question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
first-unique-character-in-a-string | Simple Python | simple-python-by-etherealoptimist-vuls | \nclass Solution:\n def firstUniqChar(self, s):\n d = {}\n seen = set()\n for idx, c in enumerate(s):\n if c not in seen:\n | etherealoptimist | NORMAL | 2018-09-11T22:16:41.899114+00:00 | 2018-09-11T22:16:41.899167+00:00 | 20,945 | false | ```\nclass Solution:\n def firstUniqChar(self, s):\n d = {}\n seen = set()\n for idx, c in enumerate(s):\n if c not in seen:\n d[c] = idx\n seen.add(c)\n elif c in d:\n del d[c]\n return min(d.values()) if d else -1\n``` | 92 | 2 | [] | 11 |
first-unique-character-in-a-string | Very Easy || 100% || Fully Explained || Java, C++, Python, JavaScript, Python3 | very-easy-100-fully-explained-java-c-pyt-0pcq | Java Solution:\n\nclass Solution {\n public int firstUniqChar(String s) {\n // Base case...\n if(s.length() == 0) return -1;\n // To ke | PratikSen07 | NORMAL | 2022-08-29T15:03:07.513946+00:00 | 2022-08-29T15:05:27.840391+00:00 | 23,712 | false | # **Java Solution:**\n```\nclass Solution {\n public int firstUniqChar(String s) {\n // Base case...\n if(s.length() == 0) return -1;\n // To keep track of the count of each character, we initialize an int[]store with size 26...\n int[] store = new int[26];\n // Traverse string to keep track number of times each character in the string appears...\n for(char ch : s.toCharArray()){\n store[ch - \'a\']++; // To access the store[] element representative of each character, we subtract \u2018a\u2019 from that character...\n }\n // Traverse string again to find a character that appears exactly one time, return it\u2019s index...\n for(int idx = 0; idx < s.length(); idx++){\n if(store[s.charAt(idx) - \'a\'] == 1){\n return idx;\n }\n }\n return -1; // if no character appeared exactly once...\n }\n}\n```\n\n# **C++ Solution:**\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n map<char, int> hmap;\n for (int idx{}; idx < s.size(); idx++) {\n // Everytime the character appears in the string, add one to its count\n hmap[s[idx]]++;\n }\n // Traverse the string from the beginning...\n for (int idx{}; idx < s.size(); idx++) {\n // If the count of the char is equal to 1, it is the first distinct character in the list.\n if (hmap[s[idx]] == 1)\n return idx;\n } \n return -1; // if no character appeared exactly once...\n }\n};\n```\n\n# **Python/Python3 Solution:**\n```\nclass Solution(object):\n def firstUniqChar(self, s):\n hset = collections.Counter(s);\n # Traverse the string from the beginning...\n for idx in range(len(s)):\n # If the count is equal to 1, it is the first distinct character in the list.\n if hset[s[idx]] == 1:\n return idx\n return -1 # If no character appeared exactly once...\n```\n \n# **JavaScript Solution:**\n```\nvar firstUniqChar = function(s) {\n for (let idx = 0; idx < s.length; idx++){\n // If same...\n if(s.indexOf(s[idx]) === s.lastIndexOf(s[idx])){\n // return the index of that unique character\n return idx\n } else {\n return -1 // If no character appeared exactly once...\n }\n }\n};\n```\n**I am working hard for you guys...\nPlease upvote if you found any help with this code...** | 86 | 0 | ['String', 'Queue', 'C', 'Python', 'Java', 'Python3', 'JavaScript'] | 13 |
first-unique-character-in-a-string | C++ O(n) 4 Lines solution, Beats 97% | c-on-4-lines-solution-beats-97-by-sherla-ul7w | \nclass Solution {\npublic:\n int firstUniqChar(string s) {\n vector<int> v(26,0);\n\t\tfor(char c : s) v[c - \'a\']++;\n\t\tfor(int i = 0; i < s.leng | sherlasd | NORMAL | 2020-02-20T15:51:16.595671+00:00 | 2020-03-05T20:09:01.717696+00:00 | 15,520 | false | ```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n vector<int> v(26,0);\n\t\tfor(char c : s) v[c - \'a\']++;\n\t\tfor(int i = 0; i < s.length(); i++){\n\t\t\tif(v[s[i] - \'a\'] == 1) return i;\n\t\t}\n\t\treturn -1;\n }\n};\n``` | 86 | 3 | ['C', 'C++'] | 12 |
first-unique-character-in-a-string | Java Simple "Another thought" | java-simple-another-thought-by-santanu_3-hudx | ```java\n\n public int firstUniqChar(String s) {\n for(char c : s.toCharArray()){\n int index = s.indexOf(c);\n int lastIndex = | santanu_33 | NORMAL | 2020-06-19T09:41:27.792520+00:00 | 2020-06-19T09:45:47.273567+00:00 | 7,646 | false | ```java\n\n public int firstUniqChar(String s) {\n for(char c : s.toCharArray()){\n int index = s.indexOf(c);\n int lastIndex = s.lastIndexOf(c);\n if(index == lastIndex)\n return index;\n }\n return -1;\n }\n//runtime O(n^2) | 80 | 2 | ['Java'] | 15 |
first-unique-character-in-a-string | 5 Lines of Code in Two Methods | 5-lines-of-code-in-two-methods-by-ganjin-3slq | \n\n# 1. Count the first occurence one and break loop\n\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i,c in enumerate(s):\n | GANJINAVEEN | NORMAL | 2023-03-21T20:50:02.833536+00:00 | 2023-03-21T20:50:02.833581+00:00 | 9,732 | false | \n\n# 1. Count the first occurence one and break loop\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i,c in enumerate(s):\n if s.count(c)==1:\n return i\n break\n return -1\n #please upvote me it would encourage me alot\n\n```\n# 2. Using Hashtable and Set\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n dic={}\n seen=set()\n for ind,let in enumerate(s):\n if let not in seen:\n dic[let]=ind\n seen.add(let)\n elif let in dic:\n del dic[let]\n return min(dic.values()) if dic else -1\n\n //please upvote me it would encourage me alot\n\n```\n# please upvote me it would encourage me alot\n | 59 | 1 | ['Python3'] | 9 |
first-unique-character-in-a-string | ✅☑Beats 100% Users || Easy Understood Solution with optimized space || 3 Approaches🔥 | beats-100-users-easy-understood-solution-6evx | \n\n# brute-force approach: \n - Initialization:\n - Get the length of the input string s and store it in the variable n.\n - Main Loop:\n - Iterate thr | MindOfshridhar | NORMAL | 2024-02-05T02:05:41.422545+00:00 | 2024-02-05T02:48:16.965286+00:00 | 10,283 | false | 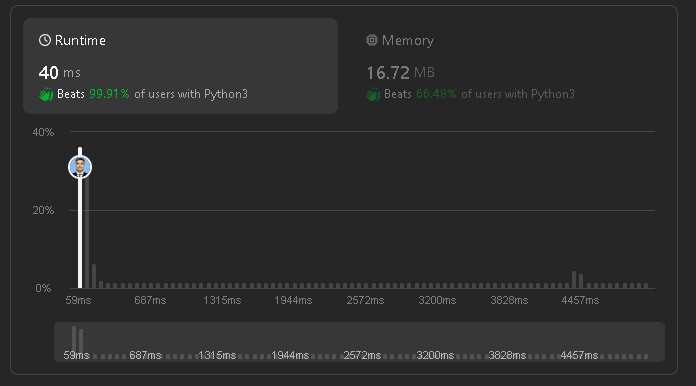\n\n# brute-force approach: \n - Initialization:\n - Get the length of the input string s and store it in the variable n.\n - Main Loop:\n - Iterate through each character in the string using the index i from 0 to n-1.\n - Duplicate Check:\n - For each character at index i, set count to 0 to track duplicate status.\n - Check for duplicates before the current index (i) by iterating through characters with index k from 0 to i-1.\n - If a duplicate is found, set count to 1 and break out of the loop.\n - Check After Current Index:\n - If no duplicates are found before the current index, check for duplicates after the current index (i) by iterating through characters with index j from i+1 to n-1.\n - If a duplicate is found, set count to 1 and break out of the loop.\n - Return First Unique:\n - If no duplicates are found, return the current index (i) as it represents the first unique character.\n - Fallback:\n - If no unique character is found in the entire string, return -1.\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1) \n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n n = len(s)\n \n # Iterate through each character in the string\n for i in range(n):\n count = 0\n \n # Check for duplicates before the current index\n for k in range(0, i):\n if s[i] == s[k]:\n count = 1\n break\n \n # If no duplicates before, check after the current index\n if count == 0:\n for j in range(i + 1, n):\n if s[i] == s[j]:\n count = 1\n break\n \n # If no duplicates found, return the current index\n if count == 0:\n return i\n \n # If no unique character is found, return -1\n return -1\n```\n# Code 2 (Map)\n\n - Initialization:\n - Create an empty dictionary freq_dict to store character frequencies.\n - Character Frequency Count:\n - Iterate through each character in the input string s.\n - For each character, update its frequency count in freq_dict.\n - If the character is not in freq_dict, set its count to 1.\n - If the character is already in freq_dict, increment its count by 1.\n - Find First Unique Character:\n - Iterate through each character and its index using enumerate(s).\n - Check if the frequency of the character in freq_dict is equal to 1.\n - If true, return the index of the first occurrence of the unique character.\n - Fallback:\n - If no unique character is found in the entire string, return -1.\n``` Python []\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n freq_dict = {}\n \n # Count character frequencies\n for char in s:\n freq_dict[char] = freq_dict.get(char, 0) + 1\n \n # Find the first unique character\n for i, char in enumerate(s):\n if freq_dict[char] == 1:\n return i\n \n # If no unique character is found\n return -1\n```\n``` C++ []\n#include <iostream>\n#include <unordered_map>\n\nclass Solution {\npublic:\n int firstUniqChar(std::string s) {\n std::unordered_map<char, int> freq_map;\n \n // Count character frequencies\n for (char c : s) {\n freq_map[c]++;\n }\n \n // Find the first unique character\n for (int i = 0; i < s.length(); ++i) {\n if (freq_map[s[i]] == 1) {\n return i;\n }\n }\n \n // If no unique character is found\n return -1;\n }\n};\n```\n``` java []\nimport java.util.HashMap;\nimport java.util.Map;\n\nclass Solution {\n public int firstUniqChar(String s) {\n Map<Character, Integer> freqMap = new HashMap<>();\n \n // Count character frequencies\n for (char c : s.toCharArray()) {\n freqMap.put(c, freqMap.getOrDefault(c, 0) + 1);\n }\n \n // Find the first unique character\n for (int i = 0; i < s.length(); ++i) {\n if (freqMap.get(s.charAt(i)) == 1) {\n return i;\n }\n }\n \n // If no unique character is found\n return -1;\n }\n}\n```\n``` JavaScript []\n/**\n * @param {string} s\n * @return {number}\n */\nvar firstUniqChar = function(s) {\n // Create an object to store character frequencies\n const freqMap = {};\n \n // Count character frequencies\n for (const char of s) {\n freqMap[char] = (freqMap[char] || 0) + 1;\n }\n \n // Find the first unique character\n for (let i = 0; i < s.length; ++i) {\n if (freqMap[s[i]] === 1) {\n return i;\n }\n }\n \n // If no unique character is found\n return -1;\n};\n```\n# Code 3 (Without Map) \uD83D\uDD25\n# Explanation\n - Initialization:\n - A pre-defined order of characters is created and stored in the variable key as \'abcdefghijklmnopqrstuvwxyz\'.\n - An initial large index, idx, is set to 10^5.\n - Character Iteration:\n - The code iterates through each character in the pre-defined order (key).\n - Character Search:\n - For each character in the iteration, it finds the first occurrence of that character in the input string s using s.find(i).\n - Uniqueness Check:\n - It checks if the character is unique in the string by verifying that its first occurrence is also its last occurrence (s.rfind(i)).\n - Update Minimum Index:\n - If the character is unique, it updates the minimum index (idx) with the minimum of its current value and the first occurrence index.\n - Result:\n - After iterating through all characters, the code returns the minimum index if a unique character is found; otherwise, it returns -1.\n\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n key = \'abcdefghijklmnopqrstuvwxyz\' # Pre-defined order of characters\n idx = 10**5 # Set an initial large index\n \n # Iterate through each character in the pre-defined order\n for i in key:\n x = s.find(i) # Find the first occurrence of the character\n # Check if the character is unique (not found after the first occurrence)\n if x != -1 and x == s.rfind(i):\n idx = min(idx, x) # Update the minimum index\n \n # Return the minimum index if found, otherwise return -1\n return idx if idx != 10**5 else -1\n```\n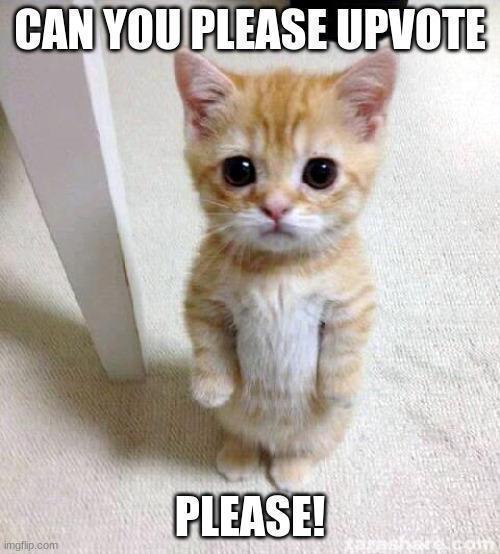\n | 56 | 2 | ['Hash Table', 'String', 'Dynamic Programming', 'Queue', 'Counting', 'Python', 'C++', 'Java', 'Python3', 'JavaScript'] | 6 |
first-unique-character-in-a-string | [ Python ] ✅✅ Simple Python Solution With Two Approach 🥳✌👍 | python-simple-python-solution-with-two-a-1bb9 | If You like the Solution, Don\'t Forget To UpVote Me, Please UpVote! \uD83D\uDD3C\uD83D\uDE4F\n# Approach 1: - Iterative \n# Runtime: 89 ms, faster than 67.59% | ashok_kumar_meghvanshi | NORMAL | 2022-02-23T06:36:33.395875+00:00 | 2024-02-05T06:20:37.386964+00:00 | 5,834 | false | # If You like the Solution, Don\'t Forget To UpVote Me, Please UpVote! \uD83D\uDD3C\uD83D\uDE4F\n# Approach 1: - Iterative \n# Runtime: 89 ms, faster than 67.59% of Python3 online submissions for First Unique Character in a String.\n# Memory Usage: 16.7 MB, less than 66.48% of Python3 online submissions for First Unique Character in a String.\n\n\tclass Solution:\n\t\tdef firstUniqChar(self, s: str) -> int:\n\n\t\t\tfor i in range(len(s)):\n\n\t\t\t\tif s[i] not in s[:i] and s[i] not in s[i+1:]:\n\n\t\t\t\t\treturn i\n\n\t\t\treturn -1\n\n# Approach 2: - HashMap or Dictionary \n# Runtime: 137 ms, faster than 71.83% of Python3 online submissions for First Unique Character in a String.\n# Memory Usage: 14.2 MB, less than 18.33% of Python3 online submissions for First Unique Character in a String.\n\n\tclass Solution:\n\t\tdef firstUniqChar(self, s: str) -> int:\n\n\t\t\tfrequency = {}\n\n\t\t\tfor char in s:\n\n\t\t\t\tif char not in frequency:\n\t\t\t\t\tfrequency[char] = 1\n\t\t\t\telse:\n\t\t\t\t\tfrequency[char] = frequency[char] + 1\n\n\t\t\tfor index in range(len(s)):\n\n\t\t\t\tif frequency[s[index]] == 1:\n\t\t\t\t\treturn index\n\n\t\t\treturn -1\n\t\t\t\n-------------------------------------------------------------------------------------------------------\n\n\tclass Solution:\n\t\tdef firstUniqChar(self, s: str) -> int:\n\n\t\t\thash_map = defaultdict(int)\n\n\t\t\tfor char in s:\n\n\t\t\t\thash_map[char] = hash_map[char] + 1\n\n\t\t\tfor index in range(len(s)):\n\n\t\t\t\tif hash_map[s[index]] == 1:\n\n\t\t\t\t\treturn index\n\n\t\t\treturn -1\n\t\n\tTime Complexity : O(N)\n\tSpace Complexity : O(N)\n# Thank You \uD83E\uDD73\u270C\uD83D\uDC4D | 53 | 0 | ['Iterator', 'Python', 'Python3'] | 2 |
first-unique-character-in-a-string | My Javascript Solution | my-javascript-solution-by-miryang-l8sy | \nvar firstUniqChar = function(s) {\n for(i=0; i<s.length; i++)\n if(s.indexOf(s[i])===s.lastIndexOf(s[i])) return i\n return -1\n};\n | miryang | NORMAL | 2019-08-29T04:12:34.628594+00:00 | 2019-08-29T04:12:34.628631+00:00 | 6,347 | false | ```\nvar firstUniqChar = function(s) {\n for(i=0; i<s.length; i++)\n if(s.indexOf(s[i])===s.lastIndexOf(s[i])) return i\n return -1\n};\n``` | 51 | 4 | ['JavaScript'] | 9 |
first-unique-character-in-a-string | Python - Simple Solution | python-simple-solution-by-nuclearoreo-xm0x | \nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n d = {}\n for l in s:\n if l not in d: d[l] = 1\n else: d[l] | nuclearoreo | NORMAL | 2019-08-10T03:52:09.292732+00:00 | 2019-08-10T03:52:09.292797+00:00 | 12,232 | false | ```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n d = {}\n for l in s:\n if l not in d: d[l] = 1\n else: d[l] += 1\n \n index = -1\n for i in range(len(s)):\n if d[s[i]] == 1:\n index = i\n break\n \n return index\n``` | 47 | 3 | ['Python', 'Python3'] | 10 |
first-unique-character-in-a-string | my 4 lines Java solution | my-4-lines-java-solution-by-lindsayling-tg39 | ```\npublic static int firstUniqChar(String s) {\n \n\t\tchar[] a = s.toCharArray();\n\t\t\n\t\tfor(int i=0; i<a.length;i++){\n\t\t\tif(s.indexOf(a[i])== | lindsayling | NORMAL | 2016-08-27T20:25:35.125000+00:00 | 2018-10-07T01:14:20.334798+00:00 | 17,756 | false | ```\npublic static int firstUniqChar(String s) {\n \n\t\tchar[] a = s.toCharArray();\n\t\t\n\t\tfor(int i=0; i<a.length;i++){\n\t\t\tif(s.indexOf(a[i])==s.lastIndexOf(a[i])){return i;}\n\t\t}\n\t\treturn -1;\n } | 45 | 10 | [] | 16 |
first-unique-character-in-a-string | ✅ 🔥 0 ms Runtime Beats 100% User confirm 🔥|| Step By Steps Solution ✅ || Beginner Friendly ✅ | | 0-ms-runtime-beats-100-user-confirm-step-09tz | \u2705 IF YOU LIKE THIS SOLUTION, PLEASE UPVOTE AT THE END \u2705 :\n\n### Intuition:\nTo find the first non-repeating character in a string, we need to determ | Letssoumen | NORMAL | 2024-11-17T02:58:25.628595+00:00 | 2024-11-17T02:58:25.628626+00:00 | 10,876 | false | \u2705 IF YOU LIKE THIS SOLUTION, PLEASE UPVOTE AT THE END \u2705 :\n\n### Intuition:\nTo find the first non-repeating character in a string, we need to determine the frequency of each character and then identify the first character with a frequency of 1. This can be efficiently achieved using a single pass to count frequencies and a second pass to find the desired character.\n\n---\n\n### Approach:\n1. Use a hash map or array of size 26 to store the frequency of each character (since the input consists of lowercase English letters).\n2. Traverse the string to count the frequency of each character.\n3. Traverse the string again to find the first character with a frequency of 1. Return its index.\n4. If no such character is found, return -1.\n\n---\n\n### Complexity:\n- **Time Complexity**: \\( O(n) \\), where \\( n \\) is the length of the string. The string is traversed twice.\n- **Space Complexity**: \\( O(1) \\), as the frequency array has a fixed size of 26.\n\n---\n\n### C++ Code:\n```cpp\n#include<string>\n#include<vector>\nusing namespace std;\nclass Solution{\npublic:\nint firstUniqChar(string s){\nvector<int>freq(26,0);\nfor(char c:s){\nfreq[c-\'a\']++;\n}\nfor(int i=0;i<s.size();i++){\nif(freq[s[i]-\'a\']==1){\nreturn i;\n}\n}\nreturn -1;\n}\n};\n\n```\n\n---\n\n### Java Code:\n```java\nclass Solution{\npublic int firstUniqChar(String s){\nint[]freq=new int[26];\nfor(char c:s.toCharArray()){\nfreq[c-\'a\']++;\n}\nfor(int i=0;i<s.length();i++){\nif(freq[s.charAt(i)-\'a\']==1){\nreturn i;\n}\n}\nreturn -1;\n}\n}\n\n```\n\n---\n\n### Python 3 Code:\n```python\nclass Solution:\ndef firstUniqChar(self,s:str)->int:\nfreq=[0]*26\nfor c in s:\nfreq[ord(c)-ord(\'a\')]+=1\nfor i,c in enumerate(s):\nif freq[ord(c)-ord(\'a\')]==1:\nreturn i\nreturn -1\n\n```\n\n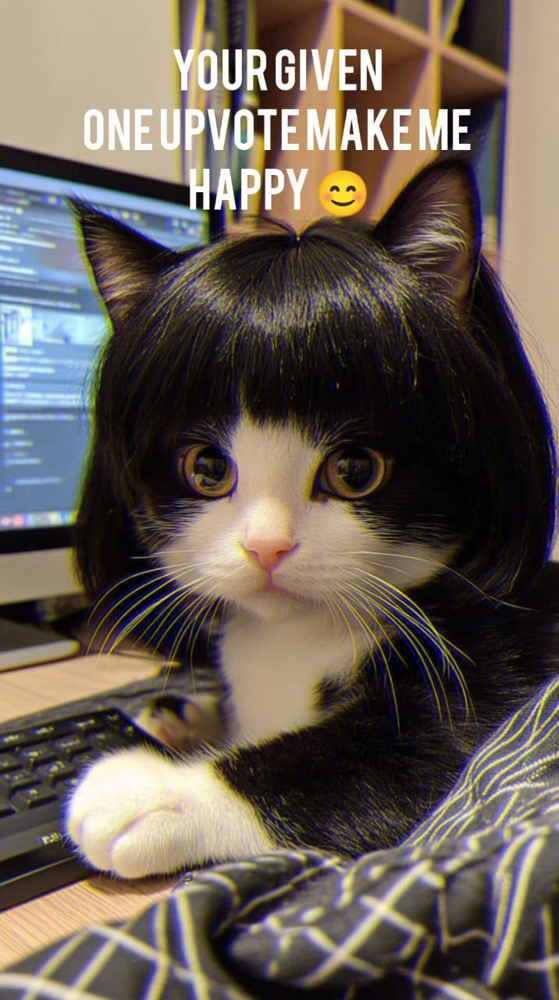\n | 43 | 0 | ['Hash Table', 'String', 'C++', 'Java', 'Python3'] | 3 |
