Spaces:
Runtime error
Runtime error
from huggingface_hub import from_pretrained_keras | |
import keras_cv | |
import gradio as gr | |
from tensorflow import keras | |
keras.mixed_precision.set_global_policy("mixed_float16") | |
# load keras model | |
resolution = 512 | |
dreambooth_model = keras_cv.models.StableDiffusion( | |
img_width=resolution, img_height=resolution, jit_compile=True, | |
) | |
loaded_diffusion_model = from_pretrained_keras("keras-dreambooth/dreambooth-galaxy-mergers") | |
dreambooth_model._diffusion_model = loaded_diffusion_model | |
def generate_images(prompt: str, negative_prompt:str, num_imgs_to_gen: int, num_steps: int, ugs: int): | |
generated_img = dreambooth_model.text_to_image( | |
prompt, | |
negative_prompt=negative_prompt, | |
batch_size=num_imgs_to_gen, | |
num_steps=num_steps, | |
unconditional_guidance_scale=ugs, | |
) | |
return generated_img | |
with gr.Blocks() as demo: | |
gr.Markdown(""" | |
# Keras-Dreambooth Galaxy Mergers πͺπ€ | |
This is a Keras Dreambooth model fine-tuned to images of galaxy mergers taken with the [Hubble Space Telescope](https://en.wikipedia.org/wiki/Hubble_Space_Telescope) (credit [ESA/Hubble](https://esahubble.org/images/)). | |
The model, part of the [Keras Dreambooth Sprint](https://github.com/huggingface/community-events/tree/main/keras-dreambooth-sprint) trained by lposti, can be found in [keras-dreambooth/dreambooth-galaxy-mergers](https://huggingface.co/keras-dreambooth/dreambooth-galaxy-mergers). | |
