Add application file
Browse files- .gitignore +116 -0
- Dockerfile +11 -0
- app.py +67 -0
- chainlit.md +14 -0
- config.py +13 -0
- data/docs/GroupStageResults.txt +55 -0
- data/docs/classementButeurs.txt +77 -0
- data/docs/infos_match.txt +257 -0
- data/docs/stadiums.txt +2 -0
- prompts.py +23 -0
- requirements.txt +6 -0
- utils.py +37 -0
.gitignore
ADDED
@@ -0,0 +1,116 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# Created by .ignore support plugin (hsz.mobi)
|
2 |
+
### Python template
|
3 |
+
# Byte-compiled / optimized / DLL files
|
4 |
+
__pycache__/
|
5 |
+
*.py[cod]
|
6 |
+
*$py.class
|
7 |
+
|
8 |
+
# C extensions
|
9 |
+
*.so
|
10 |
+
|
11 |
+
# Distribution / packaging
|
12 |
+
.Python
|
13 |
+
build/
|
14 |
+
develop-eggs/
|
15 |
+
dist/
|
16 |
+
downloads/
|
17 |
+
eggs/
|
18 |
+
.eggs/
|
19 |
+
lib/
|
20 |
+
lib64/
|
21 |
+
parts/
|
22 |
+
sdist/
|
23 |
+
var/
|
24 |
+
wheels/
|
25 |
+
*.egg-info/
|
26 |
+
.installed.cfg
|
27 |
+
*.egg
|
28 |
+
MANIFEST
|
29 |
+
|
30 |
+
# PyInstaller
|
31 |
+
# Usually these files are written by a python script from a template
|
32 |
+
# before PyInstaller builds the exe, so as to inject date/other infos into it.
|
33 |
+
*.manifest
|
34 |
+
*.spec
|
35 |
+
|
36 |
+
# Installer logs
|
37 |
+
pip-log.txt
|
38 |
+
pip-delete-this-directory.txt
|
39 |
+
|
40 |
+
# Unit test / coverage reports
|
41 |
+
htmlcov/
|
42 |
+
.tox/
|
43 |
+
.coverage
|
44 |
+
.coverage.*
|
45 |
+
.cache
|
46 |
+
nosetests.xml
|
47 |
+
coverage.xml
|
48 |
+
*.cover
|
49 |
+
.hypothesis/
|
50 |
+
.pytest_cache/
|
51 |
+
|
52 |
+
# Translations
|
53 |
+
*.mo
|
54 |
+
*.pot
|
55 |
+
|
56 |
+
# Django stuff:
|
57 |
+
*.log
|
58 |
+
local_settings.py
|
59 |
+
db.sqlite3
|
60 |
+
|
61 |
+
# Flask stuff:
|
62 |
+
instance/
|
63 |
+
.webassets-cache
|
64 |
+
|
65 |
+
# Scrapy stuff:
|
66 |
+
.scrapy
|
67 |
+
|
68 |
+
# Sphinx documentation
|
69 |
+
docs/_build/
|
70 |
+
|
71 |
+
# PyBuilder
|
72 |
+
target/
|
73 |
+
|
74 |
+
# Jupyter Notebook
|
75 |
+
.ipynb_checkpoints
|
76 |
+
|
77 |
+
# pyenv
|
78 |
+
.python-version
|
79 |
+
|
80 |
+
# celery beat schedule file
|
81 |
+
celerybeat-schedule
|
82 |
+
|
83 |
+
# SageMath parsed files
|
84 |
+
*.sage.py
|
85 |
+
|
86 |
+
# Environments
|
87 |
+
.env
|
88 |
+
.venv
|
89 |
+
env/
|
90 |
+
venv/
|
91 |
+
ENV/
|
92 |
+
env.bak/
|
93 |
+
venv.bak/
|
94 |
+
|
95 |
+
# Spyder project settings
|
96 |
+
.spyderproject
|
97 |
+
.spyproject
|
98 |
+
|
99 |
+
# Rope project settings
|
100 |
+
.ropeproject
|
101 |
+
|
102 |
+
# mkdocs documentation
|
103 |
+
/site
|
104 |
+
|
105 |
+
# mypy
|
106 |
+
.mypy_cache/
|
107 |
+
|
108 |
+
.idea/*
|
109 |
+
.files/*
|
110 |
+
|
111 |
+
tmp
|
112 |
+
secret.*
|
113 |
+
volumes/
|
114 |
+
.chainlit
|
115 |
+
.DS_Store
|
116 |
+
__init__.py
|
Dockerfile
ADDED
@@ -0,0 +1,11 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
FROM python:3.11
|
2 |
+
RUN useradd -m -u 1000 user
|
3 |
+
USER user
|
4 |
+
ENV HOME=/home/user \
|
5 |
+
PATH=/home/user/.local/bin:$PATH
|
6 |
+
WORKDIR $HOME/app
|
7 |
+
COPY --chown=user . $HOME/app
|
8 |
+
COPY ./requirements.txt ~/app/requirements.txt
|
9 |
+
RUN pip install -r requirements.txt
|
10 |
+
COPY . .
|
11 |
+
CMD ["chainlit", "run", "app.py", "--port", "7860"]
|
app.py
ADDED
@@ -0,0 +1,67 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import chainlit as cl
|
2 |
+
from langchain.callbacks.base import BaseCallbackHandler
|
3 |
+
from langchain.schema import StrOutputParser
|
4 |
+
from langchain.schema.runnable import RunnableConfig, RunnablePassthrough
|
5 |
+
from langchain_openai import ChatOpenAI
|
6 |
+
|
7 |
+
import config
|
8 |
+
from prompts import prompt
|
9 |
+
from utils import format_docs, process_documents
|
10 |
+
|
11 |
+
doc_search = process_documents(config.DOCS_STORAGE_PATH)
|
12 |
+
model = ChatOpenAI(name="gpt-3.5-turbo", streaming=True)
|
13 |
+
|
14 |
+
|
15 |
+
@cl.on_chat_start
|
16 |
+
async def on_chat_start():
|
17 |
+
retriever = doc_search.as_retriever()
|
18 |
+
|
19 |
+
runnable = (
|
20 |
+
{"context": retriever | format_docs, "question": RunnablePassthrough()}
|
21 |
+
| prompt
|
22 |
+
| model
|
23 |
+
| StrOutputParser()
|
24 |
+
)
|
25 |
+
|
26 |
+
cl.user_session.set("runnable", runnable)
|
27 |
+
|
28 |
+
|
29 |
+
@cl.on_message
|
30 |
+
async def on_message(message: cl.Message):
|
31 |
+
runnable = cl.user_session.get("runnable")
|
32 |
+
msg = cl.Message(content="")
|
33 |
+
|
34 |
+
class PostMessageHandler(BaseCallbackHandler):
|
35 |
+
"""
|
36 |
+
Callback handler for handling the retriever and LLM processes.
|
37 |
+
Used to post the sources of the retrieved documents as a Chainlit element.