first-unique-character-in-a-string | Java two pointers (slow and fast) solution (18 ms) | java-two-pointers-slow-and-fast-solution-6wgv | The idea is to use a slow pointer to point to the current unique character and a fast pointer to scan the string. The fast pointer not only just add the count o | yubad2000 | NORMAL | 2016-08-23T04:13:51.708000+00:00 | 2018-09-19T20:29:15.179815+00:00 | 30,961 | false | The idea is to use a slow pointer to point to the current unique character and a fast pointer to scan the string. The fast pointer not only just add the count of the character. Meanwhile, when fast pointer finds the identical character of the character at the current slow pointer, we move the slow pointer to the next unique character or **not visited** character. (20 ms)\n\n```\npublic class Solution {\n public int firstUniqChar(String s) {\n if (s==null || s.length()==0) return -1;\n int len = s.length();\n if (len==1) return 0;\n char[] cc = s.toCharArray();\n int slow =0, fast=1;\n int[] count = new int[256];\n count[cc[slow]]++;\n while (fast < len) {\n count[cc[fast]]++;\n // if slow pointer is not a unique character anymore, move to the next unique one\n while (slow < len && count[cc[slow]] > 1) slow++; \n if (slow >= len) return -1; // no unique character exist\n if (count[cc[slow]]==0) { // not yet visited by the fast pointer\n count[cc[slow]]++; \n fast=slow; // reset the fast pointer\n }\n fast++;\n }\n return slow;\n }\n}\n``` | 42 | 1 | [] | 16 |
first-unique-character-in-a-string | Javascript Simple 2 Iterations (hash, search) | javascript-simple-2-iterations-hash-sear-8et8 | \nvar firstUniqChar = function(s) {\n let map = {}\n \n for (let char of s) {\n map[char] ? map[char]++ : map[char] = 1\n }\n \n for (l | cu6upb | NORMAL | 2020-01-07T05:19:52.683371+00:00 | 2020-01-07T05:19:52.683422+00:00 | 4,687 | false | ```\nvar firstUniqChar = function(s) {\n let map = {}\n \n for (let char of s) {\n map[char] ? map[char]++ : map[char] = 1\n }\n \n for (let i = 0; i < s.length; i++) {\n if (map[s[i]] === 1) return i\n }\n \n return -1\n};\n``` | 36 | 0 | ['JavaScript'] | 5 |
first-unique-character-in-a-string | Python 4 Lines, beats 98% ~52ms | python-4-lines-beats-98-52ms-by-deepgosa-7kxq | \nfrom collections import OrderedDict, Counter\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n\t# Explaination: Ordered Dict will save the chara | deepgosalia1 | NORMAL | 2020-05-17T17:16:04.508983+00:00 | 2022-11-16T22:04:14.610911+00:00 | 5,425 | false | ```\nfrom collections import OrderedDict, Counter\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n\t# Explaination: Ordered Dict will save the characters it encounters in\n\t# same sequence as the original string. Hence it becomes easy to catch hold of the first\n\t#unique character. Then according to the counter variable, whenever the first 1 is encountered\n\t# the corresponding dict.key\'s index is returned from the original String.\n for i,j in OrderedDict(Counter(s)).items():\n if j == 1:\n return s.index(i)\n return -1\n```\n\nEdit (2022): With updates in Python Dict, Counter now preserves order of elements during insertions. So usage of OrderedDict is redundant now.\n```\nfrom collections import Counter\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i,j in Counter(s).items():\n if j == 1:\n return s.index(i)\n return -1\n``` | 34 | 0 | ['Python', 'Python3'] | 7 |
first-unique-character-in-a-string | ✅Short || C++ || Java || PYTHON || Explained Solution✔️ || Beginner Friendly ||🔥 🔥 BY MR CODER | short-c-java-python-explained-solution-b-iisf | Please UPVOTE if you LIKE!!\nWatch this video \uD83E\uDC83 for the better explanation of the code.\n\nhttps://www.youtube.com/watch?v=HVmq6apu3Zk\n\n\nAlso you | mrcoderrm | NORMAL | 2022-08-16T04:04:38.699089+00:00 | 2022-08-16T09:37:08.214459+00:00 | 6,965 | false | **Please UPVOTE if you LIKE!!**\n**Watch this video \uD83E\uDC83 for the better explanation of the code.**\n\nhttps://www.youtube.com/watch?v=HVmq6apu3Zk\n\n\n**Also you can SUBSCRIBE \uD83E\uDC81 \uD83E\uDC81 \uD83E\uDC81 this channel for the daily leetcode challange solution.**\n\n\n**C++**\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n \n unordered_map<char,int> ump(26);\n for(int i=0; i<s.size(); i++){\n ump[s[i]]++;\n }\n \n \n for(int i=0; i<s.size(); i++){\n if(ump[s[i]]==1) return i;\n }\n \n \n return -1;\n }\n};\n```\n\n**PYTHON**(copied)\n\n```\nclass Solution(object):\n def firstUniqChar(self, s):\n \n # Store drequency of all the characters of string in the array\n freq = Counter(s)\n \n # now check frequency of each character in the string from left-to-right\n # and whose frequency found to be 1 return the index\n for e in s :\n if freq[e] == 1 :\n return s.index(e)\n \n return -1\n```\n**JAVA**(copied)\n\n```\nclass Solution {\n public int firstUniqChar(String s) {\n \n char[] arr = s.toCharArray();\n int len = arr.length;\n \n \n int[] fre = new int[26];\n for(char c : arr) fre[c-\'a\']++;\n \n \n for(int i=0; i<len; i++) if(fre[arr[i]-\'a\'] == 1) return i;\n return -1;\n }\n}\n```\n**Please UPVOTE if you LIKE!!** | 28 | 3 | ['C', 'Python', 'C++', 'Java'] | 5 |
first-unique-character-in-a-string | 1-liners in Python, 76ms | 1-liners-in-python-76ms-by-o_sharp-mz2c | \nclass Solution(object):\n def firstUniqChar(self, s):\n return min([s.find(c) for c in string.ascii_lowercase if s.count(c)==1] or [-1])\n\nIt gave | o_sharp | NORMAL | 2016-08-22T08:04:31.003000+00:00 | 2016-08-22T08:04:31.003000+00:00 | 16,329 | false | ```\nclass Solution(object):\n def firstUniqChar(self, s):\n return min([s.find(c) for c in string.ascii_lowercase if s.count(c)==1] or [-1])\n```\nIt gave me 76ms.\n\nOr\n```\nclass Solution(object):\n def firstUniqChar(self, s):\n return min([s.find(c) for c,v in collections.Counter(s).iteritems() if v==1] or [-1])\n```\nwhich is slower. | 27 | 2 | [] | 6 |
first-unique-character-in-a-string | 🔥 Python || Easily Understood ✅ || Faster than 99.7% || 5 Lines | python-easily-understood-faster-than-997-yasj | Appreciate if you could upvote this solution\n\nCode:\n\ndef firstUniqChar(self, s: str) -> int:\n\tchr_count = Counter(s)\n\tfor x in chr_count:\n\t\tif chr_co | wingskh | NORMAL | 2022-08-16T02:56:13.381411+00:00 | 2022-08-16T03:04:33.620126+00:00 | 2,256 | false | **Appreciate if you could upvote this solution**\n\nCode:\n```\ndef firstUniqChar(self, s: str) -> int:\n\tchr_count = Counter(s)\n\tfor x in chr_count:\n\t\tif chr_count[x] == 1:\n\t\t\treturn s.index(chr_count[x])\n\treturn -1\n```\n\n**Time Complexity**: `O(n)`\n**Space Complexity**: `O(n)` | 26 | 0 | ['Python'] | 3 |
first-unique-character-in-a-string | C++✅ || Beats 100%💯|| 4 Lines Code💀💯|| EasyPizyy🔥💯 | c-beats-100-4-lines-code-easypizyy-by-ya-f9tb | 💡 IntuitionTo find the first unique character in a string, we need to determine the first character that appears only once. 🔍🛠️ Approach
📌 Use an unordered_map | yashm01 | NORMAL | 2025-02-10T03:43:38.677080+00:00 | 2025-02-10T03:43:38.677080+00:00 | 2,342 | false | # 💡 Intuition
To find the **first unique character** in a string, we need to determine the first character that appears **only once**. 🔍
# 🛠️ Approach
1. 📌 Use an **unordered_map** to store the frequency of each character.
2. 🔄 Iterate through the string to **count occurrences** of each character.
3. 🚀 Iterate again to find the **first character** with a frequency of **1** and return its index.
4. ❌ If no unique character is found, return **-1**.
# ⏳ Complexity
- **Time Complexity:** ⏱️ $$O(n)$$
- **Space Complexity:** 📦 $$O(1)$$ (Since the map stores at most 26 letters)
# 💻 Code
```cpp
class Solution {
public:
int firstUniqChar(string s) {
unordered_map<char, int> umap;
for (char c : s) umap[c]++; // 🔢 Count character frequency
for (int i = 0; i < s.length(); i++)
if (umap[s[i]] == 1) return i; // 🎯 Find the first unique character
return -1; // ❌ No unique character found
}
};
```
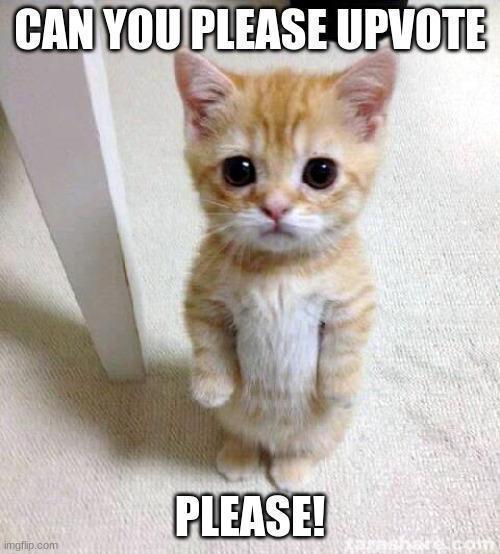
| 24 | 0 | ['Hash Table', 'String', 'Queue', 'Counting', 'C++'] | 0 |
first-unique-character-in-a-string | ✅ Three Java Simple Solution | three-java-simple-solution-by-ahmedna126-drb5 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ahmedna126 | NORMAL | 2023-10-24T19:54:25.107254+00:00 | 2023-11-07T11:30:30.517847+00:00 | 2,551 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code1\n```\nclass Solution {\n public int firstUniqChar(String s) {\n for (int i = 0; i < s.length(); i++) {\n int c = s.charAt(i);\n if (i == s.lastIndexOf(c) && i == s.indexOf(c)) return i;\n }\n\n return -1;\n\n }\n}\n```\n\n# Code2\n\n```\nimport java.util.Hashtable;\nclass Solution {\n public int firstUniqChar(String s) {\n Hashtable<Character , Integer> hashtable = new Hashtable<>();\n for (char c : s.toCharArray())\n {\n hashtable.put(c , hashtable.getOrDefault(c , 0) +1);\n }\n\n if (!hashtable.containsValue(1)) return -1;\n\n for (int i = 0; i < s.length(); i++) {\n if (hashtable.get(s.charAt(i)) == 1) return i;\n } \n \n return -1;\n }\n}\n```\n\n# Code3\n```\nclass Solution {\n public int firstUniqChar(String s) {\n HashSet<Character> hashSet = new HashSet<>();\n HashSet<Character> letters = new HashSet<>();\n\n for (char c : s.toCharArray() )\n {\n letters.add(c);\n if (hashSet.contains(c)) letters.remove(c);\n hashSet.add(c);\n }\n if (letters.isEmpty()) return -1;\n \n int i = 0;\n for (char c : s.toCharArray())\n {\n if (letters.contains(c)) return i;\n i++;\n }\n\n\n return -1;\n }\n}\n```\n## For additional problem-solving solutions, you can explore my repository on GitHub: [GitHub Repository](https://github.com/ahmedna126/java_leetcode_challenges)\n\n\n\n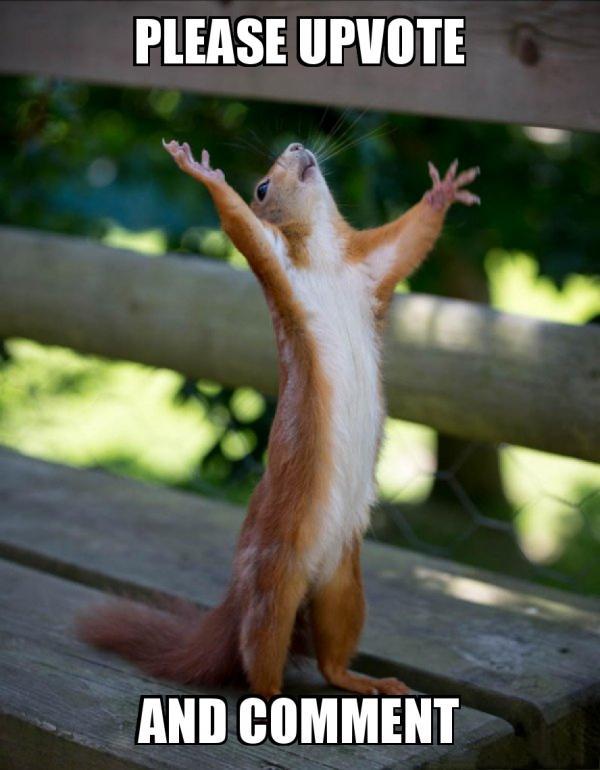\n\n | 23 | 0 | ['Hash Table', 'Java'] | 2 |
first-unique-character-in-a-string | Easy C++ Solution, beats 97% | easy-c-solution-beats-97-by-pooja0406-vqqy | Runtime: 32 ms, faster than 97.50% of C++ online submissions for First Unique Character in a String.\nMemory Usage: 12.6 MB, less than 100.00% of C++ online sub | pooja0406 | NORMAL | 2019-09-11T09:04:54.573381+00:00 | 2019-09-11T09:04:54.573416+00:00 | 3,766 | false | Runtime: 32 ms, faster than 97.50% of C++ online submissions for First Unique Character in a String.\nMemory Usage: 12.6 MB, less than 100.00% of C++ online submissions for First Unique Character in a String.\n\n```\nint firstUniqChar(string s) {\n \n int count[26] = {0};\n for(int i = 0; i<s.length(); i++)\n count[s[i] - \'a\']++;\n \n for(int i = 0; i<s.length(); i++)\n if(count[s[i] - \'a\'] == 1)\n return i;\n \n return -1;\n } | 23 | 2 | ['C'] | 4 |
first-unique-character-in-a-string | Java solution 1ms beats 100% | java-solution-1ms-beats-100-by-sameer_sa-k0q6 | \nclass Solution {\n\t\tpublic int firstUniqChar(String s) {\n int ans = Integer.MAX_VALUE;\n for (char i = \'a\'; i <= \'z\';i++) {\n | Sameer_Saraswat | NORMAL | 2021-12-16T16:19:02.967254+00:00 | 2021-12-16T16:27:21.973979+00:00 | 3,108 | false | ```\nclass Solution {\n\t\tpublic int firstUniqChar(String s) {\n int ans = Integer.MAX_VALUE;\n for (char i = \'a\'; i <= \'z\';i++) {\n int ind = s.indexOf (i);\n if (ind != -1 && ind == s.lastIndexOf (i))\n ans = Math.min (ans,ind);\n }\n if (ans == Integer.MAX_VALUE)\n return -1;\n return ans;\n }\n}\n``` | 21 | 1 | ['Java'] | 2 |
first-unique-character-in-a-string | ✔️Python🔥99% Unique & Fastest solution | Detailed Explanation | Easy understand | beginner-friendly | python99-unique-fastest-solution-detaile-7ddx | Please UPVOTE if you LIKE !!\n\nSuper fast solution:\n Instead of counting every character, we use find() & rfind() to find the first and the last index of that | luluboy168 | NORMAL | 2022-08-16T11:30:26.100903+00:00 | 2022-08-16T11:36:34.142434+00:00 | 3,015 | false | **Please UPVOTE if you LIKE !!**\n\nSuper fast solution:\n* Instead of counting every character, we use `find()` & `rfind()` to find the first and the last index of that character.\n* If both index is the same, it means that the character only appears once.\n* Store the smallest index we found, because it\'s the answer.\n\nTake `"leetcode"` for example:\n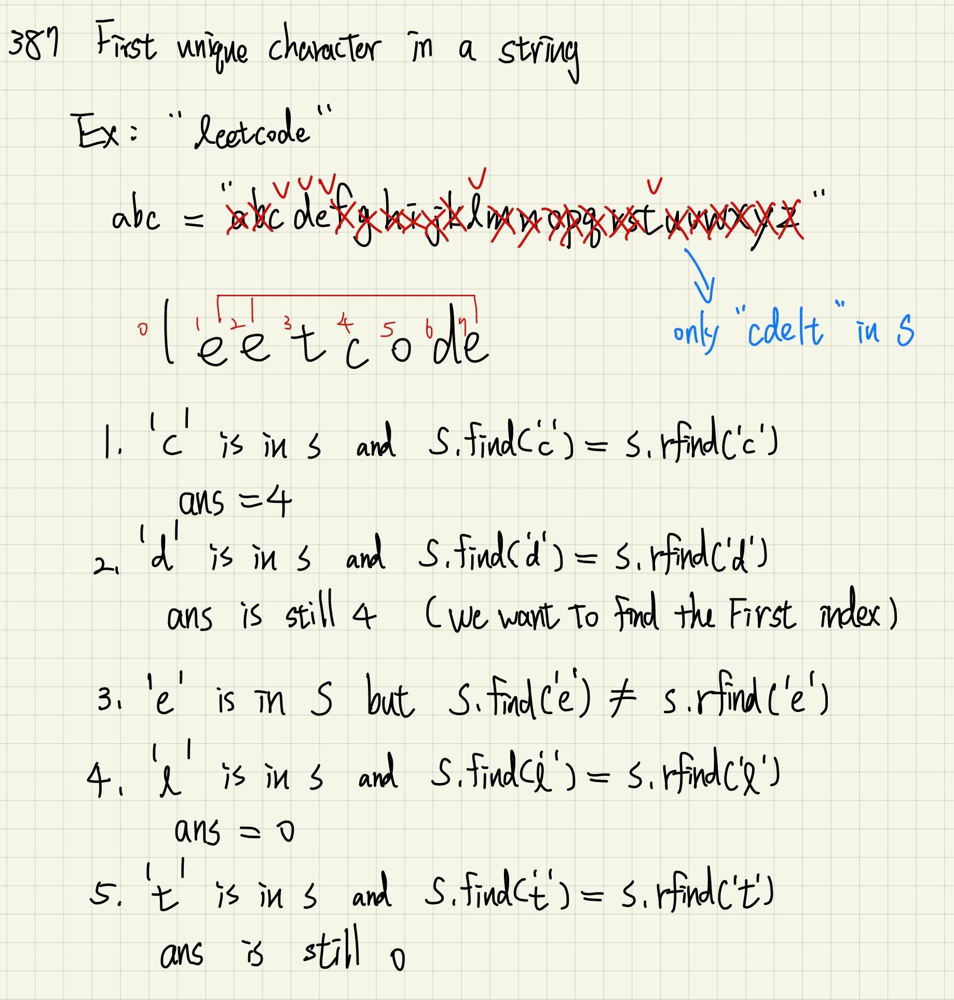\n\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n abc = "abcdefghijklmnopqrstuvwxyz"\n ans = 10**5 # since 1 <= s.length <= 105, the answer must be smaller than 10^5\n print(s.find(\'e\'))\n for c in abc:\n idx = s.find(c) # check if this word is in s\n if (idx != -1 and idx == s.rfind(c)): # check if first index == last index\n ans = min(ans, idx) # store the smallest \n \n return ans if ans < 10**5 else -1\n```\nFaster than 99%, really amazing!\n\n\nRegular solution (It\'s slower but easy to understand):\n* Just simply count how many times a character appears in s\n* If the character only appears once, we find the answer.\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n \n cnt = Counter(s)\n \n for i, c in enumerate(s):\n if cnt[c] == 1:\n return i\n \n return -1\n```\nHere\'s the easiest code for better understand:\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n \n cnt = {}\n \n for c in s:\n if c not in cnt:\n cnt[c] = 1\n else:\n cnt[c] += 1\n \n for i in range(len(s)):\n if cnt[s[i]] == 1:\n return i\n \n return -1\n```\n\n\n | 20 | 0 | ['Python'] | 6 |
first-unique-character-in-a-string | CPP || Hashmaps || | cpp-hashmaps-by-akanshapanchal21-u51z | \n#include<unordered_map>\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map<char,int> map;\n for(int i=0; i<s.length() | akanshapanchal21 | NORMAL | 2021-03-24T07:01:22.296653+00:00 | 2021-03-24T07:01:22.296694+00:00 | 2,650 | false | ```\n#include<unordered_map>\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map<char,int> map;\n for(int i=0; i<s.length(); i++){\n if(map.count(s[i])==0)\n map[s[i]] = 1;\n else\n map[s[i]]++; \n }\n for(int i=0; i<s.length(); i++)\n if(map[s.at(i)] == 1)\n return i;\n return -1;\n }\n};\n``` | 20 | 1 | ['C', 'C++'] | 2 |
first-unique-character-in-a-string | C++ 3 simple and easy solutions || concise solutions | c-3-simple-and-easy-solutions-concise-so-wqb9 | please upvote if you find it helpful\n\nclass Solution {\npublic:\n int firstUniqChar(string s)\n {\n unordered_map<char, int> letter;\n con | sailakshmi1 | NORMAL | 2022-08-16T00:03:35.411195+00:00 | 2022-08-16T00:08:19.620705+00:00 | 2,225 | false | **please upvote if you find it helpful**\n```\nclass Solution {\npublic:\n int firstUniqChar(string s)\n {\n unordered_map<char, int> letter;\n const int sSize = s.size();\n for (int i = 0; i < sSize; ++i)\n ++letter[s[i]];\n\n for (int i = 0; i < sSize; ++i)\n if (letter[s[i]] == 1)\n return i;\n return -1;\n }\n};\n```\n```\nclass Solution {\npublic:\n\tint firstUniqChar(string s) {\n unordered_map<char, pair<int, int>> m;\n int idx = s.size();\n for (int i = 0; i < s.size(); i++) {\n m[s[i]].first++;\n m[s[i]].second = i;\n }\n for (const auto& [c, p] : m) {\n if (p.first == 1) {\n idx = min(idx, p.second);\n }\n }\n return idx == s.size() ? -1 : idx;\n }\n};\n```\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) \n { \n for(int i=0;i<s.length();i++)\n {\n bool flag = true;\n for(int j=0;j<s.length();j++)\n {\n if(i!=j && s[i] == s[j])\n {\n flag = false;\n break;\n }\n }\n \n if(flag == true)\n return i;\n }\n return -1;\n }\n};\n``` | 18 | 0 | ['C', 'C++'] | 2 |
first-unique-character-in-a-string | 🗓️ Daily LeetCoding Challenge August, Day 16 | daily-leetcoding-challenge-august-day-16-oewi | This problem is the Daily LeetCoding Challenge for August, Day 16. Feel free to share anything related to this problem here! You can ask questions, discuss what | leetcode | OFFICIAL | 2022-08-16T00:00:24.739813+00:00 | 2022-08-16T00:00:24.739879+00:00 | 5,686 | false | This problem is the Daily LeetCoding Challenge for August, Day 16.
