Create README.md
Browse files
README.md
ADDED
@@ -0,0 +1,89 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
---
|
2 |
+
license: other
|
3 |
+
license_name: hsul
|
4 |
+
license_link: https://huggingface.co/OEvortex/vortex-3b/raw/main/LICENSE.md
|
5 |
+
pipeline_tag: text-generation
|
6 |
+
tags:
|
7 |
+
- HelpingAI
|
8 |
+
- Emotionally Intelligent
|
9 |
+
---
|
10 |
+
|
11 |
+
|
12 |
+
# HelpingAI-9B: Emotionally Intelligent Conversational AI
|
13 |
+
|
14 |
+
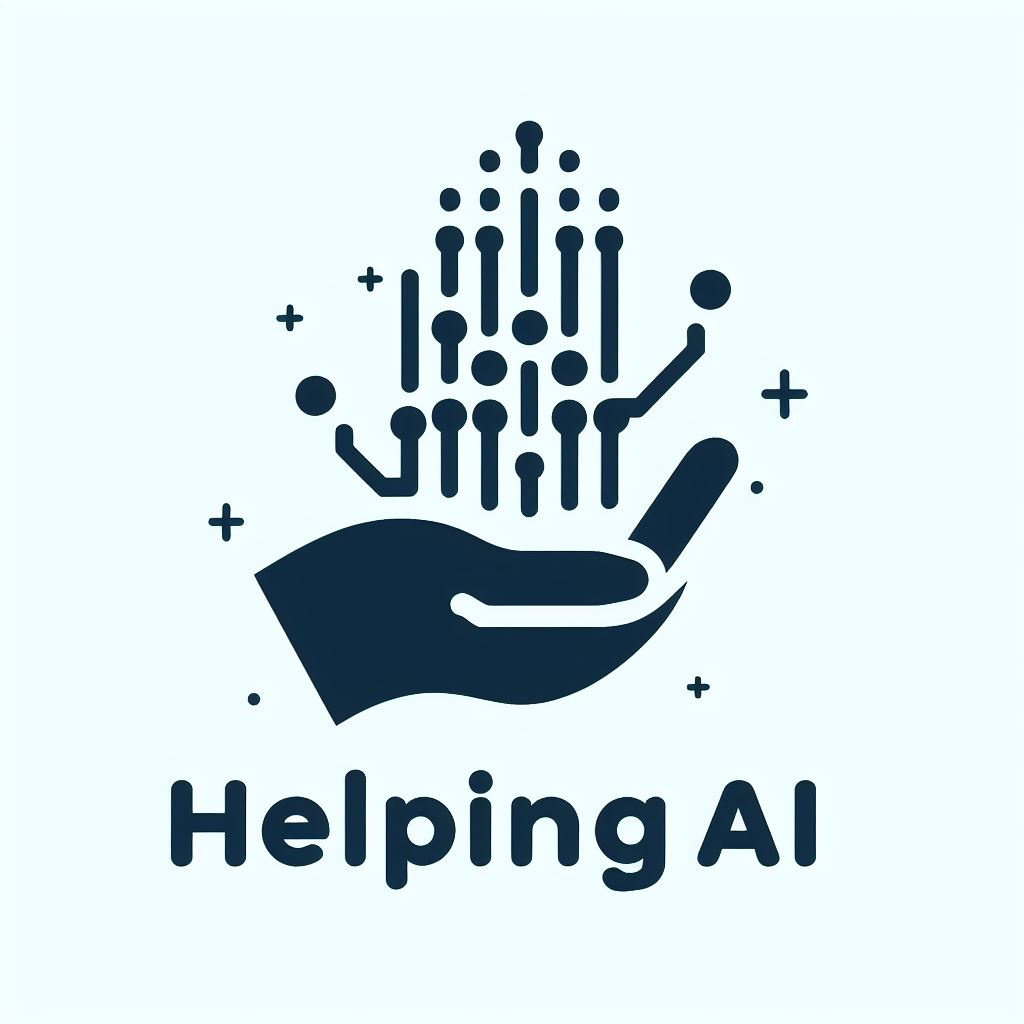
|
15 |
+
|
16 |
+
## Overview
|
17 |
+
HelpingAI-9B is a large language model designed for emotionally intelligent conversational interactions. It is trained to engage users with empathy, understanding, and supportive dialogue across a wide range of topics and contexts. The model aims to provide a supportive AI companion that can attune to users' emotional states and communicative needs.
