question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
number-of-arithmetic-triplets | ✅ A generic Dynamic Programming (A[i] - diff) || [Intuition Updated] | a-generic-dynamic-programming-ai-diff-in-y2fl | This Approach even works if the array is unsorted or elements are negative or diff is negative\n\nSimilar to 300. Longest Increasing Subsequence.\nWe simply sav | xxvvpp | NORMAL | 2022-08-07T04:15:10.106293+00:00 | 2022-08-07T09:09:42.671871+00:00 | 2,919 | false | **This Approach even works if the array is unsorted or elements are negative or diff is negative**\n\n**Similar to [300. Longest Increasing Subsequence](https://leetcode.com/problems/longest-increasing-subsequence/).**\nWe simply save the value for every we have seen till now in a array and our count will be `cnt[A[i]]= cnt[A[i]-diff]+1` which is typical Dynamic Programming,\nthat we exactly do in **Longest Increasing subsequence.**\n\nOn the way get count for `A[i]-diff` Value and increase result if sequence length till this number is `>=3`.\n\n# This Question is a simpler version of this:\n+ [1027. Longest Arithmetic Subsequence](https://leetcode.com/problems/longest-arithmetic-subsequence/)\n\n**Intuition**:\n\nWe compute answer on the way. But how??\nBasically lets say the sequence is `[a,b,c] is a AP` with `diff of k`.\nSo lets say we are at **b** element , so which element should be pick actually which will make **AP** with **b**???\nIf we know the **diff** , then its easy for us to predict the number we are looking for, and that number is **b-diff**.\n\n# So we simply do this for every element we encounter and if the AP length till that element>=3 , then we will get an extra pair.\n\n**Talking about unique Pairs**:\nWe always get unique pairs by default. \nBecause if we considered indices [1,2,3], then we are not even considering its count again in the process. \n# C++ \n\tint arithmeticTriplets(vector<int>& nums, int diff) {\n int dp[201]{};\n int res=0;\n for(auto i:nums){ \n\t\t\tdp[i]= i>=diff? dp[i-diff]+1 : 1;\n if(dp[i]>=3) res++; \n }\n return res;\n }\n\t\n# Using Hashmap\n int arithmeticTriplets(vector<int>& nums, int diff) {\n unordered_map<int,int> dp;\n int res=0;\n for(auto i:nums) \n\t if((dp[i]=dp[i-diff]+1)>=3) res++;\n return res;\n }\n**Worst Time** - O(`200`)\n**Worst Space** - O(`201`) | 23 | 2 | ['Dynamic Programming', 'C'] | 1 |
number-of-arithmetic-triplets | BRUTE FORCE 3 LOOP's && Optimized O(N) | brute-force-3-loops-optimized-on-by-up15-as5k | Contest Submission\n\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int ans=0;\n for(int i=0;i<nums.size( | up1512001 | NORMAL | 2022-08-07T04:02:53.017214+00:00 | 2022-08-08T13:06:29.695911+00:00 | 3,699 | false | **Contest Submission**\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int ans=0;\n for(int i=0;i<nums.size();i++){\n for(int j=i+1;j<nums.size();j++){\n for(int k=j+1;k<nums.size();k++){\n if((nums[j]-nums[i])==diff && (nums[k]-nums[j])==diff){\n ans++;\n }\n }\n }\n }\n return ans;\n }\n};\n```\n**After Contest Thinking**\nhere constrains are for nums[i] is 0 to 200 inclusive so let\'s create array of 201 size and mark it one in arr if it\'s present in nums.\nthen traverse nums and check if `nums[i] - diff >= 0 and nums[i] + diff <= 200` and also check for `nums[i] - diff & nums[i] + diff` is present in nums by checking marking in array.\nAnd `i < j < k` always satisfy because given nums is in sorted order.\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int arr[201] = {0};\n for(auto it : nums){\n arr[it]++;\n }\n int ans=0;\n for(auto it : nums){\n if(it-diff>=0 && it+diff<=200 && arr[it-diff] && arr[it+diff]){\n ans++;\n }\n }\n return ans;\n }\n};\n```\n```\nTime Complexity : O(N+N) ~ O(N)\nSpace Complexity : O(N)\n```\n | 20 | 0 | ['C', 'C++'] | 1 |
number-of-arithmetic-triplets | Java / C++ Brute Force to Set | java-c-brute-force-to-set-by-fllght-mwgi | The brute force method is pretty fast here, but using a set is preferable\n\n### Java Brute Force\njava\npublic int arithmeticTriplets(int[] nums, int diff) {\n | FLlGHT | NORMAL | 2022-08-07T06:07:06.808460+00:00 | 2022-08-07T07:27:36.949896+00:00 | 1,809 | false | The brute force method is pretty fast here, but using a set is preferable\n\n### Java Brute Force\n```java\npublic int arithmeticTriplets(int[] nums, int diff) {\n int count = 0;\n\n for (int i = 0; i < nums.length - 2; i++) {\n for (int j = i + 1; j < nums.length - 1; j++) {\n for (int k = j + 1; k < nums.length; k++) {\n if (nums[j] - nums[i] == diff && nums[k] - nums[j] == diff)\n count++;\n }\n }\n }\n return count;\n }\n```\n\n### C++ Brute Force\n```\nint arithmeticTriplets(vector<int>& nums, int diff) {\n int count = 0;\n\n for (int i = 0; i < nums.size() - 2; i++) {\n for (int j = i + 1; j < nums.size() - 1; j++) {\n for (int k = j + 1; k < nums.size(); k++) {\n if (nums[j] - nums[i] == diff && nums[k] - nums[j] == diff)\n count++;\n }\n }\n }\n return count;\n }\n```\n\n### Java Set\n\n```\npublic int arithmeticTriplets(int[] nums, int diff) {\n int count = 0;\n Set<Integer> set = new HashSet<>();\n for (int num : nums) {\n if (set.contains(num - diff) && set.contains(num - diff * 2))\n count++;\n \n set.add(num);\n }\n return count;\n }\n```\n\n### C++ Set\n\n```\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count = 0;\n set<int> set;\n for (int num : nums) {\n if (set.find(num - diff) != set.end() && set.find(num - diff * 2) != set.end())\n count++;\n \n set.insert(num);\n }\n return count;\n }\n``` | 16 | 0 | ['C', 'Ordered Set', 'Java'] | 3 |
number-of-arithmetic-triplets | easy hashmap solution and got 54ms ✅ | easy-hashmap-solution-and-got-54ms-by-mj-xnn4 | \nvar arithmeticTriplets = function(nums, diff) {\n \n let hash = new Map();\n let count = 0;\n\n for(let i=0; i<nums.length; i++){\n let tem | MJWHY | NORMAL | 2022-08-18T00:11:46.230923+00:00 | 2022-08-18T17:17:04.109785+00:00 | 1,179 | false | ```\nvar arithmeticTriplets = function(nums, diff) {\n \n let hash = new Map();\n let count = 0;\n\n for(let i=0; i<nums.length; i++){\n let temp = nums[i] - diff;\n \n if(hash.has(temp) && hash.has(temp - diff)){\n count++;\n }\n hash.set(nums[i] , "Hard choices easy life, Easy choices hard life.");\n }\n \n return count;\n};\n```\n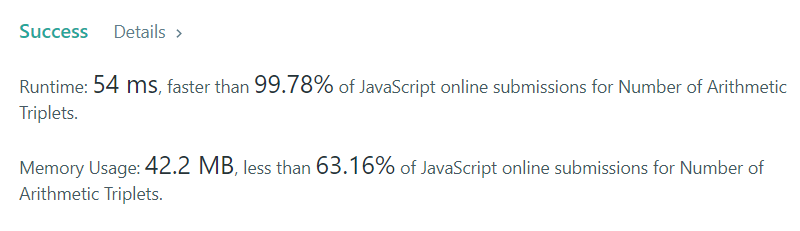\n\n | 14 | 0 | ['JavaScript'] | 2 |
number-of-arithmetic-triplets | ✔ C++ using Map with Explanation || Very Easy and Simple to understand Solution | c-using-map-with-explanation-very-easy-a-4x19 | Up Vote if you like the solution\n# Check for the nums[i] -diff and nums[i] - 2*diff, if these are present in the map or not. If present count a triplet. Then | kreakEmp | NORMAL | 2022-08-07T04:01:32.339003+00:00 | 2022-08-07T05:18:24.991141+00:00 | 2,122 | false | <b> Up Vote if you like the solution\n# Check for the nums[i] -diff and nums[i] - 2*diff, if these are present in the map or not. If present count a triplet. Then strore the current number in map for future use.\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n unordered_map<int, int> mp;\n int ans = 0;\n for(auto i: nums){\n if(mp.find(i-diff) != mp.end() && mp.find(i-2*diff) != mp.end()) ans = ans + mp[i-diff]* mp[i-2*diff];\n mp[i]++;\n }\n return ans;\n }\n};\n``` | 14 | 0 | [] | 0 |
number-of-arithmetic-triplets | Python | Easy Solution✅ | python-easy-solution-by-gmanayath-777z | \ndef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n ans = 0\n for i in range(len(nums)): # nums = [0,1,4,6,7,10], diff = 3\n | gmanayath | NORMAL | 2022-08-12T15:45:44.309663+00:00 | 2022-12-22T16:50:28.013817+00:00 | 1,712 | false | ```\ndef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n ans = 0\n for i in range(len(nums)): # nums = [0,1,4,6,7,10], diff = 3\n j = nums[i] - diff # 4 - 3 = 1 (when i ==2)\n k = diff + nums[i] # 3 + 4 = 7 (when i ==2)\n if j in nums and k in nums: # 1 and 7 is there in the list\n ans +=1\n return ans\n``` | 13 | 0 | ['Python', 'Python3'] | 4 |
number-of-arithmetic-triplets | ✅Easy Java solution||Straight Forward||Beginner Friendly🔥 | easy-java-solutionstraight-forwardbeginn-qfyc | If you really found my solution helpful please upvote it, as it motivates me to post such kind of codes and help the coding community, if you have some queries | deepVashisth | NORMAL | 2022-09-30T19:21:27.691409+00:00 | 2022-09-30T19:21:27.691448+00:00 | 1,081 | false | **If you really found my solution helpful please upvote it, as it motivates me to post such kind of codes and help the coding community, if you have some queries or some improvements please feel free to comment and share your views.**\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count = 0;\n for(int i = 0 ; i < nums.length -2 ; i++){\n for(int j = i+1; j < nums.length -1; j ++){\n for(int k = j+1; k < nums.length ; k ++){\n if(nums[j] - nums[i] == diff && nums[k] - nums[j] == diff){\n count++;\n }\n }\n }\n }\n return count;\n }\n}\n```\n**Happy Coding** | 11 | 0 | ['Java'] | 2 |
number-of-arithmetic-triplets | ( Beats 99%)3 Java Solution | Binary Search | Map | 3 Pointers | beats-993-java-solution-binary-search-ma-z9ot | 3 Different JAVA Solutions \n\nSolution Using Binary Search (Time Complexity : O(nlog(n)) , Space Complexity : O(1))\n\nclass Solution {\n public static bool | Aman__Bhardwaj | NORMAL | 2022-09-08T17:19:27.645130+00:00 | 2022-09-08T17:19:27.645171+00:00 | 740 | false | # 3 Different JAVA Solutions \n\n**Solution Using Binary Search (Time Complexity : O(nlog(n)) , Space Complexity : O(1))**\n```\nclass Solution {\n public static boolean BinarySearch(int[] arr, int key){\n int low = 0 , high = arr.length-1;\n while(low <= high){\n int mid = low + (high-low)/2;\n if(arr[mid]==key) return true;\n else if(arr[mid]<key) low = mid+1;\n else high = mid-1;\n }\n return false;\n }\n public int arithmeticTriplets(int[] nums, int diff) {\n int count = 0;\n for(int i=0;i<nums.length-2;i++){\n boolean next = BinarySearch(nums,nums[i]+diff);\n boolean nextnext = BinarySearch(nums,nums[i]+2*diff);\n if(next && nextnext) count++;\n }\n return count;\n }\n \n // Time Complexity : O(nlog(n))\n // Space Complexity : O(1)\n}\n```\n\n**Solution Using Map (Time Complexity : O(n) , Space Complexity : O(n))**\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count = 0;\n Map<Integer,Integer> map = new HashMap<>();\n for(int i=0;i<nums.length;i++) map.put(nums[i],i);\n for(int k : nums) count += (map.containsKey(k+diff) && map.containsKey(k+2*diff)) ? 1 : 0;\n return count;\n }\n \n // Time Complexity : O(log(n))\n // Space Complexity : O(n)\n\n}\n```\n\n**Solution Using 3-Pointers (Time Complexity : O(n) , Space Complexity : O(1))**\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count = 0;\n int pointer1 = 0 , pointer2 = 1 , pointer3 = 2;\n while(pointer3 < nums.length){\n int compare = nums[pointer2]-nums[pointer1];\n if(compare==diff){\n compare = nums[pointer3]-nums[pointer2];\n if(compare==diff){\n pointer1++;\n pointer2++;\n pointer3++;\n count++;\n }\n else if(compare<diff) pointer3++;\n else{\n pointer1++;pointer2++;\n pointer3 = Math.max(pointer3,pointer2+1);\n }\n }\n else if(compare<diff){\n pointer2++;\n pointer3 = Math.max(pointer3,pointer2+1);\n }\n else{\n pointer1++;\n pointer2 = Math.max(pointer2,pointer1+1);\n pointer3 = Math.max(pointer3,pointer2+1);\n }\n }\n return count;\n \n }\n \n // Time Complexity : O(n)\n // Space Complexity : O(1)\n\n}\n```\n | 10 | 0 | ['Binary Tree', 'Java'] | 1 |
number-of-arithmetic-triplets | ✅ Easy Python Solution||Binary Search Solution | easy-python-solutionbinary-search-soluti-zrt1 | Intution:-\n PLEASE UPVOTE\uD83D\uDC4D\uD83D\uDC4D IF YOU LIKE THE SOLUTION\n## Binary Search\nUse binary search to find out if nums[i]+diff and nums[i]+2diff i | amandgp | NORMAL | 2023-03-26T13:41:24.646179+00:00 | 2023-03-26T13:41:24.646222+00:00 | 506 | false | # Intution:-\n **PLEASE UPVOTE\uD83D\uDC4D\uD83D\uDC4D IF YOU LIKE THE SOLUTION**\n## Binary Search\nUse binary search to find out if nums[i]+diff and nums[i]+2*diff is present in array or not if both are present in array increment the count by 1 if any one of them is not present in array continue:\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Complexity\n- Time complexity:O(N)*O(logN)*O(logN)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def binarysearch(self,nums,val):\n s,e=0,len(nums)-1\n while s<=e:\n mid=(s+e)//2\n if nums[mid]==val:\n return True\n elif nums[mid]>val:\n e=mid-1\n else:\n s=mid+1\n return False\n\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n n=len(nums)\n cnt=0\n for i in range(n-2):\n x=self.binarysearch(nums[i+1:],nums[i]+diff)\n y=self.binarysearch(nums[i+1:],nums[i]+(2*diff))\n if x and y:\n cnt+=1\n \n return cnt\n```\n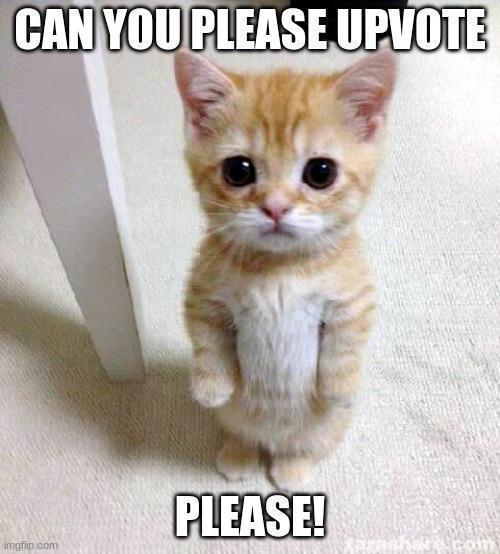\n\n\n | 8 | 0 | ['Array', 'Two Pointers', 'Binary Search', 'Enumeration', 'Python3'] | 0 |
number-of-arithmetic-triplets | C++|| Multiple Approach || Bruteforce to optimized || Easy Explanation | c-multiple-approach-bruteforce-to-optimi-hg87 | \n\t// Brute force \n\t// we will check condition for k only if nums[j]-nums[i]==diff\n\n\tclass Solution {\n\tpublic:\n\t\tint arithmeticTriplets(vector& nums, | anubhavsingh11 | NORMAL | 2022-08-09T19:47:57.117384+00:00 | 2023-01-16T05:38:00.637389+00:00 | 969 | false | \n\t// Brute force \n\t// we will check condition for k only if nums[j]-nums[i]==diff\n\n\tclass Solution {\n\tpublic:\n\t\tint arithmeticTriplets(vector<int>& nums, int diff) {\n\t\t\tint ans=0;\n\t\t\tfor(int i=0;i<nums.size()-2;i++)\n\t\t\t{\n\t\t\t\tfor(int j=i+1;j<nums.size()-1;j++)\n\t\t\t\t{\n\t\t\t\t\tif(nums[j]-nums[i]==diff)\n\t\t\t\t\tfor(int k=j+1;k<nums.size();k++)\n\t\t\t\t\t{\n\t\t\t\t\t\tif(nums[k]-nums[j]==diff)\n\t\t\t\t\t\t\tans++;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn ans ;\n\t\t}\n\t};\n\n\n\n\t// O(n) Using maps \n\t//nums[j] and nums[k] can be written as nums[j]=nums[i]+diff --(1)\n\t// and nums[k]=nums[j]+diff \n\t// nums[k]=nums[i]+2*diff from equation (1)\n\t// create a map to store element of array\n\t// check two conditon if(nums[i]+diff) is in map and nums[i]+2*diff is in map\n\n\tclass Solution {\n\tpublic:\n\t\tint arithmeticTriplets(vector<int>& nums, int diff) {\n\t\t\tint ans=0;\n\t\t\tmap<int,int>m;\n\t\t\tfor(int i=0;i<nums.size();i++)\n\t\t\t{\n\t\t\t\tm[nums[i]]++;\n\t\t\t}\n\t\t\tfor(auto &i:nums)\n\t\t\t{\n\t\t\t\tif( m[i+diff] && m[i+2*diff])\n\t\t\t\t\tans++;\n\t\t\t}\n\t\t\treturn ans;\n\t\t}\n\t\t};\n\n\t// single pass\n\t// if there is some number x for which x-diff and x-2*diff is present in map then there will always be a triplet \n\t// we can write the given equation as nums[j]=nums[k]-diff and nums[i]=nums[k]-2*diff\n\t// suppose we are having some number nums[k] and \n\t// if we can somehow find nums[k]-diff and nums[k]-2*diff \n\t// this means we have found nums[i] and nums[j] for some nums[k] which is required triplet \n\n\tclass Solution {\n\tpublic:\n\t\tint arithmeticTriplets(vector<int>& nums, int diff) {\n\t\t\tint ans=0;\n\t\t\tmap<int,int>m;\n\n\t\t\tfor(auto &i:nums)\n\t\t\t{\n\t\t\t\tif(i-diff>=0 && m[i-diff] && m[i-2*diff])\n\t\t\t\t\tans++;\n\t\t\t\tm[i]++;\n\t\t\t}\n\n\t\t\treturn ans;\n\t\t}\n\t};\n\n\n\t// O(n) Using sets \n\tclass Solution {\n\tpublic:\n\t\tint arithmeticTriplets(vector<int>& nums, int diff) {\n\t\t\tint ans=0;\n\t\t\tset<int>s(nums.begin(),nums.end());\n\t\t\tfor(auto &i:nums)\n\t\t\t{\n\t\t\t\tif(s.find(i+diff)!=s.end() && s.find(i+2*diff)!=s.end())\n\t\t\t\t\tans++;\n\t\t\t}\n\t\t\treturn ans;\n\t\t}\n\t};\n\n | 8 | 0 | ['C', 'Ordered Set'] | 2 |
