Spaces:
Running
on
Zero
Running
on
Zero
Commit
•
df86cb4
1
Parent(s):
37debce
init code
Browse files- .gitignore +1 -0
- README.md +2 -0
- app.py +419 -0
- diffusers_patches.py +541 -0
- requirements.txt +13 -0
- style.css +1 -0
.gitignore
ADDED
@@ -0,0 +1 @@
|
|
|
|
|
1 |
+
.idea
|
README.md
CHANGED
@@ -11,3 +11,5 @@ license: openrail
|
|
11 |
---
|
12 |
|
13 |
Check out the configuration reference at https://huggingface.co/docs/hub/spaces-config-reference
|
|
|
|
|
|
11 |
---
|
12 |
|
13 |
Check out the configuration reference at https://huggingface.co/docs/hub/spaces-config-reference
|
14 |
+
Inference Code: https://github.com/PixArt-alpha/PixArt-Sigma
|
15 |
+
Paper: https://arxiv.org/abs/2403.04692
|
app.py
ADDED
@@ -0,0 +1,419 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
#!/usr/bin/env python
|
2 |
+
from __future__ import annotations
|
3 |
+
import argparse
|
4 |
+
import os
|
5 |
+
import sys
|
6 |
+
from pathlib import Path
|
7 |
+
current_file_path = Path(__file__).resolve()
|
8 |
+
sys.path.insert(0, str(current_file_path.parent.parent))
|
9 |
+
import random
|
10 |
+
import gradio as gr
|
11 |
+
import numpy as np
|
12 |
+
import uuid
|
13 |
+
from diffusers import ConsistencyDecoderVAE, DPMSolverMultistepScheduler, Transformer2DModel, AutoencoderKL
|
14 |
+
import torch
|
15 |
+
from typing import Tuple
|
16 |
+
from datetime import datetime
|
17 |
+
from diffusion.sa_solver_diffusers import SASolverScheduler
|
18 |
+
from peft import PeftModel
|
19 |
+
from scripts.diffusers_patches import pixart_sigma_init_patched_inputs, PixArtSigmaPipeline
|
20 |
+
|
21 |
+
|
22 |
+
DESCRIPTION = """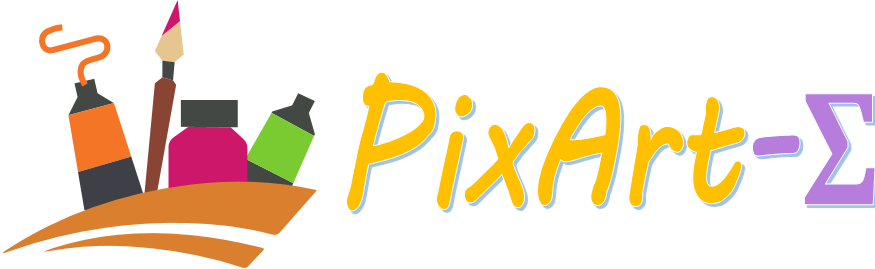
|
23 |
+
# PixArt-Sigma 1024px
|
24 |
+
#### [PixArt-Sigma 1024px](https://github.com/PixArt-alpha/PixArt-sigma) is a transformer-based text-to-image diffusion system trained on text embeddings from T5. This demo uses the [PixArt-alpha/PixArt-XL-2-1024-MS](https://huggingface.co/PixArt-alpha/PixArt-XL-2-1024-MS) checkpoint.
|
25 |
+
#### English prompts ONLY; 提示词仅限英文
|
26 |
+
### <span style='color: red;'>You may change the DPM-Solver inference steps from 14 to 20, or DPM-Solver Guidance scale from 4.5 to 3.5 if you didn't get satisfied results.
|
27 |
+
"""
|
28 |
+
if not torch.cuda.is_available():
|
29 |
+
DESCRIPTION += "\n<p>Running on CPU 🥶 This demo does not work on CPU.</p>"
|
30 |
+
|
31 |
+
MAX_SEED = np.iinfo(np.int32).max
|
32 |
+
CACHE_EXAMPLES = torch.cuda.is_available() and os.getenv("CACHE_EXAMPLES", "1") == "1"
|
33 |
+
MAX_IMAGE_SIZE = int(os.getenv("MAX_IMAGE_SIZE", "6000"))
|
34 |
+
USE_TORCH_COMPILE = os.getenv("USE_TORCH_COMPILE", "0") == "1"
|
35 |
+
ENABLE_CPU_OFFLOAD = os.getenv("ENABLE_CPU_OFFLOAD", "0") == "1"
|
36 |
+
PORT = int(os.getenv("DEMO_PORT", "15432"))
|
37 |
+
|
38 |
+
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
|
39 |
+
|
40 |
+
|
41 |
+
style_list = [
|
42 |
+
{
|
43 |
+
"name": "(No style)",
|
44 |
+
"prompt": "{prompt}",
|
45 |
+
"negative_prompt": "",
|
46 |
+
},
|
47 |
+
{
|
48 |
+
"name": "Cinematic",
|
49 |
+
"prompt": "cinematic still {prompt} . emotional, harmonious, vignette, highly detailed, high budget, bokeh, cinemascope, moody, epic, gorgeous, film grain, grainy",
|
50 |
+
"negative_prompt": "anime, cartoon, graphic, text, painting, crayon, graphite, abstract, glitch, deformed, mutated, ugly, disfigured",
|
51 |
+
},
|
52 |
+
{
|
53 |
+
"name": "Photographic",
|
54 |
+
"prompt": "cinematic photo {prompt} . 35mm photograph, film, bokeh, professional, 4k, highly detailed",
|
55 |
+
"negative_prompt": "drawing, painting, crayon, sketch, graphite, impressionist, noisy, blurry, soft, deformed, ugly",
|
56 |
+
},
|
57 |
+
{
|
58 |
+
"name": "Anime",
|
59 |
+
"prompt": "anime artwork {prompt} . anime style, key visual, vibrant, studio anime, highly detailed",
|
60 |
+
"negative_prompt": "photo, deformed, black and white, realism, disfigured, low contrast",
|
61 |
+
},
|
62 |
+
{
|
63 |
+
"name": "Manga",
|
64 |
+
"prompt": "manga style {prompt} . vibrant, high-energy, detailed, iconic, Japanese comic style",
|
65 |
+
"negative_prompt": "ugly, deformed, noisy, blurry, low contrast, realism, photorealistic, Western comic style",
|
66 |
+
},
|
67 |
+
{
|
68 |
+
"name": "Digital Art",
|
69 |
+
"prompt": "concept art {prompt} . digital artwork, illustrative, painterly, matte painting, highly detailed",
|
70 |
+
"negative_prompt": "photo, photorealistic, realism, ugly",
|
71 |
+
},
|
72 |
+
{
|
73 |
+
"name": "Pixel art",
|
74 |
+
"prompt": "pixel-art {prompt} . low-res, blocky, pixel art style, 8-bit graphics",
|
75 |
+
"negative_prompt": "sloppy, messy, blurry, noisy, highly detailed, ultra textured, photo, realistic",
|
76 |
+
},
|
77 |
+
{
|
78 |
+
"name": "Fantasy art",
|
79 |
+
