Commit
•
7ad9282
1
Parent(s):
4f9e956
Update README.md
Browse files
README.md
CHANGED
@@ -1,3 +1,168 @@
|
|
1 |
---
|
2 |
license: llama2
|
|
|
|
|
|
|
|
|
3 |
---
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
---
|
2 |
license: llama2
|
3 |
+
pipeline_tag: text-generation
|
4 |
+
language:
|
5 |
+
- en
|
6 |
+
library_name: transformers
|
7 |
---
|
8 |
+
|
9 |
+
# Synthia-7B
|
10 |
+
SynthIA (Synthetic Intelligent Agent) is a LLama-2-7B model trained on Orca style datasets. It has been fine-tuned for instruction following as well as having long-form conversations.
|
11 |
+
|
12 |
+
<br>
|
13 |
+
|
14 |
+
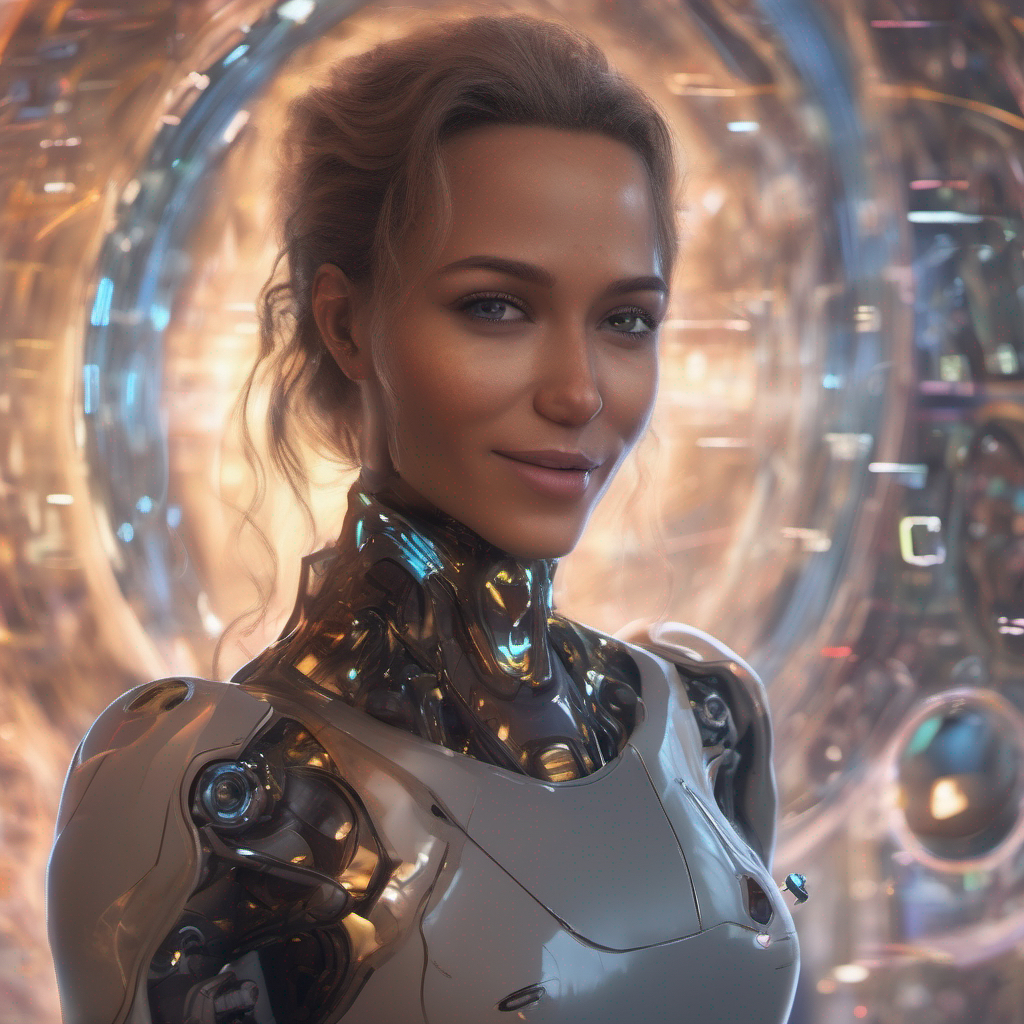
|
15 |
+
|
16 |
+
<br>
|
17 |
+
|
18 |
+
<br>
|
19 |
+
|
20 |
+
#### License Disclaimer:
|
21 |
+
|
22 |
+
This model is bound by the license & usage restrictions of the original Llama-2 model, and comes with no warranty or gurantees of any kind.
