question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
minimum-cost-to-equalize-array | Formula based on sum and max of nums | formula-based-on-sum-and-max-of-nums-by-tgghh | Approach
First, we find the maximum element and calculate required increments
We implement a cost optimization strategy:
If 2×cost1 ≤ cost2: use only single in | ivangnilomedov | NORMAL | 2024-12-18T20:08:02.121526+00:00 | 2024-12-18T20:08:02.121526+00:00 | 15 | false | # Approach\n1. First, we find the maximum element and calculate required increments\n2. We implement a cost optimization strategy:\n - If 2\xD7cost1 \u2264 cost2: use only single increments\n - Otherwise: explore efficient combinations of paired and single increments\n3. The `relax` function explores different target values by:\n - Simulating adding k increments to all elements\n - Calculating minimum required single increments for parity\n - Computing maximum possible paired increments\n - Tracking the optimal cost\n4. We search around the analytically derived optimal k value to account for parity adjustments\n\n# Complexity\n- Time complexity: $$O(n + k)$$ where n is array length and k is search range constant\n- Space complexity: $$O(1)$$ using only fixed extra space\n\nThe optimization comes from balancing between:\n- Using more paired increments (cost2) when efficient\n- Increasing the target value to reduce single increments\n- Maintaining increment parity for valid solutions\n- Analytically finding the optimal range to search\n\nKey efficiency comes from computing the theoretical optimal k value instead of searching all possibilities, then only exploring a small range around it to account for parity constraints.\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int minCostToEqualizeArray(const vector<int>& nums, int cost1, int cost2) {\n long long orig_max = *max_element(nums.begin(), nums.end());\n long long rem_sum = 0; // Calculate the total remaining increments needed to reach orig_max\n long long rem_max = 0; // and track the maximum remaining increments for any single element\n for (int i = 0; i < nums.size(); ++i) {\n rem_sum += orig_max - nums[i];\n rem_max = max(rem_max, orig_max - nums[i]);\n }\n\n // Quick exit: If increasing one element is cheaper than two => do single-element increments\n // below we assume cost2 is more efficient\n if (cost1 * 2 <= cost2) return (rem_sum * cost1) % kMod;\n\n long long res = max(cost1, cost1) * rem_sum; // Init with single-element increments\n\n // Assume we increment the origin_max by k => relax result accordingly\n auto relax = [&nums, rem_sum_cp = rem_sum, rem_max_cp = rem_max, cost1, cost2, &res](\n long long k, long long* out_max_k) {\n long long rem_sum = rem_sum_cp;\n long long rem_max = rem_max_cp;\n rem_sum += nums.size() * k; // Simulate adding k\n rem_max += k;\n\n long long rem_sum_ex_max = rem_sum - rem_max;\n // Compute how many elements need single-element increments\n // This happens when we can\'t pair up all remaining increments\n long long min_count_cost1 = max(0LL, rem_max - rem_sum_ex_max);\n\n if (out_max_k && nums.size() > 2)\n // Populate out param saying how muck it still makes sense to increment orig_max\n // to use outside lambda for optimal solution finding\n *out_max_k = min_count_cost1 / (nums.size() - 2);\n\n if ((rem_sum % 2) != (min_count_cost1 % 2))\n // Ensure rem_sum min_count_cost1 have same parity\n ++min_count_cost1;\n\n long long count_cost2 = (rem_sum - min_count_cost1) / 2;\n\n res = min(res, min_count_cost1 * cost1 + count_cost2 * cost2);\n };\n\n long long max_k = -1;\n relax(0, &max_k);\n\n // Try a small range around the optimal k to ensure we find the minimum cost\n // range needed because of parity adjustment might introduce avoidable deviation from optima\n int delta = 2;\n for (int k = max(1LL, max_k - delta); k <= max_k + delta; ++k)\n relax(k, nullptr);\n return res % kMod;\n }\n\nprivate:\n static constexpr long long kMod = 1e9 + 7;\n};\n\n``` | 0 | 0 | ['C++'] | 0 |
minimum-cost-to-equalize-array | Greedy + Enumeration | greedy-enumeration-by-up41guy-59lj | Thanks to Lee\'s answer\n\njavascript []\nvar minCostToEqualizeArray = function (arr, c1, c2) {\n // Find the maximum, minimum, and length of the array\n let | Cx1z0 | NORMAL | 2024-11-07T03:26:08.122238+00:00 | 2024-11-07T03:26:08.122276+00:00 | 12 | false | Thanks to [Lee\'s answer](https://leetcode.com/problems/minimum-cost-to-equalize-array/solutions/5114202/java-c-python-4-cases-o-n-solution/?envType=company&envId=microsoft&favoriteSlug=microsoft-thirty-days)\n\n```javascript []\nvar minCostToEqualizeArray = function (arr, c1, c2) {\n // Find the maximum, minimum, and length of the array\n let max = Math.max(...arr), min = Math.min(...arr), n = arr.length;\n\n // Calculate the total cost based on the max element in the array\n let totalCost = max * n - arr.reduce((sum, val) => sum + val, 0);\n\n // Case 1: If increment cost is less than or equal to twice the decrement cost or if there are 2 or fewer elements\n if (c1 * 2 <= c2 || n <= 2) return totalCost * c1 % (10 ** 9 + 7);\n\n // Case 2: Calculate operation costs and initial result\n let op1 = Math.max(0, (max - min) * 2 - totalCost), op2 = totalCost - op1;\n let result = (op1 + op2 % 2) * c1 + Math.floor(op2 / 2) * c2;\n\n // Case 3: Adjust total cost based on operations and find the minimum result\n totalCost += Math.floor(op1 / (n - 2)) * n;\n op1 %= n - 2;\n result = Math.min(result, (op1 + (totalCost - op1) % 2) * c1 + Math.floor((totalCost - op1) / 2) * c2);\n\n // Case 4: Further adjustments based on number of elements\n for (let i = 0; i < 2; i++) {\n totalCost += n;\n result = Math.min(result, (totalCost % 2) * c1 + Math.floor(totalCost / 2) * c2);\n }\n\n // Return the result modulo 10^9 + 7\n return result % (10 ** 9 + 7);\n};\n``` | 0 | 0 | ['Array', 'Greedy', 'Enumeration', 'JavaScript'] | 0 |
minimum-cost-to-equalize-array | Greedy + Enumeration | greedy-enumeration-by-up41guy-k2hk | Thanks to Lee\'s answer\n\njavascript []\nvar minCostToEqualizeArray = function (arr, c1, c2) {\n // Find the maximum, minimum, and length of the array\n let | Cx1z0 | NORMAL | 2024-11-07T03:24:54.591157+00:00 | 2024-11-07T03:24:54.591218+00:00 | 7 | false | Thanks to [Lee\'s answer](https://leetcode.com/problems/minimum-cost-to-equalize-array/solutions/5114202/java-c-python-4-cases-o-n-solution/?envType=company&envId=microsoft&favoriteSlug=microsoft-thirty-days)\n\n```javascript []\nvar minCostToEqualizeArray = function (arr, c1, c2) {\n // Find the maximum, minimum, and length of the array\n let max = Math.max(...arr), min = Math.min(...arr), n = arr.length;\n\n // Calculate the total cost based on the max element in the array\n let totalCost = max * n - arr.reduce((sum, val) => sum + val, 0);\n\n // Case 1: If increment cost is less than or equal to twice the decrement cost or if there are 2 or fewer elements\n if (c1 * 2 <= c2 || n <= 2) return totalCost * c1 % (10 ** 9 + 7);\n\n // Case 2: Calculate operation costs and initial result\n let op1 = Math.max(0, (max - min) * 2 - totalCost), op2 = totalCost - op1;\n let result = (op1 + op2 % 2) * c1 + Math.floor(op2 / 2) * c2;\n\n // Case 3: Adjust total cost based on operations and find the minimum result\n totalCost += Math.floor(op1 / (n - 2)) * n;\n op1 %= n - 2;\n result = Math.min(result, (op1 + (totalCost - op1) % 2) * c1 + Math.floor((totalCost - op1) / 2) * c2);\n\n // Case 4: Further adjustments based on number of elements\n for (let i = 0; i < 2; i++) {\n totalCost += n;\n result = Math.min(result, (totalCost % 2) * c1 + Math.floor(totalCost / 2) * c2);\n }\n\n // Return the result modulo 10^9 + 7\n return result % (10 ** 9 + 7);\n};\n``` | 0 | 0 | ['Array', 'Greedy', 'Enumeration', 'JavaScript'] | 0 |
minimum-cost-to-equalize-array | Very fast solution in Rust | very-fast-solution-in-rust-by-tapoafom-ymu1 | Intuition\nThe only intuition was to use TDD to progressively find out how it could be possible to solve this problem !\n\n# Approach\nFirst solve very simple c | tapoafom | NORMAL | 2024-09-19T16:03:18.850698+00:00 | 2024-09-19T16:03:18.850726+00:00 | 6 | false | # Intuition\nThe only intuition was to use TDD to progressively find out how it could be possible to solve this problem !\n\n# Approach\nFirst solve very simple case (eg. nums len equal 2, cost1 only, cost2 only)\nFind a solution to solve [1,3,4] :\n- Use the largest gap (1 to 4) = 3 to fill the remaining area (3 to 4) = 1 with cost2\n- In this example nums become [2,4,4]\n- Use cost1 only or increase max to use cost2\n- To increase, use largest gap to fill above the surface, in this case nums become [4,5,5] then it remains only one cost1 and it could be more interesting if cost1 superior to cost2\n- Identify different pattern : if remaining gap is odd and num len is odd, odd/even, even/odd, even/even imply different usage of cost2 and cost1\n- Find tune the model with all test cases : \n- if largest gap inferior to remaining area\n- if cost1 is lower than cost2\n- ...\n\n# Complexity\n- Time complexity:\nO(n) \n\n- Space complexity:\nO(n) \n\n# Code\n```rust []\n\nimpl Solution {\n\n pub fn min_cost_to_equalize_array(nums: Vec<i32>, cost1: i32, cost2: i32) -> i32 {\n\n let len = nums.len() as i64;\n let cost1 = cost1 as i64;\n let cost2 = cost2 as i64;\n\n if len <= 1 {\n return 0;\n }\n\n let mut max = match nums.iter().max() {\n Some(max) => *max as i64,\n _ => panic!("impossible to get max value from nums"),\n };\n\n let mut remaining_area = 0_i64;\n let mut largest_gap_size = 0_i64;\n\n for e in nums.iter() {\n let gap = max - *e as i64;\n if gap > largest_gap_size {\n largest_gap_size = gap;\n }\n remaining_area += gap;\n }\n remaining_area -= largest_gap_size;\n\n if len == 2 {\n return (largest_gap_size * cost1 % (10i64.pow(9) + 7)) as i32;\n }\n\n let mut result = 0;\n let mut largest_gap_remaining = 0;\n\n if remaining_area <= largest_gap_size {\n result = remaining_area * cost2;\n largest_gap_remaining = largest_gap_size - remaining_area;\n } else {\n let surface = remaining_area + largest_gap_size;\n result = (surface / 2) * cost2;\n if surface & 1 == 1 {\n largest_gap_remaining = 1;\n } \n }\n\n let largest_gap_remaining_before_increasing = largest_gap_remaining;\n let mut increase_max_by = 0;\n loop {\n let q = largest_gap_remaining / (len-1);\n\n if q == 0 {break;}\n\n increase_max_by += q;\n largest_gap_remaining = q + largest_gap_remaining % (len-1);\n }\n\n let cost_to_increase = match (largest_gap_remaining & 1, len & 1) {\n (0, 1) => \n cost2 * (((increase_max_by + 2) * len + largest_gap_remaining_before_increasing) / 2),\n (1, 0) =>\n cost1\n +\n if largest_gap_remaining == 1 {\n cost2 * (((increase_max_by) * len - 1 + largest_gap_remaining_before_increasing) / 2)\n } else {\n cost2 * (((increase_max_by + 1) * len - 1 + largest_gap_remaining_before_increasing) / 2)\n },\n (1,1) => {\n std::cmp::min(\n cost2 * (((increase_max_by + 1) * len + largest_gap_remaining_before_increasing) / 2), \n largest_gap_remaining * cost1 + cost2 * (((increase_max_by) * len + largest_gap_remaining_before_increasing) / 2), \n )},\n _ =>\n cost2 * (((increase_max_by + 1) * len + largest_gap_remaining_before_increasing) / 2), \n };\n\n result += std::cmp::min(cost_to_increase, largest_gap_remaining_before_increasing * cost1);\n \n\n (std::cmp::min(result, (largest_gap_size + remaining_area) * cost1) % (10i64.pow(9) + 7)) as i32\n\n } \n}\n``` | 0 | 0 | ['Rust'] | 0 |
minimum-cost-to-equalize-array | 💡🔧 Efficient Cost Minimization for Array Equalization in C++! 🚀✨ || Beats 100%|| | efficient-cost-minimization-for-array-eq-fxyw | Intuition\n- Determine the maximum (ma) and minimum (mi) elements in the array.\nCalculate the total sum (su) of elements in the array.\nCompute the difference | AJ_3000 | NORMAL | 2024-07-15T15:36:28.996740+00:00 | 2024-07-15T15:36:28.996773+00:00 | 13 | false | # Intuition\n- Determine the maximum (ma) and minimum (mi) elements in the array.\nCalculate the total sum (su) of elements in the array.\nCompute the difference needed to equalize the array (total).\n- Simple Cost Calculation: If the cost of two increments is less than or equal to the cost of a double operation (c1 * 2 <= c2), or if the array has two or fewer elements, the problem simplifies to using only increment operations.\nAdvanced Cost Calculation: When the double operation can be beneficial, calculate the cost using a combination of both operations.\n- Calculate the cost using different combinations of increments and double operations.\nOptimize the result by considering additional increments to adjust the total cost efficiently.\n\n\n# Approach\nThis solution combines efficient mathematical analysis and optimization techniques to achieve the minimum cost for equalizing the array.\n\n# Complexity\n- Time complexity: $$O(n)$$\n\n- Space complexity: $$O(1)$$ \n\n# Code\n```\nstatic auto fastio = [](){\n std::ios::sync_with_stdio(false);\n std::cin.tie(nullptr);\n std::cout.tie(nullptr);\n return 0;\n}();\nclass Solution {\npublic:\n int minCostToEqualizeArray(vector<int>& A, int c1, int c2) {\n int ma = *max_element(A.begin(), A.end());\n int mi = *min_element(A.begin(), A.end());\n int n = A.size(), mod = 1000000007;\n long long su = accumulate(A.begin(), A.end(), 0LL);\n long long total = 1LL * ma * n - su;\n\n if (c1 * 2 <= c2 || n <= 2) {\n return (total * c1) % mod;\n }\n\n long long op1 = max(0LL, (ma - mi) * 2 - total);\n long long op2 = total - op1;\n long long res = (op1 + op2 % 2) * c1 + op2 / 2 * c2;\n\n total += op1 / (n - 2) * n;\n op1 %= n - 2;\n op2 = total - op1;\n res = min(res, (op1 + op2 % 2) * c1 + op2 / 2 * c2);\n\n for (int i = 0; i < 2; i++) {\n total += n;\n res = min(res, total % 2 * c1 + total / 2 * c2);\n }\n\n return res % mod;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
minimum-cost-to-equalize-array | Best Solution || O(n) || Math || Greedy || 2ms | best-solution-on-math-greedy-2ms-by-priy-828j | \n\n# Complexity\n- Time complexity:\n Add your time complexity here, e.g. O(n) \nO(n)\n- Space complexity:\n Add your space complexity here, e.g. O(n) \nO(1)\n | Priyanshu_pandey15 | NORMAL | 2024-06-30T17:10:01.901495+00:00 | 2024-06-30T17:10:01.901529+00:00 | 65 | false | 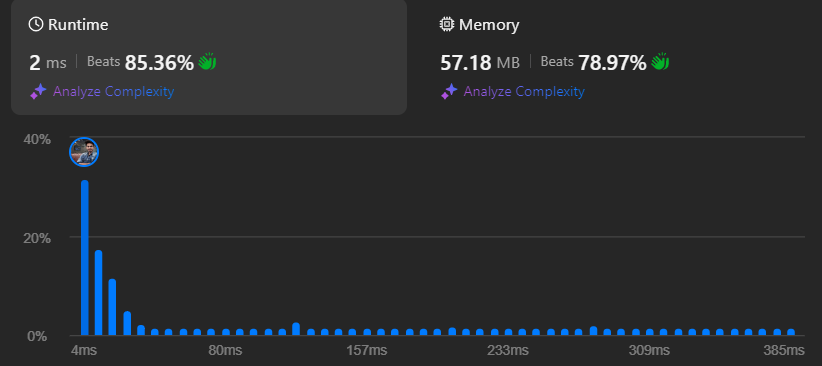\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# Code\n```\nclass Solution {\n public int minCostToEqualizeArray(int[] nums, int aa, int bb) {\n long max = -1, mi = -1, a = aa, b = bb, n = nums.length,j =0,mod = 1000000007;\n for (int i : nums)\n if (i > max)\n max = i;\n long sum = 0;\n for (int i : nums) {\n j = Math.abs(max - i);\n sum += j;\n mi = Math.max(mi, j);\n }\n if (2 * a < b) {\n return (int) ((sum * a) % 1000000007);\n }\n if ((sum - mi) >= mi) {\n if (n % 2 != 0) {\n return (int) (Math.min((((sum / 2) * b + (sum % 2) * a)), (((sum + n) / 2 * b)))\n % 1000000007);\n } else\n return (int) ((((sum / 2) % 1000000007 * b) % 1000000007 + ((sum % 2) * a) % 1000000007) % 1000000007);\n }\n long max2 = (((sum - mi) * b) % 1000000007 + (((mi + mi - sum)) * a) % 1000000007), x = 0, y = (sum - mi),\n z = (mi);\n if ((n - 1 - 1) > 0) {\n long xx = (mi + mi - sum) / (n - 1 - 1);\n y = sum - mi + (n - 1) * xx;\n z = mi + xx;\n if (xx > 0 && Math.abs(y - z) < 2) {\n max2 = Math.min(max2,\n (((n * xx + sum) / 2 * b)) % 1000000007 + (((n * xx + sum) % 2 * a)) % 1000000007);\n }\n }\n int yy = 2;\n while (yy > 0) {\n yy--;\n y += (n - 1);\n z += 1;\n x = (((z + y) / 2) * b);\n if ((z + y) % 2 != 0)\n x += a;\n x %= 1000000007;\n if (y < z)\n break;\n max2 = Math.min(x , max2 );\n }\n return (int) (max2 % 1000000007);\n }\n}\n``` | 0 | 0 | ['Math', 'Greedy', 'Java'] | 0 |
minimum-cost-to-equalize-array | ☕ Java solution ✅ || 😼 Beats 97.13% ✨ | java-solution-beats-9713-by-barakamon-j491 | \n\n\nimport java.util.Arrays;\n\nclass Solution {\n private static final int MOD = (int) 1e9 + 7;\n\n private long calcCost(long maxDelta, long sumDelta, | Barakamon | NORMAL | 2024-06-26T12:46:59.623640+00:00 | 2024-06-26T12:46:59.623671+00:00 | 43 | false | 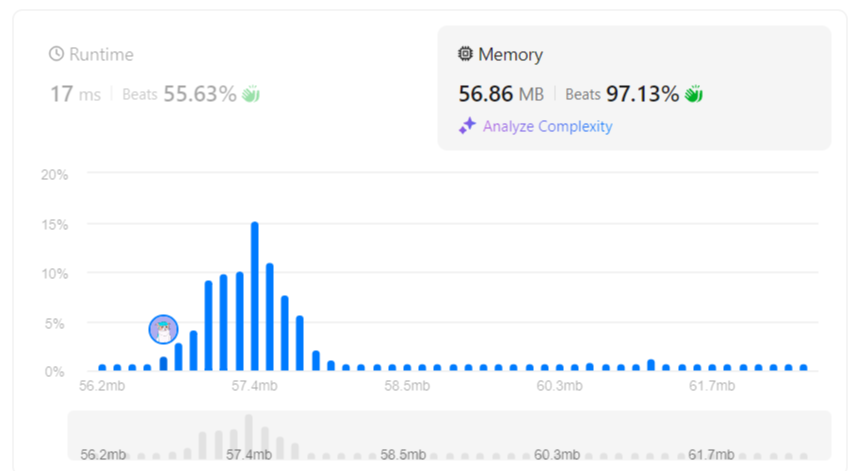\n\n```\nimport java.util.Arrays;\n\nclass Solution {\n private static final int MOD = (int) 1e9 + 7;\n\n private long calcCost(long maxDelta, long sumDelta, int cost1, int cost2) {\n if (maxDelta * 2 <= sumDelta)\n return (sumDelta / 2) * cost2 + (sumDelta & 1) * cost1;\n else\n return (2 * maxDelta - sumDelta) * cost1 + (sumDelta - maxDelta) * cost2;\n }\n\n public int minCostToEqualizeArray(int[] nums, int cost1, int cost2) {\n int n = nums.length;\n if (n <= 1) return 0;\n\n int maxVal = Arrays.stream(nums).max().getAsInt();\n long sumDelta = 0, maxDelta = 0;\n \n for (int num : nums) {\n long delta = maxVal - num;\n maxDelta = Math.max(maxDelta, delta);\n sumDelta += delta;\n }\n \n if (cost2 >= 2 * cost1) return (int) (sumDelta * cost1 % MOD);\n if (n == 2) return (int) (sumDelta * cost1 % MOD);\n\n long answer = calcCost(maxDelta, sumDelta, cost1, cost2);\n long maxDeltaTwice = maxDelta * 2;\n\n while (maxDeltaTwice > sumDelta) {\n maxDelta++;\n sumDelta += n;\n answer = Math.min(answer, calcCost(maxDelta, sumDelta, cost1, cost2));\n maxDeltaTwice += 2;\n }\n \n if (sumDelta % 2 == 1) {\n maxDelta++;\n sumDelta += n;\n answer = Math.min(answer, calcCost(maxDelta, sumDelta, cost1, cost2));\n }\n\n return (int) (answer % MOD);\n }\n}\n\n\n``` | 0 | 0 | ['Java'] | 0 |
minimum-cost-to-equalize-array | My Java Code Running | my-java-code-running-by-vivekvardhan4386-dgsk | Intuition\n\nThe problem allows two types of operations:\n\n1. Increasing a single element by 1 for a cost of \'cost1\'.\n\n2. Increasing two different elements | vivekvardhan43862 | NORMAL | 2024-06-14T09:46:20.067023+00:00 | 2024-06-14T09:46:20.067082+00:00 | 34 | false | # Intuition\n\nThe problem allows two types of operations:\n\n1. Increasing a single element by 1 for a cost of **\'cost1\'**.\n\n2. Increasing two different elements each by 1 for a combined cost of **\'cost2\'**.\n\nTo minimize the total cost, we need to consider the trade-off between using the single increment operation (**\'cost1\'**) and the double increment operation (**\'cost2\'**). Specifically, we need to determine whether it\'s more cost-effective to increase elements one by one or in pairs, especially when **\'cost2\'** is less than twice **\'cost1\'**.\n\n# Approach\n\n1. **Initial Checks**:\n\n - If **\'cost1 * 2 <= cost2\'** or the array length is less than 3, always using the single increment operation is more efficient because the double increment operation is not cost-effective or there aren\'t enough elements to pair up effectively.\n\n2. **Target Value Calculation**:\n\n - Calculate the total sum of the array.\n\n - Determine the range of possible target values. The target values are from the current maximum element to just below twice the maximum element. This ensures we consider a broad enough range to find the minimal cost.\n\n3. **Gap Calculation**:\n\n - For each potential target value, compute the total "gap", which is the sum of the differences between the target and each element in the array.\n\n - Calculate the maximum possible gap for a single element in the array, given the current minimum element.\n\n4. **Cost Calculation**:\n\n - Determine the number of pairs that can be used efficiently without exceeding the total gap.\n\n - Calculate the minimal cost using a combination of single and double increment operations.\n\n5. **Optimization**:\n\n - Iterate through possible target values to find the minimal cost and return it modulo 10^9 + 7.\n\n# Complexity\n- **Time complexity**:\n\n - Finding the minimum, maximum, and sum of the array takes O(\uD835\uDC5B).\n \n- **Space complexity**:\n\n - The space complexity is O(1) since we only use a constant amount of extra space beyond the input array.\n\n# Code\n```\nclass Solution {\n public int minCostToEqualizeArray(int[] nums, int cost1, int cost2) {\n final int kMod = 1_000_000_007;\n final int n = nums.length;\n final int minNum = Arrays.stream(nums).min().getAsInt();\n final int maxNum = Arrays.stream(nums).max().getAsInt();\n final long sum = Arrays.stream(nums).asLongStream().sum();\n long ans = Long.MAX_VALUE;\n\n if (cost1 * 2 <= cost2 || n < 3) {\n final long totalGap = 1L * maxNum * n - sum;\n return (int) ((cost1 * totalGap) % kMod);\n }\n\n for (int target = maxNum; target < 2 * maxNum; ++target) {\n final int maxGap = target - minNum;\n final long totalGap = 1L * target * n - sum;\n final long pairs = Math.min(totalGap / 2, totalGap - maxGap);\n ans = Math.min(ans, cost1 * (totalGap - 2 * pairs) + cost2 * pairs);\n }\n\n return (int) (ans % kMod);\n }\n}\n``` | 0 | 0 | ['Array', 'Greedy', 'Enumeration', 'Java'] | 0 |
minimum-cost-to-equalize-array | Minimum Cost to Equalize Array | minimum-cost-to-equalize-array-by-kenbin-6lve | Intuition\n Describe your first thoughts on how to solve this problem. \nTo solve this problem, we aim to minimize the cost required to make all elements of the | kenbinoy | NORMAL | 2024-06-12T05:54:51.560246+00:00 | 2024-06-12T05:54:51.560282+00:00 | 21 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTo solve this problem, we aim to minimize the cost required to make all elements of the array equal. We observe that if we make all elements equal to the maximum element in the array, the cost will be minimized. However, we need to consider the costs associated with the operations allowed.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe iterate through the array to find the maximum element and calculate the sum of differences between the maximum element and each element in the array. Then, we determine whether it\'s more cost-effective to use the operation with cost1 or cost2 based on the relationship between maxDelta and sumDelta. We calculate the cost accordingly.\n\nIf cost2 is significantly higher than cost1 (i.e., cost2 >= cost1 * 2), it\'s optimal to use cost1 to make all elements equal to the maximum. If the array length is 2 or less, we directly calculate and return the cost based on cost1.\n\nIf neither of these conditions holds, we use a loop to iteratively calculate the cost while adjusting maxDelta and sumDelta until maxDelta * 2 <= sumDelta. We optimize this loop by precalculating maxDelta * 2 and avoiding repeated calculations of sumDelta and maxDelta.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(1)\n\n# Code\n```\nimport java.util.Arrays;\n\nclass Solution {\n private int length, cost1, cost2;\n private final int mod = (int) 1e9 + 7;\n\n private long calculateCost(long maxDelta, long sumDelta) {\n if (maxDelta * 2 <= sumDelta)\n return (sumDelta / 2) * cost2 + (sumDelta & 1) * cost1;\n else\n return (2 * maxDelta - sumDelta) * cost1 + (sumDelta - maxDelta) * cost2;\n }\n\n public int minCostToEqualizeArray(int[] nums, int costFirst, int costSecond) {\n if (nums.length <= 1)\n return 0;\n length = nums.length;\n cost1 = costFirst;\n cost2 = costSecond;\n int maximum = Arrays.stream(nums).max().getAsInt();\n long sumDelta = 0;\n long maxDelta = 0;\n for (int num : nums) {\n long delta = maximum - num;\n maxDelta = Math.max(maxDelta, delta);\n sumDelta += delta;\n }\n if (costSecond >= costFirst * 2)\n return (int) (sumDelta * costFirst % mod);\n\n if (nums.length == 2)\n return (int) (sumDelta * costFirst % mod);\n\n long answer = calculateCost(maxDelta, sumDelta);\n long maxDeltaTwice = maxDelta * 2;\n while (maxDeltaTwice > sumDelta) {\n maxDelta++;\n sumDelta += length;\n answer = Math.min(answer, calculateCost(maxDelta, sumDelta));\n maxDeltaTwice += 2;\n }\n if (sumDelta % 2 == 1) {\n maxDelta++;\n sumDelta += length;\n answer = Math.min(answer, calculateCost(maxDelta, sumDelta));\n }\n return (int) (answer % mod);\n }\n}\n\n``` | 0 | 0 | ['Java'] | 0 |
minimum-cost-to-equalize-array | Minimize Cost to Equalize Array with Single and Double Increments | minimize-cost-to-equalize-array-with-sin-vpl4 | \n\n# Intuition\nThe goal is to make all elements in the array equal with minimum cost.\nWe can increase the elements in two ways: by a single increment (with c | afonsodemello | NORMAL | 2024-06-04T14:15:43.064157+00:00 | 2024-06-04T14:15:43.064181+00:00 | 26 | false | \n\n# Intuition\nThe goal is to make all elements in the array equal with minimum cost.\nWe can increase the elements in two ways: by a single increment (with cost c1) or by a double increment (with cost c2).\nWe need to determine the optimal combination of single and double increments to minimize the total cost.\n# Approach\nFind the maximum and minimum values in the array to determine the range of possible values.\nCalculate the total amount needed to bring all elements to the maximum value.\nCheck if using single increments (c1) exclusively or if the array size is small (less than or equal to 2) is more efficient. If so, return the total cost of using single increments.\nOtherwise, calculate the additional cost required for each element to reach the maximum value using single increments (op1) and the remaining cost (op2).\nCompute the cost of using both single and double increments and update the minimum cost accordingly.\nAdjust the total to account for remaining elements and update the result accordingly.\nIterate twice to account for additional elements and update the result.\nReturn the final result modulo 10^9 + 7.\n# Complexity\n- Time complexity:\n$$O(1)$$\n- Space complexity:\n$$O(n)$$\n# Code\n```\nstatic auto fastio = [](){\n std::ios::sync_with_stdio(false); // Improves input/output efficiency\n std::cin.tie(nullptr);\n std::cout.tie(nullptr);\n return 0;\n}();\n\nclass Solution {\npublic:\n int minCostToEqualizeArray(vector<int>& A, int c1, int c2) {\n int ma = *max_element(A.begin(), A.end()); // Find the maximum value in the array\n int mi = *min_element(A.begin(), A.end()); // Find the minimum value in the array\n int n = A.size(), mod = 1000000007; // Get the size of the array and define the modulo value\n long long su = accumulate(A.begin(), A.end(), 0LL); // Calculate the sum of all elements in the array\n long long total = 1LL * ma * n - su; // Calculate the total amount needed to make all elements equal to the maximum\n\n // Check if using single increments (c1) is more efficient or if the array size is small\n if (c1 * 2 <= c2 || n <= 2) {\n return (total * c1) % mod; // Return the total cost of using single increments\n }\n\n // Calculate the additional cost for each element to reach the maximum value with single increments (c1)\n long long op1 = max(0LL, (ma - mi) * 2 - total);\n long long op2 = total - op1;\n long long res = (op1 + op2 % 2) * c1 + op2 / 2 * c2; // Calculate the cost using both single and double increments\n\n // Adjust total to account for remaining elements and update the result accordingly\n total += op1 / (n - 2) * n;\n op1 %= n - 2;\n op2 = total - op1;\n res = min(res, (op1 + op2 % 2) * c1 + op2 / 2 * c2);\n\n // Iterate twice to account for additional elements and update the result accordingly\n for (int i = 0; i < 2; i++) {\n total += n;\n res = min(res, total % 2 * c1 + total / 2 * c2);\n }\n\n return res % mod; // Return the final result modulo the defined value\n }\n};\n\n```\nP.S.: I used a bit of chatgpt so if its simmilar to other code sorry | 0 | 0 | ['Array', 'Greedy', 'Sorting', 'C++'] | 0 |
minimum-cost-to-equalize-array | Intutive solution | intutive-solution-by-bhanu_pratap5-derz | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | bhanu_pratap5 | NORMAL | 2024-05-17T19:16:20.036504+00:00 | 2024-05-17T19:16:20.036525+00:00 | 23 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nusing ll=long long;\nclass Solution {\n ll sum=0;\n ll mn,mx;\n vector<int> arr;\n ll n,c1,c2;\n\npublic:\n ll findcost(int mxel){\n ll tot=mxel*n-sum;\n if(2*c1<=c2)return tot*c1;\n ll mxdiff=mxel-mn;\n if(mxdiff>(tot/2))return (mxdiff-(tot-mxdiff))*c1 + (tot-mxdiff)*c2;\n return (tot/2)*c2 + (tot%2)*c1;\n }\n int minCostToEqualizeArray(vector<int>& nums, int cost1, int cost2) {\n ll mod=1e9+7;\n mx=*max_element(nums.begin(),nums.end());\n mn=*min_element(nums.begin(),nums.end());\n c1=cost1;c2=cost2;\n n=nums.size();\n ll ans= 1e18;\n for(int i=0;i<n;i++){\n sum+=nums[i];\n }\n for(int c=mx;c<=2*mx;c++){\n ans=min(ans,findcost(c));\n }\n int sol= ans%mod;\n return sol;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