Feel free to share anything related to this problem here!
You can ask questions, discuss what you've learned from this problem, or show off how many days of streak you've made!
---
If you'd like to share a detailed solution to the problem, please create a new post in the discuss section and provide
- **Detailed Explanations**: Describe the algorithm you used to solve this problem. Include any insights you used to solve this problem.
- **Images** that help explain the algorithm.
- **Language and Code** you used to pass the problem.
- **Time and Space complexity analysis**.
---
**📌 Do you want to learn the problem thoroughly?**
Read [**⭐ LeetCode Official Solution⭐**](https://leetcode.com/problems/first-unique-character-in-a-string/solution) to learn the 3 approaches to the problem with detailed explanations to the algorithms, codes, and complexity analysis.
<details>
<summary> Spoiler Alert! We'll explain this 1 approach in the official solution</summary>
**Approach 1:** Linear time solution
</details>
If you're new to Daily LeetCoding Challenge, [**check out this post**](https://leetcode.com/discuss/general-discussion/655704/)!
---
<br>
<p align="center">
<a href="https://leetcode.com/subscribe/?ref=ex_dc" target="_blank">
<img src="https://assets.leetcode.com/static_assets/marketing/daily_leetcoding_banner.png" width="560px" />
</a>
</p>
<br> | 18 | 0 | [] | 93 |
first-unique-character-in-a-string | my C++ 6 lines Solution | my-c-6-lines-solution-by-iamxiaobai-aisk | \nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int list[256] = {0};\n for(auto i: s)\n list[i] ++;\n for(int i | IamXiaoBai | NORMAL | 2016-11-06T22:36:46.610000+00:00 | 2016-11-06T22:36:46.610000+00:00 | 7,171 | false | ```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int list[256] = {0};\n for(auto i: s)\n list[i] ++;\n for(int i=0; i<s.length();i++)\n if(list[s[i]]==1) return i;\n return -1;\n }\n};\n``` | 18 | 0 | [] | 9 |
first-unique-character-in-a-string | ≅ 100% Time & Space | O(N) + O(26) | Mark the ones visited only once as negative! | 100-time-space-on-o26-mark-the-ones-visi-symb | Intuition\n- If an element is visited for the first time, store the position as negative value. Else, if the value had already been visited, store the positive | astrixsanath14 | NORMAL | 2024-02-05T01:21:08.427393+00:00 | 2024-02-05T15:38:34.607296+00:00 | 4,275 | false | # Intuition\n- If an element is visited for the first time, store the position as negative value. Else, if the value had already been visited, store the positive position value. \n- Of all 26 characters, most absolute min value of negative position is the first character!\n\nPS: I didn\'t find any other post with this approach, hence shared it!\n\n# Complexity\n- Time complexity:\nO(len(s)) + O(26)\n\n- Space complexity:\nO(26)\n\n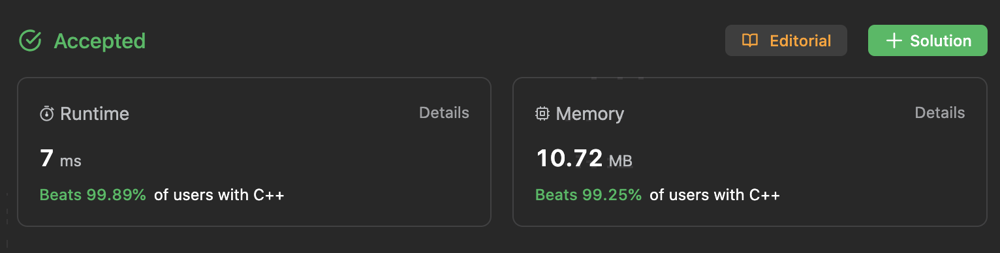\n\n\n# Code\n```\nclass Solution {\npublic:\n int firstUniqChar(string& s) {\n int position[26] = {0};\n for(int i = 1; i<=s.size(); i++)\n {\n char c = s[i-1]-\'a\';\n if(position[c] == 0)\n {\n position[c] = -i;\n }\n else\n {\n position[c] = i; // need to set some positive integer here\n }\n }\n int minPosition = INT_MAX;\n for(int i=0;i<26;i++)\n {\n if(position[i] < 0)\n minPosition = min(minPosition, -position[i]);\n }\n return minPosition == INT_MAX ? -1 : minPosition - 1;\n }\n};\n\nauto init = []()\n{ \n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n``` | 17 | 0 | ['C++'] | 4 |
first-unique-character-in-a-string | 99.56% of JavaScript online submissions ,EASY JS SOLUTION | 9956-of-javascript-online-submissions-ea-n07e | \nvar firstUniqChar = function(s) {\n for(let i=0;i<s.length;i++){\n let ch=s[i]\n if(s.indexOf(ch)==i && s.indexOf(ch,i+1)==-1){\n | sftengnr786 | NORMAL | 2021-03-28T13:12:24.657434+00:00 | 2021-03-28T13:12:24.657476+00:00 | 1,962 | false | ```\nvar firstUniqChar = function(s) {\n for(let i=0;i<s.length;i++){\n let ch=s[i]\n if(s.indexOf(ch)==i && s.indexOf(ch,i+1)==-1){\n return i\n }\n }\n return -1\n};\n``` | 17 | 2 | ['JavaScript'] | 6 |
first-unique-character-in-a-string | Python Dictionary, collections.Counter and count 3 ways | python-dictionary-collectionscounter-and-bpc7 | \nclass Solution(object):\n def firstUniqChar(self, s):\n """\n :type s: str\n :rtype: int\n """\n\n for i in range(len(s) | justinnew | NORMAL | 2017-01-20T18:53:15.032000+00:00 | 2017-01-20T18:53:15.032000+00:00 | 8,125 | false | ```\nclass Solution(object):\n def firstUniqChar(self, s):\n """\n :type s: str\n :rtype: int\n """\n\n for i in range(len(s)):\n c = s[i]\n if s.count(c)==1:\n return i\n\n return -1\n\n def firstUniqChar2(self, s):\n\n from collections import Counter\n sc = Counter(s)\n for i in range(len(s)):\n c = s[i]\n if sc.get(c,0)==1:\n return i\n\n return -1\n\n def firstUniqChar3(self, s):\n\n d = {}\n for c in s:\n if c in d.keys():\n d[c] += 1\n else:\n d[c] = 1\n\n for i in range(len(s)):\n c = s[i]\n if d[c]==1:\n return i\n\n return -1 \n``` | 17 | 0 | [] | 5 |
first-unique-character-in-a-string | Freq Count Array vs 2D array||4ms Beats 99.97% | freq-count-array-vs-2d-array4ms-beats-99-0m6q | Intuition\n Describe your first thoughts on how to solve this problem. \nThere are at most 26 alphabets, use an array for freq counting.\n\nLater try other appr | anwendeng | NORMAL | 2024-02-05T01:04:30.704430+00:00 | 2024-02-05T04:33:03.687797+00:00 | 1,682 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThere are at most 26 alphabets, use an array for `freq` counting.\n\nLater try other approach\n\n2nd approach uses 2D array. That records the info\n`freq: alphabet->(last occurrence index, count)`\nThe 2nd loop takes at most $O(26)$ time. Both of C++ &python are implemented.\n\nThanks to @DrinkJasmineTea , the negative number trick is applied to the 2nd solution to obatin 3rd version C++.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n2 pass solution.\n1 pass collects the enough info using a loop.\n2 pass use an iteration to test whether\n```\nif (freq[s[i]-\'a\']==1) \n return i;\n```\nWhen the 2nd loop goes to end, that is, there is no non-repeating character in it.\n\nLet\'s consider the test case `s ="loveleetcode"`. The process is shown as follows which is adding some output in C++ 2D array solution.\n```\nFirst loop collects info:\n0: l f=1\n1: o f=1\n2: v f=1\n3: e f=1\n4: l f=2\n5: e f=2\n6: e f=3\n7: t f=1\n8: c f=1\n9: o f=2\n10: d f=1\n11: e f=4\n------\n2D Freq Table:\nc->(8, 1)\nd->(10, 1)\ne->(11, 4)\nl->(4, 2)\no->(9, 2)\nt->(7, 1)\nv->(2, 1)\nans=2\n```\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# Code|| C++ Freq Count 4ms Beats 99.97%\n```\n#pragma GCC optimize("O3", "unroll-loops")\nclass Solution {\npublic:\n int firstUniqChar(string& s) {\n int freq[26];\n int n=s.size();\n for(int i=0; i<n; i++){\n int idx=s[i]-\'a\';\n freq[idx]++;\n }\n for (int i=0; i<n; i++)\n if (freq[s[i]-\'a\']==1) \n return i;\n return -1;\n }\n};\n\nauto init = []()\n{ \n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n```\n# 2D array solution||C++ 4ms Beats 99.97%\n\n```python []\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n freq=[[-1, 0] for _ in range(26)]\n n=len(s)\n for i, c in enumerate(s):\n idx=ord(c)-ord(\'a\')\n freq[idx][0]=i\n freq[idx][1]+=1\n\n ans=n\n for i, f in freq:\n if f==1:\n ans=min(ans, i)\n if ans==n: ans=-1\n return ans\n \n```\n```C++ []\n#pragma GCC optimize("O3", "unroll-loops")\nclass Solution {\npublic:\n int firstUniqChar(string& s) {\n int freq[26][2]; // alphabet->(last occurrence index, count)\n int n=s.size();\n for(int i=0; i<n; i++){\n int idx=s[i]-\'a\';\n freq[idx][0]=i;\n freq[idx][1]++;\n }\n int ans=n;\n for (int i=0; i<26; i++)\n if (freq[i][1]==1) \n ans=min(ans, freq[i][0]);\n return ans==n?-1:ans;\n }\n};\n\n```\n# C++ using negative number trick\n```\n#pragma GCC optimize("O3", "unroll-loops")\nclass Solution {\npublic:\n int firstUniqChar(string& s) {\n int viz[26];\n memset(viz, -1, sizeof(viz));\n int n=s.size();\n for(int i=0; i<n; i++){\n int idx=s[i]-\'a\';\n if (viz[idx]==-1) viz[idx]=i;\n else viz[idx]=-2;\n }\n // print(viz);\n\n int ans=n;\n for (int i=0; i<26; i++)\n if (viz[i]>=0) \n ans=min(ans, viz[i]);\n return ans==n?-1:ans;\n }\n};\n\n```\n | 16 | 0 | ['Array', 'C++', 'Python3'] | 4 |
first-unique-character-in-a-string | JAVA || 100 % FASTER || 3 LINE HASHMAP CODE | java-100-faster-3-line-hashmap-code-by-s-d7vl | PLEASE UPVOTE IF YOU LIKE IT;\n\n# Code\n\nclass Solution {\n public int firstUniqChar(String s) {\n HashMap<Character, Integer> map = new HashMap<>() | sharforaz_rahman | NORMAL | 2023-03-29T15:33:33.177732+00:00 | 2023-03-29T15:33:33.177768+00:00 | 5,127 | false | **PLEASE UPVOTE IF YOU LIKE IT;**\n\n# Code\n```\nclass Solution {\n public int firstUniqChar(String s) {\n HashMap<Character, Integer> map = new HashMap<>();\n for (char c : s.toCharArray()) map.put(c, map.getOrDefault(c, 0) + 1);\n\n for(int i = 0; i < s.length(); i++){\n if(map.containsKey(s.charAt(i)) && map.get(s.charAt(i)) == 1) return i;\n }\n return -1;\n }\n}\n``` | 16 | 0 | ['Hash Table', 'Counting', 'Hash Function', 'Java'] | 4 |
first-unique-character-in-a-string | 2 Method's |🧑💻 BEGINNER FREINDLY|🌟Visualization|JAVA|C++|Python | 2-methods-beginner-freindlyjavacpython-b-jfqs | ✅ IF YOU LIKE THIS SOLUTION, PLEASE UPVOTE AT THE END😊 ✅ :Approach 1: frequency counting approach using a hash map1 . Convert string s to char array st.
2 . Use | Varma5247 | NORMAL | 2025-03-10T11:51:20.896275+00:00 | 2025-03-11T18:20:19.930401+00:00 | 2,747 | false |
✅ **IF YOU LIKE THIS SOLUTION, PLEASE UPVOTE AT THE END**😊 ✅ :
# Approach 1: frequency counting approach using a hash map
<!-- Describe your approach to solving the problem. -->
1 . Convert string ```s``` to char array ```st```.
2 . Use a ```HashMap``` ```map``` to store character frequencies.
3 . Populate ```map``` by iterating through ```st``` and updating frequencies using ```map.put(c, map.getOrDefault(c, 0) + 1)```.
4 . Iterate through ```st``` again to find the first character with a frequency of 1 in```map```. Return its index if found.
5 . If no unique character is found, return ```-1```.
# ⏳Complexity Analysis
- Time complexity:$$O(n)$$ Two passes through the string, each taking $$O(n)$$ time.
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:$$O(k)$$Space required for the ```HashMap```, where ```k``` is the number of unique characters.
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
### Diagram Table:
```Example:"leetcode"```
<!-- 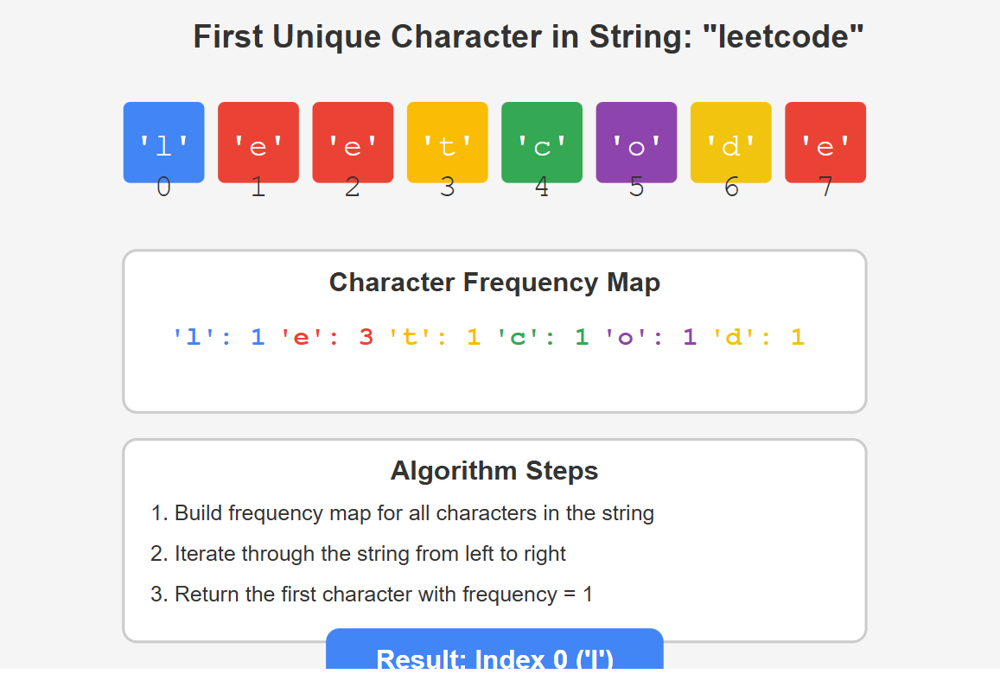 -->
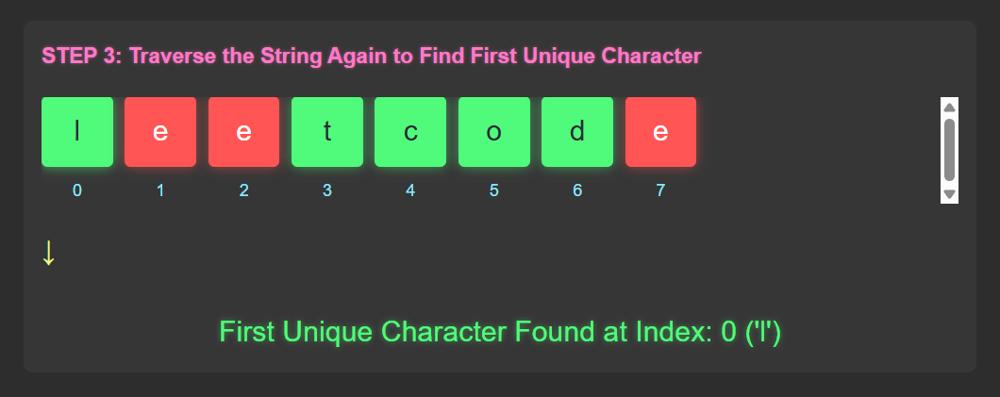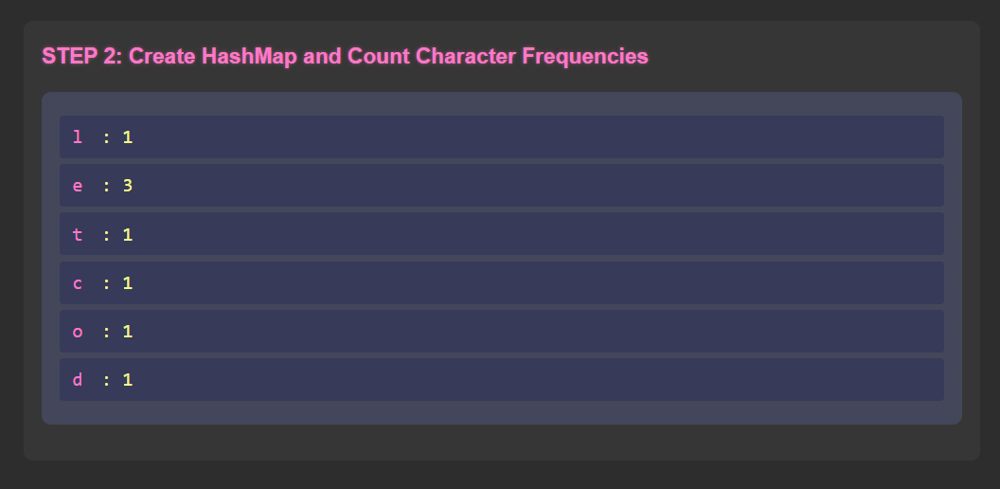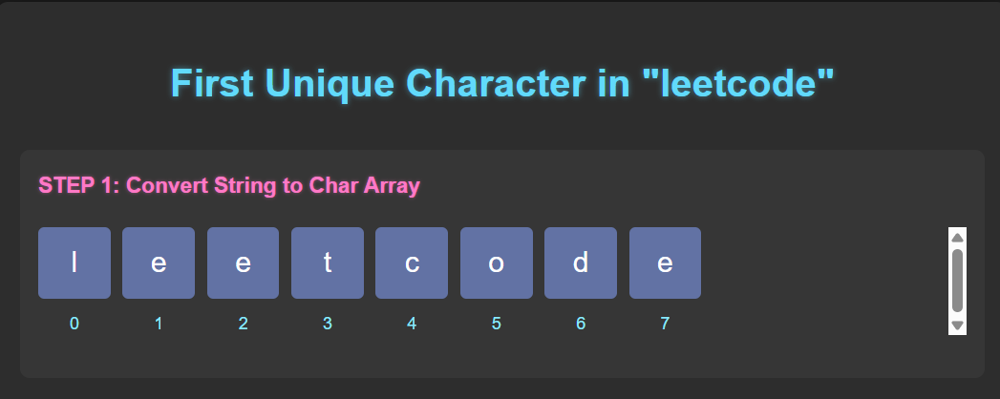
# 💻Code Implementation
```java []
class Solution {
public int firstUniqChar(String s) {
char st[]=s.toCharArray();
HashMap<Character,Integer>map=new HashMap<>();
for(char c:st){
map.put(c,map.getOrDefault(c,0)+1);
}
for (int i = 0; i < st.length; i++) {
if (map.get(st[i]) == 1) {
return i;
}
}
return -1;
}
}
```
```c++ []
class Solution {
public:
int firstUniqChar(std::string s) {
std::unordered_map<char, int> map;
// Count the frequency of each character
for (char c : s) {
map[c]++;
}
// Find the index of the first unique character
for (int i = 0; i < s.length(); i++) {
if (map[s[i]] == 1) {
return i;
}
}
return -1; // Return -1 if no unique character is found
}
};
```
```python []
class Solution:
def firstUniqChar(self, s: str) -> int:
char_count = {}
# Count the frequency of each character
for char in s:
char_count[char] = char_count.get(char, 0) + 1
# Find the index of the first unique character
for index, char in enumerate(s):
if char_count[char] == 1:
return index
return -1 # Return -1 if no unique character is found
```
____
# Approach 2:Array-Based Frequency Counting
**1.Initialize Frequency Array:**
- Create ```frequency[26]``` to count characters (```'a'``` to ```'z'``` mapped to indices 0 to 25).
**2.Count Frequencies:**
- Traverse the string and update ```frequency[c - 'a']``` for each character c.
**3.Find First Unique Character:**
Traverse the string again and return the index of the first character where ```frequency[s.charAt(i) - 'a'] == 1```.
**4.Return Result:**
- If no unique character is found, return ```-1```.
# ⏳Complexity Analysis
- Time complexity:$$O(n)$$ Two passes through the string, each taking $$O(n)$$ time.
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:$$O(1)$$ Fixed-size array of ```26``` elements, independent of input size.
