Spaces:
Sleeping
Sleeping
Update app.py
Browse files
app.py
CHANGED
@@ -1,156 +1,10 @@
|
|
1 |
import streamlit as st
|
2 |
import os
|
3 |
from PIL import Image
|
4 |
-
import json
|
5 |
-
from datetime import datetime, timedelta
|
6 |
-
|
7 |
-
# File to store click history and last update timestamp
|
8 |
-
HISTORY_FILE = 'click_history.json'
|
9 |
-
|
10 |
-
# Initialize or load click history and last update time
|
11 |
-
def init_click_history():
|
12 |
-
if os.path.exists(HISTORY_FILE):
|
13 |
-
with open(HISTORY_FILE, 'r') as file:
|
14 |
-
return json.load(file)
|
15 |
-
else:
|
16 |
-
# Initialize with all buttons set to zero clicks and current time
|
17 |
-
return {"click_counts": {button: 0 for button in all_buttons}, "last_update": datetime.now().isoformat()}
|
18 |
-
|
19 |
-
# Save click history and last update time
|
20 |
-
def save_click_history(history):
|
21 |
-
with open(HISTORY_FILE, 'w') as file:
|
22 |
-
json.dump(history, file)
|
23 |
-
|
24 |
-
# Update click count for a button and save history
|
25 |
-
def update_click_count(button_name):
|
26 |
-
history = init_click_history()
|
27 |
-
history["click_counts"][button_name] += 1
|
28 |
-
history["last_update"] = datetime.now().isoformat()
|
29 |
-
save_click_history(history)
|
30 |
-
st.experimental_rerun()
|
31 |
-
|
32 |
-
# Display buttons and handle clicks
|
33 |
-
def display_buttons():
|
34 |
-
columns = st.columns(3)
|
35 |
-
for i, category in enumerate(categories):
|
36 |
-
with columns[i % 3]:
|
37 |
-
st.markdown(f"### {category['name']}")
|
38 |
-
for button in category['buttons']:
|
39 |
-
if st.button(button["text"]):
|
40 |
-
update_click_count(button["text"])
|
41 |
-
|
42 |
-
# Calculate time since last update
|
43 |
-
def time_since_last_update():
|
44 |
-
history = init_click_history()
|
45 |
-
last_update = datetime.fromisoformat(history["last_update"])
|
46 |
-
now = datetime.now()
|
47 |
-
return int((now - last_update).total_seconds())
|
48 |
-
|
49 |
-
# Main categories and buttons
|
50 |
-
categories = [
|
51 |
-
{"name": "Inputs", "buttons": [{"text": "๐ Text"}, {"text": "๐ Read"}, {"text": "๐ท Photo"}, {"text": "๐ผ๏ธ View"}, {"text": "๐๏ธ Record"}, {"text": "๐ง Listen"}, {"text": "๐ฅ Video"}, {"text": "๐น Capture"}]},
|
52 |
-
{"name": "Outputs", "buttons": [{"text": "๐ฌ Chat"}, {"text": "โ๏ธ Write"}, {"text": "๐จ Art"}, {"text": "๐ Create"}, {"text": "๐ต Music"}, {"text": "๐ถ Compose"}, {"text": "๐ผ Watch"}, {"text": "๐ฟ Movies"}]},
|
53 |
-
{"name": "Health", "buttons": [{"text": "๐ Vaccinate"}, {"text": "๐ฉบ Diagnose"}, {"text": "๐ฅ Hospital"}, {"text": "๐ Emergency"}, {"text": "๐ Meds"}, {"text": "๐ฉน Bandage"}, {"text": "๐งฌ DNA"}, {"text": "๐ฌ Research"}, {"text": "๐ก๏ธ Temperature"}, {"text": "๐ Nutrition"}]},
|
54 |
-
{"name": "Learning", "buttons": [{"text": "๐ Study"}, {"text": "๐ง Brain"}, {"text": "๐ฉโ๐ Graduate"}, {"text": "๐ Measure"}, {"text": "๐ Search"}, {"text": "๐ Analyze"}, {"text": "๐ Plan"}, {"text": "๐๏ธ Write"}, {"text": "๐จโ๐ซ Teach"}, {"text": "๐งฉ Puzzle"}]},
|
55 |
-
{"name": "AI", "buttons": [{"text": "๐ค Robot"}, {"text": "๐พ Game"}, {"text": "๐ป Code"}, {"text": "๐งฎ Calculate"}, {"text": "๐ก Connect"}, {"text": "๐ Power"}, {"text": "๐น๏ธ Play"}, {"text": "๐ฅ๏ธ Display"}, {"text": "๐งโ๐ป Develop"}, {"text": "๐จโ๐ฌ Experiment"}]},
|
56 |
-
