text
stringlengths 2
99.9k
| meta
dict |
---|---|
import smart_imports
smart_imports.all()
@utils_jinja2.jinjaglobal
def sorted_recipients(recipients_ids, accounts):
recipients = [accounts[recipient_id] for recipient_id in recipients_ids]
recipients.sort(key=lambda account: account.nick_verbose)
return recipients
@utils_jinja2.jinjaglobal
def new_messages_number_url():
return logic.new_messages_number_url()
@utils_jinja2.jinjaglobal
def personal_messages_settings():
return conf.settings
| {
"pile_set_name": "Github"
} |
/*-
* Copyright (c) 2009-2011 Michihiro NAKAJIMA
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR(S) ``AS IS'' AND ANY EXPRESS OR
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL THE AUTHOR(S) BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#include "archive_platform.h"
__FBSDID("$FreeBSD: head/lib/libarchive/archive_read_support_compression_uu.c 201248 2009-12-30 06:12:03Z kientzle $");
#ifdef HAVE_ERRNO_H
#include <errno.h>
#endif
#ifdef HAVE_STDLIB_H
#include <stdlib.h>
#endif
#ifdef HAVE_STRING_H
#include <string.h>
#endif
#include "archive.h"
#include "archive_private.h"
#include "archive_read_private.h"
struct uudecode {
int64_t total;
unsigned char *in_buff;
#define IN_BUFF_SIZE (1024)
int in_cnt;
size_t in_allocated;
unsigned char *out_buff;
#define OUT_BUFF_SIZE (64 * 1024)
int state;
#define ST_FIND_HEAD 0
#define ST_READ_UU 1
#define ST_UUEND 2
#define ST_READ_BASE64 3
};
static int uudecode_bidder_bid(struct archive_read_filter_bidder *,
struct archive_read_filter *filter);
static int uudecode_bidder_init(struct archive_read_filter *);
static ssize_t uudecode_filter_read(struct archive_read_filter *,
const void **);
static int uudecode_filter_close(struct archive_read_filter *);
int
archive_read_support_compression_uu(struct archive *_a)
{
struct archive_read *a = (struct archive_read *)_a;
struct archive_read_filter_bidder *bidder;
bidder = __archive_read_get_bidder(a);
archive_clear_error(_a);
if (bidder == NULL)
return (ARCHIVE_FATAL);
bidder->data = NULL;
bidder->bid = uudecode_bidder_bid;
bidder->init = uudecode_bidder_init;
bidder->options = NULL;
bidder->free = NULL;
return (ARCHIVE_OK);
}
static const unsigned char ascii[256] = {
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, '\n', 0, 0, '\r', 0, 0, /* 00 - 0F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 10 - 1F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 20 - 2F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 30 - 3F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 40 - 4F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 50 - 5F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 60 - 6F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, /* 70 - 7F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 80 - 8F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 90 - 9F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* A0 - AF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* B0 - BF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* C0 - CF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* D0 - DF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* E0 - EF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* F0 - FF */
};
static const unsigned char uuchar[256] = {
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 00 - 0F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 10 - 1F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 20 - 2F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 30 - 3F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 40 - 4F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 50 - 5F */
1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 60 - 6F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 70 - 7F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 80 - 8F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 90 - 9F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* A0 - AF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* B0 - BF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* C0 - CF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* D0 - DF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* E0 - EF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* F0 - FF */
};
static const unsigned char base64[256] = {
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 00 - 0F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 10 - 1F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, /* 20 - 2F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 0, 0, /* 30 - 3F */
0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 40 - 4F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, /* 50 - 5F */
0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, /* 60 - 6F */
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, /* 70 - 7F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 80 - 8F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* 90 - 9F */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* A0 - AF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* B0 - BF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* C0 - CF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* D0 - DF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* E0 - EF */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, /* F0 - FF */
};
static const int base64num[128] = {
0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, /* 00 - 0F */
0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, /* 10 - 1F */
0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 62, 0, 0, 0, 63, /* 20 - 2F */
52, 53, 54, 55, 56, 57, 58, 59,
60, 61, 0, 0, 0, 0, 0, 0, /* 30 - 3F */
0, 0, 1, 2, 3, 4, 5, 6,
7, 8, 9, 10, 11, 12, 13, 14, /* 40 - 4F */
15, 16, 17, 18, 19, 20, 21, 22,
23, 24, 25, 0, 0, 0, 0, 0, /* 50 - 5F */
0, 26, 27, 28, 29, 30, 31, 32,
33, 34, 35, 36, 37, 38, 39, 40, /* 60 - 6F */
41, 42, 43, 44, 45, 46, 47, 48,
49, 50, 51, 0, 0, 0, 0, 0, /* 70 - 7F */
};
static ssize_t
get_line(const unsigned char *b, ssize_t avail, ssize_t *nlsize)
{
ssize_t len;
len = 0;
while (len < avail) {
switch (ascii[*b]) {
case 0: /* Non-ascii character or control character. */
if (nlsize != NULL)
*nlsize = 0;
return (-1);
case '\r':
if (avail-len > 1 && b[1] == '\n') {
if (nlsize != NULL)
*nlsize = 2;
return (len+2);
}
/* FALL THROUGH */
case '\n':
if (nlsize != NULL)
*nlsize = 1;
return (len+1);
case 1:
b++;
len++;
break;
}
}
if (nlsize != NULL)
*nlsize = 0;
return (avail);
}
static ssize_t
bid_get_line(struct archive_read_filter *filter,
const unsigned char **b, ssize_t *avail, ssize_t *ravail, ssize_t *nl)
{
ssize_t len;
int quit;
quit = 0;
if (*avail == 0) {
*nl = 0;
len = 0;
} else
len = get_line(*b, *avail, nl);
/*
* Read bytes more while it does not reach the end of line.
*/
while (*nl == 0 && len == *avail && !quit) {
ssize_t diff = *ravail - *avail;
*b = __archive_read_filter_ahead(filter, 160 + *ravail, avail);
if (*b == NULL) {
if (*ravail >= *avail)
return (0);
/* Reading bytes reaches the end of file. */
*b = __archive_read_filter_ahead(filter, *avail, avail);
quit = 1;
}
*ravail = *avail;
*b += diff;
*avail -= diff;
len = get_line(*b, *avail, nl);
}
return (len);
}
#define UUDECODE(c) (((c) - 0x20) & 0x3f)
static int
uudecode_bidder_bid(struct archive_read_filter_bidder *self,
struct archive_read_filter *filter)
{
const unsigned char *b;
ssize_t avail, ravail;
ssize_t len, nl;
int l;
int firstline;
(void)self; /* UNUSED */
b = __archive_read_filter_ahead(filter, 1, &avail);
if (b == NULL)
return (0);
firstline = 20;
ravail = avail;
for (;;) {
len = bid_get_line(filter, &b, &avail, &ravail, &nl);
if (len < 0 || nl == 0)
return (0);/* Binary data. */
if (memcmp(b, "begin ", 6) == 0 && len - nl >= 11)
l = 6;
else if (memcmp(b, "begin-base64 ", 13) == 0 && len - nl >= 18)
l = 13;
else
l = 0;
if (l > 0 && (b[l] < '0' || b[l] > '7' ||
b[l+1] < '0' || b[l+1] > '7' ||
b[l+2] < '0' || b[l+2] > '7' || b[l+3] != ' '))
l = 0;
b += len;
avail -= len;
if (l)
break;
firstline = 0;
}
if (!avail)
return (0);
len = bid_get_line(filter, &b, &avail, &ravail, &nl);
if (len < 0 || nl == 0)
return (0);/* There are non-ascii characters. */
avail -= len;
if (l == 6) {
if (!uuchar[*b])
return (0);
/* Get a length of decoded bytes. */
l = UUDECODE(*b++); len--;
if (l > 45)
/* Normally, maximum length is 45(character 'M'). */
return (0);
while (l && len-nl > 0) {
if (l > 0) {
if (!uuchar[*b++])
return (0);
if (!uuchar[*b++])
return (0);
len -= 2;
--l;
}
if (l > 0) {
if (!uuchar[*b++])
return (0);
--len;
--l;
}
if (l > 0) {
if (!uuchar[*b++])
return (0);
--len;
--l;
}
}
if (len-nl < 0)
return (0);
if (len-nl == 1 &&
(uuchar[*b] || /* Check sum. */
(*b >= 'a' && *b <= 'z'))) {/* Padding data(MINIX). */
++b;
--len;
}
b += nl;
if (avail && uuchar[*b])
return (firstline+30);
} else if (l == 13) {
/* "begin-base64 " */
while (len-nl > 0) {
if (!base64[*b++])
return (0);
--len;
}
b += nl;
if (avail >= 5 && memcmp(b, "====\n", 5) == 0)
return (firstline+40);
if (avail >= 6 && memcmp(b, "====\r\n", 6) == 0)
return (firstline+40);
if (avail > 0 && base64[*b])
return (firstline+30);
}
return (0);
}
static int
uudecode_bidder_init(struct archive_read_filter *self)
{
struct uudecode *uudecode;
void *out_buff;
void *in_buff;
self->code = ARCHIVE_COMPRESSION_UU;
self->name = "uu";
self->read = uudecode_filter_read;
self->skip = NULL; /* not supported */
self->close = uudecode_filter_close;
uudecode = (struct uudecode *)calloc(sizeof(*uudecode), 1);
out_buff = malloc(OUT_BUFF_SIZE);
in_buff = malloc(IN_BUFF_SIZE);
if (uudecode == NULL || out_buff == NULL || in_buff == NULL) {
archive_set_error(&self->archive->archive, ENOMEM,
"Can't allocate data for uudecode");
free(uudecode);
free(out_buff);
free(in_buff);
return (ARCHIVE_FATAL);
}
self->data = uudecode;
uudecode->in_buff = in_buff;
uudecode->in_cnt = 0;
uudecode->in_allocated = IN_BUFF_SIZE;
uudecode->out_buff = out_buff;
uudecode->state = ST_FIND_HEAD;
return (ARCHIVE_OK);
}
static int
ensure_in_buff_size(struct archive_read_filter *self,
struct uudecode *uudecode, size_t size)
{
if (size > uudecode->in_allocated) {
unsigned char *ptr;
size_t newsize;
/*
* Calculate a new buffer size for in_buff.
* Increase its value until it has enough size we need.
*/
newsize = uudecode->in_allocated;
do {
if (newsize < IN_BUFF_SIZE*32)
newsize <<= 1;
else
newsize += IN_BUFF_SIZE;
} while (size > newsize);
/* Allocate the new buffer. */
ptr = malloc(newsize);
if (ptr == NULL) {
free(ptr);
archive_set_error(&self->archive->archive,
ENOMEM,
"Can't allocate data for uudecode");
return (ARCHIVE_FATAL);
}
/* Move the remaining data in in_buff into the new buffer. */
if (uudecode->in_cnt)
memmove(ptr, uudecode->in_buff, uudecode->in_cnt);
/* Replace in_buff with the new buffer. */
free(uudecode->in_buff);
uudecode->in_buff = ptr;
uudecode->in_allocated = newsize;
}
return (ARCHIVE_OK);
}
static ssize_t
uudecode_filter_read(struct archive_read_filter *self, const void **buff)
{
struct uudecode *uudecode;
const unsigned char *b, *d;
unsigned char *out;
ssize_t avail_in, ravail;
ssize_t used;
ssize_t total;
ssize_t len, llen, nl;
uudecode = (struct uudecode *)self->data;
read_more:
d = __archive_read_filter_ahead(self->upstream, 1, &avail_in);
if (d == NULL && avail_in < 0)
return (ARCHIVE_FATAL);
/* Quiet a code analyzer; make sure avail_in must be zero
* when d is NULL. */
if (d == NULL)
avail_in = 0;
used = 0;
total = 0;
out = uudecode->out_buff;
ravail = avail_in;
if (uudecode->in_cnt) {
/*
* If there is remaining data which is saved by
* previous calling, use it first.
*/
if (ensure_in_buff_size(self, uudecode,
avail_in + uudecode->in_cnt) != ARCHIVE_OK)
return (ARCHIVE_FATAL);
memcpy(uudecode->in_buff + uudecode->in_cnt,
d, avail_in);
d = uudecode->in_buff;
avail_in += uudecode->in_cnt;
uudecode->in_cnt = 0;
}
for (;used < avail_in; d += llen, used += llen) {
int l, body;
b = d;
len = get_line(b, avail_in - used, &nl);
if (len < 0) {
/* Non-ascii character is found. */
archive_set_error(&self->archive->archive,
ARCHIVE_ERRNO_MISC,
"Insufficient compressed data");
return (ARCHIVE_FATAL);
}
llen = len;
if (nl == 0) {
/*
* Save remaining data which does not contain
* NL('\n','\r').
*/
if (ensure_in_buff_size(self, uudecode, len)
!= ARCHIVE_OK)
return (ARCHIVE_FATAL);
if (uudecode->in_buff != b)
memmove(uudecode->in_buff, b, len);
uudecode->in_cnt = len;
if (total == 0) {
/* Do not return 0; it means end-of-file.
* We should try to read bytes more. */
__archive_read_filter_consume(
self->upstream, ravail);
goto read_more;
}
break;
}
if (total + len * 2 > OUT_BUFF_SIZE)
break;
switch (uudecode->state) {
default:
case ST_FIND_HEAD:
if (len - nl > 13 && memcmp(b, "begin ", 6) == 0)
l = 6;
else if (len - nl > 18 &&
memcmp(b, "begin-base64 ", 13) == 0)
l = 13;
else
l = 0;
if (l != 0 && b[l] >= '0' && b[l] <= '7' &&
b[l+1] >= '0' && b[l+1] <= '7' &&
b[l+2] >= '0' && b[l+2] <= '7' && b[l+3] == ' ') {
if (l == 6)
uudecode->state = ST_READ_UU;
else
uudecode->state = ST_READ_BASE64;
}
break;
case ST_READ_UU:
body = len - nl;
if (!uuchar[*b] || body <= 0) {
archive_set_error(&self->archive->archive,
ARCHIVE_ERRNO_MISC,
"Insufficient compressed data");
return (ARCHIVE_FATAL);
}
/* Get length of undecoded bytes of curent line. */
l = UUDECODE(*b++);
body--;
if (l > body) {
archive_set_error(&self->archive->archive,
ARCHIVE_ERRNO_MISC,
"Insufficient compressed data");
return (ARCHIVE_FATAL);
}
if (l == 0) {
uudecode->state = ST_UUEND;
break;
}
while (l > 0) {
int n = 0;
if (l > 0) {
if (!uuchar[b[0]] || !uuchar[b[1]])
break;
n = UUDECODE(*b++) << 18;
n |= UUDECODE(*b++) << 12;
*out++ = n >> 16; total++;
--l;
}
if (l > 0) {
if (!uuchar[b[0]])
break;
n |= UUDECODE(*b++) << 6;
*out++ = (n >> 8) & 0xFF; total++;
--l;
}
if (l > 0) {
if (!uuchar[b[0]])
break;
n |= UUDECODE(*b++);
*out++ = n & 0xFF; total++;
--l;
}
}
if (l) {
archive_set_error(&self->archive->archive,
ARCHIVE_ERRNO_MISC,
"Insufficient compressed data");
return (ARCHIVE_FATAL);
}
break;
case ST_UUEND:
if (len - nl == 3 && memcmp(b, "end ", 3) == 0)
uudecode->state = ST_FIND_HEAD;
else {
archive_set_error(&self->archive->archive,
ARCHIVE_ERRNO_MISC,
"Insufficient compressed data");
return (ARCHIVE_FATAL);
}
break;
case ST_READ_BASE64:
l = len - nl;
if (l >= 3 && b[0] == '=' && b[1] == '=' &&
b[2] == '=') {
uudecode->state = ST_FIND_HEAD;
break;
}
while (l > 0) {
int n = 0;
if (l > 0) {
if (!base64[b[0]] || !base64[b[1]])
break;
n = base64num[*b++] << 18;
n |= base64num[*b++] << 12;
*out++ = n >> 16; total++;
l -= 2;
}
if (l > 0) {
if (*b == '=')
break;
if (!base64[*b])
break;
n |= base64num[*b++] << 6;
*out++ = (n >> 8) & 0xFF; total++;
--l;
}
if (l > 0) {
if (*b == '=')
break;
if (!base64[*b])
break;
n |= base64num[*b++];
*out++ = n & 0xFF; total++;
--l;
}
}
if (l && *b != '=') {
archive_set_error(&self->archive->archive,
ARCHIVE_ERRNO_MISC,
"Insufficient compressed data");
return (ARCHIVE_FATAL);
}
break;
}
}
__archive_read_filter_consume(self->upstream, ravail);
*buff = uudecode->out_buff;
uudecode->total += total;
return (total);
}
static int
uudecode_filter_close(struct archive_read_filter *self)
{
struct uudecode *uudecode;
uudecode = (struct uudecode *)self->data;
free(uudecode->in_buff);
free(uudecode->out_buff);
free(uudecode);
return (ARCHIVE_OK);
}
| {
"pile_set_name": "Github"
} |
// Copyright 1998-2017 Epic Games, Inc. All Rights Reserved.
#include "CocoaTextView.h"
#include "CocoaWindow.h"
#include "CocoaThread.h"
@implementation FCocoaTextView
- (id)initWithFrame:(NSRect)frame
{
SCOPED_AUTORELEASE_POOL;
self = [super initWithFrame:frame];
if (self)
{
// Initialization code here.
IMMContext = nil;
markedRange = {NSNotFound, 0};
reallyHandledEvent = false;
}
return self;
}
- (bool)imkKeyDown:(NSEvent *)theEvent
{
if (IMMContext.IsValid())
{
SCOPED_AUTORELEASE_POOL;
reallyHandledEvent = true;
return [[self inputContext] handleEvent:theEvent] && reallyHandledEvent;
}
else
{
return false;
}
}
/**
* Forward mouse events up to the window rather than through the responder chain.
*/
- (BOOL)acceptsFirstMouse:(NSEvent *)Event
{
return YES;
}
- (void)mouseDown:(NSEvent *)theEvent
{
SCOPED_AUTORELEASE_POOL;
if (IMMContext.IsValid())
{
[[self inputContext] handleEvent:theEvent];
}
FCocoaWindow* CocoaWindow = [[self window] isKindOfClass:[FCocoaWindow class]] ? (FCocoaWindow*)[self window] : nil;
if (CocoaWindow)
{
[CocoaWindow mouseDown:theEvent];
}
[NSApp preventWindowOrdering];
}
- (void)mouseDragged:(NSEvent *)theEvent
{
if (IMMContext.IsValid())
{
SCOPED_AUTORELEASE_POOL;
[[self inputContext] handleEvent:theEvent];
}
}
- (void)mouseUp:(NSEvent *)theEvent
{
SCOPED_AUTORELEASE_POOL;
if (IMMContext.IsValid())
{
[[self inputContext] handleEvent:theEvent];
}
FCocoaWindow* CocoaWindow = [[self window] isKindOfClass:[FCocoaWindow class]] ? (FCocoaWindow*)[self window] : nil;
if (CocoaWindow)
{
[CocoaWindow mouseUp:theEvent];
}
}
- (void)rightMouseDown:(NSEvent*)Event
{
SCOPED_AUTORELEASE_POOL;
FCocoaWindow* CocoaWindow = [[self window] isKindOfClass:[FCocoaWindow class]] ? (FCocoaWindow*)[self window] : nil;
if (CocoaWindow)
{
[CocoaWindow rightMouseDown:Event];
}
else
{
[super rightMouseDown:Event];
}
}
- (void)otherMouseDown:(NSEvent*)Event
{
SCOPED_AUTORELEASE_POOL;
FCocoaWindow* CocoaWindow = [[self window] isKindOfClass:[FCocoaWindow class]] ? (FCocoaWindow*)[self window] : nil;
if (CocoaWindow)
{
[CocoaWindow otherMouseDown:Event];
}
else
{
[super otherMouseDown:Event];
}
}
- (void)rightMouseUp:(NSEvent*)Event
{
SCOPED_AUTORELEASE_POOL;
FCocoaWindow* CocoaWindow = [[self window] isKindOfClass:[FCocoaWindow class]] ? (FCocoaWindow*)[self window] : nil;
if (CocoaWindow)
{
[CocoaWindow rightMouseUp:Event];
}
else
{
[super rightMouseUp:Event];
}
}
- (void)otherMouseUp:(NSEvent*)Event
{
SCOPED_AUTORELEASE_POOL;
FCocoaWindow* CocoaWindow = [[self window] isKindOfClass:[FCocoaWindow class]] ? (FCocoaWindow*)[self window] : nil;
if (CocoaWindow)
{
[CocoaWindow otherMouseUp:Event];
}
else
{
[super otherMouseUp:Event];
}
}
// Returning YES allows SlateApplication to control if window should be activated on mouse down.
// This is used for drag and drop when we don't want to activate if the mouse cursor is over a draggable item.
- (BOOL)shouldDelayWindowOrderingForEvent:(NSEvent*)Event
{
return YES;
}
- (void)activateInputMethod:(const TSharedRef<ITextInputMethodContext>&)InContext
{
SCOPED_AUTORELEASE_POOL;
if (IMMContext.IsValid())
{
[self unmarkText];
[[self inputContext] deactivate];
[[self inputContext] discardMarkedText];
}
IMMContext = InContext;
[[self inputContext] activate];
}
- (void)deactivateInputMethod
{
SCOPED_AUTORELEASE_POOL;
if (IMMContext.IsValid())
{
[self unmarkText];
IMMContext = NULL;
[[self inputContext] deactivate];
[[self inputContext] discardMarkedText];
}
}
- (bool)isActiveInputMethod:(const TSharedRef<ITextInputMethodContext>&)InContext
{
return IMMContext == InContext;
}
//@protocol NSTextInputClient
//@required
/* The receiver inserts aString replacing the content specified by replacementRange. aString can be either an NSString or NSAttributedString instance.
*/
- (void)insertText:(id)aString replacementRange:(NSRange)replacementRange
{
SCOPED_AUTORELEASE_POOL;
if (IMMContext.IsValid() && [self hasMarkedText])
{
__block uint32 SelectionLocation;
__block uint32 SelectionLength;
if (replacementRange.location == NSNotFound)
{
if (markedRange.location != NSNotFound)
{
SelectionLocation = markedRange.location;
SelectionLength = markedRange.length;
}
else
{
GameThreadCall(^{
if (IMMContext.IsValid())
{
ITextInputMethodContext::ECaretPosition CaretPosition;
IMMContext->GetSelectionRange(SelectionLocation, SelectionLength, CaretPosition);
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
}
}
else
{
SelectionLocation = replacementRange.location;
SelectionLength = replacementRange.length;
}
NSString* TheString;
if ([aString isKindOfClass:[NSAttributedString class]])
{
TheString = [(NSAttributedString*)aString string];
}
else
{
TheString = (NSString*)aString;
}
GameThreadCall(^{
SCOPED_AUTORELEASE_POOL;
if (IMMContext.IsValid())
{
FString TheFString(TheString);
IMMContext->SetTextInRange(SelectionLocation, SelectionLength, TheFString);
IMMContext->SetSelectionRange(SelectionLocation+TheFString.Len(), 0, ITextInputMethodContext::ECaretPosition::Ending);
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
[self unmarkText];
[[self inputContext] invalidateCharacterCoordinates]; // recentering
}
else
{
reallyHandledEvent = false;
}
}
/* The receiver invokes the action specified by aSelector.
*/
- (void)doCommandBySelector:(SEL)aSelector
{
reallyHandledEvent = false;
}
/* The receiver inserts aString replacing the content specified by replacementRange. aString can be either an NSString or NSAttributedString instance. selectedRange specifies the selection inside the string being inserted; hence, the location is relative to the beginning of aString. When aString is an NSString, the receiver is expected to render the marked text with distinguishing appearance (i.e. NSTextView renders with -markedTextAttributes).
*/
- (void)setMarkedText:(id)aString selectedRange:(NSRange)selectedRange replacementRange:(NSRange)replacementRange
{
if (IMMContext.IsValid())
{
SCOPED_AUTORELEASE_POOL;
__block uint32 SelectionLocation;
__block uint32 SelectionLength;
if (replacementRange.location == NSNotFound)
{
if (markedRange.location != NSNotFound)
{
SelectionLocation = markedRange.location;
SelectionLength = markedRange.length;
}
else
{
GameThreadCall(^{
if (IMMContext.IsValid())
{
ITextInputMethodContext::ECaretPosition CaretPosition;
IMMContext->GetSelectionRange(SelectionLocation, SelectionLength, CaretPosition);
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
}
}
else
{
SelectionLocation = replacementRange.location;
SelectionLength = replacementRange.length;
}
if ([aString length] == 0)
{
GameThreadCall(^{
if (IMMContext.IsValid())
{
IMMContext->SetTextInRange(SelectionLocation, SelectionLength, FString());
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
[self unmarkText];
}
else
{
if(markedRange.location == NSNotFound)
{
GameThreadCall(^{
if (IMMContext.IsValid())
{
IMMContext->BeginComposition();
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
}
markedRange = NSMakeRange(SelectionLocation, [aString length]);
__block NSRange CompositionRange = markedRange;
NSString* TheString;
if ([aString isKindOfClass:[NSAttributedString class]])
{
// While the whole string is being composed NSUnderlineStyleAttributeName is 1 to show a single line below the whole string.
// When using the pop-up glyph selection window in some IME's the NSAttributedString is broken up into separate glyph ranges, each with its own set of attributes.
// Each range specifies NSMarkedClauseSegment, incrementing the NSNumber value from 0 as well as NSUnderlineStyleAttributeName, which makes the underlining show the different ranges.
// The subrange being edited by the pop-up glyph selection window will set NSUnderlineStyleAttributeName to a value >1, while all other ranges will be set NSUnderlineStyleAttributeName to 1.
NSAttributedString* AttributedString = (NSAttributedString*)aString;
[AttributedString enumerateAttribute:NSUnderlineStyleAttributeName inRange:NSMakeRange(0, [aString length]) options:0 usingBlock:^(id Value, NSRange Range, BOOL* bStop) {
if(Value && [Value isKindOfClass:[NSNumber class]])
{
NSNumber* NumberValue = (NSNumber*)Value;
const int UnderlineValue = [NumberValue intValue];
if(UnderlineValue > 1)
{
// Found the active range, stop enumeration.
*bStop = YES;
CompositionRange.location += Range.location;
CompositionRange.length = Range.length;
}
}
}];
TheString = [AttributedString string];
}
else
{
TheString = (NSString*)aString;
}
GameThreadCall(^{
SCOPED_AUTORELEASE_POOL;
if (IMMContext.IsValid())
{
FString TheFString(TheString);
IMMContext->SetTextInRange(SelectionLocation, SelectionLength, TheFString);
IMMContext->UpdateCompositionRange(CompositionRange.location, CompositionRange.length);
IMMContext->SetSelectionRange(markedRange.location + selectedRange.location, 0, ITextInputMethodContext::ECaretPosition::Ending);
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
}
[[self inputContext] invalidateCharacterCoordinates]; // recentering
}
else
{
reallyHandledEvent = false;
}
}
/* The receiver unmarks the marked text. If no marked text, the invocation of this method has no effect.
*/
- (void)unmarkText
{
if(markedRange.location != NSNotFound)
{
markedRange = {NSNotFound, 0};
if (IMMContext.IsValid())
{
GameThreadCall(^{
if (IMMContext.IsValid())
{
IMMContext->UpdateCompositionRange(0, 0);
IMMContext->EndComposition();
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
}
}
}
/* Returns the selection range. The valid location is from 0 to the document length.
*/
- (NSRange)selectedRange
{
if (IMMContext.IsValid())
{
__block uint32 SelectionLocation;
__block uint32 SelectionLength;
__block ITextInputMethodContext::ECaretPosition CaretPosition;
GameThreadCall(^{
if (IMMContext.IsValid())
{
IMMContext->GetSelectionRange(SelectionLocation, SelectionLength, CaretPosition);
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
return {SelectionLocation, SelectionLength};
}
else
{
return {NSNotFound, 0};
}
}
/* Returns the marked range. Returns {NSNotFound, 0} if no marked range.
*/
- (NSRange)markedRange
{
if (IMMContext.IsValid())
{
return markedRange;
}
else
{
return {NSNotFound, 0};
}
}
/* Returns whether or not the receiver has marked text.
*/
- (BOOL)hasMarkedText
{
return IMMContext.IsValid() && (markedRange.location != NSNotFound);
}
/* Returns attributed string specified by aRange. It may return nil. If non-nil return value and actualRange is non-NULL, it contains the actual range for the return value. The range can be adjusted from various reasons (i.e. adjust to grapheme cluster boundary, performance optimization, etc).
*/
- (NSAttributedString *)attributedSubstringForProposedRange:(NSRange)aRange actualRange:(NSRangePointer)actualRange
{
// Deliberately no autorelease pool here - relies on the OS setting one up beforehand, otherwise the returned object won't be properly ref-counted
NSAttributedString* AttributedString = nil;
if (IMMContext.IsValid())
{
__block FString String;
GameThreadCall(^{
if (IMMContext.IsValid())
{
IMMContext->GetTextInRange(aRange.location, aRange.length, String);
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
CFStringRef CFString = FPlatformString::TCHARToCFString(*String);
if(CFString)
{
AttributedString = [[[NSAttributedString alloc] initWithString:(NSString*)CFString] autorelease];
CFRelease(CFString);
if(actualRange)
{
actualRange->location = aRange.location;
actualRange->length = String.Len();
}
}
}
return AttributedString;
}
/* Returns an array of attribute names recognized by the receiver.
*/
- (NSArray*)validAttributesForMarkedText
{
// We only allow these attributes to be set on our marked text (plus standard attributes)
// NSMarkedClauseSegmentAttributeName is important for CJK input, among other uses
// NSGlyphInfoAttributeName allows alternate forms of characters
// Deliberately no autorelease pool here - relies on the OS setting one up beforehand, otherwise the returned object won't be properly ref-counted
return [NSArray arrayWithObjects:NSMarkedClauseSegmentAttributeName, NSGlyphInfoAttributeName, nil];
}
/* Returns the first logical rectangular area for aRange. The return value is in the screen coordinate. The size value can be negative if the text flows to the left. If non-NULL, actuallRange contains the character range corresponding to the returned area.
*/
- (NSRect)firstRectForCharacterRange:(NSRange)aRange actualRange:(NSRangePointer)actualRange
{
if (IMMContext.IsValid())
{
SCOPED_AUTORELEASE_POOL;
__block FVector2D Position;
__block FVector2D Size;
GameThreadCall(^{
if (IMMContext.IsValid())
{
IMMContext->GetTextBounds(aRange.location, aRange.length, Position, Size);
}
}, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
if(actualRange)
{
*actualRange = aRange;
}
NSScreen* PrimaryScreen = [[self window] screen];
const float PrimaryScreenHeight = [PrimaryScreen visibleFrame].size.height;
Position.Y = -(Position.Y - PrimaryScreenHeight + 1);
NSRect GlyphBox = {{Position.X,Position.Y},{Size.X,Size.Y}};
return GlyphBox;
}
else
{
NSRect GlyphBox = {{0,0},{0,0}};
return GlyphBox;
}
}
/* Returns the index for character that is nearest to aPoint. aPoint is in the screen coordinate system.
*/
- (NSUInteger)characterIndexForPoint:(NSPoint)aPoint
{
if (IMMContext.IsValid())
{
FVector2D Pos(aPoint.x, aPoint.y);
int32 Index = GameThreadReturn(^{ return IMMContext.IsValid() ? IMMContext->GetCharacterIndexFromPoint(Pos) : INDEX_NONE; }, @[ NSDefaultRunLoopMode, UE4IMEEventMode ]);
NSUInteger Idx = Index == INDEX_NONE ? NSNotFound : (NSUInteger)Index;
return Idx;
}
else
{
return NSNotFound;
}
}
//@optional
/* Returns the window level of the receiver. An NSTextInputClient can implement this interface to specify its window level if it is higher than NSFloatingWindowLevel.
*/
- (NSInteger)windowLevel
{
SCOPED_AUTORELEASE_POOL;
return [[self window] level];
}
@end
| {
"pile_set_name": "Github"
} |
// Copyright 2011 Shinichiro Hamaji. All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// 1. Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// 2. Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials
// provided with the distribution.
//
// THIS SOFTWARE IS PROVIDED BY Shinichiro Hamaji ``AS IS'' AND ANY
// EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
// PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL Shinichiro Hamaji OR
// CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
// SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
// LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF
// USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
// ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
// OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT
// OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
// SUCH DAMAGE.
#include "fat.h"
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
static void fixEndian(uint32_t* p, bool be) {
if (!be) {
return;
}
uint32_t v = *p;
*p = (v << 24) | ((v << 8) & 0x00ff0000) | ((v >> 8) & 0xff00) | (v >> 24);
}
static string getArchName(uint32_t a) {
switch (a) {
case CPU_TYPE_X86:
return "i386";
case CPU_TYPE_X86_64:
return "x86-64";
case CPU_TYPE_POWERPC:
return "ppc";
case CPU_TYPE_POWERPC64:
return "ppc64";
default:
char buf[99];
sprintf(buf, "??? (%u)", a);
return buf;
}
}
bool readFatInfo(int fd, map<string, fat_arch>* fat) {
lseek(fd, 0, SEEK_SET);
fat_header header;
ssize_t len = read(fd, &header, sizeof(header));
if (len < 0) {
perror("read");
exit(1);
}
if (len != sizeof(header)) {
return false;
}
bool be = false;
if (header.magic == FAT_CIGAM) {
be = true;
} else if (header.magic != FAT_MAGIC) {
return false;
}
fixEndian(&header.nfat_arch, be);
for (uint32_t i = 0; i < header.nfat_arch; i++) {
fat_arch arch;
len = read(fd, &arch, sizeof(arch));
fixEndian(&arch.cputype, be);
fixEndian(&arch.cpusubtype, be);
fixEndian(&arch.offset, be);
fixEndian(&arch.size, be);
fixEndian(&arch.align, be);
const string& name = getArchName(arch.cputype);
fat->insert(make_pair(name, arch));
}
return true;
}
| {
"pile_set_name": "Github"
} |
java -jar Uninstaller/uninstaller.jar
| {
"pile_set_name": "Github"
} |
> eclipse skip-parents=false
> verifyScalaSettingsSuba
> verifyScalaSettingsSubb
| {
"pile_set_name": "Github"
} |
// Written by Jürgen Moßgraber - mossgrabers.de
// (c) 2017-2020
// Licensed under LGPLv3 - http://www.gnu.org/licenses/lgpl-3.0.txt
package de.mossgrabers.framework.graphics;
/**
* Interface to pre-calculated grid dimensions.
*
* @author Jürgen Moßgraber
*/
public interface IGraphicsDimensions
{
/**
* Get the width of the graphics.
*
* @return The width
*/
int getWidth ();
/**
* Get the height of the graphics.
*
* @return The height
*/
int getHeight ();
/**
* Get the size of separators.
*
* @return The size
*/
double getSeparatorSize ();
/**
* Get the height of a menu.
*
* @return The height
*/
double getMenuHeight ();
/**
* Get the size of a unit.
*
* @return The size
*/
double getUnit ();
/**
* Half of the unit.
*
* @return Half of the unit
*/
double getHalfUnit ();
/**
* Double of the unit.
*
* @return Double of the unit
*/
double getDoubleUnit ();
/**
* The height of the controls menu on top.
*
* @return The height
*/
double getControlsTop ();
/**
* Get the size of inset.
*
* @return The size
*/
double getInset ();
/**
* Get the limit for the maximum value for parameters. The value is in the range of 0 to
* upperbound-1.
*
* @return The upper bound value
*/
int getParameterUpperBound ();
}
| {
"pile_set_name": "Github"
} |
/* Community attribute related functions.
Copyright (C) 1998, 2001 Kunihiro Ishiguro
This file is part of GNU Zebra.
GNU Zebra is free software; you can redistribute it and/or modify it
under the terms of the GNU General Public License as published by the
Free Software Foundation; either version 2, or (at your option) any
later version.
GNU Zebra is distributed in the hope that it will be useful, but
WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
General Public License for more details.
You should have received a copy of the GNU General Public License
along with GNU Zebra; see the file COPYING. If not, write to the Free
Software Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA
02111-1307, USA. */
#include <zebra.h>
#include "hash.h"
#include "memory.h"
#include "bgpd/bgp_community.h"
/* Hash of community attribute. */
static struct hash *comhash;
/* Allocate a new communities value. */
static struct community *
community_new (void)
{
return (struct community *) XCALLOC (MTYPE_COMMUNITY,
sizeof (struct community));
}
/* Free communities value. */
void
community_free (struct community *com)
{
if (com->val)
XFREE (MTYPE_COMMUNITY_VAL, com->val);
if (com->str)
XFREE (MTYPE_COMMUNITY_STR, com->str);
XFREE (MTYPE_COMMUNITY, com);
}
/* Add one community value to the community. */
static void
community_add_val (struct community *com, u_int32_t val)
{
com->size++;
if (com->val)
com->val = XREALLOC (MTYPE_COMMUNITY_VAL, com->val, com_length (com));
else
com->val = XMALLOC (MTYPE_COMMUNITY_VAL, com_length (com));
val = htonl (val);
memcpy (com_lastval (com), &val, sizeof (u_int32_t));
}
/* Delete one community. */
void
community_del_val (struct community *com, u_int32_t *val)
{
int i = 0;
int c = 0;
if (! com->val)
return;
while (i < com->size)
{
if (memcmp (com->val + i, val, sizeof (u_int32_t)) == 0)
{
c = com->size -i -1;
if (c > 0)
memmove (com->val + i, com->val + (i + 1), c * sizeof (*val));
com->size--;
if (com->size > 0)
com->val = XREALLOC (MTYPE_COMMUNITY_VAL, com->val,
com_length (com));
else
{
XFREE (MTYPE_COMMUNITY_VAL, com->val);
com->val = NULL;
}
return;
}
i++;
}
}
/* Delete all communities listed in com2 from com1 */
struct community *
community_delete (struct community *com1, struct community *com2)
{
int i = 0;
while(i < com2->size)
{
community_del_val (com1, com2->val + i);
i++;
}
return com1;
}
/* Callback function from qsort(). */
static int
community_compare (const void *a1, const void *a2)
{
u_int32_t v1;
u_int32_t v2;
memcpy (&v1, a1, sizeof (u_int32_t));
memcpy (&v2, a2, sizeof (u_int32_t));
v1 = ntohl (v1);
v2 = ntohl (v2);
if (v1 < v2)
return -1;
if (v1 > v2)
return 1;
return 0;
}
int
community_include (struct community *com, u_int32_t val)
{
int i;
val = htonl (val);
for (i = 0; i < com->size; i++)
if (memcmp (&val, com_nthval (com, i), sizeof (u_int32_t)) == 0)
return 1;
return 0;
}
u_int32_t
community_val_get (struct community *com, int i)
{
u_char *p;
u_int32_t val;
p = (u_char *) com->val;
p += (i * 4);
memcpy (&val, p, sizeof (u_int32_t));
return ntohl (val);
}
/* Sort and uniq given community. */
struct community *
community_uniq_sort (struct community *com)
{
int i;
struct community *new;
u_int32_t val;
if (! com)
return NULL;
new = community_new ();;
for (i = 0; i < com->size; i++)
{
val = community_val_get (com, i);
if (! community_include (new, val))
community_add_val (new, val);
}
qsort (new->val, new->size, sizeof (u_int32_t), community_compare);
return new;
}
/* Convert communities attribute to string.
For Well-known communities value, below keyword is used.
0x0 "internet"
0xFFFFFF01 "no-export"
0xFFFFFF02 "no-advertise"
0xFFFFFF03 "local-AS"
For other values, "AS:VAL" format is used. */
static char *
community_com2str (struct community *com)
{
int i;
char *str;
char *pnt;
int len;
int first;
u_int32_t comval;
u_int16_t as;
u_int16_t val;
if (!com)
return NULL;
/* When communities attribute is empty. */
if (com->size == 0)
{
str = XMALLOC (MTYPE_COMMUNITY_STR, 1);
str[0] = '\0';
return str;
}
/* Memory allocation is time consuming work. So we calculate
required string length first. */
len = 0;
for (i = 0; i < com->size; i++)
{
memcpy (&comval, com_nthval (com, i), sizeof (u_int32_t));
comval = ntohl (comval);
switch (comval)
{
case COMMUNITY_INTERNET:
len += strlen (" internet");
break;
case COMMUNITY_NO_EXPORT:
len += strlen (" no-export");
break;
case COMMUNITY_NO_ADVERTISE:
len += strlen (" no-advertise");
break;
case COMMUNITY_LOCAL_AS:
len += strlen (" local-AS");
break;
default:
len += strlen (" 65536:65535");
break;
}
}
/* Allocate memory. */
str = pnt = XMALLOC (MTYPE_COMMUNITY_STR, len);
first = 1;
/* Fill in string. */
for (i = 0; i < com->size; i++)
{
memcpy (&comval, com_nthval (com, i), sizeof (u_int32_t));
comval = ntohl (comval);
if (first)
first = 0;
else
*pnt++ = ' ';
switch (comval)
{
case COMMUNITY_INTERNET:
strcpy (pnt, "internet");
pnt += strlen ("internet");
break;
case COMMUNITY_NO_EXPORT:
strcpy (pnt, "no-export");
pnt += strlen ("no-export");
break;
case COMMUNITY_NO_ADVERTISE:
strcpy (pnt, "no-advertise");
pnt += strlen ("no-advertise");
break;
case COMMUNITY_LOCAL_AS:
strcpy (pnt, "local-AS");
pnt += strlen ("local-AS");
break;
default:
as = (comval >> 16) & 0xFFFF;
val = comval & 0xFFFF;
sprintf (pnt, "%u:%d", as, val);
pnt += strlen (pnt);
break;
}
}
*pnt = '\0';
return str;
}
/* Intern communities attribute. */
struct community *
community_intern (struct community *com)
{
struct community *find;
/* Assert this community structure is not interned. */
assert (com->refcnt == 0);
/* Lookup community hash. */
find = (struct community *) hash_get (comhash, com, hash_alloc_intern);
/* Arguemnt com is allocated temporary. So when it is not used in
hash, it should be freed. */
if (find != com)
community_free (com);
/* Increment refrence counter. */
find->refcnt++;
/* Make string. */
if (! find->str)
find->str = community_com2str (find);
return find;
}
/* Free community attribute. */
void
community_unintern (struct community **com)
{
struct community *ret;
if ((*com)->refcnt)
(*com)->refcnt--;
/* Pull off from hash. */
if ((*com)->refcnt == 0)
{
/* Community value com must exist in hash. */
ret = (struct community *) hash_release (comhash, *com);
assert (ret != NULL);
community_free (*com);
*com = NULL;
}
}
/* Create new community attribute. */
struct community *
community_parse (u_int32_t *pnt, u_short length)
{
struct community tmp;
struct community *new;
/* If length is malformed return NULL. */
if (length % 4)
return NULL;
/* Make temporary community for hash look up. */
tmp.size = length / 4;
tmp.val = pnt;
new = community_uniq_sort (&tmp);
return community_intern (new);
}
struct community *
community_dup (struct community *com)
{
struct community *new;
new = XCALLOC (MTYPE_COMMUNITY, sizeof (struct community));
new->size = com->size;
if (new->size)
{
new->val = XMALLOC (MTYPE_COMMUNITY_VAL, com->size * 4);
memcpy (new->val, com->val, com->size * 4);
}
else
new->val = NULL;
return new;
}
/* Retrun string representation of communities attribute. */
char *
community_str (struct community *com)
{
if (!com)
return NULL;
if (! com->str)
com->str = community_com2str (com);
return com->str;
}
/* Make hash value of community attribute. This function is used by
hash package.*/
unsigned int
community_hash_make (struct community *com)
{
unsigned char *pnt = (unsigned char *)com->val;
int size = com->size * 4;
unsigned int key = 0;
int c;
for (c = 0; c < size; c += 4)
{
key += pnt[c];
key += pnt[c + 1];
key += pnt[c + 2];
key += pnt[c + 3];
}
return key;
}
int
community_match (const struct community *com1, const struct community *com2)
{
int i = 0;
int j = 0;
if (com1 == NULL && com2 == NULL)
return 1;
if (com1 == NULL || com2 == NULL)
return 0;
if (com1->size < com2->size)
return 0;
/* Every community on com2 needs to be on com1 for this to match */
while (i < com1->size && j < com2->size)
{
if (memcmp (com1->val + i, com2->val + j, sizeof (u_int32_t)) == 0)
j++;
i++;
}
if (j == com2->size)
return 1;
else
return 0;
}
/* If two aspath have same value then return 1 else return 0. This
function is used by hash package. */
int
community_cmp (const struct community *com1, const struct community *com2)
{
if (com1 == NULL && com2 == NULL)
return 1;
if (com1 == NULL || com2 == NULL)
return 0;
if (com1->size == com2->size)
if (memcmp (com1->val, com2->val, com1->size * 4) == 0)
return 1;
return 0;
}
/* Add com2 to the end of com1. */
struct community *
community_merge (struct community *com1, struct community *com2)
{
if (com1->val)
com1->val = XREALLOC (MTYPE_COMMUNITY_VAL, com1->val,
(com1->size + com2->size) * 4);
else
com1->val = XMALLOC (MTYPE_COMMUNITY_VAL, (com1->size + com2->size) * 4);
memcpy (com1->val + com1->size, com2->val, com2->size * 4);
com1->size += com2->size;
return com1;
}
/* Community token enum. */
enum community_token
{
community_token_val,
community_token_no_export,
community_token_no_advertise,
community_token_local_as,
community_token_unknown
};
/* Get next community token from string. */
static const char *
community_gettoken (const char *buf, enum community_token *token,
u_int32_t *val)
{
const char *p = buf;
/* Skip white space. */
while (isspace ((int) *p))
p++;
/* Check the end of the line. */
if (*p == '\0')
return NULL;
/* Well known community string check. */
if (isalpha ((int) *p))
{
if (strncmp (p, "internet", strlen ("internet")) == 0)
{
*val = COMMUNITY_INTERNET;
*token = community_token_no_export;
p += strlen ("internet");
return p;
}
if (strncmp (p, "no-export", strlen ("no-export")) == 0)
{
*val = COMMUNITY_NO_EXPORT;
*token = community_token_no_export;
p += strlen ("no-export");
return p;
}
if (strncmp (p, "no-advertise", strlen ("no-advertise")) == 0)
{
*val = COMMUNITY_NO_ADVERTISE;
*token = community_token_no_advertise;
p += strlen ("no-advertise");
return p;
}
if (strncmp (p, "local-AS", strlen ("local-AS")) == 0)
{
*val = COMMUNITY_LOCAL_AS;
*token = community_token_local_as;
p += strlen ("local-AS");
return p;
}
/* Unknown string. */
*token = community_token_unknown;
return NULL;
}
/* Community value. */
if (isdigit ((int) *p))
{
int separator = 0;
int digit = 0;
u_int32_t community_low = 0;
u_int32_t community_high = 0;
while (isdigit ((int) *p) || *p == ':')
{
if (*p == ':')
{
if (separator)
{
*token = community_token_unknown;
return NULL;
}
else
{
separator = 1;
digit = 0;
community_high = community_low << 16;
community_low = 0;
}
}
else
{
digit = 1;
community_low *= 10;
community_low += (*p - '0');
}
p++;
}
if (! digit)
{
*token = community_token_unknown;
return NULL;
}
*val = community_high + community_low;
*token = community_token_val;
return p;
}
*token = community_token_unknown;
return NULL;
}
/* convert string to community structure */
struct community *
community_str2com (const char *str)
{
struct community *com = NULL;
struct community *com_sort = NULL;
u_int32_t val = 0;
enum community_token token = community_token_unknown;
do
{
str = community_gettoken (str, &token, &val);
switch (token)
{
case community_token_val:
case community_token_no_export:
case community_token_no_advertise:
case community_token_local_as:
if (com == NULL)
com = community_new();
community_add_val (com, val);
break;
case community_token_unknown:
default:
if (com)
community_free (com);
return NULL;
}
} while (str);
if (! com)
return NULL;
com_sort = community_uniq_sort (com);
community_free (com);
return com_sort;
}
/* Return communities hash entry count. */
unsigned long
community_count (void)
{
return comhash->count;
}
/* Return communities hash. */
struct hash *
community_hash (void)
{
return comhash;
}
/* Initialize comminity related hash. */
void
community_init (void)
{
comhash = hash_create ((unsigned int (*) (void *))community_hash_make,
(int (*) (const void *, const void *))community_cmp);
}
void
community_finish (void)
{
hash_free (comhash);
comhash = NULL;
}
| {
"pile_set_name": "Github"
} |
using UnityEngine;
using UnityEditor;
using Crosstales.FB.EditorUtil;
namespace Crosstales.FB.EditorTask
{
/// <summary>Reminds the customer to create an UAS review.</summary>
[InitializeOnLoad]
public static class ReminderCheck
{
#region Constructor
static ReminderCheck()
{
string lastDate = EditorPrefs.GetString(EditorConstants.KEY_REMINDER_DATE);
string date = System.DateTime.Now.ToString("yyyyMMdd"); // every day
//string date = System.DateTime.Now.ToString("yyyyMMddHHmm"); // every minute (for tests)
if (!date.Equals(lastDate))
{
int count = EditorPrefs.GetInt(EditorConstants.KEY_REMINDER_COUNT) + 1;
//if (count % 1 == 0) // for testing only
if (count % 13 == 0 && EditorConfig.REMINDER_CHECK)
{
int option = EditorUtility.DisplayDialogComplex(Util.Constants.ASSET_NAME + " - Reminder",
"Please don't forget to rate " + Util.Constants.ASSET_NAME + " or even better write a little review – it would be very much appreciated!",
"Yes, let's do it!",
"Not right now",
"Don't ask again!");
if (option == 0)
{
Application.OpenURL(EditorConstants.ASSET_URL);
EditorConfig.REMINDER_CHECK = false;
Debug.LogWarning("<color=red>" + Common.Util.BaseHelper.CreateString("❤", 500) + "</color>");
Debug.LogWarning("<b>+++ Thank you for rating <color=blue>" + Util.Constants.ASSET_NAME + "</color>! +++</b>");
Debug.LogWarning("<color=red>" + Common.Util.BaseHelper.CreateString("❤", 500) + "</color>");
}
else if (option == 1)
{
// do nothing!