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
### Diagram Table: Array-Based
```Example:"leetcode"```
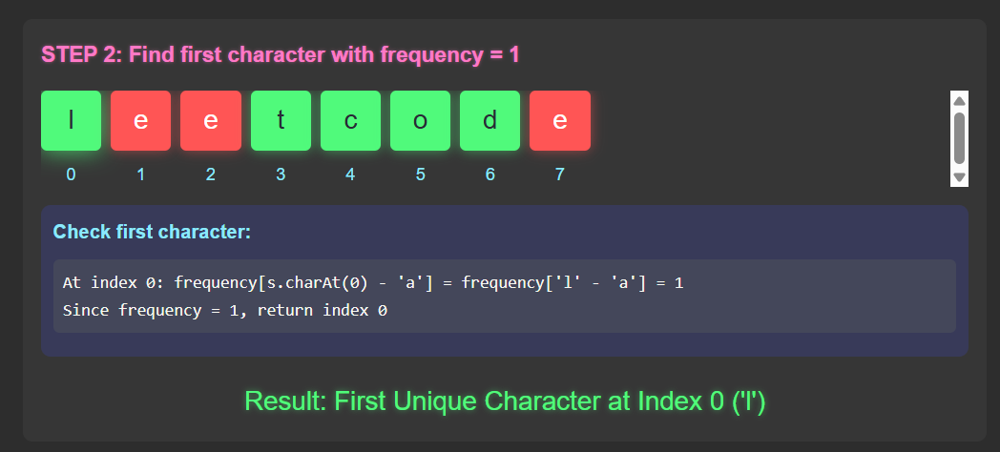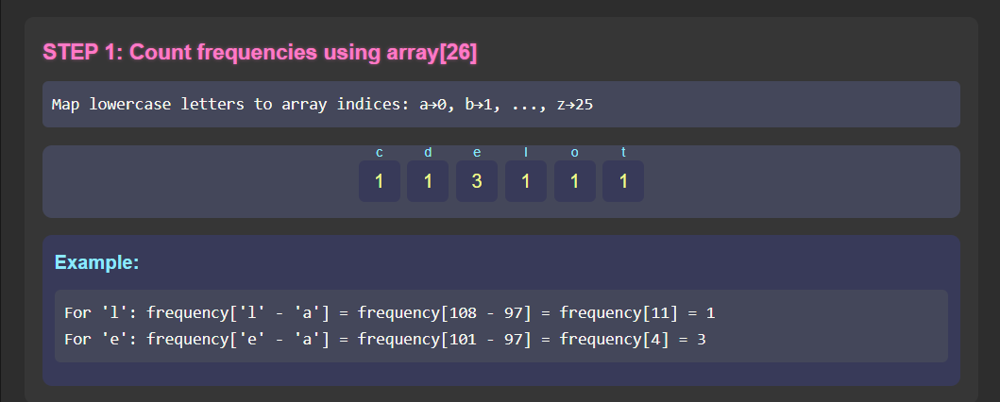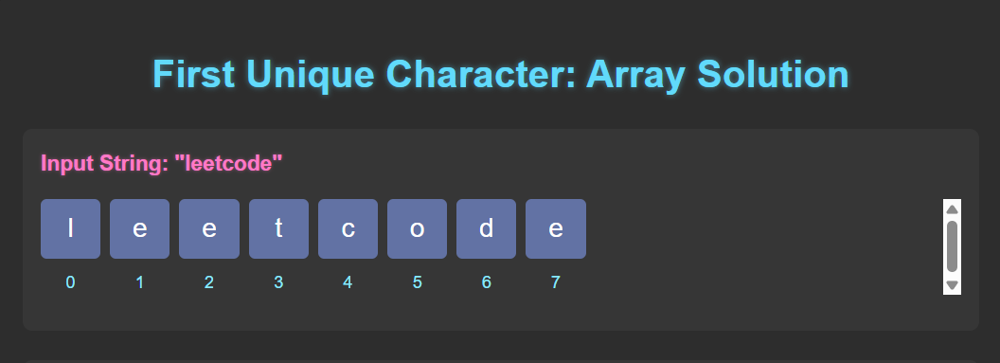
### 💻Code Implementation
```java []
class Solution {
public int firstUniqChar(String s) {
int[] frequency = new int[26];
for (char c : s.toCharArray()) {
frequency[c - 'a']++; // Map 'a' to 0, 'b' to 1, ..., 'z' to 25
}
for (int i = 0; i < s.length(); i++) {
if (frequency[s.charAt(i) - 'a'] == 1) {
return i; // Return the index of the first unique character
}
}
// If no unique character is found, return -1
return -1;
}
}
```
```c++ []
class Solution {
public:
int firstUniqChar(std::string s) {
int frequency[26] = {0}; // Assuming only lowercase letters
// Step 1: Count the frequency of each character
for (char c : s) {
frequency[c - 'a']++; // Map 'a' to 0, 'b' to 1, ..., 'z' to 25
}
// Step 2: Find the first character with a frequency of 1
for (int i = 0; i < s.length(); i++) {
if (frequency[s[i] - 'a'] == 1) {
return i; // Return the index of the first unique character
}
}
// Step 3: If no unique character is found, return -1
return -1;
}
};
```
```python []
class Solution(object):
def firstUniqChar(self, s):
# Create a list to store the frequency of each character
frequency = [0] * 26 # Assuming only lowercase letters
# Step 1: Count the frequency of each character
for char in s:
frequency[ord(char) - ord('a')] += 1 # Map 'a' to 0, 'b' to 1, ..., 'z' to 25
# Step 2: Find the first character with a frequency of 1
for index, char in enumerate(s):
if frequency[ord(char) - ord('a')] == 1:
return index # Return the index of the first unique character
# Step 3: If no unique character is found, return -1
return -1
```
___
**If you found my solution helpful, I would greatly appreciate your upvote, as it would motivate me to continue sharing more solutions.**
🔼 **Please Upvote**
🔼 **Please Upvote**
🔼 **Please Upvote**
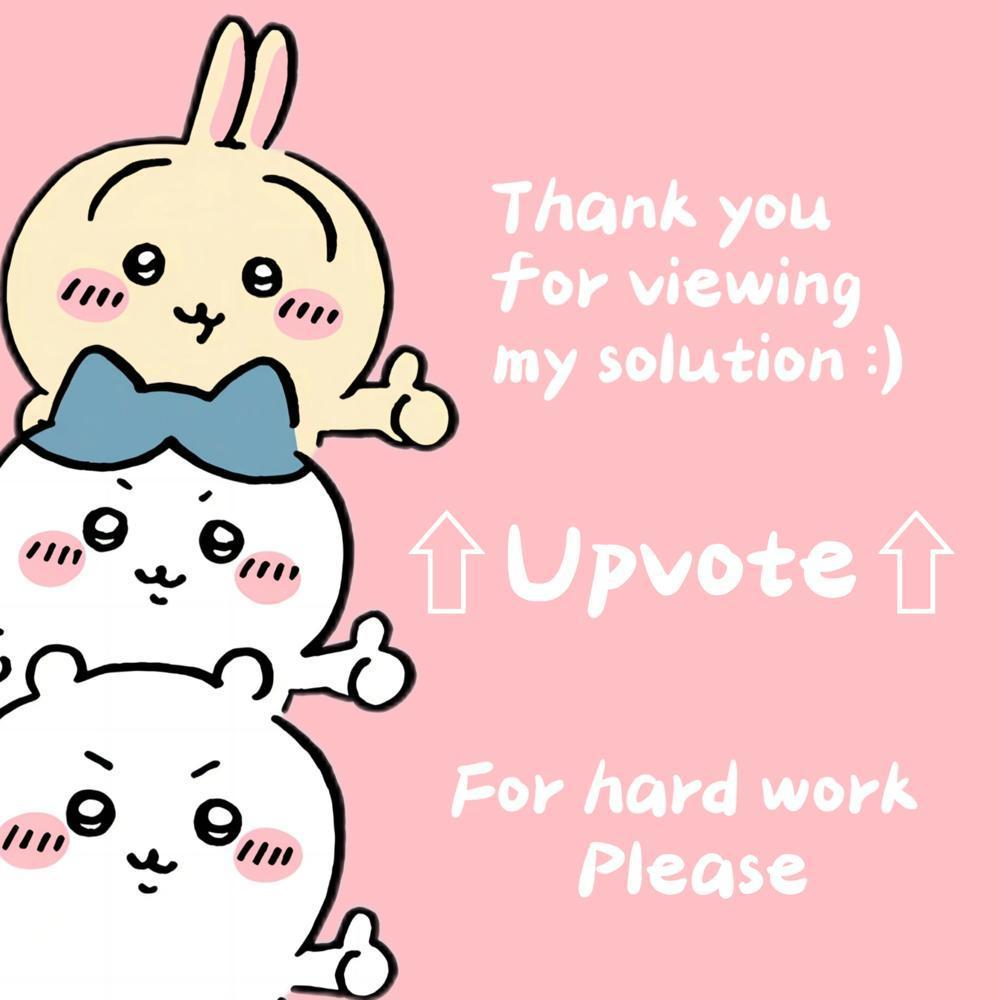
| 15 | 0 | ['Hash Table', 'String', 'Counting', 'Python', 'C++', 'Java', 'Python3'] | 1 |
first-unique-character-in-a-string | ✅✅#2 Methods || Easy & Clean Code ✅✅ | 2-methods-easy-clean-code-by-prikarshglo-248b | Idea:\nAs we need to return first unique character in the string.\nSo we need to store character and its frequency.\n\n# Complexity\n- Time complexity: O(s.size | prikarshglory | NORMAL | 2023-07-17T16:15:30.945068+00:00 | 2023-07-17T16:15:30.945093+00:00 | 1,823 | false | # Idea:\nAs we need to return first unique character in the string.\nSo we need to store character and its frequency.\n\n# Complexity\n- Time complexity: O(s.size())\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(s.size()) -> in queue\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Method #1\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n // can also use unordered_map (it will work same)\n queue<int> q;\n int arr[26]={0};\n for(int i=0;i<s.length();i++){\n char ch=s[i];\n q.push(i);\n arr[ch-\'a\']++;\n while(!q.empty()){\n if(arr[s[q.front()]-\'a\']>1)\n q.pop();\n else break;\n }\n }\n if(q.empty())\n return -1;\n return q.front(); \n }\n};\n```\n# Method #2\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map<char,int> m;\n\n for(int i=0;i<s.length();i++)\n // storing how many times a character appears in order\n // leetcode -> l e t c o d\n // 1 3 1 1 1 1\n // first time unique character apeared is -> l in string\n m[s[i]]++;\n\n // m[s[0]] -> s[0] = l and m[s[0]] =1\n // m[s[1]] -> s[1] = e and m[s[1]] =3\n // m[s[2]] -> s[2] = t and m[s[2]] =1\n // and so on\n for(int i=0;i<s.length();i++)\n {\n if(m[s[i]]==1) \n return i;\n }\n return -1; \n }\n};\n | 15 | 0 | ['Hash Table', 'String', 'Queue', 'Counting', 'C++'] | 0 |
first-unique-character-in-a-string | Easy C++ Solution | Basic Approach | Array & Loop | easy-c-solution-basic-approach-array-loo-52w6 | Intuition\nJust find the first character whose count is 1 by iterating.\n\n# Approach\nInitialised an array of 26 length to store count of alphabets.\nThen just | HariBhakt | NORMAL | 2022-12-31T17:35:17.423082+00:00 | 2022-12-31T17:35:17.423137+00:00 | 3,726 | false | # Intuition\nJust find the first character whose count is 1 by iterating.\n\n# Approach\nInitialised an array of 26 length to store count of alphabets.\nThen just iterated through the string to find 1st unique character.\n\n# Complexity\n- Time complexity: $$O(n)$$\n\n- Space complexity: $$O(n)$$\n\n# Code\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int arr[26]={0};\n int ans = -1;\n for(int i=0; i<s.length(); i++){\n int index = s[i]-\'a\';\n arr[index]++;\n }\n for(int i=0;i<s.length();i++){\n if(arr[s[i]-\'a\'] == 1) return i; \n }\n return ans;\n }\n};\n``` | 15 | 0 | ['Array', 'String', 'C++'] | 0 |
first-unique-character-in-a-string | C++ | Brute Force Approach | Efficient Approach | Easy to Understand | c-brute-force-approach-efficient-approac-07mz | Brute Force Approach - O(n^2) time complexity + 2 traversals needed \nFaster than 59.75% of cpp submissions\n\nclass Solution {\npublic:\n int firstUniqChar | manaskarlekar4 | NORMAL | 2021-06-19T06:28:24.117007+00:00 | 2021-06-19T19:05:24.433446+00:00 | 1,477 | false | * **Brute Force Approach** - O(n^2) time complexity + 2 traversals needed \nFaster than 59.75% of cpp submissions\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) \n { \n for(int i=0;i<s.length();i++)\n {\n bool flag = true;\n for(int j=0;j<s.length();j++)\n {\n if(i!=j && s[i] == s[j])\n {\n flag = false;\n break;\n }\n }\n \n if(flag == true)\n return i;\n }\n return -1;\n }\n};\n```\n\n* **Efficient Approach** - O(**len(s)** + 26) time complexity + 2 traversals needed\nFaster than 95.91% of cpp submissions\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) \n {\n \n int arr[26] = {0};\n \n for(int i=0;i<s.length();i++)\n {\n arr[s[i]-\'a\']++;\n }\n \n \n for(int i =0 ;i<s.length();i++)\n {\n if(arr[s[i]-\'a\'] ==1)\n return i;\n }\n return -1;\n }\n \n};\n```\n\n***Like it ? Upvote it !*** | 14 | 0 | ['C', 'C++'] | 1 |
first-unique-character-in-a-string | 👏🏻 Python | Two Pass & One Pass - 公瑾™ | python-two-pass-one-pass-gong-jin-tm-by-fhi5p | 387. First Unique Character in a String\n\n\n#### Two Pass \n\n> \u7C7B\u578B\uFF1AHash Table\n> Time Complexity O(2N)\n> Space Complexity O(N)\n\n\n\u626B\u4E2 | yuzhoujr | NORMAL | 2018-09-05T05:17:06.694468+00:00 | 2018-09-05T05:17:06.694516+00:00 | 2,874 | false | ### 387. First Unique Character in a String\n\n\n#### Two Pass \n```\n> \u7C7B\u578B\uFF1AHash Table\n> Time Complexity O(2N)\n> Space Complexity O(N)\n```\n\n\u626B\u4E24\u8FB9\uFF0C\u7B2C\u4E00\u904D\u5B58\u5B57\u5178\uFF0C\u7B2C\u4E8C\u904D\u67E5\u627E\uFF0C\u5982\u679C\u5B57\u5178\u91CC\u9762\u5BF9\u5E94Counter\u4E3A1\uFF0C\u5C31\u8FD4\u56DE\u3002\n\u8FD9\u91CC\u6709\u4E2A\u53EF\u4EE5\u6539\u8FDB\u7684\u5730\u65B9\uFF0C\u5982\u679C\u6211\u4EEC\u7684input\u957F\u5EA6\u7279\u522B\u7684\u957F\uFF0C\u6211\u4EEC\u7B2C\u4E8C\u6B21\u7684for loop\u5C31\u4F1A\u6BD4\u8F83\u6D6A\u8D39\u65F6\u95F4\uFF0C\u6240\u4EE5\u6211\u4EEC\u53EF\u4EE5\u5BF9\u7B2C\u4E8C\u904D\u8FED\u4EE3\u8FDB\u884C\u4F18\u5316\u3002\n\n```python\nclass Solution:\n def firstUniqChar(self, s):\n dic = {}\n for char in s:\n dic[char] = dic.get(char, 0) + 1\n \n for i, char in enumerate(s):\n if dic[char] and dic[char] == 1:\n return i\n return -1\n```\n\n#### One Pass \n```\n> \u7C7B\u578B\uFF1AHash Table\n> Time Complexity O(N * M)\n> Space Complexity O(M)\n```\n\n##### Step 1 \u626B\u63CF`s`\u5E76\u5B58\u50A8\u5B57\u5178\n\u5B58\u5B57\u5178\u65F6\u5019\uFF0C\u5C06value\u8BBE\u6210`index`\uFF0C\u5982\u679C\u5728\u5B58\u50A8\u7684\u65F6\u5019\u53D1\u73B0\u5B57\u5178\u91CC\u9762\u6709\u91CD\u590D\uFF0C\u5219\u5C06value\u8BBE\u7F6E\u6210`-1`\n\n##### Step 2 \u626B\u63CF\u5B57\u5178\uFF0C\u5B9E\u65BD\u66F4\u65B0\u6700\u5C0F\u8FD4\u56DE\u503C\u3002\n\u6211\u4EEC\u8FED\u4EE3\u5B58\u50A8\u597D\u4E86\u7684\u5B57\u5178\uFF0C\u56E0\u4E3A\u6211\u4EEC\u77E5\u9053`-1`\u4EE3\u8868\u4E00\u5B9A\u6709\u91CD\u590D\uFF0C\u6211\u4EEC\u5C31pass\u6389\u8FD9\u79CD\u60C5\u51B5\uFF0C\u53EA\u8981\u5F53\u524D`key`\u5BF9\u5E94\u7684`value`\u4E0D\u4E3A\u8D1F\u6570\uFF0C\u5C31\u8BC1\u660E`value`\u662F**unique character\u76F8\u5BF9\u5E94\u7684index**\uFF0C\u6211\u4EEC\u5728\u5916\u56F4\u8BBE\u7F6E\u4E00\u4E2A`res`\u8BB0\u5F55\u6700\u5C0F\u7684index\u503C\uFF0C\u5728\u5BF9\u5B57\u5178\u8FED\u4EE3\u7684\u65F6\u5019\uFF0C\u5B9E\u65F6\u6BD4\u8F83+\u66F4\u65B0\u5373\u53EF\u3002\n\u53E6\u5916\u5982\u679C`res`\u4ECE\u5934\u5230\u5C3E\u6CA1\u6709\u53D8\u8FC7\uFF0C\u5219\u4EE3\u8868\u5B57\u5178\u6CA1\u6709unique character\uFF0C\u8FD4\u56DE`-1`\n\n```python\nclass Solution:\n def firstUniqChar(self, s):\n dic = {}\n for i, char in enumerate(s):\n if char in dic:\n dic[char] = -1\n else:\n dic[char] = i\n \n res = float(\'inf\')\n for key, val in dic.items():\n if val == -1: continue\n res = min(res, val)\n \n return res if res != float(\'inf\') else -1\n\n``` | 14 | 2 | [] | 5 |
first-unique-character-in-a-string | Python || 3 diff way || 99% beats | python-3-diff-way-99-beats-by-vvivekyada-c82v | If you got help from this,... Plz Upvote .. it encourage me\n# Code\n> # HashMap\n\n# HASHMAP\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n | vvivekyadav | NORMAL | 2023-10-09T12:39:54.372893+00:00 | 2023-10-09T12:39:54.372983+00:00 | 1,225 | false | **If you got help from this,... Plz Upvote .. it encourage me**\n# Code\n> # HashMap\n```\n# HASHMAP\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n d = {}\n for char in s:\n d[char] = d.get(char,0) + 1\n \n for i , char in enumerate(s):\n if d[char] == 1:\n return i\n return -1\n\n\n```\n\n> # String\n``` \n# String\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i in range(len(s)):\n if s[i] not in s[i+1:] and s[i] not in s[:i] :\n return i\n return -1\n\n\n```\n\n> # Count\n```\n# Count\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i in range(len(s)):\n if s.count(s[i]) == 1:\n return i\n return -1\n``` | 13 | 0 | ['Hash Table', 'String', 'Counting', 'Python', 'Python3'] | 1 |
first-unique-character-in-a-string | OnlyCode in JAVA using HashMap ✅ || | onlycode-in-java-using-hashmap-by-saksha-y30t | \n\n# Code\n\nclass Solution {\n public int firstUniqChar(String str) {\n HashMap<Character,Integer> map = new HashMap<>();\n for(int i=0;i<str | sakshamkaushiik | NORMAL | 2023-02-03T19:10:25.247305+00:00 | 2023-02-03T19:10:25.247345+00:00 | 3,152 | false | \n\n# Code\n```\nclass Solution {\n public int firstUniqChar(String str) {\n HashMap<Character,Integer> map = new HashMap<>();\n for(int i=0;i<str.length();i++){\n if(map.containsKey(str.charAt(i))){\n map.put(str.charAt(i),map.get(str.charAt(i))+1);\n }else{\n map.put(str.charAt(i),1);\n }\n }\n for(int i=0;i<str.length();i++){\n if(map.get(str.charAt(i))==1){\n return i;\n }\n }\n return -1;\n }\n}\n``` | 13 | 0 | ['Hash Table', 'Java'] | 2 |
first-unique-character-in-a-string | ✅C beats 100%🔥| Easy to understand | beginner-friendly ^_^ | c-beats-100-easy-to-understand-beginner-j0z6v | \nint firstUniqChar(char * s){\n int i = 0, map[26], sSize = strlen(s);\n \n for(i; i < 26; i++)\n map[i] = 0;\n \n for(i=0; i < sSize; i+ | luluboy168 | NORMAL | 2022-08-16T11:54:33.147318+00:00 | 2022-08-16T11:54:33.147362+00:00 | 1,056 | false | ```\nint firstUniqChar(char * s){\n int i = 0, map[26], sSize = strlen(s);\n \n for(i; i < 26; i++)\n map[i] = 0;\n \n for(i=0; i < sSize; i++)\n map[s[i] - \'a\']++;\n \n for(i=0; i < sSize; i++)\n if (map[s[i] - \'a\'] == 1)\n return i;\n \n return -1;\n}\n```\n**Please UPVOTE if you LIKE!!**\n | 13 | 0 | ['C'] | 2 |
first-unique-character-in-a-string | PYTHON SIMPLE SOLUTION | python-simple-solution-by-coder_hash-0l4t | Input: "loveleetcode"\nOutput: 2\n\nAt position 2, if we see [lo]+[eleetcode], there is no occurance of \'v\',\nSo we return 2\n\nFor loop is finished and still | coder_hash | NORMAL | 2022-02-01T12:19:58.702966+00:00 | 2022-02-01T12:20:36.190995+00:00 | 546 | false | Input: "loveleetcode"\nOutput: 2\n\nAt position 2, if we see [lo]+[eleetcode], there is no occurance of \'v\',\nSo we return 2\n\nFor loop is finished and still nothing is returned, we return -1\n```\nclass Solution(object):\n def firstUniqChar(self, s):\n for i in range(len(s)):\n if s[i] not in s[:i]+s[i+1:]:\n return i\n return -1\n \n```\nAn upvote won\'t cost u anything :) | 13 | 0 | ['Python'] | 0 |
first-unique-character-in-a-string | Swift: First Unique Character in a String (✅ Test Cases) | swift-first-unique-character-in-a-string-1cob | swift\nclass Solution {\n func firstUniqChar(_ s: String) -> Int {\n var arr = Array(repeating: 0, count: 26)\n let a = UnicodeScalar("a").valu | AsahiOcean | NORMAL | 2021-07-09T05:36:01.397284+00:00 | 2021-07-09T05:36:01.397341+00:00 | 1,550 | false | ```swift\nclass Solution {\n func firstUniqChar(_ s: String) -> Int {\n var arr = Array(repeating: 0, count: 26)\n let a = UnicodeScalar("a").value\n s.unicodeScalars.forEach{\n arr[Int($0.value - a)] += 1\n }\n var iter = 0\n for ch in s.unicodeScalars {\n if arr[Int(ch.value - a)] == 1 { return iter }\n iter += 1\n }\n return -1\n }\n}\n```\n\n```swift\nimport XCTest\n\n// Executed 3 tests, with 0 failures (0 unexpected) in 0.045 (0.048) seconds\n\nclass Tests: XCTestCase {\n private let s = Solution()\n func test0() {\n let res = s.firstUniqChar("leetcode")\n XCTAssertEqual(res, 0)\n }\n func test1() {\n let res = s.firstUniqChar("loveleetcode")\n XCTAssertEqual(res, 2)\n }\n func test2() {\n let res = s.firstUniqChar("aabb")\n XCTAssertEqual(res, -1)\n }\n}\n\nTests.defaultTestSuite.run()\n``` | 13 | 1 | ['Swift'] | 0 |
first-unique-character-in-a-string | Easy Python Solution | easy-python-solution-by-aadit-wcfv | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | aadit_ | NORMAL | 2025-02-07T09:18:28.929807+00:00 | 2025-02-07T09:18:28.929807+00:00 | 1,299 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def firstUniqChar(self, s: str) -> int:
count = Counter(s)
for index, char in enumerate(s):
if count[char] == 1:
return index
return -1
``` | 12 | 0 | ['Python3'] | 0 |
first-unique-character-in-a-string | ✅Accepted| | ✅Easy solution || ✅Short & Simple || ✅Best Method | accepted-easy-solution-short-simple-best-k3k4 | \n# Code\n\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map<char, int> m;\n for (char& c : s) {\n m[c]++; | sanjaydwk8 | NORMAL | 2023-01-17T06:18:20.087499+00:00 | 2023-01-17T06:18:20.087553+00:00 | 2,605 | false | \n# Code\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map<char, int> m;\n for (char& c : s) {\n m[c]++;\n }\n for (int i = 0; i < s.size(); i++) {\n if (m[s[i]] == 1)\n return i;\n }\n return -1;\n }\n};\n```\nPlease **UPVOTE** if it helps \u2764\uFE0F\uD83D\uDE0A\nThank You and Happy To Help You!! | 12 | 0 | ['C++'] | 0 |
first-unique-character-in-a-string | C++ CONSTANT SPACE solution O(1) SPACE VERY FAST!! SICKEST 😷😷😷 | c-constant-space-solution-o1-space-very-dvikv | Keep a frequency map of all characters in s. \nUse a vector of size 26 for speed\nreturn first index that the count is == 1\n\nTime complexity: O(N)\nSpace com | midnightsimon | NORMAL | 2022-08-16T01:17:14.134053+00:00 | 2022-08-16T01:17:29.776335+00:00 | 2,237 | false | Keep a frequency map of all characters in s. \nUse a vector of size 26 for speed\nreturn first index that the count is == 1\n\nTime complexity: O(N)\nSpace complexity: O(1) because its just vector of size 26 everytime\n\n**SOLVED LIVE ON STREAM. link in profile. check out my sick keyboards.**\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n vector<int> freqMap(26);\n \n for(char c : s) {\n freqMap[c - \'a\']++;\n }\n \n int n = s.length();\n \n for(int i = 0; i < n; i++) {\n if(freqMap[s[i] - \'a\'] == 1) return i;\n }\n \n return -1;\n \n }\n};\n``` | 12 | 2 | [] | 6 |
first-unique-character-in-a-string | My 4 Line, O(N) Solution ! 5ms beats 100% | my-4-line-on-solution-5ms-beats-100-by-l-33vj | \npublic int firstUniqChar(String s) {\n\n int freq[] = new int[26];\n for(char i: s.toCharArray()) freq[i-\'a\']++;\n for(int i = | leetcoderkk | NORMAL | 2021-02-25T13:57:30.471643+00:00 | 2021-02-25T13:57:59.152619+00:00 | 1,983 | false | ```\npublic int firstUniqChar(String s) {\n\n int freq[] = new int[26];\n for(char i: s.toCharArray()) freq[i-\'a\']++;\n for(int i = 0; i < s.length(); i++) if(freq[s.charAt(i)-\'a\'] == 1) return i;\n return -1;\n \n }\n``` | 12 | 0 | ['Java'] | 3 |
first-unique-character-in-a-string | ✅✅✅ easy brute force||Upvote if you like ⬆️⬆️ | easy-brute-forceupvote-if-you-like-by-ra-qi8g | \n\n# Code\n\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int n=s.size();\n \n \n for(int i=0;i<n;i++)\n { | ratnesh_maurya | NORMAL | 2022-12-04T14:38:12.221630+00:00 | 2022-12-08T19:03:05.277833+00:00 | 1,175 | false | \n\n# Code\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int n=s.size();\n \n \n for(int i=0;i<n;i++)\n {\n int flag=1;\n for(int j=0;j<n;j++)\n {\n if(i!=j && s[i]==s[j])\n {\n flag=0;\n break;\n }\n \n }\n if(flag==1)\n {\n return i;\n }\n \n }\n return -1;\n \n \n }\n};\n``` | 11 | 0 | ['C++'] | 0 |
first-unique-character-in-a-string | [C++] Array-based Solutions Compared and Explained, ~100% Time, ~90% Space | c-array-based-solutions-compared-and-exp-ptpd | For this problem I decided to go straight for an array approach to build a frequency table of all the seen characters and then iterate again to find the first w | ajna | NORMAL | 2020-12-20T11:02:37.300536+00:00 | 2021-06-19T08:43:43.174370+00:00 | 1,064 | false | For this problem I decided to go straight for an array approach to build a frequency table of all the seen characters and then iterate again to find the first with a frequency of only `1`.\n\nTo do so, I created 2 support variables:\n* `len` to store the length of the string;\n* `alpha`, the aforementioned frequency table, with a size of `127`, making the char code of `\'z\'`, the last character in our range.\n\nWe will then move first of all to populate `alpha` increasing the cell matching each character by `1` as we go.\n\nWe will then loop through the string another time, this time just checking if the frequency is `1`, meaning the character is indeed unique and returning its index in case.\n\nIf we exit the loop without a match, we can then safely conclude no uniques were there and return `-1` :)\n\nThe code:\n\n```cpp\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n // support variables\n int len = s.size(), alpha[127] = {};\n\t\t// populating alpha\n for (char c: s) alpha[c]++;\n\t\t// hunting for unique elements\n for (int i = 0; i < len; i++) {\n if (alpha[s[i]] == 1) return i;\n }\n return -1;\n }\n};\n```\n\nVariation using an array of only `26` characters, making it less memory consuming, but also a bit slower due to the extra burden of normalising each character to be in the `0 - 25` range:\n\n```cpp\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n // support variables\n int len = s.size(), alpha[26] = {};\n\t\t// populating alpha\n for (char c: s) alpha[c - \'a\']++;\n\t\t// hunting for unique elements\n for (int i = 0; i < len; i++) {\n if (alpha[s[i] - \'a\'] == 1) return i;\n }\n return -1;\n }\n};\n```\n\nFurther variation where I iterate building up `alpha` while at the same time updating a `seenTwice` variable - once that hits `26` overall seen characters, it means we cannot get any matches and it does not make any sense to loop further:\n\n```cpp\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n // support variables\n int len = s.size(), alpha[127] = {}, seenTwice = 0;\n\t\t// populating alpha as long as it is needed\n for (char c: s) {\n if (alpha[c] == 1) seenTwice++;\n if (seenTwice == 26) return -1;\n alpha[c]++;\n }\n\t\t// hunting for unique elements\n for (int i = 0; i < len; i++) {\n if (alpha[s[i]] == 1) return i;\n }\n return -1;\n }\n};\n``` | 11 | 0 | ['Array', 'C', 'Counting', 'C++'] | 1 |
