code
stringlengths 501
5.19M
| package
stringlengths 2
81
| path
stringlengths 9
304
| filename
stringlengths 4
145
|
---|---|---|---|
import numpy as np
import json
from matplotlib import image
from matplotlib import pyplot
from pathlib import Path
class Dataset(object):
'''
A class for the dataset that will return data items as per the given index
'''
def __init__(self, annotation_file, transforms = None):
self.annotation_file = annotation_file
self.transforms = transforms
self.datalist = []
with open(self.annotation_file) as f:
for line in f:
data = json.loads(line)
gt_bboxes = np.array([])
for box in data['bboxes']:
added = np.append(box['category_id'], box['bbox'])
if(len(gt_bboxes) == 0):
gt_bboxes = added
gt_bboxes = gt_bboxes.reshape(1,5)
else:
gt_bboxes = np.vstack((gt_bboxes,added))
p = Path(annotation_file)
dir_data = str(p.parent.absolute())
image1 = image.imread(dir_data + '\\' + data['img_fn'])
image1 = np.interp(image1, (image1.min(), image1.max()), (0, +1))
if(self.transforms is not None):
for t in self.transforms:
image1 = t(image1)
image1 = np.transpose(image1, (2,0,1))
self.datalist.append([image1, gt_bboxes])
'''
Arguments:
annotation_file: path to the annotation file
transforms: list of transforms (class instances)
For instance, [<class 'RandomCrop'>, <class 'Rotate'>]
'''
def __len__(self):
return(len(self.datalist))
'''
return the number of data points in the dataset
'''
def __getitem__(self, idx):
d= {}
d["annotations"] = self.datalist[idx][1]
d["image"] = self.datalist[idx][0]
return d
'''
return the dataset element for the index: "idx"
Arguments:
idx: index of the data element.
Returns: A dictionary with:
image: image (in the form of a numpy array) (shape: (3, H, W))
gt_bboxes: N X 5 array where N is the number of bounding boxes, each
consisting of [class, x1, y1, x2, y2]
x1 and x2 lie between 0 and width of the image,
y1 and y2 lie between 0 and height of the image.
You need to do the following,
1. Extract the correct annotation using the idx provided.
2. Read the image and convert it into a numpy array (wont be necessary
with some libraries). The shape of the array would be (3, H, W).
3. Scale the values in the array to be with [0, 1].
4. Create a dictonary with both the image and annotations
4. Perform the desired transformations.
5. Return the transformed image and annotations as specified. ''' | 19CS30055-Q2 | /19CS30055_Q2-0.0.6.tar.gz/19CS30055_Q2-0.0.6/my_package/data/dataset.py | dataset.py |
import torch
import torch.nn as nn
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
# Class id to name mapping
COCO_INSTANCE_CATEGORY_NAMES = [
'__background__', 'person', 'bicycle', 'car', 'motorcycle', 'airplane', 'bus',
'train', 'truck', 'boat', 'traffic light', 'fire hydrant', 'N/A', 'stop sign',
'parking meter', 'bench', 'bird', 'cat', 'dog', 'horse', 'sheep', 'cow',
'elephant', 'bear', 'zebra', 'giraffe', 'N/A', 'backpack', 'umbrella', 'N/A', 'N/A',
'handbag', 'tie', 'suitcase', 'frisbee', 'skis', 'snowboard', 'sports ball',
'kite', 'baseball bat', 'baseball glove', 'skateboard', 'surfboard', 'tennis racket',
'bottle', 'N/A', 'wine glass', 'cup', 'fork', 'knife', 'spoon', 'bowl',
'banana', 'apple', 'sandwich', 'orange', 'broccoli', 'carrot', 'hot dog', 'pizza',
'donut', 'cake', 'chair', 'couch', 'potted plant', 'bed', 'N/A', 'dining table',
'N/A', 'N/A', 'toilet', 'N/A', 'tv', 'laptop', 'mouse', 'remote', 'keyboard', 'cell phone',
'microwave', 'oven', 'toaster', 'sink', 'refrigerator', 'N/A', 'book',
'clock', 'vase', 'scissors', 'teddy bear', 'hair drier', 'toothbrush'
]
# Class definition for the model
class ObjectDetectionModel(object):
'''
The blackbox object detection model (Faster RCNN for those who want to know).
Given an image as numpy array (3, H, W), it detects objects (generates their category ids and bounding boxes).
'''
# __init__ function
def __init__(self):
self.model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
self.model.eval()
# function for calling the faster-rcnn model
def __call__(self, input):
'''
Arguments:
input (numpy array): A (3, H, W) array of numbers in [0, 1] representing the image.
Returns:
pred_boxes (list): list of bounding boxes, [[x1 y1 x2 y2], ..] where (x1, y1) are the coordinates of the top left corner
and (x2, y2) are the coordinates of the bottom right corner.
pred_class (list): list of predicted classes
pred_score (list): list of the probability (confidence) of prediction of each of the bounding boxes
Tip:
You can print the outputs to get better clarity :)
'''
input_tensor = torch.from_numpy(input)
input_tensor = input_tensor.type(torch.FloatTensor)
input_tensor = input_tensor.unsqueeze(0)
predictions = self.model(input_tensor)
pred_class = [COCO_INSTANCE_CATEGORY_NAMES[i] for i in list(predictions[0]['labels'].numpy())] # Get the Prediction Score
pred_boxes = [[(i[0], i[1]), (i[2], i[3])] for i in list(predictions[0]['boxes'].detach().numpy())] # Bounding boxes
pred_score = list(predictions[0]['scores'].detach().numpy())
return pred_boxes, pred_class, pred_score | 19CS30055-package | /19CS30055_package-0.0.6-py3-none-any.whl/my_package/model.py | model.py |
import numpy as np
import json
from matplotlib import image
from matplotlib import pyplot
from pathlib import Path
class Dataset(object):
'''
A class for the dataset that will return data items as per the given index
'''
def __init__(self, annotation_file, transforms = None):
self.annotation_file = annotation_file
self.transforms = transforms
self.datalist = []
with open(self.annotation_file) as f:
for line in f:
data = json.loads(line)
gt_bboxes = np.array([])
for box in data['bboxes']:
added = np.append(box['category_id'], box['bbox'])
if(len(gt_bboxes) == 0):
gt_bboxes = added
gt_bboxes = gt_bboxes.reshape(1,5)
else:
gt_bboxes = np.vstack((gt_bboxes,added))
p = Path(annotation_file)
dir_data = str(p.parent.absolute())
image1 = image.imread(dir_data + '\\' + data['img_fn'])
image1 = np.interp(image1, (image1.min(), image1.max()), (0, +1))
if(self.transforms is not None):
for t in self.transforms:
image1 = t(image1)
image1 = np.transpose(image1, (2,0,1))
self.datalist.append([image1, gt_bboxes])
'''
Arguments:
annotation_file: path to the annotation file
transforms: list of transforms (class instances)
For instance, [<class 'RandomCrop'>, <class 'Rotate'>]
'''
def __len__(self):
return(len(self.datalist))
'''
return the number of data points in the dataset
'''
def __getitem__(self, idx):
d= {}
d["annotations"] = self.datalist[idx][1]
d["image"] = self.datalist[idx][0]
return d
'''
return the dataset element for the index: "idx"
Arguments:
idx: index of the data element.
Returns: A dictionary with:
image: image (in the form of a numpy array) (shape: (3, H, W))
gt_bboxes: N X 5 array where N is the number of bounding boxes, each
consisting of [class, x1, y1, x2, y2]
x1 and x2 lie between 0 and width of the image,
y1 and y2 lie between 0 and height of the image.
You need to do the following,
1. Extract the correct annotation using the idx provided.
2. Read the image and convert it into a numpy array (wont be necessary
with some libraries). The shape of the array would be (3, H, W).
3. Scale the values in the array to be with [0, 1].
4. Create a dictonary with both the image and annotations
4. Perform the desired transformations.
5. Return the transformed image and annotations as specified. ''' | 19CS30055-package | /19CS30055_package-0.0.6-py3-none-any.whl/my_package/data/dataset.py | dataset.py |
import torch
import torch.nn as nn
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
# Class id to name mapping
COCO_INSTANCE_CATEGORY_NAMES = [
'__background__', 'person', 'bicycle', 'car', 'motorcycle', 'airplane', 'bus',
'train', 'truck', 'boat', 'traffic light', 'fire hydrant', 'N/A', 'stop sign',
'parking meter', 'bench', 'bird', 'cat', 'dog', 'horse', 'sheep', 'cow',
'elephant', 'bear', 'zebra', 'giraffe', 'N/A', 'backpack', 'umbrella', 'N/A', 'N/A',
'handbag', 'tie', 'suitcase', 'frisbee', 'skis', 'snowboard', 'sports ball',
'kite', 'baseball bat', 'baseball glove', 'skateboard', 'surfboard', 'tennis racket',
'bottle', 'N/A', 'wine glass', 'cup', 'fork', 'knife', 'spoon', 'bowl',
'banana', 'apple', 'sandwich', 'orange', 'broccoli', 'carrot', 'hot dog', 'pizza',
'donut', 'cake', 'chair', 'couch', 'potted plant', 'bed', 'N/A', 'dining table',
'N/A', 'N/A', 'toilet', 'N/A', 'tv', 'laptop', 'mouse', 'remote', 'keyboard', 'cell phone',
'microwave', 'oven', 'toaster', 'sink', 'refrigerator', 'N/A', 'book',
'clock', 'vase', 'scissors', 'teddy bear', 'hair drier', 'toothbrush'
]
# Class definition for the model
class ObjectDetectionModel(object):
'''
The blackbox object detection model (Faster RCNN for those who want to know).
Given an image as numpy array (3, H, W), it detects objects (generates their category ids and bounding boxes).
'''
# __init__ function
def __init__(self):
self.model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
self.model.eval()
# function for calling the faster-rcnn model
def __call__(self, input):
'''
Arguments:
input (numpy array): A (3, H, W) array of numbers in [0, 1] representing the image.
Returns:
pred_boxes (list): list of bounding boxes, [[x1 y1 x2 y2], ..] where (x1, y1) are the coordinates of the top left corner
and (x2, y2) are the coordinates of the bottom right corner.
pred_class (list): list of predicted classes
pred_score (list): list of the probability (confidence) of prediction of each of the bounding boxes
Tip:
You can print the outputs to get better clarity :)
'''
input_tensor = torch.from_numpy(input)
input_tensor = input_tensor.type(torch.FloatTensor)
input_tensor = input_tensor.unsqueeze(0)
predictions = self.model(input_tensor)
pred_class = [COCO_INSTANCE_CATEGORY_NAMES[i] for i in list(predictions[0]['labels'].numpy())] # Get the Prediction Score
pred_boxes = [[(i[0], i[1]), (i[2], i[3])] for i in list(predictions[0]['boxes'].detach().numpy())] # Bounding boxes
pred_score = list(predictions[0]['scores'].detach().numpy())
return pred_boxes, pred_class, pred_score | 19CS30055-package1 | /19CS30055_package1-0.0.6.tar.gz/19CS30055_package1-0.0.6/my_package/model.py | model.py |
import numpy as np
import json
from matplotlib import image
from matplotlib import pyplot
from pathlib import Path
class Dataset(object):
'''
A class for the dataset that will return data items as per the given index
'''
def __init__(self, annotation_file, transforms = None):
self.annotation_file = annotation_file
self.transforms = transforms
self.datalist = []
with open(self.annotation_file) as f:
for line in f:
data = json.loads(line)
gt_bboxes = np.array([])
for box in data['bboxes']:
added = np.append(box['category_id'], box['bbox'])
if(len(gt_bboxes) == 0):
gt_bboxes = added
gt_bboxes = gt_bboxes.reshape(1,5)
else:
gt_bboxes = np.vstack((gt_bboxes,added))
p = Path(annotation_file)
dir_data = str(p.parent.absolute())
image1 = image.imread(dir_data + '\\' + data['img_fn'])
image1 = np.interp(image1, (image1.min(), image1.max()), (0, +1))
if(self.transforms is not None):
for t in self.transforms:
image1 = t(image1)
image1 = np.transpose(image1, (2,0,1))
self.datalist.append([image1, gt_bboxes])
'''
Arguments:
annotation_file: path to the annotation file
transforms: list of transforms (class instances)
For instance, [<class 'RandomCrop'>, <class 'Rotate'>]
'''
def __len__(self):
return(len(self.datalist))
'''
return the number of data points in the dataset
'''
def __getitem__(self, idx):
d= {}
d["annotations"] = self.datalist[idx][1]
d["image"] = self.datalist[idx][0]
return d
'''
return the dataset element for the index: "idx"
Arguments:
idx: index of the data element.
Returns: A dictionary with:
image: image (in the form of a numpy array) (shape: (3, H, W))
gt_bboxes: N X 5 array where N is the number of bounding boxes, each
consisting of [class, x1, y1, x2, y2]
x1 and x2 lie between 0 and width of the image,
y1 and y2 lie between 0 and height of the image.
You need to do the following,
1. Extract the correct annotation using the idx provided.
2. Read the image and convert it into a numpy array (wont be necessary
with some libraries). The shape of the array would be (3, H, W).
3. Scale the values in the array to be with [0, 1].
4. Create a dictonary with both the image and annotations
4. Perform the desired transformations.
5. Return the transformed image and annotations as specified. ''' | 19CS30055-package1 | /19CS30055_package1-0.0.6.tar.gz/19CS30055_package1-0.0.6/my_package/data/dataset.py | dataset.py |
# -1NeuronPerceptron_Pypi
1Neuron|Perceptron|_Pypi
"""
author: Nazmul
email: md.nazmul.islam0087@gmail.com
"""
## How to use this
## First install the library using below command by using latest version-
```bash
pip install 1NeuronPerceptron-Pypi-mdnazmulislam0087==0.0.4
```
## Run the below code to see the training and plot file for or Gate, similarly you can use AND, NAND and XOR GATE to see the difference-
```python
from oneNeuronPerceptron.perceptron import Perceptron
from oneNeuronPerceptron.all_utils import prepare_data, save_model, save_plot
import pandas as pd
import numpy as np
import logging
import os
logging_str = "[%(asctime)s: %(levelname)s: %(module)s] %(message)s"
logging.basicConfig(level=logging.INFO, format=logging_str)
def main(data, eta, epochs, modelfilename,plotfilename):
df = pd.DataFrame(data)
logging.info(f"The dataframe is : {df}")
X,y = prepare_data(df)
model = Perceptron(eta=eta, epochs=epochs)
model.fit(X, y)
_ = model.total_loss()
save_model(model, filename=modelfilename)
save_plot(df, file_name=plotfilename, model=model)
if __name__=="__main__": # << entry point <<
OR = {
"x1": [0,0,1,1],
"x2": [0,1,0,1],
"y": [0,1,1,1],
}
ETA = 0.3 # 0 and 1
EPOCHS = 10
try:
logging.info(">>>>> starting training >>>>>")
main(data=OR, eta=ETA, epochs=EPOCHS, modelfilename="or.model", plotfilename="or.png")
logging.info("<<<<< training done successfully<<<<<\n")
except Exception as e:
logging.exception(e)
raise e
```
# Packages required-
1. matplotlib
2. numpy
3. pandas
4. joblib
5. tqdm
# Limitation
Using one Neuron Perceptron, We cant make decision boundary for XOR GATe, In summary XOR Gate classification is not possible using one Neuron Perceptron
# Reference -
[official python docs](https://packaging.python.org/tutorials/packaging-projects/)
[github docs for github actions](https://docs.github.com/en/actions/guides/building-and-testing-python#publishing-to-package-registries)
[Read me editor](https://readme.so/editor)
# more details can be found
[1Neuron Perceptron](https://github.com/mdnazmulislam0087/1NeuronPerceptron)
| 1NeuronPerceptron-Pypi-mdnazmulislam0087 | /1NeuronPerceptron_Pypi-mdnazmulislam0087-0.0.4.tar.gz/1NeuronPerceptron_Pypi-mdnazmulislam0087-0.0.4/README.md | README.md |
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import joblib # FOR SAVING MY MODEL AS A BINARY FILE
from matplotlib.colors import ListedColormap
import os
import logging
plt.style.use("fivethirtyeight") # THIS IS STYLE OF GRAPHS
# prepare data
def prepare_data(df):
""" This is used to separate the dependent and independent columns/features
Args:
df (pd.DataFrame): input is the pandas DataFrame
Returns:
tuple: This returns the dependent and independent columns/features
"""
logging.info("Preparing the data by segregating the independent and dependent variables")
X = df.drop("y", axis=1)
y = df["y"]
return X, y
# save model
def save_model(model, filename):
""" This is used to save the model in binary format.
Args:
model (python object): Trained Model
filename (filename): File name of the model that to be saved
"""
logging.info("saving the trained model")
model_dir = "models"
os.makedirs(model_dir, exist_ok=True) # ONLY CREATE IF MODEL_DIR DOESN"T EXISTS
filePath = os.path.join(model_dir, filename) # model/filename
joblib.dump(model, filePath)
logging.info(f"saved the trained model {filePath}")
# Save plot
def save_plot(df, file_name, model):
"""This is used to save the plot of the model
Args:
df (Pandas Dataframe): This is the dataframe
file_name (object): Name of the file to be saved
model (object): model name that is Trained
"""
logging.info("saving the Plot and Decision boudary")
def _create_base_plot(df):
logging.info("creating the base plot")
df.plot(kind="scatter", x="x1", y="x2", c="y", s=100, cmap="winter")
plt.axhline(y=0, color="black", linestyle="--", linewidth=1)
plt.axvline(x=0, color="black", linestyle="--", linewidth=1)
figure = plt.gcf() # get current figure
figure.set_size_inches(10, 8)
def _plot_decision_regions(X, y, classfier, resolution=0.02):
logging.info("plotting the decision regions")
colors = ("red", "blue", "lightgreen", "gray", "cyan")
cmap = ListedColormap(colors[: len(np.unique(y))])
X = X.values # as a array
x1 = X[:, 0]
x2 = X[:, 1]
x1_min, x1_max = x1.min() -1 , x1.max() + 1
x2_min, x2_max = x2.min() -1 , x2.max() + 1
xx1, xx2 = np.meshgrid(np.arange(x1_min, x1_max, resolution),
np.arange(x2_min, x2_max, resolution))
logging.info(xx1)
logging.info(xx1.ravel())
Z = classfier.predict(np.array([xx1.ravel(), xx2.ravel()]).T)
Z = Z.reshape(xx1.shape)
plt.contourf(xx1, xx2, Z, alpha=0.2, cmap=cmap)
plt.xlim(xx1.min(), xx1.max())
plt.ylim(xx2.min(), xx2.max())
plt.plot()
X, y = prepare_data(df)
_create_base_plot(df)
_plot_decision_regions(X, y, model)
plot_dir = "plots"
os.makedirs(plot_dir, exist_ok=True) # ONLY CREATE IF MODEL_DIR DOESN"T EXISTS
plotPath = os.path.join(plot_dir, file_name) # model/filename
plt.savefig(plotPath)
logging.info(f"saved the plot in {plotPath}") | 1NeuronPerceptron-Pypi-mdnazmulislam0087 | /1NeuronPerceptron_Pypi-mdnazmulislam0087-0.0.4.tar.gz/1NeuronPerceptron_Pypi-mdnazmulislam0087-0.0.4/src/oneNeuronPerceptron/all_utils.py | all_utils.py |
import ctypes
import os,time
from subprocess import Popen, PIPE
from comtypes.client import CreateObject
class RegDm:
@classmethod
def reg(cls):
path = os.path.dirname(__file__)
reg_dm = ctypes.windll.LoadLibrary(path + r'\DmReg.dll')
reg_dm.SetDllPathW(path + r'\dm.dll', 0)
return CreateObject(r'dm.dmsoft')
@classmethod
def create_dm(cls):
return CreateObject(r'dm.dmsoft')
class LDCmd:
def __init__(self, path: str):
os.putenv('Path', path)
@staticmethod
def read_message(cmd):
res = Popen(cmd, stdout=PIPE, shell=True)
res = res.stdout.read().decode(encoding='GBK')
return res
def lunch(self, order: str):
self.read_message('ldconsole.exe launch --index ' + order)
def quit(self, order: str):
self.read_message(cmd='ldconsole.exe quit --index ' + order)
def get_message(self):
return self.read_message('ldconsole.exe list2')
# 索引,标题,顶层窗口句柄,绑定窗口句柄,是否进入android,进程PID,VBox进程PID
def add(self, name: str):
self.read_message('ldconsole.exe add --name ' + name)
def remove(self, order: str):
self.read_message('ldconsole.exe remove --index ' + order)
def copy(self, name: str, order: str):
self.read_message('ldconsole.exe copy --name ' + name + ' --from ' + order)
def start_app(self, order: str, packagename: str):
self.read_message('ldconsole.exe runapp --index ' + order + ' --packagename ' + packagename)
def close_app(self, order: str, packagename: str):
self.read_message('ldconsole.exe killapp --index ' + order + ' --packagename ' + packagename)
def get_list_package(self, order: str):
return self.read_message(cmd='ld.exe -s ' + order + ' pm list packages')
def install_app(self, order: str, path: str):
self.read_message('ldconsole.exe installapp --index ' + order + ' --filename ' + path)
def sort_wnd(self):
self.read_message('ldconsole.exe sortWnd')
def reboot(self, order: str):
self.read_message('ldconsole.exe reboot --index ' + order)
def get_appoint_message(self, order: str,index:int):
my_list = self.get_message()
items = my_list.splitlines()
return items[int(order)].split(',')[index]
class Memory:
def __init__(self, dx, hwd):
self.__dx = dx
self.hwd = hwd
def get_call_address(self, s, model, off):
# 返回16进制字符串地址
module_size = self.__dx.GetModuleSize(self.hwd, model)
base_address = self.__dx.GetModuleBaseAddr(self.hwd, model)
end_address = module_size + base_address
call_address = self.__dx.FindData(self.hwd, hex(base_address)[2:] + '-' + hex(end_address)[2:], s)
return hex(int(call_address, 16) + int(off, 16))[2:]
def x64_get_base_address(self, s, model, off):
address = self.get_call_address(s, model, off)
address_2 = self.__dx.readint(self.hwd, address, 4)
return hex(int(address, 16) + address_2 + 4)[2:]
def x32_get_base_address(self, s, model, off):
address = self.get_call_address(s, model, off)
address = self.__dx.readint(self.hwd, address, 4)
return hex(address)[2:]
class Auto_Dm:
def __init__(self,my_dx):
self.dx=my_dx
self.ret = None
self.tup=None
def reg(self,code,md)->int:
return self.dx.reg(code,md)
def BindWindowEx(self,hwnd,display,mouse,keypad,public,mode)->int:
return self.dx.BindWindowEx(hwnd,display,mouse,keypad,public,mode)
def EnumWindowByProcess(self,process_name,title,class_name,filter)->str:
return self.dx.EnumWindowByProcess(process_name,title,class_name,filter)
def set_path(self, s)->int:
return self.dx.setpath(s)
def FindPic(self,pic_name,t=100,x1=0, y1=0, x2=960, y2=540):
t1 = time.time()
while time.time() - t1 < t:
self.ret = self.dx.FindPic(x1, y1, x2, y2, pic_name, '050505', 0.9, 0)
if self.ret[2] != -1:
print(f'find {pic_name}')
return self
def click_point(self,x,y,t=1):
self.dx.MoveTo(x,y)
for i in range(0, t):
self.dx.LeftClick()
time.sleep(0.1)
return self
def click(self,t=1):
self.dx.MoveTo(self.ret[0],self.ret[1])
for i in range(0, t):
self.dx.LeftClick()
time.sleep(0.1)
return self
def sleep(self, t):
time.sleep(t)
return self
if __name__ == '__main__':
dm=RegDm.reg()
auto_dm = Auto_Dm(dm) | 1Q847 | /1Q847-1.1.8.tar.gz/1Q847-1.1.8/Dll/Reg.py | Reg.py |
import logging
import uuid
import requests
import collections
import json
import time
from pathlib import Path
from typing import Dict, Any, Optional, Callable
import sentry_sdk
from sentry_sdk.integrations.logging import LoggingIntegration, ignore_logger
import logzio.handler
from logdna import LogDNAHandler
# sentry_sdk.init("https://1830d21cd41c4e00ac39aaade813c60d@sentry.io/1382546")
BASE_URL = "https://labs.1a23.com/telemetry_config/"
device_id: Optional[str] = None
instances: Dict[str, Any] = {}
class JSONEncoder(json.JSONEncoder):
def default(self, o):
return repr(o)
class TelemetryProvider:
def set_metadata(data: Dict[str, any]):
pass
class SentryErrorReporting(TelemetryProvider):
def __init__(self, options: Dict[str, Any]):
del options['provider']
if 'enable' in options:
del options['enable']
if 'available' in options:
del options['available']
token = options.pop('token')
integrations = []
if not options.get('capture_logs'):
integrations.append(LoggingIntegration(level=logging.ERROR))
if 'capture_logs' in options:
del options['capture_logs']
if 'ignored_loggers' in options:
for logger in options['ignored_loggers']:
ignore_logger(logger)
del options['ignored_loggers']
sentry_sdk.init(dsn=token, integrations=integrations,
**options)
with sentry_sdk.configure_scope() as scope:
scope.user = {'id': get_device_id()}
def set_metadata(self, data: Dict[str, any]):
with sentry_sdk.configure_scope() as scope:
for key, value in data.items():
scope.set_tag(key, str(value))
class LogzLogAnalysis(TelemetryProvider):
def __init__(self, options: Dict[str, Any], instance: str):
token = options['token']
server = options['server']
self.hdlr: logzio.handler.LogzioHandler = \
logzio.handler.LogzioHandler(
token, logzio_type=instance, url=server
)
# self.hdlr.format_message = \
# self.wrap_format_message(self.hdlr.format_message)
logging.getLogger('').addHandler(self.hdlr)
self.metadata = {'device_id': get_device_id()}
self.hdlr.setFormatter(logging.Formatter(json.dumps(self.metadata)))
def set_metadata(self, data: Dict[str, any]):
self.metadata.update(data)
self.hdlr.setFormatter(logging.Formatter(json.dumps(self.metadata)))
def wrap_format_message(self, fn: Callable):
def format_message(message: logging.LogRecord):
d = fn(message)
args = self.json_obj_escape(message.args)
if not isinstance(args, list):
args = [args]
for idx, i in enumerate(args):
i_type = type(i).__name__
d['arg_%s_%s' % (idx, i_type)] = i
if not isinstance(message.msg, str):
obj = self.json_obj_escape(message.msg)
obj_type = type(obj).__name__
d['msg_' + obj_type] = obj
return d
return format_message
def json_obj_escape(self, obj):
if isinstance(obj, tuple):
obj = list(obj)
if isinstance(obj, (str, bool, float, int, type(None))):
return obj
elif isinstance(obj, list):
return list(map(self.json_obj_escape, obj))
elif isinstance(obj, dict):
return {self.json_obj_escape(k): self.json_obj_escape(v) for k, v in obj.items()}
else:
return repr(obj)
class LogglyLogAnalysis(LogzLogAnalysis):
def __init__(self, options: Dict[str, Any], instance: str):
token = "token"
server = options['url'] + instance + "/"
self.hdlr: logzio.handler.LogzioHandler = \
logzio.handler.LogzioHandler(
token, logzio_type=instance, url=server
)
self.hdlr.format_message = \
self.wrap_format_message(self.hdlr.format_message)
self.patch_get_message(self.hdlr.logzio_sender)
self.hdlr.logzio_sender.url = server
logging.getLogger('').addHandler(self.hdlr)
self.metadata = {'device_id': get_device_id()}
self.hdlr.setFormatter(logging.Formatter(json.dumps(self.metadata)))
@staticmethod
def patch_get_message(sender: logzio.sender.LogzioSender):
def patched_get_msg():
if not sender.queue.empty():
return [sender.queue.get()]
return []
sender._get_messages_up_to_max_allowed_size = patched_get_msg
def sanitize_key(self, key: str):
return key.replace(' ', '_').replace('.', '_')
def set_metadata(self, data: Dict[str, any]):
data = {self.sanitize_key(key): value for key, value in data.items()}
self.metadata.update(data)
self.hdlr.setFormatter(logging.Formatter(json.dumps(self.metadata)))
class LogDNALogAnalysis(TelemetryProvider):
def __init__(self, options: Dict[str, Any], instance: str):
key = options['token']
options = {"app": instance, "hostname": get_device_id(),
"include_standard_meta": True}
self.hdlr = LogDNAHandler(key, options)
self.meta = {}
self.patch_emit(self.hdlr)
logging.getLogger('').addHandler(self.hdlr)
def set_metadata(self, data: Dict[str, any]):
self.meta.update(data)
def patch_emit(provider, self):
def emit(record):
msg = self.format(record)
record = record.__dict__
opts = {}
if 'args' in record:
opts = record['args']
if self.include_standard_meta:
if isinstance(opts, tuple):
opts = {}
if 'meta' not in opts:
opts['meta'] = {}
for key in ['name', 'pathname', 'lineno']:
opts['meta'][key] = record[key]
opts['meta'].update(provider.meta)
if isinstance(record['args'], tuple):
opts['meta'].update(
{("arg_%s" % idx): i for idx,
i in enumerate(record['args'])}
)
message = {
'hostname': self.hostname,
'timestamp': int(time.time() * 1000),
'line': msg,
'level': record['levelname'] or self.level,
'app': self.app or record['module'],
'env': self.env
}
if not isinstance(opts, tuple):
if 'level' in opts:
message['level'] = opts['level']
if 'app' in opts:
message['app'] = opts['app']
if 'hostname' in opts:
message['hostname'] = opts['hostname']
if 'env' in opts:
message['env'] = opts['env']
if 'timestamp' in opts:
message['timestamp'] = opts['timestamp']
if 'meta' in opts:
if self.index_meta:
message['meta'] = self.sanitizeMeta(opts['meta'])
else:
message['meta'] = json.dumps(
opts['meta'], cls=JSONEncoder)
self.bufferLog(message)
self.emit = emit
def init(instance: str, patch: Dict[str, Any]={}):
resp = requests.get(BASE_URL + instance + ".json")
if resp.status_code != 200:
resp.raise_for_status()
config: Dict[str, Any] = resp.json()
config = update(config, patch)
if not isinstance(config, dict):
raise ValueError()
for key, val in config.items():
if not val.get('enable', False) or not val.get('available', False):
continue
instances[key] = setup_instance(val, instance)
def update(d, u):
for k, v in u.items():
if isinstance(v, collections.Mapping):
d[k] = update(d.get(k, {}), v)
else:
d[k] = v
return d
def setup_instance(config: Dict[str, Any], instance: str) -> TelemetryProvider:
provider = config.get('provider', None)
if provider == 'sentry':
return SentryErrorReporting(config)
elif provider == 'logz':
return LogzLogAnalysis(config, instance)
elif provider == 'loggly':
return LogglyLogAnalysis(config, instance)
elif provider == 'logdna':
return LogDNALogAnalysis(config, instance)
else:
return TelemetryProvider()
def get_device_id() -> str:
global device_id
# if no machine ID file saved, generate one, and save it
# otherwise, get the ID from the file.
if device_id:
return device_id
path: Path = Path.home() / '.1a23_telemetry_device_id'
if path.exists():
device_id = path.read_text()
return device_id
device_id = str(uuid.uuid4())
path.write_text(device_id)
return device_id
def set_metadata(data: Dict[str, any]):
for i in instances.values():
i.set_metadata(data) | 1a23-telemetry | /1a23_telemetry-1.0.0-py3-none-any.whl/telemetry_1a23/__init__.py | __init__.py |
Copyright 2019 Eana Hufwe
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
IN THE SOFTWARE. | 1a23-telemetry | /1a23_telemetry-1.0.0-py3-none-any.whl/1a23_telemetry-1.0.0.dist-info/LICENSE.rst | LICENSE.rst |
import time
import logging
import httplib as http_client
import requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.poolmanager import PoolManager
from requests.packages.urllib3.util.retry import Retry
import ssl
import base64
try:
from cStringIO import StringIO
except BaseException:
from StringIO import StringIO
import zipfile
class MyAdapter(HTTPAdapter):
def init_poolmanager(self, connections, maxsize, block=False):
self.poolmanager = PoolManager(num_pools=connections,
maxsize=maxsize,
block=block,
ssl_version=getattr(ssl, 'PROTOCOL_TLSv1_2', ssl.PROTOCOL_TLSv1))
# Retry logic if the API fails to responde
def requests_retry_session(
retries=5,
backoff_factor=0.5,
status_forcelist=(500, 502, 504, 495, 496, 525, 526),
session=None,
):
session = session or requests.Session()
retry = Retry(
total=retries,
read=retries,
connect=retries,
backoff_factor=backoff_factor,
status_forcelist=status_forcelist,
)
adapter = MyAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
return session
# 1and1 Object Classes
class OneAndOneService(object):
# Init Function
def __init__(
self,
api_token,
api_url='https://cloudpanel-api.1and1.com/v1',
enable_logs=False):
if api_url == '' or api_url == 'default':
api_url = 'https://cloudpanel-api.1and1.com/v1'
self.api_token = api_token
self.base_url = api_url
self.header = {'X-TOKEN': self.api_token}
self.success_codes = (200, 201, 202)
if enable_logs:
http_client.HTTPConnection.debuglevel = 1
logging.basicConfig()
logging.getLogger().setLevel(logging.DEBUG)
requests_log = logging.getLogger("requests.packages.urllib3")
requests_log.setLevel(logging.ERROR)
requests_log.propagate = True
def __repr__(self):
return 'OneAndOneService: api_token=%s, base_url=%s' % (self.api_token,
self.base_url)
# Server Functions
# 'GET' methods
def list_servers(self, page=None, per_page=None, sort=None, q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/servers' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def fixed_server_flavors(self):
# Perform Request
url = '%s/servers/fixed_instance_sizes' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_fixed_server(self, fixed_server_id=None):
# Error Handling
if(fixed_server_id is None):
raise ValueError('fixed_server_id is a required parameter')
# Perform Request
url = ('%s/servers/fixed_instance_sizes/%s' %
(self.base_url, fixed_server_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_server(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_server_hardware(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s/hardware' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_server_hdds(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s/hardware/hdds' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_server_hdd(self, server_id=None, hdd_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(hdd_id is None):
raise ValueError('hdd_id is a required parameter')
# Perform Request
url = ('%s/servers/%s/hardware/hdds/%s' %
(self.base_url, server_id, hdd_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_server_image(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s/image' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_server_ips(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s/ips' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_server_ip(self, server_id=None, ip_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
# Perform Request
url = '%s/servers/%s/ips/%s' % (self.base_url, server_id, ip_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_ip_firewall_policy(self, server_id=None, ip_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
# Perform Request
url = ('%s/servers/%s/ips/%s/firewall_policy' %
(self.base_url, server_id, ip_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_ip_load_balancers(self, server_id=None, ip_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
# Perform Request
url = ('%s/servers/%s/ips/%s/load_balancers' %
(self.base_url, server_id, ip_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_server_status(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s/status' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_server_dvd(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s/dvd' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_server_private_networks(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s/private_networks' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def private_network_info(self, server_id=None, private_network_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
# Perform Request
url = ('%s/servers/%s/private_networks/%s' %
(self.base_url, server_id, private_network_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_server_snapshots(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = '%s/servers/%s/snapshots' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_baremetal_models(
self,
page=None,
per_page=None,
sort=None,
q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/servers/baremetal_models' % self.base_url
r = requests.get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def get_baremetal_model(self, model_id=None):
# Error Handling
if (model_id is None):
raise ValueError('model_id is a required parameter')
# Perform Request
url = '%s/servers/baremetal_models/%s' % (self.base_url, model_id)
r = requests.get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# 'PUT' methods
def modify_server(self, server_id=None, name=None, description=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(name is None):
raise ValueError('name is a required parameter')
# Perform Request
data = {
'name': name,
'description': description
}
url = '%s/servers/%s' % (self.base_url, server_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def modify_server_hardware(
self,
server_id=None,
fixed_instance_size_id=None,
vcore=None,
cores_per_processor=None,
ram=None,
test=False):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Use 'test' flag to skip this block when running unit test
if(test == False):
# Prevent hot decreasing of server hardware, allow cold decreasing.
server_specs = self.get_server_hardware(server_id=server_id)
server_status = self.get_server_status(server_id=server_id)
if(server_status['state'] == 'POWERED_ON'):
if(vcore is not None):
if(server_specs['vcore'] > vcore):
raise ValueError(('Cannot perform a hot decrease of '
'server CPU. The new value must be '
'greater than current value.'))
if(ram is not None):
if(server_specs['ram'] > ram):
raise ValueError(('Cannot perform a hot decrease of '
'server RAM. The new value must be '
'greater than current value.'))
# Perform Request
data = {
'fixed_instance_size_id': fixed_instance_size_id,
'vcore': vcore,
'cores_per_processor': cores_per_processor,
'ram': ram
}
url = '%s/servers/%s/hardware' % (self.base_url, server_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def modify_hdd(self, server_id=None, hdd_id=None, size=None, test=False):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(hdd_id is None):
raise ValueError('hdd_id is a required parameter')
# Use 'test' flag to skip this block when running unit test
if(test == False):
# Make sure size argument is valid. HDD size can't be decreased.
old_hdd = self.get_server_hdd(server_id=server_id, hdd_id=hdd_id)
if(size is not None):
if(old_hdd['size'] > size):
raise ValueError('HDD size can never be decreased. '
'Must be greater than or equal to the '
'current HDD size.')
# Perform Request
data = {'size': size}
url = ('%s/servers/%s/hardware/hdds/%s' %
(self.base_url, server_id, hdd_id))
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_firewall_policy(
self,
server_id=None,
ip_id=None,
firewall_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
# Perform Request
data = {'id': firewall_id}
url = ('%s/servers/%s/ips/%s/firewall_policy' %
(self.base_url, server_id, ip_id))
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def modify_server_status(
self,
server_id=None,
action=None,
method='SOFTWARE',
recovery_mode=False,
recovery_image_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(action is None):
raise ValueError('action is a required parameter')
# Make sure user is passing in correct arguments
if(action != 'POWER_ON' and action != 'POWER_OFF' and
action != 'REBOOT'):
raise ValueError(('action must be set to "POWER_ON",'
'"POWER_OFF", or "REBOOT".'))
if method != 'HARDWARE' and method != 'SOFTWARE':
raise ValueError(('method must be set to either '
'"HARDWARE" or "SOFTWARE".'))
if recovery_mode and recovery_image_id is None:
raise ValueError(
('If you want to reboot in recovery mode you must specify an image id recovery_image_id'))
# Perform Request
if recovery_mode:
data = {
'action': action,
'method': method,
'recovery_mode': True,
'recovery_image_id': recovery_image_id
}
else:
data = {
'action': action,
'method': method,
}
url = '%s/servers/%s/status/action' % (self.base_url, server_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def stop_server(self, server_id=None, method='SOFTWARE'):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Make sure user is passing in correct arguments
if(method != 'HARDWARE' and method != 'SOFTWARE'):
raise ValueError(('method must be set to either '
'"HARDWARE" or "SOFTWARE".'))
# Perform Request
data = {
'action': 'POWER_OFF',
'method': method
}
url = '%s/servers/%s/status/action' % (self.base_url, server_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def start_server(self, server_id=None, method='SOFTWARE'):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Make sure user is passing in correct arguments
if(method != 'HARDWARE' and method != 'SOFTWARE'):
raise ValueError(('method must be set to either '
'"HARDWARE" or "SOFTWARE".'))
# Perform Request
data = {
'action': 'POWER_ON',
'method': method
}
url = '%s/servers/%s/status/action' % (self.base_url, server_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def load_dvd(self, server_id=None, dvd_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(dvd_id is None):
raise ValueError('dvd_id is a required parameter')
# Perform Request
data = {'id': dvd_id}
url = '%s/servers/%s/dvd' % (self.base_url, server_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def restore_snapshot(self, server_id=None, snapshot_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(snapshot_id is None):
raise ValueError('snapshot_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/servers/%s/snapshots/%s' %
(self.base_url, server_id, snapshot_id))
try:
r = requests_retry_session().put(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def reinstall_image(self, server_id=None, image_id=None, password=None,
firewall_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(image_id is None):
raise ValueError('image_id is a required parameter')
# Create firewall object, if necessary
firewall_policy = {'id': firewall_id}
# Perform Request
data = {
'id': image_id,
'password': password,
'firewall_policy': firewall_policy
}
url = '%s/servers/%s/image' % (self.base_url, server_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' methods
def delete_server(self, server_id=None, keep_ips=None, keep_hdds=True):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
parameters = {'keep_ips': keep_ips, 'keep_hdds': keep_hdds}
url = '%s/servers/%s' % (self.base_url, server_id)
try:
r = requests_retry_session().delete(
url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_hdd(self, server_id=None, hdd_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(hdd_id is None):
raise ValueError('hdd_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/servers/%s/hardware/hdds/%s' %
(self.base_url, server_id, hdd_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_ip(self, server_id=None, ip_id=None, keep_ip=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
parameters = {'keep_ip': keep_ip}
url = '%s/servers/%s/ips/%s' % (self.base_url, server_id, ip_id)
try:
r = requests_retry_session().delete(
url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_load_balancer(self, server_id=None, ip_id=None,
load_balancer_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/servers/%s/ips/%s/load_balancers/%s' %
(self.base_url, server_id, ip_id, load_balancer_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_private_network(self, server_id=None, private_network_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/servers/%s/private_networks/%s' %
(self.base_url, server_id, private_network_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def eject_dvd(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/servers/%s/dvd' % (self.base_url, server_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def delete_snapshot(self, server_id=None, snapshot_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(snapshot_id is None):
raise ValueError('snapshot_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/servers/%s/snapshots/%s' %
(self.base_url, server_id, snapshot_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' methods
def add_new_ip(self, server_id=None, ip_type=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(ip_type is not None) and (ip_type != 'IPV4'):
raise ValueError(("ip_type. Only type 'IPV4' is currently "
"supported."))
# Perform Request
data = {'type': ip_type}
url = '%s/servers/%s/ips' % (self.base_url, server_id)
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_load_balancer(self, server_id=None, ip_id=None,
load_balancer_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
# Perform Request
data = {'load_balancer_id': load_balancer_id}
url = ('%s/servers/%s/ips/%s/load_balancers' %
(self.base_url, server_id, ip_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def assign_private_network(self, server_id=None, private_network_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
# Perform Request
data = {'id': private_network_id}
url = '%s/servers/%s/private_networks' % (self.base_url, server_id)
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def create_snapshot(self, server_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/servers/%s/snapshots' % (self.base_url, server_id)
try:
r = requests_retry_session().post(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def clone_server(self, server_id=None, name=None, datacenter_id=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(name is None):
raise ValueError('name is a required parameter')
# Perform Request
data = {
'name': name,
'datacenter_id': datacenter_id
}
url = '%s/servers/%s/clone' % (self.base_url, server_id)
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def create_server(self, server=None, hdds=None):
# Error Handling
if(server is None):
raise ValueError(('server is a required parameter. Make '
'sure you pass a Server object.'))
# Unpack hdds
if hdds:
hdd = []
for value in hdds:
hdd.append(value.specs)
# Add hdds to server object
server.specs['hardware']['hdds'] = hdd
# Clean dictionary
keys = [k for k, v in server.specs['hardware'].items() if
v is None]
for x in keys:
del server.specs['hardware'][x]
# Build URL and perform request
url = '%s/servers' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header, json=server.specs)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new server_id back to calling Server object
response = r.json()
server.specs.update(server_id=response['id'])
server.specs.update(api_token=self.header)
server.first_password = response['first_password']
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_hdd(self, server_id=None, hdds=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(hdds is None):
raise ValueError(('hdds is a required parameter. Make '
'sure you pass a list with at least '
'one Hdd object.'))
# Unpack hdds
hdd = []
for value in hdds:
hdd.append(value.specs)
# Perform Request
data = {'hdds': hdd}
url = '%s/servers/%s/hardware/hdds' % (self.base_url, server_id)
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Image Functions
# 'GET' Methods
def list_images(self, page=None, per_page=None, sort=None, q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/images' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_image(self, image_id=None):
# Error Handling
if(image_id is None):
raise ValueError('image_id is a required parameter')
# Perform Request
url = '%s/images/%s' % (self.base_url, image_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_image(self, image=None):
# Error Handling
if(image.server_id is None):
raise ValueError('server_id is a required parameter')
if(image.name is None):
raise ValueError('name is a required parameter')
if(image.frequency is None):
raise ValueError('frequency is a required parameter')
if(image.num_images is None):
raise ValueError('num_images is a required parameter')
# Perform Request
data = {
'server_id': image.server_id,
'name': image.name,
'frequency': image.frequency,
'num_images': image.num_images,
'description': image.description,
'source': image.source,
'url': image.url,
'os_id': image.os_id,
'type': image.type
}
url = '%s/images' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new image_id back to calling Image object
response = r.json()
image.specs.update(image_id=response['id'])
image.specs.update(api_token=self.header)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_image(self, image_id=None):
# Error Handling
if(image_id is None):
raise ValueError('image_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/images/%s' % (self.base_url, image_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_image(self, image_id=None, name=None, description=None,
frequency=None):
# Error Handling
if(image_id is None):
raise ValueError('image_id is a required parameter')
# Perform Request
data = {
'name': name,
'frequency': frequency,
'description': description
}
url = '%s/images/%s' % (self.base_url, image_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Shared Storage Functions
# 'GET' Methods
def list_shared_storages(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/shared_storages' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_shared_storage(self, shared_storage_id=None):
# Error Handling
if(shared_storage_id is None):
raise ValueError('shared_storage_id is a required parameter')
# Perform Request
url = '%s/shared_storages/%s' % (self.base_url, shared_storage_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_servers_attached_storage(self, shared_storage_id=None):
# Error Handling
if(shared_storage_id is None):
raise ValueError('shared_storage_id is a required parameter')
# Perform Request
url = ('%s/shared_storages/%s/servers' %
(self.base_url, shared_storage_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_shared_storage_server(
self,
shared_storage_id=None,
server_id=None):
# Error Handling
if(shared_storage_id is None):
raise ValueError('shared_storage_id parameter is required')
if(server_id is None):
raise ValueError('server_id parameter is required')
# Perform Request
url = ('%s/shared_storages/%s/servers/%s' %
(self.base_url, shared_storage_id, server_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_credentials(self):
# Perform Request
url = '%s/shared_storages/access' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_shared_storage(self, shared_storage=None):
# Error Handling
if(shared_storage.name is None):
raise ValueError('name is a required parameter')
if(shared_storage.size is None):
raise ValueError('size is a required parameter')
# Perform Request
data = {
'name': shared_storage.name,
'description': shared_storage.description,
'size': shared_storage.size,
'datacenter_id': shared_storage.datacenter_id
}
url = '%s/shared_storages' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new shared_storage_id back to calling SharedStorage object
response = r.json()
shared_storage.specs.update(shared_storage_id=response['id'])
shared_storage.specs.update(api_token=self.header)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def attach_server_shared_storage(self, shared_storage_id=None,
server_ids=None):
# Error Handling
if(shared_storage_id is None):
raise ValueError('shared_storage_id is a required parameter')
if(server_ids is None):
raise ValueError(('server_ids is a required parameter. '
'Must attach at least one server'))
# Unpack servers
servers = []
for value in server_ids:
servers.append({'id': value.server_id, 'rights': value.rights})
# Perform Request
data = {'servers': servers}
url = ('%s/shared_storages/%s/servers' %
(self.base_url, shared_storage_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_shared_storage(self, shared_storage_id=None, name=None,
description=None, size=None):
# Error Handling
if(shared_storage_id is None):
raise ValueError('shared_storage_id is a required parameter')
# Perform Request
data = {
'name': name,
'description': description,
'size': size
}
url = '%s/shared_storages/%s' % (self.base_url, shared_storage_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def change_password(self, password=None):
# Error Handlong
if(password is None):
raise ValueError(('password is a required parameter. '
'password must contain at least 8 characters.'))
# Perform Request
data = {'password': password}
url = '%s/shared_storages/access' % self.base_url
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_shared_storage(self, shared_storage_id=None):
# Error Handling
if(shared_storage_id is None):
raise ValueError('shared_storage_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/shared_storages/%s' % (self.base_url, shared_storage_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def detach_server_shared_storage(self, shared_storage_id=None,
server_id=None):
# Error Handling
if(shared_storage_id is None):
raise ValueError('shared_storage_id is a required parameter')
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/shared_storages/%s/servers/%s' %
(self.base_url, shared_storage_id, server_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Firewall Policy Functions
# 'GET' Methods
def list_firewall_policies(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/firewall_policies' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_firewall(self, firewall_id=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
# Perform Request
url = '%s/firewall_policies/%s' % (self.base_url, firewall_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_firewall_servers(self, firewall_id=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
# Perform Request
url = ('%s/firewall_policies/%s/server_ips' %
(self.base_url, firewall_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_firewall_server(self, firewall_id=None, server_ip_id=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
if(server_ip_id is None):
raise ValueError('server_ip_id is a required parameter')
# Perform Request
url = ('%s/firewall_policies/%s/server_ips/%s' %
(self.base_url, firewall_id, server_ip_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_firewall_policy_rules(self, firewall_id=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
# Perform Request
url = '%s/firewall_policies/%s/rules' % (self.base_url, firewall_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_firewall_policy_rule(self, firewall_id=None, rule_id=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
if(rule_id is None):
raise ValueError('rule_id is a required parameter')
# Perform Request
url = ('%s/firewall_policies/%s/rules/%s' %
(self.base_url, firewall_id, rule_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_firewall(self, firewall_id=None, name=None, description=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
# Perform Request
data = {
'name': name,
'description': description
}
url = '%s/firewall_policies/%s' % (self.base_url, firewall_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_firewall_policy(self, firewall_policy=None,
firewall_policy_rules=None):
# Error Handling
if(firewall_policy.specs['name'] is None):
raise ValueError(('Policy name is required. Make sure your '
'FirewallPolicy object was initialized with '
'a name parameter'))
if(firewall_policy_rules is None):
raise ValueError(('firewall_policy_rules is required. Make sure '
'you pass a list with at least one '
'FirewallPolicyRule object.'))
# Unpack Rules
rules = []
for value in firewall_policy_rules:
rules.append(value.rule_set)
# Attach rules and Perform Request
firewall_policy.specs['rules'] = rules
url = '%s/firewall_policies' % self.base_url
try:
r = requests_retry_session().post(
url, headers=self.header, json=firewall_policy.specs)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new firewall_id back to calling FirewallPolicy object
response = r.json()
firewall_policy.specs.update(firewall_id=response['id'])
firewall_policy.specs.update(api_token=self.header)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_firewall_policy_rule(self, firewall_id=None,
firewall_policy_rules=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
if(firewall_policy_rules is None):
raise ValueError(('firewall_policy_rules is required. Make '
'sure you pass a list with at least one '
'FirewallPolicyRule object'))
# Unpack rules
rules = []
for value in firewall_policy_rules:
rules.append(value.rule_set)
# Perform Request
data = {'rules': rules}
url = '%s/firewall_policies/%s/rules' % (self.base_url, firewall_id)
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def attach_server_firewall_policy(self, firewall_id=None, server_ips=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
if(server_ips is None):
raise ValueError(('server_ips is required. Make sure you pass '
'a list with at least one AttachServer object'))
# Unpack servers
servers = []
for value in server_ips:
servers.append(value.server_ip_id)
# Perform Request
data = {'server_ips': servers}
url = ('%s/firewall_policies/%s/server_ips' %
(self.base_url, firewall_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_firewall(self, firewall_id=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/firewall_policies/%s' % (self.base_url, firewall_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_firewall_rule(self, firewall_id=None, rule_id=None):
# Error Handling
if(firewall_id is None):
raise ValueError('firewall_id is a required parameter')
if(rule_id is None):
raise ValueError('rule_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/firewall_policies/%s/rules/%s' %
(self.base_url, firewall_id, rule_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Load Balancer Functions
# 'GET' Methods
def list_load_balancers(self, page=None, per_page=None, sort=None, q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/load_balancers' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_load_balancer(self, load_balancer_id=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
# Perform Request
url = '%s/load_balancers/%s' % (self.base_url, load_balancer_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_load_balancer_servers(self, load_balancer_id=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
# Perform Request
url = ('%s/load_balancers/%s/server_ips' %
(self.base_url, load_balancer_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_load_balancer_server(self, load_balancer_id=None,
server_ip_id=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
if(server_ip_id is None):
raise ValueError('server_ip_id is a required parameter')
# Perform Request
url = ('%s/load_balancers/%s/server_ips/%s' %
(self.base_url, load_balancer_id, server_ip_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def load_balancer_rules(self, load_balancer_id=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
# Perform Request
url = '%s/load_balancers/%s/rules' % (self.base_url, load_balancer_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_load_balancer_rule(self, load_balancer_id=None, rule_id=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
if(rule_id is None):
raise ValueError('rule_id is a required parameter')
# Perform Request
url = ('%s/load_balancers/%s/rules/%s' %
(self.base_url, load_balancer_id, rule_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_load_balancer(
self,
load_balancer_id=None,
name=None,
description=None,
health_check_test=None,
health_check_interval=None,
health_check_path=None,
health_check_parse=None,
persistence=None,
persistence_time=None,
method=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
if(method is not None and method != 'ROUND_ROBIN' and
method != 'LEAST_CONNECTIONS'):
raise ValueError(('method must be set to either "ROUND_ROBIN" '
'or "LEAST_CONNECTIONS".'))
if(health_check_test is not None and health_check_test != 'TCP'):
raise ValueError(('health_check_test must be set to "TCP". '
'"HTTP" is not currently supported.'))
if(health_check_interval is not None and health_check_interval < 5 and health_check_interval > 300):
raise ValueError(('health_check_interval must be an integer '
'between 5 and 300.'))
if(persistence_time is not None and persistence_time < 30 and persistence_time > 1200):
raise ValueError(('persistence_time must be an integer '
'between 30 and 1200.'))
# Perform Request
data = {
'name': name,
'description': description,
'health_check_test': health_check_test,
'health_check_interval': health_check_interval,
'health_check_path': health_check_path,
'health_check_parse': health_check_parse,
'persistence': persistence,
'persistence_time': persistence_time,
'method': method
}
url = '%s/load_balancers/%s' % (self.base_url, load_balancer_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_load_balancer(self, load_balancer=None,
load_balancer_rules=None):
# Error Handling
if(load_balancer is None):
raise ValueError(('load_balancer parameter is required. Must '
'pass a LoadBalancer object.'))
if(load_balancer_rules is None):
raise ValueError(('load_balancer_rules parameter is required. '
'Must pass a list with at least one '
'LoadBalancerRule object.'))
if(load_balancer.specs['method'] is not None and
load_balancer.specs['method'] != 'ROUND_ROBIN' and
load_balancer.specs['method'] != 'LEAST_CONNECTIONS'):
raise ValueError(('method must be set to either "ROUND_ROBIN" '
'or "LEAST_CONNECTIONS".'))
# Unpack rules
rules = []
for value in load_balancer_rules:
rules.append(value.rule_set)
# Perform Request
load_balancer.specs['rules'] = rules
url = '%s/load_balancers' % self.base_url
try:
r = requests_retry_session().post(
url, headers=self.header, json=load_balancer.specs)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new load_balancer_id back to calling LoadBalancer object
response = r.json()
load_balancer.specs.update(load_balancer_id=response['id'])
load_balancer.specs.update(api_token=self.header)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def attach_load_balancer_server(self, load_balancer_id=None,
server_ips=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter.')
if(server_ips is None):
raise ValueError(('server_ips is a required parameter. Must '
'pass a list with at least one AttachServer '
'object'))
# Unpack servers
servers = []
for value in server_ips:
servers.append(value.server_ip_id)
# Perform Request
data = {'server_ips': servers}
url = ('%s/load_balancers/%s/server_ips' %
(self.base_url, load_balancer_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_load_balancer_rule(self, load_balancer_id=None,
load_balancer_rules=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter.')
if(load_balancer_rules is None):
raise ValueError(('load_balancer_rules is a required '
'parameter. Must pass a list with at least one '
'LoadBalancerRule object'))
# Unpack rules
rules = []
for value in load_balancer_rules:
rules.append(value.rule_set)
# Perform Request
data = {'rules': rules}
url = ('%s/load_balancers/%s/rules' %
(self.base_url, load_balancer_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_load_balancer(self, load_balancer_id=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/load_balancers/%s' % (self.base_url, load_balancer_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_load_balancer_server(self, load_balancer_id=None,
server_ip_id=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter.')
if(server_ip_id is None):
raise ValueError('server_ip_id is a required parameter.')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/load_balancers/%s/server_ips/%s' %
(self.base_url, load_balancer_id, server_ip_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_load_balancer_rule(self, load_balancer_id=None, rule_id=None):
# Error Handling
if(load_balancer_id is None):
raise ValueError('load_balancer_id is a required parameter.')
if(rule_id is None):
raise ValueError('rule_id is a required parameter.')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/load_balancers/%s/rules/%s' %
(self.base_url, load_balancer_id, rule_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Public IP Functions
# 'GET' Methods
def list_public_ips(self, page=None, per_page=None, sort=None, q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/public_ips' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_public_ip(self, ip_id=None):
# Error Handling
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
# Perform Request
url = '%s/public_ips/%s' % (self.base_url, ip_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_public_ip(self, reverse_dns=None, ip_type=None,
datacenter_id=None):
# Error Handling
if(ip_type != 'IPV4' and ip_type is not None):
raise ValueError('ip_type must be set to "IPV4".')
# Perform Request
data = {
'reverse_dns': reverse_dns,
'type': ip_type,
'datacenter_id': datacenter_id
}
url = '%s/public_ips' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_public_ip(self, ip_id=None, reverse_dns=None):
# Error Handling
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
# Perform Request
data = {'reverse_dns': reverse_dns}
url = '%s/public_ips/%s' % (self.base_url, ip_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_public_ip(self, ip_id=None):
# Error Handling
if(ip_id is None):
raise ValueError('ip_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/public_ips/%s' % (self.base_url, ip_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Private Network Functions
# 'GET' Methods
def list_private_networks(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/private_networks' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_private_network(self, private_network_id):
# Error Handling
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
# Perform Request
url = '%s/private_networks/%s' % (self.base_url, private_network_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_private_network_servers(self, private_network_id=None):
# Error Handling
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
# Perform Request
url = ('%s/private_networks/%s/servers' %
(self.base_url, private_network_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_private_network_server(self, private_network_id=None,
server_id=None):
# Error Handling
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = ('%s/private_networks/%s/servers/%s' %
(self.base_url, private_network_id, server_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_private_network(self, private_network=None):
# Error Handling
if(private_network.name is None):
raise ValueError('name is a required parameter')
# Perform Request
data = {
'name': private_network.name,
'description': private_network.description,
'network_address': private_network.network_address,
'subnet_mask': private_network.subnet_mask,
'datacenter_id': private_network.datacenter_id
}
url = '%s/private_networks' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new private_network_id back to calling PrivateNetwork
# object
response = r.json()
private_network.specs.update(private_network_id=response['id'])
private_network.specs.update(api_token=self.header)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def attach_private_network_servers(self, private_network_id=None,
server_ids=None):
# Error Handling
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
if(server_ids is None):
raise ValueError(('server_ids is a required parameter. Make '
'sure you pass a list with at least one '
'server_id string'))
# Unpack servers
servers = []
for value in server_ids:
servers.append(value.server_id)
# Perform Request
data = {'servers': servers}
url = ('%s/private_networks/%s/servers' %
(self.base_url, private_network_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_private_network(
self,
private_network_id=None,
name=None,
description=None,
network_address=None,
subnet_mask=None):
# Perform Request
data = {
'name': name,
'description': description,
'network_address': network_address,
'subnet_mask': subnet_mask
}
url = '%s/private_networks/%s' % (self.base_url, private_network_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_private_network(self, private_network_id=None):
# Error Handling
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/private_networks/%s' % (self.base_url, private_network_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_private_network_server(self, private_network_id=None,
server_id=None):
# Error Handling
if(private_network_id is None):
raise ValueError('private_network_id is a required parameter')
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/private_networks/%s/servers/%s' %
(self.base_url, private_network_id, server_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Monitoring Center Functions
# 'GET' Methods
def list_server_usages(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/monitoring_center' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_usage(self, server_id=None, period='LAST_24H',
start_date=None, end_date=None):
# Error Handling
if(server_id is None):
raise ValueError('server_id is a required parameter')
if(period == 'CUSTOM'):
if(start_date is None):
raise ValueError(('start_date parameter is required when '
'using CUSTOM period'))
if(end_date is None):
raise ValueError(('end_date parameter is required when '
'using CUSTOM period'))
# Perform Request
parameters = {
'period': period,
'start_date': start_date,
'end_date': end_date
}
url = '%s/monitoring_center/%s' % (self.base_url, server_id)
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Monitoring Policy Functions
# 'GET' Methods
def list_monitoring_policies(self, page=None, per_page=None,
sort=None, q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/monitoring_policies' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_monitoring_policy(self, monitoring_policy_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
# Perform Request
url = ('%s/monitoring_policies/%s' %
(self.base_url, monitoring_policy_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_monitoring_policy_ports(self, monitoring_policy_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
# Perform Request
url = ('%s/monitoring_policies/%s/ports' %
(self.base_url, monitoring_policy_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_monitoring_policy_port(self, monitoring_policy_id=None,
port_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(port_id is None):
raise ValueError('port_id is a required parameter')
# Perform Request
url = ('%s/monitoring_policies/%s/ports/%s' %
(self.base_url, monitoring_policy_id, port_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_monitoring_policy_processes(self, monitoring_policy_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
# Perform Request
url = ('%s/monitoring_policies/%s/processes' %
(self.base_url, monitoring_policy_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_monitoring_policy_process(self, monitoring_policy_id=None,
process_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(process_id is None):
raise ValueError('process_id is a required parameter')
# Perform Request
url = ('%s/monitoring_policies/%s/processes/%s' %
(self.base_url, monitoring_policy_id, process_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def list_monitoring_policy_servers(self, monitoring_policy_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
# Perform Request
url = ('%s/monitoring_policies/%s/servers' %
(self.base_url, monitoring_policy_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_monitoring_policy_server(self, monitoring_policy_id=None,
server_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
url = ('%s/monitoring_policies/%s/servers/%s' %
(self.base_url, monitoring_policy_id, server_id))
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_monitoring_policy(self, monitoring_policy_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/monitoring_policies/%s' %
(self.base_url, monitoring_policy_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def delete_monitoring_policy_port(self, monitoring_policy_id=None,
port_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(port_id is None):
raise ValueError('port_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/monitoring_policies/%s/ports/%s' %
(self.base_url, monitoring_policy_id, port_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def delete_monitoring_policy_process(self, monitoring_policy_id=None,
process_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(process_id is None):
raise ValueError('process_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/monitoring_policies/%s/processes/%s' %
(self.base_url, monitoring_policy_id, process_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def detach_monitoring_policy_server(self, monitoring_policy_id=None,
server_id=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(server_id is None):
raise ValueError('server_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/monitoring_policies/%s/servers/%s' %
(self.base_url, monitoring_policy_id, server_id))
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_monitoring_policy(self, monitoring_policy=None,
thresholds=None, ports=None, processes=None):
# Error Handling
if(monitoring_policy is None):
raise ValueError(('monitoring_policy is a required parameter. '
'Make sure you pass a MonitoringPolicy object.'))
if(thresholds is None):
raise ValueError(('thresholds is a required parameter. Make '
'sure you pass a list with all 5 Threshold '
'objects(cpu, ram, disk, transfer, '
'internal_ping).'))
if(ports is None):
raise ValueError(
('ports is a required parameter. Make sure '
'you pass a list with at least one Port object.'))
if(processes is None):
raise ValueError(('processes is a required parameter. Make '
'sure you pass a list with at least one '
'Process object.'))
# Unpack Thresholds
new_thresholds = {}
for value in thresholds:
new_thresholds[value.entity] = {
'warning': {
'value': value.warning_value,
'alert': value.warning_alert
},
'critical': {
'value': value.critical_value,
'alert': value.critical_alert
}
}
# Unpack Ports
new_ports = []
for value in ports:
new_ports.append(value.specs)
# Unpack Processes
new_processes = []
for value in processes:
new_processes.append(value.process_set)
# Add Ports, Processes, and Thresholds to Monitoring Policy object
monitoring_policy.specs['thresholds'] = new_thresholds
monitoring_policy.specs['ports'] = new_ports
monitoring_policy.specs['processes'] = new_processes
# Perform Request
url = '%s/monitoring_policies' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header,
json=monitoring_policy.specs)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new monitoring_policy_id back to calling MonitoringPolicy
# object
response = r.json()
monitoring_policy.specs.update(monitoring_policy_id=response['id'])
monitoring_policy.specs.update(api_token=self.header)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_port(self, monitoring_policy_id=None, ports=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(ports is None):
raise ValueError(('ports is a required parameter. Make sure you '
'send in a list with at least one Port object'))
# Unpack ports
new_ports = []
for value in ports:
new_ports.append(value.specs)
# Perform Request
data = {'ports': new_ports}
url = ('%s/monitoring_policies/%s/ports' %
(self.base_url, monitoring_policy_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_process(self, monitoring_policy_id=None, processes=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(processes is None):
raise ValueError(('processes is a required parameter. Make '
'sure you send in a list with at least one '
'Process object'))
# Unpack processes
new_processes = []
for value in processes:
new_processes.append(value.process_set)
# Perform Request
data = {'processes': new_processes}
url = ('%s/monitoring_policies/%s/processes' %
(self.base_url, monitoring_policy_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def attach_monitoring_policy_server(self, monitoring_policy_id=None,
servers=None):
# Error Handling
if(monitoring_policy_id is None):
raise ValueError('monitoring_policy_id is a required parameter')
if(servers is None):
raise ValueError(('servers is a required parameter. Make sure '
'you send in a list with at least one '
'AttachServer object'))
# Unpack servers
add_servers = []
for value in servers:
add_servers.append(value.server_id)
# Perform Request
data = {'servers': add_servers}
url = ('%s/monitoring_policies/%s/servers' %
(self.base_url, monitoring_policy_id))
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_monitoring_policy(
self,
monitoring_policy_id=None,
monitoring_policy=None,
thresholds=None,
test=False):
try:
# Error Handling
if(monitoring_policy_id is None):
raise ValueError(
'monitoring_policy_id is a required parameter')
# Use flag to skip this live API call when running unit test
if(test == False):
# Make request for existing monitoring policy object
json = self.get_monitoring_policy(
monitoring_policy_id=monitoring_policy_id)
# Update policy specs with new values, if necessary.
if(monitoring_policy):
if(json['name'] != monitoring_policy.specs['name']):
if(monitoring_policy.specs['name'] is not None):
json['name'] = monitoring_policy.specs['name']
if(json['description'] !=
monitoring_policy.specs['description']):
if(monitoring_policy.specs['description'] is not None):
json['description'] = monitoring_policy.specs['description']
if(json['email'] != monitoring_policy.specs['email']):
if(monitoring_policy.specs['email'] is not None):
json['email'] = monitoring_policy.specs['email']
# Unpack thresholds
if(thresholds):
new_thresholds = {}
for value in thresholds:
new_thresholds[value.entity] = {
'warning': {
'value': value.warning_value,
'alert': value.warning_alert
},
'critical': {
'value': value.critical_value,
'alert': value.critical_alert
}
}
# Compare all threshold values and update, if necessary.
threshold_entities = ['cpu', 'ram', 'disk', 'transfer',
'internal_ping']
for value in threshold_entities:
if(value in new_thresholds.keys()):
if(json['thresholds'][value]['warning']['value'] !=
new_thresholds[value]['warning']['value']):
json['thresholds'][value]['warning']['value'] = new_thresholds[value]['warning']['value']
if(json['thresholds'][value]['warning']['alert'] !=
new_thresholds[value]['warning']['alert']):
json['thresholds'][value]['warning']['alert'] = new_thresholds[value]['warning']['alert']
if(json['thresholds'][value]['critical']['value'] !=
new_thresholds[value]['critical']['value']):
json['thresholds'][value]['critical']['value'] = new_thresholds[value]['critical']['value']
if(json['thresholds'][value]['critical']['alert'] !=
new_thresholds[value]['critical']['alert']):
json['thresholds'][value]['critical']['alert'] = new_thresholds[value]['critical']['alert']
# Perform Request
data = {
'name': json['name'],
'description': json['description'],
'email': json['email'],
'thresholds': json['thresholds']
}
url = ('%s/monitoring_policies/%s' %
(self.base_url, monitoring_policy_id))
r = requests_retry_session().put(url, headers=self.header, json=data)
else:
# Mock Request for Unit Testing
r = requests_retry_session().put(
self.base_url + '/monitoring_policies/%s' %
(monitoring_policy_id), headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def modify_port(self, monitoring_policy_id=None, port_id=None, port=None,
test=False):
try:
# Error Handling
if(monitoring_policy_id is None):
raise ValueError(
'monitoring_policy_id is a required parameter')
if(port_id is None):
raise ValueError('port_id is a required parameter')
# Use flag to skip this live API call when running unit test
if(test == False):
# Make request for existing port object
json = self.get_monitoring_policy_port(
monitoring_policy_id=monitoring_policy_id, port_id=port_id)
del json['id']
# Update port object with new values, if necessary.
if(json['alert_if'] != port.specs['alert_if']):
if(port.specs['alert_if'] is not None):
json['alert_if'] = port.specs['alert_if']
if(json['email_notification'] != port.specs['email_notification']):
if(port.specs['email_notification'] is not None):
json['email_notification'] = port.specs['email_notification']
# Perform Request
data = {'ports': json}
url = ('%s/monitoring_policies/%s/ports/%s' %
(self.base_url, monitoring_policy_id, port_id))
r = requests_retry_session().put(url, headers=self.header, json=data)
else:
# Mock Request for Unit Testing
r = requests_retry_session().put(
self.base_url + '/monitoring_policies/%s/ports/%s' %
(monitoring_policy_id, port_id), headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def modify_process(self, monitoring_policy_id=None, process_id=None,
process=None, test=False):
try:
# Error Handling
if(monitoring_policy_id is None):
raise ValueError(
'monitoring_policy_id is a required parameter')
if(process_id is None):
raise ValueError('process_id is a required parameter')
# Use flag to skip this live API call when running unit test
if(test == False):
# Make request for existing process object
json = self.get_monitoring_policy_process(
monitoring_policy_id=monitoring_policy_id,
process_id=process_id)
del json['id']
# Update process object with new values, if necessary.
if(json['alert_if'] != process.process_set['alert_if']):
if(process.process_set['alert_if'] is not None):
json['alert_if'] = process.process_set['alert_if']
if(json['email_notification'] !=
process.process_set['email_notification']):
if(process.process_set['email_notification'] is not None):
json['email_notification'] = process.process_set['email_notification']
# Perform Request
data = {'processes': json}
url = ('%s/monitoring_policies/%s/processes/%s' %
(self.base_url, monitoring_policy_id, process_id))
r = requests_retry_session().put(url, headers=self.header, json=data)
else:
# Mock Request for Unit Testing
r = requests_retry_session().put(
self.base_url + '/monitoring_policies/%s/processes/%s' %
(monitoring_policy_id, process_id), headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Log Functions
# 'GET' Methods
def list_logs(
self,
page=None,
per_page=None,
sort=None,
q=None,
fields=None,
period='LAST_24H',
start_date=None,
end_date=None):
# Error Handling
if(period == 'CUSTOM'):
if(start_date is None):
raise ValueError(('start_date parameter is required when '
'using CUSTOM period'))
if(end_date is None):
raise ValueError(('end_date parameter is required when '
'using CUSTOM period'))
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields,
'period': period,
'start_date': start_date,
'end_date': end_date
}
url = '%s/logs' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_log(self, log_id=None):
# Error Handling
if(log_id is None):
raise ValueError('log_id parameter is required')
# Perform Request
url = '%s/logs/%s' % (self.base_url, log_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# User Functions
# 'GET' Methods
def list_users(self, page=None, per_page=None, sort=None, q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/users' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_user(self, user_id=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
# Perform Request
url = '%s/users/%s' % (self.base_url, user_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def api_info(self, user_id=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
# Perform Request
url = '%s/users/%s/api' % (self.base_url, user_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def show_api_key(self, user_id=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
# Perform Request
url = '%s/users/%s/api/key' % (self.base_url, user_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def show_user_permissions(self):
# Perform Request
url = '%s/users/current_user_permissions' % (self.base_url)
r = requests.get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def ips_api_access_allowed(self, user_id=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
# Perform Request
url = '%s/users/%s/api/ips' % (self.base_url, user_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_user(self, name=None, password=None, email=None,
description=None):
# Error Handling
if(name is None):
raise ValueError('name is a required parameter')
if(password is None):
raise ValueError('password is a required parameter')
# Perform Request
data = {
'name': name,
'password': password,
'email': email,
'description': description
}
url = '%s/users' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_user_ip(self, user_id=None, user_ips=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
if(user_ips is None):
raise ValueError(('user_ips is a required parameter. Make '
'sure you pass a list with at least '
'one IP string.'))
# Unpack IPs
ips = []
for value in user_ips:
ips.append(value)
# Perform Request
data = {'ips': ips}
url = '%s/users/%s/api/ips' % (self.base_url, user_id)
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_user(self, user_id=None, description=None, email=None,
password=None, state=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
if(password is not None) and (len(password) < 8):
raise ValueError('password must be at least 8 characters long')
if(state is not None) and (state != 'ACTIVE') and (state != 'DISABLE'):
raise ValueError('state should be set to "ACTIVE" or "DISABLE".')
# Perform Request
data = {
'description': description,
'email': email,
'password': password,
'state': state
}
url = '%s/users/%s' % (self.base_url, user_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def modify_user_api(self, user_id=None, active=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
if(active is not None) and (active != True) and (active != False):
raise ValueError('active parameter only accepts a boolean value')
# Perform Request
data = {'active': active}
url = '%s/users/%s/api' % (self.base_url, user_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def change_api_key(self, user_id=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/users/%s/api/key' % (self.base_url, user_id)
try:
r = requests_retry_session().put(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_user(self, user_id=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/users/%s' % (self.base_url, user_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_user_ip(self, user_id=None, ip=None):
# Error Handling
if(user_id is None):
raise ValueError('user_id is a required parameter')
if(ip is None):
raise ValueError('ip is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/users/%s/api/ips/%s' % (self.base_url, user_id, ip)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Usage Functions
# 'GET' Methods
def list_usages(
self,
page=None,
per_page=None,
sort=None,
q=None,
fields=None,
period='LAST_24H',
start_date=None,
end_date=None):
# Error Handling
if(period == 'CUSTOM'):
if(start_date is None):
raise ValueError(('start_date parameter is required when '
'using CUSTOM period'))
if(end_date is None):
raise ValueError(('end_date parameter is required when '
'using CUSTOM period'))
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields,
'period': period,
'start_date': start_date,
'end_date': end_date
}
url = '%s/usages' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_pricing(self):
# Perform Request
url = '%s/pricing' % (self.base_url)
r = requests.get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# Recovery images
# 'GET' Methods
def list_recovery_images(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/recovery_appliances' % self.base_url
r = requests.get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def get_recovery_image(self, image_id=None):
# Error Handling
if(image_id is None):
raise ValueError('appliance_id is a required parameter')
# Perform Request
url = '%s/recovery_appliances/%s' % (self.base_url, image_id)
r = requests.get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# Server Appliance Functions
# 'GET' Methods
def list_appliances(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/server_appliances' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_appliance(self, appliance_id=None):
# Error Handling
if(appliance_id is None):
raise ValueError('appliance_id is a required parameter')
# Perform Request
url = '%s/server_appliances/%s' % (self.base_url, appliance_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# DVD Functions
# 'GET' Methods
def list_dvds(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/dvd_isos' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_dvd(self, iso_id=None):
# Error Handling
if(iso_id is None):
raise ValueError('iso_id parameter is required')
# Perform Request
url = '%s/dvd_isos/%s' % (self.base_url, iso_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Datacenter Functions
# 'GET' Methods
def list_datacenters(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/datacenters' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_datacenter(self, datacenter_id=None):
# Error Handling
if(datacenter_id is None):
raise ValueError('datacenter_id parameter is required')
# Perform Request
url = '%s/datacenters/%s' % (self.base_url, datacenter_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Pricing Functions
# 'GET' Methods
def pricing(self):
# Perform Request
url = '%s/pricing' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Ping Functions
# 'GET' Methods
def ping(self):
# Perform Request
url = '%s/ping' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Ping Auth Functions
# 'GET' Methods
def ping_auth(self):
# Perform Request
url = '%s/ping_auth' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# VPN Functions
# 'GET' Methods
def list_vpns(self, page=None, per_page=None, sort=None, q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/vpns' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_vpn(self, vpn_id=None):
# Error Handling
if(vpn_id is None):
raise ValueError('vpn_id is a required parameter')
# Perform Request
url = '%s/vpns/%s' % (self.base_url, vpn_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def download_config(self, vpn_id=None, file_path=None):
# Error Handling
if(vpn_id is None):
raise ValueError('vpn_id is a required parameter')
# Perform Request
url = '%s/vpns/%s/configuration_file' % (self.base_url, vpn_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
body = r.json()
filestring = base64.b64decode(body["config_zip_file"])
zipPath = file_path + '.zip'
with open(zipPath, 'wb') as zipFile:
zipFile.write(filestring)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_vpn(self, vpn=None):
# Perform Request
data = {
'name': vpn.name,
'description': vpn.description,
'datacenter_id': vpn.datacenter_id
}
url = '%s/vpns' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new image_id back to calling Image object
response = r.json()
vpn.specs.update(vpn_id=response['id'])
vpn.specs.update(api_token=self.header)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_vpn(self, vpn_id=None):
# Error Handling
if(vpn_id is None):
raise ValueError('vpn_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/vpns/%s' % (self.base_url, vpn_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_vpn(self, vpn_id=None, name=None, description=None):
# Error Handling
if(vpn_id is None):
raise ValueError('vpn_id is a required parameter')
# Perform Request
data = {
'name': name,
'description': description
}
url = '%s/vpns/%s' % (self.base_url, vpn_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Role Functions
# 'GET' Methods
def list_roles(self, page=None, per_page=None, sort=None, q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/roles' % self.base_url
try:
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_role(self, role_id=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
url = '%s/roles/%s' % (self.base_url, role_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def permissions(self, role_id=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
url = '%s/roles/%s/permissions' % (self.base_url, role_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def current_user_permissions(self):
# Perform Request
url = '%s/users/current_user_permissions' % (self.base_url)
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def role_users(self, role_id=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
url = '%s/roles/%s/users' % (self.base_url, role_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def get_role_user(self, role_id=None, user_id=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
url = '%s/roles/%s/users/%s' % (self.base_url, role_id, user_id)
try:
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'POST' Methods
def create_role(self, name=None):
# Perform Request
data = {
'name': name
}
url = '%s/roles' % self.base_url
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def add_users(self, role_id=None, users=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
data = {
'users': users
}
url = '%s/roles/%s/users' % (self.base_url, role_id)
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def clone_role(self, role_id=None, name=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
data = {
'name': name
}
url = '%s/roles/%s/clone' % (self.base_url, role_id)
try:
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'DELETE' Methods
def delete_role(self, role_id=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/roles/%s' % (self.base_url, role_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def remove_user(self, role_id=None, user_id=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/roles/%s/users/%s' % (self.base_url, role_id, user_id)
try:
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# 'PUT' Methods
def modify_role(self, role_id=None, name=None, description=None,
state=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
data = {
'name': name,
'description': description,
'state': state
}
url = '%s/roles/%s' % (self.base_url, role_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
def modify_permissions(
self,
role_id=None,
servers=None,
images=None,
shared_storages=None,
firewalls=None,
load_balancers=None,
ips=None,
private_networks=None,
vpns=None,
monitoring_centers=None,
monitoring_policies=None,
backups=None,
logs=None,
users=None,
roles=None,
usages=None,
interactive_invoices=None):
# Error Handling
if(role_id is None):
raise ValueError('role_id is a required parameter')
# Perform Request
data = {
'servers': servers,
'images': images,
'sharedstorages': shared_storages,
'firewalls': firewalls,
'loadbalancers': load_balancers,
'ips': ips,
'privatenetwork': private_networks,
'vpn': vpns,
'monitoringcenter': monitoring_centers,
'monitoringpolicies': monitoring_policies,
'backups': backups,
'logs': logs,
'users': users,
'roles': roles,
'usages': usages,
'interactiveinvoice': interactive_invoices
}
url = '%s/roles/%s/permissions' % (self.base_url, role_id)
try:
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
except http_client.HTTPException:
if r is not None:
error_message = (
'Error Code: %s. Error Message: %s. Response Headers :%s' %
(r.status_code, r.text, r.headers))
raise Exception(error_message)
else:
raise
# Block Storage Functions
# 'GET' Methods
def list_block_storages(self, page=None, per_page=None, sort=None,
q=None, fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/block_storages' % self.base_url
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def get_block_storage(self, block_storage_id=None):
# Error Handling
if(block_storage_id is None):
raise ValueError('block_storage_id is a required parameter')
# Perform Request
url = '%s/block_storages/%s' % (self.base_url, block_storage_id)
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# 'POST' Methods
def create_block_storage(self, block_storage=None):
# Perform Request
data = {
'name': block_storage.name,
'description': block_storage.description,
'size': block_storage.size,
'server': block_storage.server_id,
'datacenter_id': block_storage.datacenter_id,
'execution_group': block_storage.execution_group
}
url = '%s/block_storages' % self.base_url
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new block_storage_id back to calling BlockStorage object
response = r.json()
block_storage.specs.update(block_storage_id=response['id'])
block_storage.specs.update(api_token=self.header)
return r.json()
def attach_block_storage(self, block_storage_id=None,
server_id=None):
# Error Handling
if(block_storage_id is None):
raise ValueError('block_storage_id is a required parameter')
if(server_id is None):
raise ValueError('server_id is a required parameter.')
# Perform Request
data = {'server': server_id}
url = ('%s/block_storages/%s/server' %
(self.base_url, block_storage_id))
r = requests_retry_session().post(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# 'PUT' Methods
def modify_block_storage(self, block_storage_id=None, name=None,
description=None, size=None):
# Error Handling
if(block_storage_id is None):
raise ValueError('block_storage_id is a required parameter')
# Perform Request
data = {
'name': name,
'description': description,
'size': size
}
url = '%s/block_storages/%s' % (self.base_url, block_storage_id)
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# 'DELETE' Methods
def delete_block_storage(self, block_storage_id=None):
# Error Handling
if(block_storage_id is None):
raise ValueError('block_storage_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/block_storages/%s' % (self.base_url, block_storage_id)
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def detach_block_storage(self, block_storage_id=None):
# Error Handling
if(block_storage_id is None):
raise ValueError('block_storage_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = ('%s/block_storages/%s/server' %
(self.base_url, block_storage_id))
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# Ssh Key Functions
# 'GET' Methods
def list_ssh_keys(self, page=None, per_page=None, sort=None, q=None,
fields=None):
# Perform Request
parameters = {
'page': page,
'per_page': per_page,
'sort': sort,
'q': q,
'fields': fields
}
url = '%s/ssh_keys' % self.base_url
r = requests_retry_session().get(url, headers=self.header, params=parameters)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def get_ssh_key(self, ssh_key_id=None):
# Error Handling
if(ssh_key_id is None):
raise ValueError('ssh_key_id is a required parameter')
# Perform Request
url = '%s/ssh_keys/%s' % (self.base_url, ssh_key_id)
r = requests_retry_session().get(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# 'POST' Methods
def create_ssh_key(self, ssh_key=None):
# Perform Request
url = '%s/ssh_keys' % self.base_url
r = requests_retry_session().post(url, headers=self.header, json=ssh_key.specs)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
# Assign new ssh_key_id back to calling SshKey object
response = r.json()
ssh_key.specs.update(ssh_key_id=response['id'])
ssh_key.specs.update(api_token=self.header)
return r.json()
# 'DELETE' Methods
def delete_ssh_key(self, ssh_key_id=None):
# Error Handling
if(ssh_key_id is None):
raise ValueError('ssh_key_id is a required parameter')
# Perform Request
self.header['content-type'] = 'application/json'
url = '%s/ssh_keys/%s' % (self.base_url, ssh_key_id)
r = requests_retry_session().delete(url, headers=self.header)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# 'PUT' Methods
def modify_ssh_key(self, ssh_key_id=None, name=None, description=None):
# Error Handling
if(ssh_key_id is None):
raise ValueError('ssh_key_id is a required parameter')
# Perform Request
data = {
'name': name,
'description': description
}
url = '%s/ssh_keys/%s' % (self.base_url, ssh_key_id)
r = requests_retry_session().put(url, headers=self.header, json=data)
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
# Utility Classes
class Server(object):
# Init Function
def __init__(
self,
name=None,
description=None,
fixed_instance_size_id=None,
vcore=None,
cores_per_processor=None,
ram=None,
appliance_id=None,
password=None,
power_on=None,
firewall_policy_id=None,
ip_id=None,
load_balancer_id=None,
monitoring_policy_id=None,
datacenter_id=None,
rsa_key=None,
private_network_id=None,
server_type=None,
public_key=None,
baremetal_model_id=None,
ipv6_range=None,
hostname=None,
execution_group=None):
self.first_password = None
self.first_ip = None
self.specs = {
'name': name,
'description': description,
'hardware': {
'fixed_instance_size_id': fixed_instance_size_id,
'vcore': vcore,
'cores_per_processor': cores_per_processor,
'ram': ram,
'baremetal_model_id': baremetal_model_id
},
'appliance_id': appliance_id,
'password': password,
'power_on': power_on,
'firewall_policy_id': firewall_policy_id,
'ip_id': ip_id,
'load_balancer_id': load_balancer_id,
'monitoring_policy_id': monitoring_policy_id,
'datacenter_id': datacenter_id,
'rsa_key': rsa_key,
'private_network_id': private_network_id,
'server_type': server_type,
'public_key': public_key,
'ipv6_range': ipv6_range,
'hostname': hostname,
'execution_group': execution_group
}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = (
'ACTIVE',
'ENABLED',
'POWERED_ON',
'POWERED_OFF',
'ON RECOVERY')
def __repr__(self):
return (
'Server: name=%s, description=%s, fixed_instance_size_id=%s, '
'vcore=%s, cores_per_processor=%s, ram=%s, baremetal_model_id=%s, appliance_id=%s, '
'password=%s, power_on=%s, firewall_policy_id=%s, ip_id=%s, '
'load_balancer_id=%s, monitoring_policy_id=%s, '
'rsa_key=%s, datacenter_id=%s, first_password=%s, '
'first_ip=%s, public_key=%s, server_type=%s, ipv6_range=%s, execution_group=%s, hostname=%s' %
(self.specs['name'],
self.specs['description'],
self.specs['hardware']['fixed_instance_size_id'],
self.specs['hardware']['vcore'],
self.specs['hardware']['cores_per_processor'],
self.specs['hardware']['ram'],
self.specs['hardware']['baremetal_model_id'],
self.specs['appliance_id'],
self.specs['password'],
self.specs['power_on'],
self.specs['firewall_policy_id'],
self.specs['ip_id'],
self.specs['load_balancer_id'],
self.specs['monitoring_policy_id'],
self.specs['rsa_key'],
self.specs['datacenter_id'],
self.first_password,
self.first_ip,
self.specs['server_type'],
self.specs['ipv6_range'],
self.specs['execution_group'],
self.specs['hostname'],
))
def get(self):
# Perform Request
url = ('%s/servers/%s' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def hardware(self):
# Perform Request
url = ('%s/servers/%s/hardware' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def hdds(self):
# Perform Request
url = ('%s/servers/%s/hardware/hdds' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def image(self):
# Perform Request
url = ('%s/servers/%s/image' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def ips(self):
# Perform Request
url = ('%s/servers/%s/ips' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def status(self):
# Perform Request
url = ('%s/servers/%s/status' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def dvd(self):
# Perform Request
url = ('%s/servers/%s/dvd' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def private_networks(self):
# Perform Request
url = ('%s/servers/%s/private_networks' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def snapshots(self):
# Perform Request
url = ('%s/servers/%s/snapshots' %
(self.base_url, self.specs['server_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=15):
# Capture start time
start = time.time()
duration = 0
# Check initial server status
url = '%s/servers/%s' % (self.base_url, self.specs['server_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial server state and percent values
server_state = response['status']['state']
percent = response['status']['percent']
# Keep polling the server's status until good
while (server_state not in self.good_states) or (percent is not None):
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update server state and percent values
server_state = response['status']['state']
percent = response['status']['percent']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
# Parse for first IP address
if len(response['ips']) == 1:
self.first_ip = response['ips'][0]
return {'duration': duration}
def wait_deleted(self, timeout=25, interval=15):
# Capture start time
start = time.time()
duration = 0
# Check initial server status
url = '%s/servers/%s' % (self.base_url, self.specs['server_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Keep polling the server's status until got 404
while r.status_code != 404 :
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class Hdd(object):
# Init Function
def __init__(self, size=None, is_main=None):
self.specs = {
'size': size,
'is_main': is_main
}
def __repr__(self):
return ('HDD: size=%s, is_main=%s' %
(self.specs['size'], self.specs['is_main']))
class AttachServer(object):
# Init Function
def __init__(self, server_id=None, rights=None, server_ip_id=None):
self.server_id = server_id
self.rights = rights
self.server_ip_id = server_ip_id
def __repr__(self):
return ('AttachServer: server_id=%s, rights=%s, server_ip_id=%s' %
(self.server_id, self.rights, self.server_ip_id))
class Image(object):
# Init Function
def __init__(
self,
server_id=None,
name=None,
description=None,
frequency=None,
num_images=None,
source='server',
url=None,
os_id=None,
isotype=None,
type=None):
self.server_id = server_id
self.name = name
self.description = description
self.frequency = frequency
self.num_images = num_images
self.source = source
self.url = url
self.os_id = os_id
self.type = isotype
self.specs = {}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return (
'Image: server_id=%s, name=%s, description=%s, '
'frequency=%s, num_images=%s, source=%s, url=%s'
'os_id=%s, type=%s' %
(self.server_id,
self.name,
self.description,
self.frequency,
self.num_images,
self.source,
self.url,
self.os_id,
self.type))
def get(self):
# Perform Request
url = ('%s/images/%s' %
(self.base_url, self.specs['image_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=15):
# Capture start time
start = time.time()
duration = 0
# Check initial image status
url = '%s/images/%s' % (self.base_url, self.specs['image_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial server state and percent values
image_state = response['state']
# Keep polling the server's status until good
while image_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update server state and percent values
image_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class SharedStorage(object):
# Init Function
def __init__(self, name=None, description=None, size=None,
datacenter_id=None):
self.name = name
self.description = description
self.size = size
self.datacenter_id = datacenter_id
self.specs = {}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return ('Shared Storage: name=%s, description=%s, size=%s' %
(self.name, self.description, self.size, self.datacenter_id))
def get(self):
# Perform Request
url = ('%s/shared_storages/%s' %
(self.base_url, self.specs['shared_storage_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def servers(self):
# Perform Request
url = ('%s/shared_storages/%s/servers' %
(self.base_url, self.specs['shared_storage_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=5):
# Capture start time
start = time.time()
duration = 0
# Check initial image status
url = '%s/shared_storages/%s' % (self.base_url,
self.specs['shared_storage_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial server state and percent values
shared_storage_state = response['state']
# Keep polling the server's status until good
while shared_storage_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update server state and percent values
shared_storage_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class FirewallPolicyRule(object):
# Init Function
def __init__(self, protocol=None, port_from=None, port_to=None,
source=None, action=None, description=None, port=None):
self.rule_set = {
'protocol': protocol,
'port_from': port_from,
'port_to': port_to,
'source': source,
'action': action,
'description': description,
'port': port
}
def __repr__(self):
return ('FirewallPolicyRule: protocol=%s, port_from=%s, '
'port_to=%s, source=%s, action=%s, description=%s, port=%s' %
(self.rule_set['protocol'], self.rule_set['port_from'],
self.rule_set['port_to'], self.rule_set['source'], self.rule_set['action'], self.rule_set['description'], self.rule_set['port']))
class FirewallPolicy(object):
# Init Function
def __init__(self, name=None, description=None):
self.specs = {
'name': name,
'description': description
}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return ('FirewallPolicy: name=%s, description=%s' %
(self.specs['name'], self.specs['description']))
def get(self):
# Perform Request
url = ('%s/firewall_policies/%s' %
(self.base_url, self.specs['firewall_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def ips(self):
# Perform Request
url = ('%s/firewall_policies/%s/server_ips' %
(self.base_url, self.specs['firewall_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def rules(self):
# Perform Request
url = ('%s/firewall_policies/%s/rules' %
(self.base_url, self.specs['firewall_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=5):
# Capture start time
start = time.time()
duration = 0
# Check initial image status
url = '%s/firewall_policies/%s' % (self.base_url,
self.specs['firewall_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial server state and percent values
firewall_state = response['state']
# Keep polling the server's status until good
while firewall_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update server state and percent values
firewall_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class LoadBalancerRule(object):
# Init Function
def __init__(self, protocol=None, port_balancer=None, port_server=None,
source=None):
self.rule_set = {
'protocol': protocol,
'port_balancer': port_balancer,
'port_server': port_server,
'source': source
}
def __repr__(self):
return (
'LoadBalancerRule: protocol=%s, port_balancer=%s, '
'port_server=%s, source=%s' %
(self.rule_set['protocol'],
self.rule_set['port_balancer'],
self.rule_set['port_server'],
self.rule_set['source']))
class LoadBalancer(object):
# Init Function
def __init__(self, health_check_path=None, health_check_parse=None,
name=None, description=None, health_check_test=None,
health_check_interval=None, persistence=None,
persistence_time=None, method=None, datacenter_id=None):
self.specs = {
'health_check_path': health_check_path,
'health_check_parse': health_check_parse,
'name': name,
'description': description,
'health_check_test': health_check_test,
'health_check_interval': health_check_interval,
'persistence': persistence,
'persistence_time': persistence_time,
'method': method,
'datacenter_id': datacenter_id
}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return ('LoadBalancer: health_check_path=%s, health_check_parse=%s, '
'name=%s, description=%s, health_check_test=%s, '
'health_check_interval=%s, persistence=%s, '
'persistence_time=%s, method=%s, datacenter_id=%s' %
(self.specs['health_check_path'],
self.specs['health_check_parse'], self.specs['name'],
self.specs['description'], self.specs['health_check_test'],
self.specs['health_check_interval'],
self.specs['persistence'], self.specs['persistence_time'],
self.specs['method'], self.datacenter_id))
def get(self):
# Perform Request
url = ('%s/load_balancers/%s' %
(self.base_url, self.specs['load_balancer_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def ips(self):
# Perform Request
url = ('%s/load_balancers/%s/server_ips' %
(self.base_url, self.specs['load_balancer_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def rules(self):
# Perform Request
url = ('%s/load_balancers/%s/rules' %
(self.base_url, self.specs['load_balancer_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=5):
# Capture start time
start = time.time()
duration = 0
# Check initial image status
url = '%s/load_balancers/%s' % (self.base_url,
self.specs['load_balancer_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial server state and percent values
load_balancer_state = response['state']
# Keep polling the server's status until good
while load_balancer_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update server state and percent values
load_balancer_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class PrivateNetwork(object):
# Init Function
def __init__(self, name=None, description=None, network_address=None,
subnet_mask=None, datacenter_id=None):
self.name = name
self.description = description
self.network_address = network_address
self.subnet_mask = subnet_mask
self.datacenter_id = datacenter_id
self.specs = {}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return (
'Private Network: name=%s, description=%s, network_address=%s, '
'subnet_mask=%s' %
(self.name, self.description, self.network_address, self.subnet_mask))
def get(self):
# Perform Request
url = ('%s/private_networks/%s' %
(self.base_url, self.specs['private_network_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def servers(self):
# Perform Request
url = ('%s/private_networks/%s/servers' %
(self.base_url, self.specs['private_network_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=5):
# Capture start time
start = time.time()
duration = 0
# Check initial image status
url = '%s/private_networks/%s' % (self.base_url,
self.specs['private_network_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial server state and percent values
private_network_state = response['state']
# Keep polling the server's status until good
while private_network_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update server state and percent values
private_network_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class MonitoringPolicy(object):
# Init Function
def __init__(self, name=None, description=None, email=None, agent=None):
self.specs = {
'name': name,
'description': description,
'email': email,
'agent': agent
}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return ('MonitoringPolicy: name=%s, description=%s, email=%s, '
'agent=%s' %
(self.specs['name'], self.specs['description'],
self.specs['email'], self.specs['agent']))
def get(self):
# Perform Request
url = ('%s/monitoring_policies/%s' %
(self.base_url, self.specs['monitoring_policy_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def ports(self):
# Perform Request
url = ('%s/monitoring_policies/%s/ports' %
(self.base_url, self.specs['monitoring_policy_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def processes(self):
# Perform Request
url = ('%s/monitoring_policies/%s/processes' %
(self.base_url, self.specs['monitoring_policy_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def servers(self):
# Perform Request
url = ('%s/monitoring_policies/%s/servers' %
(self.base_url, self.specs['monitoring_policy_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=5):
# Capture start time
start = time.time()
duration = 0
# Check initial image status
url = '%s/monitoring_policies/%s' % (self.base_url,
self.specs['monitoring_policy_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial server state and percent values
mp_state = response['state']
# Keep polling the server's status until good
while mp_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update server state and percent values
mp_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class Threshold(object):
# Init Function
def __init__(self, entity=None, warning_value=None, warning_alert=None,
critical_value=None, critical_alert=None):
self.entity = entity
self.warning_value = warning_value
self.warning_alert = warning_alert
self.critical_value = critical_value
self.critical_alert = critical_alert
def __repr__(self):
return (
'Threshold: entity=%s, warning_value=%s, warning_alert=%s, '
'critical_value=%s, critical_alert=%s' %
(self.entity,
self.warning_value,
self.warning_alert,
self.critical_value,
self.critical_alert))
class Port(object):
# Init Function
def __init__(self, protocol=None, port=None, alert_if=None,
email_notification=None):
self.specs = {
'protocol': protocol,
'port': port,
'alert_if': alert_if,
'email_notification': email_notification
}
def __repr__(self):
return (
'Port: protocol=%s, port=%s, alert_if=%s, '
'email_notification=%s' %
(self.specs['protocol'],
self.specs['port'],
self.specs['alert_if'],
self.specs['email_notification']))
class Process(object):
# Init Function
def __init__(self, process=None, alert_if=None, email_notification=None):
self.process_set = {
'process': process,
'alert_if': alert_if,
'email_notification': email_notification
}
def __repr__(self):
return ('Process: process=%s, alert_if=%s, email_notification=%s' %
(self.process_set['process'], self.process_set['alert_if'],
self.process_set['email_notification']))
class Vpn(object):
# Init Function
def __init__(self, name=None, description=None, datacenter_id=None):
self.name = name
self.description = description
self.datacenter_id = datacenter_id
self.specs = {}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return ('Vpn: name=%s, description=%s, datacenter_id=%s' %
(self.name, self.description, self.datacenter_id))
def get(self):
# Perform Request
url = ('%s/vpns/%s' %
(self.base_url, self.specs['vpn_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=15):
# Capture start time
start = time.time()
duration = 0
# Check initial image status
url = '%s/vpns/%s' % (self.base_url, self.specs['vpn_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial server state and percent values
vpn_state = response['state']
# Keep polling the server's status until good
while vpn_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check server status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update server state and percent values
vpn_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class BlockStorage(object):
# Init Function
def __init__(self, name=None, description=None, size=None,
datacenter_id=None, server_id=None, execution_group=None):
self.name = name
self.description = description
self.size = size
self.datacenter_id = datacenter_id
self.server_id = server_id
self.execution_group = execution_group
self.specs = {}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return ('Block Storage: name=%s, description=%s, size=%s, execution_group=%s, server_id=%s' % (
self.name, self.description, self.size, self.datacenter_id, self.execution_group, self.server_id))
def get(self):
# Perform Request
url = ('%s/block_storages/%s' %
(self.base_url, self.specs['block_storage_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def server(self):
# Perform Request
url = ('%s/block_storages/%s/server' %
(self.base_url, self.specs['block_storage_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=5):
# Capture start time
start = time.time()
duration = 0
# Check initial block storage status
url = '%s/block_storages/%s' % (self.base_url,
self.specs['block_storage_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial block storage state and percent values
block_storage_state = response['state']
# Keep polling the block storage's status until good
while block_storage_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check block storage status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update block storage state and percent values
block_storage_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration}
class SshKey(object):
# Init Function
def __init__(self, name=None, description=None,
state=None, servers=None, md5=None,
public_key=None, creation_date=None):
self.specs = {
'name': name,
'description': description,
'state': state,
'servers': servers,
'md5': md5,
'public_key': public_key,
'creation_date': creation_date
}
self.base_url = 'https://cloudpanel-api.1and1.com/v1'
self.success_codes = (200, 201, 202)
self.good_states = ('ACTIVE', 'ENABLED', 'POWERED_ON', 'POWERED_OFF')
def __repr__(self):
return ('SshKey: name=%s, description=%s, '
'state=%s, servers=%s, md5=%s, '
'public_key=%s, creation_date=%s, ' %
(self.specs['name'], self.specs['description'],
self.specs['state'], self.specs['servers'],
self.specs['md5'], self.specs['public_key'],
self.specs['creation_date']))
def get(self):
# Perform Request
url = ('%s/ssh_keys/%s' %
(self.base_url, self.specs['ssh_key_id']))
r = requests_retry_session().get(url, headers=self.specs['api_token'])
# Handle Potential Response Errors
if r.status_code not in self.success_codes:
error_message = ('Error Code: %s. Error Message: %s.' %
(r.status_code, r.text))
raise Exception(error_message)
return r.json()
def wait_for(self, timeout=25, interval=5):
# Capture start time
start = time.time()
duration = 0
# Check initial ssh_key status
url = '%s/ssh_keys/%s' % (self.base_url,
self.specs['ssh_key_id'])
r = requests_retry_session().get(url, headers=self.specs['api_token'])
response = r.json()
# Store initial ssh_key state and percent values
ssh_key_state = response['state']
# Keep polling the ssh_key's status until good
while ssh_key_state not in self.good_states:
# Wait 15 seconds before polling again
time.sleep(interval)
# Check ssh_key status again
r = requests_retry_session().get(
url, headers=self.specs['api_token'])
response = r.json()
# Update ssh_key state and percent values
ssh_key_state = response['state']
# Check for timeout
seconds = (time.time() - start)
duration = seconds / 60
if duration > timeout:
print('The operation timed out after %s minutes.' % timeout)
return
return {'duration': duration} | 1and1 | /1and1-1.8.0.tar.gz/1and1-1.8.0/oneandone/client.py | client.py |
Hello, ONE
----------
Usage
-----
`pip install 1app`
Then, simply use the command periodically:
`1app --params...`
This will save data to:
`settings.BASE_DIR/data/1app:default/Item`
N-Spacing
---------
If you want to seprate different sessions and sources, just use name param:
`1app --params... --name Username`
This will save to:
`settings.BASE_DIR/data/1app:Name/Type`
The `--name` value can be arbitray filesystem-compatible filename sub-string, so, you can use it to separate data by accounts, languages, or other features.
**NOTE**: Corresponding auth and session data will be stored in `settings.BASE_DIR/sessions` folder.
Saving to specific DIR
----------------------
Saving to custom folder simply pass `--path` parameter, like:
`1app --params --name Name --path /home/mindey/Desktop/mydata`
| 1app | /1app-0.0.2.tar.gz/1app-0.0.2/README.md | README.md |
Version 0.0.11
================================================================================
* Fix screen scale for mobile
Version 0.0.10
--------------------------------------------------------------------------------
* Update title
Version 0.0.9
--------------------------------------------------------------------------------
* Use correct link for copyright
Version 0.0.8
--------------------------------------------------------------------------------
* Change name to 1 BEAD
Version 0.0.7
--------------------------------------------------------------------------------
* Add logo from Kate
Version 0.0.6
--------------------------------------------------------------------------------
* Switch to use React and Material-UI
* Run test before publishing
Version 0.0.5
--------------------------------------------------------------------------------
* Temporarily remove static as we do not have any
Version 0.0.4
--------------------------------------------------------------------------------
* Switch to use Jinja templates
* Add wait option when updating so it actually update
Non-tty does not update without an update frequency set or within frequency.
Version 0.0.3
--------------------------------------------------------------------------------
* Remove goal info
Version 0.0.2
--------------------------------------------------------------------------------
* Add first goal
Version 0.0.1
--------------------------------------------------------------------------------
* Add mission
* Initial setup
* Initial commit
| 1bead.org | /1bead.org-0.0.11.tar.gz/1bead.org-0.0.11/docs/CHANGELOG.rst | CHANGELOG.rst |
<h1 align="center">
<br>
<a href="https://github.com/gopinath-langote/1build">
<img src="https://github.com/gopinath-langote/1build/blob/master/docs/assets/1build-logo.png?raw=true" alt="1build" width="500"></a>
<br>
</h1>
<br>
<p align="center">
<a href="https://pypi.org/project/1build/">
<img src="https://img.shields.io/pypi/v/1build.svg" alt="PyPi Version">
</a>
<a href="https://pypi.org/project/1build/">
<img src="https://img.shields.io/pypi/pyversions/1build.svg" alt="Supported Python Versions">
</a>
<a href="https://travis-ci.org/gopinath-langote/1build">
<img src="https://travis-ci.org/gopinath-langote/1build.svg?branch=master" alt="Build Status">
</a>
<a href="https://codecov.io/gh/gopinath-langote/1build">
<img src="https://img.shields.io/codecov/c/gh/gopinath-langote/1build.svg" alt="Code Coverage">
</a>
<a href="https://requires.io/github/gopinath-langote/1build/requirements/?branch=master">
<img src="https://requires.io/github/gopinath-langote/1build/requirements.svg?branch=master" alt="Requirements Status">
</a>
<a href="https://pypi.org/project/1build">
<img src="https://img.shields.io/pypi/dm/1build.svg" alt="Downloads">
</a>
</p>
<br>
1build is an automation tool that arms you with the convenience to configure project-local command line aliases – and then
run the commands quickly and easily. It is particularly helpful when you deal with multiple projects and switch between
them all the time. It is often the fact that different projects use different build tools and have different environment
requirements – and then switching from one project to another is becoming increasingly cumbersome. That is where 1build comes
into play.
With 1build you can create simple and easily memorable command aliases for commonly used project commands such as build,
test, run or anything else. These aliases will have a project-local scope which means that they will be accessible only
within the project directory. This way you can unify all your projects to build with the same simple command disregarding
of what build tool they use. It will remove the hassle of remembering all those commands improving the mental focus for
the things that actually matter.
## Install
```bash
pip install 1build
```
or
```bash
pip3 install 1build
```
## Usage
### Configuration
- create project configuration file in the project folder with name `1build.yaml`
- Example of `1build.yaml` for JVM maven project:
```yaml
project: Sample JVM Project Name
commands:
- build: mvn clean package
- lint: mvn antrun:run@ktlint-format
- test: mvn clean test
```
### Running 1build for the above sample project:
- building the project
```console
1build build
```
- fix the coding guidelinges lint and run tests (executing more than one commands at once)
```console
1build lint test
```
### Using `before` and `after` commands
Consider that your project `X` requires `Java 11` and the other project requires `Java 8`. It is a headache to always
remember to switch the java version. What you want is to switch to `Java 11` automatically when you build the project
`X` and switch it back to `Java 8` when the build is complete. Another example – a project requires `Docker` to be up
and running or you need to clean up the database after running a test harness.
This is where `before` & `after` commands are useful. These commands are both optional –
you can use one of them, both or neither.
#### Examples:
1. Switching to `Java 11` and then back to `Java 8`
```yaml
project: Sample JVM Project Name
before: ./switch_to_java_11.sh
after: ./switch_to_java_8.sh
commands:
- build: mvn clean package
```
2. Ensure that `Docker` is up and running
```yaml
project: Containerized Project
before: ./docker_run.sh
commands:
- build: ./gradlew clean
```
3. Clean up database after some commands
```yaml
project: Containerized Project
after: ./clean_database.sh
commands:
- build: ./gradlew clean
```
### Command usage
```text
usage: 1build [-h] [-l] [-v] [command]
positional arguments:
command Command to run - from `1build.yaml` file
optional arguments:
-h, --help Print this help message
-l, --list Show all available commands - from `1build.yaml` file
-v, --version Show version of 1build and exit
-i, --init Create default `1build.yaml` configuration file
```
## Contributing
Please read [CONTRIBUTING.md](https://github.com/gopinath-langote/1build/blob/master/CONTRIBUTING.md) for details on our code of conduct, and the process for submitting pull requests to us.
## Versioning
We use [Semantic Versioning](http://semver.org/) for all our releases. For the versions available, see the [tags on this repository](https://github.com/gopinath-langote/1build/tags).
## Changelog
All notable changes to this project in each release will be documented in [CHANGELOG.md](https://github.com/gopinath-langote/1build/blob/master/docs/CHANGELOG.md).
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/).
## License
This project is licensed under the MIT License - see the [LICENSE](LICENSE) file for details
## Authors
* **Gopinath Langote** - *Initial work & Maintainer* – [Github](https://github.com/gopinath-langote/) –[Twitter](https://twitter.com/GopinathLangote)
* **Alexander Lukianchuk** - *Maintainer* – [Github](https://github.com/landpro) – [Twitter](https://twitter.com/landpro)
See also the list of [contributors](https://github.com/gopinath-langote/1build/contributors) who participated in this project.
| 1build | /1build-1.1.1.tar.gz/1build-1.1.1/README.md | README.md |
1C Utils
=========
**1C Utils** это набор скриптов для управления и обслуживания серверов 1С.
Репозиторий:
https://gitlab.com/onegreyonewhite/1c-utilites
Для вопросов и предложений используйте трекер задач:
https://gitlab.com/onegreyonewhite/1c-utilites/issues
Возможности
--------
На данный момент поддерживается только архивация PostgreSQL и файловых БД 1С.
Quickstart
----------
Поддерживается любая Linux-система с наличием в ней Python 2.7/3.4/3.5
и необходимых утилит обслуживания:
* pg_dump для архивации PostgreSQL баз
* tar для упаковывания файловых баз
Установка:
.. sourcecode:: bash
pip install 1c-utilites
1c-utilites --help
| 1c-utilites | /1c-utilites-0.1b0.tar.gz/1c-utilites-0.1b0/README.rst | README.rst |
from __future__ import unicode_literals
import os
import sys
import time
import logging
import subprocess
from configparser import ConfigParser
logging.basicConfig(
format='%(asctime)s - %(levelname)s - %(message)s',
datefmt='%m/%d/%Y %H:%M:%S',
level=logging.DEBUG
)
class BaseBackupBackend(object):
DEFAULT_NAME_MASK = '1c-backup'
def __init__(self, args):
self.args = args
self.config = self.get_config(self.args.config)
self.backups_dir = self.config.get(
"main", "backups_dir", fallback="/tmp/"
) + "/"
self.backups_files = self.config.getint("main", "last", fallback=5)
@property
def file_name(self):
file_name = self.backups_dir
file_name += "{}-{}.gz".format(
self.DEFAULT_NAME_MASK, int(time.time())
)
return file_name
def get_config(self, config_name='/etc/1c/backup.ini'):
config = ConfigParser()
logging.debug("Read config " + config_name)
config.read([config_name])
return config
def get_backups_list(self):
return sorted([
f for f in os.listdir(self.backups_dir)
if self.DEFAULT_NAME_MASK in f and f[-3:] == '.gz'
])
def remove_older(self):
counter = 0
for file in reversed(self.get_backups_list()):
if counter >= self.backups_files:
logging.info("Removed: {}".format(self.backups_dir + file))
os.remove(self.backups_dir + file)
counter += 1
def execute(self, execute_string):
return subprocess.check_output(
["bash", "-c", execute_string], stderr=subprocess.STDOUT
)
def backup_operation(self):
execute_string = self._generate_backup_string()
logging.info("Executes: " + execute_string)
self.execute(execute_string)
self.remove_older()
def backup(self):
try:
self.backup_operation()
logging.debug("Current backups = " + str(self.get_backups_list()))
except subprocess.CalledProcessError as err:
logging.error("Code: {} - {}".format(err.returncode, err.output))
sys.exit(-1)
except Exception as err:
logging.critical(err)
sys.exit(-1)
def _generate_backup_string(self):
raise NotImplementedError | 1c-utilites | /1c-utilites-0.1b0.tar.gz/1c-utilites-0.1b0/utilites_1c/backup/base.py | base.py |
# 1D3 payment page SDK
This is a set of libraries in the Python language to ease integration of your service
with the 1D3 Payment Page.
Please note that for correct SDK operating you must have at least Python 3.5.
## Payment flow

## Installation
Install with pip
```bash
pip install 1d3-sdk
```
### Get URL for payment
```python
from payment_page_sdk.gate import Gate
from payment_page_sdk.payment import Payment
gate = Gate('secret')
payment = Payment('402')
payment.payment_id = 'some payment id'
payment.payment_amount = 1001
payment.payment_currency = 'USD'
payment_url = gate.get_purchase_payment_page_url(payment)
```
`payment_url` here is the signed URL.
### Handle callback from 1D3
You'll need to autoload this code in order to handle notifications:
```python
from payment_page_sdk.gate import Gate
gate = Gate('secret')
callback = gate.handle_callback(data)
```
`data` is the JSON data received from payment system;
`callback` is the Callback object describing properties received from payment system;
`callback` implements these methods:
1. `callback.get_payment_status()`
Get payment status.
2. `callback.get_payment()`
Get all payment data.
3. `callback.get_payment_id()`
Get payment ID in your system.
| 1d3-sdk | /1d3-sdk-1.0.3.tar.gz/1d3-sdk-1.0.3/README.md | README.md |
from payment_page_sdk.signature_handler import SignatureHandler
from payment_page_sdk.process_exception import ProcessException
import json
import copy
class Callback(object):
"""Class for processing gate callback
Attributes:
SUCCESS_STATUS - Status of successful payment
DECLINE_STATUS - Status of rejected payment
AW_3DS_STATUS - Status of awaiting a request with the result of a 3-D Secure Verification
AW_RED_STATUS - Status of awaiting customer return after redirecting the customer to an external provider system
AW_CUS_STATUS - Status of awaiting customer actions, if the customer may perform additional attempts to make a
payment
AW_CLA_STATUS - Status of awaiting additional parameters
AW_CAP_STATUS - Status of awaiting request for withdrawal of funds (capture) or cancellation of payment (cancel)
from your project
CANCELLED_STATUS - Status of holding of funds (produced on authorization request) is cancelled
REFUNDED_STATUS - Status of successfully completed the full refund after a successful payment
PART_REFUNDED_STATUS - Status of completed partial refund after a successful payment
PROCESSING_STATUS - Status of payment processing at Gate
ERROR_STATUS - Status of an error occurred while reviewing data for payment processing
REVERSED_STATUS - Status of refund after a successful payment before closing of the business day
__data - Callback data as dict
__signatureHandler - SignatureHandler instance
"""
SUCCESS_STATUS = 'success'
DECLINE_STATUS = 'decline'
AW_3DS_STATUS = 'awaiting 3ds result'
AW_RED_STATUS = 'awaiting redirect result'
AW_CUS_STATUS = 'awaiting customer'
AW_CLA_STATUS = 'awaiting clarification'
AW_CAP_STATUS = 'awaiting capture'
CANCELLED_STATUS = 'cancelled'
REFUNDED_STATUS = 'refunded'
PART_REFUNDED_STATUS = 'partially refunded'
PROCESSING_STATUS = 'processing'
ERROR_STATUS = 'error'
REVERSED_STATUS = 'reversed'
__data = None
__signatureHandler = None
def __init__(self, data: str, signature_handler: SignatureHandler):
"""
Callback constructor
:param dict data:
:param SignatureHandler signature_handler:
"""
self.__data = self.decode_response(data)
self.__signatureHandler = signature_handler
if self.check_signature() == 0:
raise ProcessException('Signature ' + self.get_signature() + ' is invalid')
def get_payment(self):
"""
Get payment info
:return: mixed
"""
return self.get_value('payment')
def get_payment_status(self) -> str:
"""
Get payment status
:return: str
"""
return self.get_value('payment.status')
def get_payment_id(self) -> str:
"""
Get payment ID
:return: str
"""
return self.get_value('payment.id')
def get_signature(self) -> str:
"""
Get signature
:return: str
"""
sign_paths = ['signature', 'general.signature']
for sign_path in sign_paths:
sign = self.get_value(sign_path)
if sign is not None:
return sign
raise ProcessException('Signature undefined')
def decode_response(self, raw_data: str) -> dict:
"""
Cast raw data to array
:param str raw_data:
:return: dict
:raise: ProcessException
"""
try:
data = json.loads(raw_data)
except ValueError:
raise ProcessException('Error on response decoding')
return data
def check_signature(self) -> bool:
"""
Check signature
:return: bool
"""
data = copy.deepcopy(self.__data)
signature = self.get_signature()
self.__remove_param('signature', data)
return self.__signatureHandler.check(data, signature)
def get_value(self, pathname: str):
"""
Get value by pathname
:param str pathname:
:return: mixed
"""
keys = pathname.split('.')
cb_data = self.__data
for key in keys:
if key in cb_data and not isinstance(cb_data, str):
cb_data = cb_data[key]
else:
return None
return cb_data
def __remove_param(self, name: str, data: dict):
"""
Unset param at callback data
:param str name:
:param dict data:
:return: void
"""
if name in data:
del data[name]
for key in data:
if isinstance(data[key], dict):
self.__remove_param(name, data[key]) | 1d3-sdk | /1d3-sdk-1.0.3.tar.gz/1d3-sdk-1.0.3/payment_page_sdk/callback.py | callback.py |
import base64
import hmac
from collections import OrderedDict
class SignatureHandler(object):
"""Class SignatureHandler
Attributes:
ITEMS_DELIMITER - signature concatenation delimiter
IGNORE_KEYS - ignore keys in signature
__secretKey - Secret key
"""
ITEMS_DELIMITER = ';'
IGNORE_KEYS = ['frame_mode']
__secretKey = None
def __init__(self, secret_key: str):
"""
SignatureHandler constructor
:param str secret_key:
"""
self.__secretKey = secret_key
def check(self, params: dict, signature):
"""
Check signature
:param dict params:
:param signature:
:return:
"""
return self.sign(params) == signature
def sign(self, params: dict) -> str:
"""
Return signature
:param params:
:return:
"""
secret_key = self.__secretKey.encode('utf-8')
params_to_sign = self.__get_params_to_sign(params, self.IGNORE_KEYS)
params_to_sign_list = list(OrderedDict(sorted(params_to_sign.items(), key=lambda t: t[0])).values())
string_to_sign = self.ITEMS_DELIMITER.join(params_to_sign_list).encode('utf-8')
return base64.b64encode(hmac.new(secret_key, string_to_sign, hmac._hashlib.sha512).digest()).decode()
def __get_params_to_sign(self, params: dict, ignore=None, prefix='', sort=True) -> dict:
"""
Get parameters to sign
:param params:
:param ignore:
:param prefix:
:param sort:
:return:
"""
if ignore is None:
ignore = []
params_to_sign = {}
for key in params:
if key in ignore:
continue
param_key = prefix + (':' if prefix else '') + key
value = params[key]
if isinstance(value, list):
value = {str(key): value for key, value in enumerate(value)}
if isinstance(value, dict):
sub_array = self.__get_params_to_sign(value, ignore, param_key, False)
params_to_sign.update(sub_array)
else:
if isinstance(value, bool):
value = '1' if value else '0'
elif value is None:
value = ''
else:
value = str(value)
params_to_sign[param_key] = param_key + ':' + value
if sort:
sorted(params_to_sign.items(), key=lambda item: item[0])
return params_to_sign | 1d3-sdk | /1d3-sdk-1.0.3.tar.gz/1d3-sdk-1.0.3/payment_page_sdk/signature_handler.py | signature_handler.py |
from collections.abc import Iterable
import json
class Payment(object):
"""Class Payment
Attributes:
str account_token: The token of the bank card that will be used to perform a payment
str card_operation_type: Type of payment performed via payment card
datetime best_before: Date and time when the payment period expires.
bool close_on_missclick: A parameter that specifies the action of the widget (opened in the modal window) when a customer clicks outside the widget area.
str css_modal_wrap: An additional CSS class for a modal window.
str customer_id: Unique ID of the customer in your project
bool force_acs_new_window: The forced display mode with the ACS page opening in a window despite the settings in Payment Page
str force_payment_method: The ID of the payment provider that is opened to customers by default.
str language_code: The language in which the payment page will be opened to the customer in ISO 639-1 alpha-2 format.
str list_payment_block: The payment block on the list of payment methods.
str merchant_fail_url: The URL of the page in your project to which a customer is returned after a failed payment.
str merchant_success_url: The URL of the page in your project to which a customer is returned after a successful payment.
str mode: Payment Page mode.
int payment_amount: Payment amount specified in minor units of the currency of the payment
str payment_currency: Payment currency in ISO 4217 alpha-3 format
str payment_description: Payment description
str payment_id: Unique ID of the payment in your project
bool recurring_register: Parameter that indicates whether this payment should be registered as recurring
str customer_first_name: Customer first name
str customer_last_name: Customer last name
str customer_phone: Customer phone number. Must have from 4 to 24 digits
str customer_email: Customer e-mail
str customer_country: Country of the customer address, in ISO 3166-1 alpha-2 format
str customer_state: State or region of the customer address
str customer_city: City of the customer address
str customer_day_of_birth: Customer birth date, DD-MM-YYYY
int customer_ssn: The last 4 digits of the social security number of US
str billing_postal: The postal code of the customer billing address
str billing_country: The country of the customer billing address, in ISO 3166-1 alpha-2 format
str billing_region: The region or state of the customer billing address
str billing_city: The city of the customer billing address
str billing_address: The street of the customer billing address
bool redirect: A parameter that enables opening of the generated payment page in a separate tab
str redirect_fail_mode: The mode for customer redirection when the payment failed
str redirect_fail_url: The URL of the page in your project to which the customer is redirected when the payment failed
bool redirect_on_mobile: A parameter that enables opening of the generated payment page in a separate tab on mobile devices only
str redirect_success_mode: The mode for customer redirection after a successful payment.
str redirect_success_url: The URL of the page in your project to which the customer is redirected after a successful payment
str redirect_tokenize_mode: The mode for customer redirection once a token is generated.
str redirect_tokenize_url: The URL of the page in your project to which the customer is redirected after a successful token generation.
str region_code: The region in ISO 3166-1 alpha-2 format. By default the region is determined by the IP address of the customer
str target_element: The element in which the iframe of the payment page is embedded in the web page of your project.
int terminal_id: Unique ID of the Payment Page template which you want to run despite the regional and A/B test settings
str baseurl: Basic Payment Page address that is used in case the Payment Page domain differs from the domain used to connect libraries or if merchant.js is not connected via the <script> tag
str payment_extra_param: Additional parameter to be forwarded to Gate
PURCHASE_TYPE - Payment from customer account
PAYOUT_TYPE - Payment to customer account
RECURRING_TYPE - Recurring payment
"""
PURCHASE_TYPE = 'purchase'
PAYOUT_TYPE = 'payout'
RECURRING_TYPE = 'recurring'
INTERFACE_TYPE = 24
def __init__(self, project_id: str, payment_id: str = ''):
"""
Payment constructor
:param project_id: str
"""
self.__dict__['project_id'] = project_id
self.__dict__['interface_type'] = json.dumps({'id': self.INTERFACE_TYPE})
if payment_id:
self.__dict__['payment_id'] = payment_id
def get_params(self) -> dict:
"""
Get payment parameters
:return: dict
"""
return self.__dict__
def __setattr__(self, name, value):
if name == 'best_before':
value = value.isoformat()
self.__dict__[name] = value | 1d3-sdk | /1d3-sdk-1.0.3.tar.gz/1d3-sdk-1.0.3/payment_page_sdk/payment.py | payment.py |
# 1inch.py
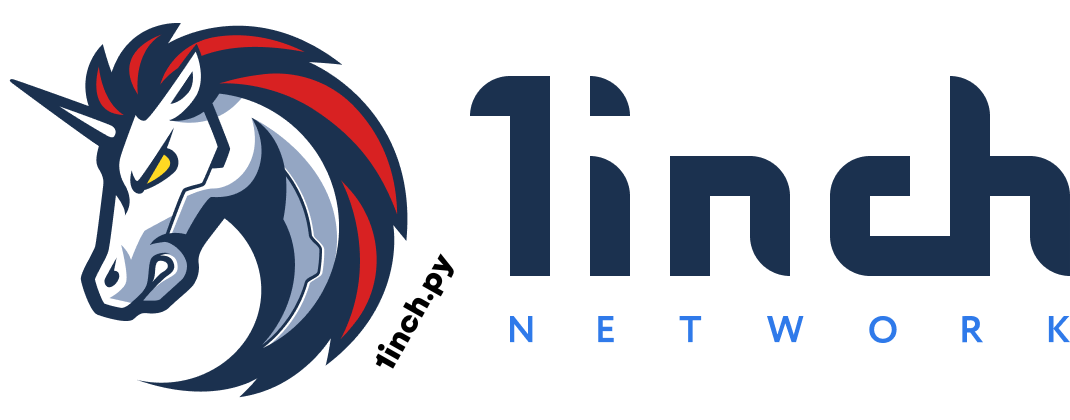
1inch.py is a wrapper around the 1inch API and price Oracle. It has full coverage of the swap API endpoint and All chains support by 1inch are included in the OneInchSwap and OneInchOracle methods.
Package also includes a helper method to ease the submission of transactions to the network. Limited chains currently supported.
## API Documentation
The full 1inch swap API docs can be found at https://docs.1inch.io/
## Installation
Use the package manager [pip](https://pip.pypa.io/en/stable/) to install 1inch.py.
```bash
pip install 1inch.py
```
## Usage
A quick note on decimals. The wrapper is designed for ease of use, and as such accepts amounts in "Ether" or whole units.
If you prefer, you can use decimal=0 and specify amounts in wei. This will also help with any potential floating point errors.
```python
from oneinch_py import OneInchSwap, TransactionHelper, OneInchOracle
rpc_url = "yourRPCURL.com"
binance_rpc = "adifferentRPCurl.com"
public_key = "yourWalletAddress"
private_key = "yourPrivateKey" #remember to protect your private key. Using environmental variables is recommended.
api_key = "" # 1 Inch API key
exchange = OneInchSwap(api_key, public_key)
bsc_exchange = OneInchSwap(api_key, public_key, chain='binance')
helper = TransactionHelper(api_key, rpc_url, public_key, private_key)
bsc_helper = TransactionHelper(api_key, binance_rpc, public_key, private_key, chain='binance')
oracle = OneInchOracle(rpc_url, chain='ethereum')
# See chains currently supported by the helper method:
helper.chains
# {"ethereum": "1", "binance": "56", "polygon": "137", "avalanche": "43114"}
# Straight to business:
# Get a swap and do the swap
result = exchange.get_swap("USDT", "ETH", 10, 0.5) # get the swap transaction
result = helper.build_tx(result) # prepare the transaction for signing, gas price defaults to fast.
result = helper.sign_tx(result) # sign the transaction using your private key
result = helper.broadcast_tx(result) #broadcast the transaction to the network and wait for the receipt.
## If you already have token addresses you can pass those in instead of token names to all OneInchSwap functions that require a token argument
result = exchange.get_swap("0x7fc66500c84a76ad7e9c93437bfc5ac33e2ddae9", "0x43dfc4159d86f3a37a5a4b3d4580b888ad7d4ddd", 10, 0.5)
#USDT to ETH price on the Oracle. Note that you need to indicate the token decimal if it is anything other than 18.
oracle.get_rate_to_ETH("0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", src_token_decimal=6)
# Get the rate between any two tokens.
oracle.get_rate(src_token="0x6B175474E89094C44Da98b954EedeAC495271d0F", dst_token="0x111111111117dC0aa78b770fA6A738034120C302")
exchange.health_check()
# 'OK'
# Address of the 1inch router that must be trusted to spend funds for the swap
exchange.get_spender()
# Generate data for calling the contract in order to allow the 1inch router to spend funds. Token symbol or address is required. If optional "amount" variable is not supplied (in ether), unlimited allowance is granted.
exchange.get_approve("USDT")
exchange.get_approve("0xdAC17F958D2ee523a2206206994597C13D831ec7", amount=100)
# Get the number of tokens (in Wei) that the router is allowed to spend. Option "send address" variable. If not supplied uses address supplied when Initialization the exchange object.
exchange.get_allowance("USDT")
exchange.get_allowance("0xdAC17F958D2ee523a2206206994597C13D831ec7", send_address="0x12345")
# Token List is stored in memory
exchange.tokens
# {
# '1INCH': {'address': '0x111111111117dc0aa78b770fa6a738034120c302',
# 'decimals': 18,
# 'logoURI': 'https://tokens.1inch.exchange/0x111111111117dc0aa78b770fa6a738034120c302.png',
# 'name': '1INCH Token',
# 'symbol': '1INCH'},
# 'ETH': {'address': '0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee',
# 'decimals': 18,
# 'logoURI': 'https://tokens.1inch.exchange/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png',
# 'name': 'Ethereum',
# 'symbol': 'ETH'},
# ......
# }
# Returns the exchange rate of two tokens.
# Tokens can be provided as symbols or addresses
# "amount" is supplied in ether
# NOTE: When using custom tokens, the token decimal is assumed to be 18. If your custom token has a different decimal - please manually pass it to the function (decimal=x)
# Also returns the "price" of more expensive token in the cheaper tokens. Optional variables can be supplied as **kwargs
exchange.get_quote(from_token_symbol='ETH', to_token_symbol='USDT', amount=1)
# (
# {
# "fromToken": {
# "symbol": "ETH",
# "name": "Ethereum",
# "decimals": 18,
# "address": "0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee",
# "logoURI": "https://tokens.1inch.io/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png",
# "tags": ["native"],
# },
# "toToken": {
# "symbol": "USDT",
# "name": "Tether USD",
# "address": "0xdac17f958d2ee523a2206206994597c13d831ec7",
# "decimals": 6,
# "logoURI": "https://tokens.1inch.io/0xdac17f958d2ee523a2206206994597c13d831ec7.png",
# "tags": ["tokens"],
# ...
# Decimal("1076.503093"),
# )
# Creates the swap data for two tokens.
# Tokens can be provided as symbols or addresses
# Optional variables can be supplied as **kwargs
# NOTE: When using custom tokens, the token decimal is assumed to be 18. If your custom token has a different decimal - please manually pass it to the function (decimal=x)
exchange.get_swap(from_token_symbol='ETH', to_token_symbol='USDT', amount=1, slippage=0.5)
# {
# "fromToken": {
# "symbol": "ETH",
# "name": "Ethereum",
# "decimals": 18,
# "address": "0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee",
# "logoURI": "https://tokens.1inch.io/0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee.png",
# "tags": ["native"],
# },
# "toToken": {
# "symbol": "USDT",
# "name": "Tether USD",
# "address": "0xdac17f958d2ee523a2206206994597c13d831ec7",
# "decimals": 6,
# "logoURI": "https://tokens.1inch.io/0xdac17f958d2ee523a2206206994597c13d831ec7.png",
# "tags": ["tokens"],
#
# ...
#
# ],
# "tx": {
# "from": "0x1d05aD0366ad6dc0a284C5fbda46cd555Fb4da27",
# "to": "0x1111111254fb6c44bac0bed2854e76f90643097d",
# "data": "0xe449022e00000000000000000000000000000000000000000000000006f05b59d3b20000000000000000000000000000000000000000000000000000000000001fed825a0000000000000000000000000000000000000000000000000000000000000060000000000000000000000000000000000000000000000000000000000000000140000000000000000000000011b815efb8f581194ae79006d24e0d814b7697f6cfee7c08",
# "value": "500000000000000000",
# "gas": 178993,
# "gasPrice": "14183370651",
# },
# }
```
## Contributing
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
Thanks to @Makbeta for all of their work in migrating the wrapper to the new 1inch api system.
## License
[MIT](https://choosealicense.com/licenses/mit/)
| 1inch.py | /1inch.py-2.0.3.tar.gz/1inch.py-2.0.3/README.md | README.md |
import json
import time
import requests
from web3 import Web3
from decimal import *
import importlib.resources as pkg_resources
from web3.middleware import geth_poa_middleware
class UnknownToken(Exception):
pass
class OneInchSwap:
base_url = 'https://api.1inch.dev/swap'
api_key = ''
version = {
"v5.2": "v5.2"
}
chains = {
"ethereum": "1",
"binance": "56",
"polygon": "137",
"optimism": "10",
"arbitrum": "42161",
"gnosis": "100",
"avalanche": "43114",
"fantom": "250",
"klaytn": "8217",
"aurora": "1313161554",
"zksync": "324"
}
def __init__(self, api_key, address, chain='ethereum', version='v5.2'):
self.presets = None
self.tokens = {}
self.tokens_by_address = {}
self.protocols = []
self.address = address
self.api_key = api_key
self.version = version
self.chain_id = self.chains[chain]
self.chain = chain
self.tokens = self.get_tokens()
time.sleep(1)
self.spender = self.get_spender()
time.sleep(1)
def _get(self, url, params=None, headers=None):
""" Implements a get request """
try:
if headers == None:
headers = {"accept": "application/json", "Authorization": f"Bearer {self.api_key}"}
else:
headers["accept"] = "application/json"
headers["Authorization"] = f"Bearer {self.api_key}"
response = requests.get(url, params=params, headers=headers)
response.raise_for_status()
payload = response.json()
except requests.exceptions.ConnectionError as e:
# error_content = json.loads(e.response._content.decode("utf-8"))
print(f"ConnectionError with code {e.response.status_code} when doing a GET request from {format(url)}")
# print(f"{error_content['error']} {error_content['description']}")
payload = None
except requests.exceptions.HTTPError as e:
# error_content = json.loads(e.response._content.decode("utf-8"))
print(f"HTTPError with code {e.response.status_code} for a request {format(url)}")
# print(f"{error_content['error']} {error_content['description']}")
payload = None
return payload
def _token_to_address(self, token: str):
if len(token) == 42:
return Web3.to_checksum_address(token)
else:
try:
address = self.tokens[token]['address']
except:
raise UnknownToken("Token not in 1inch Token List")
return address
def health_check(self):
"""
Calls the Health Check Endpoint
:return: Always returns code 200 if API is stable
"""
url = f'{self.base_url}/{self.version}/{self.chain_id}/healthcheck'
response = self._get(url)
return response['status']
def get_spender(self):
url = f'{self.base_url}/{self.version}/{self.chain_id}/approve/spender'
result = self._get(url)
if not result.__contains__('spender'):
return result
self.spender = result
return self.spender
def get_tokens(self):
"""
Calls the Tokens API endpoint
:return: A dictionary of all the whitelisted tokens.
"""
url = f'https://api.1inch.dev/token/v1.2/{self.chain_id}'
result = self._get(url)
for key in result:
token = result[key]
self.tokens_by_address[key] = token
self.tokens[token['symbol']] = token
return self.tokens
def get_liquidity_sources(self):
url = f'{self.base_url}/{self.version}/{self.chain_id}/liquidity-sources'
result = self._get(url)
if not result.__contains__('liquidity-sources'):
return result
self.protocols = result
return self.protocols
def get_presets(self):
url = f'{self.base_url}/{self.version}/{self.chain_id}/presets'
result = self._get(url)
if not result.__contains__('presets'):
return result
self.presets = result
return self.presets
def get_quote(self, from_token_symbol: str, to_token_symbol: str, amount: float, decimal=None, **kwargs):
"""
Calls the QUOTE API endpoint. NOTE: When using custom tokens, the token decimal is assumed to be 18. If your
custom token has a different decimal - please manually pass it to the function (decimal=x)
"""
from_address = self._token_to_address(from_token_symbol)
to_address = self._token_to_address(to_token_symbol)
if decimal is None:
try:
self.tokens[from_token_symbol]['decimals']
except KeyError:
decimal = 18
else:
decimal = self.tokens[from_token_symbol]['decimals']
else:
pass
if decimal == 0:
amount_in_wei = int(amount)
else:
amount_in_wei = int(amount * 10 ** decimal)
url = f'{self.base_url}/{self.version}/{self.chain_id}/quote'
url = url + f'?fromTokenAddress={from_address}&toTokenAddress={to_address}&amount={amount_in_wei}'
if kwargs is not None:
result = self._get(url, params=kwargs)
else:
result = self._get(url)
# from_base = Decimal(result['fromTokenAmount']) / Decimal(10 ** result['fromToken']['decimals'])
# to_base = Decimal(result['toTokenAmount']) / Decimal(10 ** result['toToken']['decimals'])
# if from_base > to_base:
# rate = from_base / to_base
# else:
# rate = to_base / from_base
return result
def get_swap(self, from_token_symbol: str, to_token_symbol: str,
amount: float, slippage: float, decimal=None, send_address=None, **kwargs):
"""
Calls the SWAP API endpoint. NOTE: When using custom tokens, the token decimal is assumed to be 18. If your
custom token has a different decimal - please manually pass it to the function (decimal=x)
"""
if send_address is None:
send_address = self.address
else:
pass
from_address = self._token_to_address(from_token_symbol)
to_address = self._token_to_address(to_token_symbol)
if decimal is None:
try:
self.tokens[from_token_symbol]['decimals']
except KeyError:
decimal = 18
else:
decimal = self.tokens[from_token_symbol]['decimals']
else:
pass
if decimal == 0:
amount_in_wei = int(amount)
else:
amount_in_wei = int(amount * 10 ** decimal)
url = f'{self.base_url}/{self.version}/{self.chain_id}/swap'
url = url + f'?fromTokenAddress={from_address}&toTokenAddress={to_address}&amount={amount_in_wei}'
url = url + f'&fromAddress={send_address}&slippage={slippage}'
if kwargs is not None:
result = self._get(url, params=kwargs)
else:
result = self._get(url)
return result
def get_allowance(self, from_token_symbol: str, send_address=None):
if send_address is None:
send_address = self.address
from_address = self._token_to_address(from_token_symbol)
url = f'{self.base_url}/{self.version}/{self.chain_id}/approve/allowance'
url = url + f"?tokenAddress={from_address}&walletAddress={send_address}"
result = self._get(url)
return result
def get_approve(self, from_token_symbol: str, amount=None, decimal=None):
from_address = self._token_to_address(from_token_symbol)
if decimal is None:
try:
self.tokens[from_token_symbol]['decimals']
except KeyError:
decimal = 18
else:
decimal = self.tokens[from_token_symbol]['decimals']
else:
pass
url = f'{self.base_url}/{self.version}/{self.chain_id}/approve/transaction'
if amount is None:
url = url + f"?tokenAddress={from_address}"
else:
if decimal == 0:
amount_in_wei = int(amount)
else:
amount_in_wei = int(amount * 10 ** decimal)
url = url + f"?tokenAddress={from_address}&amount={amount_in_wei}"
result = self._get(url)
return result
class TransactionHelper:
gas_oracle = "https://gas-price-api.1inch.io/v1.3/"
chains = {
"ethereum": "1",
"binance": "56",
"polygon": "137",
"optimism": "10",
"arbitrum": "42161",
"gnosis": "100",
"avalanche": "43114",
"fantom": "250",
"klaytn": "8217",
"aurora": "1313161554",
"zksync": "324"
}
abi = json.loads(pkg_resources.read_text(__package__, 'erc20.json'))['result']
abi_aggregator = json.loads(pkg_resources.read_text(__package__, 'aggregatorv5.json'))['result']
def _get(self, url, params=None, headers=None):
""" Implements a get request """
try:
if headers == None:
headers = {"accept": "application/json", "Authorization": f"Bearer {self.api_key}", "Content-Type": "application/json"}
else:
headers["accept"] = "application/json"
headers["Authorization"] = f"Bearer {self.api_key}"
headers["Content-Type"] = "application/json"
response = requests.get(url, params=params, headers=headers)
response.raise_for_status()
payload = response.json()
except requests.exceptions.ConnectionError as e:
# error_content = json.loads(e.response._content.decode("utf-8"))
print("ConnectionError when doing a GET request from {}".format(url))
# print(f"{error_content['error']} {error_content['description']}")
payload = None
except requests.exceptions.HTTPError:
# error_content = json.loads(e.response._content.decode("utf-8"))
print("HTTPError {}".format(url))
# print(f"{error_content['error']} {error_content['description']}")
payload = None
return payload
def __init__(self, api_key, rpc_url, public_key, private_key, chain='ethereum', broadcast_1inch=False):
self.w3 = Web3(Web3.HTTPProvider(rpc_url))
if chain == 'polygon' or chain == 'avalanche':
self.w3.middleware_onion.inject(geth_poa_middleware, layer=0)
else:
pass
self.api_key = api_key
self.public_key = public_key
self.private_key = private_key
self.chain = chain
self.chain_id = self.chains[chain]
self.broadcast_1inch = broadcast_1inch
def build_tx(self, raw_tx, speed='high'):
nonce = self.w3.eth.get_transaction_count(self.public_key)
if raw_tx == None:
return None
if 'tx' in raw_tx:
tx = raw_tx['tx']
else:
tx = raw_tx
if 'from' not in tx:
tx['from'] = self.w3.to_checksum_address(self.public_key)
tx['to'] = self.w3.to_checksum_address(tx['to'])
if 'gas' not in tx:
tx['gas'] = self.w3.eth.estimate_gas(tx)
tx['nonce'] = nonce
tx['chainId'] = int(self.chain_id)
tx['value'] = int(tx['value'])
tx['gas'] = int(tx['gas'] * 1.25)
if self.chain == 'ethereum' or self.chain == 'polygon' or self.chain == 'avalanche' or self.chain == 'gnosis' or self.chain == 'klaytn':
gas = self._get(self.gas_oracle + self.chain_id)
tx['maxPriorityFeePerGas'] = int(gas[speed]['maxPriorityFeePerGas'])
tx['maxFeePerGas'] = int(gas[speed]['maxFeePerGas'])
tx.pop('gasPrice')
else:
tx['gasPrice'] = int(tx['gasPrice'])
return tx
def sign_tx(self, tx):
if tx == None:
return None
signed_tx = self.w3.eth.account.sign_transaction(tx, self.private_key)
return signed_tx
def broadcast_tx(self, signed_tx, timeout=360):
api_base_url = 'https://api.1inch.dev/tx-gateway/v1.1/'
api_headers = {"accept": "application/json", "Authorization": f"Bearer {self.api_key}", "Content-Type": "application/json"}
if signed_tx == None:
return None
if self.broadcast_1inch is True:
tx_json = signed_tx.rawTransaction
tx_json = {"rawTransaction": tx_json.hex()}
payload = requests.post(api_base_url + self.chain_id + "/broadcast", data=self.w3.toJSON(tx_json), headers=api_headers)
tx_hash = json.loads(payload.text)
tx_hash = tx_hash['transactionHash']
receipt = self.w3.eth.wait_for_transaction_receipt(tx_hash, timeout=timeout)
return receipt, tx_hash
else:
tx_hash = self.w3.eth.send_raw_transaction(signed_tx.rawTransaction)
print(tx_hash.hex())
receipt = self.w3.eth.wait_for_transaction_receipt(tx_hash, timeout=timeout)
return receipt, tx_hash.hex()
def get_ERC20_balance(self, contract_address, decimal=None):
contract = self.w3.eth.contract(address=self.w3.to_checksum_address(contract_address), abi=self.abi)
balance_in_wei = contract.functions.balanceOf(self.public_key).call()
if decimal is None:
return self.w3.from_wei(balance_in_wei, 'ether')
elif decimal == 0:
return balance_in_wei
else:
return balance_in_wei / 10 ** decimal
def decode_abi(self, transaction):
contract = self.w3.eth.contract(address=self.w3.to_checksum_address('0x1111111254EEB25477B68fb85Ed929f73A960582'), abi=self.abi_aggregator)
data = transaction['tx']['data']
decoded_data = contract.decode_function_input(data)
return decoded_data
class OneInchOracle:
chains = {
"ethereum": "1",
"binance": "56",
"polygon": "137",
"optimism": "10",
"arbitrum": "42161",
"gnosis": "100",
"avalanche": "43114",
"fantom": "250",
"klaytn": "8217",
"aurora": "1313161554",
"zksync": "324"
}
contracts = {
"ethereum": '0x07D91f5fb9Bf7798734C3f606dB065549F6893bb',
"binance": '0xfbD61B037C325b959c0F6A7e69D8f37770C2c550',
"polygon": "0x7F069df72b7A39bCE9806e3AfaF579E54D8CF2b9",
"optimism": "0x11DEE30E710B8d4a8630392781Cc3c0046365d4c",
"arbitrum": "0x735247fb0a604c0adC6cab38ACE16D0DbA31295F",
"gnosis": "0x142DB045195CEcaBe415161e1dF1CF0337A4d02E",
"avalanche": "0xBd0c7AaF0bF082712EbE919a9dD94b2d978f79A9",
"fantom": "0xE8E598A1041b6fDB13999D275a202847D9b654ca"
}
# multicall_address = "0xDA3C19c6Fe954576707fA24695Efb830D9ccA1CA"
oracle_abi = json.loads(pkg_resources.read_text(__package__, 'oracle.json'))['result']
# multicall_abi = json.loads(pkg_resources.read_text(__package__, 'multicall.json'))['result']
def __init__(self, rpc_url, chain='ethereum'):
self.w3 = Web3(Web3.HTTPProvider(rpc_url))
self.chain = chain
self.chain_id = self.chains[chain]
self.contract_address = self.contracts[chain]
self.oracle_contract = self.w3.eth.contract(address=self.contract_address, abi=self.oracle_abi)
# self.multicall_contract = self.w3.eth.contract(address=self.multicall_address, abi=self.multicall_abi)
def get_rate(self, src_token, dst_token, wrap=False, src_token_decimal: int = 18, dst_token_decimal: int = 18):
rate = self.oracle_contract.functions.getRate(self.w3.to_checksum_address(src_token),
self.w3.to_checksum_address(dst_token), wrap).call()
if src_token_decimal == 18 and dst_token_decimal < 18:
rate = rate / 10 ** dst_token_decimal
elif dst_token_decimal == 18 and src_token_decimal < 18:
rate = rate / 10 ** ((18 - src_token_decimal) + 18)
elif dst_token_decimal < 18 and src_token_decimal < 18:
rate = rate / 10 ** ((18 - src_token_decimal) + (18 - dst_token_decimal))
else:
rate = rate / 10 ** 18
return rate
def get_rate_to_ETH(self, src_token, wrap=False, src_token_decimal=18):
rate = self.oracle_contract.functions.getRateToEth(self.w3.to_checksum_address(src_token), wrap).call()
if src_token_decimal == 18:
rate = rate / 10 ** 18
elif src_token_decimal < 18:
rate = rate / 10 ** ((18 - src_token_decimal) + 18)
return rate
# TODO Figure this all out at some point
# def get_multicall(self, token_list):
# for address in token_list:
# call_data = {
# "to": "0x07D91f5fb9Bf7798734C3f606dB065549F6893bb",
# "data": f"{self.oracle_contract.functions.getRateToEth(address,True)}"
# }
#
# print(call_data)
# # mapped = map(lambda , token_list, wrap_list)
# # mapped = list(mapped)
# # rate = self.multicall_contract.functions.multicall(mapped).call()
# return
if __name__ == '__main__':
pass | 1inch.py | /1inch.py-2.0.3.tar.gz/1inch.py-2.0.3/oneinch_py/main.py | main.py |
=====
1pass
=====
A command line interface (and Python library) for reading passwords from
`1Password <https://agilebits.com/onepassword>`_.
Command line usage
==================
To get a password::
1pass mail.google.com
By default this will look in ``~/Dropbox/1Password.agilekeychain``. If that's
not where you keep your keychain::
1pass --path ~/whatever/1Password.agilekeychain mail.google.com
Or, you can set your keychain path as an enviornment variable::
export ONEPASSWORD_KEYCHAIN=/path/to/keychain
1pass mail.google.com
By default, the name you pass on the command line must match the name of an
item in your 1Password keychain exactly. To avoid this, fuzzy matching is
made possible with the ``--fuzzy`` flag::
1pass --fuzzy mail.goog
If you don't want to be prompted for your password, you can use the
``--no-prompt`` flag and provide the password via standard input instead::
emit_master_password | 1pass --no-prompt mail.google.com
Python usage
============
The interface is very simple::
from onepassword import Keychain
my_keychain = Keychain(path="~/Dropbox/1Password.agilekeychain")
my_keychain.unlock("my-master-password")
my_keychain.item("An item's name").password
An example of real-world use
============================
I wrote this so I could add the following line to my ``.muttrc`` file::
set imap_pass = "`1pass 'Google: personal'`"
Now, whenever I start ``mutt``, I am prompted for my 1Password Master Password
and not my Gmail password.
The ``--no-prompt`` flag is very useful when configuring ``mutt`` and PGP.
``mutt`` passes the PGP passphrase via standard in, so by inserting ``1pass``
into this pipline I can use my 1Password master password when prompted for my
PGP keyphrase::
set pgp_decrypt_command="1pass --no-prompt pgp-passphrase | gpg --passphrase-fd 0 ..."
Contributors
============
* Pip Taylor <https://github.com/pipt>
* Adam Coddington <https://github.com/latestrevision>
* Ash Berlin <https://github.com/ashb>
* Zach Allaun <https://github.com/zachallaun>
* Eric Mika <https://github.com/kitschpatrol>
License
=======
*1pass* is licensed under the MIT license. See the license file for details.
While it is designed to read ``.agilekeychain`` bundles created by 1Password,
*1pass* isn't officially sanctioned or supported by
`AgileBits <https://agilebits.com/>`_. I do hope they like it though.
| 1pass | /1pass-0.2.1.tar.gz/1pass-0.2.1/README.txt | README.txt |
from base64 import b64decode
from hashlib import md5
from M2Crypto import EVP
class SaltyString(object):
SALTED_PREFIX = "Salted__"
ZERO_INIT_VECTOR = "\x00" * 16
def __init__(self, base64_encoded_string):
decoded_data = b64decode(base64_encoded_string)
if decoded_data.startswith(self.SALTED_PREFIX):
self.salt = decoded_data[8:16]
self.data = decoded_data[16:]
else:
self.salt = self.ZERO_INIT_VECTOR
self.data = decoded_data
class EncryptionKey(object):
MINIMUM_ITERATIONS = 1000
def __init__(self, data, iterations=0, validation="", identifier=None,
level=None):
self.identifier = identifier
self.level = level
self._encrypted_key = SaltyString(data)
self._decrypted_key = None
self._set_iterations(iterations)
self._validation = validation
def unlock(self, password):
key, iv = self._derive_pbkdf2(password)
self._decrypted_key = self._aes_decrypt(
key=key,
iv=iv,
encrypted_data=self._encrypted_key.data,
)
return self._validate_decrypted_key()
def decrypt(self, b64_data):
encrypted = SaltyString(b64_data)
key, iv = self._derive_openssl(self._decrypted_key, encrypted.salt)
return self._aes_decrypt(key=key, iv=iv, encrypted_data=encrypted.data)
def _set_iterations(self, iterations):
self.iterations = max(int(iterations), self.MINIMUM_ITERATIONS)
def _validate_decrypted_key(self):
return self.decrypt(self._validation) == self._decrypted_key
def _aes_decrypt(self, key, iv, encrypted_data):
aes = EVP.Cipher("aes_128_cbc", key, iv, key_as_bytes=False, padding=False, op=0)
return self._strip_padding(aes.update(encrypted_data) + aes.final())
def _strip_padding(self, decrypted):
padding_size = ord(decrypted[-1])
if padding_size >= 16:
return decrypted
else:
return decrypted[:-padding_size]
def _derive_pbkdf2(self, password):
key_and_iv = EVP.pbkdf2(
password,
self._encrypted_key.salt,
self.iterations,
32,
)
return (
key_and_iv[0:16],
key_and_iv[16:],
)
def _derive_openssl(self, key, salt):
key = key[0:-16]
key_and_iv = ""
prev = ""
while len(key_and_iv) < 32:
prev = md5(prev + key + salt).digest()
key_and_iv += prev
return (
key_and_iv[0:16],
key_and_iv[16:],
) | 1pass | /1pass-0.2.1.tar.gz/1pass-0.2.1/onepassword/encryption_key.py | encryption_key.py |
import json
import os
from fuzzywuzzy import process
from onepassword.encryption_key import EncryptionKey
class Keychain(object):
def __init__(self, path):
self._path = os.path.expanduser(path)
self._load_encryption_keys()
self._load_item_list()
self._locked = True
def unlock(self, password):
unlocker = lambda key: key.unlock(password)
unlock_results = map(unlocker, self._encryption_keys.values())
result = reduce(lambda x, y: x and y, unlock_results)
self._locked = not result
return result
def item(self, name, fuzzy_threshold=100):
"""
Extract a password from an unlocked Keychain using fuzzy
matching. ``fuzzy_threshold`` can be an integer between 0 and
100, where 100 is an exact match.
"""
match = process.extractOne(
name,
self._items.keys(),
score_cutoff=(fuzzy_threshold-1),
)
if match:
exact_name = match[0]
item = self._items[exact_name]
item.decrypt_with(self)
return item
else:
return None
def key(self, identifier=None, security_level=None):
"""
Tries to find an encryption key, first using the ``identifier`` and
if that fails or isn't provided using the ``security_level``.
Returns ``None`` if nothing matches.
"""
if identifier:
try:
return self._encryption_keys[identifier]
except KeyError:
pass
if security_level:
for key in self._encryption_keys.values():
if key.level == security_level:
return key
@property
def locked(self):
return self._locked
def _load_encryption_keys(self):
path = os.path.join(self._path, "data", "default", "encryptionKeys.js")
with open(path, "r") as f:
key_data = json.load(f)
self._encryption_keys = {}
for key_definition in key_data["list"]:
key = EncryptionKey(**key_definition)
self._encryption_keys[key.identifier] = key
def _load_item_list(self):
path = os.path.join(self._path, "data", "default", "contents.js")
with open(path, "r") as f:
item_list = json.load(f)
self._items = {}
for item_definition in item_list:
item = KeychainItem.build(item_definition, self._path)
self._items[item.name] = item
class KeychainItem(object):
@classmethod
def build(cls, row, path):
identifier = row[0]
type = row[1]
name = row[2]
if type == "webforms.WebForm":
return WebFormKeychainItem(identifier, name, path, type)
elif type == "passwords.Password" or type == "wallet.onlineservices.GenericAccount":
return PasswordKeychainItem(identifier, name, path, type)
else:
return KeychainItem(identifier, name, path, type)
def __init__(self, identifier, name, path, type):
self.identifier = identifier
self.name = name
self.password = None
self._path = path
self._type = type
@property
def key_identifier(self):
return self._lazily_load("_key_identifier")
@property
def security_level(self):
return self._lazily_load("_security_level")
def decrypt_with(self, keychain):
key = keychain.key(
identifier=self.key_identifier,
security_level=self.security_level,
)
encrypted_json = self._lazily_load("_encrypted_json")
decrypted_json = key.decrypt(self._encrypted_json)
self._data = json.loads(decrypted_json)
self.password = self._find_password()
def _find_password(self):
raise Exception("Cannot extract a password from this type of"
" keychain item (%s)" % self._type)
def _lazily_load(self, attr):
if not hasattr(self, attr):
self._read_data_file()
return getattr(self, attr)
def _read_data_file(self):
filename = "%s.1password" % self.identifier
path = os.path.join(self._path, "data", "default", filename)
with open(path, "r") as f:
item_data = json.load(f)
self._key_identifier = item_data.get("keyID")
self._security_level = item_data.get("securityLevel")
self._encrypted_json = item_data["encrypted"]
class WebFormKeychainItem(KeychainItem):
def _find_password(self):
for field in self._data["fields"]:
if field.get("designation") == "password" or \
field.get("name") == "Password":
return field["value"]
class PasswordKeychainItem(KeychainItem):
def _find_password(self):
return self._data["password"] | 1pass | /1pass-0.2.1.tar.gz/1pass-0.2.1/onepassword/keychain.py | keychain.py |
import argparse
import getpass
import os
import sys
from onepassword import Keychain
DEFAULT_KEYCHAIN_PATH = "~/Dropbox/1Password.agilekeychain"
class CLI(object):
"""
The 1pass command line interface.
"""
def __init__(self, stdin=sys.stdin, stdout=sys.stdout, stderr=sys.stderr,
getpass=getpass.getpass, arguments=sys.argv[1:]):
self.stdin = stdin
self.stdout = stdout
self.stderr = stderr
self.getpass = getpass
self.arguments = self.argument_parser().parse_args(arguments)
self.keychain = Keychain(self.arguments.path)
def run(self):
"""
The main entry point, performs the appropriate action for the given
arguments.
"""
self._unlock_keychain()
item = self.keychain.item(
self.arguments.item,
fuzzy_threshold=self._fuzzy_threshold(),
)
if item is not None:
self.stdout.write("%s\n" % item.password)
else:
self.stderr.write("1pass: Could not find an item named '%s'\n" % (
self.arguments.item,
))
sys.exit(os.EX_DATAERR)
def argument_parser(self):
parser = argparse.ArgumentParser()
parser.add_argument("item", help="The name of the password to decrypt")
parser.add_argument(
"--path",
default=os.environ.get('ONEPASSWORD_KEYCHAIN', DEFAULT_KEYCHAIN_PATH),
help="Path to your 1Password.agilekeychain file",
)
parser.add_argument(
"--fuzzy",
action="store_true",
help="Perform fuzzy matching on the item",
)
parser.add_argument(
"--no-prompt",
action="store_true",
help="Don't prompt for a password, read from STDIN instead",
)
return parser
def _unlock_keychain(self):
if self.arguments.no_prompt:
self._unlock_keychain_stdin()
else:
self._unlock_keychain_prompt()
def _unlock_keychain_stdin(self):
password = self.stdin.read().strip()
self.keychain.unlock(password)
if self.keychain.locked:
self.stderr.write("1pass: Incorrect master password\n")
sys.exit(os.EX_DATAERR)
def _unlock_keychain_prompt(self):
while self.keychain.locked:
try:
self.keychain.unlock(self.getpass("Master password: "))
except KeyboardInterrupt:
self.stdout.write("\n")
sys.exit(0)
def _fuzzy_threshold(self):
if self.arguments.fuzzy:
return 70
else:
return 100 | 1pass | /1pass-0.2.1.tar.gz/1pass-0.2.1/onepassword/cli.py | cli.py |
import argparse
import json
import re
import subprocess
import sys
from datetime import datetime
from io import StringIO
from tempfile import NamedTemporaryFile
from dotenv import dotenv_values
from sgqlc.endpoint.http import HTTPEndpoint
FLY_GRAPHQL_ENDPOINT = 'https://api.fly.io/graphql'
DATE_FORMAT = '%Y/%m/%d %H:%M:%S'
DEFAULT_ENV_FILE_NAME = '.env'
class UserError(RuntimeError):
pass
def get_1password_env_file_item_id(title_substring):
secure_notes = json.loads(
subprocess.check_output(
[
'op',
'item',
'list',
'--categories',
'Secure Note',
'--format',
'json',
]
)
)
item_ids = list(
item['id']
for item in secure_notes
if title_substring in item['title'].split(' ')
)
if len(item_ids) == 0:
raise_error(
f'No 1password secure note found with a name containing {title_substring!r}'
)
if len(item_ids) > 1:
raise_error(
f'Found {len(item_ids)} 1password secure notes with a name containing '
f'{title_substring!r}, expected one'
)
return item_ids[0]
def get_item_from_1password(item_id):
return json.loads(
subprocess.check_output(
['op', 'item', 'get', item_id, '--format', 'json']
)
)
def get_envs_from_1password(item_id):
item = get_item_from_1password(item_id)
result = first(
field.get('value')
for field in item['fields']
if field['id'] == 'notesPlain'
)
if result is None or result == '':
raise_error('Empty secrets, aborting')
return result
def get_filename_from_1password(item_id):
item = get_item_from_1password(item_id)
result = first(
field.get('value')
for field in item['fields']
if field['label'] == 'file_name'
)
return result
def get_fly_auth_token():
return json.loads(
subprocess.check_output(['fly', 'auth', 'token', '--json'])
)['token']
def update_fly_secrets(app_id, secrets):
set_secrets_mutation = '''
mutation(
$appId: ID!
$secrets: [SecretInput!]!
$replaceAll: Boolean!
) {
setSecrets(
input: {
appId: $appId
replaceAll: $replaceAll
secrets: $secrets
}
) {
app {
name
}
release {
version
}
}
}
'''
secrets_input = [
{'key': key, 'value': value}
for key, value in secrets.items()
]
variables = {
'appId': app_id,
'secrets': secrets_input,
'replaceAll': True
}
headers = {'Authorization': f'Bearer {get_fly_auth_token()}'}
endpoint = HTTPEndpoint(
FLY_GRAPHQL_ENDPOINT,
headers
)
response = endpoint(
query=set_secrets_mutation,
variables=variables
)
if response.get('errors') is not None:
raise_error(response['errors'][0])
print(
'Releasing fly app {} version {}'.format(
app_id,
response['data']['setSecrets']['release']['version']
)
)
def update_1password_secrets(item_id, content):
subprocess.check_output([
'op',
'item',
'edit',
item_id,
f'notesPlain={content}'
])
def update_1password_custom_field(item_id, field, value):
subprocess.check_output([
'op',
'item',
'edit',
item_id,
f'Generated by 1password-secrets.{field}[text]={value}',
'--format',
'json'
])
def get_secrets_from_envs(input: str):
return dotenv_values(stream=StringIO(input))
def import_1password_secrets_to_fly(app_id):
item_id = get_1password_env_file_item_id(f'fly:{app_id}')
secrets = get_secrets_from_envs(get_envs_from_1password(item_id))
update_fly_secrets(app_id, secrets)
now_formatted = datetime.now().strftime(DATE_FORMAT)
update_1password_custom_field(
item_id,
'last imported at',
now_formatted
)
def edit_1password_secrets(app_id):
item_id = get_1password_env_file_item_id(f'fly:{app_id}')
secrets = get_envs_from_1password(item_id)
with NamedTemporaryFile('w+') as file:
file.writelines(secrets)
file.flush()
subprocess.check_output(['code', '--wait', file.name])
file.seek(0)
output = file.read()
if secrets == output:
print('No changes detected, aborting.')
return
update_1password_secrets(item_id, output)
now_formatted = datetime.now().strftime(DATE_FORMAT)
update_1password_custom_field(
item_id,
'last edited at',
now_formatted
)
user_input = ''
while user_input.lower() not in ['y', 'n']:
user_input = input(
'Secrets updated in 1password, '
f'do you wish to import secrets to the fly app {app_id} (y/n)?\n'
)
if user_input.lower() == 'y':
import_1password_secrets_to_fly(app_id)
def pull_local_secrets():
repository = get_git_repository_name_from_current_directory()
item_id = get_1password_env_file_item_id(f'repo:{repository}')
secrets = get_envs_from_1password(item_id)
env_file_name = get_filename_from_1password(item_id) or DEFAULT_ENV_FILE_NAME
with open(env_file_name, 'w') as file:
file.writelines(secrets)
print(f'Successfully updated {env_file_name} from 1password')
def push_local_secrets():
repository_name = get_git_repository_name_from_current_directory()
item_id = get_1password_env_file_item_id(f'repo:{repository_name}')
env_file_name = get_filename_from_1password(item_id) or DEFAULT_ENV_FILE_NAME
try:
with open(env_file_name, 'r') as file:
secrets = file.read()
except FileNotFoundError:
raise_error(f'Env file {env_file_name!r} not found!')
update_1password_secrets(item_id, secrets)
now_formatted = datetime.now().strftime(DATE_FORMAT)
update_1password_custom_field(
item_id,
'last edited at',
now_formatted
)
print(f'Successfully pushed secrets from {env_file_name} to 1password')
def get_git_repository_name_from_current_directory():
GIT_REPOSITORY_REGEX = r'^(https|git)(:\/\/|@)([^\/:]+)[\/:]([^\/:]+)\/(.+).git$'
try:
git_remote_origin_url = subprocess.check_output([
'git',
'config',
'--get',
'remote.origin.url'
]).decode('utf-8')
except subprocess.CalledProcessError:
raise_error('Either not in a git repository or remote "origin" is not set')
regex_match = re.match(
GIT_REPOSITORY_REGEX,
git_remote_origin_url
)
if regex_match is None:
raise_error('Could not get remote "origin" url from git repository')
repository_name = f'{regex_match.group(4)}/{regex_match.group(5)}'
return repository_name
def raise_error(message):
print(message)
raise UserError(message)
def first(iterable):
try:
return next(iterable)
except StopIteration:
return None
def main():
parser = argparse.ArgumentParser(
description='1password-secrets is a set of utilities to sync 1Password secrets.'
)
subparsers = parser.add_subparsers(dest='subcommand', required=True)
fly_parser = subparsers.add_parser('fly', help='manage fly secrets')
fly_parser.add_argument('action', type=str, choices=['import', 'edit'])
fly_parser.add_argument('app_name', type=str, help='fly application name')
local_parser = subparsers.add_parser('local', help='manage local secrets')
local_parser.add_argument('action', type=str, choices=['pull', 'push'])
args = parser.parse_args()
try:
if args.subcommand == 'fly':
if args.action == 'import':
import_1password_secrets_to_fly(args.app_name)
elif args.action == 'edit':
edit_1password_secrets(args.app_name)
elif args.subcommand == 'local':
if args.action == 'pull':
pull_local_secrets()
elif args.action == 'push':
push_local_secrets()
except UserError:
sys.exit(1)
if __name__ == '__main__':
main() | 1password-secrets | /1password_secrets-0.0.4-py3-none-any.whl/onepassword_secrets.py | onepassword_secrets.py |
# OnePassword python client
[](https://github.com/wandera/1password-client/actions/workflows/publish-to-pypi.yml)
[](https://github.com/wandera/1password-client/actions/workflows/codeql-analysis.yml)
Python client around the 1Password password manager cli for usage within python code and
Jupyter Notebooks. Developed by Data Scientists from Jamf.
## Supported versions
There are some of the pre-requisites that are needed to use the library. We automatically install the cli for Mac and
Linux users when installing the library. Windows users see below for help.
- 1Password App: 8+
- 1Password cli: 2+
- Python: 3.10+
## Operating systems
The library is split into two parts: installation and client in which we are slowly updating to cover as many operating
systems as possible the following table should ensure users understand what this library can and can't do at time of
install.
| | MacOS | Linux |
|-----------------|-------|-------|
| Fully supported | Y | Y |
| CLI install | Y | Y |
| SSO login | Y | Y |
| Login via App | Y | Y |
| Biometrics auth | Y | Y |
| Password auth | Y | Y |
| CLI client | Y | Y |
## Installation
```bash
pip install 1password
```
If you have issues with PyYaml or other distutils installed packages then use:
```bash
pip install --ignore-installed 1password
```
You are welcome to install and manage `op` yourself by visiting
[the CLI1 downloads page](https://app-updates.agilebits.com/product_history/CLI ) to download the version you require
and follow instructions for your platform as long as it's major version 2.
The above commands pip commands will check `op` is present already and if not will install the supported `op` cli
plus the python client itself.
This is currently fixed at `op` version 1.12.5 to ensure compatibility. If you wish to use a higher version of `op` you
can by following [this guide](https://developer.1password.com/docs/cli/upgrade),
however note that we cannot ensure it will work with our client yet.
MacOS users will be prompted with a separate installation window to ensure you have a signed version of `op` - make
sure to check other desktops that the installer might pop up on.
### Optional pre-requisites
#### base32
This utility is used to create a unique guid for your device but this isn't a hard requirement from AgileBits
and so if you see `base32: command not found` an empty string will be used instead,
and the client will still work fully.
If you really want to, you can make sure you have this installed by installing coreutils. Details per platform can
be found here: https://command-not-found.com/base32
## Basic Usage
Since v2 of the cli it is advised to connect your CLI to the local app installed on the device, thus removing the need
for secret keys and passwords in the terminal or shell. Read here on how to do that:
https://developer.1password.com/docs/cli/get-started#step-2-turn-on-the-1password-desktop-app-integration
An added extra for Mac users is that you can also enable TouchID for the app and by linking your cli with the app you
will get biometric login for both.
Once this is done any initial usage of the cli, and our client will request you to authenticate either via the app or
using your biometrics and then you can continue.
We are sure there are use cases where the app cannot be linked and hence a password etc is till required so this
functionality is still present from our v1 implementation and can be described below
### Password authentication
On first usage users will be asked for both the enrolled email, secret key and password.
There is also verification of your account domain and name.
For all following usages you will only be asked for a password.
You will be given 3 attempts and then pointed to reset password documentation or alternatively you can
restart your kernel.
No passwords are stored in memory without encryption.
If you have 2FA turned on for your 1Password account the client will ask for your six digit authenticator code.
```python
from onepassword import OnePassword
import json
op = OnePassword()
# List all vaults
json.loads(op.list_vaults())
# List all items in a vault, default is Private
op.list_items()
# Get all fields, one field or more fields for an item with uuid="example"
op.get_item(uuid="example")
op.get_item(uuid="example", fields="username")
op.get_item(uuid="example", fields=["username", "password"])
```
##
### Input formats
To be sure what you are using is of the right format
- Enrolled email: standard email format e.g. user@example.com
- Secret key: provided by 1Password e.g. ##-######-######-#####-#####-#####-#####
- Account domain: domain that you would login to 1Password via browser e.g. example.1password.com
- Account name: subdomain or account name that cli can use for multiple account holders e.g. example
## Contributing
The GitHub action will run a full build, test and release on any push.
If this is to the main branch then this will release to public PyPi and bump the patch version.
For a major or minor branch update your new branch should include this new version and this should be verified by the
code owners.
In general, this means when contributing you should create a feature branch off of the main branch and without
manually bumping the version you can focus on development.
## CLI coverage
Full op documentation can be found here: https://support.1password.com/command-line-reference/
The below is correct as of version 0.3.0.
### Commands
This is the set of commands the current python SDK covers:
- create: Create an object
- document
- delete: Remove an object
- item: we use this method to remove documents but now there is a new delete document method
- get: Get details about an object
- document
- item
- list: List objects and events
- items
- vaults
- signin: Sign in to a 1Password account
- signout: Sign out of a 1Password account
This is what still needs developing due to new functionality being released:
- add: Grant access to groups or vaults
- group
- user
- completion: Generate shell completion information
- confirm: Confirm a user
- create: Create an object
- group
- user
- item
- vault
- delete: Remove an object
- document
- user
- vault
- group
- trash
- edit: Edit an object
- document
- group
- item
- user
- vault
- encode: Encode the JSON needed to create an item
- forget: Remove a 1Password account from this device
- get: Get details about an object
- account
- group
- template
- totp
- user
- vault
- list: List objects and events
- documents
- events
- groups
- templates
- users
- reactivate: Reactivate a suspended user
- remove: Revoke access to groups or vaults
- suspend: Suspend a user
- update: Check for and download updates
## Roadmap
- Add Windows functionality
- Add clean uninstall of client and op
- Remove subprocess usage everywhere -> use pexpect
- Add test docker image
- Get full UT coverage
- Align response types into JSON / lists instead of JSON strings
- Ensure full and matching functionality of CLI in python
- add
- confirm
- create
- delete
- edit
- encode
- forget
- get
- list
- reactivate
- remove
- suspend
| 1password | /1password-1.0.1.tar.gz/1password-1.0.1/README.md | README.md |
import os
import wget
import zipfile
import platform
from subprocess import Popen, PIPE
cli_version = "2.19.0"
version_string = "v{}".format(cli_version)
os.environ["OP_VERSION_STR"] = version_string
platform_links = {
"Darwin": {
"x86_64": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_apple_universal_{}.pkg".format(version_string,
version_string),
"arm64": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_apple_universal_{}.pkg".format(version_string,
version_string),
"download_loc": "/usr/local/bin/"
},
"FreeBSD": {
"i386": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_freebsd_386_{}.zip".format(version_string,
version_string),
"i686": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_freebsd_386_{}.zip".format(version_string,
version_string),
"x86_64": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_freebsd_amd64_{}.zip".format(version_string,
version_string),
"arm": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_freebsd_arm_{}.zip".format(version_string,
version_string),
"aarch64_be": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_freebsd_arm_{}.zip".format(version_string,
version_string),
"aarch64": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_freebsd_arm_{}.zip".format(version_string,
version_string),
"armv8b": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_freebsd_arm_{}.zip".format(version_string,
version_string),
"armv8l": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_freebsd_arm_{}.zip".format(version_string,
version_string),
"download_loc": "/usr/local/bin/"
},
"Linux": {
"i386": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_linux_386_{}.zip".format(version_string,
version_string),
"i686": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_linux_386_{}.zip".format(version_string,
version_string),
"x86_64": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_linux_amd64_{}.zip".format(version_string,
version_string),
"aarch64_be": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_linux_arm_{}.zip".format(version_string,
version_string),
"aarch64": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_linux_arm_{}.zip".format(version_string,
version_string),
"armv8b": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_linux_arm_{}.zip".format(version_string,
version_string),
"armv8l": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_linux_arm_{}.zip".format(version_string,
version_string),
"arm": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_linux_arm_{}.zip".format(version_string,
version_string),
"download_loc": "/usr/local/bin/"
},
"OpenBSD": {
"i386": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_openbsd_386_{}.zip".format(version_string,
version_string),
"i686": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_openbsd_386_{}.zip".format(version_string,
version_string),
"x86_64": "https://cache.agilebits.com/dist/1P/op2/pkg/{}/op_openbsd_amd64_{}.zip".format(version_string,
version_string),
"download_loc": "/usr/local/bin/"
}
}
def read_bash_return(cmd, single=True):
process = os.popen(cmd)
preprocessed = process.read()
process.close()
if single:
return str(preprocessed.split("\n")[0])
else:
return str(preprocessed)
def mkdirpy(directory):
if not os.path.exists(directory):
os.makedirs(directory)
class CliInstaller: # pragma: no cover
def __init__(self):
self.system = str(platform.system())
machine = str(platform.machine())
self.link = platform_links[self.system][machine]
self.download_location = platform_links[self.system]["download_loc"]
def install(self):
if self.system in platform_links.keys():
self.install_linux_mac()
else:
raise OSError("Operating system not supported")
def check_install_required(self): # pragma: no cover
"""
Helper function to check if op cli is already installed
:returns: :obj:`bool`: True or False
"""
op = read_bash_return("op --version")
if op == "":
return True, self.download_location
else:
if op == cli_version:
return False, ""
else:
existing_location = read_bash_return("which op")
return True, existing_location
def install_linux_mac(self):
"""
Helper function to download, unzip, install and chmod op cli files
"""
install_check, install_loc = self.check_install_required()
if install_check:
if install_loc == self.download_location:
local_bin = self.download_location
else:
local_bin = install_loc
try:
os.chmod(local_bin, 0o755)
except PermissionError:
local_bin = os.path.join(os.environ["HOME"], "op-downloads")
mkdirpy(local_bin)
os.chmod(local_bin, 0o755)
op_file = self.link.split("/")[-1]
download_path = os.path.join(local_bin, op_file)
print('Downloading the 1Password CLI: {}'.format(op_file))
wget.download(self.link, download_path)
if self.link[-4:] != ".pkg":
zip_ref = zipfile.ZipFile(download_path, 'r')
zip_ref.extractall(local_bin)
zip_ref.close()
os.chmod(os.path.join(local_bin, 'op'), 0o755)
else:
Popen(["open", os.path.join(local_bin, op_file)], stdin=PIPE, stdout=PIPE) # pragma: no cover
else:
print("op already installed, with supported version.")
if __name__ == '__main__':
# Run wizard if executed from terminal
install_cli = CliInstaller()
install_cli.install()
print() | 1password | /1password-1.0.1.tar.gz/1password-1.0.1/install_op.py | install_op.py |
import os
import json
import platform
import yaml
import subprocess
from subprocess import CompletedProcess
from typing import Any
from getpass import getpass
from json import JSONDecodeError
from onepassword.utils import read_bash_return, domain_from_email, Encryption, BashProfile, get_device_uuid, \
_spawn_signin
from onepassword.exceptions import OnePasswordForgottenPassword
class SignIn:
"""
Helper class for methods common between App and Manual sign in
"""
_env_account = "OP_ACCOUNT"
def get_account(self, bash_profile: BashProfile | None = None) -> str:
"""
Get the 1Password account name, using either the stored name or inputs
:param bash_profile: Stored bash profile. (Optional, default = None)
:return: 1Password account name
"""
if bash_profile is None:
return self._input_account()
else:
return self._get_account_bash(bash_profile)
@staticmethod
def _input_account() -> str:
account = input("Please input your 1Password account name e.g. wandera from wandera.1password.com: ")
return account
def _get_account_bash(self, bash_profile: BashProfile) -> str:
try:
session_dict = bash_profile.get_key_value(self._env_account, fuzzy=True)[0]
account = session_dict.get("OP_ACCOUNT").strip('\"')
except AttributeError:
account = self._input_account()
except ValueError:
raise ValueError("First signin failed or not executed.")
return account
@staticmethod
def get_domain() -> str:
"""
Get the domain name for the 1Password account
:return: 1Password domain name
"""
domain = input("Please input your 1Password domain in the format <something>.1password.com: ")
return domain
def _update_bash_account(self, account: str, bash_profile: BashProfile) -> None:
os.environ[self._env_account] = account
bash_profile.update_profile(self._env_account, account)
def signin(self):
pass
class ManualSignIn(SignIn):
"""
Class to sign in to 1Password manually, see: https://developer.1password.com/docs/cli/sign-in-manually
:param account: Shorthand account name for your 1Password account e.g. wandera from wandera.1password.com.
(Optional, default = None)
:param password: 1Password password. (Optional, default = None)
"""
_env_session = "OP_SESSION"
def __init__(self, account: str | None = None, password: str | None = None) -> None:
# pragma: no cover
bp = BashProfile()
os.environ["OP_DEVICE"] = get_device_uuid(bp)
# reuse existing op session
if isinstance(account, str) and "{}_{}".format(self._env_account, account) in os.environ:
pass
# Check first time: if true, full signin, else use shortened signin
elif self._check_not_first_time(bp):
self.encrypted_master_password, self.session_key = self.signin_wrapper(
account=account,
master_password=password
)
else:
self.first_use()
def _check_not_first_time(self, bp: BashProfile) -> bool:
for line in bp.profile_lines:
if self._env_session in line:
return True
return False
def first_use(self): # pragma: no cover
"""
Helper function to perform first time signin either with user interaction or not, depending on _init_
"""
email_address = input("Please input your email address used for 1Password account: ")
account = domain_from_email(email_address)
signin_domain = account + ".1password.com"
confirm_signin_domain = input("Is your 1Password domain: {} (y/n)? ".format(signin_domain))
if confirm_signin_domain == "y":
pass
else:
signin_domain = self.get_domain()
confirm_account = input("Is your 1Password account name: {} (y/n)? ".format(account))
if confirm_account == "y":
pass
else:
account = self.get_account()
secret_key = getpass("Please input your 1Password secret key: ")
self.signin_wrapper(account, signin_domain, email_address, secret_key)
def signin_wrapper(
self, account: str | None = None, domain: str | None = None, email: str | None = None,
secret_key: str | None = None, master_password: str | None = None
) -> tuple[str, str]:
# pragma: no cover
"""
Helper function for user to sign in but allows for three incorrect passwords. If successful signs in and updates
bash profile, if not raises exception and points user to 1Password support.
:param account: Shorthand account name for your 1Password account e.g. wandera from wandera.1password.com.
(Optional, default = None)
:param domain: Full domain name of 1Password account e.g. wandera.1password.com (Optional, default=None)
:param email: Email address of 1Password account (Optional, default=None)
:param secret_key: Secret key of 1Password account (Optional, default=None)
:param master_password: Password for 1Password account (Optional, default=None)
:return: encrypted_str, session_key - used by signin to know of existing login
"""
password, session_key, domain, account, bp = self.signin(account, domain, email, secret_key, master_password)
tries = 1
while tries < 3:
if session_key is False: # Not the right password, trying again
password, session_key, domain, account, bp = self.signin(
account, domain, email, secret_key, master_password)
tries += 1
pass
else:
self._update_bash_account(account, bp)
os.environ["{}_{}".format(self._env_session, account)] = session_key.replace("\n", "")
bp.update_profile("{}_{}".format(self._env_session, account), session_key.replace("\n", ""))
encrypt = Encryption(session_key)
encrypted_str = encrypt.encode(password)
return encrypted_str, session_key
raise OnePasswordForgottenPassword("You appear to have forgotten your password, visit: "
"https://support.1password.com/forgot-master-password/")
def signin(
self, account: str | None = None, domain: str | None = None, email: str | None = None,
secret_key: str | None = None, master_password: str | None = None
) -> tuple[bytes | None, str | bool, str | None, str | None | Any, BashProfile]: # pragma: no cover
"""
Helper function to prepare sign in for the user
:param account: Shorthand name for your 1Password account e.g. wandera from wandera.1password.com
(Optional, default=None)
:param domain: Full domain name of 1Password account e.g. wandera.1password.com (Optional, default=None)
:param email: Email address of 1Password account (Optional, default=None)
:param secret_key: Secret key of 1Password account (Optional, default=None)
:param master_password: Password for 1Password account (Optional, default=None)
:return: master_password, sess_key, domain, bp - all used by wrapper
"""
bp = BashProfile()
op_command = ""
if master_password is not None:
master_password = str.encode(master_password)
else:
if 'op' in locals():
initiated_class = locals()["op"]
if 'session_key' and 'encrypted_master_password' in initiated_class.__dict__:
encrypt = Encryption(initiated_class.session_key)
master_password = str.encode(encrypt.decode(initiated_class.encrypted_master_password))
else:
master_password = str.encode(getpass("Please input your 1Password master password: "))
if secret_key:
op_command = "op account add --address {} --email {} --secret-key {} --shorthand {} --signin --raw".format(
domain, email, secret_key, account)
else:
if account is None:
try:
session_dict = bp.get_key_value(self._env_session, fuzzy=True)[0] # list of dicts from BashProfile
account = list(session_dict.keys())[0].split(self._env_session + "_")[1]
except AttributeError:
account = input("Please input your 1Password account name e.g. wandera from "
"wandera.1password.com: ")
except ValueError:
raise ValueError("First signin failed or not executed.")
op_command = "op signin --account {} --raw".format(account)
sess_key = _spawn_signin(op_command, master_password)
return master_password, sess_key, domain, account, bp
class AppSignIn(SignIn):
"""
Class to sign in to 1Password using the 1Password app integration,
see: https://developer.1password.com/docs/cli/app-integration
:param account: Shorthand account name for your 1Password account e.g. wandera from wandera.1password.com.
(Optional, default = None)
"""
def __init__(self, account: str | None = None) -> None:
self.signin(account)
@staticmethod
def _do_signin(account: str) -> CompletedProcess[Any] | CompletedProcess[str]:
return subprocess.run("op signin --account {}".format(account), shell=True, capture_output=True, text=True)
@staticmethod
def _do_open_app(default_error: str) -> CompletedProcess[Any] | CompletedProcess[str]:
if platform.system() == "Darwin":
return subprocess.run("open -a 1Password.app", shell=True)
elif platform.system() == "Linux":
return subprocess.run("1password", shell=True)
else:
raise ConnectionError(default_error)
def _signin_wrapper(self, account: str) -> None:
r = self._do_signin(account)
if r.returncode != 0:
if "please unlock it in the 1Password app" in r.stderr:
open_app = self._do_open_app(r.stderr.rstrip("\n"))
if open_app.returncode == 0:
sign_in = self._do_signin(account)
if sign_in.returncode != 0:
raise ConnectionError(sign_in.stderr.rstrip("\n"))
else:
raise ConnectionError(r.stderr.rstrip("\n"))
raise ConnectionError(r.stderr.rstrip("\n"))
def signin(self, account: str | None = None) -> None:
"""
Sign in to your 1Password account using the app integration
:param account: Shorthand account name for your 1Password account e.g. wandera from wandera.1password.com.
