Create README.md
Browse files
README.md
ADDED
@@ -0,0 +1,64 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
---
|
2 |
+
language:
|
3 |
+
- en
|
4 |
+
---
|
5 |
+
```python
|
6 |
+
import paddle
|
7 |
+
from ppdiffusers import DiffusionPipeline, ControlNetModel
|
8 |
+
from ppdiffusers.utils import load_image, image_grid
|
9 |
+
import numpy as np
|
10 |
+
from PIL import Image
|
11 |
+
import cv2
|
12 |
+
|
13 |
+
class CannyDetector:
|
14 |
+
def __call__(self, img, low_threshold, high_threshold):
|
15 |
+
return cv2.Canny(img, low_threshold, high_threshold)
|
16 |
+
apply_canny = CannyDetector()
|
17 |
+
|
18 |
+
# 加载模型
|
19 |
+
controlnet = ControlNetModel.from_pretrained("lllyasviel/sd-controlnet-canny", paddle_dtype=paddle.float16)
|
20 |
+
pipe = DiffusionPipeline.from_pretrained("runwayml/stable-diffusion-v1-5",
|
21 |
+
controlnet=controlnet,
|
22 |
+
safety_checker=None,
|
23 |
+
feature_extractor=None,
|
24 |
+
requires_safety_checker=False,
|
25 |
+
paddle_dtype=paddle.float16,
|
26 |
+
custom_pipeline="webui_stable_diffusion_controlnet",
|
27 |
+
custom_revision="9aa0fcae034d99a796c3077ec6fea84808fc5875")
|
28 |
+
|
29 |
+
# 或者 # custom_pipeline="junnyu/webui_controlnet_ppdiffusers")
|
30 |
+
|
31 |
+
# 加载图片
|
32 |
+
raw_image = load_image("https://paddlenlp.bj.bcebos.com/models/community/junnyu/develop/control_bird_canny_demo.png")
|
33 |
+
canny_image = Image.fromarray(apply_canny(np.array(raw_image), low_threshold=100, high_threshold=200))
|
34 |
+
|
35 |
+
# 选择sampler
|
36 |
+
# Please choose in ['pndm', 'lms', 'euler', 'euler-ancestral', 'dpm-multi', 'dpm-single', 'unipc-multi', 'ddim', 'ddpm', 'deis-multi', 'heun', 'kdpm2-ancestral', 'kdpm2']!
|
37 |
+
pipe.switch_scheduler('euler-ancestral')
|
38 |
+
|
39 |
+
# propmpt 和 negative_prompt
|
40 |
+
prompt = "a (blue:1.5) bird"
|
41 |
+
negative_prompt = ""
|
42 |
+
# 想要返回多少张图片
|
43 |
+
num = 4
|
44 |
+
clip_skip = 2
|
45 |
+
controlnet_conditioning_scale = 1.
|
46 |
+
num_inference_steps = 50
|
47 |
+
|
48 |
+
all_images = []
|
49 |
+
print("raw_image vs canny_image")
|
50 |
+
display(image_grid([raw_image, canny_image], 1, 2))
|
51 |
+
for i in range(num):
|
52 |
+
img = pipe(
|
53 |
+
prompt=prompt,
|
54 |
+
negative_prompt = negative_prompt,
|
55 |
+
image=canny_image,
|
56 |
+
num_inference_steps=num_inference_steps,
|
57 |
+
controlnet_conditioning_scale=controlnet_conditioning_scale,
|
58 |
+
clip_skip= clip_skip,
|
59 |
+
).images[0]
|
60 |
+
all_images.append(img)
|
61 |
+
display(image_grid(all_images, 1, num))
|
62 |
+
```
|
63 |
+
|
64 |
+
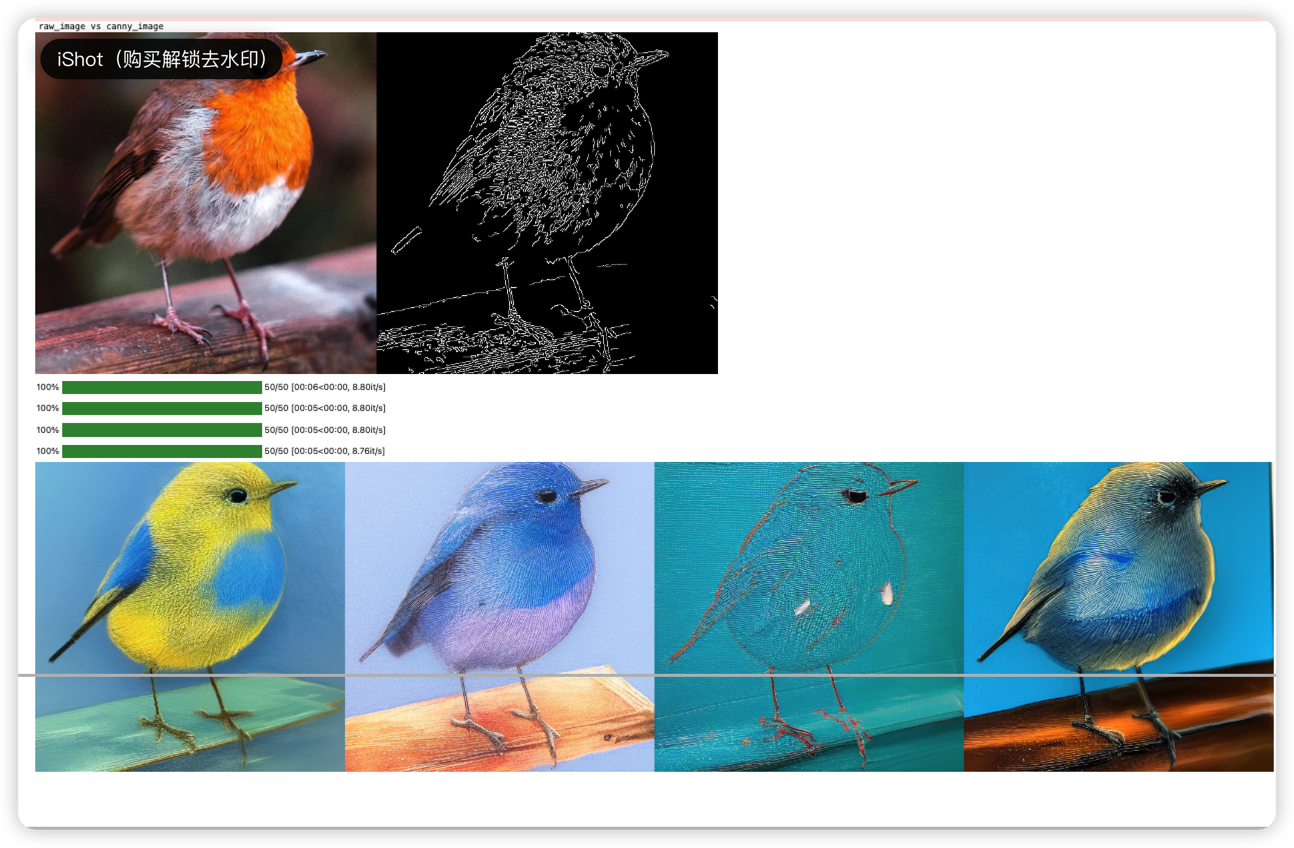
|