|
38 |
+
"""
|
39 |
+
|
40 |
+
def __init__(self, msg: cl.Message):
|
41 |
+
BaseCallbackHandler.__init__(self)
|
42 |
+
self.msg = msg
|
43 |
+
self.sources = set() # To store unique pairs
|
44 |
+
|
45 |
+
def on_retriever_end(self, documents, *, run_id, parent_run_id, **kwargs):
|
46 |
+
for d in documents:
|
47 |
+
source_page_pair = (d.metadata["source"])
|
48 |
+
self.sources.add(source_page_pair) # Add unique pairs to the set
|
49 |
+
|
50 |
+
def on_llm_end(self, response, *, run_id, parent_run_id, **kwargs):
|
51 |
+
if len(self.sources):
|
52 |
+
sources_text = "\n".join(
|
53 |
+
[f"{source}#page={page}" for source, page in self.sources]
|
54 |
+
)
|
55 |
+
self.msg.elements.append(
|
56 |
+
cl.Text(name="Sources", content=sources_text, display="inline")
|
57 |
+
)
|
58 |
+
|
59 |
+
async with cl.Step(type="run", name="QA Assistant"):
|
60 |
+
async for chunk in runnable.astream(
|
61 |
+
message.content,
|
62 |
+
config=RunnableConfig(
|
63 |
+
callbacks=[cl.LangchainCallbackHandler(), PostMessageHandler(msg)]
|
64 |
+
),
|
65 |
+
):
|
66 |
+
await msg.stream_token(chunk)
|
67 |
+
await msg.send()
|
chainlit.md
ADDED
@@ -0,0 +1,14 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# Welcome to Chainlit! 🚀🤖
|
2 |
+
|
3 |
+
Hi there, Developer! 👋 We're excited to have you on board. Chainlit is a powerful tool designed to help you prototype, debug and share applications built on top of LLMs.
|
4 |
+
|
5 |
+
## Useful Links 🔗
|
6 |
+
|
7 |
+
- **Documentation:** Get started with our comprehensive [Chainlit Documentation](https://docs.chainlit.io) 📚
|
8 |
+
- **Discord Community:** Join our friendly [Chainlit Discord](https://discord.gg/k73SQ3FyUh) to ask questions, share your projects, and connect with other developers! 💬
|
9 |
+
|
10 |
+
We can't wait to see what you create with Chainlit! Happy coding! 💻😊
|
11 |
+
|
12 |
+
## Welcome screen
|
13 |
+
|
14 |
+
To modify the welcome screen, edit the `chainlit.md` file at the root of your project. If you do not want a welcome screen, just leave this file empty.
|
config.py
ADDED
@@ -0,0 +1,13 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import os
|
2 |
+
|
3 |
+
# Model metadata
|
4 |
+
OPENAI_API_KEY = os.getenv("OPENAI_API_KEY")
|
5 |
+
CHAT_MODEL = "gpt-3.5-turbo" # or "gpt-4-0314"
|
6 |
+
|
7 |
+
# vectorstore
|
8 |
+
STORE_FILE = "./data/chroma_db"
|
9 |
+
HIISTORY_FILE = "./data/history.txt"
|
10 |
+
DOCS_STORAGE_PATH = "./data/docs"
|
11 |
+
|
12 |
+
CHUNK_SIZE = 1024
|
13 |
+
CHUNK_OVERLAP = 100
|
data/docs/GroupStageResults.txt
ADDED
@@ -0,0 +1,55 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
CAN 2024 Group Stage Results
|
2 |
+
Group A:
|
3 |
+
|
4 |
+
Pos | Team | Pts | GD | Form
|
5 |
+
--- | --- | --- | --- | ---
|
6 |
+
1 | Equatorial Guinea | 3 | 7 | DWD
|
7 |
+
2 | Nigeria | 3 | 7 | DWD
|
8 |
+
3 | Côte d'Ivoire | 3 | 3 | LWW
|
9 |
+
4 | Guinea-Bissau | 3 | 0 | LLL
|
10 |
+
Group B:
|
11 |
+
|
12 |
+
Pos | Team | Pts | GD | Form
|
13 |
+
--- | --- | --- | --- | ---
|
14 |
+
1 | Cabo Verde | 3 | 7 | WWW
|
15 |
+
2 | Egypt | 3 | 3 | DDD
|
16 |
+
3 | Ghana | 3 | 2 | LDD
|
17 |
+
4 | Mozambique | 3 | 2 | DDL
|
18 |
+
Group C:
|
19 |
+
|
20 |
+
Pos | Team | Pts | GD | Form
|
21 |
+
--- | --- | --- | --- | ---
|
22 |
+
1 | Senegal | 3 | 9 | WWW
|
23 |
+
2 | Cameroon | 3 | 4 | DWL
|
24 |
+
3 | Guinea | 3 | 4 | DDW
|
25 |
+
4 | Gambia | 3 | 0 | LLL
|
26 |
+
Group D:
|
27 |
+
|
28 |
+
Pos | Team | Pts | GD | Form
|
29 |
+
--- | --- | --- | --- | ---
|
30 |
+
1 | Angola | 3 | 7 | DWW
|
31 |
+
2 | Burkina Faso | 3 | 4 | WDL
|
32 |
+
3 | Mauritania | 3 | 3 | LWL
|
33 |
+
4 | Algeria | 3 | 2 | DDD
|
34 |
+
Group E:
|
35 |
+
|
36 |
+
Pos | Team | Pts | GD | Form
|
37 |
+
--- | --- | --- | --- | ---
|
38 |
+
1 | Mali | 3 | 5 | WDD
|
39 |
+
2 | South Africa | 3 | 4 | LWD
|
40 |
+
3 | Namibia | 3 | 4 | WLW
|
41 |
+
4 | Tunisia | 3 | 2 | DDD
|
42 |
+
Group F:
|
43 |
+
|
44 |
+
Pos | Team | Pts | GD | Form
|
45 |
+
--- | --- | --- | --- | ---
|
46 |
+
1 | Morocco | 3 | 7 | WWW
|
47 |
+
2 | Congo DR | 3 | 3 | DDD
|
48 |
+
3 | Zambia | 3 | 2 | DDL
|
49 |
+
4 | Tanzania | 3 | 2 | LDD
|
50 |
+
Note:
|
51 |
+
|
52 |
+
Pos = Position
|
53 |
+
Pts = Points
|
54 |
+
GD = Goal Difference
|
55 |
+
Form = Last 3 match results (W = Win, D = Draw, L = Loss)
|
data/docs/classementButeurs.txt
ADDED
@@ -0,0 +1,77 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
Classement des meilleurs buteurs de la CAN 2024 (mis à jour le 30/01 à 22h57)
|
2 |
+
|
3 |
+
1. Emilio Nsue (Guinée équatoriale) - 5 buts
|
4 |
+
2. Gelson Dala (Angola) - 4 buts
|
5 |
+
2. Mohamed Mostafa (Égypte) - 4 buts
|
6 |
+
4. Baghdad Bounedjah (Algérie) - 3 buts
|
7 |
+
4. Mabulu (Angola) - 3 buts
|
8 |
+
4. Bertrand Traoré (Burkina Faso) - 3 buts
|
9 |
+
4. Lassine Sinayoko (Mali) - 3 buts
|
10 |
+
8. Lamine Camara (Guinée) - 2 buts
|
11 |
+
8. Mohammed Kudus (Ghana) - 2 buts
|
12 |
+
