Spaces:
Paused
Paused
Update app.py
Browse files
app.py
CHANGED
@@ -6,16 +6,16 @@ from typing import Optional
|
|
6 |
|
7 |
import discord
|
8 |
import gradio as gr
|
|
|
9 |
from discord import Permissions
|
10 |
from discord.ext import commands
|
11 |
from discord.utils import oauth_url
|
12 |
-
|
13 |
-
import gradio_client as grc
|
14 |
from gradio_client.utils import QueueError
|
15 |
|
16 |
event = Event()
|
17 |
|
18 |
DISCORD_TOKEN = os.getenv("DISCORD_TOKEN")
|
|
|
19 |
|
20 |
|
21 |
async def wait(job):
|
@@ -24,7 +24,7 @@ async def wait(job):
|
|
24 |
|
25 |
|
26 |
def get_client(session: Optional[str] = None) -> grc.Client:
|
27 |
-
client = grc.Client("https://wop-xxx-opengpt.hf.space/", hf_token=
|
28 |
if session:
|
29 |
client.session_hash = session
|
30 |
return client
|
@@ -46,80 +46,15 @@ bot = commands.Bot(command_prefix="$", intents=intents)
|
|
46 |
@bot.event
|
47 |
async def on_ready():
|
48 |
print(f"Logged in as {bot.user} (ID: {bot.user.id})")
|
49 |
-
synced = await bot.tree.sync()
|
50 |
-
print(f"Synced commands: {', '.join([s.name for s in synced])}.")
|
51 |
event.set()
|
52 |
print("------")
|
53 |
|
54 |
|
55 |
-
thread_to_client = {}
|
56 |
-
thread_to_user = {}
|
57 |
-
|
58 |
-
|
59 |
-
@bot.hybrid_command(
|
60 |
-
name="chat",
|
61 |
-
description="Enter some text to chat with the bot! Like this: $chat Hello, how are you?",
|
62 |
-
)
|
63 |
-
async def chat(ctx, prompt: str):
|
64 |
-
if ctx.author.id == bot.user.id:
|
65 |
-
return
|
66 |
-
try:
|
67 |
-
message = await ctx.send("Creating thread...")
|
68 |
-
|
69 |
-
thread = await message.create_thread(name=prompt)
|
70 |
-
loop = asyncio.get_running_loop()
|
71 |
-
client = await loop.run_in_executor(None, get_client, None)
|
72 |
-
job = client.submit(prompt, 0.9, 2048, 0.9, 1.2, api_name="/chat")
|
73 |
-
await wait(job)
|
74 |
-
|
75 |
-
try:
|
76 |
-
job.result()
|
77 |
-
response = job.outputs()[-1]
|
78 |
-
await thread.send(truncate_response(response))
|
79 |
-
thread_to_client[thread.id] = client
|
80 |
-
thread_to_user[thread.id] = ctx.author.id
|
81 |
-
except QueueError:
|
82 |
-
await thread.send(
|
83 |
-
"The gradio space powering this bot is really busy! Please try again later!"
|
84 |
-
)
|
85 |
-
|
86 |
-
except Exception as e:
|
87 |
-
print(f"{e}")
|
88 |
-
|
89 |
-
|
90 |
-
async def continue_chat(message):
|
91 |
-
"""Continues a given conversation based on chathistory"""
|
92 |
-
try:
|
93 |
-
client = thread_to_client[message.channel.id]
|
94 |
-
prompt = message.content
|
95 |
-
job = client.submit(prompt, 0.9, 2048, 0.9, 1.2, api_name="/chat")
|
96 |
-
await wait(job)
|
97 |
-
try:
|
98 |
-
job.result()
|
99 |
-
response = job.outputs()[-1]
|
100 |
-
await message.reply(truncate_response(response))
|
101 |
-
except QueueError:
|
102 |
-
await message.reply(
|
103 |
-
"The gradio space powering this bot is really busy! Please try again later!"