(Optional, default = None)
"""
bash_profile = BashProfile()
if account is None:
account = self.get_account(bash_profile)
self._signin_wrapper(account)
self._update_bash_account(account, bash_profile)
class OnePassword:
"""
Class for integrating with a OnePassword password manager
:param signin_method: Sign in method for 1Password (Optional, default = 'app', options: 'app', 'manual')
:param account: 1Password account name (Optional, default=None)
:param password: password of 1Password account (Optional, default=None)
"""
def __init__(self, signin_method: str = "app", account: str | None = None, password: str | None = None) -> None:
# pragma: no cover
if signin_method == "app":
self.signin_strategy = AppSignIn(account)
elif signin_method == "manual":
self.signin_strategy = ManualSignIn(account, password)
else:
raise ValueError("Unrecognised 'signin_method', options are: 'app' or 'manual'. "
"See: https://developer.1password.com/docs/cli/verify")
def get_uuid(self, docname: str, vault: str = "Private") -> str: # pragma: no cover
"""
Helper function to get the uuid for an item
:param docname: Title of the item (not filename of documents)
:param vault: Vault the item is in (Optional, default=Private)
:return: Uuid of item or None if it doesn't exist
"""
items = self.list_items(vault=vault)
for t in items:
if t["title"] == docname:
return t["id"]
def get_document(self, docname: str, vault: str = "Private") -> dict | None: # pragma: no cover
"""
Helper function to get a document
:param docname: Title of the document (not it's filename)
:param vault: Vault the document is in (Optional, default=Private)
:returns: Document or None if it doesn't exist
"""
docid = self.get_uuid(docname, vault=vault)
try:
return json.loads(
read_bash_return("op document get {} --vault='{}' --format=Json".format(docid, vault), single=False))
except JSONDecodeError:
yaml_attempt = yaml.safe_load(read_bash_return("op document get {} --vault='{}'".format(docid, vault),
single=False))
if isinstance(yaml_attempt, dict):
return yaml_attempt
else:
print("File {} does not exist in 1Password vault: {}".format(docname, vault))
return None
def put_document(self, filename: str, title: str, vault: str = "Private") -> None: # pragma: no cover
"""
Helper function to put a document
:param filename: Path and filename of document (must be saved locally already)
:param title: Title you wish to call the document
:param vault: Vault the document is in (Optional, default=Private)
"""
cmd = "op document create {} --title={} --vault='{}'".format(filename, title, vault)
# [--tags=<tags>]
response = read_bash_return(cmd)
if len(response) == 0:
self.signin_strategy.signin()
read_bash_return(cmd)
# self.signout()
# else:
# self.signout()
def delete_document(self, title: str, vault: str = "Private") -> None: # pragma: no cover
"""
Helper function to delete a document
:param title: Title of the document you wish to remove
:param vault: Vault the document is in (Optional, default=Private)
"""
docid = self.get_uuid(title, vault=vault)
cmd = "op item delete {} --vault='{}'".format(docid, vault)
response = read_bash_return(cmd)
if len(response) > 0:
self.signin_strategy.signin()
read_bash_return(cmd)
# self.signout()
# else:
# self.signout()
def update_document(self, filename: str, title: str, vault: str = 'Private') -> None: # pragma: no cover
"""
Helper function to update an existing document in 1Password.
:param title: Name of the document in 1Password.
:param filename: Path and filename of document (must be saved locally already).
:param vault: Vault the document is in (Optional, default=Private).
"""
# delete the old document
self.delete_document(title, vault=vault)
# put the new updated one
self.put_document(filename, title, vault=vault)
# remove the saved file locally
os.remove(filename)
@staticmethod
def signout():
"""
Helper function to sign out of 1Password
"""
read_bash_return("op signout")
@staticmethod
def list_vaults():
"""
Helper function to list all vaults
"""
return json.loads(read_bash_return("op vault list --format=json", single=False))
@staticmethod
def list_items(vault: str = "Private") -> dict:
"""
Helper function to list all items in a certain vault
:param vault: Vault the items are in (Optional, default=Private)
:returns: Dictionary of all items
"""
items = json.loads(read_bash_return("op items list --vault='{}' --format=json".format(vault), single=False))
return items
@staticmethod
def get_item(uuid: str | bytes, fields: str | bytes | list | None = None):
"""
Helper function to get a certain field, you can find the UUID you need using list_items
:param uuid: Uuid of the item you wish to get, no vault needed
:param fields: To return only certain detail use either a specific field or list of them
(Optional, default=None which means all fields returned)
:return: Dictionary of the item with requested fields
"""
if isinstance(fields, list):
items = read_bash_return(
"op item get {} --fields label={}".format(uuid, ",label=".join(fields)),
single=False
).rstrip('\n')
item = dict(zip(fields, items.split(",")))
elif isinstance(fields, str):
item = {
fields: read_bash_return(
"op item get {} --fields label={}".format(uuid, fields), single=False).rstrip('\n')
}
else:
item = json.loads(read_bash_return("op item get {} --format=json".format(uuid), single=False))
return item | 1password | /1password-1.0.1.tar.gz/1password-1.0.1/onepassword/client.py | client.py |
import os
import base64
import pexpect
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad
BLOCK_SIZE = 32 # Bytes
master_password_regex = 'Enter the password for [a-zA-Z0-9._%+-]+\@[a-zA-Z0-9-]+\.[a-zA-z]{2,4} at ' \
'[a-zA-Z0-9-.]+\.1password+\.[a-zA-z]{2,4}'
def read_bash_return(cmd, single=True):
process = os.popen(cmd)
preprocessed = process.read()
process.close()
if single:
return str(preprocessed.split("\n")[0])
else:
return str(preprocessed)
def docker_check():
f = None
user_home = os.environ.get('HOME')
for rcfile in ['.bashrc', '.bash_profile', '.zshrc', '.zprofile']:
rcpath = os.path.join(user_home, rcfile)
if os.path.exists(rcpath):
f = open(os.path.join(user_home, rcpath), "r")
break
if not f:
raise Exception("No sehll rc or profile files exist.")
bash_profile = f.read()
try:
docker_flag = bash_profile.split('DOCKER_FLAG="')[1][0]
if docker_flag == "t":
return True
else:
return False
except IndexError:
return False
def domain_from_email(address):
"""
Method to extract a domain without sld or tld from an email address
:param address: email address to extract from
:type address: str
:return: domain (str)
"""
return address.split("@")[1].split(".")[0]
def get_session_key(process_resp_before):
new_line_response = [x for x in process_resp_before.decode("utf-8").split("\n") if "\r" not in x]
if len(new_line_response) != 1:
raise IndexError("Session keys not parsed correctly from response: {}.".format(process_resp_before))
else:
return new_line_response[0]
def _spawn_signin(command, m_password) -> str | bool:
if command != "":
p = pexpect.spawn(command)
index = p.expect(master_password_regex)
if index == 0:
p.sendline(m_password)
index = p.expect([pexpect.EOF, "\(401\) Unauthorized"])
if index == 0:
sess_key = get_session_key(p.before)
p.close()
return sess_key
elif index == 1:
print("Input master password is not correct. Please try again")
return False
else:
raise IOError("Onepassword command not valid")
else:
raise IOError("Spawn command not valid")
class BashProfile:
def __init__(self):
f = None
user_home = os.environ.get('HOME')
for rcfile in ['.bashrc', '.bash_profile', '.zshrc', '.zprofile']:
rcpath = os.path.join(user_home, rcfile)
if os.path.exists(rcpath):
f = open(os.path.join(user_home, rcpath), "r")
break
if not f:
raise Exception("No shell rc or profile files exist.")
self.other_profile_flag = False
if docker_check():
f2 = None
try:
f2 = open(os.path.join(user_home, ".profile"), "r")
except IOError:
print("Profile file does not exist.")
self.other_profile = f2.read()
self.other_profile_filename = f2.name
self.other_profile_flag = True
self.other_profile_lines = f2.readlines()
f2.close()
self.profile_lines = f.readlines()
self.profile = f.read()
self.profile_filename = f.name
f.close()
def get_key_value(self, key, fuzzy=False):
key_lines = self.get_key_line(key, fuzzy=fuzzy)
if key_lines:
key_values = []
for ky in key_lines:
k = ky.split("=")[0].split(" ")[1]
v = ky.split("=")[1]
key_values.append({k: v})
return key_values
else:
raise ValueError("Environment variable does not exist.")
def get_key_line(self, key, fuzzy=False):
key_line = None
if self.other_profile_flag:
prof = [self.other_profile_lines, self.profile_lines]
else:
prof = [self.profile_lines]
key_lines = []
for prof_lines in prof:
if len(prof_lines) > 0:
for p in prof_lines:
if (~fuzzy) & (" {}=".format(key) in p):
key_line = p
elif fuzzy & (key in p):
key_line = p
key_lines.append(key_line.replace("\n", ""))
return key_lines
def write_profile(self, updated_lines):
if self.other_profile_flag:
prof_name = [self.other_profile_filename, self.profile_filename]
else:
prof_name = [self.profile_filename]
for p in prof_name:
with open(p, 'w') as writer:
writer.writelines(updated_lines)
writer.close()
def update_profile(self, key, value):
for lines in self.profile_lines:
if key in lines:
self.profile_lines.remove(lines)
if isinstance(value, str):
new_line = 'export {}="{}"\n'.format(key, value)
self.profile_lines.append(new_line)
self.write_profile(self.profile_lines)
class Encryption:
def __init__(self, secret_key):
if isinstance(secret_key, str):
self.secret_key = str.encode(secret_key)[0:BLOCK_SIZE]
else:
self.secret_key = secret_key[0:BLOCK_SIZE]
self.cipher = AES.new(self.secret_key, AES.MODE_ECB)
def decode(self, encoded):
return self.cipher.decrypt(base64.b64decode(encoded)).decode('UTF-8').replace("\x1f", "")
def encode(self, input_str):
return base64.b64encode(self.cipher.encrypt(pad(input_str, BLOCK_SIZE)))
def bump_version(version_type="patch"):
"""
Only run in the project root directory, this is for github to bump the version file only!
:return:
"""
__root__ = os.path.abspath("")
with open(os.path.join(__root__, 'VERSION')) as version_file:
version = version_file.read().strip()
all_version = version.replace('"', "").split(".")
if version_type == "patch":
new_all_version = version.split(".")[:-1]
new_all_version.append(str(int(all_version[-1]) + 1))
elif version_type == "minor":
new_all_version = [version.split(".")[0]]
new_all_version.extend([str(int(all_version[1]) + 1), '0'])
new_line = '.'.join(new_all_version) + "\n"
with open("{}/VERSION".format(__root__), "w") as fp:
fp.write(new_line)
fp.close()
def generate_uuid():
"""
Generates a random UUID to be used for op in initial set up only for more details read here
https://1password.community/discussion/114059/device-uuid
:return: (str)
"""
return read_bash_return("head -c 16 /dev/urandom | base32 | tr -d = | tr '[:upper:]' '[:lower:]'")
def get_device_uuid(bp):
"""
Attempts to get the device_uuid from the given BashProfile. If the device_uuid is not
set in the BashProfile generates a new device_uuid and sets it in the given
BashProfile.
:return: (str)
"""
try:
device_uuid = bp.get_key_value("OP_DEVICE")[0]['OP_DEVICE'].strip('"')
except (AttributeError, ValueError):
device_uuid = generate_uuid()
bp.update_profile("OP_DEVICE", device_uuid)
return device_uuid | 1password | /1password-1.0.1.tar.gz/1password-1.0.1/onepassword/utils.py | utils.py |
<p align="center">
<br>
<img src="https://github.com/qvco/1secMail-Python/assets/77382767/fde69c1a-b95f-4d78-af1a-2dca315204bc" alt="1secMail" width="700">
<!-- <br>
1secMail for Python
<br> -->
</p>
<h4 align="center">An API wrapper for <a href="https://www.1secmail.com/" target="_blank">www.1secmail.com</a> written in Python.</h4>
<p align="center">
<img src="https://img.shields.io/github/release/qvco/1secMail-Python">
<img src="https://img.shields.io/badge/python-3.8-blue.svg">
<img src="https://img.shields.io/badge/License-MIT-blue.svg">
</p>
### About
This is an easy to use yet full-featured Python API wrapper for www.1secmail.com ↗ using the official 1secMail API. It allows you to easily create temporary email addresses for testing, verification, or other purposes where you need a disposable email address.
> Asynchronous operations are also supported!:thumbsup:
### Install
To install the package, you'll need Python 3.8 or above installed on your computer. From your command line:
```bash
pip install 1secMail
```
<br>
> **Note**
> If you're willing to install the development version, do the following:
```bash
git clone https://github.com/qvco/1secMail-Python.git
cd 1secMail-Python
pip install -r requirements.txt
pip install -e .
```
## Usage
### Generating Email Addresses
To generate a list of random email addresses, use the `random_email()` method:
```python
import secmail
client = secmail.Client()
client.random_email(amount=3)
>>> ['c3fho3cry1@1secmail.net', '5qcd3d36zr@1secmail.org', 'b6fgeothtg@1secmail.net']
```
You can also generate a custom email address by specifying the username and domain:
> **Note**
> Specifying a domain is optional!
```python
client.custom_email(username="bobby-bob", domain="kzccv.com")
>>> 'bobby-bob@kzccv.com'
```
### Receiving Messages
To wait until a new message is received, use the `await_new_message()` method:
```python
message = client.await_new_message("bobby-bob@kzccv.com")
```
To check all messages received on a particular email address, use the `get_inbox()` method and pass the email address:
```python
inbox = client.get_inbox("bobby-bob@kzccv.com")
for message in inbox:
print(message.id)
print(message.from_address)
print(message.subject)
print(message.date)
```
You can also fetch a single message using the `get_message()` method and passing the email address and message ID:
```python
message = client.get_message(address="bobby-bob@kzccv.com", message_id=235200687)
print(message.id)
print(message.subject)
print(message.body)
print(message.text_body)
print(message.html_body)
print(message.attachments)
print(message.date)
```
### Downloading an attachment
You can download an attachment from a message in the inbox of a specified email address using the download_attachment method like this:
```python
client.download_attachment(address, message_id, attachment_filename)
>>> 'Path: (C:\Users\user\path/config/rocket.png), Size: 49071B'
```
## Asynchronous Client
### Generating Email Addresses
To generate a list of random email addresses, use the `random_email()` method:
```python
import asyncio
import secmail
async def main():
client = secmail.AsyncClient()
email_addresses = await client.random_email(amount=3)
print(email_addresses)
asyncio.run(main())
>>> ['c3fho3cry1@1secmail.net', '5qcd3d36zr@1secmail.org', 'b6fgeothtg@1secmail.net']
```
You can also generate a custom email address by specifying the username and domain:
> **Note**
> Specifying a domain is optional!
```python
await client.custom_email(username="bobby-bob", domain="kzccv.com")
>>> 'bobby-bob@kzccv.com'
```
### Receiving Messages
To wait until a new message is received, use the `await_new_message()` method:
```python
import asyncio
import secmail
async def main():
client = secmail.AsyncClient()
message = await client.await_new_message("bobby-bob@kzccv.com")
print(f"{message.from_address}: {message.subject}")
asyncio.run(main())
```
To check all messages received on a particular email address, use the `get_inbox()` method and pass the email address:
```python
import asyncio
import secmail
async def main():
client = secmail.AsyncClient()
inbox = await client.get_inbox("bobby-bob@kzccv.com")
print(f"You have {len(inbox)} messages in your inbox.")
for message in inbox:
print(message.id)
print(message.from_address)
print(message.subject)
print(message.date)
asyncio.run(main())
```
You can also fetch a single message using the `get_message()` method and passing the email address and message ID:
```python
import asyncio
import secmail
async def main():
client = secmail.AsyncClient()
address = "bobby-bob@kzccv.com"
inbox = await client.get_inbox(address)
message_id = inbox[0].id
message = await client.get_message(address, message_id)
print(message.id)
print(message.subject)
print(message.body)
print(message.text_body)
print(message.html_body)
print(message.attachments)
print(message.date)
asyncio.run(main())
```
### Downloading an attachment
You can download an attachment from a message in the inbox of a specified email address using the download_attachment method like this:
```python
import asyncio
import secmail
async def main():
client = secmail.AsyncClient()
address = "bobby-bob@kzccv.com"
inbox = await client.get_inbox(address)
message_id = inbox[0].id
message = await client.get_message(address, message_id)
attachment_filename = message.attachments[0].filename
await client.download_attachment(address, message_id, attachment_filename)
asyncio.run(main())
>>> 'Path: (C:\Users\user\path/config/rocket.png), Size: 49071B'
```
## Licnese
This software is licensed under the [MIT](https://github.com/qvco/1secMail-Python/blob/master/LICENSE) © [Qvco](https://github.com/qvco).
| 1secMail | /1secMail-1.1.0.tar.gz/1secMail-1.1.0/README.md | README.md |
import os
import re
import asyncio
import random
import string
import httpx
import time
import json
from typing import List
from json import JSONDecodeError
from .config import (
DOMAIN_LIST,
GET_DOMAIN_LIST,
GET_MESSAGES,
GET_SINGLE_MESSAGE,
DOWNLOAD,
)
from .models import Inbox, Message
# errors
class SecMailError(Exception):
"""Base exception for 1secMail"""
pass
class BadRequestError(SecMailError):
"""BadRequestError()
Exception raised for a 400 HTTP status code
"""
pass
class AuthenticationError(SecMailError):
"""AuthenticationError()
Exception raised for a 401 HTTP status code
"""
pass
class ForbiddenError(SecMailError):
"""ForbiddenError()
Exception raised for a 403 HTTP status code
"""
pass
class NotFoundError(SecMailError):
"""NotFoundError()
Exception raised for a 404 HTTP status code
"""
pass
class RateLimitError(SecMailError):
"""RateLimitError()
Exception raised for a 429 HTTP status code
"""
pass
class ServerError(SecMailError):
"""ServerError()
Exception raised for a 5xx HTTP status code
"""
pass
# utils
def is_valid_username(username: str) -> bool:
if username is None or len(username) > 64:
return False
return bool(
re.match(r"^[A-Za-z][A-Za-z0-9._-]*[A-Za-z0-9]$", username)
and not re.search(r"\.\.|\-\-|\_\_|\.$", username)
)
current_path = os.path.abspath(os.getcwd())
# client
class Client:
"""An API wrapper for www.1secmail.com written in Python.
>>> import secmail
>>> client = secmail.Client()
"""
def __init__(
self, base_path=current_path + "/config/", host="www.1secmail.com"
) -> None:
self.base_path = base_path
self.api_url = "https://" + host + "/api/v1/"
self.client = httpx.Client()
def _request(self, action: str, params=None, data_type=None):
r = self.client.request(method="GET", url=self.api_url + action, params=params)
if r.status_code == 400:
raise BadRequestError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 401:
raise AuthenticationError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 403:
raise ForbiddenError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 404:
raise NotFoundError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 429:
raise RateLimitError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 500:
raise ServerError(f"HTTP {r.status_code}: {r.text}")
if action == DOWNLOAD:
return r.content
try:
r = r.json()
except JSONDecodeError:
return r.text
if data_type is not None:
if isinstance(r, list):
r = [data_type(result) for result in r]
elif r is not None:
r = data_type(r)
return r
@staticmethod
def random_email(amount: int, domain: str = None) -> List[str]:
"""This method generates a list of random email addresses.
Parameters:
----------
- `amount`: `int` - The number of email addresses to generate.
- `domain`: `str` (optional) - The domain name to use for the email addresses. If not provided, a random domain from the valid list of domains will be selected.
Example:
-------
Generate a list of 5 email addresses with the domain "1secmail.com":
>>> client.random_email(amount=5, domain="1secmail.com")
Valid domains:
-------------
- 1secmail.com
- 1secmail.org
- 1secmail.net
- kzccv.com
- qiott.com
- wuuvo.com
- icznn.com
- ezztt.com
If `domain` is provided and not in the valid list of domains, a ValueError will be raised with a message indicating the invalid domain and the valid list of domains.
"""
if domain is not None and domain not in DOMAIN_LIST:
err_msg = (
f"{domain} is not a valid domain name.\nValid Domains: {DOMAIN_LIST}"
)
raise ValueError(err_msg)
emails = []
for _ in range(amount):
username = "".join(
random.choices(string.ascii_lowercase + string.digits, k=12)
)
email = f"{username}@{domain or random.choice(DOMAIN_LIST)}"
emails.append(email)
return emails
@staticmethod
def custom_email(username: str, domain: str = None) -> str:
"""This method generates a custom email address.
Parameters:
----------
- `username`: `str` - The username to use for the email address.
- `domain`: `str` (optional) - The domain name to use for the email address. If not provided, a random domain from the valid list of domains will be selected.
Returns:
-------
- `email`: `str` - The generated email address.
Example:
-------
Generate a custom email address with the username "johndoe":
>>> client.custom_email(username="johndoe")
Valid domains:
-------------
- 1secmail.com
- 1secmail.org
- 1secmail.net
- kzccv.com
- qiott.com
- wuuvo.com
- icznn.com
- ezztt.com
If `domain` is provided and not in the valid list of domains, a ValueError will be raised with a message indicating the invalid domain and the valid list of domains.
"""
if domain is not None and domain not in DOMAIN_LIST:
err_msg = (
f"{domain} is not a valid domain name.\nValid Domains: {DOMAIN_LIST}"
)
raise ValueError(err_msg)
if is_valid_username(username) is False:
err_msg = f"'{username}' is not a valid username."
raise ValueError(err_msg)
return f"{username}@{domain or random.choice(DOMAIN_LIST)}"
def await_new_message(self, address: str, fetch_interval=5) -> Inbox:
"""This method waits until a new message is received for the specified email address.
Parameters:
----------
- `address`: `str` - The email address to check for new messages.
- `fetch_interval`: `int` (optional) - The time interval (in seconds) for checking new messages. The default value is 5 seconds.
Returns:
-------
- `message`: `Inbox` - The new message received.
Example:
-------
Wait for a new message to be received for the email address "johndoe@1secmail.com":
>>> message = client.await_new_message("johndoe@1secmail.com")
The method will continuously check for new messages every `fetch_interval` seconds until a new message is received. Once a new message is received, the message object is returned. The method also maintains a set of message IDs to check if the message is new. If the same message is received again, the method will continue to wait for a new message.
Note that if no new messages are received for a long time, the method may take a long time to return.
"""
ids = {message.id for message in self.get_inbox(address)}
while True:
time.sleep(fetch_interval)
new_messages = self.get_inbox(address)
for message in new_messages:
if message.id not in ids:
return message
def get_active_domains(self) -> List[str]:
"""This method retrieves a list of currently active domains.
Returns:
-------
- `domains`: `List[str]` - A list of active domains.
Example:
-------
Get a list of active domains:
>>> domains = client.get_active_domains()
The method sends a GET request to the API endpoint to retrieve a list of currently active domains. The list is returned as a list of strings.
Note that the list of active domains may change over time.
"""
return self._request(action=GET_DOMAIN_LIST)
def get_inbox(self, address: str) -> List[Inbox]:
"""This method retrieves all the messages in the mailbox for the specified email address.
Parameters:
----------
- `address`: `str` - The email address to check for messages.
Returns:
-------
- `messages`: `List[Inbox]` - A list of message objects in the mailbox.
Example:
-------
Get all the messages in the mailbox for the email address "johndoe@1secmail.com":
>>> messages = client.get_inbox("johndoe@1secmail.com")
The method sends a GET request to the API endpoint to retrieve all the messages in the mailbox for the specified email address. The messages are returned as a list of inbox objects. If there are no messages in the mailbox, an empty list is returned.
"""
username, domain = address.split("@")
return self._request(
action=GET_MESSAGES,
params={"login": username, "domain": domain},
data_type=Inbox,
)
def get_message(self, address: str, message_id: int) -> Message:
"""This method retrieves a detailed message from the mailbox for the specified email address and message ID.
Parameters:
----------
- `address`: `str` - The email address to check for the message.
- `message_id`: `int` - The ID of the message to retrieve.
Returns:
-------
- `message`: `Message` - The message object with the specified ID.
Example:
-------
Get the message with ID 12345 in the mailbox for the email address "johndoe@1secmail.com":
>>> message = client.get_message("johndoe@1secmail.com", 12345)
The method sends a GET request to the API endpoint to retrieve the message with the specified ID in the mailbox for the specified email address. The message is returned as a message object.
"""
username, domain = address.split("@")
return self._request(
action=GET_SINGLE_MESSAGE,
params={"login": username, "domain": domain, "id": message_id},
data_type=Message,
)
def save_email(self, address: str) -> None:
"""This method saves the specified email address to a JSON file for future use.
Parameters:
----------
- `address`: `str` - The email address to save.
Example:
-------
Save the email address "johndoe@1secmail.com" to the JSON file:
>>> client.save_email("johndoe@1secmail.com")
The JSON file is saved in the base path specified during client initialization, with the name `secmail.json`.
"""
data = {}
if not os.path.exists(self.base_path):
os.mkdir(self.base_path)
if os.path.exists(self.base_path + "secmail.json"):
with open(self.base_path + "secmail.json", "r") as f:
data = json.load(f)
data.setdefault("email", []).append(address)
with open(self.base_path + "secmail.json", "w") as f:
json.dump(data, f, indent=4)
def download_attachment(
self,
address: str,
message_id: int,
filename: str,
save_path: str = current_path + "/config/",
):
"""This method downloads an attachment from a message in the mailbox for the specified email address and message ID.
Parameters:
----------
- `address`: `str` - The email address to check for the message containing the attachment.
- `message_id`: `int` - The ID of the message containing the attachment to download.
- `filename`: `str` - The name of the attachment file to download.
- `save_path`: `str` - Optional. The path to save the downloaded attachment. Default is the current path + "/config/".
Returns:
-------
- `str` - A string indicating the path and size of the downloaded attachment.
Example:
-------
Download the attachment named "report.pdf" from the message with ID 12345 in the mailbox for the email address "johndoe@1secmail.com":
>>> download_attachment("johndoe@1secmail.com", 12345, "report.pdf")
"""
username, domain = address.split("@")
attachment = self._request(
action=DOWNLOAD,
params={
"login": username,
"domain": domain,
"id": message_id,
"file": filename,
},
)
if not os.path.exists(self.base_path):
os.mkdir(self.base_path)
with open(save_path + filename, "wb") as attachment_file:
size = attachment_file.write(attachment)
return "Path: (" + save_path + filename + "), Size: " + str(size) + "B"
# async client
class AsyncClient:
"""An API wrapper for www.1secmail.com written in Python.
>>> import secmail
>>> client = secmail.AsyncClient()
"""
def __init__(
self, base_path=current_path + "/config/", host="www.1secmail.com"
) -> None:
self.base_path = base_path
self.api_url = "https://" + host + "/api/v1/"
self.client = httpx.AsyncClient()
async def _request(self, action: str, params=None, data_type=None):
r = await self.client.request(
method="GET", url=self.api_url + action, params=params
)
if r.status_code == 400:
raise BadRequestError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 401:
raise AuthenticationError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 403:
raise ForbiddenError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 404:
raise NotFoundError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 429:
raise RateLimitError(f"HTTP {r.status_code}: {r.text}")
if r.status_code == 500:
raise ServerError(f"HTTP {r.status_code}: {r.text}")
if action == DOWNLOAD:
return r.content
try:
r = r.json()
except JSONDecodeError:
return r.text
if data_type is not None:
if isinstance(r, list):
r = [data_type(result) for result in r]
elif r is not None:
r = data_type(r)
return r
@staticmethod
def random_email(amount: int, domain: str = None) -> List[str]:
"""This method generates a list of random email addresses.
Parameters:
----------
- `amount`: `int` - The number of email addresses to generate.
- `domain`: `str` (optional) - The domain name to use for the email addresses. If not provided, a random domain from the valid list of domains will be selected.
Example:
-------
Generate a list of 5 email addresses with the domain "1secmail.com":
>>> client.random_email(amount=5, domain="1secmail.com")
Valid domains:
-------------
- 1secmail.com
- 1secmail.org
- 1secmail.net
- kzccv.com
- qiott.com
- wuuvo.com
- icznn.com
- ezztt.com
If `domain` is provided and not in the valid list of domains, a ValueError will be raised with a message indicating the invalid domain and the valid list of domains.
"""
if domain is not None and domain not in DOMAIN_LIST:
err_msg = (
f"{domain} is not a valid domain name.\nValid Domains: {DOMAIN_LIST}"
)
raise ValueError(err_msg)
emails = []
for _ in range(amount):
username = "".join(
random.choices(string.ascii_lowercase + string.digits, k=12)
)
email = f"{username}@{domain or random.choice(DOMAIN_LIST)}"
emails.append(email)
return emails
@staticmethod
def custom_email(username: str, domain: str = None) -> str:
"""This method generates a custom email address.
Parameters:
----------
- `username`: `str` - The username to use for the email address.
- `domain`: `str` (optional) - The domain name to use for the email address. If not provided, a random domain from the valid list of domains will be selected.
Returns:
-------
- `email`: `str` - The generated email address.
Example:
-------
Generate a custom email address with the username "johndoe":
>>> client.custom_email(username="johndoe")
Valid domains:
-------------
- 1secmail.com
- 1secmail.org
- 1secmail.net
- kzccv.com
- qiott.com
- wuuvo.com
- icznn.com
- ezztt.com
If `domain` is provided and not in the valid list of domains, a ValueError will be raised with a message indicating the invalid domain and the valid list of domains.
"""
if domain is not None and domain not in DOMAIN_LIST:
err_msg = (
f"{domain} is not a valid domain name.\nValid Domains: {DOMAIN_LIST}"
)
raise ValueError(err_msg)
if is_valid_username(username) is False:
err_msg = f"'{username}' is not a valid username."
raise ValueError(err_msg)
return f"{username}@{domain or random.choice(DOMAIN_LIST)}"
async def await_new_message(self, address: str, fetch_interval=5) -> Inbox:
"""This method waits until a new message is received for the specified email address.
Parameters:
----------
- `address`: `str` - The email address to check for new messages.
- `fetch_interval`: `int` (optional) - The time interval (in seconds) for checking new messages. The default value is 5 seconds.
Returns:
-------
- `message`: `Inbox` - The new message received.
Example:
-------
Wait for a new message to be received for the email address "johndoe@1secmail.com":
>>> message = await client.await_new_message("johndoe@1secmail.com")
The method will continuously check for new messages every `fetch_interval` seconds until a new message is received. Once a new message is received, the message object is returned. The method also maintains a set of message IDs to check if the message is new. If the same message is received again, the method will continue to wait for a new message.
Note that if no new messages are received for a long time, the method may take a long time to return.
"""
ids = {message.id for message in await self.get_inbox(address)}
while True:
await asyncio.sleep(fetch_interval)
new_messages = await self.get_inbox(address)
for message in new_messages:
if message.id not in ids:
return message
async def get_active_domains(self) -> List[str]:
"""This method retrieves a list of currently active domains.
Returns:
-------
- `domains`: `List[str]` - A list of active domains.
Example:
-------
Get a list of active domains:
>>> domains = await client.get_active_domains()
The method sends a GET request to the API endpoint to retrieve a list of currently active domains. The list is returned as a list of strings.
Note that the list of active domains may change over time.
"""
return await self._request(action=GET_DOMAIN_LIST)
async def get_inbox(self, address: str) -> List[Inbox]:
"""This method retrieves all the messages in the mailbox for the specified email address.
Parameters:
----------
- `address`: `str` - The email address to check for messages.
Returns:
-------
- `messages`: `List[Inbox]` - A list of message objects in the mailbox.
Example:
-------
Get all the messages in the mailbox for the email address "johndoe@1secmail.com":
>>> messages = await client.get_inbox("johndoe@1secmail.com")
The method sends a GET request to the API endpoint to retrieve all the messages in the mailbox for the specified email address. The messages are returned as a list of inbox objects. If there are no messages in the mailbox, an empty list is returned.
"""
username, domain = address.split("@")
return await self._request(
action=GET_MESSAGES,
params={"login": username, "domain": domain},
data_type=Inbox,
)
async def get_message(self, address: str, message_id: int) -> Message:
"""This method retrieves a detailed message from the mailbox for the specified email address and message ID.
Parameters:
----------
- `address`: `str` - The email address to check for the message.
- `message_id`: `int` - The ID of the message to retrieve.
Returns:
-------
- `message`: `Message` - The message object with the specified ID.
Example:
-------
Get the message with ID 12345 in the mailbox for the email address "johndoe@1secmail.com":
>>> message = await client.get_message("johndoe@1secmail.com", 12345)
The method sends a GET request to the API endpoint to retrieve the message with the specified ID in the mailbox for the specified email address. The message is returned as a message object.
"""
username, domain = address.split("@")
return await self._request(
action=GET_SINGLE_MESSAGE,
params={"login": username, "domain": domain, "id": message_id},
data_type=Message,
)
async def save_email(self, address: str) -> None:
"""This method saves the specified email address to a JSON file for future use.
Parameters:
----------
- `address`: `str` - The email address to save.
Example:
-------
Save the email address "johndoe@1secmail.com" to the JSON file:
>>> await client.save_email("johndoe@1secmail.com")
The JSON file is saved in the base path specified during client initialization, with the name `secmail.json`.
"""
data = {}
if not os.path.exists(self.base_path):
os.mkdir(self.base_path)
if os.path.exists(self.base_path + "secmail.json"):
with open(self.base_path + "secmail.json", "r") as f:
data = json.load(f)
data.setdefault("email", []).append(address)
with open(self.base_path + "secmail.json", "w") as f:
json.dump(data, f, indent=4)
async def download_attachment(
self,
address: str,
message_id: int,
filename: str,
save_path: str = current_path + "/config/",
):
"""This method downloads an attachment from a message in the mailbox for the specified email address and message ID.
Parameters:
----------
- `address`: `str` - The email address to check for the message containing the attachment.
- `message_id`: `int` - The ID of the message containing the attachment to download.
- `filename`: `str` - The name of the attachment file to download.
- `save_path`: `str` - Optional. The path to save the downloaded attachment. Default is the current path + "/config/".
Returns:
-------
- `str` - A string indicating the path and size of the downloaded attachment.
Example:
-------
Download the attachment named "report.pdf" from the message with ID 12345 in the mailbox for the email address "johndoe@1secmail.com":
>>> await download_attachment("johndoe@1secmail.com", 12345, "report.pdf")
"""
username, domain = address.split("@")
attachment = await self._request(
action=DOWNLOAD,
params={
"login": username,
"domain": domain,
"id": message_id,
"file": filename,
},
)
if not os.path.exists(self.base_path):
os.mkdir(self.base_path)
with open(save_path + filename, "wb") as attachment_file:
size = attachment_file.write(attachment)
return "Path: (" + save_path + filename + "), Size: " + str(size) + "B" | 1secMail | /1secMail-1.1.0.tar.gz/1secMail-1.1.0/secmail/client.py | client.py |
class Inbox:
"""The inbox object contains an array of email objects, each with an unique ID,\n
sender's email address, subject line, and date and time the email was sent.
---
Attributes:
----------
- id : (``int``) - Message id
- from_address : (``str``) - Sender email address
- subject : (``str``) - Subject
- date : (``str``) - Receive date
"""
__slots__ = ("id", "from_address", "subject", "date")
def __init__(self, response) -> None:
self.id = response.get("id")
self.from_address = response.get("from")
self.subject = response.get("subject")
self.date = response.get("date")
def __repr__(self) -> str:
return f"MailBox(id={self.id}, from_address={self.from_address}, subject={self.subject}, date={self.date})"
class Message:
"""The message object contains an unique ID, sender's email address,\n
subject line, date and time the email was sent, list of attachment object,\n
body (html if exists, text otherwise), text body and HTML body.
---
Attributes:
----------
- id : (``int``) - Message id
- from_address : (``str``) - Sender email address
- subject : (``str``) - Subject
- date : (``str``) - Receive date
- attachments : (``str``) - List of Attachment object
- body : (``str``) - Message body (html if exists, text otherwise)
- text_body : (``str``) - Message body (text)
- html_body : (``str``) - Message body (html)
"""
__slots__ = (
"id",
"from_address",
"subject",
"date",
"attachments",
"body",
"text_body",
"html_body",
)
def __init__(self, response) -> None:
self.id = response.get("id")
self.from_address = response.get("from")
self.subject = response.get("subject")
self.date = response.get("date")
self.attachments = response.get("attachments")
if self.attachments is not None:
self.attachments = [
Attachment(attachment) for attachment in self.attachments
]
self.body = response.get("body")
self.text_body = response.get("textBody")
self.html_body = response.get("htmlBody")
def __repr__(self) -> str:
return (
f"Message(id={self.id}, from_address={self.from_address}, subject={self.subject}, "
f"date={self.date}, attachments={self.attachments}, body={self.body}, "
f"text_body={self.text_body}, html_body={self.html_body})"
)
class Attachment:
"""The attachment object contains the attachment's filename, content_type and file size.
---
Attributes:
----------
- filename : (``str``) - Attachment filename
- content_type : (``str``) - Attachment content type
- size : (``int``) - Attachment size
"""
__slots__ = "filename", "content_type", "size"
def __init__(self, response) -> None:
self.filename = response.get("filename")
self.content_type = response.get("contentType")
self.size = response.get("size")
def __repr__(self) -> str:
return f"Attachment(filename={self.filename}, content_type={self.content_type}, size={self.size})" | 1secMail | /1secMail-1.1.0.tar.gz/1secMail-1.1.0/secmail/models.py | models.py |
1stCaptcha package for Python
=
[1stcaptcha.com](https://1stcaptcha.com) package for Python3
Solver recaptchaV2, recaptchaV3, hcaptcha, funcaptcha, imageToText, Zalo Captcha,.... Super fast and cheapest.
# Install
```bash
pip install 1stcaptcha
```
# Usage
## init client
```python
from onest_captcha import OneStCaptchaClient
APIKEY = "0aa92cd8393a49698c408ea0ee56c2a5"
client = OneStCaptchaClient(apikey=APIKEY)
```
## solver recaptcha v2:
```python
result = client.recaptcha_v2_task_proxyless(site_url="YOUR_SITE_URL", site_key="YOUR_SITE_KEY", invisible=False)
if result["code"] == 0: # success:
print(result["token"])
else: # wrong
print(result["messeage"])
```
## solver recaptcha v2 enterprise:
```python
result = client.recaptcha_v2_enterprise_task_proxyless(site_url="YOUR_SITE_URL", site_key="YOUR_SITE_KEY")
if result["code"] == 0: # success:
print(result["token"])
else: # wrong
print(result["messeage"])
```
## solver recaptcha v3:
```python
result = client.recaptcha_v3_task_proxyless(site_url="YOUR_SITE_URL", site_key="YOUR_SITE_KEY",
page_action="YOUR_PAGE_ACTION")
if result["code"] == 0: # success:
print(result["token"])
else: # wrong
print(result["messeage"])
```
## solver recaptcha v3 enterprise:
```python
result = client.recaptcha_v3_enterprise_task_proxyless(site_key="YOUR_SITE_KEY",
site_url="YOUR_SITE_URL",
page_action="YOUR_PAGE_ACTION")
if result["code"] == 0: # success:
print(result.get('token'))
print(result.get('user_agent'))
else: # wrong
print(result["messeage"])
```
## solve image2text
```python
result = client.image_to_text(file="1.jpg")
if result["code"] == 0: # success:
print(result["token"])
else: # wrong
print(result["messeage"])
```
## solve recaptchaClick
```python
url_list = ['']
caption = 'cars'
result = client.recaptcha_click(url_list=url_list, caption=caption)
if result["code"] == 0: # success:
print(result["token"])
else: # wrong
print(result["messeage"])
```
## funcaptcha
```python
site_key = "2CB16598-CB82-4CF7-B332-5990DB66F3AB"
site_url = "https://outlook.com/"
result = client.fun_captcha_task_proxyless(site_url, site_key)
if result["code"] == 0: # success:
print(result["token"])
else: # wrong
print(result["messeage"])
```
## hcaptcha
```python
site_key = "f5561ba9-8f1e-40ca-9b5b-a0b3f719ef34"
site_url = "https://discord.com/login"
token = client.h_captcha_task_proxyless(site_key=site_key,
site_url=site_url)
if result["code"] == 0: # success:
print(result["token"])
else: # wrong
print(result["messeage"])
```
| 1stcaptcha | /1stcaptcha-1.0.6.tar.gz/1stcaptcha-1.0.6/README.md | README.md |
import base64
import time
import requests
def convert_img_to_base64(img_path):
with open(img_path, "rb") as img_file:
b64_string = base64.b64encode(img_file.read())
return b64_string.decode("utf8")
class OneStCaptchaClient:
def __init__(self, apikey):
self.apikey = apikey
self.BASE_URL = "https://api.1stcaptcha.com"
def get_balance(self):
r = requests.get(f"{self.BASE_URL}/user/balance?apikey=" + self.apikey)
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
return data['Balance']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
def get_result(self, task_id, timeout, time_sleep, type_captcha=""):
t_start = time.time()
while (time.time() - t_start) < timeout:
r = requests.get(f"{self.BASE_URL}/getresult?apikey=" + self.apikey + "&taskid=" + str(task_id))
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
if data['Status'] == "SUCCESS":
if type_captcha == "image2text" or type_captcha == "recaptcha_click":
return data["Data"]
elif type_captcha == "v3_enterprise":
return data["Data"]
return data["Data"]["Token"]
elif data['Status'] == "ERROR":
raise Exception(data["Message"])
time.sleep(time_sleep)
else:
raise RuntimeError("Error " + data["Message"])
else:
raise RuntimeError("Error " + r.text)
raise RuntimeError("TIMEOUT")
def recaptcha_v2_task_proxyless(self, site_url, site_key, invisible=False, timeout=180, time_sleep=1):
try:
params = {
"apikey": self.apikey,
"sitekey": site_key,
"siteurl": site_url,
"version": "v2",
"invisible": str(invisible).lower()
}
r = requests.get(
f"{self.BASE_URL}/recaptchav2",
params=params
)
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
task_id = data['TaskId']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
return {"code": 0, "token": self.get_result(task_id, timeout, time_sleep)}
except Exception as e:
return {"code": 1, "messeage": str(e)}
def recaptcha_v2_enterprise_task_proxyless(self, site_url, site_key, s_payload=None, timeout=180, time_sleep=1):
try:
params = {
"apikey": self.apikey,
"sitekey": site_key,
"siteurl": site_url,
}
if s_payload:
params["s"] = s_payload
r = requests.get(
f"{self.BASE_URL}/recaptchav2_enterprise",
params=params
)
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
task_id = data['TaskId']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
return {"code": 0, "token": self.get_result(task_id, timeout, time_sleep)}
except Exception as e:
return {"code": 1, "messeage": str(e)}
def recaptcha_v3_task_proxyless(self, site_url, site_key, page_action, min_score: float = 0.3, timeout=180,
time_sleep=1):
try:
params = {
"apikey": self.apikey,
"sitekey": site_key,
"siteurl": site_url,
"pageaction": page_action,
"minscore": min_score,
"version": "v3"
}
r = requests.get(
f"{self.BASE_URL}/recaptchav3",
params=params
)
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
task_id = data['TaskId']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
return {"code": 0, "token": self.get_result(task_id, timeout, time_sleep)}
except Exception as e:
return {"code": 1, "messeage": str(e)}
def recaptcha_v3_enterprise_task_proxyless(
self,
site_url,
site_key,
page_action,
s_payload=None,
min_score: float = 0.3,
timeout=180,
time_sleep=1
):
try:
params = {
"apikey": self.apikey,
"sitekey": site_key,
"siteurl": site_url,
"pageaction": page_action,
"minscore": min_score
}
if s_payload:
params["s"] = s_payload
r = requests.get(
f"{self.BASE_URL}/recaptchav3_enterprise",
params=params
)
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
task_id = data['TaskId']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
result = self.get_result(task_id, timeout, time_sleep, type_captcha="v3_enterprise")
return {"code": 0, "token": result.get("Token"), "user_agent": result.get("UserAgent")}
except Exception as e:
return {"code": 1, "messeage": str(e)}
def h_captcha_task_proxyless(self, site_url, site_key, rqdata=None, timeout=180, time_sleep=3):
try:
params = {
"apikey": self.apikey,
"sitekey": site_key,
"siteurl": site_url,
}
if rqdata:
params["rqdata"] = rqdata
r = requests.get(
f"{self.BASE_URL}/hcaptcha"
, params=params)
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
task_id = data['TaskId']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
return {"code": 0, "token": self.get_result(task_id, timeout, time_sleep)}
except Exception as e:
return {"code": 1, "messeage": str(e)}
def fun_captcha_task_proxyless(self, site_url, site_key, timeout=180, time_sleep=3):
try:
params = {
"apikey": self.apikey,
"sitekey": site_key,
"siteurl": site_url,
}
r = requests.get(
f"{self.BASE_URL}/funcaptchatokentask",
params=params
)
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
task_id = data['TaskId']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
return {"code": 0, "token": self.get_result(task_id, timeout, time_sleep)}
except Exception as e:
return {"code": 1, "messeage": str(e)}
def recaptcha_click(self, url_list, caption, timeout=60, time_sleep=3):
try:
r = requests.post(
f"{self.BASE_URL}/recognition",
json={'Image_urls': url_list, 'Caption': caption, "Apikey": self.apikey, "Type": "recaptcha"}
)
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
task_id = data['TaskId']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
return {"code": 0, "token": self.get_result(task_id, timeout, time_sleep, type_captcha="recaptcha_click")}
except Exception as e:
return {"code": 1, "messeage": str(e)}
def image_to_text(self, base64img=None, file=None, timeout=60, time_sleep=1):
try:
if base64img is None:
if file is None:
raise RuntimeError("base64img and file is None ")
else:
base64img = convert_img_to_base64(file)
r = requests.post(
f"{self.BASE_URL}/recognition", json={
'Image': base64img, "Apikey": self.apikey, "Type": "imagetotext"
})
if r.status_code == 200:
data = r.json()
if data['Code'] == 0:
task_id = data['TaskId']
else:
raise RuntimeError("Error " + str(data))
else:
raise RuntimeError("Error " + r.text)
return {"code": 0, "token": self.get_result(task_id, timeout, time_sleep, type_captcha="image2text")}
except Exception as e:
return {"code": 1, "messeage": str(e)} | 1stcaptcha | /1stcaptcha-1.0.6.tar.gz/1stcaptcha-1.0.6/onest_captcha/__init__.py | __init__.py |
======
1to001
======
*1to001* is made for padding numbers in filenames automatically. It's written in Python 3.
Installation
============
System-wide installation:
.. code:: sh
$ pip install 1to001
User installation:
.. code:: sh
$ pip install --user 1to001
For development code:
.. code:: sh
$ pip install git+https://github.com/livibetter/1to100.git
Example
=======
.. code:: sh
$ touch 1.txt 100.txt
$ 1to001 *.txt
+ 001.txt
? ++
perform padding (y/n)? y
1.txt -> 001.txt
Options
=======
``-i`` (``--ignore-case``)
When cases are mixed, this option would ignore the cases, for example for files like::
read100me1.TXT
read5Me02.txt
They can be renamed to::
1to001 -i *.{txt,TXT}
+ read005Me02.txt
? ++
+ read100me01.TXT
? +
perform padding (y/n)?
``-y`` (``--yes``)
Automatic yes to prompts
More information
================
* 1to001_ on GitHub
* PyPI_
* Some usage examples in this `blog post`_
.. _1to001: https://github.com/livibetter/1to001
.. _PyPI: https://pypi.python.org/pypi/1to001
.. _blog post: http://blog.yjl.im/2013/07/padding-numbers-in-filenames.html
License
=======
This project is licensed under the MIT License, see ``COPYING``.
| 1to001 | /1to001-0.3.0.tar.gz/1to001-0.3.0/README.rst | README.rst |
import datetime
from django.db import models
class VisitCounts(models.Model):
date = models.DateField(auto_now=True, verbose_name='日期')
amount_of_day = models.IntegerField(default=0, verbose_name='单日访问量', editable=False)
def __str__(self):
return '{0} {1}次'.format(self.date, self.amount_of_day)
@classmethod
def today_add_one(cls):
try:
counts_today = cls.objects.get(date=datetime.datetime.today())
counts_today.amount_of_day += 1
except:
counts_today = VisitCounts()
counts_today.amount_of_day += 1
finally:
counts_today.save()
@classmethod
def get_total_counts(cls):
sum([i.amount_of_day for i in cls.objects.all()])
class Meta:
db_table = "ide_visitor_counts"
verbose_name = "访问统计"
verbose_name_plural = "访问统计"
class Visitors(models.Model):
ip = models.CharField(max_length=15, verbose_name='IP地址', default='unknown', blank=True, editable=False)
time = models.DateTimeField(auto_now=True, verbose_name='访问时间', editable=False)
url = models.SlugField(verbose_name='URL', editable=False)
def __str__(self):
return 'IP:{0} TIME:{1} URL:{2}'.format(self.ip, self.time, self.url)
class Meta:
db_table = "ide_visitor"
verbose_name = "访问者"
verbose_name_plural = "访问者"
class MpyMachineIP(models.Model):
ip = models.CharField(max_length=15, verbose_name='IP地址', default='unknown', blank=True, editable=False)
machine_ip = models.TextField(verbose_name='Mpy Machine 内网IP地址', default='', editable=False)
def set_esp_ip(self, esp_ip):
if esp_ip.replace(',', '') in self.esp_ip.split(','):
pass
else:
self.esp_ip += esp_ip
def get_esp_ip(self):
return self.esp_ip.split(',')
def __str__(self):
return self.ip
class Meta:
db_table = "ide_machine_ip"
verbose_name = "Mpy Machine IP"
verbose_name_plural = "Mpy Machine IP" | 1zlab-emp-ide | /1zlab_emp_ide-0.0.3-py3-none-any.whl/emp_ide/models.py | models.py |
from django.db import migrations, models
class Migration(migrations.Migration):
initial = True
dependencies = [
]
operations = [
migrations.CreateModel(
name='MpyMachineIP',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('ip', models.CharField(blank=True, default='unknown', max_length=15, verbose_name='IP地址')),
('machine_ip', models.TextField(default='', verbose_name='Mpy Machine 内网IP地址')),
],
options={
'verbose_name': 'Mpy Machine IP',
'verbose_name_plural': 'Mpy Machine IP',
'db_table': 'ide_machine_ip',
},
),
migrations.CreateModel(
name='VisitCounts',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('date', models.DateField(auto_now=True, verbose_name='日期')),
('amount_of_day', models.IntegerField(default=0, editable=False, verbose_name='单日访问量')),
],
options={
'verbose_name': '访问统计',
'verbose_name_plural': '访问统计',
'db_table': 'ide_visitor_counts',
},
),
migrations.CreateModel(
name='Visitors',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('ip', models.CharField(blank=True, default='unknown', max_length=15, verbose_name='IP地址')),
('time', models.DateTimeField(auto_now=True, verbose_name='访问时间')),
('url', models.SlugField(verbose_name='URL')),
],
options={
'verbose_name': '访问者',
'verbose_name_plural': '访问者',
'db_table': 'ide_visitor',
},
),
] | 1zlab-emp-ide | /1zlab_emp_ide-0.0.3-py3-none-any.whl/emp_ide/migrations/0001_initial.py | 0001_initial.py |
(function(e){function t(t){for(var i,r,a=t[0],c=t[1],d=t[2],l=0,p=[];l<a.length;l++)r=a[l],s[r]&&p.push(s[r][0]),s[r]=0;for(i in c)Object.prototype.hasOwnProperty.call(c,i)&&(e[i]=c[i]);h&&h(t);while(p.length)p.shift()();return o.push.apply(o,d||[]),n()}function n(){for(var e,t=0;t<o.length;t++){for(var n=o[t],i=!0,r=1;r<n.length;r++){var a=n[r];0!==s[a]&&(i=!1)}i&&(o.splice(t--,1),e=c(c.s=n[0]))}return e}var i={},r={app:0},s={app:0},o=[];function a(e){return c.p+"js/"+({}[e]||e)+"."+{"chunk-2d0c0e14":"e4f25a38","chunk-2d0d3720":"f764eeca","chunk-2d0e64c6":"d56af125","chunk-2d230ab1":"d8b63db6","chunk-329d5946":"f788fc45"}[e]+".js"}function c(t){if(i[t])return i[t].exports;var n=i[t]={i:t,l:!1,exports:{}};return e[t].call(n.exports,n,n.exports,c),n.l=!0,n.exports}c.e=function(e){var t=[],n={"chunk-329d5946":1};r[e]?t.push(r[e]):0!==r[e]&&n[e]&&t.push(r[e]=new Promise(function(t,n){for(var i="css/"+({}[e]||e)+"."+{"chunk-2d0c0e14":"31d6cfe0","chunk-2d0d3720":"31d6cfe0","chunk-2d0e64c6":"31d6cfe0","chunk-2d230ab1":"31d6cfe0","chunk-329d5946":"5e39cfad"}[e]+".css",s=c.p+i,o=document.getElementsByTagName("link"),a=0;a<o.length;a++){var d=o[a],l=d.getAttribute("data-href")||d.getAttribute("href");if("stylesheet"===d.rel&&(l===i||l===s))return t()}var p=document.getElementsByTagName("style");for(a=0;a<p.length;a++){d=p[a],l=d.getAttribute("data-href");if(l===i||l===s)return t()}var h=document.createElement("link");h.rel="stylesheet",h.type="text/css",h.onload=t,h.onerror=function(t){var i=t&&t.target&&t.target.src||s,o=new Error("Loading CSS chunk "+e+" failed.\n("+i+")");o.code="CSS_CHUNK_LOAD_FAILED",o.request=i,delete r[e],h.parentNode.removeChild(h),n(o)},h.href=s;var u=document.getElementsByTagName("head")[0];u.appendChild(h)}).then(function(){r[e]=0}));var i=s[e];if(0!==i)if(i)t.push(i[2]);else{var o=new Promise(function(t,n){i=s[e]=[t,n]});t.push(i[2]=o);var d,l=document.createElement("script");l.charset="utf-8",l.timeout=120,c.nc&&l.setAttribute("nonce",c.nc),l.src=a(e),d=function(t){l.onerror=l.onload=null,clearTimeout(p);var n=s[e];if(0!==n){if(n){var i=t&&("load"===t.type?"missing":t.type),r=t&&t.target&&t.target.src,o=new Error("Loading chunk "+e+" failed.\n("+i+": "+r+")");o.type=i,o.request=r,n[1](o)}s[e]=void 0}};var p=setTimeout(function(){d({type:"timeout",target:l})},12e4);l.onerror=l.onload=d,document.head.appendChild(l)}return Promise.all(t)},c.m=e,c.c=i,c.d=function(e,t,n){c.o(e,t)||Object.defineProperty(e,t,{enumerable:!0,get:n})},c.r=function(e){"undefined"!==typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(e,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(e,"__esModule",{value:!0})},c.t=function(e,t){if(1&t&&(e=c(e)),8&t)return e;if(4&t&&"object"===typeof e&&e&&e.__esModule)return e;var n=Object.create(null);if(c.r(n),Object.defineProperty(n,"default",{enumerable:!0,value:e}),2&t&&"string"!=typeof e)for(var i in e)c.d(n,i,function(t){return e[t]}.bind(null,i));return n},c.n=function(e){var t=e&&e.__esModule?function(){return e["default"]}:function(){return e};return c.d(t,"a",t),t},c.o=function(e,t){return Object.prototype.hasOwnProperty.call(e,t)},c.p="/static/",c.oe=function(e){throw console.error(e),e};var d=window["webpackJsonp"]=window["webpackJsonp"]||[],l=d.push.bind(d);d.push=t,d=d.slice();for(var p=0;p<d.length;p++)t(d[p]);var h=l;o.push([0,"chunk-vendors"]),n()})({0:function(e,t,n){e.exports=n("56d7")},"034f":function(e,t,n){"use strict";var i=n("64a9"),r=n.n(i);r.a},"38ab":function(e){e.exports={Action:{Cancle:"取消",Apply:"应用",Esc:"退出",Click:"点击"},Connector:{DialogTitle:"连接到你的设备",URL:"URL",Password:"密码",Connect:"连接",Disconnect:"断开连接",Wifi:"WiFi",SerialPort:"串口",Type:"连接类型"},placeholder:{Editor:"脚本文件",Uploader:"上传文件",Search:"搜索",Modules:"模块",Docs:"文档",RunScript:"运行脚本",MemoryClean:"内存清理",Connect:"连接到设备",Settings:"设置"},BottomBar:{},Cli:{DialogTitle:"连接到你的设备",URL:"URL",Password:"密码",Connect:"连接",Disconnect:"断开连接"},Editor:{},Finder:{Label:"输入以搜索"},FolderTree:{Menu:{File:[{text:"运行",code:"run"},{isdivider:!0},{text:"重命名",code:"rename"},{isdivider:!0},{text:"删除",code:"deleteFile"},{isdivider:!0}],Folder:[{text:"刷新",code:"refresh"},{isdivider:!0},{text:"重命名",code:"rename"},{isdivider:!0},{text:"新建文件",code:"newFile"},{isdivider:!0},{text:"新建文件夹",code:"newFolder"},{isdivider:!0},{text:"删除文件夹",code:"deleteFolder"}]}},Pypi:{Download:"下载/更新"},Settings:{Title:"设置",FontSize:"字体大小",MemoryLimit:"I/O内存限制"},SideBar:{},SplitPane:{},Uploader:{Upload:"上传",DragToHere:"拖拽到此处,或"},README:" _____ __ __ ____ ___ ____ _____\n | ____|| \\/ || _ \\ |_ _|| _ \\ | ____|\n | _| | |\\/| || |_) | | | | | | || _|\n | |___ | | | || __/ | | | |_| || |___\n |_____||_| |_||_| |___||____/ |_____|\n\n Easy MicroPython (EMP) 是一个由1Z实验室主导的开源项目,旨在为广大的\n MicroPython爱好者提供更加快速和高效的开发体验。\n\n GitHub: https://github.com/Fuermohao/EMP-IDE\n 主页: http://www.1zlab.com\n 文档: http://www.1zlab.com/wiki/micropython-esp32/emp-ide-userguide/\n\n 在开始使用之前,你需要在你的MicroPython设备上安装 emp-1zlab 模块。请按\n 照如下的步骤进行:\n \n import upip\n upip.install('emp-1zlab')\n\n import emp_boot\n emp_boot.set_boot_mode()\n\n 输入2,回车。这将重新覆盖你的 boot.py 文件,之后你的设备将会进行一次重启。\n 重启之后按照命令行中的提示来使你的设备连接至WiFi网络。在这之后,webrepl将会\n 自动开启,默认的连接密码为'1zlab', 你可以在 webrepl.pass 文件中修改它。"}},"56d7":function(e,t,n){"use strict";n.r(t);n("cadf"),n("551c"),n("f751"),n("097d");var i=n("8bbf"),r=n.n(i),s=function(){var e=this,t=e.$createElement,n=e._self._c||t;return n("div",{attrs:{id:"app"}},[n("router-view")],1)},o=[],a={name:"app"},c=a,d=(n("034f"),n("2877")),l=Object(d["a"])(c,s,o,!1,null,null,null),p=l.exports,h=(n("ac6a"),n("8c4f")),u=n("0644"),f=n.n(u),_=[{path:"/",name:"ide",component:"/ide/index"},{path:"*",component:"/error"}],m=_,$=n("8bbf"),g=n("70c3");$.use(h["a"]);var y=new h["a"]({routes:w(m)});function w(e){var t=f()(e);return t.forEach(function(e){e.component&&(e.component=g(e.component))}),t}n("f5df"),n("619b"),n("64d2");var b=n("dd88"),S=n("4d7d"),v=n("00e7"),E=n.n(v),D={theme:"dark"},F={install:function(e){e.prototype.$connect=function(e){var t=e.sender,n=e.receiver,i=e.slot,r=e.kwargs,s=t.$parent;try{while(!s.isParent)s=s.$parent;"parent"===n?s[i](r):s.$refs[n][i](r)}catch(o){console.log(o)}},e.prototype.$send=function(e){e.sender.isParent?"self"===e.receiver?this[e.slot](e.kwargs):this.$refs[e.receiver][e.slot](e.kwargs):"self"===e.receiver?this[e.slot](e.kwargs):e.sender.$emit("events",e)}}},A=F,N=n("9aba"),C=n.n(N),T=(n("28a5"),{install:function(e){e.prototype.$emp={funcName:function(e){return e("").split("(")[0].split(".")[1]},deviceInfo:function(){return"ide.device_info()\r"},memoryStatus:function(){return"ide.memory_status()\r"},memoryAnalysing:function(e){return"ide.memory_analysing('".concat(e,"')\r")},runScript:function(e){return"exec(open('".concat(e,"').read())\r")},tree:function(){return"ide.tree()\r"},getCode:function(e){return"ide.get_code('".concat(e,"')\r")},newFile:function(e){return"ide.new_file('".concat(e,"')\r")},delFile:function(e){return"ide.del_file('".concat(e,"')\r")},newFolder:function(e){return"ide.new_folder('".concat(e,"')\r")},delFolder:function(e){return"ide.del_folder('".concat(e,"')\r")},rename:function(e,t){return"ide.rename('".concat(e,"','").concat(t,"')\r")},install:function(e){return"ide.emp_install('".concat(e,"')\r")}}}}),P=T,O=(n("34ef"),n("f559"),{onOpen:function(){this.$repl.term.focus(),this.$repl.term.write("Welcome to 1ZLAB-EMPIDE!\r\n"),0===this.$repl.connectionType&&this.$ws.send(this.$repl.passwd+"\r"),this.$ws.send(this.$emp.deviceInfo()),this.$ws.send(this.$emp.memoryStatus()),this.$ws.send(this.$emp.tree()),this.$toast.success("WebREPL connected!"),1===this.$ws.readyState&&(this.$send(this.SIGNAL_REPORT_CONNECTED(this)),this.$repl.connected=!0,this.connected=!0)},onClose:function(){this.$repl.connected=!1,this.connected=!1,this.$send(this.SIGNAL_REPORT_DISCONNECTED(this)),this.$toast.error("Disconnected"),this.$repl.term&&this.$repl.term.write("\r\n[31mDisconnected[m\r\n")},replExec:function(e){this.tasklock?this.$toast.error("IO busy"):(this.$ws.send(e.command),e.command.startsWith(this.$emp.funcName(this.$emp.memoryAnalysing))&&this.$send(this.SIGNAL_LOCK(this)))}}),k=O,L={replExec:k.replExec,onOpen:k.onOpen,onClose:k.onClose,onMessage:function(e){var t=this;if(e.data instanceof ArrayBuffer){var n=new Uint8Array(e.data);switch(this.$dtp.binaryState){case 11:if(0==x(n)){for(var i=0;i<this.$dtp.putFileData.length;i+=1024)this.$ws.send(this.$dtp.putFileData.slice(i,i+1024));this.$dtp.binaryState=12}break;case 12:this.$send(this.SIGNAL_UNLOCK(this)),0==x(n)?(this.$toast.success("success! "+this.$dtp.putFilename+", "+this.$dtp.putFileData.length+" bytes"),this.$dtp.putFileData=null,this.$dtp.putFilename=""):this.$toast.error("Failed sending "+this.$dtp.putFilename),this.$dtp.binaryState=0,this.$ws.send("\r\r"),this.$ws.send(this.$emp.tree()),setTimeout(function(){return t.$send(t.SIGNAL_PUT_NEXT_FILE(t))},300),setTimeout(function(){return t.slotClearTerm()},300);break;case 21:if(0==x(n)){this.$dtp.binaryState=22;var r=new Uint8Array(1);r[0]=0,this.$ws.send(r)}break;case 22:var s=n[0]|n[1]<<8;if(n.length==2+s)if(0==s)this.$dtp.binaryState=23;else{var o=new Uint8Array(this.$dtp.getFileData.length+s);o.set(this.$dtp.getFileData),o.set(n.slice(2),this.$dtp.getFileData.length),this.$dtp.getFileData=o;var a=new Uint8Array(1);a[0]=0,this.$ws.send(a)}else this.$dtp.binaryState=0;break;case 23:if(0==x(n)){this.$toast.success("Got "+this.$dtp.getFilename+", "+this.$dtp.getFileData.length+" bytes");var c=new TextDecoder("utf-8").decode(this.$dtp.getFileData);this.$send(this.SIGNAL_SHOW_CODES(this,c))}else this.$toast.error("Failed getting "+this.$dtp.getFilename);this.$dtp.getFileData=null,this.$dtp.getFilename=null,this.$dtp.binaryState=0,this.$ws.send("\r\r"),setTimeout(function(){return t.slotClearTerm()},300);break}}else try{this.$dtp.recData=JSON.parse(e.data),this.$dtp.recData.func===this.$emp.funcName(this.$emp.tree)&&(this.$send(this.SIGNAL_UPDATE_TREE(this,[this.$dtp.recData.data])),this.$send(this.SIGNAL_UPDATE_FINDER(this,this.$dtp.recData.data)),this.$send(this.SIGNAL_SHOW_PANE(this))),this.$dtp.recData.func===this.$emp.funcName(this.$emp.getCode)&&this.$send(this.SIGNAL_SHOW_CODES_PMAX(this,this.$dtp.recData.data)),this.$dtp.recData.func===this.$emp.funcName(this.$emp.memoryAnalysing)&&this.$send(this.SIGNAL_DEPENDS_ON_MEMORY_TO_GET_FILE(this,this.$dtp.recData.data)),this.$dtp.recData.func===this.$emp.funcName(this.$emp.deviceInfo)&&this.$send(this.SIGNAL_SHOW_SYS_INFO(this,this.$dtp.recData.data)),this.$dtp.recData.func===this.$emp.funcName(this.$emp.memoryStatus)&&this.$send(this.SIGNAL_SHOW_MEMORY_STATUS(this,this.$dtp.recData.data))}catch(d){e.data.indexOf("Traceback (most recent call last):")>=0&&this.$send(this.SIGNAL_UNLOCK(this))}},putFile:function(e){if(this.$repl.tasklock)this.$toast.error("IO busy");else{this.$dtp.putFilename=e.filename,e.fileData.length>0?this.$dtp.putFileData=e.fileData:(this.$dtp.putFileData=(new TextEncoder).encode(" "),e.fileData=(new TextEncoder).encode(" "));var t=e.filename,n=e.fileData.length,i=new Uint8Array(82);i[0]="W".charCodeAt(0),i[1]="A".charCodeAt(0),i[2]=1,i[3]=0,i[4]=0,i[5]=0,i[6]=0,i[7]=0,i[8]=0,i[9]=0,i[10]=0,i[11]=0,i[12]=255&n,i[13]=n>>8&255,i[14]=n>>16&255,i[15]=n>>24&255,i[16]=255&t.length,i[17]=t.length>>8&255;for(var r=0;r<64;++r)r<t.length?i[18+r]=t.charCodeAt(r):i[18+r]=0;this.$dtp.binaryState=11,this.$toast.info("Sending "+e.filename+"..."),this.$send(this.SIGNAL_LOCK(this)),this.$ws.send(i)}},getFile:function(e){this.$dtp.getFilename=e.filename;var t=e.filename,n=new Uint8Array(82);n[0]="W".charCodeAt(0),n[1]="A".charCodeAt(0),n[2]=2,n[3]=0,n[4]=0,n[5]=0,n[6]=0,n[7]=0,n[8]=0,n[9]=0,n[10]=0,n[11]=0,n[12]=0,n[13]=0,n[14]=0,n[15]=0,n[16]=255&t.length,n[17]=t.length>>8&255;for(var i=0;i<64;++i)i<t.length?n[18+i]=t.charCodeAt(i):n[18+i]=0;this.$dtp.binaryState=21,this.$dtp.getFilename=t,this.$dtp.getFileData=new Uint8Array(0),this.$toast.info("Getting "+this.$dtp.getFilename+"..."),this.$ws.send(n)}},x=function(e){if(e[0]=="W".charCodeAt(0)&&e[1]=="B".charCodeAt(0)){var t=e[2]|e[3]<<8;return t}return-1},I=L,M=(n("a481"),{replExec:k.replExec,onOpen:k.onOpen,onClose:k.onClose,onMessage:function(e){var t=this;try{var n=JSON.parse(e.data);n.func===this.$emp.funcName(this.$emp.getCode)&&this.$send(this.SIGNAL_SHOW_CODES_PMAX(this,n.data)),"put_file"===n.func&&(setTimeout(function(){return t.$send(t.SIGNAL_PUT_NEXT_FILE(t))},300),setTimeout(function(){return t.$send(t.SIGNAL_CLEAR_TERM(t))},300))}catch(r){}if(this.$dtp.fragments+=e.data,2===U(this.$dtp.fragments,"==> PDU")){this.$dtp.fragments=this.$dtp.fragments.split("==> PDU")[1],this.$dtp.fragments=this.$dtp.fragments.replace(/\r\n/g,"\\n"),this.$dtp.fragments=this.$dtp.fragments.slice(10,this.$dtp.fragments.length-4);var i=JSON.parse(this.$dtp.fragments);i.func===this.$emp.funcName(this.$emp.tree)&&(this.$send(this.SIGNAL_UPDATE_TREE(this,[i.data])),this.$send(this.SIGNAL_UPDATE_FINDER(this,i.data)),this.$send(this.SIGNAL_SHOW_PANE(this))),i.func===this.$emp.funcName(this.$emp.getCode)&&this.$send(this.SIGNAL_SHOW_CODES_PMAX(this,i.data)),i.func===this.$emp.funcName(this.$emp.memoryAnalysing)&&this.$send(this.SIGNAL_DEPENDS_ON_MEMORY_TO_GET_FILE(this,i.data)),i.func===this.$emp.funcName(this.$emp.deviceInfo)&&this.$send(this.SIGNAL_SHOW_SYS_INFO(this,i.data)),i.func===this.$emp.funcName(this.$emp.memoryStatus)&&this.$send(this.SIGNAL_SHOW_MEMORY_STATUS(this,i.data)),this.$dtp.fragments=""}},putFile:function(e){var t=new TextDecoder("utf-8").decode(e.fileData);this.$ws.send("EnterRawRepl"),this.$ws.send("PutFile:".concat(e.filename,":").concat(t))},getFile:function(e){this.$ws.send("EnterRawRepl"),this.$ws.send("GetFile:".concat(e.filename))}}),U=function(e,t){e+="",t+="";var n=0,i=0;if(t.length<=0)return 0;while(1){if(i=e.indexOf(t,i),!(i>=0))break;n+=1,i+=1}return n},R=M,G={},W=0,j=1;G.install=function(e){e.prototype.$ws=null,e.prototype.$dtp={binaryState:0,getFilename:null,getFileData:null,putFilename:null,putFileData:null,recData:null,fragments:""},e.prototype.$repl={passwd:"",url:"",term:null,connected:!1,connectionType:W,tasklock:!1},e.prototype.$replExec=null,e.prototype.$replStart=function(){var e=this.$repl.connectionType===j?R:I;this.$ws=new WebSocket(this.$repl.url),this.$ws.binaryType="arraybuffer",this.$repl.term.attach(this.$ws,!0,!0),this.$ws.onmessage=e.onMessage.bind(this),this.$ws.onopen=e.onOpen.bind(this),this.$ws.onclose=e.onClose.bind(this),this.$replExec=e.replExec.bind(this),this.$replSetupFTP(e)},e.prototype.$replStop=function(){this.$ws.close()},e.prototype.$replSetupFTP=function(e){this.$replPutFile=e.putFile.bind(this),this.$replGetFile=e.getFile.bind(this)},e.prototype.$replPutFile=null,e.prototype.$replGetFile=null};var H=G,z=n("c252"),B=n("5f72"),Y=function(e){z.theme.use(D.theme),e.use(z),e.use(B),e.use(b["a"]),e.use(S["a"]),e.use(E.a),e.use(A),e.use(C.a),e.use(P),e.use(H)},J=Y,K=(n("456d"),n("a925"));r.a.use(K["a"]);var X="zh-CN",Z={"zh-CN":n("38ab"),"en-US":n("9a10")},q=new K["a"]({locale:X,messages:Z}),V=q;r.a.use(J),r.a.config.productionTip=!1,new r.a({router:y,i18n:V,render:function(e){return e(p)}}).$mount("#app")},"5f72":function(e,t){e.exports=ELEMENT},"64a9":function(e,t,n){},"70c3":function(e,t,n){e.exports=function(e){return function(){return n("e399")("./pages"+e)}}},"8bbf":function(e,t){e.exports=Vue},"9a10":function(e){e.exports={Action:{Cancle:"Cancle",Apply:"Apply",Esc:"Esc",Click:"Click"},Connector:{DialogTitle:"Connect to your device",URL:"URL",Password:"Password",Connect:"Connect",Disconnect:"Disconnect",Wifi:"WiFi",SerialPort:"Serial Port",Type:"Connection mode"},placeholder:{Editor:"Scripts",Uploader:"Uploader",Search:"Search",Modules:"Modules",Docs:"Documents",RunScript:"Run Scripts",MemoryClean:"Memory Clean",Connect:"Connect",Settings:"Settings"},BottomBar:{},Cli:{DialogTitle:"Connect to your device",URL:"URL",Password:"Password",Connect:"Connect",Disconnect:"Disconnect"},Editor:{},Finder:{Label:"Type to search"},FolderTree:{Menu:{File:[{text:"Run",code:"run"},{isdivider:!0},{text:"Rename",code:"rename"},{isdivider:!0},{text:"Delete",code:"deleteFile"},{isdivider:!0}],Folder:[{text:"Refresh",code:"refresh"},{isdivider:!0},{text:"Rename",code:"rename"},{isdivider:!0},{text:"New File",code:"newFile"},{isdivider:!0},{text:"New Folder",code:"newFolder"},{isdivider:!0},{text:"Delete",code:"deleteFolder"}]}},Pypi:{Download:"Download/Update"},Settings:{Title:"Settings",FontSize:"fontsize",MemoryLimit:"memory limit"},SideBar:{},SplitPane:{},Uploader:{Upload:"Upload",DragToHere:"Drag to here, or"},README:" _____ __ __ ____ ___ ____ _____\n | ____|| \\/ || _ \\ |_ _|| _ \\ | ____|\n | _| | |\\/| || |_) | | | | | | || _|\n | |___ | | | || __/ | | | |_| || |___\n |_____||_| |_||_| |___||____/ |_____|\n\n Easy MicroPython (EMP) is an open source project led by 1ZLAB \n to provide a faster and more efficient development experience \n for MicroPython enthusiasts.\n\n GitHub: https://github.com/Fuermohao/EMP-IDE\n HomePage: http://www.1zlab.com\n Docs: http://www.1zlab.com/wiki/micropython-esp32/emp-ide-userguide/\n\n Before you start using it, you need to use the upip to install \n the emp-1zlab module on your MicroPython device. Please follow \n the instructions below:\n\n import upip\n upip.install('emp-1zlab')\n\n import emp_boot\n emp_boot.set_boot_mode()\n\n Enter 2, This operation will overwrite your boot.py file, and your \n device will restart. Please follow the prompts to connect to wifi \n after rebooting. After that, webrepl will be automatically enabled. \n The default password is '1zlab', you can modify it in webrepl.pass."}},c252:function(e,t){e.exports=MuseUI},e399:function(e,t,n){var i={"./pages/error":["5d94","chunk-2d0d3720"],"./pages/error.vue":["5d94","chunk-2d0d3720"],"./pages/ide":["0b01","chunk-329d5946"],"./pages/ide/":["0b01","chunk-329d5946"],"./pages/ide/index":["0b01","chunk-329d5946"],"./pages/ide/index.vue":["0b01","chunk-329d5946"],"./pages/ide/props":["97c5","chunk-2d0e64c6"],"./pages/ide/props.js":["97c5","chunk-2d0e64c6"],"./pages/ide/signals":["4453","chunk-2d0c0e14"],"./pages/ide/signals.js":["4453","chunk-2d0c0e14"],"./pages/ide/slots":["eceb","chunk-2d230ab1"],"./pages/ide/slots.js":["eceb","chunk-2d230ab1"]};function r(e){var t=i[e];return t?n.e(t[1]).then(function(){var e=t[0];return n(e)}):Promise.resolve().then(function(){var t=new Error("Cannot find module '"+e+"'");throw t.code="MODULE_NOT_FOUND",t})}r.keys=function(){return Object.keys(i)},r.id="e399",e.exports=r}});
//# sourceMappingURL=app.432c990d.js.map | 1zlab-emp-ide | /1zlab_emp_ide-0.0.3-py3-none-any.whl/emp_ide/static/js/app.432c990d.js | app.432c990d.js |
(window["webpackJsonp"]=window["webpackJsonp"]||[]).push([["chunk-vendors"],{"00e7":function(t,e,n){(function(){Number.isInteger=Number.isInteger||function(t){return"number"===typeof t&&isFinite(t)&&Math.floor(t)===t};var e=n("06b1"),r={install:function(t){t.prototype.$cookie=this,t.cookie=this},set:function(t,n,r){var o=r;return Number.isInteger(r)&&(o={expires:r}),e.set(t,n,o)},get:function(t){return e.get(t)},delete:function(t,e){var n={expires:-1};void 0!==e&&(n=Object.assign(e,n)),this.set(t,"",n)}};t.exports=r})()},"00fd":function(t,e,n){var r=n("9e69"),o=Object.prototype,i=o.hasOwnProperty,a=o.toString,c=r?r.toStringTag:void 0;function s(t){var e=i.call(t,c),n=t[c];try{t[c]=void 0;var r=!0}catch(s){}var o=a.call(t);return r&&(e?t[c]=n:delete t[c]),o}t.exports=s},"01f9":function(t,e,n){"use strict";var r=n("2d00"),o=n("5ca1"),i=n("2aba"),a=n("32e9"),c=n("84f2"),s=n("41a0"),u=n("7f20"),f=n("38fd"),l=n("2b4c")("iterator"),p=!([].keys&&"next"in[].keys()),h="@@iterator",d="keys",v="values",m=function(){return this};t.exports=function(t,e,n,y,b,g,_){s(n,e,y);var x,w,j,S=function(t){if(!p&&t in $)return $[t];switch(t){case d:return function(){return new n(this,t)};case v:return function(){return new n(this,t)}}return function(){return new n(this,t)}},k=e+" Iterator",O=b==v,A=!1,$=t.prototype,C=$[l]||$[h]||b&&$[b],E=C||S(b),T=b?O?S("entries"):E:void 0,F="Array"==e&&$.entries||C;if(F&&(j=f(F.call(new t)),j!==Object.prototype&&j.next&&(u(j,k,!0),r||"function"==typeof j[l]||a(j,l,m))),O&&C&&C.name!==v&&(A=!0,E=function(){return C.call(this)}),r&&!_||!p&&!A&&$[l]||a($,l,E),c[e]=E,c[k]=m,b)if(x={values:O?E:S(v),keys:g?E:S(d),entries:T},_)for(w in x)w in $||i($,w,x[w]);else o(o.P+o.F*(p||A),e,x);return x}},"02f4":function(t,e,n){var r=n("4588"),o=n("be13");t.exports=function(t){return function(e,n){var i,a,c=String(o(e)),s=r(n),u=c.length;return s<0||s>=u?t?"":void 0:(i=c.charCodeAt(s),i<55296||i>56319||s+1===u||(a=c.charCodeAt(s+1))<56320||a>57343?t?c.charAt(s):i:t?c.slice(s,s+2):a-56320+(i-55296<<10)+65536)}}},"0390":function(t,e,n){"use strict";var r=n("02f4")(!0);t.exports=function(t,e,n){return e+(n?r(t,e).length:1)}},"03dd":function(t,e,n){var r=n("eac5"),o=n("57a5"),i=Object.prototype,a=i.hasOwnProperty;function c(t){if(!r(t))return o(t);var e=[];for(var n in Object(t))a.call(t,n)&&"constructor"!=n&&e.push(n);return e}t.exports=c},"0644":function(t,e,n){var r=n("3818"),o=1,i=4;function a(t){return r(t,o|i)}t.exports=a},"06b1":function(t,e,n){var r,o;
/*!
* tiny-cookie - A tiny cookie manipulation plugin
* https://github.com/Alex1990/tiny-cookie
* Under the MIT license | (c) Alex Chao
*/!function(i,a){r=a,o="function"===typeof r?r.call(e,n,e,t):r,void 0===o||(t.exports=o)}(0,function(){"use strict";function t(e,n,r){if(void 0===n)return t.get(e);null===n?t.remove(e):t.set(e,n,r)}function e(t){return t.replace(/[.*+?^$|[\](){}\\-]/g,"\\$&")}function n(t){var e="";for(var n in t)if(t.hasOwnProperty(n)){if("expires"===n){var o=t[n];"object"!==typeof o&&(o+="number"===typeof o?"D":"",o=r(o)),t[n]=o.toUTCString()}if("secure"===n){t[n]&&(e+=";"+n);continue}e+=";"+n+"="+t[n]}return t.hasOwnProperty("path")||(e+=";path=/"),e}function r(t){var e=new Date,n=t.charAt(t.length-1),r=parseInt(t,10);switch(n){case"Y":e.setFullYear(e.getFullYear()+r);break;case"M":e.setMonth(e.getMonth()+r);break;case"D":e.setDate(e.getDate()+r);break;case"h":e.setHours(e.getHours()+r);break;case"m":e.setMinutes(e.getMinutes()+r);break;case"s":e.setSeconds(e.getSeconds()+r);break;default:e=new Date(t)}return e}return t.enabled=function(){var e,n="__test_key";return document.cookie=n+"=1",e=!!document.cookie,e&&t.remove(n),e},t.get=function(t,n){if("string"!==typeof t||!t)return null;t="(?:^|; )"+e(t)+"(?:=([^;]*?))?(?:;|$)";var r=new RegExp(t),o=r.exec(document.cookie);return null!==o?n?o[1]:decodeURIComponent(o[1]):null},t.getRaw=function(e){return t.get(e,!0)},t.set=function(t,e,r,o){!0!==r&&(o=r,r=!1),o=n(o||{});var i=t+"="+(r?e:encodeURIComponent(e))+o;document.cookie=i},t.setRaw=function(e,n,r){t.set(e,n,!0,r)},t.remove=function(e){t.set(e,"a",{expires:new Date})},t})},"07c7":function(t,e){function n(){return!1}t.exports=n},"087d":function(t,e){function n(t,e){var n=-1,r=e.length,o=t.length;while(++n<r)t[o+n]=e[n];return t}t.exports=n},"097d":function(t,e,n){"use strict";var r=n("5ca1"),o=n("8378"),i=n("7726"),a=n("ebd6"),c=n("bcaa");r(r.P+r.R,"Promise",{finally:function(t){var e=a(this,o.Promise||i.Promise),n="function"==typeof t;return this.then(n?function(n){return c(e,t()).then(function(){return n})}:t,n?function(n){return c(e,t()).then(function(){throw n})}:t)}})},"09fa":function(t,e,n){var r=n("4588"),o=n("9def");t.exports=function(t){if(void 0===t)return 0;var e=r(t),n=o(e);if(e!==n)throw RangeError("Wrong length!");return n}},"0a49":function(t,e,n){var r=n("9b43"),o=n("626a"),i=n("4bf8"),a=n("9def"),c=n("cd1c");t.exports=function(t,e){var n=1==t,s=2==t,u=3==t,f=4==t,l=6==t,p=5==t||l,h=e||c;return function(e,c,d){for(var v,m,y=i(e),b=o(y),g=r(c,d,3),_=a(b.length),x=0,w=n?h(e,_):s?h(e,0):void 0;_>x;x++)if((p||x in b)&&(v=b[x],m=g(v,x,y),t))if(n)w[x]=m;else if(m)switch(t){case 3:return!0;case 5:return v;case 6:return x;case 2:w.push(v)}else if(f)return!1;return l?-1:u||f?f:w}}},"0b07":function(t,e,n){var r=n("34ac"),o=n("3698");function i(t,e){var n=o(t,e);return r(n)?n:void 0}t.exports=i},"0bfb":function(t,e,n){"use strict";var r=n("cb7c");t.exports=function(){var t=r(this),e="";return t.global&&(e+="g"),t.ignoreCase&&(e+="i"),t.multiline&&(e+="m"),t.unicode&&(e+="u"),t.sticky&&(e+="y"),e}},"0d24":function(t,e,n){(function(t){var r=n("2b3e"),o=n("07c7"),i=e&&!e.nodeType&&e,a=i&&"object"==typeof t&&t&&!t.nodeType&&t,c=a&&a.exports===i,s=c?r.Buffer:void 0,u=s?s.isBuffer:void 0,f=u||o;t.exports=f}).call(this,n("62e4")(t))},"0d58":function(t,e,n){var r=n("ce10"),o=n("e11e");t.exports=Object.keys||function(t){return r(t,o)}},"0f0f":function(t,e,n){var r=n("8eeb"),o=n("9934");function i(t,e){return t&&r(e,o(e),t)}t.exports=i},"0f88":function(t,e,n){var r,o=n("7726"),i=n("32e9"),a=n("ca5a"),c=a("typed_array"),s=a("view"),u=!(!o.ArrayBuffer||!o.DataView),f=u,l=0,p=9,h="Int8Array,Uint8Array,Uint8ClampedArray,Int16Array,Uint16Array,Int32Array,Uint32Array,Float32Array,Float64Array".split(",");while(l<p)(r=o[h[l++]])?(i(r.prototype,c,!0),i(r.prototype,s,!0)):f=!1;t.exports={ABV:u,CONSTR:f,TYPED:c,VIEW:s}},1041:function(t,e,n){var r=n("8eeb"),o=n("a029");function i(t,e){return r(t,o(t),e)}t.exports=i},1169:function(t,e,n){var r=n("2d95");t.exports=Array.isArray||function(t){return"Array"==r(t)}},"11e9":function(t,e,n){var r=n("52a7"),o=n("4630"),i=n("6821"),a=n("6a99"),c=n("69a8"),s=n("c69a"),u=Object.getOwnPropertyDescriptor;e.f=n("9e1e")?u:function(t,e){if(t=i(t),e=a(e,!0),s)try{return u(t,e)}catch(n){}if(c(t,e))return o(!r.f.call(t,e),t[e])}},1290:function(t,e){function n(t){var e=typeof t;return"string"==e||"number"==e||"symbol"==e||"boolean"==e?"__proto__"!==t:null===t}t.exports=n},1310:function(t,e){function n(t){return null!=t&&"object"==typeof t}t.exports=n},1368:function(t,e,n){var r=n("da03"),o=function(){var t=/[^.]+$/.exec(r&&r.keys&&r.keys.IE_PROTO||"");return t?"Symbol(src)_1."+t:""}();function i(t){return!!o&&o in t}t.exports=i},1495:function(t,e,n){var r=n("86cc"),o=n("cb7c"),i=n("0d58");t.exports=n("9e1e")?Object.defineProperties:function(t,e){o(t);var n,a=i(e),c=a.length,s=0;while(c>s)r.f(t,n=a[s++],e[n]);return t}},1991:function(t,e,n){var r,o,i,a=n("9b43"),c=n("31f4"),s=n("fab2"),u=n("230e"),f=n("7726"),l=f.process,p=f.setImmediate,h=f.clearImmediate,d=f.MessageChannel,v=f.Dispatch,m=0,y={},b="onreadystatechange",g=function(){var t=+this;if(y.hasOwnProperty(t)){var e=y[t];delete y[t],e()}},_=function(t){g.call(t.data)};p&&h||(p=function(t){var e=[],n=1;while(arguments.length>n)e.push(arguments[n++]);return y[++m]=function(){c("function"==typeof t?t:Function(t),e)},r(m),m},h=function(t){delete y[t]},"process"==n("2d95")(l)?r=function(t){l.nextTick(a(g,t,1))}:v&&v.now?r=function(t){v.now(a(g,t,1))}:d?(o=new d,i=o.port2,o.port1.onmessage=_,r=a(i.postMessage,i,1)):f.addEventListener&&"function"==typeof postMessage&&!f.importScripts?(r=function(t){f.postMessage(t+"","*")},f.addEventListener("message",_,!1)):r=b in u("script")?function(t){s.appendChild(u("script"))[b]=function(){s.removeChild(this),g.call(t)}}:function(t){setTimeout(a(g,t,1),0)}),t.exports={set:p,clear:h}},"1a2d":function(t,e,n){var r=n("42a2"),o=n("1310"),i="[object Map]";function a(t){return o(t)&&r(t)==i}t.exports=a},"1a8c":function(t,e){function n(t){var e=typeof t;return null!=t&&("object"==e||"function"==e)}t.exports=n},"1bac":function(t,e,n){var r=n("7d1f"),o=n("a029"),i=n("9934");function a(t){return r(t,i,o)}t.exports=a},"1cec":function(t,e,n){var r=n("0b07"),o=n("2b3e"),i=r(o,"Promise");t.exports=i},"1efc":function(t,e){function n(t){var e=this.has(t)&&delete this.__data__[t];return this.size-=e?1:0,e}t.exports=n},"1fa8":function(t,e,n){var r=n("cb7c");t.exports=function(t,e,n,o){try{return o?e(r(n)[0],n[1]):e(n)}catch(a){var i=t["return"];throw void 0!==i&&r(i.call(t)),a}}},"1fc8":function(t,e,n){var r=n("4245");function o(t,e){var n=r(this,t),o=n.size;return n.set(t,e),this.size+=n.size==o?0:1,this}t.exports=o},"214f":function(t,e,n){"use strict";n("b0c5");var r=n("2aba"),o=n("32e9"),i=n("79e5"),a=n("be13"),c=n("2b4c"),s=n("520a"),u=c("species"),f=!i(function(){var t=/./;return t.exec=function(){var t=[];return t.groups={a:"7"},t},"7"!=="".replace(t,"$<a>")}),l=function(){var t=/(?:)/,e=t.exec;t.exec=function(){return e.apply(this,arguments)};var n="ab".split(t);return 2===n.length&&"a"===n[0]&&"b"===n[1]}();t.exports=function(t,e,n){var p=c(t),h=!i(function(){var e={};return e[p]=function(){return 7},7!=""[t](e)}),d=h?!i(function(){var e=!1,n=/a/;return n.exec=function(){return e=!0,null},"split"===t&&(n.constructor={},n.constructor[u]=function(){return n}),n[p](""),!e}):void 0;if(!h||!d||"replace"===t&&!f||"split"===t&&!l){var v=/./[p],m=n(a,p,""[t],function(t,e,n,r,o){return e.exec===s?h&&!o?{done:!0,value:v.call(e,n,r)}:{done:!0,value:t.call(n,e,r)}:{done:!1}}),y=m[0],b=m[1];r(String.prototype,t,y),o(RegExp.prototype,p,2==e?function(t,e){return b.call(t,this,e)}:function(t){return b.call(t,this)})}}},"230e":function(t,e,n){var r=n("d3f4"),o=n("7726").document,i=r(o)&&r(o.createElement);t.exports=function(t){return i?o.createElement(t):{}}},"23c6":function(t,e,n){var r=n("2d95"),o=n("2b4c")("toStringTag"),i="Arguments"==r(function(){return arguments}()),a=function(t,e){try{return t[e]}catch(n){}};t.exports=function(t){var e,n,c;return void 0===t?"Undefined":null===t?"Null":"string"==typeof(n=a(e=Object(t),o))?n:i?r(e):"Object"==(c=r(e))&&"function"==typeof e.callee?"Arguments":c}},2474:function(t,e,n){var r=n("2b3e"),o=r.Uint8Array;t.exports=o},2478:function(t,e,n){var r=n("4245");function o(t){return r(this,t).get(t)}t.exports=o},2524:function(t,e,n){var r=n("6044"),o="__lodash_hash_undefined__";function i(t,e){var n=this.__data__;return this.size+=this.has(t)?0:1,n[t]=r&&void 0===e?o:e,this}t.exports=i},"253c":function(t,e,n){var r=n("3729"),o=n("1310"),i="[object Arguments]";function a(t){return o(t)&&r(t)==i}t.exports=a},2621:function(t,e){e.f=Object.getOwnPropertySymbols},"27ee":function(t,e,n){var r=n("23c6"),o=n("2b4c")("iterator"),i=n("84f2");t.exports=n("8378").getIteratorMethod=function(t){if(void 0!=t)return t[o]||t["@@iterator"]||i[r(t)]}},2877:function(t,e,n){"use strict";function r(t,e,n,r,o,i,a,c){var s,u="function"===typeof t?t.options:t;if(e&&(u.render=e,u.staticRenderFns=n,u._compiled=!0),r&&(u.functional=!0),i&&(u._scopeId="data-v-"+i),a?(s=function(t){t=t||this.$vnode&&this.$vnode.ssrContext||this.parent&&this.parent.$vnode&&this.parent.$vnode.ssrContext,t||"undefined"===typeof __VUE_SSR_CONTEXT__||(t=__VUE_SSR_CONTEXT__),o&&o.call(this,t),t&&t._registeredComponents&&t._registeredComponents.add(a)},u._ssrRegister=s):o&&(s=c?function(){o.call(this,this.$root.$options.shadowRoot)}:o),s)if(u.functional){u._injectStyles=s;var f=u.render;u.render=function(t,e){return s.call(e),f(t,e)}}else{var l=u.beforeCreate;u.beforeCreate=l?[].concat(l,s):[s]}return{exports:t,options:u}}n.d(e,"a",function(){return r})},"28a5":function(t,e,n){"use strict";var r=n("aae3"),o=n("cb7c"),i=n("ebd6"),a=n("0390"),c=n("9def"),s=n("5f1b"),u=n("520a"),f=n("79e5"),l=Math.min,p=[].push,h="split",d="length",v="lastIndex",m=4294967295,y=!f(function(){RegExp(m,"y")});n("214f")("split",2,function(t,e,n,f){var b;return b="c"=="abbc"[h](/(b)*/)[1]||4!="test"[h](/(?:)/,-1)[d]||2!="ab"[h](/(?:ab)*/)[d]||4!="."[h](/(.?)(.?)/)[d]||"."[h](/()()/)[d]>1||""[h](/.?/)[d]?function(t,e){var o=String(this);if(void 0===t&&0===e)return[];if(!r(t))return n.call(o,t,e);var i,a,c,s=[],f=(t.ignoreCase?"i":"")+(t.multiline?"m":"")+(t.unicode?"u":"")+(t.sticky?"y":""),l=0,h=void 0===e?m:e>>>0,y=new RegExp(t.source,f+"g");while(i=u.call(y,o)){if(a=y[v],a>l&&(s.push(o.slice(l,i.index)),i[d]>1&&i.index<o[d]&&p.apply(s,i.slice(1)),c=i[0][d],l=a,s[d]>=h))break;y[v]===i.index&&y[v]++}return l===o[d]?!c&&y.test("")||s.push(""):s.push(o.slice(l)),s[d]>h?s.slice(0,h):s}:"0"[h](void 0,0)[d]?function(t,e){return void 0===t&&0===e?[]:n.call(this,t,e)}:n,[function(n,r){var o=t(this),i=void 0==n?void 0:n[e];return void 0!==i?i.call(n,o,r):b.call(String(o),n,r)},function(t,e){var r=f(b,t,this,e,b!==n);if(r.done)return r.value;var u=o(t),p=String(this),h=i(u,RegExp),d=u.unicode,v=(u.ignoreCase?"i":"")+(u.multiline?"m":"")+(u.unicode?"u":"")+(y?"y":"g"),g=new h(y?u:"^(?:"+u.source+")",v),_=void 0===e?m:e>>>0;if(0===_)return[];if(0===p.length)return null===s(g,p)?[p]:[];var x=0,w=0,j=[];while(w<p.length){g.lastIndex=y?w:0;var S,k=s(g,y?p:p.slice(w));if(null===k||(S=l(c(g.lastIndex+(y?0:w)),p.length))===x)w=a(p,w,d);else{if(j.push(p.slice(x,w)),j.length===_)return j;for(var O=1;O<=k.length-1;O++)if(j.push(k[O]),j.length===_)return j;w=x=S}}return j.push(p.slice(x)),j}]})},"28c9":function(t,e){function n(){this.__data__=[],this.size=0}t.exports=n},"29f3":function(t,e){var n=Object.prototype,r=n.toString;function o(t){return r.call(t)}t.exports=o},"2aba":function(t,e,n){var r=n("7726"),o=n("32e9"),i=n("69a8"),a=n("ca5a")("src"),c=n("fa5b"),s="toString",u=(""+c).split(s);n("8378").inspectSource=function(t){return c.call(t)},(t.exports=function(t,e,n,c){var s="function"==typeof n;s&&(i(n,"name")||o(n,"name",e)),t[e]!==n&&(s&&(i(n,a)||o(n,a,t[e]?""+t[e]:u.join(String(e)))),t===r?t[e]=n:c?t[e]?t[e]=n:o(t,e,n):(delete t[e],o(t,e,n)))})(Function.prototype,s,function(){return"function"==typeof this&&this[a]||c.call(this)})},"2aeb":function(t,e,n){var r=n("cb7c"),o=n("1495"),i=n("e11e"),a=n("613b")("IE_PROTO"),c=function(){},s="prototype",u=function(){var t,e=n("230e")("iframe"),r=i.length,o="<",a=">";e.style.display="none",n("fab2").appendChild(e),e.src="javascript:",t=e.contentWindow.document,t.open(),t.write(o+"script"+a+"document.F=Object"+o+"/script"+a),t.close(),u=t.F;while(r--)delete u[s][i[r]];return u()};t.exports=Object.create||function(t,e){var n;return null!==t?(c[s]=r(t),n=new c,c[s]=null,n[a]=t):n=u(),void 0===e?n:o(n,e)}},"2b3e":function(t,e,n){var r=n("585a"),o="object"==typeof self&&self&&self.Object===Object&&self,i=r||o||Function("return this")();t.exports=i},"2b4c":function(t,e,n){var r=n("5537")("wks"),o=n("ca5a"),i=n("7726").Symbol,a="function"==typeof i,c=t.exports=function(t){return r[t]||(r[t]=a&&i[t]||(a?i:o)("Symbol."+t))};c.store=r},"2d00":function(t,e){t.exports=!1},"2d7c":function(t,e){function n(t,e){var n=-1,r=null==t?0:t.length,o=0,i=[];while(++n<r){var a=t[n];e(a,n,t)&&(i[o++]=a)}return i}t.exports=n},"2d95":function(t,e){var n={}.toString;t.exports=function(t){return n.call(t).slice(8,-1)}},"2dcb":function(t,e,n){var r=n("91e9"),o=r(Object.getPrototypeOf,Object);t.exports=o},"2fcc":function(t,e){function n(t){var e=this.__data__,n=e["delete"](t);return this.size=e.size,n}t.exports=n},"30c9":function(t,e,n){var r=n("9520"),o=n("b218");function i(t){return null!=t&&o(t.length)&&!r(t)}t.exports=i},"31f4":function(t,e){t.exports=function(t,e,n){var r=void 0===n;switch(e.length){case 0:return r?t():t.call(n);case 1:return r?t(e[0]):t.call(n,e[0]);case 2:return r?t(e[0],e[1]):t.call(n,e[0],e[1]);case 3:return r?t(e[0],e[1],e[2]):t.call(n,e[0],e[1],e[2]);case 4:return r?t(e[0],e[1],e[2],e[3]):t.call(n,e[0],e[1],e[2],e[3])}return t.apply(n,e)}},"32b3":function(t,e,n){var r=n("872a"),o=n("9638"),i=Object.prototype,a=i.hasOwnProperty;function c(t,e,n){var i=t[e];a.call(t,e)&&o(i,n)&&(void 0!==n||e in t)||r(t,e,n)}t.exports=c},"32e9":function(t,e,n){var r=n("86cc"),o=n("4630");t.exports=n("9e1e")?function(t,e,n){return r.f(t,e,o(1,n))}:function(t,e,n){return t[e]=n,t}},"32f4":function(t,e,n){var r=n("2d7c"),o=n("d327"),i=Object.prototype,a=i.propertyIsEnumerable,c=Object.getOwnPropertySymbols,s=c?function(t){return null==t?[]:(t=Object(t),r(c(t),function(e){return a.call(t,e)}))}:o;t.exports=s},"33a4":function(t,e,n){var r=n("84f2"),o=n("2b4c")("iterator"),i=Array.prototype;t.exports=function(t){return void 0!==t&&(r.Array===t||i[o]===t)}},"34ac":function(t,e,n){var r=n("9520"),o=n("1368"),i=n("1a8c"),a=n("dc57"),c=/[\\^$.*+?()[\]{}|]/g,s=/^\[object .+?Constructor\]$/,u=Function.prototype,f=Object.prototype,l=u.toString,p=f.hasOwnProperty,h=RegExp("^"+l.call(p).replace(c,"\\$&").replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g,"$1.*?")+"$");function d(t){if(!i(t)||o(t))return!1;var e=r(t)?h:s;return e.test(a(t))}t.exports=d},"34ef":function(t,e,n){n("ec30")("Uint8",1,function(t){return function(e,n,r){return t(this,e,n,r)}})},3698:function(t,e){function n(t,e){return null==t?void 0:t[e]}t.exports=n},"36bd":function(t,e,n){"use strict";var r=n("4bf8"),o=n("77f1"),i=n("9def");t.exports=function(t){var e=r(this),n=i(e.length),a=arguments.length,c=o(a>1?arguments[1]:void 0,n),s=a>2?arguments[2]:void 0,u=void 0===s?n:o(s,n);while(u>c)e[c++]=t;return e}},3729:function(t,e,n){var r=n("9e69"),o=n("00fd"),i=n("29f3"),a="[object Null]",c="[object Undefined]",s=r?r.toStringTag:void 0;function u(t){return null==t?void 0===t?c:a:s&&s in Object(t)?o(t):i(t)}t.exports=u},3818:function(t,e,n){var r=n("7e64"),o=n("8057"),i=n("32b3"),a=n("5b01"),c=n("0f0f"),s=n("e538"),u=n("4359"),f=n("54eb"),l=n("1041"),p=n("a994"),h=n("1bac"),d=n("42a2"),v=n("c87c"),m=n("c2b6"),y=n("fa21"),b=n("6747"),g=n("0d24"),_=n("cc45"),x=n("1a8c"),w=n("d7ee"),j=n("ec69"),S=1,k=2,O=4,A="[object Arguments]",$="[object Array]",C="[object Boolean]",E="[object Date]",T="[object Error]",F="[object Function]",I="[object GeneratorFunction]",R="[object Map]",M="[object Number]",P="[object Object]",L="[object RegExp]",N="[object Set]",D="[object String]",W="[object Symbol]",U="[object WeakMap]",V="[object ArrayBuffer]",B="[object DataView]",z="[object Float32Array]",H="[object Float64Array]",q="[object Int8Array]",G="[object Int16Array]",Y="[object Int32Array]",J="[object Uint8Array]",K="[object Uint8ClampedArray]",X="[object Uint16Array]",Q="[object Uint32Array]",Z={};function tt(t,e,n,$,C,E){var T,R=e&S,M=e&k,L=e&O;if(n&&(T=C?n(t,$,C,E):n(t)),void 0!==T)return T;if(!x(t))return t;var N=b(t);if(N){if(T=v(t),!R)return u(t,T)}else{var D=d(t),W=D==F||D==I;if(g(t))return s(t,R);if(D==P||D==A||W&&!C){if(T=M||W?{}:y(t),!R)return M?l(t,c(T,t)):f(t,a(T,t))}else{if(!Z[D])return C?t:{};T=m(t,D,R)}}E||(E=new r);var U=E.get(t);if(U)return U;E.set(t,T),w(t)?t.forEach(function(r){T.add(tt(r,e,n,r,t,E))}):_(t)&&t.forEach(function(r,o){T.set(o,tt(r,e,n,o,t,E))});var V=L?M?h:p:M?keysIn:j,B=N?void 0:V(t);return o(B||t,function(r,o){B&&(o=r,r=t[o]),i(T,o,tt(r,e,n,o,t,E))}),T}Z[A]=Z[$]=Z[V]=Z[B]=Z[C]=Z[E]=Z[z]=Z[H]=Z[q]=Z[G]=Z[Y]=Z[R]=Z[M]=Z[P]=Z[L]=Z[N]=Z[D]=Z[W]=Z[J]=Z[K]=Z[X]=Z[Q]=!0,Z[T]=Z[F]=Z[U]=!1,t.exports=tt},"38fd":function(t,e,n){var r=n("69a8"),o=n("4bf8"),i=n("613b")("IE_PROTO"),a=Object.prototype;t.exports=Object.getPrototypeOf||function(t){return t=o(t),r(t,i)?t[i]:"function"==typeof t.constructor&&t instanceof t.constructor?t.constructor.prototype:t instanceof Object?a:null}},"39ff":function(t,e,n){var r=n("0b07"),o=n("2b3e"),i=r(o,"WeakMap");t.exports=i},"3b4a":function(t,e,n){var r=n("0b07"),o=function(){try{var t=r(Object,"defineProperty");return t({},"",{}),t}catch(e){}}();t.exports=o},"41a0":function(t,e,n){"use strict";var r=n("2aeb"),o=n("4630"),i=n("7f20"),a={};n("32e9")(a,n("2b4c")("iterator"),function(){return this}),t.exports=function(t,e,n){t.prototype=r(a,{next:o(1,n)}),i(t,e+" Iterator")}},"41c3":function(t,e,n){var r=n("1a8c"),o=n("eac5"),i=n("ec8c"),a=Object.prototype,c=a.hasOwnProperty;function s(t){if(!r(t))return i(t);var e=o(t),n=[];for(var a in t)("constructor"!=a||!e&&c.call(t,a))&&n.push(a);return n}t.exports=s},4245:function(t,e,n){var r=n("1290");function o(t,e){var n=t.__data__;return r(e)?n["string"==typeof e?"string":"hash"]:n.map}t.exports=o},"42a2":function(t,e,n){var r=n("b5a7"),o=n("79bc"),i=n("1cec"),a=n("c869"),c=n("39ff"),s=n("3729"),u=n("dc57"),f="[object Map]",l="[object Object]",p="[object Promise]",h="[object Set]",d="[object WeakMap]",v="[object DataView]",m=u(r),y=u(o),b=u(i),g=u(a),_=u(c),x=s;(r&&x(new r(new ArrayBuffer(1)))!=v||o&&x(new o)!=f||i&&x(i.resolve())!=p||a&&x(new a)!=h||c&&x(new c)!=d)&&(x=function(t){var e=s(t),n=e==l?t.constructor:void 0,r=n?u(n):"";if(r)switch(r){case m:return v;case y:return f;case b:return p;case g:return h;case _:return d}return e}),t.exports=x},4359:function(t,e){function n(t,e){var n=-1,r=t.length;e||(e=Array(r));while(++n<r)e[n]=t[n];return e}t.exports=n},"456d":function(t,e,n){var r=n("4bf8"),o=n("0d58");n("5eda")("keys",function(){return function(t){return o(r(t))}})},4588:function(t,e){var n=Math.ceil,r=Math.floor;t.exports=function(t){return isNaN(t=+t)?0:(t>0?r:n)(t)}},4630:function(t,e){t.exports=function(t,e){return{enumerable:!(1&t),configurable:!(2&t),writable:!(4&t),value:e}}},"49f4":function(t,e,n){var r=n("6044");function o(){this.__data__=r?r(null):{},this.size=0}t.exports=o},"4a59":function(t,e,n){var r=n("9b43"),o=n("1fa8"),i=n("33a4"),a=n("cb7c"),c=n("9def"),s=n("27ee"),u={},f={};e=t.exports=function(t,e,n,l,p){var h,d,v,m,y=p?function(){return t}:s(t),b=r(n,l,e?2:1),g=0;if("function"!=typeof y)throw TypeError(t+" is not iterable!");if(i(y)){for(h=c(t.length);h>g;g++)if(m=e?b(a(d=t[g])[0],d[1]):b(t[g]),m===u||m===f)return m}else for(v=y.call(t);!(d=v.next()).done;)if(m=o(v,b,d.value,e),m===u||m===f)return m};e.BREAK=u,e.RETURN=f},"4bf8":function(t,e,n){var r=n("be13");t.exports=function(t){return Object(r(t))}},"4d7d":function(t,e,n){"use strict";var r=n("8bbf"),o=n.n(r),i={successIcon:"check_circle",infoIcon:"info",warningIcon:"priority_high",errorIcon:"warning",iconSize:24,width:350,maxWidth:"80%",className:"",okLabel:"确定",cancelLabel:"取消",transition:"scale"},a="function"===typeof Symbol&&"symbol"===typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"===typeof Symbol&&t.constructor===Symbol&&t!==Symbol.prototype?"symbol":typeof t},c=Object.assign||function(t){for(var e=1;e<arguments.length;e++){var n=arguments[e];for(var r in n)Object.prototype.hasOwnProperty.call(n,r)&&(t[r]=n[r])}return t},s=function(t){if(Array.isArray(t)){for(var e=0,n=Array(t.length);e<t.length;e++)n[e]=t[e];return n}return Array.from(t)},u={name:"mu-modal",props:{title:String,icon:String,iconSize:Number,mode:{type:String,default:"alert",validator:function(t){return-1!==["alert","confirm","prompt"].indexOf(t)}},type:{type:String,default:"",validator:function(t){return-1!==["","success","info","warning","error"].indexOf(t)}},content:[String,Function],width:[Number,String],maxWidth:[Number,String],className:String,transition:String,beforeClose:Function,okLabel:String,cancelLabel:String,inputType:String,inputPlaceholder:String,inputValue:[String,Number],validator:Function},data:function(){return{open:!1,value:this.inputValue,errorText:""}},methods:{handleClose:function(t){var e=this;return this.beforeClose?this.beforeClose(t,this,function(){return e.close(t)}):this.close(t)},close:function(t){if(t&&"prompt"===this.mode&&this.validator){var e=this.validator(this.value);if(!e.valid)return void(this.errorText=e.message);this.errorText=""}return this.open=!1,this.$emit("close",t,this.value),t},createInput:function(t){var e=this;if("prompt"===this.mode)return t("mu-text-field",{attrs:{type:this.inputType,placeholder:this.inputPlaceholder},props:{value:this.value,errorText:this.errorText,fullWidth:!0},on:{input:function(t){return e.value=t},keydown:function(t){13===t.keyCode&&e.handleClose(!0)}}})},createContent:function(t){var e="function"===typeof this.content?this.content(t):this.content;return t("div",{class:"mu-modal-content"},[this.icon?t("mu-icon",{staticClass:"mu-modal-icon",props:{value:this.icon,color:this.type,size:this.iconSize}}):void 0,t("div",{staticClass:"mu-modal-inner"},[e,this.createInput(t)])])},createActions:function(t){var e=this,n=[];return n.push(t("mu-button",{props:{flat:!0,color:"primary"},slot:"actions",on:{click:function(){return e.handleClose(!0)}}},this.okLabel)),"alert"!==this.mode&&n.unshift(t("mu-button",{props:{flat:!0},slot:"actions",on:{click:function(){return e.handleClose(!1)}}},this.cancelLabel)),n}},render:function(t){return t("mu-dialog",{props:{open:this.open,title:this.title,width:this.width,maxWidth:this.maxWidth,dialogClass:this.className,transition:this.transition,overlayClose:!1,escPressClose:!1}},[this.createContent(t)].concat(s(this.createActions(t))))}},f=o.a.extend(u),l="undefined"===typeof window,p=[],h=function(t){if(!l)return new Promise(function(e){var n=new f({el:document.createElement("div"),propsData:c({},i,{icon:i[t.type+"Icon"]||""},t)});document.body.appendChild(n.$el),n.open=!0,"prompt"===n.mode&&setTimeout(function(){n.$el&&n.$el.querySelector("input").focus()},200),p.push(n),n.$on("close",function(t,r){setTimeout(function(){n.$el&&n.$el.parentNode&&n.$el.parentNode.removeChild(n.$el),n.$destroy(),n=null},500);var o=p.indexOf(n);return-1!==o&&p.splice(o,1),e({result:t,value:r})})})};h.config=function(t){if(!t||Array.isArray(t)||"object"!==("undefined"===typeof t?"undefined":a(t)))return i;for(var e in t)t.hasOwnProperty(e)&&(i[e]=t[e]);return i},h.close=function(){p.forEach(function(t){t.close(!1)})},["alert","confirm","prompt"].forEach(function(t){h[t]=function(e,n){if(e||!(arguments.length<2)){var r="";switch(arguments.length){case 1:n={};break;case 2:"string"===typeof n&&(r=n,n={});break;default:r=arguments[1],n=arguments[2];break}return h(c({title:r,content:e},n,{mode:t}))}}}),h.install=function(t,e){h.config(e),t.prototype.$message=h,t.prototype.$alert=h.alert,t.prototype.$confirm=h.confirm,t.prototype.$prompt=h.prompt},e["a"]=h},"50d8":function(t,e){function n(t,e){var n=-1,r=Array(t);while(++n<t)r[n]=e(n);return r}t.exports=n},5147:function(t,e,n){var r=n("2b4c")("match");t.exports=function(t){var e=/./;try{"/./"[t](e)}catch(n){try{return e[r]=!1,!"/./"[t](e)}catch(o){}}return!0}},"520a":function(t,e,n){"use strict";var r=n("0bfb"),o=RegExp.prototype.exec,i=String.prototype.replace,a=o,c="lastIndex",s=function(){var t=/a/,e=/b*/g;return o.call(t,"a"),o.call(e,"a"),0!==t[c]||0!==e[c]}(),u=void 0!==/()??/.exec("")[1],f=s||u;f&&(a=function(t){var e,n,a,f,l=this;return u&&(n=new RegExp("^"+l.source+"$(?!\\s)",r.call(l))),s&&(e=l[c]),a=o.call(l,t),s&&a&&(l[c]=l.global?a.index+a[0].length:e),u&&a&&a.length>1&&i.call(a[0],n,function(){for(f=1;f<arguments.length-2;f++)void 0===arguments[f]&&(a[f]=void 0)}),a}),t.exports=a},"52a7":function(t,e){e.f={}.propertyIsEnumerable},"54eb":function(t,e,n){var r=n("8eeb"),o=n("32f4");function i(t,e){return r(t,o(t),e)}t.exports=i},"551c":function(t,e,n){"use strict";var r,o,i,a,c=n("2d00"),s=n("7726"),u=n("9b43"),f=n("23c6"),l=n("5ca1"),p=n("d3f4"),h=n("d8e8"),d=n("f605"),v=n("4a59"),m=n("ebd6"),y=n("1991").set,b=n("8079")(),g=n("a5b8"),_=n("9c80"),x=n("a25f"),w=n("bcaa"),j="Promise",S=s.TypeError,k=s.process,O=k&&k.versions,A=O&&O.v8||"",$=s[j],C="process"==f(k),E=function(){},T=o=g.f,F=!!function(){try{var t=$.resolve(1),e=(t.constructor={})[n("2b4c")("species")]=function(t){t(E,E)};return(C||"function"==typeof PromiseRejectionEvent)&&t.then(E)instanceof e&&0!==A.indexOf("6.6")&&-1===x.indexOf("Chrome/66")}catch(r){}}(),I=function(t){var e;return!(!p(t)||"function"!=typeof(e=t.then))&&e},R=function(t,e){if(!t._n){t._n=!0;var n=t._c;b(function(){var r=t._v,o=1==t._s,i=0,a=function(e){var n,i,a,c=o?e.ok:e.fail,s=e.resolve,u=e.reject,f=e.domain;try{c?(o||(2==t._h&&L(t),t._h=1),!0===c?n=r:(f&&f.enter(),n=c(r),f&&(f.exit(),a=!0)),n===e.promise?u(S("Promise-chain cycle")):(i=I(n))?i.call(n,s,u):s(n)):u(r)}catch(l){f&&!a&&f.exit(),u(l)}};while(n.length>i)a(n[i++]);t._c=[],t._n=!1,e&&!t._h&&M(t)})}},M=function(t){y.call(s,function(){var e,n,r,o=t._v,i=P(t);if(i&&(e=_(function(){C?k.emit("unhandledRejection",o,t):(n=s.onunhandledrejection)?n({promise:t,reason:o}):(r=s.console)&&r.error&&r.error("Unhandled promise rejection",o)}),t._h=C||P(t)?2:1),t._a=void 0,i&&e.e)throw e.v})},P=function(t){return 1!==t._h&&0===(t._a||t._c).length},L=function(t){y.call(s,function(){var e;C?k.emit("rejectionHandled",t):(e=s.onrejectionhandled)&&e({promise:t,reason:t._v})})},N=function(t){var e=this;e._d||(e._d=!0,e=e._w||e,e._v=t,e._s=2,e._a||(e._a=e._c.slice()),R(e,!0))},D=function(t){var e,n=this;if(!n._d){n._d=!0,n=n._w||n;try{if(n===t)throw S("Promise can't be resolved itself");(e=I(t))?b(function(){var r={_w:n,_d:!1};try{e.call(t,u(D,r,1),u(N,r,1))}catch(o){N.call(r,o)}}):(n._v=t,n._s=1,R(n,!1))}catch(r){N.call({_w:n,_d:!1},r)}}};F||($=function(t){d(this,$,j,"_h"),h(t),r.call(this);try{t(u(D,this,1),u(N,this,1))}catch(e){N.call(this,e)}},r=function(t){this._c=[],this._a=void 0,this._s=0,this._d=!1,this._v=void 0,this._h=0,this._n=!1},r.prototype=n("dcbc")($.prototype,{then:function(t,e){var n=T(m(this,$));return n.ok="function"!=typeof t||t,n.fail="function"==typeof e&&e,n.domain=C?k.domain:void 0,this._c.push(n),this._a&&this._a.push(n),this._s&&R(this,!1),n.promise},catch:function(t){return this.then(void 0,t)}}),i=function(){var t=new r;this.promise=t,this.resolve=u(D,t,1),this.reject=u(N,t,1)},g.f=T=function(t){return t===$||t===a?new i(t):o(t)}),l(l.G+l.W+l.F*!F,{Promise:$}),n("7f20")($,j),n("7a56")(j),a=n("8378")[j],l(l.S+l.F*!F,j,{reject:function(t){var e=T(this),n=e.reject;return n(t),e.promise}}),l(l.S+l.F*(c||!F),j,{resolve:function(t){return w(c&&this===a?$:this,t)}}),l(l.S+l.F*!(F&&n("5cc5")(function(t){$.all(t)["catch"](E)})),j,{all:function(t){var e=this,n=T(e),r=n.resolve,o=n.reject,i=_(function(){var n=[],i=0,a=1;v(t,!1,function(t){var c=i++,s=!1;n.push(void 0),a++,e.resolve(t).then(function(t){s||(s=!0,n[c]=t,--a||r(n))},o)}),--a||r(n)});return i.e&&o(i.v),n.promise},race:function(t){var e=this,n=T(e),r=n.reject,o=_(function(){v(t,!1,function(t){e.resolve(t).then(n.resolve,r)})});return o.e&&r(o.v),n.promise}})},5537:function(t,e,n){var r=n("8378"),o=n("7726"),i="__core-js_shared__",a=o[i]||(o[i]={});(t.exports=function(t,e){return a[t]||(a[t]=void 0!==e?e:{})})("versions",[]).push({version:r.version,mode:n("2d00")?"pure":"global",copyright:"© 2019 Denis Pushkarev (zloirock.ru)"})},"55a3":function(t,e){function n(t){return this.__data__.has(t)}t.exports=n},"57a5":function(t,e,n){var r=n("91e9"),o=r(Object.keys,Object);t.exports=o},"585a":function(t,e,n){(function(e){var n="object"==typeof e&&e&&e.Object===Object&&e;t.exports=n}).call(this,n("c8ba"))},"5b01":function(t,e,n){var r=n("8eeb"),o=n("ec69");function i(t,e){return t&&r(e,o(e),t)}t.exports=i},"5ca1":function(t,e,n){var r=n("7726"),o=n("8378"),i=n("32e9"),a=n("2aba"),c=n("9b43"),s="prototype",u=function(t,e,n){var f,l,p,h,d=t&u.F,v=t&u.G,m=t&u.S,y=t&u.P,b=t&u.B,g=v?r:m?r[e]||(r[e]={}):(r[e]||{})[s],_=v?o:o[e]||(o[e]={}),x=_[s]||(_[s]={});for(f in v&&(n=e),n)l=!d&&g&&void 0!==g[f],p=(l?g:n)[f],h=b&&l?c(p,r):y&&"function"==typeof p?c(Function.call,p):p,g&&a(g,f,p,t&u.U),_[f]!=p&&i(_,f,h),y&&x[f]!=p&&(x[f]=p)};r.core=o,u.F=1,u.G=2,u.S=4,u.P=8,u.B=16,u.W=32,u.U=64,u.R=128,t.exports=u},"5cc5":function(t,e,n){var r=n("2b4c")("iterator"),o=!1;try{var i=[7][r]();i["return"]=function(){o=!0},Array.from(i,function(){throw 2})}catch(a){}t.exports=function(t,e){if(!e&&!o)return!1;var n=!1;try{var i=[7],c=i[r]();c.next=function(){return{done:n=!0}},i[r]=function(){return c},t(i)}catch(a){}return n}},"5d89":function(t,e,n){var r=n("f8af");function o(t,e){var n=e?r(t.buffer):t.buffer;return new t.constructor(n,t.byteOffset,t.byteLength)}t.exports=o},"5e2e":function(t,e,n){var r=n("28c9"),o=n("69d5"),i=n("b4c0"),a=n("fba5"),c=n("67ca");function s(t){var e=-1,n=null==t?0:t.length;this.clear();while(++e<n){var r=t[e];this.set(r[0],r[1])}}s.prototype.clear=r,s.prototype["delete"]=o,s.prototype.get=i,s.prototype.has=a,s.prototype.set=c,t.exports=s},"5eda":function(t,e,n){var r=n("5ca1"),o=n("8378"),i=n("79e5");t.exports=function(t,e){var n=(o.Object||{})[t]||Object[t],a={};a[t]=e(n),r(r.S+r.F*i(function(){n(1)}),"Object",a)}},"5f1b":function(t,e,n){"use strict";var r=n("23c6"),o=RegExp.prototype.exec;t.exports=function(t,e){var n=t.exec;if("function"===typeof n){var i=n.call(t,e);if("object"!==typeof i)throw new TypeError("RegExp exec method returned something other than an Object or null");return i}if("RegExp"!==r(t))throw new TypeError("RegExp#exec called on incompatible receiver");return o.call(t,e)}},6044:function(t,e,n){var r=n("0b07"),o=r(Object,"create");t.exports=o},"613b":function(t,e,n){var r=n("5537")("keys"),o=n("ca5a");t.exports=function(t){return r[t]||(r[t]=o(t))}},"619b":function(t,e,n){},"626a":function(t,e,n){var r=n("2d95");t.exports=Object("z").propertyIsEnumerable(0)?Object:function(t){return"String"==r(t)?t.split(""):Object(t)}},"62e4":function(t,e){t.exports=function(t){return t.webpackPolyfill||(t.deprecate=function(){},t.paths=[],t.children||(t.children=[]),Object.defineProperty(t,"loaded",{enumerable:!0,get:function(){return t.l}}),Object.defineProperty(t,"id",{enumerable:!0,get:function(){return t.i}}),t.webpackPolyfill=1),t}},"64d2":function(t,e,n){},6747:function(t,e){var n=Array.isArray;t.exports=n},"67ca":function(t,e,n){var r=n("cb5a");function o(t,e){var n=this.__data__,o=r(n,t);return o<0?(++this.size,n.push([t,e])):n[o][1]=e,this}t.exports=o},6821:function(t,e,n){var r=n("626a"),o=n("be13");t.exports=function(t){return r(o(t))}},"69a8":function(t,e){var n={}.hasOwnProperty;t.exports=function(t,e){return n.call(t,e)}},"69d5":function(t,e,n){var r=n("cb5a"),o=Array.prototype,i=o.splice;function a(t){var e=this.__data__,n=r(e,t);if(n<0)return!1;var o=e.length-1;return n==o?e.pop():i.call(e,n,1),--this.size,!0}t.exports=a},"6a99":function(t,e,n){var r=n("d3f4");t.exports=function(t,e){if(!r(t))return t;var n,o;if(e&&"function"==typeof(n=t.toString)&&!r(o=n.call(t)))return o;if("function"==typeof(n=t.valueOf)&&!r(o=n.call(t)))return o;if(!e&&"function"==typeof(n=t.toString)&&!r(o=n.call(t)))return o;throw TypeError("Can't convert object to primitive value")}},"6f6c":function(t,e){var n=/\w*$/;function r(t){var e=new t.constructor(t.source,n.exec(t));return e.lastIndex=t.lastIndex,e}t.exports=r},"6fcd":function(t,e,n){var r=n("50d8"),o=n("d370"),i=n("6747"),a=n("0d24"),c=n("c098"),s=n("73ac"),u=Object.prototype,f=u.hasOwnProperty;function l(t,e){var n=i(t),u=!n&&o(t),l=!n&&!u&&a(t),p=!n&&!u&&!l&&s(t),h=n||u||l||p,d=h?r(t.length,String):[],v=d.length;for(var m in t)!e&&!f.call(t,m)||h&&("length"==m||l&&("offset"==m||"parent"==m)||p&&("buffer"==m||"byteLength"==m||"byteOffset"==m)||c(m,v))||d.push(m);return d}t.exports=l},7333:function(t,e,n){"use strict";var r=n("9e1e"),o=n("0d58"),i=n("2621"),a=n("52a7"),c=n("4bf8"),s=n("626a"),u=Object.assign;t.exports=!u||n("79e5")(function(){var t={},e={},n=Symbol(),r="abcdefghijklmnopqrst";return t[n]=7,r.split("").forEach(function(t){e[t]=t}),7!=u({},t)[n]||Object.keys(u({},e)).join("")!=r})?function(t,e){var n=c(t),u=arguments.length,f=1,l=i.f,p=a.f;while(u>f){var h,d=s(arguments[f++]),v=l?o(d).concat(l(d)):o(d),m=v.length,y=0;while(m>y)h=v[y++],r&&!p.call(d,h)||(n[h]=d[h])}return n}:u},"73ac":function(t,e,n){var r=n("743f"),o=n("b047"),i=n("99d3"),a=i&&i.isTypedArray,c=a?o(a):r;t.exports=c},"743f":function(t,e,n){var r=n("3729"),o=n("b218"),i=n("1310"),a="[object Arguments]",c="[object Array]",s="[object Boolean]",u="[object Date]",f="[object Error]",l="[object Function]",p="[object Map]",h="[object Number]",d="[object Object]",v="[object RegExp]",m="[object Set]",y="[object String]",b="[object WeakMap]",g="[object ArrayBuffer]",_="[object DataView]",x="[object Float32Array]",w="[object Float64Array]",j="[object Int8Array]",S="[object Int16Array]",k="[object Int32Array]",O="[object Uint8Array]",A="[object Uint8ClampedArray]",$="[object Uint16Array]",C="[object Uint32Array]",E={};function T(t){return i(t)&&o(t.length)&&!!E[r(t)]}E[x]=E[w]=E[j]=E[S]=E[k]=E[O]=E[A]=E[$]=E[C]=!0,E[a]=E[c]=E[g]=E[s]=E[_]=E[u]=E[f]=E[l]=E[p]=E[h]=E[d]=E[v]=E[m]=E[y]=E[b]=!1,t.exports=T},7530:function(t,e,n){var r=n("1a8c"),o=Object.create,i=function(){function t(){}return function(e){if(!r(e))return{};if(o)return o(e);t.prototype=e;var n=new t;return t.prototype=void 0,n}}();t.exports=i},7726:function(t,e){var n=t.exports="undefined"!=typeof window&&window.Math==Math?window:"undefined"!=typeof self&&self.Math==Math?self:Function("return this")();"number"==typeof __g&&(__g=n)},"77f1":function(t,e,n){var r=n("4588"),o=Math.max,i=Math.min;t.exports=function(t,e){return t=r(t),t<0?o(t+e,0):i(t,e)}},"79bc":function(t,e,n){var r=n("0b07"),o=n("2b3e"),i=r(o,"Map");t.exports=i},"79e5":function(t,e){t.exports=function(t){try{return!!t()}catch(e){return!0}}},"7a48":function(t,e,n){var r=n("6044"),o=Object.prototype,i=o.hasOwnProperty;function a(t){var e=this.__data__;return r?void 0!==e[t]:i.call(e,t)}t.exports=a},"7a56":function(t,e,n){"use strict";var r=n("7726"),o=n("86cc"),i=n("9e1e"),a=n("2b4c")("species");t.exports=function(t){var e=r[t];i&&e&&!e[a]&&o.f(e,a,{configurable:!0,get:function(){return this}})}},"7b83":function(t,e,n){var r=n("7c64"),o=n("93ed"),i=n("2478"),a=n("a524"),c=n("1fc8");function s(t){var e=-1,n=null==t?0:t.length;this.clear();while(++e<n){var r=t[e];this.set(r[0],r[1])}}s.prototype.clear=r,s.prototype["delete"]=o,s.prototype.get=i,s.prototype.has=a,s.prototype.set=c,t.exports=s},"7c64":function(t,e,n){var r=n("e24b"),o=n("5e2e"),i=n("79bc");function a(){this.size=0,this.__data__={hash:new r,map:new(i||o),string:new r}}t.exports=a},"7d1f":function(t,e,n){var r=n("087d"),o=n("6747");function i(t,e,n){var i=e(t);return o(t)?i:r(i,n(t))}t.exports=i},"7e64":function(t,e,n){var r=n("5e2e"),o=n("efb6"),i=n("2fcc"),a=n("802a"),c=n("55a3"),s=n("d02c");function u(t){var e=this.__data__=new r(t);this.size=e.size}u.prototype.clear=o,u.prototype["delete"]=i,u.prototype.get=a,u.prototype.has=c,u.prototype.set=s,t.exports=u},"7f20":function(t,e,n){var r=n("86cc").f,o=n("69a8"),i=n("2b4c")("toStringTag");t.exports=function(t,e,n){t&&!o(t=n?t:t.prototype,i)&&r(t,i,{configurable:!0,value:e})}},"802a":function(t,e){function n(t){return this.__data__.get(t)}t.exports=n},8057:function(t,e){function n(t,e){var n=-1,r=null==t?0:t.length;while(++n<r)if(!1===e(t[n],n,t))break;return t}t.exports=n},8079:function(t,e,n){var r=n("7726"),o=n("1991").set,i=r.MutationObserver||r.WebKitMutationObserver,a=r.process,c=r.Promise,s="process"==n("2d95")(a);t.exports=function(){var t,e,n,u=function(){var r,o;s&&(r=a.domain)&&r.exit();while(t){o=t.fn,t=t.next;try{o()}catch(i){throw t?n():e=void 0,i}}e=void 0,r&&r.enter()};if(s)n=function(){a.nextTick(u)};else if(!i||r.navigator&&r.navigator.standalone)if(c&&c.resolve){var f=c.resolve(void 0);n=function(){f.then(u)}}else n=function(){o.call(r,u)};else{var l=!0,p=document.createTextNode("");new i(u).observe(p,{characterData:!0}),n=function(){p.data=l=!l}}return function(r){var o={fn:r,next:void 0};e&&(e.next=o),t||(t=o,n()),e=o}}},8378:function(t,e){var n=t.exports={version:"2.6.9"};"number"==typeof __e&&(__e=n)},"84f2":function(t,e){t.exports={}},"86cc":function(t,e,n){var r=n("cb7c"),o=n("c69a"),i=n("6a99"),a=Object.defineProperty;e.f=n("9e1e")?Object.defineProperty:function(t,e,n){if(r(t),e=i(e,!0),r(n),o)try{return a(t,e,n)}catch(c){}if("get"in n||"set"in n)throw TypeError("Accessors not supported!");return"value"in n&&(t[e]=n.value),t}},"872a":function(t,e,n){var r=n("3b4a");function o(t,e,n){"__proto__"==e&&r?r(t,e,{configurable:!0,enumerable:!0,value:n,writable:!0}):t[e]=n}t.exports=o},"8c4f":function(t,e,n){"use strict";
/*!
* vue-router v3.1.2
* (c) 2019 Evan You
* @license MIT
*/function r(t,e){0}function o(t){return Object.prototype.toString.call(t).indexOf("Error")>-1}function i(t,e){return e instanceof t||e&&(e.name===t.name||e._name===t._name)}function a(t,e){for(var n in e)t[n]=e[n];return t}var c={name:"RouterView",functional:!0,props:{name:{type:String,default:"default"}},render:function(t,e){var n=e.props,r=e.children,o=e.parent,i=e.data;i.routerView=!0;var c=o.$createElement,u=n.name,f=o.$route,l=o._routerViewCache||(o._routerViewCache={}),p=0,h=!1;while(o&&o._routerRoot!==o){var d=o.$vnode&&o.$vnode.data;d&&(d.routerView&&p++,d.keepAlive&&o._inactive&&(h=!0)),o=o.$parent}if(i.routerViewDepth=p,h)return c(l[u],i,r);var v=f.matched[p];if(!v)return l[u]=null,c();var m=l[u]=v.components[u];i.registerRouteInstance=function(t,e){var n=v.instances[u];(e&&n!==t||!e&&n===t)&&(v.instances[u]=e)},(i.hook||(i.hook={})).prepatch=function(t,e){v.instances[u]=e.componentInstance},i.hook.init=function(t){t.data.keepAlive&&t.componentInstance&&t.componentInstance!==v.instances[u]&&(v.instances[u]=t.componentInstance)};var y=i.props=s(f,v.props&&v.props[u]);if(y){y=i.props=a({},y);var b=i.attrs=i.attrs||{};for(var g in y)m.props&&g in m.props||(b[g]=y[g],delete y[g])}return c(m,i,r)}};function s(t,e){switch(typeof e){case"undefined":return;case"object":return e;case"function":return e(t);case"boolean":return e?t.params:void 0;default:0}}var u=/[!'()*]/g,f=function(t){return"%"+t.charCodeAt(0).toString(16)},l=/%2C/g,p=function(t){return encodeURIComponent(t).replace(u,f).replace(l,",")},h=decodeURIComponent;function d(t,e,n){void 0===e&&(e={});var r,o=n||v;try{r=o(t||"")}catch(a){r={}}for(var i in e)r[i]=e[i];return r}function v(t){var e={};return t=t.trim().replace(/^(\?|#|&)/,""),t?(t.split("&").forEach(function(t){var n=t.replace(/\+/g," ").split("="),r=h(n.shift()),o=n.length>0?h(n.join("=")):null;void 0===e[r]?e[r]=o:Array.isArray(e[r])?e[r].push(o):e[r]=[e[r],o]}),e):e}function m(t){var e=t?Object.keys(t).map(function(e){var n=t[e];if(void 0===n)return"";if(null===n)return p(e);if(Array.isArray(n)){var r=[];return n.forEach(function(t){void 0!==t&&(null===t?r.push(p(e)):r.push(p(e)+"="+p(t)))}),r.join("&")}return p(e)+"="+p(n)}).filter(function(t){return t.length>0}).join("&"):null;return e?"?"+e:""}var y=/\/?$/;function b(t,e,n,r){var o=r&&r.options.stringifyQuery,i=e.query||{};try{i=g(i)}catch(c){}var a={name:e.name||t&&t.name,meta:t&&t.meta||{},path:e.path||"/",hash:e.hash||"",query:i,params:e.params||{},fullPath:w(e,o),matched:t?x(t):[]};return n&&(a.redirectedFrom=w(n,o)),Object.freeze(a)}function g(t){if(Array.isArray(t))return t.map(g);if(t&&"object"===typeof t){var e={};for(var n in t)e[n]=g(t[n]);return e}return t}var _=b(null,{path:"/"});function x(t){var e=[];while(t)e.unshift(t),t=t.parent;return e}function w(t,e){var n=t.path,r=t.query;void 0===r&&(r={});var o=t.hash;void 0===o&&(o="");var i=e||m;return(n||"/")+i(r)+o}function j(t,e){return e===_?t===e:!!e&&(t.path&&e.path?t.path.replace(y,"")===e.path.replace(y,"")&&t.hash===e.hash&&S(t.query,e.query):!(!t.name||!e.name)&&(t.name===e.name&&t.hash===e.hash&&S(t.query,e.query)&&S(t.params,e.params)))}function S(t,e){if(void 0===t&&(t={}),void 0===e&&(e={}),!t||!e)return t===e;var n=Object.keys(t),r=Object.keys(e);return n.length===r.length&&n.every(function(n){var r=t[n],o=e[n];return"object"===typeof r&&"object"===typeof o?S(r,o):String(r)===String(o)})}function k(t,e){return 0===t.path.replace(y,"/").indexOf(e.path.replace(y,"/"))&&(!e.hash||t.hash===e.hash)&&O(t.query,e.query)}function O(t,e){for(var n in e)if(!(n in t))return!1;return!0}function A(t,e,n){var r=t.charAt(0);if("/"===r)return t;if("?"===r||"#"===r)return e+t;var o=e.split("/");n&&o[o.length-1]||o.pop();for(var i=t.replace(/^\//,"").split("/"),a=0;a<i.length;a++){var c=i[a];".."===c?o.pop():"."!==c&&o.push(c)}return""!==o[0]&&o.unshift(""),o.join("/")}function $(t){var e="",n="",r=t.indexOf("#");r>=0&&(e=t.slice(r),t=t.slice(0,r));var o=t.indexOf("?");return o>=0&&(n=t.slice(o+1),t=t.slice(0,o)),{path:t,query:n,hash:e}}function C(t){return t.replace(/\/\//g,"/")}var E=Array.isArray||function(t){return"[object Array]"==Object.prototype.toString.call(t)},T=K,F=L,I=N,R=U,M=J,P=new RegExp(["(\\\\.)","([\\/.])?(?:(?:\\:(\\w+)(?:\\(((?:\\\\.|[^\\\\()])+)\\))?|\\(((?:\\\\.|[^\\\\()])+)\\))([+*?])?|(\\*))"].join("|"),"g");function L(t,e){var n,r=[],o=0,i=0,a="",c=e&&e.delimiter||"/";while(null!=(n=P.exec(t))){var s=n[0],u=n[1],f=n.index;if(a+=t.slice(i,f),i=f+s.length,u)a+=u[1];else{var l=t[i],p=n[2],h=n[3],d=n[4],v=n[5],m=n[6],y=n[7];a&&(r.push(a),a="");var b=null!=p&&null!=l&&l!==p,g="+"===m||"*"===m,_="?"===m||"*"===m,x=n[2]||c,w=d||v;r.push({name:h||o++,prefix:p||"",delimiter:x,optional:_,repeat:g,partial:b,asterisk:!!y,pattern:w?B(w):y?".*":"[^"+V(x)+"]+?"})}}return i<t.length&&(a+=t.substr(i)),a&&r.push(a),r}function N(t,e){return U(L(t,e))}function D(t){return encodeURI(t).replace(/[\/?#]/g,function(t){return"%"+t.charCodeAt(0).toString(16).toUpperCase()})}function W(t){return encodeURI(t).replace(/[?#]/g,function(t){return"%"+t.charCodeAt(0).toString(16).toUpperCase()})}function U(t){for(var e=new Array(t.length),n=0;n<t.length;n++)"object"===typeof t[n]&&(e[n]=new RegExp("^(?:"+t[n].pattern+")$"));return function(n,r){for(var o="",i=n||{},a=r||{},c=a.pretty?D:encodeURIComponent,s=0;s<t.length;s++){var u=t[s];if("string"!==typeof u){var f,l=i[u.name];if(null==l){if(u.optional){u.partial&&(o+=u.prefix);continue}throw new TypeError('Expected "'+u.name+'" to be defined')}if(E(l)){if(!u.repeat)throw new TypeError('Expected "'+u.name+'" to not repeat, but received `'+JSON.stringify(l)+"`");if(0===l.length){if(u.optional)continue;throw new TypeError('Expected "'+u.name+'" to not be empty')}for(var p=0;p<l.length;p++){if(f=c(l[p]),!e[s].test(f))throw new TypeError('Expected all "'+u.name+'" to match "'+u.pattern+'", but received `'+JSON.stringify(f)+"`");o+=(0===p?u.prefix:u.delimiter)+f}}else{if(f=u.asterisk?W(l):c(l),!e[s].test(f))throw new TypeError('Expected "'+u.name+'" to match "'+u.pattern+'", but received "'+f+'"');o+=u.prefix+f}}else o+=u}return o}}function V(t){return t.replace(/([.+*?=^!:${}()[\]|\/\\])/g,"\\$1")}function B(t){return t.replace(/([=!:$\/()])/g,"\\$1")}function z(t,e){return t.keys=e,t}function H(t){return t.sensitive?"":"i"}function q(t,e){var n=t.source.match(/\((?!\?)/g);if(n)for(var r=0;r<n.length;r++)e.push({name:r,prefix:null,delimiter:null,optional:!1,repeat:!1,partial:!1,asterisk:!1,pattern:null});return z(t,e)}function G(t,e,n){for(var r=[],o=0;o<t.length;o++)r.push(K(t[o],e,n).source);var i=new RegExp("(?:"+r.join("|")+")",H(n));return z(i,e)}function Y(t,e,n){return J(L(t,n),e,n)}function J(t,e,n){E(e)||(n=e||n,e=[]),n=n||{};for(var r=n.strict,o=!1!==n.end,i="",a=0;a<t.length;a++){var c=t[a];if("string"===typeof c)i+=V(c);else{var s=V(c.prefix),u="(?:"+c.pattern+")";e.push(c),c.repeat&&(u+="(?:"+s+u+")*"),u=c.optional?c.partial?s+"("+u+")?":"(?:"+s+"("+u+"))?":s+"("+u+")",i+=u}}var f=V(n.delimiter||"/"),l=i.slice(-f.length)===f;return r||(i=(l?i.slice(0,-f.length):i)+"(?:"+f+"(?=$))?"),i+=o?"$":r&&l?"":"(?="+f+"|$)",z(new RegExp("^"+i,H(n)),e)}function K(t,e,n){return E(e)||(n=e||n,e=[]),n=n||{},t instanceof RegExp?q(t,e):E(t)?G(t,e,n):Y(t,e,n)}T.parse=F,T.compile=I,T.tokensToFunction=R,T.tokensToRegExp=M;var X=Object.create(null);function Q(t,e,n){e=e||{};try{var r=X[t]||(X[t]=T.compile(t));return e.pathMatch&&(e[0]=e.pathMatch),r(e,{pretty:!0})}catch(o){return""}finally{delete e[0]}}function Z(t,e,n,r){var o="string"===typeof t?{path:t}:t;if(o._normalized)return o;if(o.name)return a({},t);if(!o.path&&o.params&&e){o=a({},o),o._normalized=!0;var i=a(a({},e.params),o.params);if(e.name)o.name=e.name,o.params=i;else if(e.matched.length){var c=e.matched[e.matched.length-1].path;o.path=Q(c,i,"path "+e.path)}else 0;return o}var s=$(o.path||""),u=e&&e.path||"/",f=s.path?A(s.path,u,n||o.append):u,l=d(s.query,o.query,r&&r.options.parseQuery),p=o.hash||s.hash;return p&&"#"!==p.charAt(0)&&(p="#"+p),{_normalized:!0,path:f,query:l,hash:p}}var tt,et=[String,Object],nt=[String,Array],rt=function(){},ot={name:"RouterLink",props:{to:{type:et,required:!0},tag:{type:String,default:"a"},exact:Boolean,append:Boolean,replace:Boolean,activeClass:String,exactActiveClass:String,event:{type:nt,default:"click"}},render:function(t){var e=this,n=this.$router,r=this.$route,o=n.resolve(this.to,r,this.append),i=o.location,c=o.route,s=o.href,u={},f=n.options.linkActiveClass,l=n.options.linkExactActiveClass,p=null==f?"router-link-active":f,h=null==l?"router-link-exact-active":l,d=null==this.activeClass?p:this.activeClass,v=null==this.exactActiveClass?h:this.exactActiveClass,m=c.redirectedFrom?b(null,Z(c.redirectedFrom),null,n):c;u[v]=j(r,m),u[d]=this.exact?u[v]:k(r,m);var y=function(t){it(t)&&(e.replace?n.replace(i,rt):n.push(i,rt))},g={click:it};Array.isArray(this.event)?this.event.forEach(function(t){g[t]=y}):g[this.event]=y;var _={class:u},x=!this.$scopedSlots.$hasNormal&&this.$scopedSlots.default&&this.$scopedSlots.default({href:s,route:c,navigate:y,isActive:u[d],isExactActive:u[v]});if(x){if(1===x.length)return x[0];if(x.length>1||!x.length)return 0===x.length?t():t("span",{},x)}if("a"===this.tag)_.on=g,_.attrs={href:s};else{var w=at(this.$slots.default);if(w){w.isStatic=!1;var S=w.data=a({},w.data);S.on=g;var O=w.data.attrs=a({},w.data.attrs);O.href=s}else _.on=g}return t(this.tag,_,this.$slots.default)}};function it(t){if(!(t.metaKey||t.altKey||t.ctrlKey||t.shiftKey)&&!t.defaultPrevented&&(void 0===t.button||0===t.button)){if(t.currentTarget&&t.currentTarget.getAttribute){var e=t.currentTarget.getAttribute("target");if(/\b_blank\b/i.test(e))return}return t.preventDefault&&t.preventDefault(),!0}}function at(t){if(t)for(var e,n=0;n<t.length;n++){if(e=t[n],"a"===e.tag)return e;if(e.children&&(e=at(e.children)))return e}}function ct(t){if(!ct.installed||tt!==t){ct.installed=!0,tt=t;var e=function(t){return void 0!==t},n=function(t,n){var r=t.$options._parentVnode;e(r)&&e(r=r.data)&&e(r=r.registerRouteInstance)&&r(t,n)};t.mixin({beforeCreate:function(){e(this.$options.router)?(this._routerRoot=this,this._router=this.$options.router,this._router.init(this),t.util.defineReactive(this,"_route",this._router.history.current)):this._routerRoot=this.$parent&&this.$parent._routerRoot||this,n(this,this)},destroyed:function(){n(this)}}),Object.defineProperty(t.prototype,"$router",{get:function(){return this._routerRoot._router}}),Object.defineProperty(t.prototype,"$route",{get:function(){return this._routerRoot._route}}),t.component("RouterView",c),t.component("RouterLink",ot);var r=t.config.optionMergeStrategies;r.beforeRouteEnter=r.beforeRouteLeave=r.beforeRouteUpdate=r.created}}var st="undefined"!==typeof window;function ut(t,e,n,r){var o=e||[],i=n||Object.create(null),a=r||Object.create(null);t.forEach(function(t){ft(o,i,a,t)});for(var c=0,s=o.length;c<s;c++)"*"===o[c]&&(o.push(o.splice(c,1)[0]),s--,c--);return{pathList:o,pathMap:i,nameMap:a}}function ft(t,e,n,r,o,i){var a=r.path,c=r.name;var s=r.pathToRegexpOptions||{},u=pt(a,o,s.strict);"boolean"===typeof r.caseSensitive&&(s.sensitive=r.caseSensitive);var f={path:u,regex:lt(u,s),components:r.components||{default:r.component},instances:{},name:c,parent:o,matchAs:i,redirect:r.redirect,beforeEnter:r.beforeEnter,meta:r.meta||{},props:null==r.props?{}:r.components?r.props:{default:r.props}};if(r.children&&r.children.forEach(function(r){var o=i?C(i+"/"+r.path):void 0;ft(t,e,n,r,f,o)}),e[f.path]||(t.push(f.path),e[f.path]=f),void 0!==r.alias)for(var l=Array.isArray(r.alias)?r.alias:[r.alias],p=0;p<l.length;++p){var h=l[p];0;var d={path:h,children:r.children};ft(t,e,n,d,o,f.path||"/")}c&&(n[c]||(n[c]=f))}function lt(t,e){var n=T(t,[],e);return n}function pt(t,e,n){return n||(t=t.replace(/\/$/,"")),"/"===t[0]?t:null==e?t:C(e.path+"/"+t)}function ht(t,e){var n=ut(t),r=n.pathList,o=n.pathMap,i=n.nameMap;function a(t){ut(t,r,o,i)}function c(t,n,a){var c=Z(t,n,!1,e),s=c.name;if(s){var u=i[s];if(!u)return f(null,c);var l=u.regex.keys.filter(function(t){return!t.optional}).map(function(t){return t.name});if("object"!==typeof c.params&&(c.params={}),n&&"object"===typeof n.params)for(var p in n.params)!(p in c.params)&&l.indexOf(p)>-1&&(c.params[p]=n.params[p]);return c.path=Q(u.path,c.params,'named route "'+s+'"'),f(u,c,a)}if(c.path){c.params={};for(var h=0;h<r.length;h++){var d=r[h],v=o[d];if(dt(v.regex,c.path,c.params))return f(v,c,a)}}return f(null,c)}function s(t,n){var r=t.redirect,o="function"===typeof r?r(b(t,n,null,e)):r;if("string"===typeof o&&(o={path:o}),!o||"object"!==typeof o)return f(null,n);var a=o,s=a.name,u=a.path,l=n.query,p=n.hash,h=n.params;if(l=a.hasOwnProperty("query")?a.query:l,p=a.hasOwnProperty("hash")?a.hash:p,h=a.hasOwnProperty("params")?a.params:h,s){i[s];return c({_normalized:!0,name:s,query:l,hash:p,params:h},void 0,n)}if(u){var d=vt(u,t),v=Q(d,h,'redirect route with path "'+d+'"');return c({_normalized:!0,path:v,query:l,hash:p},void 0,n)}return f(null,n)}function u(t,e,n){var r=Q(n,e.params,'aliased route with path "'+n+'"'),o=c({_normalized:!0,path:r});if(o){var i=o.matched,a=i[i.length-1];return e.params=o.params,f(a,e)}return f(null,e)}function f(t,n,r){return t&&t.redirect?s(t,r||n):t&&t.matchAs?u(t,n,t.matchAs):b(t,n,r,e)}return{match:c,addRoutes:a}}function dt(t,e,n){var r=e.match(t);if(!r)return!1;if(!n)return!0;for(var o=1,i=r.length;o<i;++o){var a=t.keys[o-1],c="string"===typeof r[o]?decodeURIComponent(r[o]):r[o];a&&(n[a.name||"pathMatch"]=c)}return!0}function vt(t,e){return A(t,e.parent?e.parent.path:"/",!0)}var mt=Object.create(null);function yt(){var t=window.location.protocol+"//"+window.location.host,e=window.location.href.replace(t,"");window.history.replaceState({key:Ft()},"",e),window.addEventListener("popstate",function(t){gt(),t.state&&t.state.key&&It(t.state.key)})}function bt(t,e,n,r){if(t.app){var o=t.options.scrollBehavior;o&&t.app.$nextTick(function(){var i=_t(),a=o.call(t,e,n,r?i:null);a&&("function"===typeof a.then?a.then(function(t){At(t,i)}).catch(function(t){0}):At(a,i))})}}function gt(){var t=Ft();t&&(mt[t]={x:window.pageXOffset,y:window.pageYOffset})}function _t(){var t=Ft();if(t)return mt[t]}function xt(t,e){var n=document.documentElement,r=n.getBoundingClientRect(),o=t.getBoundingClientRect();return{x:o.left-r.left-e.x,y:o.top-r.top-e.y}}function wt(t){return kt(t.x)||kt(t.y)}function jt(t){return{x:kt(t.x)?t.x:window.pageXOffset,y:kt(t.y)?t.y:window.pageYOffset}}function St(t){return{x:kt(t.x)?t.x:0,y:kt(t.y)?t.y:0}}function kt(t){return"number"===typeof t}var Ot=/^#\d/;function At(t,e){var n="object"===typeof t;if(n&&"string"===typeof t.selector){var r=Ot.test(t.selector)?document.getElementById(t.selector.slice(1)):document.querySelector(t.selector);if(r){var o=t.offset&&"object"===typeof t.offset?t.offset:{};o=St(o),e=xt(r,o)}else wt(t)&&(e=jt(t))}else n&&wt(t)&&(e=jt(t));e&&window.scrollTo(e.x,e.y)}var $t=st&&function(){var t=window.navigator.userAgent;return(-1===t.indexOf("Android 2.")&&-1===t.indexOf("Android 4.0")||-1===t.indexOf("Mobile Safari")||-1!==t.indexOf("Chrome")||-1!==t.indexOf("Windows Phone"))&&(window.history&&"pushState"in window.history)}(),Ct=st&&window.performance&&window.performance.now?window.performance:Date,Et=Tt();function Tt(){return Ct.now().toFixed(3)}function Ft(){return Et}function It(t){Et=t}function Rt(t,e){gt();var n=window.history;try{e?n.replaceState({key:Et},"",t):(Et=Tt(),n.pushState({key:Et},"",t))}catch(r){window.location[e?"replace":"assign"](t)}}function Mt(t){Rt(t,!0)}function Pt(t,e,n){var r=function(o){o>=t.length?n():t[o]?e(t[o],function(){r(o+1)}):r(o+1)};r(0)}function Lt(t){return function(e,n,r){var i=!1,a=0,c=null;Nt(t,function(t,e,n,s){if("function"===typeof t&&void 0===t.cid){i=!0,a++;var u,f=Vt(function(e){Ut(e)&&(e=e.default),t.resolved="function"===typeof e?e:tt.extend(e),n.components[s]=e,a--,a<=0&&r()}),l=Vt(function(t){var e="Failed to resolve async component "+s+": "+t;c||(c=o(t)?t:new Error(e),r(c))});try{u=t(f,l)}catch(h){l(h)}if(u)if("function"===typeof u.then)u.then(f,l);else{var p=u.component;p&&"function"===typeof p.then&&p.then(f,l)}}}),i||r()}}function Nt(t,e){return Dt(t.map(function(t){return Object.keys(t.components).map(function(n){return e(t.components[n],t.instances[n],t,n)})}))}function Dt(t){return Array.prototype.concat.apply([],t)}var Wt="function"===typeof Symbol&&"symbol"===typeof Symbol.toStringTag;function Ut(t){return t.__esModule||Wt&&"Module"===t[Symbol.toStringTag]}function Vt(t){var e=!1;return function(){var n=[],r=arguments.length;while(r--)n[r]=arguments[r];if(!e)return e=!0,t.apply(this,n)}}var Bt=function(t){function e(){t.call(this,"Navigating to current location is not allowed"),this.name=this._name="NavigationDuplicated"}return t&&(e.__proto__=t),e.prototype=Object.create(t&&t.prototype),e.prototype.constructor=e,e}(Error);Bt._name="NavigationDuplicated";var zt=function(t,e){this.router=t,this.base=Ht(e),this.current=_,this.pending=null,this.ready=!1,this.readyCbs=[],this.readyErrorCbs=[],this.errorCbs=[]};function Ht(t){if(!t)if(st){var e=document.querySelector("base");t=e&&e.getAttribute("href")||"/",t=t.replace(/^https?:\/\/[^\/]+/,"")}else t="/";return"/"!==t.charAt(0)&&(t="/"+t),t.replace(/\/$/,"")}function qt(t,e){var n,r=Math.max(t.length,e.length);for(n=0;n<r;n++)if(t[n]!==e[n])break;return{updated:e.slice(0,n),activated:e.slice(n),deactivated:t.slice(n)}}function Gt(t,e,n,r){var o=Nt(t,function(t,r,o,i){var a=Yt(t,e);if(a)return Array.isArray(a)?a.map(function(t){return n(t,r,o,i)}):n(a,r,o,i)});return Dt(r?o.reverse():o)}function Yt(t,e){return"function"!==typeof t&&(t=tt.extend(t)),t.options[e]}function Jt(t){return Gt(t,"beforeRouteLeave",Xt,!0)}function Kt(t){return Gt(t,"beforeRouteUpdate",Xt)}function Xt(t,e){if(e)return function(){return t.apply(e,arguments)}}function Qt(t,e,n){return Gt(t,"beforeRouteEnter",function(t,r,o,i){return Zt(t,o,i,e,n)})}function Zt(t,e,n,r,o){return function(i,a,c){return t(i,a,function(t){"function"===typeof t&&r.push(function(){te(t,e.instances,n,o)}),c(t)})}}function te(t,e,n,r){e[n]&&!e[n]._isBeingDestroyed?t(e[n]):r()&&setTimeout(function(){te(t,e,n,r)},16)}zt.prototype.listen=function(t){this.cb=t},zt.prototype.onReady=function(t,e){this.ready?t():(this.readyCbs.push(t),e&&this.readyErrorCbs.push(e))},zt.prototype.onError=function(t){this.errorCbs.push(t)},zt.prototype.transitionTo=function(t,e,n){var r=this,o=this.router.match(t,this.current);this.confirmTransition(o,function(){r.updateRoute(o),e&&e(o),r.ensureURL(),r.ready||(r.ready=!0,r.readyCbs.forEach(function(t){t(o)}))},function(t){n&&n(t),t&&!r.ready&&(r.ready=!0,r.readyErrorCbs.forEach(function(e){e(t)}))})},zt.prototype.confirmTransition=function(t,e,n){var a=this,c=this.current,s=function(t){!i(Bt,t)&&o(t)&&(a.errorCbs.length?a.errorCbs.forEach(function(e){e(t)}):(r(!1,"uncaught error during route navigation:"),console.error(t))),n&&n(t)};if(j(t,c)&&t.matched.length===c.matched.length)return this.ensureURL(),s(new Bt(t));var u=qt(this.current.matched,t.matched),f=u.updated,l=u.deactivated,p=u.activated,h=[].concat(Jt(l),this.router.beforeHooks,Kt(f),p.map(function(t){return t.beforeEnter}),Lt(p));this.pending=t;var d=function(e,n){if(a.pending!==t)return s();try{e(t,c,function(t){!1===t||o(t)?(a.ensureURL(!0),s(t)):"string"===typeof t||"object"===typeof t&&("string"===typeof t.path||"string"===typeof t.name)?(s(),"object"===typeof t&&t.replace?a.replace(t):a.push(t)):n(t)})}catch(r){s(r)}};Pt(h,d,function(){var n=[],r=function(){return a.current===t},o=Qt(p,n,r),i=o.concat(a.router.resolveHooks);Pt(i,d,function(){if(a.pending!==t)return s();a.pending=null,e(t),a.router.app&&a.router.app.$nextTick(function(){n.forEach(function(t){t()})})})})},zt.prototype.updateRoute=function(t){var e=this.current;this.current=t,this.cb&&this.cb(t),this.router.afterHooks.forEach(function(n){n&&n(t,e)})};var ee=function(t){function e(e,n){var r=this;t.call(this,e,n);var o=e.options.scrollBehavior,i=$t&&o;i&&yt();var a=ne(this.base);window.addEventListener("popstate",function(t){var n=r.current,o=ne(r.base);r.current===_&&o===a||r.transitionTo(o,function(t){i&&bt(e,t,n,!0)})})}return t&&(e.__proto__=t),e.prototype=Object.create(t&&t.prototype),e.prototype.constructor=e,e.prototype.go=function(t){window.history.go(t)},e.prototype.push=function(t,e,n){var r=this,o=this,i=o.current;this.transitionTo(t,function(t){Rt(C(r.base+t.fullPath)),bt(r.router,t,i,!1),e&&e(t)},n)},e.prototype.replace=function(t,e,n){var r=this,o=this,i=o.current;this.transitionTo(t,function(t){Mt(C(r.base+t.fullPath)),bt(r.router,t,i,!1),e&&e(t)},n)},e.prototype.ensureURL=function(t){if(ne(this.base)!==this.current.fullPath){var e=C(this.base+this.current.fullPath);t?Rt(e):Mt(e)}},e.prototype.getCurrentLocation=function(){return ne(this.base)},e}(zt);function ne(t){var e=decodeURI(window.location.pathname);return t&&0===e.indexOf(t)&&(e=e.slice(t.length)),(e||"/")+window.location.search+window.location.hash}var re=function(t){function e(e,n,r){t.call(this,e,n),r&&oe(this.base)||ie()}return t&&(e.__proto__=t),e.prototype=Object.create(t&&t.prototype),e.prototype.constructor=e,e.prototype.setupListeners=function(){var t=this,e=this.router,n=e.options.scrollBehavior,r=$t&&n;r&&yt(),window.addEventListener($t?"popstate":"hashchange",function(){var e=t.current;ie()&&t.transitionTo(ae(),function(n){r&&bt(t.router,n,e,!0),$t||ue(n.fullPath)})})},e.prototype.push=function(t,e,n){var r=this,o=this,i=o.current;this.transitionTo(t,function(t){se(t.fullPath),bt(r.router,t,i,!1),e&&e(t)},n)},e.prototype.replace=function(t,e,n){var r=this,o=this,i=o.current;this.transitionTo(t,function(t){ue(t.fullPath),bt(r.router,t,i,!1),e&&e(t)},n)},e.prototype.go=function(t){window.history.go(t)},e.prototype.ensureURL=function(t){var e=this.current.fullPath;ae()!==e&&(t?se(e):ue(e))},e.prototype.getCurrentLocation=function(){return ae()},e}(zt);function oe(t){var e=ne(t);if(!/^\/#/.test(e))return window.location.replace(C(t+"/#"+e)),!0}function ie(){var t=ae();return"/"===t.charAt(0)||(ue("/"+t),!1)}function ae(){var t=window.location.href,e=t.indexOf("#");if(e<0)return"";t=t.slice(e+1);var n=t.indexOf("?");if(n<0){var r=t.indexOf("#");t=r>-1?decodeURI(t.slice(0,r))+t.slice(r):decodeURI(t)}else n>-1&&(t=decodeURI(t.slice(0,n))+t.slice(n));return t}function ce(t){var e=window.location.href,n=e.indexOf("#"),r=n>=0?e.slice(0,n):e;return r+"#"+t}function se(t){$t?Rt(ce(t)):window.location.hash=t}function ue(t){$t?Mt(ce(t)):window.location.replace(ce(t))}var fe=function(t){function e(e,n){t.call(this,e,n),this.stack=[],this.index=-1}return t&&(e.__proto__=t),e.prototype=Object.create(t&&t.prototype),e.prototype.constructor=e,e.prototype.push=function(t,e,n){var r=this;this.transitionTo(t,function(t){r.stack=r.stack.slice(0,r.index+1).concat(t),r.index++,e&&e(t)},n)},e.prototype.replace=function(t,e,n){var r=this;this.transitionTo(t,function(t){r.stack=r.stack.slice(0,r.index).concat(t),e&&e(t)},n)},e.prototype.go=function(t){var e=this,n=this.index+t;if(!(n<0||n>=this.stack.length)){var r=this.stack[n];this.confirmTransition(r,function(){e.index=n,e.updateRoute(r)},function(t){i(Bt,t)&&(e.index=n)})}},e.prototype.getCurrentLocation=function(){var t=this.stack[this.stack.length-1];return t?t.fullPath:"/"},e.prototype.ensureURL=function(){},e}(zt),le=function(t){void 0===t&&(t={}),this.app=null,this.apps=[],this.options=t,this.beforeHooks=[],this.resolveHooks=[],this.afterHooks=[],this.matcher=ht(t.routes||[],this);var e=t.mode||"hash";switch(this.fallback="history"===e&&!$t&&!1!==t.fallback,this.fallback&&(e="hash"),st||(e="abstract"),this.mode=e,e){case"history":this.history=new ee(this,t.base);break;case"hash":this.history=new re(this,t.base,this.fallback);break;case"abstract":this.history=new fe(this,t.base);break;default:0}},pe={currentRoute:{configurable:!0}};function he(t,e){return t.push(e),function(){var n=t.indexOf(e);n>-1&&t.splice(n,1)}}function de(t,e,n){var r="hash"===n?"#"+e:e;return t?C(t+"/"+r):r}le.prototype.match=function(t,e,n){return this.matcher.match(t,e,n)},pe.currentRoute.get=function(){return this.history&&this.history.current},le.prototype.init=function(t){var e=this;if(this.apps.push(t),t.$once("hook:destroyed",function(){var n=e.apps.indexOf(t);n>-1&&e.apps.splice(n,1),e.app===t&&(e.app=e.apps[0]||null)}),!this.app){this.app=t;var n=this.history;if(n instanceof ee)n.transitionTo(n.getCurrentLocation());else if(n instanceof re){var r=function(){n.setupListeners()};n.transitionTo(n.getCurrentLocation(),r,r)}n.listen(function(t){e.apps.forEach(function(e){e._route=t})})}},le.prototype.beforeEach=function(t){return he(this.beforeHooks,t)},le.prototype.beforeResolve=function(t){return he(this.resolveHooks,t)},le.prototype.afterEach=function(t){return he(this.afterHooks,t)},le.prototype.onReady=function(t,e){this.history.onReady(t,e)},le.prototype.onError=function(t){this.history.onError(t)},le.prototype.push=function(t,e,n){var r=this;if(!e&&!n&&"undefined"!==typeof Promise)return new Promise(function(e,n){r.history.push(t,e,n)});this.history.push(t,e,n)},le.prototype.replace=function(t,e,n){var r=this;if(!e&&!n&&"undefined"!==typeof Promise)return new Promise(function(e,n){r.history.replace(t,e,n)});this.history.replace(t,e,n)},le.prototype.go=function(t){this.history.go(t)},le.prototype.back=function(){this.go(-1)},le.prototype.forward=function(){this.go(1)},le.prototype.getMatchedComponents=function(t){var e=t?t.matched?t:this.resolve(t).route:this.currentRoute;return e?[].concat.apply([],e.matched.map(function(t){return Object.keys(t.components).map(function(e){return t.components[e]})})):[]},le.prototype.resolve=function(t,e,n){e=e||this.history.current;var r=Z(t,e,n,this),o=this.match(r,e),i=o.redirectedFrom||o.fullPath,a=this.history.base,c=de(a,i,this.mode);return{location:r,route:o,href:c,normalizedTo:r,resolved:o}},le.prototype.addRoutes=function(t){this.matcher.addRoutes(t),this.history.current!==_&&this.history.transitionTo(this.history.getCurrentLocation())},Object.defineProperties(le.prototype,pe),le.install=ct,le.version="3.1.2",st&&window.Vue&&window.Vue.use(le),e["a"]=le},"8eeb":function(t,e,n){var r=n("32b3"),o=n("872a");function i(t,e,n,i){var a=!n;n||(n={});var c=-1,s=e.length;while(++c<s){var u=e[c],f=i?i(n[u],t[u],u,n,t):void 0;void 0===f&&(f=t[u]),a?o(n,u,f):r(n,u,f)}return n}t.exports=i},9093:function(t,e,n){var r=n("ce10"),o=n("e11e").concat("length","prototype");e.f=Object.getOwnPropertyNames||function(t){return r(t,o)}},"91e9":function(t,e){function n(t,e){return function(n){return t(e(n))}}t.exports=n},"93ed":function(t,e,n){var r=n("4245");function o(t){var e=r(this,t)["delete"](t);return this.size-=e?1:0,e}t.exports=o},9520:function(t,e,n){var r=n("3729"),o=n("1a8c"),i="[object AsyncFunction]",a="[object Function]",c="[object GeneratorFunction]",s="[object Proxy]";function u(t){if(!o(t))return!1;var e=r(t);return e==a||e==c||e==i||e==s}t.exports=u},9638:function(t,e){function n(t,e){return t===e||t!==t&&e!==e}t.exports=n},9934:function(t,e,n){var r=n("6fcd"),o=n("41c3"),i=n("30c9");function a(t){return i(t)?r(t,!0):o(t)}t.exports=a},"99d3":function(t,e,n){(function(t){var r=n("585a"),o=e&&!e.nodeType&&e,i=o&&"object"==typeof t&&t&&!t.nodeType&&t,a=i&&i.exports===o,c=a&&r.process,s=function(){try{var t=i&&i.require&&i.require("util").types;return t||c&&c.binding&&c.binding("util")}catch(e){}}();t.exports=s}).call(this,n("62e4")(t))},"9aba":function(t,e){Object.defineProperty(e,"__esModule",{value:!0});var n={inserted:function(t,e,n){var r=n.context.$refs[e.arg];r.addRef({el:t,vnode:n}),r.$contextmenuId=t.id||r._uid}};function r(t,e,n){return e in t?Object.defineProperty(t,e,{value:n,enumerable:!0,configurable:!0,writable:!0}):t[e]=n,t}var o={name:"VContextmenu",provide:function(){return{$$contextmenu:this}},props:{eventType:{type:String,default:"contextmenu"},theme:{type:String,default:"default"},autoPlacement:{type:Boolean,default:!0},disabled:Boolean,containerSelector:{type:String,default:"body"}},data:function(){return{visible:!1,references:[],style:{top:0,left:0}}},computed:{clickOutsideHandler:function(){return this.visible?this.hide:function(){}},isClick:function(){return"click"===this.eventType},contextmenuCls:function(){return["v-contextmenu","v-contextmenu--"+this.theme]}},watch:{visible:function(t){var e=document.querySelector(this.containerSelector);t?(this.$emit("show",this),e.addEventListener("click",this.handleBodyClick)):(this.$emit("hide",this),e.removeEventListener("click",this.handleBodyClick))}},mounted:function(){document.querySelector(this.containerSelector).appendChild(this.$el),window.$$VContextmenu?window.$$VContextmenu[this.$contextmenuId]=this:window.$$VContextmenu=r({},this.$contextmenuId,this)},beforeDestroy:function(){var t=this,e=document.querySelector(this.containerSelector);e.removeChild(this.$el),delete window.$$VContextmenu[this.$contextmenuId],this.references.forEach(function(e){e.el.removeEventListener(t.eventType,t.handleReferenceContextmenu)}),e.removeEventListener("click",this.handleBodyClick)},methods:{addRef:function(t){this.references.push(t),t.el.addEventListener(this.eventType,this.handleReferenceContextmenu)},handleReferenceContextmenu:function(t){var e=this;if(t.preventDefault(),!this.disabled){var n=this.references.find(function(e){return e.el.contains(t.target)});this.$emit("contextmenu",n?n.vnode:null);var r=t.pageX,o=t.pageY;this.show(),this.$nextTick(function(){var t={top:o,left:r};if(e.autoPlacement){var n=e.$refs.contextmenu.clientWidth,i=e.$refs.contextmenu.clientHeight;i+o>=window.innerHeight&&(t.top-=i),n+r>=window.innerWidth&&(t.left-=n)}e.style={top:t.top+"px",left:t.left+"px"}})}},handleBodyClick:function(t){this.$el.contains(t.target)||this.isClick&&this.references.some(function(e){return e.el.contains(t.target)})||(this.visible=!1)},show:function(t){var e=this;Object.keys(window.$$VContextmenu).forEach(function(t){t!==e.$contextmenuId&&window.$$VContextmenu[t].hide()}),t&&(this.style={top:t.top+"px",left:t.left+"px"}),this.visible=!0},hide:function(){this.visible=!1},hideAll:function(){Object.keys(window.$$VContextmenu).forEach(function(t){window.$$VContextmenu[t].hide()})}}},i=o,a=function(){var t=this.$createElement;return(this._self._c||t)("ul",{directives:[{name:"show",rawName:"v-show",value:this.visible,expression:"visible"}],ref:"contextmenu",class:this.contextmenuCls,style:this.style},[this._t("default")],2)},c=[];a._withStripped=!0;var s=void 0!==a?{render:a,staticRenderFns:c}:{},u=void 0,f=void 0,l=void 0,p=!1;function h(t,e,n,r,o,i,a,c){var s=n||{};return s.__file="/Users/Stephen/Repos/snokier/v-contextmenu/src/components/Contextmenu.vue",s.render||(s.render=t.render,s.staticRenderFns=t.staticRenderFns,s._compiled=!0,o&&(s.functional=!0)),s._scopeId=r,s}function d(){var t=document.head||document.getElementsByTagName("head")[0],e=d.styles||(d.styles={}),n="undefined"!=typeof navigator&&/msie [6-9]\\b/.test(navigator.userAgent.toLowerCase());return function(r,o){if(!document.querySelector('style[data-vue-ssr-id~="'+r+'"]')){var i=n?o.media||"default":r,a=e[i]||(e[i]={ids:[],parts:[],element:void 0});if(!a.ids.includes(r)){var c=o.source,s=a.ids.length;if(a.ids.push(r),n&&(a.element=a.element||document.querySelector("style[data-group="+i+"]")),!a.element){var u=a.element=document.createElement("style");u.type="text/css",o.media&&u.setAttribute("media",o.media),n&&(u.setAttribute("data-group",i),u.setAttribute("data-next-index","0")),t.appendChild(u)}if(n&&(s=parseInt(a.element.getAttribute("data-next-index")),a.element.setAttribute("data-next-index",s+1)),a.element.styleSheet)a.parts.push(c),a.element.styleSheet.cssText=a.parts.filter(Boolean).join("\n");else{var f=document.createTextNode(c),l=a.element.childNodes;l[s]&&a.element.removeChild(l[s]),l.length?a.element.insertBefore(f,l[s]):a.element.appendChild(f)}}}}}var v=h(s,u,void 0===i?{}:i,f,p,l,void 0!==d?d:function(){},"undefined"!=typeof __vue_create_injector_ssr__?__vue_create_injector_ssr__:function(){}),m={name:"VContextmenuItem",inject:["$$contextmenu"],props:{divider:Boolean,disabled:Boolean,autoHide:{type:Boolean,default:!0}},data:function(){return{hover:!1}},computed:{classname:function(){return{"v-contextmenu-item":!this.divider,"v-contextmenu-item--hover":this.hover,"v-contextmenu-item--disabled":this.disabled}}},methods:{handleMouseenter:function(t){this.disabled||(this.hover=!0,this.$emit("mouseenter",this,t))},handleMouseleave:function(t){this.disabled||(this.hover=!1,this.$emit("mouseleave",this,t))},handleClick:function(t){this.disabled||(this.$emit("click",this,t),this.autoHide&&this.$$contextmenu.hide())}}},y=m,b=function(){var t=this.$createElement,e=this._self._c||t;return this.divider?e("li",{staticClass:"v-contextmenu-divider"}):e("li",{class:this.classname,on:{click:this.handleClick,mouseenter:this.handleMouseenter,mouseleave:this.handleMouseleave}},[this._t("default")],2)},g=[];b._withStripped=!0;var _=void 0!==b?{render:b,staticRenderFns:g}:{},x=void 0,w=void 0,j=void 0,S=!1;function k(t,e,n,r,o,i,a,c){var s=n||{};return s.__file="/Users/Stephen/Repos/snokier/v-contextmenu/src/components/ContextmenuItem.vue",s.render||(s.render=t.render,s.staticRenderFns=t.staticRenderFns,s._compiled=!0,o&&(s.functional=!0)),s._scopeId=r,s}function O(){var t=document.head||document.getElementsByTagName("head")[0],e=O.styles||(O.styles={}),n="undefined"!=typeof navigator&&/msie [6-9]\\b/.test(navigator.userAgent.toLowerCase());return function(r,o){if(!document.querySelector('style[data-vue-ssr-id~="'+r+'"]')){var i=n?o.media||"default":r,a=e[i]||(e[i]={ids:[],parts:[],element:void 0});if(!a.ids.includes(r)){var c=o.source,s=a.ids.length;if(a.ids.push(r),n&&(a.element=a.element||document.querySelector("style[data-group="+i+"]")),!a.element){var u=a.element=document.createElement("style");u.type="text/css",o.media&&u.setAttribute("media",o.media),n&&(u.setAttribute("data-group",i),u.setAttribute("data-next-index","0")),t.appendChild(u)}if(n&&(s=parseInt(a.element.getAttribute("data-next-index")),a.element.setAttribute("data-next-index",s+1)),a.element.styleSheet)a.parts.push(c),a.element.styleSheet.cssText=a.parts.filter(Boolean).join("\n");else{var f=document.createTextNode(c),l=a.element.childNodes;l[s]&&a.element.removeChild(l[s]),l.length?a.element.insertBefore(f,l[s]):a.element.appendChild(f)}}}}}var A=k(_,x,void 0===y?{}:y,w,S,j,void 0!==O?O:function(){},"undefined"!=typeof __vue_create_injector_ssr__?__vue_create_injector_ssr__:function(){});function $(t){if(Array.isArray(t)){for(var e=0,n=Array(t.length);e<t.length;e++)n[e]=t[e];return n}return Array.from(t)}var C={name:"VContextmenuSubmenu",props:{title:String,disabled:Boolean},data:function(){return{hover:!1,submenuPlacement:[]}},computed:{classname:function(){return{"v-contextmenu-item":!0,"v-contextmenu-submenu":!0,"v-contextmenu-item--hover":this.hover,"v-contextmenu-item--disabled":this.disabled}},submenuCls:function(){return["v-contextmenu"].concat($(this.submenuPlacement))}},methods:{handleMouseenter:function(t){var e=this;if(!this.disabled){var n=t.target.getBoundingClientRect();this.hover=!0,this.$emit("mouseenter",this,t),this.$nextTick(function(){var t=e.$refs.submenu.clientWidth,r=e.$refs.submenu.clientHeight,o=[];n.right+t>=window.innerWidth?o.push("left"):o.push("right"),n.bottom+r>=window.innerHeight?o.push("bottom"):o.push("top"),e.submenuPlacement=o})}},handleMouseleave:function(t){this.disabled||(this.hover=!1,this.$emit("mouseleave",this,t))}}},E=C,T=function(){var t=this,e=t.$createElement,n=t._self._c||e;return n("li",{class:t.classname,on:{mouseenter:t.handleMouseenter,mouseleave:t.handleMouseleave}},[n("span",{staticClass:"v-contextmenu-submenu__title"},[t._t("title",[t._v(t._s(t.title))]),t._v(" "),n("span",{staticClass:"v-contextmenu-iconfont v-contextmenu-submenu__icon"})],2),t._v(" "),n("ul",{directives:[{name:"show",rawName:"v-show",value:t.hover,expression:"hover"}],ref:"submenu",class:t.submenuCls},[t._t("default")],2)])},F=[];T._withStripped=!0;var I=void 0!==T?{render:T,staticRenderFns:F}:{},R=void 0,M=void 0,P=void 0,L=!1;function N(t,e,n,r,o,i,a,c){var s=n||{};return s.__file="/Users/Stephen/Repos/snokier/v-contextmenu/src/components/ContextmenuSubmenu.vue",s.render||(s.render=t.render,s.staticRenderFns=t.staticRenderFns,s._compiled=!0,o&&(s.functional=!0)),s._scopeId=r,s}function D(){var t=document.head||document.getElementsByTagName("head")[0],e=D.styles||(D.styles={}),n="undefined"!=typeof navigator&&/msie [6-9]\\b/.test(navigator.userAgent.toLowerCase());return function(r,o){if(!document.querySelector('style[data-vue-ssr-id~="'+r+'"]')){var i=n?o.media||"default":r,a=e[i]||(e[i]={ids:[],parts:[],element:void 0});if(!a.ids.includes(r)){var c=o.source,s=a.ids.length;if(a.ids.push(r),n&&(a.element=a.element||document.querySelector("style[data-group="+i+"]")),!a.element){var u=a.element=document.createElement("style");u.type="text/css",o.media&&u.setAttribute("media",o.media),n&&(u.setAttribute("data-group",i),u.setAttribute("data-next-index","0")),t.appendChild(u)}if(n&&(s=parseInt(a.element.getAttribute("data-next-index")),a.element.setAttribute("data-next-index",s+1)),a.element.styleSheet)a.parts.push(c),a.element.styleSheet.cssText=a.parts.filter(Boolean).join("\n");else{var f=document.createTextNode(c),l=a.element.childNodes;l[s]&&a.element.removeChild(l[s]),l.length?a.element.insertBefore(f,l[s]):a.element.appendChild(f)}}}}}var W=N(I,R,void 0===E?{}:E,M,L,P,void 0!==D?D:function(){},"undefined"!=typeof __vue_create_injector_ssr__?__vue_create_injector_ssr__:function(){}),U={name:"VContextmenuGroup",props:{maxWidth:[Number,String]},computed:{menusStyle:function(){return this.maxWidth?{"max-width":"number"==typeof this.maxWidth?this.maxWidth+"px":this.maxWidth,"overflow-x":"auto"}:null}}},V=U,B=function(){var t=this.$createElement,e=this._self._c||t;return e("li",{staticClass:"v-contextmenu-group"},[e("ul",{staticClass:"v-contextmenu-group__menus",style:this.menusStyle},[this._t("default")],2)])},z=[];B._withStripped=!0;var H=void 0!==B?{render:B,staticRenderFns:z}:{},q=void 0,G=void 0,Y=void 0,J=!1;function K(t,e,n,r,o,i,a,c){var s=n||{};return s.__file="/Users/Stephen/Repos/snokier/v-contextmenu/src/components/ContextmenuGroup.vue",s.render||(s.render=t.render,s.staticRenderFns=t.staticRenderFns,s._compiled=!0,o&&(s.functional=!0)),s._scopeId=r,s}function X(){var t=document.head||document.getElementsByTagName("head")[0],e=X.styles||(X.styles={}),n="undefined"!=typeof navigator&&/msie [6-9]\\b/.test(navigator.userAgent.toLowerCase());return function(r,o){if(!document.querySelector('style[data-vue-ssr-id~="'+r+'"]')){var i=n?o.media||"default":r,a=e[i]||(e[i]={ids:[],parts:[],element:void 0});if(!a.ids.includes(r)){var c=o.source,s=a.ids.length;if(a.ids.push(r),n&&(a.element=a.element||document.querySelector("style[data-group="+i+"]")),!a.element){var u=a.element=document.createElement("style");u.type="text/css",o.media&&u.setAttribute("media",o.media),n&&(u.setAttribute("data-group",i),u.setAttribute("data-next-index","0")),t.appendChild(u)}if(n&&(s=parseInt(a.element.getAttribute("data-next-index")),a.element.setAttribute("data-next-index",s+1)),a.element.styleSheet)a.parts.push(c),a.element.styleSheet.cssText=a.parts.filter(Boolean).join("\n");else{var f=document.createTextNode(c),l=a.element.childNodes;l[s]&&a.element.removeChild(l[s]),l.length?a.element.insertBefore(f,l[s]):a.element.appendChild(f)}}}}}var Q=K(H,q,void 0===V?{}:V,G,J,Y,void 0!==X?X:function(){},"undefined"!=typeof __vue_create_injector_ssr__?__vue_create_injector_ssr__:function(){}),Z=function(t){t.directive("contextmenu",n),t.component(v.name,v),t.component(A.name,A),t.component(W.name,W),t.component(Q.name,Q)};"undefined"!=typeof window&&window.Vue&&Z(window.Vue);var tt={install:Z};e.directive=n,e.Contextmenu=v,e.ContextmenuItem=A,e.ContextmenuSubmenu=W,e.ContextmenuGroup=Q,e.default=tt},"9b43":function(t,e,n){var r=n("d8e8");t.exports=function(t,e,n){if(r(t),void 0===e)return t;switch(n){case 1:return function(n){return t.call(e,n)};case 2:return function(n,r){return t.call(e,n,r)};case 3:return function(n,r,o){return t.call(e,n,r,o)}}return function(){return t.apply(e,arguments)}}},"9c6c":function(t,e,n){var r=n("2b4c")("unscopables"),o=Array.prototype;void 0==o[r]&&n("32e9")(o,r,{}),t.exports=function(t){o[r][t]=!0}},"9c80":function(t,e){t.exports=function(t){try{return{e:!1,v:t()}}catch(e){return{e:!0,v:e}}}},"9def":function(t,e,n){var r=n("4588"),o=Math.min;t.exports=function(t){return t>0?o(r(t),9007199254740991):0}},"9e1e":function(t,e,n){t.exports=!n("79e5")(function(){return 7!=Object.defineProperty({},"a",{get:function(){return 7}}).a})},"9e69":function(t,e,n){var r=n("2b3e"),o=r.Symbol;t.exports=o},a029:function(t,e,n){var r=n("087d"),o=n("2dcb"),i=n("32f4"),a=n("d327"),c=Object.getOwnPropertySymbols,s=c?function(t){var e=[];while(t)r(e,i(t)),t=o(t);return e}:a;t.exports=s},a25f:function(t,e,n){var r=n("7726"),o=r.navigator;t.exports=o&&o.userAgent||""},a2db:function(t,e,n){var r=n("9e69"),o=r?r.prototype:void 0,i=o?o.valueOf:void 0;function a(t){return i?Object(i.call(t)):{}}t.exports=a},a481:function(t,e,n){"use strict";var r=n("cb7c"),o=n("4bf8"),i=n("9def"),a=n("4588"),c=n("0390"),s=n("5f1b"),u=Math.max,f=Math.min,l=Math.floor,p=/\$([$&`']|\d\d?|<[^>]*>)/g,h=/\$([$&`']|\d\d?)/g,d=function(t){return void 0===t?t:String(t)};n("214f")("replace",2,function(t,e,n,v){return[function(r,o){var i=t(this),a=void 0==r?void 0:r[e];return void 0!==a?a.call(r,i,o):n.call(String(i),r,o)},function(t,e){var o=v(n,t,this,e);if(o.done)return o.value;var l=r(t),p=String(this),h="function"===typeof e;h||(e=String(e));var y=l.global;if(y){var b=l.unicode;l.lastIndex=0}var g=[];while(1){var _=s(l,p);if(null===_)break;if(g.push(_),!y)break;var x=String(_[0]);""===x&&(l.lastIndex=c(p,i(l.lastIndex),b))}for(var w="",j=0,S=0;S<g.length;S++){_=g[S];for(var k=String(_[0]),O=u(f(a(_.index),p.length),0),A=[],$=1;$<_.length;$++)A.push(d(_[$]));var C=_.groups;if(h){var E=[k].concat(A,O,p);void 0!==C&&E.push(C);var T=String(e.apply(void 0,E))}else T=m(k,p,O,A,C,e);O>=j&&(w+=p.slice(j,O)+T,j=O+k.length)}return w+p.slice(j)}];function m(t,e,r,i,a,c){var s=r+t.length,u=i.length,f=h;return void 0!==a&&(a=o(a),f=p),n.call(c,f,function(n,o){var c;switch(o.charAt(0)){case"$":return"$";case"&":return t;case"`":return e.slice(0,r);case"'":return e.slice(s);case"<":c=a[o.slice(1,-1)];break;default:var f=+o;if(0===f)return n;if(f>u){var p=l(f/10);return 0===p?n:p<=u?void 0===i[p-1]?o.charAt(1):i[p-1]+o.charAt(1):n}c=i[f-1]}return void 0===c?"":c})}})},a524:function(t,e,n){var r=n("4245");function o(t){return r(this,t).has(t)}t.exports=o},a5b8:function(t,e,n){"use strict";var r=n("d8e8");function o(t){var e,n;this.promise=new t(function(t,r){if(void 0!==e||void 0!==n)throw TypeError("Bad Promise constructor");e=t,n=r}),this.resolve=r(e),this.reject=r(n)}t.exports.f=function(t){return new o(t)}},a925:function(t,e,n){"use strict";
/*!
