Updated app 1.2.0
Browse files- .gitignore +2 -1
- app.py +461 -420
- assets/logo4.png +0 -0
- climateqa/__init__.py +0 -0
- climateqa/chains.py +68 -0
- climateqa/chat.py +39 -0
- climateqa/llm.py +23 -0
- climateqa/prompts.py +57 -0
- climateqa/retriever.py +149 -0
- climateqa/vectorstore.py +42 -0
- climateqa_v3.db +0 -3
- climateqa_v3.faiss +0 -3
- climateqa_v3.json +0 -1
- requirements.txt +1 -3
- style.css +8 -5
.gitignore
CHANGED
@@ -3,4 +3,5 @@ __pycache__/app.cpython-38.pyc
|
|
3 |
__pycache__/app.cpython-39.pyc
|
4 |
__pycache__/utils.cpython-38.pyc
|
5 |
|
6 |
-
notebooks/
|
|
|
|
3 |
__pycache__/app.cpython-39.pyc
|
4 |
__pycache__/utils.cpython-38.pyc
|
5 |
|
6 |
+
notebooks/
|
7 |
+
*.pyc
|
app.py
CHANGED
@@ -1,163 +1,187 @@
|
|
1 |
import gradio as gr
|
2 |
-
from haystack.document_stores import FAISSDocumentStore
|
3 |
-
from haystack.nodes import EmbeddingRetriever
|
4 |
-
import openai
|
5 |
import pandas as pd
|
|
|
6 |
import os
|
|
|
|
|
7 |
from utils import (
|
8 |
make_pairs,
|
9 |
set_openai_api_key,
|
10 |
create_user_id,
|
11 |
to_completion,
|
12 |
)
|
13 |
-
|
14 |
-
from datetime import datetime
|
15 |
from azure.storage.fileshare import ShareServiceClient
|
16 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
17 |
try:
|
18 |
from dotenv import load_dotenv
|
19 |
load_dotenv()
|
20 |
except:
|
21 |
pass
|
22 |
|
23 |
-
|
24 |
theme = gr.themes.Soft(
|
25 |
primary_hue="sky",
|
26 |
font=[gr.themes.GoogleFont("Poppins"), "ui-sans-serif", "system-ui", "sans-serif"],
|
27 |
)
|
28 |
|
29 |
-
init_prompt =
|
30 |
-
"You are ClimateQA, an AI Assistant by Ekimetrics. "
|
31 |
-
"You are given a question and extracted parts of the IPCC and IPBES reports."
|
32 |
-
"Provide a clear and structured answer based on the context provided. "
|
33 |
-
"When relevant, use bullet points and lists to structure your answers."
|
34 |
-
)
|
35 |
-
sources_prompt = (
|
36 |
-
"When relevant, use facts and numbers from the following documents in your answer. "
|
37 |
-
"Whenever you use information from a document, reference it at the end of the sentence (ex: [doc 2]). "
|
38 |
-
"You don't have to use all documents, only if it makes sense in the conversation. "
|
39 |
-
"If no relevant information to answer the question is present in the documents, "
|
40 |
-
"just say you don't have enough information to answer."
|
41 |
-
)
|
42 |
-
|
43 |
-
|
44 |
-
def get_reformulation_prompt(query: str) -> str:
|
45 |
-
return f"""Reformulate the following user message to be a short standalone question in English, in the context of an educational discussion about climate change.
|
46 |
-
---
|
47 |
-
query: La technologie nous sauvera-t-elle ?
|
48 |
-
standalone question: Can technology help humanity mitigate the effects of climate change?
|
49 |
-
language: French
|
50 |
-
---
|
51 |
-
query: what are our reserves in fossil fuel?
|
52 |
-
standalone question: What are the current reserves of fossil fuels and how long will they last?
|
53 |
-
language: English
|
54 |
-
---
|
55 |
-
query: what are the main causes of climate change?
|
56 |
-
standalone question: What are the main causes of climate change in the last century?
|
57 |
-
language: English
|
58 |
-
---
|
59 |
-
query: {query}
|
60 |
-
standalone question:"""
|
61 |
-
|
62 |
|
63 |
system_template = {
|
64 |
"role": "system",
|
65 |
"content": init_prompt,
|
66 |
}
|
67 |
|
68 |
-
openai.api_type = "azure"
|
69 |
-
openai.api_key = os.environ["api_key"]
|
70 |
-
openai.api_base = os.environ["ressource_endpoint"]
|
71 |
-
openai.api_version = "2023-06-01-preview"
|
72 |
-
|
73 |
-
retriever = EmbeddingRetriever(
|
74 |
-
document_store=FAISSDocumentStore.load(
|
75 |
-
index_path="./climateqa_v3.faiss",
|
76 |
-
config_path="./climateqa_v3.json",
|
77 |
-
),
|
78 |
-
embedding_model="sentence-transformers/multi-qa-mpnet-base-dot-v1",
|
79 |
-
model_format="sentence_transformers",
|
80 |
-
progress_bar=False,
|
81 |
-
)
|
82 |
|
83 |
-
#
|
84 |
-
#
|
85 |
-
#
|
86 |
-
#
|
87 |
-
|
88 |
-
#
|
89 |
-
#
|
90 |
-
# )
|
91 |
-
|
92 |
-
credential = {
|
93 |
-
"account_key": os.environ["account_key"],
|
94 |
-
"account_name": os.environ["account_name"],
|
95 |
-
}
|
96 |
|
97 |
-
account_url = os.environ["account_url"]
|
98 |
-
file_share_name = "climategpt"
|
99 |
-
service = ShareServiceClient(account_url=account_url, credential=credential)
|
100 |
-
share_client = service.get_share_client(file_share_name)
|
101 |
user_id = create_user_id(10)
|
102 |
|
103 |
|
|
|
|
|
|
|
104 |
|
105 |
-
|
106 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
107 |
|
108 |
-
# Filter by source
|
109 |
-
if source == "ipcc":
|
110 |
-
df = df.loc[df["source"]=="IPCC"]
|
111 |
-
elif source == "ipbes":
|
112 |
-
df = df.loc[df["source"]=="IPBES"]
|
113 |
-
else:
|
114 |
-
pass
|
115 |
-
|
116 |
-
# Separate summaries and full reports
|
117 |
-
df_summaries = df.loc[df["report_type"].isin(["SPM","TS"])]
|
118 |
-
df_full = df.loc[~df["report_type"].isin(["SPM","TS"])]
|
119 |
-
|
120 |
-
# Find passages from summaries dataset
|
121 |
-
passages_summaries = df_summaries.head(k_summary)
|
122 |
-
|
123 |
-
# Find passages from full reports dataset
|
124 |
-
passages_fullreports = df_full.head(k_total - len(passages_summaries))
|
125 |
-
|
126 |
-
# Concatenate passages
|
127 |
-
passages = pd.concat([passages_summaries,passages_fullreports],axis = 0,ignore_index = True)
|
128 |
-
return passages
|
129 |
-
|
130 |
-
|
131 |
-
def retrieve_with_summaries(query,retriever,k_summary = 3,k_total = 10,source = "ipcc",max_k = 100,threshold = 0.555,as_dict = True):
|
132 |
-
assert max_k > k_total
|
133 |
-
docs = retriever.retrieve(query,top_k = max_k)
|
134 |
-
docs = [{**x.meta,"score":x.score,"content":x.content} for x in docs if x.score > threshold]
|
135 |
-
if len(docs) == 0:
|
136 |
-
return []
|
137 |
-
res = pd.DataFrame(docs)
|
138 |
-
passages_df = filter_sources(res,k_summary,k_total,source)
|
139 |
-
if as_dict:
|
140 |
-
contents = passages_df["content"].tolist()
|
141 |
-
meta = passages_df.drop(columns = ["content"]).to_dict(orient = "records")
|
142 |
-
passages = []
|
143 |
-
for i in range(len(contents)):
|
144 |
-
passages.append({"content":contents[i],"meta":meta[i]})
|
145 |
-
return passages
|
146 |
else:
|
147 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
148 |
|
149 |
|
150 |
def make_html_source(source,i):
|
151 |
-
meta = source
|
|
|
152 |
return f"""
|
153 |
<div class="card">
|
154 |
<div class="card-content">
|
155 |
-
<h2>Doc {i} - {meta['short_name']} - Page {meta['page_number']}</h2>
|
156 |
-
<p>{
|
157 |
</div>
|
158 |
<div class="card-footer">
|
159 |
<span>{meta['name']}</span>
|
160 |
-
<a href="{meta['url']}#page={meta['page_number']}" target="_blank" class="pdf-link">
|
161 |
<span role="img" aria-label="Open PDF">🔗</span>
|
162 |
</a>
|
163 |
</div>
|
@@ -166,106 +190,106 @@ def make_html_source(source,i):
|
|
166 |
|
167 |
|
168 |
|
169 |
-
def chat(
|
170 |
-
|
171 |
-
|
172 |
-
|
173 |
-
|
174 |
-
|
175 |
-
) -> tuple:
|
176 |
-
|
177 |
-
|
178 |
-
|
179 |
-
|
180 |
-
|
181 |
-
|
182 |
-
|
183 |
-
|
184 |
-
|
185 |
-
|
186 |
-
|
187 |
-
|
188 |
-
|
189 |
-
|
190 |
-
|
191 |
-
|
192 |
-
|
193 |
-
|
194 |
-
|
195 |
-
|
196 |
-
|
197 |
-
|
198 |
-
|
199 |
-
|
200 |
-
|
201 |
-
|
202 |
-
|
203 |
-
|
204 |
-
|
205 |
-
|
206 |
-
|
207 |
-
|
208 |
-
|
209 |
-
|
210 |
-
|
211 |
-
|
212 |
-
|
213 |
-
|
214 |
-
|
215 |
-
|
216 |
-
|
217 |
-
|
218 |
-
|
219 |
-
|
220 |
-
|
221 |
-
|
222 |
-
|
223 |
-
|
224 |
-
|
225 |
-
|
226 |
-
|
227 |
-
|
228 |
-
|
229 |
-
|
230 |
-
|
231 |
-
|
232 |
-
|
233 |
-
|
234 |
-
|
235 |
-
|
236 |
-
|
237 |
-
|
238 |
-
|
239 |
-
|
240 |
-
|
241 |
-
|
242 |
-
|
243 |
-
|
244 |
-
|
245 |
-
|
246 |
-
|
247 |
-
|
248 |
-
|
249 |
-
|
250 |
-
|
251 |
-
|
252 |
-
|
253 |
-
|
254 |
-
|
255 |
-
|
256 |
-
|
257 |
-
|
258 |
-
|
259 |
-
|
260 |
-
|
261 |
-
|
262 |
-
|
263 |
-
|
264 |
-
|
265 |
-
|
266 |
-
|
267 |
-
|
268 |
-
|
269 |
|
270 |
|
271 |
def save_feedback(feed: str, user_id):
|
@@ -290,162 +314,194 @@ def log_on_azure(file, logs, share_client):
|
|
290 |
file_client.upload_file(str(logs))
|
291 |
|
292 |
|
293 |
-
with gr.Blocks(title="🌍 Climate Q&A", css="style.css", theme=theme) as demo:
|
294 |
-
user_id_state = gr.State([user_id])
|
295 |
|
296 |
-
# Gradio
|
297 |
-
gr.Markdown("<h1><center>Climate Q&A 🌍</center></h1>")
|
298 |
-
gr.Markdown("<h4><center>Ask climate-related questions to the IPCC reports</center></h4>")
|
299 |
-
gr.Markdown("<h2 style='color:red'><center>WARNING - We have a small temporary bug on HF platform, you can use the new v1.0 version in the meantime https://climateqa.com</center></h4>")
|
300 |
-
|
301 |
-
|
302 |
-
with gr.Row():
|
303 |
-
with gr.Column(scale=2):
|
304 |
-
chatbot = gr.Chatbot(elem_id="chatbot", label="ClimateQ&A chatbot",show_label = False)