"prompt": "ethereal fantasy concept art of {prompt} . magnificent, celestial, ethereal, painterly, epic, majestic, magical, fantasy art, cover art, dreamy",
|
80 |
+
"negative_prompt": "photographic, realistic, realism, 35mm film, dslr, cropped, frame, text, deformed, glitch, noise, noisy, off-center, deformed, cross-eyed, closed eyes, bad anatomy, ugly, disfigured, sloppy, duplicate, mutated, black and white",
|
81 |
+
},
|
82 |
+
{
|
83 |
+
"name": "Neonpunk",
|
84 |
+
"prompt": "neonpunk style {prompt} . cyberpunk, vaporwave, neon, vibes, vibrant, stunningly beautiful, crisp, detailed, sleek, ultramodern, magenta highlights, dark purple shadows, high contrast, cinematic, ultra detailed, intricate, professional",
|
85 |
+
"negative_prompt": "painting, drawing, illustration, glitch, deformed, mutated, cross-eyed, ugly, disfigured",
|
86 |
+
},
|
87 |
+
{
|
88 |
+
"name": "3D Model",
|
89 |
+
"prompt": "professional 3d model {prompt} . octane render, highly detailed, volumetric, dramatic lighting",
|
90 |
+
"negative_prompt": "ugly, deformed, noisy, low poly, blurry, painting",
|
91 |
+
},
|
92 |
+
]
|
93 |
+
|
94 |
+
|
95 |
+
styles = {k["name"]: (k["prompt"], k["negative_prompt"]) for k in style_list}
|
96 |
+
STYLE_NAMES = list(styles.keys())
|
97 |
+
DEFAULT_STYLE_NAME = "(No style)"
|
98 |
+
SCHEDULE_NAME = ["DPM-Solver", "SA-Solver"]
|
99 |
+
DEFAULT_SCHEDULE_NAME = "DPM-Solver"
|
100 |
+
NUM_IMAGES_PER_PROMPT = 1
|
101 |
+
|
102 |
+
def apply_style(style_name: str, positive: str, negative: str = "") -> Tuple[str, str]:
|
103 |
+
p, n = styles.get(style_name, styles[DEFAULT_STYLE_NAME])
|
104 |
+
if not negative:
|
105 |
+
negative = ""
|
106 |
+
return p.replace("{prompt}", positive), n + negative
|
107 |
+
|
108 |
+
|
109 |
+
def get_args():
|
110 |
+
parser = argparse.ArgumentParser()
|
111 |
+
parser.add_argument('--is_lora', action='store_true', help='enable lora ckpt loading')
|
112 |
+
parser.add_argument('--repo_id', default="PixArt-alpha/PixArt-Sigma-XL-2-1024-MS", type=str)
|
113 |
+
parser.add_argument('--lora_repo_id', default=None, type=str)
|
114 |
+
parser.add_argument('--model_path', default=None, type=str)
|
115 |
+
parser.add_argument(
|
116 |
+
'--pipeline_load_from', default="PixArt-alpha/pixart_sigma_sdxlvae_T5_diffusers", type=str,
|
117 |
+
help="Download for loading text_encoder, tokenizer and vae "
|
118 |
+
"from https://huggingface.co/PixArt-alpha/PixArt-XL-2-1024-MS")
|
119 |
+
parser.add_argument('--T5_token_max_length', default=120, type=int, help='max length of tokens for T5')
|
120 |
+
return parser.parse_args()
|
121 |
+
|
122 |
+
|
123 |
+
args = get_args()
|
124 |
+
|
125 |
+
if torch.cuda.is_available():
|
126 |
+
weight_dtype = torch.float16
|
127 |
+
T5_token_max_length = args.T5_token_max_length
|
128 |
+
model_path = args.model_path
|
129 |
+
if 'Sigma' in args.model_path:
|
130 |
+
T5_token_max_length = 300
|
131 |
+
|
132 |
+
# tmp patches for diffusers PixArtSigmaPipeline Implementation
|
133 |
+
print(
|
134 |
+
"Changing _init_patched_inputs method of diffusers.models.Transformer2DModel "
|
135 |
+
"using scripts.diffusers_patches.pixart_sigma_init_patched_inputs")
|
136 |
+
setattr(Transformer2DModel, '_init_patched_inputs', pixart_sigma_init_patched_inputs)
|
137 |
+
|
138 |
+
if not args.is_lora:
|
139 |
+
transformer = Transformer2DModel.from_pretrained(
|
140 |
+
model_path,
|
141 |
+
subfolder='transformer',
|
142 |
+
torch_dtype=weight_dtype,
|
143 |
+
)
|
144 |
+
pipe = PixArtSigmaPipeline.from_pretrained(
|
145 |
+
args.pipeline_load_from,
|
146 |
+
transformer=transformer,
|
147 |
+
torch_dtype=weight_dtype,
|
148 |
+
use_safetensors=True,
|
149 |
+
)
|
150 |
+
else:
|
151 |
+
assert args.lora_repo_id is not None
|
152 |
+
transformer = Transformer2DModel.from_pretrained(args.repo_id, subfolder="transformer", torch_dtype=torch.float16)
|
153 |
+
transformer = PeftModel.from_pretrained(transformer, args.lora_repo_id)
|
154 |
+
pipe = PixArtSigmaPipeline.from_pretrained(
|
155 |
+
args.repo_id,
|
156 |
+
transformer=transformer,
|
157 |
+
torch_dtype=torch.float16,
|
158 |
+
use_safetensors=True,
|
159 |
+
)
|
160 |
+
del transformer
|
161 |
+
|
162 |
+
if os.getenv('CONSISTENCY_DECODER', False):
|
163 |
+
print("Using DALL-E 3 Consistency Decoder")
|
164 |
+
pipe.vae = ConsistencyDecoderVAE.from_pretrained("openai/consistency-decoder", torch_dtype=torch.float16)
|
165 |
+
|
166 |
+
if ENABLE_CPU_OFFLOAD:
|
167 |
+
pipe.enable_model_cpu_offload()
|
168 |
+
else:
|
169 |
+
pipe.to(device)
|
170 |
+
print("Loaded on Device!")
|
171 |
+
|
172 |
+
# speed-up T5
|
173 |
+
pipe.text_encoder.to_bettertransformer()
|
174 |
+
|
175 |
+
if USE_TORCH_COMPILE:
|
176 |
+
pipe.transformer = torch.compile(pipe.transformer, mode="reduce-overhead", fullgraph=True)
|
177 |
+
print("Model Compiled!")
