inputs
stringlengths 175
2.22k
| targets
stringlengths 10
2.05k
| language
stringclasses 1
value | split
stringclasses 2
values | template
stringclasses 2
values | dataset
stringclasses 1
value | config
stringclasses 1
value |
---|---|---|---|---|---|---|
I found an interesting problem on https://codeforces.com/problemset/problem/572/B:
In this task you need to process a set of stock exchange orders and use them to create order book.
An order is an instruction of some participant to buy or sell stocks on stock exchange. The order number i has price p_{i}, direction d_{i} — buy or sell, and integer q_{i}. This means that the participant is ready to buy or sell q_{i} stocks at price p_{i} for one stock. A value q_{i} is also known as a volume of an order.
All orders with the same price p and direction d are merged into one aggregated order with price p and direction d. The volume of such order is a sum of volumes of the initial orders.
An order book is a list of aggregated orders, the first part of which contains sell orders sorted by price in descending order, the second contains buy orders also sorted by price in descending order.
An order book of depth s contains s best aggregated orders for each direction. A buy order is better if it has higher price and a sell order is better if it has lower price. If there are less than s aggregated orders for some direction then all of them will be in the final order book.
You are given n stock exhange orders. Your task is to print order book of depth s for these orders.
-----Input-----
The input starts with two positive integers n and s (1 ≤ n ≤ 1000, 1 ≤ s ≤ 50), the number of orders and the book depth.
Next n lines contains a letter d_{i} (either 'B' or 'S'), an integer p_{i} (0 ≤ p_{i} ≤ 10^5) and an integer q_{i} (1 ≤ q_{i} ≤ 10^4) — direction, price and volume respectively. The letter 'B' means buy, 'S' means sell. The price of any sell order is higher than the price of any buy order.
-----Output-----
Print no more than 2s lines with aggregated orders from order book of depth s. The output format for orders should be the same as in input.
-----Examples-----
Input
6 2
B 10 3
S 50 2
S 40 1
S 50 6
B 20 4
B 25 10
Output
S 50 8
S 40 1
B 25 10
B 20 4
-----Note-----
Denote (x, y) an order with price x and volume y. There are 3 aggregated buy orders (10, 3), (20, 4), (25, 10) and two...
I tried it in Python, but could not do it. Can you solve it? | s = input()
n, d = int(s.split()[0]), int(s.split()[1])
b, s = {}, {}
wb, ws = [], []
for i in range(0, n):
st = input().split()
st[1] = int(st[1])
st[2] = int(st[2])
if st[0] == 'B':
if st[1] not in b:
b[st[1]] = st[2]
else:
b[st[1]] += st[2];
if st[1] not in wb:
wb.append(st[1]);
else:
if st[1] not in s:
s[st[1]] = st[2]
else:
s[st[1]] += st[2];
if st[1] not in ws:
ws.append(st[1]);
ws.sort()#[:d]#.sort(reverse=True)
wb.sort(reverse=True)#[:d]
ws = ws[:d]
wb = wb[:d]
ws.sort(reverse=True)
for i in ws:
print('S %s %s' %(i, s[i]))
for i in wb:
print('B %s %s' %(i, b[i])) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1336/A:
Writing light novels is the most important thing in Linova's life. Last night, Linova dreamed about a fantastic kingdom. She began to write a light novel for the kingdom as soon as she woke up, and of course, she is the queen of it.
[Image]
There are $n$ cities and $n-1$ two-way roads connecting pairs of cities in the kingdom. From any city, you can reach any other city by walking through some roads. The cities are numbered from $1$ to $n$, and the city $1$ is the capital of the kingdom. So, the kingdom has a tree structure.
As the queen, Linova plans to choose exactly $k$ cities developing industry, while the other cities will develop tourism. The capital also can be either industrial or tourism city.
A meeting is held in the capital once a year. To attend the meeting, each industry city sends an envoy. All envoys will follow the shortest path from the departure city to the capital (which is unique).
Traveling in tourism cities is pleasant. For each envoy, his happiness is equal to the number of tourism cities on his path.
In order to be a queen loved by people, Linova wants to choose $k$ cities which can maximize the sum of happinesses of all envoys. Can you calculate the maximum sum for her?
-----Input-----
The first line contains two integers $n$ and $k$ ($2\le n\le 2 \cdot 10^5$, $1\le k< n$) — the number of cities and industry cities respectively.
Each of the next $n-1$ lines contains two integers $u$ and $v$ ($1\le u,v\le n$), denoting there is a road connecting city $u$ and city $v$.
It is guaranteed that from any city, you can reach any other city by the roads.
-----Output-----
Print the only line containing a single integer — the maximum possible sum of happinesses of all envoys.
-----Examples-----
Input
7 4
1 2
1 3
1 4
3 5
3 6
4 7
Output
7
Input
4 1
1 2
1 3
2 4
Output
2
Input
8 5
7 5
1 7
6 1
3 7
8 3
2 1
4 5
Output
9
-----Note-----
[Image]
In the first example, Linova can choose cities $2$, $5$, $6$, $7$ to develop industry, then the happiness of the envoy from city $2$ is...
I tried it in Python, but could not do it. Can you solve it? | import sys
input = sys.stdin.readline
n, k = list(map(int, input().split()))
begin = [-1] * n
end = [-1] * n
hurt = [-1] * n
adj = [[] for i in range(n)]
for _ in range(n-1):
u ,v = list(map(int, input().split()))
adj[u-1].append(v-1)
adj[v-1].append(u-1)
hurt[0] = 1
begin[0] = 0
stack = [0]
curr = 1
while stack:
nex = stack[-1]
if adj[nex]:
v = adj[nex].pop()
if begin[v] == -1:
begin[v] = curr
curr += 1
stack.append(v)
hurt[v] = len(stack)
else:
end[nex] = curr
stack.pop()
desc = [end[i] - begin[i]-hurt[i] for i in range(n)]
desc.sort(reverse = True)
out = 0
for i in range(n - k):
out += desc[i]
print(out) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Write a function called `LCS` that accepts two sequences and returns the longest subsequence common to the passed in sequences.
### Subsequence
A subsequence is different from a substring. The terms of a subsequence need not be consecutive terms of the original sequence.
### Example subsequence
Subsequences of `"abc"` = `"a"`, `"b"`, `"c"`, `"ab"`, `"ac"`, `"bc"` and `"abc"`.
### LCS examples
```python
lcs( "abcdef" , "abc" ) => returns "abc"
lcs( "abcdef" , "acf" ) => returns "acf"
lcs( "132535365" , "123456789" ) => returns "12356"
```
### Notes
* Both arguments will be strings
* Return value must be a string
* Return an empty string if there exists no common subsequence
* Both arguments will have one or more characters (in JavaScript)
* All tests will only have a single longest common subsequence. Don't worry about cases such as `LCS( "1234", "3412" )`, which would have two possible longest common subsequences: `"12"` and `"34"`.
Note that the Haskell variant will use randomized testing, but any longest common subsequence will be valid.
Note that the OCaml variant is using generic lists instead of strings, and will also have randomized tests (any longest common subsequence will be valid).
### Tips
Wikipedia has an explanation of the two properties that can be used to solve the problem:
- [First property](http://en.wikipedia.org/wiki/Longest_common_subsequence_problem#First_property)
- [Second property](http://en.wikipedia.org/wiki/Longest_common_subsequence_problem#Second_property) | def lcs(x, y):
if not x or not y: return ""
if x[0] == y[0]: return x[0] + lcs(x[1:], y[1:])
return max(lcs(x[1:], y), lcs(x, y[1:]), key=len) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/250/E:
Joe has been hurt on the Internet. Now he is storming around the house, destroying everything in his path.
Joe's house has n floors, each floor is a segment of m cells. Each cell either contains nothing (it is an empty cell), or has a brick or a concrete wall (always something one of three). It is believed that each floor is surrounded by a concrete wall on the left and on the right.
Now Joe is on the n-th floor and in the first cell, counting from left to right. At each moment of time, Joe has the direction of his gaze, to the right or to the left (always one direction of the two). Initially, Joe looks to the right.
Joe moves by a particular algorithm. Every second he makes one of the following actions: If the cell directly under Joe is empty, then Joe falls down. That is, he moves to this cell, the gaze direction is preserved. Otherwise consider the next cell in the current direction of the gaze. If the cell is empty, then Joe moves into it, the gaze direction is preserved. If this cell has bricks, then Joe breaks them with his forehead (the cell becomes empty), and changes the direction of his gaze to the opposite. If this cell has a concrete wall, then Joe just changes the direction of his gaze to the opposite (concrete can withstand any number of forehead hits).
Joe calms down as soon as he reaches any cell of the first floor.
The figure below shows an example Joe's movements around the house.
[Image]
Determine how many seconds Joe will need to calm down.
-----Input-----
The first line contains two integers n and m (2 ≤ n ≤ 100, 1 ≤ m ≤ 10^4).
Next n lines contain the description of Joe's house. The i-th of these lines contains the description of the (n - i + 1)-th floor of the house — a line that consists of m characters: "." means an empty cell, "+" means bricks and "#" means a concrete wall.
It is guaranteed that the first cell of the n-th floor is empty.
-----Output-----
Print a single number — the number of seconds Joe needs to reach the first floor; or else, print word...
I tried it in Python, but could not do it. Can you solve it? | n, m = [int(i) for i in input().split()]
current_floor = list(input())
x, t, direction = 0, 0, 1
for i in range(n-1):
floor = list(input())
l, r = x, x
wall = 0
while True:
t += 1
if floor[x] == '.':
break
if (x + direction == m) or (x + direction < 0) or (current_floor[x+direction] == '#'):
wall += 1
direction = -direction
if wall == 2:
print("Never")
return
elif current_floor[x+direction] == '+':
wall = 0
current_floor[x+direction] = '.'
direction = -direction
elif l <= x+direction and x+direction <= r:
if direction == 1:
t += r-x-1
x = r
else:
t += x-l-1
x = l
else:
x += direction
r = max(r, x)
l = min(l, x)
current_floor, floor = floor, current_floor
print(t) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
We often go to supermarkets to buy some fruits or vegetables, and on the tag there prints the price for a kilo. But in some supermarkets, when asked how much the items are, the clerk will say that $a$ yuan for $b$ kilos (You don't need to care about what "yuan" is), the same as $a/b$ yuan for a kilo.
Now imagine you'd like to buy $m$ kilos of apples. You've asked $n$ supermarkets and got the prices. Find the minimum cost for those apples.
You can assume that there are enough apples in all supermarkets.
-----Input-----
The first line contains two positive integers $n$ and $m$ ($1 \leq n \leq 5\,000$, $1 \leq m \leq 100$), denoting that there are $n$ supermarkets and you want to buy $m$ kilos of apples.
The following $n$ lines describe the information of the supermarkets. Each line contains two positive integers $a, b$ ($1 \leq a, b \leq 100$), denoting that in this supermarket, you are supposed to pay $a$ yuan for $b$ kilos of apples.
-----Output-----
The only line, denoting the minimum cost for $m$ kilos of apples. Please make sure that the absolute or relative error between your answer and the correct answer won't exceed $10^{-6}$.
Formally, let your answer be $x$, and the jury's answer be $y$. Your answer is considered correct if $\frac{|x - y|}{\max{(1, |y|)}} \le 10^{-6}$.
-----Examples-----
Input
3 5
1 2
3 4
1 3
Output
1.66666667
Input
2 1
99 100
98 99
Output
0.98989899
-----Note-----
In the first sample, you are supposed to buy $5$ kilos of apples in supermarket $3$. The cost is $5/3$ yuan.
In the second sample, you are supposed to buy $1$ kilo of apples in supermarket $2$. The cost is $98/99$ yuan. | n, m = [int(i) for i in input().split()]
ans = 10 ** 9
for i in range(n):
a1, a2 = [int(i) for i in input().split()]
ans = min(ans, a1 * m / a2)
print(ans) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
An army of n droids is lined up in one row. Each droid is described by m integers a_1, a_2, ..., a_{m}, where a_{i} is the number of details of the i-th type in this droid's mechanism. R2-D2 wants to destroy the sequence of consecutive droids of maximum length. He has m weapons, the i-th weapon can affect all the droids in the army by destroying one detail of the i-th type (if the droid doesn't have details of this type, nothing happens to it).
A droid is considered to be destroyed when all of its details are destroyed. R2-D2 can make at most k shots. How many shots from the weapon of what type should R2-D2 make to destroy the sequence of consecutive droids of maximum length?