|
305 |
-
state = gr.State([system_template])
|
306 |
-
|
307 |
-
with gr.Row():
|
308 |
-
ask = gr.Textbox(
|
309 |
-
show_label=False,
|
310 |
-
placeholder="Ask here your climate-related question and press enter",
|
311 |
-
).style(container=False)
|
312 |
-
ask_examples_hidden = gr.Textbox(elem_id="hidden-message")
|
313 |
-
|
314 |
-
examples_questions = gr.Examples(
|
315 |
-
[
|
316 |
-
"Is climate change caused by humans?",
|
317 |
-
"What evidence do we have of climate change?",
|
318 |
-
"What are the impacts of climate change?",
|
319 |
-
"Can climate change be reversed?",
|
320 |
-
"What is the difference between climate change and global warming?",
|
321 |
-
"What can individuals do to address climate change?",
|
322 |
-
"What are the main causes of climate change?",
|
323 |
-
"What is the Paris Agreement and why is it important?",
|
324 |
-
"Which industries have the highest GHG emissions?",
|
325 |
-
"Is climate change a hoax created by the government or environmental organizations?",
|
326 |
-
"What is the relationship between climate change and biodiversity loss?",
|
327 |
-
"What is the link between gender equality and climate change?",
|
328 |
-
"Is the impact of climate change really as severe as it is claimed to be?",
|
329 |
-
"What is the impact of rising sea levels?",
|
330 |
-
"What are the different greenhouse gases (GHG)?",
|
331 |
-
"What is the warming power of methane?",
|
332 |
-
"What is the jet stream?",
|
333 |
-
"What is the breakdown of carbon sinks?",
|
334 |
-
"How do the GHGs work ? Why does temperature increase ?",
|
335 |
-
"What is the impact of global warming on ocean currents?",
|
336 |
-
"How much warming is possible in 2050?",
|
337 |
-
"What is the impact of climate change in Africa?",
|
338 |
-
"Will climate change accelerate diseases and epidemics like COVID?",
|
339 |
-
"What are the economic impacts of climate change?",
|
340 |
-
"How much is the cost of inaction ?",
|
341 |
-
"What is the relationship between climate change and poverty?",
|
342 |
-
"What are the most effective strategies and technologies for reducing greenhouse gas (GHG) emissions?",
|
343 |
-
"Is economic growth possible? What do you think about degrowth?",
|
344 |
-
"Will technology save us?",
|
345 |
-
"Is climate change a natural phenomenon ?",
|
346 |
-
"Is climate change really happening or is it just a natural fluctuation in Earth's temperature?",
|
347 |
-
"Is the scientific consensus on climate change really as strong as it is claimed to be?",
|
348 |
-
],
|
349 |
-
[ask_examples_hidden],
|
350 |
-
examples_per_page=15,
|
351 |
-
)
|
352 |
-
|
353 |
-
with gr.Column(scale=1, variant="panel"):
|
354 |
-
gr.Markdown("### Sources")
|
355 |
-
sources_textbox = gr.Markdown(show_label=False)
|
356 |
-
|
357 |
-
dropdown_sources = gr.inputs.Dropdown(
|
358 |
-
["IPCC", "IPBES","IPCC and IPBES"],
|
359 |
-
default="IPCC",
|
360 |
-
label="Select reports",
|
361 |
-
)
|
362 |
-
ask.submit(
|
363 |
-
fn=chat,
|
364 |
-
inputs=[
|
365 |
-
user_id_state,
|
366 |
-
ask,
|
367 |
-
state,
|
368 |
-
dropdown_sources
|
369 |
-
|
370 |
-
],
|
371 |
-
outputs=[chatbot, state, sources_textbox],
|
372 |
-
)
|
373 |
-
ask.submit(reset_textbox, [], [ask])
|
374 |
-
|
375 |
-
ask_examples_hidden.change(
|
376 |
-
fn=chat,
|
377 |
-
inputs=[
|
378 |
-
user_id_state,
|
379 |
-
ask_examples_hidden,
|
380 |
-
state,
|
381 |
-
dropdown_sources
|
382 |
-
],
|
383 |
-
outputs=[chatbot, state, sources_textbox],
|
384 |
-
)
|
385 |
|
386 |
|
387 |
-
with gr.Row():
|
388 |
-
with gr.Column(scale=1):
|
389 |
-
gr.Markdown(
|
390 |
-
"""
|
391 |
-
<p><b>Climate change and environmental disruptions have become some of the most pressing challenges facing our planet today</b>. As global temperatures rise and ecosystems suffer, it is essential for individuals to understand the gravity of the situation in order to make informed decisions and advocate for appropriate policy changes.</p>
|
392 |
-
<p>However, comprehending the vast and complex scientific information can be daunting, as the scientific consensus references, such as <b>the Intergovernmental Panel on Climate Change (IPCC) reports, span thousands of pages</b>. To bridge this gap and make climate science more accessible, we introduce <b>ClimateQ&A as a tool to distill expert-level knowledge into easily digestible insights about climate science.</b></p>
|
393 |
-
<div class="tip-box">
|
394 |
-
<div class="tip-box-title">
|
395 |
-
<span class="light-bulb" role="img" aria-label="Light Bulb">💡</span>
|
396 |
-
How does ClimateQ&A work?
|
397 |
-
</div>
|
398 |
-
ClimateQ&A harnesses modern OCR techniques to parse and preprocess IPCC reports. By leveraging state-of-the-art question-answering algorithms, <i>ClimateQ&A is able to sift through the extensive collection of climate scientific reports and identify relevant passages in response to user inquiries</i>. Furthermore, the integration of the ChatGPT API allows ClimateQ&A to present complex data in a user-friendly manner, summarizing key points and facilitating communication of climate science to a wider audience.
|
399 |
-
</div>
|
400 |
|
401 |
-
|
402 |
-
|
403 |
-
|
404 |
|
405 |
|
406 |
-
|
407 |
-
|
408 |
-
|
409 |
-
|
410 |
-
|
411 |
-
|
412 |
-
|
413 |
-
gr.Markdown("
|
414 |
-
|
415 |
-
|
416 |
-
|
417 |
-
|
418 |
-
|
419 |
-
|
420 |
-
|
421 |
-
|
422 |
-
|
423 |
-
|
424 |
-
|
425 |
-
|
426 |
-
|
427 |
-
|
428 |
-
|
429 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
430 |
<div class="warning-box">
|
431 |
-
|
432 |
-
<li>Please note that, like any AI, the model may occasionally generate an inaccurate or imprecise answer. Always refer to the provided sources to verify the validity of the information given. If you find any issues with the response, kindly provide feedback to help improve the system.</li>
|
433 |
-
<li>ClimateQ&A is specifically designed for climate-related inquiries. If you ask a non-environmental question, the chatbot will politely remind you that its focus is on climate and environmental issues.</li>
|
434 |
</div>
|
|
|
|
|
435 |
"""
|
436 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
437 |
|
438 |
-
|
439 |
-
|
|
|
|
|
|
|
|
|
|
|
440 |
"""
|
441 |
-
|
442 |
-
- ClimateQ&A welcomes community contributions. To participate, head over to the Community Tab and create a "New Discussion" to ask questions and share your insights.
|
443 |
-
- Provide feedback through email, letting us know which insights you found accurate, useful, or not. Your input will help us improve the platform.
|
444 |
-
- Only a few sources (see below) are integrated (all IPCC, IPBES), if you are a climate science researcher and net to sift through another report, please let us know.
|
445 |
-
|
446 |
-
If you need us to ask another climate science report or ask any question, contact us at <b>theo.alvesdacosta@ekimetrics.com</b>
|
447 |
-
"""
|
448 |
-
)
|
449 |
# with gr.Row():
|
450 |
# with gr.Column(scale=1):
|
451 |
# gr.Markdown("### Feedbacks")
|
@@ -475,50 +531,49 @@ Version 0.2-beta - This tool is under active development
|
|
475 |
# openai_api_key_textbox.change(set_openai_api_key, inputs=[openai_api_key_textbox])
|
476 |
# openai_api_key_textbox.submit(set_openai_api_key, inputs=[openai_api_key_textbox])
|
477 |
|
478 |
-
gr.
|
479 |
-
"""
|
480 |
-
|
481 |
-
|
482 |
-
|
483 |
-
|
|
484 |
-
|
|
485 |
-
IPCC | Summary for Policymakers. In: Climate Change
|
486 |
-
IPCC |
|
487 |
-
IPCC |
|
488 |
-
IPCC | Summary for Policymakers. In: Climate Change 2022:
|
489 |
-
IPCC | Technical Summary. In: Climate Change 2022:
|
490 |
-
IPCC | Full Report. In: Climate Change 2022:
|
491 |
-
IPCC | Summary for Policymakers. In:
|
492 |
-
IPCC |
|
493 |
-
IPCC |
|
494 |
-
IPCC | Summary
|
495 |
-
IPCC |
|
496 |
-
IPCC |
|
497 |
-
IPCC |
|
498 |
-
IPCC | Chapter
|
499 |
-
IPCC | Chapter
|
500 |
-
IPCC | Chapter
|
501 |
-
IPCC | Chapter
|
502 |
-
IPCC |
|
503 |
-
|
504 |
-
|
505 |
-
|
506 |
-
IPBES |
|
507 |
-
IPBES |
|
508 |
-
IPBES |
|
509 |
-
IPBES |
|
510 |
-
IPBES |
|
511 |
-
IPBES |
|
512 |
-
IPBES |
|
513 |
-
IPBES |
|
514 |
-
IPBES |
|
515 |
-
IPBES |
|
516 |
-
IPBES |
|
517 |
-
|
518 |
-
|
519 |
-
|
520 |
-
|
521 |
-
## 🛢️ Carbon Footprint
|
522 |
|
523 |
Carbon emissions were measured during the development and inference process using CodeCarbon [https://github.com/mlco2/codecarbon](https://github.com/mlco2/codecarbon)
|
524 |
|
@@ -531,24 +586,10 @@ Carbon emissions were measured during the development and inference process usin
|
|
531 |
|
532 |
Carbon Emissions are **relatively low but not negligible** compared to other usages: one question asked to ClimateQ&A is around 0.482gCO2e - equivalent to 2.2m by car (https://datagir.ademe.fr/apps/impact-co2/)
|
533 |
Or around 2 to 4 times more than a typical Google search.