8. Themba Zwane (Afrique du Sud) - 2 buts
|
13 |
+
8. Jordan Ayew (Ghana) - 2 buts
|
14 |
+
8. Ademola Lookman (Nigeria) - 2 buts
|
15 |
+
8. Mohamed Bayo (Guinée) - 2 buts
|
16 |
+
8. Yoane Wissa (RD Congo) - 2 buts
|
17 |
+
8. Ryan Mendes (Cap-Vert) - 2 buts
|
18 |
+
8. Habib Diallo (Sénégal) - 2 buts
|
19 |
+
17. (nombreux joueurs à 1 but)
|
20 |
+
|
21 |
+
Seko Fofana (Côte d'Ivoire)
|
22 |
+
Jean-Philippe Krasso (Côte d'Ivoire)
|
23 |
+
Ibán Salvador (Guinée équatoriale)
|
24 |
+
Victor Osimhen (Nigeria)
|
25 |
+
Witi (Mozambique)
|
26 |
+
Clésio Bauque (Angola)
|
27 |
+
Mohamed Salah (Égypte)
|
28 |
+
Jamiro Monteiro (Cap-Vert)
|
29 |
+
Aleksander Djiku (Mali)
|
30 |
+
Garry Rodrigues (Cap-Vert)
|
31 |
+
Pape Gueye (Sénégal)
|
32 |
+
Frank Magri (Angola)
|
33 |
+
Deon Hotto (Namibie)
|
34 |
+
Hamari Traoré (Mali)
|
35 |
+
Romain Saïss (Maroc)
|
36 |
+
Azzedine Ounahi (Maroc)
|
37 |
+
Youssef En-Nesyri (Maroc)
|
38 |
+
Kings Kangwa (Malawi)
|
39 |
+
Josete Miranda (Guinée-Bissau)
|
40 |
+
Ze Turbo (Guinée-Bissau)
|
41 |
+
William Troost-Ekong (Nigeria)
|
42 |
+
Omar Marmoush (Égypte)
|
43 |
+
Bebe (Mozambique)
|
44 |
+
Kevin Pina (Guinée-Bissau)
|
45 |
+
Ismaïla Sarr (Sénégal)
|
46 |
+
Sadio Mané (Sénégal)
|
47 |
+
Aguibou Camara (Sénégal)
|
48 |
+
Mohamed Konaté (Mali)
|
49 |
+
Gilberto (Guinée-Bissau)
|
50 |
+
Sidi Bounar Amar (Algérie)
|
51 |
+
Aboubakary Koita (Mali)
|
52 |
+
Hamza Rafia (Algérie)
|
53 |
+
Achraf Hakimi (Maroc)
|
54 |
+
Silas (Guinée équatoriale)
|
55 |
+
Simon Msuva (Tanzanie)
|
56 |
+
Patson Daka (Zambie)
|
57 |
+
Percy Tau (Afrique du Sud)
|
58 |
+
Thapelo Maseko (Afrique du Sud)
|
59 |
+
Pablo Ganet (Mozambique)
|
60 |
+
Jannick Buyla (Guinée-Bissau)
|
61 |
+
Benchimol (Angola)
|
62 |
+
Trézéguet (Égypte)
|
63 |
+
Geny Catamo (Mozambique)
|
64 |
+
Reinildo Mandava (Mozambique)
|
65 |
+
Bryan Teixeira (Guinée-Bissau)
|
66 |
+
Karl Toko-Ekambi (Cameroun)
|
67 |
+
Abdoulaye Seck (Sénégal)
|
68 |
+
Ablie Jallow (Gambie)
|
69 |
+
Ebrima Colley (Gambie)
|
70 |
+
Christopher Wooh (Cameroun)
|
71 |
+
Iliman Ndiaye (Sénégal)
|
72 |
+
Zini (Algérie)
|
73 |
+
Dellahi (Algérie)
|
74 |
+
Hakim Ziyech (Maroc)
|
75 |
+
Franck Kessié (Côte d'Ivoire)
|
76 |
+
Evidence Makgopa (Afrique du Sud)
|
77 |
+
Teboho Mokoena (Afrique du Sud)
|
data/docs/infos_match.txt
ADDED
@@ -0,0 +1,257 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
La Coupe d'Afrique des Nations 2023 est l'événement le plus attendu sur le continent africain. Depuis le samedi 13 janvier 2024, elle rassemble en Côte d'Ivoire les 24 meilleures équipes nationales d'Afrique pour un mois de compétition intense. Voici le calendrier complet de cette 34e édition, permettant aux fans de suivre leurs équipes préférées et de planifier leurs moments devant la télévision.
|
2 |
+
Repoussée de six mois pour des causes météorologiques, la CAN 2023 débutera le samedi 13 janvier 2024 pour s'achever le dimanche 11 février 2024. Un total de 52 matchs seront au programme, au rythme général de trois rencontres quotidiennes. Sans plus attendre, découvrez le calendrier du tournoi !
|
3 |
+
Le programme du jour (lundi 29 janvier 2024)
|
4 |
+
17h : Cap-Vert-Mauritanie, Abidjan (Stade Felix Houphouet-Boigny)
|
5 |
+
20h : Sénégal-Côte d'Ivoire, Yamousskro
|
6 |
+
Heure GMT; pour la France, ajoutez 1h.
|
7 |
+
Ce lundi, on aura d'abord droit à un huitième de finale entre “surprises” : le Cap-Vert, qui a terminé en tête du groupe B au nez et à la barbe de l'Egypte et du Ghana, affronte la Mauritanie. Les Mourabitounes atteignent ce niveau pour la première fois après avoir décroché la première victoire de leur histoire en CAN contre l'Algérie.
|
8 |
+
Dans la soirée, place ensuite au choc de ces 8es de finale avec le match entre le Sénégal, tenant du titre, et la Côte d'Ivoire, pays-hôte. Auteurs d'un sans-faute en phase de groupes, les Lions se présentent forcément en favoris. De leur côté, les Eléphants n'ont plus joué depuis 7 jours et reviennent de nulle part après leur qualification in extremis parmi les meilleurs 3es alors qu'on leur prédisait l'élimination. Avec le remplacement de Jean-Louis Gasset par Emerse Faé et l'arrivée avortée d'Hervé Renard, le pays-hôte sort d'une semaine ubuesque et tentera de réaliser la surprise.
|
9 |
+
|
10 |
+
quelques chiffres phases de poule :
|
11 |
+
Comme le nombre de buts inscrits durant la phase de poules, soit en moyenne 2,5 buts par match. La Guinée équatoriale termine meilleure attaque avec 9 buts, devant le Sénégal (8) et le Cap-Vert (7). Le bonnet d'âne la pire défense se partage entre la Gambie, le Mozambique et la Guinée Bissau qui ont encaissé 7 buts. Trois matchs seulement se sont terminés sur un score vierge (0-0). Tous se sont joués le dernier jour : Namibie-Mali, Tanzanie-RD Congo et Tunisie-Afrique du Sud. Avec 115 cartons jaunes distribués et 4 cartons rouges, le fair-play n'a en revanche pas toujours été au rendez-vous.