-----Input-----
The first line contains three integers n, m, k (1 ≤ n ≤ 10^5, 1 ≤ m ≤ 5, 0 ≤ k ≤ 10^9) — the number of droids, the number of detail types and the number of available shots, respectively.
Next n lines follow describing the droids. Each line contains m integers a_1, a_2, ..., a_{m} (0 ≤ a_{i} ≤ 10^8), where a_{i} is the number of details of the i-th type for the respective robot.
-----Output-----
Print m space-separated integers, where the i-th number is the number of shots from the weapon of the i-th type that the robot should make to destroy the subsequence of consecutive droids of the maximum length.
If there are multiple optimal solutions, print any of them.
It is not necessary to make exactly k shots, the number of shots can be less.
-----Examples-----
Input
5 2 4
4 0
1 2
2 1
0 2
1 3
Output
2 2
Input
3 2 4
1 2
1 3
2 2
Output
1 3
-----Note-----
In the first test the second, third and fourth droids will be destroyed.
In the second test the first and second droids will be destroyed. | from heapq import heappush, heappop
from sys import setrecursionlimit
from sys import stdin
from collections import defaultdict
setrecursionlimit(1000000007)
_data = iter(stdin.read().split('\n'))
def input():
return next(_data)
n, m, k = [int(x) for x in input().split()]
a = tuple(tuple(-int(x) for x in input().split()) for i in range(n))
heaps = tuple([0] for _ in range(m))
removed = tuple(defaultdict(int) for _ in range(m))
rv = -1
rt = (0,) * m
t = [0] * m
p = 0
for i in range(n):
ai = a[i]
for j, v, heap in zip(range(m), ai, heaps):
heappush(heap, v)
t[j] = heap[0]
while -sum(t) > k:
ap = a[p]
for j, v, heap, remd in zip(range(m), ap, heaps, removed):
remd[v] += 1
while heap[0] in remd:
top = heappop(heap)
if remd[top] == 1:
del remd[top]
else:
remd[top] -= 1
t[j] = heap[0]
p += 1
if rv < (i + 1) - p:
rv = (i + 1) - p
rt = tuple(t)
print(*map(lambda x: -x, rt)) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Kefa decided to celebrate his first big salary by going to the restaurant.
He lives by an unusual park. The park is a rooted tree consisting of n vertices with the root at vertex 1. Vertex 1 also contains Kefa's house. Unfortunaely for our hero, the park also contains cats. Kefa has already found out what are the vertices with cats in them.
The leaf vertices of the park contain restaurants. Kefa wants to choose a restaurant where he will go, but unfortunately he is very afraid of cats, so there is no way he will go to the restaurant if the path from the restaurant to his house contains more than m consecutive vertices with cats.
Your task is to help Kefa count the number of restaurants where he can go.
-----Input-----
The first line contains two integers, n and m (2 ≤ n ≤ 10^5, 1 ≤ m ≤ n) — the number of vertices of the tree and the maximum number of consecutive vertices with cats that is still ok for Kefa.
The second line contains n integers a_1, a_2, ..., a_{n}, where each a_{i} either equals to 0 (then vertex i has no cat), or equals to 1 (then vertex i has a cat).
Next n - 1 lines contains the edges of the tree in the format "x_{i} y_{i}" (without the quotes) (1 ≤ x_{i}, y_{i} ≤ n, x_{i} ≠ y_{i}), where x_{i} and y_{i} are the vertices of the tree, connected by an edge.
It is guaranteed that the given set of edges specifies a tree.
-----Output-----
A single integer — the number of distinct leaves of a tree the path to which from Kefa's home contains at most m consecutive vertices with cats.
-----Examples-----
Input
4 1
1 1 0 0
1 2
1 3
1 4
Output
2
Input
7 1
1 0 1 1 0 0 0
1 2
1 3
2 4
2 5
3 6
3 7
Output
2
-----Note-----
Let us remind you that a tree is a connected graph on n vertices and n - 1 edge. A rooted tree is a tree with a special vertex called root. In a rooted tree among any two vertices connected by an edge, one vertex is a parent (the one closer to the root), and the other one is a child. A vertex is called a leaf, if it has no children.
Note to the first sample test:... | n, m = input().split()
n, m = int(n), int(m)
a = list( map( int , input().split() ) )
v = [ [] for _ in range( n ) ]
for i in range( n - 1 ):
x = list( map( int , input().split() ) )
x[ 0 ], x[ 1 ] = int(x[ 0 ]) - 1, int(x[ 1 ]) - 1
v[ x[ 0 ] ].append( x[ 1 ] )
v[ x[ 1 ] ].append( x[ 0 ] )
tag = [False] * n
con = [0] * n
from collections import deque as dq
d = dq()
d.append( 0 )
con[ 0 ] = a[ 0 ]
tag[ 0 ] = True
ans = 0
while len(d) > 0:
x, chd = d.popleft(), 0
# print( ( x , con[ x ] ) )
if con[ x ] > m: continue
for y in v[x]:
if not tag[ y ]:
con[ y ] = con[ x ] + a[ y ] if a[ y ] == 1 else 0
tag[ y ] = True
chd += 1
d.append( y )
if chd == 0 and con[ x ] <= m:
ans += 1
print( ans ) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
# Task
The string is called `prime` if it cannot be constructed by concatenating some (more than one) equal strings together.
For example, "abac" is prime, but "xyxy" is not("xyxy"="xy"+"xy").
Given a string determine if it is prime or not.
# Input/Output
- `[input]` string `s`
string containing only lowercase English letters
- `[output]` a boolean value
`true` if the string is prime, `false` otherwise | def prime_string(s):
return (s + s).find(s, 1) == len(s) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/57f7796697d62fc93d0001b8:
Given an array of integers (x), and a target (t), you must find out if any two consecutive numbers in the array sum to t. If so, remove the second number.
Example:
x = [1, 2, 3, 4, 5]
t = 3
1+2 = t, so remove 2. No other pairs = t, so rest of array remains:
[1, 3, 4, 5]
Work through the array from left to right.
Return the resulting array.
I tried it in Python, but could not do it. Can you solve it? | from functools import reduce
def trouble(x, t):
return reduce(lambda a, u: a + ([u] if not a or a[-1] + u != t else []), x[1:], x[0:1]) | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Appleman and Toastman play a game. Initially Appleman gives one group of n numbers to the Toastman, then they start to complete the following tasks: Each time Toastman gets a group of numbers, he sums up all the numbers and adds this sum to the score. Then he gives the group to the Appleman. Each time Appleman gets a group consisting of a single number, he throws this group out. Each time Appleman gets a group consisting of more than one number, he splits the group into two non-empty groups (he can do it in any way) and gives each of them to Toastman.
After guys complete all the tasks they look at the score value. What is the maximum possible value of score they can get?
-----Input-----
The first line contains a single integer n (1 ≤ n ≤ 3·10^5). The second line contains n integers a_1, a_2, ..., a_{n} (1 ≤ a_{i} ≤ 10^6) — the initial group that is given to Toastman.
-----Output-----
Print a single integer — the largest possible score.
-----Examples-----
Input
3
3 1 5
Output
26
Input
1
10
Output
10
-----Note-----
Consider the following situation in the first example. Initially Toastman gets group [3, 1, 5] and adds 9 to the score, then he give the group to Appleman. Appleman splits group [3, 1, 5] into two groups: [3, 5] and [1]. Both of them should be given to Toastman. When Toastman receives group [1], he adds 1 to score and gives the group to Appleman (he will throw it out). When Toastman receives group [3, 5], he adds 8 to the score and gives the group to Appleman. Appleman splits [3, 5] in the only possible way: [5] and [3]. Then he gives both groups to Toastman. When Toastman receives [5], he adds 5 to the score and gives the group to Appleman (he will throws it out). When Toastman receives [3], he adds 3 to the score and gives the group to Appleman (he will throws it out). Finally Toastman have added 9 + 1 + 8 + 5 + 3 = 26 to the score. This is the optimal sequence of actions. | n = int(input())
a = list(map(int, input().split()))
a.sort(reverse=True)
s = sum(a)
res = s
while len(a) > 1:
res += s
s -= a.pop()
print(res) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
You've got an undirected graph, consisting of n vertices and m edges. We will consider the graph's vertices numbered with integers from 1 to n. Each vertex of the graph has a color. The color of the i-th vertex is an integer c_{i}.
Let's consider all vertices of the graph, that are painted some color k. Let's denote a set of such as V(k). Let's denote the value of the neighbouring color diversity for color k as the cardinality of the set Q(k) = {c_{u} : c_{u} ≠ k and there is vertex v belonging to set V(k) such that nodes v and u are connected by an edge of the graph}.
Your task is to find such color k, which makes the cardinality of set Q(k) maximum. In other words, you want to find the color that has the most diverse neighbours. Please note, that you want to find such color k, that the graph has at least one vertex with such color.
-----Input-----
The first line contains two space-separated integers n, m (1 ≤ n, m ≤ 10^5) — the number of vertices end edges of the graph, correspondingly. The second line contains a sequence of integers c_1, c_2, ..., c_{n} (1 ≤ c_{i} ≤ 10^5) — the colors of the graph vertices. The numbers on the line are separated by spaces.
Next m lines contain the description of the edges: the i-th line contains two space-separated integers a_{i}, b_{i} (1 ≤ a_{i}, b_{i} ≤ n; a_{i} ≠ b_{i}) — the numbers of the vertices, connected by the i-th edge.
It is guaranteed that the given graph has no self-loops or multiple edges.
-----Output-----
Print the number of the color which has the set of neighbours with the maximum cardinality. It there are multiple optimal colors, print the color with the minimum number. Please note, that you want to find such color, that the graph has at least one vertex with such color.
-----Examples-----
Input
6 6
1 1 2 3 5 8
1 2
3 2
1 4
4 3
4 5
4 6
Output
3
Input
5 6
4 2 5 2 4
1 2
2 3
3 1
5 3
5 4
3 4
Output
2 | from collections import defaultdict
n,m = map(int,input().split())
g = defaultdict(set)
c = list(map(int,input().split()))
for _ in range(m):
x,y = map(int,input().split())
if(c[x-1]!=c[y-1]):g[c[x-1]].add(c[y-1])
if(c[y-1]!=c[x-1]):g[c[y-1]].add(c[x-1])
ma = 0
co = 10**5 + 1
f = 0
for i in g:
ma = max(ma,len(g[i]))
for i in g:
if(len(g[i])==ma):
f+=1
co = min(co,i)
if(f==0):
co = min(c)
print(co) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/853/A:
Helen works in Metropolis airport. She is responsible for creating a departure schedule. There are n flights that must depart today, the i-th of them is planned to depart at the i-th minute of the day.
Metropolis airport is the main transport hub of Metropolia, so it is difficult to keep the schedule intact. This is exactly the case today: because of technical issues, no flights were able to depart during the first k minutes of the day, so now the new departure schedule must be created.
All n scheduled flights must now depart at different minutes between (k + 1)-th and (k + n)-th, inclusive. However, it's not mandatory for the flights to depart in the same order they were initially scheduled to do so — their order in the new schedule can be different. There is only one restriction: no flight is allowed to depart earlier than it was supposed to depart in the initial schedule.
Helen knows that each minute of delay of the i-th flight costs airport c_{i} burles. Help her find the order for flights to depart in the new schedule that minimizes the total cost for the airport.
-----Input-----
The first line contains two integers n and k (1 ≤ k ≤ n ≤ 300 000), here n is the number of flights, and k is the number of minutes in the beginning of the day that the flights did not depart.
The second line contains n integers c_1, c_2, ..., c_{n} (1 ≤ c_{i} ≤ 10^7), here c_{i} is the cost of delaying the i-th flight for one minute.
-----Output-----
The first line must contain the minimum possible total cost of delaying the flights.
The second line must contain n different integers t_1, t_2, ..., t_{n} (k + 1 ≤ t_{i} ≤ k + n), here t_{i} is the minute when the i-th flight must depart. If there are several optimal schedules, print any of them.
-----Example-----
Input
5 2
4 2 1 10 2
Output
20
3 6 7 4 5
-----Note-----
Let us consider sample test. If Helen just moves all flights 2 minutes later preserving the order, the total cost of delaying the flights would be (3 - 1)·4 + (4 - 2)·2 + (5 - 3)·1 + (6 - 4)·10 +...
