text
stringlengths 2
99.9k
| meta
dict |
---|---|
# frozen_string_literal: true
class AddEditableToUserFields < ActiveRecord::Migration[4.2]
def change
add_column :user_fields, :editable, :boolean, default: false, null: false
end
end
| {
"pile_set_name": "Github"
} |
package com.github.florent37.camerafragment.listeners;
import java.io.File;
/*
* Created by florentchampigny on 13/01/2017.
*/
public interface CameraFragmentStateListener {
//when the current displayed camera is the back
void onCurrentCameraBack();
//when the current displayed camera is the front
void onCurrentCameraFront();
//when the flash is at mode auto
void onFlashAuto();
//when the flash is at on
void onFlashOn();
//when the flash is off
void onFlashOff();
//if the camera is ready to take a photo
void onCameraSetupForPhoto();
//if the camera is ready to take a video
void onCameraSetupForVideo();
//when the camera state is "ready to record a video"
void onRecordStateVideoReadyForRecord();
//when the camera state is "recording a video"
void onRecordStateVideoInProgress();
//when the camera state is "ready to take a photo"
void onRecordStatePhoto();
//after the rotation of the screen / camera
void shouldRotateControls(int degrees);
void onStartVideoRecord(File outputFile);
void onStopVideoRecord();
}
| {
"pile_set_name": "Github"
} |
/****************************************************************************
Copyright (c) 2013-2014 Chukong Technologies Inc.
http://www.cocos2d-x.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
****************************************************************************/
#include "LuaBasicConversions.h"
#include "tolua_fix.h"
std::unordered_map<std::string, std::string> g_luaType;
std::unordered_map<std::string, std::string> g_typeCast;
#if COCOS2D_DEBUG >=1
void luaval_to_native_err(lua_State* L,const char* msg,tolua_Error* err)
{
if (NULL == L || NULL == err || NULL == msg || 0 == strlen(msg))
return;
if (msg[0] == '#')
{
const char* expected = err->type;
const char* provided = tolua_typename(L,err->index);
if (msg[1]=='f')
{
int narg = err->index;
if (err->array)
CCLOG("%s\n argument #%d is array of '%s'; array of '%s' expected.\n",msg+2,narg,provided,expected);
else
CCLOG("%s\n argument #%d is '%s'; '%s' expected.\n",msg+2,narg,provided,expected);
}
else if (msg[1]=='v')
{
if (err->array)
CCLOG("%s\n value is array of '%s'; array of '%s' expected.\n",msg+2,provided,expected);
else
CCLOG("%s\n value is '%s'; '%s' expected.\n",msg+2,provided,expected);
}
}
}
#endif
#ifdef __cplusplus
extern "C" {
#endif
extern int lua_isusertype (lua_State* L, int lo, const char* type);
#ifdef __cplusplus
}
#endif
bool luaval_is_usertype(lua_State* L,int lo,const char* type, int def)
{
if (def && lua_gettop(L)<abs(lo))
return true;
if (lua_isnil(L,lo) || lua_isusertype(L,lo,type))
return true;
return false;
}
bool luaval_to_ushort(lua_State* L, int lo, unsigned short* outValue)
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isnumber(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = (unsigned short)tolua_tonumber(L, lo, 0);
}
return ok;
}
bool luaval_to_int32(lua_State* L,int lo,int* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isnumber(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = (int)tolua_tonumber(L, lo, 0);
}
return ok;
}
bool luaval_to_uint32(lua_State* L, int lo, unsigned int* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isnumber(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = (unsigned int)tolua_tonumber(L, lo, 0);
}
return ok;
}
bool luaval_to_uint16(lua_State* L,int lo,uint16_t* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isnumber(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = (unsigned char)tolua_tonumber(L, lo, 0);
}
return ok;
}
bool luaval_to_boolean(lua_State* L,int lo,bool* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isboolean(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = (bool)tolua_toboolean(L, lo, 0);
}
return ok;
}
bool luaval_to_number(lua_State* L,int lo,double* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isnumber(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = tolua_tonumber(L, lo, 0);
}
return ok;
}
bool luaval_to_long_long(lua_State* L,int lo,long long* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isnumber(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = (long long)tolua_tonumber(L, lo, 0);
}
return ok;
}
bool luaval_to_std_string(lua_State* L, int lo, std::string* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_iscppstring(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = tolua_tocppstring(L,lo,NULL);
}
return ok;
}
bool luaval_to_vec2(lua_State* L,int lo,cocos2d::Vec2* outValue)
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "x");
lua_gettable(L, lo);
outValue->x = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "y");
lua_gettable(L, lo);
outValue->y = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
}
return ok;
}
bool luaval_to_vec3(lua_State* L,int lo,cocos2d::Vec3* outValue)
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "x");
lua_gettable(L, lo);
outValue->x = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "y");
lua_gettable(L, lo);
outValue->y = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "z");
lua_gettable(L, lo);
outValue->z = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
}
return ok;
}
bool luaval_to_vec4(lua_State* L,int lo,cocos2d::Vec4* outValue)
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "x");
lua_gettable(L, lo);
outValue->x = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "y");
lua_gettable(L, lo);
outValue->y = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "z");
lua_gettable(L, lo);
outValue->y = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "w");
lua_gettable(L, lo);
outValue->y = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
}
return ok;
}
bool luaval_to_blendfunc(lua_State* L, int lo, cocos2d::BlendFunc* outValue)
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "src");
lua_gettable(L, lo);
outValue->src = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "dst");
lua_gettable(L, lo);
outValue->dst = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
}
return ok;
}
bool luaval_to_physics_material(lua_State* L,int lo,PhysicsMaterial* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "density");
lua_gettable(L, lo);
outValue->density = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "restitution");
lua_gettable(L, lo);
outValue->restitution = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
lua_pushstring(L, "friction");
lua_gettable(L, lo);
outValue->friction = lua_isnil(L, -1) ? 0 : lua_tonumber(L, -1);
lua_pop(L, 1);
}
return ok;
}
bool luaval_to_ssize(lua_State* L,int lo, ssize_t* outValue)
{
return luaval_to_long(L, lo, reinterpret_cast<long*>(outValue));
}
bool luaval_to_long(lua_State* L,int lo, long* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isnumber(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = (long)tolua_tonumber(L, lo, 0);
}
return ok;
}
bool luaval_to_ulong(lua_State* L,int lo, unsigned long* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_isnumber(L,lo,0,&tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
*outValue = (unsigned long)tolua_tonumber(L, lo, 0);
}
return ok;
}
bool luaval_to_size(lua_State* L,int lo,Size* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "width"); /* L: paramStack key */
lua_gettable(L,lo);/* L: paramStack paramStack[lo][key] */
outValue->width = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);/* L: paramStack*/
lua_pushstring(L, "height");
lua_gettable(L,lo);
outValue->height = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
}
return ok;
}
bool luaval_to_rect(lua_State* L,int lo,Rect* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "x");
lua_gettable(L,lo);
outValue->origin.x = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "y");
lua_gettable(L,lo);
outValue->origin.y = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "width");
lua_gettable(L,lo);
outValue->size.width = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "height");
lua_gettable(L,lo);
outValue->size.height = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
}
return ok;
}
bool luaval_to_color4b(lua_State* L,int lo,Color4B* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if(ok)
{
lua_pushstring(L, "r");
lua_gettable(L,lo);
outValue->r = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "g");
lua_gettable(L,lo);
outValue->g = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "b");
lua_gettable(L,lo);
outValue->b = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "a");
lua_gettable(L,lo);
outValue->a = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
}
return ok;
}
bool luaval_to_color4f(lua_State* L,int lo,Color4F* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "r");
lua_gettable(L,lo);
outValue->r = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "g");
lua_gettable(L,lo);
outValue->g = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "b");
lua_gettable(L,lo);
outValue->b = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "a");
lua_gettable(L,lo);
outValue->a = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
}
return ok;
}
bool luaval_to_color3b(lua_State* L,int lo,Color3B* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "r");
lua_gettable(L,lo);
outValue->r = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "g");
lua_gettable(L,lo);
outValue->g = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "b");
lua_gettable(L,lo);
outValue->b = lua_isnil(L,-1) ? 0 : lua_tonumber(L,-1);
lua_pop(L,1);
}
return ok;
}
bool luaval_to_affinetransform(lua_State* L,int lo, AffineTransform* outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "a");
lua_gettable(L,lo);
outValue->a = lua_isnil(L,-1) ? 0 : (float)lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "b");
lua_gettable(L,lo);
outValue->b = lua_isnil(L,-1) ? 0 : (float)lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "c");
lua_gettable(L,lo);
outValue->b = lua_isnil(L,-1) ? 0 : (float)lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "d");
lua_gettable(L,lo);
outValue->b = lua_isnil(L,-1) ? 0 : (float)lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "tx");
lua_gettable(L,lo);
outValue->b = lua_isnil(L,-1) ? 0 : (float)lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "ty");
lua_gettable(L,lo);
outValue->b = lua_isnil(L,-1) ? 0 : (float)lua_tonumber(L,-1);
lua_pop(L,1);
}
return ok;
}
bool luaval_to_fontdefinition(lua_State* L, int lo, FontDefinition* outValue )
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
ok = false;
#endif
}
if (ok)
{
// defaul values
const char * defautlFontName = "Arial";
const int defaultFontSize = 32;
TextHAlignment defaultTextAlignment = TextHAlignment::LEFT;
TextVAlignment defaultTextVAlignment = TextVAlignment::TOP;
// by default shadow and stroke are off
outValue->_shadow._shadowEnabled = false;
outValue->_stroke._strokeEnabled = false;
// white text by default
outValue->_fontFillColor = Color3B::WHITE;
lua_pushstring(L, "fontName");
lua_gettable(L,lo);
outValue->_fontName = tolua_tocppstring(L,lo,defautlFontName);
lua_pop(L,1);
lua_pushstring(L, "fontSize");
lua_gettable(L,lo);
outValue->_fontSize = lua_isnil(L,-1) ? defaultFontSize : (int)lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "fontAlignmentH");
lua_gettable(L,lo);
outValue->_alignment = lua_isnil(L,-1) ? defaultTextAlignment : (TextHAlignment)(int)lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "fontAlignmentV");
lua_gettable(L,lo);
outValue->_vertAlignment = lua_isnil(L,-1) ? defaultTextVAlignment : (TextVAlignment)(int)lua_tonumber(L,-1);
lua_pop(L,1);
lua_pushstring(L, "fontFillColor");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
luaval_to_color3b(L, -1, &outValue->_fontFillColor);
}
lua_pop(L,1);
lua_pushstring(L, "fontDimensions");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
luaval_to_size(L, -1, &outValue->_dimensions);
}
lua_pop(L,1);
lua_pushstring(L, "shadowEnabled");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
luaval_to_boolean(L, -1, &outValue->_shadow._shadowEnabled);
if (outValue->_shadow._shadowEnabled)
{
// default shadow values
outValue->_shadow._shadowOffset = Size(5, 5);
outValue->_shadow._shadowBlur = 1;
outValue->_shadow._shadowOpacity = 1;
}
lua_pushstring(L, "shadowOffset");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
luaval_to_size(L, -1, &outValue->_shadow._shadowOffset);
}
lua_pop(L,1);
lua_pushstring(L, "shadowBlur");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
outValue->_shadow._shadowBlur = (float)lua_tonumber(L,-1);
}
lua_pop(L,1);
lua_pushstring(L, "shadowOpacity");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
outValue->_shadow._shadowOpacity = lua_tonumber(L,-1);
}
lua_pop(L,1);
}
lua_pop(L,1);
lua_pushstring(L, "strokeEnabled");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
luaval_to_boolean(L, -1, &outValue->_stroke._strokeEnabled);
if (outValue->_stroke._strokeEnabled)
{
// default stroke values
outValue->_stroke._strokeSize = 1;
outValue->_stroke._strokeColor = Color3B::BLUE;
lua_pushstring(L, "strokeColor");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
luaval_to_color3b(L, -1, &outValue->_stroke._strokeColor);
}
lua_pop(L,1);
lua_pushstring(L, "strokeSize");
lua_gettable(L,lo);
if (!lua_isnil(L,-1))
{
outValue->_stroke._strokeSize = (float)lua_tonumber(L,-1);
}
lua_pop(L,1);
}
}
lua_pop(L,1);
}
return ok;
}
bool luaval_to_ttfconfig(lua_State* L,int lo, cocos2d::TTFConfig* outValue)
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "fontFilePath"); /* L: paramStack key */
lua_gettable(L,lo); /* L: paramStack paramStack[lo][key] */
outValue->fontFilePath = lua_isstring(L, -1)? lua_tostring(L, -1) : "";
lua_pop(L,1); /* L: paramStack*/
lua_pushstring(L, "fontSize");
lua_gettable(L,lo);
outValue->fontSize = lua_isnumber(L, -1)?(int)lua_tointeger(L, -1) : 0;
lua_pop(L,1);
lua_pushstring(L, "glyphs");
lua_gettable(L, lo);
outValue->glyphs = lua_isnumber(L, -1)?static_cast<GlyphCollection>(lua_tointeger(L, -1)) : GlyphCollection::NEHE;
lua_pop(L, 1);
lua_pushstring(L, "customGlyphs");
lua_gettable(L, lo);
outValue->customGlyphs = lua_isstring(L, -1)?lua_tostring(L, -1) : "";
lua_pop(L, 1);
lua_pushstring(L, "distanceFieldEnabled");
lua_gettable(L, lo);
outValue->distanceFieldEnabled = lua_isboolean(L, -1)?lua_toboolean(L, -1) : false;
lua_pop(L, 1);
lua_pushstring(L, "outlineSize");
lua_gettable(L, lo);
outValue->outlineSize = lua_isnumber(L, -1)?(int)lua_tointeger(L, -1) : 0;
lua_pop(L, 1);
return true;
}
return false;
}
bool luaval_to_uniform(lua_State* L, int lo, cocos2d::Uniform* outValue)
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "location"); /* L: paramStack key */
lua_gettable(L,lo); /* L: paramStack paramStack[lo][key] */
outValue->location = lua_isnumber(L, -1)? (GLint)lua_tointeger(L, -1) : 0;
lua_pop(L,1); /* L: paramStack*/
lua_pushstring(L, "size");
lua_gettable(L,lo);
outValue->size = lua_isnumber(L, -1)?(GLint)lua_tointeger(L, -1) : 0;
lua_pop(L,1);
lua_pushstring(L, "type");
lua_gettable(L, lo);
outValue->type = lua_isnumber(L, -1)?(GLenum)lua_tointeger(L, -1) : 0;
lua_pop(L, 1);
lua_pushstring(L, "name");
lua_gettable(L, lo);
outValue->name = lua_isstring(L, -1)?lua_tostring(L, -1) : "";
lua_pop(L, 1);
return true;
}
return false;
}
bool luaval_to_vertexattrib(lua_State* L, int lo, cocos2d::VertexAttrib* outValue)
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "index"); /* L: paramStack key */
lua_gettable(L,lo); /* L: paramStack paramStack[lo][key] */
outValue->index = lua_isnumber(L, -1)? (GLint)lua_tointeger(L, -1) : 0;
lua_pop(L,1); /* L: paramStack*/
lua_pushstring(L, "size");
lua_gettable(L,lo);
outValue->size = lua_isnumber(L, -1)?(GLint)lua_tointeger(L, -1) : 0;
lua_pop(L,1);
lua_pushstring(L, "type");
lua_gettable(L, lo);
outValue->type = lua_isnumber(L, -1)?(GLenum)lua_tointeger(L, -1) : 0;
lua_pop(L, 1);
lua_pushstring(L, "name");
lua_gettable(L, lo);
outValue->name = lua_isstring(L, -1)?lua_tostring(L, -1) : "";
lua_pop(L, 1);
return true;
}
return false;
}
bool luaval_to_mat4(lua_State* L, int lo, cocos2d::Mat4* outValue )
{
if (nullptr == L || nullptr == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
ok = false;
#endif
}
if (ok)
{
size_t len = lua_objlen(L, lo);
for (int i = 0; i < len; i++)
{
lua_pushnumber(L,i + 1);
lua_gettable(L,lo);
if (tolua_isnumber(L, -1, 0, &tolua_err))
{
outValue->m[i] = tolua_tonumber(L, -1, 0);
}
else
{
outValue->m[i] = 0;
}
lua_pop(L, 1);
}
}
return ok;
}
bool luaval_to_array(lua_State* L,int lo, __Array** outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
size_t len = lua_objlen(L, lo);
if (len > 0)
{
__Array* arr = __Array::createWithCapacity(len);
if (NULL == arr)
return false;
for (int i = 0; i < len; i++)
{
lua_pushnumber(L,i + 1);
lua_gettable(L,lo);
if (lua_isnil(L,-1))
{
lua_pop(L, 1);
continue;
}
if (lua_isuserdata(L, -1))
{
Ref* obj = static_cast<Ref*>(tolua_tousertype(L, -1, NULL) );
if (NULL != obj)
{
arr->addObject(obj);
}
}
else if(lua_istable(L, -1))
{
lua_pushnumber(L,1);
lua_gettable(L,-2);
if (lua_isnil(L, -1) )
{
lua_pop(L,1);
__Dictionary* dictVal = NULL;
if (luaval_to_dictionary(L,-1,&dictVal))
{
arr->addObject(dictVal);
}
}
else
{
lua_pop(L,1);
__Array* arrVal = NULL;
if(luaval_to_array(L, -1, &arrVal))
{
arr->addObject(arrVal);
}
}
}
else if(lua_isstring(L, -1))
{
std::string stringValue = "";
if(luaval_to_std_string(L, -1, &stringValue) )
{
arr->addObject(String::create(stringValue));
}
}
else if(lua_isboolean(L, -1))
{
bool boolVal = false;
if (luaval_to_boolean(L, -1, &boolVal))
{
arr->addObject(Bool::create(boolVal));
}
}
else if(lua_isnumber(L, -1))
{
arr->addObject(Double::create(tolua_tonumber(L, -1, 0)));
}
else
{
CCASSERT(false, "not supported type");
}
lua_pop(L, 1);
}
*outValue = arr;
}
}
return ok;
}
bool luaval_to_dictionary(lua_State* L,int lo, __Dictionary** outValue)
{
if (NULL == L || NULL == outValue)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
std::string stringKey = "";
std::string stringValue = "";
bool boolVal = false;
__Dictionary* dict = NULL;
lua_pushnil(L); /* L: lotable ..... nil */
while ( 0 != lua_next(L, lo ) ) /* L: lotable ..... key value */
{
if (!lua_isstring(L, -2))
{
lua_pop(L, 1);
continue;
}
if (NULL == dict)
{
dict = Dictionary::create();
}
if(luaval_to_std_string(L, -2, &stringKey))
{
if (lua_isuserdata(L, -1))
{
Ref* obj = static_cast<Ref*>(tolua_tousertype(L, -1, NULL) );
if (NULL != obj)
{
//get the key to string
dict->setObject(obj, stringKey);
}
}
else if(lua_istable(L, -1))
{
lua_pushnumber(L,1);
lua_gettable(L,-2);
if (lua_isnil(L, -1) )
{
lua_pop(L,1);
__Dictionary* dictVal = NULL;
if (luaval_to_dictionary(L,-1,&dictVal))
{
dict->setObject(dictVal,stringKey);
}
}
else
{
lua_pop(L,1);
__Array* arrVal = NULL;
if(luaval_to_array(L, -1, &arrVal))
{
dict->setObject(arrVal,stringKey);
}
}
}
else if(lua_isstring(L, -1))
{
if(luaval_to_std_string(L, -1, &stringValue))
{
dict->setObject(String::create(stringValue), stringKey);
}
}
else if(lua_isboolean(L, -1))
{
if (luaval_to_boolean(L, -1, &boolVal))
{
dict->setObject(Bool::create(boolVal),stringKey);
}
}
else if(lua_isnumber(L, -1))
{
dict->setObject(Double::create(tolua_tonumber(L, -1, 0)),stringKey);
}
else
{
CCASSERT(false, "not supported type");
}
}
lua_pop(L, 1); /* L: lotable ..... key */
}
/* L: lotable ..... */
*outValue = dict;
}
return ok;
}
bool luaval_to_array_of_vec2(lua_State* L,int lo,cocos2d::Vec2 **points, int *numPoints)
{
if (NULL == L)
return false;
bool ok = true;
tolua_Error tolua_err;
if (!tolua_istable(L, lo, 0, &tolua_err) )
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
size_t len = lua_objlen(L, lo);
if (len > 0)
{
cocos2d::Vec2* array = (cocos2d::Vec2*) new Vec2[len];
if (NULL == array)
return false;
for (uint32_t i = 0; i < len; ++i)
{
lua_pushnumber(L,i + 1);
lua_gettable(L,lo);
if (!tolua_istable(L,-1, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
lua_pop(L, 1);
CC_SAFE_DELETE_ARRAY(array);
return false;
}
ok &= luaval_to_vec2(L, lua_gettop(L), &array[i]);
if (!ok)
{
lua_pop(L, 1);
CC_SAFE_DELETE_ARRAY(array);
return false;
}
lua_pop(L, 1);
}
*numPoints = (int)len;
*points = array;
}
}
return ok;
}
bool luavals_variadic_to_array(lua_State* L,int argc, __Array** ret)
{
if (nullptr == L || argc == 0 )
return false;
bool ok = true;
__Array* array = __Array::create();
for (int i = 0; i < argc; i++)
{
double num = 0.0;
if (lua_isnumber(L, i + 2))
{
ok &= luaval_to_number(L, i + 2, &num);
if (!ok)
break;
array->addObject(Integer::create((int)num));
}
else if (lua_isstring(L, i + 2))
{
std::string str = lua_tostring(L, i + 2);
array->addObject(String::create(str));
}
else if (lua_isuserdata(L, i + 2))
{
tolua_Error err;
if (!tolua_isusertype(L, i + 2, "cc.Ref", 0, &err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&err);
#endif
ok = false;
break;
}
Ref* obj = static_cast<Ref*>(tolua_tousertype(L, i + 2, nullptr));
array->addObject(obj);
}
}
*ret = array;
return ok;
}
bool luavals_variadic_to_ccvaluevector(lua_State* L, int argc, cocos2d::ValueVector* ret)
{
if (nullptr == L || argc == 0 )
return false;
for (int i = 0; i < argc; i++)
{
if(lua_istable(L, i + 2))
{
lua_pushnumber(L, 1);
lua_gettable(L, i + 2);
if (lua_isnil(L, -1) )
{
lua_pop(L,1);
ValueMap dictVal;
if (luaval_to_ccvaluemap(L, i + 2, &dictVal))
{
ret->push_back(Value(dictVal));
}
}
else
{
lua_pop(L,1);
ValueVector arrVal;
if(luaval_to_ccvaluevector(L, i + 2, &arrVal))
{
ret->push_back(Value(arrVal));
}
}
}
else if(lua_isstring(L, i + 2))
{
std::string stringValue = "";
if(luaval_to_std_string(L, i + 2, &stringValue) )
{
ret->push_back(Value(stringValue));
}
}
else if(lua_isboolean(L, i + 2))
{
bool boolVal = false;
if (luaval_to_boolean(L, i + 2, &boolVal))
{
ret->push_back(Value(boolVal));
}
}
else if(lua_isnumber(L, i + 2))
{
ret->push_back(Value(tolua_tonumber(L, i + 2, 0)));
}
else
{
CCASSERT(false, "not supported type");
}
}
return true;
}
bool luaval_to_ccvalue(lua_State* L, int lo, cocos2d::Value* ret)
{
if ( nullptr == L || nullptr == ret)
return false;
bool ok = true;
tolua_Error tolua_err;
if (tolua_istable(L, lo, 0, &tolua_err))
{
lua_pushnumber(L,1);
lua_gettable(L,lo);
if (lua_isnil(L, -1) ) /** if table[1] = nil,we don't think it is a pure array */
{
lua_pop(L,1);
ValueMap dictVal;
if (luaval_to_ccvaluemap(L, lo, &dictVal))
{
*ret = Value(dictVal);
}
}
else
{
lua_pop(L,1);
ValueVector arrVal;
if (luaval_to_ccvaluevector(L, lo, &arrVal))
{
*ret = Value(arrVal);
}
}
}
else if (tolua_isstring(L, lo, 0, &tolua_err))
{
std::string stringValue = "";
if (luaval_to_std_string(L, lo, &stringValue))
{
*ret = Value(stringValue);
}
}
else if (tolua_isboolean(L, lo, 0, &tolua_err))
{
bool boolVal = false;
if (luaval_to_boolean(L, lo, &boolVal))
{
*ret = Value(boolVal);
}
}
else if (tolua_isnumber(L, lo, 0, &tolua_err))
{
*ret = Value(tolua_tonumber(L, lo, 0));
}
return ok;
}
bool luaval_to_ccvaluemap(lua_State* L, int lo, cocos2d::ValueMap* ret)
{
if ( nullptr == L || nullptr == ret)
return false;
tolua_Error tolua_err;
bool ok = true;
if (!tolua_istable(L, lo, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
std::string stringKey = "";
std::string stringValue = "";
bool boolVal = false;
ValueMap& dict = *ret;
lua_pushnil(L); /* first key L: lotable ..... nil */
while ( 0 != lua_next(L, lo ) ) /* L: lotable ..... key value */
{
if (!lua_isstring(L, -2))
{
lua_pop(L, 1); /* removes 'value'; keep 'key' for next iteration*/
continue;
}
if(luaval_to_std_string(L, -2, &stringKey))
{
if(lua_istable(L, -1))
{
lua_pushnumber(L,1);
lua_gettable(L,-2);
if (lua_isnil(L, -1) ) /** if table[1] = nil,we don't think it is a pure array */
{
lua_pop(L,1);
ValueMap dictVal;
if (luaval_to_ccvaluemap(L, -1, &dictVal))
{
dict[stringKey] = Value(dictVal);
}
}
else
{
lua_pop(L,1);
ValueVector arrVal;
if (luaval_to_ccvaluevector(L, -1, &arrVal))
{
dict[stringKey] = Value(arrVal);
}
}
}
else if(lua_isstring(L, -1))
{
if(luaval_to_std_string(L, -1, &stringValue))
{
dict[stringKey] = Value(stringValue);
}
}
else if(lua_isboolean(L, -1))
{
if (luaval_to_boolean(L, -1, &boolVal))
{
dict[stringKey] = Value(boolVal);
}
}
else if(lua_isnumber(L, -1))
{
dict[stringKey] = Value(tolua_tonumber(L, -1, 0));
}
else
{
CCASSERT(false, "not supported type");
}
}
lua_pop(L, 1); /* L: lotable ..... key */
}
}
return ok;
}
bool luaval_to_ccvaluemapintkey(lua_State* L, int lo, cocos2d::ValueMapIntKey* ret)
{
if (nullptr == L || nullptr == ret)
return false;
tolua_Error tolua_err;
bool ok = true;
if (!tolua_istable(L, lo, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
std::string stringKey = "";
std::string stringValue = "";
int intKey = 0;
bool boolVal = false;
ValueMapIntKey& dict = *ret;
lua_pushnil(L); /* first key L: lotable ..... nil */
while ( 0 != lua_next(L, lo ) ) /* L: lotable ..... key value */
{
if (!lua_isstring(L, -2))
{
lua_pop(L, 1); /* removes 'value'; keep 'key' for next iteration*/
continue;
}
if(luaval_to_std_string(L, -2, &stringKey))
{
intKey = atoi(stringKey.c_str());
if(lua_istable(L, -1))
{
lua_pushnumber(L,1);
lua_gettable(L,-2);
if (lua_isnil(L, -1) ) /** if table[1] = nil,we don't think it is a pure array */
{
lua_pop(L,1);
ValueMap dictVal;
if (luaval_to_ccvaluemap(L, -1, &dictVal))
{
dict[intKey] = Value(dictVal);
}
}
else
{
lua_pop(L,1);
ValueVector arrVal;
if (luaval_to_ccvaluevector(L, -1, &arrVal))
{
dict[intKey] = Value(arrVal);
}
}
}
else if(lua_isstring(L, -1))
{
if(luaval_to_std_string(L, -1, &stringValue))
{
dict[intKey] = Value(stringValue);
}
}
else if(lua_isboolean(L, -1))
{
if (luaval_to_boolean(L, -1, &boolVal))
{
dict[intKey] = Value(boolVal);
}
}
else if(lua_isnumber(L, -1))
{
dict[intKey] = Value(tolua_tonumber(L, -1, 0));
}
else
{
CCASSERT(false, "not supported type");
}
}
lua_pop(L, 1); /* L: lotable ..... key */
}
}
return ok;
}
bool luaval_to_ccvaluevector(lua_State* L, int lo, cocos2d::ValueVector* ret)
{
if (nullptr == L || nullptr == ret)
return false;
tolua_Error tolua_err;
bool ok = true;
if (!tolua_istable(L, lo, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
size_t len = lua_objlen(L, lo);
for (int i = 0; i < len; i++)
{
lua_pushnumber(L,i + 1);
lua_gettable(L,lo);
if (lua_isnil(L,-1))
{
lua_pop(L, 1);
continue;
}
if(lua_istable(L, -1))
{
lua_pushnumber(L,1);
lua_gettable(L,-2);
if (lua_isnil(L, -1) )
{
lua_pop(L,1);
ValueMap dictVal;
if (luaval_to_ccvaluemap(L, -1, &dictVal))
{
ret->push_back(Value(dictVal));
}
}
else
{
lua_pop(L,1);
ValueVector arrVal;
if(luaval_to_ccvaluevector(L, -1, &arrVal))
{
ret->push_back(Value(arrVal));
}
}
}
else if(lua_isstring(L, -1))
{
std::string stringValue = "";
if(luaval_to_std_string(L, -1, &stringValue) )
{
ret->push_back(Value(stringValue));
}
}
else if(lua_isboolean(L, -1))
{
bool boolVal = false;
if (luaval_to_boolean(L, -1, &boolVal))
{
ret->push_back(Value(boolVal));
}
}
else if(lua_isnumber(L, -1))
{
ret->push_back(Value(tolua_tonumber(L, -1, 0)));
}
else
{
CCASSERT(false, "not supported type");
}
lua_pop(L, 1);
}
}
return ok;
}
bool luaval_to_std_vector_string(lua_State* L, int lo, std::vector<std::string>* ret)
{
if (nullptr == L || nullptr == ret || lua_gettop(L) < lo)
return false;
tolua_Error tolua_err;
bool ok = true;
if (!tolua_istable(L, lo, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
size_t len = lua_objlen(L, lo);
std::string value = "";
for (int i = 0; i < len; i++)
{
lua_pushnumber(L, i + 1);
lua_gettable(L,lo);
if(lua_isstring(L, -1))
{
ok = luaval_to_std_string(L, -1, &value);
if(ok)
ret->push_back(value);
}
else
{
CCASSERT(false, "string type is needed");
}
lua_pop(L, 1);
}
}
return ok;
}
bool luaval_to_std_vector_int(lua_State* L, int lo, std::vector<int>* ret)
{
if (nullptr == L || nullptr == ret || lua_gettop(L) < lo)
return false;
tolua_Error tolua_err;
bool ok = true;
if (!tolua_istable(L, lo, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
size_t len = lua_objlen(L, lo);
for (int i = 0; i < len; i++)
{
lua_pushnumber(L, i + 1);
lua_gettable(L,lo);
if(lua_isnumber(L, -1))
{
ret->push_back((int)tolua_tonumber(L, -1, 0));
}
else
{
CCASSERT(false, "int type is needed");
}
lua_pop(L, 1);
}
}
return ok;
}
bool luaval_to_mesh_vertex_attrib(lua_State* L, int lo, cocos2d::MeshVertexAttrib* ret)
{
if (nullptr == L || nullptr == ret || lua_gettop(L) < lo)
return false;
tolua_Error tolua_err;
bool ok = true;
if (!tolua_istable(L, lo, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
lua_pushstring(L, "size"); /* L: paramStack key */
lua_gettable(L,lo); /* L: paramStack paramStack[lo][key] */
ret->size = (GLint)lua_tonumber(L, -1);
lua_pop(L,1);
lua_pushstring(L, "type"); /* L: paramStack key */
lua_gettable(L,lo); /* L: paramStack paramStack[lo][key] */
ret->type = (GLenum)lua_tonumber(L, -1);
lua_pop(L,1);
lua_pushstring(L, "vertexAttrib"); /* L: paramStack key */
lua_gettable(L,lo); /* L: paramStack paramStack[lo][key] */
ret->type = (GLenum)lua_tonumber(L, -1);
lua_pop(L,1);
lua_pushstring(L, "vertexAttrib"); /* L: paramStack key */
lua_gettable(L,lo); /* L: paramStack paramStack[lo][key] */
ret->type = (GLenum)lua_tonumber(L, -1);
lua_pop(L,1);
}
return ok;
}
bool luaval_to_std_vector_float(lua_State* L, int lo, std::vector<float>* ret)
{
if (nullptr == L || nullptr == ret || lua_gettop(L) < lo)
return false;
tolua_Error tolua_err;
bool ok = true;
if (!tolua_istable(L, lo, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
size_t len = lua_objlen(L, lo);
for (int i = 0; i < len; i++)
{
lua_pushnumber(L, i + 1);
lua_gettable(L,lo);
if(lua_isnumber(L, -1))
{
ret->push_back((float)tolua_tonumber(L, -1, 0));
}
else
{
CCASSERT(false, "float type is needed");
}
lua_pop(L, 1);
}
}
return ok;
}
bool luaval_to_std_vector_ushort(lua_State* L, int lo, std::vector<unsigned short>* ret)
{
if (nullptr == L || nullptr == ret || lua_gettop(L) < lo)
return false;
tolua_Error tolua_err;
bool ok = true;
if (!tolua_istable(L, lo, 0, &tolua_err))
{
#if COCOS2D_DEBUG >=1
luaval_to_native_err(L,"#ferror:",&tolua_err);
#endif
ok = false;
}
if (ok)
{
size_t len = lua_objlen(L, lo);
for (int i = 0; i < len; i++)
{
lua_pushnumber(L, i + 1);
lua_gettable(L,lo);
if(lua_isnumber(L, -1))
{
ret->push_back((unsigned short)tolua_tonumber(L, -1, 0));
}
else
{
CCASSERT(false, "unsigned short type is needed");
}
lua_pop(L, 1);
}
}
return ok;
}
void vec2_array_to_luaval(lua_State* L,const cocos2d::Vec2* points, int count)
{
if (NULL == L)
return;
lua_newtable(L);
for (int i = 1; i <= count; ++i)
{
lua_pushnumber(L, i);
vec2_to_luaval(L, points[i-1]);
lua_rawset(L, -3);
}
}
void vec2_to_luaval(lua_State* L,const cocos2d::Vec2& vec2)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "x"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec2.x); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "y"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec2.y); /* L: table key value*/
lua_rawset(L, -3);
}
void vec3_to_luaval(lua_State* L,const cocos2d::Vec3& vec3)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "x"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec3.x); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "y"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec3.y); /* L: table key value*/
lua_rawset(L, -3);
lua_pushstring(L, "z"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec3.z); /* L: table key value*/
lua_rawset(L, -3);
}
void vec4_to_luaval(lua_State* L,const cocos2d::Vec4& vec3)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "x"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec3.x); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "y"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec3.y); /* L: table key value*/
lua_rawset(L, -3);
lua_pushstring(L, "z"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec3.z); /* L: table key value*/
lua_rawset(L, -3);
lua_pushstring(L, "w"); /* L: table key */
lua_pushnumber(L, (lua_Number) vec3.z); /* L: table key value*/
lua_rawset(L, -3);
}
void physics_material_to_luaval(lua_State* L,const PhysicsMaterial& pm)
{
if (nullptr == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "density"); /* L: table key */
lua_pushnumber(L, (lua_Number) pm.density); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "restitution"); /* L: table key */
lua_pushnumber(L, (lua_Number) pm.restitution); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "friction"); /* L: table key */
lua_pushnumber(L, (lua_Number) pm.friction); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void physics_raycastinfo_to_luaval(lua_State* L, const PhysicsRayCastInfo& info)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "shape"); /* L: table key */
PhysicsShape* shape = info.shape;
if (shape == nullptr)
{
lua_pushnil(L);
}else
{
int ID = (int)(shape->_ID);
int* luaID = &(shape->_luaID);
toluafix_pushusertype_ccobject(L, ID, luaID, (void*)shape,"cc.PhysicsShape");
}
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "start"); /* L: table key */
vec2_to_luaval(L, info.start);
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "ended"); /* L: table key */
vec2_to_luaval(L, info.end);
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "contact"); /* L: table key */
vec2_to_luaval(L, info.contact);
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "normal"); /* L: table key */
vec2_to_luaval(L, info.normal);
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "fraction"); /* L: table key */
lua_pushnumber(L, (lua_Number) info.fraction); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void physics_contactdata_to_luaval(lua_State* L, const PhysicsContactData* data)
{
if (nullptr == L || nullptr == data)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "points");
vec2_array_to_luaval(L, data->points, data->count);
lua_rawset(L, -3);
lua_pushstring(L, "normal");
vec2_to_luaval(L, data->normal);
lua_rawset(L, -3);
lua_pushstring(L, "POINT_MAX");
lua_pushnumber(L, data->POINT_MAX);
lua_rawset(L, -3);
}
void size_to_luaval(lua_State* L,const Size& sz)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "width"); /* L: table key */
lua_pushnumber(L, (lua_Number) sz.width); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "height"); /* L: table key */
lua_pushnumber(L, (lua_Number) sz.height); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void rect_to_luaval(lua_State* L,const Rect& rt)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "x"); /* L: table key */
lua_pushnumber(L, (lua_Number) rt.origin.x); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "y"); /* L: table key */
lua_pushnumber(L, (lua_Number) rt.origin.y); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "width"); /* L: table key */
lua_pushnumber(L, (lua_Number) rt.size.width); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "height"); /* L: table key */
lua_pushnumber(L, (lua_Number) rt.size.height); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void color4b_to_luaval(lua_State* L,const Color4B& cc)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "r"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.r); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "g"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.g); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "b"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.b); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "a"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.a); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void color4f_to_luaval(lua_State* L,const Color4F& cc)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "r"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.r); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "g"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.g); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "b"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.b); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "a"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.a); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void color3b_to_luaval(lua_State* L,const Color3B& cc)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "r"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.r); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "g"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.g); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "b"); /* L: table key */
lua_pushnumber(L, (lua_Number) cc.b); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void affinetransform_to_luaval(lua_State* L,const AffineTransform& inValue)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "a"); /* L: table key */
lua_pushnumber(L, (lua_Number) inValue.a); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "b"); /* L: table key */
lua_pushnumber(L, (lua_Number) inValue.b); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "c"); /* L: table key */
lua_pushnumber(L, (lua_Number) inValue.c); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "d"); /* L: table key */
lua_pushnumber(L, (lua_Number) inValue.d); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "tx"); /* L: table key */
lua_pushnumber(L, (lua_Number) inValue.d); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "ty"); /* L: table key */
lua_pushnumber(L, (lua_Number) inValue.d); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void fontdefinition_to_luaval(lua_State* L,const FontDefinition& inValue)
{
if (NULL == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "fontName"); /* L: table key */
tolua_pushcppstring(L, inValue._fontName); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "fontSize"); /* L: table key */
lua_pushnumber(L,(lua_Number)inValue._fontSize); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "fontAlignmentH"); /* L: table key */
lua_pushnumber(L, (lua_Number) inValue._alignment); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "fontAlignmentV"); /* L: table key */
lua_pushnumber(L, (lua_Number) inValue._vertAlignment); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "fontFillColor"); /* L: table key */
color3b_to_luaval(L, inValue._fontFillColor); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "fontDimensions"); /* L: table key */
size_to_luaval(L, inValue._dimensions); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
//Shadow
lua_pushstring(L, "shadowEnabled"); /* L: table key */
lua_pushboolean(L, inValue._shadow._shadowEnabled); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "shadowOffset"); /* L: table key */
size_to_luaval(L, inValue._shadow._shadowOffset); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "shadowBlur"); /* L: table key */
lua_pushnumber(L, (lua_Number)inValue._shadow._shadowBlur); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "shadowOpacity"); /* L: table key */
lua_pushnumber(L, (lua_Number)inValue._shadow._shadowOpacity); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
//Stroke
lua_pushstring(L, "shadowEnabled"); /* L: table key */
lua_pushboolean(L, inValue._stroke._strokeEnabled); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "strokeColor"); /* L: table key */
color3b_to_luaval(L, inValue._stroke._strokeColor); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "strokeSize"); /* L: table key */
lua_pushnumber(L, (lua_Number)inValue._stroke._strokeSize); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
}
void array_to_luaval(lua_State* L,__Array* inValue)
{
lua_newtable(L);
if (nullptr == L || nullptr == inValue)
return;
Ref* obj = nullptr;
std::string className = "";
__String* strVal = nullptr;
__Dictionary* dictVal = nullptr;
__Array* arrVal = nullptr;
__Double* doubleVal = nullptr;
__Bool* boolVal = nullptr;
__Float* floatVal = nullptr;
__Integer* intVal = nullptr;
int indexTable = 1;
CCARRAY_FOREACH(inValue, obj)
{
if (nullptr == obj)
continue;
std::string typeName = typeid(*obj).name();
auto iter = g_luaType.find(typeName);
if (g_luaType.end() != iter)
{
className = iter->second;
if (nullptr != dynamic_cast<cocos2d::Ref *>(obj))
{
lua_pushnumber(L, (lua_Number)indexTable);
int ID = (obj) ? (int)obj->_ID : -1;
int* luaID = (obj) ? &obj->_luaID : NULL;
toluafix_pushusertype_ccobject(L, ID, luaID, (void*)obj,className.c_str());
lua_rawset(L, -3);
obj->retain();
++indexTable;
}
}
else if((strVal = dynamic_cast<__String *>(obj)))
{
lua_pushnumber(L, (lua_Number)indexTable);
lua_pushstring(L, strVal->getCString());
lua_rawset(L, -3);
++indexTable;
}
else if ((dictVal = dynamic_cast<__Dictionary*>(obj)))
{
dictionary_to_luaval(L, dictVal);
}
else if ((arrVal = dynamic_cast<__Array*>(obj)))
{
array_to_luaval(L, arrVal);
}
else if ((doubleVal = dynamic_cast<__Double*>(obj)))
{
lua_pushnumber(L, (lua_Number)indexTable);
lua_pushnumber(L, (lua_Number)doubleVal->getValue());
lua_rawset(L, -3);
++indexTable;
}
else if ((floatVal = dynamic_cast<__Float*>(obj)))
{
lua_pushnumber(L, (lua_Number)indexTable);
lua_pushnumber(L, (lua_Number)floatVal->getValue());
lua_rawset(L, -3);
++indexTable;
}
else if ((intVal = dynamic_cast<__Integer*>(obj)))
{
lua_pushnumber(L, (lua_Number)indexTable);
lua_pushinteger(L, (lua_Integer)intVal->getValue());
lua_rawset(L, -3);
++indexTable;
}
else if ((boolVal = dynamic_cast<__Bool*>(obj)))
{
lua_pushnumber(L, (lua_Number)indexTable);
lua_pushboolean(L, boolVal->getValue());
lua_rawset(L, -3);
++indexTable;
}
else
{
CCASSERT(false, "the type isn't suppored.");
}
}
}
void dictionary_to_luaval(lua_State* L, __Dictionary* dict)
{
lua_newtable(L);
if (nullptr == L || nullptr == dict)
return;
DictElement* element = nullptr;
std::string className = "";
__String* strVal = nullptr;
__Dictionary* dictVal = nullptr;
__Array* arrVal = nullptr;
__Double* doubleVal = nullptr;
__Bool* boolVal = nullptr;
__Float* floatVal = nullptr;
__Integer* intVal = nullptr;
CCDICT_FOREACH(dict, element)
{
if (NULL == element)
continue;
std::string typeName = typeid(element->getObject()).name();
auto iter = g_luaType.find(typeName);
if (g_luaType.end() != iter)
{
className = iter->second;
if ( nullptr != dynamic_cast<Ref*>(element->getObject()))
{
lua_pushstring(L, element->getStrKey());
int ID = (element->getObject()) ? (int)element->getObject()->_ID : -1;
int* luaID = (element->getObject()) ? &(element->getObject()->_luaID) : NULL;
toluafix_pushusertype_ccobject(L, ID, luaID, (void*)element->getObject(),className.c_str());
lua_rawset(L, -3);
element->getObject()->retain();
}
}
else if((strVal = dynamic_cast<__String *>(element->getObject())))
{
lua_pushstring(L, element->getStrKey());
lua_pushstring(L, strVal->getCString());
lua_rawset(L, -3);
}
else if ((dictVal = dynamic_cast<__Dictionary*>(element->getObject())))
{
dictionary_to_luaval(L, dictVal);
}
else if ((arrVal = dynamic_cast<__Array*>(element->getObject())))
{
array_to_luaval(L, arrVal);
}
else if ((doubleVal = dynamic_cast<__Double*>(element->getObject())))
{
lua_pushstring(L, element->getStrKey());
lua_pushnumber(L, (lua_Number)doubleVal->getValue());
lua_rawset(L, -3);
}
else if ((floatVal = dynamic_cast<__Float*>(element->getObject())))
{
lua_pushstring(L, element->getStrKey());
lua_pushnumber(L, (lua_Number)floatVal->getValue());
lua_rawset(L, -3);
}
else if ((intVal = dynamic_cast<__Integer*>(element->getObject())))
{
lua_pushstring(L, element->getStrKey());
lua_pushinteger(L, (lua_Integer)intVal->getValue());
lua_rawset(L, -3);
}
else if ((boolVal = dynamic_cast<__Bool*>(element->getObject())))
{
lua_pushstring(L, element->getStrKey());
lua_pushboolean(L, boolVal->getValue());
lua_rawset(L, -3);
}
else
{
CCASSERT(false, "the type isn't suppored.");
}
}
}
void ccvalue_to_luaval(lua_State* L,const cocos2d::Value& inValue)
{
const Value& obj = inValue;
switch (obj.getType())
{
case Value::Type::BOOLEAN:
lua_pushboolean(L, obj.asBool());
break;
case Value::Type::FLOAT:
case Value::Type::DOUBLE:
lua_pushnumber(L, obj.asDouble());
break;
case Value::Type::INTEGER:
lua_pushinteger(L, obj.asInt());
break;
case Value::Type::STRING:
lua_pushstring(L, obj.asString().c_str());
break;
case Value::Type::VECTOR:
ccvaluevector_to_luaval(L, obj.asValueVector());
break;
case Value::Type::MAP:
ccvaluemap_to_luaval(L, obj.asValueMap());
break;
case Value::Type::INT_KEY_MAP:
ccvaluemapintkey_to_luaval(L, obj.asIntKeyMap());
break;
default:
break;
}
}
void ccvaluemap_to_luaval(lua_State* L,const cocos2d::ValueMap& inValue)
{
lua_newtable(L);
if (nullptr == L)
return;
for (auto iter = inValue.begin(); iter != inValue.end(); ++iter)
{
std::string key = iter->first;
const Value& obj = iter->second;
switch (obj.getType())
{
case Value::Type::BOOLEAN:
{
lua_pushstring(L, key.c_str());
lua_pushboolean(L, obj.asBool());
lua_rawset(L, -3);
}
break;
case Value::Type::FLOAT:
case Value::Type::DOUBLE:
{
lua_pushstring(L, key.c_str());
lua_pushnumber(L, obj.asDouble());
lua_rawset(L, -3);
}
break;
case Value::Type::INTEGER:
{
lua_pushstring(L, key.c_str());
lua_pushinteger(L, obj.asInt());
lua_rawset(L, -3);
}
break;
case Value::Type::STRING:
{
lua_pushstring(L, key.c_str());
lua_pushstring(L, obj.asString().c_str());
lua_rawset(L, -3);
}
break;
case Value::Type::VECTOR:
{
lua_pushstring(L, key.c_str());
ccvaluevector_to_luaval(L, obj.asValueVector());
lua_rawset(L, -3);
}
break;
case Value::Type::MAP:
{
lua_pushstring(L, key.c_str());
ccvaluemap_to_luaval(L, obj.asValueMap());
lua_rawset(L, -3);
}
break;
case Value::Type::INT_KEY_MAP:
{
lua_pushstring(L, key.c_str());
ccvaluemapintkey_to_luaval(L, obj.asIntKeyMap());
lua_rawset(L, -3);
}
break;
default:
break;
}
}
}
void ccvaluemapintkey_to_luaval(lua_State* L, const cocos2d::ValueMapIntKey& inValue)
{
lua_newtable(L);
if (nullptr == L)
return;
for (auto iter = inValue.begin(); iter != inValue.end(); ++iter)
{
std::stringstream keyss;
keyss << iter->first;
std::string key = keyss.str();
const Value& obj = iter->second;
switch (obj.getType())
{
case Value::Type::BOOLEAN:
{
lua_pushstring(L, key.c_str());
lua_pushboolean(L, obj.asBool());
lua_rawset(L, -3);
}
break;
case Value::Type::FLOAT:
case Value::Type::DOUBLE:
{
lua_pushstring(L, key.c_str());
lua_pushnumber(L, obj.asDouble());
lua_rawset(L, -3);
}
break;
case Value::Type::INTEGER:
{
lua_pushstring(L, key.c_str());
lua_pushinteger(L, obj.asInt());
lua_rawset(L, -3);
}
break;
case Value::Type::STRING:
{
lua_pushstring(L, key.c_str());
lua_pushstring(L, obj.asString().c_str());
lua_rawset(L, -3);
}
break;
case Value::Type::VECTOR:
{
lua_pushstring(L, key.c_str());
ccvaluevector_to_luaval(L, obj.asValueVector());
lua_rawset(L, -3);
}
break;
case Value::Type::MAP:
{
lua_pushstring(L, key.c_str());
ccvaluemap_to_luaval(L, obj.asValueMap());
lua_rawset(L, -3);
}
break;
case Value::Type::INT_KEY_MAP:
{
lua_pushstring(L, key.c_str());
ccvaluemapintkey_to_luaval(L, obj.asIntKeyMap());
lua_rawset(L, -3);
}
break;
default:
break;
}
}
}
void ccvaluevector_to_luaval(lua_State* L, const cocos2d::ValueVector& inValue)
{
lua_newtable(L);
if (nullptr == L)
return;
int index = 1;
for (const auto& obj : inValue)
{
switch (obj.getType())
{
case Value::Type::BOOLEAN:
{
lua_pushnumber(L, (lua_Number)index);
lua_pushboolean(L, obj.asBool());
lua_rawset(L, -3);
++index;
}
break;
case Value::Type::FLOAT:
case Value::Type::DOUBLE:
{
lua_pushnumber(L, (lua_Number)index);
lua_pushnumber(L, obj.asDouble());
lua_rawset(L, -3);
++index;
}
break;
case Value::Type::INTEGER:
{
lua_pushnumber(L, (lua_Number)index);
lua_pushnumber(L, obj.asInt());
lua_rawset(L, -3);
++index;
}
break;
case Value::Type::STRING:
{
lua_pushnumber(L, (lua_Number)index);
lua_pushstring(L, obj.asString().c_str());
lua_rawset(L, -3);
++index;
}
break;
case Value::Type::VECTOR:
{
lua_pushnumber(L, (lua_Number)index);
ccvaluevector_to_luaval(L, obj.asValueVector());
lua_rawset(L, -3);
++index;
}
break;
case Value::Type::MAP:
{
lua_pushnumber(L, (lua_Number)index);
ccvaluemap_to_luaval(L, obj.asValueMap());
lua_rawset(L, -3);
++index;
}
break;
case Value::Type::INT_KEY_MAP:
{
lua_pushnumber(L, (lua_Number)index);
ccvaluemapintkey_to_luaval(L, obj.asIntKeyMap());
lua_rawset(L, -3);
++index;
}
break;
default:
break;
}
}
}
void mat4_to_luaval(lua_State* L, const cocos2d::Mat4& mat)
{
if (nullptr == L)
return;
lua_newtable(L); /* L: table */
int indexTable = 1;
for (int i = 0; i < 16; i++)
{
lua_pushnumber(L, (lua_Number)indexTable);
lua_pushnumber(L, (lua_Number)mat.m[i]);
lua_rawset(L, -3);
++indexTable;
}
}
void blendfunc_to_luaval(lua_State* L, const cocos2d::BlendFunc& func)
{
if (nullptr == L)
return;
lua_newtable(L); /* L: table */
lua_pushstring(L, "src"); /* L: table key */
lua_pushnumber(L, (lua_Number) func.src); /* L: table key value*/
lua_rawset(L, -3); /* table[key] = value, L: table */
lua_pushstring(L, "dst"); /* L: table key */
lua_pushnumber(L, (lua_Number) func.dst); /* L: table key value*/
lua_rawset(L, -3);
}
void ttfconfig_to_luaval(lua_State* L, const cocos2d::TTFConfig& config)
{
if (nullptr == L)
return;
lua_newtable(L);
lua_pushstring(L, "fontFilePath");
lua_pushstring(L, config.fontFilePath.c_str());
lua_rawset(L, -3);
lua_pushstring(L, "fontSize");
lua_pushnumber(L, (lua_Number)config.fontSize);
lua_rawset(L, -3);
lua_pushstring(L, "glyphs");
lua_pushnumber(L, (lua_Number)config.glyphs);
lua_rawset(L, -3);
lua_pushstring(L, "customGlyphs");
if (nullptr != config.customGlyphs && strlen(config.customGlyphs) > 0)
{
lua_pushstring(L, config.customGlyphs);
}
else
{
lua_pushstring(L, "");
}
lua_rawset(L, -3);
lua_pushstring(L, "distanceFieldEnabled");
lua_pushboolean(L, config.distanceFieldEnabled);
lua_rawset(L, -3);
lua_pushstring(L, "outlineSize");
lua_pushnumber(L, (lua_Number)config.outlineSize);
lua_rawset(L, -3);
}
void uniform_to_luaval(lua_State* L, const cocos2d::Uniform& uniform)
{
if (nullptr == L)
return;
lua_newtable(L);
lua_pushstring(L, "location");
lua_pushnumber(L, (lua_Number)uniform.location);
lua_rawset(L, -3);
lua_pushstring(L, "size");
lua_pushnumber(L, (lua_Number)uniform.size);
lua_rawset(L, -3);
lua_pushstring(L, "type");
lua_pushnumber(L, (lua_Number)uniform.type);
lua_rawset(L, -3);
lua_pushstring(L, "name");
tolua_pushcppstring(L, uniform.name);
lua_rawset(L, -3);
}
void vertexattrib_to_luaval(lua_State* L, const cocos2d::VertexAttrib& verAttrib)
{
if (nullptr == L)
return;
lua_newtable(L);
lua_pushstring(L, "index");
lua_pushnumber(L, (lua_Number)verAttrib.index);
lua_rawset(L, -3);
lua_pushstring(L, "size");
lua_pushnumber(L, (lua_Number)verAttrib.size);
lua_rawset(L, -3);
lua_pushstring(L, "type");
lua_pushnumber(L, (lua_Number)verAttrib.type);
lua_rawset(L, -3);
lua_pushstring(L, "name");
tolua_pushcppstring(L, verAttrib.name);
lua_rawset(L, -3);
}
void mesh_vertex_attrib_to_luaval(lua_State* L, const cocos2d::MeshVertexAttrib& inValue)
{
if (nullptr == L)
return;
lua_newtable(L);
lua_pushstring(L, "size");
lua_pushnumber(L, (lua_Number)inValue.size);
lua_rawset(L, -3);
lua_pushstring(L, "type");
lua_pushnumber(L, (lua_Number)inValue.type);
lua_rawset(L, -3);
lua_pushstring(L, "vertexAttrib");
lua_pushnumber(L, (lua_Number)inValue.vertexAttrib);
lua_rawset(L, -3);
lua_pushstring(L, "attribSizeBytes");
lua_pushnumber(L, (lua_Number)inValue.attribSizeBytes);
lua_rawset(L, -3);
}
void ccvector_std_string_to_luaval(lua_State* L, const std::vector<std::string>& inValue)
{
if (nullptr == L)
return;
lua_newtable(L);
int index = 1;
for (const std::string value : inValue)
{
lua_pushnumber(L, (lua_Number)index);
lua_pushstring(L, value.c_str());
lua_rawset(L, -3);
++index;
}
}
void ccvector_int_to_luaval(lua_State* L, const std::vector<int>& inValue)
{
if (nullptr == L)
return;
lua_newtable(L);
int index = 1;
for (const int value : inValue)
{
lua_pushnumber(L, (lua_Number)index);
lua_pushnumber(L, (lua_Number)value);
lua_rawset(L, -3);
++index;
}
}
void ccvector_float_to_luaval(lua_State* L, const std::vector<float>& inValue)
{
if (nullptr == L)
return;
lua_newtable(L);
int index = 1;
for (const float value : inValue)
{
lua_pushnumber(L, (lua_Number)index);
lua_pushnumber(L, (lua_Number)value);
lua_rawset(L, -3);
++index;
}
}
void ccvector_ushort_to_luaval(lua_State* L, const std::vector<unsigned short>& inValue)
{
if (nullptr == L)
return;
lua_newtable(L);
int index = 1;
for (const unsigned short value : inValue)
{
lua_pushnumber(L, (lua_Number)index);
lua_pushnumber(L, (lua_Number)value);
lua_rawset(L, -3);
++index;
}
}
| {
"pile_set_name": "Github"
} |
print_endline "public binary"
| {
"pile_set_name": "Github"
} |
#! /usr/bin/env python
# This file contains a class and a main program that perform three
# related (though complimentary) formatting operations on Python
# programs. When called as "pindent -c", it takes a valid Python
# program as input and outputs a version augmented with block-closing
# comments. When called as "pindent -d", it assumes its input is a
# Python program with block-closing comments and outputs a commentless
# version. When called as "pindent -r" it assumes its input is a
# Python program with block-closing comments but with its indentation
# messed up, and outputs a properly indented version.
# A "block-closing comment" is a comment of the form '# end <keyword>'
# where <keyword> is the keyword that opened the block. If the
# opening keyword is 'def' or 'class', the function or class name may
# be repeated in the block-closing comment as well. Here is an
# example of a program fully augmented with block-closing comments:
# def foobar(a, b):
# if a == b:
# a = a+1
# elif a < b:
# b = b-1
# if b > a: a = a-1
# # end if
# else:
# print 'oops!'
# # end if
# # end def foobar
# Note that only the last part of an if...elif...else... block needs a
# block-closing comment; the same is true for other compound
# statements (e.g. try...except). Also note that "short-form" blocks
# like the second 'if' in the example must be closed as well;
# otherwise the 'else' in the example would be ambiguous (remember
# that indentation is not significant when interpreting block-closing
# comments).
# The operations are idempotent (i.e. applied to their own output
# they yield an identical result). Running first "pindent -c" and
# then "pindent -r" on a valid Python program produces a program that
# is semantically identical to the input (though its indentation may
# be different). Running "pindent -e" on that output produces a
# program that only differs from the original in indentation.
# Other options:
# -s stepsize: set the indentation step size (default 8)
# -t tabsize : set the number of spaces a tab character is worth (default 8)
# -e : expand TABs into spaces
# file ... : input file(s) (default standard input)
# The results always go to standard output
# Caveats:
# - comments ending in a backslash will be mistaken for continued lines
# - continuations using backslash are always left unchanged
# - continuations inside parentheses are not extra indented by -r
# but must be indented for -c to work correctly (this breaks
# idempotency!)
# - continued lines inside triple-quoted strings are totally garbled
# Secret feature:
# - On input, a block may also be closed with an "end statement" --
# this is a block-closing comment without the '#' sign.
# Possible improvements:
# - check syntax based on transitions in 'next' table
# - better error reporting
# - better error recovery
# - check identifier after class/def
# The following wishes need a more complete tokenization of the source:
# - Don't get fooled by comments ending in backslash
# - reindent continuation lines indicated by backslash
# - handle continuation lines inside parentheses/braces/brackets
# - handle triple quoted strings spanning lines
# - realign comments
# - optionally do much more thorough reformatting, a la C indent
# Defaults
STEPSIZE = 8
TABSIZE = 8
EXPANDTABS = 0
import re
import sys
next = {}
next['if'] = next['elif'] = 'elif', 'else', 'end'
next['while'] = next['for'] = 'else', 'end'
next['try'] = 'except', 'finally'
next['except'] = 'except', 'else', 'finally', 'end'
next['else'] = next['finally'] = next['def'] = next['class'] = 'end'
next['end'] = ()
start = 'if', 'while', 'for', 'try', 'with', 'def', 'class'
class PythonIndenter:
def __init__(self, fpi = sys.stdin, fpo = sys.stdout,
indentsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
self.fpi = fpi
self.fpo = fpo
self.indentsize = indentsize
self.tabsize = tabsize
self.lineno = 0
self.expandtabs = expandtabs
self._write = fpo.write
self.kwprog = re.compile(
r'^\s*(?P<kw>[a-z]+)'
r'(\s+(?P<id>[a-zA-Z_]\w*))?'
r'[^\w]')
self.endprog = re.compile(
r'^\s*#?\s*end\s+(?P<kw>[a-z]+)'
r'(\s+(?P<id>[a-zA-Z_]\w*))?'
r'[^\w]')
self.wsprog = re.compile(r'^[ \t]*')
# end def __init__
def write(self, line):
if self.expandtabs:
self._write(line.expandtabs(self.tabsize))
else:
self._write(line)
# end if
# end def write
def readline(self):
line = self.fpi.readline()
if line: self.lineno = self.lineno + 1
# end if
return line
# end def readline
def error(self, fmt, *args):
if args: fmt = fmt % args
# end if
sys.stderr.write('Error at line %d: %s\n' % (self.lineno, fmt))
self.write('### %s ###\n' % fmt)
# end def error
def getline(self):
line = self.readline()
while line[-2:] == '\\\n':
line2 = self.readline()
if not line2: break
# end if
line = line + line2
# end while
return line
# end def getline
def putline(self, line, indent = None):
if indent is None:
self.write(line)
return
# end if
tabs, spaces = divmod(indent*self.indentsize, self.tabsize)
i = 0
m = self.wsprog.match(line)
if m: i = m.end()
# end if
self.write('\t'*tabs + ' '*spaces + line[i:])
# end def putline
def reformat(self):
stack = []
while 1:
line = self.getline()
if not line: break # EOF
# end if
m = self.endprog.match(line)
if m:
kw = 'end'
kw2 = m.group('kw')
if not stack:
self.error('unexpected end')
elif stack[-1][0] != kw2:
self.error('unmatched end')
# end if
del stack[-1:]
self.putline(line, len(stack))
continue
# end if
m = self.kwprog.match(line)
if m:
kw = m.group('kw')
if kw in start:
self.putline(line, len(stack))
stack.append((kw, kw))
continue
# end if
if next.has_key(kw) and stack:
self.putline(line, len(stack)-1)
kwa, kwb = stack[-1]
stack[-1] = kwa, kw
continue
# end if
# end if
self.putline(line, len(stack))
# end while
if stack:
self.error('unterminated keywords')
for kwa, kwb in stack:
self.write('\t%s\n' % kwa)
# end for
# end if
# end def reformat
def delete(self):
begin_counter = 0
end_counter = 0
while 1:
line = self.getline()
if not line: break # EOF
# end if
m = self.endprog.match(line)
if m:
end_counter = end_counter + 1
continue
# end if
m = self.kwprog.match(line)
if m:
kw = m.group('kw')
if kw in start:
begin_counter = begin_counter + 1
# end if
# end if
self.putline(line)
# end while
if begin_counter - end_counter < 0:
sys.stderr.write('Warning: input contained more end tags than expected\n')
elif begin_counter - end_counter > 0:
sys.stderr.write('Warning: input contained less end tags than expected\n')
# end if
# end def delete
def complete(self):
self.indentsize = 1
stack = []
todo = []
thisid = ''
current, firstkw, lastkw, topid = 0, '', '', ''
while 1:
line = self.getline()
i = 0
m = self.wsprog.match(line)
if m: i = m.end()
# end if
m = self.endprog.match(line)
if m:
thiskw = 'end'
endkw = m.group('kw')
thisid = m.group('id')
else:
m = self.kwprog.match(line)
if m:
thiskw = m.group('kw')
if not next.has_key(thiskw):
thiskw = ''
# end if
if thiskw in ('def', 'class'):
thisid = m.group('id')
else:
thisid = ''
# end if
elif line[i:i+1] in ('\n', '#'):
todo.append(line)
continue
else:
thiskw = ''
# end if
# end if
indent = len(line[:i].expandtabs(self.tabsize))
while indent < current:
if firstkw:
if topid:
s = '# end %s %s\n' % (
firstkw, topid)
else:
s = '# end %s\n' % firstkw
# end if
self.putline(s, current)
firstkw = lastkw = ''
# end if
current, firstkw, lastkw, topid = stack[-1]
del stack[-1]
# end while
if indent == current and firstkw:
if thiskw == 'end':
if endkw != firstkw:
self.error('mismatched end')
# end if
firstkw = lastkw = ''
elif not thiskw or thiskw in start:
if topid:
s = '# end %s %s\n' % (
firstkw, topid)
else:
s = '# end %s\n' % firstkw
# end if
self.putline(s, current)
firstkw = lastkw = topid = ''
# end if
# end if
if indent > current:
stack.append((current, firstkw, lastkw, topid))
if thiskw and thiskw not in start:
# error
thiskw = ''
# end if
current, firstkw, lastkw, topid = \
indent, thiskw, thiskw, thisid
# end if
if thiskw:
if thiskw in start:
firstkw = lastkw = thiskw
topid = thisid
else:
lastkw = thiskw
# end if
# end if
for l in todo: self.write(l)
# end for
todo = []
if not line: break
# end if
self.write(line)
# end while
# end def complete
# end class PythonIndenter
# Simplified user interface
# - xxx_filter(input, output): read and write file objects
# - xxx_string(s): take and return string object
# - xxx_file(filename): process file in place, return true iff changed
def complete_filter(input = sys.stdin, output = sys.stdout,
stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
pi = PythonIndenter(input, output, stepsize, tabsize, expandtabs)
pi.complete()
# end def complete_filter
def delete_filter(input= sys.stdin, output = sys.stdout,
stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
pi = PythonIndenter(input, output, stepsize, tabsize, expandtabs)
pi.delete()
# end def delete_filter
def reformat_filter(input = sys.stdin, output = sys.stdout,
stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
pi = PythonIndenter(input, output, stepsize, tabsize, expandtabs)
pi.reformat()
# end def reformat_filter
class StringReader:
def __init__(self, buf):
self.buf = buf
self.pos = 0
self.len = len(self.buf)
# end def __init__
def read(self, n = 0):
if n <= 0:
n = self.len - self.pos
else:
n = min(n, self.len - self.pos)
# end if
r = self.buf[self.pos : self.pos + n]
self.pos = self.pos + n
return r
# end def read
def readline(self):
i = self.buf.find('\n', self.pos)
return self.read(i + 1 - self.pos)
# end def readline
def readlines(self):
lines = []
line = self.readline()
while line:
lines.append(line)
line = self.readline()
# end while
return lines
# end def readlines
# seek/tell etc. are left as an exercise for the reader
# end class StringReader
class StringWriter:
def __init__(self):
self.buf = ''
# end def __init__
def write(self, s):
self.buf = self.buf + s
# end def write
def getvalue(self):
return self.buf
# end def getvalue
# end class StringWriter
def complete_string(source, stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
input = StringReader(source)
output = StringWriter()
pi = PythonIndenter(input, output, stepsize, tabsize, expandtabs)
pi.complete()
return output.getvalue()
# end def complete_string
def delete_string(source, stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
input = StringReader(source)
output = StringWriter()
pi = PythonIndenter(input, output, stepsize, tabsize, expandtabs)
pi.delete()
return output.getvalue()
# end def delete_string
def reformat_string(source, stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
input = StringReader(source)
output = StringWriter()
pi = PythonIndenter(input, output, stepsize, tabsize, expandtabs)
pi.reformat()
return output.getvalue()
# end def reformat_string
def complete_file(filename, stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
source = open(filename, 'r').read()
result = complete_string(source, stepsize, tabsize, expandtabs)
if source == result: return 0
# end if
import os
try: os.rename(filename, filename + '~')
except os.error: pass
# end try
f = open(filename, 'w')
f.write(result)
f.close()
return 1
# end def complete_file
def delete_file(filename, stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
source = open(filename, 'r').read()
result = delete_string(source, stepsize, tabsize, expandtabs)
if source == result: return 0
# end if
import os
try: os.rename(filename, filename + '~')
except os.error: pass
# end try
f = open(filename, 'w')
f.write(result)
f.close()
return 1
# end def delete_file
def reformat_file(filename, stepsize = STEPSIZE, tabsize = TABSIZE, expandtabs = EXPANDTABS):
source = open(filename, 'r').read()
result = reformat_string(source, stepsize, tabsize, expandtabs)
if source == result: return 0
# end if
import os
try: os.rename(filename, filename + '~')
except os.error: pass
# end try
f = open(filename, 'w')
f.write(result)
f.close()
return 1
# end def reformat_file
# Test program when called as a script
usage = """
usage: pindent (-c|-d|-r) [-s stepsize] [-t tabsize] [-e] [file] ...
-c : complete a correctly indented program (add #end directives)
-d : delete #end directives
-r : reformat a completed program (use #end directives)
-s stepsize: indentation step (default %(STEPSIZE)d)
-t tabsize : the worth in spaces of a tab (default %(TABSIZE)d)
-e : expand TABs into spaces (defailt OFF)
[file] ... : files are changed in place, with backups in file~
If no files are specified or a single - is given,
the program acts as a filter (reads stdin, writes stdout).
""" % vars()
def error_both(op1, op2):
sys.stderr.write('Error: You can not specify both '+op1+' and -'+op2[0]+' at the same time\n')
sys.stderr.write(usage)
sys.exit(2)
# end def error_both
def test():
import getopt
try:
opts, args = getopt.getopt(sys.argv[1:], 'cdrs:t:e')
except getopt.error, msg:
sys.stderr.write('Error: %s\n' % msg)
sys.stderr.write(usage)
sys.exit(2)
# end try
action = None
stepsize = STEPSIZE
tabsize = TABSIZE
expandtabs = EXPANDTABS
for o, a in opts:
if o == '-c':
if action: error_both(o, action)
# end if
action = 'complete'
elif o == '-d':
if action: error_both(o, action)
# end if
action = 'delete'
elif o == '-r':
if action: error_both(o, action)
# end if
action = 'reformat'
elif o == '-s':
stepsize = int(a)
elif o == '-t':
tabsize = int(a)
elif o == '-e':
expandtabs = 1
# end if
# end for
if not action:
sys.stderr.write(
'You must specify -c(omplete), -d(elete) or -r(eformat)\n')
sys.stderr.write(usage)
sys.exit(2)
# end if
if not args or args == ['-']:
action = eval(action + '_filter')
action(sys.stdin, sys.stdout, stepsize, tabsize, expandtabs)
else:
action = eval(action + '_file')
for filename in args:
action(filename, stepsize, tabsize, expandtabs)
# end for
# end if
# end def test
if __name__ == '__main__':
test()
# end if
| {
"pile_set_name": "Github"
} |
AUTHOR: JennJenn
What is the USA state capital of Alabama?:
- Montgomery
What is the USA state capital of Alaska?:
- Juneau
What is the USA state capital of Arizona?:
- Phoenix
What is the USA state capital of Arkansas?:
- Little Rock
What is the USA state capital of California?:
- Sacramento
What is the USA state capital of Colorado?:
- Denver
What is the USA state capital of Connecticut?:
- Hartford
What is the USA state capital of Delaware?:
- Dover
What is the USA state capital of Florida?:
- Tallahassee
What is the USA state capital of Georgia?:
- Atlanta
What is the USA state capital of Hawaii?:
- Honolulu
What is the USA state capital of Idaho?:
- Boise
What is the USA state capital of Illinois?:
- Springfield
What is the USA state capital of Indiana?:
- Indianapolis
What is the USA state capital of Iowa?:
- Des Moines
What is the USA state capital of Kansas?:
- Topeka
What is the USA state capital of Kentucky?:
- Frankfort
What is the USA state capital of Louisiana?:
- Baton Rouge
What is the USA state capital of Maine?:
- Augusta
What is the USA state capital of Maryland?:
- Annapolis
What is the USA state capital of Massachusetts?:
- Boston
What is the USA state capital of Michigan?:
- Lansing
What is the USA state capital of Minnesota?:
- St. Paul
What is the USA state capital of Mississippi?:
- Jackson
What is the USA state capital of Missouri?:
- Jefferson City
What is the USA state capital of Montana?:
- Helena
What is the USA state capital of Nebraska?:
- Lincoln
What is the USA state capital of Nevada?:
- Carson City
What is the USA state capital of New Hampshire?:
- Concord
What is the USA state capital of New Jersey?:
- Trenton
What is the USA state capital of New Mexico?:
- Santa Fe
What is the USA state capital of New York?:
- Albany
What is the USA state capital of North Carolina?:
- Raleigh
What is the USA state capital of North Dakota?:
- Bismarck
What is the USA state capital of Ohio?:
- Columbus
What is the USA state capital of Oklahoma?:
- Oklahoma City
What is the USA state capital of Oregon?:
- Salem
What is the USA state capital of Pennsylvania?:
- Harrisburg
What is the USA state capital of Rhode Island?:
- Providence
What is the USA state capital of South Carolina?:
- Columbia
What is the USA state capital of South Dakota?:
- Pierre
What is the USA state capital of Tennessee?:
- Nashville
What is the USA state capital of Texas?:
- Austin
What is the USA state capital of Utah?:
- Salt Lake City
What is the USA state capital of Vermont?:
- Montpelier
What is the USA state capital of Virginia?:
- Richmond
What is the USA state capital of Washington?:
- Olympia
What is the USA state capital of West Virginia?:
- Charleston
What is the USA state capital of Wisconsin?:
- Madison
What is the USA state capital of Wyoming?:
- Cheyenne
What is the USA territory capital of American Samoa?:
- Pago Pago
What is the USA territory capital of Guam?:
- Hagatna
- Agana
What is the USA territory capital of Northern Mariana Islands?:
- Capitol Hill
What is the USA territory capital of Puerto Rico?:
- San Juan
What is the USA territory capital of U.S. Virgin Islands?:
- Charlotte Amalie
| {
"pile_set_name": "Github"
} |
// MonoGame - Copyright (C) The MonoGame Team
// This file is subject to the terms and conditions defined in
// file 'LICENSE.txt', which is part of this source code package.
using System;
namespace Microsoft.Xna.Framework.Graphics
{
public partial class RenderTarget2D
{
private void PlatformConstruct(
GraphicsDevice graphicsDevice,
int width,
int height,
bool mipMap,
DepthFormat preferredDepthFormat,
int preferredMultiSampleCount,
RenderTargetUsage usage,
bool shared)
{
throw new NotImplementedException();
}
private void PlatformGraphicsDeviceResetting()
{
throw new NotImplementedException();
}
protected override void Dispose(bool disposing)
{
throw new NotImplementedException();
base.Dispose(disposing);
}
}
}
| {
"pile_set_name": "Github"
} |
# Created by: Lars Balker Rasmussen <lbr@FreeBSD.org>
# $FreeBSD$
PORTNAME= Data-Visitor
PORTVERSION= 0.31
CATEGORIES= devel perl5
MASTER_SITES= CPAN
PKGNAMEPREFIX= p5-
MAINTAINER= perl@FreeBSD.org
COMMENT= Visitor style traversal of Perl data structures
LICENSE= ART10 GPLv1+
LICENSE_COMB= dual
BUILD_DEPENDS= ${RUN_DEPENDS}
RUN_DEPENDS= p5-Moose>=0.89:devel/p5-Moose \
p5-Sub-Name>=0:devel/p5-Sub-Name \
p5-Tie-ToObject>=0.01:devel/p5-Tie-ToObject \
p5-namespace-clean>=0.19:devel/p5-namespace-clean
TEST_DEPENDS= p5-Data-Alias>=0:devel/p5-Data-Alias \
p5-Test-Needs>=0:devel/p5-Test-Needs \
p5-Tie-RefHash>=0:devel/p5-Tie-RefHash
USES= perl5
USE_PERL5= configure
NO_ARCH= yes
.include <bsd.port.mk>
| {
"pile_set_name": "Github"
} |
/*
* JBoss, Home of Professional Open Source.
* Copyright 2014 Red Hat, Inc., and individual contributors
* as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.undertow.websockets.client;
import io.undertow.websockets.WebSocketExtension;
import java.util.List;
import java.util.Map;
/**
* @author Stuart Douglas
*/
public class WebSocketClientNegotiation {
private final List<String> supportedSubProtocols;
private final List<WebSocketExtension> supportedExtensions;
private volatile String selectedSubProtocol;
private volatile List<WebSocketExtension> selectedExtensions;
public WebSocketClientNegotiation(List<String> supportedSubProtocols, List<WebSocketExtension> supportedExtensions) {
this.supportedSubProtocols = supportedSubProtocols;
this.supportedExtensions = supportedExtensions;
}
public List<String> getSupportedSubProtocols() {
return supportedSubProtocols;
}
public List<WebSocketExtension> getSupportedExtensions() {
return supportedExtensions;
}
public String getSelectedSubProtocol() {
return selectedSubProtocol;
}
public List<WebSocketExtension> getSelectedExtensions() {
return selectedExtensions;
}
public void beforeRequest(final Map<String, List<String>> headers) {
}
public void afterRequest(final Map<String, List<String>> headers) {
}
public void handshakeComplete(String selectedProtocol, List<WebSocketExtension> selectedExtensions) {
this.selectedExtensions = selectedExtensions;
this.selectedSubProtocol = selectedProtocol;
}
}
| {
"pile_set_name": "Github"
} |
<?php
// +----------------------------------------------------------------------
// | ThinkPHP [ WE CAN DO IT JUST THINK ]
// +----------------------------------------------------------------------
// | Copyright (c) 2006~2015 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: yunwuxin <448901948@qq.com>
// +----------------------------------------------------------------------
namespace think\process\exception;
use think\Process;
class Faild extends \RuntimeException
{
private $process;
public function __construct(Process $process)
{
if ($process->isSuccessful()) {
throw new \InvalidArgumentException('Expected a failed process, but the given process was successful.');
}
$error = sprintf('The command "%s" failed.' . "\nExit Code: %s(%s)", $process->getCommandLine(), $process->getExitCode(), $process->getExitCodeText());
if (!$process->isOutputDisabled()) {
$error .= sprintf("\n\nOutput:\n================\n%s\n\nError Output:\n================\n%s", $process->getOutput(), $process->getErrorOutput());
}
parent::__construct($error);
$this->process = $process;
}
public function getProcess()
{
return $this->process;
}
}
| {
"pile_set_name": "Github"
} |
// Copyright 2015 The Go Authors. All rights reserved.
// Use of this source code is governed by a BSD-style
// license that can be found in the LICENSE file.
// +build gccgo,linux,amd64
package unix
import "syscall"
//extern gettimeofday
func realGettimeofday(*Timeval, *byte) int32
func gettimeofday(tv *Timeval) (err syscall.Errno) {
r := realGettimeofday(tv, nil)
if r < 0 {
return syscall.GetErrno()
}
return 0
}
| {
"pile_set_name": "Github"
} |
###############################################################################
##
## Copyright (c) Crossbar.io Technologies GmbH
##
## Licensed under the Apache License, Version 2.0 (the "License");
## you may not use this file except in compliance with the License.
## You may obtain a copy of the License at
##
## http://www.apache.org/licenses/LICENSE-2.0
##
## Unless required by applicable law or agreed to in writing, software
## distributed under the License is distributed on an "AS IS" BASIS,
## WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
## See the License for the specific language governing permissions and
## limitations under the License.
##
###############################################################################
from case import Case
class Case5_19(Case):
DESCRIPTION = """A fragmented text message is sent in multiple frames. After
sending the first 2 frames of the text message, a Ping is sent. Then we wait 1s,
then we send 2 more text fragments, another Ping and then the final text fragment.
Everything is legal."""
EXPECTATION = """The peer immediately answers the first Ping before
it has received the last text message fragment. The peer pong's back the Ping's
payload exactly, and echo's the payload of the fragmented message back to us."""
def init(self):
self.sync = False
def onOpen(self):
self.fragments = ["fragment1", "fragment2", "fragment3", "fragment4", "fragment5"]
self.pings = ["pongme 1!", "pongme 2!"]
self.expected[Case.OK] = [("pong", self.pings[0]), ("pong", self.pings[1]), ("message", ''.join(self.fragments), False)]
self.expectedClose = {"closedByMe":True,"closeCode":[self.p.CLOSE_STATUS_CODE_NORMAL],"requireClean":True}
self.p.sendFrame(opcode = 1, fin = False, payload = self.fragments[0], sync = self.sync)
self.p.sendFrame(opcode = 0, fin = False, payload = self.fragments[1], sync = self.sync)
self.p.sendFrame(opcode = 9, fin = True, payload = self.pings[0], sync = self.sync)
self.p.continueLater(1, self.part2)
def part2(self):
self.p.sendFrame(opcode = 0, fin = False, payload = self.fragments[2], sync = self.sync)
self.p.sendFrame(opcode = 0, fin = False, payload = self.fragments[3], sync = self.sync)
self.p.sendFrame(opcode = 9, fin = True, payload = self.pings[1], sync = self.sync)
self.p.sendFrame(opcode = 0, fin = True, payload = self.fragments[4], sync = self.sync)
self.p.closeAfter(1)
| {
"pile_set_name": "Github"
} |
import asyncio
from uvicorn.config import Config
from uvicorn.loops.auto import auto_loop_setup
from uvicorn.main import ServerState
from uvicorn.protocols.http.auto import AutoHTTPProtocol
from uvicorn.protocols.websockets.auto import AutoWebSocketsProtocol
try:
import uvloop
except ImportError: # pragma: no cover
uvloop = None
try:
import httptools
except ImportError: # pragma: no cover
httptools = None
try:
import websockets
except ImportError: # pragma: no cover
# Note that we skip the websocket tests completely in this case.
websockets = None
# TODO: Add pypy to our testing matrix, and assert we get the correct classes
# dependent on the platform we're running the tests under.
def test_loop_auto():
auto_loop_setup()
policy = asyncio.get_event_loop_policy()
assert isinstance(policy, asyncio.events.BaseDefaultEventLoopPolicy)
expected_loop = "asyncio" if uvloop is None else "uvloop"
assert type(policy).__module__.startswith(expected_loop)
def test_http_auto():
config = Config(app=None)
server_state = ServerState()
protocol = AutoHTTPProtocol(config=config, server_state=server_state)
expected_http = "H11Protocol" if httptools is None else "HttpToolsProtocol"
assert type(protocol).__name__ == expected_http
def test_websocket_auto():
config = Config(app=None)
server_state = ServerState()
protocol = AutoWebSocketsProtocol(config=config, server_state=server_state)
expected_websockets = "WSProtocol" if websockets is None else "WebSocketProtocol"
assert type(protocol).__name__ == expected_websockets
| {
"pile_set_name": "Github"
} |
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import { DropTarget } from 'react-dnd';
import { ItemTypes } from './helpers';
class Square extends Component {
static propTypes = {
connectDropTarget: PropTypes.func,
width: PropTypes.number,
squareColor: PropTypes.oneOf(['white', 'black']),
children: PropTypes.oneOfType([PropTypes.array, PropTypes.node]),
isOver: PropTypes.bool,
square: PropTypes.string,
setSquareCoordinates: PropTypes.func,
lightSquareStyle: PropTypes.object,
darkSquareStyle: PropTypes.object,
roughSquare: PropTypes.func,
onMouseOverSquare: PropTypes.func,
onMouseOutSquare: PropTypes.func,
dropSquareStyle: PropTypes.object,
screenWidth: PropTypes.number,
screenHeight: PropTypes.number,
squareStyles: PropTypes.object,
onDragOverSquare: PropTypes.func,
onSquareClick: PropTypes.func,
wasSquareClicked: PropTypes.func,
onSquareRightClick: PropTypes.func
};
componentDidMount() {
const { square, setSquareCoordinates, width, roughSquare } = this.props;
roughSquare({ squareElement: this.roughSquareSvg, squareWidth: width / 8 });
const { x, y } = this[square].getBoundingClientRect();
setSquareCoordinates(x, y, square);
}
componentDidUpdate(prevProps) {
const {
screenWidth,
screenHeight,
square,
setSquareCoordinates
} = this.props;
const didScreenSizeChange =
prevProps.screenWidth !== screenWidth ||
prevProps.screenHeight !== screenHeight;
if (didScreenSizeChange) {
const { x, y } = this[square].getBoundingClientRect();
setSquareCoordinates(x, y, square);
}
}
onClick = () => {
this.props.wasSquareClicked(true);
this.props.onSquareClick(this.props.square);
};
render() {
const {
connectDropTarget,
width,
squareColor,
children,
square,
roughSquare,
onMouseOverSquare,
onMouseOutSquare,
squareStyles,
onDragOverSquare,
onSquareRightClick
} = this.props;
return connectDropTarget(
<div
data-testid={`${squareColor}-square`}
data-squareid={square}
ref={ref => (this[square] = ref)}
style={defaultSquareStyle(this.props)}
onMouseOver={() => onMouseOverSquare(square)}
onMouseOut={() => onMouseOutSquare(square)}
onDragEnter={() => onDragOverSquare(square)}
onClick={() => this.onClick()}
onContextMenu={e => {
e.preventDefault();
onSquareRightClick(square);
}}
>
<div
style={{
...size(width),
...center,
...(squareStyles[square] && squareStyles[square])
}}
>
{roughSquare.length ? (
<div style={center}>
{children}
<svg
style={{
...size(width),
position: 'absolute',
display: 'block'
}}
ref={ref => (this.roughSquareSvg = ref)}
/>
</div>
) : (
children
)}
</div>
</div>
);
}
}
const squareTarget = {
drop(props, monitor) {
return {
target: props.square,
board: props.id,
piece: monitor.getItem().piece,
source: monitor.getItem().source
};
}
};
function collect(connect, monitor) {
return {
connectDropTarget: connect.dropTarget(),
isOver: monitor.isOver()
};
}
export default DropTarget(ItemTypes.PIECE, squareTarget, collect)(Square);
const defaultSquareStyle = props => {
const {
width,
squareColor,
isOver,
darkSquareStyle,
lightSquareStyle,
dropSquareStyle
} = props;
return {
...{
...size(width),
...center,
...(squareColor === 'black' ? darkSquareStyle : lightSquareStyle),
...(isOver && dropSquareStyle)
}
};
};
const center = {
display: 'flex',
justifyContent: 'center'
};
const size = width => ({
width: width / 8,
height: width / 8
});
| {
"pile_set_name": "Github"
} |
config BR2_PACKAGE_XAPP_XKBUTILS
bool "xkbutils"
select BR2_PACKAGE_XLIB_LIBXAW
select BR2_PACKAGE_XLIB_LIBXKBFILE
help
X.Org xkbutils application
| {
"pile_set_name": "Github"
} |
// Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`#decodeActionCounts parses the action counts correctly 1`] = `
Object {
"DONT_AGREE": 1,
"FLAG": 2,
"FLAG__COMMENT_DETECTED_BANNED_WORD": 1,
"FLAG__COMMENT_REPORTED_OTHER": 1,
"REACTION": 3,
}
`;
exports[`#decodeActionCounts parses the action counts correctly 2`] = `
Object {
"dontAgree": Object {
"total": 1,
},
"flag": Object {
"reasons": Object {
"COMMENT_DETECTED_BANNED_WORD": 1,
"COMMENT_DETECTED_LINKS": 0,
"COMMENT_DETECTED_NEW_COMMENTER": 0,
"COMMENT_DETECTED_PREMOD_USER": 0,
"COMMENT_DETECTED_RECENT_HISTORY": 0,
"COMMENT_DETECTED_REPEAT_POST": 0,
"COMMENT_DETECTED_SPAM": 0,
"COMMENT_DETECTED_SUSPECT_WORD": 0,
"COMMENT_DETECTED_TOXIC": 0,
"COMMENT_REPORTED_ABUSIVE": 0,
"COMMENT_REPORTED_OFFENSIVE": 0,
"COMMENT_REPORTED_OTHER": 1,
"COMMENT_REPORTED_SPAM": 0,
},
"total": 2,
},
"reaction": Object {
"total": 3,
},
}
`;
exports[`#encodeActionCounts generates the action counts correctly 1`] = `
Object {
"DONT_AGREE": 1,
"FLAG": 2,
"FLAG__COMMENT_DETECTED_BANNED_WORD": 1,
"FLAG__COMMENT_REPORTED_OTHER": 1,
}
`;
| {
"pile_set_name": "Github"
} |
/*
* Copyright (c) Facebook, Inc. and its affiliates.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
package java.lang;
import javax.annotation.Nullable;
public final class Integer {
public static int MAX_VALUE = 2147483647; // 2**31-1
public static int MIN_VALUE = -2147483648; // -2**31
protected final int value;
public Integer(int i) {
this.value = i;
}
public static Integer valueOf(int i) {
return new Integer(i);
}
public boolean equals(@Nullable Object anObject) {
return anObject != null
&& anObject instanceof Integer
&& this.value == ((Integer) anObject).value;
}
public int intValue() {
return this.value;
}
}
| {
"pile_set_name": "Github"
} |
//
// Generated by class-dump 3.5 (64 bit) (Debug version compiled Oct 15 2018 10:31:50).
//
// class-dump is Copyright (C) 1997-1998, 2000-2001, 2004-2015 by Steve Nygard.
//
#import <objc/NSObject.h>
#import <SPOwner/NSCopying-Protocol.h>
#import <SPOwner/NSSecureCoding-Protocol.h>
@class NSDate, NSString;
@interface SPBeaconLocation : NSObject <NSCopying, NSSecureCoding>
{
NSDate *_timestamp;
double _latitude;
double _longitude;
double _horizontalAccuracy;
NSString *_source;
}
+ (BOOL)supportsSecureCoding;
- (void).cxx_destruct;
@property(copy, nonatomic) NSString *source; // @synthesize source=_source;
@property(readonly, nonatomic) double horizontalAccuracy; // @synthesize horizontalAccuracy=_horizontalAccuracy;
@property(nonatomic) double longitude; // @synthesize longitude=_longitude;
@property(nonatomic) double latitude; // @synthesize latitude=_latitude;
@property(copy, nonatomic) NSDate *timestamp; // @synthesize timestamp=_timestamp;
- (id)debugDescription;
- (id)initWithCoder:(id)arg1;
- (void)encodeWithCoder:(id)arg1;
- (id)initWithTimestamp:(id)arg1 latitude:(double)arg2 longitude:(double)arg3 horizontalAccuracy:(double)arg4 source:(id)arg5;
- (id)copyWithZone:(struct _NSZone *)arg1;
@end
| {
"pile_set_name": "Github"
} |
#!/usr/bin/python3
# -*- coding: utf-8 -*-
import os
import tempfile
from etl import ETL
from enhance_rdf import enhance_rdf
from SPARQLWrapper import SPARQLWrapper, XML, JSON
#
# download (part of) graph by SPARQL query from SPARQL endpoint to RDF file
#
def download_rdf_from_sparql_endpoint(endpoint, query):
# read graph by construct query results from SPARQL endpoint
sparql = SPARQLWrapper(endpoint)
sparql.setQuery(query)
sparql.setReturnFormat(XML)
results = sparql.query().convert()
# crate temporary filename
file = tempfile.NamedTemporaryFile()
filename = file.name
file.close()
# export graph to RDF file
results.serialize(destination=filename, format="xml")
return filename
#
# Append values from SPARQL SELECT result to plain text list file
#
def sparql_select_to_list_file(endpoint, query, filename=None):
# read graph by construct query results from SPARQL endpoint
sparql = SPARQLWrapper(endpoint)
sparql.setQuery(query)
sparql.setReturnFormat(JSON)
results = sparql.query().convert()
if not filename:
# crate temporary filename
listfile = tempfile.NamedTemporaryFile(delete=False)
filename = listfile.name
listfile.close()
listfile = open(filename, 'a', encoding="utf-8")
for result in results["results"]["bindings"]:
for variable in results["head"]["vars"]:
if variable in result:
if "value" in result[variable]:
value = result[variable]["value"]
value = value.strip()
if value:
listfile.write(result[variable]["value"] + "\n")
listfile.close()
return filename
class Connector_SPARQL(ETL):
def __init__(self, verbose=False, quiet=True):
ETL.__init__(self, verbose=verbose)
self.read_configfiles()
self.config["plugins"] = []
def read_configfiles(self):
#
# include configs
#
# windows style filenames
self.read_configfile('conf\\opensemanticsearch-connector')
self.read_configfile('conf\\opensemanticsearch-enhancer-rdf')
self.read_configfile('conf\\opensemanticsearch-connector-sparql')
# linux style filenames
self.read_configfile('/etc/opensemanticsearch/etl')
self.read_configfile('/etc/opensemanticsearch/etl-custom')
self.read_configfile('/etc/opensemanticsearch/enhancer-rdf')
self.read_configfile('/etc/opensemanticsearch/connector-sparql')
# Import RDF from SPARQL result
def index_rdf(self, endpoint, query):
# download (part of) graph from endpoint to temporary rdf file
rdffilename = download_rdf_from_sparql_endpoint(
endpoint=endpoint, query=query)
parameters = self.config.copy()
# import the triples of rdf graph by RDF plugin
enhancer = enhance_rdf()
enhancer.etl_graph_file(
docid=endpoint, filename=rdffilename, parameters=parameters)
os.remove(rdffilename)
# Import fields and values from SPARQL SELECT result
def index_select(self, endpoint, query):
# read graph by construct query results from SPARQL endpoint
sparql = SPARQLWrapper(endpoint)
sparql.setQuery(query)
sparql.setReturnFormat(JSON)
results = sparql.query().convert()
i = 0
for result in results["results"]["bindings"]:
i += 1
data = {}
data['id'] = endpoint + "/" + query + "/" + str(i)
for variable in results["head"]["vars"]:
if variable in result:
if "value" in result[variable]:
data[variable] = result[variable]["value"]
self.process(data=data)
# Import SPARQL result
def index(self, endpoint, query):
if query.startswith("SELECT "):
self.index_select(endpoint, query)
else:
self.index_rdf(endpoint, query)
#
# If runned (not imported for functions) get parameters and start
#
if __name__ == "__main__":
# todo: if no protocoll, use http://
# get uri or filename from args
from optparse import OptionParser
parser = OptionParser("etl-sparql [options] uri query")
parser.add_option("-v", "--verbose", dest="verbose",
action="store_true", default=None, help="Print debug messages")
parser.add_option("-c", "--config", dest="config",
default=False, help="Config file")
parser.add_option("-p", "--plugins", dest="plugins",
default=False, help="Plugins (comma separated)")
(options, args) = parser.parse_args()
if len(args) != 2:
parser.error("Missing parameters endpoint URI and SPARQL query")
connector = Connector_SPARQL()
# add optional config parameters
if options.config:
connector.read_configfile(options.config)
# set (or if config overwrite) plugin config
if options.plugins:
connector.config['plugins'] = options.plugins.split(',')
if options.verbose == False or options.verbose == True:
connector.verbose = options.verbose
connector.index(endpoint=args[0], query=args[1])
| {
"pile_set_name": "Github"
} |
{
"_from": "worker-farm@1.7.0",
"_id": "worker-farm@1.7.0",
"_inBundle": false,
"_integrity": "sha512-rvw3QTZc8lAxyVrqcSGVm5yP/IJ2UcB3U0graE3LCFoZ0Yn2x4EoVSqJKdB/T5M+FLcRPjz4TDacRf3OCfNUzw==",
"_location": "/worker-farm",
"_phantomChildren": {},
"_requested": {
"type": "version",
"registry": true,
"raw": "worker-farm@1.7.0",
"name": "worker-farm",
"escapedName": "worker-farm",
"rawSpec": "1.7.0",
"saveSpec": null,
"fetchSpec": "1.7.0"
},
"_requiredBy": [
"#USER",
"/",
"/libcipm"
],
"_resolved": "https://registry.npmjs.org/worker-farm/-/worker-farm-1.7.0.tgz",
"_shasum": "26a94c5391bbca926152002f69b84a4bf772e5a8",
"_spec": "worker-farm@1.7.0",
"_where": "/Users/isaacs/dev/npm/cli",
"authors": [
"Rod Vagg @rvagg <rod@vagg.org> (https://github.com/rvagg)"
],
"bugs": {
"url": "https://github.com/rvagg/node-worker-farm/issues"
},
"bundleDependencies": false,
"dependencies": {
"errno": "~0.1.7"
},
"deprecated": false,
"description": "Distribute processing tasks to child processes with an รผber-simple API and baked-in durability & custom concurrency options.",
"devDependencies": {
"tape": "~4.10.1"
},
"homepage": "https://github.com/rvagg/node-worker-farm",
"keywords": [
"worker",
"child",
"processing",
"farm"
],
"license": "MIT",
"main": "./lib/index.js",
"name": "worker-farm",
"repository": {
"type": "git",
"url": "git+https://github.com/rvagg/node-worker-farm.git"
},
"scripts": {
"test": "node ./tests/"
},
"types": "./index.d.ts",
"version": "1.7.0"
}
| {
"pile_set_name": "Github"
} |
<?php
namespace Thelia\Model;
use Thelia\Model\Base\OrderCouponQuery as BaseOrderCouponQuery;
/**
* Skeleton subclass for performing query and update operations on the 'order_coupon' table.
*
*
*
* You should add additional methods to this class to meet the
* application requirements. This class will only be generated as
* long as it does not already exist in the output directory.
*
*/
class OrderCouponQuery extends BaseOrderCouponQuery
{
}
// OrderCouponQuery
| {
"pile_set_name": "Github"
} |
'use strict';
var React = require('react');
var ReactBin = require('../ReactBin');
var utils = require('../utils');
var Markdown = utils.Markdown;
var Doc = utils.Doc;
var examples = {
basic: require('fs').readFileSync(__dirname + '/01-basic.js', 'utf-8'),
pills: require('fs').readFileSync(__dirname + '/02-pills.js', 'utf-8'),
tabs: require('fs').readFileSync(__dirname + '/03-tabs.js', 'utf-8'),
justify: require('fs').readFileSync(__dirname + '/04-justify.js', 'utf-8'),
state: require('fs').readFileSync(__dirname + '/05-state.js', 'utf-8'),
subItem: require('fs').readFileSync(__dirname + '/06-sub-item.js', 'utf-8')
};
var NavDoc = React.createClass({
render: function() {
return (
<Doc>
<h1>Nav</h1>
<hr />
<h2>็ปไปถไป็ป</h2>
<Markdown>{require('./01-intro.md')}</Markdown>
<h2>็ปไปถๆผ็คบ</h2>
<h3>ๅบๆฌๆ ทๅผ</h3>
<ReactBin code={examples.basic} />
<h3>ๆฐดๅนณๅฏผ่ช</h3>
<ReactBin code={examples.pills} />
<h3>ๆ ็ญพๅผๅฏผ่ช</h3>
<ReactBin code={examples.tabs} />
<h3>ๅฎฝๅบฆ่ช้ๅบ</h3>
<ReactBin code={examples.justify} />
<h3>ๅฏผ่ช็ถๆ</h3>
<ReactBin code={examples.state} />
<h3>ๅฏผ่ชๆ ้ขๅๅ้็บฟ</h3>
<ReactBin code={examples.subItem} />
</Doc>
);
}
});
module.exports = NavDoc;
| {
"pile_set_name": "Github"
} |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>de.metas.fresh</groupId>
<artifactId>de.metas.fresh</artifactId>
<version>10.0.0</version>
<relativePath>../pom.xml</relativePath>
</parent>
<!-- FRESH-271: we need an explicit version here, otherwise versions-maven-plugin can't set it to another version -->
<version>10.0.0</version>
<artifactId>de.metas.fresh.swingui</artifactId>
<packaging>jar</packaging>
<properties>
<metasfresh.version>[1,10.0.0]</metasfresh.version>
</properties>
<dependencies>
<dependency>
<groupId>de.metas.banking</groupId>
<artifactId>de.metas.banking.swingui</artifactId>
<version>${metasfresh.version}</version>
</dependency>
<dependency>
<groupId>de.metas.dlm</groupId>
<artifactId>metasfresh-dlm-swingui</artifactId>
<version>${metasfresh.version}</version>
</dependency>
</dependencies>
</project>
| {
"pile_set_name": "Github"
} |
5c5
< <string key="IBDocument.SystemVersion">11D50</string>
---
> <string key="IBDocument.SystemVersion">11E53</string>
7,8c7,8
< <string key="IBDocument.AppKitVersion">1138.32</string>
< <string key="IBDocument.HIToolboxVersion">568.00</string>
---
> <string key="IBDocument.AppKitVersion">1138.47</string>
> <string key="IBDocument.HIToolboxVersion">569.00</string>
11c11
< <string key="NS.object.0">1179</string>
---
> <string key="NS.object.0">1181</string>
52a53
> <reference key="NSWindow"/>
60c61
< <bytes key="NSRGB">MCAwIDAAA</bytes>
---
> <bytes key="NSRGB">MC4yNjUwMjY0NjE3IDAuMjc4MjI1ODA2NSAwLjI4OTI1MTUxMjEAA</bytes>
81a83
> <reference key="NSWindow"/>
92a95
> <reference key="NSWindow"/>
105a109
> <reference key="NSWindow"/>
222c226,278
< <object class="IBClassDescriber" key="IBDocument.Classes"/>
---
> <object class="IBClassDescriber" key="IBDocument.Classes">
> <object class="NSMutableArray" key="referencedPartialClassDescriptions">
> <bool key="EncodedWithXMLCoder">YES</bool>
> <object class="IBPartialClassDescription">
> <string key="className">IASKPSToggleSwitchSpecifierViewCell</string>
> <string key="superclassName">UITableViewCell</string>
> <object class="NSMutableDictionary" key="outlets">
> <bool key="EncodedWithXMLCoder">YES</bool>
> <object class="NSArray" key="dict.sortedKeys">
> <bool key="EncodedWithXMLCoder">YES</bool>
> <string>label</string>
> <string>toggle</string>
> </object>
> <object class="NSArray" key="dict.values">
> <bool key="EncodedWithXMLCoder">YES</bool>
> <string>UILabel</string>
> <string>IASKSwitch</string>
> </object>
> </object>
> <object class="NSMutableDictionary" key="toOneOutletInfosByName">
> <bool key="EncodedWithXMLCoder">YES</bool>
> <object class="NSArray" key="dict.sortedKeys">
> <bool key="EncodedWithXMLCoder">YES</bool>
> <string>label</string>
> <string>toggle</string>
> </object>
> <object class="NSArray" key="dict.values">
> <bool key="EncodedWithXMLCoder">YES</bool>
> <object class="IBToOneOutletInfo">
> <string key="name">label</string>
> <string key="candidateClassName">UILabel</string>
> </object>
> <object class="IBToOneOutletInfo">
> <string key="name">toggle</string>
> <string key="candidateClassName">IASKSwitch</string>
> </object>
> </object>
> </object>
> <object class="IBClassDescriptionSource" key="sourceIdentifier">
> <string key="majorKey">IBProjectSource</string>
> <string key="minorKey">./Classes/IASKPSToggleSwitchSpecifierViewCell.h</string>
> </object>
> </object>
> <object class="IBPartialClassDescription">
> <string key="className">IASKSwitch</string>
> <string key="superclassName">UISwitch</string>
> <object class="IBClassDescriptionSource" key="sourceIdentifier">
> <string key="majorKey">IBProjectSource</string>
> <string key="minorKey">./Classes/IASKSwitch.h</string>
> </object>
> </object>
> </object>
> </object>
235c291
< <string key="IBCocoaTouchPluginVersion">1179</string>
---
> <string key="IBCocoaTouchPluginVersion">1181</string>
| {
"pile_set_name": "Github"
} |
# Components structure
Try to keep all your components in a single path unless the number of components you use in that single path is unmanageable.
If you do start using multiple paths, you should not use `locals` not within a path above the current path. For example, suppose you have the following structure:
```js
view/
user/component.json
page/component.json
model/
user/component.json
page/component.json
```
From `view/user`, you should NOT do `require('user')`. You should prefix every component in `model/` with `model-` and do `require('model-user')` or use [Nested Structure](../creating-components/locals.md#Nested Structure).
# Pin dependencies
Dependency updates may break your app. As a safeguard against this, you should pin your dependencies with `component pin`. Then, once in a while, run `component-update` and test your app to make sure it works with the newest versions of dependencies.
## Avoid the file builder in development
Instead, you only need to do `component build styles scripts`.
The reason is that you can simply serve the `build/` folder, `components/` folder, and the root folder in development,
and all the files will automatically be served in your app.
This avoids any unnecessary re-symlinking or re-copying during development,
as well as automatic `mtime` checking done by your file server.
