Spaces:
Runtime error
Runtime error
Ryan Kim
commited on
Commit
•
73f7e39
1
Parent(s):
2f9d73b
adding files for milestone 1
Browse files- .devcontainer/devcontainer.json +22 -0
- README.md +111 -0
- Screenshots/Docker_Built.png +0 -0
- Screenshots/Docker_Python_Built.png +0 -0
- Screenshots/ExtensionsForDocker.png +0 -0
- Screenshots/Install_Proof.png +0 -0
- misc_example/Dockerfile +4 -0
- misc_example/example.js +2 -0
- requirements.txt +0 -0
- src/app.py +18 -0
.devcontainer/devcontainer.json
ADDED
@@ -0,0 +1,22 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
// For format details, see https://aka.ms/devcontainer.json. For config options, see the
|
2 |
+
// README at: https://github.com/devcontainers/templates/tree/main/src/python
|
3 |
+
{
|
4 |
+
"name": "Python 3",
|
5 |
+
// Or use a Dockerfile or Docker Compose file. More info: https://containers.dev/guide/dockerfile
|
6 |
+
"image": "mcr.microsoft.com/devcontainers/python:0-3.11"
|
7 |
+
|
8 |
+
// Features to add to the dev container. More info: https://containers.dev/features.
|
9 |
+
// "features": {},
|
10 |
+
|
11 |
+
// Use 'forwardPorts' to make a list of ports inside the container available locally.
|
12 |
+
// "forwardPorts": [],
|
13 |
+
|
14 |
+
// Use 'postCreateCommand' to run commands after the container is created.
|
15 |
+
// "postCreateCommand": "pip3 install --user -r requirements.txt",
|
16 |
+
|
17 |
+
// Configure tool-specific properties.
|
18 |
+
// "customizations": {},
|
19 |
+
|
20 |
+
// Uncomment to connect as root instead. More info: https://aka.ms/dev-containers-non-root.
|
21 |
+
// "remoteUser": "root"
|
22 |
+
}
|
README.md
CHANGED
@@ -1 +1,112 @@
|
|
1 |
# cs-gy-6613-project-rk2546
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
# cs-gy-6613-project-rk2546
|
2 |
+
|
3 |
+
## Milestone 1
|
4 |
+
|
5 |
+
### Installing Necessary Prerequisites
|
6 |
+
|
7 |
+
I already have Docker installed on my system from prior projects. I recall following these specific steps:
|
8 |
+
|
9 |
+
1. Install Docker Desktop, provided via the official [Docker Website](https://www.docker.com/products/docker-desktop)
|
10 |
+
2. Installed WSL2. Unknown source.
|
11 |
+
3. Restarted PC to initialize and complete install of WSL2.
|
12 |
+
|
13 |
+
Proof of installs for both are provided in the following screenshot:
|
14 |
+
|
15 |
+

|
16 |
+
|
17 |
+
Visual Studio Code is already installed on my PC. The VS Code version was provided via the [official website](https://code.visualstudio.com/). VS Code has the recommended extensions for Docker installed for ease-of-use:
|
18 |
+
|
19 |
+

|
20 |
+
|
21 |
+
There's another list of extensions I added to my VS Code:
|
22 |
+
|
23 |
+
- **Remote - SSH**
|
24 |
+
- **Remote Development**
|
25 |
+
- **Dev Containers** <-- The REAL important one
|
26 |
+
- **Remote - Tunnels**
|
27 |
+
|
28 |
+
The third one, **Dev Containers**, will be useful for opening up folders inside the container.
|
29 |
+
|
30 |
+
The steps to get the Docker instance ready for this project follow the attached video, provided in the instructions of the milestone writeup on the course website.
|
31 |
+
|
32 |
+
[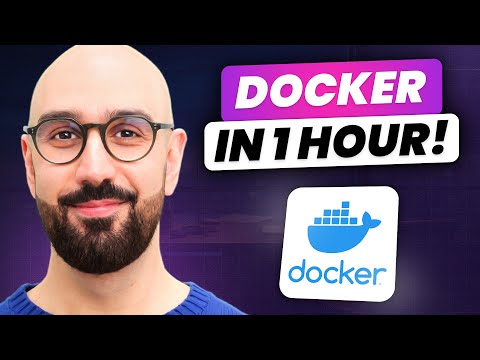](https://www.youtube.com/watch?v=pTFZFxd4hOI "Docker Tutorial for Beginners")
|
33 |
+
|
34 |
+
### Example: Creating a Docker Image
|
35 |
+
|
36 |
+
While this isn't necessarily related to setting up Docker for python development, this is here for posterity and to show how to create customized Docker images that can be used later. This example is creating a Node.JS-based image that executes a simple
|
37 |
+
|
38 |
+
The steps are as follows:
|
39 |
+
|
40 |
+
1. I created a `misc_example/` directory that will contain a sample application to run. Inside, I created an `example.js` file that has some basic code to run (ex. `console.log("Hellow World")`)
|
41 |
+
2. I created a `Dockerfile` file with the following code:
|
42 |
+
|
43 |
+
```docker
|
44 |
+
FROM node:alpine
|
45 |
+
COPY . /misc_example
|
46 |
+
WORKDIR /misc_example
|
47 |
+
CMD node example.js
|
48 |
+
```
|
49 |
+
|
50 |
+
The code above uses the "apline" linux distribution and node. It tells to copy everything in this root directory over to the `misc_example` folder, changes the working directory to the `misc_example` folder, then executes the command to run `example.js`.
|
51 |
+
|
52 |
+
To buld the docker image, we execute the following command in the CMD:
|
53 |
+
|
54 |
+
```
|
55 |
+
docker build -t csgy6613proj-rk2546 ./misc_example
|
56 |
+
```
|
57 |
+
|
58 |
+
To prove that the build was created successfully, the following command was used to list all images that Docker has access to:
|
59 |
+
|
60 |
+
```
|
61 |
+
docker image ls
|
62 |
+
```
|
63 |
+
|
64 |
+
To see if it works, we execute the following command:
|
65 |
+
|
66 |
+
```
|
67 |
+
docker run csgy6613proj-rk2546
|
68 |
+
```
|
69 |
+
|
70 |
+
We can see that the very simple console logs we wrote in `example.js` were successfully printed. Refer to the screenshot below for a full understanding of the commands executed and logs created:
|
71 |
+
|
72 |
+