{"name": "Writing", "buttons": [{"text": "โ๏ธ Author"}, {"text": "๐ Note"}, {"text": "๐๏ธ Pen"}, {"text": "๐๏ธ Sign"}, {"text": "๐ Library"}, {"text": "๐ Bookmark"}, {"text": "๐ Journal"}, {"text": "โ๏ธ Ink"}, {"text": "๐ Scroll"}]},
|
57 |
-
]
|
58 |
-
|
59 |
-
all_buttons = [button["text"] for category in categories for button in category["buttons"]]
|
60 |
-
|
61 |
-
# App title
|
62 |
-
st.markdown("# Remixable!!\n## Classifications")
|
63 |
-
|
64 |
-
# Display all buttons
|
65 |
-
display_buttons()
|
66 |
-
|
67 |
-
# Show time since last update
|
68 |
-
seconds_since_update = time_since_last_update()
|
69 |
-
st.write(f"๐ Time since last update: {seconds_since_update} seconds")
|
70 |
-
|
71 |
-
|
72 |
-
|
73 |
-
|
74 |
-
|
75 |
-
# Placeholder function for Wikipedia content fetching
|
76 |
-
# You'll need to replace this with actual fetching logic
|
77 |
-
def fetch_wikipedia_summary(keyword):
|
78 |
-
# Placeholder text, replace with actual Wikipedia fetching logic
|
79 |
-
return f"Summary about {keyword} from Wikipedia."
|
80 |
-
|
81 |
-
# Display images from the current directory and fetch related Wikipedia stories
|
82 |
-
def display_images_and_wikipedia_summaries():
|
83 |
-
st.title('Gallery with Related Stories')
|
84 |
-
|
85 |
-
# Scan current directory for PNG images
|
86 |
-
image_files = [f for f in os.listdir('.') if f.endswith('.png')]
|
87 |
-
|
88 |
-
# Check if there are any PNG images
|
89 |
-
if not image_files:
|
90 |
-
st.write("No PNG images found in the current directory.")
|
91 |
-
return
|
92 |
-
|
93 |
-
# Iterate over found images
|
94 |
-
for image_file in image_files:
|
95 |
-
# Open and display each image
|
96 |
-
image = Image.open(image_file)
|
97 |
-
st.image(image, caption=image_file, use_column_width='always')
|
98 |
-
|
99 |
-
# Extract a keyword from the image file name for Wikipedia search
|
100 |
-
# This is a simple example; adjust logic as needed for more complex titles
|
101 |
-
keyword = image_file.split('.')[0] # Assumes keyword is the file name without extension
|
102 |
-
|
103 |
-
# Fetch and display related Wikipedia content
|
104 |
-
wikipedia_summary = fetch_wikipedia_summary(keyword)
|
105 |
-
st.write(wikipedia_summary)
|
106 |
-
|
107 |
-
# Call the function to display images and summaries
|
108 |
-
display_images_and_wikipedia_summaries()
|
109 |
-
|
110 |
-
|
111 |
-
|
112 |
-
import streamlit as st
|
113 |
-
st.markdown('# Three Dragons ๐๐ Mythical Dragons Around the World by Aaron Wacker')
|
114 |
-
|
115 |
-
dragons = {
|
116 |
-
'#Fafnir #Norse': '- **Story**: Fafnir originally a dwarf, transformed into a fierce dragon due to his greed for the treasure he guarded. He was later slain by the hero Sigurd. - **Significance**: deadly sin of greed and the corrupting power of wealth.',
|
117 |
-
'#Quetzalcoatl #Aztec': '- **Story**: Quetzalcoatl, the Feathered Serpent, is not a dragon in the traditional sense but shares many similarities. He was a deity representing wind, air, and learning. - **Significance**: creator god and a symbol of death and rebirth.',
|
118 |
-
'#Tiamat #Mesopotamian': '- **Story**: Tiamat, primordial goddess of ocean, turned into a dragon-like creature in a battle against her children who threatened her authority. - **Significance**: chaos of primordial creation and is often associated with the forces of nature.'