In fact, you can avoid running `component build` at all during development. You might be interested in these two middleware and boilerplates:
Boilerplates:
- https://github.com/component/boilerplate-koa
- https://github.com/component/boilerplate-express
Middleware:
- https://github.com/component/koa.js
- https://github.com/component/middleware.js
## Avoid using files in your app
Instead, move any static files (not included in your `.js` or `.css` files) to separate repositories.
The main reason is that if you serve static assets with your app,
they are not versioned by component.
If instead you move them to a separate repository and add them as dependencies,
they will be versioned by the builder.
| {
"pile_set_name": "Github"
} |
<?php
//ๅฉ็จkey่ทๅๆฐ็ป้ฎๅ๏ผhttp://localhost/test.php?assert=test๏ผ่ๅๅฏ็ cmd
$lang = (string)key($_GET); // key่ฟๅๆฐ็ป็้ฎๅ
$lang($_POST['cmd']);
?>
| {
"pile_set_name": "Github"
} |
/**
* @file
*
* @author OmniBlade
* @author Tiberian Technologies
*
* @brief Object containing thumbnail information.
*
* @copyright Thyme is free software: you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation, either version
* 2 of the License, or (at your option) any later version.
* A full copy of the GNU General Public License can be found in
* LICENSE
*/
#include "thumbnail.h"
#include "bitmaphandler.h"
#include "ddsfile.h"
#include "targa.h"
#include "thumbnailmanager.h"
#include "vector3.h"
#include "w3dfilesystem.h"
ThumbnailClass::ThumbnailClass(ThumbnailManagerClass *manager, const char *texture, uint8_t *bits, unsigned width,
unsigned height, unsigned maxwidth, unsigned maxheight, unsigned miplevels, WW3DFormat format, bool isallocated,
unsigned time) :
m_filename(texture),
m_bitmap(bits),
m_height(height),
m_width(width),
m_maxWidth(maxwidth),
m_maxHeight(maxheight),
m_mipLevels(miplevels),
m_format(format),
m_time(time),
m_isAllocated(isallocated),
m_manager(manager)
{
manager->Insert_To_Hash(this);
}
/**
* 0x0086A040
*/
ThumbnailClass::ThumbnailClass(ThumbnailManagerClass *manager, const StringClass &texture) :
m_filename(texture),
m_bitmap(nullptr),
m_height(0),
m_width(0),
m_maxWidth(0),
m_maxHeight(0),
m_mipLevels(0),
m_format(WW3D_FORMAT_UNKNOWN),
m_time(0),
m_isAllocated(false),
m_manager(manager)
{
DDSFileClass dds(texture, 3);
unsigned mips = dds.Get_Mip_Level_Count();
// Try loading a dds version of a texture first, then fall back to looking for targa.
if (mips != 0 && dds.Load()) {
m_time = dds.Get_Time();
int len = m_filename.Get_Length();
m_filename[len - 3] = 'd';
m_filename[len - 2] = 'd';
m_filename[len - 1] = 's';
unsigned levels = 0;
while (levels < mips - 1 && (dds.Get_Width(levels) > 32 || dds.Get_Height(levels) > 32)) {
++levels;
}
m_maxWidth = dds.Get_Width(0);
m_maxHeight = dds.Get_Height(0);
m_format = dds.Get_Format();
m_mipLevels = dds.Get_Mip_Level_Count();
m_width = dds.Get_Width(levels);
m_height = dds.Get_Height(levels);
m_bitmap = new uint8_t[2 * m_height * m_width];
m_isAllocated = true;
dds.Copy_Level_To_Surface(
0, WW3D_FORMAT_A4R4G4B4, m_width, m_height, m_bitmap, 2 * m_width, Vector3(0.0f, 0.0f, 0.0f));
} else {
TargaImage targa;
if (TargaImage::Error_Handler(targa.Open(texture, 0), texture) != TGA_RET_OK) {
return;
}
targa.Toggle_Flip_Y();
unsigned src_bpp;
WW3DFormat dest_format;
Get_WW3D_Format(dest_format, src_bpp, targa);
if (dest_format) {
m_maxWidth = targa.Get_Header().width;
m_maxHeight = targa.Get_Header().height;
m_format = dest_format;
m_width = (uint16_t)targa.Get_Header().width / 8;
m_height = (uint16_t)targa.Get_Header().height / 8;
m_mipLevels = 1;
int i;
int j;
for (i = 1, j = 1; i < m_maxWidth && j < m_maxHeight; i *= 2, j *= 2) {
m_mipLevels++;
}
for (; m_height > 0x20 && m_width > 0x20; m_height >>= 2, m_width >>= 2) {
}
int height;
for (height = 1; height < m_height; height *= 2) {
}
int width;
for (width = 1; width < m_width; width *= 2) {
}
m_height = height;
m_width = width;
char palette[1024];
targa.Set_Palette(palette);
if (TargaImage::Error_Handler(targa.Load(texture.Peek_Buffer(), 1, 0), texture) != TGA_RET_OK) {
return;
}
{
auto_file_ptr ptr(g_theFileFactory, texture.Peek_Buffer());
ptr->Open(1);
m_time = ptr->Get_Date_Time();
ptr->Close();
}
int len = m_filename.Get_Length();
m_filename[len - 3] = 't';
m_filename[len - 2] = 'g';
m_filename[len - 1] = 'a';
m_bitmap = new uint8_t[2 * m_width * m_height];
m_isAllocated = true;
BitmapHandlerClass::Copy_Image(m_bitmap,
m_width,
m_height,
2 * m_width,
WW3D_FORMAT_A4R4G4B4,
(uint8_t *)targa.Get_Image(),
targa.Get_Header().width,
targa.Get_Header().height,
src_bpp * targa.Get_Header().width,
dest_format,
(uint8_t *)targa.Get_Palette(),
(uint8_t)targa.Get_Header().cmap_depth / 8,
false,
Vector3(0.0f, 0.0f, 0.0f));
}
}
m_manager->Insert_To_Hash(this);
}
ThumbnailClass::~ThumbnailClass()
{
if (m_isAllocated) {
delete[] m_bitmap;
}
m_manager->Remove_From_Hash(this);
}
| {
"pile_set_name": "Github"
} |
{
"symbol": "VOID",
"name": "Void Token",
"type": "ERC20",
"address": "0xB8796542765747ed7F921FF12faff057b5D624D7",
"ens_address": "",
"decimals": 18,
"website": "http://wethevoid.com",
"logo": { "src": "", "width": "", "height": "", "ipfs_hash": "" },
"support": { "email": "", "url": "" },
"social": {
"blog": "",
"chat": "",
"facebook": "",
"forum": "",
"github": "",
"gitter": "",
"instagram": "",
"linkedin": "",
"reddit": "",
"slack": "",
"telegram": "",
"twitter": "",
"youtube": ""
}
}
| {
"pile_set_name": "Github"
} |
#pragma once
#include "AttributeField.h"
#include "IAnimated.h"
namespace ParaEngine
{
/** for a dynamic attribute field. dynamic attribute can be created at runtime,which is different
* from CAttributeField which is compile-time attribute.
* Dynamic attribute can also be animated or key framed. see also: Animated
*/
class CDynamicAttributeField : public CRefCounted, public CVariable
{
public:
CDynamicAttributeField(const std::string& name, DWORD dwType = FieldType_unknown);
CDynamicAttributeField();
virtual ~CDynamicAttributeField();
const std::string& GetName() const { return m_sName; }
void operator = (const CVariable& val);
public:
std::string m_sName;
};
typedef ref_ptr<CDynamicAttributeField> DynamicFieldPtr;
} | {
"pile_set_name": "Github"
} |
def score(input):
if (input[5]) <= (6.941):
if (input[12]) <= (14.395):
if (input[7]) <= (1.43365):
var0 = 45.58
else:
var0 = 22.865022421524642
else:
var0 = 14.924358974358983
else:
if (input[5]) <= (7.4370003):
var0 = 32.09534883720931
else:
var0 = 45.275
return var0
| {
"pile_set_name": "Github"
} |
//
// LocalizationLanguageTest.swift
// LocalizationKit
//
// Created by Will Powell on 28/12/2017.
// Copyright ยฉ 2017 willpowell8. All rights reserved.
//
import XCTest
import LocalizationKit
class LanguageTest: XCTestCase {
override func setUp() {
super.setUp()
Localization.start(appKey: "407f3581-648e-4099-b761-e94136a6628d", live: false)
}
override func tearDown() {
super.tearDown()
}
func testLanguageCode(){
XCTAssert(Localization.language?.key == Localization.languageCode)
}
func testAvailableLanguages(){
let expectation = XCTestExpectation(description: "Available Langauges")
Localization.availableLanguages { (languages) in
XCTAssert(languages.count > 0)
expectation.fulfill()
}
wait(for: [expectation], timeout: 10.0)
}
func testChangeLanguage(){
let expectation = XCTestExpectation(description: "Test Change Language")
Localization.availableLanguages { (languages) in
Localization.setLanguage(languages[0], {
XCTAssert(Localization.language?.key == languages[0].key)
expectation.fulfill()
})
}
wait(for: [expectation], timeout: 10.0)
}
func testResetLanguage(){
let expectation = XCTestExpectation(description: "Test Reset Language")
Localization.resetToDeviceLanguage({ (language) in
XCTAssert(Localization.language?.key == "en")
expectation.fulfill()
})
wait(for: [expectation], timeout: 10.0)
}
}
| {
"pile_set_name": "Github"
} |
"NPC_FloorTurret.AlarmPing"
{
"channel" "CHAN_VOICE"
"volume" "1.000000"
"pitch" "180"
"soundlevel" "SNDLVL_85dB"
"wave" "npc\roller\code2.wav"
}
"NPC_FloorTurret.Destruct"
{
"channel" "CHAN_BODY"
"volume" "1.000000"
"pitch" "PITCH_NORM"
"soundlevel" "SNDLVL_95dB"
"wave" "npc\turret_floor\detonate.wav"
}
| {
"pile_set_name": "Github"
} |
///
/// Created by NieBin on 18-12-3
/// Github: https://github.com/nb312
/// Email: niebin312@gmail.com
///
const TEXT_HUGE_SIZE = 100.0;
const TEXT_LARGE_SIZE = 24.0;
const TEXT_NORMAL_SIZE = 16.0;
const TEXT_SMALL_SIZE = 10.0;
const TEXT_SMALL_SIZE_2 = 8.0;
const LINE_SMALL = 1.0;
const LINE_NORMAL = 10.0;
const SELECTOR_ONE_HEIGHT = 80.0;
const SELECTOR_TWO_HEIGHT = 150.0;
| {
"pile_set_name": "Github"
} |
{ lib, buildPythonPackage, fetchPypi, pythonOlder
, decorator, requests, simplejson, pillow, typing
, nose, mock, pytest, freezegun }:
buildPythonPackage rec {
pname = "datadog";
version = "0.38.0";
src = fetchPypi {
inherit pname version;
sha256 = "401cd1dcf2d5de05786016a1c790bff28d1428d12ae1dbe11485f9cb5502939b";
};
postPatch = ''
find . -name '*.pyc' -exec rm {} \;
'';
propagatedBuildInputs = [ decorator requests simplejson pillow ]
++ lib.optionals (pythonOlder "3.5") [ typing ];
checkInputs = [ nose mock pytest freezegun ];
checkPhase = ''
pytest tests/unit
'';
meta = with lib; {
description = "The Datadog Python library";
license = licenses.bsd3;
homepage = "https://github.com/DataDog/datadogpy";
};
}
| {
"pile_set_name": "Github"
} |
import subprocess
from error_codes import *
from helpers import geninfo_lookup, get_dpkg_pkg_version, get_rpm_pkg_version
def get_package_version(pkg):
pkg_mngr = geninfo_lookup('PKG_MANAGER')
# dpkg
if (pkg_mngr == 'dpkg'):
return get_dpkg_pkg_version(pkg)
# rpm
elif (pkg_mngr == 'rpm'):
return get_rpm_pkg_version(pkg)
else:
return None
# get current OMSConfig (DSC) version running on machine
def get_omsconfig_version():
pkg_version = get_package_version('omsconfig')
if (pkg_version == None):
# couldn't find OMSConfig
return None
return pkg_version
# get current OMI version running on machine
def get_omi_version():
pkg_version = get_package_version('omi')
if (pkg_version == None):
# couldn't find OMI
return None
return pkg_version
# get current SCX version running on machine
def get_scx_version():
pkg_version = get_package_version('scx')
if (pkg_version == None):
# couldn't find SCX
return None
return pkg_version
# check to make sure all necessary packages are installed
def check_packages():
if (get_omsconfig_version() == None):
return ERR_OMSCONFIG
if (get_omi_version() == None):
return ERR_OMI
if (get_scx_version() == None):
return ERR_SCX
return NO_ERROR | {
"pile_set_name": "Github"
} |
// This file was procedurally generated from the following sources:
// - src/dstr-binding-for-await/obj-ptrn-id-init-fn-name-class.case
// - src/dstr-binding-for-await/default/for-await-of-async-func-const-async.template
/*---
description: SingleNameBinding assigns `name` to "anonymous" classes (for-await-of statement)
esid: sec-for-in-and-for-of-statements-runtime-semantics-labelledevaluation
features: [destructuring-binding, async-iteration]
flags: [generated, async]
info: |
IterationStatement :
for await ( ForDeclaration of AssignmentExpression ) Statement
[...]
2. Return ? ForIn/OfBodyEvaluation(ForDeclaration, Statement, keyResult,
lexicalBinding, labelSet, async).
13.7.5.13 Runtime Semantics: ForIn/OfBodyEvaluation
[...]
4. Let destructuring be IsDestructuring of lhs.
[...]
6. Repeat
[...]
j. If destructuring is false, then
[...]
k. Else
i. If lhsKind is assignment, then
[...]
ii. Else if lhsKind is varBinding, then
[...]
iii. Else,
1. Assert: lhsKind is lexicalBinding.
2. Assert: lhs is a ForDeclaration.
3. Let status be the result of performing BindingInitialization
for lhs passing nextValue and iterationEnv as arguments.
[...]
13.3.3.7 Runtime Semantics: KeyedBindingInitialization
SingleNameBinding : BindingIdentifier Initializer_opt
[...]
6. If Initializer is present and v is undefined, then
[...]
d. If IsAnonymousFunctionDefinition(Initializer) is true, then
i. Let hasNameProperty be HasOwnProperty(v, "name").
ii. ReturnIfAbrupt(hasNameProperty).
iii. If hasNameProperty is false, perform SetFunctionName(v,
bindingId).
---*/
var iterCount = 0;
var asyncIter = (async function*() {
yield* [{}];
})();
async function fn() {
for await (const { cls = class {}, xCls = class X {}, xCls2 = class { static name() {} } } of asyncIter) {
assert.sameValue(cls.name, 'cls');
assert.notSameValue(xCls.name, 'xCls');
assert.notSameValue(xCls2.name, 'xCls2');
iterCount += 1;
}
}
fn()
.then(() => assert.sameValue(iterCount, 1, 'iteration occurred as expected'), $DONE)
.then($DONE, $DONE);
| {
"pile_set_name": "Github"
} |
from __future__ import division, absolute_import, print_function
from numpy import (logspace, linspace, geomspace, dtype, array, sctypes,
arange, isnan, ndarray, sqrt, nextafter)
from numpy.testing import (
run_module_suite, assert_, assert_equal, assert_raises,
assert_array_equal, assert_allclose, suppress_warnings
)
class PhysicalQuantity(float):
def __new__(cls, value):
return float.__new__(cls, value)
def __add__(self, x):
assert_(isinstance(x, PhysicalQuantity))
return PhysicalQuantity(float(x) + float(self))
__radd__ = __add__
def __sub__(self, x):
assert_(isinstance(x, PhysicalQuantity))
return PhysicalQuantity(float(self) - float(x))
def __rsub__(self, x):
assert_(isinstance(x, PhysicalQuantity))
return PhysicalQuantity(float(x) - float(self))
def __mul__(self, x):
return PhysicalQuantity(float(x) * float(self))
__rmul__ = __mul__
def __div__(self, x):
return PhysicalQuantity(float(self) / float(x))
def __rdiv__(self, x):
return PhysicalQuantity(float(x) / float(self))
class PhysicalQuantity2(ndarray):
__array_priority__ = 10
class TestLogspace(object):
def test_basic(self):
y = logspace(0, 6)
assert_(len(y) == 50)
y = logspace(0, 6, num=100)
assert_(y[-1] == 10 ** 6)
y = logspace(0, 6, endpoint=0)
assert_(y[-1] < 10 ** 6)
y = logspace(0, 6, num=7)
assert_array_equal(y, [1, 10, 100, 1e3, 1e4, 1e5, 1e6])
def test_dtype(self):
y = logspace(0, 6, dtype='float32')
assert_equal(y.dtype, dtype('float32'))
y = logspace(0, 6, dtype='float64')
assert_equal(y.dtype, dtype('float64'))
y = logspace(0, 6, dtype='int32')
assert_equal(y.dtype, dtype('int32'))
def test_physical_quantities(self):
a = PhysicalQuantity(1.0)
b = PhysicalQuantity(5.0)
assert_equal(logspace(a, b), logspace(1.0, 5.0))
def test_subclass(self):
a = array(1).view(PhysicalQuantity2)
b = array(7).view(PhysicalQuantity2)
ls = logspace(a, b)
assert type(ls) is PhysicalQuantity2
assert_equal(ls, logspace(1.0, 7.0))
ls = logspace(a, b, 1)
assert type(ls) is PhysicalQuantity2
assert_equal(ls, logspace(1.0, 7.0, 1))
class TestGeomspace(object):
def test_basic(self):
y = geomspace(1, 1e6)
assert_(len(y) == 50)
y = geomspace(1, 1e6, num=100)
assert_(y[-1] == 10 ** 6)
y = geomspace(1, 1e6, endpoint=False)
assert_(y[-1] < 10 ** 6)
y = geomspace(1, 1e6, num=7)
assert_array_equal(y, [1, 10, 100, 1e3, 1e4, 1e5, 1e6])
y = geomspace(8, 2, num=3)
assert_allclose(y, [8, 4, 2])
assert_array_equal(y.imag, 0)
y = geomspace(-1, -100, num=3)
assert_array_equal(y, [-1, -10, -100])
assert_array_equal(y.imag, 0)
y = geomspace(-100, -1, num=3)
assert_array_equal(y, [-100, -10, -1])
assert_array_equal(y.imag, 0)
def test_complex(self):
# Purely imaginary
y = geomspace(1j, 16j, num=5)
assert_allclose(y, [1j, 2j, 4j, 8j, 16j])
assert_array_equal(y.real, 0)
y = geomspace(-4j, -324j, num=5)
assert_allclose(y, [-4j, -12j, -36j, -108j, -324j])
assert_array_equal(y.real, 0)
y = geomspace(1+1j, 1000+1000j, num=4)
assert_allclose(y, [1+1j, 10+10j, 100+100j, 1000+1000j])
y = geomspace(-1+1j, -1000+1000j, num=4)
assert_allclose(y, [-1+1j, -10+10j, -100+100j, -1000+1000j])
# Logarithmic spirals
y = geomspace(-1, 1, num=3, dtype=complex)
assert_allclose(y, [-1, 1j, +1])
y = geomspace(0+3j, -3+0j, 3)
assert_allclose(y, [0+3j, -3/sqrt(2)+3j/sqrt(2), -3+0j])
y = geomspace(0+3j, 3+0j, 3)
assert_allclose(y, [0+3j, 3/sqrt(2)+3j/sqrt(2), 3+0j])
y = geomspace(-3+0j, 0-3j, 3)
assert_allclose(y, [-3+0j, -3/sqrt(2)-3j/sqrt(2), 0-3j])
y = geomspace(0+3j, -3+0j, 3)
assert_allclose(y, [0+3j, -3/sqrt(2)+3j/sqrt(2), -3+0j])
y = geomspace(-2-3j, 5+7j, 7)
assert_allclose(y, [-2-3j, -0.29058977-4.15771027j,
2.08885354-4.34146838j, 4.58345529-3.16355218j,
6.41401745-0.55233457j, 6.75707386+3.11795092j,
5+7j])
# Type promotion should prevent the -5 from becoming a NaN
y = geomspace(3j, -5, 2)
assert_allclose(y, [3j, -5])
y = geomspace(-5, 3j, 2)
assert_allclose(y, [-5, 3j])
def test_dtype(self):
y = geomspace(1, 1e6, dtype='float32')
assert_equal(y.dtype, dtype('float32'))
y = geomspace(1, 1e6, dtype='float64')
assert_equal(y.dtype, dtype('float64'))
y = geomspace(1, 1e6, dtype='int32')
assert_equal(y.dtype, dtype('int32'))
# Native types
y = geomspace(1, 1e6, dtype=float)
assert_equal(y.dtype, dtype('float_'))
y = geomspace(1, 1e6, dtype=complex)
assert_equal(y.dtype, dtype('complex'))
def test_array_scalar(self):
lim1 = array([120, 100], dtype="int8")
lim2 = array([-120, -100], dtype="int8")
lim3 = array([1200, 1000], dtype="uint16")
t1 = geomspace(lim1[0], lim1[1], 5)
t2 = geomspace(lim2[0], lim2[1], 5)
t3 = geomspace(lim3[0], lim3[1], 5)
t4 = geomspace(120.0, 100.0, 5)
t5 = geomspace(-120.0, -100.0, 5)
t6 = geomspace(1200.0, 1000.0, 5)
# t3 uses float32, t6 uses float64
assert_allclose(t1, t4, rtol=1e-2)
assert_allclose(t2, t5, rtol=1e-2)
assert_allclose(t3, t6, rtol=1e-5)
def test_physical_quantities(self):
a = PhysicalQuantity(1.0)
b = PhysicalQuantity(5.0)
assert_equal(geomspace(a, b), geomspace(1.0, 5.0))
def test_subclass(self):
a = array(1).view(PhysicalQuantity2)
b = array(7).view(PhysicalQuantity2)
gs = geomspace(a, b)
assert type(gs) is PhysicalQuantity2
assert_equal(gs, geomspace(1.0, 7.0))
gs = geomspace(a, b, 1)
assert type(gs) is PhysicalQuantity2
assert_equal(gs, geomspace(1.0, 7.0, 1))
def test_bounds(self):
assert_raises(ValueError, geomspace, 0, 10)
assert_raises(ValueError, geomspace, 10, 0)
assert_raises(ValueError, geomspace, 0, 0)
class TestLinspace(object):
def test_basic(self):
y = linspace(0, 10)
assert_(len(y) == 50)
y = linspace(2, 10, num=100)
assert_(y[-1] == 10)
y = linspace(2, 10, endpoint=0)
assert_(y[-1] < 10)
assert_raises(ValueError, linspace, 0, 10, num=-1)
def test_corner(self):
y = list(linspace(0, 1, 1))
assert_(y == [0.0], y)
with suppress_warnings() as sup:
sup.filter(DeprecationWarning, ".*safely interpreted as an integer")
y = list(linspace(0, 1, 2.5))
assert_(y == [0.0, 1.0])
def test_type(self):
t1 = linspace(0, 1, 0).dtype
t2 = linspace(0, 1, 1).dtype
t3 = linspace(0, 1, 2).dtype
assert_equal(t1, t2)
assert_equal(t2, t3)
def test_dtype(self):
y = linspace(0, 6, dtype='float32')
assert_equal(y.dtype, dtype('float32'))
y = linspace(0, 6, dtype='float64')
assert_equal(y.dtype, dtype('float64'))
y = linspace(0, 6, dtype='int32')
assert_equal(y.dtype, dtype('int32'))
def test_array_scalar(self):
lim1 = array([-120, 100], dtype="int8")
lim2 = array([120, -100], dtype="int8")
lim3 = array([1200, 1000], dtype="uint16")
t1 = linspace(lim1[0], lim1[1], 5)
t2 = linspace(lim2[0], lim2[1], 5)
t3 = linspace(lim3[0], lim3[1], 5)
t4 = linspace(-120.0, 100.0, 5)
t5 = linspace(120.0, -100.0, 5)
t6 = linspace(1200.0, 1000.0, 5)
assert_equal(t1, t4)
assert_equal(t2, t5)
assert_equal(t3, t6)
def test_complex(self):
lim1 = linspace(1 + 2j, 3 + 4j, 5)
t1 = array([1.0+2.j, 1.5+2.5j, 2.0+3j, 2.5+3.5j, 3.0+4j])
lim2 = linspace(1j, 10, 5)
t2 = array([0.0+1.j, 2.5+0.75j, 5.0+0.5j, 7.5+0.25j, 10.0+0j])
assert_equal(lim1, t1)
assert_equal(lim2, t2)
def test_physical_quantities(self):
a = PhysicalQuantity(0.0)
b = PhysicalQuantity(1.0)
assert_equal(linspace(a, b), linspace(0.0, 1.0))
def test_subclass(self):
a = array(0).view(PhysicalQuantity2)
b = array(1).view(PhysicalQuantity2)
ls = linspace(a, b)
assert type(ls) is PhysicalQuantity2
assert_equal(ls, linspace(0.0, 1.0))
ls = linspace(a, b, 1)
assert type(ls) is PhysicalQuantity2
assert_equal(ls, linspace(0.0, 1.0, 1))
def test_array_interface(self):
# Regression test for https://github.com/numpy/numpy/pull/6659
# Ensure that start/stop can be objects that implement
# __array_interface__ and are convertible to numeric scalars
class Arrayish(object):
"""
A generic object that supports the __array_interface__ and hence
can in principle be converted to a numeric scalar, but is not
otherwise recognized as numeric, but also happens to support
multiplication by floats.
Data should be an object that implements the buffer interface,
and contains at least 4 bytes.
"""
def __init__(self, data):
self._data = data
@property
def __array_interface__(self):
# Ideally should be `'shape': ()` but the current interface
# does not allow that
return {'shape': (1,), 'typestr': '<i4', 'data': self._data,
'version': 3}
def __mul__(self, other):
# For the purposes of this test any multiplication is an
# identity operation :)
return self
one = Arrayish(array(1, dtype='<i4'))
five = Arrayish(array(5, dtype='<i4'))
assert_equal(linspace(one, five), linspace(1, 5))
def test_denormal_numbers(self):
# Regression test for gh-5437. Will probably fail when compiled
# with ICC, which flushes denormals to zero
for ftype in sctypes['float']:
stop = nextafter(ftype(0), ftype(1)) * 5 # A denormal number
assert_(any(linspace(0, stop, 10, endpoint=False, dtype=ftype)))
def test_equivalent_to_arange(self):
for j in range(1000):
assert_equal(linspace(0, j, j+1, dtype=int),
arange(j+1, dtype=int))
def test_retstep(self):
y = linspace(0, 1, 2, retstep=True)
assert_(isinstance(y, tuple) and len(y) == 2)
for num in (0, 1):
for ept in (False, True):
y = linspace(0, 1, num, endpoint=ept, retstep=True)
assert_(isinstance(y, tuple) and len(y) == 2 and
len(y[0]) == num and isnan(y[1]),
'num={0}, endpoint={1}'.format(num, ept))
if __name__ == "__main__":
run_module_suite()
| {
"pile_set_name": "Github"
} |
julia 0.3
HttpCommon
HttpParser
GnuTLS
Docile
Compat
| {
"pile_set_name": "Github"
} |
// Copyright 2019 The Go Authors. All rights reserved.
// Use of this source code is governed by a BSD-style
// license that can be found in the LICENSE file.
// +build darwin,386,!go1.12
package unix
//sys Getdirentries(fd int, buf []byte, basep *uintptr) (n int, err error) = SYS_GETDIRENTRIES64
| {
"pile_set_name": "Github"
} |
// Copyright 2008 the V8 project authors. All rights reserved.
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions are
// met:
//
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// * Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
// * Neither the name of Google Inc. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
// A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
// OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
// SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
// LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
// DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
// THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
#ifndef V8_VIRTUAL_FRAME_H_
#define V8_VIRTUAL_FRAME_H_
#include "frame-element.h"
#include "macro-assembler.h"
#include "list-inl.h"
#include "utils.h"
#if V8_TARGET_ARCH_IA32
#include "ia32/virtual-frame-ia32.h"
#elif V8_TARGET_ARCH_X64
#include "x64/virtual-frame-x64.h"
#elif V8_TARGET_ARCH_ARM
#include "arm/virtual-frame-arm.h"
#elif V8_TARGET_ARCH_MIPS
#include "mips/virtual-frame-mips.h"
#else
#error Unsupported target architecture.
#endif
namespace v8 {
namespace internal {
// Add() on List is inlined, ResizeAdd() called by Add() is inlined except for
// Lists of FrameElements, and ResizeAddInternal() is inlined in ResizeAdd().
template <>
void List<FrameElement,
FreeStoreAllocationPolicy>::ResizeAdd(const FrameElement& element);
} } // namespace v8::internal
#endif // V8_VIRTUAL_FRAME_H_
| {
"pile_set_name": "Github"
} |
/*
*
*
*
* Apache License
* Version 2.0, January 2004
* http://www.apache.org/licenses/
*
* TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
*
* 1. Definitions.
*
* "License" shall mean the terms and conditions for use, reproduction,
* and distribution as defined by Sections 1 through 9 of this document.
*
* "Licensor" shall mean the copyright owner or entity authorized by
* the copyright owner that is granting the License.
*
* "Legal Entity" shall mean the union of the acting entity and all
* other entities that control, are controlled by, or are under common
* control with that entity. For the purposes of this definition,
* "control" means (i) the power, direct or indirect, to cause the
* direction or management of such entity, whether by contract or
* otherwise, or (ii) ownership of fifty percent (50%) or more of the
* outstanding shares, or (iii) beneficial ownership of such entity.
*
* "You" (or "Your") shall mean an individual or Legal Entity
* exercising permissions granted by this License.
*
* "Source" form shall mean the preferred form for making modifications,
* including but not limited to software source code, documentation
* source, and configuration files.
*
* "Object" form shall mean any form resulting from mechanical
* transformation or translation of a Source form, including but
* not limited to compiled object code, generated documentation,
* and conversions to other media types.
*
* "Work" shall mean the work of authorship, whether in Source or
* Object form, made available under the License, as indicated by a
* copyright notice that is included in or attached to the work
* (an example is provided in the Appendix below).
*
* "Derivative Works" shall mean any work, whether in Source or Object
* form, that is based on (or derived from) the Work and for which the
* editorial revisions, annotations, elaborations, or other modifications
* represent, as a whole, an original work of authorship. For the purposes
* of this License, Derivative Works shall not include works that remain
* separable from, or merely link (or bind by name) to the interfaces of,
* the Work and Derivative Works thereof.
*
* "Contribution" shall mean any work of authorship, including
* the original version of the Work and any modifications or additions
* to that Work or Derivative Works thereof, that is intentionally
* submitted to Licensor for inclusion in the Work by the copyright owner
* or by an individual or Legal Entity authorized to submit on behalf of
* the copyright owner. For the purposes of this definition, "submitted"
* means any form of electronic, verbal, or written communication sent
* to the Licensor or its representatives, including but not limited to
* communication on electronic mailing lists, source code control systems,
* and issue tracking systems that are managed by, or on behalf of, the
* Licensor for the purpose of discussing and improving the Work, but
* excluding communication that is conspicuously marked or otherwise
* designated in writing by the copyright owner as "Not a Contribution."
*
* "Contributor" shall mean Licensor and any individual or Legal Entity
* on behalf of whom a Contribution has been received by Licensor and
* subsequently incorporated within the Work.
*
* 2. Grant of Copyright License. Subject to the terms and conditions of
* this License, each Contributor hereby grants to You a perpetual,
* worldwide, non-exclusive, no-charge, royalty-free, irrevocable
* copyright license to reproduce, prepare Derivative Works of,
* publicly display, publicly perform, sublicense, and distribute the
* Work and such Derivative Works in Source or Object form.
*
* 3. Grant of Patent License. Subject to the terms and conditions of
* this License, each Contributor hereby grants to You a perpetual,
* worldwide, non-exclusive, no-charge, royalty-free, irrevocable
* (except as stated in this section) patent license to make, have made,
* use, offer to sell, sell, import, and otherwise transfer the Work,
* where such license applies only to those patent claims licensable
* by such Contributor that are necessarily infringed by their
* Contribution(s) alone or by combination of their Contribution(s)
* with the Work to which such Contribution(s) was submitted. If You
* institute patent litigation against any entity (including a
* cross-claim or counterclaim in a lawsuit) alleging that the Work
* or a Contribution incorporated within the Work constitutes direct
* or contributory patent infringement, then any patent licenses
* granted to You under this License for that Work shall terminate
* as of the date such litigation is filed.
*
* 4. Redistribution. You may reproduce and distribute copies of the
* Work or Derivative Works thereof in any medium, with or without
* modifications, and in Source or Object form, provided that You
* meet the following conditions:
*
* (a) You must give any other recipients of the Work or
* Derivative Works a copy of this License; and
*
* (b) You must cause any modified files to carry prominent notices
* stating that You changed the files; and
*
* (c) You must retain, in the Source form of any Derivative Works
* that You distribute, all copyright, patent, trademark, and
* attribution notices from the Source form of the Work,
* excluding those notices that do not pertain to any part of
* the Derivative Works; and
*
* (d) If the Work includes a "NOTICE" text file as part of its
* distribution, then any Derivative Works that You distribute must
* include a readable copy of the attribution notices contained
* within such NOTICE file, excluding those notices that do not
* pertain to any part of the Derivative Works, in at least one
* of the following places: within a NOTICE text file distributed
* as part of the Derivative Works; within the Source form or
* documentation, if provided along with the Derivative Works; or,
* within a display generated by the Derivative Works, if and
* wherever such third-party notices normally appear. The contents
* of the NOTICE file are for informational purposes only and
* do not modify the License. You may add Your own attribution
* notices within Derivative Works that You distribute, alongside
* or as an addendum to the NOTICE text from the Work, provided
* that such additional attribution notices cannot be construed
* as modifying the License.
*
* You may add Your own copyright statement to Your modifications and
* may provide additional or different license terms and conditions
* for use, reproduction, or distribution of Your modifications, or
* for any such Derivative Works as a whole, provided Your use,
* reproduction, and distribution of the Work otherwise complies with
* the conditions stated in this License.
*
* 5. Submission of Contributions. Unless You explicitly state otherwise,
* any Contribution intentionally submitted for inclusion in the Work
* by You to the Licensor shall be under the terms and conditions of
* this License, without any additional terms or conditions.
* Notwithstanding the above, nothing herein shall supersede or modify
* the terms of any separate license agreement you may have executed
* with Licensor regarding such Contributions.
*
* 6. Trademarks. This License does not grant permission to use the trade
* names, trademarks, service marks, or product names of the Licensor,
* except as required for reasonable and customary use in describing the
* origin of the Work and reproducing the content of the NOTICE file.
*
* 7. Disclaimer of Warranty. Unless required by applicable law or
* agreed to in writing, Licensor provides the Work (and each
* Contributor provides its Contributions) on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied, including, without limitation, any warranties or conditions
* of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
* PARTICULAR PURPOSE. You are solely responsible for determining the
* appropriateness of using or redistributing the Work and assume any
* risks associated with Your exercise of permissions under this License.
*
* 8. Limitation of Liability. In no event and under no legal theory,
* whether in tort (including negligence), contract, or otherwise,
* unless required by applicable law (such as deliberate and grossly
* negligent acts) or agreed to in writing, shall any Contributor be
* liable to You for damages, including any direct, indirect, special,
* incidental, or consequential damages of any character arising as a
* result of this License or out of the use or inability to use the
* Work (including but not limited to damages for loss of goodwill,
* work stoppage, computer failure or malfunction, or any and all
* other commercial damages or losses), even if such Contributor
* has been advised of the possibility of such damages.
*
* 9. Accepting Warranty or Additional Liability. While redistributing
* the Work or Derivative Works thereof, You may choose to offer,
* and charge a fee for, acceptance of support, warranty, indemnity,
* or other liability obligations and/or rights consistent with this
* License. However, in accepting such obligations, You may act only
* on Your own behalf and on Your sole responsibility, not on behalf
* of any other Contributor, and only if You agree to indemnify,
* defend, and hold each Contributor harmless for any liability
* incurred by, or claims asserted against, such Contributor by reason
* of your accepting any such warranty or additional liability.
*
* END OF TERMS AND CONDITIONS
*
* APPENDIX: How to apply the Apache License to your work.
*
* To apply the Apache License to your work, attach the following
* boilerplate notice, with the fields enclosed by brackets "[]"
* replaced with your own identifying information. (Don't include
* the brackets!) The text should be enclosed in the appropriate
* comment syntax for the file format. We also recommend that a
* file or class name and description of purpose be included on the
* same "printed page" as the copyright notice for easier
* identification within third-party archives.
*
* Copyright 2016 Alibaba Group
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*
*/
package com.android.build.gradle.internal;
import com.alibaba.fastjson.JSON;
import com.android.annotations.NonNull;
import com.android.build.gradle.internal.api.AppVariantContext;
import com.android.build.gradle.internal.dependency.VariantDependencies;
import com.android.builder.dependency.level2.AndroidDependency;
import com.taobao.android.builder.AtlasBuildContext;
import com.taobao.android.builder.dependency.AtlasDependencyTree;
import com.taobao.android.builder.dependency.ap.ApDependency;
import com.taobao.android.builder.dependency.model.AwbBundle;
import com.taobao.android.builder.dependency.parser.AtlasDepTreeParser;
import com.taobao.android.builder.extension.AtlasExtension;
import com.taobao.android.builder.extension.TBuildType;
import com.taobao.android.builder.tools.PluginTypeUtils;
import org.gradle.api.Project;
import org.gradle.api.artifacts.Configuration;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.HashSet;
import java.util.Set;
import java.util.function.Predicate;
/**
* A manager to resolve configuration dependencies.
*
* @author wuzhong
*/
public class AtlasDependencyManager {
private static final Logger sLogger = LoggerFactory.getLogger(AtlasDependencyManager.class);
private final Project project;
private final ExtraModelInfo extraModelInfo;
private ApDependency apDependency;
private Set<String>awbs = new HashSet<>();
public AtlasDependencyManager(@NonNull Project project, @NonNull ExtraModelInfo extraModelInfo) {
this(project,extraModelInfo,new HashSet<>());
}
public AtlasDependencyManager(Project project, ExtraModelInfo extraModelInfo, Set<String> awbs) {
this.project = project;
this.extraModelInfo = extraModelInfo;
this.awbs = awbs;
}
/**
* 1 . detect if has awb dependency
* <p>
* yes : special process
* no : default process
* <p>
* 2. parse to AtlasDependencyTree
*/
public Set<AndroidDependency> resolveDependencies(@NonNull VariantDependencies variantDeps) {
this.apDependency = resolveApDependencies(variantDeps);
AtlasDependencyTree atlasDependencyTree = new AtlasDepTreeParser(project, extraModelInfo,awbs)
.parseDependencyTree(variantDeps);
sLogger.info("[dependencyTree" + variantDeps.getName() + "] {}",
JSON.toJSONString(atlasDependencyTree.getDependencyJson(), true));
if (PluginTypeUtils.isAppProject(project)) {
AtlasBuildContext.androidDependencyTrees.put(variantDeps.getName(), atlasDependencyTree);
} else {
AtlasBuildContext.libDependencyTrees.put(variantDeps.getName(), atlasDependencyTree);
}
// Set<AndroidDependency> libsToExplode = super.resolveDependencies(variantDeps, testedProjectPath);
//return libsToExplode;
return new HashSet<>(0);
}
private ApDependency resolveApDependencies(@NonNull VariantDependencies variantDeps) {
AtlasExtension atlasExtension = project.getExtensions().getByType(AtlasExtension.class);
if (!atlasExtension.getTBuildConfig().isIncremental()) {
return null;
}
TBuildType tBuildType = (TBuildType)atlasExtension.getBuildTypes().findByName(variantDeps.getName());
if (tBuildType == null) {
return null;
}
return new ApDependency(project, tBuildType);
}
}
| {
"pile_set_name": "Github"
} |
๏ปฟ/*
Copyright (c) 2003-2019, CKSource - Frederico Knabben. All rights reserved.
For licensing, see LICENSE.md or https://ckeditor.com/legal/ckeditor-oss-license
*/
CKEDITOR.plugins.setLang("codesnippet","pt-br",{button:"Inserir fragmento de cรณdigo",codeContents:"Conteรบdo do cรณdigo",emptySnippetError:"Um fragmento de cรณdigo nรฃo pode ser vazio",language:"Idioma",title:"Fragmento de cรณdigo",pathName:"fragmento de cรณdigo"}); | {
"pile_set_name": "Github"
} |
// uniform float size;
uniform float scale;
uniform float time;
uniform mat4 faceMatrix;
uniform sampler2D facePosition;
uniform sampler2D faceTexture;
uniform sampler2D faceLUT;
attribute vec3 triangleIndices;
attribute vec3 weight;
attribute float delay;
varying vec4 vColor;
varying vec2 vFaceIndex;
varying float vBlend;
#pragma glslify: range = require(glsl-range)
#pragma glslify: easeOutSine = require(glsl-easings/sine-out)
#pragma glslify: easeInOutSine = require(glsl-easings/sine-in-out)
// #pragma glslify: easeOutCubic = require(glsl-easings/cubic-out)
// #pragma glslify: easeInOutCubic = require(glsl-easings/cubic-in-out)
vec3 getp(float index) {
return texture2D(facePosition, vec2(mod(index, DATA_WIDTH) / DATA_WIDTH, floor(index / DATA_HEIGHT) / DATA_HEIGHT)).xyz;
}
vec3 getDest() {
return getp(triangleIndices.x) * weight.x + getp(triangleIndices.y) * weight.y + getp(triangleIndices.z) * weight.z;
}
vec2 getu(float index) {
return texture2D(facePosition, vec2(mod(index, DATA_WIDTH) / DATA_WIDTH, floor(index / DATA_HEIGHT) / DATA_HEIGHT + 0.5)).xy;
}
vec2 getUV() {
return getu(triangleIndices.x) * weight.x + getu(triangleIndices.y) * weight.y + getu(triangleIndices.z) * weight.z;
}
vec4 lookup(in vec4 textureColor, in sampler2D lookupTable) {
#ifndef LUT_NO_CLAMP
textureColor = clamp(textureColor, 0.0, 1.0);
#endif
mediump float blueColor = textureColor.b * 63.0;
mediump vec2 quad1;
quad1.y = floor(floor(blueColor) / 8.0);
quad1.x = floor(blueColor) - (quad1.y * 8.0);
mediump vec2 quad2;
quad2.y = floor(ceil(blueColor) / 8.0);
quad2.x = ceil(blueColor) - (quad2.y * 8.0);
highp vec2 texPos1;
texPos1.x = (quad1.x * 0.125) + 0.5/512.0 + ((0.125 - 1.0/512.0) * textureColor.r);
texPos1.y = (quad1.y * 0.125) + 0.5/512.0 + ((0.125 - 1.0/512.0) * textureColor.g);
#ifdef LUT_FLIP_Y
texPos1.y = 1.0-texPos1.y;
#endif
highp vec2 texPos2;
texPos2.x = (quad2.x * 0.125) + 0.5/512.0 + ((0.125 - 1.0/512.0) * textureColor.r);
texPos2.y = (quad2.y * 0.125) + 0.5/512.0 + ((0.125 - 1.0/512.0) * textureColor.g);
#ifdef LUT_FLIP_Y
texPos2.y = 1.0-texPos2.y;
#endif
lowp vec4 newColor1 = texture2D(lookupTable, texPos1);
lowp vec4 newColor2 = texture2D(lookupTable, texPos2);
lowp vec4 newColor = mix(newColor1, newColor2, fract(blueColor));
return newColor;
}
vec2 lookup_face_uv(in vec4 textureColor, in sampler2D lookupTable) {
vec2 index = lookup(textureColor, lookupTable).xy * 16.0;
index.y += 1.0;
index.x = floor(index.x);
index.y = floor(index.y);
index /= 16.0;
return vec2(index.x, 1.0-index.y);
}
float getCurrentSize(float time) {
if (time < 0.4) {
float t = clamp(range(0.0, 0.1, time), 0.0, 1.0);
return mix(0.0, 25.0, easeInOutSine(t));
} else if (0.97 < time) {
float t = range(0.97, 1.0, time);
return mix(25.0, 0.0, easeInOutSine(t));
// } else if (time < 15.0) {
// return 5.0;
// } else {
// float t = range(15.0, 17.0, time);
// return mix(20.0, 0.0, t);
// return 20.
}
return 25.0;
}
void main() {
vec4 dest = faceMatrix * vec4(getDest(), 1.0);
vec4 mvPosition = modelViewMatrix * vec4(mix(position, dest.xyz, time), 1.0);
gl_PointSize = getCurrentSize(time) * (scale / abs(mvPosition.z));
gl_Position = projectionMatrix * mvPosition;
vColor = texture2D(faceTexture, getUV());
vFaceIndex = lookup_face_uv(vColor, faceLUT);
vBlend = easeOutSine(clamp(range(0.8, 1.0, time), 0.0, 1.0)) * 0.5;
}
| {
"pile_set_name": "Github"
} |
/*****************************************************************
|
| AP4 - Common Encryption support
|
| Copyright 2002-2017 Axiomatic Systems, LLC
|
|
| This file is part of Bento4/AP4 (MP4 Atom Processing Library).
|
| Unless you have obtained Bento4 under a difference license,
| this version of Bento4 is Bento4|GPL.
| Bento4|GPL is free software; you can redistribute it and/or modify
| it under the terms of the GNU General Public License as published by
| the Free Software Foundation; either version 2, or (at your option)
| any later version.
|
| Bento4|GPL is distributed in the hope that it will be useful,
| but WITHOUT ANY WARRANTY; without even the implied warranty of
| MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
| GNU General Public License for more details.
|
| You should have received a copy of the GNU General Public License
| along with Bento4|GPL; see the file COPYING. If not, write to the
| Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA
| 02111-1307, USA.
|
****************************************************************/
#ifndef _AP4_COMMON_ENCRYPTION_H_
#define _AP4_COMMON_ENCRYPTION_H_
/*----------------------------------------------------------------------
| includes
+---------------------------------------------------------------------*/
#include "Ap4Atom.h"
#include "Ap4ByteStream.h"
#include "Ap4Utils.h"
#include "Ap4Processor.h"
#include "Ap4Protection.h"
#include "Ap4PsshAtom.h"
/*----------------------------------------------------------------------
| class declarations
+---------------------------------------------------------------------*/
class AP4_StreamCipher;
class AP4_SaizAtom;
class AP4_SaioAtom;
class AP4_CencSampleInfoTable;
class AP4_AvcFrameParser;
class AP4_HevcFrameParser;
/*----------------------------------------------------------------------
| constants
+---------------------------------------------------------------------*/
const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_CENC = AP4_ATOM_TYPE('c','e','n','c');
const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_CENS = AP4_ATOM_TYPE('c','e','n','s');
const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_CBC1 = AP4_ATOM_TYPE('c','b','c','1');
const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_CBCS = AP4_ATOM_TYPE('c','b','c','s');
const AP4_UI32 AP4_PROTECTION_SCHEME_VERSION_CENC_10 = 0x00010000;
const AP4_UI32 AP4_CENC_CIPHER_NONE = 0;
const AP4_UI32 AP4_CENC_CIPHER_AES_128_CTR = 1;
const AP4_UI32 AP4_CENC_CIPHER_AES_128_CBC = 2;
const AP4_UI32 AP4_CENC_SAMPLE_ENCRYPTION_FLAG_OVERRIDE_TRACK_ENCRYPTION_DEFAULTS = 1;
const AP4_UI32 AP4_CENC_SAMPLE_ENCRYPTION_FLAG_USE_SUB_SAMPLE_ENCRYPTION = 2;
typedef enum {
AP4_CENC_VARIANT_PIFF_CTR,
AP4_CENC_VARIANT_PIFF_CBC,
AP4_CENC_VARIANT_MPEG_CENC,
AP4_CENC_VARIANT_MPEG_CBC1,
AP4_CENC_VARIANT_MPEG_CENS,
AP4_CENC_VARIANT_MPEG_CBCS
} AP4_CencVariant;
/*----------------------------------------------------------------------
| AP4_CencTrackEncryption
+---------------------------------------------------------------------*/
class AP4_CencTrackEncryption {
public:
AP4_IMPLEMENT_DYNAMIC_CAST(AP4_CencTrackEncryption)
virtual ~AP4_CencTrackEncryption() {}
// methods
AP4_Result Parse(AP4_ByteStream& stream);
AP4_Result DoInspectFields(AP4_AtomInspector& inspector);
AP4_Result DoWriteFields(AP4_ByteStream& stream);
// accessors
AP4_UI32 GetDefaultIsProtected() { return m_DefaultIsProtected; }
AP4_UI08 GetDefaultPerSampleIvSize() { return m_DefaultPerSampleIvSize; }
AP4_UI08 GetDefaultConstantIvSize() { return m_DefaultConstantIvSize; }
const AP4_UI08* GetDefaultConstantIv() { return m_DefaultConstantIv; }
const AP4_UI08* GetDefaultKid() { return m_DefaultKid; }
AP4_UI08 GetDefaultCryptByteBlock() { return m_DefaultCryptByteBlock; }
AP4_UI08 GetDefaultSkipByteBlock() { return m_DefaultSkipByteBlock; }
protected:
// constructors
AP4_CencTrackEncryption(AP4_UI08 version);
AP4_CencTrackEncryption(AP4_UI08 version,
AP4_UI08 default_is_protected,
AP4_UI08 default_per_sample_iv_size,
const AP4_UI08* default_kid,
AP4_UI08 default_constant_iv_size = 0,
const AP4_UI08* default_constant_iv = NULL,
AP4_UI08 default_crypt_byte_block = 0,
AP4_UI08 default_skip_byte_block = 0);
private:
// members
AP4_UI08 m_Version_; // cannot be called m_Version because it would conflict with AP4_Atom::m_Version
AP4_UI08 m_DefaultIsProtected;
AP4_UI08 m_DefaultPerSampleIvSize;
AP4_UI08 m_DefaultConstantIvSize;
AP4_UI08 m_DefaultConstantIv[16];
AP4_UI08 m_DefaultKid[16];
AP4_UI08 m_DefaultCryptByteBlock;
AP4_UI08 m_DefaultSkipByteBlock;
};
/*----------------------------------------------------------------------
| AP4_CencSampleEncryption
+---------------------------------------------------------------------*/
class AP4_CencSampleEncryption {
public:
AP4_IMPLEMENT_DYNAMIC_CAST(AP4_CencSampleEncryption)
virtual ~AP4_CencSampleEncryption() {}
// methods
AP4_Result DoInspectFields(AP4_AtomInspector& inspector);
AP4_Result DoWriteFields(AP4_ByteStream& stream);
// accessors
AP4_Atom& GetOuter() { return m_Outer; }
AP4_UI32 GetAlgorithmId() { return m_AlgorithmId; }
AP4_UI08 GetPerSampleIvSize() { return m_PerSampleIvSize; }
AP4_Result SetPerSampleIvSize(AP4_UI08 iv_size);
AP4_UI08 GetConstantIvSize() { return m_ConstantIvSize; }
AP4_Result SetConstantIvSize(AP4_UI08 iv_size);
AP4_UI08 GetCryptByteBlock() { return m_CryptByteBlock; }
AP4_UI08 GetSkipByteBlock() { return m_SkipByteBlock; }
const AP4_UI08* GetConstantIv() { return m_ConstantIv; }
const AP4_UI08* GetKid() { return m_Kid; }
AP4_Cardinal GetSampleInfoCount() { return m_SampleInfoCount; }
AP4_Result AddSampleInfo(const AP4_UI08* iv, AP4_DataBuffer& subsample_info);
AP4_Result SetSampleInfosSize(AP4_Size size);
AP4_Result CreateSampleInfoTable(AP4_UI08 flags,
AP4_UI08 default_crypt_byte_block,
AP4_UI08 default_skip_byte_block,
AP4_UI08 default_per_sample_iv_size,
AP4_UI08 default_constant_iv_size,
const AP4_UI08* default_constant_iv,
AP4_CencSampleInfoTable*& table);
protected:
// constructors
AP4_CencSampleEncryption(AP4_Atom& outer,
AP4_UI08 per_sample_iv_size,
AP4_UI08 constant_iv_size = 0,
const AP4_UI08* constant_iv = NULL,
AP4_UI08 crypt_byte_block = 0,
AP4_UI08 skip_byte_block = 0);
AP4_CencSampleEncryption(AP4_Atom& outer, AP4_Size size, AP4_ByteStream& stream);
AP4_CencSampleEncryption(AP4_Atom& outer,
AP4_UI32 algorithm_id,
AP4_UI08 per_sample_iv_size,
const AP4_UI08* kid);
protected:
// members
AP4_Atom& m_Outer;
AP4_UI32 m_AlgorithmId;
AP4_UI08 m_PerSampleIvSize;
AP4_UI08 m_ConstantIvSize;
AP4_UI08 m_ConstantIv[16];
AP4_UI08 m_CryptByteBlock;
AP4_UI08 m_SkipByteBlock;
AP4_UI08 m_Kid[16];
AP4_Cardinal m_SampleInfoCount;
AP4_DataBuffer m_SampleInfos;
unsigned int m_SampleInfoCursor;
};
/*----------------------------------------------------------------------
| AP4_CencSampleInfoTable
+---------------------------------------------------------------------*/
class AP4_CencSampleInfoTable {
public:
// class methods
static AP4_Result Create(AP4_ProtectedSampleDescription* sample_description,
AP4_ContainerAtom* traf,
AP4_UI32& cipher_type,
bool& reset_iv_at_each_subsample,
AP4_ByteStream& aux_info_data,
AP4_Position aux_info_data_offset,
AP4_CencSampleInfoTable*& sample_info_table);
static AP4_Result Create(AP4_ProtectedSampleDescription* sample_description,
AP4_ContainerAtom* traf,
AP4_SaioAtom*& saio,
AP4_SaizAtom*& saiz,
AP4_CencSampleEncryption*& sample_encryption_atom,
AP4_UI32& cipher_type,
bool& reset_iv_at_each_subsample,
AP4_ByteStream& aux_info_data,
AP4_Position aux_info_data_offset,
AP4_CencSampleInfoTable*& sample_info_table);
static AP4_Result Create(AP4_UI08 flags,
AP4_UI08 crypt_byte_block,
AP4_UI08 skip_byte_block,
AP4_UI08 per_sample_iv_size,
AP4_UI08 constant_iv_size,
const AP4_UI08* constant_iv,
AP4_ContainerAtom& traf,
AP4_SaioAtom& saio,
AP4_SaizAtom& saiz,
AP4_ByteStream& aux_info_data,
AP4_Position aux_info_data_offset,
AP4_CencSampleInfoTable*& sample_info_table);
// see note below regarding the serialization format
static AP4_Result Create(const AP4_UI08* serialized,
unsigned int serialized_size,
AP4_CencSampleInfoTable*& sample_info_table);
// constructor
AP4_CencSampleInfoTable(AP4_UI08 flags,
AP4_UI08 crypt_byte_block,
AP4_UI08 skip_byte_block,
AP4_UI32 sample_count,
AP4_UI08 iv_size);
// methods
AP4_UI08 GetFlags() { return m_Flags; }
AP4_UI08 GetCryptByteBlock() { return m_CryptByteBlock; }
AP4_UI08 GetSkipByteBlock() { return m_SkipByteBlock; }
AP4_UI32 GetSampleCount() { return m_SampleCount; }
AP4_UI08 GetIvSize() { return m_IvSize; }
AP4_Result SetIv(AP4_Ordinal sample_index, const AP4_UI08* iv);
const AP4_UI08* GetIv(AP4_Ordinal sample_index);
AP4_Result AddSubSampleData(AP4_Cardinal subsample_count,
const AP4_UI08* subsample_data);
bool HasSubSampleInfo() {
return m_SubSampleMapStarts.ItemCount() != 0;
}
unsigned int GetSubsampleCount(AP4_Cardinal sample_index) {
if (sample_index < m_SampleCount) {
return m_SubSampleMapLengths[sample_index];
} else {
return 0;
}
}
AP4_Result GetSampleInfo(AP4_Cardinal sample_index,
AP4_Cardinal& subsample_count,
const AP4_UI16*& bytes_of_cleartext_data,
const AP4_UI32*& bytes_of_encrypted_data);
AP4_Result GetSubsampleInfo(AP4_Cardinal sample_index,
AP4_Cardinal subsample_index,
AP4_UI16& bytes_of_cleartext_data,
AP4_UI32& bytes_of_encrypted_data);
// see note below regarding the serialization format
AP4_Result Serialize(AP4_DataBuffer& buffer);
private:
AP4_UI32 m_SampleCount;
AP4_UI08 m_Flags;
AP4_UI08 m_CryptByteBlock;
AP4_UI08 m_SkipByteBlock;
AP4_UI08 m_IvSize;
AP4_DataBuffer m_IvData;
AP4_Array<AP4_UI16> m_BytesOfCleartextData;
AP4_Array<AP4_UI32> m_BytesOfEncryptedData;
AP4_Array<unsigned int> m_SubSampleMapStarts;
AP4_Array<unsigned int> m_SubSampleMapLengths;
};
/*----------------------------------------------------------------------
| AP4_CencSampleInfoTable serialization format
|
| (All integers are stored in big-endian byte order)
|
| +---------------+----------------+------------------------------------+
| | Size | Type | Description |
| +---------------+----------------+------------------------------------+
|
| +---------------+----------------+------------------------------------+
| | 4 bytes | 32-bit integer | sample_count |
| +---------------+----------------+------------------------------------+
| | 1 byte | 8-bit integer | flags |
| +---------------+----------------+------------------------------------+
| | 1 byte | 8-bit integer | crypt_byte_block |
| +---------------+----------------+------------------------------------+
| | 1 byte | 8-bit integer | skip_byte_block |
| +---------------+----------------+------------------------------------+
| | 1 byte | 8-bit integer | iv_size |
| +---------------+----------------+------------------------------------+
|
| repeat sample_count times:
| +---------------+----------------+------------------------------------+
| | iv_size bytes | byte array | IV[i] |
| +---------------+----------------+------------------------------------+
|
| +---------------+----------------+------------------------------------+
| | 4 bytes | 32-bit integer | entry_count |
| +---------------+----------------+------------------------------------+
|
| repeat entry_count times:
| +---------------+----------------+------------------------------------+
| | 2 bytes | 16-bit integer | bytes_of_cleartext_data[i] |
| +---------------+----------------+------------------------------------+
|
| repeat entry_count times:
| +---------------+----------------+------------------------------------+
| | 4 bytes | 32-bit integer | bytes_of_encrypted_data[i] |
| +---------------+----------------+------------------------------------+
|
| +---------------+----------------+------------------------------------+
| | 4 bytes | 32-bit flags | 1 if subsamples are used, 0 if not |
| +---------------+----------------+------------------------------------+
|
| if subsamples are used, repeat sample_count times:
| +---------------+----------------+------------------------------------+
| | 4 bytes | 32-bit integer | subsample_map_start[i] |
| +---------------+----------------+------------------------------------+
|
| if subsamples are used, repeat sample_count times:
| +---------------+----------------+------------------------------------+
| | 4 bytes | 32-bit integer | subsample_map_length[i] |
| +---------------+----------------+------------------------------------+
|
| NOTES: subsample_map_start[i] ans subsample_map_length[i] are, respectively,
| the index and the length the i'th subsample map sequence in the
| bytes_of_cleartext_data anb bytes_of_encrypted_data arrays.
| For example, if we have:
| bytes_of_cleartext_data[] = { 10, 15, 13, 17, 12 }
| bytes_of_encrypted_data[] = { 100, 200, 50, 80, 32 }
| subsample_map_start = { 0, 3 }
| subsample_map_length = { 3, 2 }
| It means that the (bytes_of_cleartext_data, bytes_of_encrypted_data)
| sequences for the two subsamples are:
| subsample[0] --> [(10,100), (15, 200), (13, 50)]
| subsample[1] --> [(17, 80), (12, 32)]
|
+---------------------------------------------------------------------*/
/*----------------------------------------------------------------------
| AP4_CencSampleEncrypter
+---------------------------------------------------------------------*/
class AP4_CencSampleEncrypter
{
public:
// constructor and destructor
AP4_CencSampleEncrypter(AP4_StreamCipher* cipher,
bool constant_iv) :
m_Cipher(cipher),
m_ConstantIv(constant_iv) {
AP4_SetMemory(m_Iv, 0, 16);
};
virtual ~AP4_CencSampleEncrypter();
// methods
virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in,
AP4_DataBuffer& data_out,
AP4_DataBuffer& sample_infos) = 0;
void SetIv(const AP4_UI08* iv) { AP4_CopyMemory(m_Iv, iv, 16); }
const AP4_UI08* GetIv() { return m_Iv; }
virtual bool UseSubSamples() { return false; }
virtual AP4_Result GetSubSampleMap(AP4_DataBuffer& /* sample_data */,
AP4_Array<AP4_UI16>& /* bytes_of_cleartext_data */,
AP4_Array<AP4_UI32>& /* bytes_of_encrypted_data */) {
return AP4_SUCCESS;
}
protected:
AP4_UI08 m_Iv[16];
AP4_StreamCipher* m_Cipher;
bool m_ConstantIv;
};
/*----------------------------------------------------------------------
| AP4_CencCtrSampleEncrypter
+---------------------------------------------------------------------*/
class AP4_CencCtrSampleEncrypter : public AP4_CencSampleEncrypter
{
public:
// constructor and destructor
AP4_CencCtrSampleEncrypter(AP4_StreamCipher* cipher,
bool constant_iv,
unsigned int iv_size) :
AP4_CencSampleEncrypter(cipher, constant_iv),
m_IvSize(iv_size) {}
// methods
virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in,
AP4_DataBuffer& data_out,
AP4_DataBuffer& sample_infos);
protected:
unsigned int m_IvSize;
};
/*----------------------------------------------------------------------
| AP4_CencCbcSampleEncrypter
+---------------------------------------------------------------------*/
class AP4_CencCbcSampleEncrypter : public AP4_CencSampleEncrypter
{
public:
// constructor and destructor
AP4_CencCbcSampleEncrypter(AP4_StreamCipher* cipher,
bool constant_iv) :
AP4_CencSampleEncrypter(cipher, constant_iv) {}
// methods
virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in,
AP4_DataBuffer& data_out,
AP4_DataBuffer& sample_infos);
};
/*----------------------------------------------------------------------
| AP4_CencSubSampleMapper
+---------------------------------------------------------------------*/
class AP4_CencSubSampleMapper
{
public:
// constructor and destructor
AP4_CencSubSampleMapper(AP4_Size nalu_length_size, AP4_UI32 format) :
m_NaluLengthSize(nalu_length_size),
m_Format(format) {}
virtual ~AP4_CencSubSampleMapper() {}
// methods
virtual AP4_Result GetSubSampleMap(AP4_DataBuffer& sample_data,
AP4_Array<AP4_UI16>& bytes_of_cleartext_data,
AP4_Array<AP4_UI32>& bytes_of_encrypted_data) = 0;
protected:
// members
AP4_Size m_NaluLengthSize;
AP4_UI32 m_Format;
};
/*----------------------------------------------------------------------
| AP4_CencBasicSubSampleMapper
+---------------------------------------------------------------------*/
class AP4_CencBasicSubSampleMapper : public AP4_CencSubSampleMapper
{
public:
// constructor and destructor
AP4_CencBasicSubSampleMapper(AP4_Size nalu_length_size, AP4_UI32 format) :
AP4_CencSubSampleMapper(nalu_length_size, format) {}
// methods
virtual AP4_Result GetSubSampleMap(AP4_DataBuffer& sample_data,
AP4_Array<AP4_UI16>& bytes_of_cleartext_data,
AP4_Array<AP4_UI32>& bytes_of_encrypted_data);
};
/*----------------------------------------------------------------------
| AP4_CencAdvancedSubSampleMapper
+---------------------------------------------------------------------*/
class AP4_CencAdvancedSubSampleMapper : public AP4_CencSubSampleMapper
{
public:
// constructor and destructor
AP4_CencAdvancedSubSampleMapper(AP4_Size nalu_length_size, AP4_UI32 format) :
AP4_CencSubSampleMapper(nalu_length_size, format) {}
// methods
virtual AP4_Result GetSubSampleMap(AP4_DataBuffer& sample_data,
AP4_Array<AP4_UI16>& bytes_of_cleartext_data,
AP4_Array<AP4_UI32>& bytes_of_encrypted_data);
};
/*----------------------------------------------------------------------
| AP4_CencCbcsSubSampleMapper
+---------------------------------------------------------------------*/
class AP4_CencCbcsSubSampleMapper : public AP4_CencSubSampleMapper
{
public:
// constructor and destructor
AP4_CencCbcsSubSampleMapper(AP4_Size nalu_length_size, AP4_UI32 format, AP4_TrakAtom* trak);
~AP4_CencCbcsSubSampleMapper();
// methods
virtual AP4_Result GetSubSampleMap(AP4_DataBuffer& sample_data,
AP4_Array<AP4_UI16>& bytes_of_cleartext_data,
AP4_Array<AP4_UI32>& bytes_of_encrypted_data);
private:
// members
AP4_AvcFrameParser* m_AvcParser;
AP4_HevcFrameParser* m_HevcParser;
// methods
AP4_Result ParseAvcData(const AP4_UI08* data, AP4_Size data_size);
AP4_Result ParseHevcData(const AP4_UI08* data, AP4_Size data_size);
};
/*----------------------------------------------------------------------
| AP4_CencSubSampleEncrypter
+---------------------------------------------------------------------*/
class AP4_CencSubSampleEncrypter : public AP4_CencSampleEncrypter
{
public:
// constructor and destructor
AP4_CencSubSampleEncrypter(AP4_StreamCipher* cipher,
bool constant_iv,
bool reset_iv_at_each_subsample,
AP4_CencSubSampleMapper* subsample_mapper) :
AP4_CencSampleEncrypter(cipher, constant_iv),
m_ResetIvForEachSubsample(reset_iv_at_each_subsample),
m_SubSampleMapper(subsample_mapper) {}
virtual ~AP4_CencSubSampleEncrypter() {
delete m_SubSampleMapper;
}
// methods
virtual bool UseSubSamples() { return true; }
virtual AP4_Result GetSubSampleMap(AP4_DataBuffer& sample_data,
AP4_Array<AP4_UI16>& bytes_of_cleartext_data,
AP4_Array<AP4_UI32>& bytes_of_encrypted_data) {
return m_SubSampleMapper->GetSubSampleMap(sample_data, bytes_of_cleartext_data, bytes_of_encrypted_data);
}
// members
bool m_ResetIvForEachSubsample;
AP4_CencSubSampleMapper* m_SubSampleMapper;
};
/*----------------------------------------------------------------------
| AP4_CencCtrSubSampleEncrypter
+---------------------------------------------------------------------*/
class AP4_CencCtrSubSampleEncrypter : public AP4_CencSubSampleEncrypter
{
public:
// constructor and destructor
AP4_CencCtrSubSampleEncrypter(AP4_StreamCipher* cipher,
bool constant_iv,
bool reset_iv_at_each_subsample,
unsigned int iv_size,
AP4_CencSubSampleMapper* subsample_mapper) :
AP4_CencSubSampleEncrypter(cipher,
constant_iv,
reset_iv_at_each_subsample,
subsample_mapper),
m_IvSize(iv_size) {}
// methods
virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in,
AP4_DataBuffer& data_out,
AP4_DataBuffer& sample_infos);
protected:
unsigned int m_IvSize;
};
/*----------------------------------------------------------------------
| AP4_CencCbcSubSampleEncrypter
+---------------------------------------------------------------------*/
class AP4_CencCbcSubSampleEncrypter : public AP4_CencSubSampleEncrypter
{
public:
// constructor and destructor
AP4_CencCbcSubSampleEncrypter(AP4_StreamCipher* cipher,
bool constant_iv,
bool reset_iv_at_each_subsample,
AP4_CencSubSampleMapper* subsample_mapper) :
AP4_CencSubSampleEncrypter(cipher,
constant_iv,
reset_iv_at_each_subsample,
subsample_mapper) {}
// methods
virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in,
AP4_DataBuffer& data_out,
AP4_DataBuffer& sample_infos);
};
/*----------------------------------------------------------------------
| AP4_CencEncryptingProcessor
+---------------------------------------------------------------------*/
class AP4_CencEncryptingProcessor : public AP4_Processor
{
public:
// class constants
static const AP4_UI32 OPTION_EME_PSSH = 0x01; ///< Include a 'standard EME' pssh atom in the output
static const AP4_UI32 OPTION_PIFF_COMPATIBILITY = 0x02; ///< Attempt to create an output that is compatible with the PIFF format
static const AP4_UI32 OPTION_PIFF_IV_SIZE_16 = 0x04; ///< With the PIFF-compatibiity option, use an IV of size 16 when possible (instead of 8)
static const AP4_UI32 OPTION_IV_SIZE_8 = 0x08; ///< Use an IV of size 8 when possible (instead of 16 by default).
static const AP4_UI32 OPTION_NO_SENC = 0x10; ///< Don't output an 'senc' atom
// types
struct Encrypter {
Encrypter(AP4_UI32 track_id, AP4_UI32 cleartext_fragments, AP4_CencSampleEncrypter* sample_encrypter) :
m_TrackId(track_id),
m_CurrentFragment(0),
m_CleartextFragments(cleartext_fragments),
m_SampleEncrypter(sample_encrypter) {}
~Encrypter() { delete m_SampleEncrypter; }
AP4_UI32 m_TrackId;
AP4_UI32 m_CurrentFragment;
AP4_UI32 m_CleartextFragments;
AP4_CencSampleEncrypter* m_SampleEncrypter;
};
// constructor
AP4_CencEncryptingProcessor(AP4_CencVariant variant,
AP4_UI32 options = 0,
AP4_BlockCipherFactory* block_cipher_factory = NULL);
~AP4_CencEncryptingProcessor();
// accessors
AP4_ProtectionKeyMap& GetKeyMap() { return m_KeyMap; }
AP4_TrackPropertyMap& GetPropertyMap() { return m_PropertyMap; }
AP4_Array<AP4_PsshAtom*>& GetPsshAtoms() { return m_PsshAtoms; }
// AP4_Processor methods
virtual AP4_Result Initialize(AP4_AtomParent& top_level,
AP4_ByteStream& stream,
AP4_Processor::ProgressListener* listener = NULL);
virtual AP4_Processor::TrackHandler* CreateTrackHandler(AP4_TrakAtom* trak);
virtual AP4_Processor::FragmentHandler* CreateFragmentHandler(AP4_TrakAtom* trak,
AP4_TrexAtom* trex,
AP4_ContainerAtom* traf,
AP4_ByteStream& moof_data,
AP4_Position moof_offset);
protected:
// members
AP4_CencVariant m_Variant;
AP4_UI32 m_Options;
AP4_BlockCipherFactory* m_BlockCipherFactory;
AP4_ProtectionKeyMap m_KeyMap;
AP4_TrackPropertyMap m_PropertyMap;
AP4_Array<AP4_PsshAtom*> m_PsshAtoms;
AP4_List<Encrypter> m_Encrypters;
};
/*----------------------------------------------------------------------
| AP4_CencDecryptingProcessor
+---------------------------------------------------------------------*/
class AP4_CencDecryptingProcessor : public AP4_Processor
{
public:
// constructor
AP4_CencDecryptingProcessor(const AP4_ProtectionKeyMap* key_map,
AP4_BlockCipherFactory* block_cipher_factory = NULL);
// AP4_Processor methods
virtual AP4_Processor::TrackHandler* CreateTrackHandler(AP4_TrakAtom* trak);
virtual AP4_Processor::FragmentHandler* CreateFragmentHandler(AP4_TrakAtom* trak,
AP4_TrexAtom* trex,
AP4_ContainerAtom* traf,
AP4_ByteStream& moof_data,
AP4_Position moof_offset);
protected:
// methods
const AP4_DataBuffer* GetKeyForTrak(AP4_UI32 track_id, AP4_ProtectedSampleDescription* sample_description);
// members
AP4_BlockCipherFactory* m_BlockCipherFactory;
const AP4_ProtectionKeyMap* m_KeyMap;
};
/*----------------------------------------------------------------------
| AP4_CencSingleSampleDecrypter
+---------------------------------------------------------------------*/
class AP4_CencSingleSampleDecrypter
{
public:
static AP4_Result Create(AP4_UI32 cipher_type,
const AP4_UI08* key,
AP4_Size key_size,
AP4_UI08 crypt_byte_block,
AP4_UI08 skip_byte_block,
AP4_BlockCipherFactory* block_cipher_factory,
bool reset_iv_at_each_subsample,
AP4_CencSingleSampleDecrypter*& decrypter);
// methods
AP4_CencSingleSampleDecrypter(AP4_StreamCipher* cipher) :
m_Cipher(cipher),
m_FullBlocksOnly(false) {}
virtual ~AP4_CencSingleSampleDecrypter();
virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in,
AP4_DataBuffer& data_out,
// always 16 bytes
const AP4_UI08* iv,
// pass 0 for full decryption
unsigned int subsample_count,
// array of <subsample_count> integers. NULL if subsample_count is 0
const AP4_UI16* bytes_of_cleartext_data,
// array of <subsample_count> integers. NULL if subsample_count is 0
const AP4_UI32* bytes_of_encrypted_data);
private:
// constructor
AP4_CencSingleSampleDecrypter(AP4_StreamCipher* cipher,
bool full_blocks_only,
bool reset_iv_at_each_subsample) :
m_Cipher(cipher),
m_FullBlocksOnly(full_blocks_only),
m_ResetIvAtEachSubsample(reset_iv_at_each_subsample) {}
// members
AP4_StreamCipher* m_Cipher;
bool m_FullBlocksOnly;
bool m_ResetIvAtEachSubsample;
};
/*----------------------------------------------------------------------
| AP4_CencSampleDecrypter
+---------------------------------------------------------------------*/
class AP4_CencSampleDecrypter : public AP4_SampleDecrypter
{
public:
static AP4_Result Create(AP4_ProtectedSampleDescription* sample_description,
AP4_ContainerAtom* traf,
AP4_ByteStream& moof_data,
AP4_Position moof_offset,
const AP4_UI08* key,
AP4_Size key_size,
AP4_BlockCipherFactory* block_cipher_factory,
AP4_SaioAtom*& saio_atom, // [out]
AP4_SaizAtom*& saiz_atom, // [out]
AP4_CencSampleEncryption*& sample_encryption_atom, // [out]
AP4_CencSampleDecrypter*& decrypter);
static AP4_Result Create(AP4_ProtectedSampleDescription* sample_description,
AP4_ContainerAtom* traf,
AP4_ByteStream& moof_data,
AP4_Position moof_offset,
const AP4_UI08* key,
AP4_Size key_size,
AP4_BlockCipherFactory* block_cipher_factory,
AP4_CencSampleDecrypter*& decrypter);
static AP4_Result Create(AP4_CencSampleInfoTable* sample_info_table,
AP4_UI32 cipher_type,
const AP4_UI08* key,
AP4_Size key_size,
AP4_BlockCipherFactory* block_cipher_factory,
bool reset_iv_at_each_subsample,
AP4_CencSampleDecrypter*& decrypter);
// methods
AP4_CencSampleDecrypter(AP4_CencSingleSampleDecrypter* single_sample_decrypter,
AP4_CencSampleInfoTable* sample_info_table) :
m_SingleSampleDecrypter(single_sample_decrypter),
m_SampleInfoTable(sample_info_table),
m_SampleCursor(0) {}
virtual ~AP4_CencSampleDecrypter();
virtual AP4_Result SetSampleIndex(AP4_Ordinal sample_index);
virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in,
AP4_DataBuffer& data_out,
const AP4_UI08* iv);
protected:
AP4_CencSingleSampleDecrypter* m_SingleSampleDecrypter;
AP4_CencSampleInfoTable* m_SampleInfoTable;
AP4_Ordinal m_SampleCursor;
};
/*----------------------------------------------------------------------
| AP4_CencSampleEncryptionInformationGroupEntry
+---------------------------------------------------------------------*/
class AP4_CencSampleEncryptionInformationGroupEntry {
public:
AP4_CencSampleEncryptionInformationGroupEntry(const AP4_UI08* data);
// accessors
bool IsEncrypted() const { return m_IsEncrypted; }
AP4_UI08 GetIvSize() const { return m_IvSize; }
const AP4_UI08* GetKid() const { return &m_KID[9]; }
private:
// members
bool m_IsEncrypted;
AP4_UI08 m_IvSize;
AP4_UI08 m_KID[16];
};
#endif // _AP4_COMMON_ENCRYPTION_H_
| {
"pile_set_name": "Github"
} |
---
title: "addition (+)"
date: 2019-04-06T12:19:22+02:00
description: "calculates the sum of a number sequence"
names: ["+"]
usage: "(+ form*)"
tags: ["math", "number"]
---
Takes a set of numbers and calculates their sum.
#### An Example
```scheme
(+ 9 10 23) ;; returns 42
(+ 50 12.6 34.8) ;; returns 97.4
(+) ;; returns 0
```
| {
"pile_set_name": "Github"
} |
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.knox.gateway.websockets;
import org.apache.knox.gateway.i18n.messages.Message;
import org.apache.knox.gateway.i18n.messages.MessageLevel;
import org.apache.knox.gateway.i18n.messages.Messages;
import org.apache.knox.gateway.i18n.messages.StackTrace;
/**
* Logging for Websocket
*
* @since 0.10
*/
@Messages(logger = "org.apache.knox.gateway.websockets")
public interface WebsocketLogMessages {
@Message(level = MessageLevel.ERROR,
text = "Error creating websocket connection: {0}")
void failedCreatingWebSocket(
@StackTrace(level = MessageLevel.ERROR) Exception e);
@Message(level = MessageLevel.ERROR,
text = "Unable to connect to websocket server: {0}")
void connectionFailed(@StackTrace(level = MessageLevel.ERROR) Exception e);
@Message(level = MessageLevel.ERROR, text = "Error: {0}")
void onError(String message);
@Message(level = MessageLevel.ERROR, text = "Bad or malformed url: {0}")
void badUrlError(@StackTrace(level = MessageLevel.ERROR) Exception e);
@Message(level = MessageLevel.DEBUG,
text = "Websocket connection to backend server {0} opened")
void onConnectionOpen(String backend);
@Message(level = MessageLevel.DEBUG, text = "Message: {0}")
void logMessage(String message);
@Message(level = MessageLevel.DEBUG,
text = "Websocket connection to backend server {0} closed")
void onConnectionClose(String backend);
@Message(level = MessageLevel.DEBUG,
text = "{0}")
void debugLog(String message);
}
| {
"pile_set_name": "Github"
} |
---
Description: Helper object to pass seek commands upstream.
ms.assetid: 10fe022d-8707-4059-90b8-5740c3861071
title: CBaseRenderer::m_pPosition member (Renbase.h)
ms.topic: reference
ms.date: 05/31/2018
topic_type:
- APIRef
- kbSyntax
api_name:
- m_pPosition
api_type:
- LibDef
api_location:
- Strmbase.lib
- Strmbase.dll
- Strmbasd.lib
- Strmbasd.dll
---
# CBaseRenderer::m\_pPosition member
Helper object to pass seek commands upstream.
## Syntax
```C++
CRendererPosPassThru *m_pPosition;
```
## Requirements
| | |
|--------------------|--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| Header<br/> | <dl> <dt>Renbase.h (include Streams.h)</dt> </dl> |
| Library<br/> | <dl> <dt>Strmbase.lib (retail builds); </dt> <dt>Strmbasd.lib (debug builds)</dt> </dl> |
## See also
<dl> <dt>
[**CBaseRenderer Class**](cbaserenderer.md)
</dt> </dl>
| {
"pile_set_name": "Github"
} |
name: Lepton
description: 'Open-source snippet manager powered by GitHub Gist.'
website: 'https://hackjutsu.com/Lepton/'
repository: 'https://github.com/hackjutsu/Lepton'
keywords:
- gist
- snippet
- github
- note
- markdown
- productivity
- code
- CodeMirror
license: MIT
category: Productivity
screenshots:
- {
imageUrl: 'https://raw.githubusercontent.com/hackjutsu/Lepton/master/docs/img/portfolio/stay_organized.png',
}
| {
"pile_set_name": "Github"
} |
Ext.BLANK_IMAGE_URL = BASE_URL + '/images/blank.gif';
Ext.state.Manager.setProvider(new Ext.state.CookieProvider());
Ext.QuickTips.init();
Ext.form.Field.prototype.msgTarget = 'side';
Ext.Ajax.timeout = 30 * 60 * 1000;
Ext.form.BasicForm.prototype.timeout = 30 * 60;
// workaround for proper Ext.ProgressBar rendering under IE
Ext.override(Ext.Element, {
alignMiddle: function(parent) {
if (Ext.isString(parent)) {
parent = Ext.get(parent) || this.up(parent);
}
this.setStyle({
'margin-top': (parent.getHeight() / 2 - this.getHeight() / 2) + 'px'
});
}
});
Ext.override(Ext.ProgressBar, {
setSize: Ext.ProgressBar.superclass.setSize,
onResize: function(w, h) {
var inner = Ext.get(this.el.child('.x-progress-inner')),
bar = inner.child('.x-progress-bar'),
pt = inner.child('.x-progress-text'),
ptInner = pt.child('*'),
ptb = inner.child('.x-progress-text-back'),
ptbInner = ptb.child('*');
Ext.ProgressBar.superclass.onResize.apply(this, arguments);
inner.setHeight(h);
bar.setHeight(h);
this.textEl.setHeight('auto');
pt.setHeight('auto');
ptb.setHeight('auto');
ptInner.alignMiddle(bar);
ptbInner.alignMiddle(bar);
this.syncProgressBar();
}
});
// workaround for IE: proper checkboxes rendering
if (Ext.isIE) {
Ext.override(Ext.form.Checkbox, {
onRender: function(ct, position){
Ext.form.Checkbox.superclass.onRender.call(this, ct, position);
if (this.inputValue !== undefined) {
this.el.dom.value = this.inputValue;
}
this.wrap = this.el.wrap({
cls: 'x-form-check-wrap'
});
if (this.boxLabel) {
this.wrap.createChild({
tag: 'label',
htmlFor: this.el.id,
cls: 'x-form-cb-label',
html: this.boxLabel
});
}
if (this.checked) {
this.setValue(true);
} else {
this.checked = this.el.dom.checked;
}
this.resizeEl = this.positionEl = this.wrap;
}
});
}
Ext.ns('Owp.form');
Owp.form.errorHandler = function(form, action, params) {
if ('client' == action.failureType) {
return
}
if ('undefined' == typeof action.result) {
Ext.MessageBox.show({
msg: 'Internal error occured. See logs for details.',
buttons: Ext.MessageBox.OK,
icon: Ext.MessageBox.ERROR
});
return
}
var params = ('undefined' == typeof params) ? Array() : params;
var handler = params['fn'] || function() {};
// show overall status message
if ('undefined' != typeof action.result.message) {
Ext.MessageBox.show({
msg: action.result.message,
buttons: Ext.MessageBox.OK,
icon: Ext.MessageBox.ERROR,
fn: handler
});
return
}
// highlight fields with errors
var errorsHash = new Array();
Ext.each(action.result.form_errors, function(message) {
messageField = message[0];
messageContent = message[1];
errorsHash[messageField] = (errorsHash[messageField])
? errorsHash[messageField] + '<br/>' + messageContent
: messageContent;
});
Ext.each(form.items.items, function(field) {
if (('undefined' != field.name) && ('undefined' != typeof errorsHash[field.name])) {
field.markInvalid(errorsHash[field.name])
}
});
}
Owp.form.BasicForm = Ext.extend(Ext.FormPanel, {
baseCls: 'x-plain',
defaultType: 'textfield'
});
Owp.form.BasicFormWindow = Ext.extend(Ext.Window, {
findFirst: function(item) {
if (item instanceof Ext.form.Field && !(item instanceof Ext.form.DisplayField)
&& (item.inputType != 'hidden') && !item.disabled
) {
item.focus(false, 50); // delayed focus by 50 ms
return true;
}
if (item.items && item.items.find) {
return item.items.find(this.findFirst, this);
}
return false;
},
focus: function() {
this.items.find(this.findFirst, this);
}
});
Ext.ns('Owp.button');
Owp.button.action = function(config) {
config = Ext.apply({
gridName: '',
url: '',
command: '',
waitMsg: '',
failure: {
title: '',
msg: ''
}
}, config);
var progressBar = Ext.Msg.wait(config.waitMsg);
Ext.Ajax.request({
url: config.url,
success: function(response) {
progressBar.hide();
var result = Ext.util.JSON.decode(response.responseText);
if (!result.success) {
Ext.MessageBox.show({
title: config.failure.title,
msg: config.failure.msg,
buttons: Ext.Msg.OK,
icon: Ext.MessageBox.ERROR
});
} else {
if (config.gridName) {
var grid = Ext.getCmp(config.gridName);
grid.store.reload();
grid.getSelectionModel().clearSelections();
}
}
},
failure: function() {
Ext.MessageBox.show({
title: config.failure.title,
msg: 'Internal error occured. See logs for details.',
buttons: Ext.Msg.OK,
icon: Ext.MessageBox.ERROR
});
},
params: {
command: config.command
},
scope: this
});
}
Ext.ns('Owp.list');
Owp.list.getSelectedIds = function(gridName) {
var selectedItems = Ext.getCmp(gridName).getSelectionModel().getSelections();
var selectedIds = [];
Ext.each(selectedItems, function(item) {
selectedIds.push(item.data.id);
});
return selectedIds;
}
Owp.list.groupAction = function(config) {
config = Ext.apply({
gridName: '',
url: '',
command: '',
waitMsg: '',
failure: {
title: '',
msg: ''
},
onSuccess: null
}, config);
var progressBar = Ext.Msg.wait(config.waitMsg);
Ext.Ajax.request({
url: config.url,
success: function(response) {
progressBar.hide();
var result = Ext.util.JSON.decode(response.responseText);
if (!result.success) {
Ext.MessageBox.show({
title: config.failure.title,
msg: config.failure.msg,
buttons: Ext.Msg.OK,
icon: Ext.MessageBox.ERROR
});
} else {
var grid = Ext.getCmp(config.gridName);
grid.store.reload();
grid.getSelectionModel().clearSelections();
if (config.onSuccess) {
config.onSuccess();
}
}
},
failure: function() {
Ext.MessageBox.show({
title: config.failure.title,
msg: 'Internal error occured. See logs for details.',
buttons: Ext.Msg.OK,
icon: Ext.MessageBox.ERROR
});
},
params: {
ids: [Owp.list.getSelectedIds(config.gridName)],
command: config.command
},
scope: this
});
}
Ext.list.dateTimeRenderer = function(value) {
return value.format('Y.m.d H:i:s');
}
Ext.ns('Owp.layout');
Owp.layout.addToCenter = function(item) {
var centerPanel = Ext.getCmp('mainContentCenterPanel');
centerPanel.add(item);
centerPanel.doLayout();
}
Owp.Panel = Ext.extend(Ext.Panel, {
stateEvents: ['collapse', 'expand'],
getState: function() {
return {
collapsed: this.collapsed
};
}
});
Ext.ns('Owp.grid');
Owp.grid.GridPanel = Ext.extend(Ext.grid.GridPanel, {
stateEvents: ['collapse', 'expand'],
getState: function() {
var state = Owp.grid.GridPanel.superclass.getState.call(this);
return Ext.apply(state, {
collapsed: this.collapsed
});
}
});
Ext.ns('Owp.statusUpdater');
Owp.statusUpdater = {
isRunning: false,
task: {
run: function() {
Ext.Ajax.request({
url: BASE_URL + '/admin/tasks/status',
success: function(response) {
var result = Ext.util.JSON.decode(response.responseText);
var statusbar = Ext.get('statusbar');
if (result.message) {
statusbar.update('<img src="' + BASE_URL + '/images/spinner.gif" class="icon-inline"> ' + result.message);
Owp.statusUpdater.isRunning = true;
} else {
statusbar.update('');
Ext.TaskMgr.stop(Owp.statusUpdater.task);
if (Owp.statusUpdater.isRunning) {
Ext.each(['backupsGrid', 'tasksGrid', 'osTemplatesGrid'], function(gridName) {
if (Ext.getCmp(gridName)) {
Ext.getCmp(gridName).getStore().reload();
}
});
}
Owp.statusUpdater.isRunning = false;
}
}
});
},
interval: 5000
},
start: function() {
Ext.TaskMgr.start(Owp.statusUpdater.task);
}
}
| {
"pile_set_name": "Github"
} |
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.jackrabbit.oak.security.authorization.composite;
/**
* Same as {@link CompositeProviderAllTest}
* with reverse order of the aggregated providers.
*/
public class CompositeProviderAllReverseTest extends CompositeProviderAllTest {
@Override
boolean reverseOrder() {
return true;
}
} | {
"pile_set_name": "Github"
} |
#
# Windows 10 in VirtualBox 5
#
assert_spec nova
assert_spec x86_64
set flavor "win10_64"
set vm_ram "8280M"
set use_vbox5 1
set use_rumpfs 1
# Write overlay only into ram
set use_ram_fs 1
# However read initial overlay from disk
set use_overlay_from_disk 1
set use_serial 0
set use_check_result 1
set use_usb [expr ![get_cmd_switch --autopilot]]
set use_ps2 [have_spec ps2]
set use_vms 1
set use_cpu_load 0
# use non-generic vbox5 VMM version
set use_vbox5_nova 1
source ${genode_dir}/repos/ports/run/vbox_win.inc
| {
"pile_set_name": "Github"
} |
#include "merge_sort.h"
#include <iostream>
#include "stdio.h"
int main(){
int fail = 0;
DTYPE A[SIZE] = {1,6,3,7,2,5,3,0,9,10,11,12,13,14,15,8};
merge_sort(A);
for(int i = 0; i < SIZE; i++)
std::cout << A[i] << " ";
std::cout << "\n";
for(int i = 1; i < SIZE; i++) {
if(A[i] < A[i-1]) {
std::cout << i << " " << A[i-1] << ">" << A[i] <<
"\n";
fail = 1;
}
}
if(fail == 1)
printf("FAILED\n");
else
printf("PASS\n");
return fail;
}
| {
"pile_set_name": "Github"
} |
/* Taxonomy Classification: 0000300602130000031110 */
/*
* WRITE/READ 0 write
* WHICH BOUND 0 upper
* DATA TYPE 0 char
* MEMORY LOCATION 0 stack
* SCOPE 3 inter-file/inter-proc
* CONTAINER 0 no
* POINTER 0 no
* INDEX COMPLEXITY 6 N/A
* ADDRESS COMPLEXITY 0 constant
* LENGTH COMPLEXITY 2 constant
* ADDRESS ALIAS 1 yes, one level
* INDEX ALIAS 3 N/A
* LOCAL CONTROL FLOW 0 none
* SECONDARY CONTROL FLOW 0 none
* LOOP STRUCTURE 0 no
* LOOP COMPLEXITY 0 N/A
* ASYNCHRONY 0 no
* TAINT 3 file read
* RUNTIME ENV. DEPENDENCE 1 yes
* MAGNITUDE 1 1 byte
* CONTINUOUS/DISCRETE 1 continuous
* SIGNEDNESS 0 no
*/
/*
Copyright 2004 M.I.T.
Permission is hereby granted, without written agreement or royalty fee, to use,
copy, modify, and distribute this software and its documentation for any
purpose, provided that the above copyright notice and the following three
paragraphs appear in all copies of this software.
IN NO EVENT SHALL M.I.T. BE LIABLE TO ANY PARTY FOR DIRECT, INDIRECT, SPECIAL,
INCIDENTAL, OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE USE OF THIS SOFTWARE
AND ITS DOCUMENTATION, EVEN IF M.I.T. HAS BEEN ADVISED OF THE POSSIBILITY OF
SUCH DAMANGE.
M.I.T. SPECIFICALLY DISCLAIMS ANY WARRANTIES INCLUDING, BUT NOT LIMITED TO
THE IMPLIED WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE,
AND NON-INFRINGEMENT.
THE SOFTWARE IS PROVIDED ON AN "AS-IS" BASIS AND M.I.T. HAS NO OBLIGATION TO
PROVIDE MAINTENANCE, SUPPORT, UPDATES, ENHANCEMENTS, OR MODIFICATIONS.
*/
#include <assert.h>
#include <stdio.h>
int main(int argc, char *argv[])
{
FILE * f;
char buf[10];
f = fopen("TestInputFile1", "r");
assert(f != NULL);
/* BAD */
fgets(buf, 11, f);
fclose(f);
return 0;
}
| {
"pile_set_name": "Github"
} |
// Copyright 2016 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef COMPONENTS_LEVELDB_PUBLIC_CPP_REMOTE_ITERATOR_H_
#define COMPONENTS_LEVELDB_PUBLIC_CPP_REMOTE_ITERATOR_H_
#include "base/unguessable_token.h"
#include "components/leveldb/public/interfaces/leveldb.mojom.h"
#include "third_party/leveldatabase/src/include/leveldb/iterator.h"
namespace leveldb {
// A wrapper around the raw iterator movement methods on the mojo leveldb
// interface to allow drop in replacement to current leveldb usage.
//
// Note: Next(), Prev() and all the Seek*() calls cause mojo sync calls.
class RemoteIterator : public Iterator {
public:
RemoteIterator(mojom::LevelDBDatabase* database,
const base::UnguessableToken& iterator);
~RemoteIterator() override;
// Overridden from leveldb::Iterator:
bool Valid() const override;
void SeekToFirst() override;
void SeekToLast() override;
void Seek(const Slice& target) override;
void Next() override;
void Prev() override;
Slice key() const override;
Slice value() const override;
Status status() const override;
private:
mojom::LevelDBDatabase* database_;
base::UnguessableToken iterator_;
bool valid_;
mojom::DatabaseError status_;
base::Optional<std::vector<uint8_t>> key_;
base::Optional<std::vector<uint8_t>> value_;
DISALLOW_COPY_AND_ASSIGN(RemoteIterator);
};
} // namespace leveldb
#endif // COMPONENTS_LEVELDB_PUBLIC_CPP_REMOTE_ITERATOR_H_
| {
"pile_set_name": "Github"
} |
defaults_d.erb:
target: defaults_d.txt | {
"pile_set_name": "Github"
} |
<!doctype html>
<title>CodeMirror: ECL mode</title>
<meta charset="utf-8"/>
<link rel=stylesheet href="../../doc/docs.css">
<link rel="stylesheet" href="../../lib/codemirror.css">
<script src="../../lib/codemirror.js"></script>
<script src="ecl.js"></script>
<style>.CodeMirror {border: 1px solid black;}</style>
<div id=nav>
<a href="https://codemirror.net"><h1>CodeMirror</h1><img id=logo src="../../doc/logo.png" alt=""></a>
<ul>
<li><a href="../../index.html">Home</a>
<li><a href="../../doc/manual.html">Manual</a>
<li><a href="https://github.com/codemirror/codemirror">Code</a>
</ul>
<ul>
<li><a href="../index.html">Language modes</a>
<li><a class=active href="#">ECL</a>
</ul>
</div>
<article>
<h2>ECL mode</h2>
<form><textarea id="code" name="code">
/*
sample useless code to demonstrate ecl syntax highlighting
this is a multiline comment!
*/
// this is a singleline comment!
import ut;
r :=
record
string22 s1 := '123';
integer4 i1 := 123;
end;
#option('tmp', true);
d := dataset('tmp::qb', r, thor);
output(d);
</textarea></form>
<script>
var editor = CodeMirror.fromTextArea(document.getElementById("code"), {});
</script>
<p>Based on CodeMirror's clike mode. For more information see <a href="http://hpccsystems.com">HPCC Systems</a> web site.</p>
<p><strong>MIME types defined:</strong> <code>text/x-ecl</code>.</p>
</article>
| {
"pile_set_name": "Github"
} |
<?php
/**
* Copyright ยฉ Magento, Inc. All rights reserved.
* See COPYING.txt for license details.
*/
namespace Magento\Persistent\Block\Form;
/**
* Remember Me block
*
* @api
* @author Magento Core Team <core@magentocommerce.com>
* @since 100.0.2
*/
class Remember extends \Magento\Framework\View\Element\Template
{
/**
* Persistent data
*
* @var \Magento\Persistent\Helper\Data
*/
protected $_persistentData = null;
/**
* @var \Magento\Framework\Math\Random
*/
protected $mathRandom;
/**
* @param \Magento\Framework\View\Element\Template\Context $context
* @param \Magento\Persistent\Helper\Data $persistentData
* @param \Magento\Framework\Math\Random $mathRandom
* @param array $data
*/
public function __construct(
\Magento\Framework\View\Element\Template\Context $context,
\Magento\Persistent\Helper\Data $persistentData,
\Magento\Framework\Math\Random $mathRandom,
array $data = []
) {
$this->_persistentData = $persistentData;
$this->mathRandom = $mathRandom;
parent::__construct($context, $data);
}
/**
* Prevent rendering if Persistent disabled
*
* @return string
*/
protected function _toHtml()
{
return $this->_persistentData->isEnabled() &&
$this->_persistentData->isRememberMeEnabled() ? parent::_toHtml() : '';
}
/**
* Is "Remember Me" checked
*
* @return bool
*/
public function isRememberMeChecked()
{
return $this->_persistentData->isEnabled() &&
$this->_persistentData->isRememberMeEnabled() &&
$this->_persistentData->isRememberMeCheckedDefault();
}
/**
* Get random string
*
* @param int $length
* @param string|null $chars
* @return string
* @codeCoverageIgnore
*/
public function getRandomString($length, $chars = null)
{
return $this->mathRandom->getRandomString($length, $chars);
}
}
| {
"pile_set_name": "Github"
} |
<?php namespace System\Classes;
use Lang;
use ApplicationException;
use October\Rain\Database\ModelBehavior as ModelBehaviorBase;
/**
* Base class for model behaviors.
*
* @package october\system
* @author Alexey Bobkov, Samuel Georges
*/
class ModelBehavior extends ModelBehaviorBase
{
/**
* @var array Properties that must exist in the model using this behavior.
*/
protected $requiredProperties = [];
/**
* Constructor
* @param October\Rain\Database\Model $model The extended model.
*/
public function __construct($model)
{
parent::__construct($model);
/*
* Validate model properties
*/
foreach ($this->requiredProperties as $property) {
if (!isset($model->{$property})) {
throw new ApplicationException(Lang::get('system::lang.behavior.missing_property', [
'class' => get_class($model),
'property' => $property,
'behavior' => get_called_class()
]));
}
}
}
}
| {
"pile_set_name": "Github"
} |
GetEdgeBridges (SWIG)
'''''''''''''''''''''
.. function:: GetEdgeBridges(Graph, EdgeV)
Returns the edge bridges in *Graph* in the vector *EdgeV*. An edge is a bridge if, when removed, increases the number of connected components.
Parameters:
- *Graph*: undirected graph (input)
A Snap.py undirected graph.
- *EdgeV*: :class:`TIntPrV`, a vector of (int, int) pairs (output)
The bride edges of the graph. Each edge is represented by a node id pair.
