction: Direction,
): Sequence<Int> =
generateSequence(direction.next(row to column), direction::next)
.takeWhile { (r, c) -> r in indices && c in first().indices }
.map { (r, c) -> this[r][c] }
| 0 | Kotlin | 0 | 0 | 8c2211694111ee622ebb48f52f36547fe247be42 | 2,600 | adventofcode-2022 | Apache License 2.0 |
src/Day16.kt | sabercon | 648,989,596 | false | null | fun main() {
data class Rule(val name: String, val range1: IntRange, val range2: IntRange)
fun validRules(value: Int, rules: Set<Rule>): Set<Rule> {
return rules.filter { (_, range1, range2) -> value in range1 || value in range2 }.toSet()
}
fun buildRuleMap(candidates: List<Pair<Int, Set<Rule>>>): Map<Int, Rule> {
if (candidates.isEmpty()) return emptyMap()
val (index, rules) = candidates.minBy { (_, rules) -> rules.size }
val rule = rules.single()
return candidates.map { (i, rs) -> i to (rs - rule) }
.filter { (_, rs) -> rs.isNotEmpty() }
.let { buildRuleMap(it) }
.plus(index to rule)
}
fun buildRuleMap(rules: Set<Rule>, tickets: List<List<Int>>): Map<Int, Rule> {
return rules.indices
.map { it to tickets.fold(rules) { acc, t -> acc intersect validRules(t[it], rules) } }
.let { buildRuleMap(it) }
}
val input = readText("Day16")
val (rulesStr, myStr, nearbyStr) = """(.+)\n\nyour ticket:\n(.+)\n\nnearby tickets:\n(.+)"""
.toRegex(RegexOption.DOT_MATCHES_ALL).destructureGroups(input)
val rules = rulesStr.lines().map {
val (name, f1, l1, f2, l2) = """(.+): (\d+)-(\d+) or (\d+)-(\d+)""".toRegex().destructureGroups(it)
Rule(name, f1.toInt()..(l1.toInt()), f2.toInt()..(l2.toInt()))
}.toSet()
val myTicket = myStr.split(",").map { it.toInt() }
val nearbyTickets = nearbyStr.lines().map { line -> line.split(",").map { it.toInt() } }
nearbyTickets.flatten().filter { validRules(it, rules).isEmpty() }.sum().println()
val validTickets = nearbyTickets.filter { line -> line.all { validRules(it, rules).isNotEmpty() } }
val ruleMap = buildRuleMap(rules, validTickets)
myTicket.filterIndexed { i, _ -> ruleMap[i]!!.name.startsWith("departure") }
.fold(1L) { acc, v -> acc * v }.println()
}
| 0 | Kotlin | 0 | 0 | 81b51f3779940dde46f3811b4d8a32a5bb4534c8 | 1,911 | advent-of-code-2020 | MIT License |
2k23/aoc2k23/src/main/kotlin/19.kt | papey | 225,420,936 | false | {"Rust": 88237, "Kotlin": 63321, "Elixir": 54197, "Crystal": 47654, "Go": 44755, "Ruby": 24620, "Python": 23868, "TypeScript": 5612, "Scheme": 117} | package d19
fun main() {
val (workflows, ratings) = input.raw("19.txt").split("\n\n").let {
val workflows = it[0].split("\n")
.map { line -> Workflow(line) }.associateBy { workflow -> workflow.id }
val ratings = it[1].split("\n")
.filter { line -> line.isNotBlank() }
.map { rating -> Rating(rating) }
workflows to ratings
}
println("Part 1: ${part1(workflows, ratings)}")
println("Part 2: ${part2(workflows)}")
}
fun part1(workflows: Map<String, Workflow>, ratings: List<Rating>): Int {
return ratings.filter { rating ->
var res = workflows["in"]!!.run(rating)
while (res != "A" && res != "R") {
res = workflows[res]!!.run(rating)
}
res == "A"
}.sumOf { it.sum() }
}
fun part2(workflows: Map<String, Workflow>): Long {
return combinations(
"in",
workflows,
mapOf(
Rating.RatingKey.X to 1..4000,
Rating.RatingKey.M to 1..4000,
Rating.RatingKey.A to 1..4000,
Rating.RatingKey.S to 1..4000
)
)
}
fun combinations(id: String, workflows: Map<String, Workflow>, ranges: Map<Rating.RatingKey, IntRange>): Long =
when (id) {
"R" -> 0L
"A" -> {
ranges.values.map { it.count().toLong() }.reduce(Long::times)
}
else -> {
val newRanges = ranges.toMap().toMutableMap()
workflows[id]!!.steps.sumOf { step ->
when (step) {
is Workflow.SimpleStep -> {
combinations(step.target(), workflows, newRanges)
}
is Workflow.ConditionalStep -> {
when (step.operand) {
Workflow.ConditionalStep.Operand.LessThan -> {
val currentRange = newRanges[step.source]!!
newRanges[step.source] = currentRange.first..<step.value
combinations(step.target(), workflows, newRanges).also {
newRanges[step.source] = step.value..currentRange.last
}
}
Workflow.ConditionalStep.Operand.MoreThan -> {
val currentRange = newRanges[step.source]!!
