Spaces:
Running
Running
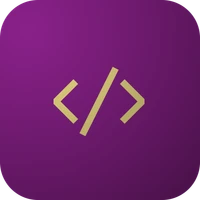
Fix Streamlit duplicate element ID error and enhance CyberOps Dashboard with graphical navigation
9a084f0
verified
import os | |
import streamlit as st | |
import pandas as pd | |
import matplotlib.pyplot as plt | |
import dask.dataframe as dd | |
from dotenv import load_dotenv | |
from itertools import combinations | |
from collections import defaultdict | |
# Load environment variables | |
load_dotenv() | |
# Configuration from environment variables | |
FILE_UPLOAD_LIMIT = int(os.getenv('FILE_UPLOAD_LIMIT', 200)) | |
EXECUTION_TIME_LIMIT = int(os.getenv('EXECUTION_TIME_LIMIT', 300)) | |
RESOURCE_LIMIT = int(os.getenv('RESOURCE_LIMIT', 1024)) # in MB | |
DATA_DIR = os.getenv('DATA_DIR', './data') | |
CONFIG_FLAG = os.getenv('CONFIG_FLAG', 'default') | |
# Main application logic | |
def main(): | |
st.title("CyberOps Dashboard") | |
# Sidebar for user inputs | |
st.sidebar.header("Options") | |
# Main frame for introduction and navigation | |
with st.container(): | |
st.subheader("Welcome to CyberOps Dashboard") | |
st.write(""" | |
The CyberOps Dashboard is designed to assist cybersecurity professionals in analyzing and visualizing data efficiently. | |
With this tool, you can upload CSV files, visualize data trends, and perform advanced data analysis. | |
""") | |
# Navigation section | |
st.subheader("Navigation") | |
st.write("Use the buttons below to navigate to different sections:") | |
col1, col2, col3 = st.columns(3) | |
with col1: | |
if st.button("Data Upload"): | |
uploaded_file = st.sidebar.file_uploader("Select a CSV file:", type=["csv"], key="file_uploader_data_upload") | |
with col2: | |
if st.button("Data Visualization"): | |
st.sidebar.selectbox('Select X-axis:', [], key="data_visualization_x") | |
st.sidebar.selectbox('Select Y-axis:', [], key="data_visualization_y") | |
with col3: | |
if st.button("Analysis Tools"): | |
st.sidebar.multiselect('Select columns for combinations:', [], key="analysis_tools_columns") | |
uploaded_file = st.sidebar.file_uploader("Select a CSV file:", type=["csv"], key="file_uploader_main") | |
if uploaded_file: | |
def load_csv(file): | |
return pd.read_csv(file) | |
def load_dask_csv(file): | |
return dd.read_csv(file) | |
if os.path.getsize(uploaded_file) < RESOURCE_LIMIT * 1024 * 1024: | |
df = load_csv(uploaded_file) | |
else: | |
df = load_dask_csv(uploaded_file) | |
if not df.empty: | |
st.write("Data Preview:") | |
st.dataframe(df.compute() if isinstance(df, dd.DataFrame) else df) | |
# Select columns for plotting | |
x_column = st.sidebar.selectbox('Select X-axis:', df.columns, key="x_column_plot") | |
y_column = st.sidebar.selectbox('Select Y-axis:', df.columns, key="y_column_plot") | |
# Plotting | |
fig, ax = plt.subplots() | |
ax.plot(df[x_column], df[y_column], marker='o') | |
ax.set_xlabel(x_column) | |
ax.set_ylabel(y_column) | |
ax.set_title(f"{y_column} vs {x_column}") | |
st.pyplot(fig) | |
# Combinatorial analysis | |
col_combinations = st.sidebar.multiselect('Select columns for combinations:', df.columns, key="col_combinations") | |
if col_combinations: | |
st.write("Column Combinations:") | |
comb = list(combinations(col_combinations, 2)) | |
st.write(comb) | |
# Grouping and aggregation | |
group_by_column = st.sidebar.selectbox('Select column to group by:', df.columns, key="group_by_column") | |
if group_by_column: | |
grouped_df = df.groupby(group_by_column).agg(list) | |
st.write("Grouped Data:") | |
st.dataframe(grouped_df.compute() if isinstance(grouped_df, dd.DataFrame) else grouped_df) | |
if __name__ == "__main__": | |
main() |