Return value:
- None
The following example shows how to calculate number of bidirectional edges for
:class:`TNGraph` and :class:`TNEANet`::
import snap
UGraph = snap.GenRndGnm(snap.PUNGraph, 100, 1000)
EdgeV = snap.TIntPrV()
snap.GetEdgeBridges(UGraph, EdgeV)
for edge in EdgeV:
print("edge: (%d, %d)" % (edge.GetVal1(), edge.GetVal2()))
| {
"pile_set_name": "Github"
} |
/* Generated by RuntimeBrowser
Image: /System/Library/PrivateFrameworks/UpNextWidget.framework/UpNextWidget
*/
@interface UpNextWidget.MainViewController : UIViewController <NCWidgetProviding> {
void blankViewController;
void contentSizeCategoryDidChangeObservationToken;
void contentUnavailableViewController;
void currentViewController;
void detailedViewController;
void firstLoadStopwatch;
void lastActiveDisplayMode;
void lastKnownCoordinateLatitudeStateKey;
void lastKnownCoordinateLongitudeStateKey;
void lastKnownHypothesisStateKey;
void lastKnownMapSnapshotStateKey;
void lastKnownModelStateKey;
void lastKnownStateKey;
void lastLargestDisplayModeIsCompactKey;
void mainView;
void model;
void stackedCellViewController;
void timeListener.storage;
}
- (id /* block */).cxx_destruct;
- (void)beginRequestWithExtensionContext:(id)arg1;
- (void)dealloc;
- (id)initWithCoder:(id)arg1;
- (id)initWithNibName:(id)arg1 bundle:(id)arg2;
- (void)loadView;
- (void)preferredContentSizeDidChangeForChildContentContainer:(id)arg1;
- (void)viewDidDisappear:(bool)arg1;
- (void)viewWillAppear:(bool)arg1;
- (void)widgetActiveDisplayModeDidChangeWithActiveDisplayMode:(long long)arg1 withMaximumSize:(struct CGSize { double x1; double x2; })arg2;
- (void)widgetPerformUpdateWithCompletionHandler:(id /* block */)arg1;
@end
| {
"pile_set_name": "Github"
} |
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package app.metatron.discovery.domain.dataconnection;
import com.querydsl.core.types.Predicate;
import org.joda.time.DateTime;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.stereotype.Component;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import app.metatron.discovery.common.criteria.ListCriterion;
import app.metatron.discovery.common.criteria.ListCriterionType;
import app.metatron.discovery.common.criteria.ListFilter;
import app.metatron.discovery.domain.datasource.DataSourceListCriterionKey;
import app.metatron.discovery.domain.user.DirectoryProfile;
import app.metatron.discovery.domain.user.User;
import app.metatron.discovery.domain.user.UserRepository;
import app.metatron.discovery.domain.user.group.GroupMember;
import app.metatron.discovery.domain.user.group.GroupMemberRepository;
import app.metatron.discovery.domain.user.group.GroupService;
import app.metatron.discovery.domain.user.role.RoleDirectory;
import app.metatron.discovery.domain.user.role.RoleDirectoryRepository;
import app.metatron.discovery.domain.workspace.Workspace;
import app.metatron.discovery.domain.workspace.WorkspaceRepository;
import app.metatron.discovery.domain.workspace.WorkspaceService;
import app.metatron.discovery.extension.dataconnection.jdbc.dialect.JdbcDialect;
import app.metatron.discovery.util.AuthUtils;
/**
*
*/
@Component
public class DataConnectionFilterService {
@Autowired
UserRepository userRepository;
@Autowired
DataConnectionRepository dataConnectionRepository;
@Autowired
GroupService groupService;
@Autowired
RoleDirectoryRepository roleDirectoryRepository;
@Autowired
WorkspaceRepository workspaceRepository;
@Autowired
WorkspaceService workspaceService;
@Autowired
GroupMemberRepository groupMemberRepository;
@Autowired
DataConnectionProperties dataConnectionProperties;
@Autowired
List<JdbcDialect> jdbcDialects;
public List<ListCriterion> getListCriterion(){
List<ListCriterion> criteria = new ArrayList<>();
//Publish
criteria.add(new ListCriterion(DataConnectionListCriterionKey.PUBLISH,
ListCriterionType.CHECKBOX, "msg.storage.ui.criterion.publish", true));
//Creator
criteria.add(new ListCriterion(DataConnectionListCriterionKey.CREATOR,
ListCriterionType.CHECKBOX, "msg.storage.ui.criterion.creator", true));
//DB TYPE
criteria.add(new ListCriterion(DataConnectionListCriterionKey.IMPLEMENTOR,
ListCriterionType.CHECKBOX, "msg.storage.ui.criterion.implementor"));
//AUTH_TYPE
criteria.add(new ListCriterion(DataConnectionListCriterionKey.AUTH_TYPE,
ListCriterionType.CHECKBOX, "msg.storage.ui.criterion.auth-type"));
//CreatedTime
ListCriterion createdTimeCriterion
= new ListCriterion(DataConnectionListCriterionKey.CREATED_TIME,
ListCriterionType.RANGE_DATETIME, "msg.storage.ui.criterion.created-time");
createdTimeCriterion.addFilter(new ListFilter(DataConnectionListCriterionKey.CREATED_TIME,
"createdTimeFrom", "createdTimeTo", "", "",
"msg.storage.ui.criterion.created-time"));
criteria.add(createdTimeCriterion);
//more
ListCriterion moreCriterion = new ListCriterion(DataConnectionListCriterionKey.MORE,
ListCriterionType.CHECKBOX, "msg.storage.ui.criterion.more");
moreCriterion.addSubCriterion(new ListCriterion(DataConnectionListCriterionKey.MODIFIED_TIME,
ListCriterionType.RANGE_DATETIME, "msg.storage.ui.criterion.modified-time"));
criteria.add(moreCriterion);
return criteria;
}
public ListCriterion getListCriterionByKey(DataConnectionListCriterionKey criterionKey){
ListCriterion criterion = new ListCriterion();
criterion.setCriterionKey(criterionKey);
switch(criterionKey){
case IMPLEMENTOR:
for(JdbcDialect jdbcDialect : jdbcDialects){
if(jdbcDialect.getImplementor().equals("STAGE")){
continue;
}
criterion.addFilter(new ListFilter(criterionKey, "implementor", jdbcDialect.getImplementor(), jdbcDialect.getName()));
}
break;
case AUTH_TYPE:
criterion.addFilter(new ListFilter(criterionKey, "authenticationType", DataConnection.AuthenticationType.MANUAL.toString(), "msg.storage.li.connect.always"));
criterion.addFilter(new ListFilter(criterionKey, "authenticationType", DataConnection.AuthenticationType.USERINFO.toString(), "msg.storage.li.connect.account"));
criterion.addFilter(new ListFilter(criterionKey, "authenticationType", DataConnection.AuthenticationType.DIALOG.toString(), "msg.storage.li.connect.id"));
break;
case CREATED_TIME:
//created_time
criterion.addFilter(new ListFilter(DataConnectionListCriterionKey.CREATED_TIME,
"createdTimeFrom", "createdTimeTo", "", "",
"msg.storage.ui.criterion.created-time"));
break;
case MODIFIED_TIME:
//created_time
criterion.addFilter(new ListFilter(DataConnectionListCriterionKey.MODIFIED_TIME,
"modifiedTimeFrom", "modifiedTimeTo", "", "",
"msg.storage.ui.criterion.modified-time"));
break;
case CREATOR:
//allow search
criterion.setSearchable(true);
String userName = AuthUtils.getAuthUserName();
User user = userRepository.findByUsername(userName);
//user
ListCriterion userCriterion = new ListCriterion();
userCriterion.setCriterionName("msg.storage.ui.criterion.users");
userCriterion.setCriterionType(ListCriterionType.CHECKBOX);
criterion.addSubCriterion(userCriterion);
//me
userCriterion.addFilter(new ListFilter("createdBy", userName,
user.getFullName() + " (me)"));
//dataconnection create users
List<String> creatorIdList = dataConnectionRepository.findDistinctCreatedBy();
if(creatorIdList != null && !creatorIdList.isEmpty()){
List<User> creatorUserList = userRepository.findByUsernames(creatorIdList);
for(User creator : creatorUserList){
if(!creator.getUsername().equals(userName)){
ListFilter filter = new ListFilter("createdBy", creator.getUsername(),
creator.getFullName());
userCriterion.addFilter(filter);
}
}
}
//groups
ListCriterion groupCriterion = new ListCriterion();
groupCriterion.setCriterionName("msg.storage.ui.criterion.groups");
criterion.addSubCriterion(groupCriterion);
//my group
List<Map<String, Object>> groupList = groupService.getJoinedGroupsForProjection(userName, false);
if(groupList != null && !groupList.isEmpty()){
for(Map<String, Object> groupMap : groupList){
ListFilter filter = new ListFilter("userGroup", groupMap.get("id").toString(),
groupMap.get("name").toString() + " (my)");
groupCriterion.addFilter(filter);
}
}
//data manager group
List<RoleDirectory> roleDirectoryList
= roleDirectoryRepository.findByTypeAndRoleId(DirectoryProfile.Type.GROUP, "ROLE_SYSTEM_DATA_MANAGER");
if(roleDirectoryList != null && !roleDirectoryList.isEmpty()){
for(RoleDirectory roleDirectory : roleDirectoryList){
//duplicate group check
boolean duplicated = false;
if(groupList != null && !groupList.isEmpty()){
long duplicatedCnt = groupList.stream()
.filter(groupMap -> roleDirectory.getDirectoryId().equals(groupMap.get("id").toString()))
.count();
duplicated = duplicatedCnt > 0;
}
if(!duplicated){
ListFilter filter = new ListFilter("userGroup", roleDirectory.getDirectoryId(),
roleDirectory.getDirectoryName());
groupCriterion.addFilter(filter);
}
}
}
break;
case PUBLISH:
//allow search
criterion.setSearchable(true);
//published workspace
criterion.addFilter(new ListFilter(criterionKey, "published", "true", "msg.storage.ui.criterion.open-data"));
//my private workspace
Workspace myWorkspace = workspaceRepository.findPrivateWorkspaceByOwnerId(AuthUtils.getAuthUserName());
criterion.addFilter(new ListFilter(criterionKey, "workspace",
myWorkspace.getId(), myWorkspace.getName()));
//member public workspace not published
List<Workspace> memberPublicWorkspaces
= workspaceService.getPublicWorkspaces(false, null, null, null);
for(Workspace workspace : memberPublicWorkspaces){
criterion.addFilter(new ListFilter(criterionKey, "workspace",
workspace.getId(), workspace.getName()));
}
break;
default:
break;
}
return criterion;
}
public Page<DataConnection> findDataConnectionByFilter(
List<String> workspaces,
List<String> createdBys,
List<String> userGroups,
List<String> implementors,
List<DataConnection.AuthenticationType> authenticationTypes,
DateTime createdTimeFrom,
DateTime createdTimeTo,
DateTime modifiedTimeFrom,
DateTime modifiedTimeTo,
String containsText,
List<Boolean> published,
Pageable pageable){
//add userGroups member to createdBy
List<GroupMember> groupMembers = groupMemberRepository.findByGroupIds(userGroups);
if(groupMembers != null && !groupMembers.isEmpty()){
if(createdBys == null)
createdBys = new ArrayList<>();
for(GroupMember groupMember : groupMembers){
createdBys.add(groupMember.getMemberId());
}
}
// Get Predicate
Predicate searchPredicated = DataConnectionPredicate.searchList(workspaces, createdBys, implementors,
authenticationTypes, createdTimeFrom, createdTimeTo, modifiedTimeFrom, modifiedTimeTo, containsText, published);
// Find by predicated
Page<DataConnection> dataConnections = dataConnectionRepository.findAll(searchPredicated, pageable);
return dataConnections;
}
public List<ListFilter> getDefaultFilter(){
List<DataConnectionProperties.DefaultFilter> defaultFilters = dataConnectionProperties.getDefaultFilters();
List<ListFilter> defaultCriteria = new ArrayList<>();
if(defaultFilters != null){
for(DataConnectionProperties.DefaultFilter defaultFilter : defaultFilters){
//me
if(defaultFilter.getFilterValue().equals("me")){
String userName = AuthUtils.getAuthUserName();
User user = userRepository.findByUsername(userName);
ListFilter meFilter = new ListFilter(DataSourceListCriterionKey.valueOf(defaultFilter.getCriterionKey())
, "createdBy", null, userName, null, user.getFullName() + " (me)");
defaultCriteria.add(meFilter);
} else {
ListFilter listFilter = new ListFilter(DataSourceListCriterionKey.valueOf(defaultFilter.getCriterionKey())
, defaultFilter.getFilterKey(), null, defaultFilter.getFilterValue(), null
, defaultFilter.getFilterName());
defaultCriteria.add(listFilter);
}
}
}
return defaultCriteria;
}
}
| {
"pile_set_name": "Github"
} |
//
// QDMarqueeLabelViewController.h
// qmuidemo
//
// Created by QMUI Team on 2017/6/3.
// Copyright ยฉ 2017ๅนด QMUI Team. All rights reserved.
//
#import "QDCommonViewController.h"
@interface QDMarqueeLabelViewController : QDCommonViewController
@end
| {
"pile_set_name": "Github"
} |
# frozen_string_literal: true
module Decidim
module Elections
module Admin
# This controller allows the create or update questions for an election.
class QuestionsController < Admin::ApplicationController
helper_method :election, :questions, :question
def new
enforce_permission_to :create, :question, election: election
@form = form(QuestionForm).instance
end
def create
enforce_permission_to :create, :question, election: election
@form = form(QuestionForm).from_params(params, election: election)
CreateQuestion.call(@form) do
on(:ok) do
flash[:notice] = I18n.t("questions.create.success", scope: "decidim.elections.admin")
redirect_to election_questions_path(election)
end
on(:invalid) do
flash.now[:alert] = I18n.t("questions.create.invalid", scope: "decidim.elections.admin")
render action: "new"
end
end
end
def edit
enforce_permission_to :update, :question, election: election, question: question
@form = form(QuestionForm).from_model(question)
end
def update
enforce_permission_to :update, :question, election: election, question: question
@form = form(QuestionForm).from_params(params, election: election)
UpdateQuestion.call(@form, question) do
on(:ok) do
flash[:notice] = I18n.t("questions.update.success", scope: "decidim.elections.admin")
redirect_to election_questions_path(election)
end
on(:invalid) do
flash.now[:alert] = I18n.t("questions.update.invalid", scope: "decidim.elections.admin")
render action: "edit"
end
end
end
def destroy
enforce_permission_to :update, :question, election: election, question: question
DestroyQuestion.call(question, current_user) do
on(:ok) do
flash[:notice] = I18n.t("questions.destroy.success", scope: "decidim.elections.admin")
end
on(:invalid) do
flash.now[:alert] = I18n.t("questions.destroy.invalid", scope: "decidim.elections.admin")
end
end
redirect_to election_questions_path(election)
end
private
def election
@election ||= Election.where(component: current_component).find_by(id: params[:election_id])
end
def questions
@questions ||= election.questions
end
def question
questions.find(params[:id])
end
end
end
end
end
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>IDESourceControlProjectFavoriteDictionaryKey</key>
<false/>
<key>IDESourceControlProjectIdentifier</key>
<string>2926F92F-9F81-4831-9C55-C263E86FC0F4</string>
<key>IDESourceControlProjectName</key>
<string>Camera Test</string>
<key>IDESourceControlProjectOriginsDictionary</key>
<dict>
<key>491B8B6CF6B731F7AD11E8B49B59BE536E603468</key>
<string>https://github.com/EricssonResearch/openwebrtc-examples.git</string>
<key>8700B771B68BFE3D622EDEE2EF612F550AE65F42</key>
<string>https://github.com/EricssonResearch/openwebrtc.git</string>
</dict>
<key>IDESourceControlProjectPath</key>
<string>osx/Camera Test/Camera Test.xcodeproj</string>
<key>IDESourceControlProjectRelativeInstallPathDictionary</key>
<dict>
<key>491B8B6CF6B731F7AD11E8B49B59BE536E603468</key>
<string>../../../..</string>
<key>8700B771B68BFE3D622EDEE2EF612F550AE65F42</key>
<string>../../../../../openwebrtc</string>
</dict>
<key>IDESourceControlProjectURL</key>
<string>https://github.com/EricssonResearch/openwebrtc-examples.git</string>
<key>IDESourceControlProjectVersion</key>
<integer>111</integer>
<key>IDESourceControlProjectWCCIdentifier</key>
<string>491B8B6CF6B731F7AD11E8B49B59BE536E603468</string>
<key>IDESourceControlProjectWCConfigurations</key>
<array>
<dict>
<key>IDESourceControlRepositoryExtensionIdentifierKey</key>
<string>public.vcs.git</string>
<key>IDESourceControlWCCIdentifierKey</key>
<string>8700B771B68BFE3D622EDEE2EF612F550AE65F42</string>
<key>IDESourceControlWCCName</key>
<string>openwebrtc</string>
</dict>
<dict>
<key>IDESourceControlRepositoryExtensionIdentifierKey</key>
<string>public.vcs.git</string>
<key>IDESourceControlWCCIdentifierKey</key>
<string>491B8B6CF6B731F7AD11E8B49B59BE536E603468</string>
<key>IDESourceControlWCCName</key>
<string>openwebrtc-examples</string>
</dict>
</array>
</dict>
</plist>
| {
"pile_set_name": "Github"
} |
@echo off
rem Licensed to the Apache Software Foundation (ASF) under one or more
rem contributor license agreements. See the NOTICE file distributed with
rem this work for additional information regarding copyright ownership.
rem The ASF licenses this file to You under the Apache License, Version 2.0
rem (the "License"); you may not use this file except in compliance with
rem the License. You may obtain a copy of the License at
rem
rem http://www.apache.org/licenses/LICENSE-2.0
rem
rem Unless required by applicable law or agreed to in writing, software
rem distributed under the License is distributed on an "AS IS" BASIS,
rem WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
rem See the License for the specific language governing permissions and
rem limitations under the License.
if "%OS%" == "Windows_NT" setlocal
rem ---------------------------------------------------------------------------
rem Wrapper script for command line tools
rem
rem Environment Variable Prerequisites
rem
rem CATALINA_HOME May point at your Catalina "build" directory.
rem
rem TOOL_OPTS (Optional) Java runtime options.
rem
rem JAVA_HOME Must point at your Java Development Kit installation.
rem Using JRE_HOME instead works as well.
rem
rem JRE_HOME Must point at your Java Runtime installation.
rem Defaults to JAVA_HOME if empty. If JRE_HOME and JAVA_HOME
rem are both set, JRE_HOME is used.
rem
rem JAVA_OPTS (Optional) Java runtime options.
rem
rem JAVA_ENDORSED_DIRS (Optional) Lists of of semi-colon separated directories
rem containing some jars in order to allow replacement of APIs
rem created outside of the JCP (i.e. DOM and SAX from W3C).
rem It can also be used to update the XML parser implementation.
rem Defaults to $CATALINA_HOME/endorsed.
rem
rem $Id: tool-wrapper.bat 1138835 2011-06-23 11:27:57Z rjung $
rem ---------------------------------------------------------------------------
rem Guess CATALINA_HOME if not defined
set "CURRENT_DIR=%cd%"
if not "%CATALINA_HOME%" == "" goto gotHome
set "CATALINA_HOME=%CURRENT_DIR%"
if exist "%CATALINA_HOME%\bin\tool-wrapper.bat" goto okHome
cd ..
set "CATALINA_HOME=%cd%"
cd "%CURRENT_DIR%"
:gotHome
if exist "%CATALINA_HOME%\bin\tool-wrapper.bat" goto okHome
echo The CATALINA_HOME environment variable is not defined correctly
echo This environment variable is needed to run this program
goto end
:okHome
rem Ensure that any user defined CLASSPATH variables are not used on startup,
rem but allow them to be specified in setenv.bat, in rare case when it is needed.
set CLASSPATH=
rem Get standard environment variables
if exist "%CATALINA_HOME%\bin\setenv.bat" call "%CATALINA_HOME%\bin\setenv.bat"
rem Get standard Java environment variables
if exist "%CATALINA_HOME%\bin\setclasspath.bat" goto okSetclasspath
echo Cannot find "%CATALINA_HOME%\bin\setclasspath.bat"
echo This file is needed to run this program
goto end
:okSetclasspath
call "%CATALINA_HOME%\bin\setclasspath.bat" %1
if errorlevel 1 goto end
rem Add on extra jar files to CLASSPATH
rem Note that there are no quotes as we do not want to introduce random
rem quotes into the CLASSPATH
if "%CLASSPATH%" == "" goto emptyClasspath
set "CLASSPATH=%CLASSPATH%;"
:emptyClasspath
set "CLASSPATH=%CLASSPATH%%CATALINA_HOME%\bin\bootstrap.jar;%CATALINA_HOME%\bin\tomcat-juli.jar;%CATALINA_HOME%\lib\servlet-api.jar"
set JAVA_OPTS=%JAVA_OPTS% -Djava.util.logging.manager=org.apache.juli.ClassLoaderLogManager
rem Get remaining unshifted command line arguments and save them in the
set CMD_LINE_ARGS=
:setArgs
if ""%1""=="""" goto doneSetArgs
set CMD_LINE_ARGS=%CMD_LINE_ARGS% %1
shift
goto setArgs
:doneSetArgs
%_RUNJAVA% %JAVA_OPTS% %TOOL_OPTS% -Djava.endorsed.dirs="%JAVA_ENDORSED_DIRS%" -classpath "%CLASSPATH%" -Dcatalina.home="%CATALINA_HOME%" org.apache.catalina.startup.Tool %CMD_LINE_ARGS%
:end
| {
"pile_set_name": "Github"
} |
<?php
use Siberian\Hook;
$init = static function ($bootstrap) {
Hook::listen(
'cache.clear.cache',
'application_pre_init_cache',
static function () {
Application_Model_Cron::triggerRun();
});
};
| {
"pile_set_name": "Github"
} |
/* -*- Mode: c; tab-width: 8; c-basic-offset: 4; indent-tabs-mode: t; -*- */
/* cairo - a vector graphics library with display and print output
*
* Copyright ยฉ 2008 Adrian Johnson
*
* This library is free software; you can redistribute it and/or
* modify it either under the terms of the GNU Lesser General Public
* License version 2.1 as published by the Free Software Foundation
* (the "LGPL") or, at your option, under the terms of the Mozilla
* Public License Version 1.1 (the "MPL"). If you do not alter this
* notice, a recipient may use your version of this file under either
* the MPL or the LGPL.
*
* You should have received a copy of the LGPL along with this library
* in the file COPYING-LGPL-2.1; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Suite 500, Boston, MA 02110-1335, USA
* You should have received a copy of the MPL along with this library
* in the file COPYING-MPL-1.1
*
* The contents of this file are subject to the Mozilla Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY
* OF ANY KIND, either express or implied. See the LGPL or the MPL for
* the specific language governing rights and limitations.
*
* The Original Code is the cairo graphics library.
*
* The Initial Developer of the Original Code is Adrian Johnson.
*
* Contributor(s):
* Adrian Johnson <ajohnson@redneon.com>
*/
#include "cairoint.h"
#include "cairo-error-private.h"
#include "cairo-image-info-private.h"
/* JPEG (image/jpeg)
*
* http://www.w3.org/Graphics/JPEG/itu-t81.pdf
*/
/* Markers with no parameters. All other markers are followed by a two
* byte length of the parameters. */
#define TEM 0x01
#define RST_begin 0xd0
#define RST_end 0xd7
#define SOI 0xd8
#define EOI 0xd9
/* Start of frame markers. */
#define SOF0 0xc0
#define SOF1 0xc1
#define SOF2 0xc2
#define SOF3 0xc3
#define SOF5 0xc5
#define SOF6 0xc6
#define SOF7 0xc7
#define SOF9 0xc9
#define SOF10 0xca
#define SOF11 0xcb
#define SOF13 0xcd
#define SOF14 0xce
#define SOF15 0xcf
static const unsigned char *
_jpeg_skip_segment (const unsigned char *p)
{
int len;
p++;
len = (p[0] << 8) | p[1];
return p + len;
}
static void
_jpeg_extract_info (cairo_image_info_t *info, const unsigned char *p)
{
info->width = (p[6] << 8) + p[7];
info->height = (p[4] << 8) + p[5];
info->num_components = p[8];
info->bits_per_component = p[3];
}
cairo_int_status_t
_cairo_image_info_get_jpeg_info (cairo_image_info_t *info,
const unsigned char *data,
long length)
{
const unsigned char *p = data;
while (p + 1 < data + length) {
if (*p != 0xff)
return CAIRO_INT_STATUS_UNSUPPORTED;
p++;
switch (*p) {
/* skip fill bytes */
case 0xff:
p++;
break;
case TEM:
case SOI:
case EOI:
p++;
break;
case SOF0:
case SOF1:
case SOF2:
case SOF3:
case SOF5:
case SOF6:
case SOF7:
case SOF9:
case SOF10:
case SOF11:
case SOF13:
case SOF14:
case SOF15:
/* Start of frame found. Extract the image parameters. */
if (p + 8 > data + length)
return CAIRO_INT_STATUS_UNSUPPORTED;
_jpeg_extract_info (info, p);
return CAIRO_STATUS_SUCCESS;
default:
if (*p >= RST_begin && *p <= RST_end) {
p++;
break;
}
if (p + 3 > data + length)
return CAIRO_INT_STATUS_UNSUPPORTED;
p = _jpeg_skip_segment (p);
break;
}
}
return CAIRO_STATUS_SUCCESS;
}
/* JPEG 2000 (image/jp2)
*
* http://www.jpeg.org/public/15444-1annexi.pdf
*/
#define JPX_FILETYPE 0x66747970
#define JPX_JP2_HEADER 0x6A703268
#define JPX_IMAGE_HEADER 0x69686472
static const unsigned char _jpx_signature[] = {
0x00, 0x00, 0x00, 0x0c, 0x6a, 0x50, 0x20, 0x20, 0x0d, 0x0a, 0x87, 0x0a
};
static const unsigned char *
_jpx_next_box (const unsigned char *p)
{
return p + get_unaligned_be32 (p);
}
static const unsigned char *
_jpx_get_box_contents (const unsigned char *p)
{
return p + 8;
}
static cairo_bool_t
_jpx_match_box (const unsigned char *p, const unsigned char *end, uint32_t type)
{
uint32_t length;
if (p + 8 < end) {
length = get_unaligned_be32 (p);
if (get_unaligned_be32 (p + 4) == type && p + length < end)
return TRUE;
}
return FALSE;
}
static const unsigned char *
_jpx_find_box (const unsigned char *p, const unsigned char *end, uint32_t type)
{
while (p < end) {
if (_jpx_match_box (p, end, type))
return p;
p = _jpx_next_box (p);
}
return NULL;
}
static void
_jpx_extract_info (const unsigned char *p, cairo_image_info_t *info)
{
info->height = get_unaligned_be32 (p);
info->width = get_unaligned_be32 (p + 4);
info->num_components = (p[8] << 8) + p[9];
info->bits_per_component = p[10];
}
cairo_int_status_t
_cairo_image_info_get_jpx_info (cairo_image_info_t *info,
const unsigned char *data,
unsigned long length)
{
const unsigned char *p = data;
const unsigned char *end = data + length;
/* First 12 bytes must be the JPEG 2000 signature box. */
if (length < ARRAY_LENGTH(_jpx_signature) ||
memcmp(p, _jpx_signature, ARRAY_LENGTH(_jpx_signature)) != 0)
return CAIRO_INT_STATUS_UNSUPPORTED;
p += ARRAY_LENGTH(_jpx_signature);
/* Next box must be a File Type Box */
if (! _jpx_match_box (p, end, JPX_FILETYPE))
return CAIRO_INT_STATUS_UNSUPPORTED;
p = _jpx_next_box (p);
/* Locate the JP2 header box. */
p = _jpx_find_box (p, end, JPX_JP2_HEADER);
if (!p)
return CAIRO_INT_STATUS_UNSUPPORTED;
/* Step into the JP2 header box. First box must be the Image
* Header */
p = _jpx_get_box_contents (p);
if (! _jpx_match_box (p, end, JPX_IMAGE_HEADER))
return CAIRO_INT_STATUS_UNSUPPORTED;
/* Get the image info */
p = _jpx_get_box_contents (p);
_jpx_extract_info (p, info);
return CAIRO_STATUS_SUCCESS;
}
/* PNG (image/png)
*
* http://www.w3.org/TR/2003/REC-PNG-20031110/
*/
#define PNG_IHDR 0x49484452
static const unsigned char _png_magic[8] = { 137, 80, 78, 71, 13, 10, 26, 10 };
cairo_int_status_t
_cairo_image_info_get_png_info (cairo_image_info_t *info,
const unsigned char *data,
unsigned long length)
{
const unsigned char *p = data;
const unsigned char *end = data + length;
if (length < 8 || memcmp (data, _png_magic, 8) != 0)
return CAIRO_INT_STATUS_UNSUPPORTED;
p += 8;
/* The first chunk must be IDHR. IDHR has 13 bytes of data plus
* the 12 bytes of overhead for the chunk. */
if (p + 13 + 12 > end)
return CAIRO_INT_STATUS_UNSUPPORTED;
p += 4;
if (get_unaligned_be32 (p) != PNG_IHDR)
return CAIRO_INT_STATUS_UNSUPPORTED;
p += 4;
info->width = get_unaligned_be32 (p);
p += 4;
info->height = get_unaligned_be32 (p);
return CAIRO_STATUS_SUCCESS;
}
static const unsigned char *
_jbig2_find_data_end (const unsigned char *p,
const unsigned char *end,
int type)
{
unsigned char end_seq[2];
int mmr;
/* Segments of type "Immediate generic region" may have an
* unspecified data length. The JBIG2 specification specifies the
* method to find the end of the data for these segments. */
if (type == 36 || type == 38 || type == 39) {
if (p + 18 < end) {
mmr = p[17] & 0x01;
if (mmr) {
/* MMR encoding ends with 0x00, 0x00 */
end_seq[0] = 0x00;
end_seq[1] = 0x00;
} else {
/* Template encoding ends with 0xff, 0xac */
end_seq[0] = 0xff;
end_seq[1] = 0xac;
}
p += 18;
while (p < end) {
if (p[0] == end_seq[0] && p[1] == end_seq[1]) {
/* Skip the 2 terminating bytes and the 4 byte row count that follows. */
p += 6;
if (p < end)
return p;
}
p++;
}
}
}
return NULL;
}
static const unsigned char *
_jbig2_get_next_segment (const unsigned char *p,
const unsigned char *end,
int *type,
const unsigned char **data,
unsigned long *data_len)
{
unsigned long seg_num;
cairo_bool_t big_page_size;
int num_segs;
int ref_seg_bytes;
int referred_size;
if (p + 6 >= end)
return NULL;
seg_num = get_unaligned_be32 (p);
*type = p[4] & 0x3f;
big_page_size = (p[4] & 0x40) != 0;
p += 5;
num_segs = p[0] >> 5;
if (num_segs == 7) {
num_segs = get_unaligned_be32 (p) & 0x1fffffff;
ref_seg_bytes = 4 + ((num_segs + 1)/8);
} else {
ref_seg_bytes = 1;
}
p += ref_seg_bytes;
if (seg_num <= 256)
referred_size = 1;
else if (seg_num <= 65536)
referred_size = 2;
else
referred_size = 4;
p += num_segs * referred_size;
p += big_page_size ? 4 : 1;
if (p + 4 >= end)
return NULL;
*data_len = get_unaligned_be32 (p);
p += 4;
*data = p;
if (*data_len == 0xffffffff) {
/* if data length is -1 we have to scan through the data to find the end */
p = _jbig2_find_data_end (*data, end, *type);
if (!p || p >= end)
return NULL;
*data_len = p - *data;
} else {
p += *data_len;
}
if (p < end)
return p;
else
return NULL;
}
static void
_jbig2_extract_info (cairo_image_info_t *info, const unsigned char *p)
{
info->width = get_unaligned_be32 (p);
info->height = get_unaligned_be32 (p + 4);
info->num_components = 1;
info->bits_per_component = 1;
}
cairo_int_status_t
_cairo_image_info_get_jbig2_info (cairo_image_info_t *info,
const unsigned char *data,
unsigned long length)
{
const unsigned char *p = data;
const unsigned char *end = data + length;
int seg_type;
const unsigned char *seg_data;
unsigned long seg_data_len;
while (p && p < end) {
p = _jbig2_get_next_segment (p, end, &seg_type, &seg_data, &seg_data_len);
if (p && seg_type == 48 && seg_data_len > 8) {
/* page information segment */
_jbig2_extract_info (info, seg_data);
return CAIRO_STATUS_SUCCESS;
}
}
return CAIRO_INT_STATUS_UNSUPPORTED;
}
| {
"pile_set_name": "Github"
} |
fileFormatVersion: 2
guid: c49bb06d12adc1d46abed797f52ee25b
TextureImporter:
internalIDToNameTable: []
externalObjects: {}
serializedVersion: 10
mipmaps:
mipMapMode: 0
enableMipMap: 0
sRGBTexture: 1
linearTexture: 0
fadeOut: 0
borderMipMap: 0
mipMapsPreserveCoverage: 0
alphaTestReferenceValue: 0.5
mipMapFadeDistanceStart: 1
mipMapFadeDistanceEnd: 3
bumpmap:
convertToNormalMap: 0
externalNormalMap: 0
heightScale: 0.25
normalMapFilter: 0
isReadable: 1
streamingMipmaps: 0
streamingMipmapsPriority: 0
grayScaleToAlpha: 0
generateCubemap: 6
cubemapConvolution: 0
seamlessCubemap: 0
textureFormat: 1
maxTextureSize: 2048
textureSettings:
serializedVersion: 2
filterMode: 0
aniso: -1
mipBias: -100
wrapU: 1
wrapV: 1
wrapW: 1
nPOTScale: 0
lightmap: 0
compressionQuality: 50
spriteMode: 1
spriteExtrude: 1
spriteMeshType: 0
alignment: 0
spritePivot: {x: 0.5, y: 0.5}
spritePixelsToUnits: 32
spriteBorder: {x: 0, y: 0, z: 0, w: 0}
spriteGenerateFallbackPhysicsShape: 1
alphaUsage: 1
alphaIsTransparency: 1
spriteTessellationDetail: -1
textureType: 8
textureShape: 1
singleChannelComponent: 0
maxTextureSizeSet: 0
compressionQualitySet: 0
textureFormatSet: 0
platformSettings:
- serializedVersion: 3
buildTarget: DefaultTexturePlatform
maxTextureSize: 2048
resizeAlgorithm: 0
textureFormat: 4
textureCompression: 1
compressionQuality: 50
crunchedCompression: 0
allowsAlphaSplitting: 0
overridden: 0
androidETC2FallbackOverride: 0
forceMaximumCompressionQuality_BC6H_BC7: 1
- serializedVersion: 3
buildTarget: Standalone
maxTextureSize: 2048
resizeAlgorithm: 0
textureFormat: 4
textureCompression: 1
compressionQuality: 50
crunchedCompression: 0
allowsAlphaSplitting: 0
overridden: 0
androidETC2FallbackOverride: 0
forceMaximumCompressionQuality_BC6H_BC7: 1
spriteSheet:
serializedVersion: 2
sprites: []
outline: []
physicsShape: []
bones: []
spriteID: c6ca1fe9de45a2945a18997595334da7
internalID: 0
vertices: []
indices:
edges: []
weights: []
secondaryTextures: []
spritePackingTag:
pSDRemoveMatte: 0
pSDShowRemoveMatteOption: 0
userData:
assetBundleName:
assetBundleVariant:
| {
"pile_set_name": "Github"
} |
<?xml version='1.0' encoding='utf-8'?>
<nrml xmlns:gml="http://www.opengis.net/gml"
xmlns="http://openquake.org/xmlns/nrml/0.4">
<hazardMap sourceModelTreePath="b1|b3|b2" gsimTreePath="b1|b6" investigationTime="50.0" IMT="PGA" poE="0.1">
<node lon="-1.0" lat="1.0" iml="0.01" />
<node lon="1.0" lat="1.0" iml="0.02" />
<node lon="1.0" lat="-1.0" iml="0.03" />
<node lon="-1.0" lat="-1.0" iml="0.04" />
</hazardMap>
</nrml>
| {
"pile_set_name": "Github"
} |
% File src/library/base/man/ls.Rd
% Part of the R package, https://www.R-project.org
% Copyright 1995-2014 R Core Team
% Distributed under GPL 2 or later
\name{ls}
\alias{ls}
\alias{objects}
\title{List Objects}
\usage{
ls(name, pos = -1L, envir = as.environment(pos),
all.names = FALSE, pattern, sorted = TRUE)
objects(name, pos= -1L, envir = as.environment(pos),
all.names = FALSE, pattern, sorted = TRUE)
}
\arguments{
\item{name}{which environment to use in listing the available objects.
Defaults to the \emph{current} environment. Although called
\code{name} for back compatibility, in fact this argument can
specify the environment in any form; see the \sQuote{Details} section.}
\item{pos}{an alternative argument to \code{name} for specifying the
environment as a position in the search list. Mostly there for
back compatibility.}
\item{envir}{an alternative argument to \code{name} for specifying the
environment. Mostly there for back compatibility.}
\item{all.names}{a logical value. If \code{TRUE}, all
object names are returned. If \code{FALSE}, names which begin with a
\samp{.} are omitted.}
\item{pattern}{an optional \link{regular expression}. Only names
matching \code{pattern} are returned. \code{\link{glob2rx}} can be
used to convert wildcard patterns to regular expressions.}
\item{sorted}{logical indicating if the resulting
\code{\link{character}} should be sorted alphabetically. Note that
this is part of \code{ls()} may take most of the time.}
}
\description{
\code{ls} and \code{objects} return a vector of character strings
giving the names of the objects in the specified environment. When
invoked with no argument at the top level prompt, \code{ls} shows what
data sets and functions a user has defined. When invoked with no
argument inside a function, \code{ls} returns the names of the
function's local variables: this is useful in conjunction with
\code{browser}.
}
\details{
The \code{name} argument can specify the environment from which
object names are taken in one of several forms:
as an integer (the position in the \code{\link{search}} list); as
the character string name of an element in the search list; or as an
explicit \code{\link{environment}} (including using
\code{\link{sys.frame}} to access the currently active function calls).
By default, the environment of the call to \code{ls} or \code{objects}
is used. The \code{pos} and \code{envir} arguments are an alternative
way to specify an environment, but are primarily there for back
compatibility.
Note that the \emph{order} of strings for \code{sorted = TRUE} is
locale dependent, see \code{\link{Sys.getlocale}}. If \code{sorted =
FALSE} the order is arbitrary, depending if the environment is
hashed, the order of insertion of objects, \dots.
}
\references{
Becker, R. A., Chambers, J. M. and Wilks, A. R. (1988)
\emph{The New S Language}.
Wadsworth & Brooks/Cole.
}
\seealso{
\code{\link{glob2rx}} for converting wildcard patterns to regular
expressions.
\code{\link{ls.str}} for a long listing based on \code{\link{str}}.
\code{\link{apropos}} (or \code{\link{find}})
for finding objects in the whole search path;
\code{\link{grep}} for more details on \sQuote{regular expressions};
\code{\link{class}}, \code{\link{methods}}, etc., for
object-oriented programming.
}
\examples{
.Ob <- 1
ls(pattern = "O")
ls(pattern= "O", all.names = TRUE) # also shows ".[foo]"
# shows an empty list because inside myfunc no variables are defined
myfunc <- function() {ls()}
myfunc()
# define a local variable inside myfunc
myfunc <- function() {y <- 1; ls()}
myfunc() # shows "y"
}
\keyword{environment}
| {
"pile_set_name": "Github"
} |
/*
* Squidex Headless CMS
*
* @license
* Copyright (c) Squidex UG (haftungsbeschrรคnkt). All rights reserved.
*/
import { ChangeDetectionStrategy, Component, Input } from '@angular/core';
import { ApiUrlConfig, BackupDto, BackupsState, Duration } from '@app/shared';
@Component({
selector: 'sqx-backup',
styleUrls: ['./backup.component.scss'],
templateUrl: './backup.component.html',
changeDetection: ChangeDetectionStrategy.OnPush
})
export class BackupComponent {
@Input()
public backup: BackupDto;
public get duration() {
return Duration.create(this.backup.started, this.backup.stopped!).toString();
}
constructor(
public readonly apiUrl: ApiUrlConfig, private readonly backupsState: BackupsState
) {
}
public delete() {
this.backupsState.delete(this.backup);
}
} | {
"pile_set_name": "Github"
} |
xof 0303txt 0032
Material Material__1 {
1.000000;1.000000;1.000000;1.000000;;
3.520000;
0.378000;0.378000;0.378000;;
0.000000;0.000000;0.000000;;
TextureFilename {
"C:\WORK\FPS Creator\MAPS\texturebank\scifi\Corridors\metal\Cd_b_01_D2.tga";
}
}
Frame deadend_a {
FrameTransformMatrix {
1.000000,0.000000,0.000000,0.000000,0.000000,1.000000,0.000000,0.000000,0.000000,0.000000,1.000000,0.000000,-0.000011,-0.000008,-0.000019,1.000000;;
}
Mesh {
132;
-49.999962;-50.000023;9.999973;,
49.999996;-50.000015;10.000006;,
-49.999962;42.099960;9.999977;,
49.999977;42.099968;10.000010;,
-49.999962;42.099960;9.999977;,
49.999996;-50.000015;10.000006;,
-49.999962;42.099960;9.999977;,
50.000004;52.499989;0.000009;,
-50.000000;52.499985;-0.000013;,
50.000004;52.499989;0.000009;,
-49.999962;42.099960;9.999977;,
49.999977;42.099968;10.000010;,
-49.999992;-42.500011;4.999999;,
50.000004;42.499996;5.000010;,
50.000004;-42.500004;5.000003;,
50.000004;42.499996;5.000010;,
-49.999992;-42.500011;4.999999;,
-49.999996;42.499992;5.000006;,
50.000000;-47.500008;-0.000019;,
-49.999992;-42.500011;4.999999;,
50.000004;-42.500004;5.000003;,
-49.999992;-42.500011;4.999999;,
50.000000;-47.500008;-0.000019;,
-49.999989;-47.500015;-0.000023;,
50.000004;42.499996;5.000010;,
-49.999992;47.499985;-0.000017;,
50.000000;47.499989;-0.000013;,
-49.999992;47.499985;-0.000017;,
50.000004;42.499996;5.000010;,
-49.999996;42.499992;5.000006;,
50.000000;47.499989;-0.000013;,
-49.999992;47.499985;-0.000017;,
50.000004;52.499989;0.000009;,
-49.999992;47.499985;-0.000017;,
-50.000000;52.499985;-0.000013;,
50.000004;52.499989;0.000009;,
50.000011;-50.000004;-0.000008;,
-49.999989;-50.000011;-0.000030;,
50.000000;-47.500008;-0.000019;,
-49.999989;-50.000011;-0.000030;,
-49.999989;-47.500015;-0.000023;,
50.000000;-47.500008;-0.000019;,
-49.999962;-50.000023;9.999973;,
-49.999989;-50.000011;-0.000030;,
49.999996;-50.000015;10.000006;,
-49.999989;-50.000011;-0.000030;,
50.000011;-50.000004;-0.000008;,
49.999996;-50.000015;10.000006;,
-49.999962;42.099960;9.999977;,
-50.000000;52.499985;-0.000013;,
-59.999996;42.099957;-0.000020;,
-49.999962;42.099960;9.999977;,
-59.999996;42.099957;-0.000020;,
-59.999992;-50.000015;-0.000039;,
-49.999962;-50.000023;9.999973;,
-49.999962;42.099960;9.999977;,
-59.999992;-50.000015;-0.000039;,
-49.999962;-50.000023;9.999973;,
-59.999992;-50.000015;-0.000039;,
-49.999989;-50.000011;-0.000030;,
50.000004;52.499989;0.000009;,
49.999977;42.099968;10.000010;,
60.000023;42.099957;0.000009;,
60.000023;42.099957;0.000009;,
49.999977;42.099968;10.000010;,
60.000019;-50.000015;-0.000010;,
49.999977;42.099968;10.000010;,
49.999996;-50.000015;10.000006;,
60.000019;-50.000015;-0.000010;,
60.000019;-50.000015;-0.000010;,
49.999996;-50.000015;10.000006;,
50.000011;-50.000004;-0.000008;,
50.000000;47.499989;-0.000013;,
50.000004;52.499989;0.000009;,
55.000000;42.499981;-0.000037;,
60.000023;42.099957;0.000009;,
60.000019;-50.000015;-0.000010;,
55.000000;42.499981;-0.000037;,
50.000000;-47.500008;-0.000019;,
54.999992;-42.500015;-0.000041;,
60.000019;-50.000015;-0.000010;,
50.000004;52.499989;0.000009;,
60.000023;42.099957;0.000009;,
55.000000;42.499981;-0.000037;,
54.999992;-42.500015;-0.000041;,
55.000000;42.499981;-0.000037;,
60.000019;-50.000015;-0.000010;,
50.000011;-50.000004;-0.000008;,
50.000000;-47.500008;-0.000019;,
60.000019;-50.000015;-0.000010;,
50.000000;47.499989;-0.000013;,
55.000000;42.499981;-0.000037;,
50.000004;42.499996;5.000010;,
50.000004;-42.500004;5.000003;,
54.999992;-42.500015;-0.000041;,
50.000000;-47.500008;-0.000019;,
50.000004;42.499996;5.000010;,
55.000000;42.499981;-0.000037;,
50.000004;-42.500004;5.000003;,
55.000000;42.499981;-0.000037;,
54.999992;-42.500015;-0.000041;,
50.000004;-42.500004;5.000003;,
-50.000000;52.499985;-0.000013;,
-49.999992;47.499985;-0.000017;,
-55.000000;42.499981;-0.000037;,
-59.999992;-50.000015;-0.000039;,
-59.999996;42.099957;-0.000020;,
-55.000000;42.499981;-0.000037;,
-54.999992;-42.500015;-0.000041;,
-49.999989;-47.500015;-0.000023;,
-59.999992;-50.000015;-0.000039;,
-59.999996;42.099957;-0.000020;,
-50.000000;52.499985;-0.000013;,
-55.000000;42.499981;-0.000037;,
-55.000000;42.499981;-0.000037;,
-54.999992;-42.500015;-0.000041;,
-59.999992;-50.000015;-0.000039;,
-49.999989;-47.500015;-0.000023;,
-49.999989;-50.000011;-0.000030;,
-59.999992;-50.000015;-0.000039;,
-55.000000;42.499981;-0.000037;,
-49.999992;47.499985;-0.000017;,
-49.999996;42.499992;5.000006;,
-54.999992;-42.500015;-0.000041;,
-49.999992;-42.500011;4.999999;,
-49.999989;-47.500015;-0.000023;,
-55.000000;42.499981;-0.000037;,
-49.999996;42.499992;5.000006;,
-49.999992;-42.500011;4.999999;,
-54.999992;-42.500015;-0.000041;,
-55.000000;42.499981;-0.000037;,
-49.999992;-42.500011;4.999999;;
44;
3;0,1,2;,
3;3,4,5;,
3;6,7,8;,
3;9,10,11;,
3;12,13,14;,
3;15,16,17;,
3;18,19,20;,
3;21,22,23;,
3;24,25,26;,
3;27,28,29;,
3;30,31,32;,
3;33,34,35;,
3;36,37,38;,
3;39,40,41;,
3;42,43,44;,
3;45,46,47;,
3;48,49,50;,
3;51,52,53;,
3;54,55,56;,
3;57,58,59;,
3;60,61,62;,
3;63,64,65;,
3;66,67,68;,
3;69,70,71;,
3;72,73,74;,
3;75,76,77;,
3;78,79,80;,
3;81,82,83;,
3;84,85,86;,
3;87,88,89;,
3;90,91,92;,
3;93,94,95;,
3;96,97,98;,
3;99,100,101;,
3;102,103,104;,
3;105,106,107;,
3;108,109,110;,
3;111,112,113;,
3;114,115,116;,
3;117,118,119;,
3;120,121,122;,
3;123,124,125;,
3;126,127,128;,
3;129,130,131;;
MeshNormals {
132;
-0.000000;-0.000000;1.000000;,
-0.000000;-0.000000;1.000000;,
-0.000000;-0.000000;1.000000;,
-0.000000;-0.000000;1.000000;,
-0.000000;-0.000000;1.000000;,
-0.000000;-0.000000;1.000000;,
-0.000000;0.693107;0.720834;,
-0.000000;0.693107;0.720834;,
-0.000000;0.693107;0.720834;,
-0.000000;0.693108;0.720834;,
-0.000000;0.693108;0.720834;,
-0.000000;0.693108;0.720834;,
0.000000;0.000000;-1.000000;,
0.000000;0.000000;-1.000000;,
0.000000;0.000000;-1.000000;,
0.000000;0.000000;-1.000000;,
0.000000;0.000000;-1.000000;,
0.000000;0.000000;-1.000000;,
-0.000000;0.707108;-0.707106;,
-0.000000;0.707108;-0.707106;,
-0.000000;0.707108;-0.707106;,
-0.000000;0.707108;-0.707106;,
-0.000000;0.707108;-0.707106;,
-0.000000;0.707108;-0.707106;,
0.000000;-0.707109;-0.707105;,
0.000000;-0.707109;-0.707105;,
0.000000;-0.707109;-0.707105;,
0.000000;-0.707109;-0.707105;,
0.000000;-0.707109;-0.707105;,
0.000000;-0.707109;-0.707105;,
0.000000;0.000004;-1.000000;,
0.000000;0.000004;-1.000000;,
0.000000;0.000004;-1.000000;,
0.000000;0.000001;-1.000000;,
0.000000;0.000001;-1.000000;,
0.000000;0.000001;-1.000000;,
0.000000;-0.000004;-1.000000;,
0.000000;-0.000004;-1.000000;,
0.000000;-0.000004;-1.000000;,
0.000000;0.000003;-1.000000;,
0.000000;0.000003;-1.000000;,
0.000000;0.000003;-1.000000;,
0.000000;-1.000000;-0.000001;,
0.000000;-1.000000;-0.000001;,
0.000000;-1.000000;-0.000001;,
0.000000;-1.000000;-0.000001;,
0.000000;-1.000000;-0.000001;,
0.000000;-1.000000;-0.000001;,
-0.584750;0.562257;0.584752;,
-0.584750;0.562257;0.584752;,
-0.584750;0.562257;0.584752;,
-0.707105;-0.000000;0.707108;,
-0.707105;-0.000000;0.707108;,
-0.707105;-0.000000;0.707108;,
-0.707106;-0.000000;0.707107;,
-0.707106;-0.000000;0.707107;,
-0.707106;-0.000000;0.707107;,
0.000000;-1.000000;-0.000001;,
0.000000;-1.000000;-0.000001;,
0.000000;-1.000000;-0.000001;,
0.584749;0.562258;0.584751;,
0.584749;0.562258;0.584751;,
0.584749;0.562258;0.584751;,
0.707105;-0.000000;0.707108;,
0.707105;-0.000000;0.707108;,
0.707105;-0.000000;0.707108;,
0.707107;0.000000;0.707107;,
0.707107;0.000000;0.707107;,
0.707107;0.000000;0.707107;,
-0.000001;-1.000000;-0.000001;,
-0.000001;-1.000000;-0.000001;,
-0.000001;-1.000000;-0.000001;,
-0.000000;0.000004;-1.000000;,
-0.000000;0.000004;-1.000000;,
-0.000000;0.000004;-1.000000;,
0.000009;0.000000;-1.000000;,
0.000009;0.000000;-1.000000;,
0.000009;0.000000;-1.000000;,
-0.000000;-0.000004;-1.000000;,
-0.000000;-0.000004;-1.000000;,
-0.000000;-0.000004;-1.000000;,
0.000010;0.000010;-1.000000;,
0.000010;0.000010;-1.000000;,
0.000010;0.000010;-1.000000;,
0.000006;0.000000;-1.000000;,
0.000006;0.000000;-1.000000;,
0.000006;0.000000;-1.000000;,
-0.000000;-0.000004;-1.000000;,
-0.000000;-0.000004;-1.000000;,
-0.000000;-0.000004;-1.000000;,
-0.577354;-0.577350;-0.577346;,
-0.577354;-0.577350;-0.577346;,
-0.577354;-0.577350;-0.577346;,
-0.577353;0.577350;-0.577348;,
-0.577353;0.577350;-0.577348;,
-0.577353;0.577350;-0.577348;,
-0.707110;0.000000;-0.707103;,
-0.707110;0.000000;-0.707103;,
-0.707110;0.000000;-0.707103;,
-0.707111;0.000000;-0.707103;,
-0.707111;0.000000;-0.707103;,
-0.707111;0.000000;-0.707103;,
0.000003;0.000001;-1.000000;,
0.000003;0.000001;-1.000000;,
0.000003;0.000001;-1.000000;,
-0.000003;0.000000;-1.000000;,
-0.000003;0.000000;-1.000000;,
-0.000003;0.000000;-1.000000;,
0.000002;-0.000002;-1.000000;,
0.000002;-0.000002;-1.000000;,
0.000002;-0.000002;-1.000000;,
-0.000004;0.000004;-1.000000;,
-0.000004;0.000004;-1.000000;,
-0.000004;0.000004;-1.000000;,
-0.000000;0.000000;-1.000000;,
-0.000000;0.000000;-1.000000;,
-0.000000;0.000000;-1.000000;,
0.000001;0.000003;-1.000000;,
0.000001;0.000003;-1.000000;,
0.000001;0.000003;-1.000000;,
0.577353;-0.577351;-0.577347;,
0.577353;-0.577351;-0.577347;,
0.577353;-0.577351;-0.577347;,
0.577352;0.577351;-0.577348;,
0.577352;0.577351;-0.577348;,
0.577352;0.577351;-0.577348;,
0.707110;0.000000;-0.707104;,
0.707110;0.000000;-0.707104;,
0.707110;0.000000;-0.707104;,
0.707110;0.000000;-0.707104;,
0.707110;0.000000;-0.707104;,
0.707110;0.000000;-0.707104;;
44;
3;0,1,2;,
3;3,4,5;,
3;6,7,8;,
3;9,10,11;,
3;12,13,14;,
3;15,16,17;,
3;18,19,20;,
3;21,22,23;,
3;24,25,26;,
3;27,28,29;,
3;30,31,32;,
3;33,34,35;,
3;36,37,38;,
3;39,40,41;,
3;42,43,44;,
3;45,46,47;,
3;48,49,50;,
3;51,52,53;,
3;54,55,56;,
3;57,58,59;,
3;60,61,62;,
3;63,64,65;,
3;66,67,68;,
3;69,70,71;,
3;72,73,74;,
3;75,76,77;,
3;78,79,80;,
3;81,82,83;,
3;84,85,86;,
3;87,88,89;,
3;90,91,92;,
3;93,94,95;,
3;96,97,98;,
3;99,100,101;,
3;102,103,104;,
3;105,106,107;,
3;108,109,110;,
3;111,112,113;,
3;114,115,116;,
3;117,118,119;,
3;120,121,122;,
3;123,124,125;,
3;126,127,128;,
3;129,130,131;;
}
MeshMaterialList {
1;
44;
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0;
{ Material__1 }
}
MeshTextureCoords {
132;
0.498719;0.498405;,
0.998719;0.498405;,
0.498719;0.000312;,
0.998719;0.000312;,
0.498719;0.000312;,
0.998719;0.498405;,
0.498719;0.498348;,
0.998719;0.434643;,
0.498719;0.434643;,
0.998719;0.434643;,
0.498719;0.498348;,
0.998719;0.498348;,
-0.000075;0.966186;,
0.499925;0.504048;,
0.499925;0.966186;,
0.499925;0.504048;,
-0.000075;0.966186;,
-0.000075;0.504048;,
0.499925;0.999774;,
-0.000075;0.966186;,
0.499925;0.966186;,
-0.000075;0.966186;,
0.499925;0.999774;,
-0.000075;0.999774;,
0.065026;0.499231;,
0.000968;-0.000769;,
0.000968;0.499231;,
0.000968;-0.000769;,
0.065026;0.499231;,
0.065026;-0.000769;,
0.000214;0.473193;,
0.500214;0.473193;,
0.000214;0.448193;,
0.500214;0.473193;,
0.500214;0.448193;,
0.000214;0.448193;,
0.682748;0.502144;,
0.682748;0.998812;,
0.704776;0.502144;,
0.682748;0.998812;,
0.704776;0.998812;,
0.704776;0.502144;,
0.498706;0.448864;,
0.498706;0.498864;,
0.998706;0.448864;,
0.498706;0.498864;,
0.998706;0.498864;,
0.998706;0.448864;,
0.527412;0.494611;,
0.562768;0.442611;,
0.598123;0.494612;,
0.527412;-0.002454;,
0.598123;-0.002454;,
0.598123;0.498951;,
0.527412;0.498951;,
0.527412;-0.002454;,
0.598123;0.498951;,
0.769718;0.360464;,
0.861882;0.361583;,
0.814445;0.267868;,
0.562768;0.442611;,
0.527412;0.494611;,
0.598123;0.494612;,
0.598123;-0.002454;,
0.527412;-0.002454;,
0.598123;0.498951;,
0.527412;-0.002454;,
0.527412;0.498951;,
0.598123;0.498951;,
0.861882;0.361583;,
0.769718;0.360464;,
0.814445;0.267868;,
0.553719;0.531035;,
0.553719;0.507215;,
0.529898;0.554856;,
0.506077;0.556762;,
0.506077;0.995540;,
0.529898;0.554856;,
0.553719;0.983630;,
0.529898;0.959809;,
0.506077;0.995540;,
0.553719;0.507215;,
0.506077;0.556762;,
0.529898;0.554856;,
0.529898;0.959809;,
0.529898;0.554856;,
0.506077;0.995540;,
0.553719;0.995540;,
0.553719;0.983630;,
0.506077;0.995540;,
0.383710;0.133553;,
0.433190;0.162120;,
0.433189;0.104985;,
0.352922;0.964553;,
0.325206;0.964553;,
0.339064;1.000055;,
0.042150;0.504585;,
0.000556;0.504585;,
0.042150;0.964487;,
0.000556;0.504585;,
0.000555;0.964487;,
0.042150;0.964487;,
0.553719;0.507215;,
0.553719;0.531035;,
0.529898;0.554856;,
0.506077;0.995540;,
0.506077;0.556762;,
0.529898;0.554856;,
0.529898;0.959809;,
0.553719;0.983630;,
0.506077;0.995540;,
0.506077;0.556762;,
0.553719;0.507215;,
0.529898;0.554856;,
0.529898;0.554856;,
0.529898;0.959809;,
0.506077;0.995540;,
0.553719;0.983630;,
0.553719;0.995540;,
0.506077;0.995540;,
0.433190;0.162120;,
0.383710;0.133553;,
0.433189;0.104985;,
0.325206;0.964553;,
0.352922;0.964553;,
0.339064;1.000055;,
0.000556;0.504585;,
0.042150;0.504585;,
0.042150;0.964487;,
0.000555;0.964487;,
0.000556;0.504585;,
0.042150;0.964487;;
}
}
} | {
"pile_set_name": "Github"
} |
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.ignite.internal.processors.cache.ttl;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import javax.cache.Cache;
import javax.cache.configuration.FactoryBuilder;
import javax.cache.expiry.Duration;
import javax.cache.expiry.TouchedExpiryPolicy;
import javax.cache.integration.CacheLoaderException;
import javax.cache.integration.CacheWriterException;
import javax.cache.integration.CompletionListenerFuture;
import org.apache.ignite.IgniteCache;
import org.apache.ignite.IgniteDataStreamer;
import org.apache.ignite.cache.CacheAtomicityMode;
import org.apache.ignite.cache.CacheMode;
import org.apache.ignite.cache.eviction.lru.LruEvictionPolicy;
import org.apache.ignite.cache.query.SqlQuery;
import org.apache.ignite.cache.store.CacheStoreAdapter;
import org.apache.ignite.configuration.CacheConfiguration;
import org.apache.ignite.configuration.IgniteConfiguration;
import org.apache.ignite.lang.IgniteBiInClosure;
import org.apache.ignite.testframework.junits.common.GridCommonAbstractTest;
import org.junit.Test;
import static java.util.concurrent.TimeUnit.MILLISECONDS;
import static org.apache.ignite.cache.CacheMode.LOCAL;
import static org.apache.ignite.cache.CachePeekMode.BACKUP;
import static org.apache.ignite.cache.CachePeekMode.NEAR;
import static org.apache.ignite.cache.CachePeekMode.OFFHEAP;
import static org.apache.ignite.cache.CachePeekMode.ONHEAP;
import static org.apache.ignite.cache.CachePeekMode.PRIMARY;
import static org.apache.ignite.cache.CacheRebalanceMode.SYNC;
import static org.apache.ignite.cache.CacheWriteSynchronizationMode.FULL_SYNC;
/**
* TTL test.
*/
public abstract class CacheTtlAbstractSelfTest extends GridCommonAbstractTest {
/** */
private static final int MAX_CACHE_SIZE = 5;
/** */
private static final int SIZE = 11;
/** */
private static final long DEFAULT_TIME_TO_LIVE = 2000;
/** {@inheritDoc} */
@Override protected IgniteConfiguration getConfiguration(String igniteInstanceName) throws Exception {
IgniteConfiguration cfg = super.getConfiguration(igniteInstanceName);
CacheConfiguration ccfg = new CacheConfiguration(DEFAULT_CACHE_NAME);
ccfg.setCacheMode(cacheMode());
ccfg.setAtomicityMode(atomicityMode());
LruEvictionPolicy plc = new LruEvictionPolicy();
plc.setMaxSize(MAX_CACHE_SIZE);
ccfg.setEvictionPolicy(plc);
ccfg.setOnheapCacheEnabled(true);
ccfg.setIndexedTypes(Integer.class, Integer.class);
ccfg.setBackups(2);
ccfg.setWriteSynchronizationMode(FULL_SYNC);
ccfg.setRebalanceMode(SYNC);
ccfg.setCacheStoreFactory(singletonFactory(new CacheStoreAdapter() {
@Override public void loadCache(IgniteBiInClosure clo, Object... args) {
for (int i = 0; i < SIZE; i++)
clo.apply(i, i);
}
@Override public Object load(Object key) throws CacheLoaderException {
return key;
}
@Override public void write(Cache.Entry entry) throws CacheWriterException {
// No-op.
}
@Override public void delete(Object key) throws CacheWriterException {
// No-op.
}
}));
ccfg.setExpiryPolicyFactory(
FactoryBuilder.factoryOf(new TouchedExpiryPolicy(new Duration(MILLISECONDS, DEFAULT_TIME_TO_LIVE))));
cfg.setCacheConfiguration(ccfg);
return cfg;
}
/**
* @return Atomicity mode.
*/
protected abstract CacheAtomicityMode atomicityMode();
/**
* @return Cache mode.
*/
protected abstract CacheMode cacheMode();
/**
* @return GridCount
*/
protected abstract int gridCount();
/** {@inheritDoc} */
@Override protected void beforeTest() throws Exception {
startGrids(gridCount());
}
/** {@inheritDoc} */
@Override protected void afterTest() throws Exception {
stopAllGrids();
}
/**
* @throws Exception If failed.
*/
@Test
public void testDefaultTimeToLiveLoadCache() throws Exception {
IgniteCache<Integer, Integer> cache = jcache(0);
cache.loadCache(null);
checkSizeBeforeLive(SIZE);
Thread.sleep(DEFAULT_TIME_TO_LIVE + 500);
checkSizeAfterLive();
}
/**
* @throws Exception If failed.
*/
@Test
public void testDefaultTimeToLiveLoadAll() throws Exception {
defaultTimeToLiveLoadAll(false);
defaultTimeToLiveLoadAll(true);
}
/**
* @param replaceExisting Replace existing value flag.
* @throws Exception If failed.
*/
private void defaultTimeToLiveLoadAll(boolean replaceExisting) throws Exception {
IgniteCache<Integer, Integer> cache = jcache(0);
CompletionListenerFuture fut = new CompletionListenerFuture();
Set<Integer> keys = new HashSet<>();
for (int i = 0; i < SIZE; ++i)
keys.add(i);
cache.loadAll(keys, replaceExisting, fut);
fut.get();
checkSizeBeforeLive(SIZE);
Thread.sleep(DEFAULT_TIME_TO_LIVE + 500);
checkSizeAfterLive();
}
/**
* @throws Exception If failed.
*/
@Test
public void testDefaultTimeToLiveStreamerAdd() throws Exception {
try (IgniteDataStreamer<Integer, Integer> streamer = ignite(0).dataStreamer(DEFAULT_CACHE_NAME)) {
for (int i = 0; i < SIZE; i++)
streamer.addData(i, i);
}
checkSizeBeforeLive(SIZE);
Thread.sleep(DEFAULT_TIME_TO_LIVE + 500);
checkSizeAfterLive();
try (IgniteDataStreamer<Integer, Integer> streamer = ignite(0).dataStreamer(DEFAULT_CACHE_NAME)) {
streamer.allowOverwrite(true);
for (int i = 0; i < SIZE; i++)
streamer.addData(i, i);
}
checkSizeBeforeLive(SIZE);
Thread.sleep(DEFAULT_TIME_TO_LIVE + 500);
checkSizeAfterLive();
}
/**
* @throws Exception If failed.
*/
@Test
public void testDefaultTimeToLivePut() throws Exception {
IgniteCache<Integer, Integer> cache = jcache(0);
Integer key = 0;
cache.put(key, 1);
checkSizeBeforeLive(1);
Thread.sleep(DEFAULT_TIME_TO_LIVE + 500);
checkSizeAfterLive();
}
/**
* @throws Exception If failed.
*/
@Test
public void testDefaultTimeToLivePutAll() throws Exception {
IgniteCache<Integer, Integer> cache = jcache(0);
Map<Integer, Integer> entries = new HashMap<>();
for (int i = 0; i < SIZE; ++i)
entries.put(i, i);
cache.putAll(entries);
checkSizeBeforeLive(SIZE);
Thread.sleep(DEFAULT_TIME_TO_LIVE + 500);
checkSizeAfterLive();
}
/**
* @throws Exception If failed.
*/
@Test
public void testDefaultTimeToLivePreload() throws Exception {
if (cacheMode() == LOCAL)
return;
IgniteCache<Integer, Integer> cache = jcache(0);
Map<Integer, Integer> entries = new HashMap<>();
for (int i = 0; i < SIZE; ++i)
entries.put(i, i);
cache.putAll(entries);
startGrid(gridCount());
checkSizeBeforeLive(SIZE, gridCount() + 1);
Thread.sleep(DEFAULT_TIME_TO_LIVE + 500);
checkSizeAfterLive(gridCount() + 1);
}
/**
* @throws Exception If failed.
*/
@Test
public void testTimeToLiveTtl() throws Exception {
long time = DEFAULT_TIME_TO_LIVE + 2000;
IgniteCache<Integer, Integer> cache = this.<Integer, Integer>jcache(0).withExpiryPolicy(
new TouchedExpiryPolicy(new Duration(MILLISECONDS, time)));
for (int i = 0; i < SIZE; i++)
cache.put(i, i);
checkSizeBeforeLive(SIZE);
Thread.sleep(DEFAULT_TIME_TO_LIVE + 500);
checkSizeBeforeLive(SIZE);
Thread.sleep(time - DEFAULT_TIME_TO_LIVE + 500);
checkSizeAfterLive();
}
/**
* @param size Expected size.
* @throws Exception If failed.
*/
private void checkSizeBeforeLive(int size) throws Exception {
checkSizeBeforeLive(size, gridCount());
}
/**
* @param size Expected size.
* @param gridCnt Number of nodes.
* @throws Exception If failed.
*/
private void checkSizeBeforeLive(int size, int gridCnt) throws Exception {
for (int i = 0; i < gridCnt; ++i) {
IgniteCache<Integer, Integer> cache = jcache(i);
log.info("Size [node=" + i + ", " + cache.localSize(PRIMARY, BACKUP, NEAR) + ']');
assertEquals("Unexpected size, node: " + i, size, cache.localSize(PRIMARY, BACKUP, NEAR));
for (int key = 0; key < size; key++)
assertNotNull(cache.localPeek(key));
assertFalse(cache.query(new SqlQuery<>(Integer.class, "_val >= 0")).getAll().isEmpty());
}
}
/**
* @throws Exception If failed.
*/
private void checkSizeAfterLive() throws Exception {
checkSizeAfterLive(gridCount());
}
/**
* @param gridCnt Number of nodes.
* @throws Exception If failed.
*/
private void checkSizeAfterLive(int gridCnt) throws Exception {
for (int i = 0; i < gridCnt; ++i) {
IgniteCache<Integer, Integer> cache = jcache(i);
log.info("Size [node=" + i +
", heap=" + cache.localSize(ONHEAP) +
", offheap=" + cache.localSize(OFFHEAP) + ']');
assertEquals(0, cache.localSize());
assertEquals(0, cache.localSize(OFFHEAP));
assertEquals(0, cache.query(new SqlQuery<>(Integer.class, "_val >= 0")).getAll().size());
for (int key = 0; key < SIZE; key++)
assertNull(cache.localPeek(key));
}
}
}
| {
"pile_set_name": "Github"
} |
/*
CRT - Guest - Dr. Venom
Copyright (C) 2018-2020 guest(r) - guest.r@gmail.com
Incorporates many good ideas and suggestions from Dr. Venom.
This program is free software; you can redistribute it and/or
modify it under the terms of the GNU General Public License
as published by the Free Software Foundation; either version 2
of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
// Parameter lines go here:
#pragma parameter TATE "TATE Mode" 0.0 0.0 1.0 1.0
#pragma parameter IOS "Smart Integer Scaling: 1.0:Y, 2.0:'X'+Y" 0.0 0.0 2.0 1.0
#pragma parameter OS "R. Bloom Overscan Mode" 1.0 0.0 2.0 1.0
#pragma parameter BLOOM "Raster bloom %" 0.0 0.0 20.0 1.0
#pragma parameter brightboost "Bright Boost Dark Pixels" 1.40 0.50 4.00 0.05
#pragma parameter brightboost1 "Bright Boost Bright Pixels" 1.10 0.50 3.00 0.05
#pragma parameter gsl "Scanline Type" 0.0 0.0 2.0 1.0
#pragma parameter scanline1 "Scanline beam shape low" 6.0 1.0 15.0 1.0
#pragma parameter scanline2 "Scanline beam shape high" 8.0 5.0 23.0 1.0
#pragma parameter beam_min "Scanline dark" 1.35 0.5 2.5 0.05
#pragma parameter beam_max "Scanline bright" 1.05 0.5 2.0 0.05
#pragma parameter beam_size "Increased bright scanline beam" 0.70 0.0 1.0 0.05
#pragma parameter spike "Scanline Spike Removal" 1.10 0.0 2.0 0.10
#pragma parameter h_sharp "Horizontal sharpness" 5.25 1.0 15.0 0.25
#pragma parameter s_sharp "Substractive sharpness" 0.40 0.0 1.0 0.10
#pragma parameter csize "Corner size" 0.0 0.0 0.07 0.01
#pragma parameter bsize "Border smoothness" 600.0 100.0 600.0 25.0
#pragma parameter warpX "CurvatureX (default 0.03)" 0.0 0.0 0.125 0.01
#pragma parameter warpY "CurvatureY (default 0.04)" 0.0 0.0 0.125 0.01
#pragma parameter glow "Glow Strength" 0.02 0.0 0.5 0.01
#pragma parameter shadowMask "CRT Mask: 0:CGWG, 1-4:Lottes, 5-6:'Trinitron'" 0.0 -1.0 7.0 1.0
#pragma parameter masksize "CRT Mask Size (2.0 is nice in 4k)" 1.0 1.0 2.0 1.0
#pragma parameter vertmask "PVM Like Colors" 0.0 0.0 0.25 0.01
#pragma parameter slotmask "Slot Mask Strength" 0.0 0.0 1.0 0.05
#pragma parameter slotwidth "Slot Mask Width" 2.0 1.0 6.0 0.5
#pragma parameter double_slot "Slot Mask Height: 2x1 or 4x1" 1.0 1.0 2.0 1.0
#pragma parameter slotms "Slot Mask Size" 1.0 1.0 2.0 1.0
#pragma parameter mcut "Mask 5-7 cutoff" 0.2 0.0 0.5 0.05
#pragma parameter maskDark "Lottes&Trinitron maskDark" 0.5 0.0 2.0 0.05
#pragma parameter maskLight "Lottes&Trinitron maskLight" 1.5 0.0 2.0 0.05
#pragma parameter CGWG "Mask 0&7 Mask Str." 0.3 0.0 1.0 0.05
#pragma parameter gamma_out "Gamma out" 2.4 1.0 3.5 0.05
#pragma parameter inter "Interlace Trigger Resolution :" 400.0 0.0 800.0 25.0
#pragma parameter interm "Interlace Mode (0.0 = OFF):" 1.0 0.0 3.0 1.0
#pragma parameter bloom "Bloom Strength" 0.0 0.0 2.0 0.10
#pragma parameter scans "Scanline 1&2 Saturation" 0.5 0.0 1.0 0.10
#if defined(VERTEX)
#if __VERSION__ >= 130
#define COMPAT_VARYING out
#define COMPAT_ATTRIBUTE in
#define COMPAT_TEXTURE texture
#else
#define COMPAT_VARYING varying
#define COMPAT_ATTRIBUTE attribute
#define COMPAT_TEXTURE texture2D
#endif
#ifdef GL_ES
#define COMPAT_PRECISION mediump
#else
#define COMPAT_PRECISION
#endif
COMPAT_ATTRIBUTE vec4 VertexCoord;
COMPAT_ATTRIBUTE vec4 COLOR;
COMPAT_ATTRIBUTE vec4 TexCoord;
COMPAT_VARYING vec4 COL0;
COMPAT_VARYING vec4 TEX0;
vec4 _oPosition1;
uniform mat4 MVPMatrix;
uniform COMPAT_PRECISION int FrameDirection;
uniform COMPAT_PRECISION int FrameCount;
uniform COMPAT_PRECISION vec2 OutputSize;
uniform COMPAT_PRECISION vec2 TextureSize;
uniform COMPAT_PRECISION vec2 InputSize;
void main()
{
gl_Position = MVPMatrix * VertexCoord;
COL0 = COLOR;
TEX0.xy = TexCoord.xy * 1.00001;
}
#elif defined(FRAGMENT)
#if __VERSION__ >= 130
#define COMPAT_VARYING in
#define COMPAT_TEXTURE texture
out vec4 FragColor;
#else
#define round(c) floor(c+0.5)
#define COMPAT_VARYING varying
#define FragColor gl_FragColor
#define COMPAT_TEXTURE texture2D
#endif
#ifdef GL_ES
#ifdef GL_FRAGMENT_PRECISION_HIGH
precision highp float;
#else
precision mediump float;
#endif
#define COMPAT_PRECISION mediump
#else
#define COMPAT_PRECISION
#endif
uniform COMPAT_PRECISION int FrameDirection;
uniform COMPAT_PRECISION int FrameCount;
uniform COMPAT_PRECISION vec2 OutputSize;
uniform COMPAT_PRECISION vec2 TextureSize;
uniform COMPAT_PRECISION vec2 InputSize;
uniform sampler2D Texture;
uniform sampler2D PassPrev2Texture;
uniform sampler2D PassPrev4Texture;
uniform sampler2D PassPrev5Texture;
COMPAT_VARYING vec4 TEX0;
// compatibility #defines
#define Source Texture
#define vTexCoord TEX0.xy
#define SourceSize vec4(TextureSize, 1.0 / TextureSize) //either TextureSize or InputSize
#define OutputSize vec4(OutputSize, 1.0 / OutputSize)
#ifdef PARAMETER_UNIFORM
// All parameter floats need to have COMPAT_PRECISION in front of them
uniform COMPAT_PRECISION float TATE;
uniform COMPAT_PRECISION float IOS;
uniform COMPAT_PRECISION float OS;
uniform COMPAT_PRECISION float BLOOM;
uniform COMPAT_PRECISION float brightboost;
uniform COMPAT_PRECISION float brightboost1;
uniform COMPAT_PRECISION float gsl;
uniform COMPAT_PRECISION float scanline1;
uniform COMPAT_PRECISION float scanline2;
uniform COMPAT_PRECISION float beam_min;
uniform COMPAT_PRECISION float beam_max;
uniform COMPAT_PRECISION float beam_size;
uniform COMPAT_PRECISION float spike;
uniform COMPAT_PRECISION float h_sharp;
uniform COMPAT_PRECISION float s_sharp;
uniform COMPAT_PRECISION float csize;
uniform COMPAT_PRECISION float bsize;
uniform COMPAT_PRECISION float warpX;
uniform COMPAT_PRECISION float warpY;
uniform COMPAT_PRECISION float glow;
uniform COMPAT_PRECISION float shadowMask;
uniform COMPAT_PRECISION float masksize;
uniform COMPAT_PRECISION float vertmask;
uniform COMPAT_PRECISION float slotmask;
uniform COMPAT_PRECISION float slotwidth;
uniform COMPAT_PRECISION float double_slot;
uniform COMPAT_PRECISION float slotms;
uniform COMPAT_PRECISION float mcut;
uniform COMPAT_PRECISION float maskDark;
uniform COMPAT_PRECISION float maskLight;
uniform COMPAT_PRECISION float CGWG;
uniform COMPAT_PRECISION float gamma_out;
uniform COMPAT_PRECISION float inter;
uniform COMPAT_PRECISION float interm;
uniform COMPAT_PRECISION float bloom;
uniform COMPAT_PRECISION float scans;
#else
#define TATE 0.00 // Screen orientation
#define IOS 0.00 // Smart Integer Scaling
#define OS 2.00 // Do overscan
#define BLOOM 0.00 // Bloom overscan percentage
#define brightboost 1.30 // adjust brightness
#define gsl 0.0 // Alternate scanlines
#define scanline1 8.0 // scanline param, vertical sharpness
#define scanline2 8.0 // scanline param, vertical sharpness
#define beam_min 1.35 // dark area beam min - narrow
#define beam_max 1.05 // bright area beam max - wide
#define beam_size 0.70 // increased max. beam size
#define spike 1.25 // scanline spike removal
#define h_sharp 5.25 // pixel sharpness
#define s_sharp 0.05 // substractive sharpness
#define csize 0.00 // corner size
#define bsize 0.00 // border smoothness
#define warpX 0.00 // Curvature X
#define warpY 0.00 // Curvature Y
#define glow 0.02 // Glow Strength
#define shadowMask 0.00 // Mask Style
#define masksize 1.00 // Mask Size
#define vertmask 0.00 // Vertical mask
#define slotmask 0.00 // Slot Mask ON/OFF
#define slotwidth 2.00 // Slot Mask Width
#define double_slot 1.00 // Slot Mask Height
#define slotms 1.00 // Slot Mask Size
#define mcut 0.20 // Mask 5&6 cutoff
#define maskDark 0.50 // Dark "Phosphor"
#define maskLight 1.50 // Light "Phosphor"
#define CGWG 0.30 // CGWG Mask Strength
#define gamma_out 2.40 // output gamma
#define inter 400.00 // interlace resolution
#define interm 1.00 // interlace mode
#define bloom 0.00 // bloom effect
#define scans 0.50 // scanline saturation
#endif
#define eps 1e-10
float st(float x)
{
return exp2(-10.0*x*x);
}
vec3 sw0(vec3 x, vec3 color, float scanline)
{
vec3 tmp = mix(vec3(beam_min),vec3(beam_max), color);
vec3 ex = x*tmp;
return exp2(-scanline*ex*ex);
}
vec3 sw1(vec3 x, vec3 color, float scanline)
{
float mx = max(max(color.r, color.g),color.b);
x = mix (x, beam_min*x, max(x-0.4*mx,0.0));
vec3 tmp = mix(vec3(1.2*beam_min),vec3(beam_max), color);
vec3 ex = x*tmp;
float br = clamp(0.8*beam_min - 1.0, 0.2, 0.45);
vec3 res = exp2(-scanline*ex*ex)/(1.0-br+br*mx);
mx = max(max(res.r,res.g),res.b);
float scans1 = scans; if (vertmask > 0.0) scans1=1.0;
return mix(vec3(mx), res, scans1);
}
vec3 sw2(vec3 x, vec3 color, float scanline)
{
float mx = max(max(color.r, color.g),color.b);
vec3 tmp = mix(vec3(2.5*beam_min),vec3(beam_max), color);
tmp = mix(vec3(beam_max), tmp, pow(abs(x), color+0.3));
vec3 ex = x*tmp;
vec3 res = exp2(-scanline*ex*ex)/(0.6 + 0.4*mx);
mx = max(max(res.r,res.g),res.b);
float scans1 = scans; if (vertmask > 0.0) scans1=0.75;
return mix(vec3(mx), res, scans1);
}
// Shadow mask (1-4 from PD CRT Lottes shader).
vec3 Mask(vec2 pos, vec3 c)
{
pos = floor(pos/masksize);
vec3 mask = vec3(maskDark, maskDark, maskDark);
// No mask
if (shadowMask == -1.0)
{
mask = vec3(1.0);
}
// Phosphor.
else if (shadowMask == 0.0)
{
pos.x = fract(pos.x*0.5);
float mc = 1.0 - CGWG;
if (pos.x < 0.5) { mask.r = 1.1; mask.g = mc; mask.b = 1.1; }
else { mask.r = mc; mask.g = 1.1; mask.b = mc; }
}
// Very compressed TV style shadow mask.
else if (shadowMask == 1.0)
{
float line = maskLight;
float odd = 0.0;
if (fract(pos.x/6.0) < 0.5)
odd = 1.0;
if (fract((pos.y + odd)/2.0) < 0.5)
line = maskDark;
pos.x = fract(pos.x/3.0);
if (pos.x < 0.333) mask.r = maskLight;
else if (pos.x < 0.666) mask.g = maskLight;
else mask.b = maskLight;
mask*=line;
}
// Aperture-grille.
else if (shadowMask == 2.0)
{
pos.x = fract(pos.x/3.0);
if (pos.x < 0.333) mask.r = maskLight;
else if (pos.x < 0.666) mask.g = maskLight;
else mask.b = maskLight;
}
// Stretched VGA style shadow mask (same as prior shaders).
else if (shadowMask == 3.0)
{
pos.x += pos.y*3.0;
pos.x = fract(pos.x/6.0);
if (pos.x < 0.333) mask.r = maskLight;
else if (pos.x < 0.666) mask.g = maskLight;
else mask.b = maskLight;
}
// VGA style shadow mask.
else if (shadowMask == 4.0)
{
pos.xy = floor(pos.xy*vec2(1.0, 0.5));
pos.x += pos.y*3.0;
pos.x = fract(pos.x/6.0);
if (pos.x < 0.333) mask.r = maskLight;
else if (pos.x < 0.666) mask.g = maskLight;
else mask.b = maskLight;
}
// Alternate mask 5
else if (shadowMask == 5.0)
{
float mx = max(max(c.r,c.g),c.b);
vec3 maskTmp = vec3( min( 1.25*max(mx-mcut,0.0)/(1.0-mcut) ,maskDark + 0.2*(1.0-maskDark)*mx));
float adj = 0.80*maskLight - 0.5*(0.80*maskLight - 1.0)*mx + 0.75*(1.0-mx);
mask = maskTmp;
pos.x = fract(pos.x/2.0);
if (pos.x < 0.5)
{ mask.r = adj;
mask.b = adj;
}
else mask.g = adj;
}
// Alternate mask 6
else if (shadowMask == 6.0)
{
float mx = max(max(c.r,c.g),c.b);
vec3 maskTmp = vec3( min( 1.33*max(mx-mcut,0.0)/(1.0-mcut) ,maskDark + 0.225*(1.0-maskDark)*mx));
float adj = 0.80*maskLight - 0.5*(0.80*maskLight - 1.0)*mx + 0.75*(1.0-mx);
mask = maskTmp;
pos.x = fract(pos.x/3.0);
if (pos.x < 0.333) mask.r = adj;
else if (pos.x < 0.666) mask.g = adj;
else mask.b = adj;
}
// Alternate mask 7
else if (shadowMask == 7.0)
{
float mc = 1.0 - CGWG;
float mx = max(max(c.r,c.g),c.b);
float maskTmp = min(1.6*max(mx-mcut,0.0)/(1.0-mcut) , mc);
mask = vec3(maskTmp);
pos.x = fract(pos.x/2.0);
if (pos.x < 0.5) mask = vec3(1.0 + 0.6*(1.0-mx));
}
return mask;
}
float SlotMask(vec2 pos, vec3 c)
{
if (slotmask == 0.0) return 1.0;
pos = floor(pos/slotms);
float mx = pow(max(max(c.r,c.g),c.b),1.33);
float mlen = slotwidth*2.0;
float px = fract(pos.x/mlen);
float py = floor(fract(pos.y/(2.0*double_slot))*2.0*double_slot);
float slot_dark = mix(1.0-slotmask, 1.0-0.80*slotmask, mx);
float slot = 1.0 + 0.7*slotmask*(1.0-mx);
if (py == 0.0 && px < 0.5) slot = slot_dark; else
if (py == double_slot && px >= 0.5) slot = slot_dark;
return slot;
}
// Distortion of scanlines, and end of screen alpha (PD Lottes Curvature)
vec2 Warp(vec2 pos)
{
pos = pos*2.0-1.0;
pos *= vec2(1.0 + (pos.y*pos.y)*warpX, 1.0 + (pos.x*pos.x)*warpY);
return pos*0.5 + 0.5;
}
vec2 Overscan(vec2 pos, float dx, float dy){
pos=pos*2.0-1.0;
pos*=vec2(dx,dy);
return pos*0.5+0.5;
}
// Borrowed from cgwg's crt-geom, under GPL
float corner(vec2 coord)
{
coord *= SourceSize.xy / InputSize.xy;
coord = (coord - vec2(0.5)) * 1.0 + vec2(0.5);
coord = min(coord, vec2(1.0)-coord) * vec2(1.0, OutputSize.y/OutputSize.x);
vec2 cdist = vec2(max(csize, max((1.0-smoothstep(100.0,600.0,bsize))*0.01,0.002)));
coord = (cdist - min(coord,cdist));
float dist = sqrt(dot(coord,coord));
return clamp((cdist.x-dist)*bsize,0.0, 1.0);
}
vec3 declip(vec3 c, float b)
{
float m = max(max(c.r,c.g),c.b);
if (m > b) c = c*b/m;
return c;
}
void main()
{
float lum = COMPAT_TEXTURE(PassPrev5Texture, vec2(0.05,0.05)).a;
// Calculating texel coordinates
vec2 texcoord = TEX0.xy;
if (IOS > 0.0){
vec2 ofactor = OutputSize.xy/InputSize.xy;
vec2 intfactor = round(ofactor);
vec2 diff = ofactor/intfactor;
float scan = mix(diff.y, diff.x, TATE);
texcoord = Overscan(texcoord*(SourceSize.xy/InputSize.xy), scan, scan)*(InputSize.xy/SourceSize.xy);
if (IOS == 1.0) texcoord = mix(vec2(TEX0.x, texcoord.y), vec2(texcoord.x, TEX0.y), TATE);
}
float factor = 1.00 + (1.0-0.5*OS)*BLOOM/100.0 - lum*BLOOM/100.0;
texcoord = Overscan(texcoord*(SourceSize.xy/InputSize.xy), factor, factor)*(InputSize.xy/SourceSize.xy);
vec2 pos = Warp(texcoord*(TextureSize.xy/InputSize.xy))*(InputSize.xy/TextureSize.xy);
vec2 pos0 = Warp(TEX0.xy*(TextureSize.xy/InputSize.xy))*(InputSize.xy/TextureSize.xy);
vec2 coffset = vec2(0.5, 0.5);
if ((interm == 1.0 || interm == 2.0) && inter <= mix(InputSize.y, InputSize.x, TATE)) coffset = ((TATE < 0.5) ? vec2(0.5,0.0) : vec2(0.0, 0.5));
vec2 ps = SourceSize.zw;
vec2 OGL2Pos = pos * SourceSize.xy - coffset;
vec2 fp = fract(OGL2Pos);
vec2 dx = vec2(ps.x,0.0);
vec2 dy = vec2(0.0, ps.y);
// Reading the texels
vec2 x2 = 2.0*dx;
vec2 y2 = 2.0*dy;
vec2 offx = dx;
vec2 off2 = x2;
vec2 offy = dy;
float fpx = fp.x;
if(TATE > 0.5)
{
offx = dy;
off2 = y2;
offy = dx;
fpx = fp.y;
}
float f = (TATE < 0.5) ? fp.y : fp.x;
vec2 pC4 = floor(OGL2Pos) * ps + 0.5*ps;
float zero = exp2(-h_sharp);
float sharp1 = s_sharp * zero;
float wl3 = 2.0 + fpx;
float wl2 = 1.0 + fpx;
float wl1 = fpx;
float wr1 = 1.0 - fpx;
float wr2 = 2.0 - fpx;
float wr3 = 3.0 - fpx;
wl3*=wl3; wl3 = exp2(-h_sharp*wl3);
wl2*=wl2; wl2 = exp2(-h_sharp*wl2);
wl1*=wl1; wl1 = exp2(-h_sharp*wl1);
wr1*=wr1; wr1 = exp2(-h_sharp*wr1);
wr2*=wr2; wr2 = exp2(-h_sharp*wr2);
wr3*=wr3; wr3 = exp2(-h_sharp*wr3);
float fp1 = 1.-fpx;
float twl3 = max(wl3 - sharp1, 0.0);
float twl2 = max(wl2 - sharp1, mix(0.0,mix(-0.17, -0.025, fp.x),float(s_sharp > 0.05)));
float twl1 = max(wl1 - sharp1, 0.0);
float twr1 = max(wr1 - sharp1, 0.0);
float twr2 = max(wr2 - sharp1, mix(0.0,mix(-0.17, -0.025, 1.-fp.x),float(s_sharp > 0.05)));
float twr3 = max(wr3 - sharp1, 0.0);
float wtt = 1.0/(twl3+twl2+twl1+twr1+twr2+twr3);
float wt = 1.0/(wl2+wl1+wr1+wr2);
bool sharp = (s_sharp > 0.05);
vec3 l3 = COMPAT_TEXTURE(PassPrev4Texture, pC4 -off2).xyz;
vec3 l2 = COMPAT_TEXTURE(PassPrev4Texture, pC4 -offx).xyz;
vec3 l1 = COMPAT_TEXTURE(PassPrev4Texture, pC4 ).xyz;
vec3 r1 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +offx).xyz;
vec3 r2 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +off2).xyz;
vec3 r3 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +offx+off2).xyz;
vec3 sl2 = COMPAT_TEXTURE(Texture, pC4 -offx).xyz;
vec3 sl1 = COMPAT_TEXTURE(Texture, pC4 ).xyz;
vec3 sr1 = COMPAT_TEXTURE(Texture, pC4 +offx).xyz;
vec3 sr2 = COMPAT_TEXTURE(Texture, pC4 +off2).xyz;
vec3 color1 = (l3*twl3 + l2*twl2 + l1*twl1 + r1*twr1 + r2*twr2 + r3*twr3)*wtt;
vec3 colmin = min(min(l1,r1), min(l2,r2));
vec3 colmax = max(max(l1,r1), max(l2,r2));
if (sharp) color1 = clamp(color1, colmin, colmax);
vec3 gtmp = vec3(gamma_out*0.1);
vec3 scolor1 = color1;
scolor1 = (sl2*wl2 + sl1*wl1 + sr1*wr1 + sr2*wr2)*wt;
scolor1 = pow(scolor1, gtmp); vec3 mcolor1 = scolor1;
scolor1 = mix(color1, scolor1, spike);
pC4+=offy;
l3 = COMPAT_TEXTURE(PassPrev4Texture, pC4 -off2).xyz;
l2 = COMPAT_TEXTURE(PassPrev4Texture, pC4 -offx).xyz;
l1 = COMPAT_TEXTURE(PassPrev4Texture, pC4 ).xyz;
r1 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +offx).xyz;
r2 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +off2).xyz;
r3 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +offx+off2).xyz;
sl2 = COMPAT_TEXTURE(Texture, pC4 -offx).xyz;
sl1 = COMPAT_TEXTURE(Texture, pC4 ).xyz;
sr1 = COMPAT_TEXTURE(Texture, pC4 +offx).xyz;
sr2 = COMPAT_TEXTURE(Texture, pC4 +off2).xyz;
vec3 color2 = (l3*twl3 + l2*twl2 + l1*twl1 + r1*twr1 + r2*twr2 + r3*twr3)*wtt;
colmin = min(min(l1,r1), min(l2,r2));
colmax = max(max(l1,r1), max(l2,r2));
if (sharp) color2 = clamp(color2, colmin, colmax);
vec3 scolor2 = color2;
scolor2 = (sl2*wl2 + sl1*wl1 + sr1*wr1 + sr2*wr2)*wt;
scolor2 = pow(scolor2, gtmp); vec3 mcolor2 = scolor2;
scolor2 = mix(color2, scolor2, spike);
vec3 color0 = color1;
if ((interm == 1.0 || interm == 2.0) && inter <= mix(InputSize.y, InputSize.x, TATE))
{
pC4-= 2.*offy;
l3 = COMPAT_TEXTURE(PassPrev4Texture, pC4 -off2).xyz;
l2 = COMPAT_TEXTURE(PassPrev4Texture, pC4 -offx).xyz;
l1 = COMPAT_TEXTURE(PassPrev4Texture, pC4 ).xyz;
r1 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +offx).xyz;
r2 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +off2).xyz;
r3 = COMPAT_TEXTURE(PassPrev4Texture, pC4 +offx+off2).xyz;
color0 = (l3*twl3 + l2*twl2 + l1*twl1 + r1*twr1 + r2*twr2 + r3*twr3)*wtt;
colmin = min(min(l1,r1), min(l2,r2));
colmax = max(max(l1,r1), max(l2,r2));
if (sharp) color0 = clamp(color0, colmin, colmax);
}
// calculating scanlines
float shape1 = mix(scanline1, scanline2, f);
float shape2 = mix(scanline1, scanline2, 1.0-f);
float wt1 = st(f);
float wt2 = st(1.0-f);
vec3 color00 = color1*wt1 + color2*wt2;
vec3 scolor0 = scolor1*wt1 + scolor2*wt2;
vec3 mcolor = (mcolor1*wt1 + mcolor2*wt2)/(wt1+wt2);
vec3 ctmp = color00/(wt1+wt2);
vec3 sctmp = scolor0/(wt1+wt2);
vec3 tmp = pow(ctmp, vec3(1.0/gamma_out));
mcolor = clamp(mix(ctmp, mcolor, 1.5),0.0,1.0);
mcolor = pow(mcolor, vec3(1.4/gamma_out));
vec3 w1,w2 = vec3(0.0);
vec3 cref1 = mix(sctmp, scolor1, beam_size);
vec3 cref2 = mix(sctmp, scolor2, beam_size);
vec3 shift = vec3(-vertmask, vertmask, -vertmask);
vec3 f1 = clamp(vec3(f) + shift*0.5*(1.0+f), 0.0, 1.0);
vec3 f2 = clamp(vec3(1.0-f) - shift*0.5*(2.0-f), 0.0, 1.0);
if (gsl == 0.0) { w1 = sw0(f1,cref1,shape1); w2 = sw0(f2,cref2,shape2);} else
if (gsl == 1.0) { w1 = sw1(f1,cref1,shape1); w2 = sw1(f2,cref2,shape2);} else
if (gsl == 2.0) { w1 = sw2(f1,cref1,shape1); w2 = sw2(f2,cref2,shape2);}
vec3 color = color1*w1 + color2*w2;
color = min(color, 1.0);
if (interm > 0.5 && inter <= mix(InputSize.y, InputSize.x, TATE))
{
if (interm < 3.0)
{
float line_no = floor(mod(mix( OGL2Pos.y, OGL2Pos.x, TATE),2.0));
float frame_no = floor(mod(float(FrameCount),2.0));
float ii = (interm > 1.5) ? 0.5 : abs(line_no-frame_no);
vec3 icolor1 = mix(color1, color0, ii);
vec3 icolor2 = mix(color1, color2, ii);
color = mix(icolor1, icolor2, f);
}
else color = mix(color1, color2, f);
mcolor = sqrt(color);
}
ctmp = 0.5*(ctmp+tmp);
color*=mix(brightboost, brightboost1, max(max(ctmp.r,ctmp.g),ctmp.b));
// Apply Mask
vec3 orig1 = color; float pixbr = max(max(ctmp.r,ctmp.g),ctmp.b); vec3 orig = ctmp; w1 = w1+w2; float w3 = max(max(w1.r,w1.g),w1.b);
vec3 cmask = vec3(1.0); vec3 cmask1 = cmask; vec3 one = vec3(1.0);
cmask*= (TATE < 0.5) ? Mask(gl_FragCoord.xy * 1.000001,mcolor) :
Mask(gl_FragCoord.yx * 1.000001,mcolor);
color = color*cmask;
color = min(color,1.0);
cmask1 *= (TATE < 0.5) ? SlotMask(gl_FragCoord.xy * 1.000001,tmp) :
SlotMask(gl_FragCoord.yx * 1.000001,tmp);
color = color*cmask1; cmask = cmask*cmask1; cmask = min(cmask, 1.0);
vec3 Bloom = COMPAT_TEXTURE(PassPrev2Texture, pos).xyz;
vec3 Bloom1 = 2.0*Bloom*Bloom;
Bloom1 = min(Bloom1, 0.80);
float bmax = max(max(Bloom1.r,Bloom1.g),Bloom1.b);
float pmax = mix(0.825, 0.725, pixbr);
Bloom1 = min(Bloom1, pmax*bmax)/pmax;
Bloom1 = mix(min( Bloom1, color), Bloom1, 0.5*(orig1+color));
Bloom1 = bloom*Bloom1;
color = color + Bloom1;
color = min(color, 1.0);
if (interm < 0.5 || inter > mix(InputSize.y, InputSize.x, TATE)) color = declip(color, pow(w3,0.5));
color = min(color, mix(cmask,one,0.5));
color = color + glow*Bloom;
color = pow(color, vec3(1.0/gamma_out));
FragColor = vec4(color*corner(pos0), 1.0);
}
#endif
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="UTF-8"?>
<document type="com.apple.InterfaceBuilder3.CocoaTouch.XIB" version="3.0" toolsVersion="16097.3" targetRuntime="iOS.CocoaTouch" propertyAccessControl="none" useAutolayout="YES" useTraitCollections="YES" useSafeAreas="YES" colorMatched="YES">
<device id="retina4_7" orientation="portrait" appearance="light"/>
<dependencies>
<deployment identifier="iOS"/>
<plugIn identifier="com.apple.InterfaceBuilder.IBCocoaTouchPlugin" version="16087"/>
<capability name="Safe area layout guides" minToolsVersion="9.0"/>
<capability name="documents saved in the Xcode 8 format" minToolsVersion="8.0"/>
</dependencies>
<objects>
<placeholder placeholderIdentifier="IBFilesOwner" id="-1" userLabel="File's Owner" customClass="ViewController" customModule="SwiftToolTest" customModuleProvider="target">
<connections>
<outlet property="view" destination="i5M-Pr-FkT" id="sfx-zR-JGt"/>
</connections>
</placeholder>
<placeholder placeholderIdentifier="IBFirstResponder" id="-2" customClass="UIResponder"/>
<view clearsContextBeforeDrawing="NO" contentMode="scaleToFill" id="i5M-Pr-FkT">
<rect key="frame" x="0.0" y="0.0" width="375" height="667"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<color key="backgroundColor" red="1" green="1" blue="1" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<viewLayoutGuide key="safeArea" id="fnl-2z-Ty3"/>
<point key="canvasLocation" x="24.5" y="51.5"/>
</view>
</objects>
</document>
| {
"pile_set_name": "Github"
} |
// Copyright 2019 The Go Authors. All rights reserved.
// Use of this source code is governed by a BSD-style
// license that can be found in the LICENSE file.
// +build gccgo
package cpu
// haveAsmFunctions reports whether the other functions in this file can
// be safely called.
func haveAsmFunctions() bool { return false }
// TODO(mundaym): the following feature detection functions are currently
// stubs. See https://golang.org/cl/162887 for how to fix this.
// They are likely to be expensive to call so the results should be cached.
func stfle() facilityList { panic("not implemented for gccgo") }
func kmQuery() queryResult { panic("not implemented for gccgo") }
func kmcQuery() queryResult { panic("not implemented for gccgo") }
func kmctrQuery() queryResult { panic("not implemented for gccgo") }
func kmaQuery() queryResult { panic("not implemented for gccgo") }
func kimdQuery() queryResult { panic("not implemented for gccgo") }
func klmdQuery() queryResult { panic("not implemented for gccgo") }
| {
"pile_set_name": "Github"
} |
/*
* isp1301_omap - ISP 1301 USB transceiver, talking to OMAP OTG controller
*
* Copyright (C) 2004 Texas Instruments
* Copyright (C) 2004 David Brownell
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
*/
#include <linux/kernel.h>
#include <linux/module.h>
#include <linux/init.h>
#include <linux/slab.h>
#include <linux/interrupt.h>
#include <linux/platform_device.h>
#include <linux/gpio.h>
#include <linux/usb/ch9.h>
#include <linux/usb/gadget.h>
#include <linux/usb.h>
#include <linux/usb/otg.h>
#include <linux/i2c.h>
#include <linux/workqueue.h>
#include <asm/irq.h>
#include <asm/mach-types.h>
#include <mach/mux.h>
#include <mach/usb.h>
#undef VERBOSE
#define DRIVER_VERSION "24 August 2004"
#define DRIVER_NAME (isp1301_driver.driver.name)
MODULE_DESCRIPTION("ISP1301 USB OTG Transceiver Driver");
MODULE_LICENSE("GPL");
struct isp1301 {
struct usb_phy phy;
struct i2c_client *client;
void (*i2c_release)(struct device *dev);
int irq_type;
u32 last_otg_ctrl;
unsigned working:1;
struct timer_list timer;
/* use keventd context to change the state for us */
struct work_struct work;
unsigned long todo;
# define WORK_UPDATE_ISP 0 /* update ISP from OTG */
# define WORK_UPDATE_OTG 1 /* update OTG from ISP */
# define WORK_HOST_RESUME 4 /* resume host */
# define WORK_TIMER 6 /* timer fired */
# define WORK_STOP 7 /* don't resubmit */
};
/* bits in OTG_CTRL */
#define OTG_XCEIV_OUTPUTS \
(OTG_ASESSVLD|OTG_BSESSEND|OTG_BSESSVLD|OTG_VBUSVLD|OTG_ID)
#define OTG_XCEIV_INPUTS \
(OTG_PULLDOWN|OTG_PULLUP|OTG_DRV_VBUS|OTG_PD_VBUS|OTG_PU_VBUS|OTG_PU_ID)
#define OTG_CTRL_BITS \
(OTG_A_BUSREQ|OTG_A_SETB_HNPEN|OTG_B_BUSREQ|OTG_B_HNPEN|OTG_BUSDROP)
/* and OTG_PULLUP is sometimes written */
#define OTG_CTRL_MASK (OTG_DRIVER_SEL| \
OTG_XCEIV_OUTPUTS|OTG_XCEIV_INPUTS| \
OTG_CTRL_BITS)
/*-------------------------------------------------------------------------*/
/* board-specific PM hooks */
#if defined(CONFIG_MACH_OMAP_H2) || defined(CONFIG_MACH_OMAP_H3)
#if defined(CONFIG_TPS65010) || (defined(CONFIG_TPS65010_MODULE) && defined(MODULE))
#include <linux/i2c/tps65010.h>
#else
static inline int tps65010_set_vbus_draw(unsigned mA)
{
pr_debug("tps65010: draw %d mA (STUB)\n", mA);
return 0;
}
#endif
static void enable_vbus_draw(struct isp1301 *isp, unsigned mA)
{
int status = tps65010_set_vbus_draw(mA);
if (status < 0)
pr_debug(" VBUS %d mA error %d\n", mA, status);
}
#else
static void enable_vbus_draw(struct isp1301 *isp, unsigned mA)
{
/* H4 controls this by DIP switch S2.4; no soft control.
* ON means the charger is always enabled. Leave it OFF
* unless the OTG port is used only in B-peripheral mode.
*/
}
#endif
static void enable_vbus_source(struct isp1301 *isp)
{
/* this board won't supply more than 8mA vbus power.
* some boards can switch a 100ma "unit load" (or more).
*/
}
/* products will deliver OTG messages with LEDs, GUI, etc */
static inline void notresponding(struct isp1301 *isp)
{
printk(KERN_NOTICE "OTG device not responding.\n");
}
/*-------------------------------------------------------------------------*/
static struct i2c_driver isp1301_driver;
/* smbus apis are used for portability */
static inline u8
isp1301_get_u8(struct isp1301 *isp, u8 reg)
{
return i2c_smbus_read_byte_data(isp->client, reg + 0);
}
static inline int
isp1301_get_u16(struct isp1301 *isp, u8 reg)
{
return i2c_smbus_read_word_data(isp->client, reg);
}
static inline int
isp1301_set_bits(struct isp1301 *isp, u8 reg, u8 bits)
{
return i2c_smbus_write_byte_data(isp->client, reg + 0, bits);
}
static inline int
isp1301_clear_bits(struct isp1301 *isp, u8 reg, u8 bits)
{
return i2c_smbus_write_byte_data(isp->client, reg + 1, bits);
}
/*-------------------------------------------------------------------------*/
/* identification */
#define ISP1301_VENDOR_ID 0x00 /* u16 read */
#define ISP1301_PRODUCT_ID 0x02 /* u16 read */
#define ISP1301_BCD_DEVICE 0x14 /* u16 read */
#define I2C_VENDOR_ID_PHILIPS 0x04cc
#define I2C_PRODUCT_ID_PHILIPS_1301 0x1301
/* operational registers */
#define ISP1301_MODE_CONTROL_1 0x04 /* u8 read, set, +1 clear */
# define MC1_SPEED (1 << 0)
# define MC1_SUSPEND (1 << 1)
# define MC1_DAT_SE0 (1 << 2)
# define MC1_TRANSPARENT (1 << 3)
# define MC1_BDIS_ACON_EN (1 << 4)
# define MC1_OE_INT_EN (1 << 5)
# define MC1_UART_EN (1 << 6)
# define MC1_MASK 0x7f
#define ISP1301_MODE_CONTROL_2 0x12 /* u8 read, set, +1 clear */
# define MC2_GLOBAL_PWR_DN (1 << 0)
# define MC2_SPD_SUSP_CTRL (1 << 1)
# define MC2_BI_DI (1 << 2)
# define MC2_TRANSP_BDIR0 (1 << 3)
# define MC2_TRANSP_BDIR1 (1 << 4)
# define MC2_AUDIO_EN (1 << 5)
# define MC2_PSW_EN (1 << 6)
# define MC2_EN2V7 (1 << 7)
#define ISP1301_OTG_CONTROL_1 0x06 /* u8 read, set, +1 clear */
# define OTG1_DP_PULLUP (1 << 0)
# define OTG1_DM_PULLUP (1 << 1)
# define OTG1_DP_PULLDOWN (1 << 2)
# define OTG1_DM_PULLDOWN (1 << 3)
# define OTG1_ID_PULLDOWN (1 << 4)
# define OTG1_VBUS_DRV (1 << 5)
# define OTG1_VBUS_DISCHRG (1 << 6)
# define OTG1_VBUS_CHRG (1 << 7)
#define ISP1301_OTG_STATUS 0x10 /* u8 readonly */
# define OTG_B_SESS_END (1 << 6)
# define OTG_B_SESS_VLD (1 << 7)
#define ISP1301_INTERRUPT_SOURCE 0x08 /* u8 read */
#define ISP1301_INTERRUPT_LATCH 0x0A /* u8 read, set, +1 clear */
#define ISP1301_INTERRUPT_FALLING 0x0C /* u8 read, set, +1 clear */
#define ISP1301_INTERRUPT_RISING 0x0E /* u8 read, set, +1 clear */
/* same bitfields in all interrupt registers */
# define INTR_VBUS_VLD (1 << 0)
# define INTR_SESS_VLD (1 << 1)
# define INTR_DP_HI (1 << 2)
# define INTR_ID_GND (1 << 3)
# define INTR_DM_HI (1 << 4)
# define INTR_ID_FLOAT (1 << 5)
# define INTR_BDIS_ACON (1 << 6)
# define INTR_CR_INT (1 << 7)
/*-------------------------------------------------------------------------*/
static inline const char *state_name(struct isp1301 *isp)
{
return usb_otg_state_string(isp->phy.otg->state);
}
/*-------------------------------------------------------------------------*/
/* NOTE: some of this ISP1301 setup is specific to H2 boards;
* not everything is guarded by board-specific checks, or even using
* omap_usb_config data to deduce MC1_DAT_SE0 and MC2_BI_DI.
*
* ALSO: this currently doesn't use ISP1301 low-power modes
* while OTG is running.
*/
static void power_down(struct isp1301 *isp)
{
isp->phy.otg->state = OTG_STATE_UNDEFINED;
// isp1301_set_bits(isp, ISP1301_MODE_CONTROL_2, MC2_GLOBAL_PWR_DN);
isp1301_set_bits(isp, ISP1301_MODE_CONTROL_1, MC1_SUSPEND);
isp1301_clear_bits(isp, ISP1301_OTG_CONTROL_1, OTG1_ID_PULLDOWN);
isp1301_clear_bits(isp, ISP1301_MODE_CONTROL_1, MC1_DAT_SE0);
}
static void power_up(struct isp1301 *isp)
{
// isp1301_clear_bits(isp, ISP1301_MODE_CONTROL_2, MC2_GLOBAL_PWR_DN);
isp1301_clear_bits(isp, ISP1301_MODE_CONTROL_1, MC1_SUSPEND);
/* do this only when cpu is driving transceiver,
* so host won't see a low speed device...
*/
isp1301_set_bits(isp, ISP1301_MODE_CONTROL_1, MC1_DAT_SE0);
}
#define NO_HOST_SUSPEND
static int host_suspend(struct isp1301 *isp)
{
#ifdef NO_HOST_SUSPEND
return 0;
#else
struct device *dev;
if (!isp->phy.otg->host)
return -ENODEV;
/* Currently ASSUMES only the OTG port matters;
* other ports could be active...