|
178 |
+
|
179 |
+
|
180 |
+
def save_image(img, seed=''):
|
181 |
+
unique_name = f"{str(uuid.uuid4())}_{seed}.png"
|
182 |
+
save_path = os.path.join(f'output/online_demo_img/{datetime.now().date()}')
|
183 |
+
os.umask(0o000) # file permission: 666; dir permission: 777
|
184 |
+
os.makedirs(save_path, exist_ok=True)
|
185 |
+
unique_name = os.path.join(save_path, unique_name)
|
186 |
+
img.save(unique_name)
|
187 |
+
return unique_name
|
188 |
+
|
189 |
+
|
190 |
+
def randomize_seed_fn(seed: int, randomize_seed: bool) -> int:
|
191 |
+
if randomize_seed:
|
192 |
+
seed = random.randint(0, MAX_SEED)
|
193 |
+
return seed
|
194 |
+
|
195 |
+
|
196 |
+
@torch.no_grad()
|
197 |
+
@torch.inference_mode()
|
198 |
+
def generate(
|
199 |
+
prompt: str,
|
200 |
+
negative_prompt: str = "",
|
201 |
+
style: str = DEFAULT_STYLE_NAME,
|
202 |
+
use_negative_prompt: bool = False,
|
203 |
+
num_imgs: int = 1,
|
204 |
+
seed: int = 0,
|
205 |
+
width: int = 1024,
|
206 |
+
height: int = 1024,
|
207 |
+
schedule: str = 'DPM-Solver',
|
208 |
+
dpms_guidance_scale: float = 4.5,
|
209 |
+
sas_guidance_scale: float = 3,
|
210 |
+
dpms_inference_steps: int = 20,
|
211 |
+
sas_inference_steps: int = 25,
|
212 |
+
randomize_seed: bool = False,
|
213 |
+
use_resolution_binning: bool = True,
|
214 |
+
progress=gr.Progress(track_tqdm=True),
|
215 |
+
):
|
216 |
+
seed = int(randomize_seed_fn(seed, randomize_seed))
|
217 |
+
generator = torch.Generator().manual_seed(seed)
|
218 |
+
print(f"{PORT}: {model_path}")
|
219 |
+
print(prompt)
|
220 |
+
|
221 |
+
if schedule == 'DPM-Solver':
|
222 |
+
if not isinstance(pipe.scheduler, DPMSolverMultistepScheduler):
|
223 |
+
pipe.scheduler = DPMSolverMultistepScheduler()
|
224 |
+
num_inference_steps = dpms_inference_steps
|
225 |
+
guidance_scale = dpms_guidance_scale
|
226 |
+
elif schedule == "SA-Solver":
|
227 |
+
if not isinstance(pipe.scheduler, SASolverScheduler):
|
228 |
+
pipe.scheduler = SASolverScheduler.from_config(pipe.scheduler.config, algorithm_type='data_prediction', tau_func=lambda t: 1 if 200 <= t <= 800 else 0, predictor_order=2, corrector_order=2)
|
229 |
+
num_inference_steps = sas_inference_steps
|
230 |
+
guidance_scale = sas_guidance_scale
|
231 |
+
else:
|
232 |
+
raise ValueError(f"Unknown schedule: {schedule}")
|
233 |
+
|
234 |
+
if not use_negative_prompt:
|
235 |
+
negative_prompt = None # type: ignore
|
236 |
+
prompt, negative_prompt = apply_style(style, prompt, negative_prompt)
|
237 |
+
|
238 |
+
images = pipe(
|
239 |
+
prompt=prompt,
|
240 |
+
width=width,
|
241 |
+
height=height,
|
242 |
+
guidance_scale=guidance_scale,
|
243 |
+
num_inference_steps=num_inference_steps,
|
244 |
+
generator=generator,
|
245 |
+
num_images_per_prompt=num_imgs,
|
246 |
+
use_resolution_binning=use_resolution_binning,
|
247 |
+
output_type="pil",
|
248 |
+
max_sequence_length=args.T5_token_max_length,
|
249 |
+
).images
|
250 |
+
|
251 |
+
image_paths = [save_image(img, seed) for img in images]
|
252 |
+
print(image_paths)
|
253 |
+
return image_paths, seed
|
254 |
+
|
255 |
+
|
256 |
+
examples = [
|
257 |
+
"A small cactus with a happy face in the Sahara desert.",
|
258 |
+
"an astronaut sitting in a diner, eating fries, cinematic, analog film",
|
259 |
+
"Pirate ship trapped in a cosmic maelstrom nebula, rendered in cosmic beach whirlpool engine, volumetric lighting, spectacular, ambient lights, light pollution, cinematic atmosphere, art nouveau style, illustration art artwork by SenseiJaye, intricate detail.",
|
260 |
+
"stars, water, brilliantly, gorgeous large scale scene, a little girl, in the style of dreamy realism, light gold and amber, blue and pink, brilliantly illuminated in the background.",
|
261 |
+
"professional portrait photo of an anthropomorphic cat wearing fancy gentleman hat and jacket walking in autumn forest.",
|
262 |
+
"beautiful lady, freckles, big smile, blue eyes, short ginger hair, dark makeup, wearing a floral blue vest top, soft light, dark grey background",
|
263 |
+
"Spectacular Tiny World in the Transparent Jar On the Table, interior of the Great Hall, Elaborate, Carved Architecture, Anatomy, Symetrical, Geometric and Parameteric Details, Precision Flat line Details, Pattern, Dark fantasy, Dark errie mood and ineffably mysterious mood, Technical design, Intricate Ultra Detail, Ornate Detail, Stylized and Futuristic and Biomorphic Details, Architectural Concept, Low contrast Details, Cinematic Lighting, 8k, by moebius, Fullshot, Epic, Fullshot, Octane render, Unreal ,Photorealistic, Hyperrealism",
|
264 |
+
"anthropomorphic profile of the white snow owl Crystal priestess , art deco painting, pretty and expressive eyes, ornate costume, mythical, ethereal, intricate, elaborate, hyperrealism, hyper detailed, 3D, 8K, Ultra Realistic, high octane, ultra resolution, amazing detail, perfection, In frame, photorealistic, cinematic lighting, visual clarity, shading , Lumen Reflections, Super-Resolution, gigapixel, color grading, retouch, enhanced, PBR, Blender, V-ray, Procreate, zBrush, Unreal Engine 5, cinematic, volumetric, dramatic, neon lighting, wide angle lens ,no digital painting blur",
|
265 |
+
"The parametric hotel lobby is a sleek and modern space with plenty of natural light. The lobby is spacious and open with a variety of seating options. The front desk is a sleek white counter with a parametric design. The walls are a light blue color with parametric patterns. The floor is a light wood color with a parametric design. There are plenty of plants and flowers throughout the space. The overall effect is a calm and relaxing space. occlusion, moody, sunset, concept art, octane rendering, 8k, highly detailed, concept art, highly detailed, beautiful scenery, cinematic, beautiful light, hyperreal, octane render, hdr, long exposure, 8K, realistic, fog, moody, fire and explosions, smoke, 50mm f2.8",
