commit files to HF hub
Browse files
README.md
ADDED
@@ -0,0 +1,68 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# regnetx_006
|
2 |
+
Implementation of RegNet proposed in [Designing Network Design
|
3 |
+
Spaces](https://arxiv.org/abs/2003.13678)
|
4 |
+
|
5 |
+
The main idea is to start with a high dimensional search space and
|
6 |
+
iteratively reduce the search space by empirically apply constrains
|
7 |
+
based on the best performing models sampled by the current search
|
8 |
+
space.
|
9 |
+
|
10 |
+
The resulting models are light, accurate, and faster than
|
11 |
+
EfficientNets (up to 5x times!)
|
12 |
+
|
13 |
+
For example, to go from $AnyNet_A$ to $AnyNet_B$ they fixed the
|
14 |
+
bottleneck ratio $b_i$ for all stage $i$. The following table shows
|
15 |
+
all the restrictions applied from one search space to the next one.
|
16 |
+
|
17 |
+
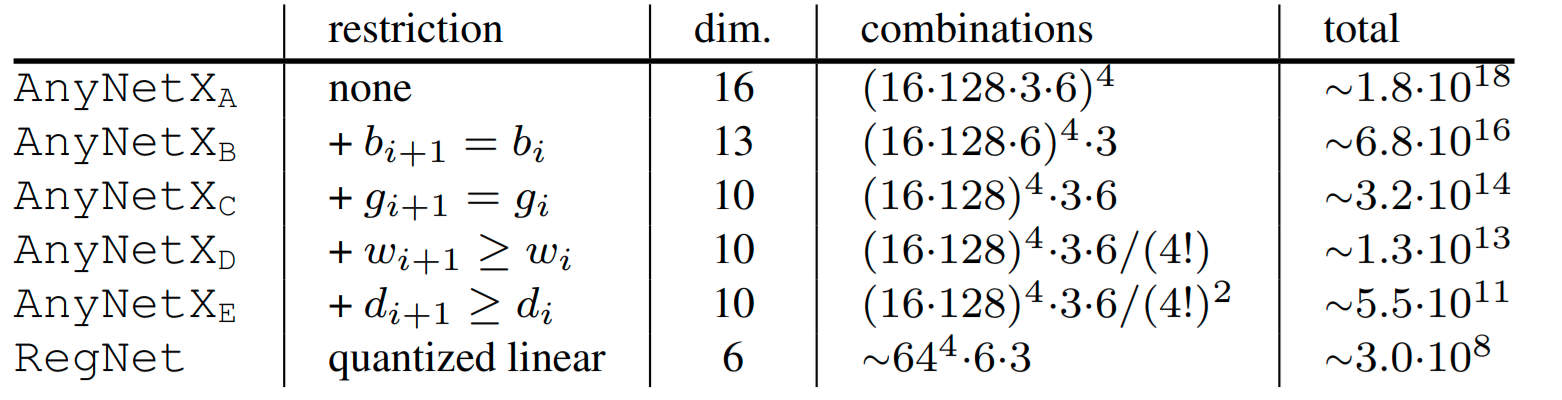
|
18 |
+
|
19 |
+
The paper is really well written and very interesting, I highly
|
20 |
+
recommended read it.
|
21 |
+
|
22 |
+
``` python
|
23 |
+
ResNet.regnetx_002()
|
24 |
+
ResNet.regnetx_004()
|
25 |
+
ResNet.regnetx_006()
|
26 |
+
ResNet.regnetx_008()
|
27 |
+
ResNet.regnetx_016()
|
28 |
+
ResNet.regnetx_040()
|
29 |
+
ResNet.regnetx_064()
|
30 |
+
ResNet.regnetx_080()
|
31 |
+
ResNet.regnetx_120()
|
32 |
+
ResNet.regnetx_160()
|
33 |
+
ResNet.regnetx_320()
|
34 |
+
# Y variants (with SE)
|
35 |
+
ResNet.regnety_002()
|
36 |
+
# ...
|
37 |
+
ResNet.regnetx_320()
|
38 |
+
|
39 |
+
You can easily customize your model
|
40 |
+
```
|
41 |
+
|
42 |
+
Examples:
|
43 |
+
|
44 |
+
``` python
|
45 |
+
# change activation
|
46 |
+
RegNet.regnetx_004(activation = nn.SELU)
|
47 |
+
# change number of classes (default is 1000 )
|
48 |
+
RegNet.regnetx_004(n_classes=100)
|
49 |
+
# pass a different block
|
50 |
+
RegNet.regnetx_004(block=RegNetYBotteneckBlock)
|
51 |
+
# change the steam
|
52 |
+
model = RegNet.regnetx_004(stem=ResNetStemC)
|
53 |
+
change shortcut
|
54 |
+
model = RegNet.regnetx_004(block=partial(RegNetYBotteneckBlock, shortcut=ResNetShorcutD))
|
55 |
+
# store each feature
|
56 |
+
x = torch.rand((1, 3, 224, 224))
|
57 |
+
# get features
|
58 |
+
model = RegNet.regnetx_004()
|
59 |
+
# first call .features, this will activate the forward hooks and tells the model you'll like to get the features
|
60 |
+
model.encoder.features
|
61 |
+
model(torch.randn((1,3,224,224)))
|
62 |
+
# get the features from the encoder
|
63 |
+
features = model.encoder.features
|
64 |
+
print([x.shape for x in features])
|
65 |
+
#[torch.Size([1, 32, 112, 112]), torch.Size([1, 32, 56, 56]), torch.Size([1, 64, 28, 28]), torch.Size([1, 160, 14, 14])]
|
66 |
+
```
|
67 |
+
|
68 |
+
|