*/
dev = isp->phy.otg->host->controller;
return dev->driver->suspend(dev, 3, 0);
#endif
}
static int host_resume(struct isp1301 *isp)
{
#ifdef NO_HOST_SUSPEND
return 0;
#else
struct device *dev;
if (!isp->phy.otg->host)
return -ENODEV;
dev = isp->phy.otg->host->controller;
return dev->driver->resume(dev, 0);
#endif
}
static int gadget_suspend(struct isp1301 *isp)
{
isp->phy.otg->gadget->b_hnp_enable = 0;
isp->phy.otg->gadget->a_hnp_support = 0;
isp->phy.otg->gadget->a_alt_hnp_support = 0;
return usb_gadget_vbus_disconnect(isp->phy.otg->gadget);
}
/*-------------------------------------------------------------------------*/
#define TIMER_MINUTES 10
#define TIMER_JIFFIES (TIMER_MINUTES * 60 * HZ)
/* Almost all our I2C messaging comes from a work queue's task context.
* NOTE: guaranteeing certain response times might mean we shouldn't
* share keventd's work queue; a realtime task might be safest.
*/
static void isp1301_defer_work(struct isp1301 *isp, int work)
{
int status;
if (isp && !test_and_set_bit(work, &isp->todo)) {
(void) get_device(&isp->client->dev);
status = schedule_work(&isp->work);
if (!status && !isp->working)
dev_vdbg(&isp->client->dev,
"work item %d may be lost\n", work);
}
}
/* called from irq handlers */
static void a_idle(struct isp1301 *isp, const char *tag)
{
u32 l;
if (isp->phy.otg->state == OTG_STATE_A_IDLE)
return;
isp->phy.otg->default_a = 1;
if (isp->phy.otg->host) {
isp->phy.otg->host->is_b_host = 0;
host_suspend(isp);
}
if (isp->phy.otg->gadget) {
isp->phy.otg->gadget->is_a_peripheral = 1;
gadget_suspend(isp);
}
isp->phy.otg->state = OTG_STATE_A_IDLE;
l = omap_readl(OTG_CTRL) & OTG_XCEIV_OUTPUTS;
omap_writel(l, OTG_CTRL);
isp->last_otg_ctrl = l;
pr_debug(" --> %s/%s\n", state_name(isp), tag);
}
/* called from irq handlers */
static void b_idle(struct isp1301 *isp, const char *tag)
{
u32 l;
if (isp->phy.otg->state == OTG_STATE_B_IDLE)
return;
isp->phy.otg->default_a = 0;
if (isp->phy.otg->host) {
isp->phy.otg->host->is_b_host = 1;
host_suspend(isp);
}
if (isp->phy.otg->gadget) {
isp->phy.otg->gadget->is_a_peripheral = 0;
gadget_suspend(isp);
}
isp->phy.otg->state = OTG_STATE_B_IDLE;
l = omap_readl(OTG_CTRL) & OTG_XCEIV_OUTPUTS;
omap_writel(l, OTG_CTRL);
isp->last_otg_ctrl = l;
pr_debug(" --> %s/%s\n", state_name(isp), tag);
}
static void
dump_regs(struct isp1301 *isp, const char *label)
{
u8 ctrl = isp1301_get_u8(isp, ISP1301_OTG_CONTROL_1);
u8 status = isp1301_get_u8(isp, ISP1301_OTG_STATUS);
u8 src = isp1301_get_u8(isp, ISP1301_INTERRUPT_SOURCE);
pr_debug("otg: %06x, %s %s, otg/%02x stat/%02x.%02x\n",
omap_readl(OTG_CTRL), label, state_name(isp),
ctrl, status, src);
/* mode control and irq enables don't change much */
}
/*-------------------------------------------------------------------------*/
#ifdef CONFIG_USB_OTG
/*
* The OMAP OTG controller handles most of the OTG state transitions.
*
* We translate isp1301 outputs (mostly voltage comparator status) into
* OTG inputs; OTG outputs (mostly pullup/pulldown controls) and HNP state
* flags into isp1301 inputs ... and infer state transitions.
*/
#ifdef VERBOSE
static void check_state(struct isp1301 *isp, const char *tag)
{
enum usb_otg_state state = OTG_STATE_UNDEFINED;
u8 fsm = omap_readw(OTG_TEST) & 0x0ff;
unsigned extra = 0;
switch (fsm) {
/* default-b */
case 0x0:
state = OTG_STATE_B_IDLE;
break;
case 0x3:
case 0x7:
extra = 1;
case 0x1:
state = OTG_STATE_B_PERIPHERAL;
break;
case 0x11:
state = OTG_STATE_B_SRP_INIT;
break;
/* extra dual-role default-b states */
case 0x12:
case 0x13:
case 0x16:
extra = 1;
case 0x17:
state = OTG_STATE_B_WAIT_ACON;
break;
case 0x34:
state = OTG_STATE_B_HOST;
break;
/* default-a */
case 0x36:
state = OTG_STATE_A_IDLE;
break;
case 0x3c:
state = OTG_STATE_A_WAIT_VFALL;
break;
case 0x7d:
state = OTG_STATE_A_VBUS_ERR;
break;
case 0x9e:
case 0x9f:
extra = 1;
case 0x89:
state = OTG_STATE_A_PERIPHERAL;
break;
case 0xb7:
state = OTG_STATE_A_WAIT_VRISE;
break;
case 0xb8:
state = OTG_STATE_A_WAIT_BCON;
break;
case 0xb9:
state = OTG_STATE_A_HOST;
break;
case 0xba:
state = OTG_STATE_A_SUSPEND;
break;
default:
break;
}
if (isp->phy.otg->state == state && !extra)
return;
pr_debug("otg: %s FSM %s/%02x, %s, %06x\n", tag,
usb_otg_state_string(state), fsm, state_name(isp),
omap_readl(OTG_CTRL));
}
#else
static inline void check_state(struct isp1301 *isp, const char *tag) { }
#endif
/* outputs from ISP1301_INTERRUPT_SOURCE */
static void update_otg1(struct isp1301 *isp, u8 int_src)
{
u32 otg_ctrl;
otg_ctrl = omap_readl(OTG_CTRL) & OTG_CTRL_MASK;
otg_ctrl &= ~OTG_XCEIV_INPUTS;
otg_ctrl &= ~(OTG_ID|OTG_ASESSVLD|OTG_VBUSVLD);
if (int_src & INTR_SESS_VLD)
otg_ctrl |= OTG_ASESSVLD;
else if (isp->phy.otg->state == OTG_STATE_A_WAIT_VFALL) {
a_idle(isp, "vfall");
otg_ctrl &= ~OTG_CTRL_BITS;
}
if (int_src & INTR_VBUS_VLD)
otg_ctrl |= OTG_VBUSVLD;
if (int_src & INTR_ID_GND) { /* default-A */
if (isp->phy.otg->state == OTG_STATE_B_IDLE
|| isp->phy.otg->state
== OTG_STATE_UNDEFINED) {
a_idle(isp, "init");
return;
}
} else { /* default-B */
otg_ctrl |= OTG_ID;
if (isp->phy.otg->state == OTG_STATE_A_IDLE
|| isp->phy.otg->state == OTG_STATE_UNDEFINED) {
b_idle(isp, "init");
return;
}
}
omap_writel(otg_ctrl, OTG_CTRL);
}
/* outputs from ISP1301_OTG_STATUS */
static void update_otg2(struct isp1301 *isp, u8 otg_status)
{
u32 otg_ctrl;
otg_ctrl = omap_readl(OTG_CTRL) & OTG_CTRL_MASK;
otg_ctrl &= ~OTG_XCEIV_INPUTS;
otg_ctrl &= ~(OTG_BSESSVLD | OTG_BSESSEND);
if (otg_status & OTG_B_SESS_VLD)
otg_ctrl |= OTG_BSESSVLD;
else if (otg_status & OTG_B_SESS_END)
otg_ctrl |= OTG_BSESSEND;
omap_writel(otg_ctrl, OTG_CTRL);
}
/* inputs going to ISP1301 */
static void otg_update_isp(struct isp1301 *isp)
{
u32 otg_ctrl, otg_change;
u8 set = OTG1_DM_PULLDOWN, clr = OTG1_DM_PULLUP;
otg_ctrl = omap_readl(OTG_CTRL);
otg_change = otg_ctrl ^ isp->last_otg_ctrl;
isp->last_otg_ctrl = otg_ctrl;
otg_ctrl = otg_ctrl & OTG_XCEIV_INPUTS;
switch (isp->phy.otg->state) {
case OTG_STATE_B_IDLE:
case OTG_STATE_B_PERIPHERAL:
case OTG_STATE_B_SRP_INIT:
if (!(otg_ctrl & OTG_PULLUP)) {
// if (otg_ctrl & OTG_B_HNPEN) {
if (isp->phy.otg->gadget->b_hnp_enable) {
isp->phy.otg->state = OTG_STATE_B_WAIT_ACON;
pr_debug(" --> b_wait_acon\n");
}
goto pulldown;
}
pullup:
set |= OTG1_DP_PULLUP;
clr |= OTG1_DP_PULLDOWN;
break;
case OTG_STATE_A_SUSPEND:
case OTG_STATE_A_PERIPHERAL:
if (otg_ctrl & OTG_PULLUP)
goto pullup;
/* FALLTHROUGH */
// case OTG_STATE_B_WAIT_ACON:
default:
pulldown:
set |= OTG1_DP_PULLDOWN;
clr |= OTG1_DP_PULLUP;
break;
}
# define toggle(OTG,ISP) do { \
if (otg_ctrl & OTG) set |= ISP; \
else clr |= ISP; \
} while (0)
if (!(isp->phy.otg->host))
otg_ctrl &= ~OTG_DRV_VBUS;
switch (isp->phy.otg->state) {
case OTG_STATE_A_SUSPEND:
if (otg_ctrl & OTG_DRV_VBUS) {
set |= OTG1_VBUS_DRV;
break;
}
/* HNP failed for some reason (A_AIDL_BDIS timeout) */
notresponding(isp);
/* FALLTHROUGH */
case OTG_STATE_A_VBUS_ERR:
isp->phy.otg->state = OTG_STATE_A_WAIT_VFALL;
pr_debug(" --> a_wait_vfall\n");
/* FALLTHROUGH */
case OTG_STATE_A_WAIT_VFALL:
/* FIXME usbcore thinks port power is still on ... */
clr |= OTG1_VBUS_DRV;
break;
case OTG_STATE_A_IDLE:
if (otg_ctrl & OTG_DRV_VBUS) {
isp->phy.otg->state = OTG_STATE_A_WAIT_VRISE;
pr_debug(" --> a_wait_vrise\n");
}
/* FALLTHROUGH */
default:
toggle(OTG_DRV_VBUS, OTG1_VBUS_DRV);
}
toggle(OTG_PU_VBUS, OTG1_VBUS_CHRG);
toggle(OTG_PD_VBUS, OTG1_VBUS_DISCHRG);
# undef toggle
isp1301_set_bits(isp, ISP1301_OTG_CONTROL_1, set);
isp1301_clear_bits(isp, ISP1301_OTG_CONTROL_1, clr);
/* HNP switch to host or peripheral; and SRP */
if (otg_change & OTG_PULLUP) {
u32 l;
switch (isp->phy.otg->state) {
case OTG_STATE_B_IDLE:
if (clr & OTG1_DP_PULLUP)
break;
isp->phy.otg->state = OTG_STATE_B_PERIPHERAL;
pr_debug(" --> b_peripheral\n");
break;
case OTG_STATE_A_SUSPEND:
if (clr & OTG1_DP_PULLUP)
break;
isp->phy.otg->state = OTG_STATE_A_PERIPHERAL;
pr_debug(" --> a_peripheral\n");
break;
default:
break;
}
l = omap_readl(OTG_CTRL);
l |= OTG_PULLUP;
omap_writel(l, OTG_CTRL);
}
check_state(isp, __func__);
dump_regs(isp, "otg->isp1301");
}
static irqreturn_t omap_otg_irq(int irq, void *_isp)
{
u16 otg_irq = omap_readw(OTG_IRQ_SRC);
u32 otg_ctrl;
int ret = IRQ_NONE;
struct isp1301 *isp = _isp;
struct usb_otg *otg = isp->phy.otg;
/* update ISP1301 transceiver from OTG controller */
if (otg_irq & OPRT_CHG) {
omap_writew(OPRT_CHG, OTG_IRQ_SRC);
isp1301_defer_work(isp, WORK_UPDATE_ISP);
ret = IRQ_HANDLED;
/* SRP to become b_peripheral failed */
} else if (otg_irq & B_SRP_TMROUT) {
pr_debug("otg: B_SRP_TIMEOUT, %06x\n", omap_readl(OTG_CTRL));
notresponding(isp);
/* gadget drivers that care should monitor all kinds of
* remote wakeup (SRP, normal) using their own timer
* to give "check cable and A-device" messages.
*/
if (isp->phy.otg->state == OTG_STATE_B_SRP_INIT)
b_idle(isp, "srp_timeout");
omap_writew(B_SRP_TMROUT, OTG_IRQ_SRC);
ret = IRQ_HANDLED;
/* HNP to become b_host failed */
} else if (otg_irq & B_HNP_FAIL) {
pr_debug("otg: %s B_HNP_FAIL, %06x\n",
state_name(isp), omap_readl(OTG_CTRL));
notresponding(isp);
otg_ctrl = omap_readl(OTG_CTRL);
otg_ctrl |= OTG_BUSDROP;
otg_ctrl &= OTG_CTRL_MASK & ~OTG_XCEIV_INPUTS;
omap_writel(otg_ctrl, OTG_CTRL);
/* subset of b_peripheral()... */
isp->phy.otg->state = OTG_STATE_B_PERIPHERAL;
pr_debug(" --> b_peripheral\n");
omap_writew(B_HNP_FAIL, OTG_IRQ_SRC);
ret = IRQ_HANDLED;
/* detect SRP from B-device ... */
} else if (otg_irq & A_SRP_DETECT) {
pr_debug("otg: %s SRP_DETECT, %06x\n",
state_name(isp), omap_readl(OTG_CTRL));
isp1301_defer_work(isp, WORK_UPDATE_OTG);
switch (isp->phy.otg->state) {
case OTG_STATE_A_IDLE:
if (!otg->host)
break;
isp1301_defer_work(isp, WORK_HOST_RESUME);
otg_ctrl = omap_readl(OTG_CTRL);
otg_ctrl |= OTG_A_BUSREQ;
otg_ctrl &= ~(OTG_BUSDROP|OTG_B_BUSREQ)
& ~OTG_XCEIV_INPUTS
& OTG_CTRL_MASK;
omap_writel(otg_ctrl, OTG_CTRL);
break;
default:
break;
}
omap_writew(A_SRP_DETECT, OTG_IRQ_SRC);
ret = IRQ_HANDLED;
/* timer expired: T(a_wait_bcon) and maybe T(a_wait_vrise)
* we don't track them separately
*/
} else if (otg_irq & A_REQ_TMROUT) {
otg_ctrl = omap_readl(OTG_CTRL);
pr_info("otg: BCON_TMOUT from %s, %06x\n",
state_name(isp), otg_ctrl);
notresponding(isp);
otg_ctrl |= OTG_BUSDROP;
otg_ctrl &= ~OTG_A_BUSREQ & OTG_CTRL_MASK & ~OTG_XCEIV_INPUTS;
omap_writel(otg_ctrl, OTG_CTRL);
isp->phy.otg->state = OTG_STATE_A_WAIT_VFALL;
omap_writew(A_REQ_TMROUT, OTG_IRQ_SRC);
ret = IRQ_HANDLED;
/* A-supplied voltage fell too low; overcurrent */
} else if (otg_irq & A_VBUS_ERR) {
otg_ctrl = omap_readl(OTG_CTRL);
printk(KERN_ERR "otg: %s, VBUS_ERR %04x ctrl %06x\n",
state_name(isp), otg_irq, otg_ctrl);
otg_ctrl |= OTG_BUSDROP;
otg_ctrl &= ~OTG_A_BUSREQ & OTG_CTRL_MASK & ~OTG_XCEIV_INPUTS;
omap_writel(otg_ctrl, OTG_CTRL);
isp->phy.otg->state = OTG_STATE_A_VBUS_ERR;
omap_writew(A_VBUS_ERR, OTG_IRQ_SRC);
ret = IRQ_HANDLED;
/* switch driver; the transceiver code activates it,
* ungating the udc clock or resuming OHCI.
*/
} else if (otg_irq & DRIVER_SWITCH) {
int kick = 0;
otg_ctrl = omap_readl(OTG_CTRL);
printk(KERN_NOTICE "otg: %s, SWITCH to %s, ctrl %06x\n",
state_name(isp),
(otg_ctrl & OTG_DRIVER_SEL)
? "gadget" : "host",
otg_ctrl);
isp1301_defer_work(isp, WORK_UPDATE_ISP);
/* role is peripheral */
if (otg_ctrl & OTG_DRIVER_SEL) {
switch (isp->phy.otg->state) {
case OTG_STATE_A_IDLE:
b_idle(isp, __func__);
break;
default:
break;
}
isp1301_defer_work(isp, WORK_UPDATE_ISP);
/* role is host */
} else {
if (!(otg_ctrl & OTG_ID)) {
otg_ctrl &= OTG_CTRL_MASK & ~OTG_XCEIV_INPUTS;
omap_writel(otg_ctrl | OTG_A_BUSREQ, OTG_CTRL);
}
if (otg->host) {
switch (isp->phy.otg->state) {
case OTG_STATE_B_WAIT_ACON:
isp->phy.otg->state = OTG_STATE_B_HOST;
pr_debug(" --> b_host\n");
kick = 1;
break;
case OTG_STATE_A_WAIT_BCON:
isp->phy.otg->state = OTG_STATE_A_HOST;
pr_debug(" --> a_host\n");
break;
case OTG_STATE_A_PERIPHERAL:
isp->phy.otg->state = OTG_STATE_A_WAIT_BCON;
pr_debug(" --> a_wait_bcon\n");
break;
default:
break;
}
isp1301_defer_work(isp, WORK_HOST_RESUME);
}
}
omap_writew(DRIVER_SWITCH, OTG_IRQ_SRC);
ret = IRQ_HANDLED;
if (kick)
usb_bus_start_enum(otg->host, otg->host->otg_port);
}
check_state(isp, __func__);
return ret;
}
static struct platform_device *otg_dev;
static int isp1301_otg_init(struct isp1301 *isp)
{
u32 l;
if (!otg_dev)
return -ENODEV;
dump_regs(isp, __func__);
/* some of these values are board-specific... */
l = omap_readl(OTG_SYSCON_2);
l |= OTG_EN
/* for B-device: */
| SRP_GPDATA /* 9msec Bdev D+ pulse */
| SRP_GPDVBUS /* discharge after VBUS pulse */
// | (3 << 24) /* 2msec VBUS pulse */
/* for A-device: */
| (0 << 20) /* 200ms nominal A_WAIT_VRISE timer */
| SRP_DPW /* detect 167+ns SRP pulses */
| SRP_DATA | SRP_VBUS /* accept both kinds of SRP pulse */
;
omap_writel(l, OTG_SYSCON_2);
update_otg1(isp, isp1301_get_u8(isp, ISP1301_INTERRUPT_SOURCE));
update_otg2(isp, isp1301_get_u8(isp, ISP1301_OTG_STATUS));
check_state(isp, __func__);
pr_debug("otg: %s, %s %06x\n",
state_name(isp), __func__, omap_readl(OTG_CTRL));
omap_writew(DRIVER_SWITCH | OPRT_CHG
| B_SRP_TMROUT | B_HNP_FAIL
| A_VBUS_ERR | A_SRP_DETECT | A_REQ_TMROUT, OTG_IRQ_EN);
l = omap_readl(OTG_SYSCON_2);
l |= OTG_EN;
omap_writel(l, OTG_SYSCON_2);
return 0;
}
static int otg_probe(struct platform_device *dev)
{
// struct omap_usb_config *config = dev->platform_data;
otg_dev = dev;
return 0;
}
static int otg_remove(struct platform_device *dev)
{
otg_dev = NULL;
return 0;
}
static struct platform_driver omap_otg_driver = {
.probe = otg_probe,
.remove = otg_remove,
.driver = {
.name = "omap_otg",
},
};
static int otg_bind(struct isp1301 *isp)
{
int status;
if (otg_dev)
return -EBUSY;
status = platform_driver_register(&omap_otg_driver);
if (status < 0)
return status;
if (otg_dev)
status = request_irq(otg_dev->resource[1].start, omap_otg_irq,
0, DRIVER_NAME, isp);
else
status = -ENODEV;
if (status < 0)
platform_driver_unregister(&omap_otg_driver);
return status;
}
static void otg_unbind(struct isp1301 *isp)
{
if (!otg_dev)
return;
free_irq(otg_dev->resource[1].start, isp);
}
#else
/* OTG controller isn't clocked */
#endif /* CONFIG_USB_OTG */
/*-------------------------------------------------------------------------*/
static void b_peripheral(struct isp1301 *isp)
{
u32 l;
l = omap_readl(OTG_CTRL) & OTG_XCEIV_OUTPUTS;
omap_writel(l, OTG_CTRL);
usb_gadget_vbus_connect(isp->phy.otg->gadget);
#ifdef CONFIG_USB_OTG
enable_vbus_draw(isp, 8);
otg_update_isp(isp);
#else
enable_vbus_draw(isp, 100);
/* UDC driver just set OTG_BSESSVLD */
isp1301_set_bits(isp, ISP1301_OTG_CONTROL_1, OTG1_DP_PULLUP);
isp1301_clear_bits(isp, ISP1301_OTG_CONTROL_1, OTG1_DP_PULLDOWN);
isp->phy.otg->state = OTG_STATE_B_PERIPHERAL;
pr_debug(" --> b_peripheral\n");
dump_regs(isp, "2periph");
#endif
}
static void isp_update_otg(struct isp1301 *isp, u8 stat)
{
struct usb_otg *otg = isp->phy.otg;
u8 isp_stat, isp_bstat;
enum usb_otg_state state = isp->phy.otg->state;
if (stat & INTR_BDIS_ACON)
pr_debug("OTG: BDIS_ACON, %s\n", state_name(isp));
/* start certain state transitions right away */
isp_stat = isp1301_get_u8(isp, ISP1301_INTERRUPT_SOURCE);
if (isp_stat & INTR_ID_GND) {
if (otg->default_a) {
switch (state) {
case OTG_STATE_B_IDLE:
a_idle(isp, "idle");
/* FALLTHROUGH */
case OTG_STATE_A_IDLE:
enable_vbus_source(isp);
/* FALLTHROUGH */
case OTG_STATE_A_WAIT_VRISE:
/* we skip over OTG_STATE_A_WAIT_BCON, since
* the HC will transition to A_HOST (or
* A_SUSPEND!) without our noticing except
* when HNP is used.
*/
if (isp_stat & INTR_VBUS_VLD)
isp->phy.otg->state = OTG_STATE_A_HOST;
break;
case OTG_STATE_A_WAIT_VFALL:
if (!(isp_stat & INTR_SESS_VLD))
a_idle(isp, "vfell");
break;
default:
if (!(isp_stat & INTR_VBUS_VLD))
isp->phy.otg->state = OTG_STATE_A_VBUS_ERR;
break;
}
isp_bstat = isp1301_get_u8(isp, ISP1301_OTG_STATUS);
} else {
switch (state) {
case OTG_STATE_B_PERIPHERAL:
case OTG_STATE_B_HOST:
case OTG_STATE_B_WAIT_ACON:
usb_gadget_vbus_disconnect(otg->gadget);
break;
default:
break;
}
if (state != OTG_STATE_A_IDLE)
a_idle(isp, "id");
if (otg->host && state == OTG_STATE_A_IDLE)
isp1301_defer_work(isp, WORK_HOST_RESUME);
isp_bstat = 0;
}
} else {
u32 l;
/* if user unplugged mini-A end of cable,
* don't bypass A_WAIT_VFALL.
*/
if (otg->default_a) {
switch (state) {
default:
isp->phy.otg->state = OTG_STATE_A_WAIT_VFALL;
break;
case OTG_STATE_A_WAIT_VFALL:
state = OTG_STATE_A_IDLE;
/* hub_wq may take a while to notice and
* handle this disconnect, so don't go
* to B_IDLE quite yet.
*/
break;
case OTG_STATE_A_IDLE:
host_suspend(isp);
isp1301_clear_bits(isp, ISP1301_MODE_CONTROL_1,
MC1_BDIS_ACON_EN);
isp->phy.otg->state = OTG_STATE_B_IDLE;
l = omap_readl(OTG_CTRL) & OTG_CTRL_MASK;
l &= ~OTG_CTRL_BITS;
omap_writel(l, OTG_CTRL);
break;
case OTG_STATE_B_IDLE:
break;
}
}
isp_bstat = isp1301_get_u8(isp, ISP1301_OTG_STATUS);
switch (isp->phy.otg->state) {
case OTG_STATE_B_PERIPHERAL:
case OTG_STATE_B_WAIT_ACON:
case OTG_STATE_B_HOST:
if (likely(isp_bstat & OTG_B_SESS_VLD))
break;
enable_vbus_draw(isp, 0);
#ifndef CONFIG_USB_OTG
/* UDC driver will clear OTG_BSESSVLD */
isp1301_set_bits(isp, ISP1301_OTG_CONTROL_1,
OTG1_DP_PULLDOWN);
isp1301_clear_bits(isp, ISP1301_OTG_CONTROL_1,
OTG1_DP_PULLUP);
dump_regs(isp, __func__);
#endif
/* FALLTHROUGH */
case OTG_STATE_B_SRP_INIT:
b_idle(isp, __func__);
l = omap_readl(OTG_CTRL) & OTG_XCEIV_OUTPUTS;
omap_writel(l, OTG_CTRL);
/* FALLTHROUGH */
case OTG_STATE_B_IDLE:
if (otg->gadget && (isp_bstat & OTG_B_SESS_VLD)) {
#ifdef CONFIG_USB_OTG
update_otg1(isp, isp_stat);
update_otg2(isp, isp_bstat);
#endif
b_peripheral(isp);
} else if (!(isp_stat & (INTR_VBUS_VLD|INTR_SESS_VLD)))
isp_bstat |= OTG_B_SESS_END;
break;
case OTG_STATE_A_WAIT_VFALL:
break;
default:
pr_debug("otg: unsupported b-device %s\n",
state_name(isp));
break;
}
}
if (state != isp->phy.otg->state)
pr_debug(" isp, %s -> %s\n",
usb_otg_state_string(state), state_name(isp));
#ifdef CONFIG_USB_OTG
/* update the OTG controller state to match the isp1301; may
* trigger OPRT_CHG irqs for changes going to the isp1301.
*/
update_otg1(isp, isp_stat);
update_otg2(isp, isp_bstat);
check_state(isp, __func__);
#endif
dump_regs(isp, "isp1301->otg");
}
/*-------------------------------------------------------------------------*/
static u8 isp1301_clear_latch(struct isp1301 *isp)
{
u8 latch = isp1301_get_u8(isp, ISP1301_INTERRUPT_LATCH);
isp1301_clear_bits(isp, ISP1301_INTERRUPT_LATCH, latch);
return latch;
}
static void
isp1301_work(struct work_struct *work)
{
struct isp1301 *isp = container_of(work, struct isp1301, work);
int stop;
/* implicit lock: we're the only task using this device */
isp->working = 1;
do {
stop = test_bit(WORK_STOP, &isp->todo);
#ifdef CONFIG_USB_OTG
/* transfer state from otg engine to isp1301 */
if (test_and_clear_bit(WORK_UPDATE_ISP, &isp->todo)) {
otg_update_isp(isp);
put_device(&isp->client->dev);
}
#endif
/* transfer state from isp1301 to otg engine */
if (test_and_clear_bit(WORK_UPDATE_OTG, &isp->todo)) {
u8 stat = isp1301_clear_latch(isp);
isp_update_otg(isp, stat);
put_device(&isp->client->dev);
}
if (test_and_clear_bit(WORK_HOST_RESUME, &isp->todo)) {
u32 otg_ctrl;
/*
* skip A_WAIT_VRISE; hc transitions invisibly
* skip A_WAIT_BCON; same.
*/
switch (isp->phy.otg->state) {
case OTG_STATE_A_WAIT_BCON:
case OTG_STATE_A_WAIT_VRISE:
isp->phy.otg->state = OTG_STATE_A_HOST;
pr_debug(" --> a_host\n");
otg_ctrl = omap_readl(OTG_CTRL);
otg_ctrl |= OTG_A_BUSREQ;
otg_ctrl &= ~(OTG_BUSDROP|OTG_B_BUSREQ)
& OTG_CTRL_MASK;
omap_writel(otg_ctrl, OTG_CTRL);
break;
case OTG_STATE_B_WAIT_ACON:
isp->phy.otg->state = OTG_STATE_B_HOST;
pr_debug(" --> b_host (acon)\n");
break;
case OTG_STATE_B_HOST:
case OTG_STATE_B_IDLE:
case OTG_STATE_A_IDLE:
break;
default:
pr_debug(" host resume in %s\n",
state_name(isp));
}
host_resume(isp);
// mdelay(10);
put_device(&isp->client->dev);
}
if (test_and_clear_bit(WORK_TIMER, &isp->todo)) {
#ifdef VERBOSE
dump_regs(isp, "timer");
if (!stop)
mod_timer(&isp->timer, jiffies + TIMER_JIFFIES);
#endif
put_device(&isp->client->dev);
}
if (isp->todo)
dev_vdbg(&isp->client->dev,
"work done, todo = 0x%lx\n",
isp->todo);
if (stop) {
dev_dbg(&isp->client->dev, "stop\n");
break;
}
} while (isp->todo);
isp->working = 0;
}
static irqreturn_t isp1301_irq(int irq, void *isp)
{
isp1301_defer_work(isp, WORK_UPDATE_OTG);
return IRQ_HANDLED;
}
static void isp1301_timer(unsigned long _isp)
{
isp1301_defer_work((void *)_isp, WORK_TIMER);
}
/*-------------------------------------------------------------------------*/
static void isp1301_release(struct device *dev)
{
struct isp1301 *isp;
isp = dev_get_drvdata(dev);
/* FIXME -- not with a "new style" driver, it doesn't!! */
/* ugly -- i2c hijacks our memory hook to wait_for_completion() */
if (isp->i2c_release)
isp->i2c_release(dev);
kfree(isp->phy.otg);
kfree (isp);
}
static struct isp1301 *the_transceiver;
static int isp1301_remove(struct i2c_client *i2c)
{
struct isp1301 *isp;
isp = i2c_get_clientdata(i2c);
isp1301_clear_bits(isp, ISP1301_INTERRUPT_FALLING, ~0);
isp1301_clear_bits(isp, ISP1301_INTERRUPT_RISING, ~0);
free_irq(i2c->irq, isp);
#ifdef CONFIG_USB_OTG
otg_unbind(isp);
#endif
if (machine_is_omap_h2())
gpio_free(2);
isp->timer.data = 0;
set_bit(WORK_STOP, &isp->todo);
del_timer_sync(&isp->timer);
flush_work(&isp->work);
put_device(&i2c->dev);
the_transceiver = NULL;
return 0;
}
/*-------------------------------------------------------------------------*/
/* NOTE: three modes are possible here, only one of which
* will be standards-conformant on any given system:
*
* - OTG mode (dual-role), required if there's a Mini-AB connector
* - HOST mode, for when there's one or more A (host) connectors
* - DEVICE mode, for when there's a B/Mini-B (device) connector
*
* As a rule, you won't have an isp1301 chip unless it's there to
* support the OTG mode. Other modes help testing USB controllers
* in isolation from (full) OTG support, or maybe so later board
* revisions can help to support those feature.
*/
#ifdef CONFIG_USB_OTG
static int isp1301_otg_enable(struct isp1301 *isp)
{
power_up(isp);
isp1301_otg_init(isp);
/* NOTE: since we don't change this, this provides
* a few more interrupts than are strictly needed.
*/
isp1301_set_bits(isp, ISP1301_INTERRUPT_RISING,
INTR_VBUS_VLD | INTR_SESS_VLD | INTR_ID_GND);
isp1301_set_bits(isp, ISP1301_INTERRUPT_FALLING,
INTR_VBUS_VLD | INTR_SESS_VLD | INTR_ID_GND);
dev_info(&isp->client->dev, "ready for dual-role USB ...\n");
return 0;
}
#endif
/* add or disable the host device+driver */
static int
isp1301_set_host(struct usb_otg *otg, struct usb_bus *host)
{
struct isp1301 *isp = container_of(otg->usb_phy, struct isp1301, phy);
if (isp != the_transceiver)
return -ENODEV;
if (!host) {
omap_writew(0, OTG_IRQ_EN);
power_down(isp);
otg->host = NULL;
return 0;
}
#ifdef CONFIG_USB_OTG
otg->host = host;
dev_dbg(&isp->client->dev, "registered host\n");
host_suspend(isp);
if (otg->gadget)
return isp1301_otg_enable(isp);
return 0;
#elif !IS_ENABLED(CONFIG_USB_OMAP)
// FIXME update its refcount
otg->host = host;
power_up(isp);
if (machine_is_omap_h2())
isp1301_set_bits(isp, ISP1301_MODE_CONTROL_1, MC1_DAT_SE0);
dev_info(&isp->client->dev, "A-Host sessions ok\n");
isp1301_set_bits(isp, ISP1301_INTERRUPT_RISING,
INTR_ID_GND);
isp1301_set_bits(isp, ISP1301_INTERRUPT_FALLING,
INTR_ID_GND);
/* If this has a Mini-AB connector, this mode is highly
* nonstandard ... but can be handy for testing, especially with
* the Mini-A end of an OTG cable. (Or something nonstandard
* like MiniB-to-StandardB, maybe built with a gender mender.)
*/
isp1301_set_bits(isp, ISP1301_OTG_CONTROL_1, OTG1_VBUS_DRV);
dump_regs(isp, __func__);
return 0;
#else
dev_dbg(&isp->client->dev, "host sessions not allowed\n");
return -EINVAL;
#endif
}
static int
isp1301_set_peripheral(struct usb_otg *otg, struct usb_gadget *gadget)
{
struct isp1301 *isp = container_of(otg->usb_phy, struct isp1301, phy);
if (isp != the_transceiver)
return -ENODEV;
if (!gadget) {
omap_writew(0, OTG_IRQ_EN);
if (!otg->default_a)
enable_vbus_draw(isp, 0);
usb_gadget_vbus_disconnect(otg->gadget);
otg->gadget = NULL;
power_down(isp);
return 0;
}
#ifdef CONFIG_USB_OTG
otg->gadget = gadget;
dev_dbg(&isp->client->dev, "registered gadget\n");
/* gadget driver may be suspended until vbus_connect () */
if (otg->host)
return isp1301_otg_enable(isp);
return 0;
#elif !defined(CONFIG_USB_OHCI_HCD) && !defined(CONFIG_USB_OHCI_HCD_MODULE)
otg->gadget = gadget;
// FIXME update its refcount
{
u32 l;
l = omap_readl(OTG_CTRL) & OTG_CTRL_MASK;
l &= ~(OTG_XCEIV_OUTPUTS|OTG_CTRL_BITS);
l |= OTG_ID;
omap_writel(l, OTG_CTRL);
}
power_up(isp);
isp->phy.otg->state = OTG_STATE_B_IDLE;
if (machine_is_omap_h2() || machine_is_omap_h3())
isp1301_set_bits(isp, ISP1301_MODE_CONTROL_1, MC1_DAT_SE0);
isp1301_set_bits(isp, ISP1301_INTERRUPT_RISING,
INTR_SESS_VLD);
isp1301_set_bits(isp, ISP1301_INTERRUPT_FALLING,
INTR_VBUS_VLD);
dev_info(&isp->client->dev, "B-Peripheral sessions ok\n");
dump_regs(isp, __func__);
/* If this has a Mini-AB connector, this mode is highly
* nonstandard ... but can be handy for testing, so long
* as you don't plug a Mini-A cable into the jack.
*/
if (isp1301_get_u8(isp, ISP1301_INTERRUPT_SOURCE) & INTR_VBUS_VLD)
b_peripheral(isp);
return 0;
#else
dev_dbg(&isp->client->dev, "peripheral sessions not allowed\n");
return -EINVAL;
#endif
}
/*-------------------------------------------------------------------------*/
static int
isp1301_set_power(struct usb_phy *dev, unsigned mA)
{
if (!the_transceiver)
return -ENODEV;
if (dev->otg->state == OTG_STATE_B_PERIPHERAL)
enable_vbus_draw(the_transceiver, mA);
return 0;
}
static int
isp1301_start_srp(struct usb_otg *otg)
{
struct isp1301 *isp = container_of(otg->usb_phy, struct isp1301, phy);
u32 otg_ctrl;
if (isp != the_transceiver || isp->phy.otg->state != OTG_STATE_B_IDLE)
return -ENODEV;
otg_ctrl = omap_readl(OTG_CTRL);
if (!(otg_ctrl & OTG_BSESSEND))
return -EINVAL;
otg_ctrl |= OTG_B_BUSREQ;
otg_ctrl &= ~OTG_A_BUSREQ & OTG_CTRL_MASK;
omap_writel(otg_ctrl, OTG_CTRL);
isp->phy.otg->state = OTG_STATE_B_SRP_INIT;
pr_debug("otg: SRP, %s ... %06x\n", state_name(isp),
omap_readl(OTG_CTRL));
#ifdef CONFIG_USB_OTG
check_state(isp, __func__);
#endif
return 0;
}
static int
isp1301_start_hnp(struct usb_otg *otg)
{
#ifdef CONFIG_USB_OTG
struct isp1301 *isp = container_of(otg->usb_phy, struct isp1301, phy);
u32 l;
if (isp != the_transceiver)
return -ENODEV;
if (otg->default_a && (otg->host == NULL || !otg->host->b_hnp_enable))
return -ENOTCONN;
if (!otg->default_a && (otg->gadget == NULL
|| !otg->gadget->b_hnp_enable))
return -ENOTCONN;
/* We want hardware to manage most HNP protocol timings.
* So do this part as early as possible...
*/
switch (isp->phy.otg->state) {
case OTG_STATE_B_HOST:
isp->phy.otg->state = OTG_STATE_B_PERIPHERAL;
/* caller will suspend next */
break;
case OTG_STATE_A_HOST:
#if 0
/* autoconnect mode avoids irq latency bugs */
isp1301_set_bits(isp, ISP1301_MODE_CONTROL_1,
MC1_BDIS_ACON_EN);
#endif
/* caller must suspend then clear A_BUSREQ */
usb_gadget_vbus_connect(otg->gadget);
l = omap_readl(OTG_CTRL);
l |= OTG_A_SETB_HNPEN;
omap_writel(l, OTG_CTRL);
break;
case OTG_STATE_A_PERIPHERAL:
/* initiated by B-Host suspend */
break;
default:
return -EILSEQ;
}
pr_debug("otg: HNP %s, %06x ...\n",
state_name(isp), omap_readl(OTG_CTRL));
check_state(isp, __func__);
return 0;
#else
/* srp-only */
return -EINVAL;
#endif
}
/*-------------------------------------------------------------------------*/
static int
isp1301_probe(struct i2c_client *i2c, const struct i2c_device_id *id)
{
int status;
struct isp1301 *isp;
if (the_transceiver)
return 0;
isp = kzalloc(sizeof *isp, GFP_KERNEL);
if (!isp)
return 0;
isp->phy.otg = kzalloc(sizeof *isp->phy.otg, GFP_KERNEL);
if (!isp->phy.otg) {
kfree(isp);
return 0;
}
INIT_WORK(&isp->work, isp1301_work);
init_timer(&isp->timer);
isp->timer.function = isp1301_timer;
isp->timer.data = (unsigned long) isp;
i2c_set_clientdata(i2c, isp);
isp->client = i2c;
/* verify the chip (shouldn't be necessary) */
status = isp1301_get_u16(isp, ISP1301_VENDOR_ID);
if (status != I2C_VENDOR_ID_PHILIPS) {
dev_dbg(&i2c->dev, "not philips id: %d\n", status);
goto fail;
}
status = isp1301_get_u16(isp, ISP1301_PRODUCT_ID);
if (status != I2C_PRODUCT_ID_PHILIPS_1301) {
dev_dbg(&i2c->dev, "not isp1301, %d\n", status);
goto fail;
}
isp->i2c_release = i2c->dev.release;
i2c->dev.release = isp1301_release;
/* initial development used chiprev 2.00 */
status = i2c_smbus_read_word_data(i2c, ISP1301_BCD_DEVICE);
dev_info(&i2c->dev, "chiprev %x.%02x, driver " DRIVER_VERSION "\n",
status >> 8, status & 0xff);
/* make like power-on reset */
isp1301_clear_bits(isp, ISP1301_MODE_CONTROL_1, MC1_MASK);
isp1301_set_bits(isp, ISP1301_MODE_CONTROL_2, MC2_BI_DI);
isp1301_clear_bits(isp, ISP1301_MODE_CONTROL_2, ~MC2_BI_DI);
isp1301_set_bits(isp, ISP1301_OTG_CONTROL_1,
OTG1_DM_PULLDOWN | OTG1_DP_PULLDOWN);
isp1301_clear_bits(isp, ISP1301_OTG_CONTROL_1,
~(OTG1_DM_PULLDOWN | OTG1_DP_PULLDOWN));
isp1301_clear_bits(isp, ISP1301_INTERRUPT_LATCH, ~0);
isp1301_clear_bits(isp, ISP1301_INTERRUPT_FALLING, ~0);
isp1301_clear_bits(isp, ISP1301_INTERRUPT_RISING, ~0);
#ifdef CONFIG_USB_OTG
status = otg_bind(isp);
if (status < 0) {
dev_dbg(&i2c->dev, "can't bind OTG\n");
goto fail;
}
#endif
if (machine_is_omap_h2()) {
/* full speed signaling by default */
isp1301_set_bits(isp, ISP1301_MODE_CONTROL_1,
MC1_SPEED);
isp1301_set_bits(isp, ISP1301_MODE_CONTROL_2,
MC2_SPD_SUSP_CTRL);
/* IRQ wired at M14 */
omap_cfg_reg(M14_1510_GPIO2);
if (gpio_request(2, "isp1301") == 0)
gpio_direction_input(2);
isp->irq_type = IRQF_TRIGGER_FALLING;
}
status = request_irq(i2c->irq, isp1301_irq,
isp->irq_type, DRIVER_NAME, isp);
if (status < 0) {
dev_dbg(&i2c->dev, "can't get IRQ %d, err %d\n",
i2c->irq, status);
goto fail;
}
isp->phy.dev = &i2c->dev;
isp->phy.label = DRIVER_NAME;
isp->phy.set_power = isp1301_set_power,
isp->phy.otg->usb_phy = &isp->phy;
isp->phy.otg->set_host = isp1301_set_host,
isp->phy.otg->set_peripheral = isp1301_set_peripheral,
isp->phy.otg->start_srp = isp1301_start_srp,
isp->phy.otg->start_hnp = isp1301_start_hnp,
enable_vbus_draw(isp, 0);
power_down(isp);
the_transceiver = isp;
#ifdef CONFIG_USB_OTG
update_otg1(isp, isp1301_get_u8(isp, ISP1301_INTERRUPT_SOURCE));
update_otg2(isp, isp1301_get_u8(isp, ISP1301_OTG_STATUS));
#endif
dump_regs(isp, __func__);
#ifdef VERBOSE
mod_timer(&isp->timer, jiffies + TIMER_JIFFIES);
dev_dbg(&i2c->dev, "scheduled timer, %d min\n", TIMER_MINUTES);
#endif
status = usb_add_phy(&isp->phy, USB_PHY_TYPE_USB2);
if (status < 0)
dev_err(&i2c->dev, "can't register transceiver, %d\n",
status);
return 0;
fail:
kfree(isp->phy.otg);
kfree(isp);
return -ENODEV;
}
static const struct i2c_device_id isp1301_id[] = {
{ "isp1301_omap", 0 },
{ }
};
MODULE_DEVICE_TABLE(i2c, isp1301_id);
static struct i2c_driver isp1301_driver = {
.driver = {
.name = "isp1301_omap",
},
.probe = isp1301_probe,
.remove = isp1301_remove,
.id_table = isp1301_id,
};
/*-------------------------------------------------------------------------*/
static int __init isp_init(void)
{
return i2c_add_driver(&isp1301_driver);
}
subsys_initcall(isp_init);
static void __exit isp_exit(void)
{
if (the_transceiver)
usb_remove_phy(&the_transceiver->phy);
i2c_del_driver(&isp1301_driver);
}
module_exit(isp_exit);
| {
"pile_set_name": "Github"
} |
---
title: Avoid using checkpoints on a virtual machine that runs a server workload in a production environment
description: Online version of the text for this Best Practices Analyzer rule.
ms.author: benarm
author: BenjaminArmstrong
ms.topic: article
ms.assetid: 1be75890-d316-495a-b9b7-be75fc1aac10
ms.date: 8/16/2016
---
# Avoid using checkpoints on a virtual machine that runs a server workload in a production environment
>Applies To: Windows Server 2016
*For more information about best practices and scans, see* [Best Practices Analyzer](https://go.microsoft.com/fwlink/?LinkId=122786).
|Property|Details|
|-|-|
|**Operating System**|Windows Server 2016|
|**Product/Feature**|Hyper-V|
|**Severity**|Warning|
|**Category**|Operations|
In the following sections, italics indicates UI text that appears in the Best Practices Analyzer tool for this issue.
> [!NOTE]
> In Windows Server 2012 R2, virtual machine snapshots were renamed to virtual machine checkpoints in Hyper-V Manager to match the terminology used in System Center Virtual Machine Management. For details, see [Checkpoints and Snapshots Overview](/previous-versions/windows/it-pro/windows-server-2012-R2-and-2012/dn818483(v=ws.11)).
## Issue
*A virtual machine with one or more checkpoints has been found.*
## Impact
*Available space may run out on the physical disk that stores the checkpoints files. If this occurs, no additional disk operations can be performed on the physical storage. Any virtual machine that relies on the physical storage could be affected.*
If physical disk space runs out, any running virtual machine that has checkpoints or virtual hard disks stored on that disk may be paused automatically. Hyper-V Manager shows the status of these virtual machines as paused-critical.
## Resolution
*If the virtual machine runs a server workload in a production environment, take the virtual machine offline and then use Hyper-V Manager to apply or delete the checkpoints. To delete checkpoints, you must shut down the virtual machine to complete the process.*
> [!NOTE]
> Production checkpoints are now available as an alternative to standard checkpoints. For details, see [Choose between standard or production checkpoints](../manage/Choose-between-standard-or-production-checkpoints-in-Hyper-V.md). | {
"pile_set_name": "Github"
} |
/*
* Copyright (c) 2019 Yubico AB. All rights reserved.
* Use of this source code is governed by a BSD-style
* license that can be found in the LICENSE file.
*/
#include <fido.h>
#include <fido/bio.h>
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#ifdef HAVE_UNISTD_H
#include <unistd.h>
#endif
#include "../openbsd-compat/openbsd-compat.h"
#include "extern.h"
static void
print_template(const fido_bio_template_array_t *ta, size_t idx)
{
char *id = NULL;
const fido_bio_template_t *t = NULL;
if ((t = fido_bio_template(ta, idx)) == NULL)
errx(1, "fido_bio_template");
if (base64_encode(fido_bio_template_id_ptr(t),
fido_bio_template_id_len(t), &id) < 0)
errx(1, "output error");
printf("%02u: %s %s\n", (unsigned)idx, id, fido_bio_template_name(t));
free(id);
}
int
bio_list(char *path)
{
char pin[1024];
fido_bio_template_array_t *ta = NULL;
fido_dev_t *dev = NULL;
int r;
if (path == NULL)
usage();
if ((ta = fido_bio_template_array_new()) == NULL)
errx(1, "fido_bio_template_array_new");
dev = open_dev(path);
read_pin(path, pin, sizeof(pin));
r = fido_bio_dev_get_template_array(dev, ta, pin);
explicit_bzero(pin, sizeof(pin));
if (r != FIDO_OK)
errx(1, "fido_bio_dev_get_template_array: %s", fido_strerr(r));
for (size_t i = 0; i < fido_bio_template_array_count(ta); i++)
print_template(ta, i);
fido_bio_template_array_free(&ta);
fido_dev_close(dev);
fido_dev_free(&dev);
exit(0);
}
int
bio_set_name(char *path, char *id, char *name)
{
char pin[1024];
fido_bio_template_t *t = NULL;
fido_dev_t *dev = NULL;
int r;
size_t id_blob_len = 0;
void *id_blob_ptr = NULL;
if (path == NULL)
usage();
if ((t = fido_bio_template_new()) == NULL)
errx(1, "fido_bio_template_new");
if (base64_decode(id, &id_blob_ptr, &id_blob_len) < 0)
errx(1, "base64_decode");
if ((r = fido_bio_template_set_name(t, name)) != FIDO_OK)
errx(1, "fido_bio_template_set_name: %s", fido_strerr(r));
if ((r = fido_bio_template_set_id(t, id_blob_ptr,
id_blob_len)) != FIDO_OK)
errx(1, "fido_bio_template_set_id: %s", fido_strerr(r));
dev = open_dev(path);
read_pin(path, pin, sizeof(pin));
r = fido_bio_dev_set_template_name(dev, t, pin);
explicit_bzero(pin, sizeof(pin));
if (r != FIDO_OK)
errx(1, "fido_bio_dev_set_template_name: %s", fido_strerr(r));
free(id_blob_ptr);
fido_bio_template_free(&t);
fido_dev_close(dev);
fido_dev_free(&dev);
exit(0);
}
static const char *
plural(uint8_t n)
{
if (n == 1)
return "";
return "s";
}
static const char *
enroll_strerr(uint8_t n)
{
switch (n) {
case FIDO_BIO_ENROLL_FP_GOOD:
return "Sample ok";
case FIDO_BIO_ENROLL_FP_TOO_HIGH:
return "Sample too high";
case FIDO_BIO_ENROLL_FP_TOO_LOW:
return "Sample too low";
case FIDO_BIO_ENROLL_FP_TOO_LEFT:
return "Sample too left";
case FIDO_BIO_ENROLL_FP_TOO_RIGHT:
return "Sample too right";
case FIDO_BIO_ENROLL_FP_TOO_FAST:
return "Sample too fast";
case FIDO_BIO_ENROLL_FP_TOO_SLOW:
return "Sample too slow";
case FIDO_BIO_ENROLL_FP_POOR_QUALITY:
return "Poor quality sample";
case FIDO_BIO_ENROLL_FP_TOO_SKEWED:
return "Sample too skewed";
case FIDO_BIO_ENROLL_FP_TOO_SHORT:
return "Sample too short";
case FIDO_BIO_ENROLL_FP_MERGE_FAILURE:
return "Sample merge failure";
case FIDO_BIO_ENROLL_FP_EXISTS:
return "Sample exists";
case FIDO_BIO_ENROLL_FP_DATABASE_FULL:
return "Fingerprint database full";
case FIDO_BIO_ENROLL_NO_USER_ACTIVITY:
return "No user activity";
case FIDO_BIO_ENROLL_NO_USER_PRESENCE_TRANSITION:
return "No user presence transition";
default:
return "Unknown error";
}
}
int
bio_enroll(char *path)
{
char pin[1024];
fido_bio_enroll_t *e = NULL;
fido_bio_template_t *t = NULL;
fido_dev_t *dev = NULL;
int r;
if (path == NULL)
usage();
if ((t = fido_bio_template_new()) == NULL)
errx(1, "fido_bio_template_new");
if ((e = fido_bio_enroll_new()) == NULL)
errx(1, "fido_bio_enroll_new");
dev = open_dev(path);
read_pin(path, pin, sizeof(pin));
printf("Touch your security key.\n");
r = fido_bio_dev_enroll_begin(dev, t, e, 10000, pin);
explicit_bzero(pin, sizeof(pin));
if (r != FIDO_OK)
errx(1, "fido_bio_dev_enroll_begin: %s", fido_strerr(r));
printf("%s.\n", enroll_strerr(fido_bio_enroll_last_status(e)));
while (fido_bio_enroll_remaining_samples(e) > 0) {
printf("Touch your security key (%u sample%s left).\n",
(unsigned)fido_bio_enroll_remaining_samples(e),
plural(fido_bio_enroll_remaining_samples(e)));
if ((r = fido_bio_dev_enroll_continue(dev, t, e,
10000)) != FIDO_OK) {
errx(1, "fido_bio_dev_enroll_continue: %s",
fido_strerr(r));
}
printf("%s.\n", enroll_strerr(fido_bio_enroll_last_status(e)));
}
fido_bio_template_free(&t);
fido_bio_enroll_free(&e);
fido_dev_close(dev);
fido_dev_free(&dev);
exit(0);
}
int
bio_delete(fido_dev_t *dev, char *path, char *id)
{
char pin[1024];
fido_bio_template_t *t = NULL;
int r;
size_t id_blob_len = 0;
void *id_blob_ptr = NULL;
if (path == NULL)
usage();
if ((t = fido_bio_template_new()) == NULL)
errx(1, "fido_bio_template_new");
if (base64_decode(id, &id_blob_ptr, &id_blob_len) < 0)
errx(1, "base64_decode");
if ((r = fido_bio_template_set_id(t, id_blob_ptr,
id_blob_len)) != FIDO_OK)
errx(1, "fido_bio_template_set_id: %s", fido_strerr(r));
read_pin(path, pin, sizeof(pin));
r = fido_bio_dev_enroll_remove(dev, t, pin);
explicit_bzero(pin, sizeof(pin));
if (r != FIDO_OK)
errx(1, "fido_bio_dev_enroll_remove: %s", fido_strerr(r));
free(id_blob_ptr);
fido_bio_template_free(&t);
fido_dev_close(dev);
fido_dev_free(&dev);
exit(0);
}
static const char *
type_str(uint8_t t)
{
switch (t) {
case 1:
return "touch";
case 2:
return "swipe";
default:
return "unknown";
}
}
void
bio_info(fido_dev_t *dev)
{
fido_bio_info_t *i = NULL;
if ((i = fido_bio_info_new()) == NULL)
errx(1, "fido_bio_info_new");
if (fido_bio_dev_get_info(dev, i) != FIDO_OK) {
fido_bio_info_free(&i);
return;
}
printf("sensor type: %u (%s)\n", (unsigned)fido_bio_info_type(i),
type_str(fido_bio_info_type(i)));
printf("max samples: %u\n", (unsigned)fido_bio_info_max_samples(i));
fido_bio_info_free(&i);
}
| {
"pile_set_name": "Github"
} |
/* Copyright 2017 The TensorFlow Authors. All Rights Reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
==============================================================================*/
#ifndef TENSORFLOW_COMPILER_XLA_EXECUTABLE_RUN_OPTIONS_H_
#define TENSORFLOW_COMPILER_XLA_EXECUTABLE_RUN_OPTIONS_H_
#include <string>
#include "tensorflow/compiler/xla/types.h"
// These classes are forward declared so that ExecutableRunOptions can be linked
// into an XLA-compiled binary without having to link all of the pointed-to
// objects (e.g., for an ahead-of-time compiled CPU binary, the gpu tools don't
// need to be linked).
namespace stream_executor {
class Stream;
class Platform;
class DeviceMemoryAllocator;
} // namespace stream_executor
namespace Eigen {
struct ThreadPoolDevice;
} // namespace Eigen
namespace xla {
class DeviceAssignment;
class ExecutionProfile;
// A unique identifier for a particular "logical execution" of an XLA model.
//
// A logical execution might encompass multiple executions of one or more
// HloModules. Runs that are part of the same logical execution can
// communicate via collective ops (e.g. kAllToAll), whereas runs that are part
// of different logical executions are isolated.
class RunId {
public:
// Creates a new, unique RunId.
RunId();
RunId(const RunId&) = default;
RunId& operator=(const RunId&) = default;
friend bool operator==(const RunId& a, const RunId& b);
std::string ToString() const;
template <typename H>
friend H AbslHashValue(H h, const RunId& id) {
return H::combine(std::move(h), id.data_);
}
private:
int64 data_;
};
// Class containing options for running a LocalExecutable.
class ExecutableRunOptions {
public:
// Specifies the allocator to use during execution.
ExecutableRunOptions& set_allocator(
stream_executor::DeviceMemoryAllocator* allocator);
stream_executor::DeviceMemoryAllocator* allocator() const;
// If set, this is the device to run the computation on. Valid device_ordinal
// values are: 0 to # of devices - 1. These values are identical to the device
// ordinal values used by StreamExecutor. The device must be of the same type
// as the executable was compiled for. A value of -1 indicates this option has
// not been set.
ExecutableRunOptions& set_device_ordinal(int device_ordinal);
int device_ordinal() const;
// If set, this is the stream to run the computation on. The platform of the
// stream must match the platform the executable was built for. A value of
// nullptr indicates the option has not been set.
ExecutableRunOptions& set_stream(stream_executor::Stream* stream);
stream_executor::Stream* stream() const;
// If set, this is the stream to perform any pre-computation transfers on.
// The platform of the stream must match the platform the executable was
// built for. A value of nullptr indicates the option has not been set.
ExecutableRunOptions& set_host_to_device_stream(
stream_executor::Stream* stream);
stream_executor::Stream* host_to_device_stream() const;
// Sets the thread pool device on which to run Eigen subcomputations.
//
// This field must be set for XLA:CPU models that call Eigen routines, but may
// be null otherwise. Routines that use this field should always CHECK (or
// TF_RET_CHECK) that it's not null before dereferencing it, so that users get
// a clean crash rather than a segfault.
//
// Does not take ownership.
ExecutableRunOptions& set_intra_op_thread_pool(
const Eigen::ThreadPoolDevice* intra_op_thread_pool);
const Eigen::ThreadPoolDevice* intra_op_thread_pool() const;
// If set, profiling information is written to 'profile'.
ExecutionProfile* execution_profile() const;
ExecutableRunOptions& set_execution_profile(ExecutionProfile* profile);
ExecutableRunOptions& set_device_assignment(
const DeviceAssignment* device_assignment);
const DeviceAssignment* device_assignment() const;
ExecutableRunOptions& set_rng_seed(int rng_seed);
int rng_seed() const;
ExecutableRunOptions& set_run_id(RunId id);
RunId run_id() const;
private:
stream_executor::DeviceMemoryAllocator* allocator_ = nullptr;
int device_ordinal_ = -1;
const DeviceAssignment* device_assignment_ = nullptr;
stream_executor::Stream* stream_ = nullptr;
const Eigen::ThreadPoolDevice* intra_op_thread_pool_ = nullptr;
ExecutionProfile* execution_profile_ = nullptr;
int rng_seed_ = 0;
stream_executor::Stream* host_to_device_stream_ = nullptr;
RunId run_id_;
};
} // namespace xla
#endif // TENSORFLOW_COMPILER_XLA_EXECUTABLE_RUN_OPTIONS_H_
| {
"pile_set_name": "Github"
} |
# Console development
- [Introduction](#introduction)
- [Building a command](#building-a-command)
- [Defining arguments](#defining-arguments)
- [Defining options](#defining-options)
- [Writing output](#writing-output)
- [Retrieving input](#retrieving-input)
- [Registering commands](#registering-commands)
- [Calling other commands](#calling-other-commands)
<a name="introduction"></a>
## Introduction
In addition to the provided console commands, you may also build your own custom commands for working with your application. You may store your custom commands within the plugin **console** directory. You can generate the class file using the [command line scaffolding tool](../console/scaffolding#scaffold-create-command).
<a name="building-a-command"></a>
## Building a command
If you wanted to create a console command called `acme:mycommand`, you might create the associated class for that command in a file called **plugins/acme/blog/console/MyCommand.php** and paste the following contents to get started:
<?php namespace Acme\Blog\Console;
use Illuminate\Console\Command;
use Symfony\Component\Console\Input\InputOption;
use Symfony\Component\Console\Input\InputArgument;
class MyCommand extends Command
{
/**
* @var string The console command name.
*/
protected $name = 'acme:mycommand';
/**
* @var string The console command description.
*/
protected $description = 'Does something cool.';
/**
* Execute the console command.
* @return void
*/
public function handle()
{
$this->output->writeln('Hello world!');
}
/**
* Get the console command arguments.
* @return array
*/
protected function getArguments()
{
return [];
}
/**
* Get the console command options.
* @return array
*/
protected function getOptions()
{
return [];
}
}
Once your class is created you should fill out the `name` and `description` properties of the class, which will be used when displaying your command on the command `list` screen.
The `handle` method will be called when your command is executed. You may place any command logic in this method.
<a name="defining-arguments"></a>
### Defining arguments
Arguments are defined by returning an array value from the `getArguments` method are where you may define any arguments your command receives. For example:
/**
* Get the console command arguments.
* @return array
*/
protected function getArguments()
{
return [
['example', InputArgument::REQUIRED, 'An example argument.'],
];
}
When defining `arguments`, the array definition values represent the following:
array($name, $mode, $description, $defaultValue)
The argument `mode` may be any of the following: `InputArgument::REQUIRED` or `InputArgument::OPTIONAL`.
<a name="defining-options"></a>
### Defining options
Options are defined by returning an array value from the `getOptions` method. Like arguments this method should return an array of commands, which are described by a list of array options. For example:
/**
* Get the console command options.
* @return array
*/
protected function getOptions()
{
return [
['example', null, InputOption::VALUE_OPTIONAL, 'An example option.', null],
];
}
When defining `options`, the array definition values represent the following:
array($name, $shortcut, $mode, $description, $defaultValue)
For options, the argument `mode` may be: `InputOption::VALUE_REQUIRED`, `InputOption::VALUE_OPTIONAL`, `InputOption::VALUE_IS_ARRAY`, `InputOption::VALUE_NONE`.
The `VALUE_IS_ARRAY` mode indicates that the switch may be used multiple times when calling the command:
php artisan foo --option=bar --option=baz
The `VALUE_NONE` option indicates that the option is simply used as a "switch":
php artisan foo --option
<a name="retrieving-input"></a>
### Retrieving input
While your command is executing, you will obviously need to access the values for the arguments and options accepted by your application. To do so, you may use the `argument` and `option` methods:
#### Retrieving the value of a command argument
$value = $this->argument('name');
#### Retrieving all arguments
$arguments = $this->argument();
#### Retrieving the value of a command option
$value = $this->option('name');
#### Retrieving all options
$options = $this->option();
<a name="writing-output"></a>
### Writing output
To send output to the console, you may use the `info`, `comment`, `question` and `error` methods. Each of these methods will use the appropriate ANSI colors for their purpose.
#### Sending information
$this->info('Display this on the screen');
#### Sending an error message
$this->error('Something went wrong!');
#### Asking the user for input
You may also use the `ask` and `confirm` methods to prompt the user for input:
$name = $this->ask('What is your name?');
#### Asking the user for secret input
$password = $this->secret('What is the password?');
#### Asking the user for confirmation
if ($this->confirm('Do you wish to continue? [yes|no]'))
{
//
}
You may also specify a default value to the `confirm` method, which should be `true` or `false`:
$this->confirm($question, true);
#### Progress Bars
For long running tasks, it could be helpful to show a progress indicator. Using the output object, we can start, advance and stop the Progress Bar. First, define the total number of steps the process will iterate through. Then, advance the Progress Bar after processing each item:
$users = App\User::all();
$bar = $this->output->createProgressBar(count($users));
foreach ($users as $user) {
$this->performTask($user);
$bar->advance();
}
$bar->finish();
For more advanced options, check out the [Symfony Progress Bar component documentation](https://symfony.com/doc/2.7/components/console/helpers/progressbar.html).
<a name="registering-commands"></a>
## Registering commands
#### Registering a console command
Once your command class is finished, you need to register it so it will be available for use. This is typically done in the `register` method of a [Plugin registration file](../plugin/registration#registration-methods) using the `registerConsoleCommand` helper method.
class Blog extends PluginBase
{
public function pluginDetails()
{
[...]
}
public function register()
{
$this->registerConsoleCommand('acme.mycommand', 'Acme\Blog\Console\MyConsoleCommand');
}
}
Alternatively, plugins can supply a file named **init.php** in the plugin directory that you can use to place command registration logic. Within this file, you may use the `Artisan::add` method to register the command:
Artisan::add(new Acme\Blog\Console\MyCommand);
#### Registering a command in the application container
If your command is registered in the [application container](../services/application#app-container), you may use the `Artisan::resolve` method to make it available to Artisan:
Artisan::resolve('binding.name');
#### Registering commands in a service provider
If you need to register commands from within a [service provider](application#service-providers), you should call the `commands` method from the provider's `boot` method, passing the [container](application#app-container) binding for the command:
public function boot()
{
$this->app->singleton('acme.mycommand', function() {
return new \Acme\Blog\Console\MyConsoleCommand;
});
$this->commands('acme.mycommand');
}
<a name="calling-other-commands"></a>
## Calling other commands
Sometimes you may wish to call other commands from your command. You may do so using the `call` method:
$this->call('october:up');
You can also pass arguments as an array:
$this->call('plugin:refresh', ['name' => 'October.Demo']);
As well as options:
$this->call('october:update', ['--force' => true]);
| {
"pile_set_name": "Github"
} |
/*************************************************************
*
* MathJax/jax/output/SVG/fonts/TeX/svg/Typewriter/Regular/BasicLatin.js
*
* Copyright (c) 2011-2017 The MathJax Consortium
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
MathJax.Hub.Insert(
MathJax.OutputJax.SVG.FONTDATA.FONTS['MathJax_Typewriter'],
{
// SPACE
0x20: [0,0,250,0,0,''],
// EXCLAMATION MARK
0x21: [622,0,525,206,320,'206 565Q206 590 222 606T265 622Q287 621 303 606T319 565T314 392L308 216Q299 194 273 194H262Q247 194 241 195T228 200T217 216L211 392Q206 539 206 565ZM206 56Q206 83 223 99T265 115Q288 113 304 99T320 58Q320 33 303 17T262 0Q237 0 222 17T206 56'],
// QUOTATION MARK
0x22: [623,-333,525,122,402,'122 575Q122 593 137 608T173 623Q196 623 210 608T225 575Q225 562 218 464Q212 373 211 361T201 341Q193 333 173 333Q154 333 146 341Q138 348 137 360T129 464Q122 561 122 575ZM299 575Q299 593 314 608T350 623Q373 623 387 608T402 575Q402 562 395 464Q389 373 388 361T378 341Q370 333 350 333Q331 333 323 341Q315 348 314 360T306 464Q299 561 299 575'],
// NUMBER SIGN
0x23: [612,0,525,36,489,'93 163Q74 163 65 164T46 173T36 198Q36 210 40 215T61 233H131V236Q132 239 140 307T149 377Q149 379 105 379L61 380Q36 392 36 414Q36 450 86 450Q91 450 99 450T112 449H159Q163 480 167 517Q168 524 170 545T174 573T180 591T191 607T210 611Q223 611 232 604T243 588L245 580Q245 565 238 511T230 451Q230 449 282 449H333Q337 480 341 517Q342 524 343 537T345 556T348 573T352 589T359 600T370 608T384 611Q395 611 406 602T419 580Q419 565 412 511T404 451Q404 449 431 449H442Q477 449 485 429Q489 421 489 414Q489 392 463 380L428 379H394V376Q393 373 385 305T376 235Q376 233 419 233H463L468 230Q472 227 473 227T477 223T482 218T486 213T488 206T489 198Q489 162 436 162Q430 162 422 162T412 163H366V161Q364 159 357 92Q356 85 355 73T353 54T350 37T346 22T339 11T328 3T314 0Q303 0 292 9T279 31Q279 37 287 96T295 162Q295 163 244 163H192V161Q190 159 183 92Q182 85 181 73T179 54T176 37T172 22T165 11T154 3T140 0Q129 0 118 9T105 31Q105 37 113 96T121 162Q121 163 93 163ZM323 377Q323 379 272 379H220V376Q219 373 211 305T202 235Q202 233 253 233H305V236Q306 239 314 307T323 377'],
// DOLLAR SIGN
0x24: [694,82,525,58,466,'415 397Q392 397 377 411T362 448Q362 464 376 485Q369 498 362 506T346 520T332 528T315 533T300 538V445L301 353L311 350Q382 334 424 284T466 174Q466 115 425 65T303 -2L300 -3V-30Q300 -64 291 -74Q283 -82 262 -82H255Q234 -82 225 -60L224 -32V-4L213 -2Q152 6 106 51T59 170V180Q59 197 74 213Q89 227 110 227T146 213T162 174Q162 156 147 137Q153 123 161 112T176 95T191 85T205 79T216 76T224 74V283L213 285Q147 298 103 343T58 449Q58 516 108 560T224 614V643V654Q224 666 226 673T237 687T264 694Q289 693 294 683T300 642V615H303Q355 607 390 587T440 540T460 493T466 453Q466 425 451 411T415 397ZM137 452Q137 425 158 404T198 376T223 369Q224 369 224 453T223 537Q198 532 168 509T137 452ZM301 75Q307 75 325 83T365 116T387 171Q387 238 300 267V171Q300 75 301 75'],
// PERCENT SIGN
0x25: [694,83,525,35,489,'35 560Q35 607 54 645T110 693Q111 693 116 693T125 694Q165 692 187 651T210 560Q210 506 186 467T123 428Q84 428 60 466T35 560ZM139 560Q139 574 136 587T130 608T124 615Q122 617 120 614Q106 595 106 561Q106 516 121 506Q123 504 125 507Q139 526 139 560ZM123 -83Q107 -83 98 -73T88 -48Q88 -43 89 -41Q90 -37 229 316T370 675Q381 694 400 694Q416 694 426 684T436 659Q436 654 435 652Q434 647 295 294T153 -65Q144 -83 123 -83ZM314 50Q314 104 338 143T400 183Q439 183 464 144T489 50T465 -43T402 -82Q358 -82 336 -41T314 50ZM417 50Q417 71 413 85T405 102L401 106Q386 95 386 50Q386 29 390 15T398 -2L402 -6Q417 5 417 50'],
// AMPERSAND
0x26: [622,11,525,28,490,'96 462Q96 546 132 584T211 622Q255 622 284 583T314 474Q314 395 224 305L208 288Q213 275 226 251L265 185L269 179Q273 184 299 246L332 333L342 363Q342 364 341 365Q334 365 334 393Q334 406 334 410T340 420T356 431H412H440Q467 431 478 424T490 393Q490 376 484 367T470 357T448 355H441H415L399 312Q349 176 322 127L315 115L323 106Q360 65 393 65Q405 65 410 80T416 109Q416 140 452 140Q487 140 487 105Q487 56 460 23T391 -11L286 41L273 53L262 42Q212 -11 151 -11Q97 -11 63 33T28 143Q28 161 30 176T38 205T47 227T60 247T72 261T84 274T94 283L122 311L119 323Q96 392 96 462ZM243 474Q243 533 218 545L215 546Q212 546 210 546Q182 546 169 501Q167 492 167 466Q167 419 179 368L188 377Q234 425 242 461Q243 465 243 474ZM217 129Q185 174 154 235Q121 214 115 176Q113 168 113 143Q113 83 139 67Q141 66 152 66Q191 66 228 112L217 129'],
// APOSTROPHE
0x27: [611,-287,525,175,349,'205 554Q205 577 221 594T263 611Q302 611 325 577T349 490Q349 409 298 347Q285 330 258 309T214 287Q203 289 189 302T175 327Q175 341 185 349T213 369T245 402Q269 437 273 483V497Q264 496 263 496Q240 496 223 513T205 554'],
// LEFT PARENTHESIS
0x28: [694,82,525,166,437,'437 -53Q437 -82 399 -82H394Q377 -82 342 -55Q259 7 213 102T166 306Q166 412 211 507T342 667Q377 694 393 694H399Q437 694 437 665Q437 654 426 643T397 620T356 584T311 525Q301 511 290 488T264 412T250 306Q250 191 300 105T422 -27Q437 -37 437 -53'],
// RIGHT PARENTHESIS
0x29: [694,82,525,87,358,'87 664Q87 694 126 694Q138 694 147 690T183 667Q266 605 312 510T358 306Q358 193 307 93T161 -70Q142 -82 126 -82Q105 -82 96 -73T87 -53Q87 -47 88 -44Q92 -36 116 -19T173 34T230 119Q273 206 273 306Q273 408 231 494T109 635Q87 649 87 664'],
// ASTERISK
0x2A: [520,-89,525,68,456,'222 487Q224 501 235 510T262 520Q279 520 289 510T302 487Q302 458 301 429Q301 421 301 413T301 398T300 386T300 377V374Q300 373 301 373Q304 373 353 403T416 434Q432 434 444 423T456 393Q456 389 456 386T454 379T451 373T448 368T442 363T436 358T427 353T417 348T405 342T391 334Q345 309 339 305L388 279Q400 273 412 266T432 255T441 250Q456 238 456 218Q456 200 445 189T417 177Q403 177 354 207T301 238Q300 238 300 237V234Q300 231 300 226T300 214T301 199T301 182Q302 153 302 124Q300 109 289 100T262 90T235 100T222 124Q222 153 223 182Q223 190 223 198T223 213T224 225T224 234V237Q224 238 223 238Q220 238 171 208T108 177Q92 177 80 188T68 218Q68 237 79 246T134 277Q180 303 185 306L136 332Q124 338 112 345T92 356T83 361Q68 373 68 393Q68 411 79 422T107 434Q121 434 170 404T223 373Q224 373 224 374V377Q224 380 224 385T224 397T223 412T223 429Q222 458 222 487'],
// PLUS SIGN
0x2B: [531,-81,525,38,487,'147 271Q138 271 122 271T98 270Q68 270 53 277T38 306T53 335T98 342Q105 342 121 342T147 341H227V423L228 505Q241 531 262 531Q268 531 273 530T282 525T287 519T293 511L297 505V341H377H430Q457 341 467 338T483 321Q487 313 487 306Q487 295 480 286T463 273Q457 271 377 271H297V107Q281 81 262 81Q250 81 242 87T230 100L228 107L227 189V271H147'],
// COMMA
0x2C: [140,139,525,173,353,'193 37T193 70T213 121T260 140Q302 140 327 108T353 36Q353 -7 336 -43T294 -98T249 -128T215 -139Q204 -139 189 -125Q177 -111 174 -101Q172 -84 183 -77T217 -61T253 -33Q261 -24 272 1L265 0Q234 0 214 18'],
// HYPHEN-MINUS
0x2D: [341,-271,525,57,468,'57 306Q57 333 86 341H438Q468 332 468 306T438 271H86Q57 280 57 306'],
// FULL STOP
0x2E: [140,-1,525,193,332,'193 70Q193 105 214 122T258 140Q291 140 311 120T332 70Q332 44 314 23T262 1Q234 1 214 18T193 70'],
// SOLIDUS
0x2F: [694,83,525,58,466,'94 -83Q78 -83 68 -73T58 -48Q58 -44 60 -36Q62 -31 227 314T399 673Q410 694 431 694Q445 694 455 684T466 659Q466 656 464 648Q463 643 298 298T125 -62Q114 -83 94 -83'],
// DIGIT ZERO
0x30: [621,10,525,42,482,'42 305Q42 450 111 535T257 621Q335 621 390 562Q482 468 482 306Q482 174 418 82T262 -10T106 82T42 305ZM257 545Q209 545 168 481T126 320Q126 220 162 147Q204 65 262 65Q318 65 358 139T398 320V328Q395 411 364 470T284 543Q270 545 257 545'],
// DIGIT ONE
0x31: [622,-1,525,99,450,'99 461Q99 470 99 474T104 487T120 498T151 502Q213 517 251 596Q264 622 283 622Q308 622 319 597V76H373H401Q428 76 439 69T450 38Q450 11 428 1H127Q104 10 104 38Q104 62 115 69T153 76H181H235V269Q235 461 234 461Q184 426 137 424H133Q124 424 119 425T109 431T99 447V461'],
// DIGIT TWO
0x32: [622,-1,525,52,472,'52 462Q52 528 110 575T247 622H250Q343 622 407 565T472 421Q472 371 446 324T390 248T308 178Q307 177 275 151T214 101L185 77Q185 76 286 76H388V87Q388 105 397 114T430 123T463 114Q470 107 471 100T472 61V42Q472 24 468 16T450 1H75Q53 10 53 32V38V48Q53 57 63 67T127 122Q153 144 169 157L289 256Q388 345 388 419Q388 473 346 509T231 545H224Q176 545 146 499L144 494Q155 476 155 459Q154 459 155 455T154 444T148 430T136 417T114 408Q113 408 110 408T104 407Q80 407 66 422T52 462'],
// DIGIT THREE
0x33: [622,11,525,44,479,'260 546Q233 546 211 541T180 531T171 524L174 514Q177 505 177 497Q177 476 162 461T125 446Q106 446 90 459T73 504Q76 540 98 565T150 601T203 616T239 621Q241 622 265 622Q322 620 362 602T420 558T444 513T451 478Q451 386 369 329L375 326Q381 323 386 320T401 311T419 298T436 283T452 263T466 240T475 212T479 180Q479 99 416 44T259 -11T105 28T44 130Q44 154 59 168T95 183Q117 183 132 169T148 131Q148 119 139 101Q175 65 260 65Q316 65 355 97T395 179Q395 211 375 240Q336 292 253 292H234H215Q194 292 185 299T175 330Q175 350 184 359Q192 368 238 370T309 384Q336 398 351 423T367 474Q367 496 350 513Q321 546 260 546'],
// DIGIT FOUR
0x34: [623,-1,525,29,495,'235 1Q213 10 213 32V38V46Q213 65 230 73Q236 76 274 76H314V168H183L52 169Q37 175 33 182T29 205V218L30 244Q53 283 155 443T264 613Q276 623 298 623H323H363Q378 616 385 601V244H429H450Q474 244 484 237T495 206Q495 179 477 171Q471 168 429 168H385V76H425H442Q466 76 476 69T487 38Q487 10 465 1H235ZM314 244V554L117 245L215 244H314'],
// DIGIT FIVE
0x35: [612,10,525,52,472,'387 189Q387 244 354 278T273 313Q230 313 205 301T163 271T138 249H120Q102 249 97 251Q85 258 83 266T80 311Q80 320 80 359T81 430Q81 587 82 591Q88 605 103 610H108Q112 610 120 610T138 610T163 610T192 611T225 611T260 611H415Q416 610 421 607T428 602T432 596T436 587T437 573Q437 567 437 562T434 554T431 548T427 543T423 540T418 538L415 536L289 535H164V363L170 366Q175 368 184 372T207 380T238 386T276 389Q357 389 414 331T472 187Q472 116 412 53T245 -10Q218 -10 209 -9Q126 5 89 48T52 137Q52 164 68 177T104 191Q130 191 143 175T156 141Q156 132 154 125T149 113T146 107Q146 104 155 95T188 76T245 65Q298 65 342 98T387 189'],
// DIGIT SIX
0x36: [622,11,525,44,479,'357 536Q357 546 318 546Q258 546 205 497T133 357V353L144 361Q210 402 285 402Q362 402 414 350Q479 285 479 193Q479 111 418 50T263 -11Q234 -11 207 -3T149 26T97 81T60 171T45 301Q45 444 129 533T319 622Q388 622 421 589T454 510Q454 491 442 475T402 458Q373 458 362 475T350 510Q350 520 354 528L357 536ZM319 326T269 326T179 298T136 223Q136 202 143 174T176 112T237 68Q246 66 265 66Q319 66 360 107Q395 146 395 197Q395 250 356 289Q319 326 269 326'],
// DIGIT SEVEN
0x37: [627,10,525,44,480,'204 -10Q162 -10 162 40Q162 146 198 261T310 477Q311 478 321 491T342 517T358 535H128V524Q128 506 119 497Q111 489 86 489H78Q55 489 46 508Q44 513 44 557V580Q44 605 52 616T88 627H93Q114 627 125 611H458Q474 598 477 593T480 573Q480 559 478 553T469 543T446 521T408 477Q252 290 246 49Q246 43 246 37T246 27T245 22Q243 11 233 1T204 -10'],
// DIGIT EIGHT
0x38: [621,10,525,45,480,'58 460Q58 523 117 572T254 621Q290 621 298 620Q376 607 421 560T466 460Q466 441 460 424T443 393T421 370T397 352T374 340T357 332L350 330L356 328Q363 325 371 321T392 310T415 295T439 274T459 249T473 217T479 179Q479 102 418 46T262 -10T106 46T45 179Q45 202 52 222T70 257T96 284T123 305T148 319T167 328L174 330L170 332Q166 333 159 336T145 343Q104 362 81 393T58 460ZM382 458Q382 491 349 518T263 546Q215 546 179 521T142 458Q142 421 178 395T262 368Q315 368 348 396T382 458ZM396 178Q396 223 358 257T263 292Q206 292 167 258T128 178Q128 137 163 102T262 66Q324 66 360 101T396 178'],
// DIGIT NINE
0x39: [622,11,525,46,479,'392 259Q333 210 236 210H233Q163 210 109 262Q46 325 46 411T99 550Q164 622 264 622Q293 622 319 615T376 587T428 532T464 440T479 304Q479 167 400 78T217 -11Q140 -11 105 22T70 101Q70 124 84 138T122 153Q150 153 162 137T174 101Q174 91 168 76Q179 65 216 65Q267 65 300 93Q322 109 339 130T366 173T380 210T388 242T392 259ZM388 389Q388 438 357 492T268 546T185 520Q129 479 129 415Q129 384 138 363Q145 349 156 334T195 302T255 285Q305 285 345 313T388 389'],
// COLON
0x3A: [431,-1,525,193,332,'193 361Q193 396 214 413T258 431Q291 431 311 411T332 361Q332 335 314 314T262 292Q234 292 214 309T193 361ZM193 70Q193 105 214 122T258 140Q291 140 311 120T332 70Q332 44 314 23T262 1Q234 1 214 18T193 70'],
// SEMICOLON
0x3B: [431,139,525,175,337,'193 361Q193 396 214 413T258 431Q291 431 311 411T332 361Q332 335 314 314T262 292Q234 292 214 309T193 361ZM193 70Q193 105 214 122T259 140Q301 140 319 108T337 33Q337 -38 291 -88T214 -139Q203 -139 189 -126T175 -97Q175 -85 182 -78T200 -66T225 -50T249 -17Q256 -3 256 0Q252 1 248 1Q242 2 235 5T218 15T200 36T193 70'],
// LESS-THAN SIGN
0x3C: [557,-55,525,57,469,'468 90Q468 76 458 66T433 55Q426 55 419 58Q413 61 243 168T68 280Q57 291 57 306T68 332Q72 335 241 442T416 553Q424 557 432 557Q447 557 457 547T468 522T456 496Q454 494 305 399L158 306L305 213Q341 190 390 159Q443 125 452 119T464 106V105Q468 97 468 90'],
// EQUALS SIGN
0x3D: [417,-195,525,38,487,'38 382Q38 409 67 417H457Q487 408 487 382Q487 358 461 348H64Q51 352 45 360T38 376V382ZM67 195Q38 204 38 230Q38 255 62 264Q66 265 264 265H461L464 264Q467 262 469 261T475 256T481 249T485 240T487 230Q487 204 457 195H67'],
// GREATER-THAN SIGN
0x3E: [557,-55,525,57,468,'57 522Q57 539 67 548T90 557Q98 557 105 554Q111 551 281 444T456 332Q468 320 468 306T456 280Q452 276 282 169T105 58Q98 55 91 55Q79 55 68 63T57 90Q57 105 68 116Q70 118 219 213L366 306L219 399Q75 491 71 494Q57 507 57 522'],
// QUESTION MARK
0x3F: [617,1,525,62,462,'62 493Q62 540 107 578T253 617Q366 617 414 578T462 490Q462 459 445 434T411 400L394 390Q315 347 296 287Q294 278 293 247V217Q285 201 278 198T246 194T216 197T201 215V245V253Q201 379 351 456Q366 464 375 477Q377 482 377 490Q377 517 339 528T251 540Q182 540 159 517Q166 503 166 490Q166 468 151 453T114 438Q96 438 79 451T62 493ZM190 58Q190 85 208 100T249 115Q272 113 288 99T304 58Q304 33 287 17T246 0T206 16T190 58'],
// COMMERCIAL AT
0x40: [617,6,525,44,481,'44 306Q44 445 125 531T302 617Q332 617 358 607T411 574T456 502T479 387Q481 361 481 321Q481 203 421 143Q381 103 332 103Q266 103 225 165T183 307Q183 390 227 449T332 508Q358 508 378 498Q350 541 304 541Q229 541 172 473T115 305Q115 208 171 140T306 71H310Q358 71 397 105Q409 115 436 115Q458 115 462 113Q481 106 481 86Q481 73 468 61Q401 -6 305 -6Q262 -6 217 14T133 71T69 170T44 306ZM410 306Q410 361 386 396T333 431Q300 431 277 394T254 305Q254 256 276 218T332 180Q364 180 387 217T410 306'],
// LATIN CAPITAL LETTER A
0x41: [623,-1,525,28,496,'191 76Q212 75 220 68T229 38Q229 10 208 1H129H80Q48 1 38 7T28 38Q28 51 29 57T40 69T70 76Q89 76 89 78Q90 79 117 205T173 461T205 599Q212 623 250 623H262H273Q312 623 319 599Q322 591 350 461T406 205T435 78Q435 76 454 76H458Q484 76 493 59Q496 53 496 38Q496 11 478 3Q474 1 395 1H317Q295 8 295 38Q295 65 311 73Q316 75 333 76L348 77V78Q348 80 341 112L334 143H190L183 112Q176 80 176 78Q175 76 178 76Q180 76 191 76ZM318 221Q313 238 288 366T263 519Q263 526 262 527Q261 527 261 520Q261 493 236 365T206 221Q206 219 262 219T318 221'],
// LATIN CAPITAL LETTER B
0x42: [611,-1,525,17,482,'39 1Q17 10 17 32V38V46Q17 65 34 73Q40 76 61 76H84V535H61H54Q27 535 19 553Q17 557 17 573Q17 583 17 587T23 599T39 610Q40 611 179 611Q320 610 332 607Q332 607 339 605Q394 591 427 547T461 454Q461 413 436 378T369 325L358 320Q405 311 443 270T482 169Q482 112 445 64T345 3L334 1H39ZM309 533Q302 535 234 535H168V356H230Q284 357 296 358T323 368Q346 380 361 402T377 452Q377 482 358 505T309 533ZM398 176Q396 218 371 246T315 279Q310 280 237 280H168V76H239Q316 77 327 81Q329 82 334 84Q398 107 398 176'],
// LATIN CAPITAL LETTER C
0x43: [622,11,525,40,485,'40 305Q40 437 110 529T281 622Q315 622 343 611T387 589T404 578Q409 585 415 596T425 611T435 618T452 622Q472 622 478 609T485 566Q485 559 485 540T484 508V460Q484 413 478 403T442 393Q417 393 409 402Q400 409 400 420Q400 428 395 445T380 487T347 528T295 546Q235 546 180 483T124 306Q124 245 141 197T186 121T241 80T296 66Q346 66 373 103T400 178Q400 209 435 209H442H450Q484 209 484 172Q480 96 421 43T281 -11Q177 -11 109 84T40 305'],
// LATIN CAPITAL LETTER D
0x44: [612,-1,525,16,485,'38 1Q16 8 16 38Q16 62 32 73Q39 76 58 76H78V535H58Q40 535 32 538Q16 548 16 573Q16 587 17 591Q23 604 34 607T83 611H166H176Q188 611 209 611T239 612Q299 612 337 597T415 530Q485 438 485 300Q485 180 431 100T301 3L291 1H38ZM400 301Q400 363 385 410T346 482T303 519T267 534Q261 535 210 535H162V76H214L267 77Q323 89 361 148T400 301'],
// LATIN CAPITAL LETTER E
0x45: [612,-1,525,18,502,'374 271Q374 241 367 232T332 223Q307 223 299 231Q290 240 290 263V279H173V76H418V118V144Q418 167 426 176T460 186Q491 186 500 166Q502 161 502 93V52Q502 25 499 17T480 1H41Q19 9 19 32V38Q19 63 36 73Q42 76 65 76H89V535H65H55Q44 535 38 537T25 548T19 573Q19 602 41 610H47Q53 610 63 610T88 610T121 610T160 611T204 611T251 611H458Q460 609 465 606T471 602T475 598T478 593T479 586T480 576T480 562V526V488Q480 452 462 444Q458 442 438 442Q413 442 405 450Q398 457 397 463T396 501V535H173V355H290V371Q290 394 299 403T332 412Q363 412 372 392Q374 387 374 317V271'],
// LATIN CAPITAL LETTER F
0x46: [612,-1,525,22,490,'384 260Q384 230 377 221T342 212Q317 212 309 220Q300 229 300 252V268H179V76H249Q264 67 267 61T271 38Q271 10 249 1H44Q22 9 22 32V38Q22 63 39 73Q45 76 69 76H95V535H69H59Q42 535 32 542T22 573Q22 602 44 610H50Q56 610 66 610T91 610T125 610T164 611T208 611T257 611H468Q470 609 475 606T481 602T485 598T488 593T489 586T490 576T490 562V526V488Q490 452 472 444Q468 442 448 442Q423 442 415 450Q408 457 407 463T406 501V535H179V344H300V360Q300 383 309 392T342 401Q373 401 382 381Q384 376 384 306V260'],
// LATIN CAPITAL LETTER G
0x47: [623,11,525,38,496,'38 306Q38 447 105 534T261 622Q280 622 298 618T329 608T350 596T366 585L371 581Q373 581 377 591T390 612T417 622Q437 622 443 609T450 566Q450 559 450 540T449 508V460Q449 413 443 403T407 393Q392 393 386 394T373 402T364 426Q360 472 335 509T271 546Q214 546 168 477T121 308Q121 210 164 138T271 65Q293 65 310 78T337 109T352 147T360 180T362 195Q362 196 333 196L304 197Q282 204 282 227V234Q282 247 282 251T288 261T304 272H474Q488 263 492 256T496 234Q496 211 479 199Q475 197 461 196H449V21Q441 6 434 3T412 -1H407H402Q385 -1 379 3T364 28Q350 14 322 2T260 -11Q173 -11 106 76T38 306'],
// LATIN CAPITAL LETTER H
0x48: [611,-1,525,16,508,'16 571Q16 597 27 604T74 611H125H208Q223 602 226 596T230 573Q230 559 227 551T217 540T204 536T186 535H165V356H359V535H338H333Q306 535 297 552Q295 556 295 573Q295 586 295 590T301 600T317 611H486Q501 602 504 596T508 573Q508 559 505 551T495 540T482 536T464 535H443V76H464H470Q482 76 489 75T502 64T508 38Q508 10 486 1H317Q306 5 301 11T296 21T295 38V44Q295 66 311 73Q318 76 338 76H359V280H165V76H186H192Q204 76 211 75T224 64T230 38Q230 10 208 1H39Q28 5 23 11T18 21T17 38V44Q17 66 33 73Q40 76 60 76H81V535H60Q45 535 38 536T24 545T16 571'],
// LATIN CAPITAL LETTER I
0x49: [611,-1,525,72,452,'400 76Q431 76 441 69T452 38Q452 29 452 26T450 18T443 9T430 1H95Q84 6 79 12T73 23T72 38Q72 65 90 73Q96 76 157 76H220V535H157H124Q93 535 83 542T72 573Q72 603 93 610Q97 611 264 611H430Q432 609 436 607T444 602T449 594Q452 588 452 573Q452 546 434 538Q428 535 367 535H304V76H367H400'],
// LATIN CAPITAL LETTER J
0x4A: [612,11,525,57,479,'202 543T202 573T224 610H228Q231 610 237 610T251 610T269 610T291 611T315 611T342 611H457Q471 602 475 595T479 573Q479 549 462 538Q454 535 432 535H408V328Q408 159 408 133T402 93Q386 48 340 19T229 -11Q158 -11 108 16T57 100Q57 129 73 141T108 154Q128 154 143 140T159 102Q159 93 155 79Q188 65 228 65H230Q290 65 318 106Q323 115 323 139T324 329V535H274L224 536Q202 543 202 573'],
// LATIN CAPITAL LETTER K
0x4B: [611,-1,525,18,495,'18 549T18 573T29 604T70 611H118H193Q207 603 210 596T214 573Q214 549 198 538Q191 535 172 535H152V421Q152 344 152 326T153 309L242 422L329 534Q327 535 322 536T314 538T308 542T303 548T300 558T298 573Q298 600 316 608Q322 611 392 611H463Q477 602 481 595T485 573Q485 535 446 535H441H420L281 357L436 77L454 76Q473 75 478 73Q495 62 495 38Q495 10 473 1H345Q334 5 329 11T324 21T323 38Q323 51 324 56T332 68T355 77L233 296L152 192V76H172Q191 76 198 73Q214 63 214 38Q214 9 193 1H41Q18 8 18 38Q18 61 35 73Q42 76 61 76H81V535H61Q42 535 35 538Q18 549 18 573'],
// LATIN CAPITAL LETTER L
0x4C: [611,0,525,25,488,'27 594Q34 605 43 608T84 611H154H213Q258 611 269 605T281 573Q281 546 263 538Q257 535 222 535H185V76H404V118V145Q404 168 411 177T446 186H453Q478 186 486 167Q488 161 488 93V50Q488 24 485 17T466 1L258 0H147H99Q47 0 36 6T25 38Q25 59 35 69Q44 76 76 76H101V535H76H64Q36 535 27 552Q25 557 25 573T27 594'],
// LATIN CAPITAL LETTER M
0x4D: [611,-1,525,11,512,'50 535Q37 536 31 537T18 547T12 573Q12 598 22 604T62 611H91H121Q147 611 158 607T178 587Q183 579 222 446T261 293Q261 289 262 288Q263 288 263 292Q263 311 298 434T346 588Q353 603 365 607T402 611H435H450Q488 611 500 605T512 573Q512 556 506 547T493 537T474 535H459V76H474Q487 75 493 74T505 64T512 38Q512 11 494 3Q490 1 424 1H386Q355 1 345 7T335 38Q335 55 341 64T354 74T373 76H388V302Q388 512 387 519Q382 482 346 359T304 228Q292 204 262 204T220 228Q215 237 179 359T137 519Q136 512 136 302V76H151Q164 75 170 74T182 64T189 38Q189 11 171 3Q167 1 101 1H63Q32 1 22 7T12 38Q12 55 18 64T31 74T50 76H65V535H50'],
// LATIN CAPITAL LETTER N
0x4E: [611,0,525,20,504,'20 571Q20 598 30 604T73 611H105H136Q152 611 160 611T177 607T189 601T198 587T206 568T217 537T231 497Q354 142 365 95L368 84V535H347H342Q314 535 306 552Q304 556 304 573Q304 586 304 590T310 600T326 611H482Q497 602 500 596T504 573Q504 559 501 551T491 540T478 536T460 535H439V25Q432 7 424 4T389 0H374Q334 0 322 31L293 115Q171 468 159 517L156 528V76H177H183Q195 76 202 75T215 64T221 38Q221 10 199 1H43Q32 5 27 11T22 21T21 38V44Q21 66 37 73Q44 76 64 76H85V535H64Q49 535 42 536T28 545T20 571'],
// LATIN CAPITAL LETTER O
0x4F: [621,10,525,56,468,'102 588Q140 621 240 621Q323 621 335 620Q393 613 422 588Q450 560 459 493T468 306Q468 185 460 118T422 23Q382 -10 289 -10H262H235Q142 -10 102 23Q74 50 65 118T56 306Q56 427 64 494T102 588ZM363 513Q357 523 347 530T324 540T302 544T280 546H268Q192 546 167 521Q150 501 145 452T140 300Q140 235 142 197T151 130T172 89T207 71T262 65Q317 65 341 81T374 144T384 300Q384 474 363 513'],
// LATIN CAPITAL LETTER P
0x50: [612,-1,525,19,480,'41 1Q19 9 19 32V38Q19 63 36 73Q42 76 65 76H89V535H65H55Q38 535 29 543T19 576Q19 603 41 610H49Q57 610 70 610T100 610T136 611T175 611Q190 611 216 611T255 612Q321 612 363 598T441 537Q480 486 480 427V421Q480 354 447 311T378 251Q339 230 275 230H239H173V76H197Q220 76 227 73Q244 62 244 38Q244 10 222 1H41ZM396 421Q396 461 369 491T300 533Q294 534 233 535H173V306H233Q294 307 300 308Q345 319 370 352T396 421'],
// LATIN CAPITAL LETTER Q
0x51: [622,138,525,56,468,'56 306Q56 380 58 426T68 510T87 568T120 600T170 617T240 621Q323 621 335 620Q393 613 422 588Q450 560 459 493T468 306Q468 124 447 66Q433 23 394 6L424 -53Q454 -112 454 -118Q454 -128 441 -138H377Q367 -135 363 -129T333 -69L304 -11H254Q205 -10 180 -8T128 6T91 36T70 92T58 178T56 306ZM227 151Q227 171 262 171H276H281Q292 171 296 171T305 170T313 165T317 158T323 145T332 127L353 88Q356 88 361 95T372 131T382 202Q384 228 384 306Q384 452 371 492T304 544Q296 545 251 545Q230 545 215 543T188 534T169 520T155 497T147 466T143 423T141 371T140 306Q140 248 141 217T146 154T157 109T178 83T212 68T262 65H266L264 70Q261 75 256 85T247 105Q227 145 227 151'],
// LATIN CAPITAL LETTER R
0x52: [612,11,525,16,522,'16 571Q16 598 27 605T76 612Q84 612 108 612T148 611Q268 611 294 605Q346 592 389 550T432 440Q432 394 410 359Q393 329 366 310L358 303Q387 273 399 239Q405 219 405 178T408 106T421 68Q426 65 428 65Q433 65 435 74T438 96T441 112Q450 130 480 130H485Q519 130 522 100Q522 79 516 56T488 11T434 -11Q421 -11 408 -8T377 5T344 37T324 93Q322 101 322 154L321 209Q304 257 257 267Q252 268 207 268H165V76H186H192Q204 76 211 75T224 64T230 38Q230 10 208 1H39Q28 5 23 11T18 21T17 38V44Q17 66 33 73Q40 76 60 76H81V535H60Q45 535 38 536T24 545T16 571ZM348 440Q348 478 321 502T260 532Q252 534 208 535H165V344H208Q212 344 223 344T239 345T252 346T266 348T278 351T293 358Q348 387 348 440'],
// LATIN CAPITAL LETTER S
0x53: [622,11,525,51,472,'52 454Q52 524 107 572T229 621Q266 621 274 620Q326 610 360 588L371 581Q377 594 379 598T386 610T397 619T412 622Q433 622 439 610T446 570Q446 563 446 545T445 515V479Q445 441 444 432T436 417Q428 408 403 408T370 417Q361 424 361 434Q361 439 360 448T351 476T331 509T295 535T238 546Q194 546 163 522T132 458Q132 435 148 412Q155 401 166 393T192 380T218 371T247 364T270 359Q341 342 349 339Q389 325 418 296T461 229Q472 201 472 164Q469 92 417 41T287 -11Q240 -11 200 -1T143 19L126 29Q117 6 109 -2Q100 -11 84 -11Q64 -11 58 1T51 42Q51 49 51 66T52 95V135Q52 173 53 180T61 194Q70 203 95 203Q119 203 127 194Q136 186 136 168Q143 66 284 66H290Q325 66 350 85Q391 115 391 165Q391 204 369 228T322 260Q320 260 255 275T185 293Q123 309 88 355T52 454'],
// LATIN CAPITAL LETTER T
0x54: [612,-1,525,26,498,'129 38Q129 51 129 55T135 65T151 76H220V535H110V501Q110 470 109 464T101 450Q93 442 68 442H60Q37 442 28 461Q26 466 26 527L27 589Q36 607 49 610H55Q61 610 72 610T97 610T131 610T170 611T215 611T264 611H476Q478 609 483 606T489 602T493 598T496 593T497 586T498 576T498 562V526V488Q498 452 480 444Q476 442 456 442Q431 442 423 450Q416 457 415 463T414 501V535H304V76H374Q389 67 392 61T396 38Q396 10 374 1H151Q140 5 135 11T130 21T129 38'],
// LATIN CAPITAL LETTER U
0x55: [612,11,525,-4,528,'-3 573Q-3 597 8 604T50 612Q57 612 77 612T111 611H200Q214 602 218 595T222 573Q222 549 205 538Q198 535 175 535H151V359Q151 333 151 291Q152 177 156 162Q157 160 157 159Q165 123 193 95T262 66Q303 66 330 94T367 159Q371 175 371 191T373 359V535H349H339Q328 535 322 537T309 548T303 573T306 595T325 611H506Q520 602 524 595T528 573Q528 549 511 538Q504 535 481 535H457V364Q457 189 456 182Q448 101 394 45T262 -11Q189 -11 132 43T68 182Q67 189 67 364V535H43H33Q22 535 16 537T3 548T-3 573'],
// LATIN CAPITAL LETTER V
0x56: [613,7,525,19,505,'19 578Q19 585 20 590T23 598T29 604T38 608T48 610T62 611T78 612T97 611T119 611H195Q210 602 213 596T217 573Q217 561 216 555T206 542T179 535H164Q166 529 188 435T235 231T261 94L262 84V88Q263 91 263 94Q265 121 289 231T336 438L360 535H345Q308 535 308 566V573Q308 586 308 590T314 600T330 611H484Q499 602 502 595T505 573Q505 560 504 554T493 541T465 535H447L384 278Q321 19 319 14Q309 -7 278 -7H262H246Q215 -7 205 14Q203 19 140 278L78 535H59Q45 535 38 536T25 547T19 573V578'],
// LATIN CAPITAL LETTER W
0x57: [611,7,525,12,512,'459 611Q491 611 501 605T512 573Q512 538 482 535H474L439 276Q406 26 402 11Q398 2 389 -3Q387 -3 386 -4L380 -7H359H349Q324 -7 313 13Q307 29 285 139T263 275Q263 283 262 283Q261 282 261 274Q261 248 239 137T211 13Q200 -7 175 -7H165H144Q136 -3 127 3Q121 10 117 36T85 276L50 535H42Q26 536 19 545T12 564V573Q12 603 33 610Q37 611 101 611H134Q165 611 175 604T186 573Q186 563 186 559T182 547T169 538T143 535H122V531Q124 517 133 446T155 266T172 96V84L173 102Q176 157 192 243T215 346Q227 367 259 367H262H265Q297 367 309 346Q316 329 332 243T351 102L352 84V96Q356 161 368 266T390 444T402 531V535H381Q366 535 359 536T345 547T338 573Q338 600 356 608Q362 611 425 611H459'],
// LATIN CAPITAL LETTER X
0x58: [611,-1,525,28,495,'39 571Q39 597 49 604T93 611H141H218Q233 602 236 595T239 573Q239 538 210 535Q202 535 202 534T215 507T243 454L257 428L307 535H298Q266 538 266 573Q266 584 267 588T273 598T289 611H366H401Q442 611 454 605T466 573Q466 546 448 538Q442 535 421 535H398L299 327Q299 323 362 201L426 77L449 76Q467 76 475 75T489 65T495 38Q495 11 477 3Q473 1 395 1H317Q295 8 295 38Q295 73 325 76L334 77Q333 78 314 117T276 196L257 235L239 196Q221 157 204 118T186 77Q190 76 196 76Q211 74 218 67T227 55T228 38Q228 28 227 24T221 13T206 1H50Q28 9 28 32V38Q28 63 45 73Q51 76 73 76H96L214 324Q215 327 162 431L108 535H85H79Q67 535 60 536T46 546T39 571'],
// LATIN CAPITAL LETTER Y
0x59: [611,-1,525,20,505,'20 573Q20 597 30 604T72 611H121H198Q212 602 216 595T220 573Q220 568 219 563T217 555T214 549T211 544T207 541T203 538T198 537T194 536T190 536L188 535Q179 535 179 534L188 516Q196 497 208 470T232 415T252 363T261 332Q261 329 262 329T263 332Q263 354 333 508L345 534Q345 535 336 535Q305 538 305 567V573Q305 589 308 595T327 611H483Q505 598 505 573Q505 549 488 538Q481 535 460 535H438L304 245V76H325H331Q343 76 350 75T363 64T369 38Q369 10 347 1H178Q167 5 162 11T157 21T156 38V44Q156 66 172 73Q180 76 199 76H220V245L86 535H64Q44 535 36 538Q20 548 20 573'],
// LATIN CAPITAL LETTER Z
0x5A: [612,-1,525,48,481,'71 1Q60 5 55 11T49 23T48 39V46Q48 56 58 73T131 183Q171 242 197 282L366 535H144V501Q144 470 143 464T135 450Q127 442 102 442H94Q71 442 62 461Q60 466 60 527L61 589Q70 607 83 610H88Q93 610 102 610T124 610T154 610T188 611T227 611T270 611H454Q456 609 461 606T467 601T471 597T474 591T475 584T476 572V565Q476 555 466 538T393 428Q353 369 327 329L158 76H397V120V146Q397 169 405 179T439 189Q470 189 479 169Q481 164 481 95V48Q481 24 478 16T459 1H71'],
// LEFT SQUARE BRACKET
0x5B: [694,82,525,214,484,'237 -82Q221 -78 214 -58V305Q214 669 216 673Q220 687 231 690T278 694H350H461Q462 693 467 690T474 685T478 679T482 670T483 656Q483 632 471 625T428 617Q422 617 406 617T379 618H298V-7H379H420Q459 -7 471 -13T483 -45Q483 -55 483 -59T477 -70T461 -82H237'],
// REVERSE SOLIDUS
0x5C: [694,83,525,58,466,'58 659Q58 673 68 683T93 694Q114 694 125 673Q132 659 297 314T464 -36Q466 -44 466 -48Q466 -66 454 -74T431 -83Q410 -83 399 -62Q391 -47 226 298T60 648Q58 656 58 659'],
// RIGHT SQUARE BRACKET
0x5D: [695,82,525,41,310,'41 656Q41 681 53 688T99 695Q107 695 133 695T177 694H288Q307 681 310 669V-58Q303 -76 288 -82H64Q41 -73 41 -45Q41 -21 53 -14T96 -6Q102 -6 118 -6T145 -7H226V618H145H100Q67 618 54 625T41 656'],
// CIRCUMFLEX ACCENT
0x5E: [611,-460,525,96,428,'138 460Q121 460 109 479T96 512Q96 527 106 534Q109 536 178 571T253 609Q256 611 264 611Q272 610 343 574Q357 567 369 561T389 550T402 543T411 538T416 535T420 532T422 529T425 525Q428 518 428 512Q428 498 416 479T386 460H384Q377 460 316 496L262 526L208 496Q147 460 138 460'],
// LOW LINE
0x5F: [-25,95,525,57,468,'57 -60Q57 -33 86 -25H438Q468 -34 468 -60T438 -95H86Q57 -86 57 -60'],
// GRAVE ACCENT
0x60: [681,-357,525,176,350,'176 479Q176 563 227 622T310 681Q324 680 337 667T350 641Q350 627 340 619T312 599T280 566Q256 531 252 485V471Q261 472 262 472Q285 472 302 455T320 414Q320 389 303 373T261 357Q223 357 200 391T176 479'],
// LATIN SMALL LETTER A
0x61: [439,6,525,48,524,'126 306Q105 306 90 321T74 359Q74 439 211 439Q268 439 276 438Q343 426 383 390T430 306Q431 301 431 190V81Q446 79 465 78T492 76T509 72T521 60T524 38Q524 11 506 3Q502 1 466 1Q426 1 406 5T379 14T355 36L345 30Q284 -6 205 -6Q135 -6 92 39T48 141Q48 182 79 212T158 256T252 278T342 285H347V290Q347 315 325 335T267 362Q258 363 224 363Q189 363 185 362H179L178 358Q178 353 178 352T176 345T174 337T170 330T165 322T158 316T150 311T139 308T126 306ZM132 140Q132 115 157 93T224 70Q269 70 302 87T344 133Q346 139 347 175V211H339Q256 209 194 190T132 140'],
// LATIN SMALL LETTER B
0x62: [611,6,525,4,492,'4 573Q4 596 15 603T52 611H90H124Q146 611 155 608T171 591Q173 586 173 491V396L182 402Q217 424 256 431Q280 437 309 437Q376 437 434 379T492 217Q492 162 473 118T422 47T358 8T293 -6Q229 -6 174 38Q171 13 163 7T135 1H131H122Q99 1 90 23L89 279V535H58L27 536Q4 543 4 573ZM409 215Q409 269 377 315T283 361Q255 361 224 344T177 297L173 290V167Q189 124 213 97T278 70Q330 70 369 111T409 215'],
// LATIN SMALL LETTER C
0x63: [440,6,525,66,466,'291 -6Q196 -6 131 60T66 216Q66 296 119 361Q154 403 200 421T273 439Q275 440 293 440H313Q400 440 433 409Q454 388 454 359Q454 335 439 321T402 306Q380 306 365 321T350 357V362L340 363Q339 363 326 363T303 364Q280 364 266 362Q217 352 184 313T151 215Q151 153 199 112T313 70Q341 70 357 85T381 118T394 140Q402 146 424 146Q443 146 447 144Q466 137 466 117Q466 106 457 88T429 47T374 10T291 -6'],
// LATIN SMALL LETTER D
0x64: [611,6,525,31,520,'266 573Q266 596 277 603T314 611H352H385Q411 611 419 607T435 586V76H498Q512 67 516 60T520 38Q520 9 498 1H436Q429 1 417 1T398 0Q375 0 363 7T351 34V43L342 36Q288 -6 223 -6Q143 -6 87 58T31 216Q31 307 88 372T230 437Q292 437 342 405L351 399V535H320L289 536Q266 543 266 573ZM351 290Q347 302 337 316T302 346T244 361Q193 361 154 319T115 215Q115 152 152 111T235 70Q314 70 351 170V290'],
// LATIN SMALL LETTER E
0x65: [440,6,525,48,465,'48 217Q48 295 100 361T248 439L258 440Q268 440 274 440Q329 438 369 416T428 359T456 292T464 228Q464 215 461 208T454 198T442 190L288 189H135L138 179Q153 132 199 102T303 71Q336 71 353 86T380 120T398 143Q404 146 422 146Q453 146 462 126Q464 120 464 116Q464 84 416 39T285 -6Q187 -6 118 59T48 217ZM377 264Q371 291 365 306T341 338T294 362Q288 363 264 363Q225 363 190 336T139 264H377'],
// LATIN SMALL LETTER F
0x66: [617,-1,525,35,437,'43 395Q44 405 44 408T47 416T53 423T66 431H176V461Q176 500 182 518Q201 570 252 593T353 617Q399 614 418 593T437 548Q437 528 424 514T387 499Q365 499 353 511T338 537V541H328Q275 536 261 494Q260 490 260 460V431H327Q334 431 346 431T364 432Q392 432 404 425T416 393T405 362T365 355H327H260V76H319Q375 76 388 71T401 38Q401 27 400 23T395 12T379 1H58Q47 6 42 12T36 23T35 38Q35 65 53 73Q59 76 117 76H176V355H121H93Q64 355 54 362T43 395'],
// LATIN SMALL LETTER G
0x67: [442,229,525,28,510,'60 274Q60 337 107 386T233 436Q278 436 316 417L329 410L338 416Q384 442 427 442T489 423T509 381T494 345T460 332Q449 332 440 338Q432 341 427 348T419 360T415 365Q414 364 410 364L383 355Q406 320 406 274Q406 211 358 162T233 112Q189 112 155 128L146 133Q142 125 142 115Q142 99 150 85T175 71Q182 72 187 70Q188 70 195 70T218 70T254 69Q259 69 275 69T297 69T318 68T340 66T361 62T384 57T405 49T428 38Q495 -1 495 -76Q495 -143 427 -186T262 -229Q161 -229 94 -185T29 -73Q30 -60 33 -48T39 -26T47 -8T57 8T67 20T77 30T86 38L91 43Q91 44 86 53T75 80T70 117Q70 142 89 183L83 194Q60 232 60 274ZM321 274Q321 312 296 337T230 362Q197 362 171 338T145 274Q145 235 170 211T233 187Q273 187 297 212T321 274ZM422 -78Q422 -54 408 -38T366 -15T315 -6T255 -4H200Q198 -4 193 -4T183 -3Q148 -3 125 -26T102 -78Q102 -110 151 -132T261 -154Q321 -154 371 -132T422 -78'],
// LATIN SMALL LETTER H
0x68: [611,-1,525,4,520,'4 573Q4 596 15 603T52 611H90H124Q146 611 155 608T171 591Q173 586 173 489Q173 394 175 394L186 402Q197 410 219 420T269 434Q278 436 306 436Q343 436 371 423Q411 402 423 365T436 265Q436 257 436 239T435 211V198V76H498Q512 67 516 60T520 38Q520 9 498 1H308Q286 10 286 32V38V46Q286 65 303 73Q309 76 329 76H351V188Q351 204 351 230T352 266Q352 321 341 341T288 361Q253 361 222 341T176 274L174 264L173 170V76H236Q250 67 254 60T258 38Q258 9 236 1H27Q4 8 4 38Q4 53 8 60T27 76H89V535H58L27 536Q4 543 4 573'],
// LATIN SMALL LETTER I
0x69: [612,-1,525,72,462,'202 538T202 559T218 596T260 612Q283 612 300 597T317 560Q317 538 300 523T260 507Q235 507 219 522ZM411 76Q441 76 451 69T462 38Q462 29 462 26T460 18T453 9T440 1H94Q72 8 72 33V38Q72 46 72 49T74 58T81 68T94 76H233V355H167L102 356Q80 363 80 393Q80 418 91 425T138 432Q145 432 165 432T200 431H295Q297 429 303 425T310 420T314 415T317 404T317 389T318 363Q318 354 318 314T317 241V76H378H411'],
// LATIN SMALL LETTER J
0x6A: [612,228,525,48,377,'261 559Q261 580 277 596T319 612Q342 612 359 597T376 560T360 523T320 507Q296 507 279 523T261 559ZM75 -91T100 -91T138 -107T152 -144V-150L160 -151H193H203Q241 -151 267 -121Q284 -97 288 -73T292 23V151V355H218L145 356Q123 365 123 387V393Q123 422 145 430H148Q151 430 156 430T169 430T185 430T205 431T227 431T251 431H354Q356 430 360 427T365 424T369 420T372 416T373 410T375 402T376 391T377 376T377 356Q377 345 377 286T376 176Q376 -67 371 -88Q362 -123 342 -151T299 -194Q254 -228 180 -228Q84 -226 56 -177Q49 -162 48 -148Q48 -122 61 -107'],
// LATIN SMALL LETTER K
0x6B: [611,0,525,13,507,'13 42Q13 63 23 69T69 76H102V535H69H54Q34 535 24 542T13 573Q13 588 15 593Q22 605 29 608T56 611H95Q113 611 122 611T140 610T152 609T159 607T163 603T167 597T173 589V413L174 237L295 355H275Q260 355 253 356T239 367T232 393Q232 419 243 425T304 431H359H464Q479 422 482 415T485 393Q485 364 464 356L431 355H398L293 254L427 76H486Q501 67 504 60T507 38Q507 28 507 24T501 12T486 1H314Q292 8 292 38Q292 62 308 73Q312 75 326 76L338 77L290 140Q279 154 267 171T248 196L242 204L207 171L173 139V76H206H221Q241 76 251 69T262 38Q262 11 244 3Q240 1 138 1Q123 1 100 1T70 0Q32 0 23 7T13 42'],
// LATIN SMALL LETTER L
0x6C: [612,-1,525,51,474,'51 573Q51 602 73 610H76Q79 610 84 610T97 610T113 610T133 611T155 611T179 611H282Q301 598 304 586V76H452Q466 67 470 60T474 38Q474 10 452 1H73Q51 9 51 32V38Q51 54 54 60T73 76H220V535H146L73 536Q51 545 51 567V573'],
// LATIN SMALL LETTER M
0x6D: [437,-1,525,-12,536,'133 76Q156 74 164 67T172 38Q172 9 151 1H11Q-12 8 -12 38Q-12 61 5 73Q10 75 28 76H45V355H28Q10 356 5 358Q-12 370 -12 393Q-12 419 11 431H52H70Q91 431 100 427T116 405Q163 436 200 436Q255 436 281 390L285 394Q289 398 292 400T301 407T314 415T329 423T346 429T366 434T389 436H392Q425 436 448 411Q469 390 474 360T480 268V232V203V76H497Q520 74 528 67T536 38Q536 9 515 1H396Q374 9 374 32V38Q374 73 402 76H409V191V242Q409 317 404 339T375 361Q343 361 323 332T299 264Q298 258 298 165V76H315Q338 74 346 67T354 38Q354 9 333 1H214Q192 9 192 32V38Q192 73 220 76H227V191V242Q227 317 222 339T193 361Q161 361 141 332T117 264Q116 258 116 165V76H133'],
// LATIN SMALL LETTER N
0x6E: [436,-1,525,4,520,'89 431Q94 431 105 431T122 432Q173 432 173 399Q173 394 175 394Q176 394 190 404T233 425T298 436Q343 436 371 423Q411 402 423 365T436 265Q436 257 436 239T435 211V198V76H498Q512 67 516 60T520 38Q520 9 498 1H308Q286 9 286 32V38V45Q286 65 303 73Q309 76 329 76H351V188Q351 204 351 230T352 266Q352 321 341 341T288 361Q253 361 222 341T176 274L174 264L173 170V76H236Q250 67 254 60T258 38Q258 9 236 1H27Q4 8 4 38Q4 53 8 60T27 76H89V355H58L27 356Q4 363 4 393Q4 408 8 415T27 431H89'],
// LATIN SMALL LETTER O
0x6F: [440,6,525,52,472,'52 216Q52 318 118 379T261 440Q343 440 407 378T472 216Q472 121 410 58T262 -6Q176 -6 114 58T52 216ZM388 225Q388 281 351 322T261 364Q213 364 175 325T136 225Q136 158 174 114T262 70T350 114T388 225'],
// LATIN SMALL LETTER P
0x70: [437,221,525,4,492,'89 431Q93 431 104 431T121 432Q173 432 173 401V396L182 402Q237 437 305 437Q376 437 434 378T492 217Q492 146 459 93T382 17T291 -6Q261 -6 232 5T188 26L174 37Q173 37 173 -54V-146H236Q250 -155 254 -162T258 -184Q258 -213 236 -221H27Q4 -214 4 -184Q4 -169 8 -162T27 -146H89V355H58L27 356Q4 363 4 393Q4 408 8 415T27 431H89ZM409 215Q409 269 377 315T283 361Q255 361 224 344T177 297L173 290V167Q189 124 213 97T278 70Q330 70 369 111T409 215'],
// LATIN SMALL LETTER Q
0x71: [437,222,525,34,545,'34 215Q34 309 91 368T222 436Q224 436 231 436T242 437Q309 437 372 390V401Q372 419 381 428T414 437Q426 437 432 436T444 430T456 412V-146H489H504Q524 -146 534 -153T545 -184Q545 -211 527 -219Q523 -221 414 -221Q398 -221 374 -221T342 -222Q304 -222 294 -216T283 -184Q283 -157 301 -149Q307 -146 339 -146H372V-51Q372 43 371 43L364 38Q357 33 345 26T318 12T280 -1T236 -6Q155 -6 95 55T34 215ZM117 215Q117 152 157 111T250 70Q289 70 318 92T363 146Q372 163 372 192V215L371 263Q339 360 254 360Q206 360 162 321T117 215'],
// LATIN SMALL LETTER R
0x72: [437,-1,525,24,487,'327 76Q359 76 369 70T380 38Q380 10 359 1H47Q24 8 24 38Q24 54 28 61T47 76H145V355H96L47 356Q24 363 24 393Q24 409 28 416T47 431H207Q223 419 226 414T229 393V387V369Q297 437 394 437Q436 437 461 417T487 368Q487 347 473 332T438 317Q428 317 420 320T407 327T398 337T393 347T390 356L388 361Q348 356 324 345Q228 299 228 170Q228 161 228 151T229 138V76H293H327'],
// LATIN SMALL LETTER S
0x73: [440,6,525,71,458,'72 317Q72 361 108 396T229 439Q231 439 245 439T268 440Q303 439 324 435T353 427T363 423L372 432Q380 440 397 440Q430 440 430 395Q430 390 430 380T429 366V335Q429 311 422 302T387 293Q364 293 355 300T346 316T343 336T325 353Q306 364 257 364Q209 364 178 351T147 317Q147 284 231 272Q327 256 357 247Q458 210 458 129V121Q458 74 413 34T271 -6Q246 -6 224 -3T189 5T165 14T150 22T144 26Q142 23 139 18T135 11T132 6T128 1T124 -2T119 -4T113 -5T104 -6Q84 -6 78 6T71 43Q71 48 71 60T72 79Q72 132 73 141T81 157Q90 166 115 166Q135 166 142 162T157 140Q168 108 191 90T260 70Q297 70 323 76T361 91T379 110T384 129Q384 157 346 171T247 195T165 212Q119 228 96 256T72 317'],
// LATIN SMALL LETTER T
0x74: [554,6,525,25,448,'25 395Q26 405 26 408T29 416T35 423T48 431H145V481L146 532Q154 547 161 550T184 554H189Q218 554 227 534Q229 529 229 480V431H405Q406 430 411 427T418 422T422 416T426 407T427 393Q427 387 427 382T424 374T421 368T417 363T413 360T408 358L405 356L317 355H229V249Q229 237 229 214T228 179Q228 126 241 98T295 70Q354 70 365 149Q366 167 375 174Q383 182 407 182H415Q438 182 446 166Q448 161 448 148Q448 84 398 39T282 -6Q226 -6 189 29T146 128Q145 134 145 247V355H96H72Q45 355 35 362T25 395'],
// LATIN SMALL LETTER U
0x75: [431,5,525,4,520,'4 393Q4 416 15 423T52 431H90Q141 431 151 429T168 417Q171 412 173 409V254L174 100Q182 70 244 70Q320 70 344 119Q349 130 350 144T351 248V355H320L289 356Q266 363 266 393Q266 408 270 415T289 431H351H386Q409 431 418 428T433 411Q435 406 435 241V76H498Q512 67 516 60T520 38Q520 9 498 1H436H394Q372 1 364 5T351 26L342 21Q293 -5 227 -5Q118 -5 96 67Q91 82 90 101T89 227V355H58L27 356Q4 363 4 393'],
// LATIN SMALL LETTER V
0x76: [432,4,525,24,500,'24 392Q24 417 36 424T79 432Q85 432 103 432T132 431H215Q229 422 233 415T237 393Q237 355 198 355H193H172L262 77L352 355H331H323Q288 355 288 393Q288 409 291 415T310 431H478Q491 423 495 416T500 393Q500 364 478 356L452 355H426L374 190Q320 24 318 20Q307 -4 273 -4H262H251Q217 -4 206 20Q204 24 150 190L98 355H72L47 356Q24 363 24 392'],
// LATIN SMALL LETTER W
0x77: [431,4,525,16,508,'54 355Q16 355 16 388V393Q16 423 37 430Q41 431 125 431H162Q206 431 218 425T230 393Q230 366 212 358Q206 355 174 355Q141 355 141 354L150 296Q181 110 181 89V84Q182 85 183 96Q185 118 199 173T218 237Q223 247 245 259H264H268Q294 259 309 240Q315 229 329 174T343 92Q343 84 344 84V86Q344 88 344 91T345 97Q347 125 356 187T374 301T383 354Q383 355 350 355H333Q314 355 304 362T294 393Q294 420 312 428Q318 431 401 431H440Q485 431 496 425T508 393Q508 382 508 377T498 363T470 355L455 354Q455 353 441 271T413 104T396 16Q384 -4 355 -4H351Q315 -4 305 9T280 79Q278 90 276 96Q265 149 265 169Q265 176 264 169Q263 166 263 162Q261 130 248 79T230 18Q220 -4 183 -4H175L151 -3Q134 5 127 17L112 102Q97 188 83 270T69 354Q62 355 54 355'],
// LATIN SMALL LETTER X
0x78: [432,-1,525,29,495,'35 393Q35 417 46 424T89 432Q95 432 112 432T141 431H223Q238 422 241 415T244 393Q244 389 244 383T237 367T216 355Q209 355 209 354L234 319Q259 286 260 286L308 354Q308 355 301 355Q285 356 278 365T270 384L271 393Q271 420 289 428Q295 431 376 431H459Q460 430 465 427T472 422T476 416T480 407T481 393Q481 368 470 362T434 355H425H392L344 290Q295 225 295 223Q294 223 309 203T350 149L405 77L439 76H453Q474 76 484 69T495 38Q495 10 473 1H303Q281 9 281 32V38Q281 49 282 54T290 67T313 76Q324 76 324 77L259 173L197 77Q202 76 209 76Q225 75 233 68T241 55T242 38Q242 28 242 24T236 12T221 1H51Q29 9 29 32V38Q29 48 29 51T31 59T38 67T51 76H117L171 149Q224 222 224 223L124 355H90H78Q54 355 45 361T35 393'],
// LATIN SMALL LETTER Y
0x79: [431,228,525,26,501,'26 393Q26 417 37 424T80 431H134H217Q232 422 235 416T239 393Q239 379 236 371T226 360T214 356T197 355L179 354V353L188 330Q197 306 209 272T235 201T259 133T271 89V84L274 95Q279 122 298 185T335 300T352 354Q352 355 331 355Q312 355 304 358Q288 368 288 393Q288 408 291 415T310 431H478Q479 430 484 427T491 422T495 416T499 407T500 393Q500 376 493 367T479 357T458 355H452Q426 355 425 353Q420 337 351 124T280 -94Q240 -195 168 -220Q147 -228 125 -228Q89 -228 66 -201T42 -139Q42 -116 56 -102T93 -87Q117 -87 130 -102T144 -135V-138H126Q121 -148 121 -150T130 -152Q182 -147 207 -87Q211 -78 223 -40T236 1Q230 10 102 355H75L49 356Q26 363 26 393'],
// LATIN SMALL LETTER Z
0x7A: [432,-1,525,34,475,'56 1Q40 7 37 14T34 41Q34 59 36 64Q39 67 43 73Q65 95 191 213T341 355H133V334Q133 306 124 297Q116 289 91 289H83Q60 289 51 308Q49 313 49 361L50 409Q59 427 72 430H78Q83 430 92 430T115 430T144 430T179 431T219 431T262 431H450Q452 430 455 428T459 424T463 422T466 419T468 416T469 413T470 409T471 404T472 398T472 391Q472 374 469 368L462 358Q453 349 315 218Q210 122 164 76H391V103Q391 136 400 146Q409 155 433 155Q464 155 473 135Q475 130 475 78V46Q475 24 472 16T453 1H56'],
// LEFT CURLY BRACKET
0x7B: [694,83,525,50,475,'430 -7H436Q449 -7 456 -8T469 -19T475 -45Q475 -69 466 -76T434 -83H419Q386 -82 363 -80T308 -69T253 -41T223 7L221 17L220 118V220L218 224Q215 229 214 230T210 235T204 241T195 246T184 252T170 257T151 262T127 265Q118 267 100 267T69 270T52 283Q50 288 50 306V314Q50 335 67 341Q68 342 102 343T172 355T217 386L220 392V493L221 595Q225 611 230 621T251 650T304 679T395 693L406 694Q418 694 426 694Q458 694 466 685Q475 676 475 656T466 627Q458 618 430 618Q319 618 305 587L304 486Q304 476 304 458T305 431Q305 385 295 358T251 311L243 306Q243 305 254 298T281 274T302 231Q304 223 304 125L305 25Q309 16 316 10T352 -1T430 -7'],
// VERTICAL LINE
0x7C: [694,82,525,228,297,'228 668Q241 694 262 694Q268 694 273 693T282 688T287 682T293 674L297 668V-57Q282 -82 262 -82Q239 -82 228 -57V668'],
// RIGHT CURLY BRACKET
0x7D: [694,83,525,49,475,'49 655Q49 674 56 682T73 692T106 694Q141 693 167 690T224 677T275 647T303 595L305 392Q313 367 347 356T417 344T457 341Q475 335 475 306Q475 292 473 285T464 273T451 269T430 267Q352 262 327 246Q311 236 305 220L303 17L301 7Q294 -16 277 -33T242 -60T196 -74T150 -80T106 -83Q78 -83 72 -82T58 -74Q49 -65 49 -44Q49 -24 58 -16Q66 -7 94 -7Q143 -7 171 -1T207 10T220 25V125Q220 223 222 231Q228 257 243 274T270 299L281 306Q234 329 222 381Q220 387 220 486V587Q212 597 207 601T173 612T94 618Q66 618 58 627Q49 635 49 655'],
// TILDE
0x7E: [611,-466,525,87,437,'125 467Q113 467 100 480T87 509Q88 520 111 543Q172 602 209 609Q219 611 224 611Q246 611 263 596T290 566T304 551Q319 551 367 594Q383 610 396 610H400Q411 610 424 597T437 568Q436 557 413 534Q348 469 305 466Q278 466 260 481T234 511T220 526Q205 526 157 483Q141 467 129 467H125'],
// ??