|
266 |
+
]
|
267 |
+
|
268 |
+
with gr.Blocks(css="scripts/style.css") as demo:
|
269 |
+
gr.Markdown(DESCRIPTION)
|
270 |
+
gr.DuplicateButton(
|
271 |
+
value="Duplicate Space for private use",
|
272 |
+
elem_id="duplicate-button",
|
273 |
+
visible=os.getenv("SHOW_DUPLICATE_BUTTON") == "1",
|
274 |
+
)
|
275 |
+
with gr.Row(equal_height=False):
|
276 |
+
with gr.Group():
|
277 |
+
with gr.Row():
|
278 |
+
prompt = gr.Text(
|
279 |
+
label="Prompt",
|
280 |
+
show_label=False,
|
281 |
+
max_lines=1,
|
282 |
+
placeholder="Enter your prompt",
|
283 |
+
container=False,
|
284 |
+
)
|
285 |
+
run_button = gr.Button("Run", scale=0)
|
286 |
+
result = gr.Gallery(label="Result", show_label=False)
|
287 |
+
# with gr.Accordion("Advanced options", open=False):
|
288 |
+
with gr.Group():
|
289 |
+
with gr.Row():
|
290 |
+
use_negative_prompt = gr.Checkbox(label="Use negative prompt", value=False, visible=True)
|
291 |
+
with gr.Row(visible=True):
|
292 |
+
schedule = gr.Radio(
|
293 |
+
show_label=True,
|
294 |
+
container=True,
|
295 |
+
interactive=True,
|
296 |
+
choices=SCHEDULE_NAME,
|
297 |
+
value=DEFAULT_SCHEDULE_NAME,
|
298 |
+
label="Sampler Schedule",
|
299 |
+
visible=True,
|
300 |
+
)
|
301 |
+
num_imgs = gr.Slider(
|
302 |
+
label="Num Images",
|
303 |
+
minimum=1,
|
304 |
+
maximum=8,
|
305 |
+
step=1,
|
306 |
+
value=1,
|
307 |
+
)
|
308 |
+
style_selection = gr.Radio(
|
309 |
+
show_label=True,
|
310 |
+
container=True,
|
311 |
+
interactive=True,
|
312 |
+
choices=STYLE_NAMES,
|
313 |
+
value=DEFAULT_STYLE_NAME,
|
314 |
+
label="Image Style",
|
315 |
+
)
|
316 |
+
negative_prompt = gr.Text(
|
317 |
+
label="Negative prompt",
|
318 |
+
max_lines=1,
|
319 |
+
placeholder="Enter a negative prompt",
|
320 |
+
visible=True,
|
321 |
+
)
|
322 |
+
seed = gr.Slider(
|
323 |
+
label="Seed",
|
324 |
+
minimum=0,
|
325 |
+
maximum=MAX_SEED,
|
326 |
+
step=1,
|
327 |
+
value=0,
|
328 |
+
)
|
329 |
+
randomize_seed = gr.Checkbox(label="Randomize seed", value=True)
|
330 |
+
with gr.Row(visible=True):
|
331 |
+
width = gr.Slider(
|
332 |
+
label="Width",
|
333 |
+
minimum=256,
|
334 |
+
maximum=MAX_IMAGE_SIZE,
|
335 |
+
step=32,
|
336 |
+
value=1024,
|
337 |
+
)
|
338 |
+
height = gr.Slider(
|
339 |
+
label="Height",
|
340 |
+
minimum=256,
|
341 |
+
maximum=MAX_IMAGE_SIZE,
|
342 |
+
step=32,
|
343 |
+
value=1024,
|
344 |
+
)
|
345 |
+
with gr.Row():
|
346 |
+
dpms_guidance_scale = gr.Slider(
|
347 |
+
label="DPM-Solver Guidance scale",
|
348 |
+
minimum=1,
|
349 |
+
maximum=10,
|
350 |
+
step=0.1,
|
351 |
+
value=4.5,
|
352 |
+
)
|
353 |
+
dpms_inference_steps = gr.Slider(
|
354 |
+
label="DPM-Solver inference steps",
|
355 |
+
minimum=5,
|
356 |
+
maximum=40,
|
357 |
+
step=1,
|
358 |
+
value=14,
|
359 |
+
)
|
360 |
+
with gr.Row():
|
361 |
+
sas_guidance_scale = gr.Slider(
|
362 |
+
label="SA-Solver Guidance scale",
|
363 |
+
minimum=1,
|
364 |
+
maximum=10,
|
365 |
+
step=0.1,
|
366 |
+
value=3,
|
367 |
+
)
|
368 |
+
sas_inference_steps = gr.Slider(
|
369 |
+
label="SA-Solver inference steps",
|
370 |
+
minimum=10,
|
371 |
+
maximum=40,
|
372 |
+
step=1,
|
373 |
+
value=25,
|
374 |
+
)
|
375 |
+
|
376 |
+
gr.Examples(
|
377 |
+
examples=examples,
|
378 |
+
inputs=prompt,
|
379 |
+
outputs=[result, seed],
|
380 |
+
fn=generate,
|
381 |
+
cache_examples=CACHE_EXAMPLES,
|
382 |
+
)
|
383 |
+
|
384 |
+
use_negative_prompt.change(
|
385 |
+
fn=lambda x: gr.update(visible=x),
|
386 |
+
inputs=use_negative_prompt,
|
387 |
+
outputs=negative_prompt,
|
388 |
+
api_name=False,
|
389 |
+
)
|
390 |
+
|
391 |
+
gr.on(
|
392 |
+
triggers=[
|
393 |
+
prompt.submit,
|
394 |
+
negative_prompt.submit,
|
395 |
+
run_button.click,
|
396 |
+
],
|
397 |
+
fn=generate,
|
398 |
+
inputs=[
|
399 |
+
prompt,
|
400 |
+
negative_prompt,
|
401 |
+
style_selection,
|
402 |
+
use_negative_prompt,
|
403 |
+
num_imgs,
|
404 |
+
seed,
|
405 |
+
width,
|
406 |
+
height,
|
407 |
+
schedule,
|
408 |
+
dpms_guidance_scale,
|
409 |
+
sas_guidance_scale,
|
410 |
+
dpms_inference_steps,
|
411 |
+
sas_inference_steps,
|
412 |
+
randomize_seed,
|
413 |
+
],
|
414 |
+
outputs=[result, seed],
|
415 |
+
api_name="run",
|
416 |
+
)
|
417 |
+
|
418 |
+
if __name__ == "__main__":
|
419 |
+
demo.queue(max_size=20).launch(server_name="0.0.0.0", server_port=PORT, debug=True)
|
diffusers_patches.py
ADDED
@@ -0,0 +1,541 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import torch
|
2 |
+
from diffusers import ImagePipelineOutput, PixArtAlphaPipeline, AutoencoderKL, Transformer2DModel, \
|
3 |
+
DPMSolverMultistepScheduler
|
4 |
+
from diffusers.image_processor import VaeImageProcessor
|
5 |
+
from diffusers.models.attention import BasicTransformerBlock
|
6 |
+
from diffusers.models.embeddings import PixArtAlphaTextProjection, PatchEmbed
|
7 |
+
from diffusers.models.normalization import AdaLayerNormSingle
|
8 |
+
from diffusers.pipelines.pixart_alpha.pipeline_pixart_alpha import retrieve_timesteps
|
9 |
+
from typing import Callable, List, Optional, Tuple, Union
|
10 |
+
|
11 |
+
from diffusers.utils import deprecate
|
12 |
+
from torch import nn
|
13 |
+
from transformers import T5Tokenizer, T5EncoderModel
|
14 |
+
|
15 |
+
ASPECT_RATIO_2048_BIN = {
|
16 |
+
"0.25": [1024.0, 4096.0],
|
17 |
+
"0.26": [1024.0, 3968.0],
|
18 |
+
"0.27": [1024.0, 3840.0],
|
19 |
+
"0.28": [1024.0, 3712.0],
|
20 |
+
"0.32": [1152.0, 3584.0],
|
21 |
+
"0.33": [1152.0, 3456.0],
|
22 |
+
"0.35": [1152.0, 3328.0],
|
23 |
+
"0.4": [1280.0, 3200.0],
|
24 |
+
"0.42": [1280.0, 3072.0],
|
25 |
+
"0.48": [1408.0, 2944.0],
|
26 |
+
"0.5": [1408.0, 2816.0],
|
27 |
+
"0.52": [1408.0, 2688.0],
|
28 |
+
"0.57": [1536.0, 2688.0],
|
29 |
+