|
104 |
-
)
|
105 |
-
|
106 |
-
except Exception as e:
|
107 |
-
print(f"Error: {e}")
|
108 |
-
|
109 |
-
|
110 |
@bot.event
|
111 |
async def on_message(message):
|
112 |
-
|
113 |
-
|
114 |
-
|
115 |
-
if message.channel.id in thread_to_user:
|
116 |
-
if thread_to_user[message.channel.id] == message.author.id:
|
117 |
-
await continue_chat(message)
|
118 |
-
else:
|
119 |
-
await bot.process_commands(message)
|
120 |
-
|
121 |
-
except Exception as e:
|
122 |
-
print(f"Error: {e}")
|
123 |
|
124 |
|
125 |
# running in thread
|
@@ -137,13 +72,10 @@ event.wait()
|
|
137 |
|
138 |
if not DISCORD_TOKEN:
|
139 |
welcome_message = """
|
140 |
-
|
141 |
## You have not specified a DISCORD_TOKEN, which means you have not created a bot account. Please follow these steps:
|
142 |
-
|
143 |
### 1. Go to https://discord.com/developers/applications and click 'New Application'
|
144 |
|
145 |
### 2. Give your bot a name 🤖
|
146 |
-
|
147 |
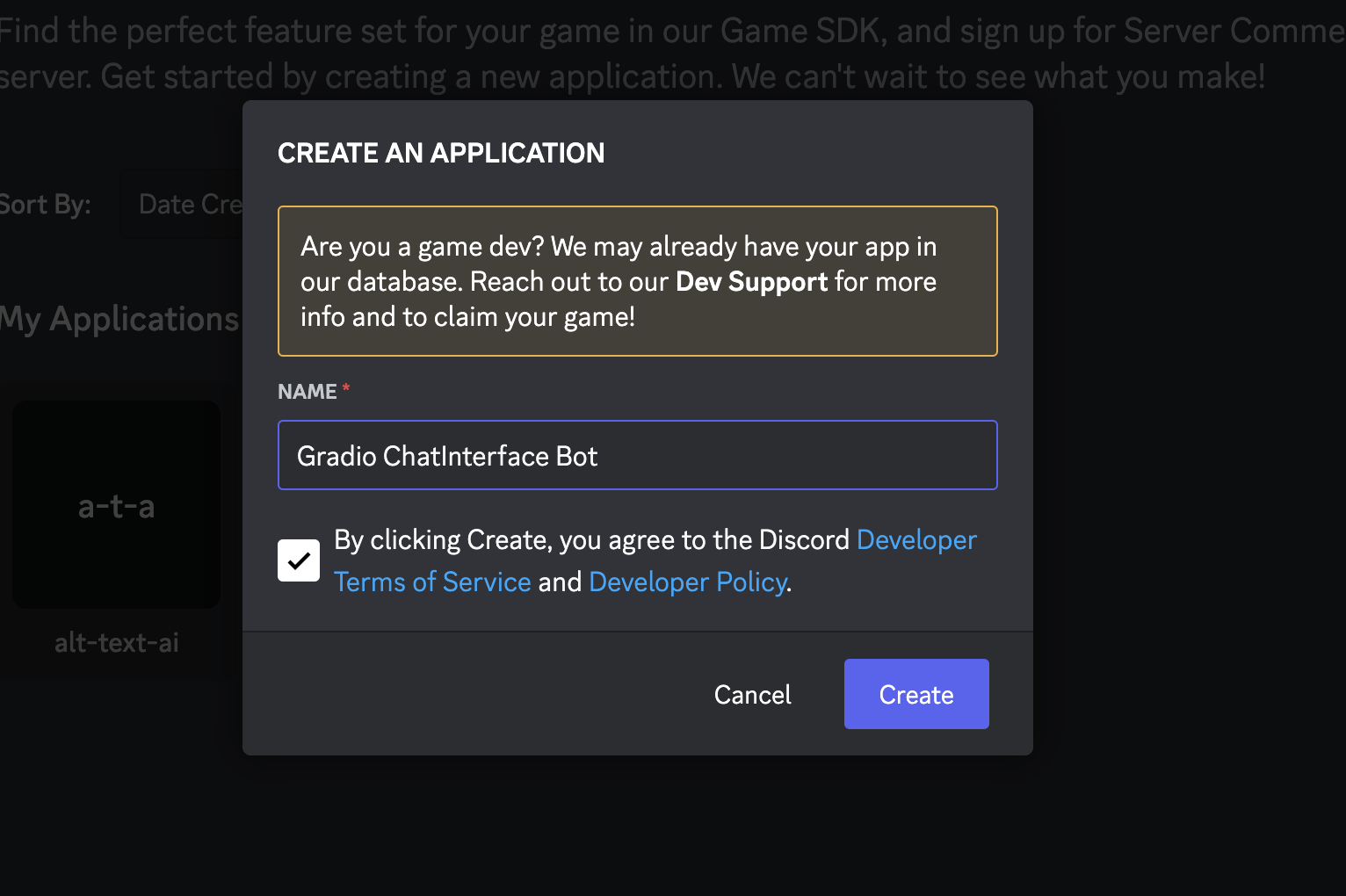
|
148 |
|
149 |
## 3. In Settings > Bot, click the 'Reset Token' button to get a new token. Write it down and keep it safe 🔐
|
@@ -155,9 +87,7 @@ if not DISCORD_TOKEN:
|
|
155 |
## 5. Scroll down and enable 'Message Content Intent' under 'Priviledged Gateway Intents'
|
156 |
|
157 |
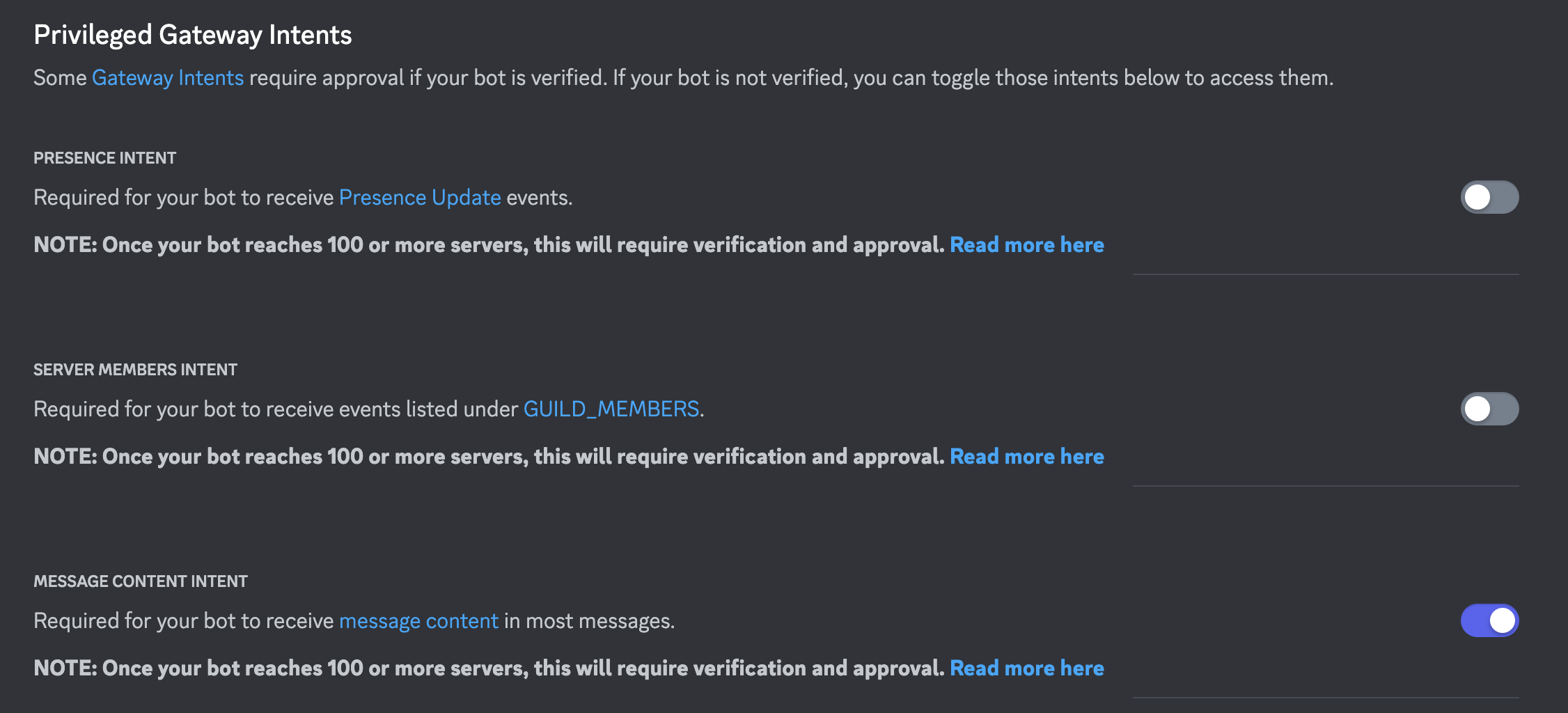
|
158 |
-
|
159 |
## 6. Save your changes!
|
160 |
-
|
161 |
## 7. The token from step 3 is the DISCORD_TOKEN. Rerun the deploy_discord command, e.g client.deploy_discord(discord_bot_token=DISCORD_TOKEN, ...), or add the token as a space secret manually.
|
162 |
"""
|
163 |
else:
|
@@ -167,15 +97,12 @@ else:
|
|
167 |
## Add this bot to your server by clicking this link:
|
168 |
|
169 |
{url}
|
170 |
-
|
171 |
## How to use it?
|
172 |
-
|
173 |
The bot can be triggered via `/chat` followed by your text prompt.
|
174 |
|
175 |
This will create a thread with the bot's response to your text prompt.
|
176 |
You can reply in the thread (without `/chat`) to continue the conversation.
|
177 |
In the thread, the bot will only reply to the original author of the command.
|
178 |
-
|
179 |
⚠️ Note ⚠️: Please make sure this bot's command does have the same name as another command in your server.
|
180 |
|
181 |
⚠️ Note ⚠️: Bot commands do not work in DMs with the bot as of now.