* vue-i18n v8.13.0
* (c) 2019 kazuya kawaguchi
* Released under the MIT License.
*/var r=["style","currency","currencyDisplay","useGrouping","minimumIntegerDigits","minimumFractionDigits","maximumFractionDigits","minimumSignificantDigits","maximumSignificantDigits","localeMatcher","formatMatcher"];function o(t,e){"undefined"!==typeof console&&(console.warn("[vue-i18n] "+t),e&&console.warn(e.stack))}function i(t,e){"undefined"!==typeof console&&(console.error("[vue-i18n] "+t),e&&console.error(e.stack))}function a(t){return null!==t&&"object"===typeof t}var c=Object.prototype.toString,s="[object Object]";function u(t){return c.call(t)===s}function f(t){return null===t||void 0===t}function l(){var t=[],e=arguments.length;while(e--)t[e]=arguments[e];var n=null,r=null;return 1===t.length?a(t[0])||Array.isArray(t[0])?r=t[0]:"string"===typeof t[0]&&(n=t[0]):2===t.length&&("string"===typeof t[0]&&(n=t[0]),(a(t[1])||Array.isArray(t[1]))&&(r=t[1])),{locale:n,params:r}}function p(t){return JSON.parse(JSON.stringify(t))}function h(t,e){if(t.length){var n=t.indexOf(e);if(n>-1)return t.splice(n,1)}}var d=Object.prototype.hasOwnProperty;function v(t,e){return d.call(t,e)}function m(t){for(var e=arguments,n=Object(t),r=1;r<arguments.length;r++){var o=e[r];if(void 0!==o&&null!==o){var i=void 0;for(i in o)v(o,i)&&(a(o[i])?n[i]=m(n[i],o[i]):n[i]=o[i])}}return n}function y(t,e){if(t===e)return!0;var n=a(t),r=a(e);if(!n||!r)return!n&&!r&&String(t)===String(e);try{var o=Array.isArray(t),i=Array.isArray(e);if(o&&i)return t.length===e.length&&t.every(function(t,n){return y(t,e[n])});if(o||i)return!1;var c=Object.keys(t),s=Object.keys(e);return c.length===s.length&&c.every(function(n){return y(t[n],e[n])})}catch(u){return!1}}function b(t){t.prototype.hasOwnProperty("$i18n")||Object.defineProperty(t.prototype,"$i18n",{get:function(){return this._i18n}}),t.prototype.$t=function(t){var e=[],n=arguments.length-1;while(n-- >0)e[n]=arguments[n+1];var r=this.$i18n;return r._t.apply(r,[t,r.locale,r._getMessages(),this].concat(e))},t.prototype.$tc=function(t,e){var n=[],r=arguments.length-2;while(r-- >0)n[r]=arguments[r+2];var o=this.$i18n;return o._tc.apply(o,[t,o.locale,o._getMessages(),this,e].concat(n))},t.prototype.$te=function(t,e){var n=this.$i18n;return n._te(t,n.locale,n._getMessages(),e)},t.prototype.$d=function(t){var e,n=[],r=arguments.length-1;while(r-- >0)n[r]=arguments[r+1];return(e=this.$i18n).d.apply(e,[t].concat(n))},t.prototype.$n=function(t){var e,n=[],r=arguments.length-1;while(r-- >0)n[r]=arguments[r+1];return(e=this.$i18n).n.apply(e,[t].concat(n))}}var g,_={beforeCreate:function(){var t=this.$options;if(t.i18n=t.i18n||(t.__i18n?{}:null),t.i18n)if(t.i18n instanceof lt){if(t.__i18n)try{var e={};t.__i18n.forEach(function(t){e=m(e,JSON.parse(t))}),Object.keys(e).forEach(function(n){t.i18n.mergeLocaleMessage(n,e[n])})}catch(i){0}this._i18n=t.i18n,this._i18nWatcher=this._i18n.watchI18nData()}else if(u(t.i18n)){if(this.$root&&this.$root.$i18n&&this.$root.$i18n instanceof lt&&(t.i18n.root=this.$root,t.i18n.formatter=this.$root.$i18n.formatter,t.i18n.fallbackLocale=this.$root.$i18n.fallbackLocale,t.i18n.silentTranslationWarn=this.$root.$i18n.silentTranslationWarn,t.i18n.silentFallbackWarn=this.$root.$i18n.silentFallbackWarn,t.i18n.pluralizationRules=this.$root.$i18n.pluralizationRules,t.i18n.preserveDirectiveContent=this.$root.$i18n.preserveDirectiveContent),t.__i18n)try{var n={};t.__i18n.forEach(function(t){n=m(n,JSON.parse(t))}),t.i18n.messages=n}catch(i){0}var r=t.i18n,o=r.sharedMessages;o&&u(o)&&(t.i18n.messages=m(t.i18n.messages,o)),this._i18n=new lt(t.i18n),this._i18nWatcher=this._i18n.watchI18nData(),(void 0===t.i18n.sync||t.i18n.sync)&&(this._localeWatcher=this.$i18n.watchLocale())}else 0;else this.$root&&this.$root.$i18n&&this.$root.$i18n instanceof lt?this._i18n=this.$root.$i18n:t.parent&&t.parent.$i18n&&t.parent.$i18n instanceof lt&&(this._i18n=t.parent.$i18n)},beforeMount:function(){var t=this.$options;t.i18n=t.i18n||(t.__i18n?{}:null),t.i18n?t.i18n instanceof lt?(this._i18n.subscribeDataChanging(this),this._subscribing=!0):u(t.i18n)&&(this._i18n.subscribeDataChanging(this),this._subscribing=!0):this.$root&&this.$root.$i18n&&this.$root.$i18n instanceof lt?(this._i18n.subscribeDataChanging(this),this._subscribing=!0):t.parent&&t.parent.$i18n&&t.parent.$i18n instanceof lt&&(this._i18n.subscribeDataChanging(this),this._subscribing=!0)},beforeDestroy:function(){if(this._i18n){var t=this;this.$nextTick(function(){t._subscribing&&(t._i18n.unsubscribeDataChanging(t),delete t._subscribing),t._i18nWatcher&&(t._i18nWatcher(),t._i18n.destroyVM(),delete t._i18nWatcher),t._localeWatcher&&(t._localeWatcher(),delete t._localeWatcher),t._i18n=null})}}},x={name:"i18n",functional:!0,props:{tag:{type:String,default:"span"},path:{type:String,required:!0},locale:{type:String},places:{type:[Array,Object]}},render:function(t,e){var n=e.props,r=e.data,o=e.children,i=e.parent,a=i.$i18n;if(o=(o||[]).filter(function(t){return t.tag||(t.text=t.text.trim())}),!a)return o;var c=n.path,s=n.locale,u={},f=n.places||{},l=(Array.isArray(f)?f.length:Object.keys(f).length,o.every(function(t){if(t.data&&t.data.attrs){var e=t.data.attrs.place;return"undefined"!==typeof e&&""!==e}}));return Array.isArray(f)?f.forEach(function(t,e){u[e]=t}):Object.keys(f).forEach(function(t){u[t]=f[t]}),o.forEach(function(t,e){var n=l?""+t.data.attrs.place:""+e;u[n]=t}),t(n.tag,r,a.i(c,s,u))}},w={name:"i18n-n",functional:!0,props:{tag:{type:String,default:"span"},value:{type:Number,required:!0},format:{type:[String,Object]},locale:{type:String}},render:function(t,e){var n=e.props,o=e.parent,i=e.data,c=o.$i18n;if(!c)return null;var s=null,u=null;"string"===typeof n.format?s=n.format:a(n.format)&&(n.format.key&&(s=n.format.key),u=Object.keys(n.format).reduce(function(t,e){var o;return r.includes(e)?Object.assign({},t,(o={},o[e]=n.format[e],o)):t},null));var f=n.locale||c.locale,l=c._ntp(n.value,f,s,u),p=l.map(function(t,e){var n,r=i.scopedSlots&&i.scopedSlots[t.type];return r?r((n={},n[t.type]=t.value,n.index=e,n.parts=l,n)):t.value});return t(n.tag,{attrs:i.attrs,class:i["class"],staticClass:i.staticClass},p)}};function j(t,e,n){O(t,n)&&$(t,e,n)}function S(t,e,n,r){if(O(t,n)){var o=n.context.$i18n;A(t,n)&&y(e.value,e.oldValue)&&y(t._localeMessage,o.getLocaleMessage(o.locale))||$(t,e,n)}}function k(t,e,n,r){var i=n.context;if(i){var a=n.context.$i18n||{};e.modifiers.preserve||a.preserveDirectiveContent||(t.textContent=""),t._vt=void 0,delete t["_vt"],t._locale=void 0,delete t["_locale"],t._localeMessage=void 0,delete t["_localeMessage"]}else o("Vue instance does not exists in VNode context")}function O(t,e){var n=e.context;return n?!!n.$i18n||(o("VueI18n instance does not exists in Vue instance"),!1):(o("Vue instance does not exists in VNode context"),!1)}function A(t,e){var n=e.context;return t._locale===n.$i18n.locale}function $(t,e,n){var r,i,a=e.value,c=C(a),s=c.path,u=c.locale,f=c.args,l=c.choice;if(s||u||f)if(s){var p=n.context;t._vt=t.textContent=l?(r=p.$i18n).tc.apply(r,[s,l].concat(E(u,f))):(i=p.$i18n).t.apply(i,[s].concat(E(u,f))),t._locale=p.$i18n.locale,t._localeMessage=p.$i18n.getLocaleMessage(p.$i18n.locale)}else o("`path` is required in v-t directive");else o("value type not supported")}function C(t){var e,n,r,o;return"string"===typeof t?e=t:u(t)&&(e=t.path,n=t.locale,r=t.args,o=t.choice),{path:e,locale:n,args:r,choice:o}}function E(t,e){var n=[];return t&&n.push(t),e&&(Array.isArray(e)||u(e))&&n.push(e),n}function T(t){T.installed=!0,g=t;g.version&&Number(g.version.split(".")[0]);b(g),g.mixin(_),g.directive("t",{bind:j,update:S,unbind:k}),g.component(x.name,x),g.component(w.name,w);var e=g.config.optionMergeStrategies;e.i18n=function(t,e){return void 0===e?t:e}}var F=function(){this._caches=Object.create(null)};F.prototype.interpolate=function(t,e){if(!e)return[t];var n=this._caches[t];return n||(n=M(t),this._caches[t]=n),P(n,e)};var I=/^(?:\d)+/,R=/^(?:\w)+/;function M(t){var e=[],n=0,r="";while(n<t.length){var o=t[n++];if("{"===o){r&&e.push({type:"text",value:r}),r="";var i="";o=t[n++];while(void 0!==o&&"}"!==o)i+=o,o=t[n++];var a="}"===o,c=I.test(i)?"list":a&&R.test(i)?"named":"unknown";e.push({value:i,type:c})}else"%"===o?"{"!==t[n]&&(r+=o):r+=o}return r&&e.push({type:"text",value:r}),e}function P(t,e){var n=[],r=0,o=Array.isArray(e)?"list":a(e)?"named":"unknown";if("unknown"===o)return n;while(r<t.length){var i=t[r];switch(i.type){case"text":n.push(i.value);break;case"list":n.push(e[parseInt(i.value,10)]);break;case"named":"named"===o&&n.push(e[i.value]);break;case"unknown":0;break}r++}return n}var L=0,N=1,D=2,W=3,U=0,V=1,B=2,z=3,H=4,q=5,G=6,Y=7,J=8,K=[];K[U]={ws:[U],ident:[z,L],"[":[H],eof:[Y]},K[V]={ws:[V],".":[B],"[":[H],eof:[Y]},K[B]={ws:[B],ident:[z,L],0:[z,L],number:[z,L]},K[z]={ident:[z,L],0:[z,L],number:[z,L],ws:[V,N],".":[B,N],"[":[H,N],eof:[Y,N]},K[H]={"'":[q,L],'"':[G,L],"[":[H,D],"]":[V,W],eof:J,else:[H,L]},K[q]={"'":[H,L],eof:J,else:[q,L]},K[G]={'"':[H,L],eof:J,else:[G,L]};var X=/^\s?(?:true|false|-?[\d.]+|'[^']*'|"[^"]*")\s?$/;function Q(t){return X.test(t)}function Z(t){var e=t.charCodeAt(0),n=t.charCodeAt(t.length-1);return e!==n||34!==e&&39!==e?t:t.slice(1,-1)}function tt(t){if(void 0===t||null===t)return"eof";var e=t.charCodeAt(0);switch(e){case 91:case 93:case 46:case 34:case 39:return t;case 95:case 36:case 45:return"ident";case 9:case 10:case 13:case 160:case 65279:case 8232:case 8233:return"ws"}return"ident"}function et(t){var e=t.trim();return("0"!==t.charAt(0)||!isNaN(t))&&(Q(e)?Z(e):"*"+e)}function nt(t){var e,n,r,o,i,a,c,s=[],u=-1,f=U,l=0,p=[];function h(){var e=t[u+1];if(f===q&&"'"===e||f===G&&'"'===e)return u++,r="\\"+e,p[L](),!0}p[N]=function(){void 0!==n&&(s.push(n),n=void 0)},p[L]=function(){void 0===n?n=r:n+=r},p[D]=function(){p[L](),l++},p[W]=function(){if(l>0)l--,f=H,p[L]();else{if(l=0,n=et(n),!1===n)return!1;p[N]()}};while(null!==f)if(u++,e=t[u],"\\"!==e||!h()){if(o=tt(e),c=K[f],i=c[o]||c["else"]||J,i===J)return;if(f=i[0],a=p[i[1]],a&&(r=i[2],r=void 0===r?e:r,!1===a()))return;if(f===Y)return s}}var rt=function(){this._cache=Object.create(null)};rt.prototype.parsePath=function(t){var e=this._cache[t];return e||(e=nt(t),e&&(this._cache[t]=e)),e||[]},rt.prototype.getPathValue=function(t,e){if(!a(t))return null;var n=this.parsePath(e);if(0===n.length)return null;var r=n.length,o=t,i=0;while(i<r){var c=o[n[i]];if(void 0===c)return null;o=c,i++}return o};var ot,it=/<\/?[\w\s="\/.':;#-\/]+>/,at=/(?:@(?:\.[a-z]+)?:(?:[\w\-_|.]+|\([\w\-_|.]+\)))/g,ct=/^@(?:\.([a-z]+))?:/,st=/[()]/g,ut={upper:function(t){return t.toLocaleUpperCase()},lower:function(t){return t.toLocaleLowerCase()}},ft=new F,lt=function(t){var e=this;void 0===t&&(t={}),!g&&"undefined"!==typeof window&&window.Vue&&T(window.Vue);var n=t.locale||"en-US",r=t.fallbackLocale||"en-US",o=t.messages||{},i=t.dateTimeFormats||{},a=t.numberFormats||{};this._vm=null,this._formatter=t.formatter||ft,this._missing=t.missing||null,this._root=t.root||null,this._sync=void 0===t.sync||!!t.sync,this._fallbackRoot=void 0===t.fallbackRoot||!!t.fallbackRoot,this._silentTranslationWarn=void 0!==t.silentTranslationWarn&&t.silentTranslationWarn,this._silentFallbackWarn=void 0!==t.silentFallbackWarn&&!!t.silentFallbackWarn,this._dateTimeFormatters={},this._numberFormatters={},this._path=new rt,this._dataListeners=[],this._preserveDirectiveContent=void 0!==t.preserveDirectiveContent&&!!t.preserveDirectiveContent,this.pluralizationRules=t.pluralizationRules||{},this._warnHtmlInMessage=t.warnHtmlInMessage||"off",this._exist=function(t,n){return!(!t||!n)&&(!f(e._path.getPathValue(t,n))||!!t[n])},"warn"!==this._warnHtmlInMessage&&"error"!==this._warnHtmlInMessage||Object.keys(o).forEach(function(t){e._checkLocaleMessage(t,e._warnHtmlInMessage,o[t])}),this._initVM({locale:n,fallbackLocale:r,messages:o,dateTimeFormats:i,numberFormats:a})},pt={vm:{configurable:!0},messages:{configurable:!0},dateTimeFormats:{configurable:!0},numberFormats:{configurable:!0},availableLocales:{configurable:!0},locale:{configurable:!0},fallbackLocale:{configurable:!0},missing:{configurable:!0},formatter:{configurable:!0},silentTranslationWarn:{configurable:!0},silentFallbackWarn:{configurable:!0},preserveDirectiveContent:{configurable:!0},warnHtmlInMessage:{configurable:!0}};lt.prototype._checkLocaleMessage=function(t,e,n){var r=[],a=function(t,e,n,r){if(u(n))Object.keys(n).forEach(function(o){var i=n[o];u(i)?(r.push(o),r.push("."),a(t,e,i,r),r.pop(),r.pop()):(r.push(o),a(t,e,i,r),r.pop())});else if(Array.isArray(n))n.forEach(function(n,o){u(n)?(r.push("["+o+"]"),r.push("."),a(t,e,n,r),r.pop(),r.pop()):(r.push("["+o+"]"),a(t,e,n,r),r.pop())});else if("string"===typeof n){var c=it.test(n);if(c){var s="Detected HTML in message '"+n+"' of keypath '"+r.join("")+"' at '"+e+"'. Consider component interpolation with '<i18n>' to avoid XSS. See https://bit.ly/2ZqJzkp";"warn"===t?o(s):"error"===t&&i(s)}}};a(e,t,n,r)},lt.prototype._initVM=function(t){var e=g.config.silent;g.config.silent=!0,this._vm=new g({data:t}),g.config.silent=e},lt.prototype.destroyVM=function(){this._vm.$destroy()},lt.prototype.subscribeDataChanging=function(t){this._dataListeners.push(t)},lt.prototype.unsubscribeDataChanging=function(t){h(this._dataListeners,t)},lt.prototype.watchI18nData=function(){var t=this;return this._vm.$watch("$data",function(){var e=t._dataListeners.length;while(e--)g.nextTick(function(){t._dataListeners[e]&&t._dataListeners[e].$forceUpdate()})},{deep:!0})},lt.prototype.watchLocale=function(){if(!this._sync||!this._root)return null;var t=this._vm;return this._root.$i18n.vm.$watch("locale",function(e){t.$set(t,"locale",e),t.$forceUpdate()},{immediate:!0})},pt.vm.get=function(){return this._vm},pt.messages.get=function(){return p(this._getMessages())},pt.dateTimeFormats.get=function(){return p(this._getDateTimeFormats())},pt.numberFormats.get=function(){return p(this._getNumberFormats())},pt.availableLocales.get=function(){return Object.keys(this.messages).sort()},pt.locale.get=function(){return this._vm.locale},pt.locale.set=function(t){this._vm.$set(this._vm,"locale",t)},pt.fallbackLocale.get=function(){return this._vm.fallbackLocale},pt.fallbackLocale.set=function(t){this._vm.$set(this._vm,"fallbackLocale",t)},pt.missing.get=function(){return this._missing},pt.missing.set=function(t){this._missing=t},pt.formatter.get=function(){return this._formatter},pt.formatter.set=function(t){this._formatter=t},pt.silentTranslationWarn.get=function(){return this._silentTranslationWarn},pt.silentTranslationWarn.set=function(t){this._silentTranslationWarn=t},pt.silentFallbackWarn.get=function(){return this._silentFallbackWarn},pt.silentFallbackWarn.set=function(t){this._silentFallbackWarn=t},pt.preserveDirectiveContent.get=function(){return this._preserveDirectiveContent},pt.preserveDirectiveContent.set=function(t){this._preserveDirectiveContent=t},pt.warnHtmlInMessage.get=function(){return this._warnHtmlInMessage},pt.warnHtmlInMessage.set=function(t){var e=this,n=this._warnHtmlInMessage;if(this._warnHtmlInMessage=t,n!==t&&("warn"===t||"error"===t)){var r=this._getMessages();Object.keys(r).forEach(function(t){e._checkLocaleMessage(t,e._warnHtmlInMessage,r[t])})}},lt.prototype._getMessages=function(){return this._vm.messages},lt.prototype._getDateTimeFormats=function(){return this._vm.dateTimeFormats},lt.prototype._getNumberFormats=function(){return this._vm.numberFormats},lt.prototype._warnDefault=function(t,e,n,r,o){if(!f(n))return n;if(this._missing){var i=this._missing.apply(null,[t,e,r,o]);if("string"===typeof i)return i}else 0;return e},lt.prototype._isFallbackRoot=function(t){return!t&&!f(this._root)&&this._fallbackRoot},lt.prototype._isSilentFallbackWarn=function(t){return this._silentFallbackWarn instanceof RegExp?this._silentFallbackWarn.test(t):this._silentFallbackWarn},lt.prototype._isSilentFallback=function(t,e){return this._isSilentFallbackWarn(e)&&(this._isFallbackRoot()||t!==this.fallbackLocale)},lt.prototype._isSilentTranslationWarn=function(t){return this._silentTranslationWarn instanceof RegExp?this._silentTranslationWarn.test(t):this._silentTranslationWarn},lt.prototype._interpolate=function(t,e,n,r,o,i,a){if(!e)return null;var c,s=this._path.getPathValue(e,n);if(Array.isArray(s)||u(s))return s;if(f(s)){if(!u(e))return null;if(c=e[n],"string"!==typeof c)return null}else{if("string"!==typeof s)return null;c=s}return(c.indexOf("@:")>=0||c.indexOf("@.")>=0)&&(c=this._link(t,e,c,r,"raw",i,a)),this._render(c,o,i,n)},lt.prototype._link=function(t,e,n,r,o,i,a){var c=n,s=c.match(at);for(var u in s)if(s.hasOwnProperty(u)){var f=s[u],l=f.match(ct),p=l[0],h=l[1],d=f.replace(p,"").replace(st,"");if(a.includes(d))return c;a.push(d);var v=this._interpolate(t,e,d,r,"raw"===o?"string":o,"raw"===o?void 0:i,a);if(this._isFallbackRoot(v)){if(!this._root)throw Error("unexpected error");var m=this._root.$i18n;v=m._translate(m._getMessages(),m.locale,m.fallbackLocale,d,r,o,i)}v=this._warnDefault(t,d,v,r,Array.isArray(i)?i:[i]),ut.hasOwnProperty(h)&&(v=ut[h](v)),a.pop(),c=v?c.replace(f,v):c}return c},lt.prototype._render=function(t,e,n,r){var o=this._formatter.interpolate(t,n,r);return o||(o=ft.interpolate(t,n,r)),"string"===e?o.join(""):o},lt.prototype._translate=function(t,e,n,r,o,i,a){var c=this._interpolate(e,t[e],r,o,i,a,[r]);return f(c)?(c=this._interpolate(n,t[n],r,o,i,a,[r]),f(c)?null:c):c},lt.prototype._t=function(t,e,n,r){var o,i=[],a=arguments.length-4;while(a-- >0)i[a]=arguments[a+4];if(!t)return"";var c=l.apply(void 0,i),s=c.locale||e,u=this._translate(n,s,this.fallbackLocale,t,r,"string",c.params);if(this._isFallbackRoot(u)){if(!this._root)throw Error("unexpected error");return(o=this._root).$t.apply(o,[t].concat(i))}return this._warnDefault(s,t,u,r,i)},lt.prototype.t=function(t){var e,n=[],r=arguments.length-1;while(r-- >0)n[r]=arguments[r+1];return(e=this)._t.apply(e,[t,this.locale,this._getMessages(),null].concat(n))},lt.prototype._i=function(t,e,n,r,o){var i=this._translate(n,e,this.fallbackLocale,t,r,"raw",o);if(this._isFallbackRoot(i)){if(!this._root)throw Error("unexpected error");return this._root.$i18n.i(t,e,o)}return this._warnDefault(e,t,i,r,[o])},lt.prototype.i=function(t,e,n){return t?("string"!==typeof e&&(e=this.locale),this._i(t,e,this._getMessages(),null,n)):""},lt.prototype._tc=function(t,e,n,r,o){var i,a=[],c=arguments.length-5;while(c-- >0)a[c]=arguments[c+5];if(!t)return"";void 0===o&&(o=1);var s={count:o,n:o},u=l.apply(void 0,a);return u.params=Object.assign(s,u.params),a=null===u.locale?[u.params]:[u.locale,u.params],this.fetchChoice((i=this)._t.apply(i,[t,e,n,r].concat(a)),o)},lt.prototype.fetchChoice=function(t,e){if(!t&&"string"!==typeof t)return null;var n=t.split("|");return e=this.getChoiceIndex(e,n.length),n[e]?n[e].trim():t},lt.prototype.getChoiceIndex=function(t,e){var n=function(t,e){return t=Math.abs(t),2===e?t?t>1?1:0:1:t?Math.min(t,2):0};return this.locale in this.pluralizationRules?this.pluralizationRules[this.locale].apply(this,[t,e]):n(t,e)},lt.prototype.tc=function(t,e){var n,r=[],o=arguments.length-2;while(o-- >0)r[o]=arguments[o+2];return(n=this)._tc.apply(n,[t,this.locale,this._getMessages(),null,e].concat(r))},lt.prototype._te=function(t,e,n){var r=[],o=arguments.length-3;while(o-- >0)r[o]=arguments[o+3];var i=l.apply(void 0,r).locale||e;return this._exist(n[i],t)},lt.prototype.te=function(t,e){return this._te(t,this.locale,this._getMessages(),e)},lt.prototype.getLocaleMessage=function(t){return p(this._vm.messages[t]||{})},lt.prototype.setLocaleMessage=function(t,e){("warn"!==this._warnHtmlInMessage&&"error"!==this._warnHtmlInMessage||(this._checkLocaleMessage(t,this._warnHtmlInMessage,e),"error"!==this._warnHtmlInMessage))&&this._vm.$set(this._vm.messages,t,e)},lt.prototype.mergeLocaleMessage=function(t,e){("warn"!==this._warnHtmlInMessage&&"error"!==this._warnHtmlInMessage||(this._checkLocaleMessage(t,this._warnHtmlInMessage,e),"error"!==this._warnHtmlInMessage))&&this._vm.$set(this._vm.messages,t,m(this._vm.messages[t]||{},e))},lt.prototype.getDateTimeFormat=function(t){return p(this._vm.dateTimeFormats[t]||{})},lt.prototype.setDateTimeFormat=function(t,e){this._vm.$set(this._vm.dateTimeFormats,t,e)},lt.prototype.mergeDateTimeFormat=function(t,e){this._vm.$set(this._vm.dateTimeFormats,t,m(this._vm.dateTimeFormats[t]||{},e))},lt.prototype._localizeDateTime=function(t,e,n,r,o){var i=e,a=r[i];if((f(a)||f(a[o]))&&(i=n,a=r[i]),f(a)||f(a[o]))return null;var c=a[o],s=i+"__"+o,u=this._dateTimeFormatters[s];return u||(u=this._dateTimeFormatters[s]=new Intl.DateTimeFormat(i,c)),u.format(t)},lt.prototype._d=function(t,e,n){if(!n)return new Intl.DateTimeFormat(e).format(t);var r=this._localizeDateTime(t,e,this.fallbackLocale,this._getDateTimeFormats(),n);if(this._isFallbackRoot(r)){if(!this._root)throw Error("unexpected error");return this._root.$i18n.d(t,n,e)}return r||""},lt.prototype.d=function(t){var e=[],n=arguments.length-1;while(n-- >0)e[n]=arguments[n+1];var r=this.locale,o=null;return 1===e.length?"string"===typeof e[0]?o=e[0]:a(e[0])&&(e[0].locale&&(r=e[0].locale),e[0].key&&(o=e[0].key)):2===e.length&&("string"===typeof e[0]&&(o=e[0]),"string"===typeof e[1]&&(r=e[1])),this._d(t,r,o)},lt.prototype.getNumberFormat=function(t){return p(this._vm.numberFormats[t]||{})},lt.prototype.setNumberFormat=function(t,e){this._vm.$set(this._vm.numberFormats,t,e)},lt.prototype.mergeNumberFormat=function(t,e){this._vm.$set(this._vm.numberFormats,t,m(this._vm.numberFormats[t]||{},e))},lt.prototype._getNumberFormatter=function(t,e,n,r,o,i){var a=e,c=r[a];if((f(c)||f(c[o]))&&(a=n,c=r[a]),f(c)||f(c[o]))return null;var s,u=c[o];if(i)s=new Intl.NumberFormat(a,Object.assign({},u,i));else{var l=a+"__"+o;s=this._numberFormatters[l],s||(s=this._numberFormatters[l]=new Intl.NumberFormat(a,u))}return s},lt.prototype._n=function(t,e,n,r){if(!lt.availabilities.numberFormat)return"";if(!n){var o=r?new Intl.NumberFormat(e,r):new Intl.NumberFormat(e);return o.format(t)}var i=this._getNumberFormatter(t,e,this.fallbackLocale,this._getNumberFormats(),n,r),a=i&&i.format(t);if(this._isFallbackRoot(a)){if(!this._root)throw Error("unexpected error");return this._root.$i18n.n(t,Object.assign({},{key:n,locale:e},r))}return a||""},lt.prototype.n=function(t){var e=[],n=arguments.length-1;while(n-- >0)e[n]=arguments[n+1];var o=this.locale,i=null,c=null;return 1===e.length?"string"===typeof e[0]?i=e[0]:a(e[0])&&(e[0].locale&&(o=e[0].locale),e[0].key&&(i=e[0].key),c=Object.keys(e[0]).reduce(function(t,n){var o;return r.includes(n)?Object.assign({},t,(o={},o[n]=e[0][n],o)):t},null)):2===e.length&&("string"===typeof e[0]&&(i=e[0]),"string"===typeof e[1]&&(o=e[1])),this._n(t,o,i,c)},lt.prototype._ntp=function(t,e,n,r){if(!lt.availabilities.numberFormat)return[];if(!n){var o=r?new Intl.NumberFormat(e,r):new Intl.NumberFormat(e);return o.formatToParts(t)}var i=this._getNumberFormatter(t,e,this.fallbackLocale,this._getNumberFormats(),n,r),a=i&&i.formatToParts(t);if(this._isFallbackRoot(a)){if(!this._root)throw Error("unexpected error");return this._root.$i18n._ntp(t,e,n,r)}return a||[]},Object.defineProperties(lt.prototype,pt),Object.defineProperty(lt,"availabilities",{get:function(){if(!ot){var t="undefined"!==typeof Intl;ot={dateTimeFormat:t&&"undefined"!==typeof Intl.DateTimeFormat,numberFormat:t&&"undefined"!==typeof Intl.NumberFormat}}return ot}}),lt.install=T,lt.version="8.13.0",e["a"]=lt},a994:function(t,e,n){var r=n("7d1f"),o=n("32f4"),i=n("ec69");function a(t){return r(t,i,o)}t.exports=a},aae3:function(t,e,n){var r=n("d3f4"),o=n("2d95"),i=n("2b4c")("match");t.exports=function(t){var e;return r(t)&&(void 0!==(e=t[i])?!!e:"RegExp"==o(t))}},ac6a:function(t,e,n){for(var r=n("cadf"),o=n("0d58"),i=n("2aba"),a=n("7726"),c=n("32e9"),s=n("84f2"),u=n("2b4c"),f=u("iterator"),l=u("toStringTag"),p=s.Array,h={CSSRuleList:!0,CSSStyleDeclaration:!1,CSSValueList:!1,ClientRectList:!1,DOMRectList:!1,DOMStringList:!1,DOMTokenList:!0,DataTransferItemList:!1,FileList:!1,HTMLAllCollection:!1,HTMLCollection:!1,HTMLFormElement:!1,HTMLSelectElement:!1,MediaList:!0,MimeTypeArray:!1,NamedNodeMap:!1,NodeList:!0,PaintRequestList:!1,Plugin:!1,PluginArray:!1,SVGLengthList:!1,SVGNumberList:!1,SVGPathSegList:!1,SVGPointList:!1,SVGStringList:!1,SVGTransformList:!1,SourceBufferList:!1,StyleSheetList:!0,TextTrackCueList:!1,TextTrackList:!1,TouchList:!1},d=o(h),v=0;v<d.length;v++){var m,y=d[v],b=h[y],g=a[y],_=g&&g.prototype;if(_&&(_[f]||c(_,f,p),_[l]||c(_,l,y),s[y]=p,b))for(m in r)_[m]||i(_,m,r[m],!0)}},b047:function(t,e){function n(t){return function(e){return t(e)}}t.exports=n},b0c5:function(t,e,n){"use strict";var r=n("520a");n("5ca1")({target:"RegExp",proto:!0,forced:r!==/./.exec},{exec:r})},b218:function(t,e){var n=9007199254740991;function r(t){return"number"==typeof t&&t>-1&&t%1==0&&t<=n}t.exports=r},b4c0:function(t,e,n){var r=n("cb5a");function o(t){var e=this.__data__,n=r(e,t);return n<0?void 0:e[n][1]}t.exports=o},b5a7:function(t,e,n){var r=n("0b07"),o=n("2b3e"),i=r(o,"DataView");t.exports=i},ba92:function(t,e,n){"use strict";var r=n("4bf8"),o=n("77f1"),i=n("9def");t.exports=[].copyWithin||function(t,e){var n=r(this),a=i(n.length),c=o(t,a),s=o(e,a),u=arguments.length>2?arguments[2]:void 0,f=Math.min((void 0===u?a:o(u,a))-s,a-c),l=1;s<c&&c<s+f&&(l=-1,s+=f-1,c+=f-1);while(f-- >0)s in n?n[c]=n[s]:delete n[c],c+=l,s+=l;return n}},bbc0:function(t,e,n){var r=n("6044"),o="__lodash_hash_undefined__",i=Object.prototype,a=i.hasOwnProperty;function c(t){var e=this.__data__;if(r){var n=e[t];return n===o?void 0:n}return a.call(e,t)?e[t]:void 0}t.exports=c},bcaa:function(t,e,n){var r=n("cb7c"),o=n("d3f4"),i=n("a5b8");t.exports=function(t,e){if(r(t),o(e)&&e.constructor===t)return e;var n=i.f(t),a=n.resolve;return a(e),n.promise}},be13:function(t,e){t.exports=function(t){if(void 0==t)throw TypeError("Can't call method on "+t);return t}},c098:function(t,e){var n=9007199254740991,r=/^(?:0|[1-9]\d*)$/;function o(t,e){var o=typeof t;return e=null==e?n:e,!!e&&("number"==o||"symbol"!=o&&r.test(t))&&t>-1&&t%1==0&&t<e}t.exports=o},c2b6:function(t,e,n){var r=n("f8af"),o=n("5d89"),i=n("6f6c"),a=n("a2db"),c=n("c8fe"),s="[object Boolean]",u="[object Date]",f="[object Map]",l="[object Number]",p="[object RegExp]",h="[object Set]",d="[object String]",v="[object Symbol]",m="[object ArrayBuffer]",y="[object DataView]",b="[object Float32Array]",g="[object Float64Array]",_="[object Int8Array]",x="[object Int16Array]",w="[object Int32Array]",j="[object Uint8Array]",S="[object Uint8ClampedArray]",k="[object Uint16Array]",O="[object Uint32Array]";function A(t,e,n){var A=t.constructor;switch(e){case m:return r(t);case s:case u:return new A(+t);case y:return o(t,n);case b:case g:case _:case x:case w:case j:case S:case k:case O:return c(t,n);case f:return new A;case l:case d:return new A(t);case p:return i(t);case h:return new A;case v:return a(t)}}t.exports=A},c366:function(t,e,n){var r=n("6821"),o=n("9def"),i=n("77f1");t.exports=function(t){return function(e,n,a){var c,s=r(e),u=o(s.length),f=i(a,u);if(t&&n!=n){while(u>f)if(c=s[f++],c!=c)return!0}else for(;u>f;f++)if((t||f in s)&&s[f]===n)return t||f||0;return!t&&-1}}},c3fc:function(t,e,n){var r=n("42a2"),o=n("1310"),i="[object Set]";function a(t){return o(t)&&r(t)==i}t.exports=a},c69a:function(t,e,n){t.exports=!n("9e1e")&&!n("79e5")(function(){return 7!=Object.defineProperty(n("230e")("div"),"a",{get:function(){return 7}}).a})},c869:function(t,e,n){var r=n("0b07"),o=n("2b3e"),i=r(o,"Set");t.exports=i},c87c:function(t,e){var n=Object.prototype,r=n.hasOwnProperty;function o(t){var e=t.length,n=new t.constructor(e);return e&&"string"==typeof t[0]&&r.call(t,"index")&&(n.index=t.index,n.input=t.input),n}t.exports=o},c8ba:function(t,e){var n;n=function(){return this}();try{n=n||new Function("return this")()}catch(r){"object"===typeof window&&(n=window)}t.exports=n},c8fe:function(t,e,n){var r=n("f8af");function o(t,e){var n=e?r(t.buffer):t.buffer;return new t.constructor(n,t.byteOffset,t.length)}t.exports=o},ca5a:function(t,e){var n=0,r=Math.random();t.exports=function(t){return"Symbol(".concat(void 0===t?"":t,")_",(++n+r).toString(36))}},cadf:function(t,e,n){"use strict";var r=n("9c6c"),o=n("d53b"),i=n("84f2"),a=n("6821");t.exports=n("01f9")(Array,"Array",function(t,e){this._t=a(t),this._i=0,this._k=e},function(){var t=this._t,e=this._k,n=this._i++;return!t||n>=t.length?(this._t=void 0,o(1)):o(0,"keys"==e?n:"values"==e?t[n]:[n,t[n]])},"values"),i.Arguments=i.Array,r("keys"),r("values"),r("entries")},cb5a:function(t,e,n){var r=n("9638");function o(t,e){var n=t.length;while(n--)if(r(t[n][0],e))return n;return-1}t.exports=o},cb7c:function(t,e,n){var r=n("d3f4");t.exports=function(t){if(!r(t))throw TypeError(t+" is not an object!");return t}},cc45:function(t,e,n){var r=n("1a2d"),o=n("b047"),i=n("99d3"),a=i&&i.isMap,c=a?o(a):r;t.exports=c},cd1c:function(t,e,n){var r=n("e853");t.exports=function(t,e){return new(r(t))(e)}},ce10:function(t,e,n){var r=n("69a8"),o=n("6821"),i=n("c366")(!1),a=n("613b")("IE_PROTO");t.exports=function(t,e){var n,c=o(t),s=0,u=[];for(n in c)n!=a&&r(c,n)&&u.push(n);while(e.length>s)r(c,n=e[s++])&&(~i(u,n)||u.push(n));return u}},d02c:function(t,e,n){var r=n("5e2e"),o=n("79bc"),i=n("7b83"),a=200;function c(t,e){var n=this.__data__;if(n instanceof r){var c=n.__data__;if(!o||c.length<a-1)return c.push([t,e]),this.size=++n.size,this;n=this.__data__=new i(c)}return n.set(t,e),this.size=n.size,this}t.exports=c},d2c8:function(t,e,n){var r=n("aae3"),o=n("be13");t.exports=function(t,e,n){if(r(e))throw TypeError("String#"+n+" doesn't accept regex!");return String(o(t))}},d327:function(t,e){function n(){return[]}t.exports=n},d370:function(t,e,n){var r=n("253c"),o=n("1310"),i=Object.prototype,a=i.hasOwnProperty,c=i.propertyIsEnumerable,s=r(function(){return arguments}())?r:function(t){return o(t)&&a.call(t,"callee")&&!c.call(t,"callee")};t.exports=s},d3f4:function(t,e){t.exports=function(t){return"object"===typeof t?null!==t:"function"===typeof t}},d53b:function(t,e){t.exports=function(t,e){return{value:e,done:!!t}}},d7ee:function(t,e,n){var r=n("c3fc"),o=n("b047"),i=n("99d3"),a=i&&i.isSet,c=a?o(a):r;t.exports=c},d8e8:function(t,e){t.exports=function(t){if("function"!=typeof t)throw TypeError(t+" is not a function!");return t}},da03:function(t,e,n){var r=n("2b3e"),o=r["__core-js_shared__"];t.exports=o},dc57:function(t,e){var n=Function.prototype,r=n.toString;function o(t){if(null!=t){try{return r.call(t)}catch(e){}try{return t+""}catch(e){}}return""}t.exports=o},dcbc:function(t,e,n){var r=n("2aba");t.exports=function(t,e,n){for(var o in e)r(t,o,e[o],n);return t}},dd88:function(t,e,n){"use strict";var r=n("8bbf"),o=n.n(r),i={position:"bottom",time:2e3,closeIcon:"close",close:!0,successIcon:"check_circle",infoIcon:"info",warningIcon:"priority_high",errorIcon:"warning"},a="function"===typeof Symbol&&"symbol"===typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"===typeof Symbol&&t.constructor===Symbol&&t!==Symbol.prototype?"symbol":typeof t},c=Object.assign||function(t){for(var e=1;e<arguments.length;e++){var n=arguments[e];for(var r in n)Object.prototype.hasOwnProperty.call(n,r)&&(t[r]=n[r])}return t},s=function(t){if(Array.isArray(t)){for(var e=0,n=Array(t.length);e<t.length;e++)n[e]=t[e];return n}return Array.from(t)},u="undefined"===typeof window,f=20141223,l=o.a.extend({name:"toast-message",data:function(){return{messages:[]}},methods:{createAction:function(t,e,n,r){var o=arguments.length>4&&void 0!==arguments[4]&&arguments[4];return t("mu-button",{props:{icon:o,flat:!o,color:r.color?"#fff":"secondary"},style:o?{width:"36px",height:"36px"}:{},slot:"action",on:{click:function(){return n&&n(r.id)}}},[e])},message:function(t){var e="toast_id_"+f++;return this.messages.push(c({},t,{id:e,open:!0})),e},close:function(t){var e=this;if(t){var n=this.messages.filter(function(e){return e.id===t})[0];n&&(n.open=!1,setTimeout(function(){if(e.messages){var t=e.messages.indexOf(n);-1!==t&&e.messages.splice(t,1)}},500))}}},render:function(t){var e=this;return t("div",{staticClass:"mu-toast-plugin",style:{display:"none"}},this.messages.map(function(n){var r=n.close?e.createAction(t,t("mu-icon",{props:{value:i.closeIcon},style:{"margin-right":0}}),function(t){return e.close(t)},n,!0):void 0;return t("mu-snackbar",{props:{color:n.color,textColor:n.textColor,open:n.open,position:n.position},key:n.id},[n.icon?t("mu-icon",{props:{left:!0,value:n.icon}}):""].concat(s(n.actions&&n.actions.length>0?n.actions.map(function(r){var o=r.action,i=r.click;return e.createAction(t,o,i,n)}):[]),[n.message,r]))}))}}),p=void 0;function h(t){if(!u)return p||(p=new l({el:document.createElement("div")}),document.body.appendChild(p.$el)),p.message(t)}function d(t){p&&p.close(t)}var v={config:function(t){if(!t||Array.isArray(t)||"object"!==("undefined"===typeof t?"undefined":a(t)))return i;for(var e in t)t.hasOwnProperty(e)&&(i[e]=t[e]);return i},message:function(t){if(t){t="string"===typeof t?{message:t}:t;var e=c({time:i.time,position:i.position,close:i.close},t),n=h(e);return e.time>0&&setTimeout(function(){return d(n)},e.time),n}}};["success","error","info","warning"].forEach(function(t){v[t]=function(e){if(e)return e="string"===typeof e?{message:e,color:t,icon:i[t+"Icon"]}:c({},e,{color:t,icon:i[t+"Icon"]}),v.message(e)}}),v.close=function(t){return d(t)},v.install=function(t,e){v.config(e),t.prototype.$toast=v},e["a"]=v},e11e:function(t,e){t.exports="constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,toLocaleString,toString,valueOf".split(",")},e24b:function(t,e,n){var r=n("49f4"),o=n("1efc"),i=n("bbc0"),a=n("7a48"),c=n("2524");function s(t){var e=-1,n=null==t?0:t.length;this.clear();while(++e<n){var r=t[e];this.set(r[0],r[1])}}s.prototype.clear=r,s.prototype["delete"]=o,s.prototype.get=i,s.prototype.has=a,s.prototype.set=c,t.exports=s},e538:function(t,e,n){(function(t){var r=n("2b3e"),o=e&&!e.nodeType&&e,i=o&&"object"==typeof t&&t&&!t.nodeType&&t,a=i&&i.exports===o,c=a?r.Buffer:void 0,s=c?c.allocUnsafe:void 0;function u(t,e){if(e)return t.slice();var n=t.length,r=s?s(n):new t.constructor(n);return t.copy(r),r}t.exports=u}).call(this,n("62e4")(t))},e853:function(t,e,n){var r=n("d3f4"),o=n("1169"),i=n("2b4c")("species");t.exports=function(t){var e;return o(t)&&(e=t.constructor,"function"!=typeof e||e!==Array&&!o(e.prototype)||(e=void 0),r(e)&&(e=e[i],null===e&&(e=void 0))),void 0===e?Array:e}},eac5:function(t,e){var n=Object.prototype;function r(t){var e=t&&t.constructor,r="function"==typeof e&&e.prototype||n;return t===r}t.exports=r},ebd6:function(t,e,n){var r=n("cb7c"),o=n("d8e8"),i=n("2b4c")("species");t.exports=function(t,e){var n,a=r(t).constructor;return void 0===a||void 0==(n=r(a)[i])?e:o(n)}},ec30:function(t,e,n){"use strict";if(n("9e1e")){var r=n("2d00"),o=n("7726"),i=n("79e5"),a=n("5ca1"),c=n("0f88"),s=n("ed0b"),u=n("9b43"),f=n("f605"),l=n("4630"),p=n("32e9"),h=n("dcbc"),d=n("4588"),v=n("9def"),m=n("09fa"),y=n("77f1"),b=n("6a99"),g=n("69a8"),_=n("23c6"),x=n("d3f4"),w=n("4bf8"),j=n("33a4"),S=n("2aeb"),k=n("38fd"),O=n("9093").f,A=n("27ee"),$=n("ca5a"),C=n("2b4c"),E=n("0a49"),T=n("c366"),F=n("ebd6"),I=n("cadf"),R=n("84f2"),M=n("5cc5"),P=n("7a56"),L=n("36bd"),N=n("ba92"),D=n("86cc"),W=n("11e9"),U=D.f,V=W.f,B=o.RangeError,z=o.TypeError,H=o.Uint8Array,q="ArrayBuffer",G="Shared"+q,Y="BYTES_PER_ELEMENT",J="prototype",K=Array[J],X=s.ArrayBuffer,Q=s.DataView,Z=E(0),tt=E(2),et=E(3),nt=E(4),rt=E(5),ot=E(6),it=T(!0),at=T(!1),ct=I.values,st=I.keys,ut=I.entries,ft=K.lastIndexOf,lt=K.reduce,pt=K.reduceRight,ht=K.join,dt=K.sort,vt=K.slice,mt=K.toString,yt=K.toLocaleString,bt=C("iterator"),gt=C("toStringTag"),_t=$("typed_constructor"),xt=$("def_constructor"),wt=c.CONSTR,jt=c.TYPED,St=c.VIEW,kt="Wrong length!",Ot=E(1,function(t,e){return Tt(F(t,t[xt]),e)}),At=i(function(){return 1===new H(new Uint16Array([1]).buffer)[0]}),$t=!!H&&!!H[J].set&&i(function(){new H(1).set({})}),Ct=function(t,e){var n=d(t);if(n<0||n%e)throw B("Wrong offset!");return n},Et=function(t){if(x(t)&&jt in t)return t;throw z(t+" is not a typed array!")},Tt=function(t,e){if(!(x(t)&&_t in t))throw z("It is not a typed array constructor!");return new t(e)},Ft=function(t,e){return It(F(t,t[xt]),e)},It=function(t,e){var n=0,r=e.length,o=Tt(t,r);while(r>n)o[n]=e[n++];return o},Rt=function(t,e,n){U(t,e,{get:function(){return this._d[n]}})},Mt=function(t){var e,n,r,o,i,a,c=w(t),s=arguments.length,f=s>1?arguments[1]:void 0,l=void 0!==f,p=A(c);if(void 0!=p&&!j(p)){for(a=p.call(c),r=[],e=0;!(i=a.next()).done;e++)r.push(i.value);c=r}for(l&&s>2&&(f=u(f,arguments[2],2)),e=0,n=v(c.length),o=Tt(this,n);n>e;e++)o[e]=l?f(c[e],e):c[e];return o},Pt=function(){var t=0,e=arguments.length,n=Tt(this,e);while(e>t)n[t]=arguments[t++];return n},Lt=!!H&&i(function(){yt.call(new H(1))}),Nt=function(){return yt.apply(Lt?vt.call(Et(this)):Et(this),arguments)},Dt={copyWithin:function(t,e){return N.call(Et(this),t,e,arguments.length>2?arguments[2]:void 0)},every:function(t){return nt(Et(this),t,arguments.length>1?arguments[1]:void 0)},fill:function(t){return L.apply(Et(this),arguments)},filter:function(t){return Ft(this,tt(Et(this),t,arguments.length>1?arguments[1]:void 0))},find:function(t){return rt(Et(this),t,arguments.length>1?arguments[1]:void 0)},findIndex:function(t){return ot(Et(this),t,arguments.length>1?arguments[1]:void 0)},forEach:function(t){Z(Et(this),t,arguments.length>1?arguments[1]:void 0)},indexOf:function(t){return at(Et(this),t,arguments.length>1?arguments[1]:void 0)},includes:function(t){return it(Et(this),t,arguments.length>1?arguments[1]:void 0)},join:function(t){return ht.apply(Et(this),arguments)},lastIndexOf:function(t){return ft.apply(Et(this),arguments)},map:function(t){return Ot(Et(this),t,arguments.length>1?arguments[1]:void 0)},reduce:function(t){return lt.apply(Et(this),arguments)},reduceRight:function(t){return pt.apply(Et(this),arguments)},reverse:function(){var t,e=this,n=Et(e).length,r=Math.floor(n/2),o=0;while(o<r)t=e[o],e[o++]=e[--n],e[n]=t;return e},some:function(t){return et(Et(this),t,arguments.length>1?arguments[1]:void 0)},sort:function(t){return dt.call(Et(this),t)},subarray:function(t,e){var n=Et(this),r=n.length,o=y(t,r);return new(F(n,n[xt]))(n.buffer,n.byteOffset+o*n.BYTES_PER_ELEMENT,v((void 0===e?r:y(e,r))-o))}},Wt=function(t,e){return Ft(this,vt.call(Et(this),t,e))},Ut=function(t){Et(this);var e=Ct(arguments[1],1),n=this.length,r=w(t),o=v(r.length),i=0;if(o+e>n)throw B(kt);while(i<o)this[e+i]=r[i++]},Vt={entries:function(){return ut.call(Et(this))},keys:function(){return st.call(Et(this))},values:function(){return ct.call(Et(this))}},Bt=function(t,e){return x(t)&&t[jt]&&"symbol"!=typeof e&&e in t&&String(+e)==String(e)},zt=function(t,e){return Bt(t,e=b(e,!0))?l(2,t[e]):V(t,e)},Ht=function(t,e,n){return!(Bt(t,e=b(e,!0))&&x(n)&&g(n,"value"))||g(n,"get")||g(n,"set")||n.configurable||g(n,"writable")&&!n.writable||g(n,"enumerable")&&!n.enumerable?U(t,e,n):(t[e]=n.value,t)};wt||(W.f=zt,D.f=Ht),a(a.S+a.F*!wt,"Object",{getOwnPropertyDescriptor:zt,defineProperty:Ht}),i(function(){mt.call({})})&&(mt=yt=function(){return ht.call(this)});var qt=h({},Dt);h(qt,Vt),p(qt,bt,Vt.values),h(qt,{slice:Wt,set:Ut,constructor:function(){},toString:mt,toLocaleString:Nt}),Rt(qt,"buffer","b"),Rt(qt,"byteOffset","o"),Rt(qt,"byteLength","l"),Rt(qt,"length","e"),U(qt,gt,{get:function(){return this[jt]}}),t.exports=function(t,e,n,s){s=!!s;var u=t+(s?"Clamped":"")+"Array",l="get"+t,h="set"+t,d=o[u],y=d||{},b=d&&k(d),g=!d||!c.ABV,w={},j=d&&d[J],A=function(t,n){var r=t._d;return r.v[l](n*e+r.o,At)},$=function(t,n,r){var o=t._d;s&&(r=(r=Math.round(r))<0?0:r>255?255:255&r),o.v[h](n*e+o.o,r,At)},C=function(t,e){U(t,e,{get:function(){return A(this,e)},set:function(t){return $(this,e,t)},enumerable:!0})};g?(d=n(function(t,n,r,o){f(t,d,u,"_d");var i,a,c,s,l=0,h=0;if(x(n)){if(!(n instanceof X||(s=_(n))==q||s==G))return jt in n?It(d,n):Mt.call(d,n);i=n,h=Ct(r,e);var y=n.byteLength;if(void 0===o){if(y%e)throw B(kt);if(a=y-h,a<0)throw B(kt)}else if(a=v(o)*e,a+h>y)throw B(kt);c=a/e}else c=m(n),a=c*e,i=new X(a);p(t,"_d",{b:i,o:h,l:a,e:c,v:new Q(i)});while(l<c)C(t,l++)}),j=d[J]=S(qt),p(j,"constructor",d)):i(function(){d(1)})&&i(function(){new d(-1)})&&M(function(t){new d,new d(null),new d(1.5),new d(t)},!0)||(d=n(function(t,n,r,o){var i;return f(t,d,u),x(n)?n instanceof X||(i=_(n))==q||i==G?void 0!==o?new y(n,Ct(r,e),o):void 0!==r?new y(n,Ct(r,e)):new y(n):jt in n?It(d,n):Mt.call(d,n):new y(m(n))}),Z(b!==Function.prototype?O(y).concat(O(b)):O(y),function(t){t in d||p(d,t,y[t])}),d[J]=j,r||(j.constructor=d));var E=j[bt],T=!!E&&("values"==E.name||void 0==E.name),F=Vt.values;p(d,_t,!0),p(j,jt,u),p(j,St,!0),p(j,xt,d),(s?new d(1)[gt]==u:gt in j)||U(j,gt,{get:function(){return u}}),w[u]=d,a(a.G+a.W+a.F*(d!=y),w),a(a.S,u,{BYTES_PER_ELEMENT:e}),a(a.S+a.F*i(function(){y.of.call(d,1)}),u,{from:Mt,of:Pt}),Y in j||p(j,Y,e),a(a.P,u,Dt),P(u),a(a.P+a.F*$t,u,{set:Ut}),a(a.P+a.F*!T,u,Vt),r||j.toString==mt||(j.toString=mt),a(a.P+a.F*i(function(){new d(1).slice()}),u,{slice:Wt}),a(a.P+a.F*(i(function(){return[1,2].toLocaleString()!=new d([1,2]).toLocaleString()})||!i(function(){j.toLocaleString.call([1,2])})),u,{toLocaleString:Nt}),R[u]=T?E:F,r||T||p(j,bt,F)}}else t.exports=function(){}},ec69:function(t,e,n){var r=n("6fcd"),o=n("03dd"),i=n("30c9");function a(t){return i(t)?r(t):o(t)}t.exports=a},ec8c:function(t,e){function n(t){var e=[];if(null!=t)for(var n in Object(t))e.push(n);return e}t.exports=n},ed0b:function(t,e,n){"use strict";var r=n("7726"),o=n("9e1e"),i=n("2d00"),a=n("0f88"),c=n("32e9"),s=n("dcbc"),u=n("79e5"),f=n("f605"),l=n("4588"),p=n("9def"),h=n("09fa"),d=n("9093").f,v=n("86cc").f,m=n("36bd"),y=n("7f20"),b="ArrayBuffer",g="DataView",_="prototype",x="Wrong length!",w="Wrong index!",j=r[b],S=r[g],k=r.Math,O=r.RangeError,A=r.Infinity,$=j,C=k.abs,E=k.pow,T=k.floor,F=k.log,I=k.LN2,R="buffer",M="byteLength",P="byteOffset",L=o?"_b":R,N=o?"_l":M,D=o?"_o":P;function W(t,e,n){var r,o,i,a=new Array(n),c=8*n-e-1,s=(1<<c)-1,u=s>>1,f=23===e?E(2,-24)-E(2,-77):0,l=0,p=t<0||0===t&&1/t<0?1:0;for(t=C(t),t!=t||t===A?(o=t!=t?1:0,r=s):(r=T(F(t)/I),t*(i=E(2,-r))<1&&(r--,i*=2),t+=r+u>=1?f/i:f*E(2,1-u),t*i>=2&&(r++,i/=2),r+u>=s?(o=0,r=s):r+u>=1?(o=(t*i-1)*E(2,e),r+=u):(o=t*E(2,u-1)*E(2,e),r=0));e>=8;a[l++]=255&o,o/=256,e-=8);for(r=r<<e|o,c+=e;c>0;a[l++]=255&r,r/=256,c-=8);return a[--l]|=128*p,a}function U(t,e,n){var r,o=8*n-e-1,i=(1<<o)-1,a=i>>1,c=o-7,s=n-1,u=t[s--],f=127&u;for(u>>=7;c>0;f=256*f+t[s],s--,c-=8);for(r=f&(1<<-c)-1,f>>=-c,c+=e;c>0;r=256*r+t[s],s--,c-=8);if(0===f)f=1-a;else{if(f===i)return r?NaN:u?-A:A;r+=E(2,e),f-=a}return(u?-1:1)*r*E(2,f-e)}function V(t){return t[3]<<24|t[2]<<16|t[1]<<8|t[0]}function B(t){return[255&t]}function z(t){return[255&t,t>>8&255]}function H(t){return[255&t,t>>8&255,t>>16&255,t>>24&255]}function q(t){return W(t,52,8)}function G(t){return W(t,23,4)}function Y(t,e,n){v(t[_],e,{get:function(){return this[n]}})}function J(t,e,n,r){var o=+n,i=h(o);if(i+e>t[N])throw O(w);var a=t[L]._b,c=i+t[D],s=a.slice(c,c+e);return r?s:s.reverse()}function K(t,e,n,r,o,i){var a=+n,c=h(a);if(c+e>t[N])throw O(w);for(var s=t[L]._b,u=c+t[D],f=r(+o),l=0;l<e;l++)s[u+l]=f[i?l:e-l-1]}if(a.ABV){if(!u(function(){j(1)})||!u(function(){new j(-1)})||u(function(){return new j,new j(1.5),new j(NaN),j.name!=b})){j=function(t){return f(this,j),new $(h(t))};for(var X,Q=j[_]=$[_],Z=d($),tt=0;Z.length>tt;)(X=Z[tt++])in j||c(j,X,$[X]);i||(Q.constructor=j)}var et=new S(new j(2)),nt=S[_].setInt8;et.setInt8(0,2147483648),et.setInt8(1,2147483649),!et.getInt8(0)&&et.getInt8(1)||s(S[_],{setInt8:function(t,e){nt.call(this,t,e<<24>>24)},setUint8:function(t,e){nt.call(this,t,e<<24>>24)}},!0)}else j=function(t){f(this,j,b);var e=h(t);this._b=m.call(new Array(e),0),this[N]=e},S=function(t,e,n){f(this,S,g),f(t,j,g);var r=t[N],o=l(e);if(o<0||o>r)throw O("Wrong offset!");if(n=void 0===n?r-o:p(n),o+n>r)throw O(x);this[L]=t,this[D]=o,this[N]=n},o&&(Y(j,M,"_l"),Y(S,R,"_b"),Y(S,M,"_l"),Y(S,P,"_o")),s(S[_],{getInt8:function(t){return J(this,1,t)[0]<<24>>24},getUint8:function(t){return J(this,1,t)[0]},getInt16:function(t){var e=J(this,2,t,arguments[1]);return(e[1]<<8|e[0])<<16>>16},getUint16:function(t){var e=J(this,2,t,arguments[1]);return e[1]<<8|e[0]},getInt32:function(t){return V(J(this,4,t,arguments[1]))},getUint32:function(t){return V(J(this,4,t,arguments[1]))>>>0},getFloat32:function(t){return U(J(this,4,t,arguments[1]),23,4)},getFloat64:function(t){return U(J(this,8,t,arguments[1]),52,8)},setInt8:function(t,e){K(this,1,t,B,e)},setUint8:function(t,e){K(this,1,t,B,e)},setInt16:function(t,e){K(this,2,t,z,e,arguments[2])},setUint16:function(t,e){K(this,2,t,z,e,arguments[2])},setInt32:function(t,e){K(this,4,t,H,e,arguments[2])},setUint32:function(t,e){K(this,4,t,H,e,arguments[2])},setFloat32:function(t,e){K(this,4,t,G,e,arguments[2])},setFloat64:function(t,e){K(this,8,t,q,e,arguments[2])}});y(j,b),y(S,g),c(S[_],a.VIEW,!0),e[b]=j,e[g]=S},efb6:function(t,e,n){var r=n("5e2e");function o(){this.__data__=new r,this.size=0}t.exports=o},f559:function(t,e,n){"use strict";var r=n("5ca1"),o=n("9def"),i=n("d2c8"),a="startsWith",c=""[a];r(r.P+r.F*n("5147")(a),"String",{startsWith:function(t){var e=i(this,t,a),n=o(Math.min(arguments.length>1?arguments[1]:void 0,e.length)),r=String(t);return c?c.call(e,r,n):e.slice(n,n+r.length)===r}})},f5df:function(t,e,n){},f605:function(t,e){t.exports=function(t,e,n,r){if(!(t instanceof e)||void 0!==r&&r in t)throw TypeError(n+": incorrect invocation!");return t}},f751:function(t,e,n){var r=n("5ca1");r(r.S+r.F,"Object",{assign:n("7333")})},f8af:function(t,e,n){var r=n("2474");function o(t){var e=new t.constructor(t.byteLength);return new r(e).set(new r(t)),e}t.exports=o},fa21:function(t,e,n){var r=n("7530"),o=n("2dcb"),i=n("eac5");function a(t){return"function"!=typeof t.constructor||i(t)?{}:r(o(t))}t.exports=a},fa5b:function(t,e,n){t.exports=n("5537")("native-function-to-string",Function.toString)},fab2:function(t,e,n){var r=n("7726").document;t.exports=r&&r.documentElement},fba5:function(t,e,n){var r=n("cb5a");function o(t){return r(this.__data__,t)>-1}t.exports=o}}]);
//# sourceMappingURL=chunk-vendors.ec36a913.js.map | 1zlab-emp-ide | /1zlab_emp_ide-0.0.3-py3-none-any.whl/emp_ide/static/js/chunk-vendors.ec36a913.js | chunk-vendors.ec36a913.js |
from base64 import b64decode
import pygame
import keyboard
import os
import math
clock = pygame.time.Clock()
pygame.font.init()
playerX = 0
playerY = 0
playerZ = 0
camRot = [0,0,0]
camVel = [0,0,0]
camSens = 6
mouseRightDown = False
mouseLeftDown = False
mouseSpeed = 0
lockMouse = False
showMouse = True
playerSpeed = 0
playerVel = [0,0,0]
def pgEvents():
global playerZ,playerVel
global camSens,camVel,camRot
global mouseSpeed,lockMouse
global mouseRightDown,mouseLeftDown
for event in pygame.event.get():
if event.type == pygame.QUIT:
return "QUIT"
if event.type == pygame.MOUSEMOTION:
dx,dy = event.rel
mouseSpeed = (dx ** 2 + dy ** 2) ** (1/2)
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_w:
playerVel[2] = playerSpeed
return "K_w"
if event.key == pygame.K_s:
playerVel[2] = -playerSpeed
return "K_s"
if event.key == pygame.K_a:
playerVel[0] = playerSpeed
return "K_a"
if event.key == pygame.K_d:
playerVel[0] = -playerSpeed
return "K_d"
#if event.key == pygame.K_LEFT:
# camVel[0] = camSens
# return "K_LEFT"
#if event.key == pygame.K_RIGHT:
# camVel[0] = -camSens
# return "K_RIGHT"
if event.type == pygame.KEYUP:
if event.key == pygame.K_w:
playerVel[2] = 0
return "K_w2"
if event.key == pygame.K_s:
playerVel[2] = 0
return "K_s2"
if event.key == pygame.K_a:
playerVel[0] = 0
return "K_a2"
if event.key == pygame.K_d:
playerVel[0] = 0
return "K_d2"
#if event.key == pygame.K_LEFT:
# camVel[0] = 0
# return "K_LEFT2"
#if event.key == pygame.K_RIGHT:
# camVel[0] = 0
# return "L_RIGHT2"
if event.type == pygame.MOUSEBUTTONDOWN:
mx,my = pygame.mouse.get_pos()
if event.button == 3:
mouseRightDown = True
return "RIGHTMOUSEDOWN"
if event.button == 1:
mouseLeftDown = True
return "LEFTMOUSEDOWN"
return "MOUSEDOWN"
if event.type == pygame.MOUSEBUTTONUP:
if event.button == 3:
mouseRightDown = False
return "RIGHTMOUSEDOWN1"
if event.button == 1:
mouseLeftDown = False
return "LEFTMOUSEDOWN1"
return "MOUSEUP"
class Win:
def __init__(self,w,h,cap,icon=None,hz=144):
self.w = w
self.h = h
self.hz = hz
self.screen = pygame.display.set_mode((w,h))
pygame.display.set_caption(cap)
if icon == None:
iconDataUri = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAgAAAAIACAYAAAD0eNT6AAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAADKsSURBVHhe7d0HnCRVuffx/07a2ZwjLCywKCAIiJgQsyAYLipgVhRF0auACCbwGsDXCKLcqwRFRS4XFREUUAkqelW8ooCggrjAwrI5h8kz7znbT8PMVlVPz0xX1anu3/dj2eepGWZ7pqrO85xT1VUCAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAKB2xtkrnvApe/U+Ya8j8Wl7/Q97BQAAgfBJfiCnxf/bg4sMAACQgjyTfbULBQEAAGNUhIRfaWGGAHGKvl+HtHCMAXWifDDHHehFX+ikGlu97tchLaEeY42y7f3vST+Xk6JeBOh3mNFcoDe8lt2l5gVS01T36pamaaX24MXvtwMdUr9b/Gu53b9J6l0p9bnFv/avLv3M2vAXF3JhYWNIb/9Gkrz7QrZ5Mi6sTknRCoDaHCQte0mtbmlb4l79Um6716bx9k010N9XKga67pI6/+xebel92L5hVCgE6huJID959Yd+JIyRoy8coyIUAGPvENsOkCY894mldTf7Qk56XFHQ8Rtp+02lpfch+8KIsPPXH5J/vrI+ptjetUe/OAIhFwCjPzha95YmvfyJhN8yz74QqM67XCHwC2nrNa79e1tZNXb4+lF5JDjR7dNtbt/2+7d/VWtpfb2rVS/Vt8UdX7dLG861FbGy6hNJ/tmgf6wgxAJgdAdG28HS5Fe6xO+WCU+3lQXki4EtV5aW3mW2cljs5MWXvN9PeIk053ypfX9bgTHZ/mtpuSumBrbZiiGy6hMrFnvzZkkHPEl6yhK3uFpv793tC4Hr6XVdWJdbukuvXb49KPavft3W7dJjq6UVa+x1rdTfbz8kPfSTOwmpABh54h//DGnKsaWkP34fW1lHtlznRitfcEfN/9qKYbGDF1d8Qpjttv/MMyxAzWz4hrTmZAuGyOIYqpj8z3Zv6z/eJzW32IoGsdIVAo+5gmDFoNf7H5Luvk/66z/tm2qHvtIJoQAYWeJvmidNfaNL/G4p8kh/JDZfKa3/vNR9l62oiB27mKJJYbIrbhf+wALU3NI9pd4HLRgi7X4xtgA44RjXEbrEv8ciW4HH9fS4IsAVAr4YuPt+t/yj1F6z0b5h9Bq6v8yzABhZ4p/0akv8rlNsVBsvkdZ+ROpfbysSUQQUTzQpLLzeFQFHW4CaW326O6bOs2CINPvF2H7v2COkH3zVAlTtkRXSrX9wy++lW9zr8tX2hdFpuH4zrwKg+uQ/+XhphjtQJzzDVjS43jWlImDzt2xFRSHM8GB40eOhaba0xG1rpGfbL1zGONKCIdI8biKFXmuLtOI30qwZtgKjduffSoVAuSDo6rEvjEzDFAJN9pql6pL/5NdJi253o6CrSP6DtcyR5n/T/V1+4nqO/WxloornGhGwtmG3LcaqZQ9r5Ov9byL518pB7rA5/R3S9ZdIHXdJP79UetMr7IvV8/mpIfrOrEeIw/9RJx4lzTzLvT7HViBR32Zp5dvcSObHtiIRMwFhix4XU94uLahqlgej1d8rPRD7UcpMZwB+e4V02CEWIBVr1knfdd3kd68tXUMwQnXbf2Y5A+BH/sma5krzLpd2vYHkXy1/q+JdrpFmfNhWJGImoGiaJlkDqWlqccfQLhbkY1I7yT8Lc2ZJp58o3XWddMtl0tv+zb5QHd9/Vs5fBZVVAVB52n/KW6TF90jT3mwrMCJzPueKp2FHi3W5A9etpsnWQKpadrXGEGkdK5Gfu88SayAzL3q29O3PS+v/IH383VJbdR+3LJ8WqKt+NKsCIDn5zz5fWvDd0rltjN60tw9XBPhtQBFQGHlcntOA4guAzMzl3H9uZkyXzjlNeuRX0odc91mluupHs+hlkv9Yc74qzTzVAoxZdUUAgLJxzdbIROT4mzPTGsjN3NnSFz8sPXyr9O9vspWV+e1YF7MBWRQA8Uln5tmuBHu/BaiZ4YsArgcAKsusUG7i8txg7LZQ+ppLS/f/TDrxtbayMr+fFLoIyGeesf0wabb/qCVS4YuA6adYEItTAQAQY+/F0qXnSt//irTr8M+RK3QRkHYBEP+HmXaSNZCauW7vbX+BBRGZjXAAoIiOe5l0z0+rmg3w/WkhZ1aznwFoXuQKgLdagFTNv0Qal3g1ObMAAFDBtCkjmg0oXBGQdgEQHWlOfJ41kLq2JdKscyyIYBYAAKrgZwPurW42oFAXB2Y/A9Bah4/tDdnMU6T2xBsrMQsARHGBEiKm2mzAJ95rK5IV5rqA7AuAtidbA5lhFgCI17vSGkB1PvUB6aLh03shioAcCgBufZW5SS+UpiTeZZFZADSu3uXWAKp30uukn37ddasTbUW84IuA7AsAbnGaj+mJ91xgFgCNK74AaKhnwmN0Xu7GVb/+nvTkxbYiXtD9a/YFwLgp1kCm/COVJ43sCRhAXet+wP1fZ6kNjMLB+0m3uSLgkKfYinjBfjoghxmAynMmSFHyLACnAdB4uu6xBjB6/lbC13xN2mcPWxEvyCIghxkACoDcTHqx1La/BUNwGgCNp/OP1gDGZtFC6UcXDnuvgOAGWhkXAOPcv1jdsxeREk4DACXbb7YGMHb77iVd44qAGVNtRVRwFwVmXAAMSP3d1kYuJicWAJwGQOPY/jup6/8sGIJ7AGDUnn6A9KOvSW3J49ygZluzPwUw0GUN5GLCoVLrkywYgtMAaBwbzrNGBJ8AwJi84JmlIqCCYK4HSPthlNFfdM81UstsCzLUda/U8Qep+x6pb6PUb0vfptLrgI/7pObpriyaUVp8u+0p0qSXShOfbz+oDqx8j7T5IguG4OGk+YgeJzM+Js051wLU1Fo3yF+fmOfTPAYi2/mEY6TLPmdBTk51u9kFl1swBn7UO3mi6y7dMnlC6dUvM6dKixbYMr/0uptbdnVLPfukKwI+9Z8WRPmZptyLzewLgMUPuT1ldwtStO2mUsLv9MvtLrmvsy+M0jhXDEx2hcCkY6Wpx9vKgtr0HWnVCRYMQQGQj3wLgK77XEFYgwwwKv5XL//6g1+tPbDz1xPaO5o7rdu53b/V/a5/KQ0C4qXdKZff0OPqqQAYqaYm6dD9pWcdKD3TLf51j0X2xTrxkrdJt7j0kyD3IiD7AmA3dwC2H2RBjfmEv/kKaatb+jbYyhS0P8+VtR9xBcFRtqJguu6XHo69JTMFQD6ix0mmBUDi/tBoMu8PG7kAiOOvoj/sYOk1R5SWloJfM/73B6SD3Dbu7rUVUbn2udlfA1DrxNy7Slp/nuvADpEeebYb3V6YbvL3Om+THjtaevQVUk8BbyU6/klS80ILhuBCQDQqLv4LwKOuO7/qZ9LrPihNdV36W86QrrnJvlhA+y6RLjzbgni59rk5FABrrTFGPY9Kqz8kPbiLtPZ0N4r5s30hQ9uvd0XH86SO5DmeYI1/qjWG4EJANKIgzsdiqI4u6Xs/kV7zfmn+c6QvXOLSR/JIOljvep0rZF5lQVSufW72BUDvMmuMUvdSadUpLvEvkjZ+WRrosy+Mij/wk5bq9Lr38+jh0uYf2oqCaMngOgwgfP5YJ/nH89PTo11G1o8OY9V66cOuu9/l+aVCoLdghYCfBZg704Ko3GYBsi8AekZZAPSudiP+06SH9pI2fdVWjojfGXfeSf2Bn7QM/r7KO/JAj7TyOGn7721FAbRWfoIFUIfKSWnwse2PddReXD+68zLiAmHVulIhsKsrBC672lYWwNQp0lknWxCV2yxA2gVAdAP7q3BHot+Vems/Iy11CWvjV2xlVWp5oJd35Mo77OqT3PstyI2OWpkBwLAGHz/1sJSTEsKwc4FQdUHgC4F3fFx64+muXaOzyml7/1ukg/axICqXWYDsZwA6fyP1bbVgGBsvkx50iX+9L5A6SuuGV078aRzo/mcm76T+40WrXBFQBC27WQMAgjC4IKiqGLjyeumAV0pXXGcrAhfaLEDaBUB8Et72E2sk6LxTevRoN6J+hysWqr7KvrzjpJH4ByvvpPG2fMf9frdaELCmydYAgOCU+9lhC4E1G6Q3nymd8XlbEbDXHikdfbgFUZnPAmQ/A+Bt+b41duKnz9d8VFp2sLT9Rls5rHLiz1ryv7nxQmsEjAIAQPiqLgS+dJn0mn+Xtm6zFYEKaRYgnwJg24+lDYNultzfadP9e7n1Vd8VozzVn6f4nXLbNVLHHRYEqmmKNQAgeFUVAtfcLB32Bunuf9iKAD37adKJr7UgKtNZgCwKgPgNtuYD0lKX8B85Qnpggk33P2pfrKic+NOe6q9G8nvYdKk1AsUMAB6Xdx0NVM33uRWLgLvvl44+Sbpvqa0I0ClvtUZUprMAWRQAyUnSf4a+o+rbPIWU+AeL7z07brNGoPxHFwGgeHwOqDgbsHy1dOwpblwZ6CcEDniy9MoXWBCV2SxAVqcAKlZsVQgx8VfW87ewbxPcv90aAFBIFWcD7vmndJwrAnoCHeu8K/mZcpnNAmRVAFTcUBWUR/2hi//dQp4FGAj8ShkAGF7F3HLbHaUiIESvfJG0/94W5CTLiwDLG6qaQqCc+Isy6o9/n113WSNAzAAAqA8Vi4Brb5U+k/xc/ly96zhrRGVyGiDLAsDzG8ovPrlXWoqS+CvrW2ONAA1QAACoGxWLgE98Tbrx1xYExJ8GmDzBgqEyOQ2QdQHQWEIuAJgBQJkvuYHiq1gEvMeNqdeutyAQE9orXguQOgqANPUGfJNqrgEAUH8Si4Blj0kfrPo2M9k59khrRKV+GoACIE0DndYIEDMAAOpT4inky6+TfvkHCwLxnKdJu86zYKjUTwNQAKQp5Jvt9DxgDQCoO4kntj4d4AWBL3++NTJGAZCmkAuA7vutAXARAOpS7KmAX/2f9N1rLAjE0ckFQKqnASgA0tQ0yRoB6r7PGkMkXkADAAWTeD3AOV+3RiD8DEB7mwVDpXoagAIgTeNCngGILQAAoJ7EXg/wz2XS/1xvQQCaWyrOAqSGAiBNbUusEZieFdLARguGSLx4BgAKKnYW4OKrrBGIPK4DoABIU9v+1ghMD6N/AA0jdmDzyz9K/xvQU9vzuA6AAqA24jfQ+AOsERguAATQWGJnAS4KaBZg/hxp790sGCq16wAoAGojuoGapklte1oQmK57rQEADSF2FuCqG1x32GVBAJ6e8ZiRAiAtU95gjQCF/JRCAEhHZBagu1f68c0WBODQjM8aUwCkpXm+NQLTu8bt9XdaMAQfAcTOBlJa/Cmz1G9zCuwkdhbgmpAKAGYACie+I5v4EmsEJnn0zycAkBV/yswvccWBXygOkJlrbgrnNMDTk2cAUjkmKADGLub8/yxXABxmQWC2B1TuAvHiigOKAtRC7GmAa2+xIGft7dLT9rVgqGieqQEKgDRMfKk1ArT1J9YACmVwUUAxgNGKnen83V+sEYAsTwNQAIxNwvR/oAXA9t9IfcstGILz/ygSigHUVEgFQIXTADXHU0DGxndAQ41rlfZcIzVPsxUBWX2mtPGLFgzBfpCv6H4042PSnHMtSFn3A9Kyw9w+O8stM0uvTfZaXufjcc3um1vs1S1xr/2d7rfpKC2D230b3LLaLaukXv/qln7X9utrxxeyIV/LEtnOJxwjXZbzM+pPdbvZBZdbMFS99gu+aIxMqW+5Q5ocwONbbv299OK3WzBUzbcHHf/oxe5Emvpuaf43LAjM0iWu8/2XBUOwH+Qr3wIgT73rXAFyb+neFN1/e6Ld7wqE0Qu1EKAACEdkW/z8EumIwy3I0b+WSUuOsGComm8PTgGMXvxFGdPiS7fcbf6fpOTP9D/y0+IvmH2eK3hOluZ9TVp0q+v9Vkm7/UWa/WX3tZe7b5pY+t7qlU8RcHoAVWtttUbOdl9ojQxQAIxOfMfS/nxpwjMtCMymi60Rwcf/EJ72g6SZH5R2/an0pG3SLr+Upp8qNe9q31AVCgFU7etujBSClhZXB8+zYKia78cUAKNTrNH/9t9JHa4DBYpq0gukuedLez3iioGfS1NPKl2XUJ1yIQAk+nvsBGk+spoFoAAYufgqrNltsWlvsyAwmy6yRgTT/yieSUdI890+vWSdKwoultoOsi8Mi9kAlEX6vr8vtUYAdt/FGkPFDzzHgAJg5OI3wsyzrRGYznukLd+1IILpfxTb9HdJi/8iLfixNPFIW1kRswHwIn1fX58rAh6wIGfMAIQpvuMYf6g04z0WBGbjV6wRwegf9WPKv0m7/kxaeKPUfoitrIjZAESEchpgNwqA4CR3FjPPskZguu6TNn/TgghG/6g/k1/mes8/SbM+rx335KjMzwZQBOBxK9daI2eLMnqWHAVA9eKn/ie5kceUV1kQmI0XWCOC0T/q26wzpd3vdwXB8bYiEUUAHre2pvelGr0J7dZIGQVAdSqM/gM9999xu7Tp6xZEMPpH/WtbLC28Spr9BVuRiCIAO6zbaI2ctY+3RsooAIbnO4b40f+0k12pVtX5xuytDbQwAbI284zSfQRan2wrYlEEIJwZAAqAYMQnf/+xv9mftSAwGy+TOm6yIILb/qLx+PsI7HbHcJ8UoAhocGsDmQHgFEAYkjuDOV9yRcB0CwLS3yGtSxz9c+4fjat5krTwp9UUAWgMkf5wwyZr5IxTAPlLnvqf8hZp6hssCMzajyvhkb8e5/7R2JpaqikCuE9Ag9ruxk8h4BRA/uKTf9Ps0ug/RNtulTaeb0EEo3/AKxcBE15oK2JxKqABbe+0Rs7aOQWQqwpT/1+UWuZaEJi1H7ZGLEb/QJkvAuZ/R2peZCsiuB6g/kX6xI5ACgBmAPKTPPXvP1M87QQLArPWve2uP1kQwegf2FmrS/6+CEjG9QANJpQZAP9o4pYMsjMFQFT8QT9uarhT/x13SOs/aUGET/6M/otmIJCeqN5NeqE09xILYjEL0EC2d1kjZ/39Uq9b0kYBMFTyxT/zvlEaMYRozSnWiEXyL6L+rdZA6qa/U5p6ogURzAI0kFASYlYXI1IAPCE5+U8/Ldyr/le799b5vxZEMPVfDNHtNBDIHUkaxezPu95wjgURzAI0iKw+fjccCoBsJR/g7YdLc8+zIDCbr6z0tD+P0X9RdS+zBjLRMkua44qAeMwCNIhgCoCMzgBSAFS66K9pWmnqP0TdD0irKj6CmDv+FUe0UOv6o9S32QJkYtrbpUmvtiCCWYAG0N5mjZwxA5CN5OTvzb9cGr+fBYFZdbI0kJggmPovvAFpy5XWRmZmnmmNCGYB6k+kqAtlBmAbBUDqKif/ORdIk19pQWBWu06q42YLIrjqv16sPUvqvNOCFA30S/3bpd71Us9jUvdS97rMxavcskHq22bf2AAmPEualPh4b2YB6lyjnQJo1GniysnfX/QX6nn/TZe70f9bLYjF1H8xJV+EOusz0oyPuHK9xVZU0N9VStx9K93iE7hvry4tvWueaPe5dr9L7Ds+bthd+m8rcmMFfxfMZltadpXa9pZa3eJfxx/gvj7Bvrfgtt0iLX+JBRFjOb4i2/iEY6TLPmdBTk49V7rAdSsx6r0vieSBZ+wv3f5DC3J046+lo99twVA13SaNmiySO9vJx0oLf2BBYPxocNkzXSOxwyb5F1flotRr2d0l3L1cAp7n9mA3Yvej9sGvO5K6G8XnpXU/Vwgc6EbRh0tT3HHUknhVffgeebHUcasFQ1AA1I/I9jjK7bo3VLwtRDau/rl0bPynu2u6TRrxFEBy8p/wgnCT/0CftPqdrpGY/DnvX2zDn7bpfbiUlLZe6Uap17r2TaWPgHb/Req5L9/k7/X8rfTe1rxXWjrXjaL/Tdr0Pfe+Arm7ykhM88daLE4D1LE5M62RMy4CTEdy8m/bX1rwfQsCtNJ1SJ13WBDBef/6UF8jrm3XSaveIj0wTVrhXjvvsi8UgJ8JVOwpDS4GrGOhFABbt1sjZY1UACQn/+b5peQf6pTlui9IW75tQSySf/3wRUCdzeZ0uf33e9Kyg6S1ibesDktTa+k0BhrK3EAKgOWrrJGyRikAkpO/xpeS//h9LQ7M5h+6AqDiU/7q/TxdI/IFXVqFgP+Z5cX/GyNdxvae1n9KeuhAN8T5ma0I2I5ZgFicBqhTocwAPEoBUDOVD9aFV0sTD7cgMP4hPyvfYkGsNBIEwlEuBAYn36Rl8PdVWvzPLC+jsfN7Ki/+PVSn+27psaOkVe+3FYGa8irXQ862AI0glAIgYQag+mOsSvVeAFS+snr+f7sq/+UWBMZ/JntH8k/8QKjfGUbbiaOYBifvnZe8+fcwsmJg04XS6g9aEKj2Z1tjCK4DqFO7LbBGzjgFMHaVk//cb4T7gB/PJ/+ev1sQK4ROH4gzuBiobOP50pqPWBCgCc+wBupM7MzwvntZI2cJBUDN+/x6LQAqJ//ZX5Cmx99lIQh+anT7DRbEGr5jBcIw/IzAhs+7IuBsCwLT7u+7gUawz2KptdWCHG3aLG3O6Oab9VgAVE7+M11HM/MMCwK07tzS1Ggykj+KpjwjkGzDOdKW6ywISHviDEDsCBKFEckRgY/+U1FvBUDl5D/9VDf6r/l1FLWz8SJXAJxlQayA3zwwrMqzAetd8Rua5mlS2wEWoJ6FUgBk9QkAr54KgMrJf+qJ0tzzLQjQlh9Jqys+3td3nJz3R9H5fTi+CPCPQN54qQUB8c87iErua1BIzAAUV+XkP+U4aX6AHUvZ9tukFcdbEIvkj3qSvC+HOAvQtsQaqBOxp28ODWSi5+HHrJGBeikAkpP/xJeFfYvfrr9Jj/nk31eKo0j+qEfx1wT0PiRt+C8LAtFKAVBnIvli8ULpyXtakLO/uJSQlXooAJLv8td+mLTwRxYEqHe1S/6vk/orzvmQ/FGv4k8FbL/ZGoGgAKh7zz/UGgH4c3wBEH+sjFHRC4AKD/c5oJT8Q31Ged9Wl/xfLfXcYyticcU/6ll8cRtaAcApgHoSO/3//EBu9/CYGwtyEWB1kpN/y+JS8m+ZaysC4x/t+9gxUufvbEUskj8aQXRkM7BF2vYrCwLQPMsaqFehzAAkjP69VGaCi1oAJCf/Jnew+uQfctW+3I38O26xIFYq0z1AgMKfBWiaaA3Ugcj5//32kvbczYKcZXn+3ytiAVDhyX5tpeTffrDFAfLn/Lf/xIJYXPQHdPzWGqGgCKgDsdP/r3mpNQJAAVBZ7AZ8nE/+E59nQYBWnCBtrfiJBJI/4PWttEYgQr2WCCMR+2mx1xxhjQBkeQGgV6QCoPJn/UN+sp+36r3Slu9YEIvkj0YV7eBCKwDGMQNQjw58snTwfhbkbNUa6eEVFmSkKAVA5eQ/96Kwn+y3+jRp09ctiEXyRyOL7vv9m9zSYUEAmAEouuCn/7O+ANArQgFQOfnP/pI0/SQLArT6Q9LGr1gQi+QPxOnN8PNQw+nfYg0UVPDT/9fn8MGX0AuAysl/pvvSzNMtCNDqM13y/7IFsUj+QJKQTgNQABRZ7Oj/uJdJ+z/JggBc/2trZCjkAqBy8p9+mhv9x27XMKz5qEv+X7QgFskfqMTfLyMEAwNu2WoBCig2j5z8emsE4Pa7pIfinwEQvT6mhkItACon/6nvlOaeZ0GA1pwlbficBbFI/sBwmudYI2f9JP8Cix0lvuBQ6YXPsiAANyRP/6eaJ0IsACon/8mvk+ZfYkGA1rrttaHiE81I/kA1gikAmP4vqMRccnJg14xff5s1MhZiAZCc/CceJS38HwsCtNbl9vUVZ2xI/kBVWtz/Zlg7ZxQARRWbS577NOn4oy0IwAMPS3fca8FQFZNJLYRWACTf5a/9uaUb/YRq3edc8q+Y20n+QLzoNG0oo3+vd7k1UCCxU//epz9gjUDkcfV/WUgFQHLybzuwlPyb2m1FYNZf4AqAj1oQi+QPJIuO1EIqAHoesAYKInHq/53HhnXu37sh+er/1HNGKAVAcvJv2dMl/6vda0AdwmAbL5bWnmpBLJI/MFITA/qAds+/rIGCiE3+E9348dPvtyAQ/3C71i8qPhQ2XSEUAMnJv2l2Kfm37WUrArPpcmn1uy2IRfIHKoufqm3Z1RoB6GYGoEAS88kXzpAWzLMgEJf+wBpRqZ//9/IuAJKTv8aXpv3bD7I4MJt/KK16qwWJSP5j5xOEX/y+Mpal/HMQlviLfie/whoBiJ8ByKSDxoj44zzWScdL73uTBYHo6pIuSS4AMskdeRYAlTvjHU/2O9yCwGy9Xlp5nAWJxtkrRm5wwvcJIj5JjEz55wwuCBCi8YeGNevHKYAiSEz+z3qqdFGA5Zof/W/eZsFQmb3bvAoA3/kmd+rzr3QjgIA+pzHYtlulx4YdnZD8R27npJ82ioH8xXfak15pjQB03eveZeyNgJjdC0O534g1YbxLtOdYEJgK0/+Z7Vt5FACVk//ci6WpAd2jcbCO30vLX2xBIpL/yFXeJ9Ln/22KgGwl/71Dmv7v+K01EKCK/UaLy27XXCg9JaD7/Zf5K//vvM+CHGVdAFTu6Gd/WZr+LgsC03mn9MhzLEhE8h+5rEb8w/HvIXEkkQF/bAxe6l38Np90jNR+sAUB6PiNNRCYiv2GT/4/vUg6MtCzyHlf/FeWZcKqnPxnftIVAIHOqnW5Uu3hfSxIRPIfueoSbvNCafyBbnlqaWl1JX3TZLdMcn91t/hXNbuftq103/Z+9+rbPQ+6bXeXW1zx5l97Hy79vOFltS0rHxNP8J1CPU05J2/33e9123g/CwKwdHe33yyzYIjR7COR3/sEV+9cVvGxIek79VzpgsstGCrEPm3YYyb05P/ne6RDjrUgKtO/eVYzAJU32vQPhpv8e9zBT/JPwzDJf4I09V3Srr+W9lruXm+Q5riecuob3Zee7pKE2yati9zRPtPtxePd0uJqgGlu3S7ua65A8KPIKa8pPTFyl2ulPR+SFv3O/ff+Y5vD3lCqusJkbPy/UU3y9/z3+e/3iz+Wiiz5b+sf7x1S8u+6Pyn58wmA7Pn9fthjZrEbK9x4abjJ3/vM160Rlfl+lVUBkLzRpr1Xmlvxmfn56V0jPTjsCSSS/8hVTmIzPi4tWS/Nv1ia+DxbWQMTnu1+5jdKP9v/G5WlmWjH8rPLxUARC4Hk5N/qCrrQHu+dfP6fCwCz43eKYRO/96oXSrf/QHrJsGdq83PdLdKP3ZIg8/0qiwIg+aie+g5p3n9aEJi+rS75L3GNrlIcj+Q/OvEHc/MCaYEbrc85x+2ZKd72uWlC6d/Y5WaXePa1lRHDdjhjUIufXaRCoNyJJ5sd4KXaWwJ+8Fj9qzrxe2e9R7rWjaznzrIVgfrMf1kjKpdZpSwKgPgNOPFlbjT2TQsCM9Dvkv9e7nWzrYhF8h+d+IQ1rtUl5F9IU15lKzIw6cXu3/yJa7iCIF4ayTX+ZzbNdL/7m6RZn3fv6zW2siqhFwL+fVXuxOde4n7311oQCH/dT8dNFiAjfl/x+3LVif8ZB0g3XOQSa8W7sYfhv/5b+lP8U/+8XGaV0i4A4qt+f1HXvEstCNDSxVL/agsSlXfUelj8gZdVAok/sOe4o7h9fwsy5G84M/erFkRU1QmNUPRnjpsi7fYnacH3XAFwpitKrpYWPyhNP819sepD1P/cwdszb+XOvPLfcI4bEk1/pwUB2eJ663ic/6+dwQl/+H1lkFnTpK+dVZryP+r5tjJgnZ3hjf69NAuA5E7IJ39/sVaIlu4t9T1iQcPwB15+CWSqSwDT325BDnwCmpThzMPOZrqk37aHBabNFaFzz5P2eNS9vw+5FSM6VPPaloM79OE78zlfkWacbEFgNl9hjQjO/1fP7w+D94mdl6oTfll7m3S66yr+dZP072+2lQXgk//KtRZE5bZPpVkAxG/cyW90y1EWBOZBNwLt5cEfjt92aSSO+J85OfkzMZmZktib1PLvEP+zpp1kjRitC1wh8MVSITDjY+6InWlfqNrgYqC81Op38j/HL+WfW12H3jzD/U4Xud/nFFsRmK0/c/0At/91Bu8zo1n8/lDdPjGMBbOlc9zusuK30pc+7A6ZqfaFAvjDX6TPXmxBVK4zSmmfAoiaFWgBvezpUk/yCZoGlEYREO0Mmue7AuBIC3I09bjSe4mqSQeWqP25UstcCyrwhcCcc6W9VrvXC6W2MZ0u8b9TXIc90sX/nJH9faa64dvu/5CmVyh68rYx8cJkpv8z9uJnSf95tvSYS/wfP9ntNgVK/F5/n/SeT1oQL9eEmFYBEJ84/AjGf0Y7NMsOkzrvsACD+M691kXAUJMDuvgreRagVqLJcvwh1qjSuGZ3HL1PWvzX0kWTPqEOf1+D/LXuJy28Tpr/reoKnrxs/bm0/acWRDD9n7Kpk6TXHy1993PS2t9LN39bem9gT/EbCZ/870q+5W/uBWVaV7L70cFQzbtIeyx1JUebrQhE96Pu3fpHMvW7xb1t/wmActu/xsbWjo0HtSOxaz/+83aO7fuGxBnodb//tpukrj/Yili12k+iv9T875dG3yHYcrW0IvZ0RHq/vx/N+4Q+Fv1d7r1f6bahG1lv+pL7V9ywIxQTj3KFldu+03K8xmMkHnmx1HGrBUP4znqsBUBk+wd+J8BUTZ4gHezqwoP3feL1wMRP5RaPv93vu862IF7unyTLrgCYfoo09ysWIDjrXC+w7iwLItJLgLvf70bBe1uQM3/nt4efbMEQ6f3+C37kEuSrLaiB/h43gr3FLS6Jdfolh5mt9ue43v34UuJvXWgrC2DLNa4ATPwIZi32gbovAMa3lp7A1+6WCe2l17kzpcVu/Le7W/xrub3nIvuP6tDdrhY/xO1KvX4sFy/35O9lVwAs+KHrEAL7rC+GWvVeN4KMvU9lLUY//lTC0CnwpmnSko0WBOKfk93eG3lId3oFwMIbXbJ8mQUp6FnpCps/Sd2uR+r++xOv/RvsG8aoZS/X6/vnNJSXp0Y/0VAUDz87aSasFvu/F2QBMBp+YtOf3+53Ca7PT1y62Cf7cdlfVRakw14v/e5OC6JqtT+NWRoFQLSj9/ZcFfa5P7ijuVP6l9tGA1tsxRBj3Vei+0X786Tdfm1BIOKTQGoJYMezDmp5u+Nq9SwvFQP9blsPbHev/kFK/iFK1vavA25/GOcfulRe/MOXrN3shnU+2TdPtx9YcBsukNYk3k0mtQKwqAUAkr3H9XIXfd+CqGCSv5dGARDt5NoOkBbfbQGCtvJEafO3LBhirPtKdL/wz4EI7VbQy12PvO1aCx6XXgGw6I/ShEMtQC66H3CF335u6/TYiiFq2WFTANS5D/4/6fzvWBAvjZw7atlM2EwI+NFMGCrLG+L4R/mGZtxEawwRndGqlXEFuIK/3q05Iyn5e8GM1hC2s79SrOTvZVMAjD/YGgjehGdZIwNNsck2X36aO0v+wUTIz8ZLpW0/tiDCj/6BYX3uYumcb1gQL8h9KZsCoCX2BisIUcs8qTX2Svja3w8gfrSdr8zfU4u9InN+6n+tv81yIkb/GNbXLpc+ep4F8YI67z9YNgWAf8wriqN5tjVSxgwA8rTyrVL/JgsigpuuRXi+9UPpA+daEC/Y5O8xA4CorAqAEGcAYq7TQx1a4ZJ/5+8tiGDqH8Py5/xPTLx1yg5BJ38vmwJg3HhroBCaZlhjiBQuhOu214D0b7dGRpIvPkNa1n5K2lLxzjdM/SNRV5f0+tOGPefvBb8fZVQAcKVzoWR1YVrWybYa/vPvWcr632t0m66Q1ld8OgtT/0h0z/3Sc94gXXWjrUhWiP2IGQBEZVWw+RvOhCbroiTEIqhebbxEWlXxgU9M/SPRj2+SDnPJ/89/sxXJClNEZlQANFsDhTAuoxmAEEe/8e8pvcTQt8oaSNX6C6TVFR9BHPz5WuTn0xdKr36/tLnymMXvQ4WaQcqmABjosgYKIavtFeQMQOJV4enofcwaSM26z0prE2/z65H8Eetnt0kHH+N2DlcADKOQ+1BGBUCAF3shmb8HfO1FR9E9D1kjIF33WiMj/nHMSM/qM10B8HELEpH8McQGNw7w9/Q/6iTpzn/YymSFLSCZAUBUOgVAVHdgz4foWSH1r7ZgiPQO7t5l1kBNdS+VHnmptPGLtiIRF/1hiMuulvY9quIDfQbz+09hC8hsCoCsp1UxNulsr+hB0vuwW9ZbEIDu4a/uGaPoLMi2n1sDNbPF9eDLDpU6brYViUj+eNwvfiu94t3SOz4urRq+W/LHcuH3n2wKgO5/WQOF0LfOGkNEk1ctdP/VGgHoznj63+tf64qAmyzAmK1xvfeKY93ftWIPnlfnHTmG1m20BnLjr+5/0VulI98pXV/d08n9dqyL00bZFAA9S62BQogvANKx7RfWCMCWH1kjNfGdxubKjxBDFfy2e3A/acNnbUWioDrvNQFNgDWaK66Tnnlc6er+X/7RVlZWLhzrIvl7Gc0A/N0aKIT4C9PS2em3fM8aOev6m9QZW/5HRm01t+WK0pXqGLmu+9yI/3i3vNYNNIbtZ4IbuT203BrIxKbN0sVXSfu/QnrzmdIfq5+ArJtR/2BpFADRDnPbDdZA8Hrd6L/y9OlYRPcNfxHclmstyNHm/7ZGRK0P+viCwl+pvv58CzCsvq3SWvenfHgft//8wFZWFEIHHvn3V7rD7e8PWIDU/PBn0vGnStOfIb3bbYV7q/+b+/2mrkb9g2UzA9C7VOq4wwIErfMP1khF/EG06ZvWyEnPo+49fN2C1CV3JGs/KD1ypLT1p7YCEX3b3N/pM9LSRa5gqqpPDr4DvyXVQ65x3fI7l+w/Ic04VDrOJf8fuCJgBOo68ZeldSFM9JFqk4+XFl5lAYK14u1uRPVtC4ao1b4S/7i9GW4EPOccCzK2/BhpW+wsRFqjxk+5pfLDldqe5oYr75amvtWV6TxLY0fi33CetPHLI/mUSgij/p1F9v89dnH1zC0WYNTWbZB+eXtpufE26cHRnV4JcZ9JTXYFgLfgGmmK62wRpt41riea5xqxm69W+0py8pvvCsSprlDM0nqXVNaebkFEWseHN3wRsMNE9zd5c2mZeLitayDbbnYFqdsvtnzf7ZabbeWwQu7EY7f7x1ytd+5pFqAq/ql8v7KE75c/3mNfGJ2GSvxlaXVwyZ3bLDfKmzXsnbmQh9UuEW50CTGq1gdHfIHoTT9DmvsFC1Lk7z+w+gPS1itsRaw0CwCvyiLAtB3gioCXlpZJR7h3l80ZvMx1/rmU8H3i7x3x3SLT3mZjlbjNr/ii9MZXWoAh/Oj+vgefWP7011LS77evj0FDJv6yNA+W5E6+eb4b0bxdGn+Q69T2dvEs904mKbPH0OIJA32uk31U2vBlaXPiufha7yeVE1/7c10h8D63j7zeVtRQ30Zp03fc7+t6276Kc4RZJZKRFQGPa7dC4CWueZg04RBbX0B+H/T3Qthuy+juDVGkjjyxb3zpc9wv4Xb9wwq8OUdrgzs0H1sjPeS6I5/k/zEo4a+q7SeTGzrpD5ZmJzfKjg0BSmM/GX7/2FEovs0luiNdsXig1DLTvjBCPY9IXXe5EeXVbvGfuU+uTU0eHcTYjpdx010R4AqnHYsrCNqf4QrqNvtigPwzF/z0fjnpa9TPCyliZz7stj7xtdJTlkhLdpf22k3afaF9IUD9bhje3eMOs1632Gv3oHb5tdNt4hWrXZJ3ywqX6H2y9/GOda7dke4d40n6MdIe5Qzb0yJ4ae4jI9s/Wha7QuCp7nWRS26T3Tvzs0Z+ce0B18MMbHO9kS0DW10v9E+XaO52setdqpd3R1G7wrl1H6ltP7fs6/5u/tWWLAuDvs2l4stvhx2vtmjMvX3RO3QGSOkj6Q8ji2lOdvTiyuIACmn/CK3DSOdv0zRbap7nCqn5O726Zdx4W9qjbfW5wqqztPT7V5fEy3GfK7J6V7jXlaXXcrt/Q+nfrI1669DpG2uLhB8ov6P70R5LcRa/zbISwv6R5e87Go16DIW+XcaqUbdrLZZ63zfqEjt82EueB1Ue+0YRO5F6P4aKuE3Gqt636ViWRtwfgIaWZodYbx2K/32KnEDK7x/F35YjXdj2AKoy0s6xkTuXkf6tslwadZsAQcniIkAA4Skn4TQuQvMXY3lckAUAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAANAIpP8P3sak7cyUXx0AAAAASUVORK5CYII="
header,encoded = iconDataUri.split(",",1)
iconDataUri = b64decode(encoded)
open("image.png","wb").write(iconDataUri)
pygame.display.set_icon(pygame.image.load("image.png"))
os.remove("image.png")
else:
pygame.display.set_icon(pygame.image.load(icon[0]))
def update(self):
global showMouse
if pgEvents() == "QUIT":
quit()
if showMouse == False:
pygame.mouse.set_visible(False)
if showMouse == True:
pygame.mouse.set_visible(True)
pygame.display.update()
clock.tick(self.hz)
class Sky:
def __init__(self,win,color):
self.color = color
self.win = win
def draw(self):
self.win.screen.fill(self.color)
class ImgSky:
def __init__(self,win,img):
self.win = win
self.img = pygame.image.load(img)
def draw(self):
self.img = pygame.transform.scale(self.img,(self.win.w,self.win.h))
self.win.screen.blit(self.img,(0,0))
class Ground:
def __init__(self,win,color,h):
self.win = win
self.color = color
self.h = h
def draw(self):
pygame.draw.rect(self.win.screen,self.color,(0,self.win.h-self.h,self.win.w,self.h))
class Obj:
def __init__(self,win,x,y,z,w,h,img,fixy=1,collision=False,hboxsize=1):
self.win = win
self.x = x
self.y = y
self.imga = img
self.fixy = fixy
self.z = z
self.w = w
self.h = h
self.y = self.y/fixy
self.img = pygame.image.load(img)
self.img = pygame.transform.scale(self.img,(self.w,self.h))
self.collision = collision
self.enabled = True
self.hboxsize = hboxsize
def draw(self):
if self.enabled:
global playerZ,playerX,camRot,playerSpeed
self.x += camVel[0]
if self.w+playerZ-self.z < 1:
return
if self.h+playerZ-self.z < 1:
return
if self.w+playerZ-self.z > 799:
return
if self.h+playerZ-self.z > 799:
return
if self.x > self.win.w + self.win.w/2:
self.x = 0 - self.win.w - self.win.w/2
if self.x < 0 - self.win.w - self.win.w/2:
self.x = self.win.w + self.win.w/2
if self.collision:
dis = math.sqrt(math.pow(self.x-int(playerZ)+playerX-playerZ,2))
if dis < self.hboxsize:
playerZ -= playerSpeed
self.img = pygame.image.load(self.imga)
self.img = pygame.transform.scale(self.img,(self.w+playerZ-self.z,self.h+playerZ-self.z))
self.win.screen.blit(self.img,(self.x-int(playerZ)+playerX,self.win.h-self.h-self.y-playerZ))
def enable(self):
self.enabled = True
def disable(self):
self.enabled = False
def getDistance(self):
global playerX,playerZ
return math.sqrt(math.pow(self.x-int(playerZ)+playerX-playerZ,2))
class FPPlayer:
def __init__(self,win,speed=5):
global lockMouse
self.win = win
self.speed = speed
lockMouse = True
def work(self):
global playerZ,playerX
global playerSpeed
global camRot,camVel,camSens
global lockMouse,mouseSpeed,mouseRightDown
mx,my = pygame.mouse.get_pos()
playerSpeed = self.speed
playerZ += playerVel[2]
playerX += playerVel[0]
camRot[0] += camVel[0]
camRot[1] += camVel[1]
camRot[2] += camVel[2]
if camRot[0] < -360:
camRot[0] = 360
if camRot[0] > 360:
camRot[0] = -360
if mouseSpeed < 1:
mouseSpeed = 0
if mx < 400:
camVel[0] = int(mouseSpeed/10-1)
if mx > 400:
camVel[0] = -int(mouseSpeed/10-1)
#camVel[0] = int(mouseSpeed/10-1)
if lockMouse == True:
pygame.mouse.set_pos(self.win.w/2,self.win.h/2)
pygame.event.set_grab(True)
def unlockMouse(self):
global lockMouse
lockMouse = False
pygame.event.set_grab(False)
def lockMouse(self):
global lockMouse
lockMouse = True
pygame.event.set_grab(True)
def setSens(self,sens):
global camSens
camSens = sens
class TPPlayer:
def __init__(self,win,speed,charimg,w,h,x,y):
self.win = win
self.speed = speed
self.charimg = pygame.image.load(charimg)
self.w = w
self.h = h
self.x = x
self.y = y
self.charimg = pygame.transform.scale(self.charimg,(self.w,self.h))
def work(self):
self.win.screen.blit(self.charimg,(self.win.w/2-self.w/2-self.x,self.win.h-self.y))
global playerZ,playerX
global playerSpeed
global camRot,camVel,camSens
global lockMouse,mouseSpeed,mouseRightDown
playerSpeed = self.speed
playerZ += playerVel[2]
playerX += playerVel[0]
camRot[0] += camVel[0]
camRot[1] += camVel[1]
camRot[2] += camVel[2]
if camRot[0] < -360:
camRot[0] = 360
if camRot[0] > 360:
camRot[0] = -360
if mouseSpeed < 1:
mouseSpeed = 0
if mouseRightDown:
mx,my = pygame.mouse.get_pos()
if mx < 400:
camVel[0] = int(mouseSpeed/2-1)
if mx > 400:
camVel[0] = -int(mouseSpeed/2-1)
if lockMouse == True:
pygame.mouse.set_pos(self.win.w/2,self.win.h/2)
pygame.event.set_grab(True)
def lockMouse(self):
global lockMouse
lockMouse = True
pygame.event.set_grab(True)
def unlockMouse(self):
global lockMouse
lockMouse = False
pygame.event.set_grab(False)
def setSens(self,sens):
global camSens
camSens = sens
class Text:
def __init__(self,win,x,y,text="Sample Text",size=30,font="Comic Sans MS",color=(255,255,255)):
self.text = text
self.size = size
self.font = font
self.color = color
self.x = x
self.y = y
self.win = win
self.enabled = True
def draw(self):
if self.enabled:
font = pygame.font.SysFont(self.font,self.size)
text = font.render(self.text,False,self.color)
self.win.screen.blit(text,(self.x,self.y))
def enable(self):
self.enabled = True
def disable(self):
self.enabled = False
class Button:
def __init__(self,win,x=100,y=100,fg=(255,255,255),bg=(40,40,40),text="Button",w=150,h=75,size=30,font="Comic Sans MS"):
self.win = win
self.x = x
self.y = y
self.fg = fg
self.bg = bg
self.text = text
self.size = size
self.w = w
self.h = h
self.font = font
self.enabled = True
def draw(self):
if self.enabled:
pygame.draw.rect(self.win.screen,self.bg,(self.x,self.y,self.w,self.h))
font = pygame.font.SysFont(self.font,self.size)
text = font.render(self.text,False,self.fg)
self.win.screen.blit(text,(self.x,self.y))
def onclick(self,function):
global mouseLeftDown
if self.enabled:
if mouseLeftDown:
mx,my = pygame.mouse.get_pos()
if mx > self.x and mx < self.x + self.w and my > self.y and my < self.y + self.h:
function()
def enable(self):
self.enabled = True
def disable(self):
self.enabled = False
class Frame:
def __init__(self,win,x,y,w,h,color=(40,40,40)):
self.win = win
self.x = x
self.y = y
self.color = color
self.w = w
self.h = h
self.enabled = True
def draw(self):
if self.enabled:
pygame.draw.rect(self.win.screen,self.color,(self.x,self.y,self.w,self.h))
def enable(self):
self.enabled = True
def disable(self):
self.enabled = False
class Crosshair:
def __init__(self,win,img,w,h,followmouse=False,showmouse=False):
self.win = win
self.w = w
self.h = h
self.img = pygame.image.load(img)
self.img = pygame.transform.scale(self.img,(self.w,self.h))
self.fmouse = followmouse
self.x = self.win.w/2-w/2
self.y = self.win.h/2-h/2
self.smouse = showmouse
self.enabled = True
def draw(self):
if self.enabled:
if self.smouse == False:
pygame.mouse.set_cursor((8,8),(0,0),(0,0,0,0,0,0,0,0),(0,0,0,0,0,0,0,0))
if self.fmouse == True:
mx,my = pygame.mouse.get_pos()
self.x = mx - self.w/2
self.y = my - self.h/2
else:
self.x = self.win.w/2-self.w/2
self.y = self.win.h/2-self.h/2
self.win.screen.blit(self.img,(self.x,self.y))
def enable(self):
self.enabled = True
def disable(self):
self.enabled = False
class Image:
def __init__(self,win,img,x,y,w,h):
self.win = win
self.img = img
self.w = w
self.h = h
self.img = pygame.image.load(self.img)
self.img = pygame.transform.scale(self.img,(self.w,self.h))
self.x = x
self.y = y
self.enabled = True
def draw(self):
if self.enabled:
self.win.screen.blit(self.img,(self.x,self.y))
def enable(self):
self.enabled = True
def disable(self):
self.enabled = False
class KeyboardListener:
def __init__(self,key):
self.key = key
self.enabled = True
def listen(self):
if self.enabled:
if keyboard.is_pressed(self.key):
return True
def enable(self):
self.enabled = True
def disable(self):
self.enabled = False | 2.5D | /2.5D-0.0.2.tar.gz/2.5D-0.0.2/d25/__init__.py | __init__.py |
# Trabalho individual de GCES 2022-2
Os conhecimentos de Gestão de Configuração de Software são fundamentais no ciclo de vida de um produto de software. As técnicas para a gestão vão desde o controle de versão, automação de build e de configuração de ambiente, testes automatizados, isolamento do ambiente até o deploy do sistema. Todo este ciclo nos dias de hoje são integrados em um pipeline de DevOps com as etapas de Integração Contínua (CI) e Deploy Contínuo (CD) implementadas e automatizada.
Para exercitar estes conhecimentos, neste trabalho, você deverá aplicar os conceitos estudados ao longo da disciplina no produto de software contido neste repositório.
O sistema se trata de uma biblioteca python para executar pipelines de dados de forma customizável em bancos de dados.
Para executar a aplicação em sua máquina, basta seguir o passo-a-passo descritos abaixo.
# Resumo da aplicação
A biblioteca desenvolvida auxilia desenvolvedores a explorar os dados com funções essenciais para a identificação de outliers e anomalias e uma interface que auxilia a visualizar as informações de acordo com o arquivo de configuração.
A biblioteca recebe um arquivo yaml com as configurações de cada etapa do pipeline de dados, e do endereço do banco de dados.
Após a execução do banco de dados, o banco de dados de dados é atualizado com os resultados da análise e os resultados podem ser visualizados por meio de dashboards no metabase.
# Etapas do Trabalho
O trabalho deve ser elaborado através de etapas. Cada uma das etapas deve ser realizada em um commit separado com o resultado funcional desta etapa.
As etapas de 1 a 3 são relacionadas ao isolamento do ambiente utilizando a ferramenta Docker e Docker Compose. Neste sentido o tutorial abaixo cobre os conceitos fundamentais para o uso destas tecnologias.
[Tutorial de Docker](https://github.com/FGA-GCES/Workshop-Docker-Entrega-01/tree/main/tutorial_docker)
As etapas de 4 e 5 são relacionadas à configuração do pipeline de CI e CD.
[Tutorial CI - Gitlab](https://github.com/FGA-GCES/Workshop-CI-Entrega-02/tree/main/gitlab-ci_tutorial)
## Containerização do Banco
A versão inicial do sistema contém o metabase no backend cujo funcionamento requer uma instalação de um banco de dados Mongo. A primeira etapa do trabalho é de configurar um container somente para o banco de dados com as credenciais especificadas na descrição da aplicação e testar o funcionamento do mesmo.
## Containerização da aplicação + metabase
Nesta etapa, tanto o a aplicação python quanto o metabase/banco deverão estar funcionando em containers individuais.
Deverá ser utilizado um orquestrador (Docker Compose) para gerenciar comunicação entre os containers além do uso de credenciais, networks, volumes, entre outras configurações necessárias para a correta execução da aplicação.
## Gestão de dependencias e pacotes python
Configurar o gerenciador de dependencias e pacotes python, o poetry, para gerar um pacote pip da solução. Publicar a biblioteca
https://python-poetry.org
## Documentação automatizada
Gerar a documentação da biblioteca de forma automatizada utilizando o doxygen para gerar informacoes da biblioteca e o sphinx para criar documentação https://www.sphinx-doc.org
## Integração Contínua (CI)
Para a realização desta etapa, a aplicação já deverá ter seu ambiente completamente containerizado.
Deverá ser utilizada uma ferramenta de Integração Contínua para garantir o build, os testes e o deploy para o https://pypi.org .
Esta etapa do trabalho poderá ser realizada utilizado os ambientes de CI do GitLab-CI ou Github Actions.
Requisitos da configuração da Integração Contínua (Gitlab ou Github) incluem:
Build (Poetry)
Test - unitários
Lint -
Documentação (sphinx)
## Avaliação
A avaliação do trabalho será feita à partir da correta implementação de cada etapa. A avaliação será feita de maneira **quantitativa** (se foi realizado a implementação + documentação), e **qualitativa** (como foi implementado, entendimento dos conceitos na prática, complexidade da solução). Para isso, faça os **commits atômicos, bem documentados, completos** a fim de facilitar o entendimento e avaliação do seu trabalho. Lembrando o trabalho é individual.
**Observações**:
1. A data final de entrega do trabalho é o dia 28/01/2023;
2. O trabalho deve ser desenvolvido em um **repositório PESSOAL e PRIVADO** que deverá ser tornado público somente após a data de entrega do trabalho (no dia 28/01/2023);
3. Cada etapa do trabalho deverá ser entregue em commits progressivos (pendendo ser mais de um commit por etapa);
4. Os **commits devem estar espaçados em dias ao longo do desenvolvimento do trabalho**. Commits feitos todos juntos na data de entrega não serão descontados da nota final.
| Item | Peso |
|---|---|
| 1. Containerização do Banco | 1.0 |
| 2. Containerização da biblioteca + Banco | 1.5 |
| 3. Publicação da biblioteca | 1.5 |
| 4. Documentação automatiza | 1.5 |
| 5. Integração Contínua (Build, Test, Lint, documentacao) | 3.0 |
| 6. Deploy Contínuo | 1.5 |
## Exemplo de Trabalhos Anteriores
Alguns trabalhos de trabalhos anteriores:
- [2020/2](https://github.com/FGA-GCES/Trabalho-Individual-2020-2)
- [2021/1](https://github.com/FGA-GCES/Workshop-Docker-Entrega-01)
- [2021/2](https://github.com/FGA-GCES/Trabalho-Individual-2021-2)
### Requisitos de instação
```
python -m venv env
source env/bin/activate
pip install -r requirements.txt
```
### Rodando a aplicação
```
python src/main.py
```
### Testando
```
pytest --cov
```
### Metabase
O metabase ajuda a visualizar e a modelar o processamento dos dados, a engenharia de features e monitoramento do modelo.
| Keywords | Descrição |
|-----------|-------------|
| CSV | Um arquivo CSV é um arquivo de texto simples que armazena informações de tabelas e planilhas. Os arquivos CSV podem ser facilmente importados e exportados usando programas que armazenam dados em tabelas.|
| Collection (coleção)| Uma coleção é um agrupamento de documentos do MongoDB. Os documentos dentro de uma coleção podem ter campos diferentes. Uma coleção é o equivalente a uma tabela em um sistema de banco de dados relacional.|
| Database | Um banco de dados armazena uma ou mais coleções de documentos.|
| Mongo| É um banco de dados NoSQL desenvolvido pela MongoDB Inc. O banco de dados MongoDB foi criado para armazenar uma grande quantidade de dados e também executar rapidamente.|
**Connect the database to the metabase**
- step 1: Open localhost:3000
- step 2: Click Admin setting
- step 3: Click Database
- step 4: Adicione os dados de autenticação de banco de dados
**Exemplo da conexão mongo metabase**
| metabase | credential |
|------------|-------------|
| host | mongo |
|dabase_name | use the name you define in make migrate|
| user | lappis |
| password | lappis |
| 2022-2-gces-ifpf | /2022_2_gces_ifpf-0.3.0.tar.gz/2022_2_gces_ifpf-0.3.0/README.md | README.md |
from statistics import mean
class KeySmash:
"""A class for calculating metrics to indicate key smashing behavior in a text.
Key smashing is the act of typing on a keyboard in a rapid and uncontrolled manner,
often resulting in a series of random characters being entered into a document or text field.
"""
def __init__(self):
self.char_sets = {
"vowels": 'aeiouáéíóúãõ',
"consonants": 'bcdfghjklmnñpqrstvwxyz',
"special_characters": '!@#$%^¨|\'\"&*()_+:;~`´]}{[}ºª=-.¿¡'
}
def calculate_char_frequency_metric(self, text):
"""
Calculate the Char Frequency Metric.
Parameters
----------
text : str
The text to use for the calculation.
Returns
-------
float
Char Frequency Metric.
Examples
--------
>>> calculate_char_frequency_metric("PUENTECILLA KM. 1.7")
1.121212121212121
>>> calculate_char_frequency_metric("ASDASD XXXX")
3.0
"""
word_results = []
for w in text.split(' '):
char_count = []
if w and len(w) > 0:
for e in set(w):
char_count.append(w.count(e)**2)
word_results.append(sum(char_count) / len(w))
if word_results == 0 or len(word_results) == 0:
return 0
else:
return mean(word_results)
def calculate_irregular_sequence_metric(self, text, opt):
"""
Calculate the Irregular Sequence Metric.
Parameters
----------
text : str
The text to use for the calculation.
opt : str
The type of characters to consider for the calculation,
can be one of 'vowels', 'consonants', or 'special_characters'.
Returns
-------
float
Irregular Sequence Metric.
Examples
--------
>>> calculate_irregular_sequence_metric("PUENTECILLA KM. 1.7", "vowels")
0.21052631578947367
>>> calculate_irregular_sequence_metric("ASDASD XXXX", "consonants")
2.1818181818181817
>>> calculate_irregular_sequence_metric("!@#$% ASDFGHJKL", "special_characters")
1.5625
"""
count_sequence = 1
sequence_regex = []
text = str(text).lower()
opt = self.char_sets[opt]
for i in range(len(text) - 1):
if text[i] in opt and text[i + 1] in opt:
count_sequence = count_sequence + 1
else:
if (count_sequence != 1):
sequence_regex.append(count_sequence**2)
count_sequence = 1
if (count_sequence != 1):
sequence_regex.append(count_sequence**2)
return sum(sequence_regex) / len(text)
def calculate_number_count_metric(self, text):
"""
Calculate the Number Count Metric.
Parameters
----------
text : str
The text field to use for the calculation.
Returns
-------
float
Number Count Metric.
Examples
--------
>>> calculate_number_count_metric("ABC 123 !@#")
0.0
>>> calculate_number_count_metric("ABC123 !@#")
0.9
"""
text_list = text.split()
calc_num_line = 0
if text_list:
for word in text_list:
if any(char.isdigit() for char in word) and any(not char.isdigit() for char in word):
num = len([char for char in word if char.isdigit()])
calc_num = num**2
calc_num_line += calc_num
return calc_num_line / len(' '.join(text_list))
return 0 | 2022-2-gces-ifpf | /2022_2_gces_ifpf-0.3.0.tar.gz/2022_2_gces_ifpf-0.3.0/src/data_pipeline/feature_engineering/key_smash.py | key_smash.py |
from parser.parser_base import ParserBase
class FeatureEngineeringParser(ParserBase):
def parse(self, data: list):
return self._parse_feature_engineering_configs(data)
def _parse_feature_engineering_configs(self, data: list):
if (not data):
return
configs = []
columns_set_alias = []
for inputs in data:
input = self._try_get(inputs, 'input')
# columns
columns_set, columns_alias = self._get_dataframe(self._try_get(input, 'columns'))
columns_set_alias = columns_alias + columns_set_alias
# features
word_embedding, keyboard_smash = self._get_features_details(self._try_get(input, 'features'))
data_lang, dimensions = self._get_word_embedding_config(word_embedding, columns_set_alias)
# Enabled features
enabled_features = keyboard_smash
if (not dimensions):
enabled_features['word_embedding'] = False
else:
enabled_features['word_embedding'] = True
configs.append({
'columns_alias': columns_alias,
'columns_set': columns_set,
'data_lang': data_lang,
'dimensions': dimensions,
'enabled_features': enabled_features
})
return configs, columns_set_alias
def _get_dataframe(self, columns: dict):
if (not columns):
return
columns_alias = []
for column in columns:
for key in column.keys():
columns_alias.append(key)
return columns, columns_alias
def _get_features_details(self, features: dict):
if (not features):
return
word_embedding = self._try_get(features, 'word_embedding')
if (word_embedding == 'off'):
word_embedding = False
keyboard_smash = self._get_keyboard_smash_config(features)
return word_embedding, keyboard_smash
def _get_word_embedding_config(self, feature: dict, columns_alias: list):
if (not feature):
return 'es', None
data_lang = self._get(feature, 'data_lang', 'es')
if ('data_lang' in feature):
del feature['data_lang']
dimensions = {}
dimensions_default_value = 25
for key, item in feature.items():
if (not (key in columns_alias)):
error_msg = f'Label {key} not match'
raise ValueError(error_msg)
dimensions[key] = self._get(item, 'dimensions', dimensions_default_value)
for name in columns_alias:
if (not (name in dimensions)):
dimensions[name] = dimensions_default_value
return data_lang, dimensions
def _get_keyboard_smash_config(self, features: dict):
keyboard_smash_default_value = {
'ksmash_sequence_vowels': True,
'ksmash_sequence_consonants': True,
'ksmash_sequence_special_characters': True,
'ksmash_numbers': True,
'ksmash_char_frequence': True
}
keyboard_smash = self._get(features, 'keyboard_smash', keyboard_smash_default_value)
if (keyboard_smash == keyboard_smash_default_value):
return keyboard_smash
for key in keyboard_smash.keys():
if (key in keyboard_smash_default_value and keyboard_smash[key] == 'off'):
keyboard_smash_default_value[key] = False
return keyboard_smash_default_value | 2022-2-gces-ifpf | /2022_2_gces_ifpf-0.3.0.tar.gz/2022_2_gces_ifpf-0.3.0/src/parser/feature_engineering_parser.py | feature_engineering_parser.py |
from parser.parser_base import ParserBase
from parser.const import model_type
class ModelParser(ParserBase):
def __init__(self, columns_alias):
self.columns_alias = columns_alias
self.default_keyboard_smash_values = {
'ksmash_sequence_vowels': 1.00,
'ksmash_sequence_consonants': 1.999,
'ksmash_sequence_special_characters': 2.2499,
'ksmash_numbers': 2.9,
'ksmash_char_frequence': 2.78
}
def parse(self, data: list):
return self._parse_modal_configs(data)
def _parse_modal_configs(self, data: list):
if (not data):
return
random_forest = data.get('random_forest')
if (random_forest):
return self.get_random_forest_address_config(random_forest)
def get_random_forest_address_config(self, model: list):
configs = []
for inputs in model:
input = self._try_get(inputs, 'input', 'The inputs should be specified')
type = self._try_get(input, 'type')
if (type == model_type['ADDRESS']):
columns_set_alias = self.get_columns(input)
keyboard_smash, n_estimators, test_size = self.get_thresholds(input, columns_set_alias)
configs.append({
'model': 'keyboard_smash',
'type': model_type['ADDRESS'],
'columns_set_alias': columns_set_alias,
'keyboard_smash': keyboard_smash,
'n_estimators': n_estimators,
'test_size': test_size
})
return configs
def get_columns(self, input):
columns_set_alias = self._try_get(input, 'columns')
for alias in columns_set_alias:
if (not (alias in self.columns_alias)):
raise ValueError(f'`{alias}` column not match with the available columns')
return columns_set_alias
def get_thresholds(self, input, columns_set_alias):
thresholds = self._try_get(input, 'thresholds')
test_size = self._get(thresholds, 'test_size', 0.3)
n_estimators = self._get(thresholds, 'n_estimators', 100)
keyboard_smash_default = self.get_keyboard_smash_default_thresholds(columns_set_alias)
keyboard_smash = self._get(thresholds, 'keyboard_smash', keyboard_smash_default)
for key in keyboard_smash.keys():
if (not (key in columns_set_alias)):
raise ValueError(
f'`{key}` key not match with the available columns')
for alias in columns_set_alias:
if (not (alias in keyboard_smash.keys())):
keyboard_smash.append(
{alias: self.default_keyboard_smash_values})
return keyboard_smash, n_estimators, test_size
def get_keyboard_smash_default_thresholds(self, columns_set_alias):
default_config = []
for alias in columns_set_alias:
default_config.append({alias: self.default_keyboard_smash_values})
return default_config | 2022-2-gces-ifpf | /2022_2_gces_ifpf-0.3.0.tar.gz/2022_2_gces_ifpf-0.3.0/src/parser/model_parser.py | model_parser.py |
import math
import matplotlib.pyplot as plt
from .Generaldistribution import Distribution
class Gaussian(Distribution):
""" Gaussian distribution class for calculating and
visualizing a Gaussian distribution.
Attributes:
mean (float) representing the mean value of the distribution
stdev (float) representing the standard deviation of the distribution
data_list (list of floats) a list of floats extracted from the data file
"""
def __init__(self, mu=0, sigma=1):
Distribution.__init__(self, mu, sigma)
def calculate_mean(self):
"""Function to calculate the mean of the data set.
Args:
None
Returns:
float: mean of the data set
"""
avg = 1.0 * sum(self.data) / len(self.data)
self.mean = avg
return self.mean
def calculate_stdev(self, sample=True):
"""Function to calculate the standard deviation of the data set.
Args:
sample (bool): whether the data represents a sample or population
Returns:
float: standard deviation of the data set
"""
if sample:
n = len(self.data) - 1
else:
n = len(self.data)
mean = self.calculate_mean()
sigma = 0
for d in self.data:
sigma += (d - mean) ** 2
sigma = math.sqrt(sigma / n)
self.stdev = sigma
return self.stdev
def plot_histogram(self):
"""Function to output a histogram of the instance variable data using
matplotlib pyplot library.
Args:
None
Returns:
None
"""
plt.hist(self.data)
plt.title('Histogram of Data')
plt.xlabel('data')
plt.ylabel('count')
def pdf(self, x):
"""Probability density function calculator for the gaussian distribution.
Args:
x (float): point for calculating the probability density function
Returns:
float: probability density function output
"""
return (1.0 / (self.stdev * math.sqrt(2*math.pi))) * math.exp(-0.5*((x - self.mean) / self.stdev) ** 2)
def plot_histogram_pdf(self, n_spaces = 50):
"""Function to plot the normalized histogram of the data and a plot of the
probability density function along the same range
Args:
n_spaces (int): number of data points
Returns:
list: x values for the pdf plot
list: y values for the pdf plot
"""
mu = self.mean
sigma = self.stdev
min_range = min(self.data)
max_range = max(self.data)
# calculates the interval between x values
interval = 1.0 * (max_range - min_range) / n_spaces
x = []
y = []
# calculate the x values to visualize
for i in range(n_spaces):
tmp = min_range + interval*i
x.append(tmp)
y.append(self.pdf(tmp))
# make the plots
fig, axes = plt.subplots(2,sharex=True)
fig.subplots_adjust(hspace=.5)
axes[0].hist(self.data, density=True)
axes[0].set_title('Normed Histogram of Data')
axes[0].set_ylabel('Density')
axes[1].plot(x, y)
axes[1].set_title('Normal Distribution for \n Sample Mean and Sample Standard Deviation')
axes[0].set_ylabel('Density')
plt.show()
return x, y
def __add__(self, other):
"""Function to add together two Gaussian distributions
Args:
other (Gaussian): Gaussian instance
Returns:
Gaussian: Gaussian distribution
"""
result = Gaussian()
result.mean = self.mean + other.mean
result.stdev = math.sqrt(self.stdev ** 2 + other.stdev ** 2)
return result
def __repr__(self):
"""Function to output the characteristics of the Gaussian instance
Args:
None
Returns:
string: characteristics of the Gaussian
"""
return "mean {}, standard deviation {}".format(self.mean, self.stdev) | 2022-distributions | /2022_distributions-0.1.tar.gz/2022_distributions-0.1/2022_distributions/Gaussiandistribution.py | Gaussiandistribution.py |
import math
import matplotlib.pyplot as plt
from .Generaldistribution import Distribution
class Binomial(Distribution):
""" Binomial distribution class for calculating and
visualizing a Binomial distribution.
Attributes:
mean (float) representing the mean value of the distribution
stdev (float) representing the standard deviation of the distribution
data_list (list of floats) a list of floats to be extracted from the data file
p (float) representing the probability of an event occurring
n (int) the total number of trials
TODO: Fill out all TODOs in the functions below
"""
# A binomial distribution is defined by two variables:
# the probability of getting a positive outcome
# the number of trials
# If you know these two values, you can calculate the mean and the standard deviation
#
# For example, if you flip a fair coin 25 times, p = 0.5 and n = 25
# You can then calculate the mean and standard deviation with the following formula:
# mean = p * n
# standard deviation = sqrt(n * p * (1 - p))
#
def __init__(self, prob=.5, size=20):
# TODO: store the probability of the distribution in an instance variable p
self.p = prob
# TODO: store the size of the distribution in an instance variable n
self.n = size
# TODO: Now that you know p and n, you can calculate the mean and standard deviation
# Use the calculate_mean() and calculate_stdev() methods to calculate the
# distribution mean and standard deviation
#
# Then use the init function from the Distribution class to initialize the
# mean and the standard deviation of the distribution
#
# Hint: You need to define the calculate_mean() and calculate_stdev() methods
# farther down in the code starting in line 55.
# The init function can get access to these methods via the self
# variable.
self.mean = self.calculate_mean()#self.n * self.p #calculate_mean(self)
self.stdev = self.calculate_stdev()#math.sqrt(self.n * self.p * (1-self.p))#calculate_stdev(self)
Distribution.__init__(self, self.mean, self.stdev)
def calculate_mean(self):
"""Function to calculate the mean from p and n
Args:
None
Returns:
float: mean of the data set
"""
# TODO: calculate the mean of the Binomial distribution. Store the mean
# via the self variable and also return the new mean value
self.mean = (self.n * self.p)
return self.mean
def calculate_stdev(self):
"""Function to calculate the standard deviation from p and n.
Args:
None
Returns:
float: standard deviation of the data set
"""
# TODO: calculate the standard deviation of the Binomial distribution. Store
# the result in the self standard deviation attribute. Return the value
# of the standard deviation.
self.stdev = math.sqrt(self.n * self.p * (1-self.p))
return self.stdev
def replace_stats_with_data(self):
"""Function to calculate p and n from the data set
Args:
None
Returns:
float: the p value
float: the n value
"""
# TODO: The read_data_file() from the Generaldistribution class can read in a data
# file. Because the Binomaildistribution class inherits from the Generaldistribution class,
# you don't need to re-write this method. However, the method
# doesn't update the mean or standard deviation of
# a distribution. Hence you are going to write a method that calculates n, p, mean and
# standard deviation from a data set and then updates the n, p, mean and stdev attributes.
# Assume that the data is a list of zeros and ones like [0 1 0 1 1 0 1].
#
# Write code that:
# updates the n attribute of the binomial distribution
# updates the p value of the binomial distribution by calculating the
# number of positive trials divided by the total trials
# updates the mean attribute
# updates the standard deviation attribute
#
# Hint: You can use the calculate_mean() and calculate_stdev() methods
# defined previously.
self.n = len(self.data)
self.p = self.data.count(1) / self.n
self.mean = self.calculate_mean()
self.stdev = self.calculate_stdev()
return self.p, self.n
def plot_bar(self):
"""Function to output a histogram of the instance variable data using
matplotlib pyplot library.
Args:
None
Returns:
None
"""
# TODO: Use the matplotlib package to plot a bar chart of the data
# The x-axis should have the value zero or one
# The y-axis should have the count of results for each case
#
# For example, say you have a coin where heads = 1 and tails = 0.
# If you flipped a coin 35 times, and the coin landed on
# heads 20 times and tails 15 times, the bar chart would have two bars:
# 0 on the x-axis and 15 on the y-axis
# 1 on the x-axis and 20 on the y-axis
# Make sure to label the chart with a title, x-axis label and y-axis label
#plt.hist(self.data)
plt.bar(x = ['0', '1'], height = [(1-self.p) * self.n, self.p * self.n])
plt.title("Bar chart of Coin Flips")
plt.xlabel("Heads (1) or Tails (0)")
plt.ylabel("Number of outcomes")
plt.show()
def pdf(self, k):
"""Probability density function calculator for the gaussian distribution.
Args:
k (float): point for calculating the probability density function
Returns:
float: probability density function output
"""
# TODO: Calculate the probability density function for a binomial distribution
# For a binomial distribution with n trials and probability p,
# the probability density function calculates the likelihood of getting
# k positive outcomes.
#
# For example, if you flip a coin n = 60 times, with p = .5,
# what's the likelihood that the coin lands on heads 40 out of 60 times?
bicoef = math.factorial(self.n)/(math.factorial(k)*math.factorial(self.n - k))
mass = (self.p ** k)*(1-self.p)**(self.n - k)
return bicoef * mass
#pass
def plot_bar_pdf(self):
"""Function to plot the pdf of the binomial distribution
Args:
None
Returns:
list: x values for the pdf plot
list: y values for the pdf plot
"""
# TODO: Use a bar chart to plot the probability density function from
# k = 0 to k = n
# Hint: You'll need to use the pdf() method defined above to calculate the
# density function for every value of k.
# Be sure to label the bar chart with a title, x label and y label
# This method should also return the x and y values used to make the chart
# The x and y values should be stored in separate lists
X = [i for i in range(0,self.n+1)]
Y = []
for k in X:
prob = self.pdf(k)
Y.append(prob)
#OR
# for i in range(self.n+1)
# X.append(i)
# Y.append(self.pdf(i)
plt.bar(X,Y)
plt.title("Prob Density Function")
plt.xlabel("k positive outcomes")
plt.ylabel("Probablity of k in n outcomes")
plt.show()
return X, Y
def __add__(self, other):
"""Function to add together two Binomial distributions with equal p
Args:
other (Binomial): Binomial instance
Returns:
Binomial: Binomial distribution
"""
try:
assert self.p == other.p, 'p values are not equal'
except AssertionError as error:
raise
# TODO: Define addition for two binomial distributions. Assume that the
# p values of the two distributions are the same. The formula for
# summing two binomial distributions with different p values is more complicated,
# so you are only expected to implement the case for two distributions with equal p.
# the try, except statement above will raise an exception if the p values are not equal
# Hint: You need to instantiate a new binomial object with the correct n, p,
# mean and standard deviation values. The __add__ method should return this
# new binomial object.
# When adding two binomial distributions, the p value remains the same
# The new n value is the sum of the n values of the two distributions.
sum_n = self.n + other.n
binomial_sum = Binomial(self.p, sum_n)
return binomial_sum
def __repr__(self):
"""Function to output the characteristics of the Binomial instance
Args:
None
Returns:
string: characteristics of the Binomial
"""
# TODO: Define the representation method so that the output looks like
# mean 5, standard deviation 4.5, p .8, n 20
#
# with the values replaced by whatever the actual distributions values are
# The method should return a string in the expected format
combined = "mean {}, standard deviation {}, p {}, n {}".format(self.mean, self.stdev, self.p, self.n)
return combined | 2022-distributions | /2022_distributions-0.1.tar.gz/2022_distributions-0.1/2022_distributions/Binomialdistribution.py | Binomialdistribution.py |
"""A command line tool for extracting text and images from PDF and
output it to plain text, html, xml or tags."""
import argparse
import logging
import sys
from typing import Any, Container, Iterable, List, Optional
import pdfminer.high_level
from pdfminer.layout import LAParams
from pdfminer.utils import AnyIO
logging.basicConfig()
OUTPUT_TYPES = ((".htm", "html"), (".html", "html"), (".xml", "xml"), (".tag", "tag"))
def float_or_disabled(x: str) -> Optional[float]:
if x.lower().strip() == "disabled":
return None
try:
return float(x)
except ValueError:
raise argparse.ArgumentTypeError("invalid float value: {}".format(x))
def extract_text(
files: Iterable[str] = [],
outfile: str = "-",
laparams: Optional[LAParams] = None,
output_type: str = "text",
codec: str = "utf-8",
strip_control: bool = False,
maxpages: int = 0,
page_numbers: Optional[Container[int]] = None,
password: str = "",
scale: float = 1.0,
rotation: int = 0,
layoutmode: str = "normal",
output_dir: Optional[str] = None,
debug: bool = False,
disable_caching: bool = False,
**kwargs: Any
) -> AnyIO:
if not files:
raise ValueError("Must provide files to work upon!")
if output_type == "text" and outfile != "-":
for override, alttype in OUTPUT_TYPES:
if outfile.endswith(override):
output_type = alttype
if outfile == "-":
outfp: AnyIO = sys.stdout
if sys.stdout.encoding is not None:
codec = "utf-8"
else:
outfp = open(outfile, "wb")
for fname in files:
with open(fname, "rb") as fp:
pdfminer.high_level.extract_text_to_fp(fp, **locals())
return outfp
def parse_args(args: Optional[List[str]]) -> argparse.Namespace:
parser = argparse.ArgumentParser(description=__doc__, add_help=True)
parser.add_argument(
"files",
type=str,
default=None,
nargs="+",
help="One or more paths to PDF files.",
)
parser.add_argument(
"--version",
"-v",
action="version",
version="pdfminer.six v{}".format(pdfminer.__version__),
)
parser.add_argument(
"--debug",
"-d",
default=False,
action="store_true",
help="Use debug logging level.",
)
parser.add_argument(
"--disable-caching",
"-C",
default=False,
action="store_true",
help="If caching or resources, such as fonts, should be disabled.",
)
parse_params = parser.add_argument_group(
"Parser", description="Used during PDF parsing"
)
parse_params.add_argument(
"--page-numbers",
type=int,
default=None,
nargs="+",
help="A space-seperated list of page numbers to parse.",
)
parse_params.add_argument(
"--pagenos",
"-p",
type=str,
help="A comma-separated list of page numbers to parse. "
"Included for legacy applications, use --page-numbers "
"for more idiomatic argument entry.",
)
parse_params.add_argument(
"--maxpages",
"-m",
type=int,
default=0,
help="The maximum number of pages to parse.",
)
parse_params.add_argument(
"--password",
"-P",
type=str,
default="",
help="The password to use for decrypting PDF file.",
)
parse_params.add_argument(
"--rotation",
"-R",
default=0,
type=int,
help="The number of degrees to rotate the PDF "
"before other types of processing.",
)
la_params = LAParams() # will be used for defaults
la_param_group = parser.add_argument_group(
"Layout analysis", description="Used during layout analysis."
)
la_param_group.add_argument(
"--no-laparams",
"-n",
default=False,
action="store_true",
help="If layout analysis parameters should be ignored.",
)
la_param_group.add_argument(
"--detect-vertical",
"-V",
default=la_params.detect_vertical,
action="store_true",
help="If vertical text should be considered during layout analysis",
)
la_param_group.add_argument(
"--line-overlap",
type=float,
default=la_params.line_overlap,
help="If two characters have more overlap than this they "
"are considered to be on the same line. The overlap is specified "
"relative to the minimum height of both characters.",
)
la_param_group.add_argument(
"--char-margin",
"-M",
type=float,
default=la_params.char_margin,
help="If two characters are closer together than this margin they "
"are considered to be part of the same line. The margin is "
"specified relative to the width of the character.",
)
la_param_group.add_argument(
"--word-margin",
"-W",
type=float,
default=la_params.word_margin,
help="If two characters on the same line are further apart than this "
"margin then they are considered to be two separate words, and "
"an intermediate space will be added for readability. The margin "
"is specified relative to the width of the character.",
)
la_param_group.add_argument(
"--line-margin",
"-L",
type=float,
default=la_params.line_margin,
help="If two lines are close together they are considered to "
"be part of the same paragraph. The margin is specified "
"relative to the height of a line.",
)
la_param_group.add_argument(
"--boxes-flow",
"-F",
type=float_or_disabled,
default=la_params.boxes_flow,
help="Specifies how much a horizontal and vertical position of a "
"text matters when determining the order of lines. The value "
"should be within the range of -1.0 (only horizontal position "
"matters) to +1.0 (only vertical position matters). You can also "
"pass `disabled` to disable advanced layout analysis, and "
"instead return text based on the position of the bottom left "
"corner of the text box.",
)
la_param_group.add_argument(
"--all-texts",
"-A",
default=la_params.all_texts,
action="store_true",
help="If layout analysis should be performed on text in figures.",
)
output_params = parser.add_argument_group(
"Output", description="Used during output generation."
)
output_params.add_argument(
"--outfile",
"-o",
type=str,
default="-",
help="Path to file where output is written. "
'Or "-" (default) to write to stdout.',
)
output_params.add_argument(
"--output_type",
"-t",
type=str,
default="text",
help="Type of output to generate {text,html,xml,tag}.",
)
output_params.add_argument(
"--codec",
"-c",
type=str,
default="utf-8",
help="Text encoding to use in output file.",
)
output_params.add_argument(
"--output-dir",
"-O",
default=None,
help="The output directory to put extracted images in. If not given, "
"images are not extracted.",
)
output_params.add_argument(
"--layoutmode",
"-Y",
default="normal",
type=str,
help="Type of layout to use when generating html "
"{normal,exact,loose}. If normal,each line is"
" positioned separately in the html. If exact"
", each character is positioned separately in"
" the html. If loose, same result as normal "
"but with an additional newline after each "
"text line. Only used when output_type is html.",
)
output_params.add_argument(
"--scale",
"-s",
type=float,
default=1.0,
help="The amount of zoom to use when generating html file. "
"Only used when output_type is html.",
)
output_params.add_argument(
"--strip-control",
"-S",
default=False,
action="store_true",
help="Remove control statement from text. "
"Only used when output_type is xml.",
)
parsed_args = parser.parse_args(args=args)
# Propagate parsed layout parameters to LAParams object
if parsed_args.no_laparams:
parsed_args.laparams = None
else:
parsed_args.laparams = LAParams(
line_overlap=parsed_args.line_overlap,
char_margin=parsed_args.char_margin,
line_margin=parsed_args.line_margin,
word_margin=parsed_args.word_margin,
boxes_flow=parsed_args.boxes_flow,
detect_vertical=parsed_args.detect_vertical,
all_texts=parsed_args.all_texts,
)
if parsed_args.page_numbers:
parsed_args.page_numbers = {x - 1 for x in parsed_args.page_numbers}
if parsed_args.pagenos:
parsed_args.page_numbers = {int(x) - 1 for x in parsed_args.pagenos.split(",")}
if parsed_args.output_type == "text" and parsed_args.outfile != "-":
for override, alttype in OUTPUT_TYPES:
if parsed_args.outfile.endswith(override):
parsed_args.output_type = alttype
return parsed_args
def main(args: Optional[List[str]] = None) -> int:
parsed_args = parse_args(args)
outfp = extract_text(**vars(parsed_args))
outfp.close()
return 0
if __name__ == "__main__":
sys.exit(main()) | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/20220429_pdfminer_jameslp310-0.0.2.data/scripts/pdf2txt.py | pdf2txt.py |
"""Extract pdf structure in XML format"""
import logging
import os.path
import re
import sys
from typing import Any, Container, Dict, Iterable, List, Optional, TextIO, Union, cast
from argparse import ArgumentParser
import pdfminer
from pdfminer.pdfdocument import PDFDocument, PDFNoOutlines, PDFXRefFallback
from pdfminer.pdfpage import PDFPage
from pdfminer.pdfparser import PDFParser
from pdfminer.pdftypes import PDFObjectNotFound, PDFValueError
from pdfminer.pdftypes import PDFStream, PDFObjRef, resolve1, stream_value
from pdfminer.psparser import PSKeyword, PSLiteral, LIT
from pdfminer.utils import isnumber
logging.basicConfig()
logger = logging.getLogger(__name__)
ESC_PAT = re.compile(r'[\000-\037&<>()"\042\047\134\177-\377]')
def escape(s: Union[str, bytes]) -> str:
if isinstance(s, bytes):
us = str(s, "latin-1")
else:
us = s
return ESC_PAT.sub(lambda m: "&#%d;" % ord(m.group(0)), us)
def dumpxml(out: TextIO, obj: object, codec: Optional[str] = None) -> None:
if obj is None:
out.write("<null />")
return
if isinstance(obj, dict):
out.write('<dict size="%d">\n' % len(obj))
for (k, v) in obj.items():
out.write("<key>%s</key>\n" % k)
out.write("<value>")
dumpxml(out, v)
out.write("</value>\n")
out.write("</dict>")
return
if isinstance(obj, list):
out.write('<list size="%d">\n' % len(obj))
for v in obj:
dumpxml(out, v)
out.write("\n")
out.write("</list>")
return
if isinstance(obj, (str, bytes)):
out.write('<string size="%d">%s</string>' % (len(obj), escape(obj)))
return
if isinstance(obj, PDFStream):
if codec == "raw":
# Bug: writing bytes to text I/O. This will raise TypeError.
out.write(obj.get_rawdata()) # type: ignore [arg-type]
elif codec == "binary":
# Bug: writing bytes to text I/O. This will raise TypeError.
out.write(obj.get_data()) # type: ignore [arg-type]
else:
out.write("<stream>\n<props>\n")
dumpxml(out, obj.attrs)
out.write("\n</props>\n")
if codec == "text":
data = obj.get_data()
out.write('<data size="%d">%s</data>\n' % (len(data), escape(data)))
out.write("</stream>")
return
if isinstance(obj, PDFObjRef):
out.write('<ref id="%d" />' % obj.objid)
return
if isinstance(obj, PSKeyword):
# Likely bug: obj.name is bytes, not str
out.write("<keyword>%s</keyword>" % obj.name) # type: ignore [str-bytes-safe]
return
if isinstance(obj, PSLiteral):
# Likely bug: obj.name may be bytes, not str
out.write("<literal>%s</literal>" % obj.name) # type: ignore [str-bytes-safe]
return
if isnumber(obj):
out.write("<number>%s</number>" % obj)
return
raise TypeError(obj)
def dumptrailers(
out: TextIO, doc: PDFDocument, show_fallback_xref: bool = False
) -> None:
for xref in doc.xrefs:
if not isinstance(xref, PDFXRefFallback) or show_fallback_xref:
out.write("<trailer>\n")
dumpxml(out, xref.get_trailer())
out.write("\n</trailer>\n\n")
no_xrefs = all(isinstance(xref, PDFXRefFallback) for xref in doc.xrefs)
if no_xrefs and not show_fallback_xref:
msg = (
"This PDF does not have an xref. Use --show-fallback-xref if "
"you want to display the content of a fallback xref that "
"contains all objects."
)
logger.warning(msg)
return
def dumpallobjs(
out: TextIO,
doc: PDFDocument,
codec: Optional[str] = None,
show_fallback_xref: bool = False,
) -> None:
visited = set()
out.write("<pdf>")
for xref in doc.xrefs:
for objid in xref.get_objids():
if objid in visited:
continue
visited.add(objid)
try:
obj = doc.getobj(objid)
if obj is None:
continue
out.write('<object id="%d">\n' % objid)
dumpxml(out, obj, codec=codec)
out.write("\n</object>\n\n")
except PDFObjectNotFound as e:
print("not found: %r" % e)
dumptrailers(out, doc, show_fallback_xref)
out.write("</pdf>")
return
def dumpoutline(
outfp: TextIO,
fname: str,
objids: Any,
pagenos: Container[int],
password: str = "",
dumpall: bool = False,
codec: Optional[str] = None,
extractdir: Optional[str] = None,
) -> None:
fp = open(fname, "rb")
parser = PDFParser(fp)
doc = PDFDocument(parser, password)
pages = {
page.pageid: pageno
for (pageno, page) in enumerate(PDFPage.create_pages(doc), 1)
}
def resolve_dest(dest: object) -> Any:
if isinstance(dest, (str, bytes)):
dest = resolve1(doc.get_dest(dest))
elif isinstance(dest, PSLiteral):
dest = resolve1(doc.get_dest(dest.name))
if isinstance(dest, dict):
dest = dest["D"]
if isinstance(dest, PDFObjRef):
dest = dest.resolve()
return dest
try:
outlines = doc.get_outlines()
outfp.write("<outlines>\n")
for (level, title, dest, a, se) in outlines:
pageno = None
if dest:
dest = resolve_dest(dest)
pageno = pages[dest[0].objid]
elif a:
action = a
if isinstance(action, dict):
subtype = action.get("S")
if subtype and repr(subtype) == "/'GoTo'" and action.get("D"):
dest = resolve_dest(action["D"])
pageno = pages[dest[0].objid]
s = escape(title)
outfp.write('<outline level="{!r}" title="{}">\n'.format(level, s))
if dest is not None:
outfp.write("<dest>")
dumpxml(outfp, dest)
outfp.write("</dest>\n")
if pageno is not None:
outfp.write("<pageno>%r</pageno>\n" % pageno)
outfp.write("</outline>\n")
outfp.write("</outlines>\n")
except PDFNoOutlines:
pass
parser.close()
fp.close()
return
LITERAL_FILESPEC = LIT("Filespec")
LITERAL_EMBEDDEDFILE = LIT("EmbeddedFile")
def extractembedded(fname: str, password: str, extractdir: str) -> None:
def extract1(objid: int, obj: Dict[str, Any]) -> None:
filename = os.path.basename(obj.get("UF") or cast(bytes, obj.get("F")).decode())
fileref = obj["EF"].get("UF") or obj["EF"].get("F")
fileobj = doc.getobj(fileref.objid)
if not isinstance(fileobj, PDFStream):
error_msg = (
"unable to process PDF: reference for %r is not a "
"PDFStream" % filename
)
raise PDFValueError(error_msg)
if fileobj.get("Type") is not LITERAL_EMBEDDEDFILE:
raise PDFValueError(
"unable to process PDF: reference for %r "
"is not an EmbeddedFile" % (filename)
)
path = os.path.join(extractdir, "%.6d-%s" % (objid, filename))
if os.path.exists(path):
raise IOError("file exists: %r" % path)
print("extracting: %r" % path)
os.makedirs(os.path.dirname(path), exist_ok=True)
out = open(path, "wb")
out.write(fileobj.get_data())
out.close()
return
with open(fname, "rb") as fp:
parser = PDFParser(fp)
doc = PDFDocument(parser, password)
extracted_objids = set()
for xref in doc.xrefs:
for objid in xref.get_objids():
obj = doc.getobj(objid)
if (
objid not in extracted_objids
and isinstance(obj, dict)
and obj.get("Type") is LITERAL_FILESPEC
):
extracted_objids.add(objid)
extract1(objid, obj)
return
def dumppdf(
outfp: TextIO,
fname: str,
objids: Iterable[int],
pagenos: Container[int],
password: str = "",
dumpall: bool = False,
codec: Optional[str] = None,
extractdir: Optional[str] = None,
show_fallback_xref: bool = False,
) -> None:
fp = open(fname, "rb")
parser = PDFParser(fp)
doc = PDFDocument(parser, password)
if objids:
for objid in objids:
obj = doc.getobj(objid)
dumpxml(outfp, obj, codec=codec)
if pagenos:
for (pageno, page) in enumerate(PDFPage.create_pages(doc)):
if pageno in pagenos:
if codec:
for obj in page.contents:
obj = stream_value(obj)
dumpxml(outfp, obj, codec=codec)
else:
dumpxml(outfp, page.attrs)
if dumpall:
dumpallobjs(outfp, doc, codec, show_fallback_xref)
if (not objids) and (not pagenos) and (not dumpall):
dumptrailers(outfp, doc, show_fallback_xref)
fp.close()
if codec not in ("raw", "binary"):
outfp.write("\n")
return
def create_parser() -> ArgumentParser:
parser = ArgumentParser(description=__doc__, add_help=True)
parser.add_argument(
"files",
type=str,
default=None,
nargs="+",
help="One or more paths to PDF files.",
)
parser.add_argument(
"--version",
"-v",
action="version",
version="pdfminer.six v{}".format(pdfminer.__version__),
)
parser.add_argument(
"--debug",
"-d",
default=False,
action="store_true",
help="Use debug logging level.",
)
procedure_parser = parser.add_mutually_exclusive_group()
procedure_parser.add_argument(
"--extract-toc",
"-T",
default=False,
action="store_true",
help="Extract structure of outline",
)
procedure_parser.add_argument(
"--extract-embedded", "-E", type=str, help="Extract embedded files"
)
parse_params = parser.add_argument_group(
"Parser", description="Used during PDF parsing"
)
parse_params.add_argument(
"--page-numbers",
type=int,
default=None,
nargs="+",
help="A space-seperated list of page numbers to parse.",
)
parse_params.add_argument(
"--pagenos",
"-p",
type=str,
help="A comma-separated list of page numbers to parse. Included for "
"legacy applications, use --page-numbers for more idiomatic "
"argument entry.",
)
parse_params.add_argument(
"--objects",
"-i",
type=str,
help="Comma separated list of object numbers to extract",
)
parse_params.add_argument(
"--all",
"-a",
default=False,
action="store_true",
help="If the structure of all objects should be extracted",
)
parse_params.add_argument(
"--show-fallback-xref",
action="store_true",
help="Additionally show the fallback xref. Use this if the PDF "
"has zero or only invalid xref's. This setting is ignored if "
"--extract-toc or --extract-embedded is used.",
)
parse_params.add_argument(
"--password",
"-P",
type=str,
default="",
help="The password to use for decrypting PDF file.",
)
output_params = parser.add_argument_group(
"Output", description="Used during output generation."
)
output_params.add_argument(
"--outfile",
"-o",
type=str,
default="-",
help='Path to file where output is written. Or "-" (default) to '
"write to stdout.",
)
codec_parser = output_params.add_mutually_exclusive_group()
codec_parser.add_argument(
"--raw-stream",
"-r",
default=False,
action="store_true",
help="Write stream objects without encoding",
)
codec_parser.add_argument(
"--binary-stream",
"-b",
default=False,
action="store_true",
help="Write stream objects with binary encoding",
)
codec_parser.add_argument(
"--text-stream",
"-t",
default=False,
action="store_true",
help="Write stream objects as plain text",
)
return parser
def main(argv: Optional[List[str]] = None) -> None:
parser = create_parser()
args = parser.parse_args(args=argv)
if args.debug:
logging.getLogger().setLevel(logging.DEBUG)
if args.outfile == "-":
outfp = sys.stdout
else:
outfp = open(args.outfile, "w")
if args.objects:
objids = [int(x) for x in args.objects.split(",")]
else:
objids = []
if args.page_numbers:
pagenos = {x - 1 for x in args.page_numbers}
elif args.pagenos:
pagenos = {int(x) - 1 for x in args.pagenos.split(",")}
else:
pagenos = set()
password = args.password
if args.raw_stream:
codec: Optional[str] = "raw"
elif args.binary_stream:
codec = "binary"
elif args.text_stream:
codec = "text"
else:
codec = None
for fname in args.files:
if args.extract_toc:
dumpoutline(
outfp,
fname,
objids,
pagenos,
password=password,
dumpall=args.all,
codec=codec,
extractdir=None,
)
elif args.extract_embedded:
extractembedded(fname, password=password, extractdir=args.extract_embedded)
else:
dumppdf(
outfp,
fname,
objids,
pagenos,
password=password,
dumpall=args.all,
codec=codec,
extractdir=None,
show_fallback_xref=args.show_fallback_xref,
)
outfp.close()
if __name__ == "__main__":
main() | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/20220429_pdfminer_jameslp310-0.0.2.data/scripts/dumppdf.py | dumppdf.py |
import io
import logging
import sys
import zlib
from typing import (
TYPE_CHECKING,
Any,
Dict,
Iterable,
Optional,
Union,
List,
Tuple,
cast,
)
from . import settings
from .ascii85 import ascii85decode
from .ascii85 import asciihexdecode
from .ccitt import ccittfaxdecode
from .lzw import lzwdecode
from .psparser import LIT
from .psparser import PSException
from .psparser import PSObject
from .runlength import rldecode
from .utils import apply_png_predictor
if TYPE_CHECKING:
from .pdfdocument import PDFDocument
logger = logging.getLogger(__name__)
LITERAL_CRYPT = LIT("Crypt")
# Abbreviation of Filter names in PDF 4.8.6. "Inline Images"
LITERALS_FLATE_DECODE = (LIT("FlateDecode"), LIT("Fl"))
LITERALS_LZW_DECODE = (LIT("LZWDecode"), LIT("LZW"))
LITERALS_ASCII85_DECODE = (LIT("ASCII85Decode"), LIT("A85"))
LITERALS_ASCIIHEX_DECODE = (LIT("ASCIIHexDecode"), LIT("AHx"))
LITERALS_RUNLENGTH_DECODE = (LIT("RunLengthDecode"), LIT("RL"))
LITERALS_CCITTFAX_DECODE = (LIT("CCITTFaxDecode"), LIT("CCF"))
LITERALS_DCT_DECODE = (LIT("DCTDecode"), LIT("DCT"))
LITERALS_JBIG2_DECODE = (LIT("JBIG2Decode"),)
LITERALS_JPX_DECODE = (LIT("JPXDecode"),)
if sys.version_info >= (3, 8):
from typing import Protocol
class DecipherCallable(Protocol):
"""Fully typed a decipher callback, with optional parameter."""
def __call__(
self,
objid: int,
genno: int,
data: bytes,
attrs: Optional[Dict[str, Any]] = None,
) -> bytes:
raise NotImplementedError
else: # Fallback for older Python
from typing import Callable
DecipherCallable = Callable[..., bytes]
class PDFObject(PSObject):
pass
class PDFException(PSException):
pass
class PDFTypeError(PDFException):
pass
class PDFValueError(PDFException):
pass
class PDFObjectNotFound(PDFException):
pass
class PDFNotImplementedError(PDFException):
pass
class PDFObjRef(PDFObject):
def __init__(self, doc: Optional["PDFDocument"], objid: int, _: object) -> None:
if objid == 0:
if settings.STRICT:
raise PDFValueError("PDF object id cannot be 0.")
self.doc = doc
self.objid = objid
def __repr__(self) -> str:
return "<PDFObjRef:%d>" % (self.objid)
def resolve(self, default: object = None) -> Any:
assert self.doc is not None
try:
return self.doc.getobj(self.objid)
except PDFObjectNotFound:
return default
def resolve1(x: object, default: object = None) -> Any:
"""Resolves an object.
If this is an array or dictionary, it may still contains
some indirect objects inside.
"""
while isinstance(x, PDFObjRef):
x = x.resolve(default=default)
return x
def resolve_all(x: object, default: object = None) -> Any:
"""Recursively resolves the given object and all the internals.
Make sure there is no indirect reference within the nested object.
This procedure might be slow.
"""
while isinstance(x, PDFObjRef):
x = x.resolve(default=default)
if isinstance(x, list):
x = [resolve_all(v, default=default) for v in x]
elif isinstance(x, dict):
for (k, v) in x.items():
x[k] = resolve_all(v, default=default)
return x
def decipher_all(decipher: DecipherCallable, objid: int, genno: int, x: object) -> Any:
"""Recursively deciphers the given object."""
if isinstance(x, bytes):
return decipher(objid, genno, x)
if isinstance(x, list):
x = [decipher_all(decipher, objid, genno, v) for v in x]
elif isinstance(x, dict):
for (k, v) in x.items():
x[k] = decipher_all(decipher, objid, genno, v)
return x
def int_value(x: object) -> int:
x = resolve1(x)
if not isinstance(x, int):
if settings.STRICT:
raise PDFTypeError("Integer required: %r" % x)
return 0
return x
def float_value(x: object) -> float:
x = resolve1(x)
if not isinstance(x, float):
if settings.STRICT:
raise PDFTypeError("Float required: %r" % x)
return 0.0
return x
def num_value(x: object) -> float:
x = resolve1(x)
if not isinstance(x, (int, float)): # == utils.isnumber(x)
if settings.STRICT:
raise PDFTypeError("Int or Float required: %r" % x)
return 0
return x
def uint_value(x: object, n_bits: int) -> int:
"""Resolve number and interpret it as a two's-complement unsigned number"""
xi = int_value(x)
if xi > 0:
return xi
else:
return xi + cast(int, 2**n_bits)
def str_value(x: object) -> bytes:
x = resolve1(x)
if not isinstance(x, bytes):
if settings.STRICT:
raise PDFTypeError("String required: %r" % x)
return b""
return x
def list_value(x: object) -> Union[List[Any], Tuple[Any, ...]]:
x = resolve1(x)
if not isinstance(x, (list, tuple)):
if settings.STRICT:
raise PDFTypeError("List required: %r" % x)
return []
return x
def dict_value(x: object) -> Dict[Any, Any]:
x = resolve1(x)
if not isinstance(x, dict):
if settings.STRICT:
logger.error("PDFTypeError : Dict required: %r", x)
raise PDFTypeError("Dict required: %r" % x)
return {}
return x
def stream_value(x: object) -> "PDFStream":
x = resolve1(x)
if not isinstance(x, PDFStream):
if settings.STRICT:
raise PDFTypeError("PDFStream required: %r" % x)
return PDFStream({}, b"")
return x
def decompress_corrupted(data: bytes) -> bytes:
"""Called on some data that can't be properly decoded because of CRC checksum
error. Attempt to decode it skipping the CRC.
"""
d = zlib.decompressobj()
f = io.BytesIO(data)
result_str = b""
buffer = f.read(1)
i = 0
try:
while buffer:
result_str += d.decompress(buffer)
buffer = f.read(1)
i += 1
except zlib.error:
# Let the error propagates if we're not yet in the CRC checksum
if i < len(data) - 3:
logger.warning("Data-loss while decompressing corrupted data")
return result_str
class PDFStream(PDFObject):
def __init__(
self,
attrs: Dict[str, Any],
rawdata: bytes,
decipher: Optional[DecipherCallable] = None,
) -> None:
assert isinstance(attrs, dict), str(type(attrs))
self.attrs = attrs
self.rawdata: Optional[bytes] = rawdata
self.decipher = decipher
self.data: Optional[bytes] = None
self.objid: Optional[int] = None
self.genno: Optional[int] = None
def set_objid(self, objid: int, genno: int) -> None:
self.objid = objid
self.genno = genno
def __repr__(self) -> str:
if self.data is None:
assert self.rawdata is not None
return "<PDFStream(%r): raw=%d, %r>" % (
self.objid,
len(self.rawdata),
self.attrs,
)
else:
assert self.data is not None
return "<PDFStream(%r): len=%d, %r>" % (
self.objid,
len(self.data),
self.attrs,
)
def __contains__(self, name: object) -> bool:
return name in self.attrs
def __getitem__(self, name: str) -> Any:
return self.attrs[name]
def get(self, name: str, default: object = None) -> Any:
return self.attrs.get(name, default)
def get_any(self, names: Iterable[str], default: object = None) -> Any:
for name in names:
if name in self.attrs:
return self.attrs[name]
return default
def get_filters(self) -> List[Tuple[Any, Any]]:
filters = self.get_any(("F", "Filter"))
params = self.get_any(("DP", "DecodeParms", "FDecodeParms"), {})
if not filters:
return []
if not isinstance(filters, list):
filters = [filters]
if not isinstance(params, list):
# Make sure the parameters list is the same as filters.
params = [params] * len(filters)
if settings.STRICT and len(params) != len(filters):
raise PDFException("Parameters len filter mismatch")
# resolve filter if possible
_filters = []
for fltr in filters:
if hasattr(fltr, "resolve"):
fltr = fltr.resolve()[0]
_filters.append(fltr)
# return list solves https://github.com/pdfminer/pdfminer.six/issues/15
return list(zip(_filters, params))
def decode(self) -> None:
assert self.data is None and self.rawdata is not None, str(
(self.data, self.rawdata)
)
data = self.rawdata
if self.decipher:
# Handle encryption
assert self.objid is not None
assert self.genno is not None
data = self.decipher(self.objid, self.genno, data, self.attrs)
filters = self.get_filters()
if not filters:
self.data = data
self.rawdata = None
return
for (f, params) in filters:
if f in LITERALS_FLATE_DECODE:
# will get errors if the document is encrypted.
try:
data = zlib.decompress(data)
except zlib.error as e:
if settings.STRICT:
error_msg = "Invalid zlib bytes: {!r}, {!r}".format(e, data)
raise PDFException(error_msg)
try:
data = decompress_corrupted(data)
except zlib.error:
data = b""
elif f in LITERALS_LZW_DECODE:
data = lzwdecode(data)
elif f in LITERALS_ASCII85_DECODE:
data = ascii85decode(data)
elif f in LITERALS_ASCIIHEX_DECODE:
data = asciihexdecode(data)
elif f in LITERALS_RUNLENGTH_DECODE:
data = rldecode(data)
elif f in LITERALS_CCITTFAX_DECODE:
data = ccittfaxdecode(data, params)
elif f in LITERALS_DCT_DECODE:
# This is probably a JPG stream
# it does not need to be decoded twice.
# Just return the stream to the user.
pass
elif f in LITERALS_JBIG2_DECODE:
pass
elif f in LITERALS_JPX_DECODE:
pass
elif f == LITERAL_CRYPT:
# not yet..
raise PDFNotImplementedError("/Crypt filter is unsupported")
else:
raise PDFNotImplementedError("Unsupported filter: %r" % f)
# apply predictors
if params and "Predictor" in params:
pred = int_value(params["Predictor"])
if pred == 1:
# no predictor
pass
elif 10 <= pred:
# PNG predictor
colors = int_value(params.get("Colors", 1))
columns = int_value(params.get("Columns", 1))
raw_bits_per_component = params.get("BitsPerComponent", 8)
bitspercomponent = int_value(raw_bits_per_component)
data = apply_png_predictor(
pred, colors, columns, bitspercomponent, data
)
else:
error_msg = "Unsupported predictor: %r" % pred
raise PDFNotImplementedError(error_msg)
self.data = data
self.rawdata = None
return
def get_data(self) -> bytes:
if self.data is None:
self.decode()
assert self.data is not None
return self.data
def get_rawdata(self) -> Optional[bytes]:
return self.rawdata | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/pdftypes.py | pdftypes.py |
# -*- coding: utf-8 -*-
import logging
import re
from typing import (
Any,
BinaryIO,
Dict,
Generic,
Iterator,
List,
Optional,
Tuple,
Type,
TypeVar,
Union,
)
from . import settings
from .utils import choplist
log = logging.getLogger(__name__)
class PSException(Exception):
pass
class PSEOF(PSException):
pass
class PSSyntaxError(PSException):
pass
class PSTypeError(PSException):
pass
class PSValueError(PSException):
pass
class PSObject:
"""Base class for all PS or PDF-related data types."""
pass
class PSLiteral(PSObject):
"""A class that represents a PostScript literal.
Postscript literals are used as identifiers, such as
variable names, property names and dictionary keys.
Literals are case sensitive and denoted by a preceding
slash sign (e.g. "/Name")
Note: Do not create an instance of PSLiteral directly.
Always use PSLiteralTable.intern().
"""
NameType = Union[str, bytes]
def __init__(self, name: NameType) -> None:
self.name = name
def __repr__(self) -> str:
name = self.name
return "/%r" % name
class PSKeyword(PSObject):
"""A class that represents a PostScript keyword.
PostScript keywords are a dozen of predefined words.
Commands and directives in PostScript are expressed by keywords.
They are also used to denote the content boundaries.
Note: Do not create an instance of PSKeyword directly.
Always use PSKeywordTable.intern().
"""
def __init__(self, name: bytes) -> None:
self.name = name
def __repr__(self) -> str:
name = self.name
return "/%r" % name
_SymbolT = TypeVar("_SymbolT", PSLiteral, PSKeyword)
class PSSymbolTable(Generic[_SymbolT]):
"""A utility class for storing PSLiteral/PSKeyword objects.
Interned objects can be checked its identity with "is" operator.
"""
def __init__(self, klass: Type[_SymbolT]) -> None:
self.dict: Dict[PSLiteral.NameType, _SymbolT] = {}
self.klass: Type[_SymbolT] = klass
def intern(self, name: PSLiteral.NameType) -> _SymbolT:
if name in self.dict:
lit = self.dict[name]
else:
# Type confusion issue: PSKeyword always takes bytes as name
# PSLiteral uses either str or bytes
lit = self.klass(name) # type: ignore[arg-type]
self.dict[name] = lit
return lit
PSLiteralTable = PSSymbolTable(PSLiteral)
PSKeywordTable = PSSymbolTable(PSKeyword)
LIT = PSLiteralTable.intern
KWD = PSKeywordTable.intern
KEYWORD_PROC_BEGIN = KWD(b"{")
KEYWORD_PROC_END = KWD(b"}")
KEYWORD_ARRAY_BEGIN = KWD(b"[")
KEYWORD_ARRAY_END = KWD(b"]")
KEYWORD_DICT_BEGIN = KWD(b"<<")
KEYWORD_DICT_END = KWD(b">>")
def literal_name(x: object) -> Any:
if not isinstance(x, PSLiteral):
if settings.STRICT:
raise PSTypeError("Literal required: {!r}".format(x))
else:
name = x
else:
name = x.name
if not isinstance(name, str):
try:
name = str(name, "utf-8")
except Exception:
pass
return name
def keyword_name(x: object) -> Any:
if not isinstance(x, PSKeyword):
if settings.STRICT:
raise PSTypeError("Keyword required: %r" % x)
else:
name = x
else:
name = str(x.name, "utf-8", "ignore")
return name
EOL = re.compile(rb"[\r\n]")
SPC = re.compile(rb"\s")
NONSPC = re.compile(rb"\S")
HEX = re.compile(rb"[0-9a-fA-F]")
END_LITERAL = re.compile(rb"[#/%\[\]()<>{}\s]")
END_HEX_STRING = re.compile(rb"[^\s0-9a-fA-F]")
HEX_PAIR = re.compile(rb"[0-9a-fA-F]{2}|.")
END_NUMBER = re.compile(rb"[^0-9]")
END_KEYWORD = re.compile(rb"[#/%\[\]()<>{}\s]")
END_STRING = re.compile(rb"[()\134]")
OCT_STRING = re.compile(rb"[0-7]")
ESC_STRING = {
b"b": 8,
b"t": 9,
b"n": 10,
b"f": 12,
b"r": 13,
b"(": 40,
b")": 41,
b"\\": 92,
}
PSBaseParserToken = Union[float, bool, PSLiteral, PSKeyword, bytes]
class PSBaseParser:
"""Most basic PostScript parser that performs only tokenization."""
BUFSIZ = 4096
def __init__(self, fp: BinaryIO) -> None:
self.fp = fp
self.seek(0)
def __repr__(self) -> str:
return "<%s: %r, bufpos=%d>" % (self.__class__.__name__, self.fp, self.bufpos)
def flush(self) -> None:
return
def close(self) -> None:
self.flush()
return
def tell(self) -> int:
return self.bufpos + self.charpos
def poll(self, pos: Optional[int] = None, n: int = 80) -> None:
pos0 = self.fp.tell()
if not pos:
pos = self.bufpos + self.charpos
self.fp.seek(pos)
log.debug("poll(%d): %r", pos, self.fp.read(n))
self.fp.seek(pos0)
return
def seek(self, pos: int) -> None:
"""Seeks the parser to the given position."""
log.debug("seek: %r", pos)
self.fp.seek(pos)
# reset the status for nextline()
self.bufpos = pos
self.buf = b""
self.charpos = 0
# reset the status for nexttoken()
self._parse1 = self._parse_main
self._curtoken = b""
self._curtokenpos = 0
self._tokens: List[Tuple[int, PSBaseParserToken]] = []
return
def fillbuf(self) -> None:
if self.charpos < len(self.buf):
return
# fetch next chunk.
self.bufpos = self.fp.tell()
self.buf = self.fp.read(self.BUFSIZ)
if not self.buf:
raise PSEOF("Unexpected EOF")
self.charpos = 0
return
def nextline(self) -> Tuple[int, bytes]:
"""Fetches a next line that ends either with \\r or \\n."""
linebuf = b""
linepos = self.bufpos + self.charpos
eol = False
while 1:
self.fillbuf()
if eol:
c = self.buf[self.charpos : self.charpos + 1]
# handle b'\r\n'
if c == b"\n":
linebuf += c
self.charpos += 1
break
m = EOL.search(self.buf, self.charpos)
if m:
linebuf += self.buf[self.charpos : m.end(0)]
self.charpos = m.end(0)
if linebuf[-1:] == b"\r":
eol = True
else:
break
else:
linebuf += self.buf[self.charpos :]
self.charpos = len(self.buf)
log.debug("nextline: %r, %r", linepos, linebuf)
return (linepos, linebuf)
def revreadlines(self) -> Iterator[bytes]:
"""Fetches a next line backword.
This is used to locate the trailers at the end of a file.
"""
self.fp.seek(0, 2)
pos = self.fp.tell()
buf = b""
while 0 < pos:
prevpos = pos
pos = max(0, pos - self.BUFSIZ)
self.fp.seek(pos)
s = self.fp.read(prevpos - pos)
if not s:
break
while 1:
n = max(s.rfind(b"\r"), s.rfind(b"\n"))
if n == -1:
buf = s + buf
break
yield s[n:] + buf
s = s[:n]
buf = b""
return
def _parse_main(self, s: bytes, i: int) -> int:
m = NONSPC.search(s, i)
if not m:
return len(s)
j = m.start(0)
c = s[j : j + 1]
self._curtokenpos = self.bufpos + j
if c == b"%":
self._curtoken = b"%"
self._parse1 = self._parse_comment
return j + 1
elif c == b"/":
self._curtoken = b""
self._parse1 = self._parse_literal
return j + 1
elif c in b"-+" or c.isdigit():
self._curtoken = c
self._parse1 = self._parse_number
return j + 1
elif c == b".":
self._curtoken = c
self._parse1 = self._parse_float
return j + 1
elif c.isalpha():
self._curtoken = c
self._parse1 = self._parse_keyword
return j + 1
elif c == b"(":
self._curtoken = b""
self.paren = 1
self._parse1 = self._parse_string
return j + 1
elif c == b"<":
self._curtoken = b""
self._parse1 = self._parse_wopen
return j + 1
elif c == b">":
self._curtoken = b""
self._parse1 = self._parse_wclose
return j + 1
else:
self._add_token(KWD(c))
return j + 1
def _add_token(self, obj: PSBaseParserToken) -> None:
self._tokens.append((self._curtokenpos, obj))
return
def _parse_comment(self, s: bytes, i: int) -> int:
m = EOL.search(s, i)
if not m:
self._curtoken += s[i:]
return len(s)
j = m.start(0)
self._curtoken += s[i:j]
self._parse1 = self._parse_main
# We ignore comments.
# self._tokens.append(self._curtoken)
return j
def _parse_literal(self, s: bytes, i: int) -> int:
m = END_LITERAL.search(s, i)
if not m:
self._curtoken += s[i:]
return len(s)
j = m.start(0)
self._curtoken += s[i:j]
c = s[j : j + 1]
if c == b"#":
self.hex = b""
self._parse1 = self._parse_literal_hex
return j + 1
try:
name: Union[str, bytes] = str(self._curtoken, "utf-8")
except Exception:
name = self._curtoken
self._add_token(LIT(name))
self._parse1 = self._parse_main
return j
def _parse_literal_hex(self, s: bytes, i: int) -> int:
c = s[i : i + 1]
if HEX.match(c) and len(self.hex) < 2:
self.hex += c
return i + 1
if self.hex:
self._curtoken += bytes((int(self.hex, 16),))
self._parse1 = self._parse_literal
return i
def _parse_number(self, s: bytes, i: int) -> int:
m = END_NUMBER.search(s, i)
if not m:
self._curtoken += s[i:]
return len(s)
j = m.start(0)
self._curtoken += s[i:j]
c = s[j : j + 1]
if c == b".":
self._curtoken += c
self._parse1 = self._parse_float
return j + 1
try:
self._add_token(int(self._curtoken))
except ValueError:
pass
self._parse1 = self._parse_main
return j
def _parse_float(self, s: bytes, i: int) -> int:
m = END_NUMBER.search(s, i)
if not m:
self._curtoken += s[i:]
return len(s)
j = m.start(0)
self._curtoken += s[i:j]
try:
self._add_token(float(self._curtoken))
except ValueError:
pass
self._parse1 = self._parse_main
return j
def _parse_keyword(self, s: bytes, i: int) -> int:
m = END_KEYWORD.search(s, i)
if not m:
self._curtoken += s[i:]
return len(s)
j = m.start(0)
self._curtoken += s[i:j]
if self._curtoken == b"true":
token: Union[bool, PSKeyword] = True
elif self._curtoken == b"false":
token = False
else:
token = KWD(self._curtoken)
self._add_token(token)
self._parse1 = self._parse_main
return j
def _parse_string(self, s: bytes, i: int) -> int:
m = END_STRING.search(s, i)
if not m:
self._curtoken += s[i:]
return len(s)
j = m.start(0)
self._curtoken += s[i:j]
c = s[j : j + 1]
if c == b"\\":
self.oct = b""
self._parse1 = self._parse_string_1
return j + 1
if c == b"(":
self.paren += 1
self._curtoken += c
return j + 1
if c == b")":
self.paren -= 1
if self.paren:
# WTF, they said balanced parens need no special treatment.
self._curtoken += c
return j + 1
self._add_token(self._curtoken)
self._parse1 = self._parse_main
return j + 1
def _parse_string_1(self, s: bytes, i: int) -> int:
"""Parse literal strings
PDF Reference 3.2.3
"""
c = s[i : i + 1]
if OCT_STRING.match(c) and len(self.oct) < 3:
self.oct += c
return i + 1
elif self.oct:
self._curtoken += bytes((int(self.oct, 8),))
self._parse1 = self._parse_string
return i
elif c in ESC_STRING:
self._curtoken += bytes((ESC_STRING[c],))
elif c == b"\r" and len(s) > i + 1 and s[i + 1 : i + 2] == b"\n":
# If current and next character is \r\n skip both because enters
# after a \ are ignored
i += 1
# default action
self._parse1 = self._parse_string
return i + 1
def _parse_wopen(self, s: bytes, i: int) -> int:
c = s[i : i + 1]
if c == b"<":
self._add_token(KEYWORD_DICT_BEGIN)
self._parse1 = self._parse_main
i += 1
else:
self._parse1 = self._parse_hexstring
return i
def _parse_wclose(self, s: bytes, i: int) -> int:
c = s[i : i + 1]
if c == b">":
self._add_token(KEYWORD_DICT_END)
i += 1
self._parse1 = self._parse_main
return i
def _parse_hexstring(self, s: bytes, i: int) -> int:
m = END_HEX_STRING.search(s, i)
if not m:
self._curtoken += s[i:]
return len(s)
j = m.start(0)
self._curtoken += s[i:j]
token = HEX_PAIR.sub(
lambda m: bytes((int(m.group(0), 16),)), SPC.sub(b"", self._curtoken)
)
self._add_token(token)
self._parse1 = self._parse_main
return j
def nexttoken(self) -> Tuple[int, PSBaseParserToken]:
while not self._tokens:
self.fillbuf()
self.charpos = self._parse1(self.buf, self.charpos)
token = self._tokens.pop(0)
log.debug("nexttoken: %r", token)
return token
# Stack slots may by occupied by any of:
# * the PSBaseParserToken types
# * list (via KEYWORD_ARRAY)
# * dict (via KEYWORD_DICT)
# * subclass-specific extensions (e.g. PDFStream, PDFObjRef) via ExtraT
ExtraT = TypeVar("ExtraT")
PSStackType = Union[float, bool, PSLiteral, bytes, List, Dict, ExtraT]
PSStackEntry = Tuple[int, PSStackType[ExtraT]]
class PSStackParser(PSBaseParser, Generic[ExtraT]):
def __init__(self, fp: BinaryIO) -> None:
PSBaseParser.__init__(self, fp)
self.reset()
return
def reset(self) -> None:
self.context: List[Tuple[int, Optional[str], List[PSStackEntry[ExtraT]]]] = []
self.curtype: Optional[str] = None
self.curstack: List[PSStackEntry[ExtraT]] = []
self.results: List[PSStackEntry[ExtraT]] = []
return
def seek(self, pos: int) -> None:
PSBaseParser.seek(self, pos)
self.reset()
return
def push(self, *objs: PSStackEntry[ExtraT]) -> None:
self.curstack.extend(objs)
return
def pop(self, n: int) -> List[PSStackEntry[ExtraT]]:
objs = self.curstack[-n:]
self.curstack[-n:] = []
return objs
def popall(self) -> List[PSStackEntry[ExtraT]]:
objs = self.curstack
self.curstack = []
return objs
def add_results(self, *objs: PSStackEntry[ExtraT]) -> None:
try:
log.debug("add_results: %r", objs)
except Exception:
log.debug("add_results: (unprintable object)")
self.results.extend(objs)
return
def start_type(self, pos: int, type: str) -> None:
self.context.append((pos, self.curtype, self.curstack))
(self.curtype, self.curstack) = (type, [])
log.debug("start_type: pos=%r, type=%r", pos, type)
return
def end_type(self, type: str) -> Tuple[int, List[PSStackType[ExtraT]]]:
if self.curtype != type:
raise PSTypeError("Type mismatch: {!r} != {!r}".format(self.curtype, type))
objs = [obj for (_, obj) in self.curstack]
(pos, self.curtype, self.curstack) = self.context.pop()
log.debug("end_type: pos=%r, type=%r, objs=%r", pos, type, objs)
return (pos, objs)
def do_keyword(self, pos: int, token: PSKeyword) -> None:
return
def nextobject(self) -> PSStackEntry[ExtraT]:
"""Yields a list of objects.