first-unique-character-in-a-string | Python simple solution 60 ms, faster than 96.16% | python-simple-solution-60-ms-faster-than-cvv9 | \nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n visited = set()\n for i in range(len(s)):\n if s[i] not in visited:\n | hemina | NORMAL | 2020-05-05T21:28:12.073448+00:00 | 2020-05-05T21:28:12.073483+00:00 | 2,283 | false | ```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n visited = set()\n for i in range(len(s)):\n if s[i] not in visited:\n visited.add(s[i])\n if s.count(s[i]) == 1:\n return i\n return -1\n``` | 11 | 3 | ['Python', 'Python3'] | 4 |
first-unique-character-in-a-string | JS Solution | js-solution-by-rano3003-j6lt | \n/**\n * @param {string} s\n * @return {number}\n */\nvar firstUniqChar = function(s) {\n for(var i =0 ; i < s.length ; i++) {\n if(s.indexOf(s[i]) = | rano3003 | NORMAL | 2020-05-05T08:00:00.923549+00:00 | 2020-05-05T08:00:00.923605+00:00 | 291 | false | ```\n/**\n * @param {string} s\n * @return {number}\n */\nvar firstUniqChar = function(s) {\n for(var i =0 ; i < s.length ; i++) {\n if(s.indexOf(s[i]) == s.lastIndexOf(s[i])) {\n return i;\n } \n }return -1\n \n};\n``` | 11 | 0 | [] | 0 |
first-unique-character-in-a-string | javascript | beats 98% | O(n) time | O(1) space | javascript-beats-98-on-time-o1-space-by-fn0zs | states is an array of 26 states (represented by an integer) corresponding to each possible character.\nIf a state is -1, the character has not been encountered | functaire | NORMAL | 2016-11-17T11:58:28.043000+00:00 | 2016-11-17T11:58:28.043000+00:00 | 2,229 | false | `states` is an array of 26 states (represented by an integer) corresponding to each possible character.\nIf a state is `-1`, the character has not been encountered yet.\nIf a state is `-2`, the character has been encountered more than once.\nOtherwise, the state represents the index at which the character was first encountered.\n\n`order` is an array containing character values.\n\nA character value is calculated by the ascii value of the character minus `97` (ascii value of `'a'`), such that the value of `'a'` is `0`, the value of `'b'` is `1`, etc.\n\nAt the end of the string traversal, `order` will contain in chronological order the value for every new character encountered. The values are unique because any character is only added on its first encounter during the string traversal.\n\nThe string traversal is as follows:\n```md\nfor (character, index) in string:\n if state of character is "not found":\n set state of character to be index\n add character to encounters\n else:\n set state of character to be "duplicate"\n```\n\nThe return value will be the index of the first character in `order` for which the state is not "not found" or "duplicate", or `-1` if none of the characters meet this requirement.\n\n```js\n/**\n * @param {string} s\n * @return {number}\n */\n\nlet firstUniqChar = s => {\n let states = Array(26).fill(-1)\n let order = []\n \n for (let i = 0; i < s.length; i++) {\n let char = s.charCodeAt(i) - 97\n \n if (states[char] === -1) {\n order.push(char)\n states[char] = i\n } else {\n states[char] = -2\n }\n }\n \n for (let i = 0; i < order.length; i++) {\n let char = order[i]\n let index = states[char]\n if (index > -1) return index\n }\n \n return -1\n};\n``` | 11 | 1 | [] | 2 |
first-unique-character-in-a-string | The Efficient First Unique Character Finder | [C++, Java, Python] | O(n) | the-efficient-first-unique-character-fin-sdj3 | Intuition:\nUtilize a fixed-size array to efficiently count the occurrences of each character in the given string.\n\n# Approach:\n1. Initialize a list of size | kartikeylapy | NORMAL | 2024-02-05T08:43:17.782339+00:00 | 2024-02-05T08:43:17.782373+00:00 | 746 | false | # Intuition:\nUtilize a fixed-size array to efficiently count the occurrences of each character in the given string.\n\n# Approach:\n1. Initialize a list of size 26 to represent the occurrences of each letter.\n2. Iterate through the string to count the occurrences of each character.\n3. Traverse the string again and return the index of the first character with a count of 1.\n\n**Note:** In the provided code, using a fixed-size array (std::array in C++) is advantageous as it ensures constant-time access with minimal memory overhead. This choice is suitable when the size of the data structure, representing character occurrences, is known at compile-time and remains constant.\n\n\n# Complexity:\n**Time Complexity: O(N)**, where N is the length of the input string.\n**Space Complexity: O(1)** (constant space for the fixed-size list).\n\n# Code\n\n**C++ Code:**\n\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n array<int, 26> k = {}; \n for(char c : s)\n k[c-\'a\']++;\n for(int i = 0; i < s.size();i++)\n if(k[s[i]-\'a\'] == 1) return i;\n return -1;\n }\n};\n```\n\n\n**Java Code:**\n\n```\nclass Solution {\n public int firstUniqChar(String s) {\n int[] abc = new int[26];\n Arrays.fill(abc, 0);\n for (char c : s.toCharArray()) {\n abc[c - \'a\'] += 1;\n }\n for (int i = 0; i < s.length(); i++) {\n if (abc[s.charAt(i) - \'a\'] == 1) {\n return i;\n }\n }\n return -1;\n }\n}\n```\n\n\n**Python Code:**\n\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n abc = [0] * 26\n for c in s:\n abc[ord(c) - ord(\'a\')] += 1\n for i in range(len(s)):\n if abc[ord(s[i]) - ord(\'a\')] == 1:\n return i\n return -1\n```\n\n# Conclusion:\nThis approach efficiently finds the index of the first non-repeating character in a string using a fixed-size array to track character occurrences. | 10 | 0 | ['String', 'Python', 'Java'] | 0 |
first-unique-character-in-a-string | Easy to Understand Java Solution using HashMap | easy-to-understand-java-solution-using-h-qwlc | \nclass Solution {\n public int firstUniqChar(String s) {\n HashMap<Character,Integer> map = new HashMap<>();//Creating a hashmap which will take aver | Abhinav_0561 | NORMAL | 2022-10-10T03:39:09.700788+00:00 | 2022-10-10T03:39:09.700864+00:00 | 1,421 | false | ```\nclass Solution {\n public int firstUniqChar(String s) {\n HashMap<Character,Integer> map = new HashMap<>();//Creating a hashmap which will take avery character and note its occurrence.\n for(int i = 0 ; i < s.length() ; i++){\n map.put(s.charAt(i), map.getOrDefault(s.charAt(i),0)+1);\n }\n for(int i = 0 ; i < s.length() ; i++){\n if(map.get(s.charAt(i))==1) return i;//If the occurrence of any char is once, then it is the required ans\n }\n return -1;//else returning -1\n }\n}\n```\nIt\'s time complexity is O(n) | 10 | 0 | ['Java'] | 0 |
first-unique-character-in-a-string | [Go] O(n) Hashmap with explanation | go-on-hashmap-with-explanation-by-maxyzl-wrbw | go\nfunc firstUniqChar(s string) int {\n\td := map[byte]int{}\n\n\t// Count each character.\n\tfor i := 0; i < len(s); i++ {\n\t\td[s[i]]++\n\t}\n\n\t// Find th | maxyzli | NORMAL | 2019-07-18T08:17:05.474115+00:00 | 2019-07-18T08:18:03.619879+00:00 | 573 | false | ```go\nfunc firstUniqChar(s string) int {\n\td := map[byte]int{}\n\n\t// Count each character.\n\tfor i := 0; i < len(s); i++ {\n\t\td[s[i]]++\n\t}\n\n\t// Find the first unique character and return.\n\tfor i := 0; i < len(s); i++ {\n\t\tif d[s[i]] == 1 {\n\t\t\treturn i\n\t\t}\n\t}\n\n\t// If there\'s no unique character then return -1.\n\treturn -1\n}\n``` | 10 | 0 | ['Go'] | 1 |
first-unique-character-in-a-string | Clean Python Solution | clean-python-solution-by-christopherwu05-bdl9 | python\nfrom collections import Counter\n\nclass Solution(object):\n def firstUniqChar(self, string):\n # counter = Counter()\n # for char in s | christopherwu0529 | NORMAL | 2019-03-20T06:18:14.538597+00:00 | 2019-09-20T01:53:04.113017+00:00 | 2,061 | false | ```python\nfrom collections import Counter\n\nclass Solution(object):\n def firstUniqChar(self, string):\n # counter = Counter()\n # for char in string:\n # counter[char]+=1\n counter = Counter(string)\n\n for i in xrange(len(string)):\n char = string[i]\n if counter[char]==1: return i\n\n return -1\n```\n\n# More Resource\nI really take time tried to make the best solution or explaination. \nBecause I wanted to help others like me. \nIf you like my answer, a star on [GitHub](https://github.com/wuduhren/leetcode-python) means a lot to me. \nhttps://github.com/wuduhren/leetcode-python | 10 | 0 | [] | 2 |
first-unique-character-in-a-string | Simple Solution, Single Pass | simple-solution-single-pass-by-fabrizio3-tuiq | \npublic int firstUniqChar(String s) {\n\tif(s==null || s.length()==0) {\n\t\treturn -1;\n\t}\n\tchar[] chars = s.toCharArray();\n\tMap<Character,Integer> chars | fabrizio3 | NORMAL | 2016-08-22T10:25:04.498000+00:00 | 2016-08-22T10:25:04.498000+00:00 | 6,141 | false | ```\npublic int firstUniqChar(String s) {\n\tif(s==null || s.length()==0) {\n\t\treturn -1;\n\t}\n\tchar[] chars = s.toCharArray();\n\tMap<Character,Integer> charsPositions = new HashMap<>();\n\tList<Integer> uniqsPositions = new ArrayList<>();\n\tfor(int i=0; i<chars.length; i++) {\n\t\tchar c = chars[i];\n\t\tif(charsPositions.containsKey(c)) {\n\t\t\tInteger charFirstPosition = charsPositions.get(c);\n\t\t\tuniqsPositions.remove(charFirstPosition);\n\t\t} else {\n\t\t\tcharsPositions.put(c,i);\n\t\t\tuniqsPositions.add(i);\n\t\t}\n\t}\n\treturn uniqsPositions.isEmpty()?-1:uniqsPositions.get(0);\n}\n``` | 10 | 3 | ['Java'] | 5 |
first-unique-character-in-a-string | TRUE O(1) extra memory with only two integers!!! using bits | true-o1-extra-memory-with-only-two-integ-r99a | Intuition\n Describe your first thoughts on how to solve this problem. \ni had initially thought about using map or array[26], or string ,ect...but then, we don | abhiram542 | NORMAL | 2024-02-06T19:08:15.785571+00:00 | 2024-02-07T09:19:33.748097+00:00 | 147 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\ni had initially thought about using map or array[26], or string ,ect...but then, we don\'t need exact frequency of each character. we need to know which of the following three states each character will be\n - 1) unvisited \n - 2) visited once\n - 3) visited twice or more\n - in binary system , we know that a bit can either be \'0\' or \'1\'.i.e one bit can represent two states. so in order to represent three states, we need atleast two bits(which can represent upto 4 states 00,01,10,11).\nso how can we use bit manipulation when there\'s only 32 bits for a int variable but need 26*2 =52 bits....?\n \n# Approach\n<!-- Describe your approach to solving the problem. -->\n ans to above question is simple, just use two integers ;) \n so now , we take two integers `first` and `second` ,initialize them to 0. (\'00000000000000000000000000000000\').\n\n in each iteration to string s, `letter` = s[i]-\'a\' . we denote our states as follows:\n- for char ch, if *letter*\'th bit in `first` and `second` respectively are\n 1. **0,0** , it was unvisited till now\n - mark it as visited once by changing the corresponding bit in `first` to 1.\n 2. **1,0** , it was visited once till now\n - mark it as visited twice by changing the corresponding bit in `second` to 1.\n 3. **1,1** , it was visited twice or more till now\n - no need to do anything as \'more than twice\' will be in same state as \'twice or more\'\n\nnow , after iterating whole string, `first` and `second` represents what states each character is in.\n\n\n\nso , to find the first unique character that was encountered in the string, iterate thw string one more time and check if that corresponding *letter*th bits are `0` and `1` in `first` and `second` respectively if yes, return the index , else continue to next iteration\n\nthe fact that we finished the above for-loop and came here means no unique character was encountered. so you know.., -1 :( \n\n \n# Code\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int first=0,second=0;\n for(int i=0;i<s.size();i++){\n\n int letter_mark=(1<<(s[i]-\'a\'));\n\n if( (first & letter_mark)==0 )\n first+=letter_mark;\n\n else if( (second & letter_mark)==0 ) \n second+=letter_mark;\n }\n for(int i=0;i<s.size();i++){\n\n int letter_mark=(1<<(s[i]-\'a\'));\n\n if( (first & letter_mark)!=0 && (second & letter_mark)==0 )return i;\n }\n return -1;\n }\n};\n```\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(n)..[two clean for loops]\nbit manipulation is so effiteint than using arrays or maps that even we iterate full string two times completely, mostly its still faster\n\nthe reason for that is you know, when we add suppose two integers x and y, whole 32 bits in both variables gets involved in processing. and so on...\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(1)..[two 32 bit integers]\nofcourse other two `i` and `letter` are inside for loop\n# tips\n->in above code, i used letter_mark directly instead of (1<<letter) every time . dont be confused (you won\'t be) .\n\nand friends! this is my first time posting solutions. hope all the people who read this post got a clear idea and also hoping that i would do more. encourage me by upvoting and sharing xDD. it motivates me so much.\n\n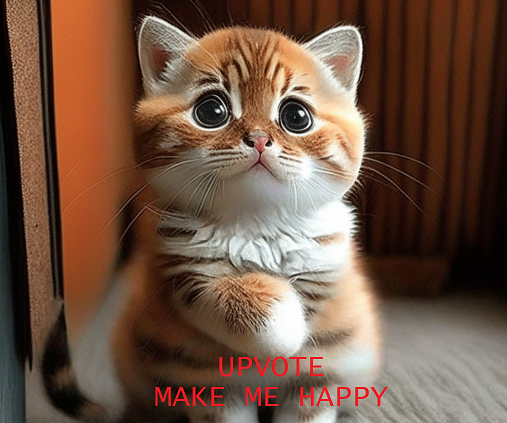\n\n-------------**-------- thank you for reading my post ----------**-------------\n\n\n\n\n | 9 | 0 | ['Bit Manipulation', 'Counting', 'Bitmask', 'C++'] | 3 |
first-unique-character-in-a-string | 🔥2 and 4 lines of code both suitable approach✅|| Easy to understand|| Beat 96% time and 97% space🚀 | 2-and-4-lines-of-code-both-suitable-appr-4exk | Intuition\n# Python and Python3 :\n1. \'for i in range(len(s)):\' : This line initiates a for loop that iterates over the indices of the characters in the strin | siddharth-kiet | NORMAL | 2024-02-05T06:03:50.825997+00:00 | 2024-02-13T19:27:40.878657+00:00 | 630 | false | # Intuition\n# **Python and Python3 :**\n1. **\'for i in range(len(s)):\' :** This line initiates a for loop that iterates over the indices of the characters in the string s. The loop variable i represents the current index.\n2. **\'if s.count(s[i]) == 1:\' :** Within the loop, it checks if the count of the current character s[i] in the entire string s is equal to 1. In other words, it checks if the character at index i is unique in the string.\n3. **return i:** If a unique character is found, it immediately returns the index i as the result. This means it returns the index of the first unique character in the string.\n4. **return -1:** If no unique character is found in the entire string, it returns -1, indicating that there is no unique character.\n\n# **C++ :**\n**we follow the hash map approach here:**\n1. **unordered_map<char, int> m; :** This line declares an unordered map (m) where the keys are characters (char) and the values are integers (int). This map is used to store the count of each character in the input string s.\n2. **for (char i : s) { m[i]++; } :** This loop iterates through each character i in the string s and increments the count of that character in the unordered map m.\n3. **for (int i = 0; i < s.length(); i++) { if (m[s[i]] == 1) { return i; } } :** This loop iterates through the string again, and for each character s[i], it checks the count in the unordered map m. If the count is 1, it means that the character is unique, and the function returns the index i as the result.\n4. **return -1; :** If no unique character is found in the entire string, the function returns -1.\n\n**I hope you understand the code \uD83D\uDE4F**\n\n# Approach : Counting and Hashing\n\n# Complexity\n- Time complexity: O(n^2)\n\n- Space complexity: O(1)\n\n# Code\n```Python3 []\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n result=next((i for i in range(len(s)) if s.count(s[i])==1),-1)\n return result\n```\n```Python []\nclass Solution(object):\n def firstUniqChar(self, s):\n """\n :type s: str\n :rtype: int\n """\n result=next((i for i in range(len(s)) if s.count(s[i])==1),-1)\n return result\n```\n\n```Python3 []\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i in range(len(s)):\n if s.count(s[i])==1:\n return i\n return -1\n```\n```python []\nclass Solution(object):\n def firstUniqChar(self, s):\n """\n :type s: str\n :rtype: int\n """\n for i in range(len(s)):\n if s.count(s[i])==1:\n return i\n return -1\n```\n```C++ []\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map<char,int> m;\n for(char i:s){\n m[i]++;\n }\n for(int i=0;i<s.length();i++){\n if(m[s[i]]==1){\n return i;\n }\n }\n return -1;\n }\n};\n```\n | 9 | 0 | ['Hash Table', 'String', 'Queue', 'Counting', 'Python', 'C++', 'Python3'] | 0 |
first-unique-character-in-a-string | Hash map solution ,C++ better and easy solution | hash-map-solution-c-better-and-easy-solu-1kqf | \nint firstUniqChar(string s) \n {\n unordered_map<char,int> map;\n for(int i=0; i<s.length(); i++)\n {\n map[s[i]]++; | vchand324 | NORMAL | 2021-06-19T17:16:59.140767+00:00 | 2021-06-19T17:16:59.140796+00:00 | 795 | false | ```\nint firstUniqChar(string s) \n {\n unordered_map<char,int> map;\n for(int i=0; i<s.length(); i++)\n {\n map[s[i]]++; \n }\n for(int i=0; i<s.length(); i++)\n { \n if(map[s[i]] == 1)\n return i;\n }\n return -1;\n \n }\n``` | 9 | 0 | ['C'] | 5 |
first-unique-character-in-a-string | Intuitive Javascript Solution | intuitive-javascript-solution-by-ishiima-3j66 | Runtime: 108 ms, faster than 81.91% of JavaScript online submissions for First Unique Character in a String.\nMemory Usage: 42.5 MB, less than 50.33% of JavaScr | ishiimatthew | NORMAL | 2021-01-06T00:08:14.329266+00:00 | 2021-01-06T00:08:29.168413+00:00 | 696 | false | Runtime: 108 ms, faster than 81.91% of JavaScript online submissions for First Unique Character in a String.\nMemory Usage: 42.5 MB, less than 50.33% of JavaScript online submissions for First Unique Character in a String.\n```\n\nvar firstUniqChar = function(s) {\n for(let i = 0; i < s.length; i++) {\n\t\t// Here we check to see if the index of the character can be found anywhere else\n\t\t// If it is indeed unique, it would only be found at the same index i\n if(s.lastIndexOf(s[i]) === i && s.indexOf(s[i]) === i) {\n return i;\n }\n } \n return -1;\n};\n``` | 9 | 0 | [] | 2 |
