|
--- |
|
datasets: |
|
- VOC2012 |
|
library_name: pytorch |
|
license: mit |
|
pipeline_tag: image-segmentation |
|
tags: |
|
- android |
|
|
|
--- |
|
|
|
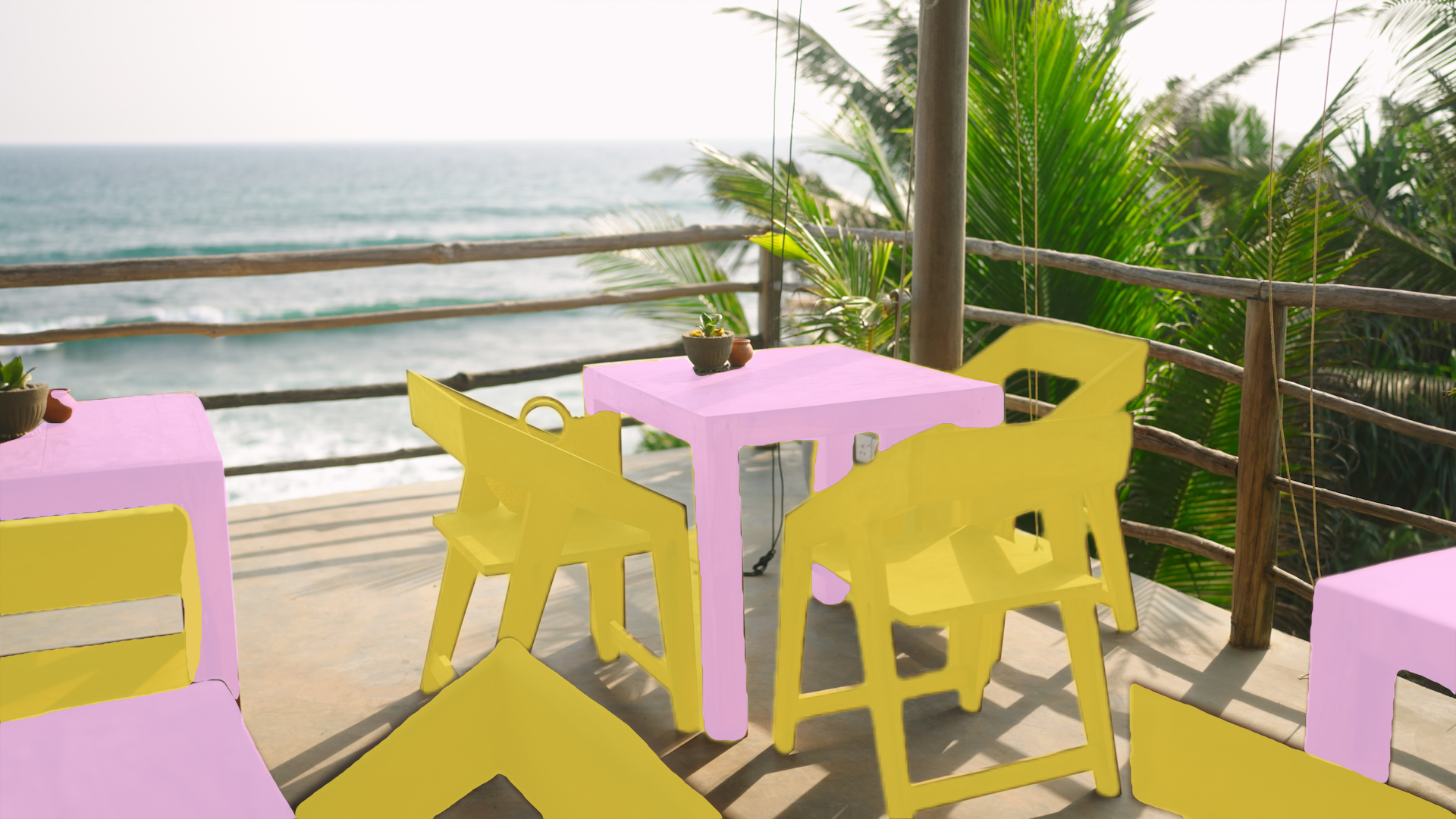 |
|
|
|
# DeepLabV3-Plus-MobileNet: Optimized for Mobile Deployment |
|
## Deep Convolutional Neural Network model for semantic segmentation |
|
|
|
DeepLabV3 is designed for semantic segmentation at multiple scales, trained on the various datasets. It uses MobileNet as a backbone. |
|
|
|
This model is an implementation of DeepLabV3-Plus-MobileNet found [here](https://github.com/jfzhang95/pytorch-deeplab-xception). |
|
This repository provides scripts to run DeepLabV3-Plus-MobileNet on Qualcomm® devices. |
|
More details on model performance across various devices, can be found |
|
[here](https://aihub.qualcomm.com/models/deeplabv3_plus_mobilenet). |
|
|
|
|
|
### Model Details |
|
|
|
- **Model Type:** Semantic segmentation |
|
- **Model Stats:** |
|
- Model checkpoint: VOC2012 |
|
- Input resolution: 513x513 |
|
- Number of parameters: 5.80M |
|
- Model size: 22.2 MB |
|
- Number of output classes: 21 |
|
|
|
|
|
|
|
|
|
| Device | Chipset | Target Runtime | Inference Time (ms) | Peak Memory Range (MB) | Precision | Primary Compute Unit | Target Model |
|
| ---|---|---|---|---|---|---|---| |
|
| Samsung Galaxy S23 Ultra (Android 13) | Snapdragon® 8 Gen 2 | TFLite | 13.088 ms | 21 - 36 MB | FP16 | NPU | [DeepLabV3-Plus-MobileNet.tflite](https://huggingface.co/qualcomm/DeepLabV3-Plus-MobileNet/blob/main/DeepLabV3-Plus-MobileNet.tflite) |
|
| Samsung Galaxy S23 Ultra (Android 13) | Snapdragon® 8 Gen 2 | QNN Model Library | 12.906 ms | 3 - 18 MB | FP16 | NPU | [DeepLabV3-Plus-MobileNet.so](https://huggingface.co/qualcomm/DeepLabV3-Plus-MobileNet/blob/main/DeepLabV3-Plus-MobileNet.so) |
|
|
|
|
|
|
|
## Installation |
|
|
|
This model can be installed as a Python package via pip. |
|
|
|
```bash |
|
pip install qai-hub-models |
|
``` |
|
|
|
|
|
## Configure Qualcomm® AI Hub to run this model on a cloud-hosted device |
|
|
|
Sign-in to [Qualcomm® AI Hub](https://app.aihub.qualcomm.com/) with your |
|
Qualcomm® ID. Once signed in navigate to `Account -> Settings -> API Token`. |
|
|
|
With this API token, you can configure your client to run models on the cloud |
|
hosted devices. |
|
```bash |
|
qai-hub configure --api_token API_TOKEN |
|
``` |
|
Navigate to [docs](https://app.aihub.qualcomm.com/docs/) for more information. |
|
|
|
|
|
|
|
## Demo off target |
|
|
|
The package contains a simple end-to-end demo that downloads pre-trained |
|
weights and runs this model on a sample input. |
|
|
|
```bash |
|
python -m qai_hub_models.models.deeplabv3_plus_mobilenet.demo |
|
``` |
|
|
|
The above demo runs a reference implementation of pre-processing, model |
|
inference, and post processing. |
|
|
|
**NOTE**: If you want running in a Jupyter Notebook or Google Colab like |
|
