Update README.md
Browse files
README.md
CHANGED
@@ -1,33 +1,98 @@
|
|
1 |
-
#
|
|
|
2 |
|
3 |
-
|
4 |
|
5 |
-
|
6 |
|
7 |
-
|
8 |
|
9 |
-
|
10 |
-
- Using T5-small size = 230 MB can be found here: https://huggingface.co/gagan3012/keytotext-small
|
11 |
|
12 |
-
|
13 |
|
|
|
|
|
|
|
|
|
|
|
14 |
```python
|
15 |
from transformers import AutoTokenizer, AutoModelWithLMHead
|
16 |
|
17 |
-
tokenizer = AutoTokenizer.from_pretrained("gagan3012/
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
18 |
|
19 |
-
|
|
|
|
|
|
|
|
|
|
|
20 |
```
|
21 |
|
22 |
-
###
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
23 |
|
24 |
-
|
25 |
|
26 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
27 |
|
28 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
29 |
|
30 |
|
31 |
-
|
|
|
|
|
|
|
32 |
|
33 |
-
|
1 |
+
# Leetcode using AI :robot:
|
2 |
+
GPT-2 Model for Leetcode Questions in python
|
3 |
|
4 |
+
**Note**: the Answers might not make sense in some cases because of the bias in GPT-2
|
5 |
|
6 |
+
**Contribtuions:** If you would like to make the model better contributions are welcome Check out [CONTRIBUTIONS.md](https://github.com/gagan3012/project-code-py/blob/master/CONTRIBUTIONS.md)
|
7 |
|
8 |
+
### 📢 Favour:
|
9 |
|
10 |
+
It would be highly motivating, if you can STAR⭐ this repo if you find it helpful.
|
|
|
11 |
|
12 |
+
## Model
|
13 |
|
14 |
+
Two models have been developed for different use cases and they can be found at https://huggingface.co/gagan3012
|
15 |
+
|
16 |
+
The model weights can be found here: [GPT-2](https://huggingface.co/gagan3012/project-code-py) and [DistilGPT-2](https://huggingface.co/gagan3012/project-code-py-small)
|
17 |
+
|
18 |
+
### Example usage:
|
19 |
```python
|
20 |
from transformers import AutoTokenizer, AutoModelWithLMHead
|
21 |
|
22 |
+
tokenizer = AutoTokenizer.from_pretrained("gagan3012/project-code-py")
|
23 |
+
|
24 |
+
model = AutoModelWithLMHead.from_pretrained("gagan3012/project-code-py")
|
25 |
+
```
|
26 |
+
|
27 |
+
## Demo
|
28 |
+
[](https://share.streamlit.io/gagan3012/project-code-py/app.py)
|
29 |
+
|
30 |
+
|
31 |
+
A streamlit webapp has been setup to use the model: https://share.streamlit.io/gagan3012/project-code-py/app.py