first-unique-character-in-a-string | C# charAndCount | c-charandcount-by-bacon-is4w | \npublic class Solution {\n public int FirstUniqChar(string s) {\n var charAndCount = new int[256];\n\n foreach (var c in s) {\n cha | bacon | NORMAL | 2019-06-23T20:36:44.040901+00:00 | 2019-06-23T20:36:44.040956+00:00 | 1,252 | false | ```\npublic class Solution {\n public int FirstUniqChar(string s) {\n var charAndCount = new int[256];\n\n foreach (var c in s) {\n charAndCount[c]++;\n }\n\n for (int i = 0; i < s.Length; i++) {\n if (charAndCount[s[i]] == 1) {\n return i;\n }\n }\n return -1;\n }\n}\n``` | 9 | 0 | [] | 2 |
first-unique-character-in-a-string | C# Linq Count Method | c-linq-count-method-by-xenoplastic-mb6d | here C# solution, using Count Method\n\npublic class Solution {\n public int FirstUniqChar(string s) {\n foreach(var itm in s.Distinct()){\n | xenoplastic | NORMAL | 2019-02-05T00:12:53.589545+00:00 | 2019-02-05T00:12:53.589585+00:00 | 931 | false | here C# solution, using Count Method\n```\npublic class Solution {\n public int FirstUniqChar(string s) {\n foreach(var itm in s.Distinct()){\n if(s.Count(x=>x==itm)==1){\n return s.IndexOf(itm);\n }\n }\n return -1;\n\n }\n}\n``` | 9 | 0 | [] | 2 |
first-unique-character-in-a-string | Python very easy solution | python-very-easy-solution-by-hayek-wdj8 | \nclass Solution(object):\n def firstUniqChar(self, str1):\n """\n :type s: str\n :rtype: int\n """\n for x in str1:\n | hayek | NORMAL | 2016-08-22T07:50:44.635000+00:00 | 2016-08-22T07:50:44.635000+00:00 | 4,407 | false | ```\nclass Solution(object):\n def firstUniqChar(self, str1):\n """\n :type s: str\n :rtype: int\n """\n for x in str1:\n if str1.find(x)==str1.rfind(x):\n return str1.find(x)\n return -1\n``` | 9 | 2 | [] | 3 |
first-unique-character-in-a-string | Golang concise solution | golang-concise-solution-by-redtree1112-h0qu | The idea is simple.\n\n1. Create a flag array that holds flags for each [a-z] characters and initialize all indexes with -1.\n2. Iterate through s and if a char | redtree1112 | NORMAL | 2017-02-14T10:43:40.726000+00:00 | 2017-02-14T10:43:40.726000+00:00 | 1,049 | false | The idea is simple.\n\n1. Create a flag array that holds flags for each [a-z] characters and initialize all indexes with `-1`.\n2. Iterate through `s` and if a character appears, set the index to the flag array. If the character appears again,\n we can set invalid index to the flag. I use `len(s)` in the code below.\n3. Iterate through the flag array and returns the least valid index found. If we cannot find the valid one, return `-1`\n\nIn this manner, we can avoid iterating through `s` itself twice. Looping through the flag array is `O(1)` because the length is limited:)\n\n```\nfunc firstUniqChar(s string) int {\n\tflags := make([]int, 26)\n\tfor i := range flags {\n\t\tflags[i] = -1\n\t}\n\tslen := len(s)\n\n\tfor i, ch := range s {\n\t\tidx := byte(ch - 'a')\n\t\tif flags[idx] == -1 {\n\t\t\tflags[idx] = i\n\t\t} else {\n\t\t\tflags[idx] = slen\n\t\t}\n\t}\n\n\tmin := slen\n\tfor i := range flags {\n\t\tif flags[i] >= 0 && flags[i] < len(s) && flags[i] < min {\n\t\t\tmin = flags[i]\n\t\t}\n\t}\n\n\tif min == slen {\n\t\treturn -1\n\t}\n\treturn min\n}\n``` | 9 | 0 | ['Go'] | 3 |
first-unique-character-in-a-string | Java || Easy to Understand ❤️❤️ || Queue || 0(N) || 2 Solutions || ❤️❤️ | java-easy-to-understand-queue-0n-2-solut-hryq | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | saurabh_kumar1 | NORMAL | 2023-09-11T07:44:11.045847+00:00 | 2023-09-11T07:44:11.045868+00:00 | 1,567 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:0(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:0(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int firstUniqChar(String s) {\n int temp[] = new int[26];\n for(int i=0; i<s.length(); i++){\n temp[s.charAt(i)-\'a\']++;\n }\n for(int i=0; i<s.length(); i++){\n if(temp[s.charAt(i)-\'a\']==1) return i;\n }\n return -1;\n\n// using Queue\n\n // int temp[] = new int [26];\n // Queue<Character> val = new LinkedList<>();\n // for(int i=0; i<s.length(); i++){\n // char ch = s.charAt(i);\n // val.add(ch);\n // temp[ch-\'a\']++;\n // while(!val.isEmpty() && temp[val.peek()-\'a\'] >1){\n // val.remove();\n // }\n // }\n // return val.isEmpty()?-1 : s.indexOf(val.peek());\n }\n}\n``` | 8 | 0 | ['String', 'Queue', 'Java'] | 2 |
first-unique-character-in-a-string | ✅Short || C++ || Java || PYTHON || Explained Solution✔️ || Beginner Friendly ||🔥 🔥 BY MR CODER | short-c-java-python-explained-solution-b-7zyv | Please UPVOTE if you LIKE!!\nWatch this video \uD83E\uDC83 for the better explanation of the code.\n\nhttps://www.youtube.com/watch?v=HVmq6apu3Zk\n\n\nAlso you | mrcoderrm | NORMAL | 2022-09-12T19:06:16.992444+00:00 | 2022-09-12T19:06:16.992491+00:00 | 1,467 | false | **Please UPVOTE if you LIKE!!**\n**Watch this video \uD83E\uDC83 for the better explanation of the code.**\n\nhttps://www.youtube.com/watch?v=HVmq6apu3Zk\n\n\n**Also you can SUBSCRIBE \uD83E\uDC81 \uD83E\uDC81 \uD83E\uDC81 this channel for the daily leetcode challange solution.**\n\n\n**C++**\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n \n unordered_map<char,int> ump(26);\n for(int i=0; i<s.size(); i++){\n ump[s[i]]++;\n }\n \n \n for(int i=0; i<s.size(); i++){\n if(ump[s[i]]==1) return i;\n }\n \n \n return -1;\n }\n};\n```\n\n**PYTHON**(copied)\n\n```\nclass Solution(object):\n def firstUniqChar(self, s):\n \n # Store drequency of all the characters of string in the array\n freq = Counter(s)\n \n # now check frequency of each character in the string from left-to-right\n # and whose frequency found to be 1 return the index\n for e in s :\n if freq[e] == 1 :\n return s.index(e)\n \n return -1\n```\n**JAVA**(copied)\n\n```\nclass Solution {\n public int firstUniqChar(String s) {\n \n char[] arr = s.toCharArray();\n int len = arr.length;\n \n \n int[] fre = new int[26];\n for(char c : arr) fre[c-\'a\']++;\n \n \n for(int i=0; i<len; i++) if(fre[arr[i]-\'a\'] == 1) return i;\n return -1;\n }\n}\n```\n**Please UPVOTE if you LIKE!!** | 8 | 0 | ['C', 'Python', 'C++', 'Java'] | 0 |
first-unique-character-in-a-string | Kotlin 1 line | kotlin-1-line-by-georgcantor-ep23 | \nfun firstUniqChar(s: String) = s.indexOfFirst { s.indexOf(it) == s.lastIndexOf(it) }\n | GeorgCantor | NORMAL | 2021-12-26T19:08:57.835133+00:00 | 2021-12-26T19:08:57.835184+00:00 | 470 | false | ```\nfun firstUniqChar(s: String) = s.indexOfFirst { s.indexOf(it) == s.lastIndexOf(it) }\n``` | 8 | 0 | ['Kotlin'] | 1 |
first-unique-character-in-a-string | Map() in JavaScript | map-in-javascript-by-danielyu74-zxtt | \nvar firstUniqChar = function(s) {\n const count = new Map();\n for(let i = 0; i < s.length; i++) {\n if(!count[s[i]]) {\n count[s[i]] | danielyu74 | NORMAL | 2021-01-15T23:34:22.331108+00:00 | 2021-01-15T23:37:36.303133+00:00 | 1,124 | false | ```\nvar firstUniqChar = function(s) {\n const count = new Map();\n for(let i = 0; i < s.length; i++) {\n if(!count[s[i]]) {\n count[s[i]] = 1;\n } else {\n count[s[i]]++\n }\n }\n for(let i = 0; i < s.length; i++) {\n if(count[s[i]] === 1) return i\n } \n return -1\n};\n``` | 8 | 3 | ['JavaScript'] | 3 |
first-unique-character-in-a-string | very easy solution with explanation c++| java | python | javascript | very-easy-solution-with-explanation-c-ja-sig8 | Intuition\nThe intuition behind this solution is to use an unordered map (M) to store the frequency of each character in the input string (s). The first loop it | prashant_71200 | NORMAL | 2024-02-05T03:36:22.819517+00:00 | 2024-02-05T03:37:58.206344+00:00 | 974 | false | # Intuition\nThe intuition behind this solution is to use an unordered map (M) to store the frequency of each character in the input string (s). The first loop iterates through the string and populates the map with the count of each character. The second loop then checks the frequency of each character in the map and returns the index of the first character with a frequency of 1, indicating it is the first unique character in the string.\n\n# Approach\n1) initialize an unordered map M to store the frequency of characters.\n\n2) Iterate through the input string s and update the frequency of each character in the map.\n\n3) Iterate through the input string s again and return the index of the first character with a frequency of 1.\n\nIf no such character is found, return -1.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n\n \n```c++ []\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map<char,int> M;\n \n for(int i=0;i<s.length();i++)\n {\n M[s[i]]++;\n \n }\n \n for(int i=0;i<s.length();i++)\n {\n if(M[s[i]]==1)\n {\n return i;\n }\n }\n return -1;\n }\n};\n```\n```python []\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n M = {}\n \n for char in s:\n M[char] = M.get(char, 0) + 1\n \n for i, char in enumerate(s):\n if M[char] == 1:\n return i\n \n return -1\n\n```\n```javascript []\n/**\n * @param {string} s\n * @return {number}\n */\nvar firstUniqChar = function(s) {\n let M = new Map();\n \n for (let i = 0; i < s.length; i++) {\n M.set(s[i], (M.get(s[i]) || 0) + 1);\n }\n \n for (let i = 0; i < s.length; i++) {\n if (M.get(s[i]) === 1) {\n return i;\n }\n }\n \n return -1;\n};\n\n```\n\n```java []\nimport java.util.HashMap;\n\nclass Solution {\n public int firstUniqChar(String s) {\n HashMap<Character, Integer> M = new HashMap<>();\n \n for (int i = 0; i < s.length(); i++) {\n M.put(s.charAt(i), M.getOrDefault(s.charAt(i), 0) + 1);\n }\n \n for (int i = 0; i < s.length(); i++) {\n if (M.get(s.charAt(i)) == 1) {\n return i;\n }\n }\n \n return -1;\n }\n}\nor (let i = 0; i < s.length; i++) {\n \n\n```\n \n```\n\nUPVOTE IF YOU FIND ITS HELPFULL | 7 | 0 | ['Python', 'C++', 'Java', 'JavaScript'] | 0 |
first-unique-character-in-a-string | Short and Easy | Using Counter | Python | short-and-easy-using-counter-python-by-p-1045 | Complexity\n- Time complexity:O(n)\n\n- Space complexity:O(n)\n\n# Code\n\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n count = Counter | pragya_2305 | NORMAL | 2024-02-05T02:12:03.915793+00:00 | 2024-02-05T02:12:03.915873+00:00 | 1,316 | false | # Complexity\n- Time complexity:O(n)\n\n- Space complexity:O(n)\n\n# Code\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n count = Counter(s)\n\n for i,c in enumerate(s):\n if count[c]==1:\n return i\n\n return -1\n``` | 7 | 0 | ['Hash Table', 'Python', 'Python3'] | 0 |
first-unique-character-in-a-string | Easy to understand || C++ | easy-to-understand-c-by-yashwardhan24_sh-rkxn | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | yashwardhan24_sharma | NORMAL | 2023-03-28T05:15:29.979825+00:00 | 2023-03-28T05:15:29.979869+00:00 | 2,169 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n \n unordered_map<char,int>mp;\n for(int i=0;i<s.size();i++){\n mp[s[i]]++; \n }\n for(int i=0;i<s.size();i++){\n if(mp.find(s[i])->second==1){\n return i;\n }\n }\n return -1;\n }\n};\n``` | 7 | 0 | ['Hash Table', 'C++'] | 0 |
first-unique-character-in-a-string | C++ ✔|| Constant Spaces o(1) || O(N) Time complexity ✅ | c-constant-spaces-o1-on-time-complexity-ic3il | \nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int n=s.size();\n vector<int> m(26,0);\n for(char a : s){\n m[ | Ayuk_05 | NORMAL | 2022-08-16T05:09:15.948348+00:00 | 2022-08-16T05:09:15.948391+00:00 | 690 | false | ```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int n=s.size();\n vector<int> m(26,0);\n for(char a : s){\n m[a-\'a\']++;\n }\n int ans=-1;\n for(int i=0;i<n;++i){\n if(m[s[i]-\'a\']==1){\n ans=i;\n break;\n }\n }\n return ans;\n }\n};\n\n``` | 7 | 2 | ['C'] | 0 |
first-unique-character-in-a-string | Easy Python Solution | easy-python-solution-by-vistrit-rj7p | \ndef firstUniqChar(self, s: str) -> int:\n c=Counter(s)\n for i,j in enumerate(s):\n if c[j]<=1:\n return i\n re | vistrit | NORMAL | 2021-12-06T04:39:24.734991+00:00 | 2021-12-06T04:39:24.735030+00:00 | 1,172 | false | ```\ndef firstUniqChar(self, s: str) -> int:\n c=Counter(s)\n for i,j in enumerate(s):\n if c[j]<=1:\n return i\n return -1\n``` | 7 | 0 | ['Python', 'Python3'] | 0 |
first-unique-character-in-a-string | Java Queue Solution | java-queue-solution-by-aatmik_coder-7c28 | ```\nclass Solution {\n public int firstUniqChar(String s) {\n Queue ch = new LinkedList();\n int len = s.length();\n char temper = 0;\n | Aatmik_Coder | NORMAL | 2021-11-09T06:05:44.096905+00:00 | 2021-11-09T06:05:44.096947+00:00 | 1,551 | false | ```\nclass Solution {\n public int firstUniqChar(String s) {\n Queue<Character> ch = new LinkedList<Character>();\n int len = s.length();\n char temper = 0;\n for(int j = 0; j < s.length(); j++)\n {\n ch.offer(s.charAt(j));\n }\n while(len > 0)\n {\n temper = ch.poll();\n if(!ch.contains(temper))\n {\n return s.indexOf(temper);\n }\n else\n {\n ch.offer(temper);\n }\n len--;\n }\n return -1;\n }\n} | 7 | 1 | ['Queue', 'Java'] | 0 |
first-unique-character-in-a-string | C simple code | c-simple-code-by-bindhushreehr-qlyh | \nint firstUniqChar(char * s){\n int alph[26]={0};\n \n if (s==NULL)\n return -1;\n \n for (int i=0;s[i]!=\'\\0\';i++)\n {\n alp | bindhushreehr | NORMAL | 2020-08-28T14:25:17.390204+00:00 | 2020-08-28T14:26:00.632599+00:00 | 720 | false | ```\nint firstUniqChar(char * s){\n int alph[26]={0};\n \n if (s==NULL)\n return -1;\n \n for (int i=0;s[i]!=\'\\0\';i++)\n {\n alph[s[i] - \'a\'] = alph[s[i] - \'a\']+1;\n }\n \n for(int i=0;s[i]!=\'\\0\';i++)\n {\n if (1 == alph[s[i] - \'a\'])\n return i;\n }\n \n return -1;\n}\n\n``` | 7 | 0 | ['Hash Table', 'C'] | 0 |
first-unique-character-in-a-string | Python | Single Pass Using Queue | python-single-pass-using-queue-by-ared25-a2wk | \nfrom collections import deque\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n d = {}\n q = deque()\n for i, x in enumerat | ared25 | NORMAL | 2020-02-27T14:41:56.994940+00:00 | 2020-02-27T14:45:04.783352+00:00 | 1,021 | false | ```\nfrom collections import deque\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n d = {}\n q = deque()\n for i, x in enumerate(s):\n d[x] = d.get(x, 0) + 1\n if d[x] == 1:\n q.append((x, i))\n while q and d[q[0][0]] > 1:\n q.popleft()\n return -1 if not q else q[0][1]\n``` | 7 | 0 | ['Python'] | 1 |
first-unique-character-in-a-string | Java using twice scans | java-using-twice-scans-by-sjhuangx-u18c | the first scan we statistics the number of each letter in string.\nthe second scan we find the first letter which number equals 1.\n\npublic class Solution {\n | sjhuangx | NORMAL | 2016-08-22T07:51:25.962000+00:00 | 2016-08-22T07:51:25.962000+00:00 | 2,778 | false | the first scan we statistics the number of each letter in string.\nthe second scan we find the first letter which number equals 1.\n```\npublic class Solution {\n public int firstUniqChar(String s) {\n if (s == null || s.isEmpty()) {\n return -1;\n }\n int[] letters = new int[26];\n for (int i = 0; i < s.length(); i++) {\n letters[s.charAt(i) - 'a']++;\n }\n for (int i = 0; i < s.length(); i++) {\n if (letters[s.charAt(i) - 'a'] == 1) {\n return i;\n }\n }\n return -1;\n }\n}\n``` | 7 | 0 | [] | 2 |
first-unique-character-in-a-string | Simple Java || C++ Codes ☠️ | simple-java-c-codes-by-abhinandannaik171-8bt1 | Bruteforce Code\njava []\nclass Solution {\n public int firstUniqChar(String s) {\n int n = s.length();\n for(int i=0;i<n;i++){\n fo | abhinandannaik1717 | NORMAL | 2024-08-23T17:18:48.920393+00:00 | 2024-08-23T17:18:48.920422+00:00 | 599 | false | # Bruteforce Code\n```java []\nclass Solution {\n public int firstUniqChar(String s) {\n int n = s.length();\n for(int i=0;i<n;i++){\n for(int j=0;j<n;j++){\n if(i==n-1 && j==n-1){\n return i;\n }\n if(i==j){\n continue;\n }\n if(s.charAt(i) == s.charAt(j)){\n break;\n }\n if(j==n-1){\n return i;\n }\n }\n }\n return -1;\n }\n}\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int n = s.length();\n for(int i=0;i<n;i++){\n for(int j=0;j<n;j++){\n if(i==n-1 && j==n-1){\n return i;\n }\n if(i==j){\n continue;\n }\n if(s[i] == s[j]){\n break;\n }\n if(j==n-1){\n return i;\n }\n }\n }\n return -1;\n }\n};\n }\n}\n```\n\n\n# Optimised Code (HashMap)\n```java []\nclass Solution {\n public int firstUniqChar(String s) {\n int n = s.length();\n Map <Character,Integer> map = new HashMap<>();\n for(int i=0;i<n;i++){\n if(!map.isEmpty() && map.containsKey(s.charAt(i))){\n map.put(s.charAt(i),map.get(s.charAt(i))+1);\n }\n else{\n map.put(s.charAt(i),1);\n }\n }\n for(int i=0;i<n;i++){\n if(map.get(s.charAt(i))==1){\n return i;\n }\n }\n return -1;\n }\n}\n```\n\n\n\n### First Code Explanation\n\n\n1. **Initialization**: The outer loop iterates through each character in the string `s` (indexed by `i`).\n\n2. **Inner Loop**: The inner loop (indexed by `j`) checks every other character in the string to see if it matches the current character from the outer loop.\n\n3. **Checking for Duplicates**:\n - The `continue` statement skips the case where `i == j` because we do not want to compare a character with itself.\n - If a match is found (`s.charAt(i) == s.charAt(j)`), the loop breaks because `s.charAt(i)` is not unique.\n - If `j` reaches the end of the string (`j == n - 1`) without finding a duplicate, it means the character `s.charAt(i)` is unique, so the index `i` is returned.\n\n4. **Final Check**:\n - If the loop completes without finding a unique character, the method returns `-1`.\n\n**Flaws and Improvements**:\n- The current implementation has several redundant checks:\n - `if (i == n - 1 && j == n - 1) { return i; }` is unnecessary. The logic can be handled more cleanly by the condition `if (j == n - 1)`.\n - The code breaks out of the inner loop as soon as it finds a duplicate, but it continues for all characters, making it O(n^2) in the worst case.\n\n### Second Code Explanation\n\n1. **Using a HashMap**:\n - A `HashMap` is used to count the frequency of each character in the string `s`.\n - The first loop iterates over each character in the string:\n - If the character is already in the map (`map.containsKey(s.charAt(i))`), its frequency is incremented by 1.\n - If the character is not in the map, it is added with a frequency of 1.\n\n2. **Finding the First Unique Character**:\n - The second loop iterates through the string again:\n - For each character, it checks if its frequency in the map is 1 (`map.get(s.charAt(i)) == 1`).\n - If a character with a frequency of 1 is found, its index is returned.\n\n3. **Returning -1**:\n - If no unique character is found after checking all characters, the method returns `-1`.\n\n**Efficiency**:\n- **Time Complexity**: The time complexity is **O(n)**. This is because both the first and second loops each run `n` times, where `n` is the length of the string. Accessing and modifying the HashMap is an O(1) operation on average.\n- **Space Complexity**: The space complexity is **O(1)** for the map since it holds a maximum of 26 keys (for lowercase English letters).\n\n### Summary\n\n- **First Code**: Uses nested loops to check each character against every other character, resulting in **O(n^2)** time complexity. It\'s less efficient due to redundant checks and unnecessary comparisons.\n \n- **Second Code**: Utilizes a `HashMap` to count the occurrences of each character, making it much more efficient with **O(n)** time complexity. It is preferred for larger inputs because it minimizes the number of checks and uses space efficiently.\n\n\n\n | 6 | 0 | ['Hash Table', 'String', 'Counting', 'C++', 'Java'] | 0 |