minimum-cost-to-equalize-array | Beats 96% of people with python.. | beats-96-of-people-with-python-by-rexton-0ffx | Code\n\nclass Solution:\n def minCostToEqualizeArray(self, A: List[int], c1: int, c2: int) -> int:\n ma, mi = max(A), min(A)\n n = len(A)\n | Rexton_George_R | NORMAL | 2024-05-17T13:41:37.589503+00:00 | 2024-05-17T13:41:37.589531+00:00 | 33 | false | # Code\n```\nclass Solution:\n def minCostToEqualizeArray(self, A: List[int], c1: int, c2: int) -> int:\n ma, mi = max(A), min(A)\n n = len(A)\n mod = 10 ** 9 + 7\n total = ma * n - sum(A)\n\n # case 1\n if c1 * 2 <= c2 or n <= 2:\n return total * c1 % mod\n\n # case 2\n op1 = max(0, (ma - mi) * 2 - total)\n op2 = total - op1\n res = (op1 + op2 % 2) * c1 + op2 // 2 * c2\n\n # case 3\n total += op1 // (n - 2) * n\n op1 %= n - 2\n op2 = total - op1\n res = min(res, (op1 + op2 % 2) * c1 + op2 // 2 * c2)\n\n # case 4\n for i in range(2):\n total += n\n res = min(res, total % 2 * c1 + total // 2 * c2)\n return res % mod\n\n``` | 0 | 0 | ['Python3'] | 1 |
minimum-cost-to-equalize-array | Easy understanding solution in O(n) time complexity | easy-understanding-solution-in-on-time-c-034x | Intuition\nConsider that the vector nums[i] is sorted ascendingly (though not required). Try best to use operation 2 if it is more valuable.\nThe vector will be | leafok | NORMAL | 2024-05-17T05:49:00.955872+00:00 | 2024-05-17T05:49:00.955908+00:00 | 15 | false | # Intuition\nConsider that the vector nums[i] is sorted ascendingly (though not required). Try best to use operation 2 if it is more valuable.\nThe vector will be converted to the following values:\n{ maxN - gap, maxN, ..., maxN }, where 0 <= gap < n - 1\nIf gap > 0, apply the opeartion 2 will convert the vector into:\n{ maxN - gap + delta + 1, maxN + 1, maxN + 1, ..., maxN + 1 }, which is equivelent to:\n{ maxN - gap + delta, maxN, maxN, ..., maxN }\nFor the remaining gap, apply operation 1.\nIt is only necessary to count the opeartion times, and calculate the answer before return.\n\n# Approach\nSee inlined comments\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution {\n enum {\n MOD = 1000000007\n };\npublic:\n int minCostToEqualizeArray(vector<int>& nums, int cost1, int cost2) {\n int n = nums.size();\n int64_t c1 = cost1;\n int64_t c2 = cost2;\n int64_t cnt1 = 0;\n int64_t cnt2 = 0;\n int maxN = nums[0];\n int minN = nums[0];\n int64_t sumN = 0L;\n\n // Get minN, maxN, sumN\n for (int i = 0; i < n; i++)\n {\n sumN += nums[i];\n if (nums[i] < minN)\n minN = nums[i];\n if (nums[i] > maxN)\n maxN = nums[i];\n }\n\n // Apply operation 1 only\n if (n == 1 || c1 * 2 <= c2) {\n for (int i = 0; i < n; i++)\n cnt1 += (maxN - nums[i]);\n\n return (c1 * cnt1 % MOD);\n }\n\n // gapRest is the sum of gaps between nums[i] (exclude minN) and maxN\n int64_t gapRest = (int64_t)maxN * (n - 1) - sumN + minN;\n int gap = maxN - minN;\n\n if (gap >= gapRest)\n {\n cnt2 += gapRest;\n gap -= gapRest;\n // minN become the only gap (with any possible size)\n }\n else\n {\n cnt2 += (gap + gapRest) / 2;\n gap = (gap + gapRest) % 2;\n // the gap of minN is reduced to 1 or 0\n }\n\n if (n > 2)\n {\n // Apply operation 2 to minN and the rest n - 1 nums\n // This will reduce the gap of minN by n - 2 effectively\n // Repeat this operation as many times as possible\n if ((n - 1) * c2 < (n - 2) * c1) // compare the cost\n {\n int times = gap / (n - 1);\n while (times > 0)\n {\n cnt2 += (n - 1) * times;\n gap -= (n - 2) * times;\n times = gap / (n - 1);\n }\n }\n\n // Apply opeartion 2 to minN (assume it is nums[0]) and the rest nums[1 ... gap] for gap (or gap + 1) times,\n // then apply the same opeartion to the rest pairs as nums[k] and nums[k + 1], where gap < k <= n - 2\n // If there is one unpaired num[n - 1], apply opeartion 2 to it together with minN once.\n // the operations could repeat (n + gap) / 2 times.\n // If n + gap is odd, the gap of minN will be reduced to 1. Otherwise, the gap will be reduced to 0.\n while (gap > 0 && gap < n - 1)\n {\n int step = (n + gap) / 2;\n int delta = ((n + gap) % 2 == 0 ? gap : gap - 1);\n if (delta == 0 || step * c2 >= delta * c1) // compare the cost\n break;\n cnt2 += step;\n gap -= delta;\n }\n }\n\n // Apply opeartion 1 to the remaining gap of minN\n cnt1 += gap;\n\n return (c1 * cnt1 + c2 * cnt2) % MOD;\n }\n};\n\n``` | 0 | 0 | ['C++'] | 0 |
minimum-cost-to-equalize-array | 👍Runtime 106 ms Beats 68.42% of users with Go | runtime-106-ms-beats-6842-of-users-with-tvg1b | Code\n\nfunc minCostToEqualizeArray(A []int, c1, c2 int) int {\n\tmod := 1000000007\n\tn := len(A)\n\tmi, ma := A[0], A[0]\n\ttotal := 0\n\n\tfor _, a := range | pvt2024 | NORMAL | 2024-05-15T10:11:54.672576+00:00 | 2024-05-15T10:11:54.672596+00:00 | 4 | false | # Code\n```\nfunc minCostToEqualizeArray(A []int, c1, c2 int) int {\n\tmod := 1000000007\n\tn := len(A)\n\tmi, ma := A[0], A[0]\n\ttotal := 0\n\n\tfor _, a := range A {\n\t\tif a < mi {\n\t\t\tmi = a\n\t\t}\n\t\tif a > ma {\n\t\t\tma = a\n\t\t}\n\t\ttotal += a\n\t}\n\n\ttotalCost := int64(ma*n - total)\n\n\t// case 1\n\tif c1*2 <= c2 || n <= 2 {\n\t\treturn int((totalCost * int64(c1)) % int64(mod))\n\t}\n\n\t// case 2\n\top1 := int64(math.Max(0, float64((ma-mi)*2)-float64(totalCost)))\n\top2 := totalCost - op1\n\tres := (op1 + op2%2) * int64(c1) + (op2 / 2) * int64(c2)\n\n\t// case 3\n\ttotalCost += op1 / int64(n-2) * int64(n)\n\top1 %= int64(n - 2)\n\top2 = totalCost - op1\n\tres = int64(math.Min(float64(res), float64((op1+op2%2)*int64(c1)+(op2/2)*int64(c2))))\n\n\t// case 4\n\tfor i := 0; i < 2; i++ {\n\t\ttotalCost += int64(n)\n\t\tres = int64(math.Min(float64(res), float64(totalCost%2*int64(c1)+(totalCost/2)*int64(c2))))\n\t}\n\n\treturn int(res % int64(mod))\n}\n``` | 0 | 0 | ['Go'] | 0 |
minimum-cost-to-equalize-array | Minimum Cost To Equalize Array 🚦 Best Performing Approach using Swift | minimum-cost-to-equalize-array-best-perf-k30u | Intuition\nTo equalize all elements in the array, the goal is to minimize the cost of operations. We have two operations available: incrementing a single elemen | lebon | NORMAL | 2024-05-15T02:09:45.173861+00:00 | 2024-05-15T02:10:34.211993+00:00 | 8 | false | # Intuition\nTo equalize all elements in the array, the goal is to minimize the cost of operations. We have two operations available: incrementing a single element at a cost (`cost1`), and incrementing two different elements at a cost (`cost2`). We need to find an optimal strategy that uses these operations to achieve the goal with minimum cost.\n\nFirst, we need to determine the total number of increments needed to make all elements equal to the maximum element in the array. Then, depending on the relative costs of the operations, we decide the optimal way to perform these increments. If `cost1` is significantly cheaper than `cost2`, we might prefer single increments. Conversely, if `cost2` is more cost-effective, we should utilize double increments where possible.\n\n# Approach\n1. **Initialization**:\n - Determine the number of elements (`n`) in the array.\n - Find the maximum (`mx`) and minimum (`minNum`) values in the array.\n - Define `mod` as `10^9 + 7` to handle large numbers.\n \n2. **Calculate Gaps**:\n - Calculate the maximum gap (`maxGap`) between the highest and lowest values.\n - Calculate the total gap (`ttlGap`) needed to equalize all numbers to the maximum value by summing the differences.\n\n3. **Cost Calculation**:\n - If using two single increments (`cost1 * 2`) is less than or equal to one double increment (`cost2`), always use single increments. The total cost will be the total gap multiplied by `cost1`.\n\n4. **Utilize Double Increments**:\n - If the maximum gap is less than or equal to half the total gap, use as many double increments as possible:\n - Calculate the cost of double increments for half the total gap.\n - If there is an odd number of increments needed, handle the last increment separately.\n\n5. **Complex Case Handling**:\n - For remaining gaps, use double increments to reduce the remaining gap.\n - If the remaining gap is greater than or equal to `n-1`, compare the costs of using single increments versus double increments and choose the cheaper option.\n - If there is still a remaining gap, compare the cost of using double increments versus single increments for the last round of increments.\n\n6. **Return Result**:\n - Ensure the result is within the modulus limit by returning `res % mod`.\n\n# Complexity\n- **Time complexity**: $$O(n)$$\n - Finding the maximum and minimum values in the array takes linear time.\n - Calculating the total gap and iterating through the array to calculate costs also takes linear time.\n \n- **Space complexity**: $$O(1)$$\n - The space used is constant and does not scale with the input size.\n \n\n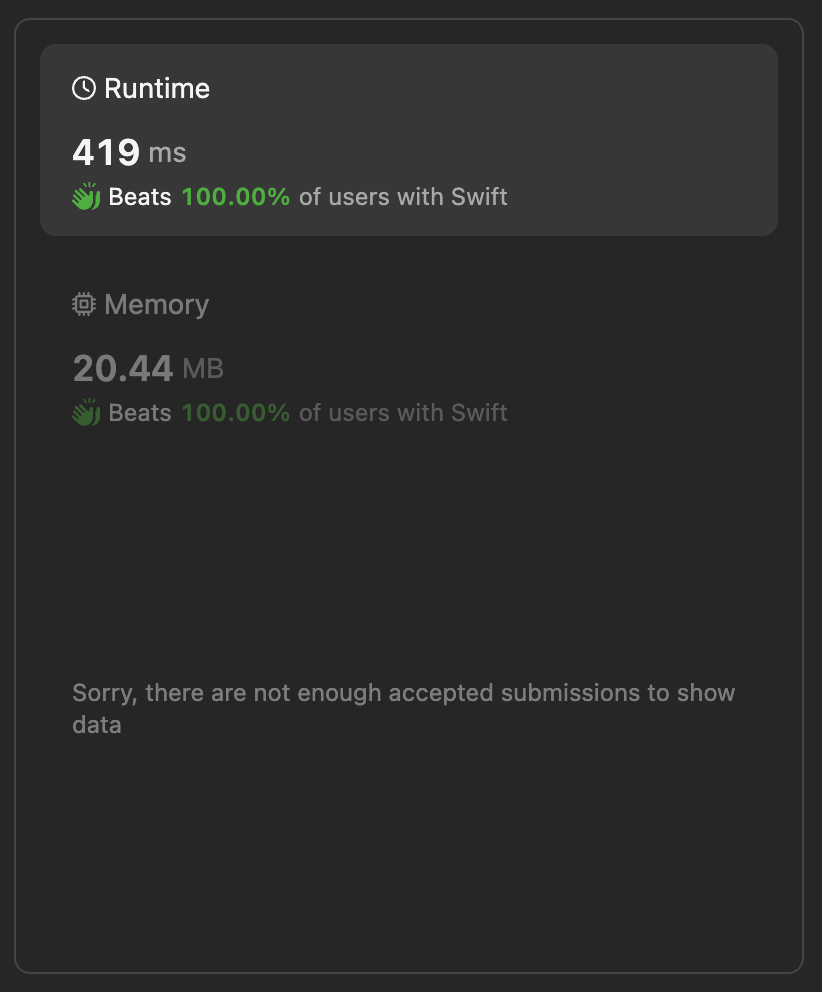\n\n\n# Code\n```swift []\nclass Solution {\n func minCostToEqualizeArray(_ nums: [Int], _ cost1: Int, _ cost2: Int) -> Int {\n let n = nums.count\n let mx = nums.max()!\n let mod = 1_000_000_007\n let minNum = nums.min()!\n \n // Maximum gap between the highest and lowest numbers\n let maxGap = mx - minNum\n // Total gap to equalize all numbers to the maximum\n let ttlGap = mx * n - nums.reduce(0, +)\n \n if cost1 * 2 <= cost2 {\n // If the cost of two single increments is less than or equal to one double increment\n // Always use single increments\n return ttlGap * cost1 % mod\n }\n \n if maxGap * 2 <= ttlGap {\n // If the maximum gap is less than or equal to half of the total gap\n // We can always find pairs to use double increments\n var res = (ttlGap / 2) * cost2\n if ttlGap % 2 == 1 {\n // If there\'s an odd number of increments needed, handle the last increment\n if n % 2 == 1 {\n res += min(cost1, cost2 * (n + 1) / 2)\n } else {\n res += cost1\n }\n }\n return res % mod\n }\n \n // Initial cost with as many double increments as possible\n var res = cost2 * (ttlGap - maxGap)\n var remainingGap = maxGap - (ttlGap - maxGap)\n \n if remainingGap >= n - 1 {\n if cost2 * (n - 1) >= cost1 * (n - 2) {\n // If it\'s cheaper to use single increments\n res += (remainingGap / (n - 1)) * (n - 1) * cost1\n remainingGap %= n - 1\n } else {\n // If it\'s cheaper to use double increments\n res += (remainingGap / (n - 2)) * (n - 1) * cost2\n remainingGap %= n - 2\n }\n }\n \n if remainingGap > 0 {\n // For the remaining gap, compare the cost of using double increments vs single increments\n let op2 = ((n + remainingGap) / 2) * cost2\n var adjustedOp2 = op2\n if (n + remainingGap) % 2 == 1 {\n if n % 2 == 1 {\n adjustedOp2 += min(cost1, cost2 * (n + 1) / 2)\n } else {\n adjustedOp2 += cost1\n }\n }\n \n res += min(adjustedOp2, remainingGap * cost1)\n }\n \n return res % mod\n }\n}\n | 0 | 0 | ['Array', 'Swift'] | 0 |
minimum-cost-to-equalize-array | Greedy O(N) | greedy-on-by-1234ankitgarg-d7s1 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | 1234ankitgarg | NORMAL | 2024-05-12T07:21:12.323935+00:00 | 2024-05-12T07:21:12.323962+00:00 | 23 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n#define ll long long\nclass Solution {\n int M=1e9+7;\npublic:\n int minCostToEqualizeArray(vector<int>& num, int cost1, int cost2) {\n \n ll maxi=0;\n ll n=num.size();\n vector<ll>nums(n);\n for(int i=0;i<n;i++) nums[i]=num[i]*1LL;\n vector<ll>diff(n,0);\n for(int i=0;i<n;i++)\n {\n maxi=max(maxi,nums[i]);\n }\n \n ll maxi1=0;\n ll sum=0;\n for(int i=0;i<n;i++)\n {\n diff[i]=abs(nums[i]-maxi);\n sum+=diff[i];\n maxi1=max(maxi1,diff[i]);\n }\n \n if(n==2)\n {\n return (cost1*maxi1)%M;\n }\n if(2*cost1<=cost2)\n {\n return (sum*cost1)%M;\n }\n if(2*maxi1<=sum){\n if(sum%2==0)\n {\n return ((sum/2)*cost2)%M;\n }\n else\n {\n if(n%2==0) return (((sum/2)*cost2 +cost1))%M;\n else return (min( (cost2*(sum+n))/2,(sum/2)*cost2 +cost1))%M;\n }\n }\n else\n {\n ll large=maxi1;\n ll temp=sum-maxi1;\n double temp_x=(double)(large-temp)/(n-2);\n ll old_sum=sum;\n ll x=ceil(temp_x);\n ll ans=0;\n ll temp_sum=sum+n*x;\n if((temp_sum)%2==0)\n {\n ans=(temp_sum/2)*cost2;\n }\n else\n {\n if(n%2==0) ans= ((temp_sum/2)*cost2 +cost1);\n else ans= min( (cost2*(temp_sum+n))/2,(temp_sum/2)*cost2 +cost1);\n }\n \n for(int i=0;i<x;i++)\n {\n ll temp_cost=(large+i-(temp+i*(n-1)))*cost1;\n temp_cost+=(temp+(i*(n-1)))*cost2;\n ans=min(ans,temp_cost);\n }\n \n return (ans)%M;\n }\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
minimum-cost-to-equalize-array | Minimum Cost To Equalize Array | minimum-cost-to-equalize-array-by-user46-lx9l | Intuition\n Describe your first thoughts on how to solve this problem. \nThe problem asks for the minimum cost required to make all elements in the array equal | user4609eh | NORMAL | 2024-05-11T09:12:02.087642+00:00 | 2024-05-11T09:12:02.087682+00:00 | 32 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem asks for the minimum cost required to make all elements in the array equal by performing two types of operations: either increase a single element or increase two different elements. We need to come up with a strategy to minimize this cost.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSort the array to identify the maximum and minimum elements.\nIf the array has only one or two elements, return the cost calculated based on the difference between them and the given costs.\nIf cost2 is greater than or equal to twice cost1, it\'s optimal to increment each element to the maximum element in the array.\nOtherwise, calculate the total sum of differences between the maximum element and each element in the array, and find the maximum difference. Based on this difference, compute the minimum cost for various scenarios:\nIf the maximum difference is less than or equal to half of the total sum, then the optimal strategy is to increase each element to the maximum element.\nIf the maximum difference is greater than half of the total sum, then we need to find an additional number to make the total sum even, and try different numbers to minimize the cost.\nFinally, return the minimum cost modulo 1e9 + 7.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nSorting the array takes **O(n log n)** time.\nCalculating the minimum cost takes **O(1)** time for each scenario, so the overall time complexity is **O(n log n)**.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is **O(1)** because we are using only a few variables regardless of the input size.\n\n# Code\n```\nclass Solution {\n private static final int MOD = (int)1e9 + 7;\n public int minCostToEqualizeArray(int[] nums, int cost1, int cost2) {\n Arrays.sort(nums);\n int n = nums.length;\n if (n == 1) return 0;\n if (n == 2) return (int)((long)(nums[1] - nums[0]) * cost1 % MOD);\n if (cost2 >= cost1 * 2) {\n long res = 0;\n for (int num: nums) {\n res = (res + (long)(nums[n-1] - num) * cost1) % MOD;\n }\n return (int)res;\n }\n \n long sum = 0;\n long max = 0;\n for (int num: nums) {\n sum += nums[n-1] - num;\n max = Math.max(max, nums[n-1] - num);\n }\n \n long res = Long.MAX_VALUE;\n for (int k = nums[n-1]; k <= nums[n-1] + 2; k++) {\n res = Math.min(res, minCost(sum + (k-nums[n-1]) * n, max + (k-nums[n-1]), cost1, cost2));\n }\n \n if (max > sum - max) {\n for (int k = (int)((2 * max - sum) / (n-2)); k <= (int)((2 * max - sum) / (n-2)) + 2; k++) {\n res = Math.min(res, minCost(sum + k * n, max + k, cost1, cost2));\n }\n }\n \n return (int)(res % MOD);\n }\n \n private long minCost(long sum, long max, int cost1, int cost2) {\n long res = 0;\n if (sum % 2 == 1) {\n res += cost1;\n max--;\n sum--;\n }\n if (max <= sum - max) {\n res = (res + sum / 2 * cost2);\n return res;\n }\n res = (res + (max - (sum-max)) * cost1);\n res = (res + (sum-max) * cost2);\n return res;\n }\n}\n``` | 0 | 0 | ['Java'] | 0 |
minimum-cost-to-equalize-array | Python solution 💯 | python-solution-by-salahww-629a | Intuition\nWe need to find the minimum cost to make all array elements equal by either increasing a single element (cost1) or increasing two elements (cost2).\n | salahww | NORMAL | 2024-05-07T14:12:49.157762+00:00 | 2024-05-07T14:12:49.157786+00:00 | 13 | false | # Intuition\nWe need to find the minimum cost to make all array elements equal by either increasing a single element (cost1) or increasing two elements (cost2).\n# Approach\nFind the range of possible target values (min to max of the array).\nTry all target values and calculate the minimum cost to make all elements equal to each target value.\nFor each target value, calculate the cost of increasing/decreasing elements individually (operation 1) and increasing pairs (operation 2).\nChoose the minimum cost between operations 1 and 2 for each element.\nReturn the minimum cost across all target values.\n\n# Complexity\n- Time complexity:\nTime complexity: O(n * (max_val - min_val)), where n is the length of the input array.\nSpace complexity: O(1), as we use constant extra space.\n\n# Code\n```\nclass Solution:\n def minCostToEqualizeArray(self, A, c1, c2):\n ma, mi = max(A), min(A)\n n = len(A)\n mod = 10 ** 9 + 7\n total = ma * n - sum(A)\n\n if c1 * 2 <= c2 or n <= 2:\n return total * c1 % mod\n\n op1 = max(0, (ma - mi) * 2 - total)\n op2 = total - op1\n res = (op1 + op2 % 2) * c1 + op2 // 2 * c2\n\n total += op1 // (n - 2) * n\n op1 %= n - 2\n op2 = total - op1\n res = min(res, (op1 + op2 % 2) * c1 + op2 // 2 * c2)\n\n for i in range(2):\n total += n\n res = min(res, total % 2 * c1 + total // 2 * c2)\n return res % mod\n\n\n``` | 0 | 0 | ['Python'] | 0 |
minimum-cost-to-equalize-array | Min Cost To equalize Array , Java Solution. Time Complexity : O(N) | min-cost-to-equalize-array-java-solution-rukp | \n\n# Code\n\nclass Solution {\n public int minCostToEqualizeArray(int[] nums, int cost1, int cost2) {\n\n int n = nums.length;\n if(n==0 | | langesicht | NORMAL | 2024-05-07T03:32:46.684882+00:00 | 2024-05-07T03:32:46.684905+00:00 | 67 | false | \n\n# Code\n```\nclass Solution {\n public int minCostToEqualizeArray(int[] nums, int cost1, int cost2) {\n\n int n = nums.length;\n if(n==0 || n==1){\n return 0;\n }\n\n int mod = 1000000007;\n long max = Long.MIN_VALUE;\n long min = Long.MAX_VALUE;\n long sum = 0;\n\n for(int i=0; i<nums.length; i++){\n sum+= nums[i];\n max = Math.max(max,nums[i]);\n min = Math.min(min,nums[i]);\n }\n\n \n long totalGaps = 1L * max*n-sum;\n long maxGap = max-min;\n\n if(2*cost1<=cost2 || n==2){\n return (int)((totalGaps*cost1)%mod);\n }\n\n long op1 = Math.max(0L, maxGap-(totalGaps-maxGap));\n long op2 = totalGaps - op1;\n\n long ans = ((((long)cost1*(op1+op2%2))+ ((long)cost2*(op2/2)))%mod);\n \n \n //1. op1 = mg-tg+mg = 2mg-tg\n // op2 = 2(tg-mg)\n\n //2. op1 = 2mg-tg\n // op2 = tg-op1 = tg-(2mg-tg) = 2(tg-mg)\n\n\n totalGaps += op1/(n-2)*n;\n op1 = op1%(n-2);\n\n op2 = totalGaps-op1;\n\n ans = Math.min(ans,(cost1*(op1+op2%2)) + (cost2*(op2/2)));\n\n\n for(int i=0; i<2; i++){\n totalGaps+=n;\n\n ans = Math.min(ans,(cost1*(totalGaps%2))+cost2*(totalGaps/2));\n }\n\n return (int)(ans)%mod;\n }\n}\n``` | 0 | 0 | ['Java'] | 0 |
minimum-cost-to-equalize-array | Python | Bruteforce | O(n) | python-bruteforce-on-by-aryonbe-xfhd | Code\n\nimport math\nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n MOD = 10**9 + 7\n ma | aryonbe | NORMAL | 2024-05-06T12:04:50.036424+00:00 | 2024-05-06T12:04:50.036454+00:00 | 39 | false | # Code\n```\nimport math\nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n MOD = 10**9 + 7\n maxv = max(nums)\n d = [maxv-num for num in nums]\n if 2*cost1 <= cost2:\n return cost1*sum(d)%MOD\n n = len(nums)\n if n == 1:\n return cost1*sum(d)%MOD\n if n == 2:\n return cost2*min(d)+cost1*(max(d)-min(d))%MOD\n maxd = max(d)\n r = sum(d)-maxd\n res = float(\'inf\')\n if maxd <= r:\n res = (r+maxd)//2*cost2 + (r+maxd)%2*cost1\n maxd += 1\n r += n-1\n res = min(res, (r+maxd)//2*cost2 + (r+maxd)%2*cost1)\n return res%MOD\n res = r*cost2 + (maxd-r)*cost1\n k = math.floor((maxd-r)/(n-2))\n maxd += k\n r += (n-1)*k\n res = min(res, r*cost2 + (maxd-r)*cost1)\n maxd += 1\n r += (n-1)\n res = min(res, (r+maxd)//2*cost2 + (r+maxd)%2*cost1)\n maxd += 1\n r += n-1\n res = min(res, (r+maxd)//2*cost2 + (r+maxd)%2*cost1)\n return res%MOD\n\n\n \n``` | 0 | 0 | ['Python3'] | 0 |
minimum-cost-to-equalize-array | Python | Bruteforce | O(n) | python-bruteforce-on-by-aryonbe-agvn | Code\n\nimport math\nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n MOD = 10**9 + 7\n ma | aryonbe | NORMAL | 2024-05-06T12:04:43.593473+00:00 | 2024-05-06T12:04:43.593501+00:00 | 19 | false | # Code\n```\nimport math\nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n MOD = 10**9 + 7\n maxv = max(nums)\n d = [maxv-num for num in nums]\n if 2*cost1 <= cost2:\n return cost1*sum(d)%MOD\n n = len(nums)\n if n == 1:\n return cost1*sum(d)%MOD\n if n == 2:\n return cost2*min(d)+cost1*(max(d)-min(d))%MOD\n maxd = max(d)\n r = sum(d)-maxd\n res = float(\'inf\')\n if maxd <= r:\n res = (r+maxd)//2*cost2 + (r+maxd)%2*cost1\n maxd += 1\n r += n-1\n res = min(res, (r+maxd)//2*cost2 + (r+maxd)%2*cost1)\n return res%MOD\n res = r*cost2 + (maxd-r)*cost1\n k = math.floor((maxd-r)/(n-2))\n maxd += k\n r += (n-1)*k\n res = min(res, r*cost2 + (maxd-r)*cost1)\n maxd += 1\n r += (n-1)\n res = min(res, (r+maxd)//2*cost2 + (r+maxd)%2*cost1)\n maxd += 1\n r += n-1\n res = min(res, (r+maxd)//2*cost2 + (r+maxd)%2*cost1)\n return res%MOD\n\n\n \n``` | 0 | 0 | ['Python3'] | 0 |
minimum-cost-to-equalize-array | Go Greedy with brief explanation | go-greedy-with-brief-explanation-by-sitl-qb6c | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n1. Find the maximum number of array\n2. Find the total difference between | sitleon | NORMAL | 2024-05-06T01:33:08.783940+00:00 | 2024-05-06T01:33:08.783961+00:00 | 30 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. Find the maximum number of array\n2. Find the total difference between maximum and each number\n3. Also, find the majority difference value\n4. Iterate to find minimum cost of each targeted value, start with the existing maximum\n 4.a Ideal case with majority difference can completely pair with others difference\n 4.b Remind to compare with the another ideal case, (cnt&1)*c1\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfunc minCostToEqualizeArray(nums []int, cost1 int, cost2 int) int {\n mx, mod := 0, int(1e9)+7\n for _, v := range nums {\n if v > mx {\n mx = v\n }\n }\n mv, cnt := 0, 0\n for _, v := range nums {\n d := mx - v\n if cnt += d; d > mv {\n mv = d // find the majority contribution\n }\n }\n ans := find(mv, cnt, cost1, cost2)\n if len(nums) <= 2 {\n return ans % mod\n }\n for (mv<<1) > cnt { // stop when the others can completely pair with majority\n mv, cnt = mv + 1, cnt + len(nums)\n ans = min(ans, find(mv, cnt, cost1, cost2))\n }\n mv, cnt = mv + 1, cnt + len(nums)\n ans = min(ans, find(mv, cnt, cost1, cost2)) // cover the another case generated with (cnt&1)*c1\n return ans % mod\n}\n\nfunc find(mv, cnt, c1, c2 int) int {\n switch {\n case c1<<1 <= c2:\n return cnt * c1 // c1 * 2 cheaper than c2\n case mv<<1 <= cnt: \n return (cnt>>1)*c2 + (cnt&1)*c1 // other * c2 + (if cnt is odd ? c1 : 0)\n }\n return (cnt-mv)*c2 + (mv<<1-cnt)*c1 // (majority - others) * c1 + others * c2\n}\n\nfunc min(x, y int) int {\n if x < y {\n return x\n }\n return y\n}\n``` | 0 | 0 | ['Greedy', 'Go'] | 0 |
minimum-cost-to-equalize-array | Easiest approach possible for this question. Human like approach! | easiest-approach-possible-for-this-quest-kpd5 | Intuition\nwe need to use cost2 as much as possible\n\n# Approach\nRead the solution with comments, they are self explanatory\n\n# Note (while reading the solut | xxfalconxx | NORMAL | 2024-05-06T00:51:32.001560+00:00 | 2024-05-06T00:51:32.001590+00:00 | 103 | false | # Intuition\nwe need to use cost2 as much as possible\n\n# Approach\nRead the solution with comments, they are self explanatory\n\n# Note (while reading the solution)\nwhen I say (i, j) I mean a c2 operation on indicies (i, j). Element at index 0 in function solveRem is always the number n. Rest are 0s because they dont need increments. \n\n# Code\n```\nlong long mod = 1000000007;\n\nclass Solution {\npublic:\n long long solveRem(long long n, long long rem, long long c1, long long c2){\n // n = last element, rem = remaining number of elements\n if(rem < 2) return (n * c1) % mod;\n if(rem%2==0) c1 = min(c1, (rem/2LL + 1LL) * c2); // for odd elements + 1 costs rem/2LL + 1LL. \n // because => (0, 1), (0, 2), (3, 4), (5,6) c2 increment operations..\n if(c1 * 2 <= c2) return (n * c1)%mod;\n\n long long minpc = n * c1; // min price if we use c1 operations\n // if we use c2 well, then operations (0, 1), (0, 2), (0, 3).. (0, rem+1), we increase 0 relatively by maxop (rem-1)\n long long tc2 = rem * c2; \n long long maxop = rem - 1;\n\n long long rn = n % maxop;\n long long ctc2 = tc2 * (n/maxop); // decrement it as much as possible by maxop, and then calculate remaining n in rn\n\n if(rem % 2 == 0){\n // now, if it is even, we can get increments of 2*i at price of rem/2 + i + 1.\n // fill up all of them with 1s, now for each of set of 2 ones in remaining\n // we can replace (2*i, 2*i+1) with (0, 2*i), (0, 2*i+1) operations for i > 0\n ctc2 += min(rn * c1, (long long)(rem/2LL + rn/2LL + 1)*c2 + ((long long)(1 - rn%2)) * c1);\n } else {\n // now, if it is odd, we can get increments of 2*i + 1 at price of rem/2 + i + 1\n ctc2 += min(rn * c1, (long long)(rem/2LL + (rn-1)/2LL + 1)*c2 + ((long long)(rn%2)) * c1);\n }\n return min(ctc2, minpc);\n }\n\n int minCostToEqualizeArray(vector<int>& numsi, int cost1i, int cost2i) {\n vector<long long> nums; for(auto i:numsi) nums.push_back((long long)i);\n long long cost1 = cost1i, cost2 = cost2i;\n sort(nums.begin(), nums.end());\n\n if(nums.size() == 1){ return 0; }\n\n for(long long i=0;i<nums.size();i++) nums[i] = nums.back() - nums[i]; // operations needed\n while(nums.size() && nums.back() == 0) nums.pop_back();\n // nums[i] = number of increments needed for i\n\n long long tc = 0;\n if(cost2 >= cost1 + cost1){ // easy case, cost1 is more effective\n for(auto i:nums) tc+=i;\n return (tc * cost1) % mod;\n } else {\n // LOGIC => create a multiset, and now pick the largest 2 elements\n // we reduce both of these (increment the 2 elements that need the most increments) by half of the min element or 1\n // half is done because there could be a case where they are not largest after incr\n // after this operation multiple times, we are left with only 1 element in the set, which we need to bring to 0\n // for the last element, refer to function solveRem\n\n multiset<long long> mst;\n for(auto i:nums) mst.insert(i);\n\n while(mst.size() > 0){\n long long n1 = *mst.rbegin(); mst.erase(mst.find(n1));\n\n if(mst.size() == 0) return (tc + solveRem(n1 , numsi.size() - 1, cost1, cost2)) % mod;\n\n long long n2 = *mst.rbegin(); mst.erase(mst.find(n2));\n long long redop = n2 / (2LL);\n if(redop == 0) redop++;\n\n tc = (tc + (redop * cost2))%mod;\n\n n1 -= redop; n2-=redop;\n if(n1) mst.insert(n1);\n if(n2) mst.insert(n2);\n }\n }\n return tc;\n }\n};\n``` | 0 | 0 | ['C++'] | 1 |
