Spaces:
Runtime error
Runtime error
import streamlit as st | |
import os | |
import json | |
from PIL import Image | |
st.set_page_config(page_title="๐ง ๐โ๏ธThree Dragons - Remixable AI๐จ๐๐จโ๐ซ", page_icon="๐", layout="wide") | |
# Directory to store scores | |
score_dir = "scores" | |
os.makedirs(score_dir, exist_ok=True) | |
# Function to generate a unique key for each button | |
def generate_key(label, header, idx): | |
return f"{header}_{label}_{idx}" | |
# Function to increment score and save it | |
def update_score(key, increment=1): | |
score_file = os.path.join(score_dir, f"{key}.json") | |
if os.path.exists(score_file): | |
with open(score_file, "r") as file: | |
score_data = json.load(file) | |
else: | |
score_data = {"clicks": 0, "score": 0} | |
score_data["clicks"] += 1 | |
score_data["score"] += increment | |
with open(score_file, "w") as file: | |
json.dump(score_data, file) | |
return score_data["score"] | |
# Function to load score | |
def load_score(key): | |
score_file = os.path.join(score_dir, f"{key}.json") | |
if os.path.exists(score_file): | |
with open(score_file, "r") as file: | |
score_data = json.load(file) | |
return score_data["score"] | |
return 0 | |
# Display headers and buttons with scores | |
def display_buttons_with_scores(): | |
headers = ["Inputs", "Outputs", "Health", "Learning", "AI", "Writing"] | |
buttons = [ | |
["๐ Text", "๐ Read", "๐ท Photo", "๐ผ๏ธ View", "๐๏ธ Record", "๐ง Listen", "๐ฅ Video", "๐น Capture"], | |
["๐ฌ Chat", "โ๏ธ Write", "๐จ Art", "๐ Create", "๐ต Music", "๐ถ Compose", "๐ผ Watch", "๐ฟ Movies"], | |
["๐ Vaccinate", "๐ฉบ Diagnose", "๐ฅ Hospital", "๐ Emergency", "๐ Meds", "๐ฉน Bandage", "๐งฌ DNA", "๐ฌ Research", "๐ก๏ธ Temperature", "๐ Nutrition"], | |
["๐ Study", "๐ง Brain", "๐ฉโ๐ Graduate", "๐ Measure", "๐ Search", "๐ Analyze", "๐ Plan", "๐๏ธ Write", "๐จโ๐ซ Teach", "๐งฉ Puzzle"], | |
["๐ค Robot", "๐พ Game", "๐ป Code", "๐งฎ Calculate", "๐ก Connect", "๐ Power", "๐น๏ธ Play", "๐ฅ๏ธ Display", "๐งโ๐ป Develop", "๐จโ๐ฌ Experiment"], | |
["โ๏ธ Author", "๐ Note", "๐๏ธ Pen", "๐๏ธ Sign", "๐ Library", "๐ Bookmark", "๐ Journal", "โ๏ธ Ink", "๐ Scroll"] | |
] | |
cols = st.columns(len(headers)) | |
for idx, (col, header, buttons_list) in enumerate(zip(cols, headers, buttons)): | |
with col: | |
st.markdown(f"### {header}") | |
for button_idx, button_label in enumerate(buttons_list, start=1): | |
key = generate_key(button_label, header, button_idx) | |
score = load_score(key) | |
if st.button(f"{button_label} {score}", key=key): | |
new_score = update_score(key) | |
# Reload the page to reflect the updated score | |
st.experimental_rerun() | |
# Main application logic | |
if __name__ == "__main__": | |
st.markdown('# Remixable!') | |
display_buttons_with_scores() | |
def fetch_wikipedia_summary(keyword): | |
# Placeholder function for fetching Wikipedia summaries | |
# In a real app, you might use requests to fetch from the Wikipedia API | |
return f"Summary for {keyword}. For more information, visit Wikipedia." | |
def create_search_url_youtube(keyword): | |
base_url = "https://www.youtube.com/results?search_query=" | |
return base_url + keyword.replace(' ', '+') | |
def create_search_url_bing(keyword): | |
base_url = "https://www.bing.com/search?q=" | |
return base_url + keyword.replace(' ', '+') | |
def create_search_url_wikipedia(keyword): | |
base_url = "https://www.wikipedia.org/search-redirect.php?family=wikipedia&language=en&search=" | |
return base_url + keyword.replace(' ', '+') | |