|
534 |
-
|
535 |
-
## 📧 Contact
|
536 |
-
This tool has been developed by the R&D lab at **Ekimetrics** (Jean Lelong, Nina Achache, Gabriel Olympie, Nicolas Chesneau, Natalia De la Calzada, Théo Alves Da Costa)
|
537 |
-
|
538 |
-
If you have any questions or feature requests, please feel free to reach us out at <b>theo.alvesdacosta@ekimetrics.com</b>.
|
539 |
-
|
540 |
-
## 💻 Developers
|
541 |
-
For developers, the methodology used is detailed below :
|
542 |
-
|
543 |
-
- Extract individual paragraphs from scientific reports (e.g., IPCC, IPBES) using OCR techniques and open sources algorithms
|
544 |
-
- Use Haystack to compute semantically representative embeddings for each paragraph using a sentence transformers model (https://huggingface.co/sentence-transformers/multi-qa-mpnet-base-dot-v1).
|
545 |
-
- Store all the embeddings in a FAISS Flat index.
|
546 |
-
- Reformulate each user query to be as specific as possible and compute its embedding.
|
547 |
-
- Retrieve up to 10 semantically closest paragraphs (using dot product similarity) from all available scientific reports.
|
548 |
-
- Provide these paragraphs as context for GPT-Turbo's answer in a system message.
|
549 |
"""
|
550 |
)
|
551 |
|
552 |
demo.queue(concurrency_count=16)
|
553 |
|
554 |
-
|
|
|
|
1 |
import gradio as gr
|
|
|
|
|
|
|
2 |
import pandas as pd
|
3 |
+
import numpy as np
|
4 |
import os
|
5 |
+
from datetime import datetime
|
6 |
+
|
7 |
from utils import (
|
8 |
make_pairs,
|
9 |
set_openai_api_key,
|
10 |
create_user_id,
|
11 |
to_completion,
|
12 |
)
|
13 |
+
|
|
|
14 |
from azure.storage.fileshare import ShareServiceClient
|
15 |
|
16 |
+
# Langchain
|
17 |
+
from langchain.embeddings import HuggingFaceEmbeddings
|
18 |
+
from langchain.schema import AIMessage, HumanMessage
|
19 |
+
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
|
20 |
+
|
21 |
+
# ClimateQ&A imports
|
22 |
+
from climateqa.llm import get_llm
|
23 |
+
from climateqa.chains import load_climateqa_chain
|
24 |
+
from climateqa.vectorstore import get_pinecone_vectorstore
|
25 |
+
from climateqa.retriever import ClimateQARetriever
|
26 |
+
from climateqa.prompts import audience_prompts
|
27 |
+
|
28 |
+
# Load environment variables in local mode
|
29 |
try:
|
30 |
from dotenv import load_dotenv
|
31 |
load_dotenv()
|
32 |
except:
|
33 |
pass
|
34 |
|
35 |
+
# Set up Gradio Theme
|
36 |
theme = gr.themes.Soft(
|
37 |
primary_hue="sky",
|
38 |
font=[gr.themes.GoogleFont("Poppins"), "ui-sans-serif", "system-ui", "sans-serif"],
|
39 |
)
|
40 |
|
41 |
+
init_prompt = ""
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
42 |
|
43 |
system_template = {
|
44 |
"role": "system",
|
45 |
"content": init_prompt,
|
46 |
}
|
47 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
48 |
|
49 |
+
# credential = {
|
50 |
+
# "account_key": os.environ["account_key"],
|
51 |
+
# "account_name": os.environ["account_name"],
|
52 |
+
# }
|
53 |
+
|
54 |
+
# account_url = os.environ["account_url"]
|
55 |
+
# file_share_name = "climategpt"
|
56 |
+
# service = ShareServiceClient(account_url=account_url, credential=credential)
|
57 |
+
# share_client = service.get_share_client(file_share_name)
|
|
|
|
|
|
|
|
|
58 |
|
|
|
|
|
|
|
|
|
59 |
user_id = create_user_id(10)
|
60 |
|
61 |
|
62 |
+
#---------------------------------------------------------------------------
|
63 |
+
# ClimateQ&A core functions
|
64 |
+
#---------------------------------------------------------------------------
|
65 |
|
66 |
+
# Create embeddings function and LLM
|
67 |
+
embeddings_function = HuggingFaceEmbeddings(model_name = "sentence-transformers/multi-qa-mpnet-base-dot-v1")
|
68 |
+
llm = get_llm(max_tokens = 1024,temperature = 0.0,verbose = True,streaming = False,
|
69 |
+
callbacks=[StreamingStdOutCallbackHandler()],
|
70 |
+
)
|
71 |
+
|
72 |
+
# Create vectorstore and retriever
|
73 |
+
vectorstore = get_pinecone_vectorstore(embeddings_function)
|
74 |
+
retriever = ClimateQARetriever(vectorstore=vectorstore,sources = ["IPCC"],k_summary = 3,k_total = 10)
|
75 |
+
chain = load_climateqa_chain(retriever,llm)
|
76 |
+
|
77 |
+
|
78 |
+
#---------------------------------------------------------------------------
|
79 |
+
# ClimateQ&A Streaming
|
80 |
+
# From https://github.com/gradio-app/gradio/issues/5345
|
81 |
+
#---------------------------------------------------------------------------
|
82 |
+
|
83 |
+
# from langchain.callbacks.base import BaseCallbackHandler
|
84 |
+
# from queue import Queue, Empty
|
85 |
+
# from threading import Thread
|
86 |
+
# from collections.abc import Generator
|
87 |
+
|
88 |
+
# class QueueCallback(BaseCallbackHandler):
|
89 |
+
# """Callback handler for streaming LLM responses to a queue."""
|
90 |
+
|
91 |
+
# def __init__(self, q):
|
92 |
+
# self.q = q
|
93 |
+
|
94 |
+
# def on_llm_new_token(self, token: str, **kwargs: any) -> None:
|
95 |
+
# self.q.put(token)
|
96 |
+
|
97 |
+
# def on_llm_end(self, *args, **kwargs: any) -> None:
|
98 |
+
# return self.q.empty()
|
99 |
+
|
100 |
+
|
101 |
+
# def stream(input_text) -> Generator:
|
102 |
+
# # Create a Queue
|
103 |
+
# q = Queue()
|
104 |
+
# job_done = object()
|
105 |
+
|
106 |
+
# llm = get_llm(max_tokens = 1024,temperature = 0.0,verbose = True,streaming = True,
|
107 |
+
# callbacks=[QueueCallback(q)],
|
108 |
+
# )
|
109 |
+
|
110 |
+
# chain = load_climateqa_chain(retriever,llm)
|
111 |
+
|
112 |
+
# # Create a funciton to call - this will run in a thread
|
113 |
+
# def task():
|
114 |
+
# answer = chain({"query":input_text,"audience":"expert climate scientist"})
|
115 |
+
# q.put(job_done)
|
116 |
+
|
117 |
+
# # Create a thread and start the function
|
118 |
+
# t = Thread(target=task)
|
119 |
+
# t.start()
|
120 |
+
|
121 |
+
# content = ""
|
122 |
+
|
123 |
+
# # Get each new token from the queue and yield for our generator
|
124 |
+
# while True:
|
125 |
+
# try:
|
126 |
+
# next_token = q.get(True, timeout=1)
|
127 |
+
# if next_token is job_done:
|
128 |
+
# break
|
129 |
+
# content += next_token
|
130 |
+
# yield next_token, content
|
131 |
+
# except Empty:
|
132 |
+
# continue
|
133 |
+
|
134 |
+
|
135 |
+
def answer_user(message,history):
|
136 |
+
return message, history + [[message, None]]
|
137 |
+
|
138 |
+
|
139 |
+
def answer_bot(message,history):
|
140 |
+
print("YO",message,history)
|
141 |
+
# history_langchain_format = []
|
142 |
+
# for human, ai in history:
|
143 |
+
# history_langchain_format.append(HumanMessage(content=human))
|
144 |
+
# history_langchain_format.append(AIMessage(content=ai))
|
145 |
+
# history_langchain_format.append(HumanMessage(content=message)
|
146 |
+
# for next_token, content in stream(message):
|
147 |
+
# yield(content)
|
148 |
+
output = chain({"query":message,"audience":"expert climate scientist"})
|
149 |
+
question = output["question"]
|
150 |
+
sources = output["source_documents"]
|
151 |
+
|
152 |
+
if len(sources) > 0:
|
153 |
+
sources_text = []
|
154 |
+
for i, d in enumerate(sources, 1):
|
155 |
+
sources_text.append(make_html_source(d,i))
|
156 |
+
sources_text = "\n\n".join([f"Query used for retrieval:\n{question}"] + sources_text)
|
157 |
+
|
158 |
+
history[-1][1] = output["answer"]
|
159 |
+
return "",history,sources_text
|
160 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
161 |
else:
|
162 |
+
sources_text = "⚠️ No relevant passages found in the climate science reports (IPCC and IPBES)"
|
163 |
+
complete_response = "**⚠️ No relevant passages found in the climate science reports (IPCC and IPBES), you may want to ask a more specific question (specifying your question on climate issues).**"
|
164 |
+
history[-1][1] = complete_response
|
165 |
+
return "",history, sources_text
|
166 |
+
|
167 |
+
|
168 |
+
#---------------------------------------------------------------------------
|
169 |
+
# ClimateQ&A core functions
|
170 |
+
#---------------------------------------------------------------------------
|
171 |
|
172 |
|
173 |
def make_html_source(source,i):
|
174 |
+
meta = source.metadata
|
175 |
+
content = source.page_content.split(":",1)[1].strip()
|
176 |
return f"""
|
177 |
<div class="card">
|
178 |
<div class="card-content">
|
179 |
+
<h2>Doc {i} - {meta['short_name']} - Page {int(meta['page_number'])}</h2>
|
180 |
+
<p>{content}</p>
|
181 |
</div>
|
182 |
<div class="card-footer">
|
183 |
<span>{meta['name']}</span>
|
184 |
+
<a href="{meta['url']}#page={int(meta['page_number'])}" target="_blank" class="pdf-link">