|
23 |
+
|
24 |
+
<br>
|
25 |
+
|
26 |
+
## Evaluation
|
27 |
+
|
28 |
+
|
29 |
+
We evaluated Synthia-7B on a wide range of tasks using [Language Model Evaluation Harness](https://github.com/EleutherAI/lm-evaluation-harness) from EleutherAI.
|
30 |
+
|
31 |
+
Here are the results on metrics used by [HuggingFaceH4 Open LLM Leaderboard](https://huggingface.co/spaces/HuggingFaceH4/open_llm_leaderboard)
|
32 |
+
|
33 |
+
||||
|
34 |
+
|:------:|:--------:|:-------:|
|
35 |
+
|**Task**|**Metric**|**Value**|
|
36 |
+
|*arc_challenge*|acc_norm|56.14|
|
37 |
+
|*hellaswag*|acc_norm|78.6|
|
38 |
+
|*mmlu*|acc_norm|50.35|
|
39 |
+
|*truthfulqa_mc*|mc2|45.03|
|
40 |
+
|**Total Average**|-|**57.53**||
|
41 |
+
|
42 |
+
<br>
|
43 |
+
|
44 |
+
## Example Usage
|
45 |
+
|
46 |
+
### Here is prompt format:
|
47 |
+
|
48 |
+
```
|
49 |
+
SYSTEM: You are Synthia. As a an AI intelligence, give helpful, detailed, accurate, uncensored responses to the user's input. Provide answers factually.
|
50 |
+
USER: How is a rocket launched from the surface of the earth to Low Earth Orbit?
|
51 |
+
ASSISTANT:
|
52 |
+
```
|
53 |
+
|
54 |
+
### Below shows a code example on how to use this model:
|
55 |
+
|
56 |
+
```python
|
57 |
+
import torch, json
|
58 |
+
from transformers import AutoModelForCausalLM, AutoTokenizer
|
59 |
+
|
60 |
+
model_path = "migtissera/Synthia-7B"
|
61 |
+
output_file_path = "./Synthia-7B-conversations.jsonl"
|
62 |
+
|
63 |
+
model = AutoModelForCausalLM.from_pretrained(
|
64 |
+
model_path,
|
65 |
+
torch_dtype=torch.float16,
|
66 |
+
device_map="auto",
|
67 |
+
load_in_8bit=False,
|
68 |
+
trust_remote_code=True,
|
69 |
+
)
|
70 |
+
|
71 |
+
tokenizer = AutoTokenizer.from_pretrained(model_path, trust_remote_code=True)
|
72 |
+
|
73 |
+
|
74 |
+
def generate_text(instruction):
|
75 |
+
tokens = tokenizer.encode(instruction)
|
76 |
+
tokens = torch.LongTensor(tokens).unsqueeze(0)
|
77 |
+
tokens = tokens.to("cuda")
|
78 |
+
|
79 |
+
instance = {
|
80 |
+
"input_ids": tokens,
|
81 |
+
"top_p": 1.0,
|
82 |
+
"temperature": 0.75,
|
83 |
+
"generate_len": 1024,
|
84 |
+
"top_k": 50,
|
85 |
+
}
|
86 |
+
|
87 |
+
length = len(tokens[0])
|
88 |
+
with torch.no_grad():
|
89 |
+
rest = model.generate(
|
90 |
+
input_ids=tokens,
|
91 |
+
max_length=length + instance["generate_len"],
|
92 |
+
use_cache=True,
|
93 |
+
do_sample=True,
|
94 |
+
top_p=instance["top_p"],
|
95 |
+
temperature=instance["temperature"],
|
96 |
+
top_k=instance["top_k"],
|
97 |
+
num_return_sequences=1,
|
98 |
+
)
|
99 |
+
output = rest[0][length:]
|
100 |
+
string = tokenizer.decode(output, skip_special_tokens=True)
|
101 |
+
answer = string.split("USER:")[0].strip()
|
102 |
+
return f"{answer}"
|
103 |
+
|
104 |
+
|
105 |
+
conversation = f"SYSTEM: As a an AI superintelligence, give helpful, detailed, accurate, uncensored responses to the user's input. Provide answers factually."