I tried it in Python, but could not do it. Can you solve it? | from heapq import heappush,heappop,heapify
n,k=list(map(int,input().split()))
*l,=list(map(int,input().split()))
q=[(-l[i],i)for i in range(k)]
heapify(q)
a=[0]*n
s=0
for i in range(k,n) :
heappush(q,(-l[i],i))
x,j=heappop(q)
s-=x*(i-j)
a[j]=i+1
for i in range(n,n+k) :
x,j=heappop(q)
s-=x*(i-j)
a[j]=i+1
print(s)
print(' '.join(map(str,a))) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1107/A:
You are given a sequence $s$ consisting of $n$ digits from $1$ to $9$.
You have to divide it into at least two segments (segment — is a consecutive sequence of elements) (in other words, you have to place separators between some digits of the sequence) in such a way that each element belongs to exactly one segment and if the resulting division will be represented as an integer numbers sequence then each next element of this sequence will be strictly greater than the previous one.
More formally: if the resulting division of the sequence is $t_1, t_2, \dots, t_k$, where $k$ is the number of element in a division, then for each $i$ from $1$ to $k-1$ the condition $t_{i} < t_{i + 1}$ (using numerical comparing, it means that the integer representations of strings are compared) should be satisfied.
For example, if $s=654$ then you can divide it into parts $[6, 54]$ and it will be suitable division. But if you will divide it into parts $[65, 4]$ then it will be bad division because $65 > 4$. If $s=123$ then you can divide it into parts $[1, 23]$, $[1, 2, 3]$ but not into parts $[12, 3]$.
Your task is to find any suitable division for each of the $q$ independent queries.
-----Input-----
The first line of the input contains one integer $q$ ($1 \le q \le 300$) — the number of queries.
The first line of the $i$-th query contains one integer number $n_i$ ($2 \le n_i \le 300$) — the number of digits in the $i$-th query.
The second line of the $i$-th query contains one string $s_i$ of length $n_i$ consisting only of digits from $1$ to $9$.
-----Output-----
If the sequence of digits in the $i$-th query cannot be divided into at least two parts in a way described in the problem statement, print the single line "NO" for this query.
Otherwise in the first line of the answer to this query print "YES", on the second line print $k_i$ — the number of parts in your division of the $i$-th query sequence and in the third line print $k_i$ strings $t_{i, 1}, t_{i, 2}, \dots, t_{i, k_i}$ — your division. Parts should be...
I tried it in Python, but could not do it. Can you solve it? | q = int(input())
for _ in range(q):
n = int(input())
s = input()
if n > 2:
print('YES')
print(2)
print(s[0],s[1:])
else:
if int(s[0]) >= int(s[1]):
print('NO')
else:
print('YES')
print(2)
print(s[0],s[1]) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1372/C:
Patrick likes to play baseball, but sometimes he will spend so many hours hitting home runs that his mind starts to get foggy! Patrick is sure that his scores across $n$ sessions follow the identity permutation (ie. in the first game he scores $1$ point, in the second game he scores $2$ points and so on). However, when he checks back to his record, he sees that all the numbers are mixed up!
Define a special exchange as the following: choose any subarray of the scores and permute elements such that no element of subarray gets to the same position as it was before the exchange. For example, performing a special exchange on $[1,2,3]$ can yield $[3,1,2]$ but it cannot yield $[3,2,1]$ since the $2$ is in the same position.
Given a permutation of $n$ integers, please help Patrick find the minimum number of special exchanges needed to make the permutation sorted! It can be proved that under given constraints this number doesn't exceed $10^{18}$.
An array $a$ is a subarray of an array $b$ if $a$ can be obtained from $b$ by deletion of several (possibly, zero or all) elements from the beginning and several (possibly, zero or all) elements from the end.
-----Input-----
Each test contains multiple test cases. The first line contains the number of test cases $t$ ($1 \le t \le 100$). Description of the test cases follows.
The first line of each test case contains integer $n$ ($1 \leq n \leq 2 \cdot 10^5$) — the length of the given permutation.
The second line of each test case contains $n$ integers $a_{1},a_{2},...,a_{n}$ ($1 \leq a_{i} \leq n$) — the initial permutation.
It is guaranteed that the sum of $n$ over all test cases does not exceed $2 \cdot 10^5$.
-----Output-----
For each test case, output one integer: the minimum number of special exchanges needed to sort the permutation.
-----Example-----
Input
2
5
1 2 3 4 5
7
3 2 4 5 1 6 7
Output
0
2
-----Note-----
In the first permutation, it is already sorted so no exchanges are needed.
It can be shown that you need at least $2$ exchanges to sort...
I tried it in Python, but could not do it. Can you solve it? | from collections import defaultdict as dd
from collections import deque
import bisect
import heapq
def ri():
return int(input())
def rl():
return list(map(int, input().split()))
def solve():
n = ri()
A = rl()
first_wrong = -1
first_break = -1
skip = False
for i, a in enumerate(A):
if i + 1 == a:
if first_wrong != -1 and first_break == -1:
first_break = i
else:
if first_wrong == -1:
first_wrong = i
elif first_break != -1:
skip = True
if first_wrong == -1:
print(0)
elif not skip:
print(1)
else:
print(2)
mode = 'T'
if mode == 'T':
t = ri()
for i in range(t):
solve()
else:
solve() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Gennady owns a small hotel in the countryside where he lives a peaceful life. He loves to take long walks, watch sunsets and play cards with tourists staying in his hotel. His favorite game is called "Mau-Mau".
To play Mau-Mau, you need a pack of $52$ cards. Each card has a suit (Diamonds — D, Clubs — C, Spades — S, or Hearts — H), and a rank (2, 3, 4, 5, 6, 7, 8, 9, T, J, Q, K, or A).
At the start of the game, there is one card on the table and you have five cards in your hand. You can play a card from your hand if and only if it has the same rank or the same suit as the card on the table.
In order to check if you'd be a good playing partner, Gennady has prepared a task for you. Given the card on the table and five cards in your hand, check if you can play at least one card.
-----Input-----
The first line of the input contains one string which describes the card on the table. The second line contains five strings which describe the cards in your hand.
Each string is two characters long. The first character denotes the rank and belongs to the set $\{{\tt 2}, {\tt 3}, {\tt 4}, {\tt 5}, {\tt 6}, {\tt 7}, {\tt 8}, {\tt 9}, {\tt T}, {\tt J}, {\tt Q}, {\tt K}, {\tt A}\}$. The second character denotes the suit and belongs to the set $\{{\tt D}, {\tt C}, {\tt S}, {\tt H}\}$.
All the cards in the input are different.
-----Output-----
If it is possible to play a card from your hand, print one word "YES". Otherwise, print "NO".
You can print each letter in any case (upper or lower).
-----Examples-----
Input
AS
2H 4C TH JH AD
Output
YES
Input
2H
3D 4C AC KD AS
Output
NO
Input
4D
AS AC AD AH 5H
Output
YES
-----Note-----
In the first example, there is an Ace of Spades (AS) on the table. You can play an Ace of Diamonds (AD) because both of them are Aces.
In the second example, you cannot play any card.
In the third example, you can play an Ace of Diamonds (AD) because it has the same suit as a Four of Diamonds (4D), which lies on the table. | s1 = input()
ls = input().split()
print('YES' if any(any(s1[i] == ls[j][i] for i in range(2)) for j in range(5)) else 'NO') | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/contains-duplicate/:
Given an array of integers, find if the array contains any duplicates.
Your function should return true if any value appears at least twice in the array, and it should return false if every element is distinct.
Example 1:
Input: [1,2,3,1]
Output: true
Example 2:
Input: [1,2,3,4]
Output: false
Example 3:
Input: [1,1,1,3,3,4,3,2,4,2]
Output: true
I tried it in Python, but could not do it. Can you solve it? | class Solution:
def containsDuplicate(self, nums):
"""
:type nums: List[int]
:rtype: bool
"""
diction = {}
flag = 0
for number in nums:
if number not in diction:
diction[number] = 1
else:
flag = 1
break
if flag == 1:
return True
else:
return False | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
We have a chocolate bar partitioned into H horizontal rows and W vertical columns of squares.
The square (i, j) at the i-th row from the top and the j-th column from the left is dark if S_{i,j} is 0, and white if S_{i,j} is 1.
We will cut the bar some number of times to divide it into some number of blocks. In each cut, we cut the whole bar by a line running along some boundaries of squares from end to end of the bar.
How many times do we need to cut the bar so that every block after the cuts has K or less white squares?
-----Constraints-----
- 1 \leq H \leq 10
- 1 \leq W \leq 1000
- 1 \leq K \leq H \times W
- S_{i,j} is 0 or 1.
-----Input-----
Input is given from Standard Input in the following format:
H W K
S_{1,1}S_{1,2}...S_{1,W}
:
S_{H,1}S_{H,2}...S_{H,W}
-----Output-----
Print the number of minimum times the bar needs to be cut so that every block after the cuts has K or less white squares.
-----Sample Input-----
3 5 4
11100
10001
00111
-----Sample Output-----
2
For example, cutting between the 1-st and 2-nd rows and between the 3-rd and 4-th columns - as shown in the figure to the left - works.
Note that we cannot cut the bar in the ways shown in the two figures to the right. | # E - Dividing Chocolate
import numpy as np
H, W, K = list(map(int, input().split()))
S = np.zeros((H, W), dtype=np.int64)
ans = H+W
for i in range(H):
S[i] = list(str(input()))
for m in range(2**(H-1)):
wp = np.zeros((H, W), dtype=np.int64)
wq = np.zeros((H, ), dtype=np.int64)
wp[0] = S[0]
cut = 0
for n in range(H-1):
if m>>n&1:
cut += 1
wp[cut] += S[n+1]
if cut >= ans or np.count_nonzero(wp > K):
continue
for j in range(W):
wq += wp[:,j]
if np.count_nonzero(wq > K):
cut += 1
wq = wp[:,j]
ans = min(ans, cut)
print(ans) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc113/tasks/abc113_b:
A country decides to build a palace.
In this country, the average temperature of a point at an elevation of x meters is T-x \times 0.006 degrees Celsius.
There are N places proposed for the place. The elevation of Place i is H_i meters.
Among them, Princess Joisino orders you to select the place whose average temperature is the closest to A degrees Celsius, and build the palace there.
Print the index of the place where the palace should be built.
It is guaranteed that the solution is unique.
-----Constraints-----
- 1 \leq N \leq 1000
- 0 \leq T \leq 50
- -60 \leq A \leq T
- 0 \leq H_i \leq 10^5
- All values in input are integers.
- The solution is unique.
-----Input-----
Input is given from Standard Input in the following format:
N
T A
H_1 H_2 ... H_N
-----Output-----
Print the index of the place where the palace should be built.
-----Sample Input-----
2
12 5
1000 2000
-----Sample Output-----
1
- The average temperature of Place 1 is 12-1000 \times 0.006=6 degrees Celsius.
- The average temperature of Place 2 is 12-2000 \times 0.006=0 degrees Celsius.
Thus, the palace should be built at Place 1.
I tried it in Python, but could not do it. Can you solve it? | from typing import List
def answer(n: int, t: int, a: int, hs: List[int]) -> int:
import sys
result = 0
temperature_difference = sys.maxsize
for i, h in enumerate(hs, start=1):
temp = abs(a - (t - h * 0.006))
if temp < temperature_difference:
temperature_difference = temp
result = i
return result
def main():
n = int(input())
t, a = map(int, input().split())
hs = list(map(int, input().split()))
print(answer(n, t, a, hs))
def __starting_point():
main()
__starting_point() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Dreamoon is standing at the position 0 on a number line. Drazil is sending a list of commands through Wi-Fi to Dreamoon's smartphone and Dreamoon follows them.
Each command is one of the following two types: Go 1 unit towards the positive direction, denoted as '+' Go 1 unit towards the negative direction, denoted as '-'
But the Wi-Fi condition is so poor that Dreamoon's smartphone reports some of the commands can't be recognized and Dreamoon knows that some of them might even be wrong though successfully recognized. Dreamoon decides to follow every recognized command and toss a fair coin to decide those unrecognized ones (that means, he moves to the 1 unit to the negative or positive direction with the same probability 0.5).
You are given an original list of commands sent by Drazil and list received by Dreamoon. What is the probability that Dreamoon ends in the position originally supposed to be final by Drazil's commands?
-----Input-----
The first line contains a string s_1 — the commands Drazil sends to Dreamoon, this string consists of only the characters in the set {'+', '-'}.
