Spaces:
Sleeping
Sleeping
File size: 6,590 Bytes
e1763bc a7ef9bd e1763bc d8cae64 e1763bc aa5098b e1763bc aa5098b e1763bc 591655a 552f561 a7ef9bd f6ab409 a7ef9bd e1763bc d8cae64 e1763bc 72e9e36 e1763bc b99353c a7ef9bd e1763bc 93efc0d 3843550 0cfc9ed e1763bc d8cae64 e1763bc 552f561 975ef7d 552f561 b88779f 32902cf d8cae64 68a42b3 e1763bc 5a38f01 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 |
# -*- coding: utf-8 -*-
"""Deploy Barcelo demo.ipynb
Automatically generated by Colaboratory.
Original file is located at
https://colab.research.google.com/drive/1FxaL8DcYgvjPrWfWruSA5hvk3J81zLY9
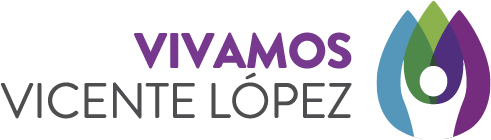
# Modelo
YOLO es una familia de modelos de detecci贸n de objetos a escala compuesta entrenados en COCO dataset, e incluye una funcionalidad simple para Test Time Augmentation (TTA), model ensembling, hyperparameter evolution, and export to ONNX, CoreML and TFLite.
## Gradio Inferencia

Este Notebook se acelera opcionalmente con un entorno de ejecuci贸n de GPU
----------------------------------------------------------------------
YOLOv5 Gradio demo
*Author: Ultralytics LLC and Gradio*
# C贸digo
"""
#!pip install -qr https://raw.githubusercontent.com/ultralytics/yolov5/master/requirements.txt gradio # install dependencies
import os
import re
import json
import pandas as pd
import gradio as gr
import torch
from PIL import Image
# Images
torch.hub.download_url_to_file('https://i.pinimg.com/originals/7f/5e/96/7f5e9657c08aae4bcd8bc8b0dcff720e.jpg', 'ejemplo1.jpg')
torch.hub.download_url_to_file('https://i.pinimg.com/originals/c2/ce/e0/c2cee05624d5477ffcf2d34ca77b47d1.jpg', 'ejemplo2.jpg')
# Model
#model = torch.hub.load('ultralytics/yolov5', 'yolov5s') # force_reload=True to update
#model = torch.hub.load('ultralytics/yolov5', 'custom', path='best.pt') # local model o google colab
#model = torch.hub.load('ultralytics/yolov5', 'custom', path='best.pt', force_reload=True, autoshape=True) # local model o google colab
#model = torch.hub.load('path/to/yolov5', 'custom', path='/content/yolov56.pt', source='local') # local repo
model = torch.hub.load('ultralytics/yolov5', 'custom', path='best.pt', force_reload=True, autoshape=True, trust_repo=True)
#HF_TOKEN = os.getenv("ZIKA_TOKEN_WRITE")
#hf_writer = gr.HuggingFaceDatasetSaver(HF_TOKEN, "demo-iazika-flags")
def getQuantity(string):
contador_raw = ''.join(string.split(" ")[3:])
resultado_especie_1 = 'Aedes'
resultado_especie_2 = 'Mosquito'
resultado_especie_3 = 'Mosca'
resultado_cantidad_1 = ''.join(re.findall(r'\d+',''.join(re.findall(r'\d+'+resultado_especie_1, contador_raw))))
resultado_cantidad_2 = ''.join(re.findall(r'\d+',''.join(re.findall(r'\d+'+resultado_especie_2, contador_raw))))
resultado_cantidad_3 = ''.join(re.findall(r'\d+',''.join(re.findall(r'\d+'+resultado_especie_3, contador_raw))))
resultado_cantidad_1 = resultado_cantidad_1 if len(resultado_cantidad_1) > 0 else "0"
resultado_cantidad_2 = resultado_cantidad_2 if len(resultado_cantidad_2) > 0 else "0"
resultado_cantidad_3 = resultado_cantidad_3 if len(resultado_cantidad_3) > 0 else "0"
resultado_lista = [[resultado_cantidad_1,resultado_especie_1],
[resultado_cantidad_2,resultado_especie_2],
[resultado_cantidad_3,resultado_especie_3]]
return resultado_lista