|
119 |
-
}
|
120 |
-
|
121 |
-
for dragon, story in dragons.items():
|
122 |
-
st.subheader(dragon)
|
123 |
-
st.markdown(f"- {story}")
|
124 |
-
|
125 |
-
|
126 |
-
st.markdown('''
|
127 |
-
|
128 |
-
https://github.com/AaronCWacker/ThreeDragons
|
129 |
-
|
130 |
-
|
131 |
-

|
132 |
-
|
133 |
-
|
134 |
-
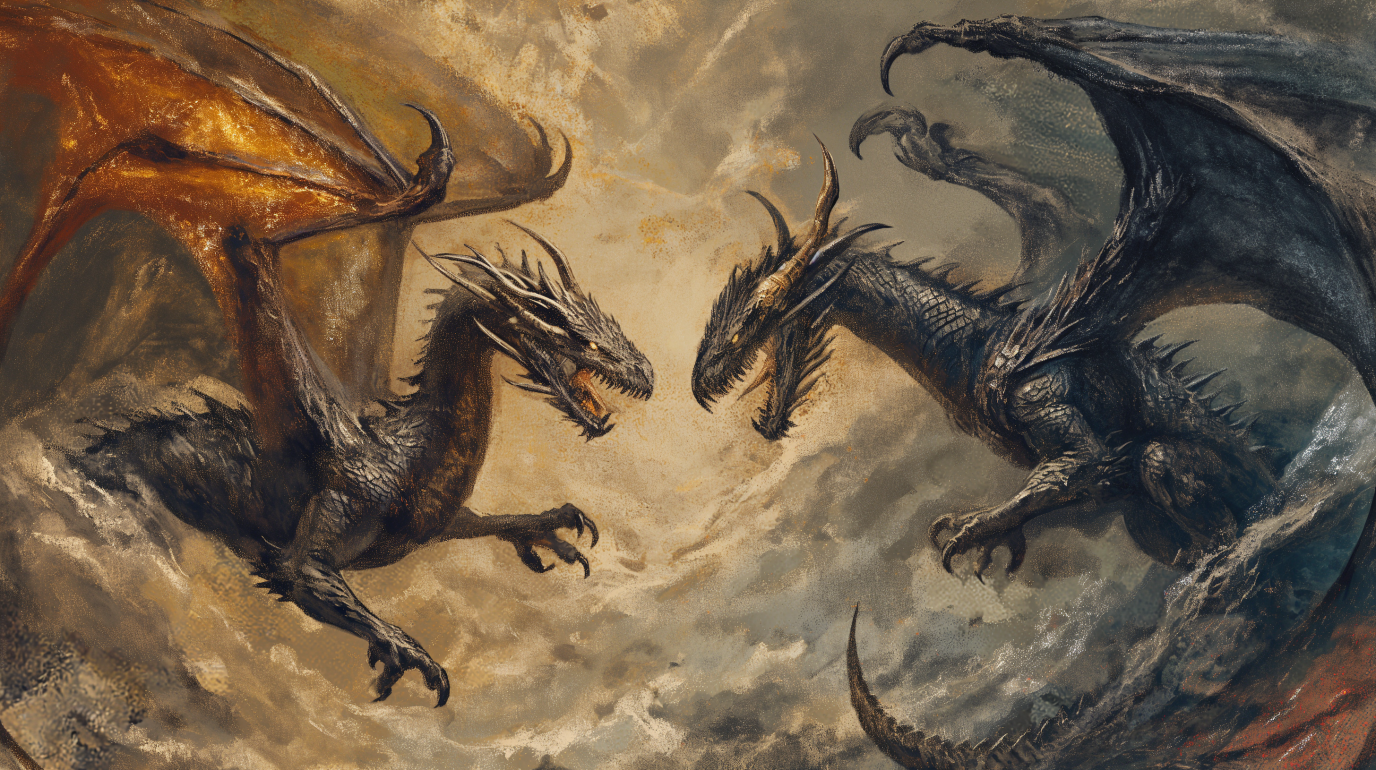
|
135 |
-
|
136 |
-
|
137 |
-

|
138 |
-
|
139 |
-
|
140 |
-

|
141 |
-
|
142 |
-
|
143 |
-

|
144 |
-
|
145 |
-
''')
|
146 |
-
|
147 |
-
|
148 |
|
149 |
st.markdown('''