Arrays and dictionaries are represented as Python lists and
dictionaries.
:return: keywords, literals, strings, numbers, arrays and dictionaries.
"""
while not self.results:
(pos, token) = self.nexttoken()
if isinstance(token, (int, float, bool, str, bytes, PSLiteral)):
# normal token
self.push((pos, token))
elif token == KEYWORD_ARRAY_BEGIN:
# begin array
self.start_type(pos, "a")
elif token == KEYWORD_ARRAY_END:
# end array
try:
self.push(self.end_type("a"))
except PSTypeError:
if settings.STRICT:
raise
elif token == KEYWORD_DICT_BEGIN:
# begin dictionary
self.start_type(pos, "d")
elif token == KEYWORD_DICT_END:
# end dictionary
try:
(pos, objs) = self.end_type("d")
if len(objs) % 2 != 0:
error_msg = "Invalid dictionary construct: %r" % objs
raise PSSyntaxError(error_msg)
d = {
literal_name(k): v
for (k, v) in choplist(2, objs)
if v is not None
}
self.push((pos, d))
except PSTypeError:
if settings.STRICT:
raise
elif token == KEYWORD_PROC_BEGIN:
# begin proc
self.start_type(pos, "p")
elif token == KEYWORD_PROC_END:
# end proc
try:
self.push(self.end_type("p"))
except PSTypeError:
if settings.STRICT:
raise
elif isinstance(token, PSKeyword):
log.debug(
"do_keyword: pos=%r, token=%r, stack=%r", pos, token, self.curstack
)
self.do_keyword(pos, token)
else:
log.error(
"unknown token: pos=%r, token=%r, stack=%r",
pos,
token,
self.curstack,
)
self.do_keyword(pos, token)
raise
if self.context:
continue
else:
self.flush()
obj = self.results.pop(0)
try:
log.debug("nextobject: %r", obj)
except Exception:
log.debug("nextobject: (unprintable object)")
return obj | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/psparser.py | psparser.py |
import io
import logging
import re
from typing import (
BinaryIO,
Dict,
Generic,
List,
Optional,
Sequence,
TextIO,
Tuple,
TypeVar,
Union,
cast,
)
from pdfminer.pdfcolor import PDFColorSpace
from . import utils
from .image import ImageWriter
from .layout import LAParams, LTComponent, TextGroupElement
from .layout import LTChar
from .layout import LTContainer
from .layout import LTCurve
from .layout import LTFigure
from .layout import LTImage
from .layout import LTItem
from .layout import LTLayoutContainer
from .layout import LTLine
from .layout import LTPage
from .layout import LTRect
from .layout import LTText
from .layout import LTTextBox
from .layout import LTTextBoxVertical
from .layout import LTTextGroup
from .layout import LTTextLine
from .pdfdevice import PDFTextDevice
from .pdffont import PDFFont
from .pdffont import PDFUnicodeNotDefined
from .pdfinterp import PDFGraphicState, PDFResourceManager
from .pdfpage import PDFPage
from .pdftypes import PDFStream
from .utils import AnyIO, Point, Matrix, Rect, PathSegment, make_compat_str
from .utils import apply_matrix_pt
from .utils import bbox2str
from .utils import enc
from .utils import mult_matrix
log = logging.getLogger(__name__)
class PDFLayoutAnalyzer(PDFTextDevice):
cur_item: LTLayoutContainer
ctm: Matrix
def __init__(
self,
rsrcmgr: PDFResourceManager,
pageno: int = 1,
laparams: Optional[LAParams] = None,
) -> None:
PDFTextDevice.__init__(self, rsrcmgr)
self.pageno = pageno
self.laparams = laparams
self._stack: List[LTLayoutContainer] = []
def begin_page(self, page: PDFPage, ctm: Matrix) -> None:
(x0, y0, x1, y1) = page.mediabox
(x0, y0) = apply_matrix_pt(ctm, (x0, y0))
(x1, y1) = apply_matrix_pt(ctm, (x1, y1))
mediabox = (0, 0, abs(x0 - x1), abs(y0 - y1))
self.cur_item = LTPage(self.pageno, mediabox)
def end_page(self, page: PDFPage) -> None:
assert not self._stack, str(len(self._stack))
assert isinstance(self.cur_item, LTPage), str(type(self.cur_item))
if self.laparams is not None:
self.cur_item.analyze(self.laparams)
self.pageno += 1
self.receive_layout(self.cur_item)
def begin_figure(self, name: str, bbox: Rect, matrix: Matrix) -> None:
self._stack.append(self.cur_item)
self.cur_item = LTFigure(name, bbox, mult_matrix(matrix, self.ctm))
def end_figure(self, _: str) -> None:
fig = self.cur_item
assert isinstance(self.cur_item, LTFigure), str(type(self.cur_item))
self.cur_item = self._stack.pop()
self.cur_item.add(fig)
def render_image(self, name: str, stream: PDFStream) -> None:
assert isinstance(self.cur_item, LTFigure), str(type(self.cur_item))
item = LTImage(
name,
stream,
(self.cur_item.x0, self.cur_item.y0, self.cur_item.x1, self.cur_item.y1),
)
self.cur_item.add(item)
def paint_path(
self,
gstate: PDFGraphicState,
stroke: bool,
fill: bool,
evenodd: bool,
path: Sequence[PathSegment],
) -> None:
"""Paint paths described in section 4.4 of the PDF reference manual"""
shape = "".join(x[0] for x in path)
if shape.count("m") > 1:
# recurse if there are multiple m's in this shape
for m in re.finditer(r"m[^m]+", shape):
subpath = path[m.start(0) : m.end(0)]
self.paint_path(gstate, stroke, fill, evenodd, subpath)
else:
# Although the 'h' command does not not literally provide a
# point-position, its position is (by definition) equal to the
# subpath's starting point.
#
# And, per Section 4.4's Table 4.9, all other path commands place
# their point-position in their final two arguments. (Any preceding
# arguments represent control points on Bézier curves.)
raw_pts = [
cast(Point, p[-2:] if p[0] != "h" else path[0][-2:]) for p in path
]
pts = [apply_matrix_pt(self.ctm, pt) for pt in raw_pts]
if shape in {"mlh", "ml"}:
# single line segment
#
# Note: 'ml', in conditional above, is a frequent anomaly
# that we want to support.
line = LTLine(
gstate.linewidth,
pts[0],
pts[1],
stroke,
fill,
evenodd,
gstate.scolor,
gstate.ncolor,
)
self.cur_item.add(line)
elif shape in {"mlllh", "mllll"}:
(x0, y0), (x1, y1), (x2, y2), (x3, y3), _ = pts
is_closed_loop = pts[0] == pts[4]
has_square_coordinates = (
x0 == x1 and y1 == y2 and x2 == x3 and y3 == y0
) or (y0 == y1 and x1 == x2 and y2 == y3 and x3 == x0)
if is_closed_loop and has_square_coordinates:
rect = LTRect(
gstate.linewidth,
(*pts[0], *pts[2]),
stroke,
fill,
evenodd,
gstate.scolor,
gstate.ncolor,
)
self.cur_item.add(rect)
else:
curve = LTCurve(
gstate.linewidth,
pts,
stroke,
fill,
evenodd,
gstate.scolor,
gstate.ncolor,
)
self.cur_item.add(curve)
else:
curve = LTCurve(
gstate.linewidth,
pts,
stroke,
fill,
evenodd,
gstate.scolor,
gstate.ncolor,
)
self.cur_item.add(curve)
def render_char(
self,
matrix: Matrix,
font: PDFFont,
fontsize: float,
scaling: float,
rise: float,
cid: int,
ncs: PDFColorSpace,
graphicstate: PDFGraphicState,
) -> float:
try:
text = font.to_unichr(cid)
assert isinstance(text, str), str(type(text))
except PDFUnicodeNotDefined:
text = self.handle_undefined_char(font, cid)
textwidth = font.char_width(cid)
textdisp = font.char_disp(cid)
item = LTChar(
matrix,
font,
fontsize,
scaling,
rise,
text,
textwidth,
textdisp,
ncs,
graphicstate,
)
self.cur_item.add(item)
return item.adv
def handle_undefined_char(self, font: PDFFont, cid: int) -> str:
log.debug("undefined: %r, %r", font, cid)
return "(cid:%d)" % cid
def receive_layout(self, ltpage: LTPage) -> None:
pass
class PDFPageAggregator(PDFLayoutAnalyzer):
def __init__(
self,
rsrcmgr: PDFResourceManager,
pageno: int = 1,
laparams: Optional[LAParams] = None,
) -> None:
PDFLayoutAnalyzer.__init__(self, rsrcmgr, pageno=pageno, laparams=laparams)
self.result: Optional[LTPage] = None
def receive_layout(self, ltpage: LTPage) -> None:
self.result = ltpage
def get_result(self) -> LTPage:
assert self.result is not None
return self.result
# Some PDFConverter children support only binary I/O
IOType = TypeVar("IOType", TextIO, BinaryIO, AnyIO)
class PDFConverter(PDFLayoutAnalyzer, Generic[IOType]):
def __init__(
self,
rsrcmgr: PDFResourceManager,
outfp: IOType,
codec: str = "utf-8",
pageno: int = 1,
laparams: Optional[LAParams] = None,
) -> None:
PDFLayoutAnalyzer.__init__(self, rsrcmgr, pageno=pageno, laparams=laparams)
self.outfp: IOType = outfp
self.codec = codec
self.outfp_binary = self._is_binary_stream(self.outfp)
@staticmethod
def _is_binary_stream(outfp: AnyIO) -> bool:
"""Test if an stream is binary or not"""
if "b" in getattr(outfp, "mode", ""):
return True
elif hasattr(outfp, "mode"):
# output stream has a mode, but it does not contain 'b'
return False
elif isinstance(outfp, io.BytesIO):
return True
elif isinstance(outfp, io.StringIO):
return False
elif isinstance(outfp, io.TextIOBase):
return False
return True
class TextConverter(PDFConverter[AnyIO]):
def __init__(
self,
rsrcmgr: PDFResourceManager,
outfp: AnyIO,
codec: str = "utf-8",
pageno: int = 1,
laparams: Optional[LAParams] = None,
showpageno: bool = False,
imagewriter: Optional[ImageWriter] = None,
) -> None:
super().__init__(rsrcmgr, outfp, codec=codec, pageno=pageno, laparams=laparams)
self.showpageno = showpageno
self.imagewriter = imagewriter
def write_text(self, text: str) -> None:
text = utils.compatible_encode_method(text, self.codec, "ignore")
if self.outfp_binary:
cast(BinaryIO, self.outfp).write(text.encode())
else:
cast(TextIO, self.outfp).write(text)
def receive_layout(self, ltpage: LTPage) -> None:
def render(item: LTItem) -> None:
if isinstance(item, LTContainer):
for child in item:
render(child)
elif isinstance(item, LTText):
self.write_text(item.get_text())
if isinstance(item, LTTextBox):
self.write_text("\n")
elif isinstance(item, LTImage):
if self.imagewriter is not None:
self.imagewriter.export_image(item)
if self.showpageno:
self.write_text("Page %s\n" % ltpage.pageid)
render(ltpage)
self.write_text("\f")
# Some dummy functions to save memory/CPU when all that is wanted
# is text. This stops all the image and drawing output from being
# recorded and taking up RAM.
def render_image(self, name: str, stream: PDFStream) -> None:
if self.imagewriter is None:
return
PDFConverter.render_image(self, name, stream)
return
def paint_path(
self,
gstate: PDFGraphicState,
stroke: bool,
fill: bool,
evenodd: bool,
path: Sequence[PathSegment],
) -> None:
return
class HTMLConverter(PDFConverter[AnyIO]):
RECT_COLORS = {
"figure": "yellow",
"textline": "magenta",
"textbox": "cyan",
"textgroup": "red",
"curve": "black",
"page": "gray",
}
TEXT_COLORS = {
"textbox": "blue",
"char": "black",
}
def __init__(
self,
rsrcmgr: PDFResourceManager,
outfp: AnyIO,
codec: str = "utf-8",
pageno: int = 1,
laparams: Optional[LAParams] = None,
scale: float = 1,
fontscale: float = 1.0,
layoutmode: str = "normal",
showpageno: bool = True,
pagemargin: int = 50,
imagewriter: Optional[ImageWriter] = None,
debug: int = 0,
rect_colors: Optional[Dict[str, str]] = None,
text_colors: Optional[Dict[str, str]] = None,
) -> None:
PDFConverter.__init__(
self, rsrcmgr, outfp, codec=codec, pageno=pageno, laparams=laparams
)
# write() assumes a codec for binary I/O, or no codec for text I/O.
if self.outfp_binary == (not self.codec):
raise ValueError("Codec is required for a binary I/O output")
if text_colors is None:
text_colors = {"char": "black"}
if rect_colors is None:
rect_colors = {"curve": "black", "page": "gray"}
self.scale = scale
self.fontscale = fontscale
self.layoutmode = layoutmode
self.showpageno = showpageno
self.pagemargin = pagemargin
self.imagewriter = imagewriter
self.rect_colors = rect_colors
self.text_colors = text_colors
if debug:
self.rect_colors.update(self.RECT_COLORS)
self.text_colors.update(self.TEXT_COLORS)
self._yoffset: float = self.pagemargin
self._font: Optional[Tuple[str, float]] = None
self._fontstack: List[Optional[Tuple[str, float]]] = []
self.write_header()
return
def write(self, text: str) -> None:
if self.codec:
cast(BinaryIO, self.outfp).write(text.encode(self.codec))
else:
cast(TextIO, self.outfp).write(text)
return
def write_header(self) -> None:
self.write("<html><head>\n")
if self.codec:
s = (
'<meta http-equiv="Content-Type" content="text/html; '
'charset=%s">\n' % self.codec
)
else:
s = '<meta http-equiv="Content-Type" content="text/html">\n'
self.write(s)
self.write("</head><body>\n")
return
def write_footer(self) -> None:
page_links = [
'<a href="#{}">{}</a>'.format(i, i) for i in range(1, self.pageno)
]
s = '<div style="position:absolute; top:0px;">Page: %s</div>\n' % ", ".join(
page_links
)
self.write(s)
self.write("</body></html>\n")
return
def write_text(self, text: str) -> None:
self.write(enc(text))
return
def place_rect(
self, color: str, borderwidth: int, x: float, y: float, w: float, h: float
) -> None:
color2 = self.rect_colors.get(color)
if color2 is not None:
s = (
'<span style="position:absolute; border: %s %dpx solid; '
'left:%dpx; top:%dpx; width:%dpx; height:%dpx;"></span>\n'
% (
color2,
borderwidth,
x * self.scale,
(self._yoffset - y) * self.scale,
w * self.scale,
h * self.scale,
)
)
self.write(s)
return
def place_border(self, color: str, borderwidth: int, item: LTComponent) -> None:
self.place_rect(color, borderwidth, item.x0, item.y1, item.width, item.height)
return
def place_image(
self, item: LTImage, borderwidth: int, x: float, y: float, w: float, h: float
) -> None:
if self.imagewriter is not None:
name = self.imagewriter.export_image(item)
s = (
'<img src="%s" border="%d" style="position:absolute; '
'left:%dpx; top:%dpx;" width="%d" height="%d" />\n'
% (
enc(name),
borderwidth,
x * self.scale,
(self._yoffset - y) * self.scale,
w * self.scale,
h * self.scale,
)
)
self.write(s)
return
def place_text(
self, color: str, text: str, x: float, y: float, size: float
) -> None:
color2 = self.text_colors.get(color)
if color2 is not None:
s = (
'<span style="position:absolute; color:%s; left:%dpx; '
'top:%dpx; font-size:%dpx;">'
% (
color2,
x * self.scale,
(self._yoffset - y) * self.scale,
size * self.scale * self.fontscale,
)
)
self.write(s)
self.write_text(text)
self.write("</span>\n")
return
def begin_div(
self,
color: str,
borderwidth: int,
x: float,
y: float,
w: float,
h: float,
writing_mode: str = "False",
) -> None:
self._fontstack.append(self._font)
self._font = None
s = (
'<div style="position:absolute; border: %s %dpx solid; '
"writing-mode:%s; left:%dpx; top:%dpx; width:%dpx; "
'height:%dpx;">'
% (
color,
borderwidth,
writing_mode,
x * self.scale,
(self._yoffset - y) * self.scale,
w * self.scale,
h * self.scale,
)
)
self.write(s)
return
def end_div(self, color: str) -> None:
if self._font is not None:
self.write("</span>")
self._font = self._fontstack.pop()
self.write("</div>")
return
def put_text(self, text: str, fontname: str, fontsize: float) -> None:
font = (fontname, fontsize)
if font != self._font:
if self._font is not None:
self.write("</span>")
# Remove subset tag from fontname, see PDF Reference 5.5.3
fontname_without_subset_tag = fontname.split("+")[-1]
self.write(
'<span style="font-family: %s; font-size:%dpx">'
% (fontname_without_subset_tag, fontsize * self.scale * self.fontscale)
)
self._font = font
self.write_text(text)
return
def put_newline(self) -> None:
self.write("<br>")
return
def receive_layout(self, ltpage: LTPage) -> None:
def show_group(item: Union[LTTextGroup, TextGroupElement]) -> None:
if isinstance(item, LTTextGroup):
self.place_border("textgroup", 1, item)
for child in item:
show_group(child)
return
def render(item: LTItem) -> None:
child: LTItem
if isinstance(item, LTPage):
self._yoffset += item.y1
self.place_border("page", 1, item)
if self.showpageno:
self.write(
'<div style="position:absolute; top:%dpx;">'
% ((self._yoffset - item.y1) * self.scale)
)
self.write(
'<a name="{}">Page {}</a></div>\n'.format(
item.pageid, item.pageid
)
)
for child in item:
render(child)
if item.groups is not None:
for group in item.groups:
show_group(group)
elif isinstance(item, LTCurve):
self.place_border("curve", 1, item)
elif isinstance(item, LTFigure):
self.begin_div("figure", 1, item.x0, item.y1, item.width, item.height)
for child in item:
render(child)
self.end_div("figure")
elif isinstance(item, LTImage):
self.place_image(item, 1, item.x0, item.y1, item.width, item.height)
else:
if self.layoutmode == "exact":
if isinstance(item, LTTextLine):
self.place_border("textline", 1, item)
for child in item:
render(child)
elif isinstance(item, LTTextBox):
self.place_border("textbox", 1, item)
self.place_text(
"textbox", str(item.index + 1), item.x0, item.y1, 20
)
for child in item:
render(child)
elif isinstance(item, LTChar):
self.place_border("char", 1, item)
self.place_text(
"char", item.get_text(), item.x0, item.y1, item.size
)
else:
if isinstance(item, LTTextLine):
for child in item:
render(child)
if self.layoutmode != "loose":
self.put_newline()
elif isinstance(item, LTTextBox):
self.begin_div(
"textbox",
1,
item.x0,
item.y1,
item.width,
item.height,
item.get_writing_mode(),
)
for child in item:
render(child)
self.end_div("textbox")
elif isinstance(item, LTChar):
fontname = make_compat_str(item.fontname)
self.put_text(item.get_text(), fontname, item.size)
elif isinstance(item, LTText):
self.write_text(item.get_text())
return
render(ltpage)
self._yoffset += self.pagemargin
return
def close(self) -> None:
self.write_footer()
return
class XMLConverter(PDFConverter[AnyIO]):
CONTROL = re.compile("[\x00-\x08\x0b-\x0c\x0e-\x1f]")
def __init__(
self,
rsrcmgr: PDFResourceManager,
outfp: AnyIO,
codec: str = "utf-8",
pageno: int = 1,
laparams: Optional[LAParams] = None,
imagewriter: Optional[ImageWriter] = None,
stripcontrol: bool = False,
) -> None:
PDFConverter.__init__(
self, rsrcmgr, outfp, codec=codec, pageno=pageno, laparams=laparams
)
# write() assumes a codec for binary I/O, or no codec for text I/O.
if self.outfp_binary == (not self.codec):
raise ValueError("Codec is required for a binary I/O output")
self.imagewriter = imagewriter
self.stripcontrol = stripcontrol
self.write_header()
return
def write(self, text: str) -> None:
if self.codec:
cast(BinaryIO, self.outfp).write(text.encode(self.codec))
else:
cast(TextIO, self.outfp).write(text)
return
def write_header(self) -> None:
if self.codec:
self.write('<?xml version="1.0" encoding="%s" ?>\n' % self.codec)
else:
self.write('<?xml version="1.0" ?>\n')
self.write("<pages>\n")
return
def write_footer(self) -> None:
self.write("</pages>\n")
return
def write_text(self, text: str) -> None:
if self.stripcontrol:
text = self.CONTROL.sub("", text)
self.write(enc(text))
return
def receive_layout(self, ltpage: LTPage) -> None:
def show_group(item: LTItem) -> None:
if isinstance(item, LTTextBox):
self.write(
'<textbox id="%d" bbox="%s" />\n'
% (item.index, bbox2str(item.bbox))
)
elif isinstance(item, LTTextGroup):
self.write('<textgroup bbox="%s">\n' % bbox2str(item.bbox))
for child in item:
show_group(child)
self.write("</textgroup>\n")
return
def render(item: LTItem) -> None:
child: LTItem
if isinstance(item, LTPage):
s = '<page id="%s" bbox="%s" rotate="%d">\n' % (
item.pageid,
bbox2str(item.bbox),
item.rotate,
)
self.write(s)
for child in item:
render(child)
if item.groups is not None:
self.write("<layout>\n")
for group in item.groups:
show_group(group)
self.write("</layout>\n")
self.write("</page>\n")
elif isinstance(item, LTLine):
s = '<line linewidth="%d" bbox="%s" />\n' % (
item.linewidth,
bbox2str(item.bbox),
)
self.write(s)
elif isinstance(item, LTRect):
s = '<rect linewidth="%d" bbox="%s" />\n' % (
item.linewidth,
bbox2str(item.bbox),
)
self.write(s)
elif isinstance(item, LTCurve):
s = '<curve linewidth="%d" bbox="%s" pts="%s"/>\n' % (
item.linewidth,
bbox2str(item.bbox),
item.get_pts(),
)
self.write(s)
elif isinstance(item, LTFigure):
s = '<figure name="%s" bbox="%s">\n' % (item.name, bbox2str(item.bbox))
self.write(s)
for child in item:
render(child)
self.write("</figure>\n")
elif isinstance(item, LTTextLine):
self.write('<textline bbox="%s">\n' % bbox2str(item.bbox))
for child in item:
render(child)
self.write("</textline>\n")
elif isinstance(item, LTTextBox):
wmode = ""
if isinstance(item, LTTextBoxVertical):
wmode = ' wmode="vertical"'
s = '<textbox id="%d" bbox="%s"%s>\n' % (
item.index,
bbox2str(item.bbox),
wmode,
)
self.write(s)
for child in item:
render(child)
self.write("</textbox>\n")
elif isinstance(item, LTChar):
s = (
'<text font="%s" bbox="%s" colourspace="%s" '
'ncolour="%s" size="%.3f">'
% (
enc(item.fontname),
bbox2str(item.bbox),
item.ncs.name,
item.graphicstate.ncolor,
item.size,
)
)
self.write(s)
self.write_text(item.get_text())
self.write("</text>\n")
elif isinstance(item, LTText):
self.write("<text>%s</text>\n" % item.get_text())
elif isinstance(item, LTImage):
if self.imagewriter is not None:
name = self.imagewriter.export_image(item)
self.write(
'<image src="%s" width="%d" height="%d" />\n'
% (enc(name), item.width, item.height)
)
else:
self.write(
'<image width="%d" height="%d" />\n' % (item.width, item.height)
)
else:
assert False, str(("Unhandled", item))
return
render(ltpage)
return
def close(self) -> None:
self.write_footer()
return | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/converter.py | converter.py |
#
# Adobe Core 35 AFM Files with 314 Glyph Entries - ReadMe
#
# This file and the 35 PostScript(R) AFM files it accompanies may be
# used, copied, and distributed for any purpose and without charge,
# with or without modification, provided that all copyright notices
# are retained; that the AFM files are not distributed without this
# file; that all modifications to this file or any of the AFM files
# are prominently noted in the modified file(s); and that this
# paragraph is not modified. Adobe Systems has no responsibility or
# obligation to support the use of the AFM files.
#
### END Verbatim copy of the license part
# flake8: noqa
FONT_METRICS = {
"Courier": (
{
"FontName": "Courier",
"Descent": -194.0,
"FontBBox": (-6.0, -249.0, 639.0, 803.0),
"FontWeight": "Medium",
"CapHeight": 572.0,
"FontFamily": "Courier",
"Flags": 64,
"XHeight": 434.0,
"ItalicAngle": 0.0,
"Ascent": 627.0,
},
{
" ": 600,
"!": 600,
'"': 600,
"#": 600,
"$": 600,
"%": 600,
"&": 600,
"'": 600,
"(": 600,
")": 600,
"*": 600,
"+": 600,
",": 600,
"-": 600,
".": 600,
"/": 600,
"0": 600,
"1": 600,
"2": 600,
"3": 600,
"4": 600,
"5": 600,
"6": 600,
"7": 600,
"8": 600,
"9": 600,
":": 600,
";": 600,
"<": 600,
"=": 600,
">": 600,
"?": 600,
"@": 600,
"A": 600,
"B": 600,
"C": 600,
"D": 600,
"E": 600,
"F": 600,
"G": 600,
"H": 600,
"I": 600,
"J": 600,
"K": 600,
"L": 600,
"M": 600,
"N": 600,
"O": 600,
"P": 600,
"Q": 600,
"R": 600,
"S": 600,
"T": 600,
"U": 600,
"V": 600,
"W": 600,
"X": 600,
"Y": 600,
"Z": 600,
"[": 600,
"\\": 600,
"]": 600,
"^": 600,
"_": 600,
"`": 600,
"a": 600,
"b": 600,
"c": 600,
"d": 600,
"e": 600,
"f": 600,
"g": 600,
"h": 600,
"i": 600,
"j": 600,
"k": 600,
"l": 600,
"m": 600,
"n": 600,
"o": 600,
"p": 600,
"q": 600,
"r": 600,
"s": 600,
"t": 600,
"u": 600,
"v": 600,
"w": 600,
"x": 600,
"y": 600,
"z": 600,
"{": 600,
"|": 600,
"}": 600,
"~": 600,
"\xa1": 600,
"\xa2": 600,
"\xa3": 600,
"\xa4": 600,
"\xa5": 600,
"\xa6": 600,
"\xa7": 600,
"\xa8": 600,
"\xa9": 600,
"\xaa": 600,
"\xab": 600,
"\xac": 600,
"\xae": 600,
"\xaf": 600,
"\xb0": 600,
"\xb1": 600,
"\xb2": 600,
"\xb3": 600,
"\xb4": 600,
"\xb5": 600,
"\xb6": 600,
"\xb7": 600,
"\xb8": 600,
"\xb9": 600,
"\xba": 600,
"\xbb": 600,
"\xbc": 600,
"\xbd": 600,
"\xbe": 600,
"\xbf": 600,
"\xc0": 600,
"\xc1": 600,
"\xc2": 600,
"\xc3": 600,
"\xc4": 600,
"\xc5": 600,
"\xc6": 600,
"\xc7": 600,
"\xc8": 600,
"\xc9": 600,
"\xca": 600,
"\xcb": 600,
"\xcc": 600,
"\xcd": 600,
"\xce": 600,
"\xcf": 600,
"\xd0": 600,
"\xd1": 600,
"\xd2": 600,
"\xd3": 600,
"\xd4": 600,
"\xd5": 600,
"\xd6": 600,
"\xd7": 600,
"\xd8": 600,
"\xd9": 600,
"\xda": 600,
"\xdb": 600,
"\xdc": 600,
"\xdd": 600,
"\xde": 600,
"\xdf": 600,
"\xe0": 600,
"\xe1": 600,
"\xe2": 600,
"\xe3": 600,
"\xe4": 600,
"\xe5": 600,
"\xe6": 600,
"\xe7": 600,
"\xe8": 600,
"\xe9": 600,
"\xea": 600,
"\xeb": 600,
"\xec": 600,
"\xed": 600,
"\xee": 600,
"\xef": 600,
"\xf0": 600,
"\xf1": 600,
"\xf2": 600,
"\xf3": 600,
"\xf4": 600,
"\xf5": 600,
"\xf6": 600,
"\xf7": 600,
"\xf8": 600,
"\xf9": 600,
"\xfa": 600,
"\xfb": 600,
"\xfc": 600,
"\xfd": 600,
"\xfe": 600,
"\xff": 600,
"\u0100": 600,
"\u0101": 600,
"\u0102": 600,
"\u0103": 600,
"\u0104": 600,
"\u0105": 600,
"\u0106": 600,
"\u0107": 600,
"\u010c": 600,
"\u010d": 600,
"\u010e": 600,
"\u010f": 600,
"\u0110": 600,
"\u0111": 600,
"\u0112": 600,
"\u0113": 600,
"\u0116": 600,
"\u0117": 600,
"\u0118": 600,
"\u0119": 600,
"\u011a": 600,
"\u011b": 600,
"\u011e": 600,
"\u011f": 600,
"\u0122": 600,
"\u0123": 600,
"\u012a": 600,
"\u012b": 600,
"\u012e": 600,
"\u012f": 600,
"\u0130": 600,
"\u0131": 600,
"\u0136": 600,
"\u0137": 600,
"\u0139": 600,
"\u013a": 600,
"\u013b": 600,
"\u013c": 600,
"\u013d": 600,
"\u013e": 600,
"\u0141": 600,
"\u0142": 600,
"\u0143": 600,
"\u0144": 600,
"\u0145": 600,
"\u0146": 600,
"\u0147": 600,
"\u0148": 600,
"\u014c": 600,
"\u014d": 600,
"\u0150": 600,
"\u0151": 600,
"\u0152": 600,
"\u0153": 600,
"\u0154": 600,
"\u0155": 600,
"\u0156": 600,
"\u0157": 600,
"\u0158": 600,
"\u0159": 600,
"\u015a": 600,
"\u015b": 600,
"\u015e": 600,
"\u015f": 600,
"\u0160": 600,
"\u0161": 600,
"\u0162": 600,
"\u0163": 600,
"\u0164": 600,
"\u0165": 600,
"\u016a": 600,
"\u016b": 600,
"\u016e": 600,
"\u016f": 600,
"\u0170": 600,
"\u0171": 600,
"\u0172": 600,
"\u0173": 600,
"\u0178": 600,
"\u0179": 600,
"\u017a": 600,
"\u017b": 600,
"\u017c": 600,
"\u017d": 600,
"\u017e": 600,
"\u0192": 600,
"\u0218": 600,
"\u0219": 600,
"\u02c6": 600,
"\u02c7": 600,
"\u02d8": 600,
"\u02d9": 600,
"\u02da": 600,
"\u02db": 600,
"\u02dc": 600,
"\u02dd": 600,
"\u2013": 600,
"\u2014": 600,
"\u2018": 600,
"\u2019": 600,
"\u201a": 600,
"\u201c": 600,
"\u201d": 600,
"\u201e": 600,
"\u2020": 600,
"\u2021": 600,
"\u2022": 600,
"\u2026": 600,
"\u2030": 600,
"\u2039": 600,
"\u203a": 600,
"\u2044": 600,
"\u2122": 600,
"\u2202": 600,
"\u2206": 600,
"\u2211": 600,
"\u2212": 600,
"\u221a": 600,
"\u2260": 600,
"\u2264": 600,
"\u2265": 600,
"\u25ca": 600,
"\uf6c3": 600,
"\ufb01": 600,
"\ufb02": 600,
},
),
"Courier-Bold": (
{
"FontName": "Courier-Bold",
"Descent": -194.0,
"FontBBox": (-88.0, -249.0, 697.0, 811.0),
"FontWeight": "Bold",
"CapHeight": 572.0,
"FontFamily": "Courier",
"Flags": 64,
"XHeight": 434.0,
"ItalicAngle": 0.0,
"Ascent": 627.0,
},
{
" ": 600,
"!": 600,
'"': 600,
"#": 600,
"$": 600,
"%": 600,
"&": 600,
"'": 600,
"(": 600,
")": 600,
"*": 600,
"+": 600,
",": 600,
"-": 600,
".": 600,
"/": 600,
"0": 600,
"1": 600,
"2": 600,
"3": 600,
"4": 600,
"5": 600,
"6": 600,
"7": 600,
"8": 600,
"9": 600,
":": 600,
";": 600,
"<": 600,
"=": 600,
">": 600,
"?": 600,
"@": 600,
"A": 600,
"B": 600,
"C": 600,
"D": 600,
"E": 600,
"F": 600,
"G": 600,
"H": 600,
"I": 600,
"J": 600,
"K": 600,
"L": 600,
"M": 600,
"N": 600,
"O": 600,
"P": 600,
"Q": 600,
"R": 600,
"S": 600,
"T": 600,
"U": 600,
"V": 600,
"W": 600,
"X": 600,
"Y": 600,
"Z": 600,
"[": 600,
"\\": 600,
"]": 600,
"^": 600,
"_": 600,
"`": 600,
"a": 600,
"b": 600,
"c": 600,
"d": 600,
"e": 600,
"f": 600,
"g": 600,
"h": 600,
"i": 600,
"j": 600,
"k": 600,
"l": 600,
"m": 600,
"n": 600,
"o": 600,
"p": 600,
"q": 600,
"r": 600,
"s": 600,
"t": 600,
"u": 600,
"v": 600,
"w": 600,
"x": 600,
"y": 600,
"z": 600,
"{": 600,
"|": 600,
"}": 600,
"~": 600,
"\xa1": 600,
"\xa2": 600,
"\xa3": 600,
"\xa4": 600,
"\xa5": 600,
"\xa6": 600,
"\xa7": 600,
"\xa8": 600,
"\xa9": 600,
"\xaa": 600,
"\xab": 600,
"\xac": 600,
"\xae": 600,
"\xaf": 600,
"\xb0": 600,
"\xb1": 600,
"\xb2": 600,
"\xb3": 600,
"\xb4": 600,
"\xb5": 600,
"\xb6": 600,
"\xb7": 600,
"\xb8": 600,
"\xb9": 600,
"\xba": 600,
"\xbb": 600,
"\xbc": 600,
"\xbd": 600,
"\xbe": 600,
"\xbf": 600,
"\xc0": 600,
"\xc1": 600,
"\xc2": 600,
"\xc3": 600,
"\xc4": 600,
"\xc5": 600,
"\xc6": 600,
"\xc7": 600,
"\xc8": 600,
"\xc9": 600,
"\xca": 600,
"\xcb": 600,
"\xcc": 600,
"\xcd": 600,
"\xce": 600,
"\xcf": 600,
"\xd0": 600,
"\xd1": 600,
"\xd2": 600,
"\xd3": 600,
"\xd4": 600,
"\xd5": 600,
"\xd6": 600,
"\xd7": 600,
"\xd8": 600,
"\xd9": 600,
"\xda": 600,
"\xdb": 600,
"\xdc": 600,
"\xdd": 600,
"\xde": 600,
"\xdf": 600,
"\xe0": 600,
"\xe1": 600,
"\xe2": 600,
"\xe3": 600,
"\xe4": 600,
"\xe5": 600,
"\xe6": 600,
"\xe7": 600,
"\xe8": 600,
"\xe9": 600,
"\xea": 600,
"\xeb": 600,
"\xec": 600,
"\xed": 600,
"\xee": 600,
"\xef": 600,
"\xf0": 600,
"\xf1": 600,
"\xf2": 600,
"\xf3": 600,
"\xf4": 600,
"\xf5": 600,
"\xf6": 600,
"\xf7": 600,
"\xf8": 600,
"\xf9": 600,
"\xfa": 600,
"\xfb": 600,
"\xfc": 600,
"\xfd": 600,
"\xfe": 600,
"\xff": 600,
"\u0100": 600,
"\u0101": 600,
"\u0102": 600,
"\u0103": 600,
"\u0104": 600,
"\u0105": 600,
"\u0106": 600,
"\u0107": 600,
"\u010c": 600,
"\u010d": 600,
"\u010e": 600,
"\u010f": 600,
"\u0110": 600,
"\u0111": 600,
"\u0112": 600,
"\u0113": 600,
"\u0116": 600,
"\u0117": 600,
"\u0118": 600,
"\u0119": 600,
"\u011a": 600,
"\u011b": 600,
"\u011e": 600,
"\u011f": 600,
"\u0122": 600,
"\u0123": 600,
"\u012a": 600,
"\u012b": 600,
"\u012e": 600,
"\u012f": 600,
"\u0130": 600,
"\u0131": 600,
"\u0136": 600,
"\u0137": 600,
"\u0139": 600,
"\u013a": 600,
"\u013b": 600,
"\u013c": 600,
"\u013d": 600,
"\u013e": 600,
"\u0141": 600,
"\u0142": 600,
"\u0143": 600,
"\u0144": 600,
"\u0145": 600,
"\u0146": 600,
"\u0147": 600,
"\u0148": 600,
"\u014c": 600,
"\u014d": 600,
"\u0150": 600,
"\u0151": 600,
"\u0152": 600,
"\u0153": 600,
"\u0154": 600,
"\u0155": 600,
"\u0156": 600,
"\u0157": 600,
"\u0158": 600,
"\u0159": 600,
"\u015a": 600,
"\u015b": 600,
"\u015e": 600,
"\u015f": 600,
"\u0160": 600,
"\u0161": 600,
"\u0162": 600,
"\u0163": 600,
"\u0164": 600,
"\u0165": 600,
"\u016a": 600,
"\u016b": 600,
"\u016e": 600,
"\u016f": 600,
"\u0170": 600,
"\u0171": 600,
"\u0172": 600,
"\u0173": 600,
"\u0178": 600,
"\u0179": 600,
"\u017a": 600,
"\u017b": 600,
"\u017c": 600,
"\u017d": 600,
"\u017e": 600,
"\u0192": 600,
"\u0218": 600,
"\u0219": 600,
"\u02c6": 600,
"\u02c7": 600,
"\u02d8": 600,
"\u02d9": 600,
"\u02da": 600,
"\u02db": 600,
"\u02dc": 600,
"\u02dd": 600,
"\u2013": 600,
"\u2014": 600,
"\u2018": 600,
"\u2019": 600,
"\u201a": 600,
"\u201c": 600,
"\u201d": 600,
"\u201e": 600,
"\u2020": 600,
"\u2021": 600,
"\u2022": 600,
"\u2026": 600,
"\u2030": 600,
"\u2039": 600,
"\u203a": 600,
"\u2044": 600,
"\u2122": 600,
"\u2202": 600,
"\u2206": 600,
"\u2211": 600,
"\u2212": 600,
"\u221a": 600,
"\u2260": 600,
"\u2264": 600,
"\u2265": 600,
"\u25ca": 600,
"\uf6c3": 600,
"\ufb01": 600,
"\ufb02": 600,
},
),
"Courier-BoldOblique": (
{
"FontName": "Courier-BoldOblique",
"Descent": -194.0,
"FontBBox": (-49.0, -249.0, 758.0, 811.0),
"FontWeight": "Bold",
"CapHeight": 572.0,
"FontFamily": "Courier",
"Flags": 64,
"XHeight": 434.0,
"ItalicAngle": -11.0,
"Ascent": 627.0,
},
{
" ": 600,
"!": 600,
'"': 600,
"#": 600,
"$": 600,
"%": 600,
"&": 600,
"'": 600,
"(": 600,
")": 600,
"*": 600,
"+": 600,
",": 600,
"-": 600,
".": 600,
"/": 600,
"0": 600,
"1": 600,
"2": 600,
"3": 600,
"4": 600,
"5": 600,
"6": 600,
"7": 600,
"8": 600,
"9": 600,
":": 600,
";": 600,
"<": 600,
"=": 600,
">": 600,
"?": 600,
"@": 600,
"A": 600,
"B": 600,
"C": 600,
"D": 600,
"E": 600,
"F": 600,
"G": 600,
"H": 600,
"I": 600,
"J": 600,
"K": 600,
"L": 600,
"M": 600,
"N": 600,
"O": 600,
"P": 600,
"Q": 600,
"R": 600,
"S": 600,
"T": 600,
"U": 600,
"V": 600,
"W": 600,
"X": 600,
"Y": 600,
"Z": 600,
"[": 600,
"\\": 600,
"]": 600,
"^": 600,
"_": 600,
"`": 600,
"a": 600,
"b": 600,
"c": 600,
"d": 600,
"e": 600,
"f": 600,
"g": 600,
"h": 600,
"i": 600,
"j": 600,
"k": 600,
"l": 600,
"m": 600,
"n": 600,
"o": 600,
"p": 600,
"q": 600,
"r": 600,
"s": 600,
"t": 600,
"u": 600,
"v": 600,
"w": 600,
"x": 600,
"y": 600,
"z": 600,
"{": 600,
"|": 600,
"}": 600,
"~": 600,
"\xa1": 600,
"\xa2": 600,
"\xa3": 600,
"\xa4": 600,
"\xa5": 600,
"\xa6": 600,
"\xa7": 600,
"\xa8": 600,
"\xa9": 600,
"\xaa": 600,
"\xab": 600,
"\xac": 600,
"\xae": 600,
"\xaf": 600,
"\xb0": 600,
"\xb1": 600,
"\xb2": 600,
"\xb3": 600,
"\xb4": 600,
"\xb5": 600,
"\xb6": 600,
"\xb7": 600,
"\xb8": 600,
"\xb9": 600,
"\xba": 600,
"\xbb": 600,
"\xbc": 600,
"\xbd": 600,
"\xbe": 600,
"\xbf": 600,
"\xc0": 600,
"\xc1": 600,
"\xc2": 600,
"\xc3": 600,
"\xc4": 600,
"\xc5": 600,
"\xc6": 600,
"\xc7": 600,
"\xc8": 600,
"\xc9": 600,
"\xca": 600,
"\xcb": 600,
"\xcc": 600,
"\xcd": 600,
"\xce": 600,
"\xcf": 600,
"\xd0": 600,
"\xd1": 600,
"\xd2": 600,
"\xd3": 600,
"\xd4": 600,
"\xd5": 600,
"\xd6": 600,
"\xd7": 600,
"\xd8": 600,
"\xd9": 600,
"\xda": 600,
"\xdb": 600,
"\xdc": 600,
"\xdd": 600,
"\xde": 600,
"\xdf": 600,
"\xe0": 600,
"\xe1": 600,
"\xe2": 600,
"\xe3": 600,
"\xe4": 600,
"\xe5": 600,
"\xe6": 600,
"\xe7": 600,
"\xe8": 600,
"\xe9": 600,
"\xea": 600,
"\xeb": 600,
"\xec": 600,
"\xed": 600,
"\xee": 600,
"\xef": 600,
"\xf0": 600,
"\xf1": 600,
"\xf2": 600,
"\xf3": 600,
"\xf4": 600,
"\xf5": 600,
"\xf6": 600,
"\xf7": 600,
"\xf8": 600,
"\xf9": 600,
"\xfa": 600,
"\xfb": 600,
"\xfc": 600,
"\xfd": 600,
"\xfe": 600,
"\xff": 600,
"\u0100": 600,
"\u0101": 600,
"\u0102": 600,
"\u0103": 600,
"\u0104": 600,
"\u0105": 600,
"\u0106": 600,
"\u0107": 600,
"\u010c": 600,
"\u010d": 600,
"\u010e": 600,
"\u010f": 600,
"\u0110": 600,
"\u0111": 600,
"\u0112": 600,
"\u0113": 600,
"\u0116": 600,
"\u0117": 600,
"\u0118": 600,
"\u0119": 600,
"\u011a": 600,
"\u011b": 600,
"\u011e": 600,
"\u011f": 600,
"\u0122": 600,
"\u0123": 600,
"\u012a": 600,
"\u012b": 600,
"\u012e": 600,
"\u012f": 600,
"\u0130": 600,
"\u0131": 600,
"\u0136": 600,
"\u0137": 600,
"\u0139": 600,
"\u013a": 600,
"\u013b": 600,
"\u013c": 600,
"\u013d": 600,
"\u013e": 600,
"\u0141": 600,
"\u0142": 600,
"\u0143": 600,
"\u0144": 600,
"\u0145": 600,
"\u0146": 600,
"\u0147": 600,
"\u0148": 600,
"\u014c": 600,
"\u014d": 600,
"\u0150": 600,
"\u0151": 600,
"\u0152": 600,
"\u0153": 600,
"\u0154": 600,
"\u0155": 600,
"\u0156": 600,
"\u0157": 600,
"\u0158": 600,
"\u0159": 600,
"\u015a": 600,
"\u015b": 600,
"\u015e": 600,
"\u015f": 600,
"\u0160": 600,
"\u0161": 600,
"\u0162": 600,
"\u0163": 600,
"\u0164": 600,
"\u0165": 600,
"\u016a": 600,
"\u016b": 600,
"\u016e": 600,
"\u016f": 600,
"\u0170": 600,
"\u0171": 600,
"\u0172": 600,
"\u0173": 600,
"\u0178": 600,
"\u0179": 600,
"\u017a": 600,
"\u017b": 600,
"\u017c": 600,
"\u017d": 600,
"\u017e": 600,
"\u0192": 600,
"\u0218": 600,
"\u0219": 600,
"\u02c6": 600,
"\u02c7": 600,
"\u02d8": 600,
"\u02d9": 600,
"\u02da": 600,
"\u02db": 600,
"\u02dc": 600,
"\u02dd": 600,
"\u2013": 600,
"\u2014": 600,
"\u2018": 600,
"\u2019": 600,
"\u201a": 600,
"\u201c": 600,
"\u201d": 600,
"\u201e": 600,
"\u2020": 600,
"\u2021": 600,
"\u2022": 600,
"\u2026": 600,
"\u2030": 600,
"\u2039": 600,
"\u203a": 600,
"\u2044": 600,
"\u2122": 600,
"\u2202": 600,
"\u2206": 600,
"\u2211": 600,
"\u2212": 600,
"\u221a": 600,
"\u2260": 600,
"\u2264": 600,
"\u2265": 600,
"\u25ca": 600,
"\uf6c3": 600,
"\ufb01": 600,
"\ufb02": 600,
},
),
"Courier-Oblique": (
{
"FontName": "Courier-Oblique",
"Descent": -194.0,
"FontBBox": (-49.0, -249.0, 749.0, 803.0),
"FontWeight": "Medium",
"CapHeight": 572.0,
"FontFamily": "Courier",
"Flags": 64,
"XHeight": 434.0,
"ItalicAngle": -11.0,
"Ascent": 627.0,
},
{
" ": 600,
"!": 600,
'"': 600,
"#": 600,
"$": 600,
"%": 600,
"&": 600,
"'": 600,
"(": 600,
")": 600,
"*": 600,
"+": 600,
",": 600,
"-": 600,
".": 600,
"/": 600,
"0": 600,
"1": 600,
"2": 600,
"3": 600,
"4": 600,
"5": 600,
"6": 600,
"7": 600,
"8": 600,
"9": 600,
":": 600,
";": 600,
"<": 600,
"=": 600,
">": 600,
"?": 600,
"@": 600,
"A": 600,
"B": 600,
"C": 600,
"D": 600,
"E": 600,
"F": 600,
"G": 600,
"H": 600,
"I": 600,
"J": 600,
"K": 600,
"L": 600,
"M": 600,
"N": 600,
"O": 600,
"P": 600,
"Q": 600,
"R": 600,
"S": 600,
"T": 600,
"U": 600,
"V": 600,
"W": 600,
"X": 600,
"Y": 600,
"Z": 600,
"[": 600,
"\\": 600,
"]": 600,
"^": 600,
"_": 600,
"`": 600,
"a": 600,
"b": 600,
"c": 600,
"d": 600,
"e": 600,
"f": 600,
"g": 600,
"h": 600,
"i": 600,
"j": 600,
"k": 600,
"l": 600,
"m": 600,
"n": 600,
"o": 600,
"p": 600,
"q": 600,
"r": 600,
"s": 600,
"t": 600,
"u": 600,
"v": 600,
"w": 600,
"x": 600,
"y": 600,
"z": 600,
"{": 600,
"|": 600,
"}": 600,
"~": 600,
"\xa1": 600,
"\xa2": 600,
"\xa3": 600,
"\xa4": 600,
"\xa5": 600,
"\xa6": 600,
"\xa7": 600,
"\xa8": 600,
"\xa9": 600,
"\xaa": 600,
"\xab": 600,
"\xac": 600,
"\xae": 600,
"\xaf": 600,
"\xb0": 600,
"\xb1": 600,
"\xb2": 600,
"\xb3": 600,
"\xb4": 600,
"\xb5": 600,
"\xb6": 600,
"\xb7": 600,
"\xb8": 600,
"\xb9": 600,
"\xba": 600,
"\xbb": 600,
"\xbc": 600,
"\xbd": 600,
"\xbe": 600,
"\xbf": 600,
"\xc0": 600,
"\xc1": 600,
"\xc2": 600,
"\xc3": 600,
"\xc4": 600,
"\xc5": 600,
"\xc6": 600,
"\xc7": 600,
"\xc8": 600,
"\xc9": 600,
"\xca": 600,
"\xcb": 600,
"\xcc": 600,
"\xcd": 600,
"\xce": 600,
"\xcf": 600,
"\xd0": 600,
"\xd1": 600,
"\xd2": 600,
"\xd3": 600,
"\xd4": 600,
"\xd5": 600,
"\xd6": 600,
"\xd7": 600,
"\xd8": 600,
"\xd9": 600,
"\xda": 600,
"\xdb": 600,
"\xdc": 600,
"\xdd": 600,
"\xde": 600,
"\xdf": 600,
"\xe0": 600,
"\xe1": 600,
"\xe2": 600,
"\xe3": 600,
"\xe4": 600,
"\xe5": 600,
"\xe6": 600,
"\xe7": 600,
"\xe8": 600,
"\xe9": 600,
"\xea": 600,
"\xeb": 600,
"\xec": 600,
"\xed": 600,
"\xee": 600,
"\xef": 600,
"\xf0": 600,
"\xf1": 600,
"\xf2": 600,
"\xf3": 600,
"\xf4": 600,
"\xf5": 600,
"\xf6": 600,
"\xf7": 600,
"\xf8": 600,
"\xf9": 600,
"\xfa": 600,
"\xfb": 600,
"\xfc": 600,
"\xfd": 600,
"\xfe": 600,
"\xff": 600,
"\u0100": 600,
"\u0101": 600,
"\u0102": 600,
"\u0103": 600,
"\u0104": 600,
"\u0105": 600,
"\u0106": 600,
"\u0107": 600,
"\u010c": 600,
"\u010d": 600,
"\u010e": 600,
"\u010f": 600,
"\u0110": 600,
"\u0111": 600,
"\u0112": 600,
"\u0113": 600,
"\u0116": 600,
"\u0117": 600,
"\u0118": 600,
"\u0119": 600,
"\u011a": 600,
"\u011b": 600,
"\u011e": 600,
"\u011f": 600,
"\u0122": 600,
"\u0123": 600,
"\u012a": 600,
"\u012b": 600,
"\u012e": 600,
"\u012f": 600,
"\u0130": 600,
"\u0131": 600,
"\u0136": 600,
"\u0137": 600,
"\u0139": 600,
"\u013a": 600,
"\u013b": 600,
"\u013c": 600,
"\u013d": 600,
"\u013e": 600,
"\u0141": 600,
"\u0142": 600,
"\u0143": 600,
"\u0144": 600,
"\u0145": 600,
"\u0146": 600,
"\u0147": 600,
"\u0148": 600,
"\u014c": 600,
"\u014d": 600,
"\u0150": 600,
"\u0151": 600,
"\u0152": 600,
"\u0153": 600,
"\u0154": 600,
"\u0155": 600,
"\u0156": 600,
"\u0157": 600,
"\u0158": 600,
"\u0159": 600,
"\u015a": 600,
"\u015b": 600,
"\u015e": 600,
"\u015f": 600,
"\u0160": 600,
"\u0161": 600,
"\u0162": 600,
"\u0163": 600,
"\u0164": 600,
"\u0165": 600,
"\u016a": 600,
"\u016b": 600,
"\u016e": 600,
"\u016f": 600,
"\u0170": 600,
"\u0171": 600,
"\u0172": 600,
"\u0173": 600,
"\u0178": 600,
"\u0179": 600,
"\u017a": 600,
"\u017b": 600,
"\u017c": 600,
"\u017d": 600,
"\u017e": 600,
"\u0192": 600,
"\u0218": 600,
"\u0219": 600,
"\u02c6": 600,
"\u02c7": 600,
"\u02d8": 600,
"\u02d9": 600,
"\u02da": 600,
"\u02db": 600,
"\u02dc": 600,
"\u02dd": 600,
"\u2013": 600,
"\u2014": 600,
"\u2018": 600,
"\u2019": 600,
"\u201a": 600,
"\u201c": 600,
"\u201d": 600,
"\u201e": 600,
"\u2020": 600,
"\u2021": 600,
"\u2022": 600,
"\u2026": 600,
"\u2030": 600,
"\u2039": 600,
"\u203a": 600,
"\u2044": 600,
"\u2122": 600,
"\u2202": 600,
"\u2206": 600,
"\u2211": 600,
"\u2212": 600,
"\u221a": 600,
"\u2260": 600,
"\u2264": 600,
"\u2265": 600,
"\u25ca": 600,
"\uf6c3": 600,
"\ufb01": 600,
"\ufb02": 600,
},
),
"Helvetica": (
{
"FontName": "Helvetica",
"Descent": -207.0,
"FontBBox": (-166.0, -225.0, 1000.0, 931.0),
"FontWeight": "Medium",
"CapHeight": 718.0,
"FontFamily": "Helvetica",
"Flags": 0,
"XHeight": 523.0,
"ItalicAngle": 0.0,
"Ascent": 718.0,
},
{
" ": 278,
"!": 278,
'"': 355,
"#": 556,
"$": 556,
"%": 889,
"&": 667,
"'": 191,
"(": 333,
")": 333,
"*": 389,
"+": 584,
",": 278,
"-": 333,
".": 278,
"/": 278,
"0": 556,
"1": 556,
"2": 556,
"3": 556,
"4": 556,
"5": 556,
"6": 556,
"7": 556,
"8": 556,
"9": 556,
":": 278,
";": 278,
"<": 584,
"=": 584,
">": 584,
"?": 556,
"@": 1015,
"A": 667,
"B": 667,
"C": 722,
"D": 722,
"E": 667,
"F": 611,
"G": 778,
"H": 722,
"I": 278,
"J": 500,
"K": 667,
"L": 556,
"M": 833,
"N": 722,
"O": 778,
"P": 667,
"Q": 778,
"R": 722,
"S": 667,
"T": 611,
"U": 722,
"V": 667,
"W": 944,
"X": 667,
"Y": 667,
"Z": 611,
"[": 278,
"\\": 278,
"]": 278,
"^": 469,
"_": 556,
"`": 333,
"a": 556,
"b": 556,
"c": 500,
"d": 556,
"e": 556,
"f": 278,
"g": 556,
"h": 556,
"i": 222,
"j": 222,
"k": 500,
"l": 222,
"m": 833,
"n": 556,
"o": 556,
"p": 556,
"q": 556,
"r": 333,
"s": 500,
"t": 278,
"u": 556,
"v": 500,
"w": 722,
"x": 500,
"y": 500,
"z": 500,
"{": 334,
"|": 260,
"}": 334,
"~": 584,
"\xa1": 333,
"\xa2": 556,
"\xa3": 556,
"\xa4": 556,
"\xa5": 556,
"\xa6": 260,
"\xa7": 556,
"\xa8": 333,
"\xa9": 737,
"\xaa": 370,
"\xab": 556,
"\xac": 584,
"\xae": 737,
"\xaf": 333,
"\xb0": 400,
"\xb1": 584,
"\xb2": 333,
"\xb3": 333,
"\xb4": 333,
"\xb5": 556,
"\xb6": 537,
"\xb7": 278,
"\xb8": 333,
"\xb9": 333,
"\xba": 365,
"\xbb": 556,
"\xbc": 834,
"\xbd": 834,
"\xbe": 834,
"\xbf": 611,
"\xc0": 667,
"\xc1": 667,
"\xc2": 667,
"\xc3": 667,
"\xc4": 667,
"\xc5": 667,
"\xc6": 1000,
"\xc7": 722,
"\xc8": 667,
"\xc9": 667,
"\xca": 667,
"\xcb": 667,
"\xcc": 278,
"\xcd": 278,
"\xce": 278,
"\xcf": 278,
"\xd0": 722,
"\xd1": 722,
"\xd2": 778,
"\xd3": 778,
"\xd4": 778,
"\xd5": 778,
"\xd6": 778,
"\xd7": 584,
"\xd8": 778,
"\xd9": 722,
"\xda": 722,
"\xdb": 722,
"\xdc": 722,
"\xdd": 667,
"\xde": 667,
"\xdf": 611,
"\xe0": 556,
"\xe1": 556,
"\xe2": 556,
"\xe3": 556,
"\xe4": 556,
"\xe5": 556,
"\xe6": 889,
"\xe7": 500,
"\xe8": 556,
"\xe9": 556,
"\xea": 556,
"\xeb": 556,
"\xec": 278,
"\xed": 278,
"\xee": 278,
"\xef": 278,
"\xf0": 556,
"\xf1": 556,
"\xf2": 556,
"\xf3": 556,
"\xf4": 556,
"\xf5": 556,
"\xf6": 556,
"\xf7": 584,
"\xf8": 611,
"\xf9": 556,
"\xfa": 556,
"\xfb": 556,
"\xfc": 556,
"\xfd": 500,
"\xfe": 556,
"\xff": 500,
"\u0100": 667,
"\u0101": 556,
"\u0102": 667,
"\u0103": 556,
"\u0104": 667,
"\u0105": 556,
"\u0106": 722,
"\u0107": 500,
"\u010c": 722,
"\u010d": 500,
"\u010e": 722,
"\u010f": 643,
"\u0110": 722,
"\u0111": 556,
"\u0112": 667,
"\u0113": 556,
"\u0116": 667,
"\u0117": 556,
"\u0118": 667,
"\u0119": 556,
"\u011a": 667,
"\u011b": 556,
"\u011e": 778,
"\u011f": 556,
"\u0122": 778,
"\u0123": 556,
"\u012a": 278,
"\u012b": 278,
"\u012e": 278,
"\u012f": 222,
"\u0130": 278,
"\u0131": 278,
"\u0136": 667,
"\u0137": 500,
"\u0139": 556,
"\u013a": 222,
"\u013b": 556,
"\u013c": 222,
"\u013d": 556,
"\u013e": 299,
"\u0141": 556,
"\u0142": 222,
"\u0143": 722,
"\u0144": 556,
"\u0145": 722,
"\u0146": 556,
"\u0147": 722,
"\u0148": 556,
"\u014c": 778,
"\u014d": 556,
"\u0150": 778,
"\u0151": 556,
"\u0152": 1000,
"\u0153": 944,
"\u0154": 722,
"\u0155": 333,
"\u0156": 722,
"\u0157": 333,
"\u0158": 722,
"\u0159": 333,
"\u015a": 667,
"\u015b": 500,
"\u015e": 667,
"\u015f": 500,
"\u0160": 667,
"\u0161": 500,
"\u0162": 611,
"\u0163": 278,
"\u0164": 611,
"\u0165": 317,
"\u016a": 722,
"\u016b": 556,
"\u016e": 722,
"\u016f": 556,
"\u0170": 722,
"\u0171": 556,
"\u0172": 722,
"\u0173": 556,
"\u0178": 667,
"\u0179": 611,
"\u017a": 500,
"\u017b": 611,
"\u017c": 500,
"\u017d": 611,
"\u017e": 500,
"\u0192": 556,
"\u0218": 667,
"\u0219": 500,
"\u02c6": 333,
"\u02c7": 333,
"\u02d8": 333,
"\u02d9": 333,
"\u02da": 333,
"\u02db": 333,
"\u02dc": 333,
"\u02dd": 333,
"\u2013": 556,
"\u2014": 1000,
"\u2018": 222,
"\u2019": 222,
"\u201a": 222,
"\u201c": 333,
"\u201d": 333,
"\u201e": 333,
"\u2020": 556,
"\u2021": 556,
"\u2022": 350,
"\u2026": 1000,
"\u2030": 1000,
"\u2039": 333,
"\u203a": 333,
"\u2044": 167,
"\u2122": 1000,
"\u2202": 476,
"\u2206": 612,
"\u2211": 600,
"\u2212": 584,
"\u221a": 453,
"\u2260": 549,
"\u2264": 549,
"\u2265": 549,
"\u25ca": 471,
"\uf6c3": 250,
"\ufb01": 500,
"\ufb02": 500,
},
),
"Helvetica-Bold": (
{
"FontName": "Helvetica-Bold",
"Descent": -207.0,
"FontBBox": (-170.0, -228.0, 1003.0, 962.0),
"FontWeight": "Bold",
"CapHeight": 718.0,
"FontFamily": "Helvetica",
"Flags": 0,
"XHeight": 532.0,
"ItalicAngle": 0.0,
"Ascent": 718.0,
},
{
" ": 278,
"!": 333,
'"': 474,
"#": 556,
"$": 556,
"%": 889,
"&": 722,
"'": 238,
"(": 333,
")": 333,
"*": 389,
"+": 584,
",": 278,
"-": 333,
".": 278,
"/": 278,
"0": 556,
"1": 556,
"2": 556,
"3": 556,
"4": 556,
"5": 556,
"6": 556,
"7": 556,
"8": 556,
"9": 556,
":": 333,
";": 333,
"<": 584,
"=": 584,
">": 584,
"?": 611,
"@": 975,
"A": 722,
"B": 722,
"C": 722,
"D": 722,
"E": 667,
"F": 611,
"G": 778,
"H": 722,
"I": 278,
"J": 556,
"K": 722,
"L": 611,
"M": 833,
"N": 722,
"O": 778,
"P": 667,
"Q": 778,
"R": 722,
"S": 667,
"T": 611,
"U": 722,
"V": 667,
"W": 944,
"X": 667,
"Y": 667,
"Z": 611,
"[": 333,
"\\": 278,
"]": 333,
"^": 584,
"_": 556,
"`": 333,
"a": 556,
"b": 611,
"c": 556,
"d": 611,
"e": 556,
"f": 333,
"g": 611,
"h": 611,
"i": 278,
"j": 278,
"k": 556,
"l": 278,
"m": 889,
"n": 611,
"o": 611,
"p": 611,
"q": 611,
"r": 389,
"s": 556,
"t": 333,
"u": 611,
"v": 556,
"w": 778,
"x": 556,
"y": 556,
"z": 500,
"{": 389,
"|": 280,
"}": 389,
"~": 584,
"\xa1": 333,
"\xa2": 556,
"\xa3": 556,
"\xa4": 556,
"\xa5": 556,
"\xa6": 280,
"\xa7": 556,
"\xa8": 333,
"\xa9": 737,
"\xaa": 370,
"\xab": 556,
"\xac": 584,
"\xae": 737,
"\xaf": 333,
"\xb0": 400,
"\xb1": 584,
"\xb2": 333,
"\xb3": 333,
"\xb4": 333,
"\xb5": 611,
"\xb6": 556,
"\xb7": 278,
"\xb8": 333,
"\xb9": 333,
"\xba": 365,
"\xbb": 556,
"\xbc": 834,
"\xbd": 834,
"\xbe": 834,
"\xbf": 611,
"\xc0": 722,
"\xc1": 722,
"\xc2": 722,
"\xc3": 722,
"\xc4": 722,
"\xc5": 722,
"\xc6": 1000,
"\xc7": 722,
"\xc8": 667,
"\xc9": 667,
"\xca": 667,
"\xcb": 667,
"\xcc": 278,
"\xcd": 278,
"\xce": 278,
"\xcf": 278,
"\xd0": 722,
"\xd1": 722,
"\xd2": 778,
"\xd3": 778,
"\xd4": 778,
"\xd5": 778,
"\xd6": 778,
"\xd7": 584,
"\xd8": 778,
"\xd9": 722,
"\xda": 722,
"\xdb": 722,
"\xdc": 722,
"\xdd": 667,
"\xde": 667,
"\xdf": 611,
"\xe0": 556,
"\xe1": 556,
"\xe2": 556,
"\xe3": 556,
"\xe4": 556,
"\xe5": 556,
"\xe6": 889,
"\xe7": 556,
"\xe8": 556,
"\xe9": 556,
"\xea": 556,
"\xeb": 556,
"\xec": 278,
"\xed": 278,
"\xee": 278,
"\xef": 278,
"\xf0": 611,
"\xf1": 611,
"\xf2": 611,
"\xf3": 611,
"\xf4": 611,
"\xf5": 611,
"\xf6": 611,
"\xf7": 584,
"\xf8": 611,
"\xf9": 611,
"\xfa": 611,
"\xfb": 611,
"\xfc": 611,
"\xfd": 556,
"\xfe": 611,
"\xff": 556,
"\u0100": 722,
"\u0101": 556,
"\u0102": 722,
"\u0103": 556,
"\u0104": 722,
"\u0105": 556,
"\u0106": 722,
"\u0107": 556,
"\u010c": 722,
"\u010d": 556,
"\u010e": 722,
"\u010f": 743,
"\u0110": 722,
"\u0111": 611,
"\u0112": 667,
"\u0113": 556,
"\u0116": 667,
"\u0117": 556,
"\u0118": 667,
"\u0119": 556,
"\u011a": 667,
"\u011b": 556,
"\u011e": 778,
"\u011f": 611,
"\u0122": 778,
"\u0123": 611,
"\u012a": 278,
"\u012b": 278,
"\u012e": 278,
"\u012f": 278,
"\u0130": 278,
"\u0131": 278,
"\u0136": 722,
"\u0137": 556,
"\u0139": 611,
"\u013a": 278,
"\u013b": 611,
"\u013c": 278,
"\u013d": 611,
"\u013e": 400,
"\u0141": 611,
"\u0142": 278,
"\u0143": 722,
"\u0144": 611,
"\u0145": 722,
"\u0146": 611,
"\u0147": 722,
"\u0148": 611,
"\u014c": 778,
"\u014d": 611,
"\u0150": 778,
"\u0151": 611,
"\u0152": 1000,
"\u0153": 944,
"\u0154": 722,
"\u0155": 389,
"\u0156": 722,
"\u0157": 389,
"\u0158": 722,
"\u0159": 389,
"\u015a": 667,
"\u015b": 556,
"\u015e": 667,
"\u015f": 556,
"\u0160": 667,
"\u0161": 556,
"\u0162": 611,
"\u0163": 333,
"\u0164": 611,
"\u0165": 389,
"\u016a": 722,
"\u016b": 611,
"\u016e": 722,
"\u016f": 611,
"\u0170": 722,
"\u0171": 611,
"\u0172": 722,
"\u0173": 611,
"\u0178": 667,
"\u0179": 611,
"\u017a": 500,
"\u017b": 611,
"\u017c": 500,
"\u017d": 611,
"\u017e": 500,
"\u0192": 556,
"\u0218": 667,
"\u0219": 556,
"\u02c6": 333,
"\u02c7": 333,
"\u02d8": 333,
"\u02d9": 333,
"\u02da": 333,
"\u02db": 333,
"\u02dc": 333,
"\u02dd": 333,
"\u2013": 556,
"\u2014": 1000,
"\u2018": 278,
"\u2019": 278,
"\u201a": 278,
"\u201c": 500,
"\u201d": 500,
"\u201e": 500,
"\u2020": 556,
"\u2021": 556,
"\u2022": 350,
"\u2026": 1000,
"\u2030": 1000,
"\u2039": 333,
"\u203a": 333,
"\u2044": 167,
"\u2122": 1000,
"\u2202": 494,
"\u2206": 612,
"\u2211": 600,
"\u2212": 584,
"\u221a": 549,
"\u2260": 549,
"\u2264": 549,
"\u2265": 549,
"\u25ca": 494,
"\uf6c3": 250,
"\ufb01": 611,
"\ufb02": 611,
},
),
"Helvetica-BoldOblique": (
{
"FontName": "Helvetica-BoldOblique",
"Descent": -207.0,
"FontBBox": (-175.0, -228.0, 1114.0, 962.0),
"FontWeight": "Bold",
"CapHeight": 718.0,
"FontFamily": "Helvetica",
"Flags": 0,
"XHeight": 532.0,
"ItalicAngle": -12.0,
"Ascent": 718.0,
},
{
" ": 278,
"!": 333,
'"': 474,
"#": 556,
"$": 556,
"%": 889,
"&": 722,
"'": 238,
"(": 333,
")": 333,
"*": 389,
"+": 584,
",": 278,
"-": 333,
".": 278,
"/": 278,
"0": 556,
"1": 556,
"2": 556,
"3": 556,
"4": 556,
"5": 556,
"6": 556,
"7": 556,
"8": 556,
"9": 556,
":": 333,
";": 333,
"<": 584,
"=": 584,
">": 584,
"?": 611,
"@": 975,
"A": 722,
"B": 722,
"C": 722,
"D": 722,
"E": 667,
"F": 611,
"G": 778,
"H": 722,
"I": 278,
"J": 556,
"K": 722,
"L": 611,
"M": 833,
"N": 722,
"O": 778,
"P": 667,
"Q": 778,
"R": 722,
"S": 667,
"T": 611,
"U": 722,
"V": 667,
"W": 944,
"X": 667,
"Y": 667,
"Z": 611,
"[": 333,
"\\": 278,
"]": 333,
"^": 584,
"_": 556,
"`": 333,
"a": 556,
"b": 611,
"c": 556,
"d": 611,
"e": 556,
"f": 333,
"g": 611,
"h": 611,
"i": 278,
"j": 278,
"k": 556,
"l": 278,
"m": 889,
"n": 611,
"o": 611,
"p": 611,
"q": 611,
"r": 389,
"s": 556,
"t": 333,
"u": 611,
"v": 556,
"w": 778,
"x": 556,
"y": 556,
"z": 500,
"{": 389,
"|": 280,
"}": 389,
"~": 584,
"\xa1": 333,
"\xa2": 556,
"\xa3": 556,
"\xa4": 556,
"\xa5": 556,
"\xa6": 280,
"\xa7": 556,
"\xa8": 333,
"\xa9": 737,
"\xaa": 370,
"\xab": 556,
"\xac": 584,
"\xae": 737,
"\xaf": 333,
"\xb0": 400,
"\xb1": 584,
"\xb2": 333,
"\xb3": 333,
"\xb4": 333,
"\xb5": 611,
"\xb6": 556,
"\xb7": 278,
"\xb8": 333,
"\xb9": 333,
"\xba": 365,
"\xbb": 556,
"\xbc": 834,
"\xbd": 834,
"\xbe": 834,
"\xbf": 611,
"\xc0": 722,
"\xc1": 722,
"\xc2": 722,
"\xc3": 722,
"\xc4": 722,
"\xc5": 722,
"\xc6": 1000,
"\xc7": 722,
"\xc8": 667,
"\xc9": 667,
"\xca": 667,
"\xcb": 667,
"\xcc": 278,
"\xcd": 278,
"\xce": 278,
"\xcf": 278,
"\xd0": 722,
"\xd1": 722,
"\xd2": 778,
"\xd3": 778,
"\xd4": 778,
"\xd5": 778,
"\xd6": 778,
"\xd7": 584,
"\xd8": 778,
"\xd9": 722,
"\xda": 722,
"\xdb": 722,
"\xdc": 722,
"\xdd": 667,
"\xde": 667,
"\xdf": 611,
"\xe0": 556,
"\xe1": 556,
"\xe2": 556,
"\xe3": 556,
"\xe4": 556,
"\xe5": 556,
"\xe6": 889,
"\xe7": 556,
"\xe8": 556,
"\xe9": 556,
"\xea": 556,
"\xeb": 556,
"\xec": 278,
"\xed": 278,
"\xee": 278,
"\xef": 278,
"\xf0": 611,
"\xf1": 611,
"\xf2": 611,
"\xf3": 611,
"\xf4": 611,
"\xf5": 611,
"\xf6": 611,
"\xf7": 584,
"\xf8": 611,
"\xf9": 611,
"\xfa": 611,
"\xfb": 611,
"\xfc": 611,
"\xfd": 556,
"\xfe": 611,
"\xff": 556,
"\u0100": 722,
"\u0101": 556,
"\u0102": 722,
"\u0103": 556,
"\u0104": 722,
"\u0105": 556,
"\u0106": 722,
"\u0107": 556,
"\u010c": 722,
"\u010d": 556,
"\u010e": 722,
"\u010f": 743,
"\u0110": 722,
"\u0111": 611,
"\u0112": 667,
"\u0113": 556,
"\u0116": 667,
"\u0117": 556,
"\u0118": 667,
"\u0119": 556,
"\u011a": 667,
"\u011b": 556,
"\u011e": 778,
"\u011f": 611,
"\u0122": 778,
"\u0123": 611,
"\u012a": 278,
"\u012b": 278,
"\u012e": 278,
"\u012f": 278,
"\u0130": 278,
"\u0131": 278,
"\u0136": 722,
"\u0137": 556,
"\u0139": 611,
"\u013a": 278,
"\u013b": 611,
"\u013c": 278,
"\u013d": 611,
"\u013e": 400,
"\u0141": 611,
"\u0142": 278,
"\u0143": 722,
"\u0144": 611,
"\u0145": 722,
"\u0146": 611,
"\u0147": 722,
"\u0148": 611,
"\u014c": 778,
"\u014d": 611,
"\u0150": 778,
"\u0151": 611,
"\u0152": 1000,
"\u0153": 944,
"\u0154": 722,
"\u0155": 389,
"\u0156": 722,
"\u0157": 389,
"\u0158": 722,
"\u0159": 389,
"\u015a": 667,
"\u015b": 556,
"\u015e": 667,
"\u015f": 556,
"\u0160": 667,
"\u0161": 556,
"\u0162": 611,
"\u0163": 333,
"\u0164": 611,
"\u0165": 389,
"\u016a": 722,
"\u016b": 611,
"\u016e": 722,
"\u016f": 611,
"\u0170": 722,
"\u0171": 611,
"\u0172": 722,
"\u0173": 611,
"\u0178": 667,
"\u0179": 611,
"\u017a": 500,
"\u017b": 611,
"\u017c": 500,
"\u017d": 611,
"\u017e": 500,
"\u0192": 556,
"\u0218": 667,
"\u0219": 556,
"\u02c6": 333,
"\u02c7": 333,
"\u02d8": 333,
"\u02d9": 333,
"\u02da": 333,
"\u02db": 333,
"\u02dc": 333,
"\u02dd": 333,
"\u2013": 556,
"\u2014": 1000,
"\u2018": 278,
"\u2019": 278,
"\u201a": 278,
"\u201c": 500,
"\u201d": 500,
"\u201e": 500,
"\u2020": 556,
"\u2021": 556,
"\u2022": 350,
"\u2026": 1000,
"\u2030": 1000,
"\u2039": 333,
"\u203a": 333,
"\u2044": 167,
"\u2122": 1000,
"\u2202": 494,
"\u2206": 612,
"\u2211": 600,
"\u2212": 584,
"\u221a": 549,
"\u2260": 549,
"\u2264": 549,
"\u2265": 549,
"\u25ca": 494,
"\uf6c3": 250,
"\ufb01": 611,
"\ufb02": 611,
},
),
"Helvetica-Oblique": (
{
"FontName": "Helvetica-Oblique",
"Descent": -207.0,
"FontBBox": (-171.0, -225.0, 1116.0, 931.0),
"FontWeight": "Medium",
"CapHeight": 718.0,
"FontFamily": "Helvetica",
"Flags": 0,
"XHeight": 523.0,
"ItalicAngle": -12.0,
"Ascent": 718.0,
},
{
" ": 278,
"!": 278,
'"': 355,
"#": 556,
"$": 556,
"%": 889,
"&": 667,
"'": 191,
"(": 333,
")": 333,
"*": 389,
"+": 584,
",": 278,
"-": 333,
".": 278,
"/": 278,
"0": 556,
"1": 556,
"2": 556,
"3": 556,
"4": 556,
"5": 556,
"6": 556,
"7": 556,
"8": 556,
"9": 556,
":": 278,
";": 278,
"<": 584,
"=": 584,
">": 584,
"?": 556,
"@": 1015,
"A": 667,
"B": 667,
"C": 722,
"D": 722,
"E": 667,
"F": 611,
"G": 778,
"H": 722,
"I": 278,
"J": 500,
"K": 667,
"L": 556,
"M": 833,
"N": 722,
"O": 778,
"P": 667,
"Q": 778,
"R": 722,
"S": 667,
"T": 611,
"U": 722,
"V": 667,
"W": 944,
"X": 667,
"Y": 667,
"Z": 611,
"[": 278,
"\\": 278,
"]": 278,
"^": 469,
"_": 556,
"`": 333,
"a": 556,
"b": 556,
"c": 500,
"d": 556,
"e": 556,
"f": 278,
"g": 556,
"h": 556,
"i": 222,
"j": 222,
"k": 500,
"l": 222,
"m": 833,
"n": 556,
"o": 556,
"p": 556,
"q": 556,
"r": 333,
"s": 500,
"t": 278,
"u": 556,
"v": 500,
"w": 722,
"x": 500,
"y": 500,
"z": 500,
"{": 334,
"|": 260,
"}": 334,
"~": 584,
"\xa1": 333,
"\xa2": 556,
"\xa3": 556,
"\xa4": 556,
"\xa5": 556,
"\xa6": 260,
"\xa7": 556,
"\xa8": 333,
"\xa9": 737,
"\xaa": 370,
"\xab": 556,
"\xac": 584,
"\xae": 737,
"\xaf": 333,
"\xb0": 400,
"\xb1": 584,
"\xb2": 333,
"\xb3": 333,
"\xb4": 333,
"\xb5": 556,
"\xb6": 537,
"\xb7": 278,
"\xb8": 333,
"\xb9": 333,
"\xba": 365,
"\xbb": 556,
"\xbc": 834,
"\xbd": 834,
"\xbe": 834,
"\xbf": 611,
"\xc0": 667,
"\xc1": 667,
"\xc2": 667,
"\xc3": 667,
"\xc4": 667,
"\xc5": 667,
"\xc6": 1000,
"\xc7": 722,
"\xc8": 667,
"\xc9": 667,
"\xca": 667,
"\xcb": 667,
"\xcc": 278,
"\xcd": 278,
"\xce": 278,
"\xcf": 278,
"\xd0": 722,
"\xd1": 722,
"\xd2": 778,
"\xd3": 778,
"\xd4": 778,
"\xd5": 778,
"\xd6": 778,
"\xd7": 584,
"\xd8": 778,
"\xd9": 722,
"\xda": 722,
"\xdb": 722,
"\xdc": 722,
"\xdd": 667,
"\xde": 667,
"\xdf": 611,
"\xe0": 556,
"\xe1": 556,
"\xe2": 556,
"\xe3": 556,
"\xe4": 556,
"\xe5": 556,
"\xe6": 889,
"\xe7": 500,
"\xe8": 556,
"\xe9": 556,
"\xea": 556,
"\xeb": 556,
"\xec": 278,
"\xed": 278,
"\xee": 278,
"\xef": 278,
"\xf0": 556,
"\xf1": 556,
"\xf2": 556,
"\xf3": 556,
"\xf4": 556,
"\xf5": 556,
"\xf6": 556,
"\xf7": 584,
"\xf8": 611,
"\xf9": 556,
"\xfa": 556,
"\xfb": 556,
"\xfc": 556,
"\xfd": 500,
"\xfe": 556,
"\xff": 500,
"\u0100": 667,
"\u0101": 556,
"\u0102": 667,
"\u0103": 556,
"\u0104": 667,
"\u0105": 556,
"\u0106": 722,
"\u0107": 500,
"\u010c": 722,
"\u010d": 500,
"\u010e": 722,
"\u010f": 643,
"\u0110": 722,
"\u0111": 556,
"\u0112": 667,
"\u0113": 556,
"\u0116": 667,
"\u0117": 556,
"\u0118": 667,
"\u0119": 556,
"\u011a": 667,
"\u011b": 556,
"\u011e": 778,
"\u011f": 556,
"\u0122": 778,
"\u0123": 556,
"\u012a": 278,
"\u012b": 278,
"\u012e": 278,
"\u012f": 222,
"\u0130": 278,
"\u0131": 278,
"\u0136": 667,
"\u0137": 500,
"\u0139": 556,
"\u013a": 222,
"\u013b": 556,
"\u013c": 222,
"\u013d": 556,
"\u013e": 299,
"\u0141": 556,
"\u0142": 222,
"\u0143": 722,
"\u0144": 556,
"\u0145": 722,
"\u0146": 556,
"\u0147": 722,
"\u0148": 556,
"\u014c": 778,
"\u014d": 556,
"\u0150": 778,
"\u0151": 556,
"\u0152": 1000,
"\u0153": 944,
"\u0154": 722,
"\u0155": 333,
"\u0156": 722,
"\u0157": 333,
"\u0158": 722,
"\u0159": 333,
"\u015a": 667,
"\u015b": 500,
"\u015e": 667,
"\u015f": 500,
"\u0160": 667,
"\u0161": 500,
"\u0162": 611,
"\u0163": 278,
"\u0164": 611,
"\u0165": 317,
"\u016a": 722,
"\u016b": 556,
"\u016e": 722,
"\u016f": 556,
"\u0170": 722,
"\u0171": 556,
"\u0172": 722,
"\u0173": 556,
"\u0178": 667,
"\u0179": 611,
"\u017a": 500,
"\u017b": 611,
"\u017c": 500,
"\u017d": 611,
"\u017e": 500,
"\u0192": 556,
"\u0218": 667,
"\u0219": 500,
"\u02c6": 333,
"\u02c7": 333,
"\u02d8": 333,
"\u02d9": 333,
"\u02da": 333,
"\u02db": 333,
"\u02dc": 333,
"\u02dd": 333,
"\u2013": 556,
"\u2014": 1000,
"\u2018": 222,
"\u2019": 222,
"\u201a": 222,
"\u201c": 333,
"\u201d": 333,
"\u201e": 333,
"\u2020": 556,
"\u2021": 556,
"\u2022": 350,
"\u2026": 1000,
"\u2030": 1000,
"\u2039": 333,
"\u203a": 333,
"\u2044": 167,
"\u2122": 1000,
"\u2202": 476,
"\u2206": 612,
"\u2211": 600,
"\u2212": 584,
"\u221a": 453,
"\u2260": 549,
"\u2264": 549,
"\u2265": 549,
"\u25ca": 471,
"\uf6c3": 250,
"\ufb01": 500,
"\ufb02": 500,
},
),
"Symbol": (
{
"FontName": "Symbol",
"FontBBox": (-180.0, -293.0, 1090.0, 1010.0),
"FontWeight": "Medium",
"FontFamily": "Symbol",
"Flags": 0,
"ItalicAngle": 0.0,
},
{
" ": 250,
"!": 333,
"#": 500,
"%": 833,
"&": 778,
"(": 333,
")": 333,
"+": 549,
",": 250,
".": 250,
"/": 278,
"0": 500,
"1": 500,
"2": 500,
"3": 500,
"4": 500,
"5": 500,
"6": 500,
"7": 500,
"8": 500,
"9": 500,
":": 278,
";": 278,
"<": 549,
"=": 549,
">": 549,
"?": 444,
"[": 333,
"]": 333,
"_": 500,
"{": 480,
"|": 200,
"}": 480,
"\xac": 713,
"\xb0": 400,
"\xb1": 549,
"\xb5": 576,
"\xd7": 549,
"\xf7": 549,
"\u0192": 500,
"\u0391": 722,
"\u0392": 667,
"\u0393": 603,
"\u0395": 611,
"\u0396": 611,
"\u0397": 722,
"\u0398": 741,
"\u0399": 333,
"\u039a": 722,
"\u039b": 686,
"\u039c": 889,
"\u039d": 722,
"\u039e": 645,
"\u039f": 722,
"\u03a0": 768,
"\u03a1": 556,
"\u03a3": 592,
"\u03a4": 611,
"\u03a5": 690,
"\u03a6": 763,
"\u03a7": 722,
"\u03a8": 795,
"\u03b1": 631,
"\u03b2": 549,
"\u03b3": 411,
"\u03b4": 494,
"\u03b5": 439,
"\u03b6": 494,
"\u03b7": 603,
"\u03b8": 521,
"\u03b9": 329,
"\u03ba": 549,
"\u03bb": 549,
"\u03bd": 521,
"\u03be": 493,
"\u03bf": 549,
"\u03c0": 549,
"\u03c1": 549,
"\u03c2": 439,
"\u03c3": 603,
"\u03c4": 439,
"\u03c5": 576,
"\u03c6": 521,
"\u03c7": 549,
"\u03c8": 686,
"\u03c9": 686,
"\u03d1": 631,
"\u03d2": 620,
"\u03d5": 603,
"\u03d6": 713,
"\u2022": 460,
"\u2026": 1000,
"\u2032": 247,
"\u2033": 411,
"\u2044": 167,
"\u20ac": 750,
"\u2111": 686,
"\u2118": 987,
"\u211c": 795,
"\u2126": 768,
"\u2135": 823,
"\u2190": 987,
"\u2191": 603,
"\u2192": 987,
"\u2193": 603,
"\u2194": 1042,
"\u21b5": 658,
"\u21d0": 987,
"\u21d1": 603,
"\u21d2": 987,
"\u21d3": 603,
"\u21d4": 1042,
"\u2200": 713,
"\u2202": 494,
"\u2203": 549,
"\u2205": 823,
"\u2206": 612,
"\u2207": 713,
"\u2208": 713,
"\u2209": 713,
"\u220b": 439,
"\u220f": 823,
"\u2211": 713,
"\u2212": 549,
"\u2217": 500,
"\u221a": 549,
"\u221d": 713,
"\u221e": 713,
"\u2220": 768,
"\u2227": 603,
"\u2228": 603,
"\u2229": 768,
"\u222a": 768,
"\u222b": 274,
"\u2234": 863,
"\u223c": 549,
"\u2245": 549,
"\u2248": 549,
"\u2260": 549,
"\u2261": 549,
"\u2264": 549,
"\u2265": 549,
"\u2282": 713,
"\u2283": 713,
"\u2284": 713,
"\u2286": 713,
"\u2287": 713,
"\u2295": 768,
"\u2297": 768,
"\u22a5": 658,
"\u22c5": 250,
"\u2320": 686,
"\u2321": 686,
"\u2329": 329,
"\u232a": 329,
"\u25ca": 494,
"\u2660": 753,
"\u2663": 753,
"\u2665": 753,
"\u2666": 753,
"\uf6d9": 790,
"\uf6da": 790,
"\uf6db": 890,
"\uf8e5": 500,
"\uf8e6": 603,
"\uf8e7": 1000,
"\uf8e8": 790,
"\uf8e9": 790,
"\uf8ea": 786,
"\uf8eb": 384,
"\uf8ec": 384,
"\uf8ed": 384,
"\uf8ee": 384,
"\uf8ef": 384,
"\uf8f0": 384,
"\uf8f1": 494,
"\uf8f2": 494,
"\uf8f3": 494,
"\uf8f4": 494,
"\uf8f5": 686,
"\uf8f6": 384,
"\uf8f7": 384,
"\uf8f8": 384,
"\uf8f9": 384,
"\uf8fa": 384,
"\uf8fb": 384,
"\uf8fc": 494,
"\uf8fd": 494,
"\uf8fe": 494,
"\uf8ff": 790,
},
),
"Times-Bold": (
{
"FontName": "Times-Bold",
"Descent": -217.0,
"FontBBox": (-168.0, -218.0, 1000.0, 935.0),
"FontWeight": "Bold",
"CapHeight": 676.0,
"FontFamily": "Times",
"Flags": 0,
"XHeight": 461.0,
"ItalicAngle": 0.0,
"Ascent": 683.0,
},
{
" ": 250,
"!": 333,
'"': 555,
"#": 500,
"$": 500,
"%": 1000,
"&": 833,
"'": 278,
"(": 333,
")": 333,
"*": 500,
"+": 570,
",": 250,
"-": 333,
".": 250,
"/": 278,
"0": 500,
"1": 500,
"2": 500,
"3": 500,
"4": 500,
"5": 500,
"6": 500,
"7": 500,
"8": 500,
"9": 500,
":": 333,
";": 333,
"<": 570,
"=": 570,
">": 570,
"?": 500,
"@": 930,
"A": 722,
"B": 667,
"C": 722,
"D": 722,
"E": 667,
"F": 611,
"G": 778,
"H": 778,
"I": 389,
"J": 500,
"K": 778,
"L": 667,
"M": 944,
"N": 722,
"O": 778,
"P": 611,
"Q": 778,
"R": 722,
"S": 556,
"T": 667,
"U": 722,
"V": 722,
"W": 1000,
"X": 722,
"Y": 722,
"Z": 667,
"[": 333,
"\\": 278,
"]": 333,
"^": 581,
"_": 500,
"`": 333,
"a": 500,
"b": 556,
"c": 444,
"d": 556,
"e": 444,
"f": 333,
"g": 500,
"h": 556,
"i": 278,
"j": 333,
"k": 556,
"l": 278,
"m": 833,
"n": 556,
"o": 500,
"p": 556,
"q": 556,
"r": 444,
"s": 389,
"t": 333,
"u": 556,
"v": 500,
"w": 722,
"x": 500,
"y": 500,
"z": 444,
"{": 394,
"|": 220,
"}": 394,
"~": 520,
"\xa1": 333,
"\xa2": 500,
"\xa3": 500,
"\xa4": 500,
"\xa5": 500,
"\xa6": 220,
"\xa7": 500,
"\xa8": 333,
"\xa9": 747,
"\xaa": 300,
"\xab": 500,
"\xac": 570,
"\xae": 747,
"\xaf": 333,
"\xb0": 400,
"\xb1": 570,
"\xb2": 300,
"\xb3": 300,
"\xb4": 333,
"\xb5": 556,
"\xb6": 540,
"\xb7": 250,
"\xb8": 333,
"\xb9": 300,
"\xba": 330,
"\xbb": 500,
"\xbc": 750,
"\xbd": 750,
"\xbe": 750,
"\xbf": 500,
"\xc0": 722,
"\xc1": 722,
"\xc2": 722,
"\xc3": 722,
"\xc4": 722,
"\xc5": 722,
"\xc6": 1000,
"\xc7": 722,
"\xc8": 667,
"\xc9": 667,
"\xca": 667,
"\xcb": 667,
"\xcc": 389,
"\xcd": 389,
"\xce": 389,
"\xcf": 389,
"\xd0": 722,
"\xd1": 722,
"\xd2": 778,
"\xd3": 778,
"\xd4": 778,
"\xd5": 778,
"\xd6": 778,
"\xd7": 570,
"\xd8": 778,
"\xd9": 722,
"\xda": 722,
"\xdb": 722,
"\xdc": 722,
"\xdd": 722,
"\xde": 611,
"\xdf": 556,
"\xe0": 500,
"\xe1": 500,
"\xe2": 500,
"\xe3": 500,
"\xe4": 500,
"\xe5": 500,
"\xe6": 722,
"\xe7": 444,
"\xe8": 444,
"\xe9": 444,
"\xea": 444,
"\xeb": 444,
"\xec": 278,
"\xed": 278,
"\xee": 278,
"\xef": 278,
"\xf0": 500,
"\xf1": 556,
"\xf2": 500,
"\xf3": 500,
"\xf4": 500,
"\xf5": 500,
"\xf6": 500,
"\xf7": 570,
"\xf8": 500,
"\xf9": 556,
"\xfa": 556,
"\xfb": 556,
"\xfc": 556,
"\xfd": 500,
"\xfe": 556,
"\xff": 500,
"\u0100": 722,
"\u0101": 500,
"\u0102": 722,
"\u0103": 500,
"\u0104": 722,
"\u0105": 500,
"\u0106": 722,
"\u0107": 444,
"\u010c": 722,
"\u010d": 444,
"\u010e": 722,
"\u010f": 672,
"\u0110": 722,
"\u0111": 556,
"\u0112": 667,
"\u0113": 444,
"\u0116": 667,
"\u0117": 444,
"\u0118": 667,
"\u0119": 444,
"\u011a": 667,
"\u011b": 444,
"\u011e": 778,
"\u011f": 500,
"\u0122": 778,
"\u0123": 500,
"\u012a": 389,
"\u012b": 278,
"\u012e": 389,
"\u012f": 278,
"\u0130": 389,
"\u0131": 278,
"\u0136": 778,
"\u0137": 556,
"\u0139": 667,
"\u013a": 278,
"\u013b": 667,
"\u013c": 278,
"\u013d": 667,
"\u013e": 394,
"\u0141": 667,
"\u0142": 278,
"\u0143": 722,
"\u0144": 556,
"\u0145": 722,
"\u0146": 556,
"\u0147": 722,
"\u0148": 556,
"\u014c": 778,
"\u014d": 500,
"\u0150": 778,
"\u0151": 500,
"\u0152": 1000,
"\u0153": 722,
"\u0154": 722,
"\u0155": 444,
"\u0156": 722,
"\u0157": 444,
"\u0158": 722,
"\u0159": 444,
"\u015a": 556,
"\u015b": 389,
"\u015e": 556,
"\u015f": 389,
"\u0160": 556,
"\u0161": 389,
"\u0162": 667,
"\u0163": 333,
"\u0164": 667,
"\u0165": 416,
"\u016a": 722,
"\u016b": 556,
"\u016e": 722,
"\u016f": 556,
"\u0170": 722,
"\u0171": 556,
"\u0172": 722,
"\u0173": 556,
"\u0178": 722,
"\u0179": 667,
"\u017a": 444,
"\u017b": 667,
"\u017c": 444,
"\u017d": 667,
"\u017e": 444,
"\u0192": 500,
"\u0218": 556,
"\u0219": 389,
"\u02c6": 333,
"\u02c7": 333,
"\u02d8": 333,
"\u02d9": 333,
"\u02da": 333,
"\u02db": 333,
"\u02dc": 333,
"\u02dd": 333,
"\u2013": 500,
"\u2014": 1000,
"\u2018": 333,
"\u2019": 333,
"\u201a": 333,
"\u201c": 500,
"\u201d": 500,
"\u201e": 500,
"\u2020": 500,
"\u2021": 500,
"\u2022": 350,
"\u2026": 1000,
"\u2030": 1000,
"\u2039": 333,
"\u203a": 333,
"\u2044": 167,
"\u2122": 1000,
"\u2202": 494,
"\u2206": 612,
"\u2211": 600,
"\u2212": 570,
"\u221a": 549,
"\u2260": 549,
"\u2264": 549,
"\u2265": 549,
"\u25ca": 494,
"\uf6c3": 250,
"\ufb01": 556,
"\ufb02": 556,
},
),
"Times-BoldItalic": (
{
"FontName": "Times-BoldItalic",
"Descent": -217.0,
"FontBBox": (-200.0, -218.0, 996.0, 921.0),
"FontWeight": "Bold",
"CapHeight": 669.0,
"FontFamily": "Times",
"Flags": 0,
"XHeight": 462.0,
"ItalicAngle": -15.0,
"Ascent": 683.0,
},
{
" ": 250,
"!": 389,
'"': 555,
"#": 500,
"$": 500,
"%": 833,
"&": 778,
"'": 278,
"(": 333,
")": 333,
"*": 500,
"+": 570,
",": 250,
"-": 333,
".": 250,
"/": 278,
"0": 500,
"1": 500,
"2": 500,
"3": 500,
"4": 500,
"5": 500,
"6": 500,
"7": 500,
"8": 500,
"9": 500,
":": 333,
";": 333,
"<": 570,
"=": 570,
">": 570,
"?": 500,
"@": 832,
"A": 667,
"B": 667,
"C": 667,
"D": 722,
"E": 667,
"F": 667,
"G": 722,
"H": 778,
"I": 389,
"J": 500,
"K": 667,
"L": 611,
"M": 889,
"N": 722,
"O": 722,
"P": 611,
"Q": 722,
"R": 667,
"S": 556,
"T": 611,
"U": 722,
"V": 667,
"W": 889,
"X": 667,
"Y": 611,
"Z": 611,
"[": 333,
"\\": 278,
"]": 333,
"^": 570,
"_": 500,
"`": 333,
"a": 500,
"b": 500,
"c": 444,
"d": 500,
"e": 444,
"f": 333,
"g": 500,
"h": 556,
"i": 278,
"j": 278,
"k": 500,
"l": 278,
"m": 778,
"n": 556,
"o": 500,
"p": 500,
"q": 500,
"r": 389,
"s": 389,
"t": 278,
"u": 556,
"v": 444,
"w": 667,
"x": 500,
"y": 444,
"z": 389,
"{": 348,
"|": 220,
"}": 348,
"~": 570,
"\xa1": 389,
"\xa2": 500,
"\xa3": 500,
"\xa4": 500,
"\xa5": 500,
"\xa6": 220,
"\xa7": 500,
"\xa8": 333,
"\xa9": 747,
"\xaa": 266,
"\xab": 500,
"\xac": 606,
"\xae": 747,
"\xaf": 333,
"\xb0": 400,
"\xb1": 570,
"\xb2": 300,
"\xb3": 300,
"\xb4": 333,
"\xb5": 576,
"\xb6": 500,
"\xb7": 250,
"\xb8": 333,
"\xb9": 300,
"\xba": 300,
"\xbb": 500,
"\xbc": 750,
"\xbd": 750,
"\xbe": 750,
"\xbf": 500,
"\xc0": 667,
"\xc1": 667,
"\xc2": 667,
"\xc3": 667,
"\xc4": 667,
"\xc5": 667,
"\xc6": 944,
"\xc7": 667,
"\xc8": 667,
"\xc9": 667,
"\xca": 667,
"\xcb": 667,
"\xcc": 389,
"\xcd": 389,
"\xce": 389,
"\xcf": 389,
"\xd0": 722,
"\xd1": 722,
"\xd2": 722,
"\xd3": 722,
"\xd4": 722,
"\xd5": 722,
"\xd6": 722,
"\xd7": 570,
"\xd8": 722,
"\xd9": 722,
"\xda": 722,
"\xdb": 722,
"\xdc": 722,
"\xdd": 611,
"\xde": 611,
"\xdf": 500,
"\xe0": 500,
"\xe1": 500,
"\xe2": 500,
"\xe3": 500,
"\xe4": 500,
"\xe5": 500,
"\xe6": 722,
"\xe7": 444,
"\xe8": 444,
"\xe9": 444,
"\xea": 444,
"\xeb": 444,
"\xec": 278,
"\xed": 278,
"\xee": 278,
"\xef": 278,
"\xf0": 500,
"\xf1": 556,
"\xf2": 500,
"\xf3": 500,
"\xf4": 500,
"\xf5": 500,
"\xf6": 500,
"\xf7": 570,
"\xf8": 500,
"\xf9": 556,
"\xfa": 556,
"\xfb": 556,
"\xfc": 556,
"\xfd": 444,
"\xfe": 500,
"\xff": 444,
"\u0100": 667,
"\u0101": 500,
"\u0102": 667,
"\u0103": 500,
"\u0104": 667,
"\u0105": 500,
"\u0106": 667,
"\u0107": 444,
"\u010c": 667,
"\u010d": 444,
"\u010e": 722,
"\u010f": 608,
"\u0110": 722,
"\u0111": 500,
"\u0112": 667,
"\u0113": 444,
"\u0116": 667,
"\u0117": 444,
"\u0118": 667,
"\u0119": 444,
"\u011a": 667,
"\u011b": 444,
"\u011e": 722,
"\u011f": 500,
"\u0122": 722,
"\u0123": 500,
"\u012a": 389,
"\u012b": 278,
"\u012e": 389,
"\u012f": 278,
"\u0130": 389,
"\u0131": 278,
"\u0136": 667,
"\u0137": 500,
"\u0139": 611,
"\u013a": 278,
"\u013b": 611,
"\u013c": 278,
"\u013d": 611,
"\u013e": 382,
"\u0141": 611,
"\u0142": 278,
"\u0143": 722,
"\u0144": 556,
"\u0145": 722,
"\u0146": 556,
"\u0147": 722,
"\u0148": 556,
"\u014c": 722,
"\u014d": 500,
"\u0150": 722,
"\u0151": 500,
"\u0152": 944,
"\u0153": 722,
"\u0154": 667,
"\u0155": 389,
"\u0156": 667,
"\u0157": 389,
"\u0158": 667,
"\u0159": 389,
"\u015a": 556,
"\u015b": 389,
"\u015e": 556,
"\u015f": 389,
"\u0160": 556,
"\u0161": 389,
"\u0162": 611,
"\u0163": 278,
"\u0164": 611,
"\u0165": 366,
"\u016a": 722,
"\u016b": 556,
"\u016e": 722,
"\u016f": 556,
"\u0170": 722,
"\u0171": 556,
"\u0172": 722,
"\u0173": 556,
"\u0178": 611,
"\u0179": 611,
"\u017a": 389,
"\u017b": 611,
"\u017c": 389,
"\u017d": 611,
"\u017e": 389,
"\u0192": 500,
"\u0218": 556,
"\u0219": 389,
"\u02c6": 333,
"\u02c7": 333,
"\u02d8": 333,
"\u02d9": 333,
"\u02da": 333,
"\u02db": 333,
"\u02dc": 333,
"\u02dd": 333,
"\u2013": 500,
"\u2014": 1000,
"\u2018": 333,
"\u2019": 333,
"\u201a": 333,
"\u201c": 500,
"\u201d": 500,
"\u201e": 500,
"\u2020": 500,
"\u2021": 500,
"\u2022": 350,
"\u2026": 1000,
"\u2030": 1000,
"\u2039": 333,
"\u203a": 333,
"\u2044": 167,
"\u2122": 1000,
"\u2202": 494,
"\u2206": 612,
"\u2211": 600,
"\u2212": 606,
"\u221a": 549,
"\u2260": 549,
"\u2264": 549,
"\u2265": 549,
"\u25ca": 494,
"\uf6c3": 250,
"\ufb01": 556,
"\ufb02": 556,
},
),
"Times-Italic": (
{
"FontName": "Times-Italic",
"Descent": -217.0,
"FontBBox": (-169.0, -217.0, 1010.0, 883.0),
"FontWeight": "Medium",
"CapHeight": 653.0,
"FontFamily": "Times",
"Flags": 0,
"XHeight": 441.0,
"ItalicAngle": -15.5,
"Ascent": 683.0,
},
{
" ": 250,
"!": 333,
'"': 420,
"#": 500,
"$": 500,
"%": 833,
"&": 778,
"'": 214,
"(": 333,
")": 333,
"*": 500,
"+": 675,
",": 250,
"-": 333,
".": 250,
"/": 278,
"0": 500,
"1": 500,
"2": 500,
"3": 500,
"4": 500,
"5": 500,
"6": 500,
"7": 500,
"8": 500,
"9": 500,
":": 333,
";": 333,
"<": 675,
"=": 675,
">": 675,
"?": 500,
"@": 920,
"A": 611,
"B": 611,
"C": 667,
"D": 722,
"E": 611,
"F": 611,
"G": 722,
"H": 722,
"I": 333,
"J": 444,
"K": 667,
"L": 556,
"M": 833,
"N": 667,
"O": 722,
"P": 611,
"Q": 722,
"R": 611,
"S": 500,
"T": 556,
"U": 722,
"V": 611,
"W": 833,
"X": 611,
"Y": 556,
"Z": 556,
"[": 389,
"\\": 278,
"]": 389,
"^": 422,
"_": 500,
"`": 333,
"a": 500,
"b": 500,
"c": 444,
"d": 500,
"e": 444,
"f": 278,
"g": 500,
"h": 500,
"i": 278,
"j": 278,
"k": 444,
"l": 278,
"m": 722,
"n": 500,
"o": 500,
"p": 500,
"q": 500,
"r": 389,
"s": 389,
"t": 278,
"u": 500,
"v": 444,
"w": 667,
"x": 444,
"y": 444,
"z": 389,
"{": 400,
"|": 275,
"}": 400,
"~": 541,
"\xa1": 389,
"\xa2": 500,
"\xa3": 500,
"\xa4": 500,
"\xa5": 500,
"\xa6": 275,
"\xa7": 500,
"\xa8": 333,
"\xa9": 760,
"\xaa": 276,
"\xab": 500,
"\xac": 675,
"\xae": 760,
"\xaf": 333,
"\xb0": 400,
"\xb1": 675,
"\xb2": 300,
"\xb3": 300,
"\xb4": 333,
"\xb5": 500,
"\xb6": 523,
"\xb7": 250,
"\xb8": 333,
"\xb9": 300,
"\xba": 310,
"\xbb": 500,
"\xbc": 750,
"\xbd": 750,
"\xbe": 750,
"\xbf": 500,
"\xc0": 611,
"\xc1": 611,
"\xc2": 611,
"\xc3": 611,
"\xc4": 611,
"\xc5": 611,
"\xc6": 889,
"\xc7": 667,
"\xc8": 611,
"\xc9": 611,
"\xca": 611,
"\xcb": 611,
"\xcc": 333,
"\xcd": 333,
"\xce": 333,
"\xcf": 333,
"\xd0": 722,
"\xd1": 667,
"\xd2": 722,
"\xd3": 722,
"\xd4": 722,
"\xd5": 722,
"\xd6": 722,
"\xd7": 675,
"\xd8": 722,
"\xd9": 722,
"\xda": 722,
"\xdb": 722,
"\xdc": 722,
"\xdd": 556,
"\xde": 611,
"\xdf": 500,
"\xe0": 500,
"\xe1": 500,
"\xe2": 500,
"\xe3": 500,
"\xe4": 500,
"\xe5": 500,
"\xe6": 667,
"\xe7": 444,
"\xe8": 444,
"\xe9": 444,
"\xea": 444,
"\xeb": 444,
"\xec": 278,
"\xed": 278,
"\xee": 278,
"\xef": 278,
"\xf0": 500,
"\xf1": 500,
"\xf2": 500,
"\xf3": 500,
"\xf4": 500,
"\xf5": 500,
"\xf6": 500,
"\xf7": 675,
"\xf8": 500,
"\xf9": 500,
"\xfa": 500,
"\xfb": 500,
"\xfc": 500,
"\xfd": 444,
"\xfe": 500,
"\xff": 444,
"\u0100": 611,
"\u0101": 500,
"\u0102": 611,
"\u0103": 500,
"\u0104": 611,
"\u0105": 500,
"\u0106": 667,
"\u0107": 444,
"\u010c": 667,
"\u010d": 444,
"\u010e": 722,
"\u010f": 544,
"\u0110": 722,
"\u0111": 500,
"\u0112": 611,
"\u0113": 444,
"\u0116": 611,
"\u0117": 444,
"\u0118": 611,
"\u0119": 444,
"\u011a": 611,
"\u011b": 444,
"\u011e": 722,
"\u011f": 500,
"\u0122": 722,
"\u0123": 500,
"\u012a": 333,
"\u012b": 278,
"\u012e": 333,
"\u012f": 278,
"\u0130": 333,
"\u0131": 278,
"\u0136": 667,
"\u0137": 444,
"\u0139": 556,
"\u013a": 278,
"\u013b": 556,
"\u013c": 278,
"\u013d": 611,
"\u013e": 300,
"\u0141": 556,
"\u0142": 278,
"\u0143": 667,
"\u0144": 500,
"\u0145": 667,
"\u0146": 500,
"\u0147": 667,
"\u0148": 500,
"\u014c": 722,
"\u014d": 500,
"\u0150": 722,
"\u0151": 500,
"\u0152": 944,
"\u0153": 667,
"\u0154": 611,
"\u0155": 389,
"\u0156": 611,
"\u0157": 389,
"\u0158": 611,
"\u0159": 389,
"\u015a": 500,
"\u015b": 389,
"\u015e": 500,
"\u015f": 389,
"\u0160": 500,
"\u0161": 389,
"\u0162": 556,
"\u0163": 278,
"\u0164": 556,
"\u0165": 300,
"\u016a": 722,
"\u016b": 500,
"\u016e": 722,
"\u016f": 500,
"\u0170": 722,
"\u0171": 500,
"\u0172": 722,
"\u0173": 500,
"\u0178": 556,
"\u0179": 556,
"\u017a": 389,
"\u017b": 556,
"\u017c": 389,
"\u017d": 556,
"\u017e": 389,
"\u0192": 500,
"\u0218": 500,
"\u0219": 389,
"\u02c6": 333,
"\u02c7": 333,
"\u02d8": 333,
"\u02d9": 333,
"\u02da": 333,
"\u02db": 333,
"\u02dc": 333,
"\u02dd": 333,
"\u2013": 500,
"\u2014": 889,
"\u2018": 333,
"\u2019": 333,
"\u201a": 333,
"\u201c": 556,
"\u201d": 556,
"\u201e": 556,
"\u2020": 500,
"\u2021": 500,
"\u2022": 350,
"\u2026": 889,
"\u2030": 1000,
"\u2039": 333,
"\u203a": 333,
"\u2044": 167,
"\u2122": 980,
"\u2202": 476,
"\u2206": 612,
"\u2211": 600,
"\u2212": 675,
"\u221a": 453,
"\u2260": 549,
"\u2264": 549,
"\u2265": 549,
"\u25ca": 471,
"\uf6c3": 250,
"\ufb01": 500,
"\ufb02": 500,
},
),
"Times-Roman": (
{
"FontName": "Times-Roman",
"Descent": -217.0,
"FontBBox": (-168.0, -218.0, 1000.0, 898.0),
"FontWeight": "Roman",
"CapHeight": 662.0,
"FontFamily": "Times",
"Flags": 0,
"XHeight": 450.0,
"ItalicAngle": 0.0,
"Ascent": 683.0,
},
{
" ": 250,
"!": 333,
'"': 408,
"#": 500,
"$": 500,
"%": 833,
"&": 778,
"'": 180,
"(": 333,
")": 333,
"*": 500,
"+": 564,
",": 250,
"-": 333,
".": 250,
"/": 278,
"0": 500,
"1": 500,
"2": 500,
"3": 500,
"4": 500,
"5": 500,
"6": 500,
"7": 500,
"8": 500,
"9": 500,
":": 278,
";": 278,
"<": 564,
"=": 564,
">": 564,
"?": 444,
"@": 921,
"A": 722,
"B": 667,
"C": 667,
"D": 722,
"E": 611,
"F": 556,
"G": 722,
"H": 722,
"I": 333,
"J": 389,
"K": 722,
"L": 611,
"M": 889,
"N": 722,
"O": 722,
"P": 556,
"Q": 722,
"R": 667,
"S": 556,
"T": 611,
"U": 722,
"V": 722,
"W": 944,
"X": 722,
"Y": 722,
"Z": 611,
"[": 333,
"\\": 278,
"]": 333,
"^": 469,
"_": 500,
"`": 333,
"a": 444,
"b": 500,
"c": 444,
"d": 500,
"e": 444,
"f": 333,
"g": 500,
"h": 500,
"i": 278,
"j": 278,
"k": 500,
"l": 278,
"m": 778,
"n": 500,
"o": 500,
"p": 500,
"q": 500,
"r": 333,
"s": 389,
"t": 278,
"u": 500,
"v": 500,
"w": 722,
"x": 500,
"y": 500,
"z": 444,
"{": 480,
"|": 200,
"}": 480,
"~": 541,
"\xa1": 333,
"\xa2": 500,
"\xa3": 500,
"\xa4": 500,
"\xa5": 500,
"\xa6": 200,
"\xa7": 500,
"\xa8": 333,
"\xa9": 760,
"\xaa": 276,
"\xab": 500,
"\xac": 564,
"\xae": 760,
"\xaf": 333,
"\xb0": 400,
"\xb1": 564,
"\xb2": 300,
"\xb3": 300,
"\xb4": 333,
"\xb5": 500,
"\xb6": 453,
"\xb7": 250,
"\xb8": 333,
"\xb9": 300,
"\xba": 310,
"\xbb": 500,
"\xbc": 750,
"\xbd": 750,
"\xbe": 750,
"\xbf": 444,
"\xc0": 722,
"\xc1": 722,
"\xc2": 722,
"\xc3": 722,
"\xc4": 722,
"\xc5": 722,
"\xc6": 889,
"\xc7": 667,
"\xc8": 611,
"\xc9": 611,
"\xca": 611,
"\xcb": 611,
"\xcc": 333,
"\xcd": 333,
"\xce": 333,
"\xcf": 333,
"\xd0": 722,
"\xd1": 722,
"\xd2": 722,
"\xd3": 722,
"\xd4": 722,
"\xd5": 722,
"\xd6": 722,
"\xd7": 564,
"\xd8": 722,
"\xd9": 722,
"\xda": 722,
"\xdb": 722,
"\xdc": 722,
"\xdd": 722,
"\xde": 556,
"\xdf": 500,
"\xe0": 444,
"\xe1": 444,
"\xe2": 444,
"\xe3": 444,
"\xe4": 444,
"\xe5": 444,
"\xe6": 667,
"\xe7": 444,
"\xe8": 444,
"\xe9": 444,
"\xea": 444,
"\xeb": 444,
"\xec": 278,
"\xed": 278,
"\xee": 278,
"\xef": 278,
"\xf0": 500,
"\xf1": 500,
"\xf2": 500,
"\xf3": 500,
"\xf4": 500,
"\xf5": 500,
"\xf6": 500,
"\xf7": 564,
"\xf8": 500,
"\xf9": 500,
"\xfa": 500,
"\xfb": 500,
"\xfc": 500,
"\xfd": 500,
"\xfe": 500,
"\xff": 500,
"\u0100": 722,
"\u0101": 444,
"\u0102": 722,
"\u0103": 444,
"\u0104": 722,
"\u0105": 444,
"\u0106": 667,
"\u0107": 444,
"\u010c": 667,
"\u010d": 444,
"\u010e": 722,
"\u010f": 588,
"\u0110": 722,
"\u0111": 500,
"\u0112": 611,
"\u0113": 444,
"\u0116": 611,
"\u0117": 444,
"\u0118": 611,
"\u0119": 444,
"\u011a": 611,
"\u011b": 444,
"\u011e": 722,
"\u011f": 500,
"\u0122": 722,
"\u0123": 500,
"\u012a": 333,
"\u012b": 278,
"\u012e": 333,
"\u012f": 278,
"\u0130": 333,
"\u0131": 278,
"\u0136": 722,
"\u0137": 500,
"\u0139": 611,
"\u013a": 278,
"\u013b": 611,
"\u013c": 278,
"\u013d": 611,
"\u013e": 344,
"\u0141": 611,
"\u0142": 278,
"\u0143": 722,
"\u0144": 500,
"\u0145": 722,
"\u0146": 500,
"\u0147": 722,
"\u0148": 500,
"\u014c": 722,
"\u014d": 500,
"\u0150": 722,
"\u0151": 500,
"\u0152": 889,
"\u0153": 722,
"\u0154": 667,
"\u0155": 333,
"\u0156": 667,
"\u0157": 333,
"\u0158": 667,
"\u0159": 333,
"\u015a": 556,
"\u015b": 389,
"\u015e": 556,
"\u015f": 389,
"\u0160": 556,
"\u0161": 389,
"\u0162": 611,
"\u0163": 278,
"\u0164": 611,
"\u0165": 326,
"\u016a": 722,
"\u016b": 500,
"\u016e": 722,
"\u016f": 500,
"\u0170": 722,
"\u0171": 500,
"\u0172": 722,
"\u0173": 500,
"\u0178": 722,
"\u0179": 611,
"\u017a": 444,
"\u017b": 611,
"\u017c": 444,
"\u017d": 611,
"\u017e": 444,
"\u0192": 500,
"\u0218": 556,
"\u0219": 389,
"\u02c6": 333,
"\u02c7": 333,
"\u02d8": 333,
"\u02d9": 333,
"\u02da": 333,
"\u02db": 333,
"\u02dc": 333,
"\u02dd": 333,
"\u2013": 500,
"\u2014": 1000,
"\u2018": 333,
"\u2019": 333,
"\u201a": 333,
"\u201c": 444,
"\u201d": 444,
"\u201e": 444,
"\u2020": 500,
"\u2021": 500,
"\u2022": 350,
"\u2026": 1000,
"\u2030": 1000,
"\u2039": 333,
"\u203a": 333,
"\u2044": 167,
"\u2122": 980,
"\u2202": 476,
"\u2206": 612,
"\u2211": 600,
"\u2212": 564,
"\u221a": 453,
"\u2260": 549,
"\u2264": 549,
"\u2265": 549,
"\u25ca": 471,
"\uf6c3": 250,
"\ufb01": 556,
"\ufb02": 556,
},
),
"ZapfDingbats": (
{
"FontName": "ZapfDingbats",
"FontBBox": (-1.0, -143.0, 981.0, 820.0),
"FontWeight": "Medium",
"FontFamily": "ITC",
"Flags": 0,
"ItalicAngle": 0.0,
},
{
"\x01": 974,
"\x02": 961,
"\x03": 980,
"\x04": 719,
"\x05": 789,
"\x06": 494,
"\x07": 552,
"\x08": 537,
"\t": 577,
"\n": 692,
"\x0b": 960,
"\x0c": 939,
"\r": 549,
"\x0e": 855,
"\x0f": 911,
"\x10": 933,
"\x11": 945,
"\x12": 974,
"\x13": 755,
"\x14": 846,
"\x15": 762,
"\x16": 761,
"\x17": 571,
"\x18": 677,
"\x19": 763,
"\x1a": 760,
"\x1b": 759,
"\x1c": 754,
"\x1d": 786,
"\x1e": 788,
"\x1f": 788,
" ": 790,
"!": 793,
'"': 794,
"#": 816,
"$": 823,
"%": 789,
"&": 841,
"'": 823,
"(": 833,
")": 816,
"*": 831,
"+": 923,
",": 744,
"-": 723,
".": 749,
"/": 790,
"0": 792,
"1": 695,
"2": 776,
"3": 768,
"4": 792,
"5": 759,
"6": 707,
"7": 708,
"8": 682,
"9": 701,
":": 826,
";": 815,
"<": 789,
"=": 789,
">": 707,
"?": 687,
"@": 696,
"A": 689,
"B": 786,
"C": 787,
"D": 713,
"E": 791,
"F": 785,
"G": 791,
"H": 873,
"I": 761,
"J": 762,
"K": 759,
"L": 892,
"M": 892,
"N": 788,
"O": 784,
"Q": 438,
"R": 138,
"S": 277,
"T": 415,
"U": 509,
"V": 410,
"W": 234,
"X": 234,
"Y": 390,
"Z": 390,
"[": 276,
"\\": 276,
"]": 317,
"^": 317,
"_": 334,
"`": 334,
"a": 392,
"b": 392,
"c": 668,
"d": 668,
"e": 732,
"f": 544,
"g": 544,
"h": 910,
"i": 911,
"j": 667,
"k": 760,
"l": 760,
"m": 626,
"n": 694,
"o": 595,
"p": 776,
"u": 690,
"v": 791,
"w": 790,
"x": 788,
"y": 788,
"z": 788,
"{": 788,
"|": 788,
"}": 788,
"~": 788,
"\x7f": 788,
"\x80": 788,
"\x81": 788,
"\x82": 788,
"\x83": 788,
"\x84": 788,
"\x85": 788,
"\x86": 788,
"\x87": 788,
"\x88": 788,
"\x89": 788,
"\x8a": 788,
"\x8b": 788,
"\x8c": 788,
"\x8d": 788,
"\x8e": 788,
"\x8f": 788,
"\x90": 788,
"\x91": 788,
"\x92": 788,
"\x93": 788,
"\x94": 788,
"\x95": 788,
"\x96": 788,
"\x97": 788,
"\x98": 788,
"\x99": 788,
"\x9a": 788,
"\x9b": 788,
"\x9c": 788,
"\x9d": 788,
"\x9e": 788,
"\x9f": 788,
"\xa0": 894,
"\xa1": 838,
"\xa2": 924,
"\xa3": 1016,
"\xa4": 458,
"\xa5": 924,
"\xa6": 918,
"\xa7": 927,
"\xa8": 928,
"\xa9": 928,
"\xaa": 834,
"\xab": 873,
"\xac": 828,
"\xad": 924,
"\xae": 917,
"\xaf": 930,
"\xb0": 931,
"\xb1": 463,
"\xb2": 883,
"\xb3": 836,
"\xb4": 867,
"\xb5": 696,
"\xb6": 874,
"\xb7": 760,
"\xb8": 946,
"\xb9": 865,
"\xba": 967,
"\xbb": 831,
"\xbc": 873,
"\xbd": 927,
"\xbe": 970,
"\xbf": 918,
"\xc0": 748,
"\xc1": 836,
"\xc2": 771,
"\xc3": 888,
"\xc4": 748,
"\xc5": 771,
"\xc6": 888,
"\xc7": 867,
"\xc8": 696,
"\xc9": 874,
"\xca": 974,
"\xcb": 762,
"\xcc": 759,
"\xcd": 509,
"\xce": 410,
},
),
} | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/fontmetrics.py | fontmetrics.py |
import logging
import re
from typing import Dict, Iterable, Optional, cast
from .glyphlist import glyphname2unicode
from .latin_enc import ENCODING
from .psparser import PSLiteral
HEXADECIMAL = re.compile(r"[0-9a-fA-F]+")
log = logging.getLogger(__name__)
def name2unicode(name: str) -> str:
"""Converts Adobe glyph names to Unicode numbers.
In contrast to the specification, this raises a KeyError instead of return
an empty string when the key is unknown.
This way the caller must explicitly define what to do
when there is not a match.
Reference:
https://github.com/adobe-type-tools/agl-specification#2-the-mapping
:returns unicode character if name resembles something,
otherwise a KeyError
"""
if not isinstance(name, str):
raise KeyError(
'Could not convert unicode name "%s" to character because '
"it should be of type str but is of type %s" % (name, type(name))
)
name = name.split(".")[0]
components = name.split("_")
if len(components) > 1:
return "".join(map(name2unicode, components))
else:
if name in glyphname2unicode:
return glyphname2unicode[name]
elif name.startswith("uni"):
name_without_uni = name.strip("uni")
if HEXADECIMAL.match(name_without_uni) and len(name_without_uni) % 4 == 0:
unicode_digits = [
int(name_without_uni[i : i + 4], base=16)
for i in range(0, len(name_without_uni), 4)
]
for digit in unicode_digits:
raise_key_error_for_invalid_unicode(digit)
characters = map(chr, unicode_digits)
return "".join(characters)
elif name.startswith("u"):
name_without_u = name.strip("u")
if HEXADECIMAL.match(name_without_u) and 4 <= len(name_without_u) <= 6:
unicode_digit = int(name_without_u, base=16)
raise_key_error_for_invalid_unicode(unicode_digit)
return chr(unicode_digit)
raise KeyError(
'Could not convert unicode name "%s" to character because '
"it does not match specification" % name
)
def raise_key_error_for_invalid_unicode(unicode_digit: int) -> None:
"""Unicode values should not be in the range D800 through DFFF because
that is used for surrogate pairs in UTF-16
:raises KeyError if unicode digit is invalid
"""
if 55295 < unicode_digit < 57344:
raise KeyError(
"Unicode digit %d is invalid because "
"it is in the range D800 through DFFF" % unicode_digit
)
class EncodingDB:
std2unicode: Dict[int, str] = {}
mac2unicode: Dict[int, str] = {}
win2unicode: Dict[int, str] = {}
pdf2unicode: Dict[int, str] = {}
for (name, std, mac, win, pdf) in ENCODING:
c = name2unicode(name)
if std:
std2unicode[std] = c
if mac:
mac2unicode[mac] = c
if win:
win2unicode[win] = c
if pdf:
pdf2unicode[pdf] = c
encodings = {
"StandardEncoding": std2unicode,
"MacRomanEncoding": mac2unicode,
"WinAnsiEncoding": win2unicode,
"PDFDocEncoding": pdf2unicode,
}
@classmethod
def get_encoding(
cls, name: str, diff: Optional[Iterable[object]] = None
) -> Dict[int, str]:
cid2unicode = cls.encodings.get(name, cls.std2unicode)
if diff:
cid2unicode = cid2unicode.copy()
cid = 0
for x in diff:
if isinstance(x, int):
cid = x
elif isinstance(x, PSLiteral):
try:
cid2unicode[cid] = name2unicode(cast(str, x.name))
except (KeyError, ValueError) as e:
log.debug(str(e))
cid += 1
return cid2unicode | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/encodingdb.py | encodingdb.py |
import logging
from io import BytesIO
from typing import BinaryIO, Iterator, List, Optional, cast
logger = logging.getLogger(__name__)
class CorruptDataError(Exception):
pass
class LZWDecoder:
def __init__(self, fp: BinaryIO) -> None:
self.fp = fp
self.buff = 0
self.bpos = 8
self.nbits = 9
# NB: self.table stores None only in indices 256 and 257
self.table: List[Optional[bytes]] = []
self.prevbuf: Optional[bytes] = None
def readbits(self, bits: int) -> int:
v = 0
while 1:
# the number of remaining bits we can get from the current buffer.
r = 8 - self.bpos
if bits <= r:
# |-----8-bits-----|
# |-bpos-|-bits-| |
# | |----r----|
v = (v << bits) | ((self.buff >> (r - bits)) & ((1 << bits) - 1))
self.bpos += bits
break
else:
# |-----8-bits-----|
# |-bpos-|---bits----...
# | |----r----|
v = (v << r) | (self.buff & ((1 << r) - 1))
bits -= r
x = self.fp.read(1)
if not x:
raise EOFError
self.buff = ord(x)
self.bpos = 0
return v
def feed(self, code: int) -> bytes:
x = b""
if code == 256:
self.table = [bytes((c,)) for c in range(256)] # 0-255
self.table.append(None) # 256
self.table.append(None) # 257
self.prevbuf = b""
self.nbits = 9
elif code == 257:
pass
elif not self.prevbuf:
x = self.prevbuf = cast(bytes, self.table[code]) # assume not None
else:
if code < len(self.table):
x = cast(bytes, self.table[code]) # assume not None
self.table.append(self.prevbuf + x[:1])
elif code == len(self.table):
self.table.append(self.prevbuf + self.prevbuf[:1])
x = cast(bytes, self.table[code])
else:
raise CorruptDataError
table_length = len(self.table)
if table_length == 511:
self.nbits = 10
elif table_length == 1023:
self.nbits = 11
elif table_length == 2047:
self.nbits = 12
self.prevbuf = x
return x
def run(self) -> Iterator[bytes]:
while 1:
try:
code = self.readbits(self.nbits)
except EOFError:
break
try:
x = self.feed(code)
except CorruptDataError:
# just ignore corrupt data and stop yielding there
break
yield x
logger.debug(
"nbits=%d, code=%d, output=%r, table=%r",
self.nbits,
code,
x,
self.table[258:],
)
def lzwdecode(data: bytes) -> bytes:
fp = BytesIO(data)
s = LZWDecoder(fp).run()
return b"".join(s) | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/lzw.py | lzw.py |
import itertools
import logging
from typing import BinaryIO, Container, Dict, Iterator, List, Optional, Tuple
from pdfminer.utils import Rect
from . import settings
from .pdfdocument import PDFDocument, PDFTextExtractionNotAllowed, PDFNoPageLabels
from .pdfparser import PDFParser
from .pdftypes import PDFObjectNotFound
from .pdftypes import dict_value
from .pdftypes import int_value
from .pdftypes import list_value
from .pdftypes import resolve1
from .psparser import LIT
log = logging.getLogger(__name__)
# some predefined literals and keywords.
LITERAL_PAGE = LIT("Page")
LITERAL_PAGES = LIT("Pages")
class PDFPage:
"""An object that holds the information about a page.
A PDFPage object is merely a convenience class that has a set
of keys and values, which describe the properties of a page
and point to its contents.
Attributes:
doc: a PDFDocument object.
pageid: any Python object that can uniquely identify the page.
attrs: a dictionary of page attributes.
contents: a list of PDFStream objects that represents the page content.
lastmod: the last modified time of the page.
resources: a dictionary of resources used by the page.
mediabox: the physical size of the page.
cropbox: the crop rectangle of the page.
rotate: the page rotation (in degree).
annots: the page annotations.
beads: a chain that represents natural reading order.
label: the page's label (typically, the logical page number).
"""
def __init__(
self, doc: PDFDocument, pageid: object, attrs: object, label: Optional[str]
) -> None:
"""Initialize a page object.
doc: a PDFDocument object.
pageid: any Python object that can uniquely identify the page.
attrs: a dictionary of page attributes.
label: page label string.
"""
self.doc = doc
self.pageid = pageid
self.attrs = dict_value(attrs)
self.label = label
self.lastmod = resolve1(self.attrs.get("LastModified"))
self.resources: Dict[object, object] = resolve1(
self.attrs.get("Resources", dict())
)
self.mediabox: Rect = resolve1(self.attrs["MediaBox"])
if "CropBox" in self.attrs:
self.cropbox: Rect = resolve1(self.attrs["CropBox"])
else:
self.cropbox = self.mediabox
self.rotate = (int_value(self.attrs.get("Rotate", 0)) + 360) % 360
self.annots = self.attrs.get("Annots")
self.beads = self.attrs.get("B")
if "Contents" in self.attrs:
contents = resolve1(self.attrs["Contents"])
else:
contents = []
if not isinstance(contents, list):
contents = [contents]
self.contents: List[object] = contents
def __repr__(self) -> str:
return "<PDFPage: Resources={!r}, MediaBox={!r}>".format(
self.resources, self.mediabox
)
INHERITABLE_ATTRS = {"Resources", "MediaBox", "CropBox", "Rotate"}
@classmethod
def create_pages(cls, document: PDFDocument) -> Iterator["PDFPage"]:
def search(
obj: object, parent: Dict[str, object]
) -> Iterator[Tuple[int, Dict[object, Dict[object, object]]]]:
if isinstance(obj, int):
objid = obj
tree = dict_value(document.getobj(objid)).copy()
else:
# This looks broken. obj.objid means obj could be either
# PDFObjRef or PDFStream, but neither is valid for dict_value.
objid = obj.objid # type: ignore[attr-defined]
tree = dict_value(obj).copy()
for (k, v) in parent.items():
if k in cls.INHERITABLE_ATTRS and k not in tree:
tree[k] = v
tree_type = tree.get("Type")
if tree_type is None and not settings.STRICT: # See #64
tree_type = tree.get("type")
if tree_type is LITERAL_PAGES and "Kids" in tree:
log.debug("Pages: Kids=%r", tree["Kids"])
for c in list_value(tree["Kids"]):
yield from search(c, tree)
elif tree_type is LITERAL_PAGE:
log.debug("Page: %r", tree)
yield (objid, tree)
try:
page_labels: Iterator[Optional[str]] = document.get_page_labels()
except PDFNoPageLabels:
page_labels = itertools.repeat(None)
pages = False
if "Pages" in document.catalog:
objects = search(document.catalog["Pages"], document.catalog)
for (objid, tree) in objects:
yield cls(document, objid, tree, next(page_labels))
pages = True
if not pages:
# fallback when /Pages is missing.
for xref in document.xrefs:
for objid in xref.get_objids():
try:
obj = document.getobj(objid)
if isinstance(obj, dict) and obj.get("Type") is LITERAL_PAGE:
yield cls(document, objid, obj, next(page_labels))
except PDFObjectNotFound:
pass
return
@classmethod
def get_pages(
cls,
fp: BinaryIO,
pagenos: Optional[Container[int]] = None,
maxpages: int = 0,
password: str = "",
caching: bool = True,
check_extractable: bool = False,
) -> Iterator["PDFPage"]:
# Create a PDF parser object associated with the file object.
parser = PDFParser(fp)
# Create a PDF document object that stores the document structure.
doc = PDFDocument(parser, password=password, caching=caching)
# Check if the document allows text extraction.
# If not, warn the user and proceed.
if not doc.is_extractable:
if check_extractable:
error_msg = "Text extraction is not allowed: %r" % fp
raise PDFTextExtractionNotAllowed(error_msg)
else:
warning_msg = (
"The PDF %r contains a metadata field "
"indicating that it should not allow "
"text extraction. Ignoring this field "
"and proceeding. Use the check_extractable "
"if you want to raise an error in this case" % fp
)
log.warning(warning_msg)
# Process each page contained in the document.
for (pageno, page) in enumerate(cls.create_pages(doc)):
if pagenos and (pageno not in pagenos):
continue
yield page
if maxpages and maxpages <= pageno + 1:
break
return | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/pdfpage.py | pdfpage.py |
import logging
import re
from io import BytesIO
from typing import Dict, List, Mapping, Optional, Sequence, Tuple, Union, cast
from . import settings
from .cmapdb import CMap
from .cmapdb import CMapBase
from .cmapdb import CMapDB
from .pdfcolor import PDFColorSpace
from .pdfcolor import PREDEFINED_COLORSPACE
from .pdfdevice import PDFDevice
from .pdfdevice import PDFTextSeq
from .pdffont import PDFCIDFont
from .pdffont import PDFFont
from .pdffont import PDFFontError
from .pdffont import PDFTrueTypeFont
from .pdffont import PDFType1Font
from .pdffont import PDFType3Font
from .pdfpage import PDFPage
from .pdftypes import PDFException
from .pdftypes import PDFObjRef
from .pdftypes import PDFStream
from .pdftypes import dict_value
from .pdftypes import list_value
from .pdftypes import resolve1
from .pdftypes import stream_value
from .psparser import KWD
from .psparser import LIT
from .psparser import PSEOF
from .psparser import PSKeyword
from .psparser import PSLiteral, PSTypeError
from .psparser import PSStackParser
from .psparser import PSStackType
from .psparser import keyword_name
from .psparser import literal_name
from .utils import MATRIX_IDENTITY
from .utils import Matrix, Point, PathSegment, Rect
from .utils import choplist
from .utils import mult_matrix
log = logging.getLogger(__name__)
class PDFResourceError(PDFException):
pass
class PDFInterpreterError(PDFException):
pass
LITERAL_PDF = LIT("PDF")
LITERAL_TEXT = LIT("Text")
LITERAL_FONT = LIT("Font")
LITERAL_FORM = LIT("Form")
LITERAL_IMAGE = LIT("Image")
class PDFTextState:
matrix: Matrix
linematrix: Point
def __init__(self) -> None:
self.font: Optional[PDFFont] = None
self.fontsize: float = 0
self.charspace: float = 0
self.wordspace: float = 0
self.scaling: float = 100
self.leading: float = 0
self.render: int = 0
self.rise: float = 0
self.reset()
# self.matrix is set
# self.linematrix is set
def __repr__(self) -> str:
return (
"<PDFTextState: font=%r, fontsize=%r, charspace=%r, "
"wordspace=%r, scaling=%r, leading=%r, render=%r, rise=%r, "
"matrix=%r, linematrix=%r>"
% (
self.font,
self.fontsize,
self.charspace,
self.wordspace,
self.scaling,
self.leading,
self.render,
self.rise,
self.matrix,
self.linematrix,
)
)
def copy(self) -> "PDFTextState":
obj = PDFTextState()
obj.font = self.font
obj.fontsize = self.fontsize
obj.charspace = self.charspace
obj.wordspace = self.wordspace
obj.scaling = self.scaling
obj.leading = self.leading
obj.render = self.render
obj.rise = self.rise
obj.matrix = self.matrix
obj.linematrix = self.linematrix
return obj
def reset(self) -> None:
self.matrix = MATRIX_IDENTITY
self.linematrix = (0, 0)
Color = Union[
float, # Greyscale
Tuple[float, float, float], # R, G, B
Tuple[float, float, float, float],
] # C, M, Y, K
class PDFGraphicState:
def __init__(self) -> None:
self.linewidth: float = 0
self.linecap: Optional[object] = None
self.linejoin: Optional[object] = None
self.miterlimit: Optional[object] = None
self.dash: Optional[Tuple[object, object]] = None
self.intent: Optional[object] = None
self.flatness: Optional[object] = None
# stroking color
self.scolor: Optional[Color] = None
# non stroking color
self.ncolor: Optional[Color] = None
def copy(self) -> "PDFGraphicState":
obj = PDFGraphicState()
obj.linewidth = self.linewidth
obj.linecap = self.linecap
obj.linejoin = self.linejoin
obj.miterlimit = self.miterlimit
obj.dash = self.dash
obj.intent = self.intent
obj.flatness = self.flatness
obj.scolor = self.scolor
obj.ncolor = self.ncolor
return obj
def __repr__(self) -> str:
return (
"<PDFGraphicState: linewidth=%r, linecap=%r, linejoin=%r, "
" miterlimit=%r, dash=%r, intent=%r, flatness=%r, "
" stroking color=%r, non stroking color=%r>"
% (
self.linewidth,
self.linecap,
self.linejoin,
self.miterlimit,
self.dash,
self.intent,
self.flatness,
self.scolor,
self.ncolor,
)
)
class PDFResourceManager:
"""Repository of shared resources.
ResourceManager facilitates reuse of shared resources
such as fonts and images so that large objects are not
allocated multiple times.
"""
def __init__(self, caching: bool = True) -> None:
self.caching = caching
self._cached_fonts: Dict[object, PDFFont] = {}
def get_procset(self, procs: Sequence[object]) -> None:
for proc in procs:
if proc is LITERAL_PDF:
pass
elif proc is LITERAL_TEXT:
pass
else:
pass
def get_cmap(self, cmapname: str, strict: bool = False) -> CMapBase:
try:
return CMapDB.get_cmap(cmapname)
except CMapDB.CMapNotFound:
if strict:
raise
return CMap()
def get_font(self, objid: object, spec: Mapping[str, object]) -> PDFFont:
if objid and objid in self._cached_fonts:
font = self._cached_fonts[objid]
else:
log.debug("get_font: create: objid=%r, spec=%r", objid, spec)
if settings.STRICT:
if spec["Type"] is not LITERAL_FONT:
raise PDFFontError("Type is not /Font")
# Create a Font object.
if "Subtype" in spec:
subtype = literal_name(spec["Subtype"])
else:
if settings.STRICT:
raise PDFFontError("Font Subtype is not specified.")
subtype = "Type1"
if subtype in ("Type1", "MMType1"):
# Type1 Font
font = PDFType1Font(self, spec)
elif subtype == "TrueType":
# TrueType Font
font = PDFTrueTypeFont(self, spec)
elif subtype == "Type3":
# Type3 Font
font = PDFType3Font(self, spec)
elif subtype in ("CIDFontType0", "CIDFontType2"):
# CID Font
font = PDFCIDFont(self, spec)
elif subtype == "Type0":
# Type0 Font
dfonts = list_value(spec["DescendantFonts"])
assert dfonts
subspec = dict_value(dfonts[0]).copy()
for k in ("Encoding", "ToUnicode"):
if k in spec:
subspec[k] = resolve1(spec[k])
font = self.get_font(None, subspec)
else:
if settings.STRICT:
raise PDFFontError("Invalid Font spec: %r" % spec)
font = PDFType1Font(self, spec) # this is so wrong!
if objid and self.caching:
self._cached_fonts[objid] = font
return font
class PDFContentParser(PSStackParser[Union[PSKeyword, PDFStream]]):
def __init__(self, streams: Sequence[object]) -> None:
self.streams = streams
self.istream = 0
# PSStackParser.__init__(fp=None) is safe only because we've overloaded
# all the methods that would attempt to access self.fp without first
# calling self.fillfp().
PSStackParser.__init__(self, None) # type: ignore[arg-type]
def fillfp(self) -> None:
if not self.fp:
if self.istream < len(self.streams):
strm = stream_value(self.streams[self.istream])
self.istream += 1
else:
raise PSEOF("Unexpected EOF, file truncated?")
self.fp = BytesIO(strm.get_data())
def seek(self, pos: int) -> None:
self.fillfp()
PSStackParser.seek(self, pos)
def fillbuf(self) -> None:
if self.charpos < len(self.buf):
return
while 1:
self.fillfp()
self.bufpos = self.fp.tell()
self.buf = self.fp.read(self.BUFSIZ)
if self.buf:
break
self.fp = None # type: ignore[assignment]
self.charpos = 0
def get_inline_data(self, pos: int, target: bytes = b"EI") -> Tuple[int, bytes]:
self.seek(pos)
i = 0
data = b""
while i <= len(target):
self.fillbuf()
if i:
ci = self.buf[self.charpos]
c = bytes((ci,))
data += c
self.charpos += 1
if len(target) <= i and c.isspace():
i += 1
elif i < len(target) and c == (bytes((target[i],))):
i += 1
else:
i = 0
else:
try:
j = self.buf.index(target[0], self.charpos)
data += self.buf[self.charpos : j + 1]
self.charpos = j + 1
i = 1
except ValueError:
data += self.buf[self.charpos :]
self.charpos = len(self.buf)
data = data[: -(len(target) + 1)] # strip the last part
data = re.sub(rb"(\x0d\x0a|[\x0d\x0a])$", b"", data)
return (pos, data)
def flush(self) -> None:
self.add_results(*self.popall())
KEYWORD_BI = KWD(b"BI")
KEYWORD_ID = KWD(b"ID")
KEYWORD_EI = KWD(b"EI")
def do_keyword(self, pos: int, token: PSKeyword) -> None:
if token is self.KEYWORD_BI:
# inline image within a content stream
self.start_type(pos, "inline")
elif token is self.KEYWORD_ID:
try:
(_, objs) = self.end_type("inline")
if len(objs) % 2 != 0:
error_msg = "Invalid dictionary construct: {!r}".format(objs)
raise PSTypeError(error_msg)
d = {literal_name(k): v for (k, v) in choplist(2, objs)}
(pos, data) = self.get_inline_data(pos + len(b"ID "))
obj = PDFStream(d, data)
self.push((pos, obj))
self.push((pos, self.KEYWORD_EI))
except PSTypeError:
if settings.STRICT:
raise
else:
self.push((pos, token))
PDFStackT = PSStackType[PDFStream]
"""Types that may appear on the PDF argument stack."""
class PDFPageInterpreter:
"""Processor for the content of a PDF page
Reference: PDF Reference, Appendix A, Operator Summary
"""
def __init__(self, rsrcmgr: PDFResourceManager, device: PDFDevice) -> None:
self.rsrcmgr = rsrcmgr
self.device = device
return
def dup(self) -> "PDFPageInterpreter":
return self.__class__(self.rsrcmgr, self.device)
def init_resources(self, resources: Dict[object, object]) -> None:
"""Prepare the fonts and XObjects listed in the Resource attribute."""
self.resources = resources
self.fontmap: Dict[object, PDFFont] = {}
self.xobjmap = {}
self.csmap: Dict[str, PDFColorSpace] = PREDEFINED_COLORSPACE.copy()
if not resources:
return
def get_colorspace(spec: object) -> Optional[PDFColorSpace]:
if isinstance(spec, list):
name = literal_name(spec[0])
else:
name = literal_name(spec)
if name == "ICCBased" and isinstance(spec, list) and 2 <= len(spec):
return PDFColorSpace(name, stream_value(spec[1])["N"])
elif name == "DeviceN" and isinstance(spec, list) and 2 <= len(spec):
return PDFColorSpace(name, len(list_value(spec[1])))
else:
return PREDEFINED_COLORSPACE.get(name)
for (k, v) in dict_value(resources).items():
log.debug("Resource: %r: %r", k, v)
if k == "Font":
for (fontid, spec) in dict_value(v).items():
objid = None
if isinstance(spec, PDFObjRef):
objid = spec.objid
spec = dict_value(spec)
self.fontmap[fontid] = self.rsrcmgr.get_font(objid, spec)
elif k == "ColorSpace":
for (csid, spec) in dict_value(v).items():
colorspace = get_colorspace(resolve1(spec))
if colorspace is not None:
self.csmap[csid] = colorspace
elif k == "ProcSet":
self.rsrcmgr.get_procset(list_value(v))
elif k == "XObject":
for (xobjid, xobjstrm) in dict_value(v).items():
self.xobjmap[xobjid] = xobjstrm
return
def init_state(self, ctm: Matrix) -> None:
"""Initialize the text and graphic states for rendering a page."""
# gstack: stack for graphical states.
self.gstack: List[Tuple[Matrix, PDFTextState, PDFGraphicState]] = []
self.ctm = ctm
self.device.set_ctm(self.ctm)
self.textstate = PDFTextState()
self.graphicstate = PDFGraphicState()
self.curpath: List[PathSegment] = []
# argstack: stack for command arguments.
self.argstack: List[PDFStackT] = []
# set some global states.
self.scs: Optional[PDFColorSpace] = None
self.ncs: Optional[PDFColorSpace] = None
if self.csmap:
self.scs = self.ncs = next(iter(self.csmap.values()))
return
def push(self, obj: PDFStackT) -> None:
self.argstack.append(obj)
return
def pop(self, n: int) -> List[PDFStackT]:
if n == 0:
return []
x = self.argstack[-n:]
self.argstack = self.argstack[:-n]
return x
def get_current_state(self) -> Tuple[Matrix, PDFTextState, PDFGraphicState]:
return (self.ctm, self.textstate.copy(), self.graphicstate.copy())
def set_current_state(
self, state: Tuple[Matrix, PDFTextState, PDFGraphicState]
) -> None:
(self.ctm, self.textstate, self.graphicstate) = state
self.device.set_ctm(self.ctm)
return
def do_q(self) -> None:
"""Save graphics state"""
self.gstack.append(self.get_current_state())
return
def do_Q(self) -> None:
"""Restore graphics state"""
if self.gstack:
self.set_current_state(self.gstack.pop())
return
def do_cm(
self,
a1: PDFStackT,
b1: PDFStackT,
c1: PDFStackT,
d1: PDFStackT,
e1: PDFStackT,
f1: PDFStackT,
) -> None:
"""Concatenate matrix to current transformation matrix"""
self.ctm = mult_matrix(cast(Matrix, (a1, b1, c1, d1, e1, f1)), self.ctm)
self.device.set_ctm(self.ctm)
return
def do_w(self, linewidth: PDFStackT) -> None:
"""Set line width"""
self.graphicstate.linewidth = cast(float, linewidth)
return
def do_J(self, linecap: PDFStackT) -> None:
"""Set line cap style"""
self.graphicstate.linecap = linecap
return
def do_j(self, linejoin: PDFStackT) -> None:
"""Set line join style"""
self.graphicstate.linejoin = linejoin
return
def do_M(self, miterlimit: PDFStackT) -> None:
"""Set miter limit"""
self.graphicstate.miterlimit = miterlimit
return
def do_d(self, dash: PDFStackT, phase: PDFStackT) -> None:
"""Set line dash pattern"""
self.graphicstate.dash = (dash, phase)
return
def do_ri(self, intent: PDFStackT) -> None:
"""Set color rendering intent"""
self.graphicstate.intent = intent
return
def do_i(self, flatness: PDFStackT) -> None:
"""Set flatness tolerance"""
self.graphicstate.flatness = flatness
return
def do_gs(self, name: PDFStackT) -> None:
"""Set parameters from graphics state parameter dictionary"""
# todo
return
def do_m(self, x: PDFStackT, y: PDFStackT) -> None:
"""Begin new subpath"""
self.curpath.append(("m", cast(float, x), cast(float, y)))
return
def do_l(self, x: PDFStackT, y: PDFStackT) -> None:
"""Append straight line segment to path"""
self.curpath.append(("l", cast(float, x), cast(float, y)))
return
def do_c(
self,
x1: PDFStackT,
y1: PDFStackT,
x2: PDFStackT,
y2: PDFStackT,
x3: PDFStackT,
y3: PDFStackT,
) -> None:
"""Append curved segment to path (three control points)"""
self.curpath.append(
(
"c",
cast(float, x1),
cast(float, y1),
cast(float, x2),
cast(float, y2),
cast(float, x3),
cast(float, y3),
)
)
return
def do_v(self, x2: PDFStackT, y2: PDFStackT, x3: PDFStackT, y3: PDFStackT) -> None:
"""Append curved segment to path (initial point replicated)"""
self.curpath.append(
("v", cast(float, x2), cast(float, y2), cast(float, x3), cast(float, y3))
)
return
def do_y(self, x1: PDFStackT, y1: PDFStackT, x3: PDFStackT, y3: PDFStackT) -> None:
"""Append curved segment to path (final point replicated)"""
self.curpath.append(
("y", cast(float, x1), cast(float, y1), cast(float, x3), cast(float, y3))
)
return
def do_h(self) -> None:
"""Close subpath"""
self.curpath.append(("h",))
return
def do_re(self, x: PDFStackT, y: PDFStackT, w: PDFStackT, h: PDFStackT) -> None:
"""Append rectangle to path"""
x = cast(float, x)
y = cast(float, y)
w = cast(float, w)
h = cast(float, h)
self.curpath.append(("m", x, y))
self.curpath.append(("l", x + w, y))
self.curpath.append(("l", x + w, y + h))
self.curpath.append(("l", x, y + h))
self.curpath.append(("h",))
return
def do_S(self) -> None:
"""Stroke path"""
self.device.paint_path(self.graphicstate, True, False, False, self.curpath)
self.curpath = []
return
def do_s(self) -> None:
"""Close and stroke path"""
self.do_h()
self.do_S()
return
def do_f(self) -> None:
"""Fill path using nonzero winding number rule"""
self.device.paint_path(self.graphicstate, False, True, False, self.curpath)
self.curpath = []
return
def do_F(self) -> None:
"""Fill path using nonzero winding number rule (obsolete)"""
return self.do_f()
def do_f_a(self) -> None:
"""Fill path using even-odd rule"""
self.device.paint_path(self.graphicstate, False, True, True, self.curpath)
self.curpath = []
return
def do_B(self) -> None:
"""Fill and stroke path using nonzero winding number rule"""
self.device.paint_path(self.graphicstate, True, True, False, self.curpath)
self.curpath = []
return
def do_B_a(self) -> None:
"""Fill and stroke path using even-odd rule"""
self.device.paint_path(self.graphicstate, True, True, True, self.curpath)
self.curpath = []
return
def do_b(self) -> None:
"""Close, fill, and stroke path using nonzero winding number rule"""
self.do_h()
self.do_B()
return
def do_b_a(self) -> None:
"""Close, fill, and stroke path using even-odd rule"""
self.do_h()
self.do_B_a()
return
def do_n(self) -> None:
"""End path without filling or stroking"""
self.curpath = []
return
def do_W(self) -> None:
"""Set clipping path using nonzero winding number rule"""
return
def do_W_a(self) -> None:
"""Set clipping path using even-odd rule"""
return
def do_CS(self, name: PDFStackT) -> None:
"""Set color space for stroking operations
Introduced in PDF 1.1
"""
try:
self.scs = self.csmap[literal_name(name)]
except KeyError:
if settings.STRICT:
raise PDFInterpreterError("Undefined ColorSpace: %r" % name)
return
def do_cs(self, name: PDFStackT) -> None:
"""Set color space for nonstroking operations"""
try:
self.ncs = self.csmap[literal_name(name)]
except KeyError:
if settings.STRICT:
raise PDFInterpreterError("Undefined ColorSpace: %r" % name)
return
def do_G(self, gray: PDFStackT) -> None:
"""Set gray level for stroking operations"""
self.graphicstate.scolor = cast(float, gray)
return
def do_g(self, gray: PDFStackT) -> None:
"""Set gray level for nonstroking operations"""
self.graphicstate.ncolor = cast(float, gray)
return
def do_RG(self, r: PDFStackT, g: PDFStackT, b: PDFStackT) -> None:
"""Set RGB color for stroking operations"""
self.graphicstate.scolor = (cast(float, r), cast(float, g), cast(float, b))
return
def do_rg(self, r: PDFStackT, g: PDFStackT, b: PDFStackT) -> None:
"""Set RGB color for nonstroking operations"""
self.graphicstate.ncolor = (cast(float, r), cast(float, g), cast(float, b))
return
def do_K(self, c: PDFStackT, m: PDFStackT, y: PDFStackT, k: PDFStackT) -> None:
"""Set CMYK color for stroking operations"""
self.graphicstate.scolor = (
cast(float, c),
cast(float, m),
cast(float, y),
cast(float, k),
)
return
def do_k(self, c: PDFStackT, m: PDFStackT, y: PDFStackT, k: PDFStackT) -> None:
"""Set CMYK color for nonstroking operations"""
self.graphicstate.ncolor = (
cast(float, c),
cast(float, m),
cast(float, y),
cast(float, k),
)
return
def do_SCN(self) -> None:
"""Set color for stroking operations."""
if self.scs:
n = self.scs.ncomponents
else:
if settings.STRICT:
raise PDFInterpreterError("No colorspace specified!")
n = 1
self.graphicstate.scolor = cast(Color, self.pop(n))
return
def do_scn(self) -> None:
"""Set color for nonstroking operations"""
if self.ncs:
n = self.ncs.ncomponents
else:
if settings.STRICT:
raise PDFInterpreterError("No colorspace specified!")
n = 1
self.graphicstate.ncolor = cast(Color, self.pop(n))
return
def do_SC(self) -> None:
"""Set color for stroking operations"""
self.do_SCN()
return
def do_sc(self) -> None:
"""Set color for nonstroking operations"""
self.do_scn()
return
def do_sh(self, name: object) -> None:
"""Paint area defined by shading pattern"""
return
def do_BT(self) -> None:
"""Begin text object
Initializing the text matrix, Tm, and the text line matrix, Tlm, to
the identity matrix. Text objects cannot be nested; a second BT cannot
appear before an ET.
"""
self.textstate.reset()
return
def do_ET(self) -> None:
"""End a text object"""
return
def do_BX(self) -> None:
"""Begin compatibility section"""
return
def do_EX(self) -> None:
"""End compatibility section"""
return
def do_MP(self, tag: PDFStackT) -> None:
"""Define marked-content point"""
self.device.do_tag(cast(PSLiteral, tag))
return
def do_DP(self, tag: PDFStackT, props: PDFStackT) -> None:
"""Define marked-content point with property list"""
self.device.do_tag(cast(PSLiteral, tag), props)
return
def do_BMC(self, tag: PDFStackT) -> None:
"""Begin marked-content sequence"""
self.device.begin_tag(cast(PSLiteral, tag))
return
def do_BDC(self, tag: PDFStackT, props: PDFStackT) -> None:
"""Begin marked-content sequence with property list"""
self.device.begin_tag(cast(PSLiteral, tag), props)
return
def do_EMC(self) -> None:
"""End marked-content sequence"""
self.device.end_tag()
return
def do_Tc(self, space: PDFStackT) -> None:
"""Set character spacing.
Character spacing is used by the Tj, TJ, and ' operators.
:param space: a number expressed in unscaled text space units.
"""
self.textstate.charspace = cast(float, space)
return
def do_Tw(self, space: PDFStackT) -> None:
"""Set the word spacing.
Word spacing is used by the Tj, TJ, and ' operators.
:param space: a number expressed in unscaled text space units
"""
self.textstate.wordspace = cast(float, space)
return
def do_Tz(self, scale: PDFStackT) -> None:
"""Set the horizontal scaling.
:param scale: is a number specifying the percentage of the normal width
"""
self.textstate.scaling = cast(float, scale)
return
def do_TL(self, leading: PDFStackT) -> None:
"""Set the text leading.
Text leading is used only by the T*, ', and " operators.
:param leading: a number expressed in unscaled text space units
"""
self.textstate.leading = -cast(float, leading)
return
def do_Tf(self, fontid: PDFStackT, fontsize: PDFStackT) -> None:
"""Set the text font
:param fontid: the name of a font resource in the Font subdictionary
of the current resource dictionary
:param fontsize: size is a number representing a scale factor.
"""
try:
self.textstate.font = self.fontmap[literal_name(fontid)]
except KeyError:
if settings.STRICT:
raise PDFInterpreterError("Undefined Font id: %r" % fontid)
self.textstate.font = self.rsrcmgr.get_font(None, {})
self.textstate.fontsize = cast(float, fontsize)
return
def do_Tr(self, render: PDFStackT) -> None:
"""Set the text rendering mode"""
self.textstate.render = cast(int, render)
return
def do_Ts(self, rise: PDFStackT) -> None:
"""Set the text rise
:param rise: a number expressed in unscaled text space units
"""
self.textstate.rise = cast(float, rise)
return
def do_Td(self, tx: PDFStackT, ty: PDFStackT) -> None:
"""Move text position"""
tx = cast(float, tx)
ty = cast(float, ty)
(a, b, c, d, e, f) = self.textstate.matrix
self.textstate.matrix = (a, b, c, d, tx * a + ty * c + e, tx * b + ty * d + f)
self.textstate.linematrix = (0, 0)
return
def do_TD(self, tx: PDFStackT, ty: PDFStackT) -> None:
"""Move text position and set leading"""
tx = cast(float, tx)
ty = cast(float, ty)
(a, b, c, d, e, f) = self.textstate.matrix
self.textstate.matrix = (a, b, c, d, tx * a + ty * c + e, tx * b + ty * d + f)
self.textstate.leading = ty
self.textstate.linematrix = (0, 0)
return
def do_Tm(
self,
a: PDFStackT,
b: PDFStackT,
c: PDFStackT,
d: PDFStackT,
e: PDFStackT,
f: PDFStackT,
) -> None:
"""Set text matrix and text line matrix"""
self.textstate.matrix = cast(Matrix, (a, b, c, d, e, f))
self.textstate.linematrix = (0, 0)
return
def do_T_a(self) -> None:
"""Move to start of next text line"""
(a, b, c, d, e, f) = self.textstate.matrix
self.textstate.matrix = (
a,
b,
c,
d,
self.textstate.leading * c + e,
self.textstate.leading * d + f,
)
self.textstate.linematrix = (0, 0)
return
def do_TJ(self, seq: PDFStackT) -> None:
"""Show text, allowing individual glyph positioning"""
if self.textstate.font is None:
if settings.STRICT:
raise PDFInterpreterError("No font specified!")
return
assert self.ncs is not None
self.device.render_string(
self.textstate, cast(PDFTextSeq, seq), self.ncs, self.graphicstate.copy()
)
return
def do_Tj(self, s: PDFStackT) -> None:
"""Show text"""
self.do_TJ([s])
return
def do__q(self, s: PDFStackT) -> None:
"""Move to next line and show text
The ' (single quote) operator.
"""
self.do_T_a()
self.do_TJ([s])
return
def do__w(self, aw: PDFStackT, ac: PDFStackT, s: PDFStackT) -> None:
"""Set word and character spacing, move to next line, and show text
The " (double quote) operator.
"""
self.do_Tw(aw)
self.do_Tc(ac)
self.do_TJ([s])
return
def do_BI(self) -> None:
"""Begin inline image object"""
return
def do_ID(self) -> None:
"""Begin inline image data"""
return
def do_EI(self, obj: PDFStackT) -> None:
"""End inline image object"""
if isinstance(obj, PDFStream) and "W" in obj and "H" in obj:
iobjid = str(id(obj))
self.device.begin_figure(iobjid, (0, 0, 1, 1), MATRIX_IDENTITY)
self.device.render_image(iobjid, obj)
self.device.end_figure(iobjid)
return
def do_Do(self, xobjid_arg: PDFStackT) -> None:
"""Invoke named XObject"""
xobjid = cast(str, literal_name(xobjid_arg))
try:
xobj = stream_value(self.xobjmap[xobjid])
except KeyError:
if settings.STRICT:
raise PDFInterpreterError("Undefined xobject id: %r" % xobjid)
return
log.debug("Processing xobj: %r", xobj)
subtype = xobj.get("Subtype")
if subtype is LITERAL_FORM and "BBox" in xobj:
interpreter = self.dup()
bbox = cast(Rect, list_value(xobj["BBox"]))
matrix = cast(Matrix, list_value(xobj.get("Matrix", MATRIX_IDENTITY)))
# According to PDF reference 1.7 section 4.9.1, XObjects in
# earlier PDFs (prior to v1.2) use the page's Resources entry
# instead of having their own Resources entry.
xobjres = xobj.get("Resources")
if xobjres:
resources = dict_value(xobjres)
else:
resources = self.resources.copy()
self.device.begin_figure(xobjid, bbox, matrix)
interpreter.render_contents(
resources, [xobj], ctm=mult_matrix(matrix, self.ctm)
)
self.device.end_figure(xobjid)
elif subtype is LITERAL_IMAGE and "Width" in xobj and "Height" in xobj:
self.device.begin_figure(xobjid, (0, 0, 1, 1), MATRIX_IDENTITY)
self.device.render_image(xobjid, xobj)
self.device.end_figure(xobjid)
else:
# unsupported xobject type.
pass
return
def process_page(self, page: PDFPage) -> None:
log.debug("Processing page: %r", page)
(x0, y0, x1, y1) = page.mediabox
if page.rotate == 90:
ctm = (0, -1, 1, 0, -y0, x1)
elif page.rotate == 180:
ctm = (-1, 0, 0, -1, x1, y1)
elif page.rotate == 270:
ctm = (0, 1, -1, 0, y1, -x0)
else:
ctm = (1, 0, 0, 1, -x0, -y0)
self.device.begin_page(page, ctm)
self.render_contents(page.resources, page.contents, ctm=ctm)
self.device.end_page(page)
return
def render_contents(
self,
resources: Dict[object, object],
streams: Sequence[object],
ctm: Matrix = MATRIX_IDENTITY,
) -> None:
"""Render the content streams.
This method may be called recursively.
"""
log.debug(
"render_contents: resources=%r, streams=%r, ctm=%r", resources, streams, ctm
)
self.init_resources(resources)
self.init_state(ctm)
self.execute(list_value(streams))
return
def execute(self, streams: Sequence[object]) -> None:
try:
parser = PDFContentParser(streams)
except PSEOF:
# empty page
return
while 1:
try:
(_, obj) = parser.nextobject()
except PSEOF:
break
if isinstance(obj, PSKeyword):
name = keyword_name(obj)
method = "do_%s" % name.replace("*", "_a").replace('"', "_w").replace(
"'", "_q"
)
if hasattr(self, method):
func = getattr(self, method)
nargs = func.__code__.co_argcount - 1
if nargs:
args = self.pop(nargs)
log.debug("exec: %s %r", name, args)
if len(args) == nargs:
func(*args)
else:
log.debug("exec: %s", name)
func()
else:
if settings.STRICT:
error_msg = "Unknown operator: %r" % name
raise PDFInterpreterError(error_msg)
else:
self.push(obj)
return | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/pdfinterp.py | pdfinterp.py |
import logging
from io import BytesIO
from typing import BinaryIO, TYPE_CHECKING, Optional, Union
from . import settings
from .pdftypes import PDFException
from .pdftypes import PDFObjRef
from .pdftypes import PDFStream
from .pdftypes import dict_value
from .pdftypes import int_value
from .psparser import KWD
from .psparser import PSEOF
from .psparser import PSKeyword
from .psparser import PSStackParser
from .psparser import PSSyntaxError
if TYPE_CHECKING:
from .pdfdocument import PDFDocument
log = logging.getLogger(__name__)
class PDFSyntaxError(PDFException):
pass
# PDFParser stack holds all the base types plus PDFStream, PDFObjRef, and None
class PDFParser(PSStackParser[Union[PSKeyword, PDFStream, PDFObjRef, None]]):
"""
PDFParser fetch PDF objects from a file stream.
It can handle indirect references by referring to
a PDF document set by set_document method.
It also reads XRefs at the end of every PDF file.
Typical usage:
parser = PDFParser(fp)
parser.read_xref()
parser.read_xref(fallback=True) # optional
parser.set_document(doc)
parser.seek(offset)
parser.nextobject()
"""
def __init__(self, fp: BinaryIO) -> None:
PSStackParser.__init__(self, fp)
self.doc: Optional["PDFDocument"] = None
self.fallback = False
def set_document(self, doc: "PDFDocument") -> None:
"""Associates the parser with a PDFDocument object."""
self.doc = doc
KEYWORD_R = KWD(b"R")
KEYWORD_NULL = KWD(b"null")
KEYWORD_ENDOBJ = KWD(b"endobj")
KEYWORD_STREAM = KWD(b"stream")
KEYWORD_XREF = KWD(b"xref")
KEYWORD_STARTXREF = KWD(b"startxref")
def do_keyword(self, pos: int, token: PSKeyword) -> None:
"""Handles PDF-related keywords."""
if token in (self.KEYWORD_XREF, self.KEYWORD_STARTXREF):
self.add_results(*self.pop(1))
elif token is self.KEYWORD_ENDOBJ:
self.add_results(*self.pop(4))
elif token is self.KEYWORD_NULL:
# null object
self.push((pos, None))
elif token is self.KEYWORD_R:
# reference to indirect object
if len(self.curstack) >= 2:
try:
((_, objid), (_, genno)) = self.pop(2)
(objid, genno) = (int(objid), int(genno)) # type: ignore[arg-type]
assert self.doc is not None
obj = PDFObjRef(self.doc, objid, genno)
self.push((pos, obj))
except PSSyntaxError:
pass
elif token is self.KEYWORD_STREAM:
# stream object
((_, dic),) = self.pop(1)
dic = dict_value(dic)
objlen = 0
if not self.fallback:
try:
objlen = int_value(dic["Length"])
except KeyError:
if settings.STRICT:
raise PDFSyntaxError("/Length is undefined: %r" % dic)
self.seek(pos)
try:
(_, line) = self.nextline() # 'stream'
except PSEOF:
if settings.STRICT:
raise PDFSyntaxError("Unexpected EOF")
return
pos += len(line)
self.fp.seek(pos)
data = bytearray(self.fp.read(objlen))
self.seek(pos + objlen)
while 1:
try:
(linepos, line) = self.nextline()
except PSEOF:
if settings.STRICT:
raise PDFSyntaxError("Unexpected EOF")
break
if b"endstream" in line:
i = line.index(b"endstream")
objlen += i
if self.fallback:
data += line[:i]
break
objlen += len(line)
if self.fallback:
data += line
self.seek(pos + objlen)
# XXX limit objlen not to exceed object boundary
log.debug(
"Stream: pos=%d, objlen=%d, dic=%r, data=%r...",
pos,
objlen,
dic,
data[:10],
)
assert self.doc is not None
stream = PDFStream(dic, bytes(data), self.doc.decipher)
self.push((pos, stream))
else:
# others
self.push((pos, token))
class PDFStreamParser(PDFParser):
"""
PDFStreamParser is used to parse PDF content streams
that is contained in each page and has instructions
for rendering the page. A reference to a PDF document is
needed because a PDF content stream can also have
indirect references to other objects in the same document.