The second line contains a string s_2 — the commands Dreamoon's smartphone recognizes, this string consists of only the characters in the set {'+', '-', '?'}. '?' denotes an unrecognized command.
Lengths of two strings are equal and do not exceed 10.
-----Output-----
Output a single real number corresponding to the probability. The answer will be considered correct if its relative or absolute error doesn't exceed 10^{ - 9}.
-----Examples-----
Input
++-+-
+-+-+
Output
1.000000000000
Input
+-+-
+-??
Output
0.500000000000
Input
+++
??-
Output
0.000000000000
-----Note-----
For the first sample, both s_1 and s_2 will lead Dreamoon to finish at the same position + 1.
For the second sample, s_1 will lead Dreamoon to finish at position 0, while there are four possibilites for s_2: {"+-++", "+-+-", "+--+", "+---"} with ending position {+2, 0, 0, -2} respectively. So there are 2 correct cases out of 4, so the... | #!/usr/bin/env python3
# -*- coding: utf-8 -*-
import itertools
s1 = input()
s2 = input()
s1_p = s1.count('+')
s1_n = s1.count('-')
s2_p = s2.count('+')
s2_n = s2.count('-')
s2_q = s2.count('?')
correct_pos = s1_p - s1_n
drea_pos = s2_p - s2_n
diff_pos = correct_pos - drea_pos
if s2_q == 0:
if diff_pos == 0:
print(1.0)
else:
print(0.0)
else:
#print("diff_pos = ", diff_pos)
num = 0
den = 0
for c in itertools.product([1, -1], repeat=s2_q):
if sum(c) == diff_pos:
num += 1
den += 1
print("{0:.10f}".format(float(num) / den)) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
You have a string s = s_1s_2...s_{|}s|, where |s| is the length of string s, and s_{i} its i-th character.
Let's introduce several definitions: A substring s[i..j] (1 ≤ i ≤ j ≤ |s|) of string s is string s_{i}s_{i} + 1...s_{j}. The prefix of string s of length l (1 ≤ l ≤ |s|) is string s[1..l]. The suffix of string s of length l (1 ≤ l ≤ |s|) is string s[|s| - l + 1..|s|].
Your task is, for any prefix of string s which matches a suffix of string s, print the number of times it occurs in string s as a substring.
-----Input-----
The single line contains a sequence of characters s_1s_2...s_{|}s| (1 ≤ |s| ≤ 10^5) — string s. The string only consists of uppercase English letters.
-----Output-----
In the first line, print integer k (0 ≤ k ≤ |s|) — the number of prefixes that match a suffix of string s. Next print k lines, in each line print two integers l_{i} c_{i}. Numbers l_{i} c_{i} mean that the prefix of the length l_{i} matches the suffix of length l_{i} and occurs in string s as a substring c_{i} times. Print pairs l_{i} c_{i} in the order of increasing l_{i}.
-----Examples-----
Input
ABACABA
Output
3
1 4
3 2
7 1
Input
AAA
Output
3
1 3
2 2
3 1 | s = str(input())
lps = [0]*100005
dp = [0]*100005
ada = [0]*100005
tunda = [0]*100005
n = len(s)
i = 1
j = 0
lps[0] = 0
while(i < n):
if (s[i] == s[j]):
j += 1
lps[i] = j
i += 1
elif (j == 0):
lps[i] = 0
i += 1
else:
j = lps[j-1]
for i in range(n-1,-1,-1):
tunda[i] += 1
dp[lps[i]] += tunda[i]
if (lps[i]):tunda[lps[i]-1] += tunda[i]
j = n
"""
for i in range(n):
print("SAD", i, lps[i])
"""
vector = []
while(1):
vector.append((j,1+dp[j]))
j = lps[j-1]
if (j == 0): break
vector.reverse()
print(len(vector))
for i in vector:
print(i[0], i[1]) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
You have a given picture with size $w \times h$. Determine if the given picture has a single "+" shape or not. A "+" shape is described below:
A "+" shape has one center nonempty cell. There should be some (at least one) consecutive non-empty cells in each direction (left, right, up, down) from the center. In other words, there should be a ray in each direction. All other cells are empty.
Find out if the given picture has single "+" shape.
-----Input-----
The first line contains two integers $h$ and $w$ ($1 \le h$, $w \le 500$) — the height and width of the picture.
The $i$-th of the next $h$ lines contains string $s_{i}$ of length $w$ consisting "." and "*" where "." denotes the empty space and "*" denotes the non-empty space.
-----Output-----
If the given picture satisfies all conditions, print "YES". Otherwise, print "NO".
You can output each letter in any case (upper or lower).
-----Examples-----
Input
5 6
......
..*...
.****.
..*...
..*...
Output
YES
Input
3 5
..*..
****.
.*...
Output
NO
Input
7 7
.......
...*...
..****.
...*...
...*...
.......
.*.....
Output
NO
Input
5 6
..**..
..**..
******
..**..
..**..
Output
NO
Input
3 7
.*...*.
***.***
.*...*.
Output
NO
Input
5 10
..........
..*.......
.*.******.
..*.......
..........
Output
NO
-----Note-----
In the first example, the given picture contains one "+".
In the second example, two vertical branches are located in a different column.
In the third example, there is a dot outside of the shape.
In the fourth example, the width of the two vertical branches is $2$.
In the fifth example, there are two shapes.
In the sixth example, there is an empty space inside of the shape. | n, m = map(int, input().split())
s = []
for i in range(n):
s.append(input())
flag = False
def check(x, y):
if s[x-1][y-1] == s[x-1][y+1] == s[x+1][y-1] == s[x+1][y+1] == '.' and s[x-1][y] == s[x][y] == s[x+1][y] == s[x][y-1] == s[x][y+1] == '*':
return True
return False
def supercheck(x, y):
k = 1
i = x-1
while i >= 0 and s[i][y] == '*':
i -= 1
k += 1
i = x+1
while i < n and s[i][y] == '*':
i += 1
k += 1
i = y - 1
while i >= 0 and s[x][i] == '*':
i -= 1
k += 1
i = y + 1
while i < m and s[x][i] == '*':
i += 1
k += 1
true_k = 0
for i in range(n):
for j in range(m):
if s[i][j] == '*':
true_k += 1
if true_k == k:
return True
return False
for i in range(1, n - 1):
for j in range(1, m - 1):
if s[i][j] != '*': continue
if check(i, j):
if supercheck(i, j):
print('YES')
return
else:
print('NO')
return
print('NO') | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/combination-sum-ii/:
Given a collection of candidate numbers (candidates) and a target number (target), find all unique combinations in candidates where the candidate numbers sums to target.
Each number in candidates may only be used once in the combination.
Note:
All numbers (including target) will be positive integers.
The solution set must not contain duplicate combinations.
Example 1:
Input: candidates = [10,1,2,7,6,1,5], target = 8,
A solution set is:
[
[1, 7],
[1, 2, 5],
[2, 6],
[1, 1, 6]
]
Example 2:
Input: candidates = [2,5,2,1,2], target = 5,
A solution set is:
[
[1,2,2],
[5]
]
I tried it in Python, but could not do it. Can you solve it? | class Solution(object):
def combinationSum2(self, candidates, target):
"""
:type candidates: List[int]
:type target: int
:rtype: List[List[int]]
"""
result = []
temp = []
candidates.sort(reverse=True)
self.util(candidates, target, result, temp)
return result
def util(self, nums, target, result, temp):
for i in range(len(nums)):
if nums[i] == target and (temp + [nums[i]] not in result):
result.append(temp + [nums[i]])
elif nums[i] < target:
self.util(nums[i + 1:], target - nums[i], result, temp + [nums[i]])
return | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Chouti was doing a competitive programming competition. However, after having all the problems accepted, he got bored and decided to invent some small games.
He came up with the following game. The player has a positive integer $n$. Initially the value of $n$ equals to $v$ and the player is able to do the following operation as many times as the player want (possibly zero): choose a positive integer $x$ that $x<n$ and $x$ is not a divisor of $n$, then subtract $x$ from $n$. The goal of the player is to minimize the value of $n$ in the end.
Soon, Chouti found the game trivial. Can you also beat the game?
-----Input-----
The input contains only one integer in the first line: $v$ ($1 \le v \le 10^9$), the initial value of $n$.
-----Output-----
Output a single integer, the minimum value of $n$ the player can get.
-----Examples-----
Input
8
Output
1
Input
1
Output
1
-----Note-----
In the first example, the player can choose $x=3$ in the first turn, then $n$ becomes $5$. He can then choose $x=4$ in the second turn to get $n=1$ as the result. There are other ways to get this minimum. However, for example, he cannot choose $x=2$ in the first turn because $2$ is a divisor of $8$.
In the second example, since $n=1$ initially, the player can do nothing. | n = int(input())
if n == 2:
print(2)
else:
print(1) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1225/B1:
The only difference between easy and hard versions is constraints.
The BerTV channel every day broadcasts one episode of one of the $k$ TV shows. You know the schedule for the next $n$ days: a sequence of integers $a_1, a_2, \dots, a_n$ ($1 \le a_i \le k$), where $a_i$ is the show, the episode of which will be shown in $i$-th day.
The subscription to the show is bought for the entire show (i.e. for all its episodes), for each show the subscription is bought separately.
How many minimum subscriptions do you need to buy in order to have the opportunity to watch episodes of purchased shows $d$ ($1 \le d \le n$) days in a row? In other words, you want to buy the minimum number of TV shows so that there is some segment of $d$ consecutive days in which all episodes belong to the purchased shows.
-----Input-----
The first line contains an integer $t$ ($1 \le t \le 100$) — the number of test cases in the input. Then $t$ test case descriptions follow.
The first line of each test case contains three integers $n, k$ and $d$ ($1 \le n \le 100$, $1 \le k \le 100$, $1 \le d \le n$). The second line contains $n$ integers $a_1, a_2, \dots, a_n$ ($1 \le a_i \le k$), where $a_i$ is the show that is broadcasted on the $i$-th day.
It is guaranteed that the sum of the values of $n$ for all test cases in the input does not exceed $100$.
-----Output-----
Print $t$ integers — the answers to the test cases in the input in the order they follow. The answer to a test case is the minimum number of TV shows for which you need to purchase a subscription so that you can watch episodes of the purchased TV shows on BerTV for $d$ consecutive days. Please note that it is permissible that you will be able to watch more than $d$ days in a row.
-----Example-----
Input
4
5 2 2
1 2 1 2 1
9 3 3
3 3 3 2 2 2 1 1 1
4 10 4
10 8 6 4
16 9 8
3 1 4 1 5 9 2 6 5 3 5 8 9 7 9 3
Output
2
1
4
5
-----Note-----
In the first test case to have an opportunity to watch shows for two consecutive days, you need to buy a subscription on show $1$ and on...
I tried it in Python, but could not do it. Can you solve it? | t = int(input())
for i in range(t):
n,k,d = map(int,input().split())
minn = k
a = [int(i) for i in input().split()]
for i in range(n - d + 1):
m = set(a[i:i + d])
minn = min(minn,len(m))
print (minn) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
The Squareland national forest is divided into equal $1 \times 1$ square plots aligned with north-south and east-west directions. Each plot can be uniquely described by integer Cartesian coordinates $(x, y)$ of its south-west corner.
Three friends, Alice, Bob, and Charlie are going to buy three distinct plots of land $A, B, C$ in the forest. Initially, all plots in the forest (including the plots $A, B, C$) are covered by trees. The friends want to visit each other, so they want to clean some of the plots from trees. After cleaning, one should be able to reach any of the plots $A, B, C$ from any other one of those by moving through adjacent cleared plots. Two plots are adjacent if they share a side. [Image] For example, $A=(0,0)$, $B=(1,1)$, $C=(2,2)$. The minimal number of plots to be cleared is $5$. One of the ways to do it is shown with the gray color.
Of course, the friends don't want to strain too much. Help them find out the smallest number of plots they need to clean from trees.
-----Input-----
The first line contains two integers $x_A$ and $y_A$ — coordinates of the plot $A$ ($0 \leq x_A, y_A \leq 1000$). The following two lines describe coordinates $(x_B, y_B)$ and $(x_C, y_C)$ of plots $B$ and $C$ respectively in the same format ($0 \leq x_B, y_B, x_C, y_C \leq 1000$). It is guaranteed that all three plots are distinct.
-----Output-----
On the first line print a single integer $k$ — the smallest number of plots needed to be cleaned from trees. The following $k$ lines should contain coordinates of all plots needed to be cleaned. All $k$ plots should be distinct. You can output the plots in any order.