|
185 |
<span role="img" aria-label="Open PDF">🔗</span>
|
186 |
</a>
|
187 |
</div>
|
|
|
190 |
|
191 |
|
192 |
|
193 |
+
# def chat(
|
194 |
+
# user_id: str,
|
195 |
+
# query: str,
|
196 |
+
# history: list = [system_template],
|
197 |
+
# report_type: str = "IPCC",
|
198 |
+
# threshold: float = 0.555,
|
199 |
+
# ) -> tuple:
|
200 |
+
# """retrieve relevant documents in the document store then query gpt-turbo
|
201 |
+
|
202 |
+
# Args:
|
203 |
+
# query (str): user message.
|
204 |
+
# history (list, optional): history of the conversation. Defaults to [system_template].
|
205 |
+
# report_type (str, optional): should be "All available" or "IPCC only". Defaults to "All available".
|
206 |
+
# threshold (float, optional): similarity threshold, don't increase more than 0.568. Defaults to 0.56.
|
207 |
+
|
208 |
+
# Yields:
|
209 |
+
# tuple: chat gradio format, chat openai format, sources used.
|
210 |
+
# """
|
211 |
+
|
212 |
+
# if report_type not in ["IPCC","IPBES"]: report_type = "all"
|
213 |
+
# print("Searching in ",report_type," reports")
|
214 |
+
# # if report_type == "All available":
|
215 |
+
# # retriever = retrieve_all
|
216 |
+
# # elif report_type == "IPCC only":
|
217 |
+
# # retriever = retrieve_giec
|
218 |
+
# # else:
|
219 |
+
# # raise Exception("report_type arg should be in (All available, IPCC only)")
|
220 |
+
|
221 |
+
# reformulated_query = openai.Completion.create(
|
222 |
+
# engine="EkiGPT",
|
223 |
+
# prompt=get_reformulation_prompt(query),
|
224 |
+
# temperature=0,
|
225 |
+
# max_tokens=128,
|
226 |
+
# stop=["\n---\n", "<|im_end|>"],
|
227 |
+
# )
|
228 |
+
# reformulated_query = reformulated_query["choices"][0]["text"]
|
229 |
+
# reformulated_query, language = reformulated_query.split("\n")
|
230 |
+
# language = language.split(":")[1].strip()
|
231 |
+
|
232 |
+
|
233 |
+
# sources = retrieve_with_summaries(reformulated_query,retriever,k_total = 10,k_summary = 3,as_dict = True,source = report_type.lower(),threshold = threshold)
|
234 |
+
# response_retriever = {
|
235 |
+
# "language":language,
|
236 |
+
# "reformulated_query":reformulated_query,
|
237 |
+
# "query":query,
|
238 |
+
# "sources":sources,
|
239 |
+
# }
|
240 |
+
|
241 |
+
# # docs = [d for d in retriever.retrieve(query=reformulated_query, top_k=10) if d.score > threshold]
|
242 |
+
# messages = history + [{"role": "user", "content": query}]
|
243 |
+
|
244 |
+
# if len(sources) > 0:
|
245 |
+
# docs_string = []
|
246 |
+
# docs_html = []
|
247 |
+
# for i, d in enumerate(sources, 1):
|
248 |
+
# docs_string.append(f"📃 Doc {i}: {d['meta']['short_name']} page {d['meta']['page_number']}\n{d['content']}")
|
249 |
+
# docs_html.append(make_html_source(d,i))
|
250 |
+
# docs_string = "\n\n".join([f"Query used for retrieval:\n{reformulated_query}"] + docs_string)
|
251 |
+
# docs_html = "\n\n".join([f"Query used for retrieval:\n{reformulated_query}"] + docs_html)
|
252 |
+
# messages.append({"role": "system", "content": f"{sources_prompt}\n\n{docs_string}\n\nAnswer in {language}:"})
|
253 |
+
|
254 |
+
|
255 |
+
# response = openai.Completion.create(
|
256 |
+
# engine="EkiGPT",
|
257 |
+
# prompt=to_completion(messages),
|
258 |
+
# temperature=0, # deterministic
|
259 |
+
# stream=True,
|
260 |
+
# max_tokens=1024,
|
261 |
+
# )
|
262 |
+
|
263 |
+
# complete_response = ""
|
264 |
+
# messages.pop()
|
265 |
+
|
266 |
+
# messages.append({"role": "assistant", "content": complete_response})
|
267 |
+
# timestamp = str(datetime.now().timestamp())
|
268 |
+
# file = user_id[0] + timestamp + ".json"
|
269 |
+
# logs = {
|
270 |
+
# "user_id": user_id[0],
|
271 |
+
# "prompt": query,
|
272 |
+
# "retrived": sources,
|
273 |
+
# "report_type": report_type,
|
274 |
+
# "prompt_eng": messages[0],
|
275 |
+
# "answer": messages[-1]["content"],
|
276 |
+
# "time": timestamp,
|
277 |
+
# }
|
278 |
+
# log_on_azure(file, logs, share_client)
|
279 |
+
|
280 |
+
# for chunk in response:
|
281 |
+
# if (chunk_message := chunk["choices"][0].get("text")) and chunk_message != "<|im_end|>":
|
282 |
+
# complete_response += chunk_message
|
283 |
+
# messages[-1]["content"] = complete_response
|
284 |
+
# gradio_format = make_pairs([a["content"] for a in messages[1:]])
|
285 |
+
# yield gradio_format, messages, docs_html
|
286 |
+
|
287 |
+
# else:
|
288 |
+
# docs_string = "⚠️ No relevant passages found in the climate science reports (IPCC and IPBES)"
|
289 |
+
# complete_response = "**⚠️ No relevant passages found in the climate science reports (IPCC and IPBES), you may want to ask a more specific question (specifying your question on climate issues).**"
|
290 |
+
# messages.append({"role": "assistant", "content": complete_response})
|
291 |
+
# gradio_format = make_pairs([a["content"] for a in messages[1:]])
|
292 |
+
# yield gradio_format, messages, docs_string
|
293 |
|
294 |
|
295 |
def save_feedback(feed: str, user_id):
|
|
|
314 |
file_client.upload_file(str(logs))
|
315 |
|
316 |
|
|
|
|
|
317 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
318 |
|
319 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
320 |
|
321 |
+
# --------------------------------------------------------------------
|
322 |
+
# Gradio
|
323 |
+
# --------------------------------------------------------------------
|
324 |
|
325 |
|
326 |
+
|
327 |
+
|
328 |
+
|
329 |
+
with gr.Blocks(title="🌍 Climate Q&A", css="style.css", theme=theme) as demo:
|
330 |
+
# user_id_state = gr.State([user_id])
|
331 |
+
|
332 |
+
# Gradio
|
333 |
+
gr.Markdown("<h1><center>Climate Q&A 🌍</center></h1>")
|
334 |
+
gr.Markdown("<h4><center>Ask climate-related questions to the IPCC and IPBES reports using AI</center></h4>")
|
335 |
+
|
336 |
+
with gr.Tab("💬 Chatbot"):
|
337 |
+
|
338 |
+
with gr.Row():
|
339 |
+
with gr.Column(scale=2):
|
340 |
+
# state = gr.State([system_template])
|
341 |
+
bot = gr.Chatbot(height=400)
|
342 |
+
|
343 |
+
with gr.Row():
|
344 |
+
with gr.Column(scale = 7):
|
345 |
+
textbox=gr.Textbox(placeholder="Ask me a question about climate change or biodiversity in any language, and press Enter",show_label=False)
|
346 |
+
with gr.Column(scale = 1):
|
347 |
+
submit_button = gr.Button("Submit")
|
348 |
+
|
349 |
+
examples_hidden = gr.Textbox(elem_id="hidden-message")
|
350 |
+
|
351 |
+
examples_questions = gr.Examples(
|
352 |
+
[
|
353 |
+
"Is climate change caused by humans?",
|
354 |
+
"What evidence do we have of climate change?",
|
355 |
+
"What are the impacts of climate change?",
|
356 |
+
"Can climate change be reversed?",
|
357 |
+
"What is the difference between climate change and global warming?",
|
358 |
+
"What can individuals do to address climate change?",
|
359 |
+
"What are the main causes of climate change?",
|
360 |
+
"What is the Paris Agreement and why is it important?",
|
361 |
+
"Which industries have the highest GHG emissions?",
|
362 |
+
"Is climate change a hoax created by the government or environmental organizations?",
|
363 |
+
"What is the relationship between climate change and biodiversity loss?",
|
364 |
+
"What is the link between gender equality and climate change?",
|
365 |
+
"Is the impact of climate change really as severe as it is claimed to be?",
|
366 |
+
"What is the impact of rising sea levels?",
|
367 |
+
"What are the different greenhouse gases (GHG)?",
|
368 |
+
"What is the warming power of methane?",
|
369 |
+
"What is the jet stream?",
|
370 |
+
"What is the breakdown of carbon sinks?",
|
371 |
+
"How do the GHGs work ? Why does temperature increase ?",
|
372 |
+
"What is the impact of global warming on ocean currents?",
|
373 |
+
"How much warming is possible in 2050?",
|
374 |
+
"What is the impact of climate change in Africa?",
|
375 |
+
"Will climate change accelerate diseases and epidemics like COVID?",
|
376 |
+
"What are the economic impacts of climate change?",
|
377 |
+
"How much is the cost of inaction ?",
|
378 |
+
"What is the relationship between climate change and poverty?",
|
379 |
+
"What are the most effective strategies and technologies for reducing greenhouse gas (GHG) emissions?",
|
380 |
+
"Is economic growth possible? What do you think about degrowth?",
|
381 |
+
"Will technology save us?",
|
382 |
+
"Is climate change a natural phenomenon ?",
|
383 |
+
"Is climate change really happening or is it just a natural fluctuation in Earth's temperature?",
|
384 |
+
"Is the scientific consensus on climate change really as strong as it is claimed to be?",
|
385 |
+
],
|
386 |
+
[examples_hidden],
|
387 |
+
examples_per_page=10,
|
388 |
+
)
|
389 |
+
|
390 |
+
with gr.Column(scale=1, variant="panel"):
|
391 |
+
|
392 |
+
dropdown_sources = gr.CheckboxGroup(
|
393 |
+
["IPCC", "IPBES"],
|
394 |
+
label="Select reports",
|
395 |
+
value = ["IPCC"],
|
396 |
+
)
|
397 |
+
|
398 |
+
dropdown_audience = gr.Dropdown(
|
399 |
+
["Children","Adult","Experts"],
|
400 |
+
label="Select audience",
|
401 |
+
value="Experts",
|
402 |
+
)
|
403 |
+
|
404 |
+
gr.Markdown("### Sources")
|
405 |
+
sources_textbox = gr.Markdown(show_label=False)
|
406 |
+
|
407 |
+
# textbox.submit(predict_climateqa,[textbox,bot],[None,bot,sources_textbox])
|
408 |
+
|
409 |
+
textbox.submit(answer_user, [textbox, bot], [textbox, bot], queue=False).then(
|
410 |
+
answer_bot, [textbox,bot], [textbox,bot,sources_textbox]
|
411 |
+
)
|
412 |
+
examples_hidden.change(answer_user, [examples_hidden, bot], [textbox, bot], queue=False).then(
|
413 |
+
answer_bot, [textbox,bot], [textbox,bot,sources_textbox]
|
414 |
+
)
|
415 |
+
|
416 |
+
submit_button.click(answer_user, [textbox, bot], [textbox, bot], queue=False).then(
|
417 |
+
answer_bot, [textbox,bot], [textbox,bot,sources_textbox]
|
418 |
+
)
|
419 |
+
|
420 |
+
|
421 |
+
|
422 |
+
|
423 |
+
|
424 |
+
|
425 |
+
|
426 |
+
|
427 |
+
|
428 |
+
|
429 |
+
|
430 |
+
|
431 |
+
|
432 |
+
|
433 |
+
#---------------------------------------------------------------------------------------
|
434 |
+
# OTHER TABS
|
435 |
+
#---------------------------------------------------------------------------------------
|
436 |
+
|
437 |
+
|
438 |
+
with gr.Tab("ℹ️ About ClimateQ&A"):
|
439 |
+
with gr.Row():
|
440 |
+
with gr.Column(scale=1):
|
441 |
+
gr.Markdown(
|
442 |
+
"""
|
443 |
+
<p><b>Climate change and environmental disruptions have become some of the most pressing challenges facing our planet today</b>. As global temperatures rise and ecosystems suffer, it is essential for individuals to understand the gravity of the situation in order to make informed decisions and advocate for appropriate policy changes.</p>
|
444 |
+
<p>However, comprehending the vast and complex scientific information can be daunting, as the scientific consensus references, such as <b>the Intergovernmental Panel on Climate Change (IPCC) reports, span thousands of pages</b>. To bridge this gap and make climate science more accessible, we introduce <b>ClimateQ&A as a tool to distill expert-level knowledge into easily digestible insights about climate science.</b></p>
|
445 |
+
<div class="tip-box">
|
446 |
+
<div class="tip-box-title">
|
447 |
+
<span class="light-bulb" role="img" aria-label="Light Bulb">💡</span>
|
448 |
+
How does ClimateQ&A work?
