commit files to HF hub
Browse files
README.md
ADDED
@@ -0,0 +1,56 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# efficientnet_b6
|
2 |
+
Implementation of EfficientNet proposed in [EfficientNet: Rethinking
|
3 |
+
Model Scaling for Convolutional Neural
|
4 |
+
Networks](https://arxiv.org/abs/1905.11946)
|
5 |
+
|
6 |
+
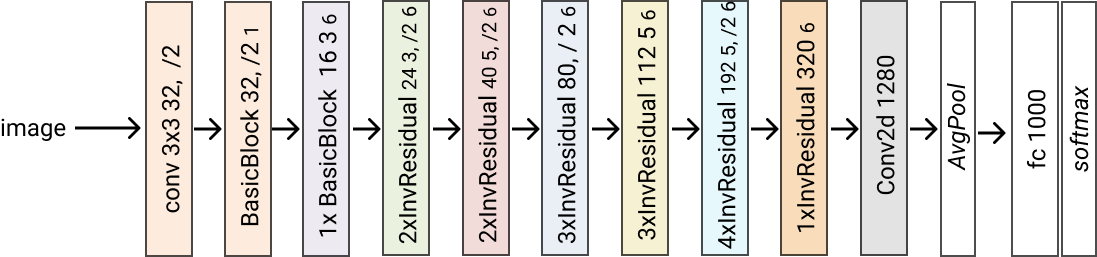
|
7 |
+
|
8 |
+
The basic architecture is similar to MobileNetV2 as was computed by
|
9 |
+
using [Progressive Neural Architecture
|
10 |
+
Search](https://arxiv.org/abs/1905.11946) .
|
11 |
+
|
12 |
+
The following table shows the basic architecture
|
13 |
+
(EfficientNet-efficientnet\_b0):
|
14 |
+
|
15 |
+

|
16 |
+
|
17 |
+
Then, the architecture is scaled up from
|
18 |
+
[-efficientnet\_b0]{.title-ref} to [-efficientnet\_b7]{.title-ref}
|
19 |
+
using compound scaling.
|
20 |
+
|
21 |
+
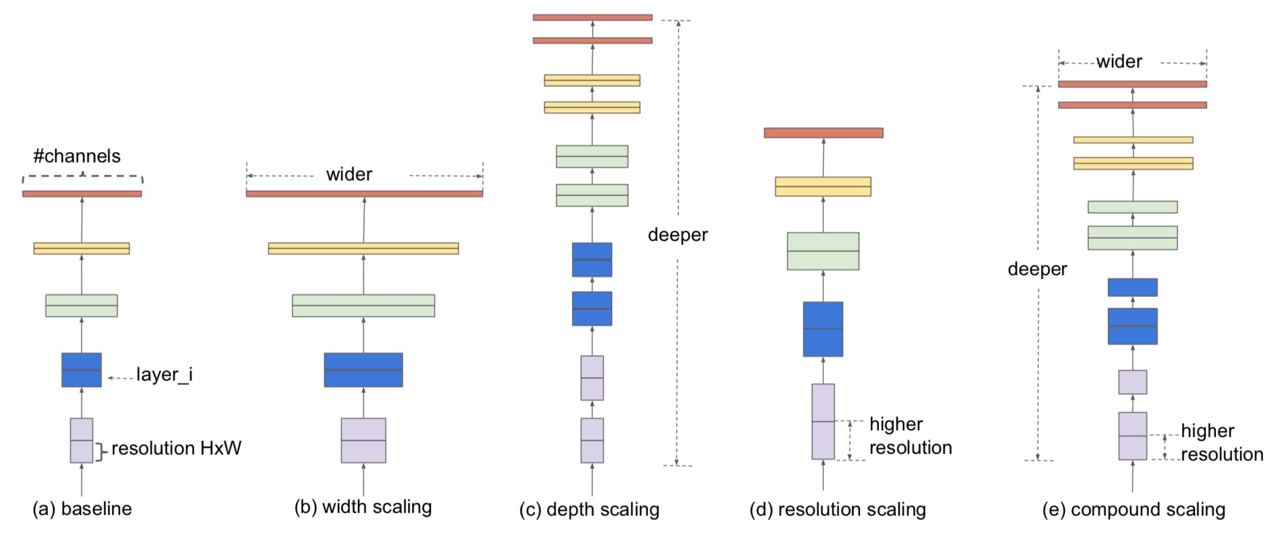
|
22 |
+
|
23 |
+
``` python
|
24 |
+
EfficientNet.efficientnet_b0()
|
25 |
+
EfficientNet.efficientnet_b1()
|
26 |
+
EfficientNet.efficientnet_b2()
|
27 |
+
EfficientNet.efficientnet_b3()
|
28 |
+
EfficientNet.efficientnet_b4()
|
29 |
+
EfficientNet.efficientnet_b5()
|
30 |
+
EfficientNet.efficientnet_b6()
|
31 |
+
EfficientNet.efficientnet_b7()
|
32 |
+
EfficientNet.efficientnet_b8()
|
33 |
+
EfficientNet.efficientnet_l2()
|
34 |
+
```
|
35 |
+
|
36 |
+
Examples:
|
37 |
+
|
38 |
+
``` python
|
39 |
+
EfficientNet.efficientnet_b0(activation = nn.SELU)
|
40 |
+
# change number of classes (default is 1000 )
|
41 |
+
EfficientNet.efficientnet_b0(n_classes=100)
|
42 |
+
# pass a different block
|
43 |
+
EfficientNet.efficientnet_b0(block=...)
|
44 |
+
# store each feature
|
45 |
+
x = torch.rand((1, 3, 224, 224))
|
46 |
+
model = EfficientNet.efficientnet_b0()
|
47 |
+
# first call .features, this will activate the forward hooks and tells the model you'll like to get the features
|
48 |
+
model.encoder.features
|
49 |
+
model(torch.randn((1,3,224,224)))
|
50 |
+
# get the features from the encoder
|
51 |
+
features = model.encoder.features
|
52 |
+
print([x.shape for x in features])
|
53 |
+
# [torch.Size([1, 32, 112, 112]), torch.Size([1, 24, 56, 56]), torch.Size([1, 40, 28, 28]), torch.Size([1, 80, 14, 14])]
|
54 |
+
```
|
55 |
+
|
56 |
+
|