newRanges[step.source] = step.value + 1..currentRange.last
combinations(step.target(), workflows, newRanges).also {
newRanges[step.source] = currentRange.first..step.value
}
}
}
}
else -> {
throw IllegalStateException("oops")
}
}
}
}
}
class Rating(input: String) {
private val ratingsRegex = Regex("""\w=(\d+)""")
val values: Map<RatingKey, Int>
init {
val rates =
ratingsRegex.findAll(input).map { rate -> rate.groupValues[1] }.map { number -> number.toInt() }.toList()
values = mapOf(
RatingKey.X to rates[0],
RatingKey.M to rates[1],
RatingKey.A to rates[2],
RatingKey.S to rates[3]
)
}
fun sum(): Int = values.values.sum()
enum class RatingKey {
X,
M,
A,
S;
companion object {
fun fromString(input: String): RatingKey =
when (input) {
"x" -> X
"m" -> M
"a" -> A
"s" -> S
else -> throw IllegalStateException("oops")
}
}
}
}
class Workflow(input: String) {
private val workflowRegex = Regex("""(\w+)\{(.*)}""")
val steps: List<StepRunner>
val id: String
init {
val matches = workflowRegex.find(input)
id = matches!!.groupValues[1]
steps = matches.groupValues[2].split(",").map { rawStep ->
if (rawStep.contains(":")) {
ConditionalStep(rawStep)
} else {
SimpleStep(rawStep)
}
}
}
fun run(rating: Rating): String {
return steps.find { step -> step.predicate(rating) }!!.target()
}
interface StepRunner {
fun target(): String
fun predicate(rating: Rating): Boolean
}
class SimpleStep(private val target: String) : StepRunner {
override fun target(): String = target
override fun predicate(rating: Rating): Boolean = true
}
class ConditionalStep(input: String) : StepRunner {
private val stepRegex = Regex("""([xmas])([<>])(\d+):(\w+)""")
enum class Operand {
LessThan,
MoreThan;
companion object {
fun fromString(input: String): Operand =
when (input) {
">" -> MoreThan
"<" -> LessThan
else -> throw IllegalStateException("oops")
}
}
}
private val target: String
val value: Int
val source: Rating.RatingKey
val operand: Operand
init {
val matches = stepRegex.find(input)
source = Rating.RatingKey.fromString(matches!!.groupValues[1])
operand = Operand.fromString(matches.groupValues[2])
value = matches.groupValues[3].toInt()
target = matches.groupValues.last()
}
override fun target(): String = target
override fun predicate(rating: Rating): Boolean =
when (operand) {
Operand.LessThan -> rating.values[source]!! < value
Operand.MoreThan -> rating.values[source]!! > value
}
}
}
| 0 | Rust | 0 | 3 | cb0ea2fc043ebef75aff6795bf6ce8a350a21aa5 | 5,996 | aoc | The Unlicense |
src/Day22/Day22.kt | martin3398 | 436,014,815 | false | {"Kotlin": 63436, "Python": 5921} | import java.lang.Long.max
import java.lang.Long.min
data class Cube(val x: Pair<Long, Long>, val y: Pair<Long, Long>, val z: Pair<Long, Long>) {
fun inSmallerCube() =
x.first >= -50 && x.second <= 50 && y.first >= -50 && y.second <= 50 && z.first >= -50 && z.second <= 50
}
fun Cube.intersect(other: Cube): Cube? {
val minX = min(x.second, other.x.second)
val maxX = max(x.first, other.x.first)
val minY = min(y.second, other.y.second)
val maxY = max(y.first, other.y.first)
val minZ = min(z.second, other.z.second)
val maxZ = max(z.first, other.z.first)
if (maxX > minX || maxY > minY || maxZ > minZ) {
return null
}
return Cube(Pair(maxX, minX), Pair(maxY, minY), Pair(maxZ, minZ))
}
fun Cube.volume() = (x.second - x.first + 1) * (y.second - y.first + 1) * (z.second - z.first + 1)
fun main() {
fun preprocess(input: List<String>): List<Pair<Boolean, Cube>> {
fun parseCube(s: String): Pair<Boolean, Cube> {
val split = s.split(" ")
val onOff = split[0] == "on"
val pos = split[1].split(",")
.map { it.split("=")[1].split("..").map { x -> x.toLong() }.zipWithNext()[0] }
return Pair(onOff, Cube(pos[0], pos[1], pos[2]))
}
return input.map { parseCube(it) }
}
fun calc(input: List<Pair<Boolean, Cube>>): Long {
var parts: Map<Cube, Long> = HashMap()
for ((onOff, cube) in input) {
val add = HashMap<Cube, Long>()
if (onOff) {
add[cube] = 1
}
for ((part, count) in parts) {
val intersection = cube.intersect(part)
if (intersection != null) {
add[intersection] = add.getOrDefault(intersection, 0) - count
}
}
parts = (parts.asSequence() + add.asSequence())
.distinct()
.groupBy({ it.key }, { it.value })
.mapValues { it.value.sum() }
}
return parts.map { it.key.volume() * it.value }.sum()
}
fun part1(input: List<Pair<Boolean, Cube>>): Long {
return calc(input.filter { it.second.inSmallerCube() })
}
fun part2(input: List<Pair<Boolean, Cube>>): Long {
return calc(input)
}
val testInput = preprocess(readInput(22, true))
val input = preprocess(readInput(22))
check(part1(testInput) == 474140L)
println(part1(input))
check(part2(testInput) == 2758514936282235L)
println(part2(input))
}