first-unique-character-in-a-string | 387. First Unique Character in a String| SC: O(1) |Bit Manipulation|TC: O(N) | 387-first-unique-character-in-a-string-s-wyem | Intuition\n Describe your first thoughts on how to solve this problem. \nTo find the first single frequency of char in a String is achieved by two interger vari | 21csa36 | NORMAL | 2024-02-05T07:00:48.603211+00:00 | 2024-02-05T07:00:48.603244+00:00 | 200 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTo find the first single frequency of char in a String is achieved by two interger varible (mask1 and mask2) used as mask to store the freq. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSince Integer has 32 bits, first 26 bits in - MASK1 VARIABLE - were used to MARK the first occurance , - MASK2 VARIABLE - used to MARK remaining occurances.\n\nmask1=0 # In the representation of 32 bits\nmask2 =0 \n\nMas1 :\n 0 0 0 0 0 0 0 | 0| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0\n_ _ _ _ _ _ _ __ z | y | x | w | v | u | t | s | r | q | p | o | n | m | l | k | j | i | h | g | f | e | d | c | b | a \n\nMask2:\n\n 0 0 0 0 0 0 0 | 0| 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0\n_ _ _ _ _ _ _ __ z | y | x | w | v | u | t | s | r | q | p | o | n | m | l | k | j | i | h | g | f | e | d | c | b | a \n\nlet s="abaa"\n------------------------------------------------------------------\n\nfor i in s : # Iterating String\n\nIteration 1 : i = a Mark \'a\' in mask1\n\nmask1:\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0mask2:\nZ Y . . . . . . C B A\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0.\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0 Z Y . . . . . . C B A\n0 0 . . . . . . 0 0 1\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA00 0 . . . . . . . 0 0 0\n-------------------------------------------------------------------\nIteration 2: i = b, Mark \'b\' in mask1\n\nmask1:\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0mask2:\nZ Y . . . . . . C B A\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0.\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0 Z Y . . . . . . C B A\n0 0 . . . . . . 0 1 1\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA00 0 . . . . . . . 0 0 0\n------------------------------------------------------------------\nIteration 3: i = a, \'a\' is already marked in mask1 so just mark \'a\' in mask2\n\nmask1:\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0mask2:\nZ Y . . . . . . C B A\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0.\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0 Z Y . . . . . . C B A\n0 0 . . . . . . 0 1 1\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA00 0 . . . . . . . 0 0 1\n------------------------------------------------------------------\nIteration 4: i = a, \'a\' is already marked in mask1 so just mark \'a\' \nin mask2, dont care about mask2\n--------------------------------------------------------------------\n\nmask1:\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0mask2:\nZ Y . . . . . . C B A\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0.\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0 Z Y . . . . . . C B A\n0 0 . . . . . . 0 1 1\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA0\xA00 0 . . . . . . . 0 0 1\n-------------------------------------------------------------------\nNow iterate the String s="abaa" , If mask2 of a any char is Zero it is the answer\n----------------------------------------------------------------------\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nTC:O(N)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```\nclass Solution(object):\n def firstUniqChar(self, s):\n """\n :type s: str\n :rtype: int\n """\n mask1,mask2=0,0\n for i in s:\n ind = ord(i)-97\n if( mask1 & 1<<ind)==0:\n mask1|=1<<ind\n else:\n mask2|=1<<ind\n for i,j in enumerate(s):\n ind=ord(j)-97\n if (mask2&1<<ind)==0:\n return i\n return -1\n \n \n``` | 6 | 0 | ['Bit Manipulation', 'Bitmask', 'Python', 'C++', 'Java', 'Python3'] | 3 |
first-unique-character-in-a-string | 🚀🚀 Beats 100% | Easiest Approach 💎🔥 | beats-100-easiest-approach-by-sanket_des-8ugk | Intuition \uD83E\uDD14:\nWe want to find the first character in the string that doesn\'t repeat. That means we need to count how many times each character appea | The_Eternal_Soul | NORMAL | 2024-02-05T01:52:49.256599+00:00 | 2024-02-05T01:52:49.256626+00:00 | 1,145 | false | **Intuition** \uD83E\uDD14:\nWe want to find the first character in the string that doesn\'t repeat. That means we need to count how many times each character appears in the string.\n\n**Approach** \uD83D\uDCA1:\n1. We\'ll use a map to count the occurrences of each character in the string.\n2. Then, we\'ll iterate through the string again to find the first character with a count of 1.\n3. Once we find such a character, we return its index. If no unique character exists, we return -1.\n\n**Time Complexity** \u23F0:\n- We iterate through the string twice, which takes O(n) time where n is the length of the string.\n- Therefore, the overall time complexity is O(n).\n\n**Space Complexity** \uD83D\uDE80:\n- We use a map to store the count of each character, which could potentially take up space proportional to the number of unique characters in the string.\n- Hence, the space complexity depends on the number of unique characters in the string, which is O(c) where c is the number of unique characters.\n\n```cpp\n// Let\'s find the first unique character in a string! \uD83D\uDE04\nint firstUniqChar(string s) {\n // We\'ll count how many times each character appears using a map! \uD83D\uDCCA\n unordered_map<char, int> m;\n \n // Let\'s count those characters! \uD83D\uDCDD\n for(auto ch : s) {\n m[ch]++;\n }\n \n // Now, let\'s find the first character that appears only once! \uD83D\uDD75\uFE0F\u200D\u2642\uFE0F\n for(int i = 0; i < s.length(); i++) {\n char ch = s[i];\n // If we find a character that appears only once, we return its index! \uD83C\uDF89\n if(m[ch] == 1)\n return i;\n }\n // If no unique character exists, we return -1! \uD83D\uDE1E\n return -1;\n}\n```\n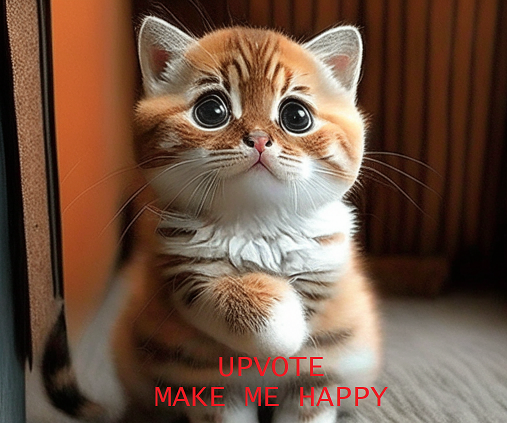\n | 6 | 1 | ['Hash Table', 'String', 'C', 'Counting', 'Python', 'C++', 'Java', 'JavaScript'] | 0 |
first-unique-character-in-a-string | simple solution for beginners | simple-solution-for-beginners-by-hrishik-nlle | \n\n# Code\n\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i in range(len(s)):\n if s.count(s[i])==1:\n r | hrishikeshprasadc | NORMAL | 2023-12-02T03:31:08.453558+00:00 | 2023-12-02T03:31:08.453635+00:00 | 333 | false | \n\n# Code\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i in range(len(s)):\n if s.count(s[i])==1:\n return i\n return -1\n\n``` | 6 | 0 | ['Python3'] | 0 |
first-unique-character-in-a-string | 387: Space 93.87%, Solution with step by step explanation | 387-space-9387-solution-with-step-by-ste-kg4z | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n1. We define a class Solution with a method firstUniqChar that takes a st | Marlen09 | NORMAL | 2023-03-04T07:02:14.391970+00:00 | 2023-03-04T07:02:14.392015+00:00 | 2,888 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. We define a class Solution with a method firstUniqChar that takes a string s as input and returns an integer.\n2. We create an empty dictionary freq to store the frequency of each character in the string.\n3. We iterate through the string s using a for loop and update the frequency of each character in the dictionary.\n - For each character c in the string s, we check if it is already present in the freq dictionary using the .get() method. If it is not present, we set its value to 0 and then add 1 to it. If it is already present, we add 1 to its existing value.\n4. We iterate through the string s again using another for loop and return the index of the first character with a frequency of 1.\n - For each character in the string s, we check if its frequency in the freq dictionary is 1. If it is 1, we return the index of that character in the string.\n5. If we do not find any character with a frequency of 1, we return -1.\n\n# Complexity\n- Time complexity:\n60.4%\n\n- Space complexity:\n93.87%\n\n# Code\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n freq = {}\n for c in s:\n freq[c] = freq.get(c, 0) + 1\n for i in range(len(s)):\n if freq[s[i]] == 1:\n return i\n return -1\n\n``` | 6 | 0 | ['Hash Table', 'String', 'Queue', 'Python', 'Python3'] | 0 |
first-unique-character-in-a-string | Python | Beats 99% | 3 Solutions | O(N) | python-beats-99-3-solutions-on-by-nadara-bdwa | \n\n\n\nThe 3rd solution beats 99%\n\n\n1. We run over s to count how many times each character appears.\n\n2. We run over s again, and whenever we meet a chara | nadaralp | NORMAL | 2022-08-16T08:34:01.272789+00:00 | 2022-08-16T08:46:22.852509+00:00 | 635 | false | 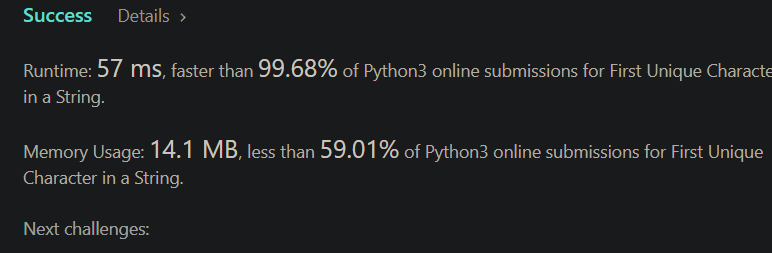\n\n<hr />\n\nThe 3rd solution beats 99%\n<hr />\n\n1. We run over `s` to count how many times each character appears.\n\n2. We run over `s` again, and whenever we meet a character that appears only once, we return the index. Since we run in order, we will meet the first non-repeating character\n\n\nWe can count letters with hashmap, like `{a: 3}` or array of length 26 to represent the a-z letters.\n\n# Counter / hashmap\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n c = Counter(s)\n for i, v in enumerate(s):\n if c[v] == 1:\n return i\n return -1\n```\n\n# Array representing english letters\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n A = [0] * 26\n for v in s: A[ord(v) - ord(\'a\')] += 1\n \n for i, v in enumerate(s):\n if A[ord(v) - ord(\'a\')] == 1:\n return i\n return -1\n```\n\n\n# 3rd solution\nIterate a-z and find the index character where the first and last occurrence match\n\n```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n best = float(\'inf\')\n for i in range(26):\n c = chr(i + ord(\'a\'))\n l = s.find(c)\n r = s.rfind(c)\n if l != -1 and l == r:\n best = min(best, l)\n return best if best != float(\'inf\') else -1\n``` | 6 | 0 | ['Python'] | 0 |
first-unique-character-in-a-string | Java / C++ Map | java-c-map-by-fllght-auer | \n\nAlso added a shorter (and slower) solution using the stream API.\n\n#### Java\n\npublic int firstUniqChar(String s) {\n Map<Character, Integer> chara | FLlGHT | NORMAL | 2022-08-16T04:56:02.296915+00:00 | 2022-08-16T06:25:45.241549+00:00 | 1,055 | false | \n\nAlso added a shorter (and slower) solution using the stream API.\n\n#### Java\n```\npublic int firstUniqChar(String s) {\n Map<Character, Integer> characterMap = new HashMap<>();\n for (char c : s.toCharArray()) {\n characterMap.put(c, characterMap.getOrDefault(c, 0) + 1);\n }\n for (int i = 0; i < s.length(); i++) {\n if (characterMap.get(s.charAt(i)) == 1)\n return i;\n }\n return -1;\n }\n```\n\n#### Java Stream\n\n```\n public int firstUniqChar(String s) {\n Map<Character, Integer> characterMap = new LinkedHashMap<>();\n s.chars().mapToObj(c -> (char) c).forEach(c -> characterMap.put(c, characterMap.getOrDefault(c, 0) + 1));\n return characterMap.entrySet().stream().filter(entry -> entry.getValue() == 1)\n .map(entry -> s.indexOf(entry.getKey())).findFirst().orElse(-1);\n }\n```\n\n#### C++\n```\npublic:\n int firstUniqChar(string s) {\n unordered_map<char, int> characterMap;\n \n for (char& c : s) {\n characterMap[c]++;\n }\n for (int i = 0; i < s.length(); i++) {\n if (characterMap[s[i]] == 1) \n return i;\n }\n return -1;\n }\n``` | 6 | 1 | ['C', 'Java'] | 1 |
first-unique-character-in-a-string | JAVA, if u want to practice HashMap | java-if-u-want-to-practice-hashmap-by-sh-qevi | class Solution {\n public int firstUniqChar(String s) {\n HashMap ans = new HashMap <>();\n \n //go through the whole string, record th | shiwenbot | NORMAL | 2022-03-16T11:25:50.005118+00:00 | 2022-03-16T11:25:50.005170+00:00 | 757 | false | class Solution {\n public int firstUniqChar(String s) {\n HashMap <Character, Integer> ans = new HashMap <>();\n \n //go through the whole string, record the frequency of each letter\n for (int i = 0; i < s.length(); i ++){\n char c = s.charAt(i);\n if(ans.containsKey(c)){\n ans.put(c,ans.get(c) + 1);\n }\n else{\n ans.put(c, 1);\n }\n }\n \n //return the first letter that has frequency of 1\n for (int i = 0; i < s.length(); i ++){\n char c = s.charAt(i);\n if (ans.get(c) == 1){\n return i;\n }\n }\n return -1;\n \n }\n} | 6 | 0 | ['Java'] | 1 |
first-unique-character-in-a-string | java easiest solution without using extra-space | java-easiest-solution-without-using-extr-538p | \n public int firstUniqChar(String s) {\n for(int i = 0; i < s.length(); i++) {\n char c = s.charAt(i);\n if(s.indexOf(c) == s.last | kanika_matta | NORMAL | 2022-02-08T18:13:19.041769+00:00 | 2022-02-08T18:13:19.041813+00:00 | 652 | false | ```\n public int firstUniqChar(String s) {\n for(int i = 0; i < s.length(); i++) {\n char c = s.charAt(i);\n if(s.indexOf(c) == s.lastIndexOf(c)) {\n return i;\n }\n }\n \n return -1;\n }\n``` | 6 | 0 | ['Array', 'Java'] | 1 |
first-unique-character-in-a-string | C++ || Short and Easy to understand solution || Using Hashmap | c-short-and-easy-to-understand-solution-7mb73 | Please Upvote if you find this solution helpful\n\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map <char,int> mp;\n f | uk1124 | NORMAL | 2022-01-24T12:23:06.150845+00:00 | 2022-01-24T12:24:28.534709+00:00 | 373 | false | **Please Upvote if you find this solution helpful**\n```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n unordered_map <char,int> mp;\n for(int i=0; i<s.size(); i++) {\n mp[s[i]]++;\n }\n for(int i=0; i<s.size(); i++) {\n if(mp[s[i]] == 1) {\n return i;\n }\n }\n return -1;\n }\n};\n``` | 6 | 0 | ['C', 'C++'] | 0 |
first-unique-character-in-a-string | Easy python solution O(n) | easy-python-solution-on-by-nirmitlakhani-z6co | \nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i in range(len(s)):\n if s[i] not in s[i+1:] and s[i] not in s[:i]:\n | nirmitlakhani314 | NORMAL | 2022-01-10T11:02:56.619866+00:00 | 2022-01-10T11:02:56.619905+00:00 | 187 | false | ```\nclass Solution:\n def firstUniqChar(self, s: str) -> int:\n for i in range(len(s)):\n if s[i] not in s[i+1:] and s[i] not in s[:i]:\n return i\n break\n return -1\n \n``` | 6 | 0 | [] | 0 |
first-unique-character-in-a-string | Easy to understand in C++ || Hashmap | easy-to-understand-in-c-hashmap-by-lucif-y6lt | class Solution {\npublic:\n\n int firstUniqChar(string s) {\n unordered_map mp;\n for(int i=0;i<s.length();i++){\n mp[s[i]]++;\n | luciferraturi | NORMAL | 2021-12-30T17:39:58.969376+00:00 | 2021-12-30T17:39:58.969418+00:00 | 156 | false | class Solution {\npublic:\n\n int firstUniqChar(string s) {\n unordered_map<char,int> mp;\n for(int i=0;i<s.length();i++){\n mp[s[i]]++;\n }\n for(int i=0;i<s.length();i++){\n if(mp[s[i]]==1){\n return i;\n }\n }\n return -1;\n }\n}; | 6 | 0 | [] | 0 |
first-unique-character-in-a-string | Most Efficient Solution in C++ with Time Complexity O(n) Space O(1) Faster than 98%. | most-efficient-solution-in-c-with-time-c-7yrw | Here I have used a 2D array with 2 rows and 26 Columns so that there occurrence can be stored in row 1 with all corresponding characters repetitions and row 2 w | diwanshubansal | NORMAL | 2021-11-03T08:37:09.124743+00:00 | 2021-11-03T09:01:21.814295+00:00 | 145 | false | Here I have used a 2D array with **2 rows and 26 Columns** so that there occurrence can be stored in **row 1** with all corresponding **characters repetitions** and **row 2** with there last **occurrence index**. Finally I iterate through the 2D array and find the **first unique char with only one occurrences**.\n\n**Time Complexity -> O(n)**\n**Space Complexity-> O(1)**(because linear array)\n\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n int arr[2][27] = {0}; \n int num=0;\n for(int i=0;i<s.length();i++)\n {\n num=s[i]-\'a\';\n arr[0][num]+=1;\n arr[1][num]=i;\n }\n int small=99999; // Storing maximum value that can possibly occur.\n for(int i=0;i<26;i++)\n {\n if(arr[0][i]==1)\n {\n if(arr[1][i]<small && arr[1][i]>=0)\n {\n small=arr[1][i];\n }\n }\n }\n if(small==99999)\n return -1;\n return small;\n }\n}; | 6 | 0 | [] | 1 |
first-unique-character-in-a-string | Javascript Solution - Simple and Clear | javascript-solution-simple-and-clear-by-8gr92 | \nconst firstUniqChar = (s) => {\n for (let i = 0; i < s.length; ++i) if (s.indexOf(s[i]) === s.lastIndexOf(s[i])) return i;\n return -1;\n};\n\n\n\nResult\nR | ahmetcetinkaya | NORMAL | 2021-01-05T15:18:31.719133+00:00 | 2021-01-05T15:18:31.719180+00:00 | 1,440 | false | ```\nconst firstUniqChar = (s) => {\n for (let i = 0; i < s.length; ++i) if (s.indexOf(s[i]) === s.lastIndexOf(s[i])) return i;\n return -1;\n};\n```\n<a href="https://gist.github.com/ahmet-cetinkaya/479fe978f9130bd09be32e6e8f063822"><img src="https://img.shields.io/badge/gist-100000?style=for-the-badge&logo=github&logoColor=white" /></a>\n\nResult\nRuntime: 96 ms, faster than 94.94% of JavaScript online submissions for First Unique Character in a String.\nMemory Usage: 42.6 MB, less than 42.64% of JavaScript online submissions for First Unique Character in a String. | 6 | 0 | ['JavaScript'] | 3 |
first-unique-character-in-a-string | C# faster than 96.38%, less than 10.00% Mem, O(n) | c-faster-than-9638-less-than-1000-mem-on-xnzm | Runtime: 80 ms\nMemory Usage: 31.9 MB\n\n```\n public int FirstUniqChar(string s) {\n int[] counts = new int[\'z\'-\'a\'+1];\n \n for(int | ve7545 | NORMAL | 2020-02-26T19:06:39.374499+00:00 | 2020-02-26T19:06:39.374533+00:00 | 413 | false | Runtime: 80 ms\nMemory Usage: 31.9 MB\n\n```\n public int FirstUniqChar(string s) {\n int[] counts = new int[\'z\'-\'a\'+1];\n \n for(int i=0; i< s.Length; i++)\n {\n counts[s[i]-\'a\']++;\n }\n \n for(int i=0; i< s.Length; i++)\n {\n if (counts[s[i]-\'a\'] == 1) { return i; }\n }\n \n return -1;\n } | 6 | 0 | [] | 0 |