}
else
{
EditorConfig.REMINDER_CHECK = false;
}
EditorConfig.Save();
}
EditorPrefs.SetString(EditorConstants.KEY_REMINDER_DATE, date);
EditorPrefs.SetInt(EditorConstants.KEY_REMINDER_COUNT, count);
}
}
#endregion
}
}
// © 2017-2019 crosstales LLC (https://www.crosstales.com) | {
"pile_set_name": "Github"
} |
/ cmp -- compare files
cmp (sp)+,$3
beq 1f
jsr r5,mesg; <Usage: cmp arg1 arg2\n\0>; .even
sys exit
1:
tst (sp)+
mov (sp)+,0f
sys open; 0:..; 0
bec 1f
jsr r5,mesg; <Can't open arg1.\n\0>; .even
sys exit
1:
mov r0,f1
mov (sp)+,0f
sys open; 0:..; 0
bec 1f
jsr r5,mesg; <Can't open arg2.\n\0>; .even
sys exit
1:
mov r0,f2
clr r2
1:
jsr r5,getw; f1
bvs eof1
mov r0,r3
jsr r5,getw; f2
bvs eof2
cmp r0,r3
beq 2f
mov r0,r4
mov r2,r0
jsr pc,octal
jsr r5,mesg; <: \0>; .even
mov r3,r0
jsr pc,octal
jsr r5,mesg; < \0>;
mov r4,r0
jsr pc,octal
jsr r5,mesg; <\n\0>
2:
add $2,r2
br 1b
eof1:
jsr r5,getw; f2
bvs 1f
jsr r5,mesg; <EOF on arg1.\n\0>; .even
sys exit
1:
sys exit
eof2:
jsr r5,mesg; <EOF on arg2.\n\0>; .even
sys exit
mesg:
movb (r5)+,ch
beq 1f
mov $1,r0
sys write; ch; 1
br mesg
1:
inc r5
bic $1,r5
rts r5
getw:
mov (r5)+,r1
cmp 2(r1),$1
bne 1f
mov *4(r1),r0
bic $!377,r0
dec 2(r1)
rts r5
1:
sub $2,2(r1)
bge 1f
mov (r1),r0
mov r1,0f
add $6,0f
sys read; 0:..; 512.
mov r0,2(r1)
bne 2f
sev
rts r5
2:
mov r1,4(r1)
add $6,4(r1)
sub $2,2(r1)
1:
mov *4(r1),r0
add $2,4(r1)
rts r5
octal:
mov r4,-(sp)
mov r0,r5
mov $6,-(sp)
1:
clr r4
alsc $1,r4
cmp (sp),$6
beq 2f
alsc $2,r4
2:
add $'0,r4
mov r4,ch
mov $1,r0
sys write; ch; 1
dec (sp)
bne 1b
tst (sp)+
mov (sp)+,r4
rts pc
.bss
ch: .=.+2
f1: .=.+6; .=.+512.
f2: .=.+6; .=.+512.
| {
"pile_set_name": "Github"
} |
/* This is a compiled file, you should be editing the file in the templates directory */
.pace {
-webkit-pointer-events: none;
pointer-events: none;
-webkit-user-select: none;
-moz-user-select: none;
user-select: none;
}
.pace.pace-inactive .pace-progress {
display: none;
}
.pace .pace-progress {
position: fixed;
z-index: 2000;
top: 0;
right: 0;
height: 5rem;
width: 5rem;
-webkit-transform: translate3d(0, 0, 0) !important;
-ms-transform: translate3d(0, 0, 0) !important;
transform: translate3d(0, 0, 0) !important;
}
.pace .pace-progress:after {
display: block;
position: absolute;
top: 0;
right: .5rem;
content: attr(data-progress-text);
font-family: "Helvetica Neue", sans-serif;
font-weight: 100;
font-size: 5rem;
line-height: 1;
text-align: right;
color: rgba(238, 49, 72, 0.19999999999999996);
}
| {
"pile_set_name": "Github"
} |
package com.itdragon.demo;
import java.util.Random;
import javax.jms.Queue;
import javax.jms.Topic;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import com.itdragon.StartApplication;
import com.itdragon.mq.ITDragonProducer;
/**
* @RunWith 它是一个运行器
* @RunWith(SpringRunner.class) 表示让测试运行于Spring测试环境,不用启动spring容器即可使用Spring环境
* @SpringBootTest(classes=StartApplication.class) 表示将StartApplication.class纳入到测试环境中,若不加这个则提示bean找不到。
*
* @author itdragon
*
*/
@RunWith(SpringRunner.class)
@SpringBootTest(classes = StartApplication.class)
public class SpringbootStudyApplicationTests {
@Autowired
private ITDragonProducer producer;
@Autowired
private Queue queue;
// @Autowired
// private Topic topic;
//
// @Autowired
// private Queue responseQueue;
/**
* 点对点(p2p)模式测试
* 包含三个角色:消息队列(Queue),发送者(Sender),接收者(Receiver)。
* 发送者将消息发送到一个特定的队列,队列保留着消息,直到接收者从队列中获取消息。
*/
/*@Test
public void testP2P(){
for (int i = 0; i < 10; i++) {
String []operators = {"+","-","*","/"};
Random random = new Random(System.currentTimeMillis());
String expression = random.nextInt(10)+operators[random.nextInt(4)]+(random.nextInt(10)+1);
producer.sendMessage(this.queue, expression);
}
}*/
/*@Test
public void testPubSub() {
for (int i = 0; i < 10; i++) {
String []operators = {"+","-","*","/"};
Random random = new Random(System.currentTimeMillis());
String expression = random.nextInt(10)+operators[random.nextInt(4)]+(random.nextInt(10)+1);
producer.sendMessage(this.topic, expression);
}
}
@Test
public void testReceiveResponse(){
for (int i = 0; i < 10; i++) {
String []operators = {"+","-","*","/"};
Random random = new Random(System.currentTimeMillis());
String expression = random.nextInt(10)+operators[random.nextInt(4)]+(random.nextInt(10)+1);
producer.sendMessage(this.responseQueue, expression);
}
}*/
}
| {
"pile_set_name": "Github"
} |
package com.gc.materialdesign.widgets;
import android.content.Context;
import android.os.Bundle;
import android.view.MotionEvent;
import android.view.View;
import android.view.View.OnTouchListener;
import android.view.Window;
import android.view.animation.Animation;
import android.view.animation.Animation.AnimationListener;
import android.view.animation.AnimationUtils;
import android.widget.RelativeLayout;
import android.widget.TextView;
import com.gc.materialdesign.R;
import com.gc.materialdesign.views.ButtonFlat;
import com.gc.materialdesign.views.ProgressBarCircularIndeterminate;
public class ProgressDialog extends android.app.Dialog{
Context context;
View view;
View backView;
String title;
TextView titleTextView;
int progressColor = -1;
public ProgressDialog(Context context,String title) {
super(context, android.R.style.Theme_Translucent);
this.title = title;
this.context = context;
}
public ProgressDialog(Context context,String title, int progressColor) {
super(context, android.R.style.Theme_Translucent);
this.title = title;
this.progressColor = progressColor;
this.context = context;
}
@Override
protected void onCreate(Bundle savedInstanceState) {
requestWindowFeature(Window.FEATURE_NO_TITLE);
super.onCreate(savedInstanceState);
setContentView(R.layout.progress_dialog);
view = (RelativeLayout)findViewById(R.id.contentDialog);
backView = (RelativeLayout)findViewById(R.id.dialog_rootView);
backView.setOnTouchListener(new OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
if (event.getX() < view.getLeft()
|| event.getX() >view.getRight()
|| event.getY() > view.getBottom()
|| event.getY() < view.getTop()) {
dismiss();
}
return false;
}
});
this.titleTextView = (TextView) findViewById(R.id.title);
setTitle(title);
if(progressColor != -1){
ProgressBarCircularIndeterminate progressBarCircularIndeterminate = (ProgressBarCircularIndeterminate) findViewById(R.id.progressBarCircularIndetermininate);
progressBarCircularIndeterminate.setBackgroundColor(progressColor);
}
}
@Override
public void show() {
// TODO 自动生成的方法存根
super.show();
// set dialog enter animations
view.startAnimation(AnimationUtils.loadAnimation(context, R.anim.dialog_main_show_amination));
backView.startAnimation(AnimationUtils.loadAnimation(context, R.anim.dialog_root_show_amin));
}
// GETERS & SETTERS
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
if(title == null)
titleTextView.setVisibility(View.GONE);
else{
titleTextView.setVisibility(View.VISIBLE);
titleTextView.setText(title);
}
}
public TextView getTitleTextView() {
return titleTextView;
}
public void setTitleTextView(TextView titleTextView) {
this.titleTextView = titleTextView;
}
@Override
public void dismiss() {
Animation anim = AnimationUtils.loadAnimation(context, R.anim.dialog_main_hide_amination);
anim.setAnimationListener(new AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
}
@Override
public void onAnimationRepeat(Animation animation) {
}
@Override
public void onAnimationEnd(Animation animation) {
view.post(new Runnable() {
@Override
public void run() {
ProgressDialog.super.dismiss();
}
});
}
});
Animation backAnim = AnimationUtils.loadAnimation(context, R.anim.dialog_root_hide_amin);
view.startAnimation(anim);
backView.startAnimation(backAnim);
}
}
| {
"pile_set_name": "Github"
} |
# Copyright (c) Facebook, Inc. and its affiliates. All Rights Reserved.
import torch
class Matcher(object):
"""
This class assigns to each predicted "element" (e.g., a box) a ground-truth
element. Each predicted element will have exactly zero or one matches; each
ground-truth element may be assigned to zero or more predicted elements.
Matching is based on the MxN match_quality_matrix, that characterizes how well
each (ground-truth, predicted)-pair match. For example, if the elements are
boxes, the matrix may contain box IoU overlap values.
The matcher returns a tensor of size N containing the index of the ground-truth
element m that matches to prediction n. If there is no match, a negative value
is returned.
"""
BELOW_LOW_THRESHOLD = -1
BETWEEN_THRESHOLDS = -2
def __init__(self, high_threshold, low_threshold, allow_low_quality_matches=False):
"""
Args:
high_threshold (float): quality values greater than or equal to
this value are candidate matches.
low_threshold (float): a lower quality threshold used to stratify
matches into three levels:
1) matches >= high_threshold
2) BETWEEN_THRESHOLDS matches in [low_threshold, high_threshold)
3) BELOW_LOW_THRESHOLD matches in [0, low_threshold)
allow_low_quality_matches (bool): if True, produce additional matches
for predictions that have only low-quality match candidates. See
set_low_quality_matches_ for more details.
"""
assert low_threshold <= high_threshold
self.high_threshold = high_threshold
self.low_threshold = low_threshold
self.allow_low_quality_matches = allow_low_quality_matches
def __call__(self, match_quality_matrix):
"""
Args:
match_quality_matrix (Tensor[float]): an MxN tensor, containing the
pairwise quality between M ground-truth elements and N predicted elements.
Returns:
matches (Tensor[int64]): an N tensor where N[i] is a matched gt in
[0, M - 1] or a negative value indicating that prediction i could not
be matched.
"""
if match_quality_matrix.numel() == 0:
# empty targets or proposals not supported during training
if match_quality_matrix.shape[0] == 0:
raise ValueError(
"No ground-truth boxes available for one of the images "
"during training")
else:
raise ValueError(
"No proposal boxes available for one of the images "
"during training")
# match_quality_matrix is M (gt) x N (predicted)
# Max over gt elements (dim 0) to find best gt candidate for each prediction
matched_vals, matches = match_quality_matrix.max(dim=0)
if self.allow_low_quality_matches:
all_matches = matches.clone()
# Assign candidate matches with low quality to negative (unassigned) values
below_low_threshold = matched_vals < self.low_threshold
between_thresholds = (matched_vals >= self.low_threshold) & (
matched_vals < self.high_threshold
)
matches[below_low_threshold] = Matcher.BELOW_LOW_THRESHOLD
matches[between_thresholds] = Matcher.BETWEEN_THRESHOLDS
if self.allow_low_quality_matches:
self.set_low_quality_matches_(matches, all_matches, match_quality_matrix)
return matches
def set_low_quality_matches_(self, matches, all_matches, match_quality_matrix):
"""
Produce additional matches for predictions that have only low-quality matches.
Specifically, for each ground-truth find the set of predictions that have
maximum overlap with it (including ties); for each prediction in that set, if
it is unmatched, then match it to the ground-truth with which it has the highest
quality value.
"""
# For each gt, find the prediction with which it has highest quality
highest_quality_foreach_gt, _ = match_quality_matrix.max(dim=1)
# Find highest quality match available, even if it is low, including ties
gt_pred_pairs_of_highest_quality = torch.nonzero(
match_quality_matrix == highest_quality_foreach_gt[:, None]
)
# Example gt_pred_pairs_of_highest_quality:
# tensor([[ 0, 39796],
# [ 1, 32055],
# [ 1, 32070],
# [ 2, 39190],
# [ 2, 40255],
# [ 3, 40390],
# [ 3, 41455],
# [ 4, 45470],
# [ 5, 45325],
# [ 5, 46390]])
# Each row is a (gt index, prediction index)
# Note how gt items 1, 2, 3, and 5 each have two ties
pred_inds_to_update = gt_pred_pairs_of_highest_quality[:, 1]
matches[pred_inds_to_update] = all_matches[pred_inds_to_update]
| {
"pile_set_name": "Github"
} |
/*
The emitter is just an object that follows the cursor and
can spawn new particle objects. It would be easier to just make
the location vector match the cursor position but I have opted
to use a velocity vector because later I will be allowing for
multiple emitters.
*/
class Emitter{
Vec3D loc;
Vec3D vel;
Vec3D velToMouse;
color myColor;
ArrayList particles;
Emitter( ){
loc = new Vec3D();
vel = new Vec3D();
velToMouse = new Vec3D();
myColor = color( 1, 1, 1 );
particles = new ArrayList();
}
void exist(){
setVelToMouse();
findVelocity();
setPosition();
iterateListExist();
render();
gl.glDisable( GL.GL_TEXTURE_2D );
if( ALLOWTRAILS )
iterateListRenderTrails();
}
void setVelToMouse(){
velToMouse.set( mouseX - loc.x, mouseY - loc.y, 0 );
}
void findVelocity(){
vel.interpolateToSelf( velToMouse, .35 );
}
void setPosition(){
loc.addSelf( vel );
if( ALLOWFLOOR ){
if( loc.y > floorLevel ){
loc.y = floorLevel;
vel.y = 0;
}
}
}
void iterateListExist(){
for( Iterator it = particles.iterator(); it.hasNext(); ){
Particle p = (Particle) it.next();
if( !p.ISDEAD ){
p.exist();
} else {
it.remove();
}
}
}
void render(){
renderImage( emitterImg, loc, 150, myColor, 1.0 );
}
void iterateListRenderTrails(){
for( Iterator it = particles.iterator(); it.hasNext(); ){
Particle p = (Particle) it.next();
p.renderTrails();
}
}
void addParticles( int _amt ){
for( int i=0; i<_amt; i++ ){
particles.add( new Particle( loc, vel ) );
}
}
}
| {
"pile_set_name": "Github"
} |
<!DOCTYPE html>
<html>
<head>
<title>C</title>
</head>
<body>
<h1>This is C</h1>
<a href="b.html">Go to B</a>
<a href="index.html">Go to Index</a>
</body>
</html> | {
"pile_set_name": "Github"
} |
'use strict';
const translators = require('../translators');
module.exports = {
meta: {
type: 'problem',
fixable: 'code',
docs: {
description: 'checks use of deprecated Translator Framework',
category: 'Possible Errors',
},
},
create: function (context) {
return {
Program: function (node) {
const header = translators.getHeaderFromAST(node);
if (!header.body) return; // if there's no file header, assume it's not a translator
const sourceCode = context.getSourceCode();
for (const comment of sourceCode.getAllComments()) {
if (comment.value.match(/^\s*FW LINE [0-9]+:/)) {
context.report({
loc: comment.loc,
message: 'uses deprecated Translator Framework'
});
}
}
}
};
},
};
| {
"pile_set_name": "Github"
} |
<form method="post" action="{$smarty.server.PHP_SELF}?action=bulkdomain" class="form-horizontal">
<input type="hidden" name="update" value="{$update}">
<input type="hidden" name="save" value="1">
{foreach from=$domainids item=domainid}
<input type="hidden" name="domids[]" value="{$domainid}" />
{/foreach}
{if $update eq "nameservers"}
{if $save}
{if $errors}
<div class="alert alert-error">
<p class="bold">
{$LANG.clientareaerrors}
</p>
<ul>
{foreach from=$errors item=error}
<li>{$error}</li>
{/foreach}
</ul>
</div>
{else}
<div class="alert alert-success">
<p>
{$LANG.changessavedsuccessfully}
</p>
</div>
{/if}
{/if}
<p>
{$LANG.domainbulkmanagementchangesaffect}
</p>
<br />
<blockquote>
<br />
{foreach from=$domains item=domain}
» {$domain}<br />
{/foreach}
<br />
</blockquote>
<p>
<label class="full control-label"><input type="radio" class="radio inline" name="nschoice" value="default" onclick="disableFields('domnsinputs',true)" checked /> {$LANG.nschoicedefault}</label>
<br />
<label class="full control-label"><input type="radio" class="radio inline" name="nschoice" value="custom" onclick="disableFields('domnsinputs','')" checked /> {$LANG.nschoicecustom}</label>
</p>
<fieldset class="control-group">
<div class="control-group">
<label class="control-label" for="ns1">{$LANG.domainnameserver1}</label>
<div class="controls">
<input class="input-xlarge domnsinputs" id="ns1" name="ns1" type="text" value="{$ns1}" />
</div>
</div>
<div class="control-group">
<label class="control-label" for="ns2">{$LANG.domainnameserver2}</label>
<div class="controls">
<input class="input-xlarge domnsinputs" id="ns2" name="ns2" type="text" value="{$ns2}" />
</div>
</div>
<div class="control-group">
<label class="control-label" for="ns3">{$LANG.domainnameserver3}</label>
<div class="controls">
<input class="input-xlarge domnsinputs" id="ns3" name="ns3" type="text" value="{$ns3}" />
</div>
</div>
<div class="control-group">
<label class="control-label" for="ns4">{$LANG.domainnameserver4}</label>
<div class="controls">
<input class="input-xlarge domnsinputs" id="ns4" name="ns4" type="text" value="{$ns4}" />
</div>
</div>
<div class="control-group">
<label class="control-label" for="ns5">{$LANG.domainnameserver5}</label>
<div class="controls">
<input class="input-xlarge domnsinputs" id="ns5" name="ns5" type="text" value="{$ns5}" />
</div>
</div>
<p align="center">
<input type="submit" class="btn btn-primary btn-large" value="{$LANG.changenameservers}" />
</p>
</fieldset>
{elseif $update eq "autorenew"}
{if $save}
<div class="alert alert-success">
<p>
{$LANG.changessavedsuccessfully}
</p>
</div>
{/if}
<p>
{$LANG.domainautorenewinfo}
</p>
<br />
<p>
{$LANG.domainautorenewrecommend}
</p>
<br />
<p>
{$LANG.domainbulkmanagementchangeaffect}
</p>
<br />
<blockquote>
<br />
{foreach from=$domains item=domain}
» {$domain}<br />
{/foreach}
<br />
</blockquote>
<br />
<p align="center">
<input type="submit" name="enable" value="{$LANG.domainsautorenewenable}" class="btn btn-success btn-large" /> <input type="submit" name="disable" value="{$LANG.domainsautorenewdisable}" class="btn btn-danger btn-large" />
</p>
{elseif $update eq "reglock"}
{if $save}
{if $errors}
<div class="alert alert-error">
<p class="bold">
{$LANG.clientareaerrors}
</p>
<ul>
{foreach from=$errors item=error}
<li>{$error}</li>
{/foreach}
</ul>
</div>
{else}
<div class="alert alert-success">
<p>
{$LANG.changessavedsuccessfully}
</p>
</div>
{/if}
{/if}
<p>
{$LANG.domainreglockinfo}
</p>
<br />
<p>
{$LANG.domainreglockrecommend}
</p>
<br />
<p>
{$LANG.domainbulkmanagementchangeaffect}
</p>
<blockquote>
<br />
{foreach from=$domains item=domain}
» {$domain}<br />
{/foreach}
<br />
</blockquote>
<br />
<p align="center">
<input type="submit" name="enable" value="{$LANG.domainreglockenable}" class="btn btn-success btn-large" /> <input type="submit" name="disable" value="{$LANG.domainreglockdisable}" class="btn btn-danger btn-large" />
</p>
{elseif $update eq "contactinfo"}
{if $save}
{if $errors}
<div class="alert alert-error">
<p class="bold">
{$LANG.clientareaerrors}
</p>
<ul>
{foreach from=$errors item=error}
<li>{$error}</li>
{/foreach}
</ul>
</div>
{else}
<div class="alert alert-success">
<p>
{$LANG.changessavedsuccessfully}
</p>
</div>
{/if}
{/if}
{literal}
<script language="javascript">
function usedefaultwhois(id) {
jQuery("."+id.substr(0,id.length-1)+"customwhois").attr("disabled", true);
jQuery("."+id.substr(0,id.length-1)+"defaultwhois").attr("disabled", false);
jQuery('#'+id.substr(0,id.length-1)+'1').attr("checked", "checked");
}
function usecustomwhois(id) {
jQuery("."+id.substr(0,id.length-1)+"customwhois").attr("disabled", false);
jQuery("."+id.substr(0,id.length-1)+"defaultwhois").attr("disabled", true);
jQuery('#'+id.substr(0,id.length-1)+'2').attr("checked", "checked");
}
</script>
{/literal}
<p>
{$LANG.domainbulkmanagementchangesaffect}
</p>
<br />
<blockquote>
{foreach from=$domains item=domain}
» {$domain}<br />
{/foreach}
</blockquote>
{foreach from=$contactdetails name=contactdetails key=contactdetail item=values}
<h3>
<a name="{$contactdetail}"></a>{$contactdetail}</strong>{if $smarty.foreach.contactdetails.first}{foreach from=$contactdetails name=contactsx key=contactdetailx item=valuesx}{if !$smarty.foreach.contactsx.first} - <a href="clientarea.php?action=bulkdomain#{$contactdetailx}">{$LANG.jumpto} {$contactdetailx}</a>{/if}{/foreach}{else} - <a href="clientarea.php?action=bulkdomain#">{$LANG.top}</a>{/if}
</h3>
<p>
</p>
<p>
<label class="full control-label"><input type="radio" class="radio inline" name="wc[{$contactdetail}]" id="{$contactdetail}1" value="contact" onclick="usedefaultwhois(id)" /> {$LANG.domaincontactusexisting}</label>
</p>
<fieldset class="onecol" id="{$contactdetail}defaultwhois">
<div class="control-group">
<label class="control-label" for="{$contactdetail}3">{$LANG.domaincontactchoose}</label>
<div class="controls">
<select class="{$contactdetail}defaultwhois" name="sel[{$contactdetail}]" id="{$contactdetail}3" onclick="usedefaultwhois(id)">
<option value="u{$clientsdetails.userid}">{$LANG.domaincontactprimary}</option>
{foreach key=num item=contact from=$contacts}
<option value="c{$contact.id}">{$contact.name}</option>
{/foreach}
</select>
</div>
</div>
</fieldset>
<p>
<label class="full control-label"><input type="radio" class="radio inline" name="wc[{$contactdetail}]" id="{$contactdetail}2" value="custom" onclick="usecustomwhois(id)" checked /> {$LANG.domaincontactusecustom}</label>
</p>
<fieldset class="onecol" id="{$contactdetail}defaultwhois">
{foreach key=name item=value from=$values}
<div class="control-group">
<label class="control-label" for="{$contactdetail}3">{$name}</label>
<div class="controls">
<input type="text" name="contactdetails[{$contactdetail}][{$name}]" value="{$value}" size="30" class="{$contactdetail}customwhois" />
</div>
</div>
{/foreach}
</fieldset>
{foreachelse}
<div class="alert alert-error">
<p>
{$LANG.domainbulkmanagementnotpossible}
</p>
</div>
{/foreach}
<p align="center">
<input type="submit" value="{$LANG.clientareasavechanges}" class="btn btn-primary" />
</p>
{/if}
<p>
<a href="clientarea.php?action=domains" class="btn btn-default">{$LANG.clientareabacklink}</a>
</p>
<br />
<br />
</form>
| {
"pile_set_name": "Github"
} |
/*
* Wazuh app - GCP sample data
* Copyright (C) 2015-2020 Wazuh, Inc.
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* Find more information about this on the LICENSE file.
*/
// GCP
export const arrayAuthAnswer = ["true", "false"];
export const arrayProtocol = ['UDP', 'TCP'];
export const arrayQueryName = ['185.5.205.124.in-addr.arpa.', '98.72.244.104.in-addr.arpa.', 'mirrors.advancedhosters.com.', '41.212.95.203.in-addr.arpa', '41.212.95.203.in-addr.arpa.'];
export const arrayQueryType = ['PTR', 'PTR', 'PTR', 'PTR', 'PTR', 'A'];
export const arrayResponseCode = ['NXDOMAIN', 'NOERROR', 'WARNING', 'CRITICAL', 'ALERT', 'EMERGENCY', 'SERVFAIL', 'INFO', 'SUCCESS', 'BADTRUNC', 'BADNAME', 'NOTAUTH'];
export const arraySourceIP = ['163.172.0.0', '1.33.213.199', '83.32.0.0', '154.84.246.205', '75.142.129.202', '171.197.217.149', '77.38.119.17'];
export const arrayLocation = ['europe-west1', 'us-central1', 'asia-east1', 'australia-southeast1', 'us-west1', 'us-west3', 'us-west2', 'us-west4', 'us-east1', 'us-east2', 'us-east3', 'southamerica-east1'];
export const arrayProject = ['wazuh-dev', 'wazuh-prod', 'wazuh-test'];
export const arraySourceType = ['gce-vm', 'internet'];
export const arraySeverity = ['ERROR', 'INFO', 'NOTICE', 'CRITICAL', 'EMERGENCY', 'ALERT'];
export const arrayType = ['dns_query', 'app_script_function', 'generic_task'];
export const arrayRules = [{
level: 12,
description: "Unable to process query due to a problem with the name server",
id: "65007",
firedtimes: 2,
mail: true,
groups: ["gcp"]
},
{
level: 5,
description: "GCP notice event",
id: "65001",
firedtimes: 1,
mail: true,
groups: ["gcp"]
},
{
level: 3,
description: "DNS external query",
id: "65032",
firedtimes: 1,
mail: true,
groups: ["gcp"]
},
{
level: 5,
description: "GCP warning event from VM 531339229531.instance-1 with source IP 83.32.0.0 from europe-west1",
id: "65034",
firedtimes: 1,
mail: true,
groups: ["gcp"]
}, {
level: 9,
description: "GCP critical event from VM 531339229531.instance-1 with source IP 83.32.0.0 from europe-west1",
id: "65036",
firedtimes: 4,
mail: true,
groups: ["gcp"]
},
{
level: 11,
description: "GCP alert event from VM 531339229531.instance-1 with source IP 83.32.0.0 from europe-west1",
id: "65037",
firedtimes: 1,
mail: true,
groups: ["gcp"]
},
{
level: 12,
description: "GCP emergency event from VM 531339229531.instance-1 with source IP 83.32.0.0 from europe-west1",
id: "65038",
firedtimes: 2,
mail: true,
groups: ["gcp"]
},
{
level: 5,
description: "GCP notice event with source IP 83.32.0.0 from europe-west1 with response code NXDOMAIN",
id: "65010",
firedtimes: 2,
mail: true,
groups: ["gcp"]
}
];
| {
"pile_set_name": "Github"
} |
/*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*
* Copyright 2011, Blender Foundation.
*/
#include "COM_BrightnessOperation.h"
BrightnessOperation::BrightnessOperation() : NodeOperation()
{
this->addInputSocket(COM_DT_COLOR);
this->addInputSocket(COM_DT_VALUE);
this->addInputSocket(COM_DT_VALUE);
this->addOutputSocket(COM_DT_COLOR);
this->m_inputProgram = NULL;
this->m_use_premultiply = false;
}
void BrightnessOperation::setUsePremultiply(bool use_premultiply)
{
this->m_use_premultiply = use_premultiply;
}
void BrightnessOperation::initExecution()
{
this->m_inputProgram = this->getInputSocketReader(0);
this->m_inputBrightnessProgram = this->getInputSocketReader(1);
this->m_inputContrastProgram = this->getInputSocketReader(2);
}
void BrightnessOperation::executePixelSampled(float output[4],
float x,
float y,
PixelSampler sampler)
{
float inputValue[4];
float a, b;
float inputBrightness[4];
float inputContrast[4];
this->m_inputProgram->readSampled(inputValue, x, y, sampler);
this->m_inputBrightnessProgram->readSampled(inputBrightness, x, y, sampler);
this->m_inputContrastProgram->readSampled(inputContrast, x, y, sampler);
float brightness = inputBrightness[0];
float contrast = inputContrast[0];
brightness /= 100.0f;
float delta = contrast / 200.0f;
/*
* The algorithm is by Werner D. Streidt
* (http://visca.com/ffactory/archives/5-99/msg00021.html)
* Extracted of OpenCV demhist.c
*/
if (contrast > 0) {
a = 1.0f - delta * 2.0f;
a = 1.0f / max_ff(a, FLT_EPSILON);
b = a * (brightness - delta);
}
else {
delta *= -1;
a = max_ff(1.0f - delta * 2.0f, 0.0f);
b = a * brightness + delta;
}
if (this->m_use_premultiply) {
premul_to_straight_v4(inputValue);
}
output[0] = a * inputValue[0] + b;
output[1] = a * inputValue[1] + b;
output[2] = a * inputValue[2] + b;
output[3] = inputValue[3];
if (this->m_use_premultiply) {
straight_to_premul_v4(output);
}
}
void BrightnessOperation::deinitExecution()
{
this->m_inputProgram = NULL;
this->m_inputBrightnessProgram = NULL;
this->m_inputContrastProgram = NULL;
}
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<Project version="2G - 1.7.5" name="{{name}}">
<Target name="Release" isCurrent="1">
<Device manufacturerId="9" manufacturerName="ST" chipId="882" chipName="STM32L053R8T6" boardId="" boardName=""/>
<BuildOption>
<Compile>
<Option name="OptimizationLevel" value="4"/>
<Option name="UseFPU" value="0"/>
<Option name="UserEditCompiler" value="-fno-common; -fmessage-length=0; -Wall; -fno-strict-aliasing; -fno-rtti; -fno-exceptions; -ffunction-sections; -fdata-sections; -std=gnu++98"/>
<Option name="FPU" value="1"/>
<Option name="SupportCPlusplus" value="1"/>
<Includepaths>
{% for path in include_paths %} <Includepath path="{{path}}"/> {% endfor %}
</Includepaths>
<DefinedSymbols>
{% for s in symbols %} <Define name="{{s}}"/> {% endfor %}
</DefinedSymbols>
</Compile>
<Link useDefault="0">
<Option name="DiscardUnusedSection" value="1"/>
<Option name="UserEditLinkder" value=""/>
<Option name="UseMemoryLayout" value="0"/>
<Option name="LTO" value="0"/>
<Option name="IsNewStartupCode" value="1"/>
<Option name="Library" value="Not use C Library"/>
<Option name="nostartfiles" value="0"/>
<Option name="UserEditLinker" value="-Wl,--wrap,main; --specs=nano.specs; -u _printf_float; -u _scanf_float; {% for file in object_files %}
${project.path}/{{file}}; {% endfor %} {% for p in library_paths %}-L${project.path}/{{p}}; {% endfor %}"/>
<LinkedLibraries>
{% for lib in libraries %}
<Libset dir="" libs="{{lib}}"/>
{% endfor %}
<Libset dir="" libs="stdc++"/>
<Libset dir="" libs="supc++"/>
<Libset dir="" libs="m"/>
<Libset dir="" libs="gcc"/>
<Libset dir="" libs="c"/>
<Libset dir="" libs="nosys"/>
</LinkedLibraries>
<MemoryAreas debugInFlashNotRAM="1">
<Memory name="IROM1" type="ReadOnly" size="0x00010000" startValue="0x08000000"/>
<Memory name="IRAM1" type="ReadWrite" size="0x00001F40" startValue="0x200000C0"/>
<Memory name="IROM2" type="ReadOnly" size="" startValue=""/>
<Memory name="IRAM2" type="ReadWrite" size="" startValue=""/>
</MemoryAreas>
<LocateLinkFile path="{{scatter_file}}" type="0"/>
</Link>
<Output>
<Option name="OutputFileType" value="0"/>
<Option name="Path" value="./"/>
<Option name="Name" value="{{name}}"/>
<Option name="HEX" value="1"/>
<Option name="BIN" value="1"/>
</Output>
<User>
<UserRun name="Run#1" type="Before" checked="0" value=""/>
<UserRun name="Run#1" type="After" checked="0" value=""/>
</User>
</BuildOption>
<DebugOption>
<Option name="org.coocox.codebugger.gdbjtag.core.adapter" value="ST-Link"/>
<Option name="org.coocox.codebugger.gdbjtag.core.debugMode" value="SWD"/>
<Option name="org.coocox.codebugger.gdbjtag.core.clockDiv" value="1M"/>
<Option name="org.coocox.codebugger.gdbjtag.corerunToMain" value="1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.jlinkgdbserver" value=""/>
<Option name="org.coocox.codebugger.gdbjtag.core.userDefineGDBScript" value=""/>
<Option name="org.coocox.codebugger.gdbjtag.core.targetEndianess" value="0"/>
<Option name="org.coocox.codebugger.gdbjtag.core.jlinkResetMode" value="Type 0: Normal"/>
<Option name="org.coocox.codebugger.gdbjtag.core.resetMode" value="SYSRESETREQ"/>
<Option name="org.coocox.codebugger.gdbjtag.core.ifSemihost" value="0"/>
<Option name="org.coocox.codebugger.gdbjtag.core.ifCacheRom" value="1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.ipAddress" value="127.0.0.1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.portNumber" value="2009"/>
<Option name="org.coocox.codebugger.gdbjtag.core.autoDownload" value="1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.verify" value="1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.downloadFuction" value="Erase Effected"/>
<Option name="org.coocox.codebugger.gdbjtag.core.defaultAlgorithm" value="./stm32l0xx_64.elf"/>
</DebugOption>
<ExcludeFile/>
</Target>
<Target name="Debug" isCurrent="0">
<Device manufacturerId="9" manufacturerName="ST" chipId="882" chipName="STM32L053R8T6" boardId="" boardName=""/>
<BuildOption>
<Compile>
<Option name="OptimizationLevel" value="0"/>
<Option name="UseFPU" value="0"/>
<Option name="UserEditCompiler" value="-fno-common; -fmessage-length=0; -Wall; -fno-strict-aliasing; -fno-rtti; -fno-exceptions; -ffunction-sections; -fdata-sections; -std=gnu++98"/>
<Option name="FPU" value="1"/>
<Option name="SupportCPlusplus" value="1"/>
<Includepaths>
{% for path in include_paths %} <Includepath path="{{path}}"/> {% endfor %}
</Includepaths>
<DefinedSymbols>
{% for s in symbols %} <Define name="{{s}}"/> {% endfor %}
</DefinedSymbols>
</Compile>
<Link useDefault="0">
<Option name="DiscardUnusedSection" value="1"/>
<Option name="UserEditLinkder" value=""/>
<Option name="UseMemoryLayout" value="0"/>
<Option name="LTO" value="0"/>
<Option name="IsNewStartupCode" value="1"/>
<Option name="Library" value="Not use C Library"/>
<Option name="nostartfiles" value="0"/>
<Option name="UserEditLinker" value="-Wl,--wrap,main; --specs=nano.specs; -u _printf_float; -u _scanf_float; {% for file in object_files %}
${project.path}/{{file}}; {% endfor %} {% for p in library_paths %}-L${project.path}/{{p}}; {% endfor %}"/>
<LinkedLibraries>
{% for lib in libraries %}
<Libset dir="" libs="{{lib}}"/>
{% endfor %}
<Libset dir="" libs="stdc++"/>
<Libset dir="" libs="supc++"/>
<Libset dir="" libs="m"/>
<Libset dir="" libs="gcc"/>
<Libset dir="" libs="c"/>
<Libset dir="" libs="nosys"/>
</LinkedLibraries>
<MemoryAreas debugInFlashNotRAM="1">
<Memory name="IROM1" type="ReadOnly" size="0x00010000" startValue="0x08000000"/>
<Memory name="IRAM1" type="ReadWrite" size="0x00001F40" startValue="0x200000C0"/>
<Memory name="IROM2" type="ReadOnly" size="" startValue=""/>
<Memory name="IRAM2" type="ReadWrite" size="" startValue=""/>
</MemoryAreas>
<LocateLinkFile path="{{scatter_file}}" type="0"/>
</Link>
<Output>
<Option name="OutputFileType" value="0"/>
<Option name="Path" value="./"/>
<Option name="Name" value="{{name}}"/>
<Option name="HEX" value="1"/>
<Option name="BIN" value="1"/>
</Output>
<User>
<UserRun name="Run#1" type="Before" checked="0" value=""/>
<UserRun name="Run#1" type="After" checked="0" value=""/>
</User>
</BuildOption>
<DebugOption>
<Option name="org.coocox.codebugger.gdbjtag.core.adapter" value="ST-Link"/>
<Option name="org.coocox.codebugger.gdbjtag.core.debugMode" value="SWD"/>
<Option name="org.coocox.codebugger.gdbjtag.core.clockDiv" value="1M"/>
<Option name="org.coocox.codebugger.gdbjtag.corerunToMain" value="1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.jlinkgdbserver" value=""/>
<Option name="org.coocox.codebugger.gdbjtag.core.userDefineGDBScript" value=""/>
<Option name="org.coocox.codebugger.gdbjtag.core.targetEndianess" value="0"/>
<Option name="org.coocox.codebugger.gdbjtag.core.jlinkResetMode" value="Type 0: Normal"/>
<Option name="org.coocox.codebugger.gdbjtag.core.resetMode" value="SYSRESETREQ"/>
<Option name="org.coocox.codebugger.gdbjtag.core.ifSemihost" value="0"/>
<Option name="org.coocox.codebugger.gdbjtag.core.ifCacheRom" value="1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.ipAddress" value="127.0.0.1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.portNumber" value="2009"/>
<Option name="org.coocox.codebugger.gdbjtag.core.autoDownload" value="1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.verify" value="1"/>
<Option name="org.coocox.codebugger.gdbjtag.core.downloadFuction" value="Erase Effected"/>
<Option name="org.coocox.codebugger.gdbjtag.core.defaultAlgorithm" value="./stm32l0xx_64.elf"/>
</DebugOption>
<ExcludeFile/>
</Target>
<Components path="./"/>
<Files>
{% for file in source_files %}
<File name="sources/{{file.path}}" path="{{file.path}}" type="{{file.type}}"/>
{% endfor %}
{% for file in header_files %}
<File name="headers/{{file.path}}" path="{{file.path}}" type="{{file.type}}"/>
{% endfor %}
</Files>
</Project>
| {
"pile_set_name": "Github"
} |
package code;
/*
* 202. Happy Number
* 题意:各位置上的数字循环求平方和,如果可以等于1,则为Happy Number
* 难度:Easy
* 分类:Hash Table, Math
* 思路:如果不会等于1,则会产生循环,用hashset记录之前是否出现过
* 也可利用判断链表是否有环的思路,一个计算一步,一个计算两步,看是会相等来判断
* Tips:
*/
import java.util.HashSet;
public class lc202 {
public boolean isHappy(int n) {
HashSet hs = new HashSet<Integer>();
hs.add(n);
int sum = 0;
while(true){
sum = 0;
while(n!=0){
sum += Math.pow(n%10,2);
n = n/10;
}
if(sum==1) return true;
else if(hs.contains(sum)) break;
hs.add(sum);
n = sum;
System.out.println(sum);
}
return false;
}
}
| {
"pile_set_name": "Github"
} |
--TEST--
\PHPUnit\Framework\MockObject\Generator::generateClassFromWsdl('3530.wsdl', 'Test')
--SKIPIF--
<?php declare(strict_types=1);
if (!extension_loaded('soap')) echo 'skip: SOAP extension is required';
--FILE--
<?php declare(strict_types=1);
require __DIR__ . '/../../../../vendor/autoload.php';
$generator = new \PHPUnit\Framework\MockObject\Generator;
print $generator->generateClassFromWsdl(
__DIR__ . '/../../../_files/3530.wsdl',
'Test'
);
--EXPECTF--
class Test extends \SoapClient
{
public function __construct($wsdl, array $options)
{
parent::__construct('%s/3530.wsdl', $options);
}
public function Contact_Information($Contact_Id)
{
}
}
| {
"pile_set_name": "Github"
} |
/* Copyright libuv project contributors. All rights reserved.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to
* deal in the Software without restriction, including without limitation the
* rights to use, copy, modify, merge, publish, distribute, sublicense, and/or
* sell copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
* IN THE SOFTWARE.
*/
#include "uv.h"
#include "task.h"
#include <stdlib.h>
#include <stdio.h>
/* Run the benchmark for this many ms */
#define TIME 5000
typedef struct {
int pongs;
int state;
uv_udp_t udp;
struct sockaddr_in server_addr;
} pinger_t;
typedef struct buf_s {
uv_buf_t uv_buf_t;
struct buf_s* next;
} buf_t;
static char PING[] = "PING\n";
static uv_loop_t* loop;
static int completed_pingers;
static unsigned long completed_pings;
static int64_t start_time;
static void buf_alloc(uv_handle_t* tcp, size_t size, uv_buf_t* buf) {
static char slab[64 * 1024];
buf->base = slab;
buf->len = sizeof(slab);
}
static void buf_free(const uv_buf_t* buf) {
}
static void pinger_close_cb(uv_handle_t* handle) {
pinger_t* pinger;
pinger = (pinger_t*)handle->data;
#if DEBUG
fprintf(stderr, "ping_pongs: %d roundtrips/s\n",
pinger->pongs / (TIME / 1000));
#endif
completed_pings += pinger->pongs;
completed_pingers++;
free(pinger);
}
static void pinger_write_ping(pinger_t* pinger) {
uv_buf_t buf;
int r;
buf = uv_buf_init(PING, sizeof(PING) - 1);
r = uv_udp_try_send(&pinger->udp, &buf, 1,
(const struct sockaddr*) &pinger->server_addr);
if (r < 0)
FATAL("uv_udp_send failed");
}
static void pinger_read_cb(uv_udp_t* udp,
ssize_t nread,
const uv_buf_t* buf,
const struct sockaddr* addr,
unsigned flags) {
ssize_t i;
pinger_t* pinger;
pinger = (pinger_t*)udp->data;
/* Now we count the pings */
for (i = 0; i < nread; i++) {
ASSERT(buf->base[i] == PING[pinger->state]);
pinger->state = (pinger->state + 1) % (sizeof(PING) - 1);
if (pinger->state == 0) {
pinger->pongs++;
if (uv_now(loop) - start_time > TIME) {
uv_close((uv_handle_t*)udp, pinger_close_cb);
break;
}
pinger_write_ping(pinger);
}
}
buf_free(buf);
}
static void udp_pinger_new(void) {
pinger_t* pinger = malloc(sizeof(*pinger));
int r;
ASSERT(0 == uv_ip4_addr("127.0.0.1", TEST_PORT, &pinger->server_addr));
pinger->state = 0;
pinger->pongs = 0;
/* Try to do NUM_PINGS ping-pongs (connection-less). */
r = uv_udp_init(loop, &pinger->udp);
ASSERT(r == 0);
pinger->udp.data = pinger;
/* Start pinging */
if (0 != uv_udp_recv_start(&pinger->udp, buf_alloc, pinger_read_cb)) {
FATAL("uv_udp_read_start failed");
}
pinger_write_ping(pinger);
}
static int ping_udp(unsigned pingers) {
unsigned i;
loop = uv_default_loop();
start_time = uv_now(loop);
for (i = 0; i < pingers; ++i) {
udp_pinger_new();
}
uv_run(loop, UV_RUN_DEFAULT);
ASSERT(completed_pingers >= 1);
fprintf(stderr, "ping_pongs: %d pingers, ~ %lu roundtrips/s\n",
completed_pingers, completed_pings / (TIME/1000));
MAKE_VALGRIND_HAPPY();
return 0;
}
#define X(PINGERS) \
BENCHMARK_IMPL(ping_udp##PINGERS) {\
return ping_udp(PINGERS); \
}
X(1)
X(10)
X(100)
#undef X
| {
"pile_set_name": "Github"
} |
/*
* Table styles
*/
table.dataTable {
width: 100%;
margin: 0 auto;
clear: both;
border-collapse: separate;
border-spacing: 0;
/*
* Header and footer styles
*/
/*
* Body styles
*/
}
table.dataTable thead th,
table.dataTable tfoot th {
font-weight: bold;
}
table.dataTable thead th,
table.dataTable thead td {
padding: 10px 18px;
border-bottom: 1px solid #111;
}
table.dataTable thead th:active,
table.dataTable thead td:active {
outline: none;
}
table.dataTable tfoot th,
table.dataTable tfoot td {
padding: 10px 18px 6px 18px;
border-top: 1px solid #111;
}
table.dataTable thead .sorting,
table.dataTable thead .sorting_asc,
table.dataTable thead .sorting_desc {
cursor: pointer;
*cursor: hand;
}
table.dataTable thead .sorting,
table.dataTable thead .sorting_asc,
table.dataTable thead .sorting_desc,
table.dataTable thead .sorting_asc_disabled,
table.dataTable thead .sorting_desc_disabled {
background-repeat: no-repeat;
background-position: center right;
}
table.dataTable thead .sorting {
background-image: url("images/sort_both.png");
}
table.dataTable thead .sorting_asc {
background-image: url("images/sort_asc.png");
}
table.dataTable thead .sorting_desc {
background-image: url("images/sort_desc.png");
}
table.dataTable thead .sorting_asc_disabled {
background-image: url("images/sort_asc_disabled.png");
}
table.dataTable thead .sorting_desc_disabled {
background-image: url("images/sort_desc_disabled.png");
}
table.dataTable tbody tr {
background-color: #ffffff;
}
table.dataTable tbody tr.selected {
background-color: #B0BED9;
}
table.dataTable tbody th,
table.dataTable tbody td {
padding: 8px 10px;
}
table.dataTable.row-border tbody th, table.dataTable.row-border tbody td, table.dataTable.display tbody th, table.dataTable.display tbody td {
border-top: 1px solid #ddd;
}
table.dataTable.row-border tbody tr:first-child th,
table.dataTable.row-border tbody tr:first-child td, table.dataTable.display tbody tr:first-child th,
table.dataTable.display tbody tr:first-child td {
border-top: none;
}
table.dataTable.cell-border tbody th, table.dataTable.cell-border tbody td {
border-top: 1px solid #ddd;
border-right: 1px solid #ddd;
}
table.dataTable.cell-border tbody tr th:first-child,
table.dataTable.cell-border tbody tr td:first-child {
border-left: 1px solid #ddd;
}
table.dataTable.cell-border tbody tr:first-child th,
table.dataTable.cell-border tbody tr:first-child td {
border-top: none;
}
table.dataTable.stripe tbody tr.odd, table.dataTable.display tbody tr.odd {
background-color: #f9f9f9;
}
table.dataTable.stripe tbody tr.odd.selected, table.dataTable.display tbody tr.odd.selected {
background-color: #abb9d3;
}
table.dataTable.hover tbody tr:hover, table.dataTable.display tbody tr:hover {
background-color: whitesmoke;
}
table.dataTable.hover tbody tr:hover.selected, table.dataTable.display tbody tr:hover.selected {
background-color: #a9b7d1;
}
table.dataTable.order-column tbody tr > .sorting_1,
table.dataTable.order-column tbody tr > .sorting_2,
table.dataTable.order-column tbody tr > .sorting_3, table.dataTable.display tbody tr > .sorting_1,
table.dataTable.display tbody tr > .sorting_2,
table.dataTable.display tbody tr > .sorting_3 {
background-color: #f9f9f9;
}
table.dataTable.order-column tbody tr.selected > .sorting_1,
table.dataTable.order-column tbody tr.selected > .sorting_2,
table.dataTable.order-column tbody tr.selected > .sorting_3, table.dataTable.display tbody tr.selected > .sorting_1,
table.dataTable.display tbody tr.selected > .sorting_2,
table.dataTable.display tbody tr.selected > .sorting_3 {
background-color: #acbad4;
}
table.dataTable.display tbody tr.odd > .sorting_1, table.dataTable.order-column.stripe tbody tr.odd > .sorting_1 {
background-color: #f1f1f1;
}
table.dataTable.display tbody tr.odd > .sorting_2, table.dataTable.order-column.stripe tbody tr.odd > .sorting_2 {
background-color: #f3f3f3;
}
table.dataTable.display tbody tr.odd > .sorting_3, table.dataTable.order-column.stripe tbody tr.odd > .sorting_3 {
background-color: whitesmoke;
}
table.dataTable.display tbody tr.odd.selected > .sorting_1, table.dataTable.order-column.stripe tbody tr.odd.selected > .sorting_1 {
background-color: #a6b3cd;
}
table.dataTable.display tbody tr.odd.selected > .sorting_2, table.dataTable.order-column.stripe tbody tr.odd.selected > .sorting_2 {
background-color: #a7b5ce;
}
table.dataTable.display tbody tr.odd.selected > .sorting_3, table.dataTable.order-column.stripe tbody tr.odd.selected > .sorting_3 {
background-color: #a9b6d0;
}
table.dataTable.display tbody tr.even > .sorting_1, table.dataTable.order-column.stripe tbody tr.even > .sorting_1 {
background-color: #f9f9f9;
}
table.dataTable.display tbody tr.even > .sorting_2, table.dataTable.order-column.stripe tbody tr.even > .sorting_2 {
background-color: #fbfbfb;
}
table.dataTable.display tbody tr.even > .sorting_3, table.dataTable.order-column.stripe tbody tr.even > .sorting_3 {
background-color: #fdfdfd;
}
table.dataTable.display tbody tr.even.selected > .sorting_1, table.dataTable.order-column.stripe tbody tr.even.selected > .sorting_1 {
background-color: #acbad4;
}
table.dataTable.display tbody tr.even.selected > .sorting_2, table.dataTable.order-column.stripe tbody tr.even.selected > .sorting_2 {
background-color: #adbbd6;
}
table.dataTable.display tbody tr.even.selected > .sorting_3, table.dataTable.order-column.stripe tbody tr.even.selected > .sorting_3 {
background-color: #afbdd8;
}
table.dataTable.display tbody tr:hover > .sorting_1, table.dataTable.order-column.hover tbody tr:hover > .sorting_1 {
background-color: #eaeaea;
}
table.dataTable.display tbody tr:hover > .sorting_2, table.dataTable.order-column.hover tbody tr:hover > .sorting_2 {
background-color: #ebebeb;
}
table.dataTable.display tbody tr:hover > .sorting_3, table.dataTable.order-column.hover tbody tr:hover > .sorting_3 {
background-color: #eeeeee;
}
table.dataTable.display tbody tr:hover.selected > .sorting_1, table.dataTable.order-column.hover tbody tr:hover.selected > .sorting_1 {
background-color: #a1aec7;
}
table.dataTable.display tbody tr:hover.selected > .sorting_2, table.dataTable.order-column.hover tbody tr:hover.selected > .sorting_2 {
background-color: #a2afc8;
}
table.dataTable.display tbody tr:hover.selected > .sorting_3, table.dataTable.order-column.hover tbody tr:hover.selected > .sorting_3 {
background-color: #a4b2cb;
}
table.dataTable.no-footer {
border-bottom: 1px solid #111;
}
table.dataTable.nowrap th, table.dataTable.nowrap td {
white-space: nowrap;
}
table.dataTable.compact thead th,
table.dataTable.compact thead td {
padding: 4px 17px 4px 4px;
}
table.dataTable.compact tfoot th,
table.dataTable.compact tfoot td {
padding: 4px;
}
table.dataTable.compact tbody th,
table.dataTable.compact tbody td {
padding: 4px;
}
table.dataTable th.dt-left,
table.dataTable td.dt-left {
text-align: left;
}
table.dataTable th.dt-center,
table.dataTable td.dt-center,
table.dataTable td.dataTables_empty {
text-align: center;
}
table.dataTable th.dt-right,
table.dataTable td.dt-right {
text-align: right;
}
table.dataTable th.dt-justify,
table.dataTable td.dt-justify {
text-align: justify;
}
table.dataTable th.dt-nowrap,
table.dataTable td.dt-nowrap {
white-space: nowrap;
}
table.dataTable thead th.dt-head-left,
table.dataTable thead td.dt-head-left,
table.dataTable tfoot th.dt-head-left,
table.dataTable tfoot td.dt-head-left {
text-align: left;
}
table.dataTable thead th.dt-head-center,
table.dataTable thead td.dt-head-center,
table.dataTable tfoot th.dt-head-center,
table.dataTable tfoot td.dt-head-center {
text-align: center;
}
table.dataTable thead th.dt-head-right,
table.dataTable thead td.dt-head-right,
table.dataTable tfoot th.dt-head-right,
table.dataTable tfoot td.dt-head-right {
text-align: right;
}
table.dataTable thead th.dt-head-justify,
table.dataTable thead td.dt-head-justify,
table.dataTable tfoot th.dt-head-justify,
table.dataTable tfoot td.dt-head-justify {
text-align: justify;
}
table.dataTable thead th.dt-head-nowrap,
table.dataTable thead td.dt-head-nowrap,
table.dataTable tfoot th.dt-head-nowrap,
table.dataTable tfoot td.dt-head-nowrap {
white-space: nowrap;
}
table.dataTable tbody th.dt-body-left,
table.dataTable tbody td.dt-body-left {
text-align: left;
}
table.dataTable tbody th.dt-body-center,
table.dataTable tbody td.dt-body-center {
text-align: center;
}
table.dataTable tbody th.dt-body-right,
table.dataTable tbody td.dt-body-right {
text-align: right;
}
table.dataTable tbody th.dt-body-justify,
table.dataTable tbody td.dt-body-justify {
text-align: justify;
}
table.dataTable tbody th.dt-body-nowrap,
table.dataTable tbody td.dt-body-nowrap {
white-space: nowrap;
}
table.dataTable,
table.dataTable th,
table.dataTable td {
-webkit-box-sizing: content-box;
-moz-box-sizing: content-box;
box-sizing: content-box;
}
/*
* Control feature layout
*/
.dataTables_wrapper {
position: relative;
clear: both;
*zoom: 1;
zoom: 1;
}
.dataTables_wrapper .dataTables_length {
float: left;
}
.dataTables_wrapper .dataTables_filter {
float: right;
text-align: right;
}
.dataTables_wrapper .dataTables_filter input {
margin-left: 0.5em;
}
.dataTables_wrapper .dataTables_info {
clear: both;
float: left;
padding-top: 0.755em;
}
.dataTables_wrapper .dataTables_paginate {
float: right;
text-align: right;
padding-top: 0.25em;
}
.dataTables_wrapper .dataTables_paginate .paginate_button {
box-sizing: border-box;
display: inline-block;
min-width: 1.5em;
padding: 0.5em 1em;
margin-left: 2px;
text-align: center;
text-decoration: none !important;
cursor: pointer;
*cursor: hand;
color: #333 !important;
border: 1px solid transparent;
}
.dataTables_wrapper .dataTables_paginate .paginate_button.current, .dataTables_wrapper .dataTables_paginate .paginate_button.current:hover {
color: #333 !important;
border: 1px solid #cacaca;
background-color: white;
background: -webkit-gradient(linear, left top, left bottom, color-stop(0%, white), color-stop(100%, #dcdcdc));
/* Chrome,Safari4+ */
background: -webkit-linear-gradient(top, white 0%, #dcdcdc 100%);
/* Chrome10+,Safari5.1+ */
background: -moz-linear-gradient(top, white 0%, #dcdcdc 100%);
/* FF3.6+ */
background: -ms-linear-gradient(top, white 0%, #dcdcdc 100%);
/* IE10+ */
background: -o-linear-gradient(top, white 0%, #dcdcdc 100%);
/* Opera 11.10+ */
background: linear-gradient(to bottom, white 0%, #dcdcdc 100%);
/* W3C */
}
.dataTables_wrapper .dataTables_paginate .paginate_button.disabled, .dataTables_wrapper .dataTables_paginate .paginate_button.disabled:hover, .dataTables_wrapper .dataTables_paginate .paginate_button.disabled:active {
cursor: default;
color: #666 !important;
border: 1px solid transparent;
background: transparent;
box-shadow: none;
}
.dataTables_wrapper .dataTables_paginate .paginate_button:hover {
color: white !important;
border: 1px solid #111;
background-color: #585858;
background: -webkit-gradient(linear, left top, left bottom, color-stop(0%, #585858), color-stop(100%, #111));
/* Chrome,Safari4+ */
background: -webkit-linear-gradient(top, #585858 0%, #111 100%);
/* Chrome10+,Safari5.1+ */
background: -moz-linear-gradient(top, #585858 0%, #111 100%);
/* FF3.6+ */
background: -ms-linear-gradient(top, #585858 0%, #111 100%);
/* IE10+ */
background: -o-linear-gradient(top, #585858 0%, #111 100%);
/* Opera 11.10+ */
background: linear-gradient(to bottom, #585858 0%, #111 100%);
/* W3C */
}
.dataTables_wrapper .dataTables_paginate .paginate_button:active {
outline: none;
background-color: #2b2b2b;
background: -webkit-gradient(linear, left top, left bottom, color-stop(0%, #2b2b2b), color-stop(100%, #0c0c0c));
/* Chrome,Safari4+ */
background: -webkit-linear-gradient(top, #2b2b2b 0%, #0c0c0c 100%);
/* Chrome10+,Safari5.1+ */
background: -moz-linear-gradient(top, #2b2b2b 0%, #0c0c0c 100%);
/* FF3.6+ */
background: -ms-linear-gradient(top, #2b2b2b 0%, #0c0c0c 100%);
/* IE10+ */
background: -o-linear-gradient(top, #2b2b2b 0%, #0c0c0c 100%);
/* Opera 11.10+ */
background: linear-gradient(to bottom, #2b2b2b 0%, #0c0c0c 100%);
/* W3C */
box-shadow: inset 0 0 3px #111;
}
.dataTables_wrapper .dataTables_paginate .ellipsis {
padding: 0 1em;
}
.dataTables_wrapper .dataTables_processing {
position: absolute;
top: 50%;
left: 50%;
width: 100%;
height: 40px;
margin-left: -50%;
margin-top: -25px;
padding-top: 20px;
text-align: center;
font-size: 1.2em;
background-color: white;
background: -webkit-gradient(linear, left top, right top, color-stop(0%, rgba(255, 255, 255, 0)), color-stop(25%, rgba(255, 255, 255, 0.9)), color-stop(75%, rgba(255, 255, 255, 0.9)), color-stop(100%, rgba(255, 255, 255, 0)));
/* Chrome,Safari4+ */
background: -webkit-linear-gradient(left, rgba(255, 255, 255, 0) 0%, rgba(255, 255, 255, 0.9) 25%, rgba(255, 255, 255, 0.9) 75%, rgba(255, 255, 255, 0) 100%);
/* Chrome10+,Safari5.1+ */
background: -moz-linear-gradient(left, rgba(255, 255, 255, 0) 0%, rgba(255, 255, 255, 0.9) 25%, rgba(255, 255, 255, 0.9) 75%, rgba(255, 255, 255, 0) 100%);
/* FF3.6+ */
background: -ms-linear-gradient(left, rgba(255, 255, 255, 0) 0%, rgba(255, 255, 255, 0.9) 25%, rgba(255, 255, 255, 0.9) 75%, rgba(255, 255, 255, 0) 100%);
/* IE10+ */
background: -o-linear-gradient(left, rgba(255, 255, 255, 0) 0%, rgba(255, 255, 255, 0.9) 25%, rgba(255, 255, 255, 0.9) 75%, rgba(255, 255, 255, 0) 100%);
/* Opera 11.10+ */
background: linear-gradient(to right, rgba(255, 255, 255, 0) 0%, rgba(255, 255, 255, 0.9) 25%, rgba(255, 255, 255, 0.9) 75%, rgba(255, 255, 255, 0) 100%);
/* W3C */
}
.dataTables_wrapper .dataTables_length,
.dataTables_wrapper .dataTables_filter,
.dataTables_wrapper .dataTables_info,
.dataTables_wrapper .dataTables_processing,
.dataTables_wrapper .dataTables_paginate {
color: #333;
}
.dataTables_wrapper .dataTables_scroll {
clear: both;
}
.dataTables_wrapper .dataTables_scroll div.dataTables_scrollBody {
*margin-top: -1px;
-webkit-overflow-scrolling: touch;
}
.dataTables_wrapper .dataTables_scroll div.dataTables_scrollBody th > div.dataTables_sizing,
.dataTables_wrapper .dataTables_scroll div.dataTables_scrollBody td > div.dataTables_sizing {
height: 0;
overflow: hidden;
margin: 0 !important;
padding: 0 !important;
}
.dataTables_wrapper.no-footer .dataTables_scrollBody {
border-bottom: 1px solid #111;
}
.dataTables_wrapper.no-footer div.dataTables_scrollHead table,
.dataTables_wrapper.no-footer div.dataTables_scrollBody table {
border-bottom: none;
}
.dataTables_wrapper:after {
visibility: hidden;
display: block;
content: "";
clear: both;
height: 0;
}
@media screen and (max-width: 767px) {
.dataTables_wrapper .dataTables_info,
.dataTables_wrapper .dataTables_paginate {
float: none;
text-align: center;
}
.dataTables_wrapper .dataTables_paginate {
margin-top: 0.5em;
}
}
@media screen and (max-width: 640px) {
.dataTables_wrapper .dataTables_length,
.dataTables_wrapper .dataTables_filter {
float: none;
text-align: center;
}
.dataTables_wrapper .dataTables_filter {
margin-top: 0.5em;
}
}
| {
"pile_set_name": "Github"
} |
瞳最新番号
【SQTE-192】S-Cute制服エッチコレクション 8時間
【AMGZ-056】寝トリ寝トラレ vol.7 巨乳むっちむちパイズリ奉仕!糸引き名器マ○コ変態人妻!巨乳不倫妻厳選5人
【RVG-043】AV女優が撮影現場に来たらいきなりSEX BEST vol.1
【SQTE-095】S-Cute レズリレー 白咲碧 瞳 有本沙世 彩城ゆりな
【CNZ-022】スレンダージュニアのイク瞬間 4時間
【SQTE-089】S-Cute 女の子ランキング 2015 TOP10
【IRCP-020】フェラガール ホットカプセル5
【SQTE-065】卒業Memories 瞳
【SQTE-060】S-Cute 女の子ランキング 2014 TOP10
【PSD-918】日本女性のアナル 50人6時間
【NEO-335】女子校生のおいしいオシッコ 放尿・よだれ・唾・鼻水・マン汁 女子校生ホルモン 4
【SQTE-053】S-Cute 年間売上ランキング2013 TOP30
【SS-026】AV女優が撮影現場に来たらいきなりSEX 即ハメ 生中出し
【SERO-209】巨根ハメまくりFUCK 瞳
【SQTE-049】S-Cute Girls 瞳 保坂えり 朝倉ことみ
【IRCP-005】ジュニアぺっと2 瞳
【EKDV-316】INSTANT LOVE 43
【GG-207】出会い系サイトで知り合った素人娘 ひとみ
【HAVD-861】初めてのレズロリ温泉 ~ママには内緒のネコとタチ~ 南梨央奈×瞳
【CND-036】初体験!キモメンさんたちと楽しいセックス 瞳
【UFD-038】白衣の天使と性交 瞳</a>2013-05-17ドリームチケット$$$ドリームチケット123分钟 | {
"pile_set_name": "Github"
} |
# Copyright (c) Facebook, Inc. and its affiliates.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
from thrift.py3.server import ServiceInterface
class CppHandler(ServiceInterface):
def __init__(self) -> None: ...