| 0 | Kotlin | 0 | 0 | 085b1f2995e13233ade9cbde9cd506cafe64e1b5 | 2,550 | advent-of-code-2021 | Apache License 2.0 |
src/main/kotlin/Path.kt | alebedev | 573,733,821 | false | {"Kotlin": 82424} | fun main() = Path.solve()
private typealias Matrix = List<List<Int>>
private object Path {
const val START = 0
const val TARGET = ('z'.code - 'a'.code) + 1
fun solve() {
val input = readInput()
val starts = findAll(input, START)
val target = findAll(input, TARGET).first()
val graph = buildGraph(input)
println("Shorted max elevation path: ${starts.map { shortestPath(graph, it, target) }.filter { it > 0 }.min()}")
}
private fun readInput(): Matrix {
return generateSequence(::readLine).map {
it.splitToSequence("").filter { !it.isEmpty() }.map {
when (val char = it.first()) {
in 'a'..'z' -> char.code - 'a'.code
'S' -> START
'E' -> TARGET
else -> throw Error("Unexpected input char $char")
}
}.toList()
}.toList()
}
private fun findAll(matrix: Matrix, target: Int): List<Pos> {
val result = mutableListOf<Pos>()
for (y in matrix.indices) {
for (x in matrix[y].indices) {
if (matrix[y][x] == target) {
result.add(Pos(x, y))
}
}
}
return result
}
private fun buildGraph(matrix: Matrix): Map<Pos, Node> {
val height = matrix.size
val width = matrix.first().size
fun neighbours(pos: Pos) = listOf(
Pos(pos.x - 1, pos.y),
Pos(pos.x + 1, pos.y),
Pos(pos.x, pos.y - 1),
Pos(pos.x, pos.y + 1)
).filter { it.x in 0 until width && it.y in 0 until height }
val result = mutableMapOf<Pos, Node>()
for (y in matrix.indices) {
for (x in matrix[y].indices) {
val pos = Pos(x, y)
val node = result.getOrPut(pos) { Node(pos, matrix[y][x]) }
for (nPos in neighbours(pos)) {
val nNode = result.getOrPut(nPos) { Node(nPos, matrix[nPos.y][nPos.x]) }
if (nNode.height <= node.height + 1) {
node.edges.add(nPos)
}
}
}
}
return result
}
private fun shortestPath(graph: Map<Pos, Node>, start: Pos, target: Pos): Int {
val paths = mutableMapOf<Pos, Int>()
val queue = mutableListOf(Pair(start, 0))
while (queue.size > 0) {
val (pos, pathLen) = queue.removeAt(0)
if (pos in paths) continue
paths[pos] = pathLen
for (edge in graph[pos]!!.edges) {
if (edge in paths) continue
if (edge == target) {
return pathLen + 1
}
queue.add(Pair(edge, pathLen + 1))
}
}
return -1
}
data class Pos(val x: Int, val y: Int)
data class Node(val pos: Pos, val height: Int, val edges: MutableList<Pos> = mutableListOf())
}
| 0 | Kotlin | 0 | 0 | d6ba46bc414c6a55a1093f46a6f97510df399cd1 | 3,014 | aoc2022 | MIT License |
2023/src/day07/day07.kt | Bridouille | 433,940,923 | false | {"Kotlin": 171124, "Go": 37047} | package day07
import GREEN
import RESET
import printTimeMillis
import readInput
data class CamelCards(
val hand: String,
val occurences: Map<Char, Int>,
val occurencesWithJoker: Map<Char, Int>,
val bid: Int
)
private fun typeScore(occurences: Map<Char, Int>): Int {
if (occurences.values.contains(5)) return 1_000_000 // Five of a kind
if (occurences.values.contains(4)) return 100_000 // Four of a kind
if (occurences.values.contains(3)) {
if (occurences.values.contains(2)) return 10_000 // Full house
return 1_000 // Three of a kind
}
val nbPairs = occurences.values.filter { it == 2 }.size
if (nbPairs == 2) return 100 // Two pairs
if (nbPairs == 1) return 10 // One pair
return 1 // High card
}
private fun List<String>.toCards(): List<CamelCards> = map { str ->
val occurences = str.split(" ")[0].groupingBy { it }.eachCount()
val occurencesWithJoker = buildList {
// Replace each 'J' card with a potential card
// Calculate the score and sort them to get the best possible score
listOf('A', 'K', 'Q', 'T', '9', '8', '7', '6', '5', '4', '3', '2').forEach {
val replaced = str.split(" ")[0].replace('J', it)
add(replaced.groupingBy { it }.eachCount())
}
}
.distinct()
.sortedByDescending { typeScore(it) }
.first()
CamelCards(
str.split(" ")[0],
occurences,
occurencesWithJoker,
str.split(" ")[1].toInt()
)
}
private fun sortCards(
cards: List<CamelCards>,
withJokers: Boolean
): List<CamelCards> {
val cardScore = if (withJokers) {
mapOf(
'A' to 13, 'K' to 12, 'Q' to 11, 'T' to 10,
'9' to 9, '8' to 8, '7' to 7, '6' to 6, '5' to 5, '4' to 4, '3' to 3, '2' to 2, 'J' to 1,
)
} else {
mapOf(
'A' to 13, 'K' to 12, 'Q' to 11, 'J' to 10, 'T' to 9,
'9' to 8, '8' to 7, '7' to 6, '6' to 5, '5' to 4, '4' to 3, '3' to 2, '2' to 1
)
}
val c = Comparator<CamelCards> { a, b ->
val scoreA = typeScore(if (withJokers) a.occurencesWithJoker else a.occurences)
val scoreB = typeScore(if (withJokers) b.occurencesWithJoker else b.occurences)
if (scoreA == scoreB) { // Same type, compare card by card
for (i in 0 until 5) {
if (a.hand[i] != b.hand[i]) {
return@Comparator cardScore[a.hand[i]]!! - cardScore[b.hand[i]]!!