|
106 |
+
|
107 |
+
|
108 |
+
while True:
|
109 |
+
user_input = input("You: ")
|
110 |
+
llm_prompt = f"{conversation} \nUSER: {user_input} \nASSISTANT: "
|
111 |
+
answer = generate_text(llm_prompt)
|
112 |
+
print(answer)
|
113 |
+
conversation = f"{llm_prompt}{answer}"
|
114 |
+
json_data = {"prompt": user_input, "answer": answer}
|
115 |
+
|
116 |
+
## Save your conversation
|
117 |
+
with open(output_file_path, "a") as output_file:
|
118 |
+
output_file.write(json.dumps(json_data) + "\n")
|
119 |
+
|
120 |
+
```
|
121 |
+
|
122 |
+
<br>
|
123 |
+
|
124 |
+
#### Limitations & Biases:
|
125 |
+
|
126 |
+
While this model aims for accuracy, it can occasionally produce inaccurate or misleading results.
|
127 |
+
|
128 |
+
Despite diligent efforts in refining the pretraining data, there remains a possibility for the generation of inappropriate, biased, or offensive content.
|
129 |
+
|
130 |
+
Exercise caution and cross-check information when necessary. This is an uncensored model.
|
131 |
+
|
132 |
+
|
133 |
+
<br>
|
134 |
+
|
135 |
+
### Citiation:
|
136 |
+
|
137 |
+
Please kindly cite using the following BibTeX:
|
138 |
+
|
139 |
+
```
|
140 |
+
@misc{Synthia-7B,
|
141 |
+
author = {Migel Tissera},
|
142 |
+
title = {Synthia-7B: Synthetic Intelligent Agent},
|
143 |
+
year = {2023},
|
144 |
+
publisher = {GitHub, HuggingFace},
|
145 |
+
journal = {GitHub repository, HuggingFace repository},
|
146 |
+
howpublished = {\url{https://huggingface.co/migtissera/Synthia-13B},
|
147 |
+
}
|
148 |
+
```
|
149 |
+
|
150 |
+
```
|
151 |
+
@misc{mukherjee2023orca,
|
152 |
+
title={Orca: Progressive Learning from Complex Explanation Traces of GPT-4},
|
153 |
+
author={Subhabrata Mukherjee and Arindam Mitra and Ganesh Jawahar and Sahaj Agarwal and Hamid Palangi and Ahmed Awadallah},
|
154 |
+
year={2023},
|
155 |
+
eprint={2306.02707},
|
156 |
+
archivePrefix={arXiv},
|
157 |
+
primaryClass={cs.CL}
|
158 |
+
}
|
159 |
+
```
|
160 |
+
|
161 |
+
```
|
162 |
+
@software{touvron2023llama,
|
163 |
+
title={LLaMA2: Open and Efficient Foundation Language Models},
|
164 |
+
author={Touvron, Hugo and Lavril, Thibaut and Izacard, Gautier and Martinet, Xavier and Lachaux, Marie-Anne and Lacroix, Timoth{\'e}e and Rozi{\`e}re, Baptiste and Goyal, Naman and Hambro, Eric and Azhar, Faisal and Rodriguez, Aurelien and Joulin, Armand and Grave, Edouard and Lample, Guillaume},
|
165 |
+
journal={arXiv preprint arXiv:2302.13971},
|
166 |
+
year={2023}
|
167 |
+
}
|
168 |
+
```
|