number-of-arithmetic-triplets | Python easy understand solution O(n) space, O(n) time | python-easy-understand-solution-on-space-dzdk | Use a set to memorize each interger is exist or not. \npython\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n s | amikai | NORMAL | 2022-08-07T13:49:28.493723+00:00 | 2022-08-07T14:45:40.890742+00:00 | 1,517 | false | Use a set to memorize each interger is exist or not. \n```python\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n s = set(nums)\n count = 0\n for num in nums:\n if (num + diff) in s and (num + diff + diff) in s:\n count += 1\n return count\n``` | 8 | 0 | ['Ordered Set', 'Python', 'Python3'] | 5 |
number-of-arithmetic-triplets | Java Easy solution using HashMap | java-easy-solution-using-hashmap-by-basi-kc53 | For each element check element + diff && element + 2 * diff exist in map.\n```\npublic int arithmeticTriplets(int[] nums, int diff) {\n HashMap map = new | basitimam | NORMAL | 2022-08-07T04:01:23.409876+00:00 | 2022-08-07T04:08:56.653024+00:00 | 963 | false | For each element check element + diff && element + 2 * diff exist in map.\n```\npublic int arithmeticTriplets(int[] nums, int diff) {\n HashMap<Integer, Integer> map = new HashMap<>();\n for(int i : nums){\n map.put(i, map.getOrDefault(i, 0) + 1);\n }\n int count = 0;\n for(int i : nums){\n if(map.containsKey(i+diff) && map.containsKey(i+diff+diff)){\n count++;\n }\n }\n return count;\n } | 7 | 0 | ['Java'] | 3 |
number-of-arithmetic-triplets | Two (Three) Pointers. Beates 100 % | two-three-pointers-beates-100-by-sberik-gq2s | Approach\nThe main idea of the code is to use three pointers to search for arithmetic triplets in thenumsarray, where the difference between adjacent elements i | SBerik | NORMAL | 2023-10-31T04:53:08.569549+00:00 | 2023-10-31T05:03:58.697126+00:00 | 185 | false | # Approach\nThe main idea of the code is to use three pointers to search for arithmetic triplets in the`nums`array, where the difference between adjacent elements is equal to the given `diff`. The pointers `i`, `j`, and `k` move through the array.\n\n# Complexity\n- Time complexity: $$O(N)$$\n\n- Space complexity: $$O(1)$$\n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int n = nums.length;\n int count = 0;\n int i = 0, j = 1, k = 2;\n \n while (i < n && j < n && k < n) {\n if (nums[j] - nums[i] < diff) {\n j++;\n } else if (nums[j] - nums[i] > diff) {\n i++;\n } else {\n if (nums[k] - nums[j] < diff) {\n k++;\n } else if (nums[k] - nums[j] > diff) {\n j++;\n } else {\n count++;\n k++;\n }\n }\n }\n return count;\n }\n}\n\n```\n\n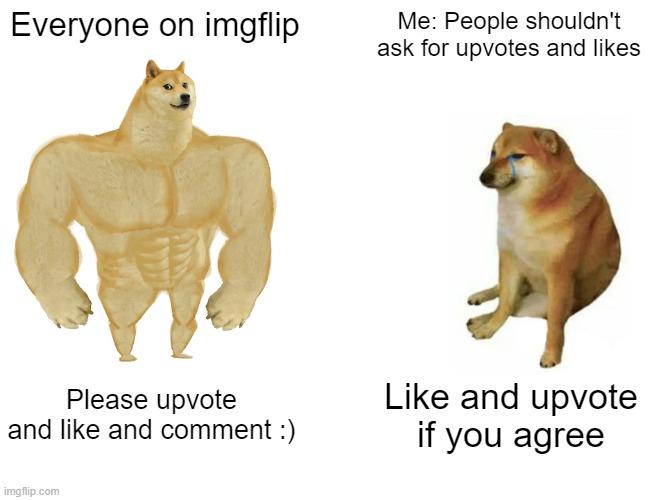\n\n | 6 | 0 | ['Two Pointers', 'Java'] | 0 |
number-of-arithmetic-triplets | C++ || Unordered-map || Easy-to-Understand || no of arithmetic tripplet. | c-unordered-map-easy-to-understand-no-of-yuil | # Intuition \n\n\n\n\n\n\n\n\n\n\n\n\n# Code\n\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int n=nums.size( | m_isha_125 | NORMAL | 2022-11-10T20:55:26.502303+00:00 | 2022-11-10T20:55:26.502344+00:00 | 567 | false | <!-- # Intuition -->\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n<!-- # Approach -->\n<!-- Describe your approach to solving the problem. -->\n\n<!-- # Complexity -->\n<!-- - Time complexity: -->\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n<!-- - Space complexity: -->\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int n=nums.size();\n unordered_map<int,bool>mp;\n int count=0;\n\n for(int i=0;i<n;i++){\n mp[nums[i]]=true;\n }\n\n for(int i=0;i<n;i++){\n if(mp[nums[i]-diff] && mp[nums[i]+diff]){\n count++;\n }\n }\n return count;\n }\n};\nif it helps plz. don\'t forget to upvote it :)\n``` | 6 | 0 | ['Hash Table', 'C++'] | 0 |
number-of-arithmetic-triplets | Python O(n) Two Pointers Approach | python-on-two-pointers-approach-by-brent-e45q | We can take advantage of the fact that the array is already sorted to find arithmetic triples quickly.\nThe big idea is that if either nums[j] - nums[i] or nums | brent_pappas | NORMAL | 2022-10-08T15:05:42.229714+00:00 | 2022-10-08T15:05:42.229746+00:00 | 661 | false | We can take advantage of the fact that the array is already sorted to find arithmetic triples quickly.\nThe big idea is that if either `nums[j] - nums[i]` or `nums[k] - nums[j]` is not equal to diff, we increment the appropriate index.\n\n```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n n = len(nums)\n count = 0\n i, j, k = 0, 1, 2\n while i < n and j < n and k < n:\n if nums[j] - nums[i] < diff:\n\t\t\t\t# diff is too small: increment j so that we get a larger diff\n j += 1\n elif nums[j] - nums[i] > diff:\n\t\t\t\t# diff is too big: increment i so that we get a smaller diff\n i += 1\n else:\n\t\t\t\t# we might have found a triple, so now check nums[k] - nums[j]\n if nums[k] - nums[j] < diff:\n\t\t\t\t\t# diff is too small\n k += 1\n elif nums[k] - nums[j] > diff:\n\t\t\t\t\t# diff is too big\n j += 1\n else:\n\t\t\t\t\t# found a triple\n count += 1\n\t\t\t\t\t# increment k so that we get closer to breaking the loop\n\t\t\t\t\t# i\'m actually not sure if this has to be k,\n\t\t\t\t\t# or if we could increment j instead\n k += 1\n return count\n``` | 6 | 0 | ['Two Pointers', 'Python'] | 1 |
number-of-arithmetic-triplets | python 95% fast and 97% memory | python-95-fast-and-97-memory-by-tul-jo37 | \nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n dic = {} # store nums[i]\n quest = {} # store require nu | TUL | NORMAL | 2022-08-29T04:52:21.911732+00:00 | 2022-08-29T04:52:21.911789+00:00 | 899 | false | ```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n dic = {} # store nums[i]\n quest = {} # store require number you possible find after nums[i]\n count = 0 # count answer\n for i in nums:\n dic[i] = True \n if i in quest: count += 1 # meet damand \n if i - diff in dic: quest[i + diff] = True\n return count\n``` | 6 | 0 | ['Hash Table', 'Python', 'Python3'] | 1 |
number-of-arithmetic-triplets | ✅SIMPLE AND EASY C++ SOLUTION USING HASHMAP | simple-and-easy-c-solution-using-hashmap-uq8n | Time Complexity: O(n)\n\nCode:\n\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count=0;\n unordered_ | ke4e | NORMAL | 2022-08-21T07:08:20.426713+00:00 | 2022-08-21T07:08:20.426759+00:00 | 458 | false | Time Complexity: O(n)\n\nCode:\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count=0;\n unordered_map<int, bool> data;\n for (int num:nums)\n data[num]=true;\n \n for (int num: nums)\n if (data[num-diff] && data[num+diff])\n count++;\n \n return count;\n }\n};\n``` | 6 | 0 | ['C'] | 0 |
number-of-arithmetic-triplets | Common Difference A.P||C++ | common-difference-apc-by-priyanshu3237-obvv | class Solution {\npublic:\n int arithmeticTriplets(vector& nums, int diff) {\n int ans = 0;\n for(int j = 1;j=0 && k<nums.size()){\n | priyanshu3237 | NORMAL | 2022-08-07T04:06:04.059362+00:00 | 2022-08-07T06:49:19.591583+00:00 | 526 | false | class Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int ans = 0;\n for(int j = 1;j<nums.size()-1;j++){\n int i = j-1, k = j+1;\n \n while(i>=0 && k<nums.size()){\n \n if(nums[j]-nums[i] == diff && nums[k]-nums[j] == diff)\n {\n ans++;\n i--;\n k++;\n }\n else if(nums[k]-nums[j]<diff){\n k++;\n }\n else\n i--;\n }\n }\n return ans;\n }\n}; | 6 | 0 | [] | 2 |
number-of-arithmetic-triplets | JAVA || 10000% beats ❤️ || 3 Solution || HashSet || ArrayList || ❤️ | java-10000-beats-3-solution-hashset-arra-06tc | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | saurabh_kumar1 | NORMAL | 2023-08-30T16:22:01.360415+00:00 | 2023-08-30T16:22:01.360434+00:00 | 653 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:0(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:0(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int low = 0 , high = 1 , count = 0;\n Set<Integer> temp = new HashSet<>();\n for(int i : nums) temp.add(i);\n while(nums.length>high){\n if(nums[high]-nums[low]==2*diff && temp.contains(diff+nums[low])) count++;\n if(nums[high] - nums[low] < 2 * diff) high++;\n else low++; \n }\n return count;\n\n\n// Using HASHSET (Another Solution)\n\n // int count = 0;\n // Set<Integer> temp = new HashSet<>();\n // for(int i : nums) temp.add(i); // UPVOTE ME(PLEASE)...\n // for(int i : nums){\n // if(temp.contains(i+diff) && temp.contains((i+diff)+diff)) count++;\n // }\n // return count;\n }\n}\n\n// Another solutions (Using ARRAYLIST) --> ( Try this one also)\n\n // int count = 0;\n // List<Integer> temp = new ArrayList<>();\n // for(int i=0; i<nums.length; i++) temp.add(nums[i]);\n // for(int i : nums) if(temp.contains(i+diff) && temp.contains((i+diff)+diff)) count++;\n // return count;\n``` | 5 | 0 | ['Array', 'Hash Table', 'C++', 'Java'] | 1 |
number-of-arithmetic-triplets | my_arithmeticTriplets | my_arithmetictriplets-by-rinatmambetov-4ww3 | # Intuition \n\n\n\n\n\n\n\n\n\n\n\n# Code\n\n/**\n * @param {number[]} nums\n * @param {number} diff\n * @return {number}\n */\nvar arithmeticTriplets = func | RinatMambetov | NORMAL | 2023-05-14T14:23:07.857122+00:00 | 2023-05-14T14:23:07.857163+00:00 | 881 | false | <!-- # Intuition -->\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n<!-- # Approach -->\n<!-- Describe your approach to solving the problem. -->\n\n<!-- # Complexity\n- Time complexity: -->\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n<!-- - Space complexity: -->\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n/**\n * @param {number[]} nums\n * @param {number} diff\n * @return {number}\n */\nvar arithmeticTriplets = function (nums, diff) {\n let count = 0;\n\n for (let j = 1; j < nums.length - 1; j++) {\n for (let i = 0; i < j; i++) {\n for (let k = j + 1; k < nums.length; k++) {\n if (nums[j] - nums[i] === diff && nums[k] - nums[j] === diff) count++;\n }\n }\n }\n \n return count++;\n};\n``` | 5 | 0 | ['JavaScript'] | 0 |
number-of-arithmetic-triplets | Two simple and fast Map and Array based solutions | two-simple-and-fast-map-and-array-based-wy2n2 | Approach\nEach number can have only one potenial triplet and the numbers should go in sequential order. So we just need to check that. If array we can check for | balfin | NORMAL | 2023-02-11T09:50:15.872915+00:00 | 2023-02-12T05:26:56.603744+00:00 | 804 | false | # Approach\nEach number can have only one potenial triplet and the numbers should go in sequential order. So we just need to check that. If array we can check forward values, if map we need to fill it with data and check backwards. Map solution is a bit slower per leetcode tests, looks like map.has is not that optimized as array.includes. Array version beats up to 90% by speed and memory as well. \n\n\n# Array solution\n```\n/**\n * @param {number[]} nums\n * @param {number} diff\n * @return {number}\n */\nvar arithmeticTriplets = function (nums, diff) {\n let count = 0;\n //The third triplet element\n let diff2 = 2 * diff;\n for (let i = 0; i < nums.length; i++) {\n /**\n * Checking if there is second and third triplet elements in array\n * Starting from the next element in array for the second \n * And from the +2 for the third to optimize search\n * && excludes second check if first failed\n */\n if (nums.includes(nums[i] + diff, i +1) && nums.includes(nums[i] + diff2, i + 2)) {\n count++\n }\n }\n return count;\n};\n```\n\n# MAP solution\n```\n/**\n * @param {number[]} nums\n * @param {number} diff\n * @return {number}\n */\nvar arithmeticTriplets = function (nums, diff) {\n let map = new Map();\n let count = 0;\n\n for(let i = 0; i < nums.length; i++){\n /**\n * For Map we need to fill it with the data so we check two \n * previous numbers backwards\n */\n let prev = nums[i] - diff;\n \n if(map.has(prev) && map.has(prev - diff)){\n count++;\n }\n map.set(nums[i] , 1);\n }\n \n return count;\n};\n``` | 5 | 0 | ['JavaScript'] | 0 |
number-of-arithmetic-triplets | using binary_search | using-binary_search-by-bhupendraj9-9zev | \nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count=0;\n for(int i=0;i<nums.size();i++)\n {\n | bhupendraj9 | NORMAL | 2022-11-08T09:14:00.153022+00:00 | 2023-11-01T12:20:05.427694+00:00 | 438 | false | ```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count=0;\n for(int i=0;i<nums.size();i++)\n {\n if(binary_search(nums.begin(),nums.end(),nums[i]+diff) && binary_search(nums.begin(),nums.end(),nums[i]+2*diff))\n count++;\n }\n return count;\n }\n};\n``` | 5 | 0 | ['Binary Search', 'C++'] | 3 |
number-of-arithmetic-triplets | Swift One-Liner | Also: Optimized Alternates | swift-one-liner-also-optimized-alternate-weos | One-Liner (accepted answer)\n\nclass Solution {\n func arithmeticTriplets(_ nums: [Int], _ diff: Int) -> Int {\n nums.filter { nums.contains($0+diff) | UpvoteThisPls | NORMAL | 2022-08-08T23:19:36.474617+00:00 | 2022-08-08T23:19:36.474660+00:00 | 102 | false | **One-Liner (accepted answer)**\n```\nclass Solution {\n func arithmeticTriplets(_ nums: [Int], _ diff: Int) -> Int {\n nums.filter { nums.contains($0+diff) && nums.contains($0+diff*2) }.count\n }\n}\n```\n\n**Two-Liner, much faster approach (accepted answer)**\n```\nclass Solution {\n func arithmeticTriplets(_ nums: [Int], _ diff: Int) -> Int {\n let set = Set(nums)\n return nums.filter { set.contains($0+diff) && set.contains($0+diff*2) }.count\n }\n}\n```\nThe complexity of `.contains()` is improved from **O(n)** to **O(1)** between the One-Liner and Two-Liner. Much better! \n\n---\n\n**More Optimized approach (accepted answer)** \nBreak out of loop early when it becomes impossible to create Arithmetic Triplet.\n```\nclass Solution {\n func arithmeticTriplets(_ nums: [Int], _ diff: Int) -> Int {\n // Constraints:\n // 1) 3 <= nums.length <= 200\n // 2) 0 <= nums[i] <= 200\n // 3) 1 <= diff <= 50\n // 4) nums is strictly increasing.\n let set = Set(nums)\n var counter = 0, max = nums.last! - diff * 2 + 1\n for n in nums {\n guard n < max else { break }\n if set.contains(n + diff) && set.contains(n + diff*2) {\n counter += 1\n }\n }\n return counter\n }\n}\n``` | 5 | 0 | [] | 1 |
number-of-arithmetic-triplets | Python easy solution for beginners using slightly optimized brute force | python-easy-solution-for-beginners-using-ova9 | This solution is slightly better than pure brute force as it calls the 3rd loop only if a condition is met.\n\n```\nclass Solution:\n def arithmeticTriplets( | elefant1805 | NORMAL | 2022-08-07T07:08:51.665627+00:00 | 2022-08-07T07:13:07.895189+00:00 | 438 | false | ##### This solution is slightly better than pure brute force as it calls the 3rd loop only if a condition is met.\n\n```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n res = 0\n for i in range(len(nums)):\n for j in range(i+1, len(nums)):\n if nums[j] - nums[i] == diff:\n for k in range(j+1, len(nums)):\n if nums[k] - nums[j] == diff:\n res += 1\n return res | 5 | 0 | ['Python', 'Python3'] | 0 |