}
}
}
scoreA - scoreB
}
return cards.sortedWith(c)
}
fun part1(input: List<String>): Int {
val sorted = sortCards(input.toCards(), withJokers = false)
return sorted.mapIndexed { index, camelCards -> (index + 1) * camelCards.bid }.sum()
}
fun part2(input: List<String>): Int {
val sorted = sortCards(input.toCards(), withJokers = true)
return sorted.mapIndexed { index, camelCards -> (index + 1) * camelCards.bid }.sum()
}
fun main() {
val testInput = readInput("day07_example.txt")
printTimeMillis { print("part1 example = $GREEN" + part1(testInput) + RESET) }
printTimeMillis { print("part2 example = $GREEN" + part2(testInput) + RESET) }
val input = readInput("day07.txt")
printTimeMillis { print("part1 input = $GREEN" + part1(input) + RESET) }
printTimeMillis { print("part2 input = $GREEN" + part2(input) + RESET) }
}
| 0 | Kotlin | 0 | 2 | 8ccdcce24cecca6e1d90c500423607d411c9fee2 | 3,403 | advent-of-code | Apache License 2.0 |
src/Day07.kt | palex65 | 572,937,600 | false | {"Kotlin": 68582} |
sealed class Entry(val name: String)
class File(name: String, val size: Int): Entry(name) {
override fun toString() = "File($name,$size)"
}
class Dir(name: String, val parent: Dir?, var entries: List<Entry> = emptyList(), var size: Int=0): Entry(name) {
override fun toString() = "Dir($name,$size {$entries} )"
fun processSize() {
size = entries.sumOf {
when (it) {
is Dir -> { it.processSize(); it.size}
is File -> it.size
}
}
}
fun allSizes(): List<Int> {
val dirs: List<Dir> = entries.filterIsInstance<Dir>()
return if (dirs.isEmpty()) listOf(size) else
dirs.map { it.allSizes() }.flatten() + size
}
}
fun processCommands(cmds: List<Command>): Dir {
val root = Dir("/",null)
var dir = root
for (cmd in cmds) {
when(cmd) {
is LS -> dir.entries = cmd.entries.map {
val (a,b) = it.split(' ')
if (a=="dir") Dir(b,dir) else File(b,a.toInt())
}
is CD -> dir =
if (cmd.name=="..") dir.parent!!
else dir.entries.first { cmd.name == it.name } as Dir
}
}
return root
}
sealed class Command()
class CD(val name: String) : Command()
class LS(val entries: List<String>) : Command()
fun List<String>.toCommands(): List<Command> {
val lst = mutableListOf<Command>()
var idx = 0
while (idx < size) {
val cmd = this[idx++].substring(2).split(' ')
when( cmd[0] ) {
"ls" -> {
var idxLast = idx
while (idxLast < size && this[idxLast][0]!='$') ++idxLast
lst.add( LS(subList(idx, idxLast) ) )
idx = idxLast
}
"cd" -> lst.add( CD( cmd[1] ) )
}
}
return lst
}
private fun part1(lines: List<String>): Int {
val root = processCommands(lines.toCommands().drop(1))
root.processSize()
return root.allSizes().sumOf { if (it<=100000) it else 0 }
}
private fun part2(lines: List<String>): Int {
val root = processCommands(lines.toCommands().drop(1))
root.processSize()
val toFree = 30000000 - (70000000-root.size)
val allSizes = root.allSizes().sorted()
return allSizes.first { it >= toFree }
}
fun main() {
val testInput = readInput("Day07_test")
check(part1(testInput) == 95437)
check(part2(testInput) == 24933642) // 8381165
val input = readInput("Day07")
println(part1(input)) // 306611
println(part2(input)) // 13210366
}
| 0 | Kotlin | 0 | 2 | 35771fa36a8be9862f050496dba9ae89bea427c5 | 2,568 | aoc2022 | Apache License 2.0 |
src/year2023/day07/Day07.kt | lukaslebo | 573,423,392 | false | {"Kotlin": 222221} | package year2023.day07
import check
import readInput
import kotlin.math.pow
fun main() {
val testInput = readInput("2023", "Day07_test")
check(part1(testInput), 6440)
check(part2(testInput), 5905)
val input = readInput("2023", "Day07")