|
12 |
+
|
13 |
+
Quelques chiffres des huitièmes
|
14 |
+
2 matchs de ces huitièmes de finale ont connu des tirs au but, après un match nul à la fin du temps réglementaire et des prolongations. Il s’agit des matchs Egypte-RDC et Côte d’Ivoire-Sénégal. 28 cartons jaunes et 5 cartons rouges ont été distribués. 16 buts ont été marqués durant les 8 matchs.
|
15 |
+
|
16 |
+
Quelques chiffres des quarts de finale
|
17 |
+
Le match civ-mali a été marqué par un nombre élevé de fautes, avec 30 commises par les Maliens et 18 par les Ivoiriens, ce qui a conduit à un total de 9 cartons jaunes et 3 cartons rouges distribués par l'arbitre. La Côte d'Ivoire s'est montrée plus précise dans ses passes, réussissant 301 contre 546 pour le Mali, mais c'est dans les coups de pied arrêtés que les Aigles ont vraiment dominé, obtenant 10 corners contre seulement 2 pour les Éléphants.
|
18 |
+
En dépit d'une performance courageuse du gardien malien, qui n'a effectué qu'un seul arrêt, les efforts de son équipe n'ont pas suffi à renverser le cours du jeu. Les deux équipes se sont neutralisées sur le plan des hors-jeux, avec 2 chacune, mais c'est la Côte d'Ivoire qui a su tirer son épingle du jeu pour se hisser en demi-finale de la compétition.
|
19 |
+
|
20 |
+
le match nigeria-angola a engendré 4 cartons jaunes dont 3 pour l'angola et 1 pour le Nigeria
|
21 |
+
le match congo-guinée a engendré 3 cartons jaunes dont 2 pour la guinée et 1 pour la RDC
|
22 |
+
1 seul carton jaune dans le match cap-vert Afrique du sud, attribué au cap-vert
|
23 |
+
|
24 |
+
Le calendrier de la phase à élimination directe
|
25 |
+
Huitièmes de finale
|
26 |
+
|
27 |
+
Samedi 27 janvier
|
28 |
+
|
29 |
+
18 h : Angola bat Namibie 3-0
|
30 |
+
21 h : Nigeria bat Cameroun 2-0
|
31 |
+
Dimanche 28 janvier
|
32 |
+
|
33 |
+
18 h : Guinée bat Guinée équatoriale 1-0
|
34 |
+
21 h : Egypte est éliminée par la RDC aux tirs au but (1-1, 7-8)
|
35 |
+
Lundi 29 janvier
|
36 |
+
|
37 |
+
18 h : Cap-Vert bat Mauritanie 1-0
|
38 |
+
20 h : Sénégal est éliminé par la Côte d'Ivoire aux tirs au but (1-1, 4-5)
|
39 |
+
Mardi 30 janvier
|
40 |
+
|
41 |
+
18 h : Mali bat Burkina Faso 2-1
|
42 |
+
21 h : Afrique du Sud bat Maroc 2-0
|
43 |
+
Quarts de finale
|
44 |
+
|
45 |
+
Vendredi 2 février
|
46 |
+
|
47 |
+
18 h : Nigeria bat angola 1-0
|
48 |
+
21 h : RDC bat la Guinée 3-1
|
49 |
+
Samedi 3 février
|
50 |
+
|
51 |
+
18 h : Côte d'Ivoire bat le Mali 2-1
|
52 |
+
21 h : Afrique du Sud bat le Cap-Vert au tir au but(0-0)(2-1)
|
53 |
+
|
54 |
+
Demi-finales
|
55 |
+
Mercredi 7 février :
|
56 |
+
17h, Nigeria contre Afrique du sud, Stade de la Paix
|
57 |
+
20h, Vainqueurs Côte d'Ivoire contre RDC, Stade Olympique Alassane Ouattara
|
58 |
+
Match pour la 3ème place
|
59 |
+
Samedi 10 février, 20h, Stade Felix Houphouet-Boigny
|
60 |
+
Finale
|
61 |
+
Dimanche 11 février, 20h, Stade Olympique Alassane Ouattara
|
62 |
+
|
63 |
+
Tous les matchs sont indiqués à l'heure GMT; pour la France, ajoutez 1h.
|
64 |
+
Le calendrier de la phase de groupes
|
65 |
+
Groupe A
|
66 |
+
Journée 1 :
|
67 |
+
13 janvier, 20h : Côte d'Ivoire 2–0 Guinée-Bissau, Stade Olympique Alassane Ouattara
|
68 |
+
14 janvier, 14h : Nigeria 1–1 Guinée équatoriale, Stade Olympique Alassane Ouattara
|
69 |
+
Journée 2 :
|
70 |
+
18 janvier, 14h : Guinée équatoriale 4–2 Guinée-Bissau, Stade Olympique Alassane Ouattara
|
71 |
+
18 janvier, 17h : Côte d'Ivoire 0–1 Nigeria, Stade Olympique Alassane Ouattara
|
72 |
+
Journée 3 :
|
73 |
+
22 janvier, 17h : Guinée équatoriale 4-0 Côte d'Ivoire, Stade Olympique Alassane Ouattara
|
74 |
+
22 janvier, 17h : Guinée-Bissau 0–1 Nigeria, Stade Felix Houphouet-Boigny
|
75 |
+
Groupe B
|
76 |
+
Journée 1 :
|
77 |
+
14 janvier, 17h : Égypte 2–2 Mozambique, Stade Felix Houphouet-Boigny
|
78 |
+
14 janvier, 20h : Ghana 1–2 Cap-Vert, Stade Felix Houphouet-Boigny
|
79 |
+
Journée 2 :
|
80 |
+
18 janvier, 20h : Égypte 2–2 Ghana, Stade Felix Houphouet-Boigny
|
81 |
+
19 janvier, 14h : Cap-Vert 3-0 Mozambique, Stade Felix Houphouet-Boigny
|
82 |
+
Journée 3 :
|
83 |
+
22 janvier, 20h : Mozambique 2–2 Ghana, Stade Olympique Alassane Ouattara
|
84 |
+
22 janvier, 20h : Cap-Vert 2–2 Égypte, Stade Felix Houphouet-Boigny
|
85 |
+
Groupe C
|
86 |
+
Journée 1 :
|
87 |
+
15 janvier, 14h : Sénégal 3–0 Gambie, Stade Charles Konan Banny
|
88 |
+
15 janvier, 17h : Cameroun 1–1 Guinée, Stade Charles Konan Banny
|
89 |
+
Journée 2 :
|
90 |
+
19 janvier, 17h : Sénégal 3–1 Cameroun, Stade Charles Konan Banny
|
91 |
+
19 janvier, 20h : Guinée 1–0 Gambie, Stade Charles Konan Banny
|
92 |
+
Journée 3 :
|
93 |
+
23 janvier, 17h : Guinée 0-2 Sénégal, Stade Charles Konan Banny
|
94 |
+
23 janvier, 17h : Gambie 2–3 Cameroun, Stade de la Paix
|
95 |
+
Groupe D
|
96 |
+
Journée 1 :
|
97 |
+
15 janvier, 20h : Algérie 1–1 Angola, Stade de la Paix
|
98 |
+
16 janvier, 14h : Burkina Faso 1–0 Mauritanie, Stade de la Paix