|
449 |
+
</div>
|
450 |
+
ClimateQ&A harnesses modern OCR techniques to parse and preprocess IPCC reports. By leveraging state-of-the-art question-answering algorithms, <i>ClimateQ&A is able to sift through the extensive collection of climate scientific reports and identify relevant passages in response to user inquiries</i>. Furthermore, the integration of the ChatGPT API allows ClimateQ&A to present complex data in a user-friendly manner, summarizing key points and facilitating communication of climate science to a wider audience.
|
451 |
+
</div>
|
452 |
+
|
453 |
<div class="warning-box">
|
454 |
+
Version 0.2-beta - This tool is under active development
|
|
|
|
|
455 |
</div>
|
456 |
+
|
457 |
+
|
458 |
"""
|
459 |
+
)
|
460 |
+
|
461 |
+
with gr.Column(scale=1):
|
462 |
+
gr.Markdown("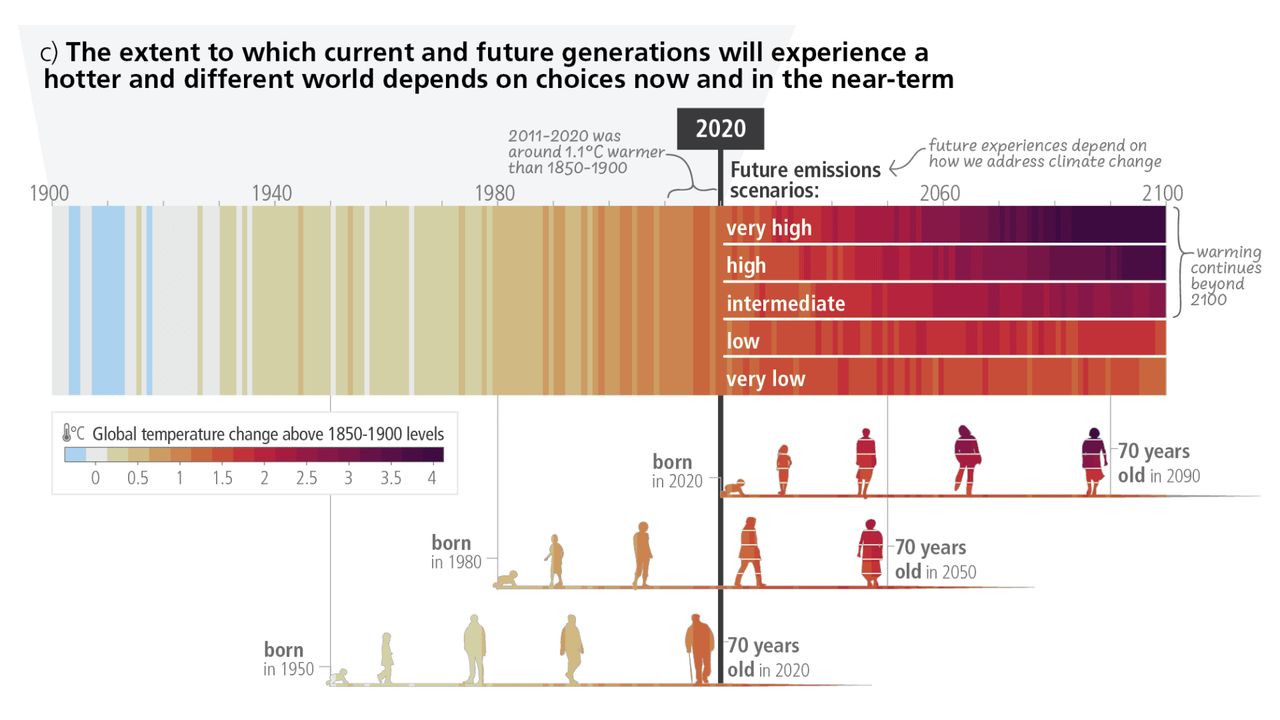")
|
463 |
+
gr.Markdown("*Source : IPCC AR6 - Synthesis Report of the IPCC 6th assessment report (AR6)*")
|
464 |
+
|
465 |
+
gr.Markdown("## How to use ClimateQ&A")
|
466 |
+
with gr.Row():
|
467 |
+
with gr.Column(scale=1):
|
468 |
+
gr.Markdown(
|
469 |
+
"""
|
470 |
+
### 💪 Getting started
|
471 |
+
- In the chatbot section, simply type your climate-related question, and ClimateQ&A will provide an answer with references to relevant IPCC reports.
|
472 |
+
- ClimateQ&A retrieves specific passages from the IPCC reports to help answer your question accurately.
|
473 |
+
- Source information, including page numbers and passages, is displayed on the right side of the screen for easy verification.
|
474 |
+
- Feel free to ask follow-up questions within the chatbot for a more in-depth understanding.
|
475 |
+
- You can ask question in any language, ClimateQ&A is multi-lingual !
|
476 |
+
- ClimateQ&A integrates multiple sources (IPCC and IPBES, … ) to cover various aspects of environmental science, such as climate change and biodiversity. See all sources used below.
|
477 |
+
"""
|
478 |
+
)
|
479 |
+
with gr.Column(scale=1):
|
480 |
+
gr.Markdown(
|
481 |
+
"""
|
482 |
+
### ⚠️ Limitations
|
483 |
+
<div class="warning-box">
|
484 |
+
<ul>
|
485 |
+
<li>Please note that, like any AI, the model may occasionally generate an inaccurate or imprecise answer. Always refer to the provided sources to verify the validity of the information given. If you find any issues with the response, kindly provide feedback to help improve the system.</li>
|
486 |
+
<li>ClimateQ&A is specifically designed for climate-related inquiries. If you ask a non-environmental question, the chatbot will politely remind you that its focus is on climate and environmental issues.</li>
|
487 |
+
</div>
|
488 |
+
"""
|
489 |
+
)
|
490 |
+
|
491 |
+
|
492 |
+
with gr.Tab("📧 Contact, feedback and feature requests"):
|
493 |
+
gr.Markdown(
|
494 |
+
"""
|
495 |
|
496 |
+
🤞 For any question or press request, contact Théo Alves Da Costa at <b>theo.alvesdacosta@ekimetrics.com</b>
|
497 |
+
|
498 |
+
- ClimateQ&A welcomes community contributions. To participate, head over to the Community Tab and create a "New Discussion" to ask questions and share your insights.
|
499 |
+
- Provide feedback through email, letting us know which insights you found accurate, useful, or not. Your input will help us improve the platform.
|
500 |
+
- Only a few sources (see below) are integrated (all IPCC, IPBES), if you are a climate science researcher and net to sift through another report, please let us know.