println(part1(input))
println(part2(input))
}
private fun part1(input: List<String>): Int {
return input.map { it.toHand() }
.sortedWith(compareBy<Hand> { it.type }.thenBy { it.strength })
.mapIndexed { index, hand -> (index + 1) * hand.bid }
.sum()
}
private fun part2(input: List<String>): Int {
return input.map { it.toHand(withJokers = true) }
.sortedWith(compareBy<Hand> { it.type }.thenBy { it.strength })
.mapIndexed { index, hand -> (index + 1) * hand.bid }
.sum()
}
private data class Hand(
val cards: String,
val bid: Int,
val type: Int,
val strength: Int,
)
private fun String.toHand(withJokers: Boolean = false): Hand {
val (cards, bid) = split(" ")
return Hand(cards, bid.toInt(), cards.getType(withJokers), cards.getStrength(withJokers))
}
private fun String.getType(withJokers: Boolean = false): Int {
val jokers = count { it == 'J' && withJokers }
val groups = groupBy { it }.filter { it.key != 'J' || !withJokers }.map { it.value.size }
val maxGroup = groups.maxOrNull() ?: 0
val pairs = groups.count { it == 2 }
return when {
maxGroup + jokers == 5 -> 7
maxGroup + jokers == 4 -> 6
(maxGroup == 3 && pairs == 1) || (pairs == 2 && jokers == 1) -> 5
maxGroup + jokers == 3 -> 4
pairs == 2 -> 3
maxGroup + jokers == 2 -> 2
else -> 1
}
}
private fun String.getStrength(withJokers: Boolean): Int {
return reversed().mapIndexed { i, c ->
val points = if (withJokers) cardsWithJokers.indexOf(c) else cards.indexOf(c)
(points + 1) * 13.0.pow(i).toInt()
}.sum()
}
private const val cardsWithJokers = "J23456789TQKA"
private const val cards = "23456789TJQKA"
| 0 | Kotlin | 0 | 1 | f3cc3e935bfb49b6e121713dd558e11824b9465b | 2,021 | AdventOfCode | Apache License 2.0 |
src/day07/Day07.kt | pnavais | 727,416,570 | false | {"Kotlin": 17859} | package day07
import readInput
val cardValueMapping = mapOf('2' to 2, '3' to 3, '4' to 4, '5' to 5, '6' to 6, '7' to 7, '8' to 8,
'9' to 9, 'T' to 10, 'J' to 11, 'Q' to 12, 'K' to 13, 'A' to 14)
enum class Type(val value: Int) {
FIVE_OF_A_KIND(7), FOUR_OF_A_KIND(6), FULL_HOUSE(5), THREE_OF_A_KIND(4), TWO_PAIR(3), ONE_PAIR(2), HIGH_CARD(1), WRONG_CARDS(0);
companion object {
fun fromCards(cards: String, useJokers: Boolean = false) : Type {
val cardsProcessed = if (useJokers) { translateJokers(cards) } else cards
val cardGroups = cardsProcessed.groupingBy { it }.eachCount()
return when (cardGroups.size) {
5 -> HIGH_CARD
4 -> ONE_PAIR
3 -> if (cardGroups.maxBy { it.value }.value == 3) THREE_OF_A_KIND else TWO_PAIR // 3, 1, 1 or 2, 2, 1
2 -> if (cardGroups.maxBy { it.value }.value == 4) FOUR_OF_A_KIND else FULL_HOUSE // 4, 1 or 3, 2
1 -> FIVE_OF_A_KIND
else -> WRONG_CARDS
}
}
private fun translateJokers(cards: String): String {
return if (cards != "JJJJJ") {
// Transform jokers into the highest grouped card
val maxCard = cards.replace("J", "").groupingBy { it }.eachCount()
.map { it.value to it.key }.groupBy { it.first }.maxBy { it.key }
.value
.map { it.second }
.sortedWith(Comparator { o1, o2 ->
cardValueMapping[o2]?.compareTo(cardValueMapping[o1] ?: -1)!!
})[0]
cards.replace('J', maxCard)
} else { cards }
}
}
}
data class Hand(val cards: String, val bid: Long, val useJokers: Boolean = false): Comparable<Hand> {
private val type : Type = Type.fromCards(cards, useJokers)
override fun compareTo(other: Hand): Int {
var res = type.compareTo(other.type)
// Compare equal hands by pos value
if (res == 0) {
for (i in 0..other.cards.length) {
val aux = getCardValue(other.cards[i], useJokers)?.compareTo(getCardValue(cards[i], useJokers) ?: -1)!!