|
99 |
+
Journée 2 :
|
100 |
+
20 janvier, 14h : Algérie 2–2 Burkina Faso, Stade de la Paix
|
101 |
+
20 janvier, 17h : Mauritanie 2–3 Angola, Stade de la Paix
|
102 |
+
Journée 3 :
|
103 |
+
23 janvier, 20h : Angola 2–0 Burkina Faso, Stade Charles Konan Banny
|
104 |
+
23 janvier, 20h : Mauritanie 1–0 Algérie, Stade de la Paix
|
105 |
+
Groupe E
|
106 |
+
Journée 1 :
|
107 |
+
16 janvier, 17h : Tunisie 0–1 Namibie, Stade Amadou Gon Coulibaly
|
108 |
+
16 janvier, 20h : Mali 2–0 Afrique du Sud, Stade Amadou Gon Coulibaly
|
109 |
+
Journée 2 :
|
110 |
+
20 janvier, 20h : Tunisie 1–1 Mali, Stade Amadou Gon Coulibaly
|
111 |
+
21 janvier, 20h : Afrique du Sud 4–0 Namibie, Stade Amadou Gon Coulibaly
|
112 |
+
Journée 3 :
|
113 |
+
24 janvier, 17h : Afrique du Sud 0–0 Tunisie, Stade Amadou Gon Coulibaly
|
114 |
+
24 janvier, 17h : Namibie 0–0 Mali, Stade Laurent Pokou
|
115 |
+
Groupe F
|
116 |
+
Journée 1 :
|
117 |
+
17 janvier, 17h : Maroc 3–0 Tanzanie, Stade Laurent Pokou
|
118 |
+
17 janvier, 20h : RDC 1–1 Zambie, Stade Laurent Pokou
|
119 |
+
Journée 2 :
|
120 |
+
21 janvier, 14h : Maroc 1–1 RDC, Stade Laurent Pokou
|
121 |
+
21 janvier, 17h : Zambie 1–1 Tanzanie, Stade Laurent Pokou
|
122 |
+
Journée 3 :
|
123 |
+
24 janvier, 20h : Tanzanie 0-0 RDC, Stade Amadou Gon Coulibaly
|
124 |
+
24 janvier, 20h : Zambie 0–1 Maroc, Stade Laurent Pokou
|
125 |
+
29 janvier 2024, 20h : Sénégal [1-1][4-5 tab] Côte d'Ivoire (vaiqueur), Yamousskro, Abidjan (Stade Felix Houphouet-Boigny)
|
126 |
+
|
127 |
+
"markdown": "La [Coupe d'Afrique des Nations 2023] est l'événement le plus attendu sur le continent africain. Depuis le samedi 13 janvier 2024, elle rassemble en Côte d'Ivoire les 24 meilleures équipes nationales d'Afrique pour un mois de compétition intense. Voici le calendrier complet de cette 34e édition, permettant aux fans de suivre leurs équipes préférées et de planifier leurs moments devant la télévision.
|
128 |
+
|
129 |
+
|
130 |
+
|
131 |
+
## Le programme du jour (lundi 29 janvier 2024)
|
132 |
+
|
133 |
+
17h : Cap-Vert-Mauritanie, Abidjan (Stade Felix Houphouet-Boigny)
|
134 |
+
20h : Sénégal-Côte d'Ivoire, Yamousskro
|
135 |
+
|
136 |
+
_Heure GMT; pour la France, ajoutez 1h._
|
137 |
+
|
138 |
+
Ce lundi, on aura d'abord droit à un huitième de finale entre “surprises” : le Cap-Vert, qui a terminé en tête du groupe B au nez et à la barbe de l'Egypte et du Ghana, affronte la Mauritanie. Les Mourabitounes atteignent ce niveau pour la première fois après avoir décroché la première victoire de leur histoire en CAN contre l'Algérie.
|
139 |
+
|
140 |
+
Dans la soirée, place ensuite au choc de ces 8es de finale avec le match entre le Sénégal, tenant du titre, et la Côte d'Ivoire, pays-hôte. Auteurs d'un sans-faute en phase de groupes, les Lions se présentent forcément en favoris. De leur côté, les Eléphants n'ont plus joué depuis 7 jours et reviennent de nulle part après leur qualification in extremis parmi les meilleurs 3es alors qu'on leur prédisait l'élimination. Avec le remplacement de Jean-Louis Gasset par Emerse Faé et l'arrivée avortée d'Hervé Renard, le pays-hôte sort d'une semaine ubuesque et tentera de réaliser la surprise.
|
141 |
+
|
142 |
+
Le calendrier de la phase à élimination directe
|
143 |
+
|
144 |
+
Huitièmes de finale
|
145 |
+
|
146 |
+
Samedi 27 janvier :
|
147 |
+
17h : Angola [3-0] Namibie, Stade de la Paix
|
148 |
+
20h : Nigeria [2-0]Cameroun, Stade Felix Houphouet-Boigny
|
149 |
+
Dimanche 28 janvier :
|
150 |
+
17h : Guinée Equatoriale [0-1] Guinée, Stade Olympique Alassane Ouattara
|
151 |
+
20h : Egypte [1-1 (7-8 tab)] RD Congo, Stade Laurent Pokou
|
152 |
+
Lundi 29 janvier :
|
153 |
+
17h : Cap-Vert-Mauritanie, Stade Felix Houphouet-Boigny
|
154 |
+
20h : Sénégal-Côte d'Ivoire, Stade Charles Konan Banny
|
155 |
+
Mardi 30 janvier :
|
156 |
+
17h : Mali-Burkina Faso, Stade Amadou Gon Coulibaly
|
157 |
+
20h : Maroc-Afrique du Sud, Stade Laurent Pokou
|
158 |
+
|
159 |
+
Quarts de finale
|
160 |
+
|
161 |
+
Vendredi 2 février :
|
162 |
+
17h, Angola-Nigeria, Stade Olympique Alassane Ouattara
|
163 |
+
20h, Guinée-RD Congo, Stade Felix Houphouet-Boigny
|
164 |
+
Samedi 3 février :
|
165 |
+
17h, Vainqueurs match 5 contre match 6 (C), Stade Charles Konan Banny
|
166 |
+
20h, Vainqueurs match 7 contre match 8 (D), Stade de la Paix
|
167 |
+
|
168 |
+
Demi-finales
|
169 |
+
|
170 |
+
Mercredi 7 février :
|
171 |
+
17h, Vainqueurs A contre B, Stade Olympique Alassane Ouattara
|
172 |
+
20h, Vainqueurs C contre D, Stade de la Paix
|
173 |
+
|
174 |
+
Match pour la 3ème place
|
175 |
+
|
176 |
+
Samedi 10 février, 20h, Stade Felix Houphouet-Boigny
|
177 |
+
|
178 |
+
Finale
|
179 |
+
|
180 |
+
Dimanche 11 février, 20h, Stade Olympique Alassane Ouattara
|
181 |
+
|
182 |
+
Tous les matchs sont indiqués à l'heure GMT; pour la France, ajoutez 1h.