| {
"pile_set_name": "Github"
} |
//=======================================================================
// Copyright 2007 Aaron Windsor
//
// Distributed under the Boost Software License, Version 1.0. (See
// accompanying file LICENSE_1_0.txt or copy at
// http://www.boost.org/LICENSE_1_0.txt)
//=======================================================================
#ifndef __IS_KURATOWSKI_SUBGRAPH_HPP__
#define __IS_KURATOWSKI_SUBGRAPH_HPP__
#include <boost/config.hpp>
#include <boost/tuple/tuple.hpp> //for tie
#include <boost/property_map/property_map.hpp>
#include <boost/graph/properties.hpp>
#include <boost/graph/isomorphism.hpp>
#include <boost/graph/adjacency_list.hpp>
#include <algorithm>
#include <vector>
#include <set>
namespace boost
{
namespace detail
{
template <typename Graph>
Graph make_K_5()
{
typename graph_traits<Graph>::vertex_iterator vi, vi_end, inner_vi;
Graph K_5(5);
for(boost::tie(vi,vi_end) = vertices(K_5); vi != vi_end; ++vi)
for(inner_vi = next(vi); inner_vi != vi_end; ++inner_vi)
add_edge(*vi, *inner_vi, K_5);
return K_5;
}
template <typename Graph>
Graph make_K_3_3()
{
typename graph_traits<Graph>::vertex_iterator
vi, vi_end, bipartition_start, inner_vi;
Graph K_3_3(6);
bipartition_start = next(next(next(vertices(K_3_3).first)));
for(boost::tie(vi, vi_end) = vertices(K_3_3); vi != bipartition_start; ++vi)
for(inner_vi= bipartition_start; inner_vi != vi_end; ++inner_vi)
add_edge(*vi, *inner_vi, K_3_3);
return K_3_3;
}
template <typename AdjacencyList, typename Vertex>
void contract_edge(AdjacencyList& neighbors, Vertex u, Vertex v)
{
// Remove u from v's neighbor list
neighbors[v].erase(std::remove(neighbors[v].begin(),
neighbors[v].end(), u
),
neighbors[v].end()
);
// Replace any references to u with references to v
typedef typename AdjacencyList::value_type::iterator
adjacency_iterator_t;
adjacency_iterator_t u_neighbor_end = neighbors[u].end();
for(adjacency_iterator_t u_neighbor_itr = neighbors[u].begin();
u_neighbor_itr != u_neighbor_end; ++u_neighbor_itr
)
{
Vertex u_neighbor(*u_neighbor_itr);
std::replace(neighbors[u_neighbor].begin(),
neighbors[u_neighbor].end(), u, v
);
}
// Remove v from u's neighbor list
neighbors[u].erase(std::remove(neighbors[u].begin(),
neighbors[u].end(), v
),
neighbors[u].end()
);
// Add everything in u's neighbor list to v's neighbor list
std::copy(neighbors[u].begin(),
neighbors[u].end(),
std::back_inserter(neighbors[v])
);
// Clear u's neighbor list
neighbors[u].clear();
}
enum target_graph_t { tg_k_3_3, tg_k_5};
} // namespace detail
template <typename Graph, typename ForwardIterator, typename VertexIndexMap>
bool is_kuratowski_subgraph(const Graph& g,
ForwardIterator begin,
ForwardIterator end,
VertexIndexMap vm
)
{
typedef typename graph_traits<Graph>::vertex_descriptor vertex_t;
typedef typename graph_traits<Graph>::vertex_iterator vertex_iterator_t;
typedef typename graph_traits<Graph>::edge_descriptor edge_t;
typedef typename graph_traits<Graph>::edges_size_type e_size_t;
typedef typename graph_traits<Graph>::vertices_size_type v_size_t;
typedef typename std::vector<vertex_t> v_list_t;
typedef typename v_list_t::iterator v_list_iterator_t;
typedef iterator_property_map
<typename std::vector<v_list_t>::iterator, VertexIndexMap>
vertex_to_v_list_map_t;
typedef adjacency_list<vecS, vecS, undirectedS> small_graph_t;
detail::target_graph_t target_graph = detail::tg_k_3_3; //unless we decide otherwise later
static small_graph_t K_5(detail::make_K_5<small_graph_t>());
static small_graph_t K_3_3(detail::make_K_3_3<small_graph_t>());
v_size_t n_vertices(num_vertices(g));
v_size_t max_num_edges(3*n_vertices - 5);
std::vector<v_list_t> neighbors_vector(n_vertices);
vertex_to_v_list_map_t neighbors(neighbors_vector.begin(), vm);
e_size_t count = 0;
for(ForwardIterator itr = begin; itr != end; ++itr)
{
if (count++ > max_num_edges)
return false;
edge_t e(*itr);
vertex_t u(source(e,g));
vertex_t v(target(e,g));
neighbors[u].push_back(v);
neighbors[v].push_back(u);
}
for(v_size_t max_size = 2; max_size < 5; ++max_size)
{
vertex_iterator_t vi, vi_end;
for(boost::tie(vi,vi_end) = vertices(g); vi != vi_end; ++vi)
{
vertex_t v(*vi);
//a hack to make sure we don't contract the middle edge of a path
//of four degree-3 vertices
if (max_size == 4 && neighbors[v].size() == 3)
{
if (neighbors[neighbors[v][0]].size() +
neighbors[neighbors[v][1]].size() +
neighbors[neighbors[v][2]].size()
< 11 // so, it has two degree-3 neighbors
)
continue;
}
while (neighbors[v].size() > 0 && neighbors[v].size() < max_size)
{
// Find one of v's neighbors u such that v and u
// have no neighbors in common. We'll look for such a
// neighbor with a naive cubic-time algorithm since the
// max size of any of the neighbor sets we'll consider
// merging is 3
bool neighbor_sets_intersect = false;
vertex_t min_u = graph_traits<Graph>::null_vertex();
vertex_t u;
v_list_iterator_t v_neighbor_end = neighbors[v].end();
for(v_list_iterator_t v_neighbor_itr = neighbors[v].begin();
v_neighbor_itr != v_neighbor_end;
++v_neighbor_itr
)
{
neighbor_sets_intersect = false;
u = *v_neighbor_itr;
v_list_iterator_t u_neighbor_end = neighbors[u].end();
for(v_list_iterator_t u_neighbor_itr =
neighbors[u].begin();
u_neighbor_itr != u_neighbor_end &&
!neighbor_sets_intersect;
++u_neighbor_itr
)
{
for(v_list_iterator_t inner_v_neighbor_itr =
neighbors[v].begin();
inner_v_neighbor_itr != v_neighbor_end;
++inner_v_neighbor_itr
)
{
if (*u_neighbor_itr == *inner_v_neighbor_itr)
{
neighbor_sets_intersect = true;
break;
}
}
}
if (!neighbor_sets_intersect &&
(min_u == graph_traits<Graph>::null_vertex() ||
neighbors[u].size() < neighbors[min_u].size())
)
{
min_u = u;
}
}
if (min_u == graph_traits<Graph>::null_vertex())
// Exited the loop without finding an appropriate neighbor of
// v, so v must be a lost cause. Move on to other vertices.
break;
else
u = min_u;
detail::contract_edge(neighbors, u, v);
}//end iteration over v's neighbors
}//end iteration through vertices v
if (max_size == 3)
{
// check to see whether we should go on to find a K_5
for(boost::tie(vi,vi_end) = vertices(g); vi != vi_end; ++vi)
if (neighbors[*vi].size() == 4)
{
target_graph = detail::tg_k_5;
break;
}
if (target_graph == detail::tg_k_3_3)
break;
}
}//end iteration through max degree 2,3, and 4
//Now, there should only be 5 or 6 vertices with any neighbors. Find them.
v_list_t main_vertices;
vertex_iterator_t vi, vi_end;
for(boost::tie(vi,vi_end) = vertices(g); vi != vi_end; ++vi)
{
if (!neighbors[*vi].empty())
main_vertices.push_back(*vi);
}
// create a graph isomorphic to the contracted graph to test
// against K_5 and K_3_3
small_graph_t contracted_graph(main_vertices.size());
std::map<vertex_t,typename graph_traits<small_graph_t>::vertex_descriptor>
contracted_vertex_map;
typename v_list_t::iterator itr, itr_end;
itr_end = main_vertices.end();
typename graph_traits<small_graph_t>::vertex_iterator
si = vertices(contracted_graph).first;
for(itr = main_vertices.begin(); itr != itr_end; ++itr, ++si)
{
contracted_vertex_map[*itr] = *si;
}
typename v_list_t::iterator jtr, jtr_end;
for(itr = main_vertices.begin(); itr != itr_end; ++itr)
{
jtr_end = neighbors[*itr].end();
for(jtr = neighbors[*itr].begin(); jtr != jtr_end; ++jtr)
{
if (get(vm,*itr) < get(vm,*jtr))
{
add_edge(contracted_vertex_map[*itr],
contracted_vertex_map[*jtr],
contracted_graph
);
}
}
}
if (target_graph == detail::tg_k_5)
{
return boost::isomorphism(K_5,contracted_graph);
}
else //target_graph == tg_k_3_3
{
return boost::isomorphism(K_3_3,contracted_graph);
}
}
template <typename Graph, typename ForwardIterator>
bool is_kuratowski_subgraph(const Graph& g,
ForwardIterator begin,
ForwardIterator end
)
{
return is_kuratowski_subgraph(g, begin, end, get(vertex_index,g));
}
}
#endif //__IS_KURATOWSKI_SUBGRAPH_HPP__
| {
"pile_set_name": "Github"
} |
/* SPDX-License-Identifier: GPL-2.0 */
/*
* hfc_usb.h
*
* $Id: hfc_usb.h,v 1.1.2.5 2007/08/20 14:36:03 mbachem Exp $
*/
#ifndef __HFC_USB_H__
#define __HFC_USB_H__
#define DRIVER_AUTHOR "Peter Sprenger (sprenger@moving-byters.de)"
#define DRIVER_DESC "HFC-S USB based HiSAX ISDN driver"
#define HFC_CTRL_TIMEOUT 20 /* 5ms timeout writing/reading regs */
#define HFC_TIMER_T3 8000 /* timeout for l1 activation timer */
#define HFC_TIMER_T4 500 /* time for state change interval */
#define HFCUSB_L1_STATECHANGE 0 /* L1 state changed */
#define HFCUSB_L1_DRX 1 /* D-frame received */
#define HFCUSB_L1_ERX 2 /* E-frame received */
#define HFCUSB_L1_DTX 4 /* D-frames completed */
#define MAX_BCH_SIZE 2048 /* allowed B-channel packet size */
#define HFCUSB_RX_THRESHOLD 64 /* threshold for fifo report bit rx */
#define HFCUSB_TX_THRESHOLD 64 /* threshold for fifo report bit tx */
#define HFCUSB_CHIP_ID 0x16 /* Chip ID register index */
#define HFCUSB_CIRM 0x00 /* cirm register index */
#define HFCUSB_USB_SIZE 0x07 /* int length register */
#define HFCUSB_USB_SIZE_I 0x06 /* iso length register */
#define HFCUSB_F_CROSS 0x0b /* bit order register */
#define HFCUSB_CLKDEL 0x37 /* bit delay register */
#define HFCUSB_CON_HDLC 0xfa /* channel connect register */
#define HFCUSB_HDLC_PAR 0xfb
#define HFCUSB_SCTRL 0x31 /* S-bus control register (tx) */
#define HFCUSB_SCTRL_E 0x32 /* same for E and special funcs */
#define HFCUSB_SCTRL_R 0x33 /* S-bus control register (rx) */
#define HFCUSB_F_THRES 0x0c /* threshold register */
#define HFCUSB_FIFO 0x0f /* fifo select register */
#define HFCUSB_F_USAGE 0x1a /* fifo usage register */
#define HFCUSB_MST_MODE0 0x14
#define HFCUSB_MST_MODE1 0x15
#define HFCUSB_P_DATA 0x1f
#define HFCUSB_INC_RES_F 0x0e
#define HFCUSB_STATES 0x30
#define HFCUSB_CHIPID 0x40 /* ID value of HFC-S USB */
/* fifo registers */
#define HFCUSB_NUM_FIFOS 8 /* maximum number of fifos */
#define HFCUSB_B1_TX 0 /* index for B1 transmit bulk/int */
#define HFCUSB_B1_RX 1 /* index for B1 receive bulk/int */
#define HFCUSB_B2_TX 2
#define HFCUSB_B2_RX 3
#define HFCUSB_D_TX 4
#define HFCUSB_D_RX 5
#define HFCUSB_PCM_TX 6
#define HFCUSB_PCM_RX 7
/*
* used to switch snd_transfer_mode for different TA modes e.g. the Billion USB TA just
* supports ISO out, while the Cologne Chip EVAL TA just supports BULK out
*/
#define USB_INT 0
#define USB_BULK 1
#define USB_ISOC 2
#define ISOC_PACKETS_D 8
#define ISOC_PACKETS_B 8
#define ISO_BUFFER_SIZE 128
/* Fifo flow Control for TX ISO */
#define SINK_MAX 68
#define SINK_MIN 48
#define SINK_DMIN 12
#define SINK_DMAX 18
#define BITLINE_INF (-64 * 8)
/* HFC-S USB register access by Control-URSs */
#define write_usb(a, b, c) usb_control_msg((a)->dev, (a)->ctrl_out_pipe, 0, 0x40, (c), (b), NULL, 0, HFC_CTRL_TIMEOUT)
#define read_usb(a, b, c) usb_control_msg((a)->dev, (a)->ctrl_in_pipe, 1, 0xC0, 0, (b), (c), 1, HFC_CTRL_TIMEOUT)
#define HFC_CTRL_BUFSIZE 32
/* entry and size of output/input control buffer */
typedef struct {
__u8 hfc_reg; /* register number */
__u8 reg_val; /* value to be written (or read) */
int action; /* data for action handler */
} ctrl_buft;
/* Debugging Flags */
#define HFCUSB_DBG_INIT 0x0001
#define HFCUSB_DBG_STATES 0x0002
#define HFCUSB_DBG_DCHANNEL 0x0080
#define HFCUSB_DBG_FIFO_ERR 0x4000
#define HFCUSB_DBG_VERBOSE_USB 0x8000
/*
* URB error codes:
* Used to represent a list of values and their respective symbolic names
*/
struct hfcusb_symbolic_list {
const int num;
const char *name;
};
static struct hfcusb_symbolic_list urb_errlist[] = {
{-ENOMEM, "No memory for allocation of internal structures"},
{-ENOSPC, "The host controller's bandwidth is already consumed"},
{-ENOENT, "URB was canceled by unlink_urb"},
{-EXDEV, "ISO transfer only partially completed"},
{-EAGAIN, "Too match scheduled for the future"},
{-ENXIO, "URB already queued"},
{-EFBIG, "Too much ISO frames requested"},
{-ENOSR, "Buffer error (overrun)"},
{-EPIPE, "Specified endpoint is stalled (device not responding)"},
{-EOVERFLOW, "Babble (bad cable?)"},
{-EPROTO, "Bit-stuff error (bad cable?)"},
{-EILSEQ, "CRC/Timeout"},
{-ETIMEDOUT, "NAK (device does not respond)"},
{-ESHUTDOWN, "Device unplugged"},
{-1, NULL}
};
/*
* device dependent information to support different
* ISDN Ta's using the HFC-S USB chip
*/
/* USB descriptor need to contain one of the following EndPoint combination: */
#define CNF_4INT3ISO 1 // 4 INT IN, 3 ISO OUT
#define CNF_3INT3ISO 2 // 3 INT IN, 3 ISO OUT
#define CNF_4ISO3ISO 3 // 4 ISO IN, 3 ISO OUT
#define CNF_3ISO3ISO 4 // 3 ISO IN, 3 ISO OUT
#define EP_NUL 1 // Endpoint at this position not allowed
#define EP_NOP 2 // all type of endpoints allowed at this position
#define EP_ISO 3 // Isochron endpoint mandatory at this position
#define EP_BLK 4 // Bulk endpoint mandatory at this position
#define EP_INT 5 // Interrupt endpoint mandatory at this position
/*
* List of all supported endpoint configuration sets, used to find the
* best matching endpoint configuration within a devices' USB descriptor.
* We need at least 3 RX endpoints, and 3 TX endpoints, either
* INT-in and ISO-out, or ISO-in and ISO-out)
* with 4 RX endpoints even E-Channel logging is possible
*/
static int validconf[][19] = {
// INT in, ISO out config
{EP_NUL, EP_INT, EP_NUL, EP_INT, EP_NUL, EP_INT, EP_NOP, EP_INT,
EP_ISO, EP_NUL, EP_ISO, EP_NUL, EP_ISO, EP_NUL, EP_NUL, EP_NUL,
CNF_4INT3ISO, 2, 1},
{EP_NUL, EP_INT, EP_NUL, EP_INT, EP_NUL, EP_INT, EP_NUL, EP_NUL,
EP_ISO, EP_NUL, EP_ISO, EP_NUL, EP_ISO, EP_NUL, EP_NUL, EP_NUL,
CNF_3INT3ISO, 2, 0},
// ISO in, ISO out config
{EP_NUL, EP_NUL, EP_NUL, EP_NUL, EP_NUL, EP_NUL, EP_NUL, EP_NUL,
EP_ISO, EP_ISO, EP_ISO, EP_ISO, EP_ISO, EP_ISO, EP_NOP, EP_ISO,
CNF_4ISO3ISO, 2, 1},
{EP_NUL, EP_NUL, EP_NUL, EP_NUL, EP_NUL, EP_NUL, EP_NUL, EP_NUL,
EP_ISO, EP_ISO, EP_ISO, EP_ISO, EP_ISO, EP_ISO, EP_NUL, EP_NUL,
CNF_3ISO3ISO, 2, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0} // EOL element
};
#ifdef CONFIG_HISAX_DEBUG
// string description of chosen config
static char *conf_str[] = {
"4 Interrupt IN + 3 Isochron OUT",
"3 Interrupt IN + 3 Isochron OUT",
"4 Isochron IN + 3 Isochron OUT",
"3 Isochron IN + 3 Isochron OUT"
};
#endif
typedef struct {
int vendor; // vendor id
int prod_id; // product id
char *vend_name; // vendor string
__u8 led_scheme; // led display scheme
signed short led_bits[8]; // array of 8 possible LED bitmask settings
} vendor_data;
#define LED_OFF 0 // no LED support
#define LED_SCHEME1 1 // LED standard scheme
#define LED_SCHEME2 2 // not used yet...
#define LED_POWER_ON 1
#define LED_POWER_OFF 2
#define LED_S0_ON 3
#define LED_S0_OFF 4
#define LED_B1_ON 5
#define LED_B1_OFF 6
#define LED_B1_DATA 7
#define LED_B2_ON 8
#define LED_B2_OFF 9
#define LED_B2_DATA 10
#define LED_NORMAL 0 // LEDs are normal
#define LED_INVERTED 1 // LEDs are inverted
#endif // __HFC_USB_H__
| {
"pile_set_name": "Github"
} |
/*
* Copyright (C) 2012 Indragie Karunaratne <i@indragie.com>
* All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* - Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* - Neither the name of Indragie Karunaratne nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#import "SNRQueueCoordinator.h"
#import "SNRQueueAnimationContainer.h"
#import "SNRQueueScrollView.h"
#import "SPMediaKeyTap.h"
#import "SNRWindow.h"
#import "SNRVolumeViewController.h"
#import "SNRSaveMixWindowController.h"
#import "SNRBlockMenuItem.h"
#import "SNRLastFMEngine.h"
#import "SNRMix.h"
#import "NSUserDefaults-SNRAdditions.h"
#import "NSObject+SNRAdditions.h"
#import "NSManagedObjectContext-SNRAdditions.h"
#define kQueueCoordinatorEqualizerdBMultiplier 20
#define kQueueCoordinatorMediaKeySeekRepeatMultiplier 0.05f
#define kQueueCoordinatorVolumeButtonIncrement 0.1f
#define kQueueCoordinatorAnimationDuration 0.5f
static NSString* const kImageRepeat = @"repeat-icon";
static NSString* const kImageRepeatAll = @"repeat-all";
static NSString* const kImageRepeatOne = @"repeat-one";
typedef enum {
SNRQueueCoordinatorMediaButtonNone,
SNRQueueCoordinatorMediaButtonPlayPause,
SNRQueueCoordinatorMediaButtonNext,
SNRQueueCoordinatorMediaButtonPrevious,
SNRQueueCoordinatorMediaButtonVolumeUp,
SNRQueueCoordinatorMediaButtonVolumeDown
} SNRQueueCoordinatorMediaButton;
@interface SNRQueueCoordinator ()
- (SNRQueueController *)newQueueController;
- (void)registerForKVONotifications;
- (void)registerForNCNotifications;
+ (void)configureEqualizerForPlayer:(SNRAudioPlayer*)player;
- (void)windowPlayPause:(NSNotification*)notification;
- (void)windowClear:(NSNotification*)notification;
- (void)windowPrevious:(NSNotification*)notification;
- (void)windowNext:(NSNotification*)notification;
- (void)workspaceWillSleep:(NSNotification*)notification;
- (void)handleMediaButton:(SNRQueueCoordinatorMediaButton)button state:(BOOL)state repeated:(BOOL)repeated;
- (void)resetRepeatButtonImage;
@property (nonatomic, assign) SNRQueueControllerRepeatMode repeatMode;
@end
@implementation SNRQueueCoordinator {
NSMutableArray *_queueControllers;
SNRQueueController *_mainQueueController;
SNRQueueController *_mixQueueController;
NSPoint _savedScrollPosition;
SPMediaKeyTap *_mediaKeyTap;
NSUInteger _mediaKeySeekRepeatCount;
NSPopover *_volumePopover;
SNRSaveMixWindowController *_saveMixWindowController;
}
@synthesize repeatButton = _repeatButton;
@synthesize queueControllers = _queueControllers;
@synthesize repeatMode = _repeatMode;
@synthesize mainQueueView = _mainQueueView;
// --------------------
// Register the media event handlers
// --------------------
- (id)init
{
if ((self = [super init])) {
_queueControllers = [NSMutableArray array];
_mediaKeyTap = [[SPMediaKeyTap alloc] initWithDelegate:self];
[_mediaKeyTap startWatchingMediaKeys];
HIDRemote *remote = [HIDRemote sharedHIDRemote];
[remote setDelegate:self];
[remote startRemoteControl:kHIDRemoteModeExclusive];
self.repeatMode = [[NSUserDefaults standardUserDefaults] repeatMode];
[self registerForKVONotifications];
[self registerForNCNotifications];
[self mainQueueController]; // create it
}
return self;
}
// --------------------
// Create & configure a new queue controller
// Set it as the active queue controller if there isn't one already
// --------------------
- (SNRQueueController*)newQueueController
{
SNRQueueController *controller = [SNRQueueController new];
SNRAudioPlayer *player = [controller audioPlayer];
[[self class] configureEqualizerForPlayer:player];
if (!self.activeQueueController) {
self.activeQueueController = controller;
}
[_queueControllers addObject:controller];
[controller addObserver:self forKeyPath:@"playerState" options:0 context:NULL];
return controller;
}
// --------------------
// Get the main queue controller, creating it if needed
// --------------------
- (SNRQueueController*)mainQueueController
{
if (!_mainQueueController) {
_mainQueueController = [self newQueueController];
_mainQueueController.identifier = @"SNRMainQueue";
// The main queue is restorable, so register it with the restoration manager
[[SNRRestorationManager sharedManager] registerRestorationObject:_mainQueueController];
}
return _mainQueueController;
}
// --------------------
// Get the mix queue controller (for mix editing)
// --------------------
- (SNRQueueController*)mixQueueController
{
if (!_mixQueueController) {
_mixQueueController = [self newQueueController];
_mixQueueController.identifier = @"SNRMixQueue";
}
return _mixQueueController;
}
// --------------------
// Creates the mix queue controller, loads content, and shows it
// --------------------
- (void)showMixEditingQueueControllerForMix:(SNRMix*)mix
{
SNRQueueController *mixQueueController = [self mixQueueController];
if ([mixQueueController representedObject]) { return; }
[mixQueueController setRepresentedObject:mix];
[mixQueueController enqueueSongs:[mix.songs array]];
[self setActiveQueueController:mixQueueController];
}
// --------------------
// Set up the outlets for the active queue controller
// --------------------
- (void)awakeFromNib
{
[super awakeFromNib];
self.mainQueueView.delegate = _activeQueueController;
self.mainQueueView.dataSource = _activeQueueController;
[_activeQueueController setQueueView:self.mainQueueView];
[self resetRepeatButtonImage];
}
// --------------------
// Bunch of methods to handle notification registration
// --------------------
- (void)registerForKVONotifications
{
NSUserDefaultsController *controller = [NSUserDefaultsController sharedUserDefaultsController];
[controller addObserver:self forKeyPath:@"values.eqBas" options:0 context:NULL];
[controller addObserver:self forKeyPath:@"values.eqMid" options:0 context:NULL];
[controller addObserver:self forKeyPath:@"values.eqTre" options:0 context:NULL];
[controller addObserver:self forKeyPath:@"values.repeatMode" options:0 context:NULL];
}
- (void)registerForNCNotifications
{
NSNotificationCenter *nc = [NSNotificationCenter defaultCenter];
[nc addObserver:self selector:@selector(windowPlayPause:) name:SNRWindowSpaceKeyPressedNotification object:nil];
[nc addObserver:self selector:@selector(windowClear:) name:SNRWindowEscapeKeyPressedNotification object:nil];
[nc addObserver:self selector:@selector(windowPrevious:) name:SNRWindowLeftKeyPressedNotification object:nil];
[nc addObserver:self selector:@selector(windowNext:) name:SNRWindowRightKeyPressedNotification object:nil];
NSNotificationCenter *wnc = [[NSWorkspace sharedWorkspace] notificationCenter];
[wnc addObserver:self selector:@selector(workspaceWillSleep:) name:NSWorkspaceWillSleepNotification object:nil];
}
- (void)dealloc
{
NSUserDefaultsController *controller = [NSUserDefaultsController sharedUserDefaultsController];
[controller removeObserver:self forKeyPath:@"values.eqBas"];
[controller removeObserver:self forKeyPath:@"values.eqMid"];
[controller removeObserver:self forKeyPath:@"values.eqTre"];
[controller removeObserver:self forKeyPath:@"values.repeatMode"];
[[[NSWorkspace sharedWorkspace] notificationCenter] removeObserver:self];
[[NSNotificationCenter defaultCenter] removeObserver:self];
}
// --------------------
// Configures the equalizer for the specified audio player
// using the values from user defaults
// --------------------
+ (void)configureEqualizerForPlayer:(SNRAudioPlayer *)player
{
NSUserDefaults *ud = [NSUserDefaults standardUserDefaults];
float bas = [ud floatForKey:@"eqBas"] * kQueueCoordinatorEqualizerdBMultiplier;
float mid = [ud floatForKey:@"eqMid"] * kQueueCoordinatorEqualizerdBMultiplier;
float tre = [ud floatForKey:@"eqTre"] * kQueueCoordinatorEqualizerdBMultiplier;
[player setEQValue:bas forEQBand:0];
[player setEQValue:bas*(1/2) + mid*(1/8) forEQBand:1];
[player setEQValue:bas*(1/4) + mid*(1/4) forEQBand:2];
[player setEQValue:bas*(1/8) + mid*(1/2) forEQBand:3];
[player setEQValue:mid forEQBand:4];
[player setEQValue:mid forEQBand:5];
[player setEQValue:mid*(1/2) + tre*(1/8) forEQBand:6];
[player setEQValue:mid*(1/4) + tre*(1/4) forEQBand:7];
[player setEQValue:mid*(1/8) + tre*(1/2) forEQBand:8];
[player setEQValue:tre forEQBand:9];
}
#pragma mark - Notifications
// --------------------
// Forward the notifications to the active queue controller
// --------------------
- (void)windowPlayPause:(NSNotification*)notification
{
[self.activeQueueController playPause];
}
- (void)windowClear:(NSNotification*)notification
{
[self.activeQueueController clearQueueImmediately:NO];
}
- (void)windowPrevious:(NSNotification*)notification
{
[self.activeQueueController previous];
}
- (void)windowNext:(NSNotification*)notification
{
[self.activeQueueController next];
}
- (void)workspaceWillSleep:(NSNotification*)notification
{
[self.activeQueueController pause];
}
#pragma mark - Button Actions
// --------------------
// Change the repeat mode
// --------------------
- (IBAction)repeat:(id)sender
{
switch (self.repeatMode) {
case SNRQueueControllerRepeatModeNone:
self.repeatMode = SNRQueueControllerRepeatModeAll;
break;
case SNRQueueControllerRepeatModeAll:
self.repeatMode = SNRQueueControllerRepeatModeOne;
break;
case SNRQueueControllerRepeatModeOne:
self.repeatMode = SNRQueueControllerRepeatModeNone;
break;
default:
break;
}
[[NSUserDefaults standardUserDefaults] setRepeatMode:self.repeatMode];
}
// --------------------
// Show the volume & EQ popover
// --------------------
- (IBAction)volume:(id)sender
{
if (_volumePopover) {
[_volumePopover close];
_volumePopover = nil;
} else {
SNRVolumeViewController *vol = [[SNRVolumeViewController alloc] init];
_volumePopover = [[NSPopover alloc] init];
_volumePopover.delegate = self;
_volumePopover.contentViewController = vol;
_volumePopover.behavior = NSPopoverBehaviorSemitransient;
[_volumePopover showRelativeToRect:[sender bounds] ofView:sender preferredEdge:NSMaxYEdge];
}
}
// --------------------
// Save the contents of the active queue controller as a mix
// --------------------
- (IBAction)save:(id)sender
{
_saveMixWindowController = [[SNRSaveMixWindowController alloc] initWithSongs:[self.activeQueueController queue]];
[[_saveMixWindowController window] setDelegate:self];
[_saveMixWindowController showWindow:nil];
}
// --------------------
// Clear the active queue controller
// --------------------
- (IBAction)clear:(id)sender
{
[self.activeQueueController clearQueueImmediately:NO];
}
// --------------------
// Shuffle the active queue controller
// --------------------
- (IBAction)shuffle:(id)sender
{
[self.activeQueueController shuffle];
}
// --------------------
// Save the mix in the mix queue
// --------------------
- (IBAction)saveMix:(id)sender
{
SNRMix *mix = [[self mixQueueController] representedObject];
NSArray *songs = [[self mixQueueController] queue];
[mix setSongs:[NSOrderedSet orderedSetWithArray:songs]];
[[mix managedObjectContext] saveChanges];
[self setActiveQueueController:[self mainQueueController]];
}
// --------------------
// Cancel saving the mix
// --------------------
- (IBAction)cancelMix:(id)sender
{
[self setActiveQueueController:[self mainQueueController]];
}
#pragma mark - NSWindowDelegate
- (void)windowWillClose:(NSNotification *)notification
{
_saveMixWindowController = nil;
}
#pragma mark - NSPopoverDelegate
- (void)popoverDidClose:(NSNotification *)notification
{
_volumePopover = nil;
}
#pragma mark - Accessors
// --------------------
// Show a new active queue controller with animation
// --------------------
- (void)setActiveQueueController:(SNRQueueController *)activeQueueController
{
if (_activeQueueController != activeQueueController) {
NSScrollView *currentScrollView = [self.mainQueueView enclosingScrollView];
NSView *parentView = [currentScrollView superview];
BOOL showingMainQueue = _activeQueueController == _mainQueueController;
if (showingMainQueue) {
_savedScrollPosition = [currentScrollView documentVisibleRect].origin;
}
// Show the animation container with a rendering of the view in its current state
SNRQueueAnimationContainer *container = [[SNRQueueAnimationContainer alloc] initWithFrame:[currentScrollView frame]];
[container setAnimationView:currentScrollView];
// When we're moving from the main queue to the mix queue, we want the mix queue to slide
// over the main queue from left to right. The steps for this look like this:
//
// 1. Take a snapshot of the view in its current state (showing the main queue)
// 2. Render that into the bottom layer
// 3. Place the animation container over the actual view
// 4. Set the active queue controller to the mix queue
// 5. Render that state into the top layer
// 6. Position the top layer to the right of the visible rect and animate left
// Conversely, when moving from the mix queue to the main queue (right to left) the steps look like this:
//
// 1. Take a snapshot of the view in its current state (showing the mix queue)
// 2. Render that into the TOP layer (the top layer is always the one that will be animated)
// 3. Place the animation container over the view
// 4. Set the active queue controller to the main queue
// 5. Render the main queue into the bottom layer
// 6. Animate the top layer (containing the mix queue) off to the right and off screen
//
[parentView addSubview:container positioned:NSWindowAbove relativeTo:currentScrollView];
[container setDirection:showingMainQueue ? SNRQueueAnimationDirectionLeft : SNRQueueAnimationDirectionRight];
if (showingMainQueue) {
[container renderBottomLayer];
} else {
[container renderTopLayer];
}
// Detach the queue view from the current queue controller
// and set the new queue controller for it to reload the queue view
[_activeQueueController setQueueView:nil];
_activeQueueController = activeQueueController;
[_activeQueueController setLoadArtworkSynchronously:YES];
[_activeQueueController setQueueView:self.mainQueueView];
// Do the second rendering with the new queue controller
if (!showingMainQueue) {
[self.mainQueueView scrollPoint:_savedScrollPosition];
[container renderBottomLayer];
} else {
[container renderTopLayer];
}
// Perform the animation (moving the top layer)
[CATransaction begin];
[CATransaction setAnimationDuration:kQueueCoordinatorAnimationDuration];
[CATransaction setCompletionBlock:^{
if (!showingMainQueue) {
SNRQueueController *controller = [self mixQueueController];
[controller clearQueueImmediately:YES];
[controller setRepresentedObject:nil];
}
[_activeQueueController setLoadArtworkSynchronously:NO];
[container removeFromSuperview];
}];
[container animate];
[CATransaction commit];
// Animate the buttons view
NSView *oldButtonsView = showingMainQueue ? self.mainButtonsContainer : self.mixButtonsContainer;
NSView *newButtonsView = showingMainQueue ? self.mixButtonsContainer : self.mainButtonsContainer;
[newButtonsView setAlphaValue:0.f];
[newButtonsView setFrame:[oldButtonsView frame]];
[[oldButtonsView superview] addSubview:newButtonsView];
[NSAnimationContext beginGrouping];
[[NSAnimationContext currentContext] setCompletionHandler:^{
[oldButtonsView removeFromSuperview];
}];
[[NSAnimationContext currentContext] setDuration:kQueueCoordinatorAnimationDuration];
[[newButtonsView animator] setAlphaValue:1.f];
[[oldButtonsView animator] setAlphaValue:0.f];
[NSAnimationContext endGrouping];
}
}
#pragma mark - SPMediaKeyTapDelegate
- (void)mediaKeyTap:(SPMediaKeyTap*)keyTap receivedMediaKeyEvent:(NSEvent*)event
{
int keyCode = (([event data1] & 0xFFFF0000) >> 16);
int keyFlags = ([event data1] & 0x0000FFFF);
int keyState = (((keyFlags & 0xFF00) >> 8)) == 0xA;
int keyRepeat = (keyFlags & 0x1);
SNRQueueCoordinatorMediaButton button = SNRQueueCoordinatorMediaButtonNone;
switch (keyCode) {
case NX_KEYTYPE_PLAY:
button = SNRQueueCoordinatorMediaButtonPlayPause;
break;
case NX_KEYTYPE_FAST:
button = SNRQueueCoordinatorMediaButtonNext;
break;
case NX_KEYTYPE_REWIND:
button = SNRQueueCoordinatorMediaButtonPrevious;
break;
}
if (button != SNRQueueCoordinatorMediaButtonNone) {
[self handleMediaButton:button state:keyState repeated:keyRepeat];
}
}
#pragma mark - HIDRemoteDelegate
- (void)hidRemote:(HIDRemote *)hidRemote eventWithButton:(HIDRemoteButtonCode)buttonCode isPressed:(BOOL)isPressed fromHardwareWithAttributes:(NSMutableDictionary *)attributes
{
HIDRemoteButtonCode button = buttonCode & kHIDRemoteButtonCodeCodeMask;
int repeat = buttonCode & kHIDRemoteButtonCodeHoldMask;
SNRQueueCoordinatorMediaButton mediaButton = SNRQueueCoordinatorMediaButtonNone;
switch (button) {
case kHIDRemoteButtonCodeCenter:
case kHIDRemoteButtonCodePlay:
mediaButton = SNRQueueCoordinatorMediaButtonPlayPause;
break;
case kHIDRemoteButtonCodeUp:
mediaButton = SNRQueueCoordinatorMediaButtonVolumeUp;
break;
case kHIDRemoteButtonCodeDown:
mediaButton = SNRQueueCoordinatorMediaButtonVolumeDown;
break;
case kHIDRemoteButtonCodeLeft:
mediaButton = SNRQueueCoordinatorMediaButtonPrevious;
break;
case kHIDRemoteButtonCodeRight:
mediaButton = SNRQueueCoordinatorMediaButtonNext;
break;
default:
break;
}
if (button != SNRQueueCoordinatorMediaButtonNone) {
[self handleMediaButton:mediaButton state:isPressed repeated:repeat];
}
}
#pragma mark - Control Events
- (void)handleMediaButton:(SNRQueueCoordinatorMediaButton)button state:(BOOL)state repeated:(BOOL)repeated
{
NSUserDefaults *ud = [NSUserDefaults standardUserDefaults];
switch (button) {
case SNRQueueCoordinatorMediaButtonPlayPause: {
if (state) { [self.activeQueueController playPause]; }
break;
} case SNRQueueCoordinatorMediaButtonNext: {
if (!repeated && !state && !_mediaKeySeekRepeatCount) {
[self.activeQueueController next];
} else if (!repeated && !state && _mediaKeySeekRepeatCount) {
_mediaKeySeekRepeatCount = 0;
} else if (repeated && state) {
_mediaKeySeekRepeatCount++;
[self.activeQueueController seekForward:kQueueCoordinatorMediaKeySeekRepeatMultiplier * _mediaKeySeekRepeatCount];
}
break;
} case SNRQueueCoordinatorMediaButtonPrevious: {
if (!repeated && !state && !_mediaKeySeekRepeatCount) {
[self.activeQueueController previous];
} else if (!repeated && !state && _mediaKeySeekRepeatCount) {
_mediaKeySeekRepeatCount = 0;
} else if (repeated && state) {
_mediaKeySeekRepeatCount++;
[self.activeQueueController seekBackward:kQueueCoordinatorMediaKeySeekRepeatMultiplier * _mediaKeySeekRepeatCount];
}
break;
} case SNRQueueCoordinatorMediaButtonVolumeUp: {
float volume = ud.volume;
if (volume < 1.f) {
ud.volume = MIN(volume + kQueueCoordinatorVolumeButtonIncrement, 1.f);
}
break;
} case SNRQueueCoordinatorMediaButtonVolumeDown: {
float volume = ud.volume;
if (volume > 0.f) {
ud.volume = MAX(volume - kQueueCoordinatorVolumeButtonIncrement, 0.f);
}
} default:
break;
}
}
#pragma mark - NSMenuDelegate
- (void)menuNeedsUpdate:(NSMenu *)menu
{
__weak SNRQueueCoordinator *weakSelf = self;
[menu removeAllItems];
NSMenuItem *repeatContainer = [[NSMenuItem alloc] initWithTitle:NSLocalizedString(@"Repeat", nil) action:nil keyEquivalent:@""];
NSMenu *repeatMenu = [[NSMenu alloc] initWithTitle:@"Repeat"];
NSUserDefaults *ud = [NSUserDefaults standardUserDefaults];
SNRBlockMenuItem *repeatNone = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(@"RepeatNone", nil) keyEquivalent:@"" block:^(NSMenuItem *item) {
[ud setRepeatMode:SNRQueueControllerRepeatModeNone];
}];
repeatNone.state = self.repeatMode == SNRQueueControllerRepeatModeNone;
[repeatMenu addItem:repeatNone];
SNRBlockMenuItem *repeatAll = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(@"RepeatAll", nil) keyEquivalent:@"" block:^(NSMenuItem *item) {
[ud setRepeatMode:SNRQueueControllerRepeatModeAll];
}];
repeatAll.state = self.repeatMode == SNRQueueControllerRepeatModeAll;
[repeatMenu addItem:repeatAll];
SNRBlockMenuItem *repeatOne = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(@"RepeatOne", nil) keyEquivalent:@"" block:^(NSMenuItem *item) {
[ud setRepeatMode:SNRQueueControllerRepeatModeOne];
}];
repeatOne.state = self.repeatMode == SNRQueueControllerRepeatModeOne;
[repeatMenu addItem:repeatOne];
repeatContainer.submenu = repeatMenu;
[menu addItem:repeatContainer];
if ([[self.activeQueueController queue] count] > 1) {
SNRBlockMenuItem *shuffle = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(@"Shuffle", nil) keyEquivalent:@"" block:^(NSMenuItem *item) {
SNRQueueCoordinator *strongSelf = weakSelf;
[strongSelf.activeQueueController shuffle];
}];
[menu addItem:shuffle];
}
[menu addItem:[NSMenuItem separatorItem]];
if ([[SNRLastFMEngine sharedInstance] isAuthenticated]) {
NSUserDefaults *ud = [NSUserDefaults standardUserDefaults];
SNRBlockMenuItem *scrobble = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(@"ScrobbleTracks", nil) keyEquivalent:@"" block:^(NSMenuItem *item) {
[item setState:!(BOOL)[item state]];
[ud setScrobble:[item state]];
}];
[scrobble setState:[ud scrobble]];
[menu addItem:scrobble];
if ([self.activeQueueController currentQueueItem]) {
SNRBlockMenuItem *love = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(@"LoveTrack", nil) keyEquivalent:@"l" block:^(NSMenuItem *item) {
SNRQueueCoordinator *strongSelf = weakSelf;
[[strongSelf.activeQueueController currentQueueItem] lastFMLoveTrack];
}];
[menu addItem:love];
}
[menu addItem:[NSMenuItem separatorItem]];
}
BOOL playing = [self.activeQueueController playerState] == SNRQueueControllerPlayerStatePlaying;
SNRBlockMenuItem *play = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(playing ? @"Pause" : @"Play", nil) keyEquivalent:@"" block:^(NSMenuItem *item) {
SNRQueueCoordinator *strongSelf = weakSelf;
[strongSelf.activeQueueController playPause];
}];
if (!playing && ![[self.activeQueueController queue] count]) {
play.target = nil;
play.action = nil;
}
[menu addItem:play];
SNRBlockMenuItem *previous = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(@"Previous", nil) keyEquivalent:@"" block:^(NSMenuItem *item) {
SNRQueueCoordinator *strongSelf = weakSelf;
[strongSelf.activeQueueController previous];
}];
[menu addItem:previous];
SNRBlockMenuItem *next = [[SNRBlockMenuItem alloc] initWithTitle:NSLocalizedString(@"Next", nil) keyEquivalent:@"" block:^(NSMenuItem *item) {
SNRQueueCoordinator *strongSelf = weakSelf;
[strongSelf.activeQueueController next];
}];
[menu addItem:next];
}
#pragma mark - Key Value Observation
- (void)observeValueForKeyPath:(NSString *)keyPath ofObject:(id)object change:(NSDictionary *)change context:(void *)context
{
if ([keyPath hasPrefix:@"values.eq"]) {
for (SNRQueueController *controller in self.queueControllers) {
[[self class] configureEqualizerForPlayer:[controller audioPlayer]];
}
} else if ([keyPath isEqualToString:@"values.repeatMode"]) {
self.repeatMode = [[NSUserDefaults standardUserDefaults] repeatMode];
[self resetRepeatButtonImage];
} else if ([keyPath isEqualToString:@"playerState"]) {
SNRQueueController *controller = object;
if (controller.playerState == SNRQueueControllerPlayerStatePlaying) {
for (SNRQueueController *c in self.queueControllers) {
if (c != controller) { [c pause]; }
}
}
}
}
- (void)resetRepeatButtonImage
{
NSString *imageName = nil;
switch (self.repeatMode) {
case SNRQueueControllerRepeatModeAll:
imageName = kImageRepeatAll;
break;
case SNRQueueControllerRepeatModeOne:
imageName = kImageRepeatOne;
break;
default:
imageName = kImageRepeat;
break;
}
[self.repeatButton setImage:[NSImage imageNamed:imageName]];
}
@end
| {
"pile_set_name": "Github"
} |
/*
Copyright 2019 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package transformers
import (
"fmt"
"regexp"
"strings"
"sigs.k8s.io/kustomize/pkg/image"
"sigs.k8s.io/kustomize/pkg/resmap"
)
// imageTransformer replace image names and tags
type imageTransformer struct {
images []image.Image
}
var _ Transformer = &imageTransformer{}
// NewImageTransformer constructs an imageTransformer.
func NewImageTransformer(slice []image.Image) (Transformer, error) {
return &imageTransformer{slice}, nil
}
// Transform finds the matching images and replaces name, tag and/or digest
func (pt *imageTransformer) Transform(resources resmap.ResMap) error {
if len(pt.images) == 0 {
return nil
}
for _, res := range resources {
err := pt.findAndReplaceImage(res.Map())
if err != nil {
return err
}
}
return nil
}
/*
findAndReplaceImage replaces the image name and tags inside one object
It searches the object for container session
then loops though all images inside containers session,
finds matched ones and update the image name and tag name
*/
func (pt *imageTransformer) findAndReplaceImage(obj map[string]interface{}) error {
paths := []string{"containers", "initContainers"}
found := false
for _, path := range paths {
_, found = obj[path]
if found {
err := pt.updateContainers(obj, path)
if err != nil {
return err
}
}
}
if !found {
return pt.findContainers(obj)
}
return nil
}
func (pt *imageTransformer) updateContainers(obj map[string]interface{}, path string) error {
containers, ok := obj[path].([]interface{})
if !ok {
return fmt.Errorf("containers path is not of type []interface{} but %T", obj[path])
}
for i := range containers {
container := containers[i].(map[string]interface{})
containerImage, found := container["image"]
if !found {
continue
}
imageName := containerImage.(string)
for _, img := range pt.images {
if !isImageMatched(imageName, img.Name) {
continue
}
name, tag := split(imageName)
if img.NewName != "" {
name = img.NewName
}
if img.NewTag != "" {
tag = ":" + img.NewTag
}
if img.Digest != "" {
tag = "@" + img.Digest
}
container["image"] = name + tag
break
}
}
return nil
}
func (pt *imageTransformer) findContainers(obj map[string]interface{}) error {
for key := range obj {
switch typedV := obj[key].(type) {
case map[string]interface{}:
err := pt.findAndReplaceImage(typedV)
if err != nil {
return err
}
case []interface{}:
for i := range typedV {
item := typedV[i]
typedItem, ok := item.(map[string]interface{})
if ok {
err := pt.findAndReplaceImage(typedItem)
if err != nil {
return err
}
}
}
}
}
return nil
}
func isImageMatched(s, t string) bool {
// Tag values are limited to [a-zA-Z0-9_.-].
pattern, _ := regexp.Compile("^" + t + "(:[a-zA-Z0-9_.-]*)?$")
return pattern.MatchString(s)
}
// split separates and returns the name and tag parts
// from the image string using either colon `:` or at `@` separators.