|
150 |
-
|
151 |
# Remixable!!
|
152 |
-
|
153 |
-
## Classifications''')
|
154 |
|
155 |
# Create three columns for Inputs, Outputs, and Health
|
156 |
col1, col2, col3 = st.columns(3)
|
@@ -231,6 +85,68 @@ with col6:
|
|
231 |
st.button("โ๏ธ Ink")
|
232 |
st.button("๐ Scroll")
|
233 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
234 |
# Similarly, create columns for Coding, Remix, Movies, Video, and Audio as needed
|
235 |
# You can continue this pattern for organizing the rest of your buttons into columns
|
236 |
|
|
|
1 |
import streamlit as st
|
2 |
import os
|
3 |
from PIL import Image
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
4 |
|
5 |
st.markdown('''
|
|
|
6 |
# Remixable!!
|
7 |
+
''')
|
|
|
8 |
|
9 |
# Create three columns for Inputs, Outputs, and Health
|
10 |
col1, col2, col3 = st.columns(3)
|
|
|
85 |
st.button("โ๏ธ Ink")
|
86 |
st.button("๐ Scroll")
|
87 |
|
88 |
+
|
89 |
+
# Placeholder function for Wikipedia content fetching
|
90 |
+
# You'll need to replace this with actual fetching logic
|
91 |
+
def fetch_wikipedia_summary(keyword):
|
92 |
+
# Placeholder text, replace with actual Wikipedia fetching logic
|
93 |
+
return f"Summary about {keyword} from Wikipedia."
|
94 |
+
|
95 |
+
# Display images from the current directory and fetch related Wikipedia stories
|
96 |
+
def display_images_and_wikipedia_summaries():
|
97 |
+
st.title('Gallery with Related Stories')
|
98 |
+
|
99 |
+
# Scan current directory for PNG images
|
100 |
+
image_files = [f for f in os.listdir('.') if f.endswith('.png')]
|
101 |
+
|
102 |
+
# Check if there are any PNG images
|
103 |
+
if not image_files:
|
104 |
+
st.write("No PNG images found in the current directory.")
|
105 |
+
return
|
106 |
+
|
107 |
+
# Iterate over found images
|
108 |
+
for image_file in image_files:
|
109 |
+
# Open and display each image
|
110 |
+
image = Image.open(image_file)
|
111 |
+
st.image(image, caption=image_file, use_column_width='always')
|
112 |
+
|
113 |
+
# Extract a keyword from the image file name for Wikipedia search
|
114 |
+
# This is a simple example; adjust logic as needed for more complex titles
|
115 |
+
keyword = image_file.split('.')[0] # Assumes keyword is the file name without extension
|
116 |
+
|
117 |
+
# Fetch and display related Wikipedia content
|
118 |
+
wikipedia_summary = fetch_wikipedia_summary(keyword)
|
119 |
+
st.write(wikipedia_summary)
|
120 |
+
|
121 |
+
# Call the function to display images and summaries
|
122 |
+
display_images_and_wikipedia_summaries()
|
123 |
+
|
124 |
+
|
125 |
+
|
126 |
+
import streamlit as st
|
127 |
+
st.markdown('# Three Dragons ๐๐ Mythical Dragons Around the World by Aaron Wacker')
|
128 |
+
|
129 |
+
dragons = {
|
130 |
+
'#Fafnir #Norse': '- **Story**: Fafnir originally a dwarf, transformed into a fierce dragon due to his greed for the treasure he guarded. He was later slain by the hero Sigurd. - **Significance**: deadly sin of greed and the corrupting power of wealth.',
|
131 |
+
'#Quetzalcoatl #Aztec': '- **Story**: Quetzalcoatl, the Feathered Serpent, is not a dragon in the traditional sense but shares many similarities. He was a deity representing wind, air, and learning. - **Significance**: creator god and a symbol of death and rebirth.',
|
132 |
+
'#Tiamat #Mesopotamian': '- **Story**: Tiamat, primordial goddess of ocean, turned into a dragon-like creature in a battle against her children who threatened her authority. - **Significance**: chaos of primordial creation and is often associated with the forces of nature.'
