Spaces:
Running
Running
import streamlit as st | |
import os | |
from PIL import Image | |
st.markdown(''' | |
# Remixable! | |
''') | |
col1, col2, col3 = st.columns(3) | |
with col1: | |
st.markdown('''### Inputs''') | |
st.button("๐ Text") | |
st.button("๐ Read") | |
st.button("๐ท Photo") | |
st.button("๐ผ๏ธ View") | |
st.button("๐๏ธ Record") | |
st.button("๐ง Listen") | |
st.button("๐ฅ Video") | |
st.button("๐น Capture") | |
with col2: | |
st.markdown('''### Outputs''') | |
st.button("๐ฌ Chat") | |
st.button("โ๏ธ Write") | |
st.button("๐จ Art") | |
st.button("๐ Create") | |
st.button("๐ต Music") | |
st.button("๐ถ Compose") | |
st.button("๐ผ Watch") | |
st.button("๐ฟ Movies") | |
with col3: | |
st.markdown('''### Health''') | |
st.button("๐ Vaccinate") | |
st.button("๐ฉบ Diagnose") | |
st.button("๐ฅ Hospital") | |
st.button("๐ Emergency") | |
st.button("๐ Meds") | |
st.button("๐ฉน Bandage") | |
st.button("๐งฌ DNA") | |
st.button("๐ฌ Research") | |
st.button("๐ก๏ธ Temperature") | |
st.button("๐ Nutrition") | |
col4, col5, col6 = st.columns(3) | |
with col4: | |
st.markdown('''### Learning''') | |
st.button("๐ Study") | |
st.button("๐ง Brain") | |
st.button("๐ฉโ๐ Graduate") | |
st.button("๐ Measure") | |
st.button("๐ Search") | |
st.button("๐ Analyze") | |
st.button("๐ Plan") | |
st.button("๐๏ธ Write") | |
st.button("๐จโ๐ซ Teach") | |
st.button("๐งฉ Puzzle") | |
with col5: | |
st.markdown('''### AI''') | |
st.button("๐ค Robot") | |
st.button("๐พ Game") | |
st.button("๐ป Code") | |
st.button("๐งฎ Calculate") | |
st.button("๐ก Connect") | |
st.button("๐ Power") | |
st.button("๐น๏ธ Play") | |
st.button("๐ฅ๏ธ Display") | |
st.button("๐งโ๐ป Develop") | |
st.button("๐จโ๐ฌ Experiment") | |
with col6: | |
st.markdown('''### Writing''') | |
st.button("โ๏ธ Author") | |
st.button("๐ Note") | |
st.button("๐๏ธ Pen") | |
st.button("๐๏ธ Sign") | |
st.button("๐ Library") | |