// Note that the returned tag keeps its separator.
func split(imageName string) (name string, tag string) {
// check if image name contains a domain
// if domain is present, ignore domain and check for `:`
ic := -1
if slashIndex := strings.Index(imageName, "/"); slashIndex < 0 {
ic = strings.LastIndex(imageName, ":")
} else {
lastIc := strings.LastIndex(imageName[slashIndex:], ":")
// set ic only if `:` is present
if lastIc > 0 {
ic = slashIndex + lastIc
}
}
ia := strings.LastIndex(imageName, "@")
if ic < 0 && ia < 0 {
return imageName, ""
}
i := ic
if ic < 0 {
i = ia
}
name = imageName[:i]
tag = imageName[i:]
return
}
| {
"pile_set_name": "Github"
} |
# Copyright (c) 2017-present, Facebook, Inc. All rights reserved.
#
# You are hereby granted a non-exclusive, worldwide, royalty-free license to use,
# copy, modify, and distribute this software in source code or binary form for use
# in connection with the web services and APIs provided by Facebook.
#
# As with any software that integrates with the Facebook platform, your use of
# this software is subject to the Facebook Platform Policy
# [http://developers.facebook.com/policy/]. This copyright notice shall be
# included in all copies or substantial portions of the software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
# FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
# COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
# IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
# CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
# FB:AUTOGEN
module FacebookAds
# This class is auto-generated.
# For any issues or feature requests related to this class, please let us know
# on github and we'll fix in our codegen framework. We'll not be able to accept
# pull request for this class.
class AdAccountMaxBid < AdObject
field :max_bid, 'int'
has_no_id
has_no_get
has_no_post
has_no_delete
end
end
| {
"pile_set_name": "Github"
} |
<?php
class ControllerExtensionModuleLatest extends Controller {
private $error = array();
public function index() {
$this->load->language('extension/module/latest');
$this->document->setTitle($this->language->get('heading_title'));
$this->load->model('extension/module');
if (($this->request->server['REQUEST_METHOD'] == 'POST') && $this->validate()) {
if (!isset($this->request->get['module_id'])) {
$this->model_extension_module->addModule('latest', $this->request->post);
} else {
$this->model_extension_module->editModule($this->request->get['module_id'], $this->request->post);
}
$this->cache->delete('product');
$this->session->data['success'] = $this->language->get('text_success');
$this->response->redirect($this->url->link('extension/extension', 'token=' . $this->session->data['token'] . '&type=module', true));
}
$data['heading_title'] = $this->language->get('heading_title');
$data['text_edit'] = $this->language->get('text_edit');
$data['text_enabled'] = $this->language->get('text_enabled');
$data['text_disabled'] = $this->language->get('text_disabled');
$data['entry_name'] = $this->language->get('entry_name');
$data['entry_limit'] = $this->language->get('entry_limit');
$data['entry_width'] = $this->language->get('entry_width');
$data['entry_height'] = $this->language->get('entry_height');
$data['entry_status'] = $this->language->get('entry_status');
$data['button_save'] = $this->language->get('button_save');
$data['button_cancel'] = $this->language->get('button_cancel');
if (isset($this->error['warning'])) {
$data['error_warning'] = $this->error['warning'];
} else {
$data['error_warning'] = '';
}
if (isset($this->error['name'])) {
$data['error_name'] = $this->error['name'];
} else {
$data['error_name'] = '';
}
if (isset($this->error['width'])) {
$data['error_width'] = $this->error['width'];
} else {
$data['error_width'] = '';
}
if (isset($this->error['height'])) {
$data['error_height'] = $this->error['height'];
} else {
$data['error_height'] = '';
}
$data['breadcrumbs'] = array();
$data['breadcrumbs'][] = array(
'text' => $this->language->get('text_home'),
'href' => $this->url->link('common/dashboard', 'token=' . $this->session->data['token'], true)
);
$data['breadcrumbs'][] = array(
'text' => $this->language->get('text_extension'),
'href' => $this->url->link('extension/extension', 'token=' . $this->session->data['token'] . '&type=module', true)
);
if (!isset($this->request->get['module_id'])) {
$data['breadcrumbs'][] = array(
'text' => $this->language->get('heading_title'),
'href' => $this->url->link('extension/module/latest', 'token=' . $this->session->data['token'], true)
);
} else {
$data['breadcrumbs'][] = array(
'text' => $this->language->get('heading_title'),
'href' => $this->url->link('extension/module/latest', 'token=' . $this->session->data['token'] . '&module_id=' . $this->request->get['module_id'], true)
);
}
if (!isset($this->request->get['module_id'])) {
$data['action'] = $this->url->link('extension/module/latest', 'token=' . $this->session->data['token'], true);
} else {
$data['action'] = $this->url->link('extension/module/latest', 'token=' . $this->session->data['token'] . '&module_id=' . $this->request->get['module_id'], true);
}
$data['cancel'] = $this->url->link('extension/extension', 'token=' . $this->session->data['token'] . '&type=module', true);
if (isset($this->request->get['module_id']) && ($this->request->server['REQUEST_METHOD'] != 'POST')) {
$module_info = $this->model_extension_module->getModule($this->request->get['module_id']);
}
if (isset($this->request->post['name'])) {
$data['name'] = $this->request->post['name'];
} elseif (!empty($module_info)) {
$data['name'] = $module_info['name'];
} else {
$data['name'] = '';
}
if (isset($this->request->post['limit'])) {
$data['limit'] = $this->request->post['limit'];
} elseif (!empty($module_info)) {
$data['limit'] = $module_info['limit'];
} else {
$data['limit'] = 5;
}
if (isset($this->request->post['width'])) {
$data['width'] = $this->request->post['width'];
} elseif (!empty($module_info)) {
$data['width'] = $module_info['width'];
} else {
$data['width'] = 200;
}
if (isset($this->request->post['height'])) {
$data['height'] = $this->request->post['height'];
} elseif (!empty($module_info)) {
$data['height'] = $module_info['height'];
} else {
$data['height'] = 200;
}
if (isset($this->request->post['status'])) {
$data['status'] = $this->request->post['status'];
} elseif (!empty($module_info)) {
$data['status'] = $module_info['status'];
} else {
$data['status'] = '';
}
$data['header'] = $this->load->controller('common/header');
$data['column_left'] = $this->load->controller('common/column_left');
$data['footer'] = $this->load->controller('common/footer');
$this->response->setOutput($this->load->view('extension/module/latest', $data));
}
protected function validate() {
if (!$this->user->hasPermission('modify', 'extension/module/latest')) {
$this->error['warning'] = $this->language->get('error_permission');
}
if ((utf8_strlen($this->request->post['name']) < 3) || (utf8_strlen($this->request->post['name']) > 64)) {
$this->error['name'] = $this->language->get('error_name');
}
if (!$this->request->post['width']) {
$this->error['width'] = $this->language->get('error_width');
}
if (!$this->request->post['height']) {
$this->error['height'] = $this->language->get('error_height');
}
return !$this->error;
}
} | {
"pile_set_name": "Github"
} |
/*
* Copyright (C) Igor Sysoev
* Copyright (C) Nginx, Inc.
*/
#include <ngx_config.h>
#include <ngx_core.h>
#include <ngx_http.h>
#define ngx_http_upstream_tries(p) ((p)->number \
+ ((p)->next ? (p)->next->number : 0))
static ngx_http_upstream_rr_peer_t *ngx_http_upstream_get_peer(
ngx_http_upstream_rr_peer_data_t *rrp);
#if (NGX_HTTP_SSL)
static ngx_int_t ngx_http_upstream_empty_set_session(ngx_peer_connection_t *pc,
void *data);
static void ngx_http_upstream_empty_save_session(ngx_peer_connection_t *pc,
void *data);
#endif
ngx_int_t
ngx_http_upstream_init_round_robin(ngx_conf_t *cf,
ngx_http_upstream_srv_conf_t *us)
{
ngx_url_t u;
ngx_uint_t i, j, n, w;
ngx_http_upstream_server_t *server;
ngx_http_upstream_rr_peer_t *peer, **peerp;
ngx_http_upstream_rr_peers_t *peers, *backup;
us->peer.init = ngx_http_upstream_init_round_robin_peer;
if (us->servers) {
server = us->servers->elts;
n = 0;
w = 0;
for (i = 0; i < us->servers->nelts; i++) {
if (server[i].backup) {
continue;
}
n += server[i].naddrs;
w += server[i].naddrs * server[i].weight;
}
if (n == 0) {
ngx_log_error(NGX_LOG_EMERG, cf->log, 0,
"no servers in upstream \"%V\" in %s:%ui",
&us->host, us->file_name, us->line);
return NGX_ERROR;
}
peers = ngx_pcalloc(cf->pool, sizeof(ngx_http_upstream_rr_peers_t));
if (peers == NULL) {
return NGX_ERROR;
}
peer = ngx_pcalloc(cf->pool, sizeof(ngx_http_upstream_rr_peer_t) * n);
if (peer == NULL) {
return NGX_ERROR;
}
peers->single = (n == 1);
peers->number = n;
peers->weighted = (w != n);
peers->total_weight = w;
peers->name = &us->host;
n = 0;
peerp = &peers->peer;
for (i = 0; i < us->servers->nelts; i++) {
if (server[i].backup) {
continue;
}
for (j = 0; j < server[i].naddrs; j++) {
peer[n].sockaddr = server[i].addrs[j].sockaddr;
peer[n].socklen = server[i].addrs[j].socklen;
peer[n].name = server[i].addrs[j].name;
peer[n].weight = server[i].weight;
peer[n].effective_weight = server[i].weight;
peer[n].current_weight = 0;
peer[n].max_conns = server[i].max_conns;
peer[n].max_fails = server[i].max_fails;
peer[n].fail_timeout = server[i].fail_timeout;
peer[n].down = server[i].down;
peer[n].server = server[i].name;
*peerp = &peer[n];
peerp = &peer[n].next;
n++;
}
}
us->peer.data = peers;
/* backup servers */
n = 0;
w = 0;
for (i = 0; i < us->servers->nelts; i++) {
if (!server[i].backup) {
continue;
}
n += server[i].naddrs;
w += server[i].naddrs * server[i].weight;
}
if (n == 0) {
return NGX_OK;
}
backup = ngx_pcalloc(cf->pool, sizeof(ngx_http_upstream_rr_peers_t));
if (backup == NULL) {
return NGX_ERROR;
}
peer = ngx_pcalloc(cf->pool, sizeof(ngx_http_upstream_rr_peer_t) * n);
if (peer == NULL) {
return NGX_ERROR;
}
peers->single = 0;
backup->single = 0;
backup->number = n;
backup->weighted = (w != n);
backup->total_weight = w;
backup->name = &us->host;
n = 0;
peerp = &backup->peer;
for (i = 0; i < us->servers->nelts; i++) {
if (!server[i].backup) {
continue;
}
for (j = 0; j < server[i].naddrs; j++) {
peer[n].sockaddr = server[i].addrs[j].sockaddr;
peer[n].socklen = server[i].addrs[j].socklen;
peer[n].name = server[i].addrs[j].name;
peer[n].weight = server[i].weight;
peer[n].effective_weight = server[i].weight;
peer[n].current_weight = 0;
peer[n].max_conns = server[i].max_conns;
peer[n].max_fails = server[i].max_fails;
peer[n].fail_timeout = server[i].fail_timeout;
peer[n].down = server[i].down;
peer[n].server = server[i].name;
*peerp = &peer[n];
peerp = &peer[n].next;
n++;
}
}
peers->next = backup;
return NGX_OK;
}
/* an upstream implicitly defined by proxy_pass, etc. */
if (us->port == 0) {
ngx_log_error(NGX_LOG_EMERG, cf->log, 0,
"no port in upstream \"%V\" in %s:%ui",
&us->host, us->file_name, us->line);
return NGX_ERROR;
}
ngx_memzero(&u, sizeof(ngx_url_t));
u.host = us->host;
u.port = us->port;
if (ngx_inet_resolve_host(cf->pool, &u) != NGX_OK) {
if (u.err) {
ngx_log_error(NGX_LOG_EMERG, cf->log, 0,
"%s in upstream \"%V\" in %s:%ui",
u.err, &us->host, us->file_name, us->line);
}
return NGX_ERROR;
}
n = u.naddrs;
peers = ngx_pcalloc(cf->pool, sizeof(ngx_http_upstream_rr_peers_t));
if (peers == NULL) {
return NGX_ERROR;
}
peer = ngx_pcalloc(cf->pool, sizeof(ngx_http_upstream_rr_peer_t) * n);
if (peer == NULL) {
return NGX_ERROR;
}
peers->single = (n == 1);
peers->number = n;
peers->weighted = 0;
peers->total_weight = n;
peers->name = &us->host;
peerp = &peers->peer;
for (i = 0; i < u.naddrs; i++) {
peer[i].sockaddr = u.addrs[i].sockaddr;
peer[i].socklen = u.addrs[i].socklen;
peer[i].name = u.addrs[i].name;
peer[i].weight = 1;
peer[i].effective_weight = 1;
peer[i].current_weight = 0;
peer[i].max_conns = 0;
peer[i].max_fails = 1;
peer[i].fail_timeout = 10;
*peerp = &peer[i];
peerp = &peer[i].next;
}
us->peer.data = peers;
/* implicitly defined upstream has no backup servers */
return NGX_OK;
}
ngx_int_t
ngx_http_upstream_init_round_robin_peer(ngx_http_request_t *r,
ngx_http_upstream_srv_conf_t *us)
{
ngx_uint_t n;
ngx_http_upstream_rr_peer_data_t *rrp;
rrp = r->upstream->peer.data;
if (rrp == NULL) {
rrp = ngx_palloc(r->pool, sizeof(ngx_http_upstream_rr_peer_data_t));
if (rrp == NULL) {
return NGX_ERROR;
}
r->upstream->peer.data = rrp;
}
rrp->peers = us->peer.data;
rrp->current = NULL;
rrp->config = 0;
n = rrp->peers->number;
if (rrp->peers->next && rrp->peers->next->number > n) {
n = rrp->peers->next->number;
}
if (n <= 8 * sizeof(uintptr_t)) {
rrp->tried = &rrp->data;
rrp->data = 0;
} else {
n = (n + (8 * sizeof(uintptr_t) - 1)) / (8 * sizeof(uintptr_t));
rrp->tried = ngx_pcalloc(r->pool, n * sizeof(uintptr_t));
if (rrp->tried == NULL) {
return NGX_ERROR;
}
}
r->upstream->peer.get = ngx_http_upstream_get_round_robin_peer;
r->upstream->peer.free = ngx_http_upstream_free_round_robin_peer;
r->upstream->peer.tries = ngx_http_upstream_tries(rrp->peers);
#if (NGX_HTTP_SSL)
r->upstream->peer.set_session =
ngx_http_upstream_set_round_robin_peer_session;
r->upstream->peer.save_session =
ngx_http_upstream_save_round_robin_peer_session;
#endif
return NGX_OK;
}
ngx_int_t
ngx_http_upstream_create_round_robin_peer(ngx_http_request_t *r,
ngx_http_upstream_resolved_t *ur)
{
u_char *p;
size_t len;
socklen_t socklen;
ngx_uint_t i, n;
struct sockaddr *sockaddr;
ngx_http_upstream_rr_peer_t *peer, **peerp;
ngx_http_upstream_rr_peers_t *peers;
ngx_http_upstream_rr_peer_data_t *rrp;
rrp = r->upstream->peer.data;
if (rrp == NULL) {
rrp = ngx_palloc(r->pool, sizeof(ngx_http_upstream_rr_peer_data_t));
if (rrp == NULL) {
return NGX_ERROR;
}
r->upstream->peer.data = rrp;
}
peers = ngx_pcalloc(r->pool, sizeof(ngx_http_upstream_rr_peers_t));
if (peers == NULL) {
return NGX_ERROR;
}
peer = ngx_pcalloc(r->pool, sizeof(ngx_http_upstream_rr_peer_t)
* ur->naddrs);
if (peer == NULL) {
return NGX_ERROR;
}
peers->single = (ur->naddrs == 1);
peers->number = ur->naddrs;
peers->name = &ur->host;
if (ur->sockaddr) {
peer[0].sockaddr = ur->sockaddr;
peer[0].socklen = ur->socklen;
peer[0].name = ur->name.data ? ur->name : ur->host;
peer[0].weight = 1;
peer[0].effective_weight = 1;
peer[0].current_weight = 0;
peer[0].max_conns = 0;
peer[0].max_fails = 1;
peer[0].fail_timeout = 10;
peers->peer = peer;
} else {
peerp = &peers->peer;
for (i = 0; i < ur->naddrs; i++) {
socklen = ur->addrs[i].socklen;
sockaddr = ngx_palloc(r->pool, socklen);
if (sockaddr == NULL) {
return NGX_ERROR;
}
ngx_memcpy(sockaddr, ur->addrs[i].sockaddr, socklen);
ngx_inet_set_port(sockaddr, ur->port);
p = ngx_pnalloc(r->pool, NGX_SOCKADDR_STRLEN);
if (p == NULL) {
return NGX_ERROR;
}
len = ngx_sock_ntop(sockaddr, socklen, p, NGX_SOCKADDR_STRLEN, 1);
peer[i].sockaddr = sockaddr;
peer[i].socklen = socklen;
peer[i].name.len = len;
peer[i].name.data = p;
peer[i].weight = 1;
peer[i].effective_weight = 1;
peer[i].current_weight = 0;
peer[i].max_conns = 0;
peer[i].max_fails = 1;
peer[i].fail_timeout = 10;
*peerp = &peer[i];
peerp = &peer[i].next;
}
}
rrp->peers = peers;
rrp->current = NULL;
rrp->config = 0;
if (rrp->peers->number <= 8 * sizeof(uintptr_t)) {
rrp->tried = &rrp->data;
rrp->data = 0;
} else {
n = (rrp->peers->number + (8 * sizeof(uintptr_t) - 1))
/ (8 * sizeof(uintptr_t));
rrp->tried = ngx_pcalloc(r->pool, n * sizeof(uintptr_t));
if (rrp->tried == NULL) {
return NGX_ERROR;
}
}
r->upstream->peer.get = ngx_http_upstream_get_round_robin_peer;
r->upstream->peer.free = ngx_http_upstream_free_round_robin_peer;
r->upstream->peer.tries = ngx_http_upstream_tries(rrp->peers);
#if (NGX_HTTP_SSL)
r->upstream->peer.set_session = ngx_http_upstream_empty_set_session;
r->upstream->peer.save_session = ngx_http_upstream_empty_save_session;
#endif
return NGX_OK;
}
ngx_int_t
ngx_http_upstream_get_round_robin_peer(ngx_peer_connection_t *pc, void *data)
{
ngx_http_upstream_rr_peer_data_t *rrp = data;
ngx_int_t rc;
ngx_uint_t i, n;
ngx_http_upstream_rr_peer_t *peer;
ngx_http_upstream_rr_peers_t *peers;
ngx_log_debug1(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"get rr peer, try: %ui", pc->tries);
pc->cached = 0;
pc->connection = NULL;
peers = rrp->peers;
ngx_http_upstream_rr_peers_wlock(peers);
if (peers->single) {
peer = peers->peer;
if (peer->down) {
goto failed;
}
if (peer->max_conns && peer->conns >= peer->max_conns) {
goto failed;
}
rrp->current = peer;
} else {
/* there are several peers */
peer = ngx_http_upstream_get_peer(rrp);
if (peer == NULL) {
goto failed;
}
ngx_log_debug2(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"get rr peer, current: %p %i",
peer, peer->current_weight);
}
pc->sockaddr = peer->sockaddr;
pc->socklen = peer->socklen;
pc->name = &peer->name;
peer->conns++;
ngx_http_upstream_rr_peers_unlock(peers);
return NGX_OK;
failed:
if (peers->next) {
ngx_log_debug0(NGX_LOG_DEBUG_HTTP, pc->log, 0, "backup servers");
rrp->peers = peers->next;
n = (rrp->peers->number + (8 * sizeof(uintptr_t) - 1))
/ (8 * sizeof(uintptr_t));
for (i = 0; i < n; i++) {
rrp->tried[i] = 0;
}
ngx_http_upstream_rr_peers_unlock(peers);
rc = ngx_http_upstream_get_round_robin_peer(pc, rrp);
if (rc != NGX_BUSY) {
return rc;
}
ngx_http_upstream_rr_peers_wlock(peers);
}
ngx_http_upstream_rr_peers_unlock(peers);
pc->name = peers->name;
return NGX_BUSY;
}
static ngx_http_upstream_rr_peer_t *
ngx_http_upstream_get_peer(ngx_http_upstream_rr_peer_data_t *rrp)
{
time_t now;
uintptr_t m;
ngx_int_t total;
ngx_uint_t i, n, p;
ngx_http_upstream_rr_peer_t *peer, *best;
now = ngx_time();
best = NULL;
total = 0;
#if (NGX_SUPPRESS_WARN)
p = 0;
#endif
for (peer = rrp->peers->peer, i = 0;
peer;
peer = peer->next, i++)
{
n = i / (8 * sizeof(uintptr_t));
m = (uintptr_t) 1 << i % (8 * sizeof(uintptr_t));
if (rrp->tried[n] & m) {
continue;
}
if (peer->down) {
continue;
}
if (peer->max_fails
&& peer->fails >= peer->max_fails
&& now - peer->checked <= peer->fail_timeout)
{
continue;
}
if (peer->max_conns && peer->conns >= peer->max_conns) {
continue;
}
peer->current_weight += peer->effective_weight;
total += peer->effective_weight;
if (peer->effective_weight < peer->weight) {
peer->effective_weight++;
}
if (best == NULL || peer->current_weight > best->current_weight) {
best = peer;
p = i;
}
}
if (best == NULL) {
return NULL;
}
rrp->current = best;
n = p / (8 * sizeof(uintptr_t));
m = (uintptr_t) 1 << p % (8 * sizeof(uintptr_t));
rrp->tried[n] |= m;
best->current_weight -= total;
if (now - best->checked > best->fail_timeout) {
best->checked = now;
}
return best;
}
void
ngx_http_upstream_free_round_robin_peer(ngx_peer_connection_t *pc, void *data,
ngx_uint_t state)
{
ngx_http_upstream_rr_peer_data_t *rrp = data;
time_t now;
ngx_http_upstream_rr_peer_t *peer;
ngx_log_debug2(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"free rr peer %ui %ui", pc->tries, state);
/* TODO: NGX_PEER_KEEPALIVE */
peer = rrp->current;
ngx_http_upstream_rr_peers_rlock(rrp->peers);
ngx_http_upstream_rr_peer_lock(rrp->peers, peer);
if (rrp->peers->single) {
peer->conns--;
ngx_http_upstream_rr_peer_unlock(rrp->peers, peer);
ngx_http_upstream_rr_peers_unlock(rrp->peers);
pc->tries = 0;
return;
}
if (state & NGX_PEER_FAILED) {
now = ngx_time();
peer->fails++;
peer->accessed = now;
peer->checked = now;
if (peer->max_fails) {
peer->effective_weight -= peer->weight / peer->max_fails;
if (peer->fails >= peer->max_fails) {
ngx_log_error(NGX_LOG_WARN, pc->log, 0,
"upstream server temporarily disabled");
}
}
ngx_log_debug2(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"free rr peer failed: %p %i",
peer, peer->effective_weight);
if (peer->effective_weight < 0) {
peer->effective_weight = 0;
}
} else {
/* mark peer live if check passed */
if (peer->accessed < peer->checked) {
peer->fails = 0;
}
}
peer->conns--;
ngx_http_upstream_rr_peer_unlock(rrp->peers, peer);
ngx_http_upstream_rr_peers_unlock(rrp->peers);
if (pc->tries) {
pc->tries--;
}
}
#if (NGX_HTTP_SSL)
ngx_int_t
ngx_http_upstream_set_round_robin_peer_session(ngx_peer_connection_t *pc,
void *data)
{
ngx_http_upstream_rr_peer_data_t *rrp = data;
ngx_int_t rc;
ngx_ssl_session_t *ssl_session;
ngx_http_upstream_rr_peer_t *peer;
#if (NGX_HTTP_UPSTREAM_ZONE)
int len;
#if OPENSSL_VERSION_NUMBER >= 0x0090707fL
const
#endif
u_char *p;
ngx_http_upstream_rr_peers_t *peers;
u_char buf[NGX_SSL_MAX_SESSION_SIZE];
#endif
peer = rrp->current;
#if (NGX_HTTP_UPSTREAM_ZONE)
peers = rrp->peers;
if (peers->shpool) {
ngx_http_upstream_rr_peers_rlock(peers);
ngx_http_upstream_rr_peer_lock(peers, peer);
if (peer->ssl_session == NULL) {
ngx_http_upstream_rr_peer_unlock(peers, peer);
ngx_http_upstream_rr_peers_unlock(peers);
return NGX_OK;
}
len = peer->ssl_session_len;
ngx_memcpy(buf, peer->ssl_session, len);
ngx_http_upstream_rr_peer_unlock(peers, peer);
ngx_http_upstream_rr_peers_unlock(peers);
p = buf;
ssl_session = d2i_SSL_SESSION(NULL, &p, len);
rc = ngx_ssl_set_session(pc->connection, ssl_session);
ngx_log_debug1(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"set session: %p", ssl_session);
ngx_ssl_free_session(ssl_session);
return rc;
}
#endif
ssl_session = peer->ssl_session;
rc = ngx_ssl_set_session(pc->connection, ssl_session);
ngx_log_debug1(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"set session: %p", ssl_session);
return rc;
}
void
ngx_http_upstream_save_round_robin_peer_session(ngx_peer_connection_t *pc,
void *data)
{
ngx_http_upstream_rr_peer_data_t *rrp = data;
ngx_ssl_session_t *old_ssl_session, *ssl_session;
ngx_http_upstream_rr_peer_t *peer;
#if (NGX_HTTP_UPSTREAM_ZONE)
int len;
u_char *p;
ngx_http_upstream_rr_peers_t *peers;
u_char buf[NGX_SSL_MAX_SESSION_SIZE];
#endif
#if (NGX_HTTP_UPSTREAM_ZONE)
peers = rrp->peers;
if (peers->shpool) {
ssl_session = SSL_get0_session(pc->connection->ssl->connection);
if (ssl_session == NULL) {
return;
}
ngx_log_debug1(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"save session: %p", ssl_session);
len = i2d_SSL_SESSION(ssl_session, NULL);
/* do not cache too big session */
if (len > NGX_SSL_MAX_SESSION_SIZE) {
return;
}
p = buf;
(void) i2d_SSL_SESSION(ssl_session, &p);
peer = rrp->current;
ngx_http_upstream_rr_peers_rlock(peers);
ngx_http_upstream_rr_peer_lock(peers, peer);
if (len > peer->ssl_session_len) {
ngx_shmtx_lock(&peers->shpool->mutex);
if (peer->ssl_session) {
ngx_slab_free_locked(peers->shpool, peer->ssl_session);
}
peer->ssl_session = ngx_slab_alloc_locked(peers->shpool, len);
ngx_shmtx_unlock(&peers->shpool->mutex);
if (peer->ssl_session == NULL) {
peer->ssl_session_len = 0;
ngx_http_upstream_rr_peer_unlock(peers, peer);
ngx_http_upstream_rr_peers_unlock(peers);
return;
}
peer->ssl_session_len = len;
}
ngx_memcpy(peer->ssl_session, buf, len);
ngx_http_upstream_rr_peer_unlock(peers, peer);
ngx_http_upstream_rr_peers_unlock(peers);
return;
}
#endif
ssl_session = ngx_ssl_get_session(pc->connection);
if (ssl_session == NULL) {
return;
}
ngx_log_debug1(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"save session: %p", ssl_session);
peer = rrp->current;
old_ssl_session = peer->ssl_session;
peer->ssl_session = ssl_session;
if (old_ssl_session) {
ngx_log_debug1(NGX_LOG_DEBUG_HTTP, pc->log, 0,
"old session: %p", old_ssl_session);
/* TODO: may block */
ngx_ssl_free_session(old_ssl_session);
}
}
static ngx_int_t
ngx_http_upstream_empty_set_session(ngx_peer_connection_t *pc, void *data)
{
return NGX_OK;
}
static void
ngx_http_upstream_empty_save_session(ngx_peer_connection_t *pc, void *data)
{
return;
}
#endif
| {
"pile_set_name": "Github"
} |
/*
QBImagePicker.strings
QBImagePicker
Created by Katsuma Tanaka on 2015/04/03.
Copyright (c) 2015 Katsuma Tanaka. All rights reserved.
*/
"albums.title" = "照片";
"assets.footer.photo" = "共%ld张照片";
"assets.footer.photos" = "共%ld张照片";
"assets.footer.video" = "共%ld个视频";
"assets.footer.videos" = "共%ld个视频";
"assets.footer.photo-and-video" = "共%ld 张照片, %ld 个视频";
"assets.footer.photos-and-video" = "共%ld 张照片, %ld 个视频";
"assets.footer.photo-and-videos" = "共%ld 张照片, %ld 个视频";
"assets.footer.photos-and-videos" = "共%ld 张照片, %ld 个视频";
"assets.toolbar.item-selected" = "选择了%ld项";
"assets.toolbar.items-selected" = "选择了%ld项";
| {
"pile_set_name": "Github"
} |
;FinalCountdown
M300 S987 P120
M300 S880 P120
M300 S987 P480
M300 S1318 P480
M300 S0 P480
M300 S0 P240
M300 S2093 P120
M300 S987 P120
M300 S2093 P240
M300 S987 P240
M300 S880 P480
M300 S0 P480
M300 S0 P240
M300 S2093 P120
M300 S987 P120
M300 S2093 P480
M300 S1318 P480
M300 S0 P480
M300 S0 P240
M300 S880 P120
M300 S1567 P120
M300 S880 P240
M300 S1567 P240
M300 S1479 P240
M300 S880 P240
M300 S1567 P720
M300 S1479 P120
M300 S1567 P120
M300 S880 P720
M300 S1567 P120
M300 S880 P120
M300 S987 P240
M300 S880 P240
M300 S1567 P240
M300 S1479 P240
M300 S1318 P480
M300 S2093 P480
M300 S987 P1440
M300 S987 P120
M300 S2093 P120
M300 S987 P120
M300 S880 P120
M300 S987 P1920
| {
"pile_set_name": "Github"
} |
[
"id",
"id",
"id",
"frag",
"foo",
"if",
"include",
"first",
"foo",
"after",
"field1",
"alias",
"field2",
"defer",
"User",
"id",
"foo",
"unless",
"skip",
"id",
"id",
"node",
"whoever123is",
"ComplexType",
"foo",
"Site",
"site",
"queryName",
"id",
"story",
"defer",
"story",
"like",
"likeStory",
"count",
"likers",
"text",
"likeSentence",
"story",
"input",
"input",
"storyLikeSubscribe",
"StoryLikeSubscribeInput",
"input",
"StoryLikeSubscription",
"size",
"size",
"b",
"bar",
"key",
"block",
"obj",
"foo",
"Friend",
"frag",
"truthy",
"falsey",
"nullish",
"unnamed",
"query"
]
| {
"pile_set_name": "Github"
} |
/**
* Copyright (c) 2010-2020 Contributors to the openHAB project
*
* See the NOTICE file(s) distributed with this work for additional
* information.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0
*
* SPDX-License-Identifier: EPL-2.0
*/
package org.openhab.binding.plugwise.protocol;
import java.util.HashMap;
import java.util.Map;
/**
* Plugwise message types - many are still missing, and require further protocol analysis
*
* @author Karel Goderis
* @since 1.1.0
*/
public enum MessageType {
ACKNOWLEDGEMENT(0),
NODE_AVAILABLE(6),
NODE_AVAILABLE_RESPONSE(7),
NETWORK_RESET_REQUEST(8),
INITIALISE_REQUEST(10),
INITIALISE_RESPONSE(17),
POWER_INFORMATION_REQUEST(18),
POWER_INFORMATION_RESPONSE(19),
CLOCK_SET_REQUEST(22),
POWER_CHANGE_REQUEST(23),
DEVICE_ROLECALL_REQUEST(24),
DEVICE_ROLECALL_RESPONSE(25),
DEVICE_INFORMATION_REQUEST(35),
DEVICE_INFORMATION_RESPONSE(36),
DEVICE_CALIBRATION_REQUEST(38),
DEVICE_CALIBRATION_RESPONSE(39),
REALTIMECLOCK_GET_REQUEST(41),
REALTIMECLOCK_GET_RESPONSE(58),
CLOCK_GET_REQUEST(62),
CLOCK_GET_RESPONSE(63),
POWER_BUFFER_REQUEST(72),
POWER_BUFFER_RESPONSE(73),
ANNOUNCE_AWAKE_REQUEST(79),
BROADCAST_GROUP_SWITCH_RESPONSE(86),
MODULE_JOINED_NETWORK_REQUEST(97),
SENSE_REPORT_REQUEST(261);
private int identifier;
private MessageType(int value) {
identifier = value;
}
private static final Map<Integer, MessageType> typesByValue = new HashMap<Integer, MessageType>();
static {
for (MessageType type : MessageType.values()) {
typesByValue.put(type.identifier, type);
}
}
public static MessageType forValue(int value) {
return typesByValue.get(value);
}
public int toInt() {
return identifier;
}
};
| {
"pile_set_name": "Github"
} |
# For a deeper introduction, see
# "DAPPER/tutorials/T4 - Dynamical systems, chaos, Lorenz.ipynb"
from dapper import *
from core import step, x0
##
simulator = with_recursion(step, prog="Simulating")
xx = simulator(x0, k=5*10**3, t0=0, dt=0.01)
##
fig, ax = plt.subplots(subplot_kw={'projection':'3d'})
ax.plot(*xx.T, lw=1)
ax.plot(*np.atleast_2d(x0 ).T, '*g', ms=14)
ax.plot(*np.atleast_2d(xx[-1]).T, '*r', ms=14)
fig.suptitle('Phase space evolution')
ax.set_facecolor('w')
[eval("ax.set_%slabel('%s')"%(s,s)) for s in "xyz"]
##
| {
"pile_set_name": "Github"
} |
/*
Legal Notice: Some portions of the source code contained in this file were
derived from the source code of TrueCrypt 7.1a, which is
Copyright (c) 2003-2012 TrueCrypt Developers Association and which is
governed by the TrueCrypt License 3.0, also from the source code of
Encryption for the Masses 2.02a, which is Copyright (c) 1998-2000 Paul Le Roux
and which is governed by the 'License Agreement for Encryption for the Masses'
Modifications and additions to the original source code (contained in this file)
and all other portions of this file are Copyright (c) 2013-2017 IDRIX
and are governed by the Apache License 2.0 the full text of which is
contained in the file License.txt included in VeraCrypt binary and source
code distribution packages. */
#ifndef PASSWORD_H
#define PASSWORD_H
// User text input limits
#define MIN_PASSWORD 1 // Minimum possible password length
#if defined(TC_WINDOWS_BOOT) || defined(_UEFI)
#define MAX_PASSWORD 64 // Maximum possible password length
#else
#define MAX_LEGACY_PASSWORD 64 // Maximum possible legacy password length
#define MAX_PASSWORD 128 // Maximum possible password length
#endif
#define MAX_PIM 7 // Maximum allowed digits in a PIM (enough for maximum value)
#define MAX_PIM_VALUE 2147468 // Maximum value to have a positive 32-bit result for formula 15000 + (PIM x 1000)
#define MAX_BOOT_PIM 5 // Maximum allowed digits in a PIM for boot (enough for 16-bit value)
#define MAX_BOOT_PIM_VALUE 65535
#define PASSWORD_LEN_WARNING 20 // Display a warning when a password is shorter than this
#ifdef __cplusplus
extern "C" {
#endif
typedef struct
{
// Modifying this structure can introduce incompatibility with previous versions
unsigned __int32 Length;
unsigned char Text[MAX_PASSWORD + 1];
char Pad[3]; // keep 64-bit alignment
} Password;
#if defined(TC_WINDOWS_BOOT) || defined(_UEFI)
#define PasswordLegacy Password
#else
typedef struct
{
// Modifying this structure can introduce incompatibility with previous versions
unsigned __int32 Length;
unsigned char Text[MAX_LEGACY_PASSWORD + 1];
char Pad[3]; // keep 64-bit alignment
} PasswordLegacy;
#endif
#if defined(_WIN32) && !defined(TC_WINDOWS_DRIVER) && !defined(_UEFI)
void VerifyPasswordAndUpdate ( HWND hwndDlg , HWND hButton , HWND hPassword , HWND hVerify , unsigned char *szPassword , char *szVerify, BOOL keyFilesEnabled );
BOOL CheckPasswordLength (HWND hwndDlg, unsigned __int32 passwordLength, int pim, BOOL bForBoot, int bootPRF, BOOL bSkipPasswordWarning, BOOL bSkipPimWarning);
BOOL CheckPasswordCharEncoding (HWND hPassword, Password *ptrPw);
int ChangePwd (const wchar_t *lpszVolume, Password *oldPassword, int old_pkcs5, int old_pim, BOOL truecryptMode, Password *newPassword, int pkcs5, int pim, int wipePassCount, HWND hwndDlg);
#endif // defined(_WIN32) && !defined(TC_WINDOWS_DRIVER) && !defined(_UEFI)
#ifdef __cplusplus
}
#endif
#endif // PASSWORD_H
| {
"pile_set_name": "Github"
} |
/* $NetBSD: __aeabi_fcmple.c,v 1.1 2013/04/16 10:37:39 matt Exp $ */
/** @file
*
* Copyright (c) 2013 - 2014, ARM Limited. All rights reserved.
*
* This program and the accompanying materials
* are licensed and made available under the terms and conditions of the BSD License
* which accompanies this distribution. The full text of the license may be found at
* http://opensource.org/licenses/bsd-license.php
*
* THE PROGRAM IS DISTRIBUTED UNDER THE BSD LICENSE ON AN "AS IS" BASIS,
* WITHOUT WARRANTIES OR REPRESENTATIONS OF ANY KIND, EITHER EXPRESS OR IMPLIED.
*
**/
/*
* Written by Ben Harris, 2000. This file is in the Public Domain.
*/
#include "softfloat-for-gcc.h"
#include "milieu.h"
#include "softfloat.h"
#include <sys/cdefs.h>
#if defined(LIBC_SCCS) && !defined(lint)
__RCSID("$NetBSD: __aeabi_fcmple.c,v 1.1 2013/04/16 10:37:39 matt Exp $");
#endif /* LIBC_SCCS and not lint */
int __aeabi_fcmple(float32, float32);
int
__aeabi_fcmple(float32 a, float32 b)
{
return float32_le(a, b);
}
| {
"pile_set_name": "Github"
} |
{
"nome": "Tufino",
"codice": "063085",
"zona": {
"codice": "4",
"nome": "Sud"
},
"regione": {
"codice": "15",
"nome": "Campania"
},
"provincia": {
"codice": "063",
"nome": "Napoli"
},
"sigla": "NA",
"codiceCatastale": "L460",
"cap": [
"80030"
],
"popolazione": 3785
}
| {
"pile_set_name": "Github"
} |
/*
* This file is part of the UCB release of Plan 9. It is subject to the license
* terms in the LICENSE file found in the top-level directory of this
* distribution and at http://akaros.cs.berkeley.edu/files/Plan9License. No
* part of the UCB release of Plan 9, including this file, may be copied,
* modified, propagated, or distributed except according to the terms contained
* in the LICENSE file.
*/
#include <u.h>
#include <libc.h>
#include <authsrv.h>
#define CHAR(x) *p++ = f->x
#define SHORT(x) p[0] = f->x; p[1] = f->x>>8; p += 2
#define VLONG(q) p[0] = (q); p[1] = (q)>>8; p[2] = (q)>>16; p[3] = (q)>>24; p += 4
#define LONG(x) VLONG(f->x)
#define STRING(x,n) memmove(p, f->x, n); p += n
int
convPR2M(Passwordreq *f, char *ap, char *key)
{
int n;
uchar *p;
p = (uchar*)ap;
CHAR(num);
STRING(old, ANAMELEN);
STRING(new, ANAMELEN);
CHAR(changesecret);
STRING(secret, SECRETLEN);
n = p - (uchar*)ap;
if(key)
encrypt(key, ap, n);
return n;
}
| {
"pile_set_name": "Github"
} |
(function (App) {
'use strict';
var SCROLL_MORE = 0.7; // 70% of window height
var NUM_MOVIES_IN_ROW = 7;
var _this;
function elementInViewport(container, element) {
if (element.length === 0) {
return;
}
var $container = $(container),
$el = $(element);
var docViewTop = $container.offset().top;
var docViewBottom = docViewTop + $container.height();
var elemTop = $el.offset().top;
var elemBottom = elemTop + $el.height();
return ((elemBottom >= docViewTop) && (elemTop <= docViewBottom) && (elemBottom <= docViewBottom) && (elemTop >= docViewTop));
}
var ErrorView = Backbone.Marionette.ItemView.extend({
template: '#movie-error-tpl',
ui: {
retryButton: '.retry-button',
onlineSearch: '.online-search'
},
onBeforeRender: function () {
this.model.set('error', this.error);
},
onRender: function () {
if (this.retry) {
switch (App.currentview) {
case 'movies':
case 'shows':
case 'anime':
this.ui.onlineSearch.css('visibility', 'visible');
this.ui.retryButton.css('visibility', 'visible');
break;
case 'Watchlist':
this.ui.retryButton.css('visibility', 'visible');
this.ui.retryButton.css('margin-left', 'calc(50% - 100px)');
break;
default:
}
}
}
});
var List = Backbone.Marionette.CompositeView.extend({
template: '#list-tpl',
tagName: 'ul',
className: 'list',
childView: App.View.Item,
childViewContainer: '.items',
events: {
'scroll': 'onScroll',
'mousewheel': 'onScroll',
'keydown': 'onScroll'
},
ui: {
spinner: '.spinner'
},
isEmpty: function () {
return !this.collection.length && this.collection.state !== 'loading';
},
getEmptyView: function () {
switch (App.currentview) {
case 'movies':
case 'shows':
case 'anime':
if (this.collection.state === 'error') {
return ErrorView.extend({
retry: true,
error: i18n.__('The remote ' + App.currentview + ' API failed to respond, please check %s and try again later', '<a class="links" href="' + Settings.statusUrl + '">' + Settings.statusUrl + '</a>')
});
} else if (this.collection.state !== 'loading') {
return ErrorView.extend({
error: i18n.__('No ' + App.currentview + ' found...')
});
}
break;
case 'Favorites':
if (this.collection.state === 'error') {
return ErrorView.extend({
retry: true,
error: i18n.__('Error, database is probably corrupted. Try flushing the bookmarks in settings.')
});
} else if (this.collection.state !== 'loading') {
return ErrorView.extend({
error: i18n.__('No ' + App.currentview + ' found...')
});
}
break;
case 'Watchlist':
if (this.collection.state === 'error') {
return ErrorView.extend({
retry: true,
error: i18n.__('This feature only works if you have your TraktTv account synced. Please go to Settings and enter your credentials.')
});
} else if (this.collection.state !== 'loading') {
return ErrorView.extend({
error: i18n.__('No ' + App.currentview + ' found...')
});
}
break;
}
},
initialize: function () {
_this = this;
this.listenTo(this.collection, 'loading', this.onLoading);
this.listenTo(this.collection, 'loaded', this.onLoaded);
App.vent.on('shortcuts:list', _this.initKeyboardShortcuts);
_this.initKeyboardShortcuts();
_this.initPosterResizeKeys();
},
initKeyboardShortcuts: function () {
Mousetrap.bind('up', _this.moveUp);
Mousetrap.bind('down', _this.moveDown);
Mousetrap.bind('left', _this.moveLeft);
Mousetrap.bind('right', _this.moveRight);
Mousetrap.bind('f', _this.toggleSelectedFavourite);
Mousetrap.bind('w', _this.toggleSelectedWatched);
Mousetrap.bind(['enter', 'space'], _this.selectItem);
Mousetrap.bind(['ctrl+f', 'command+f'], _this.focusSearch);
Mousetrap(document.querySelector('input')).bind(['ctrl+f', 'command+f', 'esc'], function (e, combo) {
$('.search input').blur();
});
Mousetrap.bind(['tab', 'shift+tab'], function (e, combo) {
if ((App.PlayerView === undefined || App.PlayerView.isDestroyed) && $('#about-container').children().length <= 0 && $('#player').children().length <= 0) {
if (combo === 'tab') {
switch (App.currentview) {
case 'movies':
App.currentview = 'shows';
break;
case 'shows':
App.currentview = 'anime';
break;
default:
App.currentview = 'movies';
}
} else if (combo === 'shift+tab') {
switch (App.currentview) {
case 'movies':
App.currentview = 'anime';
break;
case 'anime':
App.currentview = 'shows';
break;
default:
App.currentview = 'movies';
}
}
App.vent.trigger('torrentCollection:close');
App.vent.trigger(App.currentview + ':list', []);
$('.filter-bar').find('.active').removeClass('active');
$('.source.show' + App.currentview.charAt(0).toUpperCase() + App.currentview.slice(1)).addClass('active');
}
});
Mousetrap.bind(['ctrl+1', 'ctrl+2', 'ctrl+3'], function (e, combo) {
if ((App.PlayerView === undefined || App.PlayerView.isDestroyed) && $('#about-container').children().length <= 0 && $('#player').children().length <= 0) {
switch (combo) {
case 'ctrl+1':
App.currentview = 'movies';
break;
case 'ctrl+2':
App.currentview = 'shows';
break;
case 'ctrl+3':
App.currentview = 'anime';
break;
}
App.vent.trigger('torrentCollection:close');
App.vent.trigger(App.currentview + ':list', []);
$('.filter-bar').find('.active').removeClass('active');
$('.source.show' + App.currentview.charAt(0).toUpperCase() + App.currentview.slice(1)).addClass('active');
}
});
Mousetrap.bind(['`', 'b'], function () {
if ((App.PlayerView === undefined || App.PlayerView.isDestroyed) && $('#about-container').children().length <= 0 && $('#player').children().length <= 0) {
$('.favorites').click();
}
});
Mousetrap.bind('i', function () {
if ((App.PlayerView === undefined || App.PlayerView.isDestroyed) && $('#player').children().length <= 0) {
$('.about').click();
}
});
},
initPosterResizeKeys: function () {
$(window)
.on('mousewheel', function (event) { // Ctrl + wheel doesnt seems to be working on node-webkit (works just fine on chrome)
if (event.altKey === true) {
event.preventDefault();
if (event.originalEvent.wheelDelta > 0) {
_this.increasePoster();
} else {
_this.decreasePoster();
}
}
})
.on('keydown', function (event) {
if (event.ctrlKey === true || event.metaKey === true) {
if ($.inArray(event.keyCode, [107, 187]) !== -1) {
_this.increasePoster();
return false;
} else if ($.inArray(event.keyCode, [109, 189]) !== -1) {
_this.decreasePoster();
return false;
}
}
});
},
onShow: function () {
if (this.collection.state === 'loading') {
this.onLoading();
}
},
onLoading: function () {
$('.status-loadmore').hide();
$('#loading-more-animi').show();
},
onLoaded: function () {
App.vent.trigger('list:loaded');
this.checkEmpty();
var self = this;
this.addloadmore();
this.AddGhostsToBottomRow();
$(window).resize(function () {
var addghost;
clearTimeout(addghost);
addghost = setTimeout(function () {
self.AddGhostsToBottomRow();
}, 100);
});
if (typeof (this.ui.spinner) === 'object') {
this.ui.spinner.hide();
}
$('.filter-bar').on('mousedown', function (e) {
if (e.target.localName !== 'div') {
return;
}
_.defer(function () {
self.$('.items:first').focus();
});
});
$('.items').attr('tabindex', '1');
_.defer(function () {
self.checkFetchMore();
self.$('.items:first').focus();
});
},
checkFetchMore: function () {
// if load more is visible onLoaded, fetch more results
if (elementInViewport(this.$el, $('#load-more-item'))) {
this.collection.fetchMore();
}
},
addloadmore: function () {
var self = this;
// maxResults to hide load-more on providers that return hasMore=true no matter what.
var currentPage = Math.ceil(this.collection.length / 50);
var maxResults = currentPage * 50;
switch (App.currentview) {
case 'movies':
case 'shows':
case 'anime':
$('#load-more-item').remove();
// we add a load more
if (this.collection.hasMore && !this.collection.filter.keywords && this.collection.state !== 'error' && this.collection.length !== 0 && this.collection.length >= maxResults) {
$('.items').append('<div id="load-more-item" class="load-more"><span class="status-loadmore">' + i18n.__('Load More') + '</span><div id="loading-more-animi" class="loading-container"><div class="ball"></div><div class="ball1"></div></div></div>');
$('#load-more-item').click(function () {
$('#load-more-item').off('click');
self.collection.fetchMore();
});
$('#loading-more-animi').hide();
$('.status-loadmore').show();
}
break;
case 'Favorites':
break;
case 'Watchlist':
break;
}
},
AddGhostsToBottomRow: function () {
$('.ghost').remove();
var listWidth = $('.items').width();
var itemWidth = $('.items .item').width() + (2 * parseInt($('.items .item').css('margin')));
var itemsPerRow = parseInt(listWidth / itemWidth);
/* in case we .hide() items at some point:
var visibleItems = 0;
var hiddenItems = 0;
$('.item').each(function () {
$(this).is(':visible') ? visibleItems++ : hiddenItems++;
});
var itemsInLastRow = visibleItems % itemsPerRow;*/
NUM_MOVIES_IN_ROW = itemsPerRow;
var itemsInLastRow = $('.items .item').length % itemsPerRow;
var ghostsToAdd = itemsPerRow - itemsInLastRow;
while (ghostsToAdd > 0) {
$('.items').append($('<li/>').addClass('item ghost'));
ghostsToAdd--;
}
},
onScroll: function () {
if (!this.collection.hasMore) {
return;
}
var totalHeight = this.$el.prop('scrollHeight');
var currentPosition = this.$el.scrollTop() + this.$el.height();
if (this.collection.state === 'loaded' &&
(currentPosition / totalHeight) > SCROLL_MORE) {
this.collection.fetchMore();
}
},
focusSearch: function (e) {
$('.search input').focus();
},
increasePoster: function (e) {
var postersWidthIndex = Settings.postersJump.indexOf(parseInt(Settings.postersWidth));
if (postersWidthIndex !== -1 && postersWidthIndex + 1 in Settings.postersJump) {
App.db.writeSetting({
key: 'postersWidth',
value: Settings.postersJump[postersWidthIndex + 1]
})
.then(function () {
App.vent.trigger('updatePostersSizeStylesheet');
});
} else {
// do nothing for now
}
},
decreasePoster: function (e) {
var postersWidth;
var postersWidthIndex = Settings.postersJump.indexOf(parseInt(Settings.postersWidth));
if (postersWidthIndex !== -1 && postersWidthIndex - 1 in Settings.postersJump) {
postersWidth = Settings.postersJump[postersWidthIndex - 1];
} else {
postersWidth = Settings.postersJump[0];
}
App.db.writeSetting({
key: 'postersWidth',
value: postersWidth
})
.then(function () {
App.vent.trigger('updatePostersSizeStylesheet');
});
},
selectItem: function (e) {
if (e.type) {
e.preventDefault();
e.stopPropagation();
}
$('.item.selected .cover').trigger('click');
},
selectIndex: function (index) {
if ($('.items .item').eq(index).length === 0 || $('.items .item').eq(index).children().length === 0) {
return;
}
$('.item.selected').removeClass('selected');
$('.items .item').eq(index).addClass('selected');
var $movieEl = $('.item.selected')[0];
if (!elementInViewport(this.$el, $movieEl)) {
$movieEl.scrollIntoView(false);
this.onScroll();
}
},
moveUp: function (e) {
if (e.type) {
e.preventDefault();
e.stopPropagation();
}
var index = $('.item.selected').index();
if (index === -1) {
index = 0;
} else {
index = index - NUM_MOVIES_IN_ROW;
}
if (index < 0) {
return;
}
_this.selectIndex(index);
},
moveDown: function (e) {
if (e.type) {
e.preventDefault();
e.stopPropagation();
}
var index = $('.item.selected').index();
if (index === -1) {
index = 0;
} else {
index = index + NUM_MOVIES_IN_ROW;
}
_this.selectIndex(index);
},
moveLeft: function (e) {
if (e.type) {
e.preventDefault();
e.stopPropagation();
}
var index = $('.item.selected').index();
if (index === -1) {
index = 0;
} else if (index === 0) {
index = 0;
} else {
index = index - 1;
}
_this.selectIndex(index);
},
moveRight: function (e) {
if (e.type) {
e.preventDefault();
e.stopPropagation();
}
var index = $('.item.selected').index();
if (index === -1) {
index = 0;
} else {
index = index + 1;
}
_this.selectIndex(index);
},
toggleSelectedFavourite: function (e) {
$('.item.selected .actions-favorites').click();
},
toggleSelectedWatched: function (e) {
$('.item.selected .actions-watched').click();
},
});
App.View.List = List;
})(window.App);
| {
"pile_set_name": "Github"
} |
# Add project specific ProGuard rules here.
# By default, the flags in this file are appended to flags specified
# in /Users/jaychang/Library/Android/sdk/tools/proguard/proguard-android.txt
# You can edit the include path and order by changing the proguardFiles
# directive in build.gradle.
#
# For more details, see
# http://developer.android.com/guide/developing/tools/proguard.html
# Add any project specific keep options here:
# If your project uses WebView create JS, uncomment the following
# and specify the fully qualified class name to the JavaScript interface
# class:
#-keepclassmembers class fqcn.of.javascript.interface.for.webview {
# public *;
#}
# Uncomment this to preserve the line number information for
# debugging stack traces.
#-keepattributes SourceFile,LineNumberTable
# If you keep the line number information, uncomment this to
# hide the original source file name.
#-renamesourcefileattribute SourceFile
| {
"pile_set_name": "Github"
} |
/*
Unix SMB/CIFS implementation.
routines for marshalling/unmarshalling DCOM string arrays
Copyright (C) Jelmer Vernooij 2004
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
*/
//#define NDR_CHECK_DEBUG
#include "includes.h"
#include "librpc/gen_ndr/ndr_dcom.h"
#include "librpc/gen_ndr/ndr_wmi.h"
#include "librpc/ndr/ndr_wmi.h"
// Just for debugging
int NDR_CHECK_depth = 0;
int NDR_CHECK_shift = 0x18;
enum ndr_err_code ndr_push_BSTR(struct ndr_push *ndr, int ndr_flags, const struct BSTR *r)
{
uint32_t len;
uint32_t flags;
enum ndr_err_code status;
len = strlen(r->data);
if (ndr_flags & NDR_SCALARS) {
NDR_CHECK(ndr_push_align(ndr, 4));
NDR_CHECK(ndr_push_uint32(ndr, NDR_SCALARS, 0x72657355));
NDR_CHECK(ndr_push_uint32(ndr, NDR_SCALARS, len));
NDR_CHECK(ndr_push_uint32(ndr, NDR_SCALARS, 2*len));
flags = ndr->flags;
ndr_set_flags(&ndr->flags, LIBNDR_FLAG_STR_NOTERM | LIBNDR_FLAG_STR_SIZE4);
status = ndr_push_string(ndr, NDR_SCALARS, r->data);
ndr->flags = flags;
return status;
}
return NDR_ERR_SUCCESS;
}
enum ndr_err_code ndr_pull_BSTR(struct ndr_pull *ndr, int ndr_flags, struct BSTR *r)
{
return NDR_ERR_BAD_SWITCH;
}
void ndr_print_BSTR(struct ndr_print *ndr, const char *name, const struct BSTR *r)
{
ndr->print(ndr, "%-25s: BSTR(\"%s\")", name, r->data);
}
| {
"pile_set_name": "Github"
} |
/**
* GAS - Google Analytics on Steroids
*
* Multi-Domain Tracking Plugin
*
* Copyright 2011, Cardinal Path and Direct Performance
* Licensed under the GPLv3 license.
*
* @author Eduardo Cereto <eduardocereto@gmail.com>
*/
/**
* Private variable to store allowAnchor choice
*/
_gas._allowAnchor = false;
/**
* _setAllowAnchor Hook to store choice for easier use of Anchor
*
* This stored value is used on _getLinkerUrl, _link and _linkByPost so it's
* used the same by default
*/
_gas.push(['_addHook', '_setAllowAnchor', function (val) {
_gas._allowAnchor = !!val;
}]);
/**
* _link Hook to use stored allowAnchor value.
*/
_gas.push(['_addHook', '_link', function (url, use_anchor) {
if (use_anchor === undefined) {
use_anchor = _gas._allowAnchor;
}
return [url, use_anchor];
}]);
/**
* _linkByPost Hook to use stored allowAnchor value.
*/
_gas.push(['_addHook', '_linkByPost', function (url, use_anchor) {
if (use_anchor === undefined) {
use_anchor = _gas._allowAnchor;
}
return [url, use_anchor];
}]);
/**
* Store all domains pushed by _setDomainName that don't match current domain.
*
* @type {Array.<string>}
*/
var _external_domains = [];
/**
* Store the internal domain name
*
* @type string
*/
var _internal_domain;
/**
* _setDomainName Hook to add pushed domains to _external_domains if it doesn't
* match current domain.
*
* This Hook let you call _setDomainName multiple times. So _gas will only
* apply the one that matches the current domain and the other ones will be
* used to track external domains with cookie data.
*/
_gas.push(['_addHook', '_setDomainName', function (domainName) {
if (sindexOf.call('.' + document.location.hostname, domainName) < 0) {
_external_domains.push(domainName);
return false;
}
_internal_domain = domainName;
}]);
/**
* _addExternalDomainName Hook.