environment, please add the following to your cell (instead of the above). |
|
``` |
|
%run -m qai_hub_models.models.deeplabv3_plus_mobilenet.demo |
|
``` |
|
|
|
|
|
### Run model on a cloud-hosted device |
|
|
|
In addition to the demo, you can also run the model on a cloud-hosted Qualcomm® |
|
device. This script does the following: |
|
* Performance check on-device on a cloud-hosted device |
|
* Downloads compiled assets that can be deployed on-device for Android. |
|
* Accuracy check between PyTorch and on-device outputs. |
|
|
|
```bash |
|
python -m qai_hub_models.models.deeplabv3_plus_mobilenet.export |
|
``` |
|
|
|
``` |
|
Profile Job summary of DeepLabV3-Plus-MobileNet |
|
-------------------------------------------------- |
|
Device: SA8255 (Proxy) (13) |
|
Estimated Inference Time: 12.94 ms |
|
Estimated Peak Memory Range: 3.05-18.20 MB |
|
Compute Units: NPU (124) | Total (124) |
|
|
|
|
|
``` |
|
|
|
|
|
## How does this work? |
|
|
|
This [export script](https://aihub.qualcomm.com/models/deeplabv3_plus_mobilenet/qai_hub_models/models/DeepLabV3-Plus-MobileNet/export.py) |
|
leverages [Qualcomm® AI Hub](https://aihub.qualcomm.com/) to optimize, validate, and deploy this model |
|
on-device. Lets go through each step below in detail: |
|
|
|
Step 1: **Compile model for on-device deployment** |
|
|
|
To compile a PyTorch model for on-device deployment, we first trace the model |
|
in memory using the `jit.trace` and then call the `submit_compile_job` API. |
|
|
|
```python |
|
import torch |
|
|
|
import qai_hub as hub |
|
from qai_hub_models.models.deeplabv3_plus_mobilenet import Model |
|
|
|
# Load the model |
|
torch_model = Model.from_pretrained() |
|
|
|
# Device |
|
device = hub.Device("Samsung Galaxy S23") |
|
|
|
# Trace model |
|
input_shape = torch_model.get_input_spec() |
|
sample_inputs = torch_model.sample_inputs() |
|
|
|
pt_model = torch.jit.trace(torch_model, [torch.tensor(data[0]) for _, data in sample_inputs.items()]) |
|
|
|
# Compile model on a specific device |
|
compile_job = hub.submit_compile_job( |
|
model=pt_model, |
|
device=device, |
|
input_specs=torch_model.get_input_spec(), |
|
) |
|
|
|
# Get target model to run on-device |
|
target_model = compile_job.get_target_model() |
|
|
|
``` |
|
|
|
|
|
Step 2: **Performance profiling on cloud-hosted device** |
|
|
|
After compiling models from step 1. Models can be profiled model on-device using the |
|
`target_model`. Note that this scripts runs the model on a device automatically |
|
provisioned in the cloud. Once the job is submitted, you can navigate to a |
|
provided job URL to view a variety of on-device performance metrics. |
|
```python |
|
profile_job = hub.submit_profile_job( |
|
model=target_model, |
|
device=device, |
|
) |
|
|
|
``` |
|
|
|
Step 3: **Verify on-device accuracy** |
|
|
|