minimum-cost-to-equalize-array | [Python] All relevant preimages of cost function | python-all-relevant-preimages-of-cost-fu-txfs | Let\'s call the moves single and double. First, c2 = min(c2, 2*c1) if two single moves are cheaper than a double.\n\nSuppose the final position has every elemen | awice | NORMAL | 2024-05-05T23:39:27.605534+00:00 | 2024-05-05T23:39:27.605551+00:00 | 51 | false | Let\'s call the moves single and double. First, `c2 = min(c2, 2*c1)` if two single moves are cheaper than a double.\n\nSuppose the final position has every element equal to `height`, what will be the `cost(height)`?\n\n- Say we have a total of `volume` increments to do. If no element requires a lot of work, we could pair everything up efficiently.\n- Otherwise, some element `biggest` requires a lot of work, we can\'t clear it with just pairing it among the remaining `volume - biggest increments`, there will be `biggest - remaining` unpaired elements that require single moves.\n\nNow let\'s analyze which inputs of `cost` are sufficient to look at in order to have found the minimum of this function.\n- The inequality `biggest <= volume - biggest` reduces to `height * (N-2) >= S - 2 * mi`, so `alpha` is one inflection point.\n- If you have unpaired elements all in one column, you can make N-1 pair moves that will put a `1` in every column plus another `N-2` in one column. So then to clear that unpaired stack of `E = max(2 * biggest - volume, 0)`, you\'ll need `E / (N-2)` moves.\n- Sometimes you\'ll need to add 1 because of the extra parity for avoiding triggering a `+c1` term.\n\n# Code\n```\nclass Solution:\n def minCostToEqualizeArray(self, A: List[int], c1: int, c2: int) -> int:\n MOD = 10 ** 9 + 7\n c2 = min(c2, 2 * c1)\n N, S, mi, ma = len(A), sum(A), min(A), max(A)\n\n def cost(height):\n if height < ma:\n return inf\n \n biggest = height - mi\n volume = height * N - S\n if biggest <= volume - biggest:\n return c2 * (volume//2) + c1 * (volume%2)\n return c2 * (volume - biggest) + c1 * (2 * biggest - volume)\n\n biggest = ma - mi\n volume = ma * N - S\n if N <= 2:\n return volume * c1 % MOD\n\n alpha = (S - 2*mi) // (N - 2)\n beta = ma + ceil(max(2 * biggest - volume, 0) / (N - 2))\n return min(cost(p) for p in [ma, ma+1, alpha, beta, beta+1]) % MOD\n``` | 0 | 0 | ['Python3'] | 0 |
minimum-cost-to-equalize-array | Python Hard | python-hard-by-lucasschnee-cnbt | \nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n MOD = 10 ** 9 + 7\n INF = 10 ** 20\n | lucasschnee | NORMAL | 2024-05-05T21:19:43.771801+00:00 | 2024-05-05T21:20:07.813876+00:00 | 22 | false | ```\nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n MOD = 10 ** 9 + 7\n INF = 10 ** 20\n N = len(nums)\n mx = max(nums)\n mn = min(nums)\n c1, c2 = cost1, cost2\n\n if c2 >= 2 * c1:\n return (sum(mx - n for n in nums) * c1) % MOD\n\n total_diff = sum(mx - n for n in nums)\n\n def calc(mxx):\n total = total_diff + N * (mxx - mx)\n biggest_diff = mxx - mn\n other_sm = total - biggest_diff\n\n if biggest_diff - 1 > other_sm:\n \n extra = biggest_diff - other_sm\n return other_sm * c2 + extra * c1\n\n\n return (total // 2) * c2 + (total % 2) * c1 \n\n ans = INF\n j = mx\n while True:\n v = calc(j)\n if v < ans: # linearly decreasing\n ans = v\n j += 1\n else:\n return ans % MOD\n \n \n\n \n\n\n\n \n \n``` | 0 | 0 | ['Python3'] | 0 |
minimum-cost-to-equalize-array | Clear Solution with Easy-to-Understand and Intuitive Explanation | O(N) Time and O(1) Space | clear-solution-with-easy-to-understand-a-oyau | Intuition\n Describe your first thoughts on how to solve this problem. \n\nIn this problem, we need to equalize the nums array with either single operations or | machinescholar01 | NORMAL | 2024-05-05T19:49:04.945914+00:00 | 2024-05-05T20:57:46.340718+00:00 | 34 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nIn this problem, we need to equalize the nums array with either <b>single operations</b> or <b>pair operations</b> for an amount of <b>work = max(nums) * len(nums) - sum(nums)</b>. The amount of work is due to that we can greedily solve this problem by applying the cheapest operations to make all elements equal to the max item.\n\n* <b>Case 1</b>: If the "pair" operation costs more than twice "single" operations, we always use single operations.\n\n* <b>Case 2</b>: Since "pair" operations are cheaper, we want to apply them a maximum number of times, i.e., <b>2[max(nums) - min(nums)]</b>. Unforunately, being greedy, we might incur an <b>extra work = 2[max(nums) - min(nums)] - work</b> if this term is greater than zero. We handle the extra work in 3 different ways then take the one with the minimum cost.\n\n * <i>Scheme a</i>: Finishing extra work with only single operations.\n \n * <i>Scheme c</i> Finishing extra work ith "only" pair operations. An important fact is optimal repeated application of pair operations would eventually give an array with all equal elements except one. For example, [58, 60, 60, 60], [58, 60, 60] and [59, 60, 60, 60].\n * [58, 60, 60, 60] and [58, 60, 60] can be then continued to be equalized with only pairs. Note that the above "only" is in quotation marks because only-pair-operation scheme is <b>not</b> always possible. For instance, [59, 60, 60, 60] can not be equalized with pair operations. \n * <b>The next insight</b> is that to maximize the number of pair operations, we need to "pair-off" all the leftover work units. \n * For example [58, 60, 60, 60], the leftover work units are 2 and can be pair-off with 4 more additional work units. For example [58, 60, 60], we can pair-off with 6 additional work units. The 4 or 6 depends on whether the length of the nums array is even or odd.\n * For [59, 60, 60, 60], we can only finish off with a single operation.\n\n * <i>Scheme b</i> Finish extra work with a mixture of "pair" and "single" operations. Compared to <b>Scheme c</b>, we use single operations to finish the leftover work units after reaching the state of all equal elements except one. \n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n n = len(nums)\n mod = 10 ** 9 + 7\n work = max(nums) * n - sum(nums) # work to be done\n\n # CASE 1\n if cost1 * 2 <= cost2 or n <= 2: \n return work * cost1 % mod\n\n # CASE 2\n max_pair_ctns = (max(nums) - min(nums)) * 2 # max contribution by greedy pairing scheme\n extra_work = max(0, max_pair_ctns - work)\n\n\n # 2.a: do extra work with only single operations\n lone_ctn = extra_work\n pair_ctn = work - lone_ctn\n scha = (lone_ctn + pair_ctn % 2) * cost1 + pair_ctn // 2 * cost2 \n\n # adjust extra work for optimal pairs\n # - 2 are the item with leftover units and max item\n work += extra_work // (n - 2) * n \n \n # 2.b: do extra work with a mixture of pair and single operations \n lone_ctn = lone_ctn % (n - 2)\n pair_ctn = work - lone_ctn\n schb = (lone_ctn + pair_ctn % 2) * cost1 + pair_ctn // 2 * cost2\n\n # 2.c: do extra with maximum number pair operations\n schc = maxsize\n for i in range(2):\n work += n\n schc = min(schc, work % 2 * cost1 + work // 2 * cost2)\n \n return min(scha, schb, schc) % mod\n``` | 0 | 0 | ['Greedy', 'Python3'] | 0 |
minimum-cost-to-equalize-array | O(N) | 2 or 3 Case Discussion with some math. | on-2-or-3-case-discussion-with-some-math-cevz | Intuition\nSeveral case discussion and need some carefullness for each case. Can expand more details if needed. For now, I put some comments in the code.\n\n# C | popzkk | NORMAL | 2024-05-05T17:48:16.464868+00:00 | 2024-05-06T00:50:17.362092+00:00 | 53 | false | # Intuition\nSeveral case discussion and need some carefullness for each case. Can expand more details if needed. For now, I put some comments in the code.\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```python3\nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n n = len(nums)\n # Trivial case.\n if n == 1: return 0\n t = max(nums)\n delta = t * n - sum(nums)\n # Trivial case.\n if cost2 >= cost1 * 2 or n == 2: return delta * cost1 % 1000000007\n\n top1, top2 = t + 1, t + 2\n for num in nums:\n if num < top1: top1, top2 = num, top1\n elif num < top2: top2 = num\n top1, top2 = t - top1, t - top2\n delta -= top1 + top2\n\n if delta + top2 >= top1:\n ans = (top1 + (delta - top1 + top2) // 2) * cost2 % 1000000007\n delta = (delta - top1 + top2) % 2\n else:\n ans = (top2 + delta) * cost2 % 1000000007\n delta = top1 - top2 - delta\n if delta >= n - 2:\n ans = (ans + delta // (n - 2) * min((n - 2) * cost1, (n - 1) * cost2)) % 1000000007\n delta %= n - 2\n if delta == 0: return ans\n\n # At this point, what we are facing is equal to [0, d, d, d ...], and d < n-2.\n\n # Case#1: use pure Op#1 to make it [d, d, ...].\n case1 = delta * cost1\n # Case#2: use some Op#2 to make it [d+1, d+1, ...],\n # or [d, d+1, d+1, d+1, ...], then apply at most one Op#1.\n case2 = (delta + (n - delta) // 2) * cost2 + (n - delta) % 2 * cost1\n # Case#3, only when Case#2 involves Op#1 and we can\n # replace the only Op#1 in Case#2 with a bunch of Op#2.\n if n % 2 == 1 and delta % 2 == 0:\n case3 = (n + delta // 2) * cost2\n return (ans + min(case1, case2, case3)) % 1000000007\n return (ans + min(case1, case2)) % 1000000007\n``` | 0 | 0 | ['Math', 'Greedy', 'Python3'] | 0 |
minimum-cost-to-equalize-array | Solution By Vivek Gupta || Linear Time | solution-by-vivek-gupta-linear-time-by-t-z2iv | Youtube Explaination\n\n# Code\n\nusing lli = long long int;\nclass Solution {\npublic:\n int minCostToEqualizeArray(vector<int>& nums, int cost1, int cost2) | paardarshee | NORMAL | 2024-05-05T14:17:55.328001+00:00 | 2024-05-05T14:17:55.328029+00:00 | 57 | false | [Youtube Explaination](https://www.youtube.com/live/6N_gzgld8PI?t=2254s)\n\n# Code\n```\nusing lli = long long int;\nclass Solution {\npublic:\n int minCostToEqualizeArray(vector<int>& nums, int cost1, int cost2) {\n cost2 = min(cost2,2*cost1);\n lli n = nums.size(), minVal = 1e9,maxVal = 0,sumVal=0,finalAns=2e18;\n for(lli ele:nums){\n sumVal += ele;\n minVal = min(minVal,ele);\n maxVal = max(maxVal,ele);\n }\n for(int x = maxVal;x<=maxVal + 1e6; x++){\n lli sum = n*x - sumVal;\n lli maxi = x - minVal;\n if(maxi > (sum-maxi) ){\n lli pairs = (sum-maxi);\n lli left = maxi*2 - sum;\n finalAns = min(finalAns,pairs*cost2+left*cost1);\n }\n else{\n lli pairs = sum/2;\n lli left = sum%2;\n finalAns = min(finalAns,pairs*cost2+left*cost1);\n }\n }\n return finalAns%(1000000007);\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
minimum-cost-to-equalize-array | C++ || Bruteforce + Greedy + sorting || Easy approach | c-bruteforce-greedy-sorting-easy-approac-ft5t | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Yuvraj161 | NORMAL | 2024-05-05T12:39:01.581111+00:00 | 2024-05-05T12:39:01.581153+00:00 | 49 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(n*logn)\n\n- Space complexity: O(n)\n\n# Code\n```\nclass Solution {\npublic:\n #define ll long long\n ll mod=1e9+7;\n int minCostToEqualizeArray(vector<int>& nums, int cost1, int cost2) {\n sort(nums.begin(),nums.end());\n ll sum=0,n=nums.size(),maxi=nums.back()-nums[0],l=nums.back();\n \n for(int i=0;i<n;i++){\n sum +=l-nums[i];\n }\n\n ll ans=sum*cost1;\n for(ll i=l;i<=2*l;i++){\n if(sum-maxi<maxi){\n ans = min(ans,cost2*(sum-maxi)+ (2*maxi-sum)*cost1);\n } else {\n if((sum&1)) ans=min({ans,cost2*(sum/2) + cost1});\n else ans=min({ans,cost2*(sum/2)});\n }\n\n sum+=n;maxi++;\n }\n \n return ans%mod;\n }\n};\n``` | 0 | 0 | ['Array', 'Math', 'Sorting', 'C++'] | 0 |
minimum-cost-to-equalize-array | My Solution | my-solution-by-hope_ma-mima | \n/**\n * let `n` be the length of the vector `nums`\n * 1. find the maximum number `max_num`, minimum number `min_num` from the vector `nums`\n * 2. calculate | hope_ma | NORMAL | 2024-05-05T12:12:02.806274+00:00 | 2024-05-06T15:46:47.385704+00:00 | 9 | false | ```\n/**\n * let `n` be the length of the vector `nums`\n * 1. find the maximum number `max_num`, minimum number `min_num` from the vector `nums`\n * 2. calculate the sum `total` of the numbers of the vector `nums`\n * let\'s construct a vector `stones` of size `n`,\n * each element of which is (`max_num` - `num`),\n * where `num` is the corresponding element of the vector `nums`,\n * that is, `stones[i]` = `max_num` - `nums[i]`\n * let `max_stone` is the maximum element of the vector `stones`\n * let `total_stone` is the sum of the elements of the vector `stones`\n * \n * if `n` < 3 or `cost1` * 2 <= cost2,\n * just use the operation1 to make every element of the vector `nums` be equal to `max_num`,\n * the cost is `total_stone` * cost1\n *\n * otherwise,\n * 1. if `max_stone` > `total_stone` - `max_stone`,\n * the cost `cost` should be\n * (`total_stone` - `max_stone`) * cost2 + (`max_stone` - (`total_stone` - `max_stone`)) * cost1\n * let\'s add some value `x` to `max_stone`, then\n * `new_max_stone` = `max_stone` + `x`\n * `new_total_stone` = `total_stone` + `n` * `x`\n * 1.1 if `new_max_stone` > `new_total_stone` - `new_max_stone`, that is,\n * `max_stone` + `x` > `total_stone` + `n` * `x` - (`max_stone` + `x`), that is,\n * `x` < (2 * `max_stone` - `total_stone`) / (n - 2)\n * the cost `new_cost` should be\n * (`new_total_stone` - `new_max_stone`) * cost2 + (`new_max_stone` - (`new_total_stone` - `new_max_stone`)) * cost1\n * that is,\n * (`total_stone` + `n` * `x` - (`max_stone` + `x`)) * cost2 +\n * ((`max_stone` + `x`) - ((`total_stone` + `n` * `x`) - (`max_stone` + `x`))) * cost1\n * that is,\n * `cost` + ((`n` - 1) * cost2 + (2 - n) * cost1) * `x`\n * this value could be increasing, keeping still, or decreasing with the `x`\'s increasing\n * 1.2 if `new_max_stone` <= `new_total_stone` - `new_max_stone`, that is,\n * `x` >= (2 * `max_stone` - `total_stone`) / (n - 2)\n * the cost `new_cost` should be\n * (`new_total_stone` / 2) * cost2 + (`new_total_stone` % 2) * cost1, that is\n * ((`total_stone` + `x`) / 2) * cost2 + ((`total_stone` + `x`) % 2) * cost1\n * this value is increasing with the `x`\'s increasing when `x` keeps the odd or the even\n * 2. if `max_stone` <= `total_stone` - `max_stone`\n * the cost `cost` should be\n * (`total_stone` / 2) * cost2 + (`total_stone` % 2) * cost1\n * if some value `x` is added to `max_stone`, the conclusion is the same with `1.2`\n * so the following conclusion can be drawn,\n * the minimum cost could be one of the following cases,\n * 1. x = 0\n * 2. x = 1\n * 3. x = (2 * `max_stone` - `total_stone`) / (n - 2) - 1\n * 4. x = (2 * `max_stone` - `total_stone`) / (n - 2)\n * 5. x = (2 * `max_stone` - `total_stone`) / (n - 2) + 1\n * 6. x = (2 * `max_stone` - `total_stone`) / (n - 2) + 2\n *\n * Time Complexity: O(n)\n * Space Complexity: O(1)\n */\nclass Solution {\n public:\n int minCostToEqualizeArray(const vector<int> &nums, const int cost1, const int cost2) {\n constexpr int mod = 1000000007;\n constexpr int min_length_needed_to_use_operation2 = 3;\n const int n = static_cast<int>(nums.size());\n int min_num = numeric_limits<int>::max();\n int max_num = numeric_limits<int>::min();\n long long total = 0LL;\n for (const int num : nums) {\n min_num = min(min_num, num);\n max_num = max(max_num, num);\n total += num;\n }\n const int max_stone = max_num - min_num;\n const long long total_stone = static_cast<long long>(max_num) * n - total;\n \n if (n < min_length_needed_to_use_operation2 || (cost1 << 1) <= cost2) {\n return static_cast<int>(total_stone * cost1 % mod);\n }\n \n const int separator = ((max_stone << 1) - total_stone) / (n - 2);\n const vector<int> xs{0, 1, separator - 1, separator, separator + 1, separator + 2};\n long long ret = numeric_limits<long long>::max();\n for (const int x : xs) {\n if (x < 0) {\n continue;\n }\n\n const int new_max_stone = max_stone + x;\n const long long new_total_stone = total_stone + static_cast<long long>(n) * x;\n ret = min(ret, calculate_cost(new_max_stone, new_total_stone, cost1, cost2));\n }\n return static_cast<int>(ret % mod);\n }\n \n private:\n long long calculate_cost(const int max_stone, const long long total_stone, const int cost1, const int cost2) {\n if (max_stone > total_stone - max_stone) {\n return (total_stone - max_stone) * cost2 + (max_stone - (total_stone - max_stone)) * cost1;\n }\n return (total_stone >> 1) * cost2 + (total_stone & 1) * cost1;\n }\n};\n``` | 0 | 0 | [] | 0 |
minimum-cost-to-equalize-array | O(maxValue) simple solution | omaxvalue-simple-solution-by-dmitrii_bok-wdjo | Just go through all possible valuse from [maxValue, 2 * maxValue] and fast check each of them simple math\n\n int minCostToEqualizeArray(const vector<int>& n | dmitrii_bokovikov | NORMAL | 2024-05-05T11:26:12.251776+00:00 | 2024-05-05T11:30:39.444105+00:00 | 38 | false | Just go through all possible valuse from [maxValue, 2 * maxValue] and fast check each of them simple math\n```\n int minCostToEqualizeArray(const vector<int>& nums, int c1, int c2) {\n using ll = long long;\n constexpr ll MOD = 1\'000\'000\'007;\n const ll sum = accumulate(cbegin(nums), cend(nums), 0ll);\n const auto [minIt, maxIt] = ranges::minmax_element(nums);\n const ll maxValue = *maxIt, minValue = *minIt;\n const ll n = size(nums);\n if (2 * c1 <= c2 || size(nums) <= 2) {\n return c1 * (n * maxValue - sum) % MOD;\n }\n \n ll ans = numeric_limits<ll>::max();\n for (ll i = maxValue; i <= 2 * maxValue; ++i) {\n const ll steps = n * i - sum;\n ll res = 0;\n if (ll c = steps - i + minValue; c < steps / 2) {\n res = c * c2 + (i - minValue - c) * c1; \n } else {\n res = steps / 2 * c2 + ((steps % 2) ? c1 : 0);\n }\n ans = min(ans, res);\n }\n \n return ans % MOD;\n }\n``` | 0 | 0 | ['C++'] | 1 |
minimum-cost-to-equalize-array | Tricky Greedy Solution | tricky-greedy-solution-by-ritiksharma_09-ia9e | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\nwe use window of 4maxi due to constraint \n Describe your approach to sol | Ritiksharma_09 | NORMAL | 2024-05-05T09:42:11.861786+00:00 | 2024-05-05T09:43:28.056263+00:00 | 77 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nwe use window of 4*maxi due to constraint \n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:O(4*MAXI)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n#define ll long long\nclass Solution {\npublic:\n\nll solve(vector<int>&nums,ll c1,ll c2,ll maxi,ll mini,ll sum){\n int n=nums.size();\n ll maxdiff=maxi-mini;\n ll opneed=maxi*n-sum;\n if(2*c1<=c2){\n return opneed*c1;\n }\n ll operationinc2=opneed-maxdiff;\n if(operationinc2<maxdiff){\n return (operationinc2*c2)+(c1*(maxdiff-operationinc2));\n }\n\n ll pair=(opneed/2);\n if(opneed%2==0){\n return pair*c2;\n }\n else{\n return (pair*c2)+c1;\n }\n\n}\n int minCostToEqualizeArray(vector<int>& nums, int cost1, int cost2) {\n ll maxi=*max_element(nums.begin(),nums.end());\n ll mini=*min_element(nums.begin(),nums.end());\n ll sum=0;\n for(int i=0;i<nums.size();i++){\n sum+=nums[i];\n }\nll ans=1e18;\nll mod=1000000007;\n for(int i=maxi;i<=4*maxi;i++){\n ans=min(ans,solve(nums,cost1,cost2,i,mini,sum));\n }\n return ans%mod;\n }\n};\n``` | 0 | 0 | ['Greedy', 'C++'] | 0 |
minimum-cost-to-equalize-array | Just a runnable solution | just-a-runnable-solution-by-ssrlive-6xlb | \n\nimpl Solution {\n pub fn min_cost_to_equalize_array(nums: Vec<i32>, cost1: i32, cost2: i32) -> i32 {\n let nums = nums.iter().map(|&x| x as i64).c | ssrlive | NORMAL | 2024-05-05T05:56:34.591674+00:00 | 2024-05-05T05:56:34.591707+00:00 | 25 | false | \n```\nimpl Solution {\n pub fn min_cost_to_equalize_array(nums: Vec<i32>, cost1: i32, cost2: i32) -> i32 {\n let nums = nums.iter().map(|&x| x as i64).collect::<Vec<i64>>();\n let n = nums.len();\n let mut maxi = 0;\n let mut tot = 0;\n let mut mini = 1_000_000_000_i64;\n for &nums_i in nums.iter().take(n) {\n maxi = maxi.max(nums_i);\n mini = mini.min(nums_i);\n tot += nums_i;\n }\n\n let mut ans = 100_000_000_000_000_000;\n\n for eq in (maxi..=maxi * 2).rev() {\n let mut rem = eq * n as i64 - tot;\n let minadd = eq - mini;\n let mut curcost = 0;\n\n if minadd * 2 >= rem {\n let extra = minadd - (rem - minadd);\n curcost += extra * cost1 as i64;\n rem -= extra;\n }\n\n if rem % 2 == 1 {\n rem -= 1;\n curcost += cost1 as i64;\n }\n\n curcost += (cost1 as i64 * rem).min(cost2 as i64 * (rem / 2));\n ans = ans.min(curcost);\n }\n\n (ans % 1_000_000_007) as i32\n }\n}\n``` | 0 | 0 | ['Rust'] | 0 |
minimum-cost-to-equalize-array | JS Solution - Greedy + Math | js-solution-greedy-math-by-cutetn-ml25 | Pro tip: I LOVE JUSTINA XIE \uD83D\uDC9C\uD83D\uDC9C\uD83D\uDC9C\nYEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEE | CuteTN | NORMAL | 2024-05-05T05:33:38.820150+00:00 | 2024-05-05T16:33:14.504117+00:00 | 57 | false | Pro tip: I LOVE JUSTINA XIE \uD83D\uDC9C\uD83D\uDC9C\uD83D\uDC9C\nYEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEEET.\n\n# Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(1)$$\n\n# Code\n```js\nlet MOD = 1000000007;\n\n/**\n * @param {number[]} nums\n * @param {number} cost1\n * @param {number} cost2\n * @return {number}\n */\nvar minCostToEqualizeArray = function (nums, cost1, cost2) {\n let n = nums.length;\n cost2 = Math.min(cost2, cost1 << 1);\n\n if (n == 1) return 0;\n if (n == 2) return (Math.abs(nums[1] - nums[0]) * cost1) % MOD;\n\n let s = 0;\n let f = nums[0];\n let minTarget = f;\n for (let x of nums) {\n s += x;\n f = Math.min(f, x);\n minTarget = Math.max(minTarget, x);\n }\n let maxTarget = Math.max(minTarget, Math.floor((s - 2 * f) / (n - 2)));\n\n function yeet(m) {\n let n1 = s - 2 * f - m * (n - 2);\n if (n1 < 0) n1 = ((n1 % 2) + 2) % 2;\n let n2 = (m * n - s - n1) / 2;\n\n return cost1 * n1 + cost2 * n2;\n }\n\n return (\n Math.min(\n yeet(minTarget),\n yeet(maxTarget),\n yeet(maxTarget + 1),\n yeet(maxTarget + 2)\n ) % MOD\n );\n};\n``` | 0 | 0 | ['Math', 'Greedy', 'JavaScript'] | 0 |
minimum-cost-to-equalize-array | Javascript greedy 1318ms | javascript-greedy-1318ms-by-henrychen222-b1hk | \nconst mod = 1e9 + 7, ll = BigInt, bmod = ll(mod)\n\nconst minCostToEqualizeArray = (a, b, c) => {\n b = ll(b), c = ll(c)\n let n = ll(a.length), limit = | henrychen222 | NORMAL | 2024-05-05T05:29:06.570963+00:00 | 2024-05-05T05:29:06.570979+00:00 | 24 | false | ```\nconst mod = 1e9 + 7, ll = BigInt, bmod = ll(mod)\n\nconst minCostToEqualizeArray = (a, b, c) => {\n b = ll(b), c = ll(c)\n let n = ll(a.length), limit = 0;\n for (const x of a) limit = Math.max(limit, x);\n\n let sum = 0n, max = 0n;\n for (const x of a) {\n let remove = ll(limit - x);\n sum += remove;\n if (remove > max) max = remove;\n }\n if (n <= 2 || b * 2n <= c) return ll(sum) * ll(b) % bmod;\n\n let res = ll(1e18);\n for (let i = limit; i <= limit * 2; i++) {\n let tmp;\n if (max > sum - max) {\n tmp = (sum - max) * c;\n let rem = max - (sum - max);\n tmp += rem * b;\n } else {\n tmp = sum / 2n * c;\n if (sum % 2n != 0) tmp += b;\n }\n if (tmp < res) res = tmp;\n sum += n;\n max++;\n }\n return res % bmod;\n};\n``` | 0 | 0 | ['Math', 'Greedy', 'JavaScript'] | 0 |
minimum-cost-to-equalize-array | Python solution, fairly simple | python-solution-fairly-simple-by-treehou-owy7 | Intuition\nFirst sort nums. Now we\'re trying to get all the values to max(nums). Think of it as filling holes. For example if the smallest num is 3 and max is | TreeHouseCoding | NORMAL | 2024-05-05T05:25:51.104073+00:00 | 2024-05-05T06:16:35.349252+00:00 | 67 | false | # Intuition\nFirst sort nums. Now we\'re trying to get all the values to max(nums). Think of it as filling holes. For example if the smallest num is 3 and max is 10, then we need to fill 7 holes to get that to target.\n\nIf there are at least three elements in nums and the number of holes for the leftmost (smallest) number is less than or equal to the holes for all the other numbers combined, then we can just max out our cost2 additions and possibly use a single cost1 addition for parity.\n\nHowever, we want to choose other targets than just max(nums) since we want to allow the possibility that it\'s better to overshoot if we get to use less cost1 additions (by increasing cost2 additions). I used the following to figure out how much to add to max(nums) to choose other targets. To max out cost2 additions, we can try to get the leftmost holes to be smaller than the rest of the holes:\nleftholes + n <= restholes + n * (len(nums) - 1)\n==>\nn >= (leftholes - restholes) / (len(nums) - 2)\n\nHowever we want to actually round down because we don\'t want to overshoot\nn = (leftholes - restholes) // (len(nums) - 2)\n\nNote that if we increase n by 1 or by 2, we\'re guaranteed to be able to use only cost2 additions (since we\'re guaranteed to be filling up an even number of holes in at least one of the cases). So also try those values as well.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python\n\nclass Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n MOD = 10**9 + 7\n if len(nums)==1:\n return 0\n if len(nums)==2 or cost2 >= cost1 * 2:\n return (max(nums) * len(nums) - sum(nums)) * cost1 % MOD\n nums = sorted(nums)\n maxn = nums[-1]\n left, rest = nums[0], sum(nums[1:])\n leftholes = maxn - left\n restholes = (maxn * (len(nums) - 1) - rest)\n n_add = max(0, (leftholes - restholes)) // (len(nums) - 2)\n targets = [maxn, maxn + n_add, maxn + n_add + 1, maxn + n_add + 2]\n cost = inf\n for target in targets:\n leftholes = target - left\n restholes = (target * (len(nums) - 1) - rest)\n if leftholes <= restholes:\n cost2_adds = (leftholes + restholes) // 2\n cost1_adds = (leftholes + restholes) % 2\n else:\n cost2_adds = restholes\n cost1_adds = leftholes - restholes\n cost = min(cost, cost2_adds * cost2 + cost1_adds * cost1)\n return cost % MOD\n``` | 0 | 0 | ['Python3'] | 0 |
minimum-cost-to-equalize-array | java solution | java-solution-by-prakharvsrivastava-vk7t | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | prakharvsrivastava | NORMAL | 2024-05-05T05:13:34.986272+00:00 | 2024-05-05T05:13:34.986305+00:00 | 101 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n private static final int MOD = (int)1e9 + 7;\n public int minCostToEqualizeArray(int[] nums, int cost1, int cost2) {\n Arrays.sort(nums);\n int n = nums.length;\n if (n == 1) return 0;\n if (n == 2) return (int)((long)(nums[1] - nums[0]) * cost1 % MOD);\n if (cost2 >= cost1 * 2) {\n long res = 0;\n for (int num: nums) {\n res = (res + (long)(nums[n-1] - num) * cost1) % MOD;\n }\n return (int)res;\n }\n long sum = 0;\n long max = 0;\n for (int num: nums) {\n sum += nums[n-1] - num;\n max = Math.max(max, nums[n-1] - num);\n }\n long res = Long.MAX_VALUE;\n for (int k = nums[n-1]; k <= nums[n-1] + 2; k++) {\n res = Math.min(res, minCost(sum + (k-nums[n-1]) * n, max + (k-nums[n-1]), cost1, cost2));\n }\n if (max > sum - max) {\n for (int k = (int)((2 * max - sum) / (n-2)); k <= (int)((2 * max - sum) / (n-2)) + 2; k++) {\n res = Math.min(res, minCost(sum + k * n, max + k, cost1, cost2));\n }\n }\n return (int)(res % MOD);\n }\n private long minCost(long sum, long max, int cost1, int cost2) {\n long res = 0;\n if (sum % 2 == 1) {\n res += cost1;\n max--;\n sum--;\n }\n if (max <= sum - max) {\n res = (res + sum / 2 * cost2);\n return res;\n }\n res = (res + (max - (sum-max)) * cost1);\n res = (res + (sum-max) * cost2);\n return res;\n }\n}\n``` | 0 | 0 | ['Array', 'Java'] | 0 |
minimum-cost-to-equalize-array | [Go] Greedy algorithm (106 ms) | go-greedy-algorithm-106-ms-by-andvt-r2ej | Intuition\nhttps://leetcode.com/problems/minimum-cost-to-equalize-array/submissions/1249630464/\n\n# Approach\n Describe your approach to solving the problem. \ | AnDVT | NORMAL | 2024-05-05T04:43:26.082516+00:00 | 2024-05-05T04:43:26.082543+00:00 | 77 | false | # Intuition\nhttps://leetcode.com/problems/minimum-cost-to-equalize-array/submissions/1249630464/\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nconst mo7 = 1000000007\nfunc abs(x int) int {\n if x > 0 {\n return x\n }\n return -x\n}\n\nfunc minCostToEqualizeArray(nums []int, cost1 int, cost2 int) int {\n if len(nums) == 2 {\n return abs(nums[0]-nums[1]) * cost1 % mo7\n }\n maxNum := 0\n for _, v := range nums {\n maxNum = max(maxNum, v)\n }\n l := []int{}\n maxL := 0\n for _, v := range nums {\n vl := maxNum - v\n maxL = max(maxL, vl)\n l = append(l, vl)\n }\n sum := 0\n for _, v := range l {\n sum += v\n }\n // fmt.Println(sum)\n if cost1*2 <= cost2 || len(l) == 1 {\n return sum * cost1 % mo7\n }\n res := math.MaxInt64\n fmt.Println(res)\n rest := sum - maxL\n newRes := 0\n if maxL >= rest {\n newRes = (maxL - rest) * cost1 + rest*cost2\n } else {\n sum = rest + maxL\n newRes = sum/2*cost2 + (sum%2)*cost1\n }\n for res > newRes {\n res = newRes\n maxL += 1\n rest += len(l)-1\n if maxL >= rest {\n newRes = (maxL - rest) * cost1 + rest*cost2\n } else {\n sum = rest + maxL\n newRes = sum/2*cost2 + (sum%2)*cost1\n }\n }\n return res % mo7\n}\n``` | 0 | 0 | ['Go'] | 0 |
minimum-operations-to-make-character-frequencies-equal | It's no more hard if you read this solution | its-no-more-hard-if-you-read-this-soluti-lgvj | Intuition
Count Character Frequencies – Analyze how frequently each character appears.
