Upload README.md
Browse files
README.md
ADDED
@@ -0,0 +1,65 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
---
|
2 |
+
license: apache-2.0
|
3 |
+
tags:
|
4 |
+
- vision
|
5 |
+
- image-classification
|
6 |
+
|
7 |
+
datasets:
|
8 |
+
- imagenet-1k
|
9 |
+
|
10 |
+
widget:
|
11 |
+
- src: https://huggingface.co/datasets/mishig/sample_images/resolve/main/tiger.jpg
|
12 |
+
example_title: Tiger
|
13 |
+
- src: https://huggingface.co/datasets/mishig/sample_images/resolve/main/teapot.jpg
|
14 |
+
example_title: Teapot
|
15 |
+
- src: https://huggingface.co/datasets/mishig/sample_images/resolve/main/palace.jpg
|
16 |
+
example_title: Palace
|
17 |
+
|
18 |
+
---
|
19 |
+
|
20 |
+
# ResNet
|
21 |
+
|
22 |
+
ResNet model trained on imagenet-1k. It was introduced in the paper [Deep Residual Learning for Image Recognition](https://arxiv.org/abs/1512.03385) and first released in [this repository](https://github.com/KaimingHe/deep-residual-networks).
|
23 |
+
|
24 |
+
Disclaimer: The team releasing ResNet did not write a model card for this model so this model card has been written by the Hugging Face team.
|
25 |
+
|
26 |
+
## Model description
|
27 |
+
|
28 |
+
ResNet introduced residual connections, they allow to train networks with an unseen number of layers (up to 1000). ResNet won the 2015 ILSVRC & COCO competition, one important milestone in deep computer vision.
|
29 |
+
|
30 |
+
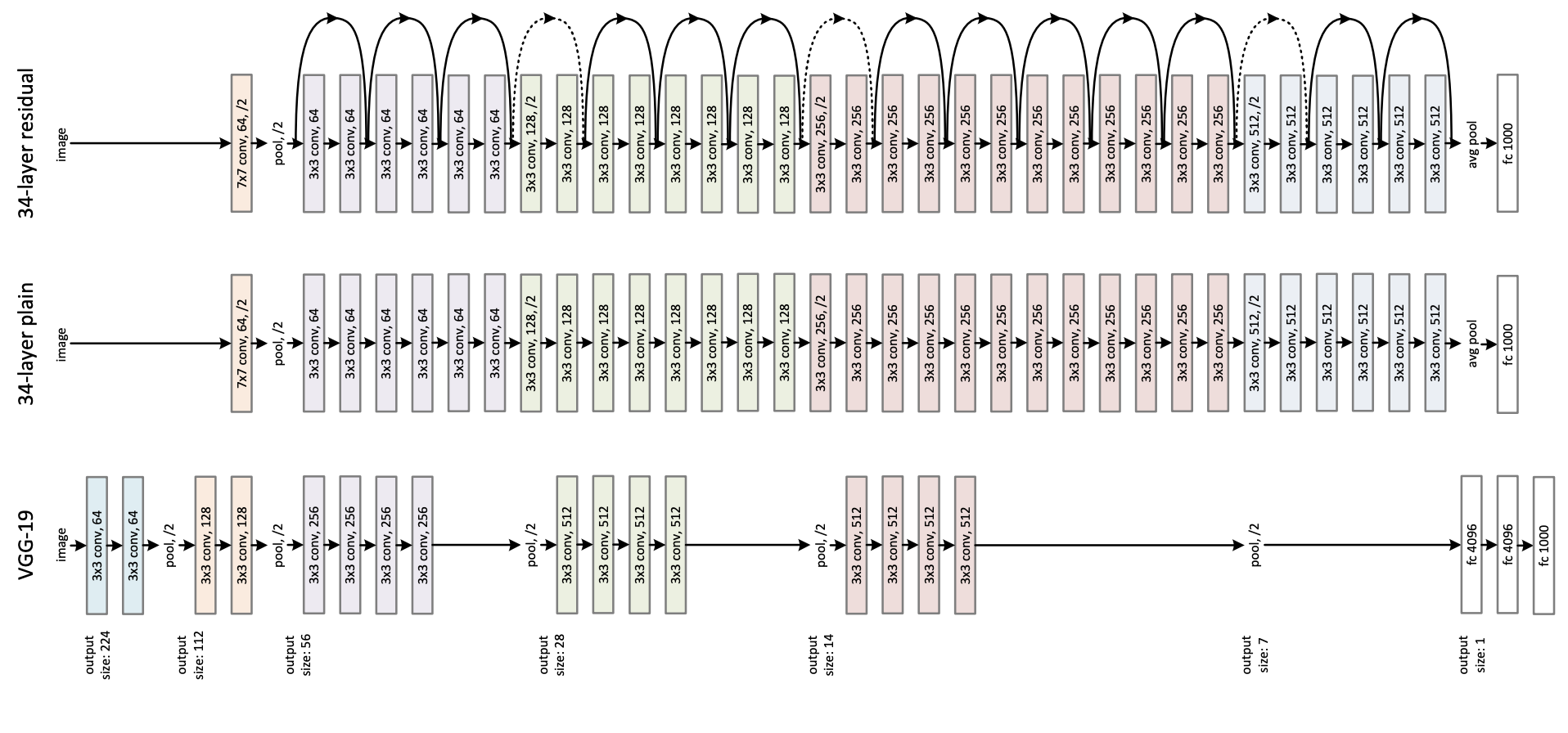
|
31 |
+
|
32 |
+
## Intended uses & limitations
|
33 |
+
|
34 |
+
You can use the raw model for image classification. See the [model hub](https://huggingface.co/models?search=resnet) to look for
|
35 |
+
fine-tuned versions on a task that interests you.
|
36 |
+
|
37 |
+
### How to use
|
38 |
+
|
39 |
+
Here is how to use this model:
|
40 |
+
|
41 |
+
```python
|
42 |
+
>>> from transformers import AutoFeatureExtractor, ResNetForImageClassification
|
43 |
+
>>> import torch
|
44 |
+
>>> from datasets import load_dataset
|
45 |
+
|
46 |
+
>>> dataset = load_dataset("huggingface/cats-image")
|
47 |
+
>>> image = dataset["test"]["image"][0]
|
48 |
+
|
49 |
+
>>> feature_extractor = AutoFeatureExtractor.from_pretrained("microsoft/resnet-50")
|
50 |
+
>>> model = ResNetForImageClassification.from_pretrained("microsoft/resnet-50")
|
51 |
+
|
52 |
+
>>> inputs = feature_extractor(image, return_tensors="pt")
|
53 |
+
|
54 |
+
>>> with torch.no_grad():
|
55 |
+
... logits = model(**inputs).logits
|
56 |
+
|
57 |
+
>>> # model predicts one of the 1000 ImageNet classes
|
58 |
+
>>> predicted_label = logits.argmax(-1).item()
|
59 |
+
>>> print(model.config.id2label[predicted_label])
|
60 |
+
tiger cat
|
61 |
+
```
|
62 |
+
|
63 |
+
|
64 |
+
|
65 |
+
For more code examples, we refer to the [documentation](https://huggingface.co/docs/transformers/master/en/model_doc/resnet).
|