*
* This hook let you add external domains so that urls on current page to this
* domain are marked to send cookies.
* You should use _setDomainName for this in most of the cases.
*/
_gas.push(['_addHook', '_addExternalDomainName', function (domainName) {
_external_domains.push(domainName);
return false;
}]);
/**
* Function to mark links on the current pages to send links
*
* This function is used to make it easy to implement multi-domain-tracking.
* @param {string} event_used Should be 'now', 'click' or 'mousedown'. Default
* 'click'.
* @this {GasHelper} GAS Helper functions
* @return {boolean} Returns false to avoid this is puhed to _gaq.
*/
function track_links(event_used) {
if (!this._multidomainTracked) {
this._multidomainTracked = true;
} else {
//Oops double tracking detected.
return;
}
var internal = document.location.hostname,
gh = this,
i, j, el,
links = document.getElementsByTagName('a');
if (event_used !== 'now' && event_used !== 'mousedown') {
event_used = 'click';
}
for (i = 0; i < links.length; i++) {
el = links[i];
if (sindexOf.call(el.href, 'http') === 0) {
// Check to see if it's a internal link
if (el.hostname === internal ||
sindexOf.call(el.hostname, _internal_domain) >= 0) {
continue;
}
// Tag external Links either now or on mouse event.
for (j = 0; j < _external_domains.length; j++) {
if (sindexOf.call(el.hostname, _external_domains[j]) >= 0) {
if (event_used === 'now') {
el.href = gh['tracker']['_getLinkerUrl'](
el.href,
_gas._allowAnchor
);
} else {
if (event_used === 'click') {
this._addEventListener(el, event_used, function (e) {
if (this.target && this.target === '_blank') {
window.open(
gh['tracker']['_getLinkerUrl'](
this.href, _gas._allowAnchor
)
);
} else {
_gas.push(
['_link', this.href, _gas._allowAnchor]
);
}
if (e.preventDefault)
e.preventDefault();
else
e.returnValue = false;
return false; //needed for ie7
});
} else {
this._addEventListener(el, event_used, function () {
this.href = gh['tracker']['_getLinkerUrl'](
this.href,
_gas._allowAnchor
);
});
}
}
}
}
}
}
return false;
}
var _gasMultiDomain = function () {
var gh = this;
var args = slice.call(arguments);
if (gh && gh._DOMReady) {
gh._DOMReady(function () {
track_links.apply(gh, args);
});
}
return false;
};
/**
* Registers Hook to _setMultiDomain
*/
_gas.push(['_addHook', '_gasMultiDomain', _gasMultiDomain]);
// Old API to be deprecated on v2.0
_gas.push(['_addHook', '_setMultiDomain', _gasMultiDomain]);
| {
"pile_set_name": "Github"
} |
<?php
/*
* Copyright 2014 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
class Google_Service_TPU_ReimageNodeRequest extends Google_Model
{
public $tensorflowVersion;
public function setTensorflowVersion($tensorflowVersion)
{
$this->tensorflowVersion = $tensorflowVersion;
}
public function getTensorflowVersion()
{
return $this->tensorflowVersion;
}
}
| {
"pile_set_name": "Github"
} |
var c = require('./common');
exports.a = 1;
exports.b = c;
| {
"pile_set_name": "Github"
} |
type: php
build:
flavor: drupal
| {
"pile_set_name": "Github"
} |
<%--
DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
Copyright (c) 2007 Sun Microsystems Inc. All Rights Reserved
The contents of this file are subject to the terms
of the Common Development and Distribution License
(the License). You may not use this file except in
compliance with the License.
You can obtain a copy of the License at
https://opensso.dev.java.net/public/CDDLv1.0.html or
opensso/legal/CDDLv1.0.txt
See the License for the specific language governing
permission and limitations under the License.
When distributing Covered Code, include this CDDL
Header Notice in each file and include the License file
at opensso/legal/CDDLv1.0.txt.
If applicable, add the following below the CDDL Header,
with the fields enclosed by brackets [] replaced by
your own identifying information:
"Portions Copyrighted [year] [name of copyright owner]"
$Id: ServerEditAdvanced.jsp,v 1.4 2008/09/16 23:43:27 asyhuang Exp $
--%>
<%@ page info="ServerEditAdvanced" language="java" %>
<%@taglib uri="/WEB-INF/jato.tld" prefix="jato" %>
<%@taglib uri="/WEB-INF/cc.tld" prefix="cc" %>
<jato:useViewBean
className="com.sun.identity.console.service.ServerEditAdvancedViewBean"
fireChildDisplayEvents="true" >
<cc:i18nbundle baseName="amConsole" id="amConsole"
locale="<%=((com.sun.identity.console.base.AMViewBeanBase)viewBean).getUserLocale()%>"/>
<cc:header name="hdrCommon" pageTitle="webconsole.title" bundleID="amConsole" copyrightYear="2007" fireDisplayEvents="true">
<script language="javascript" src="../console/js/am.js"></script>
<script language="javascript">
function consolidateProperties() {
var form = document.getElementsByName("ServerEditAdvanced")[0];
var elm = form.elements['ServerEditAdvanced.properties'];
var propertiesValues = '';
var table = getActionTable();
var rows = table.getElementsByTagName('tr');
if (rows.length > 2) {
for (i = 2; i < rows.length; i++) {
var row = rows[i];
var inputs = row.getElementsByTagName('input');
propertiesValues += inputs[2].value + "=" + inputs[3].value + "\n";
}
}
elm.value = propertiesValues;
}
function deletePropertyRow() {
var table = getActionTable();
var rows = table.getElementsByTagName('tr');
for (i = rows.length-1; i >= 2; --i) {
var row = rows[i];
var inputs = row.getElementsByTagName('input');
var cb = inputs[0];
if (cb.checked) {
table.deleteRow(i);
}
}
rows = table.getElementsByTagName('tr');
if (rows.length == 2) {
addPropertyRow();
}
tblBtnCounter['tblButton'] = 0;
ccSetButtonDisabled('ServerEditAdvanced.tblButtonDelete', 'ServerEditAdvanced', true);
return false;
}
function addPropertyRow() {
var table = getActionTable();
var tBody = table.getElementsByTagName("TBODY").item(0);
var row = document.createElement("TR");
var cell1 = document.createElement("TD");
cell1.setAttribute("align", "center");
cell1.setAttribute("valign", "top");
var cell2 = document.createElement("TD");
var cell3 = document.createElement("TD");
var cb = document.createElement("input");
var hidden = document.createElement("input");
var textnode1 = document.createElement("input");
var textnode2 = document.createElement("input");
hidden.setAttribute("type", "hidden");
cb.setAttribute("type", "checkbox");
cb.setAttribute("onclick", "toggleTblButtonState('ServerEditAdvanced', 'ServerEditAdvanced.tblAdvancedProperties', 'tblButton', 'ServerEditAdvanced.tblButtonDelete', this)");
textnode1.setAttribute("size", "50");
textnode2.setAttribute("size", "50");
cell1.appendChild(cb);
cell1.appendChild(hidden);
cell2.appendChild(textnode1);
cell3.appendChild(textnode2);
row.appendChild(cell1);
row.appendChild(cell2);
row.appendChild(cell3);
tBody.appendChild(row);
scrollToObject(row);
return false;
}
function scrollToObject(elt) {
var posX = 0;
var posY = 0;
while (elt != null) {
posX += elt.offsetLeft;
posY += elt.offsetTop;
elt = elt.offsetParent;
}
window.scrollTo(posX, posY);
}
function getActionTable() {
var nodes = document.getElementsByTagName("table");
var len = nodes.length;
for (var i = 0; i < len; i++) {
if (nodes[i].className == 'Tbl') {
return nodes[i];
}
}
}
</script>
<cc:form name="ServerEditAdvanced" method="post" defaultCommandChild="/button1"
onSubmit="consolidateProperties();">
<script language="javascript">
function confirmLogout() {
return confirm("<cc:text name="txtLogout" defaultValue="masthead.logoutMessage" bundleID="amConsole"/>");
}
</script>
<cc:primarymasthead name="mhCommon" bundleID="amConsole" logoutOnClick="return confirmLogout();" locale="<%=((com.sun.identity.console.base.AMViewBeanBase)viewBean).getUserLocale()%>"/>
<cc:breadcrumbs name="breadCrumb" bundleID="amConsole" />
<cc:tabs name="tabCommon" bundleID="amConsole" />
<table border="0" cellpadding="10" cellspacing="0" width="100%">
<tr>
<td><cc:alertinline name="ialertCommon" bundleID="amConsole" /></td>
</tr>
</table>
<%-- PAGE CONTENT --------------------------------------------------------- --%>
<cc:pagetitle name="pgtitleThreeBtns" bundleID="amConsole" pageTitleText="page.title.server.edit" showPageTitleSeparator="true" viewMenuLabel="" pageTitleHelpMessage="" showPageButtonsTop="true" showPageButtonsBottom="false" />
<jato:hidden name="szCache" />
<jato:hidden name="properties" />
<cc:spacer name="spacer" height="10" newline="true" />
<cc:actiontable
name="tblAdvancedProperties"
title="table.serverconfig.advanced.property.title.name"
bundleID="amConsole"
summary="table.serverconfig.advanced.property.summary"
empty="table.serverconfig.advanced.property.empty.message"
selectionType="multiple"
showAdvancedSortingIcon="false"
showLowerActions="false"
showPaginationControls="false"
showPaginationIcon="false"
showSelectionIcons="false"
showSelectionSortIcon="false"
selectionJavascript="toggleTblButtonState('ServerEditAdvanced', 'ServerEditAdvanced.tblAdvancedProperties', 'tblButton', 'ServerEditAdvanced.tblButtonDelete', this)"
showSortingRow="false">
<attribute name="extrahtml" value="name=\"propertyTable\"" />
</cc:actiontable>
</cc:form>
</cc:header>
</jato:useViewBean>
| {
"pile_set_name": "Github"
} |
+-----------+-------------------+
| housing | R Documentation |
+-----------+-------------------+
Frequency Table from a Copenhagen Housing Conditions Survey
-----------------------------------------------------------
Description
~~~~~~~~~~~
The ``housing`` data frame has 72 rows and 5 variables.
Usage
~~~~~
::
housing
Format
~~~~~~
``Sat``
Satisfaction of householders with their present housing
circumstances, (High, Medium or Low, ordered factor).
``Infl``
Perceived degree of influence householders have on the management of
the property (High, Medium, Low).
``Type``
Type of rental accommodation, (Tower, Atrium, Apartment, Terrace).
``Cont``
Contact residents are afforded with other residents, (Low, High).
``Freq``
Frequencies: the numbers of residents in each class.
Source
~~~~~~
Madsen, M. (1976) Statistical analysis of multiple contingency tables.
Two examples. *Scand. J. Statist.* **3**, 97–106.
Cox, D. R. and Snell, E. J. (1984) *Applied Statistics, Principles and
Examples*. Chapman & Hall.
References
~~~~~~~~~~
Venables, W. N. and Ripley, B. D. (2002) *Modern Applied Statistics with
S.* Fourth edition. Springer.
Examples
~~~~~~~~
::
options(contrasts = c("contr.treatment", "contr.poly"))
# Surrogate Poisson models
house.glm0 <- glm(Freq ~ Infl*Type*Cont + Sat, family = poisson,
data = housing)
summary(house.glm0, cor = FALSE)
addterm(house.glm0, ~. + Sat:(Infl+Type+Cont), test = "Chisq")
house.glm1 <- update(house.glm0, . ~ . + Sat*(Infl+Type+Cont))
summary(house.glm1, cor = FALSE)
1 - pchisq(deviance(house.glm1), house.glm1$df.residual)
dropterm(house.glm1, test = "Chisq")
addterm(house.glm1, ~. + Sat:(Infl+Type+Cont)^2, test = "Chisq")
hnames <- lapply(housing[, -5], levels) # omit Freq
newData <- expand.grid(hnames)
newData$Sat <- ordered(newData$Sat)
house.pm <- predict(house.glm1, newData,
type = "response") # poisson means
house.pm <- matrix(house.pm, ncol = 3, byrow = TRUE,
dimnames = list(NULL, hnames[[1]]))
house.pr <- house.pm/drop(house.pm %*% rep(1, 3))
cbind(expand.grid(hnames[-1]), round(house.pr, 2))
# Iterative proportional scaling
loglm(Freq ~ Infl*Type*Cont + Sat*(Infl+Type+Cont), data = housing)
# multinomial model
library(nnet)
(house.mult<- multinom(Sat ~ Infl + Type + Cont, weights = Freq,
data = housing))
house.mult2 <- multinom(Sat ~ Infl*Type*Cont, weights = Freq,
data = housing)
anova(house.mult, house.mult2)
house.pm <- predict(house.mult, expand.grid(hnames[-1]), type = "probs")
cbind(expand.grid(hnames[-1]), round(house.pm, 2))
# proportional odds model
house.cpr <- apply(house.pr, 1, cumsum)
logit <- function(x) log(x/(1-x))
house.ld <- logit(house.cpr[2, ]) - logit(house.cpr[1, ])
(ratio <- sort(drop(house.ld)))
mean(ratio)
(house.plr <- polr(Sat ~ Infl + Type + Cont,
data = housing, weights = Freq))
house.pr1 <- predict(house.plr, expand.grid(hnames[-1]), type = "probs")
cbind(expand.grid(hnames[-1]), round(house.pr1, 2))
Fr <- matrix(housing$Freq, ncol = 3, byrow = TRUE)
2*sum(Fr*log(house.pr/house.pr1))
house.plr2 <- stepAIC(house.plr, ~.^2)
house.plr2$anova
| {
"pile_set_name": "Github"
} |
int fun(x, y)
{
asm(""); // Don't inline me, bro!
int i;
for (i = 0; i < 0; ++i)
{
}
return x + y;
}
void main()
{
int i;
for (i = 0; i < 200000000; i++)
{
fun(i, 1);
}
}
| {
"pile_set_name": "Github"
} |
/*
* Copyright 1995-2016 The OpenSSL Project Authors. All Rights Reserved.
*
* Licensed under the OpenSSL license (the "License"). You may not use
* this file except in compliance with the License. You can obtain a copy
* in the file LICENSE in the source distribution or at
* https://www.openssl.org/source/license.html
*/
#ifndef HEADER_MD4_H
# define HEADER_MD4_H
# include <openssl/opensslconf.h>
# ifndef OPENSSL_NO_MD4
# include <openssl/e_os2.h>
# include <stddef.h>
# ifdef __cplusplus
extern "C" {
# endif
/*-
* !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
* ! MD4_LONG has to be at least 32 bits wide. !
* !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
*/
# define MD4_LONG unsigned int
# define MD4_CBLOCK 64
# define MD4_LBLOCK (MD4_CBLOCK/4)
# define MD4_DIGEST_LENGTH 16
typedef struct MD4state_st {
MD4_LONG A, B, C, D;
MD4_LONG Nl, Nh;
MD4_LONG data[MD4_LBLOCK];
unsigned int num;
} MD4_CTX;
int MD4_Init(MD4_CTX *c);
int MD4_Update(MD4_CTX *c, const void *data, size_t len);
int MD4_Final(unsigned char *md, MD4_CTX *c);
unsigned char *MD4(const unsigned char *d, size_t n, unsigned char *md);
void MD4_Transform(MD4_CTX *c, const unsigned char *b);
# ifdef __cplusplus
}
# endif
# endif
#endif
| {
"pile_set_name": "Github"
} |
libimagequant is derived from code by Jef Poskanzer and Greg Roelofs
licensed under pngquant's original license (at the end of this file),
and contains extensive changes and additions by Kornel Lesiński
licensed under GPL v3 or later.
- - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - -
libimagequant © 2009-2018 by Kornel Lesiński.
GNU GENERAL PUBLIC LICENSE
Version 3, 29 June 2007
Copyright (C) 2007 Free Software Foundation, Inc. <http://fsf.org/>
Everyone is permitted to copy and distribute verbatim copies
of this license document, but changing it is not allowed.
Preamble
The GNU General Public License is a free, copyleft license for
software and other kinds of works.
The licenses for most software and other practical works are designed
to take away your freedom to share and change the works. By contrast,
the GNU General Public License is intended to guarantee your freedom to
share and change all versions of a program--to make sure it remains free
software for all its users. We, the Free Software Foundation, use the
GNU General Public License for most of our software; it applies also to
any other work released this way by its authors. You can apply it to
your programs, too.
When we speak of free software, we are referring to freedom, not
price. Our General Public Licenses are designed to make sure that you
have the freedom to distribute copies of free software (and charge for
them if you wish), that you receive source code or can get it if you
want it, that you can change the software or use pieces of it in new
free programs, and that you know you can do these things.
To protect your rights, we need to prevent others from denying you
these rights or asking you to surrender the rights. Therefore, you have
certain responsibilities if you distribute copies of the software, or if
you modify it: responsibilities to respect the freedom of others.
For example, if you distribute copies of such a program, whether
gratis or for a fee, you must pass on to the recipients the same
freedoms that you received. You must make sure that they, too, receive
or can get the source code. And you must show them these terms so they
know their rights.
Developers that use the GNU GPL protect your rights with two steps:
(1) assert copyright on the software, and (2) offer you this License
giving you legal permission to copy, distribute and/or modify it.
For the developers' and authors' protection, the GPL clearly explains
that there is no warranty for this free software. For both users' and
authors' sake, the GPL requires that modified versions be marked as
changed, so that their problems will not be attributed erroneously to
authors of previous versions.
Some devices are designed to deny users access to install or run
modified versions of the software inside them, although the manufacturer
can do so. This is fundamentally incompatible with the aim of
protecting users' freedom to change the software. The systematic
pattern of such abuse occurs in the area of products for individuals to
use, which is precisely where it is most unacceptable. Therefore, we
have designed this version of the GPL to prohibit the practice for those
products. If such problems arise substantially in other domains, we
stand ready to extend this provision to those domains in future versions
of the GPL, as needed to protect the freedom of users.
Finally, every program is threatened constantly by software patents.
States should not allow patents to restrict development and use of
software on general-purpose computers, but in those that do, we wish to
avoid the special danger that patents applied to a free program could
make it effectively proprietary. To prevent this, the GPL assures that
patents cannot be used to render the program non-free.
The precise terms and conditions for copying, distribution and
modification follow.
TERMS AND CONDITIONS
0. Definitions.
"This License" refers to version 3 of the GNU General Public License.
"Copyright" also means copyright-like laws that apply to other kinds of
works, such as semiconductor masks.
"The Program" refers to any copyrightable work licensed under this
License. Each licensee is addressed as "you". "Licensees" and
"recipients" may be individuals or organizations.
To "modify" a work means to copy from or adapt all or part of the work
in a fashion requiring copyright permission, other than the making of an
exact copy. The resulting work is called a "modified version" of the
earlier work or a work "based on" the earlier work.
A "covered work" means either the unmodified Program or a work based
on the Program.
To "propagate" a work means to do anything with it that, without
permission, would make you directly or secondarily liable for
infringement under applicable copyright law, except executing it on a
computer or modifying a private copy. Propagation includes copying,
distribution (with or without modification), making available to the
public, and in some countries other activities as well.
To "convey" a work means any kind of propagation that enables other
parties to make or receive copies. Mere interaction with a user through
a computer network, with no transfer of a copy, is not conveying.
An interactive user interface displays "Appropriate Legal Notices"
to the extent that it includes a convenient and prominently visible
feature that (1) displays an appropriate copyright notice, and (2)
tells the user that there is no warranty for the work (except to the
extent that warranties are provided), that licensees may convey the
work under this License, and how to view a copy of this License. If
the interface presents a list of user commands or options, such as a
menu, a prominent item in the list meets this criterion.
1. Source Code.
The "source code" for a work means the preferred form of the work
for making modifications to it. "Object code" means any non-source
form of a work.
A "Standard Interface" means an interface that either is an official
standard defined by a recognized standards body, or, in the case of
interfaces specified for a particular programming language, one that
is widely used among developers working in that language.
The "System Libraries" of an executable work include anything, other
than the work as a whole, that (a) is included in the normal form of
packaging a Major Component, but which is not part of that Major
Component, and (b) serves only to enable use of the work with that
Major Component, or to implement a Standard Interface for which an
implementation is available to the public in source code form. A
"Major Component", in this context, means a major essential component
(kernel, window system, and so on) of the specific operating system
(if any) on which the executable work runs, or a compiler used to
produce the work, or an object code interpreter used to run it.
The "Corresponding Source" for a work in object code form means all
the source code needed to generate, install, and (for an executable
work) run the object code and to modify the work, including scripts to
control those activities. However, it does not include the work's
System Libraries, or general-purpose tools or generally available free
programs which are used unmodified in performing those activities but
which are not part of the work. For example, Corresponding Source
includes interface definition files associated with source files for
the work, and the source code for shared libraries and dynamically
linked subprograms that the work is specifically designed to require,
such as by intimate data communication or control flow between those
subprograms and other parts of the work.
The Corresponding Source need not include anything that users
can regenerate automatically from other parts of the Corresponding
Source.
The Corresponding Source for a work in source code form is that
same work.
2. Basic Permissions.
All rights granted under this License are granted for the term of
copyright on the Program, and are irrevocable provided the stated
conditions are met. This License explicitly affirms your unlimited
permission to run the unmodified Program. The output from running a
covered work is covered by this License only if the output, given its
content, constitutes a covered work. This License acknowledges your
rights of fair use or other equivalent, as provided by copyright law.
You may make, run and propagate covered works that you do not
convey, without conditions so long as your license otherwise remains
in force. You may convey covered works to others for the sole purpose
of having them make modifications exclusively for you, or provide you
with facilities for running those works, provided that you comply with
the terms of this License in conveying all material for which you do
not control copyright. Those thus making or running the covered works
for you must do so exclusively on your behalf, under your direction
and control, on terms that prohibit them from making any copies of
your copyrighted material outside their relationship with you.
Conveying under any other circumstances is permitted solely under
the conditions stated below. Sublicensing is not allowed; section 10
makes it unnecessary.
3. Protecting Users' Legal Rights From Anti-Circumvention Law.
No covered work shall be deemed part of an effective technological
measure under any applicable law fulfilling obligations under article
11 of the WIPO copyright treaty adopted on 20 December 1996, or
similar laws prohibiting or restricting circumvention of such
measures.
When you convey a covered work, you waive any legal power to forbid
circumvention of technological measures to the extent such circumvention
is effected by exercising rights under this License with respect to
the covered work, and you disclaim any intention to limit operation or
modification of the work as a means of enforcing, against the work's
users, your or third parties' legal rights to forbid circumvention of
technological measures.
4. Conveying Verbatim Copies.
You may convey verbatim copies of the Program's source code as you
receive it, in any medium, provided that you conspicuously and
appropriately publish on each copy an appropriate copyright notice;
keep intact all notices stating that this License and any
non-permissive terms added in accord with section 7 apply to the code;
keep intact all notices of the absence of any warranty; and give all
recipients a copy of this License along with the Program.
You may charge any price or no price for each copy that you convey,
and you may offer support or warranty protection for a fee.
5. Conveying Modified Source Versions.
You may convey a work based on the Program, or the modifications to
produce it from the Program, in the form of source code under the
terms of section 4, provided that you also meet all of these conditions:
a) The work must carry prominent notices stating that you modified
it, and giving a relevant date.
b) The work must carry prominent notices stating that it is
released under this License and any conditions added under section
7. This requirement modifies the requirement in section 4 to
"keep intact all notices".
c) You must license the entire work, as a whole, under this
License to anyone who comes into possession of a copy. This
License will therefore apply, along with any applicable section 7
additional terms, to the whole of the work, and all its parts,
regardless of how they are packaged. This License gives no
permission to license the work in any other way, but it does not
invalidate such permission if you have separately received it.
d) If the work has interactive user interfaces, each must display
Appropriate Legal Notices; however, if the Program has interactive
interfaces that do not display Appropriate Legal Notices, your
work need not make them do so.
A compilation of a covered work with other separate and independent
works, which are not by their nature extensions of the covered work,
and which are not combined with it such as to form a larger program,
in or on a volume of a storage or distribution medium, is called an
"aggregate" if the compilation and its resulting copyright are not
used to limit the access or legal rights of the compilation's users
beyond what the individual works permit. Inclusion of a covered work
in an aggregate does not cause this License to apply to the other
parts of the aggregate.
6. Conveying Non-Source Forms.
You may convey a covered work in object code form under the terms
of sections 4 and 5, provided that you also convey the
machine-readable Corresponding Source under the terms of this License,
in one of these ways:
a) Convey the object code in, or embodied in, a physical product
(including a physical distribution medium), accompanied by the
Corresponding Source fixed on a durable physical medium
customarily used for software interchange.
b) Convey the object code in, or embodied in, a physical product
(including a physical distribution medium), accompanied by a
written offer, valid for at least three years and valid for as
long as you offer spare parts or customer support for that product
model, to give anyone who possesses the object code either (1) a
copy of the Corresponding Source for all the software in the
product that is covered by this License, on a durable physical
medium customarily used for software interchange, for a price no
more than your reasonable cost of physically performing this
conveying of source, or (2) access to copy the
Corresponding Source from a network server at no charge.
c) Convey individual copies of the object code with a copy of the
written offer to provide the Corresponding Source. This
alternative is allowed only occasionally and noncommercially, and
only if you received the object code with such an offer, in accord
with subsection 6b.
d) Convey the object code by offering access from a designated
place (gratis or for a charge), and offer equivalent access to the
Corresponding Source in the same way through the same place at no
further charge. You need not require recipients to copy the
Corresponding Source along with the object code. If the place to
copy the object code is a network server, the Corresponding Source
may be on a different server (operated by you or a third party)
that supports equivalent copying facilities, provided you maintain
clear directions next to the object code saying where to find the
Corresponding Source. Regardless of what server hosts the
Corresponding Source, you remain obligated to ensure that it is
available for as long as needed to satisfy these requirements.
e) Convey the object code using peer-to-peer transmission, provided
you inform other peers where the object code and Corresponding
Source of the work are being offered to the general public at no
charge under subsection 6d.
A separable portion of the object code, whose source code is excluded
from the Corresponding Source as a System Library, need not be
included in conveying the object code work.
A "User Product" is either (1) a "consumer product", which means any
tangible personal property which is normally used for personal, family,
or household purposes, or (2) anything designed or sold for incorporation
into a dwelling. In determining whether a product is a consumer product,
doubtful cases shall be resolved in favor of coverage. For a particular
product received by a particular user, "normally used" refers to a
typical or common use of that class of product, regardless of the status
of the particular user or of the way in which the particular user
actually uses, or expects or is expected to use, the product. A product
is a consumer product regardless of whether the product has substantial
commercial, industrial or non-consumer uses, unless such uses represent
the only significant mode of use of the product.
"Installation Information" for a User Product means any methods,
procedures, authorization keys, or other information required to install
and execute modified versions of a covered work in that User Product from
a modified version of its Corresponding Source. The information must
suffice to ensure that the continued functioning of the modified object
code is in no case prevented or interfered with solely because
modification has been made.
If you convey an object code work under this section in, or with, or
specifically for use in, a User Product, and the conveying occurs as
part of a transaction in which the right of possession and use of the
User Product is transferred to the recipient in perpetuity or for a
fixed term (regardless of how the transaction is characterized), the
Corresponding Source conveyed under this section must be accompanied
by the Installation Information. But this requirement does not apply
if neither you nor any third party retains the ability to install
modified object code on the User Product (for example, the work has
been installed in ROM).
The requirement to provide Installation Information does not include a
requirement to continue to provide support service, warranty, or updates
for a work that has been modified or installed by the recipient, or for
the User Product in which it has been modified or installed. Access to a
network may be denied when the modification itself materially and
adversely affects the operation of the network or violates the rules and
protocols for communication across the network.
Corresponding Source conveyed, and Installation Information provided,
in accord with this section must be in a format that is publicly
documented (and with an implementation available to the public in
source code form), and must require no special password or key for
unpacking, reading or copying.
7. Additional Terms.
"Additional permissions" are terms that supplement the terms of this
License by making exceptions from one or more of its conditions.
Additional permissions that are applicable to the entire Program shall
be treated as though they were included in this License, to the extent
that they are valid under applicable law. If additional permissions
apply only to part of the Program, that part may be used separately
under those permissions, but the entire Program remains governed by
this License without regard to the additional permissions.
When you convey a copy of a covered work, you may at your option
remove any additional permissions from that copy, or from any part of
it. (Additional permissions may be written to require their own
removal in certain cases when you modify the work.) You may place
additional permissions on material, added by you to a covered work,
for which you have or can give appropriate copyright permission.
Notwithstanding any other provision of this License, for material you
add to a covered work, you may (if authorized by the copyright holders of
that material) supplement the terms of this License with terms:
a) Disclaiming warranty or limiting liability differently from the
terms of sections 15 and 16 of this License; or
b) Requiring preservation of specified reasonable legal notices or
author attributions in that material or in the Appropriate Legal
Notices displayed by works containing it; or
c) Prohibiting misrepresentation of the origin of that material, or
requiring that modified versions of such material be marked in
reasonable ways as different from the original version; or
d) Limiting the use for publicity purposes of names of licensors or
authors of the material; or
e) Declining to grant rights under trademark law for use of some
trade names, trademarks, or service marks; or
f) Requiring indemnification of licensors and authors of that
material by anyone who conveys the material (or modified versions of
it) with contractual assumptions of liability to the recipient, for
any liability that these contractual assumptions directly impose on
those licensors and authors.
All other non-permissive additional terms are considered "further
restrictions" within the meaning of section 10. If the Program as you
received it, or any part of it, contains a notice stating that it is
governed by this License along with a term that is a further
restriction, you may remove that term. If a license document contains
a further restriction but permits relicensing or conveying under this
License, you may add to a covered work material governed by the terms
of that license document, provided that the further restriction does
not survive such relicensing or conveying.
If you add terms to a covered work in accord with this section, you
must place, in the relevant source files, a statement of the
additional terms that apply to those files, or a notice indicating
where to find the applicable terms.
Additional terms, permissive or non-permissive, may be stated in the
form of a separately written license, or stated as exceptions;
the above requirements apply either way.
8. Termination.
You may not propagate or modify a covered work except as expressly
provided under this License. Any attempt otherwise to propagate or
modify it is void, and will automatically terminate your rights under
this License (including any patent licenses granted under the third
paragraph of section 11).
However, if you cease all violation of this License, then your
license from a particular copyright holder is reinstated (a)
provisionally, unless and until the copyright holder explicitly and
finally terminates your license, and (b) permanently, if the copyright
holder fails to notify you of the violation by some reasonable means
prior to 60 days after the cessation.
Moreover, your license from a particular copyright holder is
reinstated permanently if the copyright holder notifies you of the
violation by some reasonable means, this is the first time you have
received notice of violation of this License (for any work) from that
copyright holder, and you cure the violation prior to 30 days after
your receipt of the notice.
Termination of your rights under this section does not terminate the
licenses of parties who have received copies or rights from you under
this License. If your rights have been terminated and not permanently
reinstated, you do not qualify to receive new licenses for the same
material under section 10.
9. Acceptance Not Required for Having Copies.
You are not required to accept this License in order to receive or
run a copy of the Program. Ancillary propagation of a covered work
occurring solely as a consequence of using peer-to-peer transmission
to receive a copy likewise does not require acceptance. However,
nothing other than this License grants you permission to propagate or
modify any covered work. These actions infringe copyright if you do
not accept this License. Therefore, by modifying or propagating a
covered work, you indicate your acceptance of this License to do so.
10. Automatic Licensing of Downstream Recipients.
Each time you convey a covered work, the recipient automatically
receives a license from the original licensors, to run, modify and
propagate that work, subject to this License. You are not responsible
for enforcing compliance by third parties with this License.
An "entity transaction" is a transaction transferring control of an
organization, or substantially all assets of one, or subdividing an
organization, or merging organizations. If propagation of a covered
work results from an entity transaction, each party to that
transaction who receives a copy of the work also receives whatever
licenses to the work the party's predecessor in interest had or could
give under the previous paragraph, plus a right to possession of the
Corresponding Source of the work from the predecessor in interest, if
the predecessor has it or can get it with reasonable efforts.
You may not impose any further restrictions on the exercise of the
rights granted or affirmed under this License. For example, you may
not impose a license fee, royalty, or other charge for exercise of
rights granted under this License, and you may not initiate litigation
(including a cross-claim or counterclaim in a lawsuit) alleging that
any patent claim is infringed by making, using, selling, offering for
sale, or importing the Program or any portion of it.
11. Patents.
A "contributor" is a copyright holder who authorizes use under this
License of the Program or a work on which the Program is based. The
work thus licensed is called the contributor's "contributor version".
A contributor's "essential patent claims" are all patent claims
owned or controlled by the contributor, whether already acquired or
hereafter acquired, that would be infringed by some manner, permitted
by this License, of making, using, or selling its contributor version,
but do not include claims that would be infringed only as a
consequence of further modification of the contributor version. For
purposes of this definition, "control" includes the right to grant
patent sublicenses in a manner consistent with the requirements of
this License.
Each contributor grants you a non-exclusive, worldwide, royalty-free
patent license under the contributor's essential patent claims, to
make, use, sell, offer for sale, import and otherwise run, modify and
propagate the contents of its contributor version.
In the following three paragraphs, a "patent license" is any express
agreement or commitment, however denominated, not to enforce a patent
(such as an express permission to practice a patent or covenant not to
sue for patent infringement). To "grant" such a patent license to a
party means to make such an agreement or commitment not to enforce a
patent against the party.
If you convey a covered work, knowingly relying on a patent license,
and the Corresponding Source of the work is not available for anyone
to copy, free of charge and under the terms of this License, through a
publicly available network server or other readily accessible means,
then you must either (1) cause the Corresponding Source to be so
available, or (2) arrange to deprive yourself of the benefit of the
patent license for this particular work, or (3) arrange, in a manner
consistent with the requirements of this License, to extend the patent
license to downstream recipients. "Knowingly relying" means you have
actual knowledge that, but for the patent license, your conveying the
covered work in a country, or your recipient's use of the covered work
in a country, would infringe one or more identifiable patents in that
country that you have reason to believe are valid.
If, pursuant to or in connection with a single transaction or
arrangement, you convey, or propagate by procuring conveyance of, a
covered work, and grant a patent license to some of the parties
receiving the covered work authorizing them to use, propagate, modify
or convey a specific copy of the covered work, then the patent license
you grant is automatically extended to all recipients of the covered
work and works based on it.
A patent license is "discriminatory" if it does not include within
the scope of its coverage, prohibits the exercise of, or is
conditioned on the non-exercise of one or more of the rights that are
specifically granted under this License. You may not convey a covered
work if you are a party to an arrangement with a third party that is
in the business of distributing software, under which you make payment
to the third party based on the extent of your activity of conveying
the work, and under which the third party grants, to any of the
parties who would receive the covered work from you, a discriminatory
patent license (a) in connection with copies of the covered work
conveyed by you (or copies made from those copies), or (b) primarily
for and in connection with specific products or compilations that
contain the covered work, unless you entered into that arrangement,
or that patent license was granted, prior to 28 March 2007.
Nothing in this License shall be construed as excluding or limiting
any implied license or other defenses to infringement that may
otherwise be available to you under applicable patent law.
12. No Surrender of Others' Freedom.
If conditions are imposed on you (whether by court order, agreement or
otherwise) that contradict the conditions of this License, they do not
excuse you from the conditions of this License. If you cannot convey a
covered work so as to satisfy simultaneously your obligations under this
License and any other pertinent obligations, then as a consequence you may
not convey it at all. For example, if you agree to terms that obligate you
to collect a royalty for further conveying from those to whom you convey
the Program, the only way you could satisfy both those terms and this
License would be to refrain entirely from conveying the Program.
13. Use with the GNU Affero General Public License.
Notwithstanding any other provision of this License, you have
permission to link or combine any covered work with a work licensed
under version 3 of the GNU Affero General Public License into a single
combined work, and to convey the resulting work. The terms of this
License will continue to apply to the part which is the covered work,
but the special requirements of the GNU Affero General Public License,
section 13, concerning interaction through a network will apply to the
combination as such.
14. Revised Versions of this License.
The Free Software Foundation may publish revised and/or new versions of
the GNU General Public License from time to time. Such new versions will
be similar in spirit to the present version, but may differ in detail to
address new problems or concerns.
Each version is given a distinguishing version number. If the
Program specifies that a certain numbered version of the GNU General
Public License "or any later version" applies to it, you have the
option of following the terms and conditions either of that numbered
version or of any later version published by the Free Software
Foundation. If the Program does not specify a version number of the
GNU General Public License, you may choose any version ever published
by the Free Software Foundation.
If the Program specifies that a proxy can decide which future
versions of the GNU General Public License can be used, that proxy's
public statement of acceptance of a version permanently authorizes you
to choose that version for the Program.
Later license versions may give you additional or different
permissions. However, no additional obligations are imposed on any
author or copyright holder as a result of your choosing to follow a
later version.
15. Disclaimer of Warranty.
THERE IS NO WARRANTY FOR THE PROGRAM, TO THE EXTENT PERMITTED BY
APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT
HOLDERS AND/OR OTHER PARTIES PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY
OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO,
THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE PROGRAM
IS WITH YOU. SHOULD THE PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF
ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
16. Limitation of Liability.
IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING
WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MODIFIES AND/OR CONVEYS
THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY
GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE
USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED TO LOSS OF
DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD
PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER PROGRAMS),
EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF
SUCH DAMAGES.
17. Interpretation of Sections 15 and 16.
If the disclaimer of warranty and limitation of liability provided
above cannot be given local legal effect according to their terms,
reviewing courts shall apply local law that most closely approximates
an absolute waiver of all civil liability in connection with the
Program, unless a warranty or assumption of liability accompanies a
copy of the Program in return for a fee.
- - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - -
© 1989, 1991 by Jef Poskanzer.
© 1997, 2000, 2002 by Greg Roelofs.
Permission to use, copy, modify, and distribute this software and its
documentation for any purpose and without fee is hereby granted, provided
that the above copyright notice appear in all copies and that both that
copyright notice and this permission notice appear in supporting
documentation. This software is provided "as is" without express or
implied warranty.
| {
"pile_set_name": "Github"
} |
//
// serial_port_service.hpp
// ~~~~~~~~~~~~~~~~~~~~~~~
//
// Copyright (c) 2003-2016 Christopher M. Kohlhoff (chris at kohlhoff dot com)
//
// Distributed under the Boost Software License, Version 1.0. (See accompanying
// file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
//
#ifndef BOOST_ASIO_SERIAL_PORT_SERVICE_HPP
#define BOOST_ASIO_SERIAL_PORT_SERVICE_HPP
#if defined(_MSC_VER) && (_MSC_VER >= 1200)
# pragma once
#endif // defined(_MSC_VER) && (_MSC_VER >= 1200)
#include <boost/asio/detail/config.hpp>
#if defined(BOOST_ASIO_HAS_SERIAL_PORT) \
|| defined(GENERATING_DOCUMENTATION)
#include <cstddef>
#include <string>
#include <boost/asio/async_result.hpp>
#include <boost/asio/detail/reactive_serial_port_service.hpp>
#include <boost/asio/detail/win_iocp_serial_port_service.hpp>
#include <boost/asio/error.hpp>
#include <boost/asio/io_service.hpp>
#include <boost/asio/serial_port_base.hpp>
#include <boost/asio/detail/push_options.hpp>
namespace boost {
namespace asio {
/// Default service implementation for a serial port.
class serial_port_service
#if defined(GENERATING_DOCUMENTATION)
: public boost::asio::io_service::service
#else
: public boost::asio::detail::service_base<serial_port_service>
#endif
{
public:
#if defined(GENERATING_DOCUMENTATION)
/// The unique service identifier.
static boost::asio::io_service::id id;
#endif
private:
// The type of the platform-specific implementation.
#if defined(BOOST_ASIO_HAS_IOCP)
typedef detail::win_iocp_serial_port_service service_impl_type;
#else
typedef detail::reactive_serial_port_service service_impl_type;
#endif
public:
/// The type of a serial port implementation.
#if defined(GENERATING_DOCUMENTATION)
typedef implementation_defined implementation_type;
#else
typedef service_impl_type::implementation_type implementation_type;
#endif
/// (Deprecated: Use native_handle_type.) The native handle type.
#if defined(GENERATING_DOCUMENTATION)
typedef implementation_defined native_type;
#else
typedef service_impl_type::native_handle_type native_type;
#endif
/// The native handle type.
#if defined(GENERATING_DOCUMENTATION)
typedef implementation_defined native_handle_type;
#else
typedef service_impl_type::native_handle_type native_handle_type;
#endif
/// Construct a new serial port service for the specified io_service.
explicit serial_port_service(boost::asio::io_service& io_service)
: boost::asio::detail::service_base<serial_port_service>(io_service),
service_impl_(io_service)
{
}
/// Construct a new serial port implementation.
void construct(implementation_type& impl)
{
service_impl_.construct(impl);
}
#if defined(BOOST_ASIO_HAS_MOVE) || defined(GENERATING_DOCUMENTATION)
/// Move-construct a new serial port implementation.
void move_construct(implementation_type& impl,
implementation_type& other_impl)
{
service_impl_.move_construct(impl, other_impl);
}
/// Move-assign from another serial port implementation.
void move_assign(implementation_type& impl,
serial_port_service& other_service,
implementation_type& other_impl)
{
service_impl_.move_assign(impl, other_service.service_impl_, other_impl);
}
#endif // defined(BOOST_ASIO_HAS_MOVE) || defined(GENERATING_DOCUMENTATION)
/// Destroy a serial port implementation.
void destroy(implementation_type& impl)
{
service_impl_.destroy(impl);
}
/// Open a serial port.
boost::system::error_code open(implementation_type& impl,
const std::string& device, boost::system::error_code& ec)
{
return service_impl_.open(impl, device, ec);
}
/// Assign an existing native handle to a serial port.
boost::system::error_code assign(implementation_type& impl,
const native_handle_type& handle, boost::system::error_code& ec)
{
return service_impl_.assign(impl, handle, ec);
}
/// Determine whether the handle is open.
bool is_open(const implementation_type& impl) const
{
return service_impl_.is_open(impl);
}
/// Close a serial port implementation.
boost::system::error_code close(implementation_type& impl,
boost::system::error_code& ec)
{
return service_impl_.close(impl, ec);
}
/// (Deprecated: Use native_handle().) Get the native handle implementation.
native_type native(implementation_type& impl)
{
return service_impl_.native_handle(impl);
}
/// Get the native handle implementation.
native_handle_type native_handle(implementation_type& impl)
{
return service_impl_.native_handle(impl);
}
/// Cancel all asynchronous operations associated with the handle.
boost::system::error_code cancel(implementation_type& impl,
boost::system::error_code& ec)
{
return service_impl_.cancel(impl, ec);
}
/// Set a serial port option.
template <typename SettableSerialPortOption>
boost::system::error_code set_option(implementation_type& impl,
const SettableSerialPortOption& option, boost::system::error_code& ec)
{
return service_impl_.set_option(impl, option, ec);
}
/// Get a serial port option.
template <typename GettableSerialPortOption>
boost::system::error_code get_option(const implementation_type& impl,
GettableSerialPortOption& option, boost::system::error_code& ec) const
{
return service_impl_.get_option(impl, option, ec);
}
/// Send a break sequence to the serial port.
boost::system::error_code send_break(implementation_type& impl,
boost::system::error_code& ec)
{
return service_impl_.send_break(impl, ec);
}
/// Write the given data to the stream.
template <typename ConstBufferSequence>
std::size_t write_some(implementation_type& impl,
const ConstBufferSequence& buffers, boost::system::error_code& ec)
{
return service_impl_.write_some(impl, buffers, ec);
}
/// Start an asynchronous write.
template <typename ConstBufferSequence, typename WriteHandler>
BOOST_ASIO_INITFN_RESULT_TYPE(WriteHandler,
void (boost::system::error_code, std::size_t))
async_write_some(implementation_type& impl,
const ConstBufferSequence& buffers,
BOOST_ASIO_MOVE_ARG(WriteHandler) handler)
{
detail::async_result_init<
WriteHandler, void (boost::system::error_code, std::size_t)> init(
BOOST_ASIO_MOVE_CAST(WriteHandler)(handler));
service_impl_.async_write_some(impl, buffers, init.handler);
return init.result.get();
}
/// Read some data from the stream.
template <typename MutableBufferSequence>
std::size_t read_some(implementation_type& impl,
const MutableBufferSequence& buffers, boost::system::error_code& ec)
{
return service_impl_.read_some(impl, buffers, ec);
}
/// Start an asynchronous read.
template <typename MutableBufferSequence, typename ReadHandler>
BOOST_ASIO_INITFN_RESULT_TYPE(ReadHandler,
void (boost::system::error_code, std::size_t))
async_read_some(implementation_type& impl,
const MutableBufferSequence& buffers,
BOOST_ASIO_MOVE_ARG(ReadHandler) handler)
{
detail::async_result_init<
ReadHandler, void (boost::system::error_code, std::size_t)> init(
BOOST_ASIO_MOVE_CAST(ReadHandler)(handler));
service_impl_.async_read_some(impl, buffers, init.handler);
return init.result.get();
}
private:
// Destroy all user-defined handler objects owned by the service.
void shutdown_service()
{
service_impl_.shutdown_service();
}
// The platform-specific implementation.
service_impl_type service_impl_;
};
} // namespace asio
} // namespace boost
#include <boost/asio/detail/pop_options.hpp>
#endif // defined(BOOST_ASIO_HAS_SERIAL_PORT)
// || defined(GENERATING_DOCUMENTATION)
#endif // BOOST_ASIO_SERIAL_PORT_SERVICE_HPP
| {
"pile_set_name": "Github"
} |
'use strict'
const fs = require('graceful-fs')
const BB = require('bluebird')
const chmod = BB.promisify(fs.chmod)
const unlink = BB.promisify(fs.unlink)
let move
let pinflight
module.exports = moveFile
function moveFile (src, dest) {
// This isn't quite an fs.rename -- the assumption is that
// if `dest` already exists, and we get certain errors while
// trying to move it, we should just not bother.
//
// In the case of cache corruption, users will receive an
// EINTEGRITY error elsewhere, and can remove the offending
// content their own way.
//
// Note that, as the name suggests, this strictly only supports file moves.
return BB.fromNode(cb => {
fs.link(src, dest, err => {
if (err) {
if (err.code === 'EEXIST' || err.code === 'EBUSY') {
// file already exists, so whatever
} else if (err.code === 'EPERM' && process.platform === 'win32') {
// file handle stayed open even past graceful-fs limits
} else {
return cb(err)
}
}
return cb()
})
}).then(() => {
// content should never change for any reason, so make it read-only
return BB.join(unlink(src), process.platform !== 'win32' && chmod(dest, '0444'))
}).catch(err => {
if (process.platform !== 'win32') {
throw err
} else {
if (!pinflight) { pinflight = require('promise-inflight') }
return pinflight('cacache-move-file:' + dest, () => {
return BB.promisify(fs.stat)(dest).catch(err => {
if (err !== 'ENOENT') {
// Something else is wrong here. Bail bail bail
throw err
}
// file doesn't already exist! let's try a rename -> copy fallback
if (!move) { move = require('move-concurrently') }
return move(src, dest, { BB, fs })
})
})
}
})
}
| {
"pile_set_name": "Github"
} |
import React from 'react';
function connect(WrappedComponent, select) {
return function(props) {
const selectors = select(props);
return <WrappedComponent {...props} {...selectors} />;
};
}
export default connect;
| {
"pile_set_name": "Github"
} |
module.exports.hello = (event, context, callback) => {
const response = {
/* Status code required for default lambda integration */
statusCode: 200,
body: JSON.stringify({
message: 'Hi ⊂◉‿◉つ',
event: event,
}),
}
/** callback(error, response) */
return callback(null, response)
}
| {
"pile_set_name": "Github"
} |
;;; calc-aent.el --- algebraic entry functions for Calc
;; Copyright (C) 1990, 1991, 1992, 1993, 2001, 2002, 2003, 2004,
;; 2005, 2006, 2007, 2008, 2009 Free Software Foundation, Inc.
;; Author: Dave Gillespie <daveg@synaptics.com>
;; Maintainer: Jay Belanger <jay.p.belanger@gmail.com>
;; This file is part of GNU Emacs.
;; GNU Emacs is free software: you can redistribute it and/or modify
;; it under the terms of the GNU General Public License as published by
;; the Free Software Foundation, either version 3 of the License, or
;; (at your option) any later version.
;; GNU Emacs is distributed in the hope that it will be useful,
;; but WITHOUT ANY WARRANTY; without even the implied warranty of
;; MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
;; GNU General Public License for more details.
;; You should have received a copy of the GNU General Public License
;; along with GNU Emacs. If not, see <http://www.gnu.org/licenses/>.
;;; Commentary:
;;; Code:
;; This file is autoloaded from calc.el.
(require 'calc)
(require 'calc-macs)
;; Declare functions which are defined elsewhere.
(declare-function calc-refresh-evaltos "calc-ext" (&optional which-var))
(declare-function calc-execute-kbd-macro "calc-prog" (mac arg &rest prefix))
(declare-function math-is-true "calc-ext" (expr))
(declare-function calc-explain-why "calc-stuff" (why &optional more))
(declare-function calc-alg-edit "calc-yank" (str))
(declare-function math-composite-inequalities "calc-prog" (x op))
(declare-function math-flatten-lands "calc-rewr" (expr))
(declare-function math-multi-subst "calc-map" (expr olds news))
(declare-function calcFunc-vmatches "calc-rewr" (expr pat))
(declare-function math-simplify "calc-alg" (top-expr))
(declare-function math-known-matrixp "calc-arith" (a))
(declare-function math-parse-fortran-subscr "calc-lang" (sym args))
(declare-function math-to-radians-2 "calc-math" (a))
(declare-function math-read-string "calc-ext" ())
(declare-function math-read-brackets "calc-vec" (space-sep math-rb-close))
(declare-function math-read-angle-brackets "calc-forms" ())
(declare-function math-to-percentsigns "calccomp" (x))
(defvar calc-quick-calc-history nil
"The history list for quick-calc.")
;;;###autoload
(defun calc-do-quick-calc ()
(require 'calc-ext)
(calc-check-defines)
(if (eq major-mode 'calc-mode)
(calc-algebraic-entry t)
(let (buf shortbuf)
(save-excursion
(calc-create-buffer)
(let* ((calc-command-flags nil)
(calc-dollar-values calc-quick-prev-results)
(calc-dollar-used 0)
(enable-recursive-minibuffers t)
(calc-language (if (memq calc-language '(nil big))
'flat calc-language))
(entry (calc-do-alg-entry "" "Quick calc: " t 'calc-quick-calc-history))
(alg-exp (mapcar 'math-evaluate-expr entry)))
(when (and (= (length alg-exp) 1)
(eq (car-safe (car alg-exp)) 'calcFunc-assign)
(= (length (car alg-exp)) 3)
(eq (car-safe (nth 1 (car alg-exp))) 'var))
(set (nth 2 (nth 1 (car alg-exp))) (nth 2 (car alg-exp)))
(calc-refresh-evaltos (nth 2 (nth 1 (car alg-exp))))
(setq alg-exp (list (nth 2 (car alg-exp)))))
(setq calc-quick-prev-results alg-exp
buf (mapconcat (function (lambda (x)
(math-format-value x 1000)))
alg-exp
" ")
shortbuf buf)
(if (and (= (length alg-exp) 1)
(memq (car-safe (car alg-exp)) '(nil bigpos bigneg))
(< (length buf) 20)
(= calc-number-radix 10))
(setq buf (concat buf " ("
(let ((calc-number-radix 16))
(math-format-value (car alg-exp) 1000))
", "
(let ((calc-number-radix 8))
(math-format-value (car alg-exp) 1000))
", "
(let ((calc-number-radix 2))
(math-format-value (car alg-exp) 1000))
(if (and (integerp (car alg-exp))
(> (car alg-exp) 0)
(< (car alg-exp) 127))
(format ", \"%c\"" (car alg-exp))
"")
")")))
(if (and (< (length buf) (frame-width)) (= (length entry) 1)
(featurep 'calc-ext))
(let ((long (concat (math-format-value (car entry) 1000)
" => " buf)))
(if (<= (length long) (- (frame-width) 8))
(setq buf long))))
(calc-handle-whys)
(message "Result: %s" buf)))
(if (eq last-command-event 10)
(insert shortbuf)
(kill-new shortbuf)))))
;;;###autoload
(defun calc-do-calc-eval (str separator args)
(calc-check-defines)
(catch 'calc-error
(save-excursion
(calc-create-buffer)
(cond
((and (consp str) (not (symbolp (car str))))
(let ((calc-language nil)
(math-expr-opers (math-standard-ops))
(calc-internal-prec 12)
(calc-word-size 32)
(calc-symbolic-mode nil)
(calc-matrix-mode nil)
(calc-angle-mode 'deg)
(calc-number-radix 10)
(calc-twos-complement-mode nil)
(calc-leading-zeros nil)
(calc-group-digits nil)
(calc-point-char ".")