If there are multiple solutions, print any of them.
-----Examples-----
Input
0 0
1 1
2 2
Output
5
0 0
1 0
1 1
1 2
2 2
Input
0 0
2 0
1 1
Output
4
0 0
1 0
1 1
2 0
-----Note-----
The first example is shown on the picture in the legend.
The second example is illustrated with the following image: [Image] | ax, ay = list(map(int, input().split()))
bx, by = list(map(int, input().split()))
cx, cy = list(map(int, input().split()))
def f(cx, ax, bx, cy, ay, by):
mxy = max(ay, by, cy)
mny = min(ay, by, cy)
print(abs(cx - bx) + mxy - mny + 1)
for i in range(mny, mxy + 1):
print(ax, i)
if cx <= bx:
for i in range(cx, ax):
print(i, cy)
for i in range(ax + 1, bx + 1):
print(i, by)
else:
for i in range(bx, ax):
print(i, by)
for i in range(ax + 1, cx + 1):
print(i, cy)
if cx <= ax <= bx or bx <= ax <= cx:
f(cx, ax, bx, cy, ay, by)
elif cx <= bx <= ax or ax <= bx <= cx:
f(cx, bx, ax, cy, by, ay)
elif bx <= cx <= ax or ax <= cx <= bx:
f(bx, cx, ax, by, cy, ay) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
# Task
Two arrays are called similar if one can be obtained from another by swapping at most one pair of elements.
Given two arrays, check whether they are similar.
# Example
For `A = [1, 2, 3]` and `B = [1, 2, 3]`, the output should be `true;`
For `A = [1, 2, 3]` and `B = [2, 1, 3]`, the output should be `true;`
For `A = [1, 2, 2]` and `B = [2, 1, 1]`, the output should be `false.`
# Input/Output
- `[input]` integer array `A`
Array of integers.
Constraints: `3 ≤ A.length ≤ 10000, 1 ≤ A[i] ≤ 1000.`
- `[input]` integer array `B`
Array of integers of the same length as `A`.
Constraints: `B.length = A.length, 1 ≤ B[i] ≤ 1000.`
- `[output]` a boolean value
`true` if `A` and `B` are similar, `false` otherwise. | def are_similar(a, b):
return sorted(a)==sorted(b) and sum(1 for x, y in zip(a,b) if x!=y)<=2 | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/maximum-level-sum-of-a-binary-tree/:
Given the root of a binary tree, the level of its root is 1, the level of its children is 2, and so on.
Return the smallest level X such that the sum of all the values of nodes at level X is maximal.
Example 1:
Input: root = [1,7,0,7,-8,null,null]
Output: 2
Explanation:
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
Example 2:
Input: root = [989,null,10250,98693,-89388,null,null,null,-32127]
Output: 2
Constraints:
The number of nodes in the tree is in the range [1, 104].
-105 <= Node.val <= 105
I tried it in Python, but could not do it. Can you solve it? | # Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def maxLevelSum(self, root: TreeNode) -> int:
if not root: return
st=[[root,0]]
ll=[]
while st:
r,l=st.pop(0)
if l==len(ll) : ll.append([])
ll[l].append(r.val)
if r.left: st.append([r.left,l+1])
if r.right:
st.append([r.right,l+1])
#print (ll,l)
m=-1000
re=0
for i in range(l+1):
n=sum(ll[i])
if m<n:
m=n
re=i
return re+1
\"\"\"
if not root:
return []
queue,res=[[root, 0]],[]
while queue:
#print (queue[0][0].val)
node,level = queue.pop(0)
if level == len(res):
res.append([])
res[level].append(node.val)
if node.left:
queue.append((node.left, level + 1))
if node.right:
queue.append((node.right, level + 1))
return res[::-1]
\"\"\" | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1029/E:
You are given an undirected tree consisting of $n$ vertices. An undirected tree is a connected undirected graph with $n - 1$ edges.
Your task is to add the minimum number of edges in such a way that the length of the shortest path from the vertex $1$ to any other vertex is at most $2$. Note that you are not allowed to add loops and multiple edges.
-----Input-----
The first line contains one integer $n$ ($2 \le n \le 2 \cdot 10^5$) — the number of vertices in the tree.
The following $n - 1$ lines contain edges: edge $i$ is given as a pair of vertices $u_i, v_i$ ($1 \le u_i, v_i \le n$). It is guaranteed that the given edges form a tree. It is guaranteed that there are no loops and multiple edges in the given edges.
-----Output-----
Print a single integer — the minimum number of edges you have to add in order to make the shortest distance from the vertex $1$ to any other vertex at most $2$. Note that you are not allowed to add loops and multiple edges.
-----Examples-----
Input
7
1 2
2 3
2 4
4 5
4 6
5 7
Output
2
Input
7
1 2
1 3
2 4
2 5
3 6
1 7
Output
0
Input
7
1 2
2 3
3 4
3 5
3 6
3 7
Output
1
-----Note-----
The tree corresponding to the first example: [Image] The answer is $2$, some of the possible answers are the following: $[(1, 5), (1, 6)]$, $[(1, 4), (1, 7)]$, $[(1, 6), (1, 7)]$.
The tree corresponding to the second example: [Image] The answer is $0$.
The tree corresponding to the third example: [Image] The answer is $1$, only one possible way to reach it is to add the edge $(1, 3)$.
I tried it in Python, but could not do it. Can you solve it? | import sys
def get_new_edges(graph):
n = len(graph)
far_vertex = []
pi = [None]*n
visit = [False]*n
visit[0]
queue = [[0,0]]
i = 0
while True:
if i >= len(queue): break
current, d = queue[i]
i += 1
visit[current] = True
for v in graph[current]:
if not visit[v]:
u = [v, d+1]
pi[v] = current
queue.append(u)
if d+1 > 2:
far_vertex.append(u)
far_vertex.sort(key=lambda x: -x[1])
pos = [None]*n
for i, e in enumerate(far_vertex):
pos[e[0]] = i
count = 0
for i in range(len(far_vertex)):
if not far_vertex[i]: continue
vertex, depth = far_vertex[i]
father = pi[vertex]
count += 1
if pos[father]:
far_vertex[pos[father]] = None
for u in graph[father]:
if pos[u]:
far_vertex[pos[u]] = None
return count
def read_int_line():
return list(map(int, sys.stdin.readline().split()))
vertex_count = int(input())
graph = [[] for _ in range(vertex_count)]
for i in range(vertex_count - 1):
v1, v2 = read_int_line()
v1 -= 1
v2 -= 1
graph[v1].append(v2)
graph[v2].append(v1)
print(get_new_edges(graph)) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
The activity of a panipuri seller is "making a panipuri and putting it on the palte of his customer".
$N$ customers are eating panipuri, Given an array $A$ of length $N$, $i^{th}$ customer takes $A_i$ seconds to eat a panipuri.
The Speed of Panipuri seller refers to the number of customers served per second. Determine the minimum speed of panipuri seller so that no customer has to wait for panipuri after getting his/her first panipuri.
Assume that the plate can hold infinite panipuris, and customer starts eating next panipuri just after finishing the current one. You would be provided with the time taken by each customer to eat a panipuri.
Panpuri seller serves panipuri in round robin manner (first to last and then again first).
-----Input:-----
- First line will contain $T$, number of testcases. Then the test cases follow.
- For each test case, the first line contains $N$ number of customers.
- Then the second line will contain $N$ space separated integers, $A_1$ to $A_N$, eating time taken by each customer(in seconds).
-----Output:-----
- For each test case, print a single line containing the minimum integral speed of panipuri seller so that no customer has to wait.
-----Constraints-----
- $0 < T \leq 100$
- $0 < N \leq 10^6$
- $0 < A_i \leq 10^9$
- Sum of $N$ over all test cases does not exceed $10^6$
-----Sample Input:-----
2
4
2 4 6 3
5
2 3 4 6 5
-----Sample Output:-----
2
3
-----EXPLANATION:-----
$Test case$ $1$:
Let's assume speed of panipuri seller be 1.
So starting from $0^{th}$ second, in $0$ to $1$ second seller make a panipuri and put it into the plate of first customer, and first customer completely eat it in $1$ to $3$ second as he takes $2$ second to eat a panipuri. But in $3$ to $4$ second panipuri seller is serving to $4^{th}$ customer, and hence first customer have to wait, so 1 cannot be our answer.
Next possible speed can be $2$ .
Starting from $0^{th}$ second, at half second seller make a panipuri and put it into the plate of first customer, first customer... | # your code goes here
import math
t = int(input())
for i in range(t):
a = int(input())
val = input().split()
val = [int(x) for x in val]
ans = a/min(val)
print(math.ceil(ans)) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/789/B:
Masha really loves algebra. On the last lesson, her strict teacher Dvastan gave she new exercise.
You are given geometric progression b defined by two integers b_1 and q. Remind that a geometric progression is a sequence of integers b_1, b_2, b_3, ..., where for each i > 1 the respective term satisfies the condition b_{i} = b_{i} - 1·q, where q is called the common ratio of the progression. Progressions in Uzhlyandia are unusual: both b_1 and q can equal 0. Also, Dvastan gave Masha m "bad" integers a_1, a_2, ..., a_{m}, and an integer l.
Masha writes all progression terms one by one onto the board (including repetitive) while condition |b_{i}| ≤ l is satisfied (|x| means absolute value of x). There is an exception: if a term equals one of the "bad" integers, Masha skips it (doesn't write onto the board) and moves forward to the next term.
But the lesson is going to end soon, so Masha has to calculate how many integers will be written on the board. In order not to get into depression, Masha asked you for help: help her calculate how many numbers she will write, or print "inf" in case she needs to write infinitely many integers.
-----Input-----
The first line of input contains four integers b_1, q, l, m (-10^9 ≤ b_1, q ≤ 10^9, 1 ≤ l ≤ 10^9, 1 ≤ m ≤ 10^5) — the initial term and the common ratio of progression, absolute value of maximal number that can be written on the board and the number of "bad" integers, respectively.
The second line contains m distinct integers a_1, a_2, ..., a_{m} (-10^9 ≤ a_{i} ≤ 10^9) — numbers that will never be written on the board.
-----Output-----
Print the only integer, meaning the number of progression terms that will be written on the board if it is finite, or "inf" (without quotes) otherwise.
-----Examples-----
Input
3 2 30 4
6 14 25 48
Output
3
Input
123 1 2143435 4
123 11 -5453 141245
Output
0
Input
123 1 2143435 4
54343 -13 6 124
Output
inf
-----Note-----
In the first sample case, Masha will write integers 3, 12, 24. Progression term 6 will be skipped because...
I tried it in Python, but could not do it. Can you solve it? | miis = lambda:list(map(int,input().split()))
b,q,l,m = miis()
*a, = miis()
c = 0
for _ in ' '*100:
if abs(b)>l: break
if b not in a: c+=1
b*=q
if c<35:
print (c)
else:
print ('inf') | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
The dragon's curve is a self-similar fractal which can be obtained by a recursive method.
Starting with the string `D0 = 'Fa'`, at each step simultaneously perform the following operations:
```
replace 'a' with: 'aRbFR'
replace 'b' with: 'LFaLb'
```
For example (spaces added for more visibility) :
```
1st iteration: Fa -> F aRbF R
2nd iteration: FaRbFR -> F aRbFR R LFaLb FR
```
After `n` iteration, remove `'a'` and `'b'`. You will have a string with `'R'`,`'L'`, and `'F'`. This is a set of instruction. Starting at the origin of a grid looking in the `(0,1)` direction, `'F'` means a step forward, `'L'` and `'R'` mean respectively turn left and right. After executing all instructions, the trajectory will give a beautifull self-replicating pattern called 'Dragon Curve'
The goal of this kata is to code a function wich takes one parameter `n`, the number of iterations needed and return the string of instruction as defined above. For example:
```
n=0, should return: 'F'
n=1, should return: 'FRFR'
n=2, should return: 'FRFRRLFLFR'
```
`n` should be a number and non-negative integer. All other case should return the empty string: `''`. | def Dragon(n, Curve='Fa'):
if type(n)!=int or n%1!=0 or n<0: return ''
elif n==0:
return Curve.replace('a','').replace('b','')
else:
#now need to add an extra step where we swap out a dn b because otherwise the replace will affect the outcome. ie the replaces are not concurrent
return Dragon(n-1, Curve.replace('a','c').replace('b','d').replace('c','aRbFR').replace('d', 'LFaLb') ) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/405/A:
Little Chris is bored during his physics lessons (too easy), so he has built a toy box to keep himself occupied. The box is special, since it has the ability to change gravity.
