Patrick von Platen
commited on
Commit
•
dbeae06
1
Parent(s):
95fcac5
up
Browse files- README.md +117 -0
- alphabet.json +1 -0
- config.json +95 -0
- language_model/4-gram.bin +3 -0
- language_model/attrs.json +1 -0
- language_model/unigrams.txt +0 -0
- preprocessor_config.json +10 -0
- pytorch_model.bin +3 -0
- special_tokens_map.json +1 -0
- tokenizer_config.json +1 -0
- vocab.json +1 -0
README.md
ADDED
@@ -0,0 +1,117 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
---
|
2 |
+
language: en
|
3 |
+
datasets:
|
4 |
+
- librispeech_asr
|
5 |
+
tags:
|
6 |
+
- speech
|
7 |
+
- hf-asr-leaderboard
|
8 |
+
|
9 |
+
license: apache-2.0
|
10 |
+
widget:
|
11 |
+
- example_title: Librispeech sample 1
|
12 |
+
src: https://cdn-media.huggingface.co/speech_samples/sample1.flac
|
13 |
+
- example_title: Librispeech sample 2
|
14 |
+
src: https://cdn-media.huggingface.co/speech_samples/sample2.flac
|
15 |
+
model-index:
|
16 |
+
- name: data2vec-audio-base-960h
|
17 |
+
results:
|
18 |
+
- task:
|
19 |
+
name: Automatic Speech Recognition
|
20 |
+
type: automatic-speech-recognition
|
21 |
+
dataset:
|
22 |
+
name: Librispeech (clean)
|
23 |
+
type: librispeech_asr
|
24 |
+
args: en
|
25 |
+
metrics:
|
26 |
+
- name: Test WER
|
27 |
+
type: wer
|
28 |
+
value: 2.8
|
29 |
+
---
|
30 |
+
|
31 |
+
# Data2Vec-Audio-Base-960h
|
32 |
+
|
33 |
+
[Facebook's Data2Vec](https://ai.facebook.com/research/data2vec-a-general-framework-for-self-supervised-learning-in-speech-vision-and-language/)
|
34 |
+
|
35 |
+
The base model pretrained and fine-tuned on 960 hours of Librispeech on 16kHz sampled speech audio. When using the model
|
36 |
+
make sure that your speech input is also sampled at 16Khz.
|
37 |
+
|
38 |
+
[Paper](https://arxiv.org/abs/2202.03555)
|
39 |
+
|
40 |
+
Authors: Alexei Baevski, Wei-Ning Hsu, Qiantong Xu, Arun Babu, Jiatao Gu, Michael Auli
|
41 |
+
|
42 |
+
**Abstract**
|
43 |
+
|
44 |
+
While the general idea of self-supervised learning is identical across modalities, the actual algorithms and objectives differ widely because they were developed with a single modality in mind. To get us closer to general self-supervised learning, we present data2vec, a framework that uses the same learning method for either speech, NLP or computer vision. The core idea is to predict latent representations of the full input data based on a masked view of the input in a self-distillation setup using a standard Transformer architecture. Instead of predicting modality-specific targets such as words, visual tokens or units of human speech which are local in nature, data2vec predicts contextualized latent representations that contain information from the entire input. Experiments on the major benchmarks of speech recognition, image classification, and natural language understanding demonstrate a new state of the art or competitive performance to predominant approaches.
|
45 |
+
|
46 |
+
The original model can be found under https://github.com/pytorch/fairseq/tree/main/examples/data2vec .
|
47 |
+
|
48 |
+
# Pre-Training method
|
49 |
+
|
50 |
+
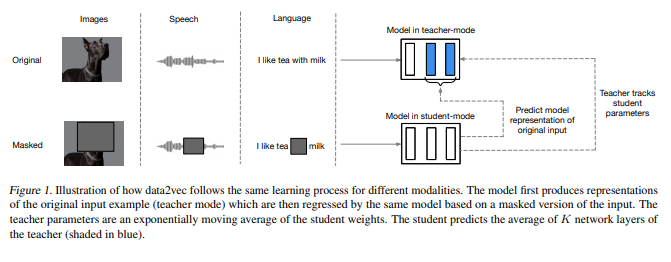
|
51 |
+
|
52 |
+
For more information, please take a look at the [official paper](https://arxiv.org/abs/2202.03555).