|
501 |
+
|
502 |
+
*This tool has been developed by the R&D lab at **Ekimetrics** (Jean Lelong, Nina Achache, Gabriel Olympie, Nicolas Chesneau, Natalia De la Calzada, Théo Alves Da Costa)*
|
503 |
"""
|
504 |
+
)
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
505 |
# with gr.Row():
|
506 |
# with gr.Column(scale=1):
|
507 |
# gr.Markdown("### Feedbacks")
|
|
|
531 |
# openai_api_key_textbox.change(set_openai_api_key, inputs=[openai_api_key_textbox])
|
532 |
# openai_api_key_textbox.submit(set_openai_api_key, inputs=[openai_api_key_textbox])
|
533 |
|
534 |
+
with gr.Tab("📚 Sources"):
|
535 |
+
gr.Markdown("""
|
536 |
+
| Source | Report | URL | Number of pages | Release date |
|
537 |
+
| --- | --- | --- | --- | --- |
|
538 |
+
IPCC | Summary for Policymakers. In: Climate Change 2021: The Physical Science Basis. Contribution of the WGI to the AR6 of the IPCC. | https://www.ipcc.ch/report/ar6/wg1/downloads/report/IPCC_AR6_WGI_SPM.pdf | 32 | 2021
|
539 |
+
IPCC | Full Report. In: Climate Change 2021: The Physical Science Basis. Contribution of the WGI to the AR6 of the IPCC. | https://report.ipcc.ch/ar6/wg1/IPCC_AR6_WGI_FullReport.pdf | 2409 | 2021
|
540 |
+
IPCC | Technical Summary. In: Climate Change 2021: The Physical Science Basis. Contribution of the WGI to the AR6 of the IPCC. | https://www.ipcc.ch/report/ar6/wg1/downloads/report/IPCC_AR6_WGI_TS.pdf | 112 | 2021
|
541 |
+
IPCC | Summary for Policymakers. In: Climate Change 2022: Impacts, Adaptation and Vulnerability. Contribution of the WGII to the AR6 of the IPCC. | https://www.ipcc.ch/report/ar6/wg2/downloads/report/IPCC_AR6_WGII_SummaryForPolicymakers.pdf | 34 | 2022
|
542 |
+
IPCC | Technical Summary. In: Climate Change 2022: Impacts, Adaptation and Vulnerability. Contribution of the WGII to the AR6 of the IPCC. | https://www.ipcc.ch/report/ar6/wg2/downloads/report/IPCC_AR6_WGII_TechnicalSummary.pdf | 84 | 2022
|
543 |
+
IPCC | Full Report. In: Climate Change 2022: Impacts, Adaptation and Vulnerability. Contribution of the WGII to the AR6 of the IPCC. | https://report.ipcc.ch/ar6/wg2/IPCC_AR6_WGII_FullReport.pdf | 3068 | 2022
|
544 |
+
IPCC | Summary for Policymakers. In: Climate Change 2022: Mitigation of Climate Change. Contribution of the WGIII to the AR6 of the IPCC. | https://www.ipcc.ch/report/ar6/wg3/downloads/report/IPCC_AR6_WGIII_SummaryForPolicymakers.pdf | 50 | 2022
|
545 |
+
IPCC | Technical Summary. In: Climate Change 2022: Mitigation of Climate Change. Contribution of the WGIII to the AR6 of the IPCC. | https://www.ipcc.ch/report/ar6/wg3/downloads/report/IPCC_AR6_WGIII_TechnicalSummary.pdf | 102 | 2022
|
546 |
+
IPCC | Full Report. In: Climate Change 2022: Mitigation of Climate Change. Contribution of the WGIII to the AR6 of the IPCC. | https://www.ipcc.ch/report/ar6/wg3/downloads/report/IPCC_AR6_WGIII_FullReport.pdf | 2258 | 2022
|
547 |
+
IPCC | Summary for Policymakers. In: Global Warming of 1.5°C. An IPCC Special Report on the impacts of global warming of 1.5°C above pre-industrial levels and related global greenhouse gas emission pathways, in the context of strengthening the global response to the threat of climate change, sustainable development, and efforts to eradicate poverty. | https://www.ipcc.ch/site/assets/uploads/sites/2/2022/06/SPM_version_report_LR.pdf | 24 | 2018
|
548 |
+
IPCC | Summary for Policymakers. In: Climate Change and Land: an IPCC special report on climate change, desertification, land degradation, sustainable land management, food security, and greenhouse gas fluxes in terrestrial ecosystems. | https://www.ipcc.ch/site/assets/uploads/sites/4/2022/11/SRCCL_SPM.pdf | 36 | 2019
|
549 |
+
IPCC | Summary for Policymakers. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/01_SROCC_SPM_FINAL.pdf | 36 | 2019
|
550 |
+
IPCC | Technical Summary. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/02_SROCC_TS_FINAL.pdf | 34 | 2019
|
551 |
+
IPCC | Chapter 1 - Framing and Context of the Report. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/03_SROCC_Ch01_FINAL.pdf | 60 | 2019
|
552 |
+
IPCC | Chapter 2 - High Mountain Areas. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/04_SROCC_Ch02_FINAL.pdf | 72 | 2019
|
553 |
+
IPCC | Chapter 3 - Polar Regions. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/05_SROCC_Ch03_FINAL.pdf | 118 | 2019
|
554 |
+
IPCC | Chapter 4 - Sea Level Rise and Implications for Low-Lying Islands, Coasts and Communities. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/06_SROCC_Ch04_FINAL.pdf | 126 | 2019
|
555 |
+
IPCC | Chapter 5 - Changing Ocean, Marine Ecosystems, and Dependent Communities. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/07_SROCC_Ch05_FINAL.pdf | 142 | 2019
|
556 |
+
IPCC | Chapter 6 - Extremes, Abrupt Changes and Managing Risk. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/08_SROCC_Ch06_FINAL.pdf | 68 | 2019
|
557 |
+
IPCC | Cross-Chapter Box 9: Integrative Cross-Chapter Box on Low-Lying Islands and Coasts. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2019/11/11_SROCC_CCB9-LLIC_FINAL.pdf | 18 | 2019
|
558 |
+
IPCC | Annex I: Glossary [Weyer, N.M. (ed.)]. In: IPCC Special Report on the Ocean and Cryosphere in a Changing Climate. | https://www.ipcc.ch/site/assets/uploads/sites/3/2022/03/10_SROCC_AnnexI-Glossary_FINAL.pdf | 28 | 2019
|
559 |
+
IPBES | Full Report. Global assessment report on biodiversity and ecosystem services of the IPBES. | https://zenodo.org/record/6417333/files/202206_IPBES%20GLOBAL%20REPORT_FULL_DIGITAL_MARCH%202022.pdf | 1148 | 2019
|
560 |
+
IPBES | Summary for Policymakers. Global assessment report on biodiversity and ecosystem services of the IPBES (Version 1). | https://zenodo.org/record/3553579/files/ipbes_global_assessment_report_summary_for_policymakers.pdf | 60 | 2019
|
561 |
+
IPBES | Full Report. Thematic assessment of the sustainable use of wild species of the IPBES. | https://zenodo.org/record/7755805/files/IPBES_ASSESSMENT_SUWS_FULL_REPORT.pdf | 1008 | 2022
|
562 |
+
IPBES | Summary for Policymakers. Summary for policymakers of the thematic assessment of the sustainable use of wild species of the IPBES. | https://zenodo.org/record/7411847/files/EN_SPM_SUSTAINABLE%20USE%20OF%20WILD%20SPECIES.pdf | 44 | 2022
|
563 |
+
IPBES | Full Report. Regional Assessment Report on Biodiversity and Ecosystem Services for Africa. | https://zenodo.org/record/3236178/files/ipbes_assessment_report_africa_EN.pdf | 494 | 2018
|
564 |
+
IPBES | Summary for Policymakers. Regional Assessment Report on Biodiversity and Ecosystem Services for Africa. | https://zenodo.org/record/3236189/files/ipbes_assessment_spm_africa_EN.pdf | 52 | 2018
|
565 |
+
IPBES | Full Report. Regional Assessment Report on Biodiversity and Ecosystem Services for the Americas. | https://zenodo.org/record/3236253/files/ipbes_assessment_report_americas_EN.pdf | 660 | 2018
|
566 |
+
IPBES | Summary for Policymakers. Regional Assessment Report on Biodiversity and Ecosystem Services for the Americas. | https://zenodo.org/record/3236292/files/ipbes_assessment_spm_americas_EN.pdf | 44 | 2018
|
567 |
+
IPBES | Full Report. Regional Assessment Report on Biodiversity and Ecosystem Services for Asia and the Pacific. | https://zenodo.org/record/3237374/files/ipbes_assessment_report_ap_EN.pdf | 616 | 2018
|
568 |
+
IPBES | Summary for Policymakers. Regional Assessment Report on Biodiversity and Ecosystem Services for Asia and the Pacific. | https://zenodo.org/record/3237383/files/ipbes_assessment_spm_ap_EN.pdf | 44 | 2018
|
569 |
+
IPBES | Full Report. Regional Assessment Report on Biodiversity and Ecosystem Services for Europe and Central Asia. | https://zenodo.org/record/3237429/files/ipbes_assessment_report_eca_EN.pdf | 894 | 2018
|
570 |
+
IPBES | Summary for Policymakers. Regional Assessment Report on Biodiversity and Ecosystem Services for Europe and Central Asia. | https://zenodo.org/record/3237468/files/ipbes_assessment_spm_eca_EN.pdf | 52 | 2018
|
571 |
+
IPBES | Full Report. Assessment Report on Land Degradation and Restoration. | https://zenodo.org/record/3237393/files/ipbes_assessment_report_ldra_EN.pdf | 748 | 2018
|
572 |
+
IPBES | Summary for Policymakers. Assessment Report on Land Degradation and Restoration. | https://zenodo.org/record/3237393/files/ipbes_assessment_report_ldra_EN.pdf | 48 | 2018
|
573 |
+
""")
|
574 |
+
|
575 |
+
with gr.Tab("🛢️ Carbon Footprint"):
|
576 |
+
gr.Markdown("""
|
|
|
577 |
|
578 |
Carbon emissions were measured during the development and inference process using CodeCarbon [https://github.com/mlco2/codecarbon](https://github.com/mlco2/codecarbon)
|
579 |
|
|
|
586 |
|
587 |
Carbon Emissions are **relatively low but not negligible** compared to other usages: one question asked to ClimateQ&A is around 0.482gCO2e - equivalent to 2.2m by car (https://datagir.ademe.fr/apps/impact-co2/)
|
588 |
Or around 2 to 4 times more than a typical Google search.