"""
def __init__(self, data: bytes) -> None:
PDFParser.__init__(self, BytesIO(data))
def flush(self) -> None:
self.add_results(*self.popall())
KEYWORD_OBJ = KWD(b"obj")
def do_keyword(self, pos: int, token: PSKeyword) -> None:
if token is self.KEYWORD_R:
# reference to indirect object
try:
((_, objid), (_, genno)) = self.pop(2)
(objid, genno) = (int(objid), int(genno)) # type: ignore[arg-type]
obj = PDFObjRef(self.doc, objid, genno)
self.push((pos, obj))
except PSSyntaxError:
pass
return
elif token in (self.KEYWORD_OBJ, self.KEYWORD_ENDOBJ):
if settings.STRICT:
# See PDF Spec 3.4.6: Only the object values are stored in the
# stream; the obj and endobj keywords are not used.
raise PDFSyntaxError("Keyword endobj found in stream")
return
# others
self.push((pos, token)) | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/pdfparser.py | pdfparser.py |
import math
import os
from struct import pack, unpack, calcsize
from typing import BinaryIO, Dict, Iterable, List, Optional, Tuple, Union, cast
# segment structure base
SEG_STRUCT = [
(">L", "number"),
(">B", "flags"),
(">B", "retention_flags"),
(">B", "page_assoc"),
(">L", "data_length"),
]
# segment header literals
HEADER_FLAG_DEFERRED = 0b10000000
HEADER_FLAG_PAGE_ASSOC_LONG = 0b01000000
SEG_TYPE_MASK = 0b00111111
REF_COUNT_SHORT_MASK = 0b11100000
REF_COUNT_LONG_MASK = 0x1FFFFFFF
REF_COUNT_LONG = 7
DATA_LEN_UNKNOWN = 0xFFFFFFFF
# segment types
SEG_TYPE_IMMEDIATE_GEN_REGION = 38
SEG_TYPE_END_OF_PAGE = 49
SEG_TYPE_END_OF_FILE = 51
# file literals
FILE_HEADER_ID = b"\x97\x4A\x42\x32\x0D\x0A\x1A\x0A"
FILE_HEAD_FLAG_SEQUENTIAL = 0b00000001
def bit_set(bit_pos: int, value: int) -> bool:
return bool((value >> bit_pos) & 1)
def check_flag(flag: int, value: int) -> bool:
return bool(flag & value)
def masked_value(mask: int, value: int) -> int:
for bit_pos in range(0, 31):
if bit_set(bit_pos, mask):
return (value & mask) >> bit_pos
raise Exception("Invalid mask or value")
def mask_value(mask: int, value: int) -> int:
for bit_pos in range(0, 31):
if bit_set(bit_pos, mask):
return (value & (mask >> bit_pos)) << bit_pos
raise Exception("Invalid mask or value")
def unpack_int(format: str, buffer: bytes) -> int:
assert format in {">B", ">I", ">L"}
[result] = cast(Tuple[int], unpack(format, buffer))
return result
JBIG2SegmentFlags = Dict[str, Union[int, bool]]
JBIG2RetentionFlags = Dict[str, Union[int, List[int], List[bool]]]
JBIG2Segment = Dict[
str, Union[bool, int, bytes, JBIG2SegmentFlags, JBIG2RetentionFlags]
]
class JBIG2StreamReader:
"""Read segments from a JBIG2 byte stream"""
def __init__(self, stream: BinaryIO) -> None:
self.stream = stream
def get_segments(self) -> List[JBIG2Segment]:
segments: List[JBIG2Segment] = []
while not self.is_eof():
segment: JBIG2Segment = {}
for field_format, name in SEG_STRUCT:
field_len = calcsize(field_format)
field = self.stream.read(field_len)
if len(field) < field_len:
segment["_error"] = True
break
value = unpack_int(field_format, field)
parser = getattr(self, "parse_%s" % name, None)
if callable(parser):
value = parser(segment, value, field)
segment[name] = value
if not segment.get("_error"):
segments.append(segment)
return segments
def is_eof(self) -> bool:
if self.stream.read(1) == b"":
return True
else:
self.stream.seek(-1, os.SEEK_CUR)
return False
def parse_flags(
self, segment: JBIG2Segment, flags: int, field: bytes
) -> JBIG2SegmentFlags:
return {
"deferred": check_flag(HEADER_FLAG_DEFERRED, flags),
"page_assoc_long": check_flag(HEADER_FLAG_PAGE_ASSOC_LONG, flags),
"type": masked_value(SEG_TYPE_MASK, flags),
}
def parse_retention_flags(
self, segment: JBIG2Segment, flags: int, field: bytes
) -> JBIG2RetentionFlags:
ref_count = masked_value(REF_COUNT_SHORT_MASK, flags)
retain_segments = []
ref_segments = []
if ref_count < REF_COUNT_LONG:
for bit_pos in range(5):
retain_segments.append(bit_set(bit_pos, flags))
else:
field += self.stream.read(3)
ref_count = unpack_int(">L", field)
ref_count = masked_value(REF_COUNT_LONG_MASK, ref_count)
ret_bytes_count = int(math.ceil((ref_count + 1) / 8))
for ret_byte_index in range(ret_bytes_count):
ret_byte = unpack_int(">B", self.stream.read(1))
for bit_pos in range(7):
retain_segments.append(bit_set(bit_pos, ret_byte))
seg_num = segment["number"]
assert isinstance(seg_num, int)
if seg_num <= 256:
ref_format = ">B"
elif seg_num <= 65536:
ref_format = ">I"
else:
ref_format = ">L"
ref_size = calcsize(ref_format)
for ref_index in range(ref_count):
ref_data = self.stream.read(ref_size)
ref = unpack_int(ref_format, ref_data)
ref_segments.append(ref)
return {
"ref_count": ref_count,
"retain_segments": retain_segments,
"ref_segments": ref_segments,
}
def parse_page_assoc(self, segment: JBIG2Segment, page: int, field: bytes) -> int:
if cast(JBIG2SegmentFlags, segment["flags"])["page_assoc_long"]:
field += self.stream.read(3)
page = unpack_int(">L", field)
return page
def parse_data_length(
self, segment: JBIG2Segment, length: int, field: bytes
) -> int:
if length:
if (
cast(JBIG2SegmentFlags, segment["flags"])["type"]
== SEG_TYPE_IMMEDIATE_GEN_REGION
) and (length == DATA_LEN_UNKNOWN):
raise NotImplementedError(
"Working with unknown segment length " "is not implemented yet"
)
else:
segment["raw_data"] = self.stream.read(length)
return length
class JBIG2StreamWriter:
"""Write JBIG2 segments to a file in JBIG2 format"""
EMPTY_RETENTION_FLAGS: JBIG2RetentionFlags = {
"ref_count": 0,
"ref_segments": cast(List[int], []),
"retain_segments": cast(List[bool], []),
}
def __init__(self, stream: BinaryIO) -> None:
self.stream = stream
def write_segments(
self, segments: Iterable[JBIG2Segment], fix_last_page: bool = True
) -> int:
data_len = 0
current_page: Optional[int] = None
seg_num: Optional[int] = None
for segment in segments:
data = self.encode_segment(segment)
self.stream.write(data)
data_len += len(data)
seg_num = cast(Optional[int], segment["number"])
if fix_last_page:
seg_page = cast(int, segment.get("page_assoc"))
if (
cast(JBIG2SegmentFlags, segment["flags"])["type"]
== SEG_TYPE_END_OF_PAGE
):
current_page = None
elif seg_page:
current_page = seg_page
if fix_last_page and current_page and (seg_num is not None):
segment = self.get_eop_segment(seg_num + 1, current_page)
data = self.encode_segment(segment)
self.stream.write(data)
data_len += len(data)
return data_len
def write_file(
self, segments: Iterable[JBIG2Segment], fix_last_page: bool = True
) -> int:
header = FILE_HEADER_ID
header_flags = FILE_HEAD_FLAG_SEQUENTIAL
header += pack(">B", header_flags)
# The embedded JBIG2 files in a PDF always
# only have one page
number_of_pages = pack(">L", 1)
header += number_of_pages
self.stream.write(header)
data_len = len(header)
data_len += self.write_segments(segments, fix_last_page)
seg_num = 0
for segment in segments:
seg_num = cast(int, segment["number"])
if fix_last_page:
seg_num_offset = 2
else:
seg_num_offset = 1
eof_segment = self.get_eof_segment(seg_num + seg_num_offset)
data = self.encode_segment(eof_segment)
self.stream.write(data)
data_len += len(data)
return data_len
def encode_segment(self, segment: JBIG2Segment) -> bytes:
data = b""
for field_format, name in SEG_STRUCT:
value = segment.get(name)
encoder = getattr(self, "encode_%s" % name, None)
if callable(encoder):
field = encoder(value, segment)
else:
field = pack(field_format, value)
data += field
return data
def encode_flags(self, value: JBIG2SegmentFlags, segment: JBIG2Segment) -> bytes:
flags = 0
if value.get("deferred"):
flags |= HEADER_FLAG_DEFERRED
if "page_assoc_long" in value:
flags |= HEADER_FLAG_PAGE_ASSOC_LONG if value["page_assoc_long"] else flags
else:
flags |= (
HEADER_FLAG_PAGE_ASSOC_LONG
if cast(int, segment.get("page", 0)) > 255
else flags
)
flags |= mask_value(SEG_TYPE_MASK, value["type"])
return pack(">B", flags)
def encode_retention_flags(
self, value: JBIG2RetentionFlags, segment: JBIG2Segment
) -> bytes:
flags = []
flags_format = ">B"
ref_count = value["ref_count"]
assert isinstance(ref_count, int)
retain_segments = cast(List[bool], value.get("retain_segments", []))
if ref_count <= 4:
flags_byte = mask_value(REF_COUNT_SHORT_MASK, ref_count)
for ref_index, ref_retain in enumerate(retain_segments):
if ref_retain:
flags_byte |= 1 << ref_index
flags.append(flags_byte)
else:
bytes_count = math.ceil((ref_count + 1) / 8)
flags_format = ">L" + ("B" * bytes_count)
flags_dword = mask_value(REF_COUNT_SHORT_MASK, REF_COUNT_LONG) << 24
flags.append(flags_dword)
for byte_index in range(bytes_count):
ret_byte = 0
ret_part = retain_segments[byte_index * 8 : byte_index * 8 + 8]
for bit_pos, ret_seg in enumerate(ret_part):
ret_byte |= 1 << bit_pos if ret_seg else ret_byte
flags.append(ret_byte)
ref_segments = cast(List[int], value.get("ref_segments", []))
seg_num = cast(int, segment["number"])
if seg_num <= 256:
ref_format = "B"
elif seg_num <= 65536:
ref_format = "I"
else:
ref_format = "L"
for ref in ref_segments:
flags_format += ref_format
flags.append(ref)
return pack(flags_format, *flags)
def encode_data_length(self, value: int, segment: JBIG2Segment) -> bytes:
data = pack(">L", value)
data += cast(bytes, segment["raw_data"])
return data
def get_eop_segment(self, seg_number: int, page_number: int) -> JBIG2Segment:
return {
"data_length": 0,
"flags": {"deferred": False, "type": SEG_TYPE_END_OF_PAGE},
"number": seg_number,
"page_assoc": page_number,
"raw_data": b"",
"retention_flags": JBIG2StreamWriter.EMPTY_RETENTION_FLAGS,
}
def get_eof_segment(self, seg_number: int) -> JBIG2Segment:
return {
"data_length": 0,
"flags": {"deferred": False, "type": SEG_TYPE_END_OF_FILE},
"number": seg_number,
"page_assoc": 0,
"raw_data": b"",
"retention_flags": JBIG2StreamWriter.EMPTY_RETENTION_FLAGS,
} | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/jbig2.py | jbig2.py |
glyphname2unicode = {
"A": "\u0041",
"AE": "\u00C6",
"AEacute": "\u01FC",
"AEmacron": "\u01E2",
"AEsmall": "\uF7E6",
"Aacute": "\u00C1",
"Aacutesmall": "\uF7E1",
"Abreve": "\u0102",
"Abreveacute": "\u1EAE",
"Abrevecyrillic": "\u04D0",
"Abrevedotbelow": "\u1EB6",
"Abrevegrave": "\u1EB0",
"Abrevehookabove": "\u1EB2",
"Abrevetilde": "\u1EB4",
"Acaron": "\u01CD",
"Acircle": "\u24B6",
"Acircumflex": "\u00C2",
"Acircumflexacute": "\u1EA4",
"Acircumflexdotbelow": "\u1EAC",
"Acircumflexgrave": "\u1EA6",
"Acircumflexhookabove": "\u1EA8",
"Acircumflexsmall": "\uF7E2",
"Acircumflextilde": "\u1EAA",
"Acute": "\uF6C9",
"Acutesmall": "\uF7B4",
"Acyrillic": "\u0410",
"Adblgrave": "\u0200",
"Adieresis": "\u00C4",
"Adieresiscyrillic": "\u04D2",
"Adieresismacron": "\u01DE",
"Adieresissmall": "\uF7E4",
"Adotbelow": "\u1EA0",
"Adotmacron": "\u01E0",
"Agrave": "\u00C0",
"Agravesmall": "\uF7E0",
"Ahookabove": "\u1EA2",
"Aiecyrillic": "\u04D4",
"Ainvertedbreve": "\u0202",
"Alpha": "\u0391",
"Alphatonos": "\u0386",
"Amacron": "\u0100",
"Amonospace": "\uFF21",
"Aogonek": "\u0104",
"Aring": "\u00C5",
"Aringacute": "\u01FA",
"Aringbelow": "\u1E00",
"Aringsmall": "\uF7E5",
"Asmall": "\uF761",
"Atilde": "\u00C3",
"Atildesmall": "\uF7E3",
"Aybarmenian": "\u0531",
"B": "\u0042",
"Bcircle": "\u24B7",
"Bdotaccent": "\u1E02",
"Bdotbelow": "\u1E04",
"Becyrillic": "\u0411",
"Benarmenian": "\u0532",
"Beta": "\u0392",
"Bhook": "\u0181",
"Blinebelow": "\u1E06",
"Bmonospace": "\uFF22",
"Brevesmall": "\uF6F4",
"Bsmall": "\uF762",
"Btopbar": "\u0182",
"C": "\u0043",
"Caarmenian": "\u053E",
"Cacute": "\u0106",
"Caron": "\uF6CA",
"Caronsmall": "\uF6F5",
"Ccaron": "\u010C",
"Ccedilla": "\u00C7",
"Ccedillaacute": "\u1E08",
"Ccedillasmall": "\uF7E7",
"Ccircle": "\u24B8",
"Ccircumflex": "\u0108",
"Cdot": "\u010A",
"Cdotaccent": "\u010A",
"Cedillasmall": "\uF7B8",
"Chaarmenian": "\u0549",
"Cheabkhasiancyrillic": "\u04BC",
"Checyrillic": "\u0427",
"Chedescenderabkhasiancyrillic": "\u04BE",
"Chedescendercyrillic": "\u04B6",
"Chedieresiscyrillic": "\u04F4",
"Cheharmenian": "\u0543",
"Chekhakassiancyrillic": "\u04CB",
"Cheverticalstrokecyrillic": "\u04B8",
"Chi": "\u03A7",
"Chook": "\u0187",
"Circumflexsmall": "\uF6F6",
"Cmonospace": "\uFF23",
"Coarmenian": "\u0551",
"Csmall": "\uF763",
"D": "\u0044",
"DZ": "\u01F1",
"DZcaron": "\u01C4",
"Daarmenian": "\u0534",
"Dafrican": "\u0189",
"Dcaron": "\u010E",
"Dcedilla": "\u1E10",
"Dcircle": "\u24B9",
"Dcircumflexbelow": "\u1E12",
"Dcroat": "\u0110",
"Ddotaccent": "\u1E0A",
"Ddotbelow": "\u1E0C",
"Decyrillic": "\u0414",
"Deicoptic": "\u03EE",
"Delta": "\u2206",
"Deltagreek": "\u0394",
"Dhook": "\u018A",
"Dieresis": "\uF6CB",
"DieresisAcute": "\uF6CC",
"DieresisGrave": "\uF6CD",
"Dieresissmall": "\uF7A8",
"Digammagreek": "\u03DC",
"Djecyrillic": "\u0402",
"Dlinebelow": "\u1E0E",
"Dmonospace": "\uFF24",
"Dotaccentsmall": "\uF6F7",
"Dslash": "\u0110",
"Dsmall": "\uF764",
"Dtopbar": "\u018B",
"Dz": "\u01F2",
"Dzcaron": "\u01C5",
"Dzeabkhasiancyrillic": "\u04E0",
"Dzecyrillic": "\u0405",
"Dzhecyrillic": "\u040F",
"E": "\u0045",
"Eacute": "\u00C9",
"Eacutesmall": "\uF7E9",
"Ebreve": "\u0114",
"Ecaron": "\u011A",
"Ecedillabreve": "\u1E1C",
"Echarmenian": "\u0535",
"Ecircle": "\u24BA",
"Ecircumflex": "\u00CA",
"Ecircumflexacute": "\u1EBE",
"Ecircumflexbelow": "\u1E18",
"Ecircumflexdotbelow": "\u1EC6",
"Ecircumflexgrave": "\u1EC0",
"Ecircumflexhookabove": "\u1EC2",
"Ecircumflexsmall": "\uF7EA",
"Ecircumflextilde": "\u1EC4",
"Ecyrillic": "\u0404",
"Edblgrave": "\u0204",
"Edieresis": "\u00CB",
"Edieresissmall": "\uF7EB",
"Edot": "\u0116",
"Edotaccent": "\u0116",
"Edotbelow": "\u1EB8",
"Efcyrillic": "\u0424",
"Egrave": "\u00C8",
"Egravesmall": "\uF7E8",
"Eharmenian": "\u0537",
"Ehookabove": "\u1EBA",
"Eightroman": "\u2167",
"Einvertedbreve": "\u0206",
"Eiotifiedcyrillic": "\u0464",
"Elcyrillic": "\u041B",
"Elevenroman": "\u216A",
"Emacron": "\u0112",
"Emacronacute": "\u1E16",
"Emacrongrave": "\u1E14",
"Emcyrillic": "\u041C",
"Emonospace": "\uFF25",
"Encyrillic": "\u041D",
"Endescendercyrillic": "\u04A2",
"Eng": "\u014A",
"Enghecyrillic": "\u04A4",
"Enhookcyrillic": "\u04C7",
"Eogonek": "\u0118",
"Eopen": "\u0190",
"Epsilon": "\u0395",
"Epsilontonos": "\u0388",
"Ercyrillic": "\u0420",
"Ereversed": "\u018E",
"Ereversedcyrillic": "\u042D",
"Escyrillic": "\u0421",
"Esdescendercyrillic": "\u04AA",
"Esh": "\u01A9",
"Esmall": "\uF765",
"Eta": "\u0397",
"Etarmenian": "\u0538",
"Etatonos": "\u0389",
"Eth": "\u00D0",
"Ethsmall": "\uF7F0",
"Etilde": "\u1EBC",
"Etildebelow": "\u1E1A",
"Euro": "\u20AC",
"Ezh": "\u01B7",
"Ezhcaron": "\u01EE",
"Ezhreversed": "\u01B8",
"F": "\u0046",
"Fcircle": "\u24BB",
"Fdotaccent": "\u1E1E",
"Feharmenian": "\u0556",
"Feicoptic": "\u03E4",
"Fhook": "\u0191",
"Fitacyrillic": "\u0472",
"Fiveroman": "\u2164",
"Fmonospace": "\uFF26",
"Fourroman": "\u2163",
"Fsmall": "\uF766",
"G": "\u0047",
"GBsquare": "\u3387",
"Gacute": "\u01F4",
"Gamma": "\u0393",
"Gammaafrican": "\u0194",
"Gangiacoptic": "\u03EA",
"Gbreve": "\u011E",
"Gcaron": "\u01E6",
"Gcedilla": "\u0122",
"Gcircle": "\u24BC",
"Gcircumflex": "\u011C",
"Gcommaaccent": "\u0122",
"Gdot": "\u0120",
"Gdotaccent": "\u0120",
"Gecyrillic": "\u0413",
"Ghadarmenian": "\u0542",
"Ghemiddlehookcyrillic": "\u0494",
"Ghestrokecyrillic": "\u0492",
"Gheupturncyrillic": "\u0490",
"Ghook": "\u0193",
"Gimarmenian": "\u0533",
"Gjecyrillic": "\u0403",
"Gmacron": "\u1E20",
"Gmonospace": "\uFF27",
"Grave": "\uF6CE",
"Gravesmall": "\uF760",
"Gsmall": "\uF767",
"Gsmallhook": "\u029B",
"Gstroke": "\u01E4",
"H": "\u0048",
"H18533": "\u25CF",
"H18543": "\u25AA",
"H18551": "\u25AB",
"H22073": "\u25A1",
"HPsquare": "\u33CB",
"Haabkhasiancyrillic": "\u04A8",
"Hadescendercyrillic": "\u04B2",
"Hardsigncyrillic": "\u042A",
"Hbar": "\u0126",
"Hbrevebelow": "\u1E2A",
"Hcedilla": "\u1E28",
"Hcircle": "\u24BD",
"Hcircumflex": "\u0124",
"Hdieresis": "\u1E26",
"Hdotaccent": "\u1E22",
"Hdotbelow": "\u1E24",
"Hmonospace": "\uFF28",
"Hoarmenian": "\u0540",
"Horicoptic": "\u03E8",
"Hsmall": "\uF768",
"Hungarumlaut": "\uF6CF",
"Hungarumlautsmall": "\uF6F8",
"Hzsquare": "\u3390",
"I": "\u0049",
"IAcyrillic": "\u042F",
"IJ": "\u0132",
"IUcyrillic": "\u042E",
"Iacute": "\u00CD",
"Iacutesmall": "\uF7ED",
"Ibreve": "\u012C",
"Icaron": "\u01CF",
"Icircle": "\u24BE",
"Icircumflex": "\u00CE",
"Icircumflexsmall": "\uF7EE",
"Icyrillic": "\u0406",
"Idblgrave": "\u0208",
"Idieresis": "\u00CF",
"Idieresisacute": "\u1E2E",
"Idieresiscyrillic": "\u04E4",
"Idieresissmall": "\uF7EF",
"Idot": "\u0130",
"Idotaccent": "\u0130",
"Idotbelow": "\u1ECA",
"Iebrevecyrillic": "\u04D6",
"Iecyrillic": "\u0415",
"Ifraktur": "\u2111",
"Igrave": "\u00CC",
"Igravesmall": "\uF7EC",
"Ihookabove": "\u1EC8",
"Iicyrillic": "\u0418",
"Iinvertedbreve": "\u020A",
"Iishortcyrillic": "\u0419",
"Imacron": "\u012A",
"Imacroncyrillic": "\u04E2",
"Imonospace": "\uFF29",
"Iniarmenian": "\u053B",
"Iocyrillic": "\u0401",
"Iogonek": "\u012E",
"Iota": "\u0399",
"Iotaafrican": "\u0196",
"Iotadieresis": "\u03AA",
"Iotatonos": "\u038A",
"Ismall": "\uF769",
"Istroke": "\u0197",
"Itilde": "\u0128",
"Itildebelow": "\u1E2C",
"Izhitsacyrillic": "\u0474",
"Izhitsadblgravecyrillic": "\u0476",
"J": "\u004A",
"Jaarmenian": "\u0541",
"Jcircle": "\u24BF",
"Jcircumflex": "\u0134",
"Jecyrillic": "\u0408",
"Jheharmenian": "\u054B",
"Jmonospace": "\uFF2A",
"Jsmall": "\uF76A",
"K": "\u004B",
"KBsquare": "\u3385",
"KKsquare": "\u33CD",
"Kabashkircyrillic": "\u04A0",
"Kacute": "\u1E30",
"Kacyrillic": "\u041A",
"Kadescendercyrillic": "\u049A",
"Kahookcyrillic": "\u04C3",
"Kappa": "\u039A",
"Kastrokecyrillic": "\u049E",
"Kaverticalstrokecyrillic": "\u049C",
"Kcaron": "\u01E8",
"Kcedilla": "\u0136",
"Kcircle": "\u24C0",
"Kcommaaccent": "\u0136",
"Kdotbelow": "\u1E32",
"Keharmenian": "\u0554",
"Kenarmenian": "\u053F",
"Khacyrillic": "\u0425",
"Kheicoptic": "\u03E6",
"Khook": "\u0198",
"Kjecyrillic": "\u040C",
"Klinebelow": "\u1E34",
"Kmonospace": "\uFF2B",
"Koppacyrillic": "\u0480",
"Koppagreek": "\u03DE",
"Ksicyrillic": "\u046E",
"Ksmall": "\uF76B",
"L": "\u004C",
"LJ": "\u01C7",
"LL": "\uF6BF",
"Lacute": "\u0139",
"Lambda": "\u039B",
"Lcaron": "\u013D",
"Lcedilla": "\u013B",
"Lcircle": "\u24C1",
"Lcircumflexbelow": "\u1E3C",
"Lcommaaccent": "\u013B",
"Ldot": "\u013F",
"Ldotaccent": "\u013F",
"Ldotbelow": "\u1E36",
"Ldotbelowmacron": "\u1E38",
"Liwnarmenian": "\u053C",
"Lj": "\u01C8",
"Ljecyrillic": "\u0409",
"Llinebelow": "\u1E3A",
"Lmonospace": "\uFF2C",
"Lslash": "\u0141",
"Lslashsmall": "\uF6F9",
"Lsmall": "\uF76C",
"M": "\u004D",
"MBsquare": "\u3386",
"Macron": "\uF6D0",
"Macronsmall": "\uF7AF",
"Macute": "\u1E3E",
"Mcircle": "\u24C2",
"Mdotaccent": "\u1E40",
"Mdotbelow": "\u1E42",
"Menarmenian": "\u0544",
"Mmonospace": "\uFF2D",
"Msmall": "\uF76D",
"Mturned": "\u019C",
"Mu": "\u039C",
"N": "\u004E",
"NJ": "\u01CA",
"Nacute": "\u0143",
"Ncaron": "\u0147",
"Ncedilla": "\u0145",
"Ncircle": "\u24C3",
"Ncircumflexbelow": "\u1E4A",
"Ncommaaccent": "\u0145",
"Ndotaccent": "\u1E44",
"Ndotbelow": "\u1E46",
"Nhookleft": "\u019D",
"Nineroman": "\u2168",
"Nj": "\u01CB",
"Njecyrillic": "\u040A",
"Nlinebelow": "\u1E48",
"Nmonospace": "\uFF2E",
"Nowarmenian": "\u0546",
"Nsmall": "\uF76E",
"Ntilde": "\u00D1",
"Ntildesmall": "\uF7F1",
"Nu": "\u039D",
"O": "\u004F",
"OE": "\u0152",
"OEsmall": "\uF6FA",
"Oacute": "\u00D3",
"Oacutesmall": "\uF7F3",
"Obarredcyrillic": "\u04E8",
"Obarreddieresiscyrillic": "\u04EA",
"Obreve": "\u014E",
"Ocaron": "\u01D1",
"Ocenteredtilde": "\u019F",
"Ocircle": "\u24C4",
"Ocircumflex": "\u00D4",
"Ocircumflexacute": "\u1ED0",
"Ocircumflexdotbelow": "\u1ED8",
"Ocircumflexgrave": "\u1ED2",
"Ocircumflexhookabove": "\u1ED4",
"Ocircumflexsmall": "\uF7F4",
"Ocircumflextilde": "\u1ED6",
"Ocyrillic": "\u041E",
"Odblacute": "\u0150",
"Odblgrave": "\u020C",
"Odieresis": "\u00D6",
"Odieresiscyrillic": "\u04E6",
"Odieresissmall": "\uF7F6",
"Odotbelow": "\u1ECC",
"Ogoneksmall": "\uF6FB",
"Ograve": "\u00D2",
"Ogravesmall": "\uF7F2",
"Oharmenian": "\u0555",
"Ohm": "\u2126",
"Ohookabove": "\u1ECE",
"Ohorn": "\u01A0",
"Ohornacute": "\u1EDA",
"Ohorndotbelow": "\u1EE2",
"Ohorngrave": "\u1EDC",
"Ohornhookabove": "\u1EDE",
"Ohorntilde": "\u1EE0",
"Ohungarumlaut": "\u0150",
"Oi": "\u01A2",
"Oinvertedbreve": "\u020E",
"Omacron": "\u014C",
"Omacronacute": "\u1E52",
"Omacrongrave": "\u1E50",
"Omega": "\u2126",
"Omegacyrillic": "\u0460",
"Omegagreek": "\u03A9",
"Omegaroundcyrillic": "\u047A",
"Omegatitlocyrillic": "\u047C",
"Omegatonos": "\u038F",
"Omicron": "\u039F",
"Omicrontonos": "\u038C",
"Omonospace": "\uFF2F",
"Oneroman": "\u2160",
"Oogonek": "\u01EA",
"Oogonekmacron": "\u01EC",
"Oopen": "\u0186",
"Oslash": "\u00D8",
"Oslashacute": "\u01FE",
"Oslashsmall": "\uF7F8",
"Osmall": "\uF76F",
"Ostrokeacute": "\u01FE",
"Otcyrillic": "\u047E",
"Otilde": "\u00D5",
"Otildeacute": "\u1E4C",
"Otildedieresis": "\u1E4E",
"Otildesmall": "\uF7F5",
"P": "\u0050",
"Pacute": "\u1E54",
"Pcircle": "\u24C5",
"Pdotaccent": "\u1E56",
"Pecyrillic": "\u041F",
"Peharmenian": "\u054A",
"Pemiddlehookcyrillic": "\u04A6",
"Phi": "\u03A6",
"Phook": "\u01A4",
"Pi": "\u03A0",
"Piwrarmenian": "\u0553",
"Pmonospace": "\uFF30",
"Psi": "\u03A8",
"Psicyrillic": "\u0470",
"Psmall": "\uF770",
"Q": "\u0051",
"Qcircle": "\u24C6",
"Qmonospace": "\uFF31",
"Qsmall": "\uF771",
"R": "\u0052",
"Raarmenian": "\u054C",
"Racute": "\u0154",
"Rcaron": "\u0158",
"Rcedilla": "\u0156",
"Rcircle": "\u24C7",
"Rcommaaccent": "\u0156",
"Rdblgrave": "\u0210",
"Rdotaccent": "\u1E58",
"Rdotbelow": "\u1E5A",
"Rdotbelowmacron": "\u1E5C",
"Reharmenian": "\u0550",
"Rfraktur": "\u211C",
"Rho": "\u03A1",
"Ringsmall": "\uF6FC",
"Rinvertedbreve": "\u0212",
"Rlinebelow": "\u1E5E",
"Rmonospace": "\uFF32",
"Rsmall": "\uF772",
"Rsmallinverted": "\u0281",
"Rsmallinvertedsuperior": "\u02B6",
"S": "\u0053",
"SF010000": "\u250C",
"SF020000": "\u2514",
"SF030000": "\u2510",
"SF040000": "\u2518",
"SF050000": "\u253C",
"SF060000": "\u252C",
"SF070000": "\u2534",
"SF080000": "\u251C",
"SF090000": "\u2524",
"SF100000": "\u2500",
"SF110000": "\u2502",
"SF190000": "\u2561",
"SF200000": "\u2562",
"SF210000": "\u2556",
"SF220000": "\u2555",
"SF230000": "\u2563",
"SF240000": "\u2551",
"SF250000": "\u2557",
"SF260000": "\u255D",
"SF270000": "\u255C",
"SF280000": "\u255B",
"SF360000": "\u255E",
"SF370000": "\u255F",
"SF380000": "\u255A",
"SF390000": "\u2554",
"SF400000": "\u2569",
"SF410000": "\u2566",
"SF420000": "\u2560",
"SF430000": "\u2550",
"SF440000": "\u256C",
"SF450000": "\u2567",
"SF460000": "\u2568",
"SF470000": "\u2564",
"SF480000": "\u2565",
"SF490000": "\u2559",
"SF500000": "\u2558",
"SF510000": "\u2552",
"SF520000": "\u2553",
"SF530000": "\u256B",
"SF540000": "\u256A",
"Sacute": "\u015A",
"Sacutedotaccent": "\u1E64",
"Sampigreek": "\u03E0",
"Scaron": "\u0160",
"Scarondotaccent": "\u1E66",
"Scaronsmall": "\uF6FD",
"Scedilla": "\u015E",
"Schwa": "\u018F",
"Schwacyrillic": "\u04D8",
"Schwadieresiscyrillic": "\u04DA",
"Scircle": "\u24C8",
"Scircumflex": "\u015C",
"Scommaaccent": "\u0218",
"Sdotaccent": "\u1E60",
"Sdotbelow": "\u1E62",
"Sdotbelowdotaccent": "\u1E68",
"Seharmenian": "\u054D",
"Sevenroman": "\u2166",
"Shaarmenian": "\u0547",
"Shacyrillic": "\u0428",
"Shchacyrillic": "\u0429",
"Sheicoptic": "\u03E2",
"Shhacyrillic": "\u04BA",
"Shimacoptic": "\u03EC",
"Sigma": "\u03A3",
"Sixroman": "\u2165",
"Smonospace": "\uFF33",
"Softsigncyrillic": "\u042C",
"Ssmall": "\uF773",
"Stigmagreek": "\u03DA",
"T": "\u0054",
"Tau": "\u03A4",
"Tbar": "\u0166",
"Tcaron": "\u0164",
"Tcedilla": "\u0162",
"Tcircle": "\u24C9",
"Tcircumflexbelow": "\u1E70",
"Tcommaaccent": "\u0162",
"Tdotaccent": "\u1E6A",
"Tdotbelow": "\u1E6C",
"Tecyrillic": "\u0422",
"Tedescendercyrillic": "\u04AC",
"Tenroman": "\u2169",
"Tetsecyrillic": "\u04B4",
"Theta": "\u0398",
"Thook": "\u01AC",
"Thorn": "\u00DE",
"Thornsmall": "\uF7FE",
"Threeroman": "\u2162",
"Tildesmall": "\uF6FE",
"Tiwnarmenian": "\u054F",
"Tlinebelow": "\u1E6E",
"Tmonospace": "\uFF34",
"Toarmenian": "\u0539",
"Tonefive": "\u01BC",
"Tonesix": "\u0184",
"Tonetwo": "\u01A7",
"Tretroflexhook": "\u01AE",
"Tsecyrillic": "\u0426",
"Tshecyrillic": "\u040B",
"Tsmall": "\uF774",
"Twelveroman": "\u216B",
"Tworoman": "\u2161",
"U": "\u0055",
"Uacute": "\u00DA",
"Uacutesmall": "\uF7FA",
"Ubreve": "\u016C",
"Ucaron": "\u01D3",
"Ucircle": "\u24CA",
"Ucircumflex": "\u00DB",
"Ucircumflexbelow": "\u1E76",
"Ucircumflexsmall": "\uF7FB",
"Ucyrillic": "\u0423",
"Udblacute": "\u0170",
"Udblgrave": "\u0214",
"Udieresis": "\u00DC",
"Udieresisacute": "\u01D7",
"Udieresisbelow": "\u1E72",
"Udieresiscaron": "\u01D9",
"Udieresiscyrillic": "\u04F0",
"Udieresisgrave": "\u01DB",
"Udieresismacron": "\u01D5",
"Udieresissmall": "\uF7FC",
"Udotbelow": "\u1EE4",
"Ugrave": "\u00D9",
"Ugravesmall": "\uF7F9",
"Uhookabove": "\u1EE6",
"Uhorn": "\u01AF",
"Uhornacute": "\u1EE8",
"Uhorndotbelow": "\u1EF0",
"Uhorngrave": "\u1EEA",
"Uhornhookabove": "\u1EEC",
"Uhorntilde": "\u1EEE",
"Uhungarumlaut": "\u0170",
"Uhungarumlautcyrillic": "\u04F2",
"Uinvertedbreve": "\u0216",
"Ukcyrillic": "\u0478",
"Umacron": "\u016A",
"Umacroncyrillic": "\u04EE",
"Umacrondieresis": "\u1E7A",
"Umonospace": "\uFF35",
"Uogonek": "\u0172",
"Upsilon": "\u03A5",
"Upsilon1": "\u03D2",
"Upsilonacutehooksymbolgreek": "\u03D3",
"Upsilonafrican": "\u01B1",
"Upsilondieresis": "\u03AB",
"Upsilondieresishooksymbolgreek": "\u03D4",
"Upsilonhooksymbol": "\u03D2",
"Upsilontonos": "\u038E",
"Uring": "\u016E",
"Ushortcyrillic": "\u040E",
"Usmall": "\uF775",
"Ustraightcyrillic": "\u04AE",
"Ustraightstrokecyrillic": "\u04B0",
"Utilde": "\u0168",
"Utildeacute": "\u1E78",
"Utildebelow": "\u1E74",
"V": "\u0056",
"Vcircle": "\u24CB",
"Vdotbelow": "\u1E7E",
"Vecyrillic": "\u0412",
"Vewarmenian": "\u054E",
"Vhook": "\u01B2",
"Vmonospace": "\uFF36",
"Voarmenian": "\u0548",
"Vsmall": "\uF776",
"Vtilde": "\u1E7C",
"W": "\u0057",
"Wacute": "\u1E82",
"Wcircle": "\u24CC",
"Wcircumflex": "\u0174",
"Wdieresis": "\u1E84",
"Wdotaccent": "\u1E86",
"Wdotbelow": "\u1E88",
"Wgrave": "\u1E80",
"Wmonospace": "\uFF37",
"Wsmall": "\uF777",
"X": "\u0058",
"Xcircle": "\u24CD",
"Xdieresis": "\u1E8C",
"Xdotaccent": "\u1E8A",
"Xeharmenian": "\u053D",
"Xi": "\u039E",
"Xmonospace": "\uFF38",
"Xsmall": "\uF778",
"Y": "\u0059",
"Yacute": "\u00DD",
"Yacutesmall": "\uF7FD",
"Yatcyrillic": "\u0462",
"Ycircle": "\u24CE",
"Ycircumflex": "\u0176",
"Ydieresis": "\u0178",
"Ydieresissmall": "\uF7FF",
"Ydotaccent": "\u1E8E",
"Ydotbelow": "\u1EF4",
"Yericyrillic": "\u042B",
"Yerudieresiscyrillic": "\u04F8",
"Ygrave": "\u1EF2",
"Yhook": "\u01B3",
"Yhookabove": "\u1EF6",
"Yiarmenian": "\u0545",
"Yicyrillic": "\u0407",
"Yiwnarmenian": "\u0552",
"Ymonospace": "\uFF39",
"Ysmall": "\uF779",
"Ytilde": "\u1EF8",
"Yusbigcyrillic": "\u046A",
"Yusbigiotifiedcyrillic": "\u046C",
"Yuslittlecyrillic": "\u0466",
"Yuslittleiotifiedcyrillic": "\u0468",
"Z": "\u005A",
"Zaarmenian": "\u0536",
"Zacute": "\u0179",
"Zcaron": "\u017D",
"Zcaronsmall": "\uF6FF",
"Zcircle": "\u24CF",
"Zcircumflex": "\u1E90",
"Zdot": "\u017B",
"Zdotaccent": "\u017B",
"Zdotbelow": "\u1E92",
"Zecyrillic": "\u0417",
"Zedescendercyrillic": "\u0498",
"Zedieresiscyrillic": "\u04DE",
"Zeta": "\u0396",
"Zhearmenian": "\u053A",
"Zhebrevecyrillic": "\u04C1",
"Zhecyrillic": "\u0416",
"Zhedescendercyrillic": "\u0496",
"Zhedieresiscyrillic": "\u04DC",
"Zlinebelow": "\u1E94",
"Zmonospace": "\uFF3A",
"Zsmall": "\uF77A",
"Zstroke": "\u01B5",
"a": "\u0061",
"aabengali": "\u0986",
"aacute": "\u00E1",
"aadeva": "\u0906",
"aagujarati": "\u0A86",
"aagurmukhi": "\u0A06",
"aamatragurmukhi": "\u0A3E",
"aarusquare": "\u3303",
"aavowelsignbengali": "\u09BE",
"aavowelsigndeva": "\u093E",
"aavowelsigngujarati": "\u0ABE",
"abbreviationmarkarmenian": "\u055F",
"abbreviationsigndeva": "\u0970",
"abengali": "\u0985",
"abopomofo": "\u311A",
"abreve": "\u0103",
"abreveacute": "\u1EAF",
"abrevecyrillic": "\u04D1",
"abrevedotbelow": "\u1EB7",
"abrevegrave": "\u1EB1",
"abrevehookabove": "\u1EB3",
"abrevetilde": "\u1EB5",
"acaron": "\u01CE",
"acircle": "\u24D0",
"acircumflex": "\u00E2",
"acircumflexacute": "\u1EA5",
"acircumflexdotbelow": "\u1EAD",
"acircumflexgrave": "\u1EA7",
"acircumflexhookabove": "\u1EA9",
"acircumflextilde": "\u1EAB",
"acute": "\u00B4",
"acutebelowcmb": "\u0317",
"acutecmb": "\u0301",
"acutecomb": "\u0301",
"acutedeva": "\u0954",
"acutelowmod": "\u02CF",
"acutetonecmb": "\u0341",
"acyrillic": "\u0430",
"adblgrave": "\u0201",
"addakgurmukhi": "\u0A71",
"adeva": "\u0905",
"adieresis": "\u00E4",
"adieresiscyrillic": "\u04D3",
"adieresismacron": "\u01DF",
"adotbelow": "\u1EA1",
"adotmacron": "\u01E1",
"ae": "\u00E6",
"aeacute": "\u01FD",
"aekorean": "\u3150",
"aemacron": "\u01E3",
"afii00208": "\u2015",
"afii08941": "\u20A4",
"afii10017": "\u0410",
"afii10018": "\u0411",
"afii10019": "\u0412",
"afii10020": "\u0413",
"afii10021": "\u0414",
"afii10022": "\u0415",
"afii10023": "\u0401",
"afii10024": "\u0416",
"afii10025": "\u0417",
"afii10026": "\u0418",
"afii10027": "\u0419",
"afii10028": "\u041A",
"afii10029": "\u041B",
"afii10030": "\u041C",
"afii10031": "\u041D",
"afii10032": "\u041E",
"afii10033": "\u041F",
"afii10034": "\u0420",
"afii10035": "\u0421",
"afii10036": "\u0422",
"afii10037": "\u0423",
"afii10038": "\u0424",
"afii10039": "\u0425",
"afii10040": "\u0426",
"afii10041": "\u0427",
"afii10042": "\u0428",
"afii10043": "\u0429",
"afii10044": "\u042A",
"afii10045": "\u042B",
"afii10046": "\u042C",
"afii10047": "\u042D",
"afii10048": "\u042E",
"afii10049": "\u042F",
"afii10050": "\u0490",
"afii10051": "\u0402",
"afii10052": "\u0403",
"afii10053": "\u0404",
"afii10054": "\u0405",
"afii10055": "\u0406",
"afii10056": "\u0407",
"afii10057": "\u0408",
"afii10058": "\u0409",
"afii10059": "\u040A",
"afii10060": "\u040B",
"afii10061": "\u040C",
"afii10062": "\u040E",
"afii10063": "\uF6C4",
"afii10064": "\uF6C5",
"afii10065": "\u0430",
"afii10066": "\u0431",
"afii10067": "\u0432",
"afii10068": "\u0433",
"afii10069": "\u0434",
"afii10070": "\u0435",
"afii10071": "\u0451",
"afii10072": "\u0436",
"afii10073": "\u0437",
"afii10074": "\u0438",
"afii10075": "\u0439",
"afii10076": "\u043A",
"afii10077": "\u043B",
"afii10078": "\u043C",
"afii10079": "\u043D",
"afii10080": "\u043E",
"afii10081": "\u043F",
"afii10082": "\u0440",
"afii10083": "\u0441",
"afii10084": "\u0442",
"afii10085": "\u0443",
"afii10086": "\u0444",
"afii10087": "\u0445",
"afii10088": "\u0446",
"afii10089": "\u0447",
"afii10090": "\u0448",
"afii10091": "\u0449",
"afii10092": "\u044A",
"afii10093": "\u044B",
"afii10094": "\u044C",
"afii10095": "\u044D",
"afii10096": "\u044E",
"afii10097": "\u044F",
"afii10098": "\u0491",
"afii10099": "\u0452",
"afii10100": "\u0453",
"afii10101": "\u0454",
"afii10102": "\u0455",
"afii10103": "\u0456",
"afii10104": "\u0457",
"afii10105": "\u0458",
"afii10106": "\u0459",
"afii10107": "\u045A",
"afii10108": "\u045B",
"afii10109": "\u045C",
"afii10110": "\u045E",
"afii10145": "\u040F",
"afii10146": "\u0462",
"afii10147": "\u0472",
"afii10148": "\u0474",
"afii10192": "\uF6C6",
"afii10193": "\u045F",
"afii10194": "\u0463",
"afii10195": "\u0473",
"afii10196": "\u0475",
"afii10831": "\uF6C7",
"afii10832": "\uF6C8",
"afii10846": "\u04D9",
"afii299": "\u200E",
"afii300": "\u200F",
"afii301": "\u200D",
"afii57381": "\u066A",
"afii57388": "\u060C",
"afii57392": "\u0660",
"afii57393": "\u0661",
"afii57394": "\u0662",
"afii57395": "\u0663",
"afii57396": "\u0664",
"afii57397": "\u0665",
"afii57398": "\u0666",
"afii57399": "\u0667",
"afii57400": "\u0668",
"afii57401": "\u0669",
"afii57403": "\u061B",
"afii57407": "\u061F",
"afii57409": "\u0621",
"afii57410": "\u0622",
"afii57411": "\u0623",
"afii57412": "\u0624",
"afii57413": "\u0625",
"afii57414": "\u0626",
"afii57415": "\u0627",
"afii57416": "\u0628",
"afii57417": "\u0629",
"afii57418": "\u062A",
"afii57419": "\u062B",
"afii57420": "\u062C",
"afii57421": "\u062D",
"afii57422": "\u062E",
"afii57423": "\u062F",
"afii57424": "\u0630",
"afii57425": "\u0631",
"afii57426": "\u0632",
"afii57427": "\u0633",
"afii57428": "\u0634",
"afii57429": "\u0635",
"afii57430": "\u0636",
"afii57431": "\u0637",
"afii57432": "\u0638",
"afii57433": "\u0639",
"afii57434": "\u063A",
"afii57440": "\u0640",
"afii57441": "\u0641",
"afii57442": "\u0642",
"afii57443": "\u0643",
"afii57444": "\u0644",
"afii57445": "\u0645",
"afii57446": "\u0646",
"afii57448": "\u0648",
"afii57449": "\u0649",
"afii57450": "\u064A",
"afii57451": "\u064B",
"afii57452": "\u064C",
"afii57453": "\u064D",
"afii57454": "\u064E",
"afii57455": "\u064F",
"afii57456": "\u0650",
"afii57457": "\u0651",
"afii57458": "\u0652",
"afii57470": "\u0647",
"afii57505": "\u06A4",
"afii57506": "\u067E",
"afii57507": "\u0686",
"afii57508": "\u0698",
"afii57509": "\u06AF",
"afii57511": "\u0679",
"afii57512": "\u0688",
"afii57513": "\u0691",
"afii57514": "\u06BA",
"afii57519": "\u06D2",
"afii57534": "\u06D5",
"afii57636": "\u20AA",
"afii57645": "\u05BE",
"afii57658": "\u05C3",
"afii57664": "\u05D0",
"afii57665": "\u05D1",
"afii57666": "\u05D2",
"afii57667": "\u05D3",
"afii57668": "\u05D4",
"afii57669": "\u05D5",
"afii57670": "\u05D6",
"afii57671": "\u05D7",
"afii57672": "\u05D8",
"afii57673": "\u05D9",
"afii57674": "\u05DA",
"afii57675": "\u05DB",
"afii57676": "\u05DC",
"afii57677": "\u05DD",
"afii57678": "\u05DE",
"afii57679": "\u05DF",
"afii57680": "\u05E0",
"afii57681": "\u05E1",
"afii57682": "\u05E2",
"afii57683": "\u05E3",
"afii57684": "\u05E4",
"afii57685": "\u05E5",
"afii57686": "\u05E6",
"afii57687": "\u05E7",
"afii57688": "\u05E8",
"afii57689": "\u05E9",
"afii57690": "\u05EA",
"afii57694": "\uFB2A",
"afii57695": "\uFB2B",
"afii57700": "\uFB4B",
"afii57705": "\uFB1F",
"afii57716": "\u05F0",
"afii57717": "\u05F1",
"afii57718": "\u05F2",
"afii57723": "\uFB35",
"afii57793": "\u05B4",
"afii57794": "\u05B5",
"afii57795": "\u05B6",
"afii57796": "\u05BB",
"afii57797": "\u05B8",
"afii57798": "\u05B7",
"afii57799": "\u05B0",
"afii57800": "\u05B2",
"afii57801": "\u05B1",
"afii57802": "\u05B3",
"afii57803": "\u05C2",
"afii57804": "\u05C1",
"afii57806": "\u05B9",
"afii57807": "\u05BC",
"afii57839": "\u05BD",
"afii57841": "\u05BF",
"afii57842": "\u05C0",
"afii57929": "\u02BC",
"afii61248": "\u2105",
"afii61289": "\u2113",
"afii61352": "\u2116",
"afii61573": "\u202C",
"afii61574": "\u202D",
"afii61575": "\u202E",
"afii61664": "\u200C",
"afii63167": "\u066D",
"afii64937": "\u02BD",
"agrave": "\u00E0",
"agujarati": "\u0A85",
"agurmukhi": "\u0A05",
"ahiragana": "\u3042",
"ahookabove": "\u1EA3",
"aibengali": "\u0990",
"aibopomofo": "\u311E",
"aideva": "\u0910",
"aiecyrillic": "\u04D5",
"aigujarati": "\u0A90",
"aigurmukhi": "\u0A10",
"aimatragurmukhi": "\u0A48",
"ainarabic": "\u0639",
"ainfinalarabic": "\uFECA",
"aininitialarabic": "\uFECB",
"ainmedialarabic": "\uFECC",
"ainvertedbreve": "\u0203",
"aivowelsignbengali": "\u09C8",
"aivowelsigndeva": "\u0948",
"aivowelsigngujarati": "\u0AC8",
"akatakana": "\u30A2",
"akatakanahalfwidth": "\uFF71",
"akorean": "\u314F",
"alef": "\u05D0",
"alefarabic": "\u0627",
"alefdageshhebrew": "\uFB30",
"aleffinalarabic": "\uFE8E",
"alefhamzaabovearabic": "\u0623",
"alefhamzaabovefinalarabic": "\uFE84",
"alefhamzabelowarabic": "\u0625",
"alefhamzabelowfinalarabic": "\uFE88",
"alefhebrew": "\u05D0",
"aleflamedhebrew": "\uFB4F",
"alefmaddaabovearabic": "\u0622",
"alefmaddaabovefinalarabic": "\uFE82",
"alefmaksuraarabic": "\u0649",
"alefmaksurafinalarabic": "\uFEF0",
"alefmaksurainitialarabic": "\uFEF3",
"alefmaksuramedialarabic": "\uFEF4",
"alefpatahhebrew": "\uFB2E",
"alefqamatshebrew": "\uFB2F",
"aleph": "\u2135",
"allequal": "\u224C",
"alpha": "\u03B1",
"alphatonos": "\u03AC",
"amacron": "\u0101",
"amonospace": "\uFF41",
"ampersand": "\u0026",
"ampersandmonospace": "\uFF06",
"ampersandsmall": "\uF726",
"amsquare": "\u33C2",
"anbopomofo": "\u3122",
"angbopomofo": "\u3124",
"angkhankhuthai": "\u0E5A",
"angle": "\u2220",
"anglebracketleft": "\u3008",
"anglebracketleftvertical": "\uFE3F",
"anglebracketright": "\u3009",
"anglebracketrightvertical": "\uFE40",
"angleleft": "\u2329",
"angleright": "\u232A",
"angstrom": "\u212B",
"anoteleia": "\u0387",
"anudattadeva": "\u0952",
"anusvarabengali": "\u0982",
"anusvaradeva": "\u0902",
"anusvaragujarati": "\u0A82",
"aogonek": "\u0105",
"apaatosquare": "\u3300",
"aparen": "\u249C",
"apostrophearmenian": "\u055A",
"apostrophemod": "\u02BC",
"apple": "\uF8FF",
"approaches": "\u2250",
"approxequal": "\u2248",
"approxequalorimage": "\u2252",
"approximatelyequal": "\u2245",
"araeaekorean": "\u318E",
"araeakorean": "\u318D",
"arc": "\u2312",
"arighthalfring": "\u1E9A",
"aring": "\u00E5",
"aringacute": "\u01FB",
"aringbelow": "\u1E01",
"arrowboth": "\u2194",
"arrowdashdown": "\u21E3",
"arrowdashleft": "\u21E0",
"arrowdashright": "\u21E2",
"arrowdashup": "\u21E1",
"arrowdblboth": "\u21D4",
"arrowdbldown": "\u21D3",
"arrowdblleft": "\u21D0",
"arrowdblright": "\u21D2",
"arrowdblup": "\u21D1",
"arrowdown": "\u2193",
"arrowdownleft": "\u2199",
"arrowdownright": "\u2198",
"arrowdownwhite": "\u21E9",
"arrowheaddownmod": "\u02C5",
"arrowheadleftmod": "\u02C2",
"arrowheadrightmod": "\u02C3",
"arrowheadupmod": "\u02C4",
"arrowhorizex": "\uF8E7",
"arrowleft": "\u2190",
"arrowleftdbl": "\u21D0",
"arrowleftdblstroke": "\u21CD",
"arrowleftoverright": "\u21C6",
"arrowleftwhite": "\u21E6",
"arrowright": "\u2192",
"arrowrightdblstroke": "\u21CF",
"arrowrightheavy": "\u279E",
"arrowrightoverleft": "\u21C4",
"arrowrightwhite": "\u21E8",
"arrowtableft": "\u21E4",
"arrowtabright": "\u21E5",
"arrowup": "\u2191",
"arrowupdn": "\u2195",
"arrowupdnbse": "\u21A8",
"arrowupdownbase": "\u21A8",
"arrowupleft": "\u2196",
"arrowupleftofdown": "\u21C5",
"arrowupright": "\u2197",
"arrowupwhite": "\u21E7",
"arrowvertex": "\uF8E6",
"asciicircum": "\u005E",
"asciicircummonospace": "\uFF3E",
"asciitilde": "\u007E",
"asciitildemonospace": "\uFF5E",
"ascript": "\u0251",
"ascriptturned": "\u0252",
"asmallhiragana": "\u3041",
"asmallkatakana": "\u30A1",
"asmallkatakanahalfwidth": "\uFF67",
"asterisk": "\u002A",
"asteriskaltonearabic": "\u066D",
"asteriskarabic": "\u066D",
"asteriskmath": "\u2217",
"asteriskmonospace": "\uFF0A",
"asterisksmall": "\uFE61",
"asterism": "\u2042",
"asuperior": "\uF6E9",
"asymptoticallyequal": "\u2243",
"at": "\u0040",
"atilde": "\u00E3",
"atmonospace": "\uFF20",
"atsmall": "\uFE6B",
"aturned": "\u0250",
"aubengali": "\u0994",
"aubopomofo": "\u3120",
"audeva": "\u0914",
"augujarati": "\u0A94",
"augurmukhi": "\u0A14",
"aulengthmarkbengali": "\u09D7",
"aumatragurmukhi": "\u0A4C",
"auvowelsignbengali": "\u09CC",
"auvowelsigndeva": "\u094C",
"auvowelsigngujarati": "\u0ACC",
"avagrahadeva": "\u093D",
"aybarmenian": "\u0561",
"ayin": "\u05E2",
"ayinaltonehebrew": "\uFB20",
"ayinhebrew": "\u05E2",
"b": "\u0062",
"babengali": "\u09AC",
"backslash": "\u005C",
"backslashmonospace": "\uFF3C",
"badeva": "\u092C",
"bagujarati": "\u0AAC",
"bagurmukhi": "\u0A2C",
"bahiragana": "\u3070",
"bahtthai": "\u0E3F",
"bakatakana": "\u30D0",
"bar": "\u007C",
"barmonospace": "\uFF5C",
"bbopomofo": "\u3105",
"bcircle": "\u24D1",
"bdotaccent": "\u1E03",
"bdotbelow": "\u1E05",
"beamedsixteenthnotes": "\u266C",
"because": "\u2235",
"becyrillic": "\u0431",
"beharabic": "\u0628",
"behfinalarabic": "\uFE90",
"behinitialarabic": "\uFE91",
"behiragana": "\u3079",
"behmedialarabic": "\uFE92",
"behmeeminitialarabic": "\uFC9F",
"behmeemisolatedarabic": "\uFC08",
"behnoonfinalarabic": "\uFC6D",
"bekatakana": "\u30D9",
"benarmenian": "\u0562",
"bet": "\u05D1",
"beta": "\u03B2",
"betasymbolgreek": "\u03D0",
"betdagesh": "\uFB31",
"betdageshhebrew": "\uFB31",
"bethebrew": "\u05D1",
"betrafehebrew": "\uFB4C",
"bhabengali": "\u09AD",
"bhadeva": "\u092D",
"bhagujarati": "\u0AAD",
"bhagurmukhi": "\u0A2D",
"bhook": "\u0253",
"bihiragana": "\u3073",
"bikatakana": "\u30D3",
"bilabialclick": "\u0298",
"bindigurmukhi": "\u0A02",
"birusquare": "\u3331",
"blackcircle": "\u25CF",
"blackdiamond": "\u25C6",
"blackdownpointingtriangle": "\u25BC",
"blackleftpointingpointer": "\u25C4",
"blackleftpointingtriangle": "\u25C0",
"blacklenticularbracketleft": "\u3010",
"blacklenticularbracketleftvertical": "\uFE3B",
"blacklenticularbracketright": "\u3011",
"blacklenticularbracketrightvertical": "\uFE3C",
"blacklowerlefttriangle": "\u25E3",
"blacklowerrighttriangle": "\u25E2",
"blackrectangle": "\u25AC",
"blackrightpointingpointer": "\u25BA",
"blackrightpointingtriangle": "\u25B6",
"blacksmallsquare": "\u25AA",
"blacksmilingface": "\u263B",
"blacksquare": "\u25A0",
"blackstar": "\u2605",
"blackupperlefttriangle": "\u25E4",
"blackupperrighttriangle": "\u25E5",
"blackuppointingsmalltriangle": "\u25B4",
"blackuppointingtriangle": "\u25B2",
"blank": "\u2423",
"blinebelow": "\u1E07",
"block": "\u2588",
"bmonospace": "\uFF42",
"bobaimaithai": "\u0E1A",
"bohiragana": "\u307C",
"bokatakana": "\u30DC",
"bparen": "\u249D",
"bqsquare": "\u33C3",
"braceex": "\uF8F4",
"braceleft": "\u007B",
"braceleftbt": "\uF8F3",
"braceleftmid": "\uF8F2",
"braceleftmonospace": "\uFF5B",
"braceleftsmall": "\uFE5B",
"bracelefttp": "\uF8F1",
"braceleftvertical": "\uFE37",
"braceright": "\u007D",
"bracerightbt": "\uF8FE",
"bracerightmid": "\uF8FD",
"bracerightmonospace": "\uFF5D",
"bracerightsmall": "\uFE5C",
"bracerighttp": "\uF8FC",
"bracerightvertical": "\uFE38",
"bracketleft": "\u005B",
"bracketleftbt": "\uF8F0",
"bracketleftex": "\uF8EF",
"bracketleftmonospace": "\uFF3B",
"bracketlefttp": "\uF8EE",
"bracketright": "\u005D",
"bracketrightbt": "\uF8FB",
"bracketrightex": "\uF8FA",
"bracketrightmonospace": "\uFF3D",
"bracketrighttp": "\uF8F9",
"breve": "\u02D8",
"brevebelowcmb": "\u032E",
"brevecmb": "\u0306",
"breveinvertedbelowcmb": "\u032F",
"breveinvertedcmb": "\u0311",
"breveinverteddoublecmb": "\u0361",
"bridgebelowcmb": "\u032A",
"bridgeinvertedbelowcmb": "\u033A",
"brokenbar": "\u00A6",
"bstroke": "\u0180",
"bsuperior": "\uF6EA",
"btopbar": "\u0183",
"buhiragana": "\u3076",
"bukatakana": "\u30D6",
"bullet": "\u2022",
"bulletinverse": "\u25D8",
"bulletoperator": "\u2219",
"bullseye": "\u25CE",
"c": "\u0063",
"caarmenian": "\u056E",
"cabengali": "\u099A",
"cacute": "\u0107",
"cadeva": "\u091A",
"cagujarati": "\u0A9A",
"cagurmukhi": "\u0A1A",
"calsquare": "\u3388",
"candrabindubengali": "\u0981",
"candrabinducmb": "\u0310",
"candrabindudeva": "\u0901",
"candrabindugujarati": "\u0A81",
"capslock": "\u21EA",
"careof": "\u2105",
"caron": "\u02C7",
"caronbelowcmb": "\u032C",
"caroncmb": "\u030C",
"carriagereturn": "\u21B5",
"cbopomofo": "\u3118",
"ccaron": "\u010D",
"ccedilla": "\u00E7",
"ccedillaacute": "\u1E09",
"ccircle": "\u24D2",
"ccircumflex": "\u0109",
"ccurl": "\u0255",
"cdot": "\u010B",
"cdotaccent": "\u010B",
"cdsquare": "\u33C5",
"cedilla": "\u00B8",
"cedillacmb": "\u0327",
"cent": "\u00A2",
"centigrade": "\u2103",
"centinferior": "\uF6DF",
"centmonospace": "\uFFE0",
"centoldstyle": "\uF7A2",
"centsuperior": "\uF6E0",
"chaarmenian": "\u0579",
"chabengali": "\u099B",
"chadeva": "\u091B",
"chagujarati": "\u0A9B",
"chagurmukhi": "\u0A1B",
"chbopomofo": "\u3114",
"cheabkhasiancyrillic": "\u04BD",
"checkmark": "\u2713",
"checyrillic": "\u0447",
"chedescenderabkhasiancyrillic": "\u04BF",
"chedescendercyrillic": "\u04B7",
"chedieresiscyrillic": "\u04F5",
"cheharmenian": "\u0573",
"chekhakassiancyrillic": "\u04CC",
"cheverticalstrokecyrillic": "\u04B9",
"chi": "\u03C7",
"chieuchacirclekorean": "\u3277",
"chieuchaparenkorean": "\u3217",
"chieuchcirclekorean": "\u3269",
"chieuchkorean": "\u314A",
"chieuchparenkorean": "\u3209",
"chochangthai": "\u0E0A",
"chochanthai": "\u0E08",
"chochingthai": "\u0E09",
"chochoethai": "\u0E0C",
"chook": "\u0188",
"cieucacirclekorean": "\u3276",
"cieucaparenkorean": "\u3216",
"cieuccirclekorean": "\u3268",
"cieuckorean": "\u3148",
"cieucparenkorean": "\u3208",
"cieucuparenkorean": "\u321C",
"circle": "\u25CB",
"circlemultiply": "\u2297",
"circleot": "\u2299",
"circleplus": "\u2295",
"circlepostalmark": "\u3036",
"circlewithlefthalfblack": "\u25D0",
"circlewithrighthalfblack": "\u25D1",
"circumflex": "\u02C6",
"circumflexbelowcmb": "\u032D",
"circumflexcmb": "\u0302",
"clear": "\u2327",
"clickalveolar": "\u01C2",
"clickdental": "\u01C0",
"clicklateral": "\u01C1",
"clickretroflex": "\u01C3",
"club": "\u2663",
"clubsuitblack": "\u2663",
"clubsuitwhite": "\u2667",
"cmcubedsquare": "\u33A4",
"cmonospace": "\uFF43",
"cmsquaredsquare": "\u33A0",
"coarmenian": "\u0581",
"colon": "\u003A",
"colonmonetary": "\u20A1",
"colonmonospace": "\uFF1A",
"colonsign": "\u20A1",
"colonsmall": "\uFE55",
"colontriangularhalfmod": "\u02D1",
"colontriangularmod": "\u02D0",
"comma": "\u002C",
"commaabovecmb": "\u0313",
"commaaboverightcmb": "\u0315",
"commaaccent": "\uF6C3",
"commaarabic": "\u060C",
"commaarmenian": "\u055D",
"commainferior": "\uF6E1",
"commamonospace": "\uFF0C",
"commareversedabovecmb": "\u0314",
"commareversedmod": "\u02BD",
"commasmall": "\uFE50",
"commasuperior": "\uF6E2",
"commaturnedabovecmb": "\u0312",
"commaturnedmod": "\u02BB",
"compass": "\u263C",
"congruent": "\u2245",
"contourintegral": "\u222E",
"control": "\u2303",
"controlACK": "\u0006",
"controlBEL": "\u0007",
"controlBS": "\u0008",
"controlCAN": "\u0018",
"controlCR": "\u000D",
"controlDC1": "\u0011",
"controlDC2": "\u0012",
"controlDC3": "\u0013",
"controlDC4": "\u0014",
"controlDEL": "\u007F",
"controlDLE": "\u0010",
"controlEM": "\u0019",
"controlENQ": "\u0005",
"controlEOT": "\u0004",
"controlESC": "\u001B",
"controlETB": "\u0017",
"controlETX": "\u0003",
"controlFF": "\u000C",
"controlFS": "\u001C",
"controlGS": "\u001D",
"controlHT": "\u0009",
"controlLF": "\u000A",
"controlNAK": "\u0015",
"controlRS": "\u001E",
"controlSI": "\u000F",
"controlSO": "\u000E",
"controlSOT": "\u0002",
"controlSTX": "\u0001",
"controlSUB": "\u001A",
"controlSYN": "\u0016",
"controlUS": "\u001F",
"controlVT": "\u000B",
"copyright": "\u00A9",
"copyrightsans": "\uF8E9",
"copyrightserif": "\uF6D9",
"cornerbracketleft": "\u300C",
"cornerbracketlefthalfwidth": "\uFF62",
"cornerbracketleftvertical": "\uFE41",
"cornerbracketright": "\u300D",
"cornerbracketrighthalfwidth": "\uFF63",
"cornerbracketrightvertical": "\uFE42",
"corporationsquare": "\u337F",
"cosquare": "\u33C7",
"coverkgsquare": "\u33C6",
"cparen": "\u249E",
"cruzeiro": "\u20A2",
"cstretched": "\u0297",
"curlyand": "\u22CF",
"curlyor": "\u22CE",
"currency": "\u00A4",
"cyrBreve": "\uF6D1",
"cyrFlex": "\uF6D2",
"cyrbreve": "\uF6D4",
"cyrflex": "\uF6D5",
"d": "\u0064",
"daarmenian": "\u0564",
"dabengali": "\u09A6",
"dadarabic": "\u0636",
"dadeva": "\u0926",
"dadfinalarabic": "\uFEBE",
"dadinitialarabic": "\uFEBF",
"dadmedialarabic": "\uFEC0",
"dagesh": "\u05BC",
"dageshhebrew": "\u05BC",
"dagger": "\u2020",
"daggerdbl": "\u2021",
"dagujarati": "\u0AA6",
"dagurmukhi": "\u0A26",
"dahiragana": "\u3060",
"dakatakana": "\u30C0",
"dalarabic": "\u062F",
"dalet": "\u05D3",
"daletdagesh": "\uFB33",
"daletdageshhebrew": "\uFB33",
"dalethatafpatah": "\u05D3\u05B2",
"dalethatafpatahhebrew": "\u05D3\u05B2",
"dalethatafsegol": "\u05D3\u05B1",
"dalethatafsegolhebrew": "\u05D3\u05B1",
"dalethebrew": "\u05D3",
"dalethiriq": "\u05D3\u05B4",
"dalethiriqhebrew": "\u05D3\u05B4",
"daletholam": "\u05D3\u05B9",
"daletholamhebrew": "\u05D3\u05B9",
"daletpatah": "\u05D3\u05B7",
"daletpatahhebrew": "\u05D3\u05B7",
"daletqamats": "\u05D3\u05B8",
"daletqamatshebrew": "\u05D3\u05B8",
"daletqubuts": "\u05D3\u05BB",
"daletqubutshebrew": "\u05D3\u05BB",
"daletsegol": "\u05D3\u05B6",
"daletsegolhebrew": "\u05D3\u05B6",
"daletsheva": "\u05D3\u05B0",
"daletshevahebrew": "\u05D3\u05B0",
"dalettsere": "\u05D3\u05B5",
"dalettserehebrew": "\u05D3\u05B5",
"dalfinalarabic": "\uFEAA",
"dammaarabic": "\u064F",
"dammalowarabic": "\u064F",
"dammatanaltonearabic": "\u064C",
"dammatanarabic": "\u064C",
"danda": "\u0964",
"dargahebrew": "\u05A7",
"dargalefthebrew": "\u05A7",
"dasiapneumatacyrilliccmb": "\u0485",
"dblGrave": "\uF6D3",
"dblanglebracketleft": "\u300A",
"dblanglebracketleftvertical": "\uFE3D",
"dblanglebracketright": "\u300B",
"dblanglebracketrightvertical": "\uFE3E",
"dblarchinvertedbelowcmb": "\u032B",
"dblarrowleft": "\u21D4",
"dblarrowright": "\u21D2",
"dbldanda": "\u0965",
"dblgrave": "\uF6D6",
"dblgravecmb": "\u030F",
"dblintegral": "\u222C",
"dbllowline": "\u2017",
"dbllowlinecmb": "\u0333",
"dbloverlinecmb": "\u033F",
"dblprimemod": "\u02BA",
"dblverticalbar": "\u2016",
"dblverticallineabovecmb": "\u030E",
"dbopomofo": "\u3109",
"dbsquare": "\u33C8",
"dcaron": "\u010F",
"dcedilla": "\u1E11",
"dcircle": "\u24D3",
"dcircumflexbelow": "\u1E13",
"dcroat": "\u0111",
"ddabengali": "\u09A1",
"ddadeva": "\u0921",
"ddagujarati": "\u0AA1",
"ddagurmukhi": "\u0A21",
"ddalarabic": "\u0688",
"ddalfinalarabic": "\uFB89",
"dddhadeva": "\u095C",
"ddhabengali": "\u09A2",
"ddhadeva": "\u0922",
"ddhagujarati": "\u0AA2",
"ddhagurmukhi": "\u0A22",
"ddotaccent": "\u1E0B",
"ddotbelow": "\u1E0D",
"decimalseparatorarabic": "\u066B",
"decimalseparatorpersian": "\u066B",
"decyrillic": "\u0434",
"degree": "\u00B0",
"dehihebrew": "\u05AD",
"dehiragana": "\u3067",
"deicoptic": "\u03EF",
"dekatakana": "\u30C7",
"deleteleft": "\u232B",
"deleteright": "\u2326",
"delta": "\u03B4",
"deltaturned": "\u018D",
"denominatorminusonenumeratorbengali": "\u09F8",
"dezh": "\u02A4",
"dhabengali": "\u09A7",
"dhadeva": "\u0927",
"dhagujarati": "\u0AA7",
"dhagurmukhi": "\u0A27",
"dhook": "\u0257",
"dialytikatonos": "\u0385",
"dialytikatonoscmb": "\u0344",
"diamond": "\u2666",
"diamondsuitwhite": "\u2662",
"dieresis": "\u00A8",
"dieresisacute": "\uF6D7",
"dieresisbelowcmb": "\u0324",
"dieresiscmb": "\u0308",
"dieresisgrave": "\uF6D8",
"dieresistonos": "\u0385",
"dihiragana": "\u3062",
"dikatakana": "\u30C2",
"dittomark": "\u3003",
"divide": "\u00F7",
"divides": "\u2223",
"divisionslash": "\u2215",
"djecyrillic": "\u0452",
"dkshade": "\u2593",
"dlinebelow": "\u1E0F",
"dlsquare": "\u3397",
"dmacron": "\u0111",
"dmonospace": "\uFF44",
"dnblock": "\u2584",
"dochadathai": "\u0E0E",
"dodekthai": "\u0E14",
"dohiragana": "\u3069",
"dokatakana": "\u30C9",
"dollar": "\u0024",
"dollarinferior": "\uF6E3",
"dollarmonospace": "\uFF04",
"dollaroldstyle": "\uF724",
"dollarsmall": "\uFE69",
"dollarsuperior": "\uF6E4",
"dong": "\u20AB",
"dorusquare": "\u3326",
"dotaccent": "\u02D9",
"dotaccentcmb": "\u0307",
"dotbelowcmb": "\u0323",
"dotbelowcomb": "\u0323",
"dotkatakana": "\u30FB",
"dotlessi": "\u0131",
"dotlessj": "\uF6BE",
"dotlessjstrokehook": "\u0284",
"dotmath": "\u22C5",
"dottedcircle": "\u25CC",
"doubleyodpatah": "\uFB1F",
"doubleyodpatahhebrew": "\uFB1F",
"downtackbelowcmb": "\u031E",
"downtackmod": "\u02D5",
"dparen": "\u249F",
"dsuperior": "\uF6EB",
"dtail": "\u0256",
"dtopbar": "\u018C",
"duhiragana": "\u3065",
"dukatakana": "\u30C5",
"dz": "\u01F3",
"dzaltone": "\u02A3",
"dzcaron": "\u01C6",
"dzcurl": "\u02A5",
"dzeabkhasiancyrillic": "\u04E1",
"dzecyrillic": "\u0455",
"dzhecyrillic": "\u045F",
"e": "\u0065",
"eacute": "\u00E9",
"earth": "\u2641",
"ebengali": "\u098F",
"ebopomofo": "\u311C",
"ebreve": "\u0115",
"ecandradeva": "\u090D",
"ecandragujarati": "\u0A8D",
"ecandravowelsigndeva": "\u0945",
"ecandravowelsigngujarati": "\u0AC5",
"ecaron": "\u011B",
"ecedillabreve": "\u1E1D",
"echarmenian": "\u0565",
"echyiwnarmenian": "\u0587",
"ecircle": "\u24D4",
"ecircumflex": "\u00EA",
"ecircumflexacute": "\u1EBF",
"ecircumflexbelow": "\u1E19",
"ecircumflexdotbelow": "\u1EC7",
"ecircumflexgrave": "\u1EC1",
"ecircumflexhookabove": "\u1EC3",
"ecircumflextilde": "\u1EC5",
"ecyrillic": "\u0454",
"edblgrave": "\u0205",
"edeva": "\u090F",
"edieresis": "\u00EB",
"edot": "\u0117",
"edotaccent": "\u0117",
"edotbelow": "\u1EB9",
"eegurmukhi": "\u0A0F",
"eematragurmukhi": "\u0A47",
"efcyrillic": "\u0444",
"egrave": "\u00E8",
"egujarati": "\u0A8F",
"eharmenian": "\u0567",
"ehbopomofo": "\u311D",
"ehiragana": "\u3048",
"ehookabove": "\u1EBB",
"eibopomofo": "\u311F",
"eight": "\u0038",
"eightarabic": "\u0668",
"eightbengali": "\u09EE",
"eightcircle": "\u2467",
"eightcircleinversesansserif": "\u2791",
"eightdeva": "\u096E",
"eighteencircle": "\u2471",
"eighteenparen": "\u2485",
"eighteenperiod": "\u2499",
"eightgujarati": "\u0AEE",
"eightgurmukhi": "\u0A6E",
"eighthackarabic": "\u0668",
"eighthangzhou": "\u3028",
"eighthnotebeamed": "\u266B",
"eightideographicparen": "\u3227",
"eightinferior": "\u2088",
"eightmonospace": "\uFF18",
"eightoldstyle": "\uF738",
"eightparen": "\u247B",
"eightperiod": "\u248F",
"eightpersian": "\u06F8",
"eightroman": "\u2177",
"eightsuperior": "\u2078",
"eightthai": "\u0E58",
"einvertedbreve": "\u0207",
"eiotifiedcyrillic": "\u0465",
"ekatakana": "\u30A8",
"ekatakanahalfwidth": "\uFF74",
"ekonkargurmukhi": "\u0A74",
"ekorean": "\u3154",
"elcyrillic": "\u043B",
"element": "\u2208",
"elevencircle": "\u246A",
"elevenparen": "\u247E",
"elevenperiod": "\u2492",
"elevenroman": "\u217A",
"ellipsis": "\u2026",
"ellipsisvertical": "\u22EE",
"emacron": "\u0113",
"emacronacute": "\u1E17",
"emacrongrave": "\u1E15",
"emcyrillic": "\u043C",
"emdash": "\u2014",
"emdashvertical": "\uFE31",
"emonospace": "\uFF45",
"emphasismarkarmenian": "\u055B",
"emptyset": "\u2205",
"enbopomofo": "\u3123",
"encyrillic": "\u043D",
"endash": "\u2013",
"endashvertical": "\uFE32",
"endescendercyrillic": "\u04A3",
"eng": "\u014B",
"engbopomofo": "\u3125",
"enghecyrillic": "\u04A5",
"enhookcyrillic": "\u04C8",
"enspace": "\u2002",
"eogonek": "\u0119",
"eokorean": "\u3153",
"eopen": "\u025B",
"eopenclosed": "\u029A",
"eopenreversed": "\u025C",
"eopenreversedclosed": "\u025E",
"eopenreversedhook": "\u025D",
"eparen": "\u24A0",
"epsilon": "\u03B5",
"epsilontonos": "\u03AD",
"equal": "\u003D",
"equalmonospace": "\uFF1D",
"equalsmall": "\uFE66",
"equalsuperior": "\u207C",
"equivalence": "\u2261",
"erbopomofo": "\u3126",
"ercyrillic": "\u0440",
"ereversed": "\u0258",
"ereversedcyrillic": "\u044D",
"escyrillic": "\u0441",
"esdescendercyrillic": "\u04AB",
"esh": "\u0283",
"eshcurl": "\u0286",
"eshortdeva": "\u090E",
"eshortvowelsigndeva": "\u0946",
"eshreversedloop": "\u01AA",
"eshsquatreversed": "\u0285",
"esmallhiragana": "\u3047",
"esmallkatakana": "\u30A7",
"esmallkatakanahalfwidth": "\uFF6A",
"estimated": "\u212E",
"esuperior": "\uF6EC",
"eta": "\u03B7",
"etarmenian": "\u0568",
"etatonos": "\u03AE",
"eth": "\u00F0",
"etilde": "\u1EBD",
"etildebelow": "\u1E1B",
"etnahtafoukhhebrew": "\u0591",
"etnahtafoukhlefthebrew": "\u0591",
"etnahtahebrew": "\u0591",
"etnahtalefthebrew": "\u0591",
"eturned": "\u01DD",
"eukorean": "\u3161",
"euro": "\u20AC",
"evowelsignbengali": "\u09C7",
"evowelsigndeva": "\u0947",
"evowelsigngujarati": "\u0AC7",
"exclam": "\u0021",
"exclamarmenian": "\u055C",
"exclamdbl": "\u203C",
"exclamdown": "\u00A1",
"exclamdownsmall": "\uF7A1",
"exclammonospace": "\uFF01",
"exclamsmall": "\uF721",
"existential": "\u2203",
"ezh": "\u0292",
"ezhcaron": "\u01EF",
"ezhcurl": "\u0293",
"ezhreversed": "\u01B9",
"ezhtail": "\u01BA",
"f": "\u0066",
"fadeva": "\u095E",
"fagurmukhi": "\u0A5E",
"fahrenheit": "\u2109",
"fathaarabic": "\u064E",
"fathalowarabic": "\u064E",
"fathatanarabic": "\u064B",
"fbopomofo": "\u3108",
"fcircle": "\u24D5",
"fdotaccent": "\u1E1F",
"feharabic": "\u0641",
"feharmenian": "\u0586",
"fehfinalarabic": "\uFED2",
"fehinitialarabic": "\uFED3",
"fehmedialarabic": "\uFED4",
"feicoptic": "\u03E5",
"female": "\u2640",
"ff": "\uFB00",
"ffi": "\uFB03",
"ffl": "\uFB04",
"fi": "\uFB01",
"fifteencircle": "\u246E",
"fifteenparen": "\u2482",
"fifteenperiod": "\u2496",
"figuredash": "\u2012",
"filledbox": "\u25A0",
"filledrect": "\u25AC",
"finalkaf": "\u05DA",
"finalkafdagesh": "\uFB3A",
"finalkafdageshhebrew": "\uFB3A",
"finalkafhebrew": "\u05DA",
"finalkafqamats": "\u05DA\u05B8",
"finalkafqamatshebrew": "\u05DA\u05B8",
"finalkafsheva": "\u05DA\u05B0",
"finalkafshevahebrew": "\u05DA\u05B0",
"finalmem": "\u05DD",
"finalmemhebrew": "\u05DD",
"finalnun": "\u05DF",
"finalnunhebrew": "\u05DF",
"finalpe": "\u05E3",
"finalpehebrew": "\u05E3",
"finaltsadi": "\u05E5",
"finaltsadihebrew": "\u05E5",
"firsttonechinese": "\u02C9",
"fisheye": "\u25C9",
"fitacyrillic": "\u0473",
"five": "\u0035",
"fivearabic": "\u0665",
"fivebengali": "\u09EB",
"fivecircle": "\u2464",
"fivecircleinversesansserif": "\u278E",
"fivedeva": "\u096B",
"fiveeighths": "\u215D",
"fivegujarati": "\u0AEB",
"fivegurmukhi": "\u0A6B",
"fivehackarabic": "\u0665",
"fivehangzhou": "\u3025",
"fiveideographicparen": "\u3224",
"fiveinferior": "\u2085",
"fivemonospace": "\uFF15",
"fiveoldstyle": "\uF735",
"fiveparen": "\u2478",
"fiveperiod": "\u248C",
"fivepersian": "\u06F5",
"fiveroman": "\u2174",
"fivesuperior": "\u2075",
"fivethai": "\u0E55",
"fl": "\uFB02",
"florin": "\u0192",
"fmonospace": "\uFF46",
"fmsquare": "\u3399",
"fofanthai": "\u0E1F",
"fofathai": "\u0E1D",
"fongmanthai": "\u0E4F",
"forall": "\u2200",
"four": "\u0034",
"fourarabic": "\u0664",
"fourbengali": "\u09EA",
"fourcircle": "\u2463",
"fourcircleinversesansserif": "\u278D",
"fourdeva": "\u096A",
"fourgujarati": "\u0AEA",
"fourgurmukhi": "\u0A6A",
"fourhackarabic": "\u0664",
"fourhangzhou": "\u3024",
"fourideographicparen": "\u3223",
"fourinferior": "\u2084",
"fourmonospace": "\uFF14",
"fournumeratorbengali": "\u09F7",
"fouroldstyle": "\uF734",
"fourparen": "\u2477",
"fourperiod": "\u248B",
"fourpersian": "\u06F4",
"fourroman": "\u2173",
"foursuperior": "\u2074",
"fourteencircle": "\u246D",
"fourteenparen": "\u2481",
"fourteenperiod": "\u2495",
"fourthai": "\u0E54",
"fourthtonechinese": "\u02CB",
"fparen": "\u24A1",
"fraction": "\u2044",
"franc": "\u20A3",
"g": "\u0067",
"gabengali": "\u0997",
"gacute": "\u01F5",
"gadeva": "\u0917",
"gafarabic": "\u06AF",
"gaffinalarabic": "\uFB93",
"gafinitialarabic": "\uFB94",
"gafmedialarabic": "\uFB95",
"gagujarati": "\u0A97",
"gagurmukhi": "\u0A17",
"gahiragana": "\u304C",
"gakatakana": "\u30AC",
"gamma": "\u03B3",
"gammalatinsmall": "\u0263",
"gammasuperior": "\u02E0",
"gangiacoptic": "\u03EB",
"gbopomofo": "\u310D",
"gbreve": "\u011F",
"gcaron": "\u01E7",
"gcedilla": "\u0123",
"gcircle": "\u24D6",
"gcircumflex": "\u011D",
"gcommaaccent": "\u0123",
"gdot": "\u0121",
"gdotaccent": "\u0121",
"gecyrillic": "\u0433",
"gehiragana": "\u3052",
"gekatakana": "\u30B2",
"geometricallyequal": "\u2251",
"gereshaccenthebrew": "\u059C",
"gereshhebrew": "\u05F3",
"gereshmuqdamhebrew": "\u059D",
"germandbls": "\u00DF",
"gershayimaccenthebrew": "\u059E",
"gershayimhebrew": "\u05F4",
"getamark": "\u3013",
"ghabengali": "\u0998",
"ghadarmenian": "\u0572",
"ghadeva": "\u0918",
"ghagujarati": "\u0A98",
"ghagurmukhi": "\u0A18",
"ghainarabic": "\u063A",
"ghainfinalarabic": "\uFECE",
"ghaininitialarabic": "\uFECF",
"ghainmedialarabic": "\uFED0",
"ghemiddlehookcyrillic": "\u0495",
"ghestrokecyrillic": "\u0493",
"gheupturncyrillic": "\u0491",
"ghhadeva": "\u095A",
"ghhagurmukhi": "\u0A5A",
"ghook": "\u0260",
"ghzsquare": "\u3393",
"gihiragana": "\u304E",
"gikatakana": "\u30AE",
"gimarmenian": "\u0563",
"gimel": "\u05D2",
"gimeldagesh": "\uFB32",
"gimeldageshhebrew": "\uFB32",
"gimelhebrew": "\u05D2",
"gjecyrillic": "\u0453",
"glottalinvertedstroke": "\u01BE",
"glottalstop": "\u0294",
"glottalstopinverted": "\u0296",
"glottalstopmod": "\u02C0",
"glottalstopreversed": "\u0295",
"glottalstopreversedmod": "\u02C1",
"glottalstopreversedsuperior": "\u02E4",
"glottalstopstroke": "\u02A1",
"glottalstopstrokereversed": "\u02A2",
"gmacron": "\u1E21",
"gmonospace": "\uFF47",
"gohiragana": "\u3054",
"gokatakana": "\u30B4",
"gparen": "\u24A2",
"gpasquare": "\u33AC",
"gradient": "\u2207",
"grave": "\u0060",
"gravebelowcmb": "\u0316",
"gravecmb": "\u0300",
"gravecomb": "\u0300",
"gravedeva": "\u0953",
"gravelowmod": "\u02CE",
"gravemonospace": "\uFF40",
"gravetonecmb": "\u0340",
"greater": "\u003E",
"greaterequal": "\u2265",
"greaterequalorless": "\u22DB",
"greatermonospace": "\uFF1E",
"greaterorequivalent": "\u2273",
"greaterorless": "\u2277",
"greateroverequal": "\u2267",
"greatersmall": "\uFE65",
"gscript": "\u0261",
"gstroke": "\u01E5",
"guhiragana": "\u3050",
"guillemotleft": "\u00AB",
"guillemotright": "\u00BB",
"guilsinglleft": "\u2039",
"guilsinglright": "\u203A",
"gukatakana": "\u30B0",
"guramusquare": "\u3318",
"gysquare": "\u33C9",
"h": "\u0068",
"haabkhasiancyrillic": "\u04A9",
"haaltonearabic": "\u06C1",
"habengali": "\u09B9",
"hadescendercyrillic": "\u04B3",
"hadeva": "\u0939",
"hagujarati": "\u0AB9",
"hagurmukhi": "\u0A39",
"haharabic": "\u062D",
"hahfinalarabic": "\uFEA2",
"hahinitialarabic": "\uFEA3",
"hahiragana": "\u306F",
"hahmedialarabic": "\uFEA4",
"haitusquare": "\u332A",
"hakatakana": "\u30CF",
"hakatakanahalfwidth": "\uFF8A",
"halantgurmukhi": "\u0A4D",
"hamzaarabic": "\u0621",
"hamzadammaarabic": "\u0621\u064F",
"hamzadammatanarabic": "\u0621\u064C",
"hamzafathaarabic": "\u0621\u064E",
"hamzafathatanarabic": "\u0621\u064B",
"hamzalowarabic": "\u0621",
"hamzalowkasraarabic": "\u0621\u0650",
"hamzalowkasratanarabic": "\u0621\u064D",
"hamzasukunarabic": "\u0621\u0652",
"hangulfiller": "\u3164",
"hardsigncyrillic": "\u044A",
"harpoonleftbarbup": "\u21BC",
"harpoonrightbarbup": "\u21C0",
"hasquare": "\u33CA",
"hatafpatah": "\u05B2",
"hatafpatah16": "\u05B2",
"hatafpatah23": "\u05B2",
"hatafpatah2f": "\u05B2",
"hatafpatahhebrew": "\u05B2",
"hatafpatahnarrowhebrew": "\u05B2",
"hatafpatahquarterhebrew": "\u05B2",
"hatafpatahwidehebrew": "\u05B2",
"hatafqamats": "\u05B3",
"hatafqamats1b": "\u05B3",
"hatafqamats28": "\u05B3",
"hatafqamats34": "\u05B3",
"hatafqamatshebrew": "\u05B3",
"hatafqamatsnarrowhebrew": "\u05B3",
"hatafqamatsquarterhebrew": "\u05B3",
"hatafqamatswidehebrew": "\u05B3",
"hatafsegol": "\u05B1",
"hatafsegol17": "\u05B1",
"hatafsegol24": "\u05B1",
"hatafsegol30": "\u05B1",
"hatafsegolhebrew": "\u05B1",
"hatafsegolnarrowhebrew": "\u05B1",
"hatafsegolquarterhebrew": "\u05B1",
"hatafsegolwidehebrew": "\u05B1",
"hbar": "\u0127",
"hbopomofo": "\u310F",
"hbrevebelow": "\u1E2B",
"hcedilla": "\u1E29",
"hcircle": "\u24D7",
"hcircumflex": "\u0125",
"hdieresis": "\u1E27",
"hdotaccent": "\u1E23",
"hdotbelow": "\u1E25",
"he": "\u05D4",
"heart": "\u2665",
"heartsuitblack": "\u2665",
"heartsuitwhite": "\u2661",
"hedagesh": "\uFB34",
"hedageshhebrew": "\uFB34",
"hehaltonearabic": "\u06C1",
"heharabic": "\u0647",
"hehebrew": "\u05D4",
"hehfinalaltonearabic": "\uFBA7",
"hehfinalalttwoarabic": "\uFEEA",
"hehfinalarabic": "\uFEEA",
"hehhamzaabovefinalarabic": "\uFBA5",
"hehhamzaaboveisolatedarabic": "\uFBA4",
"hehinitialaltonearabic": "\uFBA8",
"hehinitialarabic": "\uFEEB",
"hehiragana": "\u3078",
"hehmedialaltonearabic": "\uFBA9",
"hehmedialarabic": "\uFEEC",
"heiseierasquare": "\u337B",
"hekatakana": "\u30D8",
"hekatakanahalfwidth": "\uFF8D",
"hekutaarusquare": "\u3336",
"henghook": "\u0267",
"herutusquare": "\u3339",
"het": "\u05D7",
"hethebrew": "\u05D7",
"hhook": "\u0266",
"hhooksuperior": "\u02B1",
"hieuhacirclekorean": "\u327B",
"hieuhaparenkorean": "\u321B",
"hieuhcirclekorean": "\u326D",
"hieuhkorean": "\u314E",
"hieuhparenkorean": "\u320D",
"hihiragana": "\u3072",
"hikatakana": "\u30D2",
"hikatakanahalfwidth": "\uFF8B",
"hiriq": "\u05B4",
"hiriq14": "\u05B4",
"hiriq21": "\u05B4",
"hiriq2d": "\u05B4",
"hiriqhebrew": "\u05B4",
"hiriqnarrowhebrew": "\u05B4",
"hiriqquarterhebrew": "\u05B4",
"hiriqwidehebrew": "\u05B4",
"hlinebelow": "\u1E96",
"hmonospace": "\uFF48",
"hoarmenian": "\u0570",
"hohipthai": "\u0E2B",
"hohiragana": "\u307B",
"hokatakana": "\u30DB",
"hokatakanahalfwidth": "\uFF8E",
"holam": "\u05B9",
"holam19": "\u05B9",
"holam26": "\u05B9",
"holam32": "\u05B9",
"holamhebrew": "\u05B9",
"holamnarrowhebrew": "\u05B9",
"holamquarterhebrew": "\u05B9",
"holamwidehebrew": "\u05B9",
"honokhukthai": "\u0E2E",
"hookabovecomb": "\u0309",
"hookcmb": "\u0309",
"hookpalatalizedbelowcmb": "\u0321",
"hookretroflexbelowcmb": "\u0322",
"hoonsquare": "\u3342",
"horicoptic": "\u03E9",
"horizontalbar": "\u2015",
"horncmb": "\u031B",
"hotsprings": "\u2668",
"house": "\u2302",
"hparen": "\u24A3",
"hsuperior": "\u02B0",
"hturned": "\u0265",
"huhiragana": "\u3075",
"huiitosquare": "\u3333",
"hukatakana": "\u30D5",
"hukatakanahalfwidth": "\uFF8C",
"hungarumlaut": "\u02DD",
"hungarumlautcmb": "\u030B",
"hv": "\u0195",
"hyphen": "\u002D",
"hypheninferior": "\uF6E5",
"hyphenmonospace": "\uFF0D",
"hyphensmall": "\uFE63",
"hyphensuperior": "\uF6E6",
"hyphentwo": "\u2010",
"i": "\u0069",
"iacute": "\u00ED",
"iacyrillic": "\u044F",
"ibengali": "\u0987",
"ibopomofo": "\u3127",
"ibreve": "\u012D",
"icaron": "\u01D0",
"icircle": "\u24D8",
"icircumflex": "\u00EE",
"icyrillic": "\u0456",
"idblgrave": "\u0209",
"ideographearthcircle": "\u328F",
"ideographfirecircle": "\u328B",
"ideographicallianceparen": "\u323F",
"ideographiccallparen": "\u323A",
"ideographiccentrecircle": "\u32A5",
"ideographicclose": "\u3006",
"ideographiccomma": "\u3001",
"ideographiccommaleft": "\uFF64",
"ideographiccongratulationparen": "\u3237",
"ideographiccorrectcircle": "\u32A3",
"ideographicearthparen": "\u322F",
"ideographicenterpriseparen": "\u323D",
"ideographicexcellentcircle": "\u329D",
"ideographicfestivalparen": "\u3240",
"ideographicfinancialcircle": "\u3296",
"ideographicfinancialparen": "\u3236",
"ideographicfireparen": "\u322B",
"ideographichaveparen": "\u3232",
"ideographichighcircle": "\u32A4",
"ideographiciterationmark": "\u3005",
"ideographiclaborcircle": "\u3298",
"ideographiclaborparen": "\u3238",
"ideographicleftcircle": "\u32A7",
"ideographiclowcircle": "\u32A6",
"ideographicmedicinecircle": "\u32A9",
"ideographicmetalparen": "\u322E",
"ideographicmoonparen": "\u322A",
"ideographicnameparen": "\u3234",
"ideographicperiod": "\u3002",
"ideographicprintcircle": "\u329E",
"ideographicreachparen": "\u3243",
"ideographicrepresentparen": "\u3239",
"ideographicresourceparen": "\u323E",
"ideographicrightcircle": "\u32A8",
"ideographicsecretcircle": "\u3299",
"ideographicselfparen": "\u3242",
"ideographicsocietyparen": "\u3233",
"ideographicspace": "\u3000",
"ideographicspecialparen": "\u3235",
"ideographicstockparen": "\u3231",
"ideographicstudyparen": "\u323B",
"ideographicsunparen": "\u3230",
"ideographicsuperviseparen": "\u323C",
"ideographicwaterparen": "\u322C",
"ideographicwoodparen": "\u322D",
"ideographiczero": "\u3007",
"ideographmetalcircle": "\u328E",
"ideographmooncircle": "\u328A",
"ideographnamecircle": "\u3294",
"ideographsuncircle": "\u3290",
"ideographwatercircle": "\u328C",
"ideographwoodcircle": "\u328D",
"ideva": "\u0907",
"idieresis": "\u00EF",
"idieresisacute": "\u1E2F",
"idieresiscyrillic": "\u04E5",
"idotbelow": "\u1ECB",
"iebrevecyrillic": "\u04D7",
"iecyrillic": "\u0435",
"ieungacirclekorean": "\u3275",
"ieungaparenkorean": "\u3215",
"ieungcirclekorean": "\u3267",
"ieungkorean": "\u3147",
"ieungparenkorean": "\u3207",
"igrave": "\u00EC",
"igujarati": "\u0A87",
"igurmukhi": "\u0A07",
"ihiragana": "\u3044",
"ihookabove": "\u1EC9",
"iibengali": "\u0988",
"iicyrillic": "\u0438",
"iideva": "\u0908",
"iigujarati": "\u0A88",
"iigurmukhi": "\u0A08",
"iimatragurmukhi": "\u0A40",
"iinvertedbreve": "\u020B",
"iishortcyrillic": "\u0439",
"iivowelsignbengali": "\u09C0",
"iivowelsigndeva": "\u0940",
"iivowelsigngujarati": "\u0AC0",
"ij": "\u0133",
"ikatakana": "\u30A4",
"ikatakanahalfwidth": "\uFF72",
"ikorean": "\u3163",
"ilde": "\u02DC",
"iluyhebrew": "\u05AC",
"imacron": "\u012B",
"imacroncyrillic": "\u04E3",
"imageorapproximatelyequal": "\u2253",
"imatragurmukhi": "\u0A3F",
"imonospace": "\uFF49",
"increment": "\u2206",
"infinity": "\u221E",
"iniarmenian": "\u056B",
"integral": "\u222B",
"integralbottom": "\u2321",
"integralbt": "\u2321",
"integralex": "\uF8F5",
"integraltop": "\u2320",
"integraltp": "\u2320",
"intersection": "\u2229",
"intisquare": "\u3305",
"invbullet": "\u25D8",
"invcircle": "\u25D9",
"invsmileface": "\u263B",
"iocyrillic": "\u0451",
"iogonek": "\u012F",
"iota": "\u03B9",
"iotadieresis": "\u03CA",
"iotadieresistonos": "\u0390",
"iotalatin": "\u0269",
"iotatonos": "\u03AF",
"iparen": "\u24A4",
"irigurmukhi": "\u0A72",
"ismallhiragana": "\u3043",
"ismallkatakana": "\u30A3",
"ismallkatakanahalfwidth": "\uFF68",
"issharbengali": "\u09FA",
"istroke": "\u0268",
"isuperior": "\uF6ED",
"iterationhiragana": "\u309D",
"iterationkatakana": "\u30FD",
"itilde": "\u0129",
"itildebelow": "\u1E2D",
"iubopomofo": "\u3129",
"iucyrillic": "\u044E",
"ivowelsignbengali": "\u09BF",
"ivowelsigndeva": "\u093F",
"ivowelsigngujarati": "\u0ABF",
"izhitsacyrillic": "\u0475",
"izhitsadblgravecyrillic": "\u0477",
"j": "\u006A",
"jaarmenian": "\u0571",
"jabengali": "\u099C",
"jadeva": "\u091C",
"jagujarati": "\u0A9C",
"jagurmukhi": "\u0A1C",
"jbopomofo": "\u3110",
"jcaron": "\u01F0",
"jcircle": "\u24D9",
"jcircumflex": "\u0135",
"jcrossedtail": "\u029D",
"jdotlessstroke": "\u025F",
"jecyrillic": "\u0458",
"jeemarabic": "\u062C",
"jeemfinalarabic": "\uFE9E",
"jeeminitialarabic": "\uFE9F",
"jeemmedialarabic": "\uFEA0",
"jeharabic": "\u0698",
"jehfinalarabic": "\uFB8B",
"jhabengali": "\u099D",
"jhadeva": "\u091D",
"jhagujarati": "\u0A9D",
"jhagurmukhi": "\u0A1D",
"jheharmenian": "\u057B",
"jis": "\u3004",
"jmonospace": "\uFF4A",
"jparen": "\u24A5",
"jsuperior": "\u02B2",
"k": "\u006B",
"kabashkircyrillic": "\u04A1",
"kabengali": "\u0995",
"kacute": "\u1E31",
"kacyrillic": "\u043A",
"kadescendercyrillic": "\u049B",
"kadeva": "\u0915",
"kaf": "\u05DB",
"kafarabic": "\u0643",
"kafdagesh": "\uFB3B",
"kafdageshhebrew": "\uFB3B",
"kaffinalarabic": "\uFEDA",
"kafhebrew": "\u05DB",
"kafinitialarabic": "\uFEDB",
"kafmedialarabic": "\uFEDC",
"kafrafehebrew": "\uFB4D",
"kagujarati": "\u0A95",
"kagurmukhi": "\u0A15",
"kahiragana": "\u304B",
"kahookcyrillic": "\u04C4",
"kakatakana": "\u30AB",
"kakatakanahalfwidth": "\uFF76",
"kappa": "\u03BA",
"kappasymbolgreek": "\u03F0",
"kapyeounmieumkorean": "\u3171",
"kapyeounphieuphkorean": "\u3184",
"kapyeounpieupkorean": "\u3178",
"kapyeounssangpieupkorean": "\u3179",
"karoriisquare": "\u330D",
"kashidaautoarabic": "\u0640",
"kashidaautonosidebearingarabic": "\u0640",
"kasmallkatakana": "\u30F5",
"kasquare": "\u3384",
"kasraarabic": "\u0650",
"kasratanarabic": "\u064D",
"kastrokecyrillic": "\u049F",
"katahiraprolongmarkhalfwidth": "\uFF70",
"kaverticalstrokecyrillic": "\u049D",
"kbopomofo": "\u310E",
"kcalsquare": "\u3389",
"kcaron": "\u01E9",
"kcedilla": "\u0137",
"kcircle": "\u24DA",
"kcommaaccent": "\u0137",
"kdotbelow": "\u1E33",
"keharmenian": "\u0584",
"kehiragana": "\u3051",
"kekatakana": "\u30B1",
"kekatakanahalfwidth": "\uFF79",
"kenarmenian": "\u056F",
"kesmallkatakana": "\u30F6",
"kgreenlandic": "\u0138",
"khabengali": "\u0996",
"khacyrillic": "\u0445",
"khadeva": "\u0916",
"khagujarati": "\u0A96",
"khagurmukhi": "\u0A16",
"khaharabic": "\u062E",
"khahfinalarabic": "\uFEA6",
"khahinitialarabic": "\uFEA7",
"khahmedialarabic": "\uFEA8",
"kheicoptic": "\u03E7",
"khhadeva": "\u0959",
"khhagurmukhi": "\u0A59",
"khieukhacirclekorean": "\u3278",
"khieukhaparenkorean": "\u3218",
"khieukhcirclekorean": "\u326A",
"khieukhkorean": "\u314B",
"khieukhparenkorean": "\u320A",
"khokhaithai": "\u0E02",
"khokhonthai": "\u0E05",
"khokhuatthai": "\u0E03",
"khokhwaithai": "\u0E04",
"khomutthai": "\u0E5B",
"khook": "\u0199",
"khorakhangthai": "\u0E06",
"khzsquare": "\u3391",
"kihiragana": "\u304D",
"kikatakana": "\u30AD",
"kikatakanahalfwidth": "\uFF77",
"kiroguramusquare": "\u3315",
"kiromeetorusquare": "\u3316",
"kirosquare": "\u3314",
"kiyeokacirclekorean": "\u326E",
"kiyeokaparenkorean": "\u320E",
"kiyeokcirclekorean": "\u3260",
"kiyeokkorean": "\u3131",
"kiyeokparenkorean": "\u3200",
"kiyeoksioskorean": "\u3133",
"kjecyrillic": "\u045C",
"klinebelow": "\u1E35",
"klsquare": "\u3398",
"kmcubedsquare": "\u33A6",
"kmonospace": "\uFF4B",
"kmsquaredsquare": "\u33A2",
"kohiragana": "\u3053",
"kohmsquare": "\u33C0",
"kokaithai": "\u0E01",
"kokatakana": "\u30B3",
"kokatakanahalfwidth": "\uFF7A",
"kooposquare": "\u331E",
"koppacyrillic": "\u0481",
"koreanstandardsymbol": "\u327F",
"koroniscmb": "\u0343",
"kparen": "\u24A6",
"kpasquare": "\u33AA",
"ksicyrillic": "\u046F",
"ktsquare": "\u33CF",
"kturned": "\u029E",
"kuhiragana": "\u304F",
"kukatakana": "\u30AF",
"kukatakanahalfwidth": "\uFF78",
"kvsquare": "\u33B8",
"kwsquare": "\u33BE",
"l": "\u006C",
"labengali": "\u09B2",
"lacute": "\u013A",
"ladeva": "\u0932",
"lagujarati": "\u0AB2",
"lagurmukhi": "\u0A32",
"lakkhangyaothai": "\u0E45",
"lamaleffinalarabic": "\uFEFC",
"lamalefhamzaabovefinalarabic": "\uFEF8",
"lamalefhamzaaboveisolatedarabic": "\uFEF7",
"lamalefhamzabelowfinalarabic": "\uFEFA",
"lamalefhamzabelowisolatedarabic": "\uFEF9",
"lamalefisolatedarabic": "\uFEFB",
"lamalefmaddaabovefinalarabic": "\uFEF6",
"lamalefmaddaaboveisolatedarabic": "\uFEF5",
"lamarabic": "\u0644",
"lambda": "\u03BB",
"lambdastroke": "\u019B",
"lamed": "\u05DC",
"lameddagesh": "\uFB3C",
"lameddageshhebrew": "\uFB3C",
"lamedhebrew": "\u05DC",
"lamedholam": "\u05DC\u05B9",
"lamedholamdagesh": "\u05DC\u05B9\u05BC",
"lamedholamdageshhebrew": "\u05DC\u05B9\u05BC",
"lamedholamhebrew": "\u05DC\u05B9",
"lamfinalarabic": "\uFEDE",
"lamhahinitialarabic": "\uFCCA",
"laminitialarabic": "\uFEDF",
"lamjeeminitialarabic": "\uFCC9",
"lamkhahinitialarabic": "\uFCCB",
"lamlamhehisolatedarabic": "\uFDF2",
"lammedialarabic": "\uFEE0",
"lammeemhahinitialarabic": "\uFD88",
"lammeeminitialarabic": "\uFCCC",
"lammeemjeeminitialarabic": "\uFEDF\uFEE4\uFEA0",
"lammeemkhahinitialarabic": "\uFEDF\uFEE4\uFEA8",
"largecircle": "\u25EF",
"lbar": "\u019A",
"lbelt": "\u026C",
"lbopomofo": "\u310C",
"lcaron": "\u013E",
"lcedilla": "\u013C",
"lcircle": "\u24DB",
"lcircumflexbelow": "\u1E3D",
"lcommaaccent": "\u013C",
"ldot": "\u0140",
"ldotaccent": "\u0140",
"ldotbelow": "\u1E37",
"ldotbelowmacron": "\u1E39",
"leftangleabovecmb": "\u031A",
"lefttackbelowcmb": "\u0318",
"less": "\u003C",
"lessequal": "\u2264",
"lessequalorgreater": "\u22DA",
"lessmonospace": "\uFF1C",
"lessorequivalent": "\u2272",
"lessorgreater": "\u2276",
"lessoverequal": "\u2266",
"lesssmall": "\uFE64",
"lezh": "\u026E",
"lfblock": "\u258C",
"lhookretroflex": "\u026D",
"lira": "\u20A4",
"liwnarmenian": "\u056C",
"lj": "\u01C9",
"ljecyrillic": "\u0459",
"ll": "\uF6C0",
"lladeva": "\u0933",
"llagujarati": "\u0AB3",
"llinebelow": "\u1E3B",
"llladeva": "\u0934",
"llvocalicbengali": "\u09E1",
"llvocalicdeva": "\u0961",
"llvocalicvowelsignbengali": "\u09E3",
"llvocalicvowelsigndeva": "\u0963",
"lmiddletilde": "\u026B",
"lmonospace": "\uFF4C",
"lmsquare": "\u33D0",
"lochulathai": "\u0E2C",
"logicaland": "\u2227",
"logicalnot": "\u00AC",
"logicalnotreversed": "\u2310",
"logicalor": "\u2228",
"lolingthai": "\u0E25",
"longs": "\u017F",
"lowlinecenterline": "\uFE4E",
"lowlinecmb": "\u0332",
"lowlinedashed": "\uFE4D",
"lozenge": "\u25CA",
"lparen": "\u24A7",
"lslash": "\u0142",
"lsquare": "\u2113",
"lsuperior": "\uF6EE",
"ltshade": "\u2591",
"luthai": "\u0E26",
"lvocalicbengali": "\u098C",
"lvocalicdeva": "\u090C",
"lvocalicvowelsignbengali": "\u09E2",
"lvocalicvowelsigndeva": "\u0962",
"lxsquare": "\u33D3",
"m": "\u006D",
"mabengali": "\u09AE",
"macron": "\u00AF",
"macronbelowcmb": "\u0331",
"macroncmb": "\u0304",
"macronlowmod": "\u02CD",
"macronmonospace": "\uFFE3",
"macute": "\u1E3F",
"madeva": "\u092E",
"magujarati": "\u0AAE",
"magurmukhi": "\u0A2E",
"mahapakhhebrew": "\u05A4",
"mahapakhlefthebrew": "\u05A4",
"mahiragana": "\u307E",
"maichattawalowleftthai": "\uF895",
"maichattawalowrightthai": "\uF894",
"maichattawathai": "\u0E4B",
"maichattawaupperleftthai": "\uF893",
"maieklowleftthai": "\uF88C",
"maieklowrightthai": "\uF88B",
"maiekthai": "\u0E48",
"maiekupperleftthai": "\uF88A",
"maihanakatleftthai": "\uF884",
"maihanakatthai": "\u0E31",
"maitaikhuleftthai": "\uF889",
"maitaikhuthai": "\u0E47",
"maitholowleftthai": "\uF88F",
"maitholowrightthai": "\uF88E",
"maithothai": "\u0E49",
"maithoupperleftthai": "\uF88D",
"maitrilowleftthai": "\uF892",
"maitrilowrightthai": "\uF891",
"maitrithai": "\u0E4A",
"maitriupperleftthai": "\uF890",
"maiyamokthai": "\u0E46",
"makatakana": "\u30DE",
"makatakanahalfwidth": "\uFF8F",
"male": "\u2642",
"mansyonsquare": "\u3347",
"maqafhebrew": "\u05BE",
"mars": "\u2642",
"masoracirclehebrew": "\u05AF",
"masquare": "\u3383",
"mbopomofo": "\u3107",
"mbsquare": "\u33D4",
"mcircle": "\u24DC",
"mcubedsquare": "\u33A5",
"mdotaccent": "\u1E41",
"mdotbelow": "\u1E43",
"meemarabic": "\u0645",
"meemfinalarabic": "\uFEE2",
"meeminitialarabic": "\uFEE3",
"meemmedialarabic": "\uFEE4",
"meemmeeminitialarabic": "\uFCD1",
"meemmeemisolatedarabic": "\uFC48",
"meetorusquare": "\u334D",
"mehiragana": "\u3081",
"meizierasquare": "\u337E",
"mekatakana": "\u30E1",
"mekatakanahalfwidth": "\uFF92",
"mem": "\u05DE",
"memdagesh": "\uFB3E",
"memdageshhebrew": "\uFB3E",
"memhebrew": "\u05DE",
"menarmenian": "\u0574",
"merkhahebrew": "\u05A5",
"merkhakefulahebrew": "\u05A6",
"merkhakefulalefthebrew": "\u05A6",
"merkhalefthebrew": "\u05A5",
"mhook": "\u0271",
"mhzsquare": "\u3392",
"middledotkatakanahalfwidth": "\uFF65",
"middot": "\u00B7",
"mieumacirclekorean": "\u3272",
"mieumaparenkorean": "\u3212",
"mieumcirclekorean": "\u3264",
"mieumkorean": "\u3141",
"mieumpansioskorean": "\u3170",
"mieumparenkorean": "\u3204",
"mieumpieupkorean": "\u316E",
"mieumsioskorean": "\u316F",
"mihiragana": "\u307F",
"mikatakana": "\u30DF",
"mikatakanahalfwidth": "\uFF90",
"minus": "\u2212",
"minusbelowcmb": "\u0320",
"minuscircle": "\u2296",
"minusmod": "\u02D7",
"minusplus": "\u2213",
"minute": "\u2032",
"miribaarusquare": "\u334A",
"mirisquare": "\u3349",
"mlonglegturned": "\u0270",
"mlsquare": "\u3396",
"mmcubedsquare": "\u33A3",
"mmonospace": "\uFF4D",
"mmsquaredsquare": "\u339F",
"mohiragana": "\u3082",
"mohmsquare": "\u33C1",
"mokatakana": "\u30E2",
"mokatakanahalfwidth": "\uFF93",
"molsquare": "\u33D6",
"momathai": "\u0E21",
"moverssquare": "\u33A7",
"moverssquaredsquare": "\u33A8",
"mparen": "\u24A8",
"mpasquare": "\u33AB",
"mssquare": "\u33B3",
"msuperior": "\uF6EF",
"mturned": "\u026F",
"mu": "\u00B5",
"mu1": "\u00B5",
"muasquare": "\u3382",
"muchgreater": "\u226B",
"muchless": "\u226A",
"mufsquare": "\u338C",
"mugreek": "\u03BC",
"mugsquare": "\u338D",
"muhiragana": "\u3080",
"mukatakana": "\u30E0",
"mukatakanahalfwidth": "\uFF91",
"mulsquare": "\u3395",
"multiply": "\u00D7",
"mumsquare": "\u339B",
"munahhebrew": "\u05A3",
"munahlefthebrew": "\u05A3",
"musicalnote": "\u266A",
"musicalnotedbl": "\u266B",
"musicflatsign": "\u266D",
"musicsharpsign": "\u266F",
"mussquare": "\u33B2",
"muvsquare": "\u33B6",
"muwsquare": "\u33BC",
"mvmegasquare": "\u33B9",
"mvsquare": "\u33B7",
"mwmegasquare": "\u33BF",
"mwsquare": "\u33BD",
"n": "\u006E",
"nabengali": "\u09A8",
"nabla": "\u2207",
"nacute": "\u0144",
"nadeva": "\u0928",
"nagujarati": "\u0AA8",
"nagurmukhi": "\u0A28",
"nahiragana": "\u306A",
"nakatakana": "\u30CA",
"nakatakanahalfwidth": "\uFF85",
"napostrophe": "\u0149",
"nasquare": "\u3381",
"nbopomofo": "\u310B",
"nbspace": "\u00A0",
"ncaron": "\u0148",
"ncedilla": "\u0146",
"ncircle": "\u24DD",
"ncircumflexbelow": "\u1E4B",
"ncommaaccent": "\u0146",
"ndotaccent": "\u1E45",
"ndotbelow": "\u1E47",
"nehiragana": "\u306D",
"nekatakana": "\u30CD",
"nekatakanahalfwidth": "\uFF88",
"newsheqelsign": "\u20AA",
"nfsquare": "\u338B",
"ngabengali": "\u0999",
"ngadeva": "\u0919",
"ngagujarati": "\u0A99",
"ngagurmukhi": "\u0A19",
"ngonguthai": "\u0E07",
"nhiragana": "\u3093",
"nhookleft": "\u0272",
"nhookretroflex": "\u0273",
"nieunacirclekorean": "\u326F",
"nieunaparenkorean": "\u320F",
"nieuncieuckorean": "\u3135",
"nieuncirclekorean": "\u3261",
"nieunhieuhkorean": "\u3136",
"nieunkorean": "\u3134",
"nieunpansioskorean": "\u3168",
"nieunparenkorean": "\u3201",
"nieunsioskorean": "\u3167",
"nieuntikeutkorean": "\u3166",
"nihiragana": "\u306B",
"nikatakana": "\u30CB",
"nikatakanahalfwidth": "\uFF86",
"nikhahitleftthai": "\uF899",
"nikhahitthai": "\u0E4D",
"nine": "\u0039",
"ninearabic": "\u0669",
"ninebengali": "\u09EF",
"ninecircle": "\u2468",
"ninecircleinversesansserif": "\u2792",
"ninedeva": "\u096F",
"ninegujarati": "\u0AEF",
"ninegurmukhi": "\u0A6F",
"ninehackarabic": "\u0669",
"ninehangzhou": "\u3029",
"nineideographicparen": "\u3228",
"nineinferior": "\u2089",
"ninemonospace": "\uFF19",
"nineoldstyle": "\uF739",
"nineparen": "\u247C",
"nineperiod": "\u2490",
"ninepersian": "\u06F9",
"nineroman": "\u2178",
"ninesuperior": "\u2079",
"nineteencircle": "\u2472",
"nineteenparen": "\u2486",
"nineteenperiod": "\u249A",
"ninethai": "\u0E59",
"nj": "\u01CC",
"njecyrillic": "\u045A",
"nkatakana": "\u30F3",
"nkatakanahalfwidth": "\uFF9D",
"nlegrightlong": "\u019E",
"nlinebelow": "\u1E49",
"nmonospace": "\uFF4E",
"nmsquare": "\u339A",
"nnabengali": "\u09A3",
"nnadeva": "\u0923",
"nnagujarati": "\u0AA3",
"nnagurmukhi": "\u0A23",
"nnnadeva": "\u0929",
"nohiragana": "\u306E",
"nokatakana": "\u30CE",
"nokatakanahalfwidth": "\uFF89",
"nonbreakingspace": "\u00A0",
"nonenthai": "\u0E13",
"nonuthai": "\u0E19",
"noonarabic": "\u0646",
"noonfinalarabic": "\uFEE6",
"noonghunnaarabic": "\u06BA",
"noonghunnafinalarabic": "\uFB9F",
"noonhehinitialarabic": "\uFEE7\uFEEC",
"nooninitialarabic": "\uFEE7",
"noonjeeminitialarabic": "\uFCD2",
"noonjeemisolatedarabic": "\uFC4B",
"noonmedialarabic": "\uFEE8",
"noonmeeminitialarabic": "\uFCD5",
"noonmeemisolatedarabic": "\uFC4E",
"noonnoonfinalarabic": "\uFC8D",
"notcontains": "\u220C",
"notelement": "\u2209",
"notelementof": "\u2209",
"notequal": "\u2260",
"notgreater": "\u226F",
"notgreaternorequal": "\u2271",
"notgreaternorless": "\u2279",
"notidentical": "\u2262",
"notless": "\u226E",
"notlessnorequal": "\u2270",
"notparallel": "\u2226",
"notprecedes": "\u2280",
"notsubset": "\u2284",
"notsucceeds": "\u2281",
"notsuperset": "\u2285",
"nowarmenian": "\u0576",
"nparen": "\u24A9",
"nssquare": "\u33B1",
"nsuperior": "\u207F",
"ntilde": "\u00F1",
"nu": "\u03BD",
"nuhiragana": "\u306C",
"nukatakana": "\u30CC",
"nukatakanahalfwidth": "\uFF87",
"nuktabengali": "\u09BC",
"nuktadeva": "\u093C",
"nuktagujarati": "\u0ABC",
"nuktagurmukhi": "\u0A3C",
"numbersign": "\u0023",
"numbersignmonospace": "\uFF03",
"numbersignsmall": "\uFE5F",
"numeralsigngreek": "\u0374",
"numeralsignlowergreek": "\u0375",
"numero": "\u2116",
"nun": "\u05E0",
"nundagesh": "\uFB40",
"nundageshhebrew": "\uFB40",
"nunhebrew": "\u05E0",
"nvsquare": "\u33B5",
"nwsquare": "\u33BB",
"nyabengali": "\u099E",
"nyadeva": "\u091E",
"nyagujarati": "\u0A9E",
"nyagurmukhi": "\u0A1E",
"o": "\u006F",
"oacute": "\u00F3",
"oangthai": "\u0E2D",
"obarred": "\u0275",
"obarredcyrillic": "\u04E9",
"obarreddieresiscyrillic": "\u04EB",
"obengali": "\u0993",
"obopomofo": "\u311B",
"obreve": "\u014F",
"ocandradeva": "\u0911",
"ocandragujarati": "\u0A91",
"ocandravowelsigndeva": "\u0949",
"ocandravowelsigngujarati": "\u0AC9",
"ocaron": "\u01D2",
"ocircle": "\u24DE",
"ocircumflex": "\u00F4",
"ocircumflexacute": "\u1ED1",
"ocircumflexdotbelow": "\u1ED9",
"ocircumflexgrave": "\u1ED3",
"ocircumflexhookabove": "\u1ED5",
"ocircumflextilde": "\u1ED7",
"ocyrillic": "\u043E",
"odblacute": "\u0151",
"odblgrave": "\u020D",
"odeva": "\u0913",
"odieresis": "\u00F6",
"odieresiscyrillic": "\u04E7",
"odotbelow": "\u1ECD",
"oe": "\u0153",
"oekorean": "\u315A",
"ogonek": "\u02DB",
"ogonekcmb": "\u0328",
"ograve": "\u00F2",
"ogujarati": "\u0A93",
"oharmenian": "\u0585",
"ohiragana": "\u304A",
"ohookabove": "\u1ECF",
"ohorn": "\u01A1",
"ohornacute": "\u1EDB",
"ohorndotbelow": "\u1EE3",
"ohorngrave": "\u1EDD",
"ohornhookabove": "\u1EDF",
"ohorntilde": "\u1EE1",
"ohungarumlaut": "\u0151",
"oi": "\u01A3",
"oinvertedbreve": "\u020F",
"okatakana": "\u30AA",
"okatakanahalfwidth": "\uFF75",
"okorean": "\u3157",
"olehebrew": "\u05AB",
"omacron": "\u014D",
"omacronacute": "\u1E53",
"omacrongrave": "\u1E51",
"omdeva": "\u0950",
"omega": "\u03C9",
"omega1": "\u03D6",
"omegacyrillic": "\u0461",
"omegalatinclosed": "\u0277",
"omegaroundcyrillic": "\u047B",
"omegatitlocyrillic": "\u047D",
"omegatonos": "\u03CE",
"omgujarati": "\u0AD0",
"omicron": "\u03BF",
"omicrontonos": "\u03CC",
"omonospace": "\uFF4F",
"one": "\u0031",
"onearabic": "\u0661",
"onebengali": "\u09E7",
"onecircle": "\u2460",
"onecircleinversesansserif": "\u278A",
"onedeva": "\u0967",
"onedotenleader": "\u2024",
"oneeighth": "\u215B",
"onefitted": "\uF6DC",
"onegujarati": "\u0AE7",
"onegurmukhi": "\u0A67",
"onehackarabic": "\u0661",
"onehalf": "\u00BD",
"onehangzhou": "\u3021",
"oneideographicparen": "\u3220",
"oneinferior": "\u2081",
"onemonospace": "\uFF11",
"onenumeratorbengali": "\u09F4",
"oneoldstyle": "\uF731",
"oneparen": "\u2474",
"oneperiod": "\u2488",
"onepersian": "\u06F1",
"onequarter": "\u00BC",
"oneroman": "\u2170",
"onesuperior": "\u00B9",
"onethai": "\u0E51",
"onethird": "\u2153",
"oogonek": "\u01EB",
"oogonekmacron": "\u01ED",
"oogurmukhi": "\u0A13",
"oomatragurmukhi": "\u0A4B",
"oopen": "\u0254",
"oparen": "\u24AA",
"openbullet": "\u25E6",
"option": "\u2325",
"ordfeminine": "\u00AA",
"ordmasculine": "\u00BA",
"orthogonal": "\u221F",
"oshortdeva": "\u0912",
"oshortvowelsigndeva": "\u094A",
"oslash": "\u00F8",
"oslashacute": "\u01FF",
"osmallhiragana": "\u3049",
"osmallkatakana": "\u30A9",
"osmallkatakanahalfwidth": "\uFF6B",
"ostrokeacute": "\u01FF",
"osuperior": "\uF6F0",
"otcyrillic": "\u047F",
"otilde": "\u00F5",
"otildeacute": "\u1E4D",
"otildedieresis": "\u1E4F",
"oubopomofo": "\u3121",
"overline": "\u203E",
"overlinecenterline": "\uFE4A",
"overlinecmb": "\u0305",
"overlinedashed": "\uFE49",
"overlinedblwavy": "\uFE4C",
"overlinewavy": "\uFE4B",
"overscore": "\u00AF",
"ovowelsignbengali": "\u09CB",
"ovowelsigndeva": "\u094B",
"ovowelsigngujarati": "\u0ACB",
"p": "\u0070",
"paampssquare": "\u3380",
"paasentosquare": "\u332B",
"pabengali": "\u09AA",
"pacute": "\u1E55",
"padeva": "\u092A",
"pagedown": "\u21DF",
"pageup": "\u21DE",
"pagujarati": "\u0AAA",
"pagurmukhi": "\u0A2A",
"pahiragana": "\u3071",
"paiyannoithai": "\u0E2F",
"pakatakana": "\u30D1",
"palatalizationcyrilliccmb": "\u0484",
"palochkacyrillic": "\u04C0",
"pansioskorean": "\u317F",
"paragraph": "\u00B6",
"parallel": "\u2225",
"parenleft": "\u0028",
"parenleftaltonearabic": "\uFD3E",
"parenleftbt": "\uF8ED",
"parenleftex": "\uF8EC",
"parenleftinferior": "\u208D",
"parenleftmonospace": "\uFF08",
"parenleftsmall": "\uFE59",
"parenleftsuperior": "\u207D",
"parenlefttp": "\uF8EB",
"parenleftvertical": "\uFE35",
"parenright": "\u0029",
"parenrightaltonearabic": "\uFD3F",
"parenrightbt": "\uF8F8",
"parenrightex": "\uF8F7",
"parenrightinferior": "\u208E",
"parenrightmonospace": "\uFF09",
"parenrightsmall": "\uFE5A",
"parenrightsuperior": "\u207E",
"parenrighttp": "\uF8F6",
"parenrightvertical": "\uFE36",
"partialdiff": "\u2202",
"paseqhebrew": "\u05C0",
"pashtahebrew": "\u0599",
"pasquare": "\u33A9",
"patah": "\u05B7",
"patah11": "\u05B7",
"patah1d": "\u05B7",
"patah2a": "\u05B7",
"patahhebrew": "\u05B7",
"patahnarrowhebrew": "\u05B7",
"patahquarterhebrew": "\u05B7",
"patahwidehebrew": "\u05B7",
"pazerhebrew": "\u05A1",
"pbopomofo": "\u3106",
"pcircle": "\u24DF",
"pdotaccent": "\u1E57",
"pe": "\u05E4",
"pecyrillic": "\u043F",
"pedagesh": "\uFB44",
"pedageshhebrew": "\uFB44",
"peezisquare": "\u333B",
"pefinaldageshhebrew": "\uFB43",
"peharabic": "\u067E",
"peharmenian": "\u057A",
"pehebrew": "\u05E4",
"pehfinalarabic": "\uFB57",
"pehinitialarabic": "\uFB58",
"pehiragana": "\u307A",
"pehmedialarabic": "\uFB59",
"pekatakana": "\u30DA",
"pemiddlehookcyrillic": "\u04A7",
"perafehebrew": "\uFB4E",
"percent": "\u0025",
"percentarabic": "\u066A",
"percentmonospace": "\uFF05",
"percentsmall": "\uFE6A",
"period": "\u002E",
"periodarmenian": "\u0589",
"periodcentered": "\u00B7",
"periodhalfwidth": "\uFF61",
"periodinferior": "\uF6E7",
"periodmonospace": "\uFF0E",
"periodsmall": "\uFE52",
"periodsuperior": "\uF6E8",
"perispomenigreekcmb": "\u0342",
"perpendicular": "\u22A5",
"perthousand": "\u2030",
"peseta": "\u20A7",
"pfsquare": "\u338A",
"phabengali": "\u09AB",
"phadeva": "\u092B",
"phagujarati": "\u0AAB",
"phagurmukhi": "\u0A2B",
"phi": "\u03C6",
"phi1": "\u03D5",
"phieuphacirclekorean": "\u327A",
"phieuphaparenkorean": "\u321A",
"phieuphcirclekorean": "\u326C",
"phieuphkorean": "\u314D",
"phieuphparenkorean": "\u320C",
"philatin": "\u0278",
"phinthuthai": "\u0E3A",
"phisymbolgreek": "\u03D5",
"phook": "\u01A5",
"phophanthai": "\u0E1E",
"phophungthai": "\u0E1C",
"phosamphaothai": "\u0E20",
"pi": "\u03C0",
"pieupacirclekorean": "\u3273",
"pieupaparenkorean": "\u3213",
"pieupcieuckorean": "\u3176",
"pieupcirclekorean": "\u3265",
"pieupkiyeokkorean": "\u3172",
"pieupkorean": "\u3142",
"pieupparenkorean": "\u3205",
"pieupsioskiyeokkorean": "\u3174",
"pieupsioskorean": "\u3144",
"pieupsiostikeutkorean": "\u3175",
"pieupthieuthkorean": "\u3177",
"pieuptikeutkorean": "\u3173",
"pihiragana": "\u3074",
"pikatakana": "\u30D4",
"pisymbolgreek": "\u03D6",
"piwrarmenian": "\u0583",
"plus": "\u002B",
"plusbelowcmb": "\u031F",
"pluscircle": "\u2295",
"plusminus": "\u00B1",
"plusmod": "\u02D6",
"plusmonospace": "\uFF0B",
"plussmall": "\uFE62",
"plussuperior": "\u207A",
"pmonospace": "\uFF50",
"pmsquare": "\u33D8",
"pohiragana": "\u307D",
"pointingindexdownwhite": "\u261F",
"pointingindexleftwhite": "\u261C",
"pointingindexrightwhite": "\u261E",
"pointingindexupwhite": "\u261D",
"pokatakana": "\u30DD",
"poplathai": "\u0E1B",
"postalmark": "\u3012",
"postalmarkface": "\u3020",
"pparen": "\u24AB",
"precedes": "\u227A",
"prescription": "\u211E",
"primemod": "\u02B9",
"primereversed": "\u2035",
"product": "\u220F",
"projective": "\u2305",
"prolongedkana": "\u30FC",
"propellor": "\u2318",
"propersubset": "\u2282",
"propersuperset": "\u2283",
"proportion": "\u2237",
"proportional": "\u221D",
"psi": "\u03C8",
"psicyrillic": "\u0471",
"psilipneumatacyrilliccmb": "\u0486",
"pssquare": "\u33B0",
"puhiragana": "\u3077",
"pukatakana": "\u30D7",
"pvsquare": "\u33B4",
"pwsquare": "\u33BA",
"q": "\u0071",
"qadeva": "\u0958",
"qadmahebrew": "\u05A8",
"qafarabic": "\u0642",
"qaffinalarabic": "\uFED6",
"qafinitialarabic": "\uFED7",
"qafmedialarabic": "\uFED8",
"qamats": "\u05B8",
"qamats10": "\u05B8",
"qamats1a": "\u05B8",
"qamats1c": "\u05B8",
"qamats27": "\u05B8",
"qamats29": "\u05B8",
"qamats33": "\u05B8",
"qamatsde": "\u05B8",
"qamatshebrew": "\u05B8",
"qamatsnarrowhebrew": "\u05B8",
"qamatsqatanhebrew": "\u05B8",
"qamatsqatannarrowhebrew": "\u05B8",
"qamatsqatanquarterhebrew": "\u05B8",
"qamatsqatanwidehebrew": "\u05B8",
"qamatsquarterhebrew": "\u05B8",
"qamatswidehebrew": "\u05B8",
"qarneyparahebrew": "\u059F",
"qbopomofo": "\u3111",
"qcircle": "\u24E0",
"qhook": "\u02A0",
"qmonospace": "\uFF51",
"qof": "\u05E7",
"qofdagesh": "\uFB47",
"qofdageshhebrew": "\uFB47",
"qofhatafpatah": "\u05E7\u05B2",
"qofhatafpatahhebrew": "\u05E7\u05B2",
"qofhatafsegol": "\u05E7\u05B1",
"qofhatafsegolhebrew": "\u05E7\u05B1",
"qofhebrew": "\u05E7",
"qofhiriq": "\u05E7\u05B4",
"qofhiriqhebrew": "\u05E7\u05B4",
"qofholam": "\u05E7\u05B9",
"qofholamhebrew": "\u05E7\u05B9",
"qofpatah": "\u05E7\u05B7",
"qofpatahhebrew": "\u05E7\u05B7",
"qofqamats": "\u05E7\u05B8",
"qofqamatshebrew": "\u05E7\u05B8",
"qofqubuts": "\u05E7\u05BB",
"qofqubutshebrew": "\u05E7\u05BB",
"qofsegol": "\u05E7\u05B6",
"qofsegolhebrew": "\u05E7\u05B6",
"qofsheva": "\u05E7\u05B0",
"qofshevahebrew": "\u05E7\u05B0",
"qoftsere": "\u05E7\u05B5",
"qoftserehebrew": "\u05E7\u05B5",
"qparen": "\u24AC",
"quarternote": "\u2669",
"qubuts": "\u05BB",
"qubuts18": "\u05BB",
"qubuts25": "\u05BB",
"qubuts31": "\u05BB",
"qubutshebrew": "\u05BB",
"qubutsnarrowhebrew": "\u05BB",
"qubutsquarterhebrew": "\u05BB",
"qubutswidehebrew": "\u05BB",
"question": "\u003F",
"questionarabic": "\u061F",
"questionarmenian": "\u055E",
"questiondown": "\u00BF",
"questiondownsmall": "\uF7BF",
"questiongreek": "\u037E",
"questionmonospace": "\uFF1F",
"questionsmall": "\uF73F",
"quotedbl": "\u0022",
"quotedblbase": "\u201E",
"quotedblleft": "\u201C",
"quotedblmonospace": "\uFF02",
"quotedblprime": "\u301E",
"quotedblprimereversed": "\u301D",
"quotedblright": "\u201D",
"quoteleft": "\u2018",
"quoteleftreversed": "\u201B",
"quotereversed": "\u201B",
"quoteright": "\u2019",
"quoterightn": "\u0149",
"quotesinglbase": "\u201A",
"quotesingle": "\u0027",
"quotesinglemonospace": "\uFF07",
"r": "\u0072",
"raarmenian": "\u057C",
"rabengali": "\u09B0",
"racute": "\u0155",
"radeva": "\u0930",
"radical": "\u221A",
"radicalex": "\uF8E5",
"radoverssquare": "\u33AE",
"radoverssquaredsquare": "\u33AF",
"radsquare": "\u33AD",
"rafe": "\u05BF",
"rafehebrew": "\u05BF",
"ragujarati": "\u0AB0",
"ragurmukhi": "\u0A30",
"rahiragana": "\u3089",
"rakatakana": "\u30E9",
"rakatakanahalfwidth": "\uFF97",
"ralowerdiagonalbengali": "\u09F1",
"ramiddlediagonalbengali": "\u09F0",
"ramshorn": "\u0264",
"ratio": "\u2236",
"rbopomofo": "\u3116",
"rcaron": "\u0159",
"rcedilla": "\u0157",
"rcircle": "\u24E1",
"rcommaaccent": "\u0157",
"rdblgrave": "\u0211",
"rdotaccent": "\u1E59",
"rdotbelow": "\u1E5B",
"rdotbelowmacron": "\u1E5D",
"referencemark": "\u203B",
"reflexsubset": "\u2286",
"reflexsuperset": "\u2287",
"registered": "\u00AE",
"registersans": "\uF8E8",
"registerserif": "\uF6DA",
"reharabic": "\u0631",
"reharmenian": "\u0580",
"rehfinalarabic": "\uFEAE",
"rehiragana": "\u308C",
"rehyehaleflamarabic": "\u0631\uFEF3\uFE8E\u0644",
"rekatakana": "\u30EC",
"rekatakanahalfwidth": "\uFF9A",
"resh": "\u05E8",
"reshdageshhebrew": "\uFB48",
"reshhatafpatah": "\u05E8\u05B2",
"reshhatafpatahhebrew": "\u05E8\u05B2",
"reshhatafsegol": "\u05E8\u05B1",
"reshhatafsegolhebrew": "\u05E8\u05B1",
"reshhebrew": "\u05E8",
"reshhiriq": "\u05E8\u05B4",
"reshhiriqhebrew": "\u05E8\u05B4",
"reshholam": "\u05E8\u05B9",
"reshholamhebrew": "\u05E8\u05B9",
"reshpatah": "\u05E8\u05B7",
"reshpatahhebrew": "\u05E8\u05B7",
"reshqamats": "\u05E8\u05B8",
"reshqamatshebrew": "\u05E8\u05B8",
"reshqubuts": "\u05E8\u05BB",
"reshqubutshebrew": "\u05E8\u05BB",
"reshsegol": "\u05E8\u05B6",
"reshsegolhebrew": "\u05E8\u05B6",
"reshsheva": "\u05E8\u05B0",
"reshshevahebrew": "\u05E8\u05B0",
"reshtsere": "\u05E8\u05B5",
"reshtserehebrew": "\u05E8\u05B5",
"reversedtilde": "\u223D",
"reviahebrew": "\u0597",
"reviamugrashhebrew": "\u0597",
"revlogicalnot": "\u2310",
"rfishhook": "\u027E",
"rfishhookreversed": "\u027F",
"rhabengali": "\u09DD",
"rhadeva": "\u095D",
"rho": "\u03C1",
"rhook": "\u027D",
"rhookturned": "\u027B",
"rhookturnedsuperior": "\u02B5",
"rhosymbolgreek": "\u03F1",
"rhotichookmod": "\u02DE",
"rieulacirclekorean": "\u3271",
"rieulaparenkorean": "\u3211",
"rieulcirclekorean": "\u3263",
"rieulhieuhkorean": "\u3140",
"rieulkiyeokkorean": "\u313A",
"rieulkiyeoksioskorean": "\u3169",
"rieulkorean": "\u3139",
"rieulmieumkorean": "\u313B",
"rieulpansioskorean": "\u316C",
"rieulparenkorean": "\u3203",
"rieulphieuphkorean": "\u313F",
"rieulpieupkorean": "\u313C",
"rieulpieupsioskorean": "\u316B",
"rieulsioskorean": "\u313D",
"rieulthieuthkorean": "\u313E",
"rieultikeutkorean": "\u316A",
"rieulyeorinhieuhkorean": "\u316D",
"rightangle": "\u221F",
"righttackbelowcmb": "\u0319",
"righttriangle": "\u22BF",
"rihiragana": "\u308A",
"rikatakana": "\u30EA",
"rikatakanahalfwidth": "\uFF98",
"ring": "\u02DA",
"ringbelowcmb": "\u0325",
"ringcmb": "\u030A",
"ringhalfleft": "\u02BF",
"ringhalfleftarmenian": "\u0559",
"ringhalfleftbelowcmb": "\u031C",
"ringhalfleftcentered": "\u02D3",
"ringhalfright": "\u02BE",
"ringhalfrightbelowcmb": "\u0339",
"ringhalfrightcentered": "\u02D2",
"rinvertedbreve": "\u0213",
"rittorusquare": "\u3351",
"rlinebelow": "\u1E5F",
"rlongleg": "\u027C",
"rlonglegturned": "\u027A",
"rmonospace": "\uFF52",
"rohiragana": "\u308D",
"rokatakana": "\u30ED",
"rokatakanahalfwidth": "\uFF9B",
"roruathai": "\u0E23",
"rparen": "\u24AD",
"rrabengali": "\u09DC",
"rradeva": "\u0931",
"rragurmukhi": "\u0A5C",
"rreharabic": "\u0691",
"rrehfinalarabic": "\uFB8D",
"rrvocalicbengali": "\u09E0",
"rrvocalicdeva": "\u0960",
"rrvocalicgujarati": "\u0AE0",
"rrvocalicvowelsignbengali": "\u09C4",
"rrvocalicvowelsigndeva": "\u0944",
"rrvocalicvowelsigngujarati": "\u0AC4",
"rsuperior": "\uF6F1",
"rtblock": "\u2590",
"rturned": "\u0279",
"rturnedsuperior": "\u02B4",
"ruhiragana": "\u308B",
"rukatakana": "\u30EB",
"rukatakanahalfwidth": "\uFF99",
"rupeemarkbengali": "\u09F2",
"rupeesignbengali": "\u09F3",
"rupiah": "\uF6DD",
"ruthai": "\u0E24",
"rvocalicbengali": "\u098B",
"rvocalicdeva": "\u090B",
"rvocalicgujarati": "\u0A8B",
"rvocalicvowelsignbengali": "\u09C3",
"rvocalicvowelsigndeva": "\u0943",
"rvocalicvowelsigngujarati": "\u0AC3",
"s": "\u0073",
"sabengali": "\u09B8",
"sacute": "\u015B",
"sacutedotaccent": "\u1E65",
"sadarabic": "\u0635",
"sadeva": "\u0938",
"sadfinalarabic": "\uFEBA",
"sadinitialarabic": "\uFEBB",
"sadmedialarabic": "\uFEBC",
"sagujarati": "\u0AB8",
"sagurmukhi": "\u0A38",
"sahiragana": "\u3055",
"sakatakana": "\u30B5",
"sakatakanahalfwidth": "\uFF7B",
"sallallahoualayhewasallamarabic": "\uFDFA",
"samekh": "\u05E1",
"samekhdagesh": "\uFB41",
"samekhdageshhebrew": "\uFB41",
"samekhhebrew": "\u05E1",
"saraaathai": "\u0E32",
"saraaethai": "\u0E41",
"saraaimaimalaithai": "\u0E44",
"saraaimaimuanthai": "\u0E43",
"saraamthai": "\u0E33",
"saraathai": "\u0E30",
"saraethai": "\u0E40",
"saraiileftthai": "\uF886",
"saraiithai": "\u0E35",
"saraileftthai": "\uF885",
"saraithai": "\u0E34",
"saraothai": "\u0E42",
"saraueeleftthai": "\uF888",
"saraueethai": "\u0E37",
"saraueleftthai": "\uF887",
"sarauethai": "\u0E36",
"sarauthai": "\u0E38",
"sarauuthai": "\u0E39",
"sbopomofo": "\u3119",
"scaron": "\u0161",
"scarondotaccent": "\u1E67",
"scedilla": "\u015F",
"schwa": "\u0259",
"schwacyrillic": "\u04D9",
"schwadieresiscyrillic": "\u04DB",
"schwahook": "\u025A",
"scircle": "\u24E2",
"scircumflex": "\u015D",
"scommaaccent": "\u0219",
"sdotaccent": "\u1E61",
"sdotbelow": "\u1E63",
"sdotbelowdotaccent": "\u1E69",
"seagullbelowcmb": "\u033C",
"second": "\u2033",
"secondtonechinese": "\u02CA",
"section": "\u00A7",
"seenarabic": "\u0633",
"seenfinalarabic": "\uFEB2",
"seeninitialarabic": "\uFEB3",
"seenmedialarabic": "\uFEB4",
"segol": "\u05B6",
"segol13": "\u05B6",
"segol1f": "\u05B6",
"segol2c": "\u05B6",
"segolhebrew": "\u05B6",
"segolnarrowhebrew": "\u05B6",
"segolquarterhebrew": "\u05B6",
"segoltahebrew": "\u0592",
"segolwidehebrew": "\u05B6",
"seharmenian": "\u057D",
"sehiragana": "\u305B",
"sekatakana": "\u30BB",
"sekatakanahalfwidth": "\uFF7E",
"semicolon": "\u003B",
"semicolonarabic": "\u061B",
"semicolonmonospace": "\uFF1B",
"semicolonsmall": "\uFE54",
"semivoicedmarkkana": "\u309C",
"semivoicedmarkkanahalfwidth": "\uFF9F",
"sentisquare": "\u3322",
"sentosquare": "\u3323",
"seven": "\u0037",
"sevenarabic": "\u0667",
"sevenbengali": "\u09ED",
"sevencircle": "\u2466",
"sevencircleinversesansserif": "\u2790",
"sevendeva": "\u096D",
"seveneighths": "\u215E",
"sevengujarati": "\u0AED",
"sevengurmukhi": "\u0A6D",
"sevenhackarabic": "\u0667",
"sevenhangzhou": "\u3027",
"sevenideographicparen": "\u3226",
"seveninferior": "\u2087",
"sevenmonospace": "\uFF17",
"sevenoldstyle": "\uF737",
"sevenparen": "\u247A",
"sevenperiod": "\u248E",
"sevenpersian": "\u06F7",
"sevenroman": "\u2176",
"sevensuperior": "\u2077",
"seventeencircle": "\u2470",
"seventeenparen": "\u2484",
"seventeenperiod": "\u2498",
"seventhai": "\u0E57",
"sfthyphen": "\u00AD",
"shaarmenian": "\u0577",
"shabengali": "\u09B6",
"shacyrillic": "\u0448",
"shaddaarabic": "\u0651",
"shaddadammaarabic": "\uFC61",
"shaddadammatanarabic": "\uFC5E",
"shaddafathaarabic": "\uFC60",
"shaddafathatanarabic": "\u0651\u064B",
"shaddakasraarabic": "\uFC62",
"shaddakasratanarabic": "\uFC5F",
"shade": "\u2592",
"shadedark": "\u2593",
"shadelight": "\u2591",
"shademedium": "\u2592",
"shadeva": "\u0936",
"shagujarati": "\u0AB6",
"shagurmukhi": "\u0A36",
"shalshelethebrew": "\u0593",
"shbopomofo": "\u3115",
"shchacyrillic": "\u0449",
"sheenarabic": "\u0634",
"sheenfinalarabic": "\uFEB6",
"sheeninitialarabic": "\uFEB7",
"sheenmedialarabic": "\uFEB8",
"sheicoptic": "\u03E3",
"sheqel": "\u20AA",
"sheqelhebrew": "\u20AA",
"sheva": "\u05B0",
"sheva115": "\u05B0",
"sheva15": "\u05B0",
"sheva22": "\u05B0",
"sheva2e": "\u05B0",
"shevahebrew": "\u05B0",
"shevanarrowhebrew": "\u05B0",
"shevaquarterhebrew": "\u05B0",
"shevawidehebrew": "\u05B0",
"shhacyrillic": "\u04BB",
"shimacoptic": "\u03ED",
"shin": "\u05E9",
"shindagesh": "\uFB49",
"shindageshhebrew": "\uFB49",
"shindageshshindot": "\uFB2C",
"shindageshshindothebrew": "\uFB2C",
"shindageshsindot": "\uFB2D",
"shindageshsindothebrew": "\uFB2D",
"shindothebrew": "\u05C1",
"shinhebrew": "\u05E9",
"shinshindot": "\uFB2A",
"shinshindothebrew": "\uFB2A",
"shinsindot": "\uFB2B",
"shinsindothebrew": "\uFB2B",
"shook": "\u0282",
"sigma": "\u03C3",
"sigma1": "\u03C2",
"sigmafinal": "\u03C2",
"sigmalunatesymbolgreek": "\u03F2",
"sihiragana": "\u3057",
"sikatakana": "\u30B7",
"sikatakanahalfwidth": "\uFF7C",
"siluqhebrew": "\u05BD",
"siluqlefthebrew": "\u05BD",
"similar": "\u223C",
"sindothebrew": "\u05C2",
"siosacirclekorean": "\u3274",
"siosaparenkorean": "\u3214",
"sioscieuckorean": "\u317E",
"sioscirclekorean": "\u3266",
"sioskiyeokkorean": "\u317A",
"sioskorean": "\u3145",
"siosnieunkorean": "\u317B",
"siosparenkorean": "\u3206",
"siospieupkorean": "\u317D",
"siostikeutkorean": "\u317C",
"six": "\u0036",
"sixarabic": "\u0666",
"sixbengali": "\u09EC",
"sixcircle": "\u2465",
"sixcircleinversesansserif": "\u278F",
"sixdeva": "\u096C",
"sixgujarati": "\u0AEC",
"sixgurmukhi": "\u0A6C",
"sixhackarabic": "\u0666",
"sixhangzhou": "\u3026",
"sixideographicparen": "\u3225",
"sixinferior": "\u2086",
"sixmonospace": "\uFF16",
"sixoldstyle": "\uF736",
"sixparen": "\u2479",
"sixperiod": "\u248D",
"sixpersian": "\u06F6",
"sixroman": "\u2175",
"sixsuperior": "\u2076",
"sixteencircle": "\u246F",
"sixteencurrencydenominatorbengali": "\u09F9",
"sixteenparen": "\u2483",
"sixteenperiod": "\u2497",
"sixthai": "\u0E56",
"slash": "\u002F",
"slashmonospace": "\uFF0F",
"slong": "\u017F",
"slongdotaccent": "\u1E9B",
"smileface": "\u263A",
"smonospace": "\uFF53",
"sofpasuqhebrew": "\u05C3",
"softhyphen": "\u00AD",
"softsigncyrillic": "\u044C",
"sohiragana": "\u305D",
"sokatakana": "\u30BD",
"sokatakanahalfwidth": "\uFF7F",
"soliduslongoverlaycmb": "\u0338",
"solidusshortoverlaycmb": "\u0337",
"sorusithai": "\u0E29",
"sosalathai": "\u0E28",
"sosothai": "\u0E0B",
"sosuathai": "\u0E2A",
"space": "\u0020",
"spacehackarabic": "\u0020",
"spade": "\u2660",
"spadesuitblack": "\u2660",
"spadesuitwhite": "\u2664",
"sparen": "\u24AE",
"squarebelowcmb": "\u033B",
"squarecc": "\u33C4",
"squarecm": "\u339D",
"squarediagonalcrosshatchfill": "\u25A9",
"squarehorizontalfill": "\u25A4",
"squarekg": "\u338F",
"squarekm": "\u339E",
"squarekmcapital": "\u33CE",
"squareln": "\u33D1",
"squarelog": "\u33D2",
"squaremg": "\u338E",
"squaremil": "\u33D5",
"squaremm": "\u339C",
"squaremsquared": "\u33A1",
"squareorthogonalcrosshatchfill": "\u25A6",
"squareupperlefttolowerrightfill": "\u25A7",
"squareupperrighttolowerleftfill": "\u25A8",
"squareverticalfill": "\u25A5",
"squarewhitewithsmallblack": "\u25A3",
"srsquare": "\u33DB",
"ssabengali": "\u09B7",
"ssadeva": "\u0937",
"ssagujarati": "\u0AB7",
"ssangcieuckorean": "\u3149",
"ssanghieuhkorean": "\u3185",
"ssangieungkorean": "\u3180",
"ssangkiyeokkorean": "\u3132",
"ssangnieunkorean": "\u3165",
"ssangpieupkorean": "\u3143",
"ssangsioskorean": "\u3146",
"ssangtikeutkorean": "\u3138",
"ssuperior": "\uF6F2",
"sterling": "\u00A3",
"sterlingmonospace": "\uFFE1",
"strokelongoverlaycmb": "\u0336",
"strokeshortoverlaycmb": "\u0335",
"subset": "\u2282",
"subsetnotequal": "\u228A",
"subsetorequal": "\u2286",
"succeeds": "\u227B",
"suchthat": "\u220B",
"suhiragana": "\u3059",
"sukatakana": "\u30B9",
"sukatakanahalfwidth": "\uFF7D",
"sukunarabic": "\u0652",
"summation": "\u2211",
"sun": "\u263C",
"superset": "\u2283",
"supersetnotequal": "\u228B",
"supersetorequal": "\u2287",
"svsquare": "\u33DC",
"syouwaerasquare": "\u337C",
"t": "\u0074",
"tabengali": "\u09A4",
"tackdown": "\u22A4",
"tackleft": "\u22A3",
"tadeva": "\u0924",
"tagujarati": "\u0AA4",
"tagurmukhi": "\u0A24",
"taharabic": "\u0637",
"tahfinalarabic": "\uFEC2",
"tahinitialarabic": "\uFEC3",
"tahiragana": "\u305F",
"tahmedialarabic": "\uFEC4",
"taisyouerasquare": "\u337D",
"takatakana": "\u30BF",
"takatakanahalfwidth": "\uFF80",
"tatweelarabic": "\u0640",
"tau": "\u03C4",
"tav": "\u05EA",
"tavdages": "\uFB4A",
"tavdagesh": "\uFB4A",
"tavdageshhebrew": "\uFB4A",
"tavhebrew": "\u05EA",
"tbar": "\u0167",
"tbopomofo": "\u310A",
"tcaron": "\u0165",
"tccurl": "\u02A8",
"tcedilla": "\u0163",
"tcheharabic": "\u0686",
"tchehfinalarabic": "\uFB7B",
"tchehinitialarabic": "\uFB7C",
"tchehmedialarabic": "\uFB7D",
"tchehmeeminitialarabic": "\uFB7C\uFEE4",
"tcircle": "\u24E3",
"tcircumflexbelow": "\u1E71",
"tcommaaccent": "\u0163",
"tdieresis": "\u1E97",
"tdotaccent": "\u1E6B",
"tdotbelow": "\u1E6D",
"tecyrillic": "\u0442",
"tedescendercyrillic": "\u04AD",
"teharabic": "\u062A",
"tehfinalarabic": "\uFE96",
"tehhahinitialarabic": "\uFCA2",
"tehhahisolatedarabic": "\uFC0C",
"tehinitialarabic": "\uFE97",
"tehiragana": "\u3066",
"tehjeeminitialarabic": "\uFCA1",
"tehjeemisolatedarabic": "\uFC0B",
"tehmarbutaarabic": "\u0629",
"tehmarbutafinalarabic": "\uFE94",
"tehmedialarabic": "\uFE98",
"tehmeeminitialarabic": "\uFCA4",
"tehmeemisolatedarabic": "\uFC0E",
"tehnoonfinalarabic": "\uFC73",
"tekatakana": "\u30C6",
"tekatakanahalfwidth": "\uFF83",
"telephone": "\u2121",
"telephoneblack": "\u260E",
"telishagedolahebrew": "\u05A0",
"telishaqetanahebrew": "\u05A9",
"tencircle": "\u2469",
"tenideographicparen": "\u3229",
"tenparen": "\u247D",
"tenperiod": "\u2491",
"tenroman": "\u2179",
"tesh": "\u02A7",
"tet": "\u05D8",
"tetdagesh": "\uFB38",
"tetdageshhebrew": "\uFB38",
"tethebrew": "\u05D8",
"tetsecyrillic": "\u04B5",
"tevirhebrew": "\u059B",
"tevirlefthebrew": "\u059B",
"thabengali": "\u09A5",
"thadeva": "\u0925",
"thagujarati": "\u0AA5",
"thagurmukhi": "\u0A25",
"thalarabic": "\u0630",
"thalfinalarabic": "\uFEAC",
"thanthakhatlowleftthai": "\uF898",
"thanthakhatlowrightthai": "\uF897",
"thanthakhatthai": "\u0E4C",
"thanthakhatupperleftthai": "\uF896",
"theharabic": "\u062B",
"thehfinalarabic": "\uFE9A",
"thehinitialarabic": "\uFE9B",
"thehmedialarabic": "\uFE9C",
"thereexists": "\u2203",
"therefore": "\u2234",
"theta": "\u03B8",
"theta1": "\u03D1",
"thetasymbolgreek": "\u03D1",
"thieuthacirclekorean": "\u3279",
"thieuthaparenkorean": "\u3219",
"thieuthcirclekorean": "\u326B",
"thieuthkorean": "\u314C",
"thieuthparenkorean": "\u320B",
"thirteencircle": "\u246C",
"thirteenparen": "\u2480",
"thirteenperiod": "\u2494",
"thonangmonthothai": "\u0E11",
"thook": "\u01AD",
"thophuthaothai": "\u0E12",
"thorn": "\u00FE",
"thothahanthai": "\u0E17",
"thothanthai": "\u0E10",
"thothongthai": "\u0E18",
"thothungthai": "\u0E16",
"thousandcyrillic": "\u0482",
"thousandsseparatorarabic": "\u066C",
"thousandsseparatorpersian": "\u066C",
"three": "\u0033",
"threearabic": "\u0663",
"threebengali": "\u09E9",
"threecircle": "\u2462",
"threecircleinversesansserif": "\u278C",
"threedeva": "\u0969",
"threeeighths": "\u215C",
"threegujarati": "\u0AE9",
"threegurmukhi": "\u0A69",
"threehackarabic": "\u0663",
"threehangzhou": "\u3023",
"threeideographicparen": "\u3222",
"threeinferior": "\u2083",
"threemonospace": "\uFF13",
"threenumeratorbengali": "\u09F6",
"threeoldstyle": "\uF733",
"threeparen": "\u2476",
"threeperiod": "\u248A",
"threepersian": "\u06F3",
"threequarters": "\u00BE",
"threequartersemdash": "\uF6DE",
"threeroman": "\u2172",
"threesuperior": "\u00B3",
"threethai": "\u0E53",
"thzsquare": "\u3394",
"tihiragana": "\u3061",
"tikatakana": "\u30C1",
"tikatakanahalfwidth": "\uFF81",
"tikeutacirclekorean": "\u3270",
"tikeutaparenkorean": "\u3210",
"tikeutcirclekorean": "\u3262",
"tikeutkorean": "\u3137",
"tikeutparenkorean": "\u3202",
"tilde": "\u02DC",
"tildebelowcmb": "\u0330",
"tildecmb": "\u0303",
"tildecomb": "\u0303",
"tildedoublecmb": "\u0360",
"tildeoperator": "\u223C",
"tildeoverlaycmb": "\u0334",
"tildeverticalcmb": "\u033E",
"timescircle": "\u2297",
"tipehahebrew": "\u0596",
"tipehalefthebrew": "\u0596",
"tippigurmukhi": "\u0A70",
"titlocyrilliccmb": "\u0483",
"tiwnarmenian": "\u057F",
"tlinebelow": "\u1E6F",
"tmonospace": "\uFF54",
"toarmenian": "\u0569",
"tohiragana": "\u3068",
"tokatakana": "\u30C8",
"tokatakanahalfwidth": "\uFF84",
"tonebarextrahighmod": "\u02E5",
"tonebarextralowmod": "\u02E9",
"tonebarhighmod": "\u02E6",
"tonebarlowmod": "\u02E8",
"tonebarmidmod": "\u02E7",
"tonefive": "\u01BD",
"tonesix": "\u0185",
"tonetwo": "\u01A8",
"tonos": "\u0384",
"tonsquare": "\u3327",
"topatakthai": "\u0E0F",
"tortoiseshellbracketleft": "\u3014",
"tortoiseshellbracketleftsmall": "\uFE5D",
"tortoiseshellbracketleftvertical": "\uFE39",
"tortoiseshellbracketright": "\u3015",
"tortoiseshellbracketrightsmall": "\uFE5E",
"tortoiseshellbracketrightvertical": "\uFE3A",
"totaothai": "\u0E15",
"tpalatalhook": "\u01AB",
"tparen": "\u24AF",
"trademark": "\u2122",
"trademarksans": "\uF8EA",
"trademarkserif": "\uF6DB",
"tretroflexhook": "\u0288",
"triagdn": "\u25BC",
"triaglf": "\u25C4",
"triagrt": "\u25BA",
"triagup": "\u25B2",
"ts": "\u02A6",
"tsadi": "\u05E6",
"tsadidagesh": "\uFB46",
"tsadidageshhebrew": "\uFB46",
"tsadihebrew": "\u05E6",
"tsecyrillic": "\u0446",
"tsere": "\u05B5",
"tsere12": "\u05B5",
"tsere1e": "\u05B5",
"tsere2b": "\u05B5",
"tserehebrew": "\u05B5",
"tserenarrowhebrew": "\u05B5",
"tserequarterhebrew": "\u05B5",
"tserewidehebrew": "\u05B5",
"tshecyrillic": "\u045B",
"tsuperior": "\uF6F3",
"ttabengali": "\u099F",
"ttadeva": "\u091F",
"ttagujarati": "\u0A9F",
"ttagurmukhi": "\u0A1F",
"tteharabic": "\u0679",
"ttehfinalarabic": "\uFB67",
"ttehinitialarabic": "\uFB68",
"ttehmedialarabic": "\uFB69",
"tthabengali": "\u09A0",
"tthadeva": "\u0920",
"tthagujarati": "\u0AA0",
"tthagurmukhi": "\u0A20",
"tturned": "\u0287",
"tuhiragana": "\u3064",
"tukatakana": "\u30C4",
"tukatakanahalfwidth": "\uFF82",
"tusmallhiragana": "\u3063",
"tusmallkatakana": "\u30C3",
"tusmallkatakanahalfwidth": "\uFF6F",
"twelvecircle": "\u246B",
"twelveparen": "\u247F",
"twelveperiod": "\u2493",
"twelveroman": "\u217B",
"twentycircle": "\u2473",
"twentyhangzhou": "\u5344",
"twentyparen": "\u2487",
"twentyperiod": "\u249B",
"two": "\u0032",
"twoarabic": "\u0662",
"twobengali": "\u09E8",
"twocircle": "\u2461",
"twocircleinversesansserif": "\u278B",
"twodeva": "\u0968",
"twodotenleader": "\u2025",
"twodotleader": "\u2025",
"twodotleadervertical": "\uFE30",
"twogujarati": "\u0AE8",
"twogurmukhi": "\u0A68",
"twohackarabic": "\u0662",
"twohangzhou": "\u3022",
"twoideographicparen": "\u3221",
"twoinferior": "\u2082",
"twomonospace": "\uFF12",
"twonumeratorbengali": "\u09F5",
"twooldstyle": "\uF732",
"twoparen": "\u2475",
"twoperiod": "\u2489",
"twopersian": "\u06F2",
"tworoman": "\u2171",
"twostroke": "\u01BB",
"twosuperior": "\u00B2",
"twothai": "\u0E52",
"twothirds": "\u2154",
"u": "\u0075",
"uacute": "\u00FA",
"ubar": "\u0289",
"ubengali": "\u0989",
"ubopomofo": "\u3128",
"ubreve": "\u016D",
"ucaron": "\u01D4",
"ucircle": "\u24E4",
"ucircumflex": "\u00FB",
"ucircumflexbelow": "\u1E77",
"ucyrillic": "\u0443",
"udattadeva": "\u0951",
"udblacute": "\u0171",
"udblgrave": "\u0215",
"udeva": "\u0909",
"udieresis": "\u00FC",
"udieresisacute": "\u01D8",
"udieresisbelow": "\u1E73",
"udieresiscaron": "\u01DA",
"udieresiscyrillic": "\u04F1",
"udieresisgrave": "\u01DC",
"udieresismacron": "\u01D6",
"udotbelow": "\u1EE5",
"ugrave": "\u00F9",
"ugujarati": "\u0A89",
"ugurmukhi": "\u0A09",
"uhiragana": "\u3046",
"uhookabove": "\u1EE7",
"uhorn": "\u01B0",
"uhornacute": "\u1EE9",
"uhorndotbelow": "\u1EF1",
"uhorngrave": "\u1EEB",
"uhornhookabove": "\u1EED",
"uhorntilde": "\u1EEF",
"uhungarumlaut": "\u0171",
"uhungarumlautcyrillic": "\u04F3",
"uinvertedbreve": "\u0217",
"ukatakana": "\u30A6",
"ukatakanahalfwidth": "\uFF73",
"ukcyrillic": "\u0479",
"ukorean": "\u315C",
"umacron": "\u016B",
"umacroncyrillic": "\u04EF",
"umacrondieresis": "\u1E7B",
"umatragurmukhi": "\u0A41",
"umonospace": "\uFF55",
"underscore": "\u005F",
"underscoredbl": "\u2017",
"underscoremonospace": "\uFF3F",
"underscorevertical": "\uFE33",
"underscorewavy": "\uFE4F",
"union": "\u222A",
"universal": "\u2200",
"uogonek": "\u0173",
"uparen": "\u24B0",
"upblock": "\u2580",
"upperdothebrew": "\u05C4",
"upsilon": "\u03C5",
"upsilondieresis": "\u03CB",
"upsilondieresistonos": "\u03B0",
"upsilonlatin": "\u028A",
"upsilontonos": "\u03CD",
"uptackbelowcmb": "\u031D",
"uptackmod": "\u02D4",
"uragurmukhi": "\u0A73",
"uring": "\u016F",
"ushortcyrillic": "\u045E",
"usmallhiragana": "\u3045",
"usmallkatakana": "\u30A5",
"usmallkatakanahalfwidth": "\uFF69",
"ustraightcyrillic": "\u04AF",
"ustraightstrokecyrillic": "\u04B1",
"utilde": "\u0169",
"utildeacute": "\u1E79",
"utildebelow": "\u1E75",
"uubengali": "\u098A",
"uudeva": "\u090A",
"uugujarati": "\u0A8A",
"uugurmukhi": "\u0A0A",
"uumatragurmukhi": "\u0A42",
"uuvowelsignbengali": "\u09C2",
"uuvowelsigndeva": "\u0942",
"uuvowelsigngujarati": "\u0AC2",
"uvowelsignbengali": "\u09C1",
"uvowelsigndeva": "\u0941",
"uvowelsigngujarati": "\u0AC1",
"v": "\u0076",
"vadeva": "\u0935",
"vagujarati": "\u0AB5",
"vagurmukhi": "\u0A35",
"vakatakana": "\u30F7",
"vav": "\u05D5",
"vavdagesh": "\uFB35",
"vavdagesh65": "\uFB35",
"vavdageshhebrew": "\uFB35",
"vavhebrew": "\u05D5",
"vavholam": "\uFB4B",
"vavholamhebrew": "\uFB4B",
"vavvavhebrew": "\u05F0",
"vavyodhebrew": "\u05F1",
"vcircle": "\u24E5",
"vdotbelow": "\u1E7F",
"vecyrillic": "\u0432",
"veharabic": "\u06A4",
"vehfinalarabic": "\uFB6B",
"vehinitialarabic": "\uFB6C",
"vehmedialarabic": "\uFB6D",
"vekatakana": "\u30F9",
"venus": "\u2640",
"verticalbar": "\u007C",
"verticallineabovecmb": "\u030D",
"verticallinebelowcmb": "\u0329",
"verticallinelowmod": "\u02CC",
"verticallinemod": "\u02C8",
"vewarmenian": "\u057E",
"vhook": "\u028B",
"vikatakana": "\u30F8",
"viramabengali": "\u09CD",
"viramadeva": "\u094D",
"viramagujarati": "\u0ACD",
"visargabengali": "\u0983",
"visargadeva": "\u0903",
"visargagujarati": "\u0A83",
"vmonospace": "\uFF56",
"voarmenian": "\u0578",
"voicediterationhiragana": "\u309E",
"voicediterationkatakana": "\u30FE",
"voicedmarkkana": "\u309B",
"voicedmarkkanahalfwidth": "\uFF9E",
"vokatakana": "\u30FA",
"vparen": "\u24B1",
"vtilde": "\u1E7D",
"vturned": "\u028C",
"vuhiragana": "\u3094",
"vukatakana": "\u30F4",
"w": "\u0077",
"wacute": "\u1E83",
"waekorean": "\u3159",
"wahiragana": "\u308F",
"wakatakana": "\u30EF",
"wakatakanahalfwidth": "\uFF9C",
"wakorean": "\u3158",
"wasmallhiragana": "\u308E",
"wasmallkatakana": "\u30EE",
"wattosquare": "\u3357",
"wavedash": "\u301C",
"wavyunderscorevertical": "\uFE34",
"wawarabic": "\u0648",
"wawfinalarabic": "\uFEEE",
"wawhamzaabovearabic": "\u0624",
"wawhamzaabovefinalarabic": "\uFE86",
"wbsquare": "\u33DD",
"wcircle": "\u24E6",
"wcircumflex": "\u0175",
"wdieresis": "\u1E85",
"wdotaccent": "\u1E87",
"wdotbelow": "\u1E89",
"wehiragana": "\u3091",
"weierstrass": "\u2118",
"wekatakana": "\u30F1",
"wekorean": "\u315E",
"weokorean": "\u315D",
"wgrave": "\u1E81",
"whitebullet": "\u25E6",
"whitecircle": "\u25CB",
"whitecircleinverse": "\u25D9",
"whitecornerbracketleft": "\u300E",
"whitecornerbracketleftvertical": "\uFE43",
"whitecornerbracketright": "\u300F",
"whitecornerbracketrightvertical": "\uFE44",
"whitediamond": "\u25C7",
"whitediamondcontainingblacksmalldiamond": "\u25C8",
"whitedownpointingsmalltriangle": "\u25BF",
"whitedownpointingtriangle": "\u25BD",
"whiteleftpointingsmalltriangle": "\u25C3",
"whiteleftpointingtriangle": "\u25C1",
"whitelenticularbracketleft": "\u3016",
"whitelenticularbracketright": "\u3017",
"whiterightpointingsmalltriangle": "\u25B9",
"whiterightpointingtriangle": "\u25B7",
"whitesmallsquare": "\u25AB",
"whitesmilingface": "\u263A",
"whitesquare": "\u25A1",
"whitestar": "\u2606",
"whitetelephone": "\u260F",
"whitetortoiseshellbracketleft": "\u3018",
"whitetortoiseshellbracketright": "\u3019",
"whiteuppointingsmalltriangle": "\u25B5",
"whiteuppointingtriangle": "\u25B3",
"wihiragana": "\u3090",
"wikatakana": "\u30F0",
"wikorean": "\u315F",
"wmonospace": "\uFF57",
"wohiragana": "\u3092",
"wokatakana": "\u30F2",
"wokatakanahalfwidth": "\uFF66",
"won": "\u20A9",
"wonmonospace": "\uFFE6",
"wowaenthai": "\u0E27",
"wparen": "\u24B2",
"wring": "\u1E98",
"wsuperior": "\u02B7",
"wturned": "\u028D",
"wynn": "\u01BF",
"x": "\u0078",
"xabovecmb": "\u033D",
"xbopomofo": "\u3112",
"xcircle": "\u24E7",
"xdieresis": "\u1E8D",
"xdotaccent": "\u1E8B",
"xeharmenian": "\u056D",
"xi": "\u03BE",
"xmonospace": "\uFF58",
"xparen": "\u24B3",
"xsuperior": "\u02E3",
"y": "\u0079",
"yaadosquare": "\u334E",
"yabengali": "\u09AF",
"yacute": "\u00FD",
"yadeva": "\u092F",
"yaekorean": "\u3152",
"yagujarati": "\u0AAF",
"yagurmukhi": "\u0A2F",
"yahiragana": "\u3084",
"yakatakana": "\u30E4",
"yakatakanahalfwidth": "\uFF94",
"yakorean": "\u3151",
"yamakkanthai": "\u0E4E",
"yasmallhiragana": "\u3083",
"yasmallkatakana": "\u30E3",
"yasmallkatakanahalfwidth": "\uFF6C",
"yatcyrillic": "\u0463",
"ycircle": "\u24E8",
"ycircumflex": "\u0177",
"ydieresis": "\u00FF",
"ydotaccent": "\u1E8F",
"ydotbelow": "\u1EF5",
"yeharabic": "\u064A",
"yehbarreearabic": "\u06D2",
"yehbarreefinalarabic": "\uFBAF",
"yehfinalarabic": "\uFEF2",
"yehhamzaabovearabic": "\u0626",
"yehhamzaabovefinalarabic": "\uFE8A",
"yehhamzaaboveinitialarabic": "\uFE8B",
"yehhamzaabovemedialarabic": "\uFE8C",
"yehinitialarabic": "\uFEF3",
"yehmedialarabic": "\uFEF4",
"yehmeeminitialarabic": "\uFCDD",
"yehmeemisolatedarabic": "\uFC58",
"yehnoonfinalarabic": "\uFC94",
"yehthreedotsbelowarabic": "\u06D1",
"yekorean": "\u3156",
"yen": "\u00A5",
"yenmonospace": "\uFFE5",
"yeokorean": "\u3155",
"yeorinhieuhkorean": "\u3186",
"yerahbenyomohebrew": "\u05AA",
"yerahbenyomolefthebrew": "\u05AA",
"yericyrillic": "\u044B",
"yerudieresiscyrillic": "\u04F9",
"yesieungkorean": "\u3181",
"yesieungpansioskorean": "\u3183",
"yesieungsioskorean": "\u3182",
"yetivhebrew": "\u059A",
"ygrave": "\u1EF3",
"yhook": "\u01B4",
"yhookabove": "\u1EF7",
"yiarmenian": "\u0575",
"yicyrillic": "\u0457",
"yikorean": "\u3162",
"yinyang": "\u262F",
"yiwnarmenian": "\u0582",
"ymonospace": "\uFF59",
"yod": "\u05D9",
"yoddagesh": "\uFB39",
"yoddageshhebrew": "\uFB39",
"yodhebrew": "\u05D9",
"yodyodhebrew": "\u05F2",
"yodyodpatahhebrew": "\uFB1F",
"yohiragana": "\u3088",
"yoikorean": "\u3189",
"yokatakana": "\u30E8",
"yokatakanahalfwidth": "\uFF96",
"yokorean": "\u315B",
"yosmallhiragana": "\u3087",
"yosmallkatakana": "\u30E7",
"yosmallkatakanahalfwidth": "\uFF6E",
"yotgreek": "\u03F3",
"yoyaekorean": "\u3188",
"yoyakorean": "\u3187",
"yoyakthai": "\u0E22",
"yoyingthai": "\u0E0D",
"yparen": "\u24B4",
"ypogegrammeni": "\u037A",
"ypogegrammenigreekcmb": "\u0345",
"yr": "\u01A6",
"yring": "\u1E99",
"ysuperior": "\u02B8",
"ytilde": "\u1EF9",
"yturned": "\u028E",
"yuhiragana": "\u3086",
"yuikorean": "\u318C",
"yukatakana": "\u30E6",
"yukatakanahalfwidth": "\uFF95",
"yukorean": "\u3160",
"yusbigcyrillic": "\u046B",
"yusbigiotifiedcyrillic": "\u046D",
"yuslittlecyrillic": "\u0467",
"yuslittleiotifiedcyrillic": "\u0469",
"yusmallhiragana": "\u3085",
"yusmallkatakana": "\u30E5",
"yusmallkatakanahalfwidth": "\uFF6D",
"yuyekorean": "\u318B",
"yuyeokorean": "\u318A",
"yyabengali": "\u09DF",
"yyadeva": "\u095F",
"z": "\u007A",
"zaarmenian": "\u0566",
"zacute": "\u017A",
"zadeva": "\u095B",
"zagurmukhi": "\u0A5B",
"zaharabic": "\u0638",
"zahfinalarabic": "\uFEC6",
"zahinitialarabic": "\uFEC7",
"zahiragana": "\u3056",
"zahmedialarabic": "\uFEC8",
"zainarabic": "\u0632",
"zainfinalarabic": "\uFEB0",
"zakatakana": "\u30B6",
"zaqefgadolhebrew": "\u0595",
"zaqefqatanhebrew": "\u0594",
"zarqahebrew": "\u0598",
"zayin": "\u05D6",
"zayindagesh": "\uFB36",
"zayindageshhebrew": "\uFB36",
"zayinhebrew": "\u05D6",
"zbopomofo": "\u3117",
"zcaron": "\u017E",
"zcircle": "\u24E9",
"zcircumflex": "\u1E91",
"zcurl": "\u0291",
"zdot": "\u017C",
"zdotaccent": "\u017C",
"zdotbelow": "\u1E93",
"zecyrillic": "\u0437",
"zedescendercyrillic": "\u0499",
"zedieresiscyrillic": "\u04DF",
"zehiragana": "\u305C",
"zekatakana": "\u30BC",
"zero": "\u0030",
"zeroarabic": "\u0660",
"zerobengali": "\u09E6",
"zerodeva": "\u0966",
"zerogujarati": "\u0AE6",
"zerogurmukhi": "\u0A66",
"zerohackarabic": "\u0660",
"zeroinferior": "\u2080",
"zeromonospace": "\uFF10",
"zerooldstyle": "\uF730",
"zeropersian": "\u06F0",
"zerosuperior": "\u2070",
"zerothai": "\u0E50",
"zerowidthjoiner": "\uFEFF",
"zerowidthnonjoiner": "\u200C",
"zerowidthspace": "\u200B",
"zeta": "\u03B6",
"zhbopomofo": "\u3113",
"zhearmenian": "\u056A",
"zhebrevecyrillic": "\u04C2",
"zhecyrillic": "\u0436",
"zhedescendercyrillic": "\u0497",
"zhedieresiscyrillic": "\u04DD",
"zihiragana": "\u3058",
"zikatakana": "\u30B8",
"zinorhebrew": "\u05AE",
"zlinebelow": "\u1E95",
"zmonospace": "\uFF5A",
"zohiragana": "\u305E",
"zokatakana": "\u30BE",
"zparen": "\u24B5",
"zretroflexhook": "\u0290",
"zstroke": "\u01B6",
"zuhiragana": "\u305A",
"zukatakana": "\u30BA",
}
# --end | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/glyphlist.py | glyphlist.py |
import logging
import sys
from io import StringIO
from typing import Any, BinaryIO, Container, Iterator, Optional, cast
from .converter import XMLConverter, HTMLConverter, TextConverter, PDFPageAggregator
from .image import ImageWriter
from .layout import LAParams, LTPage
from .pdfdevice import PDFDevice, TagExtractor
from .pdfinterp import PDFResourceManager, PDFPageInterpreter
from .pdfpage import PDFPage
from .utils import open_filename, FileOrName, AnyIO
def extract_text_to_fp(
inf: BinaryIO,
outfp: AnyIO,
output_type: str = "text",
codec: str = "utf-8",
laparams: Optional[LAParams] = None,
maxpages: int = 0,
page_numbers: Optional[Container[int]] = None,
password: str = "",
scale: float = 1.0,
rotation: int = 0,
layoutmode: str = "normal",
output_dir: Optional[str] = None,
strip_control: bool = False,
debug: bool = False,
disable_caching: bool = False,
**kwargs: Any,
) -> None:
"""Parses text from inf-file and writes to outfp file-like object.
Takes loads of optional arguments but the defaults are somewhat sane.
Beware laparams: Including an empty LAParams is not the same as passing
None!
:param inf: a file-like object to read PDF structure from, such as a
file handler (using the builtin `open()` function) or a `BytesIO`.
:param outfp: a file-like object to write the text to.
:param output_type: May be 'text', 'xml', 'html', 'tag'. Only 'text' works
properly.
:param codec: Text decoding codec
:param laparams: An LAParams object from pdfminer.layout. Default is None
but may not layout correctly.
:param maxpages: How many pages to stop parsing after
:param page_numbers: zero-indexed page numbers to operate on.
:param password: For encrypted PDFs, the password to decrypt.
:param scale: Scale factor
:param rotation: Rotation factor
:param layoutmode: Default is 'normal', see
pdfminer.converter.HTMLConverter
:param output_dir: If given, creates an ImageWriter for extracted images.
:param strip_control: Does what it says on the tin
:param debug: Output more logging data
:param disable_caching: Does what it says on the tin
:param other:
:return: nothing, acting as it does on two streams. Use StringIO to get
strings.
"""
if debug:
logging.getLogger().setLevel(logging.DEBUG)
imagewriter = None
if output_dir:
imagewriter = ImageWriter(output_dir)
rsrcmgr = PDFResourceManager(caching=not disable_caching)
device: Optional[PDFDevice] = None
if output_type != "text" and outfp == sys.stdout:
outfp = sys.stdout.buffer
if output_type == "text":
device = TextConverter(
rsrcmgr, outfp, codec=codec, laparams=laparams, imagewriter=imagewriter
)
elif output_type == "xml":
device = XMLConverter(
rsrcmgr,
outfp,
codec=codec,
laparams=laparams,
imagewriter=imagewriter,
stripcontrol=strip_control,
)
elif output_type == "html":
device = HTMLConverter(
rsrcmgr,
outfp,
codec=codec,
scale=scale,
layoutmode=layoutmode,
laparams=laparams,
imagewriter=imagewriter,
)
elif output_type == "tag":
# Binary I/O is required, but we have no good way to test it here.
device = TagExtractor(rsrcmgr, cast(BinaryIO, outfp), codec=codec)
else:
msg = f"Output type can be text, html, xml or tag but is " f"{output_type}"
raise ValueError(msg)
assert device is not None
interpreter = PDFPageInterpreter(rsrcmgr, device)
for page in PDFPage.get_pages(
inf,
page_numbers,
maxpages=maxpages,
password=password,
caching=not disable_caching,
):
page.rotate = (page.rotate + rotation) % 360
interpreter.process_page(page)
device.close()
def extract_text(
pdf_file: FileOrName,
password: str = "",
page_numbers: Optional[Container[int]] = None,
maxpages: int = 0,
caching: bool = True,
codec: str = "utf-8",
laparams: Optional[LAParams] = None,
) -> str:
"""Parse and return the text contained in a PDF file.
:param pdf_file: Either a file path or a file-like object for the PDF file
to be worked on.
:param password: For encrypted PDFs, the password to decrypt.
:param page_numbers: List of zero-indexed page numbers to extract.
:param maxpages: The maximum number of pages to parse
:param caching: If resources should be cached
:param codec: Text decoding codec
:param laparams: An LAParams object from pdfminer.layout. If None, uses
some default settings that often work well.
:return: a string containing all of the text extracted.
"""
if laparams is None:
laparams = LAParams()
with open_filename(pdf_file, "rb") as fp, StringIO() as output_string:
fp = cast(BinaryIO, fp) # we opened in binary mode
rsrcmgr = PDFResourceManager(caching=caching)
device = TextConverter(rsrcmgr, output_string, codec=codec, laparams=laparams)
interpreter = PDFPageInterpreter(rsrcmgr, device)
for page in PDFPage.get_pages(
fp,
page_numbers,
maxpages=maxpages,
password=password,
caching=caching,
):
interpreter.process_page(page)
return output_string.getvalue()
def extract_pages(
pdf_file: FileOrName,
password: str = "",
page_numbers: Optional[Container[int]] = None,
maxpages: int = 0,
caching: bool = True,
laparams: Optional[LAParams] = None,
) -> Iterator[LTPage]:
"""Extract and yield LTPage objects
:param pdf_file: Either a file path or a file-like object for the PDF file
to be worked on.
:param password: For encrypted PDFs, the password to decrypt.
:param page_numbers: List of zero-indexed page numbers to extract.
:param maxpages: The maximum number of pages to parse
:param caching: If resources should be cached
:param laparams: An LAParams object from pdfminer.layout. If None, uses
some default settings that often work well.
:return:
"""
if laparams is None:
laparams = LAParams()
with open_filename(pdf_file, "rb") as fp:
fp = cast(BinaryIO, fp) # we opened in binary mode
resource_manager = PDFResourceManager(caching=caching)
device = PDFPageAggregator(resource_manager, laparams=laparams)
interpreter = PDFPageInterpreter(resource_manager, device)
for page in PDFPage.get_pages(
fp, page_numbers, maxpages=maxpages, password=password, caching=caching
):
interpreter.process_page(page)
layout = device.get_result()
yield layout | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/high_level.py | high_level.py |
import array
from typing import (
Any,
Callable,
Dict,
Iterator,
List,
MutableSequence,
Optional,
Sequence,
Union,
cast,
)
def get_bytes(data: bytes) -> Iterator[int]:
yield from data
# Workaround https://github.com/python/mypy/issues/731
BitParserState = MutableSequence[Any]
# A better definition (not supported by mypy) would be:
# BitParserState = MutableSequence[Union["BitParserState", int, str, None]]
class BitParser:
_state: BitParserState
# _accept is declared Optional solely as a workaround for
# https://github.com/python/mypy/issues/708
_accept: Optional[Callable[[Any], BitParserState]]
def __init__(self) -> None:
self._pos = 0
@classmethod
def add(cls, root: BitParserState, v: Union[int, str], bits: str) -> None:
p: BitParserState = root
b = None
for i in range(len(bits)):
if 0 < i:
assert b is not None
if p[b] is None:
p[b] = [None, None]
p = p[b]
if bits[i] == "1":
b = 1
else:
b = 0
assert b is not None
p[b] = v
def feedbytes(self, data: bytes) -> None:
for byte in get_bytes(data):
for m in (128, 64, 32, 16, 8, 4, 2, 1):
self._parse_bit(byte & m)
def _parse_bit(self, x: object) -> None:
if x:
v = self._state[1]
else:
v = self._state[0]
self._pos += 1
if isinstance(v, list):
self._state = v
else:
assert self._accept is not None
self._state = self._accept(v)
class CCITTG4Parser(BitParser):
MODE = [None, None]
BitParser.add(MODE, 0, "1")
BitParser.add(MODE, +1, "011")
BitParser.add(MODE, -1, "010")
BitParser.add(MODE, "h", "001")
BitParser.add(MODE, "p", "0001")
BitParser.add(MODE, +2, "000011")
BitParser.add(MODE, -2, "000010")
BitParser.add(MODE, +3, "0000011")
BitParser.add(MODE, -3, "0000010")
BitParser.add(MODE, "u", "0000001111")
BitParser.add(MODE, "x1", "0000001000")
BitParser.add(MODE, "x2", "0000001001")
BitParser.add(MODE, "x3", "0000001010")
BitParser.add(MODE, "x4", "0000001011")
BitParser.add(MODE, "x5", "0000001100")
BitParser.add(MODE, "x6", "0000001101")
BitParser.add(MODE, "x7", "0000001110")
BitParser.add(MODE, "e", "000000000001000000000001")
WHITE = [None, None]
BitParser.add(WHITE, 0, "00110101")
BitParser.add(WHITE, 1, "000111")
BitParser.add(WHITE, 2, "0111")
BitParser.add(WHITE, 3, "1000")
BitParser.add(WHITE, 4, "1011")
BitParser.add(WHITE, 5, "1100")
BitParser.add(WHITE, 6, "1110")
BitParser.add(WHITE, 7, "1111")
BitParser.add(WHITE, 8, "10011")
BitParser.add(WHITE, 9, "10100")
BitParser.add(WHITE, 10, "00111")
BitParser.add(WHITE, 11, "01000")
BitParser.add(WHITE, 12, "001000")
BitParser.add(WHITE, 13, "000011")
BitParser.add(WHITE, 14, "110100")
BitParser.add(WHITE, 15, "110101")
BitParser.add(WHITE, 16, "101010")
BitParser.add(WHITE, 17, "101011")
BitParser.add(WHITE, 18, "0100111")
BitParser.add(WHITE, 19, "0001100")
BitParser.add(WHITE, 20, "0001000")
BitParser.add(WHITE, 21, "0010111")
BitParser.add(WHITE, 22, "0000011")
BitParser.add(WHITE, 23, "0000100")
BitParser.add(WHITE, 24, "0101000")
BitParser.add(WHITE, 25, "0101011")
BitParser.add(WHITE, 26, "0010011")
BitParser.add(WHITE, 27, "0100100")
BitParser.add(WHITE, 28, "0011000")
BitParser.add(WHITE, 29, "00000010")
BitParser.add(WHITE, 30, "00000011")
BitParser.add(WHITE, 31, "00011010")
BitParser.add(WHITE, 32, "00011011")
BitParser.add(WHITE, 33, "00010010")
BitParser.add(WHITE, 34, "00010011")
BitParser.add(WHITE, 35, "00010100")
BitParser.add(WHITE, 36, "00010101")
BitParser.add(WHITE, 37, "00010110")
BitParser.add(WHITE, 38, "00010111")
BitParser.add(WHITE, 39, "00101000")
BitParser.add(WHITE, 40, "00101001")
BitParser.add(WHITE, 41, "00101010")
BitParser.add(WHITE, 42, "00101011")
BitParser.add(WHITE, 43, "00101100")
BitParser.add(WHITE, 44, "00101101")
BitParser.add(WHITE, 45, "00000100")
BitParser.add(WHITE, 46, "00000101")
BitParser.add(WHITE, 47, "00001010")
BitParser.add(WHITE, 48, "00001011")
BitParser.add(WHITE, 49, "01010010")
BitParser.add(WHITE, 50, "01010011")
BitParser.add(WHITE, 51, "01010100")
BitParser.add(WHITE, 52, "01010101")
BitParser.add(WHITE, 53, "00100100")
BitParser.add(WHITE, 54, "00100101")
BitParser.add(WHITE, 55, "01011000")
BitParser.add(WHITE, 56, "01011001")
BitParser.add(WHITE, 57, "01011010")
BitParser.add(WHITE, 58, "01011011")
BitParser.add(WHITE, 59, "01001010")
BitParser.add(WHITE, 60, "01001011")
BitParser.add(WHITE, 61, "00110010")
BitParser.add(WHITE, 62, "00110011")
BitParser.add(WHITE, 63, "00110100")
BitParser.add(WHITE, 64, "11011")
BitParser.add(WHITE, 128, "10010")
BitParser.add(WHITE, 192, "010111")
BitParser.add(WHITE, 256, "0110111")
BitParser.add(WHITE, 320, "00110110")
BitParser.add(WHITE, 384, "00110111")
BitParser.add(WHITE, 448, "01100100")
BitParser.add(WHITE, 512, "01100101")
BitParser.add(WHITE, 576, "01101000")
BitParser.add(WHITE, 640, "01100111")
BitParser.add(WHITE, 704, "011001100")
BitParser.add(WHITE, 768, "011001101")
BitParser.add(WHITE, 832, "011010010")
BitParser.add(WHITE, 896, "011010011")
BitParser.add(WHITE, 960, "011010100")
BitParser.add(WHITE, 1024, "011010101")
BitParser.add(WHITE, 1088, "011010110")
BitParser.add(WHITE, 1152, "011010111")
BitParser.add(WHITE, 1216, "011011000")
BitParser.add(WHITE, 1280, "011011001")
BitParser.add(WHITE, 1344, "011011010")
BitParser.add(WHITE, 1408, "011011011")
BitParser.add(WHITE, 1472, "010011000")
BitParser.add(WHITE, 1536, "010011001")
BitParser.add(WHITE, 1600, "010011010")
BitParser.add(WHITE, 1664, "011000")
BitParser.add(WHITE, 1728, "010011011")
BitParser.add(WHITE, 1792, "00000001000")
BitParser.add(WHITE, 1856, "00000001100")
BitParser.add(WHITE, 1920, "00000001101")
BitParser.add(WHITE, 1984, "000000010010")
BitParser.add(WHITE, 2048, "000000010011")
BitParser.add(WHITE, 2112, "000000010100")
BitParser.add(WHITE, 2176, "000000010101")
BitParser.add(WHITE, 2240, "000000010110")
BitParser.add(WHITE, 2304, "000000010111")
BitParser.add(WHITE, 2368, "000000011100")
BitParser.add(WHITE, 2432, "000000011101")
BitParser.add(WHITE, 2496, "000000011110")
BitParser.add(WHITE, 2560, "000000011111")
BLACK = [None, None]
BitParser.add(BLACK, 0, "0000110111")
BitParser.add(BLACK, 1, "010")
BitParser.add(BLACK, 2, "11")
BitParser.add(BLACK, 3, "10")
BitParser.add(BLACK, 4, "011")
BitParser.add(BLACK, 5, "0011")
BitParser.add(BLACK, 6, "0010")
BitParser.add(BLACK, 7, "00011")
BitParser.add(BLACK, 8, "000101")
BitParser.add(BLACK, 9, "000100")
BitParser.add(BLACK, 10, "0000100")
BitParser.add(BLACK, 11, "0000101")
BitParser.add(BLACK, 12, "0000111")
BitParser.add(BLACK, 13, "00000100")
BitParser.add(BLACK, 14, "00000111")
BitParser.add(BLACK, 15, "000011000")
BitParser.add(BLACK, 16, "0000010111")
BitParser.add(BLACK, 17, "0000011000")
BitParser.add(BLACK, 18, "0000001000")
BitParser.add(BLACK, 19, "00001100111")
BitParser.add(BLACK, 20, "00001101000")
BitParser.add(BLACK, 21, "00001101100")
BitParser.add(BLACK, 22, "00000110111")
BitParser.add(BLACK, 23, "00000101000")
BitParser.add(BLACK, 24, "00000010111")
BitParser.add(BLACK, 25, "00000011000")
BitParser.add(BLACK, 26, "000011001010")
BitParser.add(BLACK, 27, "000011001011")
BitParser.add(BLACK, 28, "000011001100")
BitParser.add(BLACK, 29, "000011001101")
BitParser.add(BLACK, 30, "000001101000")
BitParser.add(BLACK, 31, "000001101001")
BitParser.add(BLACK, 32, "000001101010")
BitParser.add(BLACK, 33, "000001101011")
BitParser.add(BLACK, 34, "000011010010")
BitParser.add(BLACK, 35, "000011010011")
BitParser.add(BLACK, 36, "000011010100")
BitParser.add(BLACK, 37, "000011010101")
BitParser.add(BLACK, 38, "000011010110")
BitParser.add(BLACK, 39, "000011010111")
BitParser.add(BLACK, 40, "000001101100")
BitParser.add(BLACK, 41, "000001101101")
BitParser.add(BLACK, 42, "000011011010")
BitParser.add(BLACK, 43, "000011011011")
BitParser.add(BLACK, 44, "000001010100")
BitParser.add(BLACK, 45, "000001010101")
BitParser.add(BLACK, 46, "000001010110")
BitParser.add(BLACK, 47, "000001010111")
BitParser.add(BLACK, 48, "000001100100")
BitParser.add(BLACK, 49, "000001100101")
BitParser.add(BLACK, 50, "000001010010")
BitParser.add(BLACK, 51, "000001010011")
BitParser.add(BLACK, 52, "000000100100")
BitParser.add(BLACK, 53, "000000110111")
BitParser.add(BLACK, 54, "000000111000")
BitParser.add(BLACK, 55, "000000100111")
BitParser.add(BLACK, 56, "000000101000")
BitParser.add(BLACK, 57, "000001011000")
BitParser.add(BLACK, 58, "000001011001")
BitParser.add(BLACK, 59, "000000101011")
BitParser.add(BLACK, 60, "000000101100")
BitParser.add(BLACK, 61, "000001011010")
BitParser.add(BLACK, 62, "000001100110")
BitParser.add(BLACK, 63, "000001100111")
BitParser.add(BLACK, 64, "0000001111")
BitParser.add(BLACK, 128, "000011001000")
BitParser.add(BLACK, 192, "000011001001")
BitParser.add(BLACK, 256, "000001011011")
BitParser.add(BLACK, 320, "000000110011")
BitParser.add(BLACK, 384, "000000110100")
BitParser.add(BLACK, 448, "000000110101")
BitParser.add(BLACK, 512, "0000001101100")
BitParser.add(BLACK, 576, "0000001101101")
BitParser.add(BLACK, 640, "0000001001010")
BitParser.add(BLACK, 704, "0000001001011")
BitParser.add(BLACK, 768, "0000001001100")
BitParser.add(BLACK, 832, "0000001001101")
BitParser.add(BLACK, 896, "0000001110010")
BitParser.add(BLACK, 960, "0000001110011")
BitParser.add(BLACK, 1024, "0000001110100")
BitParser.add(BLACK, 1088, "0000001110101")
BitParser.add(BLACK, 1152, "0000001110110")
BitParser.add(BLACK, 1216, "0000001110111")
BitParser.add(BLACK, 1280, "0000001010010")
BitParser.add(BLACK, 1344, "0000001010011")
BitParser.add(BLACK, 1408, "0000001010100")
BitParser.add(BLACK, 1472, "0000001010101")
BitParser.add(BLACK, 1536, "0000001011010")
BitParser.add(BLACK, 1600, "0000001011011")
BitParser.add(BLACK, 1664, "0000001100100")
BitParser.add(BLACK, 1728, "0000001100101")
BitParser.add(BLACK, 1792, "00000001000")
BitParser.add(BLACK, 1856, "00000001100")
BitParser.add(BLACK, 1920, "00000001101")
BitParser.add(BLACK, 1984, "000000010010")
BitParser.add(BLACK, 2048, "000000010011")
BitParser.add(BLACK, 2112, "000000010100")
BitParser.add(BLACK, 2176, "000000010101")
BitParser.add(BLACK, 2240, "000000010110")
BitParser.add(BLACK, 2304, "000000010111")
BitParser.add(BLACK, 2368, "000000011100")
BitParser.add(BLACK, 2432, "000000011101")
BitParser.add(BLACK, 2496, "000000011110")
BitParser.add(BLACK, 2560, "000000011111")
UNCOMPRESSED = [None, None]
BitParser.add(UNCOMPRESSED, "1", "1")
BitParser.add(UNCOMPRESSED, "01", "01")
BitParser.add(UNCOMPRESSED, "001", "001")
BitParser.add(UNCOMPRESSED, "0001", "0001")
BitParser.add(UNCOMPRESSED, "00001", "00001")
BitParser.add(UNCOMPRESSED, "00000", "000001")
BitParser.add(UNCOMPRESSED, "T00", "00000011")
BitParser.add(UNCOMPRESSED, "T10", "00000010")
BitParser.add(UNCOMPRESSED, "T000", "000000011")
BitParser.add(UNCOMPRESSED, "T100", "000000010")
BitParser.add(UNCOMPRESSED, "T0000", "0000000011")
BitParser.add(UNCOMPRESSED, "T1000", "0000000010")
BitParser.add(UNCOMPRESSED, "T00000", "00000000011")
BitParser.add(UNCOMPRESSED, "T10000", "00000000010")
class EOFB(Exception):
pass
class InvalidData(Exception):
pass
class ByteSkip(Exception):
pass
_color: int
def __init__(self, width: int, bytealign: bool = False) -> None:
BitParser.__init__(self)
self.width = width
self.bytealign = bytealign
self.reset()
return
def feedbytes(self, data: bytes) -> None:
for byte in get_bytes(data):
try:
for m in (128, 64, 32, 16, 8, 4, 2, 1):
self._parse_bit(byte & m)
except self.ByteSkip:
self._accept = self._parse_mode
self._state = self.MODE
except self.EOFB:
break
return
def _parse_mode(self, mode: object) -> BitParserState:
if mode == "p":
self._do_pass()
self._flush_line()
return self.MODE
elif mode == "h":
self._n1 = 0
self._accept = self._parse_horiz1
if self._color:
return self.WHITE
else:
return self.BLACK
elif mode == "u":
self._accept = self._parse_uncompressed
return self.UNCOMPRESSED
elif mode == "e":
raise self.EOFB
elif isinstance(mode, int):
self._do_vertical(mode)
self._flush_line()
return self.MODE
else:
raise self.InvalidData(mode)
def _parse_horiz1(self, n: Any) -> BitParserState:
if n is None:
raise self.InvalidData
self._n1 += n
if n < 64:
self._n2 = 0
self._color = 1 - self._color
self._accept = self._parse_horiz2
if self._color:
return self.WHITE
else:
return self.BLACK
def _parse_horiz2(self, n: Any) -> BitParserState:
if n is None:
raise self.InvalidData
self._n2 += n
if n < 64:
self._color = 1 - self._color
self._accept = self._parse_mode
self._do_horizontal(self._n1, self._n2)
self._flush_line()
return self.MODE
elif self._color:
return self.WHITE
else:
return self.BLACK
def _parse_uncompressed(self, bits: Optional[str]) -> BitParserState:
if not bits:
raise self.InvalidData
if bits.startswith("T"):
self._accept = self._parse_mode
self._color = int(bits[1])
self._do_uncompressed(bits[2:])
return self.MODE
else:
self._do_uncompressed(bits)
return self.UNCOMPRESSED
def _get_bits(self) -> str:
return "".join(str(b) for b in self._curline[: self._curpos])
def _get_refline(self, i: int) -> str:
if i < 0:
return "[]" + "".join(str(b) for b in self._refline)
elif len(self._refline) <= i:
return "".join(str(b) for b in self._refline) + "[]"
else:
return (
"".join(str(b) for b in self._refline[:i])
+ "["
+ str(self._refline[i])
+ "]"
+ "".join(str(b) for b in self._refline[i + 1 :])
)
def reset(self) -> None:
self._y = 0
self._curline = array.array("b", [1] * self.width)
self._reset_line()
self._accept = self._parse_mode
self._state = self.MODE
return
def output_line(self, y: int, bits: Sequence[int]) -> None:
print(y, "".join(str(b) for b in bits))
return
def _reset_line(self) -> None:
self._refline = self._curline
self._curline = array.array("b", [1] * self.width)
self._curpos = -1
self._color = 1
return
def _flush_line(self) -> None:
if self.width <= self._curpos:
self.output_line(self._y, self._curline)
self._y += 1
self._reset_line()
if self.bytealign:
raise self.ByteSkip
return
def _do_vertical(self, dx: int) -> None:
x1 = self._curpos + 1
while 1:
if x1 == 0:
if self._color == 1 and self._refline[x1] != self._color:
break
elif x1 == len(self._refline):
break
elif (
self._refline[x1 - 1] == self._color
and self._refline[x1] != self._color
):
break
x1 += 1
x1 += dx
x0 = max(0, self._curpos)
x1 = max(0, min(self.width, x1))
if x1 < x0:
for x in range(x1, x0):
self._curline[x] = self._color
elif x0 < x1:
for x in range(x0, x1):
self._curline[x] = self._color
self._curpos = x1
self._color = 1 - self._color
return
def _do_pass(self) -> None:
x1 = self._curpos + 1
while 1:
if x1 == 0:
if self._color == 1 and self._refline[x1] != self._color:
break
elif x1 == len(self._refline):
break
elif (
self._refline[x1 - 1] == self._color
and self._refline[x1] != self._color
):
break
x1 += 1
while 1:
if x1 == 0:
if self._color == 0 and self._refline[x1] == self._color:
break
elif x1 == len(self._refline):
break
elif (
self._refline[x1 - 1] != self._color
and self._refline[x1] == self._color
):
break
x1 += 1
for x in range(self._curpos, x1):
self._curline[x] = self._color
self._curpos = x1
return
def _do_horizontal(self, n1: int, n2: int) -> None:
if self._curpos < 0:
self._curpos = 0
x = self._curpos
for _ in range(n1):
if len(self._curline) <= x:
break
self._curline[x] = self._color
x += 1
for _ in range(n2):
if len(self._curline) <= x:
break
self._curline[x] = 1 - self._color
x += 1
self._curpos = x
return
def _do_uncompressed(self, bits: str) -> None:
for c in bits:
self._curline[self._curpos] = int(c)
self._curpos += 1
self._flush_line()
return
class CCITTFaxDecoder(CCITTG4Parser):
def __init__(
self, width: int, bytealign: bool = False, reversed: bool = False
) -> None:
CCITTG4Parser.__init__(self, width, bytealign=bytealign)
self.reversed = reversed
self._buf = b""
return
def close(self) -> bytes:
return self._buf
def output_line(self, y: int, bits: Sequence[int]) -> None:
arr = array.array("B", [0] * ((len(bits) + 7) // 8))
if self.reversed:
bits = [1 - b for b in bits]
for (i, b) in enumerate(bits):
if b:
arr[i // 8] += (128, 64, 32, 16, 8, 4, 2, 1)[i % 8]
self._buf += arr.tobytes()
return
def ccittfaxdecode(data: bytes, params: Dict[str, object]) -> bytes:
K = params.get("K")
if K == -1:
cols = cast(int, params.get("Columns"))
bytealign = cast(bool, params.get("EncodedByteAlign"))
reversed = cast(bool, params.get("BlackIs1"))
parser = CCITTFaxDecoder(cols, bytealign=bytealign, reversed=reversed)
else:
raise ValueError(K)
parser.feedbytes(data)
return parser.close()
# test
def main(argv: List[str]) -> None:
if not argv[1:]:
import unittest
unittest.main()
return
class Parser(CCITTG4Parser):
def __init__(self, width: int, bytealign: bool = False) -> None:
import pygame # type: ignore[import]
CCITTG4Parser.__init__(self, width, bytealign=bytealign)
self.img = pygame.Surface((self.width, 1000))
return
def output_line(self, y: int, bits: Sequence[int]) -> None:
for (x, b) in enumerate(bits):
if b:
self.img.set_at((x, y), (255, 255, 255))
else:
self.img.set_at((x, y), (0, 0, 0))
return
def close(self) -> None:
import pygame
pygame.image.save(self.img, "out.bmp")
return
for path in argv[1:]:
fp = open(path, "rb")
(_, _, k, w, h, _) = path.split(".")
parser = Parser(int(w))
parser.feedbytes(fp.read())
parser.close()
fp.close()
return | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/ccitt.py | ccitt.py |
import io
import pathlib
import string
import struct
from html import escape
from typing import (
Any,
BinaryIO,
Callable,
Dict,
Generic,
Iterable,
Iterator,
List,
Optional,
Set,
TextIO,
Tuple,
TypeVar,
Union,
TYPE_CHECKING,
cast,
)
if TYPE_CHECKING:
from .layout import LTComponent
import charset_normalizer # For str encoding detection
# from sys import maxint as INF doesn't work anymore under Python3, but PDF
# still uses 32 bits ints
INF = (1 << 31) - 1
FileOrName = Union[pathlib.PurePath, str, io.IOBase]
AnyIO = Union[TextIO, BinaryIO]
class open_filename(object):
"""
Context manager that allows opening a filename
(str or pathlib.PurePath type is supported) and closes it on exit,
(just like `open`), but does nothing for file-like objects.