def listJSON(resultado):
resultado_lista = getQuantity(resultado)
img_name = " ".join(resultado.split(" ")[0:2])
img_size = "".join(resultado.split(" ")[2])
strlista = ""
for resultado_lista, description in resultado_lista:
strlista += '{"quantity":"'+resultado_lista+'","description":"'+description+'"},'
strlista = strlista[:-1]
str_resultado_lista = '{"image":"'+str(img_name)+'","size":"'+str(img_size)+'","detail":['+strlista+']}'
json_string = json.loads(str_resultado_lista)
return json_string
def arrayLista(resultado):
resultado_lista = getQuantity(resultado)
df = pd.DataFrame(resultado_lista,columns=['Cantidad','Especie'])
return df
def yolo(size, iou, conf, im):
'''Wrapper fn for gradio'''
g = (int(size) / max(im.size)) # gain
im = im.resize((int(x * g) for x in im.size), Image.LANCZOS) # resize with antialiasing
model.iou = iou
model.conf = conf
results2 = model(im) # inference
#print(type(results2))
results2.render() # updates results.imgs with boxes and labels
results_detail = str(results2)
lista = listJSON(results_detail)
lista2 = arrayLista(results_detail)
return Image.fromarray(results2.ims[0]), lista2, lista
#------------ Interface-------------
in1 = gr.Radio(['640', '1280'], label="Tama帽o de la imagen", type='value')
in2 = gr.Slider(minimum=0, maximum=1, step=0.05, label='NMS IoU threshold')
in3 = gr.Slider(minimum=0, maximum=1, step=0.05, label='Umbral o threshold')
in4 = gr.Image(type='pil', label="Original Image")
out2 = gr.Image(type="pil", label="YOLOv5")
out3 = gr.Dataframe(label="Cantidad_especie", headers=['Cantidad','Especie'], type="pandas")
out4 = gr.JSON(label="JSON")
#-------------- Text-----
title = 'Trampas Barcel贸'
description = """
<p>
<center>
Sistemas de Desarrollado por Subsecretar铆a de Modernizaci贸n del Municipio de Vicente L贸pez. Advertencia solo usar fotos provenientes de las trampas Barcel贸, no de celular o foto de internet.
<img src="https://www.vicentelopez.gov.ar/assets/images/logo-mvl.png" alt="logo" width="250"/>
</center>
</p>
"""
article ="<p style='text-align: center'><a href='https://docs.google.com/presentation/d/1T5CdcLSzgRe8cQpoi_sPB4U170551NGOrZNykcJD0xU/edit?usp=sharing' target='_blank'>Para mas info, clik para ir al white paper</a></p><p style='text-align: center'><a href='https://drive.google.com/drive/folders/1owACN3HGIMo4zm2GQ_jf-OhGNeBVRS7l?usp=sharing ' target='_blank'>Google Colab Demo</a></p><p style='text-align: center'><a href='https://github.com/Municipalidad-de-Vicente-Lopez/Trampa_Barcelo' target='_blank'>Repo Github</a></p></center></p>"
examples = [['640',0.45, 0.75,'ejemplo1.jpg'], ['640',0.45, 0.75,'ejemplo2.jpg']]
iface = gr.Interface(yolo,
inputs=[in1, in2, in3, in4],
outputs=[out2,out3,out4], title=title,
description=description,
article=article,
examples=examples,
analytics_enabled=False,
allow_flagging="manual",
flagging_options=["Correcto", "Incorrecto", "Casi correcto", "Error", "Otro"],
#flagging_callback=hf_writer
)
iface.launch(debug=True)
"""For YOLOv5 PyTorch Hub inference with **PIL**, **OpenCV**, **Numpy** or **PyTorch** inputs please see the full [YOLOv5 PyTorch Hub Tutorial](https://github.com/ultralytics/yolov5/issues/36).
## Citation
[](https://zenodo.org/badge/latestdoi/264818686)
""" |