|
133 |
+
}
|
134 |
+
|
135 |
+
for dragon, story in dragons.items():
|
136 |
+
st.subheader(dragon)
|
137 |
+
st.markdown(f"- {story}")
|
138 |
+
|
139 |
+
|
140 |
+
st.markdown('''
|
141 |
+
https://github.com/AaronCWacker/ThreeDragons
|
142 |
+

|
143 |
+
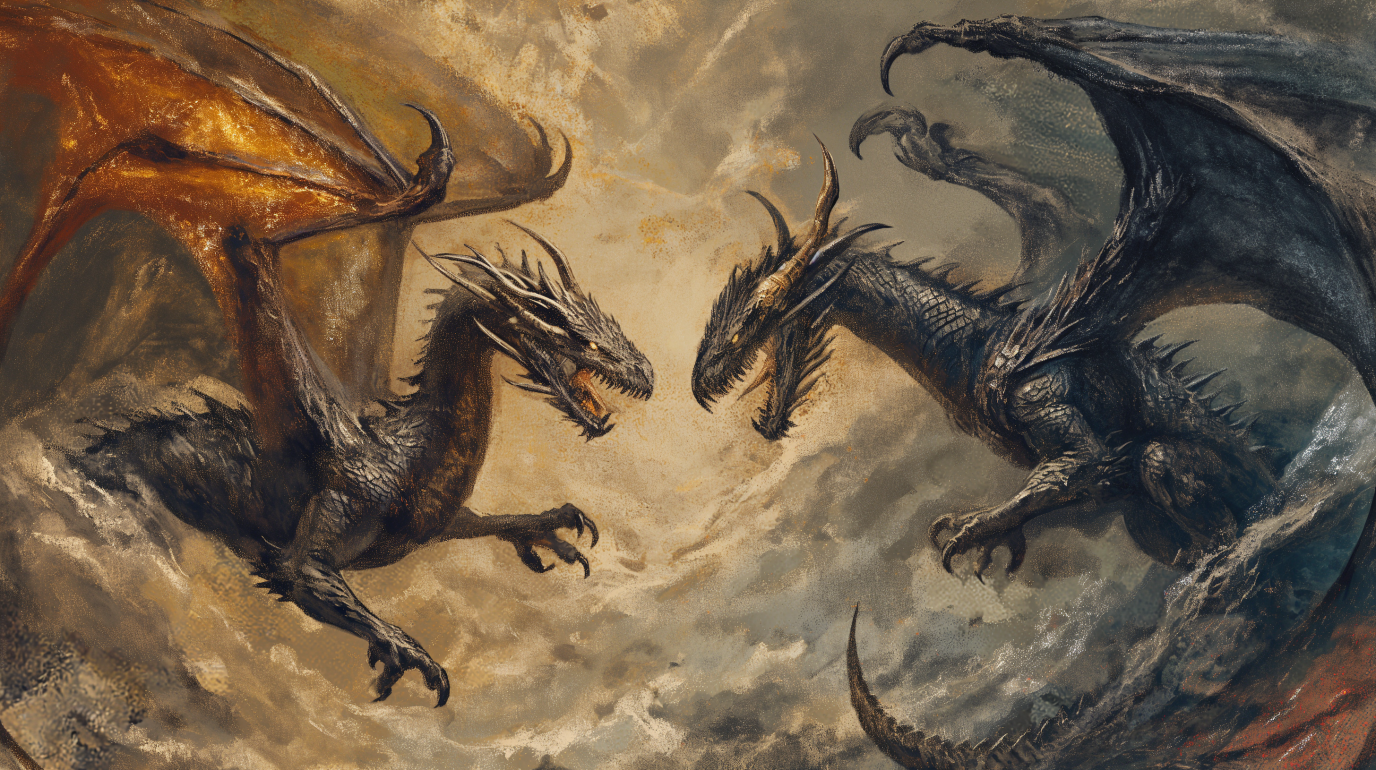
|
144 |
+

|
145 |
+

|
146 |
+

|
147 |
+
''')
|
148 |
+
|
149 |
+
|
150 |
# Similarly, create columns for Coding, Remix, Movies, Video, and Audio as needed
|
151 |
# You can continue this pattern for organizing the rest of your buttons into columns
|
152 |
|