(calc-frac-format '(":" nil))
(calc-prefer-frac nil)
(calc-hms-format "%s@ %s' %s\"")
(calc-date-format '((H ":" mm C SS pp " ")
Www " " Mmm " " D ", " YYYY))
(calc-float-format '(float 0))
(calc-full-float-format '(float 0))
(calc-complex-format nil)
(calc-matrix-just nil)
(calc-full-vectors t)
(calc-break-vectors nil)
(calc-vector-commas ",")
(calc-vector-brackets "[]")
(calc-matrix-brackets '(R O))
(calc-complex-mode 'cplx)
(calc-infinite-mode nil)
(calc-display-strings nil)
(calc-simplify-mode nil)
(calc-display-working-message 'lots)
(strp (cdr str)))
(while strp
(set (car strp) (nth 1 strp))
(setq strp (cdr (cdr strp))))
(calc-do-calc-eval (car str) separator args)))
((eq separator 'eval)
(eval str))
((eq separator 'macro)
(require 'calc-ext)
(let* ((calc-buffer (current-buffer))
(calc-window (get-buffer-window calc-buffer))
(save-window (selected-window)))
(if calc-window
(unwind-protect
(progn
(select-window calc-window)
(calc-execute-kbd-macro str nil (car args)))
(and (window-point save-window)
(select-window save-window)))
(save-window-excursion
(select-window (get-largest-window))
(switch-to-buffer calc-buffer)
(calc-execute-kbd-macro str nil (car args)))))
nil)
((eq separator 'pop)
(or (not (integerp str))
(= str 0)
(calc-pop (min str (calc-stack-size))))
(calc-stack-size))
((eq separator 'top)
(and (integerp str)
(> str 0)
(<= str (calc-stack-size))
(math-format-value (calc-top-n str (car args)) 1000)))
((eq separator 'rawtop)
(and (integerp str)
(> str 0)
(<= str (calc-stack-size))
(calc-top-n str (car args))))
(t
(let* ((calc-command-flags nil)
(calc-next-why nil)
(calc-language (if (memq calc-language '(nil big))
'flat calc-language))
(calc-dollar-values (mapcar
(function
(lambda (x)
(if (stringp x)
(progn
(setq x (math-read-exprs x))
(if (eq (car-safe x)
'error)
(throw 'calc-error
(calc-eval-error
(cdr x)))
(car x)))
x)))
args))
(calc-dollar-used 0)
(res (if (stringp str)
(math-read-exprs str)
(list str)))
buf)
(if (eq (car res) 'error)
(calc-eval-error (cdr res))
(setq res (mapcar 'calc-normalize res))
(and (memq 'clear-message calc-command-flags)
(message ""))
(cond ((eq separator 'pred)
(require 'calc-ext)
(if (= (length res) 1)
(math-is-true (car res))
(calc-eval-error '(0 "Single value expected"))))
((eq separator 'raw)
(if (= (length res) 1)
(car res)
(calc-eval-error '(0 "Single value expected"))))
((eq separator 'list)
res)
((memq separator '(num rawnum))
(if (= (length res) 1)
(if (math-constp (car res))
(if (eq separator 'num)
(math-format-value (car res) 1000)
(car res))
(calc-eval-error
(list 0
(if calc-next-why
(calc-explain-why (car calc-next-why))
"Number expected"))))
(calc-eval-error '(0 "Single value expected"))))
((eq separator 'push)
(calc-push-list res)
nil)
(t (while res
(setq buf (concat buf
(and buf (or separator ", "))
(math-format-value (car res) 1000))
res (cdr res)))
buf)))))))))
(defvar calc-eval-error nil
"Determines how calc handles errors.
If nil, return a list containing the character position of error.
STRING means return error message as string rather than list.
The value t means abort and give an error message.")
(defun calc-eval-error (msg)
(if calc-eval-error
(if (eq calc-eval-error 'string)
(nth 1 msg)
(error "%s" (nth 1 msg)))
msg))
;;;; Reading an expression in algebraic form.
;;;###autoload
(defun calc-auto-algebraic-entry (&optional prefix)
(interactive "P")
(calc-algebraic-entry prefix t))
;;;###autoload
(defun calc-algebraic-entry (&optional prefix auto)
(interactive "P")
(calc-wrapper
(let ((calc-language (if prefix nil calc-language))
(math-expr-opers (if prefix (math-standard-ops) (math-expr-ops))))
(calc-alg-entry (and auto (char-to-string last-command-event))))))
(defvar calc-alg-entry-history nil
"History for algebraic entry.")
;;;###autoload
(defun calc-alg-entry (&optional initial prompt)
(let* ((sel-mode nil)
(calc-dollar-values (mapcar 'calc-get-stack-element
(nthcdr calc-stack-top calc-stack)))
(calc-dollar-used 0)
(calc-plain-entry t)
(alg-exp (calc-do-alg-entry initial prompt t 'calc-alg-entry-history)))
(if (stringp alg-exp)
(progn
(require 'calc-ext)
(calc-alg-edit alg-exp))
(let* ((calc-simplify-mode (if (eq last-command-event ?\C-j)
'none
calc-simplify-mode))
(nvals (mapcar 'calc-normalize alg-exp)))
(while alg-exp
(calc-record (if (featurep 'calc-ext) (car alg-exp) (car nvals))
"alg'")
(calc-pop-push-record-list calc-dollar-used
(and (not (equal (car alg-exp)
(car nvals)))
(featurep 'calc-ext)
"")
(list (car nvals)))
(setq alg-exp (cdr alg-exp)
nvals (cdr nvals)
calc-dollar-used 0)))
(calc-handle-whys))))
(defvar calc-alg-ent-map nil
"The keymap used for algebraic entry.")
(defvar calc-alg-ent-esc-map nil
"The keymap used for escapes in algebraic entry.")
(defvar calc-alg-exp)
;;;###autoload
(defun calc-do-alg-entry (&optional initial prompt no-normalize history)
(let* ((calc-buffer (current-buffer))
(blink-paren-function 'calcAlg-blink-matching-open)
(calc-alg-exp 'error))
(unless calc-alg-ent-map
(setq calc-alg-ent-map (copy-keymap minibuffer-local-map))
(define-key calc-alg-ent-map "'" 'calcAlg-previous)
(define-key calc-alg-ent-map "`" 'calcAlg-edit)
(define-key calc-alg-ent-map "\C-m" 'calcAlg-enter)
(define-key calc-alg-ent-map "\C-j" 'calcAlg-enter)
(let ((i 33))
(setq calc-alg-ent-esc-map (copy-keymap esc-map))
(while (< i 127)
(aset (nth 1 calc-alg-ent-esc-map) i 'calcAlg-escape)
(setq i (1+ i)))))
(define-key calc-alg-ent-map "\e" nil)
(if (eq calc-algebraic-mode 'total)
(define-key calc-alg-ent-map "\e" calc-alg-ent-esc-map)
(define-key calc-alg-ent-map "\e+" 'calcAlg-plus-minus)
(define-key calc-alg-ent-map "\em" 'calcAlg-mod)
(define-key calc-alg-ent-map "\e=" 'calcAlg-equals)
(define-key calc-alg-ent-map "\e\r" 'calcAlg-equals)
(define-key calc-alg-ent-map "\ep" 'previous-history-element)
(define-key calc-alg-ent-map "\en" 'next-history-element)
(define-key calc-alg-ent-map "\e%" 'self-insert-command))
(setq calc-aborted-prefix nil)
(let ((buf (read-from-minibuffer (or prompt "Algebraic: ")
(or initial "")
calc-alg-ent-map nil history)))
(when (eq calc-alg-exp 'error)
(when (eq (car-safe (setq calc-alg-exp (math-read-exprs buf))) 'error)
(setq calc-alg-exp nil)))
(setq calc-aborted-prefix "alg'")
(or no-normalize
(and calc-alg-exp (setq calc-alg-exp (mapcar 'calc-normalize calc-alg-exp))))
calc-alg-exp)))
(defun calcAlg-plus-minus ()
(interactive)
(if (calc-minibuffer-contains ".* \\'")
(insert "+/- ")
(insert " +/- ")))
(defun calcAlg-mod ()
(interactive)
(if (not (calc-minibuffer-contains ".* \\'"))
(insert " "))
(if (calc-minibuffer-contains ".* mod +\\'")
(if calc-previous-modulo
(insert (math-format-flat-expr calc-previous-modulo 0))
(beep))
(insert "mod ")))
(defun calcAlg-previous ()
(interactive)
(if (calc-minibuffer-contains "\\'")
(previous-history-element 1)
(insert "'")))
(defun calcAlg-equals ()
(interactive)
(unwind-protect
(calcAlg-enter)
(if (consp calc-alg-exp)
(progn (setq prefix-arg (length calc-alg-exp))
(calc-unread-command ?=)))))
(defun calcAlg-escape ()
(interactive)
(calc-unread-command)
(save-excursion
(calc-select-buffer)
(use-local-map calc-mode-map))
(calcAlg-enter))
(defvar calc-plain-entry nil)
(defun calcAlg-edit ()
(interactive)
(if (or (not calc-plain-entry)
(calc-minibuffer-contains
"\\`\\([^\"]*\"[^\"]*\"\\)*[^\"]*\"[^\"]*\\'"))
(insert "`")
(setq calc-alg-exp (minibuffer-contents))
(exit-minibuffer)))
(defvar calc-buffer)
(defun calcAlg-enter ()
(interactive)
(let* ((str (minibuffer-contents))
(exp (and (> (length str) 0)
(with-current-buffer calc-buffer
(math-read-exprs str)))))
(if (eq (car-safe exp) 'error)
(progn
(goto-char (minibuffer-prompt-end))
(forward-char (nth 1 exp))
(beep)
(calc-temp-minibuffer-message
(concat " [" (or (nth 2 exp) "Error") "]"))
(calc-clear-unread-commands))
(setq calc-alg-exp (if (calc-minibuffer-contains "\\` *\\[ *\\'")
'((incomplete vec))
exp))
(exit-minibuffer))))
(defun calcAlg-blink-matching-open ()
(let ((rightpt (point))
(leftpt nil)
(rightchar (preceding-char))
leftchar
rightsyntax
leftsyntax)
(save-excursion
(condition-case ()
(setq leftpt (scan-sexps rightpt -1)
leftchar (char-after leftpt))
(error nil)))
(if (and leftpt
(or (and (= rightchar ?\))
(= leftchar ?\[))
(and (= rightchar ?\])
(= leftchar ?\()))
(save-excursion
(goto-char leftpt)
(looking-at ".+\\(\\.\\.\\|\\\\dots\\|\\\\ldots\\)")))
(let ((leftsaved (aref (syntax-table) leftchar))
(rightsaved (aref (syntax-table) rightchar)))
(unwind-protect
(progn
(cond ((= leftchar ?\[)
(aset (syntax-table) leftchar (cons 4 ?\)))
(aset (syntax-table) rightchar (cons 5 ?\[)))
(t
(aset (syntax-table) leftchar (cons 4 ?\]))
(aset (syntax-table) rightchar (cons 5 ?\())))
(blink-matching-open))
(aset (syntax-table) leftchar leftsaved)
(aset (syntax-table) rightchar rightsaved)))
(blink-matching-open))))
;;;###autoload
(defun calc-alg-digit-entry ()
(calc-alg-entry
(cond ((eq last-command-event ?e)
(if (> calc-number-radix 14) (format "%d.^" calc-number-radix) "1e"))
((eq last-command-event ?#) (format "%d#" calc-number-radix))
((eq last-command-event ?_) "-")
((eq last-command-event ?@) "0@ ")
(t (char-to-string last-command-event)))))
;; The variable calc-digit-value is initially declared in calc.el,
;; but can be set by calcDigit-algebraic and calcDigit-edit.
(defvar calc-digit-value)
;;;###autoload
(defun calcDigit-algebraic ()
(interactive)
(if (calc-minibuffer-contains ".*[@oh] *[^'m ]+[^'m]*\\'")
(calcDigit-key)
(setq calc-digit-value (minibuffer-contents))
(exit-minibuffer)))
;;;###autoload
(defun calcDigit-edit ()
(interactive)
(calc-unread-command)
(setq calc-digit-value (minibuffer-contents))
(exit-minibuffer))
;;; Algebraic expression parsing. [Public]
(defvar math-read-replacement-list
'(;; Misc symbols
("±" "+/-") ; plus or minus
("×" "*") ; multiplication sign
("÷" ":") ; division sign
("−" "-") ; subtraction sign
("∕" "/") ; division sign
("∗" "*") ; asterisk multiplication
("∞" "inf") ; infinity symbol
("≤" "<=")
("≥" ">=")
("≦" "<=")
("≧" ">=")
;; fractions
("¼" "(1:4)") ; 1/4
("½" "(1:2)") ; 1/2
("¾" "(3:4)") ; 3/4
("⅓" "(1:3)") ; 1/3
("⅔" "(2:3)") ; 2/3
("⅕" "(1:5)") ; 1/5
("⅖" "(2:5)") ; 2/5
("⅗" "(3:5)") ; 3/5
("⅘" "(4:5)") ; 4/5
("⅙" "(1:6)") ; 1/6
("⅚" "(5:6)") ; 5/6
("⅛" "(1:8)") ; 1/8
("⅜" "(3:8)") ; 3/8
("⅝" "(5:8)") ; 5/8
("⅞" "(7:8)") ; 7/8
("⅟" "1:") ; 1/...
;; superscripts
("⁰" "0") ; 0
("¹" "1") ; 1
("²" "2") ; 2
("³" "3") ; 3
("⁴" "4") ; 4
("⁵" "5") ; 5
("⁶" "6") ; 6
("⁷" "7") ; 7
("⁸" "8") ; 8
("⁹" "9") ; 9
("⁺" "+") ; +
("⁻" "-") ; -
("⁽" "(") ; (
("⁾" ")") ; )
("ⁿ" "n") ; n
("ⁱ" "i") ; i
;; subscripts
("₀" "0") ; 0
("₁" "1") ; 1
("₂" "2") ; 2
("₃" "3") ; 3
("₄" "4") ; 4
("₅" "5") ; 5
("₆" "6") ; 6
("₇" "7") ; 7
("₈" "8") ; 8
("₉" "9") ; 9
("₊" "+") ; +
("₋" "-") ; -
("₍" "(") ; (
("₎" ")")) ; )
"A list whose elements (old new) indicate replacements to make
in Calc algebraic input.")
(defvar math-read-superscripts
"⁰¹²³⁴⁵⁶⁷⁸⁹⁺⁻⁽⁾ⁿⁱ" ; 0123456789+-()ni
"A string consisting of the superscripts allowed by Calc.")
(defvar math-read-subscripts
"₀₁₂₃₄₅₆₇₈₉₊₋₍₎" ; 0123456789+-()
"A string consisting of the subscripts allowed by Calc.")
;;;###autoload
(defun math-read-preprocess-string (str)
"Replace some substrings of STR by Calc equivalents."
(setq str
(replace-regexp-in-string (concat "[" math-read-superscripts "]+")
"^(\\&)" str))
(setq str
(replace-regexp-in-string (concat "[" math-read-subscripts "]+")
"_(\\&)" str))
(let ((rep-list math-read-replacement-list))
(while rep-list
(setq str
(replace-regexp-in-string (nth 0 (car rep-list))
(nth 1 (car rep-list)) str))
(setq rep-list (cdr rep-list))))
str)
;; The next few variables are local to math-read-exprs (and math-read-expr
;; in calc-ext.el), but are set in functions they call.
(defvar math-exp-pos)
(defvar math-exp-str)
(defvar math-exp-old-pos)
(defvar math-exp-token)
(defvar math-exp-keep-spaces)
(defvar math-expr-data)
;;;###autoload
(defun math-read-exprs (math-exp-str)
(let ((math-exp-pos 0)
(math-exp-old-pos 0)
(math-exp-keep-spaces nil)
math-exp-token math-expr-data)
(setq math-exp-str (math-read-preprocess-string math-exp-str))
(if (memq calc-language calc-lang-allow-percentsigns)
(setq math-exp-str (math-remove-percentsigns math-exp-str)))
(if calc-language-input-filter
(setq math-exp-str (funcall calc-language-input-filter math-exp-str)))
(while (setq math-exp-token
(string-match "\\.\\.\\([^.]\\|.[^.]\\)" math-exp-str))
(setq math-exp-str
(concat (substring math-exp-str 0 math-exp-token) "\\dots"
(substring math-exp-str (+ math-exp-token 2)))))
(math-build-parse-table)
(math-read-token)
(let ((val (catch 'syntax (math-read-expr-list))))
(if (stringp val)
(list 'error math-exp-old-pos val)
(if (equal math-exp-token 'end)
val
(list 'error math-exp-old-pos "Syntax error"))))))
;;;###autoload
(defun math-read-expr-list ()
(let* ((math-exp-keep-spaces nil)
(val (list (math-read-expr-level 0)))
(last val))
(while (equal math-expr-data ",")
(math-read-token)
(let ((rest (list (math-read-expr-level 0))))
(setcdr last rest)
(setq last rest)))
val))
(defvar calc-user-parse-table nil)
(defvar calc-last-main-parse-table nil)
(defvar calc-last-user-lang-parse-table nil)
(defvar calc-last-lang-parse-table nil)
(defvar calc-user-tokens nil)
(defvar calc-user-token-chars nil)
(defvar math-toks nil
"Tokens to pass between math-build-parse-table and math-find-user-tokens.")
;;;###autoload
(defun math-build-parse-table ()
(let ((mtab (cdr (assq nil calc-user-parse-tables)))
(ltab (cdr (assq calc-language calc-user-parse-tables)))
(lltab (get calc-language 'math-parse-table)))
(or (and (eq mtab calc-last-main-parse-table)
(eq ltab calc-last-user-lang-parse-table)
(eq lltab calc-last-lang-parse-table))
(let ((p (append mtab ltab lltab))
(math-toks nil))
(setq calc-user-parse-table p)
(setq calc-user-token-chars nil)
(while p
(math-find-user-tokens (car (car p)))
(setq p (cdr p)))
(setq calc-user-tokens (mapconcat 'identity
(sort (mapcar 'car math-toks)
(function (lambda (x y)
(> (length x)
(length y)))))
"\\|")
calc-last-main-parse-table mtab
calc-last-user-lang-parse-table ltab
calc-last-lang-parse-table lltab)))))
;;;###autoload
(defun math-find-user-tokens (p)
(while p
(cond ((and (stringp (car p))
(or (> (length (car p)) 1) (equal (car p) "$")
(equal (car p) "\""))
(string-match "[^a-zA-Z0-9]" (car p)))
(let ((s (regexp-quote (car p))))
(if (string-match "\\`[a-zA-Z0-9]" s)
(setq s (concat "\\<" s)))
(if (string-match "[a-zA-Z0-9]\\'" s)
(setq s (concat s "\\>")))
(or (assoc s math-toks)
(progn
(setq math-toks (cons (list s) math-toks))
(or (memq (aref (car p) 0) calc-user-token-chars)
(setq calc-user-token-chars
(cons (aref (car p) 0)
calc-user-token-chars)))))))
((consp (car p))
(math-find-user-tokens (nth 1 (car p)))
(or (eq (car (car p)) '\?)
(math-find-user-tokens (nth 2 (car p))))))
(setq p (cdr p))))
;;;###autoload
(defun math-read-token ()
(if (>= math-exp-pos (length math-exp-str))
(setq math-exp-old-pos math-exp-pos
math-exp-token 'end
math-expr-data "\000")
(let (adfn
(ch (aref math-exp-str math-exp-pos)))
(setq math-exp-old-pos math-exp-pos)
(cond ((memq ch '(32 10 9))
(setq math-exp-pos (1+ math-exp-pos))
(if math-exp-keep-spaces
(setq math-exp-token 'space
math-expr-data " ")
(math-read-token)))
((and (memq ch calc-user-token-chars)
(let ((case-fold-search nil))
(eq (string-match
calc-user-tokens math-exp-str math-exp-pos)
math-exp-pos)))
(setq math-exp-token 'punc
math-expr-data (math-match-substring math-exp-str 0)
math-exp-pos (match-end 0)))
((or (and (>= ch ?a) (<= ch ?z))
(and (>= ch ?A) (<= ch ?Z)))
(string-match
(cond
((and (memq calc-language calc-lang-allow-underscores)
(memq calc-language calc-lang-allow-percentsigns))
"[a-zA-Z0-9_'#]*")
((memq calc-language calc-lang-allow-underscores)
"[a-zA-Z0-9_#]*")
(t "[a-zA-Z0-9'#]*"))
math-exp-str math-exp-pos)
(setq math-exp-token 'symbol
math-exp-pos (match-end 0)
math-expr-data (math-restore-dashes
(math-match-substring math-exp-str 0)))
(if (setq adfn (get calc-language 'math-lang-adjust-words))
(funcall adfn)))
((or (and (>= ch ?0) (<= ch ?9))
(and (eq ch '?\.)
(eq (string-match "\\.[0-9]" math-exp-str math-exp-pos)
math-exp-pos))
(and (eq ch '?_)
(eq (string-match "_\\.?[0-9]" math-exp-str math-exp-pos)
math-exp-pos)
(or (eq math-exp-pos 0)
(and (not (memq calc-language
calc-lang-allow-underscores))
(eq (string-match "[^])}\"a-zA-Z0-9'$]_"
math-exp-str (1- math-exp-pos))
(1- math-exp-pos))))))
(or (and (memq calc-language calc-lang-c-type-hex)
(string-match "0[xX][0-9a-fA-F]+" math-exp-str math-exp-pos))
(string-match "_?\\([0-9]+.?0*@ *\\)?\\([0-9]+.?0*' *\\)?\\(0*\\([2-9]\\|1[0-4]\\)\\(#[#]?\\|\\^\\^\\)[0-9a-dA-D.]+[eE][-+_]?[0-9]+\\|0*\\([2-9]\\|[0-2][0-9]\\|3[0-6]\\)\\(#[#]?\\|\\^\\^\\)[0-9a-zA-Z:.]+\\|[0-9]+:[0-9:]+\\|[0-9.]+\\([eE][-+_]?[0-9]+\\)?\"?\\)?"
math-exp-str math-exp-pos))
(setq math-exp-token 'number
math-expr-data (math-match-substring math-exp-str 0)
math-exp-pos (match-end 0)))
((and (setq adfn
(assq ch (get calc-language 'math-lang-read-symbol)))
(eval (nth 1 adfn)))
(eval (nth 2 adfn)))
((eq ch ?\$)
(if (eq (string-match "\\$\\([1-9][0-9]*\\)" math-exp-str math-exp-pos)
math-exp-pos)
(setq math-expr-data (- (string-to-number (math-match-substring
math-exp-str 1))))
(string-match "\\$+" math-exp-str math-exp-pos)
(setq math-expr-data (- (match-end 0) (match-beginning 0))))
(setq math-exp-token 'dollar
math-exp-pos (match-end 0)))
((eq ch ?\#)
(if (eq (string-match "#\\([1-9][0-9]*\\)" math-exp-str math-exp-pos)
math-exp-pos)
(setq math-expr-data (string-to-number
(math-match-substring math-exp-str 1))
math-exp-pos (match-end 0))
(setq math-expr-data 1
math-exp-pos (1+ math-exp-pos)))
(setq math-exp-token 'hash))
((eq (string-match "~=\\|<=\\|>=\\|<>\\|/=\\|\\+/-\\|\\\\dots\\|\\\\ldots\\|\\*\\*\\|<<\\|>>\\|==\\|!=\\|&&&\\||||\\|!!!\\|&&\\|||\\|!!\\|:=\\|::\\|=>"
math-exp-str math-exp-pos)
math-exp-pos)
(setq math-exp-token 'punc
math-expr-data (math-match-substring math-exp-str 0)
math-exp-pos (match-end 0)))
((and (eq ch ?\")
(string-match "\\(\"\\([^\"\\]\\|\\\\.\\)*\\)\\(\"\\|\\'\\)"
math-exp-str math-exp-pos))
(setq math-exp-token 'string
math-expr-data (math-match-substring math-exp-str 1)
math-exp-pos (match-end 0)))
((and (setq adfn (get calc-language 'math-lang-read))
(eval (nth 0 adfn))
(eval (nth 1 adfn))))
((eq (string-match "%%.*$" math-exp-str math-exp-pos) math-exp-pos)
(setq math-exp-pos (match-end 0))
(math-read-token))
(t
(if (setq adfn (assq ch (get calc-language 'math-punc-table)))
(setq ch (cdr adfn)))
(setq math-exp-token 'punc
math-expr-data (char-to-string ch)
math-exp-pos (1+ math-exp-pos)))))))
(defconst math-alg-inequalities
'(calcFunc-lt calcFunc-gt calcFunc-leq calcFunc-geq
calcFunc-eq calcFunc-neq))
(defun math-read-expr-level (exp-prec &optional exp-term)
(let* ((math-expr-opers (math-expr-ops))
(x (math-read-factor))
(first t)
op op2)
(while (and (or (and calc-user-parse-table
(setq op (calc-check-user-syntax x exp-prec))
(setq x op
op '("2x" ident 999999 -1)))
(and (setq op (assoc math-expr-data math-expr-opers))
(/= (nth 2 op) -1)
(or (and (setq op2 (assoc
math-expr-data
(cdr (memq op math-expr-opers))))
(eq (= (nth 3 op) -1)
(/= (nth 3 op2) -1))
(eq (= (nth 3 op2) -1)
(not (math-factor-after)))
(setq op op2))
t))
(and (or (eq (nth 2 op) -1)
(memq math-exp-token '(symbol number dollar hash))
(equal math-expr-data "(")
(and (equal math-expr-data "[")
(not (equal
(get calc-language
'math-function-open) "["))
(not (and math-exp-keep-spaces
(eq (car-safe x) 'vec)))))
(or (not (setq op (assoc math-expr-data math-expr-opers)))
(/= (nth 2 op) -1))
(or (not calc-user-parse-table)
(not (eq math-exp-token 'symbol))
(let ((p calc-user-parse-table))
(while (and p
(or (not (integerp
(car (car (car p)))))
(not (equal
(nth 1 (car (car p)))
math-expr-data))))
(setq p (cdr p)))
(not p)))
(setq op (assoc "2x" math-expr-opers))))
(not (and exp-term (equal math-expr-data exp-term)))
(>= (nth 2 op) exp-prec))
(if (not (equal (car op) "2x"))
(math-read-token))
(and (memq (nth 1 op) '(sdev mod))
(require 'calc-ext))
(setq x (cond ((consp (nth 1 op))
(funcall (car (nth 1 op)) x op))
((eq (nth 3 op) -1)
(if (eq (nth 1 op) 'ident)
x
(if (eq (nth 1 op) 'closing)
(if (eq (nth 2 op) exp-prec)
(progn
(setq exp-prec 1000)
x)
(throw 'syntax "Mismatched delimiters"))
(list (nth 1 op) x))))
((and (not first)
(memq (nth 1 op) math-alg-inequalities)
(memq (car-safe x) math-alg-inequalities))
(require 'calc-ext)
(math-composite-inequalities x op))
(t (list (nth 1 op)
x
(math-read-expr-level (nth 3 op) exp-term))))
first nil))
x))
;; calc-arg-values is defined in calc-ext.el, but is used here.
(defvar calc-arg-values)
;;;###autoload
(defun calc-check-user-syntax (&optional x prec)
(let ((p calc-user-parse-table)
(matches nil)
match rule)
(while (and p
(or (not (progn
(setq rule (car (car p)))
(if x
(and (integerp (car rule))
(>= (car rule) prec)
(equal math-expr-data
(car (setq rule (cdr rule)))))
(equal math-expr-data (car rule)))))
(let ((save-exp-pos math-exp-pos)
(save-exp-old-pos math-exp-old-pos)
(save-exp-token math-exp-token)
(save-exp-data math-expr-data))
(or (not (listp
(setq matches (calc-match-user-syntax rule))))
(let ((args (progn
(require 'calc-ext)
calc-arg-values))
(conds nil)
temp)
(if x
(setq matches (cons x matches)))
(setq match (cdr (car p)))
(while (and (eq (car-safe match)
'calcFunc-condition)
(= (length match) 3))
(setq conds (append (math-flatten-lands
(nth 2 match))
conds)
match (nth 1 match)))
(while (and conds match)
(require 'calc-ext)
(cond ((eq (car-safe (car conds))
'calcFunc-let)
(setq temp (car conds))
(or (= (length temp) 3)
(and (= (length temp) 2)
(eq (car-safe (nth 1 temp))
'calcFunc-assign)
(= (length (nth 1 temp)) 3)
(setq temp (nth 1 temp)))
(setq match nil))
(setq matches (cons
(math-normalize
(math-multi-subst
(nth 2 temp)
args matches))
matches)
args (cons (nth 1 temp)
args)))
((and (eq (car-safe (car conds))
'calcFunc-matches)
(= (length (car conds)) 3))
(setq temp (calcFunc-vmatches
(math-multi-subst
(nth 1 (car conds))
args matches)
(nth 2 (car conds))))
(if (eq temp 0)
(setq match nil)
(while (setq temp (cdr temp))
(setq matches (cons (nth 2 (car temp))
matches)
args (cons (nth 1 (car temp))
args)))))
(t
(or (math-is-true (math-simplify
(math-multi-subst
(car conds)
args matches)))
(setq match nil))))
(setq conds (cdr conds)))
(if match
(not (setq match (math-multi-subst
match args matches)))
(setq math-exp-old-pos save-exp-old-pos
math-exp-token save-exp-token
math-expr-data save-exp-data
math-exp-pos save-exp-pos)))))))
(setq p (cdr p)))
(and p match)))
;;;###autoload
(defun calc-match-user-syntax (p &optional term)
(let ((matches nil)
(save-exp-pos math-exp-pos)
(save-exp-old-pos math-exp-old-pos)
(save-exp-token math-exp-token)
(save-exp-data math-expr-data)
m)
(while (and p
(cond ((stringp (car p))
(and (equal math-expr-data (car p))
(progn
(math-read-token)
t)))
((integerp (car p))
(and (setq m (catch 'syntax
(math-read-expr-level
(car p)
(if (cdr p)
(if (consp (nth 1 p))
(car (nth 1 (nth 1 p)))
(nth 1 p))
term))))
(not (stringp m))
(setq matches (nconc matches (list m)))))
((eq (car (car p)) '\?)
(setq m (calc-match-user-syntax (nth 1 (car p))))
(or (nth 2 (car p))
(setq matches
(nconc matches
(list
(cons 'vec (and (listp m) m))))))
(or (listp m) (not (nth 2 (car p)))
(not (eq (aref (car (nth 2 (car p))) 0) ?\$))
(eq math-exp-token 'end)))
(t
(setq m (calc-match-user-syntax (nth 1 (car p))
(car (nth 2 (car p)))))
(if (listp m)
(let ((vec (cons 'vec m))
opos mm)
(while (and (listp
(setq opos math-exp-pos
mm (calc-match-user-syntax
(or (nth 2 (car p))
(nth 1 (car p)))
(car (nth 2 (car p))))))
(> math-exp-pos opos))
(setq vec (nconc vec mm)))
(setq matches (nconc matches (list vec))))
(and (eq (car (car p)) '*)
(setq matches (nconc matches (list '(vec)))))))))
(setq p (cdr p)))
(if p
(setq math-exp-pos save-exp-pos
math-exp-old-pos save-exp-old-pos
math-exp-token save-exp-token
math-expr-data save-exp-data
matches "Failed"))
matches))
;;;###autoload
(defun math-remove-dashes (x)
(if (string-match "\\`\\(.*\\)-\\(.*\\)\\'" x)
(math-remove-dashes
(concat (math-match-substring x 1) "#" (math-match-substring x 2)))
x))
(defun math-remove-percentsigns (x)
(if (string-match "\\`\\(.*\\)%\\(.*\\)\\'" x)
(math-remove-percentsigns
(concat (math-match-substring x 1) "o'o" (math-match-substring x 2)))
x))
(defun math-restore-dashes (x)
(if (string-match "\\`\\(.*\\)[#_]\\(.*\\)\\'" x)
(math-restore-dashes
(concat (math-match-substring x 1) "-" (math-match-substring x 2)))
x))
(defun math-restore-placeholders (x)
"Replace placeholders by the proper characters in the symbol x.
This includes `#' for `_' and `'' for `%'.
If the current Calc language does not use placeholders, return nil."
(if (or (memq calc-language calc-lang-allow-underscores)
(memq calc-language calc-lang-allow-percentsigns))
(let ((sx (symbol-name x)))
(when (memq calc-language calc-lang-allow-percentsigns)
(require 'calccomp)
(setq sx (math-to-percentsigns sx)))
(if (memq calc-language calc-lang-allow-underscores)
(setq sx (math-string-restore-underscores sx)))
(intern-soft sx))))
(defun math-string-restore-underscores (x)
"Replace pound signs by underscores in the string x."
(if (string-match "\\`\\(.*\\)#\\(.*\\)\\'" x)
(math-string-restore-underscores
(concat (math-match-substring x 1) "_" (math-match-substring x 2)))
x))
;;;###autoload
(defun math-read-if (cond op)
(let ((then (math-read-expr-level 0)))
(or (equal math-expr-data ":")
(throw 'syntax "Expected ':'"))
(math-read-token)
(list 'calcFunc-if cond then (math-read-expr-level (nth 3 op)))))
(defun math-factor-after ()
(let ((math-exp-pos math-exp-pos)
math-exp-old-pos math-exp-token math-expr-data)
(math-read-token)
(or (memq math-exp-token '(number symbol dollar hash string))
(and (assoc math-expr-data '(("-") ("+") ("!") ("|") ("/")))
(assoc (concat "u" math-expr-data) math-expr-opers))
(eq (nth 2 (assoc math-expr-data math-expr-opers)) -1)
(assoc math-expr-data '(("(") ("[") ("{"))))))
(defun math-read-factor ()
(let ((math-expr-opers (math-expr-ops))
op)
(cond ((eq math-exp-token 'number)
(let ((num (math-read-number math-expr-data)))
(if (not num)
(progn
(setq math-exp-old-pos math-exp-pos)
(throw 'syntax "Bad format")))
(math-read-token)
(if (and math-read-expr-quotes
(consp num))
(list 'quote num)
num)))
((and calc-user-parse-table
(setq op (calc-check-user-syntax)))
op)
((or (equal math-expr-data "-")
(equal math-expr-data "+")
(equal math-expr-data "!")
(equal math-expr-data "|")
(equal math-expr-data "/"))
(setq math-expr-data (concat "u" math-expr-data))
(math-read-factor))
((and (setq op (assoc math-expr-data math-expr-opers))
(eq (nth 2 op) -1))
(if (consp (nth 1 op))
(funcall (car (nth 1 op)) op)
(math-read-token)
(let ((val (math-read-expr-level (nth 3 op))))
(cond ((eq (nth 1 op) 'ident)
val)
((and (Math-numberp val)
(equal (car op) "u-"))
(math-neg val))
(t (list (nth 1 op) val))))))
((eq math-exp-token 'symbol)
(let ((sym (intern math-expr-data)))
(math-read-token)
(if (equal math-expr-data calc-function-open)
(let ((f (assq sym math-expr-function-mapping)))
(math-read-token)
(if (consp (cdr f))
(funcall (car (cdr f)) f sym)
(let ((args (if (or (equal math-expr-data calc-function-close)
(eq math-exp-token 'end))
nil
(math-read-expr-list))))
(if (not (or (equal math-expr-data calc-function-close)
(eq math-exp-token 'end)))
(throw 'syntax "Expected `)'"))
(math-read-token)
(if (and (memq calc-language
calc-lang-parens-are-subscripts)
args
(require 'calc-ext)
(let ((calc-matrix-mode 'scalar))
(math-known-matrixp
(list 'var sym
(intern
(concat "var-"
(symbol-name sym)))))))
(math-parse-fortran-subscr sym args)
(if f
(setq sym (cdr f))
(and (= (aref (symbol-name sym) 0) ?\\)
(< (prefix-numeric-value calc-language-option)
0)
(setq sym (intern (substring (symbol-name sym)
1))))
(or (string-match "-" (symbol-name sym))
(setq sym (intern
(concat "calcFunc-"
(symbol-name sym))))))
(cons sym args)))))
(if math-read-expr-quotes
sym
(let ((val (list 'var
(intern (math-remove-dashes
(symbol-name sym)))
(if (string-match "-" (symbol-name sym))
sym
(intern (concat "var-"
(symbol-name sym)))))))
(let ((v (or
(assq (nth 1 val) math-expr-variable-mapping)
(assq (math-restore-placeholders (nth 1 val))
math-expr-variable-mapping))))
(and v (setq val (if (consp (cdr v))
(funcall (car (cdr v)) v val)
(list 'var
(intern
(substring (symbol-name (cdr v))
4))
(cdr v))))))
(while (and (memq calc-language
calc-lang-brackets-are-subscripts)
(equal math-expr-data "["))
(math-read-token)
(let ((el (math-read-expr-list)))
(while el
(setq val (append (list 'calcFunc-subscr val)
(list (car el))))
(setq el (cdr el))))
(if (equal math-expr-data "]")
(math-read-token)
(throw 'syntax "Expected ']'")))
val)))))
((eq math-exp-token 'dollar)
(let ((abs (if (> math-expr-data 0) math-expr-data (- math-expr-data))))
(if (>= (length calc-dollar-values) abs)
(let ((num math-expr-data))
(math-read-token)
(setq calc-dollar-used (max calc-dollar-used num))
(math-check-complete (nth (1- abs) calc-dollar-values)))
(throw 'syntax (if calc-dollar-values
"Too many $'s"
"$'s not allowed in this context")))))
((eq math-exp-token 'hash)
(or calc-hashes-used
(throw 'syntax "#'s not allowed in this context"))
(require 'calc-ext)
(if (<= math-expr-data (length calc-arg-values))
(let ((num math-expr-data))
(math-read-token)
(setq calc-hashes-used (max calc-hashes-used num))
(nth (1- num) calc-arg-values))
(throw 'syntax "Too many # arguments")))
((equal math-expr-data "(")
(let* ((exp (let ((math-exp-keep-spaces nil))
(math-read-token)
(if (or (equal math-expr-data "\\dots")
(equal math-expr-data "\\ldots"))
'(neg (var inf var-inf))
(math-read-expr-level 0)))))
(let ((math-exp-keep-spaces nil))
(cond
((equal math-expr-data ",")
(progn
(math-read-token)
(let ((exp2 (math-read-expr-level 0)))
(setq exp
(if (and exp2 (Math-realp exp) (Math-realp exp2))
(math-normalize (list 'cplx exp exp2))
(list '+ exp (list '* exp2 '(var i var-i))))))))
((equal math-expr-data ";")
(progn
(math-read-token)
(let ((exp2 (math-read-expr-level 0)))
(setq exp (if (and exp2 (Math-realp exp)
(Math-anglep exp2))
(math-normalize (list 'polar exp exp2))
(require 'calc-ext)
(list '* exp
(list 'calcFunc-exp
(list '*
(math-to-radians-2 exp2)
'(var i var-i)))))))))
((or (equal math-expr-data "\\dots")
(equal math-expr-data "\\ldots"))
(progn
(math-read-token)
(let ((exp2 (if (or (equal math-expr-data ")")
(equal math-expr-data "]")
(eq math-exp-token 'end))
'(var inf var-inf)
(math-read-expr-level 0))))
(setq exp
(list 'intv
(if (equal math-expr-data ")") 0 1)
exp
exp2)))))))
(if (not (or (equal math-expr-data ")")
(and (equal math-expr-data "]") (eq (car-safe exp) 'intv))
(eq math-exp-token 'end)))
(throw 'syntax "Expected `)'"))
(math-read-token)
exp))
((eq math-exp-token 'string)
(require 'calc-ext)
(math-read-string))
((equal math-expr-data "[")
(require 'calc-ext)
(math-read-brackets t "]"))
((equal math-expr-data "{")
(require 'calc-ext)
(math-read-brackets nil "}"))
((equal math-expr-data "<")
(require 'calc-ext)
(math-read-angle-brackets))
(t (throw 'syntax "Expected a number")))))
(provide 'calc-aent)
;; Local variables:
;; generated-autoload-file: "calc-loaddefs.el"
;; End:
;; arch-tag: 5599e45d-e51e-44bb-9a20-9f4ed8c96c32
;;; calc-aent.el ends here
| {
"pile_set_name": "Github"
} |
import { NAME_BUTTON, NAME_BUTTON_GROUP } from '../../constants/components'
import Vue, { mergeData } from '../../utils/vue'
import { getComponentConfig } from '../../utils/config'
export const props = {
vertical: {
type: Boolean,
default: false
},
size: {
type: String,
default: () => getComponentConfig(NAME_BUTTON, 'size')
},
tag: {
type: String,
default: 'div'
},
ariaRole: {
type: String,
default: 'group'
}
}
// @vue/component
export const BButtonGroup = /*#__PURE__*/ Vue.extend({
name: NAME_BUTTON_GROUP,
functional: true,
props,
render(h, { props, data, children }) {
return h(
props.tag,
mergeData(data, {
class: {
'btn-group': !props.vertical,
'btn-group-vertical': props.vertical,
[`btn-group-${props.size}`]: props.size
},
attrs: { role: props.ariaRole }
}),
children
)
}
})
| {
"pile_set_name": "Github"
} |
//
// detail/descriptor_read_op.hpp
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
//
// Copyright (c) 2003-2015 Christopher M. Kohlhoff (chris at kohlhoff dot com)
//
// Distributed under the Boost Software License, Version 1.0. (See accompanying
// file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
//
#ifndef ASIO_DETAIL_DESCRIPTOR_READ_OP_HPP
#define ASIO_DETAIL_DESCRIPTOR_READ_OP_HPP
#if defined(_MSC_VER) && (_MSC_VER >= 1200)
# pragma once
#endif // defined(_MSC_VER) && (_MSC_VER >= 1200)
#include "asio/detail/config.hpp"
#if !defined(ASIO_WINDOWS) && !defined(__CYGWIN__)
#include "asio/detail/bind_handler.hpp"
#include "asio/detail/buffer_sequence_adapter.hpp"
#include "asio/detail/descriptor_ops.hpp"
#include "asio/detail/fenced_block.hpp"
#include "asio/detail/handler_work.hpp"
#include "asio/detail/memory.hpp"
#include "asio/detail/reactor_op.hpp"
#include "asio/detail/push_options.hpp"
namespace asio {
namespace detail {
template <typename MutableBufferSequence>
class descriptor_read_op_base : public reactor_op
{
public:
descriptor_read_op_base(int descriptor,
const MutableBufferSequence& buffers, func_type complete_func)
: reactor_op(&descriptor_read_op_base::do_perform, complete_func),
descriptor_(descriptor),
buffers_(buffers)
{
}
static bool do_perform(reactor_op* base)
{
descriptor_read_op_base* o(static_cast<descriptor_read_op_base*>(base));
buffer_sequence_adapter<asio::mutable_buffer,
MutableBufferSequence> bufs(o->buffers_);
return descriptor_ops::non_blocking_read(o->descriptor_,
bufs.buffers(), bufs.count(), o->ec_, o->bytes_transferred_);
}
private:
int descriptor_;
MutableBufferSequence buffers_;
};
template <typename MutableBufferSequence, typename Handler>
class descriptor_read_op
: public descriptor_read_op_base<MutableBufferSequence>
{
public:
ASIO_DEFINE_HANDLER_PTR(descriptor_read_op);
descriptor_read_op(int descriptor,
const MutableBufferSequence& buffers, Handler& handler)
: descriptor_read_op_base<MutableBufferSequence>(
descriptor, buffers, &descriptor_read_op::do_complete),
handler_(ASIO_MOVE_CAST(Handler)(handler))
{
handler_work<Handler>::start(handler_);
}
static void do_complete(void* owner, operation* base,
const asio::error_code& /*ec*/,
std::size_t /*bytes_transferred*/)
{
// Take ownership of the handler object.
descriptor_read_op* o(static_cast<descriptor_read_op*>(base));
ptr p = { asio::detail::addressof(o->handler_), o, o };
handler_work<Handler> w(o->handler_);
ASIO_HANDLER_COMPLETION((o));
// Make a copy of the handler so that the memory can be deallocated before
// the upcall is made. Even if we're not about to make an upcall, a
// sub-object of the handler may be the true owner of the memory associated
// with the handler. Consequently, a local copy of the handler is required
// to ensure that any owning sub-object remains valid until after we have
// deallocated the memory here.
detail::binder2<Handler, asio::error_code, std::size_t>
handler(o->handler_, o->ec_, o->bytes_transferred_);
p.h = asio::detail::addressof(handler.handler_);
p.reset();
// Make the upcall if required.
if (owner)
{
fenced_block b(fenced_block::half);
ASIO_HANDLER_INVOCATION_BEGIN((handler.arg1_, handler.arg2_));
w.complete(handler, handler.handler_);
ASIO_HANDLER_INVOCATION_END;
}
}
private:
Handler handler_;
};
} // namespace detail
} // namespace asio
#include "asio/detail/pop_options.hpp"
#endif // !defined(ASIO_WINDOWS) && !defined(__CYGWIN__)
#endif // ASIO_DETAIL_DESCRIPTOR_READ_OP_HPP
| {
"pile_set_name": "Github"
} |
// (C) Copyright Gennadiy Rozental 2001.
// Distributed under the Boost Software License, Version 1.0.
// (See accompanying file LICENSE_1_0.txt or copy at
// http://www.boost.org/LICENSE_1_0.txt)
// See http://www.boost.org/libs/test for the library home page.
//
//!@file
//!@brief suppress some warnings
// ***************************************************************************
#ifdef BOOST_MSVC
# pragma warning(push)
# pragma warning(disable: 4511) // copy constructor can't not be generated
# pragma warning(disable: 4512) // assignment operator can't not be generated
# pragma warning(disable: 4100) // unreferenced formal parameter
# pragma warning(disable: 4996) // <symbol> was declared deprecated
# pragma warning(disable: 4355) // 'this' : used in base member initializer list
# pragma warning(disable: 4706) // assignment within conditional expression
# pragma warning(disable: 4251) // class 'A<T>' needs to have dll-interface to be used by clients of class 'B'
# pragma warning(disable: 4127) // conditional expression is constant
# pragma warning(disable: 4290) // C++ exception specification ignored except to ...
# pragma warning(disable: 4180) // qualifier applied to function type has no meaning; ignored
# pragma warning(disable: 4275) // non dll-interface class ... used as base for dll-interface class ...
# pragma warning(disable: 4267) // 'var' : conversion from 'size_t' to 'type', possible loss of data
# pragma warning(disable: 4511) // 'class' : copy constructor could not be generated
#endif
#if BOOST_CLANG
# pragma clang diagnostic push
# pragma clang diagnostic ignored "-Wvariadic-macros"
#endif
#if defined(BOOST_GCC) && (BOOST_GCC >= 4 * 10000 + 6 * 100)
# pragma GCC diagnostic push
# pragma GCC diagnostic ignored "-Wvariadic-macros"
#endif
| {
"pile_set_name": "Github"
} |
["0.4.0"]
git-tree-sha1 = "ff1a2323cb468ec5f201838fcbe3c232266b1f95"
["0.5.0"]
git-tree-sha1 = "afccf037da52fa596223e5a0e331ff752e0e845c"
| {
"pile_set_name": "Github"
} |
/* Serbian i18n for the jQuery UI date picker plugin. */
/* Written by Dejan Dimić. */
( function( factory ) {
if ( typeof define === "function" && define.amd ) {
// AMD. Register as an anonymous module.
define( [ "../widgets/datepicker" ], factory );
} else {
// Browser globals
factory( jQuery.datepicker );
}
}( function( datepicker ) {
datepicker.regional.sr = {
closeText: "Затвори",
prevText: "<",
nextText: ">",
currentText: "Данас",
monthNames: [ "Јануар","Фебруар","Март","Април","Мај","Јун",
"Јул","Август","Септембар","Октобар","Новембар","Децембар" ],
monthNamesShort: [ "Јан","Феб","Мар","Апр","Мај","Јун",
"Јул","Авг","Сеп","Окт","Нов","Дец" ],
dayNames: [ "Недеља","Понедељак","Уторак","Среда","Четвртак","Петак","Субота" ],
dayNamesShort: [ "Нед","Пон","Уто","Сре","Чет","Пет","Суб" ],
dayNamesMin: [ "Не","По","Ут","Ср","Че","Пе","Су" ],
weekHeader: "Сед",
dateFormat: "dd.mm.yy",
firstDay: 1,
isRTL: false,
showMonthAfterYear: false,
yearSuffix: "" };
datepicker.setDefaults( datepicker.regional.sr );
return datepicker.regional.sr;
} ) );
| {
"pile_set_name": "Github"
} |
# Cavium Octeon III CN7300
Please contact wolfSSL at info@wolfssl.com to request an evaluation.
| {
"pile_set_name": "Github"
} |
marketsession -- Market sessions
================================
.. automodule:: pyalgotrade.marketsession
:members:
:member-order: bysource
:show-inheritance:
| {
"pile_set_name": "Github"
} |
namespace JexusManager.Features.Main
{
using System.ComponentModel;
using System.Windows.Forms;
partial class ServerPage
{
/// <summary>
/// Required designer variable.
/// </summary>
private IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Component Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.listView1 = new System.Windows.Forms.ListView();
this.imageList1 = new System.Windows.Forms.ImageList(this.components);
this.splitContainer1 = new System.Windows.Forms.SplitContainer();
this.txtTitle = new System.Windows.Forms.Label();
this.panel2 = new System.Windows.Forms.Panel();
this.toolStrip2 = new System.Windows.Forms.ToolStrip();
this.toolStripLabel2 = new System.Windows.Forms.ToolStripLabel();
this.cbFilter = new System.Windows.Forms.ToolStripComboBox();
this.btnGo = new System.Windows.Forms.ToolStripSplitButton();
this.protocolToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.btnShowAll = new System.Windows.Forms.ToolStripButton();
this.toolStripSeparator4 = new System.Windows.Forms.ToolStripSeparator();
this.toolStripLabel3 = new System.Windows.Forms.ToolStripLabel();
this.cbGroup = new System.Windows.Forms.ToolStripComboBox();
this.btnView = new System.Windows.Forms.ToolStripSplitButton();
this.detailsToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.iconsToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.tilesToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.listToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.pictureBox1 = new System.Windows.Forms.PictureBox();
this.tableLayoutPanel1 = new System.Windows.Forms.TableLayoutPanel();
this.panel1 = new System.Windows.Forms.Panel();
this.label1 = new System.Windows.Forms.Label();
this.tsActionPanel = new System.Windows.Forms.ToolStrip();
this.cmsActionPanel = new System.Windows.Forms.ContextMenuStrip(this.components);
((System.ComponentModel.ISupportInitialize)(this.splitContainer1)).BeginInit();
this.splitContainer1.Panel1.SuspendLayout();
this.splitContainer1.Panel2.SuspendLayout();
this.splitContainer1.SuspendLayout();
this.panel2.SuspendLayout();
this.toolStrip2.SuspendLayout();
((System.ComponentModel.ISupportInitialize)(this.pictureBox1)).BeginInit();
this.tableLayoutPanel1.SuspendLayout();
this.panel1.SuspendLayout();
this.SuspendLayout();
//
// listView1
//
this.listView1.Dock = System.Windows.Forms.DockStyle.Fill;
this.listView1.LargeImageList = this.imageList1;
this.listView1.Location = new System.Drawing.Point(0, 25);
this.listView1.Name = "listView1";
this.listView1.Size = new System.Drawing.Size(560, 515);
this.listView1.TabIndex = 0;
this.listView1.UseCompatibleStateImageBehavior = false;
this.listView1.MouseDoubleClick += new System.Windows.Forms.MouseEventHandler(this.listView1_MouseDoubleClick);
//
// imageList1
//
this.imageList1.ColorDepth = System.Windows.Forms.ColorDepth.Depth32Bit;
this.imageList1.ImageSize = new System.Drawing.Size(36, 36);
this.imageList1.TransparentColor = System.Drawing.Color.Transparent;
//
// splitContainer1
//
this.splitContainer1.Dock = System.Windows.Forms.DockStyle.Fill;
this.splitContainer1.Location = new System.Drawing.Point(0, 0);
this.splitContainer1.Name = "splitContainer1";
//
// splitContainer1.Panel1
//
this.splitContainer1.Panel1.BackColor = System.Drawing.Color.White;
this.splitContainer1.Panel1.ContextMenuStrip = this.cmsActionPanel;
this.splitContainer1.Panel1.Controls.Add(this.txtTitle);
this.splitContainer1.Panel1.Controls.Add(this.panel2);
this.splitContainer1.Panel1.Controls.Add(this.pictureBox1);
//
// splitContainer1.Panel2
//
this.splitContainer1.Panel2.Controls.Add(this.tableLayoutPanel1);
this.splitContainer1.Panel2MinSize = 200;
this.splitContainer1.Size = new System.Drawing.Size(800, 600);
this.splitContainer1.SplitterDistance = 580;
this.splitContainer1.TabIndex = 2;
this.splitContainer1.SplitterMoved += new System.Windows.Forms.SplitterEventHandler(this.splitContainer1_SplitterMoved);
//
// txtTitle
//
this.txtTitle.AutoSize = true;
this.txtTitle.Font = new System.Drawing.Font("Microsoft Sans Serif", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.txtTitle.Location = new System.Drawing.Point(48, 10);
this.txtTitle.Name = "txtTitle";
this.txtTitle.Size = new System.Drawing.Size(62, 24);
this.txtTitle.TabIndex = 4;
this.txtTitle.Text = "Home";
//
// panel2
//
this.panel2.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.panel2.Controls.Add(this.listView1);
this.panel2.Controls.Add(this.toolStrip2);
this.panel2.Location = new System.Drawing.Point(10, 50);
this.panel2.Name = "panel2";
this.panel2.Size = new System.Drawing.Size(560, 540);
this.panel2.TabIndex = 5;
//
// toolStrip2
//
this.toolStrip2.GripStyle = System.Windows.Forms.ToolStripGripStyle.Hidden;
this.toolStrip2.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.toolStripLabel2,
this.cbFilter,
this.btnGo,
this.btnShowAll,
this.toolStripSeparator4,
this.toolStripLabel3,
this.cbGroup,
this.btnView});
this.toolStrip2.Location = new System.Drawing.Point(0, 0);
this.toolStrip2.Name = "toolStrip2";
this.toolStrip2.Size = new System.Drawing.Size(560, 25);
this.toolStrip2.TabIndex = 3;
this.toolStrip2.Text = "toolStrip2";
//
// toolStripLabel2
//
this.toolStripLabel2.Name = "toolStripLabel2";
this.toolStripLabel2.Size = new System.Drawing.Size(36, 22);
this.toolStripLabel2.Text = "Filter:";
//
// cbFilter
//
this.cbFilter.Name = "cbFilter";
this.cbFilter.Size = new System.Drawing.Size(121, 25);
//
// btnGo
//
this.btnGo.DropDownItems.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.protocolToolStripMenuItem});
this.btnGo.ImageTransparentColor = System.Drawing.Color.Magenta;
this.btnGo.Name = "btnGo";
this.btnGo.Size = new System.Drawing.Size(54, 22);
this.btnGo.Text = "Go";
this.btnGo.ToolTipText = "Go";
//
// protocolToolStripMenuItem
//
this.protocolToolStripMenuItem.Name = "protocolToolStripMenuItem";
this.protocolToolStripMenuItem.Size = new System.Drawing.Size(88, 22);
this.protocolToolStripMenuItem.Text = "All";
//
// btnShowAll
//
this.btnShowAll.ImageTransparentColor = System.Drawing.Color.Magenta;
this.btnShowAll.Name = "btnShowAll";
this.btnShowAll.Size = new System.Drawing.Size(73, 22);
this.btnShowAll.Text = "Show All";
//
// toolStripSeparator4
//
this.toolStripSeparator4.Name = "toolStripSeparator4";
this.toolStripSeparator4.Size = new System.Drawing.Size(6, 25);
//
// toolStripLabel3
//
this.toolStripLabel3.Name = "toolStripLabel3";
this.toolStripLabel3.Size = new System.Drawing.Size(59, 22);
this.toolStripLabel3.Text = "Group by:";
//
// cbGroup
//
this.cbGroup.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList;
this.cbGroup.Items.AddRange(new object[] {
"No Grouping",
"Category",
"Area"});
this.cbGroup.Name = "cbGroup";
this.cbGroup.Size = new System.Drawing.Size(121, 25);
//
// btnView
//
this.btnView.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.btnView.DropDownItems.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.detailsToolStripMenuItem,
this.iconsToolStripMenuItem,
this.tilesToolStripMenuItem,
this.listToolStripMenuItem});
this.btnView.ImageTransparentColor = System.Drawing.Color.Magenta;
this.btnView.Name = "btnView";
this.btnView.Size = new System.Drawing.Size(32, 22);
this.btnView.Text = "toolStripButton1";
//
// detailsToolStripMenuItem
//
this.detailsToolStripMenuItem.Name = "detailsToolStripMenuItem";
this.detailsToolStripMenuItem.Size = new System.Drawing.Size(109, 22);
this.detailsToolStripMenuItem.Text = "Details";
//
// iconsToolStripMenuItem
//
this.iconsToolStripMenuItem.Name = "iconsToolStripMenuItem";
this.iconsToolStripMenuItem.Size = new System.Drawing.Size(109, 22);
this.iconsToolStripMenuItem.Text = "Icons";
//
// tilesToolStripMenuItem
//
this.tilesToolStripMenuItem.Name = "tilesToolStripMenuItem";
this.tilesToolStripMenuItem.Size = new System.Drawing.Size(109, 22);
this.tilesToolStripMenuItem.Text = "Tiles";
//
// listToolStripMenuItem
//
this.listToolStripMenuItem.Name = "listToolStripMenuItem";
this.listToolStripMenuItem.Size = new System.Drawing.Size(109, 22);
this.listToolStripMenuItem.Text = "List";
//
// pictureBox1
//
this.pictureBox1.Location = new System.Drawing.Point(10, 10);
this.pictureBox1.Name = "pictureBox1";
this.pictureBox1.Size = new System.Drawing.Size(32, 32);
this.pictureBox1.TabIndex = 3;
this.pictureBox1.TabStop = false;
//
// tableLayoutPanel1
//
this.tableLayoutPanel1.ColumnCount = 1;
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F));
this.tableLayoutPanel1.Controls.Add(this.panel1, 0, 0);
this.tableLayoutPanel1.Controls.Add(this.tsActionPanel, 0, 1);
this.tableLayoutPanel1.Dock = System.Windows.Forms.DockStyle.Fill;
this.tableLayoutPanel1.Location = new System.Drawing.Point(0, 0);
this.tableLayoutPanel1.Name = "tableLayoutPanel1";
this.tableLayoutPanel1.RowCount = 2;
this.tableLayoutPanel1.RowStyles.Add(new System.Windows.Forms.RowStyle(System.Windows.Forms.SizeType.Absolute, 23F));
this.tableLayoutPanel1.RowStyles.Add(new System.Windows.Forms.RowStyle(System.Windows.Forms.SizeType.Percent, 100F));
this.tableLayoutPanel1.Size = new System.Drawing.Size(216, 600);
this.tableLayoutPanel1.TabIndex = 2;
//
// panel1
//
this.panel1.BackColor = System.Drawing.SystemColors.ActiveBorder;
this.panel1.Controls.Add(this.label1);
this.panel1.Dock = System.Windows.Forms.DockStyle.Fill;
this.panel1.Location = new System.Drawing.Point(0, 0);
this.panel1.Margin = new System.Windows.Forms.Padding(0);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(216, 23);
this.panel1.TabIndex = 1;
//
// label1
//
this.label1.AutoSize = true;
this.label1.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label1.Location = new System.Drawing.Point(3, 5);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(49, 13);
this.label1.TabIndex = 0;
this.label1.Text = "Actions";
//
// tsActionPanel
//
this.tsActionPanel.CanOverflow = false;
this.tsActionPanel.Dock = System.Windows.Forms.DockStyle.Fill;
this.tsActionPanel.GripStyle = System.Windows.Forms.ToolStripGripStyle.Hidden;
this.tsActionPanel.LayoutStyle = System.Windows.Forms.ToolStripLayoutStyle.VerticalStackWithOverflow;
this.tsActionPanel.Location = new System.Drawing.Point(0, 23);
this.tsActionPanel.Name = "tsActionPanel";
this.tsActionPanel.Size = new System.Drawing.Size(216, 577);
this.tsActionPanel.TabIndex = 2;
this.tsActionPanel.Text = "toolStrip1";
//
// cmsActionPanel
//
this.cmsActionPanel.Name = "cmsActionPanel";
this.cmsActionPanel.Size = new System.Drawing.Size(61, 4);
//
// ServerPage
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.Controls.Add(this.splitContainer1);
this.Name = "ServerPage";
this.Size = new System.Drawing.Size(800, 600);
this.splitContainer1.Panel1.ResumeLayout(false);
this.splitContainer1.Panel1.PerformLayout();
this.splitContainer1.Panel2.ResumeLayout(false);
((System.ComponentModel.ISupportInitialize)(this.splitContainer1)).EndInit();
this.splitContainer1.ResumeLayout(false);
this.panel2.ResumeLayout(false);
this.panel2.PerformLayout();
this.toolStrip2.ResumeLayout(false);
this.toolStrip2.PerformLayout();
((System.ComponentModel.ISupportInitialize)(this.pictureBox1)).EndInit();
this.tableLayoutPanel1.ResumeLayout(false);
this.tableLayoutPanel1.PerformLayout();
this.panel1.ResumeLayout(false);
this.panel1.PerformLayout();
this.ResumeLayout(false);
}
#endregion
private ListView listView1;
private SplitContainer splitContainer1;
private ImageList imageList1;
private TableLayoutPanel tableLayoutPanel1;
private Panel panel1;
private Label label1;
private ToolStrip tsActionPanel;
private Panel panel2;
private ToolStrip toolStrip2;
private ToolStripLabel toolStripLabel2;
private ToolStripComboBox cbFilter;
private ToolStripSplitButton btnGo;
private ToolStripMenuItem protocolToolStripMenuItem;
private ToolStripButton btnShowAll;
private ToolStripSeparator toolStripSeparator4;
private ToolStripLabel toolStripLabel3;
private ToolStripComboBox cbGroup;
private Label txtTitle;
private PictureBox pictureBox1;
private ToolStripSplitButton btnView;
private ToolStripMenuItem detailsToolStripMenuItem;
private ToolStripMenuItem iconsToolStripMenuItem;
private ToolStripMenuItem tilesToolStripMenuItem;
private ToolStripMenuItem listToolStripMenuItem;
private ContextMenuStrip cmsActionPanel;
}
}
| {
"pile_set_name": "Github"
} |
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { BarComponent } from './bar.component';
import { BarLeftDirective } from './directives/bar-left.directive';
import { BarMiddleDirective } from './directives/bar-middle.directive';
import { BarRightDirective } from './directives/bar-right.directive';
import { BarElementDirective } from './directives/bar-element.directive';
@NgModule({
declarations: [BarComponent, BarLeftDirective, BarMiddleDirective, BarRightDirective, BarElementDirective],
imports: [CommonModule],
exports: [BarComponent, BarLeftDirective, BarMiddleDirective, BarRightDirective, BarElementDirective]
})
export class BarModule {}
| {
"pile_set_name": "Github"
} |
{
"": [
"--------------------------------------------------------------------------------------------",
"Copyright (c) Microsoft Corporation. All rights reserved.",
"Licensed under the Source EULA. See License.txt in the project root for license information.",
"--------------------------------------------------------------------------------------------",
"Do not edit this file. It is machine generated."