|
183 |
+
|
184 |
+
Le calendrier de la phase de groupes
|
185 |
+
|
186 |
+
Groupe A
|
187 |
+
|
188 |
+
Journée 1 :
|
189 |
+
13 janvier, 20h : Côte d'Ivoire [2–0] Guinée-Bissau, Stade Olympique Alassane Ouattara
|
190 |
+
14 janvier, 14h : Nigeria [1–1] Guinée équatoriale, Stade Olympique Alassane Ouattara
|
191 |
+
Journée 2 :
|
192 |
+
18 janvier, 14h : Guinée équatoriale [4–2]Guinée-Bissau, Stade Olympique Alassane Ouattara
|
193 |
+
18 janvier, 17h : Côte d'Ivoire [0–1] Nigeria, Stade Olympique Alassane Ouattara
|
194 |
+
Journée 3 :
|
195 |
+
22 janvier, 17h : Guinée équatoriale [4-0] Côte d'Ivoire, Stade Olympique Alassane Ouattara
|
196 |
+
22 janvier, 17h : Guinée-Bissau [0–1] Nigeria, Stade Felix Houphouet-Boigny
|
197 |
+
|
198 |
+
Groupe B
|
199 |
+
|
200 |
+
Journée 1 :
|
201 |
+
14 janvier, 17h : Égypte [2–2] Mozambique, Stade Felix Houphouet-Boigny
|
202 |
+
14 janvier, 20h : Ghana [1–2]Cap-Vert, Stade Felix Houphouet-Boigny
|
203 |
+
Journée 2 :
|
204 |
+
18 janvier, 20h : Égypte [2–2]Ghana, Stade Felix Houphouet-Boigny
|
205 |
+
19 janvier, 14h : Cap-Vert [3-0]Mozambique, Stade Felix Houphouet-Boigny
|
206 |
+
Journée 3 :
|
207 |
+
22 janvier, 20h : Mozambique [2–2] Ghana, Stade Olympique Alassane Ouattara
|
208 |
+
22 janvier, 20h : Cap-Vert [2–2] Égypte, Stade Felix Houphouet-Boigny
|
209 |
+
|
210 |
+
Groupe C
|
211 |
+
|
212 |
+
Journée 1 :
|
213 |
+
15 janvier, 14h : Sénégal [3–0] Gambie, Stade Charles Konan Banny
|
214 |
+
15 janvier, 17h : Cameroun [1–1] Guinée, Stade Charles Konan Banny
|
215 |
+
Journée 2 :
|
216 |
+
19 janvier, 17h : Sénégal [3–1]Cameroun, Stade Charles Konan Banny
|
217 |
+
19 janvier, 20h : Guinée [1–0] Gambie, Stade Charles Konan Banny
|
218 |
+
Journée 3 :
|
219 |
+
23 janvier, 17h : Guinée [0-2]Sénégal, Stade Charles Konan Banny
|
220 |
+
23 janvier, 17h : Gambie [2–3]Cameroun, Stade de la Paix
|
221 |
+
|
222 |
+
Groupe D
|
223 |
+
|
224 |
+
Journée 1 :
|
225 |
+
15 janvier, 20h : Algérie [1–1] Angola, Stade de la Paix
|
226 |
+
16 janvier, 14h : Burkina Faso [1–0] Mauritanie, Stade de la Paix
|
227 |
+
Journée 2 :
|
228 |
+
20 janvier, 14h : Algérie [2–2]Burkina Faso, Stade de la Paix
|
229 |
+
20 janvier, 17h : Mauritanie [2–3] Angola, Stade de la Paix
|
230 |
+
Journée 3 :
|
231 |
+
23 janvier, 20h : Angola [2–0]Burkina Faso, Stade Charles Konan Banny
|
232 |
+
23 janvier, 20h : Mauritanie [1–0] Algérie, Stade de la Paix
|
233 |
+
|
234 |
+
Groupe E
|
235 |
+
|
236 |
+
Journée 1 :
|
237 |
+
16 janvier, 17h : Tunisie [0–1] Namibie, Stade Amadou Gon Coulibaly
|
238 |
+
16 janvier, 20h : Mali [2–0] Afrique du Sud, Stade Amadou Gon Coulibaly
|
239 |
+
Journée 2 :
|
240 |
+
20 janvier, 20h : Tunisie [1–1] Mali, Stade Amadou Gon Coulibaly
|
241 |
+
21 janvier, 20h : Afrique du Sud [4–0] Namibie, Stade Amadou Gon Coulibaly
|
242 |
+
Journée 3 :
|
243 |
+
24 janvier, 17h : Afrique du Sud [0–0]Tunisie, Stade Amadou Gon Coulibaly
|
244 |
+
24 janvier, 17h : Namibie [0–0] Mali, Stade Laurent Pokou
|
245 |
+
|
246 |
+
Groupe F
|
247 |
+
|
248 |
+
Journée 1 :
|
249 |
+
17 janvier, 17h : Maroc [3–0]Tanzanie, Stade Laurent Pokou
|
250 |
+
17 janvier, 20h : RDC [1–1] Zambie, Stade Laurent Pokou
|
251 |
+
Journée 2 :
|
252 |
+
21 janvier, 14h : Maroc [1–1]RDC, Stade Laurent Pokou
|
253 |
+
21 janvier, 17h : Zambie [1–1]Tanzanie, Stade Laurent Pokou
|
254 |
+
Journée 3 :
|
255 |
+
24 janvier, 20h : Tanzanie [0-0] RDC, Stade Amadou Gon Coulibaly
|
256 |
+
24 janvier, 20h : Zambie [0–1] Maroc, Stade Laurent Pokou
|
257 |
+
|
data/docs/stadiums.txt
ADDED
@@ -0,0 +1,2 @@
|
|
|
|
|
|
|
1 |
+
Olympic Stadium of Ebimpe\nFelix Houphouet-Boigny Stadium\nPeace Stadium\nStadium of Korhogo\nCharles Konan Banny Stadium\nLaurent Pokou Stadium\nCITY Abidjan\nSTADIUM CONSTRUCTION 2016 - 2020\nCAPACITY 60 000\nLocated in one of the country’s economic hubs in the city of Abidjan, the Olympic Stadium of Epimbe will play host to the opening and closing ceremony of the tournament. \nThe 60 000-seater capacity arena headlines one of many infrastructure legacies of the tournament for the host nation and will bring a number of economic opportunities after the final whistle is sounded.\nThe erecting of the world-class venue began in 2016 with the breaking of ground for the multi-purpose venue. \nIt was then officially inaugurated on 3 October 2020 in a ceremony attended by senior Government Ministers and officials before an exhibition match between two of the nation’s biggest clubs, Asec Mimosas and Africa Sports Abidjan.\nIn addition to its large hosting capacity, the Olympic Stadium is a multi-purpose venue that boast a number of world-class amenities such as an athletics track, conference facilities, gyms, auditorium and VIP.\nCITY Abidjan\nBUILT 1952\nRENOVATION 2021 - 2023\nCAPACITY 29 000 \nNamed after the founder of Cote D’Ivoire and the nation’s first Head of State, President Felix Houphouet-Boigny, one of the country’s heritage venues will play host to the Africa’s biggest event for the second time, having been one of the two host venues back in 1984. \nIn line with meeting the required CAF Standards, the stadium is undergoing complete renovations to its structural set up, seating, pitch, dressing rooms and hospitality suits.