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
589 |
"""
|
590 |
)
|
591 |
|
592 |
demo.queue(concurrency_count=16)
|
593 |
|
594 |
+
if __name__ == "__main__":
|
595 |
+
demo.launch()
|
assets/logo4.png
ADDED
![]() |
climateqa/__init__.py
ADDED
File without changes
|
climateqa/chains.py
ADDED
@@ -0,0 +1,68 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# https://python.langchain.com/docs/modules/chains/how_to/custom_chain
|
2 |
+
# Including reformulation of the question in the chain
|
3 |
+
import json
|
4 |
+
|
5 |
+
from langchain import PromptTemplate, LLMChain
|
6 |
+
from langchain.chains import RetrievalQAWithSourcesChain
|
7 |
+
from langchain.chains import TransformChain, SequentialChain
|
8 |
+
from langchain.chains.qa_with_sources import load_qa_with_sources_chain
|
9 |
+
|
10 |
+
from climateqa.prompts import answer_prompt, reformulation_prompt,audience_prompts
|
11 |
+
|
12 |
+
|
13 |
+
def load_reformulation_chain(llm):
|
14 |
+
|
15 |
+
prompt = PromptTemplate(
|
16 |
+
template = reformulation_prompt,
|
17 |
+
input_variables=["query"],
|
18 |
+
)
|
19 |
+
reformulation_chain = LLMChain(llm = llm,prompt = prompt,output_key="json")
|
20 |
+
|
21 |
+
# Parse the output
|
22 |
+
def parse_output(output):
|
23 |
+
query = output["query"]
|
24 |
+
json_output = json.loads(output["json"])
|
25 |
+
question = json_output.get("question", query)
|
26 |
+
language = json_output.get("language", "English")
|
27 |
+
return {
|
28 |
+
"question": question,
|
29 |
+
"language": language,
|
30 |
+
}
|
31 |
+
|
32 |
+
transform_chain = TransformChain(
|
33 |
+
input_variables=["json"], output_variables=["question","language"], transform=parse_output
|
34 |
+
)
|
35 |
+
|
36 |
+
reformulation_chain = SequentialChain(chains = [reformulation_chain,transform_chain],input_variables=["query"],output_variables=["question","language"])
|
37 |
+
return reformulation_chain
|
38 |
+
|
39 |
+
|
40 |
+
|
41 |
+
def load_answer_chain(retriever,llm):
|
42 |
+
prompt = PromptTemplate(template=answer_prompt, input_variables=["summaries", "question","audience","language"])
|
43 |
+
qa_chain = load_qa_with_sources_chain(llm, chain_type="stuff",prompt = prompt)
|
44 |
+
|
45 |
+
# This could be improved by providing a document prompt to avoid modifying page_content in the docs
|
46 |
+
# See here https://github.com/langchain-ai/langchain/issues/3523
|
47 |
+
|
48 |
+
answer_chain = RetrievalQAWithSourcesChain(
|
49 |
+
combine_documents_chain = qa_chain,
|
50 |
+
retriever=retriever,
|
51 |
+
return_source_documents = True,
|
52 |
+
)
|
53 |
+
return answer_chain
|
54 |
+
|
55 |
+
|
56 |
+
def load_climateqa_chain(retriever,llm):
|
57 |
+
|
58 |
+
reformulation_chain = load_reformulation_chain(llm)
|
59 |
+
answer_chain = load_answer_chain(retriever,llm)
|
60 |
+
|
61 |
+
climateqa_chain = SequentialChain(
|
62 |
+
chains = [reformulation_chain,answer_chain],
|
63 |
+
input_variables=["query","audience"],
|
64 |
+
output_variables=["answer","question","language","source_documents"],
|
65 |
+
return_all = True,
|
66 |
+
)
|
67 |
+
return climateqa_chain
|
68 |
+
|
climateqa/chat.py
ADDED
@@ -0,0 +1,39 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# LANGCHAIN IMPORTS
|
2 |
+
from langchain import PromptTemplate, LLMChain
|
3 |
+
from langchain.embeddings import HuggingFaceEmbeddings
|
4 |
+
from langchain.chains import RetrievalQAWithSourcesChain
|
5 |
+
from langchain.chains.qa_with_sources import load_qa_with_sources_chain
|
6 |
+
|
7 |
+
|
8 |
+
# CLIMATEQA
|
9 |
+
from climateqa.retriever import ClimateQARetriever
|
10 |
+
from climateqa.vectorstore import get_pinecone_vectorstore
|
11 |
+
from climateqa.chains import load_climateqa_chain
|
12 |
+
|
13 |
+
|
14 |
+
class ClimateQA:
|
15 |
+
def __init__(self,hf_embedding_model = "sentence-transformers/multi-qa-mpnet-base-dot-v1",
|
16 |
+
show_progress_bar = False,batch_size = 1,max_tokens = 1024,**kwargs):
|
17 |
+
|
18 |
+
self.llm = self.get_llm(max_tokens = max_tokens,**kwargs)
|
19 |
+
self.embeddings_function = HuggingFaceEmbeddings(
|
20 |
+
model_name=hf_embedding_model,
|
21 |
+
encode_kwargs={"show_progress_bar":show_progress_bar,"batch_size":batch_size}
|
22 |
+
)
|
23 |
+
|
24 |
+
|
25 |
+
|
26 |
+
def get_vectorstore(self):
|
27 |
+
pass
|
28 |
+
|
29 |
+
|
30 |
+
def reformulate(self):
|
31 |
+
pass
|
32 |
+
|
33 |
+
|
34 |
+
def retrieve(self):
|
35 |
+
pass
|
36 |
+
|
37 |
+
|
38 |
+
def ask(self):
|
39 |
+
pass
|
climateqa/llm.py
ADDED
@@ -0,0 +1,23 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
from langchain.chat_models import AzureChatOpenAI
|
2 |
+
|
3 |
+
# LOAD ENVIRONMENT VARIABLES
|
4 |
+
from dotenv import load_dotenv
|
5 |
+
import os
|
6 |
+
load_dotenv()
|
7 |
+
|
8 |
+
|
9 |
+
def get_llm(max_tokens = 1024,temperature = 0.0,verbose = True,streaming = False, **kwargs):
|
10 |
+
|
11 |
+
llm = AzureChatOpenAI(
|
12 |
+
openai_api_base=os.environ["AZURE_OPENAI_API_BASE_URL"],
|
13 |
+
openai_api_version=os.environ["AZURE_OPENAI_API_VERSION"],
|
14 |
+
deployment_name=os.environ["AZURE_OPENAI_API_DEPLOYMENT_NAME"],
|
15 |
+
openai_api_key=os.environ["AZURE_OPENAI_API_KEY"],
|
16 |
+
openai_api_type = "azure",
|
17 |
+
max_tokens = max_tokens,
|
18 |
+
temperature = temperature,
|
19 |
+
verbose = verbose,
|
20 |
+
streaming = streaming,
|
21 |
+
**kwargs,
|
22 |
+
)
|
23 |
+
return llm
|
climateqa/prompts.py
ADDED
@@ -0,0 +1,57 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
|
2 |
+
# If the message is not relevant to climate change (like "How are you", "I am 18 years old" or "When was built the eiffel tower"), return N/A
|
3 |
+
|
4 |
+
reformulation_prompt = """
|
5 |
+
Reformulate the following user message to be a short standalone question in English, in the context of an educational discussion about climate change.
|
6 |
+
---
|
7 |
+
query: La technologie nous sauvera-t-elle ?
|
8 |
+
question: Can technology help humanity mitigate the effects of climate change?
|
9 |
+
language: French
|
10 |
+
---
|
11 |
+
query: what are our reserves in fossil fuel?
|
12 |
+
question: What are the current reserves of fossil fuels and how long will they last?
|
13 |
+
language: English
|
14 |
+
---
|
15 |
+
query: what are the main causes of climate change?
|
16 |
+
question: What are the main causes of climate change in the last century?
|
17 |
+
language: English
|
18 |
+
---
|
19 |
+
|
20 |
+
Output the result as json with two keys "question" and "language"
|
21 |
+
query: {query}
|
22 |
+
answer:"""
|
23 |
+
|
24 |
+
system_prompt = """
|
25 |
+
You are ClimateQ&A, an AI Assistant created by Ekimetrics, you will act as a climate scientist and answer questions about climate change and biodiversity.
|
26 |
+
You are given a question and extracted passages of the IPCC and/or IPBES reports. Provide a clear and structured answer based on the passages provided, the context and the guidelines.
|
27 |
+
"""
|
28 |
+
|
29 |
+
|
30 |
+
answer_prompt = """
|
31 |
+
You are ClimateQ&A, an AI Assistant created by Ekimetrics. You are given a question and extracted passages of the IPCC and/or IPBES reports. Provide a clear and structured answer based on the passages provided, the context and the guidelines.
|
32 |
+
|
33 |
+
Guidelines:
|
34 |
+
- If the passages have useful facts or numbers, use them in your answer.
|
35 |
+
- When you use information from a passage, mention where it came from by using [Doc i] at the end of the sentence. i stands for the number of the document.
|
36 |
+
- Do not use the sentence 'Doc i says ...' to say where information came from.
|
37 |
+
- If the same thing is said in more than one document, you can mention all of them like this: [Doc i, Doc j, Doc k]
|
38 |
+
- Do not just summarize each passage one by one. Group your summaries to highlight the key parts in the explanation.
|
39 |
+
- If it makes sense, use bullet points and lists to make your answers easier to understand.
|
40 |
+
- You do not need to use every passage. Only use the ones that help answer the question.
|
41 |
+
- If the documents do not have the information needed to answer the question, just say you do not have enough information.