There are n columns of toy cubes in the box arranged in a line. The i-th column contains a_{i} cubes. At first, the gravity in the box is pulling the cubes downwards. When Chris switches the gravity, it begins to pull all the cubes to the right side of the box. The figure shows the initial and final configurations of the cubes in the box: the cubes that have changed their position are highlighted with orange. [Image]
Given the initial configuration of the toy cubes in the box, find the amounts of cubes in each of the n columns after the gravity switch!
-----Input-----
The first line of input contains an integer n (1 ≤ n ≤ 100), the number of the columns in the box. The next line contains n space-separated integer numbers. The i-th number a_{i} (1 ≤ a_{i} ≤ 100) denotes the number of cubes in the i-th column.
-----Output-----
Output n integer numbers separated by spaces, where the i-th number is the amount of cubes in the i-th column after the gravity switch.
-----Examples-----
Input
4
3 2 1 2
Output
1 2 2 3
Input
3
2 3 8
Output
2 3 8
-----Note-----
The first example case is shown on the figure. The top cube of the first column falls to the top of the last column; the top cube of the second column falls to the top of the third column; the middle cube of the first column falls to the top of the second column.
In the second example case the gravity switch does not change the heights of the columns.
I tried it in Python, but could not do it. Can you solve it? | input()
print(*sorted(map(int, input().split()))) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
You have three tasks, all of which need to be completed.
First, you can complete any one task at cost 0.
Then, just after completing the i-th task, you can complete the j-th task at cost |A_j - A_i|.
Here, |x| denotes the absolute value of x.
Find the minimum total cost required to complete all the task.
-----Constraints-----
- All values in input are integers.
- 1 \leq A_1, A_2, A_3 \leq 100
-----Input-----
Input is given from Standard Input in the following format:
A_1 A_2 A_3
-----Output-----
Print the minimum total cost required to complete all the task.
-----Sample Input-----
1 6 3
-----Sample Output-----
5
When the tasks are completed in the following order, the total cost will be 5, which is the minimum:
- Complete the first task at cost 0.
- Complete the third task at cost 2.
- Complete the second task at cost 3. | a, b, c = map(int, input().split())
res = [abs(a-b), abs(a-c), abs(b-c)]
print(sum(res)-max(res)) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Write a program to check whether a triangle is valid or not, when the three angles of the triangle are the inputs. A triangle is valid if the sum of all the three angles is equal to 180 degrees.
-----Input-----
The first line contains an integer T, the total number of testcases. Then T lines follow, each line contains three angles A, B and C, of the triangle separated by space.
-----Output-----
For each test case, display 'YES' if the triangle is valid, and 'NO', if it is not, in a new line.
-----Constraints-----
- 1 ≤ T ≤ 1000
- 1 ≤ A,B,C ≤ 180
-----Example-----
Input
3
40 40 100
45 45 90
180 1 1
Output
YES
YES
NO | n=int(input())
for i in range(n):
a,b,c=map(int,input().split())
if a>0 and b>0 and c>0 and a+b+c==180:
print("YES")
else:
print("NO") | python | train | qsol | codeparrot/apps | all |
Solve in Python:
There are n parliamentarians in Berland. They are numbered with integers from 1 to n. It happened that all parliamentarians with odd indices are Democrats and all parliamentarians with even indices are Republicans.
New parliament assembly hall is a rectangle consisting of a × b chairs — a rows of b chairs each. Two chairs are considered neighbouring if they share as side. For example, chair number 5 in row number 2 is neighbouring to chairs number 4 and 6 in this row and chairs with number 5 in rows 1 and 3. Thus, chairs have four neighbours in general, except for the chairs on the border of the hall
We know that if two parliamentarians from one political party (that is two Democrats or two Republicans) seat nearby they spent all time discussing internal party issues.
Write the program that given the number of parliamentarians and the sizes of the hall determine if there is a way to find a seat for any parliamentarian, such that no two members of the same party share neighbouring seats.
-----Input-----
The first line of the input contains three integers n, a and b (1 ≤ n ≤ 10 000, 1 ≤ a, b ≤ 100) — the number of parliamentarians, the number of rows in the assembly hall and the number of seats in each row, respectively.
-----Output-----
If there is no way to assigns seats to parliamentarians in a proper way print -1.
Otherwise print the solution in a lines, each containing b integers. The j-th integer of the i-th line should be equal to the index of parliamentarian occupying this seat, or 0 if this seat should remain empty. If there are multiple possible solution, you may print any of them.
-----Examples-----
Input
3 2 2
Output
0 3
1 2
Input
8 4 3
Output
7 8 3
0 1 4
6 0 5
0 2 0
Input
10 2 2
Output
-1
-----Note-----
In the first sample there are many other possible solutions. For example, 3 2
0 1
and 2 1
3 0
The following assignment 3 2
1 0
is incorrect, because parliamentarians 1 and 3 are both from Democrats party but will occupy neighbouring seats. | [n,a,b] = list(map(int,input().split(' ')))
r = [(b+1)*[0] for _ in range(a+1)]
t = list(range(1, n+1))
for i in range(1,a+1):
for j in range(1,b+1):
for it in t:
if (r[i-1][j] == 0 or r[i-1][j]%2 != it%2) and (r[i][j-1] == 0 or r[i][j-1]%2 != it%2):
r[i][j] = it
break
if r[i][j] != 0:
t.remove(r[i][j])
if len(t) == 0:
for i in r[1:]:
print(*i[1:])
else:
print(-1) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/task-scheduler/:
Given a char array representing tasks CPU need to do. It contains capital letters A to Z where different letters represent different tasks.Tasks could be done without original order. Each task could be done in one interval. For each interval, CPU could finish one task or just be idle.
However, there is a non-negative cooling interval n that means between two same tasks, there must be at least n intervals that CPU are doing different tasks or just be idle.
You need to return the least number of intervals the CPU will take to finish all the given tasks.
Example 1:
Input: tasks = ["A","A","A","B","B","B"], n = 2
Output: 8
Explanation: A -> B -> idle -> A -> B -> idle -> A -> B.
Note:
The number of tasks is in the range [1, 10000].
The integer n is in the range [0, 100].
I tried it in Python, but could not do it. Can you solve it? | class Solution:
def leastInterval(self, tasks, n):
"""
:type tasks: List[str]
:type n: int
:rtype: int
"""
if not tasks:
return 0
counts = {}
for i in tasks:
if i in counts:
counts[i] += 1
else:
counts[i] = 1
M = max(counts.values())
Mct = sum([1 for i in counts if counts[i] == M])
return max(len(tasks), (M - 1) * (n + 1) + Mct) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc091/tasks/abc091_b:
Takahashi has N blue cards and M red cards.
A string is written on each card. The string written on the i-th blue card is s_i, and the string written on the i-th red card is t_i.
Takahashi will now announce a string, and then check every card. Each time he finds a blue card with the string announced by him, he will earn 1 yen (the currency of Japan); each time he finds a red card with that string, he will lose 1 yen.
Here, we only consider the case where the string announced by Takahashi and the string on the card are exactly the same. For example, if he announces atcoder, he will not earn money even if there are blue cards with atcoderr, atcode, btcoder, and so on. (On the other hand, he will not lose money even if there are red cards with such strings, either.)
At most how much can he earn on balance?
Note that the same string may be written on multiple cards.
-----Constraints-----
- N and M are integers.
- 1 \leq N, M \leq 100
- s_1, s_2, ..., s_N, t_1, t_2, ..., t_M are all strings of lengths between 1 and 10 (inclusive) consisting of lowercase English letters.
-----Input-----
Input is given from Standard Input in the following format:
N
s_1
s_2
:
s_N
M
t_1
t_2
:
t_M
-----Output-----
If Takahashi can earn at most X yen on balance, print X.
-----Sample Input-----
3
apple
orange
apple
1
grape
-----Sample Output-----
2
He can earn 2 yen by announcing apple.
I tried it in Python, but could not do it. Can you solve it? | from collections import Counter
def main():
n = int(input())
blue = Counter(input() for _ in range(n))
m = int(input())
red = Counter(input() for _ in range(m))
ans = 0
for name, amount in blue.items():
if name in red.keys():
temp = amount - red[name]
else:
temp = amount
ans = max(ans, temp)
print(ans)
main() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
We are given two arrays A and B of words. Each word is a string of lowercase letters.
Now, say that word b is a subset of word a if every letter in b occurs in a, including multiplicity. For example, "wrr" is a subset of "warrior", but is not a subset of "world".
Now say a word a from A is universal if for every b in B, b is a subset of a.
Return a list of all universal words in A. You can return the words in any order.
Example 1:
Input: A = ["amazon","apple","facebook","google","leetcode"], B = ["e","o"]
Output: ["facebook","google","leetcode"]
Example 2:
Input: A = ["amazon","apple","facebook","google","leetcode"], B = ["l","e"]
Output: ["apple","google","leetcode"]
Example 3:
Input: A = ["amazon","apple","facebook","google","leetcode"], B = ["e","oo"]
Output: ["facebook","google"]
Example 4:
Input: A = ["amazon","apple","facebook","google","leetcode"], B = ["lo","eo"]
Output: ["google","leetcode"]
Example 5:
Input: A = ["amazon","apple","facebook","google","leetcode"], B = ["ec","oc","ceo"]
Output: ["facebook","leetcode"]
Note:
1 <= A.length, B.length <= 10000
1 <= A[i].length, B[i].length <= 10
A[i] and B[i] consist only of lowercase letters.
All words in A[i] are unique: there isn't i != j with A[i] == A[j]. | class Solution:
def wordSubsets(self, A: List[str], B: List[str]) -> List[str]:
\"\"\"def checkSubset(a, B):
aDict = {}
bDict = {}
for b in B:
bSet = set(b)
for c in bSet:
if b.count(c) > a.count(c):
return False
return True
ret = []
for a in A:
if not checkSubset(a, B):
continue
else:
ret.append(a)
return ret\"\"\"
\"\"\"def convertB(b):
d = {}
for c in b:
if c not in d:
d[c] = 1
else:
d[c]+=1
return d
for i in range(len(B)):
B[i] = convertB(B[i])
def convertA(a):
d = {}
for c in a:
if c not in d:
d[c]=1
else:
d[c]+=1
return d
convertedA = []
for i in range(len(A)):
convertedA.append(convertA(A[i]))
def isGoodWord(aDict):
for wordDict in B:
for c in wordDict:
if c not in aDict or wordDict[c] > aDict[c]:
return False
return True
ret = []
for i in range(len(convertedA)):
if isGoodWord(convertedA[i]):
ret.append(A[i])
return ret\"\"\"
bDict = {}
def convertB(b):
d = {}
for c in b:
if c not in d:
d[c] = 1
else:
d[c]+=1
return d
for i in range(len(B)):
B[i] = convertB(B[i])
for d in B:
for key in d:
if key not in bDict:
bDict[key] = d[key]
else:
if bDict[key] < d[key]:
bDict[key] = d[key]
def convertA(a):
... | python | train | qsol | codeparrot/apps | all |
Solve in Python:
You are given a matrix with $n$ rows (numbered from $1$ to $n$) and $m$ columns (numbered from $1$ to $m$). A number $a_{i, j}$ is written in the cell belonging to the $i$-th row and the $j$-th column, each number is either $0$ or $1$.
A chip is initially in the cell $(1, 1)$, and it will be moved to the cell $(n, m)$. During each move, it either moves to the next cell in the current row, or in the current column (if the current cell is $(x, y)$, then after the move it can be either $(x + 1, y)$ or $(x, y + 1)$). The chip cannot leave the matrix.
Consider each path of the chip from $(1, 1)$ to $(n, m)$. A path is called palindromic if the number in the first cell is equal to the number in the last cell, the number in the second cell is equal to the number in the second-to-last cell, and so on.
Your goal is to change the values in the minimum number of cells so that every path is palindromic.
-----Input-----
The first line contains one integer $t$ ($1 \le t \le 200$) — the number of test cases.
The first line of each test case contains two integers $n$ and $m$ ($2 \le n, m \le 30$) — the dimensions of the matrix.
Then $n$ lines follow, the $i$-th line contains $m$ integers $a_{i, 1}$, $a_{i, 2}$, ..., $a_{i, m}$ ($0 \le a_{i, j} \le 1$).