"0.6": [1536.0, 2560.0],
|
30 |
+
"0.68": [1664.0, 2432.0],
|
31 |
+
"0.72": [1664.0, 2304.0],
|
32 |
+
"0.78": [1792.0, 2304.0],
|
33 |
+
"0.82": [1792.0, 2176.0],
|
34 |
+
"0.88": [1920.0, 2176.0],
|
35 |
+
"0.94": [1920.0, 2048.0],
|
36 |
+
"1.0": [2048.0, 2048.0],
|
37 |
+
"1.07": [2048.0, 1920.0],
|
38 |
+
"1.13": [2176.0, 1920.0],
|
39 |
+
"1.21": [2176.0, 1792.0],
|
40 |
+
"1.29": [2304.0, 1792.0],
|
41 |
+
"1.38": [2304.0, 1664.0],
|
42 |
+
"1.46": [2432.0, 1664.0],
|
43 |
+
"1.67": [2560.0, 1536.0],
|
44 |
+
"1.75": [2688.0, 1536.0],
|
45 |
+
"2.0": [2816.0, 1408.0],
|
46 |
+
"2.09": [2944.0, 1408.0],
|
47 |
+
"2.4": [3072.0, 1280.0],
|
48 |
+
"2.5": [3200.0, 1280.0],
|
49 |
+
"2.89": [3328.0, 1152.0],
|
50 |
+
"3.0": [3456.0, 1152.0],
|
51 |
+
"3.11": [3584.0, 1152.0],
|
52 |
+
"3.62": [3712.0, 1024.0],
|
53 |
+
"3.75": [3840.0, 1024.0],
|
54 |
+
"3.88": [3968.0, 1024.0],
|
55 |
+
"4.0": [4096.0, 1024.0]
|
56 |
+
}
|
57 |
+
|
58 |
+
ASPECT_RATIO_256_BIN = {
|
59 |
+
"0.25": [128.0, 512.0],
|
60 |
+
"0.28": [128.0, 464.0],
|
61 |
+
"0.32": [144.0, 448.0],
|
62 |
+
"0.33": [144.0, 432.0],
|
63 |
+
"0.35": [144.0, 416.0],
|
64 |
+
"0.4": [160.0, 400.0],
|
65 |
+
"0.42": [160.0, 384.0],
|
66 |
+
"0.48": [176.0, 368.0],
|
67 |
+
"0.5": [176.0, 352.0],
|
68 |
+
"0.52": [176.0, 336.0],
|
69 |
+
"0.57": [192.0, 336.0],
|
70 |
+
"0.6": [192.0, 320.0],
|
71 |
+
"0.68": [208.0, 304.0],
|
72 |
+
"0.72": [208.0, 288.0],
|
73 |
+
"0.78": [224.0, 288.0],
|
74 |
+
"0.82": [224.0, 272.0],
|
75 |
+
"0.88": [240.0, 272.0],
|
76 |
+
"0.94": [240.0, 256.0],
|
77 |
+
"1.0": [256.0, 256.0],
|
78 |
+
"1.07": [256.0, 240.0],
|
79 |
+
"1.13": [272.0, 240.0],
|
80 |
+
"1.21": [272.0, 224.0],
|
81 |
+
"1.29": [288.0, 224.0],
|
82 |
+
"1.38": [288.0, 208.0],
|
83 |
+
"1.46": [304.0, 208.0],
|
84 |
+
"1.67": [320.0, 192.0],
|
85 |
+
"1.75": [336.0, 192.0],
|
86 |
+
"2.0": [352.0, 176.0],
|
87 |
+
"2.09": [368.0, 176.0],
|
88 |
+
"2.4": [384.0, 160.0],
|
89 |
+
"2.5": [400.0, 160.0],
|
90 |
+
"3.0": [432.0, 144.0],
|
91 |
+
"4.0": [512.0, 128.0]
|
92 |
+
}
|
93 |
+
|
94 |
+
ASPECT_RATIO_1024_BIN = {
|
95 |
+
"0.25": [512.0, 2048.0],
|
96 |
+
"0.28": [512.0, 1856.0],
|
97 |
+
"0.32": [576.0, 1792.0],
|
98 |
+
"0.33": [576.0, 1728.0],
|
99 |
+
"0.35": [576.0, 1664.0],
|
100 |
+
"0.4": [640.0, 1600.0],
|
101 |
+
"0.42": [640.0, 1536.0],
|
102 |
+
"0.48": [704.0, 1472.0],
|
103 |
+
"0.5": [704.0, 1408.0],
|
104 |
+
"0.52": [704.0, 1344.0],
|
105 |
+
"0.57": [768.0, 1344.0],
|
106 |
+
"0.6": [768.0, 1280.0],
|
107 |
+
"0.68": [832.0, 1216.0],
|
108 |
+
"0.72": [832.0, 1152.0],
|
109 |
+
"0.78": [896.0, 1152.0],
|
110 |
+
"0.82": [896.0, 1088.0],
|
111 |
+
"0.88": [960.0, 1088.0],
|
112 |
+
"0.94": [960.0, 1024.0],
|
113 |
+
"1.0": [1024.0, 1024.0],
|
114 |
+
"1.07": [1024.0, 960.0],
|
115 |
+
"1.13": [1088.0, 960.0],
|
116 |
+
"1.21": [1088.0, 896.0],
|
117 |
+
"1.29": [1152.0, 896.0],
|
118 |
+
"1.38": [1152.0, 832.0],
|
119 |
+
"1.46": [1216.0, 832.0],
|
120 |
+
"1.67": [1280.0, 768.0],
|
121 |
+
"1.75": [1344.0, 768.0],
|
122 |
+
"2.0": [1408.0, 704.0],
|
123 |
+
"2.09": [1472.0, 704.0],
|
124 |
+
"2.4": [1536.0, 640.0],
|
125 |
+
"2.5": [1600.0, 640.0],
|
126 |
+
"3.0": [1728.0, 576.0],
|
127 |
+
"4.0": [2048.0, 512.0],
|
128 |
+
}
|
129 |
+
|
130 |
+
ASPECT_RATIO_512_BIN = {
|
131 |
+
"0.25": [256.0, 1024.0],
|
132 |
+
"0.28": [256.0, 928.0],
|
133 |
+
"0.32": [288.0, 896.0],
|
134 |
+
"0.33": [288.0, 864.0],
|
135 |
+
"0.35": [288.0, 832.0],
|
136 |
+
"0.4": [320.0, 800.0],
|
137 |
+
"0.42": [320.0, 768.0],
|
138 |
+
"0.48": [352.0, 736.0],
|
139 |
+
"0.5": [352.0, 704.0],
|
140 |
+
"0.52": [352.0, 672.0],
|
141 |
+
"0.57": [384.0, 672.0],
|
142 |
+
"0.6": [384.0, 640.0],
|
143 |
+
"0.68": [416.0, 608.0],
|
144 |
+
"0.72": [416.0, 576.0],
|
145 |
+
"0.78": [448.0, 576.0],
|
146 |
+
"0.82": [448.0, 544.0],
|
147 |
+
"0.88": [480.0, 544.0],
|
148 |
+
"0.94": [480.0, 512.0],
|
149 |
+
"1.0": [512.0, 512.0],
|
150 |
+
"1.07": [512.0, 480.0],
|
151 |
+
"1.13": [544.0, 480.0],
|
152 |
+
"1.21": [544.0, 448.0],
|
153 |
+
"1.29": [576.0, 448.0],
|
154 |
+
"1.38": [576.0, 416.0],
|
155 |
+
"1.46": [608.0, 416.0],
|
156 |
+
"1.67": [640.0, 384.0],
|
157 |
+
"1.75": [672.0, 384.0],
|
158 |
+
"2.0": [704.0, 352.0],
|
159 |
+
"2.09": [736.0, 352.0],
|
160 |
+
"2.4": [768.0, 320.0],
|
161 |
+
"2.5": [800.0, 320.0],
|
162 |
+
"3.0": [864.0, 288.0],
|
163 |
+
"4.0": [1024.0, 256.0],
|
164 |
+
}
|
165 |
+
|
166 |
+
|
167 |
+
def pipeline_pixart_alpha_call(
|
168 |
+
self,
|
169 |
+
prompt: Union[str, List[str]] = None,
|
170 |
+
negative_prompt: str = "",
|
171 |
+
num_inference_steps: int = 20,
|
172 |
+
timesteps: List[int] = None,
|
173 |
+
guidance_scale: float = 4.5,
|
174 |
+
num_images_per_prompt: Optional[int] = 1,
|
175 |
+
height: Optional[int] = None,
|
176 |
+
width: Optional[int] = None,
|
177 |
+
eta: float = 0.0,
|
178 |
+
generator: Optional[Union[torch.Generator, List[torch.Generator]]] = None,
|
179 |
+
latents: Optional[torch.FloatTensor] = None,
|
180 |
+
prompt_embeds: Optional[torch.FloatTensor] = None,
|
181 |
+
prompt_attention_mask: Optional[torch.FloatTensor] = None,
|
182 |
+
negative_prompt_embeds: Optional[torch.FloatTensor] = None,
|
183 |
+
negative_prompt_attention_mask: Optional[torch.FloatTensor] = None,
|
184 |
+
output_type: Optional[str] = "pil",
|
185 |
+
return_dict: bool = True,
|
186 |
+
callback: Optional[Callable[[int, int, torch.FloatTensor], None]] = None,
|
187 |
+
callback_steps: int = 1,
|
188 |
+
clean_caption: bool = True,
|
189 |
+
use_resolution_binning: bool = True,
|
190 |
+
max_sequence_length: int = 120,
|
191 |
+
**kwargs,
|
192 |
+
) -> Union[ImagePipelineOutput, Tuple]:
|
193 |
+
"""
|
194 |
+
Function invoked when calling the pipeline for generation.