|
|
|
6 |
|
7 |
import discord
|
8 |
import gradio as gr
|
9 |
+
import gradio_client as grc
|
10 |
from discord import Permissions
|
11 |
from discord.ext import commands
|
12 |
from discord.utils import oauth_url
|
|
|
|
|
13 |
from gradio_client.utils import QueueError
|
14 |
|
15 |
event = Event()
|
16 |
|
17 |
DISCORD_TOKEN = os.getenv("DISCORD_TOKEN")
|
18 |
+
HF_TOKEN = os.getenv("HF_TOKEN")
|
19 |
|
20 |
|
21 |
async def wait(job):
|
|
|
24 |
|
25 |
|
26 |
def get_client(session: Optional[str] = None) -> grc.Client:
|
27 |
+
client = grc.Client("https://wop-xxx-opengpt.hf.space/", hf_token=HF_TOKEN)
|
28 |
if session:
|
29 |
client.session_hash = session
|
30 |
return client
|
|
|
46 |
@bot.event
|
47 |
async def on_ready():
|
48 |
print(f"Logged in as {bot.user} (ID: {bot.user.id})")
|
|
|
|
|
49 |
event.set()
|
50 |
print("------")
|
51 |
|
52 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
53 |
@bot.event
|
54 |
async def on_message(message):
|
55 |
+
if message.channel.id == 1210559547874222083 and not message.author.bot:
|
56 |
+
await message.channel.send(f"Hello {message.author.mention}!")