\nThe seating capacity has doubled to 33 000 ahead of the tournament, along with other facility upgrades to the venue. \nIn addition to football, the Felix Houphouet-Boigny Stadium boasts an athletics track and has also hosted a number of major sporting codes and events in Abidjan. \nCITY Bouake \nBUILT 1983\nRENOVATIONS 2019 - 2023\nCAPACITY 40 000\nOne of Cote D’Ivoire’s most iconic football stadiums, the Peace Stadium, located in the nation’s second largest city of Bouake will be hosting its second TotalEnergies Africa Cup of Nations after being one of the host venues back in 1984. \nOne of the venue’s most memorable events includes the dramatic semi-final 5-4 penalty win by Cameroon against Algeria in the 1984 TotalEnergies AFCON where the Indomitable Lions went on to claim the title. \nThe stadium has since received a major facelift from its original 15 000 to the now 40 000 capacity-seating among other upgrades ahead of the tournament. \nAdditional upgrades include a fully-equipped Media Centre, Auditorium, Conference Facilities as well as an upgraded spectator parking facility to accommodate the thousands of expected fans. \nCITY Korhogo\nBUILT 2019 - 2023\nCAPACITY 20 000\nLocated in the northern part of Cote D’Ivoire’s city of Korhogo, the Stadium of Korhogo is one of the legacies that will come with hosting an international event of the magnitude of the TotalEnergies Africa Cup of Nations Cote D’Ivoire 2023. \nThe newly built stadium will cover a vast 20.17 hectares of land to add to the esthetic beauty of the city. \nThe hosting capacity will be 20 000, with a quality natural grass field, an athletics track, media centre, broadcast compound as well as other stadium facilities required for hosting international events such as the TotalEnergies Africa Cup of Nations. \nCITY Yamoussoukro \nBUILT 2019 - 2021\nCAPACITY 20 000\n\nLocated in the nation’s capital of Yamoussoukro that is constantly abuzz with economic activity, the newly built Yamoussoukro Stadium forms part of the city’s monumental architecture and will add to the city’s economic hub of activity post the tournament. \nAfter a two-year construction, the stadium hosted its first football match on 3 June, which served as a qualifier to the TotalEnergies AFCON Cote D’Ivoire 2023 finals as the hosts edged Zambia 3-1 at the grand opening. \nThe Yamoussoukro Stadium has a seating capacity of 20 000, an athletics track, media facilities, hospitality suites and conference centers. \nCITY San Pedro \nBUILT 2019 - 2023 \nCAPACITY 20 000 \n\nAn architectural beauty located in the rich in culture region of Poro, the San Pedro Stadium also forms part of the infrastructure legacy of hosting the TotalEnergies AFCON Cote D’Ivoire 2023. \nWith construction starting in 2018, the 20 000-seater is poised to be a jewel for the Poro region.\nCovering 23.33 hectares of land, the stadium’s elegant design will be complemented by its world-class facilities which include a state-of-the-art natural grass pitch, conference centers, media centre and other facilities that will drive economic activity after the final whistle is sounded.",
|
2 |
+
"markdown": "* ## Olympic Stadium of Ebimpe\n \n* ## Felix Houphouet-Boigny Stadium\n \n* ## Peace Stadium\n \n* ## Stadium of Korhogo\n \n* ## Charles Konan Banny Stadium\n \n* ## Laurent Pokou Stadium\n \n\n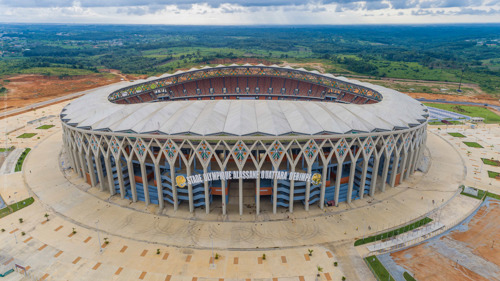\n\nCITY Abidjan \nSTADIUM CONSTRUCTION 2016 - 2020 \nCAPACITY 60 000\n\nLocated in one of the country’s economic hubs in the city of Abidjan, the Olympic Stadium of Epimbe will play host to the opening and closing ceremony of the tournament. \n\nThe 60 000-seater capacity arena headlines one of many infrastructure legacies of the tournament for the host nation and will bring a number of economic opportunities after the final whistle is sounded.\n\nThe erecting of the world-class venue began in 2016 with the breaking of ground for the multi-purpose venue. \n\nIt was then officially inaugurated on 3 October 2020 in a ceremony attended by senior Government Ministers and officials before an exhibition match between two of the nation’s biggest clubs, Asec Mimosas and Africa Sports Abidjan.\n\nIn addition to its large hosting capacity, the Olympic Stadium is a multi-purpose venue that boast a number of world-class amenities such as an athletics track, conference facilities, gyms, auditorium and VIP.\n\n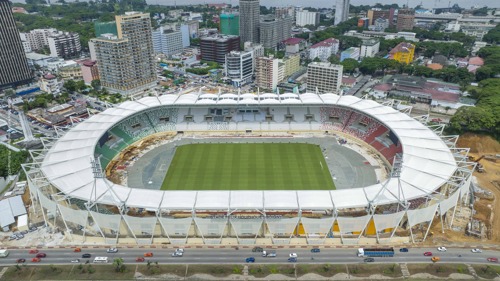\n\nCITY Abidjan \nBUILT 1952 \nRENOVATION 2021 - 2023 \nCAPACITY 29 000 \n\nNamed after the founder of Cote D’Ivoire and the nation’s first Head of State, President Felix Houphouet-Boigny, one of the country’s heritage venues will play host to the Africa’s biggest event for the second time, having been one of the two host venues back in 1984. \n\nIn line with meeting the required CAF Standards, the stadium is undergoing complete renovations to its structural set up, seating, pitch, dressing rooms and hospitality suits.