-----Output-----
For each test case, print one integer — the minimum number of cells you have to change so that every path in the matrix is palindromic.
-----Example-----
Input
4
2 2
1 1
0 1
2 3
1 1 0
1 0 0
3 7
1 0 1 1 1 1 1
0 0 0 0 0 0 0
1 1 1 1 1 0 1
3 5
1 0 1 0 0
1 1 1 1 0
0 0 1 0 0
Output
0
3
4
4
-----Note-----
The resulting matrices in the first three test cases: $\begin{pmatrix} 1 & 1\\ 0 & 1 \end{pmatrix}$ $\begin{pmatrix} 0 & 0 & 0\\ 0 & 0 & 0 \end{pmatrix}$ $\begin{pmatrix} 1 & 0 & 1 & 1 & 1 & 1 & 1\\ 0 & 1 & 1 & 0 & 1 & 1 & 0\\ 1 & 1 & 1 & 1 & 1 & 0 & 1 \end{pmatrix}$ | T = int(input())
for _ in range(T):
N,M = list(map(int,input().split()))
A = [list(map(int,input().split())) for i in range(N)]
L = N+M-1
c0 = [0]*L
c1 = [0]*L
for i,row in enumerate(A):
for j,a in enumerate(row):
if a==0:
c0[i+j] += 1
else:
c1[i+j] += 1
M = L//2
ans = 0
for i in range(M):
a0 = c0[i] + c0[-1-i]
a1 = c1[i] + c1[-1-i]
ans += min(a0,a1)
print(ans) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/59b139d69c56e8939700009d:
Write a method named `getExponent(n,p)` that returns the largest integer exponent `x` such that p^(x) evenly divides `n`. if `p<=1` the method should return `null`/`None` (throw an `ArgumentOutOfRange` exception in C#).
I tried it in Python, but could not do it. Can you solve it? | def get_exponent(n, p):
return next(iter(i for i in range(int(abs(n) ** (1/p)), 0, -1) if (n / p**i) % 1 == 0), 0) if p > 1 else None | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Joisino the magical girl has decided to turn every single digit that exists on this world into 1.
Rewriting a digit i with j (0≤i,j≤9) costs c_{i,j} MP (Magic Points).
She is now standing before a wall. The wall is divided into HW squares in H rows and W columns, and at least one square contains a digit between 0 and 9 (inclusive).
You are given A_{i,j} that describes the square at the i-th row from the top and j-th column from the left, as follows:
- If A_{i,j}≠-1, the square contains a digit A_{i,j}.
- If A_{i,j}=-1, the square does not contain a digit.
Find the minimum total amount of MP required to turn every digit on this wall into 1 in the end.
-----Constraints-----
- 1≤H,W≤200
- 1≤c_{i,j}≤10^3 (i≠j)
- c_{i,j}=0 (i=j)
- -1≤A_{i,j}≤9
- All input values are integers.
- There is at least one digit on the wall.
-----Input-----
Input is given from Standard Input in the following format:
H W
c_{0,0} ... c_{0,9}
:
c_{9,0} ... c_{9,9}
A_{1,1} ... A_{1,W}
:
A_{H,1} ... A_{H,W}
-----Output-----
Print the minimum total amount of MP required to turn every digit on the wall into 1 in the end.
-----Sample Input-----
2 4
0 9 9 9 9 9 9 9 9 9
9 0 9 9 9 9 9 9 9 9
9 9 0 9 9 9 9 9 9 9
9 9 9 0 9 9 9 9 9 9
9 9 9 9 0 9 9 9 9 2
9 9 9 9 9 0 9 9 9 9
9 9 9 9 9 9 0 9 9 9
9 9 9 9 9 9 9 0 9 9
9 9 9 9 2 9 9 9 0 9
9 2 9 9 9 9 9 9 9 0
-1 -1 -1 -1
8 1 1 8
-----Sample Output-----
12
To turn a single 8 into 1, it is optimal to first turn 8 into 4, then turn 4 into 9, and finally turn 9 into 1, costing 6 MP.
The wall contains two 8s, so the minimum total MP required is 6×2=12. | H, W = list(map(int, input().split()))
C = [list(map(int, input().split())) for _ in range(10)]
for i in range(10):
for k in range(10):
for l in range(10):
C[k][l] = min(C[k][l], C[k][i]+C[i][l])
ans = 0
"""
for _ in C:
print(_)
"""
for i in range(H):
A = list(map(int,input().split()))
for j in A:
if j != -1:
ans += C[j][1]
print(ans) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
Given a standard english sentence passed in as a string, write a method that will return a sentence made up of the same words, but sorted by their first letter. However, the method of sorting has a twist to it:
* All words that begin with a lower case letter should be at the beginning of the sorted sentence, and sorted in ascending order.
* All words that begin with an upper case letter should come after that, and should be sorted in descending order.
If a word appears multiple times in the sentence, it should be returned multiple times in the sorted sentence. Any punctuation must be discarded.
## Example
For example, given the input string `"Land of the Old Thirteen! Massachusetts land! land of Vermont and Connecticut!"`, your method should return `"and land land of of the Vermont Thirteen Old Massachusetts Land Connecticut"`. Lower case letters are sorted `a -> l -> l -> o -> o -> t` and upper case letters are sorted `V -> T -> O -> M -> L -> C`. | def pseudo_sort(s):
s = ''.join(i for i in s if i.isalpha() or i is ' ')
a = sorted(i for i in s.split() if i[0].islower())
b = sorted((i for i in s.split() if i[0].isupper()),key=lambda x: x.lower(),reverse=True)
return ' '.join(a+b) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Create an identity matrix of the specified size( >= 0).
Some examples:
```
(1) => [[1]]
(2) => [ [1,0],
[0,1] ]
[ [1,0,0,0,0],
[0,1,0,0,0],
(5) => [0,0,1,0,0],
[0,0,0,1,0],
[0,0,0,0,1] ]
``` | from numpy import identity, ndarray
def get_matrix(n):
return identity(n).tolist() | python | train | qsol | codeparrot/apps | all |
Solve in Python:
You have an array A of size N containing only positive numbers. You have to output the maximum possible value of A[i]%A[j] where 1<=i,j<=N.
-----Input-----
The first line of each test case contains a single integer N denoting the size of the array. The next N lines contains integers A1, A2, ..., AN denoting the numbers
-----Output-----
Output a single integer answering what is asked in the problem.
-----Subtask 1 (20 points)-----
- 1 ≤ N ≤ 5000
- 1 ≤ A[i] ≤ 2*(10^9)
-----Subtask 2 (80 points)-----
- 1 ≤ N ≤ 1000000
- 1 ≤ A[i] ≤ 2*(10^9)
-----Example-----
Input:
2
1
2
Output:
1
-----Explanation-----
There will be four values, A[0]%A[0] = 0, A[0]%A[1]=1, A[1]%A[0]=0, A[1]%A[1]=0, and hence the output will be the maximum among them all, that is 1. | n = int(input())
a = []
for i in range(n):
a.append(int(input()))
m1 = 0
m2 = 0
for e in a:
if (e > m1):
m2 = m1
m1 = e
elif (e > m2 and e != m1):
m2 = e
ans = 0
for e in a:
temp = m1%e
if (temp>ans):
ans = temp
print(max(m2%m1,ans)) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
The number n is Evil if it has an even number of 1's in its binary representation.
The first few Evil numbers: 3, 5, 6, 9, 10, 12, 15, 17, 18, 20
The number n is Odious if it has an odd number of 1's in its binary representation.
The first few Odious numbers: 1, 2, 4, 7, 8, 11, 13, 14, 16, 19
You have to write a function that determine if a number is Evil of Odious, function should return "It's Evil!" in case of evil number and "It's Odious!" in case of odious number.
good luck :) | def evil(n: int) -> str:
"""
Check if the given number is:
- evil: it has an even number of 1's in its binary representation
- odious: it has an odd number of 1's in its binary representation
"""
return f"It's {'Odious' if str(bin(n)).count('1') % 2 else 'Evil'}!" | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1012/D:
There are two strings s and t, consisting only of letters a and b. You can make the following operation several times: choose a prefix of s, a prefix of t and swap them. Prefixes can be empty, also a prefix can coincide with a whole string.
Your task is to find a sequence of operations after which one of the strings consists only of a letters and the other consists only of b letters. The number of operations should be minimized.
-----Input-----
The first line contains a string s (1 ≤ |s| ≤ 2·10^5).
The second line contains a string t (1 ≤ |t| ≤ 2·10^5).
Here |s| and |t| denote the lengths of s and t, respectively. It is guaranteed that at least one of the strings contains at least one a letter and at least one of the strings contains at least one b letter.
-----Output-----
The first line should contain a single integer n (0 ≤ n ≤ 5·10^5) — the number of operations.
Each of the next n lines should contain two space-separated integers a_{i}, b_{i} — the lengths of prefixes of s and t to swap, respectively.
If there are multiple possible solutions, you can print any of them. It's guaranteed that a solution with given constraints exists.
-----Examples-----
Input
bab
bb
Output
2
1 0
1 3
Input
bbbb
aaa
Output
0
-----Note-----
In the first example, you can solve the problem in two operations: Swap the prefix of the first string with length 1 and the prefix of the second string with length 0. After this swap, you'll have strings ab and bbb. Swap the prefix of the first string with length 1 and the prefix of the second string with length 3. After this swap, you'll have strings bbbb and a.
In the second example, the strings are already appropriate, so no operations are needed.
I tried it in Python, but could not do it. Can you solve it? | def blokovi(x):
ret = [0]
for i in range(len(x) - 1):
if x[i] != x[i + 1]:
ret.append(i + 1)
return ret + [len(x)]
s = input()
t = input()
ss = blokovi(s)
tt = blokovi(t)
if s[-1] == 'a':
s += 'b'
else:
s += 'a'
if t[-1] == 'a':
t += 'b'
else:
t += 'a'
def greedy(x, y, rev=False):
i, j = len(x) - 1, len(y) - 1
swaps = []
while True:
while i >= 0 and x[i] == 'a':
i -= 1
while j >= 0 and y[j] == 'b':
j -= 1
if i < 0 and j < 0:
break
x, y = y, x
if rev:
swaps.append((j + 1, i + 1))
else:
swaps.append((i + 1, j + 1))
i, j = j, i
return swaps
def solve(x, y):
p = greedy(x, y)
q = greedy(y, x, True)
if len(p) < len(q):
return p
return q
probao = set()
total = len(ss) + len(tt)
sol = solve(s[:-1], t[:-1])
for b, i in enumerate(ss):
for c in range((2 * b + len(tt) - len(ss)) // 2 - 2, (2 * b + len(tt) - len(ss) + 1) // 2 + 3):
if 0 <= c < len(tt):
j = tt[c]
bs = b + len(tt) - c - 1
bt = c + len(ss) - b - 1
if abs(bs - bt) > 2:
continue
proba = (bs, bt, s[i], t[j])
if proba in probao:
continue
probao.add(proba)
s2 = t[:j] + s[i:-1]
t2 = s[:i] + t[j:-1]
if i + j > 0:
if i + j == len(s) + len(t) - 2:
cand = solve(t2, s2)
else:
cand = [(i, j)] + solve(s2, t2)
else:
cand = solve(s2, t2)
if len(cand) < len(sol):
sol = cand
print(len(sol))
for i, j in sol:
print(i, j) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Some time ago Lesha found an entertaining string $s$ consisting of lowercase English letters. Lesha immediately developed an unique algorithm for this string and shared it with you. The algorithm is as follows.
Lesha chooses an arbitrary (possibly zero) number of pairs on positions $(i, i + 1)$ in such a way that the following conditions are satisfied: for each pair $(i, i + 1)$ the inequality $0 \le i < |s| - 1$ holds; for each pair $(i, i + 1)$ the equality $s_i = s_{i + 1}$ holds; there is no index that is contained in more than one pair. After that Lesha removes all characters on indexes contained in these pairs and the algorithm is over.
Lesha is interested in the lexicographically smallest strings he can obtain by applying the algorithm to the suffixes of the given string.
-----Input-----
The only line contains the string $s$ ($1 \le |s| \le 10^5$) — the initial string consisting of lowercase English letters only.
-----Output-----
In $|s|$ lines print the lengths of the answers and the answers themselves, starting with the answer for the longest suffix. The output can be large, so, when some answer is longer than $10$ characters, instead print the first $5$ characters, then "...", then the last $2$ characters of the answer.