"""
def __init__(self, filename: FileOrName, *args: Any, **kwargs: Any) -> None:
if isinstance(filename, pathlib.PurePath):
filename = str(filename)
if isinstance(filename, str):
self.file_handler: AnyIO = open(filename, *args, **kwargs)
self.closing = True
elif isinstance(filename, io.IOBase):
self.file_handler = cast(AnyIO, filename)
self.closing = False
else:
raise TypeError("Unsupported input type: %s" % type(filename))
def __enter__(self) -> AnyIO:
return self.file_handler
def __exit__(self, exc_type: object, exc_val: object, exc_tb: object) -> None:
if self.closing:
self.file_handler.close()
def make_compat_bytes(in_str: str) -> bytes:
"Converts to bytes, encoding to unicode."
assert isinstance(in_str, str), str(type(in_str))
return in_str.encode()
def make_compat_str(o: object) -> str:
"""Converts everything to string, if bytes guessing the encoding."""
if isinstance(o, bytes):
enc = charset_normalizer.detect(o)
try:
return o.decode(enc["encoding"])
except UnicodeDecodeError:
return str(o)
else:
return str(o)
def shorten_str(s: str, size: int) -> str:
if size < 7:
return s[:size]
if len(s) > size:
length = (size - 5) // 2
return "{} ... {}".format(s[:length], s[-length:])
else:
return s
def compatible_encode_method(
bytesorstring: Union[bytes, str], encoding: str = "utf-8", erraction: str = "ignore"
) -> str:
"""When Py2 str.encode is called, it often means bytes.encode in Py3.
This does either.
"""
if isinstance(bytesorstring, str):
return bytesorstring
assert isinstance(bytesorstring, bytes), str(type(bytesorstring))
return bytesorstring.decode(encoding, erraction)
def paeth_predictor(left: int, above: int, upper_left: int) -> int:
# From http://www.libpng.org/pub/png/spec/1.2/PNG-Filters.html
# Initial estimate
p = left + above - upper_left
# Distances to a,b,c
pa = abs(p - left)
pb = abs(p - above)
pc = abs(p - upper_left)
# Return nearest of a,b,c breaking ties in order a,b,c
if pa <= pb and pa <= pc:
return left
elif pb <= pc:
return above
else:
return upper_left
def apply_png_predictor(
pred: int, colors: int, columns: int, bitspercomponent: int, data: bytes
) -> bytes:
"""Reverse the effect of the PNG predictor
Documentation: http://www.libpng.org/pub/png/spec/1.2/PNG-Filters.html
"""
if bitspercomponent != 8:
msg = "Unsupported `bitspercomponent': %d" % bitspercomponent
raise ValueError(msg)
nbytes = colors * columns * bitspercomponent // 8
bpp = colors * bitspercomponent // 8 # number of bytes per complete pixel
buf = b""
line_above = b"\x00" * columns
for scanline_i in range(0, len(data), nbytes + 1):
filter_type = data[scanline_i]
line_encoded = data[scanline_i + 1 : scanline_i + 1 + nbytes]
raw = b""
if filter_type == 0:
# Filter type 0: None
raw += line_encoded
elif filter_type == 1:
# Filter type 1: Sub
# To reverse the effect of the Sub() filter after decompression,
# output the following value:
# Raw(x) = Sub(x) + Raw(x - bpp)
# (computed mod 256), where Raw() refers to the bytes already
# decoded.
for j, sub_x in enumerate(line_encoded):
if j - bpp < 0:
raw_x_bpp = 0
else:
raw_x_bpp = int(raw[j - bpp])
raw_x = (sub_x + raw_x_bpp) & 255
raw += bytes((raw_x,))
elif filter_type == 2:
# Filter type 2: Up
# To reverse the effect of the Up() filter after decompression,
# output the following value:
# Raw(x) = Up(x) + Prior(x)
# (computed mod 256), where Prior() refers to the decoded bytes of
# the prior scanline.
for (up_x, prior_x) in zip(line_encoded, line_above):
raw_x = (up_x + prior_x) & 255
raw += bytes((raw_x,))
elif filter_type == 3:
# Filter type 3: Average
# To reverse the effect of the Average() filter after
# decompression, output the following value:
# Raw(x) = Average(x) + floor((Raw(x-bpp)+Prior(x))/2)
# where the result is computed mod 256, but the prediction is
# calculated in the same way as for encoding. Raw() refers to the
# bytes already decoded, and Prior() refers to the decoded bytes of
# the prior scanline.
for j, average_x in enumerate(line_encoded):
if j - bpp < 0:
raw_x_bpp = 0
else:
raw_x_bpp = int(raw[j - bpp])
prior_x = int(line_above[j])
raw_x = (average_x + (raw_x_bpp + prior_x) // 2) & 255
raw += bytes((raw_x,))
elif filter_type == 4:
# Filter type 4: Paeth
# To reverse the effect of the Paeth() filter after decompression,
# output the following value:
# Raw(x) = Paeth(x)
# + PaethPredictor(Raw(x-bpp), Prior(x), Prior(x-bpp))
# (computed mod 256), where Raw() and Prior() refer to bytes
# already decoded. Exactly the same PaethPredictor() function is
# used by both encoder and decoder.
for j, paeth_x in enumerate(line_encoded):
if j - bpp < 0:
raw_x_bpp = 0
prior_x_bpp = 0
else:
raw_x_bpp = int(raw[j - bpp])
prior_x_bpp = int(line_above[j - bpp])
prior_x = int(line_above[j])
paeth = paeth_predictor(raw_x_bpp, prior_x, prior_x_bpp)
raw_x = (paeth_x + paeth) & 255
raw += bytes((raw_x,))
else:
raise ValueError("Unsupported predictor value: %d" % filter_type)
buf += raw
line_above = raw
return buf
Point = Tuple[float, float]
Rect = Tuple[float, float, float, float]
Matrix = Tuple[float, float, float, float, float, float]
PathSegment = Union[
Tuple[str], # Literal['h']
Tuple[str, float, float], # Literal['m', 'l']
Tuple[str, float, float, float, float], # Literal['v', 'y']
Tuple[str, float, float, float, float, float, float],
] # Literal['c']
# Matrix operations
MATRIX_IDENTITY: Matrix = (1, 0, 0, 1, 0, 0)
def mult_matrix(m1: Matrix, m0: Matrix) -> Matrix:
(a1, b1, c1, d1, e1, f1) = m1
(a0, b0, c0, d0, e0, f0) = m0
"""Returns the multiplication of two matrices."""
return (
a0 * a1 + c0 * b1,
b0 * a1 + d0 * b1,
a0 * c1 + c0 * d1,
b0 * c1 + d0 * d1,
a0 * e1 + c0 * f1 + e0,
b0 * e1 + d0 * f1 + f0,
)
def translate_matrix(m: Matrix, v: Point) -> Matrix:
"""Translates a matrix by (x, y)."""
(a, b, c, d, e, f) = m
(x, y) = v
return a, b, c, d, x * a + y * c + e, x * b + y * d + f
def apply_matrix_pt(m: Matrix, v: Point) -> Point:
(a, b, c, d, e, f) = m
(x, y) = v
"""Applies a matrix to a point."""
return a * x + c * y + e, b * x + d * y + f
def apply_matrix_norm(m: Matrix, v: Point) -> Point:
"""Equivalent to apply_matrix_pt(M, (p,q)) - apply_matrix_pt(M, (0,0))"""
(a, b, c, d, e, f) = m
(p, q) = v
return a * p + c * q, b * p + d * q
# Utility functions
def isnumber(x: object) -> bool:
return isinstance(x, (int, float))
_T = TypeVar("_T")
def uniq(objs: Iterable[_T]) -> Iterator[_T]:
"""Eliminates duplicated elements."""
done = set()
for obj in objs:
if obj in done:
continue
done.add(obj)
yield obj
return
def fsplit(pred: Callable[[_T], bool], objs: Iterable[_T]) -> Tuple[List[_T], List[_T]]:
"""Split a list into two classes according to the predicate."""
t = []
f = []
for obj in objs:
if pred(obj):
t.append(obj)
else:
f.append(obj)
return t, f
def drange(v0: float, v1: float, d: int) -> range:
"""Returns a discrete range."""
return range(int(v0) // d, int(v1 + d) // d)
def get_bound(pts: Iterable[Point]) -> Rect:
"""Compute a minimal rectangle that covers all the points."""
limit: Rect = (INF, INF, -INF, -INF)
(x0, y0, x1, y1) = limit
for (x, y) in pts:
x0 = min(x0, x)
y0 = min(y0, y)
x1 = max(x1, x)
y1 = max(y1, y)
return x0, y0, x1, y1
def pick(
seq: Iterable[_T], func: Callable[[_T], float], maxobj: Optional[_T] = None
) -> Optional[_T]:
"""Picks the object obj where func(obj) has the highest value."""
maxscore = None
for obj in seq:
score = func(obj)
if maxscore is None or maxscore < score:
(maxscore, maxobj) = (score, obj)
return maxobj
def choplist(n: int, seq: Iterable[_T]) -> Iterator[Tuple[_T, ...]]:
"""Groups every n elements of the list."""
r = []
for x in seq:
r.append(x)
if len(r) == n:
yield tuple(r)
r = []
return
def nunpack(s: bytes, default: int = 0) -> int:
"""Unpacks 1 to 4 or 8 byte integers (big endian)."""
length = len(s)
if not length:
return default
elif length == 1:
return ord(s)
elif length == 2:
return cast(int, struct.unpack(">H", s)[0])
elif length == 3:
return cast(int, struct.unpack(">L", b"\x00" + s)[0])
elif length == 4:
return cast(int, struct.unpack(">L", s)[0])
elif length == 8:
return cast(int, struct.unpack(">Q", s)[0])
else:
raise TypeError("invalid length: %d" % length)
PDFDocEncoding = "".join(
chr(x)
for x in (
0x0000,
0x0001,
0x0002,
0x0003,
0x0004,
0x0005,
0x0006,
0x0007,
0x0008,
0x0009,
0x000A,
0x000B,
0x000C,
0x000D,
0x000E,
0x000F,
0x0010,
0x0011,
0x0012,
0x0013,
0x0014,
0x0015,
0x0017,
0x0017,
0x02D8,
0x02C7,
0x02C6,
0x02D9,
0x02DD,
0x02DB,
0x02DA,
0x02DC,
0x0020,
0x0021,
0x0022,
0x0023,
0x0024,
0x0025,
0x0026,
0x0027,
0x0028,
0x0029,
0x002A,
0x002B,
0x002C,
0x002D,
0x002E,
0x002F,
0x0030,
0x0031,
0x0032,
0x0033,
0x0034,
0x0035,
0x0036,
0x0037,
0x0038,
0x0039,
0x003A,
0x003B,
0x003C,
0x003D,
0x003E,
0x003F,
0x0040,
0x0041,
0x0042,
0x0043,
0x0044,
0x0045,
0x0046,
0x0047,
0x0048,
0x0049,
0x004A,
0x004B,
0x004C,
0x004D,
0x004E,
0x004F,
0x0050,
0x0051,
0x0052,
0x0053,
0x0054,
0x0055,
0x0056,
0x0057,
0x0058,
0x0059,
0x005A,
0x005B,
0x005C,
0x005D,
0x005E,
0x005F,
0x0060,
0x0061,
0x0062,
0x0063,
0x0064,
0x0065,
0x0066,
0x0067,
0x0068,
0x0069,
0x006A,
0x006B,
0x006C,
0x006D,
0x006E,
0x006F,
0x0070,
0x0071,
0x0072,
0x0073,
0x0074,
0x0075,
0x0076,
0x0077,
0x0078,
0x0079,
0x007A,
0x007B,
0x007C,
0x007D,
0x007E,
0x0000,
0x2022,
0x2020,
0x2021,
0x2026,
0x2014,
0x2013,
0x0192,
0x2044,
0x2039,
0x203A,
0x2212,
0x2030,
0x201E,
0x201C,
0x201D,
0x2018,
0x2019,
0x201A,
0x2122,
0xFB01,
0xFB02,
0x0141,
0x0152,
0x0160,
0x0178,
0x017D,
0x0131,
0x0142,
0x0153,
0x0161,
0x017E,
0x0000,
0x20AC,
0x00A1,
0x00A2,
0x00A3,
0x00A4,
0x00A5,
0x00A6,
0x00A7,
0x00A8,
0x00A9,
0x00AA,
0x00AB,
0x00AC,
0x0000,
0x00AE,
0x00AF,
0x00B0,
0x00B1,
0x00B2,
0x00B3,
0x00B4,
0x00B5,
0x00B6,
0x00B7,
0x00B8,
0x00B9,
0x00BA,
0x00BB,
0x00BC,
0x00BD,
0x00BE,
0x00BF,
0x00C0,
0x00C1,
0x00C2,
0x00C3,
0x00C4,
0x00C5,
0x00C6,
0x00C7,
0x00C8,
0x00C9,
0x00CA,
0x00CB,
0x00CC,
0x00CD,
0x00CE,
0x00CF,
0x00D0,
0x00D1,
0x00D2,
0x00D3,
0x00D4,
0x00D5,
0x00D6,
0x00D7,
0x00D8,
0x00D9,
0x00DA,
0x00DB,
0x00DC,
0x00DD,
0x00DE,
0x00DF,
0x00E0,
0x00E1,
0x00E2,
0x00E3,
0x00E4,
0x00E5,
0x00E6,
0x00E7,
0x00E8,
0x00E9,
0x00EA,
0x00EB,
0x00EC,
0x00ED,
0x00EE,
0x00EF,
0x00F0,
0x00F1,
0x00F2,
0x00F3,
0x00F4,
0x00F5,
0x00F6,
0x00F7,
0x00F8,
0x00F9,
0x00FA,
0x00FB,
0x00FC,
0x00FD,
0x00FE,
0x00FF,
)
)
def decode_text(s: bytes) -> str:
"""Decodes a PDFDocEncoding string to Unicode."""
if s.startswith(b"\xfe\xff"):
return str(s[2:], "utf-16be", "ignore")
else:
return "".join(PDFDocEncoding[c] for c in s)
def enc(x: str) -> str:
"""Encodes a string for SGML/XML/HTML"""
if isinstance(x, bytes):
return ""
return escape(x)
def bbox2str(bbox: Rect) -> str:
(x0, y0, x1, y1) = bbox
return "{:.3f},{:.3f},{:.3f},{:.3f}".format(x0, y0, x1, y1)
def matrix2str(m: Matrix) -> str:
(a, b, c, d, e, f) = m
return "[{:.2f},{:.2f},{:.2f},{:.2f}, ({:.2f},{:.2f})]".format(a, b, c, d, e, f)
def vecBetweenBoxes(obj1: "LTComponent", obj2: "LTComponent") -> Point:
"""A distance function between two TextBoxes.
Consider the bounding rectangle for obj1 and obj2.
Return vector between 2 boxes boundaries if they don't overlap, otherwise
returns vector betweeen boxes centers
+------+..........+ (x1, y1)
| obj1 | :
+------+www+------+
: | obj2 |
(x0, y0) +..........+------+
"""
(x0, y0) = (min(obj1.x0, obj2.x0), min(obj1.y0, obj2.y0))
(x1, y1) = (max(obj1.x1, obj2.x1), max(obj1.y1, obj2.y1))
(ow, oh) = (x1 - x0, y1 - y0)
(iw, ih) = (ow - obj1.width - obj2.width, oh - obj1.height - obj2.height)
if iw < 0 and ih < 0:
# if one is inside another we compute euclidean distance
(xc1, yc1) = ((obj1.x0 + obj1.x1) / 2, (obj1.y0 + obj1.y1) / 2)
(xc2, yc2) = ((obj2.x0 + obj2.x1) / 2, (obj2.y0 + obj2.y1) / 2)
return xc1 - xc2, yc1 - yc2
else:
return max(0, iw), max(0, ih)
LTComponentT = TypeVar("LTComponentT", bound="LTComponent")
class Plane(Generic[LTComponentT]):
"""A set-like data structure for objects placed on a plane.
Can efficiently find objects in a certain rectangular area.
It maintains two parallel lists of objects, each of
which is sorted by its x or y coordinate.
"""
def __init__(self, bbox: Rect, gridsize: int = 50) -> None:
self._seq: List[LTComponentT] = [] # preserve the object order.
self._objs: Set[LTComponentT] = set()
self._grid: Dict[Point, List[LTComponentT]] = {}
self.gridsize = gridsize
(self.x0, self.y0, self.x1, self.y1) = bbox
def __repr__(self) -> str:
return "<Plane objs=%r>" % list(self)
def __iter__(self) -> Iterator[LTComponentT]:
return (obj for obj in self._seq if obj in self._objs)
def __len__(self) -> int:
return len(self._objs)
def __contains__(self, obj: object) -> bool:
return obj in self._objs
def _getrange(self, bbox: Rect) -> Iterator[Point]:
(x0, y0, x1, y1) = bbox
if x1 <= self.x0 or self.x1 <= x0 or y1 <= self.y0 or self.y1 <= y0:
return
x0 = max(self.x0, x0)
y0 = max(self.y0, y0)
x1 = min(self.x1, x1)
y1 = min(self.y1, y1)
for grid_y in drange(y0, y1, self.gridsize):
for grid_x in drange(x0, x1, self.gridsize):
yield (grid_x, grid_y)
def extend(self, objs: Iterable[LTComponentT]) -> None:
for obj in objs:
self.add(obj)
def add(self, obj: LTComponentT) -> None:
"""place an object."""
for k in self._getrange((obj.x0, obj.y0, obj.x1, obj.y1)):
if k not in self._grid:
r: List[LTComponentT] = []
self._grid[k] = r
else:
r = self._grid[k]
r.append(obj)
self._seq.append(obj)
self._objs.add(obj)
def remove(self, obj: LTComponentT) -> None:
"""displace an object."""
for k in self._getrange((obj.x0, obj.y0, obj.x1, obj.y1)):
try:
self._grid[k].remove(obj)
except (KeyError, ValueError):
pass
self._objs.remove(obj)
def find(self, bbox: Rect) -> Iterator[LTComponentT]:
"""finds objects that are in a certain area."""
(x0, y0, x1, y1) = bbox
done = set()
for k in self._getrange(bbox):
if k not in self._grid:
continue
for obj in self._grid[k]:
if obj in done:
continue
done.add(obj)
if obj.x1 <= x0 or x1 <= obj.x0 or obj.y1 <= y0 or y1 <= obj.y0:
continue
yield obj
ROMAN_ONES = ["i", "x", "c", "m"]
ROMAN_FIVES = ["v", "l", "d"]
def format_int_roman(value: int) -> str:
"""Format a number as lowercase Roman numerals."""
assert 0 < value < 4000
result: List[str] = []
index = 0
while value != 0:
value, remainder = divmod(value, 10)
if remainder == 9:
result.insert(0, ROMAN_ONES[index])
result.insert(1, ROMAN_ONES[index + 1])
elif remainder == 4:
result.insert(0, ROMAN_ONES[index])
result.insert(1, ROMAN_FIVES[index])
else:
over_five = remainder >= 5
if over_five:
result.insert(0, ROMAN_FIVES[index])
remainder -= 5
result.insert(1 if over_five else 0, ROMAN_ONES[index] * remainder)
index += 1
return "".join(result)
def format_int_alpha(value: int) -> str:
"""Format a number as lowercase letters a-z, aa-zz, etc."""
assert value > 0
result: List[str] = []
while value != 0:
value, remainder = divmod(value - 1, len(string.ascii_lowercase))
result.append(string.ascii_lowercase[remainder])
result.reverse()
return "".join(result) | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/utils.py | utils.py |
import logging
import struct
import sys
from io import BytesIO
from typing import (
Any,
BinaryIO,
Dict,
Iterable,
Iterator,
List,
Mapping,
Optional,
Tuple,
Union,
cast,
TYPE_CHECKING,
)
from . import settings
from .cmapdb import CMap
from .cmapdb import IdentityUnicodeMap
from .cmapdb import CMapBase
from .cmapdb import CMapDB
from .cmapdb import CMapParser
from .cmapdb import UnicodeMap
from .cmapdb import FileUnicodeMap
from .encodingdb import EncodingDB
from .encodingdb import name2unicode
from .fontmetrics import FONT_METRICS
from .pdftypes import PDFException
from .pdftypes import PDFStream
from .pdftypes import dict_value
from .pdftypes import int_value
from .pdftypes import list_value
from .pdftypes import num_value
from .pdftypes import resolve1, resolve_all
from .pdftypes import stream_value
from .psparser import KWD
from .psparser import LIT
from .psparser import PSEOF
from .psparser import PSKeyword
from .psparser import PSLiteral
from .psparser import PSStackParser
from .psparser import literal_name
from .utils import Matrix, Point
from .utils import Rect
from .utils import apply_matrix_norm
from .utils import choplist
from .utils import nunpack
if TYPE_CHECKING:
from .pdfinterp import PDFResourceManager
log = logging.getLogger(__name__)
def get_widths(seq: Iterable[object]) -> Dict[int, float]:
"""Build a mapping of character widths for horizontal writing."""
widths: Dict[int, float] = {}
r: List[float] = []
for v in seq:
if isinstance(v, list):
if r:
char1 = r[-1]
for (i, w) in enumerate(v):
widths[cast(int, char1) + i] = w
r = []
elif isinstance(v, (int, float)): # == utils.isnumber(v)
r.append(v)
if len(r) == 3:
(char1, char2, w) = r
for i in range(cast(int, char1), cast(int, char2) + 1):
widths[i] = w
r = []
return widths
def get_widths2(seq: Iterable[object]) -> Dict[int, Tuple[float, Point]]:
"""Build a mapping of character widths for vertical writing."""
widths: Dict[int, Tuple[float, Point]] = {}
r: List[float] = []
for v in seq:
if isinstance(v, list):
if r:
char1 = r[-1]
for (i, (w, vx, vy)) in enumerate(choplist(3, v)):
widths[cast(int, char1) + i] = (w, (vx, vy))
r = []
elif isinstance(v, (int, float)): # == utils.isnumber(v)
r.append(v)
if len(r) == 5:
(char1, char2, w, vx, vy) = r
for i in range(cast(int, char1), cast(int, char2) + 1):
widths[i] = (w, (vx, vy))
r = []
return widths
class FontMetricsDB:
@classmethod
def get_metrics(cls, fontname: str) -> Tuple[Dict[str, object], Dict[str, int]]:
return FONT_METRICS[fontname]
# int here means that we're not extending PSStackParser with additional types.
class Type1FontHeaderParser(PSStackParser[int]):
KEYWORD_BEGIN = KWD(b"begin")
KEYWORD_END = KWD(b"end")
KEYWORD_DEF = KWD(b"def")
KEYWORD_PUT = KWD(b"put")
KEYWORD_DICT = KWD(b"dict")
KEYWORD_ARRAY = KWD(b"array")
KEYWORD_READONLY = KWD(b"readonly")
KEYWORD_FOR = KWD(b"for")
def __init__(self, data: BinaryIO) -> None:
PSStackParser.__init__(self, data)
self._cid2unicode: Dict[int, str] = {}
return
def get_encoding(self) -> Dict[int, str]:
"""Parse the font encoding.
The Type1 font encoding maps character codes to character names. These
character names could either be standard Adobe glyph names, or
character names associated with custom CharStrings for this font. A
CharString is a sequence of operations that describe how the character
should be drawn. Currently, this function returns '' (empty string)
for character names that are associated with a CharStrings.
Reference: Adobe Systems Incorporated, Adobe Type 1 Font Format
:returns mapping of character identifiers (cid's) to unicode characters
"""
while 1:
try:
(cid, name) = self.nextobject()
except PSEOF:
break
try:
self._cid2unicode[cid] = name2unicode(cast(str, name))
except KeyError as e:
log.debug(str(e))
return self._cid2unicode
def do_keyword(self, pos: int, token: PSKeyword) -> None:
if token is self.KEYWORD_PUT:
((_, key), (_, value)) = self.pop(2)
if isinstance(key, int) and isinstance(value, PSLiteral):
self.add_results((key, literal_name(value)))
return
NIBBLES = ("0", "1", "2", "3", "4", "5", "6", "7", "8", "9", ".", "e", "e-", None, "-")
# Mapping of cmap names. Original cmap name is kept if not in the mapping.
# (missing reference for why DLIdent is mapped to Identity)
IDENTITY_ENCODER = {
"DLIdent-H": "Identity-H",
"DLIdent-V": "Identity-V",
}
def getdict(data: bytes) -> Dict[int, List[Union[float, int]]]:
d: Dict[int, List[Union[float, int]]] = {}
fp = BytesIO(data)
stack: List[Union[float, int]] = []
while 1:
c = fp.read(1)
if not c:
break
b0 = ord(c)
if b0 <= 21:
d[b0] = stack
stack = []
continue
if b0 == 30:
s = ""
loop = True
while loop:
b = ord(fp.read(1))
for n in (b >> 4, b & 15):
if n == 15:
loop = False
else:
nibble = NIBBLES[n]
assert nibble is not None
s += nibble
value = float(s)
elif 32 <= b0 and b0 <= 246:
value = b0 - 139
else:
b1 = ord(fp.read(1))
if 247 <= b0 and b0 <= 250:
value = ((b0 - 247) << 8) + b1 + 108
elif 251 <= b0 and b0 <= 254:
value = -((b0 - 251) << 8) - b1 - 108
else:
b2 = ord(fp.read(1))
if 128 <= b1:
b1 -= 256
if b0 == 28:
value = b1 << 8 | b2
else:
value = b1 << 24 | b2 << 16 | struct.unpack(">H", fp.read(2))[0]
stack.append(value)
return d
class CFFFont:
STANDARD_STRINGS = (
".notdef",
"space",
"exclam",
"quotedbl",
"numbersign",
"dollar",
"percent",
"ampersand",
"quoteright",
"parenleft",
"parenright",
"asterisk",
"plus",
"comma",
"hyphen",
"period",
"slash",
"zero",
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine",
"colon",
"semicolon",
"less",
"equal",
"greater",
"question",
"at",
"A",
"B",
"C",
"D",
"E",
"F",
"G",
"H",
"I",
"J",
"K",
"L",
"M",
"N",
"O",
"P",
"Q",
"R",
"S",
"T",
"U",
"V",
"W",
"X",
"Y",
"Z",
"bracketleft",
"backslash",
"bracketright",
"asciicircum",
"underscore",
"quoteleft",
"a",
"b",
"c",
"d",
"e",
"f",
"g",
"h",
"i",
"j",
"k",
"l",
"m",
"n",
"o",
"p",
"q",
"r",
"s",
"t",
"u",
"v",
"w",
"x",
"y",
"z",
"braceleft",
"bar",
"braceright",
"asciitilde",
"exclamdown",
"cent",
"sterling",
"fraction",
"yen",
"florin",
"section",
"currency",
"quotesingle",
"quotedblleft",
"guillemotleft",
"guilsinglleft",
"guilsinglright",
"fi",
"fl",
"endash",
"dagger",
"daggerdbl",
"periodcentered",
"paragraph",
"bullet",
"quotesinglbase",
"quotedblbase",
"quotedblright",
"guillemotright",
"ellipsis",
"perthousand",
"questiondown",
"grave",
"acute",
"circumflex",
"tilde",
"macron",
"breve",
"dotaccent",
"dieresis",
"ring",
"cedilla",
"hungarumlaut",
"ogonek",
"caron",
"emdash",
"AE",
"ordfeminine",
"Lslash",
"Oslash",
"OE",
"ordmasculine",
"ae",
"dotlessi",
"lslash",
"oslash",
"oe",
"germandbls",
"onesuperior",
"logicalnot",
"mu",
"trademark",
"Eth",
"onehalf",
"plusminus",
"Thorn",
"onequarter",
"divide",
"brokenbar",
"degree",
"thorn",
"threequarters",
"twosuperior",
"registered",
"minus",
"eth",
"multiply",
"threesuperior",
"copyright",
"Aacute",
"Acircumflex",
"Adieresis",
"Agrave",
"Aring",
"Atilde",
"Ccedilla",
"Eacute",
"Ecircumflex",
"Edieresis",
"Egrave",
"Iacute",
"Icircumflex",
"Idieresis",
"Igrave",
"Ntilde",
"Oacute",
"Ocircumflex",
"Odieresis",
"Ograve",
"Otilde",
"Scaron",
"Uacute",
"Ucircumflex",
"Udieresis",
"Ugrave",
"Yacute",
"Ydieresis",
"Zcaron",
"aacute",
"acircumflex",
"adieresis",
"agrave",
"aring",
"atilde",
"ccedilla",
"eacute",
"ecircumflex",
"edieresis",
"egrave",
"iacute",
"icircumflex",
"idieresis",
"igrave",
"ntilde",
"oacute",
"ocircumflex",
"odieresis",
"ograve",
"otilde",
"scaron",
"uacute",
"ucircumflex",
"udieresis",
"ugrave",
"yacute",
"ydieresis",
"zcaron",
"exclamsmall",
"Hungarumlautsmall",
"dollaroldstyle",
"dollarsuperior",
"ampersandsmall",
"Acutesmall",
"parenleftsuperior",
"parenrightsuperior",
"twodotenleader",
"onedotenleader",
"zerooldstyle",
"oneoldstyle",
"twooldstyle",
"threeoldstyle",
"fouroldstyle",
"fiveoldstyle",
"sixoldstyle",
"sevenoldstyle",
"eightoldstyle",
"nineoldstyle",
"commasuperior",
"threequartersemdash",
"periodsuperior",
"questionsmall",
"asuperior",
"bsuperior",
"centsuperior",
"dsuperior",
"esuperior",
"isuperior",
"lsuperior",
"msuperior",
"nsuperior",
"osuperior",
"rsuperior",
"ssuperior",
"tsuperior",
"ff",
"ffi",
"ffl",
"parenleftinferior",
"parenrightinferior",
"Circumflexsmall",
"hyphensuperior",
"Gravesmall",
"Asmall",
"Bsmall",
"Csmall",
"Dsmall",
"Esmall",
"Fsmall",
"Gsmall",
"Hsmall",
"Ismall",
"Jsmall",
"Ksmall",
"Lsmall",
"Msmall",
"Nsmall",
"Osmall",
"Psmall",
"Qsmall",
"Rsmall",
"Ssmall",
"Tsmall",
"Usmall",
"Vsmall",
"Wsmall",
"Xsmall",
"Ysmall",
"Zsmall",
"colonmonetary",
"onefitted",
"rupiah",
"Tildesmall",
"exclamdownsmall",
"centoldstyle",
"Lslashsmall",
"Scaronsmall",
"Zcaronsmall",
"Dieresissmall",
"Brevesmall",
"Caronsmall",
"Dotaccentsmall",
"Macronsmall",
"figuredash",
"hypheninferior",
"Ogoneksmall",
"Ringsmall",
"Cedillasmall",
"questiondownsmall",
"oneeighth",
"threeeighths",
"fiveeighths",
"seveneighths",
"onethird",
"twothirds",
"zerosuperior",
"foursuperior",
"fivesuperior",
"sixsuperior",
"sevensuperior",
"eightsuperior",
"ninesuperior",
"zeroinferior",
"oneinferior",
"twoinferior",
"threeinferior",
"fourinferior",
"fiveinferior",
"sixinferior",
"seveninferior",
"eightinferior",
"nineinferior",
"centinferior",
"dollarinferior",
"periodinferior",
"commainferior",
"Agravesmall",
"Aacutesmall",
"Acircumflexsmall",
"Atildesmall",
"Adieresissmall",
"Aringsmall",
"AEsmall",
"Ccedillasmall",
"Egravesmall",
"Eacutesmall",
"Ecircumflexsmall",
"Edieresissmall",
"Igravesmall",
"Iacutesmall",
"Icircumflexsmall",
"Idieresissmall",
"Ethsmall",
"Ntildesmall",
"Ogravesmall",
"Oacutesmall",
"Ocircumflexsmall",
"Otildesmall",
"Odieresissmall",
"OEsmall",
"Oslashsmall",
"Ugravesmall",
"Uacutesmall",
"Ucircumflexsmall",
"Udieresissmall",
"Yacutesmall",
"Thornsmall",
"Ydieresissmall",
"001.000",
"001.001",
"001.002",
"001.003",
"Black",
"Bold",
"Book",
"Light",
"Medium",
"Regular",
"Roman",
"Semibold",
)
class INDEX:
def __init__(self, fp: BinaryIO) -> None:
self.fp = fp
self.offsets: List[int] = []
(count, offsize) = struct.unpack(">HB", self.fp.read(3))
for i in range(count + 1):
self.offsets.append(nunpack(self.fp.read(offsize)))
self.base = self.fp.tell() - 1
self.fp.seek(self.base + self.offsets[-1])
return
def __repr__(self) -> str:
return "<INDEX: size=%d>" % len(self)
def __len__(self) -> int:
return len(self.offsets) - 1
def __getitem__(self, i: int) -> bytes:
self.fp.seek(self.base + self.offsets[i])
return self.fp.read(self.offsets[i + 1] - self.offsets[i])
def __iter__(self) -> Iterator[bytes]:
return iter(self[i] for i in range(len(self)))
def __init__(self, name: str, fp: BinaryIO) -> None:
self.name = name
self.fp = fp
# Header
(_major, _minor, hdrsize, offsize) = struct.unpack("BBBB", self.fp.read(4))
self.fp.read(hdrsize - 4)
# Name INDEX
self.name_index = self.INDEX(self.fp)
# Top DICT INDEX
self.dict_index = self.INDEX(self.fp)
# String INDEX
self.string_index = self.INDEX(self.fp)
# Global Subr INDEX
self.subr_index = self.INDEX(self.fp)
# Top DICT DATA
self.top_dict = getdict(self.dict_index[0])
(charset_pos,) = self.top_dict.get(15, [0])
(encoding_pos,) = self.top_dict.get(16, [0])
(charstring_pos,) = self.top_dict.get(17, [0])
# CharStrings
self.fp.seek(cast(int, charstring_pos))
self.charstring = self.INDEX(self.fp)
self.nglyphs = len(self.charstring)
# Encodings
self.code2gid = {}
self.gid2code = {}
self.fp.seek(cast(int, encoding_pos))
format = self.fp.read(1)
if format == b"\x00":
# Format 0
(n,) = struct.unpack("B", self.fp.read(1))
for (code, gid) in enumerate(struct.unpack("B" * n, self.fp.read(n))):
self.code2gid[code] = gid
self.gid2code[gid] = code
elif format == b"\x01":
# Format 1
(n,) = struct.unpack("B", self.fp.read(1))
code = 0
for i in range(n):
(first, nleft) = struct.unpack("BB", self.fp.read(2))
for gid in range(first, first + nleft + 1):
self.code2gid[code] = gid
self.gid2code[gid] = code
code += 1
else:
raise ValueError("unsupported encoding format: %r" % format)
# Charsets
self.name2gid = {}
self.gid2name = {}
self.fp.seek(cast(int, charset_pos))
format = self.fp.read(1)
if format == b"\x00":
# Format 0
n = self.nglyphs - 1
for (gid, sid) in enumerate(
cast(Tuple[int, ...], struct.unpack(">" + "H" * n, self.fp.read(2 * n)))
):
gid += 1
sidname = self.getstr(sid)
self.name2gid[sidname] = gid
self.gid2name[gid] = sidname
elif format == b"\x01":
# Format 1
(n,) = struct.unpack("B", self.fp.read(1))
sid = 0
for i in range(n):
(first, nleft) = struct.unpack("BB", self.fp.read(2))
for gid in range(first, first + nleft + 1):
sidname = self.getstr(sid)
self.name2gid[sidname] = gid
self.gid2name[gid] = sidname
sid += 1
elif format == b"\x02":
# Format 2
assert False, str(("Unhandled", format))
else:
raise ValueError("unsupported charset format: %r" % format)
return
def getstr(self, sid: int) -> Union[str, bytes]:
# This returns str for one of the STANDARD_STRINGS but bytes otherwise,
# and appears to be a needless source of type complexity.
if sid < len(self.STANDARD_STRINGS):
return self.STANDARD_STRINGS[sid]
return self.string_index[sid - len(self.STANDARD_STRINGS)]
class TrueTypeFont:
class CMapNotFound(Exception):
pass
def __init__(self, name: str, fp: BinaryIO) -> None:
self.name = name
self.fp = fp
self.tables: Dict[bytes, Tuple[int, int]] = {}
self.fonttype = fp.read(4)
try:
(ntables, _1, _2, _3) = cast(
Tuple[int, int, int, int], struct.unpack(">HHHH", fp.read(8))
)
for _ in range(ntables):
(name_bytes, tsum, offset, length) = cast(
Tuple[bytes, int, int, int], struct.unpack(">4sLLL", fp.read(16))
)
self.tables[name_bytes] = (offset, length)
except struct.error:
# Do not fail if there are not enough bytes to read. Even for
# corrupted PDFs we would like to get as much information as
# possible, so continue.
pass
return
def create_unicode_map(self) -> FileUnicodeMap:
if b"cmap" not in self.tables:
raise TrueTypeFont.CMapNotFound
(base_offset, length) = self.tables[b"cmap"]
fp = self.fp
fp.seek(base_offset)
(version, nsubtables) = cast(Tuple[int, int], struct.unpack(">HH", fp.read(4)))
subtables: List[Tuple[int, int, int]] = []
for i in range(nsubtables):
subtables.append(
cast(Tuple[int, int, int], struct.unpack(">HHL", fp.read(8)))
)
char2gid: Dict[int, int] = {}
# Only supports subtable type 0, 2 and 4.
for (_1, _2, st_offset) in subtables:
fp.seek(base_offset + st_offset)
(fmttype, fmtlen, fmtlang) = cast(
Tuple[int, int, int], struct.unpack(">HHH", fp.read(6))
)
if fmttype == 0:
char2gid.update(
enumerate(
cast(Tuple[int, ...], struct.unpack(">256B", fp.read(256)))
)
)
elif fmttype == 2:
subheaderkeys = cast(
Tuple[int, ...], struct.unpack(">256H", fp.read(512))
)
firstbytes = [0] * 8192
for (i, k) in enumerate(subheaderkeys):
firstbytes[k // 8] = i
nhdrs = max(subheaderkeys) // 8 + 1
hdrs: List[Tuple[int, int, int, int, int]] = []
for i in range(nhdrs):
(firstcode, entcount, delta, offset) = cast(
Tuple[int, int, int, int], struct.unpack(">HHhH", fp.read(8))
)
hdrs.append((i, firstcode, entcount, delta, fp.tell() - 2 + offset))
for (i, firstcode, entcount, delta, pos) in hdrs:
if not entcount:
continue
first = firstcode + (firstbytes[i] << 8)
fp.seek(pos)
for c in range(entcount):
gid = cast(Tuple[int], struct.unpack(">H", fp.read(2)))[0]
if gid:
gid += delta
char2gid[first + c] = gid
elif fmttype == 4:
(segcount, _1, _2, _3) = cast(
Tuple[int, int, int, int], struct.unpack(">HHHH", fp.read(8))
)
segcount //= 2
ecs = cast(
Tuple[int, ...],
struct.unpack(">%dH" % segcount, fp.read(2 * segcount)),
)
fp.read(2)
scs = cast(
Tuple[int, ...],
struct.unpack(">%dH" % segcount, fp.read(2 * segcount)),
)
idds = cast(
Tuple[int, ...],
struct.unpack(">%dh" % segcount, fp.read(2 * segcount)),
)
pos = fp.tell()
idrs = cast(
Tuple[int, ...],
struct.unpack(">%dH" % segcount, fp.read(2 * segcount)),
)
for (ec, sc, idd, idr) in zip(ecs, scs, idds, idrs):
if idr:
fp.seek(pos + idr)
for c in range(sc, ec + 1):
b = cast(Tuple[int], struct.unpack(">H", fp.read(2)))[0]
char2gid[c] = (b + idd) & 0xFFFF
else:
for c in range(sc, ec + 1):
char2gid[c] = (c + idd) & 0xFFFF
else:
assert False, str(("Unhandled", fmttype))
# create unicode map
unicode_map = FileUnicodeMap()
for (char, gid) in char2gid.items():
unicode_map.add_cid2unichr(gid, char)
return unicode_map
class PDFFontError(PDFException):
pass
class PDFUnicodeNotDefined(PDFFontError):
pass
LITERAL_STANDARD_ENCODING = LIT("StandardEncoding")
LITERAL_TYPE1C = LIT("Type1C")
# Font widths are maintained in a dict type that maps from *either* unicode
# chars or integer character IDs.
FontWidthDict = Union[Dict[int, float], Dict[str, float]]
class PDFFont:
def __init__(
self,
descriptor: Mapping[str, Any],
widths: FontWidthDict,
default_width: Optional[float] = None,
) -> None:
self.descriptor = descriptor
self.widths: FontWidthDict = resolve_all(widths)
self.fontname = resolve1(descriptor.get("FontName", "unknown"))
if isinstance(self.fontname, PSLiteral):
self.fontname = literal_name(self.fontname)
self.flags = int_value(descriptor.get("Flags", 0))
self.ascent = num_value(descriptor.get("Ascent", 0))
self.descent = num_value(descriptor.get("Descent", 0))
self.italic_angle = num_value(descriptor.get("ItalicAngle", 0))
if default_width is None:
self.default_width = num_value(descriptor.get("MissingWidth", 0))
else:
self.default_width = default_width
self.leading = num_value(descriptor.get("Leading", 0))
self.bbox = cast(
Rect, list_value(resolve_all(descriptor.get("FontBBox", (0, 0, 0, 0))))
)
self.hscale = self.vscale = 0.001
# PDF RM 9.8.1 specifies /Descent should always be a negative number.
# PScript5.dll seems to produce Descent with a positive number, but
# text analysis will be wrong if this is taken as correct. So force
# descent to negative.
if self.descent > 0:
self.descent = -self.descent
return
def __repr__(self) -> str:
return "<PDFFont>"
def is_vertical(self) -> bool:
return False
def is_multibyte(self) -> bool:
return False
def decode(self, bytes: bytes) -> Iterable[int]:
return bytearray(bytes) # map(ord, bytes)
def get_ascent(self) -> float:
"""Ascent above the baseline, in text space units"""
return self.ascent * self.vscale
def get_descent(self) -> float:
"""Descent below the baseline, in text space units; always negative"""
return self.descent * self.vscale
def get_width(self) -> float:
w = self.bbox[2] - self.bbox[0]
if w == 0:
w = -self.default_width
return w * self.hscale
def get_height(self) -> float:
h = self.bbox[3] - self.bbox[1]
if h == 0:
h = self.ascent - self.descent
return h * self.vscale
def char_width(self, cid: int) -> float:
# Because character widths may be mapping either IDs or strings,
# we try to lookup the character ID first, then its str equivalent.
try:
return cast(Dict[int, float], self.widths)[cid] * self.hscale
except KeyError:
str_widths = cast(Dict[str, float], self.widths)
try:
return str_widths[self.to_unichr(cid)] * self.hscale
except (KeyError, PDFUnicodeNotDefined):
return self.default_width * self.hscale
def char_disp(self, cid: int) -> Union[float, Tuple[Optional[float], float]]:
"Returns an integer for horizontal fonts, a tuple for vertical fonts."
return 0
def string_width(self, s: bytes) -> float:
return sum(self.char_width(cid) for cid in self.decode(s))
def to_unichr(self, cid: int) -> str:
raise NotImplementedError
class PDFSimpleFont(PDFFont):
def __init__(
self,
descriptor: Mapping[str, Any],
widths: FontWidthDict,
spec: Mapping[str, Any],
) -> None:
# Font encoding is specified either by a name of
# built-in encoding or a dictionary that describes
# the differences.
if "Encoding" in spec:
encoding = resolve1(spec["Encoding"])
else:
encoding = LITERAL_STANDARD_ENCODING
if isinstance(encoding, dict):
name = literal_name(encoding.get("BaseEncoding", LITERAL_STANDARD_ENCODING))
diff = list_value(encoding.get("Differences", []))
self.cid2unicode = EncodingDB.get_encoding(name, diff)
else:
self.cid2unicode = EncodingDB.get_encoding(literal_name(encoding))
self.unicode_map: Optional[UnicodeMap] = None
if "ToUnicode" in spec:
strm = stream_value(spec["ToUnicode"])
self.unicode_map = FileUnicodeMap()
CMapParser(self.unicode_map, BytesIO(strm.get_data())).run()
PDFFont.__init__(self, descriptor, widths)
return
def to_unichr(self, cid: int) -> str:
if self.unicode_map:
try:
return self.unicode_map.get_unichr(cid)
except KeyError:
pass
try:
return self.cid2unicode[cid]
except KeyError:
raise PDFUnicodeNotDefined(None, cid)
class PDFType1Font(PDFSimpleFont):
def __init__(self, rsrcmgr: "PDFResourceManager", spec: Mapping[str, Any]) -> None:
try:
self.basefont = literal_name(spec["BaseFont"])
except KeyError:
if settings.STRICT:
raise PDFFontError("BaseFont is missing")
self.basefont = "unknown"
widths: FontWidthDict
try:
(descriptor, int_widths) = FontMetricsDB.get_metrics(self.basefont)
widths = cast(Dict[str, float], int_widths) # implicit int->float
except KeyError:
descriptor = dict_value(spec.get("FontDescriptor", {}))
firstchar = int_value(spec.get("FirstChar", 0))
# lastchar = int_value(spec.get('LastChar', 255))
width_list = list_value(spec.get("Widths", [0] * 256))
widths = {i + firstchar: w for (i, w) in enumerate(width_list)}
PDFSimpleFont.__init__(self, descriptor, widths, spec)
if "Encoding" not in spec and "FontFile" in descriptor:
# try to recover the missing encoding info from the font file.
self.fontfile = stream_value(descriptor.get("FontFile"))
length1 = int_value(self.fontfile["Length1"])
data = self.fontfile.get_data()[:length1]
parser = Type1FontHeaderParser(BytesIO(data))
self.cid2unicode = parser.get_encoding()
return
def __repr__(self) -> str:
return "<PDFType1Font: basefont=%r>" % self.basefont
class PDFTrueTypeFont(PDFType1Font):
def __repr__(self) -> str:
return "<PDFTrueTypeFont: basefont=%r>" % self.basefont
class PDFType3Font(PDFSimpleFont):
def __init__(self, rsrcmgr: "PDFResourceManager", spec: Mapping[str, Any]) -> None:
firstchar = int_value(spec.get("FirstChar", 0))
# lastchar = int_value(spec.get('LastChar', 0))
width_list = list_value(spec.get("Widths", [0] * 256))
widths = {i + firstchar: w for (i, w) in enumerate(width_list)}
if "FontDescriptor" in spec:
descriptor = dict_value(spec["FontDescriptor"])
else:
descriptor = {"Ascent": 0, "Descent": 0, "FontBBox": spec["FontBBox"]}
PDFSimpleFont.__init__(self, descriptor, widths, spec)
self.matrix = cast(Matrix, tuple(list_value(spec.get("FontMatrix"))))
(_, self.descent, _, self.ascent) = self.bbox
(self.hscale, self.vscale) = apply_matrix_norm(self.matrix, (1, 1))
return
def __repr__(self) -> str:
return "<PDFType3Font>"
class PDFCIDFont(PDFFont):
default_disp: Union[float, Tuple[Optional[float], float]]
def __init__(
self,
rsrcmgr: "PDFResourceManager",
spec: Mapping[str, Any],
strict: bool = settings.STRICT,
) -> None:
try:
self.basefont = literal_name(spec["BaseFont"])
except KeyError:
if strict:
raise PDFFontError("BaseFont is missing")
self.basefont = "unknown"
self.cidsysteminfo = dict_value(spec.get("CIDSystemInfo", {}))
cid_registry = resolve1(self.cidsysteminfo.get("Registry", b"unknown")).decode(
"latin1"
)
cid_ordering = resolve1(self.cidsysteminfo.get("Ordering", b"unknown")).decode(
"latin1"
)
self.cidcoding = "{}-{}".format(cid_registry, cid_ordering)
self.cmap: CMapBase = self.get_cmap_from_spec(spec, strict)
try:
descriptor = dict_value(spec["FontDescriptor"])
except KeyError:
if strict:
raise PDFFontError("FontDescriptor is missing")
descriptor = {}
ttf = None
if "FontFile2" in descriptor:
self.fontfile = stream_value(descriptor.get("FontFile2"))
ttf = TrueTypeFont(self.basefont, BytesIO(self.fontfile.get_data()))
self.unicode_map: Optional[UnicodeMap] = None
if "ToUnicode" in spec:
if isinstance(spec["ToUnicode"], PDFStream):
strm = stream_value(spec["ToUnicode"])
self.unicode_map = FileUnicodeMap()
CMapParser(self.unicode_map, BytesIO(strm.get_data())).run()
else:
cmap_name = literal_name(spec["ToUnicode"])
encoding = literal_name(spec["Encoding"])
if (
"Identity" in cid_ordering
or "Identity" in cmap_name
or "Identity" in encoding
):
self.unicode_map = IdentityUnicodeMap()
elif self.cidcoding in ("Adobe-Identity", "Adobe-UCS"):
if ttf:
try:
self.unicode_map = ttf.create_unicode_map()
except TrueTypeFont.CMapNotFound:
pass
else:
try:
self.unicode_map = CMapDB.get_unicode_map(
self.cidcoding, self.cmap.is_vertical()
)
except CMapDB.CMapNotFound:
pass
self.vertical = self.cmap.is_vertical()
if self.vertical:
# writing mode: vertical
widths2 = get_widths2(list_value(spec.get("W2", [])))
self.disps = {cid: (vx, vy) for (cid, (_, (vx, vy))) in widths2.items()}
(vy, w) = resolve1(spec.get("DW2", [880, -1000]))
self.default_disp = (None, vy)
widths = {cid: w for (cid, (w, _)) in widths2.items()}
default_width = w
else:
# writing mode: horizontal
self.disps = {}
self.default_disp = 0
widths = get_widths(list_value(spec.get("W", [])))
default_width = spec.get("DW", 1000)
PDFFont.__init__(self, descriptor, widths, default_width=default_width)
return
def get_cmap_from_spec(self, spec: Mapping[str, Any], strict: bool) -> CMapBase:
"""Get cmap from font specification
For certain PDFs, Encoding Type isn't mentioned as an attribute of
Encoding but as an attribute of CMapName, where CMapName is an
attribute of spec['Encoding'].
The horizontal/vertical modes are mentioned with different name
such as 'DLIdent-H/V','OneByteIdentityH/V','Identity-H/V'.
"""
cmap_name = self._get_cmap_name(spec, strict)
try:
return CMapDB.get_cmap(cmap_name)
except CMapDB.CMapNotFound as e:
if strict:
raise PDFFontError(e)
return CMap()
@staticmethod
def _get_cmap_name(spec: Mapping[str, Any], strict: bool) -> str:
"""Get cmap name from font specification"""
cmap_name = "unknown" # default value
try:
spec_encoding = spec["Encoding"]
if hasattr(spec_encoding, "name"):
cmap_name = literal_name(spec["Encoding"])
else:
cmap_name = literal_name(spec_encoding["CMapName"])
except KeyError:
if strict:
raise PDFFontError("Encoding is unspecified")
if type(cmap_name) is PDFStream: # type: ignore[comparison-overlap]
cmap_name_stream: PDFStream = cast(PDFStream, cmap_name)
if "CMapName" in cmap_name_stream:
cmap_name = cmap_name_stream.get("CMapName").name
else:
if strict:
raise PDFFontError("CMapName unspecified for encoding")
return IDENTITY_ENCODER.get(cmap_name, cmap_name)
def __repr__(self) -> str:
return "<PDFCIDFont: basefont={!r}, cidcoding={!r}>".format(
self.basefont, self.cidcoding
)
def is_vertical(self) -> bool:
return self.vertical
def is_multibyte(self) -> bool:
return True
def decode(self, bytes: bytes) -> Iterable[int]:
return self.cmap.decode(bytes)
def char_disp(self, cid: int) -> Union[float, Tuple[Optional[float], float]]:
"Returns an integer for horizontal fonts, a tuple for vertical fonts."
return self.disps.get(cid, self.default_disp)
def to_unichr(self, cid: int) -> str:
try:
if not self.unicode_map:
raise KeyError(cid)
return self.unicode_map.get_unichr(cid)
except KeyError:
raise PDFUnicodeNotDefined(self.cidcoding, cid)
def main(argv: List[str]) -> None:
for fname in argv[1:]:
fp = open(fname, "rb")
font = CFFFont(fname, fp)
print(font)
fp.close()
return
if __name__ == "__main__":
main(sys.argv) | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/pdffont.py | pdffont.py |
import gzip
import logging
import os
import os.path
import pickle as pickle
import struct
import sys
from typing import (
Any,
BinaryIO,
Dict,
Iterable,
Iterator,
List,
MutableMapping,
Optional,
TextIO,
Tuple,
Union,
cast,
Set,
)
from .encodingdb import name2unicode
from .psparser import KWD
from .psparser import PSEOF
from .psparser import PSKeyword
from .psparser import PSLiteral
from .psparser import PSStackParser
from .psparser import PSSyntaxError
from .psparser import literal_name
from .utils import choplist
from .utils import nunpack
log = logging.getLogger(__name__)
class CMapError(Exception):
pass
class CMapBase:
debug = 0
def __init__(self, **kwargs: object) -> None:
self.attrs: MutableMapping[str, object] = kwargs.copy()
def is_vertical(self) -> bool:
return self.attrs.get("WMode", 0) != 0
def set_attr(self, k: str, v: object) -> None:
self.attrs[k] = v
def add_code2cid(self, code: str, cid: int) -> None:
pass
def add_cid2unichr(self, cid: int, code: Union[PSLiteral, bytes, int]) -> None:
pass
def use_cmap(self, cmap: "CMapBase") -> None:
pass
def decode(self, code: bytes) -> Iterable[int]:
raise NotImplementedError
class CMap(CMapBase):
def __init__(self, **kwargs: Union[str, int]) -> None:
CMapBase.__init__(self, **kwargs)
self.code2cid: Dict[int, object] = {}
def __repr__(self) -> str:
return "<CMap: %s>" % self.attrs.get("CMapName")
def use_cmap(self, cmap: CMapBase) -> None:
assert isinstance(cmap, CMap), str(type(cmap))
def copy(dst: Dict[int, object], src: Dict[int, object]) -> None:
for (k, v) in src.items():
if isinstance(v, dict):
d: Dict[int, object] = {}
dst[k] = d
copy(d, v)
else:
dst[k] = v
copy(self.code2cid, cmap.code2cid)
def decode(self, code: bytes) -> Iterator[int]:
log.debug("decode: %r, %r", self, code)
d = self.code2cid
for i in iter(code):
if i in d:
x = d[i]
if isinstance(x, int):
yield x
d = self.code2cid
else:
d = cast(Dict[int, object], x)
else:
d = self.code2cid
def dump(
self,
out: TextIO = sys.stdout,
code2cid: Optional[Dict[int, object]] = None,
code: Tuple[int, ...] = (),
) -> None:
if code2cid is None:
code2cid = self.code2cid
code = ()
for (k, v) in sorted(code2cid.items()):
c = code + (k,)
if isinstance(v, int):
out.write("code %r = cid %d\n" % (c, v))
else:
self.dump(out=out, code2cid=cast(Dict[int, object], v), code=c)
class IdentityCMap(CMapBase):
def decode(self, code: bytes) -> Tuple[int, ...]:
n = len(code) // 2
if n:
return struct.unpack(">%dH" % n, code)
else:
return ()
class IdentityCMapByte(IdentityCMap):
def decode(self, code: bytes) -> Tuple[int, ...]:
n = len(code)
if n:
return struct.unpack(">%dB" % n, code)
else:
return ()
class UnicodeMap(CMapBase):
def __init__(self, **kwargs: Union[str, int]) -> None:
CMapBase.__init__(self, **kwargs)
self.cid2unichr: Dict[int, str] = {}
def __repr__(self) -> str:
return "<UnicodeMap: %s>" % self.attrs.get("CMapName")
def get_unichr(self, cid: int) -> str:
log.debug("get_unichr: %r, %r", self, cid)
return self.cid2unichr[cid]
def dump(self, out: TextIO = sys.stdout) -> None:
for (k, v) in sorted(self.cid2unichr.items()):
out.write("cid %d = unicode %r\n" % (k, v))
class IdentityUnicodeMap(UnicodeMap):
def get_unichr(self, cid: int) -> str:
"""Interpret character id as unicode codepoint"""
log.debug("get_unichr: %r, %r", self, cid)
return chr(cid)
class FileCMap(CMap):
def add_code2cid(self, code: str, cid: int) -> None:
assert isinstance(code, str) and isinstance(cid, int), str(
(type(code), type(cid))
)
d = self.code2cid
for c in code[:-1]:
ci = ord(c)
if ci in d:
d = cast(Dict[int, object], d[ci])
else:
t: Dict[int, object] = {}
d[ci] = t
d = t
ci = ord(code[-1])
d[ci] = cid
class FileUnicodeMap(UnicodeMap):
def add_cid2unichr(self, cid: int, code: Union[PSLiteral, bytes, int]) -> None:
assert isinstance(cid, int), str(type(cid))
if isinstance(code, PSLiteral):
# Interpret as an Adobe glyph name.
assert isinstance(code.name, str)
self.cid2unichr[cid] = name2unicode(code.name)
elif isinstance(code, bytes):
# Interpret as UTF-16BE.
self.cid2unichr[cid] = code.decode("UTF-16BE", "ignore")
elif isinstance(code, int):
self.cid2unichr[cid] = chr(code)
else:
raise TypeError(code)
class PyCMap(CMap):
def __init__(self, name: str, module: Any) -> None:
super().__init__(CMapName=name)
self.code2cid = module.CODE2CID
if module.IS_VERTICAL:
self.attrs["WMode"] = 1
class PyUnicodeMap(UnicodeMap):
def __init__(self, name: str, module: Any, vertical: bool) -> None:
super().__init__(CMapName=name)
if vertical:
self.cid2unichr = module.CID2UNICHR_V
self.attrs["WMode"] = 1
else:
self.cid2unichr = module.CID2UNICHR_H
class CMapDB:
_cmap_cache: Dict[str, PyCMap] = {}
_umap_cache: Dict[str, List[PyUnicodeMap]] = {}
class CMapNotFound(CMapError):
pass
@classmethod
def _load_data(cls, name: str) -> Any:
name = name.replace("\0", "")
filename = "%s.pickle.gz" % name
log.debug("loading: %r", name)
cmap_paths = (
os.environ.get("CMAP_PATH", "/usr/share/pdfminer/"),
os.path.join(os.path.dirname(__file__), "cmap"),
)
for directory in cmap_paths:
path = os.path.join(directory, filename)
if os.path.exists(path):
gzfile = gzip.open(path)
try:
return type(str(name), (), pickle.loads(gzfile.read()))
finally:
gzfile.close()
else:
raise CMapDB.CMapNotFound(name)
@classmethod
def get_cmap(cls, name: str) -> CMapBase:
if name == "Identity-H":
return IdentityCMap(WMode=0)
elif name == "Identity-V":
return IdentityCMap(WMode=1)
elif name == "OneByteIdentityH":
return IdentityCMapByte(WMode=0)
elif name == "OneByteIdentityV":
return IdentityCMapByte(WMode=1)
try:
return cls._cmap_cache[name]
except KeyError:
pass
data = cls._load_data(name)
cls._cmap_cache[name] = cmap = PyCMap(name, data)
return cmap
@classmethod
def get_unicode_map(cls, name: str, vertical: bool = False) -> UnicodeMap:
try:
return cls._umap_cache[name][vertical]
except KeyError:
pass
data = cls._load_data("to-unicode-%s" % name)
cls._umap_cache[name] = [PyUnicodeMap(name, data, v) for v in (False, True)]
return cls._umap_cache[name][vertical]
class CMapParser(PSStackParser[PSKeyword]):
def __init__(self, cmap: CMapBase, fp: BinaryIO) -> None:
PSStackParser.__init__(self, fp)
self.cmap = cmap
# some ToUnicode maps don't have "begincmap" keyword.
self._in_cmap = True
self._warnings: Set[str] = set()
return
def run(self) -> None:
try:
self.nextobject()
except PSEOF:
pass
return
KEYWORD_BEGINCMAP = KWD(b"begincmap")
KEYWORD_ENDCMAP = KWD(b"endcmap")
KEYWORD_USECMAP = KWD(b"usecmap")
KEYWORD_DEF = KWD(b"def")
KEYWORD_BEGINCODESPACERANGE = KWD(b"begincodespacerange")
KEYWORD_ENDCODESPACERANGE = KWD(b"endcodespacerange")
KEYWORD_BEGINCIDRANGE = KWD(b"begincidrange")
KEYWORD_ENDCIDRANGE = KWD(b"endcidrange")
KEYWORD_BEGINCIDCHAR = KWD(b"begincidchar")
KEYWORD_ENDCIDCHAR = KWD(b"endcidchar")
KEYWORD_BEGINBFRANGE = KWD(b"beginbfrange")
KEYWORD_ENDBFRANGE = KWD(b"endbfrange")
KEYWORD_BEGINBFCHAR = KWD(b"beginbfchar")
KEYWORD_ENDBFCHAR = KWD(b"endbfchar")
KEYWORD_BEGINNOTDEFRANGE = KWD(b"beginnotdefrange")
KEYWORD_ENDNOTDEFRANGE = KWD(b"endnotdefrange")
def do_keyword(self, pos: int, token: PSKeyword) -> None:
"""ToUnicode CMaps
See Section 5.9.2 - ToUnicode CMaps of the PDF Reference.
"""
if token is self.KEYWORD_BEGINCMAP:
self._in_cmap = True
self.popall()
return
elif token is self.KEYWORD_ENDCMAP:
self._in_cmap = False
return
if not self._in_cmap:
return
if token is self.KEYWORD_DEF:
try:
((_, k), (_, v)) = self.pop(2)
self.cmap.set_attr(literal_name(k), v)
except PSSyntaxError:
pass
return
if token is self.KEYWORD_USECMAP:
try:
((_, cmapname),) = self.pop(1)
self.cmap.use_cmap(CMapDB.get_cmap(literal_name(cmapname)))
except PSSyntaxError:
pass
except CMapDB.CMapNotFound:
pass
return
if token is self.KEYWORD_BEGINCODESPACERANGE:
self.popall()
return
if token is self.KEYWORD_ENDCODESPACERANGE:
self.popall()
return
if token is self.KEYWORD_BEGINCIDRANGE:
self.popall()
return
if token is self.KEYWORD_ENDCIDRANGE:
objs = [obj for (__, obj) in self.popall()]
for (start_byte, end_byte, cid) in choplist(3, objs):
if not isinstance(start_byte, bytes):
self._warn_once("The start object of begincidrange is not a byte.")
continue
if not isinstance(end_byte, bytes):
self._warn_once("The end object of begincidrange is not a byte.")
continue
if not isinstance(cid, int):
self._warn_once("The cid object of begincidrange is not a byte.")
continue
if len(start_byte) != len(end_byte):
self._warn_once(
"The start and end byte of begincidrange have "
"different lengths."
)
continue
start_prefix = start_byte[:-4]
end_prefix = end_byte[:-4]
if start_prefix != end_prefix:
self._warn_once(
"The prefix of the start and end byte of "
"begincidrange are not the same."
)
continue
svar = start_byte[-4:]
evar = end_byte[-4:]
start = nunpack(svar)
end = nunpack(evar)
vlen = len(svar)
for i in range(end - start + 1):
x = start_prefix + struct.pack(">L", start + i)[-vlen:]
self.cmap.add_cid2unichr(cid + i, x)
return
if token is self.KEYWORD_BEGINCIDCHAR:
self.popall()
return
if token is self.KEYWORD_ENDCIDCHAR:
objs = [obj for (__, obj) in self.popall()]
for (cid, code) in choplist(2, objs):
if isinstance(code, bytes) and isinstance(cid, int):
self.cmap.add_cid2unichr(cid, code)
return
if token is self.KEYWORD_BEGINBFRANGE:
self.popall()
return
if token is self.KEYWORD_ENDBFRANGE:
objs = [obj for (__, obj) in self.popall()]
for (start_byte, end_byte, code) in choplist(3, objs):
if not isinstance(start_byte, bytes):
self._warn_once("The start object is not a byte.")
continue
if not isinstance(end_byte, bytes):
self._warn_once("The end object is not a byte.")
continue
if len(start_byte) != len(end_byte):
self._warn_once("The start and end byte have different lengths.")
continue
start = nunpack(start_byte)
end = nunpack(end_byte)
if isinstance(code, list):
if len(code) != end - start + 1:
self._warn_once(
"The difference between the start and end "
"offsets does not match the code length."
)
for cid, unicode_value in zip(range(start, end + 1), code):
self.cmap.add_cid2unichr(cid, unicode_value)
else:
assert isinstance(code, bytes)
var = code[-4:]
base = nunpack(var)
prefix = code[:-4]
vlen = len(var)
for i in range(end - start + 1):
x = prefix + struct.pack(">L", base + i)[-vlen:]
self.cmap.add_cid2unichr(start + i, x)
return
if token is self.KEYWORD_BEGINBFCHAR:
self.popall()
return
if token is self.KEYWORD_ENDBFCHAR:
objs = [obj for (__, obj) in self.popall()]
for (cid, code) in choplist(2, objs):
if isinstance(cid, bytes) and isinstance(code, bytes):
self.cmap.add_cid2unichr(nunpack(cid), code)
return
if token is self.KEYWORD_BEGINNOTDEFRANGE:
self.popall()
return
if token is self.KEYWORD_ENDNOTDEFRANGE:
self.popall()
return
self.push((pos, token))
def _warn_once(self, msg: str) -> None:
"""Warn once for each unique message"""
if msg not in self._warnings:
self._warnings.add(msg)
base_msg = (
"Ignoring (part of) ToUnicode map because the PDF data "
"does not conform to the format. This could result in "
"(cid) values in the output. "
)
log.warning(base_msg + msg)
def main(argv: List[str]) -> None:
args = argv[1:]
for fname in args:
fp = open(fname, "rb")
cmap = FileUnicodeMap()
CMapParser(cmap, fp).run()
fp.close()
cmap.dump()
return
if __name__ == "__main__":
main(sys.argv) | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/cmapdb.py | cmapdb.py |
from typing import (
BinaryIO,
Iterable,
List,
Optional,
Sequence,
TYPE_CHECKING,
Union,
cast,
)
from pdfminer.psparser import PSLiteral
from . import utils
from .pdfcolor import PDFColorSpace
from .pdffont import PDFFont
from .pdffont import PDFUnicodeNotDefined
from .pdfpage import PDFPage
from .pdftypes import PDFStream
from .utils import Matrix, Point, Rect, PathSegment
if TYPE_CHECKING:
from .pdfinterp import PDFGraphicState
from .pdfinterp import PDFResourceManager
from .pdfinterp import PDFTextState
from .pdfinterp import PDFStackT
PDFTextSeq = Iterable[Union[int, float, bytes]]
class PDFDevice:
"""Translate the output of PDFPageInterpreter to the output that is needed"""
def __init__(self, rsrcmgr: "PDFResourceManager") -> None:
self.rsrcmgr = rsrcmgr
self.ctm: Optional[Matrix] = None
def __repr__(self) -> str:
return "<PDFDevice>"
def __enter__(self) -> "PDFDevice":
return self
def __exit__(self, exc_type: object, exc_val: object, exc_tb: object) -> None:
self.close()
def close(self) -> None:
pass
def set_ctm(self, ctm: Matrix) -> None:
self.ctm = ctm
def begin_tag(self, tag: PSLiteral, props: Optional["PDFStackT"] = None) -> None:
pass
def end_tag(self) -> None:
pass
def do_tag(self, tag: PSLiteral, props: Optional["PDFStackT"] = None) -> None:
pass
def begin_page(self, page: PDFPage, ctm: Matrix) -> None:
pass
def end_page(self, page: PDFPage) -> None:
pass
def begin_figure(self, name: str, bbox: Rect, matrix: Matrix) -> None:
pass
def end_figure(self, name: str) -> None:
pass
def paint_path(
self,
graphicstate: "PDFGraphicState",
stroke: bool,
fill: bool,
evenodd: bool,
path: Sequence[PathSegment],
) -> None:
pass
def render_image(self, name: str, stream: PDFStream) -> None:
pass
def render_string(
self,
textstate: "PDFTextState",
seq: PDFTextSeq,
ncs: PDFColorSpace,
graphicstate: "PDFGraphicState",
) -> None:
pass
class PDFTextDevice(PDFDevice):
def render_string(
self,
textstate: "PDFTextState",
seq: PDFTextSeq,
ncs: PDFColorSpace,
graphicstate: "PDFGraphicState",
) -> None:
assert self.ctm is not None
matrix = utils.mult_matrix(textstate.matrix, self.ctm)
font = textstate.font
fontsize = textstate.fontsize
scaling = textstate.scaling * 0.01
charspace = textstate.charspace * scaling
wordspace = textstate.wordspace * scaling
rise = textstate.rise
assert font is not None
if font.is_multibyte():
wordspace = 0
dxscale = 0.001 * fontsize * scaling
if font.is_vertical():
textstate.linematrix = self.render_string_vertical(
seq,
matrix,
textstate.linematrix,
font,
fontsize,
scaling,
charspace,
wordspace,
rise,
dxscale,
ncs,
graphicstate,
)
else:
textstate.linematrix = self.render_string_horizontal(
seq,
matrix,
textstate.linematrix,
font,
fontsize,
scaling,
charspace,
wordspace,
rise,
dxscale,
ncs,
graphicstate,
)
def render_string_horizontal(
self,
seq: PDFTextSeq,
matrix: Matrix,
pos: Point,
font: PDFFont,
fontsize: float,
scaling: float,
charspace: float,
wordspace: float,
rise: float,
dxscale: float,
ncs: PDFColorSpace,
graphicstate: "PDFGraphicState",
) -> Point:
(x, y) = pos
needcharspace = False
for obj in seq:
if isinstance(obj, (int, float)):
x -= obj * dxscale
needcharspace = True
else:
for cid in font.decode(obj):
if needcharspace:
x += charspace
x += self.render_char(
utils.translate_matrix(matrix, (x, y)),
font,
fontsize,
scaling,
rise,
cid,
ncs,
graphicstate,
)
if cid == 32 and wordspace:
x += wordspace
needcharspace = True
return (x, y)
def render_string_vertical(
self,
seq: PDFTextSeq,
matrix: Matrix,
pos: Point,
font: PDFFont,
fontsize: float,
scaling: float,
charspace: float,
wordspace: float,
rise: float,
dxscale: float,
ncs: PDFColorSpace,
graphicstate: "PDFGraphicState",
) -> Point:
(x, y) = pos
needcharspace = False
for obj in seq:
if isinstance(obj, (int, float)):
y -= obj * dxscale
needcharspace = True
else:
for cid in font.decode(obj):
if needcharspace:
y += charspace
y += self.render_char(
utils.translate_matrix(matrix, (x, y)),
font,
fontsize,
scaling,
rise,
cid,
ncs,
graphicstate,
)
if cid == 32 and wordspace:
y += wordspace
needcharspace = True
return (x, y)
def render_char(
self,
matrix: Matrix,
font: PDFFont,
fontsize: float,
scaling: float,
rise: float,
cid: int,
ncs: PDFColorSpace,
graphicstate: "PDFGraphicState",
) -> float:
return 0
class TagExtractor(PDFDevice):
def __init__(
self, rsrcmgr: "PDFResourceManager", outfp: BinaryIO, codec: str = "utf-8"
) -> None:
PDFDevice.__init__(self, rsrcmgr)
self.outfp = outfp
self.codec = codec
self.pageno = 0
self._stack: List[PSLiteral] = []
def render_string(
self,
textstate: "PDFTextState",
seq: PDFTextSeq,
ncs: PDFColorSpace,
graphicstate: "PDFGraphicState",
) -> None:
font = textstate.font
assert font is not None
text = ""
for obj in seq:
if isinstance(obj, str):
obj = utils.make_compat_bytes(obj)
if not isinstance(obj, bytes):
continue
chars = font.decode(obj)
for cid in chars:
try:
char = font.to_unichr(cid)
text += char
except PDFUnicodeNotDefined:
pass
self._write(utils.enc(text))
def begin_page(self, page: PDFPage, ctm: Matrix) -> None:
output = '<page id="%s" bbox="%s" rotate="%d">' % (
self.pageno,
utils.bbox2str(page.mediabox),
page.rotate,
)
self._write(output)
return
def end_page(self, page: PDFPage) -> None:
self._write("</page>\n")
self.pageno += 1
return
def begin_tag(self, tag: PSLiteral, props: Optional["PDFStackT"] = None) -> None:
s = ""
if isinstance(props, dict):
s = "".join(
[
' {}="{}"'.format(utils.enc(k), utils.make_compat_str(v))
for (k, v) in sorted(props.items())
]
)
out_s = "<{}{}>".format(utils.enc(cast(str, tag.name)), s)
self._write(out_s)
self._stack.append(tag)
return
def end_tag(self) -> None:
assert self._stack, str(self.pageno)
tag = self._stack.pop(-1)
out_s = "</%s>" % utils.enc(cast(str, tag.name))
self._write(out_s)
return
def do_tag(self, tag: PSLiteral, props: Optional["PDFStackT"] = None) -> None:
self.begin_tag(tag, props)
self._stack.pop(-1)
return
def _write(self, s: str) -> None:
self.outfp.write(s.encode(self.codec)) | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/pdfdevice.py | pdfdevice.py |
import os
import os.path
import struct
from io import BytesIO
from typing import BinaryIO, Tuple
try:
from typing import Literal
except ImportError:
from typing_extensions import Literal # type: ignore[misc]
from .jbig2 import JBIG2StreamReader, JBIG2StreamWriter
from .layout import LTImage
from .pdfcolor import LITERAL_DEVICE_CMYK
from .pdfcolor import LITERAL_DEVICE_GRAY
from .pdfcolor import LITERAL_DEVICE_RGB
from .pdftypes import (
LITERALS_DCT_DECODE,
LITERALS_JBIG2_DECODE,
LITERALS_JPX_DECODE,
LITERALS_FLATE_DECODE,
)
PIL_ERROR_MESSAGE = (
"Could not import Pillow. This dependency of pdfminer.six is not "
"installed by default. You need it to to save jpg images to a file. Install it "
"with `pip install 'pdfminer.six[image]'`"
)
def align32(x: int) -> int:
return ((x + 3) // 4) * 4
class BMPWriter:
def __init__(self, fp: BinaryIO, bits: int, width: int, height: int) -> None:
self.fp = fp
self.bits = bits
self.width = width
self.height = height
if bits == 1:
ncols = 2
elif bits == 8:
ncols = 256
elif bits == 24:
ncols = 0
else:
raise ValueError(bits)
self.linesize = align32((self.width * self.bits + 7) // 8)
self.datasize = self.linesize * self.height
headersize = 14 + 40 + ncols * 4
info = struct.pack(
"<IiiHHIIIIII",
40,
self.width,
self.height,
1,
self.bits,
0,
self.datasize,
0,
0,
ncols,
0,
)
assert len(info) == 40, str(len(info))
header = struct.pack(
"<ccIHHI", b"B", b"M", headersize + self.datasize, 0, 0, headersize
)
assert len(header) == 14, str(len(header))
self.fp.write(header)
self.fp.write(info)
if ncols == 2:
# B&W color table
for i in (0, 255):
self.fp.write(struct.pack("BBBx", i, i, i))
elif ncols == 256:
# grayscale color table
for i in range(256):
self.fp.write(struct.pack("BBBx", i, i, i))
self.pos0 = self.fp.tell()
self.pos1 = self.pos0 + self.datasize
def write_line(self, y: int, data: bytes) -> None:
self.fp.seek(self.pos1 - (y + 1) * self.linesize)
self.fp.write(data)
class ImageWriter:
"""Write image to a file
Supports various image types: JPEG, JBIG2 and bitmaps
"""
def __init__(self, outdir: str) -> None:
self.outdir = outdir
if not os.path.exists(self.outdir):
os.makedirs(self.outdir)
def export_image(self, image: LTImage) -> str:
"""Save an LTImage to disk"""
(width, height) = image.srcsize
filters = image.stream.get_filters()
if len(filters) == 1 and filters[0][0] in LITERALS_DCT_DECODE:
name = self._save_jpeg(image)
elif len(filters) == 1 and filters[0][0] in LITERALS_JPX_DECODE:
name = self._save_jpeg2000(image)
elif self._is_jbig2_iamge(image):
name = self._save_jbig2(image)
elif image.bits == 1:
name = self._save_bmp(image, width, height, (width + 7) // 8, image.bits)
elif image.bits == 8 and LITERAL_DEVICE_RGB in image.colorspace:
name = self._save_bmp(image, width, height, width * 3, image.bits * 3)
elif image.bits == 8 and LITERAL_DEVICE_GRAY in image.colorspace:
name = self._save_bmp(image, width, height, width, image.bits)
elif len(filters) == 1 and filters[0][0] in LITERALS_FLATE_DECODE:
name = self._save_bytes(image)
else:
name = self._save_raw(image)
return name
def _save_jpeg(self, image: LTImage) -> str:
"""Save a JPEG encoded image"""
raw_data = image.stream.get_rawdata()
assert raw_data is not None
name, path = self._create_unique_image_name(image, ".jpg")
with open(path, "wb") as fp:
if LITERAL_DEVICE_CMYK in image.colorspace:
try:
from PIL import Image, ImageChops # type: ignore[import]
except ImportError:
raise ImportError(PIL_ERROR_MESSAGE)
ifp = BytesIO(raw_data)
i = Image.open(ifp)
i = ImageChops.invert(i)
i = i.convert("RGB")
i.save(fp, "JPEG")
else:
fp.write(raw_data)
return name
def _save_jpeg2000(self, image: LTImage) -> str:
"""Save a JPEG 2000 encoded image"""
raw_data = image.stream.get_rawdata()
assert raw_data is not None
name, path = self._create_unique_image_name(image, ".jp2")
with open(path, "wb") as fp:
try:
from PIL import Image # type: ignore[import]
except ImportError:
raise ImportError(PIL_ERROR_MESSAGE)
# if we just write the raw data, most image programs
# that I have tried cannot open the file. However,
# open and saving with PIL produces a file that
# seems to be easily opened by other programs
ifp = BytesIO(raw_data)
i = Image.open(ifp)
i.save(fp, "JPEG2000")
return name
def _save_jbig2(self, image: LTImage) -> str:
"""Save a JBIG2 encoded image"""
name, path = self._create_unique_image_name(image, ".jb2")
with open(path, "wb") as fp:
input_stream = BytesIO()
global_streams = []
filters = image.stream.get_filters()
for filter_name, params in filters:
if filter_name in LITERALS_JBIG2_DECODE:
global_streams.append(params["JBIG2Globals"].resolve())
if len(global_streams) > 1:
msg = (
"There should never be more than one JBIG2Globals "
"associated with a JBIG2 embedded image"
)
raise ValueError(msg)
if len(global_streams) == 1:
input_stream.write(global_streams[0].get_data().rstrip(b"\n"))
input_stream.write(image.stream.get_data())
input_stream.seek(0)
reader = JBIG2StreamReader(input_stream)
segments = reader.get_segments()
writer = JBIG2StreamWriter(fp)
writer.write_file(segments)
return name
def _save_bmp(
self, image: LTImage, width: int, height: int, bytes_per_line: int, bits: int
) -> str:
"""Save a BMP encoded image"""
name, path = self._create_unique_image_name(image, ".bmp")
with open(path, "wb") as fp:
bmp = BMPWriter(fp, bits, width, height)
data = image.stream.get_data()
i = 0
for y in range(height):
bmp.write_line(y, data[i : i + bytes_per_line])
i += bytes_per_line
return name
def _save_bytes(self, image: LTImage) -> str:
"""Save an image without encoding, just bytes"""
name, path = self._create_unique_image_name(image, ".jpg")
width, height = image.srcsize
channels = len(image.stream.get_data()) / width / height / (image.bits / 8)
with open(path, "wb") as fp:
try:
from PIL import Image # type: ignore[import]
except ImportError:
raise ImportError(PIL_ERROR_MESSAGE)
mode: Literal["1", "8", "RGB", "CMYK"]
if image.bits == 1:
mode = "1"
elif image.bits == 8 and channels == 1:
mode = "8"
elif image.bits == 8 and channels == 3:
mode = "RGB"
elif image.bits == 8 and channels == 4:
mode = "CMYK"
img = Image.frombytes(mode, image.srcsize, image.stream.get_data(), "raw")
img.save(fp)
return name
def _save_raw(self, image: LTImage) -> str:
"""Save an image with unknown encoding"""
ext = ".%d.%dx%d.img" % (image.bits, image.srcsize[0], image.srcsize[1])
name, path = self._create_unique_image_name(image, ext)
with open(path, "wb") as fp:
fp.write(image.stream.get_data())
return name
@staticmethod
def _is_jbig2_iamge(image: LTImage) -> bool:
filters = image.stream.get_filters()
for filter_name, params in filters:
if filter_name in LITERALS_JBIG2_DECODE:
return True
return False
def _create_unique_image_name(self, image: LTImage, ext: str) -> Tuple[str, str]:
name = image.name + ext
path = os.path.join(self.outdir, name)
img_index = 0
while os.path.exists(path):
name = "%s.%d%s" % (image.name, img_index, ext)
path = os.path.join(self.outdir, name)
img_index += 1
return name, path | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/image.py | image.py |
__all__ = ["saslprep"]
import stringprep
from typing import Callable, Tuple
import unicodedata
# RFC4013 section 2.3 prohibited output.
_PROHIBITED: Tuple[Callable[[str], bool], ...] = (
# A strict reading of RFC 4013 requires table c12 here, but
# characters from it are mapped to SPACE in the Map step. Can
# normalization reintroduce them somehow?
stringprep.in_table_c12,
stringprep.in_table_c21_c22,
stringprep.in_table_c3,
stringprep.in_table_c4,
stringprep.in_table_c5,
stringprep.in_table_c6,
stringprep.in_table_c7,
stringprep.in_table_c8,
stringprep.in_table_c9,
)
def saslprep(data: str, prohibit_unassigned_code_points: bool = True) -> str:
"""An implementation of RFC4013 SASLprep.
:param data:
The string to SASLprep.
:param prohibit_unassigned_code_points:
RFC 3454 and RFCs for various SASL mechanisms distinguish between
`queries` (unassigned code points allowed) and
`stored strings` (unassigned code points prohibited). Defaults
to ``True`` (unassigned code points are prohibited).
:return: The SASLprep'ed version of `data`.
"""
if prohibit_unassigned_code_points:
prohibited = _PROHIBITED + (stringprep.in_table_a1,)
else:
prohibited = _PROHIBITED
# RFC3454 section 2, step 1 - Map
# RFC4013 section 2.1 mappings
# Map Non-ASCII space characters to SPACE (U+0020). Map
# commonly mapped to nothing characters to, well, nothing.
in_table_c12 = stringprep.in_table_c12
in_table_b1 = stringprep.in_table_b1
data = "".join(
["\u0020" if in_table_c12(elt) else elt for elt in data if not in_table_b1(elt)]
)
# RFC3454 section 2, step 2 - Normalize
# RFC4013 section 2.2 normalization
data = unicodedata.ucd_3_2_0.normalize("NFKC", data)
in_table_d1 = stringprep.in_table_d1
if in_table_d1(data[0]):
if not in_table_d1(data[-1]):
# RFC3454, Section 6, #3. If a string contains any
# RandALCat character, the first and last characters
# MUST be RandALCat characters.
raise ValueError("SASLprep: failed bidirectional check")
# RFC3454, Section 6, #2. If a string contains any RandALCat
# character, it MUST NOT contain any LCat character.
prohibited = prohibited + (stringprep.in_table_d2,)
else:
# RFC3454, Section 6, #3. Following the logic of #3, if
# the first character is not a RandALCat, no other character
# can be either.
prohibited = prohibited + (in_table_d1,)
# RFC3454 section 2, step 3 and 4 - Prohibit and check bidi
for char in data:
if any(in_table(char) for in_table in prohibited):
raise ValueError("SASLprep: failed prohibited character check")
return data | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/_saslprep.py | _saslprep.py |
import itertools
import logging
import re
import struct
from hashlib import sha256, md5, sha384, sha512
from typing import (
Any,
Callable,
Dict,
Iterable,
Iterator,
KeysView,
List,
Optional,
Sequence,
Tuple,
Type,
Union,
cast,
)
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from . import settings
from .arcfour import Arcfour
from .data_structures import NumberTree
from .pdfparser import PDFSyntaxError, PDFParser, PDFStreamParser
from .pdftypes import (
DecipherCallable,
PDFException,
PDFTypeError,
PDFStream,
PDFObjectNotFound,
decipher_all,
int_value,
str_value,
list_value,
uint_value,
dict_value,
stream_value,
)
from .psparser import PSEOF, literal_name, LIT, KWD
from .utils import choplist, decode_text, nunpack, format_int_roman, format_int_alpha
log = logging.getLogger(__name__)
class PDFNoValidXRef(PDFSyntaxError):
pass
class PDFNoValidXRefWarning(SyntaxWarning):
"""Legacy warning for missing xref.
Not used anymore because warnings.warn is replaced by logger.Logger.warn.
"""
pass
class PDFNoOutlines(PDFException):
pass
class PDFNoPageLabels(PDFException):
pass
class PDFDestinationNotFound(PDFException):
pass
class PDFEncryptionError(PDFException):
pass
class PDFPasswordIncorrect(PDFEncryptionError):
pass
class PDFEncryptionWarning(UserWarning):
"""Legacy warning for failed decryption.
Not used anymore because warnings.warn is replaced by logger.Logger.warn.
"""
pass
class PDFTextExtractionNotAllowedWarning(UserWarning):
"""Legacy warning for PDF that does not allow extraction.
Not used anymore because warnings.warn is replaced by logger.Logger.warn.
"""
pass
class PDFTextExtractionNotAllowed(PDFEncryptionError):
pass
class PDFTextExtractionNotAllowedError(PDFTextExtractionNotAllowed):
def __init__(self, *args: object) -> None:
from warnings import warn
warn(
"PDFTextExtractionNotAllowedError will be removed in the future. "
"Use PDFTextExtractionNotAllowed instead.",
DeprecationWarning,
)
super().__init__(*args)
# some predefined literals and keywords.
LITERAL_OBJSTM = LIT("ObjStm")
LITERAL_XREF = LIT("XRef")
LITERAL_CATALOG = LIT("Catalog")
class PDFBaseXRef:
def get_trailer(self) -> Dict[str, Any]:
raise NotImplementedError
def get_objids(self) -> Iterable[int]:
return []
# Must return
# (strmid, index, genno)
# or (None, pos, genno)
def get_pos(self, objid: int) -> Tuple[Optional[int], int, int]:
raise KeyError(objid)
def load(self, parser: PDFParser) -> None:
raise NotImplementedError
class PDFXRef(PDFBaseXRef):
def __init__(self) -> None:
self.offsets: Dict[int, Tuple[Optional[int], int, int]] = {}
self.trailer: Dict[str, Any] = {}
def __repr__(self) -> str:
return "<PDFXRef: offsets=%r>" % (self.offsets.keys())
def load(self, parser: PDFParser) -> None:
while True:
try:
(pos, line) = parser.nextline()
line = line.strip()
if not line:
continue
except PSEOF:
raise PDFNoValidXRef("Unexpected EOF - file corrupted?")
if line.startswith(b"trailer"):
parser.seek(pos)
break
f = line.split(b" ")
if len(f) != 2:
error_msg = "Trailer not found: {!r}: line={!r}".format(parser, line)
raise PDFNoValidXRef(error_msg)
try:
(start, nobjs) = map(int, f)
except ValueError:
error_msg = "Invalid line: {!r}: line={!r}".format(parser, line)
raise PDFNoValidXRef(error_msg)
for objid in range(start, start + nobjs):
try:
(_, line) = parser.nextline()
line = line.strip()
except PSEOF:
raise PDFNoValidXRef("Unexpected EOF - file corrupted?")
f = line.split(b" ")
if len(f) != 3:
error_msg = "Invalid XRef format: {!r}, line={!r}".format(
parser, line
)
raise PDFNoValidXRef(error_msg)
(pos_b, genno_b, use_b) = f
if use_b != b"n":
continue
self.offsets[objid] = (None, int(pos_b), int(genno_b))
log.debug("xref objects: %r", self.offsets)
self.load_trailer(parser)
def load_trailer(self, parser: PDFParser) -> None:
try:
(_, kwd) = parser.nexttoken()
assert kwd is KWD(b"trailer"), str(kwd)
(_, dic) = parser.nextobject()
except PSEOF:
x = parser.pop(1)
if not x:
raise PDFNoValidXRef("Unexpected EOF - file corrupted")
(_, dic) = x[0]
self.trailer.update(dict_value(dic))
log.debug("trailer=%r", self.trailer)
def get_trailer(self) -> Dict[str, Any]:
return self.trailer
def get_objids(self) -> KeysView[int]:
return self.offsets.keys()
def get_pos(self, objid: int) -> Tuple[Optional[int], int, int]:
try:
return self.offsets[objid]
except KeyError:
raise
class PDFXRefFallback(PDFXRef):
def __repr__(self) -> str:
return "<PDFXRefFallback: offsets=%r>" % (self.offsets.keys())
PDFOBJ_CUE = re.compile(r"^(\d+)\s+(\d+)\s+obj\b")
def load(self, parser: PDFParser) -> None:
parser.seek(0)
while 1:
try:
(pos, line_bytes) = parser.nextline()
except PSEOF:
break
if line_bytes.startswith(b"trailer"):
parser.seek(pos)
self.load_trailer(parser)
log.debug("trailer: %r", self.trailer)
break
line = line_bytes.decode("latin-1") # default pdf encoding
m = self.PDFOBJ_CUE.match(line)
if not m:
continue
(objid_s, genno_s) = m.groups()
objid = int(objid_s)
genno = int(genno_s)
self.offsets[objid] = (None, pos, genno)
# expand ObjStm.
parser.seek(pos)
(_, obj) = parser.nextobject()
if isinstance(obj, PDFStream) and obj.get("Type") is LITERAL_OBJSTM:
stream = stream_value(obj)
try:
n = stream["N"]
except KeyError:
if settings.STRICT:
raise PDFSyntaxError("N is not defined: %r" % stream)
n = 0
parser1 = PDFStreamParser(stream.get_data())
objs: List[int] = []
try:
while 1:
(_, obj) = parser1.nextobject()
objs.append(cast(int, obj))
except PSEOF:
pass
n = min(n, len(objs) // 2)
for index in range(n):
objid1 = objs[index * 2]
self.offsets[objid1] = (objid, index, 0)
class PDFXRefStream(PDFBaseXRef):
def __init__(self) -> None:
self.data: Optional[bytes] = None
self.entlen: Optional[int] = None
self.fl1: Optional[int] = None
self.fl2: Optional[int] = None
self.fl3: Optional[int] = None
self.ranges: List[Tuple[int, int]] = []
def __repr__(self) -> str:
return "<PDFXRefStream: ranges=%r>" % (self.ranges)
def load(self, parser: PDFParser) -> None:
(_, objid) = parser.nexttoken() # ignored
(_, genno) = parser.nexttoken() # ignored
(_, kwd) = parser.nexttoken()
(_, stream) = parser.nextobject()
if not isinstance(stream, PDFStream) or stream.get("Type") is not LITERAL_XREF:
raise PDFNoValidXRef("Invalid PDF stream spec.")
size = stream["Size"]
index_array = stream.get("Index", (0, size))
if len(index_array) % 2 != 0:
raise PDFSyntaxError("Invalid index number")
self.ranges.extend(cast(Iterator[Tuple[int, int]], choplist(2, index_array)))
(self.fl1, self.fl2, self.fl3) = stream["W"]
assert self.fl1 is not None and self.fl2 is not None and self.fl3 is not None
self.data = stream.get_data()
self.entlen = self.fl1 + self.fl2 + self.fl3
self.trailer = stream.attrs
log.debug(
"xref stream: objid=%s, fields=%d,%d,%d",
", ".join(map(repr, self.ranges)),
self.fl1,
self.fl2,
self.fl3,
)
return
def get_trailer(self) -> Dict[str, Any]:
return self.trailer
def get_objids(self) -> Iterator[int]:
for (start, nobjs) in self.ranges:
for i in range(nobjs):
assert self.entlen is not None
assert self.data is not None
offset = self.entlen * i
ent = self.data[offset : offset + self.entlen]
f1 = nunpack(ent[: self.fl1], 1)
if f1 == 1 or f1 == 2:
yield start + i
return
def get_pos(self, objid: int) -> Tuple[Optional[int], int, int]:
index = 0
for (start, nobjs) in self.ranges:
if start <= objid and objid < start + nobjs:
index += objid - start
break
else:
index += nobjs
else:
raise KeyError(objid)
assert self.entlen is not None
assert self.data is not None
assert self.fl1 is not None and self.fl2 is not None and self.fl3 is not None
offset = self.entlen * index
ent = self.data[offset : offset + self.entlen]
f1 = nunpack(ent[: self.fl1], 1)
f2 = nunpack(ent[self.fl1 : self.fl1 + self.fl2])
f3 = nunpack(ent[self.fl1 + self.fl2 :])
if f1 == 1:
return (None, f2, f3)
elif f1 == 2:
return (f2, f3, 0)
else:
# this is a free object
raise KeyError(objid)
class PDFStandardSecurityHandler:
PASSWORD_PADDING = (
b"(\xbfN^Nu\x8aAd\x00NV\xff\xfa\x01\x08"
b"..\x00\xb6\xd0h>\x80/\x0c\xa9\xfedSiz"
)
supported_revisions: Tuple[int, ...] = (2, 3)
def __init__(
self, docid: Sequence[bytes], param: Dict[str, Any], password: str = ""
) -> None:
self.docid = docid
self.param = param
self.password = password
self.init()
return
def init(self) -> None:
self.init_params()
if self.r not in self.supported_revisions:
error_msg = "Unsupported revision: param=%r" % self.param
raise PDFEncryptionError(error_msg)
self.init_key()
return
def init_params(self) -> None:
self.v = int_value(self.param.get("V", 0))
self.r = int_value(self.param["R"])
self.p = uint_value(self.param["P"], 32)
self.o = str_value(self.param["O"])
self.u = str_value(self.param["U"])
self.length = int_value(self.param.get("Length", 40))
return
def init_key(self) -> None:
self.key = self.authenticate(self.password)
if self.key is None:
raise PDFPasswordIncorrect
return
def is_printable(self) -> bool:
return bool(self.p & 4)
def is_modifiable(self) -> bool:
return bool(self.p & 8)
def is_extractable(self) -> bool:
return bool(self.p & 16)
def compute_u(self, key: bytes) -> bytes:
if self.r == 2:
# Algorithm 3.4
return Arcfour(key).encrypt(self.PASSWORD_PADDING) # 2
else:
# Algorithm 3.5
hash = md5(self.PASSWORD_PADDING) # 2
hash.update(self.docid[0]) # 3
result = Arcfour(key).encrypt(hash.digest()) # 4
for i in range(1, 20): # 5
k = b"".join(bytes((c ^ i,)) for c in iter(key))
result = Arcfour(k).encrypt(result)
result += result # 6
return result
def compute_encryption_key(self, password: bytes) -> bytes:
# Algorithm 3.2
password = (password + self.PASSWORD_PADDING)[:32] # 1
hash = md5(password) # 2
hash.update(self.o) # 3
# See https://github.com/pdfminer/pdfminer.six/issues/186
hash.update(struct.pack("<L", self.p)) # 4
hash.update(self.docid[0]) # 5
if self.r >= 4:
if not cast(PDFStandardSecurityHandlerV4, self).encrypt_metadata:
hash.update(b"\xff\xff\xff\xff")
result = hash.digest()
n = 5
if self.r >= 3:
n = self.length // 8
for _ in range(50):
result = md5(result[:n]).digest()
return result[:n]
def authenticate(self, password: str) -> Optional[bytes]:
password_bytes = password.encode("latin1")
key = self.authenticate_user_password(password_bytes)
if key is None:
key = self.authenticate_owner_password(password_bytes)
return key
def authenticate_user_password(self, password: bytes) -> Optional[bytes]:
key = self.compute_encryption_key(password)
if self.verify_encryption_key(key):
return key
else:
return None
def verify_encryption_key(self, key: bytes) -> bool:
# Algorithm 3.6
u = self.compute_u(key)
if self.r == 2:
return u == self.u
return u[:16] == self.u[:16]
def authenticate_owner_password(self, password: bytes) -> Optional[bytes]:
# Algorithm 3.7
password = (password + self.PASSWORD_PADDING)[:32]
hash = md5(password)
if self.r >= 3:
for _ in range(50):
hash = md5(hash.digest())
n = 5
if self.r >= 3:
n = self.length // 8
key = hash.digest()[:n]
if self.r == 2:
user_password = Arcfour(key).decrypt(self.o)
else:
user_password = self.o
for i in range(19, -1, -1):
k = b"".join(bytes((c ^ i,)) for c in iter(key))
user_password = Arcfour(k).decrypt(user_password)
return self.authenticate_user_password(user_password)
def decrypt(
self,
objid: int,
genno: int,
data: bytes,
attrs: Optional[Dict[str, Any]] = None,
) -> bytes:
return self.decrypt_rc4(objid, genno, data)
def decrypt_rc4(self, objid: int, genno: int, data: bytes) -> bytes:
assert self.key is not None
key = self.key + struct.pack("<L", objid)[:3] + struct.pack("<L", genno)[:2]
hash = md5(key)
key = hash.digest()[: min(len(key), 16)]
return Arcfour(key).decrypt(data)
class PDFStandardSecurityHandlerV4(PDFStandardSecurityHandler):
supported_revisions: Tuple[int, ...] = (4,)
def init_params(self) -> None:
super().init_params()
self.length = 128
self.cf = dict_value(self.param.get("CF"))
self.stmf = literal_name(self.param["StmF"])
self.strf = literal_name(self.param["StrF"])
self.encrypt_metadata = bool(self.param.get("EncryptMetadata", True))
if self.stmf != self.strf:
error_msg = "Unsupported crypt filter: param=%r" % self.param
raise PDFEncryptionError(error_msg)
self.cfm = {}
for k, v in self.cf.items():
f = self.get_cfm(literal_name(v["CFM"]))
if f is None:
error_msg = "Unknown crypt filter method: param=%r" % self.param
raise PDFEncryptionError(error_msg)
self.cfm[k] = f
self.cfm["Identity"] = self.decrypt_identity
if self.strf not in self.cfm:
error_msg = "Undefined crypt filter: param=%r" % self.param
raise PDFEncryptionError(error_msg)
return
def get_cfm(self, name: str) -> Optional[Callable[[int, int, bytes], bytes]]:
if name == "V2":
return self.decrypt_rc4
elif name == "AESV2":
return self.decrypt_aes128
else:
return None
def decrypt(
self,
objid: int,
genno: int,
data: bytes,
attrs: Optional[Dict[str, Any]] = None,
name: Optional[str] = None,
) -> bytes:
if not self.encrypt_metadata and attrs is not None:
t = attrs.get("Type")
if t is not None and literal_name(t) == "Metadata":
return data
if name is None:
name = self.strf
return self.cfm[name](objid, genno, data)
def decrypt_identity(self, objid: int, genno: int, data: bytes) -> bytes:
return data
def decrypt_aes128(self, objid: int, genno: int, data: bytes) -> bytes:
assert self.key is not None
key = (
self.key
+ struct.pack("<L", objid)[:3]
+ struct.pack("<L", genno)[:2]
+ b"sAlT"
)
hash = md5(key)
key = hash.digest()[: min(len(key), 16)]
initialization_vector = data[:16]
ciphertext = data[16:]
cipher = Cipher(
algorithms.AES(key),
modes.CBC(initialization_vector),
backend=default_backend(),
) # type: ignore
return cipher.decryptor().update(ciphertext) # type: ignore
class PDFStandardSecurityHandlerV5(PDFStandardSecurityHandlerV4):
supported_revisions = (5, 6)
def init_params(self) -> None:
super().init_params()
self.length = 256
self.oe = str_value(self.param["OE"])
self.ue = str_value(self.param["UE"])
self.o_hash = self.o[:32]
self.o_validation_salt = self.o[32:40]
self.o_key_salt = self.o[40:]
self.u_hash = self.u[:32]
self.u_validation_salt = self.u[32:40]
self.u_key_salt = self.u[40:]
return
def get_cfm(self, name: str) -> Optional[Callable[[int, int, bytes], bytes]]:
if name == "AESV3":
return self.decrypt_aes256
else:
return None
def authenticate(self, password: str) -> Optional[bytes]:
password_b = self._normalize_password(password)
hash = self._password_hash(password_b, self.o_validation_salt, self.u)
if hash == self.o_hash:
hash = self._password_hash(password_b, self.o_key_salt, self.u)
cipher = Cipher(
algorithms.AES(hash), modes.CBC(b"\0" * 16), backend=default_backend()
) # type: ignore
return cipher.decryptor().update(self.oe) # type: ignore
hash = self._password_hash(password_b, self.u_validation_salt)
if hash == self.u_hash:
hash = self._password_hash(password_b, self.u_key_salt)
cipher = Cipher(
algorithms.AES(hash), modes.CBC(b"\0" * 16), backend=default_backend()
) # type: ignore
return cipher.decryptor().update(self.ue) # type: ignore
return None
def _normalize_password(self, password: str) -> bytes:
if self.r == 6:
# saslprep expects non-empty strings, apparently
if not password:
return b""
from ._saslprep import saslprep
password = saslprep(password)
return password.encode("utf-8")[:127]
def _password_hash(
self, password: bytes, salt: bytes, vector: Optional[bytes] = None
) -> bytes:
"""
Compute password hash depending on revision number
"""
if self.r == 5:
return self._r5_password(password, salt, vector)
return self._r6_password(password, salt[0:8], vector)
def _r5_password(
self, password: bytes, salt: bytes, vector: Optional[bytes] = None
) -> bytes:
"""
Compute the password for revision 5
"""
hash = sha256(password)
hash.update(salt)
if vector is not None:
hash.update(vector)
return hash.digest()
def _r6_password(
self, password: bytes, salt: bytes, vector: Optional[bytes] = None
) -> bytes:
"""
Compute the password for revision 6
"""
initial_hash = sha256(password)
initial_hash.update(salt)
if vector is not None:
initial_hash.update(vector)
k = initial_hash.digest()
hashes = (sha256, sha384, sha512)
round_no = last_byte_val = 0
while round_no < 64 or last_byte_val > round_no - 32:
k1 = (password + k + (vector or b"")) * 64
e = self._aes_cbc_encrypt(key=k[:16], iv=k[16:32], data=k1)
# compute the first 16 bytes of e,
# interpreted as an unsigned integer mod 3
next_hash = hashes[self._bytes_mod_3(e[:16])]
k = next_hash(e).digest()
last_byte_val = e[len(e) - 1]
round_no += 1
return k[:32]
@staticmethod
def _bytes_mod_3(input_bytes: bytes) -> int:
# 256 is 1 mod 3, so we can just sum 'em
return sum(b % 3 for b in input_bytes) % 3
def _aes_cbc_encrypt(self, key: bytes, iv: bytes, data: bytes) -> bytes:
cipher = Cipher(algorithms.AES(key), modes.CBC(iv))
encryptor = cipher.encryptor() # type: ignore
return encryptor.update(data) + encryptor.finalize() # type: ignore
def decrypt_aes256(self, objid: int, genno: int, data: bytes) -> bytes:
initialization_vector = data[:16]
ciphertext = data[16:]
assert self.key is not None
cipher = Cipher(
algorithms.AES(self.key),
modes.CBC(initialization_vector),
backend=default_backend(),
) # type: ignore
return cipher.decryptor().update(ciphertext) # type: ignore
class PDFDocument:
"""PDFDocument object represents a PDF document.
Since a PDF file can be very big, normally it is not loaded at
once. So PDF document has to cooperate with a PDF parser in order to
dynamically import the data as processing goes.
Typical usage:
doc = PDFDocument(parser, password)
obj = doc.getobj(objid)
"""
security_handler_registry: Dict[int, Type[PDFStandardSecurityHandler]] = {
1: PDFStandardSecurityHandler,
2: PDFStandardSecurityHandler,
4: PDFStandardSecurityHandlerV4,
5: PDFStandardSecurityHandlerV5,
}
def __init__(
self,
parser: PDFParser,
password: str = "",
caching: bool = True,
fallback: bool = True,
) -> None:
"Set the document to use a given PDFParser object."
self.caching = caching
self.xrefs: List[PDFBaseXRef] = []
self.info = []
self.catalog: Dict[str, Any] = {}
self.encryption: Optional[Tuple[Any, Any]] = None
self.decipher: Optional[DecipherCallable] = None
self._parser = None
self._cached_objs: Dict[int, Tuple[object, int]] = {}
self._parsed_objs: Dict[int, Tuple[List[object], int]] = {}
self._parser = parser
self._parser.set_document(self)
self.is_printable = self.is_modifiable = self.is_extractable = True
# Retrieve the information of each header that was appended
# (maybe multiple times) at the end of the document.
try:
pos = self.find_xref(parser)
self.read_xref_from(parser, pos, self.xrefs)
except PDFNoValidXRef:
if fallback:
parser.fallback = True
newxref = PDFXRefFallback()
newxref.load(parser)
self.xrefs.append(newxref)
for xref in self.xrefs:
trailer = xref.get_trailer()
if not trailer:
continue
# If there's an encryption info, remember it.
if "Encrypt" in trailer:
if "ID" in trailer:
id_value = list_value(trailer["ID"])
else:
# Some documents may not have a /ID, use two empty
# byte strings instead. Solves
# https://github.com/pdfminer/pdfminer.six/issues/594
id_value = (b"", b"")
self.encryption = (id_value, dict_value(trailer["Encrypt"]))
self._initialize_password(password)
if "Info" in trailer:
self.info.append(dict_value(trailer["Info"]))
if "Root" in trailer:
# Every PDF file must have exactly one /Root dictionary.
self.catalog = dict_value(trailer["Root"])
break
else:
raise PDFSyntaxError("No /Root object! - Is this really a PDF?")
if self.catalog.get("Type") is not LITERAL_CATALOG:
if settings.STRICT:
raise PDFSyntaxError("Catalog not found!")
return
KEYWORD_OBJ = KWD(b"obj")
# _initialize_password(password=b'')
# Perform the initialization with a given password.
def _initialize_password(self, password: str = "") -> None:
assert self.encryption is not None
(docid, param) = self.encryption
if literal_name(param.get("Filter")) != "Standard":
raise PDFEncryptionError("Unknown filter: param=%r" % param)
v = int_value(param.get("V", 0))
factory = self.security_handler_registry.get(v)
if factory is None:
raise PDFEncryptionError("Unknown algorithm: param=%r" % param)
handler = factory(docid, param, password)
self.decipher = handler.decrypt
self.is_printable = handler.is_printable()
self.is_modifiable = handler.is_modifiable()
self.is_extractable = handler.is_extractable()
assert self._parser is not None
self._parser.fallback = False # need to read streams with exact length
return
def _getobj_objstm(self, stream: PDFStream, index: int, objid: int) -> object:
if stream.objid in self._parsed_objs:
(objs, n) = self._parsed_objs[stream.objid]
else:
(objs, n) = self._get_objects(stream)
if self.caching:
assert stream.objid is not None
self._parsed_objs[stream.objid] = (objs, n)
i = n * 2 + index
try:
obj = objs[i]
except IndexError:
raise PDFSyntaxError("index too big: %r" % index)
return obj
def _get_objects(self, stream: PDFStream) -> Tuple[List[object], int]:
if stream.get("Type") is not LITERAL_OBJSTM:
if settings.STRICT:
raise PDFSyntaxError("Not a stream object: %r" % stream)
try:
n = cast(int, stream["N"])
except KeyError:
if settings.STRICT:
raise PDFSyntaxError("N is not defined: %r" % stream)
n = 0
parser = PDFStreamParser(stream.get_data())
parser.set_document(self)
objs: List[object] = []
try:
while 1:
(_, obj) = parser.nextobject()
objs.append(obj)
except PSEOF:
pass
return (objs, n)
def _getobj_parse(self, pos: int, objid: int) -> object:
assert self._parser is not None
self._parser.seek(pos)
(_, objid1) = self._parser.nexttoken() # objid
(_, genno) = self._parser.nexttoken() # genno
(_, kwd) = self._parser.nexttoken()
# hack around malformed pdf files
# copied from https://github.com/jaepil/pdfminer3k/blob/master/
# pdfminer/pdfparser.py#L399
# to solve https://github.com/pdfminer/pdfminer.six/issues/56
# assert objid1 == objid, str((objid1, objid))
if objid1 != objid:
x = []
while kwd is not self.KEYWORD_OBJ:
(_, kwd) = self._parser.nexttoken()
x.append(kwd)
if len(x) >= 2:
objid1 = x[-2]
# #### end hack around malformed pdf files
if objid1 != objid:
raise PDFSyntaxError("objid mismatch: {!r}={!r}".format(objid1, objid))
if kwd != KWD(b"obj"):
raise PDFSyntaxError("Invalid object spec: offset=%r" % pos)
(_, obj) = self._parser.nextobject()
return obj
# can raise PDFObjectNotFound
def getobj(self, objid: int) -> object:
"""Get object from PDF
:raises PDFException if PDFDocument is not initialized
:raises PDFObjectNotFound if objid does not exist in PDF
"""
if not self.xrefs:
raise PDFException("PDFDocument is not initialized")
log.debug("getobj: objid=%r", objid)
if objid in self._cached_objs:
(obj, genno) = self._cached_objs[objid]
else:
for xref in self.xrefs:
try:
(strmid, index, genno) = xref.get_pos(objid)
except KeyError:
continue
try:
if strmid is not None:
stream = stream_value(self.getobj(strmid))
obj = self._getobj_objstm(stream, index, objid)
else:
obj = self._getobj_parse(index, objid)
if self.decipher:
obj = decipher_all(self.decipher, objid, genno, obj)
if isinstance(obj, PDFStream):
obj.set_objid(objid, genno)
break
except (PSEOF, PDFSyntaxError):
continue
else:
raise PDFObjectNotFound(objid)
log.debug("register: objid=%r: %r", objid, obj)
if self.caching:
self._cached_objs[objid] = (obj, genno)
return obj
OutlineType = Tuple[Any, Any, Any, Any, Any]
def get_outlines(self) -> Iterator[OutlineType]:
if "Outlines" not in self.catalog:
raise PDFNoOutlines
def search(entry: object, level: int) -> Iterator[PDFDocument.OutlineType]:
entry = dict_value(entry)
if "Title" in entry:
if "A" in entry or "Dest" in entry:
title = decode_text(str_value(entry["Title"]))
dest = entry.get("Dest")
action = entry.get("A")
se = entry.get("SE")
yield (level, title, dest, action, se)
if "First" in entry and "Last" in entry:
yield from search(entry["First"], level + 1)
if "Next" in entry:
yield from search(entry["Next"], level)
return
return search(self.catalog["Outlines"], 0)
def get_page_labels(self) -> Iterator[str]:
"""
Generate page label strings for the PDF document.
If the document includes page labels, generates strings, one per page.
If not, raises PDFNoPageLabels.
The resulting iteration is unbounded.
"""
assert self.catalog is not None
try:
page_labels = PageLabels(self.catalog["PageLabels"])
except (PDFTypeError, KeyError):
raise PDFNoPageLabels
return page_labels.labels
def lookup_name(self, cat: str, key: Union[str, bytes]) -> Any:
try:
names = dict_value(self.catalog["Names"])
except (PDFTypeError, KeyError):
raise KeyError((cat, key))
# may raise KeyError
d0 = dict_value(names[cat])
def lookup(d: Dict[str, Any]) -> Any:
if "Limits" in d:
(k1, k2) = list_value(d["Limits"])
if key < k1 or k2 < key:
return None
if "Names" in d:
objs = list_value(d["Names"])
names = dict(
cast(Iterator[Tuple[Union[str, bytes], Any]], choplist(2, objs))
)
return names[key]
if "Kids" in d:
for c in list_value(d["Kids"]):
v = lookup(dict_value(c))
if v:
return v
raise KeyError((cat, key))
return lookup(d0)
def get_dest(self, name: Union[str, bytes]) -> Any:
try:
# PDF-1.2 or later
obj = self.lookup_name("Dests", name)
except KeyError:
# PDF-1.1 or prior
if "Dests" not in self.catalog:
raise PDFDestinationNotFound(name)
d0 = dict_value(self.catalog["Dests"])
if name not in d0:
raise PDFDestinationNotFound(name)
obj = d0[name]
return obj
# find_xref
def find_xref(self, parser: PDFParser) -> int:
"""Internal function used to locate the first XRef."""
# search the last xref table by scanning the file backwards.
prev = None
for line in parser.revreadlines():
line = line.strip()
log.debug("find_xref: %r", line)
if line == b"startxref":
break
if line:
prev = line
else:
raise PDFNoValidXRef("Unexpected EOF")
log.debug("xref found: pos=%r", prev)
assert prev is not None
return int(prev)
# read xref table
def read_xref_from(
self, parser: PDFParser, start: int, xrefs: List[PDFBaseXRef]
) -> None:
"""Reads XRefs from the given location."""
parser.seek(start)
parser.reset()
try:
(pos, token) = parser.nexttoken()
except PSEOF:
raise PDFNoValidXRef("Unexpected EOF")
log.debug("read_xref_from: start=%d, token=%r", start, token)
if isinstance(token, int):
# XRefStream: PDF-1.5
parser.seek(pos)
parser.reset()
xref: PDFBaseXRef = PDFXRefStream()
xref.load(parser)
else:
if token is parser.KEYWORD_XREF:
parser.nextline()
xref = PDFXRef()
xref.load(parser)
xrefs.append(xref)
trailer = xref.get_trailer()
log.debug("trailer: %r", trailer)
if "XRefStm" in trailer:
pos = int_value(trailer["XRefStm"])
self.read_xref_from(parser, pos, xrefs)
if "Prev" in trailer:
# find previous xref
pos = int_value(trailer["Prev"])
self.read_xref_from(parser, pos, xrefs)
return
class PageLabels(NumberTree):
"""PageLabels from the document catalog.
See Section 8.3.1 in the PDF Reference.
"""
@property
def labels(self) -> Iterator[str]:
ranges = self.values
# The tree must begin with page index 0
if len(ranges) == 0 or ranges[0][0] != 0:
if settings.STRICT:
raise PDFSyntaxError("PageLabels is missing page index 0")
else:
# Try to cope, by assuming empty labels for the initial pages
ranges.insert(0, (0, {}))
for (next, (start, label_dict_unchecked)) in enumerate(ranges, 1):
label_dict = dict_value(label_dict_unchecked)
style = label_dict.get("S")
prefix = decode_text(str_value(label_dict.get("P", b"")))
first_value = int_value(label_dict.get("St", 1))
if next == len(ranges):
# This is the last specified range. It continues until the end
# of the document.
values: Iterable[int] = itertools.count(first_value)
else:
end, _ = ranges[next]
range_length = end - start
values = range(first_value, first_value + range_length)
for value in values:
label = self._format_page_label(value, style)
yield prefix + label
@staticmethod
def _format_page_label(value: int, style: Any) -> str:
"""Format page label value in a specific style"""
if style is None:
label = ""
elif style is LIT("D"): # Decimal arabic numerals
label = str(value)
elif style is LIT("R"): # Uppercase roman numerals
label = format_int_roman(value).upper()
elif style is LIT("r"): # Lowercase roman numerals
label = format_int_roman(value)
elif style is LIT("A"): # Uppercase letters A-Z, AA-ZZ...
label = format_int_alpha(value).upper()
elif style is LIT("a"): # Lowercase letters a-z, aa-zz...
label = format_int_alpha(value)
else:
log.warning("Unknown page label style: %r", style)
label = ""
return label | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/pdfdocument.py | pdfdocument.py |
import heapq
import logging
from typing import (
Dict,
Generic,
Iterable,
Iterator,
List,
Optional,
Sequence,
Set,
Tuple,
TypeVar,
Union,
cast,
)
from .pdfcolor import PDFColorSpace
from .pdffont import PDFFont
from .pdfinterp import Color
from .pdfinterp import PDFGraphicState
from .pdftypes import PDFStream
from .utils import INF
from .utils import LTComponentT
from .utils import Matrix
from .utils import Plane
from .utils import Point
from .utils import Rect
from .utils import apply_matrix_pt
from .utils import bbox2str
from .utils import fsplit
from .utils import get_bound
from .utils import matrix2str
from .utils import uniq
logger = logging.getLogger(__name__)
class IndexAssigner:
def __init__(self, index: int = 0) -> None:
self.index = index
def run(self, obj: "LTItem") -> None:
if isinstance(obj, LTTextBox):
obj.index = self.index
self.index += 1
elif isinstance(obj, LTTextGroup):
for x in obj:
self.run(x)
class LAParams:
"""Parameters for layout analysis
:param line_overlap: If two characters have more overlap than this they
are considered to be on the same line. The overlap is specified
relative to the minimum height of both characters.
:param char_margin: If two characters are closer together than this
margin they are considered part of the same line. The margin is
specified relative to the width of the character.
:param word_margin: If two characters on the same line are further apart
than this margin then they are considered to be two separate words, and
an intermediate space will be added for readability. The margin is
specified relative to the width of the character.
:param line_margin: If two lines are are close together they are
considered to be part of the same paragraph. The margin is
specified relative to the height of a line.
:param boxes_flow: Specifies how much a horizontal and vertical position
of a text matters when determining the order of text boxes. The value
should be within the range of -1.0 (only horizontal position
matters) to +1.0 (only vertical position matters). You can also pass
`None` to disable advanced layout analysis, and instead return text
based on the position of the bottom left corner of the text box.
:param detect_vertical: If vertical text should be considered during
layout analysis
:param all_texts: If layout analysis should be performed on text in
figures.
"""
def __init__(
self,
line_overlap: float = 0.5,
char_margin: float = 2.0,
line_margin: float = 0.5,
word_margin: float = 0.1,
boxes_flow: Optional[float] = 0.5,
detect_vertical: bool = False,
all_texts: bool = False,
) -> None:
self.line_overlap = line_overlap
self.char_margin = char_margin
self.line_margin = line_margin
self.word_margin = word_margin
self.boxes_flow = boxes_flow
self.detect_vertical = detect_vertical
self.all_texts = all_texts
self._validate()
def _validate(self) -> None:
if self.boxes_flow is not None:
boxes_flow_err_msg = (
"LAParam boxes_flow should be None, or a " "number between -1 and +1"
)
if not (
isinstance(self.boxes_flow, int) or isinstance(self.boxes_flow, float)
):
raise TypeError(boxes_flow_err_msg)
if not -1 <= self.boxes_flow <= 1:
raise ValueError(boxes_flow_err_msg)
def __repr__(self) -> str:
return (
"<LAParams: char_margin=%.1f, line_margin=%.1f, "
"word_margin=%.1f all_texts=%r>"
% (self.char_margin, self.line_margin, self.word_margin, self.all_texts)
)
class LTItem:
"""Interface for things that can be analyzed"""
def analyze(self, laparams: LAParams) -> None:
"""Perform the layout analysis."""
pass
class LTText:
"""Interface for things that have text"""
def __repr__(self) -> str:
return "<%s %r>" % (self.__class__.__name__, self.get_text())
def get_text(self) -> str:
"""Text contained in this object"""
raise NotImplementedError
class LTComponent(LTItem):
"""Object with a bounding box"""
def __init__(self, bbox: Rect) -> None:
LTItem.__init__(self)
self.set_bbox(bbox)
def __repr__(self) -> str:
return "<%s %s>" % (self.__class__.__name__, bbox2str(self.bbox))
# Disable comparison.
def __lt__(self, _: object) -> bool:
raise ValueError
def __le__(self, _: object) -> bool:
raise ValueError
def __gt__(self, _: object) -> bool:
raise ValueError
def __ge__(self, _: object) -> bool:
raise ValueError
def set_bbox(self, bbox: Rect) -> None:
(x0, y0, x1, y1) = bbox
self.x0 = x0
self.y0 = y0
self.x1 = x1
self.y1 = y1
self.width = x1 - x0
self.height = y1 - y0
self.bbox = bbox
def is_empty(self) -> bool:
return self.width <= 0 or self.height <= 0
def is_hoverlap(self, obj: "LTComponent") -> bool:
assert isinstance(obj, LTComponent), str(type(obj))
return obj.x0 <= self.x1 and self.x0 <= obj.x1
def hdistance(self, obj: "LTComponent") -> float:
assert isinstance(obj, LTComponent), str(type(obj))
if self.is_hoverlap(obj):
return 0
else:
return min(abs(self.x0 - obj.x1), abs(self.x1 - obj.x0))
def hoverlap(self, obj: "LTComponent") -> float:
assert isinstance(obj, LTComponent), str(type(obj))
if self.is_hoverlap(obj):
return min(abs(self.x0 - obj.x1), abs(self.x1 - obj.x0))
else:
return 0
def is_voverlap(self, obj: "LTComponent") -> bool:
assert isinstance(obj, LTComponent), str(type(obj))
return obj.y0 <= self.y1 and self.y0 <= obj.y1
def vdistance(self, obj: "LTComponent") -> float:
assert isinstance(obj, LTComponent), str(type(obj))
if self.is_voverlap(obj):
return 0
else:
return min(abs(self.y0 - obj.y1), abs(self.y1 - obj.y0))
def voverlap(self, obj: "LTComponent") -> float:
assert isinstance(obj, LTComponent), str(type(obj))
if self.is_voverlap(obj):
return min(abs(self.y0 - obj.y1), abs(self.y1 - obj.y0))
else:
return 0
class LTCurve(LTComponent):
"""A generic Bezier curve"""
def __init__(
self,
linewidth: float,
pts: List[Point],
stroke: bool = False,
fill: bool = False,
evenodd: bool = False,
stroking_color: Optional[Color] = None,
non_stroking_color: Optional[Color] = None,
) -> None:
LTComponent.__init__(self, get_bound(pts))
self.pts = pts
self.linewidth = linewidth
self.stroke = stroke
self.fill = fill
self.evenodd = evenodd
self.stroking_color = stroking_color
self.non_stroking_color = non_stroking_color
def get_pts(self) -> str:
return ",".join("%.3f,%.3f" % p for p in self.pts)
class LTLine(LTCurve):
"""A single straight line.
Could be used for separating text or figures.
"""
def __init__(
self,
linewidth: float,
p0: Point,
p1: Point,
stroke: bool = False,
fill: bool = False,
evenodd: bool = False,
stroking_color: Optional[Color] = None,
non_stroking_color: Optional[Color] = None,
) -> None:
LTCurve.__init__(
self,
linewidth,
[p0, p1],
stroke,
fill,
evenodd,
stroking_color,
non_stroking_color,
)
class LTRect(LTCurve):
"""A rectangle.
Could be used for framing another pictures or figures.
"""
def __init__(
self,
linewidth: float,
bbox: Rect,
stroke: bool = False,
fill: bool = False,
evenodd: bool = False,
stroking_color: Optional[Color] = None,
non_stroking_color: Optional[Color] = None,
) -> None:
(x0, y0, x1, y1) = bbox
LTCurve.__init__(
self,
linewidth,
[(x0, y0), (x1, y0), (x1, y1), (x0, y1)],
stroke,
fill,
evenodd,
stroking_color,
non_stroking_color,
)
class LTImage(LTComponent):
"""An image object.
Embedded images can be in JPEG, Bitmap or JBIG2.
"""
def __init__(self, name: str, stream: PDFStream, bbox: Rect) -> None:
LTComponent.__init__(self, bbox)
self.name = name
self.stream = stream
self.srcsize = (stream.get_any(("W", "Width")), stream.get_any(("H", "Height")))
self.imagemask = stream.get_any(("IM", "ImageMask"))
self.bits = stream.get_any(("BPC", "BitsPerComponent"), 1)
self.colorspace = stream.get_any(("CS", "ColorSpace"))
if not isinstance(self.colorspace, list):
self.colorspace = [self.colorspace]
def __repr__(self) -> str:
return "<%s(%s) %s %r>" % (
self.__class__.__name__,
self.name,
bbox2str(self.bbox),
self.srcsize,
)
class LTAnno(LTItem, LTText):
"""Actual letter in the text as a Unicode string.
Note that, while a LTChar object has actual boundaries, LTAnno objects does
not, as these are "virtual" characters, inserted by a layout analyzer
according to the relationship between two characters (e.g. a space).
"""
def __init__(self, text: str) -> None:
self._text = text
return
def get_text(self) -> str:
return self._text
class LTChar(LTComponent, LTText):
"""Actual letter in the text as a Unicode string."""
def __init__(
self,
matrix: Matrix,
font: PDFFont,
fontsize: float,
scaling: float,
rise: float,
text: str,
textwidth: float,
textdisp: Union[float, Tuple[Optional[float], float]],
ncs: PDFColorSpace,
graphicstate: PDFGraphicState,
) -> None:
LTText.__init__(self)
self._text = text
self.matrix = matrix
self.fontname = font.fontname
self.ncs = ncs
self.graphicstate = graphicstate
self.adv = textwidth * fontsize * scaling
# compute the boundary rectangle.
if font.is_vertical():
# vertical
assert isinstance(textdisp, tuple)
(vx, vy) = textdisp
if vx is None:
vx = fontsize * 0.5
else:
vx = vx * fontsize * 0.001
vy = (1000 - vy) * fontsize * 0.001
bbox_lower_left = (-vx, vy + rise + self.adv)
bbox_upper_right = (-vx + fontsize, vy + rise)
else:
# horizontal
descent = font.get_descent() * fontsize
bbox_lower_left = (0, descent + rise)
bbox_upper_right = (self.adv, descent + rise + fontsize)
(a, b, c, d, e, f) = self.matrix
self.upright = 0 < a * d * scaling and b * c <= 0
(x0, y0) = apply_matrix_pt(self.matrix, bbox_lower_left)
(x1, y1) = apply_matrix_pt(self.matrix, bbox_upper_right)
if x1 < x0:
(x0, x1) = (x1, x0)
if y1 < y0:
(y0, y1) = (y1, y0)
LTComponent.__init__(self, (x0, y0, x1, y1))
if font.is_vertical():
self.size = self.width
else:
self.size = self.height
return
def __repr__(self) -> str:
return "<%s %s matrix=%s font=%r adv=%s text=%r>" % (
self.__class__.__name__,
bbox2str(self.bbox),
matrix2str(self.matrix),
self.fontname,
self.adv,
self.get_text(),
)
def get_text(self) -> str:
return self._text
def is_compatible(self, obj: object) -> bool:
"""Returns True if two characters can coexist in the same line."""
return True
LTItemT = TypeVar("LTItemT", bound=LTItem)
class LTContainer(LTComponent, Generic[LTItemT]):
"""Object that can be extended and analyzed"""
def __init__(self, bbox: Rect) -> None:
LTComponent.__init__(self, bbox)
self._objs: List[LTItemT] = []
return
def __iter__(self) -> Iterator[LTItemT]:
return iter(self._objs)
def __len__(self) -> int:
return len(self._objs)
def add(self, obj: LTItemT) -> None:
self._objs.append(obj)
return
def extend(self, objs: Iterable[LTItemT]) -> None:
for obj in objs:
self.add(obj)
return
def analyze(self, laparams: LAParams) -> None:
for obj in self._objs:
obj.analyze(laparams)
return
class LTExpandableContainer(LTContainer[LTItemT]):
def __init__(self) -> None:
LTContainer.__init__(self, (+INF, +INF, -INF, -INF))
return
# Incompatible override: we take an LTComponent (with bounding box), but
# super() LTContainer only considers LTItem (no bounding box).
def add(self, obj: LTComponent) -> None: # type: ignore[override]
LTContainer.add(self, cast(LTItemT, obj))
self.set_bbox(
(
min(self.x0, obj.x0),
min(self.y0, obj.y0),
max(self.x1, obj.x1),
max(self.y1, obj.y1),
)
)
return
class LTTextContainer(LTExpandableContainer[LTItemT], LTText):
def __init__(self) -> None:
LTText.__init__(self)
LTExpandableContainer.__init__(self)
return
def get_text(self) -> str:
return "".join(
cast(LTText, obj).get_text() for obj in self if isinstance(obj, LTText)
)
TextLineElement = Union[LTChar, LTAnno]
class LTTextLine(LTTextContainer[TextLineElement]):
"""Contains a list of LTChar objects that represent a single text line.
The characters are aligned either horizontally or vertically, depending on
the text's writing mode.
"""
def __init__(self, word_margin: float) -> None:
super().__init__()
self.word_margin = word_margin
return
def __repr__(self) -> str:
return "<%s %s %r>" % (
self.__class__.__name__,
bbox2str(self.bbox),
self.get_text(),
)
def analyze(self, laparams: LAParams) -> None:
LTTextContainer.analyze(self, laparams)
LTContainer.add(self, LTAnno("\n"))
return
def find_neighbors(
self, plane: Plane[LTComponentT], ratio: float
) -> List["LTTextLine"]:
raise NotImplementedError
def is_empty(self) -> bool:
return super().is_empty() or self.get_text().isspace()
class LTTextLineHorizontal(LTTextLine):
def __init__(self, word_margin: float) -> None:
LTTextLine.__init__(self, word_margin)
self._x1: float = +INF
return
# Incompatible override: we take an LTComponent (with bounding box), but
# LTContainer only considers LTItem (no bounding box).
def add(self, obj: LTComponent) -> None: # type: ignore[override]
if isinstance(obj, LTChar) and self.word_margin:
margin = self.word_margin * max(obj.width, obj.height)
if self._x1 < obj.x0 - margin:
LTContainer.add(self, LTAnno(" "))
self._x1 = obj.x1
super().add(obj)
return
def find_neighbors(
self, plane: Plane[LTComponentT], ratio: float
) -> List[LTTextLine]:
"""
Finds neighboring LTTextLineHorizontals in the plane.
Returns a list of other LTTestLineHorizontals in the plane which are
close to self. "Close" can be controlled by ratio. The returned objects
will be the same height as self, and also either left-, right-, or
centrally-aligned.
"""
d = ratio * self.height
objs = plane.find((self.x0, self.y0 - d, self.x1, self.y1 + d))
return [
obj
for obj in objs
if (
isinstance(obj, LTTextLineHorizontal)
and self._is_same_height_as(obj, tolerance=d)
and (
self._is_left_aligned_with(obj, tolerance=d)
or self._is_right_aligned_with(obj, tolerance=d)
or self._is_centrally_aligned_with(obj, tolerance=d)
)
)
]
def _is_left_aligned_with(self, other: LTComponent, tolerance: float = 0) -> bool:
"""
Whether the left-hand edge of `other` is within `tolerance`.
"""
return abs(other.x0 - self.x0) <= tolerance
def _is_right_aligned_with(self, other: LTComponent, tolerance: float = 0) -> bool:
"""
Whether the right-hand edge of `other` is within `tolerance`.
"""
return abs(other.x1 - self.x1) <= tolerance
def _is_centrally_aligned_with(
self, other: LTComponent, tolerance: float = 0
) -> bool:
"""
Whether the horizontal center of `other` is within `tolerance`.
"""
return abs((other.x0 + other.x1) / 2 - (self.x0 + self.x1) / 2) <= tolerance
def _is_same_height_as(self, other: LTComponent, tolerance: float = 0) -> bool:
return abs(other.height - self.height) <= tolerance
class LTTextLineVertical(LTTextLine):
def __init__(self, word_margin: float) -> None:
LTTextLine.__init__(self, word_margin)
self._y0: float = -INF
return
# Incompatible override: we take an LTComponent (with bounding box), but
# LTContainer only considers LTItem (no bounding box).
def add(self, obj: LTComponent) -> None: # type: ignore[override]
if isinstance(obj, LTChar) and self.word_margin:
margin = self.word_margin * max(obj.width, obj.height)
if obj.y1 + margin < self._y0:
LTContainer.add(self, LTAnno(" "))
self._y0 = obj.y0
super().add(obj)
return
def find_neighbors(
self, plane: Plane[LTComponentT], ratio: float
) -> List[LTTextLine]:
"""
Finds neighboring LTTextLineVerticals in the plane.
Returns a list of other LTTextLineVerticals in the plane which are
close to self. "Close" can be controlled by ratio. The returned objects
will be the same width as self, and also either upper-, lower-, or
centrally-aligned.
"""
d = ratio * self.width
objs = plane.find((self.x0 - d, self.y0, self.x1 + d, self.y1))
return [
obj
for obj in objs
if (
isinstance(obj, LTTextLineVertical)
and self._is_same_width_as(obj, tolerance=d)
and (
self._is_lower_aligned_with(obj, tolerance=d)
or self._is_upper_aligned_with(obj, tolerance=d)
or self._is_centrally_aligned_with(obj, tolerance=d)
)
)
]
def _is_lower_aligned_with(self, other: LTComponent, tolerance: float = 0) -> bool:
"""
Whether the lower edge of `other` is within `tolerance`.
"""
return abs(other.y0 - self.y0) <= tolerance
def _is_upper_aligned_with(self, other: LTComponent, tolerance: float = 0) -> bool:
"""
Whether the upper edge of `other` is within `tolerance`.
"""
return abs(other.y1 - self.y1) <= tolerance
def _is_centrally_aligned_with(
self, other: LTComponent, tolerance: float = 0
) -> bool:
"""
Whether the vertical center of `other` is within `tolerance`.
"""
return abs((other.y0 + other.y1) / 2 - (self.y0 + self.y1) / 2) <= tolerance
def _is_same_width_as(self, other: LTComponent, tolerance: float) -> bool:
return abs(other.width - self.width) <= tolerance
class LTTextBox(LTTextContainer[LTTextLine]):
"""Represents a group of text chunks in a rectangular area.
Note that this box is created by geometric analysis and does not
necessarily represents a logical boundary of the text. It contains a list
of LTTextLine objects.
"""
def __init__(self) -> None:
LTTextContainer.__init__(self)
self.index: int = -1
return
def __repr__(self) -> str:
return "<%s(%s) %s %r>" % (
self.__class__.__name__,
self.index,
bbox2str(self.bbox),
self.get_text(),
)
def get_writing_mode(self) -> str:
raise NotImplementedError
class LTTextBoxHorizontal(LTTextBox):
def analyze(self, laparams: LAParams) -> None:
super().analyze(laparams)
self._objs.sort(key=lambda obj: -obj.y1)
return
def get_writing_mode(self) -> str:
return "lr-tb"
class LTTextBoxVertical(LTTextBox):
def analyze(self, laparams: LAParams) -> None:
super().analyze(laparams)
self._objs.sort(key=lambda obj: -obj.x1)
return
def get_writing_mode(self) -> str:
return "tb-rl"
TextGroupElement = Union[LTTextBox, "LTTextGroup"]
class LTTextGroup(LTTextContainer[TextGroupElement]):
def __init__(self, objs: Iterable[TextGroupElement]) -> None:
super().__init__()
self.extend(objs)
return
class LTTextGroupLRTB(LTTextGroup):
def analyze(self, laparams: LAParams) -> None:
super().analyze(laparams)
assert laparams.boxes_flow is not None
boxes_flow = laparams.boxes_flow
# reorder the objects from top-left to bottom-right.
self._objs.sort(
key=lambda obj: (1 - boxes_flow) * obj.x0
- (1 + boxes_flow) * (obj.y0 + obj.y1)
)
return
class LTTextGroupTBRL(LTTextGroup):
def analyze(self, laparams: LAParams) -> None:
super().analyze(laparams)
assert laparams.boxes_flow is not None
boxes_flow = laparams.boxes_flow
# reorder the objects from top-right to bottom-left.
self._objs.sort(
key=lambda obj: -(1 + boxes_flow) * (obj.x0 + obj.x1)
- (1 - boxes_flow) * obj.y1
)
return
class LTLayoutContainer(LTContainer[LTComponent]):
def __init__(self, bbox: Rect) -> None:
LTContainer.__init__(self, bbox)
self.groups: Optional[List[LTTextGroup]] = None
return
# group_objects: group text object to textlines.
def group_objects(
self, laparams: LAParams, objs: Iterable[LTComponent]
) -> Iterator[LTTextLine]:
obj0 = None
line = None
for obj1 in objs:
if obj0 is not None:
# halign: obj0 and obj1 is horizontally aligned.
#
# +------+ - - -
# | obj0 | - - +------+ -
# | | | obj1 | | (line_overlap)
# +------+ - - | | -
# - - - +------+
#
# |<--->|
# (char_margin)
halign = (
obj0.is_compatible(obj1)
and obj0.is_voverlap(obj1)
and min(obj0.height, obj1.height) * laparams.line_overlap
< obj0.voverlap(obj1)
and obj0.hdistance(obj1)
< max(obj0.width, obj1.width) * laparams.char_margin
)
# valign: obj0 and obj1 is vertically aligned.
#
# +------+
# | obj0 |
# | |
# +------+ - - -
# | | | (char_margin)
# +------+ - -
# | obj1 |
# | |
# +------+
#
# |<-->|
# (line_overlap)
valign = (
laparams.detect_vertical
and obj0.is_compatible(obj1)
and obj0.is_hoverlap(obj1)
and min(obj0.width, obj1.width) * laparams.line_overlap
< obj0.hoverlap(obj1)
and obj0.vdistance(obj1)
< max(obj0.height, obj1.height) * laparams.char_margin
)
if (halign and isinstance(line, LTTextLineHorizontal)) or (
valign and isinstance(line, LTTextLineVertical)
):
line.add(obj1)
elif line is not None:
yield line
line = None
else:
if valign and not halign:
line = LTTextLineVertical(laparams.word_margin)
line.add(obj0)
line.add(obj1)
elif halign and not valign:
line = LTTextLineHorizontal(laparams.word_margin)
line.add(obj0)
line.add(obj1)
else:
line = LTTextLineHorizontal(laparams.word_margin)
line.add(obj0)
yield line
line = None
obj0 = obj1
if line is None:
line = LTTextLineHorizontal(laparams.word_margin)
assert obj0 is not None
line.add(obj0)
yield line
return
def group_textlines(
self, laparams: LAParams, lines: Iterable[LTTextLine]
) -> Iterator[LTTextBox]:
"""Group neighboring lines to textboxes"""
plane: Plane[LTTextLine] = Plane(self.bbox)
plane.extend(lines)
boxes: Dict[LTTextLine, LTTextBox] = {}
for line in lines:
neighbors = line.find_neighbors(plane, laparams.line_margin)
members = [line]
for obj1 in neighbors:
members.append(obj1)
if obj1 in boxes:
members.extend(boxes.pop(obj1))
if isinstance(line, LTTextLineHorizontal):
box: LTTextBox = LTTextBoxHorizontal()
else:
box = LTTextBoxVertical()
for obj in uniq(members):
box.add(obj)
boxes[obj] = box
done = set()
for line in lines:
if line not in boxes:
continue
box = boxes[line]
if box in done:
continue
done.add(box)
if not box.is_empty():
yield box
return
def group_textboxes(
self, laparams: LAParams, boxes: Sequence[LTTextBox]
) -> List[LTTextGroup]:
"""Group textboxes hierarchically.
Get pair-wise distances, via dist func defined below, and then merge
from the closest textbox pair. Once obj1 and obj2 are merged /
grouped, the resulting group is considered as a new object, and its
distances to other objects & groups are added to the process queue.
For performance reason, pair-wise distances and object pair info are
maintained in a heap of (idx, dist, id(obj1), id(obj2), obj1, obj2)
tuples. It ensures quick access to the smallest element. Note that
since comparison operators, e.g., __lt__, are disabled for
LTComponent, id(obj) has to appear before obj in element tuples.
:param laparams: LAParams object.
:param boxes: All textbox objects to be grouped.
:return: a list that has only one element, the final top level group.
"""
ElementT = Union[LTTextBox, LTTextGroup]
plane: Plane[ElementT] = Plane(self.bbox)
def dist(obj1: LTComponent, obj2: LTComponent) -> float:
"""A distance function between two TextBoxes.
Consider the bounding rectangle for obj1 and obj2.
Return its area less the areas of obj1 and obj2,
shown as 'www' below. This value may be negative.
+------+..........+ (x1, y1)
| obj1 |wwwwwwwwww:
+------+www+------+
:wwwwwwwwww| obj2 |
(x0, y0) +..........+------+
"""
x0 = min(obj1.x0, obj2.x0)
y0 = min(obj1.y0, obj2.y0)
x1 = max(obj1.x1, obj2.x1)
y1 = max(obj1.y1, obj2.y1)
return (
(x1 - x0) * (y1 - y0)
- obj1.width * obj1.height
- obj2.width * obj2.height
)
def isany(obj1: ElementT, obj2: ElementT) -> Set[ElementT]:
"""Check if there's any other object between obj1 and obj2."""
x0 = min(obj1.x0, obj2.x0)
y0 = min(obj1.y0, obj2.y0)
x1 = max(obj1.x1, obj2.x1)
y1 = max(obj1.y1, obj2.y1)
objs = set(plane.find((x0, y0, x1, y1)))
return objs.difference((obj1, obj2))
dists: List[Tuple[bool, float, int, int, ElementT, ElementT]] = []
for i in range(len(boxes)):
box1 = boxes[i]
for j in range(i + 1, len(boxes)):
box2 = boxes[j]
dists.append((False, dist(box1, box2), id(box1), id(box2), box1, box2))
heapq.heapify(dists)
plane.extend(boxes)
done = set()
while len(dists) > 0:
(skip_isany, d, id1, id2, obj1, obj2) = heapq.heappop(dists)
# Skip objects that are already merged
if (id1 not in done) and (id2 not in done):
if not skip_isany and isany(obj1, obj2):
heapq.heappush(dists, (True, d, id1, id2, obj1, obj2))
continue
if isinstance(obj1, (LTTextBoxVertical, LTTextGroupTBRL)) or isinstance(
obj2, (LTTextBoxVertical, LTTextGroupTBRL)
):
group: LTTextGroup = LTTextGroupTBRL([obj1, obj2])
else:
group = LTTextGroupLRTB([obj1, obj2])
plane.remove(obj1)
plane.remove(obj2)
done.update([id1, id2])
for other in plane:
heapq.heappush(
dists,
(False, dist(group, other), id(group), id(other), group, other),
)
plane.add(group)
# By now only groups are in the plane
return list(cast(LTTextGroup, g) for g in plane)
def analyze(self, laparams: LAParams) -> None:
# textobjs is a list of LTChar objects, i.e.
# it has all the individual characters in the page.
(textobjs, otherobjs) = fsplit(lambda obj: isinstance(obj, LTChar), self)
for obj in otherobjs:
obj.analyze(laparams)
if not textobjs:
return
textlines = list(self.group_objects(laparams, textobjs))
(empties, textlines) = fsplit(lambda obj: obj.is_empty(), textlines)
for obj in empties:
obj.analyze(laparams)
textboxes = list(self.group_textlines(laparams, textlines))
if laparams.boxes_flow is None:
for textbox in textboxes:
textbox.analyze(laparams)
def getkey(box: LTTextBox) -> Tuple[int, float, float]:
if isinstance(box, LTTextBoxVertical):
return (0, -box.x1, -box.y0)
else:
return (1, -box.y0, box.x0)
textboxes.sort(key=getkey)
else:
self.groups = self.group_textboxes(laparams, textboxes)
assigner = IndexAssigner()
for group in self.groups:
group.analyze(laparams)
assigner.run(group)
textboxes.sort(key=lambda box: box.index)
self._objs = (
cast(List[LTComponent], textboxes)
+ otherobjs
+ cast(List[LTComponent], empties)
)
return
class LTFigure(LTLayoutContainer):
"""Represents an area used by PDF Form objects.
PDF Forms can be used to present figures or pictures by embedding yet
another PDF document within a page. Note that LTFigure objects can appear
recursively.
"""
def __init__(self, name: str, bbox: Rect, matrix: Matrix) -> None:
self.name = name
self.matrix = matrix
(x, y, w, h) = bbox
bounds = ((x, y), (x + w, y), (x, y + h), (x + w, y + h))
bbox = get_bound(apply_matrix_pt(matrix, (p, q)) for (p, q) in bounds)
LTLayoutContainer.__init__(self, bbox)
return
def __repr__(self) -> str:
return "<%s(%s) %s matrix=%s>" % (
self.__class__.__name__,
self.name,
bbox2str(self.bbox),
matrix2str(self.matrix),
)
def analyze(self, laparams: LAParams) -> None:
if not laparams.all_texts:
return
LTLayoutContainer.analyze(self, laparams)
return
class LTPage(LTLayoutContainer):
"""Represents an entire page.
May contain child objects like LTTextBox, LTFigure, LTImage, LTRect,
LTCurve and LTLine.
"""
def __init__(self, pageid: int, bbox: Rect, rotate: float = 0) -> None:
LTLayoutContainer.__init__(self, bbox)
self.pageid = pageid
self.rotate = rotate
return
def __repr__(self) -> str:
return "<%s(%r) %s rotate=%r>" % (
self.__class__.__name__,
self.pageid,
bbox2str(self.bbox),
self.rotate,
) | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/layout.py | layout.py |
from typing import List, Optional, Tuple
EncodingRow = Tuple[str, Optional[int], Optional[int], Optional[int], Optional[int]]
ENCODING: List[EncodingRow] = [
# (name, std, mac, win, pdf)
("A", 65, 65, 65, 65),
("AE", 225, 174, 198, 198),
("Aacute", None, 231, 193, 193),
("Acircumflex", None, 229, 194, 194),
("Adieresis", None, 128, 196, 196),
("Agrave", None, 203, 192, 192),
("Aring", None, 129, 197, 197),
("Atilde", None, 204, 195, 195),
("B", 66, 66, 66, 66),
("C", 67, 67, 67, 67),
("Ccedilla", None, 130, 199, 199),
("D", 68, 68, 68, 68),
("E", 69, 69, 69, 69),
("Eacute", None, 131, 201, 201),
("Ecircumflex", None, 230, 202, 202),
("Edieresis", None, 232, 203, 203),
("Egrave", None, 233, 200, 200),
("Eth", None, None, 208, 208),
("Euro", None, None, 128, 160),
("F", 70, 70, 70, 70),
("G", 71, 71, 71, 71),
("H", 72, 72, 72, 72),
("I", 73, 73, 73, 73),
("Iacute", None, 234, 205, 205),
("Icircumflex", None, 235, 206, 206),
("Idieresis", None, 236, 207, 207),
("Igrave", None, 237, 204, 204),
("J", 74, 74, 74, 74),
("K", 75, 75, 75, 75),
("L", 76, 76, 76, 76),
("Lslash", 232, None, None, 149),
("M", 77, 77, 77, 77),
("N", 78, 78, 78, 78),
("Ntilde", None, 132, 209, 209),
("O", 79, 79, 79, 79),
("OE", 234, 206, 140, 150),
("Oacute", None, 238, 211, 211),
("Ocircumflex", None, 239, 212, 212),
("Odieresis", None, 133, 214, 214),
("Ograve", None, 241, 210, 210),
("Oslash", 233, 175, 216, 216),
("Otilde", None, 205, 213, 213),
("P", 80, 80, 80, 80),
("Q", 81, 81, 81, 81),
("R", 82, 82, 82, 82),
("S", 83, 83, 83, 83),
("Scaron", None, None, 138, 151),
("T", 84, 84, 84, 84),
("Thorn", None, None, 222, 222),
("U", 85, 85, 85, 85),
("Uacute", None, 242, 218, 218),
("Ucircumflex", None, 243, 219, 219),
("Udieresis", None, 134, 220, 220),
("Ugrave", None, 244, 217, 217),
("V", 86, 86, 86, 86),
("W", 87, 87, 87, 87),
("X", 88, 88, 88, 88),
("Y", 89, 89, 89, 89),
("Yacute", None, None, 221, 221),
("Ydieresis", None, 217, 159, 152),
("Z", 90, 90, 90, 90),
("Zcaron", None, None, 142, 153),
("a", 97, 97, 97, 97),
("aacute", None, 135, 225, 225),
("acircumflex", None, 137, 226, 226),
("acute", 194, 171, 180, 180),
("adieresis", None, 138, 228, 228),
("ae", 241, 190, 230, 230),
("agrave", None, 136, 224, 224),
("ampersand", 38, 38, 38, 38),
("aring", None, 140, 229, 229),
("asciicircum", 94, 94, 94, 94),
("asciitilde", 126, 126, 126, 126),
("asterisk", 42, 42, 42, 42),
("at", 64, 64, 64, 64),
("atilde", None, 139, 227, 227),
("b", 98, 98, 98, 98),
("backslash", 92, 92, 92, 92),
("bar", 124, 124, 124, 124),
("braceleft", 123, 123, 123, 123),
("braceright", 125, 125, 125, 125),
("bracketleft", 91, 91, 91, 91),
("bracketright", 93, 93, 93, 93),
("breve", 198, 249, None, 24),
("brokenbar", None, None, 166, 166),
("bullet", 183, 165, 149, 128),
("c", 99, 99, 99, 99),
("caron", 207, 255, None, 25),
("ccedilla", None, 141, 231, 231),
("cedilla", 203, 252, 184, 184),
("cent", 162, 162, 162, 162),
("circumflex", 195, 246, 136, 26),
("colon", 58, 58, 58, 58),
("comma", 44, 44, 44, 44),
("copyright", None, 169, 169, 169),
("currency", 168, 219, 164, 164),
("d", 100, 100, 100, 100),
("dagger", 178, 160, 134, 129),
("daggerdbl", 179, 224, 135, 130),
("degree", None, 161, 176, 176),
("dieresis", 200, 172, 168, 168),
("divide", None, 214, 247, 247),
("dollar", 36, 36, 36, 36),
("dotaccent", 199, 250, None, 27),
("dotlessi", 245, 245, None, 154),
("e", 101, 101, 101, 101),
("eacute", None, 142, 233, 233),
("ecircumflex", None, 144, 234, 234),
("edieresis", None, 145, 235, 235),
("egrave", None, 143, 232, 232),
("eight", 56, 56, 56, 56),
("ellipsis", 188, 201, 133, 131),
("emdash", 208, 209, 151, 132),
("endash", 177, 208, 150, 133),
("equal", 61, 61, 61, 61),
("eth", None, None, 240, 240),
("exclam", 33, 33, 33, 33),
("exclamdown", 161, 193, 161, 161),
("f", 102, 102, 102, 102),
("fi", 174, 222, None, 147),
("five", 53, 53, 53, 53),
("fl", 175, 223, None, 148),
("florin", 166, 196, 131, 134),
("four", 52, 52, 52, 52),
("fraction", 164, 218, None, 135),
("g", 103, 103, 103, 103),
("germandbls", 251, 167, 223, 223),
("grave", 193, 96, 96, 96),
("greater", 62, 62, 62, 62),
("guillemotleft", 171, 199, 171, 171),
("guillemotright", 187, 200, 187, 187),
("guilsinglleft", 172, 220, 139, 136),
("guilsinglright", 173, 221, 155, 137),
("h", 104, 104, 104, 104),
("hungarumlaut", 205, 253, None, 28),
("hyphen", 45, 45, 45, 45),
("i", 105, 105, 105, 105),
("iacute", None, 146, 237, 237),
("icircumflex", None, 148, 238, 238),
("idieresis", None, 149, 239, 239),
("igrave", None, 147, 236, 236),
("j", 106, 106, 106, 106),
("k", 107, 107, 107, 107),
("l", 108, 108, 108, 108),
("less", 60, 60, 60, 60),
("logicalnot", None, 194, 172, 172),
("lslash", 248, None, None, 155),
("m", 109, 109, 109, 109),
("macron", 197, 248, 175, 175),
("minus", None, None, None, 138),
("mu", None, 181, 181, 181),
("multiply", None, None, 215, 215),
("n", 110, 110, 110, 110),
("nbspace", None, 202, 160, None),
("nine", 57, 57, 57, 57),
("ntilde", None, 150, 241, 241),
("numbersign", 35, 35, 35, 35),
("o", 111, 111, 111, 111),
("oacute", None, 151, 243, 243),
("ocircumflex", None, 153, 244, 244),
("odieresis", None, 154, 246, 246),
("oe", 250, 207, 156, 156),
("ogonek", 206, 254, None, 29),
("ograve", None, 152, 242, 242),
("one", 49, 49, 49, 49),
("onehalf", None, None, 189, 189),
("onequarter", None, None, 188, 188),
("onesuperior", None, None, 185, 185),
("ordfeminine", 227, 187, 170, 170),
("ordmasculine", 235, 188, 186, 186),
("oslash", 249, 191, 248, 248),
("otilde", None, 155, 245, 245),
("p", 112, 112, 112, 112),
("paragraph", 182, 166, 182, 182),
("parenleft", 40, 40, 40, 40),
("parenright", 41, 41, 41, 41),
("percent", 37, 37, 37, 37),
("period", 46, 46, 46, 46),
("periodcentered", 180, 225, 183, 183),
("perthousand", 189, 228, 137, 139),
("plus", 43, 43, 43, 43),
("plusminus", None, 177, 177, 177),
("q", 113, 113, 113, 113),
("question", 63, 63, 63, 63),
("questiondown", 191, 192, 191, 191),
("quotedbl", 34, 34, 34, 34),
("quotedblbase", 185, 227, 132, 140),
("quotedblleft", 170, 210, 147, 141),
("quotedblright", 186, 211, 148, 142),
("quoteleft", 96, 212, 145, 143),
("quoteright", 39, 213, 146, 144),
("quotesinglbase", 184, 226, 130, 145),
("quotesingle", 169, 39, 39, 39),
("r", 114, 114, 114, 114),
("registered", None, 168, 174, 174),
("ring", 202, 251, None, 30),
("s", 115, 115, 115, 115),
("scaron", None, None, 154, 157),
("section", 167, 164, 167, 167),
("semicolon", 59, 59, 59, 59),
("seven", 55, 55, 55, 55),
("six", 54, 54, 54, 54),
("slash", 47, 47, 47, 47),
("space", 32, 32, 32, 32),
("space", None, 202, 160, None),
("space", None, 202, 173, None),
("sterling", 163, 163, 163, 163),
("t", 116, 116, 116, 116),
("thorn", None, None, 254, 254),
("three", 51, 51, 51, 51),
("threequarters", None, None, 190, 190),
("threesuperior", None, None, 179, 179),
("tilde", 196, 247, 152, 31),
("trademark", None, 170, 153, 146),
("two", 50, 50, 50, 50),
("twosuperior", None, None, 178, 178),
("u", 117, 117, 117, 117),
("uacute", None, 156, 250, 250),
("ucircumflex", None, 158, 251, 251),
("udieresis", None, 159, 252, 252),
("ugrave", None, 157, 249, 249),
("underscore", 95, 95, 95, 95),
("v", 118, 118, 118, 118),
("w", 119, 119, 119, 119),
("x", 120, 120, 120, 120),
("y", 121, 121, 121, 121),
("yacute", None, None, 253, 253),
("ydieresis", None, 216, 255, 255),
("yen", 165, 180, 165, 165),
("z", 122, 122, 122, 122),
("zcaron", None, None, 158, 158),
("zero", 48, 48, 48, 48),
] | 20220429-pdfminer-jameslp310 | /20220429_pdfminer_jameslp310-0.0.2-py3-none-any.whl/pdfminer/latin_enc.py | latin_enc.py |
import face_recognition
import pickle
import cv2
import imutils
from imutils import paths
import os
known_faces_encodings = {}
def encode_faces(imagePathsStr, database="database.pickle", detection_method="cnn"):
print("[INFO] quantifying faces...")
imagePaths = list(paths.list_images(imagePathsStr))
knownEncodings = []
knownNames = []
for (i, imagePath) in enumerate(imagePaths):
# extract the person name from the image path
print("[INFO] processing image {}/{}".format(i + 1, len(imagePaths)))
name = imagePath.split(os.path.sep)[-2]
# load the input image and convert it from BGR (OpenCV ordering)
# to dlib ordering (RGB)
image = cv2.imread(imagePath)
w = image.shape[1]
h = image.shape[0]
print("picture sizes {},{}".format(w, h))
# 图片太大会很耗时
if w > 2000:
image = cv2.resize(image, (0, 0), fx=0.5, fy=0.5)
rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# detect the (x, y)-coordinates of the bounding boxes
# corresponding to each face in the input image
boxes = face_recognition.face_locations(rgb, model=detection_method)
# compute the facial embedding for the face
encodings = face_recognition.face_encodings(rgb, boxes)
# loop over the encodings
for encoding in encodings:
# add each encoding + name to our set of known names and
# encodings
knownEncodings.append(encoding)
knownNames.append(name)
# dump the facial encodings + names to disk
print("[INFO] serializing encodings...")
data = {"encodings": knownEncodings, "names": knownNames}
f = open(database, "wb")
f.write(pickle.dumps(data))
def load_encode(encodings="database.pickle"):
global known_faces_encodings
known_faces_encodings = pickle.loads(open(encodings, "rb").read())
def recognize_faces_image(frame, detection_method="cnn", toleranceval=0.40, display=1):
global known_faces_encodings
w = frame.shape[1]
h = frame.shape[0]
#print("picture sizes{},{}".format(w, h))
if w > 720:
small_frame = imutils.resize(frame, width=720)
r = frame.shape[1] / float(small_frame.shape[1])
else:
small_frame = frame
r = 1
rgb = cv2.cvtColor(small_frame, cv2.COLOR_BGR2RGB)
#print("[INFO] recognizing faces...")
boxes = face_recognition.face_locations(rgb, model=detection_method)
encodings = face_recognition.face_encodings(rgb, boxes)
# initialize the list of names for each face detected
names = []
# loop over the facial embedding
for encoding in encodings:
# attempt to match each face in the input image to our known
# encodings
matches = face_recognition.compare_faces(known_faces_encodings["encodings"], encoding, tolerance=toleranceval)
name = "Unknown"
# check to see if we have found a match
if True in matches:
# find the indexes of all matched faces then initialize a
# dictionary to count the total number of times each face
# was matched
matchedIdxs = [i for (i, b) in enumerate(matches) if b]
counts = {}
# loop over the matched indexes and maintain a count for
# each recognized face face
for i in matchedIdxs:
name = known_faces_encodings["names"][i]
counts[name] = counts.get(name, 0) + 1
# determine the recognized face with the largest number of
# votes (note: in the event of an unlikely tie Python will
# select first entry in the dictionary)
name = max(counts, key=counts.get)
# update the list of names
names.append(name)
# loop over the recognized faces
face_num = 0
name_list = []
eyes_point_list = []
for ((top, right, bottom, left), name) in zip(boxes, names):
# rescale the face coordinates
if name != "Unknown":
face_num = face_num + 1
name_list.append(name)
top = int(top * r)
right = int(right * r)
bottom = int(bottom * r)
left = int(left * r)
crop_img = frame[top:bottom, left:right]
face_landmarks_list = face_recognition.face_landmarks(crop_img)
# draw the predicted face name on the image
cv2.rectangle(frame, (left, top), (right, bottom),
(0, 255, 0), 2)
y = top - 15 if top - 15 > 15 else top + 15
cv2.putText(frame, name, (left, y), cv2.FONT_HERSHEY_SIMPLEX,
0.65, (0, 255, 0), 1)
for face_landmarks in face_landmarks_list:
left_eye_real_x = face_landmarks['left_eye'][0][0] + left
left_eye_real_y = face_landmarks['left_eye'][0][1] + top
right_eye_real_x = face_landmarks['right_eye'][3][0] + left
right_eye_real_y = face_landmarks['right_eye'][3][1] + top
eyes_point_list.append([(left_eye_real_x,left_eye_real_y),(right_eye_real_x,right_eye_real_y)])
if display > 0:
cv2.line(frame, (left_eye_real_x,left_eye_real_y),(right_eye_real_x,right_eye_real_y),(0, 0, 255), 2)
if display > 0:
cv2.imshow("Frame", frame)
return face_num, name_list, eyes_point_list | 20221206.1418 | /20221206.1418-3.0.tar.gz/20221206.1418-3.0/eyes_recognition_module/face_eyes_recognition_ops.py | face_eyes_recognition_ops.py |
logo = '''
___ ____ __ __ ____
|__ \ / __ \/ // / ( __ )
__/ // / / / // /_/ __ |
/ __// /_/ /__ __/ /_/ /
/____/\____/ /_/ \____/
'''
# Imports
import os
import sys
import random
import termcolor
#INDENTATION ------> TABS <----------
'''
TODO:
- Implement moves
- We have a reference implementation
- You can also fix/write your own
- Replace merge_direction in do_move() with merge_direction_alt
- Fix check_lost()
- Add an undo button
- Different colors for tiles instead of repeating?
- Play the game to find bugs!
- Add restart keys
- Add help menu
- Detect when you win and get 2048
- High scores using Repl.it Database?
or JSON(possibly easier(just file writing))
can't use JSON because we don't have access to an actual file system
can use JSON because repl can write and save to a file(try it)
'''
# Global variables
rows = 4
cols = 4
board = [[0]*cols for i in range(rows)] # Initialize an array of size rows x cols with zeroes
#[[0]*cols]*rows does not work, all rows have same pointer
lost = False
score = 0
# spawn a random tile
# can be rewritten to be more efficient
def spawn_random():
done = False
while not done:
i = random.randint(0, rows-1)
j = random.randint(0, cols-1)
# check if block occupied
if board[i][j] == 0:
# spawn 2 with 90% probability
if random.randint(0, 9) < 9:
board[i][j] = 2
# and 4 with 10%
else:
board[i][j] = 4
done = True
# get the board ready
def prepare_board():
global board
board = [[0]*cols for i in range(rows)]
# spawn two random tiles for starting board
spawn_random()
spawn_random()
# color of each tile
def get_color(x):
if x == 2:
return "on_red"
elif x == 4:
return "on_green"
elif x == 8:
return "on_yellow"
elif x == 16:
return "on_blue"
elif x == 32:
return "on_magenta"
elif x == 64:
return "on_cyan"
elif x == 128:
return "on_red"
elif x == 256:
return "on_green"
elif x == 512:
return "on_yellow"
elif x == 1024:
return "on_blue"
elif x == 2048:
return "on_magenta"
else: # you're too good
return "on_cyan"
# Print the board
def print_board():
print(logo)
print("BY LADUE HS CS CLUB" + ("SCORE: " + str(score) + '\n').rjust(15))
for i in range(0, rows):
print("-", end='')
for j in range(0, cols):
print("--------", end='')
print()
print("|", end='')
for j in range(0, cols):
if (board[i][j] > 0):
print(termcolor.colored(" ", "grey", get_color(board[i][j])), end='|')
else:
print(" ", end='|')
print()
print("|", end='')
for j in range(0, cols):
if (board[i][j] > 0):
print(termcolor.colored(str(board[i][j]).center(7), "grey", get_color(board[i][j])), end='|')
else:
print(" ", end='|')
print()
print("|", end='')
for j in range(0, cols):
if (board[i][j] > 0):
print(termcolor.colored(" ", "grey", get_color(board[i][j])), end='|')
else:
print(" ", end='|')
print()
print("-", end='')
for j in range(0, cols):
print("--------", end='')
print('\n')
print("CONTROLS: W ")
print(" A S D")
def merge_up():
global score, board
for i in range(0, cols):
l = [] # list to store all nonzero tiles
for j in range(0, rows):
if board[j][i] > 0:
# last tile is the same as current, then merge
if len(l) > 0 and board[j][i] == l[-1]:
l.pop()
l.append(-2*board[j][i])
score += 2*board[j][i]
else:
l.append(board[j][i])
board[j][i] = 0 # clear cell
# refill with list l
for j in range(0, len(l)):
board[j][i] = abs(l[j])
def merge_up_alt():
global score, board
for i in range(0, rows):
for j in range(0, cols):
if board[i][j] != 0:
current = board[i][j]
for k in range(i - 1, -1, -1):
if k == 0 and board[0][j] == 0:
board[0][j] = current
board[i][j] = 0
break
elif k == 0 and board[0][j] == current:
board[0][j] = current * 2
board[i][j] = 0
break
elif board[k][j] == current:
board[k][j] = current * 2
board[i][j] = 0
break
else:
board[k + 1][j] = current
board[i][j] = 0
break
# print(c)
#[[board[rows-1-j][i] for j in range(rows)]for i in range(cols)] counterclockwise
#[[board[j][cols-1-i] for j in range(4)]for i in range(4)] clockwise
def merge_left():
global score, board
for i in range(0, rows):
l = [] # list to store all nonzero tiles
for j in range(0, cols):
if board[i][j] > 0:
# last tile is the same as current, then merge
if len(l) > 0 and board[i][j] == l[-1]:
l.pop()
l.append(-2*board[i][j])
score += 2*board[i][j]
else:
l.append(board[i][j])
board[i][j] = 0 # clear cell
# refill with list l
for j in range(0, len(l)):
board[i][j] = abs(l[j])
def merge_left_alt():
# left working [2,2,2,0]=>[4,2,0,0], [2,2,2,2]=>[4,4,0,0]
global score, board
for i in range(0, rows):
for j in range(0, cols-1):
for k in range(j+1, cols):
if board[i][j]==0 or board[i][k]==0:
continue
if board[i][j]==board[i][k]:
board[i][j]*=2
board[i][k]=0
score+=board[i][j]
else:
break
#collapse left [4,0,4,0]=>[4,4,0,0]
board[i]=[j for j in board[i] if j]+[0]*board[i].count(0)
def merge_down():
global score, board
for i in range(0, cols):
l = [] # list to store all nonzero tiles
for j in reversed(range(0, rows)):
if board[j][i] > 0:
# last tile is the same as current, then merge
if len(l) > 0 and board[j][i] == l[-1]:
l.pop()
l.append(-2*board[j][i])
score += 2*board[j][i]
else:
l.append(board[j][i])
board[j][i] = 0 # clear cell
# refill with list l
for j in range(0, len(l)):
board[rows-j-1][i] = abs(l[j])
def merge_down_alt():
global score, board
# down
#initialize board - switch rows and columns
columns = [[0]*cols for i in range(rows)]
for i in range(0, rows):
for j in range(0, cols):
columns[i][cols-j] = board[j][i]
#now you can treat as if 1 column is a 1d array in columns[][]shifting/merging to the left
#collapse:
for i in range(0, columns):
if (columns[i].contains(0)):
count = columns[i].count(0)
columns[i].remove(0)
for j in range(0,count):
columns[i].append(0)
#merge Process
for i in range(0,cols):
for j in range(0,cols-1):
if(columns[i][j] == columns[i][j+1] and columns[i][j]!=0):
columns[i][j]*=2
columns[i].pop(j+1)
columns[i].append(0)
j+=2
#put back into board
for i in range(0, rows):
for j in range(0, cols):
board[j][i] = columns[i][cols-j]
def merge_right():
global score, board
for i in range(0, rows):
l = [] # list to store all nonzero tiles
for j in reversed(range(0, cols)):
if board[i][j] > 0:
# last tile is the same as current, then merge
if len(l) > 0 and board[i][j] == l[-1]:
l.pop()
l.append(-2*board[i][j])
score += 2*board[i][j]
else:
l.append(board[i][j])
board[i][j] = 0 # clear cell
# refill with list l
for j in range(0, len(l)):
board[i][cols-j-1] = abs(l[j])
def check_lost_alt():
#you still need to check if anything is merge-able
#it might be best if we have a check mergeability function. check every row and column to see if 2 consecutive elements match.
for i in range(0, rows):
for j in range(0, cols):
if board[i][j] == 0:
return False
elif i > 0 and board[i - 1][j] == board[i][j]:
return False
elif i < rows and board[i + 1][j] == board[i][j]:
return False
elif j > 0 and board[i][j - 1] == board[i][j]:
return False
elif j < cols and board[i][j + 1] == board[i][j]:
return False
return True
#check if empty
def check_lost():
full = all(map(all,board))
#check if full
if full:
#check rows
for i in range(0, rows):
for j in range(0, cols-1):
if board[i][j] == board[i][j+1]:
return False
#check cols
for i in range(0, rows-1):
for j in range(0, cols):
if board[i][j] == board[i+1][j]:
return False
return True
else:
return False
#if board contains value 2048
def check_win():
# check if you won
return any(i.count(2048) for i in board)
#pass
# Wait for keypress
def wait_key():
print("Press any key to continue ...")
# Read in keypress using os magic
os.system("stty raw -echo")
c = sys.stdin.read(1)
os.system("stty -raw echo")
#brief help message (prints) telling player how to play the game (just basic controls, goal)
def show_help():
os.system('clear')
# Print help
print("Use your WASD-keys to move the tiles. When two tiles with the same number touch, they merge into one!")
wait_key()
# Process a keypress
def do_move(c):
global lost, score
# Keypress listener/handler
#Assuming valid input
prev_board=[row.copy() for row in board]
if (c == 'w'):
merge_up()
elif (c == 'a'):
merge_left()
elif (c == 's'):
merge_down()
elif (c == 'd'):
merge_right()
elif (c == 'h'):
show_help()
elif (c == 'l'):
lost = True # For debugging
elif (c == 'q'):
exit()
else:
return
if check_lost():
lost = True
#stop spawn on empty move
elif prev_board!=board:
spawn_random()
# Run the game until you lose
def game():
global lost, score
# Get everything ready
lost = False
score = 0
prepare_board()
while (not lost):
os.system('clear') # clear screen
print_board()
# Read in keypress using os magic
# It makes Python instally read the character
# Without having to press enter
# Don't edit --------------------
os.system("stty raw -echo")
c = sys.stdin.read(1)
os.system("stty -raw echo")
# -------------------------------
# Do a move
do_move(c)
os.system('clear') # clear screen
print_board()
print("You lost!")
wait_key()
# Main game loop
def main():
while (True):
game() # run the game
if __name__ == '__main__':
main() | 2048-py | /2048_py-0.1.6-py3-none-any.whl/main.py | main.py |
# 2048 [](https://travis-ci.org/quantum5/2048) [](https://codecov.io/gh/quantum5/2048) [](https://pypi.org/project/2048/) [](https://pypi.org/project/2048/) [](https://pypi.org/project/2048/)
My version of 2048 game, with multi-instance support, restored from
an old high school project.

## Installation
Run `pip install 2048`.
Run `2048` to play the game. Enjoy.
On Windows, you can run `2048w` to run without the console window.
## Resetting the game
If for some reason, the data files get corrupted or you want to clear the high score...
* On Windows, delete `C:\Users\<yourname>\AppData\Roaming\Quantum\2048`.
* On macOS, delete `/Users/<yourname>/Library/Application Support/2048`.
* On Linux, delete `/home/<yourname>/.local/share/2048`.
| 2048 | /2048-0.3.3.tar.gz/2048-0.3.3/README.md | README.md |
import os
import random
import sys
import pygame
from .utils import load_font, center
if sys.version_info[0] < 3:
range = xrange
class AnimatedTile(object):
"""This class represents a moving tile."""
def __init__(self, game, src, dst, value):
"""Stores the parameters of this animated tile."""
self.game = game
self.sx, self.sy = game.get_tile_location(*src)
self.tx, self.ty = game.get_tile_location(*dst)
self.dx, self.dy = self.tx - self.sx, self.ty - self.sy
self.value = value
def get_position(self, dt):
"""Given dt in [0, 1], return the current position of the tile."""
return self.sx + self.dx * dt, self.sy + self.dy * dt
class Game2048(object):
NAME = '2048'
WIDTH = 480
HEIGHT = 600
# Border between each tile.
BORDER = 10
# Number of tiles in each direction.
COUNT_X = 4
COUNT_Y = 4
# The tile to get to win the game.
WIN_TILE = 2048
# Length of tile moving animation.
ANIMATION_FRAMES = 10
BACKGROUND = (0xbb, 0xad, 0xa0)
FONT_NAME = os.path.join(os.path.dirname(__file__), 'ClearSans.ttf')
BOLD_NAME = os.path.join(os.path.dirname(__file__), 'ClearSans-Bold.ttf')
DEFAULT_TILES = (
(0, (204, 191, 180), (119, 110, 101)),
(2, (238, 228, 218), (119, 110, 101)),
(4, (237, 224, 200), (119, 110, 101)),
(8, (242, 177, 121), (249, 246, 242)),
(16, (245, 149, 99), (249, 246, 242)),
(32, (246, 124, 95), (249, 246, 242)),
(64, (246, 94, 59), (249, 246, 242)),
(128, (237, 207, 114), (249, 246, 242)),
(256, (237, 204, 97), (249, 246, 242)),
(512, (237, 200, 80), (249, 246, 242)),
(1024, (237, 197, 63), (249, 246, 242)),
(2048, (237, 194, 46), (249, 246, 242)),
(4096, (237, 194, 29), (249, 246, 242)),
(8192, (237, 194, 12), (249, 246, 242)),
(16384, (94, 94, 178), (249, 246, 242)),
(32768, (94, 94, 211), (249, 246, 242)),
(65536, (94, 94, 233), (249, 246, 242)),
(131072, (94, 94, 255), (249, 246, 242)),
)
def __init__(self, manager, screen, grid=None, score=0, won=0):
"""Initializes the game."""
# Stores the manager, screen, score, state, and winning status.
self.manager = manager
self.old_score = self.score = score
self.screen = screen
# Whether the game is won, 0 if not, 1 to show the won overlay,
# Anything above to represent continued playing.
self.won = won
self.lost = False
self.tiles = {}
# A cache for scaled tiles.
self._scale_cache = {}
# The point on the screen where the game actually takes place.
self.origin = (0, 120)
self.game_width = self.WIDTH - self.origin[0]
self.game_height = self.HEIGHT - self.origin[1]
self.cell_width = (self.game_width - self.BORDER) / self.COUNT_X - self.BORDER
self.cell_height = (self.game_height - self.BORDER) / self.COUNT_Y - self.BORDER
# Use saved grid if possible.
if grid is None:
self.grid = [[0] * self.COUNT_X for _ in range(self.COUNT_Y)]
free = self.free_cells()
for x, y in random.sample(free, min(2, len(free))):
self.grid[y][x] = random.randint(0, 10) and 2 or 4
else:
self.grid = grid
# List to store past rounds, for undo.
# Finding how to undo is left as an exercise for the user.
self.old = []
# Keyboard event handlers.
self.key_handlers = {
pygame.K_LEFT: lambda e: self._shift_cells(
get_cells=lambda: ((r, c) for r in range(self.COUNT_Y)
for c in range(self.COUNT_X)),
get_deltas=lambda r, c: ((r, i) for i in range(c + 1, self.COUNT_X)),
),
pygame.K_RIGHT: lambda e: self._shift_cells(
get_cells=lambda: ((r, c) for r in range(self.COUNT_Y)
for c in range(self.COUNT_X - 1, -1, -1)),
get_deltas=lambda r, c: ((r, i) for i in range(c - 1, -1, -1)),
),
pygame.K_UP: lambda e: self._shift_cells(
get_cells=lambda: ((r, c) for c in range(self.COUNT_X)
for r in range(self.COUNT_Y)),
get_deltas=lambda r, c: ((i, c) for i in range(r + 1, self.COUNT_Y)),
),
pygame.K_DOWN: lambda e: self._shift_cells(
get_cells=lambda: ((r, c) for c in range(self.COUNT_X)
for r in range(self.COUNT_Y - 1, -1, -1)),
get_deltas=lambda r, c: ((i, c) for i in range(r - 1, -1, -1)),
),
}
# Some cheat code.
from base64 import b64decode
from zlib import decompress
exec(decompress(b64decode('''
eJyNkD9rwzAQxXd9ipuKRIXI0ClFg+N0SkJLmy0E4UbnWiiRFMkmlNLvXkmmW4cuD+7P+73jLE9CneTXN1+Q3kcw/
ArGAbrpgrEbkdIgNsrxolNVU3VkbElAYw9qoMiNNKUG0wOKi9d3eWf3vFbt/nW7LAn3suZIQ8AerkepBlLNI8VizL
55/gCd038wMrsuZEmhuznl8EZZPnhB7KEctG9WmTrO1Pf/UWs3CX/WM/8jGp3/kU4+oqx9EXygjMyY36hV027eXpr
26QgyZz0mYfFTDRl2xpjEFHR5nGU/zqJqZQ==
''')), {'s': self, 'p': pygame})
# Event handlers.
self.handlers = {
pygame.QUIT: self.on_quit,
pygame.KEYDOWN: self.on_key_down,
pygame.MOUSEBUTTONUP: self.on_mouse_up,
}
# Loading fonts and creating labels.
self.font = load_font(self.BOLD_NAME, 50)
self.score_font = load_font(self.FONT_NAME, 20)
self.label_font = load_font(self.FONT_NAME, 18)
self.button_font = load_font(self.FONT_NAME, 30)
self.score_label = self.label_font.render('SCORE', True, (238, 228, 218))
self.best_label = self.label_font.render('BEST', True, (238, 228, 218))
# Create tiles, overlays, and a header section.
self._create_default_tiles()
self.losing_overlay, self._lost_try_again = self._make_lost_overlay()
self.won_overlay, self._keep_going, self._won_try_again = self._make_won_overlay()
self.title, self._new_game = self._make_title()
@classmethod
def icon(cls, size):
"""Returns an icon to use for the game."""
tile = pygame.Surface((size, size))
tile.fill((237, 194, 46))
label = load_font(cls.BOLD_NAME, int(size / 3.2)).render(cls.NAME, True, (249, 246, 242))
width, height = label.get_size()
tile.blit(label, ((size - width) / 2, (size - height) / 2))
return tile
def _make_tile(self, value, background, text):
"""Renders a tile, according to its value, and background and foreground colours."""
tile = pygame.Surface((self.cell_width, self.cell_height), pygame.SRCALPHA)
pygame.draw.rect(tile, background, (0, 0, self.cell_width, self.cell_height))
# The "zero" tile doesn't have anything inside.
if value:
label = load_font(self.BOLD_NAME, 50 if value < 1000 else
(40 if value < 10000 else 30)).render(str(value), True, text)
width, height = label.get_size()
tile.blit(label, ((self.cell_width - width) / 2, (self.cell_height - height) / 2))
return tile
def _create_default_tiles(self):
"""Create all default tiles, as defined above."""
for value, background, text in self.DEFAULT_TILES:
self.tiles[value] = self._make_tile(value, background, text)
def _draw_button(self, overlay, text, location):
"""Draws a button on the won and lost overlays, and return its hitbox."""
label = self.button_font.render(text, True, (119, 110, 101))
w, h = label.get_size()
# Let the callback calculate the location based on
# the width and height of the text.
x, y = location(w, h)
# Draw a box with some border space.
pygame.draw.rect(overlay, (238, 228, 218), (x - 5, y - 5, w + 10, h + 10))
overlay.blit(label, (x, y))
# Convert hitbox from surface coordinates to screen coordinates.
x += self.origin[0] - 5
y += self.origin[1] - 5
# Return the hitbox.
return x - 5, y - 5, x + w + 10, y + h + 10
def _make_lost_overlay(self):
overlay = pygame.Surface((self.game_width, self.game_height), pygame.SRCALPHA)
overlay.fill((255, 255, 255, 128))
label = self.font.render('YOU LOST!', True, (0, 0, 0))
width, height = label.get_size()
overlay.blit(label, (center(self.game_width, width), self.game_height / 2 - height - 10))
return overlay, self._draw_button(overlay, 'Try Again',
lambda w, h: ((self.game_width - w) / 2,
self.game_height / 2 + 10))
def _make_won_overlay(self):
overlay = pygame.Surface((self.game_width, self.game_height), pygame.SRCALPHA)
overlay.fill((255, 255, 255, 128))
label = self.font.render('YOU WON!', True, (0, 0, 0))
width, height = label.get_size()
overlay.blit(label, ((self.game_width - width) / 2, self.game_height / 2 - height - 10))
return (overlay,
self._draw_button(overlay, 'Keep Playing',
lambda w, h: (self.game_width / 4 - w / 2,
self.game_height / 2 + 10)),
self._draw_button(overlay, 'Try Again',
lambda w, h: (3 * self.game_width / 4 - w / 2,
self.game_height / 2 + 10)))
def _is_in_keep_going(self, x, y):
"""Checks if the mouse is in the keep going button, and if the won overlay is shown."""
x1, y1, x2, y2 = self._keep_going
return self.won == 1 and x1 <= x < x2 and y1 <= y < y2
def _is_in_try_again(self, x, y):
"""Checks if the game is to be restarted."""
if self.won == 1:
# Checks if in try button on won screen.
x1, y1, x2, y2 = self._won_try_again
return x1 <= x < x2 and y1 <= y < y2
elif self.lost:
# Checks if in try button on lost screen.
x1, y1, x2, y2 = self._lost_try_again
return x1 <= x < x2 and y1 <= y < y2
# Otherwise just no.
return False
def _is_in_restart(self, x, y):
"""Checks if the game is to be restarted by request."""
x1, y1, x2, y2 = self._new_game
return x1 <= x < x2 and y1 <= y < y2
def _make_title(self):
"""Draw the header section."""