number-of-arithmetic-triplets | C++ ✅ using SET O(n2) ✅ ✅ | c-using-set-on2-by-pratapsingha264-vob1 | class Solution {\npublic:\n int arithmeticTriplets(vector& nums, int diff) \n {\n int count=0;\n int n= nums.size();\n set s;\n | Pratapsingha264 | NORMAL | 2022-08-07T04:17:52.085132+00:00 | 2022-08-07T04:20:56.378463+00:00 | 586 | false | class Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) \n {\n int count=0;\n int n= nums.size();\n set<int> s;\n \n \n for(int i=0;i<n;i++)\n {\n s.insert(nums[i]);\n for(int j=i+1;j<n;j++)\n {\n if(nums[j]-nums[i]==diff && s.find(nums[i]-diff)!=s.end())\n count++;\n }\n }\n return count;\n\t\t}\n}; | 5 | 1 | ['C', 'Ordered Set'] | 0 |
number-of-arithmetic-triplets | SIMPLE SET COMMENTED C++ SOLUTION WITH EXPLANATION | simple-set-commented-c-solution-with-exp-wd9i | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Jeffrin2005 | NORMAL | 2024-07-18T12:39:01.981499+00:00 | 2024-07-18T12:39:01.981523+00:00 | 107 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:o(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n\n/*\nnums = [0, 1, 4, 6, 7, 10] and diff = 3.\nset = {0, 1, 4, 6, 7, 10}\nWhen i = 1 (nums[i] = 1):\nnums[i] + diff = 1 + 3 = 4 (exists in the set)\nnums[i] + 2 * diff = 1 + 6 = 7 (exists in the set)\nBoth conditions are true, so this is a valid arithmetic triplet: (1, 4, 7) corresponding to indices (1, 2, 4).\n\n\nThe condition numSet.count(nums[i] + diff) checks if the number nums[i] + diff exists in the set.\nSimilarly, numSet.count(nums[i] + 2 * diff) checks for the existence of the number nums[i] + 2 * diff.\nThe && operator ensures that both conditions must be true for the if statement to execute its body. \nThis means both nums[i] + diff and nums[i] + 2 * diff must be present in the set for the triplet to be considered valid.\n\n\n*/\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n set<int>numSet(nums.begin(), nums.end());\n int count = 0;\n for(int i = 0; i < nums.size(); i++){\n if(numSet.count(nums[i] + diff) and numSet.count(nums[i] + 2 * diff)){\n count++;\n }\n }\n \n return count;\n }\n};\n``` | 4 | 0 | ['C++'] | 0 |
number-of-arithmetic-triplets | Easiest way | easiest-way-by-nurzhansultanov-1wg5 | \n\nclass Solution(object):\n def arithmeticTriplets(self, nums, diff):\n result = []\n for i in range(len(nums)-1, 1, -1):\n for j | NurzhanSultanov | NORMAL | 2024-01-30T14:55:26.936789+00:00 | 2024-01-30T14:55:26.936824+00:00 | 174 | false | \n```\nclass Solution(object):\n def arithmeticTriplets(self, nums, diff):\n result = []\n for i in range(len(nums)-1, 1, -1):\n for j in range(i-1, 0, -1):\n for k in range(j-1, -1, -1):\n if nums[i] - nums[j] == nums[j] - nums[k] == diff:\n a = [i, j, k]\n result.append(a)\n return len(set(tuple(sorted(a)) for a in result))\n``` | 4 | 0 | ['Python'] | 1 |
number-of-arithmetic-triplets | TypeScript/JavaScript O(n) Solution | typescriptjavascript-on-solution-by-hals-xjn3 | \n# Complexity\n- Time complexity:\n Add your time complexity here, e.g. O(n) \n\n- Space complexity:\n Add your space complexity here, e.g. O(n) \n\n# Code\n\n | halshar | NORMAL | 2023-09-22T18:48:12.736640+00:00 | 2023-09-26T14:38:33.860133+00:00 | 312 | false | \n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfunction arithmeticTriplets(nums: number[], diff: number): number {\n const hash = new Set<number>();\n let ans = 0;\n\n // what we need to find are the triplets\n // let\'s say if 1,4,7 are the triplets and\n // the diff is 3, so we need to find 3 numbers\n // who have difference of 3 between them\n // let\'s assume we\'re on number 7, so we would\n // neet to find 4(num-diff) and 1(num - diff - diff)\n // if we find both the numbers then increment the count\n // else we add the current number in the hash\n for (const num of nums) {\n if (hash.has(num - diff) && hash.has(num - diff * 2)) ans++;\n hash.add(num);\n }\n return ans;\n}\n``` | 4 | 0 | ['TypeScript', 'JavaScript'] | 1 |
number-of-arithmetic-triplets | 📍||USING SET ||2 Liner ||🔥🔥🔥🔥|| OPTIMISED SOLUTION | using-set-2-liner-optimised-solution-by-jr0bn | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Sautramani | NORMAL | 2023-08-20T05:54:31.523936+00:00 | 2023-08-20T05:57:32.374694+00:00 | 802 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(N)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(N) CAUSE WE HAVE USED SET DATA STRUCTURE\n\n# Code\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n\n int count =0;\n\n // JUST CONVERTING ARRAY TO SET\n set<int>s(nums.begin(),nums.end());\n\n for(int i=0;i<s.size();i++)\n {\n if(s.find(nums[i]-diff)!=s.end() && s.find(nums[i]-2*diff)!=s.end())\n // SET .END HONE SE PEHLE (nums[i]-diff) YE VALUE SET MEIN MILI YA NAHI\n {\n count++;\n }\n } \n return count; \n }\n};\n``` | 4 | 0 | ['Array', 'Ordered Set', 'Python', 'C++', 'Java'] | 1 |
number-of-arithmetic-triplets | Go. Two pointers. Time complexity O(n) and Space complexity O(1) | go-two-pointers-time-complexity-on-and-s-trtu | Intuition\n\n##### Analyzing the problem, the following points can be emphasized:\n1. We are given an array of integers sorted in ascending order.\n1. We need t | sergei-m | NORMAL | 2023-08-01T15:54:07.222484+00:00 | 2023-08-05T23:22:40.020599+00:00 | 264 | false | # Intuition\n\n##### Analyzing the problem, the following points can be emphasized:\n1. We are given an array of integers sorted in ascending order.\n1. We need to find pairs of values that will match the condition `y - x = diff && z - y = diff`.\n1. This means that there is no need to look for a pair `z - y = diff` if we did not find `y - x = diff`.\nThe way to find the pairs is identical, so we can use the same approach to confirm the finding of the desired pair.\n\n##### From the description, I can suggest the following solutions:\n- Brute force with complexity: time $$O(n^3)$$, space $$O(1)$$\n- Using a hash map with complexity: time $$O(n)$$, space $$O(n)$$\n- Using the two pointer method with complexity: time $$O(n)$$, space $$O(1)$$\n\nPersonally, I like the option with complexity time $$O(n)$$ and space $$O(1)$$ aka the two pointer method.\nIf you want me to describe other implementations, let me know and I\'ll add them.\n\n# Approach\nMethod of two pointers, about the fact that we have pointers and we move them (masterful explanation).Usually these pointers are called `r`ight & `l`eft And that\'s what we\'re going to do!\n\nInstead of parsing the whole code, I want to focus on how we move the pointers to find all the `unique arithmetic triplets`.\n\nSince the search for the first pair of values \u200B\u200Bis no different from the search for another pair. Then, having figured out the rules for finding the first pair, we can apply them to the search for the next pair.\n\n##### We remember that we have a sorted array. Therefore, we can offer:\n\n- If the statement `diff > r - l` is true, then\nwe can assume that the value of `r` is not large enough and we need to move the right pointer `r` forward.\n- If the statement `diff < r - l` is true, then the value of `r` has become larger than necessary and must be reduced by shifting the left pointer `l` forward.\n\nPseudocode:\n```\nif diff > nums[r] - nums[l]\n r++\nelse if diff < nums[r] - nums[l]\n l++\nelse \n // we have found a pair that matches the condition\n```\n\nUsing our pseudocode, check the condition for finding the first pair:\n```\n// example\nnums[0,1,4]; diff=3\nl = 0; r = 1\n\n// The first check will shift the right pointer\nif diff > nums[r] - nums[l] // 3 > 1\n r++ // r = 2\n\n// The second check will shift the left pointer\nif diff < nums[r] - nums[l] // 3 < 4\n l++ // l = 1\n\n// The last check will fulfill the condition\nif diff == nums[r] - nums[l] // 3 == 3\n // we can start looking for the next pair that matches the condition\n```\n\nAs you can see, we found the first pair in O(n). And since the further search will continue from the current pointers, the time complexity will remain $$O(n)$$.\n\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\nThis algorithm can be better, if you know how, then let me know, I\'ll take it into account with pleasure.\n\n```\nfunc arithmeticTriplets(nums []int, diff int) int {\n triplets := 0\n i, j, k := 0, 1, 2\n\n for i < len(nums) - 2 && j < len(nums) - 1 && k < len(nums) {\n // Search for the first pair. Left pointer = i, right pointer = j\n if !foundPair(diff, &i, &j, &nums) {\n continue\n }\n // We found the first pair\n\n // Search for the second pair. Left pointer = j, right pointer = k\n if !foundPair(diff, &j, &k, &nums) {\n continue\n }\n // We found the second pair\n\n // Which means we can move the pointers to the right and increase the value of triplets\n i++\n j++\n k++\n triplets++\n } \n\n return triplets\n}\n\nfunc foundPair(diff int, l, r *int, nums *[]int) bool {\n value := (*nums)[*r] - (*nums)[*l]\n\n if diff > value {\n *r++\n\n return false\n }\n\n if diff < value {\n *l++\n\n return false\n }\n\n return true\n}\n```\n | 4 | 0 | ['Array', 'Two Pointers', 'Go'] | 0 |
number-of-arithmetic-triplets | FASTEST solution with JAVA(1ms) | fastest-solution-with-java1ms-by-amin_az-ygj2 | Code\n\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count = 0; \n Set<Integer> list = new HashSet<>();\n | amin_aziz | NORMAL | 2023-04-12T09:05:48.679776+00:00 | 2023-04-12T18:07:18.237541+00:00 | 1,893 | false | # Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count = 0; \n Set<Integer> list = new HashSet<>();\n for(int a : nums){\n if(list.contains(a-diff) && list.contains(a-diff*2)) count++;\n list.add(a);\n }\n return count;\n }\n}\n``` | 4 | 0 | ['Java'] | 3 |
number-of-arithmetic-triplets | C++ easy solution with loops. | c-easy-solution-with-loops-by-aloneguy-e06b | \n# Complexity\n- Time complexity: 6 ms.Beats 65.9% solutions.\n Add your time complexity here, e.g. O(n) \n\n- Space complexity:8.6 Mb.Beats 88.98% solutions.\ | aloneguy | NORMAL | 2023-03-23T07:03:21.747706+00:00 | 2023-03-23T07:03:21.747748+00:00 | 454 | false | \n# Complexity\n- Time complexity: 6 ms.Beats 65.9% solutions.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:8.6 Mb.Beats 88.98% solutions.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count=0;\n for(int i=0;i<nums.size()-2;i++)\n for(int j=i+1;j<nums.size()-1;j++){\n if(nums[j]-nums[i]==diff){\n for(int k=j+1;k<nums.size();k++){\n if(nums[j]-nums[i]==diff and nums[k]-nums[j]==diff)\n count++;\n }\n }\n }\n return count;\n }\n};\n``` | 4 | 0 | ['C++'] | 0 |
number-of-arithmetic-triplets | C# easy solution. | c-easy-solution-by-aloneguy-vux7 | \n# Complexity\n- Time complexity: 89 ms.Beats 66.24% of other solutions.\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: 38.1 Mb.Beats 85.99 | aloneguy | NORMAL | 2023-03-23T06:57:44.832885+00:00 | 2023-03-23T06:57:44.832925+00:00 | 373 | false | \n# Complexity\n- Time complexity: 89 ms.Beats 66.24% of other solutions.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: 38.1 Mb.Beats 85.99% of others.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\npublic class Solution {\n public int count=0;\n public int ArithmeticTriplets(int[] nums, int diff) {\n for(int i=0;i<nums.Length-2;i++)\n for(int j=i+1;j<nums.Length-1;j++){\n if(nums[j]-nums[i]==diff){\n for(int k=j+1;k<nums.Length;k++){\n if(nums[k]-nums[j]==diff)\n count++;\n }\n }\n }\n return count;\n }\n}\n``` | 4 | 0 | ['Math', 'C#'] | 0 |
number-of-arithmetic-triplets | java solution using HashSet !! | java-solution-using-hashset-by-aanshi_07-kg9o | \n# Code\n\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n Set<Integer> set = new HashSet<>();\n for(int n: nums){ | aanshi_07 | NORMAL | 2022-12-10T10:14:36.778815+00:00 | 2022-12-10T10:18:10.855162+00:00 | 797 | false | \n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n Set<Integer> set = new HashSet<>();\n for(int n: nums){\n set.add(n);\n }\n int count =0;\n for(int n:nums){\n if(set.contains(n+diff) && set.contains(n+(2*diff)))\n count++;\n }\n return count;\n }\n}\n``` | 4 | 0 | ['Hash Table', 'Java'] | 3 |
number-of-arithmetic-triplets | 100% Faster || TypeScript || Easy to understand | 100-faster-typescript-easy-to-understand-4btn | Let me know in comments if you have any doubts. I will be happy to answer.\nPlease upvote if you found the solution useful.\n\n\nconst arithmeticTriplets = (num | viniciusteixeiradias | NORMAL | 2022-11-14T02:36:28.105429+00:00 | 2022-11-16T01:48:43.163198+00:00 | 106 | false | Let me know in comments if you have any doubts. I will be happy to answer.\nPlease upvote if you found the solution useful.\n\n```\nconst arithmeticTriplets = (nums: number[], diff: number): number => {\n let sum = 0;\n let hash = new Set();\n \n for(let i = 0; i < nums.length; i++) {\n if (hash.has(nums[i] - diff) && hash.has(nums[i] - diff - diff)) {\n sum++;\n }\n \n hash.add(nums[i]);\n }\n \n return sum;\n};\n``` | 4 | 0 | ['TypeScript'] | 0 |
number-of-arithmetic-triplets | C++ Naive Approach Solution | c-naive-approach-solution-by-king_of_kin-ugy0 | int arithmeticTriplets(vector& nums, int diff) {\n\n int ans = 0 ;\n for(int i = 0 ; i < nums.size()-2 ; i++)\n {\n for(int j = | King_of_Kings101 | NORMAL | 2022-09-23T07:03:31.293751+00:00 | 2022-09-23T07:06:36.055976+00:00 | 623 | false | int arithmeticTriplets(vector<int>& nums, int diff) {\n\n int ans = 0 ;\n for(int i = 0 ; i < nums.size()-2 ; i++)\n {\n for(int j = i+1 ; j < nums.size()-1 ; j++)\n {\n if(nums[i]+diff==nums[j])\n {\n for(int k = j +1; k < nums.size(); k++)\n {\n if(nums[j]+diff==nums[k])\n ans++;\n }\n }\n }\n }\n return ans;\n } | 4 | 0 | ['Array', 'C', 'C++'] | 0 |
number-of-arithmetic-triplets | C Sharp Solution | c-sharp-solution-by-fadydev123-3n1c | \nint count = 0;\nHashSet<int> hash_num = new HashSet<int>();\nforeach(int num in nums)\n{\n\tif(hash_num.Contains(num - diff) && hash_num.Contains(num - 2 * di | fadydev123 | NORMAL | 2022-09-11T07:08:23.635368+00:00 | 2022-09-11T07:08:39.984015+00:00 | 271 | false | ```\nint count = 0;\nHashSet<int> hash_num = new HashSet<int>();\nforeach(int num in nums)\n{\n\tif(hash_num.Contains(num - diff) && hash_num.Contains(num - 2 * diff))\n\t\tcount++;\n\thash_num.Add(num); \n}\nreturn count;\n\n``` | 4 | 0 | ['C#'] | 0 |
number-of-arithmetic-triplets | JavaScript | Hashmap solution | O(n) | javascript-hashmap-solution-on-by-zhe1ka-megg | ```\nfunction arithmeticTriplets(nums: number[], diff: number): number {\n let sum = 0;\n let hash = new Set();\n \n for (let numIndex = 0; numIndex | Zhe1ka | NORMAL | 2022-08-29T06:34:27.142829+00:00 | 2022-08-29T06:34:27.142854+00:00 | 533 | false | ```\nfunction arithmeticTriplets(nums: number[], diff: number): number {\n let sum = 0;\n let hash = new Set();\n \n for (let numIndex = 0; numIndex < nums.length; numIndex++) {\n const currentNum = nums[numIndex];\n \n if (hash.has(currentNum - diff) && hash.has(currentNum - diff - diff)) {\n sum++;\n }\n \n hash.add(currentNum);\n }\n \n return sum;\n}; | 4 | 0 | ['TypeScript', 'JavaScript'] | 0 |
number-of-arithmetic-triplets | Three Pointers, one pass O(n) time and O(1) space | three-pointers-one-pass-on-time-and-o1-s-osqq | Intuition"Strictly increasing" is the key.Complexity
Time complexity:O(n)
Space complexity:O(1)
Code | SoulMan | NORMAL | 2025-02-09T08:55:22.860188+00:00 | 2025-02-19T09:10:59.236542+00:00 | 227 | false | # Intuition
"Strictly increasing" is the key.