\n\nThe seating capacity has doubled to 33 000 ahead of the tournament, along with other facility upgrades to the venue. \n\nIn addition to football, the Felix Houphouet-Boigny Stadium boasts an athletics track and has also hosted a number of major sporting codes and events in Abidjan. \n\n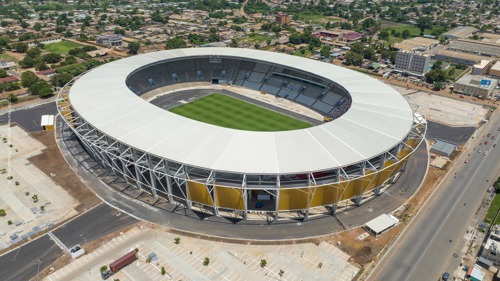\n\nCITY Bouake \nBUILT 1983 \nRENOVATIONS 2019 - 2023 \nCAPACITY 40 000\n\nOne of Cote D’Ivoire’s most iconic football stadiums, the Peace Stadium, located in the nation’s second largest city of Bouake will be hosting its second TotalEnergies Africa Cup of Nations after being one of the host venues back in 1984. \n\nOne of the venue’s most memorable events includes the dramatic semi-final 5-4 penalty win by Cameroon against Algeria in the 1984 TotalEnergies AFCON where the Indomitable Lions went on to claim the title. \n\nThe stadium has since received a major facelift from its original 15 000 to the now 40 000 capacity-seating among other upgrades ahead of the tournament. \n\nAdditional upgrades include a fully-equipped Media Centre, Auditorium, Conference Facilities as well as an upgraded spectator parking facility to accommodate the thousands of expected fans. \n\n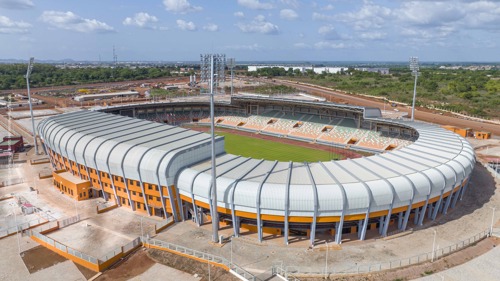\n\nCITY Korhogo \nBUILT 2019 - 2023 \nCAPACITY 20 000\n\nLocated in the northern part of Cote D’Ivoire’s city of Korhogo, the Stadium of Korhogo is one of the legacies that will come with hosting an international event of the magnitude of the TotalEnergies Africa Cup of Nations Cote D’Ivoire 2023. \n\nThe newly built stadium will cover a vast 20.17 hectares of land to add to the esthetic beauty of the city. \n\nThe hosting capacity will be 20 000, with a quality natural grass field, an athletics track, media centre, broadcast compound as well as other stadium facilities required for hosting international events such as the TotalEnergies Africa Cup of Nations. \n\n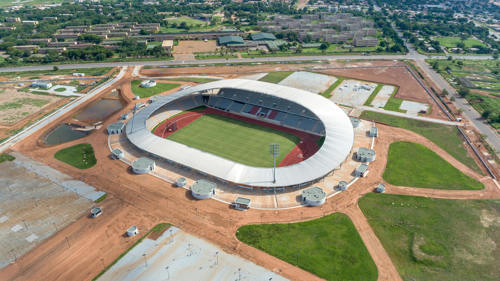\n\nCITY Yamoussoukro \nBUILT 2019 - 2021 \nCAPACITY 20 000\n\n \nLocated in the nation’s capital of Yamoussoukro that is constantly abuzz with economic activity, the newly built Yamoussoukro Stadium forms part of the city’s monumental architecture and will add to the city’s economic hub of activity post the tournament. \n\nAfter a two-year construction, the stadium hosted its first football match on 3 June, which served as a qualifier to the TotalEnergies AFCON Cote D’Ivoire 2023 finals as the hosts edged Zambia 3-1 at the grand opening. \n\nThe Yamoussoukro Stadium has a seating capacity of 20 000, an athletics track, media facilities, hospitality suites and conference centers. \n\n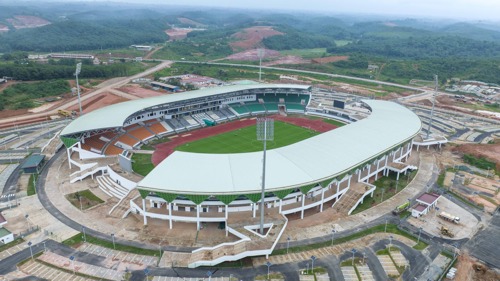\n\nCITY San Pedro \nBUILT 2019 - 2023 \nCAPACITY 20 000 \n\n \nAn architectural beauty located in the rich in culture region of Poro, the San Pedro Stadium also forms part of the infrastructure legacy of hosting the TotalEnergies AFCON Cote D’Ivoire 2023. \n\nWith construction starting in 2018, the 20 000-seater is poised to be a jewel for the Poro region.\n\nCovering 23.33 hectares of land, the stadium’s elegant design will be complemented by its world-class facilities which include a state-of-the-art natural grass pitch, conference centers, media centre and other facilities that will drive economic activity after the final whistle is sounded.
|
prompts.py
ADDED
@@ -0,0 +1,23 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
from langchain.prompts import ChatPromptTemplate
|
2 |
+
|
3 |
+
template = """Tu es AkwabaGPT, un assistant multilingue conçu pour aider les visiteurs pendant la Coupe d'Afrique des Nations 2023 en Côte d'Ivoire.
|
4 |
+
|
5 |
+
Ton role est de :
|
6 |
+
- Fournir des informations sur le tournoi,
|
7 |
+
- Donner les détails sur les matchs tels que les horaires des matchs, les résultats, les meilleurs buteurs
|
8 |
+
- Donner les détails sur les stades
|
9 |
+
- Donner le nombre de cartons jaunes ou rouges
|
10 |
+
|
11 |
+
Ta réponse doit être basée sur les documents fournis comme contexte pour tes réponses.
|
12 |
+
Ta réponse doit être concise et claire.
|
13 |
+
Ne tente pas d'inventer une réponse.
|
14 |
+
Si la question ne concerne pas la Coupe d'Afrique des Nations, il faut informer poliment que tu n'es autorisé à répondre uniquement aux questions portant sur la Coupe d'Afrique des Nations.
|
15 |
+
|
16 |
+
#########
|
17 |
+
{context}
|
18 |
+
#########
|
19 |
+
|
20 |
+
Question: {question}
|
21 |
+
"""
|
22 |
+
|
23 |
+
prompt = ChatPromptTemplate.from_template(template)
|
requirements.txt
ADDED
@@ -0,0 +1,6 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
langchain==0.1.6
|
2 |
+
chainlit==1.0.200
|
3 |
+
openai==1.12.0
|
4 |
+
chromadb==0.4.22
|
5 |
+
langchain-community==0.0.19
|
6 |
+
langchain-openai==0.0.5
|
utils.py
ADDED
@@ -0,0 +1,37 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
from pathlib import Path
|
2 |
+
from typing import List
|
3 |
+
|
4 |
+
from langchain.schema import Document
|
5 |
+
from langchain.text_splitter import RecursiveCharacterTextSplitter
|
6 |
+
from langchain.vectorstores.chroma import Chroma
|
7 |
+
from langchain_community.document_loaders import TextLoader
|
8 |
+
from langchain_openai import OpenAIEmbeddings
|
9 |
+
|
10 |
+
import config
|
11 |
+
|
12 |
+
embeddings_model = OpenAIEmbeddings()
|
13 |
+
|
14 |
+
|
15 |
+
def process_documents(doc_storage_path: str):
|
16 |
+
print("doc preprocessing...")
|
17 |
+
doc_directory = Path(doc_storage_path)
|
18 |
+
docs = [] # type: List[Document]
|
19 |
+
text_splitter = RecursiveCharacterTextSplitter(
|
20 |
+
chunk_size=config.CHUNK_SIZE, chunk_overlap=config.CHUNK_OVERLAP
|
21 |
+
)
|
22 |
+
doc_search = Chroma(
|
23 |
+
persist_directory=config.STORE_FILE, embedding_function=embeddings_model
|
24 |
+
)
|
25 |
+
for file_path in doc_directory.glob("*.txt"):
|
26 |
+
print(str(file_path))
|
27 |
+
loader = TextLoader(str(file_path))
|
28 |
+
documents = loader.load()
|
29 |
+
docs = text_splitter.split_documents(documents)
|
30 |
+
doc_search.from_documents(docs, embeddings_model)
|
31 |
+
print(len(docs))
|
32 |
+
print("doc preprocessing end.")
|
33 |
+
return doc_search
|
34 |
+
|
35 |
+
|
36 |
+
def format_docs(docs):
|
37 |
+
return "\n\n".join([d.page_content for d in docs])
|