def create_search_url_google(keyword): | |
base_url = "https://www.google.com/search?q=" | |
return base_url + keyword.replace(' ', '+') | |
def display_images_and_wikipedia_summaries(): | |
st.title('Gallery with Related Stories') | |
image_files = [f for f in os.listdir('.') if f.endswith('.png')] | |
if not image_files: | |
st.write("No PNG images found in the current directory.") | |
return | |
for image_file in image_files: | |
image = Image.open(image_file) | |
st.image(image, caption=image_file, use_column_width=True) | |
keyword = image_file.split('.')[0] # Assumes keyword is the file name without extension | |
# Display Wikipedia and Google search links | |
wikipedia_url = create_search_url_wikipedia(keyword) | |
google_url = create_search_url_google(keyword) | |
youtube_url = create_search_url_youtube(keyword) | |
bing_url = create_search_url_bing(keyword) | |
links_md = f""" | |
[Wikipedia]({wikipedia_url}) | | |
[Google]({google_url}) | | |
[YouTube]({youtube_url}) | | |
[Bing]({bing_url}) | |
""" | |
st.markdown(links_md) | |
display_images_and_wikipedia_summaries() | |
st.markdown('# Three Dragons ๐๐ Mythical Dragons Around the World by Aaron Wacker') | |
dragons = { | |
'#Fafnir #Norse': '- **Story**: Fafnir originally a dwarf, transformed into a fierce dragon due to his greed for the treasure he guarded. He was later slain by the hero Sigurd. - **Significance**: deadly sin of greed and the corrupting power of wealth.', | |
'#Quetzalcoatl #Aztec': '- **Story**: Quetzalcoatl, the Feathered Serpent, is not a dragon in the traditional sense but shares many similarities. He was a deity representing wind, air, and learning. - **Significance**: creator god and a symbol of death and rebirth.', | |
'#Tiamat #Mesopotamian': '- **Story**: Tiamat, primordial goddess of ocean, turned into a dragon-like creature in a battle against her children who threatened her authority. - **Significance**: chaos of primordial creation and is often associated with the forces of nature.' | |
} | |
for dragon, story in dragons.items(): | |
st.subheader(dragon) | |
st.markdown(f"- {story}") | |
st.markdown(''' | |
https://github.com/AaronCWacker/ThreeDragons | |
 | |
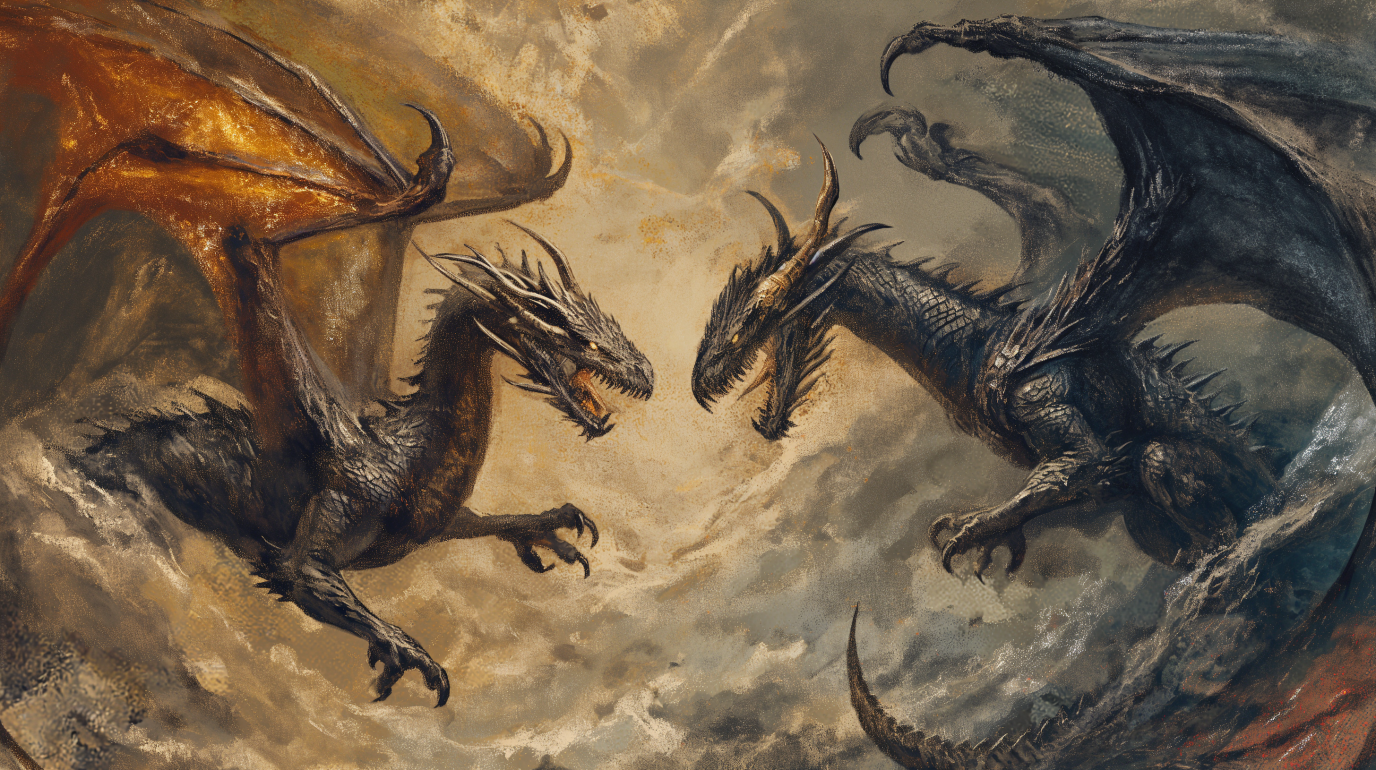 | |
 | |
 | |
 | |
''') | |
# Function to handle repeated query parameters | |
def get_all_query_params(key): | |
return st.query_params().get(key, []) | |
# Function to clear all query parameters | |
def clear_query_params(): | |
st.query_params() # Clear by setting to empty | |
st.title("Query Parameters Demo") | |
# Display current query parameters | |
st.write("Current Query Parameters:", st.query_params) | |
# Example: Using query parameters to navigate or trigger functionalities | |
if 'action' in st.query_params: | |
action = st.query_params()['action'][0] # Get the first (or only) 'action' parameter | |
if action == 'show_message': | |
st.success("Showing a message because 'action=show_message' was found in the URL.") | |
elif action == 'clear': | |
clear_query_params() | |
st.experimental_rerun() | |
# Handling repeated keys | |
if 'multi' in st.query_params: | |
multi_values = get_all_query_params('multi') | |
st.write("Values for 'multi':", multi_values) | |
# Manual entry for demonstration | |
st.write("Enter query parameters in the URL like this: ?action=show_message&multi=1&multi=2") | |
# Clear query parameters button | |
if st.button("Clear Query Parameters"): | |
clear_query_params() | |
st.experimental_rerun() |