if (aux != 0) {
res = aux
break
}
}
}
return res
}
private fun getCardValue(card: Char, useJokers: Boolean): Int? {
return if ((useJokers) && (card == 'J')) { 1 } else { cardValueMapping[card] }
}
}
fun part1(input: List<String>): Long {
return input.map { s -> val (cards, bid) = s.split(" "); Hand(cards, bid.toLong()) }
.sortedDescending()
.mapIndexed { index,hand -> hand.bid.times(index+1) }
.sum()
}
fun part2(input: List<String>): Long {
return input.map { s -> val (cards, bid) = s.split(" "); Hand(cards, bid.toLong(), useJokers = true) }
.sortedDescending()
.mapIndexed { index,hand -> hand.bid.times(index+1) }
.sum()
}
fun main() {
val testInput = readInput("input/day07")
println(part1(testInput))
println(part2(testInput))
}
| 0 | Kotlin | 0 | 0 | f5b1f7ac50d5c0c896d00af83e94a423e984a6b1 | 3,181 | advent-of-code-2k3 | Apache License 2.0 |
src/Day12.kt | coolcut69 | 572,865,721 | false | {"Kotlin": 36853} | import java.util.*
fun main() {
fun part1(input: List<String>): Int {
val heightMap = parseInput(input)
return heightMap.shortestPath(
begin = heightMap.start,
isGoal = { it == heightMap.end },
canMove = { from, to -> to - from <= 1 })
}
fun part2(input: List<String>): Int {
val heightMap = parseInput(input)
return heightMap.shortestPath(
begin = heightMap.end,
isGoal = { heightMap.elevations[it] == 0 },
canMove = { from, to -> from - to <= 1 })
}
// test if implementation meets criteria from the description, like:
val testInput = readInput("Day12_test")
check(part1(testInput) == 31)
check(part2(testInput) == 29)
val input = readInput("Day12")
println(part1(input))
check(part1(input) == 394)
println(part2(input))
check(part2(input) == 388)
}
private class HeightMap(val elevations: Map<Point2D, Int>, val start: Point2D, val end: Point2D) {
fun shortestPath(
begin: Point2D,
isGoal: (Point2D) -> Boolean,
canMove: (Int, Int) -> Boolean
): Int {
val seen = mutableSetOf<Point2D>()
val queue = PriorityQueue<PathCost>().apply { add(PathCost(begin, 0)) }
while (queue.isNotEmpty()) {
val nextPoint = queue.poll()
if (nextPoint.point !in seen) {
seen += nextPoint.point
val neighbors = nextPoint.point.cardinalNeighbors()
.filter { it in elevations }
.filter { canMove(elevations.getValue(nextPoint.point), elevations.getValue(it)) }
if (neighbors.any { isGoal(it) }) return nextPoint.cost + 1
queue.addAll(neighbors.map { PathCost(it, nextPoint.cost + 1) })
}
}
throw IllegalStateException("No valid path from $start to $end")
}
}
data class Point2D(val x: Int = 0, val y: Int = 0) {
fun cardinalNeighbors(): Set<Point2D> =
setOf(
copy(x = x - 1),
copy(x = x + 1),
copy(y = y - 1),
copy(y = y + 1)
)
}
private fun parseInput(input: List<String>): HeightMap {
var start: Point2D? = null
var end: Point2D? = null
val elevations = input.flatMapIndexed { y, row ->
row.mapIndexed { x, char ->
val here = Point2D(x, y)
here to when (char) {
'S' -> 0.also { start = here }
'E' -> 25.also { end = here }
else -> char - 'a'
}
}
}.toMap()
return HeightMap(elevations, start!!, end!!)
}
private data class PathCost(val point: Point2D, val cost: Int) : Comparable<PathCost> {
override fun compareTo(other: PathCost): Int =
this.cost.compareTo(other.cost)
}
| 0 | Kotlin | 0 | 0 | 031301607c2e1c21a6d4658b1e96685c4135fd44 | 2,838 | aoc-2022-in-kotlin | Apache License 2.0 |
src/Day19.kt | amelentev | 573,120,350 | false | {"Kotlin": 87839} | fun main() {
val input = readInput("Day19")
data class Rule(val c: Char, val cmp: Char, val v: Int, val to: String)
data class Workflow(val name: String, val rules: List<Rule>, val elseTo: String)
val workflows = input.take(input.indexOfFirst { it.isEmpty() }).map {
val name = it.substringBefore('{')
val rules = it.substringAfter('{').substringBefore('}').split(",")
Workflow(
name = name,
rules = rules.dropLast(1).map { rule ->
Rule(
c = rule[0],
cmp = rule[1],
v = rule.substring(2).substringBefore(':').toInt(),
to = rule.substringAfter(':')
)
},
elseTo = rules.last()
)
}.associateBy { it.name }
val parts = input.drop(input.indexOfFirst { it.isEmpty() }+1).map { part ->
part.trim('{', '}').split(',').associate {
it[0] to it.substring(2).toLong()
}
}
fun process(part: Map<Char, Long>, w: Workflow): String {
for (r in w.rules) {
var diff = part[r.c]!! - r.v
if (r.cmp == '<') diff = -diff
if (diff > 0) return r.to
}
return w.elseTo
}
fun solve1(part: Map<Char, Long>): Boolean {
var w = workflows["in"]!!
while (true) {
val next = process(part, w)
when (next) {
"A" -> return true
"R" -> return false
}
w = workflows[next]!!
}
}
var res1 = 0L
for (part in parts) {
if (solve1(part)) {
res1 += part.values.sum()
}
}
println(res1)
var res2 = 0L
fun dfs(workflow: String, range: Map<Char, Pair<Int, Int>>) {
if (workflow == "A") {
res2 += range.values.fold(1L) { a, x -> a * (x.second - x.first + 1) }
return
} else if (workflow == "R" || range.values.any { it.first > it.second }) {
return
}
val w = workflows[workflow]!!
var r = range
for (rule in w.rules) {
val (x1, x2) = r[rule.c]!!