<!-- Describe your first thoughts on how to solve this problem. -->
# Complexity
- Time complexity: $$O(n)$$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: $$O(1)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int arithmeticTriplets(vector<int>& nums, int diff) {
int l = 0, r = 2, ans = 0;
for(int i=1; i<nums.size()-1; i++){
while(nums[i] - nums[l] > diff) l++;
while(r < nums.size()-1 && nums[r] - nums[i] < diff) r++;
if(nums[i] - nums[l] == diff && nums[r] - nums[i] == diff) ans++;
}
return ans;
}
};
```
```java []
class Solution {
public int arithmeticTriplets(int[] nums, int diff) {
int l = 0, r = 2, ans = 0;
for(int i=1; i<nums.length-1; i++){
while(nums[i] - nums[l] > diff) l++;
while(r < nums.length-1 && nums[r] - nums[i] < diff) r++;
if(nums[i] - nums[l] == diff && nums[r] - nums[i] == diff) ans++;
}
return ans;
}
}
``` | 3 | 0 | ['C++', 'Java'] | 0 |
number-of-arithmetic-triplets | 💢☠💫Easiest👾Faster✅💯 Lesser🧠 🎯 C++✅Python3🐍✅Java✅C✅Python🐍✅C#✅💥🔥💫Explained☠💥🔥 Beats 💯 | easiestfaster-lesser-cpython3javacpython-vnsh | Intuition\n\n\nThese are the almost same questions which are similar to this one which are as follows :-\n\n---\nQ - 1460 --> https://leetcode.com/problems/make | Edwards310 | NORMAL | 2024-07-26T13:19:02.822977+00:00 | 2024-07-26T14:15:15.201707+00:00 | 357 | false | # Intuition\n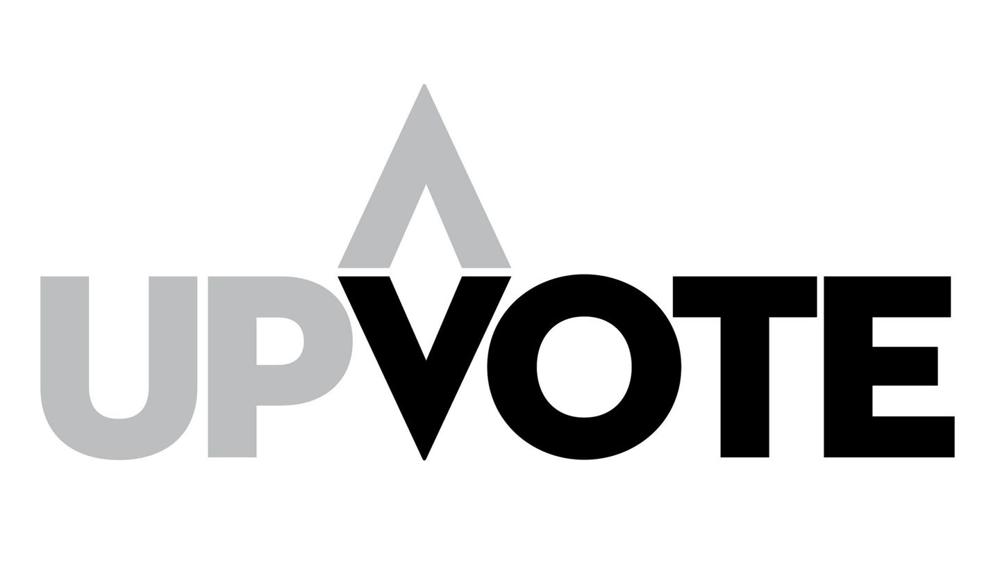\n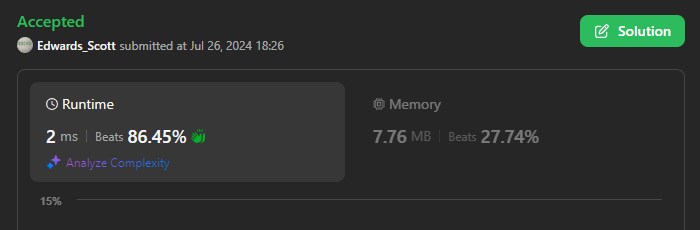\nThese are the almost same questions which are similar to this one which are as follows :-\n\n---\n***Q - 1460 -->*** https://leetcode.com/problems/make-two-arrays-equal-by-reversing-subarrays/solutions/5538697/easiestfaster-lesser-cpython3javacpythoncexplained-beats\n\n***Q - 1534 -->*** https://leetcode.com/problems/count-good-triplets/solutions/5538845/easiestfaster-lesser-cpython3javacpythoncexplained-beats\n\n***Q - 2475-->*** https://leetcode.com/problems/number-of-unequal-triplets-in-array/solutions/5538953/easiestfaster-lesser-cpython3javacpythoncexplained-beats\n\n***Q - 2908 -->*** https://leetcode.com/problems/minimum-sum-of-mountain-triplets-i/solutions/5539207/easiestfaster-lesser-cpython3javacpythoncexplained-beats\n\n\n---\n\n\n```C++ []\n// Start Of Anuj Bhati aka Edwards310\'s Template...\n#pragma GCC optimize("O2")\n#include <bits/stdc++.h>\nusing namespace std;\n\n\n#define FOR(a, b, c) for (int a = b; a < c; a++)\n#define FOR1(a, b, c) for (int a = b; a <= c; ++a)\n#define Rep(i, n) FOR(i, 0, n)\n\n// end of Anuj Bhati\'s aka Edwards310\'s Template\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int n = nums.size();\n int cnt = 0;\n Rep(i, n - 2){\n FOR(j, i + 1, n - 1){\n if(nums[j] - nums[i] == diff){\n FOR(k, j + 1, n){\n if(nums[k] - nums[j] == diff)\n ++cnt;\n }\n }\n }\n }\n return cnt;\n }\n};\n```\n```python3 []\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n n = len(nums)\n cnt = 0\n for i in range(n - 2):\n for j in range(i + 1, n - 1):\n if nums[j] - nums[i] == diff:\n for k in range(j + 1, n):\n if nums[k] - nums[j] == diff:\n cnt += 1\n \n return cnt\n```\n```C# []\npublic class Solution {\n public int ArithmeticTriplets(int[] nums, int diff) {\n int n = nums.Length;\n int cnt = 0;\n for(int i = 0; i < n - 2; ++i)\n for(int j = i + 1; j < n - 1; ++j)\n if(nums[j] - nums[i] == diff)\n for(int k = j + 1; k < n; k++)\n if(nums[k] - nums[j] == diff)\n ++cnt;\n \n return cnt;\n }\n}\n```\n```Java []\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int n = nums.length;\n int cnt = 0;\n for(int i = 0; i < n - 2; ++i)\n for(int j = i + 1; j < n - 1; ++j)\n if(nums[j] - nums[i] == diff)\n for(int k = j + 1; k < n; k++)\n if(nums[k] - nums[j] == diff)\n ++cnt;\n \n return cnt;\n }\n}\n```\n```python []\nclass Solution(object):\n def arithmeticTriplets(self, nums, diff):\n """\n :type nums: List[int]\n :type diff: int\n :rtype: int\n """\n n = len(nums)\n cnt = 0\n for i in range(n - 2):\n for j in range(i + 1, n - 1):\n if nums[j] - nums[i] == diff:\n for k in range(j + 1, n):\n if nums[k] - nums[j] == diff:\n cnt += 1\n \n return cnt\n```\n```C []\nint arithmeticTriplets(int* nums, int numsSize, int diff) {\n int n = numsSize;\n int cnt = 0;\n for (int i = 0; i < n - 2; ++i)\n for (int j = i + 1; j < n - 1; ++j)\n if (nums[j] - nums[i] == diff)\n for (int k = j + 1; k < n; k++)\n if (nums[k] - nums[j] == diff)\n ++cnt;\n\n return cnt;\n}\n```\n\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(N^3)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(1)\n# Code\n```\nint arithmeticTriplets(int* nums, int numsSize, int diff) {\n int n = numsSize;\n int cnt = 0;\n for (int i = 0; i < n - 2; ++i)\n for (int j = i + 1; j < n - 1; ++j)\n if (nums[j] - nums[i] == diff)\n for (int k = j + 1; k < n; k++)\n if (nums[k] - nums[j] == diff)\n ++cnt;\n\n return cnt;\n}\n```\n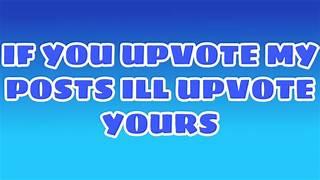\n | 3 | 0 | ['Array', 'Hash Table', 'Two Pointers', 'C', 'Enumeration', 'Python', 'C++', 'Java', 'Python3', 'C#'] | 0 |
number-of-arithmetic-triplets | Easy to understand - Java | easy-to-understand-java-by-ungureanu_ovi-o5g5 | Upvote if you like the solution\n\n# Intuition\n\nThe problem requires finding unique arithmetic triplets in an array, where the difference between consecutive | Ungureanu_Ovidiu | NORMAL | 2024-07-02T10:09:52.126395+00:00 | 2024-07-02T10:09:52.126431+00:00 | 142 | false | ##### Upvote if you like the solution\n\n# Intuition\n\nThe problem requires finding unique arithmetic triplets in an array, where the difference between consecutive elements in the triplet is a given value `diff`.\n\n## Approach\n\n1. **Set for Lookup**:\n - Use a `HashSet` called `presentNums` to store all the integers from the array `nums`. This allows for O(1) average time complexity for lookups.\n - Traverse the array `nums` and add each element to the `HashSet`.\n\n2. **Finding Triplets**:\n - Initialize a counter `result` to zero to keep track of the number of valid triplets.\n - Iterate through the `HashSet`:\n - For each element `key` in the `HashSet`, check if `key + diff` and `key + 2 * diff` are also in the `HashSet`.\n - If both are present, increment the `result` counter.\n\n3. **Return**:\n - Return the `result` counter, which represents the number of unique arithmetic triplets in the array.\n\n\n## Complexity\n\n- **Time Complexity**: O(n), where n is the length of the array `nums`. This includes the time to add elements to the `HashSet` and the time to check for triplets.\n- **Space Complexity**: O(n), where n is the length of the array `nums`. This space is used by the `HashSet`.\n\n## Code\n\n```Java\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n Set<Integer> presentNums = new HashSet<>();\n int i, result = 0;\n\n for(i = 0 ; i < nums.length; i = i + 1) {\n presentNums.add(nums[i]);\n }\n\n for(Integer key: presentNums) {\n if(presentNums.contains(key + diff) && presentNums.contains(key + diff + diff)) {\n result++;\n }\n }\n\n return result;\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
number-of-arithmetic-triplets | Easy solution java | easy-solution-java-by-abhinav29code-zoz2 | \n\n# Code\n\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n if(nums.length < 3) return 0;\n\n int count = 0;\n | abhinav29code | NORMAL | 2023-12-26T04:22:39.315944+00:00 | 2023-12-26T04:22:39.315983+00:00 | 364 | false | \n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n if(nums.length < 3) return 0;\n\n int count = 0;\n HashSet<Integer> set = new HashSet<>();\n for(int num : nums) {\n if(set.contains(num - diff) && set.contains(num - diff*2)) {\n count++;\n }\n set.add(num);\n }\n return count;\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
number-of-arithmetic-triplets | Easy to understand for beginner using "HashMap" | easy-to-understand-for-beginner-using-ha-2u6h | Approach\n\nfirst of all you should be aware about hashmap and its functions.\nOne such function which I have used is "containsKey" it return true if a key is p | Priyansh_max | NORMAL | 2023-12-17T15:44:08.631449+00:00 | 2023-12-17T15:44:08.631470+00:00 | 387 | false | # Approach\n\nfirst of all you should be aware about hashmap and its functions.\nOne such function which I have used is "containsKey" it return true if a key is present in the hashmap that you have inputed.\n\n1 --> creating a hashmap\n2 --> create a <key , value> pair \n\n3 --> check if "diff + current index is present" and (diff + current index) + diff\n\nif yes increase the count and return count at the end \n\nlets understand with a test case\n\nInput: nums = [0,1,4,6,7,10], diff = 3\n\nhere 1 , 4, 7 is one of the solution \n\nhashmap has <key : value> pair as\n[0,0] , [1,1] , [4,2] , [6,3] , [7,4] , [10,5] \nkey is nums[i]\nvalue is index "i"\n\nthen we check:\nlike for an existing pair 1 , 4 , 7 we run the test case\niteration 1\ni = 0 \nwe diff + nums[0] = 3 + 0 = 3 we dont have 3 as key present in the hashmap\n\niteration 2\ni = 1\ndiff + nums[1] = 3 + 1 = 4 we have 4 as a key i.e [4,2]\ntherefore we check again \n(diff + nums[i]) + diff --> (3+1)+3 = 7 we have 7 also present a key \ni.e [7,4]\n\ntherefore we increase the count\n\nthis way this algorithm works... :)\n\nhope you got it....\n\n\n\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n\n HashMap<Integer , Integer> hashmap = new HashMap<>();\n\n int count = 0;\n\n for(int i = 0 ; i < nums.length ; i++){\n hashmap.put(nums[i] , i);\n }\n\n for(int j = 0 ; j < nums.length ; j++){\n if(hashmap.containsKey(diff + nums[j]) && hashmap.containsKey((diff + nums[j])+diff)){\n count++;\n\n }\n }\n\n return count;\n \n }\n}\n``` | 3 | 0 | ['Java'] | 2 |
number-of-arithmetic-triplets | Better than nested loops. | better-than-nested-loops-by-drugged_monk-c7i3 | Intuition\nProblem should be solved in one loop.\n\n# Approach\nFirst I convert array nums to HashSet. Then I traverse array nums to find every triplet nums[i], | Drugged_Monkey | NORMAL | 2023-12-01T20:20:53.173227+00:00 | 2024-06-08T15:09:40.389792+00:00 | 102 | false | # Intuition\nProblem should be solved in one loop.\n\n# Approach\nFirst I convert array `nums` to `HashSet`. Then I traverse array `nums` to find every triplet `nums[i]`, `nums[i] - diff` and `nums[i] + diff`. That\'s it.\n\n# Complexity\n- Time complexity:\n$$O(n)$$. Despite `HashSet.Contains()` implementation isn\'t realy $$O(1)$$ it\'s way faster than nested loop to search `2nd` and another nested loop to search `3rd` triplet\'s members.\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\npublic sealed class Solution \n{\n public int ArithmeticTriplets(int[] nums, int diff) \n {\n HashSet<int> s = new HashSet<int>(nums);\n\n int r = 0;\n\n for(int i = 1; i < nums.Length - 1; i++)\n {\n if(s.Contains(nums[i] - diff) && s.Contains(nums[i] + diff))\n {\n r++;\n }\n }\n\n return r;\n }\n}\n``` | 3 | 0 | ['C#'] | 2 |
number-of-arithmetic-triplets | ✅Iteratively||🔥Simple Solution 🔥|| JAVA || C++|| Python || 🚀Clean Codes🚀 ||Easy to Understand✅✅ | iterativelysimple-solution-java-c-python-xpng | \n# Approach\n Describe your approach to solving the problem. \nHere\'s a breakdown of the code:\n\n1. int ans = 0;: This initializes a variable ans to store th | preans1412 | NORMAL | 2023-08-11T16:47:23.178415+00:00 | 2023-08-11T16:47:23.178434+00:00 | 497 | false | \n# Approach\n<!-- Describe your approach to solving the problem. -->\nHere\'s a breakdown of the code:\n\n1. int ans = 0;: This initializes a variable ans to store the count of arithmetic triplets found in the vector.\n\n2. The code uses three nested for loops to iterate through all possible combinations of three indices i, j, and k within the nums vector.\n3. The outermost loop (i) iterates from the beginning of the vector (0) up to the third-to-last element (nums.size() - 2). This is because the code is looking for triplets, and the last two elements can\'t form a triplet with any other element.\n4. The middle loop (j) iterates from the next index after i (i + 1) up to the second-to-last element (nums.size() - 1). This ensures that j is always ahead of i.\n5. The innermost loop (k) iterates from the index after j (j + 1) up to the last element (nums.size()). This ensures that k is always ahead of both i and j.\n6. The code checks whether the differences between nums[j] and nums[i] and between nums[k] and nums[j] are both equal to the given diff. If this condition is met, it means that the current combination of i, j, and k forms an arithmetic triplet with the specified difference.\n7. If the condition in step 3 is satisfied, the ans variable is incremented, indicating that a valid arithmetic triplet has been found.\n8. After all possible combinations have been checked, the function returns the final value of ans, which represents the total count of arithmetic triplets found in the nums vector.\n\n\n\n\n\n\n\n\n# Complexity\n- Time complexity:$$O(n^3)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n`However, it\'s important to note that this code has a time complexity of O(n^3), where n is the size of the nums vector. This is because it uses three nested loops, resulting in a cubic time complexity, which could be quite slow for larger input vectors. There are more efficient algorithms to solve this problem with better time complexity.`\n\n\n\n```C++ []\nclass Solution \n{\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) \n {\n int ans=0;\n for(int i=0;i<nums.size()-2;i++)\n {\n for(int j=i+1;j<nums.size()-1;j++)\n {\n for(int k=j+1;k<nums.size();k++)\n {\n if((nums[j]-nums[i]==diff) && (nums[k]-nums[j]==diff))\n {\n ans++;\n }\n }\n }\n }\n return ans;\n }\n};\n```\n```JAVA []\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) \n {\n int ans=0;\n for(int i=0;i<nums.length-2;i++)\n {\n for(int j=i+1;j<nums.length-1;j++)\n {\n for(int k=j+1;k<nums.length;k++)\n {\n if((nums[j]-nums[i]==diff) && (nums[k]-nums[j]==diff))\n ans++;\n }\n }\n } \n \n return ans;\n }\n\n}\n```\n```Python []\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n ans=0\n for i in range(len(nums)):\n for j in range(len(nums)):\n for k in range(len(nums)):\n if i<j and j<k and nums[j]-nums[i]==diff and nums[k]-nums[j]==diff:\n ans+=1 \n #in python, ans++ don\'t work\n return ans\n\n```\n | 3 | 0 | ['Array', 'Python', 'C++', 'Java', 'Python3'] | 1 |
number-of-arithmetic-triplets | Easy C++ Solution | easy-c-solution-by-adityagarg217-wmqq | class Solution {\npublic:\n\n int arithmeticTriplets(vector& nums, int diff) {\n int ans = 0 ;\n \n for(int i=0 ;i<nums.size()-2 ;i++){\n | adityagarg217 | NORMAL | 2023-06-04T10:39:36.839663+00:00 | 2023-06-05T11:49:43.064449+00:00 | 1,475 | false | class Solution {\npublic:\n\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int ans = 0 ;\n \n for(int i=0 ;i<nums.size()-2 ;i++){\n int count = 0 ;\n for(int j=i+1 ; j<nums.size() ;j++){\n \n if(nums[j]-nums[i]==diff || nums[j]-nums[i]==2*diff){\n count++;\n }\n }\n if(count==2){\n ans++;\n }\n }\n return ans ;\n }\n}; | 3 | 0 | ['Array', 'C'] | 0 |
number-of-arithmetic-triplets | [Java] Easy set solution 100% O(n) | java-easy-set-solution-100-on-by-ytchoua-mu5m | java\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n Set<Integer> set = new HashSet<>();\n int i = 0, k = 1, result | YTchouar | NORMAL | 2023-04-01T07:04:11.285197+00:00 | 2023-04-01T07:04:25.892014+00:00 | 2,685 | false | ```java\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n Set<Integer> set = new HashSet<>();\n int i = 0, k = 1, result = 0;\n\n for(int num : nums)\n set.add(num);\n\n while(k < nums.length) {\n if(nums[k] - nums[i] == 2 * diff && set.contains(diff + nums[i]))\n ++result;\n \n if(nums[k] - nums[i] < 2 * diff)\n ++k;\n else\n ++i;\n }\n\n return result;\n }\n}\n\n// nums[j] - nums[i] == diff\n// nums[k] - nums[j] == diff\n// num[j] = diff + num[i]\n// num[j] = num[k] - diff\n// diff + num[i] = num[k] - diff\n// 2diff = num[k] - num[i]\n``` | 3 | 0 | ['Java'] | 3 |
number-of-arithmetic-triplets | single pass | single-pass-by-rajsiddi-r2ed | \n\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int trip = 0;\n for(int i=0;i<nums.size();i++){\n | rajsiddi | NORMAL | 2023-03-05T09:35:59.011537+00:00 | 2023-03-05T09:44:41.552036+00:00 | 1,060 | false | \n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int trip = 0;\n for(int i=0;i<nums.size();i++){\n int find = nums[i]+diff;\n if(count(nums.begin(),nums.end(),find)>0){\n int find2 = find+diff;\n if(count(nums.begin(),nums.end(),find2)>0){\ntrip++;\n }\n }\n }\n return trip;\n }\n};\n``` | 3 | 0 | ['C++'] | 1 |
number-of-arithmetic-triplets | Runtime 52 ms Beats 57.18% Memory 13.9 MB Beats 55.96%, beginner easy to understand | runtime-52-ms-beats-5718-memory-139-mb-b-xzlv | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | NEWYORK2009 | NORMAL | 2023-02-09T17:48:08.180089+00:00 | 2023-02-09T17:48:08.180144+00:00 | 697 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n res = 0\n\n for i in range(len(nums)):\n if nums[i] +diff in nums and nums[i]+diff*2 in nums:\n res+=1\n\n return res\n``` | 3 | 0 | ['Python3'] | 1 |
number-of-arithmetic-triplets | Beats 97.36% || Number of Arithmetic Triplets | beats-9736-number-of-arithmetic-triplets-9x8p | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | himshrivas | NORMAL | 2023-01-29T17:23:05.418261+00:00 | 2023-01-29T17:23:05.418307+00:00 | 1,779 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n count=0\n for i in range(len(nums)):\n for j in range(len(nums)):\n for k in range(len(nums)):\n if i<j and j<k and nums[j]-nums[i]==diff and nums[k]-nums[j]==diff:\n count+=1\n return count\n\n``` | 3 | 0 | ['Python3'] | 4 |