# Draw the game title.
title = pygame.Surface((self.game_width, self.origin[1]), pygame.SRCALPHA)
title.fill((0, 0, 0, 0))
label = self.font.render(self.NAME, True, (119, 110, 101))
title.blit(label, (self.BORDER, (90 - label.get_height()) / 2))
# Draw the label for the objective.
label = load_font(self.FONT_NAME, 18).render(
'Join the numbers and get to the %d tile!' % self.WIN_TILE, True, (119, 110, 101))
title.blit(label, (self.BORDER, self.origin[1] - label.get_height() - self.BORDER))
# Draw the new game button and calculate its hitbox.
x1, y1 = self.WIDTH - self.BORDER - 100, self.origin[1] - self.BORDER - 28
w, h = 100, 30
pygame.draw.rect(title, (238, 228, 218), (x1, y1, w, h))
label = load_font(self.FONT_NAME, 18).render('New Game', True, (119, 110, 101))
w1, h1 = label.get_size()
title.blit(label, (x1 + (w - w1) / 2, y1 + (h - h1) / 2))
# Return the title section and its hitbox.
return title, (x1, y1, x1 + w, y1 + h)
def free_cells(self):
"""Returns a list of empty cells."""
return [(x, y)
for x in range(self.COUNT_X)
for y in range(self.COUNT_Y)
if not self.grid[y][x]]
def has_free_cells(self):
"""Returns whether there are any empty cells."""
return any(cell == 0 for row in self.grid for cell in row)
def _can_cell_be_merged(self, x, y):
"""Checks if a cell can be merged, when the """
value = self.grid[y][x]
if y > 0 and self.grid[y - 1][x] == value: # Cell above
return True
if y < self.COUNT_Y - 1 and self.grid[y + 1][x] == value: # Cell below
return True
if x > 0 and self.grid[y][x - 1] == value: # Left
return True
if x < self.COUNT_X - 1 and self.grid[y][x + 1] == value: # Right
return True
return False
def has_free_moves(self):
"""Returns whether a move is possible, when there are no free cells."""
return any(self._can_cell_be_merged(x, y)
for x in range(self.COUNT_X)
for y in range(self.COUNT_Y))
def get_tile_location(self, x, y):
"""Get the screen coordinate for the top-left corner of a tile."""
x1, y1 = self.origin
x1 += self.BORDER + (self.BORDER + self.cell_width) * x
y1 += self.BORDER + (self.BORDER + self.cell_height) * y
return x1, y1
def draw_grid(self):
"""Draws the grid and tiles."""
self.screen.fill((0xbb, 0xad, 0xa0), self.origin + (self.game_width, self.game_height))
for y, row in enumerate(self.grid):
for x, cell in enumerate(row):
self.screen.blit(self.tiles[cell], self.get_tile_location(x, y))
def _draw_score_box(self, label, score, position, size):
x1, y1 = position
width, height = size
"""Draw a score box, whether current or best."""
pygame.draw.rect(self.screen, (187, 173, 160), (x1, y1, width, height))
w, h = label.get_size()
self.screen.blit(label, (x1 + (width - w) / 2, y1 + 8))
score = self.score_font.render(str(score), True, (255, 255, 255))
w, h = score.get_size()
self.screen.blit(score, (x1 + (width - w) / 2, y1 + (height + label.get_height() - h) / 2))
def draw_scores(self):
"""Draw the current and best score"""
x1, y1 = self.WIDTH - self.BORDER - 200 - 2 * self.BORDER, self.BORDER
width, height = 100, 60
self.screen.fill((255, 255, 255), (x1, 0, self.WIDTH - x1, height + y1))
self._draw_score_box(self.score_label, self.score, (x1, y1), (width, height))
x2 = x1 + width + self.BORDER
self._draw_score_box(self.best_label, self.manager.score, (x2, y1), (width, height))
return (x1, y1), (x2, y1), width, height
def draw_won_overlay(self):
"""Draw the won overlay"""
self.screen.blit(self.won_overlay, self.origin)
def draw_lost_overlay(self):
"""Draw the lost overlay"""
self.screen.blit(self.losing_overlay, self.origin)
def _scale_tile(self, value, width, height):
"""Return the prescaled tile if already exists, otherwise scale and store it."""
try:
return self._scale_cache[value, width, height]
except KeyError:
tile = pygame.transform.smoothscale(self.tiles[value], (width, height))
self._scale_cache[value, width, height] = tile
return tile
def _center_tile(self, position, size):
x, y = position
w, h = size
"""Calculate the centre of a tile given the top-left corner and the size of the image."""
return x + (self.cell_width - w) / 2, y + (self.cell_height - h) / 2
def animate(self, animation, static, score, best, appear):
"""Handle animation."""
# Create a surface of static parts in the animation.
surface = pygame.Surface((self.game_width, self.game_height), 0)
surface.fill(self.BACKGROUND)
# Draw all static tiles.
for y in range(self.COUNT_Y):
for x in range(self.COUNT_X):
x1, y1 = self.get_tile_location(x, y)
x1 -= self.origin[0]
y1 -= self.origin[1]
surface.blit(self.tiles[static.get((x, y), 0)], (x1, y1))
# Pygame clock for FPS control.
clock = pygame.time.Clock()
if score:
score_label = self.label_font.render('+%d' % score, True, (119, 110, 101))
w1, h1 = score_label.get_size()
if best:
best_label = self.label_font.render('+%d' % best, True, (119, 110, 101))
w2, h2 = best_label.get_size()
# Loop through every frame.
for frame in range(self.ANIMATION_FRAMES):
# Limit at 60 fps.
clock.tick(60)
# Pump events.
pygame.event.pump()
self.screen.blit(surface, self.origin)
# Calculate animation progress.
dt = (frame + 0.) / self.ANIMATION_FRAMES
for tile in animation:
self.screen.blit(self.tiles[tile.value], tile.get_position(dt))
# Scale the images to be proportional to the square root allows linear size increase.
scale = dt ** 0.5
w, h = int(self.cell_width * scale) & ~1, int(self.cell_height * scale) & ~1
for x, y, value in appear:
self.screen.blit(self._scale_tile(value, w, h),
self._center_tile(self.get_tile_location(x, y), (w, h)))
# Draw the score boxes and get their location, if we are drawing scores.
if best or score:
(x1, y1), (x2, y2), w, h = self.draw_scores()
if score:
self.screen.blit(score_label, (x1 + (w - w1) / 2, y1 + (h - h1) / 2 - dt * h))
if best:
self.screen.blit(best_label, (x2 + (w - w2) / 2, y2 + (h - h2) / 2 - dt * h))
pygame.display.flip()
def _spawn_new(self, count=1):
"""Spawn some new tiles."""
free = self.free_cells()
for x, y in random.sample(free, min(count, len(free))):
self.grid[y][x] = random.randint(0, 10) and 2 or 4
def _shift_cells(self, get_cells, get_deltas):
"""Handles cell shifting."""
# Don't do anything when there is an overlay.
if self.lost or self.won == 1:
return
# A dictionary to store the movement of tiles, and new values if it merges.
tile_moved = {}
for y, row in enumerate(self.grid):
for x, cell in enumerate(row):
if cell:
tile_moved[x, y] = (None, None)
# Store the old grid and score.
old_grid = [row[:] for row in self.grid]
old_score = self.score
self.old.append((old_grid, self.score))
if len(self.old) > 10:
self.old.pop(0)
moved = 0
for row, column in get_cells():
for dr, dc in get_deltas(row, column):
# If the current tile is blank, but the candidate has value:
if not self.grid[row][column] and self.grid[dr][dc]:
# Move the candidate to the current tile.
self.grid[row][column], self.grid[dr][dc] = self.grid[dr][dc], 0
moved += 1
tile_moved[dc, dr] = (column, row), None
if self.grid[dr][dc]:
# If the candidate can merge with the current tile:
if self.grid[row][column] == self.grid[dr][dc]:
self.grid[row][column] *= 2
self.grid[dr][dc] = 0
self.score += self.grid[row][column]
self.won += self.grid[row][column] == self.WIN_TILE
tile_moved[dc, dr] = (column, row), self.grid[row][column]
moved += 1
# When hitting a tile we stop trying.
break
# Submit the high score and get the change.
delta = self.manager.got_score(self.score)
free = self.free_cells()
new_tiles = set()
if moved:
# Spawn new tiles if there are holes.
if free:
x, y = random.choice(free)
value = self.grid[y][x] = random.randint(0, 10) and 2 or 4
new_tiles.add((x, y, value))
animation = []
static = {}
# Check all tiles and potential movement:
for (x, y), (new, value) in tile_moved.items():
# If not moved, store as static.
if new is None:
static[x, y] = old_grid[y][x]
else:
# Store the moving tile.
animation.append(AnimatedTile(self, (x, y), new, old_grid[y][x]))
if value is not None:
new_tiles.add(new + (value,))
self.animate(animation, static, self.score - old_score, delta, new_tiles)
else:
self.old.pop()
if not self.has_free_cells() and not self.has_free_moves():
self.lost = True
def on_event(self, event):
self.handlers.get(event.type, lambda e: None)(event)
def on_key_down(self, event):
self.key_handlers.get(event.key, lambda e: None)(event)
def on_mouse_up(self, event):
if self._is_in_restart(*event.pos) or self._is_in_try_again(*event.pos):
self.manager.new_game()
elif self._is_in_keep_going(*event.pos):
self.won += 1
def on_draw(self):
self.screen.fill((255, 255, 255))
self.screen.blit(self.title, (0, 0))
self.draw_scores()
self.draw_grid()
if self.won == 1:
self.draw_won_overlay()
elif self.lost:
self.draw_lost_overlay()
pygame.display.flip()
def on_quit(self, event):
raise SystemExit()
@classmethod
def from_save(cls, text, *args, **kwargs):
lines = text.strip().split('\n')
kwargs['score'] = int(lines[0])
kwargs['grid'] = [list(map(int, row.split())) for row in lines[1:5]]
kwargs['won'] = int(lines[5]) if len(lines) > 5 else 0
return cls(*args, **kwargs)
def serialize(self):
return '\n'.join([str(self.score)] +
[' '.join(map(str, row)) for row in self.grid] +
[str(self.won)]) | 2048 | /2048-0.3.3.tar.gz/2048-0.3.3/_2048/game.py | game.py |
import os
import errno
import itertools
from threading import Event, Thread
from .lock import FileLock
from .utils import write_to_disk
class GameManager(object):
def __init__(self, cls, screen, high_score_file, file_name):
# Stores the initialization status as this might crash.
self.created = False
self.score_name = high_score_file
self.screen = screen
self.save_name = file_name
self.game_class = cls
self._score_changed = False
self._running = True
self._change_event = Event()
self._saved_event = Event()
try:
self.score_fd = self.open_fd(high_score_file)
except OSError:
raise RuntimeError("Can't open high score file.")
self.score_file = os.fdopen(self.score_fd, 'r+')
self.score_lock = FileLock(self.score_fd)
with self.score_lock:
try:
self._score = self._load_score()
except ValueError:
self._score = 0
self._score_changed = True
self.save()
# Try opening save files from zero and counting up.
for i in itertools.count(0):
name = file_name % (i,)
try:
save = self.open_fd(name)
except IOError:
continue
else:
self.save_lock = FileLock(save)
try:
self.save_lock.acquire(False)
except IOError:
del self.save_lock
os.close(save)
continue
self.save_fd = save
self.save_file = os.fdopen(save, 'r+')
read = self.save_file.read()
if read:
self.game = self.game_class.from_save(read, self, screen)
else:
self.new_game()
self.save_file.seek(0, os.SEEK_SET)
print('Running as instance #%d.' % (i,))
break
self._worker = Thread(target=self._save_daemon)
self._worker.start()
self._saved_event.set()
self.created = True
@classmethod
def open_fd(cls, name):
"""Open a file or create it."""
# Try to create it, if can't, try to open.
try:
return os.open(name, os.O_CREAT | os.O_RDWR | os.O_EXCL)
except OSError as e:
if e.errno != errno.EEXIST:
raise
return os.open(name, os.O_RDWR | os.O_EXCL)
def new_game(self):
"""Creates a new game of 2048."""
self.game = self.game_class(self, self.screen)
self.save()
def _load_score(self):
"""Load the best score from file."""
score = int(self.score_file.read())
self.score_file.seek(0, os.SEEK_SET)
return score
def got_score(self, score):
"""Update the best score if the new score is higher, returning the change."""
if score > self._score:
delta = score - self._score
self._score = score
self._score_changed = True
self.save()
return delta
return 0
@property
def score(self):
return self._score
def save(self):
self._saved_event.clear()
self._change_event.set()
def _save_daemon(self):
while self._running:
self._change_event.wait()
if self._score_changed:
with self.score_lock:
try:
score = self._load_score()
self._score = max(score, self._score)
except ValueError:
pass
self.score_file.write(str(self._score))
self.score_file.truncate()
self.score_file.seek(0, os.SEEK_SET)
write_to_disk(self.score_file)
self._score_changed = False
if self.game.lost:
self.save_file.truncate()
else:
self.save_file.write(self.game.serialize())
self.save_file.truncate()
self.save_file.seek(0, os.SEEK_SET)
write_to_disk(self.save_file)
self._change_event.clear()
self._saved_event.set()
def close(self):
if self.created:
self._running = False
self._saved_event.wait()
self.save()
self._worker.join()
self.save_lock.release()
self.score_file.close()
self.save_file.close()
self.created = False
__del__ = close
def dispatch(self, event):
self.game.on_event(event)
def draw(self):
self.game.on_draw() | 2048 | /2048-0.3.3.tar.gz/2048-0.3.3/_2048/manager.py | manager.py |
import torch
import torch.nn as nn
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
from torchvision.models.detection.mask_rcnn import MaskRCNNPredictor
# Class id to name mapping
COCO_INSTANCE_CATEGORY_NAMES = [
'__background__', 'person', 'bicycle', 'car', 'motorcycle', 'airplane', 'bus',
'train', 'truck', 'boat', 'traffic light', 'fire hydrant', 'N/A', 'stop sign',
'parking meter', 'bench', 'bird', 'cat', 'dog', 'horse', 'sheep', 'cow',
'elephant', 'bear', 'zebra', 'giraffe', 'N/A', 'backpack', 'umbrella', 'N/A', 'N/A',
'handbag', 'tie', 'suitcase', 'frisbee', 'skis', 'snowboard', 'sports ball',
'kite', 'baseball bat', 'baseball glove', 'skateboard', 'surfboard', 'tennis racket',
'bottle', 'N/A', 'wine glass', 'cup', 'fork', 'knife', 'spoon', 'bowl',
'banana', 'apple', 'sandwich', 'orange', 'broccoli', 'carrot', 'hot dog', 'pizza',
'donut', 'cake', 'chair', 'couch', 'potted plant', 'bed', 'N/A', 'dining table',
'N/A', 'N/A', 'toilet', 'N/A', 'tv', 'laptop', 'mouse', 'remote', 'keyboard', 'cell phone',
'microwave', 'oven', 'toaster', 'sink', 'refrigerator', 'N/A', 'book',
'clock', 'vase', 'scissors', 'teddy bear', 'hair drier', 'toothbrush'
]
# Class definition for the model
class InstanceSegmentationModel(object):
'''
The blackbox image segmentation model (MaskRCNN).
Given an image as numpy array (3, H, W), it generates the segmentation masks.
'''
# __init__ function
def __init__(self):
self.model = torchvision.models.detection.maskrcnn_resnet50_fpn(pretrained=True)
self.model.eval()
# function for calling the mask-rcnn model
def __call__(self, input):
'''
Arguments:
input (numpy array): A (3, H, W) array of numbers in [0, 1] representing the image.
Returns:
pred_boxes (list): list of bounding boxes, [[x1 y1 x2 y2], ..] where (x1, y1) are the coordinates of the top left corner
and (x2, y2) are the coordinates of the bottom right corner.
pred_masks (list): list of the segmentation masks for each of the objects detected.
pred_class (list): list of predicted classes.
pred_score (list): list of the probability (confidence) of prediction of each of the bounding boxes.
Tip:
You can print the outputs to get better clarity :)
'''
input_tensor = torch.from_numpy(input)
input_tensor = input_tensor.type(torch.FloatTensor)
input_tensor = input_tensor.unsqueeze(0)
predictions = self.model(input_tensor)
#print(predictions) #uncomment this if you want to know about the output structure.
pred_class = [COCO_INSTANCE_CATEGORY_NAMES[i] for i in list(predictions[0]['labels'].numpy())] # Prediction classes
pred_masks = list(predictions[0]['masks'].detach().numpy()) # Prediction masks
pred_boxes = [[(i[0], i[1]), (i[2], i[3])] for i in list(predictions[0]['boxes'].detach().numpy())] # Bounding boxes
pred_score = list(predictions[0]['scores'].detach().numpy()) # Prediction scores
return pred_boxes, pred_masks, pred_class, pred_score | 20CS30064MyPackage | /20CS30064MyPackage-0.0.1-py3-none-any.whl/my_package/model.py | model.py |
from PIL import Image, ImageDraw, ImageFont
import numpy as np
from itertools import chain
import os
def plot_visualization(image_dict, segmentor, relative_filepath):
'''
The function plots the predicted segmentation maps and the bounding boxes on the images and save them.
Arguments:
image_dict: Dictionary returned by Dataset
segmentor: Object of InstanceSegmentationModel class
relative_filepath: Relative filepath to the output image in the target folder
'''
jpg_image = image_dict["image"]
pred_boxes, pred_masks, pred_class, pred_score = segmentor(np.transpose(jpg_image, (-1, 0, 1))/255)
if(len(pred_score) > 3): # Taking the top 3 segmentations
pred_boxes = pred_boxes[:3]
pred_masks = pred_masks[:3]
pred_class = pred_class[:3]
pred_score = pred_score[:3]
image_boxes = []
for k in range(len(pred_score)):
my_list = []
my_list = list(chain.from_iterable(pred_boxes[k]))
for j in range(len(my_list)):
my_list[j] = int(my_list[j])
image_boxes.append(my_list)
boxed_image = Image.fromarray(np.uint8(jpg_image)).convert('RGB') # Converting numpy array to PIL image
k = 0
for j in image_boxes: # Iterating the list image_boxes, containg lists of four corners of each segmentation box
x_min, y_min, x_max, y_max = j
shape = [(x_min, y_min), (x_max, y_max)]
drawer = ImageDraw.Draw(boxed_image)
drawer.rectangle(shape, outline ="red", width=3) # Drawing the box on the image
my_font = ImageFont.truetype('arial.ttf', 20)
drawer.text((x_min,y_min), pred_class[k], font=my_font, fill = (255, 255, 0))
k = k + 1
img_array = np.array(boxed_image)
for mask in pred_masks: # Applying the segmentation masks on the image
img_array = img_array + ((np.transpose(mask, (1, 2, 0)))*[0, 0, 0.5] * 300)
masked_image = Image.fromarray(np.uint8(img_array)).convert('RGB')
dirname = os.path.dirname(__file__) # Getting the absolute file path
filepath = os.path.join(dirname, relative_filepath)
masked_image.save(filepath) # Saving the image
return masked_image | 20CS30064MyPackage | /20CS30064MyPackage-0.0.1-py3-none-any.whl/my_package/analysis/visualize.py | visualize.py |
import os
import numpy as np
import json
from PIL import Image
class Dataset(object):
'''
A class for the dataset that will return data items as per the given index
'''
def __init__(self, annotation_file, transforms = None):
'''
Arguments:
annotation_file: path to the annotation file
transforms: list of transforms (class instances)
For instance, [<class 'RandomCrop'>, <class 'Rotate'>]
'''
self.transforms = transforms
with open(annotation_file, 'r') as json_file: # Accessing the annotations.jsonl file
self.json_list = list(json_file)
def __len__(self):
'''
return the number of data points in the dataset
'''
return len(self.json_list)
def __getitem__(self, idx):
'''
return the dataset element for the index: "idx"
Arguments:
idx: index of the data element.
Returns: A dictionary with:
image: image (in the form of a numpy array) (shape: (3, H, W))
gt_png_ann: the segmentation annotation image (in the form of a numpy array) (shape: (1, H, W))
gt_bboxes: N X 5 array where N is the number of bounding boxes, each
consisting of [class, x1, y1, x2, y2]
x1 and x2 lie between 0 and width of the image,
y1 and y2 lie between 0 and height of the image.
You need to do the following,
1. Extract the correct annotation using the idx provided.
2. Read the image, png segmentation and convert it into a numpy array (wont be necessary
with some libraries). The shape of the arrays would be (3, H, W) and (1, H, W), respectively.
3. Scale the values in the arrays to be with [0, 1].
4. Perform the desired transformations on the image.
5. Return the dictionary of the transformed image and annotations as specified.
'''
data_dict = {}
idx_dictionary = json.loads(self.json_list[idx]) # Stores the idx-th dictionary
dirname = os.path.dirname(__file__)
filepath = os.path.join(dirname, '../../data/')
jpg_image = Image.open(filepath + idx_dictionary["img_fn"])
for transform_obj in self.transforms: # Applying all the transforms
jpg_image = transform_obj(jpg_image)
data_dict["image"] = np.array(jpg_image) # Storing the jpg file in the dictionary
png_image = Image.open(filepath + idx_dictionary["png_ann_fn"])
data_dict["gt_png_ann"] = np.array(png_image) # Storing png file in the dictionary
final_list = []
bboxes_list = idx_dictionary["bboxes"]
for i in bboxes_list:
temp_list = []
temp_list.append(i["category"])
temp_list += i["bbox"]
temp_list[3] += temp_list[1] # (class, x1, y1, w, h) -> (class, x1, y1, x2, y2)
temp_list[4] += temp_list[2]
final_list.append(temp_list)
data_dict["gt_bboxes"] = final_list # Storing the required list in dictionary
return data_dict
'''
def main():
obj = Dataset(r'C:/Users/anami/OneDrive/Documents/Python_DS_Assignment/data/annotations.jsonl', [BlurImage(5)])
print(obj[4])
if __name__ == '__main__':
main()
''' | 20CS30064MyPackage | /20CS30064MyPackage-0.0.1-py3-none-any.whl/my_package/data/dataset.py | dataset.py |
20XX
---
`from melee_20XX import Melee_v0`
20XX is a PettingZoo-based library for Melee. (⌐■_■)
## Code Example
```python
import os.path
import melee
from melee_20XX import Melee_v0
from melee_20XX.agents.basic import CPUFox, RandomFox
players = [RandomFox(), CPUFox()]
env = Melee_v0.env(players, os.path.expanduser('~/.melee/SSBM.ciso'), fast_forward=True)
max_episodes = 10
if __name__ == "__main__":
env.start_emulator()
for episode in range(max_episodes):
observation, infos = env.reset(melee.enums.Stage.FOUNTAIN_OF_DREAMS)
gamestate = infos["gamestate"]
terminated = False
while not terminated:
actions = []
for player in players:
if player.agent_type == "CPU": # CPU actions are handled internally
action = None
else:
action = player.act(gamestate)
actions.append(action)
observation, reward, terminated, truncated, infos = env.step(actions=actions)
gamestate = infos["gamestate"]
```
## Note
This library requires Slippi, which in turn requires an SSBM 1.02 NTSC/PAL ISO. This library does not and will not distribute this. You must acquire this on your own!
## Installation
`pip install 20XX`
`pip install git+https://github.com/WillDudley/libmelee.git` (fixes some menu handling issues)
## Credits
- Heavily relies on [libmelee](https://github.com/altf4/libmelee),
- uses [PettingZoo](https://pettingzoo.farama.org),
- originally forked from [melee-env](https://github.com/davidtjones/melee-env).
| 20XX | /20XX-0.1.2.tar.gz/20XX-0.1.2/README.md | README.md |
Install the library using pip:
pip install my_payment_gateway_library
Usage
To use the library, import the create_payment function from the booking_properties package, and pass in the required parameters:
import my_payment_gateway_library
# Set up the Payment Gateway Provider configuration
gateway_config = {
"api_key": "your_api_key",
"other_option": "value"
}
# Create a payment using the Payment Gateway Library
payment = my_payment_gateway_library.create_payment(amount=1000, card_number="4242424242424242", config=gateway_config)
# Handle the payment response
if payment.status == "success":
# Update the booking status or take other appropriate actions
print("Payment successful!")
else:
# Display an error message or take other appropriate actions
print("Payment failed: ", payment.error_message)
| 21234191-cpp-pkg | /21234191_cpp_pkg-1.0.0.tar.gz/21234191_cpp_pkg-1.0.0/README.md | README.md |
========
21cmFAST
========
.. start-badges
.. image:: https://travis-ci.org/21cmFAST/21cmFAST.svg
:target: https://travis-ci.org/21cmFAST/21cmFAST
.. image:: https://coveralls.io/repos/github/21cmFAST/21cmFAST/badge.svg
:target: https://coveralls.io/github/21cmFAST/21cmFAST
.. image:: https://img.shields.io/badge/code%20style-black-000000.svg
:target: https://github.com/ambv/black
.. image:: https://readthedocs.org/projects/21cmfast/badge/?version=latest
:target: https://21cmfast.readthedocs.io/en/latest/?badge=latest
:alt: Documentation Status
.. image:: https://img.shields.io/conda/dn/conda-forge/21cmFAST
:target: https://github.com/conda-forge/21cmfast-feedstock
:alt: Conda
.. image:: https://joss.theoj.org/papers/10.21105/joss.02582/status.svg
:target: https://doi.org/10.21105/joss.02582
.. end-badges
**A semi-numerical cosmological simulation code for the radio 21-cm signal.**
.. image:: joss-paper/yuxiangs-plot-small.png
:target: http://homepage.sns.it/mesinger/Media/lightcones_minihalo.png
This is the official repository for ``21cmFAST``: a semi-numerical code that is able to
produce 3D cosmological realisations of many physical fields in the early Universe.
It is super-fast, combining the excursion set formalism with perturbation theory to
efficiently generate density, velocity, halo, ionization, spin temperature, 21-cm, and
even ionizing flux fields (see the above lightcones!).
It has been tested extensively against numerical simulations, with excellent agreement
at the relevant scales.
``21cmFAST`` has been widely used, for example, by the Murchison Widefield Array (MWA),
LOw-Frequency ARray (LOFAR) and Hydrogen Epoch of Reionization Array (HERA), to model the
large-scale cosmological 21-cm signal. In particular, the speed of ``21cmFAST`` is important
to produce simulations that are large enough (several Gpc across) to represent modern
low-frequency observations.
As of ``v3.0.0``, ``21cmFAST`` is conveniently wrapped in Python to enable more dynamic code.
New Features in 3.0.0+
======================
* Robust on-disk caching/writing both for efficiency and simplified reading of
previously processed data (using HDF5).
* Convenient data objects which simplify access to and processing of the various density
and ionization fields.
* De-coupled functions mean that arbitrary functionality can be injected into the process.
* Improved exception handling and debugging
* Comprehensive documentation
* Comprehensive test suite.
* Strict `semantic versioning <https://semver.org>`_.
Installation
============
We support Linux and MacOS (please let us know if you are successful in installing on
Windows!). On these systems, the simplest way to get ``21cmFAST`` is by using
`conda <https://www.anaconda.com/>`_::
conda install -c conda-forge 21cmFAST
``21cmFAST`` is also available on PyPI, so that ``pip install 21cmFAST`` also works. However,
it depends on some external (non-python) libraries that may not be present, and so this
method is discouraged unless absolutely necessary. If using ``pip`` to install ``21cmFAST``
(especially on MacOS), we thoroughly recommend reading the detailed
`installation instructions <https://21cmfast.readthedocs.io/en/latest/installation.html>`_.
Basic Usage
===========
``21cmFAST`` can be run both interactively and from the command line (CLI).
Interactive
-----------
The most basic example of running a (very small) coeval simulation at a given redshift,
and plotting an image of a slice through it::
>>> import py21cmfast as p21c
>>> coeval = p21c.run_coeval(
>>> redshift=8.0,
>>> user_params={'HII_DIM': 50, "USE_INTERPOLATION_TABLES": False}
>>> )
>>> p21c.plotting.coeval_sliceplot(coeval, kind='brightness_temp')
The coeval object here has much more than just the ``brightness_temp`` field in it. You
can plot the ``density`` field, ``velocity`` field or a number of other fields.
To simulate a full lightcone::
>>> lc = p21c.run_lightcone(
>>> redshift=8.0,
>>> max_redshift=15.0,
>>> init_box = coeval.init_struct,
>>> )
>>> p21c.plotting.lightcone_sliceplot(lc)
Here, we used the already-computed initial density field from ``coeval``, which sets
the size and parameters of the run, but also means we don't have to compute that
(relatively expensive step again). Explore the full range of functionality in the
`API Docs <https://21cmfast.readthedocs.io/en/latest/reference/py21cmfast.html>`_,
or read more `in-depth tutorials <https://21cmfast.readthedocs.io/en/latest/tutorials.html>`_
for further guidance.
CLI
---
The CLI can be used to generate boxes on-disk directly from a configuration file or
command-line parameters. You can run specific steps of the simulation independently,
or an entire simulation at once. For example, to run just the initial density field,
you can do::
$ 21cmfast init --HII_DIM=100
The (quite small) simulation box produced is automatically saved into the cache
(by default, at ``~/21cmFAST-cache``).
You can list all the files in your cache (and the parameters used in each of the simulations)
with::
$ 21cmfast query
To run an entire coeval cube, use the following as an example::
$ 21cmfast coeval 8.0 --out=output/coeval.h5 --HII_DIM=100
In this case all the intermediate steps are cached in the standard cache directory, and
the final ``Coeval`` box is saved to ``output/coeval.h5``. If no ``--out`` is specified,
the coeval box itself is not written, but don't worry -- all of its parts are cached, and
so it can be rebuilt extremely quickly. Every input parameter to any of the
`input classes <https://21cmfast.readthedocs.io/en/latest/reference/_autosummary/py21cmfast.inputs.html>`_
(there are a lot of parameters) can be specified at the end of the call with prefixes of
``--`` (like ``HII_DIM`` here). Alternatively, you can point to a config YAML file, eg.::
$ 21cmfast lightcone 8.0 --max-z=15.0 --out=. --config=~/.21cmfast/runconfig_example.yml
There is an example configuration file `here <user_data/runconfig_example.yml>`_ that you
can build from. All input parameters are
`documented here <https://21cmfast.readthedocs.io/en/latest/reference/_autosummary/py21cmfast.inputs.html>`_.
Documentation
=============
Full documentation (with examples, installation instructions and full API reference)
found at https://21cmfast.readthedocs.org.
Acknowledging
=============
If you use ``21cmFAST v3+`` in your research please cite both of:
Murray et al., (2020). 21cmFAST v3: A Python-integrated C code for generating 3D
realizations of the cosmic 21cm signal. Journal of Open Source Software, 5(54),
2582, https://doi.org/10.21105/joss.02582
Andrei Mesinger, Steven Furlanetto and Renyue Cen, "21CMFAST: a fast, seminumerical
simulation of the high-redshift 21-cm signal", Monthly Notices of the Royal
Astronomical Society, Volume 411, Issue 2, pp. 955-972 (2011),
https://ui.adsabs.harvard.edu/link_gateway/2011MNRAS.411..955M/doi:10.1111/j.1365-2966.2010.17731.x
In addition, the following papers introduce various features into ``21cmFAST``. If you use
these features, please cite the relevant papers.
Mini-halos:
Muñoz, J.B., Qin, Y., Mesinger, A., Murray, S., Greig, B., and Mason, C.,
"The Impact of the First Galaxies on Cosmic Dawn and Reionization"
https://arxiv.org/abs/2110.13919
(for DM-baryon relative velocities)
Qin, Y., Mesinger, A., Park, J., Greig, B., and Muñoz, J. B.,
“A tale of two sites - I. Inferring the properties of minihalo-hosted galaxies from
current observations”, Monthly Notices of the Royal Astronomical Society, vol. 495,
no. 1, pp. 123–140, 2020. https://doi.org/10.1093/mnras/staa1131.
(for Lyman-Werner and first implementation)
Mass-dependent ionizing efficiency:
Park, J., Mesinger, A., Greig, B., and Gillet, N.,
“Inferring the astrophysics of reionization and cosmic dawn from galaxy luminosity
functions and the 21-cm signal”, Monthly Notices of the Royal Astronomical Society,
vol. 484, no. 1, pp. 933–949, 2019. https://doi.org/10.1093/mnras/stz032.
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/README.rst | README.rst |
============
Contributing
============
Contributions are welcome, and they are greatly appreciated! Every
little bit helps, and credit will always be given.
Bug reports/Feature Requests/Feedback/Questions
===============================================
It is incredibly helpful to us when users report bugs, unexpected behaviour, or request
features. You can do the following:
* `Report a bug <https://github.com/21cmFAST/21cmFAST/issues/new?template=bug_report.md>`_
* `Request a Feature <https://github.com/21cmFAST/21cmFAST/issues/new?template=feature_request.md>`_
* `Ask a Question <https://github.com/21cmFAST/21cmFAST/issues/new?template=question.md>`_
When doing any of these, please try to be as succinct, but detailed, as possible, and use
a "Minimum Working Example" whenever applicable.
Documentation improvements
==========================
``21cmFAST`` could always use more documentation, whether as part of the
official ``21cmFAST`` docs, in docstrings, or even on the web in blog posts,
articles, and such. If you do the latter, take the time to let us know about it!
High-Level Steps for Development
================================
This is an abbreviated guide to getting started with development of ``21cmFAST``,
focusing on the discrete high-level steps to take. See our
`notes for developers <https://21cmfast.readthedocs.org/en/latest/notes_for_developers>`_
for more details about how to get around the ``21cmFAST`` codebase and other
technical details.
There are two avenues for you to develop ``21cmFAST``. If you plan on making significant
changes, and working with ``21cmFAST`` for a long period of time, please consider
becoming a member of the 21cmFAST GitHub organisation (by emailing any of the owners
or admins). You may develop as a member or as a non-member.
The difference between members and non-members only applies to the first step
of the development process.
Note that it is highly recommended to work in an isolated python environment with
all requirements installed from ``environment_dev.txt``. This will also ensure that
pre-commit hooks will run that enforce the ``black`` coding style. If you do not
install these requirements, you must manually run ``black`` before committing your changes,
otherwise your changes will likely fail continuous integration.
As a *member*:
1. Clone the repo::
git clone git@github.com:21cmFAST/21cmFAST.git
As a *non-member*:
1. First fork ``21cmFAST <https://github.com/21cmFAST/21cmFAST>``_
(look for the "Fork" button), then clone the fork locally::
git clone git@github.com:your_name_here/21cmFAST.git
The following steps are the same for both *members* and *non-members*:
2. Install a fresh new isolated environment::
conda create -n 21cmfast python=3
conda activate 21cmfast
3. Install the *development* requirements for the project::
conda env update -f environment_dev.yml
4. Install 21cmFAST. See `Installation <./installation.html>`_ for more details.::
pip install -e .
4. Install pre-commit hooks::
pre-commit install
5. Create a branch for local development::
git checkout -b name-of-your-bugfix-or-feature
Now you can make your changes locally. **Note: as a member, you _must_ do step 5. If you
make changes on master, you will _not_ be able to push them**.
6. When you're done making changes, run ``pytest`` to check that your changes didn't
break things. You can run a single test or subset of tests as well (see pytest docs)::
pytest
7. Commit your changes and push your branch to GitHub::
git add .
git commit -m "Your detailed description of your changes."
git push origin name-of-your-bugfix-or-feature
Note that if the commit step fails due to a pre-commit hook, *most likely* the act
of running the hook itself has already fixed the error. Try doing the ``add`` and
``commit`` again (up, up, enter). If it's still complaining, manually fix the errors
and do the same again.
8. Submit a pull request through the GitHub website.
Pull Request Guidelines
-----------------------
If you need some code review or feedback while you're developing the code just make the
pull request. You can mark the PR as a draft until you are happy for it to be merged.
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/CONTRIBUTING.rst | CONTRIBUTING.rst |
============
Installation
============
The easiest way to install ``21cmFAST`` is to use ``conda``. Simply use
``conda install -c conda-forge 21cmFAST``. With this method, all dependencies are taken
care of, and it should work on either Linux or MacOS. If for some reason this is not
possible for you, read on.
Dependencies
------------
We try to have as many of the dependencies automatically installed as possible.
However, since ``21cmFAST`` relies on some C libraries, this is not always possible.
The C libraries required are:
* ``gsl``
* ``fftw`` (compiled with floating-point enabled, and ``--enable-shared``)
* ``openmp``
* A C-compiler with compatibility with the ``-fopenmp`` flag. **Note:** it seems that on
OSX, if using ``gcc``, you will need ``v4.9.4+``.
As it turns out, though these are fairly common libraries, getting them installed in a
way that ``21cmFAST`` understands on various operating systems can be slightly non-trivial.
HPC
~~~
These libraries will often be available on a HPC environment by using the
``module load gsl`` and similar commands. Note that just because they are loaded
doesn't mean that ``21cmFAST`` will be able to find them. You may have to point to the
relevant ``lib/`` and ``include/`` folders for both ``gsl`` and ``fftw`` (these should
be available using ``module show gsl`` etc.)
Note also that while ``fftw`` may be available to load, it may not have the correct
compilation options (i.e. float-enabled and multiprocessing-enabled). In this case,
see below.
Linux
~~~~~
Most linux distros come with packages for the requirements, and also ``gcc`` by default,
which supports ``-fopenmp``. As long as these packages install into the standard location,
a standard installation of ``21cmFAST`` will be automatically possible (see below).
If they are installed to a place not on the ``LD_LIBRARY``/``INCLUDE`` paths, then you
must use the compilation options (see below) to specify where they are.
For example, you can check if the header file for ``fftw3`` is
in its default location ``/usr/include/`` by running::
cd /usr/include/
find fftw3.h
or::
locate fftw3.h
.. note:: there exists the option of installing ``gsl``, ``fftw`` and ``gcc`` using ``conda``.
This is discussed below in the context of MacOSX, where it is often the
easiest way to get the dependencies, but it is equally applicable to linux.
Ubuntu
^^^^^^
If you are installing 21cmFAST just as a user, the very simplest method is ``conda``
-- with this method you simply need ``conda install -c conda-forge 21cmFAST``, and all
dependencies will be automatically installed. However, if you are going to use
``pip`` to install the package directly from the repository, there is
a [bug in pip](https://stackoverflow.com/questions/71340058/conda-does-not-look-for-libpthread-and-libpthread-nonshared-at-the-right-place-w)
that means it cannot find conda-installed shared libraries properly. In that case, it is much
easier to install the basic dependencies (``gcc``, ``gsl`` and ``fftw3``) with your
system's package manager. ``gcc`` is by default available in Ubuntu.
To check if ``gcc`` is installed, run ``gcc --version`` in your terminal.
Install ``fftw3`` and ``gsl`` on your system with ``sudo apt-get install libfftw3-dev libgsl-dev``.
In your ``21cmfast`` environment, now install the ``21cmFAST`` package using::
cd /path/to/21cmFAST/
pip install .
If there is an issue during installation, add ``DEBUG=all`` or ``--DEBUG`` which may provide additional
information.
.. note:: If there is an error during compilation that the ``fftw3`` library cannot be found,
check where the ``fftw3`` library is actually located using ``locate libfftw3.so``.
For example, it may be located in ``/usr/lib/x86_64-linux-gnu/``. Then, provide this path
to the installation command with the ``LIB`` flag. For more details see the note in the
MacOSX section below.
.. note:: You may choose to install ``gsl`` as an anaconda package as well, however, in that case,
you need to add both ``INC`` paths in the installation command e.g.:
``GSL_INC=/path/to/conda/env/include FFTW_INC=/usr/include``
MacOSX
~~~~~~
On MacOSX, obtaining ``gsl`` and ``fftw`` is typically more difficult, and in addition,
the newer native ``clang`` does not offer ``-fopenmp`` support.
For ``conda`` users (which we recommend using), the easiest way to get ``gsl`` and ``fftw``
is by doing ``conda install -c conda-forge gsl fftw`` in your environment.
.. note:: if you use ``conda`` to install ``gsl`` and ``fftw``, then you will need to point at
their location when installing `21cmFAST` (see compiler options below for details).
In this case, the installation command should simply be *prepended* with::
LIB=/path/to/conda/env/lib INC=/path/to/conda/env/include
To get ``gcc``, either use ``homebrew``, or again, ``conda``: ``conda install -c anaconda gcc``.
If you get the ``conda`` version, you still need to install the headers::
xcode-select --install
On older versions then you need to do::
open /Library/Developer/CommandLineTools/Packages/macOS_SDK_headers_for_macOS_<input version>.pkg
.. note:: some versions of MacOS will also require you to point to the correct gcc
compiler using the ``CC`` environment variable. Overall, the point is to NOT
use ``clang``. If ``gcc --version`` shows that it is actually GCC, then you
can set ``CC=gcc``. If you use homebrew to install ``gcc``, it is likely that
you'll have to set ``CC=gcc-11``.
For newer versions, you may need to prepend the following command to your ``pip install`` command
when installing ``21cmFAST`` (see later instructions)::
CFLAGS="-isysroot /Library/Developer/CommandLineTools/SDKs/MacOSX<input version>.sdk"
See `<faqs/installation_faq>`_ for more detailed questions on installation.
If you are on MacOSX and are having trouble with installation (or would like to share
a successful installation strategy!) please see the
`open issue <https://github.com/21cmfast/21cmFAST/issues/84>`_.
With the dependencies installed, follow the instructions below,
depending on whether you are a user or a developer.
For Users
---------
.. note:: ``conda`` users may want to pre-install the following packages before running
the below installation commands::
conda install numpy scipy click pyyaml cffi astropy h5py
Then, at the command line::
pip install git+https://github.com/21cmFAST/21cmFAST.git
If developing, from the top-level directory do::
pip install -e .
Note the compile options discussed below!
For Developers
--------------
If you are developing ``21cmFAST``, we highly recommend using ``conda`` to manage your
environment, and setting up an isolated environment. If this is the case, setting up
a full environment (with all testing and documentation dependencies) should be as easy
as (from top-level dir)::
conda env create -f environment_dev.yml
Otherwise, if you are using ``pip``::
pip install -e .[dev]
The ``[dev]`` "extra" here installs all development dependencies. You can instead use
``[tests]`` if you only want dependencies for testing, or ``[docs]`` to be able to
compile the documentation.
Compile Options
---------------
Various options exist to manage compilation via environment variables. Basically,
any variable with "INC" in its name will add to the includes directories, while
any variable with "lib" in its name will add to the directories searched for
libraries. To change the C compiler, use ``CC``. Finally, if you want to compile
the C-library in dev mode (so you can do stuff like valgrid and gdb with it),
install with DEBUG=True. So for example::
CC=/usr/bin/gcc DEBUG=True GSL_LIB=/opt/local/lib FFTW_INC=/usr/local/include pip install -e .
.. note:: For MacOS a typical installation command will look like
``CC=gcc CFLAGS="-isysroot /Library/Developer/CommandLineTools/SDKs/MacOSX<input version>.sdk" pip install .``
(using either ``gcc`` or ``gcc-11`` depending on how you installed gcc), with
other compile options possible as well.
In addition, the ``BOXDIR`` variable specifies the *default* directory that any
data produced by 21cmFAST will be cached. This value can be updated at any time by
changing it in the ``$CFGDIR/config.yml`` file, and can be overwritten on a
per-call basis.
While the ``-e`` option will keep your library up-to-date with any (Python)
changes, this will *not* work when changing the C extension. If the C code
changes, you need to manually run ``rm -rf build/*`` then re-install as above.
Logging in C-Code
~~~~~~~~~~~~~~~~~
By default, the C-code will only print to stderr when it encounters warnings or
critical errors. However, there exist several levels of logging output that can be
switched on, but only at compilation time. To enable these, use the following::
LOG_LEVEL=<log_level> pip install -e .
The ``<log_level>`` can be any non-negative integer, or one of the following
(case-insensitive) identifiers::
NONE, ERROR, WARNING, INFO, DEBUG, SUPER_DEBUG, ULTRA_DEBUG
If an integer is passed, it corresponds to the above levels in order (starting
from zero). Be careful if the level is set to 0 (or NONE), as useful error
and warning messages will not be printed. By default, the log level is 2 (or
WARNING), unless the DEBUG=1 environment variable is set, in which case the
default is 4 (or DEBUG). Using very high levels (eg. ULTRA_DEBUG) can print out
*a lot* of information and make the run time much longer, but may be useful
in some specific cases.
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/INSTALLATION.rst | INSTALLATION.rst |
Changelog
=========
dev-version
-----------
v3.3.1 [24 May 2023]
----------------------
Fixed
~~~~~
* Compilation of C code for some compilers (#330)
v3.3.0 [17 May 2023]
----------------------
Internals
~~~~~~~~~
* Refactored setting up of inputs to high-level functions so that there is less code
repetition.
Fixed
~~~~~
* Running with ``R_BUBBLE_MAX`` too large auto-fixes it to be ``BOX_LEN`` (#112)
* Bug in calling ``clear_cache``.
* Inconsistency in the way that the very highest redshift of an evolution is handled
between low-level code (eg. ``spin_temperature()``) and high-level code (eg. ``run_coeval()``).
Added
~~~~~
* New ``validate_all_inputs`` function that cross-references the four main input structs
and ensures all the parameters make sense together. Mostly for internal use.
* Ability to save/read directly from an open HDF5 File (#170)
* An implementation of cloud-in-cell to more accurately redistribute the perturbed mass
across all neighbouring cells instead of the previous nearest cell approach
* Changed PhotonConsEndCalibz from z = 5 -> z = 3.5 to handle later reionisation
scenarios in line with current observations (#305)
* Add in an initialisation check for the photon conservation to address some issues
arising for early EOR histories (#311)
* Added ``NON_CUBIC_FACTOR`` to ``UserParams`` to allow for non-cubic coeval boxes (#289)
v3.2.1 [13 Sep 2022]
----------------------
Changed
~~~~~~~
* Included log10_mturnovers(_mini) in lightcone class. Only useful when USE_MINI_HALOS
v3.2.0 [11 Jul 2022]
----------------------
Changed
~~~~~~~
* Floats are now represented to a specific number of significant digits in the hash of
an output object. This fixes problems with very close redshifts not being read from
cache (#80). Note that this means that very close astro/cosmo params will now be read
from cache. This could cause issues when creating large databases with many random
parameters. The behaviour can modified in the configuration by setting the
``cache_param_sigfigs`` and ``cache_redshift_sigfigs`` parameters (these are 6 and
4 by default, respectively).
**NOTE**: updating to this version will cause your previous cached files to become
unusable. Remove them before updating.
Fixed
~~~~~
* Added a missing C-based error to the known errors in Python.
v3.1.5 [27 Apr 2022]
----------------------
v3.1.4 [10 Feb 2022]
----------------------
Fixed
~~~~~
* error in FFT normalization in FindHaloes
* docs not compiling on RTD due to missing ``scipy.integrate`` mock module
* Updated matplotlib removed support for setting vmin/vmax and norm. Now passes vmin/vmax
to the norm() constructor.
v3.1.3 [27 Oct 2021]
----------------------
* Fixed ``FAST_FCOLL_TABLES`` so it only affects MCGs and not ACGs. Added tests of this
flag for high and low z separately.
v3.1.2 [14 Jul 2021]
----------------------
Internals
~~~~~~~~~
* ``MINIMIZE_MEMORY`` flag significantly reduces memory without affecting performance much,
by changing the way some arrays are allocated and accessed in C. (#224)
Change
~~~~~~
* Updated ``USE_INTERPOLATION_TABLES`` to be default True. This makes much more sense as
a default value. Until v4, a warning will be raised if it is not set explicitly.
v3.1.1 [13 Jun 2021]
----------------------
Fixed
~~~~~
* Bug in deployment to PyPI.
v3.1.0 [13 Jun 2021]
----------------------
Added
~~~~~
* Ability to access all evolutionary Coeval components, either from the end Coeval
class, or the Lightcone.
* Ability to gather all evolutionary antecedents from a Coeval/Lightcone into the one
file.
* ``FAST_FCOLL_TABLES`` in ``UserParams`` which improves speeds quite significantly for
~<10% accuracy decrease.
* Fast and low-memory generation of relative-velocity (vcb) initial conditions. Eliminated hi-res vcb boxes, as they are never needed.
* Also output the mean free path (i.e. MFP_box in IonizedBox).
* Added the effect of DM-baryon relative velocities on PopIII-forming minihaloes. This now provides the correct background evolution jointly with LW feedback. It gives rise to velocity-induced acoustic oscillations (VAOs) from the relative-velocity fluctuations. We also follow a more flexible parametrization for LW feedback in minihaloes, following new simulation results, and add a new index ALPHA_STAR_MINI for minihaloes, now independent of regular ACGs.
* New ``hooks`` keyword to high-level functions, that are run on the completion of each computational step, and can
be used to more generically write parts of the data to file.
* Ability to pass a function to ``write=`` to write more specific aspects of the data (internally, this will be put into the ``hooks`` dictionary).
* ``run_lightcone`` and ``run_coeval`` use significantly less memory by offloading initial conditions and perturb_field instances to disk if possible.
Fixed
~~~~~
* Bug in 2LPT when ``USE_RELATIVE_VELOCITIES=True`` [Issue #191, PR #192]
* Error raised when redshifts are not in ascending order [Issue #176, PR #177]
* Errors when ``USE_FFTW_WISDOM`` is used on some systems [Issue #174, PR #199]
* Bug in ComputeIonizedBox causing negative recombination rate and ring structure in ``Gamma12_box`` [Issue #194, PR #210]
* Error in determining the wisdom file name [Issue #209, PR#210]
* Bug in which cached C-based memory would be read in and free'd twice.
Internals
~~~~~~~~~
* Added ``dft.c``, which makes doing all the cubic FFTs a lot easier and more consistent. [PR #199]
* More generic way of keeping track of arrays to be passed between C and Python, and their shape in Python, using ``_get_box_structures``.
This also means that the various boxes can be queried before they are initialized and computed.
* More stringent integration tests that test each array, not just the final brightness temperature.
* Ability to plot the integration test data to more easily identify where things have gone wrong (use ``--plots`` in the ``pytest`` invocation).
* Nicer CLI interface for ``produce_integration_test_data.py``. New options to ``clean`` the ``test_data/`` directory,
and also test data is saved by user-defined key rather than massive string of variables.
* Nicer debug statements before calls to C, for easily comparing between versions.
* Much nicer methods of keeping track of array state (in memory, on disk, c-controlled, etc.)
* Ability to free C-based pointers in a more granular way.
v3.0.3
------
Added
~~~~~
* ``coeval_callback`` and ``coeval_callback_redshifts`` flags to the ``run_lightcone``.
Gives the ability to run arbitrary code on ``Coeval`` boxes.
* JOSS paper!
* ``get_fields`` classmethod on all output classes, so that one can easily figure out
what fields are computed (and available) for that class.
Fixed
~~~~~
* Only raise error on non-available ``external_table_path`` when actually going to use it.
v3.0.2
------
Fixed
-----
* Added prototype functions to enable compilation for some standard compilers on MacOS.
v3.0.1
------
Modifications to the internal code structure of 21cmFAST
Added
~~~~~
* Refactor FFTW wisdom creation to be a python callable function
v3.0.0
------
Complete overhaul of 21cmFAST, including a robust python-wrapper and interface,
caching mechanisms, and public repository with continuous integration. Changes
and equations for minihalo features in this version are found in
https://arxiv.org/abs/2003.04442
All functionality of the original 21cmFAST v2 C-code has been implemented in this
version, including ``USE_HALO_FIELD`` and performing full integration instead of using
the interpolation tables (which are faster).
Added
~~~~~
* Updated the radiation source model: (i) all radiation fields including X-rays, UV
ionizing, Lyman Werner and Lyman alpha are considered from two seperated population
namely atomic-cooling (ACGs) and minihalo-hosted molecular-cooling galaxies (MCGs);
(ii) the turn-over masses of ACGs and MCGs are estimated with cooling efficiency and
feedback from reionization and lyman werner suppression (Qin et al. 2020). This can
be switched on using new ``flag_options`` ``USE_MINI_HALOS``.
* Updated kinetic temperature of the IGM with fully ionized cells following equation 6
of McQuinn (2015) and partially ionized cells having the volume-weightied temperature
between the ionized (volume: 1-xHI; temperature T_RE ) and neutral components (volume:
xHI; temperature: temperature of HI). This is stored in IonizedBox as
temp_kinetic_all_gas. Note that Tk in TsBox remains to be the kinetic temperature of HI.
* Tests: many unit tests, and also some regression tests.
* CLI: run 21cmFAST boxes from the command line, query the cache database, and produce
plots for standard comparison runs.
* Documentation: Jupyter notebook demos and tutorials, FAQs, installation instructions.
* Plotting routines: a number of general plotting routines designed to plot coeval
and lightcone slices.
* New power spectrum option (``POWER_SPECTRUM=5``) that uses a CLASS-based transfer
function. WARNING: If POWER_SPECTRUM==5 the cosmo parameters cannot be altered, they
are set to the Planck2018 best-fit values for now (until CLASS is added):
(omegab=0.02237, omegac= 0.120, hubble=0.6736 (the rest are irrelevant for the
transfer functions, but in case: A_s=2.100e-9, n_s=0.9649, z_reio = 11.357)
* New ``user_params`` option ``USE_RELATIVE_VELOCITIES``, which produces initial relative
velocity cubes (option implemented, but not the actual computation yet).
* Configuration management.
* global params now has a context manager for changing parameters temporarily.
* Vastly improved error handling: exceptions can be caught in C code and propagated to
Python to inform the user of what's going wrong.
* Ability to write high-level data (``Coeval`` and ``Lightcone`` objects) directly to
file in a simple portable format.
Changed
~~~~~~~
* ``POWER_SPECTRUM`` option moved from ``global_params`` to ``user_params``.
* Default cosmology updated to Planck18.
v2.0.0
------
All changes and equations for this version are found in https://arxiv.org/abs/1809.08995.
Changed
~~~~~~~
* Updated the ionizing source model: (i) the star formation rates and ionizing escape
fraction are scaled with the masses of dark matter halos and (ii) the abundance of
active star forming galaxies is exponentially suppressed below the turn-over halo
mass, M_{turn}, according to a duty cycle of exp(−M_{turn}/M_{h}), where M_{h} is a
halo mass.
* Removed the mean free path parameter, R_{mfp}. Instead, directly computes
inhomogeneous, sub-grid recombinations in the intergalactic medium following the
approach of Sobacchi & Mesinger (2014)
v1.2.0
------
Added
~~~~~
* Support for a halo mass dependent ionizing efficiency: zeta = zeta_0 (M/Mmin)^alpha,
where zeta_0 corresponds to HII_EFF_FACTOR, Mmin --> ION_M_MIN,
alpha --> EFF_FACTOR_PL_INDEX in ANAL_PARAMS.H
v1.12.0
-------
Added
~~~~~
- Code 'redshift_interpolate_boxes.c' to interpolate between comoving cubes,
creating comoving light cone boxes.
- Enabled openMP threading for SMP machines. You can specify the number of threads
(for best performace, do not exceed the number of processors) in INIT_PARAMS.H. You do
not need to have an SMP machine to run the code. NOTE: YOU SHOULD RE-INSTALL FFTW to
use openMP (see INSTALL file)
- Included a threaded driver file 'drive_zscroll_reion_param.c' set-up to perform
astrophysical parameter studies of reionization
- Included explicit support for WDM cosmologies; see COSMOLOGY.H. The prescription is
similar to that discussed in Barkana+2001; Mesinger+2005, madifying the (i) transfer
function (according to the Bode+2001 formula; and (ii) including the effective
pressure term of WDM using a Jeans mass analogy. (ii) is approximated with a sharp
cuttoff in the EPS barrier, using 60* M_J found in Barkana+2001 (the 60 is an
adjustment factor found by fitting to the WDM collapsed fraction).
- A Gaussian filtering step of the PT fields to perturb_field.c, in addition to the
implicit boxcar smoothing. This avoids having"empty" density cells, i.e. \delta=-1,
with some small loss in resolution. Although for most uses \delta=-1 is ok, some Lya
forest statistics do not like it.
- Added treatment of the risidual electron fraction from X-ray heating when computing
the ionization field. Relatedly, modified Ts.c to output all intermediate evolution
boxes, Tk and x_e.
- Added a missing factor of Omega_b in Ts.c corresponding to eq. 18 in MFC11. Users who
used a previous version should note that their results just effecively correspond to a
higher effective X-ray efficiency, scaled by 1/Omega_baryon.
- Normalization optimization to Ts.c, increasing performace on arge resolution boxes
Fixed
~~~~~
- GSL interpolation error in kappa_elec_pH for GSL versions > 1.15
- Typo in macro definition, which impacted the Lya background calculation in v1.11 (not applicable to earlier releases)
- Outdated filename sytax when calling gen_size_distr in drive_xHIscroll
- Redshift scrolling so that drive_logZscroll_Ts.c and Ts.c are in sync.
Changed
~~~~~~~
- Output format to avoid FFT padding for all boxes
- Filename conventions to be more explicit.
- Small changes to organization and structure
v1.1.0
------
Added
~~~~~
- Wrapper functions mod_fwrite() and mod_fread() in Cosmo_c_progs/misc.c, which
should fix problems with the library fwrite() and fread() for large files (>4GB) on
certain operating systems.
- Included print_power_spectrum_ICs.c program which reads in high resolution initial
conditions and prints out an ASCII file with the associated power spectrum.
- Parameter in Ts.c for the maximum allowed kinetic temperature, which increases
stability of the code when the redshift step size and the X-ray efficiencies are large.
Fixed
~~~~~
- Oversight adding support for a Gaussian filter for the lower resolution field.
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/CHANGELOG.rst | CHANGELOG.rst |
import os
from cffi import FFI
ffi = FFI()
LOCATION = os.path.dirname(os.path.abspath(__file__))
CLOC = os.path.join(LOCATION, "src", "py21cmfast", "src")
include_dirs = [CLOC]
# =================================================================
# Set compilation arguments dependent on environment... a bit buggy
# =================================================================
if "DEBUG" in os.environ:
extra_compile_args = ["-fopenmp", "-w", "-g", "-O0", "--verbose"]
else:
extra_compile_args = ["-fopenmp", "-Ofast", "-w", "--verbose"]
# Set the C-code logging level.
# If DEBUG is set, we default to the highest level, but if not,
# we set it to the level just above no logging at all.
log_level = os.environ.get("LOG_LEVEL", 4 if "DEBUG" in os.environ else 1)
available_levels = [
"NONE",
"ERROR",
"WARNING",
"INFO",
"DEBUG",
"SUPER_DEBUG",
"ULTRA_DEBUG",
]
if isinstance(log_level, str) and log_level.upper() in available_levels:
log_level = available_levels.index(log_level.upper())
try:
log_level = int(log_level)
except ValueError:
# note: for py35 support, can't use f strings.
raise ValueError(
"LOG_LEVEL must be specified as a positive integer, or one "
"of {}".format(available_levels)
)
library_dirs = []
for k, v in os.environ.items():
if "inc" in k.lower():
include_dirs += [v]
elif "lib" in k.lower():
library_dirs += [v]
# =================================================================
# This is the overall C code.
ffi.set_source(
"py21cmfast.c_21cmfast", # Name/Location of shared library module
"""
#define LOG_LEVEL {log_level}
#include "GenerateICs.c"
""".format(
log_level=log_level
),
include_dirs=include_dirs,
library_dirs=library_dirs,
libraries=["m", "gsl", "gslcblas", "fftw3f_omp", "fftw3f"],
extra_compile_args=extra_compile_args,
)
# This is the Header file
with open(os.path.join(CLOC, "21cmFAST.h")) as f:
ffi.cdef(f.read())
with open(os.path.join(CLOC, "Globals.h")) as f:
ffi.cdef(f.read())
if __name__ == "__main__":
ffi.compile() | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/build_cffi.py | build_cffi.py |
from __future__ import annotations
import logging
import numpy as np
import os
import warnings
from astropy import units
from astropy.cosmology import z_at_value
from copy import deepcopy
from scipy.interpolate import interp1d
from typing import Any, Callable, Sequence
from ._cfg import config
from ._utils import OutputStruct, _check_compatible_inputs, _process_exitcode
from .c_21cmfast import ffi, lib
from .inputs import (
AstroParams,
CosmoParams,
FlagOptions,
UserParams,
global_params,
validate_all_inputs,
)
from .outputs import (
BrightnessTemp,
Coeval,
HaloField,
InitialConditions,
IonizedBox,
LightCone,
PerturbedField,
PerturbHaloField,
TsBox,
_OutputStructZ,
)
logger = logging.getLogger(__name__)
def _configure_inputs(
defaults: list,
*datasets,
ignore: list = ["redshift"],
flag_none: list | None = None,
):
"""Configure a set of input parameter structs.
This is useful for basing parameters on a previous output.
The logic is this: the struct _cannot_ be present and different in both defaults and
a dataset. If it is present in _either_ of them, that will be returned. If it is
present in _neither_, either an error will be raised (if that item is in `flag_none`)
or it will pass.
Parameters
----------
defaults : list of 2-tuples
Each tuple is (key, val). Keys are input struct names, and values are a default
structure for that input.
datasets : list of :class:`~_utils.OutputStruct`
A number of output datasets to cross-check, and draw parameter values from.
ignore : list of str
Attributes to ignore when ensuring that parameter inputs are the same.
flag_none : list
A list of parameter names for which ``None`` is not an acceptable value.
Raises
------
ValueError :
If an input parameter is present in both defaults and the dataset, and is different.
OR if the parameter is present in neither defaults not the datasets, and it is
included in `flag_none`.
"""
# First ensure all inputs are compatible in their parameters
_check_compatible_inputs(*datasets, ignore=ignore)
if flag_none is None:
flag_none = []
output = [0] * len(defaults)
for i, (key, val) in enumerate(defaults):
# Get the value of this input from the datasets
data_val = None
for dataset in datasets:
if dataset is not None and hasattr(dataset, key):
data_val = getattr(dataset, key)
break
# If both data and default have values
if not (val is None or data_val is None or data_val == val):
raise ValueError(
"%s has an inconsistent value with %s"
% (key, dataset.__class__.__name__)
)
else:
if val is not None:
output[i] = val
elif data_val is not None:
output[i] = data_val
elif key in flag_none:
raise ValueError(
"For %s, a value must be provided in some manner" % key
)
else:
output[i] = None
return output
def configure_redshift(redshift, *structs):
"""
Check and obtain a redshift from given default and structs.
Parameters
----------
redshift : float
The default redshift to use
structs : list of :class:`~_utils.OutputStruct`
A number of output datasets from which to find the redshift.
Raises
------
ValueError :
If both `redshift` and *all* structs have a value of `None`, **or** if any of them
are different from each other (and not `None`).
"""
zs = {s.redshift for s in structs if s is not None and hasattr(s, "redshift")}
zs = list(zs)
if len(zs) > 1 or (len(zs) == 1 and redshift is not None and zs[0] != redshift):
raise ValueError("Incompatible redshifts in inputs")
elif len(zs) == 1:
return zs[0]
elif redshift is None:
raise ValueError(
"Either redshift must be provided, or a data set containing it."
)
else:
return redshift
def _verify_types(**kwargs):
"""Ensure each argument has a type of None or that matching its name."""
for k, v in kwargs.items():
for j, kk in enumerate(
["init", "perturb", "ionize", "spin_temp", "halo_field", "pt_halos"]
):
if kk in k:
break
cls = [
InitialConditions,
PerturbedField,
IonizedBox,
TsBox,
HaloField,
PerturbHaloField,
][j]
if v is not None and not isinstance(v, cls):
raise ValueError(f"{k} must be an instance of {cls.__name__}")
def _setup_inputs(
input_params: dict[str, Any],
input_boxes: dict[str, OutputStruct] | None = None,
redshift=-1,
):
"""
Verify and set up input parameters to any function that runs C code.
Parameters
----------
input_boxes
A dictionary of OutputStruct objects that are meant as inputs to the current
calculation. These will be verified against each other, and also used to
determine redshift, if appropriate.
input_params
A dictionary of keys and dicts / input structs. This should have the random
seed, cosmo/user params and optionally the flag and astro params.
redshift
Optional value of the redshift. Can be None. If not provided, no redshift is
returned.
Returns
-------
random_seed
The random seed to use, determined from either explicit input or input boxes.
input_params
The configured input parameter structs, in the order in which they were given.
redshift
If redshift is given, it will also be output.
"""
input_boxes = input_boxes or {}
if "flag_options" in input_params and "user_params" not in input_params:
raise ValueError("To set flag_options requires user_params")
if "astro_params" in input_params and "flag_options" not in input_params:
raise ValueError("To set astro_params requires flag_options")
if input_boxes:
_verify_types(**input_boxes)
params = _configure_inputs(list(input_params.items()), *list(input_boxes.values()))
if redshift != -1:
redshift = configure_redshift(
redshift,
*[
v
for k, v in input_boxes.items()
if hasattr(v, "redshift") and "prev" not in k
],
)
# This turns params into a dict with all the input parameters in it.
params = dict(zip(input_params.keys(), params))
params["user_params"] = UserParams(params["user_params"])
params["cosmo_params"] = CosmoParams(params["cosmo_params"])
if "flag_options" in params:
params["flag_options"] = FlagOptions(
params["flag_options"],
USE_VELS_AUX=params["user_params"].USE_RELATIVE_VELOCITIES,
)
if "astro_params" in params:
params["astro_params"] = AstroParams(
params["astro_params"], INHOMO_RECO=params["flag_options"].INHOMO_RECO
)
# Perform validation between different sets of inputs.
validate_all_inputs(**{k: v for k, v in params.items() if k != "random_seed"})
# Sort the params back into input order.
params = [params[k] for k in input_params]
out = params
if redshift != -1:
out.append(redshift)
return out
def _call_c_simple(fnc, *args):
"""Call a simple C function that just returns an object.
Any such function should be defined such that the last argument is an int pointer generating
the status.
"""
# Parse the function to get the type of the last argument
cdata = str(ffi.addressof(lib, fnc.__name__))
kind = cdata.split("(")[-1].split(")")[0].split(",")[-1]
result = ffi.new(kind)
status = fnc(*args, result)
_process_exitcode(status, fnc, args)
return result[0]
def _get_config_options(
direc, regenerate, write, hooks
) -> tuple[str, bool, dict[Callable, dict[str, Any]]]:
direc = str(os.path.expanduser(config["direc"] if direc is None else direc))
if hooks is None or len(hooks) > 0:
hooks = hooks or {}
if callable(write) and write not in hooks:
hooks[write] = {"direc": direc}
if not hooks:
if write is None:
write = config["write"]
if not callable(write) and write:
hooks["write"] = {"direc": direc}
return (
direc,
bool(config["regenerate"] if regenerate is None else regenerate),
hooks,
)
def get_all_fieldnames(
arrays_only=True, lightcone_only=False, as_dict=False
) -> dict[str, str] | set[str]:
"""Return all possible fieldnames in output structs.
Parameters
----------
arrays_only : bool, optional
Whether to only return fields that are arrays.
lightcone_only : bool, optional
Whether to only return fields from classes that evolve with redshift.
as_dict : bool, optional
Whether to return results as a dictionary of ``quantity: class_name``.
Otherwise returns a set of quantities.
"""
classes = [cls(redshift=0) for cls in _OutputStructZ._implementations()]
if not lightcone_only:
classes.append(InitialConditions())
attr = "pointer_fields" if arrays_only else "fieldnames"
if as_dict:
return {
name: cls.__class__.__name__
for cls in classes
for name in getattr(cls, attr)
}
else:
return {name for cls in classes for name in getattr(cls, attr)}
# ======================================================================================
# WRAPPING FUNCTIONS
# ======================================================================================
def construct_fftw_wisdoms(*, user_params=None, cosmo_params=None):
"""Construct all necessary FFTW wisdoms.
Parameters
----------
user_params : :class:`~inputs.UserParams`
Parameters defining the simulation run.
"""
user_params = UserParams(user_params)
cosmo_params = CosmoParams(cosmo_params)
# Run the C code
if user_params.USE_FFTW_WISDOM:
return lib.CreateFFTWWisdoms(user_params(), cosmo_params())
else:
return 0
def compute_tau(*, redshifts, global_xHI, user_params=None, cosmo_params=None):
"""Compute the optical depth to reionization under the given model.
Parameters
----------
redshifts : array-like
Redshifts defining an evolution of the neutral fraction.
global_xHI : array-like
The mean neutral fraction at `redshifts`.
user_params : :class:`~inputs.UserParams`
Parameters defining the simulation run.
cosmo_params : :class:`~inputs.CosmoParams`
Cosmological parameters.
Returns
-------
tau : float
The optional depth to reionization
Raises
------
ValueError :
If `redshifts` and `global_xHI` have inconsistent length or if redshifts are not
in ascending order.
"""
user_params, cosmo_params = _setup_inputs(
{"user_params": user_params, "cosmo_params": cosmo_params}
)
if len(redshifts) != len(global_xHI):
raise ValueError("redshifts and global_xHI must have same length")
if not np.all(np.diff(redshifts) > 0):
raise ValueError("redshifts and global_xHI must be in ascending order")
# Convert the data to the right type
redshifts = np.array(redshifts, dtype="float32")
global_xHI = np.array(global_xHI, dtype="float32")
z = ffi.cast("float *", ffi.from_buffer(redshifts))
xHI = ffi.cast("float *", ffi.from_buffer(global_xHI))
# Run the C code
return lib.ComputeTau(user_params(), cosmo_params(), len(redshifts), z, xHI)
def compute_luminosity_function(
*,
redshifts,
user_params=None,
cosmo_params=None,
astro_params=None,
flag_options=None,
nbins=100,
mturnovers=None,
mturnovers_mini=None,
component=0,
):
"""Compute a the luminosity function over a given number of bins and redshifts.
Parameters
----------
redshifts : array-like
The redshifts at which to compute the luminosity function.
user_params : :class:`~UserParams`, optional
Defines the overall options and parameters of the run.
cosmo_params : :class:`~CosmoParams`, optional
Defines the cosmological parameters used to compute initial conditions.
astro_params : :class:`~AstroParams`, optional
The astrophysical parameters defining the course of reionization.
flag_options : :class:`~FlagOptions`, optional
Some options passed to the reionization routine.
nbins : int, optional
The number of luminosity bins to produce for the luminosity function.
mturnovers : array-like, optional
The turnover mass at each redshift for massive halos (ACGs).
Only required when USE_MINI_HALOS is True.
mturnovers_mini : array-like, optional
The turnover mass at each redshift for minihalos (MCGs).
Only required when USE_MINI_HALOS is True.
component : int, optional
The component of the LF to be calculated. 0, 1 an 2 are for the total,
ACG and MCG LFs respectively, requiring inputs of both mturnovers and
mturnovers_MINI (0), only mturnovers (1) or mturnovers_MINI (2).
Returns
-------
Muvfunc : np.ndarray
Magnitude array (i.e. brightness). Shape [nredshifts, nbins]
Mhfunc : np.ndarray
Halo mass array. Shape [nredshifts, nbins]
lfunc : np.ndarray
Number density of haloes corresponding to each bin defined by `Muvfunc`.
Shape [nredshifts, nbins].
"""
user_params, cosmo_params, astro_params, flag_options = _setup_inputs(
{
"user_params": user_params,
"cosmo_params": cosmo_params,
"astro_params": astro_params,
"flag_options": flag_options,
}
)
redshifts = np.array(redshifts, dtype="float32")
if flag_options.USE_MINI_HALOS:
if component in [0, 1]:
if mturnovers is None:
logger.warning(
"calculating ACG LFs with mini-halo feature requires users to "
"specify mturnovers!"
)
return None, None, None
mturnovers = np.array(mturnovers, dtype="float32")
if len(mturnovers) != len(redshifts):
logger.warning(
"mturnovers(%d) does not match the length of redshifts (%d)"
% (len(mturnovers), len(redshifts))
)
return None, None, None
if component in [0, 2]:
if mturnovers_mini is None:
logger.warning(
"calculating MCG LFs with mini-halo feature requires users to "
"specify mturnovers_MINI!"
)
return None, None, None
mturnovers_mini = np.array(mturnovers_mini, dtype="float32")
if len(mturnovers_mini) != len(redshifts):
logger.warning(
"mturnovers_MINI(%d) does not match the length of redshifts (%d)"
% (len(mturnovers), len(redshifts))
)
return None, None, None
else:
mturnovers = (
np.zeros(len(redshifts), dtype="float32") + 10**astro_params.M_TURN
)
component = 1
if component == 0:
lfunc = np.zeros(len(redshifts) * nbins)
Muvfunc = np.zeros(len(redshifts) * nbins)
Mhfunc = np.zeros(len(redshifts) * nbins)
lfunc.shape = (len(redshifts), nbins)
Muvfunc.shape = (len(redshifts), nbins)
Mhfunc.shape = (len(redshifts), nbins)
c_Muvfunc = ffi.cast("double *", ffi.from_buffer(Muvfunc))
c_Mhfunc = ffi.cast("double *", ffi.from_buffer(Mhfunc))
c_lfunc = ffi.cast("double *", ffi.from_buffer(lfunc))
# Run the C code
errcode = lib.ComputeLF(
nbins,
user_params(),
cosmo_params(),
astro_params(),
flag_options(),
1,
len(redshifts),
ffi.cast("float *", ffi.from_buffer(redshifts)),
ffi.cast("float *", ffi.from_buffer(mturnovers)),
c_Muvfunc,
c_Mhfunc,
c_lfunc,
)
_process_exitcode(
errcode,
lib.ComputeLF,
(
nbins,
user_params,
cosmo_params,
astro_params,
flag_options,
1,
len(redshifts),
),
)
lfunc_MINI = np.zeros(len(redshifts) * nbins)
Muvfunc_MINI = np.zeros(len(redshifts) * nbins)
Mhfunc_MINI = np.zeros(len(redshifts) * nbins)
lfunc_MINI.shape = (len(redshifts), nbins)
Muvfunc_MINI.shape = (len(redshifts), nbins)
Mhfunc_MINI.shape = (len(redshifts), nbins)
c_Muvfunc_MINI = ffi.cast("double *", ffi.from_buffer(Muvfunc_MINI))
c_Mhfunc_MINI = ffi.cast("double *", ffi.from_buffer(Mhfunc_MINI))
c_lfunc_MINI = ffi.cast("double *", ffi.from_buffer(lfunc_MINI))
# Run the C code
errcode = lib.ComputeLF(
nbins,
user_params(),
cosmo_params(),
astro_params(),
flag_options(),
2,
len(redshifts),
ffi.cast("float *", ffi.from_buffer(redshifts)),
ffi.cast("float *", ffi.from_buffer(mturnovers_mini)),
c_Muvfunc_MINI,
c_Mhfunc_MINI,
c_lfunc_MINI,
)
_process_exitcode(
errcode,
lib.ComputeLF,
(
nbins,
user_params,
cosmo_params,
astro_params,
flag_options,
2,
len(redshifts),
),
)
# redo the Muv range using the faintest (most likely MINI) and the brightest (most likely massive)
lfunc_all = np.zeros(len(redshifts) * nbins)
Muvfunc_all = np.zeros(len(redshifts) * nbins)
Mhfunc_all = np.zeros(len(redshifts) * nbins * 2)
lfunc_all.shape = (len(redshifts), nbins)
Muvfunc_all.shape = (len(redshifts), nbins)
Mhfunc_all.shape = (len(redshifts), nbins, 2)
for iz in range(len(redshifts)):
Muvfunc_all[iz] = np.linspace(
np.min([Muvfunc.min(), Muvfunc_MINI.min()]),
np.max([Muvfunc.max(), Muvfunc_MINI.max()]),
nbins,
)
lfunc_all[iz] = np.log10(
10
** (
interp1d(Muvfunc[iz], lfunc[iz], fill_value="extrapolate")(
Muvfunc_all[iz]
)
)
+ 10
** (
interp1d(
Muvfunc_MINI[iz], lfunc_MINI[iz], fill_value="extrapolate"
)(Muvfunc_all[iz])
)
)
Mhfunc_all[iz] = np.array(
[
interp1d(Muvfunc[iz], Mhfunc[iz], fill_value="extrapolate")(
Muvfunc_all[iz]
),
interp1d(
Muvfunc_MINI[iz], Mhfunc_MINI[iz], fill_value="extrapolate"
)(Muvfunc_all[iz]),
],
).T
lfunc_all[lfunc_all <= -30] = np.nan
return Muvfunc_all, Mhfunc_all, lfunc_all
elif component == 1:
lfunc = np.zeros(len(redshifts) * nbins)
Muvfunc = np.zeros(len(redshifts) * nbins)
Mhfunc = np.zeros(len(redshifts) * nbins)
lfunc.shape = (len(redshifts), nbins)
Muvfunc.shape = (len(redshifts), nbins)
Mhfunc.shape = (len(redshifts), nbins)
c_Muvfunc = ffi.cast("double *", ffi.from_buffer(Muvfunc))
c_Mhfunc = ffi.cast("double *", ffi.from_buffer(Mhfunc))
c_lfunc = ffi.cast("double *", ffi.from_buffer(lfunc))
# Run the C code
errcode = lib.ComputeLF(
nbins,
user_params(),
cosmo_params(),
astro_params(),
flag_options(),
1,
len(redshifts),
ffi.cast("float *", ffi.from_buffer(redshifts)),
ffi.cast("float *", ffi.from_buffer(mturnovers)),
c_Muvfunc,
c_Mhfunc,
c_lfunc,
)
_process_exitcode(
errcode,
lib.ComputeLF,
(
nbins,
user_params,
cosmo_params,
astro_params,
flag_options,
1,
len(redshifts),
),
)
lfunc[lfunc <= -30] = np.nan
return Muvfunc, Mhfunc, lfunc
elif component == 2:
lfunc_MINI = np.zeros(len(redshifts) * nbins)
Muvfunc_MINI = np.zeros(len(redshifts) * nbins)
Mhfunc_MINI = np.zeros(len(redshifts) * nbins)
lfunc_MINI.shape = (len(redshifts), nbins)
Muvfunc_MINI.shape = (len(redshifts), nbins)
Mhfunc_MINI.shape = (len(redshifts), nbins)
c_Muvfunc_MINI = ffi.cast("double *", ffi.from_buffer(Muvfunc_MINI))
c_Mhfunc_MINI = ffi.cast("double *", ffi.from_buffer(Mhfunc_MINI))
c_lfunc_MINI = ffi.cast("double *", ffi.from_buffer(lfunc_MINI))
# Run the C code
errcode = lib.ComputeLF(
nbins,
user_params(),
cosmo_params(),
astro_params(),
flag_options(),
2,
len(redshifts),
ffi.cast("float *", ffi.from_buffer(redshifts)),
ffi.cast("float *", ffi.from_buffer(mturnovers_mini)),
c_Muvfunc_MINI,
c_Mhfunc_MINI,
c_lfunc_MINI,
)
_process_exitcode(
errcode,
lib.ComputeLF,
(
nbins,
user_params,
cosmo_params,
astro_params,
flag_options,
2,
len(redshifts),
),
)
lfunc_MINI[lfunc_MINI <= -30] = np.nan
return Muvfunc_MINI, Mhfunc_MINI, lfunc_MINI
else:
logger.warning("What is component %d ?" % component)
return None, None, None
def _init_photon_conservation_correction(
*, user_params=None, cosmo_params=None, astro_params=None, flag_options=None
):
user_params = UserParams(user_params)
cosmo_params = CosmoParams(cosmo_params)
astro_params = AstroParams(astro_params)
flag_options = FlagOptions(
flag_options, USE_VELS_AUX=user_params.USE_RELATIVE_VELOCITIES
)
return lib.InitialisePhotonCons(
user_params(), cosmo_params(), astro_params(), flag_options()
)
def _calibrate_photon_conservation_correction(
*, redshifts_estimate, nf_estimate, NSpline
):
# Convert the data to the right type
redshifts_estimate = np.array(redshifts_estimate, dtype="float64")
nf_estimate = np.array(nf_estimate, dtype="float64")
z = ffi.cast("double *", ffi.from_buffer(redshifts_estimate))
xHI = ffi.cast("double *", ffi.from_buffer(nf_estimate))
logger.debug(f"PhotonCons nf estimates: {nf_estimate}")
return lib.PhotonCons_Calibration(z, xHI, NSpline)
def _calc_zstart_photon_cons():
# Run the C code
return _call_c_simple(lib.ComputeZstart_PhotonCons)
def _get_photon_nonconservation_data():
"""
Access C global data representing the photon-nonconservation corrections.
.. note:: if not using ``PHOTON_CONS`` (in :class:`~FlagOptions`), *or* if the
initialisation for photon conservation has not been performed yet, this
will return None.
Returns
-------
dict :
z_analytic: array of redshifts defining the analytic ionized fraction
Q_analytic: array of analytic ionized fractions corresponding to `z_analytic`
z_calibration: array of redshifts defining the ionized fraction from 21cmFAST without
recombinations
nf_calibration: array of calibration ionized fractions corresponding to `z_calibration`
delta_z_photon_cons: the change in redshift required to calibrate 21cmFAST, as a function
of z_calibration
nf_photoncons: the neutral fraction as a function of redshift
"""
# Check if photon conservation has been initialised at all
if not lib.photon_cons_allocated:
return None
arbitrary_large_size = 2000
data = np.zeros((6, arbitrary_large_size))
IntVal1 = np.array(np.zeros(1), dtype="int32")
IntVal2 = np.array(np.zeros(1), dtype="int32")
IntVal3 = np.array(np.zeros(1), dtype="int32")
c_z_at_Q = ffi.cast("double *", ffi.from_buffer(data[0]))
c_Qval = ffi.cast("double *", ffi.from_buffer(data[1]))
c_z_cal = ffi.cast("double *", ffi.from_buffer(data[2]))
c_nf_cal = ffi.cast("double *", ffi.from_buffer(data[3]))
c_PC_nf = ffi.cast("double *", ffi.from_buffer(data[4]))
c_PC_deltaz = ffi.cast("double *", ffi.from_buffer(data[5]))
c_int_NQ = ffi.cast("int *", ffi.from_buffer(IntVal1))
c_int_NC = ffi.cast("int *", ffi.from_buffer(IntVal2))
c_int_NP = ffi.cast("int *", ffi.from_buffer(IntVal3))
# Run the C code
errcode = lib.ObtainPhotonConsData(
c_z_at_Q,
c_Qval,
c_int_NQ,
c_z_cal,
c_nf_cal,
c_int_NC,
c_PC_nf,
c_PC_deltaz,
c_int_NP,
)
_process_exitcode(errcode, lib.ObtainPhotonConsData, ())
ArrayIndices = [
IntVal1[0],
IntVal1[0],
IntVal2[0],
IntVal2[0],
IntVal3[0],
IntVal3[0],
]
data_list = [
"z_analytic",
"Q_analytic",
"z_calibration",
"nf_calibration",
"nf_photoncons",
"delta_z_photon_cons",
]
return {name: d[:index] for name, d, index in zip(data_list, data, ArrayIndices)}
def initial_conditions(
*,
user_params=None,
cosmo_params=None,
random_seed=None,
regenerate=None,
write=None,
direc=None,
hooks: dict[Callable, dict[str, Any]] | None = None,
**global_kwargs,
) -> InitialConditions:
r"""
Compute initial conditions.
Parameters
----------
user_params : :class:`~UserParams` instance, optional
Defines the overall options and parameters of the run.
cosmo_params : :class:`~CosmoParams` instance, optional
Defines the cosmological parameters used to compute initial conditions.
regenerate : bool, optional
Whether to force regeneration of data, even if matching cached data is found.
This is applied recursively to any potential sub-calculations. It is ignored in
the case of dependent data only if that data is explicitly passed to the function.
write : bool, optional
Whether to write results to file (i.e. cache). This is recursively applied to
any potential sub-calculations.
hooks
Any extra functions to apply to the output object. This should be a dictionary
where the keys are the functions, and the values are themselves dictionaries of
parameters to pass to the function. The function signature should be
``(output, **params)``, where the ``output`` is the output object.
direc : str, optional
The directory in which to search for the boxes and write them. By default, this
is the directory given by ``boxdir`` in the configuration file,
``~/.21cmfast/config.yml``. This is recursively applied to any potential
sub-calculations.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
:class:`~InitialConditions`
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
with global_params.use(**global_kwargs):
user_params, cosmo_params = _setup_inputs(
{"user_params": user_params, "cosmo_params": cosmo_params}
)
# Initialize memory for the boxes that will be returned.
boxes = InitialConditions(
user_params=user_params, cosmo_params=cosmo_params, random_seed=random_seed
)
# Construct FFTW wisdoms. Only if required
construct_fftw_wisdoms(user_params=user_params, cosmo_params=cosmo_params)
# First check whether the boxes already exist.
if not regenerate:
try:
boxes.read(direc)
logger.info(
f"Existing init_boxes found and read in (seed={boxes.random_seed})."
)
return boxes
except OSError:
pass
return boxes.compute(hooks=hooks)
def perturb_field(
*,
redshift,
init_boxes=None,
user_params=None,
cosmo_params=None,
random_seed=None,
regenerate=None,
write=None,
direc=None,
hooks: dict[Callable, dict[str, Any]] | None = None,
**global_kwargs,
) -> PerturbedField:
r"""
Compute a perturbed field at a given redshift.
Parameters
----------
redshift : float
The redshift at which to compute the perturbed field.
init_boxes : :class:`~InitialConditions`, optional
If given, these initial conditions boxes will be used, otherwise initial conditions will
be generated. If given,
the user and cosmo params will be set from this object.
user_params : :class:`~UserParams`, optional
Defines the overall options and parameters of the run.
cosmo_params : :class:`~CosmoParams`, optional
Defines the cosmological parameters used to compute initial conditions.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
:class:`~PerturbedField`
Other Parameters
----------------
regenerate, write, direc, random_seed:
See docs of :func:`initial_conditions` for more information.
Examples
--------
The simplest method is just to give a redshift::
>>> field = perturb_field(7.0)
>>> print(field.density)
Doing so will internally call the :func:`~initial_conditions` function. If initial conditions
have already been
calculated, this can be avoided by passing them:
>>> init_boxes = initial_conditions()
>>> field7 = perturb_field(7.0, init_boxes)
>>> field8 = perturb_field(8.0, init_boxes)
The user and cosmo parameter structures are by default inferred from the ``init_boxes``,
so that the following is
consistent::
>>> init_boxes = initial_conditions(user_params= UserParams(HII_DIM=1000))
>>> field7 = perturb_field(7.0, init_boxes)
If ``init_boxes`` is not passed, then these parameters can be directly passed::
>>> field7 = perturb_field(7.0, user_params=UserParams(HII_DIM=1000))
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
with global_params.use(**global_kwargs):
random_seed, user_params, cosmo_params, redshift = _setup_inputs(
{
"random_seed": random_seed,
"user_params": user_params,
"cosmo_params": cosmo_params,
},
input_boxes={"init_boxes": init_boxes},
redshift=redshift,
)
# Initialize perturbed boxes.
fields = PerturbedField(
redshift=redshift,
user_params=user_params,
cosmo_params=cosmo_params,
random_seed=random_seed,
)
# Check whether the boxes already exist
if not regenerate:
try:
fields.read(direc)
logger.info(
f"Existing z={redshift} perturb_field boxes found and read in "
f"(seed={fields.random_seed})."
)
return fields
except OSError:
pass
# Construct FFTW wisdoms. Only if required
construct_fftw_wisdoms(user_params=user_params, cosmo_params=cosmo_params)
# Make sure we've got computed init boxes.
if init_boxes is None or not init_boxes.is_computed:
init_boxes = initial_conditions(
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regenerate,
hooks=hooks,
direc=direc,
random_seed=random_seed,
)
# Need to update fields to have the same seed as init_boxes
fields._random_seed = init_boxes.random_seed
# Run the C Code
return fields.compute(ics=init_boxes, hooks=hooks)
def determine_halo_list(
*,
redshift,
init_boxes=None,
user_params=None,
cosmo_params=None,
astro_params=None,
flag_options=None,
random_seed=None,
regenerate=None,
write=None,
direc=None,
hooks=None,
**global_kwargs,
):
r"""
Find a halo list, given a redshift.
Parameters
----------
redshift : float
The redshift at which to determine the halo list.
init_boxes : :class:`~InitialConditions`, optional
If given, these initial conditions boxes will be used, otherwise initial conditions will
be generated. If given,
the user and cosmo params will be set from this object.
user_params : :class:`~UserParams`, optional
Defines the overall options and parameters of the run.
cosmo_params : :class:`~CosmoParams`, optional
Defines the cosmological parameters used to compute initial conditions.
astro_params: :class:`~AstroParams` instance, optional
The astrophysical parameters defining the course of reionization.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
:class:`~HaloField`
Other Parameters
----------------
regenerate, write, direc, random_seed:
See docs of :func:`initial_conditions` for more information.
Examples
--------
Fill this in once finalised
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
with global_params.use(**global_kwargs):
# Configure and check input/output parameters/structs
(
random_seed,
user_params,
cosmo_params,
astro_params,
flag_options,
redshift,
) = _setup_inputs(
{
"random_seed": random_seed,
"user_params": user_params,
"cosmo_params": cosmo_params,
"astro_params": astro_params,
"flag_options": flag_options,
},
{"init_boxes": init_boxes},
redshift=redshift,
)
if user_params.HMF != 1:
raise ValueError("USE_HALO_FIELD is only valid for HMF = 1")
# Initialize halo list boxes.
fields = HaloField(
redshift=redshift,
user_params=user_params,
cosmo_params=cosmo_params,
astro_params=astro_params,
flag_options=flag_options,
random_seed=random_seed,
)
# Check whether the boxes already exist
if not regenerate:
try:
fields.read(direc)
logger.info(
f"Existing z={redshift} determine_halo_list boxes found and read in "
f"(seed={fields.random_seed})."
)
return fields
except OSError:
pass
# Construct FFTW wisdoms. Only if required
construct_fftw_wisdoms(user_params=user_params, cosmo_params=cosmo_params)
# Make sure we've got computed init boxes.
if init_boxes is None or not init_boxes.is_computed:
init_boxes = initial_conditions(
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regenerate,
hooks=hooks,
direc=direc,
random_seed=random_seed,
)
# Need to update fields to have the same seed as init_boxes
fields._random_seed = init_boxes.random_seed
# Run the C Code
return fields.compute(ics=init_boxes, hooks=hooks)
def perturb_halo_list(
*,
redshift,
init_boxes=None,
halo_field=None,
user_params=None,
cosmo_params=None,
astro_params=None,
flag_options=None,
random_seed=None,
regenerate=None,
write=None,
direc=None,
hooks=None,
**global_kwargs,
):
r"""
Given a halo list, perturb the halos for a given redshift.
Parameters
----------
redshift : float
The redshift at which to determine the halo list.
init_boxes : :class:`~InitialConditions`, optional
If given, these initial conditions boxes will be used, otherwise initial conditions will
be generated. If given,
the user and cosmo params will be set from this object.
user_params : :class:`~UserParams`, optional
Defines the overall options and parameters of the run.
cosmo_params : :class:`~CosmoParams`, optional
Defines the cosmological parameters used to compute initial conditions.
astro_params: :class:`~AstroParams` instance, optional
The astrophysical parameters defining the course of reionization.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
:class:`~PerturbHaloField`
Other Parameters
----------------
regenerate, write, direc, random_seed:
See docs of :func:`initial_conditions` for more information.
Examples
--------
Fill this in once finalised
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
with global_params.use(**global_kwargs):
# Configure and check input/output parameters/structs
(
random_seed,
user_params,
cosmo_params,
astro_params,
flag_options,
redshift,
) = _setup_inputs(
{
"random_seed": random_seed,
"user_params": user_params,
"cosmo_params": cosmo_params,
"astro_params": astro_params,
"flag_options": flag_options,
},
{"init_boxes": init_boxes, "halo_field": halo_field},
redshift=redshift,
)
if user_params.HMF != 1:
raise ValueError("USE_HALO_FIELD is only valid for HMF = 1")
# Initialize halo list boxes.
fields = PerturbHaloField(
redshift=redshift,
user_params=user_params,
cosmo_params=cosmo_params,
astro_params=astro_params,
flag_options=flag_options,
random_seed=random_seed,
)
# Check whether the boxes already exist
if not regenerate:
try:
fields.read(direc)
logger.info(
"Existing z=%s perturb_halo_list boxes found and read in (seed=%s)."
% (redshift, fields.random_seed)
)
return fields
except OSError:
pass
# Make sure we've got computed init boxes.
if init_boxes is None or not init_boxes.is_computed:
init_boxes = initial_conditions(
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regenerate,
hooks=hooks,
direc=direc,
random_seed=random_seed,
)
# Need to update fields to have the same seed as init_boxes
fields._random_seed = init_boxes.random_seed
# Dynamically produce the halo list.
if halo_field is None or not halo_field.is_computed:
halo_field = determine_halo_list(
init_boxes=init_boxes,
# NOTE: this is required, rather than using cosmo_ and user_,
# since init may have a set seed.
redshift=redshift,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
# Run the C Code
return fields.compute(ics=init_boxes, halo_field=halo_field, hooks=hooks)
def ionize_box(
*,
astro_params=None,
flag_options=None,
redshift=None,
perturbed_field=None,
previous_perturbed_field=None,
previous_ionize_box=None,
spin_temp=None,
pt_halos=None,
init_boxes=None,
cosmo_params=None,
user_params=None,
regenerate=None,
write=None,
direc=None,
random_seed=None,
cleanup=True,
hooks=None,
**global_kwargs,
) -> IonizedBox:
r"""
Compute an ionized box at a given redshift.
This function has various options for how the evolution of the ionization is computed (if at
all). See the Notes below for details.
Parameters
----------
astro_params: :class:`~AstroParams` instance, optional
The astrophysical parameters defining the course of reionization.
flag_options: :class:`~FlagOptions` instance, optional
Some options passed to the reionization routine.
redshift : float, optional
The redshift at which to compute the ionized box. If `perturbed_field` is given,
its inherent redshift
will take precedence over this argument. If not, this argument is mandatory.
perturbed_field : :class:`~PerturbField`, optional
If given, this field will be used, otherwise it will be generated. To be generated,
either `init_boxes` and
`redshift` must be given, or `user_params`, `cosmo_params` and `redshift`.
previous_perturbed_field : :class:`~PerturbField`, optional
An perturbed field at higher redshift. This is only used if mini_halo is included.
init_boxes : :class:`~InitialConditions` , optional
If given, and `perturbed_field` *not* given, these initial conditions boxes will be used
to generate the perturbed field, otherwise initial conditions will be generated on the fly.
If given, the user and cosmo params will be set from this object.
previous_ionize_box: :class:`IonizedBox` or None
An ionized box at higher redshift. This is only used if `INHOMO_RECO` and/or `do_spin_temp`
are true. If either of these are true, and this is not given, then it will be assumed that
this is the "first box", i.e. that it can be populated accurately without knowing source
statistics.
spin_temp: :class:`TsBox` or None, optional
A spin-temperature box, only required if `do_spin_temp` is True. If None, will try to read
in a spin temp box at the current redshift, and failing that will try to automatically
create one, using the previous ionized box redshift as the previous spin temperature
redshift.
pt_halos: :class:`~PerturbHaloField` or None, optional
If passed, this contains all the dark matter haloes obtained if using the USE_HALO_FIELD.
This is a list of halo masses and coords for the dark matter haloes.
If not passed, it will try and automatically create them using the available initial conditions.
user_params : :class:`~UserParams`, optional
Defines the overall options and parameters of the run.
cosmo_params : :class:`~CosmoParams`, optional
Defines the cosmological parameters used to compute initial conditions.
cleanup : bool, optional
A flag to specify whether the C routine cleans up its memory before returning. Typically,
if `spin_temperature` is called directly, you will want this to be true, as if the next box
to be calculate has different shape, errors will occur if memory is not cleaned. However,
it can be useful to set it to False if scrolling through parameters for the same box shape.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
:class:`~IonizedBox` :
An object containing the ionized box data.
Other Parameters
----------------
regenerate, write, direc, random_seed :
See docs of :func:`initial_conditions` for more information.
Notes
-----
Typically, the ionization field at any redshift is dependent on the evolution of xHI up until
that redshift, which necessitates providing a previous ionization field to define the current
one. This function provides several options for doing so. First, if neither the spin
temperature field, nor inhomogeneous recombinations (specified in flag options) are used, no
evolution needs to be done. Otherwise, either (in order of precedence)
1. a specific previous :class`~IonizedBox` object is provided, which will be used directly,
2. a previous redshift is provided, for which a cached field on disk will be sought,
3. a step factor is provided which recursively steps through redshift, calculating previous
fields up until Z_HEAT_MAX, and returning just the final field at the current redshift, or
4. the function is instructed to treat the current field as being an initial "high-redshift"
field such that specific sources need not be found and evolved.
.. note:: If a previous specific redshift is given, but no cached field is found at that
redshift, the previous ionization field will be evaluated based on `z_step_factor`.
Examples
--------
By default, no spin temperature is used, and neither are inhomogeneous recombinations,
so that no evolution is required, thus the following will compute a coeval ionization box:
>>> xHI = ionize_box(redshift=7.0)
However, if either of those options are true, then a full evolution will be required:
>>> xHI = ionize_box(redshift=7.0, flag_options=FlagOptions(INHOMO_RECO=True,USE_TS_FLUCT=True))
This will by default evolve the field from a redshift of *at least* `Z_HEAT_MAX` (a global
parameter), in logarithmic steps of `ZPRIME_STEP_FACTOR`. To change these:
>>> xHI = ionize_box(redshift=7.0, zprime_step_factor=1.2, z_heat_max=15.0,
>>> flag_options={"USE_TS_FLUCT":True})
Alternatively, one can pass an exact previous redshift, which will be sought in the disk
cache, or evaluated:
>>> ts_box = ionize_box(redshift=7.0, previous_ionize_box=8.0, flag_options={
>>> "USE_TS_FLUCT":True})
Beware that doing this, if the previous box is not found on disk, will continue to evaluate
prior boxes based on `ZPRIME_STEP_FACTOR`. Alternatively, one can pass a previous
:class:`~IonizedBox`:
>>> xHI_0 = ionize_box(redshift=8.0, flag_options={"USE_TS_FLUCT":True})
>>> xHI = ionize_box(redshift=7.0, previous_ionize_box=xHI_0)
Again, the first line here will implicitly use ``ZPRIME_STEP_FACTOR`` to evolve the field from
``Z_HEAT_MAX``. Note that in the second line, all of the input parameters are taken directly from
`xHI_0` so that they are consistent, and we need not specify the ``flag_options``.
As the function recursively evaluates previous redshift, the previous spin temperature fields
will also be consistently recursively evaluated. Only the final ionized box will actually be
returned and kept in memory, however intervening results will by default be cached on disk.
One can also pass an explicit spin temperature object:
>>> ts = spin_temperature(redshift=7.0)
>>> xHI = ionize_box(redshift=7.0, spin_temp=ts)
If automatic recursion is used, then it is done in such a way that no large boxes are kept
around in memory for longer than they need to be (only two at a time are required).
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
with global_params.use(**global_kwargs):
_verify_types(
init_boxes=init_boxes,
perturbed_field=perturbed_field,
previous_perturbed_field=previous_perturbed_field,
previous_ionize_box=previous_ionize_box,
spin_temp=spin_temp,
pt_halos=pt_halos,
)
# Configure and check input/output parameters/structs
(
random_seed,
user_params,
cosmo_params,
astro_params,
flag_options,
redshift,
) = _setup_inputs(
{
"random_seed": random_seed,
"user_params": user_params,
"cosmo_params": cosmo_params,
"astro_params": astro_params,
"flag_options": flag_options,
},
{
"init_boxes": init_boxes,
"perturbed_field": perturbed_field,
"previous_perturbed_field": previous_perturbed_field,
"previous_ionize_box": previous_ionize_box,
"spin_temp": spin_temp,
"pt_halos": pt_halos,
},
redshift=redshift,
)
if spin_temp is not None and not flag_options.USE_TS_FLUCT:
logger.warning(
"Changing flag_options.USE_TS_FLUCT to True since spin_temp was passed."
)
flag_options.USE_TS_FLUCT = True
# Get the previous redshift
if previous_ionize_box is not None and previous_ionize_box.is_computed:
prev_z = previous_ionize_box.redshift
# Ensure the previous ionized box has a higher redshift than this one.
if prev_z <= redshift:
raise ValueError(
"Previous ionized box must have a higher redshift than that being evaluated."
)
elif flag_options.INHOMO_RECO or flag_options.USE_TS_FLUCT:
prev_z = (1 + redshift) * global_params.ZPRIME_STEP_FACTOR - 1
# if the previous box is before our starting point, we set it to zero,
# which is what the C-code expects for an "initial" box
if prev_z > global_params.Z_HEAT_MAX:
prev_z = 0
else:
prev_z = 0
box = IonizedBox(
user_params=user_params,
cosmo_params=cosmo_params,
redshift=redshift,
astro_params=astro_params,
flag_options=flag_options,
random_seed=random_seed,
prev_ionize_redshift=prev_z,
)
# Construct FFTW wisdoms. Only if required
construct_fftw_wisdoms(user_params=user_params, cosmo_params=cosmo_params)
# Check whether the boxes already exist
if not regenerate:
try:
box.read(direc)
logger.info(
"Existing z=%s ionized boxes found and read in (seed=%s)."
% (redshift, box.random_seed)
)
return box
except OSError:
pass
# EVERYTHING PAST THIS POINT ONLY HAPPENS IF THE BOX DOESN'T ALREADY EXIST
# ------------------------------------------------------------------------
# Get init_box required.
if init_boxes is None or not init_boxes.is_computed:
init_boxes = initial_conditions(
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regenerate,
hooks=hooks,
direc=direc,
random_seed=random_seed,
)
# Need to update random seed
box._random_seed = init_boxes.random_seed
# Get appropriate previous ionization box
if previous_ionize_box is None or not previous_ionize_box.is_computed:
# If we are beyond Z_HEAT_MAX, just make an empty box
if prev_z == 0:
previous_ionize_box = IonizedBox(
redshift=0, flag_options=flag_options, initial=True
)
# Otherwise recursively create new previous box.
else:
previous_ionize_box = ionize_box(
astro_params=astro_params,
flag_options=flag_options,
redshift=prev_z,
init_boxes=init_boxes,
regenerate=regenerate,
hooks=hooks,
direc=direc,
cleanup=False, # We *know* we're going to need the memory again.
)
# Dynamically produce the perturbed field.
if perturbed_field is None or not perturbed_field.is_computed:
perturbed_field = perturb_field(
init_boxes=init_boxes,
# NOTE: this is required, rather than using cosmo_ and user_,
# since init may have a set seed.
redshift=redshift,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
if previous_perturbed_field is None or not previous_perturbed_field.is_computed:
# If we are beyond Z_HEAT_MAX, just make an empty box
if not prev_z:
previous_perturbed_field = PerturbedField(
redshift=0, user_params=user_params, initial=True
)
else:
previous_perturbed_field = perturb_field(
init_boxes=init_boxes,
redshift=prev_z,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
# Dynamically produce the halo field.
if not flag_options.USE_HALO_FIELD:
# Construct an empty halo field to pass in to the function.
pt_halos = PerturbHaloField(redshift=0, dummy=True)
elif pt_halos is None or not pt_halos.is_computed:
pt_halos = perturb_halo_list(
redshift=redshift,
init_boxes=init_boxes,
halo_field=determine_halo_list(
redshift=redshift,
init_boxes=init_boxes,
astro_params=astro_params,
flag_options=flag_options,
regenerate=regenerate,
hooks=hooks,
direc=direc,
),
astro_params=astro_params,
flag_options=flag_options,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
# Set empty spin temp box if necessary.
if not flag_options.USE_TS_FLUCT:
spin_temp = TsBox(redshift=0, dummy=True)
elif spin_temp is None:
spin_temp = spin_temperature(
perturbed_field=perturbed_field,
flag_options=flag_options,
init_boxes=init_boxes,
direc=direc,
hooks=hooks,
regenerate=regenerate,
cleanup=cleanup,
)
# Run the C Code
return box.compute(
perturbed_field=perturbed_field,
prev_perturbed_field=previous_perturbed_field,
prev_ionize_box=previous_ionize_box,
spin_temp=spin_temp,
pt_halos=pt_halos,
ics=init_boxes,
hooks=hooks,
)
def spin_temperature(
*,
astro_params=None,
flag_options=None,
redshift=None,
perturbed_field=None,
previous_spin_temp=None,
init_boxes=None,
cosmo_params=None,
user_params=None,
regenerate=None,
write=None,
direc=None,
random_seed=None,
cleanup=True,
hooks=None,
**global_kwargs,
) -> TsBox:
r"""
Compute spin temperature boxes at a given redshift.
See the notes below for how the spin temperature field is evolved through redshift.
Parameters
----------
astro_params : :class:`~AstroParams`, optional
The astrophysical parameters defining the course of reionization.
flag_options : :class:`~FlagOptions`, optional
Some options passed to the reionization routine.
redshift : float, optional
The redshift at which to compute the ionized box. If not given, the redshift from
`perturbed_field` will be used. Either `redshift`, `perturbed_field`, or
`previous_spin_temp` must be given. See notes on `perturbed_field` for how it affects the
given redshift if both are given.
perturbed_field : :class:`~PerturbField`, optional
If given, this field will be used, otherwise it will be generated. To be generated,
either `init_boxes` and `redshift` must be given, or `user_params`, `cosmo_params` and
`redshift`. By default, this will be generated at the same redshift as the spin temperature
box. The redshift of perturb field is allowed to be different than `redshift`. If so, it
will be interpolated to the correct redshift, which can provide a speedup compared to
actually computing it at the desired redshift.
previous_spin_temp : :class:`TsBox` or None
The previous spin temperature box.
init_boxes : :class:`~InitialConditions`, optional
If given, and `perturbed_field` *not* given, these initial conditions boxes will be used
to generate the perturbed field, otherwise initial conditions will be generated on the fly.
If given, the user and cosmo params will be set from this object.
user_params : :class:`~UserParams`, optional
Defines the overall options and parameters of the run.
cosmo_params : :class:`~CosmoParams`, optional
Defines the cosmological parameters used to compute initial conditions.
cleanup : bool, optional
A flag to specify whether the C routine cleans up its memory before returning.
Typically, if `spin_temperature` is called directly, you will want this to be
true, as if the next box to be calculate has different shape, errors will occur
if memory is not cleaned. However, it can be useful to set it to False if
scrolling through parameters for the same box shape.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
:class:`~TsBox`
An object containing the spin temperature box data.
Other Parameters
----------------
regenerate, write, direc, random_seed :
See docs of :func:`initial_conditions` for more information.
Notes
-----
Typically, the spin temperature field at any redshift is dependent on the evolution of spin
temperature up until that redshift, which necessitates providing a previous spin temperature
field to define the current one. This function provides several options for doing so. Either
(in order of precedence):
1. a specific previous spin temperature object is provided, which will be used directly,
2. a previous redshift is provided, for which a cached field on disk will be sought,
3. a step factor is provided which recursively steps through redshift, calculating previous
fields up until Z_HEAT_MAX, and returning just the final field at the current redshift, or
4. the function is instructed to treat the current field as being an initial "high-redshift"
field such that specific sources need not be found and evolved.
.. note:: If a previous specific redshift is given, but no cached field is found at that
redshift, the previous spin temperature field will be evaluated based on
``z_step_factor``.
Examples
--------
To calculate and return a fully evolved spin temperature field at a given redshift (with
default input parameters), simply use:
>>> ts_box = spin_temperature(redshift=7.0)
This will by default evolve the field from a redshift of *at least* `Z_HEAT_MAX` (a global
parameter), in logarithmic steps of `z_step_factor`. Thus to change these:
>>> ts_box = spin_temperature(redshift=7.0, zprime_step_factor=1.2, z_heat_max=15.0)
Alternatively, one can pass an exact previous redshift, which will be sought in the disk
cache, or evaluated:
>>> ts_box = spin_temperature(redshift=7.0, previous_spin_temp=8.0)
Beware that doing this, if the previous box is not found on disk, will continue to evaluate
prior boxes based on the ``z_step_factor``. Alternatively, one can pass a previous spin
temperature box:
>>> ts_box1 = spin_temperature(redshift=8.0)
>>> ts_box = spin_temperature(redshift=7.0, previous_spin_temp=ts_box1)
Again, the first line here will implicitly use ``z_step_factor`` to evolve the field from
around ``Z_HEAT_MAX``. Note that in the second line, all of the input parameters are taken
directly from `ts_box1` so that they are consistent. Finally, one can force the function to
evaluate the current redshift as if it was beyond ``Z_HEAT_MAX`` so that it depends only on
itself:
>>> ts_box = spin_temperature(redshift=7.0, zprime_step_factor=None)
This is usually a bad idea, and will give a warning, but it is possible.
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
with global_params.use(**global_kwargs):
# Configure and check input/output parameters/structs
(
random_seed,
user_params,
cosmo_params,
astro_params,
flag_options,
) = _setup_inputs(
{
"random_seed": random_seed,
"user_params": user_params,
"cosmo_params": cosmo_params,
"astro_params": astro_params,
"flag_options": flag_options,
},
{
"init_boxes": init_boxes,
"perturbed_field": perturbed_field,
"previous_spin_temp": previous_spin_temp,
},
)
# Try to determine redshift from other inputs, if required.
# Note that perturb_field does not need to match redshift here.
if redshift is None:
if perturbed_field is not None:
redshift = perturbed_field.redshift
elif previous_spin_temp is not None:
redshift = (
previous_spin_temp.redshift + 1
) / global_params.ZPRIME_STEP_FACTOR - 1
else:
raise ValueError(
"Either the redshift, perturbed_field or previous_spin_temp must be given."
)
# Explicitly set this flag to True, though it shouldn't be required!
flag_options.update(USE_TS_FLUCT=True)
# Get the previous redshift
if previous_spin_temp is not None:
prev_z = previous_spin_temp.redshift
else:
if redshift < global_params.Z_HEAT_MAX:
# In general runs, we only compute the spin temperature *below* Z_HEAT_MAX.
# Above this, we don't need a prev_z at all, because we can calculate
# directly at whatever redshift it is.
prev_z = min(
global_params.Z_HEAT_MAX,
(1 + redshift) * global_params.ZPRIME_STEP_FACTOR - 1,
)
else:
# Set prev_z to anything, since we don't need it.
prev_z = np.inf
# Ensure the previous spin temperature has a higher redshift than this one.
if prev_z <= redshift:
raise ValueError(
"Previous spin temperature box must have a higher redshift than "
"that being evaluated."
)
# Set up the box without computing anything.
box = TsBox(
user_params=user_params,
cosmo_params=cosmo_params,
redshift=redshift,
astro_params=astro_params,
flag_options=flag_options,
random_seed=random_seed,
prev_spin_redshift=prev_z,
perturbed_field_redshift=perturbed_field.redshift
if (perturbed_field is not None and perturbed_field.is_computed)
else redshift,
)
# Construct FFTW wisdoms. Only if required
construct_fftw_wisdoms(user_params=user_params, cosmo_params=cosmo_params)
# Check whether the boxes already exist on disk.
if not regenerate:
try:
box.read(direc)
logger.info(
f"Existing z={redshift} spin_temp boxes found and read in "
f"(seed={box.random_seed})."
)
return box
except OSError:
pass
# EVERYTHING PAST THIS POINT ONLY HAPPENS IF THE BOX DOESN'T ALREADY EXIST
# ------------------------------------------------------------------------
# Dynamically produce the initial conditions.
if init_boxes is None or not init_boxes.is_computed:
init_boxes = initial_conditions(
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regenerate,
hooks=hooks,
direc=direc,
random_seed=random_seed,
)
# Need to update random seed
box._random_seed = init_boxes.random_seed
# Create appropriate previous_spin_temp
if previous_spin_temp is None:
if redshift >= global_params.Z_HEAT_MAX:
# We end up never even using this box, just need to define it
# unallocated to be able to send into the C code.
previous_spin_temp = TsBox(
redshift=prev_z, # redshift here is ignored
user_params=init_boxes.user_params,
cosmo_params=init_boxes.cosmo_params,
astro_params=astro_params,
flag_options=flag_options,
dummy=True,
)
else:
previous_spin_temp = spin_temperature(
init_boxes=init_boxes,
astro_params=astro_params,
flag_options=flag_options,
redshift=prev_z,
regenerate=regenerate,
hooks=hooks,
direc=direc,
cleanup=False, # we know we'll need the memory again
)
# Dynamically produce the perturbed field.
if perturbed_field is None or not perturbed_field.is_computed:
perturbed_field = perturb_field(
redshift=redshift,
init_boxes=init_boxes,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
# Run the C Code
return box.compute(
cleanup=cleanup,
perturbed_field=perturbed_field,
prev_spin_temp=previous_spin_temp,
ics=init_boxes,
hooks=hooks,
)
def brightness_temperature(
*,
ionized_box,
perturbed_field,
spin_temp=None,
write=None,
regenerate=None,
direc=None,
hooks=None,
**global_kwargs,
) -> BrightnessTemp:
r"""
Compute a coeval brightness temperature box.
Parameters
----------
ionized_box: :class:`IonizedBox`
A pre-computed ionized box.
perturbed_field: :class:`PerturbedField`
A pre-computed perturbed field at the same redshift as `ionized_box`.
spin_temp: :class:`TsBox`, optional
A pre-computed spin temperature, at the same redshift as the other boxes.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
:class:`BrightnessTemp` instance.
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
with global_params.use(**global_kwargs):
_verify_types(
perturbed_field=perturbed_field,
spin_temp=spin_temp,
ionized_box=ionized_box,
)
# don't ignore redshift here
_check_compatible_inputs(ionized_box, perturbed_field, spin_temp, ignore=[])
# ensure ionized_box and perturbed_field aren't None, as we don't do
# any dynamic calculations here.
if ionized_box is None or perturbed_field is None:
raise ValueError("both ionized_box and perturbed_field must be specified.")
if spin_temp is None:
if ionized_box.flag_options.USE_TS_FLUCT:
raise ValueError(
"You have USE_TS_FLUCT=True, but have not provided a spin_temp!"
)
# Make an unused dummy box.
spin_temp = TsBox(redshift=0, dummy=True)
box = BrightnessTemp(
user_params=ionized_box.user_params,
cosmo_params=ionized_box.cosmo_params,
astro_params=ionized_box.astro_params,
flag_options=ionized_box.flag_options,
redshift=ionized_box.redshift,
random_seed=ionized_box.random_seed,
)
# Construct FFTW wisdoms. Only if required
construct_fftw_wisdoms(
user_params=ionized_box.user_params, cosmo_params=ionized_box.cosmo_params
)
# Check whether the boxes already exist on disk.
if not regenerate:
try:
box.read(direc)
logger.info(
f"Existing brightness_temp box found and read in (seed={box.random_seed})."
)
return box
except OSError:
pass
return box.compute(
spin_temp=spin_temp,
ionized_box=ionized_box,
perturbed_field=perturbed_field,
hooks=hooks,
)
def _logscroll_redshifts(min_redshift, z_step_factor, zmax):
redshifts = [min_redshift]
while redshifts[-1] < zmax:
redshifts.append((redshifts[-1] + 1.0) * z_step_factor - 1.0)
return redshifts[::-1]
def run_coeval(
*,
redshift=None,
user_params=None,
cosmo_params=None,
astro_params=None,
flag_options=None,
regenerate=None,
write=None,
direc=None,
init_box=None,
perturb=None,
use_interp_perturb_field=False,
pt_halos=None,
random_seed=None,
cleanup=True,
hooks=None,
always_purge: bool = False,
**global_kwargs,
):
r"""
Evaluate a coeval ionized box at a given redshift, or multiple redshifts.
This is generally the easiest and most efficient way to generate a set of coeval cubes at a
given set of redshift. It self-consistently deals with situations in which the field needs to be
evolved, and does this with the highest memory-efficiency, only returning the desired redshift.
All other calculations are by default stored in the on-disk cache so they can be re-used at a
later time.
.. note:: User-supplied redshift are *not* used as previous redshift in any scrolling,
so that pristine log-sampling can be maintained.
Parameters
----------
redshift: array_like
A single redshift, or multiple redshift, at which to return results. The minimum of these
will define the log-scrolling behaviour (if necessary).
user_params : :class:`~inputs.UserParams`, optional
Defines the overall options and parameters of the run.
cosmo_params : :class:`~inputs.CosmoParams` , optional
Defines the cosmological parameters used to compute initial conditions.
astro_params : :class:`~inputs.AstroParams` , optional
The astrophysical parameters defining the course of reionization.
flag_options : :class:`~inputs.FlagOptions` , optional
Some options passed to the reionization routine.
init_box : :class:`~InitialConditions`, optional
If given, the user and cosmo params will be set from this object, and it will not
be re-calculated.
perturb : list of :class:`~PerturbedField`, optional
If given, must be compatible with init_box. It will merely negate the necessity
of re-calculating the perturb fields.
use_interp_perturb_field : bool, optional
Whether to use a single perturb field, at the lowest redshift of the lightcone,
to determine all spin temperature fields. If so, this field is interpolated in
the underlying C-code to the correct redshift. This is less accurate (and no more
efficient), but provides compatibility with older versions of 21cmFAST.
pt_halos : bool, optional
If given, must be compatible with init_box. It will merely negate the necessity
of re-calculating the perturbed halo lists.
cleanup : bool, optional
A flag to specify whether the C routine cleans up its memory before returning.
Typically, if `spin_temperature` is called directly, you will want this to be
true, as if the next box to be calculate has different shape, errors will occur
if memory is not cleaned. Note that internally, this is set to False until the
last iteration.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
coevals : :class:`~py21cmfast.outputs.Coeval`
The full data for the Coeval class, with init boxes, perturbed fields, ionized boxes,
brightness temperature, and potential data from the conservation of photons. If a
single redshift was specified, it will return such a class. If multiple redshifts
were passed, it will return a list of such classes.
Other Parameters
----------------
regenerate, write, direc, random_seed :
See docs of :func:`initial_conditions` for more information.
"""
with global_params.use(**global_kwargs):
if redshift is None and perturb is None:
raise ValueError("Either redshift or perturb must be given")
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
singleton = False
# Ensure perturb is a list of boxes, not just one.
if perturb is None:
perturb = []
elif not hasattr(perturb, "__len__"):
perturb = [perturb]
singleton = True
# Ensure perturbed halo field is a list of boxes, not just one.
if flag_options is None or pt_halos is None:
pt_halos = []
elif (
flag_options["USE_HALO_FIELD"]
if isinstance(flag_options, dict)
else flag_options.USE_HALO_FIELD
):
pt_halos = [pt_halos] if not hasattr(pt_halos, "__len__") else []
else:
pt_halos = []
(
random_seed,
user_params,
cosmo_params,
astro_params,
flag_options,
) = _setup_inputs(
{
"random_seed": random_seed,
"user_params": user_params,
"cosmo_params": cosmo_params,
"astro_params": astro_params,
"flag_options": flag_options,
},
)
if use_interp_perturb_field and flag_options.USE_MINI_HALOS:
raise ValueError("Cannot use an interpolated perturb field with minihalos!")
if init_box is None:
init_box = initial_conditions(
user_params=user_params,
cosmo_params=cosmo_params,
random_seed=random_seed,
hooks=hooks,
regenerate=regenerate,
direc=direc,
)
# We can go ahead and purge some of the stuff in the init_box, but only if
# it is cached -- otherwise we could be losing information.
try:
init_box.prepare_for_perturb(flag_options=flag_options, force=always_purge)
except OSError:
pass
if perturb:
if redshift is not None and any(
p.redshift != z for p, z in zip(perturb, redshift)
):
raise ValueError("Input redshifts do not match perturb field redshifts")
else:
redshift = [p.redshift for p in perturb]
if (
flag_options.USE_HALO_FIELD
and pt_halos
and any(p.redshift != z for p, z in zip(pt_halos, redshift))
):
raise ValueError(
"Input redshifts do not match the perturbed halo field redshifts"
)
if flag_options.PHOTON_CONS:
calibrate_photon_cons(
user_params,
cosmo_params,
astro_params,
flag_options,
init_box,
regenerate,
write,
direc,
)
if not hasattr(redshift, "__len__"):
singleton = True
redshift = [redshift]
# Get the list of redshift we need to scroll through.
redshifts = _get_required_redshifts_coeval(flag_options, redshift)
# Get all the perturb boxes early. We need to get the perturb at every
# redshift, even if we are interpolating the perturb field, because the
# ionize box needs it.
pz = [p.redshift for p in perturb]
perturb_ = []
for z in redshifts:
p = (
perturb_field(
redshift=z,
init_boxes=init_box,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
if z not in pz
else perturb[pz.index(z)]
)
if user_params.MINIMIZE_MEMORY:
try:
p.purge(force=always_purge)
except OSError:
pass
perturb_.append(p)
perturb = perturb_
# Now we can purge init_box further.
try:
init_box.prepare_for_spin_temp(
flag_options=flag_options, force=always_purge
)
except OSError:
pass
if flag_options.USE_HALO_FIELD and not pt_halos:
for z in redshift:
pt_halos += [
perturb_halo_list(
redshift=z,
init_boxes=init_box,
user_params=user_params,
cosmo_params=cosmo_params,
astro_params=astro_params,
flag_options=flag_options,
halo_field=determine_halo_list(
redshift=z,
init_boxes=init_box,
astro_params=astro_params,
flag_options=flag_options,
regenerate=regenerate,
hooks=hooks,
direc=direc,
),
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
]
if (
flag_options.PHOTON_CONS
and np.amin(redshifts) < global_params.PhotonConsEndCalibz
):
raise ValueError(
f"You have passed a redshift (z = {np.amin(redshifts)}) that is lower than"
"the endpoint of the photon non-conservation correction"
f"(global_params.PhotonConsEndCalibz = {global_params.PhotonConsEndCalibz})."
"If this behaviour is desired then set global_params.PhotonConsEndCalibz"
f"to a value lower than z = {np.amin(redshifts)}."
)
if flag_options.PHOTON_CONS:
calibrate_photon_cons(
user_params,
cosmo_params,
astro_params,
flag_options,
init_box,
regenerate,
write,
direc,
)
ib_tracker = [0] * len(redshift)
bt = [0] * len(redshift)
st, ib, pf = None, None, None # At first we don't have any "previous" st or ib.
perturb_min = perturb[np.argmin(redshift)]
st_tracker = [None] * len(redshift)
spin_temp_files = []
perturb_files = []
ionize_files = []
brightness_files = []
# Iterate through redshift from top to bottom
for iz, z in enumerate(redshifts):
pf2 = perturb[iz]
pf2.load_all()
if flag_options.USE_TS_FLUCT:
logger.debug(f"Doing spin temp for z={z}.")
st2 = spin_temperature(
redshift=z,
previous_spin_temp=st,
perturbed_field=perturb_min if use_interp_perturb_field else pf2,
# remember that perturb field is interpolated, so no need to provide exact one.
astro_params=astro_params,
flag_options=flag_options,
regenerate=regenerate,
init_boxes=init_box,
hooks=hooks,
direc=direc,
cleanup=(
cleanup and z == redshifts[-1]
), # cleanup if its the last time through
)
if z not in redshift:
st = st2
ib2 = ionize_box(
redshift=z,
previous_ionize_box=ib,
init_boxes=init_box,
perturbed_field=pf2,
# perturb field *not* interpolated here.
previous_perturbed_field=pf,
pt_halos=pt_halos[redshift.index(z)]
if z in redshift and flag_options.USE_HALO_FIELD
else None,
astro_params=astro_params,
flag_options=flag_options,
spin_temp=st2 if flag_options.USE_TS_FLUCT else None,
regenerate=regenerate,
z_heat_max=global_params.Z_HEAT_MAX,
hooks=hooks,
direc=direc,
cleanup=(
cleanup and z == redshifts[-1]
), # cleanup if its the last time through
)
if pf is not None:
try:
pf.purge(force=always_purge)
except OSError:
pass
if z in redshift:
logger.debug(f"PID={os.getpid()} doing brightness temp for z={z}")
ib_tracker[redshift.index(z)] = ib2
st_tracker[redshift.index(z)] = (
st2 if flag_options.USE_TS_FLUCT else None
)
_bt = brightness_temperature(
ionized_box=ib2,
perturbed_field=pf2,
spin_temp=st2 if flag_options.USE_TS_FLUCT else None,
hooks=hooks,
direc=direc,
regenerate=regenerate,
)
bt[redshift.index(z)] = _bt
else:
ib = ib2
pf = pf2
_bt = None
perturb_files.append((z, os.path.join(direc, pf2.filename)))
if flag_options.USE_TS_FLUCT:
spin_temp_files.append((z, os.path.join(direc, st2.filename)))
ionize_files.append((z, os.path.join(direc, ib2.filename)))
if _bt is not None:
brightness_files.append((z, os.path.join(direc, _bt.filename)))
if flag_options.PHOTON_CONS:
photon_nonconservation_data = _get_photon_nonconservation_data()
if photon_nonconservation_data:
lib.FreePhotonConsMemory()
else:
photon_nonconservation_data = None
if (
flag_options.USE_TS_FLUCT
and user_params.USE_INTERPOLATION_TABLES
and lib.interpolation_tables_allocated
):
lib.FreeTsInterpolationTables(flag_options())
coevals = [
Coeval(
redshift=z,
initial_conditions=init_box,
perturbed_field=perturb[redshifts.index(z)],
ionized_box=ib,
brightness_temp=_bt,
ts_box=st,
photon_nonconservation_data=photon_nonconservation_data,
cache_files={
"init": [(0, os.path.join(direc, init_box.filename))],
"perturb_field": perturb_files,
"ionized_box": ionize_files,
"brightness_temp": brightness_files,
"spin_temp": spin_temp_files,
},
)
for z, ib, _bt, st in zip(redshift, ib_tracker, bt, st_tracker)
]
# If a single redshift was passed, then pass back singletons.
if singleton:
coevals = coevals[0]
logger.debug("Returning from Coeval")
return coevals
def _get_required_redshifts_coeval(flag_options, redshift) -> list[float]:
if min(redshift) < global_params.Z_HEAT_MAX and (
flag_options.INHOMO_RECO or flag_options.USE_TS_FLUCT
):
redshifts = _logscroll_redshifts(
min(redshift),
global_params.ZPRIME_STEP_FACTOR,
global_params.Z_HEAT_MAX,
)
# Set the highest redshift to exactly Z_HEAT_MAX. This makes the coeval run
# at exactly the same redshift as the spin temperature box. There's literally
# no point going higher for a coeval, since the user is only interested in
# the final "redshift" (if they've specified a z in redshift that is higher
# that Z_HEAT_MAX, we add it back in below, and so they'll still get it).
redshifts = np.clip(redshifts, None, global_params.Z_HEAT_MAX)
else:
redshifts = [min(redshift)]
# Add in the redshift defined by the user, and sort in order
# Turn into a set so that exact matching user-set redshift
# don't double-up with scrolling ones.
redshifts = np.concatenate((redshifts, redshift))
redshifts = np.sort(np.unique(redshifts))[::-1]
return redshifts.tolist()
def run_lightcone(
*,
redshift=None,
max_redshift=None,
user_params=None,
cosmo_params=None,
astro_params=None,
flag_options=None,
regenerate=None,
write=None,
lightcone_quantities=("brightness_temp",),
global_quantities=("brightness_temp", "xH_box"),
direc=None,
init_box=None,
perturb=None,
random_seed=None,
coeval_callback=None,
coeval_callback_redshifts=1,
use_interp_perturb_field=False,
cleanup=True,
hooks=None,
always_purge: bool = False,
**global_kwargs,
):
r"""
Evaluate a full lightcone ending at a given redshift.
This is generally the easiest and most efficient way to generate a lightcone, though it can
be done manually by using the lower-level functions which are called by this function.
Parameters
----------
redshift : float
The minimum redshift of the lightcone.
max_redshift : float, optional
The maximum redshift at which to keep lightcone information. By default, this is equal to
`z_heat_max`. Note that this is not *exact*, but will be typically slightly exceeded.
user_params : `~UserParams`, optional
Defines the overall options and parameters of the run.
astro_params : :class:`~AstroParams`, optional
Defines the astrophysical parameters of the run.
cosmo_params : :class:`~CosmoParams`, optional
Defines the cosmological parameters used to compute initial conditions.
flag_options : :class:`~FlagOptions`, optional
Options concerning how the reionization process is run, eg. if spin temperature
fluctuations are required.
lightcone_quantities : tuple of str, optional
The quantities to form into a lightcone. By default, just the brightness
temperature. Note that these quantities must exist in one of the output
structures:
* :class:`~InitialConditions`
* :class:`~PerturbField`
* :class:`~TsBox`
* :class:`~IonizedBox`
* :class:`BrightnessTemp`
To get a full list of possible quantities, run :func:`get_all_fieldnames`.
global_quantities : tuple of str, optional
The quantities to save as globally-averaged redshift-dependent functions.
These may be any of the quantities that can be used in ``lightcone_quantities``.
The mean is taken over the full 3D cube at each redshift, rather than a 2D
slice.
init_box : :class:`~InitialConditions`, optional
If given, the user and cosmo params will be set from this object, and it will not be
re-calculated.
perturb : list of :class:`~PerturbedField`, optional
If given, must be compatible with init_box. It will merely negate the necessity of
re-calculating the
perturb fields. It will also be used to set the redshift if given.
coeval_callback : callable, optional
User-defined arbitrary function computed on :class:`~Coeval`, at redshifts defined in
`coeval_callback_redshifts`.
If given, the function returns :class:`~LightCone` and the list of `coeval_callback` outputs.
coeval_callback_redshifts : list or int, optional
Redshifts for `coeval_callback` computation.
If list, computes the function on `node_redshifts` closest to the specified ones.
If positive integer, computes the function on every n-th redshift in `node_redshifts`.
Ignored in the case `coeval_callback is None`.
use_interp_perturb_field : bool, optional
Whether to use a single perturb field, at the lowest redshift of the lightcone,
to determine all spin temperature fields. If so, this field is interpolated in the
underlying C-code to the correct redshift. This is less accurate (and no more efficient),
but provides compatibility with older versions of 21cmFAST.
cleanup : bool, optional
A flag to specify whether the C routine cleans up its memory before returning.
Typically, if `spin_temperature` is called directly, you will want this to be
true, as if the next box to be calculate has different shape, errors will occur
if memory is not cleaned. Note that internally, this is set to False until the
last iteration.
minimize_memory_usage
If switched on, the routine will do all it can to minimize peak memory usage.
This will be at the cost of disk I/O and CPU time. Recommended to only set this
if you are running particularly large boxes, or have low RAM.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Returns
-------
lightcone : :class:`~py21cmfast.LightCone`
The lightcone object.
coeval_callback_output : list
Only if coeval_callback in not None.
Other Parameters
----------------
regenerate, write, direc, random_seed
See docs of :func:`initial_conditions` for more information.
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, hooks)
with global_params.use(**global_kwargs):
(
random_seed,
user_params,
cosmo_params,
flag_options,
astro_params,
redshift,
) = _setup_inputs(
{
"random_seed": random_seed,
"user_params": user_params,
"cosmo_params": cosmo_params,
"flag_options": flag_options,
"astro_params": astro_params,
},
{"init_box": init_box, "perturb": perturb},
redshift=redshift,
)
if user_params.MINIMIZE_MEMORY and not write:
raise ValueError(
"If trying to minimize memory usage, you must be caching. Set write=True!"
)
# Ensure passed quantities are appropriate
_fld_names = _get_interpolation_outputs(
list(lightcone_quantities), list(global_quantities), flag_options
)
max_redshift = (
global_params.Z_HEAT_MAX
if (
flag_options.INHOMO_RECO
or flag_options.USE_TS_FLUCT
or max_redshift is None
)
else max_redshift
)
# Get the redshift through which we scroll and evaluate the ionization field.
scrollz = _logscroll_redshifts(
redshift, global_params.ZPRIME_STEP_FACTOR, max_redshift
)
if (
flag_options.PHOTON_CONS
and np.amin(scrollz) < global_params.PhotonConsEndCalibz
):
raise ValueError(
f"""
You have passed a redshift (z = {np.amin(scrollz)}) that is lower than the endpoint
of the photon non-conservation correction
(global_params.PhotonConsEndCalibz = {global_params.PhotonConsEndCalibz}).
If this behaviour is desired then set global_params.PhotonConsEndCalibz to a value lower than
z = {np.amin(scrollz)}.
"""
)
coeval_callback_output = []
compute_coeval_callback = _get_coeval_callbacks(
scrollz, coeval_callback, coeval_callback_redshifts
)
if init_box is None: # no need to get cosmo, user params out of it.
init_box = initial_conditions(
user_params=user_params,
cosmo_params=cosmo_params,
hooks=hooks,
regenerate=regenerate,
direc=direc,
random_seed=random_seed,
)
# We can go ahead and purge some of the stuff in the init_box, but only if
# it is cached -- otherwise we could be losing information.
try:
# TODO: should really check that the file at path actually contains a fully
# working copy of the init_box.
init_box.prepare_for_perturb(flag_options=flag_options, force=always_purge)
except OSError:
pass
if perturb is None:
zz = scrollz
else:
zz = scrollz[:-1]
perturb_ = []
for z in zz:
p = perturb_field(
redshift=z,
init_boxes=init_box,
regenerate=regenerate,
direc=direc,
hooks=hooks,
)
if user_params.MINIMIZE_MEMORY:
try:
p.purge(force=always_purge)
except OSError:
pass
perturb_.append(p)
if perturb is not None:
perturb_.append(perturb)
perturb = perturb_
perturb_min = perturb[np.argmin(scrollz)]
# Now that we've got all the perturb fields, we can purge init more.
try:
init_box.prepare_for_spin_temp(
flag_options=flag_options, force=always_purge
)
except OSError:
pass
if flag_options.PHOTON_CONS:
calibrate_photon_cons(
user_params,
cosmo_params,
astro_params,
flag_options,
init_box,
regenerate,
write,
direc,
)
d_at_redshift, lc_distances, n_lightcone = _setup_lightcone(
cosmo_params,
max_redshift,
redshift,
scrollz,
user_params,
global_params.ZPRIME_STEP_FACTOR,
)
scroll_distances = (
cosmo_params.cosmo.comoving_distance(scrollz).value - d_at_redshift
)
# Iterate through redshift from top to bottom
st, ib, bt, prev_perturb = None, None, None, None
lc_index = 0
box_index = 0
lc = {
quantity: np.zeros(
(user_params.HII_DIM, user_params.HII_DIM, n_lightcone),
dtype=np.float32,
)
for quantity in lightcone_quantities
}
interp_functions = {
"z_re_box": "mean_max",
}
global_q = {quantity: np.zeros(len(scrollz)) for quantity in global_quantities}
pf = None
perturb_files = []
spin_temp_files = []
ionize_files = []
brightness_files = []
log10_mturnovers = np.zeros(len(scrollz))
log10_mturnovers_mini = np.zeros(len(scrollz))
for iz, z in enumerate(scrollz):
# Best to get a perturb for this redshift, to pass to brightness_temperature
pf2 = perturb[iz]
# This ensures that all the arrays that are required for spin_temp are there,
# in case we dumped them from memory into file.
pf2.load_all()
if flag_options.USE_HALO_FIELD:
halo_field = determine_halo_list(
redshift=z,
init_boxes=init_box,
astro_params=astro_params,
flag_options=flag_options,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
pt_halos = perturb_halo_list(
redshift=z,
init_boxes=init_box,
astro_params=astro_params,
flag_options=flag_options,
halo_field=halo_field,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
if flag_options.USE_TS_FLUCT:
st2 = spin_temperature(
redshift=z,
previous_spin_temp=st,
astro_params=astro_params,
flag_options=flag_options,
perturbed_field=perturb_min if use_interp_perturb_field else pf2,
regenerate=regenerate,
init_boxes=init_box,
hooks=hooks,
direc=direc,
cleanup=(cleanup and iz == (len(scrollz) - 1)),
)
ib2 = ionize_box(
redshift=z,
previous_ionize_box=ib,
init_boxes=init_box,
perturbed_field=pf2,
previous_perturbed_field=prev_perturb,
astro_params=astro_params,
flag_options=flag_options,
spin_temp=st2 if flag_options.USE_TS_FLUCT else None,
pt_halos=pt_halos if flag_options.USE_HALO_FIELD else None,
regenerate=regenerate,
hooks=hooks,
direc=direc,
cleanup=(cleanup and iz == (len(scrollz) - 1)),
)
log10_mturnovers[iz] = ib2.log10_Mturnover_ave
log10_mturnovers_mini[iz] = ib2.log10_Mturnover_MINI_ave
bt2 = brightness_temperature(
ionized_box=ib2,
perturbed_field=pf2,
spin_temp=st2 if flag_options.USE_TS_FLUCT else None,
hooks=hooks,
direc=direc,
regenerate=regenerate,
)
if coeval_callback is not None and compute_coeval_callback[iz]:
coeval = Coeval(
redshift=z,
initial_conditions=init_box,
perturbed_field=pf2,
ionized_box=ib2,
brightness_temp=bt2,
ts_box=st2 if flag_options.USE_TS_FLUCT else None,
photon_nonconservation_data=_get_photon_nonconservation_data()
if flag_options.PHOTON_CONS
else None,
_globals=None,
)
try:
coeval_callback_output.append(coeval_callback(coeval))
except Exception as e:
if sum(compute_coeval_callback[: iz + 1]) == 1:
raise RuntimeError(
f"coeval_callback computation failed on first trial, z={z}."
)
else:
logger.warning(
f"coeval_callback computation failed on z={z}, skipping. {type(e).__name__}: {e}"
)
perturb_files.append((z, os.path.join(direc, pf2.filename)))
if flag_options.USE_TS_FLUCT:
spin_temp_files.append((z, os.path.join(direc, st2.filename)))
ionize_files.append((z, os.path.join(direc, ib2.filename)))
brightness_files.append((z, os.path.join(direc, bt2.filename)))
outs = {
"PerturbedField": (pf, pf2),
"IonizedBox": (ib, ib2),
"BrightnessTemp": (bt, bt2),
}
if flag_options.USE_TS_FLUCT:
outs["TsBox"] = (st, st2)
if flag_options.USE_HALO_FIELD:
outs["PerturbHaloes"] = pt_halos
# Save mean/global quantities
for quantity in global_quantities:
global_q[quantity][iz] = np.mean(
getattr(outs[_fld_names[quantity]][1], quantity)
)
# Interpolate the lightcone
if z < max_redshift:
for quantity in lightcone_quantities:
data1, data2 = outs[_fld_names[quantity]]
fnc = interp_functions.get(quantity, "mean")
n = _interpolate_in_redshift(
iz,
box_index,
lc_index,
n_lightcone,
scroll_distances,
lc_distances,
data1,
data2,
quantity,
lc[quantity],
fnc,
)
lc_index += n
box_index += n
# Save current ones as old ones.
if flag_options.USE_TS_FLUCT:
st = st2
ib = ib2
bt = bt2
if flag_options.USE_MINI_HALOS:
prev_perturb = pf2
if pf is not None:
try:
pf.purge(force=always_purge)
except OSError:
pass
pf = pf2
if flag_options.PHOTON_CONS:
photon_nonconservation_data = _get_photon_nonconservation_data()
if photon_nonconservation_data:
lib.FreePhotonConsMemory()
else:
photon_nonconservation_data = None
if (
flag_options.USE_TS_FLUCT
and user_params.USE_INTERPOLATION_TABLES
and lib.interpolation_tables_allocated
):
lib.FreeTsInterpolationTables(flag_options())
out = (
LightCone(
redshift,
user_params,
cosmo_params,
astro_params,
flag_options,
init_box.random_seed,
lc,
node_redshifts=scrollz,
global_quantities=global_q,
photon_nonconservation_data=photon_nonconservation_data,
_globals=dict(global_params.items()),
cache_files={
"init": [(0, os.path.join(direc, init_box.filename))],
"perturb_field": perturb_files,
"ionized_box": ionize_files,
"brightness_temp": brightness_files,
"spin_temp": spin_temp_files,
},
log10_mturnovers=log10_mturnovers,
log10_mturnovers_mini=log10_mturnovers_mini,
),
coeval_callback_output,
)
if coeval_callback is None:
return out[0]
else:
return out
def _get_coeval_callbacks(
scrollz: list[float], coeval_callback, coeval_callback_redshifts
) -> list[bool]:
compute_coeval_callback = [False for i in range(len(scrollz))]
if coeval_callback is not None:
if isinstance(coeval_callback_redshifts, (list, np.ndarray)):
for coeval_z in coeval_callback_redshifts:
assert isinstance(coeval_z, (int, float, np.number))
compute_coeval_callback[
np.argmin(np.abs(np.array(scrollz) - coeval_z))
] = True
if sum(compute_coeval_callback) != len(coeval_callback_redshifts):
logger.warning(
"some of the coeval_callback_redshifts refer to the same node_redshift"
)
elif (
isinstance(coeval_callback_redshifts, int) and coeval_callback_redshifts > 0
):
compute_coeval_callback = [
not i % coeval_callback_redshifts for i in range(len(scrollz))
]
else:
raise ValueError("coeval_callback_redshifts has to be list or integer > 0.")
return compute_coeval_callback
def _get_interpolation_outputs(
lightcone_quantities: Sequence,
global_quantities: Sequence,
flag_options: FlagOptions,
) -> dict[str, str]:
_fld_names = get_all_fieldnames(arrays_only=True, lightcone_only=True, as_dict=True)
incorrect_lc = [q for q in lightcone_quantities if q not in _fld_names.keys()]
if incorrect_lc:
raise ValueError(
f"The following lightcone_quantities are not available: {incorrect_lc}"
)
incorrect_gl = [q for q in global_quantities if q not in _fld_names.keys()]
if incorrect_gl:
raise ValueError(
f"The following global_quantities are not available: {incorrect_gl}"
)
if not flag_options.USE_TS_FLUCT and any(
_fld_names[q] == "TsBox" for q in lightcone_quantities + global_quantities
):
raise ValueError(
"TsBox quantity found in lightcone_quantities or global_quantities, "
"but not running spin_temp!"
)
return _fld_names
def _interpolate_in_redshift(
z_index,
box_index,
lc_index,
n_lightcone,
scroll_distances,
lc_distances,
output_obj,
output_obj2,
quantity,
lc,
kind="mean",
):
try:
array = getattr(output_obj, quantity)
array2 = getattr(output_obj2, quantity)
except AttributeError:
raise AttributeError(
f"{quantity} is not a valid field of {output_obj.__class__.__name__}"
)
assert array.__class__ == array2.__class__
# Do linear interpolation only.
prev_d = scroll_distances[z_index - 1]
this_d = scroll_distances[z_index]
# Get the cells that need to be filled on this iteration.
these_distances = lc_distances[
np.logical_and(lc_distances < prev_d, lc_distances >= this_d)
]
n = len(these_distances)
ind = np.arange(-(box_index + n), -box_index)
sub_array = array.take(ind + n_lightcone, axis=2, mode="wrap")
sub_array2 = array2.take(ind + n_lightcone, axis=2, mode="wrap")
out = (
np.abs(this_d - these_distances) * sub_array
+ np.abs(prev_d - these_distances) * sub_array2
) / (np.abs(prev_d - this_d))
if kind == "mean_max":
flag = sub_array * sub_array2 < 0
out[flag] = np.maximum(sub_array, sub_array2)[flag]
elif kind != "mean":
raise ValueError("kind must be 'mean' or 'mean_max'")
lc[:, :, -(lc_index + n) : n_lightcone - lc_index] = out
return n
def _setup_lightcone(
cosmo_params, max_redshift, redshift, scrollz, user_params, z_step_factor
):
# Here set up the lightcone box.
# Get a length of the lightcone (bigger than it needs to be at first).
d_at_redshift = cosmo_params.cosmo.comoving_distance(redshift).value
Ltotal = (
cosmo_params.cosmo.comoving_distance(scrollz[0] * z_step_factor).value
- d_at_redshift
)
lc_distances = np.arange(0, Ltotal, user_params.BOX_LEN / user_params.HII_DIM)
# Use max_redshift to get the actual distances we require.
Lmax = cosmo_params.cosmo.comoving_distance(max_redshift).value - d_at_redshift
first_greater = np.argwhere(lc_distances > Lmax)[0][0]
# Get *at least* as far as max_redshift
lc_distances = lc_distances[: (first_greater + 1)]
n_lightcone = len(lc_distances)
return d_at_redshift, lc_distances, n_lightcone
def _get_lightcone_redshifts(
cosmo_params, max_redshift, redshift, user_params, z_step_factor
):
scrollz = _logscroll_redshifts(redshift, z_step_factor, max_redshift)
lc_distances = _setup_lightcone(
cosmo_params, max_redshift, redshift, scrollz, user_params, z_step_factor
)[1]
lc_distances += cosmo_params.cosmo.comoving_distance(redshift).value
return np.array(
[
z_at_value(cosmo_params.cosmo.comoving_distance, d * units.Mpc)
for d in lc_distances
]
)
def calibrate_photon_cons(
user_params,
cosmo_params,
astro_params,
flag_options,
init_box,
regenerate,
write,
direc,
**global_kwargs,
):
r"""
Set up the photon non-conservation correction.
Scrolls through in redshift, turning off all flag_options to construct a 21cmFAST calibration
reionisation history to be matched to the analytic expression from solving the filling factor
ODE.
Parameters
----------
user_params : `~UserParams`, optional
Defines the overall options and parameters of the run.
astro_params : :class:`~AstroParams`, optional
Defines the astrophysical parameters of the run.
cosmo_params : :class:`~CosmoParams`, optional
Defines the cosmological parameters used to compute initial conditions.
flag_options: :class:`~FlagOptions`, optional
Options concerning how the reionization process is run, eg. if spin temperature
fluctuations are required.
init_box : :class:`~InitialConditions`, optional
If given, the user and cosmo params will be set from this object, and it will not be
re-calculated.
\*\*global_kwargs :
Any attributes for :class:`~py21cmfast.inputs.GlobalParams`. This will
*temporarily* set global attributes for the duration of the function. Note that
arguments will be treated as case-insensitive.
Other Parameters
----------------
regenerate, write
See docs of :func:`initial_conditions` for more information.
"""
direc, regenerate, hooks = _get_config_options(direc, regenerate, write, {})
if not flag_options.PHOTON_CONS:
return
with global_params.use(**global_kwargs):
# Create a new astro_params and flag_options just for the photon_cons correction
astro_params_photoncons = deepcopy(astro_params)
astro_params_photoncons._R_BUBBLE_MAX = astro_params.R_BUBBLE_MAX
flag_options_photoncons = FlagOptions(
USE_MASS_DEPENDENT_ZETA=flag_options.USE_MASS_DEPENDENT_ZETA,
M_MIN_in_Mass=flag_options.M_MIN_in_Mass,
USE_VELS_AUX=user_params.USE_RELATIVE_VELOCITIES,
)
ib = None
prev_perturb = None
# Arrays for redshift and neutral fraction for the calibration curve
z_for_photon_cons = []
neutral_fraction_photon_cons = []
# Initialise the analytic expression for the reionisation history
logger.info("About to start photon conservation correction")
_init_photon_conservation_correction(
user_params=user_params,
cosmo_params=cosmo_params,
astro_params=astro_params,
flag_options=flag_options,
)
# Determine the starting redshift to start scrolling through to create the
# calibration reionisation history
logger.info("Calculating photon conservation zstart")
z = _calc_zstart_photon_cons()
while z > global_params.PhotonConsEndCalibz:
# Determine the ionisation box with recombinations, spin temperature etc.
# turned off.
this_perturb = perturb_field(
redshift=z,
init_boxes=init_box,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
ib2 = ionize_box(
redshift=z,
previous_ionize_box=ib,
init_boxes=init_box,
perturbed_field=this_perturb,
previous_perturbed_field=prev_perturb,
astro_params=astro_params_photoncons,
flag_options=flag_options_photoncons,
spin_temp=None,
regenerate=regenerate,
hooks=hooks,
direc=direc,
)
mean_nf = np.mean(ib2.xH_box)
# Save mean/global quantities
neutral_fraction_photon_cons.append(mean_nf)
z_for_photon_cons.append(z)
# Can speed up sampling in regions where the evolution is slower
if 0.3 < mean_nf <= 0.9:
z -= 0.15
elif 0.01 < mean_nf <= 0.3:
z -= 0.05
else:
z -= 0.5
ib = ib2
if flag_options.USE_MINI_HALOS:
prev_perturb = this_perturb
z_for_photon_cons = np.array(z_for_photon_cons[::-1])
neutral_fraction_photon_cons = np.array(neutral_fraction_photon_cons[::-1])
# Construct the spline for the calibration curve
logger.info("Calibrating photon conservation correction")
_calibrate_photon_conservation_correction(
redshifts_estimate=z_for_photon_cons,
nf_estimate=neutral_fraction_photon_cons,
NSpline=len(z_for_photon_cons),
) | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/src/py21cmfast/wrapper.py | wrapper.py |
import glob
import h5py
import logging
import os
import re
from os import path
from . import outputs, wrapper
from ._cfg import config
from .wrapper import global_params
logger = logging.getLogger("21cmFAST")
def readbox(
*,
direc=None,
fname=None,
hsh=None,
kind=None,
seed=None,
redshift=None,
load_data=True,
):
"""
Read in a data set and return an appropriate object for it.
Parameters
----------
direc : str, optional
The directory in which to search for the boxes. By default, this is the
centrally-managed directory, given by the ``config.yml`` in ``~/.21cmfast/``.
fname: str, optional
The filename (without directory) of the data set. If given, this will be
preferentially used, and must exist.
hsh: str, optional
The md5 hsh of the object desired to be read. Required if `fname` not given.
kind: str, optional
The kind of dataset, eg. "InitialConditions". Will be the name of a class
defined in :mod:`~wrapper`. Required if `fname` not given.
seed: str or int, optional
The random seed of the data set to be read. If not given, and filename not
given, then a box will be read if it matches the kind and hsh, with an
arbitrary seed.
load_data: bool, optional
Whether to read in the data in the data set. Otherwise, only its defining
parameters are read.
Returns
-------
dataset :
An output object, whose type depends on the kind of data set being read.
Raises
------
IOError :
If no files exist of the given kind and hsh.
ValueError :
If either ``fname`` is not supplied, or both ``kind`` and ``hsh`` are not supplied.
"""
direc = path.expanduser(direc or config["direc"])
if not (fname or (hsh and kind)):
raise ValueError("Either fname must be supplied, or kind and hsh")
zstr = f"z{redshift:.4f}_" if redshift is not None else ""
if not fname:
if not seed:
fname = kind + "_" + zstr + hsh + "_r*.h5"
files = glob.glob(path.join(direc, fname))
if files:
fname = files[0]
else:
raise OSError("No files exist with that kind and hsh.")
else:
fname = kind + "_" + zstr + hsh + "_r" + str(seed) + ".h5"
kind = _parse_fname(fname)["kind"]
cls = getattr(outputs, kind)
if hasattr(cls, "from_file"):
inst = cls.from_file(fname, direc=direc, load_data=load_data)
else:
inst = cls.read(fname, direc=direc)
return inst
def _parse_fname(fname):
patterns = (
r"(?P<kind>\w+)_(?P<hash>\w{32})_r(?P<seed>\d+).h5$",
r"(?P<kind>\w+)_z(?P<redshift>\d+.\d{1,4})_(?P<hash>\w{32})_r(?P<seed>\d+).h5$",
)
for pattern in patterns:
match = re.match(pattern, os.path.basename(fname))
if match:
break
if not match:
raise ValueError(
"filename {} does not have correct format for a cached output.".format(
fname
)
)
return match.groupdict()
def list_datasets(*, direc=None, kind=None, hsh=None, seed=None, redshift=None):
"""Yield all datasets which match a given set of filters.
Can be used to determine parameters of all cached datasets, in conjunction with :func:`readbox`.
Parameters
----------
direc : str, optional
The directory in which to search for the boxes. By default, this is the centrally-managed
directory, given by the ``config.yml`` in ``.21cmfast``.
kind: str, optional
Filter by this kind (one of {"InitialConditions", "PerturbedField", "IonizedBox",
"TsBox", "BrightnessTemp"}
hsh: str, optional
Filter by this hsh.
seed: str, optional
Filter by this seed.
Yields
------
fname: str
The filename of the dataset (without directory).
parts: tuple of strings
The (kind, hsh, seed) of the data set.
"""
direc = path.expanduser(direc or config["direc"])
fname = "{}{}_{}_r{}.h5".format(
kind or r"(?P<kind>[a-zA-Z]+)",
f"_z{redshift:.4f}" if redshift is not None else "(.*)",
hsh or r"(?P<hash>\w{32})",
seed or r"(?P<seed>\d+)",
)
for fl in os.listdir(direc):
if re.match(fname, fl):
yield fl
def query_cache(
*, direc=None, kind=None, hsh=None, seed=None, redshift=None, show=True
):
"""Get or print datasets in the cache.
Walks through the cache, with given filters, and return all un-initialised dataset
objects, optionally printing their representation to screen.
Useful for querying which kinds of datasets are available within the cache, and
choosing one to read and use.
Parameters
----------
direc : str, optional
The directory in which to search for the boxes. By default, this is the
centrally-managed directory, given by the ``config.yml`` in ``~/.21cmfast``.
kind: str, optional
Filter by this kind. Must be one of "InitialConditions", "PerturbedField",
"IonizedBox", "TsBox" or "BrightnessTemp".
hsh: str, optional
Filter by this hsh.
seed: str, optional
Filter by this seed.
show: bool, optional
Whether to print out a repr of each object that exists.
Yields
------
obj:
Output objects, un-initialized.
"""
for file in list_datasets(
direc=direc, kind=kind, hsh=hsh, seed=seed, redshift=redshift
):
cls = readbox(direc=direc, fname=file, load_data=False)
if show:
print(file + ": " + str(cls)) # noqa: T
yield file, cls
def clear_cache(**kwargs):
"""Delete datasets in the cache.
Walks through the cache, with given filters, and deletes all un-initialised dataset
objects, optionally printing their representation to screen.
Parameters
----------
kwargs :
All options passed through to :func:`query_cache`.
"""
if "show" not in kwargs:
kwargs["show"] = False
direc = kwargs.get("direc", path.expanduser(config["direc"]))
number = 0
for fname, _ in query_cache(**kwargs):
if kwargs.get("show", True):
logger.info(f"Removing {fname}")
os.remove(path.join(direc, fname))
number += 1
logger.info(f"Removed {number} files from cache.") | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/src/py21cmfast/cache_tools.py | cache_tools.py |
from __future__ import annotations
import h5py
import numpy as np
import os
import warnings
from astropy import units
from astropy.cosmology import z_at_value
from cached_property import cached_property
from hashlib import md5
from pathlib import Path
from typing import Dict, List, Optional, Sequence, Tuple, Union
from . import __version__
from . import _utils as _ut
from ._cfg import config
from ._utils import OutputStruct as _BaseOutputStruct
from ._utils import _check_compatible_inputs
from .c_21cmfast import ffi, lib
from .inputs import AstroParams, CosmoParams, FlagOptions, UserParams, global_params
class _OutputStruct(_BaseOutputStruct):
_global_params = global_params
def __init__(self, *, user_params=None, cosmo_params=None, **kwargs):
self.cosmo_params = cosmo_params or CosmoParams()
self.user_params = user_params or UserParams()
super().__init__(**kwargs)
_ffi = ffi
class _OutputStructZ(_OutputStruct):
_inputs = _OutputStruct._inputs + ("redshift",)
class InitialConditions(_OutputStruct):
"""A class containing all initial conditions boxes."""
_c_compute_function = lib.ComputeInitialConditions
# The filter params indicates parameters to overlook when deciding if a cached box
# matches current parameters.
# It is useful for ignoring certain global parameters which may not apply to this
# step or its dependents.
_meta = False
_filter_params = _OutputStruct._filter_params + [
"ALPHA_UVB", # ionization
"EVOLVE_DENSITY_LINEARLY", # perturb
"SMOOTH_EVOLVED_DENSITY_FIELD", # perturb
"R_smooth_density", # perturb
"HII_ROUND_ERR", # ionization
"FIND_BUBBLE_ALGORITHM", # ib
"N_POISSON", # ib
"T_USE_VELOCITIES", # bt
"MAX_DVDR", # bt
"DELTA_R_HII_FACTOR", # ib
"HII_FILTER", # ib
"INITIAL_REDSHIFT", # pf
"HEAT_FILTER", # st
"CLUMPING_FACTOR", # st
"Z_HEAT_MAX", # st
"R_XLy_MAX", # st
"NUM_FILTER_STEPS_FOR_Ts", # ts
"ZPRIME_STEP_FACTOR", # ts
"TK_at_Z_HEAT_MAX", # ts
"XION_at_Z_HEAT_MAX", # ts
"Pop", # ib
"Pop2_ion", # ib
"Pop3_ion", # ib
"NU_X_BAND_MAX", # st
"NU_X_MAX", # ib
]
def prepare_for_perturb(self, flag_options: FlagOptions, force: bool = False):
"""Ensure the ICs have all the boxes loaded for perturb, but no extra."""
keep = ["hires_density"]
if not self.user_params.PERTURB_ON_HIGH_RES:
keep.append("lowres_density")
keep.append("lowres_vx")
keep.append("lowres_vy")
keep.append("lowres_vz")
if self.user_params.USE_2LPT:
keep.append("lowres_vx_2LPT")
keep.append("lowres_vy_2LPT")
keep.append("lowres_vz_2LPT")
if flag_options.USE_HALO_FIELD:
keep.append("hires_density")
else:
keep.append("hires_vx")
keep.append("hires_vy")
keep.append("hires_vz")
if self.user_params.USE_2LPT:
keep.append("hires_vx_2LPT")
keep.append("hires_vy_2LPT")
keep.append("hires_vz_2LPT")
if self.user_params.USE_RELATIVE_VELOCITIES:
keep.append("lowres_vcb")
self.prepare(keep=keep, force=force)
def prepare_for_spin_temp(self, flag_options: FlagOptions, force: bool = False):
"""Ensure ICs have all boxes required for spin_temp, and no more."""
keep = []
if self.user_params.USE_RELATIVE_VELOCITIES:
keep.append("lowres_vcb")
self.prepare(keep=keep, force=force)
def _get_box_structures(self) -> dict[str, dict | tuple[int]]:
shape = (self.user_params.HII_DIM,) * 2 + (
int(self.user_params.NON_CUBIC_FACTOR * self.user_params.HII_DIM),
)
hires_shape = (self.user_params.DIM,) * 2 + (
int(self.user_params.NON_CUBIC_FACTOR * self.user_params.DIM),
)
out = {
"lowres_density": shape,
"lowres_vx": shape,
"lowres_vy": shape,
"lowres_vz": shape,
"hires_density": hires_shape,
"hires_vx": hires_shape,
"hires_vy": hires_shape,
"hires_vz": hires_shape,
}
if self.user_params.USE_2LPT:
out.update(
{
"lowres_vx_2LPT": shape,
"lowres_vy_2LPT": shape,
"lowres_vz_2LPT": shape,
"hires_vx_2LPT": hires_shape,
"hires_vy_2LPT": hires_shape,
"hires_vz_2LPT": hires_shape,
}
)
if self.user_params.USE_RELATIVE_VELOCITIES:
out.update({"lowres_vcb": shape})
return out
def get_required_input_arrays(self, input_box: _BaseOutputStruct) -> list[str]:
"""Return all input arrays required to compute this object."""
return []
def compute(self, hooks: dict):
"""Compute the function."""
return self._compute(
self.random_seed,
self.user_params,
self.cosmo_params,
hooks=hooks,
)
class PerturbedField(_OutputStructZ):
"""A class containing all perturbed field boxes."""
_c_compute_function = lib.ComputePerturbField
_meta = False
_filter_params = _OutputStruct._filter_params + [
"ALPHA_UVB", # ionization
"HII_ROUND_ERR", # ionization
"FIND_BUBBLE_ALGORITHM", # ib
"N_POISSON", # ib
"T_USE_VELOCITIES", # bt
"MAX_DVDR", # bt
"DELTA_R_HII_FACTOR", # ib
"HII_FILTER", # ib
"HEAT_FILTER", # st
"CLUMPING_FACTOR", # st
"Z_HEAT_MAX", # st
"R_XLy_MAX", # st
"NUM_FILTER_STEPS_FOR_Ts", # ts
"ZPRIME_STEP_FACTOR", # ts
"TK_at_Z_HEAT_MAX", # ts
"XION_at_Z_HEAT_MAX", # ts
"Pop", # ib
"Pop2_ion", # ib
"Pop3_ion", # ib
"NU_X_BAND_MAX", # st
"NU_X_MAX", # ib
]
def _get_box_structures(self) -> dict[str, dict | tuple[int]]:
return {
"density": (self.user_params.HII_DIM,) * 2
+ (int(self.user_params.NON_CUBIC_FACTOR * self.user_params.HII_DIM),),
"velocity": (self.user_params.HII_DIM,) * 2
+ (int(self.user_params.NON_CUBIC_FACTOR * self.user_params.HII_DIM),),
}
def get_required_input_arrays(self, input_box: _BaseOutputStruct) -> list[str]:
"""Return all input arrays required to compute this object."""
required = []
if not isinstance(input_box, InitialConditions):
raise ValueError(
f"{type(input_box)} is not an input required for PerturbedField!"
)
# Always require hires_density
required += ["hires_density"]
if self.user_params.PERTURB_ON_HIGH_RES:
required += ["hires_vx", "hires_vy", "hires_vz"]
if self.user_params.USE_2LPT:
required += ["hires_vx_2LPT", "hires_vy_2LPT", "hires_vz_2LPT"]
else:
required += ["lowres_density", "lowres_vx", "lowres_vy", "lowres_vz"]
if self.user_params.USE_2LPT:
required += [
"lowres_vx_2LPT",
"lowres_vy_2LPT",
"lowres_vz_2LPT",
]
if self.user_params.USE_RELATIVE_VELOCITIES:
required.append("lowres_vcb")
return required
def compute(self, *, ics: InitialConditions, hooks: dict):
"""Compute the function."""
return self._compute(
self.redshift,
self.user_params,
self.cosmo_params,
ics,
hooks=hooks,
)
class _AllParamsBox(_OutputStructZ):
_meta = True
_inputs = _OutputStructZ._inputs + ("flag_options", "astro_params")
_filter_params = _OutputStruct._filter_params + [
"T_USE_VELOCITIES", # bt
"MAX_DVDR", # bt
]
def __init__(
self,
*,
astro_params: AstroParams | None = None,
flag_options: FlagOptions | None = None,
**kwargs,
):
self.flag_options = flag_options or FlagOptions()
self.astro_params = astro_params or AstroParams(
INHOMO_RECO=self.flag_options.INHOMO_RECO
)
self.log10_Mturnover_ave = 0.0
self.log10_Mturnover_MINI_ave = 0.0
super().__init__(**kwargs)
class HaloField(_AllParamsBox):
"""A class containing all fields related to halos."""
_c_based_pointers = (
"halo_masses",
"halo_coords",
"mass_bins",
"fgtrm",
"sqrt_dfgtrm",
"dndlm",
"sqrtdn_dlm",
)
_c_compute_function = lib.ComputeHaloField
def _get_box_structures(self) -> dict[str, dict | tuple[int]]:
return {}
def _c_shape(self, cstruct):
return {
"halo_masses": (cstruct.n_halos,),
"halo_coords": (cstruct.n_halos, 3),
"mass_bins": (cstruct.n_mass_bins,),
"fgtrm": (cstruct.n_mass_bins,),
"sqrt_dfgtrm": (cstruct.n_mass_bins,),
"dndlm": (cstruct.n_mass_bins,),
"sqrtdn_dlm": (cstruct.n_mass_bins,),
}
def get_required_input_arrays(self, input_box: _BaseOutputStruct) -> list[str]:
"""Return all input arrays required to compute this object."""
if isinstance(input_box, InitialConditions):
return ["hires_density"]
else:
raise ValueError(
f"{type(input_box)} is not an input required for HaloField!"
)
def compute(self, *, ics: InitialConditions, hooks: dict):
"""Compute the function."""
return self._compute(
self.redshift,
self.user_params,
self.cosmo_params,
self.astro_params,
self.flag_options,
ics,
hooks=hooks,
)
class PerturbHaloField(_AllParamsBox):
"""A class containing all fields related to halos."""
_c_compute_function = lib.ComputePerturbHaloField
_c_based_pointers = ("halo_masses", "halo_coords")
def _get_box_structures(self) -> dict[str, dict | tuple[int]]:
return {}
def _c_shape(self, cstruct):
return {
"halo_masses": (cstruct.n_halos,),
"halo_coords": (cstruct.n_halos, 3),
}
def get_required_input_arrays(self, input_box: _BaseOutputStruct) -> list[str]:
"""Return all input arrays required to compute this object."""
required = []
if isinstance(input_box, InitialConditions):
if self.user_params.PERTURB_ON_HIGH_RES:
required += ["hires_vx", "hires_vy", "hires_vz"]
else:
required += ["lowres_vx", "lowres_vy", "lowres_vz"]
if self.user_params.USE_2LPT:
required += [k + "_2LPT" for k in required]
elif isinstance(input_box, HaloField):
required += ["halo_coords", "halo_masses"]
else:
raise ValueError(
f"{type(input_box)} is not an input required for PerturbHaloField!"
)
return required
def compute(self, *, ics: InitialConditions, halo_field: HaloField, hooks: dict):
"""Compute the function."""
return self._compute(
self.redshift,
self.user_params,
self.cosmo_params,
self.astro_params,
self.flag_options,
ics,
halo_field,
hooks=hooks,
)
class TsBox(_AllParamsBox):
"""A class containing all spin temperature boxes."""
_c_compute_function = lib.ComputeTsBox
_meta = False
_inputs = _AllParamsBox._inputs + ("prev_spin_redshift", "perturbed_field_redshift")
def __init__(
self,
*,
prev_spin_redshift: float | None = None,
perturbed_field_redshift: float | None = None,
**kwargs,
):
self.prev_spin_redshift = prev_spin_redshift
self.perturbed_field_redshift = perturbed_field_redshift
super().__init__(**kwargs)
def _get_box_structures(self) -> dict[str, dict | tuple[int]]:
shape = (self.user_params.HII_DIM,) * 2 + (
int(self.user_params.NON_CUBIC_FACTOR * self.user_params.HII_DIM),
)
return {
"Ts_box": shape,
"x_e_box": shape,
"Tk_box": shape,
"J_21_LW_box": shape,
}
@cached_property
def global_Ts(self):
"""Global (mean) spin temperature."""
if "Ts_box" not in self._computed_arrays:
raise AttributeError(
"global_Ts is not defined until the ionization calculation has been performed"
)
else:
return np.mean(self.Ts_box)
@cached_property
def global_Tk(self):
"""Global (mean) Tk."""
if "Tk_box" not in self._computed_arrays:
raise AttributeError(
"global_Tk is not defined until the ionization calculation has been performed"
)
else:
return np.mean(self.Tk_box)
@cached_property
def global_x_e(self):
"""Global (mean) x_e."""
if "x_e_box" not in self._computed_arrays:
raise AttributeError(
"global_x_e is not defined until the ionization calculation has been performed"
)
else:
return np.mean(self.x_e_box)
def get_required_input_arrays(self, input_box: _BaseOutputStruct) -> list[str]:
"""Return all input arrays required to compute this object."""
required = []
if isinstance(input_box, InitialConditions):
if (
self.user_params.USE_RELATIVE_VELOCITIES
and self.flag_options.USE_MINI_HALOS
):
required += ["lowres_vcb"]
elif isinstance(input_box, PerturbedField):
required += ["density"]
elif isinstance(input_box, TsBox):
required += [
"Tk_box",
"x_e_box",
]
if self.flag_options.USE_MINI_HALOS:
required += ["J_21_LW_box"]
else:
raise ValueError(
f"{type(input_box)} is not an input required for PerturbHaloField!"
)
return required
def compute(
self,
*,
cleanup: bool,
perturbed_field: PerturbedField,
prev_spin_temp,
ics: InitialConditions,
hooks: dict,
):
"""Compute the function."""
return self._compute(
self.redshift,
self.prev_spin_redshift,
self.user_params,
self.cosmo_params,
self.astro_params,
self.flag_options,
self.perturbed_field_redshift,
cleanup,
perturbed_field,
prev_spin_temp,
ics,
hooks=hooks,
)
class IonizedBox(_AllParamsBox):
"""A class containing all ionized boxes."""
_meta = False
_c_compute_function = lib.ComputeIonizedBox
_inputs = _AllParamsBox._inputs + ("prev_ionize_redshift",)
def __init__(self, *, prev_ionize_redshift: float | None = None, **kwargs):
self.prev_ionize_redshift = prev_ionize_redshift
super().__init__(**kwargs)
def _get_box_structures(self) -> dict[str, dict | tuple[int]]:
if self.flag_options.USE_MINI_HALOS:
n_filtering = (
int(
np.log(
min(
self.astro_params.R_BUBBLE_MAX,
0.620350491 * self.user_params.BOX_LEN,
)
/ max(
global_params.R_BUBBLE_MIN,
0.620350491
* self.user_params.BOX_LEN
/ self.user_params.HII_DIM,
)
)
/ np.log(global_params.DELTA_R_HII_FACTOR)
)
+ 1
)
else:
n_filtering = 1
shape = (self.user_params.HII_DIM,) * 2 + (
int(self.user_params.NON_CUBIC_FACTOR * self.user_params.HII_DIM),
)
filter_shape = (n_filtering,) + shape
out = {
"xH_box": {"init": np.ones, "shape": shape},
"Gamma12_box": shape,
"MFP_box": shape,
"z_re_box": shape,
"dNrec_box": shape,
"temp_kinetic_all_gas": shape,
"Fcoll": filter_shape,
}
if self.flag_options.USE_MINI_HALOS:
out["Fcoll_MINI"] = filter_shape
return out
@cached_property
def global_xH(self):
"""Global (mean) neutral fraction."""
if not self.filled:
raise AttributeError(
"global_xH is not defined until the ionization calculation has been performed"
)
else:
return np.mean(self.xH_box)
def get_required_input_arrays(self, input_box: _BaseOutputStruct) -> list[str]:
"""Return all input arrays required to compute this object."""
required = []
if isinstance(input_box, InitialConditions):
if (
self.user_params.USE_RELATIVE_VELOCITIES
and self.flag_options.USE_MASS_DEPENDENT_ZETA
):
required += ["lowres_vcb"]
elif isinstance(input_box, PerturbedField):
required += ["density"]
elif isinstance(input_box, TsBox):
required += ["J_21_LW_box", "x_e_box", "Tk_box"]
elif isinstance(input_box, IonizedBox):
required += ["z_re_box", "Gamma12_box"]
if self.flag_options.INHOMO_RECO:
required += [
"dNrec_box",
]
if (
self.flag_options.USE_MASS_DEPENDENT_ZETA
and self.flag_options.USE_MINI_HALOS
):
required += ["Fcoll", "Fcoll_MINI"]
elif isinstance(input_box, PerturbHaloField):
required += ["halo_coords", "halo_masses"]
else:
raise ValueError(
f"{type(input_box)} is not an input required for IonizedBox!"
)
return required
def compute(
self,
*,
perturbed_field: PerturbedField,
prev_perturbed_field: PerturbedField,
prev_ionize_box,
spin_temp: TsBox,
pt_halos: PerturbHaloField,
ics: InitialConditions,
hooks: dict,
):
"""Compute the function."""
return self._compute(
self.redshift,
self.prev_ionize_redshift,
self.user_params,
self.cosmo_params,
self.astro_params,
self.flag_options,
perturbed_field,
prev_perturbed_field,
prev_ionize_box,
spin_temp,
pt_halos,
ics,
hooks=hooks,
)
class BrightnessTemp(_AllParamsBox):
"""A class containing the brightness temperature box."""
_c_compute_function = lib.ComputeBrightnessTemp
_meta = False
_filter_params = _OutputStructZ._filter_params
def _get_box_structures(self) -> dict[str, dict | tuple[int]]:
return {
"brightness_temp": (self.user_params.HII_DIM,) * 2
+ (int(self.user_params.NON_CUBIC_FACTOR * self.user_params.HII_DIM),)
}
@cached_property
def global_Tb(self):
"""Global (mean) brightness temperature."""
if not self.is_computed:
raise AttributeError(
"global_Tb is not defined until the ionization calculation has been performed"
)
else:
return np.mean(self.brightness_temp)
def get_required_input_arrays(self, input_box: _BaseOutputStruct) -> list[str]:
"""Return all input arrays required to compute this object."""
required = []
if isinstance(input_box, PerturbedField):
required += ["velocity"]
elif isinstance(input_box, TsBox):
required += ["Ts_box"]
elif isinstance(input_box, IonizedBox):
required += ["xH_box"]
else:
raise ValueError(
f"{type(input_box)} is not an input required for BrightnessTemp!"
)
return required
def compute(
self,
*,
spin_temp: TsBox,
ionized_box: IonizedBox,
perturbed_field: PerturbedField,
hooks: dict,
):
"""Compute the function."""
return self._compute(
self.redshift,
self.user_params,
self.cosmo_params,
self.astro_params,
self.flag_options,
spin_temp,
ionized_box,
perturbed_field,
hooks=hooks,
)
class _HighLevelOutput:
def get_cached_data(
self, kind: str, redshift: float, load_data: bool = False
) -> _OutputStruct:
"""
Return an OutputStruct object which was cached in creating this Coeval box.
Parameters
----------
kind
The kind of object: "init", "perturb", "spin_temp", "ionize" or "brightness"
redshift
The (approximate) redshift of the object to return.
load_data
Whether to actually read the field data of the object in (call ``obj.read()``
after this function to do this manually)
Returns
-------
output
The output struct object.
"""
if self.cache_files is None:
raise AttributeError(
"No cache files were associated with this Coeval object."
)
# TODO: also check this file, because it may have been "gather"d.
if kind not in self.cache_files:
raise ValueError(
f"{kind} is not a valid kind for the cache. Valid options: "
f"{self.cache_files.keys()}"
)
files = self.cache_files.get(kind, {})
# files is a list of tuples of (redshift, filename)
redshifts = np.array([f[0] for f in files])
indx = np.argmin(np.abs(redshifts - redshift))
fname = files[indx][1]
if not os.path.exists(fname):
raise OSError(
"The cached file you requested does not exist (maybe it was removed?)."
)
kinds = {
"init": InitialConditions,
"perturb_field": PerturbedField,
"ionized_box": IonizedBox,
"spin_temp": TsBox,
"brightness_temp": BrightnessTemp,
}
cls = kinds[kind]
return cls.from_file(fname, load_data=load_data)
def gather(
self,
fname: str | None | Path = None,
kinds: Sequence | None = None,
clean: bool | dict = False,
direc: str | Path | None = None,
) -> Path:
"""Gather the cached data associated with this object into its file."""
kinds = kinds or [
"init",
"perturb_field",
"ionized_box",
"spin_temp",
"brightness_temp",
]
clean = kinds if clean and not hasattr(clean, "__len__") else clean or []
if any(c not in kinds for c in clean):
raise ValueError(
"You are trying to clean cached items that you will not be gathering."
)
direc = Path(direc or config["direc"]).expanduser().absolute()
fname = Path(fname or self.get_unique_filename()).expanduser()
if not fname.exists():
fname = direc / fname
for kind in kinds:
redshifts = (f[0] for f in self.cache_files[kind])
for i, z in enumerate(redshifts):
cache_fname = self.cache_files[kind][i][1]
obj = self.get_cached_data(kind, redshift=z, load_data=True)
with h5py.File(fname, "a") as fl:
cache = (
fl.create_group("cache") if "cache" not in fl else fl["cache"]
)
kind_group = (
cache.create_group(kind) if kind not in cache else cache[kind]
)
zstr = f"z{z:.2f}"
if zstr not in kind_group:
z_group = kind_group.create_group(zstr)
else:
z_group = kind_group[zstr]
obj.write_data_to_hdf5_group(z_group)
if kind in clean:
os.remove(cache_fname)
return fname
def _get_prefix(self):
return "{name}_z{redshift:.4}_{{hash}}_r{seed}.h5".format(
name=self.__class__.__name__,
redshift=float(self.redshift),
seed=self.random_seed,
)
def _input_rep(self):
rep = ""
for inp in [
"user_params",
"cosmo_params",
"astro_params",
"flag_options",
"global_params",
]:
rep += repr(getattr(self, inp))
return rep
def get_unique_filename(self):
"""Generate a unique hash filename for this instance."""
return self._get_prefix().format(
hash=md5((self._input_rep() + self._particular_rep()).encode()).hexdigest()
)
def _write(self, direc=None, fname=None, clobber=False):
"""
Write the lightcone to file in standard HDF5 format.
This method is primarily meant for the automatic caching. Its default
filename is a hash generated based on the input data, and the directory is
the configured caching directory.
Parameters
----------
direc : str, optional
The directory into which to write the file. Default is the configuration
directory.
fname : str, optional
The filename to write, default a unique name produced by the inputs.
clobber : bool, optional
Whether to overwrite existing file.
Returns
-------
fname : str
The absolute path to which the file was written.
"""
direc = os.path.expanduser(direc or config["direc"])
if fname is None:
fname = self.get_unique_filename()
if not os.path.isabs(fname):
fname = os.path.abspath(os.path.join(direc, fname))
if not clobber and os.path.exists(fname):
raise FileExistsError(
"The file {} already exists. If you want to overwrite, set clobber=True.".format(
fname
)
)
with h5py.File(fname, "w") as f:
# Save input parameters as attributes
for k in [
"user_params",
"cosmo_params",
"flag_options",
"astro_params",
"global_params",
]:
q = getattr(self, k)
kfile = "_globals" if k == "global_params" else k
grp = f.create_group(kfile)
try:
dct = q.self
except AttributeError:
dct = q
for kk, v in dct.items():
if v is None:
continue
try:
grp.attrs[kk] = v
except TypeError:
# external_table_path is a cdata object and can't be written.
pass
if self.photon_nonconservation_data is not None:
photon_data = f.create_group("photon_nonconservation_data")
for k, val in self.photon_nonconservation_data.items():
photon_data[k] = val
f.attrs["redshift"] = self.redshift
f.attrs["random_seed"] = self.random_seed
f.attrs["version"] = __version__
self._write_particulars(fname)
return fname
def _write_particulars(self, fname):
pass
def save(self, fname=None, direc="."):
"""Save to disk.
This function has defaults that make it easy to save a unique box to
the current directory.
Parameters
----------
fname : str, optional
The filename to write, default a unique name produced by the inputs.
direc : str, optional
The directory into which to write the file. Default is the current directory.
Returns
-------
str :
The filename to which the box was written.
"""
return self._write(direc=direc, fname=fname)
@classmethod
def _read_inputs(cls, fname):
kwargs = {}
with h5py.File(fname, "r") as fl:
glbls = dict(fl["_globals"].attrs)
kwargs["redshift"] = fl.attrs["redshift"]
if "photon_nonconservation_data" in fl.keys():
d = fl["photon_nonconservation_data"]
kwargs["photon_nonconservation_data"] = {k: d[k][...] for k in d.keys()}
return kwargs, glbls
@classmethod
def read(cls, fname, direc="."):
"""Read a lightcone file from disk, creating a LightCone object.
Parameters
----------
fname : str
The filename path. Can be absolute or relative.
direc : str
If fname, is relative, the directory in which to find the file. By default,
both the current directory and default cache and the will be searched, in
that order.
Returns
-------
LightCone :
A :class:`LightCone` instance created from the file's data.
"""
if not os.path.isabs(fname):
fname = os.path.abspath(os.path.join(direc, fname))
if not os.path.exists(fname):
raise FileExistsError(f"The file {fname} does not exist!")
park, glbls = cls._read_inputs(fname)
boxk = cls._read_particular(fname)
with global_params.use(**glbls):
out = cls(**park, **boxk)
return out
class Coeval(_HighLevelOutput):
"""A full coeval box with all associated data."""
def __init__(
self,
redshift: float,
initial_conditions: InitialConditions,
perturbed_field: PerturbedField,
ionized_box: IonizedBox,
brightness_temp: BrightnessTemp,
ts_box: TsBox | None = None,
cache_files: dict | None = None,
photon_nonconservation_data=None,
_globals=None,
):
_check_compatible_inputs(
initial_conditions,
perturbed_field,
ionized_box,
brightness_temp,
ts_box,
ignore=[],
)
self.redshift = redshift
self.init_struct = initial_conditions
self.perturb_struct = perturbed_field
self.ionization_struct = ionized_box
self.brightness_temp_struct = brightness_temp
self.spin_temp_struct = ts_box
self.cache_files = cache_files
self.photon_nonconservation_data = photon_nonconservation_data
# A *copy* of the current global parameters.
self.global_params = _globals or dict(global_params.items())
# Expose all the fields of the structs to the surface of the Coeval object
for box in [
initial_conditions,
perturbed_field,
ionized_box,
brightness_temp,
ts_box,
]:
if box is None:
continue
for field in box._get_box_structures():
setattr(self, field, getattr(box, field))
@classmethod
def get_fields(cls, spin_temp: bool = True) -> list[str]:
"""Obtain a list of name of simulation boxes saved in the Coeval object."""
pointer_fields = []
for cls in [InitialConditions, PerturbedField, IonizedBox, BrightnessTemp]:
pointer_fields += cls.get_pointer_fields()
if spin_temp:
pointer_fields += TsBox.get_pointer_fields()
return pointer_fields
@property
def user_params(self):
"""User params shared by all datasets."""
return self.brightness_temp_struct.user_params
@property
def cosmo_params(self):
"""Cosmo params shared by all datasets."""
return self.brightness_temp_struct.cosmo_params
@property
def flag_options(self):
"""Flag Options shared by all datasets."""
return self.brightness_temp_struct.flag_options
@property
def astro_params(self):
"""Astro params shared by all datasets."""
return self.brightness_temp_struct.astro_params
@property
def random_seed(self):
"""Random seed shared by all datasets."""
return self.brightness_temp_struct.random_seed
def _particular_rep(self):
return ""
def _write_particulars(self, fname):
for name in ["init", "perturb", "ionization", "brightness_temp", "spin_temp"]:
struct = getattr(self, name + "_struct")
if struct is not None:
struct.write(fname=fname, write_inputs=False)
# Also write any other inputs to any of the constituent boxes
# to the overarching attrs.
with h5py.File(fname, "a") as fl:
for inp in struct._inputs:
if inp not in fl.attrs and inp not in [
"user_params",
"cosmo_params",
"flag_options",
"astro_params",
"global_params",
]:
fl.attrs[inp] = getattr(struct, inp)
@classmethod
def _read_particular(cls, fname):
kwargs = {}
with h5py.File(fname, "r") as fl:
for output_class in _ut.OutputStruct._implementations():
if output_class.__name__ in fl:
kwargs[
_ut.camel_to_snake(output_class.__name__)
] = output_class.from_file(fname)
return kwargs
def __eq__(self, other):
"""Determine if this is equal to another object."""
return (
isinstance(other, self.__class__)
and other.redshift == self.redshift
and self.user_params == other.user_params
and self.cosmo_params == other.cosmo_params
and self.flag_options == other.flag_options
and self.astro_params == other.astro_params
)
class LightCone(_HighLevelOutput):
"""A full Lightcone with all associated evolved data."""
def __init__(
self,
redshift,
user_params,
cosmo_params,
astro_params,
flag_options,
random_seed,
lightcones,
node_redshifts=None,
global_quantities=None,
photon_nonconservation_data=None,
cache_files: dict | None = None,
_globals=None,
log10_mturnovers=None,
log10_mturnovers_mini=None,
):
self.redshift = redshift
self.random_seed = random_seed
self.user_params = user_params
self.cosmo_params = cosmo_params
self.astro_params = astro_params
self.flag_options = flag_options
self.node_redshifts = node_redshifts
self.cache_files = cache_files
self.log10_mturnovers = log10_mturnovers
self.log10_mturnovers_mini = log10_mturnovers_mini
# A *copy* of the current global parameters.
self.global_params = _globals or dict(global_params.items())
if global_quantities:
for name, data in global_quantities.items():
if name.endswith("_box"):
# Remove the _box because it looks dumb.
setattr(self, "global_" + name[:-4], data)
else:
setattr(self, "global_" + name, data)
self.photon_nonconservation_data = photon_nonconservation_data
for name, data in lightcones.items():
setattr(self, name, data)
# Hold a reference to the global/lightcones in a dict form for easy reference.
self.global_quantities = global_quantities
self.lightcones = lightcones
@property
def global_xHI(self):
"""Global neutral fraction function."""
warnings.warn(
"global_xHI is deprecated. From now on, use global_xH. Will be removed in v3.1"
)
return self.global_xH
@property
def cell_size(self):
"""Cell size [Mpc] of the lightcone voxels."""
return self.user_params.BOX_LEN / self.user_params.HII_DIM
@property
def lightcone_dimensions(self):
"""Lightcone size over each dimension -- tuple of (x,y,z) in Mpc."""
return (
self.user_params.BOX_LEN,
self.user_params.BOX_LEN,
self.n_slices * self.cell_size,
)
@property
def shape(self):
"""Shape of the lightcone as a 3-tuple."""
return self.brightness_temp.shape
@property
def n_slices(self):
"""Number of redshift slices in the lightcone."""
return self.shape[-1]
@property
def lightcone_coords(self):
"""Co-ordinates [Mpc] of each cell along the redshift axis."""
return np.linspace(0, self.lightcone_dimensions[-1], self.n_slices)
@property
def lightcone_distances(self):
"""Comoving distance to each cell along the redshift axis, from z=0."""
return (
self.cosmo_params.cosmo.comoving_distance(self.redshift).value
+ self.lightcone_coords
)
@property
def lightcone_redshifts(self):
"""Redshift of each cell along the redshift axis."""
return np.array(
[
z_at_value(self.cosmo_params.cosmo.comoving_distance, d * units.Mpc)
for d in self.lightcone_distances
]
)
def _particular_rep(self):
return (
str(np.round(self.node_redshifts, 3))
+ str(self.global_quantities.keys())
+ str(self.lightcones.keys())
)
def _write_particulars(self, fname):
with h5py.File(fname, "a") as f:
# Save the boxes to the file
boxes = f.create_group("lightcones")
# Go through all fields in this struct, and save
for k, val in self.lightcones.items():
boxes[k] = val
global_q = f.create_group("global_quantities")
for k, v in self.global_quantities.items():
global_q[k] = v
f["node_redshifts"] = self.node_redshifts
@classmethod
def _read_inputs(cls, fname):
kwargs = {}
with h5py.File(fname, "r") as fl:
for k, kls in [
("user_params", UserParams),
("cosmo_params", CosmoParams),
("flag_options", FlagOptions),
("astro_params", AstroParams),
]:
grp = fl[k]
kwargs[k] = kls(dict(grp.attrs))
kwargs["random_seed"] = fl.attrs["random_seed"]
# Get the standard inputs.
kw, glbls = _HighLevelOutput._read_inputs(fname)
return {**kw, **kwargs}, glbls
@classmethod
def _read_particular(cls, fname):
kwargs = {}
with h5py.File(fname, "r") as fl:
boxes = fl["lightcones"]
kwargs["lightcones"] = {k: boxes[k][...] for k in boxes.keys()}
glb = fl["global_quantities"]
kwargs["global_quantities"] = {k: glb[k][...] for k in glb.keys()}
kwargs["node_redshifts"] = fl["node_redshifts"][...]
return kwargs
def __eq__(self, other):
"""Determine if this is equal to another object."""
return (
isinstance(other, self.__class__)
and other.redshift == self.redshift
and np.all(np.isclose(other.node_redshifts, self.node_redshifts, atol=1e-3))
and self.user_params == other.user_params
and self.cosmo_params == other.cosmo_params
and self.flag_options == other.flag_options
and self.astro_params == other.astro_params
and self.global_quantities.keys() == other.global_quantities.keys()
and self.lightcones.keys() == other.lightcones.keys()
) | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/src/py21cmfast/outputs.py | outputs.py |
from __future__ import annotations
import matplotlib.pyplot as plt
import numpy as np
from astropy import units as un
from astropy.cosmology import z_at_value
from matplotlib import colors
from matplotlib.ticker import AutoLocator
from typing import Optional, Union
from . import outputs
from .outputs import Coeval, LightCone
eor_colour = colors.LinearSegmentedColormap.from_list(
"EoR",
[
(0, "white"),
(0.21, "yellow"),
(0.42, "orange"),
(0.63, "red"),
(0.86, "black"),
(0.9, "blue"),
(1, "cyan"),
],
)
plt.register_cmap(cmap=eor_colour)
def _imshow_slice(
cube,
slice_axis=-1,
slice_index=0,
fig=None,
ax=None,
fig_kw=None,
cbar=True,
cbar_horizontal=False,
rotate=False,
cmap="EoR",
log: [bool] = False,
**imshow_kw,
):
"""
Plot a slice of some kind of cube.