first-unique-character-in-a-string | cpp solution that beats 97.96% | cpp-solution-that-beats-9796-by-leondele-tuq9 | \nclass Solution {\npublic:\n int firstUniqChar(string s) {\n vector<int> record(26, 0);\n for(char c: s) {\n int tmp = c - \'a\';\n | leondelee | NORMAL | 2019-01-21T16:25:42.129543+00:00 | 2019-01-21T16:25:42.129604+00:00 | 810 | false | ```\nclass Solution {\npublic:\n int firstUniqChar(string s) {\n vector<int> record(26, 0);\n for(char c: s) {\n int tmp = c - \'a\';\n record[tmp]++;\n }\n for(int i = 0; i < s.size(); i ++) {\n int tmp = s[i] - \'a\';\n if(record[tmp] == 1) return i;\n }\n return - 1;\n }\n};\n``` | 6 | 0 | [] | 3 |
number-of-arithmetic-triplets | Check [n - diff] and [n - 2 * diff] | check-n-diff-and-n-2-diff-by-votrubac-v83k | C++\ncpp\nint arithmeticTriplets(vector<int>& nums, int diff) {\n int cnt[201] = {}, res = 0;\n for (auto n : nums) {\n if (n >= 2 * diff)\n | votrubac | NORMAL | 2022-08-07T04:04:54.423643+00:00 | 2022-08-07T04:04:54.423673+00:00 | 12,780 | false | **C++**\n```cpp\nint arithmeticTriplets(vector<int>& nums, int diff) {\n int cnt[201] = {}, res = 0;\n for (auto n : nums) {\n if (n >= 2 * diff)\n res += cnt[n - diff] && cnt[n - 2 * diff];\n cnt[n] = true;\n }\n return res;\n}\n``` | 134 | 2 | [] | 25 |
number-of-arithmetic-triplets | ✅ C++ Easy Hashmap solution | with explanation | c-easy-hashmap-solution-with-explanation-s46l | Hashing Technique.\n\nO(N) space and O(N) Time :\n\n1. Simply first store all the elements of array in a map or a set.\n2. Now again traverse the array and see | avaneeshyadav | NORMAL | 2022-08-07T08:38:43.751622+00:00 | 2022-08-07T11:43:52.899542+00:00 | 10,501 | false | # Hashing Technique.\n\n**O(N) space and O(N) Time :**\n\n1. Simply first store all the elements of array in a map or a set.\n2. Now again traverse the array and see for every element say \'x\', if there are two more elements present in map i.e x+diff and x-diff.\n3. If present then increment the count by 1.\n4. Return the count variable.\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n \n int cnt = 0;\n \n unordered_map<int,bool> mp;\n \n // Mark every elem presence in map.\n for(int i=0;i<nums.size();i++)\n mp[nums[i]] = true;\n \n \n // For every element say \'elm\' check if there exist both numbers, (elm + diff) and (elm - diff) inside map. If yes then increment cnt\n for(int i=0;i<nums.size();i++)\n {\n if(mp[nums[i]-diff] && mp[nums[i]+diff])\n cnt++;\n }\n \n\t\t\n\t\t// Happy return :)\n return cnt;\n }\n};\n``` | 127 | 1 | ['Array', 'C++'] | 10 |
number-of-arithmetic-triplets | ✅5 Method's || C++ || JAVA || PYTHON || Beginner Friendly🔥🔥🔥 | 5-methods-c-java-python-beginner-friendl-1i26 | Intuition\n Describe your first thoughts on how to solve this problem. \nThe "Number of Arithmetic Triplets" problem requires finding triplets in a given sorted | datpham0412 | NORMAL | 2024-04-20T07:57:35.904420+00:00 | 2024-04-20T07:57:35.904449+00:00 | 4,398 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe "Number of Arithmetic Triplets" problem requires finding triplets in a given sorted array nums such that two consecutive differences match a given value diff. This problem tests our ability to efficiently find specific sequences in an array that meet certain numerical criteria. Given the constraints and the strictly increasing nature of the array, multiple methods can be employed, each with different trade-offs in terms of time and space efficiency.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n## 1. Brute force\n The most straightforward approach is a brute force method using three nested loops. This method iteratively checks every possible triplet to see if the consecutive differences match \'**diff**\'. However, this approach has a cubic time complexity O(n^3), making it inefficient for larger arrays.\n1. Iterate over each element as a potential first element of the triplet\n2. Use a second loop to find a middle element that is greater than the first element by \'**diff**\'\n3. Use a third loop to find a third element that is greater than the second element by \'**diff**\'.\n4. Count each valid triple.\n\n## 2. Array \nA slightly more refined approach involves using the \'**find**\' function for the array. This reduces the nesting of llops to one, but each call to \'**find**\' is O(n), leading to overall complexity of O(n^2)\n\n1. For each element in the array, use \'**find**\' to check if there exists another element exactly \'diff\' away.\n2. If such an element exists, use \'**find**\' again to check for a third element another \'diff\' away.\n3. Count valid sequences.\n\n## 3. Hashmap\nUsing a hashmap significantly speeds up the search processes by allowing constant-time complexity lookups. This method achieves O(n) time complexity by trading off with O(n) space complexity.\n\n1. First, map each number to its index in the array.\n2. For each number in the array, check if the next two sequential differences of \'**diff**\' exist in the map.\n3. If both exist, count this as a valid triplet.\n\n## 4. Unordered Set\nSimilarly to the hashmap, using an unordered set allows for constant-time complexity lookups for existence but does not concern itself with indices. It also operates in O(n) time and O(n) space.\n1. Store all numbers in an unordered set for quick lookup.\n2. or each number, check if it is the first in a valid triplet by looking up the two necessary succeeding numbers formed by adding \'**diff**\' twice.\n3. Count each valid sequence.\n\n## 5. Binary Search\nUsing binary search leverages the sorted nature of the array to check for the existence of required numbers with O(log n) complexity per search, resulting in an overall complexity of O(n log n).\n1. For each number, perform a binary search to find the next number in the sequence.\n2. If found, perform another binary search for the third number.\n3. Count each valid triplet found this way.\n\nThis approach is particularly useful when space is at a premium, as it avoids the need for additional data structures beyond the input array itself, making it ideal for scenarios where maintaining a low memory footprint is crucial.\n\n\n# Complexity\n## 1. Brute Force\n- Time complexity: O(n^3)\n- Space complexity: O(1)\n## 2. Array\n- Time complexity: O(n^2)\n- Space complexity: O(1)\n## 3. Hashmap\n- Time complexity: O(n)\n- Space complexity: O(n)\n## 4. Unordered Set\n- Time complexity: O(n)\n- Space complexity: O(n)\n## 5. Binary Search\n- Time complexity: O(n log n)\n- Space complexity: O(1)\n# Code\n## 1. Brute Force\n```C++ []\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int counter = 0;\n for (int i = 0; i < nums.size(); i ++){\n for (int j = i + 1; j < nums.size(); j++){\n for (int k = j + 1; k < nums.size(); k++){\n if (nums[k] - nums[j] == diff && nums[j] - nums[i] == diff)\n counter ++;\n }\n }\n } \n return counter;\n }\n};\n```\n```Java []\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int counter = 0;\n for (int i = 0; i < nums.length; i++) {\n for (int j = i + 1; j < nums.length; j++) {\n for (int k = j + 1; k < nums.length; k++) {\n if (nums[k] - nums[j] == diff && nums[j] - nums[i] == diff) {\n counter++;\n }\n }\n }\n }\n return counter;\n }\n}\n```\n```Python3 []\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n counter = 0\n n = len(nums)\n for i in range(n):\n for j in range(i + 1, n):\n for k in range(j + 1, n):\n if nums[k] - nums[j] == diff and nums[j] - nums[i] == diff:\n counter += 1\n return counter\n```\n\n## 2. Array\n```C++ []\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int counter = 0;\n for (int i = 0; i < nums.size(); i++){\n if ((find(nums.begin(), nums.end(), nums[i] + diff) != nums.end()) &&\n (find(nums.begin(), nums.end(), nums[i] + (2*diff))!= nums.end())){\n counter ++;\n }\n }\n return counter;\n }\n};\n```\n```Java []\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int counter = 0;\n for (int i = 0; i < nums.length; i++) {\n if (contains(nums, nums[i] + diff) && contains(nums, nums[i] + 2 * diff)) {\n counter++;\n }\n }\n return counter;\n }\n\n private boolean contains(int[] nums, int target) {\n for (int num : nums) {\n if (num == target) {\n return true;\n }\n }\n return false;\n }\n}\n```\n```Python3 []\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n counter = 0\n for i in range(len(nums)):\n if self.contains(nums, nums[i] + diff) and self.contains(nums, nums[i] + 2 * diff):\n counter += 1\n return counter\n\n def contains(self, nums: List[int], target: int) -> bool:\n return target in nums\n```\n\n## 3. Hashmap\n```C++ []\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n unordered_map <int, int> seen;\n int counter = 0;\n for (int i = 0; i < nums.size(); i++){\n seen[nums[i]] = i;\n }\n for (int i = 0; i < nums.size(); i++){\n if(seen.count(nums[i] + diff) && seen.count(nums[i] + (2*diff))){\n counter++;\n }\n }\n return counter;\n }\n};\n```\n```Java []\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n HashMap<Integer, Integer> seen = new HashMap<>();\n int counter = 0;\n for (int i = 0; i < nums.length; i++) {\n seen.put(nums[i], i);\n }\n for (int num : nums) {\n if (seen.containsKey(num + diff) && seen.containsKey(num + 2 * diff)) {\n counter++;\n }\n }\n return counter;\n }\n}\n```\n```Python3 []\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n seen = {}\n counter = 0\n for i in range(len(nums)):\n seen[nums[i]] = i\n for num in nums:\n if (num + diff) in seen and (num + 2 * diff) in seen:\n counter += 1\n return counter\n```\n## 4. Unordered Set\n```C++ []\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int counter = 0;\n unordered_set <int> numSet (nums.begin(), nums.end());\n for (int i = 0; i < nums.size(); i++){\n if (numSet.count(nums[i] + diff) && numSet.count(nums[i] + (2*diff))){\n counter ++;\n }\n }\n return counter;\n }\n};\n```\n```Java []\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n int counter = 0;\n Set<Integer> numSet = new HashSet<>();\n for (int num : nums) {\n numSet.add(num);\n }\n for (int num : nums) {\n if (numSet.contains(num + diff) && numSet.contains(num + 2 * diff)) {\n counter++;\n }\n }\n return counter;\n }\n}\n```\n```Python3 []\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n counter = 0\n numSet = set(nums) \n for num in nums:\n if (num + diff) in numSet and (num + 2 * diff) in numSet:\n counter += 1\n \n return counter\n```\n## 5. Binary Search\n```C++ []\nclass Solution {\n private:\n bool binarySearch(vector<int>&nums, int target){\n int low = 0;\n int high = nums.size() - 1;\n while (low <= high){\n int mid = low + (high - low)/2;\n if (nums[mid] == target){\n return true;\n }\n else if (nums[mid] < target){\n low = mid + 1;\n }\n else{\n high = mid - 1;\n }\n }\n return false;\n }\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) {\n int counter = 0;\n for (int i = 0; i < nums.size(); i++){\n if(binarySearch(nums,nums[i] + diff) && binarySearch(nums, nums[i] + (2*diff))){\n counter ++;\n }\n }\n return counter;\n }\n};\n```\n```Java []\nclass Solution {\n private boolean binarySearch(int[] nums, int target) {\n int low = 0;\n int high = nums.length - 1;\n while (low <= high) {\n int mid = low + (high - low) / 2;\n if (nums[mid] == target) {\n return true;\n } else if (nums[mid] < target) {\n low = mid + 1;\n } else {\n high = mid - 1;\n }\n }\n return false;\n }\n public int arithmeticTriplets(int[] nums, int diff) {\n int counter = 0;\n for (int num : nums) {\n if (binarySearch(nums, num + diff) && binarySearch(nums, num + 2 * diff)) {\n counter++;\n }\n }\n return counter;\n }\n}\n```\n```Python3 []\nclass Solution:\n def binarySearch(self, nums: List[int], target: int) -> bool:\n low, high = 0, len(nums) - 1\n while low <= high:\n mid = low + (high - low) // 2\n if nums[mid] == target:\n return True\n elif nums[mid] < target:\n low = mid + 1\n else:\n high = mid - 1\n return False\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n counter = 0\n for num in nums:\n if self.binarySearch(nums, num + diff) and self.binarySearch(nums, num + 2 * diff):\n counter += 1\n return counter\n```\n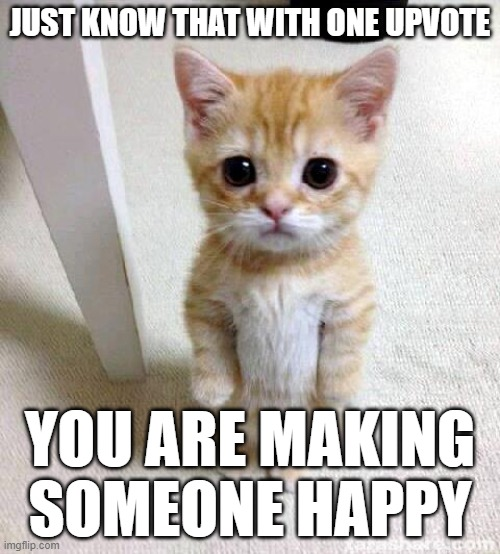\n\n### If you found my solution useful, I\'d truly appreciate your upvote. Your support encourages me to keep sharing more solutions. Thank you!!!\n\n\n\n\n\n\n\n\n\n\n | 126 | 0 | ['C++', 'Java', 'Python3'] | 9 |
number-of-arithmetic-triplets | [Java/Python 3] HashSet O(n) codes w/ analysis. | javapython-3-hashset-on-codes-w-analysis-n8mh | Credit to @dms6 for the improvement from 2 pass to 1 pass.\n\njava\n public int arithmeticTriplets(int[] nums, int diff) {\n int cnt = 0;\n Set | rock | NORMAL | 2022-08-07T04:04:49.226082+00:00 | 2023-05-29T12:49:15.485887+00:00 | 12,480 | false | Credit to **@dms6** for the improvement from 2 pass to 1 pass.\n\n```java\n public int arithmeticTriplets(int[] nums, int diff) {\n int cnt = 0;\n Set<Integer> seen = new HashSet<>();\n for (int num : nums) {\n if (seen.contains(num - diff) && seen.contains(num - diff * 2)) {\n ++cnt;\n }\n seen.add(num);\n }\n return cnt;\n }\n```\n\n```python\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n seen = set()\n cnt = 0\n for num in nums:\n if num - diff in seen and num - diff * 2 in seen:\n cnt += 1\n seen.add(num)\n return cnt\n```\n\nMake the above shorter: - Credit to **@SunnyvaleCA**\n\n```python\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n seen = set(nums)\n return sum(num - diff in seen and num - diff * 2 in seen for num in seen)\n```\n\nOr 1 liner (time `O(n ^ 2)`:\n```python\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n return sum( n+diff in nums and n+diff*2 in nums for n in nums )\n```\n**Analysis:**\n\nTime & space: `O(n)`, where `n = nums.length`. | 83 | 0 | ['Java', 'Python3'] | 11 |
number-of-arithmetic-triplets | ✅Python || Easy Approach | python-easy-approach-by-chuhonghao01-or6g | \nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n \n ans = 0\n n = len(nums)\n for i in rang | chuhonghao01 | NORMAL | 2022-08-07T22:01:06.148020+00:00 | 2022-08-19T01:56:40.485393+00:00 | 7,128 | false | ```\nclass Solution:\n def arithmeticTriplets(self, nums: List[int], diff: int) -> int:\n \n ans = 0\n n = len(nums)\n for i in range(n):\n if nums[i] + diff in nums and nums[i] + 2 * diff in nums:\n ans += 1\n \n return ans\n``` | 40 | 0 | ['Python', 'Python3'] | 8 |
number-of-arithmetic-triplets | Beats 100% of users || Step By Step Explain || Using HashMap || Easy to Understand || | beats-100-of-users-step-by-step-explain-m8bu4 | Abhiraj Pratap Singh \n\n---\n\n# if you like the solution Please UPVOTE it....\n\n---\n\n# Intuition\n Describe your first thoughts on how to solve this proble | abhirajpratapsingh | NORMAL | 2024-01-31T14:57:36.277233+00:00 | 2024-01-31T14:57:36.277263+00:00 | 1,233 | false | # Abhiraj Pratap Singh \n\n---\n\n# if you like the solution Please UPVOTE it....\n\n---\n\n# Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n- The intuition behind this code is to find arithmetic triplets in a given vector nums with a specified difference diff. An arithmetic triplet is a sequence of three elements in an array such that the difference between the consecutive elements is the same.\n\n----\n\n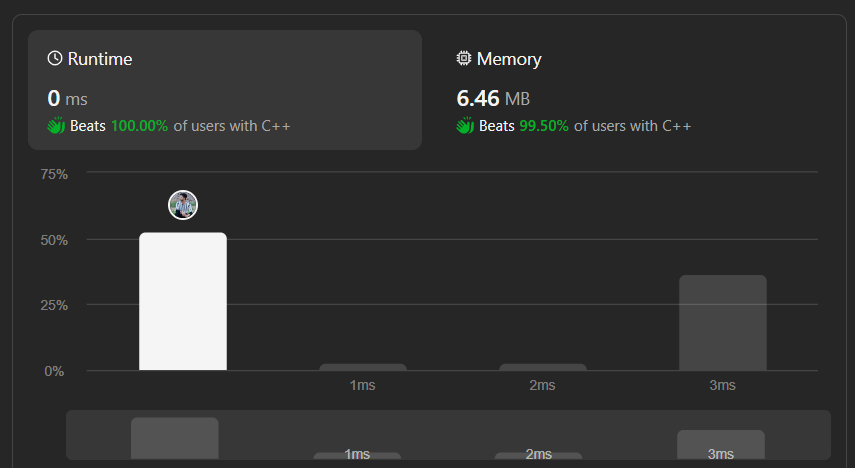\n\n---\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- First, the code initializes a map mp to store the frequency of each element in the input vector nums.\n- Then, it iterates through the elements of nums and updates the frequency count in the map mp.\n- Next, it again iterates through the elements of nums and checks for each element nums[i] whether there exist two other elements in the array, namely nums[i]+diff and nums[i]+diff+diff, with the same difference diff.\n- If such elements exist (i.e., mp[nums[i]+diff] and mp[nums[i]+diff+diff] are both non-zero), it increments the count variable.\n- Finally, it returns the count of arithmetic triplets found in the array.\n\n\n---\n# Complexity\n\n- **Time complexity:** The time complexity of this approach is O(n), where n is the size of the input vector nums. This is because the code iterates through the elements of nums twice, and each iteration takes O(n) time.\n\n- **Space complexity:** The space complexity is also O(n) as the code uses a map mp to store the frequency of elements, and in the worst case, all elements of nums could be distinct.\n\n---\n# Code\n```\nclass Solution {\npublic:\n int arithmeticTriplets(vector<int>& nums, int diff) \n {\n map<int,int>mp;\n int count = 0 ;\n for( int i = 0 ; i < nums.size() ; i++ )\n mp[ nums[i] ]++;\n for( int i = 0 ; i < nums.size() ; i++ )\n {\n if ( mp[nums[i]+diff] && mp[nums[i]+diff+diff] )\n count++;\n }\n return count ;\n }\n};\n```\n\n---\n# if you like the solution please UPVOTE it....\n\n---\n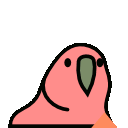\n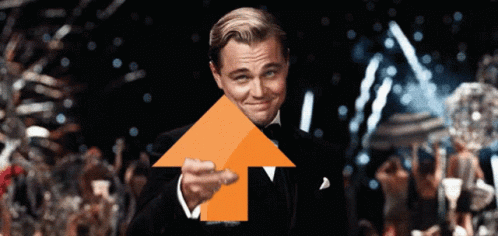\n\n---\n--- | 24 | 0 | ['Array', 'Hash Table', 'Two Pointers', 'Enumeration', 'C++'] | 4 |
number-of-arithmetic-triplets | Java| Easy| HashSet | java-easy-hashset-by-sachinapex-9jvk | \nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n \n\n Set<Integer> set = new HashSet<>();\n \n for( i | sachinapex | NORMAL | 2022-08-07T04:03:17.107346+00:00 | 2022-08-07T10:05:09.273439+00:00 | 2,674 | false | ```\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n \n\n Set<Integer> set = new HashSet<>();\n \n for( int num : nums){\n set.add(num);\n }\n \n int ans = 0;\n for( int num : nums){\n if(( set.contains(num + diff ) && set.contains(num + 2 * diff) ) ){\n ans++;\n \n }\n }\n \n return ans;\n \n }\n}\n\n``` | 24 | 0 | [] | 6 |
number-of-arithmetic-triplets | Beginner friendly [Python/JavaScript/Java] solutions | beginner-friendly-pythonjavascriptjava-s-fdi9 | \npython []\nclass Solution(object):\n def arithmeticTriplets(self, nums, diff):\n count = 0\n for num in nums:\n if num + diff in n | HimanshuBhoir | NORMAL | 2022-08-22T13:53:05.761215+00:00 | 2023-01-04T06:40:06.129627+00:00 | 3,568 | false | \n```python []\nclass Solution(object):\n def arithmeticTriplets(self, nums, diff):\n count = 0\n for num in nums:\n if num + diff in nums and num + diff*2 in nums:\n count += 1\n return count\n```\n\n```javascript []\nvar arithmeticTriplets = function(nums, diff) {\n let count = 0\n for(let num of nums){\n if(nums.includes(num + diff) && nums.includes(num + diff*2)){\n count++\n }\n }\n return count;\n};\n```\n\n```java []\nclass Solution {\n public int arithmeticTriplets(int[] nums, int diff) {\n Set set = new HashSet();\n for(int num: nums) set.add(num);\n int count = 0;\n for(int num : nums){\n if(set.contains(num + diff) && set.contains(num + diff*2)){\n count++;\n }\n }\n return count;\n }\n}\n``` | 23 | 0 | ['Python', 'Java', 'JavaScript'] | 3 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.