number-of-arithmetic-triplets | O(N) Set + reduce solution | on-set-reduce-solution-by-ods967-xhzm | Code\n\n/**\n * @param {number[]} nums\n * @param {number} diff\n * @return {number}\n */\nvar arithmeticTriplets = function(nums, diff) {\n const set = new | ods967 | NORMAL | 2023-01-05T06:52:29.348880+00:00 | 2023-01-05T06:52:29.348926+00:00 | 417 | false | # Code\n```\n/**\n * @param {number[]} nums\n * @param {number} diff\n * @return {number}\n */\nvar arithmeticTriplets = function(nums, diff) {\n const set = new Set();\n\n return nums.reduce((acc, num) => {\n set.add(num);\n\n if (set.has(num - diff) && set.has(num - 2 * diff)) {\n return acc + 1;\n }\n\n return acc;\n }, 0);\n};\n``` | 3 | 0 | ['JavaScript'] | 0 |
number-of-arithmetic-triplets | 3 loops || JAVA || Easy to understand | 3-loops-java-easy-to-understand-by-im_ob-0iqq | Intuition\n\nDon\'t forget to upvote if you found it useful\n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time complexity:\ | im_obid | NORMAL | 2022-11-21T08:58:00.843601+00:00 | 2022-11-21T08:58:00.843633+00:00 | 1,012 | false | # Intuition\n\nDon\'t forget to upvote if you found it useful\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\nO(n^3)\n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count=0;\n for(int i=0;i<nums.length;i++){\n for(int j=i+1;j<nums.length;j++){\n if(nums[j]-nums[i]==diff){\n for(int k=j+1;k<nums.length;k++){\n if(nums[k]-nums[j]==diff)\n count++;\n }\n }\n }\n }\n return count;\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
number-of-arithmetic-triplets | O(n) Solution | Java | on-solution-java-by-pawan300-7681 | ```\n public int arithmeticTriplets(int[] nums, int diff) {\n int n = nums.length;\n int res =0;\n boolean arr[] = new boolean[nums[n-1]+di | pawan300 | NORMAL | 2022-09-30T18:09:30.960908+00:00 | 2022-09-30T18:09:30.960941+00:00 | 369 | false | ```\n public int arithmeticTriplets(int[] nums, int diff) {\n int n = nums.length;\n int res =0;\n boolean arr[] = new boolean[nums[n-1]+diff+1];\n for(int num:nums){\n arr[num]= true;\n }\n for(int i=0;i<n-2;i++){\n int val = nums[i];\n int val2 = val+ diff;\n int val3 = val2+diff;\n if(arr[val2] && arr[val3]){\n res++;\n }\n }\n return res; \n }\n} | 3 | 0 | ['Java'] | 0 |
number-of-arithmetic-triplets | First SC - O(1) Solution | TC - O(n) | CPP, C, JAVA | first-sc-o1-solution-tc-on-cpp-c-java-by-plt8 | Please upvote If you like\n\nCPP\n\n\tunsigned short int count = 0,\n\ti = 0,\n\tj = i + 1,\n\tk = j + 1;\n\twhile (i < nums.size() && j < nums.size() && k < nu | rushil12 | NORMAL | 2022-09-13T03:41:59.724695+00:00 | 2022-09-15T03:59:31.908930+00:00 | 112 | false | **Please upvote If you like**\n\n**CPP**\n\n\tunsigned short int count = 0,\n\ti = 0,\n\tj = i + 1,\n\tk = j + 1;\n\twhile (i < nums.size() && j < nums.size() && k < nums.size()) {\n\n\t\tif (nums[j] - nums[i] == diff && nums[k] - nums[j] == diff) {\n\t\t\tcount++;\n\t\t\ti++;\n\t\t\tj++;\n\t\t\tk++;\n\t\t\tcontinue;\n\t\t}\n\n\t\tif (i == j) {\n\t\t\tj++;\n\t\t} else if (nums[j] - nums[i] < diff) {\n\t\t\tj++;\n\t\t} else if (nums[j] - nums[i] > diff) {\n\t\t\ti++;\n\t\t} else if (j == k) {\n\t\t\tk++;\n\t\t} else if (nums[k] - nums[j] < diff) {\n\t\t\tk++;\n\t\t} else if (nums[k] - nums[j] > diff) {\n\t\t\tj++;\n\t\t}\n\t}\n\n\treturn count;\n\n\n\n**Java**\n\n\n\tint count = 0,\n\ti = 0,\n\tj = i + 1,\n\tk = j + 1;\n\twhile (i < nums.length && j < nums.length && k < nums.length) {\n\n\t\tif (nums[j] - nums[i] == diff && nums[k] - nums[j] == diff) {\n\t\t\tcount++;\n\t\t\ti++;\n\t\t\tj++;\n\t\t\tk++;\n\t\t\tcontinue;\n\t\t}\n\n\t\tif (i == j) {\n\t\t\tj++;\n\t\t} else if (nums[j] - nums[i] < diff) {\n\t\t\tj++;\n\t\t} else if (nums[j] - nums[i] > diff) {\n\t\t\ti++;\n\t\t} else if (j == k) {\n\t\t\tk++;\n\t\t} else if (nums[k] - nums[j] < diff) {\n\t\t\tk++;\n\t\t} else if (nums[k] - nums[j] > diff) {\n\t\t\tj++;\n\t\t}\n\t}\n\n\treturn count;\n\t\n\t\n**C**\n\n\tunsigned short int count = 0,\n\ti = 0,\n\tj = i + 1,\n\tk = j + 1;\n\twhile (i < numsSize && j < numsSize && k < numsSize) {\n\n\t\tif (nums[j] - nums[i] == diff && nums[k] - nums[j] == diff) {\n\t\t\tcount++;\n\t\t\ti++;\n\t\t\tj++;\n\t\t\tk++;\n\t\t\tcontinue;\n\t\t}\n\n\t\tif (i == j) {\n\t\t\tj++;\n\t\t} else if (nums[j] - nums[i] < diff) {\n\t\t\tj++;\n\t\t} else if (nums[j] - nums[i] > diff) {\n\t\t\ti++;\n\t\t} else if (j == k) {\n\t\t\tk++;\n\t\t} else if (nums[k] - nums[j] < diff) {\n\t\t\tk++;\n\t\t} else if (nums[k] - nums[j] > diff) {\n\t\t\tj++;\n\t\t}\n\t}\n\n\treturn count; | 3 | 0 | [] | 0 |
number-of-arithmetic-triplets | [Java] Most easiest solution with explanation. | java-most-easiest-solution-with-explanat-trle | //This is a two pointer simple approach. Take a variable count to keep a count of the triplets.\n//initialiaze i=0; and then take a while loop which will run ti | vishady7 | NORMAL | 2022-08-20T19:53:29.879137+00:00 | 2022-08-20T19:53:29.879169+00:00 | 520 | false | //This is a two pointer simple approach. Take a variable count to keep a count of the triplets.\n//initialiaze i=0; and then take a while loop which will run till i is less than nums.length-2.\n//inside this while loop take two variable j = i+1 and k = j+1;\n\n\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count = 0;\n int i=0;\n \n while(i<nums.length-2){\n int j = i+1, k=j+1;\n \n\t\t //run the loop till the element on j gives the required difference.\n while(j<nums.length-1 && nums[j]-nums[i]<diff){\n j++;\n }\n //run the loop till the element on k gives the required difference.\n while(k<nums.length && nums[k]-nums[j]<diff){\n k++;\n }\n\t\t\t\n\t\t\t//now if all i,j and k satisfy the requirement increase count and also the value of i.\n if(j<nums.length-1 && k<nums.length && nums[j]-nums[i]==diff && nums[k]-nums[j]==diff){\n count++;\n i++;\n }\n\t\t\t// if all the above conditions are false then just increase i.\n else{\n i++;\n }\n }\n \n // return count to get the answer.\n return count;\n }\n} | 3 | 0 | ['Two Pointers', 'Java'] | 0 |
number-of-arithmetic-triplets | ✅✅Faster || Easy To Understand || C++ Code | faster-easy-to-understand-c-code-by-__kr-qbb3 | Using Unordered Set\n\n Time Complexity :- O(N)\n\n Space Complexity :- O(N)\n\n\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int d | __KR_SHANU_IITG | NORMAL | 2022-08-17T07:10:16.977521+00:00 | 2022-08-17T07:10:16.977552+00:00 | 399 | false | * ***Using Unordered Set***\n\n* ***Time Complexity :- O(N)***\n\n* ***Space Complexity :- O(N)***\n\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n \n int n = nums.size();\n \n // insert all the elements into the set\n \n unordered_set<int> s;\n \n for(int i = 0; i < n; i++)\n {\n s.insert(nums[i]);\n }\n \n int count = 0;\n \n // iterate over array\n \n for(int i = 0; i < n - 2; i++)\n {\n int a = nums[i];\n \n int b = nums[i] + diff;\n \n int c = nums[i] + 2 * diff;\n \n // if b, c are present in set then sequence is found\n \n if(s.count(b) && s.count(c))\n count++;\n }\n \n return count;\n }\n};\n``` | 3 | 0 | ['C', 'C++'] | 1 |
number-of-arithmetic-triplets | C++ 0ms Faster than 100% | c-0ms-faster-than-100-by-husain_267-6i8r | class Solution {\npublic:\n int arithmeticTriplets(vector& nums, int diff) {\n int cnt = 0;\n unordered_setset;\n for(int i=0;i<nums.siz | husain_267 | NORMAL | 2022-08-13T10:28:36.635983+00:00 | 2022-08-13T10:28:36.636025+00:00 | 241 | false | class Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int cnt = 0;\n unordered_set<int>set;\n for(int i=0;i<nums.size();i++){\n set.insert(nums[i]);\n }\n for(int i=0;i<nums.size();i++){\n if(set.find(nums[i]+diff)!=set.end() && set.find(nums[i]+diff+diff)!=set.end()){\n cnt++;\n }\n }\n return cnt;\n }\n};\n\n\n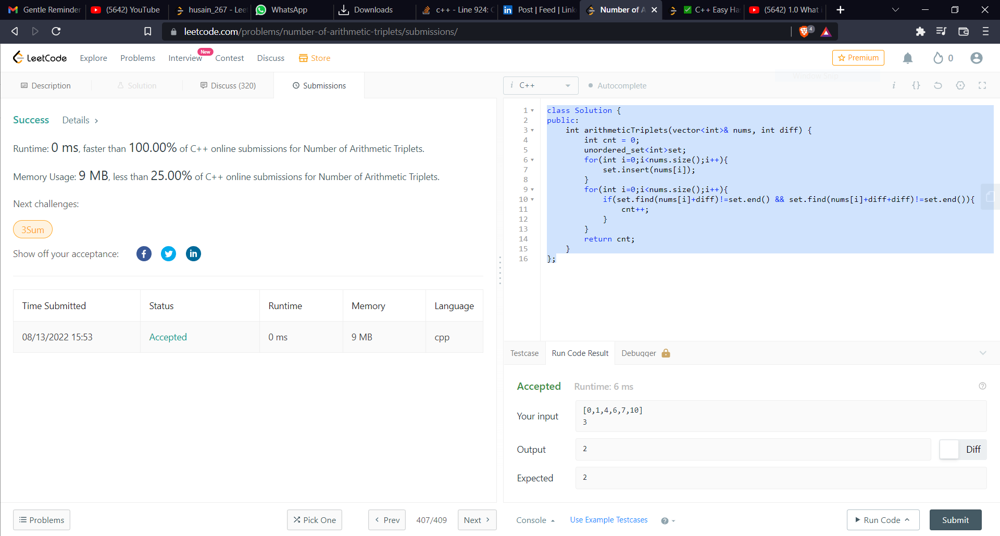\n | 3 | 0 | ['C', 'Ordered Set'] | 0 |
number-of-arithmetic-triplets | Python Elegant & Short | Two solutions | O(n) and O(n*log(n)) | Binary search | python-elegant-short-two-solutions-on-an-1ekf | \tclass Solution:\n\t\t"""\n\t\tTime: O(n*log(n))\n\t\tMemory: O(1)\n\t\t"""\n\n\t\tdef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n\t\t\tco | Kyrylo-Ktl | NORMAL | 2022-08-12T10:27:36.955680+00:00 | 2022-08-12T10:35:41.767537+00:00 | 381 | false | \tclass Solution:\n\t\t"""\n\t\tTime: O(n*log(n))\n\t\tMemory: O(1)\n\t\t"""\n\n\t\tdef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n\t\t\tcount = 0\n\t\t\tleft, right = 0, len(nums) - 1\n\n\t\t\tfor j, num in enumerate(nums):\n\t\t\t\tif self.binary_search(nums, num - diff, left, j - 1) != -1 and \\\n\t\t\t\t self.binary_search(nums, num + diff, j + 1, right) != -1:\n\t\t\t\t\tcount += 1\n\n\t\t\treturn count\n\n\t\t@staticmethod\n\t\tdef binary_search(nums: List[int], target: int, left: int, right: int) -> int:\n\t\t\twhile left <= right:\n\t\t\t\tmid = (left + right) // 2\n\n\t\t\t\tif nums[mid] == target:\n\t\t\t\t\treturn mid\n\n\t\t\t\tif nums[mid] > target:\n\t\t\t\t\tright = mid - 1\n\t\t\t\telse:\n\t\t\t\t\tleft = mid + 1\n\n\t\t\treturn -1\n\n\n\tclass Solution:\n\t\t"""\n\t\tTime: O(n)\n\t\tMemory: O(n)\n\t\t"""\n\n\t\tdef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n\t\t\tuniq_nums = set(nums)\n\t\t\treturn sum(num + diff in uniq_nums and num + 2 * diff in uniq_nums for num in nums) | 3 | 0 | ['Binary Tree', 'Python', 'Python3'] | 1 |
number-of-arithmetic-triplets | O(n^3) Solution for Beginners | on3-solution-for-beginners-by-ssbaviskar-ufvb | \n\nlet arr = []\n for (let i=0;i<=nums.length-3;i++){\n for(let j=i+1;j<=nums.length-2;j++){\n for(let k = j+1;k<=nums.length-1;k++){\n | SSBaviskar | NORMAL | 2022-08-09T13:38:29.314767+00:00 | 2022-09-25T18:03:59.899868+00:00 | 289 | false | ```\n\nlet arr = []\n for (let i=0;i<=nums.length-3;i++){\n for(let j=i+1;j<=nums.length-2;j++){\n for(let k = j+1;k<=nums.length-1;k++){\n if(nums[j]-nums[i]==diff && nums[k]-nums[j]==diff){\n arr.push([nums[i],nums[j],nums[k]]);\n }\n }\n }\n }\n return arr.length;\n\t\n``` | 3 | 0 | ['JavaScript'] | 1 |
number-of-arithmetic-triplets | C++ Simple 2 pointer approach | c-simple-2-pointer-approach-by-hi2406-hnef | \nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count=0;\n for(int i=0;i<nums.size()-2;i++){\n int sta | hi2406 | NORMAL | 2022-08-07T21:14:47.401703+00:00 | 2022-08-07T21:14:47.401732+00:00 | 381 | false | ```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count=0;\n for(int i=0;i<nums.size()-2;i++){\n int start = i+1,end = nums.size()-1;\n while(start < end){\n if(nums[start]-nums[i] == diff && nums[end]-nums[start] == diff){\n count++;\n\n break;\n }\n if(nums[start]-nums[i] < diff) start++;\n else if(nums[start]-nums[i] == diff && nums[end]-nums[start]>diff) end--;\n else break;\n }\n }\n return count;\n }\n};\n``` | 3 | 0 | ['Two Pointers', 'C'] | 0 |
number-of-arithmetic-triplets | C# Hashset | c-hashset-by-zloytvoy-595a | \tpublic int ArithmeticTriplets(int[] nums, int diff) \n\t\t{\n\t\t\tvar hashset = new HashSet();\n\t\t\tfor(int i = 0; i < nums.Length; ++i)\n\t\t\t{\n\t\t\t\t | zloytvoy | NORMAL | 2022-08-07T20:27:41.355796+00:00 | 2022-08-07T20:27:41.355829+00:00 | 57 | false | \tpublic int ArithmeticTriplets(int[] nums, int diff) \n\t\t{\n\t\t\tvar hashset = new HashSet<int>();\n\t\t\tfor(int i = 0; i < nums.Length; ++i)\n\t\t\t{\n\t\t\t\thashset.Add(nums[i]); \n\t\t\t}\n\n\t\t\tint result = 0;\n\t\t\tfor(int i = 0; i < nums.Length; ++i)\n\t\t\t{\n\t\t\t\tif(hashset.Contains(nums[i] + diff) && hashset.Contains(nums[i] + 2*diff))\n\t\t\t\t{\n\t\t\t\t\t++result;\n\t\t\t\t}\n\t\t\t}\n\n\t\t\treturn result;\n\t\t} | 3 | 0 | [] | 0 |
number-of-arithmetic-triplets | C++ | O(n) | Map | Easy to understand | Commented & Readable | c-on-map-easy-to-understand-commented-re-p89e | \nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n unordered_map<int, int> mp;\n int ans = 0;\n \n// | shreyanshxyz | NORMAL | 2022-08-07T19:24:29.844077+00:00 | 2022-08-07T19:24:29.844117+00:00 | 60 | false | ```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n unordered_map<int, int> mp;\n int ans = 0;\n \n// we check in the map if the number with the current number\'s difference & its 2x difference exists or not, if it does not, we simply push the current number into the map. If they do, we have successfully found a triplet so we update our answer.\n for(int i = 0; i < nums.size(); i++){\n if(mp[nums[i] - diff] && mp[nums[i] - 2 * diff]){ \n ans++;\n }\n mp[nums[i]]++;\n }\n return ans;\n }\n};\n``` | 3 | 0 | ['C'] | 0 |
number-of-arithmetic-triplets | C++ solution || Using unordered map || 💯Faster | c-solution-using-unordered-map-faster-by-7qxt | \n int cnt = 0;\n unordered_map<int,bool> mp;\n for(int i=0;i<nums.size();i++)\n mp[nums[i]] = true;\n for(int i=0;i<nums.size( | Amanmalviya | NORMAL | 2022-08-07T11:14:36.337316+00:00 | 2022-08-07T11:14:36.337356+00:00 | 402 | false | ```\n int cnt = 0;\n unordered_map<int,bool> mp;\n for(int i=0;i<nums.size();i++)\n mp[nums[i]] = true;\n for(int i=0;i<nums.size();i++)\n {\n if(mp[nums[i]-diff] && mp[nums[i]+diff])\n cnt++;\n } \n return cnt;\n``` | 3 | 0 | ['C++'] | 0 |
number-of-arithmetic-triplets | Latest Solution || Detailed Explanation || 2 Approaches | latest-solution-detailed-explanation-2-a-eoio | BIG IDEA: SAY YOU ARE GIVEN SINGLE NUMBER 6 AND DIFF = 3 , WHAT OTHER TWO NUMBERS DO YOU NEED TO SATISY CONDITIONS GIVEN IN THE PROBLEM? YOU NEED 6- DIFF AND 6 | progressivefailure | NORMAL | 2022-08-07T07:36:50.976604+00:00 | 2022-08-07T07:36:50.976640+00:00 | 188 | false | # BIG IDEA: SAY YOU ARE GIVEN SINGLE NUMBER 6 AND DIFF = 3 , WHAT OTHER TWO NUMBERS DO YOU NEED TO SATISY CONDITIONS GIVEN IN THE PROBLEM? YOU NEED 6- DIFF AND 6 + DIFF. SO WE ARE SIMPLY DOING THAT, THE THING IS YOU ONLY NEED TO IDENTIFY THIS AND THEN THE QUESTION IS A CAKEWALK.\n# FIRST APPROACH \n# NOTE: AS IT IS GIVEN ITS A STRICTLY INCREASING SEQUENCE, WE KNOW BINARY SEARCH COULD BE APPLIED, SO FOR EVERY ELEMENT X IN ARRAY WE SEARCH FOR *X+DIFF AND X-DIFF*.\n\n```\nclass Solution:\n\n \n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n \n def binarysearch(array, start, end , target):\n \n while start <= end:\n \n \n \n mid = start + (end-start)//2\n \n if len(nums) == mid:\n return \n \n \n if array[mid] == target:\n return True\n \n elif array[mid] > target:\n return binarysearch(array,start,mid-1,target)\n \n elif array[mid] < target:\n return binarysearch (array, mid +1 , end , target)\n \n \n return False\n \n count = 0 \n \n for num in nums:\n x_plus_diff = binarysearch(nums,0 , len(nums), num +diff)\n x_minus_diff = binarysearch(nums,0, len(nums), num - diff)\n # print(x_plus_diff, x_minus_diff)\n \n if x_plus_diff == True and x_minus_diff == True:\n count +=1\n \n return count\n```\n\n# SECOND APPROACH\n# THIS APPROACH USES EXTRA SPACE OF O(N) AND WE AGAIN SIMPLY SEARCH FOR IF "X+DIFF" AND "X-DIFF" EXIST.\n```\n hashmap = {}\n \n for i in nums :\n \n hashmap[i] = 1\n \n count = 0\n \n for num in nums:\n \n if num + diff in hashmap and num - diff in hashmap:\n count = count +1\n \n return count\n``` | 3 | 0 | ['Binary Tree', 'Python'] | 1 |
number-of-arithmetic-triplets | JAVA | easy to understand | for loop | java-easy-to-understand-for-loop-by-pazu-413s | Assume all number can be the first number of triplet\n2. if map[num - diff] == 1, it means that num is the second number of triplet\n\tor map[num - diff] == 2, | PazuC | NORMAL | 2022-08-07T04:13:40.750241+00:00 | 2022-08-07T04:19:50.375160+00:00 | 706 | false | 1. Assume all number can be the first number of triplet\n2. if map[num - diff] == 1, it means that num is the second number of triplet\n\tor map[num - diff] == 2, it means that num is the third number of triplet\n\t...\n3. so, just need to count how many map[num] >= 3 (inside a triplet)\n\nin case 1, 2 triplets are [1,4,7] & [4, 7, 10], map[10] == 4 > 3, so 10 is also inside a triplet\n(map[1] == 1, map[4] == 2, map[7] == 3, map[10] == 4)\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int result = 0;\n int[] map = new int[201];\n \n for(int num: nums) {\n map[num] = 1;\n \n if(num - diff >= 0) {\n map[num] += map[num - diff];\n }\n \n if(map[num] >= 3) result += 1;\n }\n \n return result;\n }\n}\n``` | 3 | 0 | ['Java'] | 1 |
number-of-arithmetic-triplets | ✅Easy C++ || Looop || Basic Approach || No extra space needed! | easy-c-looop-basic-approach-no-extra-spa-jwac | ```\nclass Solution {\npublic:\n int arithmeticTriplets(vector& nums, int diff) {\n int n = nums.size();\n int c=0;\n for(int i=0;i<n-2; | gittyAditya | NORMAL | 2022-08-07T04:08:17.716726+00:00 | 2022-08-07T04:08:17.716753+00:00 | 153 | false | ```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int n = nums.size();\n int c=0;\n for(int i=0;i<n-2;++i)\n for(int j=i+1;j<n-1;++j)\n for(int k=j+1;k<n;++k)\n if(nums[j]-nums[i] == diff && nums[k]-nums[j] == diff)\n c++;\n return c;\n }\n}; | 3 | 0 | ['C'] | 0 |
number-of-arithmetic-triplets | Python Solution | python-solution-by-swanand_joshi-flt9 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Swanand_Joshi | NORMAL | 2025-02-21T18:32:09.251736+00:00 | 2025-02-21T18:32:09.251736+00:00 | 61 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def arithmeticTriplets(self, nums: List[int], diff: int) -> int:
count = 0
for i in range(len(nums)):
for j in range(i+1,len(nums)):
for k in range(j+1,len(nums)):
if nums[j] - nums[i] == diff and nums[k] - nums[j] == diff:
count += 1
return count
``` | 2 | 0 | ['Python3'] | 0 |
number-of-arithmetic-triplets | Number of Arithmetic Triplets || Python || Easy Solution | number-of-arithmetic-triplets-python-eas-db42 | Step-by-Step Explanation1. Initialize a Counter
Start with triplet_count set to 0. This variable will store the number of valid arithmetic triplets.