Try Different Targets – Assume that the final "good" string will have cha | senorita143 | NORMAL | 2024-12-22T15:26:16.112850+00:00 | 2024-12-22T15:26:16.112850+00:00 | 1,287 | false | # Intuition
1. Count Character Frequencies – Analyze how frequently each character appears.
2. Try Different Targets – Assume that the final "good" string will have characters appearing at some uniform frequency (target).
3. Minimize Operations – For each target, calculate the minimum number of deletions/insertions needed to adjust character frequencies.
4. Memoization – Cache results of subproblems to avoid redundant calculations and improve efficiency.
# Approach
**STEP-1**: Count Character Frequencies – Track how often each character appears.
**STEP-2**: Dynamic Programming – Use a recursive function f(i, target, deleted) to adjust character frequencies by:
1. if frequency==target or frequency:
- Jump to next character
2. if(frequency>target):
- Deletion
3. else:
- Minimum(Insertion,Deletion,Changing)
[NOTE: Changing can be if deletion of previous character and insertion of current character can be taken as a single operation]
**STEP-3**: Memoization – Store results to avoid recalculating the same subproblems.
**STEP-4**: Iterate Over Targets – Try all target frequencies (from 0 to max frequency) and pick the one that minimizes operations.
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O($$ -->
O(26*T)(where T is the max character frequency).
- Space complexity:
O(26*T)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def makeStringGood(self, s: str) -> int:
count=[0]*26
for i in s:
count[ord(i)-ord('a')]+=1
@cache
def f(i,target,deleted):
if(i==26):
return 0
x=count[i]
if(x==target or x==0):
return f(i+1,target,0)
if(x>target):
#Adjusting to Target if has greater frequency
delete=x-target+f(i+1,target,x-target)
return delete
else:
need = target-x
#Increasing frequency towards target
insert = f(i+1,target,0)+need
#Drop to zero
delete = f(i+1,target,x)+x
#Changing ch-1 character to ch like deleting ch-1 character and incrementing ch character is far more better than converting ch-1 to ch
change = f(i+1,target,0)+ (need-min(need,deleted))
return min(insert,delete,change)
mini=float('inf')
for i in range(0,max(count)+1):
mini=min(mini,f(0,i,0))
return mini
```
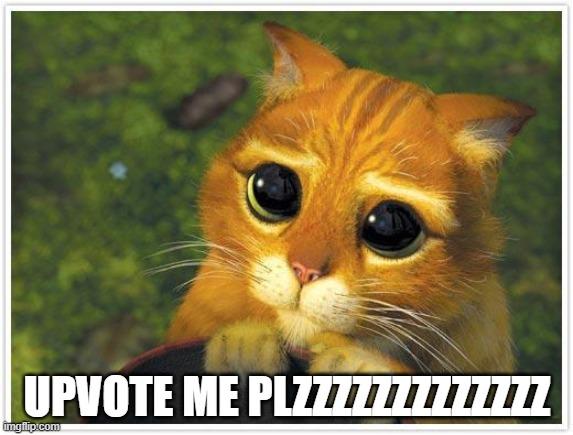
| 25 | 1 | ['Hash Table', 'Dynamic Programming', 'Python3'] | 8 |
minimum-operations-to-make-character-frequencies-equal | [Python] DP | python-dp-by-awice-kkdr | Count the characters and say all characters in the final string have common frequency target. Then all characters have count either 0 or target. Also, a chara | awice | NORMAL | 2024-12-15T04:46:43.451931+00:00 | 2024-12-15T04:46:43.451931+00:00 | 1,550 | false | Count the characters and say all characters in the final string have common frequency `target`. Then all characters have count either `0` or `target`. Also, a character is never moved 2 or more times, otherwise you could just delete and insert it.\n\nSo compared to only being allowed to insert/delete characters, cost savings happen only if you make an insertion immediately following a deletion. For example, if you have `count[\'a\']` deleted `3` times and `count[\'b\']` increased `5` times, then you can save `min(3, 5)` moves.\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n count = [0] * 26\n for c in s:\n count[ord(c) - ord("a")] += 1\n\n @cache\n def dp(i, deleted, target):\n if i == 26:\n return 0\n\n x = count[i]\n if x <= target:\n ans = dp(i + 1, x, target) + x\n increase = min(deleted, target - x)\n cand = dp(i + 1, 0, target) + target - x - increase\n ans = min(ans, cand)\n else:\n ans = dp(i + 1, x - target, target) + x - target\n return ans\n\n return min(dp(0, 0, target) for target in range(max(count) + 1))\n``` | 19 | 1 | ['Python3'] | 5 |
minimum-operations-to-make-character-frequencies-equal | Those who know 👀 | those-who-know-by-etian6795-q9nd | IntuitionTry every frequencyApproachUse DP to find the min operations to get all characters to the current frequencydp1 is min ops if previous character was del | fluffyunicorn1125 | NORMAL | 2024-12-15T04:12:12.651306+00:00 | 2024-12-15T04:23:58.672735+00:00 | 1,784 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTry every frequency\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUse DP to find the min operations to get all characters to the current frequency\n\ndp1 is min ops if previous character was deleted\ndp2 is min ops if previous character was added to\nfree is the number of free operations if the previous character was deleted (using op 3)\n\nIf the character frequency is greater than the current frequency,\nadd the difference to min of previous dp\n\nIf the character frequency is less than the current frequency, either delete all of current character, or add characters until we get the frequency either using dp1 and free, or just dp2\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public int makeStringGood(String s) {\n int n = s.length();\n int[] f = new int[26];\n for(int i = 0; i < n; i++) f[s.charAt(i)-\'a\']++;\n\n int ans = n;\n for(int m = 1; m <= n; m++){\n int res = 0;\n int dp1 = 0;\n int dp2 = 0;\n int free = 0;\n for(int i = 0; i < 26; i++){\n int ndp1, ndp2, nfree;\n if(f[i] >= m){\n ndp1 = Math.min(dp1, dp2) + (f[i] - m);\n ndp2 = n;\n nfree = f[i] - m;\n }\n else{\n ndp1 = Math.min(dp1, dp2) + f[i];\n ndp2 = Math.min(dp1 + Math.max(0, m - f[i] - free), dp2 + m - f[i]);\n nfree = f[i];\n }\n dp1 = ndp1;\n dp2 = ndp2;\n free = nfree;\n }\n res = Math.min(dp1, dp2);\n ans = Math.min(ans, res);\n }\n return ans;\n }\n}\n``` | 11 | 2 | ['Java'] | 1 |
minimum-operations-to-make-character-frequencies-equal | C++, brute force final good string character count | c-brute-force-final-good-string-characte-knlg | IntuitionIterate over each possible character count value in the final good string.
Let dp[j] be the number of operations to make the string good from character | chasey | NORMAL | 2024-12-15T14:31:13.415933+00:00 | 2024-12-15T14:31:13.415933+00:00 | 462 | false | # Intuition\nIterate over each possible character count value in the final good string.\nLet dp[j] be the number of operations to make the string good from character j to \'z\'.\n\nGoing backwards from \'z\' to \'a\' for each character you have three options:\n1. Remove all characters:\ndp[j] = c + dp[j+1])\n2. Make characters size equal to target:\ndp[j] = abs(count[j]-target) + dp[j+1]\n3. Pass some amount of it to the next character (It only makes sense if the next character count is less than target. It doens\'t make sense to pass it so that the next character will be over target)\na) If the current amount less than target move all of it\nb) If the current amount greater than target move only extra count\nll moveNext = count[j] > target ? count[j]-target : count[j]\ndp[j] = moveNext + max(target-count[j+1]-moveNext, 0) + dp[j+2] \n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n*26)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```cpp []\n#define ll int\n#define REP(i,n) FOR(i,0,n)\n#define SORT(v) sort((v).begin(),(v).end())\n#define FOR(i,a,b) for(ll i=(a);i<(b);i++)\n\nclass Solution {\npublic:\n int makeStringGood(string s) {\n vector<ll> count(26, 0); for (auto c : s) count[c-\'a\']++;\n ll mx = 0; for (auto x : count) mx = max(x, mx);\n ll res = INT_MAX;\n FOR(target, 1, mx+1) {\n vector<ll> dp(27, 0);\n for (ll j = 25; j >=0; j--) {\n dp[j] = count[j] + dp[j+1]; // delete all \n dp[j] = min(dp[j], abs(count[j]-target) + dp[j+1]); // insert or remove to have exactly target\n ll moveNext = count[j] > target ? count[j]-target : count[j]; // move next\n if (j+1 < 26 && count[j+1] < target)\n dp[j] = min(dp[j], moveNext + max(target-count[j+1]-moveNext, 0) + dp[j+2]); \n }\n res = min(res, dp[0]);\n }\n return res;\n }\n};\n``` | 5 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | [C++] DP Solution | c-dp-solution-by-junbinliang-il18 | Brief Idea :
We can think about the problem as we have an array of 26 piles of stones, in 1 operation you can either increase/decrease each piles by 1, or decre | junbinliang | NORMAL | 2024-12-15T04:17:44.775548+00:00 | 2024-12-15T04:21:12.006175+00:00 | 1,084 | false | Brief Idea :\n1. We can think about the problem as we have an array of 26 piles of stones, in 1 operation you can either increase/decrease each piles by 1, or decrease by 1 and increase the next pile by 1. You need to make them all euqal.\n2. There is no need to use the move to adjecent operation more than once. You only change \'a\' to \'b\' but would not do \'a\' to \'c\'.\n3. Use dp and fix the frequency as `k`. You can either make the current number to k or 0. If you move some to next, dont\' move it again.\n\n```\nconst int INF = 1e6;\nclass Solution {\npublic:\n int makeStringGood(string s) {\n int n = s.size();\n vector<int> a(26);\n for(char c : s) {\n a[c - \'a\']++;\n }\n \n int ans = n;\n for(int x : a) {\n ans = min(ans, n - x);\n }\n\n //The most operations needed\n for(int k = 1; k <= n; k++) { //average frequency\n vector<int> dp(26, -1);\n ans = min(ans, dfs(dp, a, k, 0));\n }\n \n return ans;\n }\n \n int dfs(vector<int>& dp, vector<int>& a, int k, int i) {\n if(i >= a.size()) {\n return 0;\n }\n \n if(dp[i] != -1) {\n return dp[i];\n }\n \n int ans = INF;\n if(a[i] >= k) {\n int more = a[i] - k;\n ans = min(ans, a[i] - k + dfs(dp, a, k, i + 1));\n if(i + 1 < a.size() && a[i + 1] <= k) {\n if(a[i + 1] + more >= k) {\n ans = min(ans, more + dfs(dp, a, k, i + 2));\n } else {\n //move all\n ans = min(ans, min(more + k - (a[i + 1] + more), more + a[i + 1]) + dfs(dp, a, k, i + 2)); \n }\n }\n \n } else {\n ans = min(ans, min(k - a[i], a[i]) + dfs(dp, a, k, i + 1));\n if(i + 1 < a.size() && a[i + 1] <= k) {\n if(a[i + 1] + a[i] >= k) {\n ans = min(ans, a[i] + dfs(dp, a, k, i + 2));\n } else {\n ans = min(ans, a[i] + (k - (a[i] + a[i + 1])) + dfs(dp, a, k, i + 2));\n }\n }\n }\n \n return dp[i] = ans;\n }\n};\n``` | 5 | 0 | ['C++'] | 1 |
minimum-operations-to-make-character-frequencies-equal | Heavily Commented Code using Dynamic Programming | heavily-commented-code-using-dynamic-pro-90r9 | Well yet again I was unable to solve the 4th Question so here is the most understandable beautiful solution I could find from the leaderboard.
This solution is | LeadingTheAbyss | NORMAL | 2024-12-15T05:04:00.165521+00:00 | 2024-12-15T05:04:19.650237+00:00 | 513 | false | - Well yet again I was unable to solve the 4th Question so here is the most understandable beautiful solution I could find from the leaderboard.\n- This solution is by [h_bugw7](https://leetcode.com/u/h_bugw7/) , so all credits to them for this beautiful Solution.\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int makeStringGood(string s) {\n // Initialize a count array for each character in the alphabet\n int cnt[26] = {0};\n \n // Count the frequency of each character in the string\n for (char c : s) cnt[c - \'a\']++;\n \n // Define the range of n (substrings\' length) from 1 to the length of the string\n int l = 1;\n int r = s.length();\n \n // Initialize the result with a large value, as we are looking for a minimum\n int res = INT_MAX;\n\n // Loop through all possible lengths of substrings (from 1 to n)\n for (int n = l; n <= r; n++) {\n // dp[i][j] stores the minimum number of changes required for the first i letters of the alphabet\n // j = 0: when the character is not included in the substring of length n\n // j = 1: when the character is included in the substring of length n\n int dp[27][2] = {0}; // dp for 26 letters + extra index for base case\n // cy[i][j] stores the number of characters that have been used in the conversion\n int cy[27][2] = {0};\n \n // Loop through all characters of the alphabet (0 to 25 for \'a\' to \'z\')\n for (int i = 0; i < 26; i++) {\n // For the case where the character is not included in the substring of length n\n dp[i + 1][0] = min(dp[i][0], dp[i][1]) + cnt[i];\n cy[i + 1][0] = cnt[i];\n \n // If the current character\'s frequency is greater than or equal to n, it can be included\n if (cnt[i] >= n) {\n dp[i + 1][1] = min(dp[i][0], dp[i][1]) + cnt[i] - n;\n cy[i + 1][1] = cnt[i] - n;\n } else {\n // If the current character\'s frequency is less than n, we have to make up for the deficit\n dp[i + 1][1] = min(\n dp[i][0] + max(0, n - cnt[i] - cy[i][0]), // Case where we take from the previous non-included state\n dp[i][1] + max(0, n - cnt[i] - cy[i][1]) // Case where we take from the previous included state\n );\n cy[i + 1][1] = 0; // Reset the cycle count for this state\n }\n }\n \n // Update the result with the minimum number of changes needed for the current substring length n\n res = min(res, min(dp[26][0], dp[26][1]));\n }\n\n // Return the final result, which is the minimum number of changes required\n return res;\n }\n};\n\n``` | 4 | 0 | ['Dynamic Programming', 'C++'] | 1 |
minimum-operations-to-make-character-frequencies-equal | O(26^2) | memoization of a recursive solution for freq balancing using an ordered map | o262-memoization-of-a-recursive-solution-23jq | Complexity
Time complexity: O(262)
Space complexity: O(263)
Code | lokeshwar777 | NORMAL | 2025-01-09T06:50:30.097702+00:00 | 2025-01-09T06:50:30.097702+00:00 | 181 | false | # Complexity
- Time complexity: $$O(26^2)$$
- Space complexity: $$O(26^3)$$
# Code
```cpp []
// curfreq-current frequency, expfreq-expected frequency,
// ulimit-upper limit, prevops- previous operations
class Solution {
private:
int freq[26];
map<tuple<int,int,int>,int>dp;
int getMinOps(int i,int expfreq,int prevops){
if(i==26)return 0;
tuple<int,int,int>key=make_tuple(i,expfreq,prevops);
if(dp.find(key)!=dp.end())return dp[key];
int curfreq=freq[i];
// reset prevops
if(curfreq==0||curfreq==expfreq)return dp[key]=getMinOps(i+1,expfreq,0);
// deleting some chars
int curops=curfreq-expfreq;
if(curfreq>expfreq) return dp[key]=curops+getMinOps(i+1,expfreq,curops);
// take advantage of previous operations
if((curfreq+prevops)>=expfreq)return dp[key]=getMinOps(i+1,expfreq,0);
// inserting some chars or deleting all of chars and taking advantage in the next freq
return dp[key]=min(curfreq+getMinOps(i+1,expfreq,curfreq),(expfreq-(curfreq+prevops))+getMinOps(i+1,expfreq,0));
}
public:
int makeStringGood(string s) {
int n=s.size();
for(char c:s)freq[c-'a']++;
int ulimit=*max_element(freq,freq+26);
int minops=n-ulimit;
for(int target=0;target<=ulimit;++target){
minops=min(minops,getMinOps(0,target,0));
}
return minops;
}
};
``` | 3 | 0 | ['Hash Table', 'String', 'Dynamic Programming', 'Recursion', 'Counting', 'C++'] | 1 |
minimum-operations-to-make-character-frequencies-equal | C++ DP Recursive & 4ms Tabulation (2 Solutions) | c-dp-recursive-tabulations-2-solutions-b-4xzq | 0ms Submission LinkDP State: [char, hasSaveFromPreviousChar]Complexity
Time complexity: O(n∗26∗2)=O(n)
Space complexity: O(1) (tabulation)
CodeVersion 1: R | bramar2 | NORMAL | 2024-12-18T09:07:59.554400+00:00 | 2024-12-18T09:11:14.884936+00:00 | 431 | false | 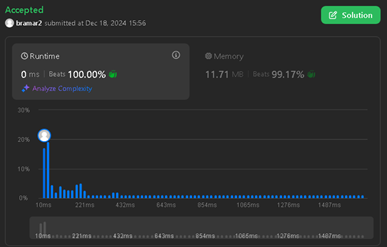\n[0ms Submission Link](https://leetcode.com/problems/minimum-operations-to-make-character-frequencies-equal/submissions/1481861167/)\n\n**DP State: [char, hasSaveFromPreviousChar]**\n\n# Complexity\n- Time complexity: $O(n*26*2) = O(n)$\n\n- Space complexity: $O(1)$ (tabulation)\n\n# Code\n\n---\n## Version 1: Recursive\n```cpp [Recursive]\n#include <bits/stdc++.h>\nusing namespace std;\n\nclass Solution {\npublic:\n int makeStringGood(const string& s) {\n int n = s.size();\n int freq[26] {};\n \tfor(char c : s) freq[c-\'a\']++;\n \tint ans = n;\n for(int x = 1; x <= n; x++) {\n vector memo(26, vector(2, -1));\n auto go = [&](this auto&& go, int ch, bool hasSave) -> int {\n if(ch >= 26) return 0;\n if(memo[ch][hasSave] >= 0) return memo[ch][hasSave];\n if(freq[ch] == 0) return memo[ch][hasSave] = go(ch + 1, false);\n int ans = INT_MAX;\n if(!hasSave) {\n if(freq[ch] >= x) {\n // del until freq[ch] = x\n ans = min(ans, (freq[ch] - x) + go(ch + 1, true));\n }else {\n // del until freq[ch] = 0\n ans = min(ans, freq[ch] + go(ch + 1, true));\n // insert until freq[ch] = x\n ans = min(ans, (x - freq[ch]) + go(ch + 1, false));\n }\n }else {\n if(freq[ch] >= x) {\n // del until freq[ch] = x\n ans = min(ans, (freq[ch] - x) + go(ch + 1, true));\n }else {\n // del until freq[ch] = 0\n ans = min(ans, freq[ch] + go(ch + 1, true));\n\n int save = freq[ch - 1];\n if(save >= x) save -= x;\n // using save from previous, insert until freq[ch] = x\n ans = min(ans, (x - min(freq[ch] + save, x)) + go(ch + 1, false));\n }\n }\n return memo[ch][hasSave] = ans;\n };\n ans = min(ans, go(0, false));\n }\n return ans;\n }\n};\nauto init = [](){ cin.tie(0)->sync_with_stdio(0); return true; }();\n```\n---\n## Version 2: Tabulation\n```cpp []\n#include <bits/stdc++.h>\nusing namespace std;\nint dp[27][2];\nclass Solution {\npublic:\n int makeStringGood(const string& s) {\n int n = s.size();\n int freq[26] {};\n for(char c : s) freq[c-\'a\']++;\n int ans = n;\n for(int x = 1, mx = *max_element(freq, freq + 26); x <= mx; x++) {\n for(int ch = 25; ch >= 0; ch--) {\n for(int hasSave = 0; hasSave <= 1; hasSave++) {\n if(ch == 0 && hasSave == 1) break;\n if(freq[ch] == 0) {\n dp[ch][hasSave] = dp[ch + 1][false];\n continue;\n }\n if(freq[ch] >= x) {\n // del until freq[ch] = x\n dp[ch][hasSave] = (freq[ch] - x) + dp[ch + 1][true];\n }else {\n // del until freq[ch] = 0\n dp[ch][hasSave] = (freq[ch]) + dp[ch + 1][true];\n int save = (hasSave) ? freq[ch - 1] : 0;\n if(save >= x) save -= x;\n // using save from previous (if has it), insert until freq[ch] = x\n dp[ch][hasSave] = min(dp[ch][hasSave],\n (x - min(freq[ch] + save, x)) + dp[ch + 1][false]);\n }\n }\n }\n ans = min(ans, dp[0][false]);\n }\n return ans;\n }\n};\n\nauto init = [](){ cin.tie(0)->sync_with_stdio(0); dp[26][0] = dp[26][1] = 0; return true; }();\n``` | 3 | 0 | ['String', 'Dynamic Programming', 'C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | O(n) 28 ms | on-28-ms-by-votrubac-b9du | We count characters using cnt, and consider all character frequencies within [min(cnt), max(cnt)] range.\n\n> It can be shown that we do not need to consider fr | votrubac | NORMAL | 2024-12-15T07:30:18.430488+00:00 | 2024-12-15T08:34:26.229767+00:00 | 197 | false | We count characters using `cnt`, and consider all character frequencies within `[min(cnt), max(cnt)]` range.\n\n> It can be shown that we do not need to consider frequencies outside of that range. \n\nThen, for each frequency, we can compute the minimum number of operations in a linear time (26 * 2) using take-not take DP.\n\n### Transition Function\n`dp[i][take]` is the minimum number of operations to make the frequency of the first `i` characters either zero or `freq`.\n\nWhen `take` is true, the frequency of the previos (`i - 1`) character is `freq`, or zero otherwise.\n\nThus, the `take` parameter determines how many characters we can `borrow`, if needed, from the previous character. \n\nTo compute the current state, we take the minimum of two operations:\n- zero the frequency.\n- make the frequency equal `freq`.\n\t- for this operation, we can `borrow` available previous characters. \n\n**C++**\n```cpp\nint dp[26][2], cnt[26] = {}, res = INT_MAX;\nint dfs(int i, bool take, int freq) {\n if (i == 26)\n return 0;\n if (dp[i][take] < 0) {\n int borrow = i ? (take ? max(0, cnt[i - 1] - freq) : cnt[i - 1]) : 0; \n if (cnt[i] <= freq)\n dp[i][take] = min(cnt[i] + dfs(i + 1, false, freq), \n max(0, freq - cnt[i] - borrow) + dfs(i + 1, true, freq));\n else\n dp[i][take] = cnt[i] - freq + dfs(i + 1, true, freq);\n }\n return dp[i][take];\n}\nint makeStringGood(string s) {\n for (char ch : s)\n ++cnt[ch - \'a\'];\n auto [min_p, max_p] = minmax_element(begin(cnt), end(cnt));\n for (int freq = *min_p; freq <= *max_p; ++freq) {\n memset(dp, -1, sizeof(dp));\n res = min(res, dfs(0, 0, freq));\n }\n return res;\n}\n``` | 2 | 0 | [] | 0 |
minimum-operations-to-make-character-frequencies-equal | C++ dp memo with intuition of dp memo explanation | c-dp-memo-with-intuition-of-dp-memo-expl-wnp9 | IntuitionTry to find the optimal frequency each character should be with a loop. Use DP memo to find the amount of moves required for each frequency in O(1).For | user5976fh | NORMAL | 2024-12-15T07:07:30.171096+00:00 | 2024-12-15T07:12:26.541854+00:00 | 227 | false | # Intuition\nTry to find the optimal frequency each character should be with a loop. Use DP memo to find the amount of moves required for each frequency in O(1).\n\nFor dp memo, after taking a look at other discussion posts I figured out the logic behind why I could memoize based on current index and a boolean value representing if I could use the previous index for a donation.\n\nThe trick was that if canUsePrev is true, I could use the previous column for a donation, and I could infer how much I could donate to the current column based on if the previous column was > t. If prev was < t that means we deleted it to allow for a donation; if it is > t that means we got rid of the difference (prev - t) to allow for a donation.\n\nThe donation should never occur past 1 column as once we reach 2 columns the cost is the same as deleting and replacing, which allows for the dp memo code to be simple.\n# Code\n```cpp []\nclass Solution {\npublic:\n vector<int> v;\n \n int findCost(int t) {\n vector<vector<int>> dp(26, vector<int>(2, -1));\n return dfs(t, dp, 0, 0);\n }\n\n int dfs(int t, vector<vector<int>>& dp, int i, bool canUsePrev){\n if (i == v.size()) return 0;\n if (dp[i][canUsePrev] == -1){\n int ans = dfs(t, dp, i + 1, 1) + v[i];\n if (v[i] < t && canUsePrev){\n int canTake = v[i - 1] > t ? v[i - 1] - t : v[i - 1];\n ans = min(ans, dfs(t, dp, i + 1, 0) + t - min(t, v[i] + canTake));\n }\n else{\n if (v[i] > t){\n ans = min(ans, dfs(t, dp, i + 1, 1) + v[i] - t);\n }\n else{\n ans = min(ans, dfs(t, dp, i + 1, 0) + t - v[i]);\n }\n }\n\n dp[i][canUsePrev] = ans;\n }\n return dp[i][canUsePrev];\n\n }\n\n int makeStringGood(string s) {\n v.resize(26);\n int l = 1, r = 0;\n for (auto& c : s)\n r = max(r, ++v[c - \'a\']);\n int ans = INT_MAX;\n for (int i = 1; i <= r; ++i)\n ans = min(ans, findCost(i));\n return ans;\n }\n};\n``` | 2 | 0 | ['C++'] | 1 |
minimum-operations-to-make-character-frequencies-equal | BEATS 100% || Java Solution || Optimum Solution | beats-100-java-solution-optimum-solution-9io9 | Complexity
Time complexity: O(N + K)
Space complexity: O(1)
Code | yallavamsipavan1234 | NORMAL | 2024-12-15T04:57:54.265280+00:00 | 2024-12-15T04:57:54.265280+00:00 | 508 | false | # Complexity\n- Time complexity: `O(N + K)`\n\n- Space complexity: `O(1)`\n\n# Code\n```java []\nclass Solution {\n public int makeStringGood(String s) {\n char[] str = s.toCharArray();\n int n = str.length;\n int[] cnt = new int[26];\n for (char c : str) {\n cnt[c - \'a\']++;\n }\n int mn = n, mx = 0;\n for (int c : cnt) {\n if (c > 0) {\n mn = Math.min(mn, c);\n mx = Math.max(mx, c);\n }\n }\n if (mn == mx) {\n return 0;\n }\n int[][] dp = new int[26][2];\n int ans = n - 1;\n for (int i = mn; i <= mx; i++) {\n dp[0][0] = cnt[0];\n dp[0][1] = cnt[0] <= i ? i - cnt[0] : cnt[0] - i;\n for (int j = 1; j < 26; j++) {\n dp[j][0] = Math.min(dp[j - 1][0], dp[j - 1][1]) + cnt[j];\n if (cnt[j] >= i) {\n dp[j][1] = Math.min(dp[j - 1][0], dp[j - 1][1]) + (cnt[j] - i);\n } else {\n dp[j][1] = Math.min(dp[j - 1][0] + i - Math.min(i, cnt[j] + cnt[j - 1]), dp[j - 1][1] + i - Math.min(i, cnt[j] + Math.max(0, cnt[j - 1] - i)));\n }\n }\n ans = Math.min(ans, Math.min(dp[25][0], dp[25][1]));\n }\n return ans;\n }\n}\n``` | 2 | 0 | ['Array', 'Math', 'String', 'Dynamic Programming', 'Greedy', 'Matrix', 'Counting', 'Java'] | 1 |