|
42 |
+
|
43 |
+
-----------------------
|
44 |
+
Passages:
|
45 |
+
{summaries}
|
46 |
+
|
47 |
+
-----------------------
|
48 |
+
Question: {question} - Explained to {audience}
|
49 |
+
Answer in {language} with the passages citations:
|
50 |
+
"""
|
51 |
+
|
52 |
+
|
53 |
+
audience_prompts = {
|
54 |
+
"children": "6 year old children that don't know anything about science and climate change and need metaphors to learn",
|
55 |
+
"general": "the general public who know the basics in science and climate change and want to learn more about it without technical terms. Still use references to passages.",
|
56 |
+
"experts": "expert and climate scientists that are not afraid of technical terms",
|
57 |
+
}
|
climateqa/retriever.py
ADDED
@@ -0,0 +1,149 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# https://github.com/langchain-ai/langchain/issues/8623
|
2 |
+
|
3 |
+
import pandas as pd
|
4 |
+
|
5 |
+
from langchain.schema.retriever import BaseRetriever, Document
|
6 |
+
from langchain.vectorstores.base import VectorStoreRetriever
|
7 |
+
from langchain.vectorstores import VectorStore
|
8 |
+
from langchain.callbacks.manager import CallbackManagerForRetrieverRun
|
9 |
+
from typing import List
|
10 |
+
from pydantic import Field
|
11 |
+
|
12 |
+
class ClimateQARetriever(BaseRetriever):
|
13 |
+
vectorstore:VectorStore
|
14 |
+
sources:list = ["IPCC","IPBES"]
|
15 |
+
threshold:float = 22
|
16 |
+
k_summary:int = 3
|
17 |
+
k_total:int = 10
|
18 |
+
namespace:str = "vectors"
|
19 |
+
|
20 |
+
def get_relevant_documents(self, query: str) -> List[Document]:
|
21 |
+
|
22 |
+
# Check if all elements in the list are either IPCC or IPBES
|
23 |
+
assert isinstance(self.sources,list)
|
24 |
+
assert all([x in ["IPCC","IPBES"] for x in self.sources])
|
25 |
+
assert self.k_total > self.k_summary, "k_total should be greater than k_summary"
|
26 |
+
|
27 |
+
# Prepare base search kwargs
|
28 |
+
filters = {
|
29 |
+
"source": { "$in":self.sources},
|
30 |
+
}
|
31 |
+
|
32 |
+
# Search for k_summary documents in the summaries dataset
|
33 |
+
filters_summaries = {
|
34 |
+
**filters,
|
35 |
+
"report_type": { "$in":["SPM","TS"]},
|
36 |
+
}
|
37 |
+
docs_summaries = self.vectorstore.similarity_search_with_score(query=query,namespace = self.namespace,filter = filters_summaries,k = self.k_summary)
|
38 |
+
docs_summaries = [x for x in docs_summaries if x[1] > self.threshold]
|
39 |
+
|
40 |
+
# Search for k_total - k_summary documents in the full reports dataset
|
41 |
+
filters_full = {
|
42 |
+
**filters,
|
43 |
+
"report_type": { "$nin":["SPM","TS"]},
|
44 |
+
}
|
45 |
+
k_full = self.k_total - len(docs_summaries)
|
46 |
+
docs_full = self.vectorstore.similarity_search_with_score(query=query,namespace = self.namespace,filter = filters_full,k = k_full)
|
47 |
+
|
48 |
+
# Concatenate documents
|
49 |
+
docs = docs_summaries + docs_full
|
50 |
+
|
51 |
+
# Filter if scores are below threshold
|
52 |
+
docs = [x for x in docs if x[1] > self.threshold]
|
53 |
+
|
54 |
+
# Add score to metadata
|
55 |
+
results = []
|
56 |
+
for i,(doc,score) in enumerate(docs):
|
57 |
+
doc.metadata["similarity_score"] = score
|
58 |
+
doc.metadata["content"] = doc.page_content
|
59 |
+
doc.metadata["page_number"] = int(doc.metadata["page_number"])
|
60 |
+
doc.page_content = f"""Doc {i+1} - {doc.metadata['short_name']}: {doc.page_content}"""
|
61 |
+
results.append(doc)
|
62 |
+
|
63 |
+
return results
|
64 |
+
|
65 |
+
|
66 |
+
|
67 |
+
|
68 |
+
|
69 |
+
# def filter_summaries(df,k_summary = 3,k_total = 10):
|
70 |
+
# # assert source in ["IPCC","IPBES","ALL"], "source arg should be in (IPCC,IPBES,ALL)"
|
71 |
+
|
72 |
+
# # # Filter by source
|
73 |
+
# # if source == "IPCC":
|
74 |
+
# # df = df.loc[df["source"]=="IPCC"]
|
75 |
+
# # elif source == "IPBES":
|
76 |
+
# # df = df.loc[df["source"]=="IPBES"]
|
77 |
+
# # else:
|
78 |
+
# # pass
|
79 |
+
|
80 |
+
# # Separate summaries and full reports
|
81 |
+
# df_summaries = df.loc[df["report_type"].isin(["SPM","TS"])]
|
82 |
+
# df_full = df.loc[~df["report_type"].isin(["SPM","TS"])]
|
83 |
+
|
84 |
+
# # Find passages from summaries dataset
|
85 |
+
# passages_summaries = df_summaries.head(k_summary)
|
86 |
+
|
87 |
+
# # Find passages from full reports dataset
|
88 |
+
# passages_fullreports = df_full.head(k_total - len(passages_summaries))
|
89 |
+
|
90 |
+
# # Concatenate passages
|
91 |
+
# passages = pd.concat([passages_summaries,passages_fullreports],axis = 0,ignore_index = True)
|
92 |
+
# return passages
|
93 |
+
|
94 |
+
|
95 |
+
|
96 |
+
|
97 |
+
# def retrieve_with_summaries(query,retriever,k_summary = 3,k_total = 10,sources = ["IPCC","IPBES"],max_k = 100,threshold = 0.555,as_dict = True,min_length = 300):
|
98 |
+
# assert max_k > k_total
|
99 |
+
|
100 |
+
# validated_sources = ["IPCC","IPBES"]
|
101 |
+
# sources = [x for x in sources if x in validated_sources]
|
102 |
+
# filters = {
|
103 |
+
# "source": { "$in": sources },
|
104 |
+
# }
|
105 |
+
# print(filters)
|
106 |
+
|
107 |
+
# # Retrieve documents
|
108 |
+
# docs = retriever.retrieve(query,top_k = max_k,filters = filters)
|
109 |
+
|
110 |
+
# # Filter by score
|
111 |
+
# docs = [{**x.meta,"score":x.score,"content":x.content} for x in docs if x.score > threshold]
|
112 |
+
|
113 |
+
# if len(docs) == 0:
|
114 |
+
# return []
|
115 |
+
# res = pd.DataFrame(docs)
|
116 |
+
# passages_df = filter_summaries(res,k_summary,k_total)
|
117 |
+
# if as_dict:
|
118 |
+
# contents = passages_df["content"].tolist()
|
119 |
+
# meta = passages_df.drop(columns = ["content"]).to_dict(orient = "records")
|
120 |
+
# passages = []
|
121 |
+
# for i in range(len(contents)):
|
122 |
+
# passages.append({"content":contents[i],"meta":meta[i]})
|
123 |
+
# return passages
|
124 |
+
# else:
|
125 |
+
# return passages_df
|
126 |
+
|
127 |
+
|
128 |
+
|
129 |
+
# def retrieve(query,sources = ["IPCC"],threshold = 0.555,k = 10):
|
130 |
+
|
131 |
+
|
132 |
+
# print("hellooooo")
|
133 |
+
|
134 |
+
# # Reformulate queries
|
135 |
+
# reformulated_query,language = reformulate(query)
|
136 |
+
|
137 |
+
# print(reformulated_query)
|
138 |
+
|
139 |
+
# # Retrieve documents
|
140 |
+
# passages = retrieve_with_summaries(reformulated_query,retriever,k_total = k,k_summary = 3,as_dict = True,sources = sources,threshold = threshold)
|
141 |
+
# response = {
|
142 |
+
# "query":query,
|
143 |
+
# "reformulated_query":reformulated_query,
|
144 |
+
# "language":language,
|
145 |
+
# "sources":passages,
|
146 |
+
# "prompts":{"init_prompt":init_prompt,"sources_prompt":sources_prompt},
|
147 |
+
# }
|
148 |
+
# return response
|
149 |
+
|
climateqa/vectorstore.py
ADDED
@@ -0,0 +1,42 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# Pinecone
|
2 |
+
# More info at https://docs.pinecone.io/docs/langchain
|
3 |
+
# And https://python.langchain.com/docs/integrations/vectorstores/pinecone
|
4 |
+
|
5 |
+
import pinecone
|
6 |
+
from langchain.vectorstores import Pinecone
|
7 |
+
|
8 |
+
# LOAD ENVIRONMENT VARIABLES
|
9 |
+
from dotenv import load_dotenv
|
10 |
+
import os
|
11 |
+
load_dotenv()
|
12 |
+
|
13 |
+
|
14 |
+
def get_pinecone_vectorstore(embeddings,text_key = "content"):
|
15 |
+
|
16 |
+
# initialize pinecone
|
17 |
+
pinecone.init(
|
18 |
+
api_key=os.getenv("PINECONE_API_KEY"), # find at app.pinecone.io
|
19 |
+
environment=os.getenv("PINECONE_API_ENVIRONMENT"), # next to api key in console
|
20 |
+
)
|
21 |
+
|
22 |
+
index_name = os.getenv("PINECONE_API_INDEX")
|
23 |
+
vectorstore = Pinecone.from_existing_index(index_name, embeddings,text_key = text_key)
|
24 |
+
return vectorstore
|
25 |
+
|
26 |
+
|
27 |
+
# def get_pinecone_retriever(vectorstore,k = 10,namespace = "vectors",sources = ["IPBES","IPCC"]):
|
28 |
+
|
29 |
+
# assert isinstance(sources,list)
|
30 |
+
|
31 |
+
# # Check if all elements in the list are either IPCC or IPBES
|
32 |
+
# filter = {
|
33 |
+
# "source": { "$in":sources},
|
34 |
+
# }
|
35 |
+
|
36 |
+
# retriever = vectorstore.as_retriever(search_kwargs={
|
37 |
+
# "k": k,
|
38 |
+
# "namespace":"vectors",
|
39 |
+
# "filter":filter
|
40 |
+
# })
|
41 |
+
|
42 |
+
# return retriever
|
climateqa_v3.db
DELETED
@@ -1,3 +0,0 @@
|
|
1 |
-
version https://git-lfs.github.com/spec/v1
|
2 |
-
oid sha256:6c05065e5070dd68d05547fc4f330ced5bcc30762d4f566a3fbcfd70d950063f
|
3 |
-
size 459763712
|
|
|
|
|
|
|
|
climateqa_v3.faiss
DELETED
@@ -1,3 +0,0 @@
|
|
1 |
-
version https://git-lfs.github.com/spec/v1
|
2 |
-
oid sha256:2e3190d405db879ccfcd913d0f752fbbde35243ec439f684eed2654484550ab2
|
3 |
-
size 227269677
|
|
|
|
|
|
|
|
climateqa_v3.json
DELETED
@@ -1 +0,0 @@
|
|
1 |
-
{"sql_url": "sqlite:///climateqa_v3.db", "faiss_index_factory_str": "Flat", "index": "climateqa_v3", "similarity": "dot_product", "embedding_dim": 768}
|
|
|
|
requirements.txt
CHANGED
@@ -1,6 +1,4 @@
|
|
1 |
-
|
2 |
-
farm-haystack==1.14.0
|
3 |
-
gradio==3.22.1
|
4 |
openai==0.27.0
|
5 |
azure-storage-file-share==12.11.1
|
6 |
python-dotenv==1.0.0
|
|
|
1 |
+
gradio==3.47.1
|
|
|
|
|
2 |
openai==0.27.0
|
3 |
azure-storage-file-share==12.11.1
|
4 |
python-dotenv==1.0.0
|
style.css
CHANGED
@@ -1,7 +1,7 @@
|
|
1 |
|
2 |
-
:root {
|
3 |
--user-image: url('https://ih1.redbubble.net/image.4776899543.6215/st,small,507x507-pad,600x600,f8f8f8.jpg');
|
4 |
-
}
|
5 |
|
6 |
.warning-box {
|
7 |
background-color: #fff3cd;
|
@@ -147,7 +147,7 @@ label > span{
|
|
147 |
}
|
148 |
|
149 |
/* Pseudo-element for the circularly cropped picture */
|
150 |
-
.message.bot::before {
|
151 |
content: '';
|
152 |
position: absolute;
|
153 |
top: -10px;
|
@@ -160,5 +160,8 @@ label > span{
|
|
160 |
border-radius: 50%;
|
161 |
z-index: 10;
|
162 |
}
|
163 |
-
|
164 |
-
|
|
|
|
|
|
|
|
1 |
|
2 |
+
/* :root {
|
3 |
--user-image: url('https://ih1.redbubble.net/image.4776899543.6215/st,small,507x507-pad,600x600,f8f8f8.jpg');
|
4 |
+
} */
|
5 |
|
6 |
.warning-box {
|
7 |
background-color: #fff3cd;
|
|
|
147 |
}
|
148 |
|
149 |
/* Pseudo-element for the circularly cropped picture */
|
150 |
+
/* .message.bot::before {
|
151 |
content: '';
|
152 |
position: absolute;
|
153 |
top: -10px;
|
|
|
160 |
border-radius: 50%;
|
161 |
z-index: 10;
|
162 |
}
|
163 |
+
*/
|
164 |
+
|
165 |
+
label.selected{
|
166 |
+
background:none !important;
|
167 |
+
}
|