|
195 |
+
|
196 |
+
Args:
|
197 |
+
prompt (`str` or `List[str]`, *optional*):
|
198 |
+
The prompt or prompts to guide the image generation. If not defined, one has to pass `prompt_embeds`.
|
199 |
+
instead.
|
200 |
+
negative_prompt (`str` or `List[str]`, *optional*):
|
201 |
+
The prompt or prompts not to guide the image generation. If not defined, one has to pass
|
202 |
+
`negative_prompt_embeds` instead. Ignored when not using guidance (i.e., ignored if `guidance_scale` is
|
203 |
+
less than `1`).
|
204 |
+
num_inference_steps (`int`, *optional*, defaults to 100):
|
205 |
+
The number of denoising steps. More denoising steps usually lead to a higher quality image at the
|
206 |
+
expense of slower inference.
|
207 |
+
timesteps (`List[int]`, *optional*):
|
208 |
+
Custom timesteps to use for the denoising process. If not defined, equal spaced `num_inference_steps`
|
209 |
+
timesteps are used. Must be in descending order.
|
210 |
+
guidance_scale (`float`, *optional*, defaults to 4.5):
|
211 |
+
Guidance scale as defined in [Classifier-Free Diffusion Guidance](https://arxiv.org/abs/2207.12598).
|
212 |
+
`guidance_scale` is defined as `w` of equation 2. of [Imagen
|
213 |
+
Paper](https://arxiv.org/pdf/2205.11487.pdf). Guidance scale is enabled by setting `guidance_scale >
|
214 |
+
1`. Higher guidance scale encourages to generate images that are closely linked to the text `prompt`,
|
215 |
+
usually at the expense of lower image quality.
|
216 |
+
num_images_per_prompt (`int`, *optional*, defaults to 1):
|
217 |
+
The number of images to generate per prompt.
|
218 |
+
height (`int`, *optional*, defaults to self.unet.config.sample_size):
|
219 |
+
The height in pixels of the generated image.
|
220 |
+
width (`int`, *optional*, defaults to self.unet.config.sample_size):
|
221 |
+
The width in pixels of the generated image.
|
222 |
+
eta (`float`, *optional*, defaults to 0.0):
|
223 |
+
Corresponds to parameter eta (η) in the DDIM paper: https://arxiv.org/abs/2010.02502. Only applies to
|
224 |
+
[`schedulers.DDIMScheduler`], will be ignored for others.
|
225 |
+
generator (`torch.Generator` or `List[torch.Generator]`, *optional*):
|
226 |
+
One or a list of [torch generator(s)](https://pytorch.org/docs/stable/generated/torch.Generator.html)
|
227 |
+
to make generation deterministic.
|
228 |
+
latents (`torch.FloatTensor`, *optional*):
|
229 |
+
Pre-generated noisy latents, sampled from a Gaussian distribution, to be used as inputs for image
|
230 |
+
generation. Can be used to tweak the same generation with different prompts. If not provided, a latents
|
231 |
+
tensor will ge generated by sampling using the supplied random `generator`.
|
232 |
+
prompt_embeds (`torch.FloatTensor`, *optional*):
|
233 |
+
Pre-generated text embeddings. Can be used to easily tweak text inputs, *e.g.* prompt weighting. If not
|
234 |
+
provided, text embeddings will be generated from `prompt` input argument.
|
235 |
+
prompt_attention_mask (`torch.FloatTensor`, *optional*): Pre-generated attention mask for text embeddings.
|
236 |
+
negative_prompt_embeds (`torch.FloatTensor`, *optional*):
|
237 |
+
Pre-generated negative text embeddings. For PixArt-Alpha this negative prompt should be "". If not
|
238 |
+
provided, negative_prompt_embeds will be generated from `negative_prompt` input argument.
|
239 |
+
negative_prompt_attention_mask (`torch.FloatTensor`, *optional*):
|
240 |
+
Pre-generated attention mask for negative text embeddings.
|
241 |
+
output_type (`str`, *optional*, defaults to `"pil"`):
|
242 |
+
The output format of the generate image. Choose between
|
243 |
+
[PIL](https://pillow.readthedocs.io/en/stable/): `PIL.Image.Image` or `np.array`.
|
244 |
+
return_dict (`bool`, *optional*, defaults to `True`):
|
245 |
+
Whether or not to return a [`~pipelines.stable_diffusion.IFPipelineOutput`] instead of a plain tuple.
|
246 |
+
callback (`Callable`, *optional*):
|
247 |
+
A function that will be called every `callback_steps` steps during inference. The function will be
|
248 |
+
called with the following arguments: `callback(step: int, timestep: int, latents: torch.FloatTensor)`.
|
249 |
+
callback_steps (`int`, *optional*, defaults to 1):
|
250 |
+
The frequency at which the `callback` function will be called. If not specified, the callback will be
|
251 |
+
called at every step.
|
252 |
+
clean_caption (`bool`, *optional*, defaults to `True`):
|
253 |
+
Whether or not to clean the caption before creating embeddings. Requires `beautifulsoup4` and `ftfy` to
|
254 |
+
be installed. If the dependencies are not installed, the embeddings will be created from the raw
|
255 |
+
prompt.
|
256 |
+
use_resolution_binning (`bool` defaults to `True`):
|
257 |
+
If set to `True`, the requested height and width are first mapped to the closest resolutions using
|
258 |
+
`ASPECT_RATIO_1024_BIN`. After the produced latents are decoded into images, they are resized back to
|
259 |
+
the requested resolution. Useful for generating non-square images.
|
260 |
+
|
261 |
+
Examples:
|
262 |
+
|
263 |
+
Returns:
|
264 |
+
[`~pipelines.ImagePipelineOutput`] or `tuple`:
|
265 |
+
If `return_dict` is `True`, [`~pipelines.ImagePipelineOutput`] is returned, otherwise a `tuple` is
|
266 |
+
returned where the first element is a list with the generated images
|
267 |
+
"""
|
268 |
+
if "mask_feature" in kwargs:
|
269 |
+
deprecation_message = "The use of `mask_feature` is deprecated. It is no longer used in any computation and that doesn't affect the end results. It will be removed in a future version."