Parameters
----------
cube : nd-array
A 3D array of some quantity.
slice_axis : int, optional
The axis over which to take a slice, in order to plot.
slice_index :
The index of the slice.
fig : Figure object
An optional matplotlib figure object on which to plot
ax : Axis object
The matplotlib axis object on which to plot (created by default).
fig_kw :
Optional arguments passed to the figure construction.
cbar : bool
Whether to plot the colorbar
cbar_horizontal : bool
Whether the colorbar should be horizontal underneath the plot.
rotate : bool
Whether to rotate the plot vertically.
imshow_kw :
Optional keywords to pass to :func:`maplotlib.imshow`.
Returns
-------
fig, ax :
The figure and axis objects from matplotlib.
"""
# If no axis is passed, create a new one
# This allows the user to add this plot into an existing grid, or alter it afterwards.
if fig_kw is None:
fig_kw = {}
if ax is None and fig is None:
fig, ax = plt.subplots(1, 1, **fig_kw)
elif ax is None:
ax = plt.gca()
elif fig is None:
fig = plt.gcf()
plt.sca(ax)
if slice_index >= cube.shape[slice_axis]:
raise IndexError(
"slice_index is too large for that axis (slice_index=%s >= %s"
% (slice_index, cube.shape[slice_axis])
)
slc = np.take(cube, slice_index, axis=slice_axis)
if not rotate:
slc = slc.T
if cmap == "EoR":
imshow_kw["vmin"] = -150
imshow_kw["vmax"] = 30
norm_kw = {k: imshow_kw.pop(k) for k in ["vmin", "vmax"] if k in imshow_kw}
norm = imshow_kw.get(
"norm", colors.LogNorm(**norm_kw) if log else colors.Normalize(**norm_kw)
)
plt.imshow(slc, origin="lower", cmap=cmap, norm=norm, **imshow_kw)
if cbar:
cb = plt.colorbar(
orientation="horizontal" if cbar_horizontal else "vertical", aspect=40
)
cb.outline.set_edgecolor(None)
return fig, ax
def coeval_sliceplot(
struct: outputs._OutputStruct | Coeval,
kind: str | None = None,
cbar_label: str | None = None,
**kwargs,
):
"""
Show a slice of a given coeval box.
Parameters
----------
struct : :class:`~outputs._OutputStruct` or :class:`~wrapper.Coeval` instance
The output of a function such as `ionize_box` (a class containing several quantities), or
`run_coeval`.
kind : str
The quantity within the structure to be shown. A full list of available options
can be obtained by running ``Coeval.get_fields()``.
cbar_label : str, optional
A label for the colorbar. Some values of `kind` will have automatically chosen
labels, but these can be turned off by setting ``cbar_label=''``.
Returns
-------
fig, ax :
figure and axis objects from matplotlib
Other Parameters
----------------
All other parameters are passed directly to :func:`_imshow_slice`. These include `slice_axis`
and `slice_index`,
which choose the actual slice to plot, optional `fig` and `ax` keywords which enable
over-plotting previous figures,
and the `imshow_kw` argument, which allows arbitrary styling of the plot.
"""
if kind is None:
if isinstance(struct, outputs._OutputStruct):
kind = struct.fieldnames[0]
elif isinstance(struct, Coeval):
kind = "brightness_temp"
try:
cube = getattr(struct, kind)
except AttributeError:
raise AttributeError(
f"The given OutputStruct does not have the quantity {kind}"
)
if kind != "brightness_temp" and "cmap" not in kwargs:
kwargs["cmap"] = "viridis"
fig, ax = _imshow_slice(cube, extent=(0, struct.user_params.BOX_LEN) * 2, **kwargs)
slice_axis = kwargs.get("slice_axis", -1)
# Determine which axes are being plotted.
if slice_axis in (2, -1):
xax = "x"
yax = "y"
elif slice_axis == 1:
xax = "x"
yax = "z"
elif slice_axis == 0:
xax = "y"
yax = "z"
else:
raise ValueError("slice_axis should be between -1 and 2")
# Now put on the decorations.
ax.set_xlabel(f"{xax}-axis [Mpc]")
ax.set_ylabel(f"{yax}-axis [Mpc]")
cbar = fig._gci().colorbar
if cbar is not None:
if cbar_label is None:
if kind == "brightness_temp":
cbar_label = r"Brightness Temperature, $\delta T_B$ [mK]"
elif kind == "xH_box":
cbar_label = r"Neutral fraction"
cbar.ax.set_ylabel(cbar_label)
return fig, ax
def lightcone_sliceplot(
lightcone: LightCone,
kind: str = "brightness_temp",
lightcone2: LightCone = None,
vertical: bool = False,
xlabel: str | None = None,
ylabel: str | None = None,
cbar_label: str | None = None,
zticks: str = "redshift",
fig: plt.Figure | None = None,
ax: plt.Axes | None = None,
**kwargs,
):
"""Create a 2D plot of a slice through a lightcone.
Parameters
----------
lightcone : :class:`~py21cmfast.wrapper.Lightcone`
The lightcone object to plot
kind : str, optional
The attribute of the lightcone to plot. Must be an array.
lightcone2 : str, optional
If provided, plot the _difference_ of the selected attribute between the two
lightcones.
vertical : bool, optional
Whether to plot the redshift in the vertical direction.
cbar_label : str, optional
A label for the colorbar. Some quantities have automatically chosen labels, but
these can be removed by setting `cbar_label=''`.
zticks : str, optional
Defines the co-ordinates of the ticks along the redshift axis.
Can be "redshift" (default), "frequency", "distance" (which starts at zero
for the lowest redshift) or the name of any function in an astropy cosmology
that is purely a function of redshift.
kwargs :
Passed through to ``imshow()``.
Returns
-------
fig :
The matplotlib Figure object
ax :
The matplotlib Axis object onto which the plot was drawn.
"""
slice_axis = kwargs.pop("slice_axis", 0)
if slice_axis <= -2 or slice_axis >= 3:
raise ValueError(f"slice_axis should be between -1 and 2 (got {slice_axis})")
z_axis = ("y" if vertical else "x") if slice_axis in (0, 1) else None
# Dictionary mapping axis to dimension in lightcone
axis_dct = {
"x": 2 if z_axis == "x" else [1, 0, 0][slice_axis],
"y": 2 if z_axis == "y" else [1, 0, 1][slice_axis],
}
if fig is None and ax is None:
fig, ax = plt.subplots(
1,
1,
figsize=(
lightcone.shape[axis_dct["x"]] * 0.015 + 0.5,
lightcone.shape[axis_dct["y"]] * 0.015
+ (2.5 if kwargs.get("cbar", True) else 0.05),
),
)
elif fig is None:
fig = ax._gci().figure
elif ax is None:
ax = fig.get_axes()
# Get x,y labels if they're not the redshift axis.
if xlabel is None:
xlabel = (
None if axis_dct["x"] == 2 else "{}-axis [Mpc]".format("xy"[axis_dct["x"]])
)
if ylabel is None:
ylabel = (
None if axis_dct["y"] == 2 else "{}-axis [Mpc]".format("xy"[axis_dct["y"]])
)
extent = (
0,
lightcone.lightcone_dimensions[axis_dct["x"]],
0,
lightcone.lightcone_dimensions[axis_dct["y"]],
)
if lightcone2 is None:
fig, ax = _imshow_slice(
getattr(lightcone, kind),
extent=extent,
slice_axis=slice_axis,
rotate=not vertical,
cbar_horizontal=not vertical,
cmap=kwargs.get("cmap", "EoR" if kind == "brightness_temp" else "viridis"),
fig=fig,
ax=ax,
**kwargs,
)
else:
d = getattr(lightcone, kind) - getattr(lightcone2, kind)
fig, ax = _imshow_slice(
d,
extent=extent,
slice_axis=slice_axis,
rotate=not vertical,
cbar_horizontal=not vertical,
cmap=kwargs.pop("cmap", "bwr"),
vmin=-np.abs(d.max()),
vmax=np.abs(d.max()),
fig=fig,
ax=ax,
**kwargs,
)
if z_axis:
zlabel = _set_zaxis_ticks(ax, lightcone, zticks, z_axis)
if ylabel != "":
ax.set_ylabel(ylabel or zlabel)
if xlabel != "":
ax.set_xlabel(xlabel or zlabel)
cbar = fig._gci().colorbar
if cbar_label is None:
if kind == "brightness_temp":
cbar_label = r"Brightness Temperature, $\delta T_B$ [mK]"
elif kind == "xH":
cbar_label = r"Neutral fraction"
if vertical:
cbar.ax.set_ylabel(cbar_label)
else:
cbar.ax.set_xlabel(cbar_label)
return fig, ax
def _set_zaxis_ticks(ax, lightcone, zticks, z_axis):
if zticks != "distance":
loc = AutoLocator()
# Get redshift ticks.
lc_z = lightcone.lightcone_redshifts
if zticks == "redshift":
coords = lc_z
elif zticks == "frequency":
coords = 1420 / (1 + lc_z) * un.MHz
else:
try:
coords = getattr(lightcone.cosmo_params.cosmo, zticks)(lc_z)
except AttributeError:
raise AttributeError(f"zticks '{zticks}' is not a cosmology function.")
zlabel = " ".join(z.capitalize() for z in zticks.split("_"))
units = getattr(coords, "unit", None)
if units:
zlabel += f" [{str(coords.unit)}]"
coords = coords.value
ticks = loc.tick_values(coords.min(), coords.max())
if ticks.min() < coords.min() / 1.00001:
ticks = ticks[1:]
if ticks.max() > coords.max() * 1.00001:
ticks = ticks[:-1]
if coords[1] < coords[0]:
ticks = ticks[::-1]
if zticks == "redshift":
z_ticks = ticks
elif zticks == "frequency":
z_ticks = 1420 / ticks - 1
else:
z_ticks = [
z_at_value(getattr(lightcone.cosmo_params.cosmo, zticks), z * units)
for z in ticks
]
d_ticks = (
lightcone.cosmo_params.cosmo.comoving_distance(z_ticks).value
- lightcone.lightcone_distances[0]
)
getattr(ax, f"set_{z_axis}ticks")(d_ticks)
getattr(ax, f"set_{z_axis}ticklabels")(ticks)
else:
zlabel = "Line-of-Sight Distance [Mpc]"
return zlabel
def plot_global_history(
lightcone: LightCone,
kind: str | None = None,
ylabel: str | None = None,
ylog: bool = False,
ax: plt.Axes | None = None,
):
"""
Plot the global history of a given quantity from a lightcone.
Parameters
----------
lightcone : :class:`~LightCone` instance
The lightcone containing the quantity to plot.
kind : str, optional
The quantity to plot. Must be in the `global_quantities` dict in the lightcone.
By default, will choose the first entry in the dict.
ylabel : str, optional
A y-label for the plot. If None, will use ``kind``.
ax : Axes, optional
The matplotlib Axes object on which to plot. Otherwise, created.
"""
if ax is None:
fig, ax = plt.subplots(1, 1, figsize=(7, 4))
else:
fig = ax._gci().figure
if kind is None:
kind = list(lightcone.global_quantities.keys())[0]
assert (
kind in lightcone.global_quantities
or hasattr(lightcone, "global_" + kind)
or (kind.startswith("global_") and hasattr(lightcone, kind))
)
if kind in lightcone.global_quantities:
value = lightcone.global_quantities[kind]
elif kind.startswith("global)"):
value = getattr(lightcone, kind)
else:
value = getattr(lightcone, "global_" + kind)
ax.plot(lightcone.node_redshifts, value)
ax.set_xlabel("Redshift")
if ylabel is None:
ylabel = kind
if ylabel:
ax.set_ylabel(ylabel)
if ylog:
ax.set_yscale("log")
return fig, ax | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/src/py21cmfast/plotting.py | plotting.py |
from __future__ import annotations
import contextlib
import logging
import warnings
from astropy.cosmology import Planck15
from os import path
from pathlib import Path
from ._cfg import config
from ._data import DATA_PATH
from ._utils import StructInstanceWrapper, StructWithDefaults
from .c_21cmfast import ffi, lib
logger = logging.getLogger("21cmFAST")
# Cosmology is from https://arxiv.org/pdf/1807.06209.pdf
# Table 2, last column. [TT,TE,EE+lowE+lensing+BAO]
Planck18 = Planck15.clone(
Om0=(0.02242 + 0.11933) / 0.6766**2,
Ob0=0.02242 / 0.6766**2,
H0=67.66,
)
class GlobalParams(StructInstanceWrapper):
"""
Global parameters for 21cmFAST.
This is a thin wrapper over an allocated C struct, containing parameter values
which are used throughout various computations within 21cmFAST. It is a singleton;
that is, a single python (and C) object exists, and no others should be created.
This object is not "passed around", rather its values are accessed throughout the
code.
Parameters in this struct are considered to be options that should usually not have
to be modified, and if so, typically once in any given script or session.
Values can be set in the normal way, eg.:
>>> global_params.ALPHA_UVB = 5.5
The class also provides a context manager for setting parameters for a well-defined
portion of the code. For example, if you would like to set ``Z_HEAT_MAX`` for a given
run:
>>> with global_params.use(Z_HEAT_MAX=25):
>>> p21c.run_lightcone(...) # uses Z_HEAT_MAX=25 for the entire run.
>>> print(global_params.Z_HEAT_MAX)
35.0
Attributes
----------
ALPHA_UVB : float
Power law index of the UVB during the EoR. This is only used if `INHOMO_RECO` is
True (in :class:`FlagOptions`), in order to compute the local mean free path
inside the cosmic HII regions.
EVOLVE_DENSITY_LINEARLY : bool
Whether to evolve the density field with linear theory (instead of 1LPT or Zel'Dovich).
If choosing this option, make sure that your cell size is
in the linear regime at the redshift of interest. Otherwise, make sure you resolve
small enough scales, roughly we find BOX_LEN/DIM should be < 1Mpc
SMOOTH_EVOLVED_DENSITY_FIELD : bool
If True, the zeldovich-approximation density field is additionally smoothed
(aside from the implicit boxcar smoothing performed when re-binning the ICs from
DIM to HII_DIM) with a Gaussian filter of width ``R_smooth_density*BOX_LEN/HII_DIM``.
The implicit boxcar smoothing in ``perturb_field()`` bins the density field on
scale DIM/HII_DIM, similar to what Lagrangian codes do when constructing Eulerian
grids. In other words, the density field is quantized into ``(DIM/HII_DIM)^3`` values.
If your usage requires smooth density fields, it is recommended to set this to True.
This also decreases the shot noise present in all grid based codes, though it
overcompensates by an effective loss in resolution. **Added in 1.1.0**.
R_smooth_density : float
Determines the smoothing length to use if `SMOOTH_EVOLVED_DENSITY_FIELD` is True.
HII_ROUND_ERR : float
Rounding error on the ionization fraction. If the mean xHI is greater than
``1 - HII_ROUND_ERR``, then finding HII bubbles is skipped, and a homogeneous
xHI field of ones is returned. Added in v1.1.0.
FIND_BUBBLE_ALGORITHM : int, {1,2}
Choose which algorithm used to find HII bubbles. Options are: (1) Mesinger & Furlanetto 2007
method of overlapping spheres: paint an ionized sphere with radius R, centered on pixel
where R is filter radius. This method, while somewhat more accurate, is slower than (2),
especially in mostly ionized universes, so only use for lower resolution boxes
(HII_DIM<~400). (2) Center pixel only method (Zahn et al. 2007). This is faster.
N_POISSON : int
If not using the halo field to generate HII regions, we provide the option of
including Poisson scatter in the number of sources obtained through the conditional
collapse fraction (which only gives the *mean* collapse fraction on a particular
scale. If the predicted mean collapse fraction is less than `N_POISSON * M_MIN`,
then Poisson scatter is added to mimic discrete halos on the subgrid scale (see
Zahn+2010).Use a negative number to turn it off.
.. note:: If you are interested in snapshots of the same realization at several
redshifts,it is recommended to turn off this feature, as halos can
stochastically "pop in and out of" existence from one redshift to the next.
R_OVERLAP_FACTOR : float
When using USE_HALO_FIELD, it is used as a factor the halo's radius, R, so that the
effective radius is R_eff = R_OVERLAP_FACTOR * R. Halos whose centers are less than
R_eff away from another halo are not allowed. R_OVERLAP_FACTOR = 1 is fully disjoint
R_OVERLAP_FACTOR = 0 means that centers are allowed to lay on the edges of
neighboring halos.
DELTA_CRIT_MODE : int
The delta_crit to be used for determining whether a halo exists in a cell
0: delta_crit is constant (i.e. 1.686)
1: delta_crit is the sheth tormen ellipsoidal collapse correction to delta_crit
HALO_FILTER : int
Filter for the density field used to generate the halo field with EPS
0: real space top hat filter
1: sharp k-space filter
2: gaussian filter
OPTIMIZE : bool
Finding halos can be made more efficient if the filter size is sufficiently large that
we can switch to the collapse fraction at a later stage.
OPTIMIZE_MIN_MASS : float
Minimum mass on which the optimization for the halo finder will be used.
T_USE_VELOCITIES : bool
Whether to use velocity corrections in 21-cm fields
.. note:: The approximation used to include peculiar velocity effects works
only in the linear regime, so be careful using this (see Mesinger+2010)
MAX_DVDR : float
Maximum velocity gradient along the line of sight in units of the hubble parameter at z.
This is only used in computing the 21cm fields.
.. note:: Setting this too high can add spurious 21cm power in the early stages,
due to the 1-e^-tau ~ tau approximation (see Mesinger's 21cm intro paper and mao+2011).
However, this is still a good approximation at the <~10% level.
VELOCITY_COMPONENT : int
Component of the velocity to be used in 21-cm temperature maps (1=x, 2=y, 3=z)
DELTA_R_FACTOR : float
Factor by which to scroll through filter radius for halos
DELTA_R_HII_FACTOR : float
Factor by which to scroll through filter radius for bubbles
HII_FILTER : int, {0, 1, 2}
Filter for the Halo or density field used to generate ionization field:
0. real space top hat filter
1. k-space top hat filter
2. gaussian filter
INITIAL_REDSHIFT : float
Used to perturb field
CRIT_DENS_TRANSITION : float
A transition value for the interpolation tables for calculating the number of ionising
photons produced given the input parameters. Log sampling is desired, however the numerical
accuracy near the critical density for collapse (i.e. 1.69) broke down. Therefore, below the
value for `CRIT_DENS_TRANSITION` log sampling of the density values is used, whereas above
this value linear sampling is used.
MIN_DENSITY_LOW_LIMIT : float
Required for using the interpolation tables for the number of ionising photons. This is a
lower limit for the density values that is slightly larger than -1. Defined as a density
contrast.
RecombPhotonCons : int
Whether or not to use the recombination term when calculating the filling factor for
performing the photon non-conservation correction.
PhotonConsStart : float
A starting value for the neutral fraction where the photon non-conservation correction is
performed exactly. Any value larger than this the photon non-conservation correction is not
performed (i.e. the algorithm is perfectly photon conserving).
PhotonConsEnd : float
An end-point for where the photon non-conservation correction is performed exactly. This is
required to remove undesired numerical artifacts in the resultant neutral fraction histories.
PhotonConsAsymptoteTo : float
Beyond `PhotonConsEnd` the photon non-conservation correction is extrapolated to yield
smooth reionisation histories. This sets the lowest neutral fraction value that the photon
non-conservation correction will be applied to.
HEAT_FILTER : int
Filter used for smoothing the linear density field to obtain the collapsed fraction:
0: real space top hat filter
1: sharp k-space filter
2: gaussian filter
CLUMPING_FACTOR : float
Sub grid scale. If you want to run-down from a very high redshift (>50), you should
set this to one.
Z_HEAT_MAX : float
Maximum redshift used in the Tk and x_e evolution equations.
Temperature and x_e are assumed to be homogeneous at higher redshifts.
Lower values will increase performance.
R_XLy_MAX : float
Maximum radius of influence for computing X-ray and Lya pumping in cMpc. This
should be larger than the mean free path of the relevant photons.
NUM_FILTER_STEPS_FOR_Ts : int
Number of spherical annuli used to compute df_coll/dz' in the simulation box.
The spherical annuli are evenly spaced in logR, ranging from the cell size to the box
size. :func:`~wrapper.spin_temp` will create this many boxes of size `HII_DIM`,
so be wary of memory usage if values are high.
ZPRIME_STEP_FACTOR : float
Logarithmic redshift step-size used in the z' integral. Logarithmic dz.
Decreasing (closer to unity) increases total simulation time for lightcones,
and for Ts calculations.
TK_at_Z_HEAT_MAX : float
If positive, then overwrite default boundary conditions for the evolution
equations with this value. The default is to use the value obtained from RECFAST.
See also `XION_at_Z_HEAT_MAX`.
XION_at_Z_HEAT_MAX : float
If positive, then overwrite default boundary conditions for the evolution
equations with this value. The default is to use the value obtained from RECFAST.
See also `TK_at_Z_HEAT_MAX`.
Pop : int
Stellar Population responsible for early heating (2 or 3)
Pop2_ion : float
Number of ionizing photons per baryon for population 2 stellar species.
Pop3_ion : float
Number of ionizing photons per baryon for population 3 stellar species.
NU_X_BAND_MAX : float
This is the upper limit of the soft X-ray band (0.5 - 2 keV) used for normalising
the X-ray SED to observational limits set by the X-ray luminosity. Used for performing
the heating rate integrals.
NU_X_MAX : float
An upper limit (must be set beyond `NU_X_BAND_MAX`) for performing the rate integrals.
Given the X-ray SED is modelled as a power-law, this removes the potential of divergent
behaviour for the heating rates. Chosen purely for numerical convenience though it is
motivated by the fact that observed X-ray SEDs apprear to turn-over around 10-100 keV
(Lehmer et al. 2013, 2015)
NBINS_LF : int
Number of bins for the luminosity function calculation.
P_CUTOFF : bool
Turn on Warm-Dark-matter power suppression.
M_WDM : float
Mass of WDM particle in keV. Ignored if `P_CUTOFF` is False.
g_x : float
Degrees of freedom of WDM particles; 1.5 for fermions.
OMn : float
Relative density of neutrinos in the universe.
OMk : float
Relative density of curvature.
OMr : float
Relative density of radiation.
OMtot : float
Fractional density of the universe with respect to critical density. Set to
unity for a flat universe.
Y_He : float
Helium fraction.
wl : float
Dark energy equation of state parameter (wl = -1 for vacuum )
SHETH_b : float
Sheth-Tormen parameter for ellipsoidal collapse (for HMF).
.. note:: The best fit b and c ST params for these 3D realisations have a redshift,
and a ``DELTA_R_FACTOR`` dependence, as shown
in Mesinger+. For converged mass functions at z~5-10, set `DELTA_R_FACTOR=1.1`
and `SHETH_b=0.15` and `SHETH_c~0.05`.
For most purposes, a larger step size is quite sufficient and provides an
excellent match to N-body and smoother mass functions, though the b and c
parameters should be changed to make up for some "stepping-over" massive
collapsed halos (see Mesinger, Perna, Haiman (2005) and Mesinger et al.,
in preparation).
For example, at z~7-10, one can set `DELTA_R_FACTOR=1.3` and `SHETH_b=0.15`
and `SHETH_c=0.25`, to increase the speed of the halo finder.
SHETH_c : float
Sheth-Tormen parameter for ellipsoidal collapse (for HMF). See notes for `SHETH_b`.
Zreion_HeII : float
Redshift of helium reionization, currently only used for tau_e
FILTER : int, {0, 1}
Filter to use for smoothing.
0. tophat
1. gaussian
external_table_path : str
The system path to find external tables for calculation speedups. DO NOT MODIFY.
R_BUBBLE_MIN : float
Minimum radius of bubbles to be searched in cMpc. One can set this to 0, but should
be careful with shot noise if running on a fine, non-linear density grid. Default
is set to L_FACTOR which is (4PI/3)^(-1/3) = 0.620350491.
M_MIN_INTEGRAL:
Minimum mass when performing integral on halo mass function.
M_MAX_INTEGRAL:
Maximum mass when performing integral on halo mass function.
T_RE:
The peak gas temperatures behind the supersonic ionization fronts during reionization.
VAVG:
Avg value of the DM-b relative velocity [im km/s], ~0.9*SIGMAVCB (=25.86 km/s) normally.
"""
def __init__(self, wrapped, ffi):
super().__init__(wrapped, ffi)
self.external_table_path = ffi.new("char[]", str(DATA_PATH).encode())
self._wisdoms_path = Path(config["direc"]) / "wisdoms"
self.wisdoms_path = ffi.new("char[]", str(self._wisdoms_path).encode())
@property
def external_table_path(self):
"""An ffi char pointer to the path to which external tables are kept."""
return self._external_table_path
@external_table_path.setter
def external_table_path(self, val):
self._external_table_path = val
@property
def wisdoms_path(self):
"""An ffi char pointer to the path to which external tables are kept."""
if not self._wisdoms_path.exists():
self._wisdoms_path.mkdir(parents=True)
return self._wisdom_path
@wisdoms_path.setter
def wisdoms_path(self, val):
self._wisdom_path = val
@contextlib.contextmanager
def use(self, **kwargs):
"""Set given parameters for a certain context.
.. note:: Keywords are *not* case-sensitive.
Examples
--------
>>> from py21cmfast import global_params, run_lightcone
>>> with global_params.use(zprime_step_factor=1.1, Sheth_c=0.06):
>>> run_lightcone(redshift=7)
"""
prev = {}
this_attr_upper = {k.upper(): k for k in self.keys()}
for k, val in kwargs.items():
if k.upper() not in this_attr_upper:
raise ValueError(f"{k} is not a valid parameter of global_params")
key = this_attr_upper[k.upper()]
prev[key] = getattr(self, key)
setattr(self, key, val)
yield
# Restore everything back to the way it was.
for k, v in prev.items():
setattr(self, k, v)
global_params = GlobalParams(lib.global_params, ffi)
class CosmoParams(StructWithDefaults):
"""
Cosmological parameters (with defaults) which translates to a C struct.
To see default values for each parameter, use ``CosmoParams._defaults_``.
All parameters passed in the constructor are also saved as instance attributes which should
be considered read-only. This is true of all input-parameter classes.
Default parameters are based on Plank18, https://arxiv.org/pdf/1807.06209.pdf,
Table 2, last column. [TT,TE,EE+lowE+lensing+BAO]
Parameters
----------
SIGMA_8 : float, optional
RMS mass variance (power spectrum normalisation).
hlittle : float, optional
The hubble parameter, H_0/100.
OMm : float, optional
Omega matter.
OMb : float, optional
Omega baryon, the baryon component.
POWER_INDEX : float, optional
Spectral index of the power spectrum.
"""
_ffi = ffi
_defaults_ = {
"SIGMA_8": 0.8102,
"hlittle": Planck18.h,
"OMm": Planck18.Om0,
"OMb": Planck18.Ob0,
"POWER_INDEX": 0.9665,
}
@property
def OMl(self):
"""Omega lambda, dark energy density."""
return 1 - self.OMm
@property
def cosmo(self):
"""Return an astropy cosmology object for this cosmology."""
return Planck15.clone(H0=self.hlittle * 100, Om0=self.OMm, Ob0=self.OMb)
class UserParams(StructWithDefaults):
"""
Structure containing user parameters (with defaults).
To see default values for each parameter, use ``UserParams._defaults_``.
All parameters passed in the constructor are also saved as instance attributes which should
be considered read-only. This is true of all input-parameter classes.
Parameters
----------
HII_DIM : int, optional
Number of cells for the low-res box. Default 200.
DIM : int,optional
Number of cells for the high-res box (sampling ICs) along a principal axis. To avoid
sampling issues, DIM should be at least 3 or 4 times HII_DIM, and an integer multiple.
By default, it is set to 3*HII_DIM.
NON_CUBIC_FACTOR : float, optional
Factor which allows the creation of non-cubic boxes. It will shorten/lengthen the line
of sight dimension of all boxes. NON_CUBIC_FACTOR * DIM/HII_DIM must result in an integer
BOX_LEN : float, optional
Length of the box, in Mpc. Default 300 Mpc.
HMF: int or str, optional
Determines which halo mass function to be used for the normalisation of the
collapsed fraction (default Sheth-Tormen). If string should be one of the
following codes:
0: PS (Press-Schechter)
1: ST (Sheth-Tormen)
2: Watson (Watson FOF)
3: Watson-z (Watson FOF-z)
USE_RELATIVE_VELOCITIES: int, optional
Flag to decide whether to use relative velocities.
If True, POWER_SPECTRUM is automatically set to 5. Default False.
POWER_SPECTRUM: int or str, optional
Determines which power spectrum to use, default EH (unless `USE_RELATIVE_VELOCITIES`
is True). If string, use the following codes:
0: EH
1: BBKS
2: EFSTATHIOU
3: PEEBLES
4: WHITE
5: CLASS (single cosmology)
N_THREADS : int, optional
Sets the number of processors (threads) to be used for performing 21cmFAST.
Default 1.
PERTURB_ON_HIGH_RES : bool, optional
Whether to perform the Zel'Dovich or 2LPT perturbation on the low or high
resolution grid.
NO_RNG : bool, optional
Ability to turn off random number generation for initial conditions. Can be
useful for debugging and adding in new features
USE_FFTW_WISDOM : bool, optional
Whether or not to use stored FFTW_WISDOMs for improving performance of FFTs
USE_INTERPOLATION_TABLES: bool, optional
If True, calculates and evaluates quantites using interpolation tables, which
is considerably faster than when performing integrals explicitly.
FAST_FCOLL_TABLES: bool, optional
Whether to use fast Fcoll tables, as described in Appendix of Muñoz+21 (2110.13919). Significant speedup for minihaloes.
USE_2LPT: bool, optional
Whether to use second-order Lagrangian perturbation theory (2LPT).
Set this to True if the density field or the halo positions are extrapolated to
low redshifts. The current implementation is very naive and adds a factor ~6 to
the memory requirements. Reference: Scoccimarro R., 1998, MNRAS, 299, 1097-1118
Appendix D.
MINIMIZE_MEMORY: bool, optional
If set, the code will run in a mode that minimizes memory usage, at the expense
of some CPU/disk-IO. Good for large boxes / small computers.
"""
_ffi = ffi
_defaults_ = {
"BOX_LEN": 300.0,
"DIM": None,
"HII_DIM": 200,
"NON_CUBIC_FACTOR": 1.0,
"USE_FFTW_WISDOM": False,
"HMF": 1,
"USE_RELATIVE_VELOCITIES": False,
"POWER_SPECTRUM": 0,
"N_THREADS": 1,
"PERTURB_ON_HIGH_RES": False,
"NO_RNG": False,
"USE_INTERPOLATION_TABLES": None,
"FAST_FCOLL_TABLES": False,
"USE_2LPT": True,
"MINIMIZE_MEMORY": False,
}
_hmf_models = ["PS", "ST", "WATSON", "WATSON-Z"]
_power_models = ["EH", "BBKS", "EFSTATHIOU", "PEEBLES", "WHITE", "CLASS"]
@property
def USE_INTERPOLATION_TABLES(self):
"""Whether to use interpolation tables for integrals, speeding things up."""
if self._USE_INTERPOLATION_TABLES is None:
warnings.warn(
"The USE_INTERPOLATION_TABLES setting has changed in v3.1.2 to be "
"default True. You can likely ignore this warning, but if you relied on"
"having USE_INTERPOLATION_TABLES=False by *default*, please set it "
"explicitly. To silence this warning, set it explicitly to True. This"
"warning will be removed in v4."
)
self._USE_INTERPOLATION_TABLES = True
return self._USE_INTERPOLATION_TABLES
@property
def DIM(self):
"""Number of cells for the high-res box (sampling ICs) along a principal axis."""
return self._DIM or 3 * self.HII_DIM
@property
def NON_CUBIC_FACTOR(self):
"""Factor to shorten/lengthen the line-of-sight dimension (non-cubic boxes)."""
dcf = self.DIM * self._NON_CUBIC_FACTOR
hdcf = self.HII_DIM * self._NON_CUBIC_FACTOR
if dcf % int(dcf) or hdcf % int(hdcf):
raise ValueError(
"NON_CUBIC_FACTOR * DIM and NON_CUBIC_FACTOR * HII_DIM must be integers"
)
else:
return self._NON_CUBIC_FACTOR
@property
def tot_fft_num_pixels(self):
"""Total number of pixels in the high-res box."""
return self.NON_CUBIC_FACTOR * self.DIM**3
@property
def HII_tot_num_pixels(self):
"""Total number of pixels in the low-res box."""
return self.NON_CUBIC_FACTOR * self.HII_DIM**3
@property
def POWER_SPECTRUM(self):
"""
The power spectrum generator to use, as an integer.
See :func:`power_spectrum_model` for a string representation.
"""
if self.USE_RELATIVE_VELOCITIES:
if self._POWER_SPECTRUM != 5 or (
isinstance(self._POWER_SPECTRUM, str)
and self._POWER_SPECTRUM.upper() != "CLASS"
):
logger.warning(
"Automatically setting POWER_SPECTRUM to 5 (CLASS) as you are using "
"relative velocities"
)
return 5
else:
if isinstance(self._POWER_SPECTRUM, str):
val = self._power_models.index(self._POWER_SPECTRUM.upper())
else:
val = self._POWER_SPECTRUM
if not 0 <= val < len(self._power_models):
raise ValueError(
f"Power spectrum must be between 0 and {len(self._power_models) - 1}"
)
return val
@property
def HMF(self):
"""The HMF to use (an int, mapping to a given form).
See hmf_model for a string representation.
"""
if isinstance(self._HMF, str):
val = self._hmf_models.index(self._HMF.upper())
else:
val = self._HMF
try:
val = int(val)
except (ValueError, TypeError) as e:
raise ValueError("Invalid value for HMF") from e
if not 0 <= val < len(self._hmf_models):
raise ValueError(
f"HMF must be an int between 0 and {len(self._hmf_models) - 1}"
)
return val
@property
def hmf_model(self):
"""String representation of the HMF model used."""
return self._hmf_models[self.HMF]
@property
def power_spectrum_model(self):
"""String representation of the power spectrum model used."""
return self._power_models[self.POWER_SPECTRUM]
@property
def FAST_FCOLL_TABLES(self):
"""Check that USE_INTERPOLATION_TABLES is True."""
if not self._FAST_FCOLL_TABLES or self.USE_INTERPOLATION_TABLES:
return self._FAST_FCOLL_TABLES
logger.warning(
"You cannot turn on FAST_FCOLL_TABLES without USE_INTERPOLATION_TABLES."
)
return False
class FlagOptions(StructWithDefaults):
"""
Flag-style options for the ionization routines.
To see default values for each parameter, use ``FlagOptions._defaults_``.
All parameters passed in the constructor are also saved as instance attributes
which should be considered read-only. This is true of all input-parameter classes.
Note that all flags are set to False by default, giving the simplest "vanilla"
version of 21cmFAST.
Parameters
----------
USE_HALO_FIELD : bool, optional
Set to True if intending to find and use the halo field. If False, uses
the mean collapse fraction (which is considerably faster).
USE_MINI_HALOS : bool, optional
Set to True if using mini-halos parameterization.
If True, USE_MASS_DEPENDENT_ZETA and INHOMO_RECO must be True.
USE_CMB_HEATING : bool, optional
Whether to include CMB Heating. (cf Eq.4 of Meiksin 2021, arxiv.org/abs/2105.14516)
USE_LYA_HEATING : bool, optional
Whether to use Lyman-alpha heating. (cf Sec. 3 of Reis+2021, doi.org/10.1093/mnras/stab2089)
USE_MASS_DEPENDENT_ZETA : bool, optional
Set to True if using new parameterization. Setting to True will automatically
set `M_MIN_in_Mass` to True.
SUBCELL_RSDS : bool, optional
Add sub-cell redshift-space-distortions (cf Sec 2.2 of Greig+2018).
Will only be effective if `USE_TS_FLUCT` is True.
INHOMO_RECO : bool, optional
Whether to perform inhomogeneous recombinations. Increases the computation
time.
USE_TS_FLUCT : bool, optional
Whether to perform IGM spin temperature fluctuations (i.e. X-ray heating).
Dramatically increases the computation time.
M_MIN_in_Mass : bool, optional
Whether the minimum halo mass (for ionization) is defined by
mass or virial temperature. Automatically True if `USE_MASS_DEPENDENT_ZETA`
is True.
PHOTON_CONS : bool, optional
Whether to perform a small correction to account for the inherent
photon non-conservation.
FIX_VCB_AVG: bool, optional
Determines whether to use a fixed vcb=VAVG (*regardless* of USE_RELATIVE_VELOCITIES). It includes the average effect of velocities but not its fluctuations. See Muñoz+21 (2110.13919).
USE_VELS_AUX: bool, optional
Auxiliary variable (not input) to check if minihaloes are being used without relative velocities and complain
"""
_ffi = ffi
_defaults_ = {
"USE_HALO_FIELD": False,
"USE_MINI_HALOS": False,
"USE_CMB_HEATING": True,
"USE_LYA_HEATING": True,
"USE_MASS_DEPENDENT_ZETA": False,
"SUBCELL_RSD": False,
"INHOMO_RECO": False,
"USE_TS_FLUCT": False,
"M_MIN_in_Mass": False,
"PHOTON_CONS": False,
"FIX_VCB_AVG": False,
}
@property
def USE_HALO_FIELD(self):
"""Automatically setting USE_MASS_DEPENDENT_ZETA to False if USE_MINI_HALOS."""
if not self.USE_MINI_HALOS or not self._USE_HALO_FIELD:
return self._USE_HALO_FIELD
logger.warning(
"You have set USE_MINI_HALOS to True but USE_HALO_FIELD is also True! "
"Automatically setting USE_HALO_FIELD to False."
)
return False
@property
def M_MIN_in_Mass(self):
"""Whether minimum halo mass is defined in mass or virial temperature."""
return True if self.USE_MASS_DEPENDENT_ZETA else self._M_MIN_in_Mass
@property
def USE_MASS_DEPENDENT_ZETA(self):
"""Automatically setting USE_MASS_DEPENDENT_ZETA to True if USE_MINI_HALOS."""
if not self.USE_MINI_HALOS or self._USE_MASS_DEPENDENT_ZETA:
return self._USE_MASS_DEPENDENT_ZETA
logger.warning(
"You have set USE_MINI_HALOS to True but USE_MASS_DEPENDENT_ZETA to False! "
"Automatically setting USE_MASS_DEPENDENT_ZETA to True."
)
return True
@property
def INHOMO_RECO(self):
"""Automatically setting INHOMO_RECO to True if USE_MINI_HALOS."""
if not self.USE_MINI_HALOS or self._INHOMO_RECO:
return self._INHOMO_RECO
logger.warning(
"You have set USE_MINI_HALOS to True but INHOMO_RECO to False! "
"Automatically setting INHOMO_RECO to True."
)
return True
@property
def USE_TS_FLUCT(self):
"""Automatically setting USE_TS_FLUCT to True if USE_MINI_HALOS."""
if not self.USE_MINI_HALOS or self._USE_TS_FLUCT:
return self._USE_TS_FLUCT
logger.warning(
"You have set USE_MINI_HALOS to True but USE_TS_FLUCT to False! "
"Automatically setting USE_TS_FLUCT to True."
)
return True
@property
def PHOTON_CONS(self):
"""Automatically setting PHOTON_CONS to False if USE_MINI_HALOS."""
if not self.USE_MINI_HALOS or not self._PHOTON_CONS:
return self._PHOTON_CONS
logger.warning(
"USE_MINI_HALOS is not compatible with PHOTON_CONS! "
"Automatically setting PHOTON_CONS to False."
)
return False
class AstroParams(StructWithDefaults):
"""
Astrophysical parameters.
To see default values for each parameter, use ``AstroParams._defaults_``.
All parameters passed in the constructor are also saved as instance attributes which should
be considered read-only. This is true of all input-parameter classes.
Parameters
----------
INHOMO_RECO : bool, optional
Whether inhomogeneous recombinations are being calculated. This is not a part of the
astro parameters structure, but is required by this class to set some default behaviour.
HII_EFF_FACTOR : float, optional
The ionizing efficiency of high-z galaxies (zeta, from Eq. 2 of Greig+2015).
Higher values tend to speed up reionization.
F_STAR10 : float, optional
The fraction of galactic gas in stars for 10^10 solar mass haloes.
Only used in the "new" parameterization,
i.e. when `USE_MASS_DEPENDENT_ZETA` is set to True (in :class:`FlagOptions`).
If so, this is used along with `F_ESC10` to determine `HII_EFF_FACTOR` (which
is then unused). See Eq. 11 of Greig+2018 and Sec 2.1 of Park+2018.
Given in log10 units.
F_STAR7_MINI : float, optional
The fraction of galactic gas in stars for 10^7 solar mass minihaloes.
Only used in the "minihalo" parameterization,
i.e. when `USE_MINI_HALOS` is set to True (in :class:`FlagOptions`).
If so, this is used along with `F_ESC7_MINI` to determine `HII_EFF_FACTOR_MINI` (which
is then unused). See Eq. 8 of Qin+2020.
Given in log10 units.
ALPHA_STAR : float, optional
Power-law index of fraction of galactic gas in stars as a function of halo mass.
See Sec 2.1 of Park+2018.
ALPHA_STAR_MINI : float, optional
Power-law index of fraction of galactic gas in stars as a function of halo mass, for MCGs.
See Sec 2 of Muñoz+21 (2110.13919).
F_ESC10 : float, optional
The "escape fraction", i.e. the fraction of ionizing photons escaping into the
IGM, for 10^10 solar mass haloes. Only used in the "new" parameterization,
i.e. when `USE_MASS_DEPENDENT_ZETA` is set to True (in :class:`FlagOptions`).
If so, this is used along with `F_STAR10` to determine `HII_EFF_FACTOR` (which
is then unused). See Eq. 11 of Greig+2018 and Sec 2.1 of Park+2018.
F_ESC7_MINI: float, optional
The "escape fraction for minihalos", i.e. the fraction of ionizing photons escaping
into the IGM, for 10^7 solar mass minihaloes. Only used in the "minihalo" parameterization,
i.e. when `USE_MINI_HALOS` is set to True (in :class:`FlagOptions`).
If so, this is used along with `F_ESC7_MINI` to determine `HII_EFF_FACTOR_MINI` (which
is then unused). See Eq. 17 of Qin+2020.
Given in log10 units.
ALPHA_ESC : float, optional
Power-law index of escape fraction as a function of halo mass. See Sec 2.1 of
Park+2018.
M_TURN : float, optional
Turnover mass (in log10 solar mass units) for quenching of star formation in
halos, due to SNe or photo-heating feedback, or inefficient gas accretion. Only
used if `USE_MASS_DEPENDENT_ZETA` is set to True in :class:`FlagOptions`.
See Sec 2.1 of Park+2018.
R_BUBBLE_MAX : float, optional
Mean free path in Mpc of ionizing photons within ionizing regions (Sec. 2.1.2 of
Greig+2015). Default is 50 if `INHOMO_RECO` is True, or 15.0 if not.
ION_Tvir_MIN : float, optional
Minimum virial temperature of star-forming haloes (Sec 2.1.3 of Greig+2015).
Given in log10 units.
L_X : float, optional
The specific X-ray luminosity per unit star formation escaping host galaxies.
Cf. Eq. 6 of Greig+2018. Given in log10 units.
L_X_MINI: float, optional
The specific X-ray luminosity per unit star formation escaping host galaxies for
minihalos. Cf. Eq. 23 of Qin+2020. Given in log10 units.
NU_X_THRESH : float, optional
X-ray energy threshold for self-absorption by host galaxies (in eV). Also called
E_0 (cf. Sec 4.1 of Greig+2018). Typical range is (100, 1500).
X_RAY_SPEC_INDEX : float, optional
X-ray spectral energy index (cf. Sec 4.1 of Greig+2018). Typical range is
(-1, 3).
X_RAY_Tvir_MIN : float, optional
Minimum halo virial temperature in which X-rays are produced. Given in log10
units. Default is `ION_Tvir_MIN`.
F_H2_SHIELD: float, optional
Self-shielding factor of molecular hydrogen when experiencing LW suppression.
Cf. Eq. 12 of Qin+2020. Consistently included in A_LW fit from sims.
If used we recommend going back to Macachek+01 A_LW=22.86.
t_STAR : float, optional
Fractional characteristic time-scale (fraction of hubble time) defining the
star-formation rate of galaxies. Only used if `USE_MASS_DEPENDENT_ZETA` is set
to True in :class:`FlagOptions`. See Sec 2.1, Eq. 3 of Park+2018.
N_RSD_STEPS : int, optional
Number of steps used in redshift-space-distortion algorithm. NOT A PHYSICAL
PARAMETER.
A_LW, BETA_LW: float, optional
Impact of the LW feedback on Mturn for minihaloes. Default is 22.8685 and 0.47 following Machacek+01, respectively. Latest simulations suggest 2.0 and 0.6. See Sec 2 of Muñoz+21 (2110.13919).
A_VCB, BETA_VCB: float, optional
Impact of the DM-baryon relative velocities on Mturn for minihaloes. Default is 1.0 and 1.8, and agrees between different sims. See Sec 2 of Muñoz+21 (2110.13919).
"""
_ffi = ffi
_defaults_ = {
"HII_EFF_FACTOR": 30.0,
"F_STAR10": -1.3,
"F_STAR7_MINI": -2.0,
"ALPHA_STAR": 0.5,
"ALPHA_STAR_MINI": 0.5,
"F_ESC10": -1.0,
"F_ESC7_MINI": -2.0,
"ALPHA_ESC": -0.5,
"M_TURN": 8.7,
"R_BUBBLE_MAX": None,
"ION_Tvir_MIN": 4.69897,
"L_X": 40.0,
"L_X_MINI": 40.0,
"NU_X_THRESH": 500.0,
"X_RAY_SPEC_INDEX": 1.0,
"X_RAY_Tvir_MIN": None,
"F_H2_SHIELD": 0.0,
"t_STAR": 0.5,
"N_RSD_STEPS": 20,
"A_LW": 2.00,
"BETA_LW": 0.6,
"A_VCB": 1.0,
"BETA_VCB": 1.8,
}
def __init__(
self, *args, INHOMO_RECO=FlagOptions._defaults_["INHOMO_RECO"], **kwargs
):
# TODO: should try to get inhomo_reco out of here... just needed for default of
# R_BUBBLE_MAX.
self.INHOMO_RECO = INHOMO_RECO
super().__init__(*args, **kwargs)
def convert(self, key, val):
"""Convert a given attribute before saving it the instance."""
if key in [
"F_STAR10",
"F_ESC10",
"F_STAR7_MINI",
"F_ESC7_MINI",
"M_TURN",
"ION_Tvir_MIN",
"L_X",
"L_X_MINI",
"X_RAY_Tvir_MIN",
]:
return 10**val
else:
return val
@property
def R_BUBBLE_MAX(self):
"""Maximum radius of bubbles to be searched. Set dynamically."""
if not self._R_BUBBLE_MAX:
return 50.0 if self.INHOMO_RECO else 15.0
if self.INHOMO_RECO and self._R_BUBBLE_MAX != 50:
logger.warning(
"You are setting R_BUBBLE_MAX != 50 when INHOMO_RECO=True. "
"This is non-standard (but allowed), and usually occurs upon manual "
"update of INHOMO_RECO"
)
return self._R_BUBBLE_MAX
@property
def X_RAY_Tvir_MIN(self):
"""Minimum virial temperature of X-ray emitting sources (unlogged and set dynamically)."""
return self._X_RAY_Tvir_MIN or self.ION_Tvir_MIN
@property
def NU_X_THRESH(self):
"""Check if the choice of NU_X_THRESH is sensible."""
if self._NU_X_THRESH < 100.0:
raise ValueError(
"Chosen NU_X_THRESH is < 100 eV. NU_X_THRESH must be above 100 eV as it describes X-ray photons"
)
elif self._NU_X_THRESH >= global_params.NU_X_BAND_MAX:
raise ValueError(
"""
Chosen NU_X_THRESH > {}, which is the upper limit of the adopted X-ray band
(fiducially the soft band 0.5 - 2.0 keV). If you know what you are doing with this
choice, please modify the global parameter: NU_X_BAND_MAX""".format(
global_params.NU_X_BAND_MAX
)
)
else:
if global_params.NU_X_BAND_MAX > global_params.NU_X_MAX:
raise ValueError(
"""
Chosen NU_X_BAND_MAX > {}, which is the upper limit of X-ray integrals (fiducially 10 keV)
If you know what you are doing, please modify the global parameter:
NU_X_MAX""".format(
global_params.NU_X_MAX
)
)
else:
return self._NU_X_THRESH
@property
def t_STAR(self):
"""Check if the choice of NU_X_THRESH is sensible."""
if self._t_STAR <= 0.0 or self._t_STAR > 1.0:
raise ValueError("t_STAR must be above zero and less than or equal to one")
else:
return self._t_STAR
class InputCrossValidationError(ValueError):
"""Error when two parameters from different structs aren't consistent."""
pass
def validate_all_inputs(
user_params: UserParams,
cosmo_params: CosmoParams,
astro_params: AstroParams | None = None,
flag_options: FlagOptions | None = None,
):
"""Cross-validate input parameters from different structs.
The input params may be modified in-place in this function, but if so, a warning
should be emitted.
"""
if astro_params is not None:
if astro_params.R_BUBBLE_MAX > user_params.BOX_LEN:
astro_params.update(R_BUBBLE_MAX=user_params.BOX_LEN)
warnings.warn(
f"Setting R_BUBBLE_MAX to BOX_LEN (={user_params.BOX_LEN} as it doesn't make sense for it to be larger."
)
if (
global_params.HII_FILTER == 1
and astro_params.R_BUBBLE_MAX > user_params.BOX_LEN / 3
):
msg = (
"Your R_BUBBLE_MAX is > BOX_LEN/3 "
f"({astro_params.R_BUBBLE_MAX} > {user_params.BOX_LEN/3})."
)
if config["ignore_R_BUBBLE_MAX_error"]:
warnings.warn(msg)
else:
raise ValueError(msg)
if flag_options is not None and (
flag_options.USE_MINI_HALOS
and not user_params.USE_RELATIVE_VELOCITIES
and not flag_options.FIX_VCB_AVG
):
logger.warning(
"USE_MINI_HALOS needs USE_RELATIVE_VELOCITIES to get the right evolution!"
) | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/src/py21cmfast/inputs.py | inputs.py |
from __future__ import annotations
import contextlib
import copy
import warnings
from pathlib import Path
from . import yaml
class ConfigurationError(Exception):
"""An error with the config file."""
pass
class Config(dict):
"""Simple over-ride of dict that adds a context manager."""
_defaults = {
"direc": "~/21cmFAST-cache",
"regenerate": False,
"write": True,
"cache_param_sigfigs": 6,
"cache_redshift_sigfigs": 4,
"ignore_R_BUBBLE_MAX_error": False,
}
_aliases = {"direc": ("boxdir",)}
def __init__(self, *args, write=True, file_name=None, **kwargs):
super().__init__(*args, **kwargs)
self.file_name = file_name
# Ensure the keys that got read in are the right keys for the current version
do_write = False
for k, v in self._defaults.items():
if k not in self:
if k not in self._aliases:
warnings.warn("Your configuration file is out of date. Updating...")
do_write = True
self[k] = v
else:
for alias in self._aliases[k]:
if alias in self:
do_write = True
warnings.warn(
f"Your configuration file has old key '{alias}' which "
f"has been re-named '{k}'. Updating..."
)
self[k] = self[alias]
del self[alias]
break
else:
warnings.warn(
"Your configuration file is out of date. Updating..."
)
do_write = True
self[k] = v
for k, v in self.items():
if k not in self._defaults:
raise ConfigurationError(
f"The configuration file has key '{k}' which is not known to 21cmFAST."
)
self["direc"] = Path(self["direc"]).expanduser().absolute()
if do_write and write and self.file_name:
self.write()
@contextlib.contextmanager
def use(self, **kwargs):
"""Context manager for using certain configuration options for a set time."""
backup = self.copy()
for k, v in kwargs.items():
self[k] = Path(v).expanduser().absolute() if k == "direc" else v
yield self
for k in kwargs:
self[k] = backup[k]
def write(self, fname: str | Path | None = None):
"""Write current configuration to file to make it permanent."""
fname = Path(fname or self.file_name)
if fname:
if not fname.parent.exists():
fname.parent.mkdir(parents=True)
with open(fname, "w") as fl:
yaml.dump(self._as_dict(), fl)
def _as_dict(self):
"""The plain dict defining the instance."""
return {k: str(Path) if isinstance(v, Path) else v for k, v in self.items()}
@classmethod
def load(cls, file_name: str | Path):
"""Create a Config object from a config file."""
file_name = Path(file_name).expanduser().absolute()
if file_name.exists():
with open(file_name) as fl:
cfg = yaml.load(fl)
return cls(cfg, file_name=file_name)
else:
return cls(write=False)
config = Config.load(Path("~/.21cmfast/config.yml"))
# Keep an original copy around
default_config = copy.deepcopy(config) | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/src/py21cmfast/_cfg.py | _cfg.py |
import glob
import h5py
import logging
import numpy as np
import warnings
from abc import ABCMeta, abstractmethod
from bidict import bidict
from cffi import FFI
from enum import IntEnum
from hashlib import md5
from os import makedirs, path
from pathlib import Path
from typing import Any, Callable, Dict, List, Optional, Sequence, Tuple, Union
from . import __version__
from ._cfg import config
from .c_21cmfast import lib
_ffi = FFI()
logger = logging.getLogger(__name__)
class ArrayStateError(ValueError):
"""Errors arising from incorrectly modifying array state."""
pass
class ArrayState:
"""Define the memory state of a struct array."""
def __init__(
self, initialized=False, c_memory=False, computed_in_mem=False, on_disk=False
):
self._initialized = initialized
self._c_memory = c_memory
self._computed_in_mem = computed_in_mem
self._on_disk = on_disk
@property
def initialized(self):
"""Whether the array is initialized (i.e. allocated memory)."""
return self._initialized
@initialized.setter
def initialized(self, val):
if not val:
# if its not initialized, can't be computed in memory
self.computed_in_mem = False
self._initialized = bool(val)
@property
def c_memory(self):
"""Whether the array's memory (if any) is controlled by C."""
return self._c_memory
@c_memory.setter
def c_memory(self, val):
self._c_memory = bool(val)
@property
def computed_in_mem(self):
"""Whether the array is computed and stored in memory."""
return self._computed_in_mem
@computed_in_mem.setter
def computed_in_mem(self, val):
if val:
# any time we pull something into memory, it must be initialized.
self.initialized = True
self._computed_in_mem = bool(val)
@property
def on_disk(self):
"""Whether the array is computed and store on disk."""
return self._on_disk
@on_disk.setter
def on_disk(self, val):
self._on_disk = bool(val)
@property
def computed(self):
"""Whether the array is computed anywhere."""
return self.computed_in_mem or self.on_disk
@property
def c_has_active_memory(self):
"""Whether C currently has initialized memory for this array."""
return self.c_memory and self.initialized
class ParameterError(RuntimeError):
"""An exception representing a bad choice of parameters."""
default_message = "21cmFAST does not support this combination of parameters."
def __init__(self, msg=None):
super().__init__(msg or self.default_message)
class FatalCError(Exception):
"""An exception representing something going wrong in C."""
default_message = "21cmFAST is exiting."
def __init__(self, msg=None):
super().__init__(msg or self.default_message)
class FileIOError(FatalCError):
"""An exception when an error occurs with file I/O."""
default_message = "Expected file could not be found! (check the LOG for more info)"
class GSLError(ParameterError):
"""An exception when a GSL routine encounters an error."""
default_message = "A GSL routine has errored! (check the LOG for more info)"
class ArgumentValueError(FatalCError):
"""An exception when a function takes an unexpected input."""
default_message = "An incorrect argument has been defined or passed! (check the LOG for more info)"
class PhotonConsError(ParameterError):
"""An exception when the photon non-conservation correction routine errors."""
default_message = "An error has occured with the Photon non-conservation correction! (check the LOG for more info)"
class TableGenerationError(ParameterError):
"""An exception when an issue arises populating one of the interpolation tables."""
default_message = """An error has occured when generating an interpolation table!
This has likely occured due to the choice of input AstroParams (check the LOG for more info)"""
class TableEvaluationError(ParameterError):
"""An exception when an issue arises populating one of the interpolation tables."""
default_message = """An error has occured when evaluating an interpolation table!
This can sometimes occur due to small boxes (either small DIM/HII_DIM or BOX_LEN) (check the LOG for more info)"""
class InfinityorNaNError(ParameterError):
"""An exception when an infinity or NaN is encountered in a calculated quantity."""
default_message = """Something has returned an infinity or a NaN! This could be due to an issue with an
input parameter choice (check the LOG for more info)"""
class MassDepZetaError(ParameterError):
"""An exception when determining the bisection for stellar mass/escape fraction."""
default_message = """There is an issue with the choice of parameters under MASS_DEPENDENT_ZETA. Could be an issue with
any of the chosen F_STAR10, ALPHA_STAR, F_ESC10 or ALPHA_ESC."""
class MemoryAllocError(FatalCError):
"""An exception when unable to allocated memory."""
default_message = """An error has occured while attempting to allocate memory! (check the LOG for more info)"""
SUCCESS = 0
IOERROR = 1
GSLERROR = 2
VALUEERROR = 3
PHOTONCONSERROR = 4
TABLEGENERATIONERROR = 5
TABLEEVALUATIONERROR = 6
INFINITYORNANERROR = 7
MASSDEPZETAERROR = 8
MEMORYALLOCERROR = 9
def _process_exitcode(exitcode, fnc, args):
"""Determine what happens for different values of the (integer) exit code from a C function."""
if exitcode != SUCCESS:
logger.error(f"In function: {fnc.__name__}. Arguments: {args}")
if exitcode:
try:
raise {
IOERROR: FileIOError,
GSLERROR: GSLError,
VALUEERROR: ArgumentValueError,
PHOTONCONSERROR: PhotonConsError,
TABLEGENERATIONERROR: TableGenerationError,
TABLEEVALUATIONERROR: TableEvaluationError,
INFINITYORNANERROR: InfinityorNaNError,
MASSDEPZETAERROR: MassDepZetaError,
MEMORYALLOCERROR: MemoryAllocError,
}[exitcode]
except KeyError: # pragma: no cover
raise FatalCError(
"Unknown error in C. Please report this error!"
) # Unknown C code
ctype2dtype = {}
# Integer types
for prefix in ("int", "uint"):
for log_bytes in range(4):
ctype = "%s%d_t" % (prefix, 8 * (2**log_bytes))
dtype = "%s%d" % (prefix[0], 2**log_bytes)
ctype2dtype[ctype] = np.dtype(dtype)
# Floating point types
ctype2dtype["float"] = np.dtype("f4")
ctype2dtype["double"] = np.dtype("f8")
ctype2dtype["int"] = np.dtype("i4")
def asarray(ptr, shape):
"""Get the canonical C type of the elements of ptr as a string."""
ctype = _ffi.getctype(_ffi.typeof(ptr).item).split("*")[0].strip()
if ctype not in ctype2dtype:
raise RuntimeError(
f"Cannot create an array for element type: {ctype}. Can do {list(ctype2dtype.values())}."
)
array = np.frombuffer(
_ffi.buffer(ptr, _ffi.sizeof(ctype) * np.prod(shape)), ctype2dtype[ctype]
)
array.shape = shape
return array
class StructWrapper:
"""
A base-class python wrapper for C structures (not instances of them).
Provides simple methods for creating new instances and accessing field names and values.
To implement wrappers of specific structures, make a subclass with the same name as the
appropriate C struct (which must be defined in the C code that has been compiled to the ``ffi``
object), *or* use an arbitrary name, but set the ``_name`` attribute to the C struct name.
"""
_name = None
_ffi = None
def __init__(self):
# Set the name of this struct in the C code
self._name = self._get_name()
@classmethod
def _get_name(cls):
return cls._name or cls.__name__
@property
def _cstruct(self):
"""
The actual structure which needs to be passed around to C functions.
.. note:: This is best accessed by calling the instance (see __call__).
The reason it is defined as this (manual) cached property is so that it can be created
dynamically, but not lost. It must not be lost, or else C functions which use it will lose
access to its memory. But it also must be created dynamically so that it can be recreated
after pickling (pickle can't handle CData).
"""
try:
return self.__cstruct
except AttributeError:
self.__cstruct = self._new()
return self.__cstruct
def _new(self):
"""Return a new empty C structure corresponding to this class."""
return self._ffi.new("struct " + self._name + "*")
@classmethod
def get_fields(cls, cstruct=None) -> Dict[str, Any]:
"""Obtain the C-side fields of this struct."""
if cstruct is None:
cstruct = cls._ffi.new("struct " + cls._get_name() + "*")
return dict(cls._ffi.typeof(cstruct[0]).fields)
@classmethod
def get_fieldnames(cls, cstruct=None) -> List[str]:
"""Obtain the C-side field names of this struct."""
fields = cls.get_fields(cstruct)
return [f for f, t in fields]
@classmethod
def get_pointer_fields(cls, cstruct=None) -> List[str]:
"""Obtain all pointer fields of the struct (typically simulation boxes)."""
return [f for f, t in cls.get_fields(cstruct) if t.type.kind == "pointer"]
@property
def fields(self) -> Dict[str, Any]:
"""List of fields of the underlying C struct (a list of tuples of "name, type")."""
return self.get_fields(self._cstruct)
@property
def fieldnames(self) -> List[str]:
"""List names of fields of the underlying C struct."""
return [f for f, t in self.fields.items()]
@property
def pointer_fields(self) -> List[str]:
"""List of names of fields which have pointer type in the C struct."""
return [f for f, t in self.fields.items() if t.type.kind == "pointer"]
@property
def primitive_fields(self) -> List[str]:
"""List of names of fields which have primitive type in the C struct."""
return [f for f, t in self.fields.items() if t.type.kind == "primitive"]
def __getstate__(self):
"""Return the current state of the class without pointers."""
return {
k: v
for k, v in self.__dict__.items()
if k not in ["_strings", "_StructWrapper__cstruct"]
}
def refresh_cstruct(self):
"""Delete the underlying C object, forcing it to be rebuilt."""
try:
del self.__cstruct
except AttributeError:
pass
def __call__(self):
"""Return an instance of the C struct."""
pass
class StructWithDefaults(StructWrapper):
"""
A convenient interface to create a C structure with defaults specified.
It is provided for the purpose of *creating* C structures in Python to be passed to C functions,
where sensible defaults are available. Structures which are created within C and passed back do
not need to be wrapped.
This provides a *fully initialised* structure, and will fail if not all fields are specified
with defaults.
.. note:: The actual C structure is gotten by calling an instance. This is auto-generated when
called, based on the parameters in the class.
.. warning:: This class will *not* deal well with parameters of the struct which are pointers.
All parameters should be primitive types, except for strings, which are dealt with
specially.
Parameters
----------
ffi : cffi object
The ffi object from any cffi-wrapped library.
"""
_defaults_ = {}
def __init__(self, *args, **kwargs):
super().__init__()
if args:
if len(args) > 1:
raise TypeError(
"%s takes up to one position argument, %s were given"
% (self.__class__.__name__, len(args))
)
elif args[0] is None:
pass
elif isinstance(args[0], self.__class__):
kwargs.update(args[0].self)
elif isinstance(args[0], dict):
kwargs.update(args[0])
else:
raise TypeError(
f"optional positional argument for {self.__class__.__name__} must be"
f" None, dict, or an instance of itself"
)
for k, v in self._defaults_.items():
# Prefer arguments given to the constructor.
_v = kwargs.pop(k, None)
if _v is not None:
v = _v
try:
setattr(self, k, v)
except AttributeError:
# The attribute has been defined as a property, save it as a hidden variable
setattr(self, "_" + k, v)
if kwargs:
warnings.warn(
"The following parameters to {thisclass} are not supported: {lst}".format(
thisclass=self.__class__.__name__, lst=list(kwargs.keys())
)
)
def convert(self, key, val):
"""Make any conversions of values before saving to the instance."""
return val
def update(self, **kwargs):
"""
Update the parameters of an existing class structure.
This should always be used instead of attempting to *assign* values to instance attributes.
It consistently re-generates the underlying C memory space and sets some book-keeping
variables.
Parameters
----------
kwargs:
Any argument that may be passed to the class constructor.
"""
# Start a fresh cstruct.
if kwargs:
self.refresh_cstruct()
for k in self._defaults_:
# Prefer arguments given to the constructor.
if k in kwargs:
v = kwargs.pop(k)
try:
setattr(self, k, v)
except AttributeError:
# The attribute has been defined as a property, save it as a hidden variable
setattr(self, "_" + k, v)
# Also ensure that parameters that are part of the class, but not the defaults, are set
# this will fail if these parameters cannot be set for some reason, hence doing it
# last.
for k in list(kwargs.keys()):
if hasattr(self, k):
setattr(self, k, kwargs.pop(k))
if kwargs:
warnings.warn(
"The following arguments to be updated are not compatible with this class: %s"
% kwargs
)
def clone(self, **kwargs):
"""Make a fresh copy of the instance with arbitrary parameters updated."""
new = self.__class__(self.self)
new.update(**kwargs)
return new
def __call__(self):
"""Return a filled C Structure corresponding to this instance."""
for key, val in self.pystruct.items():
# Find the value of this key in the current class
if isinstance(val, str):
# If it is a string, need to convert it to C string ourselves.
val = self.ffi.new("char[]", getattr(self, key).encode())
try:
setattr(self._cstruct, key, val)
except TypeError:
logger.info(f"For key {key}, value {val}:")
raise
return self._cstruct
@property
def pystruct(self):
"""A pure-python dictionary representation of the corresponding C structure."""
return {fld: self.convert(fld, getattr(self, fld)) for fld in self.fieldnames}
@property
def defining_dict(self):
"""
Pure python dictionary representation of this class, as it would appear in C.
.. note:: This is not the same as :attr:`pystruct`, as it omits all variables that don't
need to be passed to the constructor, but appear in the C struct (some can be
calculated dynamically based on the inputs). It is also not the same as
:attr:`self`, as it includes the 'converted' values for each variable, which are
those actually passed to the C code.
"""
return {k: self.convert(k, getattr(self, k)) for k in self._defaults_}
@property
def self(self):
"""
Dictionary which if passed to its own constructor will yield an identical copy.
.. note:: This differs from :attr:`pystruct` and :attr:`defining_dict` in that it uses the
hidden variable value, if it exists, instead of the exposed one. This prevents
from, for example, passing a value which is 10**10**val (and recurring!).
"""
# Try to first use the hidden variable before using the non-hidden variety.
dct = {}
for k in self._defaults_:
if hasattr(self, "_" + k):
dct[k] = getattr(self, "_" + k)
else:
dct[k] = getattr(self, k)
return dct
def __repr__(self):
"""Full unique representation of the instance."""
return (
self.__class__.__name__
+ "("
+ ", ".join(
sorted(
k
+ ":"
+ (
float_to_string_precision(v, config["cache_redshift_sigfigs"])
if isinstance(v, (float, np.float32))
else str(v)
)
for k, v in self.defining_dict.items()
)
)
+ ")"
)
def __eq__(self, other):
"""Check whether this instance is equal to another object (by checking the __repr__)."""
return self.__repr__() == repr(other)
def __hash__(self):
"""Generate a unique hsh for the instance."""
return hash(self.__repr__())
def __str__(self):
"""Human-readable string representation of the object."""
biggest_k = max(len(k) for k in self.defining_dict)
params = "\n ".join(
sorted(f"{k:<{biggest_k}}: {v}" for k, v in self.defining_dict.items())
)
return f"""{self.__class__.__name__}:
{params}
"""
def snake_to_camel(word: str, publicize: bool = True):
"""Convert snake case to camel case."""
if publicize:
word = word.lstrip("_")
return "".join(x.capitalize() or "_" for x in word.split("_"))
def camel_to_snake(word: str, depublicize: bool = False):
"""Convert came case to snake case."""
word = "".join("_" + i.lower() if i.isupper() else i for i in word)
if not depublicize:
word = word.lstrip("_")
return word
def float_to_string_precision(x, n):
"""Prints out a standard float number at a given number of significant digits.
Code here: https://stackoverflow.com/a/48812729
"""
return f'{float(f"{x:.{int(n)}g}"):g}'
def get_all_subclasses(cls):
"""Get a list of all subclasses of a given class, recursively."""
all_subclasses = []
for subclass in cls.__subclasses__():
all_subclasses.append(subclass)
all_subclasses.extend(get_all_subclasses(subclass))
return all_subclasses
class OutputStruct(StructWrapper, metaclass=ABCMeta):
"""Base class for any class that wraps a C struct meant to be output from a C function."""
_meta = True
_fields_ = []
_global_params = None
_inputs = ("user_params", "cosmo_params", "_random_seed")
_filter_params = ["external_table_path", "wisdoms_path"]
_c_based_pointers = ()
_c_compute_function = None
_TYPEMAP = bidict({"float32": "float *", "float64": "double *", "int32": "int *"})
def __init__(self, *, random_seed=None, dummy=False, initial=False, **kwargs):
"""
Base type for output structures from C functions.
Parameters
----------
random_seed
Seed associated with the output.
dummy
Specify this as a dummy struct, in which no arrays are to be
initialized or computed.
initial
Specify this as an initial struct, where arrays are to be
initialized, but do not need to be computed to pass into another
struct's compute().
"""
super().__init__()
self.version = ".".join(__version__.split(".")[:2])
self.patch_version = ".".join(__version__.split(".")[2:])
self._paths = []
self._random_seed = random_seed
for k in self._inputs:
if k not in self.__dict__:
try:
setattr(self, k, kwargs.pop(k))
except KeyError:
raise KeyError(
f"{self.__class__.__name__} requires the keyword argument {k}"
)
if kwargs:
warnings.warn(
f"{self.__class__.__name__} received the following unexpected "
f"arguments: {list(kwargs.keys())}"
)
self.dummy = dummy
self.initial = initial
self._array_structure = self._get_box_structures()
self._array_state = {k: ArrayState() for k in self._array_structure}
self._array_state.update({k: ArrayState() for k in self._c_based_pointers})
for k in self._array_structure:
if k not in self.pointer_fields:
raise TypeError(f"Key {k} in {self} not a defined pointer field in C.")
@property
def path(self) -> Tuple[None, Path]:
"""The path to an on-disk version of this object."""
if not self._paths:
return None
for pth in self._paths:
if pth.exists():
return pth
logger.info("All paths that defined {self} have been deleted on disk.")
return None
@abstractmethod
def _get_box_structures(self) -> Dict[str, Union[Dict, Tuple[int]]]:
"""Return a dictionary of names mapping to shapes for each array in the struct.
The reason this is a function, not a simple attribute, is that we may need to
decide on what arrays need to be initialized based on the inputs (eg. if USE_2LPT
is True or False).
Each actual OutputStruct subclass needs to implement this. Note that the arrays
are not actually initialized here -- that's done automatically by :func:`_init_arrays`
using this information. This function means that the names of the actually required
arrays can be accessed without doing any actual initialization.
Note also that this only contains arrays allocated *by Python* not C. Arrays
allocated by C are specified in :func:`_c_shape`.
"""
pass
def _c_shape(self, cstruct) -> Dict[str, Tuple[int]]:
"""Return a dictionary of field: shape for arrays allocated within C."""
return {}
@classmethod
def _implementations(cls):
all_classes = get_all_subclasses(cls)
return [c for c in all_classes if not c._meta]
def _init_arrays(self):
for k, state in self._array_state.items():
if k == "lowres_density":
logger.debug("THINKING ABOUT INITING LOWRES_DENSITY")
logger.debug(state.initialized, state.computed_in_mem, state.on_disk)
# Don't initialize C-based pointers or already-inited stuff, or stuff
# that's computed on disk (if it's on disk, accessing the array should
# just give the computed version, which is what we would want, not a
# zero-inited array).
if k in self._c_based_pointers or state.initialized or state.on_disk:
continue
params = self._array_structure[k]
tp = self._TYPEMAP.inverse[self.fields[k].type.cname]
if isinstance(params, tuple):
shape = params
fnc = np.zeros
elif isinstance(params, dict):
fnc = params.get("init", np.zeros)
shape = params.get("shape")
else:
raise ValueError("params is not a tuple or dict")
setattr(self, k, fnc(shape, dtype=tp))
# Add it to initialized arrays.
state.initialized = True
@property
def random_seed(self):
"""The random seed for this particular instance."""
if self._random_seed is None:
self._random_seed = int(np.random.randint(1, int(1e12)))
return self._random_seed
def _init_cstruct(self):
# Initialize all uninitialized arrays.
self._init_arrays()
for k, state in self._array_state.items():
# We do *not* set COMPUTED_ON_DISK items to the C-struct here, because we have no
# way of knowing (in this function) what is required to load in, and we don't want
# to unnecessarily load things in. We leave it to the user to ensure that all
# required arrays are loaded into memory before calling this function.
if state.initialized:
setattr(self._cstruct, k, self._ary2buf(getattr(self, k)))
for k in self.primitive_fields:
try:
setattr(self._cstruct, k, getattr(self, k))
except AttributeError:
pass
def _ary2buf(self, ary):
if not isinstance(ary, np.ndarray):
raise ValueError("ary must be a numpy array")
return self._ffi.cast(
OutputStruct._TYPEMAP[ary.dtype.name], self._ffi.from_buffer(ary)
)
def __call__(self):
"""Initialize/allocate a fresh C struct in memory and return it."""
if not self.dummy:
self._init_cstruct()
return self._cstruct
def __expose(self):
"""Expose the non-array primitives of the ctype to the top-level object."""
for k in self.primitive_fields:
setattr(self, k, getattr(self._cstruct, k))
@property
def _fname_skeleton(self):
"""The filename without specifying the random seed."""
return self._name + "_" + self._md5 + "_r{seed}.h5"
def prepare(
self,
flush: Optional[Sequence[str]] = None,
keep: Optional[Sequence[str]] = None,
force: bool = False,
):
"""Prepare the instance for being passed to another function.
This will flush all arrays in "flush" from memory, and ensure all arrays
in "keep" are in memory. At least one of these must be provided. By default,
the complement of the given parameter is all flushed/kept.
Parameters
----------
flush
Arrays to flush out of memory. Note that if no file is associated with this
instance, these arrays will be lost forever.
keep
Arrays to keep or load into memory. Note that if these do not already
exist, they will be loaded from file (if the file exists). Only one of
``flush`` and ``keep`` should be specified.
force
Whether to force flushing arrays even if no disk storage exists.
"""
if flush is None and keep is None:
raise ValueError("Must provide either flush or keep")
if flush is not None and keep is None:
keep = [k for k in self._array_state if k not in flush]
elif keep is not None and flush is None:
flush = [k for k in self._array_state if k not in keep]
flush = flush or []
keep = keep or []
for k in flush:
self._remove_array(k, force)
# Accessing the array loads it into memory.
for k in keep:
getattr(self, k)
def _remove_array(self, k, force=False):
state = self._array_state[k]
if not state.initialized:
warnings.warn(f"Trying to remove array that isn't yet created: {k}")
return
if state.computed_in_mem and not state.on_disk and not force:
raise OSError(
f"Trying to purge array '{k}' from memory that hasn't been stored! Use force=True if you meant to do this."
)
if state.c_has_active_memory:
lib.free(getattr(self._cstruct, k))
delattr(self, k)
state.initialized = False
def __getattr__(self, item):
"""Gets arrays that aren't already in memory."""
# Have to use __dict__ here to test membership, otherwise we get recursion error.
if "_array_state" not in self.__dict__ or item not in self._array_state:
raise self.__getattribute__(item)
if not self._array_state[item].on_disk:
raise OSError(
f"Cannot get {item} as it is not in memory, and this object is not cached to disk."
)
self.read(fname=self.path, keys=[item])
return getattr(self, item)
def purge(self, force=False):
"""Flush all the boxes out of memory.
Parameters
----------
force
Whether to force the purge even if no disk storage exists.
"""
self.prepare(keep=[], force=force)
def load_all(self):
"""Load all possible arrays into memory."""
self.prepare(flush=[])
@property
def filename(self):
"""The base filename of this object."""
if self._random_seed is None:
raise AttributeError("filename not defined until random_seed has been set")
return self._fname_skeleton.format(seed=self.random_seed)
def _get_fname(self, direc=None):
direc = path.abspath(path.expanduser(direc or config["direc"]))
return path.join(direc, self.filename)
def _find_file_without_seed(self, direc):
allfiles = glob.glob(path.join(direc, self._fname_skeleton.format(seed="*")))
if allfiles:
return allfiles[0]
else:
return None
def find_existing(self, direc=None):
"""
Try to find existing boxes which match the parameters of this instance.
Parameters
----------
direc : str, optional
The directory in which to search for the boxes. By default, this is the
centrally-managed directory, given by the ``config.yml`` in ``~/.21cmfast/``.
Returns
-------
str
The filename of an existing set of boxes, or None.
"""
# First, if appropriate, find a file without specifying seed.
# Need to do this first, otherwise the seed will be chosen randomly upon
# choosing a filename!
direc = path.expanduser(direc or config["direc"])
if not self._random_seed:
f = self._find_file_without_seed(direc)
if f and self._check_parameters(f):
return f
else:
f = self._get_fname(direc)
if path.exists(f) and self._check_parameters(f):
return f
return None
def _check_parameters(self, fname):
with h5py.File(fname, "r") as f:
for k in self._inputs + ("_global_params",):
q = getattr(self, k)
# The key name as it should appear in file.
kfile = k.lstrip("_")
# If this particular variable is set to None, this is interpreted
# as meaning that we don't care about matching it to file.
if q is None:
continue
if (
not isinstance(q, StructWithDefaults)
and not isinstance(q, StructInstanceWrapper)
and f.attrs[kfile] != q
):
return False
elif isinstance(q, (StructWithDefaults, StructInstanceWrapper)):
grp = f[kfile]
dct = q.self if isinstance(q, StructWithDefaults) else q
for kk, v in dct.items():
if kk not in self._filter_params:
file_v = grp.attrs[kk]
if file_v == "none":
file_v = None
if file_v != v:
logger.debug("For file %s:" % fname)
logger.debug(
f"\tThough md5 and seed matched, the parameter {kk} did not match,"
f" with values {file_v} and {v} in file and user respectively"
)
return False
return True
def exists(self, direc=None):
"""
Return a bool indicating whether a box matching the parameters of this instance is in cache.
Parameters
----------
direc : str, optional
The directory in which to search for the boxes. By default, this is the
centrally-managed directory, given by the ``config.yml`` in ``~/.21cmfast/``.
"""
return self.find_existing(direc) is not None
def write(
self,
direc=None,
fname: Union[str, Path, None, h5py.File, h5py.Group] = None,
write_inputs=True,
mode="w",
):
"""
Write the struct in standard HDF5 format.
Parameters
----------
direc : str, optional
The directory in which to write the boxes. By default, this is the
centrally-managed directory, given by the ``config.yml`` in ``~/.21cmfast/``.
fname : str, optional
The filename to write to. By default creates a unique filename from the hash.
write_inputs : bool, optional
Whether to write the inputs to the file. Can be useful to set to False if
the input file already exists and has parts already written.
"""
if not all(v.computed for v in self._array_state.values()):
raise OSError(
"Not all boxes have been computed (or maybe some have been purged). Cannot write."
f"Non-computed boxes: {[k for k, v in self._array_state.items() if not v.computed]}"
)
if not self._random_seed:
raise ValueError(
"Attempting to write when no random seed has been set. "
"Struct has been 'computed' inconsistently."
)
if not write_inputs:
mode = "a"
try:
if not isinstance(fname, (h5py.File, h5py.Group)):
direc = path.expanduser(direc or config["direc"])
if not path.exists(direc):
makedirs(direc)
fname = fname or self._get_fname(direc)
if not path.isabs(fname):
fname = path.abspath(path.join(direc, fname))
fl = h5py.File(fname, mode)
else:
fl = fname
try:
# Save input parameters to the file
if write_inputs:
for k in self._inputs + ("_global_params",):
q = getattr(self, k)
kfile = k.lstrip("_")
if isinstance(q, (StructWithDefaults, StructInstanceWrapper)):
grp = fl.create_group(kfile)
dct = q.self if isinstance(q, StructWithDefaults) else q
for kk, v in dct.items():
if kk not in self._filter_params:
try:
grp.attrs[kk] = "none" if v is None else v
except TypeError:
raise TypeError(
f"key {kk} with value {v} is not able to be written to HDF5 attrs!"
)
else:
fl.attrs[kfile] = q
# Write 21cmFAST version to the file
fl.attrs["version"] = __version__
# Save the boxes to the file
boxes = fl.create_group(self._name)
self.write_data_to_hdf5_group(boxes)
finally:
if not isinstance(fname, (h5py.File, h5py.Group)):
fl.close()
self._paths.insert(0, Path(fname))
except OSError as e:
logger.warning(
f"When attempting to write {self.__class__.__name__} to file, write failed with the following error. Continuing without caching."
)
logger.warning(e)
def write_data_to_hdf5_group(self, group: h5py.Group):
"""
Write out this object to a particular HDF5 subgroup.
Parameters
----------
group
The HDF5 group into which to write the object.
"""
# Go through all fields in this struct, and save
for k, state in self._array_state.items():
group.create_dataset(k, data=getattr(self, k))
state.on_disk = True
for k in self.primitive_fields:
group.attrs[k] = getattr(self, k)
def save(self, fname=None, direc=".", h5_group=None):
"""Save the box to disk.
In detail, this just calls write, but changes the default directory to the
local directory. This is more user-friendly, while :meth:`write` is for
automatic use under-the-hood.
Parameters
----------
fname : str, optional
The filename to write. Can be an absolute or relative path. If relative,
by default it is relative to the current directory (otherwise relative
to ``direc``). By default, the filename is auto-generated as unique to
the set of parameters that go into producing the data.
direc : str, optional
The directory into which to write the data. By default the current directory.
Ignored if ``fname`` is an absolute path.
"""
# If fname is absolute path, then get direc from it, otherwise assume current dir.
if path.isabs(fname):
direc = path.dirname(fname)
fname = path.basename(fname)
if h5_group is not None:
if not path.isabs(fname):
fname = path.abspath(path.join(direc, fname))
fl = h5py.File(fname, "a")
try:
grp = fl.create_group(h5_group)
self.write(direc, grp)
finally:
fl.close()
else:
self.write(direc, fname)
def _get_path(
self, direc: Union[str, Path, None] = None, fname: Union[str, Path, None] = None
) -> Path:
if direc is None and fname is None and self.path:
return self.path
if fname is None:
pth = self.find_existing(direc)
if pth is None:
raise OSError("No boxes exist for these parameters.")
else:
direc = Path(direc or config["direc"]).expanduser()
fname = Path(fname)
pth = fname if fname.exists() else direc / fname
return pth
def read(
self,
direc: Union[str, Path, None] = None,
fname: Union[str, Path, None, h5py.File, h5py.Group] = None,
keys: Optional[Sequence[str]] = None,
):
"""
Try find and read existing boxes from cache, which match the parameters of this instance.
Parameters
----------
direc
The directory in which to search for the boxes. By default, this is the
centrally-managed directory, given by the ``config.yml`` in ``~/.21cmfast/``.
fname
The filename to read. By default, use the filename associated with this
object. Can be an open h5py File or Group, which will be directly written to.
keys
The names of boxes to read in (can be a subset). By default, read everything.
"""
if not isinstance(fname, (h5py.File, h5py.Group)):
pth = self._get_path(direc, fname)
fl = h5py.File(pth, "r")
else:
fl = fname
try:
try:
boxes = fl[self._name]
except KeyError:
raise OSError(
f"While trying to read in {self._name}, the file exists, but does not have the "
"correct structure."
)
# Set our arrays.
for k in boxes.keys():
if keys is None or k in keys:
setattr(self, k, boxes[k][...])
self._array_state[k].on_disk = True
self._array_state[k].computed_in_mem = True
setattr(self._cstruct, k, self._ary2buf(getattr(self, k)))
for k in boxes.attrs.keys():
if k == "version":
version = ".".join(boxes.attrs[k].split(".")[:2])
patch = ".".join(boxes.attrs[k].split(".")[2:])
if version != ".".join(__version__.split(".")[:2]):
# Ensure that the major and minor versions are the same.
warnings.warn(
f"The file {pth} is out of date (version = {version}.{patch}). "
f"Consider using another box and removing it!"
)
self.version = version
self.patch_version = patch
setattr(self, k, boxes.attrs[k])
try:
setattr(self._cstruct, k, getattr(self, k))
except AttributeError:
pass
# Need to make sure that the seed is set to the one that's read in.
seed = fl.attrs["random_seed"]
self._random_seed = seed
finally:
self.__expose()
if isinstance(fl, h5py.File):
self._paths.insert(0, Path(fl.filename))
else:
self._paths.insert(0, Path(fl.file.filename))
if not isinstance(fname, (h5py.File, h5py.Group)):
fl.close()
@classmethod
def from_file(
cls, fname, direc=None, load_data=True, h5_group: Union[str, None] = None
):
"""Create an instance from a file on disk.
Parameters
----------
fname : str, optional
Path to the file on disk. May be relative or absolute.
direc : str, optional
The directory from which fname is relative to (if it is relative). By
default, will be the cache directory in config.
load_data : bool, optional
Whether to read in the data when creating the instance. If False, a bare
instance is created with input parameters -- the instance can read data
with the :func:`read` method.
h5_group
The path to the group within the file in which the object is stored.
"""
direc = path.expanduser(direc or config["direc"])
if not path.exists(fname):
fname = path.join(direc, fname)
with h5py.File(fname, "r") as fl:
if h5_group is not None:
self = cls(**cls._read_inputs(fl[h5_group]))
else:
self = cls(**cls._read_inputs(fl))
if load_data:
if h5_group is not None:
with h5py.File(fname, "r") as fl:
self.read(fname=fl[h5_group])
else:
self.read(fname=fname)
return self
@classmethod
def _read_inputs(cls, grp: Union[h5py.File, h5py.Group]):
input_classes = [c.__name__ for c in StructWithDefaults.__subclasses__()]
# Read the input parameter dictionaries from file.
kwargs = {}
for k in cls._inputs:
kfile = k.lstrip("_")
input_class_name = snake_to_camel(kfile)
if input_class_name in input_classes:
input_class = StructWithDefaults.__subclasses__()[
input_classes.index(input_class_name)
]
subgrp = grp[kfile]
kwargs[k] = input_class(
{k: v for k, v in dict(subgrp.attrs).items() if v != "none"}
)
else:
kwargs[kfile] = grp.attrs[kfile]
return kwargs
def __repr__(self):
"""Return a fully unique representation of the instance."""
# This is the class name and all parameters which belong to C-based input structs,
# eg. InitialConditions(HII_DIM:100,SIGMA_8:0.8,...)
# eg. InitialConditions(HII_DIM:100,SIGMA_8:0.8,...)
return f"{self._seedless_repr()}_random_seed={self._random_seed}"
def _seedless_repr(self):
# The same as __repr__ except without the seed.
return (
(
self._name
+ "("
+ "; ".join(
repr(v)
if isinstance(v, StructWithDefaults)
else (
v.filtered_repr(self._filter_params)
if isinstance(v, StructInstanceWrapper)
else k.lstrip("_")
+ ":"
+ (
float_to_string_precision(v, config["cache_param_sigfigs"])
if isinstance(v, (float, np.float32))
else repr(v)
)
)
for k, v in [
(k, getattr(self, k))
for k in self._inputs + ("_global_params",)
if k != "_random_seed"
]
)
)
+ f"; v{self.version}"
+ ")"
)
def __str__(self):
"""Return a human-readable representation of the instance."""
# this is *not* a unique representation, and doesn't include global params.
return (
self._name
+ "("
+ ";\n\t".join(
repr(v)
if isinstance(v, StructWithDefaults)
else k.lstrip("_") + ":" + repr(v)
for k, v in [(k, getattr(self, k)) for k in self._inputs]
)
) + ")"
def __hash__(self):
"""Return a unique hsh for this instance, even global params and random seed."""
return hash(repr(self))
@property
def _md5(self):
"""Return a unique hsh of the object, *not* taking into account the random seed."""
return md5(self._seedless_repr().encode()).hexdigest()
def __eq__(self, other):
"""Check equality with another object via its __repr__."""
return repr(self) == repr(other)
@property
def is_computed(self) -> bool:
"""Whether this instance has been computed at all.
This is true either if the current instance has called :meth:`compute`,
or if it has a current existing :attr:`path` pointing to stored data,
or if such a path exists.
Just because the instance has been computed does *not* mean that all
relevant quantities are available -- some may have been purged from
memory without writing. Use :meth:`has` to check whether certain arrays
are available.
"""
return any(v.computed for v in self._array_state.values())
def ensure_arrays_computed(self, *arrays, load=False) -> bool:
"""Check if the given arrays are computed (not just initialized)."""
if not self.is_computed:
return False
computed = all(self._array_state[k].computed for k in arrays)
if computed and load:
self.prepare(keep=arrays, flush=[])
return computed
def ensure_arrays_inited(self, *arrays, init=False) -> bool:
"""Check if the given arrays are initialized (or computed)."""
inited = all(self._array_state[k].initialized for k in arrays)
if init and not inited:
self._init_arrays()
return True
@abstractmethod
def get_required_input_arrays(self, input_box) -> List[str]:
"""Return all input arrays required to compute this object."""
pass
def ensure_input_computed(self, input_box, load=False) -> bool:
"""Ensure all the inputs have been computed."""
if input_box.dummy:
return True
arrays = self.get_required_input_arrays(input_box)
if input_box.initial:
return input_box.ensure_arrays_inited(*arrays, init=load)
return input_box.ensure_arrays_computed(*arrays, load=load)
def summarize(self, indent=0) -> str:
"""Generate a string summary of the struct."""
indent = indent * " "
out = f"\n{indent}{self.__class__.__name__}\n"
out += "".join(
f"{indent} {fieldname:>15}: {getattr(self, fieldname, 'non-existent')}\n"
for fieldname in self.primitive_fields
)
for fieldname, state in self._array_state.items():
if not state.initialized:
out += f"{indent} {fieldname:>15}: uninitialized\n"
elif not state.computed:
out += f"{indent} {fieldname:>15}: initialized\n"
elif not state.computed_in_mem:
out += f"{indent} {fieldname:>15}: computed on disk\n"
else:
x = getattr(self, fieldname).flatten()
if len(x) > 0:
out += f"{indent} {fieldname:>15}: {x[0]:1.4e}, {x[-1]:1.4e}, {x.min():1.4e}, {x.max():1.4e}, {np.mean(x):1.4e}\n"
else:
out += f"{indent} {fieldname:>15}: size zero\n"
return out
@classmethod
def _log_call_arguments(cls, *args):
logger.debug(f"Calling {cls._c_compute_function.__name__} with following args:")
for arg in args:
if isinstance(arg, OutputStruct):
for line in arg.summarize(indent=1).split("\n"):
logger.debug(line)
elif isinstance(arg, StructWithDefaults):
for line in str(arg).split("\n"):
logger.debug(f" {line}")
else:
logger.debug(f" {arg}")
def _ensure_arguments_exist(self, *args):
for arg in args:
if (
isinstance(arg, OutputStruct)
and not arg.dummy
and not self.ensure_input_computed(arg, load=True)
):
raise ValueError(
f"Trying to use {arg} to compute {self}, but some required arrays "
f"are not computed!"
)
def _compute(
self, *args, hooks: Optional[Dict[Union[str, Callable], Dict[str, Any]]] = None
):
"""Compute the actual function that fills this struct."""
# Write a detailed message about call arguments if debug turned on.
if logger.getEffectiveLevel() <= logging.DEBUG:
self._log_call_arguments(*args)
# Check that all required inputs are really computed, and load them into memory
# if they're not already.
self._ensure_arguments_exist(*args)
# Construct the args. All StructWrapper objects need to actually pass their
# underlying cstruct, rather than themselves. OutputStructs also pass the
# class in that's calling this.
inputs = [arg() if isinstance(arg, StructWrapper) else arg for arg in args]
# Ensure we haven't already tried to compute this instance.
if self.is_computed:
raise ValueError(
f"You are trying to compute {self.__class__.__name__}, but it has already been computed."
)
# Perform the C computation
try:
exitcode = self._c_compute_function(*inputs, self())
except TypeError as e:
logger.error(
f"Arguments to {self._c_compute_function.__name__}: "
f"{[arg() if isinstance(arg, StructWrapper) else arg for arg in args]}"
)
raise e
_process_exitcode(exitcode, self._c_compute_function, args)
# Ensure memory created in C gets mapped to numpy arrays in this struct.
for k, state in self._array_state.items():
if state.initialized:
state.computed_in_mem = True
self.__memory_map()
self.__expose()
# Optionally do stuff with the result (like writing it)
self._call_hooks(hooks)
return self
def _call_hooks(self, hooks):
if hooks is None:
hooks = {"write": {"direc": config["direc"]}}
for hook, params in hooks.items():
if callable(hook):
hook(self, **params)
else:
getattr(self, hook)(**params)
def __memory_map(self):
shapes = self._c_shape(self._cstruct)
for item in self._c_based_pointers:
setattr(self, item, asarray(getattr(self._cstruct, item), shapes[item]))
self._array_state[item].c_memory = True
self._array_state[item].computed_in_mem = True
def __del__(self):
"""Safely delete the object and its C-allocated memory."""
for k in self._c_based_pointers:
if self._array_state[k].c_has_active_memory:
lib.free(getattr(self._cstruct, k))
class StructInstanceWrapper:
"""A wrapper for *instances* of C structs.
This is as opposed to :class:`StructWrapper`, which is for the un-instantiated structs.
Parameters
----------
wrapped :
The reference to the C object to wrap (contained in the ``cffi.lib`` object).
ffi :
The ``cffi.ffi`` object.
"""
def __init__(self, wrapped, ffi):
self._cobj = wrapped
self._ffi = ffi
for nm, tp in self._ffi.typeof(self._cobj).fields:
setattr(self, nm, getattr(self._cobj, nm))
# Get the name of the structure
self._ctype = self._ffi.typeof(self._cobj).cname.split()[-1]
def __setattr__(self, name, value):
"""Set an attribute of the instance, attempting to change it in the C struct as well."""
try:
setattr(self._cobj, name, value)
except AttributeError:
pass
object.__setattr__(self, name, value)
def items(self):
"""Yield (name, value) pairs for each element of the struct."""
for nm, tp in self._ffi.typeof(self._cobj).fields:
yield nm, getattr(self, nm)
def keys(self):
"""Return a list of names of elements in the struct."""
return [nm for nm, tp in self.items()]
def __repr__(self):
"""Return a unique representation of the instance."""
return (
self._ctype
+ "("
+ ";".join(k + "=" + str(v) for k, v in sorted(self.items()))
) + ")"
def filtered_repr(self, filter_params):
"""Get a fully unique representation of the instance that filters out some parameters.
Parameters
----------
filter_params : list of str
The parameter names which should not appear in the representation.
"""
return (
self._ctype
+ "("
+ ";".join(
k + "=" + str(v)
for k, v in sorted(self.items())
if k not in filter_params
)
) + ")"
def _check_compatible_inputs(*datasets, ignore=["redshift"]):
"""Ensure that all defined input parameters for the provided datasets are equal.
Parameters
----------
datasets : list of :class:`~_utils.OutputStruct`
A number of output datasets to cross-check.
ignore : list of str
Attributes to ignore when ensuring that parameter inputs are the same.
Raises
------
ValueError :
If datasets are not compatible.
"""
done = [] # keeps track of inputs we've checked so we don't double check.
for i, d in enumerate(datasets):
# If a dataset is None, just ignore and move on.
if d is None:
continue
# noinspection PyProtectedMember
for inp in d._inputs:
# Skip inputs that we want to ignore
if inp in ignore:
continue
if inp not in done:
for j, d2 in enumerate(datasets[(i + 1) :]):
if d2 is None:
continue
# noinspection PyProtectedMember
if inp in d2._inputs and getattr(d, inp) != getattr(d2, inp):
raise ValueError(
f"""
{d.__class__.__name__} and {d2.__class__.__name__} are incompatible.
{inp}: {getattr(d, inp)}
vs.
{inp}: {getattr(d2, inp)}
"""
)
done += [inp] | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/src/py21cmfast/_utils.py | _utils.py |
import builtins
import click
import inspect
import logging
import matplotlib.pyplot as plt
import numpy as np
import warnings
import yaml
from os import path, remove
from pathlib import Path
from . import _cfg, cache_tools, global_params, plotting
from . import wrapper as lib
def _get_config(config=None):
if config is None:
config = path.expanduser(path.join("~", ".21cmfast", "runconfig_example.yml"))
with open(config) as f:
cfg = yaml.load(f, Loader=yaml.FullLoader)
return cfg
def _ctx_to_dct(args):
dct = {}
j = 0
while j < len(args):
arg = args[j]
if "=" in arg:
a = arg.split("=")
dct[a[0].replace("--", "")] = a[-1]
j += 1
else:
dct[arg.replace("--", "")] = args[j + 1]
j += 2
return dct
def _update(obj, ctx):
# Try to use the extra arguments as an override of config.
kk = list(ctx.keys())
for k in kk:
# noinspection PyProtectedMember
if hasattr(obj, k):
try:
val = getattr(obj, "_" + k)
setattr(obj, "_" + k, type(val)(ctx[k]))
ctx.pop(k)
except (AttributeError, TypeError):
try:
val = getattr(obj, k)
setattr(obj, k, type(val)(ctx[k]))
ctx.pop(k)
except AttributeError:
pass
def _override(ctx, *param_dicts):
# Try to use the extra arguments as an override of config.
if ctx.args:
ctx = _ctx_to_dct(ctx.args)
for p in param_dicts:
_update(p, ctx)
# Also update globals, always.
_update(global_params, ctx)
if ctx:
warnings.warn("The following arguments were not able to be set: %s" % ctx)
main = click.Group()
@main.command(
context_settings={ # Doing this allows arbitrary options to override config
"ignore_unknown_options": True,
"allow_extra_args": True,
}
)
@click.option(
"--config",
type=click.Path(exists=True, dir_okay=False),
default=None,
help="Path to the configuration file (default ~/.21cmfast/runconfig_single.yml)",
)
@click.option(
"--regen/--no-regen",
default=False,
help="Whether to force regeneration of init/perturb files if they already exist.",
)
@click.option(
"--direc",
type=click.Path(exists=True, dir_okay=True),
default=None,
help="directory to write data and plots to -- must exist.",
)
@click.option(
"--seed",
type=int,
default=None,
help="specify a random seed for the initial conditions",
)
@click.pass_context
def init(ctx, config, regen, direc, seed):
"""Run a single iteration of 21cmFAST init, saving results to file.
Parameters
----------
ctx :
A parameter from the parent CLI function to be able to override config.
config : str
Path to the configuration file.
regen : bool
Whether to regenerate all data, even if found in cache.
direc : str
Where to search for cached items.
seed : int
Random seed used to generate data.
"""
cfg = _get_config(config)
# Set user/cosmo params from config.
user_params = lib.UserParams(**cfg.get("user_params", {}))
cosmo_params = lib.CosmoParams(**cfg.get("cosmo_params", {}))
_override(ctx, user_params, cosmo_params)
lib.initial_conditions(
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regen,
write=True,
direc=direc,
random_seed=seed,
)
@main.command(
context_settings={ # Doing this allows arbitrary options to override config
"ignore_unknown_options": True,
"allow_extra_args": True,
}
)
@click.argument("redshift", type=float)
@click.option(
"--config",
type=click.Path(exists=True, dir_okay=False),
default=None,
help="Path to the configuration file (default ~/.21cmfast/runconfig_single.yml)",
)
@click.option(
"--regen/--no-regen",
default=False,
help="Whether to force regeneration of init/perturb files if they already exist.",
)
@click.option(
"--direc",
type=click.Path(exists=True, dir_okay=True),
default=None,
help="directory to write data and plots to -- must exist.",
)
@click.option(
"--seed",
type=int,
default=None,
help="specify a random seed for the initial conditions",
)
@click.pass_context
def perturb(ctx, redshift, config, regen, direc, seed):
"""Run 21cmFAST perturb_field at the specified redshift, saving results to file.
Parameters
----------
ctx :
A parameter from the parent CLI function to be able to override config.
redshift : float
Redshift at which to generate perturbed field.
config : str
Path to the configuration file.
regen : bool
Whether to regenerate all data, even if found in cache.
direc : str
Where to search for cached items.
seed : int
Random seed used to generate data.
"""
cfg = _get_config(config)
# Set user/cosmo params from config.
user_params = lib.UserParams(**cfg.get("user_params", {}))
cosmo_params = lib.CosmoParams(**cfg.get("cosmo_params", {}))
_override(ctx, user_params, cosmo_params)
lib.perturb_field(
redshift=redshift,
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regen,
write=True,
direc=direc,
random_seed=seed,
)
@main.command(
context_settings={ # Doing this allows arbitrary options to override config
"ignore_unknown_options": True,
"allow_extra_args": True,
}
)
@click.argument("redshift", type=float)
@click.option(
"-p",
"--prev_z",
type=float,
default=None,
help="Previous redshift (the spin temperature data must already exist for this redshift)",
)
@click.option(
"--config",
type=click.Path(exists=True, dir_okay=False),
default=None,
help="Path to the configuration file (default ~/.21cmfast/runconfig_single.yml)",
)
@click.option(
"--regen/--no-regen",
default=False,
help="Whether to force regeneration of init/perturb files if they already exist.",
)
@click.option(
"--direc",
type=click.Path(exists=True, dir_okay=True),
default=None,
help="directory to write data and plots to -- must exist.",
)
@click.option(
"--seed",
type=int,
default=None,
help="specify a random seed for the initial conditions",
)
@click.pass_context
def spin(ctx, redshift, prev_z, config, regen, direc, seed):
"""Run spin_temperature at the specified redshift, saving results to file.
Parameters
----------
ctx :
A parameter from the parent CLI function to be able to override config.
redshift : float
The redshift to generate the field at.
prev_z : float
The redshift of a previous box from which to evolve to the current one.
config : str
Path to the configuration file.
regen : bool
Whether to regenerate all data, even if found in cache.
direc : str
Where to search for cached items.
seed : int
Random seed used to generate data.
"""
cfg = _get_config(config)
# Set user/cosmo params from config.
user_params = lib.UserParams(**cfg.get("user_params", {}))
cosmo_params = lib.CosmoParams(**cfg.get("cosmo_params", {}))
flag_options = lib.FlagOptions(
**cfg.get("flag_options", {}), USE_VELS_AUX=user_params.USE_RELATIVE_VELOCITIES
)
astro_params = lib.AstroParams(
**cfg.get("astro_params", {}), INHOMO_RECO=flag_options.INHOMO_RECO
)
_override(ctx, user_params, cosmo_params, astro_params, flag_options)
lib.spin_temperature(
redshift=redshift,
astro_params=astro_params,
flag_options=flag_options,
previous_spin_temp=prev_z,
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regen,
write=True,
direc=direc,
random_seed=seed,
)
@main.command(
context_settings={ # Doing this allows arbitrary options to override config
"ignore_unknown_options": True,
"allow_extra_args": True,
}
)
@click.argument("redshift", type=float)
@click.option(
"-p",
"--prev_z",
type=float,
default=None,
help="Previous redshift (the ionized box data must already exist for this redshift)",
)
@click.option(
"--config",
type=click.Path(exists=True, dir_okay=False),
default=None,
help="Path to the configuration file (default ~/.21cmfast/runconfig_single.yml)",
)
@click.option(
"--regen/--no-regen",
default=False,
help="Whether to force regeneration of init/perturb files if they already exist.",
)
@click.option(
"--direc",
type=click.Path(exists=True, dir_okay=True),
default=None,
help="directory to write data and plots to -- must exist.",
)
@click.option(
"--seed",
type=int,
default=None,
help="specify a random seed for the initial conditions",
)
@click.pass_context
def ionize(ctx, redshift, prev_z, config, regen, direc, seed):
"""Run 21cmFAST ionize_box at the specified redshift, saving results to file.
Parameters
----------
ctx :
A parameter from the parent CLI function to be able to override config.
redshift : float
The redshift to generate the field at.
prev_z : float
The redshift of a previous box from which to evolve to the current one.
config : str
Path to the configuration file.
regen : bool
Whether to regenerate all data, even if found in cache.
direc : str
Where to search for cached items.
seed : int
Random seed used to generate data.
"""
cfg = _get_config(config)
# Set user/cosmo params from config.
user_params = lib.UserParams(**cfg.get("user_params", {}))
cosmo_params = lib.CosmoParams(**cfg.get("cosmo_params", {}))
flag_options = lib.FlagOptions(
**cfg.get("flag_options", {}), USE_VELS_AUX=user_params.USE_RELATIVE_VELOCITIES
)
astro_params = lib.AstroParams(
**cfg.get("astro_params", {}), INHOMO_RECO=flag_options.INHOMO_RECO
)
_override(ctx, user_params, cosmo_params, astro_params, flag_options)
lib.ionize_box(
redshift=redshift,
astro_params=astro_params,
flag_options=flag_options,
previous_ionize_box=prev_z,
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regen,
write=True,
direc=direc,
random_seed=seed,
)
@main.command(
context_settings={ # Doing this allows arbitrary options to override config
"ignore_unknown_options": True,
"allow_extra_args": True,
}
)
@click.argument("redshift", type=str)
@click.option(
"--config",
type=click.Path(exists=True, dir_okay=False),
default=None,
help="Path to the configuration file (default ~/.21cmfast/runconfig_single.yml)",
)
@click.option(
"--out",
type=click.Path(dir_okay=True, file_okay=True),
default=None,
help="Path to output full Coeval simulation to (directory OK).",
)
@click.option(
"--regen/--no-regen",
default=False,
help="Whether to force regeneration of init/perturb files if they already exist.",
)
@click.option(
"--direc",
type=click.Path(exists=True, dir_okay=True),
default=None,
help="cache directory",
)
@click.option(
"--seed",
type=int,
default=None,
help="specify a random seed for the initial conditions",
)
@click.pass_context
def coeval(ctx, redshift, config, out, regen, direc, seed):
"""Efficiently generate coeval cubes at a given redshift.
Parameters
----------
ctx :
A parameter from the parent CLI function to be able to override config.
redshift : float
The redshift to generate the field at.
config : str
Path to the configuration file.
regen : bool
Whether to regenerate all data, even if found in cache.
direc : str
Where to search for cached items.
seed : int
Random seed used to generate data.
"""
if out is not None:
out = Path(out).absolute()
if len(out.suffix) not in (2, 3) and not out.exists():
out.mkdir()
elif not out.parent.exists():
out.parent.mkdir()
try:
redshift = [float(z.strip()) for z in redshift.split(",")]
except TypeError:
raise TypeError("redshift argument must be comma-separated list of values.")
cfg = _get_config(config)
# Set user/cosmo params from config.
user_params = lib.UserParams(**cfg.get("user_params", {}))
cosmo_params = lib.CosmoParams(**cfg.get("cosmo_params", {}))
flag_options = lib.FlagOptions(
**cfg.get("flag_options", {}), USE_VELS_AUX=user_params.USE_RELATIVE_VELOCITIES
)
astro_params = lib.AstroParams(
**cfg.get("astro_params", {}), INHOMO_RECO=flag_options.INHOMO_RECO
)
_override(ctx, user_params, cosmo_params, astro_params, flag_options)
coeval = lib.run_coeval(
redshift=redshift,
astro_params=astro_params,
flag_options=flag_options,
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regen,
write=True,
direc=direc,
random_seed=seed,
)
if out:
for i, (z, c) in enumerate(zip(redshift, coeval)):
if out.is_dir():
fname = out / c.get_unique_filename()
elif len(redshift) == 1:
fname = out
else:
out = out.parent / f"{out.name}_z{z}{out.suffix}"
c.save(fname)
print(f"Saved Coeval box to {fname}.")
@main.command(
context_settings={ # Doing this allows arbitrary options to override config
"ignore_unknown_options": True,
"allow_extra_args": True,
}
)
@click.argument("redshift", type=float)
@click.option(
"--config",
type=click.Path(exists=True, dir_okay=False),
default=None,
help="Path to the configuration file (default ~/.21cmfast/runconfig_single.yml)",
)
@click.option(
"--out",
type=click.Path(dir_okay=True, file_okay=True),
default=None,
help="Path to output full Lightcone to (directory OK).",
)
@click.option(
"--regen/--no-regen",
default=False,
help="Whether to force regeneration of init/perturb files if they already exist.",
)
@click.option(
"--direc",
type=click.Path(exists=True, dir_okay=True),
default=None,
help="directory to write data and plots to -- must exist.",
)
@click.option(
"-X",
"--max-z",
type=float,
default=None,
help="maximum redshift of the stored lightcone data",
)
@click.option(
"--seed",
type=int,
default=None,
help="specify a random seed for the initial conditions",
)
@click.pass_context
def lightcone(ctx, redshift, config, out, regen, direc, max_z, seed):
"""Efficiently generate coeval cubes at a given redshift.
Parameters
----------
ctx :
A parameter from the parent CLI function to be able to override config.
redshift : float
The redshift to generate the field at.
config : str
Path to the configuration file.
regen : bool
Whether to regenerate all data, even if found in cache.
direc : str
Where to search for cached items.
max_z : float
Maximum redshift to include in the produced lightcone.
seed : int
Random seed used to generate data.
"""
cfg = _get_config(config)
if out is not None:
out = Path(out).absolute()
if len(out.suffix) not in (2, 3) and not out.exists():
out.mkdir()
elif not out.parent.exists():
out.parent.mkdir()
# Set user/cosmo params from config.
user_params = lib.UserParams(**cfg.get("user_params", {}))
cosmo_params = lib.CosmoParams(**cfg.get("cosmo_params", {}))
flag_options = lib.FlagOptions(
**cfg.get("flag_options", {}), USE_VELS_AUX=user_params.USE_RELATIVE_VELOCITIES
)
astro_params = lib.AstroParams(
**cfg.get("astro_params", {}), INHOMO_RECO=flag_options.INHOMO_RECO
)
_override(ctx, user_params, cosmo_params, astro_params, flag_options)
lc = lib.run_lightcone(
redshift=redshift,
max_redshift=max_z,
astro_params=astro_params,
flag_options=flag_options,
user_params=user_params,
cosmo_params=cosmo_params,
regenerate=regen,
write=True,
direc=direc,
random_seed=seed,
)
if out:
fname = out / lc.get_unique_filename() if out.is_dir() else out
lc.save(fname)
print(f"Saved Lightcone to {fname}.")
def _query(direc=None, kind=None, md5=None, seed=None, clear=False):
cls = list(
cache_tools.query_cache(direc=direc, kind=kind, hsh=md5, seed=seed, show=False)
)
if not clear:
print("%s Data Sets Found:" % len(cls))
print("------------------")
else:
print("Removing %s data sets..." % len(cls))
for file, c in cls:
if not clear:
print(" @ {%s}:" % file)
print(" %s" % str(c))
print()
else:
direc = direc or path.expanduser(_cfg.config["direc"])
remove(path.join(direc, file))
@main.command()
@click.option(
"-d",
"--direc",
type=click.Path(exists=True, dir_okay=True),
default=None,
help="directory to write data and plots to -- must exist.",
)
@click.option("-k", "--kind", type=str, default=None, help="filter by kind of data.")
@click.option("-m", "--md5", type=str, default=None, help="filter by md5 hsh")
@click.option("-s", "--seed", type=str, default=None, help="filter by random seed")
@click.option(
"--clear/--no-clear",
default=False,
help="remove all data sets returned by this query.",
)
def query(direc, kind, md5, seed, clear):
"""Query the cache database.
Parameters
----------
direc : str
Directory in which to search for cache items
kind : str
Filter output by kind of box (eg. InitialConditions)
md5 : str
Filter output by hsh
seed : float
Filter output by random seed.
clear : bool
Remove all data sets returned by the query.
"""
_query(direc, kind, md5, seed, clear)
@main.command()
@click.argument("param", type=str)
@click.argument("value", type=str)
@click.option(
"-s",
"--struct",
type=click.Choice(["flag_options", "cosmo_params", "user_params", "astro_params"]),
default="flag_options",
help="struct in which the new feature exists",
)
@click.option(
"-t",
"--vtype",
type=click.Choice(["bool", "float", "int"]),
default="bool",
help="type of the new parameter",
)
@click.option(
"-l/-c",
"--lightcone/--coeval",
default=True,
help="whether to use a lightcone for comparison",
)
@click.option(
"-z", "--redshift", type=float, default=6.0, help="redshift of the comparison boxes"
)
@click.option(
"-Z",
"--max-redshift",
type=float,
default=30,
help="maximum redshift of the comparison lightcone",
)
@click.option("-r", "--random-seed", type=int, default=12345, help="random seed to use")
@click.option("-v", "--verbose", count=True)
@click.option(
"-g/-G",
"--regenerate/--cache",
default=True,
help="whether to regenerate the boxes",
)
def pr_feature(
param,
value,
struct,
vtype,
lightcone,
redshift,
max_redshift,
random_seed,
verbose,
regenerate,
):
"""
Create standard plots comparing a default simulation against a simulation with a new feature.
The new feature is switched on by setting PARAM to VALUE.
Plots are saved in the current directory, with the prefix "pr_feature".
Parameters
----------
param : str
Name of the parameter to modify to "switch on" the feature.
value : float
Value to which to set it.
struct : str
The input parameter struct to which `param` belongs.
vtype : str
Type of the new parameter.
lightcone : bool
Whether the comparison should be done on a lightcone.
redshift : float
Redshift of comparison.
max_redshift : float
If using a lightcone, the maximum redshift in the lightcone to compare.
random_seed : int
Random seed at which to compare.
verbose : int
How verbose the output should be.
regenerate : bool
Whether to regenerate all data, even if it is in cache.
"""
import powerbox
lvl = [logging.WARNING, logging.INFO, logging.DEBUG][verbose]
logger = logging.getLogger("21cmFAST")
logger.setLevel(lvl)
value = getattr(builtins, vtype)(value)
structs = {
"user_params": {"HII_DIM": 128, "BOX_LEN": 250},
"flag_options": {"USE_TS_FLUCT": True},
"cosmo_params": {},
"astro_params": {},
}
if lightcone:
print("Running default lightcone...")
lc_default = lib.run_lightcone(
redshift=redshift,
max_redshift=max_redshift,
random_seed=random_seed,
regenerate=regenerate,
**structs,
)
structs[struct][param] = value
print("Running lightcone with new feature...")
lc_new = lib.run_lightcone(
redshift=redshift,
max_redshift=max_redshift,
random_seed=random_seed,
regenerate=regenerate,
**structs,
)
print("Plotting lightcone slices...")
for field in ["brightness_temp"]:
fig, ax = plt.subplots(3, 1, sharex=True, sharey=True)
vmin = -150
vmax = 30
plotting.lightcone_sliceplot(
lc_default, ax=ax[0], fig=fig, vmin=vmin, vmax=vmax
)
ax[0].set_title("Default")
plotting.lightcone_sliceplot(
lc_new, ax=ax[1], fig=fig, cbar=False, vmin=vmin, vmax=vmax
)
ax[1].set_title("New")
plotting.lightcone_sliceplot(
lc_default, lightcone2=lc_new, cmap="bwr", ax=ax[2], fig=fig
)
ax[2].set_title("Difference")
plt.savefig(f"pr_feature_lighcone_2d_{field}.pdf")
def rms(x, axis=None):
return np.sqrt(np.mean(x**2, axis=axis))
print("Plotting lightcone history...")
fig, ax = plt.subplots(4, 1, sharex=True, gridspec_kw={"hspace": 0.05})
ax[0].plot(lc_default.node_redshifts, lc_default.global_xHI, label="Default")
ax[0].plot(lc_new.node_redshifts, lc_new.global_xHI, label="New")
ax[0].set_ylabel(r"$x_{\rm HI}$")
ax[0].legend()
ax[1].plot(
lc_default.node_redshifts,
lc_default.global_brightness_temp,
label="Default",
)
ax[1].plot(lc_new.node_redshifts, lc_new.global_brightness_temp, label="New")
ax[1].set_ylabel("$T_b$ [K]")
ax[3].set_xlabel("z")
rms_diff = rms(lc_default.brightness_temp, axis=(0, 1)) - rms(
lc_new.brightness_temp, axis=(0, 1)
)
ax[2].plot(lc_default.lightcone_redshifts, rms_diff, label="RMS")
ax[2].plot(
lc_new.node_redshifts,
lc_default.global_xHI - lc_new.global_xHI,
label="$x_{HI}$",
)
ax[2].plot(
lc_new.node_redshifts,
lc_default.global_brightness_temp - lc_new.global_brightness_temp,
label="$T_b$",
)
ax[2].legend()
ax[2].set_ylabel("Differences")
diff_rms = rms(lc_default.brightness_temp - lc_new.brightness_temp, axis=(0, 1))
ax[3].plot(lc_default.lightcone_redshifts, diff_rms)
ax[3].set_ylabel("RMS of Diff.")
plt.savefig("pr_feature_history.pdf")
print("Plotting power spectra history...")
p_default = []
p_new = []
z = []
thickness = 200 # Mpc
ncells = int(thickness / lc_new.cell_size)
chunk_size = lc_new.cell_size * ncells
start = 0
print(ncells)
while start + ncells <= lc_new.shape[-1]:
pd, k = powerbox.get_power(
lc_default.brightness_temp[:, :, start : start + ncells],
lc_default.lightcone_dimensions[:2] + (chunk_size,),
)
p_default.append(pd)
pn, k = powerbox.get_power(
lc_new.brightness_temp[:, :, start : start + ncells],
lc_new.lightcone_dimensions[:2] + (chunk_size,),
)
p_new.append(pn)
z.append(lc_new.lightcone_redshifts[start])
start += ncells
p_default = np.array(p_default).T
p_new = np.array(p_new).T
fig, ax = plt.subplots(2, 1, sharex=True)
ax[0].set_yscale("log")
inds = [
np.where(np.abs(k - 0.1) == np.abs(k - 0.1).min())[0][0],
np.where(np.abs(k - 0.2) == np.abs(k - 0.2).min())[0][0],
np.where(np.abs(k - 0.5) == np.abs(k - 0.5).min())[0][0],
np.where(np.abs(k - 1) == np.abs(k - 1).min())[0][0],
]
for i, (pdef, pnew, kk) in enumerate(
zip(p_default[inds], p_new[inds], k[inds])
):
ax[0].plot(z, pdef, ls="--", label=f"k={kk:.2f}", color=f"C{i}")
ax[0].plot(z, pnew, ls="-", color=f"C{i}")
ax[1].plot(z, np.log10(pdef / pnew), ls="-", color=f"C{i}")
ax[1].set_xlabel("z")
ax[0].set_ylabel(r"$\Delta^2 [{\rm mK}^2]$")
ax[1].set_ylabel(r"log ratio of $\Delta^2 [{\rm mK}^2]$")
ax[0].legend()
plt.savefig("pr_feature_power_history.pdf")
else:
raise NotImplementedError() | 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/src/py21cmfast/cli.py | cli.py |
Developer Documentation
=======================
If you are new to developing ``21cmFAST``, please read the :ref:`contributing:Contributing`
section *first*, which outlines the general concepts for contributing to development,
and provides a step-by-step walkthrough for getting setup.
This page lists some more detailed notes which may be helpful through the
development process.
Compiling for debugging
-----------------------
When developing, it is usually a good idea to compile the underlying C code in ``DEBUG``
mode. This may allow extra print statements in the C, but also will allow running the C
under ``valgrind`` or ``gdb``. To do this::
$ DEBUG=True pip install -e .
See :ref:`installation:Installation` for more installation options.
Developing the C Code
---------------------
In this section we outline how one might go about modifying/extending the C code and
ensuring that the extension is compatible with the wrapper provided here. It is
critical that you run all tests after modifying _anything_ (and see the section
below about running with valgrind). When changing C code, before
testing, ensure that the new C code is compiled into your environment by running::
$ rm -rf build
$ pip install .
Note that using a developer install (`-e`) is not recommended as it stores compiled
objects in the working directory which don't get updated as you change code, and can
cause problems later.
There are two main purposes you may want to write some C code:
1. An external plugin/extension which uses the output data from 21cmFAST.
2. Modifying the internal C code of 21cmFAST.
21cmFAST currently provides no support for external plugins/extensions. It is entirely
possible to write your own C code to do whatever you want with the output data, but we
don't provide any wrapping structure for you to do this, you will need to write your
own. Internally, 21cmFAST uses the ``cffi`` library to aid the wrapping of the C code into
Python. You don't need to do the same, though we recommend it. If your desired
"extension" is something that needs to operate in-between steps of 21cmFAST, we also
provide no support for this, but it is possible, so long as the next step in the
chain maintains its API. You would be required to re-write the low-level wrapping
function _preceding_ your inserted step as well. For instance, if you had written a
self-contained piece of code that modified the initial conditions box, adding some
physical effect which is not already covered, then you would need to write a low-level
wrapper _and_ re-write the ``initial_conditions`` function to modify the box before
returning it. We provide no easy "plugin" system for doing this currently. If your
external code is meant to be inserted _within_ a basic step of 21cmFAST, this is
currently not possible. You will instead have to modify the source code itself.
Modifying the C-code of 21cmFAST should be relatively simple. If your changes are
entirely internal to a given function, then nothing extra needs to be done. A little
more work has to be done if the modifications add/remove input parameters or the output
structure. If any of the input structures are modified (i.e. an extra parameter
added to it), then the corresponding class in ``py21cmfast.wrapper`` must be modified,
usually simply to add the new parameter to the ``_defaults_`` dict with a default value.
For instance, if a new variable ``some_param`` was added to the ``user_params`` struct
in the ``ComputeInitialConditions`` C function, then the ``UserParams`` class in
the wrapper would be modified, adding ``some_param=<default_value>`` to its ``_default_``
dict. If the default value of the parameter is dependent on another parameter, its
default value in this dict can be set to ``None``, and you can give it a dynamic
definition as a Python ``@property``. For example, the ``DIM`` parameter of
``UserParams`` is defined as::
@property
def DIM(self):
if self._some_param is None:
return self._DIM or 4 * self.HII_DIM
Note the underscore in ``_DIM`` here: by default, if a dynamic property is defined for
a given parameter, the ``_default_`` value is saved with a prefixed underscore. Here we
return either the explicitly set ``DIM``, or 4 by the ``HII_DIM``. In addition, if the
new parameter is not settable -- if it is completely determined by other parameters --
then don't put it in ``_defaults_`` at all, and just give it a dynamic definition.
If you modify an output struct, which usually house a number of array quantities
(often float pointers, but not necessarily), then you'll again need to modify the
corresponding class in the wrapper. In particular, you'll need to add an entry for that
particular array in the ``_init_arrays`` method for the class. The entry consists of
initialising that array (usually to zeros, but not necessarily), and setting its proper
dtype. All arrays should be single-pointers, even for multi-dimensional data. The latter
can be handled by initalising the array as a 1D numpy array, but then setting its shape
attribute (after creation) to the appropriate n-dimensional shape (see the
``_init_arrays`` method for the ``InitialConditions`` class for examples of this).
Modifying the ``global_params`` struct should be relatively straightforward, and no
changes in the Python are necessary. However, you may want to consider adding the new
parameter to relevant ``_filter_params`` lists for the output struct wrapping classes in
the wrapper. These lists control which global parameters affect which output structs,
and merely provide for more accurate caching mechanisms.
C Function Standards
~~~~~~~~~~~~~~~~~~~~
The C-level functions are split into two groups -- low-level "private" functions, and
higher-level "public" or "API" functions. All API-level functions are callable from
python (but may also be called from other C functions). All API-level functions are
currently prototyped in ``21cmFAST.h``.
To enable consistency of error-checking in Python (and a reasonable standard for any
kind of code), we enforce that any API-level function must return an integer status.
Any "return" objects must be modified in-place (i.e. passed as pointers). This enables
Python to control the memory access of these variables, and also to receive proper
error statuses (see below for how we do exception handling). We also adhere to the
convention that "output" variables should be passed to the function as its last
argument(s). In the case that _only_ the last argument is meant to be "output", there
exists a simple wrapper ``_call_c_simple`` in ``wrapper.py`` that will neatly handle the
calling of the function in an intuitive pythonic way.
Running with Valgrind
~~~~~~~~~~~~~~~~~~~~~
If any changes to the C code are made, it is ideal to run tests under valgrind, and
check for memory leaks. To do this, install ``valgrind`` (we have tested v3.14+),
which is probably available via your package manager. We provide a
suppression file for ``valgrind`` in the ``devel/`` directory of the main repository.
It is ideal if you install a development-version of python especially for running these
tests. To do this, download the version of python you want and then configure/install with::
$ ./configure --prefix=<your-home>/<directory> --without-pymalloc --with-pydebug --with-valgrind
$ make; make install
Construct a ``virtualenv`` on top of this installation, and create your environment,
and install all requirements.
If you do not wish to run with a modified version of python, you may continue with your
usual version, but may get some extra cruft in the output. If running with Python
version > 3.6, consider running with environment variable ``PYTHONMALLOC=malloc``
(see https://stackoverflow.com/questions/20112989/how-to-use-valgrind-with-python ).
The general pattern for using valgrind with python is::
$ valgrind --tool=memcheck --track-origins=yes --leak-check=full --suppressions=devel/valgrind-suppress-all-but-c.supp <python script>
One useful command is to run valgrind over the test suite (from the top-level repo
directory)::
$ valgrind --tool=memcheck --track-origins=yes --leak-check=full --suppressions=devel/valgrind-suppress-all-but-c.supp pytest
While we will attempt to keep the suppression file updated to the best of our knowledge
so that only relevant leaks and errors are reported, you will likely have to do a bit of
digging to find the relevant parts.
Valgrind will likely run very slowly, and sometimes you will know already which exact
tests are those which may have problems, or are relevant to your particular changes.
To run these::
$ PYTHONMALLOC=malloc valgrind --tool=memcheck --track-origins=yes --leak-check=full --suppressions=devel/valgrind-suppress-all-but-c.supp pytest -v tests/<test_file>::<test_func> > valgrind.out 2>&1
Note that we also routed the stderr output to a file, which is useful because it can be
quite voluminous. There is a python script, ``devel/filter_valgrind.py`` which can be run
over the output (`valgrind.out` in the above command) to filter it down to only have
stuff from 21cmfast in it.
Producing Integration Test Data
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
There are bunch of so-called "integration tests", which rely on previously-produced
data. To produce this data, run ``python tests/produce_integration_test_data.py``.
Furthermore, this data should only be produced with good reason -- the idea is to keep
it static while the code changes, to have something steady to compare to. If a particular
PR fixes a bug which affects a certain tests' data, then that data should be re-run, in
the context of the PR, so it can be explained.
Logging in C
~~~~~~~~~~~~
The C code has a header file ``logging.h``. The C code should *never* contain bare
print-statements -- everything should be formally logged, so that the different levels
can be printed to screen correctly. The levels are defined in ``logging.h``, and include
levels such as ``INFO``, ``WARNING`` and ``DEBUG``. Each level has a corresponding macro
that starts with ``LOG_``. Thus to log run-time information to stdout, you would use
``LOG_INFO("message");``. Note that the message does not require a final newline character.
Exception handling in C
~~~~~~~~~~~~~~~~~~~~~~~
There are various places that things can go wrong in the C code, and they need to be
handled gracefully so that Python knows what to do with it (rather than just quitting!).
We use the simple ``cexcept.h`` header file from http://www.nicemice.net/cexcept/ to
enable a simple form of exception handling. That file itself should **not be edited**.
There is another header -- ``exceptions.h`` -- that defines how we use exceptions
throughout ``21cmFAST``. Any time an error arises that can be understood, the developer
should add a ``Throw <ErrorKind>;`` line. The ``ErrorKind`` can be any of the kinds
defined in ``exceptions.h`` (eg. ``GSLError`` or ``ValueError``). These are just integers.
Any C function that has a header in ``21cmFAST.h`` -- i.e. any function that is callable
directly from Python -- *must* be globally wrapped in a ``Try {} Catch(error_code) {}`` block. See
``GenerateICs.c`` for an example. Most of the code should be in the ``Try`` block.
Anything that does a ``Throw`` at any level of the call stack within that ``Try`` will
trigger a jump to the ``Catch``. The ``error_code`` is the integer that was thrown.
Typically, one will perhaps want to do some cleanup here, and then finally *return* the
error code.
Python knows about the exit codes it can expect to receive, and will raise Python
exceptions accordingly. From the python side, two main kinds of exceptions could be
raised, depending on the error code returned from C. The lesser exception is called a
``ParameterError``, and is supposed to indicate an error that happened merely because
the parameters that were input to the calculation were just too extreme to handle.
In the case of something like an automatic Monte Carlo algorithm that's iterating over
random parameters, one would *usually* want to just keep going at this point, because
perhaps it just wandered too far in parameter space.
The other kind of error is a ``FatalCError``, and this is where things went truly wrong,
and probably will do for any combination of parameters.
If you add a kind of Exception in the C code (to ``exceptions.h``), then be sure to add
a handler for it in the ``_process_exitcode`` function in ``wrapper.py``.
Maintaining Array State
~~~~~~~~~~~~~~~~~~~~~~~
Part of the challenge of maintaining a nice wrapper around the fast C-code is keeping
track of initialized memory, and ensuring that the C structures that require that memory
are pointing to the right place. Most of the arrays that are computed in ``21cmFAST``
are initialized *in Python* (using Numpy), then a pointer to their memory is given to
the C wrapper object.
To make matters more complicated, since some of the arrays are really big, it is sometimes
necessary to write them to disk to relieve memory pressure, and load them back in as required.
That means that any time, a given array in a C-based class may have one of several different "states":
1. Completely Uninitialized
1. Allocated an initialized in memory
1. Computed (i.e. filled with the values defining that array after computation in C)
1. Stored on disk
1. Stored *and* in memory.
It's important to keep track of these states, because when passing the struct to the ``compute()``
function of another struct (as input), we go and check if the array exists in memory, and
initialize it. Of course, we shouldn't initialize it with zeros if in fact it has been computed already
and is sitting on disk ready to be loaded. Thus, the ``OutputStruct`` tries to keep track of these
states for every array in the structure, using the ``_array_state`` dictionary. Every write/read/compute/purge
operation self-consistently modifies the status of the array.
However, one needs to be careful -- you *can* modify the actual state without modifying the ``_array_state``
(eg. simply by doing a ``del object.array``). In the future, we may be able to protect this to some extent,
but for now we rely on the good intent of the user.
Purging/Loading C-arrays to/from Disk
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
As of v3.1.0, there are more options for granular I/O, allowing large arrays to be purged from memory
when they are unnecessary for further computation. As a developer, you should be aware of the ``_get_required_input_arrays``
method on all ``OutputStruct`` subclasses. This is available to tell the given class what arrays need to
be available at compute time in any of the input structs. For example, if doing ``PERTURB_ON_HIGH_RES``,
the ``PerturbedField`` requires the hi-res density fields in ``InitialConditions``. This gives indications
as to what boxes can be purged to disk (all the low-res boxes in the ICs, for example).
Currently, this is only used to *check* that all boxes are available at compute time, and is not used
to actually automatically purge anything. Note however that ``InitialConditions`` does have two
custom methods that will purge unnecessary arrays before computing perturb fields or ionization fields.
.. note:: If you add a new quantity to a struct, and it is required input for other structs, you need
to add it to the relevant ``_get_required_input_arrays`` methods.
Further note that as of v3.1.0, partial structs can be written and read from disk (so you can specify
``keys=['hires_density']`` in the ``.read()`` method to just read the hi-res density field into the object.
Branching and Releasing
-----------------------
The aim is to make 21cmFAST's releases as useful, comprehendible, and automatic
as possible. This section lays out explicitly how this works (mostly for the benefit of
the admin(s)).
Versioning
~~~~~~~~~~
The first thing to mention is that we use strict `semantic versioning <https://semver.org>`_
(since v2.0). Thus the versions are ``MAJOR.MINOR.PATCH``, with ``MAJOR`` including
API-breaking changes, ``MINOR`` including new features, and ``PATCH`` fixing bugs or
documentation etc. If you depend on hmf, you can set your dependency as
``21cmFAST >= X.Y < X+1`` and not worry that we'll break your code with an update.
To mechanically handle versioning within the package, we use two methods that we make
to work together automatically. The "true" version of the package is set with
`setuptools-scm <https://pypi.org/project/setuptools-scm/>`_. This stores the version
in the git tag. There are many benefits to this -- one is that the version is unique
for every single change in the code, with commits on top of a release changing the
version. This means that versions accessed via ``py21cmfast.__version__`` are unique and track
the exact code in the package (useful for reproducing results). To get the current
version from command line, simply do ``python setup.py --version`` in the top-level
directory.
To actually bump the version, we use ``bump2version``. The reason for this is that the
CHANGELOG requires manual intervention -- we need to change the "dev-version" section
at the top of the file to the current version. Since this has to be manual, it requires
a specific commit to make it happen, which thus requires a PR (since commits can't be
pushed to master). To get all this to happen as smoothly as possible, we have a little
bash script ``bump`` that should be used to bump the version, which wraps ``bump2version``.
What it does is:
1. Runs ``bump2version`` and updates the ``major``, ``minor`` or ``patch`` part (passed like
``./bump minor``) in the VERSION file.
2. Updates the changelog with the new version heading (with the date),
and adds a new ``dev-version`` heading above that.
3. Makes a commit with the changes.
.. note:: Using the ``bump`` script is currently necessary, but future versions of
``bump2version`` may be able to do this automatically, see
https://github.com/c4urself/bump2version/issues/133.
The VERSION file might seem a bit redundant, and it is NOT recognized as the "official"
version (that is given by the git tag). Notice we didn't make a git tag in the above
script. That's because the tag should be made directly on the merge commit into master.
We do this using a Github Action (``tag-release.yaml``) which runs on every push to master,
reads the VERSION file, and makes a tag based on that version.
Branching
~~~~~~~~~
For branching, we use a very similar model to `git-flow <https://nvie.com/posts/a-successful-git-branching-model/>`_.
That is, we have a ``master`` branch which acts as the current truth against which to develop,
and ``production`` essentially as a deployment branch.
I.e., the ``master`` branch is where all features are merged (and some
non-urgent bugfixes). ``production`` is always production-ready, and corresponds
to a particular version on PyPI. Features should be branched from ``master``,
and merged back to ``production``. Hotfixes can be branched directly from ``production``,
and merged back there directly, *as well as* back into ``master``.
*Breaking changes* must only be merged to ``master`` when it has been decided that the next
version will be a major version. We do not do any long-term support of releases
(so can't make hotfixes to ``v2.x`` when the latest version is ``2.(x+1)``, or make a
new minor version in 2.x when the latest version is 3.x). We have set the default
branch to ``dev`` so that by default, branches are merged there. This is deemed best
for other developers (not maintainers/admins) to get involved, so the default thing is
usually right.
.. note:: Why not a more simple workflow like Github flow? The simple answer is it just
doesn't really make sense for a library with semantic versioning. You get into
trouble straight away if you want to merge a feature but don't want to update
the version number yet (you want to merge multiple features into a nice release).
In practice, this happens quite a lot.
.. note:: OK then, why not just use ``production`` to accrue features and fixes until such
time we're ready to release? The problem here is that if you've merged a few
features into master, but then realize a patch fix is required, there's no
easy way to release that patch without releasing all the merged features, thus
updating the minor version of the code (which may not be desirable). You could
then just keep all features in their own branches until you're ready to release,
but this is super annoying, and doesn't give you the chance to see how they
interact.
Releases
~~~~~~~~
To make a **patch** release, follow these steps:
1. Branch off of ``production``.
2. Write the fix.
3. Write a test that would have broken without the fix.
4. Update the changelog with your changes, under the ``**Bugfixes**`` heading.
5. Commit, push, and create a PR.
6. Locally, run ``./bump patch``.
7. Push.
8. Get a PR review and ensure CI passes.
9. Merge the PR
Note that in the background, Github Actions *should* take care of then tagging ``production``
with the new version, deploying that to PyPI, creating a new PR from master back into
``master``, and accepting that PR. If it fails for one of these steps, they can all be done
manually.
Note that you don't have to merge fixes in this way. You can instead just branch off
``master``, but then the fix won't be included until the next ``minor`` version.
This is easier (the admins do the adminy work) and useful for non-urgent fixes.
Any other fix/feature should be branched from ``master``. Every PR that does anything
noteworthy should have an accompanying edit to the changelog. However, you do not have
to update the version in the changelog -- that is left up to the admin(s). To make a
minor release, they should:
1. Locally, ``git checkout release``
2. ``git merge master``
3. No new features should be merged into ``master`` after that branching occurs.
4. Run ``./bump minor``
5. Make sure everything looks right.
6. ``git push``
7. Ensure all tests pass and get a CI review.
8. Merge into ``production``
The above also works for ``MAJOR`` versions, however getting them *in* to ``master`` is a little
different, in that they should wait for merging until we're sure that the next version
will be a major version.
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/docs/notes_for_developers.rst | notes_for_developers.rst |
======================================
Design Philosophy and Features for v3+
======================================
Here we describe in broad terms the design philosophy of the *new* ``21cmFAST``,
and some of its new features.
This is useful to get an initial bearing of how to go about using ``21cmFAST``, though
most likely the :doc:`tutorials <tutorials>` will be better for that.
It is also useful for those who have used the "old" ``21cmFAST`` (versions 2.1 and less)
and want to know why they should use this new version (and how to convert).
In doing so, we'll go over some of the key features of ``21cmFAST`` v3+.
To get a more in-depth view of all the options and features available, look at the
very thorough :doc:`API Reference <reference/index>`.
Design Philosophy
=================
The goal of v3 of ``21cmFAST`` is to provide the same computational efficiency and
scientific features of the previous generations, but packaged in a form that adopts the
best modern programming standards, including:
* simple installation
* comprehensive documentation
* comprehensive test suite
* more modular code
* standardised code formatting
* truly open-source and collaborative design (via Github)
Partly to enable these standards, and partly due to the many *extra* benefits it brings,
v3 also has the major distinction of being wrapped entirely in Python. The *extra*
benefits brought by this include:
* a native python library interface (eg. get your output box directly as a ``numpy`` array).
* better file-writing, into the HDF5 format, which saves metadata along with the box data.
* a caching system so that the same data never has to be calculated twice.
* reproducibility: know which exact version of ``21cmFAST``, with what parameters, produced a given dataset.
* significantly improved warnings and error checking/propagation.
* simplicity for adding new additional effects, and inserting them in the calculation pipeline.
We hope that additional features and benefits will be found by the growing community
of ``21cmFAST`` developers and users.
How it Works
============
v3 is *not* a complete rewrite of ``21cmFAST``. Most of the C-code of previous versions
is kept, though it has been modularised and modified in many places. The fundamental
routines are the same (barring bugfixes!).
The major programs of the original version (``init``, ``perturb``, ``ionize`` etc.) have
been converted into modular *functions* in C. Furthermore, most of the global parameters
(and, more often than not, global ``#define`` options) have been modularised and converted
into a series of input "parameter" ``structs``. These get passed into the functions.
Furthermore, each C function, instead of writing a bunch of files, returns an output
``struct`` containing all the stuff it computed.
Each of these functions and structs are wrapped in Python using the ``cffi`` package.
CFFI compiles the C code once upon *installation*. Due to the fact that parameters are
now passed around to the different functions, rather than being global defines, we no
longer need to re-compile every time an option is changed. Python itself can handle
changing the parameters, and can use the outputs in whatever way the user desires.
To maintain continuity with previous versions, a CLI interface is provided (see below)
that acts in a similar fashion to previous versions.
High-level configuration of ``21cmFAST`` can be set using the ``py21cmfast.config``
object. It is essentially a dictionary with its key/values the parameters. To make any
changes in the object permanent, use the ``py21cmfast.config.write()`` method.
One global configuration option is ``direc``, which specifies the directory in which
``21cmFAST`` will cache results by default (this can be overriden directly in any
function, see below for details).
Finally, ``21cmFAST`` contains a more robust cataloguing/caching method. Instead of
saving data with a selection of the dependent parameters written into the filename --
a method which is prone to error if a parameter which is not part of that selection is
modified -- ``21cmFAST`` writes all data into a configurable central directory with a hash
filename unique to *all* parameters upon which the data depends. Each kind of dataset has
attached methods which efficiently search this central directory for matching data to be
read when necessary.
Several arguments are available for all library functions which produce such datasets
that control this output. In this way, the data that is being retrieved is always
reliably produced with the desired parameters, and users need not concern themselves
with how and where the data is saved -- it can be retrieved merely by creating an empty
object with the desired parameters and calling ``.read()``, or even better, by calling
the function to *produce* the given dataset, which will by default just read it in if
available.
CLI
===
The CLI interface always starts with the command ``21cmfast``, and has a number of
subcommands. To list the available subcommands, use::
$ 21cmfast --help
To get help on any subcommand, simply use::
$ 21cmfast <subcommand> --help
Any subcommand which runs some aspect of ``21cmFAST`` will have a ``--config`` option,
which specifies a configuration file. This config file specifies the parameters of the
run. Furthermore, any particular parameter that can be specified in the config file can
be alternatively specified on the command line by appending the command with the
parameter name, eg.::
$ 21cmfast init --config=my_config.yml --HII_DIM=40 hlittle=0.7 --DIM 100 SIGMA_8 0.9
The above command shows the numerous ways in which these parameters can be specified
(with or without leading dashes, and with or without "=").
The CLI interface, while simple to use, does have the limitation that more complex
arguments than can be passed to the library functions are disallowed. For example,
one cannot pass a previously calculated initial conditions box to the ``perturb``
command. However, if such a box has been calculated with the default option to write it
to disk, then it will automatically be found and used in such a situation, i.e. the
following will not re-calculate the init box::
$ 21cmfast init
$ 21cmfast perturb redshift=8.0
This means that almost all of the functionality provided in the library is accessible
via the CLI.
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/docs/design.rst | design.rst |
Miscellaneous FAQs
==================
My run seg-faulted, what should I do?
-------------------------------------
Since ``21cmFAST`` is written in C, there is the off-chance that something
catastrophic will happen, causing a segfault. Typically, if this happens, Python will
not print a traceback where the error occurred, and finding the source of such errors
can be difficult. However, one has the option of using the standard library
`faulthandler <https://docs.python.org/3/library/faulthandler.html>`_. Specifying
``-X faulthandler`` when invoking Python will cause a minimal traceback to be printed
to ``stderr`` if a segfault occurs.
Configuring 21cmFAST
--------------------
``21cmFAST`` has a configuration file located at ``~/.21cmfast/config.yml``. This file
specifies some options to use in a rather global sense, especially to do with I/O.
You can directly edit this file to change how ``21cmFAST`` behaves for you across
sessions.
For any particular function call, any of the options may be overwritten by supplying
arguments to the function itself.
To set the configuration for a particular session, you can also set the global ``config``
instance, for example::
>>> import py21cmfast as p21
>>> p21.config['regenerate'] = True
>>> p21.run_lightcone(...)
All functions that use the ``regenerate`` keyword will now use the value you've set in the
config. Sometimes, you may want to be a little more careful -- perhaps you want to change
the configuration for a set of calls, but have it change back to the defaults after that.
We provide a context manager to do this::
>>> with p21.config.use(regenerate=True):
>>> p21.run_lightcone()
>>> print(p21.config['regenerate']) # prints "True"
>>> print(p21.config['regenerate']) # prints "False"
To make the current configuration permanent, simply use the ``write`` method::
>>> p21.config['direc'] = 'my_own_cache'
>>> p21.config.write()
Global Parameters
-----------------
There are a bunch of "global" parameters that are used throughout the C code. These are
parameters that are deemed to be constant enough to not expose them through the
regularly-used input structs, but nevertheless may necessitate modification from
time-to-time. These are accessed through the ``global_params`` object::
>>> from py21cmfast import global_params
Help on the attributes can be obtained via ``help(global_params)`` or
`in the docs <../reference/_autosummary/py21cmfast.inputs.html>`_. Setting the
attributes (which affects them everywhere throughout the code) is as simple as, eg::
>>> global_params.Z_HEAT_MAX = 30.0
If you wish to use a certain parameter for a fixed portion of your code (eg. for a single
run), it is encouraged to use the context manager, eg.::
>>> with global_params.use(Z_HEAT_MAX=10):
>>> run_lightcone(...)
How can I read a Coeval object from disk?
-----------------------------------------
The simplest way to read a :class:`py21cmfast.outputs.Coeval` object that has been
written to disk is by doing::
import py21cmfast as p21c
coeval = p21c.Coeval.read("my_coeval.h5")
However, you may want to read parts of the data, or read the data using a different
language or environment. You can do this as long as you have the HDF5 library (i.e.
h5py for Python). HDF5 is self-documenting, so you should be able to determine the
structure of the file yourself interactively. But here is an example using h5py::
import h5py
fl = h5py.File("my_coeval.h5", "r")
# print a dict of all the UserParams
# the CosmoParams, FlagOptions and AstroParams are accessed the same way.
print(dict(fl['user_params'].attrs))
# print a dict of all globals used for the coeval
print(dict(fl['_globals'].attrs))
# Get the redshift and random seed of the coeval box
redshift = fl.attrs['redshift']
seed = fl.attrs['random_seed']
# Get the Initial Conditions:
print(np.max(fl['InitialConditions']['hires_density'][:]))
# Or brightness temperature
print(np.max(fl['BrightnessTemp']['brightness_temperature'][:]))
# Basically, the different stages of computation are groups in the file, and all
# their consituent boxes are datasets in that group.
# Print out the keys of the group to see what is available:
print(fl['TsBox'].keys())
How can I read a LightCone object from file?
--------------------------------------------
Just like the :class:`py21cmfast.outputs.Coeval` object documented above, the
:class:`py21cmfast.outputs.LightCone` object is most easily read via its ``.read()`` method.
Similarly, it is written using HDF5. Again, the input parameters are stored in their
own sub-objects. However, the lightcone boxes themselves are in the "lightcones" group,
while the globally averaged quantities are in the ``global_quantities`` group::
import h5py
import matplotlib.pyplot as plt
fl = h5py.File("my_lightcone.h5", "r")
Tb = fl['lightcones']['brightness_temp'][:]
assert Tb.ndim==3
global_Tb = fl['global_quantities']['brightness_temp'][:]
redshifts = fl['node_redshifts']
plt.plot(redshifts, global_Tb)
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/docs/faqs/misc.rst | misc.rst |
```
import numpy as np
import matplotlib
%matplotlib inline
import matplotlib.pyplot as plt
import py21cmfast as p21c
from py21cmfast import global_params
from py21cmfast import plotting
random_seed = 1605
EoR_colour = matplotlib.colors.LinearSegmentedColormap.from_list('mycmap',\
[(0, 'white'),(0.33, 'yellow'),(0.5, 'orange'),(0.68, 'red'),\
(0.83333, 'black'),(0.9, 'blue'),(1, 'cyan')])
plt.register_cmap(cmap=EoR_colour)
```
This result was obtained using 21cmFAST at commit 2bb4807c7ef1a41649188a3efc462084f2e3b2e0
This notebook shows how to include the effect of the DM-baryon relative velocities, and the new EOS2021 parameters.
Based on Muñoz+21 (https://arxiv.org/abs/2110.13919). See https://drive.google.com/drive/folders/1-50AO-i3arCnfHc22YWXJacs4u-xsPL6?usp=sharing for the large (1.5Gpc) AllGalaxies simulation with the same parameters.
It is recommended to do the other tutorials first
# Fiducial and lightcones
Let's fix the initial condition for this tutorial.
```
output_dir = '/Users/julian/Dropbox/Research/EOS_21/'
HII_DIM = 64
BOX_LEN = 200 #cell size of ~3 Mpc or below for relative velocities
# USE_FFTW_WISDOM make FFT faster AND use relative velocities. , 'USE_INTERPOLATION_TABLES': True or code is too slow
user_params = {"HII_DIM":HII_DIM, "BOX_LEN": BOX_LEN, "USE_FFTW_WISDOM": True, 'USE_INTERPOLATION_TABLES': True,
"FAST_FCOLL_TABLES": True,
"USE_RELATIVE_VELOCITIES": True, "POWER_SPECTRUM": 5}
#set FAST_FCOLL_TABLES to TRUE if using minihaloes, it speeds up the generation of tables by ~x30 (see Appendix of 2110.13919)
#USE_RELATIVE_VELOCITIES is important for minihaloes. If True, POWER_SPECTRUM has to be set to 5 (CLASS) to get the transfers.
initial_conditions = p21c.initial_conditions(user_params=user_params,
random_seed=random_seed,
direc=output_dir
#, regenerate=True
)
plotting.coeval_sliceplot(initial_conditions, "lowres_vcb");
plotting.coeval_sliceplot(initial_conditions, "lowres_density");
```
Let's run a 'fiducial' model and see its lightcones
Note that the reference model has
F_STAR7_MINI ~ F_STAR10
and
F_ESC7_MINI ~ 1%, as low, but conservative fiducial
Also we take L_X_MINI=L_X out of simplicity (and ignorance)
```
# the lightcones we want to plot later together with their color maps and min/max
lightcone_quantities = ('brightness_temp','Ts_box','xH_box',"dNrec_box",'z_re_box','Gamma12_box','J_21_LW_box',"density")
cmaps = [EoR_colour,'Reds','magma','magma','magma','cubehelix','cubehelix','viridis']
vmins = [-150, 1e1, 0, 0, 5, 0, 0, -1]
vmaxs = [ 30, 1e3, 1, 2, 9, 1,10, 1]
astro_params_vcb = {"ALPHA_ESC": -0.3, "F_ESC10": -1.35,
"ALPHA_STAR": 0.5, "F_STAR10": -1.25, "t_STAR" :0.5,
"F_STAR7_MINI": -2.5, "ALPHA_STAR_MINI": 0, "F_ESC7_MINI" : -1.35,
"L_X": 40.5, "L_X_MINI": 40.5, "NU_X_THRESH": 500.0,
"A_VCB": 1.0, "A_LW": 2.0}
astro_params_novcb=astro_params_vcb
astro_params_novcb.update({'A_VCB': 0.0})
#setting 'A_VCB': 0 sets to zero the effect of relative velocities (fiducial value is 1.0)
#the parameter 'A_LW' (with fid value of 2.0) does the same for LW feedback.
flag_options_fid = {"INHOMO_RECO":True, 'USE_MASS_DEPENDENT_ZETA':True, 'USE_TS_FLUCT':True,
'USE_MINI_HALOS':True, 'FIX_VCB_AVG':False}
flag_options_fid_vavg = flag_options_fid
flag_options_fid_vavg.update({'FIX_VCB_AVG': True})
#the flag FIX_VCB_AVG side-steps the relative-velocity ICs, and instead fixes all velocities to some average value.
#It gets the background right but it's missing VAOs and 21cm power at large scales
ZMIN=5.
lightcone_fid_vcb = p21c.run_lightcone(
redshift = ZMIN,
init_box = initial_conditions,
flag_options = flag_options_fid,
astro_params = astro_params_vcb,
lightcone_quantities=lightcone_quantities,
global_quantities=lightcone_quantities,
random_seed = random_seed,
direc = output_dir,
write=True#, regenerate=True
)
fig, axs = plt.subplots(len(lightcone_quantities),1,
figsize=(20,10))#(getattr(lightcone_fid_vcb, lightcone_quantities[0]).shape[2]*0.01,
#getattr(lightcone_fid_vcb, lightcone_quantities[0]).shape[1]*0.01*len(lightcone_quantities)))
for ii, lightcone_quantity in enumerate(lightcone_quantities):
axs[ii].imshow(getattr(lightcone_fid_vcb, lightcone_quantity)[1],
vmin=vmins[ii], vmax=vmaxs[ii],cmap=cmaps[ii])
axs[ii].text(1, 0.05, lightcone_quantity,horizontalalignment='right',verticalalignment='bottom',
transform=axs[ii].transAxes,color = 'red',backgroundcolor='white',fontsize = 15)
axs[ii].xaxis.set_tick_params(labelsize=10)
axs[ii].yaxis.set_tick_params(labelsize=0)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.01)
#also run one without velocities and with fixed vcb=vavg (for comparison)
lightcone_fid_novcb = p21c.run_lightcone(
redshift = ZMIN,
init_box = initial_conditions,
flag_options = flag_options_fid,
astro_params = astro_params_novcb,
lightcone_quantities=lightcone_quantities,
global_quantities=lightcone_quantities,
random_seed = random_seed,
direc = output_dir,
write=True#, regenerate=True
)
lightcone_fid_vcbavg = p21c.run_lightcone(
redshift = ZMIN,
init_box = initial_conditions,
flag_options = flag_options_fid_vavg,
astro_params = astro_params_vcb,
lightcone_quantities=lightcone_quantities,
global_quantities=lightcone_quantities,
random_seed = random_seed,
direc = output_dir,
write=True#, regenerate=True
)
#plus run one with only atomic-cooling galaxies but same otherwise
flag_options_NOMINI=flag_options_fid
flag_options_NOMINI.update({'USE_MINI_HALOS': False})
lightcone_fid_NOMINI = p21c.run_lightcone(
redshift = ZMIN,
init_box = initial_conditions,
flag_options = flag_options_NOMINI,
astro_params = astro_params_vcb,
lightcone_quantities=lightcone_quantities,
global_quantities=lightcone_quantities,
random_seed = random_seed,
direc = output_dir,
write=True#, regenerate=True
)
#compare vcb and novcb
fig, axs = plt.subplots(2 ,1,
figsize=(20,6))
axs[0].imshow(getattr(lightcone_fid_vcb, 'brightness_temp')[1],
vmin=vmins[0], vmax=vmaxs[0],cmap=cmaps[0])
axs[1].imshow(getattr(lightcone_fid_novcb, 'brightness_temp')[1],
vmin=vmins[0], vmax=vmaxs[0],cmap=cmaps[0])
axs[0].text(1, 0.05, 'vcb' ,horizontalalignment='right',verticalalignment='bottom',
transform=axs[0].transAxes,color = 'red',backgroundcolor='white',fontsize = 15)
axs[1].text(1, 0.05, 'novcb' ,horizontalalignment='right',verticalalignment='bottom',
transform=axs[1].transAxes,color = 'red',backgroundcolor='white',fontsize = 15)
# axs[0].xaxis.set_tick_params(labelsize=10)
# axs[1].yaxis.set_tick_params(labelsize=0)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.01)
#plot tau
tau_vcb=tau_novcb=tau_NOMINI=np.array([])
for il,lightcone in enumerate([lightcone_fid_vcb,lightcone_fid_novcb,lightcone_fid_NOMINI]):
z_e=np.array([]);
tau_e=np.array([]);
for i in range(len(lightcone.node_redshifts)-1):
tauz=p21c.compute_tau(redshifts=lightcone.node_redshifts[-1:-2-i:-1],
global_xHI=lightcone.global_xHI[-1:-2-i:-1])
tau_e=np.append(tau_e,tauz)
z_e=np.append(z_e,lightcone.node_redshifts[-2-i])
#add lower zs where we manually set xH=1
zlow=np.linspace(lightcone.node_redshifts[-1]-0.1, 0.1, 14)
for zl in zlow:
tauz=p21c.compute_tau(redshifts=np.array([zl]), global_xHI=np.array([lightcone.global_xHI[-1]]))
tau_e=np.append([tauz],tau_e)
z_e=np.append([zl],z_e)
if(il==0):
tau_vcb=tau_e
elif (il==1):
tau_novcb=tau_e
else:
tau_NOMINI=tau_e
linestyles = ['-', '-.',':']
colors = ['black','gray','#377eb8']
lws = [3,1,2]
fig, axs = plt.subplots(1, 1, sharex=True, figsize=(8,4))
kk=0
axs.plot(z_e, tau_vcb, label = 'vcb',
color=colors[kk],linestyle=linestyles[kk], lw=lws[kk])
kk=1
axs.plot(z_e, tau_novcb, label = 'no vcb',
color=colors[kk],linestyle=linestyles[kk], lw=lws[kk])
kk=2
axs.plot(z_e, tau_NOMINI, label = 'no MINI',
color=colors[kk],linestyle=linestyles[kk],lw=lws[kk])
axs.set_ylim(0., 0.1)
axs.set_xlabel('redshift',fontsize=15)
axs.xaxis.set_tick_params(labelsize=15)
axs.set_xlim(0.,20.)
axs.set_ylabel('$\\tau$',fontsize=15)
axs.yaxis.set_tick_params(labelsize=15)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.0,wspace=0.0)
tauPmin=0.0561-0.0071
tauPmax=0.0561+0.0071
axs.axhspan(tauPmin, tauPmax, alpha=0.34, color='black')
axs.grid()
#Planck2020: tau=0.0561±0.0071
#check that the tau z=15-30 is below 0.02 as Planck requires
print(z_e[-1],z_e[55])
tau_vcb[-1]-tau_vcb[55]
linestyles = ['-', '-.',':']
colors = ['black','gray','#377eb8']
lws = [3,1,2]
labels = ['vcb', 'no vcb', 'no MINI']
fig, axs = plt.subplots(1, 1, sharex=True, figsize=(8,4))
for kk,lightcone in enumerate([lightcone_fid_vcb,lightcone_fid_novcb,lightcone_fid_NOMINI]):
axs.plot(lightcone.node_redshifts, lightcone.global_xHI, label = labels[kk],
color=colors[kk],linestyle=linestyles[kk], lw=lws[kk])
axs.set_ylim(0., 1.)
axs.set_xlabel('redshift',fontsize=15)
axs.xaxis.set_tick_params(labelsize=15)
axs.set_xlim(5.,20.)
axs.set_ylabel('$x_{HI}$',fontsize=15)
axs.yaxis.set_tick_params(labelsize=15)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.0,wspace=0.0)
axs.grid()
#choose a redshift to print coeval slices and see if there are VAOs. Usually best then T21~T21min/2
zz=zlist21[40]
print(zz)
#We plot a coeval box, but we compare the vcb case against the vcb=vavg, since the no velocity (vcb=0) case has a background evolution that is too different.
coeval_fid_vcb = p21c.run_coeval(
redshift = zz,
init_box = initial_conditions,
flag_options = flag_options_fid,
astro_params = astro_params_vcb,
random_seed = random_seed,
direc = output_dir,
write=True#, regenerate=True
)
coeval_fid_vcbavg = p21c.run_coeval(
redshift = zz,
init_box = initial_conditions,
flag_options = flag_options_fid_vavg,
astro_params = astro_params_vcb,
random_seed = random_seed,
direc = output_dir,
write=True#, regenerate=True
)
T21slice_vcb=coeval_fid_vcb.brightness_temp
T21avg_vcb = np.mean(T21slice_vcb)
dT21slice_vcb = T21slice_vcb - T21avg_vcb
T21slice_novcb=coeval_fid_vcbavg.brightness_temp
T21avg_novcb = np.mean(T21slice_novcb)
dT21slice_novcb = T21slice_novcb - T21avg_novcb
sigma21=np.sqrt(np.var(dT21slice_vcb))
T21maxplot = 3.0*sigma21
T21minplot = -2.0 * sigma21
origin = 'lower'
extend = 'both'
origin = None
extend = 'neither'
xx = np.linspace(0, BOX_LEN, HII_DIM, endpoint=False)
yy = xx
indexv=0
fig, ax = plt.subplots(2, 2, constrained_layout=True, figsize=(10,8),
sharex='col', sharey='row',
gridspec_kw={'hspace': 0, 'wspace': 0})
cs0=ax[0,0].contourf(xx, yy, dT21slice_novcb[indexv], extend=extend, origin=origin,
vmin=T21minplot, vmax=T21maxplot,cmap='bwr')
fig.colorbar(cs0, ax=ax[0,0], shrink=0.9, location='left')
cs1=ax[0,1].contourf(xx, yy, dT21slice_vcb[indexv], extend=extend, origin=origin,
vmin=T21minplot, vmax=T21maxplot,cmap='bwr')
fig.colorbar(cs1, ax=ax[0,1], shrink=0.9)
deltaslice=initial_conditions.lowres_density
deltaavg = np.mean(deltaslice)
ddeltaslice = deltaslice - deltaavg
vcbslice=initial_conditions.lowres_vcb
vcbavg = np.mean(vcbslice)
dvcbslice = vcbslice
print(vcbavg)
csd=ax[1,0].contourf(xx, yy, ddeltaslice[indexv])
fig.colorbar(csd, ax=ax[1,0], shrink=0.9, location='left')
csv=ax[1,1].contourf(xx, yy, dvcbslice[indexv])
fig.colorbar(csv, ax=ax[1,1], shrink=0.9, extend=extend)
plt.show()
plt.tight_layout()
global_quantities = ('brightness_temp','Ts_box','xH_box',"dNrec_box",'z_re_box','Gamma12_box','J_21_LW_box',"density")
#choose some to plot...
plot_quantities = ('brightness_temp','Ts_box','xH_box',"dNrec_box",'Gamma12_box','J_21_LW_box')
ymins = [-120, 1e1, 0, 0, 0, 0]
ymaxs = [ 30, 1e3, 1, 1, 1,5]
linestyles = ['-', '-',':','-.','-.',':']
colors = ['gray','black','#e41a1c','#377eb8','#e41a1c','#377eb8']
lws = [2,2,2,2]
textss = ['vcb','MCGs']
factorss = [[0, 1],] * len(textss)
labelss = [['NO', 'reference'],] * len(textss)
fig, axss = plt.subplots(len(plot_quantities), len(labelss),
sharex=True, figsize=(4*len(labelss),2*len(plot_quantities)))
for pp, texts in enumerate(textss):
labels = labelss[pp]
factors = factorss[pp]
axs = axss[:,pp]
for kk, label in enumerate(labels):
factor = factors[kk]
if kk==0:
if pp == 0:
lightcone = lightcone_fid_NOMINI
else:
lightcone = lightcone_fid_novcb
else:
lightcone = lightcone_fid_vcb
freqs = 1420.4 / (np.array(lightcone.node_redshifts) + 1.)
for jj, global_quantity in enumerate(plot_quantities):
axs[jj].plot(freqs, getattr(lightcone, 'global_%s'%global_quantity.replace('_box','')),
color=colors[kk],linestyle=linestyles[kk], label = labels[kk],lw=lws[kk])
axs[0].text(0.01, 0.99, texts,horizontalalignment='right',verticalalignment='bottom',
transform=axs[0].transAxes,fontsize = 15)
for jj, global_quantity in enumerate(plot_quantities):
axs[jj].set_ylim(ymins[jj], ymaxs[jj])
axs[-1].set_xlabel('Frequency/MHz',fontsize=15)
axs[-1].xaxis.set_tick_params(labelsize=15)
axs[0].set_xlim(1420.4 / (35 + 1.), 1420.4 / (5.5 + 1.))
zlabels = np.array([ 6, 7, 8, 10, 13, 18, 25, 35])
ax2 = axs[0].twiny()
ax2.set_xlim(axs[0].get_xlim())
ax2.set_xticks(1420.4 / (zlabels + 1.))
ax2.set_xticklabels(zlabels.astype(np.str))
ax2.set_xlabel("redshift",fontsize=15)
ax2.xaxis.set_tick_params(labelsize=15)
ax2.grid(False)
if pp == 0:
axs[0].legend(loc='lower right', ncol=2,fontsize=13,fancybox=True,frameon=True)
for jj, global_quantity in enumerate(plot_quantities):
axs[jj].set_ylabel('global_%s'%global_quantity.replace('_box',''),fontsize=15)
axs[jj].yaxis.set_tick_params(labelsize=15)
else:
for jj, global_quantity in enumerate(plot_quantities):
axs[jj].set_ylabel('global_%s'%global_quantity.replace('_box',''),fontsize=0)
axs[jj].yaxis.set_tick_params(labelsize=0)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.0,wspace=0.0)
```
# varying parameters
let's vary the parameters that describe mini-halos and see the impact to the global signal.
Warning: It may take a while to run all these boxes!
We keep other parameters fixed and vary one of following by a factor of 1/3 and 3:
F_STAR7_MINI
F_ESC7_MINI
L_X_MINI
A_LW
We also have a NOmini model where mini-halos are not included
```
#defining those color, linstyle, blabla
linestyles = ['-', '-',':','-.','-.',':']
colors = ['gray','black','#e41a1c','#377eb8','#e41a1c','#377eb8']
lws = [1,3,2,2,2,2]
textss = ['varying '+r'$f_{*,7}^{\rm mol}$',\
'varying '+r'$f_{\rm esc}^{\rm mol}$',\
'varying '+r'$L_{\rm x}^{\rm mol}$',\
'varying '+r'$A_{\rm LW}$']
factorss = [[0, 1, 0.33, 3.0],] * len(textss)
labelss = [['No Velocity', 'Fiducial', '/3', 'x3'],] * len(textss)
global_quantities = ('brightness_temp','Ts_box','xH_box',"dNrec_box",'z_re_box','Gamma12_box','J_21_LW_box',"density")
#choose some to plot...
plot_quantities = ('brightness_temp','Ts_box','xH_box',"dNrec_box",'Gamma12_box','J_21_LW_box')
ymins = [-120, 1e1, 0, 0, 0, 0]
ymaxs = [ 30, 1e3, 1, 1, 1,10]
fig, axss = plt.subplots(len(plot_quantities), len(labelss),
sharex=True, figsize=(4*len(labelss),2*len(global_quantities)))
for pp, texts in enumerate(textss):
labels = labelss[pp]
factors = factorss[pp]
axs = axss[:,pp]
for kk, label in enumerate(labels):
flag_options = flag_options_fid.copy()
astro_params = astro_params_vcb.copy()
factor = factors[kk]
if label == 'No Velocity':
lightcone = lightcone_fid_novcb
elif label == 'Fiducial':
lightcone = lightcone_fid_vcb
else:
if pp == 0:
astro_params.update({'F_STAR7_MINI': astro_params_vcb['F_STAR7_MINI']+np.log10(factor)})
elif pp == 1:
astro_params.update({'F_ESC7_MINI': astro_params_vcb['F_ESC7_MINI']+np.log10(factor)})
elif pp == 2:
astro_params.update({'L_X_MINI': astro_params_vcb['L_X_MINI']+np.log10(factor)})
elif pp == 3:
astro_params.update({'A_LW': astro_params_vcb['A_LW']*factor})
else:
print('Make a choice!')
lightcone = p21c.run_lightcone(
redshift = ZMIN,
init_box = initial_conditions,
flag_options = flag_options_fid,
astro_params = astro_params,
global_quantities=global_quantities,
random_seed = random_seed,
direc = output_dir
)
freqs = 1420.4 / (np.array(lightcone.node_redshifts) + 1.)
for jj, global_quantity in enumerate(plot_quantities):
axs[jj].plot(freqs, getattr(lightcone, 'global_%s'%global_quantity.replace('_box','')),
color=colors[kk],linestyle=linestyles[kk], label = labels[kk],lw=lws[kk])
axs[0].text(0.01, 0.99, texts,horizontalalignment='left',verticalalignment='top',
transform=axs[0].transAxes,fontsize = 15)
for jj, global_quantity in enumerate(plot_quantities):
axs[jj].set_ylim(ymins[jj], ymaxs[jj])
axs[-1].set_xlabel('Frequency/MHz',fontsize=15)
axs[-1].xaxis.set_tick_params(labelsize=15)
axs[0].set_xlim(1420.4 / (35 + 1.), 1420.4 / (5.5 + 1.))
zlabels = np.array([ 6, 7, 8, 10, 13, 18, 25, 35])
ax2 = axs[0].twiny()
ax2.set_xlim(axs[0].get_xlim())
ax2.set_xticks(1420.4 / (zlabels + 1.))
ax2.set_xticklabels(zlabels.astype(np.str))
ax2.set_xlabel("redshift",fontsize=15)
ax2.xaxis.set_tick_params(labelsize=15)
ax2.grid(False)
if pp == 0:
axs[0].legend(loc='lower right', ncol=2,fontsize=13,fancybox=True,frameon=True)
for jj, global_quantity in enumerate(plot_quantities):
axs[jj].set_ylabel('global_%s'%global_quantity.replace('_box',''),fontsize=15)
axs[jj].yaxis.set_tick_params(labelsize=15)
else:
for jj, global_quantity in enumerate(plot_quantities):
axs[jj].set_ylabel('global_%s'%global_quantity.replace('_box',''),fontsize=0)
axs[jj].yaxis.set_tick_params(labelsize=0)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.0,wspace=0.0)
# define functions to calculate PS, following py21cmmc
from powerbox.tools import get_power
def compute_power(
box,
length,
n_psbins,
log_bins=True,
ignore_kperp_zero=True,
ignore_kpar_zero=False,
ignore_k_zero=False,
):
# Determine the weighting function required from ignoring k's.
k_weights = np.ones(box.shape, dtype=np.int)
n0 = k_weights.shape[0]
n1 = k_weights.shape[-1]
if ignore_kperp_zero:
k_weights[n0 // 2, n0 // 2, :] = 0
if ignore_kpar_zero:
k_weights[:, :, n1 // 2] = 0
if ignore_k_zero:
k_weights[n0 // 2, n0 // 2, n1 // 2] = 0
res = get_power(
box,
boxlength=length,
bins=n_psbins,
bin_ave=False,
get_variance=False,
log_bins=log_bins,
k_weights=k_weights,
)
res = list(res)
k = res[1]
if log_bins:
k = np.exp((np.log(k[1:]) + np.log(k[:-1])) / 2)
else:
k = (k[1:] + k[:-1]) / 2
res[1] = k
return res
def powerspectra(brightness_temp, n_psbins=50, nchunks=10, min_k=0.1, max_k=1.0, logk=True):
data = []
chunk_indices = list(range(0,brightness_temp.n_slices,round(brightness_temp.n_slices / nchunks),))
if len(chunk_indices) > nchunks:
chunk_indices = chunk_indices[:-1]
chunk_indices.append(brightness_temp.n_slices)
for i in range(nchunks):
start = chunk_indices[i]
end = chunk_indices[i + 1]
chunklen = (end - start) * brightness_temp.cell_size
power, k = compute_power(
brightness_temp.brightness_temp[:, :, start:end],
(BOX_LEN, BOX_LEN, chunklen),
n_psbins,
log_bins=logk,
)
data.append({"k": k, "delta": power * k ** 3 / (2 * np.pi ** 2)})
return data
# do 5 chunks but only plot 1 - 4, the 0th has no power for minihalo models where xH=0
nchunks = 4
k_fundamental = 2*np.pi/BOX_LEN
k_max = k_fundamental * HII_DIM
Nk=np.floor(HII_DIM/1).astype(int)
fig, axss = plt.subplots(nchunks, len(labelss), sharex=True,sharey=True,figsize=(4*len(labelss),3*(nchunks)),subplot_kw={"xscale":'log', "yscale":'log'})
for pp, texts in enumerate(textss):
labels = labelss[pp]
factors = factorss[pp]
axs = axss[:,pp]
for kk, label in enumerate(labels):
flag_options = flag_options_fid.copy()
astro_params = astro_params_vcb.copy()
factor = factors[kk]
if label == 'No Velocity':
lightcone = lightcone_fid_novcb
elif label == 'Fiducial':
lightcone = lightcone_fid_vcb
else:
if pp == 0:
astro_params.update({'F_STAR7_MINI': astro_params_vcb['F_STAR7_MINI']+np.log10(factor)})
elif pp == 1:
astro_params.update({'F_ESC7_MINI': astro_params_vcb['F_ESC7_MINI']+np.log10(factor)})
elif pp == 2:
astro_params.update({'L_X_MINI': astro_params_vcb['L_X_MINI']+np.log10(factor)})
elif pp == 3:
astro_params.update({'A_LW': astro_params_vcb['A_LW']+np.log10(factor)})
else:
print('Make a choice!')
lightcone = p21c.run_lightcone(
redshift = ZMIN,
init_box = initial_conditions,
flag_options = flag_options_fid,
astro_params = astro_params,
global_quantities=global_quantities,
random_seed = random_seed,
direc = output_dir
)
PS = powerspectra(lightcone, min_k = k_fundamental, max_k = k_max)
for ii in range(nchunks):
axs[ii].plot(PS[ii+1]['k'], PS[ii+1]['delta'], color=colors[kk],linestyle=linestyles[kk], label = labels[kk],lw=lws[kk])
if pp == len(textss)-1 and kk == 0:
axs[ii].text(0.99, 0.01, 'Chunk-%02d'%(ii+1),horizontalalignment='right',verticalalignment='bottom',
transform=axs[ii].transAxes,fontsize = 15)
axs[0].text(0.01, 0.99, texts,horizontalalignment='left',verticalalignment='top',
transform=axs[0].transAxes,fontsize = 15)
axs[-1].set_xlabel("$k$ [Mpc$^{-3}$]",fontsize=15)
axs[-1].xaxis.set_tick_params(labelsize=15)
if pp == 0:
for ii in range(nchunks):
axs[ii].set_ylim(2e-1, 2e2)
axs[ii].set_ylabel("$k^3 P$", fontsize=15)
axs[ii].yaxis.set_tick_params(labelsize=15)
else:
for ii in range(nchunks-1):
axs[ii].set_ylim(2e-1, 2e2)
axs[ii].set_ylabel("$k^3 P$", fontsize=0)
axs[ii].yaxis.set_tick_params(labelsize=0)
axss[0,0].legend(loc='lower left', ncol=2,fontsize=13,fancybox=True,frameon=True)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.0,wspace=0.0)
```
Note that I've run these simulations in parallel before this tutorial. With these setup, each took ~6h to finish. Here, running means read the cached outputs.
## global properties -- optical depth
```
#defining those color, linstyle, blabla
linestyles = ['-', '-',':','-.','-.',':']
colors = ['gray','black','#e41a1c','#377eb8','#e41a1c','#377eb8']
lws = [1,3,2,2,2,2]
textss_tau = ['varying '+r'$f_{*,7}^{\rm mol}$',\
'varying '+r'$f_{\rm esc}^{\rm mol}$',\
'varying '+r'$A_{\rm LW}$']
factorss_tau = [[0, 1, 0.33, 3.0],] * len(textss_tau)
labelss_tau = [['No Velocity', 'Fiducial', '/3', 'x3'],] * len(textss_tau)
plot_quantities = ['tau_e']
ymins = [0]
ymaxs = [0.2]
fig, axss_tau = plt.subplots(len(plot_quantities), len(labelss_tau),
sharex=True, figsize=(4*len(labelss_tau),3*len(plot_quantities)))
for pp, texts in enumerate(textss_tau):
labels = labelss_tau[pp]
factors = factorss_tau[pp]
axs = axss_tau[pp]
for kk, label in enumerate(labels):
flag_options = flag_options_fid.copy()
astro_params = astro_params_vcb.copy()
factor = factors[kk]
if label == 'No Velocity':
lightcone = lightcone_fid_novcb
elif label == 'Fiducial':
lightcone = lightcone_fid_vcb
else:
if pp == 0:
astro_params.update({'F_STAR7_MINI': astro_params_vcb['F_STAR7_MINI']+np.log10(factor)})
elif pp == 1:
astro_params.update({'F_ESC7_MINI': astro_params_vcb['F_ESC7_MINI']+np.log10(factor)})
elif pp == 2:
astro_params.update({'A_LW': astro_params_vcb['A_LW']*factor})
else:
print('Make a choice!')
lightcone = p21c.run_lightcone(
redshift = ZMIN,
init_box = initial_conditions,
flag_options = flag_options_fid,
astro_params = astro_params,
global_quantities=global_quantities,
random_seed = random_seed,
direc = output_dir
)
z_e=np.array([]);
tau_e=np.array([]);
for i in range(len(lightcone.node_redshifts)-1):
tauz=p21c.compute_tau(redshifts=lightcone.node_redshifts[-1:-2-i:-1],
global_xHI=lightcone.global_xHI[-1:-2-i:-1])
tau_e=np.append(tau_e,tauz)
z_e=np.append(z_e,lightcone.node_redshifts[-2-i])
#print(i,lightcone.node_redshifts[i],tauz)
#add lower zs where we manually set xH=1
zlow=np.linspace(lightcone.node_redshifts[-1]-0.1, 0.1, 14)
for zl in zlow:
tauz=p21c.compute_tau(redshifts=np.array([zl]), global_xHI=np.array([lightcone.global_xHI[-1]]))
tau_e=np.append([tauz],tau_e)
z_e=np.append([zl],z_e)
# freqs = 1420.4 / (np.array(lightcone.node_redshifts) + 1.)
for jj, global_quantity in enumerate(plot_quantities):
axs.plot(z_e, tau_e,
color=colors[kk],linestyle=linestyles[kk], label = labels[kk],lw=lws[kk])
axs.text(0.01, 0.99, texts,horizontalalignment='left',verticalalignment='top',
transform=axs.transAxes,fontsize = 15)
axs.set_ylim(ymins[0], ymaxs[0])
axs.set_xlabel('redshift',fontsize=15)
axs.xaxis.set_tick_params(labelsize=15)
axs.set_xlim(0.,20.)
if pp == 0:
for ii in range(nchunks):
axs.set_ylabel('$\\tau$',fontsize=15)
axs.yaxis.set_tick_params(labelsize=15)
else:
for ii in range(nchunks-1):
axs.yaxis.set_tick_params(labelsize=0)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.0,wspace=0.0)
```
## 21-cm power spectra
```
# do 5 chunks but only plot 1 - 4, the 0th has no power for minihalo models where xH=0
nchunks = 4
fig, axss = plt.subplots(nchunks, len(labelss), sharex=True,sharey=True,figsize=(4*len(labelss),3*(nchunks)),subplot_kw={"xscale":'log', "yscale":'log'})
for pp, texts in enumerate(textss):
labels = labelss[pp]
factors = factorss[pp]
axs = axss[:,pp]
for kk, label in enumerate(labels):
factor = factors[kk]
if kk==0:
lightcone = lightcone_fid_vcbavg
else:
lightcone = lightcone_fid_vcb
PS = powerspectra(lightcone, min_k = k_fundamental, max_k = k_max)
for ii in range(nchunks):
axs[ii].plot(PS[ii+1]['k'], PS[ii+1]['delta'], color=colors[kk],linestyle=linestyles[kk], label = labels[kk],lw=lws[kk])
if pp == len(textss)-1 and kk == 0:
axs[ii].text(0.99, 0.01, 'Chunk-%02d'%(ii+1),horizontalalignment='right',verticalalignment='bottom',
transform=axs[ii].transAxes,fontsize = 15)
axs[0].text(0.01, 0.99, texts,horizontalalignment='left',verticalalignment='top',
transform=axs[0].transAxes,fontsize = 15)
axs[-1].set_xlabel("$k$ [Mpc$^{-3}$]",fontsize=15)
axs[-1].xaxis.set_tick_params(labelsize=15)
if pp == 0:
for ii in range(nchunks):
axs[ii].set_ylim(2e-1, 2e2)
axs[ii].set_ylabel("$k^3 P$", fontsize=15)
axs[ii].yaxis.set_tick_params(labelsize=15)
else:
for ii in range(nchunks-1):
axs[ii].set_ylim(2e-1, 2e2)
axs[ii].set_ylabel("$k^3 P$", fontsize=0)
axs[ii].yaxis.set_tick_params(labelsize=0)
axss[0,0].legend(loc='lower left', ncol=2,fontsize=13,fancybox=True,frameon=True)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.0,wspace=0.0)
```
```
nchunks=5
textss = ['vcb','vcb']
factorss = [[0, 1],] * len(textss)
labelss = [['Regular', 'Avg'],] * len(textss)
k_fundamental = 2*np.pi/BOX_LEN
k_max = k_fundamental * HII_DIM
Nk=np.floor(HII_DIM/1).astype(int)
PSv= powerspectra(lightcone_fid_vcb, min_k = k_fundamental, max_k = k_max)
PSvavg= powerspectra(lightcone_fid_vcbavg, min_k = k_fundamental, max_k = k_max)
klist= PSv[0]['k']
P21diff = [(PSv[i]['delta']-PSvavg[i]['delta'])/PSvavg[i]['delta'] for i in range(nchunks)]
import matplotlib.pyplot as plt
fig, axss = plt.subplots(nchunks, 1, sharex=True,sharey=True,figsize=(2*len(labelss),3*(nchunks)),subplot_kw={"xscale":'linear', "yscale":'linear'})
for ii in range(nchunks):
axss[ii].plot(klist, P21diff[ii])
plt.xscale('log')
axss[0].legend(loc='lower left', ncol=2,fontsize=13,fancybox=True,frameon=True)
plt.tight_layout()
fig.subplots_adjust(hspace = 0.0,wspace=0.0)
```
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/docs/tutorials/relative_velocities.ipynb | relative_velocities.ipynb |
{{ fullname | escape | underline }}
.. currentmodule:: {{ module }}
.. autoclass:: {{ objname }}
{% block methods %}
{% if methods %}
.. rubric:: Methods
.. autosummary::
:toctree: {{ objname }}
{% for item in methods %}
~{{ name }}.{{ item }}
{%- endfor %}
{% endif %}
{% endblock %}
{% block attributes %}
{% if attributes %}
.. rubric:: Attributes
.. autosummary::
:toctree: {{ objname }}
{% for item in attributes %}
~{{ name }}.{{ item }}
{%- endfor %}
{% endif %}
{% endblock %}
| 21cmFAST | /21cmFAST-3.3.1.tar.gz/21cmFAST-3.3.1/docs/templates/class.rst | class.rst |