The model should be used with a prompt containing `sks galaxies merging`. A typical prompt for this model is `image of sks galaxies merging in space`. | |
""") | |
with gr.Row(): | |
with gr.Column(): | |
prompt = gr.Textbox(lines=1, value="image of sks galaxies merging in space", label="Base Prompt") | |
negative_prompt = gr.Textbox(lines=1, value="deformed", label="Negative Prompt") | |
samples = gr.Slider(minimum=1, maximum=5, value=1, step=1, label="Number of Image") | |
num_steps = gr.Slider(label="Inference Steps",value=40) | |
ugs = gr.Slider(value=15, minimum=5, maximum=25, step=1, label="Unconditional Guidance Scale") | |
run = gr.Button(value="Run") | |
with gr.Column(): | |
gallery = gr.Gallery(label="Outputs").style(grid=(1,2)) | |
run.click(generate_images, inputs=[prompt,negative_prompt, samples, num_steps, ugs], outputs=gallery) | |
gr.Examples([["image of sks galaxies merging in space, 8k, high quality","deformed, blurry, grain, artifacts, low quality", 1, 40, 12], | |
["painting of sks galaxies merging in van gogh style, 8k, high quality, trending on artstation","deformed, blurry, grain, artifacts, low quality", 1, 40, 12], | |
], | |
[prompt,negative_prompt, samples,num_steps, ugs], gallery, generate_images) | |
gr.Markdown(""" | |
## Output samples | |
> image of sks galaxies merging in space | |
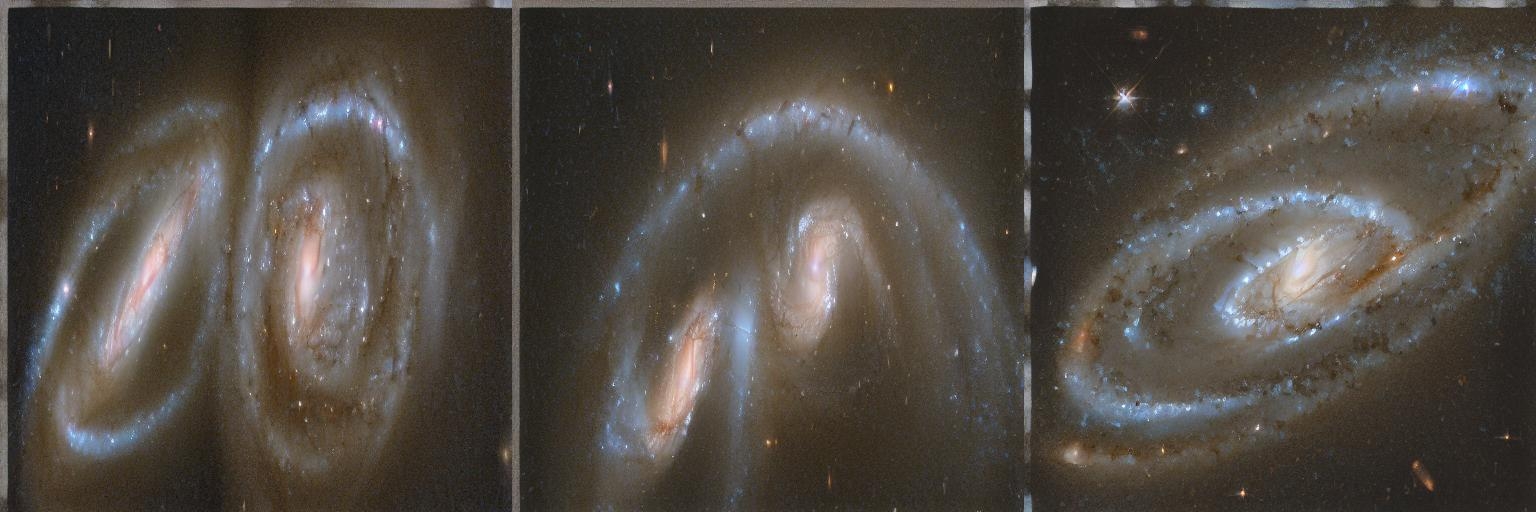 | |
> painting of sks galaxies merging in van gogh style | |
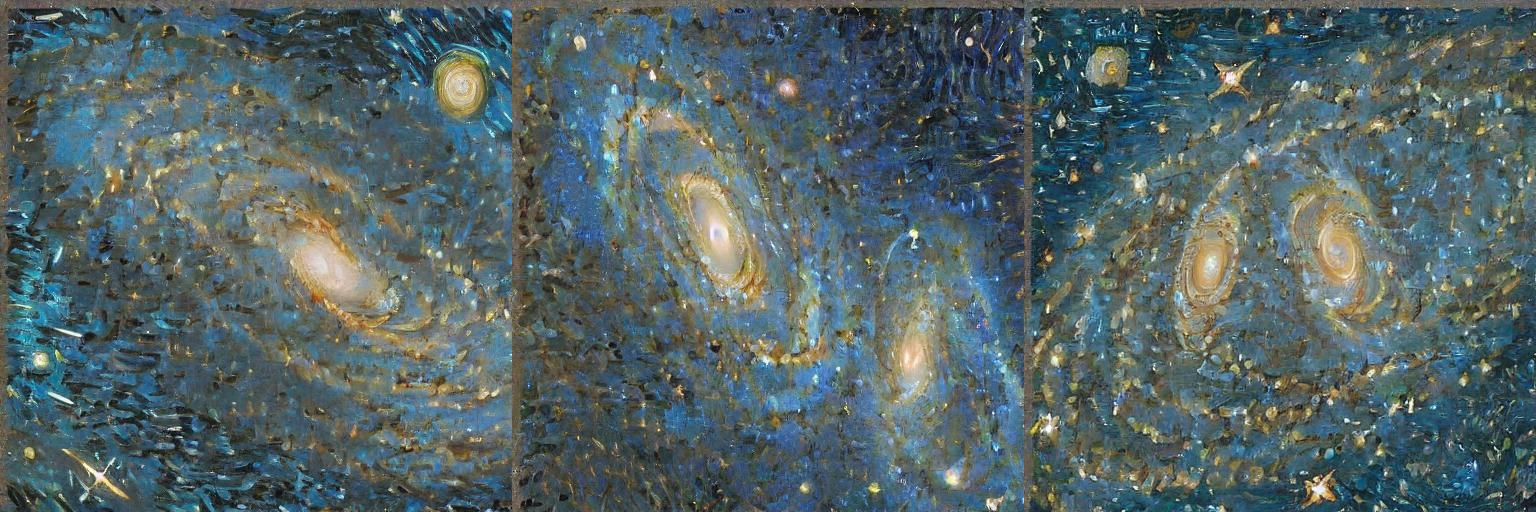 | |
## Cosmic Hugs π€: an artistic rendition of galaxy collisions | |
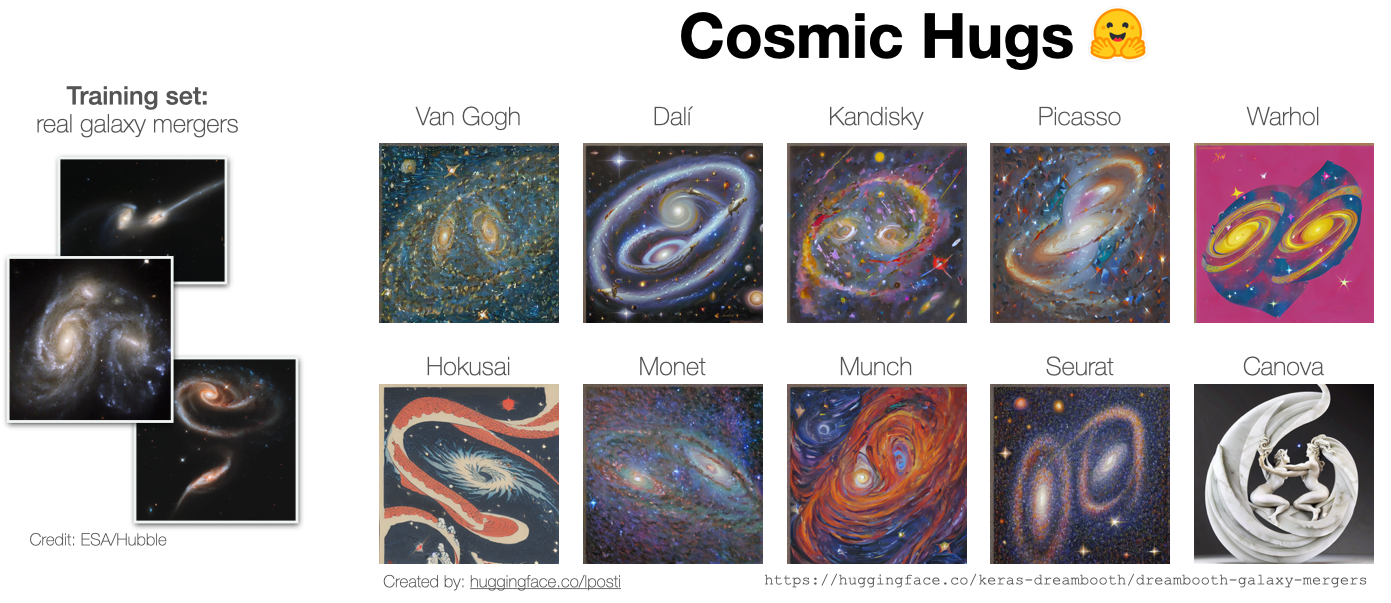 | |
""") | |
demo.launch(debug=True) |