|
270 |
+
deprecate("mask_feature", "1.0.0", deprecation_message, standard_warn=False)
|
271 |
+
# 1. Check inputs. Raise error if not correct
|
272 |
+
height = height or self.transformer.config.sample_size * self.vae_scale_factor
|
273 |
+
width = width or self.transformer.config.sample_size * self.vae_scale_factor
|
274 |
+
if use_resolution_binning:
|
275 |
+
if self.transformer.config.sample_size == 32:
|
276 |
+
aspect_ratio_bin = ASPECT_RATIO_256_BIN
|
277 |
+
elif self.transformer.config.sample_size == 64:
|
278 |
+
aspect_ratio_bin = ASPECT_RATIO_512_BIN
|
279 |
+
elif self.transformer.config.sample_size == 128:
|
280 |
+
aspect_ratio_bin = ASPECT_RATIO_1024_BIN
|
281 |
+
elif self.transformer.config.sample_size == 256:
|
282 |
+
aspect_ratio_bin = ASPECT_RATIO_2048_BIN
|
283 |
+
else:
|
284 |
+
raise ValueError("Invalid sample size")
|
285 |
+
orig_height, orig_width = height, width
|
286 |
+
height, width = self.classify_height_width_bin(height, width, ratios=aspect_ratio_bin)
|
287 |
+
|
288 |
+
self.check_inputs(
|
289 |
+
prompt,
|
290 |
+
height,
|
291 |
+
width,
|
292 |
+
negative_prompt,
|
293 |
+
callback_steps,
|
294 |
+
prompt_embeds,
|
295 |
+
negative_prompt_embeds,
|
296 |
+
prompt_attention_mask,
|
297 |
+
negative_prompt_attention_mask,
|
298 |
+
)
|
299 |
+
|
300 |
+
# 2. Default height and width to transformer
|
301 |
+
if prompt is not None and isinstance(prompt, str):
|
302 |
+
batch_size = 1
|
303 |
+
elif prompt is not None and isinstance(prompt, list):
|
304 |
+
batch_size = len(prompt)
|
305 |
+
else:
|
306 |
+
batch_size = prompt_embeds.shape[0]
|
307 |
+
|
308 |
+
device = self._execution_device
|
309 |
+
|
310 |
+
# here `guidance_scale` is defined analog to the guidance weight `w` of equation (2)
|
311 |
+
# of the Imagen paper: https://arxiv.org/pdf/2205.11487.pdf . `guidance_scale = 1`
|
312 |
+
# corresponds to doing no classifier free guidance.
|
313 |
+
do_classifier_free_guidance = guidance_scale > 1.0
|
314 |
+
|
315 |
+
# 3. Encode input prompt
|
316 |
+
(
|
317 |
+
prompt_embeds,
|
318 |
+
prompt_attention_mask,
|
319 |
+
negative_prompt_embeds,
|
320 |
+
negative_prompt_attention_mask,
|
321 |
+
) = self.encode_prompt(
|
322 |
+
prompt,
|
323 |
+
do_classifier_free_guidance,
|
324 |
+
negative_prompt=negative_prompt,
|
325 |
+
num_images_per_prompt=num_images_per_prompt,
|
326 |
+
device=device,
|
327 |
+
prompt_embeds=prompt_embeds,
|
328 |
+
negative_prompt_embeds=negative_prompt_embeds,
|
329 |
+
prompt_attention_mask=prompt_attention_mask,
|
330 |
+
negative_prompt_attention_mask=negative_prompt_attention_mask,
|
331 |
+
clean_caption=clean_caption,
|
332 |
+
max_sequence_length=max_sequence_length,
|
333 |
+
)
|
334 |
+
if do_classifier_free_guidance:
|
335 |
+
prompt_embeds = torch.cat([negative_prompt_embeds, prompt_embeds], dim=0)
|
336 |
+
prompt_attention_mask = torch.cat([negative_prompt_attention_mask, prompt_attention_mask], dim=0)
|
337 |
+
|
338 |
+
# 4. Prepare timesteps
|
339 |
+
timesteps, num_inference_steps = retrieve_timesteps(self.scheduler, num_inference_steps, device, timesteps)
|
340 |
+
|
341 |
+
# 5. Prepare latents.
|
342 |
+
latent_channels = self.transformer.config.in_channels
|
343 |
+
latents = self.prepare_latents(
|
344 |
+
batch_size * num_images_per_prompt,
|
345 |
+
latent_channels,
|
346 |
+
height,
|
347 |
+
width,
|
348 |
+
prompt_embeds.dtype,
|
349 |
+
device,
|
350 |
+
generator,
|
351 |
+
latents,
|
352 |
+
)
|
353 |
+
|
354 |
+
# 6. Prepare extra step kwargs. TODO: Logic should ideally just be moved out of the pipeline
|
355 |
+
extra_step_kwargs = self.prepare_extra_step_kwargs(generator, eta)
|
356 |
+
|
357 |
+
# 6.1 Prepare micro-conditions.
|
358 |
+
added_cond_kwargs = {"resolution": None, "aspect_ratio": None}
|
359 |
+
if self.transformer.config.sample_size == 128:
|
360 |
+
resolution = torch.tensor([height, width]).repeat(batch_size * num_images_per_prompt, 1)
|
361 |
+
aspect_ratio = torch.tensor([float(height / width)]).repeat(batch_size * num_images_per_prompt, 1)
|
362 |
+
resolution = resolution.to(dtype=prompt_embeds.dtype, device=device)
|
363 |
+
aspect_ratio = aspect_ratio.to(dtype=prompt_embeds.dtype, device=device)
|
364 |
+
|
365 |
+
if do_classifier_free_guidance:
|
366 |
+
resolution = torch.cat([resolution, resolution], dim=0)
|
367 |
+
aspect_ratio = torch.cat([aspect_ratio, aspect_ratio], dim=0)
|
368 |
+
|
369 |
+
added_cond_kwargs = {"resolution": resolution, "aspect_ratio": aspect_ratio}
|
370 |
+
|
371 |
+
# 7. Denoising loop
|
372 |
+
num_warmup_steps = max(len(timesteps) - num_inference_steps * self.scheduler.order, 0)
|
373 |
+
|
374 |
+
with self.progress_bar(total=num_inference_steps) as progress_bar:
|
375 |
+
for i, t in enumerate(timesteps):
|
376 |
+
latent_model_input = torch.cat([latents] * 2) if do_classifier_free_guidance else latents
|
377 |
+
latent_model_input = self.scheduler.scale_model_input(latent_model_input, t)
|
378 |
+
|
379 |
+
current_timestep = t
|
380 |
+
if not torch.is_tensor(current_timestep):
|
381 |
+
# TODO: this requires sync between CPU and GPU. So try to pass timesteps as tensors if you can
|
382 |
+
# This would be a good case for the `match` statement (Python 3.10+)
|
383 |
+
is_mps = latent_model_input.device.type == "mps"
|
384 |
+
if isinstance(current_timestep, float):
|
385 |
+
dtype = torch.float32 if is_mps else torch.float64
|
386 |
+
else:
|
387 |
+
dtype = torch.int32 if is_mps else torch.int64
|
388 |
+
current_timestep = torch.tensor([current_timestep], dtype=dtype, device=latent_model_input.device)
|
389 |
+
elif len(current_timestep.shape) == 0:
|
390 |
+
current_timestep = current_timestep[None].to(latent_model_input.device)
|
391 |
+
# broadcast to batch dimension in a way that's compatible with ONNX/Core ML
|
392 |
+
current_timestep = current_timestep.expand(latent_model_input.shape[0])
|
393 |
+
|
394 |
+
# predict noise model_output
|
395 |
+
noise_pred = self.transformer(
|
396 |
+
latent_model_input,
|
397 |
+
encoder_hidden_states=prompt_embeds,
|
398 |
+
encoder_attention_mask=prompt_attention_mask,
|
399 |
+
timestep=current_timestep,
|
400 |
+
added_cond_kwargs=added_cond_kwargs,
|
401 |
+
return_dict=False,
|
402 |
+
)[0]
|
403 |
+
|
404 |
+
# perform guidance
|
405 |
+
if do_classifier_free_guidance:
|
406 |
+
noise_pred_uncond, noise_pred_text = noise_pred.chunk(2)
|
407 |
+
noise_pred = noise_pred_uncond + guidance_scale * (noise_pred_text - noise_pred_uncond)
|
408 |
+
|
409 |
+
# learned sigma
|
410 |
+
if self.transformer.config.out_channels // 2 == latent_channels:
|
411 |
+
noise_pred = noise_pred.chunk(2, dim=1)[0]
|
412 |
+
else:
|
413 |
+
noise_pred = noise_pred
|
414 |
+
|
415 |
+
# compute previous image: x_t -> x_t-1
|
416 |
+
if num_inference_steps == 1:
|
417 |
+
latents = self.scheduler.step(noise_pred, t, latents, **extra_step_kwargs).pred_original_sample
|
418 |
+
else:
|
419 |
+
latents = self.scheduler.step(noise_pred, t, latents, **extra_step_kwargs, return_dict=False)[0]
|
420 |
+
|
421 |
+
# call the callback, if provided
|
422 |
+
if i == len(timesteps) - 1 or ((i + 1) > num_warmup_steps and (i + 1) % self.scheduler.order == 0):
|
423 |
+
progress_bar.update()
|
424 |
+
if callback is not None and i % callback_steps == 0:
|
425 |
+
step_idx = i // getattr(self.scheduler, "order", 1)
|
426 |
+
callback(step_idx, t, latents)
|
427 |
+
|
428 |
+
if not output_type == "latent":
|
429 |
+
image = self.vae.decode(latents / self.vae.config.scaling_factor, return_dict=False)[0]
|
430 |
+
if use_resolution_binning:
|
431 |
+
image = self.resize_and_crop_tensor(image, orig_width, orig_height)
|
432 |
+
else:
|
433 |
+
image = latents
|
434 |
+
|
435 |
+
if not output_type == "latent":
|
436 |
+
image = self.image_processor.postprocess(image, output_type=output_type)
|
437 |
+
|
438 |
+
# Offload all models
|
439 |
+
self.maybe_free_model_hooks()
|
440 |
+
|
441 |
+
if not return_dict:
|
442 |
+
return (image,)
|
443 |
+
|
444 |
+
return ImagePipelineOutput(images=image)
|
445 |
+
|
446 |
+
|
447 |
+
class PixArtSigmaPipeline(PixArtAlphaPipeline):
|
448 |
+
r"""
|
449 |
+
tmp Pipeline for text-to-image generation using PixArt-Sigma.