val (rt,rf) = if (rule.cmp == '<') {
(x1 to minOf(x2, rule.v-1)) to (maxOf(minOf(x2, rule.v-1)+1, x1) to x2)
} else {
(maxOf(x1, rule.v+1) to x2) to (x1 to minOf(maxOf(x1, rule.v+1)-1, x2))
}
dfs(rule.to, r + mapOf(rule.c to rt))
r = r + mapOf(rule.c to rf)
}
dfs(w.elseTo, r)
}
dfs("in", mapOf(
'x' to (1 to 4000),
'm' to (1 to 4000),
'a' to (1 to 4000),
's' to (1 to 4000),
))
println(res2)
}
| 0 | Kotlin | 0 | 0 | a137d895472379f0f8cdea136f62c106e28747d5 | 2,725 | advent-of-code-kotlin | Apache License 2.0 |
src/day05/Day05.kt | mherda64 | 512,106,270 | false | {"Kotlin": 10058} | package day05
import readInput
fun main() {
val pattern = Regex("(\\d*),(\\d*) -> (\\d*),(\\d*)")
fun processInput(input: List<String>): List<Pair<Point, Point>> {
return input.map {
val (x1, y1, x2, y2) = pattern.find(it)!!.destructured
Pair(Point(x1.toInt(), y1.toInt()), Point(x2.toInt(), y2.toInt()))
}
}
fun part1(input: List<Pair<Point, Point>>): Int {
val max = input.maxOf { maxOf(maxOf(it.first.x, it.first.y), maxOf(it.second.x, it.second.y)) }
val map = Map(max + 1)
map.mark(input.filter { (first, second) -> first.x == second.x || first.y == second.y })
return map.countOverlapping()
}
fun part2(input: List<Pair<Point, Point>>): Int {
val max = input.maxOf { maxOf(maxOf(it.first.x, it.first.y), maxOf(it.second.x, it.second.y)) }
val map = Map(max + 1)
map.mark(input)
return map.countOverlapping()
}
val testInput = readInput("Day05_test")
check(part1(processInput(testInput)) == 5)
check(part2(processInput(testInput)) == 12)
val input = readInput("Day05")
println(part1(processInput(input)))
println(part2(processInput(input)))
}
class Map(val size: Int) {
val map: Array<Array<Int>> = Array(size) { Array(size) { 0 } }
fun mark(pairs: List<Pair<Point, Point>>) {
pairs.map { (first, second) ->
when {
first.x == second.x ->
(if (first.y > second.y) first.y downTo second.y else first.y..second.y)
.map { map[first.x][it] += 1 }
first.y == second.y ->
(if (first.x > second.x) first.x downTo second.x else first.x..second.x)
.map { map[it][first.y] += 1 }
else -> {
val xRange = if (first.x > second.x) first.x downTo second.x else first.x..second.x
val yRange = if (first.y > second.y) first.y downTo second.y else first.y..second.y
xRange.zip(yRange).map { map[it.first][it.second] += 1 }
}
}
}
}
fun countOverlapping(): Int {
var counter = 0
for ((rowIndex, row) in map.withIndex()) {
for (column in row.indices) {
counter += if (map[rowIndex][column] > 1) 1 else 0
}
}
return counter
}
}
data class Point(val x: Int, val y: Int)
| 0 | Kotlin | 0 | 0 | d04e179f30ad6468b489d2f094d6973b3556de1d | 2,464 | AoC2021_kotlin | Apache License 2.0 |
src/main/kotlin/days/y2023/day23/Day23.kt | jewell-lgtm | 569,792,185 | false | {"Kotlin": 161272, "Jupyter Notebook": 103410, "TypeScript": 78635, "JavaScript": 123} | package days.y2023.day23
import util.InputReader
class Day23(val input: List<String>) {
private val start = Position(0, input.first().indexOfOnly('.'))
private val end = Position(input.size - 1, input.last().indexOfOnly('.'))
private val neighbors = input.flatMapIndexed { y, row ->
row.indices.map { x ->
Position(y, x) to input.neighbors(Position(y, x))
}
}.toMap()
private val cells = input.flatMapIndexed { y, row ->
row.indices.map { x ->
Position(y, x) to row[x]
}
}.toMap()
fun partOne(): Int = longestPath(true, start, setOf(start))
fun partTwo(): Int = longestPath(false, start, setOf(start))
private fun longestPath(slipperyHills: Boolean, from: Position, visited: Set<Position>): Int {
if (from == end) return visited.size - 1
val unvisited = from.neighbors().filter { it !in visited }
if (unvisited.isEmpty()) return -1
return if (!slipperyHills) {
unvisited.maxOfOrNull { longestPath(false, it, visited + it) } ?: -1
} else {
unvisited.mapNotNull { next ->
when (cells.getValue(next)) {
'.' -> longestPath(true, next, visited + next)
'>' -> if (next.right() !in visited) longestPath(
true,
next.right(),
visited + next + next.right()
) else null
'<' -> if (next.left() !in visited) longestPath(
true,
next.left(),
visited + next + next.left()
) else null
'^' -> if (next.up() !in visited) longestPath(
true,
next.up(),
visited + next + next.up()
) else null
'v' -> if (next.down() !in visited) longestPath(
true,
next.down(),
visited + next + next.down()
) else null
else -> null
}
}.max()
}
}
data class Position(val y: Int, val x: Int)
private fun String.indexOfOnly(char: Char): Int {
require(count { it == char } == 1) { "The character $char should only appear once." }
return indexOf(char)
}
private fun Position.neighbors(): Set<Position> = neighbors.getValue(this)
private fun Position.down() = copy(y = y + 1)
private fun Position.up() = copy(y = y - 1)
private fun Position.left() = copy(x = x - 1)
private fun Position.right() = copy(x = x + 1)
private fun List<String>.neighbors(position: Position): Set<Position> {
return listOf(
position.up(), position.down(), position.left(), position.right()
).filter {
it.y in indices && it.x in this[it.y].indices && this[it.y][it.x] != '#'
}.toSet()
}
}
fun main() {
val year = 2023
val day = 23
val exampleInput = InputReader.getExampleLines(year, day)
val puzzleInput = InputReader.getPuzzleLines(year, day)
fun partOne(input: List<String>) = Day23(input).partOne()
fun partTwo(input: List<String>) = Day23(input).partTwo()
println("Example 1: ${partOne(exampleInput)}")
println("Puzzle 1: ${partOne(puzzleInput)}")
println("Example 2: ${partTwo(exampleInput)}")
println("Puzzle 2: ${partTwo(puzzleInput)}")
}
| 0 | Kotlin | 0 | 0 | b274e43441b4ddb163c509ed14944902c2b011ab | 3,534 | AdventOfCode | Creative Commons Zero v1.0 Universal |
src/Day24.kt | Flame239 | 570,094,570 | false | {"Kotlin": 60685} | private fun map(): List<String> {
val m = readInput("Day24")
h = m.size
w = m[0].length
cycle = lcm(h - 2, w - 2)
start = CC(0, m[0].indexOf("."))