],
"dashboard.container.gridtab.items": "La liste des widgets ou webviews qui s’afficheront dans cet onglet.",
"gridContainer.invalidInputs": "les widgets ou les webviews doivent être dans un conteneur de widgets pour l'extension."
} | {
"pile_set_name": "Github"
} |
%PARSC Parse classifier
%
% PARSC(W)
%
% Displays the type and, for combining classifiers, the structure of the
% mapping W.
%
% See also MAPPINGS
% Copyright: R.P.W. Duin, duin@ph.tn.tudelft.nl
% Faculty of Applied Physics, Delft University of Technology
% P.O. Box 5046, 2600 GA Delft, The Netherlands
% $Id: parsc.m,v 1.2 2006/03/08 22:06:58 duin Exp $
function parsc(w,space)
% If W is not a mapping, do not generate an error but simply return.
if (~isa(w,'prmapping')) return; end
if (nargin == 1)
space = '';
end
% Display W's type.
display(w,space);
% If W is a combining classifier, recursively call PARSC to plot the
% combined classier.
pars = getdata(w);
if (iscell(pars))
space = [space ' '];
for i=1:length(pars)
parsc(pars{i},space)
end
end
return
| {
"pile_set_name": "Github"
} |
// Copyright 2011-2016 Google Inc. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
#ifndef WINCESOCKET_HPP
#define WINCESOCKET_HPP
#include <windows.h>
#include <Psapi.h>
#include <winsock.h>
#include "../SocketConnection.hpp"
SOCKET Bind_To_Socket(unsigned int port);
SOCKET Wait_For_Connection(SOCKET sock);
/**
* Connection policy class for WinCE sockets.
*/
class WinCESocket : public SocketConnection {
private:
/**
* The server socket on which the debug client listens for a BinNavi
* connection.
*/
SOCKET localSocket;
/**
* The remote socket that is used to communicate with a connected BinNavi.
*/
SOCKET remoteSocket;
protected:
NaviError bindSocket();
NaviError closeSocket();
NaviError send(const char* buffer, unsigned int size) const;
NaviError read(char* buffer, unsigned int size) const;
public:
/**
* Creates a new WinCESocket object at a given port.
*
* @param port The port on which the debug client listens for BinNavi
* connections.
*/
WinCESocket(unsigned int port)
: SocketConnection(port) {
}
/**
* Converts a value from host to network byte order.
*
* @param val The value in host order.
*
* @return The same value in network order.
*/
int htonl(int val) const {
return ::htonl(val);
}
/**
* Converts a value from network to host byte order.
*
* @param val The value in network order.
*
* @return The same value in byte order.
*/
int ntohl(int val) const {
return ::ntohl(val);
}
bool hasData() const;
NaviError waitForConnection();
void printConnectionInfo();
};
#endif
| {
"pile_set_name": "Github"
} |
<div class="ngb-track-header-action-buttons" layout="row" layout-align="start center">
<div class="ngb-track-header-action-button" ng-repeat="action in ctrl.actions">
<div ng-if="action.enabled()">
<div ng-if="action.type === 'checkbox'"
layout="row"
layout-align="start center">
<md-checkbox class="ngb-track-header-checkbox md-warn"
aria-label="action"
md-no-ink
ng-mousedown="$event.stopImmediatePropagation();"
ng-click="ctrl.handle(action);"
ng-checked="action.value()"
>
</md-checkbox>
{{action.label}}
</div>
<div ng-if="action.type === 'groupLinks'"
class="ngb-track-header-action-group-links"
layout="row"
layout-align="start center">
{{action.label}}:
<div ng-repeat="link in action.links">
<a class="link-action" href="" ng-click="ctrl.handle(link);">{{link.label}}</a>
</div>
</div>
</div>
<div ng-hide="action.enabled()"
class="ngb-track-header-message"
layout="row"
layout-align="start center">{{action.disabledMessage()}}
</div>
</div>
</div> | {
"pile_set_name": "Github"
} |
#include <cmath>
#include "mex.h"
#include "segment-image.h"
#define UInt8 char
/*
* Segment an image
*
* Matlab Wrapper around the code of Felzenszwalb and Huttenlocher created
* by Jasper Uijlings, 2012
*
* Returns a color image representing the segmentation.
* JASPER: Random is replaced by just an index.
*
* im: image to segment.
* sigma: to smooth the image.
* c: constant for treshold function.
* min_size: minimum component size (enforced by post-processing stage).
* num_ccs: number of connected components in the segmentation.
*/
double *segment_image_index(image<rgb> *im, float sigma, float c, int min_size,
int *num_ccs) {
int width = im->width();
int height = im->height();
image<float> *r = new image<float>(width, height);
image<float> *g = new image<float>(width, height);
image<float> *b = new image<float>(width, height);
// smooth each color channel
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
imRef(r, x, y) = imRef(im, x, y).r;
imRef(g, x, y) = imRef(im, x, y).g;
imRef(b, x, y) = imRef(im, x, y).b;
}
}
image<float> *smooth_r = smooth(r, sigma);
image<float> *smooth_g = smooth(g, sigma);
image<float> *smooth_b = smooth(b, sigma);
delete r;
delete g;
delete b;
// build graph
edge *edges = new edge[width*height*4];
int num = 0;
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
if (x < width-1) {
edges[num].a = y * width + x;
edges[num].b = y * width + (x+1);
edges[num].w = diff(smooth_r, smooth_g, smooth_b, x, y, x+1, y);
num++;
}
if (y < height-1) {
edges[num].a = y * width + x;
edges[num].b = (y+1) * width + x;
edges[num].w = diff(smooth_r, smooth_g, smooth_b, x, y, x, y+1);
num++;
}
if ((x < width-1) && (y < height-1)) {
edges[num].a = y * width + x;
edges[num].b = (y+1) * width + (x+1);
edges[num].w = diff(smooth_r, smooth_g, smooth_b, x, y, x+1, y+1);
num++;
}
if ((x < width-1) && (y > 0)) {
edges[num].a = y * width + x;
edges[num].b = (y-1) * width + (x+1);
edges[num].w = diff(smooth_r, smooth_g, smooth_b, x, y, x+1, y-1);
num++;
}
}
}
delete smooth_r;
delete smooth_g;
delete smooth_b;
// segment
universe *u = segment_graph(width*height, num, edges, c);
// post process small components
for (int i = 0; i < num; i++) {
int a = u->find(edges[i].a);
int b = u->find(edges[i].b);
if ((a != b) && ((u->size(a) < min_size) || (u->size(b) < min_size)))
u->join(a, b);
}
delete [] edges;
*num_ccs = u->num_sets();
//image<rgb> *output = new image<rgb>(width, height);
// pick random colors for each component
double *colors = new double[width*height];
for (int i = 0; i < width*height; i++)
colors[i] = 0;
int idx = 1;
double* indexmap = new double[width * height];
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int comp = u->find(y * width + x);
if (!(colors[comp])){
colors[comp] = idx;
idx = idx + 1;
}
//imRef(output, x, y) = colors[comp];
indexmap[x * height + y] = colors[comp];
}
}
//mexPrintf("indexmap 0: %f\n", indexmap[0]);
//mexPrintf("indexmap 1: %f\n", indexmap[1]);
delete [] colors;
delete u;
return indexmap;
}
void mexFunction(int nlhs, mxArray *out[], int nrhs, const mxArray *input[])
{
// Checking number of arguments
if(nlhs > 3){
mexErrMsgTxt("Function has three return values");
return;
}
if(nrhs != 4){
mexErrMsgTxt("Usage: mexFelzenSegment(UINT8 im, double sigma, double c, int minSize)");
return;
}
if(!mxIsClass(input[0], "uint8")){
mexErrMsgTxt("Only image arrays of the UINT8 class are allowed.");
return;
}
// Load in arrays and parameters
UInt8* matIm = (UInt8*) mxGetPr(input[0]);
int nrDims = (int) mxGetNumberOfDimensions(input[0]);
int* dims = (int*) mxGetDimensions(input[0]);
double* sigma = mxGetPr(input[1]);
double* c = mxGetPr(input[2]);
double* minSize = mxGetPr(input[3]);
int min_size = (int) *minSize;
int height = dims[0];
int width = dims[1];
int imSize = height * width;
// Convert to image.
int idx;
image<rgb>* theIm = new image<rgb>(width, height);
for (int x = 0; x < width; x++){
for (int y = 0; y < height; y++){
idx = x * height + y;
imRef(theIm, x, y).r = matIm[idx];
imRef(theIm, x, y).g = matIm[idx + imSize];
imRef(theIm, x, y).b = matIm[idx + 2 * imSize];
}
}
// KOEN: Disable randomness of the algorithm
srand(12345);
// Call Felzenswalb segmentation algorithm
int num_css;
//image<rgb>* segIm = segment_image(theIm, *sigma, *c, min_size, &num_css);
double* segIndices = segment_image_index(theIm, *sigma, *c, min_size, &num_css);
//mexPrintf("numCss: %d\n", num_css);
// The segmentation index image
out[0] = mxCreateDoubleMatrix(dims[0], dims[1], mxREAL);
double* outSegInd = mxGetPr(out[0]);
// Keep track of minimum and maximum of each blob
out[1] = mxCreateDoubleMatrix(num_css, 4, mxREAL);
double* minmax = mxGetPr(out[1]);
for (int i=0; i < num_css; i++)
minmax[i] = dims[0];
for (int i= num_css; i < 2 * num_css; i++)
minmax[i] = dims[1];
// Keep track of neighbouring blobs using square matrix
out[2] = mxCreateDoubleMatrix(num_css, num_css, mxREAL);
double* nn = mxGetPr(out[2]);
// Copy the contents of segIndices
// Keep track of neighbours
// Get minimum and maximum
// These actually comprise of the bounding boxes
double currDouble;
int mprev, curr, prevHori, mcurr;
for(int x = 0; x < width; x++){
mprev = segIndices[x * height]-1;
for(int y=0; y < height; y++){
//mexPrintf("x: %d y: %d\n", x, y);
idx = x * height + y;
//mexPrintf("idx: %d\n", idx);
//currDouble = segIndices[idx];
//mexPrintf("currDouble: %d\n", currDouble);
curr = segIndices[idx];
//mexPrintf("curr: %d\n", curr);
outSegInd[idx] = curr; // copy contents
//mexPrintf("outSegInd: %f\n", outSegInd[idx]);
mcurr = curr-1;
// Get neighbours (vertical)
//mexPrintf("idx: %d", curr * num_css + mprev);
//mexPrintf(" %d\n", curr + num_css * mprev);
//mexPrintf("mprev: %d\n", mprev);
nn[(mcurr) * num_css + mprev] = 1;
nn[(mcurr) + num_css * mprev] = 1;
// Get horizontal neighbours
//mexPrintf("Get horizontal neighbours\n");
if (x > 0){
prevHori = outSegInd[(x-1) * height + y] - 1;
nn[mcurr * num_css + prevHori] = 1;
nn[mcurr + num_css * prevHori] = 1;
}
// Keep track of min and maximum index of blobs
//mexPrintf("Keep track of min and maximum index\n");
if (minmax[mcurr] > y)
minmax[mcurr] = y;
if (minmax[mcurr + num_css] > x)
minmax[mcurr + num_css] = x;
if (minmax[mcurr + 2 * num_css] < y)
minmax[mcurr + 2 * num_css] = y;
if (minmax[mcurr + 3 * num_css] < x)
minmax[mcurr + 3 * num_css] = x;
//mexPrintf("Mprev = mcurr");
mprev = mcurr;
}
}
// Do minmax plus one for Matlab
for (int i=0; i < 4 * num_css; i++)
minmax[i] += 1;
delete theIm;
delete [] segIndices;
return;
}
| {
"pile_set_name": "Github"
} |
set(LLVM_LINK_COMPONENTS
${LLVM_TARGETS_TO_BUILD}
CodeGen
Core
MC
MIRParser
Support
Target
)
add_llvm_unittest(MITests
LiveIntervalTest.cpp
)
| {
"pile_set_name": "Github"
} |
import { mount } from "enzyme"
import React from "react"
import {
ArtworkFilterContextProvider,
useArtworkFilterContext,
} from "../../ArtworkFilterContext"
import { FollowedArtistsFilter } from "../FollowedArtistsFilter"
describe("FollowedArtistsFilter", () => {
let context
const getWrapper = () => {
return mount(
<ArtworkFilterContextProvider>
<FollowedArtistsFilterTest />
</ArtworkFilterContextProvider>
)
}
const FollowedArtistsFilterTest = () => {
context = useArtworkFilterContext()
return <FollowedArtistsFilter />
}
it("updates context on filter change", done => {
const wrapper = getWrapper()
wrapper.find("Checkbox").simulate("click")
setTimeout(() => {
expect(context.filters.includeArtworksByFollowedArtists).toEqual(true)
done()
}, 0)
})
})
| {
"pile_set_name": "Github"
} |
# 自定义语言的实现——解释器模式(二)
18.2 文法规则和抽象语法树
解释器模式描述了如何为简单的语言定义一个文法,如何在该语言中表示一个句子,以及如何解释这些句子。在正式分析解释器模式结构之前,我们先来学习如何表示一个语言的文法规则以及如何构造一棵抽象语法树。
在前面所提到的加法/减法解释器中,每一个输入表达式,例如“1 + 2 + 3 – 4 + 1”,都包含了三个语言单位,可以使用如下文法规则来定义:
```
expression ::= value | operation
operation ::= expression '+' expression | expression '-' expression
value ::= an integer //一个整数值
```
该文法规则包含三条语句,第一条表示表达式的组成方式,其中value和operation是后面两个语言单位的定义,每一条语句所定义的字符串如operation和value称为语言构造成分或语言单位,符号“::=”表示“定义为”的意思,其左边的语言单位通过右边来进行说明和定义,语言单位对应终结符表达式和非终结符表达式。如本规则中的operation是非终结符表达式,它的组成元素仍然可以是表达式,可以进一步分解,而value是终结符表达式,它的组成元素是最基本的语言单位,不能再进行分解。
在文法规则定义中可以使用一些符号来表示不同的含义,如使用“|”表示或,使用“{”和“}”表示组合,使用“*”表示出现0次或多次等,其中使用频率最高的符号是表示“或”关系的“|”,如文法规则“boolValue ::= 0 | 1”表示终结符表达式boolValue的取值可以为0或者1。
除了使用文法规则来定义一个语言,在解释器模式中还可以通过一种称之为抽象语法树(Abstract Syntax Tree, AST)的图形方式来直观地表示语言的构成,每一棵抽象语法树对应一个语言实例,如加法/减法表达式语言中的语句“1+ 2 + 3 – 4 + 1”,可以通过如图18-2所示抽象语法树来表示:
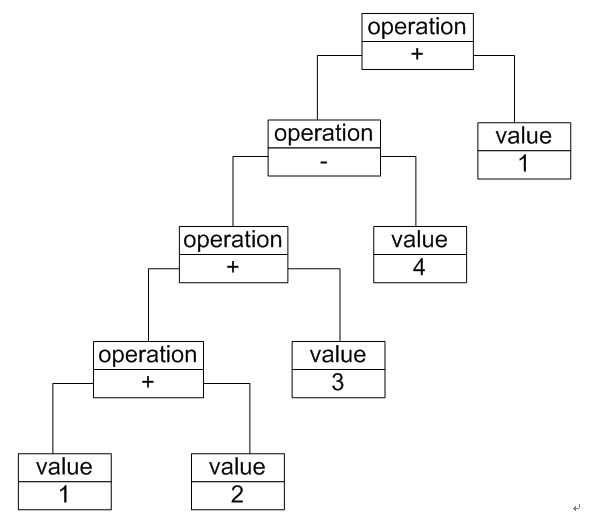
图18-2 抽象语法树示意图
在该抽象语法树中,可以通过终结符表达式value和非终结符表达式operation组成复杂的语句,每个文法规则的语言实例都可以表示为一个抽象语法树,即每一条具体的语句都可以用类似图18-2所示的抽象语法树来表示,在图中终结符表达式类的实例作为树的叶子节点,而非终结符表达式类的实例作为非叶子节点,它们可以将终结符表达式类的实例以及包含终结符和非终结符实例的子表达式作为其子节点。抽象语法树描述了如何构成一个复杂的句子,通过对抽象语法树的分析,可以识别出语言中的终结符类和非终结符类。 | {
"pile_set_name": "Github"
} |
0 0 0 1 0.34308 -0.364099
0 0 6.5 1 0.29569 0.0422474
10 0 0 1 -0.319517 -0.492328
10 0 6.5 1 -0.349241 0.00608137
10 10 0 1 -0.308696 -0.331868
10 10 6.5 1 -0.32638 -0.0437467
0 10 0 1 0.103389 -0.27011
0 10 6.5 1 0.078673 -0.0153531
0 5 10 1 0.145793 0.168739
10 5 10 1 -0.346208 0.162066
0 6 0 1 0.173789 -0.297716
0 8 0 1 0.135725 -0.28279
0 8 5 1 0.1145 -0.0698657
0 6 5 1 0.149968 -0.0660103
0 2 2.5 1 0.258691 -0.194832
0 4 2.5 1 0.205567 -0.187089
0 4 5 1 0.192179 -0.0614218
0 2 5 1 0.243259 -0.0558692
0.5 0.5 -0.5 1 0.301311 -0.393645
0.5 0.5 6 1 0.256162 0.00665129
10.5 0.5 -0.5 1 -0.355884 -0.525502
10.5 0.5 6 1 -0.383492 -0.0361903
10.5 10.5 -0.5 1 -0.329961 -0.353181
10.5 10.5 6 1 -0.34691 -0.0681165
0.5 10.5 -0.5 1 0.0798623 -0.289726
0.5 10.5 6 1 0.0559269 -0.0373583
0.5 5.5 9.5 1 0.117483 0.139766
10.5 5.5 9.5 1 -0.371293 0.128771
0.5 6.5 -0.5 1 0.145216 -0.320394
0.5 8.5 -0.5 1 0.109913 -0.303828
0.5 8.5 4.5 1 0.0894072 -0.0930739
0.5 6.5 4.5 1 0.122263 -0.0912573
0.5 2.5 2 1 0.223131 -0.223619
0.5 4.5 2 1 0.174139 -0.213261
0.5 4.5 4.5 1 0.161278 -0.0891003
0.5 2.5 4.5 1 0.208361 -0.086497
| {
"pile_set_name": "Github"
} |
//
// Generated by class-dump 3.5 (64 bit) (Debug version compiled Oct 15 2018 10:31:50).
//
// class-dump is Copyright (C) 1997-1998, 2000-2001, 2004-2015 by Steve Nygard.
//
#import <objc/NSObject.h>
@class NSArray, NSBundle, NSLock, NSMutableDictionary, NSString;
@protocol WFApplicationContextProvider;
@interface WFApplicationContext : NSObject
{
BOOL _canLoadShortcutsDatabase;
BOOL _inactive;
BOOL _inBackground;
BOOL _canLoadShortcutsDatabaseEntitlementChecked;
id <WFApplicationContextProvider> _provider;
NSBundle *_applicationBundle;
NSMutableDictionary *_applicationStateObserversByEvent;
NSLock *_observersLock;
}
+ (id)sharedContext;
+ (id)imageNamed:(id)arg1;
- (void).cxx_destruct;
@property(nonatomic) BOOL canLoadShortcutsDatabaseEntitlementChecked; // @synthesize canLoadShortcutsDatabaseEntitlementChecked=_canLoadShortcutsDatabaseEntitlementChecked;
@property(nonatomic) BOOL inBackground; // @synthesize inBackground=_inBackground;
@property(nonatomic) BOOL inactive; // @synthesize inactive=_inactive;
@property(readonly, nonatomic) NSLock *observersLock; // @synthesize observersLock=_observersLock;
@property(retain, nonatomic) NSMutableDictionary *applicationStateObserversByEvent; // @synthesize applicationStateObserversByEvent=_applicationStateObserversByEvent;
@property(readonly, nonatomic) BOOL canLoadShortcutsDatabase; // @synthesize canLoadShortcutsDatabase=_canLoadShortcutsDatabase;
@property(nonatomic, getter=isIdleTimerDisabled) BOOL idleTimerDisabled;
@property(readonly, nonatomic) BOOL shouldReverseLayoutDirection;
@property(readonly, nonatomic) NSBundle *applicationBundle; // @synthesize applicationBundle=_applicationBundle;
@property(readonly, nonatomic) NSString *currentUserInterfaceType;
@property(readonly, nonatomic) NSBundle *bundle;
@property(readonly, nonatomic) NSArray *applicationBundleURLSchemes;
- (void)openURL:(id)arg1 withBundleIdentifier:(id)arg2 userInterface:(id)arg3 completionHandler:(CDUnknownBlockType)arg4;
- (void)openURL:(id)arg1 userInterface:(id)arg2 completionHandler:(CDUnknownBlockType)arg3;
- (void)openURL:(id)arg1 completionHandler:(CDUnknownBlockType)arg2;
- (void)openURL:(id)arg1;
- (BOOL)canOpenURL:(id)arg1;
@property(readonly, nonatomic) long long applicationState;
- (void)removeApplicationStateObserver:(id)arg1 forEvent:(long long)arg2;
- (void)addApplicationStateObserver:(id)arg1 forEvent:(long long)arg2;
- (void)handleApplicationStateEvent:(long long)arg1;
- (void)applicationWillEnterForeground;
- (void)applicationDidEnterBackground;
- (void)applicationWillResignActive;
- (void)applicationDidBecomeActive;
- (void)dealloc;
@property(retain, nonatomic) id <WFApplicationContextProvider> provider; // @synthesize provider=_provider;
- (id)init;
@end
| {
"pile_set_name": "Github"
} |
#pragma warning disable 0649
//------------------------------------------------------------------------------
// <auto-generated>This code was generated by LLBLGen Pro v4.1.</auto-generated>
//------------------------------------------------------------------------------
using System;
using System.Data.Linq;
using System.Data.Linq.Mapping;
using System.ComponentModel;
namespace L2S.Bencher.EntityClasses
{
/// <summary>Class which represents the entity 'ProductModelIllustration', mapped on table 'AdventureWorks.Production.ProductModelIllustration'.</summary>
[Table(Name="[Production].[ProductModelIllustration]")]
public partial class ProductModelIllustration : INotifyPropertyChanging, INotifyPropertyChanged
{
#region Events
/// <summary>Event which is raised when a property value is changing.</summary>
public event PropertyChangingEventHandler PropertyChanging;
/// <summary>Event which is raised when a property value changes.</summary>
public event PropertyChangedEventHandler PropertyChanged;
#endregion
#region Class Member Declarations
private System.Int32 _illustrationId;
private System.DateTime _modifiedDate;
private System.Int32 _productModelId;
private EntityRef <Illustration> _illustration;
private EntityRef <ProductModel> _productModel;
#endregion
#region Extensibility Method Definitions
partial void OnLoaded();
partial void OnValidate(System.Data.Linq.ChangeAction action);
partial void OnCreated();
partial void OnIllustrationIdChanging(System.Int32 value);
partial void OnIllustrationIdChanged();
partial void OnModifiedDateChanging(System.DateTime value);
partial void OnModifiedDateChanged();
partial void OnProductModelIdChanging(System.Int32 value);
partial void OnProductModelIdChanged();
#endregion
/// <summary>Initializes a new instance of the <see cref="ProductModelIllustration"/> class.</summary>
public ProductModelIllustration()
{
_illustration = default(EntityRef<Illustration>);
_productModel = default(EntityRef<ProductModel>);
OnCreated();
}
/// <summary>Raises the PropertyChanging event</summary>
/// <param name="propertyName">name of the property which is changing</param>
protected virtual void SendPropertyChanging(string propertyName)
{
if((this.PropertyChanging != null))
{
this.PropertyChanging(this, new PropertyChangingEventArgs(propertyName));
}
}
/// <summary>Raises the PropertyChanged event for the property specified</summary>
/// <param name="propertyName">name of the property which was changed</param>
protected virtual void SendPropertyChanged(string propertyName)
{
if((this.PropertyChanged != null))
{
this.PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
#region Class Property Declarations
/// <summary>Gets or sets the IllustrationId field. Mapped on target field 'IllustrationID'. </summary>
[Column(Name="IllustrationID", Storage="_illustrationId", CanBeNull=false, DbType="int NOT NULL", IsPrimaryKey=true)]
public System.Int32 IllustrationId
{
get { return _illustrationId; }
set
{
if((_illustrationId != value))
{
if(_illustration.HasLoadedOrAssignedValue)
{
throw new System.Data.Linq.ForeignKeyReferenceAlreadyHasValueException();
}
OnIllustrationIdChanging(value);
SendPropertyChanging("IllustrationId");
_illustrationId = value;
SendPropertyChanged("IllustrationId");
OnIllustrationIdChanged();
}
}
}
/// <summary>Gets or sets the ModifiedDate field. Mapped on target field 'ModifiedDate'. </summary>
[Column(Name="ModifiedDate", Storage="_modifiedDate", CanBeNull=false, DbType="datetime NOT NULL")]
public System.DateTime ModifiedDate
{
get { return _modifiedDate; }
set
{
if((_modifiedDate != value))
{
OnModifiedDateChanging(value);
SendPropertyChanging("ModifiedDate");
_modifiedDate = value;
SendPropertyChanged("ModifiedDate");
OnModifiedDateChanged();
}
}
}
/// <summary>Gets or sets the ProductModelId field. Mapped on target field 'ProductModelID'. </summary>
[Column(Name="ProductModelID", Storage="_productModelId", CanBeNull=false, DbType="int NOT NULL", IsPrimaryKey=true)]
public System.Int32 ProductModelId
{
get { return _productModelId; }
set
{
if((_productModelId != value))
{
if(_productModel.HasLoadedOrAssignedValue)
{
throw new System.Data.Linq.ForeignKeyReferenceAlreadyHasValueException();
}
OnProductModelIdChanging(value);
SendPropertyChanging("ProductModelId");
_productModelId = value;
SendPropertyChanged("ProductModelId");
OnProductModelIdChanged();
}
}
}
/// <summary>Represents the navigator which is mapped onto the association 'ProductModelIllustration.Illustration - Illustration.ProductModelIllustrations (m:1)'</summary>
[Association(Name="ProductModelIllustration_Illustrationa5a27066452c47558be7c9d862a501ce", Storage="_illustration", ThisKey="IllustrationId", IsForeignKey=true)]
public Illustration Illustration
{
get { return _illustration.Entity; }
set
{
Illustration previousValue = _illustration.Entity;
if((previousValue != value) || (_illustration.HasLoadedOrAssignedValue == false))
{
this.SendPropertyChanging("Illustration");
if(previousValue != null)
{
_illustration.Entity = null;
previousValue.ProductModelIllustrations.Remove(this);
}
_illustration.Entity = value;
if(value == null)
{
_illustrationId = default(System.Int32);
}
else
{
value.ProductModelIllustrations.Add(this);
_illustrationId = value.IllustrationId;
}
this.SendPropertyChanged("Illustration");
}
}
}
/// <summary>Represents the navigator which is mapped onto the association 'ProductModelIllustration.ProductModel - ProductModel.ProductModelIllustrations (m:1)'</summary>
[Association(Name="ProductModelIllustration_ProductModel3a9db1ea24f94a64ae6d1ae9345271f3", Storage="_productModel", ThisKey="ProductModelId", IsForeignKey=true)]
public ProductModel ProductModel
{
get { return _productModel.Entity; }
set
{
ProductModel previousValue = _productModel.Entity;
if((previousValue != value) || (_productModel.HasLoadedOrAssignedValue == false))
{
this.SendPropertyChanging("ProductModel");
if(previousValue != null)
{
_productModel.Entity = null;
previousValue.ProductModelIllustrations.Remove(this);
}
_productModel.Entity = value;
if(value == null)
{
_productModelId = default(System.Int32);
}
else
{
value.ProductModelIllustrations.Add(this);
_productModelId = value.ProductModelId;
}
this.SendPropertyChanged("ProductModel");
}
}
}
#endregion
}
}
#pragma warning restore 0649 | {
"pile_set_name": "Github"
} |
[android-components](../../index.md) / [mozilla.components.concept.sync](../index.md) / [SyncableStore](./index.md)
# SyncableStore
`interface SyncableStore` [(source)](https://github.com/mozilla-mobile/android-components/blob/master/components/concept/sync/src/main/java/mozilla/components/concept/sync/Sync.kt#L48)
Describes a "sync" entry point for a storage layer.
### Functions
| Name | Summary |
|---|---|
| [getHandle](get-handle.md) | `abstract fun getHandle(): `[`Long`](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin/-long/index.html)<br>This should be removed. See: https://github.com/mozilla/application-services/issues/1877 |
### Extension Functions
| Name | Summary |
|---|---|
| [loadResourceAsString](../../mozilla.components.support.test.file/kotlin.-any/load-resource-as-string.md) | `fun `[`Any`](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin/-any/index.html)`.loadResourceAsString(path: `[`String`](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin/-string/index.html)`): `[`String`](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin/-string/index.html)<br>Loads a file from the resources folder and returns its content as a string object. |
### Inheritors
| Name | Summary |
|---|---|
| [PlacesBookmarksStorage](../../mozilla.components.browser.storage.sync/-places-bookmarks-storage/index.md) | `open class PlacesBookmarksStorage : `[`PlacesStorage`](../../mozilla.components.browser.storage.sync/-places-storage/index.md)`, `[`BookmarksStorage`](../../mozilla.components.concept.storage/-bookmarks-storage/index.md)`, `[`SyncableStore`](./index.md)<br>Implementation of the [BookmarksStorage](../../mozilla.components.concept.storage/-bookmarks-storage/index.md) which is backed by a Rust Places lib via [PlacesApi](#). |
| [PlacesHistoryStorage](../../mozilla.components.browser.storage.sync/-places-history-storage/index.md) | `open class PlacesHistoryStorage : `[`PlacesStorage`](../../mozilla.components.browser.storage.sync/-places-storage/index.md)`, `[`HistoryStorage`](../../mozilla.components.concept.storage/-history-storage/index.md)`, `[`SyncableStore`](./index.md)<br>Implementation of the [HistoryStorage](../../mozilla.components.concept.storage/-history-storage/index.md) which is backed by a Rust Places lib via [PlacesApi](#). |
| [PlacesStorage](../../mozilla.components.browser.storage.sync/-places-storage/index.md) | `abstract class PlacesStorage : `[`Storage`](../../mozilla.components.concept.storage/-storage/index.md)`, `[`SyncableStore`](./index.md)<br>A base class for concrete implementations of PlacesStorages |
| [RemoteTabsStorage](../../mozilla.components.browser.storage.sync/-remote-tabs-storage/index.md) | `open class RemoteTabsStorage : `[`Storage`](../../mozilla.components.concept.storage/-storage/index.md)`, `[`SyncableStore`](./index.md)<br>An interface which defines read/write methods for remote tabs data. |
| [SyncableLoginsStorage](../../mozilla.components.service.sync.logins/-syncable-logins-storage/index.md) | `class SyncableLoginsStorage : `[`LoginsStorage`](../../mozilla.components.concept.storage/-logins-storage/index.md)`, `[`SyncableStore`](./index.md)`, `[`AutoCloseable`](http://docs.oracle.com/javase/7/docs/api/java/lang/AutoCloseable.html)<br>An implementation of [LoginsStorage](../../mozilla.components.concept.storage/-logins-storage/index.md) backed by application-services' `logins` library. Synchronization support is provided both directly (via [sync](../../mozilla.components.service.sync.logins/-syncable-logins-storage/sync.md)) when only syncing this storage layer, or via [getHandle](../../mozilla.components.service.sync.logins/-syncable-logins-storage/get-handle.md) when syncing multiple stores. Use the latter in conjunction with [FxaAccountManager](#). |
| {
"pile_set_name": "Github"
} |
{% extends "pretixcontrol/event/base.html" %}
{% load i18n %}
{% block title %}
{% trans "Approve order" %}
{% endblock %}
{% block content %}
<h1>
{% trans "Approve order" %}
</h1>
<p>{% blocktrans trimmed %}
Do you really want to approve this order?
{% endblocktrans %}</p>
<form method="post" href="">
{% csrf_token %}
<div class="row checkout-button-row">
<div class="col-md-4">
<a class="btn btn-block btn-default btn-lg"
href="{% url "control:event.order" event=request.event.slug organizer=request.event.organizer.slug code=order.code %}">
{% trans "No, take me back" %}
</a>
</div>
<div class="col-md-4 col-md-offset-4">
<button class="btn btn-block btn-primary btn-lg" type="submit">
{% trans "Yes, approve order" %}
</button>
</div>
<div class="clearfix"></div>
</div>
</form>
{% endblock %}
| {
"pile_set_name": "Github"
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Node Url Shortener</title>
<link rel="apple-touch-icon" sizes="180x180" href="/apple-touch-icon.png">
<link rel="shortcut icon" href="/favicon.ico">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" crossorigin="anonymous">
<link rel="stylesheet" href="/css/nus.css" type="text/css">
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div id="header" class="container">
<header class="header">
<h1>NUS</h1>
<p class="lead">A modern, minimalist, and lightweight URL shortener using Node.js and Redis.</p>
</header><!-- /.header -->
</div>
<div class="container">
<section class="row">
<div class="col-md-12 form-group url-form">
<form class="form" id="nus">
<div class="row">
<div class="col-lg-10 input-group url-input">
<input id="link" name="long_url" type="text" class="form-control input-lg" placeholder="Paste a link here">
<span class="input-group-btn">
<button class="btn btn-default btn-lg" type="submit">
<span class="glyphicon glyphicon-link"></span>
Shorten
</button>
<button class="btn btn-default btn-lg" data-clipboard-target="#link" type="button">
<span class="glyphicon glyphicon-share-alt"></span>
Copy
</button>
</span>
</div><!-- /.input-group -->
</div><!--/.row-->
<div class="row flex-group">
<div class='col-lg-9 input-group date url-input flex-item' id='datetimepicker1'>
<input id="start_date" name="start_date" type="date" class="form-control" >
<span class="input-group-addon">
<span class="glyphicon glyphicon-calendar"></span>
</span>
</div>
<div class='col-lg-9 input-group date url-input flex-item' id='datetimepicker2'>
<input id="end_date" name="end_date" type="date" class="form-control" >
<span class="input-group-addon">
<span class="glyphicon glyphicon-calendar"></span>
</span>
</div>
</div><!--/.row-->
</form>
</div><!--/.form-group-->
</section>
</div><!-- /.container -->
<!-- GitHub Corners http://tholman.com/github-corners -->
<a href="https://github.com/dotzero/node-url-shortener" class="github-corner"><svg width="80" height="80" viewBox="0 0 250 250" style="fill:#151513; color:#fff; position: absolute; top: 0; border: 0; right: 0;"><path d="M0,0 L115,115 L130,115 L142,142 L250,250 L250,0 Z"></path><path d="M128.3,109.0 C113.8,99.7 119.0,89.6 119.0,89.6 C122.0,82.7 120.5,78.6 120.5,78.6 C119.2,72.0 123.4,76.3 123.4,76.3 C127.3,80.9 125.5,87.3 125.5,87.3 C122.9,97.6 130.6,101.9 134.4,103.2" fill="currentColor" style="transform-origin: 130px 106px;" class="octo-arm"></path><path d="M115.0,115.0 C114.9,115.1 118.7,116.5 119.8,115.4 L133.7,101.6 C136.9,99.2 139.9,98.4 142.2,98.6 C133.8,88.0 127.5,74.4 143.8,58.0 C148.5,53.4 154.0,51.2 159.7,51.0 C160.3,49.4 163.2,43.6 171.4,40.1 C171.4,40.1 176.1,42.5 178.8,56.2 C183.1,58.6 187.2,61.8 190.9,65.4 C194.5,69.0 197.7,73.2 200.1,77.6 C213.8,80.2 216.3,84.9 216.3,84.9 C212.7,93.1 206.9,96.0 205.4,96.6 C205.1,102.4 203.0,107.8 198.3,112.5 C181.9,128.9 168.3,122.5 157.7,114.1 C157.9,116.9 156.7,120.9 152.7,124.9 L141.0,136.5 C139.8,137.7 141.6,141.9 141.8,141.8 Z" fill="currentColor" class="octo-body"></path></svg></a><style>.github-corner:hover .octo-arm{animation:octocat-wave 560ms ease-in-out}@keyframes octocat-wave{0%,100%{transform:rotate(0)}20%,60%{transform:rotate(-25deg)}40%,80%{transform:rotate(10deg)}}@media (max-width:500px){.github-corner:hover .octo-arm{animation:none}.github-corner .octo-arm{animation:octocat-wave 560ms ease-in-out}}</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/clipboard.js/1.5.10/clipboard.min.js"></script>
<script src="/js/nus.js" type="text/javascript"></script>
</body>
</html>
| {
"pile_set_name": "Github"
} |
"""
Name : c9_55_end_of_chapter_problem_update_yanMonthly.py
Book : Python for Finance (2nd ed.)
Publisher: Packt Publishing Ltd.
Author : Yuxing Yan
Date : 6/6/2017
email : yany@canisius.edu
paulyxy@hotmail.com
"""
import pandas as pd
x=pd.read_pickle('c:/temp/yanMonthly.pkl')
print(x.head(2))
print(x.tail(3))
| {
"pile_set_name": "Github"
} |
package com.jeesuite.mybatis.crud.builder;
import org.apache.ibatis.executor.keygen.Jdbc3KeyGenerator;
import org.apache.ibatis.executor.keygen.KeyGenerator;
import org.apache.ibatis.executor.keygen.NoKeyGenerator;
import org.apache.ibatis.mapping.MappedStatement;
import org.apache.ibatis.mapping.SqlCommandType;
import org.apache.ibatis.mapping.SqlSource;
import org.apache.ibatis.scripting.LanguageDriver;
import org.apache.ibatis.session.Configuration;
import com.jeesuite.mybatis.crud.helper.EntityHelper;
import com.jeesuite.mybatis.crud.helper.EntityMapper;
import com.jeesuite.mybatis.parser.EntityInfo;
/**
*
* <br>
* Class Name : AbstractMethodBuilder
*
* @author jiangwei
* @version 1.0.0
* @date 2020年5月9日
*/
public abstract class AbstractMethodBuilder {
public void build(Configuration configuration, LanguageDriver languageDriver,EntityInfo entity) {
for (String name : methodNames()) {
String msId = entity.getMapperClass().getName() + "." + name;
// 从参数对象里提取注解信息
EntityMapper entityMapper = EntityHelper.getEntityMapper(entity.getEntityClass());
// 生成sql
String sql = buildSQL(entityMapper,name.endsWith("Selective"));
SqlSource sqlSource = languageDriver.createSqlSource(configuration, sql, entity.getEntityClass());
MappedStatement.Builder statementBuilder = new MappedStatement.Builder(configuration, msId, sqlSource,sqlCommandType());
//主键策略
if(sqlCommandType() == SqlCommandType.INSERT){
KeyGenerator keyGenerator = entityMapper.autoId() ? new Jdbc3KeyGenerator() : new NoKeyGenerator();
statementBuilder.keyGenerator(keyGenerator)//
.keyProperty(entityMapper.getIdColumn().getProperty())//
.keyColumn(entityMapper.getIdColumn().getColumn());
}
MappedStatement statement = statementBuilder.build();
//
setResultType(configuration, statement, entity.getEntityClass());
configuration.addMappedStatement(statement);
}
}
abstract SqlCommandType sqlCommandType();
abstract String[] methodNames();
abstract String buildSQL(EntityMapper entityMapper,boolean selective);
abstract void setResultType(Configuration configuration, MappedStatement statement,Class<?> entityClass);
}
| {
"pile_set_name": "Github"
} |
/*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
*
* Copyright (c) 2015-2017 Oracle and/or its affiliates. All rights reserved.
*
* The contents of this file are subject to the terms of either the GNU
* General Public License Version 2 only ("GPL") or the Common Development
* and Distribution License("CDDL") (collectively, the "License"). You
* may not use this file except in compliance with the License. You can
* obtain a copy of the License at
* https://oss.oracle.com/licenses/CDDL+GPL-1.1
* or LICENSE.txt. See the License for the specific
* language governing permissions and limitations under the License.
*
* When distributing the software, include this License Header Notice in each
* file and include the License file at LICENSE.txt.
*
* GPL Classpath Exception:
* Oracle designates this particular file as subject to the "Classpath"
* exception as provided by Oracle in the GPL Version 2 section of the License
* file that accompanied this code.
*
* Modifications:
* If applicable, add the following below the License Header, with the fields
* enclosed by brackets [] replaced by your own identifying information:
* "Portions Copyright [year] [name of copyright owner]"
*
* Contributor(s):
* If you wish your version of this file to be governed by only the CDDL or
* only the GPL Version 2, indicate your decision by adding "[Contributor]
* elects to include this software in this distribution under the [CDDL or GPL
* Version 2] license." If you don't indicate a single choice of license, a
* recipient has the option to distribute your version of this file under
* either the CDDL, the GPL Version 2 or to extend the choice of license to
* its licensees as provided above. However, if you add GPL Version 2 code
* and therefore, elected the GPL Version 2 license, then the option applies
* only if the new code is made subject to such option by the copyright
* holder.
*/
package org.glassfish.jersey.server.internal.inject;
import javax.ws.rs.core.MultivaluedMap;
import org.glassfish.jersey.internal.inject.ExtractorException;
import org.glassfish.jersey.server.internal.LocalizationMessages;
/**
* Value extractor for {@link java.lang.Character} and {@code char} parameters.
*
* @author Pavel Bucek (pavel.bucek at oracle.com)
*/
class PrimitiveCharacterExtractor implements MultivaluedParameterExtractor<Object> {
final String parameter;
final String defaultStringValue;
final Object defaultPrimitiveTypeValue;
public PrimitiveCharacterExtractor(String parameter, String defaultStringValue, Object defaultPrimitiveTypeValue) {
this.parameter = parameter;
this.defaultStringValue = defaultStringValue;
this.defaultPrimitiveTypeValue = defaultPrimitiveTypeValue;
}
@Override
public String getName() {
return parameter;
}
@Override
public String getDefaultValueString() {
return defaultStringValue;
}
@Override
public Object extract(MultivaluedMap<String, String> parameters) {
String v = parameters.getFirst(parameter);
if (v != null && !v.trim().isEmpty()) {
if (v.length() == 1) {
return v.charAt(0);
} else {
throw new ExtractorException(LocalizationMessages.ERROR_PARAMETER_INVALID_CHAR_VALUE(v));
}
} else if (defaultStringValue != null && !defaultStringValue.trim().isEmpty()) {
if (defaultStringValue.length() == 1) {
return defaultStringValue.charAt(0);
} else {
throw new ExtractorException(LocalizationMessages.ERROR_PARAMETER_INVALID_CHAR_VALUE(defaultStringValue));
}
}
return defaultPrimitiveTypeValue;
}
}
| {
"pile_set_name": "Github"
} |
//
// Generated by class-dump 3.5 (64 bit).
//
// class-dump is Copyright (C) 1997-1998, 2000-2001, 2004-2013 by Steve Nygard.
//
#import "CDStructures.h"
@class IDEArchive, IDEDistributionItem, NSString;
@protocol IDEDistributionAppleProvidedContent
@property(readonly) NSString *mainAppStubCopySubpath;
@property(readonly) NSString *packagePath;
@property(readonly) NSString *archivePath;
@property(readonly) NSString *archiveOperationDestinationBuildSettingName;
- (BOOL)isAppleProvidedContent:(IDEDistributionItem *)arg1;
- (BOOL)shouldCopySidecarContentFromArchive:(IDEArchive *)arg1;
- (BOOL)shouldRemoveForAppStoreDistributionDistributionItem:(IDEDistributionItem *)arg1 shouldRemoveParentDirectory:(char *)arg2;
- (BOOL)shouldCodeSignDistributionItem:(IDEDistributionItem *)arg1;
@end
| {
"pile_set_name": "Github"
} |
/*
* Licensed to Elasticsearch under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.elasticsearch.client.indices;
import java.util.Arrays;
import java.util.List;
/**
* A request to check for the existence of index templates
*/
public class IndexTemplatesExistRequest extends GetIndexTemplatesRequest {
/**
* Create a request to check for the existence of index templates. At least one template index name must be provided
*
* @param names the names of templates to check for the existence of
*/
public IndexTemplatesExistRequest(String... names) {
this(Arrays.asList(names));
}
/**
* Create a request to check for the existence of index templates. At least one template index name must be provided
*
* @param names the names of templates to check for the existence of
*/
public IndexTemplatesExistRequest(List<String> names) {
super(names);
if (names().isEmpty()) {
throw new IllegalArgumentException("must provide at least one index template name");
}
}
}
| {
"pile_set_name": "Github"
} |
--TEST--
Test krsort() function : usage variations - sort octal values
--FILE--
<?php
/* Prototype : bool krsort ( array &$array [, int $sort_flags] )
* Description: Sort an array by key in reverse order, maintaining key to data correlation.