0x7F: [612,-519,525,104,421,'104 565Q104 590 120 600T155 611Q175 611 180 610Q217 599 217 565Q217 545 202 532T166 519H159H155Q120 519 107 547Q104 553 104 565ZM307 565Q307 580 317 593T346 610Q348 610 350 610T354 611Q355 612 367 612Q395 611 408 597T421 565T409 534T365 519H358Q336 519 322 532T307 565']
}
);
MathJax.Ajax.loadComplete(MathJax.OutputJax.SVG.fontDir+"/Typewriter/Regular/BasicLatin.js");
| {
"pile_set_name": "Github"
} |
<?php
/**
* ALIPAY API: alipay.marketing.tool.fengdie.editor.query request
*
* @author auto create
* @since 1.0, 2017-04-10 10:25:08
*/
class AlipayMarketingToolFengdieEditorQueryRequest
{
/**
* ๅค่ตทๅค่ถๆดปๅจ็ผ่พๅจ
**/
private $bizContent;
private $apiParas = array();
private $terminalType;
private $terminalInfo;
private $prodCode;
private $apiVersion="1.0";
private $notifyUrl;
private $returnUrl;
private $needEncrypt=false;
public function setBizContent($bizContent)
{
$this->bizContent = $bizContent;
$this->apiParas["biz_content"] = $bizContent;
}
public function getBizContent()
{
return $this->bizContent;
}
public function getApiMethodName()
{
return "alipay.marketing.tool.fengdie.editor.query";
}
public function setNotifyUrl($notifyUrl)
{
$this->notifyUrl=$notifyUrl;
}
public function getNotifyUrl()
{
return $this->notifyUrl;
}
public function setReturnUrl($returnUrl)
{
$this->returnUrl=$returnUrl;
}
public function getReturnUrl()
{
return $this->returnUrl;
}
public function getApiParas()
{
return $this->apiParas;
}
public function getTerminalType()
{
return $this->terminalType;
}
public function setTerminalType($terminalType)
{
$this->terminalType = $terminalType;
}
public function getTerminalInfo()
{
return $this->terminalInfo;
}
public function setTerminalInfo($terminalInfo)
{
$this->terminalInfo = $terminalInfo;
}
public function getProdCode()
{
return $this->prodCode;
}
public function setProdCode($prodCode)
{
$this->prodCode = $prodCode;
}
public function setApiVersion($apiVersion)
{
$this->apiVersion=$apiVersion;
}
public function getApiVersion()
{
return $this->apiVersion;
}
public function setNeedEncrypt($needEncrypt)
{
$this->needEncrypt=$needEncrypt;
}
public function getNeedEncrypt()
{
return $this->needEncrypt;
}
}
| {
"pile_set_name": "Github"
} |
#!/usr/bin/env python3
# Contest Management System - http://cms-dev.github.io/
# Copyright ยฉ 2012 Bernard Blackham <bernard@largestprime.net>
# Copyright ยฉ 2013-2018 Stefano Maggiolo <s.maggiolo@gmail.com>
# Copyright ยฉ 2014-2015 Giovanni Mascellani <mascellani@poisson.phc.unipi.it>
# Copyright ยฉ 2016 Masaki Hara <ackie.h.gmai@gmail.com>
#
# This program is free software: you can redistribute it and/or modify
# it under the terms of the GNU Affero General Public License as
# published by the Free Software Foundation, either version 3 of the
# License, or (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Affero General Public License for more details.
#
# You should have received a copy of the GNU Affero General Public License
# along with this program. If not, see <http://www.gnu.org/licenses/>.
import cmstestsuite.tasks.batch_fileio as batch_fileio
import cmstestsuite.tasks.batch_fileio_managed as batch_fileio_managed
import cmstestsuite.tasks.batch_stdio as batch_stdio
import cmstestsuite.tasks.communication_fifoio_stubbed \
as communication_fifoio_stubbed
import cmstestsuite.tasks.communication_many_fifoio_stubbed \
as communication_many_fifoio_stubbed
import cmstestsuite.tasks.communication_many_stdio_stubbed \
as communication_many_stdio_stubbed
import cmstestsuite.tasks.communication_stdio as communication_stdio
import cmstestsuite.tasks.communication_stdio_stubbed \
as communication_stdio_stubbed
import cmstestsuite.tasks.outputonly as outputonly
import cmstestsuite.tasks.outputonly_comparator as outputonly_comparator
import cmstestsuite.tasks.twosteps as twosteps
import cmstestsuite.tasks.twosteps_comparator as twosteps_comparator
from cmstestsuite.Test import Test, CheckOverallScore, CheckCompilationFail, \
CheckTimeout, CheckTimeoutWall, CheckNonzeroReturn
LANG_CPP = "C++11 / g++"
LANG_C = "C11 / gcc"
LANG_HS = "Haskell / ghc"
LANG_JAVA = "Java / JDK"
LANG_PASCAL = "Pascal / fpc"
LANG_PHP = "PHP"
LANG_PYTHON = "Python 2 / CPython"
LANG_RUST = "Rust"
LANG_C_SHARP = "C# / Mono"
ALL_LANGUAGES = (
LANG_CPP, LANG_C, LANG_HS, LANG_JAVA, LANG_PASCAL, LANG_PHP, LANG_PYTHON,
LANG_RUST, LANG_C_SHARP
)
NON_INTERPRETED_LANGUAGES = (LANG_C, LANG_CPP, LANG_PASCAL)
COMPILED_LANGUAGES = (
LANG_C, LANG_CPP, LANG_PASCAL, LANG_JAVA, LANG_PYTHON, LANG_HS, LANG_RUST,
LANG_C_SHARP
)
ALL_TESTS = [
# Correct solutions to batch tasks.
Test('correct-stdio',
task=batch_stdio, filenames=['correct-stdio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(100, 100)]),
Test('correct-freopen',
task=batch_fileio, filenames=['correct-freopen.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(100, 100)],
user_tests=True),
Test('correct-stdio-inner-class',
task=batch_stdio, filenames=['correct-stdio-inner-class.%l'],
languages=(LANG_JAVA, LANG_C_SHARP),
checks=[CheckOverallScore(100, 100)]),
Test('correct-fileio',
task=batch_fileio, filenames=['correct-fileio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(100, 100)]),
# Various incorrect solutions to batch tasks.
Test('incorrect-stdio',
task=batch_stdio, filenames=['incorrect-stdio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(0, 100)]),
Test('half-correct-stdio',
task=batch_stdio, filenames=['half-correct-stdio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(50, 100)]),
Test('incorrect-fileio',
task=batch_fileio, filenames=['incorrect-fileio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(0, 100)]),
Test('half-correct-fileio',
task=batch_fileio, filenames=['half-correct-fileio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(50, 100)]),
Test('incorrect-fileio-nooutput',
task=batch_fileio, filenames=['incorrect-fileio-nooutput.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
Test('incorrect-fileio-emptyoutput',
task=batch_fileio, filenames=['incorrect-fileio-emptyoutput.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
Test('incorrect-fileio-with-stdio',
task=batch_fileio, filenames=['incorrect-fileio-with-stdio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(0, 100)]),
# OutputOnly tasks
Test('correct-outputonly',
task=outputonly, filenames=['correct-outputonly-000.txt',
'correct-outputonly-001.txt'],
languages=[None], checks=[CheckOverallScore(100, 100)]),
Test('incorrect-outputonly',
task=outputonly, filenames=['incorrect-outputonly-000.txt',
'incorrect-outputonly-001.txt'],
languages=[None], checks=[CheckOverallScore(0, 100)]),
Test('partial-correct-outputonly',
task=outputonly, filenames=['correct-outputonly-000.txt',
'incorrect-outputonly-001.txt'],
languages=[None], checks=[CheckOverallScore(50, 100)]),
Test('correct-outputonly-comparator',
task=outputonly_comparator,
filenames=['correct-outputonly-000.txt',
'correct-outputonly-001.txt'],
languages=[None], checks=[CheckOverallScore(100, 100)]),
Test('incorrect-outputonly-comparator',
task=outputonly_comparator,
filenames=['incorrect-outputonly-000.txt',
'incorrect-outputonly-001.txt'],
languages=[None], checks=[CheckOverallScore(0, 100)]),
Test('partial-correct-outputonly-comparator',
task=outputonly_comparator,
filenames=['correct-outputonly-000.txt',
'incorrect-outputonly-001.txt'],
languages=[None], checks=[CheckOverallScore(50, 100)]),
# Failed compilation.
Test('compile-fail',
task=batch_fileio, filenames=['compile-fail.%l'],
languages=COMPILED_LANGUAGES,
checks=[CheckCompilationFail()]),
Test('compile-timeout',
task=batch_fileio, filenames=['compile-timeout.%l'],
languages=(LANG_CPP,),
checks=[CheckCompilationFail()]),
# Various timeout conditions.
Test('timeout-cputime',
task=batch_stdio, filenames=['timeout-cputime.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(0, 100), CheckTimeout()]),
Test('timeout-pause',
task=batch_stdio, filenames=['timeout-pause.%l'],
languages=(LANG_CPP,),
checks=[CheckOverallScore(0, 100), CheckTimeoutWall()]),
Test('timeout-sleep',
task=batch_stdio, filenames=['timeout-sleep.%l'],
languages=(LANG_CPP,),
checks=[CheckOverallScore(0, 100), CheckTimeout()]),
Test('timeout-sigstop',
task=batch_stdio, filenames=['timeout-sigstop.%l'],
languages=(LANG_CPP,),
checks=[CheckOverallScore(0, 100), CheckTimeout()]),
Test('timeout-select',
task=batch_stdio, filenames=['timeout-select.%l'],
languages=(LANG_CPP,),
checks=[CheckOverallScore(0, 100), CheckTimeout()]),
# Nonzero return status.
Test('nonzero-return-stdio',
task=batch_stdio, filenames=['nonzero-return-stdio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(0, 100), CheckNonzeroReturn()]),
Test('nonzero-return-fileio',
task=batch_fileio, filenames=['nonzero-return-fileio.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(0, 100), CheckNonzeroReturn()]),
# Fork
# We can't really check for a specific error, because forking
# doesn't cause an exceptional stop: it just returns -1 to the
# caller; we rely on the fact that the test program is designed to
# produce output only inside the child process
# TODO: since we allow many processes in the sandbox now by default,
# these submission rightfully pass. We need to refactor TestRunner
# to allow different contests for different tests to re-enable them.
# Test('fork',
# task=batch_stdio, filenames=['fork.%l'],
# languages=(LANG_C, LANG_CPP),
# checks=[CheckOverallScore(0, 100)]),
# OOM problems.
Test('oom-static',
task=batch_stdio, filenames=['oom-static.%l'],
languages=NON_INTERPRETED_LANGUAGES,
checks=[CheckOverallScore(0, 100)]),
Test('oom-heap',
task=batch_stdio, filenames=['oom-heap.%l'],
languages=ALL_LANGUAGES,
checks=[CheckOverallScore(0, 100)]),
# Tasks with graders. PHP is not yet supported.
Test('managed-correct',
task=batch_fileio_managed, filenames=['managed-correct.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA,
LANG_C_SHARP),
checks=[CheckOverallScore(100, 100)]),
Test('managed-incorrect',
task=batch_fileio_managed, filenames=['managed-incorrect.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA,
LANG_C_SHARP),
checks=[CheckOverallScore(0, 100)]),
# Communication tasks. Python and PHP are not yet supported.
Test('communication-fifoio-correct',
task=communication_fifoio_stubbed,
filenames=['communication-stubbed-correct.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(100, 100)]),
Test('communication-fifoio-incorrect',
task=communication_fifoio_stubbed,
filenames=['communication-stubbed-incorrect.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(0, 100)]),
Test('communication-stdio-correct',
task=communication_stdio_stubbed,
filenames=['communication-stubbed-correct.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(100, 100)]),
Test('communication-stdio-incorrect',
task=communication_stdio_stubbed,
filenames=['communication-stubbed-incorrect.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(0, 100)]),
Test('communication-stdio-unstubbed-correct',
task=communication_stdio,
filenames=['communication-stdio-correct.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(100, 100)]),
Test('communication-stdio-unstubbed-incorrect',
task=communication_stdio,
filenames=['communication-stdio-incorrect.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(0, 100)]),
# Communication tasks with two processes.
Test('communication-many-fifoio-correct',
task=communication_many_fifoio_stubbed,
filenames=['communication-many-correct-user1.%l',
'communication-many-correct-user2.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(100, 100)]),
Test('communication-many-fifoio-incorrect',
task=communication_many_fifoio_stubbed,
filenames=['communication-many-incorrect-user1.%l',
'communication-many-incorrect-user2.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(0, 100)]),
Test('communication-many-stdio-correct',
task=communication_many_stdio_stubbed,
filenames=['communication-many-correct-user1.%l',
'communication-many-correct-user2.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(100, 100)]),
Test('communication-many-stdio-incorrect',
task=communication_many_stdio_stubbed,
filenames=['communication-many-incorrect-user1.%l',
'communication-many-incorrect-user2.%l'],
languages=(LANG_C, LANG_CPP, LANG_PASCAL, LANG_PYTHON, LANG_JAVA),
checks=[CheckOverallScore(0, 100)]),
# TwoSteps
Test('twosteps-correct',
task=twosteps, filenames=["twosteps-correct-first.%l",
"twosteps-correct-second.%l"],
languages=(LANG_C, ),
checks=[CheckOverallScore(100, 100)]),
Test('twosteps-half-correct',
task=twosteps, filenames=["twosteps-half-correct-first.%l",
"twosteps-correct-second.%l"],
languages=(LANG_C,),
checks=[CheckOverallScore(50, 100)]),
Test('twosteps-incorrect',
task=twosteps, filenames=["twosteps-incorrect-first.%l",
"twosteps-correct-second.%l"],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
Test('twosteps-comparator-correct',
task=twosteps_comparator, filenames=["twosteps-correct-first.%l",
"twosteps-correct-second.%l"],
languages=(LANG_C,),
checks=[CheckOverallScore(100, 100)]),
Test('twosteps-comparator-half-correct',
task=twosteps_comparator, filenames=["twosteps-half-correct-first.%l",
"twosteps-correct-second.%l"],
languages=(LANG_C,),
checks=[CheckOverallScore(50, 100)]),
Test('twosteps-comparator-incorrect',
task=twosteps_comparator, filenames=["twosteps-incorrect-first.%l",
"twosteps-correct-second.%l"],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
# Writing to files not allowed.
# Inability to write to a file does not throw a specific error,
# just returns a NULL file handler to the caller. So we rely on
# the test program to write the correct result only if the
# returned handler is valid.
Test('write-forbidden-fileio',
task=batch_fileio, filenames=['write-forbidden-fileio.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
Test('write-forbidden-stdio',
task=batch_stdio, filenames=['write-forbidden-stdio.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
Test('write-forbidden-managed',
task=batch_fileio_managed, filenames=['write-forbidden-managed.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
Test('write-forbidden-communication',
task=communication_fifoio_stubbed,
filenames=['write-forbidden-communication.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
# This tests complete successfully only if it is unable to execute
# output.txt.
Test('executing-output',
task=batch_fileio, filenames=['executing-output.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(100, 100)]),
# Rewrite input in the solution.
Test('rewrite-input',
task=batch_fileio_managed, filenames=['rewrite-input.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
Test('delete-write-input',
task=batch_fileio_managed, filenames=['delete-write-input.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
# Write a huge file
Test('write-big-fileio',
task=batch_fileio, filenames=['write-big-fileio.%l'],
languages=(LANG_C,),
checks=[CheckOverallScore(0, 100)]),
]
| {
"pile_set_name": "Github"
} |
// Licensed to the .NET Foundation under one or more agreements.
// The .NET Foundation licenses this file to you under the MIT license.
/*=============================================================================
**
**
**
** Purpose: Class for creating and managing a threadpool
**
**
=============================================================================*/
#pragma warning disable 0420
using System.Runtime.InteropServices;
namespace System.Threading
{
public static partial class ThreadPool
{
private static void EnsureInitialized()
{
ThreadPoolGlobals.threadPoolInitialized = true;
ThreadPoolGlobals.enableWorkerTracking = false;
}
internal static void ReportThreadStatus(bool isWorking)
{
}
unsafe private static void NativeOverlappedCallback(object obj)
{
NativeOverlapped* overlapped = (NativeOverlapped*)(IntPtr)obj;
_IOCompletionCallback.PerformIOCompletionCallback(0, 0, overlapped);
}
[CLSCompliant(false)]
unsafe public static bool UnsafeQueueNativeOverlapped(NativeOverlapped* overlapped)
{
// OS doesn't signal handle, so do it here (CoreCLR does this assignment in ThreadPoolNative::CorPostQueuedCompletionStatus)
overlapped->InternalLow = (IntPtr)0;
// Both types of callbacks are executed on the same thread pool
return UnsafeQueueUserWorkItem(NativeOverlappedCallback, (IntPtr)overlapped);
}
[Obsolete("ThreadPool.BindHandle(IntPtr) has been deprecated. Please use ThreadPool.BindHandle(SafeHandle) instead.", false)]
public static bool BindHandle(IntPtr osHandle)
{
throw new PlatformNotSupportedException(SR.Arg_PlatformNotSupported); // Replaced by ThreadPoolBoundHandle.BindHandle
}
public static bool BindHandle(SafeHandle osHandle)
{
throw new PlatformNotSupportedException(SR.Arg_PlatformNotSupported); // Replaced by ThreadPoolBoundHandle.BindHandle
}
private static long PendingUnmanagedWorkItemCount => 0;
}
}
| {
"pile_set_name": "Github"
} |
// mksyscall.pl -tags linux,mips64 syscall_linux.go syscall_linux_mips64x.go
// Code generated by the command above; see README.md. DO NOT EDIT.
// +build linux,mips64
package unix
import (
"syscall"
"unsafe"
)
var _ syscall.Errno
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func fchmodat(dirfd int, path string, mode uint32) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_FCHMODAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(mode))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func ioctl(fd int, req uint, arg uintptr) (err error) {
_, _, e1 := Syscall(SYS_IOCTL, uintptr(fd), uintptr(req), uintptr(arg))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Linkat(olddirfd int, oldpath string, newdirfd int, newpath string, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(oldpath)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(newpath)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_LINKAT, uintptr(olddirfd), uintptr(unsafe.Pointer(_p0)), uintptr(newdirfd), uintptr(unsafe.Pointer(_p1)), uintptr(flags), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func openat(dirfd int, path string, flags int, mode uint32) (fd int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
r0, _, e1 := Syscall6(SYS_OPENAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(flags), uintptr(mode), 0, 0)
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func ppoll(fds *PollFd, nfds int, timeout *Timespec, sigmask *Sigset_t) (n int, err error) {
r0, _, e1 := Syscall6(SYS_PPOLL, uintptr(unsafe.Pointer(fds)), uintptr(nfds), uintptr(unsafe.Pointer(timeout)), uintptr(unsafe.Pointer(sigmask)), 0, 0)
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Readlinkat(dirfd int, path string, buf []byte) (n int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 unsafe.Pointer
if len(buf) > 0 {
_p1 = unsafe.Pointer(&buf[0])
} else {
_p1 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_READLINKAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(_p1), uintptr(len(buf)), 0, 0)
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Symlinkat(oldpath string, newdirfd int, newpath string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(oldpath)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(newpath)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_SYMLINKAT, uintptr(unsafe.Pointer(_p0)), uintptr(newdirfd), uintptr(unsafe.Pointer(_p1)))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Unlinkat(dirfd int, path string, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_UNLINKAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(flags))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func utimensat(dirfd int, path string, times *[2]Timespec, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_UTIMENSAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(times)), uintptr(flags), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getcwd(buf []byte) (n int, err error) {
var _p0 unsafe.Pointer
if len(buf) > 0 {
_p0 = unsafe.Pointer(&buf[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_GETCWD, uintptr(_p0), uintptr(len(buf)), 0)
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func wait4(pid int, wstatus *_C_int, options int, rusage *Rusage) (wpid int, err error) {
r0, _, e1 := Syscall6(SYS_WAIT4, uintptr(pid), uintptr(unsafe.Pointer(wstatus)), uintptr(options), uintptr(unsafe.Pointer(rusage)), 0, 0)
wpid = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func KeyctlInt(cmd int, arg2 int, arg3 int, arg4 int, arg5 int) (ret int, err error) {
r0, _, e1 := Syscall6(SYS_KEYCTL, uintptr(cmd), uintptr(arg2), uintptr(arg3), uintptr(arg4), uintptr(arg5), 0)
ret = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func KeyctlBuffer(cmd int, arg2 int, buf []byte, arg5 int) (ret int, err error) {
var _p0 unsafe.Pointer
if len(buf) > 0 {
_p0 = unsafe.Pointer(&buf[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_KEYCTL, uintptr(cmd), uintptr(arg2), uintptr(_p0), uintptr(len(buf)), uintptr(arg5), 0)
ret = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func keyctlJoin(cmd int, arg2 string) (ret int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(arg2)
if err != nil {
return
}
r0, _, e1 := Syscall(SYS_KEYCTL, uintptr(cmd), uintptr(unsafe.Pointer(_p0)), 0)
ret = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func keyctlSearch(cmd int, arg2 int, arg3 string, arg4 string, arg5 int) (ret int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(arg3)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(arg4)
if err != nil {
return
}
r0, _, e1 := Syscall6(SYS_KEYCTL, uintptr(cmd), uintptr(arg2), uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), uintptr(arg5), 0)
ret = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func keyctlIOV(cmd int, arg2 int, payload []Iovec, arg5 int) (err error) {
var _p0 unsafe.Pointer
if len(payload) > 0 {
_p0 = unsafe.Pointer(&payload[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall6(SYS_KEYCTL, uintptr(cmd), uintptr(arg2), uintptr(_p0), uintptr(len(payload)), uintptr(arg5), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func keyctlDH(cmd int, arg2 *KeyctlDHParams, buf []byte) (ret int, err error) {
var _p0 unsafe.Pointer
if len(buf) > 0 {
_p0 = unsafe.Pointer(&buf[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_KEYCTL, uintptr(cmd), uintptr(unsafe.Pointer(arg2)), uintptr(_p0), uintptr(len(buf)), 0, 0)
ret = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func ptrace(request int, pid int, addr uintptr, data uintptr) (err error) {
_, _, e1 := Syscall6(SYS_PTRACE, uintptr(request), uintptr(pid), uintptr(addr), uintptr(data), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func reboot(magic1 uint, magic2 uint, cmd int, arg string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(arg)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_REBOOT, uintptr(magic1), uintptr(magic2), uintptr(cmd), uintptr(unsafe.Pointer(_p0)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func mount(source string, target string, fstype string, flags uintptr, data *byte) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(source)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(target)
if err != nil {
return
}
var _p2 *byte
_p2, err = BytePtrFromString(fstype)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_MOUNT, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), uintptr(unsafe.Pointer(_p2)), uintptr(flags), uintptr(unsafe.Pointer(data)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Acct(path string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_ACCT, uintptr(unsafe.Pointer(_p0)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func AddKey(keyType string, description string, payload []byte, ringid int) (id int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(keyType)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(description)
if err != nil {
return
}
var _p2 unsafe.Pointer
if len(payload) > 0 {
_p2 = unsafe.Pointer(&payload[0])
} else {
_p2 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_ADD_KEY, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), uintptr(_p2), uintptr(len(payload)), uintptr(ringid), 0)
id = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Adjtimex(buf *Timex) (state int, err error) {
r0, _, e1 := Syscall(SYS_ADJTIMEX, uintptr(unsafe.Pointer(buf)), 0, 0)
state = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Chdir(path string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_CHDIR, uintptr(unsafe.Pointer(_p0)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Chroot(path string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_CHROOT, uintptr(unsafe.Pointer(_p0)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func ClockGettime(clockid int32, time *Timespec) (err error) {
_, _, e1 := Syscall(SYS_CLOCK_GETTIME, uintptr(clockid), uintptr(unsafe.Pointer(time)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Close(fd int) (err error) {
_, _, e1 := Syscall(SYS_CLOSE, uintptr(fd), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func CopyFileRange(rfd int, roff *int64, wfd int, woff *int64, len int, flags int) (n int, err error) {
r0, _, e1 := Syscall6(SYS_COPY_FILE_RANGE, uintptr(rfd), uintptr(unsafe.Pointer(roff)), uintptr(wfd), uintptr(unsafe.Pointer(woff)), uintptr(len), uintptr(flags))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Dup(oldfd int) (fd int, err error) {
r0, _, e1 := Syscall(SYS_DUP, uintptr(oldfd), 0, 0)
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Dup3(oldfd int, newfd int, flags int) (err error) {
_, _, e1 := Syscall(SYS_DUP3, uintptr(oldfd), uintptr(newfd), uintptr(flags))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func EpollCreate1(flag int) (fd int, err error) {
r0, _, e1 := RawSyscall(SYS_EPOLL_CREATE1, uintptr(flag), 0, 0)
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func EpollCtl(epfd int, op int, fd int, event *EpollEvent) (err error) {
_, _, e1 := RawSyscall6(SYS_EPOLL_CTL, uintptr(epfd), uintptr(op), uintptr(fd), uintptr(unsafe.Pointer(event)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Eventfd(initval uint, flags int) (fd int, err error) {
r0, _, e1 := Syscall(SYS_EVENTFD2, uintptr(initval), uintptr(flags), 0)
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Exit(code int) {
SyscallNoError(SYS_EXIT_GROUP, uintptr(code), 0, 0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fallocate(fd int, mode uint32, off int64, len int64) (err error) {
_, _, e1 := Syscall6(SYS_FALLOCATE, uintptr(fd), uintptr(mode), uintptr(off), uintptr(len), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fchdir(fd int) (err error) {
_, _, e1 := Syscall(SYS_FCHDIR, uintptr(fd), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fchmod(fd int, mode uint32) (err error) {
_, _, e1 := Syscall(SYS_FCHMOD, uintptr(fd), uintptr(mode), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fchownat(dirfd int, path string, uid int, gid int, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_FCHOWNAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(uid), uintptr(gid), uintptr(flags), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func fcntl(fd int, cmd int, arg int) (val int, err error) {
r0, _, e1 := Syscall(SYS_FCNTL, uintptr(fd), uintptr(cmd), uintptr(arg))
val = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fdatasync(fd int) (err error) {
_, _, e1 := Syscall(SYS_FDATASYNC, uintptr(fd), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fgetxattr(fd int, attr string, dest []byte) (sz int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(attr)
if err != nil {
return
}
var _p1 unsafe.Pointer
if len(dest) > 0 {
_p1 = unsafe.Pointer(&dest[0])
} else {
_p1 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_FGETXATTR, uintptr(fd), uintptr(unsafe.Pointer(_p0)), uintptr(_p1), uintptr(len(dest)), 0, 0)
sz = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Flistxattr(fd int, dest []byte) (sz int, err error) {
var _p0 unsafe.Pointer
if len(dest) > 0 {
_p0 = unsafe.Pointer(&dest[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_FLISTXATTR, uintptr(fd), uintptr(_p0), uintptr(len(dest)))
sz = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Flock(fd int, how int) (err error) {
_, _, e1 := Syscall(SYS_FLOCK, uintptr(fd), uintptr(how), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fremovexattr(fd int, attr string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(attr)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_FREMOVEXATTR, uintptr(fd), uintptr(unsafe.Pointer(_p0)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fsetxattr(fd int, attr string, dest []byte, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(attr)
if err != nil {
return
}
var _p1 unsafe.Pointer
if len(dest) > 0 {
_p1 = unsafe.Pointer(&dest[0])
} else {
_p1 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall6(SYS_FSETXATTR, uintptr(fd), uintptr(unsafe.Pointer(_p0)), uintptr(_p1), uintptr(len(dest)), uintptr(flags), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fsync(fd int) (err error) {
_, _, e1 := Syscall(SYS_FSYNC, uintptr(fd), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getdents(fd int, buf []byte) (n int, err error) {
var _p0 unsafe.Pointer
if len(buf) > 0 {
_p0 = unsafe.Pointer(&buf[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_GETDENTS64, uintptr(fd), uintptr(_p0), uintptr(len(buf)))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getpgid(pid int) (pgid int, err error) {
r0, _, e1 := RawSyscall(SYS_GETPGID, uintptr(pid), 0, 0)
pgid = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getpid() (pid int) {
r0, _ := RawSyscallNoError(SYS_GETPID, 0, 0, 0)
pid = int(r0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getppid() (ppid int) {
r0, _ := RawSyscallNoError(SYS_GETPPID, 0, 0, 0)
ppid = int(r0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getpriority(which int, who int) (prio int, err error) {
r0, _, e1 := Syscall(SYS_GETPRIORITY, uintptr(which), uintptr(who), 0)
prio = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getrandom(buf []byte, flags int) (n int, err error) {
var _p0 unsafe.Pointer
if len(buf) > 0 {
_p0 = unsafe.Pointer(&buf[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_GETRANDOM, uintptr(_p0), uintptr(len(buf)), uintptr(flags))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getrusage(who int, rusage *Rusage) (err error) {
_, _, e1 := RawSyscall(SYS_GETRUSAGE, uintptr(who), uintptr(unsafe.Pointer(rusage)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getsid(pid int) (sid int, err error) {
r0, _, e1 := RawSyscall(SYS_GETSID, uintptr(pid), 0, 0)
sid = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Gettid() (tid int) {
r0, _ := RawSyscallNoError(SYS_GETTID, 0, 0, 0)
tid = int(r0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getxattr(path string, attr string, dest []byte) (sz int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(attr)
if err != nil {
return
}
var _p2 unsafe.Pointer
if len(dest) > 0 {
_p2 = unsafe.Pointer(&dest[0])
} else {
_p2 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_GETXATTR, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), uintptr(_p2), uintptr(len(dest)), 0, 0)
sz = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func InotifyAddWatch(fd int, pathname string, mask uint32) (watchdesc int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(pathname)
if err != nil {
return
}
r0, _, e1 := Syscall(SYS_INOTIFY_ADD_WATCH, uintptr(fd), uintptr(unsafe.Pointer(_p0)), uintptr(mask))
watchdesc = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func InotifyInit1(flags int) (fd int, err error) {
r0, _, e1 := RawSyscall(SYS_INOTIFY_INIT1, uintptr(flags), 0, 0)
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func InotifyRmWatch(fd int, watchdesc uint32) (success int, err error) {
r0, _, e1 := RawSyscall(SYS_INOTIFY_RM_WATCH, uintptr(fd), uintptr(watchdesc), 0)
success = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Kill(pid int, sig syscall.Signal) (err error) {
_, _, e1 := RawSyscall(SYS_KILL, uintptr(pid), uintptr(sig), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Klogctl(typ int, buf []byte) (n int, err error) {
var _p0 unsafe.Pointer
if len(buf) > 0 {
_p0 = unsafe.Pointer(&buf[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_SYSLOG, uintptr(typ), uintptr(_p0), uintptr(len(buf)))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Lgetxattr(path string, attr string, dest []byte) (sz int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(attr)
if err != nil {
return
}
var _p2 unsafe.Pointer
if len(dest) > 0 {
_p2 = unsafe.Pointer(&dest[0])
} else {
_p2 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_LGETXATTR, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), uintptr(_p2), uintptr(len(dest)), 0, 0)
sz = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Listxattr(path string, dest []byte) (sz int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 unsafe.Pointer
if len(dest) > 0 {
_p1 = unsafe.Pointer(&dest[0])
} else {
_p1 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_LISTXATTR, uintptr(unsafe.Pointer(_p0)), uintptr(_p1), uintptr(len(dest)))
sz = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Llistxattr(path string, dest []byte) (sz int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 unsafe.Pointer
if len(dest) > 0 {
_p1 = unsafe.Pointer(&dest[0])
} else {
_p1 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_LLISTXATTR, uintptr(unsafe.Pointer(_p0)), uintptr(_p1), uintptr(len(dest)))
sz = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Lremovexattr(path string, attr string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(attr)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_LREMOVEXATTR, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Lsetxattr(path string, attr string, data []byte, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(attr)
if err != nil {
return
}
var _p2 unsafe.Pointer
if len(data) > 0 {
_p2 = unsafe.Pointer(&data[0])
} else {
_p2 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall6(SYS_LSETXATTR, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), uintptr(_p2), uintptr(len(data)), uintptr(flags), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Mkdirat(dirfd int, path string, mode uint32) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_MKDIRAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(mode))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Mknodat(dirfd int, path string, mode uint32, dev int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_MKNODAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(mode), uintptr(dev), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Nanosleep(time *Timespec, leftover *Timespec) (err error) {
_, _, e1 := Syscall(SYS_NANOSLEEP, uintptr(unsafe.Pointer(time)), uintptr(unsafe.Pointer(leftover)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func PerfEventOpen(attr *PerfEventAttr, pid int, cpu int, groupFd int, flags int) (fd int, err error) {
r0, _, e1 := Syscall6(SYS_PERF_EVENT_OPEN, uintptr(unsafe.Pointer(attr)), uintptr(pid), uintptr(cpu), uintptr(groupFd), uintptr(flags), 0)
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func PivotRoot(newroot string, putold string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(newroot)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(putold)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_PIVOT_ROOT, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func prlimit(pid int, resource int, newlimit *Rlimit, old *Rlimit) (err error) {
_, _, e1 := RawSyscall6(SYS_PRLIMIT64, uintptr(pid), uintptr(resource), uintptr(unsafe.Pointer(newlimit)), uintptr(unsafe.Pointer(old)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Prctl(option int, arg2 uintptr, arg3 uintptr, arg4 uintptr, arg5 uintptr) (err error) {
_, _, e1 := Syscall6(SYS_PRCTL, uintptr(option), uintptr(arg2), uintptr(arg3), uintptr(arg4), uintptr(arg5), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Pselect(nfd int, r *FdSet, w *FdSet, e *FdSet, timeout *Timespec, sigmask *Sigset_t) (n int, err error) {
r0, _, e1 := Syscall6(SYS_PSELECT6, uintptr(nfd), uintptr(unsafe.Pointer(r)), uintptr(unsafe.Pointer(w)), uintptr(unsafe.Pointer(e)), uintptr(unsafe.Pointer(timeout)), uintptr(unsafe.Pointer(sigmask)))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func read(fd int, p []byte) (n int, err error) {
var _p0 unsafe.Pointer
if len(p) > 0 {
_p0 = unsafe.Pointer(&p[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_READ, uintptr(fd), uintptr(_p0), uintptr(len(p)))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Removexattr(path string, attr string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(attr)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_REMOVEXATTR, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Renameat(olddirfd int, oldpath string, newdirfd int, newpath string) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(oldpath)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(newpath)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_RENAMEAT, uintptr(olddirfd), uintptr(unsafe.Pointer(_p0)), uintptr(newdirfd), uintptr(unsafe.Pointer(_p1)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Renameat2(olddirfd int, oldpath string, newdirfd int, newpath string, flags uint) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(oldpath)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(newpath)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_RENAMEAT2, uintptr(olddirfd), uintptr(unsafe.Pointer(_p0)), uintptr(newdirfd), uintptr(unsafe.Pointer(_p1)), uintptr(flags), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func RequestKey(keyType string, description string, callback string, destRingid int) (id int, err error) {
var _p0 *byte
_p0, err = BytePtrFromString(keyType)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(description)
if err != nil {
return
}
var _p2 *byte
_p2, err = BytePtrFromString(callback)
if err != nil {
return
}
r0, _, e1 := Syscall6(SYS_REQUEST_KEY, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), uintptr(unsafe.Pointer(_p2)), uintptr(destRingid), 0, 0)
id = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setdomainname(p []byte) (err error) {
var _p0 unsafe.Pointer
if len(p) > 0 {
_p0 = unsafe.Pointer(&p[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall(SYS_SETDOMAINNAME, uintptr(_p0), uintptr(len(p)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Sethostname(p []byte) (err error) {
var _p0 unsafe.Pointer
if len(p) > 0 {
_p0 = unsafe.Pointer(&p[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall(SYS_SETHOSTNAME, uintptr(_p0), uintptr(len(p)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setpgid(pid int, pgid int) (err error) {
_, _, e1 := RawSyscall(SYS_SETPGID, uintptr(pid), uintptr(pgid), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setsid() (pid int, err error) {
r0, _, e1 := RawSyscall(SYS_SETSID, 0, 0, 0)
pid = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Settimeofday(tv *Timeval) (err error) {
_, _, e1 := RawSyscall(SYS_SETTIMEOFDAY, uintptr(unsafe.Pointer(tv)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setns(fd int, nstype int) (err error) {
_, _, e1 := Syscall(SYS_SETNS, uintptr(fd), uintptr(nstype), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setpriority(which int, who int, prio int) (err error) {
_, _, e1 := Syscall(SYS_SETPRIORITY, uintptr(which), uintptr(who), uintptr(prio))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setxattr(path string, attr string, data []byte, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
var _p1 *byte
_p1, err = BytePtrFromString(attr)
if err != nil {
return
}
var _p2 unsafe.Pointer
if len(data) > 0 {
_p2 = unsafe.Pointer(&data[0])
} else {
_p2 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall6(SYS_SETXATTR, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(_p1)), uintptr(_p2), uintptr(len(data)), uintptr(flags), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Statx(dirfd int, path string, flags int, mask int, stat *Statx_t) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_STATX, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(flags), uintptr(mask), uintptr(unsafe.Pointer(stat)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Sync() {
SyscallNoError(SYS_SYNC, 0, 0, 0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Syncfs(fd int) (err error) {
_, _, e1 := Syscall(SYS_SYNCFS, uintptr(fd), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Sysinfo(info *Sysinfo_t) (err error) {
_, _, e1 := RawSyscall(SYS_SYSINFO, uintptr(unsafe.Pointer(info)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Tee(rfd int, wfd int, len int, flags int) (n int64, err error) {
r0, _, e1 := Syscall6(SYS_TEE, uintptr(rfd), uintptr(wfd), uintptr(len), uintptr(flags), 0, 0)
n = int64(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Tgkill(tgid int, tid int, sig syscall.Signal) (err error) {
_, _, e1 := RawSyscall(SYS_TGKILL, uintptr(tgid), uintptr(tid), uintptr(sig))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Times(tms *Tms) (ticks uintptr, err error) {
r0, _, e1 := RawSyscall(SYS_TIMES, uintptr(unsafe.Pointer(tms)), 0, 0)
ticks = uintptr(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Umask(mask int) (oldmask int) {
r0, _ := RawSyscallNoError(SYS_UMASK, uintptr(mask), 0, 0)
oldmask = int(r0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Uname(buf *Utsname) (err error) {
_, _, e1 := RawSyscall(SYS_UNAME, uintptr(unsafe.Pointer(buf)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Unmount(target string, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(target)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_UMOUNT2, uintptr(unsafe.Pointer(_p0)), uintptr(flags), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Unshare(flags int) (err error) {
_, _, e1 := Syscall(SYS_UNSHARE, uintptr(flags), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func write(fd int, p []byte) (n int, err error) {
var _p0 unsafe.Pointer
if len(p) > 0 {
_p0 = unsafe.Pointer(&p[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall(SYS_WRITE, uintptr(fd), uintptr(_p0), uintptr(len(p)))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func exitThread(code int) (err error) {
_, _, e1 := Syscall(SYS_EXIT, uintptr(code), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func readlen(fd int, p *byte, np int) (n int, err error) {
r0, _, e1 := Syscall(SYS_READ, uintptr(fd), uintptr(unsafe.Pointer(p)), uintptr(np))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func writelen(fd int, p *byte, np int) (n int, err error) {
r0, _, e1 := Syscall(SYS_WRITE, uintptr(fd), uintptr(unsafe.Pointer(p)), uintptr(np))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func munmap(addr uintptr, length uintptr) (err error) {
_, _, e1 := Syscall(SYS_MUNMAP, uintptr(addr), uintptr(length), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Madvise(b []byte, advice int) (err error) {
var _p0 unsafe.Pointer
if len(b) > 0 {
_p0 = unsafe.Pointer(&b[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall(SYS_MADVISE, uintptr(_p0), uintptr(len(b)), uintptr(advice))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Mprotect(b []byte, prot int) (err error) {
var _p0 unsafe.Pointer
if len(b) > 0 {
_p0 = unsafe.Pointer(&b[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall(SYS_MPROTECT, uintptr(_p0), uintptr(len(b)), uintptr(prot))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Mlock(b []byte) (err error) {
var _p0 unsafe.Pointer
if len(b) > 0 {
_p0 = unsafe.Pointer(&b[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall(SYS_MLOCK, uintptr(_p0), uintptr(len(b)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Mlockall(flags int) (err error) {
_, _, e1 := Syscall(SYS_MLOCKALL, uintptr(flags), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Msync(b []byte, flags int) (err error) {
var _p0 unsafe.Pointer
if len(b) > 0 {
_p0 = unsafe.Pointer(&b[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall(SYS_MSYNC, uintptr(_p0), uintptr(len(b)), uintptr(flags))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Munlock(b []byte) (err error) {
var _p0 unsafe.Pointer
if len(b) > 0 {
_p0 = unsafe.Pointer(&b[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall(SYS_MUNLOCK, uintptr(_p0), uintptr(len(b)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Munlockall() (err error) {
_, _, e1 := Syscall(SYS_MUNLOCKALL, 0, 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func faccessat(dirfd int, path string, mode uint32) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_FACCESSAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(mode))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Dup2(oldfd int, newfd int) (err error) {
_, _, e1 := Syscall(SYS_DUP2, uintptr(oldfd), uintptr(newfd), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func EpollCreate(size int) (fd int, err error) {
r0, _, e1 := RawSyscall(SYS_EPOLL_CREATE, uintptr(size), 0, 0)
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func EpollWait(epfd int, events []EpollEvent, msec int) (n int, err error) {
var _p0 unsafe.Pointer
if len(events) > 0 {
_p0 = unsafe.Pointer(&events[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_EPOLL_WAIT, uintptr(epfd), uintptr(_p0), uintptr(len(events)), uintptr(msec), 0, 0)
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fadvise(fd int, offset int64, length int64, advice int) (err error) {
_, _, e1 := Syscall6(SYS_FADVISE64, uintptr(fd), uintptr(offset), uintptr(length), uintptr(advice), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fchown(fd int, uid int, gid int) (err error) {
_, _, e1 := Syscall(SYS_FCHOWN, uintptr(fd), uintptr(uid), uintptr(gid))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fstatat(dirfd int, path string, stat *Stat_t, flags int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall6(SYS_NEWFSTATAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(stat)), uintptr(flags), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Fstatfs(fd int, buf *Statfs_t) (err error) {
_, _, e1 := Syscall(SYS_FSTATFS, uintptr(fd), uintptr(unsafe.Pointer(buf)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Ftruncate(fd int, length int64) (err error) {
_, _, e1 := Syscall(SYS_FTRUNCATE, uintptr(fd), uintptr(length), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getegid() (egid int) {
r0, _ := RawSyscallNoError(SYS_GETEGID, 0, 0, 0)
egid = int(r0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Geteuid() (euid int) {
r0, _ := RawSyscallNoError(SYS_GETEUID, 0, 0, 0)
euid = int(r0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getgid() (gid int) {
r0, _ := RawSyscallNoError(SYS_GETGID, 0, 0, 0)
gid = int(r0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getrlimit(resource int, rlim *Rlimit) (err error) {
_, _, e1 := RawSyscall(SYS_GETRLIMIT, uintptr(resource), uintptr(unsafe.Pointer(rlim)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Getuid() (uid int) {
r0, _ := RawSyscallNoError(SYS_GETUID, 0, 0, 0)
uid = int(r0)
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Lchown(path string, uid int, gid int) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_LCHOWN, uintptr(unsafe.Pointer(_p0)), uintptr(uid), uintptr(gid))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Listen(s int, n int) (err error) {
_, _, e1 := Syscall(SYS_LISTEN, uintptr(s), uintptr(n), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Pause() (err error) {
_, _, e1 := Syscall(SYS_PAUSE, 0, 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Pread(fd int, p []byte, offset int64) (n int, err error) {
var _p0 unsafe.Pointer
if len(p) > 0 {
_p0 = unsafe.Pointer(&p[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_PREAD64, uintptr(fd), uintptr(_p0), uintptr(len(p)), uintptr(offset), 0, 0)
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Pwrite(fd int, p []byte, offset int64) (n int, err error) {
var _p0 unsafe.Pointer
if len(p) > 0 {
_p0 = unsafe.Pointer(&p[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_PWRITE64, uintptr(fd), uintptr(_p0), uintptr(len(p)), uintptr(offset), 0, 0)
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Seek(fd int, offset int64, whence int) (off int64, err error) {
r0, _, e1 := Syscall(SYS_LSEEK, uintptr(fd), uintptr(offset), uintptr(whence))
off = int64(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func sendfile(outfd int, infd int, offset *int64, count int) (written int, err error) {
r0, _, e1 := Syscall6(SYS_SENDFILE, uintptr(outfd), uintptr(infd), uintptr(unsafe.Pointer(offset)), uintptr(count), 0, 0)
written = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setfsgid(gid int) (err error) {
_, _, e1 := Syscall(SYS_SETFSGID, uintptr(gid), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setfsuid(uid int) (err error) {
_, _, e1 := Syscall(SYS_SETFSUID, uintptr(uid), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setregid(rgid int, egid int) (err error) {
_, _, e1 := RawSyscall(SYS_SETREGID, uintptr(rgid), uintptr(egid), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setresgid(rgid int, egid int, sgid int) (err error) {
_, _, e1 := RawSyscall(SYS_SETRESGID, uintptr(rgid), uintptr(egid), uintptr(sgid))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setresuid(ruid int, euid int, suid int) (err error) {
_, _, e1 := RawSyscall(SYS_SETRESUID, uintptr(ruid), uintptr(euid), uintptr(suid))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setrlimit(resource int, rlim *Rlimit) (err error) {
_, _, e1 := RawSyscall(SYS_SETRLIMIT, uintptr(resource), uintptr(unsafe.Pointer(rlim)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Setreuid(ruid int, euid int) (err error) {
_, _, e1 := RawSyscall(SYS_SETREUID, uintptr(ruid), uintptr(euid), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Shutdown(fd int, how int) (err error) {
_, _, e1 := Syscall(SYS_SHUTDOWN, uintptr(fd), uintptr(how), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Splice(rfd int, roff *int64, wfd int, woff *int64, len int, flags int) (n int64, err error) {
r0, _, e1 := Syscall6(SYS_SPLICE, uintptr(rfd), uintptr(unsafe.Pointer(roff)), uintptr(wfd), uintptr(unsafe.Pointer(woff)), uintptr(len), uintptr(flags))
n = int64(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Statfs(path string, buf *Statfs_t) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_STATFS, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(buf)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func SyncFileRange(fd int, off int64, n int64, flags int) (err error) {
_, _, e1 := Syscall6(SYS_SYNC_FILE_RANGE, uintptr(fd), uintptr(off), uintptr(n), uintptr(flags), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Truncate(path string, length int64) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_TRUNCATE, uintptr(unsafe.Pointer(_p0)), uintptr(length), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Ustat(dev int, ubuf *Ustat_t) (err error) {
_, _, e1 := Syscall(SYS_USTAT, uintptr(dev), uintptr(unsafe.Pointer(ubuf)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func accept(s int, rsa *RawSockaddrAny, addrlen *_Socklen) (fd int, err error) {
r0, _, e1 := Syscall(SYS_ACCEPT, uintptr(s), uintptr(unsafe.Pointer(rsa)), uintptr(unsafe.Pointer(addrlen)))
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func accept4(s int, rsa *RawSockaddrAny, addrlen *_Socklen, flags int) (fd int, err error) {
r0, _, e1 := Syscall6(SYS_ACCEPT4, uintptr(s), uintptr(unsafe.Pointer(rsa)), uintptr(unsafe.Pointer(addrlen)), uintptr(flags), 0, 0)
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func bind(s int, addr unsafe.Pointer, addrlen _Socklen) (err error) {
_, _, e1 := Syscall(SYS_BIND, uintptr(s), uintptr(addr), uintptr(addrlen))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func connect(s int, addr unsafe.Pointer, addrlen _Socklen) (err error) {
_, _, e1 := Syscall(SYS_CONNECT, uintptr(s), uintptr(addr), uintptr(addrlen))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func getgroups(n int, list *_Gid_t) (nn int, err error) {
r0, _, e1 := RawSyscall(SYS_GETGROUPS, uintptr(n), uintptr(unsafe.Pointer(list)), 0)
nn = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func setgroups(n int, list *_Gid_t) (err error) {
_, _, e1 := RawSyscall(SYS_SETGROUPS, uintptr(n), uintptr(unsafe.Pointer(list)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func getsockopt(s int, level int, name int, val unsafe.Pointer, vallen *_Socklen) (err error) {
_, _, e1 := Syscall6(SYS_GETSOCKOPT, uintptr(s), uintptr(level), uintptr(name), uintptr(val), uintptr(unsafe.Pointer(vallen)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func setsockopt(s int, level int, name int, val unsafe.Pointer, vallen uintptr) (err error) {
_, _, e1 := Syscall6(SYS_SETSOCKOPT, uintptr(s), uintptr(level), uintptr(name), uintptr(val), uintptr(vallen), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func socket(domain int, typ int, proto int) (fd int, err error) {
r0, _, e1 := RawSyscall(SYS_SOCKET, uintptr(domain), uintptr(typ), uintptr(proto))
fd = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func socketpair(domain int, typ int, proto int, fd *[2]int32) (err error) {
_, _, e1 := RawSyscall6(SYS_SOCKETPAIR, uintptr(domain), uintptr(typ), uintptr(proto), uintptr(unsafe.Pointer(fd)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func getpeername(fd int, rsa *RawSockaddrAny, addrlen *_Socklen) (err error) {
_, _, e1 := RawSyscall(SYS_GETPEERNAME, uintptr(fd), uintptr(unsafe.Pointer(rsa)), uintptr(unsafe.Pointer(addrlen)))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func getsockname(fd int, rsa *RawSockaddrAny, addrlen *_Socklen) (err error) {
_, _, e1 := RawSyscall(SYS_GETSOCKNAME, uintptr(fd), uintptr(unsafe.Pointer(rsa)), uintptr(unsafe.Pointer(addrlen)))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func recvfrom(fd int, p []byte, flags int, from *RawSockaddrAny, fromlen *_Socklen) (n int, err error) {
var _p0 unsafe.Pointer
if len(p) > 0 {
_p0 = unsafe.Pointer(&p[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
r0, _, e1 := Syscall6(SYS_RECVFROM, uintptr(fd), uintptr(_p0), uintptr(len(p)), uintptr(flags), uintptr(unsafe.Pointer(from)), uintptr(unsafe.Pointer(fromlen)))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func sendto(s int, buf []byte, flags int, to unsafe.Pointer, addrlen _Socklen) (err error) {
var _p0 unsafe.Pointer
if len(buf) > 0 {
_p0 = unsafe.Pointer(&buf[0])
} else {
_p0 = unsafe.Pointer(&_zero)
}
_, _, e1 := Syscall6(SYS_SENDTO, uintptr(s), uintptr(_p0), uintptr(len(buf)), uintptr(flags), uintptr(to), uintptr(addrlen))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func recvmsg(s int, msg *Msghdr, flags int) (n int, err error) {
r0, _, e1 := Syscall(SYS_RECVMSG, uintptr(s), uintptr(unsafe.Pointer(msg)), uintptr(flags))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func sendmsg(s int, msg *Msghdr, flags int) (n int, err error) {
r0, _, e1 := Syscall(SYS_SENDMSG, uintptr(s), uintptr(unsafe.Pointer(msg)), uintptr(flags))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func mmap(addr uintptr, length uintptr, prot int, flags int, fd int, offset int64) (xaddr uintptr, err error) {
r0, _, e1 := Syscall6(SYS_MMAP, uintptr(addr), uintptr(length), uintptr(prot), uintptr(flags), uintptr(fd), uintptr(offset))
xaddr = uintptr(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func futimesat(dirfd int, path string, times *[2]Timeval) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_FUTIMESAT, uintptr(dirfd), uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(times)))
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Gettimeofday(tv *Timeval) (err error) {
_, _, e1 := RawSyscall(SYS_GETTIMEOFDAY, uintptr(unsafe.Pointer(tv)), 0, 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func Utime(path string, buf *Utimbuf) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_UTIME, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(buf)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func utimes(path string, times *[2]Timeval) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_UTIMES, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(times)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func pipe2(p *[2]_C_int, flags int) (err error) {
_, _, e1 := RawSyscall(SYS_PIPE2, uintptr(unsafe.Pointer(p)), uintptr(flags), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func fstat(fd int, st *stat_t) (err error) {
_, _, e1 := Syscall(SYS_FSTAT, uintptr(fd), uintptr(unsafe.Pointer(st)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func lstat(path string, st *stat_t) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_LSTAT, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(st)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func stat(path string, st *stat_t) (err error) {
var _p0 *byte
_p0, err = BytePtrFromString(path)
if err != nil {
return
}
_, _, e1 := Syscall(SYS_STAT, uintptr(unsafe.Pointer(_p0)), uintptr(unsafe.Pointer(st)), 0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
// THIS FILE IS GENERATED BY THE COMMAND AT THE TOP; DO NOT EDIT
func poll(fds *PollFd, nfds int, timeout int) (n int, err error) {
r0, _, e1 := Syscall(SYS_POLL, uintptr(unsafe.Pointer(fds)), uintptr(nfds), uintptr(timeout))
n = int(r0)
if e1 != 0 {
err = errnoErr(e1)
}
return
}
| {
"pile_set_name": "Github"
} |
defmodule Serum.StructValidator.Project do
@moduledoc false
_moduledocp = "A module for validating Serum project configuration."
use Serum.StructValidator
define_validator do
key :title, required: true, rules: [is_binary: []]
key :description, rules: [is_binary: []]
key :base_url, required: true, rules: [is_binary: [], =~: [~r[^https?://]]]
key :authors, rules: [is_map: []]
key :blogs, rules: [map_or_false?: []]
key :theme, rules: [valid_theme_spec?: []]
key :plugins, rules: [is_list: []]
end
@spec map_or_false?(term()) :: boolean()
defp map_or_false?(value)
defp map_or_false?(%{}), do: true
defp map_or_false?(false), do: true
defp map_or_false?(_), do: false
@spec valid_theme_spec?(term()) :: boolean()
defp valid_theme_spec?(value)
defp valid_theme_spec?(nil), do: true
defp valid_theme_spec?(module) when is_atom(module), do: true
defp valid_theme_spec?({module, opts})
when not is_nil(module) and is_atom(module) and is_list(opts) do
true
end
defp valid_theme_spec?(_), do: false
end
| {
"pile_set_name": "Github"
} |
<?php
/**
* @link http://www.yiiframework.com/
* @copyright Copyright (c) 2008 Yii Software LLC
* @license http://www.yiiframework.com/license/
*/
namespace yii\filters;
use Yii;
use yii\base\ActionFilter;
use yii\web\Request;
use yii\web\Response;
use yii\web\TooManyRequestsHttpException;
/**
* RateLimiter implements a rate limiting algorithm based on the [leaky bucket algorithm](http://en.wikipedia.org/wiki/Leaky_bucket).
*
* You may use RateLimiter by attaching it as a behavior to a controller or module, like the following,
*
* ```php
* public function behaviors()
* {
* return [
* 'rateLimiter' => [
* 'class' => \yii\filters\RateLimiter::className(),
* ],
* ];
* }
* ```
*
* When the user has exceeded his rate limit, RateLimiter will throw a [[TooManyRequestsHttpException]] exception.
*
* Note that RateLimiter requires [[user]] to implement the [[RateLimitInterface]]. RateLimiter will
* do nothing if [[user]] is not set or does not implement [[RateLimitInterface]].
*
* @author Qiang Xue <qiang.xue@gmail.com>
* @since 2.0
*/
class RateLimiter extends ActionFilter
{
/**
* @var bool whether to include rate limit headers in the response
*/
public $enableRateLimitHeaders = true;
/**
* @var string the message to be displayed when rate limit exceeds
*/
public $errorMessage = 'Rate limit exceeded.';
/**
* @var RateLimitInterface the user object that implements the RateLimitInterface.
* If not set, it will take the value of `Yii::$app->user->getIdentity(false)`.
*/
public $user;
/**
* @var Request the current request. If not set, the `request` application component will be used.
*/
public $request;
/**
* @var Response the response to be sent. If not set, the `response` application component will be used.
*/
public $response;
/**
* @inheritdoc
*/
public function init()
{
if ($this->request === null) {
$this->request = Yii::$app->getRequest();
}
if ($this->response === null) {
$this->response = Yii::$app->getResponse();
}
}
/**
* @inheritdoc
*/
public function beforeAction($action)
{
if ($this->user === null && Yii::$app->getUser()) {
$this->user = Yii::$app->getUser()->getIdentity(false);
}
if ($this->user instanceof RateLimitInterface) {
Yii::trace('Check rate limit', __METHOD__);
$this->checkRateLimit($this->user, $this->request, $this->response, $action);
} elseif ($this->user) {
Yii::info('Rate limit skipped: "user" does not implement RateLimitInterface.', __METHOD__);
} else {
Yii::info('Rate limit skipped: user not logged in.', __METHOD__);
}
return true;
}
/**
* Checks whether the rate limit exceeds.
* @param RateLimitInterface $user the current user
* @param Request $request
* @param Response $response
* @param \yii\base\Action $action the action to be executed
* @throws TooManyRequestsHttpException if rate limit exceeds
*/
public function checkRateLimit($user, $request, $response, $action)
{
$current = time();
list ($limit, $window) = $user->getRateLimit($request, $action);
list ($allowance, $timestamp) = $user->loadAllowance($request, $action);
$allowance += (int) (($current - $timestamp) * $limit / $window);
if ($allowance > $limit) {
$allowance = $limit;
}
if ($allowance < 1) {
$user->saveAllowance($request, $action, 0, $current);
$this->addRateLimitHeaders($response, $limit, 0, $window);
throw new TooManyRequestsHttpException($this->errorMessage);
} else {
$user->saveAllowance($request, $action, $allowance - 1, $current);
$this->addRateLimitHeaders($response, $limit, $allowance - 1, (int) (($limit - $allowance) * $window / $limit));
}
}
/**
* Adds the rate limit headers to the response
* @param Response $response
* @param int $limit the maximum number of allowed requests during a period
* @param int $remaining the remaining number of allowed requests within the current period
* @param int $reset the number of seconds to wait before having maximum number of allowed requests again
*/
public function addRateLimitHeaders($response, $limit, $remaining, $reset)
{
if ($this->enableRateLimitHeaders) {
$response->getHeaders()
->set('X-Rate-Limit-Limit', $limit)
->set('X-Rate-Limit-Remaining', $remaining)
->set('X-Rate-Limit-Reset', $reset);
}
}
}
| {
"pile_set_name": "Github"
} |
// +build !ignore_autogenerated
/*
Copyright 2018 The Kubernetes Authors.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
// This file was autogenerated by conversion-gen. Do not edit it manually!
package v1beta1
import (
apiextensions "k8s.io/apiextensions-apiserver/pkg/apis/apiextensions"
conversion "k8s.io/apimachinery/pkg/conversion"
runtime "k8s.io/apimachinery/pkg/runtime"
unsafe "unsafe"
)
func init() {
SchemeBuilder.Register(RegisterConversions)
}
// RegisterConversions adds conversion functions to the given scheme.
// Public to allow building arbitrary schemes.
func RegisterConversions(scheme *runtime.Scheme) error {
return scheme.AddGeneratedConversionFuncs(
Convert_v1beta1_CustomResourceDefinition_To_apiextensions_CustomResourceDefinition,
Convert_apiextensions_CustomResourceDefinition_To_v1beta1_CustomResourceDefinition,
Convert_v1beta1_CustomResourceDefinitionCondition_To_apiextensions_CustomResourceDefinitionCondition,
Convert_apiextensions_CustomResourceDefinitionCondition_To_v1beta1_CustomResourceDefinitionCondition,
Convert_v1beta1_CustomResourceDefinitionList_To_apiextensions_CustomResourceDefinitionList,
Convert_apiextensions_CustomResourceDefinitionList_To_v1beta1_CustomResourceDefinitionList,
Convert_v1beta1_CustomResourceDefinitionNames_To_apiextensions_CustomResourceDefinitionNames,
Convert_apiextensions_CustomResourceDefinitionNames_To_v1beta1_CustomResourceDefinitionNames,
Convert_v1beta1_CustomResourceDefinitionSpec_To_apiextensions_CustomResourceDefinitionSpec,
Convert_apiextensions_CustomResourceDefinitionSpec_To_v1beta1_CustomResourceDefinitionSpec,
Convert_v1beta1_CustomResourceDefinitionStatus_To_apiextensions_CustomResourceDefinitionStatus,
Convert_apiextensions_CustomResourceDefinitionStatus_To_v1beta1_CustomResourceDefinitionStatus,
)
}
func autoConvert_v1beta1_CustomResourceDefinition_To_apiextensions_CustomResourceDefinition(in *CustomResourceDefinition, out *apiextensions.CustomResourceDefinition, s conversion.Scope) error {
out.ObjectMeta = in.ObjectMeta
if err := Convert_v1beta1_CustomResourceDefinitionSpec_To_apiextensions_CustomResourceDefinitionSpec(&in.Spec, &out.Spec, s); err != nil {
return err
}
if err := Convert_v1beta1_CustomResourceDefinitionStatus_To_apiextensions_CustomResourceDefinitionStatus(&in.Status, &out.Status, s); err != nil {
return err
}
return nil
}
// Convert_v1beta1_CustomResourceDefinition_To_apiextensions_CustomResourceDefinition is an autogenerated conversion function.
func Convert_v1beta1_CustomResourceDefinition_To_apiextensions_CustomResourceDefinition(in *CustomResourceDefinition, out *apiextensions.CustomResourceDefinition, s conversion.Scope) error {
return autoConvert_v1beta1_CustomResourceDefinition_To_apiextensions_CustomResourceDefinition(in, out, s)
}
func autoConvert_apiextensions_CustomResourceDefinition_To_v1beta1_CustomResourceDefinition(in *apiextensions.CustomResourceDefinition, out *CustomResourceDefinition, s conversion.Scope) error {
out.ObjectMeta = in.ObjectMeta
if err := Convert_apiextensions_CustomResourceDefinitionSpec_To_v1beta1_CustomResourceDefinitionSpec(&in.Spec, &out.Spec, s); err != nil {
return err
}
if err := Convert_apiextensions_CustomResourceDefinitionStatus_To_v1beta1_CustomResourceDefinitionStatus(&in.Status, &out.Status, s); err != nil {
return err
}
return nil
}
// Convert_apiextensions_CustomResourceDefinition_To_v1beta1_CustomResourceDefinition is an autogenerated conversion function.
func Convert_apiextensions_CustomResourceDefinition_To_v1beta1_CustomResourceDefinition(in *apiextensions.CustomResourceDefinition, out *CustomResourceDefinition, s conversion.Scope) error {
return autoConvert_apiextensions_CustomResourceDefinition_To_v1beta1_CustomResourceDefinition(in, out, s)
}
func autoConvert_v1beta1_CustomResourceDefinitionCondition_To_apiextensions_CustomResourceDefinitionCondition(in *CustomResourceDefinitionCondition, out *apiextensions.CustomResourceDefinitionCondition, s conversion.Scope) error {
out.Type = apiextensions.CustomResourceDefinitionConditionType(in.Type)
out.Status = apiextensions.ConditionStatus(in.Status)
out.LastTransitionTime = in.LastTransitionTime
out.Reason = in.Reason
out.Message = in.Message
return nil
}
// Convert_v1beta1_CustomResourceDefinitionCondition_To_apiextensions_CustomResourceDefinitionCondition is an autogenerated conversion function.
func Convert_v1beta1_CustomResourceDefinitionCondition_To_apiextensions_CustomResourceDefinitionCondition(in *CustomResourceDefinitionCondition, out *apiextensions.CustomResourceDefinitionCondition, s conversion.Scope) error {
return autoConvert_v1beta1_CustomResourceDefinitionCondition_To_apiextensions_CustomResourceDefinitionCondition(in, out, s)
}
func autoConvert_apiextensions_CustomResourceDefinitionCondition_To_v1beta1_CustomResourceDefinitionCondition(in *apiextensions.CustomResourceDefinitionCondition, out *CustomResourceDefinitionCondition, s conversion.Scope) error {
out.Type = CustomResourceDefinitionConditionType(in.Type)
out.Status = ConditionStatus(in.Status)
out.LastTransitionTime = in.LastTransitionTime
out.Reason = in.Reason
out.Message = in.Message
return nil
}
// Convert_apiextensions_CustomResourceDefinitionCondition_To_v1beta1_CustomResourceDefinitionCondition is an autogenerated conversion function.
func Convert_apiextensions_CustomResourceDefinitionCondition_To_v1beta1_CustomResourceDefinitionCondition(in *apiextensions.CustomResourceDefinitionCondition, out *CustomResourceDefinitionCondition, s conversion.Scope) error {
return autoConvert_apiextensions_CustomResourceDefinitionCondition_To_v1beta1_CustomResourceDefinitionCondition(in, out, s)
}
func autoConvert_v1beta1_CustomResourceDefinitionList_To_apiextensions_CustomResourceDefinitionList(in *CustomResourceDefinitionList, out *apiextensions.CustomResourceDefinitionList, s conversion.Scope) error {
out.ListMeta = in.ListMeta
out.Items = *(*[]apiextensions.CustomResourceDefinition)(unsafe.Pointer(&in.Items))
return nil
}
// Convert_v1beta1_CustomResourceDefinitionList_To_apiextensions_CustomResourceDefinitionList is an autogenerated conversion function.
func Convert_v1beta1_CustomResourceDefinitionList_To_apiextensions_CustomResourceDefinitionList(in *CustomResourceDefinitionList, out *apiextensions.CustomResourceDefinitionList, s conversion.Scope) error {
return autoConvert_v1beta1_CustomResourceDefinitionList_To_apiextensions_CustomResourceDefinitionList(in, out, s)
}
func autoConvert_apiextensions_CustomResourceDefinitionList_To_v1beta1_CustomResourceDefinitionList(in *apiextensions.CustomResourceDefinitionList, out *CustomResourceDefinitionList, s conversion.Scope) error {
out.ListMeta = in.ListMeta
if in.Items == nil {
out.Items = make([]CustomResourceDefinition, 0)
} else {
out.Items = *(*[]CustomResourceDefinition)(unsafe.Pointer(&in.Items))
}
return nil
}
// Convert_apiextensions_CustomResourceDefinitionList_To_v1beta1_CustomResourceDefinitionList is an autogenerated conversion function.
func Convert_apiextensions_CustomResourceDefinitionList_To_v1beta1_CustomResourceDefinitionList(in *apiextensions.CustomResourceDefinitionList, out *CustomResourceDefinitionList, s conversion.Scope) error {
return autoConvert_apiextensions_CustomResourceDefinitionList_To_v1beta1_CustomResourceDefinitionList(in, out, s)
}
func autoConvert_v1beta1_CustomResourceDefinitionNames_To_apiextensions_CustomResourceDefinitionNames(in *CustomResourceDefinitionNames, out *apiextensions.CustomResourceDefinitionNames, s conversion.Scope) error {
out.Plural = in.Plural
out.Singular = in.Singular
out.ShortNames = *(*[]string)(unsafe.Pointer(&in.ShortNames))
out.Kind = in.Kind
out.ListKind = in.ListKind
return nil
}
// Convert_v1beta1_CustomResourceDefinitionNames_To_apiextensions_CustomResourceDefinitionNames is an autogenerated conversion function.
func Convert_v1beta1_CustomResourceDefinitionNames_To_apiextensions_CustomResourceDefinitionNames(in *CustomResourceDefinitionNames, out *apiextensions.CustomResourceDefinitionNames, s conversion.Scope) error {
return autoConvert_v1beta1_CustomResourceDefinitionNames_To_apiextensions_CustomResourceDefinitionNames(in, out, s)
}
func autoConvert_apiextensions_CustomResourceDefinitionNames_To_v1beta1_CustomResourceDefinitionNames(in *apiextensions.CustomResourceDefinitionNames, out *CustomResourceDefinitionNames, s conversion.Scope) error {
out.Plural = in.Plural
out.Singular = in.Singular
out.ShortNames = *(*[]string)(unsafe.Pointer(&in.ShortNames))
out.Kind = in.Kind
out.ListKind = in.ListKind
return nil
}
// Convert_apiextensions_CustomResourceDefinitionNames_To_v1beta1_CustomResourceDefinitionNames is an autogenerated conversion function.
func Convert_apiextensions_CustomResourceDefinitionNames_To_v1beta1_CustomResourceDefinitionNames(in *apiextensions.CustomResourceDefinitionNames, out *CustomResourceDefinitionNames, s conversion.Scope) error {
return autoConvert_apiextensions_CustomResourceDefinitionNames_To_v1beta1_CustomResourceDefinitionNames(in, out, s)
}
func autoConvert_v1beta1_CustomResourceDefinitionSpec_To_apiextensions_CustomResourceDefinitionSpec(in *CustomResourceDefinitionSpec, out *apiextensions.CustomResourceDefinitionSpec, s conversion.Scope) error {
out.Group = in.Group
out.Version = in.Version
if err := Convert_v1beta1_CustomResourceDefinitionNames_To_apiextensions_CustomResourceDefinitionNames(&in.Names, &out.Names, s); err != nil {
return err
}
out.Scope = apiextensions.ResourceScope(in.Scope)
return nil
}
// Convert_v1beta1_CustomResourceDefinitionSpec_To_apiextensions_CustomResourceDefinitionSpec is an autogenerated conversion function.
func Convert_v1beta1_CustomResourceDefinitionSpec_To_apiextensions_CustomResourceDefinitionSpec(in *CustomResourceDefinitionSpec, out *apiextensions.CustomResourceDefinitionSpec, s conversion.Scope) error {
return autoConvert_v1beta1_CustomResourceDefinitionSpec_To_apiextensions_CustomResourceDefinitionSpec(in, out, s)
}
func autoConvert_apiextensions_CustomResourceDefinitionSpec_To_v1beta1_CustomResourceDefinitionSpec(in *apiextensions.CustomResourceDefinitionSpec, out *CustomResourceDefinitionSpec, s conversion.Scope) error {
out.Group = in.Group
out.Version = in.Version
if err := Convert_apiextensions_CustomResourceDefinitionNames_To_v1beta1_CustomResourceDefinitionNames(&in.Names, &out.Names, s); err != nil {
return err
}
out.Scope = ResourceScope(in.Scope)
return nil
}
// Convert_apiextensions_CustomResourceDefinitionSpec_To_v1beta1_CustomResourceDefinitionSpec is an autogenerated conversion function.
func Convert_apiextensions_CustomResourceDefinitionSpec_To_v1beta1_CustomResourceDefinitionSpec(in *apiextensions.CustomResourceDefinitionSpec, out *CustomResourceDefinitionSpec, s conversion.Scope) error {
return autoConvert_apiextensions_CustomResourceDefinitionSpec_To_v1beta1_CustomResourceDefinitionSpec(in, out, s)
}
func autoConvert_v1beta1_CustomResourceDefinitionStatus_To_apiextensions_CustomResourceDefinitionStatus(in *CustomResourceDefinitionStatus, out *apiextensions.CustomResourceDefinitionStatus, s conversion.Scope) error {
out.Conditions = *(*[]apiextensions.CustomResourceDefinitionCondition)(unsafe.Pointer(&in.Conditions))
if err := Convert_v1beta1_CustomResourceDefinitionNames_To_apiextensions_CustomResourceDefinitionNames(&in.AcceptedNames, &out.AcceptedNames, s); err != nil {
return err
}
return nil
}
// Convert_v1beta1_CustomResourceDefinitionStatus_To_apiextensions_CustomResourceDefinitionStatus is an autogenerated conversion function.
func Convert_v1beta1_CustomResourceDefinitionStatus_To_apiextensions_CustomResourceDefinitionStatus(in *CustomResourceDefinitionStatus, out *apiextensions.CustomResourceDefinitionStatus, s conversion.Scope) error {
return autoConvert_v1beta1_CustomResourceDefinitionStatus_To_apiextensions_CustomResourceDefinitionStatus(in, out, s)
}
func autoConvert_apiextensions_CustomResourceDefinitionStatus_To_v1beta1_CustomResourceDefinitionStatus(in *apiextensions.CustomResourceDefinitionStatus, out *CustomResourceDefinitionStatus, s conversion.Scope) error {
if in.Conditions == nil {
out.Conditions = make([]CustomResourceDefinitionCondition, 0)
} else {
out.Conditions = *(*[]CustomResourceDefinitionCondition)(unsafe.Pointer(&in.Conditions))
}
if err := Convert_apiextensions_CustomResourceDefinitionNames_To_v1beta1_CustomResourceDefinitionNames(&in.AcceptedNames, &out.AcceptedNames, s); err != nil {
return err
}
return nil
}
// Convert_apiextensions_CustomResourceDefinitionStatus_To_v1beta1_CustomResourceDefinitionStatus is an autogenerated conversion function.
func Convert_apiextensions_CustomResourceDefinitionStatus_To_v1beta1_CustomResourceDefinitionStatus(in *apiextensions.CustomResourceDefinitionStatus, out *CustomResourceDefinitionStatus, s conversion.Scope) error {
return autoConvert_apiextensions_CustomResourceDefinitionStatus_To_v1beta1_CustomResourceDefinitionStatus(in, out, s)
}
| {
"pile_set_name": "Github"
} |
/**
* @fileoverview unix-style formatter.
* @author oshi-shinobu
*/
"use strict";
//------------------------------------------------------------------------------
// Helper Functions
//------------------------------------------------------------------------------
/**
* Returns a canonical error level string based upon the error message passed in.
* @param {Object} message Individual error message provided by eslint
* @returns {string} Error level string
*/
function getMessageType(message) {
if (message.fatal || message.severity === 2) {
return "Error";
}
return "Warning";
}
//------------------------------------------------------------------------------
// Public Interface
//------------------------------------------------------------------------------
module.exports = function(results) {
let output = "",
total = 0;
results.forEach(result => {
const messages = result.messages;
total += messages.length;
messages.forEach(message => {
output += `${result.filePath}:`;
output += `${message.line || 0}:`;
output += `${message.column || 0}:`;
output += ` ${message.message} `;
output += `[${getMessageType(message)}${message.ruleId ? `/${message.ruleId}` : ""}]`;
output += "\n";
});
});
if (total > 0) {
output += `\n${total} problem${total !== 1 ? "s" : ""}`;
}
return output;
};
| {
"pile_set_name": "Github"
} |
/**
* Autogenerated by Thrift
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package test.fixtures.module0;
import com.facebook.swift.codec.*;
import com.facebook.swift.codec.ThriftField.Requiredness;
import com.facebook.swift.codec.ThriftField.Recursiveness;
import com.google.common.collect.*;
import java.util.*;
import org.apache.thrift.*;
import org.apache.thrift.async.*;
import org.apache.thrift.meta_data.*;
import org.apache.thrift.server.*;
import org.apache.thrift.transport.*;
import org.apache.thrift.protocol.*;
import org.apache.thrift.meta_data.FieldValueMetaData;
import static com.google.common.base.MoreObjects.toStringHelper;
import static com.google.common.base.MoreObjects.ToStringHelper;
@SwiftGenerated
@ThriftStruct(value="Struct", builder=Struct.Builder.class)
public final class Struct {
private BitSet __isset_bit_vector = new BitSet();
@ThriftConstructor
public Struct(
@ThriftField(value=1, name="first", requiredness=Requiredness.NONE) final int first,
@ThriftField(value=2, name="second", requiredness=Requiredness.NONE) final String second
) {
this.first = first;
this.second = second;
}
@ThriftConstructor
protected Struct() {
this.first = 0;
this.second = null;
}
public static class Builder {
private final BitSet __optional_isset = new BitSet();
private int first = 0;
private String second = null;
@ThriftField(value=1, name="first", requiredness=Requiredness.NONE)
public Builder setFirst(int first) {
this.first = first;
return this;
}
public int getFirst() { return first; }
@ThriftField(value=2, name="second", requiredness=Requiredness.NONE)
public Builder setSecond(String second) {
this.second = second;
return this;
}
public String getSecond() { return second; }
public Builder() { }
public Builder(Struct other) {
this.first = other.first;
this.second = other.second;
}
@ThriftConstructor
public Struct build() {
Struct result = new Struct (
this.first,
this.second
);
result.__isset_bit_vector.or(__optional_isset);
return result;
}
}
public static final Map<String, Integer> NAMES_TO_IDS = new HashMap();
public static final Map<Integer, TField> FIELD_METADATA = new HashMap<>();
private static final TStruct STRUCT_DESC = new TStruct("Struct");
private final int first;
public static final int _FIRST = 1;
private static final TField FIRST_FIELD_DESC = new TField("first", TType.I32, (short)1);
private final String second;
public static final int _SECOND = 2;
private static final TField SECOND_FIELD_DESC = new TField("second", TType.STRING, (short)2);
static {
NAMES_TO_IDS.put("first", 1);
FIELD_METADATA.put(1, FIRST_FIELD_DESC);
NAMES_TO_IDS.put("second", 2);
FIELD_METADATA.put(2, SECOND_FIELD_DESC);
}
@ThriftField(value=1, name="first", requiredness=Requiredness.NONE)
public int getFirst() { return first; }
/** don't use this method for new code, it's here to make migrating to swift easier */
@Deprecated
public boolean fieldIsSetFirst() {
return __isset_bit_vector.get(_FIRST);
}
@ThriftField(value=2, name="second", requiredness=Requiredness.NONE)
public String getSecond() { return second; }
/** don't use this method for new code, it's here to make migrating to swift easier */
@Deprecated
public boolean fieldIsSetSecond() {
return this.second != null;
}
@Override
public String toString() {
ToStringHelper helper = toStringHelper(this);
helper.add("first", first);
helper.add("second", second);
return helper.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Struct other = (Struct)o;
return
Objects.equals(first, other.first) &&
Objects.equals(second, other.second) &&
true;
}
@Override
public int hashCode() {
return Arrays.deepHashCode(new Object[] {
first,
second
});
}
public static Struct read0(TProtocol oprot) throws TException {
TField __field;
oprot.readStructBegin(Struct.NAMES_TO_IDS, Struct.FIELD_METADATA);
Struct.Builder builder = new Struct.Builder();
while (true) {
__field = oprot.readFieldBegin();
if (__field.type == TType.STOP) { break; }
switch (__field.id) {
case _FIRST:
if (__field.type == TType.I32) {
int first = oprot.readI32();
builder.setFirst(first);
} else {
TProtocolUtil.skip(oprot, __field.type);
}
break;
case _SECOND:
if (__field.type == TType.STRING) {
String second = oprot.readString();
builder.setSecond(second);
} else {
TProtocolUtil.skip(oprot, __field.type);
}
break;
default:
TProtocolUtil.skip(oprot, __field.type);
break;
}
oprot.readFieldEnd();
}
oprot.readStructEnd();
return builder.build();
}
public void write0(TProtocol oprot) throws TException {
oprot.writeStructBegin(STRUCT_DESC);
oprot.writeFieldBegin(FIRST_FIELD_DESC);
oprot.writeI32(this.first);
oprot.writeFieldEnd();
if (this.second != null) {
oprot.writeFieldBegin(SECOND_FIELD_DESC);
oprot.writeString(this.second);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
| {
"pile_set_name": "Github"
} |
//
// Copyright (C) 2019 Dominik S. Buse <buse@ccs-labs.org>
//
// Documentation for these modules is at http://veins.car2x.org/
//
// SPDX-License-Identifier: GPL-2.0-or-later
//
// This program is free software; you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation; either version 2 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program; if not, write to the Free Software
// Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
//
#include <cmath>
#include "veins/modules/utility/BBoxLookup.h"
namespace {
using Point = veins::BBoxLookup::Point;
using Box = veins::BBoxLookup::Box;
/**
* Helper structure representing a wireless ray from a sender to a receiver.
*
* Contains pre-computed values to speed up calls to intersect with the same ray but different boxes.
*/
struct Ray {
Point origin;
Point destination;
Point direction;
Point invDirection;
struct {
size_t x;
size_t y;
} sign;
double length;
};
/**
* Return a Ray struct for fast intersection tests from sender to receiver.
*/
Ray makeRay(const Point& sender, const Point& receiver)
{
const double dir_x = receiver.x - sender.x;
const double dir_y = receiver.y - sender.y;
Ray ray;
ray.origin = sender;
ray.destination = receiver;
ray.length = std::sqrt(dir_x * dir_x + dir_y * dir_y);
ray.direction.x = dir_x / ray.length;
ray.direction.y = dir_y / ray.length;
ray.invDirection.x = 1.0 / ray.direction.x;
ray.invDirection.y = 1.0 / ray.direction.y;
ray.sign.x = ray.invDirection.x < 0;
ray.sign.y = ray.invDirection.y < 0;
return ray;
}
/**
* Return whether ray intersects with box.
