Spaces:
Runtime error
Runtime error
Commit
•
383b0d8
1
Parent(s):
9c0622a
initial test
Browse files- app.py +91 -4
- requirements.txt +1 -0
- unsafe.png +0 -0
app.py
CHANGED
@@ -1,7 +1,94 @@
|
|
1 |
import gradio as gr
|
|
|
|
|
2 |
|
3 |
-
|
4 |
-
return "Hello " + name + "!!"
|
5 |
|
6 |
-
|
7 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
import gradio as gr
|
2 |
+
import torch
|
3 |
+
from PIL import Image
|
4 |
|
5 |
+
from lambda_diffusers import StableDiffusionImageEmbedPipeline
|
|
|
6 |
|
7 |
+
def main(
|
8 |
+
input_im,
|
9 |
+
scale=3.0,
|
10 |
+
n_samples=4,
|
11 |
+
seed=0,
|
12 |
+
steps=25,
|
13 |
+
):
|
14 |
+
generator = torch.Generator(device=device).manual_seed(seed)
|
15 |
+
|
16 |
+
images_list = pipe(
|
17 |
+
n_samples*[input_im],
|
18 |
+
guidance_scale=scale,
|
19 |
+
num_inference_steps=steps,
|
20 |
+
generator=generator,
|
21 |
+
)
|
22 |
+
|
23 |
+
images = []
|
24 |
+
safe_image = Image.open(r"unsafe.png")
|
25 |
+
for i, image in enumerate(images_list["sample"]):
|
26 |
+
if(images_list["nsfw_content_detected"][i]):
|
27 |
+
images.append(safe_image)
|
28 |
+
else:
|
29 |
+
images.append(image)
|
30 |
+
return images
|
31 |
+
|
32 |
+
|
33 |
+
description = \
|
34 |
+
"""Generate variations on an input image using a fine-tuned version of Stable Diffision.
|
35 |
+
Trained by [Justin Pinkney](https://www.justinpinkney.com) ([@Buntworthy](https://twitter.com/Buntworthy)) at [Lambda](https://lambdalabs.com/)
|
36 |
+
|
37 |
+
__Get the [code](https://github.com/justinpinkney/stable-diffusion) and [model](https://huggingface.co/lambdalabs/stable-diffusion-image-conditioned).__
|
38 |
+
|
39 |
+
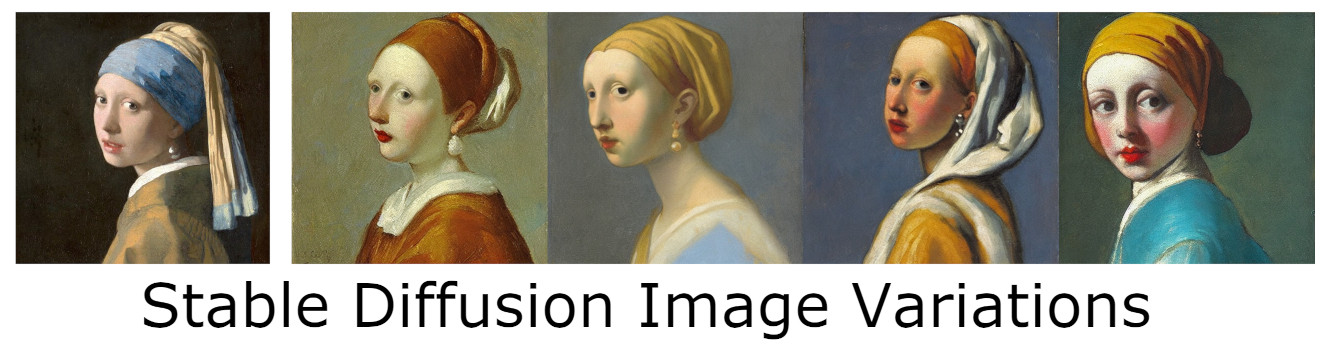
|
40 |
+
|
41 |
+
"""
|
42 |
+
|
43 |
+
article = \
|
44 |
+
"""
|
45 |
+
## How does this work?
|
46 |
+
|
47 |
+
The normal Stable Diffusion model is trained to be conditioned on text input. This version has had the original text encoder (from CLIP) removed, and replaced with
|
48 |
+
the CLIP _image_ encoder instead. So instead of generating images based a text input, images are generated to match CLIP's embedding of the image.
|
49 |
+
This creates images which have the same rough style and content, but different details, in particular the composition is generally quite different.
|
50 |
+
This is a totally different approach to the img2img script of the original Stable Diffusion and gives very different results.
|
51 |
+
|
52 |
+
The model was fine tuned on the [LAION aethetics v2 6+ dataset](https://laion.ai/blog/laion-aesthetics/) to accept the new conditioning.
|
53 |
+
Training was done on 4xA6000 GPUs on [Lambda GPU Cloud](https://lambdalabs.com/service/gpu-cloud).
|
54 |
+
More details on the method and training will come in a future blog post.
|
55 |
+
"""
|
56 |
+
|
57 |
+
device = "cpu"
|
58 |
+
pipe = StableDiffusionImageEmbedPipeline.from_pretrained(
|
59 |
+
"lambdalabs/sd-image-variations-diffusers",
|
60 |
+
revision="273115e88df42350019ef4d628265b8c29ef4af5",
|
61 |
+
)
|
62 |
+
pipe = pipe.to(device)
|
63 |
+
|
64 |
+
inputs = [
|
65 |
+
gr.Image(),
|
66 |
+
gr.Slider(0, 25, value=3, step=1, label="Guidance scale"),
|
67 |
+
gr.Slider(1, 4, value=1, step=1, label="Number images"),
|
68 |
+
gr.Slider(5, 50, value=25, step=5, label="Steps"),
|
69 |
+
gr.Slider(
|
70 |
+
label="Seed",
|
71 |
+
minimum=0,
|
72 |
+
maximum=2147483647,
|
73 |
+
step=1,
|
74 |
+
randomize=True,
|
75 |
+
)
|
76 |
+
]
|
77 |
+
output = gr.Gallery(label="Generated variations")
|
78 |
+
output.style(grid=2)
|
79 |
+
|
80 |
+
examples = [
|
81 |
+
["assets/im-examples/vermeer.jpg", 3, 1, True, 25],
|
82 |
+
["assets/im-examples/matisse.jpg", 3, 1, True, 25],
|
83 |
+
]
|
84 |
+
|
85 |
+
demo = gr.Interface(
|
86 |
+
fn=main,
|
87 |
+
title="Stable Diffusion Image Variations",
|
88 |
+
description=description,
|
89 |
+
article=article,
|
90 |
+
inputs=inputs,
|
91 |
+
outputs=output,
|
92 |
+
examples=examples,
|
93 |
+
)
|
94 |
+
demo.launch()
|
requirements.txt
ADDED
@@ -0,0 +1 @@
|
|
|
|
|
1 |
+
git+git://github.com/LambdaLabsML/lambda-diffusers.git#egg=lambda-diffusers
|
unsafe.png
ADDED
![]() |