minimum-operations-to-make-character-frequencies-equal | [C++] DP | c-dp-by-projectcoder-qih6 | Let f(i, 0/1/2) represent the minimum cost when considering the first i elements and applying operation 0/1/2 (delete all/fill the deficit/transfer the excess) | projectcoder | NORMAL | 2024-12-15T06:40:07.979697+00:00 | 2024-12-15T06:40:07.979729+00:00 | 80 | false | Let f(i, 0/1/2) represent the minimum cost when considering the first i elements and applying operation 0/1/2 (delete all/fill the deficit/transfer the excess) to element i:\n\n f(i,0) = min{ f(i-1,0), f(i-1,1), f(i-1,2) } + ai\n f(i,1) = min{ f(i-1,0) + max(avg-aj-ai,0), f(i-1,1) + (avg-ai), f(i-1,2) + max(avg-(aj-avg)-ai,0) }\n f(i,2) = min{ f(i-1,0) + f(i-1,1) + f(i-1,2) } + (ai-avg) \n\t\n**CODE:**\n```\nclass Solution {\npublic:\n int makeStringGood(string s) { int n = s.size();\n int a[110] = {0};\n for (auto& ch : s) a[ch-\'a\'+1]++;\n\n int f[30][3];\n\n int ans = 0x3f3f3f3f, inf = 0x3f3f3f3f;\n for (int avg = 1; avg <= n; avg++) {\n memset(f, 0x3f, sizeof(f));\n f[0][0] = 0;\n for (int i = 1; i <= 26; i++) {\n f[i][0] = min({f[i-1][0], f[i-1][1], f[i-1][2]}) + a[i] ;\n f[i][1] = a[i] > avg ? inf : min({f[i-1][0]+max(avg-a[i-1]-a[i],0), f[i-1][1]+(avg-a[i]), f[i-1][2]+max(avg-(a[i-1]-avg)-a[i],0)});\n f[i][2] = a[i] < avg ? inf : min({f[i-1][0], f[i-1][1], f[i-1][2]}) + (a[i]-avg) ;\n }\n ans = min({ans, f[26][0], f[26][1], f[26][2]});\n }\n return ans;\n }\n};\n``` | 1 | 0 | [] | 0 |
minimum-operations-to-make-character-frequencies-equal | How it feels working with legacy codebase... | how-it-feels-working-with-legacy-codebas-jrqk | Don\'t feel like cleaning up the code right now, but the logic is as follows:\n\nNotice we can only increment the letter, not decrement.\n\nwe can use greedy to | JJZin | NORMAL | 2024-12-15T04:26:17.923784+00:00 | 2024-12-15T04:33:29.828534+00:00 | 77 | false | Don\'t feel like cleaning up the code right now, but the logic is as follows:\n\nNotice we can only increment the letter, not decrement.\n\nwe can use greedy to compute in O(n) time if we have a predefined target\n\n\nThis is the important part: if the letter count is GREATER than predefined target, you can either remove until meeting target, or increment the character. That\'s what the parameter \'adds\' represents, the amount of carryover from characters over the given count. Note these carryovers can only be used at idx + 1, they cannot be carried over between multiple indices\n\nif the given letter count is LESS than predefined target you can either decrement to 0 or in increase to meet target (if you have carryover from previous character you may apply that carryover to this character \n\n\nNotice how bounds is 10^4, not 10^5, this allows our trash O(n^2) slop to pass\n\n```\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n \n global moxom\n moxom = 0\n alpha = \'abcdefghijklmnopqrstuvwxyz\'\n \n counter = defaultdict(int)\n \n for i in s:\n counter[alpha.index(i)] += 1\n moxom = max(moxom, counter[alpha.index(i)])\n \n \n \n @cache\n def recourse(idx, goal, adds, subs):\n global moxom\n subs = 0\n \n \n if idx == 26:\n return 0\n \n if counter[idx] == 0:\n return recourse(idx + 1, goal, 0, 0)\n \n if goal == -1:\n ans = 10**20\n anew = 0\n for i in range(0, moxom + 1):\n \n if counter[idx] > i:\n ans = min(ans, counter[idx] - i + recourse(idx + 1, i, counter[idx] - i, 0))\n continue\n if counter[idx] == i:\n \n ans = min(ans, recourse(idx + 1, i, 0, 0))\n continue\n \n anew = counter[idx] + recourse(idx + 1, i, counter[idx], 0)\n \n anew = min(anew, min(counter[idx], i - counter[idx]) + recourse(idx + 1, i, 0, i - counter[idx]))\n # if anew > ans and i > 500:\n # break\n ans = min(ans, anew)\n \n return ans\n if counter[idx] == goal:\n return recourse(idx + 1, goal, 0, 0)\n \n if counter[idx] > goal:\n \n return (counter[idx] - goal - subs) + recourse(idx + 1, goal, counter[idx] - goal, 0)\n if counter[idx] < goal:\n # if (adds) > (goal - counter[idx]):\n # return recourse(idx + 1, goal, 0, 0)\n \n return min(counter[idx] + recourse(idx + 1, goal, counter[idx], 0), max(0, goal - counter[idx] - adds) + recourse(idx + 1, goal, 0, 0))\n \n return ans\n \n \n ans = recourse(0, -1, 0, 0)\n recourse.cache_clear()\n return ans\n | 1 | 0 | [] | 0 |
minimum-operations-to-make-character-frequencies-equal | Using Bottom-up DP with TC O(26 * N) + explanation | Simple code, easy to understand! | using-bottom-up-dp-with-tc-o26-n-explana-sfm5 | Complexity
Time complexity: O(26∗n)
Space complexity: O(n)
Code | phutruonnttn | NORMAL | 2024-12-24T15:55:39.302455+00:00 | 2024-12-24T15:55:39.302455+00:00 | 54 | false | 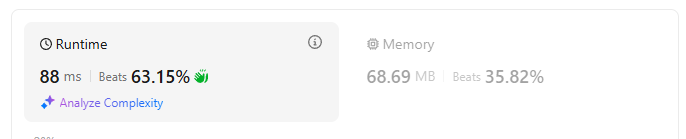
# Complexity
- Time complexity: $$O(26 * n)$$
- Space complexity: $$O(n)$$
# Code
```cpp []
class Solution {
public:
int makeStringGood(string s) {
int n = s.size();
vector<int> freq(26, 0);
for (int i = 0; i < n; i++) {
freq[s[i] - 'a']++;
}
int ans = n;
for (int targetFreq = 0; targetFreq <= n; targetFreq++) {
vector<int> dp(26, 0);
for (int i = 0; i < 26; i++) {
if (freq[i] == 0) {
if (i) dp[i] = dp[i - 1];
} else {
// Using delete or insert characters (to make the frequency of the character 'i' equal to 0 or targetFreq)
int preDp = i ? dp[i-1] : 0;
dp[i] = preDp + min(abs(targetFreq - freq[i]), freq[i]);
// Using the third operation
if (i && freq[i - 1] > 0 && freq[i] < targetFreq) {
int prevDp2 = i >= 2 ? dp[i-2] : 0;
// Number of character i-1 will be change to i
int numChange = min(freq[i - 1] > targetFreq ? freq[i - 1] - targetFreq : freq[i - 1], targetFreq - freq[i]);
// Cost for make the frequency of the character 'i - 1' (after using the third operation) equal to 0 or targetFreq
int cost1 = min(abs(targetFreq - freq[i - 1] + numChange), freq[i - 1] - numChange);
// Cost for make the frequency of the character 'i' (after using the third operation) equal to 0 or targetFreq
int cost2 = targetFreq - (freq[i] + numChange);
dp[i] = min(dp[i], prevDp2 + cost1 + cost2 + numChange);
}
}
}
ans = min(ans, dp[25]);
}
return ans;
}
};
``` | 1 | 0 | ['Dynamic Programming', 'C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | [C++] iterate through the frequency O(26*n) | c-iterate-through-the-frequency-o26n-by-w0hmh | IntuitionApproachFixed the target number per letter, and then figure out where to insert/delete/bump to meet the condition.Once the number is fixed, the 3 opera | ahimawan | NORMAL | 2024-12-15T08:43:12.466194+00:00 | 2024-12-15T08:43:12.466194+00:00 | 140 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nFixed the target number per letter, and then figure out where to insert/delete/bump to meet the condition.\n\n Once the number is fixed, the 3 operations:\n 1. bump -- this is moving frequency from one character to the frequency of the next character. Notice that bump operation is never used consecutively, e.g: instead of bumping \'a\' -> \'b\', then \'b\' -> \'c\', it\'s just simpler to delete \'a\' and add \'c\', and just as cheap or cheaper for longer chain. So, we\'ll just need to keep another variable, representing a carryover \'credit\', that can be used to increase frequency of the next character.\n 2. deletion -- deleting is almost useless. Just bump, store it as carryover credit, and ignore the credit if not needed in the next character\n 3. insertion -- insertion cost can use the credit, if available from previous character processing\n\n\n# Complexity\n- Time complexity: $$O(26*n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\n/**\n* Fixed the target numbers per letter, and then figure out where to insert/delete/bump to meet the condition\n*\n* Once the number is fixed, the 3 operations:\n* 1. bump -- this is moving frequency from one character to the frequency of the next character. Notice\n* that bump operation is never used consecutively, e.g: instead of bumping \'a\' -> \'b\', then \'b\' -> \'c\'\n* it\'s just simpler to delete \'a\' and add \'c\', and just as cheap or cheaper for longer chain. So,\n* we\'ll just need to keep another variable, representing a carryover \'credit\', that can be used to\n* increase frequency of the next character.\n* 2. deletion -- deleting is almost useless. Just bump, store it as carryover credit, and ignore the credit\n* if not needed in the next character\n* 3. insertion -- insertion cost can use the credit, if available from previous character processing\n*/\nclass Solution {\npublic:\n int makeStringGood(string s) {\n int a[26];\n memset(a, 0, sizeof(a));\n for (auto &c: s) {\n a[c - \'a\']++;\n }\n \n int ret = s.length() - 1;\n for (int le = 1; le <= s.length(); le++) {\n // r2 is for the scenario when we have carryover credit.\n int r1 = 0, r2 = 0;\n int c = 0;\n for (int i = 0; i < 26; i++) {\n assert(r1 <= r2);\n if (a[i] == 0 || a[i] == le) {\n c = 0;\n r2 = r1;\n }\n else if (a[i] > le) {\n r2 = r1 + a[i] - le;\n c = a[i] - le;\n r1 = r2;\n } else {\n // decreasing a[i] to 0\n int r4 = r1 + a[i];\n int c2 = a[i];\n\n // increasing a[i] to le\n int r3 = min(r2 + max(0, le - a[i] - c),\n r1 + le - a[i]);\n \n r2 = r4;\n c = c2;\n r1 = min(r3, r2);\n } \n }\n ret = min(ret, r1);\n }\n return ret;\n }\n};\n``` | 1 | 0 | ['C++'] | 2 |
minimum-operations-to-make-character-frequencies-equal | Simple O(max frequency) time and O(1) space solution 3ms | simple-omax-frequency-time-and-o1-space-mkbrs | IntuitionWe first notice that the order of the string does not matter and so we can convert it into a 26 dimensional frequency array freq. Our operations become | lovesbumblebees | NORMAL | 2024-12-15T07:03:09.225561+00:00 | 2024-12-15T07:03:09.225561+00:00 | 422 | false | # Intuition\nWe first notice that the order of the string does not matter and so we can convert it into a 26 dimensional frequency array <code>freq</code>. Our operations become fixing an index i and performing one of the following: <code>freq[i]++</code>, <code>freq[i]--</code>, <code>freq[i]--, freq[i+1]++</code>. The objective is to find the number $k$ such that the number of operations required to make <code>freq</code> take values in $\\{0,k\\}$ is minimized. \n\nWe simplify the problem conceptually by combining the second and third operation into "carrying" where the quantity carried to the next index is considered optional and any amount can be ignored. \n\nSince I am not sure of how we can determine an optimal value of $k$ I simply consider each value between 0 and the maximum frequency present and then pick the one that minimizes the total operations. Looking at the test cases, the function mapping $k$ to minimal operations does not always have monotonicity (although it eventually forms a well around the optimal value). \n\nWith $k$ fixed our goal is to find the number of operations it takes to make <code>freq</code> $\\{0, k\\}$ valued. We use dynamic programming where our states are pairs $\\{(i,j)\\; |\\; 0 \\leq i < 25, j \\in \\{\\text{carry}, \\text{no carry}\\} \\}$ and the value of our variable at each of these states is the minimal number of operations required to to fix the characters between $0$ and $i$, along with the value of the carry itself. Since <code>dp[i]</code> depends only on the values of <code>dp[i-1]</code> we do not have to allocate a vector and instead use three values: the minimal operations from the previous step without carry, the minimal operations from the previous step with carry, and finally the carry amount. \n\n# Approach\nIf <code>freq[i] >= k</code> then the incoming carry can be ignored. We require <code>delta = freq[i] - k</code> additional operations to make this value equal to $k$. <code>delta</code> will also be our new carry amount. Since we do not need the previous carry, we will take the minimum of the two operation total from the previous step and increase it by </code>delta</code>.\n\nTo produce a carry result we simply carry all of <code>freq[i]</code>. This costs <code>freq[i]</code> operations plus the minimum of the two previous steps. \n\nProducing a no carry result is slightly more complicated. If <code>freq[i] + carry >= k</code> then we can use the carry operations from the previous step without any additional operations. Alternatively we can ignore the carry and instead use <code> k - freq[i]</code> additional operations. If the carry does not put us over the $k$ then we need to consider the difference when using the carry. \n\n# Complexity\n- Time complexity:\n$$O(m)$$ where $m$ is the greatest frequency among characters. Measuring the number of operations to make a string $k$ good is constant time (26 iterations) \n\n- Space complexity:\n$$O(1)$$ we use 26 integers to represent a string of arbitrary length, we use a variable to keep track of the minimal number of operations, then our function requires us to keep track of three integers representing minimal no carry operations, minimal carry operations, and the carry value (along with a temporary variable and a loop counter)\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int makeStringGood(string s) {\n int freq[26] = {};\n int m = 0;\n for(const char c: s) {\n freq[c-\'a\']++;\n m = max(m, freq[c-\'a\']); \n }\n int best = s.size();\n for(int i = m; i >= 0; i--) {\n int result = operations(freq, i);\n best = min(best, result);\n }\n return best;\n }\n int operations(int* freq, int k) {\n int prev_carry = 0;\n int prev_nocarry = 0;\n int carry = 0;\n for(size_t i = 0; i < 26; i++) {\n if(freq[i] >= k) {\n // if we are already larger than k, then the incoming carry does not matter\n carry = freq[i] - k;\n // both results are the same\n prev_carry = freq[i] - k + min(prev_carry, prev_nocarry); \n prev_nocarry = prev_carry; \n } else {\n if(freq[i] + carry >= k) {\n int prev = prev_nocarry;\n // either we accept the carry or we have to fill in the gap upwards\n prev_nocarry = min(prev_carry, k - freq[i] + prev_nocarry); \n // to get a carry we always decrease and ignore the incoming carry\n prev_carry = freq[i] + min(prev_carry, prev);\n carry = freq[i];\n } else {\n int prev = prev_carry;\n // to get a carry we always decrease and ignore the incoming carry\n prev_carry = freq[i] + min(prev_carry, prev_nocarry); \n // for the no carry option we increase up until k using the cary or not\n prev_nocarry = min(k - (freq[i] + carry) + prev, k - freq[i] + prev_nocarry);\n carry = freq[i]; \n }\n \n }\n }\n return min(prev_carry, prev_nocarry); \n }\n};\n``` | 1 | 0 | ['Dynamic Programming', 'C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | iterate over finally frequency of each character | iterate-over-finally-frequency-of-each-c-eohh | Intuitioniterate over finally frequency of each character,
use dp to find best way to make all characters have same frequencyApproachiterate over finally freque | c337134154 | NORMAL | 2024-12-15T06:04:59.606615+00:00 | 2024-12-15T06:04:59.606615+00:00 | 310 | false | # Intuition\niterate over finally frequency of each character,\nuse dp to find best way to make all characters have same frequency\n\n# Approach\niterate over finally frequency of each character,\nuse dp to find best way to make all characters have same frequency\n\n# Complexity\n- Time complexity:\nO(n) x 26\n\n- Space complexity:\n26\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n freq = {}\n for c in s:\n freq[ord(c)-ord(\'a\')] = freq.get(ord(c)-ord(\'a\'), 0) + 1\n \n ans = len(s)\n vals = sorted(freq.keys())\n print(\'freq:\', freq, \'vals:\', vals)\n for k in range(0, max(freq.values()) + 10):\n ops = [[0,0] for _ in range(len(vals))]\n carry = [[0,0] for _ in range(len(vals))]\n f = freq[vals[0]]\n if f > k:\n carry[0][0] = f - k\n carry[0][1] = f - k\n ops[0][0] = f - k\n ops[0][1] = f - k\n else:\n carry[0][0] = f\n carry[0][1] = 0\n ops[0][0] = f\n ops[0][1] = k-f\n for idx in range(1, len(vals)):\n f = freq[vals[idx]]\n \n if vals[idx]==vals[idx-1]+1:\n if f > k:\n carry[idx][0] = f - k\n carry[idx][1] = f - k\n ops[idx][0] = min(ops[idx-1][0],ops[idx-1][1])+f - k\n ops[idx][1] = min(ops[idx-1][0],ops[idx-1][1])+f - k\n else:\n ops[idx][1] = min(ops[idx-1][0] + max(k-f-carry[idx-1][0],0),\n ops[idx-1][1] + max(k-f-carry[idx-1][1],0))\n ops[idx][0] = min(ops[idx-1][0],ops[idx-1][1]) + f\n carry[idx][0] = f\n carry[idx][1] = 0\n else:\n if f > k:\n carry[idx][0] = f - k\n carry[idx][1] = f - k\n ops[idx][0] = min(ops[idx-1][0],ops[idx-1][1]) + f - k\n ops[idx][1] = min(ops[idx-1][0],ops[idx-1][1]) + f - k\n else:\n carry[idx][0] = f\n carry[idx][1] = 0\n ops[idx][0] = min(ops[idx-1][0],ops[idx-1][1]) + f\n ops[idx][1] = min(ops[idx-1][0],ops[idx-1][1]) + k - f\n \n # print(\'k:\',k, \'ops:\',ops)\n ans = min(ans, min(ops[-1][0], ops[-1][1]))\n \n return ans\n``` | 1 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | DP | O(N * 26) | O(26*2) DP | dp-on-26-o262-dp-by-then00bprogrammer-ff0m | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | then00bprogrammer | NORMAL | 2024-12-15T05:07:19.091188+00:00 | 2024-12-15T05:07:19.091188+00:00 | 488 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\n int dp[26][2];\n \n int rec(int i,bool surplus,vector <int> &nf, int &target) {\n if(i>=26) return 0;\n \n if(dp[i][surplus]!=-1) return dp[i][surplus];\n \n int curr = nf[i] + (surplus? (nf[i-1]<target ? nf[i-1] : nf[i-1] - target):0);\n \n if(nf[i]>=target) {\n int needed = target - nf[i+1];\n int extra = nf[i] - target;\n \n if(nf[i+1]>=target) return dp[i][surplus] = extra + rec(i+1, false, nf, target);\n \n if(extra>=needed) return dp[i][surplus] = extra + rec(i+2, false, nf, target);\n \n return dp[i][surplus] = extra + min({\n rec(i+1, true, nf, target),\n rec(i+1, false, nf, target)\n \n });\n }\n \n int ans=min(target-curr, curr) + rec(i+1, false, nf, target);\n \n if(nf[i+1]<target) {\n if(nf[i]+nf[i+1]>=target) ans=min(ans, nf[i]+rec(i+2, false, nf,target));\n else ans=min(ans, nf[i]+rec(i+1, true, nf, target));\n } \n \n return dp[i][surplus]=ans;\n }\npublic:\n int makeStringGood(string s) {\n int n = s.size();\n \n vector <int> fre(27);\n for(auto ch:s) fre[ch-\'a\']++;\n \n int res = n;\n \n for(int i=1;i<=n;i++) {\n memset(dp, -1, sizeof(dp));\n res = min(res, rec(0,0,fre,i));\n }\n \n return res;\n }\n};\n``` | 1 | 0 | ['Dynamic Programming', 'C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | DP on all possible target frequencies. | dp-on-all-possible-target-frequencies-by-4fp4 | IntuitionEvery deletion can be used to make free incrment for next character in alphabetApproachfor all possible target frequency [0,max_frequency].
dp for min | sandeep_p | NORMAL | 2024-12-15T04:49:32.973489+00:00 | 2024-12-15T06:08:33.635411+00:00 | 218 | false | # Intuition\nEvery deletion can be used to make free incrment for next character in alphabet\n\n# Approach\nfor all possible target frequency [0,max_frequency].\ndp for min cost.\ndp(i,carry)=min cost to make all chars [i,26] same freq as target.\ncarry is the number of free increments we can make( carried over from previous deletions.)\n\n# Complexity\n- Time complexity:\nO(n * 26 * 26) ~ O(n)\n- Space complexity:\nO(26 * 26) ~ O(1)\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n c=Counter(s)\n c=[c[ch] for ch in ascii_lowercase]\n ans=inf\n for target in range(max(c)+1):\n @cache\n def dp(i,carry):\n if i==26:\n return 0\n cur=c[i]\n if cur==0:\n return dp(i+1,0)\n ans=dp(i+1,cur)+cur\n if cur<target:\n cur+=carry\n cost=max(0,(target-cur))\n ans=min(ans,dp(i+1,0)+cost)\n elif cur>=target:\n diff=cur-target\n ans=min(ans,dp(i+1,diff)+diff)\n return ans\n ans=min(ans,dp(0,0))\n dp.cache_clear()\n return ans\n``` | 1 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | without dp | without-dp-by-mustafaansari-fo7h | ApproachInstead of using dynamic programming with multiple variables (dp1, dp2, free), we can break the problem into simpler steps by using frequency counts and | mustafaansari | NORMAL | 2024-12-15T04:17:25.175875+00:00 | 2024-12-15T04:17:25.175875+00:00 | 241 | false | \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nInstead of using dynamic programming with multiple variables (dp1, dp2, free), we can break the problem into simpler steps by using frequency counts and performing operations directly on those counts. This can simplify the solution while maintaining the same structure.\n\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n n = len(s)\n f = [0] * 26\n for char in s:\n f[ord(char) - ord(\'a\')] += 1\n ans = n\n for m in range(1, n + 1):\n res = 0\n dp1 = 0\n dp2 = 0\n free = 0\n for i in range(26):\n if f[i] >= m:\n ndp1 = min(dp1, dp2) + (f[i] - m)\n ndp2 = n\n nfree = f[i] - m\n else:\n ndp1 = min(dp1, dp2) + f[i]\n ndp2 = min(dp1 + max(0, m - f[i] - free), dp2 + m - f[i])\n nfree = f[i] \n dp1 = ndp1\n dp2 = ndp2\n free = nfree\n res = min(dp1, dp2)\n ans = min(ans, res)\n return ans\n\n``` | 1 | 3 | ['Python3'] | 1 |
minimum-operations-to-make-character-frequencies-equal | python dp | python-dp-by-mykono-n7gj | Code | Mykono | NORMAL | 2025-02-12T04:58:14.522412+00:00 | 2025-02-12T04:58:14.522412+00:00 | 12 | false | # Code
```python3 []
class Solution:
def makeStringGood(self, s: str) -> int:
# Count frequency of each letter a-z
freq = [0] * 26
for char in s:
freq[ord(char) - ord("a")] += 1
@cache
def dp(letter_idx: int, carry: int, target: int) -> int:
# Base case: all letters processed
if letter_idx == 26:
return 0
count = freq[letter_idx]
if count <= target:
# Option 1: Delete all occurrences of this letter.
cost_delete = dp(letter_idx + 1, count, target) + count
# Option 2: Fill missing occurrences using available carry.
fillable = min(carry, target - count)
cost_fill = dp(letter_idx + 1, 0, target) + (target - count - fillable)
return min(cost_delete, cost_fill)
else:
# If count > target, delete extras and pass them as carry.
extra = count - target
return dp(letter_idx + 1, extra, target) + extra
# Try all possible target frequencies from 0 up to max(freq)
return min(dp(0, 0, target) for target in range(max(freq) + 1))
``` | 0 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | DP | Kotlin [EXPLAINED] | dp-kotlin-explained-by-r9n-gx0c | IntuitionMake all characters in the string appear with the same frequency using the minimum number of operations. The operations allowed are inserting or removi | r9n | NORMAL | 2025-02-11T17:00:41.656148+00:00 | 2025-02-11T17:00:41.656148+00:00 | 6 | false | # Intuition
Make all characters in the string appear with the same frequency using the minimum number of operations. The operations allowed are inserting or removing characters to balance the frequencies.
# Approach
I start by counting the frequency of each character in the string. Then, I use recursion with memoization to explore different ways of adjusting the frequencies, either by removing extra characters or inserting new ones, until all frequencies match a target value.