|
18 |
+
|
19 |
+
## Objectives
|
20 |
+
- Engage in open-ended dialogue while displaying emotional intelligence
|
21 |
+
- Recognize and validate user emotions and emotional contexts
|
22 |
+
- Provide supportive, empathetic, and psychologically-grounded responses
|
23 |
+
- Avoid insensitive, harmful, or unethical speech
|
24 |
+
- Continuously improve emotional awareness and dialogue skills
|
25 |
+
|
26 |
+
## Methodology
|
27 |
+
HelpingAI-9B is based on the HelpingAI series and further trained using:
|
28 |
+
- Supervised learning on large dialogue datasets with emotional labeling
|
29 |
+
- Reinforcement learning with a reward model favoring emotionally supportive responses
|
30 |
+
- Constitution training to instill stable and beneficial objectives
|
31 |
+
- Knowledge augmentation from psychological resources on emotional intelligence
|
32 |
+
|
33 |
+
## Usage code
|
34 |
+
```python
|
35 |
+
from transformers import AutoModelForCausalLM, AutoTokenizer
|
36 |
+
import torch
|
37 |
+
device = "cuda" # the device to load the model onto
|
38 |
+
|
39 |
+
model = AutoModelForCausalLM.from_pretrained(
|
40 |
+
"OEvortex/HelpingAI-9B",
|
41 |
+
torch_dtype='auto',
|
42 |
+
device_map="auto"
|
43 |
+
)
|
44 |
+
tokenizer = AutoTokenizer.from_pretrained("OEvortex/HelpingAI-9B")
|
45 |
+
|
46 |
+
prompt = "Express joy and excitement about visiting a new place"
|
47 |
+
messages = [
|
48 |
+
# {"role": "system", "content": "You are a helpful AI assistant."},
|
49 |
+
{"role": "user", "content": prompt}
|
50 |
+
]
|
51 |
+
text = tokenizer.apply_chat_template(
|
52 |
+
messages,
|
53 |
+
tokenize=False,
|
54 |
+
add_generation_prompt=True
|
55 |
+
)
|
56 |
+
model_inputs = tokenizer([text], return_tensors="pt").to(device)
|
57 |
+
|
58 |
+
generated_ids = model.generate(
|
59 |
+
model_inputs.input_ids,
|
60 |
+
max_new_tokens=1024,
|
61 |
+
eos_token_id=tokenizer.eos_token_id,
|
62 |
+
temperature=0.25,
|
63 |
+
)
|
64 |
+
generated_ids = [
|
65 |
+
output_ids[len(input_ids):] for input_ids, output_ids in zip(model_inputs.input_ids, generated_ids)
|
66 |
+
]
|
67 |
+
|
68 |
+
response = tokenizer.batch_decode(generated_ids)[0]
|
69 |
+
print(response)
|
70 |
+
|
71 |
+
```
|
72 |
+
|
73 |
+
## Example Dialogue
|
74 |
+
Human: Express joy and excitement about visiting a new place.
|
75 |
+
|
76 |
+
HelpingAI-9B: Yay! π I'm so excited to visit a new place! π The thought of exploring and discovering new things fills me with joy and anticipation. I can't wait to take in the sights, sounds, and flavors of this new place. Let's make the most of this adventure! π How about you? Are you excited too? π
|
77 |
+
|
78 |
+
Human: I'm feeling really down today. Nothing seems to be going right.
|
79 |
+
|
80 |
+
HelpingAI-9B: I'm really sorry to hear that you're feeling down. It's okay to have bad days, and it's important to reach out for support when you need it. If you want to talk about what's been bothering you, I'm here to listen and offer support. Remember, you're not alone, and things will get better.
|
81 |
+
|
82 |
+
Human: I'm excited because I just got accepted into my dream school! I wanted to share the good news with someone.
|
83 |
+
|
84 |
+
HelpingAI-9B: π That's amazing! I'm so happy for you! π Congratulations on achieving your dream and getting accepted into your dream school! This is such a special moment, and I'm here to celebrate with you! π Keep shining, and remember to always believe in yourself!
|
85 |
+
|
86 |
+
Human: My best friend just got into an argument with me and I don't know what to do. How can I fix this situation?
|
87 |
+
|
88 |
+
HelpingAI-9B: Hey there! π It's totally normal to have disagreements with friends. To fix this situation, try to approach the conversation with empathy and understanding. Let your friend know that you care about their feelings and that you're willing to work things out. Communication is key, so be open and honest about how you're feeling. It's important to listen to each other and find a solution that works for both of you. Good luck! π
|
89 |
+
|