2. Iterate | aishvgandhi | NORMAL | 2025-01-23T06:14:17.230517+00:00 | 2025-01-23T06:14:17.230517+00:00 | 113 | false | # Step-by-Step Explanation
## 1. **Initialize a Counter**
```python
triplet_count = 0
```
- Start with `triplet_count` set to 0. This variable will store the number of valid arithmetic triplets.
## 2. **Iterate Over `nums` Using a Set**
```python
for i in set(nums):
```
- Convert `nums` into a set for efficient O(1) lookups.
- Iterate over each unique number `i` in `nums`.
## 3. **Check for Triplet Conditions**
```python
if i + diff in nums and i + 2 * diff in nums:
```
- For the current number `i`:
- Check if `i + diff` exists in `nums` (middle element of the triplet).
- Check if `i + 2 * diff` exists in `nums` (last element of the triplet).
- If both conditions are satisfied, it means `(i, i + diff, i + 2 * diff)` forms an arithmetic triplet.
## 4. **Increment the Counter**
```python
triplet_count += 1
```
- If the conditions are satisfied, increment the `triplet_count`.
## 5. **Return the Result**
```python
return triplet_count
```
- After iterating through all numbers, return the total count of arithmetic triplets.
---
# If you find my solution helpful, Do Upvote 😀
---
# Code
```python3 []
class Solution:
def arithmeticTriplets(self, nums: List[int], diff: int) -> int:
triplet_count = 0
for i in set(nums):
if i + diff in nums and i + 2 * diff in nums:
triplet_count += 1
return triplet_count
``` | 2 | 0 | ['Array', 'Two Pointers', 'Python3'] | 1 |
number-of-arithmetic-triplets | SIMPLE BRUTEFORCE C++ SOLUTION | simple-bruteforce-c-solution-by-jeffrin2-5ce3 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Jeffrin2005 | NORMAL | 2024-07-18T12:39:56.990900+00:00 | 2024-07-18T12:39:56.990932+00:00 | 150 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:o(n^3)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:o(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff){\n int n=nums.size();\n int cnt=0; \n for(int i=0; i < n; i++){\n for(int j = i + 1;j < n; j++){\n for(int k = j + 1; k < n; k++){\n if(nums[k] - nums[j] == diff && nums[j] - nums[i] == diff){\n cnt++;\n }\n }\n }\n }\n return cnt;\n\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
number-of-arithmetic-triplets | ⭐Creative solution using HashMap (w/ detailed explanation) | creative-solution-using-hashmap-w-detail-2lse | Observation:\n\nIn an arithmetic sequence of length n, there are n - 3 + 1 arithmetic triplets. For example, the sequence [2, 4, 6, 8] contains 2 arithmetic tri | Nature711 | NORMAL | 2024-05-25T07:56:33.402993+00:00 | 2024-05-25T07:56:33.403013+00:00 | 124 | false | **Observation**:\n\nIn an arithmetic sequence of length `n`, there are `n - 3 + 1` arithmetic triplets. For example, the sequence `[2, 4, 6, 8]` contains 2 arithmetic triplets: `[2, 4, 6]` and `[4, 6, 8]`.\n\nGiven that the input array is sorted, we can scan from the start to find all possible "longest" arithmetic sequences.\n\n**Initial Approach**:\n\n*Initialize Variables*: \nFor each starting index i, initialize len to 0 to track the current arithmetic sequence length, and set curr to nums[i] as the start of the sequence.\n\n*Extend the Sequence*: \nTry to extend this sequence by checking if `curr + diff` exists in the array. If yes, update `curr` to `curr + diff` and increment `len` by 1. Given the array is sorted, once we encounter an element greater than `nums[i] + diff`, we terminate this round as we know there can\'t be any element smaller than this. \n\n*Time Complexity Issue*:\nConsider the input [1, 2, 3, 4, 5, 6]:\nStarting from the first element 1, we find the sequence [1, 3, 5]. During this process, we\'ve also scanned elements 2, 4, 6, which are not used in this sequence.\nTo actually find the sequence [2, 4, 6], we need to scan the input again starting from the number 2.\nIn the worst case, we end up with `O(n^2)` time complexity. \n\n**Optimized Approach with HashMap**:\n\nWhat if we could remember the numbers we\'ve seen and use them to form sequences more efficiently? Enter HashMap:\n\nWe use a HashMap where the keys are the "next expected number" of all possible arithmetic sequences, and the values are the corresponding length of that sequence. This allows us to efficiently keep track of potential sequences without needing to rescan the array multiple times.\n\n*Scan the Input*: \nScan the input array once. For each number `num`, store the "expected next number" in the map. E.g., if we encounter 1 and `diff == 3`, the expected next number is `1 + 3 = 4`. This will help us quickly check if a number can be used to extend an existing sequence later.\n\n*Form Sequencee*:\nCheck if the current number exists in the map. If yes, this means it can form an arithmetic sequence with a previous number. Extend the sequence and update the map with the next expected number. If no, we store the next expected number in the map, effectively starting a new sequence from that number. \n\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n HashMap<Integer, Integer> map = new HashMap<>();\n for (int num: nums) {\n if (map.containsKey(num)) {\n\t\t\t // the current number forms an arithmetic sequence with some previous number\n\t\t\t\t// increment the length of this sequence and update the starting point\n map.put(num + diff, map.get(num) + 1);\n map.remove(num);\n } else {\n\t\t\t // start a new arithmetic sequence, storing the next number we expect to see\n map.put(num + diff, 1);\n }\n }\n\t\t\n\t\t// count the total number of arithmetic tuples from all the arithmetic sequences found\n int res = 0;\n for (int key: map.keySet()) {\n res += Math.max(0, map.get(key) - 3 + 1);\n }\n return res;\n }\n}\n``` | 2 | 0 | ['Java'] | 1 |
number-of-arithmetic-triplets | Efficient O(n) Solution for Counting Arithmetic Triplets in TypeScript | JavaScript | efficient-on-solution-for-counting-arith-e5jb | Intuition\n Describe your first thoughts on how to solve this problem. \n\n1. Create a Counter Hash:\nInitialize a hash to keep track of the number of arithmeti | sameeneeti | NORMAL | 2023-09-22T18:54:43.905645+00:00 | 2023-09-26T14:40:47.650834+00:00 | 314 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n1. Create a Counter Hash:\nInitialize a hash to keep track of the number of arithmetic triplets found so far.\n\n2. Iterate Through the Array:\nIterate through the array nums. For each element nums[j], calculate the potential previous elements nums[i] and nums[k] in the arithmetic triplet.\nIn an arithmetic triplet, nums[j] - nums[i] == diff and nums[k] - nums[j] == diff. Hence, for the current element nums[j], the potential previous elements are nums[j] - diff and nums[j] - 2 * diff.\n\n3. Update Counter:\nUpdate the counter for arithmetic triplets by considering the count of potential previous elements for each nums[j].\n\n4. Return the Total Count:\nReturn the total count of unique arithmetic triplets.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(n)\n\n- Space complexity: O(n)\n\n# Code\n```\nfunction arithmeticTriplets(nums: number[], diff: number): number {\nconst n = nums.length \nlet count = 0 // Total count of arithmetic triplets\nconst hashMap = new Map()\n\nfor(let i=0; i<n; i++){\n const val1 = nums[i] - diff // First potential previous element\n const val2 = nums[i] - 2 * diff; // Second potential previous element\n if(hashMap.has(val1) && hashMap.has(val2)){\n count++\n }\n hashMap.set(nums[i],nums[i])\n}\n\nreturn count\n};\n``` | 2 | 0 | ['TypeScript', 'JavaScript'] | 0 |
number-of-arithmetic-triplets | [C++] check out this simple constant space solution. | c-check-out-this-simple-constant-space-s-3d8a | Code - 1 (Using Binary Search)\n- Time Complexity : O(nlogn)\n- Space Complexity : O(1)\n\n\nclass Solution {\npublic:\n int bs (vector<int> &nums, int &key, | vanshdhawan60 | NORMAL | 2023-09-07T22:43:47.953613+00:00 | 2023-09-07T22:43:47.953629+00:00 | 485 | false | # Code - 1 (Using Binary Search)\n- **Time Complexity :** $$O(n*logn)$$\n- **Space Complexity :** $$O(1)$$\n\n```\nclass Solution {\npublic:\n int bs (vector<int> &nums, int &key, int start, int end) {\n int low = start, high = end;\n while (low <= high) {\n int mid = low+(high-low)/2;\n if (nums[mid] == key) return mid;\n else if (nums[mid] > key) high = mid-1;\n else low = mid+1;\n }\n return -1;\n }\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int n = nums.size();\n int cnt = 0;\n for (int i=0; i<n-2; i++) {\n int key = diff + nums[i];\n int j = bs(nums, key, i+1, n-2);\n if (j==-1) continue;\n key += diff;\n int k = bs(nums, key, j+1, n-1);\n if (k!=-1) cnt++;\n }\n return cnt;\n }\n};\n```\n\n\n# Code - 2 (Using HashMap)\n\n- **Time Complexity :** $$O(n)$$\n- **Space Complexity :** $$O(n)$$\n\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n \n int cnt = 0;\n unordered_map<int,bool> mp;\n \n for(int i=0;i<nums.size();i++)\n mp[nums[i]] = true;\n\n for(int i=0;i<nums.size();i++)\n {\n if(mp[nums[i]-diff] && mp[nums[i]+diff])\n cnt++;\n }\n return cnt;\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
number-of-arithmetic-triplets | Binary Search approach in C++ | binary-search-approach-in-c-by-hustling_-5yww | Intuition\n Describe your first thoughts on how to solve this problem. \nIntution is all about arithmatic mean concept . We should check wheather nums[i]+diff & | Hustling_lad | NORMAL | 2023-07-23T07:53:56.210954+00:00 | 2023-07-23T07:53:56.210987+00:00 | 293 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIntution is all about arithmatic mean concept . We should check wheather nums[i]+diff & nums[i]+2*diff is present in the vector or not.If true then increase count then return count\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nAs the test cases depicts that vector contains increasing elements . So we can apply binary search concept on this problem \n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n) --- one loop\nO(logn) --- Binary search function\n\noverall ---- O(n).O(logn).O(logn)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->O(1)\n\n# Code\n```\nclass Solution {\npublic:\n bool BS (vector<int>& nums , int value)\n {\n int start = 0;\n int end = nums.size()-1;\n int mid = start+(end-start)/2;\n\n while(start<=end)\n {\n if(nums[mid] == value)\n {\n return true;\n }\n\n else if(nums[mid] > value)\n {\n end = mid-1;\n }\n\n else\n {\n start = mid+1;\n }\n\n mid = start+(end-start)/2;\n }\n return false;\n }\n\n int arithmeticTriplets(vector<int>& nums, int diff) \n {\n int count = 0;\n\n for(int i=0;i<nums.size();i++)\n {\n if(BS(nums , nums[i]+diff) && BS(nums, nums[i]+2*diff))\n {\n count++;\n }\n }\n\n return count;\n\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
number-of-arithmetic-triplets | Easy-Simple Java Solution in O(n^2) | easy-simple-java-solution-in-on2-by-tauq-dju4 | \n# Approach\n Describe your approach to solving the problem. \nWe have to find a triplet of (i, j, k) such that \ni<j=2 we add 1 to ans and at last return it.\ | tauqueeralam42 | NORMAL | 2023-07-02T09:58:16.819238+00:00 | 2023-07-02T09:58:16.819264+00:00 | 492 | false | \n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe have to find a triplet of (i, j, k) such that \ni<j<k , \nnums[j] - nums[i] == diff, and \nnums[k] - nums[j] == diff\n\nin other word we can also say nums[k]-nums[i]== 2*diff. To reduce the time complexity I use only 2 loop remove the loop for k and include it in j iterartion such that nums[j]-nums[i] == 2*diff. So in every iterartion of j we will get 2 condition nums[j]-nums[i] == diff and nums[j]-nums[i] == 2*diff when these 2 condition get satisfied in j iterartion, We get 1 count as answer. So for every iteration when count>=2 we add 1 to ans and at last return it.\n\n# Complexity\n- Time complexity: O(n^2)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int ans =0;\n for(int i=0; i<nums.length-2;i++){\n int count =0;\n for(int j=i+1; j<nums.length; j++){\n if(nums[j]-nums[i]==diff || nums[j]-nums[i]==2*diff){\n count++;\n }\n }\n if(count >= 2){\n ans++;\n }\n }\n\n return ans;\n \n }\n}\n``` | 2 | 0 | ['Array', 'Java'] | 0 |
number-of-arithmetic-triplets | Simple-Easy Brute Force Approach | simple-easy-brute-force-approach-by-tauq-f8hy | \n\n# Approach\n Describe your approach to solving the problem. \nHere I use simple brute force approach. Use 3 for loop iterarte i, j, k one by one and search | tauqueeralam42 | NORMAL | 2023-07-02T09:36:23.699190+00:00 | 2023-07-02T09:36:23.699218+00:00 | 694 | false | \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nHere I use simple brute force approach. Use 3 for loop iterarte i, j, k one by one and search a combinantion of i, j, k which can satisfied these given conditions and count that combinations.\n\n# Complexity\n- Time complexity: O(n^3)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count =0;\n for(int i=0; i<nums.length-2;i++){\n for(int j=i+1; j<nums.length-1; j++){\n for(int k=j+1; k<nums.length; k++){\n if(nums[k]-nums[j] == diff && nums[j]-nums[i]== diff){\n count++;\n }\n }\n }\n }\n\n return count;\n \n }\n}\n``` | 2 | 0 | ['Array', 'Java'] | 0 |
number-of-arithmetic-triplets | Faster than 100%✅✅ || C++ || Very easy-logic with explanation | faster-than-100-c-very-easy-logic-with-e-oklg | Proof :please upvote if you liked my solution\n\n\nLogic: \nIf you have attempted 2sum leetcode question this code will be quite easy for you.The simple logic b | 40metersdeep | NORMAL | 2023-05-29T17:44:39.908014+00:00 | 2023-05-29T17:52:01.092773+00:00 | 437 | false | **Proof :**_please upvote if you liked my solution_\n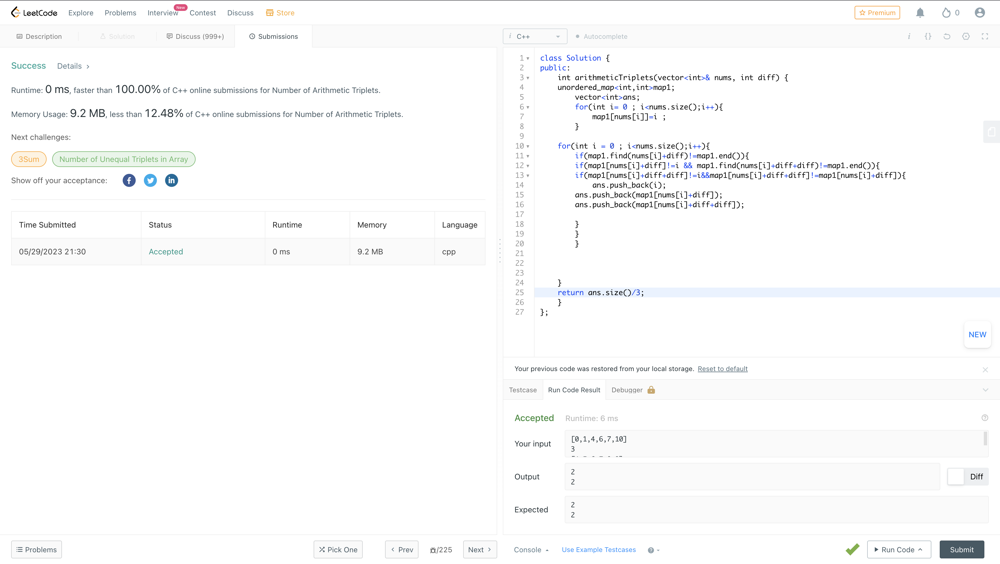\n\n**Logic:** \nIf you have attempted 2sum leetcode question this code will be quite easy for you.The simple logic behind the code is that there for every element **i**, if there are elements **i+diff** and **i+diff+diff** in the array then, they form an arithmetic triplet.we only need to make sure that all the three elements do not share same indexes in the array.There is no chance of triplets repeating as we are not iterating over the array again.\n\n**Code:**\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n unordered_map<int, int> map1; // Map to store the index of each number\n vector<int> ans; // Vector to store the indices of the arithmetic triplets\n\n // Populating the map with the index of each number\n for (int i = 0; i < nums.size(); i++) {\n map1[nums[i]] = i;\n }\n\n // Finding arithmetic triplets\n for (int i = 0; i < nums.size(); i++) {\n if (map1.find(nums[i] + diff) != map1.end()) { // If nums[i]+diff exists in the map\n if (map1[nums[i] + diff] != i && map1.find(nums[i] + diff + diff) != map1.end()) { // If nums[i]+diff and nums[i]+diff+diff exist in the map\n if (map1[nums[i] + diff + diff] != i && map1[nums[i] + diff + diff] != map1[nums[i] + diff]) { // If the indices are distinct\n ans.push_back(i); // Pushing the indices of the arithmetic triplet\n ans.push_back(map1[nums[i] + diff]);\n ans.push_back(map1[nums[i] + diff + diff]);\n }\n }\n }\n }\n \n return ans.size() / 3; // Dividing the total number of indices by 3 to get the count of arithmetic triplets\n }\n};\n```\n**Flowchart:**\n\n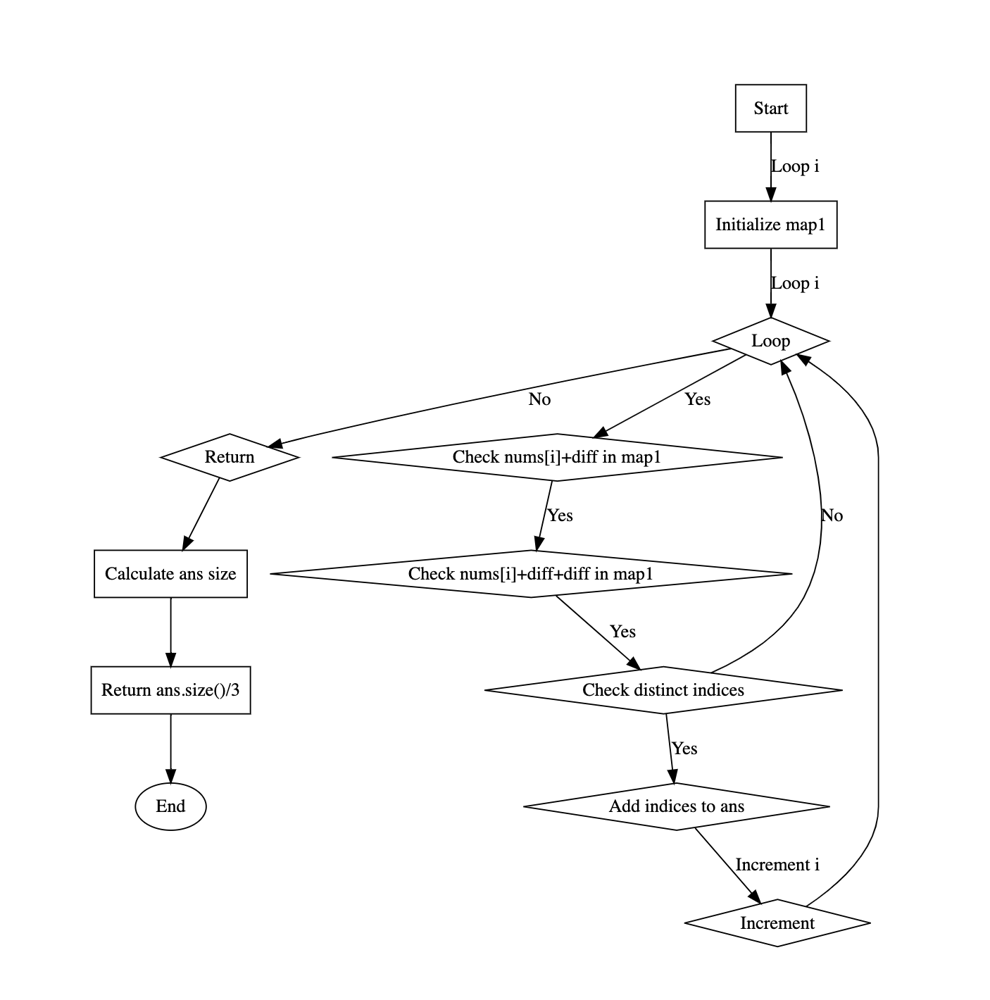\n | 2 | 0 | ['C'] | 0 |
number-of-arithmetic-triplets | Fastest at 1ms | fastest-at-1ms-by-mohammad__irfan-5rqu | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | mohammad__irfan | NORMAL | 2023-05-02T11:33:19.669124+00:00 | 2023-05-02T11:33:19.669166+00:00 | 1,134 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count = 0; \n Set<Integer> list = new HashSet<>();\n for(int a : nums){\n if(list.contains(a-diff) && list.contains(a-diff*2)) count++;\n list.add(a);\n }\n return count;\n }\n}\n``` | 2 | 0 | ['Java'] | 3 |