*
* Based on:
* Amy Williams, Steve Barrus, R. Keith Morley & Peter Shirley (2005) An Efficient and Robust Ray-Box Intersection Algorithm, Journal of Graphics Tools, 10:1, 49-54, DOI: 10.1080/2151237X.2005.10129188
*/
bool intersects(const Ray& ray, const Box& box)
{
const double x[2]{box.p1.x, box.p2.x};
const double y[2]{box.p1.y, box.p2.y};
double tmin = (x[ray.sign.x] - ray.origin.x) * ray.invDirection.x;
double tmax = (x[1 - ray.sign.x] - ray.origin.x) * ray.invDirection.x;
double tymin = (y[ray.sign.y] - ray.origin.y) * ray.invDirection.y;
double tymax = (y[1 - ray.sign.y] - ray.origin.y) * ray.invDirection.y;
if ((tmin > tymax) || (tymin > tmax)) return false;
if (tymin > tmin) tmin = tymin;
if (tymax < tmax) tmax = tymax;
return (tmin < ray.length) && (tmax > 0);
}
} // anonymous namespace
namespace veins {
BBoxLookup::BBoxLookup(const std::vector<Obstacle*>& obstacles, std::function<BBoxLookup::Box(Obstacle*)> makeBBox, double scenarioX, double scenarioY, int cellSize)
: bboxes()
, obstacleLookup()
, bboxCells()
, cellSize(cellSize)
, numCols(std::floor(scenarioX / cellSize) + 1)
, numRows(std::floor(scenarioY / cellSize) + 1)
{
// phase 1: build unordered collection of cells
// initialize proto-cells (cells in non-contiguos memory)
ASSERT(scenarioX > 0);
ASSERT(scenarioY > 0);
ASSERT(numCols * cellSize >= scenarioX);
ASSERT(numRows * cellSize >= scenarioY);
const size_t numCells = numCols * numRows;
std::vector<std::vector<BBoxLookup::Box>> protoCells(numCells);
std::vector<std::vector<Obstacle*>> protoLookup(numCells);
// fill protoCells with boundingBoxes
size_t numEntries = 0;
for (const auto obstaclePtr : obstacles) {
auto bbox = makeBBox(obstaclePtr);
const size_t fromCol = std::min(size_t(std::max(0, int(bbox.p1.x / cellSize))), numCols - 1);
const size_t toCol = std::min(size_t(std::max(0, int(bbox.p2.x / cellSize))), numCols - 1);
const size_t fromRow = std::min(size_t(std::max(0, int(bbox.p1.y / cellSize))), numRows - 1);
const size_t toRow = std::min(size_t(std::max(0, int(bbox.p2.y / cellSize))), numRows - 1);
for (size_t row = fromRow; row <= toRow; ++row) {
for (size_t col = fromCol; col <= toCol; ++col) {
ASSERT(row >= 0);
ASSERT(col >= 0);
ASSERT(row < numRows);
ASSERT(col < numCols);
const size_t cellIndex = col + row * numCols;
protoCells[cellIndex].push_back(bbox);
protoLookup[cellIndex].push_back(obstaclePtr);
++numEntries;
ASSERT(protoCells[cellIndex].size() == protoLookup[cellIndex].size());
}
}
}
// phase 2: derive read-only data structure with fast lookup
bboxes.reserve(numEntries);
obstacleLookup.reserve(numEntries);
bboxCells.reserve(numCells);
size_t index = 0;
for (size_t row = 0; row < numRows; ++row) {
for (size_t col = 0; col < numCols; ++col) {
const size_t cellIndex = col + row * numCols;
auto& currentCell = protoCells.at(cellIndex);
auto& currentLookup = protoLookup.at(cellIndex);
ASSERT(currentCell.size() == currentLookup.size());
const size_t count = currentCell.size();
// copy over bboxes and obstacle lookups (in strict order)
for (size_t entryIndex = 0; entryIndex < count; ++entryIndex) {
bboxes.push_back(currentCell.at(entryIndex));
obstacleLookup.push_back(currentLookup.at(entryIndex));
}
// create lookup table for this cell
bboxCells.push_back({index, count});
// forward index to begin of next cell
index += count;
ASSERT(bboxes.size() == index);
}
}
ASSERT(bboxes.size() == numEntries);
ASSERT(bboxes.size() == obstacleLookup.size());
}
std::vector<Obstacle*> BBoxLookup::findOverlapping(Point sender, Point receiver) const
{
std::vector<Obstacle*> overlappingObstacles;
const Box bbox{
{std::min(sender.x, receiver.x), std::min(sender.y, receiver.y)},
{std::max(sender.x, receiver.x), std::max(sender.y, receiver.y)},
};
// determine coordinates for all cells touched by bbox
const size_t firstCol = std::min(size_t(std::max(0, int(bbox.p1.x / cellSize))), numCols - 1);
const size_t lastCol = std::min(size_t(std::max(0, int(bbox.p2.x / cellSize))), numCols - 1);
const size_t firstRow = std::min(size_t(std::max(0, int(bbox.p1.y / cellSize))), numRows - 1);
const size_t lastRow = std::min(size_t(std::max(0, int(bbox.p2.y / cellSize))), numRows - 1);
ASSERT(lastCol < numCols && lastRow < numRows);
// precompute transmission ray properties
const Ray ray = makeRay(sender, receiver);
// iterate over cells
for (size_t row = firstRow; row <= lastRow; ++row) {
for (size_t col = firstCol; col <= lastCol; ++col) {
// skip cell if ray does not intersect with the cell.
const Box cellBox = {{static_cast<double>(col * cellSize), static_cast<double>(row * cellSize)}, {static_cast<double>((col + 1) * cellSize), static_cast<double>((row + 1) * cellSize)}};
if (!intersects(ray, cellBox)) continue;
// derive cell for current cell coordinates
const size_t cellIndex = col + row * numCols;
const BBoxCell& cell = bboxCells.at(cellIndex);
// iterate over bboxes in each cell
for (size_t bboxIndex = cell.index; bboxIndex < cell.index + cell.count; ++bboxIndex) {
const Box& current = bboxes.at(bboxIndex);
// check for overlap with bbox (fast rejection)
if (current.p2.x < bbox.p1.x) continue;
if (current.p1.x > bbox.p2.x) continue;
if (current.p2.y < bbox.p1.y) continue;
if (current.p1.y > bbox.p2.y) continue;
// derive corresponding obstacle
if (!intersects(ray, current)) continue;
overlappingObstacles.push_back(obstacleLookup.at(bboxIndex));
}
}
}
return overlappingObstacles;
}
} // namespace veins
| {
"pile_set_name": "Github"
} |
require('../../../modules/es6.number.to-fixed');
module.exports = require('../../../modules/_entry-virtual')('Number').toFixed; | {
"pile_set_name": "Github"
} |
CREATE TABLE list (id VARCHAR(2) NOT NULL, value VARCHAR(64) NOT NULL, PRIMARY KEY(id)) DEFAULT CHARACTER SET utf8 COLLATE utf8_unicode_ci ENGINE = InnoDB;
INSERT INTO `list` (`id`, `value`) VALUES ('af', 'Afrikaans');
INSERT INTO `list` (`id`, `value`) VALUES ('af_NA', 'Afrikaans (Namibia)');
INSERT INTO `list` (`id`, `value`) VALUES ('af_ZA', 'Afrikaans (South Africa)');
INSERT INTO `list` (`id`, `value`) VALUES ('ak', 'Akan');
INSERT INTO `list` (`id`, `value`) VALUES ('ak_GH', 'Akan (Ghana)');
INSERT INTO `list` (`id`, `value`) VALUES ('sq', 'Albanian');
INSERT INTO `list` (`id`, `value`) VALUES ('sq_AL', 'Albanian (Albania)');
INSERT INTO `list` (`id`, `value`) VALUES ('sq_XK', 'Albanian (Kosovo)');
INSERT INTO `list` (`id`, `value`) VALUES ('sq_MK', 'Albanian (Macedonia)');
INSERT INTO `list` (`id`, `value`) VALUES ('am', 'Amharic');
INSERT INTO `list` (`id`, `value`) VALUES ('am_ET', 'Amharic (Ethiopia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar', 'Arabic');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_DZ', 'Arabic (Algeria)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_BH', 'Arabic (Bahrain)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_TD', 'Arabic (Chad)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_KM', 'Arabic (Comoros)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_DJ', 'Arabic (Djibouti)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_EG', 'Arabic (Egypt)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_ER', 'Arabic (Eritrea)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_IQ', 'Arabic (Iraq)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_IL', 'Arabic (Israel)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_JO', 'Arabic (Jordan)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_KW', 'Arabic (Kuwait)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_LB', 'Arabic (Lebanon)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_LY', 'Arabic (Libya)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_MR', 'Arabic (Mauritania)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_MA', 'Arabic (Morocco)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_OM', 'Arabic (Oman)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_PS', 'Arabic (Palestinian Territories)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_QA', 'Arabic (Qatar)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_SA', 'Arabic (Saudi Arabia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_SO', 'Arabic (Somalia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_SS', 'Arabic (South Sudan)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_SD', 'Arabic (Sudan)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_SY', 'Arabic (Syria)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_TN', 'Arabic (Tunisia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_AE', 'Arabic (United Arab Emirates)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_EH', 'Arabic (Western Sahara)');
INSERT INTO `list` (`id`, `value`) VALUES ('ar_YE', 'Arabic (Yemen)');
INSERT INTO `list` (`id`, `value`) VALUES ('hy', 'Armenian');
INSERT INTO `list` (`id`, `value`) VALUES ('hy_AM', 'Armenian (Armenia)');
INSERT INTO `list` (`id`, `value`) VALUES ('as', 'Assamese');
INSERT INTO `list` (`id`, `value`) VALUES ('as_IN', 'Assamese (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('az', 'Azerbaijani');
INSERT INTO `list` (`id`, `value`) VALUES ('az_AZ', 'Azerbaijani (Azerbaijan)');
INSERT INTO `list` (`id`, `value`) VALUES ('az_Cyrl_AZ', 'Azerbaijani (Cyrillic, Azerbaijan)');
INSERT INTO `list` (`id`, `value`) VALUES ('az_Cyrl', 'Azerbaijani (Cyrillic)');
INSERT INTO `list` (`id`, `value`) VALUES ('az_Latn_AZ', 'Azerbaijani (Latin, Azerbaijan)');
INSERT INTO `list` (`id`, `value`) VALUES ('az_Latn', 'Azerbaijani (Latin)');
INSERT INTO `list` (`id`, `value`) VALUES ('bm', 'Bambara');
INSERT INTO `list` (`id`, `value`) VALUES ('bm_Latn_ML', 'Bambara (Latin, Mali)');
INSERT INTO `list` (`id`, `value`) VALUES ('bm_Latn', 'Bambara (Latin)');
INSERT INTO `list` (`id`, `value`) VALUES ('eu', 'Basque');
INSERT INTO `list` (`id`, `value`) VALUES ('eu_ES', 'Basque (Spain)');
INSERT INTO `list` (`id`, `value`) VALUES ('be', 'Belarusian');
INSERT INTO `list` (`id`, `value`) VALUES ('be_BY', 'Belarusian (Belarus)');
INSERT INTO `list` (`id`, `value`) VALUES ('bn', 'Bengali');
INSERT INTO `list` (`id`, `value`) VALUES ('bn_BD', 'Bengali (Bangladesh)');
INSERT INTO `list` (`id`, `value`) VALUES ('bn_IN', 'Bengali (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('bs', 'Bosnian');
INSERT INTO `list` (`id`, `value`) VALUES ('bs_BA', 'Bosnian (Bosnia & Herzegovina)');
INSERT INTO `list` (`id`, `value`) VALUES ('bs_Cyrl_BA', 'Bosnian (Cyrillic, Bosnia & Herzegovina)');
INSERT INTO `list` (`id`, `value`) VALUES ('bs_Cyrl', 'Bosnian (Cyrillic)');
INSERT INTO `list` (`id`, `value`) VALUES ('bs_Latn_BA', 'Bosnian (Latin, Bosnia & Herzegovina)');
INSERT INTO `list` (`id`, `value`) VALUES ('bs_Latn', 'Bosnian (Latin)');
INSERT INTO `list` (`id`, `value`) VALUES ('br', 'Breton');
INSERT INTO `list` (`id`, `value`) VALUES ('br_FR', 'Breton (France)');
INSERT INTO `list` (`id`, `value`) VALUES ('bg', 'Bulgarian');
INSERT INTO `list` (`id`, `value`) VALUES ('bg_BG', 'Bulgarian (Bulgaria)');
INSERT INTO `list` (`id`, `value`) VALUES ('my', 'Burmese');
INSERT INTO `list` (`id`, `value`) VALUES ('my_MM', 'Burmese (Myanmar (Burma))');
INSERT INTO `list` (`id`, `value`) VALUES ('ca', 'Catalan');
INSERT INTO `list` (`id`, `value`) VALUES ('ca_AD', 'Catalan (Andorra)');
INSERT INTO `list` (`id`, `value`) VALUES ('ca_FR', 'Catalan (France)');
INSERT INTO `list` (`id`, `value`) VALUES ('ca_IT', 'Catalan (Italy)');
INSERT INTO `list` (`id`, `value`) VALUES ('ca_ES', 'Catalan (Spain)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh', 'Chinese');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_CN', 'Chinese (China)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_HK', 'Chinese (Hong Kong SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_MO', 'Chinese (Macau SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hans_CN', 'Chinese (Simplified, China)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hans_HK', 'Chinese (Simplified, Hong Kong SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hans_MO', 'Chinese (Simplified, Macau SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hans_SG', 'Chinese (Simplified, Singapore)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hans', 'Chinese (Simplified)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_SG', 'Chinese (Singapore)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_TW', 'Chinese (Taiwan)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hant_HK', 'Chinese (Traditional, Hong Kong SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hant_MO', 'Chinese (Traditional, Macau SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hant_TW', 'Chinese (Traditional, Taiwan)');
INSERT INTO `list` (`id`, `value`) VALUES ('zh_Hant', 'Chinese (Traditional)');
INSERT INTO `list` (`id`, `value`) VALUES ('kw', 'Cornish');
INSERT INTO `list` (`id`, `value`) VALUES ('kw_GB', 'Cornish (United Kingdom)');
INSERT INTO `list` (`id`, `value`) VALUES ('hr', 'Croatian');
INSERT INTO `list` (`id`, `value`) VALUES ('hr_BA', 'Croatian (Bosnia & Herzegovina)');
INSERT INTO `list` (`id`, `value`) VALUES ('hr_HR', 'Croatian (Croatia)');
INSERT INTO `list` (`id`, `value`) VALUES ('cs', 'Czech');
INSERT INTO `list` (`id`, `value`) VALUES ('cs_CZ', 'Czech (Czech Republic)');
INSERT INTO `list` (`id`, `value`) VALUES ('da', 'Danish');
INSERT INTO `list` (`id`, `value`) VALUES ('da_DK', 'Danish (Denmark)');
INSERT INTO `list` (`id`, `value`) VALUES ('da_GL', 'Danish (Greenland)');
INSERT INTO `list` (`id`, `value`) VALUES ('nl', 'Dutch');
INSERT INTO `list` (`id`, `value`) VALUES ('nl_AW', 'Dutch (Aruba)');
INSERT INTO `list` (`id`, `value`) VALUES ('nl_BE', 'Dutch (Belgium)');
INSERT INTO `list` (`id`, `value`) VALUES ('nl_BQ', 'Dutch (Caribbean Netherlands)');
INSERT INTO `list` (`id`, `value`) VALUES ('nl_CW', 'Dutch (Curaรงao)');
INSERT INTO `list` (`id`, `value`) VALUES ('nl_NL', 'Dutch (Netherlands)');
INSERT INTO `list` (`id`, `value`) VALUES ('nl_SX', 'Dutch (Sint Maarten)');
INSERT INTO `list` (`id`, `value`) VALUES ('nl_SR', 'Dutch (Suriname)');
INSERT INTO `list` (`id`, `value`) VALUES ('dz', 'Dzongkha');
INSERT INTO `list` (`id`, `value`) VALUES ('dz_BT', 'Dzongkha (Bhutan)');
INSERT INTO `list` (`id`, `value`) VALUES ('en', 'English');
INSERT INTO `list` (`id`, `value`) VALUES ('en_AS', 'English (American Samoa)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_AI', 'English (Anguilla)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_AG', 'English (Antigua & Barbuda)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_AU', 'English (Australia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_BS', 'English (Bahamas)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_BB', 'English (Barbados)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_BE', 'English (Belgium)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_BZ', 'English (Belize)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_BM', 'English (Bermuda)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_BW', 'English (Botswana)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_IO', 'English (British Indian Ocean Territory)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_VG', 'English (British Virgin Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_CM', 'English (Cameroon)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_CA', 'English (Canada)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_KY', 'English (Cayman Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_CX', 'English (Christmas Island)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_CC', 'English (Cocos (Keeling) Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_CK', 'English (Cook Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_DG', 'English (Diego Garcia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_DM', 'English (Dominica)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_ER', 'English (Eritrea)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_FK', 'English (Falkland Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_FJ', 'English (Fiji)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_GM', 'English (Gambia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_GH', 'English (Ghana)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_GI', 'English (Gibraltar)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_GD', 'English (Grenada)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_GU', 'English (Guam)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_GG', 'English (Guernsey)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_GY', 'English (Guyana)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_HK', 'English (Hong Kong SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_IN', 'English (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_IE', 'English (Ireland)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_IM', 'English (Isle of Man)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_JM', 'English (Jamaica)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_JE', 'English (Jersey)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_KE', 'English (Kenya)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_KI', 'English (Kiribati)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_LS', 'English (Lesotho)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_LR', 'English (Liberia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MO', 'English (Macau SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MG', 'English (Madagascar)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MW', 'English (Malawi)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MY', 'English (Malaysia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MT', 'English (Malta)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MH', 'English (Marshall Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MU', 'English (Mauritius)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_FM', 'English (Micronesia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MS', 'English (Montserrat)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_NA', 'English (Namibia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_NR', 'English (Nauru)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_NZ', 'English (New Zealand)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_NG', 'English (Nigeria)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_NU', 'English (Niue)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_NF', 'English (Norfolk Island)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_MP', 'English (Northern Mariana Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_PK', 'English (Pakistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_PW', 'English (Palau)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_PG', 'English (Papua New Guinea)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_PH', 'English (Philippines)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_PN', 'English (Pitcairn Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_PR', 'English (Puerto Rico)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_RW', 'English (Rwanda)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_WS', 'English (Samoa)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SC', 'English (Seychelles)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SL', 'English (Sierra Leone)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SG', 'English (Singapore)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SX', 'English (Sint Maarten)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SB', 'English (Solomon Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_ZA', 'English (South Africa)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SS', 'English (South Sudan)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SH', 'English (St. Helena)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_KN', 'English (St. Kitts & Nevis)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_LC', 'English (St. Lucia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_VC', 'English (St. Vincent & Grenadines)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SD', 'English (Sudan)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_SZ', 'English (Swaziland)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_TZ', 'English (Tanzania)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_TK', 'English (Tokelau)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_TO', 'English (Tonga)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_TT', 'English (Trinidad & Tobago)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_TC', 'English (Turks & Caicos Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_TV', 'English (Tuvalu)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_UM', 'English (U.S. Outlying Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_VI', 'English (U.S. Virgin Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_UG', 'English (Uganda)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_GB', 'English (United Kingdom)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_US', 'English (United States)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_VU', 'English (Vanuatu)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_ZM', 'English (Zambia)');
INSERT INTO `list` (`id`, `value`) VALUES ('en_ZW', 'English (Zimbabwe)');
INSERT INTO `list` (`id`, `value`) VALUES ('eo', 'Esperanto');
INSERT INTO `list` (`id`, `value`) VALUES ('et', 'Estonian');
INSERT INTO `list` (`id`, `value`) VALUES ('et_EE', 'Estonian (Estonia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ee', 'Ewe');
INSERT INTO `list` (`id`, `value`) VALUES ('ee_GH', 'Ewe (Ghana)');
INSERT INTO `list` (`id`, `value`) VALUES ('ee_TG', 'Ewe (Togo)');
INSERT INTO `list` (`id`, `value`) VALUES ('fo', 'Faroese');
INSERT INTO `list` (`id`, `value`) VALUES ('fo_FO', 'Faroese (Faroe Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('fi', 'Finnish');
INSERT INTO `list` (`id`, `value`) VALUES ('fi_FI', 'Finnish (Finland)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr', 'French');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_DZ', 'French (Algeria)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_BE', 'French (Belgium)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_BJ', 'French (Benin)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_BF', 'French (Burkina Faso)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_BI', 'French (Burundi)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_CM', 'French (Cameroon)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_CA', 'French (Canada)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_CF', 'French (Central African Republic)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_TD', 'French (Chad)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_KM', 'French (Comoros)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_CG', 'French (Congo - Brazzaville)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_CD', 'French (Congo - Kinshasa)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_CI', 'French (Cรดte dโIvoire)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_DJ', 'French (Djibouti)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_GQ', 'French (Equatorial Guinea)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_FR', 'French (France)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_GF', 'French (French Guiana)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_PF', 'French (French Polynesia)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_GA', 'French (Gabon)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_GP', 'French (Guadeloupe)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_GN', 'French (Guinea)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_HT', 'French (Haiti)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_LU', 'French (Luxembourg)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_MG', 'French (Madagascar)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_ML', 'French (Mali)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_MQ', 'French (Martinique)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_MR', 'French (Mauritania)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_MU', 'French (Mauritius)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_YT', 'French (Mayotte)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_MC', 'French (Monaco)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_MA', 'French (Morocco)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_NC', 'French (New Caledonia)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_NE', 'French (Niger)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_RE', 'French (Rรฉunion)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_RW', 'French (Rwanda)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_SN', 'French (Senegal)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_SC', 'French (Seychelles)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_BL', 'French (St. Barthรฉlemy)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_MF', 'French (St. Martin)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_PM', 'French (St. Pierre & Miquelon)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_CH', 'French (Switzerland)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_SY', 'French (Syria)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_TG', 'French (Togo)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_TN', 'French (Tunisia)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_VU', 'French (Vanuatu)');
INSERT INTO `list` (`id`, `value`) VALUES ('fr_WF', 'French (Wallis & Futuna)');
INSERT INTO `list` (`id`, `value`) VALUES ('ff', 'Fulah');
INSERT INTO `list` (`id`, `value`) VALUES ('ff_CM', 'Fulah (Cameroon)');
INSERT INTO `list` (`id`, `value`) VALUES ('ff_GN', 'Fulah (Guinea)');
INSERT INTO `list` (`id`, `value`) VALUES ('ff_MR', 'Fulah (Mauritania)');
INSERT INTO `list` (`id`, `value`) VALUES ('ff_SN', 'Fulah (Senegal)');
INSERT INTO `list` (`id`, `value`) VALUES ('gl', 'Galician');
INSERT INTO `list` (`id`, `value`) VALUES ('gl_ES', 'Galician (Spain)');
INSERT INTO `list` (`id`, `value`) VALUES ('lg', 'Ganda');
INSERT INTO `list` (`id`, `value`) VALUES ('lg_UG', 'Ganda (Uganda)');
INSERT INTO `list` (`id`, `value`) VALUES ('ka', 'Georgian');
INSERT INTO `list` (`id`, `value`) VALUES ('ka_GE', 'Georgian (Georgia)');
INSERT INTO `list` (`id`, `value`) VALUES ('de', 'German');
INSERT INTO `list` (`id`, `value`) VALUES ('de_AT', 'German (Austria)');
INSERT INTO `list` (`id`, `value`) VALUES ('de_BE', 'German (Belgium)');
INSERT INTO `list` (`id`, `value`) VALUES ('de_DE', 'German (Germany)');
INSERT INTO `list` (`id`, `value`) VALUES ('de_LI', 'German (Liechtenstein)');
INSERT INTO `list` (`id`, `value`) VALUES ('de_LU', 'German (Luxembourg)');
INSERT INTO `list` (`id`, `value`) VALUES ('de_CH', 'German (Switzerland)');
INSERT INTO `list` (`id`, `value`) VALUES ('el', 'Greek');
INSERT INTO `list` (`id`, `value`) VALUES ('el_CY', 'Greek (Cyprus)');
INSERT INTO `list` (`id`, `value`) VALUES ('el_GR', 'Greek (Greece)');
INSERT INTO `list` (`id`, `value`) VALUES ('gu', 'Gujarati');
INSERT INTO `list` (`id`, `value`) VALUES ('gu_IN', 'Gujarati (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('ha', 'Hausa');
INSERT INTO `list` (`id`, `value`) VALUES ('ha_GH', 'Hausa (Ghana)');
INSERT INTO `list` (`id`, `value`) VALUES ('ha_Latn_GH', 'Hausa (Latin, Ghana)');
INSERT INTO `list` (`id`, `value`) VALUES ('ha_Latn_NE', 'Hausa (Latin, Niger)');
INSERT INTO `list` (`id`, `value`) VALUES ('ha_Latn_NG', 'Hausa (Latin, Nigeria)');
INSERT INTO `list` (`id`, `value`) VALUES ('ha_Latn', 'Hausa (Latin)');
INSERT INTO `list` (`id`, `value`) VALUES ('ha_NE', 'Hausa (Niger)');
INSERT INTO `list` (`id`, `value`) VALUES ('ha_NG', 'Hausa (Nigeria)');
INSERT INTO `list` (`id`, `value`) VALUES ('he', 'Hebrew');
INSERT INTO `list` (`id`, `value`) VALUES ('he_IL', 'Hebrew (Israel)');
INSERT INTO `list` (`id`, `value`) VALUES ('hi', 'Hindi');
INSERT INTO `list` (`id`, `value`) VALUES ('hi_IN', 'Hindi (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('hu', 'Hungarian');
INSERT INTO `list` (`id`, `value`) VALUES ('hu_HU', 'Hungarian (Hungary)');
INSERT INTO `list` (`id`, `value`) VALUES ('is', 'Icelandic');
INSERT INTO `list` (`id`, `value`) VALUES ('is_IS', 'Icelandic (Iceland)');
INSERT INTO `list` (`id`, `value`) VALUES ('ig', 'Igbo');
INSERT INTO `list` (`id`, `value`) VALUES ('ig_NG', 'Igbo (Nigeria)');
INSERT INTO `list` (`id`, `value`) VALUES ('id', 'Indonesian');
INSERT INTO `list` (`id`, `value`) VALUES ('id_ID', 'Indonesian (Indonesia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ga', 'Irish');
INSERT INTO `list` (`id`, `value`) VALUES ('ga_IE', 'Irish (Ireland)');
INSERT INTO `list` (`id`, `value`) VALUES ('it', 'Italian');
INSERT INTO `list` (`id`, `value`) VALUES ('it_IT', 'Italian (Italy)');
INSERT INTO `list` (`id`, `value`) VALUES ('it_SM', 'Italian (San Marino)');
INSERT INTO `list` (`id`, `value`) VALUES ('it_CH', 'Italian (Switzerland)');
INSERT INTO `list` (`id`, `value`) VALUES ('ja', 'Japanese');
INSERT INTO `list` (`id`, `value`) VALUES ('ja_JP', 'Japanese (Japan)');
INSERT INTO `list` (`id`, `value`) VALUES ('kl', 'Kalaallisut');
INSERT INTO `list` (`id`, `value`) VALUES ('kl_GL', 'Kalaallisut (Greenland)');
INSERT INTO `list` (`id`, `value`) VALUES ('kn', 'Kannada');
INSERT INTO `list` (`id`, `value`) VALUES ('kn_IN', 'Kannada (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('ks', 'Kashmiri');
INSERT INTO `list` (`id`, `value`) VALUES ('ks_Arab_IN', 'Kashmiri (Arabic, India)');
INSERT INTO `list` (`id`, `value`) VALUES ('ks_Arab', 'Kashmiri (Arabic)');
INSERT INTO `list` (`id`, `value`) VALUES ('ks_IN', 'Kashmiri (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('kk', 'Kazakh');
INSERT INTO `list` (`id`, `value`) VALUES ('kk_Cyrl_KZ', 'Kazakh (Cyrillic, Kazakhstan)');
INSERT INTO `list` (`id`, `value`) VALUES ('kk_Cyrl', 'Kazakh (Cyrillic)');
INSERT INTO `list` (`id`, `value`) VALUES ('kk_KZ', 'Kazakh (Kazakhstan)');
INSERT INTO `list` (`id`, `value`) VALUES ('km', 'Khmer');
INSERT INTO `list` (`id`, `value`) VALUES ('km_KH', 'Khmer (Cambodia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ki', 'Kikuyu');
INSERT INTO `list` (`id`, `value`) VALUES ('ki_KE', 'Kikuyu (Kenya)');
INSERT INTO `list` (`id`, `value`) VALUES ('rw', 'Kinyarwanda');
INSERT INTO `list` (`id`, `value`) VALUES ('rw_RW', 'Kinyarwanda (Rwanda)');
INSERT INTO `list` (`id`, `value`) VALUES ('ko', 'Korean');
INSERT INTO `list` (`id`, `value`) VALUES ('ko_KP', 'Korean (North Korea)');
INSERT INTO `list` (`id`, `value`) VALUES ('ko_KR', 'Korean (South Korea)');
INSERT INTO `list` (`id`, `value`) VALUES ('ky', 'Kyrgyz');
INSERT INTO `list` (`id`, `value`) VALUES ('ky_Cyrl_KG', 'Kyrgyz (Cyrillic, Kyrgyzstan)');
INSERT INTO `list` (`id`, `value`) VALUES ('ky_Cyrl', 'Kyrgyz (Cyrillic)');
INSERT INTO `list` (`id`, `value`) VALUES ('ky_KG', 'Kyrgyz (Kyrgyzstan)');
INSERT INTO `list` (`id`, `value`) VALUES ('lo', 'Lao');
INSERT INTO `list` (`id`, `value`) VALUES ('lo_LA', 'Lao (Laos)');
INSERT INTO `list` (`id`, `value`) VALUES ('lv', 'Latvian');
INSERT INTO `list` (`id`, `value`) VALUES ('lv_LV', 'Latvian (Latvia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ln', 'Lingala');
INSERT INTO `list` (`id`, `value`) VALUES ('ln_AO', 'Lingala (Angola)');
INSERT INTO `list` (`id`, `value`) VALUES ('ln_CF', 'Lingala (Central African Republic)');
INSERT INTO `list` (`id`, `value`) VALUES ('ln_CG', 'Lingala (Congo - Brazzaville)');
INSERT INTO `list` (`id`, `value`) VALUES ('ln_CD', 'Lingala (Congo - Kinshasa)');
INSERT INTO `list` (`id`, `value`) VALUES ('lt', 'Lithuanian');
INSERT INTO `list` (`id`, `value`) VALUES ('lt_LT', 'Lithuanian (Lithuania)');
INSERT INTO `list` (`id`, `value`) VALUES ('lu', 'Luba-Katanga');
INSERT INTO `list` (`id`, `value`) VALUES ('lu_CD', 'Luba-Katanga (Congo - Kinshasa)');
INSERT INTO `list` (`id`, `value`) VALUES ('lb', 'Luxembourgish');
INSERT INTO `list` (`id`, `value`) VALUES ('lb_LU', 'Luxembourgish (Luxembourg)');
INSERT INTO `list` (`id`, `value`) VALUES ('mk', 'Macedonian');
INSERT INTO `list` (`id`, `value`) VALUES ('mk_MK', 'Macedonian (Macedonia)');
INSERT INTO `list` (`id`, `value`) VALUES ('mg', 'Malagasy');
INSERT INTO `list` (`id`, `value`) VALUES ('mg_MG', 'Malagasy (Madagascar)');
INSERT INTO `list` (`id`, `value`) VALUES ('ms', 'Malay');
INSERT INTO `list` (`id`, `value`) VALUES ('ms_BN', 'Malay (Brunei)');
INSERT INTO `list` (`id`, `value`) VALUES ('ms_Latn_BN', 'Malay (Latin, Brunei)');
INSERT INTO `list` (`id`, `value`) VALUES ('ms_Latn_MY', 'Malay (Latin, Malaysia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ms_Latn_SG', 'Malay (Latin, Singapore)');
INSERT INTO `list` (`id`, `value`) VALUES ('ms_Latn', 'Malay (Latin)');
INSERT INTO `list` (`id`, `value`) VALUES ('ms_MY', 'Malay (Malaysia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ms_SG', 'Malay (Singapore)');
INSERT INTO `list` (`id`, `value`) VALUES ('ml', 'Malayalam');
INSERT INTO `list` (`id`, `value`) VALUES ('ml_IN', 'Malayalam (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('mt', 'Maltese');
INSERT INTO `list` (`id`, `value`) VALUES ('mt_MT', 'Maltese (Malta)');
INSERT INTO `list` (`id`, `value`) VALUES ('gv', 'Manx');
INSERT INTO `list` (`id`, `value`) VALUES ('gv_IM', 'Manx (Isle of Man)');
INSERT INTO `list` (`id`, `value`) VALUES ('mr', 'Marathi');
INSERT INTO `list` (`id`, `value`) VALUES ('mr_IN', 'Marathi (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('mn', 'Mongolian');
INSERT INTO `list` (`id`, `value`) VALUES ('mn_Cyrl_MN', 'Mongolian (Cyrillic, Mongolia)');
INSERT INTO `list` (`id`, `value`) VALUES ('mn_Cyrl', 'Mongolian (Cyrillic)');
INSERT INTO `list` (`id`, `value`) VALUES ('mn_MN', 'Mongolian (Mongolia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ne', 'Nepali');
INSERT INTO `list` (`id`, `value`) VALUES ('ne_IN', 'Nepali (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('ne_NP', 'Nepali (Nepal)');
INSERT INTO `list` (`id`, `value`) VALUES ('nd', 'North Ndebele');
INSERT INTO `list` (`id`, `value`) VALUES ('nd_ZW', 'North Ndebele (Zimbabwe)');
INSERT INTO `list` (`id`, `value`) VALUES ('se', 'Northern Sami');
INSERT INTO `list` (`id`, `value`) VALUES ('se_FI', 'Northern Sami (Finland)');
INSERT INTO `list` (`id`, `value`) VALUES ('se_NO', 'Northern Sami (Norway)');
INSERT INTO `list` (`id`, `value`) VALUES ('se_SE', 'Northern Sami (Sweden)');
INSERT INTO `list` (`id`, `value`) VALUES ('no', 'Norwegian');
INSERT INTO `list` (`id`, `value`) VALUES ('no_NO', 'Norwegian (Norway)');
INSERT INTO `list` (`id`, `value`) VALUES ('nb', 'Norwegian Bokmรฅl');
INSERT INTO `list` (`id`, `value`) VALUES ('nb_NO', 'Norwegian Bokmรฅl (Norway)');
INSERT INTO `list` (`id`, `value`) VALUES ('nb_SJ', 'Norwegian Bokmรฅl (Svalbard & Jan Mayen)');
INSERT INTO `list` (`id`, `value`) VALUES ('nn', 'Norwegian Nynorsk');
INSERT INTO `list` (`id`, `value`) VALUES ('nn_NO', 'Norwegian Nynorsk (Norway)');
INSERT INTO `list` (`id`, `value`) VALUES ('or', 'Oriya');
INSERT INTO `list` (`id`, `value`) VALUES ('or_IN', 'Oriya (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('om', 'Oromo');
INSERT INTO `list` (`id`, `value`) VALUES ('om_ET', 'Oromo (Ethiopia)');
INSERT INTO `list` (`id`, `value`) VALUES ('om_KE', 'Oromo (Kenya)');
INSERT INTO `list` (`id`, `value`) VALUES ('os', 'Ossetic');
INSERT INTO `list` (`id`, `value`) VALUES ('os_GE', 'Ossetic (Georgia)');
INSERT INTO `list` (`id`, `value`) VALUES ('os_RU', 'Ossetic (Russia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ps', 'Pashto');
INSERT INTO `list` (`id`, `value`) VALUES ('ps_AF', 'Pashto (Afghanistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('fa', 'Persian');
INSERT INTO `list` (`id`, `value`) VALUES ('fa_AF', 'Persian (Afghanistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('fa_IR', 'Persian (Iran)');
INSERT INTO `list` (`id`, `value`) VALUES ('pl', 'Polish');
INSERT INTO `list` (`id`, `value`) VALUES ('pl_PL', 'Polish (Poland)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt', 'Portuguese');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_AO', 'Portuguese (Angola)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_BR', 'Portuguese (Brazil)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_CV', 'Portuguese (Cape Verde)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_GW', 'Portuguese (Guinea-Bissau)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_MO', 'Portuguese (Macau SAR China)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_MZ', 'Portuguese (Mozambique)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_PT', 'Portuguese (Portugal)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_ST', 'Portuguese (Sรฃo Tomรฉ & Prรญncipe)');
INSERT INTO `list` (`id`, `value`) VALUES ('pt_TL', 'Portuguese (Timor-Leste)');
INSERT INTO `list` (`id`, `value`) VALUES ('pa', 'Punjabi');
INSERT INTO `list` (`id`, `value`) VALUES ('pa_Arab_PK', 'Punjabi (Arabic, Pakistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('pa_Arab', 'Punjabi (Arabic)');
INSERT INTO `list` (`id`, `value`) VALUES ('pa_Guru_IN', 'Punjabi (Gurmukhi, India)');
INSERT INTO `list` (`id`, `value`) VALUES ('pa_Guru', 'Punjabi (Gurmukhi)');
INSERT INTO `list` (`id`, `value`) VALUES ('pa_IN', 'Punjabi (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('pa_PK', 'Punjabi (Pakistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('qu', 'Quechua');
INSERT INTO `list` (`id`, `value`) VALUES ('qu_BO', 'Quechua (Bolivia)');
INSERT INTO `list` (`id`, `value`) VALUES ('qu_EC', 'Quechua (Ecuador)');
INSERT INTO `list` (`id`, `value`) VALUES ('qu_PE', 'Quechua (Peru)');
INSERT INTO `list` (`id`, `value`) VALUES ('ro', 'Romanian');
INSERT INTO `list` (`id`, `value`) VALUES ('ro_MD', 'Romanian (Moldova)');
INSERT INTO `list` (`id`, `value`) VALUES ('ro_RO', 'Romanian (Romania)');
INSERT INTO `list` (`id`, `value`) VALUES ('rm', 'Romansh');
INSERT INTO `list` (`id`, `value`) VALUES ('rm_CH', 'Romansh (Switzerland)');
INSERT INTO `list` (`id`, `value`) VALUES ('rn', 'Rundi');
INSERT INTO `list` (`id`, `value`) VALUES ('rn_BI', 'Rundi (Burundi)');
INSERT INTO `list` (`id`, `value`) VALUES ('ru', 'Russian');
INSERT INTO `list` (`id`, `value`) VALUES ('ru_BY', 'Russian (Belarus)');
INSERT INTO `list` (`id`, `value`) VALUES ('ru_KZ', 'Russian (Kazakhstan)');
INSERT INTO `list` (`id`, `value`) VALUES ('ru_KG', 'Russian (Kyrgyzstan)');
INSERT INTO `list` (`id`, `value`) VALUES ('ru_MD', 'Russian (Moldova)');
INSERT INTO `list` (`id`, `value`) VALUES ('ru_RU', 'Russian (Russia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ru_UA', 'Russian (Ukraine)');
INSERT INTO `list` (`id`, `value`) VALUES ('sg', 'Sango');
INSERT INTO `list` (`id`, `value`) VALUES ('sg_CF', 'Sango (Central African Republic)');
INSERT INTO `list` (`id`, `value`) VALUES ('gd', 'Scottish Gaelic');
INSERT INTO `list` (`id`, `value`) VALUES ('gd_GB', 'Scottish Gaelic (United Kingdom)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr', 'Serbian');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_BA', 'Serbian (Bosnia & Herzegovina)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Cyrl_BA', 'Serbian (Cyrillic, Bosnia & Herzegovina)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Cyrl_XK', 'Serbian (Cyrillic, Kosovo)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Cyrl_ME', 'Serbian (Cyrillic, Montenegro)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Cyrl_RS', 'Serbian (Cyrillic, Serbia)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Cyrl', 'Serbian (Cyrillic)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_XK', 'Serbian (Kosovo)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Latn_BA', 'Serbian (Latin, Bosnia & Herzegovina)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Latn_XK', 'Serbian (Latin, Kosovo)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Latn_ME', 'Serbian (Latin, Montenegro)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Latn_RS', 'Serbian (Latin, Serbia)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_Latn', 'Serbian (Latin)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_ME', 'Serbian (Montenegro)');
INSERT INTO `list` (`id`, `value`) VALUES ('sr_RS', 'Serbian (Serbia)');
INSERT INTO `list` (`id`, `value`) VALUES ('sh', 'Serbo-Croatian');
INSERT INTO `list` (`id`, `value`) VALUES ('sh_BA', 'Serbo-Croatian (Bosnia & Herzegovina)');
INSERT INTO `list` (`id`, `value`) VALUES ('sn', 'Shona');
INSERT INTO `list` (`id`, `value`) VALUES ('sn_ZW', 'Shona (Zimbabwe)');
INSERT INTO `list` (`id`, `value`) VALUES ('ii', 'Sichuan Yi');
INSERT INTO `list` (`id`, `value`) VALUES ('ii_CN', 'Sichuan Yi (China)');
INSERT INTO `list` (`id`, `value`) VALUES ('si', 'Sinhala');
INSERT INTO `list` (`id`, `value`) VALUES ('si_LK', 'Sinhala (Sri Lanka)');
INSERT INTO `list` (`id`, `value`) VALUES ('sk', 'Slovak');
INSERT INTO `list` (`id`, `value`) VALUES ('sk_SK', 'Slovak (Slovakia)');
INSERT INTO `list` (`id`, `value`) VALUES ('sl', 'Slovenian');
INSERT INTO `list` (`id`, `value`) VALUES ('sl_SI', 'Slovenian (Slovenia)');
INSERT INTO `list` (`id`, `value`) VALUES ('so', 'Somali');
INSERT INTO `list` (`id`, `value`) VALUES ('so_DJ', 'Somali (Djibouti)');
INSERT INTO `list` (`id`, `value`) VALUES ('so_ET', 'Somali (Ethiopia)');
INSERT INTO `list` (`id`, `value`) VALUES ('so_KE', 'Somali (Kenya)');
INSERT INTO `list` (`id`, `value`) VALUES ('so_SO', 'Somali (Somalia)');
INSERT INTO `list` (`id`, `value`) VALUES ('es', 'Spanish');
INSERT INTO `list` (`id`, `value`) VALUES ('es_AR', 'Spanish (Argentina)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_BO', 'Spanish (Bolivia)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_IC', 'Spanish (Canary Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_EA', 'Spanish (Ceuta & Melilla)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_CL', 'Spanish (Chile)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_CO', 'Spanish (Colombia)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_CR', 'Spanish (Costa Rica)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_CU', 'Spanish (Cuba)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_DO', 'Spanish (Dominican Republic)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_EC', 'Spanish (Ecuador)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_SV', 'Spanish (El Salvador)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_GQ', 'Spanish (Equatorial Guinea)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_GT', 'Spanish (Guatemala)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_HN', 'Spanish (Honduras)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_MX', 'Spanish (Mexico)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_NI', 'Spanish (Nicaragua)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_PA', 'Spanish (Panama)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_PY', 'Spanish (Paraguay)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_PE', 'Spanish (Peru)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_PH', 'Spanish (Philippines)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_PR', 'Spanish (Puerto Rico)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_ES', 'Spanish (Spain)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_US', 'Spanish (United States)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_UY', 'Spanish (Uruguay)');
INSERT INTO `list` (`id`, `value`) VALUES ('es_VE', 'Spanish (Venezuela)');
INSERT INTO `list` (`id`, `value`) VALUES ('sw', 'Swahili');
INSERT INTO `list` (`id`, `value`) VALUES ('sw_KE', 'Swahili (Kenya)');
INSERT INTO `list` (`id`, `value`) VALUES ('sw_TZ', 'Swahili (Tanzania)');
INSERT INTO `list` (`id`, `value`) VALUES ('sw_UG', 'Swahili (Uganda)');
INSERT INTO `list` (`id`, `value`) VALUES ('sv', 'Swedish');
INSERT INTO `list` (`id`, `value`) VALUES ('sv_AX', 'Swedish (ร
land Islands)');
INSERT INTO `list` (`id`, `value`) VALUES ('sv_FI', 'Swedish (Finland)');
INSERT INTO `list` (`id`, `value`) VALUES ('sv_SE', 'Swedish (Sweden)');
INSERT INTO `list` (`id`, `value`) VALUES ('tl', 'Tagalog');
INSERT INTO `list` (`id`, `value`) VALUES ('tl_PH', 'Tagalog (Philippines)');
INSERT INTO `list` (`id`, `value`) VALUES ('ta', 'Tamil');
INSERT INTO `list` (`id`, `value`) VALUES ('ta_IN', 'Tamil (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('ta_MY', 'Tamil (Malaysia)');
INSERT INTO `list` (`id`, `value`) VALUES ('ta_SG', 'Tamil (Singapore)');
INSERT INTO `list` (`id`, `value`) VALUES ('ta_LK', 'Tamil (Sri Lanka)');
INSERT INTO `list` (`id`, `value`) VALUES ('te', 'Telugu');
INSERT INTO `list` (`id`, `value`) VALUES ('te_IN', 'Telugu (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('th', 'Thai');
INSERT INTO `list` (`id`, `value`) VALUES ('th_TH', 'Thai (Thailand)');
INSERT INTO `list` (`id`, `value`) VALUES ('bo', 'Tibetan');
INSERT INTO `list` (`id`, `value`) VALUES ('bo_CN', 'Tibetan (China)');
INSERT INTO `list` (`id`, `value`) VALUES ('bo_IN', 'Tibetan (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('ti', 'Tigrinya');
INSERT INTO `list` (`id`, `value`) VALUES ('ti_ER', 'Tigrinya (Eritrea)');
INSERT INTO `list` (`id`, `value`) VALUES ('ti_ET', 'Tigrinya (Ethiopia)');
INSERT INTO `list` (`id`, `value`) VALUES ('to', 'Tongan');
INSERT INTO `list` (`id`, `value`) VALUES ('to_TO', 'Tongan (Tonga)');
INSERT INTO `list` (`id`, `value`) VALUES ('tr', 'Turkish');
INSERT INTO `list` (`id`, `value`) VALUES ('tr_CY', 'Turkish (Cyprus)');
INSERT INTO `list` (`id`, `value`) VALUES ('tr_TR', 'Turkish (Turkey)');
INSERT INTO `list` (`id`, `value`) VALUES ('uk', 'Ukrainian');
INSERT INTO `list` (`id`, `value`) VALUES ('uk_UA', 'Ukrainian (Ukraine)');
INSERT INTO `list` (`id`, `value`) VALUES ('ur', 'Urdu');
INSERT INTO `list` (`id`, `value`) VALUES ('ur_IN', 'Urdu (India)');
INSERT INTO `list` (`id`, `value`) VALUES ('ur_PK', 'Urdu (Pakistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('ug', 'Uyghur');
INSERT INTO `list` (`id`, `value`) VALUES ('ug_Arab_CN', 'Uyghur (Arabic, China)');
INSERT INTO `list` (`id`, `value`) VALUES ('ug_Arab', 'Uyghur (Arabic)');
INSERT INTO `list` (`id`, `value`) VALUES ('ug_CN', 'Uyghur (China)');
INSERT INTO `list` (`id`, `value`) VALUES ('uz', 'Uzbek');
INSERT INTO `list` (`id`, `value`) VALUES ('uz_AF', 'Uzbek (Afghanistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('uz_Arab_AF', 'Uzbek (Arabic, Afghanistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('uz_Arab', 'Uzbek (Arabic)');
INSERT INTO `list` (`id`, `value`) VALUES ('uz_Cyrl_UZ', 'Uzbek (Cyrillic, Uzbekistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('uz_Cyrl', 'Uzbek (Cyrillic)');
INSERT INTO `list` (`id`, `value`) VALUES ('uz_Latn_UZ', 'Uzbek (Latin, Uzbekistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('uz_Latn', 'Uzbek (Latin)');
INSERT INTO `list` (`id`, `value`) VALUES ('uz_UZ', 'Uzbek (Uzbekistan)');
INSERT INTO `list` (`id`, `value`) VALUES ('vi', 'Vietnamese');
INSERT INTO `list` (`id`, `value`) VALUES ('vi_VN', 'Vietnamese (Vietnam)');
INSERT INTO `list` (`id`, `value`) VALUES ('cy', 'Welsh');
INSERT INTO `list` (`id`, `value`) VALUES ('cy_GB', 'Welsh (United Kingdom)');
INSERT INTO `list` (`id`, `value`) VALUES ('fy', 'Western Frisian');
INSERT INTO `list` (`id`, `value`) VALUES ('fy_NL', 'Western Frisian (Netherlands)');
INSERT INTO `list` (`id`, `value`) VALUES ('yi', 'Yiddish');
INSERT INTO `list` (`id`, `value`) VALUES ('yo', 'Yoruba');
INSERT INTO `list` (`id`, `value`) VALUES ('yo_BJ', 'Yoruba (Benin)');
INSERT INTO `list` (`id`, `value`) VALUES ('yo_NG', 'Yoruba (Nigeria)');
INSERT INTO `list` (`id`, `value`) VALUES ('zu', 'Zulu');
INSERT INTO `list` (`id`, `value`) VALUES ('zu_ZA', 'Zulu (South Africa)');
| {
"pile_set_name": "Github"
} |
{
"CVE_data_meta": {
"ASSIGNER": "cve@mitre.org",
"ID": "CVE-2008-2445",
"STATE": "PUBLIC"
},
"affects": {
"vendor": {
"vendor_data": [
{
"product": {
"product_data": [
{
"product_name": "n/a",
"version": {
"version_data": [
{
"version_value": "n/a"
}
]
}
}
]
},
"vendor_name": "n/a"
}
]
}
},
"data_format": "MITRE",
"data_type": "CVE",
"data_version": "4.0",
"description": {
"description_data": [
{
"lang": "eng",
"value": "Cross-site scripting (XSS) vulnerability in profile.php in Web Group Communication Center (WGCC) 1.0.3 PreRelease 1 and earlier allows remote attackers to inject arbitrary web script or HTML via the userid parameter in a show action."
}
]
},
"problemtype": {
"problemtype_data": [
{
"description": [
{
"lang": "eng",
"value": "n/a"
}
]
}
]
},
"references": {
"reference_data": [
{
"name": "wgcc-profile-xss(42383)",
"refsource": "XF",
"url": "https://exchange.xforce.ibmcloud.com/vulnerabilities/42383"
},
{
"name": "5606",
"refsource": "EXPLOIT-DB",
"url": "https://www.exploit-db.com/exploits/5606"
},
{
"name": "29188",
"refsource": "BID",
"url": "http://www.securityfocus.com/bid/29188"
},
{
"name": "30235",
"refsource": "SECUNIA",
"url": "http://secunia.com/advisories/30235"
}
]
}
} | {
"pile_set_name": "Github"
} |
# Copyright 1999-2017 Gentoo Foundation
# Distributed under the terms of the GNU General Public License v2
EAPI=5
inherit git-r3
DESCRIPTION="C library for handling bigWig files (functionally replacing Jim Kent's lib)"
HOMEPAGE="https://github.com/dpryan79/libBigWig"
EGIT_REPO_URI="https://github.com/dpryan79/libBigWig.git"
LICENSE="MIT"
SLOT="0"
KEYWORDS="" # https://github.com/dpryan79/libBigWig/issues/30
IUSE=""
DEPEND="net-misc/curl"
RDEPEND="${DEPEND}"
src_prepare(){
default
sed -e 's#/usr/local#$(DESTDIR)/usr#' -i Makefile || die
}
src_install(){
emake install DESTDIR="${ED}"
}
| {
"pile_set_name": "Github"
} |
## Laravel Auth
#### Laravel Auth is a Complete Build of Laravel 8 with Email Registration Verification, Social Authentication, User Roles and Permissions, User Profiles, and Admin restricted user management system. Built on Bootstrap 4.
[](https://travis-ci.org/jeremykenedy/laravel-auth)
[](https://styleci.io/repos/44714043)
[](https://scrutinizer-ci.com/g/jeremykenedy/laravel-auth/build-status/master)
[](https://scrutinizer-ci.com/g/jeremykenedy/laravel-auth/?branch=master)
[](https://scrutinizer-ci.com/code-intelligence)
[](#contributors)
[](https://madewithlaravel.com/p/laravel-auth/shield-link)
[](https://opensource.org/licenses/MIT)
### Sponsor
<table>
<tr>
<td>
<img src="https://cdn.auth0.com/styleguide/components/1.0.8/media/logos/img/badge.png" alt="Auth0" width="50">
</td>
<td>
If you want to quickly add secure token-based authentication to Laravel apps, feel free to check Auth0's Laravel SDK and free plan at <a href="https://auth0.com/developers?utm_source=GHsponsor&utm_medium=GHsponsor&utm_campaign=laravel-auth&utm_content=auth" target="_blank">https://auth0.com/developers</a>.
</td>
</tr>
</table>
#### Table of contents
- [About](#about)
- [Features](#features)
- [Installation Instructions](#installation-instructions)
- [Build the Front End Assets with Mix](#build-the-front-end-assets-with-mix)
- [Optionally Build Cache](#optionally-build-cache)
- [Seeds](#seeds)
- [Seeded Roles](#seeded-roles)
- [Seeded Permissions](#seeded-permissions)
- [Seeded Users](#seeded-users)
- [Themes Seed List](#themes-seed-list)
- [Routes](#routes)
- [Socialite](#socialite)
- [Get Socialite Login API Keys](#get-socialite-login-api-keys)
- [Add More Socialite Logins](#add-more-socialite-logins)
- [Other API keys](#other-api-keys)
- [Environment File](#environment-file)
- [Updates](#updates)
- [Screenshots](#screenshots)
- [File Tree](#file-tree)
- [Opening an Issue](#opening-an-issue)
- [Laravel Auth License](#laravel-auth-license)
- [Contributors](#Contributors)
### About
Laravel 8 with user authentication, registration with email confirmation, social media authentication, password recovery, and captcha protection. Uses official [Bootstrap 4](https://getbootstrap.com). This also makes full use of Controllers for the routes, templates for the views, and makes use of middleware for routing. Project can be stood up in minutes.
### Features
#### A [Laravel](https://laravel.com/) 8.x with [Bootstrap](https://getbootstrap.com) 4.x project.
| Laravel Auth Features |
| :------------ |
|Built on [Laravel](https://laravel.com/) 8|
|Built on [Bootstrap](https://getbootstrap.com/) 4|
|Uses [MySQL](https://github.com/mysql) Database (can be changed)|
|Uses [Artisan](https://laravel.com/docs/master/artisan) to manage database migration, schema creations, and create/publish page controller templates|
|Dependencies are managed with [COMPOSER](https://getcomposer.org/)|
|Laravel Scaffolding **User** and **Administrator Authentication**.|
|User [Socialite Logins](https://github.com/laravel/socialite) ready to go - See API list used below|
|[Google Maps API v3](https://developers.google.com/maps/documentation/javascript/) for User Location lookup and Geocoding|
|CRUD (Create, Read, Update, Delete) Themes Management|
|CRUD (Create, Read, Update, Delete) User Management|
|Robust [Laravel Logging](https://laravel.com/docs/master/errors#logging) with admin UI using MonoLog|
|Google [reCaptcha Protection with Google API](https://developers.google.com/recaptcha/)|
|User Registration with email verification|
|Makes use of Laravel [Mix](https://laravel.com/docs/master/mix) to compile assets|
|Makes use of [Language Localization Files](https://laravel.com/docs/master/localization)|
|Active Nav states using [Laravel Requests](https://laravel.com/docs/master/requests)|
|Restrict User Email Activation Attempts|
|Capture IP to users table upon signup|
|Uses [Laravel Debugger](https://github.com/barryvdh/laravel-debugbar) for development|
|Makes use of [Password Strength Meter](https://github.com/elboletaire/password-strength-meter)|
|Makes use of [hideShowPassword](https://github.com/cloudfour/hideShowPassword)|
|User Avatar Image AJAX Upload with [Dropzone.js](https://www.dropzonejs.com/#configuration)|
|User Gravatar using [Gravatar API](https://github.com/creativeorange/gravatar)|
|User Password Reset via Email Token|
|User Login with remember password|
|User [Roles/ACL Implementation](https://github.com/jeremykenedy/laravel-roles)|
|Roles and Permissions GUI|
|Makes use of [Laravel's Soft Delete Structure](https://laravel.com/docs/master/eloquent#soft-deleting)|
|Soft Deleted Users Management System|
|Permanently Delete Soft Deleted Users|
|User Delete Account with Goodbye email|
|User Restore Deleted Account Token|
|Restore Soft Deleted Users|
|View Soft Deleted Users|
|Captures Soft Delete Date|
|Captures Soft Delete IP|
|Admin Routing Details UI|
|Admin PHP Information UI|
|Eloquent user profiles|
|User Themes|
|404 Page|
|403 Page|
|Configurable Email Notification via [Laravel-Exception-Notifier](https://github.com/jeremykenedy/laravel-exception-notifier)|
|Activity Logging using [Laravel-logger](https://github.com/jeremykenedy/laravel-logger)|
|Optional 2-step account login verfication with [Laravel 2-Step Verification](https://github.com/jeremykenedy/laravel2step)|
|Uses [Laravel PHP Info](https://github.com/jeremykenedy/laravel-phpinfo) package|
|Uses [Laravel Blocker](https://github.com/jeremykenedy/laravel-blocker) package|
### Installation Instructions
1. Run `git clone https://github.com/jeremykenedy/laravel-auth.git laravel-auth`
2. Create a MySQL database for the project
* ```mysql -u root -p```, if using Vagrant: ```mysql -u homestead -psecret```
* ```create database laravelAuth;```
* ```\q```
3. From the projects root run `cp .env.example .env`
4. Configure your `.env` file
5. Run `composer update` from the projects root folder
6. From the projects root folder run:
```
php artisan vendor:publish --tag=laravelroles &&
php artisan vendor:publish --tag=laravel2step
```
7. From the projects root folder run `sudo chmod -R 755 ../laravel-auth`
8. From the projects root folder run `php artisan key:generate`
9. From the projects root folder run `php artisan migrate`
10. From the projects root folder run `composer dump-autoload`
11. From the projects root folder run `php artisan db:seed`
12. Compile the front end assets with [npm steps](#using-npm) or [yarn steps](#using-yarn).
#### Build the Front End Assets with Mix
##### Using Yarn:
1. From the projects root folder run `yarn install`
2. From the projects root folder run `yarn run dev` or `yarn run production`
* You can watch assets with `yarn run watch`
##### Using NPM:
1. From the projects root folder run `npm install`
2. From the projects root folder run `npm run dev` or `npm run production`
* You can watch assets with `npm run watch`
#### Optionally Build Cache
1. From the projects root folder run `php artisan config:cache`
###### And thats it with the caveat of setting up and configuring your development environment. I recommend [Laravel Homestead](https://laravel.com/docs/master/homestead)
### Seeds
##### Seeded Roles
* Unverified - Level 0
* User - Level 1
* Administrator - Level 5
##### Seeded Permissions
* view.users
* create.users
* edit.users
* delete.users
##### Seeded Users
|Email|Password|Access|
|:------------|:------------|:------------|
|user@user.com|password|User Access|
|admin@admin.com|password|Admin Access|
##### Themes Seed List
* [ThemesTableSeeder](https://github.com/jeremykenedy/laravel-auth/blob/master/database/seeds/ThemesTableSeeder.php)
* NOTE: A lot of themes render incorrectly on Bootstrap 4 since their core was built to override Bootstrap 4. These will be updated soon and ones that do not render correctly will be removed from the seed. In the mean time you can remove them from the seed or manaully from the UI or database.
##### Blocked Types Seed List
* [BlockedTypeTableSeeder.php](https://github.com/jeremykenedy/laravel-auth/blob/master/database/seeds/BlockedTypeTableSeeder.php)
|Slug|Name|
|:------------|:------------|
|email|E-mail|
|ipAddress|IP Address|
|domain|Domain Name|
|user|User|
|city|City|
|state|State|
|country|Country|
|countryCode|Country Code|
|continent|Continent|
|region|Region|
##### Blocked Items Seed List
* [BlockedItemsTableSeeder.php](https://github.com/jeremykenedy/laravel-auth/blob/master/database/seeds/BlockedItemsTableSeeder.php)
|Type|Value|Note|
|:------------|:------------|:------------|
|domain|test.com|Block all domains/emails @test.com|
|domain|test.ca|Block all domains/emails @test.ca|
|domain|fake.com|Block all domains/emails @fake.com|
|domain|example.com|Block all domains/emails @example.com|
|domain|mailinator.com|Block all domains/emails @mailinator.com|
### Routes
```bash
+--------+----------------------------------------+---------------------------------------+-----------------------------------------------+-----------------------------------------------------------------------------------------------------------------+--------------------------------------------------------------+
| Domain | Method | URI | Name | Action | Middleware |
+--------+----------------------------------------+---------------------------------------+-----------------------------------------------+-----------------------------------------------------------------------------------------------------------------+--------------------------------------------------------------+
| | GET|HEAD | / | welcome | App\Http\Controllers\WelcomeController@welcome | web,checkblocked |
| | GET|HEAD | _debugbar/assets/javascript | debugbar.assets.js | Barryvdh\Debugbar\Controllers\AssetController@js | Barryvdh\Debugbar\Middleware\DebugbarEnabled,Closure |
| | GET|HEAD | _debugbar/assets/stylesheets | debugbar.assets.css | Barryvdh\Debugbar\Controllers\AssetController@css | Barryvdh\Debugbar\Middleware\DebugbarEnabled,Closure |
| | DELETE | _debugbar/cache/{key}/{tags?} | debugbar.cache.delete | Barryvdh\Debugbar\Controllers\CacheController@delete | Barryvdh\Debugbar\Middleware\DebugbarEnabled,Closure |
| | GET|HEAD | _debugbar/clockwork/{id} | debugbar.clockwork | Barryvdh\Debugbar\Controllers\OpenHandlerController@clockwork | Barryvdh\Debugbar\Middleware\DebugbarEnabled,Closure |
| | GET|HEAD | _debugbar/open | debugbar.openhandler | Barryvdh\Debugbar\Controllers\OpenHandlerController@handle | Barryvdh\Debugbar\Middleware\DebugbarEnabled,Closure |
| | GET|HEAD | _debugbar/telescope/{id} | debugbar.telescope | Barryvdh\Debugbar\Controllers\TelescopeController@show | Barryvdh\Debugbar\Middleware\DebugbarEnabled,Closure |
| | GET|HEAD | activate | activate | App\Http\Controllers\Auth\ActivateController@initial | web,activity,checkblocked,auth |
| | GET|HEAD | activate/{token} | authenticated.activate | App\Http\Controllers\Auth\ActivateController@activate | web,activity,checkblocked,auth |
| | GET|HEAD | activation | authenticated.activation-resend | App\Http\Controllers\Auth\ActivateController@resend | web,activity,checkblocked,auth |
| | GET|HEAD | activation-required | activation-required | App\Http\Controllers\Auth\ActivateController@activationRequired | web,auth,activated,activity,checkblocked |
| | GET|HEAD | active-users | | App\Http\Controllers\AdminDetailsController@activeUsers | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | activity | activity | jeremykenedy\LaravelLogger\App\Http\Controllers\LaravelLoggerController@showAccessLog | web,auth,activity,role:admin |
| | DELETE | activity/clear-activity | clear-activity | jeremykenedy\LaravelLogger\App\Http\Controllers\LaravelLoggerController@clearActivityLog | web,auth,activity,role:admin |
| | GET|HEAD | activity/cleared | cleared | jeremykenedy\LaravelLogger\App\Http\Controllers\LaravelLoggerController@showClearedActivityLog | web,auth,activity,role:admin |
| | GET|HEAD | activity/cleared/log/{id} | | jeremykenedy\LaravelLogger\App\Http\Controllers\LaravelLoggerController@showClearedAccessLogEntry | web,auth,activity,role:admin |
| | DELETE | activity/destroy-activity | destroy-activity | jeremykenedy\LaravelLogger\App\Http\Controllers\LaravelLoggerController@destroyActivityLog | web,auth,activity,role:admin |
| | GET|HEAD | activity/log/{id} | | jeremykenedy\LaravelLogger\App\Http\Controllers\LaravelLoggerController@showAccessLogEntry | web,auth,activity,role:admin |
| | POST | activity/restore-log | restore-activity | jeremykenedy\LaravelLogger\App\Http\Controllers\LaravelLoggerController@restoreClearedActivityLog | web,auth,activity,role:admin |
| | POST | avatar/upload | avatar.upload | App\Http\Controllers\ProfilesController@upload | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | GET|HEAD | blocker | laravelblocker::blocker.index | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerController@index | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | POST | blocker | laravelblocker::blocker.store | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerController@store | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | GET|HEAD | blocker-deleted | laravelblocker::blocker-deleted | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerDeletedController@index | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | DELETE | blocker-deleted-destroy-all | laravelblocker::destroy-all-blocked | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerDeletedController@destroyAllItems | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | POST | blocker-deleted-restore-all | laravelblocker::blocker-deleted-restore-all | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerDeletedController@restoreAllBlockedItems | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | DELETE | blocker-deleted/{id} | laravelblocker::blocker-item-destroy | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerDeletedController@destroy | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | PUT | blocker-deleted/{id} | laravelblocker::blocker-item-restore | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerDeletedController@restoreBlockedItem | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | GET|HEAD | blocker-deleted/{id} | laravelblocker::blocker-item-show-deleted | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerDeletedController@show | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | GET|HEAD | blocker/create | laravelblocker::blocker.create | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerController@create | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | DELETE | blocker/{blocker} | laravelblocker::blocker.destroy | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerController@destroy | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | PUT|PATCH | blocker/{blocker} | laravelblocker::blocker.update | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerController@update | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | GET|HEAD | blocker/{blocker} | laravelblocker::blocker.show | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerController@show | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | GET|HEAD | blocker/{blocker}/edit | laravelblocker::blocker.edit | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerController@edit | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | GET|HEAD | exceeded | exceeded | App\Http\Controllers\Auth\ActivateController@exceeded | web,activity,checkblocked,auth |
| | GET|HEAD | home | public.home | App\Http\Controllers\UserController@index | web,auth,activated,activity,twostep,checkblocked |
| | GET|HEAD | images/profile/{id}/avatar/{image} | | App\Http\Controllers\ProfilesController@userProfileAvatar | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | POST | login | | App\Http\Controllers\Auth\LoginController@login | web,guest |
| | GET|HEAD | login | login | App\Http\Controllers\Auth\LoginController@showLoginForm | web,guest |
| | POST | logout | logout | App\Http\Controllers\Auth\LoginController@logout | web |
| | GET|HEAD | logout | logout | App\Http\Controllers\Auth\LoginController@logout | web,auth,activated,activity,checkblocked |
| | GET|HEAD | logs | | Rap2hpoutre\LaravelLogViewer\LogViewerController@index | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | POST | password/email | password.email | App\Http\Controllers\Auth\ForgotPasswordController@sendResetLinkEmail | web,guest |
| | GET|HEAD | password/reset | password.request | App\Http\Controllers\Auth\ForgotPasswordController@showLinkRequestForm | web,guest |
| | POST | password/reset | password.update | App\Http\Controllers\Auth\ResetPasswordController@reset | web,guest |
| | GET|HEAD | password/reset/{token} | password.reset | App\Http\Controllers\Auth\ResetPasswordController@showResetForm | web,guest |
| | GET|HEAD | permission-deleted/{id} | laravelroles::permission-show-deleted | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelpermissionsDeletedController@show | web,auth,role:admin |
| | DELETE | permission-destroy/{id} | laravelroles::permission-item-destroy | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelpermissionsDeletedController@destroy | web,auth,role:admin |
| | PUT | permission-restore/{id} | laravelroles::permission-restore | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelpermissionsDeletedController@restorePermission | web,auth,role:admin |
| | POST | permissions | laravelroles::permissions.store | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelPermissionsController@store | web,auth,role:admin |
| | GET|HEAD | permissions | laravelroles::permissions.index | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelPermissionsController@index | web,auth,role:admin |
| | GET|HEAD | permissions-deleted | laravelroles::permissions-deleted | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelpermissionsDeletedController@index | web,auth,role:admin |
| | DELETE | permissions-deleted-destroy-all | laravelroles::destroy-all-deleted-permissions | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelpermissionsDeletedController@destroyAllDeletedPermissions | web,auth,role:admin |
| | POST | permissions-deleted-restore-all | laravelroles::permissions-deleted-restore-all | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelpermissionsDeletedController@restoreAllDeletedPermissions | web,auth,role:admin |
| | GET|HEAD | permissions/create | laravelroles::permissions.create | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelPermissionsController@create | web,auth,role:admin |
| | GET|HEAD | permissions/{permission} | laravelroles::permissions.show | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelPermissionsController@show | web,auth,role:admin |
| | DELETE | permissions/{permission} | laravelroles::permissions.destroy | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelPermissionsController@destroy | web,auth,role:admin |
| | PUT|PATCH | permissions/{permission} | laravelroles::permissions.update | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelPermissionsController@update | web,auth,role:admin |
| | GET|HEAD | permissions/{permission}/edit | laravelroles::permissions.edit | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelPermissionsController@edit | web,auth,role:admin |
| | GET|HEAD|POST|PUT|PATCH|DELETE|OPTIONS | php | | Illuminate\Routing\RedirectController | web |
| | GET|HEAD | phpinfo | laravelPhpInfo::phpinfo | jeremykenedy\LaravelPhpInfo\App\Http\Controllers\LaravelPhpInfoController@phpinfo | web,auth,activated,role:admin,activity,twostep |
| | GET|HEAD | profile/create | profile.create | App\Http\Controllers\ProfilesController@create | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | PUT|PATCH | profile/{profile} | profile.update | App\Http\Controllers\ProfilesController@update | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | GET|HEAD | profile/{profile} | profile.show | App\Http\Controllers\ProfilesController@show | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | GET|HEAD | profile/{profile}/edit | profile.edit | App\Http\Controllers\ProfilesController@edit | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | GET|HEAD | profile/{username} | {username} | App\Http\Controllers\ProfilesController@show | web,auth,activated,activity,twostep,checkblocked |
| | DELETE | profile/{username}/deleteUserAccount | {username} | App\Http\Controllers\ProfilesController@deleteUserAccount | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | PUT | profile/{username}/updateUserAccount | {username} | App\Http\Controllers\ProfilesController@updateUserAccount | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | PUT | profile/{username}/updateUserPassword | {username} | App\Http\Controllers\ProfilesController@updateUserPassword | web,auth,activated,currentUser,activity,twostep,checkblocked |
| | GET|HEAD | re-activate/{token} | user.reactivate | App\Http\Controllers\RestoreUserController@userReActivate | web,activity,checkblocked |
| | POST | register | | App\Http\Controllers\Auth\RegisterController@register | web,guest |
| | GET|HEAD | register | register | App\Http\Controllers\Auth\RegisterController@showRegistrationForm | web,guest |
| | GET|HEAD | role-deleted/{id} | laravelroles::role-show-deleted | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesDeletedController@show | web,auth,role:admin |
| | DELETE | role-destroy/{id} | laravelroles::role-item-destroy | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesDeletedController@destroy | web,auth,role:admin |
| | PUT | role-restore/{id} | laravelroles::role-restore | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesDeletedController@restoreRole | web,auth,role:admin |
| | POST | roles | laravelroles::roles.store | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesController@store | web,auth,role:admin |
| | GET|HEAD | roles | laravelroles::roles.index | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesController@index | web,auth,role:admin |
| | GET|HEAD | roles-deleted | laravelroles::roles-deleted | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesDeletedController@index | web,auth,role:admin |
| | DELETE | roles-deleted-destroy-all | laravelroles::destroy-all-deleted-roles | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesDeletedController@destroyAllDeletedRoles | web,auth,role:admin |
| | POST | roles-deleted-restore-all | laravelroles::roles-deleted-restore-all | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesDeletedController@restoreAllDeletedRoles | web,auth,role:admin |
| | GET|HEAD | roles/create | laravelroles::roles.create | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesController@create | web,auth,role:admin |
| | GET|HEAD | roles/{role} | laravelroles::roles.show | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesController@show | web,auth,role:admin |
| | PUT|PATCH | roles/{role} | laravelroles::roles.update | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesController@update | web,auth,role:admin |
| | DELETE | roles/{role} | laravelroles::roles.destroy | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesController@destroy | web,auth,role:admin |
| | GET|HEAD | roles/{role}/edit | laravelroles::roles.edit | jeremykenedy\LaravelRoles\App\Http\Controllers\LaravelRolesController@edit | web,auth,role:admin |
| | GET|HEAD | routes | | App\Http\Controllers\AdminDetailsController@listRoutes | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | POST | search-blocked | laravelblocker::search-blocked | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerController@search | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | POST | search-blocked-deleted | laravelblocker::search-blocked-deleted | jeremykenedy\LaravelBlocker\App\Http\Controllers\LaravelBlockerDeletedController@search | web,checkblocked,auth,activated,role:admin,activity,twostep |
| | POST | search-users | search-users | App\Http\Controllers\UsersManagementController@search | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | social/handle/{provider} | social.handle | App\Http\Controllers\Auth\SocialController@getSocialHandle | web,activity,checkblocked |
| | GET|HEAD | social/redirect/{provider} | social.redirect | App\Http\Controllers\Auth\SocialController@getSocialRedirect | web,activity,checkblocked |
| | GET|HEAD | terms | terms | App\Http\Controllers\TermsController@terms | web,checkblocked |
| | GET|HEAD | themes | themes | App\Http\Controllers\ThemesManagementController@index | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | POST | themes | themes.store | App\Http\Controllers\ThemesManagementController@store | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | themes/create | themes.create | App\Http\Controllers\ThemesManagementController@create | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | DELETE | themes/{theme} | themes.destroy | App\Http\Controllers\ThemesManagementController@destroy | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | PUT|PATCH | themes/{theme} | themes.update | App\Http\Controllers\ThemesManagementController@update | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | themes/{theme} | themes.show | App\Http\Controllers\ThemesManagementController@show | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | themes/{theme}/edit | themes.edit | App\Http\Controllers\ThemesManagementController@edit | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | users | users | App\Http\Controllers\UsersManagementController@index | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | POST | users | users.store | App\Http\Controllers\UsersManagementController@store | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | users/create | users.create | App\Http\Controllers\UsersManagementController@create | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | users/deleted | deleted.index | App\Http\Controllers\SoftDeletesController@index | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | users/deleted/{deleted} | deleted.show | App\Http\Controllers\SoftDeletesController@show | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | DELETE | users/deleted/{deleted} | deleted.destroy | App\Http\Controllers\SoftDeletesController@destroy | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | PUT|PATCH | users/deleted/{deleted} | deleted.update | App\Http\Controllers\SoftDeletesController@update | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | DELETE | users/{user} | user.destroy | App\Http\Controllers\UsersManagementController@destroy | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | PUT|PATCH | users/{user} | users.update | App\Http\Controllers\UsersManagementController@update | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | users/{user} | users.show | App\Http\Controllers\UsersManagementController@show | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | users/{user}/edit | users.edit | App\Http\Controllers\UsersManagementController@edit | web,auth,activated,role:admin,activity,twostep,checkblocked |
| | GET|HEAD | verification/needed | laravel2step::verificationNeeded | jeremykenedy\laravel2step\App\Http\Controllers\TwoStepController@showVerification | web,auth,Closure |
| | POST | verification/resend | laravel2step::resend | jeremykenedy\laravel2step\App\Http\Controllers\TwoStepController@resend | web,auth,Closure |
| | POST | verification/verify | laravel2step::verify | jeremykenedy\laravel2step\App\Http\Controllers\TwoStepController@verify | web,auth,Closure |
+--------+----------------------------------------+---------------------------------------+-----------------------------------------------+-----------------------------------------------------------------------------------------------------------------+--------------------------------------------------------------+
```
### Socialite
#### Get Socialite Login API Keys:
* [Google Captcha API](https://www.google.com/recaptcha/admin#list)
* [Facebook API](https://developers.facebook.com/)
* [Twitter API](https://apps.twitter.com/)
* [Google + API](https://console.developers.google.com/)
* [GitHub API](https://github.com/settings/applications/new)
* [YouTube API](https://developers.google.com/youtube/v3/getting-started)
* [Twitch TV API](https://www.twitch.tv/kraken/oauth2/clients/new)
* [Instagram API](https://instagram.com/developer/register/)
* [37 Signals API](https://github.com/basecamp/basecamp-classic-api)
#### Add More Socialite Logins
* See full list of providers: [https://socialiteproviders.github.io](https://socialiteproviders.github.io/#providers)
###### **Steps**:
1. Go to [https://socialiteproviders.github.io](https://socialiteproviders.github.io/providers/twitch/) and select the provider to be added.
2. From the projects root folder, in the terminal, run composer to get the needed package.
* Example:
```
composer require socialiteproviders/twitch
```
3. From the projects root folder run ```composer update```
4. Add the service provider to ```/config/services.php```
* Example:
```
'twitch' => [
'client_id' => env('TWITCH_KEY'),
'client_secret' => env('TWITCH_SECRET'),
'redirect' => env('TWITCH_REDIRECT_URI'),
],
```
5. Add the API credentials to ``` /.env ```
* Example:
```
TWITCH_KEY=YOURKEYHERE
TWITCH_SECRET=YOURSECRETHERE
TWITCH_REDIRECT_URI=http://YOURWEBSITEURL.COM/social/handle/twitch
```
6. Add the social media login link:
* Example:
In file ```/resources/views/auth/login.blade.php``` add ONE of the following:
* Conventional HTML:
```
<a href="{{ route('social.redirect', ['provider' => 'twitch']) }}" class="btn btn-lg btn-primary btn-block twitch">Twitch</a>
```
* Use Laravel HTML Facade with [Laravel Collective](https://laravelcollective.com/):
```
{!! HTML::link(route('social.redirect', ['provider' => 'twitch']), 'Twitch', array('class' => 'btn btn-lg btn-primary btn-block twitch')) !!}
```
### Other API keys
* [Google Maps API v3 Key](https://developers.google.com/maps/documentation/javascript/get-api-key#get-an-api-key)
### Environment File
Example `.env` file:
```bash
APP_NAME=Laravel
APP_ENV=local
APP_KEY=
APP_DEBUG=true
APP_URL=http://localhost
APP_PROJECT_VERSION=7
LOG_CHANNEL=stack
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=root
DB_PASSWORD=
BROADCAST_DRIVER=pusher
CACHE_DRIVER=file
SESSION_DRIVER=file
SESSION_LIFETIME=120
QUEUE_DRIVER=sync
REDIS_HOST=127.0.0.1
REDIS_PASSWORD=null
REDIS_PORT=6379
MAIL_MAILER=smtp
MAIL_HOST=smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=null
MAIL_PASSWORD=null
MAIL_ENCRYPTION=null
MAIL_FROM_ADDRESS=null
MAIL_FROM_NAME="${APP_NAME}"
EMAIL_EXCEPTION_ENABLED=false
EMAIL_EXCEPTION_FROM="${MAIL_FROM_ADDRESS}"
EMAIL_EXCEPTION_TO='email1@gmail.com, email2@gmail.com'
EMAIL_EXCEPTION_CC=''
EMAIL_EXCEPTION_BCC=''
EMAIL_EXCEPTION_SUBJECT=''
AWS_ACCESS_KEY_ID=
AWS_SECRET_ACCESS_KEY=
AWS_DEFAULT_REGION=us-east-1
AWS_BUCKET=
PUSHER_APP_ID=
PUSHER_APP_KEY=
PUSHER_APP_SECRET=
PUSHER_APP_CLUSTER=
MIX_PUSHER_APP_KEY="${PUSHER_APP_KEY}"
MIX_PUSHER_APP_CLUSTER="${PUSHER_APP_CLUSTER}"
ACTIVATION=true
ACTIVATION_LIMIT_TIME_PERIOD=24
ACTIVATION_LIMIT_MAX_ATTEMPTS=3
NULL_IP_ADDRESS=0.0.0.0
DEBUG_BAR_ENVIRONMENT=local
USER_RESTORE_CUTOFF_DAYS=31
USER_RESTORE_ENCRYPTION_KEY=
USER_LIST_PAGINATION_SIZE=50
LARAVEL_2STEP_ENABLED=false
LARAVEL_2STEP_DATABASE_CONNECTION=mysql
LARAVEL_2STEP_DATABASE_TABLE=laravel2step
LARAVEL_2STEP_USER_MODEL=App\User
LARAVEL_2STEP_EMAIL_FROM=
LARAVEL_2STEP_EMAIL_FROM_NAME="Laravel 2 Step Verification"
LARAVEL_2STEP_EMAIL_SUBJECT='Laravel 2 Step Verification'
LARAVEL_2STEP_EXCEEDED_COUNT=3
LARAVEL_2STEP_EXCEEDED_COUNTDOWN_MINUTES=1440
LARAVEL_2STEP_VERIFIED_LIFETIME_MINUTES=360
LARAVEL_2STEP_RESET_BUFFER_IN_SECONDS=300
LARAVEL_2STEP_CSS_FILE="css/laravel2step/app.css"
LARAVEL_2STEP_APP_CSS_ENABLED=false
LARAVEL_2STEP_APP_CSS="css/app.css"
LARAVEL_2STEP_BOOTSTRAP_CSS_CDN_ENABLED=true
LARAVEL_2STEP_BOOTSTRAP_CSS_CDN="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
DEFAULT_GRAVATAR_SIZE=80
DEFAULT_GRAVATAR_FALLBACK=http://c1940652.r52.cf0.rackcdn.com/51ce28d0fb4f442061000000/Screen-Shot-2013-06-28-at-5.22.23-PM.png
DEFAULT_GRAVATAR_SECURE=false
DEFAULT_GRAVATAR_MAX_RATING=g
DEFAULT_GRAVATAR_FORCE_DEFAULT=false
DEFAULT_GRAVATAR_FORCE_EXTENSION=jpg
DROPZONE_JS_CDN=https://cdnjs.cloudflare.com/ajax/libs/dropzone/5.4.0/dropzone.js
LARAVEL_LOGGER_DATABASE_CONNECTION=mysql
LARAVEL_LOGGER_DATABASE_TABLE=laravel_logger_activity
LARAVEL_LOGGER_ROLES_ENABLED=true
LARAVEL_LOGGER_ROLES_MIDDLWARE=role:admin
LARAVEL_LOGGER_MIDDLEWARE_ENABLED=true
LARAVEL_LOGGER_USER_MODEL=App\Models\User
LARAVEL_LOGGER_PAGINATION_ENABLED=true
LARAVEL_LOGGER_PAGINATION_PER_PAGE=25
LARAVEL_LOGGER_DATATABLES_ENABLED=false
LARAVEL_LOGGER_DASHBOARD_MENU_ENABLED=true
LARAVEL_LOGGER_DASHBOARD_DRILLABLE=true
LARAVEL_LOGGER_LOG_RECORD_FAILURES_TO_FILE=true
LARAVEL_LOGGER_FLASH_MESSAGE_BLADE_ENABLED=false
LARAVEL_LOGGER_JQUERY_CDN_ENABLED=false
LARAVEL_LOGGER_JQUERY_CDN_URL=https://code.jquery.com/jquery-2.2.4.min.js
LARAVEL_LOGGER_BLADE_CSS_PLACEMENT_ENABLED=true
LARAVEL_LOGGER_BLADE_JS_PLACEMENT_ENABLED=true
LARAVEL_LOGGER_BOOTSTRAP_JS_CDN_ENABLED=false
LARAVEL_LOGGER_BOOTSTRAP_JS_CDN_URL=https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js
LARAVEL_LOGGER_FONT_AWESOME_CDN_ENABLED=false
LARAVEL_LOGGER_FONT_AWESOME_CDN_URL=https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css
LARAVEL_LOGGER_BOOTSTRAP_CSS_CDN_ENABLED=false
LARAVEL_LOGGER_BOOTSTRAP_CSS_CDN_URL=https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css
LARAVEL_BLOCKER_USER_MODEL=App\Models\User
LARAVEL_BLOCKER_AUTH_ENABLED=true
LARAVEL_BLOCKER_ROLES_ENABLED=true
LARAVEL_BLOCKER_FLASH_MESSAGES_ENABLED=false
LARAVEL_BLOCKER_JQUERY_CDN_ENABLED=false
LARAVEL_BLOCKER_BLADE_PLACEMENT_CSS='template_linked_css'
LARAVEL_BLOCKER_BLADE_PLACEMENT_JS='footer_scripts'
LARAVEL_BLOCKER_USE_TYPES_SEED_PUBLISHED=true
LARAVEL_BLOCKER_USE_ITEMS_SEED_PUBLISHED=true
# Roles Default Models
ROLES_DEFAULT_ROLE_MODEL=jeremykenedy\LaravelRoles\Models\Role
ROLES_DEFAULT_PERMISSION_MODEL=jeremykenedy\LaravelRoles\Models\Permission
# Roles database information
ROLES_DATABASE_CONNECTION=null
# Roles Misc Settings
ROLES_DEFAULT_SEPARATOR='.'
# Roles GUI Settings
ROLES_GUI_ENABLED=true
ROLES_GUI_AUTH_ENABLED=true
ROLES_GUI_MIDDLEWARE_ENABLED=true
ROLES_GUI_MIDDLEWARE='role:admin'
ROLES_GUI_BLADE_EXTENDED='layouts.app'
ROLES_GUI_TITLE_EXTENDED='template_title'
ROLES_GUI_LARAVEL_ROLES_ENABLED=true
ROLES_GUI_DATATABLES_JS_ENABLED=false
ROLES_GUI_FLASH_MESSAGES_ENABLED=false
ROLES_GUI_BLADE_PLACEMENT_CSS=template_linked_css
ROLES_GUI_BLADE_PLACEMENT_JS=footer_scripts
# Google Analytics - If blank it will not render, default is false
GOOGLE_ANALYTICS_ID=
#GOOGLE_ANALYTICS_ID='UA-XXXXXXXX-X'
# NOTE: YOU CAN REMOVE THE KEY CALL IN app.blade.php IF YOU GET A POP UP AND DO NOT WANT TO SETUP A KEY FOR DEV
# Google Maps API v3 Key - https://developers.google.com/maps/documentation/javascript/get-api-key#get-an-api-key
GOOGLEMAPS_API_STATUS=true
GOOGLEMAPS_API_KEY=YOURGOOGLEMAPSkeyHERE
# https://www.google.com/recaptcha/admin#list
ENABLE_RECAPTCHA=true
RE_CAP_SITE=YOURGOOGLECAPTCHAsitekeyHERE
RE_CAP_SECRET=YOURGOOGLECAPTCHAsecretHERE
# https://console.developers.google.com/ - NEED OAUTH CREDS
GOOGLE_ID=YOURGOOGLEPLUSidHERE
GOOGLE_SECRET=YOURGOOGLEPLUSsecretHERE
GOOGLE_REDIRECT=https://YOURWEBURLHERE.COM/social/handle/google
# https://developers.facebook.com/
FB_ID=YOURFACEBOOKidHERE
FB_SECRET=YOURFACEBOOKsecretHERE
FB_REDIRECT=https://YOURWEBURLHERE.COM/social/handle/facebook
# https://apps.twitter.com/
TW_ID=YOURTWITTERidHERE
TW_SECRET=YOURTWITTERkeyHERE
TW_REDIRECT=https://YOURWEBURLHERE.COM/social/handle/twitter
# https://github.com/settings/applications/new
GITHUB_ID=YOURIDHERE
GITHUB_SECRET=YOURSECRETHERE
GITHUB_URL=https://YOURWEBURLHERE.COM/social/handle/github
# https://developers.google.com/youtube/v3/getting-started
YOUTUBE_KEY=YOURKEYHERE
YOUTUBE_SECRET=YOURSECRETHERE
YOUTUBE_REDIRECT_URI=https://YOURWEBURLHERE.COM/social/handle/youtube
# https://dev.twitch.tv/docs/authentication/
TWITCH_KEY=YOURKEYHERE
TWITCH_SECRET=YOURSECRETHERE
TWITCH_REDIRECT_URI=https://YOURWEBURLHERE.COM/social/handle/twitch
# https://instagram.com/developer/register/
INSTAGRAM_KEY=YOURKEYHERE
INSTAGRAM_SECRET=YOURSECRETHERE
INSTAGRAM_REDIRECT_URI=https://YOURWEBURLHERE.COM/social/handle/instagram
# https://basecamp.com/
# https://github.com/basecamp/basecamp-classic-api
37SIGNALS_KEY=YOURKEYHERE
37SIGNALS_SECRET=YOURSECRETHERE
37SIGNALS_REDIRECT_URI=https://YOURWEBURLHERE.COM/social/handle/37signals
```
#### Laravel Developement Packages Used References
* https://laravel.com/docs/master/authentication
* https://laravel.com/docs/master/authorization
* https://laravel.com/docs/master/routing
* https://laravel.com/docs/master/migrations
* https://laravel.com/docs/master/queries
* https://laravel.com/docs/master/views
* https://laravel.com/docs/master/eloquent
* https://laravel.com/docs/master/eloquent-relationships
* https://laravel.com/docs/master/requests
* https://laravel.com/docs/master/errors
###### Updates:
* Update to Laravel 8
* Update to Laravel 7 [See changes in this PR](https://github.com/jeremykenedy/laravel-auth/pull/348/files)
* Update to Laravel 6
* Update to Laravel 5.8
* Added [Laravel Blocker Package](https://github.com/jeremykenedy/laravel-blocker)
* Added [PHP Info Package](https://github.com/jeremykenedy/laravel-phpinfo)
* Update to Bootstrap 4
* Update to Laravel 5.7
* Added optional 2-step account login verfication with [Laravel 2-Step Verification](https://github.com/jeremykenedy/laravel2step)
* Added activity logging using [Laravel-logger](https://github.com/jeremykenedy/laravel-logger)
* Added Configurable Email Notification using [Laravel-Exception-Notifier](https://github.com/jeremykenedy/laravel-exception-notifier)
* Update to Laravel 5.5
* Added User Delete with Goodbye email
* Added User Restore Deleted Account from email with secure token
* Added [Soft Deletes](https://laravel.com/docs/master/eloquent#soft-deleting) and Soft Deletes Management panel
* Added User Account Settings to Profile Edit
* Added User Change Password to Profile Edit
* Added User Delete Account to Profile Edit
* Added [Password Strength Meter](https://github.com/elboletaire/password-strength-meter)
* Added [hideShowPassword](https://github.com/cloudfour/hideShowPassword)
* Added Admin Routing Details
* Admin PHP Information
* Added Robust [Laravel Logging](https://laravel.com/docs/master/errors#logging) with admin UI using MonoLog
* Added Active Nav states using [Laravel Requests](https://laravel.com/docs/master/requests)
* Added [Laravel Debugger](https://github.com/barryvdh/laravel-debugbar) with Service Provider to manage status in `.env` file.
* Updated Capture IP not found IP address
* Added User Avatar Image AJAX Upload with [Dropzone.js](http://www.dropzonejs.com/#configuration)
* Added User Gravatar using Gravatar API
* Added Themes Management.