finish = CC(m.size - 1, m[m.size - 1].indexOf("."))
return m
}
private var h = -1
private var w = -1
private var cycle = -1
private lateinit var start: CC
private lateinit var finish: CC
/*
Just bfs, but 'visited' is tricky. Map repeats every 'cycle = lcm(h - 2, w - 2)' steps,
so we should check if we visited cell on (step % cycle) before.
e.g. if we visited a cell 600 steps before, we should skip it, as map looks exactly the same
*/
private fun shortestPathTo(map: List<String>, initStep: Int, start: CC, finish: CC): Int {
val visited = HashMap<CC, BooleanArray>()
var step = initStep
val q = ArrayDeque(listOf(start))
while (q.isNotEmpty()) {
repeat(q.size) {
val pos = q.removeFirst()
if (pos == finish) return step - 1
listOf(pos, pos.up(), pos.down(), pos.left(), pos.right())
.filter { p -> shouldVisit(map, visited, p, step) }
.forEach(q::add)
}
step++
}
return -1
}
private fun shouldVisit(map: List<String>, visited: HashMap<CC, BooleanArray>, p: CC, step: Int): Boolean {
if (p.i < 0 || p.i >= h || map[p.i][p.j] == '#') return false
val pVis = visited.getOrPut(p) { BooleanArray(cycle) }
if (pVis[step % cycle]) return false
pVis[step % cycle] = true
// IMPROVEMENT: pre-calculate all possible maps (there will be `cycle` of them)
if (map[p.i][(p.j - 1 - step).mod(w - 2) + 1] == '>') return false
if (map[p.i][(p.j - 1 + step).mod(w - 2) + 1] == '<') return false
if (map[(p.i - 1 - step).mod(h - 2) + 1][p.j] == 'v') return false
if (map[(p.i - 1 + step).mod(h - 2) + 1][p.j] == '^') return false
return true
}
private fun part1(map: List<String>): Int = shortestPathTo(map, 1, start, finish)
private fun part2(map: List<String>): Int {
var step = shortestPathTo(map, 1, start, finish)
step = shortestPathTo(map, step, finish, start)
return shortestPathTo(map, step, start, finish)
}
fun main() {
measure { part1(map()) }
measure { part2(map()) }
}
| 0 | Kotlin | 0 | 0 | 27f3133e4cd24b33767e18777187f09e1ed3c214 | 2,231 | advent-of-code-2022 | Apache License 2.0 |
Dataset Summary
The dataset contains 25,000 Kotlin code samples selected from the KStack dataset. The selection is performed based on the value of the code for learning algorithmic concepts in Kotlin. In total, the dataset contains about 23M CodeLlama-7b tokens (vocab size 32,016).
Column description
The dataset contains the following columns:
size
— size of the file in bytescontent
— text (content) of the file after removing personal identifiable informationrepo_id
— GitHub ID of the repositorypath
— path to a fileowner
— repo owner on GitHubname
— repo name on GitHubcommit_sha
— hash of the commit, from which the revision of the file is takenstars
— number of stars in the repo at the moment of collectionforks
— number of forks in the repo at the moment of collectionissues
— number of issues in the repo at the moment of collectionis_fork
—true
if the repo is a fork or not as defined by GitHubmain_language
— main language of the repo as defined by GitHublanguages_distribution
— JSON with the distribution of languages by size in bytes in the repolicense
— permissive license of the repository
Dataset Collection
The filtering from KStack is performed using zero-shot quality estimation based on Mistral-7B-Instruct-v0.2. The model is prompted to determine which of two files has higher "educational value for learning algorithms in Kotlin". The results of the comparisons are averaged and used to train a binary classifier based on CodeT5p-220m. The binary classifier is then applied to the entire KStack to obtain scores for each sample in the dataset. The log-probability of the classifier prediction is used as a criterion of the selection.
Opt-out
If you want your data to be removed from dataset, or have any other questions, please reach out to Sergey Titov: sergey.titov@jetbrains.com
- Downloads last month
- 104
Models trained or fine-tuned on JetBrains/KStack-clean
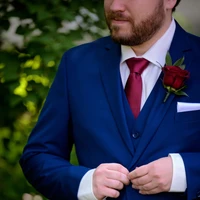