|
53 |
+
|
54 |
+
# Usage
|
55 |
+
|
56 |
+
To transcribe audio files the model can be used as a standalone acoustic model as follows:
|
57 |
+
|
58 |
+
```python
|
59 |
+
from transformers import Wav2Vec2Processor, Data2VecForCTC
|
60 |
+
from datasets import load_dataset
|
61 |
+
import torch
|
62 |
+
|
63 |
+
# load model and processor
|
64 |
+
processor = Wav2Vec2Processor.from_pretrained("facebook/data2vec-audio-base-960h")
|
65 |
+
model = Data2VecForCTC.from_pretrained("facebook/data2vec-audio-base-960h")
|
66 |
+
|
67 |
+
# load dummy dataset and read soundfiles
|
68 |
+
ds = load_dataset("patrickvonplaten/librispeech_asr_dummy", "clean", split="validation")
|
69 |
+
|
70 |
+
# tokenize
|
71 |
+
input_values = processor(ds[0]["audio"]["array"],, return_tensors="pt", padding="longest").input_values # Batch size 1
|
72 |
+
|
73 |
+
# retrieve logits
|
74 |
+
logits = model(input_values).logits
|
75 |
+
|
76 |
+
# take argmax and decode
|
77 |
+
predicted_ids = torch.argmax(logits, dim=-1)
|
78 |
+
transcription = processor.batch_decode(predicted_ids)
|
79 |
+
```
|
80 |
+
|
81 |
+
## Evaluation
|
82 |
+
|
83 |
+
This code snippet shows how to evaluate **facebook/data2vec-audio-base-960h** on LibriSpeech's "clean" and "other" test data.
|
84 |
+
|
85 |
+
```python
|
86 |
+
from transformers import Wav2Vec2Processor, Data2VecForCTC
|
87 |
+
from datasets import load_dataset
|
88 |
+
import torch
|
89 |
+
from jiwer import wer
|
90 |
+
|
91 |
+
# load model and processor
|
92 |
+
processor = Wav2Vec2Processor.from_pretrained("facebook/data2vec-audio-base-960h").to("cuda")
|
93 |
+
model = Data2VecForCTC.from_pretrained("facebook/data2vec-audio-base-960h")
|
94 |
+
|
95 |
+
|
96 |
+
librispeech_eval = load_dataset("librispeech_asr", "clean", split="test")
|
97 |
+
|
98 |
+
def map_to_pred(batch):
|
99 |
+
input_values = processor(batch["audio"]["array"], return_tensors="pt", padding="longest").input_values
|
100 |
+
with torch.no_grad():
|
101 |
+
logits = model(input_values.to("cuda")).logits
|
102 |
+
|
103 |
+
predicted_ids = torch.argmax(logits, dim=-1)
|
104 |
+
transcription = processor.batch_decode(predicted_ids)
|
105 |
+
batch["transcription"] = transcription
|
106 |
+
return batch
|
107 |
+
|
108 |
+
result = librispeech_eval.map(map_to_pred, batched=True, batch_size=1, remove_columns=["audio"])
|
109 |
+
|
110 |
+
print("WER:", wer(result["text"], result["transcription"]))
|
111 |
+
```
|
112 |
+
|
113 |
+
*Result (WER)*:
|
114 |
+
|
115 |
+
| "clean" | "other" |
|
116 |
+
|---|---|
|
117 |
+
| 2.77 | 7.08 |
|
alphabet.json
ADDED
@@ -0,0 +1 @@
|
|
|
1 |
+
{"labels": ["", "<s>", "</s>", "\u2047", " ", "E", "T", "A", "O", "N", "I", "H", "S", "R", "D", "L", "U", "M", "W", "C", "F", "G", "Y", "P", "B", "V", "K", "'", "X", "J", "Q", "Z"], "is_bpe": false}
|
config.json
ADDED
@@ -0,0 +1,95 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"activation_dropout": 0.1,
|
3 |
+
"adapter_kernel_size": 3,
|
4 |
+
"adapter_stride": 2,
|
5 |
+
"add_adapter": false,
|
6 |
+
"architectures": [
|
7 |
+
"Data2VecAudioForCTC"
|
8 |
+
],
|
9 |
+
"attention_dropout": 0.1,
|
10 |
+
"bos_token_id": 1,
|
11 |
+
"classifier_proj_size": 256,
|
12 |
+
"conv_bias": false,
|
13 |
+
"conv_dim": [
|
14 |
+
512,
|
15 |
+
512,
|
16 |
+
512,
|
17 |
+
512,
|
18 |
+
512,
|
19 |
+
512,
|
20 |
+
512
|
21 |
+
],
|
22 |
+
"conv_kernel": [
|
23 |
+
10,
|
24 |
+
3,
|
25 |
+
3,
|
26 |
+
3,
|
27 |
+
3,
|
28 |
+
2,
|
29 |
+
2
|
30 |
+
],
|
31 |
+
"conv_pos_kernel_size": 19,
|
32 |
+
"conv_stride": [
|
33 |
+
5,
|
34 |
+
2,
|
35 |
+
2,
|
36 |
+
2,
|
37 |
+
2,