st.button("๐ Bookmark") | |
st.button("๐ Journal") | |
st.button("โ๏ธ Ink") | |
st.button("๐ Scroll") | |
def display_images_and_wikipedia_summaries(): | |
st.title('Gallery with Related Stories') | |
image_files = [f for f in os.listdir('.') if f.endswith('.png')] | |
if not image_files: | |
st.write("No PNG images found in the current directory.") | |
return | |
for image_file in image_files: | |
image = Image.open(image_file) | |
st.image(image, caption=image_file, use_column_width='always') | |
keyword = image_file.split('.')[0] # Assumes keyword is the file name without extension | |
wikipedia_summary = fetch_wikipedia_summary(keyword) | |
st.write(wikipedia_summary) | |
display_images_and_wikipedia_summaries() | |
st.markdown('# Three Dragons ๐๐ Mythical Dragons Around the World by Aaron Wacker') | |
dragons = { | |
'#Fafnir #Norse': '- **Story**: Fafnir originally a dwarf, transformed into a fierce dragon due to his greed for the treasure he guarded. He was later slain by the hero Sigurd. - **Significance**: deadly sin of greed and the corrupting power of wealth.', | |
'#Quetzalcoatl #Aztec': '- **Story**: Quetzalcoatl, the Feathered Serpent, is not a dragon in the traditional sense but shares many similarities. He was a deity representing wind, air, and learning. - **Significance**: creator god and a symbol of death and rebirth.', | |
'#Tiamat #Mesopotamian': '- **Story**: Tiamat, primordial goddess of ocean, turned into a dragon-like creature in a battle against her children who threatened her authority. - **Significance**: chaos of primordial creation and is often associated with the forces of nature.' | |
} | |
for dragon, story in dragons.items(): | |
st.subheader(dragon) | |
st.markdown(f"- {story}") | |
st.markdown(''' | |
https://github.com/AaronCWacker/ThreeDragons | |
 | |
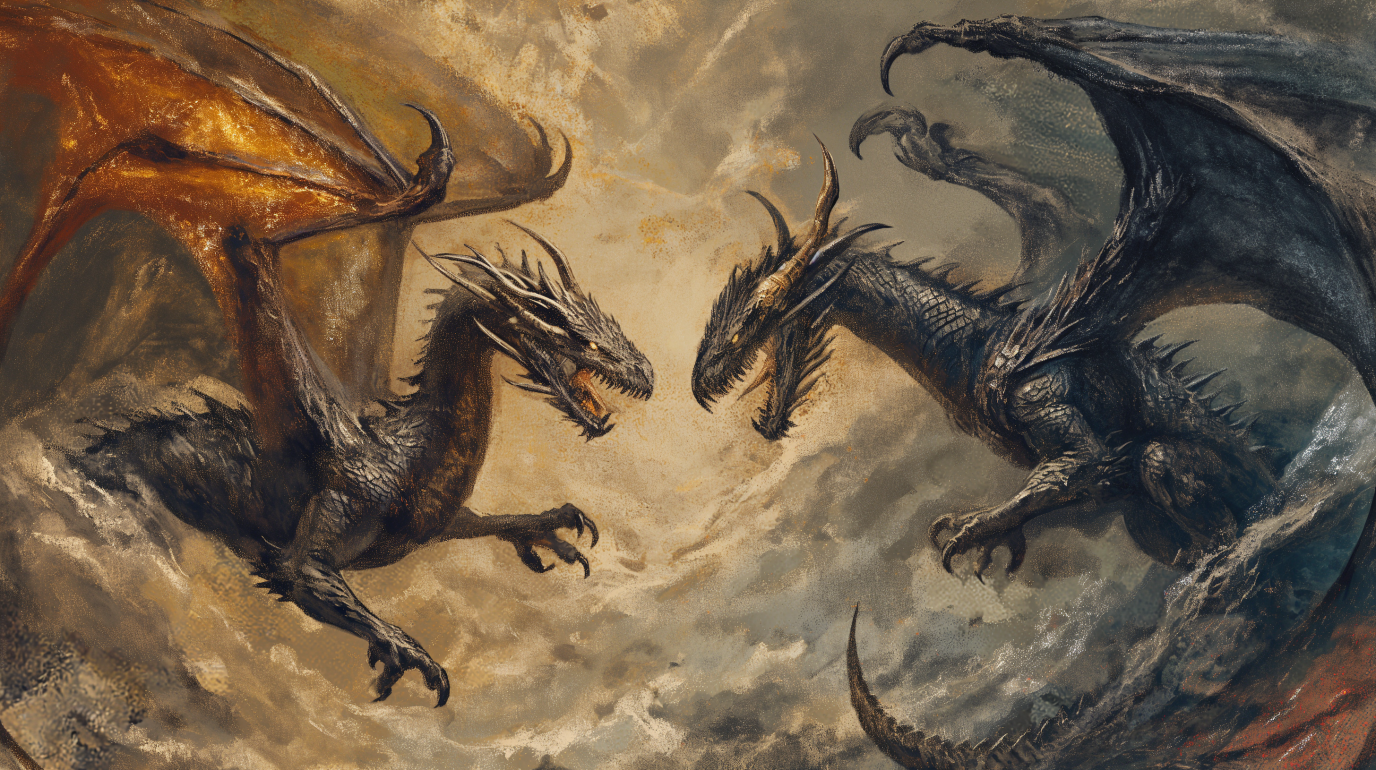 | |
 | |
 | |
 | |
''') | |