Spaces:
Runtime error
Runtime error
Upload app.py
Browse files
app.py
ADDED
@@ -0,0 +1,52 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import streamlit as st
|
2 |
+
import os
|
3 |
+
import random
|
4 |
+
|
5 |
+
def get_gifs(directory):
|
6 |
+
return [f for f in os.listdir(directory) if f.endswith('.gif')]
|
7 |
+
|
8 |
+
def showAnimatedGif(gif):
|
9 |
+
import streamlit as st
|
10 |
+
import base64
|
11 |
+
#st.markdown("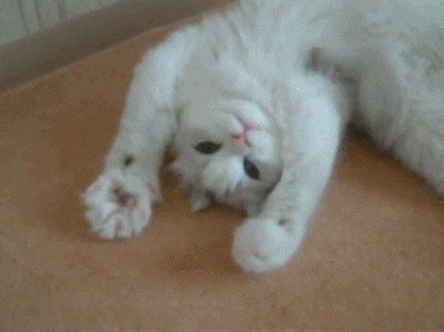")
|
12 |
+
st.write('Loading: ' + gif)
|
13 |
+
file_ = open(gif, "rb")
|
14 |
+
contents = file_.read()
|
15 |
+
data_url = base64.b64encode(contents).decode("utf-8")
|
16 |
+
file_.close()
|
17 |
+
st.write(data_url)
|
18 |
+
|
19 |
+
st.markdown(
|
20 |
+
f'<img src="data:image/gif;base64,{data_url}" alt="gif">',
|
21 |
+
unsafe_allow_html=True,
|
22 |
+
)
|
23 |
+
|
24 |
+
def main():
|
25 |
+
st.title('Animated GIFs in Streamlit')
|
26 |
+
|
27 |
+
directory = './gifs' # Replace with your directory of GIFs
|
28 |
+
gif_files = get_gifs(directory)
|
29 |
+
|
30 |
+
num_rows = len(gif_files) // 3
|
31 |
+
if len(gif_files) % 3:
|
32 |
+
num_rows += 1
|
33 |
+
|
34 |
+
cols = [st.columns(3) for _ in range(num_rows)]
|
35 |
+
|
36 |
+
for i in range(num_rows):
|
37 |
+
for j in range(3):
|
38 |
+
idx = i*3 + j
|
39 |
+
if idx < len(gif_files):
|
40 |
+
#showAnimatedGif(os.path.join(directory, gif_files[idx]))
|
41 |
+
cols[i][j].image(os.path.join(directory, gif_files[idx]), width=200)
|
42 |
+
|
43 |
+
if st.button('Randomize'):
|
44 |
+
random.shuffle(gif_files)
|
45 |
+
for i in range(num_rows):
|
46 |
+
for j in range(3):
|
47 |
+
idx = i*3 + j
|
48 |
+
if idx < len(gif_files):
|
49 |
+
cols[i][j].image(os.path.join(directory, gif_files[idx]), width=200)
|
50 |
+
|
51 |
+
if __name__ == "__main__":
|
52 |
+
main()
|