|
32 |
+
|
33 |
+
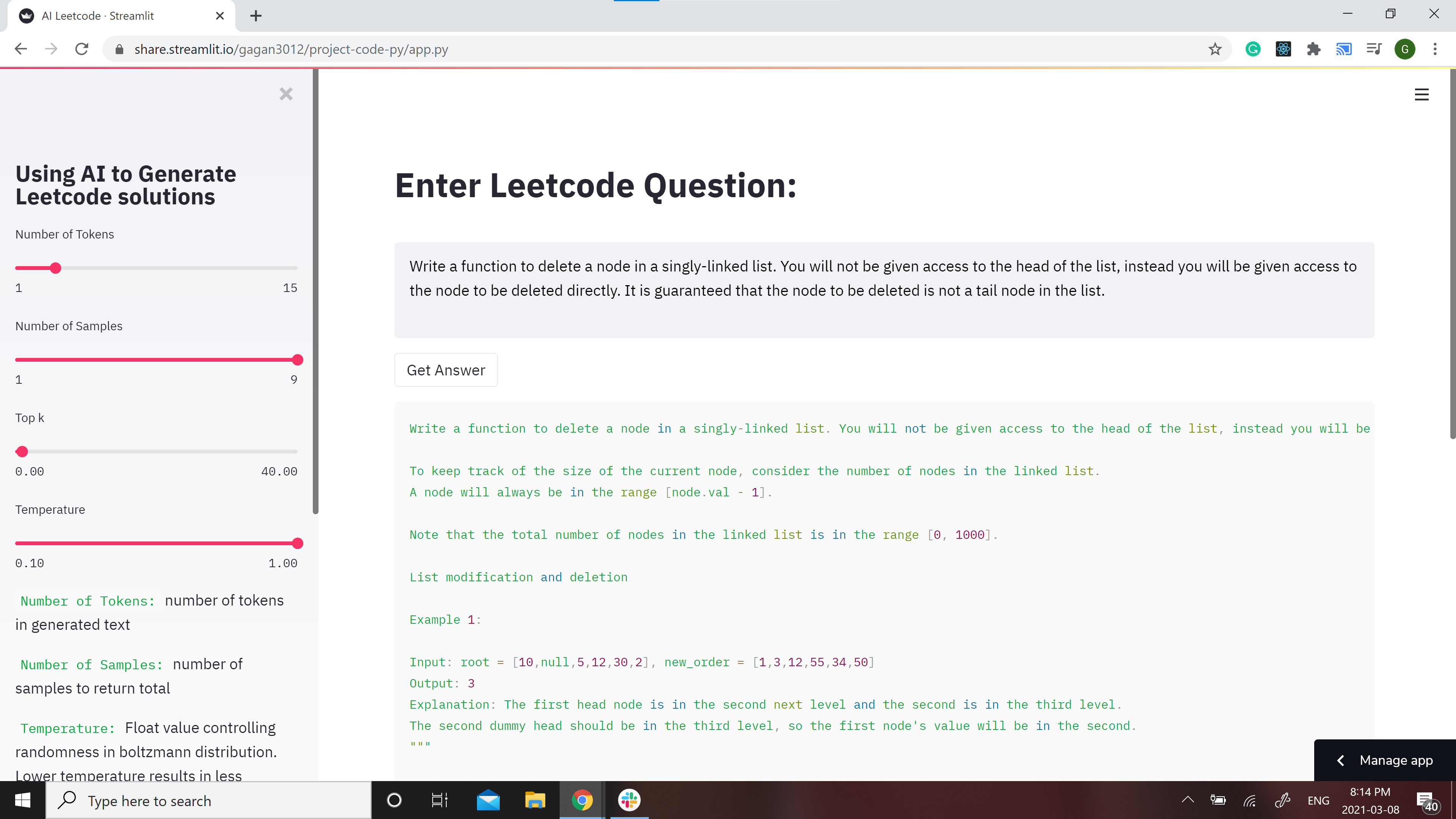
|
34 |
+
|
35 |
|
36 |
+
## Example results:
|
37 |
+
|
38 |
+
### Question:
|
39 |
+
|
40 |
+
```
|
41 |
+
Write a function to delete a node in a singly-linked list. You will not be given access to the head of the list, instead you will be given access to the node to be deleted directly. It is guaranteed that the node to be deleted is not a tail node in the list.
|
42 |
```
|
43 |
|
44 |
+
### Answer:
|
45 |
+
|
46 |
+
```python
|
47 |
+
""" Write a function to delete a node in a singly-linked list. You will not be given access to the head of the list, instead you will be given access to the node to be deleted directly. It is guaranteed that the node to be deleted is not a tail node in the list.
|
48 |
+
|
49 |
+
For example,
|
50 |
+
a = 1->2->3
|
51 |
+
b = 3->1->2
|
52 |
+
t = ListNode(-1, 1)
|
53 |
+
|
54 |
+
Note: The lexicographic ordering of the nodes in a tree matters. Do not assign values to nodes in a tree.
|
55 |
+
Example 1:
|
56 |
+
|
57 |
+
Input: [1,2,3]
|
58 |
+
Output: 1->2->5
|
59 |
+
Explanation: 1->2->3->3->4, then 1->2->5[2] and then 5->1->3->4.
|
60 |
+
|
61 |
|
62 |
+
Note:
|
63 |
|
64 |
+
The length of a linked list will be in the range [1, 1000].
|
65 |
+
Node.val must be a valid LinkedListNode type.
|
66 |
+
Both the length and the value of the nodes in a linked list will be in the range [-1000, 1000].
|
67 |
+
All nodes are distinct.
|
68 |
+
"""
|
69 |
+
# Definition for singly-linked list.
|
70 |
+
# class ListNode:
|
71 |
+
# def __init__(self, x):
|
72 |
+
# self.val = x
|
73 |
+
# self.next = None
|
74 |
|
75 |
+
class Solution:
|
76 |
+
def deleteNode(self, head: ListNode, val: int) -> None:
|
77 |
+
"""
|
78 |
+
BFS
|
79 |
+
Linked List
|
80 |
+
:param head: ListNode
|
81 |
+
:param val: int
|
82 |
+
:return: ListNode
|
83 |
+
"""
|
84 |
+
if head is not None:
|
85 |
+
return head
|
86 |
+
dummy = ListNode(-1, 1)
|
87 |
+
dummy.next = head
|
88 |
+
dummy.next.val = val
|
89 |
+
dummy.next.next = head
|
90 |
+
dummy.val = ""
|
91 |
|
92 |
|
93 |
+
s1 = Solution()
|
94 |
+
print(s1.deleteNode(head))
|
95 |
+
print(s1.deleteNode(-1))
|
96 |
+
print(s1.deleteNode(-1))
|
97 |
|
98 |
+
```
|