# Complexity
- Time complexity:
Approximately O(26 × n²), where 26 is the number of characters and n is the length of the string. This is due to the recursion and memoization.
- Space complexity:
O(26 × n) because of the memoization storage and the frequency array.
# Code
```kotlin []
class Solution {
private val freq = IntArray(26) // Array to store frequency of each character
private val dp = mutableMapOf<Triple<Int, Int, Int>, Int>() // Map to store previously calculated results for memoization
// Recursive function to calculate the minimum number of operations required
private fun getMinOps(i: Int, expfreq: Int, prevops: Int): Int {
if (i == 26) return 0 // Base case: If we have processed all characters, no more operations are needed
val key = Triple(i, expfreq, prevops) // Create a unique key for memoization
if (dp.containsKey(key)) return dp[key]!! // Return the memoized result if available
val curfreq = freq[i] // Get the current frequency of the character
// If current frequency is either 0 or already matches expected frequency, move to next character
if (curfreq == 0 || curfreq == expfreq) return dp.getOrPut(key) { getMinOps(i + 1, expfreq, 0) }
// If the current frequency is greater than the expected frequency, remove the excess characters
val curops = curfreq - expfreq
if (curfreq > expfreq) return dp.getOrPut(key) { curops + getMinOps(i + 1, expfreq, curops) }
// If previous operations allow us to match the expected frequency, move to the next character
if ((curfreq + prevops) >= expfreq) return dp.getOrPut(key) { getMinOps(i + 1, expfreq, 0) }
// If not, we either insert new characters or remove all of the current characters and adjust with the next frequency
return dp.getOrPut(key) {
minOf(curfreq + getMinOps(i + 1, expfreq, curfreq),
(expfreq - (curfreq + prevops)) + getMinOps(i + 1, expfreq, 0))
}
}
// Function to determine the minimum operations required to make the string "good"
fun makeStringGood(s: String): Int {
val n = s.length // Get the length of the string
for (c in s) freq[c - 'a']++ // Update frequency count for each character in the string
val ulimit = freq.maxOrNull() ?: 0 // Find the maximum frequency of any character
var minops = n - ulimit // Calculate the minimum operations needed based on the max frequency
for (target in 0..ulimit) {
minops = minOf(minops, getMinOps(0, target, 0)) // Find the optimal solution by iterating over all possible target frequencies
}
return minops // Return the minimum number of operations required
}
}
``` | 0 | 0 | ['Array', 'Hash Table', 'Math', 'Binary Search', 'Dynamic Programming', 'Memoization', 'Matrix', 'Counting', 'Kotlin'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Optimizing Character Frequencies with Recursive DP+Memo | O(26 * N) Solution | optimizing-character-frequencies-with-re-d4u1 | Intuition
Count Frequencies:
First, we count the frequency of each character in the string.
Store these frequencies in a sorted order (based on characters). | cpriyanshug00 | NORMAL | 2025-01-30T14:04:05.607763+00:00 | 2025-01-30T14:04:05.607763+00:00 | 10 | false | # Intuition
- Count Frequencies:
- First, we count the frequency of each character in the string.
Store these frequencies in a sorted order (based on characters).
Dynamic Programming + Recursion (fxn function):
- Try making every possible frequency i (from 0 to mx, the max frequency in s) the standard frequency.
Recursively decide the minimum cost to adjust the frequency of each character.
# Approach
Recursive DP + Memo
# Complexity
- Time complexity: O(26 X 2 X 5e4)
- Space complexity:O(26*2)
# Code
```cpp []
class Solution {
public:
int fxn(int i, int n, vector<pair<char, int>>& v, int length, int lastTake, vector<vector<int>>& dp) {
if (i == n) return 0; // Base case: if we reach the end, no more cost
if (dp[i][lastTake] != -1) return dp[i][lastTake]; // Memoization check
int ans = 1e9; // Initialize with a large value
if (i + 1 != n) { // If not the last character
if (!lastTake) { // If the previous character was not adjusted
if (v[i + 1].first - v[i].first == 1) { // If consecutive characters
if (v[i].second > length) {
// Change i-th character length equal to length
ans = min(ans, v[i].second - length + fxn(i + 1, n, v, length, 1, dp));
// 1 in fxn is for using some frequencies of previous character
ans = min(ans, v[i].second - length + fxn(i + 1, n, v, length, lastTake, dp));
} else {
// Change i-th character length equal to length
ans = min(ans, length - v[i].second + fxn(i + 1, n, v, length, lastTake, dp));
ans = min(ans, v[i].second + fxn(i + 1, n, v, length, lastTake, dp));
// 1 in fxn is for using some frequencies of previous character
ans = min(ans, v[i].second + fxn(i + 1, n, v, length, 1, dp));
}
} else {
// If not consecutive characters, simply change i-th character length equal to length
if (v[i].second > length) {
ans = min(ans, v[i].second - length + fxn(i + 1, n, v, length, lastTake, dp));
} else {
ans = min(ans, length - v[i].second + fxn(i + 1, n, v, length, lastTake, dp));
ans = min(ans, v[i].second + fxn(i + 1, n, v, length, lastTake, dp));
}
}
} else { // If the previous character was adjusted
if (v[i - 1].second > length) {
// Extra is the remaining characters coming from previous characters
int extra = v[i - 1].second - length;
// If already the i-th character length is greater than length, we have to equalize it
if (v[i].second > length) {
ans = min(ans, v[i].second - length + fxn(i + 1, n, v, length, 0, dp));
}
// If i-th character's length + extra is greater than length, it means it's already equal to the length
else if (v[i].second + extra > length) {
ans = min(ans, fxn(i + 1, n, v, length, 0, dp));
}
// If i-th character's length + extra is less than length, we need to make it equal to length
else {
ans = min(ans, length - (v[i].second + extra) + fxn(i + 1, n, v, length, 0, dp));
}
} else {
int extra = v[i - 1].second;
if (v[i].second > length) {
ans = min(ans, v[i].second - length + fxn(i + 1, n, v, length, 0, dp));
} else if (v[i].second + extra > length) {
ans = min(ans, fxn(i + 1, n, v, length, 0, dp));
} else {
ans = min(ans, length - (v[i].second + extra) + fxn(i + 1, n, v, length, 0, dp));
}
}
}
} else { // If it's the last character
if (!lastTake) {
// If not consecutive characters, simply change i-th character length equal to length
if (v[i].second > length) {
ans = min(ans, v[i].second - length + fxn(i + 1, n, v, length, lastTake, dp));
} else {
ans = min(ans, length - v[i].second + fxn(i + 1, n, v, length, lastTake, dp));
ans = min(ans, v[i].second + fxn(i + 1, n, v, length, lastTake, dp));
}
} else {
if (v[i - 1].second > length) {
// Extra is the remaining characters coming from previous characters
int extra = v[i - 1].second - length;
// If already the i-th character length is greater than length, we have to equalize it
if (v[i].second > length) {
ans = min(ans, v[i].second - length + fxn(i + 1, n, v, length, 0, dp));
}
// If i-th character's length + extra is greater than length, it means it's already equal to the length
else if (v[i].second + extra > length) {
ans = min(ans, fxn(i + 1, n, v, length, 0, dp));
}
// If i-th character's length + extra is less than length, we need to make it equal to length
else {
ans = min(ans, length - (v[i].second + extra) + fxn(i + 1, n, v, length, 0, dp));
}
} else {
int extra = v[i - 1].second;
if (v[i].second > length) {
ans = min(ans, v[i].second - length + fxn(i + 1, n, v, length, 0, dp));
} else if (v[i].second + extra > length) {
ans = min(ans, fxn(i + 1, n, v, length, 0, dp));
} else {
ans = min(ans, length - (v[i].second + extra) + fxn(i + 1, n, v, length, 0, dp));
}
}
}
}
return dp[i][lastTake] = ans; // Store result in DP table
}
int makeStringGood(string s) {
map<char, int> m;
for (auto &i : s) m[i]++;
vector<pair<char, int>> v;
int mx = 0;
for (auto &i : m) {
v.push_back({i.first, i.second});
mx = max(mx, i.second);
}
int n = v.size();
int ans = 1e9;
for (int i = 0; i <= mx; i++) {
vector<vector<int>> dp(n, vector<int>(2, -1));
ans = min(ans, fxn(0, n, v, i, 0, dp));
}
return ans;
}
};
``` | 0 | 0 | ['Hash Table', 'String', 'Dynamic Programming', 'C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Minimum Operations to Make Character Frequencies Equal | minimum-operations-to-make-character-fre-j5nh | IntuitionApproachDynamic ProgrammingComplexity
Time complexity:O(n * 26 *2)
Space complexity:O(26 * 2)
Code | Subham_Pujari23 | NORMAL | 2025-01-17T03:57:27.065617+00:00 | 2025-01-17T03:57:27.065617+00:00 | 27 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
Dynamic Programming
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:O(n * 26 *2)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(26 * 2)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int makeStringGood(String s) {
int n = s.length();
int[] count = new int[26];
int[][] dp = new int[27][2];
for(int i = 0; i < n; ++i) count[s.charAt(i)-'a']++;
int res = n;
for(int target = 1; target <= n; ++target){
for(int i = 25; i >= 0; --i){
for(int deleted = 0; deleted <= 1; ++deleted){
if(i == 0 && deleted == 1) break;
int x = count[i];
if(x == target){
dp[i][deleted] = dp[i+1][0];
}
else if(x > target){
dp[i][deleted] = x - target + dp[i+1][1];
}
else{
int delete_cost = x + dp[i+1][1];
int deleted_amount = deleted>0?(count[i-1]>target?count[i-1]-target:(count[i-1]==target?0:count[i-1])):0;
int insert_cost = Math.max(target-x-deleted_amount, 0)+dp[i+1][0];
dp[i][deleted] = Math.min(delete_cost, insert_cost);
}
}
}
res = Math.min(res, dp[0][0]);
}
return res;
}
}
``` | 0 | 0 | ['Java'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Simple Java solution that beats 97% | simple-java-solution-that-beats-97-by-yu-t6ek | IntuitionApproachComplexity
Time complexity:
O(N + K)
N being length of the string
K being maximum count of a char in a stringcreating the count by char array i | yunkook | NORMAL | 2025-01-09T16:19:24.647474+00:00 | 2025-01-09T16:19:24.647474+00:00 | 41 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
O(N + K)
N being length of the string
K being maximum count of a char in a string
creating the count by char array is N +
calculating number of operations needs by going thru each character
26 * K
= N + 26K
which can be reduced to N + K
- Space complexity:
O(1) = array to store count per char, which is constant size = 26
# Code
```java []
class Solution {
public int makeStringGood(String s) {
int[] cntsByChar = new int[26];
int maxCount=0;
for(int i=0;i<s.length();i++){
cntsByChar[s.charAt(i)-'a']++;
maxCount = Math.max(maxCount, cntsByChar[s.charAt(i)-'a']);
}
int ans = Integer.MAX_VALUE;
for(int targetCount=1;targetCount<=maxCount;targetCount++){
ans = Math.min(ans,
getNumberOfOperationsNeeded(cntsByChar,targetCount));
}
return ans;
}
public int getNumberOfOperationsNeeded( int[] cntsByChar, int target){
int carry = 0;
int prevAdd= 0;
int prevDelete = 0;
for(int i =0;i<26;i++){
if(cntsByChar[i]==0){
carry=0;
continue;
}
int curAdd = 0;
int curDelete = 0;
//if current char count is less than target
if(target > cntsByChar[i]){
// insert to reach target
// we can simply insert
// or we could change char if previous delete operation was done
// minimum of those two count would be the number of operation for this
curAdd= Math.min( target-cntsByChar[i] + Math.min(prevAdd, prevDelete),
Math.max(target-cntsByChar[i]-carry,0)+prevDelete);
// or delete to make it zero
curDelete = cntsByChar[i] + Math.min(prevAdd,prevDelete);
//this carry will be to reduce operations when next char is inserted
carry = cntsByChar[i];
}else {
//only delete to reach the target
curDelete = cntsByChar[i] - target + Math.min(prevAdd,prevDelete);
carry = cntsByChar[i]- target;
//add is not an option so we just put same number for add
curAdd = curDelete;
}
prevDelete = curDelete;
prevAdd = curAdd;
}
return Math.min(prevAdd,prevDelete);
}
}
``` | 0 | 0 | ['Java'] | 0 |
minimum-operations-to-make-character-frequencies-equal | C++ Working Dp Solution | c-working-dp-solution-by-learner_11-tu5o | Working Approach:\nThe task is to transform a given string into a "Good String", where all characters appear with the same frequency. This frequency can range f | Learner_11 | NORMAL | 2024-12-17T14:02:47.706423+00:00 | 2024-12-18T06:01:19.471421+00:00 | 31 | false | Working Approach:\nThe task is to transform a given string into a "Good String", where all characters appear with the same frequency. This frequency can range from 0 to n (where n is the length of the string). To determine the minimal number of operations required to make the string "Good," we identify the global minimum operations needed across all possible target frequencies.\n\nApproach:\nFrequency Map:\nFirst, calculate the frequency of each character in the given string and store it in a map (charFreq).\n\nIterating Over Target Frequencies:\nFor every possible target frequency (from 0 to n), calculate the minimum operations required to make all characters in the string conform to this target frequency.\n\nRecursive Helper Function:\nA helper function (makeStringGoodHelper) is used to compute the number of operations required for a specific target frequency. It works character by character (from \'a\' to \'z\'), considering three types of operations:\n\nInsertions: Adding characters to match the target frequency.\nDeletions: Removing extra characters to match the target frequency.\nTranslations: Changing one character into another.\nThe function uses memoization (dp) to avoid recomputing results for overlapping subproblems.\n\nParameters of the Helper Function:\n\ncurChar: The current character being processed.\ncharFreq: A map containing the frequency of each character in the original string.\ntargetFreq: The target frequency for each character to achieve.\nprevOperations: Tracks surplus characters from previous steps, which can either be reused (as translations) or removed (deletions).\ndp: A 2D vector used for memoization to store previously computed results.\ndpSecondDimension -> for space optimization of second dimension\nBase Case:\n\nIf curChar > \'z\', all characters have been processed, so return 0 (no further operations needed).\nTransition Logic:\n\nIf the current character\'s frequency (including prevOperations(i.e change of previous letter to current letter operations) is greater than or equal to the target frequency:\nCase 1: If the frequency is already greater, excess characters are deleted.\nCase 2: If it\'s equal, no further operations are needed, and we move to the next character.\nIf the current character\'s frequency is less than the target frequency:\nCase 1: All existing characters are deleted or translated, moving to the next character.\nCase 2: Add missing characters to meet the target frequency.\nFinal Answer:\nFor each possible target frequency, compute the minimum operations using the helper function. The final answer is the minimum across all these results.\n\n```\nclass Solution {\npublic:\n int minimumOperations=INT_MAX;\n int makeStringGoodHelper(char curChar,unordered_map<char,int> &charFreq,int targetFreq,int prevOperations,\n vector<vector<int>> &dp,int dpSecondDimension)\n {\n if(curChar>\'z\')\n {\n return 0;\n }\n if(dp[curChar-\'a\'][dpSecondDimension]!=-1)\n {\n return dp[curChar-\'a\'][dpSecondDimension];\n }\n int operations=INT_MAX;\n if(charFreq[curChar]+prevOperations>=targetFreq)\n {\n operations=0;\n if(charFreq[curChar]>=targetFreq)\n {\n operations=(charFreq[curChar]-targetFreq);\n operations+=makeStringGoodHelper(curChar+1,charFreq,targetFreq,operations,dp,2);\n }\n else\n {\n operations+=makeStringGoodHelper(curChar+1,charFreq,targetFreq,0,dp,1);\n }\n }\n else if(charFreq[curChar]<targetFreq)\n {\n //two cases\n //1 case to convert all elements to next or delete state\n //2 case add same character to make it to equal frequency of targetFreq\n \n //case 1:\n \n int miniCaseOp=INT_MAX;\n \n int case1Operations=charFreq[curChar];\n case1Operations+=makeStringGoodHelper(curChar+1,charFreq,targetFreq,case1Operations,dp,3);\n \n int case2Operations=targetFreq-charFreq[curChar]-prevOperations;\n case2Operations+=makeStringGoodHelper(curChar+1,charFreq,targetFreq,0,dp,4);\n \n operations=min(case2Operations,case1Operations);\n \n }\n return dp[curChar-\'a\'][dpSecondDimension]=operations;\n }\n int makeStringGood(string s) {\n minimumOperations=INT_MAX;\n unordered_map<char,int> charFreq;\n for(auto x:s)\n {\n charFreq[x]++;\n }\n for(int i=0;i<=s.size();i++)\n {\n vector<vector<int>> dp(30,vector<int>(10,-1));\n minimumOperations=min(minimumOperations,makeStringGoodHelper(\'a\',charFreq,i,0,dp,0));\n }\n return minimumOperations;\n }\n};\n``` | 0 | 0 | ['Dynamic Programming', 'Memoization'] | 0 |
minimum-operations-to-make-character-frequencies-equal | My Solution | my-solution-by-hope_ma-keh7 | \n/**\n * Time Complexity: O(n + max_count)\n * Space Complexity: O(1)\n * where `n` is the length of the string `s`\n * `max_count` is the maximum number | hope_ma | NORMAL | 2024-12-16T07:03:10.938845+00:00 | 2024-12-16T07:03:10.938877+00:00 | 9 | false | ```\n/**\n * Time Complexity: O(n + max_count)\n * Space Complexity: O(1)\n * where `n` is the length of the string `s`\n * `max_count` is the maximum number of all letters of the string `s`\n */\nclass Solution {\n public:\n int makeStringGood(const string &s) {\n /**\n * name the following operations\n * operation 1. delete `letter` from `s`\n * operation 2. insert `letter` into `s`\n * operation 3. change `letter` to (`letter` + 1) in `s`\n */\n constexpr int letters = 26;\n constexpr char a = \'a\';\n int letter_to_count[letters]{};\n int max_count = 0;\n for (const char c : s) {\n max_count = max(max_count, ++letter_to_count[c - a]);\n }\n \n int ret = numeric_limits<int>::max();\n for (int count = 0; count < max_count + 1; ++count) {\n int dp[letters + 1]{};\n for (int letter = letters - 1; letter > -1; --letter) {\n if (letter_to_count[letter] == 0) {\n dp[letter] = dp[letter + 1];\n continue;\n }\n\n /**\n * option 1, handle `letter` alone\n */\n dp[letter] = min(\n /**\n * option i,\n * perform the operation 1 `letter_to_count[letter]` times on `letter`\n * to make the number of it be `0`\n */\n letter_to_count[letter],\n /*\n * option ii,\n * perform the operation 1 or the operation 2\n * `abs(count - letter_to_count[letter])` times on `letter`\n * to make the number of it be `count`\n */\n abs(count - letter_to_count[letter])\n ) + dp[letter + 1];\n \n /**\n * option 2, handle both `letter` and (`letter` + 1)\n * 1. perform the operation 3 as many as times on `letter`\n * 2. perform the operation 1 or operation 2 on `letter` and (`letter` + 1) \n * if necessary\n */\n if (letter + 1 < letters && letter_to_count[letter + 1] < count) {\n if (letter_to_count[letter] > count) {\n /**\n * make the number of both `letter` and (`letter` + 1) be `count`\n */\n dp[letter] = min(\n dp[letter],\n max(\n letter_to_count[letter] - count,\n count - letter_to_count[letter + 1]\n ) + dp[letter + 2]\n );\n } else {\n /**\n * 1. make the number of `letter` be `0`\n * 2. make the number of (`letter` + 1) be `count`\n */\n dp[letter] = min(\n dp[letter],\n max(\n letter_to_count[letter],\n count - letter_to_count[letter + 1]\n ) + dp[letter + 2]\n );\n }\n }\n }\n ret = min(ret, dp[0]);\n }\n return ret;\n }\n};\n``` | 0 | 0 | [] | 0 |
minimum-operations-to-make-character-frequencies-equal | T: O(N), M: O(N) | t-on-m-on-by-vladislav_zavadski-zcpx | Iterating over all posibble amount of character occuring c . And check how many operations needed. \nCase 1: Symbol occurs c or 0 times, then do nothing, just s | vladislav_zavadski | NORMAL | 2024-12-15T14:12:23.397973+00:00 | 2024-12-15T14:22:33.505737+00:00 | 42 | false | Iterating over all posibble amount of character occuring `c` . And check how many operations needed. \nCase 1: Symbol occurs `c` or `0` times, then do nothing, just switching to the next one\nCase 2: Symbol occurs more than `c` times, then dicrease amount to `c` and pass deleted amount (`from_prev`) next (see Case 3).\nCase 3: Symbol occurs less than `c` times, then we have 2 options:\n1) Decrease occurance of this symbol to `0` \n2) Increase occurance to `c` by inserting characters and/or changing character (using `from_prev` variable)\n\n```\nclass Solution:\n \n def __init__(self):\n self.alphabet = "abcdefghijklmnopqrstuvwxyz"\n \n def makeStringGood(self, s: str) -> int:\n count = Counter(s)\n \n def ops_amount(c):\n @cache\n def dp(i, from_prev):\n if i >= 26:\n return 0\n\n if count[self.alphabet[i]] == c or count[self.alphabet[i]] == 0:\n return dp(i + 1, 0)\n elif count[self.alphabet[i]] > c:\n return dp(i + 1, count[self.alphabet[i]] - c) + count[self.alphabet[i]] - c\n else:\n return min(dp(i + 1, count[self.alphabet[i]]) + count[self.alphabet[i]], dp(i + 1, 0) + c - count[self.alphabet[i]] - min(from_prev, c - count[self.alphabet[i]]))\n \n return dp(0,0)\n \n return min(ops_amount(c) for c in range(len(s) + 1))\n``` | 0 | 0 | ['Dynamic Programming', 'Memoization', 'Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Easy Solving | easy-solving-by-siam01f-i8eq | Let\'s break this down as simply as possible and focus on the core logic.\n\nProblem Understanding:\nWe need to balance the frequency of characters in the strin | siam01f | NORMAL | 2024-12-15T05:18:15.253599+00:00 | 2024-12-15T05:18:15.253625+00:00 | 79 | false | Let\'s break this down as simply as possible and focus on the core logic.\n\nProblem Understanding:\nWe need to balance the frequency of characters in the string s to meet a predefined target frequency. The trick is we can only add characters (increment), not remove them, but we can adjust counts as we move through the string.\n\nKey Points:\nYou have 26 letters in the alphabet.\nFor each character, we can either:\nIncrease the count (add more of that letter).\nRemove the excess if it\'s greater than a predefined target.\nThe problem is to calculate the minimum operations to balance the string by either adding or "carrying over" extra characters.\n\nParameters:\nadds: This represents the leftover or carryover of excess characters from the previous index.\nThis carryover can be used for the next character. If you have more characters than the target for one letter, you can carry them over to the next letter to adjust its frequency.\nImportant rule: You cannot carry over excess from one letter (say \'a\') to a far-off letter (say \'f\'). Each letter\'s carryover only applies to the next letter.\nSimplified Approach:\nCount the occurrences of each character in the string s.\nStart with the first character (say \'a\') and try to balance its count to a target.\nIf the count of the character is more than the target, you have two choices:\nRemove excess characters to meet the target.\nIncrease the next character\'s count to absorb this excess.\nIf the count is less than the target:\nYou can either:\nDecrease to zero (but we can\'t really remove characters, so this option is typically for balancing purposes).\nIncrease the character\'s count to match the target using the carryover from previous characters.\nGreedy Strategy:\nFor each character, if its count is greater than the target, you remove the excess or pass the carryover to the next character.\nIf its count is less than the target, use the carryover to make up the deficit.\nEfficiency: Since the problem allows up to 10^4 characters, the greedy approach works in linear time O(n), where n is the length of the string, making it efficient enough.\nKey Idea:\nThe trick is that you carry over the excess from one character to the next, but only within adjacent letters. You adjust each character greedily, either removing excess or increasing the character using carryover from the previous letter.\n\nSimple Example:\nSuppose we have the string "aabcc". Here\'s the process:\n\nCount occurrences:\n\'a\' appears 2 times.\n\'b\' appears 1 time.\n\'c\' appears 2 times.\nNow, let\'s say the target frequency for each character is 2:\nFor \'a\': No change needed. It has 2 occurrences (which is the target).\nFor \'b\': It\'s less than 2 (only 1 \'b\'), so we need 1 more \'b\'. We can use the carryover from \'a\' (if there was any extra \'a\'s from a previous step, though in this case there was none).\nFor \'c\': It also has 2 occurrences (which is the target), so no change needed here.\n```\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n \n global moxom\n moxom = 0\n alpha = \'abcdefghijklmnopqrstuvwxyz\'\n \n counter = defaultdict(int)\n \n for i in s:\n counter[alpha.index(i)] += 1\n moxom = max(moxom, counter[alpha.index(i)])\n \n \n \n @cache\n def recourse(idx, goal, adds, subs):\n global moxom\n subs = 0\n \n \n if idx == 26:\n return 0\n \n if counter[idx] == 0:\n return recourse(idx + 1, goal, 0, 0)\n \n if goal == -1:\n ans = 10**20\n anew = 0\n for i in range(0, moxom + 1):\n \n if counter[idx] > i:\n ans = min(ans, counter[idx] - i + recourse(idx + 1, i, counter[idx] - i, 0))\n continue\n if counter[idx] == i:\n \n ans = min(ans, recourse(idx + 1, i, 0, 0))\n continue\n \n anew = counter[idx] + recourse(idx + 1, i, counter[idx], 0)\n \n anew = min(anew, min(counter[idx], i - counter[idx]) + recourse(idx + 1, i, 0, i - counter[idx]))\n # if anew > ans and i > 500:\n # break\n ans = min(ans, anew)\n \n return ans\n if counter[idx] == goal:\n return recourse(idx + 1, goal, 0, 0)\n \n if counter[idx] > goal:\n \n return (counter[idx] - goal - subs) + recourse(idx + 1, goal, counter[idx] - goal, 0)\n if counter[idx] < goal:\n # if (adds) > (goal - counter[idx]):\n # return recourse(idx + 1, goal, 0, 0)\n \n return min(counter[idx] + recourse(idx + 1, goal, counter[idx], 0), max(0, goal - counter[idx] - adds) + recourse(idx + 1, goal, 0, 0))\n \n return ans\n \n \n ans = recourse(0, -1, 0, 0)\n recourse.cache_clear()\n return ans\n | 0 | 0 | ['Python3'] | 1 |
minimum-operations-to-make-character-frequencies-equal | Python Hard | python-hard-by-lucasschnee-0uls | null | lucasschnee | NORMAL | 2024-12-27T17:35:23.319486+00:00 | 2024-12-27T17:42:44.266605+00:00 | 26 | false | ```
class Solution:
def makeStringGood(self, s: str) -> int:
'''
two cases:
1. all of s is the same
2. all of s is 0 or the same
leftover only carries one time, because delete + add is better for 2 or more times
'''
N = len(s)
INF = 10 ** 10
A = [0] * 26
for c in s:
A[ord(c) - ord('a')] += 1
def go(target):
@cache
def calc(index, leftover):
if index == 26:
return 0
amt = A[index]
# make 0
best = calc(index + 1, 0) + amt
# delete
if amt > target:
# make 0
diff = amt - target
best = min(best, calc(index + 1, 0) + diff)
# equal
if amt == target:
best = min(best, calc(index + 1, 0))
# less
if amt < target:
diff = target - amt
if diff <= leftover:
# use leftover to pay for extra
best = min(best, calc(index + 1, 0))
else:
diff2 = diff - leftover
# use leftover then add rest
best = min(best, calc(index + 1, 0) + diff2)
if amt > target:
# change amt - target to next char
best = min(best, calc(index + 1, amt - target) + amt - target)
else:
# change all amt to next char, leaving amt at 0
best = min(best, calc(index + 1, amt) + amt)
return best
best = calc(0, 0)
calc.cache_clear()
return best
mx = max(A)
best = INF
for i in range(0, mx + 1):
best = min(best, go(i))
return best
``` | 0 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | DP Recursive Solution | dp-recursive-solution-by-ankuraj_27-fpx2 | Code | ankuraj_27 | NORMAL | 2024-12-26T15:35:18.676070+00:00 | 2024-12-26T15:35:18.676070+00:00 | 22 | false | # Code
```cpp []
class Solution {
public:
int f(int &n,int ind,int extra,int &th,vector<int> &v,vector<vector<int>> &dp) {
if(ind==26) return 0;
if(dp[ind][extra]!=-1) return dp[ind][extra];
int mn=1e9,a=v[ind];
if(extra) {
if(v[ind]<th) {
if(v[ind-1]<=th) a=min(a+v[ind-1],th);
else a=min(a+v[ind-1]-th,th);
}
}
if(a<=th) {
mn=min(mn,th-a+f(n,ind+1,0,th,v,dp));
mn=min(mn,a+f(n,ind+1,0,th,v,dp));
if(ind!=25) mn=min(mn,a+f(n,ind+1,1,th,v,dp));
}
else {
mn=min(mn,a-th+f(n,ind+1,0,th,v,dp));
if(ind!=25) mn=min(mn,a-th+f(n,ind+1,1,th,v,dp));
}
return dp[ind][extra] = mn;
}
int makeStringGood(string s) {
int n=s.size();
vector<int> v(26);
for(int i=0;i<n;i++) {
v[s[i]-'a']++;
}
int mn=1e9;
for(int i=0;i<=n;i++) {
vector<vector<int>> dp(26,vector<int>(2,-1));
int x=f(n,0,0,i,v,dp);
mn=min(mn,x);
}
return mn;
}
};
``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Java | DP | O(n^2) | java-dp-on2-by-aryonbe-rj2h | Code | aryonbe | NORMAL | 2024-12-24T06:03:31.374029+00:00 | 2024-12-24T06:03:31.374029+00:00 | 48 | false | # Code
```java []
class Solution {
public int makeStringGood(String s) {
int n = s.length();
int[] count = new int[26];
int[][] dp = new int[27][2];
for(int i = 0; i < n; ++i) count[s.charAt(i)-'a']++;
int res = n;
for(int target = 1; target <= n; ++target){
for(int i = 25; i >= 0; --i){
for(int deleted = 0; deleted <= 1; ++deleted){
if(i == 0 && deleted == 1) break;
int x = count[i];
if(x == target){
dp[i][deleted] = dp[i+1][0];
}
else if(x > target){
dp[i][deleted] = x - target + dp[i+1][1];
}
else{
int delete_cost = x + dp[i+1][1];
int deleted_amount = deleted>0?(count[i-1]>target?count[i-1]-target:(count[i-1]==target?0:count[i-1])):0;
int insert_cost = Math.max(target-x-deleted_amount, 0)+dp[i+1][0];
dp[i][deleted] = Math.min(delete_cost, insert_cost);
}
}
}
res = Math.min(res, dp[0][0]);
}
return res;
}
}
``` | 0 | 0 | ['Java'] | 0 |
minimum-operations-to-make-character-frequencies-equal | C++ | DP | O(n^2) | c-dp-on2-by-aryonbe-sx8m | Code | aryonbe | NORMAL | 2024-12-24T05:48:55.575145+00:00 | 2024-12-24T05:48:55.575145+00:00 | 16 | false | # Code
```cpp []
class Solution {
public:
int makeStringGood(string s) {
int n = s.size();
int count[26];
int dp[27][2];
for(char c: s) count[c-'a']++;
int res = n;
for(int target = 1, mx = *max_element(count,count+26); target <= mx; target++){
dp[26][0] = 0;
dp[26][1] = 0;
for(int i = 25; i>=0; --i){
for(int deleted = 0; deleted <= 1; ++deleted){
if(i == 0 && deleted == 1) break;
int x = count[i];
if(x == target){
dp[i][deleted] = dp[i+1][0];
}
else if(x > target){
dp[i][deleted] = x-target+dp[i+1][1];
}
else{
int delete_cost = x + dp[i+1][1];
int deleted_amount = deleted?(count[i-1]>target?count[i-1]-target:(count[i-1]==target?0:count[i-1])):0;
int insert_cost = max(target-x-deleted_amount, 0) + dp[i+1][0];
dp[i][deleted] = min(delete_cost, insert_cost);
}
}
}
res = min(res, dp[0][0]);
}
return res;
}
};
``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Python | DP | O(n^2) | python-dp-on2-by-aryonbe-14f7 | Code | aryonbe | NORMAL | 2024-12-24T04:16:16.304296+00:00 | 2024-12-24T04:16:16.304296+00:00 | 21 | false | # Code
```python3 []
class Solution:
def makeStringGood(self, s: str) -> int:
count = [0]*26
for c in s:
count[ord(c)-ord('a')] += 1
@cache
def dp(i, deleted, target):
if i == 26: return 0
if count[i] == target:
return dp(i+1, 0, target)
x = count[i]
if x > target:
return x-target + dp(i+1, x-target, target)
delete = x + dp(i+1, x, target)
insert = max(target-x-deleted,0)+dp(i+1, 0, target)
return min(delete,insert)
return min(dp(0,0,target) for target in range(max(count)+1))
``` | 0 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Key Idea Explained | key-idea-explained-by-sarthakbhandari-9b80 | Intuition
Find X such that sum(abs(X - A[i])) is minimised.