|
57 |
+
await bot.process_commands(message)
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
58 |
|
59 |
|
60 |
# running in thread
|
|
|
72 |
|
73 |
if not DISCORD_TOKEN:
|
74 |
welcome_message = """
|
|
|
75 |
## You have not specified a DISCORD_TOKEN, which means you have not created a bot account. Please follow these steps:
|
|
|
76 |
### 1. Go to https://discord.com/developers/applications and click 'New Application'
|
77 |
|
78 |
### 2. Give your bot a name 🤖
|
|
|
79 |
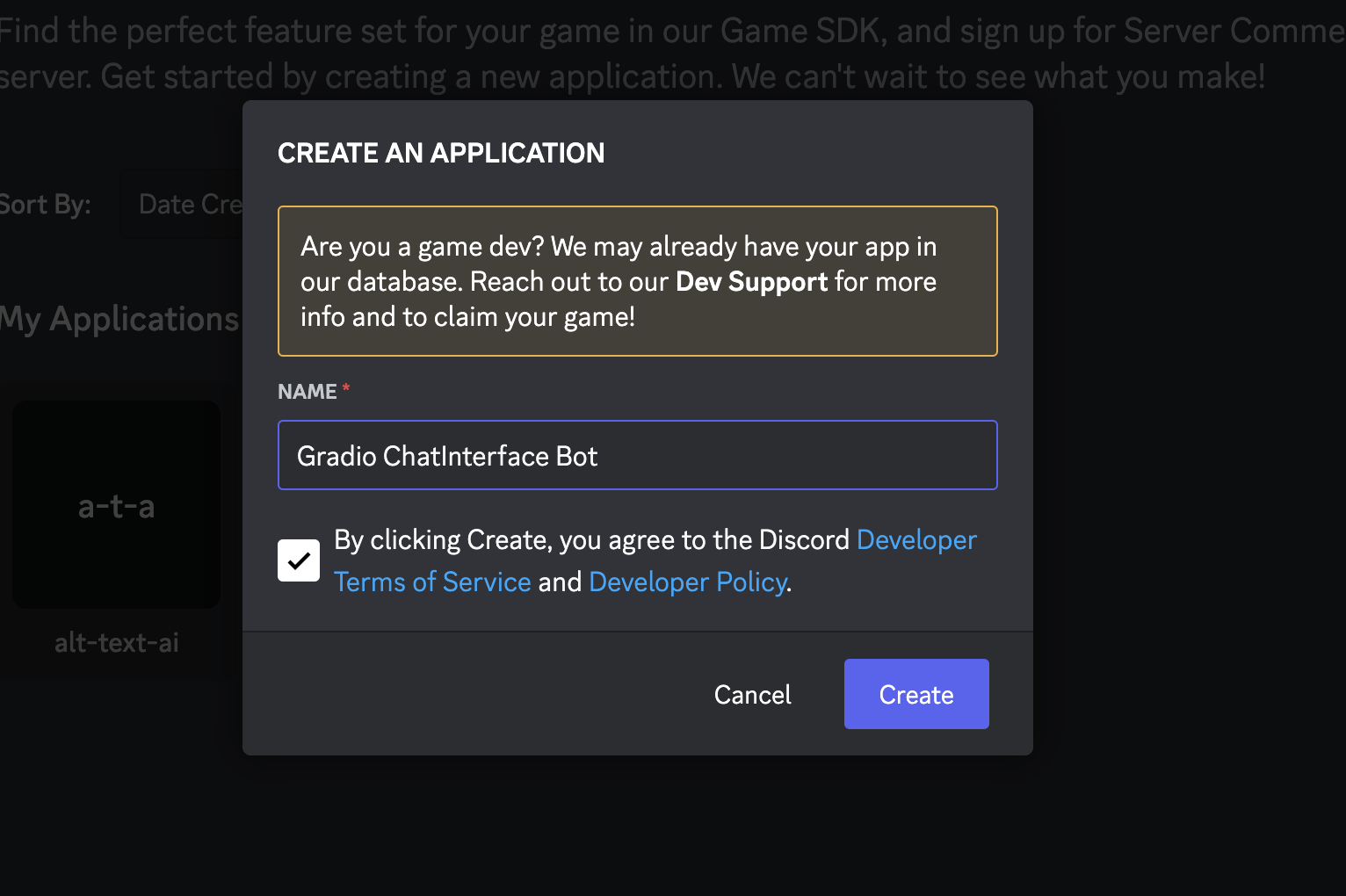
|
80 |
|
81 |
## 3. In Settings > Bot, click the 'Reset Token' button to get a new token. Write it down and keep it safe 🔐
|
|
|
87 |
## 5. Scroll down and enable 'Message Content Intent' under 'Priviledged Gateway Intents'
|
88 |
|
89 |
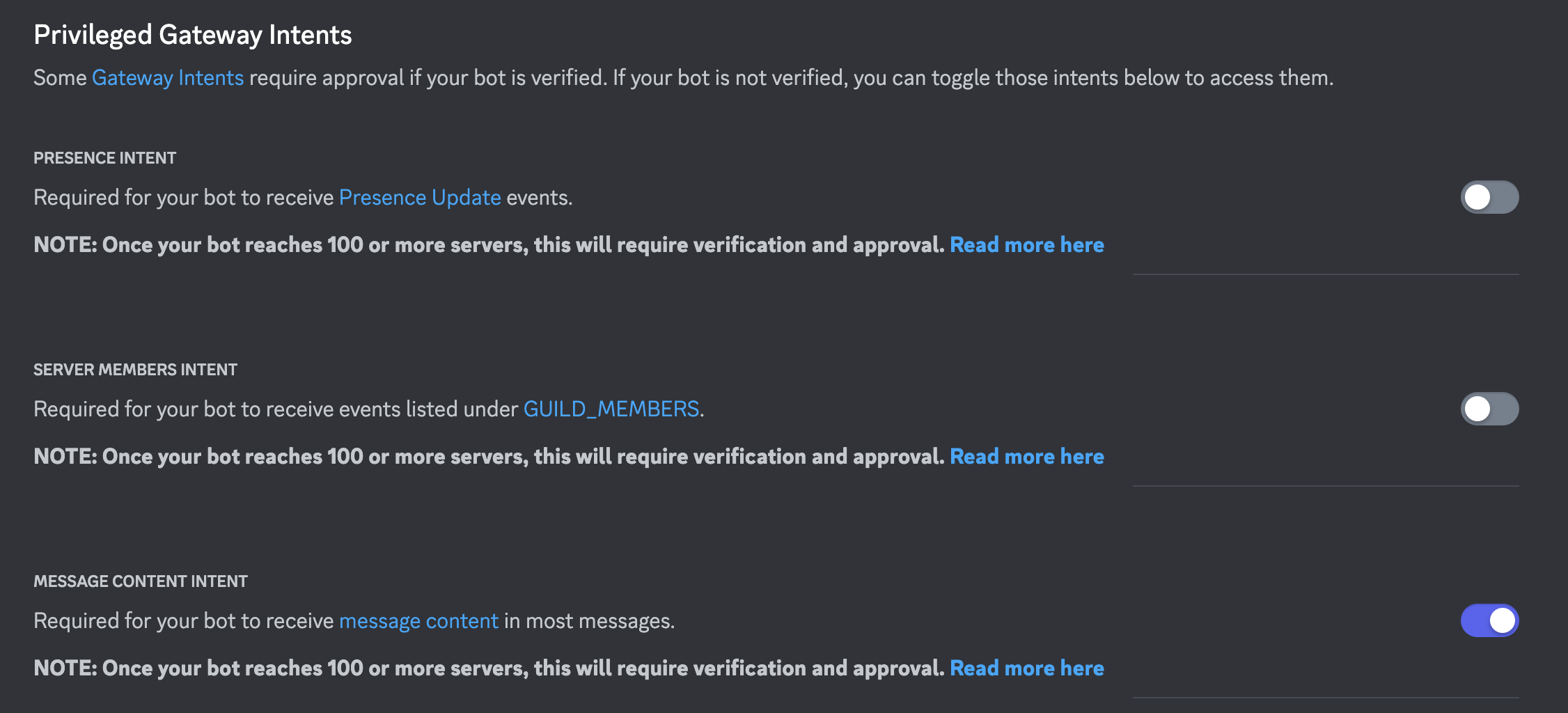
|
|
|
90 |
## 6. Save your changes!
|
|
|
91 |
## 7. The token from step 3 is the DISCORD_TOKEN. Rerun the deploy_discord command, e.g client.deploy_discord(discord_bot_token=DISCORD_TOKEN, ...), or add the token as a space secret manually.
|
92 |
"""
|
93 |
else:
|
|
|
97 |
## Add this bot to your server by clicking this link:
|
98 |
|
99 |
{url}
|
|
|
100 |
## How to use it?
|
|
|
101 |
The bot can be triggered via `/chat` followed by your text prompt.
|
102 |
|
103 |
This will create a thread with the bot's response to your text prompt.
|
104 |
You can reply in the thread (without `/chat`) to continue the conversation.
|
105 |
In the thread, the bot will only reply to the original author of the command.
|
|
|
106 |
⚠️ Note ⚠️: Please make sure this bot's command does have the same name as another command in your server.
|
107 |
|
108 |
⚠️ Note ⚠️: Bot commands do not work in DMs with the bot as of now.
|