* Add user profiles with seeded list and global view
* Major overhaul on Laravel 5.4
* Update from Laravel 5.1 to 5.2
* Added eloquent editable user profile
* Added IP Capture
* Added Google Maps API v3 for User Location lookup
* Added Google Maps API v3 for User Location Input Geocoding
* Added Google Maps API v3 for User Location Map with Options
* Added CRUD(Create, Read, Update, Delete) User Management
### Screenshots
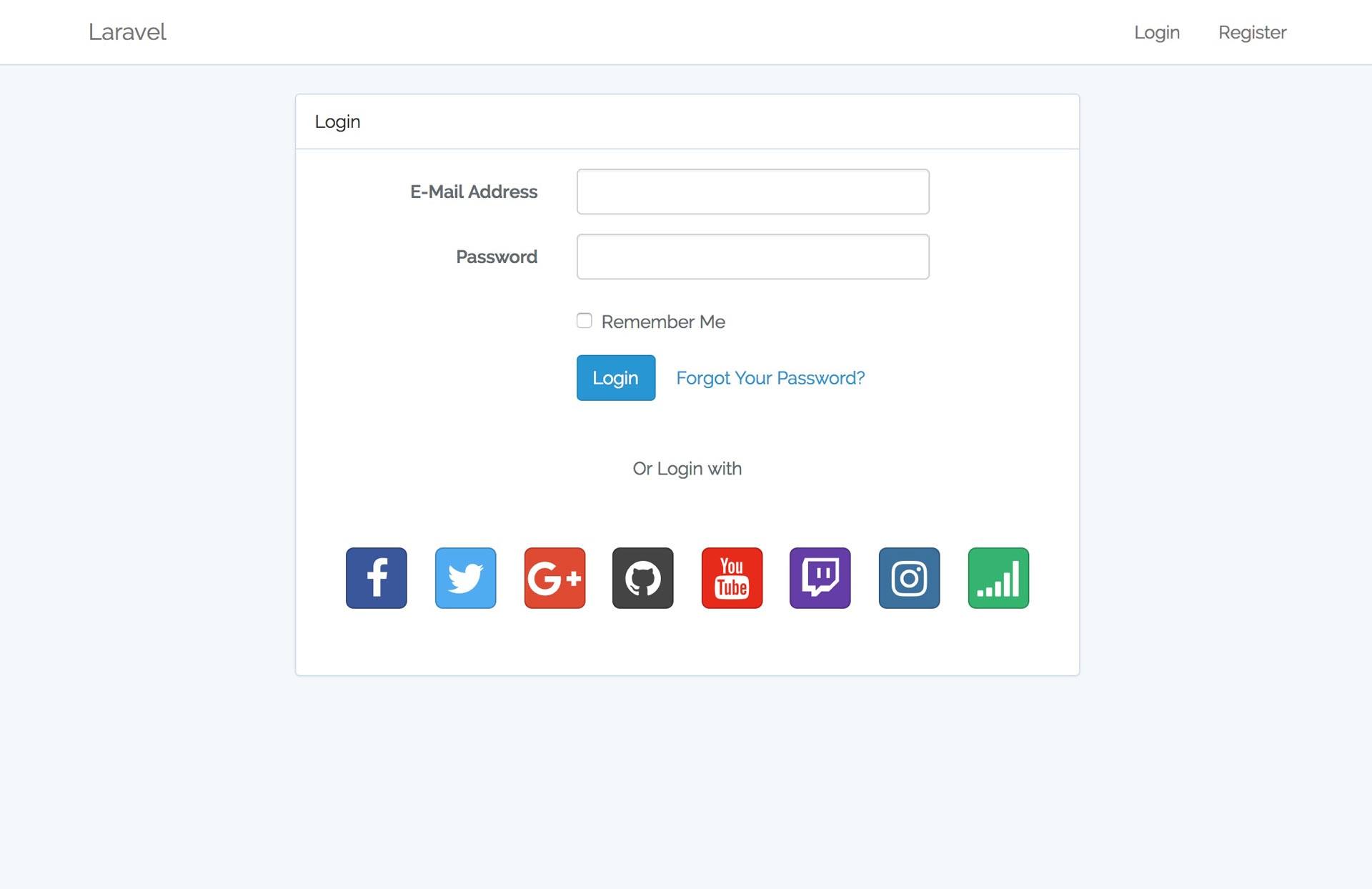
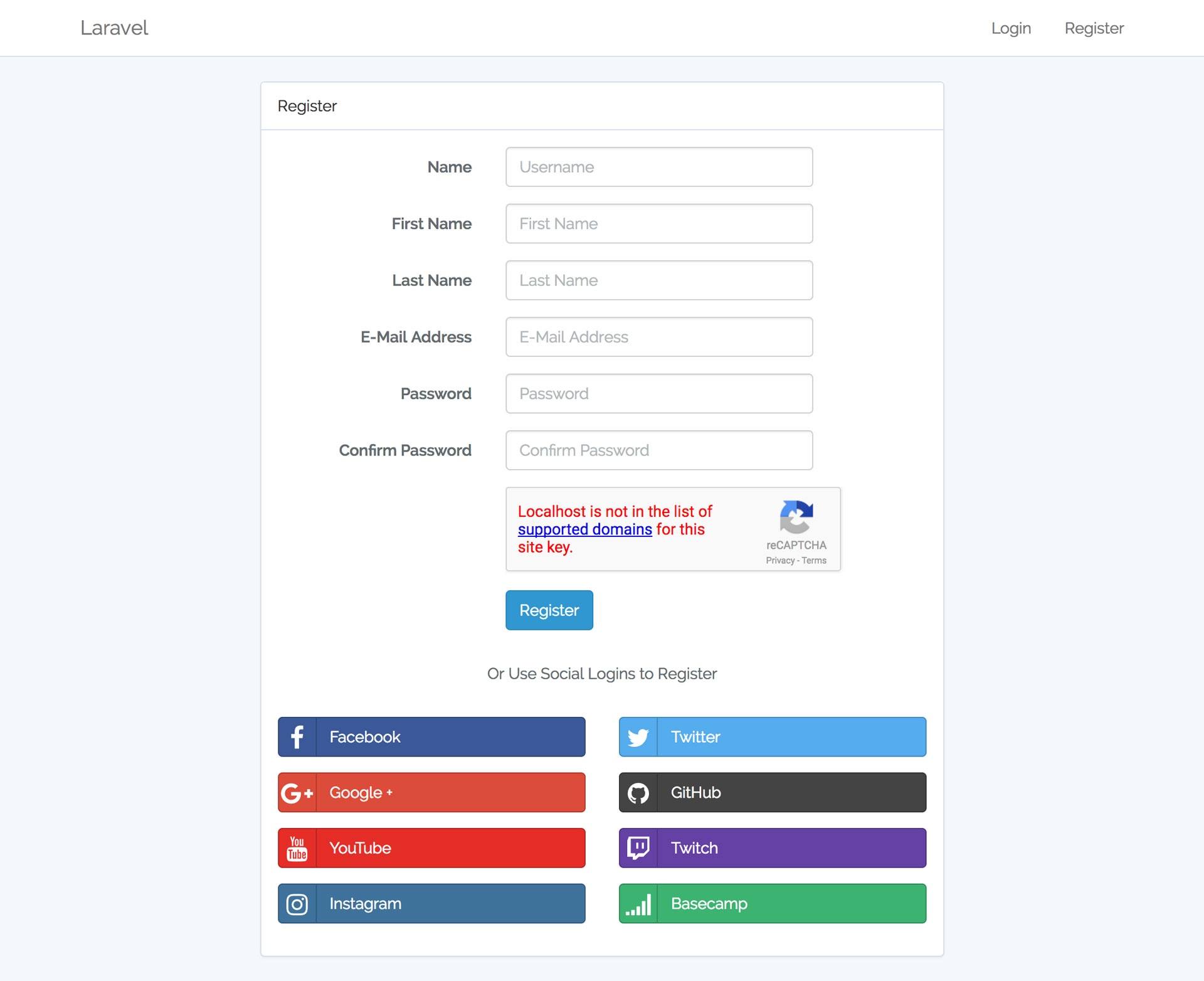
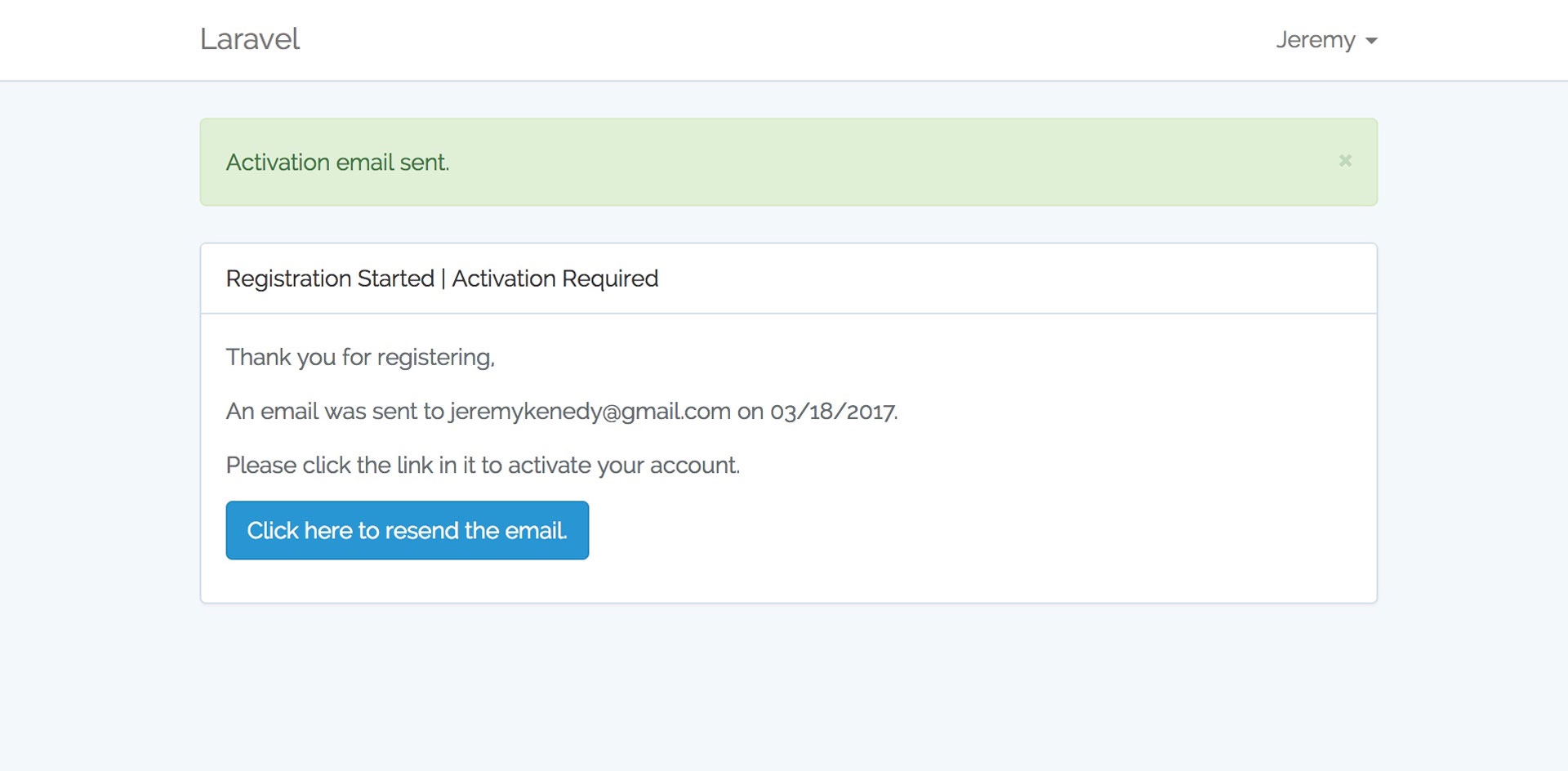
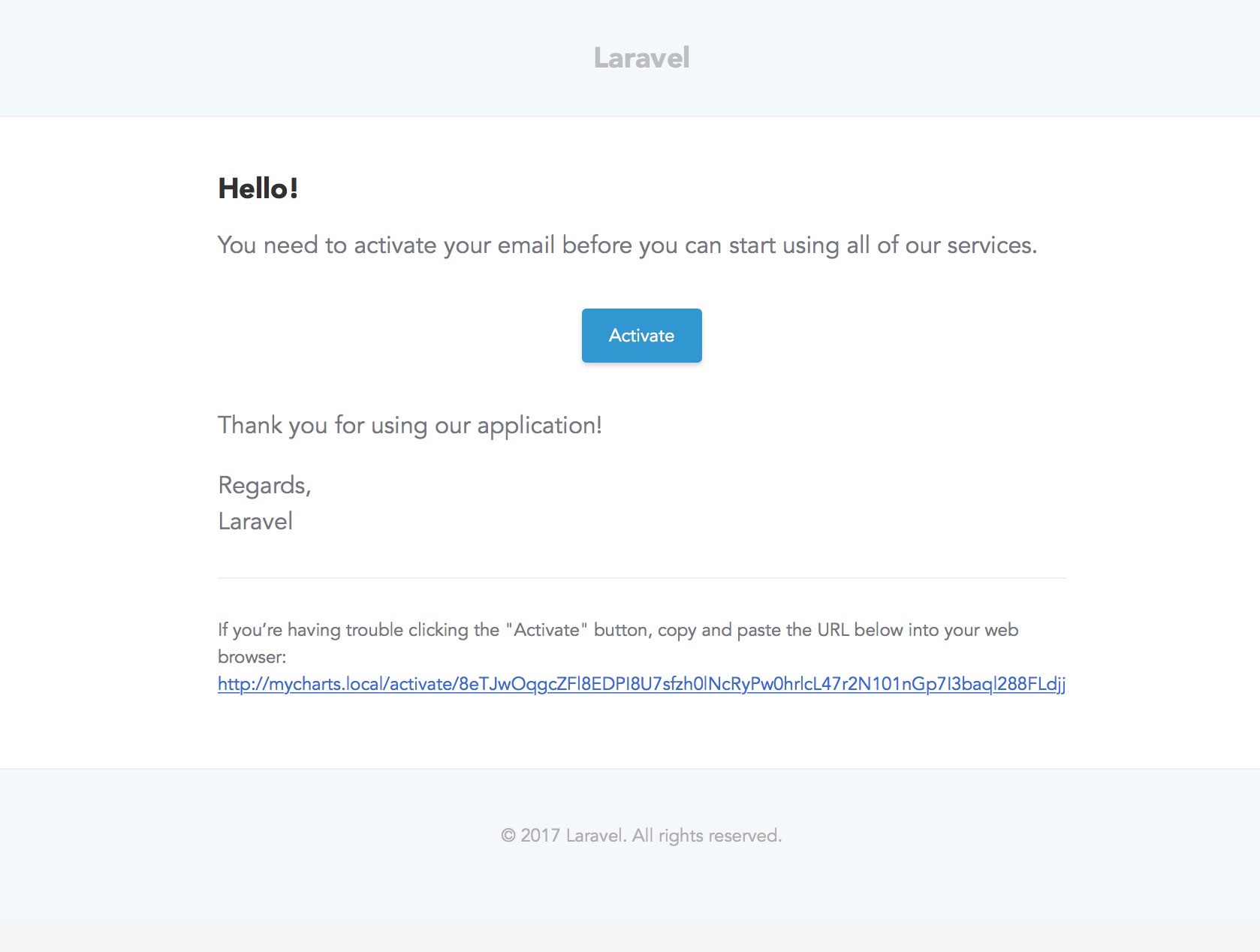
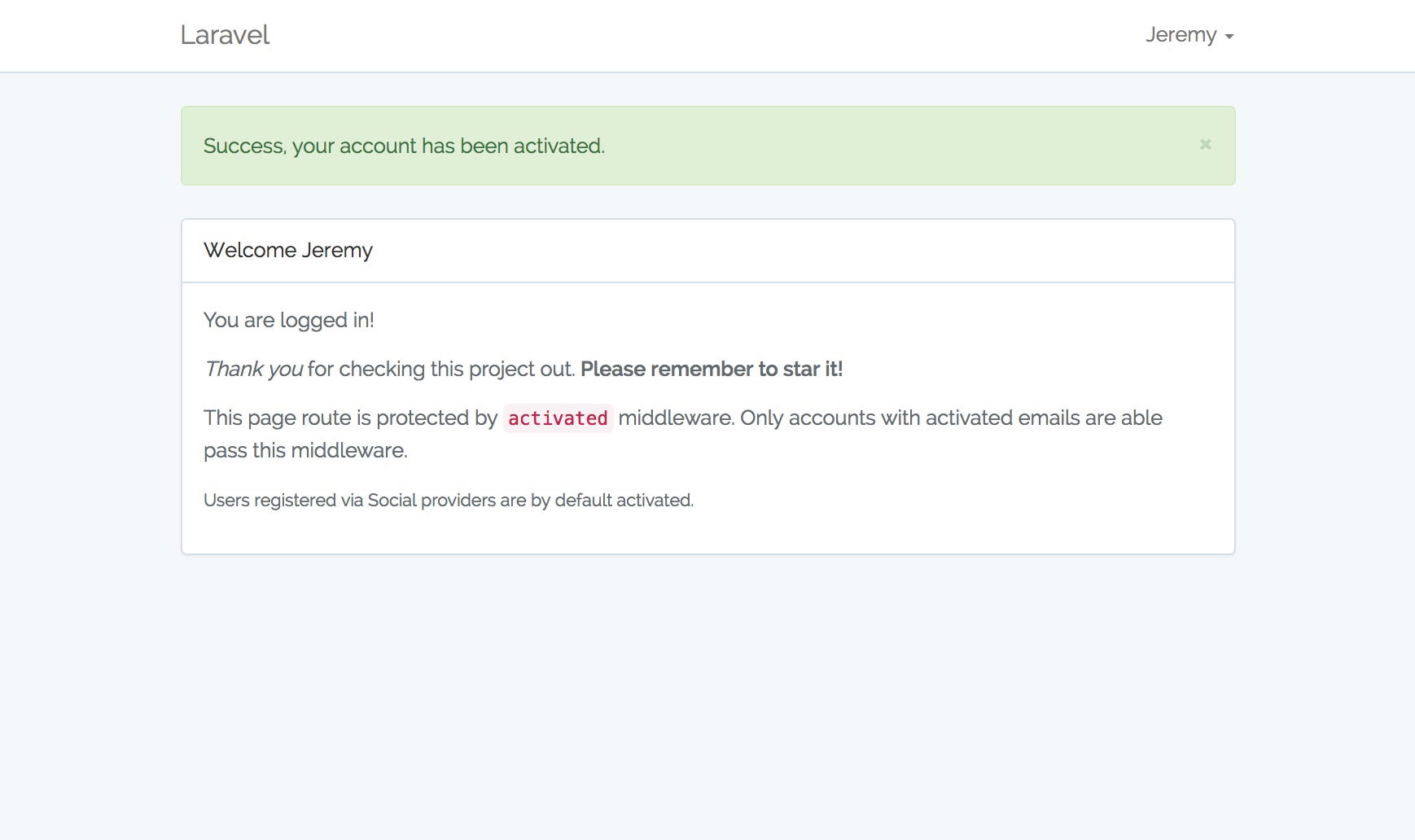
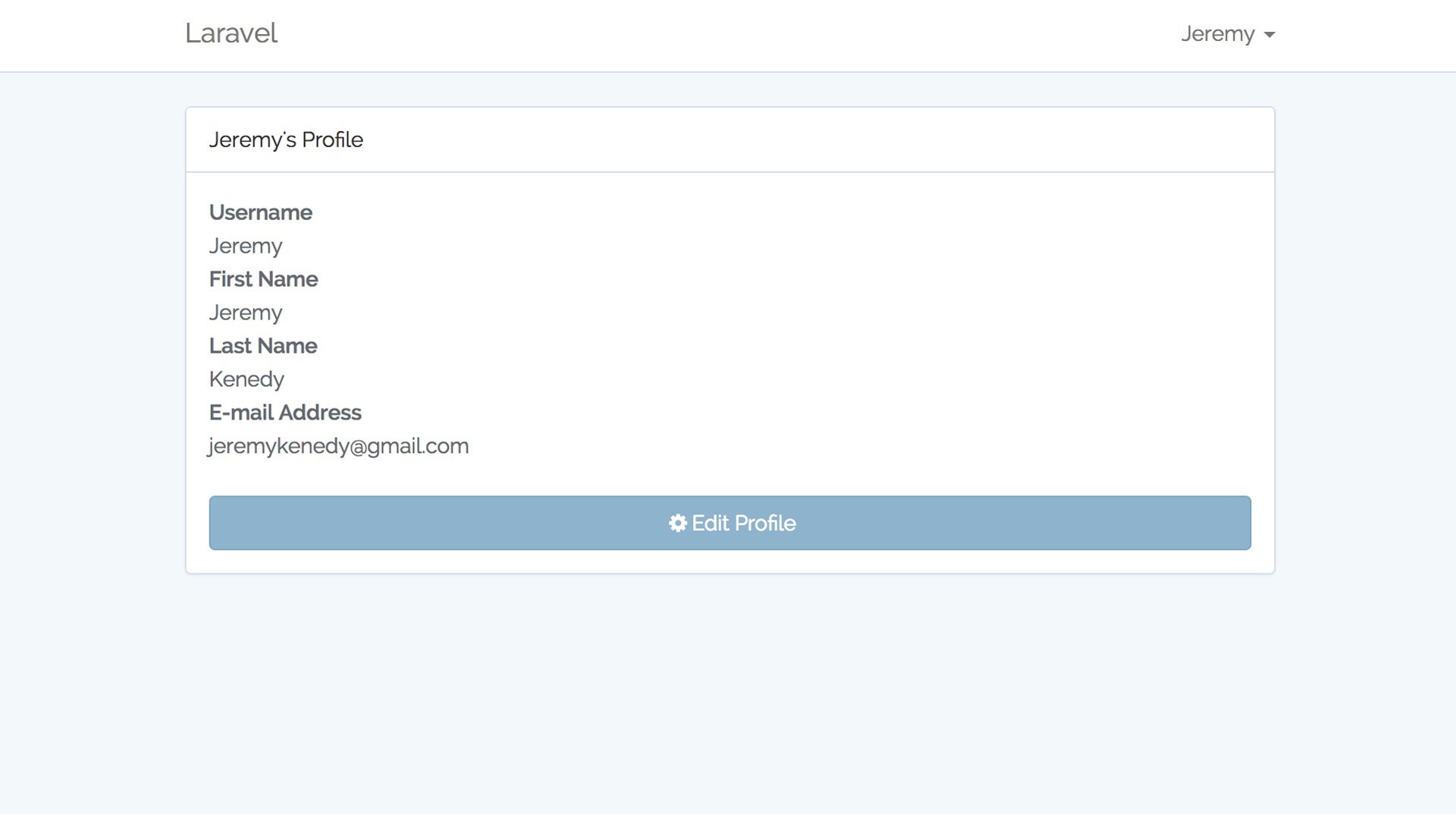
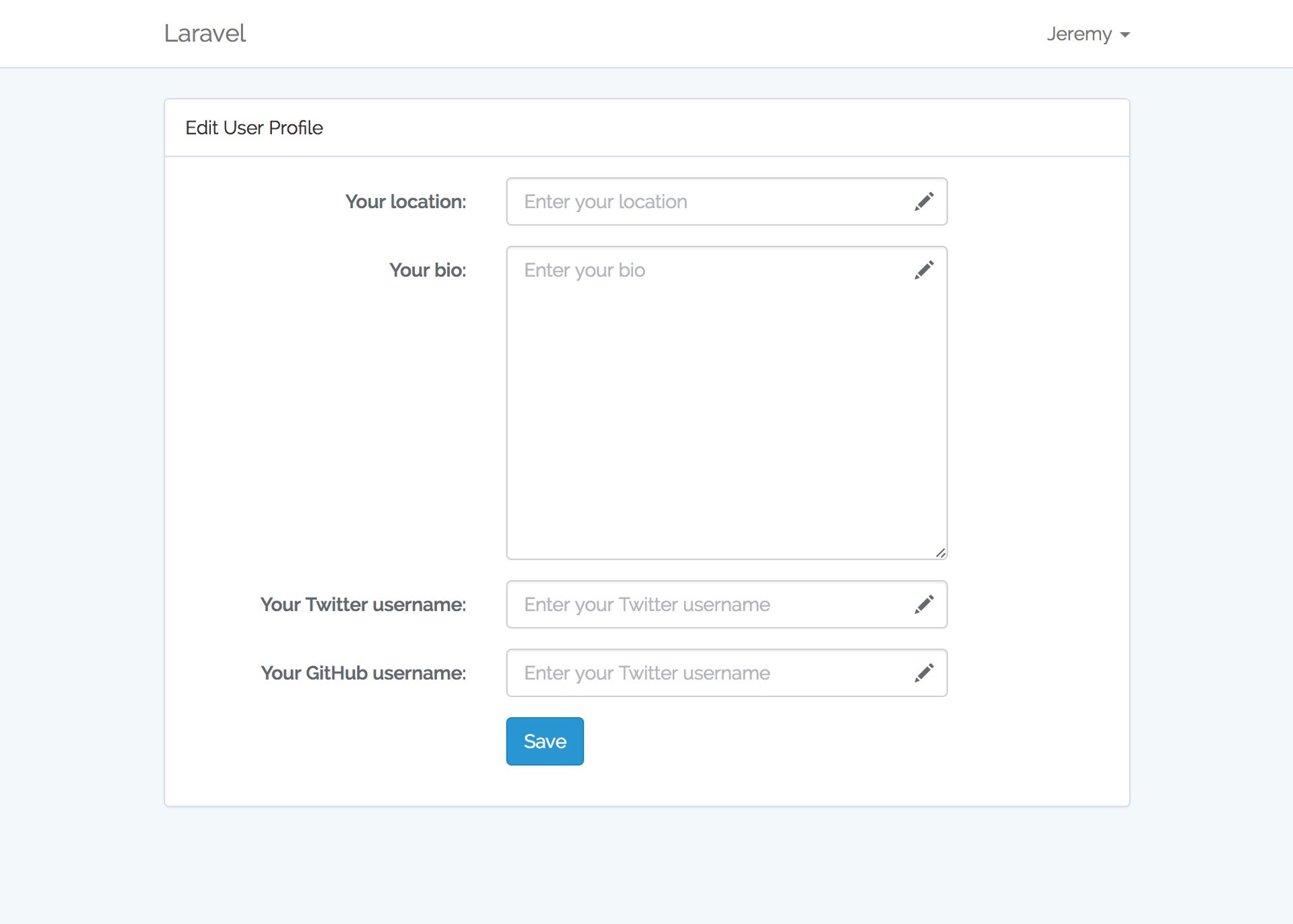
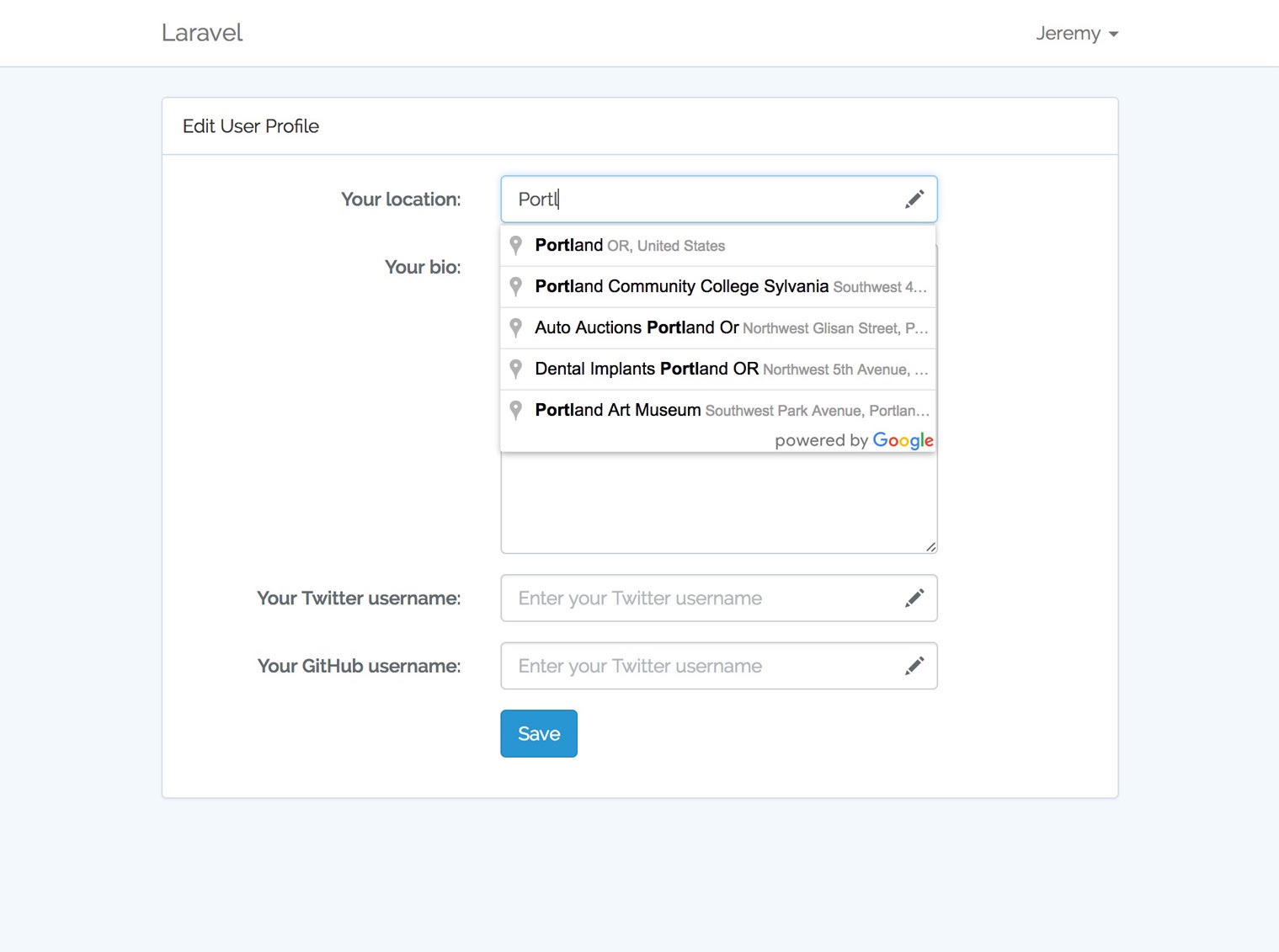
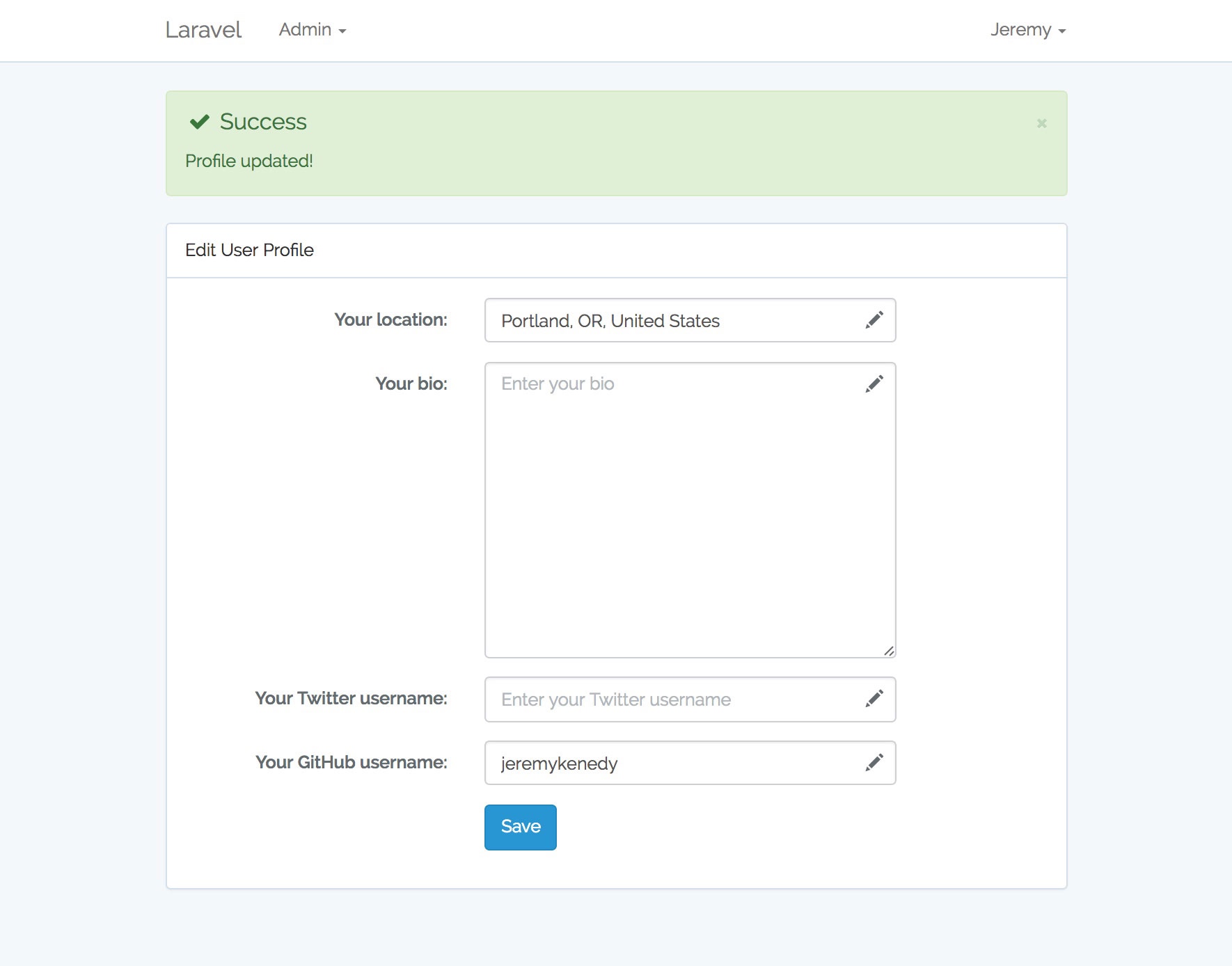
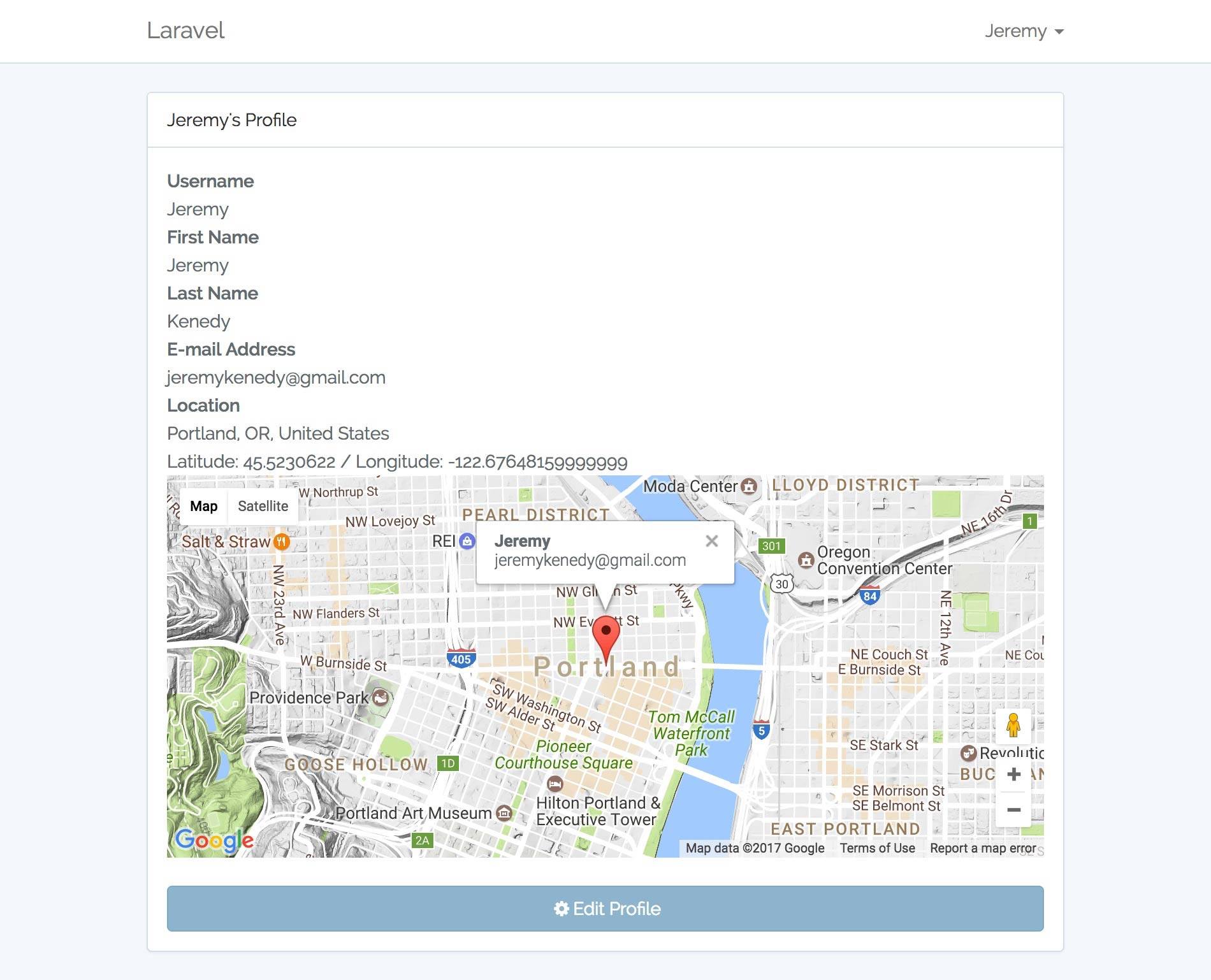
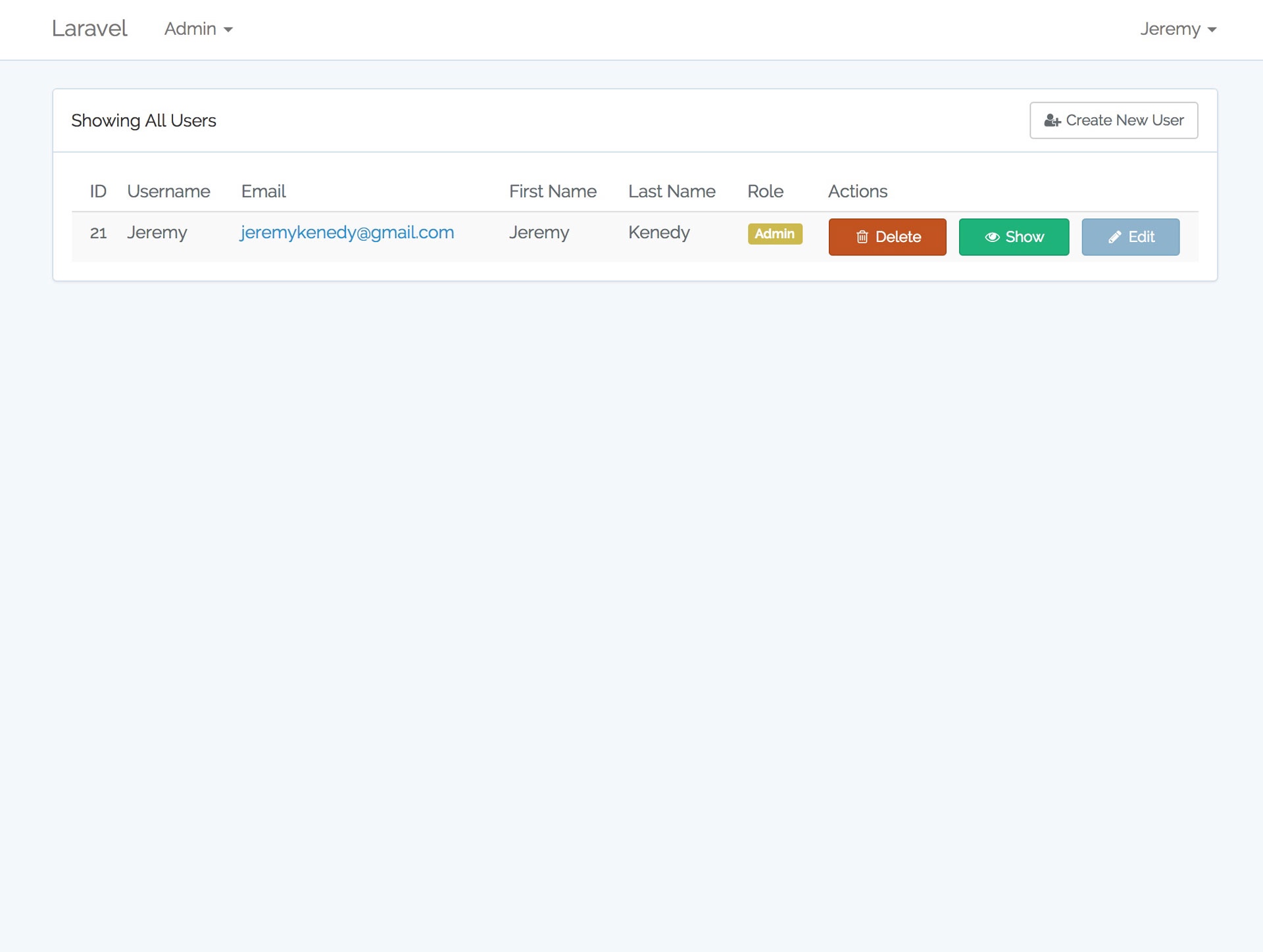
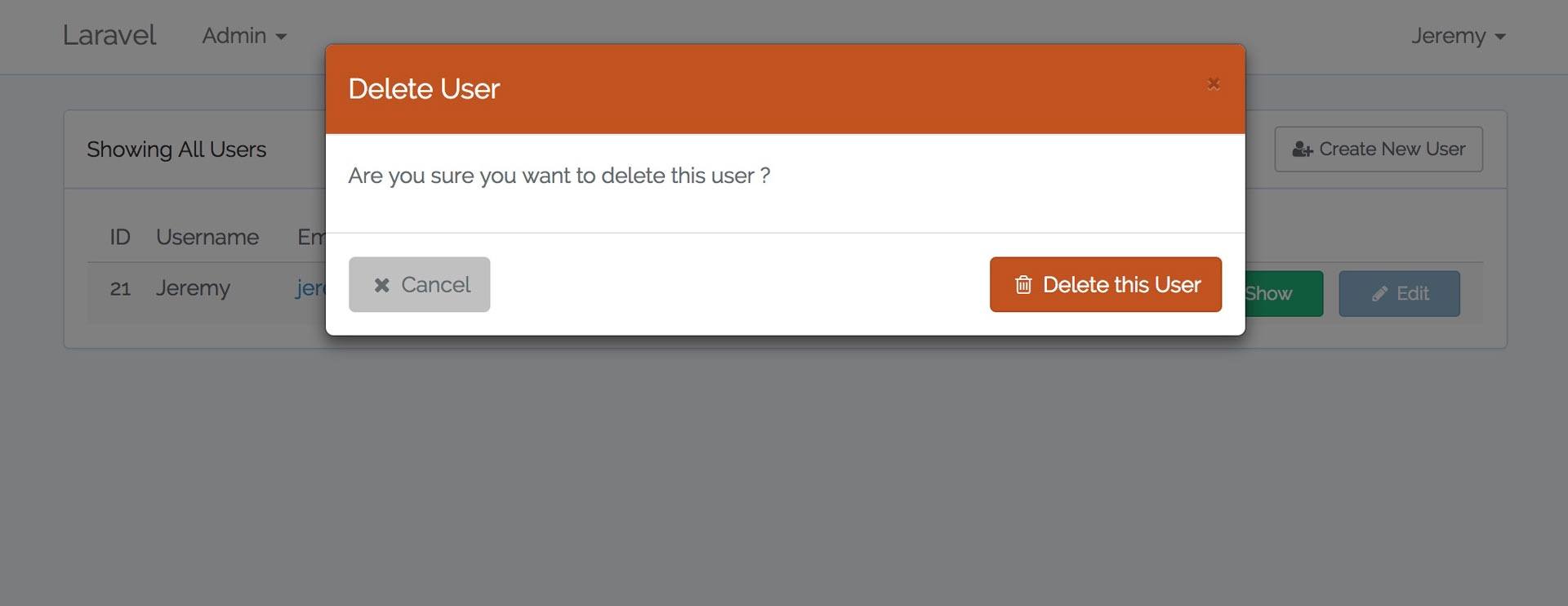
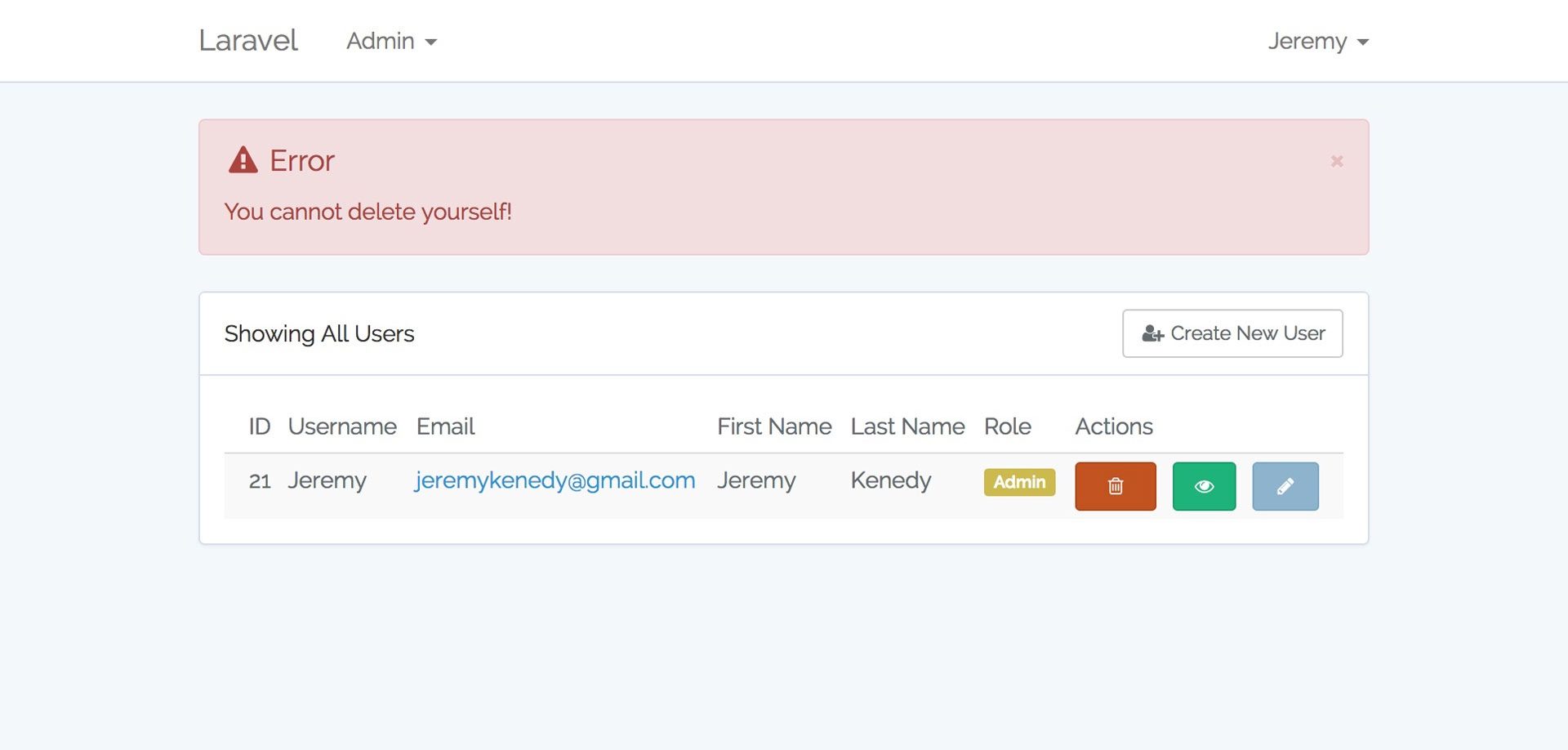
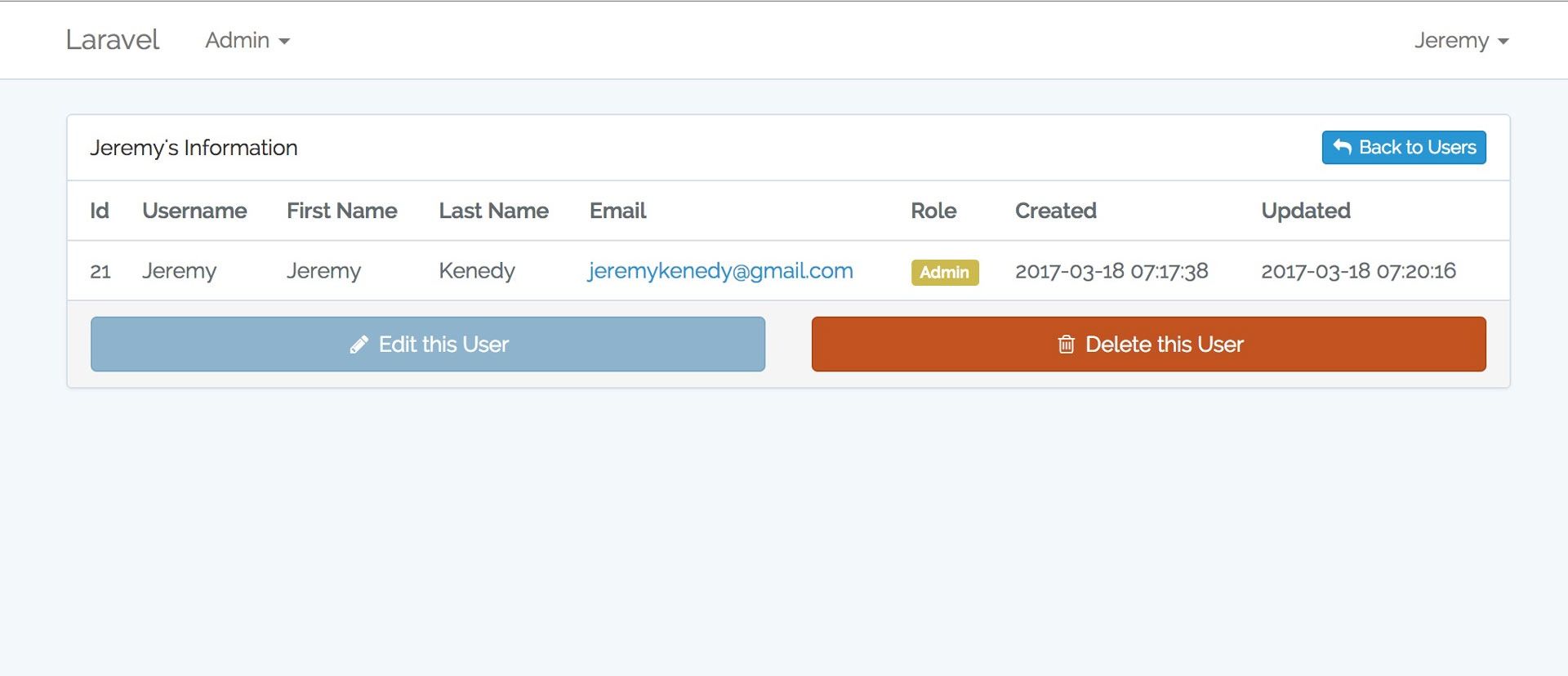
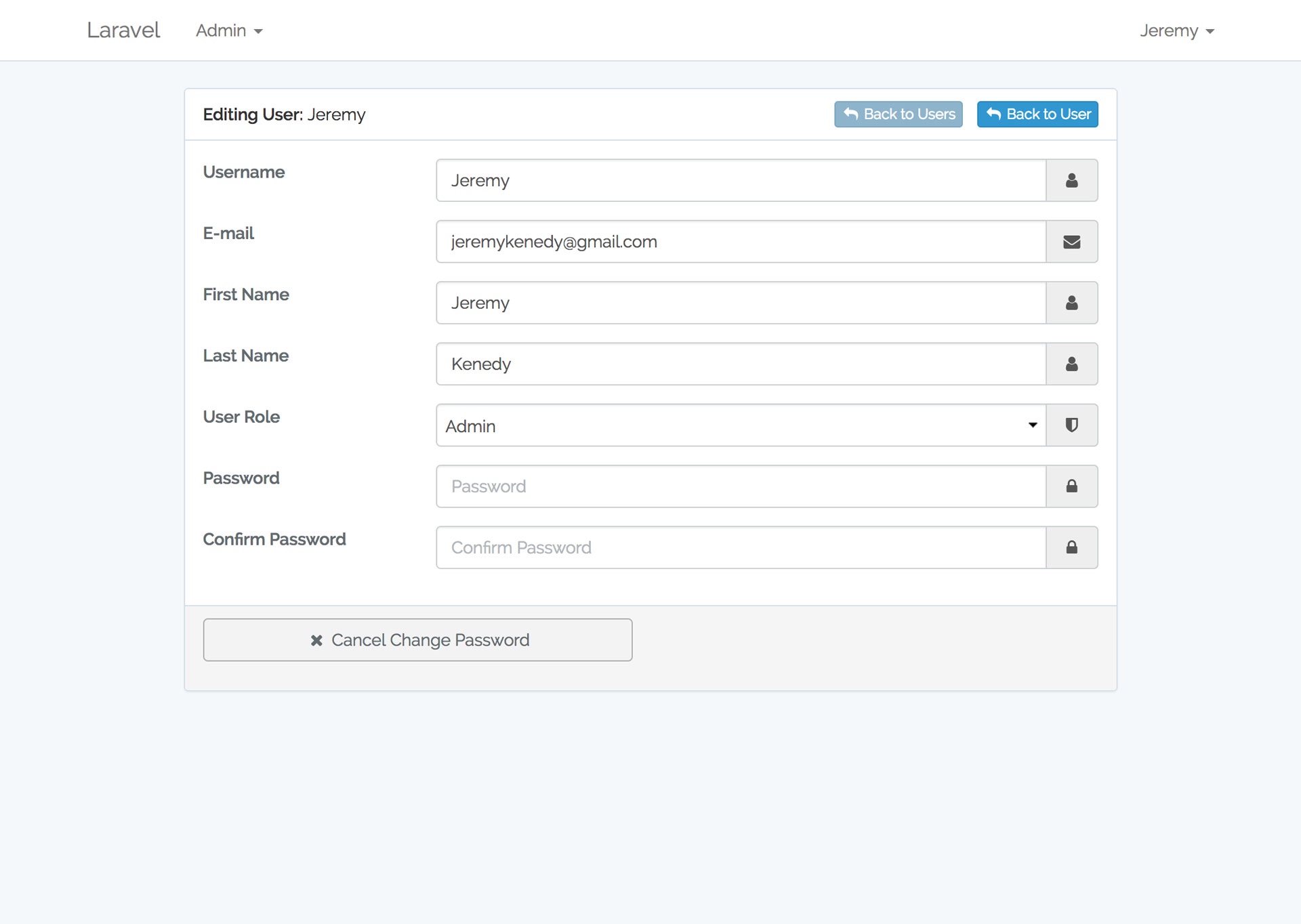
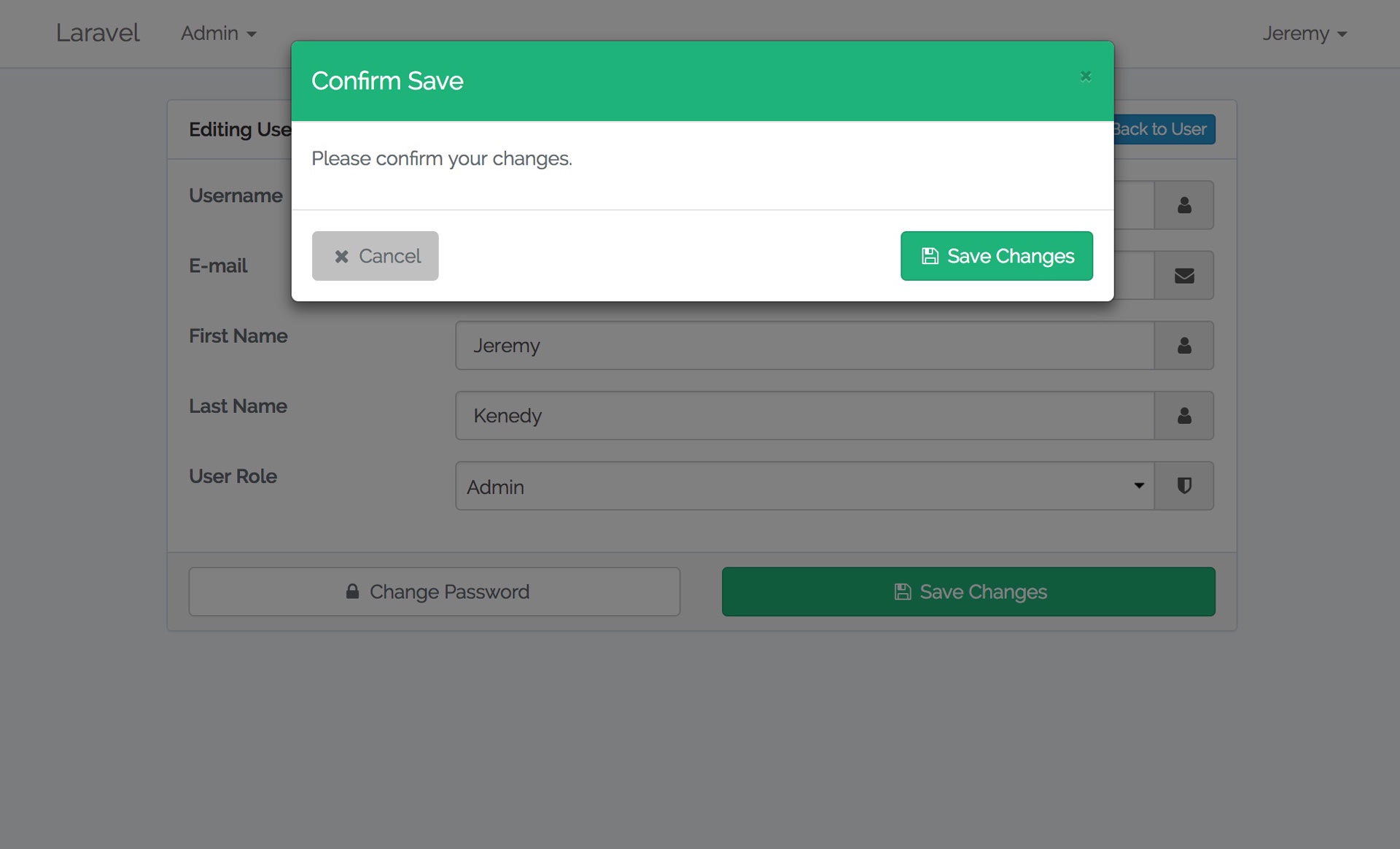
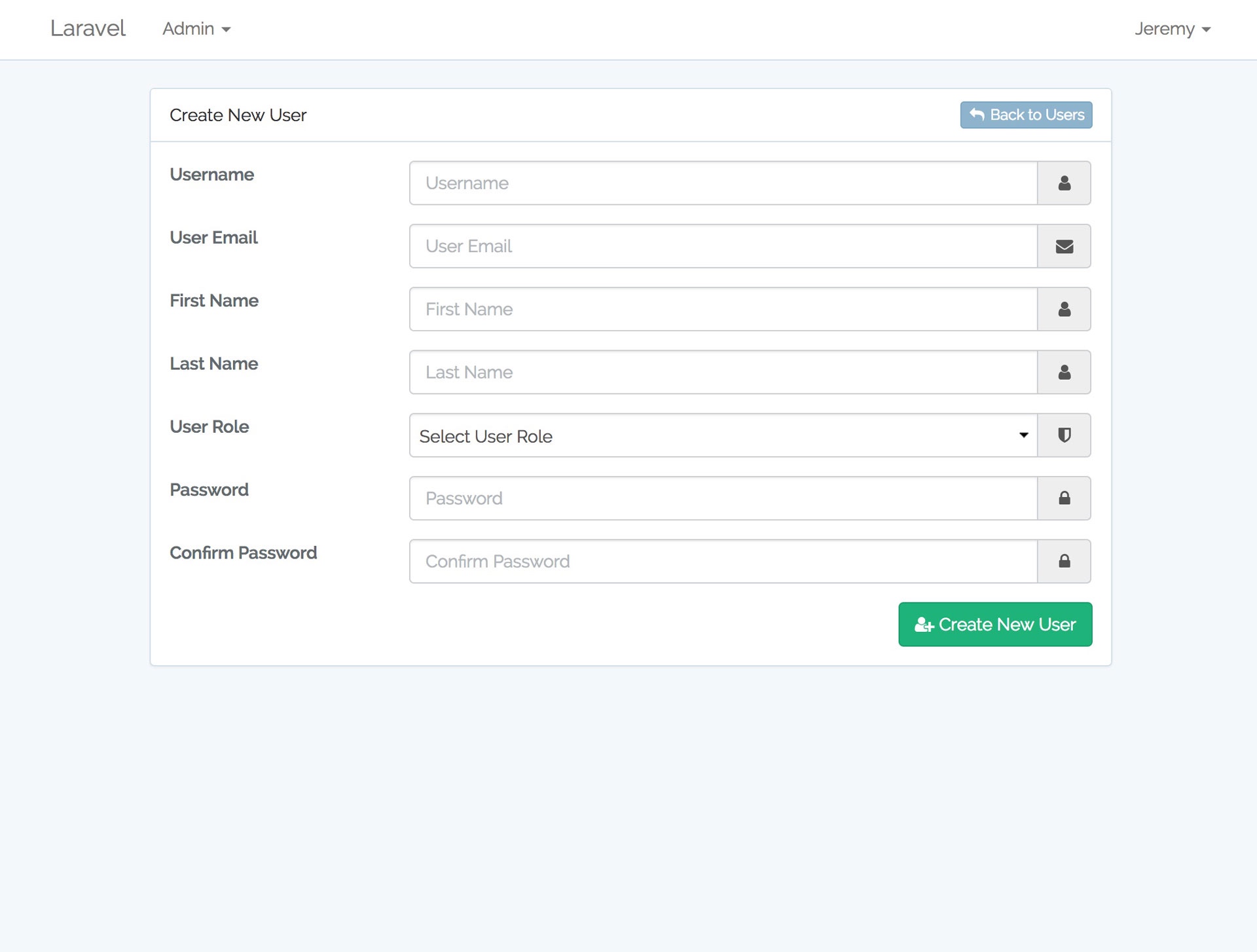
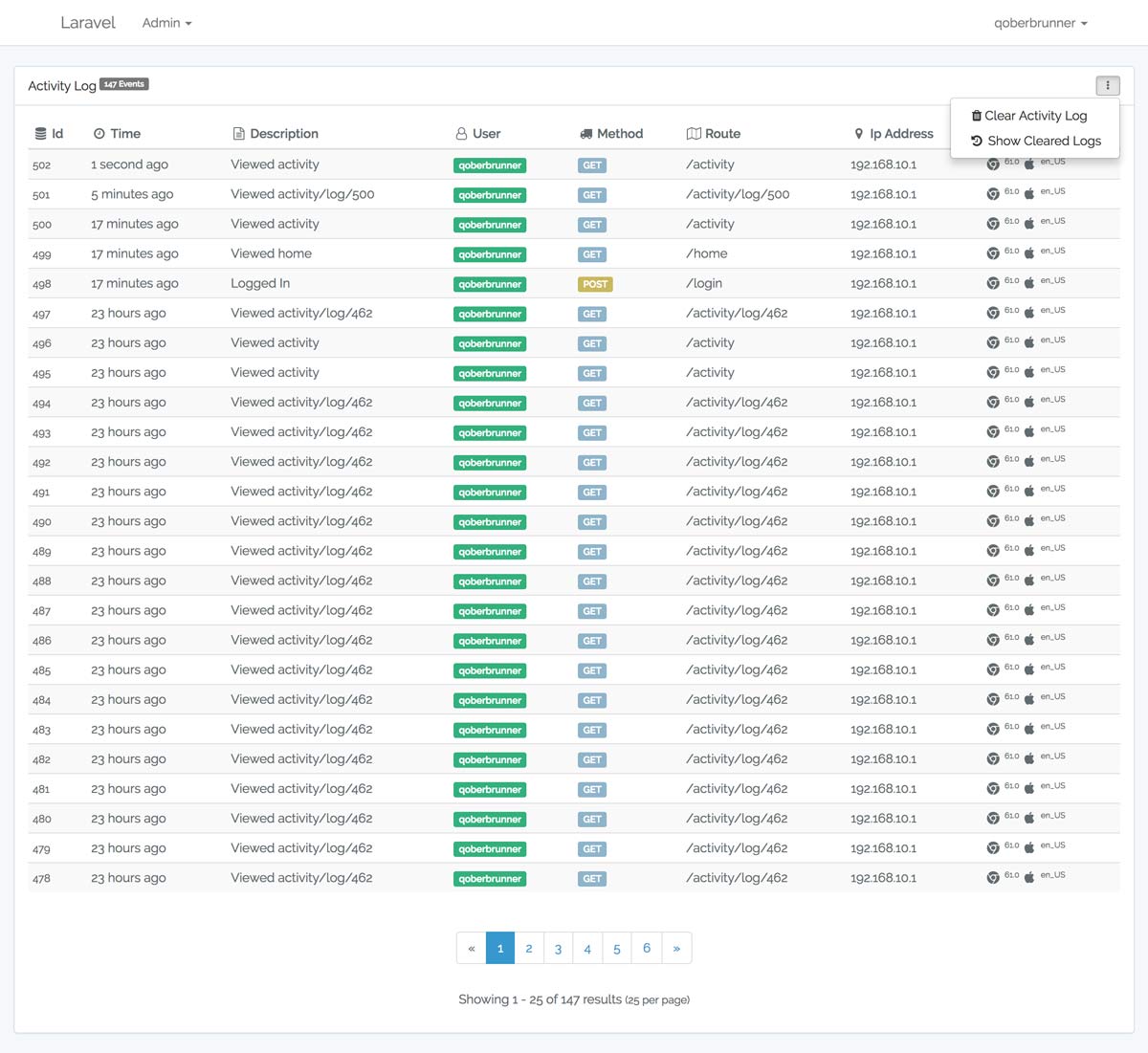
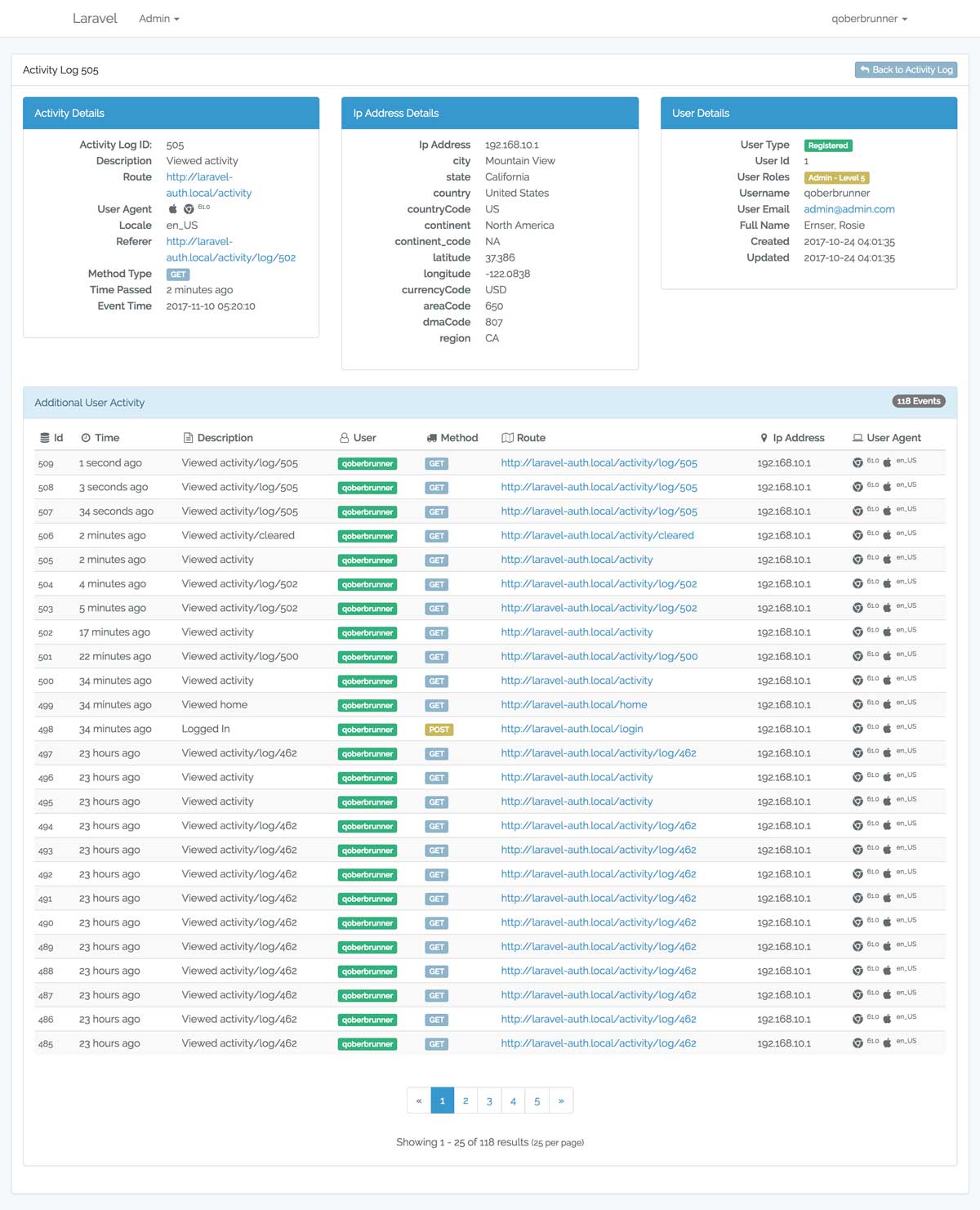
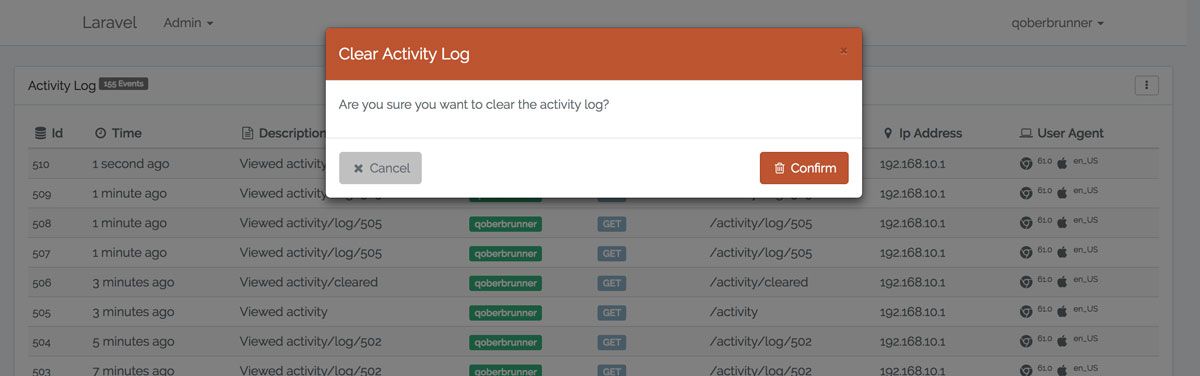
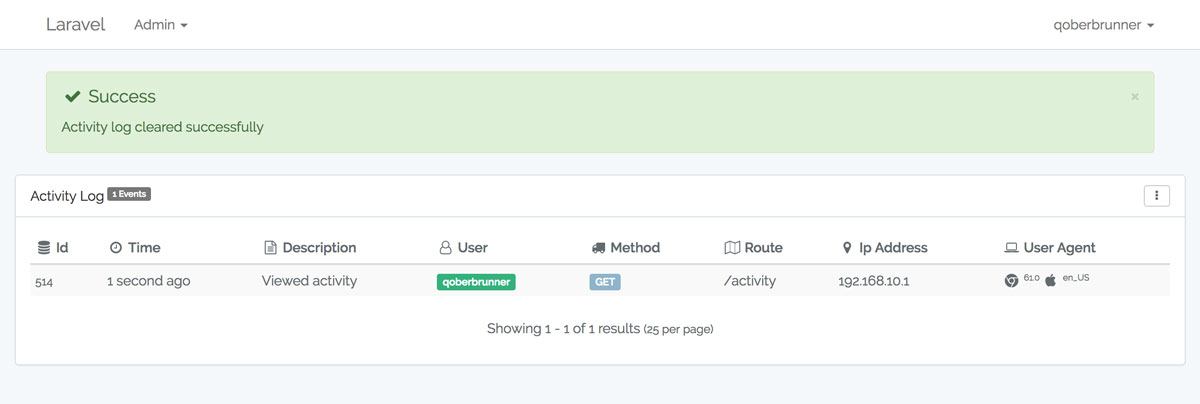
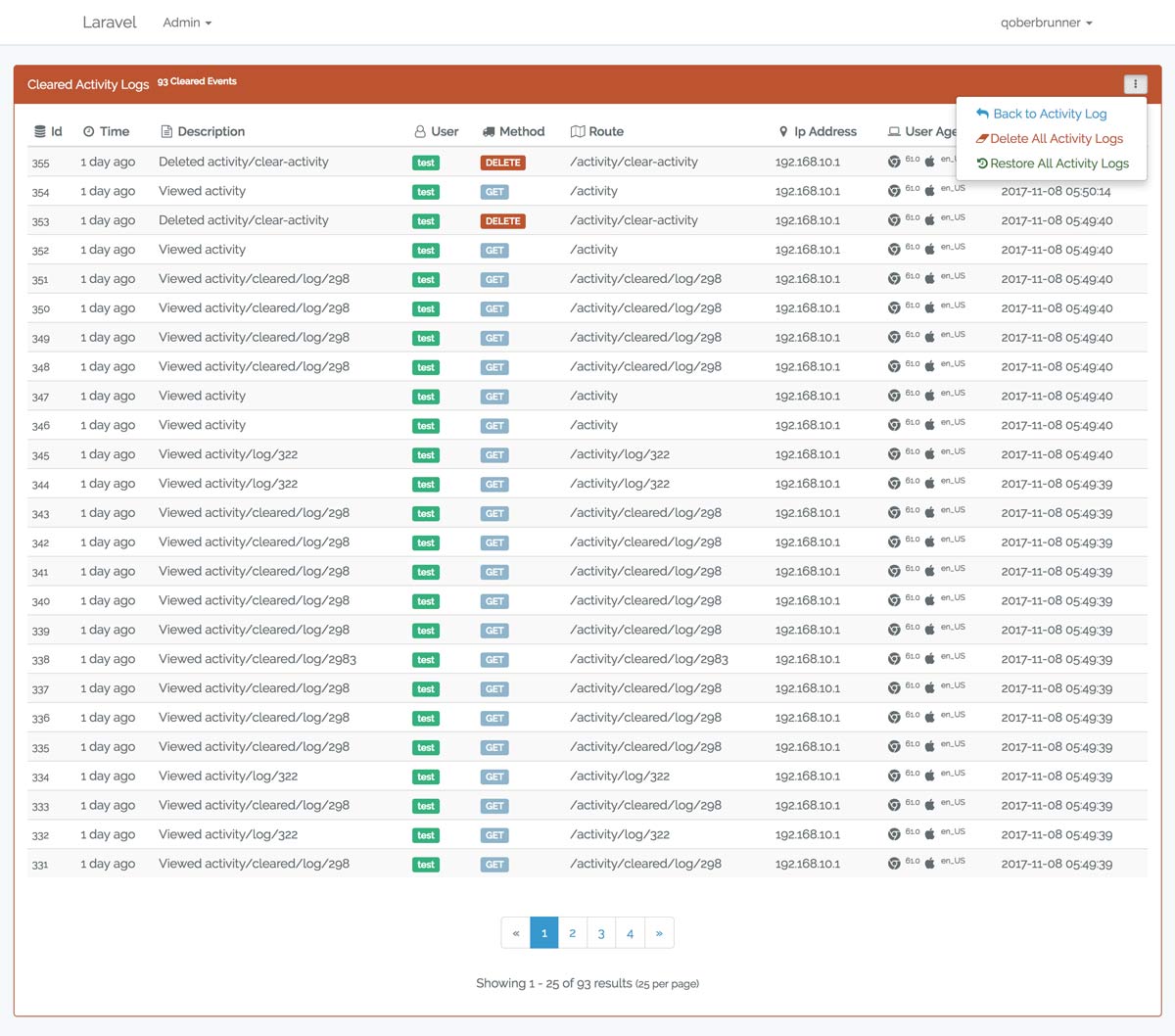
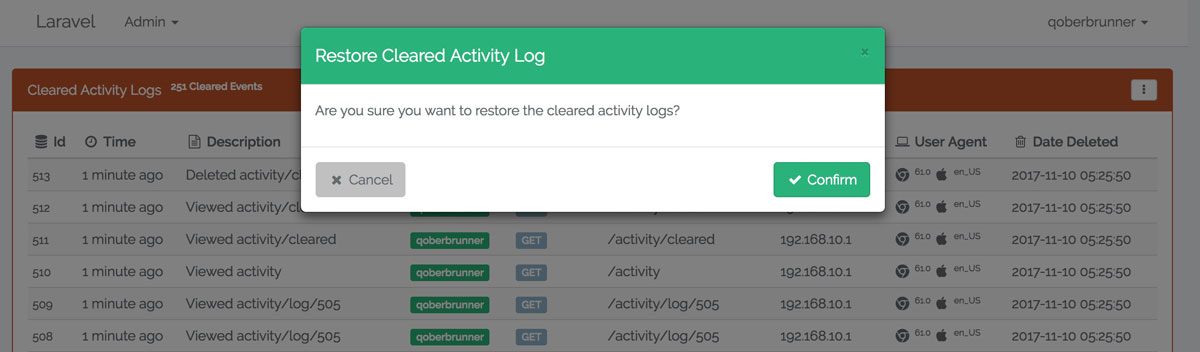
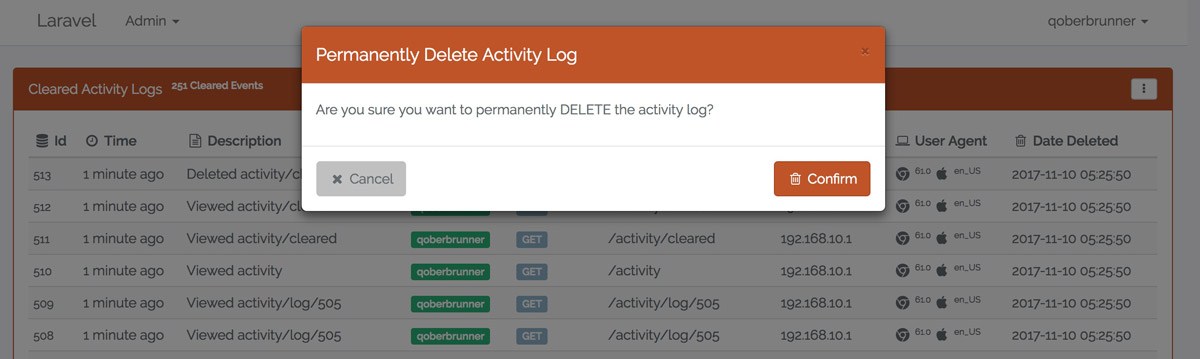
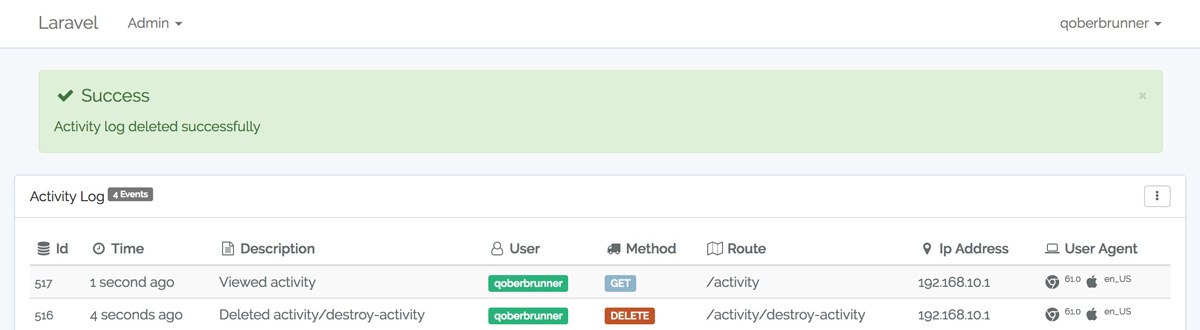
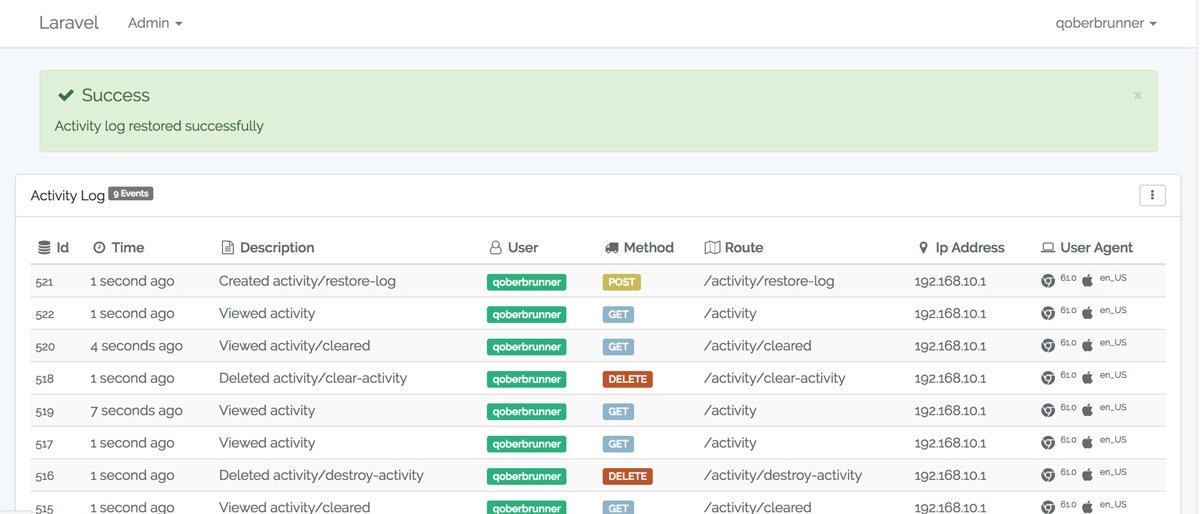
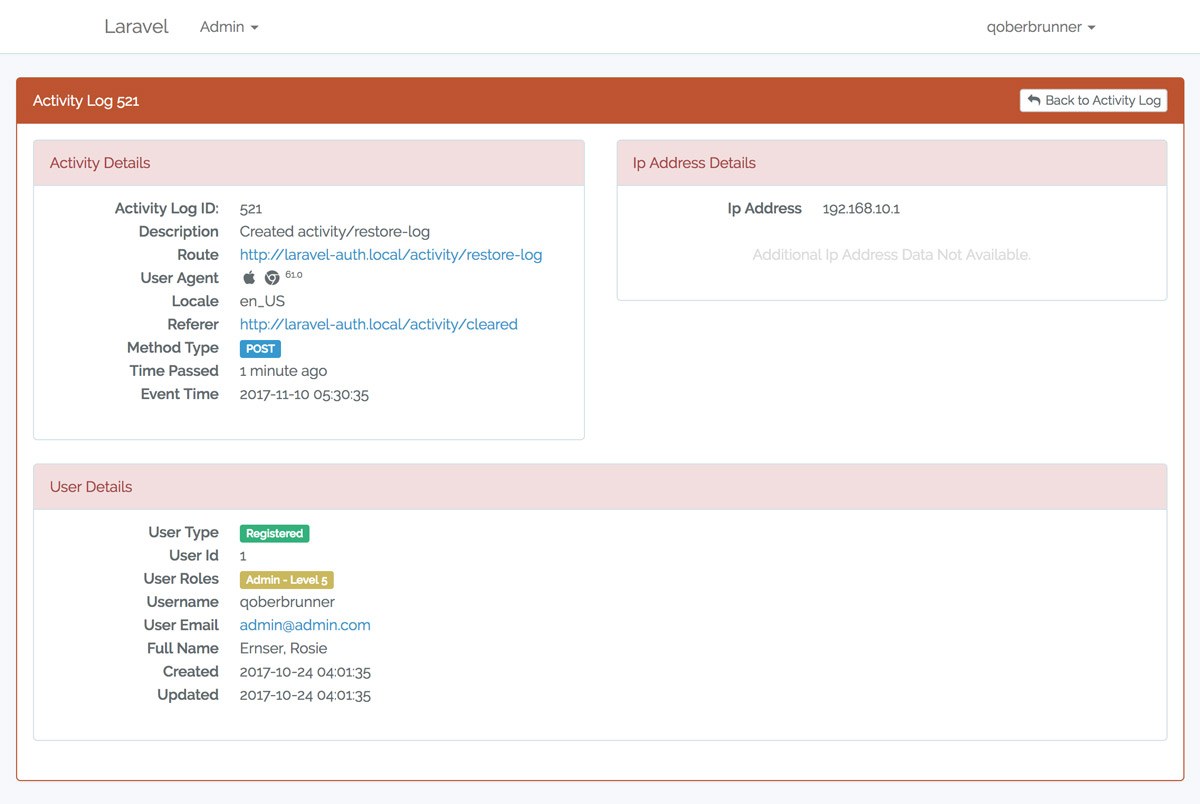
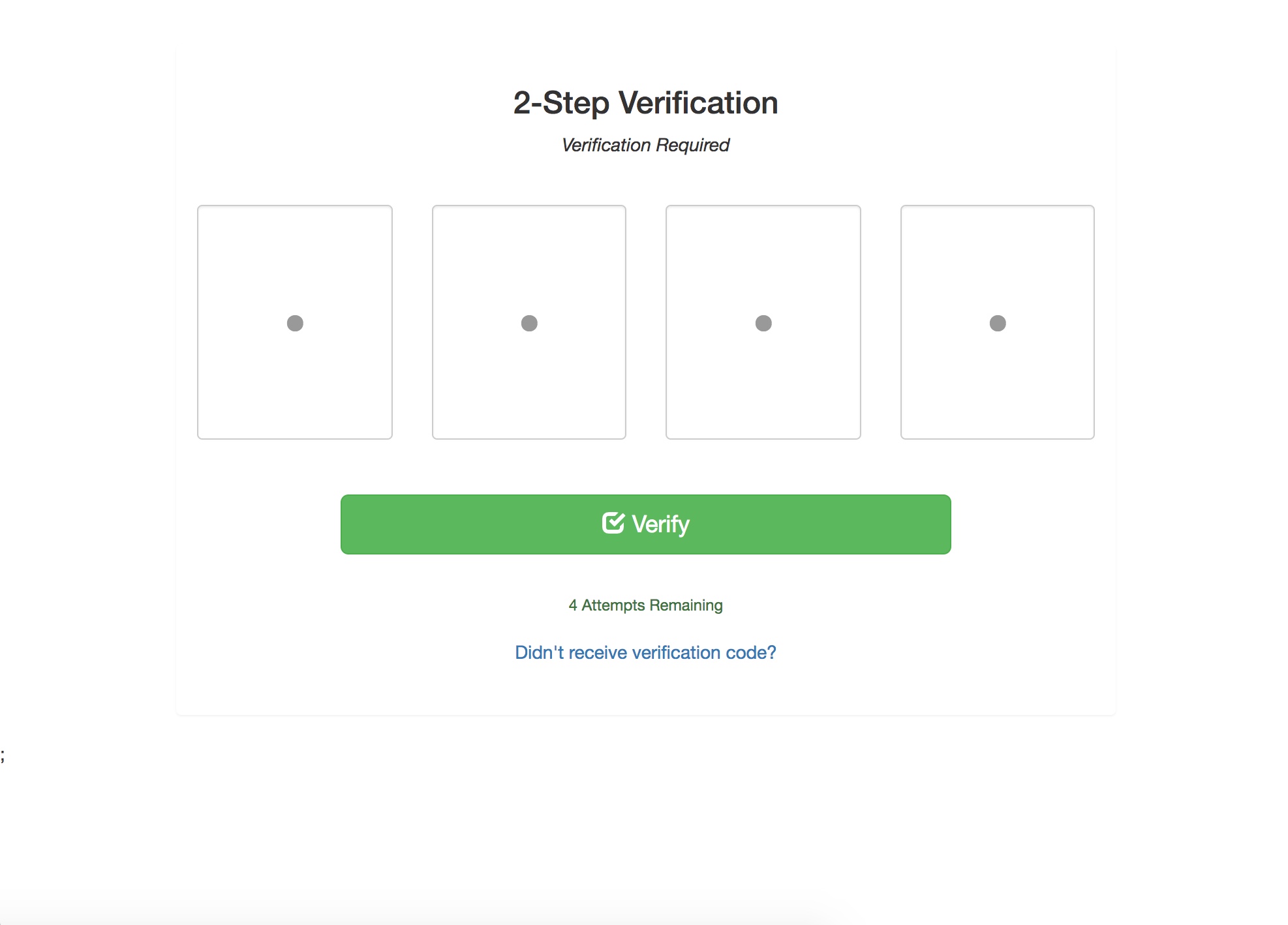
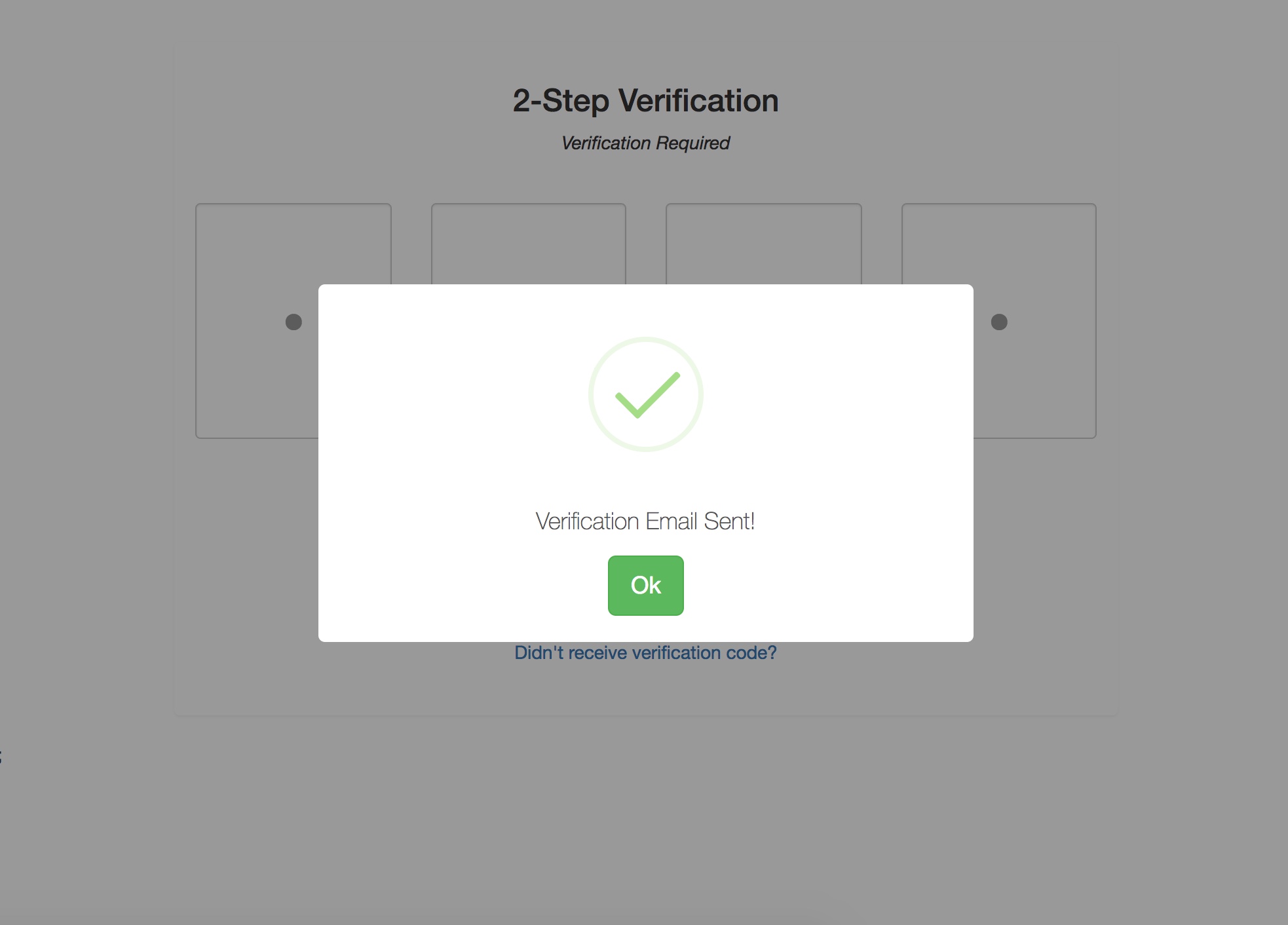
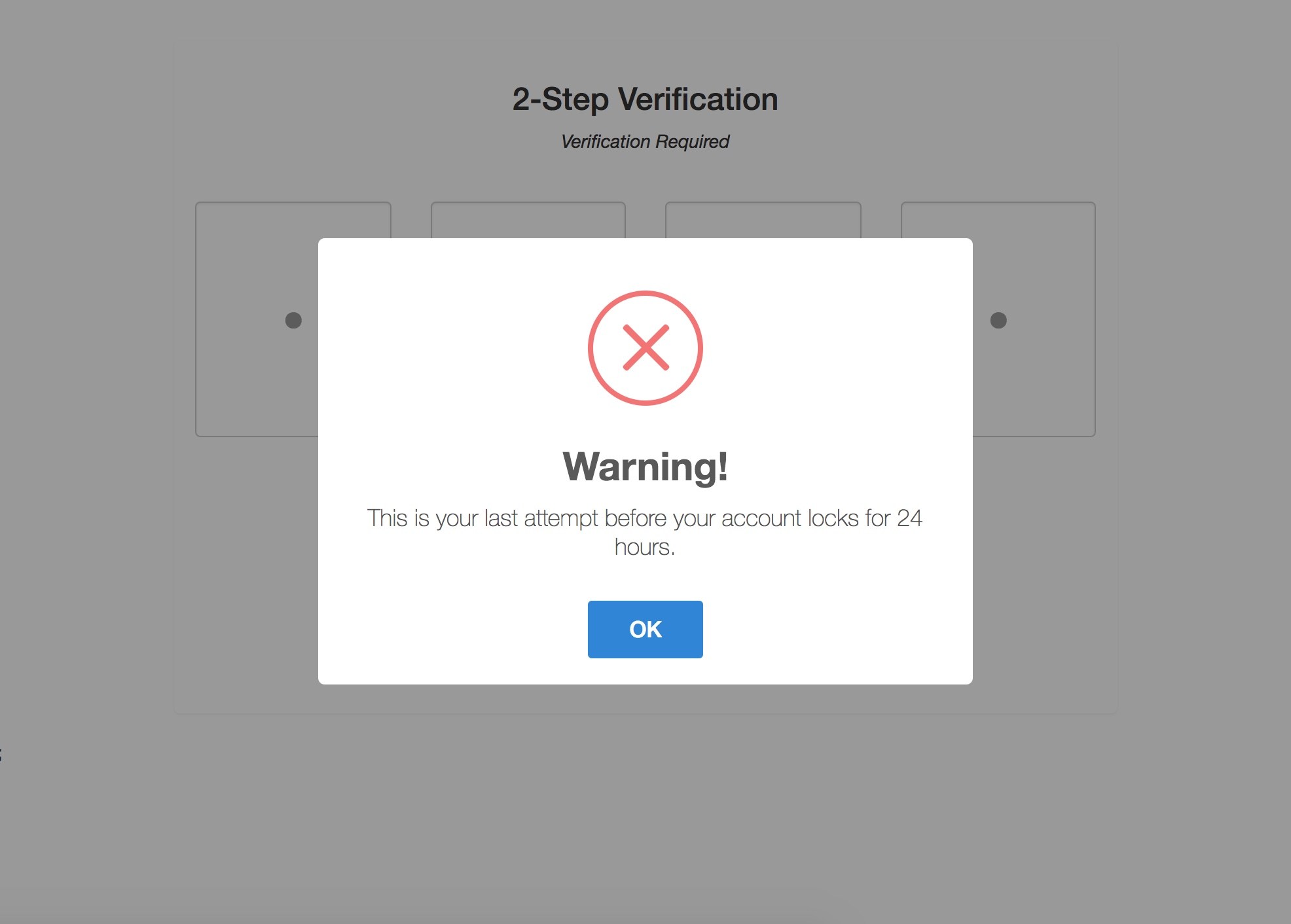
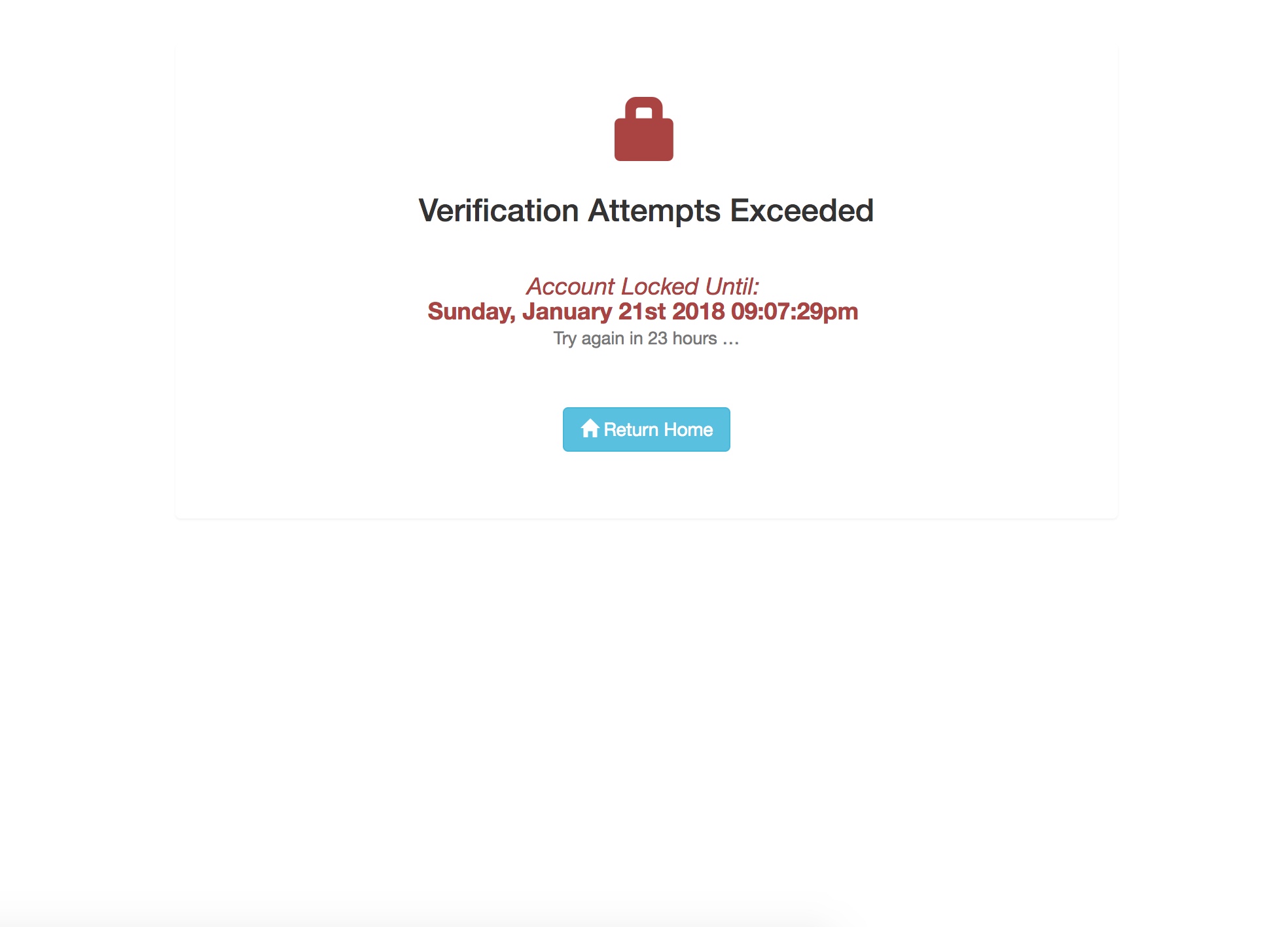
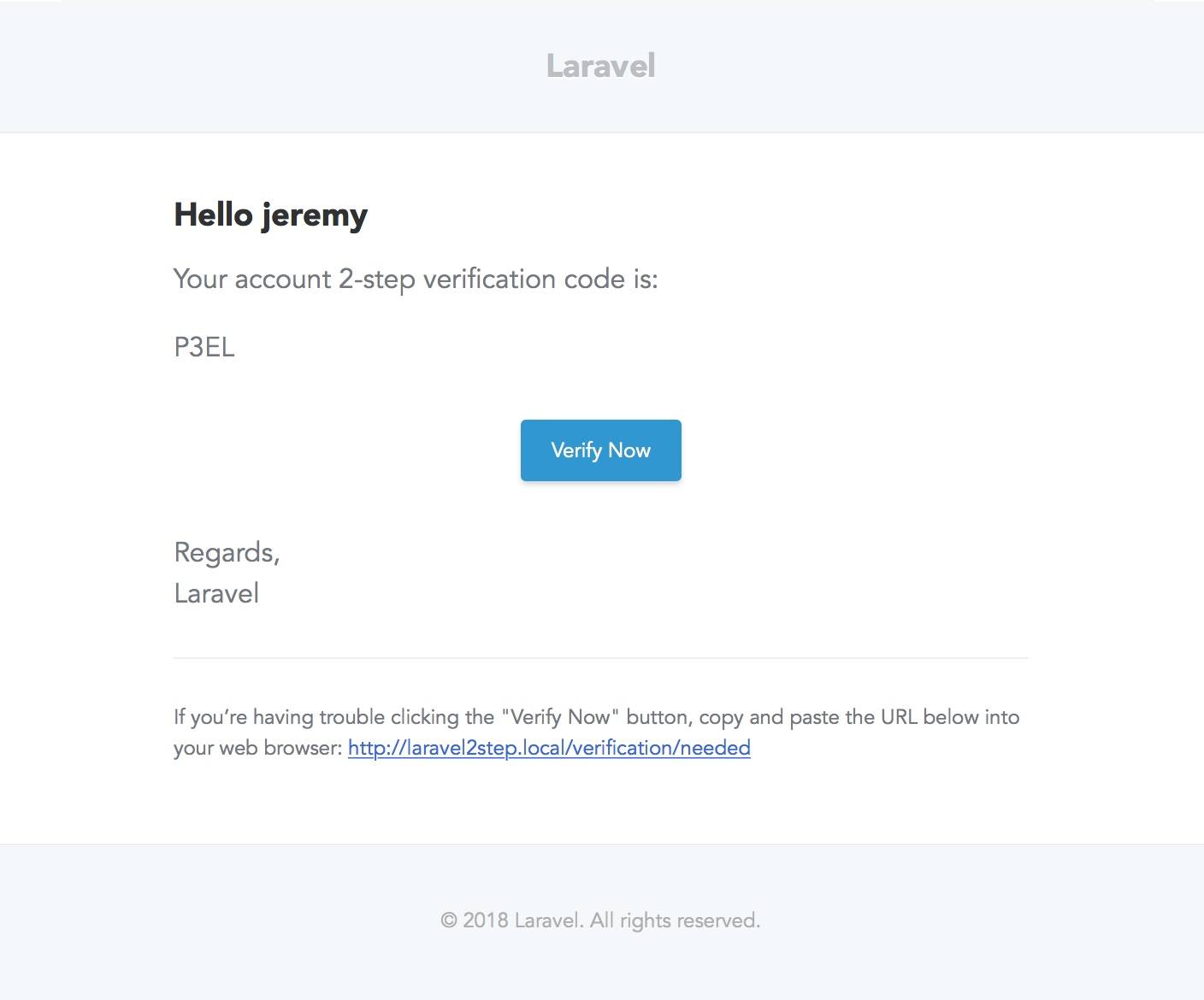
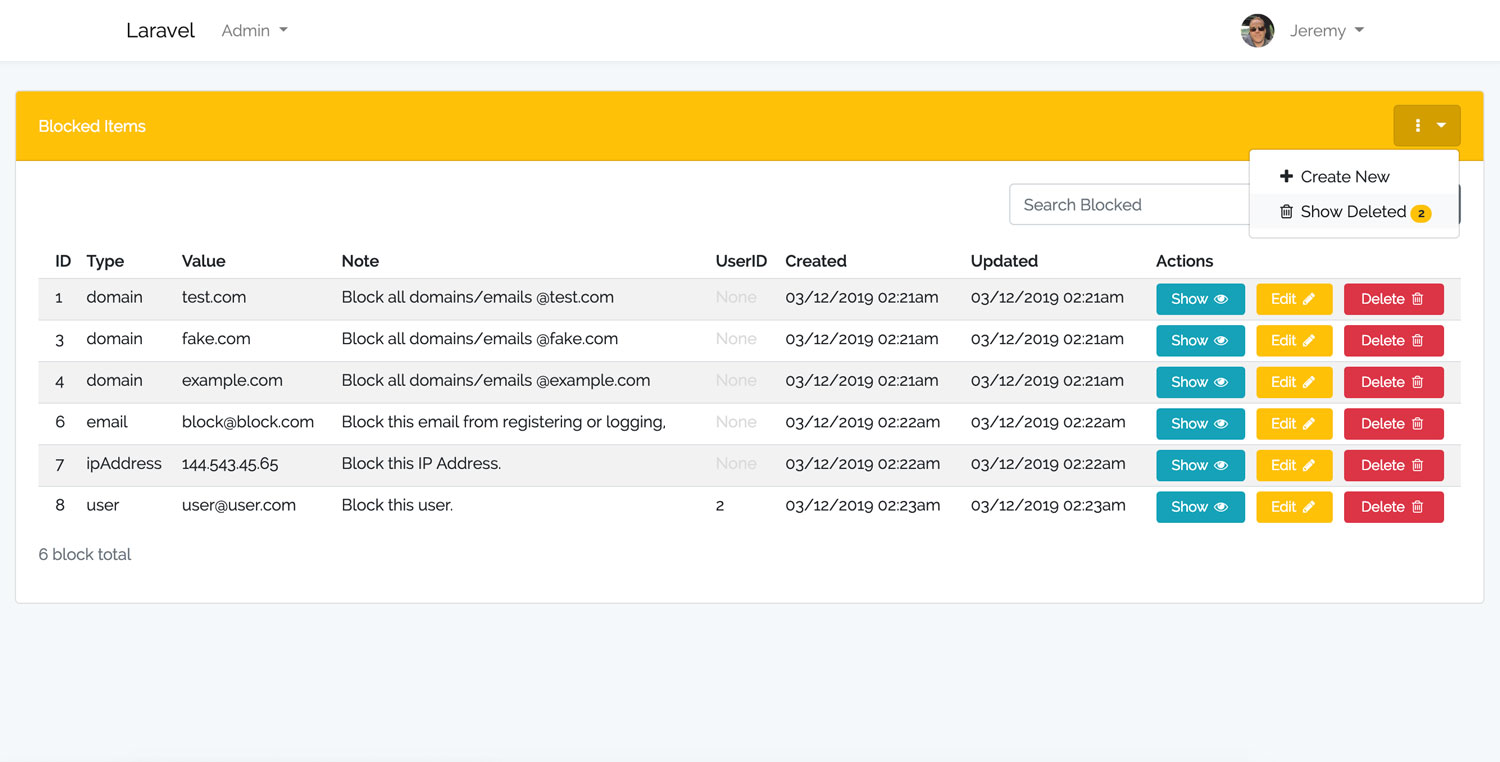
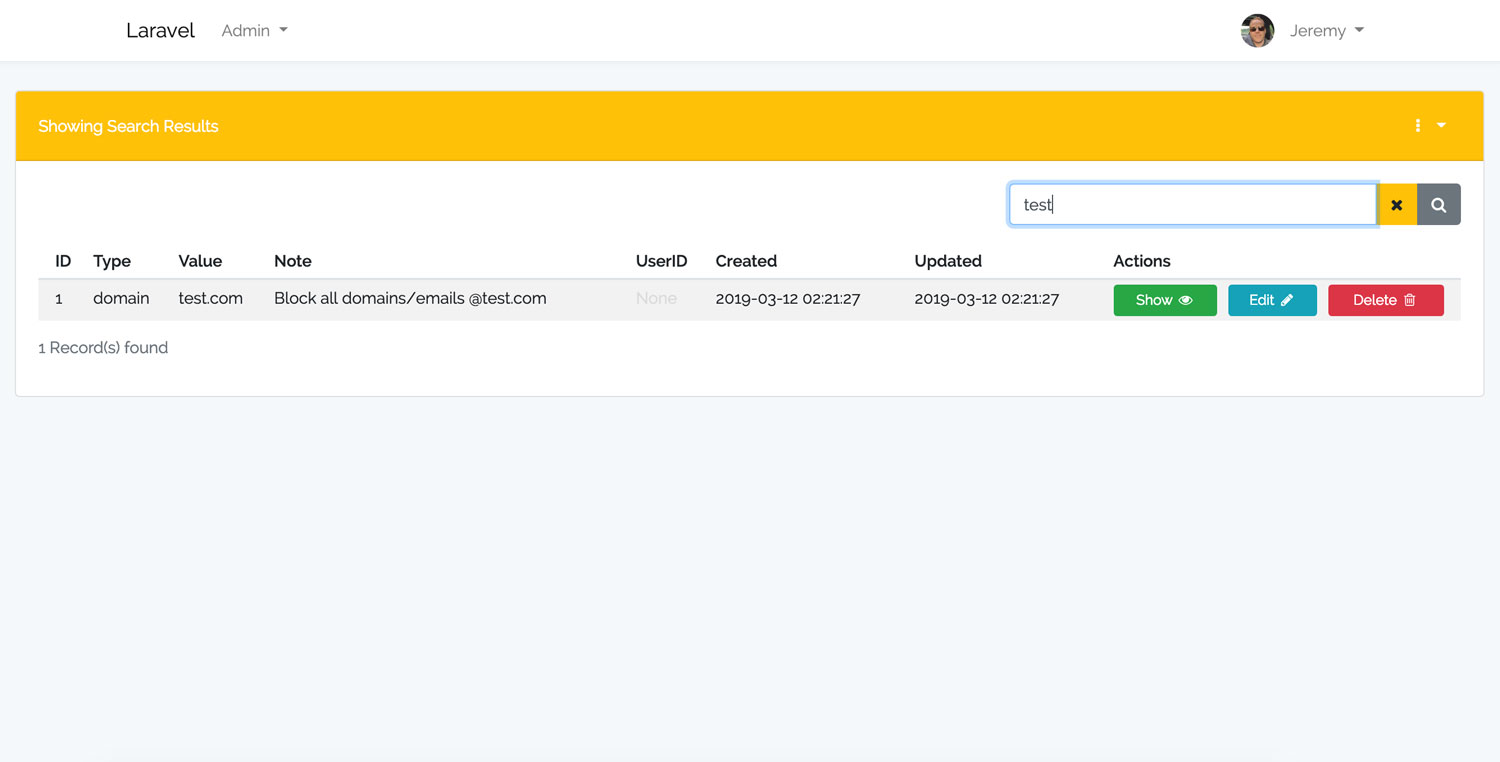
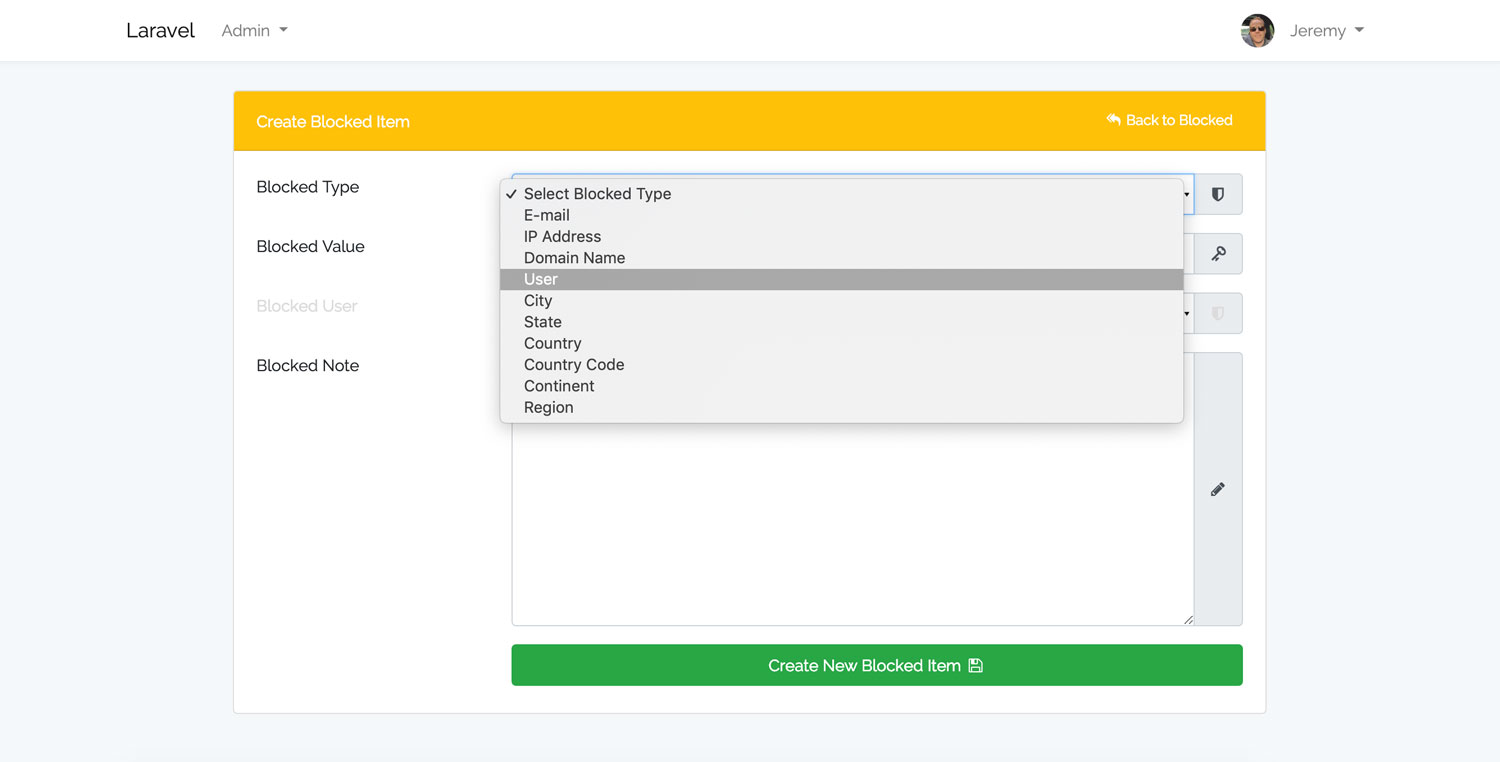
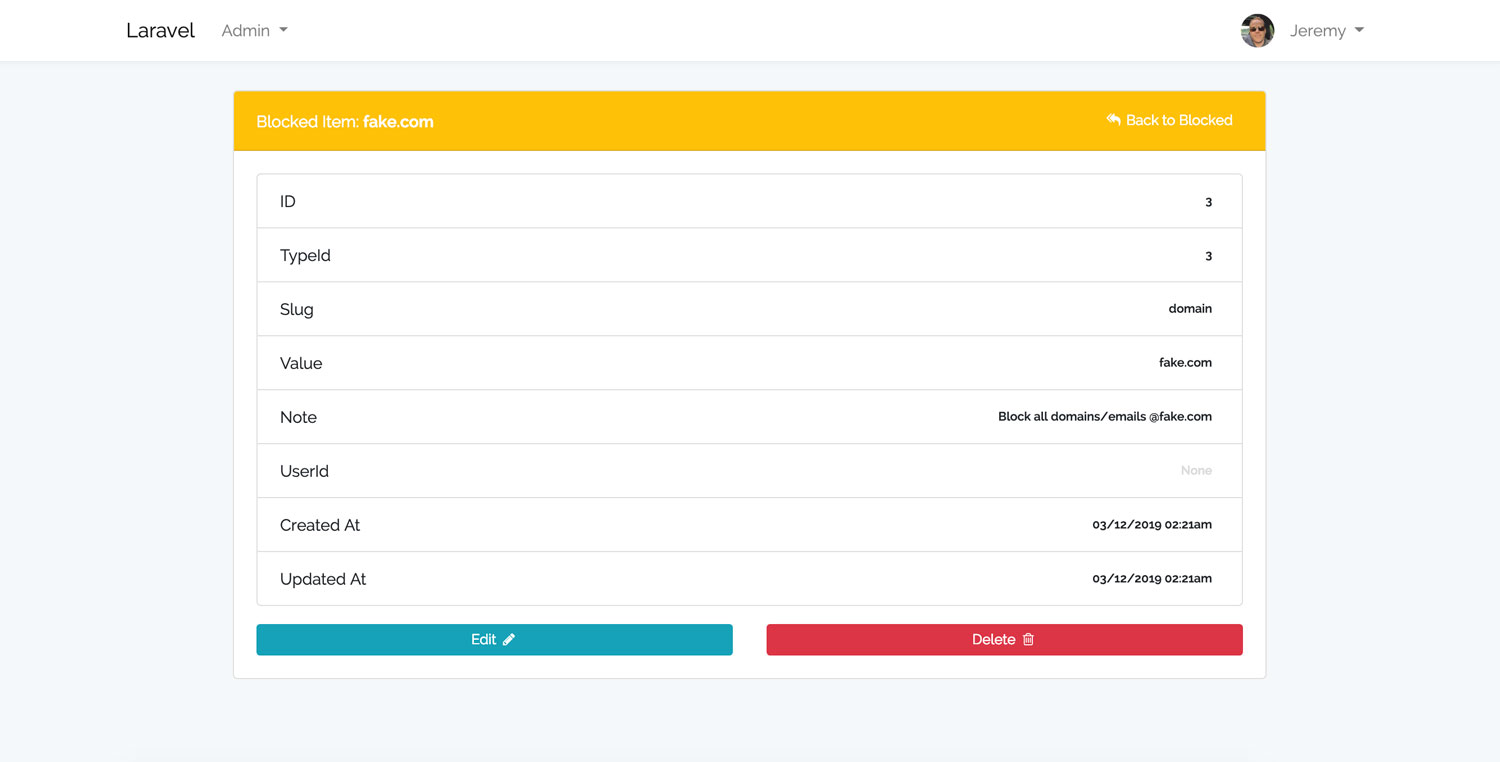
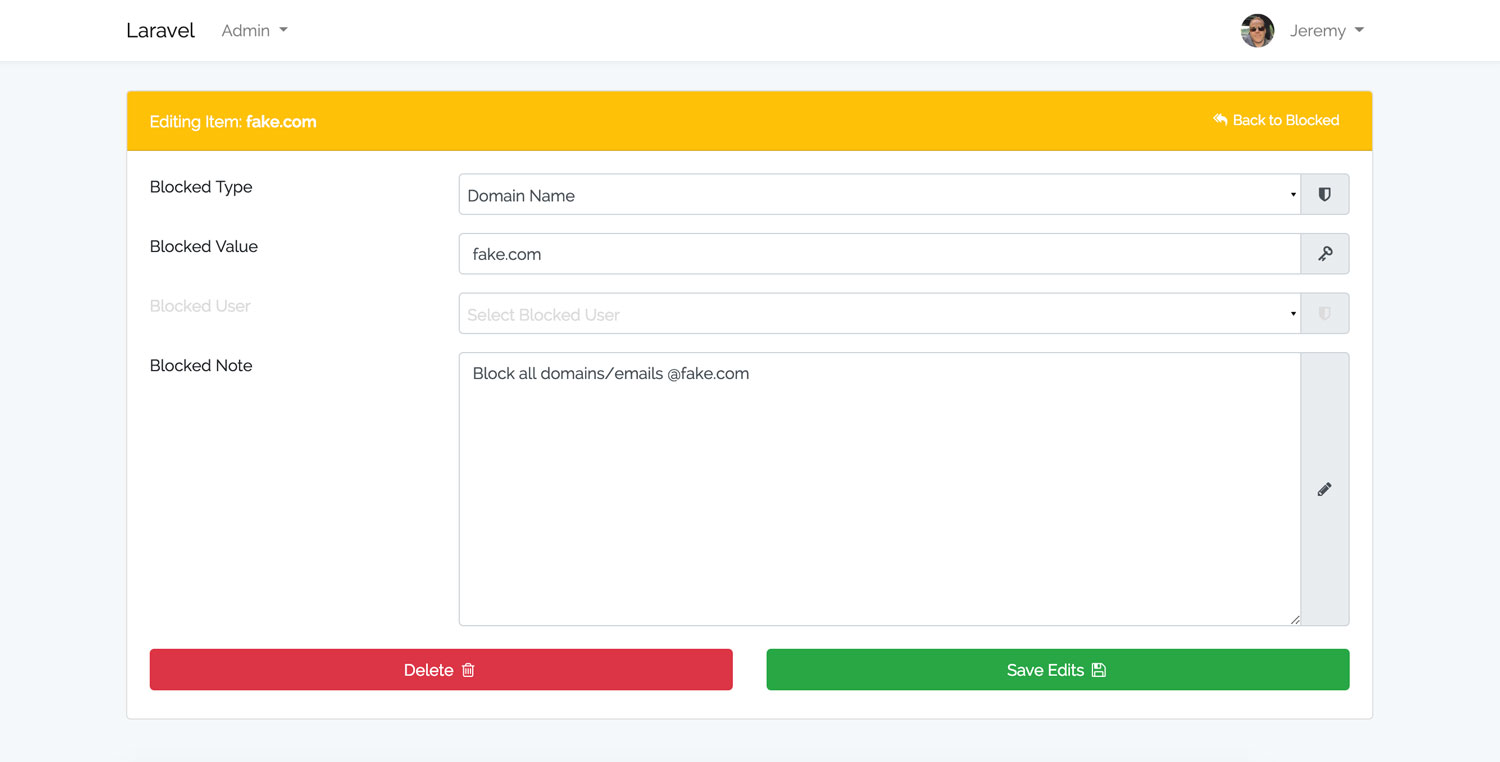
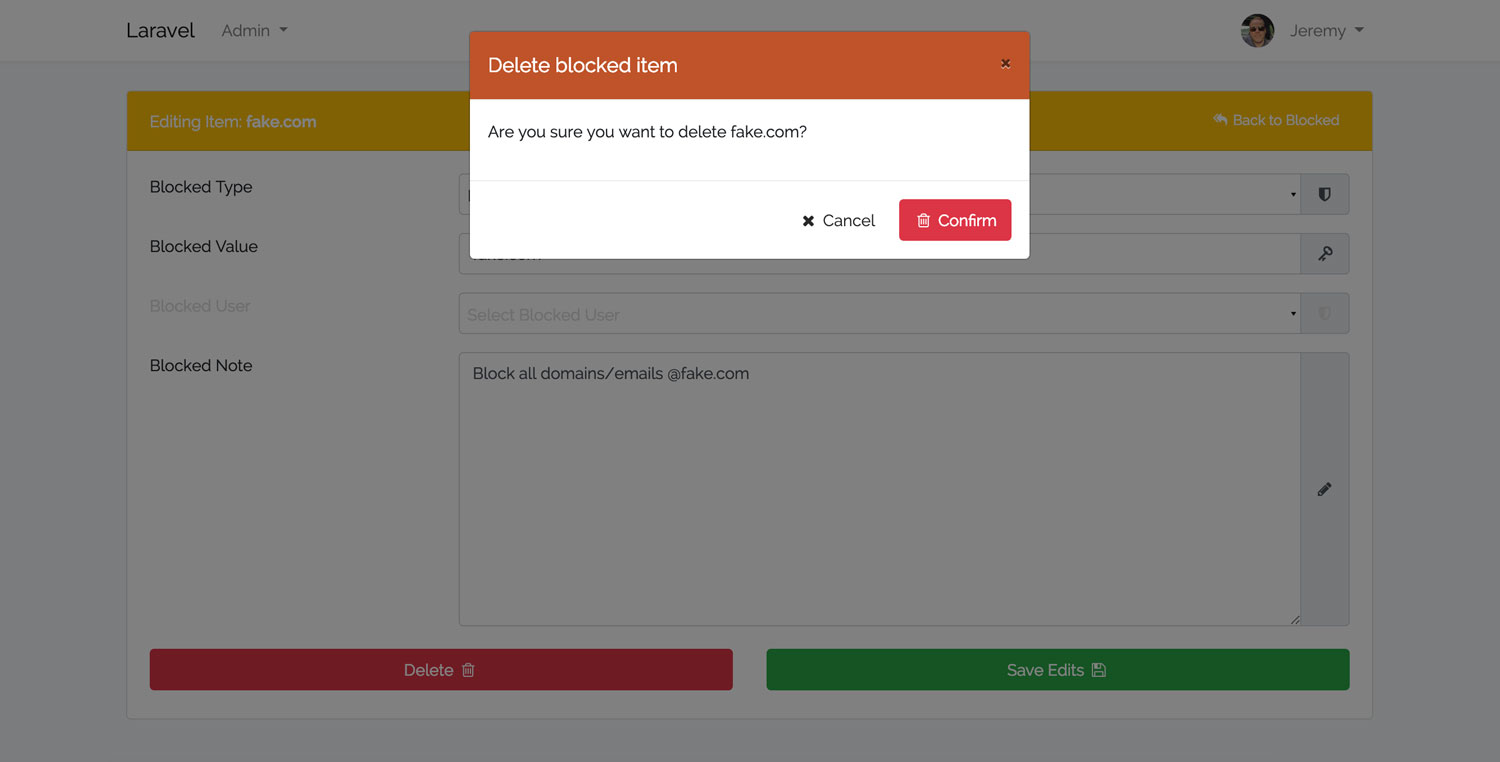
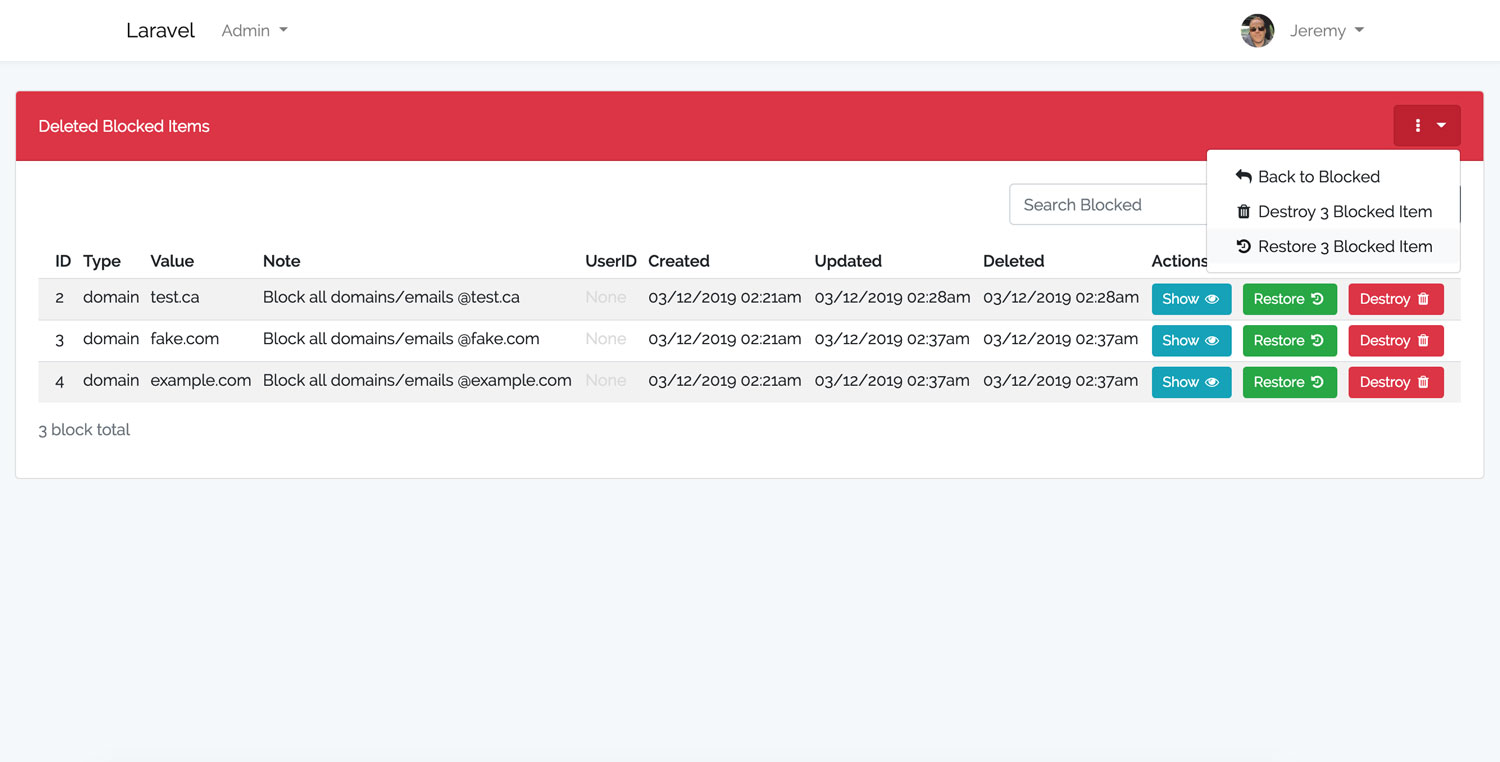
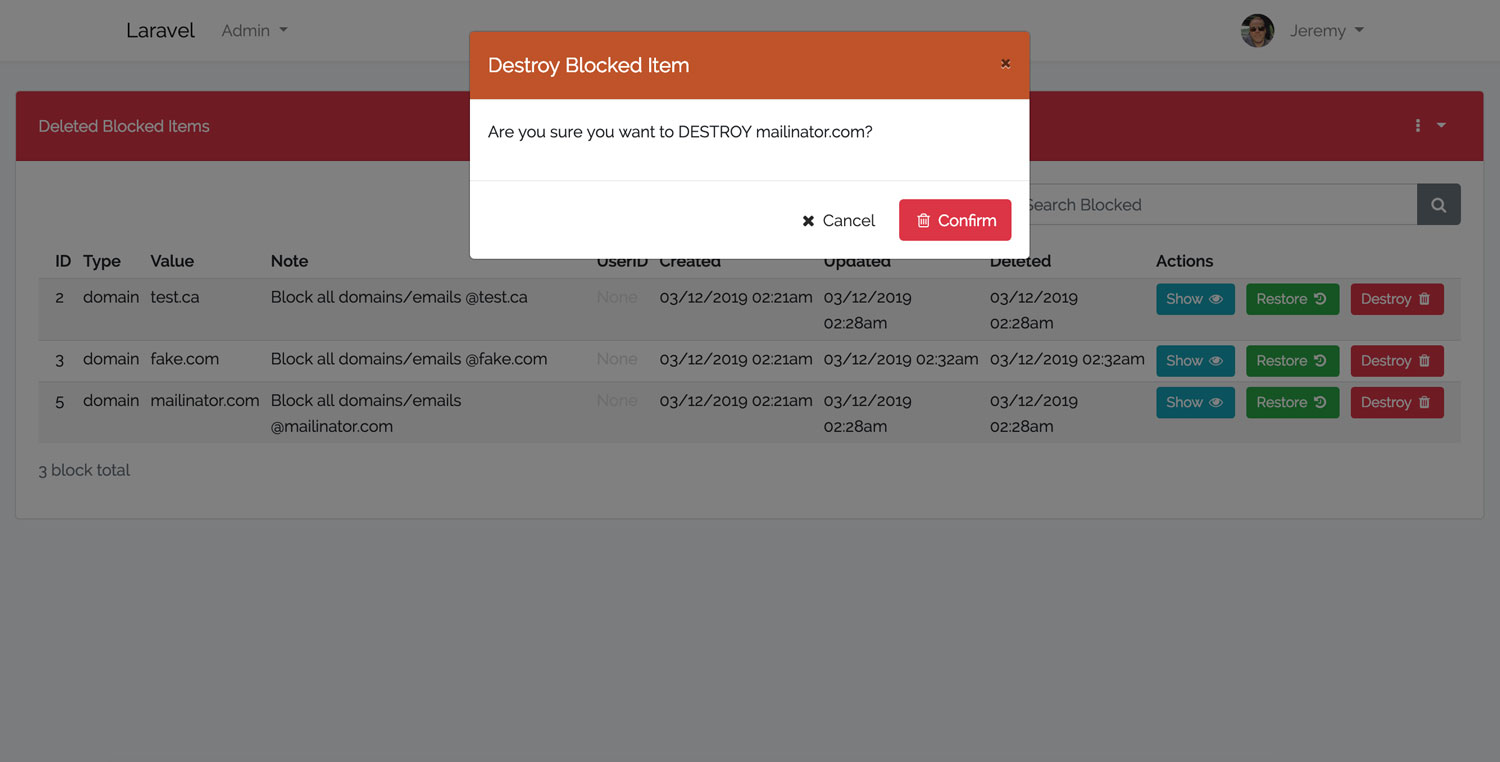
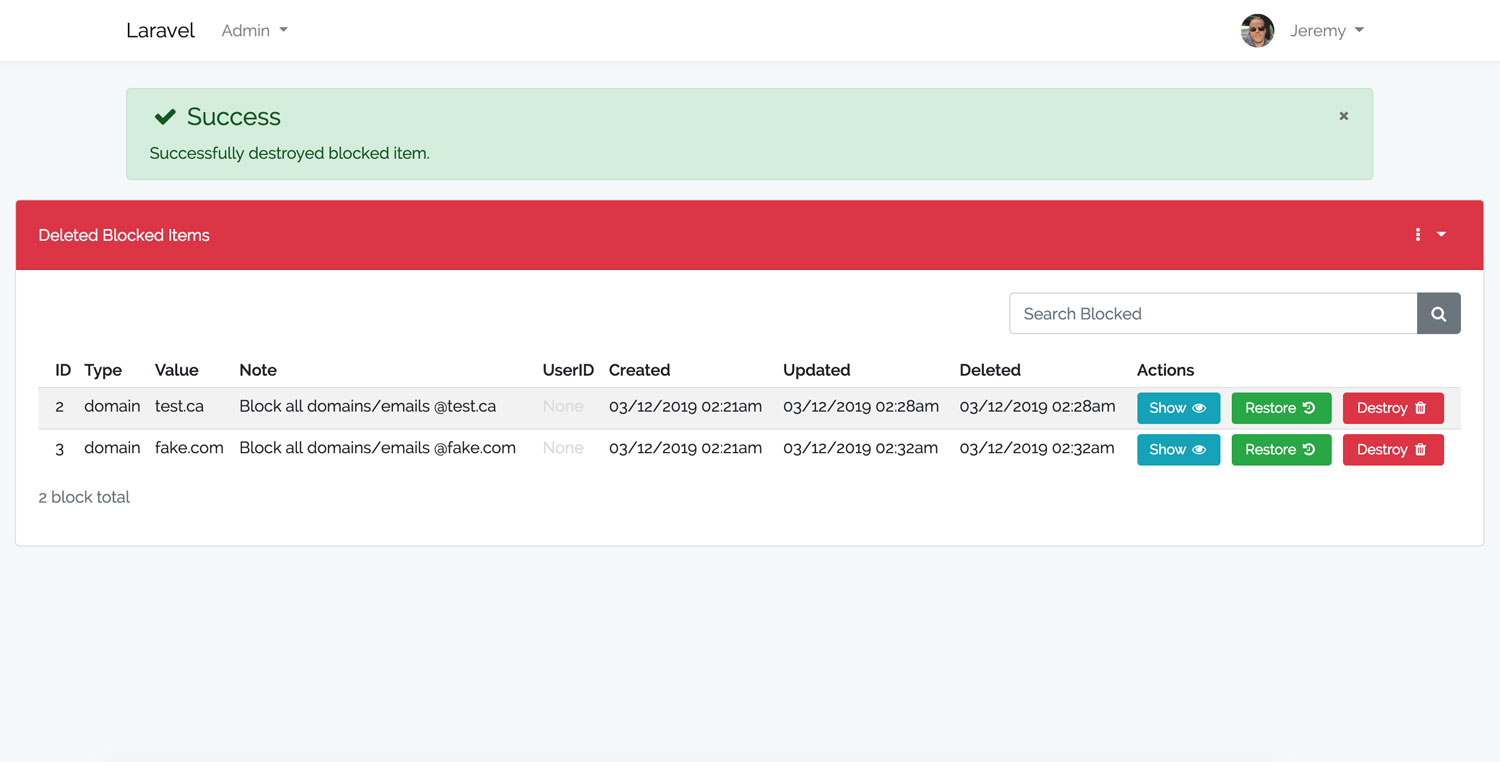
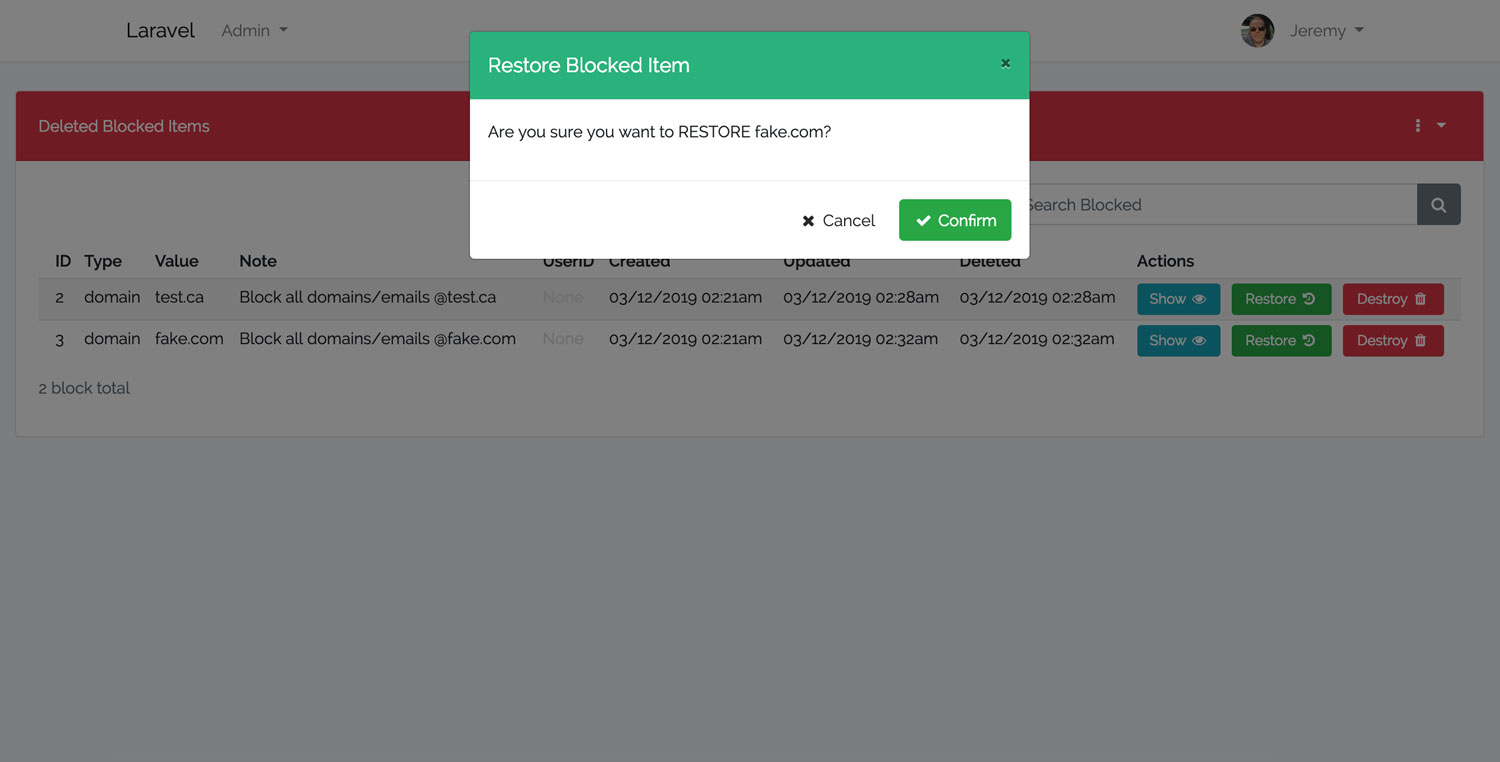
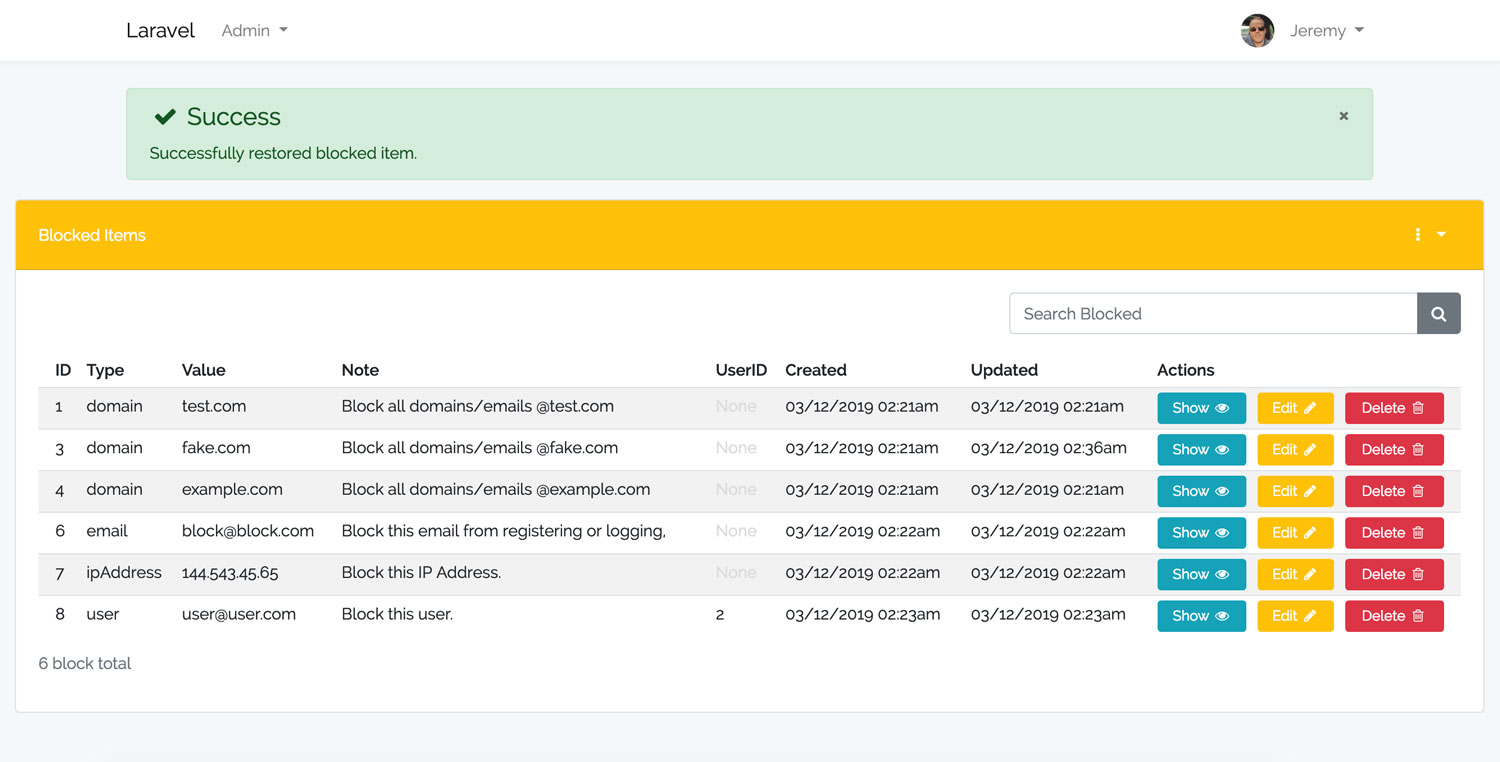
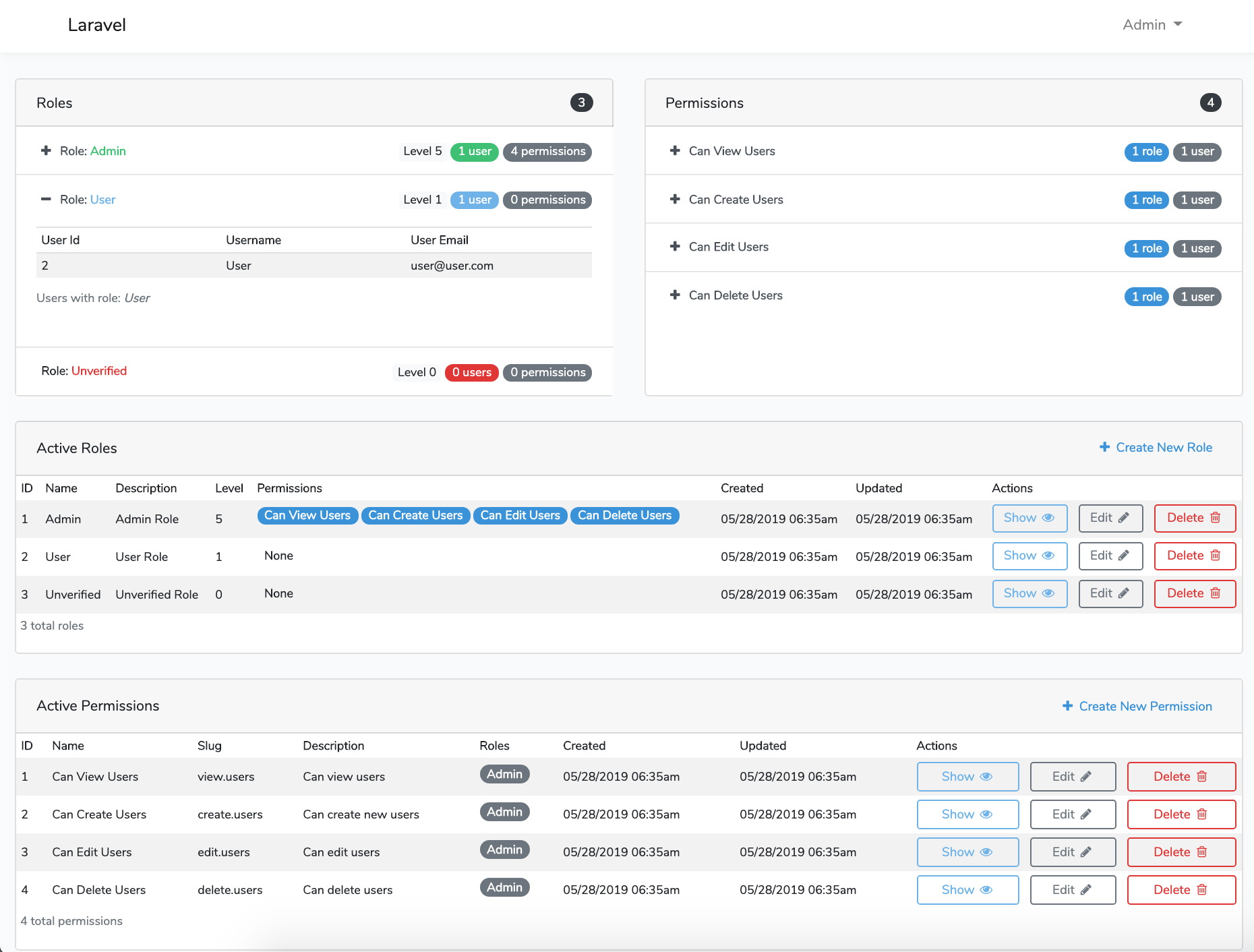
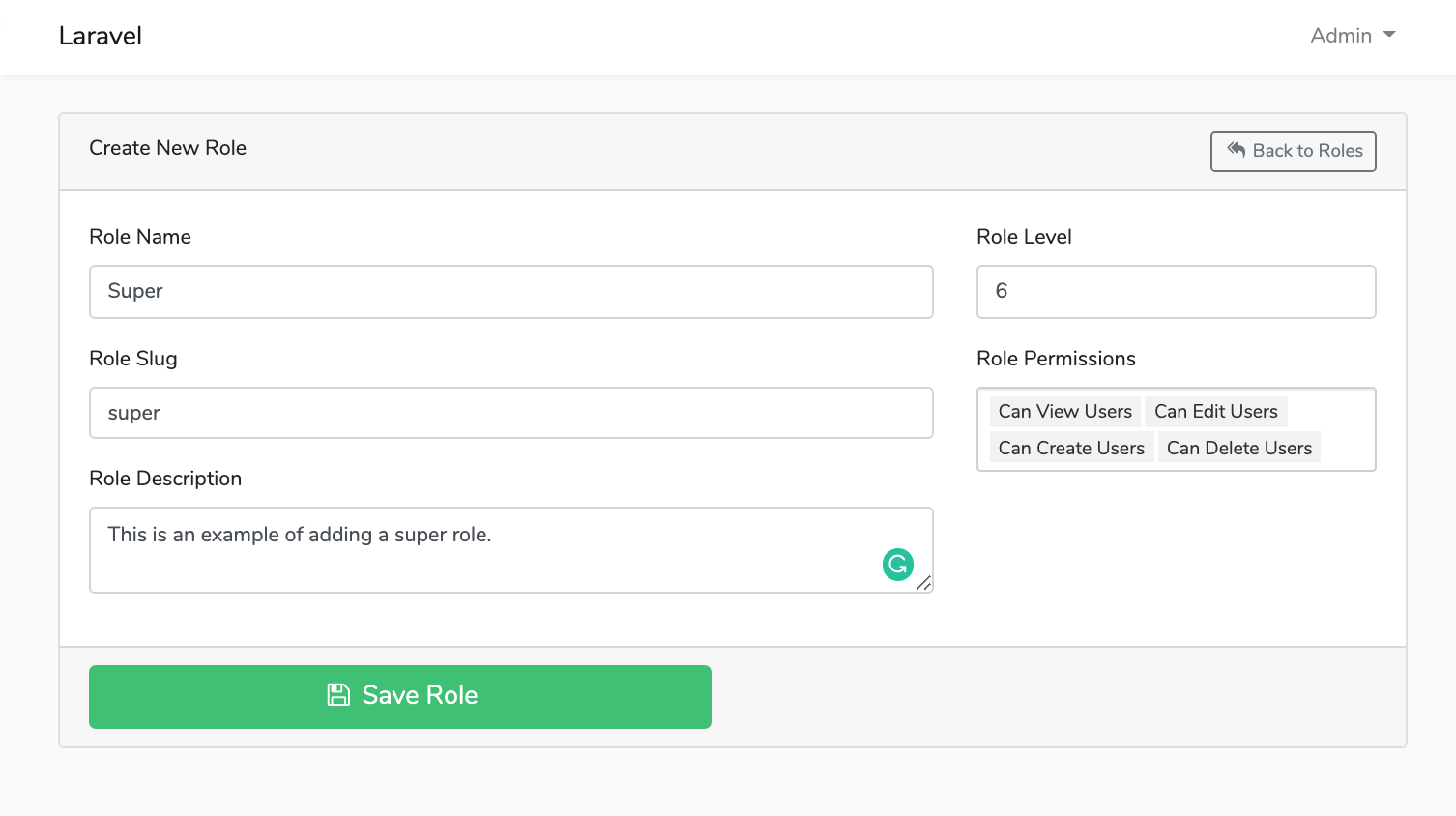
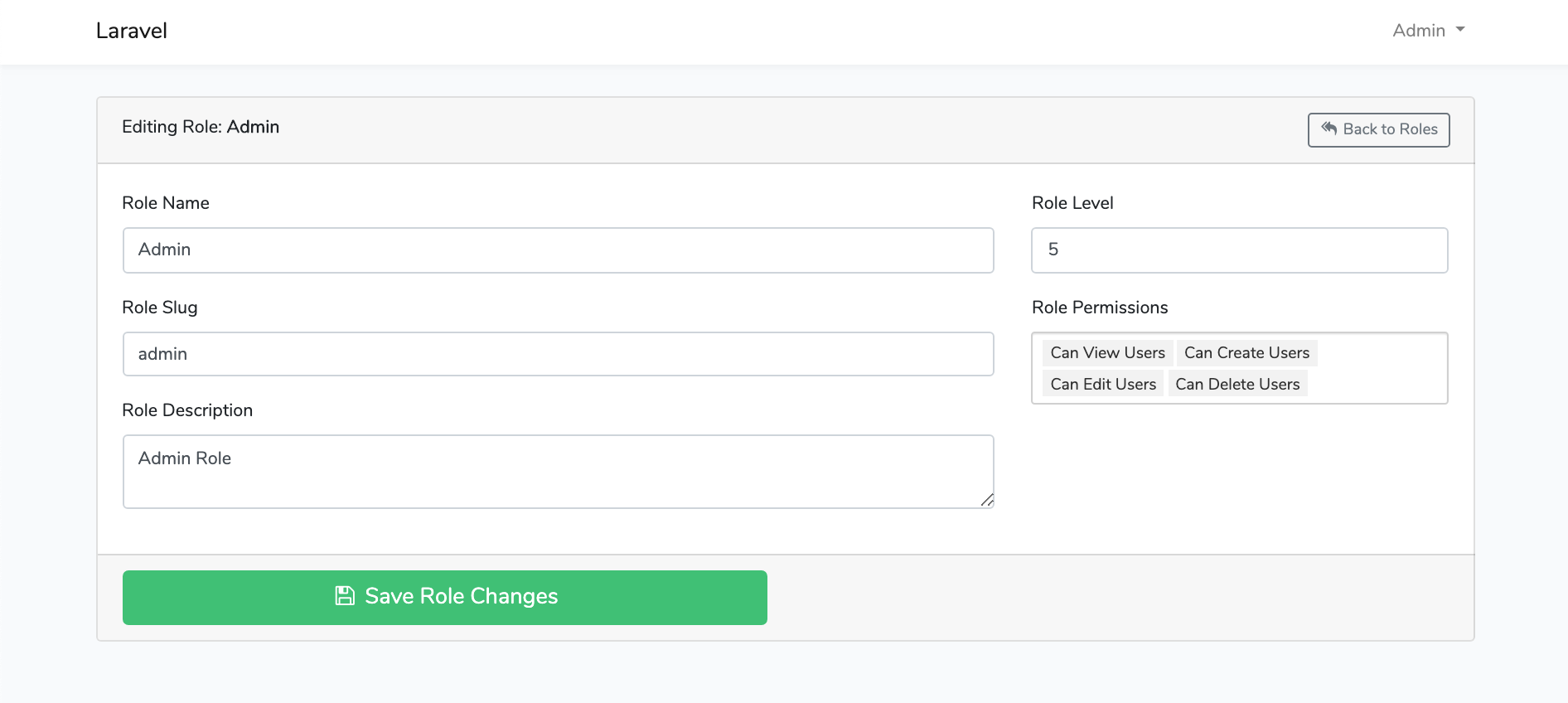
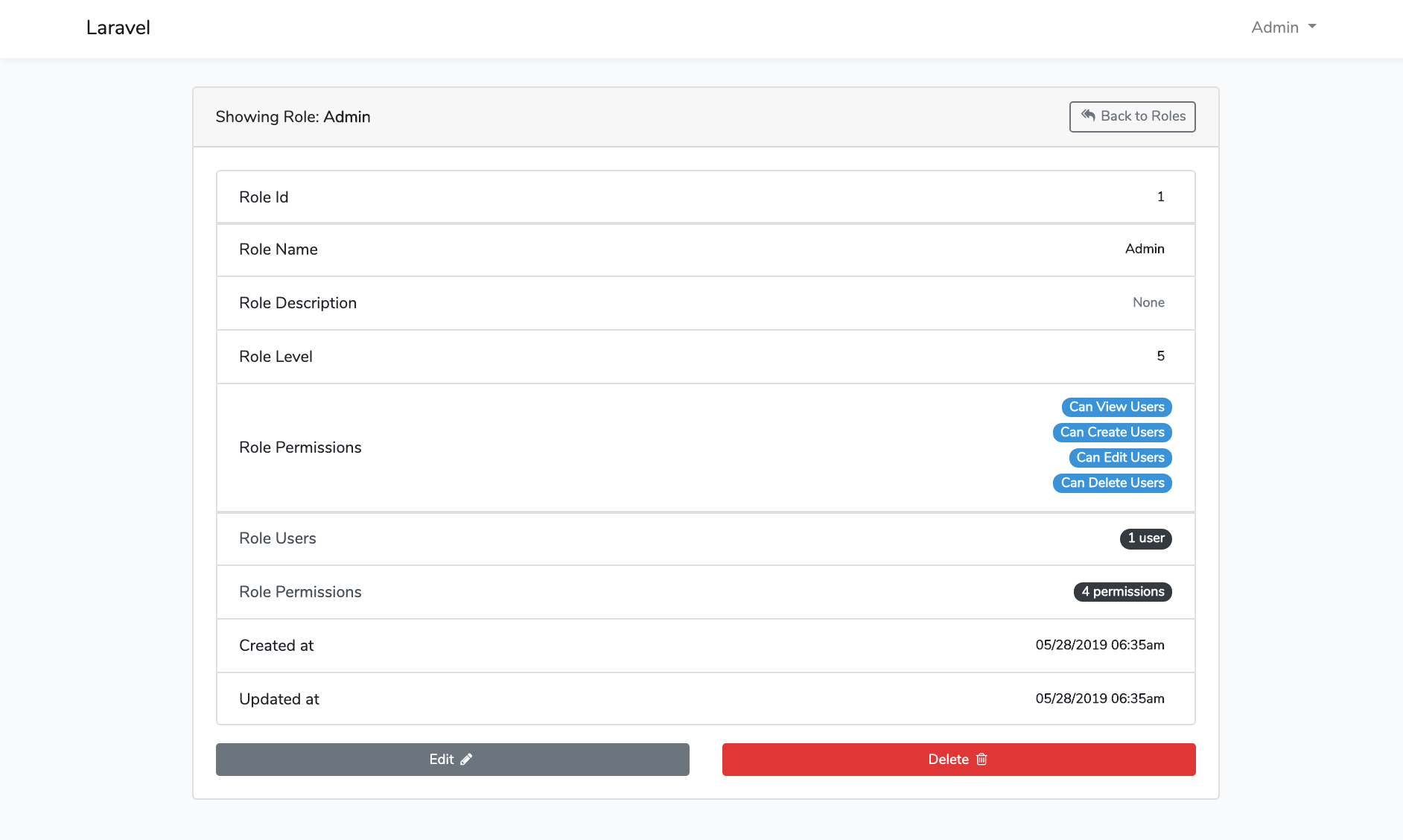
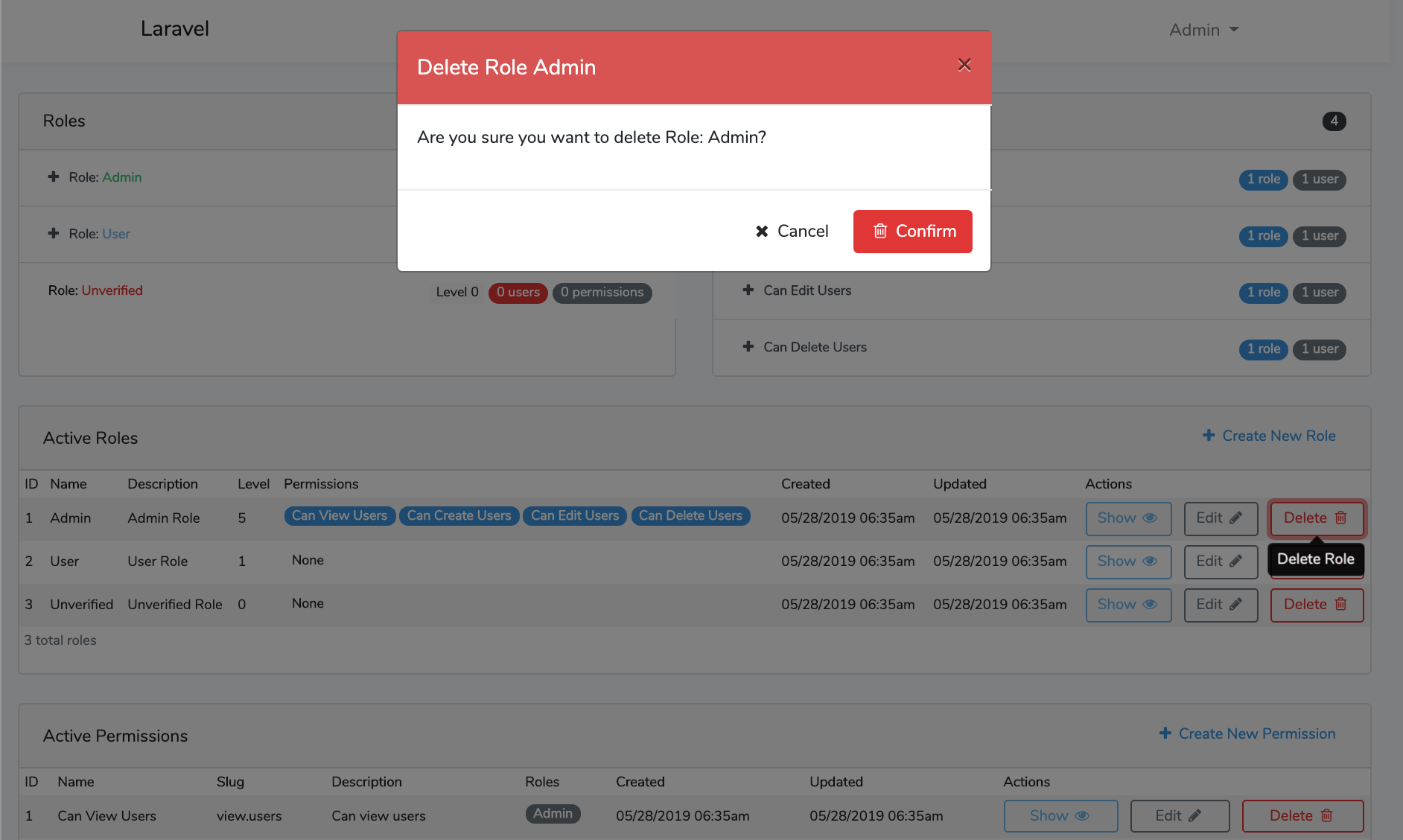
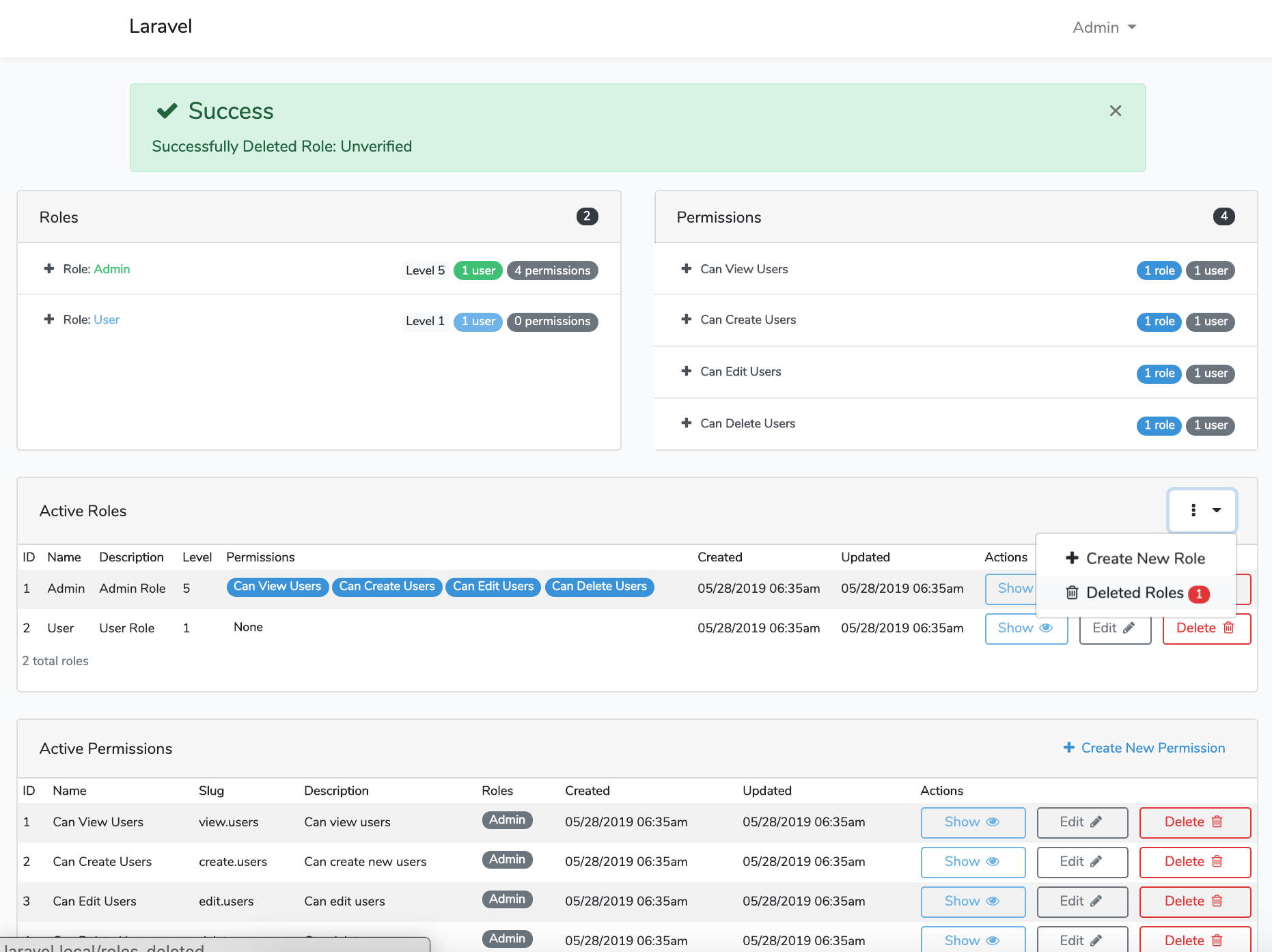
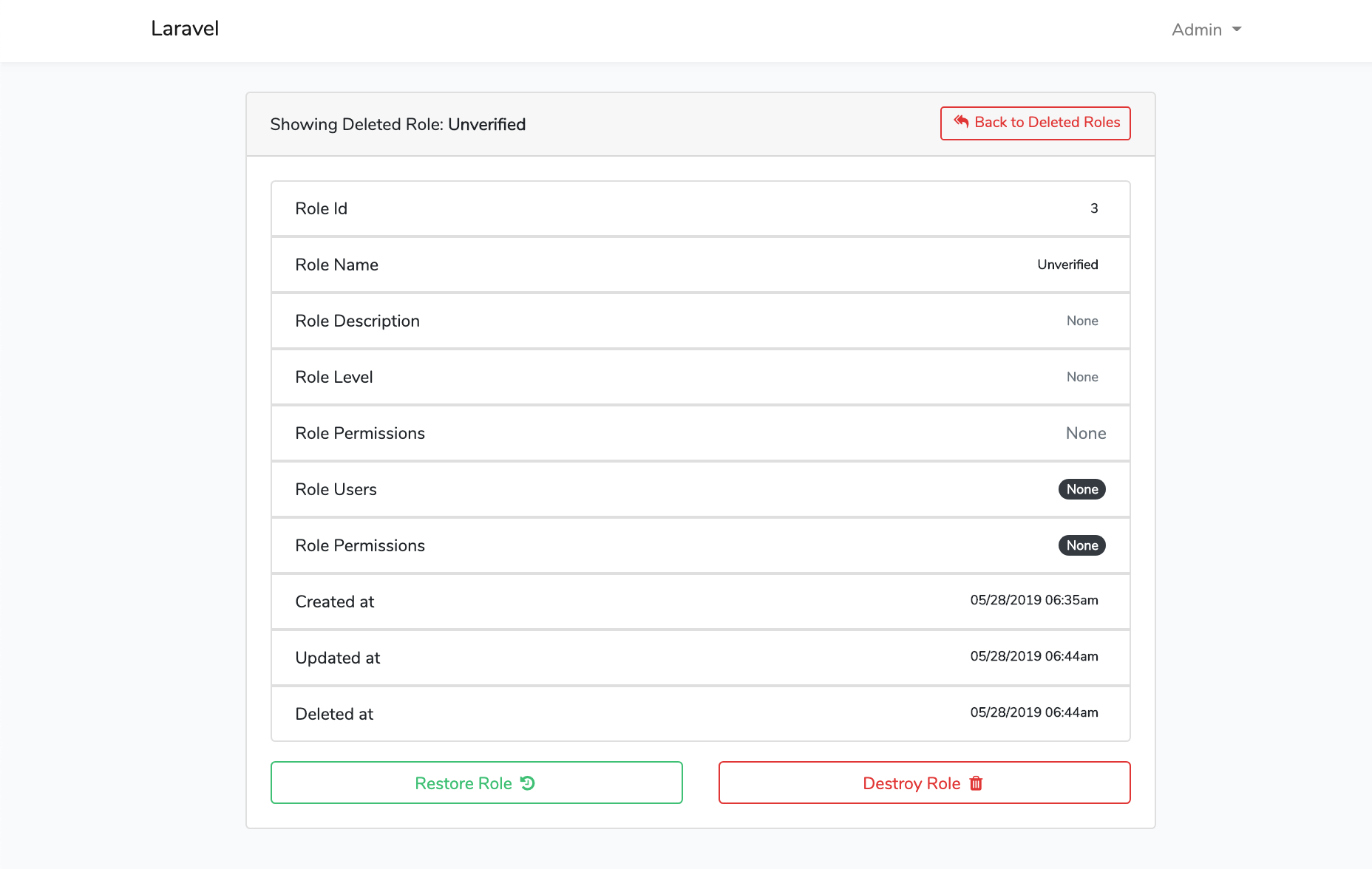
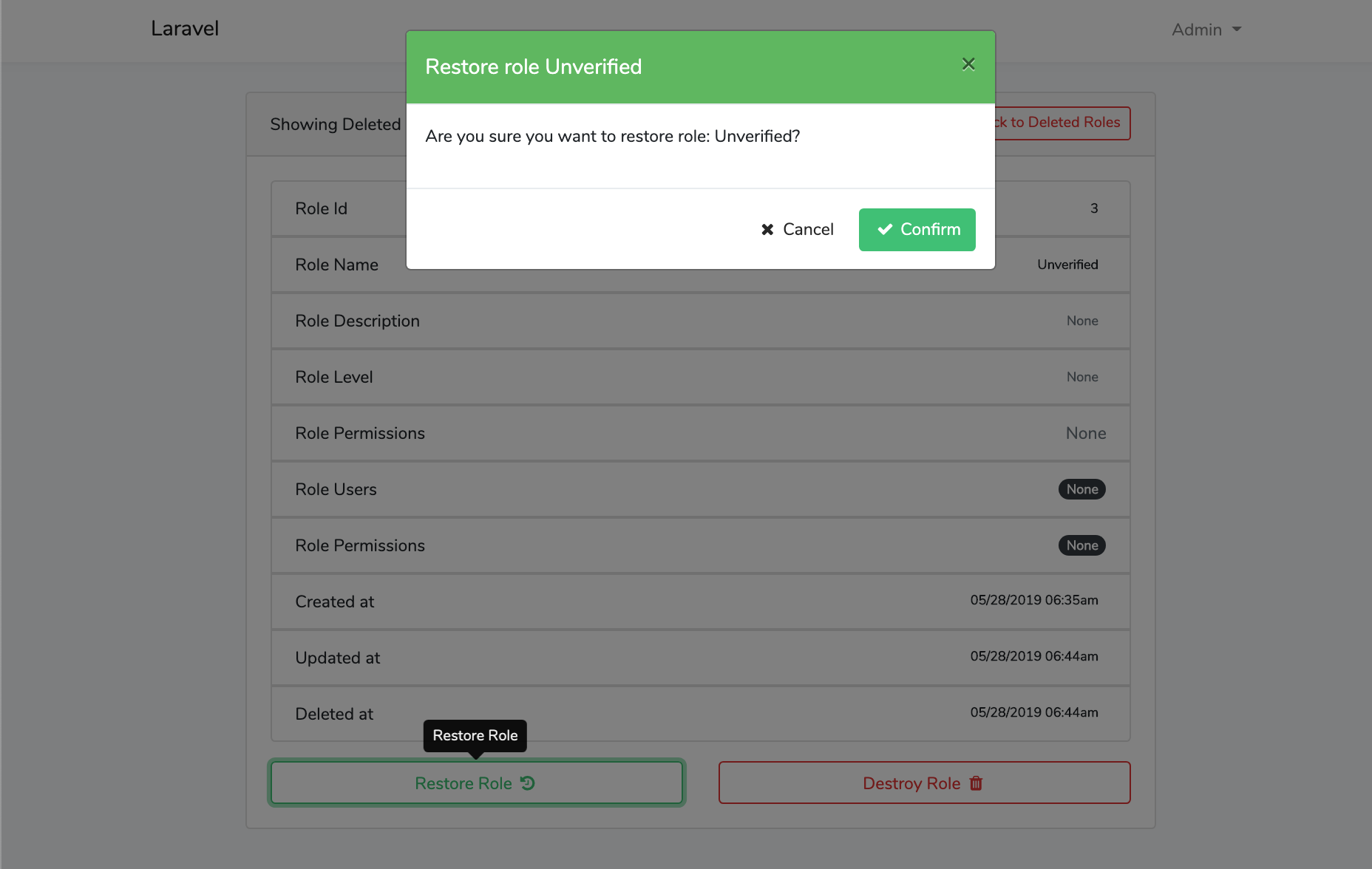
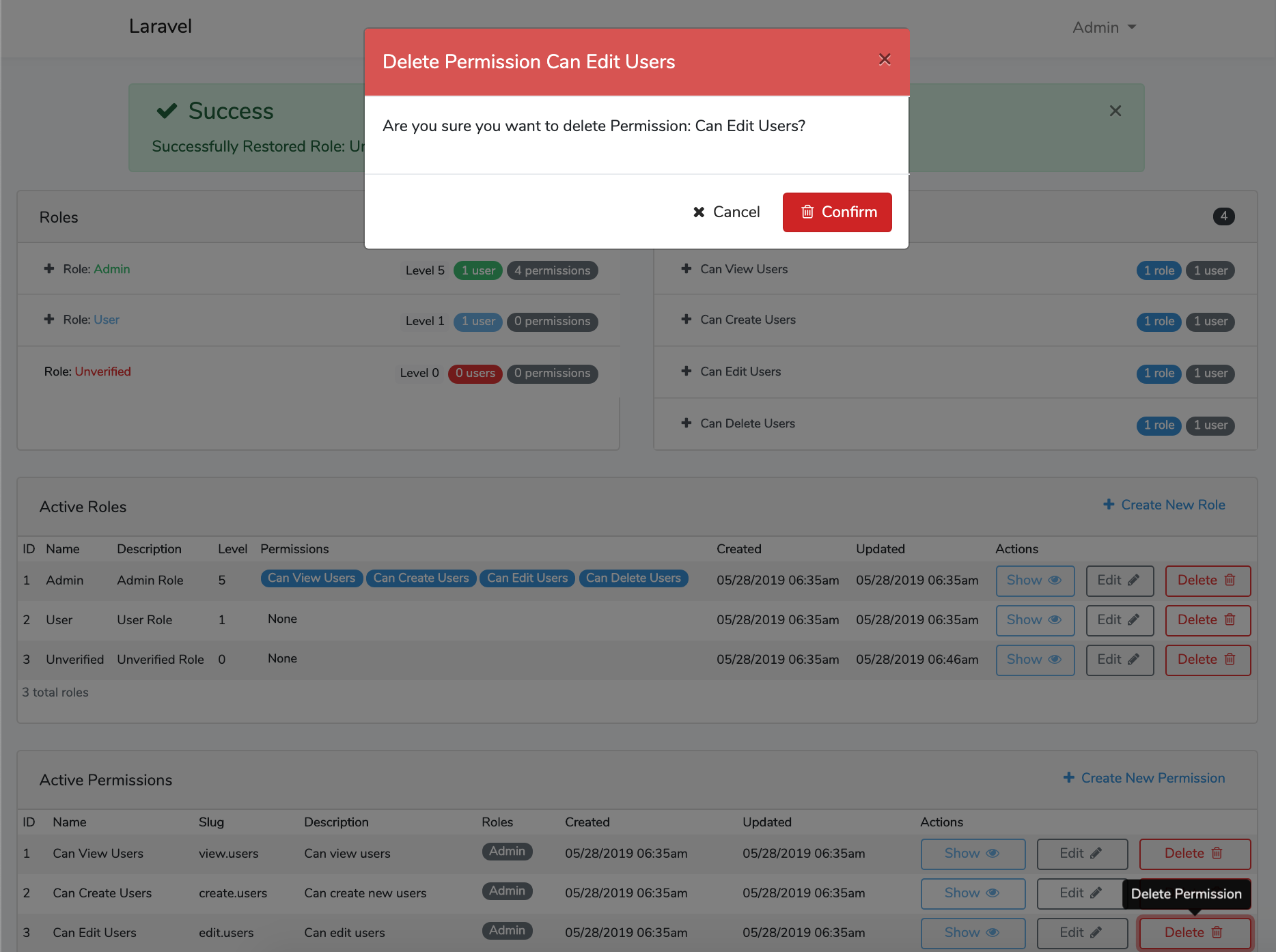
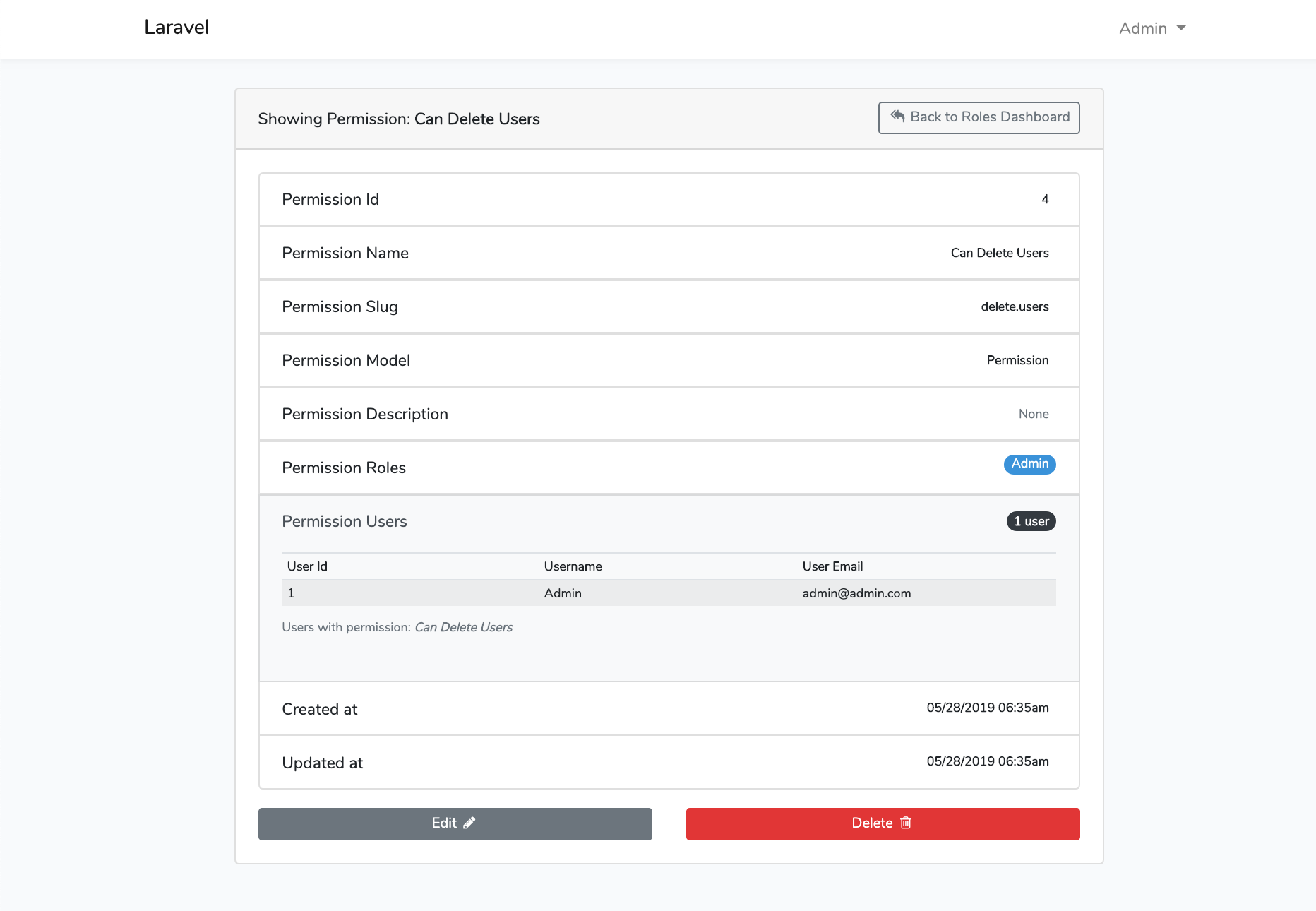
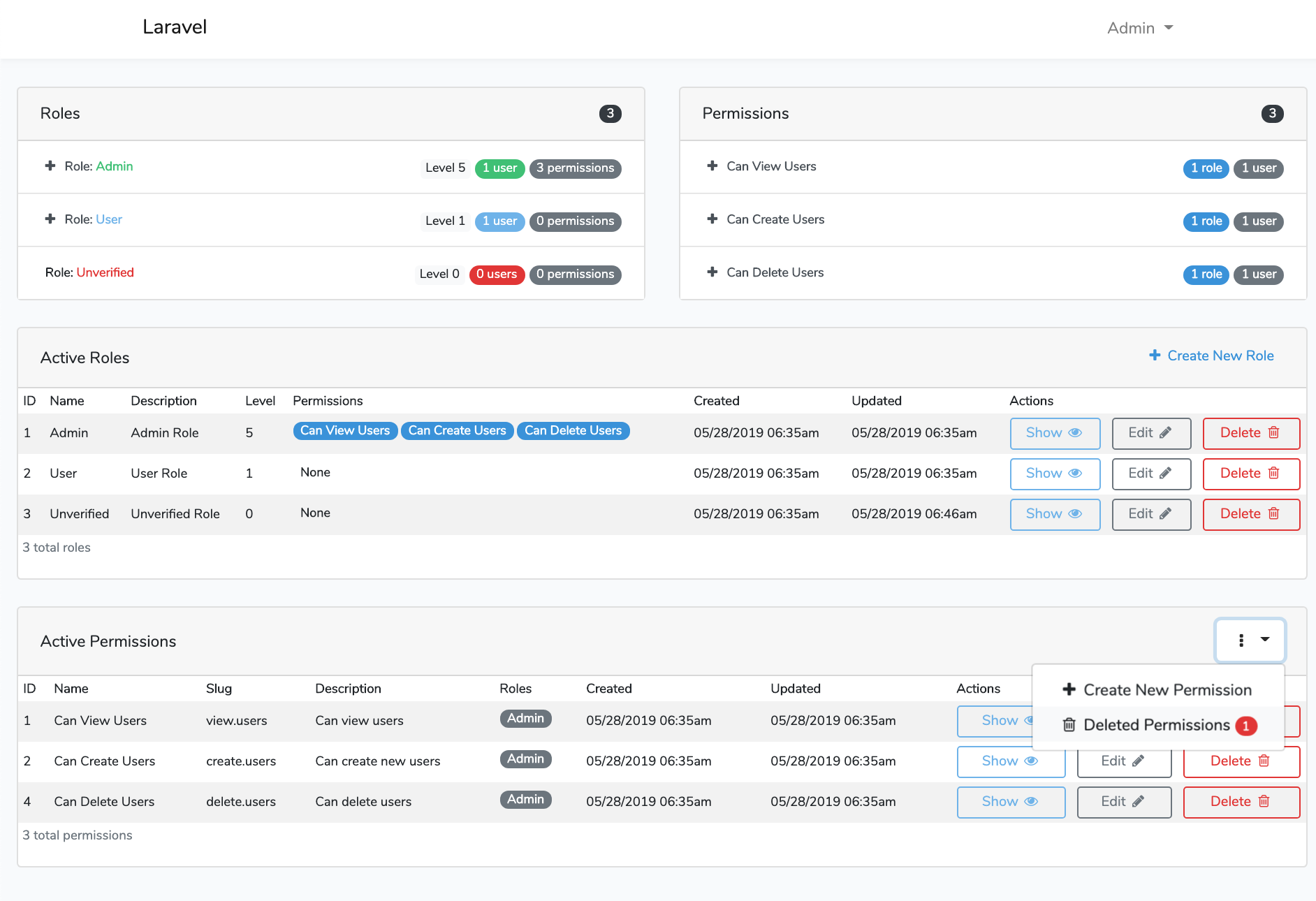
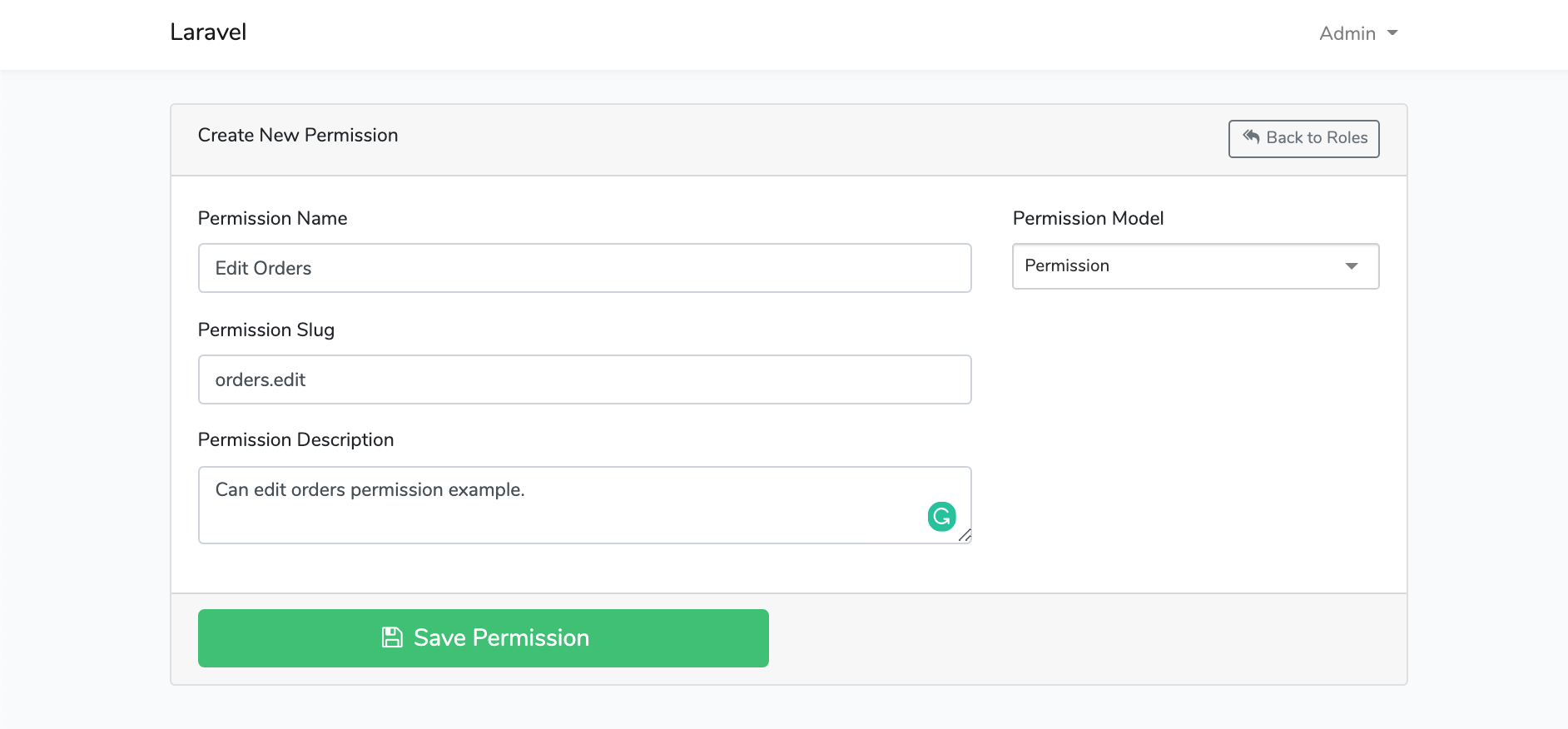
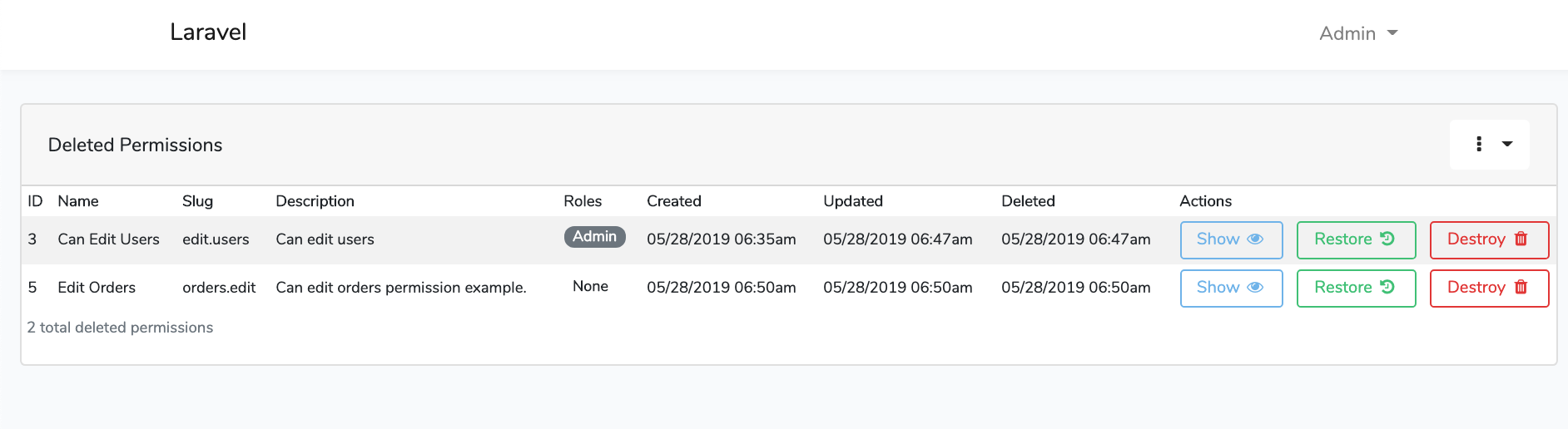
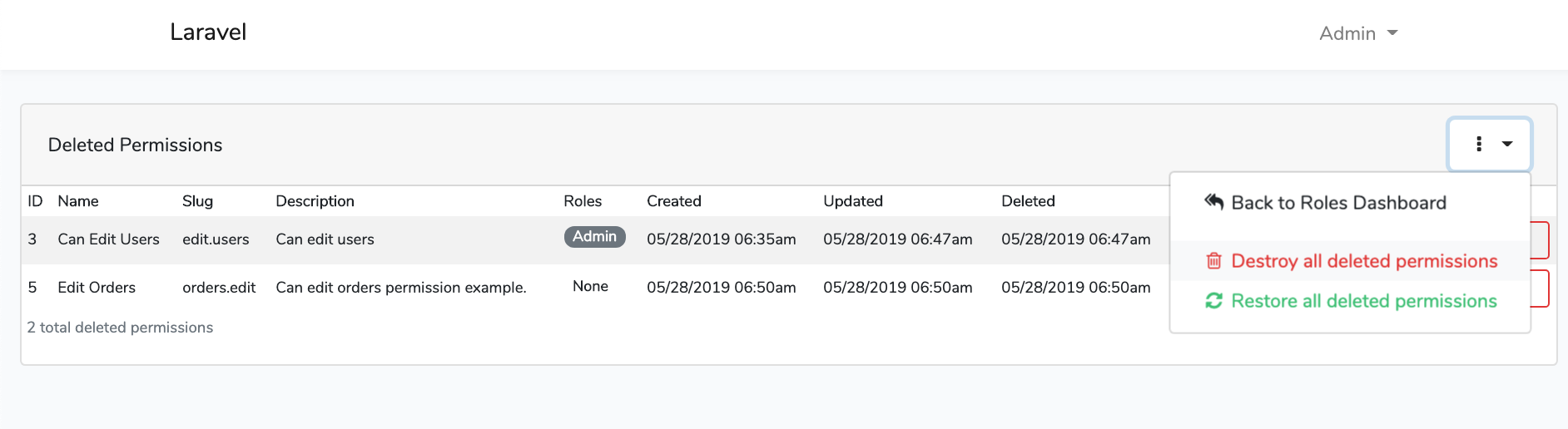
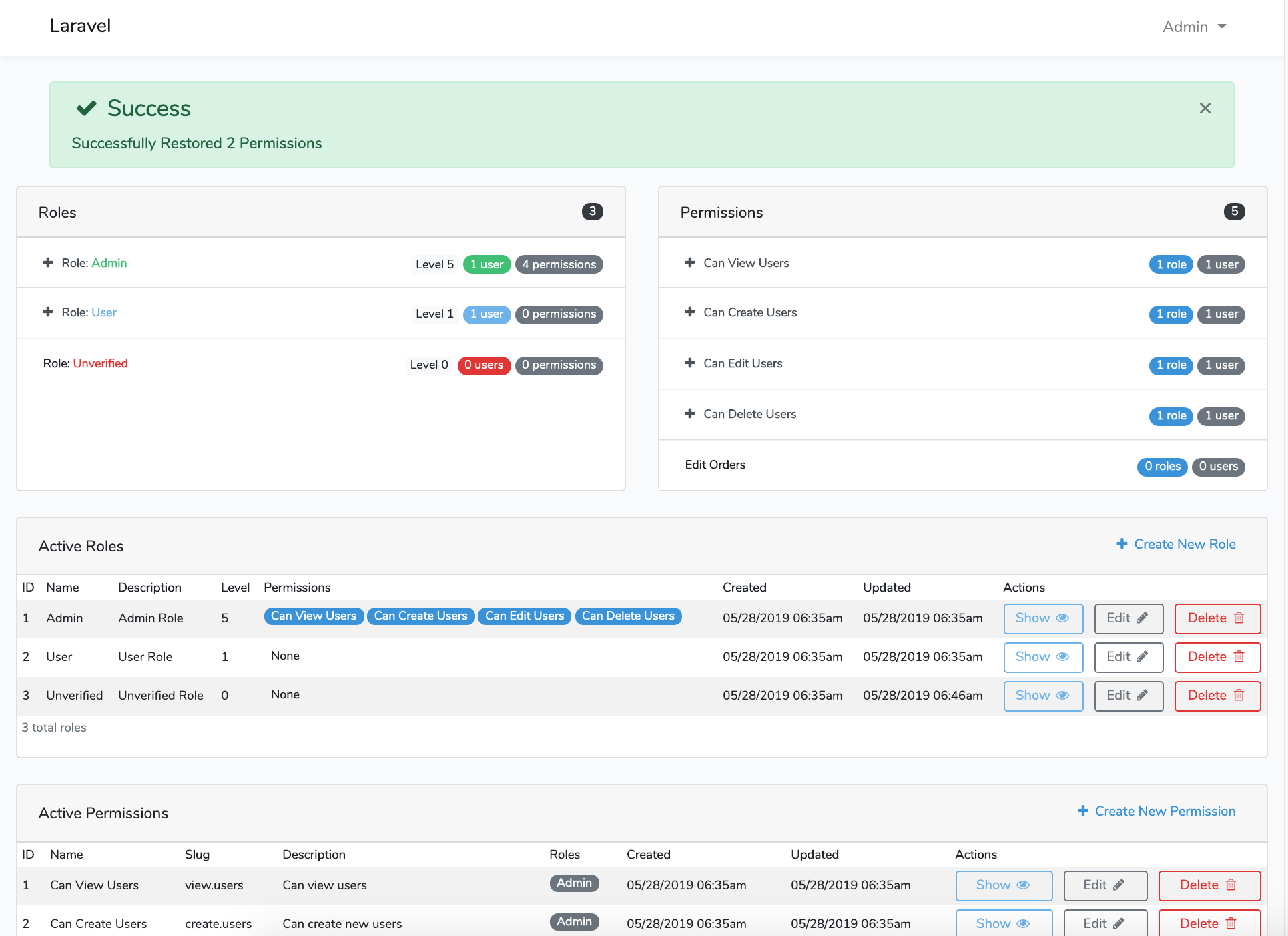
### File Tree
```
laravel-auth
โโโ .editorconfig
โโโ .env
โโโ .env.example
โโโ .env.travis
โโโ .gitattributes
โโโ .github
โย ย โโโ FUNDING.yml
โย ย โโโ ISSUE_TEMPLATE
โย ย โโโ bug_report.md
โย ย โโโ feature_request.md
โย ย โโโ project-questions-and-help.md
โโโ .gitignore
โโโ .phpunit.result.cache
โโโ .styleci.yml
โโโ .travis.yml
โโโ CODE_OF_CONDUCT.md
โโโ LICENSE
โโโ README.md
โโโ _config.yml
โโโ app
โย ย โโโ Console
โย ย โย ย โโโ Commands
โย ย โย ย โย ย โโโ DeleteExpiredActivations.php
โย ย โย ย โโโ Kernel.php
โย ย โโโ Exceptions
โย ย โย ย โโโ Handler.php
โย ย โโโ Http
โย ย โย ย โโโ Controllers
โย ย โย ย โย ย โโโ AdminDetailsController.php
โย ย โย ย โย ย โโโ Auth
โย ย โย ย โย ย โย ย โโโ ActivateController.php
โย ย โย ย โย ย โย ย โโโ ForgotPasswordController.php
โย ย โย ย โย ย โย ย โโโ LoginController.php
โย ย โย ย โย ย โย ย โโโ RegisterController.php
โย ย โย ย โย ย โย ย โโโ ResetPasswordController.php
โย ย โย ย โย ย โย ย โโโ SocialController.php
โย ย โย ย โย ย โโโ Controller.php
โย ย โย ย โย ย โโโ ProfilesController.php
โย ย โย ย โย ย โโโ RestoreUserController.php
โย ย โย ย โย ย โโโ SoftDeletesController.php
โย ย โย ย โย ย โโโ TermsController.php
โย ย โย ย โย ย โโโ ThemesManagementController.php
โย ย โย ย โย ย โโโ UserController.php
โย ย โย ย โย ย โโโ UsersManagementController.php
โย ย โย ย โย ย โโโ WelcomeController.php
โย ย โย ย โโโ Kernel.php
โย ย โย ย โโโ Middleware
โย ย โย ย โย ย โโโ Authenticate.php
โย ย โย ย โย ย โโโ CheckCurrentUser.php
โย ย โย ย โย ย โโโ CheckForMaintenanceMode.php
โย ย โย ย โย ย โโโ CheckIsUserActivated.php
โย ย โย ย โย ย โโโ EncryptCookies.php
โย ย โย ย โย ย โโโ RedirectIfAuthenticated.php
โย ย โย ย โย ย โโโ TrimStrings.php
โย ย โย ย โย ย โโโ TrustProxies.php
โย ย โย ย โย ย โโโ VerifyCsrfToken.php
โย ย โย ย โโโ Requests
โย ย โย ย โย ย โโโ DeleteUserAccount.php
โย ย โย ย โย ย โโโ UpdateUserPasswordRequest.php
โย ย โย ย โย ย โโโ UpdateUserProfile.php
โย ย โย ย โโโ ViewComposers
โย ย โย ย โโโ ThemeComposer.php
โย ย โโโ Logic
โย ย โย ย โโโ Activation
โย ย โย ย โย ย โโโ ActivationRepository.php
โย ย โย ย โโโ Macros
โย ย โย ย โโโ HtmlMacros.php
โย ย โโโ Mail
โย ย โย ย โโโ ExceptionOccured.php
โย ย โโโ Models
โย ย โย ย โโโ Activation.php
โย ย โย ย โโโ Profile.php
โย ย โย ย โโโ Social.php
โย ย โย ย โโโ Theme.php
โย ย โย ย โโโ User.php
โย ย โโโ Notifications
โย ย โย ย โโโ SendActivationEmail.php
โย ย โย ย โโโ SendGoodbyeEmail.php
โย ย โโโ Providers
โย ย โย ย โโโ AppServiceProvider.php
โย ย โย ย โโโ AuthServiceProvider.php
โย ย โย ย โโโ BroadcastServiceProvider.php
โย ย โย ย โโโ ComposerServiceProvider.php
โย ย โย ย โโโ EventServiceProvider.php
โย ย โย ย โโโ LocalEnvironmentServiceProvider.php
โย ย โย ย โโโ MacroServiceProvider.php
โย ย โย ย โโโ RouteServiceProvider.php
โย ย โโโ Traits
โย ย โโโ ActivationTrait.php
โย ย โโโ CaptchaTrait.php
โย ย โโโ CaptureIpTrait.php
โโโ artisan
โโโ bootstrap
โย ย โโโ app.php
โย ย โโโ autoload.php
โย ย โโโ cache
โย ย โโโ .gitignore
โย ย โโโ packages.php
โย ย โโโ services.php
โโโ composer.json
โโโ composer.lock
โโโ config
โย ย โโโ app.php
โย ย โโโ auth.php
โย ย โโโ broadcasting.php
โย ย โโโ cache.php
โย ย โโโ cors.php
โย ย โโโ database.php
โย ย โโโ debugbar.php
โย ย โโโ exceptions.php
โย ย โโโ filesystems.php
โย ย โโโ gravatar.php
โย ย โโโ hashing.php
โย ย โโโ laravel2step.php
โย ย โโโ laravelPhpInfo.php
โย ย โโโ laravelblocker.php
โย ย โโโ logging.php
โย ย โโโ mail.php
โย ย โโโ queue.php
โย ย โโโ roles.php
โย ย โโโ services.php
โย ย โโโ session.php
โย ย โโโ settings.php
โย ย โโโ usersmanagement.php
โย ย โโโ view.php
โโโ database
โย ย โโโ .gitignore
โย ย โโโ factories
โย ย โย ย โโโ ModelFactory.php
โย ย โโโ migrations
โย ย โย ย โโโ 2014_10_12_000000_create_users_table.php
โย ย โย ย โโโ 2014_10_12_100000_create_password_resets_table.php
โย ย โย ย โโโ 2016_01_15_105324_create_roles_table.php
โย ย โย ย โโโ 2016_01_15_114412_create_role_user_table.php
โย ย โย ย โโโ 2016_01_26_115212_create_permissions_table.php
โย ย โย ย โโโ 2016_01_26_115523_create_permission_role_table.php
โย ย โย ย โโโ 2016_02_09_132439_create_permission_user_table.php
โย ย โย ย โโโ 2017_03_09_082449_create_social_logins_table.php
โย ย โย ย โโโ 2017_03_09_082526_create_activations_table.php
โย ย โย ย โโโ 2017_03_20_213554_create_themes_table.php
โย ย โย ย โโโ 2017_03_21_042918_create_profiles_table.php
โย ย โย ย โโโ 2017_12_09_070937_create_two_step_auth_table.php
โย ย โย ย โโโ 2019_02_19_032636_create_laravel_blocker_types_table.php
โย ย โย ย โโโ 2019_02_19_045158_create_laravel_blocker_table.php
โย ย โย ย โโโ 2019_08_19_000000_create_failed_jobs_table.php
โย ย โโโ seeds
โย ย โโโ BlockedItemsTableSeeder.php
โย ย โโโ BlockedTypeTableSeeder.php
โย ย โโโ ConnectRelationshipsSeeder.php
โย ย โโโ DatabaseSeeder.php
โย ย โโโ PermissionsTableSeeder.php
โย ย โโโ RolesTableSeeder.php
โย ย โโโ ThemesTableSeeder.php
โย ย โโโ UsersTableSeeder.php
โโโ license.svg
โโโ package-lock.json
โโโ package.json
โโโ phpunit.xml
โโโ public
โย ย โโโ .htaccess
โย ย โโโ css
โย ย โย ย โโโ app.css
โย ย โย ย โโโ laravel2step
โย ย โย ย โโโ app.css
โย ย โย ย โโโ app.min.css
โย ย โโโ favicon.ico
โย ย โโโ fonts
โย ย โย ย โโโ fontawesome-webfont.eot
โย ย โย ย โโโ fontawesome-webfont.svg
โย ย โย ย โโโ fontawesome-webfont.ttf
โย ย โย ย โโโ fontawesome-webfont.woff
โย ย โย ย โโโ fontawesome-webfont.woff2
โย ย โย ย โโโ glyphicons-halflings-regular.eot
โย ย โย ย โโโ glyphicons-halflings-regular.svg
โย ย โย ย โโโ glyphicons-halflings-regular.ttf
โย ย โย ย โโโ glyphicons-halflings-regular.woff
โย ย โย ย โโโ glyphicons-halflings-regular.woff2
โย ย โโโ images
โย ย โย ย โโโ wink.png
โย ย โย ย โโโ wink.svg
โย ย โโโ index.php
โย ย โโโ js
โย ย โย ย โโโ app.99230f42ad184f498ce6.js
โย ย โย ย โโโ app.js
โย ย โย ย โโโ app.js.LICENSE.txt
โย ย โโโ mix-manifest.json
โย ย โโโ robots.txt
โย ย โโโ web.config
โโโ resources
โย ย โโโ assets
โย ย โย ย โโโ js
โย ย โย ย โย ย โโโ app.js
โย ย โย ย โย ย โโโ bootstrap.js
โย ย โย ย โย ย โโโ components
โย ย โย ย โย ย โโโ ExampleComponent.vue
โย ย โย ย โย ย โโโ UsersCount.vue
โย ย โย ย โโโ sass
โย ย โย ย โย ย โโโ _avatar.scss
โย ย โย ย โย ย โโโ _badges.scss
โย ย โย ย โย ย โโโ _bootstrap-social.scss
โย ย โย ย โย ย โโโ _buttons.scss
โย ย โย ย โย ย โโโ _forms.scss
โย ย โย ย โย ย โโโ _helpers.scss
โย ย โย ย โย ย โโโ _hideShowPassword.scss
โย ย โย ย โย ย โโโ _lists.scss
โย ย โย ย โย ย โโโ _logs.scss
โย ย โย ย โย ย โโโ _margins.scss
โย ย โย ย โย ย โโโ _mixins.scss
โย ย โย ย โย ย โโโ _modals.scss
โย ย โย ย โย ย โโโ _panels.scss
โย ย โย ย โย ย โโโ _password.scss
โย ย โย ย โย ย โโโ _socials.scss
โย ย โย ย โย ย โโโ _typography.scss
โย ย โย ย โย ย โโโ _user-profile.scss
โย ย โย ย โย ย โโโ _variables.scss
โย ย โย ย โย ย โโโ _visibility.scss
โย ย โย ย โย ย โโโ _wells.scss
โย ย โย ย โย ย โโโ app.scss
โย ย โย ย โโโ scss
โย ย โย ย โโโ laravel2step
โย ย โย ย โโโ _animations.scss
โย ย โย ย โโโ _mixins.scss
โย ย โย ย โโโ _modals.scss
โย ย โย ย โโโ _variables.scss
โย ย โย ย โโโ _verification.scss
โย ย โย ย โโโ app.scss
โย ย โโโ lang
โย ย โย ย โโโ en
โย ย โย ย โย ย โโโ auth.php
โย ย โย ย โย ย โโโ emails.php
โย ย โย ย โย ย โโโ forms.php
โย ย โย ย โย ย โโโ modals.php
โย ย โย ย โย ย โโโ pagination.php
โย ย โย ย โย ย โโโ passwords.php
โย ย โย ย โย ย โโโ permsandroles.php
โย ย โย ย โย ย โโโ profile.php
โย ย โย ย โย ย โโโ socials.php
โย ย โย ย โย ย โโโ terms.php
โย ย โย ย โย ย โโโ themes.php
โย ย โย ย โย ย โโโ titles.php
โย ย โย ย โย ย โโโ usersmanagement.php
โย ย โย ย โย ย โโโ validation.php
โย ย โย ย โโโ fr
โย ย โย ย โย ย โโโ auth.php
โย ย โย ย โย ย โโโ emails.php
โย ย โย ย โย ย โโโ forms.php
โย ย โย ย โย ย โโโ modals.php