|
450 |
+
"""
|
451 |
+
|
452 |
+
def __init__(
|
453 |
+
self,
|
454 |
+
tokenizer: T5Tokenizer,
|
455 |
+
text_encoder: T5EncoderModel,
|
456 |
+
vae: AutoencoderKL,
|
457 |
+
transformer: Transformer2DModel,
|
458 |
+
scheduler: DPMSolverMultistepScheduler,
|
459 |
+
):
|
460 |
+
super().__init__(tokenizer, text_encoder, vae, transformer, scheduler)
|
461 |
+
|
462 |
+
self.register_modules(
|
463 |
+
tokenizer=tokenizer, text_encoder=text_encoder, vae=vae, transformer=transformer, scheduler=scheduler
|
464 |
+
)
|
465 |
+
|
466 |
+
self.vae_scale_factor = 2 ** (len(self.vae.config.block_out_channels) - 1)
|
467 |
+
self.image_processor = VaeImageProcessor(vae_scale_factor=self.vae_scale_factor)
|
468 |
+
|
469 |
+
|
470 |
+
def pixart_sigma_init_patched_inputs(self, norm_type):
|
471 |
+
assert self.config.sample_size is not None, "Transformer2DModel over patched input must provide sample_size"
|
472 |
+
|
473 |
+
self.height = self.config.sample_size
|
474 |
+
self.width = self.config.sample_size
|
475 |
+
|
476 |
+
self.patch_size = self.config.patch_size
|
477 |
+
interpolation_scale = (
|
478 |
+
self.config.interpolation_scale
|
479 |
+
if self.config.interpolation_scale is not None
|
480 |
+
else max(self.config.sample_size // 64, 1)
|
481 |
+
)
|
482 |
+
self.pos_embed = PatchEmbed(
|
483 |
+
height=self.config.sample_size,
|
484 |
+
width=self.config.sample_size,
|
485 |
+
patch_size=self.config.patch_size,
|
486 |
+
in_channels=self.in_channels,
|
487 |
+
embed_dim=self.inner_dim,
|
488 |
+
interpolation_scale=interpolation_scale,
|
489 |
+
)
|
490 |
+
|
491 |
+
self.transformer_blocks = nn.ModuleList(
|
492 |
+
[
|
493 |
+
BasicTransformerBlock(
|
494 |
+
self.inner_dim,
|
495 |
+
self.config.num_attention_heads,
|
496 |
+
self.config.attention_head_dim,
|
497 |
+
dropout=self.config.dropout,
|
498 |
+
cross_attention_dim=self.config.cross_attention_dim,
|
499 |
+
activation_fn=self.config.activation_fn,
|
500 |
+
num_embeds_ada_norm=self.config.num_embeds_ada_norm,
|
501 |
+
attention_bias=self.config.attention_bias,
|
502 |
+
only_cross_attention=self.config.only_cross_attention,
|
503 |
+
double_self_attention=self.config.double_self_attention,
|
504 |
+
upcast_attention=self.config.upcast_attention,
|
505 |
+
norm_type=norm_type,
|
506 |
+
norm_elementwise_affine=self.config.norm_elementwise_affine,
|
507 |
+
norm_eps=self.config.norm_eps,
|
508 |
+
attention_type=self.config.attention_type,
|
509 |
+
)
|
510 |
+
for _ in range(self.config.num_layers)
|
511 |
+
]
|
512 |
+
)
|
513 |
+
|
514 |
+
if self.config.norm_type != "ada_norm_single":
|
515 |
+
self.norm_out = nn.LayerNorm(self.inner_dim, elementwise_affine=False, eps=1e-6)
|
516 |
+
self.proj_out_1 = nn.Linear(self.inner_dim, 2 * self.inner_dim)
|
517 |
+
self.proj_out_2 = nn.Linear(
|
518 |
+
self.inner_dim, self.config.patch_size * self.config.patch_size * self.out_channels
|
519 |
+
)
|
520 |
+
elif self.config.norm_type == "ada_norm_single":
|
521 |
+
self.norm_out = nn.LayerNorm(self.inner_dim, elementwise_affine=False, eps=1e-6)
|
522 |
+
self.scale_shift_table = nn.Parameter(torch.randn(2, self.inner_dim) / self.inner_dim ** 0.5)
|
523 |
+
self.proj_out = nn.Linear(
|
524 |
+
self.inner_dim, self.config.patch_size * self.config.patch_size * self.out_channels
|
525 |
+
)
|
526 |
+
|
527 |
+
# PixArt-Sigma blocks.
|
528 |
+
self.adaln_single = None
|
529 |
+
self.use_additional_conditions = False
|
530 |
+
if self.config.norm_type == "ada_norm_single":
|
531 |
+
# TODO(Sayak, PVP) clean this, PixArt-Sigma doesn't use additional_conditions anymore
|
532 |
+
# additional conditions until we find better name
|
533 |
+
self.adaln_single = AdaLayerNormSingle(
|
534 |
+
self.inner_dim, use_additional_conditions=self.use_additional_conditions
|
535 |
+
)
|
536 |
+
|
537 |
+
self.caption_projection = None
|
538 |
+
if self.caption_channels is not None:
|
539 |
+
self.caption_projection = PixArtAlphaTextProjection(
|
540 |
+
in_features=self.caption_channels, hidden_size=self.inner_dim
|
541 |
+
)
|
requirements.txt
ADDED
@@ -0,0 +1,13 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
git+https://github.com/huggingface/diffusers
|
2 |
+
accelerate
|
3 |
+
transformers
|
4 |
+
gradio==4.1.1
|
5 |
+
Pillow==10.1.0
|
6 |
+
sentencepiece==0.1.99
|
7 |
+
optimum
|
8 |
+
beautifulsoup4
|
9 |
+
ftfy
|
10 |
+
--extra-index-url https://download.pytorch.org/whl/cu118
|
11 |
+
torch==2.0.1
|
12 |
+
|
13 |
+
|
style.css
ADDED
@@ -0,0 +1 @@
|
|
|
|
|
1 |
+
.gradio-container{width:680px!important}
|