Commit
•
66b5d62
1
Parent(s):
6f6245f
Create README.md
Browse files
README.md
ADDED
@@ -0,0 +1,62 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
---
|
2 |
+
language: en
|
3 |
+
license: mit
|
4 |
+
tags:
|
5 |
+
- vision
|
6 |
+
- image-captioning
|
7 |
+
pipeline_tag: image-to-text
|
8 |
+
---
|
9 |
+
|
10 |
+
Quantization done with [bitsandbytes](https://github.com/TimDettmers/bitsandbytes) - _Mediocre_
|
11 |
+
|
12 |
+
# InstructBLIP model
|
13 |
+
|
14 |
+
InstructBLIP model using [Flan-T5-xl](https://huggingface.co/google/flan-t5-xl) as language model. InstructBLIP was introduced in the paper [InstructBLIP: Towards General-purpose Vision-Language Models with Instruction Tuning](https://arxiv.org/abs/2305.06500) by Dai et al.
|
15 |
+
|
16 |
+
Disclaimer: The team releasing InstructBLIP did not write a model card for this model so this model card has been written by the Hugging Face team.
|
17 |
+
|
18 |
+
## Model description
|
19 |
+
|
20 |
+
InstructBLIP is a visual instruction tuned version of [BLIP-2](https://huggingface.co/docs/transformers/main/model_doc/blip-2). Refer to the paper for details.
|
21 |
+
|
22 |
+
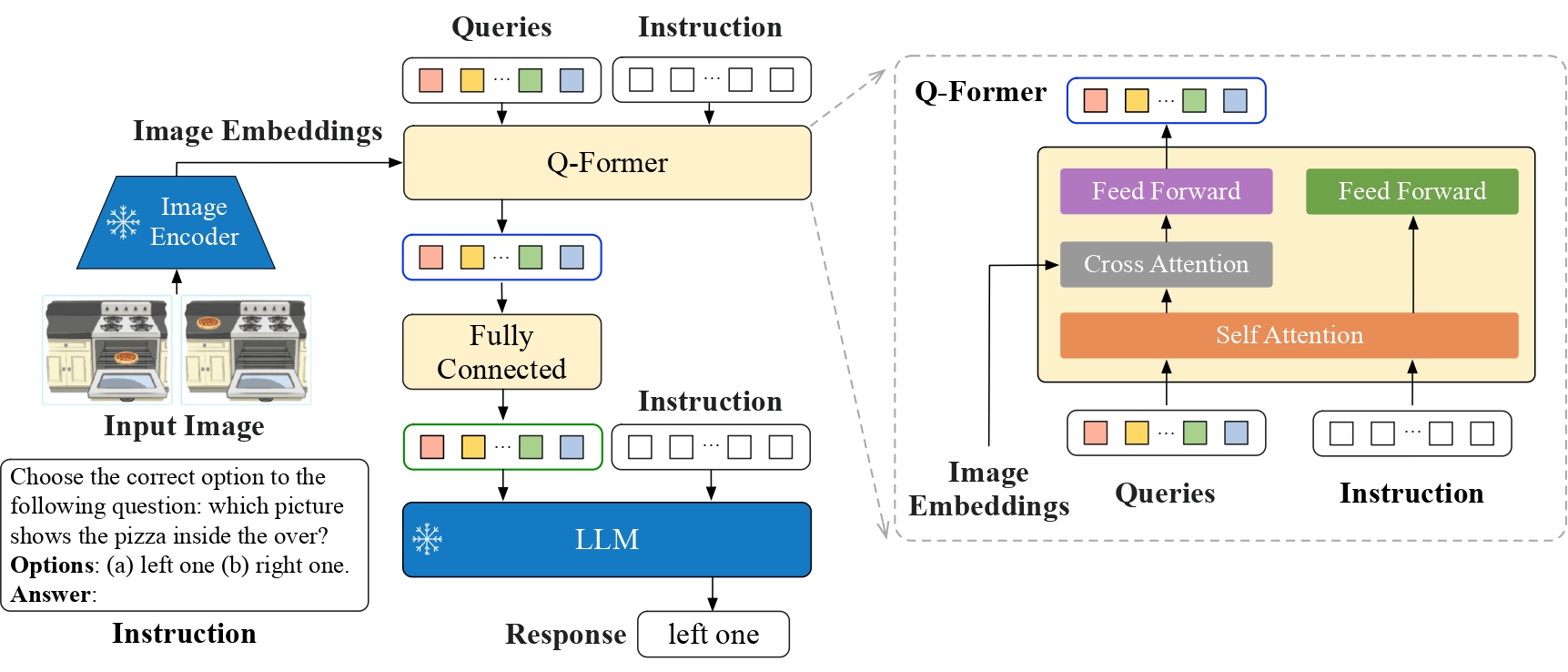
|
23 |
+
|
24 |
+
## Intended uses & limitations
|
25 |
+
|
26 |
+
Usage is as follows:
|
27 |
+
|
28 |
+
```
|
29 |
+
from transformers import InstructBlipProcessor, InstructBlipForConditionalGeneration
|
30 |
+
import torch
|
31 |
+
from PIL import Image
|
32 |
+
import requests
|
33 |
+
|
34 |
+
model = InstructBlipForConditionalGeneration.from_pretrained("Salesforce/instructblip-flan-t5-xl")
|
35 |
+
processor = InstructBlipProcessor.from_pretrained("Salesforce/instructblip-flan-t5-xl")
|
36 |
+
|
37 |
+
device = "cuda" if torch.cuda.is_available() else "cpu"
|
38 |
+
model.to(device)
|
39 |
+
|
40 |
+
url = "https://raw.githubusercontent.com/salesforce/LAVIS/main/docs/_static/Confusing-Pictures.jpg"
|
41 |
+
image = Image.open(requests.get(url, stream=True).raw).convert("RGB")
|
42 |
+
prompt = "What is unusual about this image?"
|
43 |
+
inputs = processor(images=image, text=prompt, return_tensors="pt").to(device)
|
44 |
+
|
45 |
+
outputs = model.generate(
|
46 |
+
**inputs,
|
47 |
+
do_sample=False,
|
48 |
+
num_beams=5,
|
49 |
+
max_length=256,
|
50 |
+
min_length=1,
|
51 |
+
top_p=0.9,
|
52 |
+
repetition_penalty=1.5,
|
53 |
+
length_penalty=1.0,
|
54 |
+
temperature=1,
|
55 |
+
)
|
56 |
+
generated_text = processor.batch_decode(outputs, skip_special_tokens=True)[0].strip()
|
57 |
+
print(generated_text)
|
58 |
+
```
|
59 |
+
|
60 |
+
### How to use
|
61 |
+
|
62 |
+
For code examples, we refer to the [documentation](https://huggingface.co/docs/transformers/main/en/model_doc/instructblip).
|