number-of-arithmetic-triplets | JAVA | 1ms | O(N^2) | java-1ms-on2-by-firdavs06-ivdd | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Firdavs06 | NORMAL | 2023-02-27T07:19:04.038382+00:00 | 2023-02-27T07:19:04.038426+00:00 | 826 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int x = 0;\n int l = 0;\n int k;\n for (int i = 0; i < nums.length; i++) {\n k = nums[i];\n for (int j = i + 1; j < nums.length; j++) {\n if (nums[j] - k == diff) {\n x++;\n k = nums[j];\n }\n if(x == 2){\n l++;\n break;\n }\n }\n x = 0;\n }\n return l;\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
number-of-arithmetic-triplets | pointer manipulation in C | pointer-manipulation-in-c-by-fantsai8686-hhdp | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \nex:\n[0,1,4,6,7,10]\ntr | fantsai8686 | NORMAL | 2023-02-21T11:27:39.885087+00:00 | 2023-03-29T08:21:09.288216+00:00 | 468 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nex:\n[0,1,4,6,7,10]\ntraversing nums in for loop from index1= 0~numsSize-3 (0,1,4,6).\n\nThere is a while loop in each for loop starting from index2= 1~numsSize-1 to check if nums[index2]-nums[index1]==diff.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n0 ms\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n5.9 MB\n# Code\n```\nint arithmeticTriplets(int* nums, int numsSize, int diff){\n \n int* numPtr=nums;\n int cnt=1, total=0;\n for(int i=0; i<numsSize-2; i++){\n numPtr=nums+i+1;\n cnt=1;\n while(numPtr!=nums+numsSize){ \n if(*numPtr-nums[i]==diff*cnt) cnt++;\n if(cnt==3){\n total++;\n break;\n }\n *numPtr++;\n } \n }\n return total;\n}\n``` | 2 | 0 | ['C'] | 0 |
number-of-arithmetic-triplets | Solution in python easy to understand | solution-in-python-easy-to-understand-by-3prr | Input: nums = [0,1,4,6,7,10], diff = 3\nOutput: 2\nExplanation:\n(1, 2, 4) is an arithmetic triplet because both 7 - 4 == 3 and 4 - 1 == 3.\n(2, 4, 5) is an ari | mittalaparna | NORMAL | 2023-01-31T19:45:13.189850+00:00 | 2023-01-31T19:45:13.189887+00:00 | 83 | false | Input: nums = [0,1,4,6,7,10], diff = 3\nOutput: 2\nExplanation:\n(1, 2, 4) is an arithmetic triplet because both 7 - 4 == 3 and 4 - 1 == 3.\n(2, 4, 5) is an arithmetic triplet because both 10 - 7 == 3 and 7 - 4 == 3.\n\nCode explaination:\n((nums[i] + diff) in arr) and ((nums[i] + 2 * diff) in arr)\n = 1 + 3 in arr and 1 + 2 * 3 in arr\n = 4 in arr and 7 in arr \nthen count +1\n\n# Code\n```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n count=0\n arr=set(nums)\n for i in range(len(nums)):\n if ((nums[i]+diff) in arr) and ((nums[i]+2*diff) in arr):\n count +=1\n return count\n``` | 2 | 0 | ['Hash Table', 'Python3'] | 0 |
number-of-arithmetic-triplets | Hashset n-diff and n-2*diff | hashset-n-diff-and-n-2diff-by-mohan_66-a6xg | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Mohan_66 | NORMAL | 2023-01-28T05:26:46.572858+00:00 | 2023-01-28T05:26:46.572903+00:00 | 318 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n seen=set()\n c=0\n for n in nums:\n seen.add(n)\n if n-diff in seen and n-2*diff in seen:\n c+=1\n return c\n \n \n``` | 2 | 0 | ['Python3'] | 0 |
number-of-arithmetic-triplets | Binary Search || by searching || without any space🔥 | binary-search-by-searching-without-any-s-8l31 | INTUTION: \n\n1. search no+diff and no+2diff by the help of lower bound. \n1. if both numbers present in array then just count++\n2. same thing is done for each | suyashdewangan9 | NORMAL | 2022-10-18T16:40:41.814627+00:00 | 2022-10-18T16:51:54.188030+00:00 | 195 | false | ##### INTUTION: \n\n1. search no+diff and no+2diff by the help of lower bound. \n1. if both numbers present in array then just count++\n2. same thing is done for each element of nums\n\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int count=0;\n for(int i=0;i<nums.size();i++){\n int ind=lower_bound(nums.begin(),nums.end(),nums[i]+diff)-nums.begin();\n if(ind>=nums.size())continue;\n else if(nums[ind]!=nums[i]+diff)continue;\n else{\n int ind2=lower_bound(nums.begin(),nums.end(),nums[ind]+diff)-nums.begin();\n if(ind2>=nums.size())continue;\n else if(nums[ind2]!=nums[ind]+diff)continue;\n else{\n count++;\n }\n }\n }\n return count;\n }\n};\n```\n\t\t\t\t | 2 | 0 | ['Binary Search', 'C'] | 0 |
number-of-arithmetic-triplets | 100% faster || c++ || simple | 100-faster-c-simple-by-recursive_coder-xvhd | \n\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n \n vector<int> hash(305,0); // for storing cnt of each | recursive_coder | NORMAL | 2022-10-08T07:03:09.372355+00:00 | 2022-10-08T07:03:48.649782+00:00 | 908 | false | 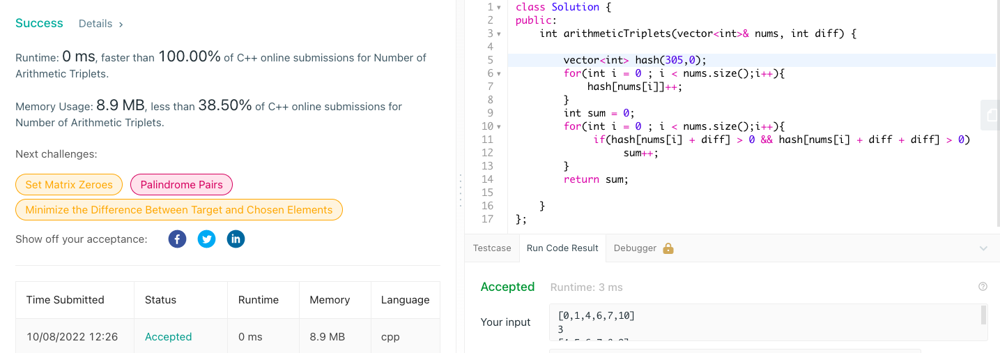\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n \n vector<int> hash(305,0); // for storing cnt of each element in the array\n for(int i = 0 ; i < nums.size();i++){\n hash[nums[i]]++; // storing cnt\n }\n int sum = 0;\n for(int i = 0 ; i < nums.size();i++){\n if(hash[nums[i] + diff] > 0 && hash[nums[i] + diff + diff] > 0) // as array is sorted - > 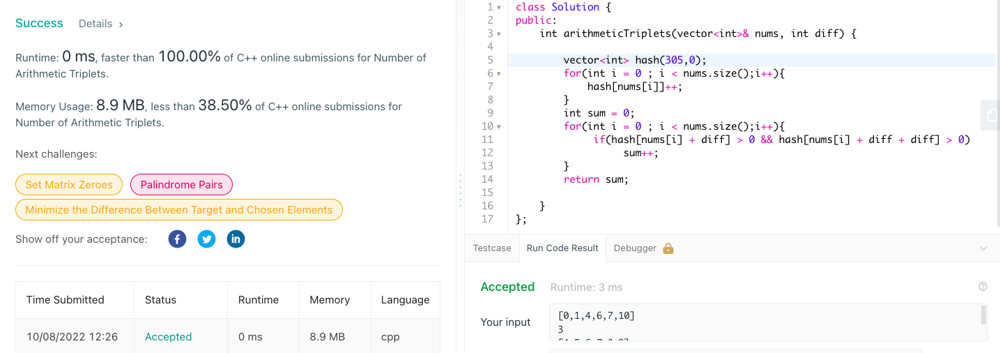\ni < j < k for all nums[i] < nums[j] < nums[j] \n sum++; \n }\n return sum;\n \n }\n};\n``` | 2 | 0 | ['C'] | 0 |
number-of-arithmetic-triplets | Python O(n log n) time, O(1) space solution with comments | python-on-log-n-time-o1-space-solution-w-36fh | Here is my optimized code that has:\nTime: O(n log n)\nSpace: O(1)\n\npython\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n n = | trpaslik | NORMAL | 2022-09-16T10:53:06.383950+00:00 | 2022-09-16T10:53:40.646376+00:00 | 279 | false | Here is my optimized code that has:\nTime: O(n log n)\nSpace: O(1)\n\n```python\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n n = len(nums)\n ans = 0\n for i in range(n-2):\n num0 = nums[i] # current num\n num1 = num0 + diff # expected nums[j]\n j = bisect_left(nums, num1, i+1, n-1) # time cost O(log n)\n if num1 != nums[j]: # num1 not found\n continue\n num2 = num1 + diff # expected nums[k]\n k = bisect_left(nums, num2, j+1, n)\n if k==n or num2 != nums[k]: # num2 not found\n continue\n ans += 1 # triplet i,j,k is good\n return ans\n``` | 2 | 0 | ['Python'] | 1 |
number-of-arithmetic-triplets | SIMPLE JAVA CODE | simple-java-code-by-priyankan_23-8gp2 | \nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count=0;\n for(int i=0;i<nums.length-2;i++){\n int | priyankan_23 | NORMAL | 2022-09-11T14:05:06.728079+00:00 | 2022-09-11T14:05:06.728114+00:00 | 188 | false | ```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int count=0;\n for(int i=0;i<nums.length-2;i++){\n int j=i+1,check=0;\n while(j<nums.length){\n if(nums[j]-nums[i]==diff){\n check=1;\n break;\n }\n j++;\n }\n if(check==1){\n int temp =nums[j];\n j=j+1;\n while(j<nums.length){\n if(nums[j]-temp==diff)count++;\n j++;\n }\n \n }\n }\n return count;\n }\n}\n``` | 2 | 0 | [] | 0 |
number-of-arithmetic-triplets | Python | Linear | Fast | Explained with Comments | python-linear-fast-explained-with-commen-p3t9 | \nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n \n # a variable to store number of triplets\n # i | rohitsh26 | NORMAL | 2022-09-08T04:06:30.264182+00:00 | 2022-09-08T04:23:09.232989+00:00 | 160 | false | ```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n \n # a variable to store number of triplets\n # initializing with 0\n counterOfUniqueArithmeticTriplet = 0\n \n # create a set of number in the nums list\n _set = set(nums)\n \n # for each num in nums \n # we will try to find if we have num - diff in the _set\n # and we also have num + diff in the set\n # if we have both that makes a triplet for us\n # Example from Input: nums = [0,1,4,6,7,10], diff = 3\n # when num = 4,\n # remaining = 4 - diff => 4 - 3 = 1\n # nextToFind = 4 + diff => 4 + 3 = 7\n # and both are present in nums, we can increment counterOfUniqueArithmeticTriplet\n # as we have found a unique triplet\n for num in nums:\n remaining = num - diff\n nextToFind = num + diff\n \n # if both are in nums we increment counterOfUniqueArithmeticTriplet\n if remaining in nums and nextToFind in nums:\n counterOfUniqueArithmeticTriplet += 1\n return counterOfUniqueArithmeticTriplet\n```\n | 2 | 0 | ['Python'] | 1 |
number-of-arithmetic-triplets | Brute force solution of java | brute-force-solution-of-java-by-annshu_8-vq6z | \tclass Solution {\n\t\tpublic int arithmeticTriplets(int[] nums, int diff) {\n\t\t int count = 0;\n\t\t\tfor(int i= 0; i< nums.length ; i++){\n\t\t\t\tfor( i | annshu_8410 | NORMAL | 2022-09-07T19:25:58.885742+00:00 | 2022-09-07T19:25:58.885780+00:00 | 53 | false | \tclass Solution {\n\t\tpublic int arithmeticTriplets(int[] nums, int diff) {\n\t\t int count = 0;\n\t\t\tfor(int i= 0; i< nums.length ; i++){\n\t\t\t\tfor( int j = i+1; j < nums.length ; j++){\n\t\t\t\t\tfor( int k = j+1; k < nums.length ; k++){\n\t\t\t\t\t\tif(i < j && j < k &&\n\t\t\t\t\t\t nums[j] - nums[i] == diff &&\n\t\t\t\t\t\t nums[k] - nums[j] == diff){\n\t\t\t\t\t\t count++; \n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn count;\n\t\t}\n\t} | 2 | 0 | ['Java'] | 0 |
number-of-arithmetic-triplets | FULLY EXPLAINED - O(n^3), O(n^2) and O(n) Solutions | fully-explained-on3-on2-and-on-solutions-3ccu | 1. O(n^3)\n\nThe straightforward way is to have three nested loops and then check the conditions. But ofcourse this won\'t be an efficient approach and will giv | itsarvindhere | NORMAL | 2022-09-07T13:13:49.275515+00:00 | 2022-09-07T13:54:05.495455+00:00 | 223 | false | ## **1. O(n^3)**\n\nThe straightforward way is to have three nested loops and then check the conditions. But ofcourse this won\'t be an efficient approach and will give TLE in case of large input. \n\n```\ndef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n count = 0;\n for i,num1 in enumerate(nums):\n for j,num2 in enumerate(nums):\n for k, num3 in enumerate(nums):\n if(i < j and j < k): \n if(num2 - num1 == diff and num3 - num2 == diff): count += 1\n \n return count\n```\n\n## **2. O(n^2)**\n\nTo make the code more efficient, what we can do is maintain a set of all the elements.\n\ne.g. nums = [0,1,4,6,7,10], diff = 3\n\nSo we will have a set = {0,1,4,6,7,10}\n\t\nAnd now, instead of three nested loops, we can have only two. Such that when we got a pair with a difference of 3, then we can check if a set contains any element that can be the third number to make it a triplet. \n\t\ne.g. if first loop currently points to number = 1 And second loop points to number = 4 then we see their diff is 3 which is same as the diff we want\n\t\t So now, all we want is to find a third number such that \n\t\t\t 4 - third number = diff\n\t\tSo, rearranging this equation, we get \n\t\t\t4 + diff = thirdNumber\n\t\t\t\nHence, we seach in the set whether (4 + diff) is present or not.\n\t\t\n```\ndef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n\tcount = 0\n\ts = set(nums)\n\n\tfor i, num1 in enumerate(nums):\n\t\tfor j, num2 in enumerate(nums):\n\t\t\tif(i != j and num2 - num1 == diff):\n\t\t\t\tif((diff + num2) in s): count += 1\n\treturn count\n```\n\n## **3. O(n) - Using Largest value of triplet to find other two values**\n\nI couldn\'t think of this solution so had to head over to the dicussion page to see how can we do this problem with O(n) complexity. \n\n[votrubac](https://leetcode.com/votrubac) shared a beautiful approach where we are using the largest value of a triplet to find the other two values and if both exist in the array, then we have a valid triplet.\n\nSo, we will use arr[k] to get back arr[j] and arr[i]\n\nWE are given that :\n\t\n\t\tarr[j] - arr[i] = diff && arr[k] - arr[j] = diff\n\t\nSo, from arr[k] - arr[j] = diff, we get: \n\t\t\n\t\tarr[j] = arr[k] - diff // This will be the formula to get arr[j] using arr[k] ---- (a)\n\nNow, if we substitute this value of arr[j] in arr[j] - arr[i] = diff:\n\tarr[k] - diff - arr[i] = diff\n\tarr[i] = arr[k] - diff - diff\n\t\n\t\tarr[i] = arr[k] - (2 * diff) // This will be the formula to get arr[i] using arr[k] ---- (b)\n\t\n\nSo we will use (a) and (b) to get values of other two values of a triplet for a particular number.\n\n```\ndef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n count = 0\n s = set(nums)\n \n for i, num3 in enumerate(nums):\n num2 = num3 - diff\n num1 = num3 - (2 * diff)\n if(num1 in s and num2 in s): count += 1\n \n return count\n```\n\n\n\n## **4. O(n) - Using smallest value of triplet to find other two values**\n\nSo, if we can use the largest number to get back the other two numbers, can we do the other way around as well? Lets try to use the smallest number of a triplet to find other two numbers i.e., using a[i] to find a[j] and a[k]\n\nFirst condition is \n\t\t\n\t\tarr[j] - arr[i] = diff\n\nFrom this we get\n\t\n\t\tarr[j] = arr[i] + diff //This is how we will find arr[j] using arr[i] ----- (a)\n\t\nAnd the second condition is \n\t\n\t\tarr[k] - arr[j] = diff\n\t\t\nSubstituting the value of arr[j] from (a) \n\t\n\t\tarr[k] - (arr[i] + diff) = diff\n\t\tarr[k] - arr[i] - diff = diff\n\t\tarr[k] = diff + arr[i] + diff\n\t\tarr[k] = (2 * diff) + arr[i] //This is how we will find arr[k] using arr[i] ------ (b)\n\t\t\nSo, we will use (a) and (b) to get the other two values in the triplet using the smallest value of triplet.\n\n```\ndef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n count = 0\n s = set(nums)\n \n for i, num1 in enumerate(nums):\n num2 = num1 + diff\n num3 = num1 + (2 * diff)\n if(num2 in s and num3 in s): count += 1\n\t\t\t\n return count\n```\n\n\n## **5. O(n) - Using middle value of triplet to find other two values**\n\nSo we were able to use the smallest and the largest value in a triplet to find other two values. Can we now use the middle element i.e., arr[j] to get back other two numbers in triplet? Let\'s see.\n\nFirst condition is \n\t\t\n\t\tarr[j] - arr[i] = diff\n\nFrom this we get\n\t\n\t\tarr[i] = arr[j] - diff //This is how we will find arr[i] using arr[j] ----- (a)\n\t\nAnd the second condition is \n\t\n\t\tarr[k] - arr[j] = diff\n\t\t\nThis gives us: \n\t\n\t\tarr[k] = arr[j] + diff //This is how we will find arr[k] using arr[j] ----- (b)\n\t\t\nSo, we will use (a) and (b) to get the other two values in the triplet using the middle value of triplet.\n\n```\ndef arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n count = 0\n s = set(nums)\n \n for i, num2 in enumerate(nums):\n num1 = num2 - diff\n num3 = num2 + diff\n if(num1 in s and num3 in s): count += 1\n \n return count\n```\n\n\nPHEW! That was a lot to write but all thanks to [votrubac](https://leetcode.com/votrubac) for the O(n) approach. Here is his post -> https://leetcode.com/problems/number-of-arithmetic-triplets/discuss/2390637/Check-n-diff-and-n-2-*-diff | 2 | 0 | ['Python'] | 0 |
number-of-arithmetic-triplets | C+++ || 0ms Solution || Using Constant Space | c-0ms-solution-using-constant-space-by-k-hnzj | \nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n \n int count = 0;\n \n for(int i = 0 ; i<num | Khwaja_Abdul_Samad | NORMAL | 2022-09-02T13:04:59.492129+00:00 | 2022-09-02T13:04:59.492168+00:00 | 245 | false | ```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n \n int count = 0;\n \n for(int i = 0 ; i<nums.size()-2 ; i++)\n {\n int j = i+1 ;\n while(j<nums.size()-1 && nums[j]-nums[i] < diff)\n j++; \n \n if( j== nums.size()-1 || nums[j]-nums[i] != diff)\n continue;\n \n int k = j+1;\n \n while(k<nums.size() && nums[k]-nums[j] < diff)\n k++;\n \n if(k < nums.size() && nums[k] - nums[j] == diff)\n count++;\n }\n \n return count;\n }\n};\n```\n\n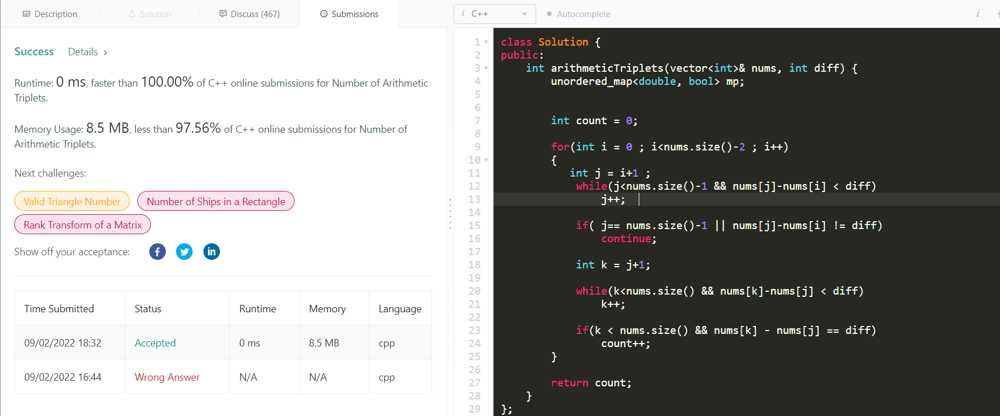\n | 2 | 0 | ['C'] | 1 |