To verify the accuracy of the model on-device, you can run on-device inference |
|
on sample input data on the same cloud hosted device. |
|
```python |
|
input_data = torch_model.sample_inputs() |
|
inference_job = hub.submit_inference_job( |
|
model=target_model, |
|
device=device, |
|
inputs=input_data, |
|
) |
|
|
|
on_device_output = inference_job.download_output_data() |
|
|
|
``` |
|
With the output of the model, you can compute like PSNR, relative errors or |
|
spot check the output with expected output. |
|
|
|
**Note**: This on-device profiling and inference requires access to Qualcomm® |
|
AI Hub. [Sign up for access](https://myaccount.qualcomm.com/signup). |
|
|
|
|
|
|
|
## Run demo on a cloud-hosted device |
|
|
|
You can also run the demo on-device. |
|
|
|
```bash |
|
python -m qai_hub_models.models.deeplabv3_plus_mobilenet.demo --on-device |
|
``` |
|
|
|
**NOTE**: If you want running in a Jupyter Notebook or Google Colab like |
|
environment, please add the following to your cell (instead of the above). |
|
``` |
|
%run -m qai_hub_models.models.deeplabv3_plus_mobilenet.demo -- --on-device |
|
``` |
|
|
|
|
|
## Deploying compiled model to Android |
|
|
|
|
|
The models can be deployed using multiple runtimes: |
|
- TensorFlow Lite (`.tflite` export): [This |
|
tutorial](https://www.tensorflow.org/lite/android/quickstart) provides a |
|
guide to deploy the .tflite model in an Android application. |
|
|
|
|
|
- QNN (`.so` export ): This [sample |
|
app](https://docs.qualcomm.com/bundle/publicresource/topics/80-63442-50/sample_app.html) |
|
provides instructions on how to use the `.so` shared library in an Android application. |
|
|
|
|
|
## View on Qualcomm® AI Hub |
|
Get more details on DeepLabV3-Plus-MobileNet's performance across various devices [here](https://aihub.qualcomm.com/models/deeplabv3_plus_mobilenet). |
|
Explore all available models on [Qualcomm® AI Hub](https://aihub.qualcomm.com/) |
|
|
|
## License |
|
- The license for the original implementation of DeepLabV3-Plus-MobileNet can be found |
|
[here](https://github.com/jfzhang95/pytorch-deeplab-xception/blob/master/LICENSE). |
|
- The license for the compiled assets for on-device deployment can be found [here](https://qaihub-public-assets.s3.us-west-2.amazonaws.com/qai-hub-models/Qualcomm+AI+Hub+Proprietary+License.pdf) |
|
|
|
## References |
|
* [Rethinking Atrous Convolution for Semantic Image Segmentation](https://arxiv.org/abs/1706.05587) |
|
* [Source Model Implementation](https://github.com/jfzhang95/pytorch-deeplab-xception) |
|
|
|
## Community |
|
* Join [our AI Hub Slack community](https://qualcomm-ai-hub.slack.com/join/shared_invite/zt-2d5zsmas3-Sj0Q9TzslueCjS31eXG2UA#/shared-invite/email) to collaborate, post questions and learn more about on-device AI. |
|
* For questions or feedback please [reach out to us](mailto:ai-hub-support@qti.qualcomm.com). |
|
|
|
|
|
|