X is median of A[], this is the proof
Approach
Try all X values in [MIN_CHAR_FREQ, MAX_CHAR_FREQ] an | sarthakBhandari | NORMAL | 2024-12-22T22:52:58.652372+00:00 | 2024-12-22T22:52:58.652372+00:00 | 24 | false | **Intuition**
Find `X` such that `sum(abs(X - A[i]))` is minimised.
- `X` is median of `A[]`, this is the [proof](https://www.youtube.com/watch?v=yiZlbK_t_9o)
**Approach**
Try all `X` values in `[MIN_CHAR_FREQ, MAX_CHAR_FREQ]` and choose the best one
How to solve for particular `X`? Problem would have been quite easy if only remove and add operations were allowed. But the third operation has the ability to do first two operations with singular cost.
You can also completely remove an element `ch` by driving its frequency to 0. That might be a better choice if `freq[ch] < x - freq[ch]`
About third kind of operation:
- If `freq[ch] > x`, then you can use the residual `freq[ch] - x` to help `freq[ch+1] -> x`
- If `freq[ch] < x` and you choose to `freq[ch] -> 0`, then residual `freq[ch]` can be used to help `freq[ch+1] -> x`
So for each character in alphabet, try all possibilities.
```
# Time: O(maxFreq * 26 * 2)
# Space: O(maxFreq * 26 * 2)
def makeStringGood(self, s: str) -> int:
freq = Counter(s)
MIN = min(freq.values())
MAX = max(freq.values())
def prev(ch):
return chr(ord(ch) - 1)
def nex(ch):
return chr(ord(ch) + 1)
@lru_cache(None)
def dp(x, ch, prevZerod):
if ch > 'z':
return 0
if ch not in freq:
return dp(x, nex(ch), 0)
prevFrq = freq.get(prev(ch), 0)
best = inf
if freq[ch] < x:
# -> 0
best = dp(x, nex(ch), 1) + freq[ch]
# -> x
cand = dp(x, nex(ch), 0) + x - freq[ch]
if prevZerod:
cand -= min(x - freq[ch], prevFrq)
elif prevFrq > x:
cand -= min(prevFrq - x, x - freq[ch])
best = min(best, cand)
else:
# -> x
best = dp(x, nex(ch), 0) + freq[ch] - x
return best
return min(dp(x, 'a', 0) for x in range(MIN, MAX + 1))
``` | 0 | 0 | ['Hash Table', 'String', 'Dynamic Programming', 'Greedy', 'Counting', 'Python'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Java Solution || Dynamic Programming | java-solution-dynamic-programming-by-roh-s83e | IntuitionTo make the string s "good," we need to ensure that the frequency of all characters in the string becomes equal. This is done by considering all possib | rohit-motwani1313 | NORMAL | 2024-12-22T05:33:36.749818+00:00 | 2024-12-22T05:33:36.749818+00:00 | 61 | false | # Intuition
To make the string s "good," we need to ensure that the frequency of all characters in the string becomes equal. This is done by considering all possible target frequencies (tar) from 1 to the maximum frequency of any character in the string.
For each target frequency, we calculate the minimum operations required to adjust the frequency of each character to either match the target frequency or drop it to 0.
The possible operations include:
1. Deleting characters to reduce frequency.
2. Inserting characters to increase frequency.
3. Passing surplus characters from one character to the next to adjust the frequencies optimally (using a passed variable to track this).
By exploring all possibilities, the algorithm identifies the minimum number of operations required for each target frequency and returns the global minimum.
# Approach
1. Frequency Array Calculation:
- Calculate the frequency of each character in the string and store it in an array v of size 26 (one for each letter of the alphabet).
- Also, determine the maximum frequency (maxi) of any character in the string.
2. Dynamic Programming:
- Use a recursive function f(i, passed, tar, v, dp) to calculate the minimum operations required to make the substring starting from character i (where i ranges from 0 to 26) "good" for a given target frequency tar.
- Use a memoization table dp[i][passed] to avoid recalculating results for the same states.
3. Handling Three Cases:
- If the current frequency v[i] > tar:
- Either delete the extra characters or pass them to the next character (set passed = 1).
- If v[i] == tar:
- No operation is needed; move to the next character.
- If v[i] < tar:
- If passed == 1: Try to balance the deficit by borrowing from the previous character.
- Otherwise, consider inserting characters, deleting all characters, or passing all characters to the next.
4. Iterate Over All Target Frequencies:
- For each target frequency from 1 to maxi, calculate the minimum operations using the recursive function and memoization.
- Track the global minimum across all target frequencies.
5. Return the Result:
- Return the global minimum as the result.
# Code
```java []
class Solution {
private int f(int i, int passed, int tar, int[] v, int[][] dp) {
if (i >= 26) return 0;
if (dp[i][passed] != -1) return dp[i][passed];
int ans = Integer.MAX_VALUE;
if (v[i] > tar) {
ans = Math.min(ans, (v[i] - tar) + f(i + 1, 0, tar, v, dp)); //delete the characters
ans = Math.min(ans, (v[i] - tar) + f(i + 1, 1, tar, v, dp)); //pass the characters
} else if (v[i] == tar) {
ans = Math.min(ans, f(i + 1, 0, tar, v, dp)); //simply move to next character in string
} else {
// v[i] < tar
if (passed == 1) {
int req = 0;
if (v[i - 1] < tar) {
req = (tar - v[i] - v[i - 1]);
} else {
req = (tar - v[i] - (v[i - 1] - tar));
}
req = Math.max(req, 0);
ans = Math.min(ans, req + f(i + 1, 0, tar, v, dp));
} else {
ans = Math.min(ans, (tar - v[i]) + f(i + 1, 0, tar, v, dp)); // insert the character
ans = Math.min(ans, v[i] + f(i + 1, 0, tar, v, dp)); // delete all the characters
ans = Math.min(ans, v[i] + f(i + 1, 1, tar, v, dp)); // pass all the characters
}
}
return dp[i][passed] = ans;
}
public int makeStringGood(String s) {
int n = s.length(), ans = Integer.MAX_VALUE;
int[] v = new int[26];
int maxi = 0;
// Frequency array
for (char ch : s.toCharArray()) {
v[ch - 'a']++;
maxi = Math.max(maxi, v[ch - 'a']);
}
// Try every frequency from 1 to maximum frequency
for (int freq = 1; freq <= maxi; freq++) {
int[][] dp = new int[26][2];
for (int i = 0; i < 26; i++) {
dp[i][0] = -1;
dp[i][1] = -1;
}
ans = Math.min(ans, f(0, 0, freq, v, dp));
}
return ans;
}
}
``` | 0 | 0 | ['String', 'Dynamic Programming', 'Java'] | 0 |
minimum-operations-to-make-character-frequencies-equal | easy dp solution | easy-dp-solution-by-barkaaat-m6m6 | Code | Barkaaat | NORMAL | 2024-12-21T22:14:04.651023+00:00 | 2024-12-21T22:14:04.651023+00:00 | 17 | false | # Code
```cpp []
class Solution {
public:
int dp[27][20009], vis[27][20009], k, id;
int v[27];
int solve(int i=0, int rem=0) {
if (i == 26) return rem;
int &ans=dp[i][rem];
if (vis[i][rem] == id) return ans;
vis[i][rem]=id;
ans=solve(i+1, v[i])+rem;
if (v[i] >= k) {
ans=min(ans, solve(i+1, v[i]-k)+rem);
} else {
ans=min(ans, solve(i+1, 0)+max(rem, k-v[i]));
}
return ans;
}
int makeStringGood(string s) {
map<char, int> mp;
for (auto it:s) v[it-'a']++;
int ans=s.size();
for (int i=0; i<=s.size(); i++) {
k=i;
id++;
ans=min(ans, solve());
}
return ans;
}
};
``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | C++ Dynamic Programming With Memoization (n * 26 * 2) Complexity | c-dynamic-programming-with-memoization-n-lo7p | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | smitvavliya | NORMAL | 2024-12-20T15:05:34.607656+00:00 | 2024-12-20T15:05:34.607656+00:00 | 21 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int recursion(int pos, int isPrevReplaceable, int occ, vector<int> &freq, vector<vector<int>> &dp) {
if(pos == 26) return 0;
if(dp[pos][isPrevReplaceable] != -1) {
return dp[pos][isPrevReplaceable];
}
if(freq[pos] == -1) {
return dp[pos][isPrevReplaceable] = recursion(pos + 1, 0, occ, freq, dp);
}
int ans = freq[pos] + recursion(pos + 1, 1, occ, freq, dp);
if(freq[pos] < occ) {
ans = min(ans, occ - freq[pos] + recursion(pos + 1, 0, occ, freq, dp));
if(isPrevReplaceable) {
int maxReplaceable = pos - 1 >= 0 ? freq[pos - 1] < occ ? freq[pos - 1] : freq[pos - 1] - occ : 0;
ans = min(ans, max(0, occ - freq[pos] - maxReplaceable) + recursion(pos + 1, 0, occ, freq, dp));
}
}
if(freq[pos] == occ) {
ans = min(ans, recursion(pos + 1, 0, occ, freq, dp));
}
if(freq[pos] > occ) {
ans = min(ans, freq[pos] - occ + recursion(pos + 1, 1, occ, freq, dp));
}
return dp[pos][isPrevReplaceable] = ans;
}
int makeStringGood(string s) {
int n = s.size(), mx = 25;
vector<int> freq(26, -1);
for(auto &ch: s) {
if(freq[ch - 'a'] == -1) {
freq[ch - 'a'] = 1;
} else {
freq[ch - 'a']++;
}
}
int ans = INT_MAX;
for(int occ = 0; occ <= n; occ++) {
vector<vector<int>> dp(26, vector<int> (2, -1));
int res = recursion(0, 0, occ, freq, dp);
ans = min(ans, res);
}
return ans;
}
};
``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | 4 Hour to solve it :<<, C++ | 4-hour-to-solve-it-c-by-nguyenbaphuchung-dof0 | It took me 4 hours to realize thisIntuitionAfter reading the topic, I see that there are 3 states that are suspicious of using dynamic programming. I tried comb | nguyenbaphuchung2 | NORMAL | 2024-12-20T11:34:49.860001+00:00 | 2024-12-20T11:34:49.860001+00:00 | 23 | false |
**It took me 4 hours to realize this**
--
# Intuition
------------
After reading the topic, I see that there are 3 states that are suspicious of using dynamic programming. I tried combining the case of decreasing and increasing into one, because when decreasing, it can decrease to the frequency k under consideration, or transform it. to 0, as for element state transition, it can only happen when the previous word has a number of elements greater than the k currently being considered or makes the frequency go to 0, then build the formula
--------------
# Code
```cpp []
class Solution {
public:
int makeStringGood(string s) {
map<int,int> c;
int m=0;
for(int i=0; i <s.size(); i++)
{
c[s[i]-'a'+1]++;
m=max(m,c[s[i]-'a'+1]);
}
vector<vector<int>> dp(m+1,vector<int>(c.size()+1));
int vv=1;
vector<int> kc;
int pre=0;
kc.push_back(0);
for(auto i: c)
{
dp[0][vv]=i.second;
kc.push_back(i.first-pre);
pre=i.first;
vv++;
}
for(int i=0; i< kc.size(); i++) cout<<kc[i]<<" ";
cout<<endl<<endl;
for(int j=1; j<=c.size(); j++)
{
for(int i=1; i<=m ;i++)
{
//them, xoa
dp[i][j]=min(dp[0][j],abs(i-dp[0][j]))+dp[i][j-1];
// change to next
if(j>1 && i>dp[0][j] && kc[j]==1 )
{
int a=dp[0][j-1]-0;
int need=i-dp[0][j];
dp[i][j]=min(dp[i][j],max(a,need)+dp[i][j-2]);
if(dp[0][j-1]>i)
{
int b=dp[0][j-1]-i;
dp[i][j]=min(dp[i][j],max(b,need)+dp[i][j-2]);
}
}
}
}
int ans=INT_MAX;
for(int i=1; i<=m ;i++)
{
ans=min(ans,dp[i][c.size()]);
}
return ans;
}
};
``` | 0 | 0 | ['Dynamic Programming', 'C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Minimum Operations to Make Character Frequencies Equal | minimum-operations-to-make-character-fre-1kuu | Code | Ansh1707 | NORMAL | 2024-12-20T10:39:56.905936+00:00 | 2024-12-20T10:39:56.905936+00:00 | 15 | false |
# Code
```python []
class Solution(object):
def makeStringGood(self, s):
"""
:type s: str
:rtype: int
"""
char_frequency = [0] * 26
for char in s:
char_frequency[ord(char) - 97] += 1
max_frequency = max(char_frequency)
min_operations = len(s)
for target_frequency in range(1, max_frequency + 1):
increase_cost = decrease_cost = 0
for char_index in range(26):
if char_frequency[char_index] == 0:
continue
current_freq = char_frequency[char_index]
previous_increase = increase_cost
previous_decrease = decrease_cost
if target_frequency <= current_freq:
diff = current_freq - target_frequency
increase_cost = diff + min(previous_increase, previous_decrease)
decrease_cost = diff + min(previous_increase, previous_decrease)
else:
increase_cost = target_frequency - current_freq + min(previous_increase, previous_decrease)
if char_index > 0:
adjustment = 0
if char_frequency[char_index - 1] >= target_frequency:
adjustment = char_frequency[char_index - 1] - target_frequency
else:
adjustment = char_frequency[char_index - 1]
extra_cost = max(0, target_frequency - current_freq - adjustment) + previous_decrease
increase_cost = min(increase_cost, extra_cost)
decrease_cost = min(previous_increase, previous_decrease) + current_freq
min_operations = min(min_operations, increase_cost, decrease_cost)
return min_operations
``` | 0 | 0 | ['String', 'Python'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Python DP Solution with Key Comments | python-dp-solution-following-hints-by-xi-qjob | IntuitionI did not come up with this solution on my own during the contest. I followed all the 7 hints and succeeded after some trial and error.I did peek at ot | geemaple | NORMAL | 2024-12-19T16:32:48.174639+00:00 | 2024-12-19T16:51:46.052063+00:00 | 35 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
I did not come up with this solution on my own during the contest. I followed all the 7 hints and succeeded after some trial and error.
I did peek at other contestant’s answer and knew I should use 2D DP.
# Approach
<!-- Describe your approach to solving the problem. -->
1. Count all the characters, get `low` and `high` count values
2. Loop through [`low`, `high`], so we get constant `c` in each iteration (hint 4)
3. Define dp as 2d array, which `dp[i][0]` represents `+characters`, while `dp[i][1]` represents `-cahracters`. loop through all 26 alphabets
- op1 delete, if count > k we decrease it to k, otherwise we decrease it to zero
- op2 insert, only if count < k, we can increase it to k.
- op3 change, only if previous is `-cahracters` operation. it can contribute to reducing further.
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
O(N)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
O(1)
# Code
```python3 []
class Solution:
def makeStringGood(self, s: str) -> int:
n = len(s)
occ = [0 for i in range(26)]
for x in s:
index = ord(x) - ord('a')
occ[index] += 1
low = min(x for x in occ if x != 0)
high = max(occ)
res = float('inf')
for k in range(low, high + 1):
op = self.helper(s, k, occ)
res = min(res, op)
return res
def helper(self, s: str, k: int, occ: list) -> int:
dp = [[float('inf'), float('inf')] for i in range(27)]
dp[0] = [0, 0]
for i in range(1, 27):
if occ[i - 1] == 0 or occ[i - 1] == k:
dp[i] = dp[i - 1]
continue
# op1
dp[i][1] = min(dp[i - 1]) + (occ[i - 1] - k if occ[i - 1] > k else occ[i - 1])
if occ[i - 1] < k:
# op2
dp[i][0] = min(dp[i - 1]) + k - occ[i - 1]
if i - 2 >= 0:
# op3
op = occ[i - 2] - k if occ[i - 2] >= k else occ[i - 2]
res = dp[i - 1][1] + max(k - occ[i - 1] - op, 0)
dp[i][0] = min(dp[i][0], res)
return min(dp[-1])
``` | 0 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Minimum Operations to Make Character Frequencies Equal | minimum-operations-to-make-character-fre-l39i | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | AryanSaraf | NORMAL | 2024-12-18T09:15:03.270273+00:00 | 2024-12-18T09:15:03.270273+00:00 | 117 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public int makeStringGood(String s) {\n char[] str = s.toCharArray();\n int n = str.length;\n int[] cnt = new int[26];\n for (char c : str) {\n cnt[c - \'a\']++;\n }\n int mn = n, mx = 0;\n for (int c : cnt) {\n if (c > 0) {\n mn = Math.min(mn, c);\n mx = Math.max(mx, c);\n }\n }\n if (mn == mx) {\n return 0;\n }\n int[][] dp = new int[26][2];\n int ans = n - 1;\n for (int i = mn; i <= mx; i++) {\n dp[0][0] = cnt[0];\n dp[0][1] = cnt[0] <= i ? i - cnt[0] : cnt[0] - i;\n for (int j = 1; j < 26; j++) {\n dp[j][0] = Math.min(dp[j - 1][0], dp[j - 1][1]) + cnt[j];\n if (cnt[j] >= i) {\n dp[j][1] = Math.min(dp[j - 1][0], dp[j - 1][1]) + (cnt[j] - i);\n } else {\n dp[j][1] = Math.min(dp[j - 1][0] + i - Math.min(i, cnt[j] + cnt[j - 1]), dp[j - 1][1] + i - Math.min(i, cnt[j] + Math.max(0, cnt[j - 1] - i)));\n }\n }\n ans = Math.min(ans, Math.min(dp[25][0], dp[25][1]));\n }\n return ans;\n }\n}\n``` | 0 | 0 | ['Java'] | 1 |
minimum-operations-to-make-character-frequencies-equal | Optimizing String Goodness: A Dynamic Programming Adventure!" 🚀✨ | optimizing-string-goodness-a-dynamic-pro-rulv | IntuitionThe problem requires modifying the frequency of characters in a string to make it "good" under specific conditions. My first thought was to find an opt | jerryjacob09 | NORMAL | 2024-12-17T15:52:21.858619+00:00 | 2024-12-17T15:52:21.858619+00:00 | 30 | false | # Intuition \nThe problem requires modifying the frequency of characters in a string to make it "good" under specific conditions. My first thought was to find an optimal method to adjust character frequencies while minimizing the cost. Since the problem involves adjacent relationships, dynamic programming seems like a reasonable approach to efficiently solve this issue.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nFirst, count the frequency of each character in the string and store it in a vector (freq) of size 26.\nDynamic Programming Setup to solve the characters of the good used solve function like the min() which was iterating all over the target and the outer loop tries to target the frequencies and rack the minimum cost across all possible values.\n# Complexity\n- Time complexity: O(N)\n\n\n\n\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n\n\n\n\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n //ooperation 3 is optimal for only chnaging adjancent elements\n int solve(vector<int> &cnt,int x){\n vector<int> dp(26,INT_MAX);\n dp[0]=min(abs(cnt[0]),abs(x-cnt[0]));\n\n for(int i=1;i<26;i++){\n dp[i]=dp[i-1]+min(cnt[i],abs(x-cnt[i]));\n int prev=0;\n\n if(i-2>=0){\n prev=dp[i-2];\n }\n\n if(cnt[i]<x){\n int carry=cnt[i-1];\n if(cnt[i-1]>=x){\n carry=cnt[i-1]-x; \n }\n dp[i]=min(dp[i],prev+carry+max(0,x-(cnt[i]+carry)));\n \n }\n\n }\n return dp[25];\n }\n int makeStringGood(string s) {\n vector<int> freq(26,0);\n int n=s.size();\n for(auto ch:s){\n freq[ch-\'a\']++;\n }\n\n int ans=INT_MAX;\n for(int i=0;i<=n;i++){\n ans=min(ans,solve(freq,i));\n }\n\n return ans;\n\n\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | DP + Brute Force | Python | dp-brute-force-python-by-justinleung0204-wg0c | Intuitionquota is the number of free quotas to increase cnt[i] without extra cost(operations).At each index i, if cnt[i] has to be decreased, we always assume i | justinleung0204 | NORMAL | 2024-12-17T12:22:51.701894+00:00 | 2024-12-17T12:23:25.645144+00:00 | 45 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n$$quota$$ is the number of free quotas to increase cnt[i] without extra cost(operations). \n\nAt each index $$i$$, if $$cnt[i]$$ has to be decreased, we always assume it is a Operation 3 and save quota for $$cnt[i+1]$$ to be increase. \n\n$$i+1$$ can use any amount of the quota, or not to use it (which degenerates back to Operation 2. But who cares? Both are cost 1)\n\nNote that quota will only propagate to the immediate next element, since for $$i+1<j$$, the cost of propagating wont be better than doing $$cnt[i]--$$ and $$cnt[j]++$$ separately.\n\nThe rest are decision dp and brute force on the value to fix.\n\n# Complexity\n- Time complexity: $$O(max(cnt) * 26*avg(cnt))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n orda = ord(\'a\')\n cnt = [0 for _ in range(26)]\n for c in s:\n cnt[ord(c)-orda]+=1\n maxf = max(cnt) #must be set all to [0,maxf]\n INF = 10**20\n\n def count(k):\n """\n i: ith char , form \'a\' to \'z\'\n quota: #chars passed from i-1\n """\n @cache\n def dfs(i,quota): #26*avg(cnt)\n if i==26:\n return 0\n res = INF\n if cnt[i]<k: #2 choices\n #drop to 0\n cost = cnt[i]\n res = min(res, cost + dfs(i+1, cnt[i])) #default quotafor i+1\n #raise to k\n cost = max(0,k-cnt[i] - quota)\n res = min(res, cost + dfs(i+1, 0))\n elif cnt[i]>k: #1 choice\n #drop to k\n cost = cnt[i]-k\n res = min(res, cost + dfs(i+1, cnt[i]-k)) #default quota i+1\n else: #do nothing\n res = min(res, dfs(i+1, 0)) \n\n return res\n\n return dfs(0,0)\n\n\n ans = sum(cnt) #base case: del all\n for k in range(maxf+1):\n #try fix all to k\n ans = min(ans, count(k))\n\n return ans\n\n\n``` | 0 | 0 | ['Dynamic Programming', 'Greedy', 'Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Python3 Solution | Using @cache DP --- (Upsolved after the Contest) | python3-solution-using-cache-dp-upsolved-igaf | Code | apurvdwivedi518 | NORMAL | 2024-12-16T18:30:03.363410+00:00 | 2024-12-16T18:30:03.363410+00:00 | 71 | false | # Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n freq = Counter(s)\n\n @cache\n def dfs(i, expectedOcc, leftoverChars):\n if i==26:\n return 0\n\n char=chr(i+ord(\'a\'))\n occurance=freq.get(char,0)\n\n # Case 1: If the current character does not appear in the string\n # Skip this character and move to the next\n if occurance==0:\n return dfs(i+1, expectedOcc, 0)\n\n # Case 2: If the frequency of the character is greater than the expectedOcc\n # Remove the extra characters and carry any excess to the next step\n if occurance>expectedOcc:\n return occurance-expectedOcc+dfs(i+1, expectedOcc, occurance-expectedOcc)\n\n # Case 3: If the frequency of the character is less than the expectedOcc\n # Consider two options:\n # 1. Add characters to reach the expectedOcc, then reset leftoverChars\n # 2. Use all available characters and carry them over\n if occurance+leftoverChars<expectedOcc:\n return min(\n expectedOcc-occurance-leftoverChars+dfs(i + 1, expectedOcc, 0), # Add characters\n occurance+dfs(i + 1, expectedOcc, occurance) # Use all available\n )\n\n # Case 4: If the frequency matches the expectedOcc or can be balanced by leftoverChars\n # No need to add or delete characters, just proceed\n return dfs(i+1, expectedOcc, 0)\n\n # Step 2: Try all possible expectedOcc frequencies from 0 to the maximum frequency\n # The result is the minimum cost across all possible expectedOccs\n return min(dfs(0, expectedOcc, 0) for expectedOcc in range(max(freq.values()) + 1))\n``` | 0 | 0 | ['Hash Table', 'Dynamic Programming', 'Memoization', 'Python', 'Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | O(n * 26) | on-26-by-user9754iq-crbj | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | user9754IQ | NORMAL | 2024-12-16T05:54:40.565450+00:00 | 2024-12-16T05:54:40.565450+00:00 | 57 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n alpha_dict = [0] * 27\n for char in s:\n alpha_dict[ord(char) - ord(\'a\') + 1] += 1\n\n max_freq = max(alpha_dict[1:])\n min_freq = min(alpha_dict[1:])\n \n #line = 5\n min_val = float(\'inf\')\n for line in range(min_freq, max_freq + 1):\n dp = [[0 for i in range(2)] for j in range(27)]\n for i in range(1, 27):\n if alpha_dict[i] >= line:\n dp[i][1] = min(dp[i - 1][0], dp[i - 1][1]) + alpha_dict[i] - line\n else:\n if (alpha_dict[i - 1] + alpha_dict[i]) < line: #this implies that alpha_dict[i - 1] < line\n a = dp[i - 1][0] + line - (alpha_dict[i] + alpha_dict[i - 1]) # make prev 0\n b = dp[i - 1][1] + line - alpha_dict[i] # make prev 1 \n dp[i][1] = min(a, b)\n else:\n diff = alpha_dict[i - 1] - line\n if (alpha_dict[i - 1] > line):\n if (diff >= line - alpha_dict[i]):\n dp[i][1] = dp[i - 1][1]\n else:\n dp[i][1] = min(dp[i - 1][0], dp[i - 1][1] + line - (diff +alpha_dict[i]))\n else:\n dp[i][1] = min(dp[i - 1][0], dp[i - 1][1] + line - (alpha_dict[i]))\n dp[i][0] = min(dp[i - 1][0], dp[i - 1][1]) + alpha_dict[i]\n min_val = min(min_val, min(dp[-1]))\n return(min_val)\n \n\n\n``` | 0 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | DP | O(n^1.5) time, O(n^1.5) space | Python3 | dp-on15-time-on15-space-python3-by-haris-drpw | Complexity
Time complexity: O(n1.5)
Space complexity: O(n1.5)