number-of-arithmetic-triplets | Java | beats 69% lol | easy and simple solution | | java-beats-69-lol-easy-and-simple-soluti-vz3y | ```\nint ans = 0;\n //we take an answer that we will be returning ;\n \n for(int i = nums.length-1 ; i >=0 ; i--){//for the first element | k_io | NORMAL | 2022-08-21T09:22:54.137440+00:00 | 2022-08-21T09:22:54.137467+00:00 | 214 | false | ```\nint ans = 0;\n //we take an answer that we will be returning ;\n \n for(int i = nums.length-1 ; i >=0 ; i--){//for the first element of the triplet\n \n meow :\n for(int j = nums.length-1 ; j >=0 ; j--){//for the second element of the triplet ;\n if(nums[i] - nums[j] == diff){\n for(int k = j ; k >= 0 ; k --){//for the third element of triplet ;\n if(nums[j] - nums[k] == diff){\n ans++ ;\n break meow ;//breaks and starts for another first element because we want triplet only ;\n }\n }\n }\n }\n }\n return ans; | 2 | 0 | ['Array', 'Java'] | 0 |
number-of-arithmetic-triplets | Java solution | O(n) | java-solution-on-by-sourin_bruh-gt5i | \nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n \n Set<Integer> set = new HashSet<>();\n \n for (int | sourin_bruh | NORMAL | 2022-08-21T08:03:11.503787+00:00 | 2022-08-21T09:36:28.388880+00:00 | 115 | false | ```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n \n Set<Integer> set = new HashSet<>();\n \n for (int x : nums) set.add(x);\n \n int ans = 0;\n \n for (int x : nums) {\n if (set.contains(x - diff) && set.contains(x + diff)) ans++;\n }\n\t\t\n\t\t// OR\n\t\t// for (int i = nums.length - 1; i >= 0; i--) {\n // if (set.contains(nums[i] - diff) && set.contains(nums[i] - 2 * diff)) ans++;\n // }\n \n return ans;\n }\n}\n\n// TC: O(n), SC: O(n)\n``` | 2 | 0 | ['Java'] | 2 |
number-of-arithmetic-triplets | Java Solution | Simple solution | java-solution-simple-solution-by-shiladi-149f | class Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n Set set = new HashSet<>();\n for (int x : nums) {\n set.a | shiladityaroy | NORMAL | 2022-08-14T20:01:26.366631+00:00 | 2022-08-14T20:01:26.366660+00:00 | 293 | false | class Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n Set<Integer> set = new HashSet<>();\n for (int x : nums) {\n set.add(x);\n }\n \n int ans = 0;\n for (int x : nums) {\n if (set.contains(x - diff) && set.contains(x + diff)) {\n ans++;\n }\n }\n return ans;\n }\n} | 2 | 0 | ['Java'] | 1 |
reordered-power-of-2 | [C++/Java/Python] Straight Forward | cjavapython-straight-forward-by-lee215-5nse | counter will counter the number of digits 9876543210 in the given number.\nThen I just compare counter(N) with all counter(power of 2).\n1 <= N <= 10^9, so up t | lee215 | NORMAL | 2018-07-15T03:07:40.854887+00:00 | 2018-10-25T02:53:21.915764+00:00 | 23,923 | false | `counter` will counter the number of digits 9876543210 in the given number.\nThen I just compare `counter(N)` with all `counter(power of 2)`.\n`1 <= N <= 10^9`, so up to 8 same digits.\nIf `N > 10^9`, we can use a hash map.\n\n**C++:**\n```\n bool reorderedPowerOf2(int N) {\n long c = counter(N);\n for (int i = 0; i < 32; i++)\n if (counter(1 << i) == c) return true;\n return false;\n }\n\n long counter(int N) {\n long res = 0;\n for (; N; N /= 10) res += pow(10, N % 10);\n return res;\n }\n```\n\n**Java:**\n```\n public boolean reorderedPowerOf2(int N) {\n long c = counter(N);\n for (int i = 0; i < 32; i++)\n if (counter(1 << i) == c) return true;\n return false;\n }\n public long counter(int N) {\n long res = 0;\n for (; N > 0; N /= 10) res += (int)Math.pow(10, N % 10);\n return res;\n }\n```\n**Python:**\n```\n def reorderedPowerOf2(self, N):\n c = collections.Counter(str(N))\n return any(c == collections.Counter(str(1 << i)) for i in xrange(30))\n```\n\n\n**Python 1-line**\nsuggested by @urashima9616, bests 80%\n```\n def reorderedPowerOf2(self, N):\n return sorted(str(N)) in [sorted(str(1 << i)) for i in range(30)]\n``` | 325 | 4 | [] | 47 |
reordered-power-of-2 | Simple Java Solution Based on String Sorting | simple-java-solution-based-on-string-sor-cg66 | The idea here is similar to that of group Anagrams problem (Leetcode #49). \n\nFirst, we convert the input number (N) into a string and sort the string. Next, w | trotro | NORMAL | 2018-07-20T23:27:49.802904+00:00 | 2018-07-20T23:27:49.802904+00:00 | 5,807 | false | The idea here is similar to that of group Anagrams problem (Leetcode #49). \n\nFirst, we convert the input number (N) into a string and sort the string. Next, we get the digits that form the power of 2 (by using 1 << i and vary i), convert them into a string, and then sort them. As we convert the powers of 2 (and there are only 31 that are <= 10^9), for each power of 2, we compare if the string is equal to that of string based on N. If the two strings are equal, then we return true.\n\n```\nclass Solution {\n public boolean reorderedPowerOf2(int N) {\n char[] a1 = String.valueOf(N).toCharArray();\n Arrays.sort(a1);\n String s1 = new String(a1);\n \n for (int i = 0; i < 31; i++) {\n char[] a2 = String.valueOf((int)(1 << i)).toCharArray();\n Arrays.sort(a2);\n String s2 = new String(a2);\n if (s1.equals(s2)) return true;\n }\n \n return false;\n }\n}\n``` | 143 | 0 | [] | 12 |
reordered-power-of-2 | C++ Super Simple and Short Solution, 0 ms, Faster than 100% | c-super-simple-and-short-solution-0-ms-f-h8kl | \nclass Solution {\npublic:\n bool reorderedPowerOf2(int N) {\n string N_str = sorted_num(N);\n for (int i = 0; i < 32; i++)\n if (N | yehudisk | NORMAL | 2021-03-21T08:35:30.265019+00:00 | 2021-03-21T08:35:36.558397+00:00 | 7,636 | false | ```\nclass Solution {\npublic:\n bool reorderedPowerOf2(int N) {\n string N_str = sorted_num(N);\n for (int i = 0; i < 32; i++)\n if (N_str == sorted_num(1 << i)) return true;\n return false;\n }\n \n string sorted_num(int n) {\n string res = to_string(n);\n sort(res.begin(), res.end());\n return res;\n }\n};\n```\n**Like it? please upvote...** | 108 | 13 | ['C'] | 12 |
reordered-power-of-2 | JS, Python, Java, C++ | Easy, Short Solution w/ Explanation | js-python-java-c-easy-short-solution-w-e-hcjo | (Note: This is part of a series of Leetcode solution explanations. If you like this solution or find it useful, please upvote this post.)\n\n---\n\n#### Idea:\n | sgallivan | NORMAL | 2021-03-21T08:05:47.270873+00:00 | 2021-03-21T09:04:21.120695+00:00 | 3,967 | false | *(Note: This is part of a series of Leetcode solution explanations. If you like this solution or find it useful,* ***please upvote*** *this post.)*\n\n---\n\n#### ***Idea:***\n\nThe easiest way to check if two things are shuffled versions of each other, which is what this problem is asking us to do, is to sort them both and the compare the result.\n\nIn that sense, the easiest solution here is to do exactly that: we can convert **N** to an array of its digits, sort it, then compare that result to the result of the same process on each power of **2**.\n\nSince the constraint upon **N** is **10e9**, we only need to check powers in the range **[0,29]**.\n\nTo make things easier to compare, we can always **join()** the resulting digit arrays into strings before comparison.\n\nThere are ways to very slightly improve the run time and memory here, but with an operation this small, it\'s honestly not very necessary.\n\n---\n\n#### ***Implementation:***\n\nPython can directly compare the lists and Java can directly compare the char arrays without needing to join them into strings. C++ can sort the strings in-place without needing to convert to an array.\n\n---\n\n#### ***Javascript Code:***\n\nThe best result for the code below is **72ms / 38.8MB** (beats 100% / 44).\n```javascript\nvar reorderedPowerOf2 = function(N) {\n let res = N.toString().split("").sort().join("")\n for (let i = 0; i < 30; i++)\n if ((1 << i).toString().split("").sort().join("") === res) return true\n return false\n};\n```\n\n---\n\n#### ***Python Code:***\n\nThe best result for the code below is **24ms / 14.1MB** (beats 98% / 76%).\n```python\nclass Solution:\n def reorderedPowerOf2(self, N: int) -> bool:\n res = sorted([int(x) for x in str(N)])\n for i in range(30):\n if sorted([int(x) for x in str(1 << i)]) == res: return True\n return False\n```\n\n---\n\n#### ***Java Code:***\n\nThe best result for the code below is **1ms / 35.8MB** (beats 97% / 88%).\n```java\nclass Solution {\n public boolean reorderedPowerOf2(int N) {\n char[] res1 = String.valueOf(N).toCharArray();\n Arrays.sort(res1);\n for (int i = 0; i < 30; i++) {\n char[] res2 = String.valueOf(1 << i).toCharArray();\n Arrays.sort(res2);\n if (Arrays.equals(res1, res2)) return true;\n }\n return false;\n }\n}\n```\n\n---\n\n#### ***C++ Code:***\n\nThe best result for the code below is **0ms / 5.8MB** (beats 100% / 99%).\n```c++\nclass Solution {\npublic:\n bool reorderedPowerOf2(int N) {\n string res1 = to_string(N);\n sort(res1.begin(), res1.end());\n for (int i = 0; i < 30; i++) {\n string res2 = to_string(1 << i);\n sort(res2.begin(), res2.end());\n if (res1 == res2) return true;\n }\n return false;\n }\n};\n``` | 71 | 12 | ['C', 'Python', 'Java', 'JavaScript'] | 2 |
reordered-power-of-2 | C++ || 100% fast || 0ms | c-100-fast-0ms-by-manuraj_tomar-q8im | \nbool reorderedPowerOf2(int n) {\n string s = to_string(n);\n sort(s.begin(),s.end());\n\t\t\n vector<string> power;\n for(int i=0; | Manuraj_Tomar | NORMAL | 2022-08-26T00:25:33.346170+00:00 | 2022-08-26T00:25:33.346214+00:00 | 11,209 | false | ```\nbool reorderedPowerOf2(int n) {\n string s = to_string(n);\n sort(s.begin(),s.end());\n\t\t\n vector<string> power;\n for(int i=0;i<=30;i++){\n int p = pow(2,i);\n power.push_back(to_string(p));\n }\n \n for(int i=0;i<=30;i++){\n sort(power[i].begin(),power[i].end());\n }\n \n for(int i=0;i<=30;i++){\n if(power[i] == s ) return true;\n }\n return false;\n }\n``` | 70 | 0 | ['C'] | 17 |
reordered-power-of-2 | Quick Math | quick-math-by-fllght-8pw1 | The main idea is what powers of 2 look like in binary form:\n\n\n\nthis will help easily iterate over all powers of two\n\nSo all we need is to convert n to sor | FLlGHT | NORMAL | 2022-08-26T03:07:13.630261+00:00 | 2023-05-12T05:19:51.907428+00:00 | 6,415 | false | The main idea is what powers of 2 look like in binary form:\n\n<img width="200" src="https://assets.leetcode.com/users/images/ae9964b4-ffbd-40a5-a934-e3dc1de49d28_1661482426.3498342.png">\n\nthis will help easily iterate over all powers of two\n\nSo all we need is to convert n to sorted digits and then compare them with the sorted digits for each power of two.\n`n <= 10e9`, we only need to check powers in the range `[0,29]`\n\na little explanation of how the shift operation `<< `works: \n\n<img src="https://assets.leetcode.com/users/images/df39060e-624d-4fb8-abe2-e3f1da6d0bf9_1661486107.3084147.png">\n\n\n##### Java\n\n```java\npublic boolean reorderedPowerOf2(int n) {\n char[] number = sortedDigits(n);\n\n for (int i = 0; i < 30; ++i) {\n char[] powerOfTwo = sortedDigits(1 << i);\n if (Arrays.equals(number, powerOfTwo))\n return true;\n }\n return false;\n }\n\n private char[] sortedDigits(int n) {\n char[] digits = String.valueOf(n).toCharArray();\n Arrays.sort(digits);\n return digits;\n }\n```\n\n##### C++\n\n```\npublic:\n bool reorderedPowerOf2(int n) {\n string number = sortedDigits(n);\n\n for (int i = 0; i < 30; ++i) {\n string powerOfTwo = sortedDigits(1 << i);\n if (number == powerOfTwo)\n return true;\n }\n\n return false;\n }\n\nprivate:\n string sortedDigits(int n) {\n string digits = to_string(n);\n sort(digits.begin(), digits.end());\n return digits;\n }\n```\n\n##### Python\n```python\ndef reorderedPowerOf2(self, n: int) -> bool:\n digits = Counter(str(n))\n \n for i in range(30):\n powerOfTwo = str(1 << i)\n if digits == Counter(powerOfTwo):\n return True\n return False\n```\n\nMy repositories with leetcode problems solving - [Java](https://github.com/FLlGHT/algorithms/tree/master/j-algorithms/src/main/java), [C++](https://github.com/FLlGHT/algorithms/tree/master/c-algorithms/src/main/c%2B%2B)\n | 59 | 0 | ['C', 'Python', 'Java'] | 14 |
reordered-power-of-2 | ✅Reordered Power of 2 | Short & Easy w/ Explanation | Beats 100% | reordered-power-of-2-short-easy-w-explan-sn76 | Solution - I (Counting Digits Frequency)\n\nA simple solution is to check if frequency of digits in N and all powers of 2 less than 10^9 are equal. In our case, | archit91 | NORMAL | 2021-03-21T09:16:40.942964+00:00 | 2021-03-21T14:52:30.030476+00:00 | 3,360 | false | ***Solution - I (Counting Digits Frequency)***\n\nA simple solution is to check if frequency of digits in N and all powers of 2 less than `10^9` are equal. In our case, we need to check for all powers of 2 from `2^0` to `2^29` and if any of them matches with digits in `N`, return true.\n\n```\n// counts frequency of each digit in given number N and returns it as vector\nvector<int> countDigits(int N){\n\tvector<int>digitsInN(10);\n\twhile(N)\n\t\tdigitsInN[N % 10]++, N /= 10;\n\treturn digitsInN;\n}\nbool reorderedPowerOf2(int N) {\n\tvector<int> digitsInN = countDigits(N); // freq of digits in N\n\t// powOf2 goes from 2^0 to 2^29 and each time freq of digits in powOf2 is compared with digitsInN\n\tfor(int i = 0, powOf2 = 1; i < 30; i++, powOf2 <<= 1)\n\t\tif(digitsInN == countDigits(powOf2)) return true; // return true if both have same frequency of each digits\n\treturn false;\n}\n```\n\n**Time Complexity :** **`O(logn)`**, where `n` is maximum power of 2 for which digits are counted (2^30). More specifically the time complexity can be written as `O(logN + log2 + log4 + ... + log(2^30))` which after ignoring the constant factors and lower order terms comes out to `O(logn)`.\n**Time Complexity :** **`O(1)`**. We are using vector to store digits of `N` and powers of 2 but they are taking constant space and don\'t depend on the input `N`.\n\n---------\n---------\n\n***Solution - II (Convert to string & sort)***\n\nWe can convert `N` to string, sort it and compare it with every power of 2 by converting and sorting that as well.\n\n```\nbool reorderedPowerOf2(int N) {\n\tstring n = to_string(N);\n\tsort(begin(n), end(n));\n\tfor(int i = 0, powOf2 = 1; i < 30; i++, powOf2 <<= 1){\n\t\tstring pow2_str = to_string(powOf2);\n\t\tsort(begin(pow2_str), end(pow2_str));\n\t\tif(n == pow2_str) return true; \n\t}\n\treturn false;\n}\n```\n\n-------\n\nBoth solutions have the same run-time -\n\n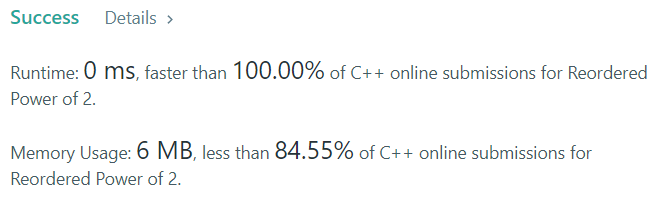\n\n-------\n------- | 42 | 1 | ['C'] | 5 |
reordered-power-of-2 | ✅Easy Java Solution || 100% Faster 1ms 🔥🔥 || With Basic Explanation | easy-java-solution-100-faster-1ms-with-b-appb | If you really found my solution helpful please upvote it, as it motivates me to post such kind of codes and help the coding community, if you have some queries | ganajayant | NORMAL | 2022-08-26T05:22:19.464149+00:00 | 2022-08-26T06:04:02.776417+00:00 | 2,943 | false | **If you really found my solution helpful please upvote it, as it motivates me to post such kind of codes and help the coding community, if you have some queries or some improvements please feel free to comment and share your views.**\n\nbasically we are finding occurrence of each digit in n after that we are finding same occurrence of each digit of each power of 2 which is in range between (2^0 to 2^30) if any power of 2 matches same occurence than we can return true if none of the power of 2 matches with occurence of our number n we return false\n\n```\npublic boolean reorderedPowerOf2(int n) {\n int Count[] = Count(n);\n int power = 1;\n for (int i = 0; i < 31; i++) {\n int[] PowerCount = Count(power);\n if (Equal(Count, PowerCount)) {\n return true;\n }\n power *= 2;\n }\n return false;\n }\n\n private int[] Count(int n) { // Counting Occurence of each digit\n int Count[] = new int[10];\n while (n != 0) {\n Count[n % 10]++;\n n /= 10;\n }\n return Count;\n }\n\n private boolean Equal(int ar1[], int ar2[]) {\n for (int i = 0; i < ar2.length; i++) {\n if (ar1[i] != ar2[i]) {\n return false;\n }\n }\n return true;\n }\n``` | 36 | 0 | ['Math', 'Counting', 'Java'] | 12 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.