|
73 |
+
|
74 |
+
### Initializing a Docker Development Environment for Python
|
75 |
+
|
76 |
+
A part of this milestone will probably involve a lot of dev work in python and Docker. We will save time by having our project code open in a pre-existing Docker Container specifically designed for python work.
|
77 |
+
|
78 |
+
To that end, I have a basic `src` directory that will be used to store all dev work in the repo. Inside is a `app.py` file that does some basic operations and prints them to console. We will open this repo inside of a python-specific Docker container and see if we can work from there.
|
79 |
+
|
80 |
+
Here are the steps to follow:
|
81 |
+
|
82 |
+
1. Generate a `requirements.txt` file. We will palce this inside of the root of this repo. I generated it using the following a single command (shown below). This was inspired by [this article](https://learnpython.com/blog/python-requirements-file/) that describes how to generate the new `requirements.txt` file. This file contains all important packages that are key for python development in modern use cases.
|
83 |
+
|
84 |
+
```
|
85 |
+
pip freeze > requirements.txt
|
86 |
+
```
|
87 |
+
|
88 |
+
2. Have the project open in VS Code.
|
89 |
+
3. Make sure that the **Dev Containers** extension is installed.
|
90 |
+
4. In the bottom-left corner, there is a `><` button. Click it, and select the option `Reopen in Container`.
|
91 |
+
5. In the popup window, type `Python` and select `Python 3`, whichever version you want (I go with the default, 3.11).
|
92 |
+
6. No need to add any additional features. Simply click "OK".
|
93 |
+
|
94 |
+
You will have VS Code reopen a new window, this time with the folder opened inside a new `Python 3` container. You can confirm this by looking at the bottom-left corner of the window and checking if it says "Dev Container: Python 3". You should also see an additional new `.devcontainer` directory that was added. This is a very convenient step that actually makes the process simpler the next go around - instead of designating which container to open the folder in and all that, this directory caches our selections and simply skips a lot of the above steps.
|
95 |
+
|
96 |
+
One last step is to install some key packages that will be helpful for dev work in Python. These are recorded in `requirements.txt`, and we can install them all using a one-line command in the terminal:
|
97 |
+
|
98 |
+
```
|
99 |
+
pip install -r requirements.txt
|
100 |
+
```
|
101 |
+
|
102 |
+
### Example: Hello World Python Test
|
103 |
+
|
104 |
+
For example, we'll create a new `src` folder. The new `src` folder will contain a simple python script `app.py` that spits out some lists of numbers of varying dimensionalities.
|
105 |
+
|
106 |
+
We can simply run this command by clicking the "Play" button on the top-right corner of the window, assuming you have `src/app.py` open in the window.
|
107 |
+
|
108 |
+
An example of the output is provided below as proof that we have this working:
|
109 |
+
|
110 |
+

|
111 |
+
|
112 |
+
The main benefit is that any changes we make to these files will be automatically saved to our local files as well, speeding up any logistics behind how the Docker container is interacting with our original files.
|
Screenshots/Docker_Built.png
ADDED
![]() |
Screenshots/Docker_Python_Built.png
ADDED
![]() |
Screenshots/ExtensionsForDocker.png
ADDED
![]() |
Screenshots/Install_Proof.png
ADDED
![]() |
misc_example/Dockerfile
ADDED
@@ -0,0 +1,4 @@
|
|
|
|
|
|
|
|
|
|
|
1 |
+
FROM node:alpine
|
2 |
+
COPY . /misc_example
|
3 |
+
WORKDIR /misc_example
|
4 |
+
CMD node example.js
|
misc_example/example.js
ADDED
@@ -0,0 +1,2 @@
|
|
|
|
|
|
|
1 |
+
console.log("Hello World!")
|
2 |
+
console.log("- Ryan Kim (rk2546)")
|
requirements.txt
ADDED
Binary file (882 Bytes). View file
|
|
src/app.py
ADDED
@@ -0,0 +1,18 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import random
|
2 |
+
import numpy as np
|
3 |
+
|
4 |
+
random_vals = random.sample(range(10, 30), 5)
|
5 |
+
print(random_vals)
|
6 |
+
|
7 |
+
arr = np.array( [[ 1, 2, 3],
|
8 |
+
[ 4, 2, 5]] )
|
9 |
+
print(arr)
|
10 |
+
|
11 |
+
arr2 = np.array(
|
12 |
+
[
|
13 |
+
[2,5],
|
14 |
+
[2,6],
|
15 |
+
[100,34]
|
16 |
+
]
|
17 |
+
)
|
18 |
+
print(arr2)
|