Code | HariShankarKarthik | NORMAL | 2024-12-16T05:30:17.001379+00:00 | 2024-12-16T05:30:17.001379+00:00 | 29 | false | # Complexity\n- Time complexity: $$O(n^{1.5})$$\n- Space complexity: $$O(n^{1.5})$$\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n hash = [0] * 26\n for c in s:\n hash[ord(c) - ord("a")] += 1\n\n max_freq = max(hash)\n memo = [dict() for _ in range(26)]\n\n def dp(i, f, dropped):\n if i >= 26:\n return 0\n\n if (f, dropped) in memo[i]:\n return memo[i][(f, dropped)]\n\n res = (\n dp(i + 1, f, 0)\n if hash[i] in {0, f}\n else (\n # drop excess\n hash[i] - f + dp(i + 1, f, hash[i] - f)\n if hash[i] > f\n else min(\n # drop all\n hash[i] + dp(i + 1, f, hash[i]),\n # add deficit\n max(f - (hash[i] + dropped), 0) + dp(i + 1, f, 0),\n )\n )\n )\n\n memo[i][(f, dropped)] = res\n return res\n\n return min([dp(0, f, 0) for f in range(1, 1 + max(hash))])\n\n # O(n^1.5) time\n # O(n^1.5) space\n\n``` | 0 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | simple dp | simple-dp-by-chen3933-yxst | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | chen3933 | NORMAL | 2024-12-16T01:07:58.058695+00:00 | 2024-12-16T01:07:58.058695+00:00 | 49 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n w_cnt = [0]*26\n for c in s:\n w_cnt[ord(c) - ord(\'a\')] += 1\n\n @cache\n def dfs(i, target, carry_in):\n if i == 26:\n return 0\n if w_cnt[i] == 0:\n return dfs(i+1, target, 0)\n if w_cnt[i] > target:\n return w_cnt[i] - target + dfs(i+1, target, w_cnt[i]-target)\n if w_cnt[i] + carry_in < target:\n return min(target - w_cnt[i] - carry_in + dfs(i+1, target, 0), \n w_cnt[i] + dfs(i+1, target, w_cnt[i]))\n return dfs(i+1, target, 0)\n\n return min(dfs(0, target,0) for target in range(max(w_cnt) + 1))\n \n\n \n\n``` | 0 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | just a variant | just-a-variant-by-igormsc-383v | Code | igormsc | NORMAL | 2024-12-15T20:06:16.385369+00:00 | 2024-12-15T20:06:16.385369+00:00 | 22 | false | # Code\n```kotlin []\nclass Solution {\n fun makeStringGood(s: String): Int =\n s.fold(IntArray(\'z\'.code + 1)) { r, c -> r[c.code]++; r }.slice(\'a\'.code..\'z\'.code).let { cnt ->\n (1..s.length).minOf { j ->\n cnt.fold(IntArray(3)) { r, c ->\n if (c >= j) intArrayOf(minOf(r[0], r[1]) + c - j, s.length, c - j)\n else intArrayOf(minOf(r[0], r[1]) + c, minOf(r[0] + maxOf(0, j - c - r[2]), r[1] + j - c), c)\n }.dropLast(1).min()\n }\n }\n}\n``` | 0 | 0 | ['Kotlin'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Unique O(26^3 * log N + N) Ternary Search + DP in Python3 | unique-o263-log-n-ternary-search-dp-in-p-xfoy | IntuitionMy solution explores the number of distinct characters to use. For using k distinct characters, I apply ternary search to find the best length L, which | metaphysicalist | NORMAL | 2024-12-15T19:08:35.258789+00:00 | 2024-12-16T08:31:35.623297+00:00 | 74 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nMy solution explores the number of distinct characters to use. For using $k$ distinct characters, I apply ternary search to find the best length $L$, which is a multiple of $k$. \n\nTo compute the cost of $(L, k)$, a dynamic programming within $O(2\\times 26^2)$ is employed. The DP is to find $k$ letters from all the 26 ones to distribute all the $L$ characters. \n\nIn this way, the overall complexity is $O(26^3 \\times \\log N + N)$, superior for even larger $N$.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe dp is to explore to or not to select the $i$-th letter. A state is defined by three parameters $(i, j, d)$, denoting that $j$ letters are selected from the first $i$ letters, where the boolean value $d$ denotes if the $(i-1)$-th letter is not selected. \n\nIf we want not to select the $i$-th letter, we just remove all the occurrences of the letter with a cost of its occurrences in `s`.\nOtherwise, we have to make the occurrences of the letter in `s` equal to $w = \\frac{L}{k}$. Let $c_i$ is the occurrences of the $i$-th letter in original `s`. If $c_i > w$, we need to remove $c_i - w$ occurrences with a cost of $c_i - w$. If $c_i < w$, we need to add $w - c_i$ occurrences of $i$-th letter. In this case, we can use the $i-1$-th letters that are removed from the previous stage $(i-1, j-1)$ because the operation of substitution costs only 1 instead of a removal and an addition (cost 2). The DP can be done in $O(2 \\times 26^2)$. \n\n# Complexity\n- Time complexity: $O(26^3 \\times \\log N + N)$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n cnt = Counter([ord(c) - ord(\'a\') for c in s])\n n = len(s)\n\n @cache\n def dp(i, j, width, d=False):\n if j < 0:\n return inf\n if i >= 26:\n if j == 0:\n return 0\n return inf\n s1 = dp(i+1, j, width, True) + cnt[i]\n s2 = dp(i+1, j-1, width, False)\n if cnt[i] > width:\n s2 += cnt[i] - width\n elif cnt[i] < width:\n if i > 0:\n if d:\n q = cnt[i-1]\n else:\n q = max(0, cnt[i-1] - width)\n else:\n q = 0\n q = min(q, width - cnt[i])\n s2 += width - cnt[i] - q\n return min(s1, s2)\n\n def check(l, k):\n return dp(0, k, l // k) \n\n def search(k):\n lo, hi = 0, 2 * n // k + 1\n while hi - lo >= 3:\n m1 = lo + (hi - lo) // 3\n m2 = hi - (hi - lo) // 3\n f1 = -check(m1 * k, k)\n f2 = -check(m2 * k, k)\n if f1 < f2:\n lo = m1\n else:\n hi = m2\n return min(check(j * k, k) for j in range(lo, hi+1))\n \n ans = min(search(i) for i in range(1, len(set(s))+1))\n dp.cache_clear()\n return ans\n``` | 0 | 0 | ['Binary Search', 'Dynamic Programming', 'Memoization', 'Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Recursive O(n) DP with comments | recursive-on-dp-with-comments-by-ac12110-rikb | Complexity
Time complexity:
O(n)
Space complexity:
O(1)
Code | ac121102 | NORMAL | 2024-12-15T16:35:23.875078+00:00 | 2024-12-15T16:35:23.875078+00:00 | 64 | false | # Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int work(vector<int> &freq, int &tar, int i, int gave, vector<array<int, 2>> &dp) {\n if (i == freq.size()) return 0; // Base case\n bool gv = (gave > 0);\n if (dp[i][gv] != -1) return dp[i][gv]; // Memoized result\n\n int ans = INT_MAX;\n\n if (freq[i] == 0 || freq[i] == tar) {\n ans = work(freq, tar, i + 1, 0, dp); // No change needed\n } else if (freq[i] < tar) {\n int new_freq = min(tar, freq[i] + gave);\n ans = min(ans, (tar - new_freq) + work(freq, tar, i + 1, 0, dp)); // Increase\n ans = min(ans, freq[i] + work(freq, tar, i + 1, freq[i], dp)); // Decrease to 0\n } else {\n ans = min(ans, (freq[i] - tar) + work(freq, tar, i + 1, freq[i] - tar, dp)); // Decrease to target\n }\n\n return dp[i][gv] = ans;\n }\n\n int makeStringGood(string s) {\n vector<int> freq(26, 0); \n vector<array<int, 2>> dp(26, {-1, -1}); \n for (char &c : s) freq[c - \'a\']++;\n\n int ans = INT_MAX;\n\n for (int tar = 0; tar <= s.size(); tar++) {\n fill(dp.begin(), dp.end(), array<int, 2>{-1, -1});\n ans = min(ans, work(freq, tar, 0, 0, dp));\n }\n\n return ans;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Nothing to say | nothing-to-say-by-yatin2004-raqh | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | yatin2004 | NORMAL | 2024-12-15T12:17:28.503045+00:00 | 2024-12-15T12:17:28.503045+00:00 | 110 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public int makeStringGood(String s) {\n char[] chars = s.toCharArray();\n int length = chars.length;\n int[] freq = new int[26];\n\n // Count frequency of each character\n for (char c : chars) {\n freq[c - \'a\']++;\n }\n\n int minFreq = length, maxFreq = 0;\n for (int count : freq) {\n if (count > 0) {\n minFreq = Math.min(minFreq, count);\n maxFreq = Math.max(maxFreq, count);\n }\n }\n\n if (minFreq == maxFreq) {\n return 0;\n }\n\n int[][] dp = new int[26][2];\n int result = length - 1;\n\n for (int i = minFreq; i <= maxFreq; i++) {\n dp[0][0] = freq[0];\n dp[0][1] = freq[0] <= i ? i - freq[0] : freq[0] - i;\n\n for (int j = 1; j < 26; j++) {\n dp[j][0] = Math.min(dp[j - 1][0], dp[j - 1][1]) + freq[j];\n\n if (freq[j] >= i) {\n dp[j][1] = Math.min(dp[j - 1][0], dp[j - 1][1]) + (freq[j] - i);\n } else {\n dp[j][1] = Math.min(dp[j - 1][0] + i - Math.min(i, freq[j] + freq[j - 1]),\n dp[j - 1][1] + i - Math.min(i, freq[j] + Math.max(0, freq[j - 1] - i)));\n }\n }\n\n result = Math.min(result, Math.min(dp[25][0], dp[25][1]));\n }\n\n return result;\n }\n}\n\n``` | 0 | 0 | ['Java'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Dp + hash table | dp-hash-table-by-maxorgus-lb2t | count s into freq - freq[i] is the frequency of the ith lettertry every target within min(freq) (non-zero) and max(freq)do the dp, for every target, at index i | MaxOrgus | NORMAL | 2024-12-15T11:26:10.862585+00:00 | 2024-12-15T11:26:10.862585+00:00 | 73 | false | count s into freq - freq[i] is the frequency of the ith letter\n\ntry every target within min(freq) (non-zero) and max(freq)\n\ndo the dp, for every target, at index i\n\nwhen freq[i] is zero, go over it\n\nelse, first try increase it to target or decrease it to zero, get the one that would cost the minimum steps.\n\nthen if i >= 0 and freq[i-1]>0 we can consider using the third case on the base of dp(i-2,target).\n\nwe can either use operation3 to get freq[i-1] = target or freq[i] = i, in both cases there are some finer branches to explore.\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n FREQ = Counter(s)\n freq = [FREQ[chr(ord(\'a\')+i)] for i in range(26)]\n m = min([f for f in freq if f > 0])\n M = max(freq)\n @cache\n def dp(i,target):\n if i == -1:\n return 0\n if freq[i] == 0:return dp(i-1,target)\n preres = dp(i-1,target)\n res = min(abs(target-freq[i]),freq[i])+preres\n \n if freq[i] < target and i > 0 and freq[i-1]>0:\n D1 = dp(i-2,target)\n if freq[i-1] >= target - freq[i]:\n D2 = target - freq[i]\n D3 = freq[i-1] - D2\n D4 = min(abs(target - D3),D3)\n D = D2+D4\n else:\n D2 = freq[i-1] + target - freq[i] - freq[i-1]\n D = D2\n D += D1\n res = min(res,D)\n if i > 0 and freq[i-1]>target:\n D0 = dp(i-2,target)\n D1 = freq[i-1] - target\n D2 = min(freq[i] + D1,abs(target - (freq[i] + D1)))\n res = min(res,D1+D2+D0)\n return res\n return min(dp(25,t) for t in range(m,M+1)) \n\n\n \n\n``` | 0 | 0 | ['Hash Table', 'Dynamic Programming', 'Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Simple 2 understand Memorization DP solution | simple-2-understand-memorization-dp-solu-71jv | Code | user7634ri | NORMAL | 2024-12-15T11:02:30.750135+00:00 | 2024-12-15T11:02:30.750135+00:00 | 77 | false | \n\n# Code\n```cpp []\nclass Solution {\npublic:\n int recur(vector<int> & dp, vector<int> &v, int c, int i) {\n if(i == 26) {\n return 0;\n }\n\n if(dp[i] != -1) {\n return dp[i];\n }\n\n int ans = v[i] + recur(dp, v, c, i + 1);\n // basically handle i and i + 1 together.\n\n if(i <= 24) {\n // do transfers as well.\n // we want to keep both of them and both of them have c occurence.\n // we wish to make c occurence of i + 1 for sure.\n\n int diff = c - v[i + 1];\n \n if(diff > 0) {\n // best to transfer v[i] - c to i + 1 and move forward.\n\n int avail = max(v[i] - diff, 0);\n // remaining available for ith\n\n int way1 = avail; // remove all current.\n if(avail > c) {\n way1 = min(way1, avail - c);\n } else {\n way1 = min(way1, c - avail); // insert remaining for first.\n }\n\n ans = min(ans, diff + way1 + recur(dp, v, c, i + 2));\n\n // another way is to first ensure that current is sorted then transfer access there.\n\n if(v[i] > c && v[i + 1] < c) {\n int avail = v[i] - c;\n int need = c - v[i + 1];\n\n int transfer = min(need, avail);\n int extra = c - (transfer + v[i + 1]);\n\n ans = min(ans, avail + extra + recur(dp, v, c, i + 2));\n }\n }\n }\n \n if(v[i] > c) {\n ans = min(ans, v[i] - c + recur(dp, v, c, i + 1));\n } else {\n ans = min(ans, c - v[i] + recur(dp, v, c, i + 1));\n }\n\n return dp[i] = ans;\n }\n\n int makeStringGood(string s) {\n vector<int> v(26);\n\n\n\n for(auto c: s) {\n v[c - \'a\'] ++;\n }\n\n int ans = INT_MAX;\n\n for(int i = 0; i <= 2*s.length(); i ++) {\n vector<int> dp(26, -1);\n ans = min(ans, recur(dp, v, i, 0));\n }\n\n return ans;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | C++. Brute-Force DP. Iterating over all possible occurrences. | c-brute-force-dp-iterating-over-all-poss-oak9 | Intuition
Ordering does not matter. Simply count up the characters in an array.
Try every possible occurence for each character. Thus a solution can be done | cakuang1 | NORMAL | 2024-12-15T08:55:16.382735+00:00 | 2024-12-15T08:55:16.382735+00:00 | 62 | false | # Intuition\n\n1. Ordering does not matter. Simply count up the characters in an array.\n2. Try every possible occurence for each character. Thus a solution can be done in O(26n).\n3. Now for some fixed occurrence x, we determine the minimum number of operations needed to make each character\'s occurrence x or 0.\n4. This can be done using dp, where we define dp[i] equal to the minimum number of operations needed to make each character\'s occurrence x or 0 from A[0 ... i].\n5. State transitions.\n\n **DP[i] = DP[i - 1] if A[i] == 0**\n Let Z be the maximum number of operation 3 you can use on A[i - 1] and A[i]. \n **DP[i] = MIN(DP[i - 1] + MIN(A[i] ,ABS(A[i] - x)), DP[i - 2] + Z + MIN(A[i - 1] - Z , ABS((A[i - 1] - Z) - x)) + MIN(A[i] + Z , ABS((A[i] + Z) - x))) IF A[i] != 0**\n\n\n\n \n\n\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int makeStringGood(string s) {\n vector<int> counter(26, 0);\n int n = s.size();\n\n // Count frequencies of each character\n for (int i = 0; i < n; i++) {\n counter[s[i] - \'a\']++;\n }\n int minele = 0, maxele = n;\n int currmin = INT_MAX;\n for (int target = minele; target <= maxele; target++) {\n vector<int> dp(26, INT_MAX);\n dp[0] = min(counter[0], abs(target - counter[0])); \n for (int c = 1; c < 26; c++) { \n if (counter[c] == 0) {\n dp[c] = dp[c - 1]; \n } else {\n dp[c] = dp[c - 1] + min(counter[c], abs(target - counter[c]));\n if (c > 0 && counter[c] < target && counter[c - 1]) {\n int adjust;\n if (counter[c - 1] > target) {\n adjust = min(abs(counter[c - 1] - target) , abs(counter[c] - target));\n } else {\n adjust = min(counter[c - 1], abs(counter[c] - target));\n }\n int newbefore = counter[c - 1] - adjust;\n int newafter = counter[c] + adjust;\n int b1 = min(newbefore,abs(target - newbefore));\n int a1 = min(newafter,abs(target - newafter));\n if (c > 1) {\n dp[c] = min(dp[c], dp[c - 2] + adjust + b1 + a1);\n } else {\n dp[c] = min(dp[c],adjust + b1 + a1 );\n }\n }\n }\n\n }\n }\n currmin = min(currmin, dp[25]); \n }\n return currmin;\n }\n};\n\n``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | Iterating over the final frequency, use DP to count | iterating-over-the-final-frequency-use-d-qwm9 | IntuitionFor each character, we have 2 choices:
increase / decrease to the target frequency
decrease to 0
For the operation decrease, we have 2 choices:
decreas | nguyenquocthao00 | NORMAL | 2024-12-15T06:24:05.622588+00:00 | 2024-12-15T06:30:40.584476+00:00 | 62 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFor each character, we have 2 choices:\n- increase / decrease to the target frequency\n- decrease to 0 \n\nFor the operation decrease, we have 2 choices:\n- decrease current character\n- decrease current character, increase the next character\n\nSo if we decrease the current character by x; we can increase the next character by any amount in [0..x]\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python3 []\nclass Solution:\n def makeStringGood(self, s: str) -> int:\n c=Counter(s)\n keys=sorted(ord(ch) for ch in c.keys())\n vals=[c[chr(v)] for v in keys]\n def count(target):\n @lru_cache(None)\n def dp(i, maxop2):\n if i>=len(keys): return 0\n useop2 = (i+1 < len(keys) and keys[i+1]-keys[i]==1)\n v = vals[i]\n if v>=target: \n added = v-target\n return added + dp(i+1, added if useop2 else 0)\n return min(v + dp(i+1, v if useop2 else 0), target-min(target, v + maxop2) + dp(i+1,0))\n return dp(0,0)\n return min(count(t) for t in range(min(vals), max(vals)+1))\n \n``` | 0 | 0 | ['Python3'] | 0 |
minimum-operations-to-make-character-frequencies-equal | [c++] DFS + DP + Greedy | c-dfs-dp-greedy-by-lyronly-c072 | IntuitionApproach1 similar framework as binary search (but it is not binary search), fix one dimension : frequency of each char, then caculate minimum number of | lyronly | NORMAL | 2024-12-15T06:18:14.288282+00:00 | 2024-12-15T06:35:25.372461+00:00 | 82 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1 similar framework as binary search (but it is not binary search), fix one dimension : frequency of each char, then caculate minimum number of operations based on this fixed frequency. \n\n2 The tricky part is for Operation: Change a character in s to its next letter in the alphabet.\n\nWe can greedly predict the number of letter moving to next alphabet, this will further reduce the time complexity.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n\nTime compelexity O(K * 26 * k) K is the max frequency of letter.\n \n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\ntypedef long long ll;\nclass Solution {\npublic:\n vector<int> transform(string& s)\n {\n vector<int> cnt(26, 0);\n for (auto c : s) cnt[c - \'a\']++;\n return cnt;\n }\n unordered_map<ll, int> cache;\n int n;\n int dfs(vector<int>& dp, int i, int k)\n {\n if (i >= 26) return 0;\n ll cur = dp[i];\n ll key = i * (n + 1) + cur;\n if (cache.contains(key)) return cache[key];\n if (dp[i] == 0 || dp[i] == k) return dfs(dp, i + 1, k);\n int x = dp[i];\n int y = (i + 1 < 26) ? dp[i + 1] : -1;\n int ans = INT_MAX;\n if (dp[i] > k) \n ans = min(ans, dp[i] - k + dfs(dp, i + 1, k));\n else\n ans = min(ans, min(dp[i], k - dp[i]) + dfs(dp, i + 1, k));\n\n if (i < 25 && y > 0 && y < k && dp[i] != k)\n {\n int c = (dp[i] > k) ? dp[i] - k : dp[i];\n int d = k - y;\n int inc = min(c, d);\n dp[i + 1] += inc;\n int b = c + dfs(dp, i + 1, k);\n dp[i + 1] = y;\n ans = min(ans, b);\n }\n cache[key] = ans;\n return ans;\n }\n int makeStringGood(string s) {\n n = s.size();\n vector<int> cnt = transform(s);\n \n int mx = 0;\n for (auto c : cnt) mx = max(mx, c);\n int ans = s.size();\n for (int k = 0; k <= mx; k++)\n {\n cache.clear();\n int a = dfs(cnt, 0, k);\n ans = min(ans, a);\n }\n return ans;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
minimum-operations-to-make-character-frequencies-equal | C# DP | c-dp-by-user8702ha-o2kg | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | user8702Ha | NORMAL | 2024-12-15T05:23:37.548965+00:00 | 2024-12-15T05:23:37.548965+00:00 | 24 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```csharp []\npublic class Solution {\n private int[] count;\n private Dictionary<(int i, int deleted, int target), int> memo;\n \n public int MakeStringGood(string s) {\n count = new int[26];\n foreach (char c in s) {\n count[c - \'a\']++;\n }\n \n memo = new Dictionary<(int i, int deleted, int target), int>();\n \n int maxCount = 0;\n foreach (int x in count) {\n maxCount = Math.Max(maxCount, x);\n }\n \n int minOperations = int.MaxValue;\n for (int target = 0; target <= maxCount; target++) {\n minOperations = Math.Min(minOperations, DP(0, 0, target));\n }\n \n return minOperations;\n }\n \n private int DP(int i, int deleted, int target) {\n if (i == 26) {\n return 0;\n }\n \n var key = (i, deleted, target);\n if (memo.ContainsKey(key)) {\n return memo[key];\n }\n \n int x = count[i];\n int ans;\n \n if (x <= target) {\n ans = DP(i + 1, x, target) + x;\n int increase = Math.Min(deleted, target - x);\n int cand = DP(i + 1, 0, target) + target - x - increase;\n ans = Math.Min(ans, cand);\n } else {\n ans = DP(i + 1, x - target, target) + x - target;\n }\n \n memo[key] = ans;\n return ans;\n }\n}\n\n``` | 0 | 0 | ['C#'] | 0 |
course-schedule-iv | [Java/Python] Floyd–Warshall Algorithm - Clean code - O(n^3) | javapython-floyd-warshall-algorithm-clea-9q2v | Idea\n- This problem is to check if 2 vertices are connected in directed graph. \n- Floyd-Warshall O(n^3) is an algorithm that will output the minium distance o | hiepit | NORMAL | 2020-05-30T16:01:24.436586+00:00 | 2021-09-10T02:09:33.542465+00:00 | 19,547 | false | **Idea**\n- This problem is to check if 2 vertices are connected in directed graph. \n- Floyd-Warshall `O(n^3)` is an algorithm that will output the minium distance of any vertices. We can modified it to output if any vertices is connected or not.\n\n**Complexity:**\n- Time: `O(n^3)`\n- Space: `O(n^2)`\n\n**More Floy-warshall problems:**\n- [1334. Find the City With the Smallest Number of Neighbors at a Threshold Distance](https://leetcode.com/problems/find-the-city-with-the-smallest-number-of-neighbors-at-a-threshold-distance/discuss/491446/)\n\n**Java**\n```java\nclass Solution {\n public List<Boolean> checkIfPrerequisite(int n, int[][] prerequisites, int[][] queries) {\n boolean[][] connected = new boolean[n][n];\n for (int[] p : prerequisites)\n connected[p[0]][p[1]] = true; // p[0] -> p[1]\n for (int k = 0; k < n; k++)\n for (int i = 0; i < n; i++)\n for (int j = 0; j < n; j++)\n connected[i][j] = connected[i][j] || connected[i][k] && connected[k][j];\n List<Boolean> ans = new ArrayList<>();\n for (int[] q : queries)\n ans.add(connected[q[0]][q[1]]);\n return ans;\n }\n}\n```\n\n**Python**\n```\nclass Solution:\n def checkIfPrerequisite(self, n, prerequisites, queries):\n connected = [[False] * n for i in xrange(n)]\n\n for i, j in prerequisites:\n connected[i][j] = True\n\n for k in range(n):\n for i in range(n):\n for j in range(n):\n connected[i][j] = connected[i][j] or (connected[i][k] and connected[k][j])\n\n return [connected[i][j] for i, j in queries]\n``` | 302 | 4 | [] | 40 |
course-schedule-iv | [Java] Topological Sorting | java-topological-sorting-by-asuka-soryu-5zyk | A -> B -> C\nC is prerequisites of B\nB is prerequisites of A\nSo when we do a topology Sort , just simply add A\'s direct prerequisite B to A and also all prer | ASUKA-Soryu | NORMAL | 2020-05-30T17:18:05.583822+00:00 | 2020-05-31T13:57:30.344798+00:00 | 11,944 | false | **A -> B -> C**\nC is prerequisites of B\nB is prerequisites of A\nSo when we do a topology Sort , just simply add A\'s direct prerequisite B to A and also all prerequisites of B to A. \nBesides this part, everything is same as course schedule I and course schedule II\n\nTopology sort can transfer the prerequisites conditons from a prerequisites node to all the successors, \nThis trick can also use for Leetcode 851 as well https://leetcode.com/problems/loud-and-rich/\n\n\n```\n class Solution {\n public List<Boolean> checkIfPrerequisite(int n, int[][] prerequisites, int[][] queries) {\n int[] indegree = new int[n];\n Map<Integer, Set<Integer>> adj = new HashMap<>(); \n Map<Integer, Set<Integer>> prerequisitesMap = new HashMap<>(); \n \n for (int i = 0 ; i < n; i++) {\n prerequisitesMap.put(i, new HashSet<>());\n adj.put(i, new HashSet<>());\n }\n \n for (int[] pre : prerequisites) {\n indegree[pre[1]]++;\n adj.get(pre[0]).add(pre[1]);\n }\n \n Queue<Integer> queue = new LinkedList<>();\n for (int i = 0; i < n; i++) {\n if (indegree[i] == 0) {\n queue.offer(i);\n }\n }\n \n while (!queue.isEmpty()) {\n int node = queue.poll(); \n Set<Integer> set = adj.get(node);\n for (int next : set) {\n prerequisitesMap.get(next).add(node);\n prerequisitesMap.get(next).addAll(prerequisitesMap.get(node));\n indegree[next]--;\n if (indegree[next] == 0) {\n queue.offer(next);\n }\n }\n }\n \n List<Boolean> res = new ArrayList<>();\n for (int[] pair : queries) {\n Set<Integer> set = prerequisitesMap.get(pair[1]);\n if (set.contains(pair[0])) {\n res.add(true);\n }\n else {\n res.add(false);\n }\n }\n return res;\n\t}\n}\n``` | 119 | 1 | [] | 19 |
course-schedule-iv | C++ | Kahn's Algorithm | Short and simple | Explained | c-kahns-algorithm-short-and-simple-expla-ebp9 | This problem is a direct application of kahn\'s algorithm with addition to that, we need to maintain a table which represents if two courses are prerequisites o | shield75_ | NORMAL | 2021-10-22T18:12:28.101777+00:00 | 2021-10-22T18:12:28.101819+00:00 | 7,102 | false | This problem is a direct application of kahn\'s algorithm with addition to that, we need to maintain a table which represents if two courses are prerequisites of one another.\n\n1. Push all the vertices with indegree 0 to the queue.\n2. For all such vertices, decrease their adjacent vertex\'s indegree by 1 since we are removing current vertex from the queue.\n3. for table[i][j] such that `i ` is prerequsite of ` j` , make it true for current vertex & it\'s adjacent, and also if current vertex had a prerequisite, that will apply to current vertex\'s neighbour also `for(int i=0;i<n;i++) if(table[i][cur]==true) table[i][it] = true;`\n```\nclass Solution {\npublic:\n vector<bool> checkIfPrerequisite(int n, vector<vector<int>>& prerequisites, vector<vector<int>>& queries) {\n vector<bool> ans;\n vector<vector<int>> graph(n); \n vector<vector<bool>> table(n,vector<bool>(n,false));\n vector<int> indegree(n,0);\n for(int i=0;i<prerequisites.size();i++)\n {\n graph[prerequisites[i][0]].push_back(prerequisites[i][1]);\n indegree[prerequisites[i][1]]++;\n }\n queue<int> q;\n for(int i=0;i<n;i++) if(indegree[i]==0) q.push(i);\n while(!q.empty())\n {\n int cur=q.front();\n q.pop();\n for(auto &it:graph[cur])\n {\n table[cur][it]=true;\n for(int i=0;i<n;i++) if(table[i][cur]==true) table[i][it] = true;\n indegree[it]--;\n if(indegree[it]==0) q.push(it);\n }\n }\n for(int i=0;i<queries.size();i++) ans.push_back(table[queries[i][0]][queries[i][1]]);\n return ans;\n }\n};\n``` | 66 | 0 | ['Topological Sort', 'C', 'C++'] | 2 |
course-schedule-iv | Transitive closure - Floyd Warshall with detailed explaination - python ,c++, java | transitive-closure-floyd-warshall-with-d-1mha | Brute force :\n for each i th query \n start dfs from queries[i][0]\n if you reach queries[i][1] return True \n else False\n \n\n | sankethbk7777 | NORMAL | 2020-05-30T16:04:17.217352+00:00 | 2020-05-30T16:19:25.100342+00:00 | 7,583 | false | Brute force :\n for each i th query \n start dfs from queries[i][0]\n if you reach queries[i][1] return True \n else False\n \n\n\nSince there can be 10^4 queries, we cannot do dfs every time for each query to\nfind if there is a path between queries[i][0] and queries[i][1]\n\nWe must answer each query in O(1) time \n\nWhat is transitive closure of a graph \n\nIt is a matrix m in which \nm[i][j] is True if there j is reachable from i (can be a more than 1 edge path)\nm[i][j] is False if j cannot be reached from i\n\n\nOnce we get the matrix of transitive closure, each query can be answered in O(1) time \neg: query = (x,y) , answer will be m[x][y] \n\nTo compute the matrix of transitive closure we use Floyd Warshall\'s algorithm which takes O(n^3) time and O(n^2) space.\n\nPython3 \n\n```\nclass Solution:\n def checkIfPrerequisite(self, n: int, prerequisites: List[List[int]], queries: List[List[int]]) -> List[bool]:\n \n def floydWarshall(reachable):\n for k in range(n):\n for i in range(n):\n for j in range(n):\n reachable[i][j] = reachable[i][j] or (reachable[i][k] and reachable[k][j])\n \n return reachable\n \n \n adjMatrix = [[ 0 for i in range(n)] for j in range(n)]\n for i in prerequisites:\n adjMatrix[i[0]][i[1]] = 1\n \n #print(adjMatrix)\n ans = []\n floydWarshall(adjMatrix)\n #print(adjMatrix)\n for i in queries:\n ans.append(bool(adjMatrix[i[0]][i[1]]))\n \n return ans\n```\n\nC++\n```\nclass Solution {\npublic:\n vector<bool> checkIfPrerequisite(int n, vector<vector<int>>& prerequisites, vector<vector<int>>& queries) {\n \n vector<vector<int>> adjMatrix(n, vector<int>(n));\n for (int i = 0; i < prerequisites.size(); ++i){\n adjMatrix[prerequisites[i][0]][prerequisites[i][1]] = 1;\n }\n \n FloydWarshall(n, adjMatrix);\n vector<bool> ans;\n \n for (int i = 0; i < queries.size(); ++i){\n ans.push_back(adjMatrix[queries[i][0]][queries[i][1]]);\n }\n \n return ans;\n \n }\n \n void FloydWarshall(int n, vector<vector<int>>& m){\n \n for (int k = 0; k < n ; ++k){\n for (int i = 0; i < n ; ++i){\n for (int j = 0; j < n ; ++j){\n m[i][j] = m[i][j] or (m[i][k] and m[k][j]);\n }\n }\n }\n }\n};\n```\n\nJava\n\n```\n\nclass Solution {\n public List<Boolean> checkIfPrerequisite(int n, int[][] prerequisites, int[][] queries) {\n \n boolean adjMatrix[][] = new boolean[n][n];\n \n for (int[] i : prerequisites){\n adjMatrix[i[0]][i[1]] = true;\n }\n \n for (int k = 0; k < n; ++k){\n for (int i = 0; i < n ; ++i){\n for (int j = 0; j < n ; ++j){\n adjMatrix[i][j] = adjMatrix[i][j] || (adjMatrix[i][k] && adjMatrix[k][j]);\n }\n }\n }\n \n List<Boolean> ans = new ArrayList<Boolean>();\n \n for (int i = 0; i < queries.length; ++i){\n ans.add(adjMatrix[queries[i][0]][queries[i][1]]);\n }\n \n return ans;\n \n \n }\n}\n\n```\n\n | 59 | 0 | ['C', 'Python', 'Java'] | 2 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.