* Source code: ext/standard/array.c
*/
/*
* testing krsort() by providing array of octal values for $array argument
* with following flag values:
* 1.flag value as defualt
* 2.SORT_REGULAR - compare items normally
* 3.SORT_NUMERIC - compare items numerically
*/
echo "*** Testing krsort() : usage variations ***\n";
// an array containing unsorted octal values
$unsorted_oct_array = array (
01235 => 01, 0321 => 02, 0345 => 03, 066 => 04, 0772 => 05,
077 => 06, -066 => -01, -0345 => -02, 0 => 0
);
echo "\n-- Testing krsort() by supplying octal value array, 'flag' value is defualt --\n";
$temp_array = $unsorted_oct_array;
var_dump( krsort($temp_array) ); // expecting : bool(true)
var_dump($temp_array);
echo "\n-- Testing krsort() by supplying octal value array, 'flag' value is SORT_REGULAR --\n";
$temp_array = $unsorted_oct_array;
var_dump( krsort($temp_array, SORT_REGULAR) ); // expecting : bool(true)
var_dump($temp_array);
echo "\n-- Testing krsort() by supplying octal value array, 'flag' value is SORT_NUMERIC --\n";
$temp_array = $unsorted_oct_array;
var_dump( krsort($temp_array, SORT_NUMERIC) ); // expecting : bool(true)
var_dump($temp_array);
echo "Done\n";
?>
--EXPECTF--
*** Testing krsort() : usage variations ***
-- Testing krsort() by supplying octal value array, 'flag' value is defualt --
bool(true)
array(9) {
[669]=>
int(1)
[506]=>
int(5)
[229]=>
int(3)
[209]=>
int(2)
[63]=>
int(6)
[54]=>
int(4)
[0]=>
int(0)
[-54]=>
int(-1)
[-229]=>
int(-2)
}
-- Testing krsort() by supplying octal value array, 'flag' value is SORT_REGULAR --
bool(true)
array(9) {
[669]=>
int(1)
[506]=>
int(5)
[229]=>
int(3)
[209]=>
int(2)
[63]=>
int(6)
[54]=>
int(4)
[0]=>
int(0)
[-54]=>
int(-1)
[-229]=>
int(-2)
}
-- Testing krsort() by supplying octal value array, 'flag' value is SORT_NUMERIC --
bool(true)
array(9) {
[669]=>
int(1)
[506]=>
int(5)
[229]=>
int(3)
[209]=>
int(2)
[63]=>
int(6)
[54]=>
int(4)
[0]=>
int(0)
[-54]=>
int(-1)
[-229]=>
int(-2)
}
Done
| {
"pile_set_name": "Github"
} |
package acmpca
import (
"bytes"
"encoding/json"
"fmt"
"github.com/awslabs/goformation/v4/cloudformation/policies"
)
// CertificateAuthorityActivation AWS CloudFormation Resource (AWS::ACMPCA::CertificateAuthorityActivation)
// See: http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-acmpca-certificateauthorityactivation.html
type CertificateAuthorityActivation struct {
// Certificate AWS CloudFormation Property
// Required: true
// See: http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-acmpca-certificateauthorityactivation.html#cfn-acmpca-certificateauthorityactivation-certificate
Certificate string `json:"Certificate,omitempty"`
// CertificateAuthorityArn AWS CloudFormation Property
// Required: true
// See: http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-acmpca-certificateauthorityactivation.html#cfn-acmpca-certificateauthorityactivation-certificateauthorityarn
CertificateAuthorityArn string `json:"CertificateAuthorityArn,omitempty"`
// CertificateChain AWS CloudFormation Property
// Required: false
// See: http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-acmpca-certificateauthorityactivation.html#cfn-acmpca-certificateauthorityactivation-certificatechain
CertificateChain string `json:"CertificateChain,omitempty"`
// Status AWS CloudFormation Property
// Required: false
// See: http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-acmpca-certificateauthorityactivation.html#cfn-acmpca-certificateauthorityactivation-status
Status string `json:"Status,omitempty"`
// AWSCloudFormationDeletionPolicy represents a CloudFormation DeletionPolicy
AWSCloudFormationDeletionPolicy policies.DeletionPolicy `json:"-"`
// AWSCloudFormationUpdateReplacePolicy represents a CloudFormation UpdateReplacePolicy
AWSCloudFormationUpdateReplacePolicy policies.UpdateReplacePolicy `json:"-"`
// AWSCloudFormationDependsOn stores the logical ID of the resources to be created before this resource
AWSCloudFormationDependsOn []string `json:"-"`
// AWSCloudFormationMetadata stores structured data associated with this resource
AWSCloudFormationMetadata map[string]interface{} `json:"-"`
// AWSCloudFormationCondition stores the logical ID of the condition that must be satisfied for this resource to be created
AWSCloudFormationCondition string `json:"-"`
}
// AWSCloudFormationType returns the AWS CloudFormation resource type
func (r *CertificateAuthorityActivation) AWSCloudFormationType() string {
return "AWS::ACMPCA::CertificateAuthorityActivation"
}
// MarshalJSON is a custom JSON marshalling hook that embeds this object into
// an AWS CloudFormation JSON resource's 'Properties' field and adds a 'Type'.
func (r CertificateAuthorityActivation) MarshalJSON() ([]byte, error) {
type Properties CertificateAuthorityActivation
return json.Marshal(&struct {
Type string
Properties Properties
DependsOn []string `json:"DependsOn,omitempty"`
Metadata map[string]interface{} `json:"Metadata,omitempty"`
DeletionPolicy policies.DeletionPolicy `json:"DeletionPolicy,omitempty"`
UpdateReplacePolicy policies.UpdateReplacePolicy `json:"UpdateReplacePolicy,omitempty"`
Condition string `json:"Condition,omitempty"`
}{
Type: r.AWSCloudFormationType(),
Properties: (Properties)(r),
DependsOn: r.AWSCloudFormationDependsOn,
Metadata: r.AWSCloudFormationMetadata,
DeletionPolicy: r.AWSCloudFormationDeletionPolicy,
UpdateReplacePolicy: r.AWSCloudFormationUpdateReplacePolicy,
Condition: r.AWSCloudFormationCondition,
})
}
// UnmarshalJSON is a custom JSON unmarshalling hook that strips the outer
// AWS CloudFormation resource object, and just keeps the 'Properties' field.
func (r *CertificateAuthorityActivation) UnmarshalJSON(b []byte) error {
type Properties CertificateAuthorityActivation
res := &struct {
Type string
Properties *Properties
DependsOn []string
Metadata map[string]interface{}
DeletionPolicy string
UpdateReplacePolicy string
Condition string
}{}
dec := json.NewDecoder(bytes.NewReader(b))
dec.DisallowUnknownFields() // Force error if unknown field is found
if err := dec.Decode(&res); err != nil {
fmt.Printf("ERROR: %s\n", err)
return err
}
// If the resource has no Properties set, it could be nil
if res.Properties != nil {
*r = CertificateAuthorityActivation(*res.Properties)
}
if res.DependsOn != nil {
r.AWSCloudFormationDependsOn = res.DependsOn
}
if res.Metadata != nil {
r.AWSCloudFormationMetadata = res.Metadata
}
if res.DeletionPolicy != "" {
r.AWSCloudFormationDeletionPolicy = policies.DeletionPolicy(res.DeletionPolicy)
}
if res.UpdateReplacePolicy != "" {
r.AWSCloudFormationUpdateReplacePolicy = policies.UpdateReplacePolicy(res.UpdateReplacePolicy)
}
if res.Condition != "" {
r.AWSCloudFormationCondition = res.Condition
}
return nil
}
| {
"pile_set_name": "Github"
} |
import io.reactivex.rxjava3.core.Completable;
import io.reactivex.rxjava3.core.Notification;
import io.reactivex.rxjava3.core.Single;
import io.reactivex.rxjava3.functions.Consumer;
import io.reactivex.rxjava3.functions.Function;
import utils.BigFraction;
import utils.BigFractionUtils;
import java.math.BigInteger;
import java.util.concurrent.Callable;
import static utils.BigFractionUtils.*;
/**
* This class shows how to apply Project Reactor features
* synchronously to to reduce and display BigFractions via
* basic Mono operations, including fromCallable(), map(),
* doOnSuccess(), and then().
*/
public class SingleEx {
/**
* Test synchronous BigFraction reduction using a mono and a
* pipeline of operations that run on the calling thread.
*/
public static Completable testFractionReductionSync1() {
StringBuilder sb =
new StringBuilder(">> Calling testFractionReductionSync1()\n");
// Create a new unreduced big fraction.
BigFraction unreducedFraction = BigFraction
.valueOf(new BigInteger(sBI1),
new BigInteger(sBI2),
false);
return Single
// Use fromCallable() to begin synchronously reducing a
// big fraction in the calling thread.
.fromCallable(() -> BigFraction.reduce(unreducedFraction))
// After big fraction is reduced return a mono and use
// map() to call a function that converts the reduced
// fraction to a mixed fraction string.
.map(BigFraction::toMixedString)
// Use doOnSuccess() to print the result after it's been
// successfully converted to a mixed fraction. If an
// exception is thrown doOnSuccess() will be skipped.
.doOnSuccess(result -> {
sb.append(" mixed reduced fraction = " + result + "\n");
// Display the result.
display(sb.toString());
})
// Return a Completable to synchronize with the
// AsyncTester framework.
.ignoreElement();
}
/**
* Test synchronous BigFraction reduction using a mono and a
* pipeline of operations that run on the calling thread.
* Combines mono with Java functional programming features.
*/
public static Completable testFractionReductionSync2() {
StringBuilder sb =
new StringBuilder(">> Calling testFractionReductionSync2()\n");
// Create a new unreduced big fraction.
BigFraction unreducedFraction = BigFraction
.valueOf(new BigInteger(sBI1),
new BigInteger(sBI2),
false);
// Create a callable lambda expression that
// reduces an unreduced big fraction.
Callable<BigFraction> reduceFraction = () -> {
// Reduce the big fraction.
BigFraction reducedFraction = BigFraction
.reduce(unreducedFraction);
sb.append(" unreducedFraction "
+ unreducedFraction.toString()
+ "\n reduced improper fraction = "
+ reducedFraction.toString());
// Return the reduced big fraction.
return reducedFraction;
};
// Create a lambda function that converts a reduced improper
// big fraction to a mixed big fraction.
Function<BigFraction, String> convertToMixedString = result -> {
sb.append("\n calling BigFraction::toMixedString\n");
return result.toMixedString();
};
// Create a consumer to print the mixed big fraction result.
Consumer<String> printResult = result -> {
sb.append(" mixed reduced fraction = " + result + "\n");
// Display the result.
BigFractionUtils.display(sb.toString());
};
return Single
// Use fromCallable() to begin synchronously reducing a
// big fraction in the calling thread.
.fromCallable(reduceFraction)
// After big fraction is reduced return a mono and use
// map() to call a function that converts the reduced
// fraction to a mixed fraction string.
.map(convertToMixedString)
// Use doOnSuccess() to print the result after it's been
// successfully converted to a mixed fraction. If
// something goes wrong doOnSuccess() will be skipped.
.doOnSuccess(printResult)
// Return a Completable to synchronize with the
// AsyncTester framework.
.ignoreElement();
}
}
| {
"pile_set_name": "Github"
} |
/**
@file Ray.h
Ray class
@maintainer Morgan McGuire, http://graphics.cs.williams.edu
@created 2002-07-12
@edited 2009-06-29
*/
#ifndef G3D_Ray_h
#define G3D_Ray_h
#include "G3D/platform.h"
#include "G3D/Vector3.h"
#include "G3D/Triangle.h"
namespace G3D {
/**
A 3D Ray.
*/
class Ray {
private:
friend class Intersect;
Vector3 m_origin;
/** Unit length */
Vector3 m_direction;
/** 1.0 / direction */
Vector3 m_invDirection;
// The following are for the "ray slope" optimization from
// "Fast Ray / Axis-Aligned Bounding Box Overlap Tests using Ray Slopes"
// by Martin Eisemann, Thorsten Grosch, Stefan Müller and Marcus Magnor
// Computer Graphics Lab, TU Braunschweig, Germany and
// University of Koblenz-Landau, Germany*/
enum Classification {MMM, MMP, MPM, MPP, PMM, PMP, PPM, PPP, POO, MOO, OPO, OMO, OOP, OOM, OMM, OMP, OPM, OPP, MOM, MOP, POM, POP, MMO, MPO, PMO, PPO}; Classification classification;
// ray slope
float ibyj, jbyi, kbyj, jbyk, ibyk, kbyi;
// Precomputed components
float c_xy, c_xz, c_yx, c_yz, c_zx, c_zy;
public:
void set(const Vector3& origin, const Vector3& direction);
inline const Vector3& origin() const {
return m_origin;
}
/** Unit direction vector. */
inline const Vector3& direction() const {
return m_direction;
}
/** Component-wise inverse of direction vector. May have inf() components */
inline const Vector3& invDirection() const {
return m_invDirection;
}
inline Ray() {
set(Vector3::zero(), Vector3::unitX());
}
inline Ray(const Vector3& origin, const Vector3& direction) {
set(origin, direction);
}
Ray(class BinaryInput& b);
void serialize(class BinaryOutput& b) const;
void deserialize(class BinaryInput& b);
/**
Creates a Ray from a origin and a (nonzero) unit direction.
*/
static Ray fromOriginAndDirection(const Vector3& point, const Vector3& direction) {
return Ray(point, direction);
}
/** Advances the origin along the direction by @a distance */
inline Ray bump(float distance) const {
return Ray(m_origin + m_direction * distance, m_direction);
}
/** Advances the origin along the @a bumpDirection by @a distance and returns the new ray*/
inline Ray bump(float distance, const Vector3& bumpDirection) const {
return Ray(m_origin + bumpDirection * distance, m_direction);
}
/**
Returns the closest point on the Ray to point.
*/
Vector3 closestPoint(const Vector3& point) const {
float t = m_direction.dot(point - m_origin);
if (t < 0) {
return m_origin;
} else {
return m_origin + m_direction * t;
}
}
/**
Returns the closest distance between point and the Ray
*/
float distance(const Vector3& point) const {
return (closestPoint(point) - point).magnitude();
}
/**
Returns the point where the Ray and plane intersect. If there
is no intersection, returns a point at infinity.
Planes are considered one-sided, so the ray will not intersect
a plane where the normal faces in the traveling direction.
*/
Vector3 intersection(const class Plane& plane) const;
/**
Returns the distance until intersection with the sphere or the (solid) ball bounded by the sphere.
Will be 0 if inside the sphere, inf if there is no intersection.
The ray direction is <B>not</B> normalized. If the ray direction
has unit length, the distance from the origin to intersection
is equal to the time. If the direction does not have unit length,
the distance = time * direction.length().
See also the G3D::CollisionDetection "movingPoint" methods,
which give more information about the intersection.
\param solid If true, rays inside the sphere immediately intersect (good for collision detection). If false, they hit the opposite side of the sphere (good for ray tracing).
*/
float intersectionTime(const class Sphere& sphere, bool solid = false) const;
float intersectionTime(const class Plane& plane) const;
float intersectionTime(const class Box& box) const;
float intersectionTime(const class AABox& box) const;
/**
The three extra arguments are the weights of vertices 0, 1, and 2
at the intersection point; they are useful for texture mapping
and interpolated normals.
*/
float intersectionTime(
const Vector3& v0, const Vector3& v1, const Vector3& v2,
const Vector3& edge01, const Vector3& edge02,
double& w0, double& w1, double& w2) const;
/**
Ray-triangle intersection for a 1-sided triangle. Fastest version.
@cite http://www.acm.org/jgt/papers/MollerTrumbore97/
http://www.graphics.cornell.edu/pubs/1997/MT97.html
*/
inline float intersectionTime(
const Vector3& vert0,
const Vector3& vert1,
const Vector3& vert2,
const Vector3& edge01,
const Vector3& edge02) const;
inline float intersectionTime(
const Vector3& vert0,
const Vector3& vert1,
const Vector3& vert2) const {
return intersectionTime(vert0, vert1, vert2, vert1 - vert0, vert2 - vert0);
}
inline float intersectionTime(
const Vector3& vert0,
const Vector3& vert1,
const Vector3& vert2,
double& w0,
double& w1,
double& w2) const {
return intersectionTime(vert0, vert1, vert2, vert1 - vert0, vert2 - vert0, w0, w1, w2);
}
/* One-sided triangle
*/
inline float intersectionTime(const Triangle& triangle) const {
return intersectionTime(
triangle.vertex(0), triangle.vertex(1), triangle.vertex(2),
triangle.edge01(), triangle.edge02());
}
inline float intersectionTime(
const Triangle& triangle,
double& w0,
double& w1,
double& w2) const {
return intersectionTime(triangle.vertex(0), triangle.vertex(1), triangle.vertex(2),
triangle.edge01(), triangle.edge02(), w0, w1, w2);
}
/** Refracts about the normal
using G3D::Vector3::refractionDirection
and bumps the ray slightly from the newOrigin. */
Ray refract(
const Vector3& newOrigin,
const Vector3& normal,
float iInside,
float iOutside) const;
/** Reflects about the normal
using G3D::Vector3::reflectionDirection
and bumps the ray slightly from
the newOrigin. */
Ray reflect(
const Vector3& newOrigin,
const Vector3& normal) const;
};
#define EPSILON 0.000001
#define CROSS(dest,v1,v2) \
dest[0]=v1[1]*v2[2]-v1[2]*v2[1]; \
dest[1]=v1[2]*v2[0]-v1[0]*v2[2]; \
dest[2]=v1[0]*v2[1]-v1[1]*v2[0];
#define DOT(v1,v2) (v1[0]*v2[0]+v1[1]*v2[1]+v1[2]*v2[2])
#define SUB(dest,v1,v2) \
dest[0]=v1[0]-v2[0]; \
dest[1]=v1[1]-v2[1]; \
dest[2]=v1[2]-v2[2];
inline float Ray::intersectionTime(
const Vector3& vert0,
const Vector3& vert1,
const Vector3& vert2,
const Vector3& edge1,
const Vector3& edge2) const {
(void)vert1;
(void)vert2;
// Barycenteric coords
float u, v;
float tvec[3], pvec[3], qvec[3];
// begin calculating determinant - also used to calculate U parameter
CROSS(pvec, m_direction, edge2);
// if determinant is near zero, ray lies in plane of triangle
const float det = DOT(edge1, pvec);
if (det < EPSILON) {
return finf();
}
// calculate distance from vert0 to ray origin
SUB(tvec, m_origin, vert0);
// calculate U parameter and test bounds
u = DOT(tvec, pvec);
if ((u < 0.0f) || (u > det)) {
// Hit the plane outside the triangle
return finf();
}
// prepare to test V parameter
CROSS(qvec, tvec, edge1);
// calculate V parameter and test bounds
v = DOT(m_direction, qvec);
if ((v < 0.0f) || (u + v > det)) {
// Hit the plane outside the triangle
return finf();
}
// Case where we don't need correct (u, v):
const float t = DOT(edge2, qvec);
if (t >= 0.0f) {
// Note that det must be positive
return t / det;
} else {
// We had to travel backwards in time to intersect
return finf();
}
}
inline float Ray::intersectionTime(
const Vector3& vert0,
const Vector3& vert1,
const Vector3& vert2,
const Vector3& edge1,
const Vector3& edge2,
double& w0,
double& w1,
double& w2) const {
(void)vert1;
(void)vert2;
// Barycenteric coords
float u, v;
float tvec[3], pvec[3], qvec[3];
// begin calculating determinant - also used to calculate U parameter
CROSS(pvec, m_direction, edge2);
// if determinant is near zero, ray lies in plane of triangle
const float det = DOT(edge1, pvec);
if (det < EPSILON) {
return finf();
}
// calculate distance from vert0 to ray origin
SUB(tvec, m_origin, vert0);
// calculate U parameter and test bounds
u = DOT(tvec, pvec);
if ((u < 0.0f) || (u > det)) {
// Hit the plane outside the triangle
return finf();
}
// prepare to test V parameter
CROSS(qvec, tvec, edge1);
// calculate V parameter and test bounds
v = DOT(m_direction, qvec);
if ((v < 0.0f) || (u + v > det)) {
// Hit the plane outside the triangle
return finf();
}
float t = DOT(edge2, qvec);
if (t >= 0) {
const float inv_det = 1.0f / det;
t *= inv_det;
u *= inv_det;
v *= inv_det;
w0 = (1.0f - u - v);
w1 = u;
w2 = v;
return t;
} else {
// We had to travel backwards in time to intersect
return finf();
}
}
#undef EPSILON
#undef CROSS
#undef DOT
#undef SUB
}// namespace
#endif
| {
"pile_set_name": "Github"
} |
/*
* Copyright © 2019 Lisk Foundation
*
* See the LICENSE file at the top-level directory of this distribution
* for licensing information.
*
* Unless otherwise agreed in a custom licensing agreement with the Lisk Foundation,
* no part of this software, including this file, may be copied, modified,
* propagated, or distributed except according to the terms contained in the
* LICENSE file.
*
* Removal or modification of this copyright notice is prohibited.
*
*/
import { EventEmitter } from 'events';
import * as socketClusterClient from 'socketcluster-client';
import { SCServerSocket } from 'socketcluster-server';
import { codec } from '@liskhq/lisk-codec';
import {
DEFAULT_PRODUCTIVITY,
DEFAULT_PRODUCTIVITY_RESET_INTERVAL,
FORBIDDEN_CONNECTION,
FORBIDDEN_CONNECTION_REASON,
INTENTIONAL_DISCONNECT_CODE,
INVALID_PEER_INFO_PENALTY,
INVALID_PEER_LIST_PENALTY,
} from '../constants';
import {
InvalidPeerInfoError,
InvalidPeerInfoListError,
RPCResponseError,
InvalidNodeInfoError,
} from '../errors';
import {
EVENT_BAN_PEER,
EVENT_DISCOVERED_PEER,
EVENT_FAILED_PEER_INFO_UPDATE,
EVENT_FAILED_TO_FETCH_PEERS,
EVENT_FAILED_TO_FETCH_PEER_INFO,
EVENT_INVALID_MESSAGE_RECEIVED,
EVENT_INVALID_REQUEST_RECEIVED,
EVENT_MESSAGE_RECEIVED,
EVENT_REQUEST_RECEIVED,
EVENT_UPDATED_PEER_INFO,
PROTOCOL_EVENTS_TO_RATE_LIMIT,
REMOTE_EVENT_POST_NODE_INFO,
REMOTE_EVENT_RPC_GET_NODE_INFO,
REMOTE_EVENT_RPC_GET_PEERS_LIST,
REMOTE_SC_EVENT_MESSAGE,
REMOTE_SC_EVENT_RPC_REQUEST,
} from '../events';
import { P2PRequest } from '../p2p_request';
import {
P2PInternalState,
P2PMessagePacket,
P2PNodeInfo,
P2PPeerInfo,
P2PRequestPacket,
P2PResponsePacket,
RPCSchemas,
P2PSharedState,
} from '../types';
import {
assignInternalInfo,
sanitizeIncomingPeerInfo,
validatePeerCompatibility,
validatePeerInfo,
validatePeerInfoList,
validateProtocolMessage,
validateRPCRequest,
validateNodeInfo,
} from '../utils';
import { decodePeerInfo, decodeNodeInfo } from '../utils/codec';
// eslint-disable-next-line @typescript-eslint/no-unsafe-assignment
export const socketErrorStatusCodes: { [key: number]: string | undefined } = {
// eslint-disable-next-line @typescript-eslint/no-unsafe-member-access,@typescript-eslint/no-explicit-any
...(socketClusterClient.SCClientSocket as any).errorStatuses,
1000: 'Intentionally disconnected',
};
// Can be used to convert a rate which is based on the rateCalculationInterval into a per-second rate.
const RATE_NORMALIZATION_FACTOR = 1000;
// Peer status message rate to be checked in every 10 seconds and reset
const PEER_STATUS_MESSAGE_RATE_INTERVAL = 10000;
export type SCClientSocket = socketClusterClient.SCClientSocket;
export type SCServerSocketUpdated = {
// eslint-disable-next-line @typescript-eslint/method-signature-style
destroy(code?: number, data?: string | object): void;
// eslint-disable-next-line @typescript-eslint/method-signature-style
on(event: string | unknown, listener: (packet?: unknown) => void): void;
// eslint-disable-next-line @typescript-eslint/method-signature-style,@typescript-eslint/no-explicit-any
on(event: string, listener: (packet: any, respond: any) => void): void;
} & SCServerSocket;
export enum ConnectionState {
CONNECTING = 'connecting',
OPEN = 'open',
CLOSED = 'closed',
}
export interface ConnectedPeerInfo extends P2PPeerInfo {
internalState: P2PInternalState;
}
export interface PeerConfig {
readonly hostPort: number;
readonly connectTimeout?: number;
readonly ackTimeout?: number;
readonly rateCalculationInterval: number;
readonly wsMaxMessageRate: number;
readonly wsMaxMessageRatePenalty: number;
readonly wsMaxPayload?: number;
readonly maxPeerInfoSize: number;
readonly maxPeerDiscoveryResponseLength: number;
readonly secret: number;
readonly serverNodeInfo?: P2PNodeInfo;
readonly rpcSchemas: RPCSchemas;
readonly peerStatusMessageRate: number;
}
interface GetPeersResponseData {
readonly data: {
readonly success: boolean;
readonly peers: ReadonlyArray<string>;
};
}
export class Peer extends EventEmitter {
protected readonly _handleRawRPC: (
packet: unknown,
respond: (responseError?: Error, responseData?: unknown) => void,
) => void;
protected readonly _handleWSMessage: (message: string) => void;
protected readonly _handleRawMessage: (packet: unknown) => void;
protected _socket: SCServerSocketUpdated | SCClientSocket | undefined;
protected _peerInfo: ConnectedPeerInfo;
protected readonly _peerConfig: PeerConfig;
protected _serverNodeInfo: P2PNodeInfo | undefined;
protected _rateInterval: number;
private readonly _rpcSchemas: RPCSchemas;
private readonly _discoveryMessageCounter: {
getPeers: number;
getNodeInfo: number;
postNodeInfo: number;
};
private readonly _peerStatusMessageRate: number;
private readonly _peerStatusRateInterval: NodeJS.Timer;
private readonly _counterResetInterval: NodeJS.Timer;
private readonly _productivityResetInterval: NodeJS.Timer;
public constructor(peerInfo: P2PPeerInfo, peerConfig: PeerConfig) {
super();
this._peerConfig = peerConfig;
this._rpcSchemas = peerConfig.rpcSchemas;
codec.addSchema(this._rpcSchemas.peerInfo);
codec.addSchema(this._rpcSchemas.nodeInfo);
this._peerInfo = this._initializeInternalState(peerInfo) as ConnectedPeerInfo;
this._rateInterval = this._peerConfig.rateCalculationInterval;
this._counterResetInterval = setInterval(() => {
this._resetCounters();
}, this._rateInterval);
this._productivityResetInterval = setInterval(() => {
this._resetProductivity();
}, DEFAULT_PRODUCTIVITY_RESET_INTERVAL);
this._serverNodeInfo = peerConfig.serverNodeInfo;
this._discoveryMessageCounter = {
getPeers: 0,
getNodeInfo: 0,
postNodeInfo: 0,
};
this._peerStatusMessageRate = peerConfig.peerStatusMessageRate;
this._peerStatusRateInterval = setInterval(() => {
this._resetStatusMessageRate();
}, PEER_STATUS_MESSAGE_RATE_INTERVAL);
// This needs to be an arrow function so that it can be used as a listener.
this._handleRawRPC = (
packet: unknown,
respond: (responseError?: Error, responseData?: unknown) => void,
): void => {
let rawRequest;
try {
rawRequest = validateRPCRequest(packet);
} catch (error) {
respond(error);
this.emit(EVENT_INVALID_REQUEST_RECEIVED, {
packet,
peerId: this._peerInfo.peerId,
});
return;
}
// Apply penalty when you receive getNodeInfo RPC more than once
if (rawRequest.procedure === REMOTE_EVENT_RPC_GET_NODE_INFO) {
this._discoveryMessageCounter.getNodeInfo += 1;
if (this._discoveryMessageCounter.getNodeInfo > 1) {
this.applyPenalty(10);
}
}
// Apply penalty when you receive getPeers RPC more than once
if (rawRequest.procedure === REMOTE_EVENT_RPC_GET_PEERS_LIST) {
this._discoveryMessageCounter.getPeers += 1;
if (this._discoveryMessageCounter.getPeers > 1) {
this.applyPenalty(10);
}
}
if (
PROTOCOL_EVENTS_TO_RATE_LIMIT.has(rawRequest.procedure) &&
this._peerInfo.internalState.rpcCounter.has(rawRequest.procedure)
) {
this._updateRPCCounter(rawRequest);
return;
}
// Protocol RCP request limiter LIP-0004
this._updateRPCCounter(rawRequest);
const rate = this._getRPCRate(rawRequest);
// Each P2PRequest contributes to the Peer's productivity.
// A P2PRequest can mutate this._productivity from the current Peer instance.
const request = new P2PRequest(
{
procedure: rawRequest.procedure,
data: rawRequest.data,
id: this.peerInfo.peerId,
rate,
productivity: this.internalState.productivity,
},
respond,
);
this.emit(EVENT_REQUEST_RECEIVED, request);
};
this._handleWSMessage = (): void => {
this._peerInfo.internalState.wsMessageCount += 1;
};
// This needs to be an arrow function so that it can be used as a listener.
this._handleRawMessage = (packet: unknown): void => {
let message;
try {
message = validateProtocolMessage(packet);
} catch (error) {
this.emit(EVENT_INVALID_MESSAGE_RECEIVED, {
packet,
peerId: this._peerInfo.peerId,
});
return;
}
this._updateMessageCounter(message);
const rate = this._getMessageRate(message);
const messageWithRateInfo = {
...message,
peerId: this._peerInfo.peerId,
rate,
};
if (message.event === REMOTE_EVENT_POST_NODE_INFO) {
this._discoveryMessageCounter.postNodeInfo += 1;
if (this._discoveryMessageCounter.postNodeInfo > this._peerStatusMessageRate) {
this.applyPenalty(10);
}
this._handleUpdateNodeInfo(message);
}
this.emit(EVENT_MESSAGE_RECEIVED, messageWithRateInfo);
};
}
public get id(): string {
return this._peerInfo.peerId;
}
public get ipAddress(): string {
return this._peerInfo.ipAddress;
}
public get port(): number {
return this._peerInfo.port;
}
public get internalState(): P2PInternalState {
return this.peerInfo.internalState;
}
public get state(): ConnectionState {
// eslint-disable-next-line no-nested-ternary
const state = this._socket
? this._socket.state === this._socket.OPEN
? ConnectionState.OPEN
: ConnectionState.CLOSED
: ConnectionState.CLOSED;
return state;
}
public updateInternalState(internalState: P2PInternalState): void {
this._peerInfo = {
...this._peerInfo,
internalState,
};
}
public get peerInfo(): ConnectedPeerInfo {
return this._peerInfo;
}
public updatePeerInfo(newPeerInfo: P2PPeerInfo): void {
// The ipAddress and port properties cannot be updated after the initial discovery.
this._peerInfo = {
sharedState: newPeerInfo.sharedState,
internalState: this._peerInfo.internalState,
ipAddress: this.ipAddress,
port: this.port,
peerId: this.id,
};
}
public connect(): void {
if (!this._socket) {
throw new Error('Peer socket does not exist');
}
}
public disconnect(code: number = INTENTIONAL_DISCONNECT_CODE, reason?: string): void {
clearInterval(this._counterResetInterval);
clearInterval(this._productivityResetInterval);
clearInterval(this._peerStatusRateInterval);
if (this._socket) {
this._socket.destroy(code, reason);
}
}
public send(packet: P2PMessagePacket): void {
if (!this._socket) {
throw new Error('Peer socket does not exist');
}
this._socket.emit(REMOTE_SC_EVENT_MESSAGE, {
event: packet.event,
data: packet.data,
});
}
public async request(packet: P2PRequestPacket): Promise<P2PResponsePacket> {
return new Promise<P2PResponsePacket>(
(resolve: (result: P2PResponsePacket) => void, reject: (result: Error) => void): void => {
if (!this._socket) {
throw new Error('Peer socket does not exist');
}
this._socket.emit(
REMOTE_SC_EVENT_RPC_REQUEST,
{
procedure: packet.procedure,
data: packet.data,
},
(error: Error | undefined, responseData: unknown) => {
if (error) {
reject(error);
return;
}
if (responseData) {
resolve(responseData as P2PResponsePacket);
return;
}
reject(
new RPCResponseError(
`Failed to handle response for procedure ${packet.procedure}`,
`${this.ipAddress}:${this.port}`,
),
);
},
);
},
);
}
public async fetchPeers(): Promise<ReadonlyArray<P2PPeerInfo>> {
try {
const response = (await this.request({
procedure: REMOTE_EVENT_RPC_GET_PEERS_LIST,
})) as GetPeersResponseData;
const { peers, success } = response.data;
const decodedPeers = peers.map((peer: string) =>
decodePeerInfo(this._rpcSchemas.peerInfo, peer),
);
const validatedPeers = validatePeerInfoList(
{ peers: decodedPeers, success },
this._peerConfig.maxPeerDiscoveryResponseLength,
this._peerConfig.maxPeerInfoSize,
);
return validatedPeers.map(peerInfo => ({
...peerInfo,
sourceAddress: this.ipAddress,
}));
} catch (error) {
if (error instanceof InvalidPeerInfoError || error instanceof InvalidPeerInfoListError) {
this.applyPenalty(INVALID_PEER_LIST_PENALTY);
}
this.emit(EVENT_FAILED_TO_FETCH_PEERS, error);
throw new RPCResponseError('Failed to fetch peer list of peer', this.ipAddress);
}
}
public async discoverPeers(): Promise<ReadonlyArray<P2PPeerInfo>> {
const discoveredPeerInfoList = await this.fetchPeers();
discoveredPeerInfoList.forEach(peerInfo => {
this.emit(EVENT_DISCOVERED_PEER, peerInfo);
});
return discoveredPeerInfoList;
}
public async fetchAndUpdateStatus(): Promise<P2PPeerInfo> {
let response: P2PResponsePacket;
try {
response = await this.request({
procedure: REMOTE_EVENT_RPC_GET_NODE_INFO,
});
} catch (error) {
this.emit(EVENT_FAILED_TO_FETCH_PEER_INFO, error);
throw new RPCResponseError(
'Failed to fetch peer info of peer',
`${this.ipAddress}:${this.port}`,
);
}
try {
const receivedNodeInfo = decodeNodeInfo(this._rpcSchemas.nodeInfo, response.data);
this._updateFromProtocolPeerInfo(receivedNodeInfo);
} catch (error) {
this.emit(EVENT_FAILED_PEER_INFO_UPDATE, error);
// Apply penalty for malformed PeerInfo
if (error instanceof InvalidNodeInfoError) {
this.applyPenalty(INVALID_PEER_INFO_PENALTY);
}
throw new RPCResponseError(
'Failed to update peer info of peer due to validation of peer compatibility',
`${this.ipAddress}:${this.port}`,
);
}
this.emit(EVENT_UPDATED_PEER_INFO, this._peerInfo);
// Return the updated detailed peer info.
return this._peerInfo;
}
public applyPenalty(penalty: number): void {
this.peerInfo.internalState.reputation -= penalty;
if (this.internalState.reputation <= 0) {
this._banPeer();
}
}
private _resetCounters(): void {
this._peerInfo.internalState.wsMessageRate =
(this.peerInfo.internalState.wsMessageCount * RATE_NORMALIZATION_FACTOR) / this._rateInterval;
this._peerInfo.internalState.wsMessageCount = 0;
if (this.peerInfo.internalState.wsMessageRate > this._peerConfig.wsMaxMessageRate) {
// Allow to increase penalty based on message rate limit exceeded
const messageRateExceedCoefficient = Math.floor(
this.peerInfo.internalState.wsMessageRate / this._peerConfig.wsMaxMessageRate,
);
const penaltyRateMultiplier =
messageRateExceedCoefficient > 1 ? messageRateExceedCoefficient : 1;
this.applyPenalty(this._peerConfig.wsMaxMessageRatePenalty * penaltyRateMultiplier);
}
this._peerInfo.internalState.rpcRates = new Map(
[...this.internalState.rpcCounter.entries()].map(([key, value]) => {
const rate = value / this._rateInterval;
// Protocol RCP request limiter LIP-0004
if (PROTOCOL_EVENTS_TO_RATE_LIMIT.has(key) && value > 1) {
this.applyPenalty(this._peerConfig.wsMaxMessageRatePenalty);
}
// eslint-disable-next-line @typescript-eslint/no-unsafe-return,@typescript-eslint/no-explicit-any
return [key, rate] as any;
}),
);
this._peerInfo.internalState.rpcCounter = new Map<string, number>();
this._peerInfo.internalState.messageRates = new Map(
[...this.internalState.messageCounter.entries()].map(([key, value]) => {
const rate = value / this._rateInterval;
// eslint-disable-next-line @typescript-eslint/no-unsafe-return,@typescript-eslint/no-explicit-any
return [key, rate] as any;
}),
);
this._peerInfo.internalState.messageCounter = new Map<string, number>();
}
private _resetProductivity(): void {
// If peer has not recently responded, reset productivity to 0
if (
this.peerInfo.internalState.productivity.lastResponded <
Date.now() - DEFAULT_PRODUCTIVITY_RESET_INTERVAL
) {
this._peerInfo.internalState.productivity = { ...DEFAULT_PRODUCTIVITY };
}
}
private _resetStatusMessageRate(): void {
// Reset only postNodeInfo counter to zero after every 10 seconds
this._discoveryMessageCounter.postNodeInfo = 0;
// Reset getPeers RPC request count to zero
this._discoveryMessageCounter.getPeers = 0;
}
private _updateFromProtocolPeerInfo(rawPeerInfo: unknown): void {
if (!this._serverNodeInfo) {
throw new Error('Missing server node info.');
}
// Sanitize and validate PeerInfo
const peerInfo = validatePeerInfo(
sanitizeIncomingPeerInfo({
...(rawPeerInfo as object),
ipAddress: this.ipAddress,
port: this.port,
}),
this._peerConfig.maxPeerInfoSize,
);
const result = validatePeerCompatibility(peerInfo, this._serverNodeInfo);
if (!result.success && result.error) {
throw new Error(`${result.error} : ${peerInfo.ipAddress}:${peerInfo.port}`);
}
this.updatePeerInfo(peerInfo);
}
private _handleUpdateNodeInfo(message: P2PMessagePacket): void {
// Update peerInfo with the latest values from the remote peer.
try {
const nodeInfoBuffer = Buffer.from(message.data as string, 'hex');
// Check incoming nodeInfo size before deocoding
validateNodeInfo(nodeInfoBuffer, this._peerConfig.maxPeerInfoSize);
const decodedNodeInfo = decodeNodeInfo(this._rpcSchemas.nodeInfo, message.data);
// Only update options object
const { options } = decodedNodeInfo;
// Only update options property
this._peerInfo = {
...this._peerInfo,
sharedState: {
...this._peerInfo.sharedState,
options: { ...this._peerInfo.sharedState?.options, ...options },
} as P2PSharedState,
};
} catch (error) {
// Apply penalty for malformed nodeInfo update
if (error instanceof InvalidNodeInfoError) {
this.applyPenalty(INVALID_PEER_INFO_PENALTY);
}
this.emit(EVENT_FAILED_PEER_INFO_UPDATE, error);
return;
}
this.emit(EVENT_UPDATED_PEER_INFO, this.peerInfo);
}
private _banPeer(): void {
this.emit(EVENT_BAN_PEER, this.id);
this.disconnect(FORBIDDEN_CONNECTION, FORBIDDEN_CONNECTION_REASON);
}
private _updateRPCCounter(packet: P2PRequestPacket): void {
const key = packet.procedure;
const count = (this.internalState.rpcCounter.get(key) ?? 0) + 1;
this.peerInfo.internalState.rpcCounter.set(key, count);
}
private _getRPCRate(packet: P2PRequestPacket): number {
const rate = this.peerInfo.internalState.rpcRates.get(packet.procedure) ?? 0;
return rate * RATE_NORMALIZATION_FACTOR;
}
private _updateMessageCounter(packet: P2PMessagePacket): void {
const key = packet.event;
const count = (this.internalState.messageCounter.get(key) ?? 0) + 1;
this.peerInfo.internalState.messageCounter.set(key, count);
}
private _getMessageRate(packet: P2PMessagePacket): number {
const rate = this.internalState.messageRates.get(packet.event) ?? 0;
return rate * RATE_NORMALIZATION_FACTOR;
}
private _initializeInternalState(peerInfo: P2PPeerInfo): P2PPeerInfo {
return peerInfo.internalState
? peerInfo
: {
...peerInfo,
internalState: assignInternalInfo(peerInfo, this._peerConfig.secret),
};
}
}
| {
"pile_set_name": "Github"
} |
/**
* plugin.js
*
* Released under LGPL License.
* Copyright (c) 1999-2015 Ephox Corp. All rights reserved
*
* License: http://www.tinymce.com/license
* Contributing: http://www.tinymce.com/contributing
*/
/*global tinymce:true */
(function() {
tinymce.create('tinymce.plugins.BBCodePlugin', {
init: function(ed) {
var self = this, dialect = ed.getParam('bbcode_dialect', 'punbb').toLowerCase();
ed.on('beforeSetContent', function(e) {
e.content = self['_' + dialect + '_bbcode2html'](e.content);
});
ed.on('postProcess', function(e) {
if (e.set) {
e.content = self['_' + dialect + '_bbcode2html'](e.content);
}
if (e.get) {
e.content = self['_' + dialect + '_html2bbcode'](e.content);
}
});
},
getInfo: function() {
return {
longname: 'BBCode Plugin',
author: 'Ephox Corp',
authorurl: 'http://www.tinymce.com',
infourl: 'http://www.tinymce.com/wiki.php/Plugin:bbcode'
};
},
// Private methods
// HTML -> BBCode in PunBB dialect
_punbb_html2bbcode: function(s) {
s = tinymce.trim(s);
function rep(re, str) {
s = s.replace(re, str);
}
// example: <strong> to [b]
rep(/<a.*?href=\"(.*?)\".*?>(.*?)<\/a>/gi, "[url=$1]$2[/url]");
rep(/<font.*?color=\"(.*?)\".*?class=\"codeStyle\".*?>(.*?)<\/font>/gi, "[code][color=$1]$2[/color][/code]");
rep(/<font.*?color=\"(.*?)\".*?class=\"quoteStyle\".*?>(.*?)<\/font>/gi, "[quote][color=$1]$2[/color][/quote]");
rep(/<font.*?class=\"codeStyle\".*?color=\"(.*?)\".*?>(.*?)<\/font>/gi, "[code][color=$1]$2[/color][/code]");
rep(/<font.*?class=\"quoteStyle\".*?color=\"(.*?)\".*?>(.*?)<\/font>/gi, "[quote][color=$1]$2[/color][/quote]");
rep(/<span style=\"color: ?(.*?);\">(.*?)<\/span>/gi, "[color=$1]$2[/color]");
rep(/<font.*?color=\"(.*?)\".*?>(.*?)<\/font>/gi, "[color=$1]$2[/color]");
rep(/<span style=\"font-size:(.*?);\">(.*?)<\/span>/gi, "[size=$1]$2[/size]");
rep(/<font>(.*?)<\/font>/gi, "$1");
rep(/<img.*?src=\"(.*?)\".*?\/>/gi, "[img]$1[/img]");
rep(/<span class=\"codeStyle\">(.*?)<\/span>/gi, "[code]$1[/code]");
rep(/<span class=\"quoteStyle\">(.*?)<\/span>/gi, "[quote]$1[/quote]");
rep(/<strong class=\"codeStyle\">(.*?)<\/strong>/gi, "[code][b]$1[/b][/code]");
rep(/<strong class=\"quoteStyle\">(.*?)<\/strong>/gi, "[quote][b]$1[/b][/quote]");
rep(/<em class=\"codeStyle\">(.*?)<\/em>/gi, "[code][i]$1[/i][/code]");
rep(/<em class=\"quoteStyle\">(.*?)<\/em>/gi, "[quote][i]$1[/i][/quote]");
rep(/<u class=\"codeStyle\">(.*?)<\/u>/gi, "[code][u]$1[/u][/code]");
rep(/<u class=\"quoteStyle\">(.*?)<\/u>/gi, "[quote][u]$1[/u][/quote]");
rep(/<\/(strong|b)>/gi, "[/b]");
rep(/<(strong|b)>/gi, "[b]");
rep(/<\/(em|i)>/gi, "[/i]");
rep(/<(em|i)>/gi, "[i]");
rep(/<\/u>/gi, "[/u]");
rep(/<span style=\"text-decoration: ?underline;\">(.*?)<\/span>/gi, "[u]$1[/u]");
rep(/<u>/gi, "[u]");
rep(/<blockquote[^>]*>/gi, "[quote]");
rep(/<\/blockquote>/gi, "[/quote]");
rep(/<br \/>/gi, "\n");
rep(/<br\/>/gi, "\n");
rep(/<br>/gi, "\n");
rep(/<p>/gi, "");
rep(/<\/p>/gi, "\n");
rep(/ |\u00a0/gi, " ");
rep(/"/gi, "\"");
rep(/</gi, "<");
rep(/>/gi, ">");
rep(/&/gi, "&");
return s;
},
// BBCode -> HTML from PunBB dialect
_punbb_bbcode2html: function(s) {
s = tinymce.trim(s);
function rep(re, str) {
s = s.replace(re, str);
}
// example: [b] to <strong>
rep(/\n/gi, "<br />");
rep(/\[b\]/gi, "<strong>");
rep(/\[\/b\]/gi, "</strong>");
rep(/\[i\]/gi, "<em>");
rep(/\[\/i\]/gi, "</em>");
rep(/\[u\]/gi, "<u>");
rep(/\[\/u\]/gi, "</u>");
rep(/\[url=([^\]]+)\](.*?)\[\/url\]/gi, "<a href=\"$1\">$2</a>");
rep(/\[url\](.*?)\[\/url\]/gi, "<a href=\"$1\">$1</a>");
rep(/\[img\](.*?)\[\/img\]/gi, "<img src=\"$1\" />");
rep(/\[color=(.*?)\](.*?)\[\/color\]/gi, "<font color=\"$1\">$2</font>");
rep(/\[code\](.*?)\[\/code\]/gi, "<span class=\"codeStyle\">$1</span> ");
rep(/\[quote.*?\](.*?)\[\/quote\]/gi, "<span class=\"quoteStyle\">$1</span> ");
return s;
}
});
// Register plugin
tinymce.PluginManager.add('bbcode', tinymce.plugins.BBCodePlugin);
})(); | {
"pile_set_name": "Github"
} |
/*
20021118-2.c from the execute part of the gcc torture suite.
*/
#include <testfwk.h>
#ifdef __SDCC
#pragma std_c99
#endif
/* Since these tests use function pointers with multiple parameters */
/* we can only proceed if we are compiling reentrant functions. */
#ifdef __SDCC_STACK_AUTO
/* Originally added to test SH constant pool layout. t1() failed for
non-PIC and t2() failed for PIC. */
int t1 (float *f, int i,
void (*f1) (double),
void (*f2) (float, float))
{
f1 (3.0);
f[i] = f[i + 1];
f2 (2.5f, 3.5f);
}
int t2 (float *f, int i,
void (*f1) (double),
void (*f2) (float, float),
void (*f3) (float))
{
f3 (6.0f);
f1 (3.0);
f[i] = f[i + 1];
f2 (2.5f, 3.5f);
}
void f1 (double d)
{
if (d != 3.0)
ASSERT (0);
}
void f2 (float f1, float f2)
{
if (f1 != 2.5f || f2 != 3.5f)
ASSERT (0);
}
void f3 (float f)
{
if (f != 6.0f)
ASSERT (0);
}
#endif
void
testTortureExecute (void)
{
#if __SDCC_STACK_AUTO
float f[3] = { 2.0f, 3.0f, 4.0f };
t1 (f, 0, f1, f2);
t2 (f, 1, f1, f2, f3);
if (f[0] != 3.0f && f[1] != 4.0f)
ASSERT (0);
return;
#endif
}
| {
"pile_set_name": "Github"
} |
com/itranswarp/learnjava/Application.class
com/itranswarp/learnjava/Application$1.class
| {
"pile_set_name": "Github"
} |
<?php
declare(strict_types=1);
namespace spec\Akeneo\Connectivity\Connection\Application\Settings\Command;
use Akeneo\Connectivity\Connection\Application\Settings\Command\DeleteConnectionCommand;
use PhpSpec\ObjectBehavior;
/**
* @author Pierre Jolly <pierre.jolly@akeneo.com>
* @copyright 2019 Akeneo SAS (http://www.akeneo.com)
* @license http://opensource.org/licenses/osl-3.0.php Open Software License (OSL 3.0)
*/
class DeleteConnectionCommandSpec extends ObjectBehavior
{
public function let()
{
$this->beConstructedWith('magento');
}
public function it_is_initializable()
{
$this->shouldHaveType(DeleteConnectionCommand::class);
}
public function it_returns_the_code()
{
$this->code()->shouldReturn('magento');
}
}
| {
"pile_set_name": "Github"
} |
package okhttp3.internal.http2;
import android.support.v4.widget.ExploreByTouchHelper;
import java.io.Closeable;
import java.io.IOException;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import okhttp3.internal.Util;
import okio.Buffer;
import okio.BufferedSink;
final class Http2Writer implements Closeable {
private static final Logger logger = Logger.getLogger(Http2.class.getName());
private final boolean client;
private boolean closed;
private final Buffer hpackBuffer = new Buffer();
final Writer hpackWriter = new Writer(this.hpackBuffer);
private int maxFrameSize = 16384;
private final BufferedSink sink;
public Http2Writer(BufferedSink sink, boolean client) {
this.sink = sink;
this.client = client;
}
public synchronized void connectionPreface() throws IOException {
if (this.closed) {
throw new IOException("closed");
} else if (this.client) {
if (logger.isLoggable(Level.FINE)) {
logger.fine(Util.format(">> CONNECTION %s", Http2.CONNECTION_PREFACE.hex()));
}
this.sink.write(Http2.CONNECTION_PREFACE.toByteArray());
this.sink.flush();
}
}
public synchronized void applyAndAckSettings(Settings peerSettings) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
this.maxFrameSize = peerSettings.getMaxFrameSize(this.maxFrameSize);
if (peerSettings.getHeaderTableSize() != -1) {
this.hpackWriter.setHeaderTableSizeSetting(peerSettings.getHeaderTableSize());
}
frameHeader(0, 0, (byte) 4, (byte) 1);
this.sink.flush();
}
public synchronized void pushPromise(int streamId, int promisedStreamId, List<Header> requestHeaders) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
this.hpackWriter.writeHeaders(requestHeaders);
long byteCount = this.hpackBuffer.size();
int length = (int) Math.min((long) (this.maxFrameSize - 4), byteCount);
frameHeader(streamId, length + 4, (byte) 5, byteCount == ((long) length) ? (byte) 4 : (byte) 0);
this.sink.writeInt(Integer.MAX_VALUE & promisedStreamId);
this.sink.write(this.hpackBuffer, (long) length);
if (byteCount > ((long) length)) {
writeContinuationFrames(streamId, byteCount - ((long) length));
}
}
public synchronized void flush() throws IOException {
if (this.closed) {
throw new IOException("closed");
}
this.sink.flush();
}
public synchronized void synStream(boolean outFinished, int streamId, int associatedStreamId, List<Header> headerBlock) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
headers(outFinished, streamId, headerBlock);
}
public synchronized void synReply(boolean outFinished, int streamId, List<Header> headerBlock) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
headers(outFinished, streamId, headerBlock);
}
public synchronized void headers(int streamId, List<Header> headerBlock) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
headers(false, streamId, headerBlock);
}
public synchronized void rstStream(int streamId, ErrorCode errorCode) throws IOException {
if (this.closed) {
throw new IOException("closed");
} else if (errorCode.httpCode == -1) {
throw new IllegalArgumentException();
} else {
frameHeader(streamId, 4, (byte) 3, (byte) 0);
this.sink.writeInt(errorCode.httpCode);
this.sink.flush();
}
}
public int maxDataLength() {
return this.maxFrameSize;
}
public synchronized void data(boolean outFinished, int streamId, Buffer source, int byteCount) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
byte flags = (byte) 0;
if (outFinished) {
flags = (byte) 1;
}
dataFrame(streamId, flags, source, byteCount);
}
void dataFrame(int streamId, byte flags, Buffer buffer, int byteCount) throws IOException {
frameHeader(streamId, byteCount, (byte) 0, flags);
if (byteCount > 0) {
this.sink.write(buffer, (long) byteCount);
}
}
public synchronized void settings(Settings settings) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
frameHeader(0, settings.size() * 6, (byte) 4, (byte) 0);
for (int i = 0; i < 10; i++) {
if (settings.isSet(i)) {
int id = i;
if (id == 4) {
id = 3;
} else if (id == 7) {
id = 4;
}
this.sink.writeShort(id);
this.sink.writeInt(settings.get(i));
}
}
this.sink.flush();
}
public synchronized void ping(boolean ack, int payload1, int payload2) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
frameHeader(0, 8, (byte) 6, ack ? (byte) 1 : (byte) 0);
this.sink.writeInt(payload1);
this.sink.writeInt(payload2);
this.sink.flush();
}
public synchronized void goAway(int lastGoodStreamId, ErrorCode errorCode, byte[] debugData) throws IOException {
if (this.closed) {
throw new IOException("closed");
} else if (errorCode.httpCode == -1) {
throw Http2.illegalArgument("errorCode.httpCode == -1", new Object[0]);
} else {
frameHeader(0, debugData.length + 8, (byte) 7, (byte) 0);
this.sink.writeInt(lastGoodStreamId);
this.sink.writeInt(errorCode.httpCode);
if (debugData.length > 0) {
this.sink.write(debugData);
}
this.sink.flush();
}
}
public synchronized void windowUpdate(int streamId, long windowSizeIncrement) throws IOException {
if (this.closed) {
throw new IOException("closed");
} else if (windowSizeIncrement == 0 || windowSizeIncrement > 2147483647L) {
throw Http2.illegalArgument("windowSizeIncrement == 0 || windowSizeIncrement > 0x7fffffffL: %s", Long.valueOf(windowSizeIncrement));
} else {
frameHeader(streamId, 4, (byte) 8, (byte) 0);
this.sink.writeInt((int) windowSizeIncrement);
this.sink.flush();
}
}
public void frameHeader(int streamId, int length, byte type, byte flags) throws IOException {
if (logger.isLoggable(Level.FINE)) {
logger.fine(Http2.frameLog(false, streamId, length, type, flags));
}
if (length > this.maxFrameSize) {
throw Http2.illegalArgument("FRAME_SIZE_ERROR length > %d: %d", Integer.valueOf(this.maxFrameSize), Integer.valueOf(length));
} else if ((ExploreByTouchHelper.INVALID_ID & streamId) != 0) {
throw Http2.illegalArgument("reserved bit set: %s", Integer.valueOf(streamId));
} else {
writeMedium(this.sink, length);
this.sink.writeByte(type & 255);
this.sink.writeByte(flags & 255);
this.sink.writeInt(Integer.MAX_VALUE & streamId);
}
}
public synchronized void close() throws IOException {
this.closed = true;
this.sink.close();
}
private static void writeMedium(BufferedSink sink, int i) throws IOException {
sink.writeByte((i >>> 16) & 255);
sink.writeByte((i >>> 8) & 255);
sink.writeByte(i & 255);
}
private void writeContinuationFrames(int streamId, long byteCount) throws IOException {
while (byteCount > 0) {
int length = (int) Math.min((long) this.maxFrameSize, byteCount);
byteCount -= (long) length;
frameHeader(streamId, length, (byte) 9, byteCount == 0 ? (byte) 4 : (byte) 0);
this.sink.write(this.hpackBuffer, (long) length);
}
}
void headers(boolean outFinished, int streamId, List<Header> headerBlock) throws IOException {
if (this.closed) {
throw new IOException("closed");
}
this.hpackWriter.writeHeaders(headerBlock);
long byteCount = this.hpackBuffer.size();
int length = (int) Math.min((long) this.maxFrameSize, byteCount);
byte flags = byteCount == ((long) length) ? (byte) 4 : (byte) 0;
if (outFinished) {
flags = (byte) (flags | 1);
}
frameHeader(streamId, length, (byte) 1, flags);
this.sink.write(this.hpackBuffer, (long) length);
if (byteCount > ((long) length)) {
writeContinuationFrames(streamId, byteCount - ((long) length));
}
}
}
| {
"pile_set_name": "Github"
} |
zipcmp=${pc_top_builddir}/${pcfiledir}/src/zipcmp
Name: libzip uninstalled
Description: library for handling zip archvies, uninstalled
Version: @VERSION@
Libs: ${pc_top_builddir}/${pcfiledir}/lib/libzip.la @LIBS@
Cflags: -I${pc_top_builddir}/${pcfiledir}/@srcdir@/lib
| {
"pile_set_name": "Github"
} |
#!/usr/bin/env ruby
# encoding: ascii-8bit
# Copyright 2015 Ball Aerospace & Technologies Corp.
# All Rights Reserved.
#
# This program is free software; you can modify and/or redistribute it
# under the terms of the GNU General Public License
# as published by the Free Software Foundation; version 3 with
# attribution addendums as found in the LICENSE.txt
require_relative 'tool_launch'
tool_launch do
require 'cosmos/tools/tlm_grapher/tlm_grapher'
Cosmos::TlmGrapher.run
end
| {
"pile_set_name": "Github"
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.