added README.md
Browse files
README.md
ADDED
@@ -0,0 +1,50 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
---
|
2 |
+
license: apache-2.0
|
3 |
+
library_name: pytorch
|
4 |
+
---
|
5 |
+
|
6 |
+
# identity
|
7 |
+
|
8 |
+
A neuron that performs the IDENTITY logical computation.
|
9 |
+
|
10 |
+
It is inspired by McCulloch & Pitts' 1943 paper 'A Logical Calculus of the Ideas Immanent in Nervous Activity'.
|
11 |
+
|
12 |
+
It doesn't contain any parameters.
|
13 |
+
|
14 |
+
It takes as input a single column vector of zeros and ones. It outputs a single column vector of zeros and ones.
|
15 |
+
|
16 |
+
Its mechanism is outlined in Figure 10-3 of Aurelien Geron's book 'Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow'.
|
17 |
+
|
18 |
+
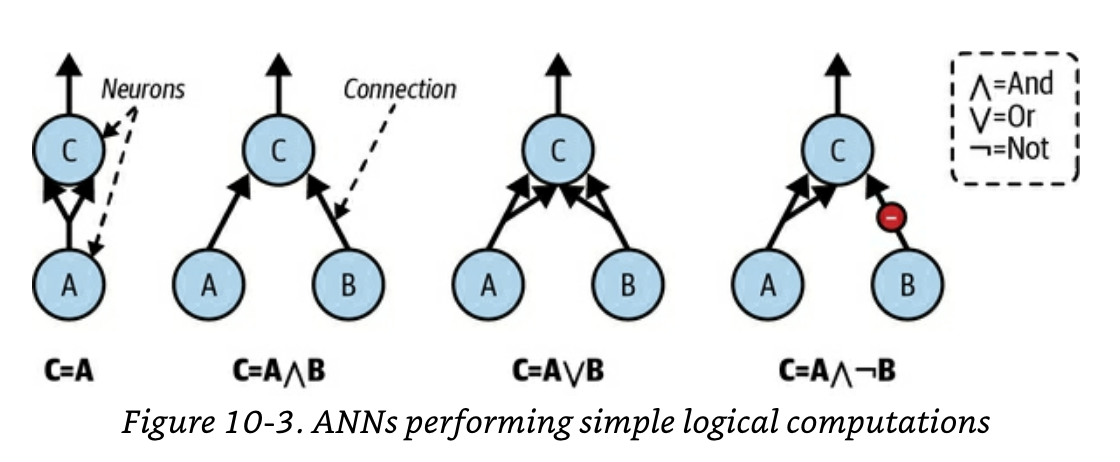
|
19 |
+
|
20 |
+
Like all the other neurons in Figure 10-3, it is activated when at least two of its input connections are active.
|
21 |
+
|
22 |
+
## Usage
|
23 |
+
|
24 |
+
```
|
25 |
+
import torch
|
26 |
+
import torch.nn as nn
|
27 |
+
from huggingface_hub import PyTorchModelHubMixin
|
28 |
+
|
29 |
+
# Let's create a column vector containing two values - `0` and `1`:
|
30 |
+
batch = torch.tensor([[0], [1]])
|
31 |
+
|
32 |
+
class IDENTITY(nn.Module, PyTorchModelHubMixin):
|
33 |
+
def __init__(self):
|
34 |
+
super().__init__()
|
35 |
+
self.operation = "C = A"
|
36 |
+
|
37 |
+
def forward(self, x):
|
38 |
+
a = x
|
39 |
+
a = torch.cat([a, a], dim=1)
|
40 |
+
column_sum = torch.sum(a, dim=1, keepdim=True)
|
41 |
+
output = (column_sum >= 2).long()
|
42 |
+
return output
|
43 |
+
|
44 |
+
# Instantiate:
|
45 |
+
identity = IDENTITY.from_pretrained("sadhaklal/identity")
|
46 |
+
|
47 |
+
# Forward pass:
|
48 |
+
output = identity(batch)
|
49 |
+
print(output)
|
50 |
+
```
|