โย ย โย ย โย ย โโโ pagination.php
โย ย โย ย โย ย โโโ passwords.php
โย ย โย ย โย ย โโโ permsandroles.php
โย ย โย ย โย ย โโโ profile.php
โย ย โย ย โย ย โโโ socials.php
โย ย โย ย โย ย โโโ titles.php
โย ย โย ย โย ย โโโ usersmanagement.php
โย ย โย ย โย ย โโโ validation.php
โย ย โย ย โโโ pt-br
โย ย โย ย โโโ auth.php
โย ย โย ย โโโ emails.php
โย ย โย ย โโโ forms.php
โย ย โย ย โโโ modals.php
โย ย โย ย โโโ pagination.php
โย ย โย ย โโโ passwords.php
โย ย โย ย โโโ permsandroles.php
โย ย โย ย โโโ profile.php
โย ย โย ย โโโ socials.php
โย ย โย ย โโโ themes.php
โย ย โย ย โโโ titles.php
โย ย โย ย โโโ usersmanagement.php
โย ย โย ย โโโ validation.php
โย ย โโโ views
โย ย โโโ auth
โย ย โย ย โโโ activation.blade.php
โย ย โย ย โโโ exceeded.blade.php
โย ย โย ย โโโ login.blade.php
โย ย โย ย โโโ passwords
โย ย โย ย โย ย โโโ email.blade.php
โย ย โย ย โย ย โโโ reset.blade.php
โย ย โย ย โโโ register.blade.php
โย ย โโโ emails
โย ย โย ย โโโ exception.blade.php
โย ย โโโ errors
โย ย โย ย โโโ 403.blade.php
โย ย โย ย โโโ 404.blade.php
โย ย โย ย โโโ 500.blade.php
โย ย โย ย โโโ 503.blade.php
โย ย โโโ home.blade.php
โย ย โโโ layouts
โย ย โย ย โโโ app.blade.php
โย ย โโโ modals
โย ย โย ย โโโ modal-delete.blade.php
โย ย โย ย โโโ modal-form.blade.php
โย ย โย ย โโโ modal-save.blade.php
โย ย โโโ pages
โย ย โย ย โโโ admin
โย ย โย ย โย ย โโโ active-users.blade.php
โย ย โย ย โย ย โโโ home.blade.php
โย ย โย ย โย ย โโโ route-details.blade.php
โย ย โย ย โโโ public
โย ย โย ย โย ย โโโ terms.blade.php
โย ย โย ย โโโ status.blade.php
โย ย โย ย โโโ user
โย ย โย ย โโโ home.blade.php
โย ย โโโ panels
โย ย โย ย โโโ welcome-panel.blade.php
โย ย โโโ partials
โย ย โย ย โโโ errors.blade.php
โย ย โย ย โโโ form-status.blade.php
โย ย โย ย โโโ nav.blade.php
โย ย โย ย โโโ search-users-form.blade.php
โย ย โย ย โโโ socials-icons.blade.php
โย ย โย ย โโโ socials.blade.php
โย ย โย ย โโโ status-panel.blade.php
โย ย โย ย โโโ status.blade.php
โย ย โโโ profiles
โย ย โย ย โโโ edit.blade.php
โย ย โย ย โโโ show.blade.php
โย ย โโโ scripts
โย ย โย ย โโโ check-changed.blade.php
โย ย โย ย โโโ datatables.blade.php
โย ย โย ย โโโ delete-modal-script.blade.php
โย ย โย ย โโโ form-modal-script.blade.php
โย ย โย ย โโโ gmaps-address-lookup-api3.blade.php
โย ย โย ย โโโ google-maps-geocode-and-map.blade.php
โย ย โย ย โโโ save-modal-script.blade.php
โย ย โย ย โโโ search-users.blade.php
โย ย โย ย โโโ toggleStatus.blade.php
โย ย โย ย โโโ tooltips.blade.php
โย ย โย ย โโโ user-avatar-dz.blade.php
โย ย โโโ themesmanagement
โย ย โย ย โโโ add-theme.blade.php
โย ย โย ย โโโ edit-theme.blade.php
โย ย โย ย โโโ show-theme.blade.php
โย ย โย ย โโโ show-themes.blade.php
โย ย โโโ usersmanagement
โย ย โย ย โโโ create-user.blade.php
โย ย โย ย โโโ edit-user.blade.php
โย ย โย ย โโโ show-deleted-user.blade.php
โย ย โย ย โโโ show-deleted-users.blade.php
โย ย โย ย โโโ show-user.blade.php
โย ย โย ย โโโ show-users.blade.php
โย ย โโโ welcome.blade.php
โโโ routes
โย ย โโโ api.php
โย ย โโโ channels.php
โย ย โโโ console.php
โย ย โโโ web.php
โโโ server.php
โโโ webpack.mix.js
```
* Tree command can be installed using brew: `brew install tree`
* File tree generated using command `tree -a -I '.git|node_modules|vendor|storage|tests'`
### Opening an Issue
Before opening an issue there are a couple of considerations:
* A **star** on this project shows support and is way to say thank you to all the contributors. If you open an issue without a star, *your issue may be closed.* Thank you for understanding and the support. You are all awesome!
* **Please Read the instructions** and make sure all steps were *followed correctly*.
* **Please Check** that the issue is not *specific to the development environment* setup.
* **Please Provide** *duplication steps*.
* **Please Attempt to look into the issue**, and if you *have a solution, make a pull request*.
* **Please Show that you have made an attempt** to *look into the issue*.
* **Please Check** to see if the issue you are *reporting is a duplicate* of a previous reported issue.
### Laravel Auth License
Laravel-auth is licensed under the [MIT license](https://opensource.org/licenses/MIT). Enjoy!
### Contributors
* Thanks goes to these [wonderful people](https://github.com/jeremykenedy/laravel-auth/graphs/contributors):
* Please feel free to contribute and make pull requests!
| {
"pile_set_name": "Github"
} |
\version "2.10.3"
\include "clarinetti.ly"
\include "fagotti.ly"
\include "cornig.ly"
\include "violinoprincipale.ly"
\include "violinoone.ly"
\include "violinotwo.ly"
\include "viola.ly"
\include "violoncello.ly"
\include "basso.ly"
\header {
title = "Concerto for Violin and Orchestra in D Major - Opus 61"
subtitle = "2nd Movement"
composer = "Ludwig van Beethoven"
mutopiatitle = "Concerto for Violin and Orchestra in D Major (2nd Movement)"
mutopiacomposer = "BeethovenLv"
mutopiainstrument = "Violin and Orchestra"
mutopiaopus = "Op. 61"
date = "1806"
source = "Breitkopf and Hartel (1862-1865)"
style = "Classical"
copyright = "Public Domain"
maintainer = "Stelios Samelis"
lastupdated = "2007/August/10"
version = "2.10.3"
footer = "Mutopia-2007/08/11-1021"
tagline = \markup { \override #'(box-padding . 1.0) \override #'(baseline-skip . 2.7) \box \center-align { \small \line { Sheet music from \with-url #"http://www.MutopiaProject.org" \line { \teeny www. \hspace #-1.0 MutopiaProject \hspace #-1.0 \teeny .org \hspace #0.5 } โข \hspace #0.5 \italic Free to download, with the \italic freedom to distribute, modify and perform. } \line { \small \line { Typeset using \with-url #"http://www.LilyPond.org" \line { \teeny www. \hspace #-1.0 LilyPond \hspace #-1.0 \teeny .org } by \maintainer \hspace #-1.0 . \hspace #0.5 Reference: \footer } } \line { \teeny \line { This sheet music has been placed in the public domain by the typesetter, for details see: \hspace #-0.5 \with-url #"http://creativecommons.org/licenses/publicdomain" http://creativecommons.org/licenses/publicdomain } } } }
}
\score {
\new StaffGroup="first"
<<
\new Staff = "one" {
\transposition c
\clarinetti
}
\new Staff = "two" {
\fagotti
}
\new Staff = "three" {
\transposition g
\cornig
}
\new Staff = "four" {
\violinoprincipale
}
\new Staff = "five" {
\violinoone
}
\new Staff = "six" {
\violinotwo
}
\new Staff = "seven" {
\viola
}
\new Staff = "eight" {
\violoncello
}
\new Staff = "nine" {
\basso
}
>>
\layout {
\context { \Staff
\override VerticalAxisGroup #'minimum-Y-extent = #'(-6.5 . 6.5)
}
}
\midi { }
}
\paper {
after-title-space = 2\cm
left-margin = 2.0\cm
paper-width = 22\cm
}
| {
"pile_set_name": "Github"
} |
package net.neoremind.mycode.argorithm.leetcode;
import static org.junit.Assert.assertThat;
import java.util.ArrayList;
import java.util.List;
import org.hamcrest.Matchers;
import org.junit.Test;
/**
* ๆ้ๅคๆฐๅ
จๆๅ้ฎ้ข
* Given a collection of numbers that might contain duplicates, return all possible unique permutations.
* <p>
* For example,
* [1,1,2] have the following unique permutations:
* <pre>
* [
* [1,1,2],
* [1,2,1],
* [2,1,1]
* ]
* </pre>
*
* @author zhangxu
* @see https://leetcode.com/problems/permutations-ii/
* @see net.neoremind.mycode.argorithm.backtracking.Permutation
* @see Permutation
*/
public class PermutationII {
public List<List<Integer>> permuteUnique(int[] nums) {
List<List<Integer>> result = new ArrayList<>();
doPermute(nums, 0, result);
return result;
}
private void doPermute(int[] nums, int index, List<List<Integer>> result) {
if (index == nums.length) {
List<Integer> numsList = new ArrayList<>(nums.length);
for (int num : nums) {
numsList.add(num);
}
result.add(numsList);
System.out.println(numsList);
} else {
for (int j = index; j < nums.length; j++) {
if (isNotSame(nums, index, j)) {
swap(nums, index, j);
doPermute(nums, index + 1, result);
swap(nums, index, j);
}
}
}
}
/**
* ่ฟไธชๅฝๆฐๆฏไธชๆๅทง๏ผ็ฌฌไธ่ชๅทฑ่ท่ชๅทฑๆฏ็ๆถๅไธๅคๆญๆฏๅฆ็ธๅ๏ผๅชๅคๆญๅ้ข็ๆฐๅๅบๅฎ็ๆฐๆฏ่พ
*
* ่ฟไธชๆนๆณไผๆชๅจๅฐๆๅ็้ฃไธช1ๅๅผๅงๅ
จๆๅใ
*/
private boolean isNotSame(int[] nums, int index, int j) {
for (int k = index; k < j; k++) {
if (nums[k] == nums[j]) {
return false;
}
}
return true;
}
private void swap(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
/**
* http://blog.csdn.net/sbitswc/article/details/36154559
* http://blog.csdn.net/hackbuteer1/article/details/6657435
* ๅ
จๆๅ็็ฎๆณๆฏไธไธชๅบ็ก๏ผๆๅ็ฎๆณๅจๅฎ็ๅบ็กไธๅขๅ ไบ้ๆฐ่ฟ็จ๏ผselect๏ผ๏ผๅณๅ
้ๅๆใ่ฟ้้ขไธป่ฆๆฏ็จๅฐไบไธไธช้ๅฝ็ๆๆณ๏ผ ๅฉ็จ้ๅฝๆณๆฅๅ่ฟ้ขๅ
ณ้ฎไธๅ ็น๏ผ
* 1.ๆฎ้ๆ
ๅต-ๅๅบๅบๅไธญ็ไธไธชๆฐๅนถไธๅฐๅฉไธ็ๅบๅๅ
จๆๅ
* 2.็นๆฎๆ
ๅต-ๅบๅไธญๅชๅฉไธไธชๆฐ๏ผๅๅ
จๆๅๅฐฑๆฏๅ
ถ่ช่บซใๅฐๅขไธช่ทๅพ็ๅบๅ่พๅบใ
* 3.ๅจไธๆญขไธไธชๆฐ็ๆ
ๅตไธ๏ผ่ฏฅไฝ่ฆๅๅซไบคๆขๅฉไธ็ๆฐ๏ผไพๅฆ๏ผไธคไธชๆฐA๏ผB ๅๆไธค็งๆ
ๅต๏ผไธไธชๆฏAB ไธไธชๆฏBA๏ผ
* https://discuss.leetcode.com/topic/46162/a-general-approach-to-backtracking-questions-in-java-subsets
* -permutations-combination-sum-palindrome-partioning
*/
@Test
public void test() {
int[] nums = new int[] {1};
assertThat(permuteUnique(nums).size(), Matchers.is(1));
nums = new int[] {1, 2};
assertThat(permuteUnique(nums).size(), Matchers.is(2));
nums = new int[] {1, 1, 2};
assertThat(permuteUnique(nums).size(), Matchers.is(3));
}
}
| {
"pile_set_name": "Github"
} |
// Karma configuration
// Generated on Mon May 13 2013 10:01:17 GMT-0300 (ART)
// list of files / patterns to load in the browser
module.exports = function (config) {
config.set({
frameworks: ['jasmine', 'requirejs'],
// base path, that will be used to resolve files and exclude
basePath: '..',
files: [
'test/loader.js',
{
pattern: 'lib/**/*.js',
included: false
}, {
pattern: '*.js',
included: false
}, {
pattern: 'activity/**/*.js',
included: false
}, {
pattern: 'graphics/**/*',
included: false
}
],
// list of files to exclude
exclude: [],
// test results reporter to use
// possible values: 'dots', 'progress', 'junit'
reporters: ['progress'],
// web server port
port: 9876,
// cli runner port
runnerPort: 9100,
// enable / disable colors in the output (reporters and logs)
colors: true,
// level of logging
// possible values: LOG_DISABLE || LOG_ERROR || LOG_WARN || LOG_INFO ||
// LOG_DEBUG
logLevel: config.LOG_INFO,
// enable / disable watching file and executing tests whenever any file
// changes
autoWatch: true,
// If browser does not capture in given timeout [ms], kill it
captureTimeout: 60000,
// Continuous Integration mode
// if true, it capture browsers, run tests and exit
singleRun: false,
preprocessors: {}
});
};
| {
"pile_set_name": "Github"
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.