|
38 |
+
2,
|
39 |
+
2
|
40 |
+
],
|
41 |
+
"ctc_loss_reduction": "sum",
|
42 |
+
"ctc_zero_infinity": false,
|
43 |
+
"eos_token_id": 2,
|
44 |
+
"feat_extract_activation": "gelu",
|
45 |
+
"feat_proj_dropout": 0.0,
|
46 |
+
"final_dropout": 0.1,
|
47 |
+
"hidden_act": "gelu",
|
48 |
+
"hidden_dropout": 0.1,
|
49 |
+
"hidden_size": 768,
|
50 |
+
"initializer_range": 0.02,
|
51 |
+
"intermediate_size": 3072,
|
52 |
+
"layer_norm_eps": 1e-05,
|
53 |
+
"layerdrop": 0.1,
|
54 |
+
"mask_feature_length": 10,
|
55 |
+
"mask_feature_min_masks": 0,
|
56 |
+
"mask_feature_prob": 0.0,
|
57 |
+
"mask_time_length": 10,
|
58 |
+
"mask_time_min_masks": 2,
|
59 |
+
"mask_time_prob": 0.05,
|
60 |
+
"model_type": "data2vec-audio",
|
61 |
+
"num_adapter_layers": 3,
|
62 |
+
"num_attention_heads": 12,
|
63 |
+
"num_conv_pos_embedding_groups": 16,
|
64 |
+
"num_conv_pos_embeddings": 5,
|
65 |
+
"num_feat_extract_layers": 7,
|
66 |
+
"num_hidden_layers": 12,
|
67 |
+
"output_hidden_size": 768,
|
68 |
+
"pad_token_id": 0,
|
69 |
+
"tdnn_dilation": [
|
70 |
+
1,
|
71 |
+
2,
|
72 |
+
3,
|
73 |
+
1,
|
74 |
+
1
|
75 |
+
],
|
76 |
+
"tdnn_dim": [
|
77 |
+
512,
|
78 |
+
512,
|
79 |
+
512,
|
80 |
+
512,
|
81 |
+
1500
|
82 |
+
],
|
83 |
+
"tdnn_kernel": [
|
84 |
+
5,
|
85 |
+
3,
|
86 |
+
3,
|
87 |
+
1,
|
88 |
+
1
|
89 |
+
],
|
90 |
+
"torch_dtype": "float32",
|
91 |
+
"transformers_version": "4.17.0.dev0",
|
92 |
+
"use_weighted_layer_sum": false,
|
93 |
+
"vocab_size": 32,
|
94 |
+
"xvector_output_dim": 512
|
95 |
+
}
|
language_model/4-gram.bin
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:e674d4a61df15bef37cd49183dc4fb087aaa3d7819d0ff8347068a880f033c61
|
3 |
+
size 3124591979
|
language_model/attrs.json
ADDED
@@ -0,0 +1 @@
|
|
|
1 |
+
{"alpha": 0.5, "beta": 1.5, "unk_score_offset": -10.0, "score_boundary": true}
|
language_model/unigrams.txt
ADDED
The diff for this file is too large to render.
See raw diff
|
preprocessor_config.json
ADDED
@@ -0,0 +1,10 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"do_normalize": true,
|
3 |
+
"feature_extractor_type": "Wav2Vec2FeatureExtractor",
|
4 |
+
"feature_size": 1,
|
5 |
+
"padding_side": "right",
|
6 |
+
"padding_value": 0.0,
|
7 |
+
"processor_class": "Wav2Vec2ProcessorWithLM",
|
8 |
+
"return_attention_mask": true,
|
9 |
+
"sampling_rate": 16000
|
10 |
+
}
|
pytorch_model.bin
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:ba0f29b6b713f88df166a3edfe8db2657fcc9f728d32d68793de5109efacc52d
|
3 |
+
size 372850161
|
special_tokens_map.json
ADDED
@@ -0,0 +1 @@
|
|
|
1 |
+
{"bos_token": "<s>", "eos_token": "</s>", "unk_token": "<unk>", "pad_token": "<pad>"}
|
tokenizer_config.json
ADDED
@@ -0,0 +1 @@
|
|
|
1 |
+
{"unk_token": "<unk>", "bos_token": "<s>", "eos_token": "</s>", "pad_token": "<pad>", "do_lower_case": false, "word_delimiter_token": "|", "replace_word_delimiter_char": " ", "return_attention_mask": false, "do_normalize": true, "special_tokens_map_file": "/home/patrick/.cache/huggingface/transformers/60230682499b8486f2a3109ba26ac7395fd4eba61426f05432329ccbfac7c190.9d6cd81ef646692fb1c169a880161ea1cb95f49694f220aced9b704b457e51dd", "name_or_path": "facebook/wav2vec2-large-lv60", "tokenizer_class": "Wav2Vec2CTCTokenizer", "processor_class": "Wav2Vec2Processor"}
|
vocab.json
ADDED
@@ -0,0 +1 @@
|
|
|
1 |
+
{"<pad>": 0, "<s>": 1, "</s>": 2, "<unk>": 3, "|": 4, "E": 5, "T": 6, "A": 7, "O": 8, "N": 9, "I": 10, "H": 11, "S": 12, "R": 13, "D": 14, "L": 15, "U": 16, "M": 17, "W": 18, "C": 19, "F": 20, "G": 21, "Y": 22, "P": 23, "B": 24, "V": 25, "K": 26, "'": 27, "X": 28, "J": 29, "Q": 30, "Z": 31}
|