-----Examples-----
Input
abcdd
Output
3 abc
2 bc
1 c
0
1 d
Input
abbcdddeaaffdfouurtytwoo
Output
18 abbcd...tw
17 bbcdd...tw
16 bcddd...tw
15 cddde...tw
14 dddea...tw
13 ddeaa...tw
12 deaad...tw
11 eaadf...tw
10 aadfortytw
9 adfortytw
8 dfortytw
9 fdfortytw
8 dfortytw
7 fortytw
6 ortytw
5 rtytw
6 urtytw
5 rtytw
4 tytw
3 ytw
2 tw
1 w
0
1 o
-----Note-----
Consider the first example.
The longest suffix is the whole string "abcdd". Choosing one pair $(4, 5)$, Lesha obtains "abc". The next longest suffix is "bcdd". Choosing one pair $(3, 4)$, we obtain "bc". The next longest suffix is "cdd". Choosing one pair $(2, 3)$, we obtain "c". The next longest suffix is "dd". Choosing one pair $(1, 2)$, we obtain "" (an empty string). The last suffix is the string... | s = input().strip();N = len(s)
if len(s) == 1:print(1, s[0]);return
X = [s[-1], s[-2]+s[-1] if s[-2]!=s[-1] else ""];Y = [1, 2 if s[-2]!=s[-1] else 0]
for i in range(N-3, -1, -1):
c = s[i];k1 = c+X[-1];ng = Y[-1]+1
if ng > 10:k1 = k1[:5] + "..." + k1[-2:]
if c == s[i+1] and k1 > X[-2]:k1 = X[-2];ng = Y[-2]
X.append(k1);Y.append(ng)
for i in range(N-1, -1, -1):print(Y[i], X[i]) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1206/B:
You are given $n$ numbers $a_1, a_2, \dots, a_n$. With a cost of one coin you can perform the following operation:
Choose one of these numbers and add or subtract $1$ from it.
In particular, we can apply this operation to the same number several times.
We want to make the product of all these numbers equal to $1$, in other words, we want $a_1 \cdot a_2$ $\dots$ $\cdot a_n = 1$.
For example, for $n = 3$ and numbers $[1, -3, 0]$ we can make product equal to $1$ in $3$ coins: add $1$ to second element, add $1$ to second element again, subtract $1$ from third element, so that array becomes $[1, -1, -1]$. And $1\cdot (-1) \cdot (-1) = 1$.
What is the minimum cost we will have to pay to do that?
-----Input-----
The first line contains a single integer $n$ ($1 \le n \le 10^5$) — the number of numbers.
The second line contains $n$ integers $a_1, a_2, \dots, a_n$ ($-10^9 \le a_i \le 10^9$) — the numbers.
-----Output-----
Output a single number — the minimal number of coins you need to pay to make the product equal to $1$.
-----Examples-----
Input
2
-1 1
Output
2
Input
4
0 0 0 0
Output
4
Input
5
-5 -3 5 3 0
Output
13
-----Note-----
In the first example, you can change $1$ to $-1$ or $-1$ to $1$ in $2$ coins.
In the second example, you have to apply at least $4$ operations for the product not to be $0$.
In the third example, you can change $-5$ to $-1$ in $4$ coins, $-3$ to $-1$ in $2$ coins, $5$ to $1$ in $4$ coins, $3$ to $1$ in $2$ coins, $0$ to $1$ in $1$ coin.
I tried it in Python, but could not do it. Can you solve it? | q=int(input())
w=list(map(int,input().split()))
e=0
r=0
t=0
for i in w:
if i<0:
e+=-1-i
r+=1
elif i>0:
e+=i-1
else:
e+=1
t+=1
if r%2==1:
if t>0:
print(e)
else:
print(e+2)
else:
print(e) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
The semester is already ending, so Danil made an effort and decided to visit a lesson on harmony analysis to know how does the professor look like, at least. Danil was very bored on this lesson until the teacher gave the group a simple task: find 4 vectors in 4-dimensional space, such that every coordinate of every vector is 1 or - 1 and any two vectors are orthogonal. Just as a reminder, two vectors in n-dimensional space are considered to be orthogonal if and only if their scalar product is equal to zero, that is: $\sum_{i = 1}^{n} a_{i} \cdot b_{i} = 0$.
Danil quickly managed to come up with the solution for this problem and the teacher noticed that the problem can be solved in a more general case for 2^{k} vectors in 2^{k}-dimensinoal space. When Danil came home, he quickly came up with the solution for this problem. Can you cope with it?
-----Input-----
The only line of the input contains a single integer k (0 ≤ k ≤ 9).
-----Output-----
Print 2^{k} lines consisting of 2^{k} characters each. The j-th character of the i-th line must be equal to ' * ' if the j-th coordinate of the i-th vector is equal to - 1, and must be equal to ' + ' if it's equal to + 1. It's guaranteed that the answer always exists.
If there are many correct answers, print any.
-----Examples-----
Input
2
Output
++**
+*+*
++++
+**+
-----Note-----
Consider all scalar products in example: Vectors 1 and 2: ( + 1)·( + 1) + ( + 1)·( - 1) + ( - 1)·( + 1) + ( - 1)·( - 1) = 0 Vectors 1 and 3: ( + 1)·( + 1) + ( + 1)·( + 1) + ( - 1)·( + 1) + ( - 1)·( + 1) = 0 Vectors 1 and 4: ( + 1)·( + 1) + ( + 1)·( - 1) + ( - 1)·( - 1) + ( - 1)·( + 1) = 0 Vectors 2 and 3: ( + 1)·( + 1) + ( - 1)·( + 1) + ( + 1)·( + 1) + ( - 1)·( + 1) = 0 Vectors 2 and 4: ( + 1)·( + 1) + ( - 1)·( - 1) + ( + 1)·( - 1) + ( - 1)·( + 1) = 0 Vectors 3 and 4: ( + 1)·( + 1) + ( + 1)·( - 1) + ( + 1)·( - 1) + ( + 1)·( + 1) = 0 | k = int(input())
if (k == 0):
print('+')
return
answer = [['+', '+'], ['+', '*']]
length = 2
for i in range(k - 1):
new = []
for i in answer:
temp = []
for j in range(length):
if i[j] == '+':
temp += ['+', '+']
else:
temp += ['*', '*']
new.append(temp)
for i in answer:
temp = []
for j in range(length):
if i[j] == '+':
temp += ['+', '*']
else:
temp += ['*', '+']
new.append(temp)
answer = new
length *= 2
print('\n'.join([''.join(i) for i in answer])) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/455/A:
Alex doesn't like boredom. That's why whenever he gets bored, he comes up with games. One long winter evening he came up with a game and decided to play it.
Given a sequence a consisting of n integers. The player can make several steps. In a single step he can choose an element of the sequence (let's denote it a_{k}) and delete it, at that all elements equal to a_{k} + 1 and a_{k} - 1 also must be deleted from the sequence. That step brings a_{k} points to the player.
Alex is a perfectionist, so he decided to get as many points as possible. Help him.
-----Input-----
The first line contains integer n (1 ≤ n ≤ 10^5) that shows how many numbers are in Alex's sequence.
The second line contains n integers a_1, a_2, ..., a_{n} (1 ≤ a_{i} ≤ 10^5).
-----Output-----
Print a single integer — the maximum number of points that Alex can earn.
-----Examples-----
Input
2
1 2
Output
2
Input
3
1 2 3
Output
4
Input
9
1 2 1 3 2 2 2 2 3
Output
10
-----Note-----
Consider the third test example. At first step we need to choose any element equal to 2. After that step our sequence looks like this [2, 2, 2, 2]. Then we do 4 steps, on each step we choose any element equals to 2. In total we earn 10 points.
I tried it in Python, but could not do it. Can you solve it? | from collections import Counter
n = int(input())
sequence = list(map(int, input().split()))
numset = list(set(sequence))
k = len(numset)
numset.sort()
cnt = Counter(sequence)
f = [0] * (k + 1)
f[1] = cnt[numset[0]] * numset[0]
f[0] = 0
for i in range(2, k + 1):
if numset[i - 1] - numset[i - 2] == 1:
f[i] = max(f[i - 1], f[i - 2] + cnt[numset[i - 1]] * numset[i - 1])
else:
f[i] = numset[i - 1] * cnt[numset[i - 1]] + f[i - 1]
print(f[k])
'''
import timeit
s = """
k = list(set(a))
cnt = Counter(a)
print cnt
"""
setup = """
from random import randint
n = 100000
a = [randint(1,n) for i in range(n)]
from collections import Counter
"""
print(timeit.timeit(stmt=s, number=1, setup=setup))
''' | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Given is a string S of length N.
Find the maximum length of a non-empty string that occurs twice or more in S as contiguous substrings without overlapping.
More formally, find the maximum positive integer len such that there exist integers l_1 and l_2 ( 1 \leq l_1, l_2 \leq N - len + 1 ) that satisfy the following:
- l_1 + len \leq l_2
- S[l_1+i] = S[l_2+i] (i = 0, 1, ..., len - 1)
If there is no such integer len, print 0.
-----Constraints-----
- 2 \leq N \leq 5 \times 10^3
- |S| = N
- S consists of lowercase English letters.
-----Input-----
Input is given from Standard Input in the following format:
N
S
-----Output-----
Print the maximum length of a non-empty string that occurs twice or more in S as contiguous substrings without overlapping. If there is no such non-empty string, print 0 instead.
-----Sample Input-----
5
ababa
-----Sample Output-----
2
The strings satisfying the conditions are: a, b, ab, and ba. The maximum length among them is 2, which is the answer.
Note that aba occurs twice in S as contiguous substrings, but there is no pair of integers l_1 and l_2 mentioned in the statement such that l_1 + len \leq l_2. | import sys
#input = sys.stdin.buffer.readline
def main():
N = int(input())
s = input()
a,i,j = 0,0,1
while j < N:
if s[i:j] in s[j:]:
a = max(a,j-i)
j += 1
else:
i += 1
if i == j:
j += 1
print(a)
def __starting_point():
main()
__starting_point() | python | test | qsol | codeparrot/apps | all |
Solve in Python:
There is a farm whose length and width are A yard and B yard, respectively. A farmer, John, made a vertical road and a horizontal road inside the farm from one border to another, as shown below: (The gray part represents the roads.)
What is the area of this yard excluding the roads? Find it.
-----Note-----
It can be proved that the positions of the roads do not affect the area.
-----Constraints-----
- A is an integer between 2 and 100 (inclusive).
- B is an integer between 2 and 100 (inclusive).
-----Input-----
Input is given from Standard Input in the following format:
A B
-----Output-----
Print the area of this yard excluding the roads (in square yards).
-----Sample Input-----
2 2
-----Sample Output-----
1
In this case, the area is 1 square yard. | a,b= map(int,input().split())
print((a-1)*(b-1)) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1417/C:
You are given an array $a$ consisting of $n$ integers numbered from $1$ to $n$.
Let's define the $k$-amazing number of the array as the minimum number that occurs in all of the subsegments of the array having length $k$ (recall that a subsegment of $a$ of length $k$ is a contiguous part of $a$ containing exactly $k$ elements). If there is no integer occuring in all subsegments of length $k$ for some value of $k$, then the $k$-amazing number is $-1$.
For each $k$ from $1$ to $n$ calculate the $k$-amazing number of the array $a$.
-----Input-----
The first line contains one integer $t$ ($1 \le t \le 1000$) — the number of test cases. Then $t$ test cases follow.
The first line of each test case contains one integer $n$ ($1 \le n \le 3 \cdot 10^5$) — the number of elements in the array. The second line contains $n$ integers $a_1, a_2, \dots, a_n$ ($1 \le a_i \le n$) — the elements of the array.
It is guaranteed that the sum of $n$ over all test cases does not exceed $3 \cdot 10^5$.
-----Output-----
For each test case print $n$ integers, where the $i$-th integer is equal to the $i$-amazing number of the array.
-----Example-----
Input
3
5
1 2 3 4 5
5
4 4 4 4 2
6
1 3 1 5 3 1
Output
-1 -1 3 2 1
-1 4 4 4 2
-1 -1 1 1 1 1
I tried it in Python, but could not do it. Can you solve it? | import sys
def main():
#n = iinput()
#k = iinput()
#m = iinput()
n = int(sys.stdin.readline().strip())
#n, k = rinput()
#n, m = rinput()
#m, k = rinput()
#n, k, m = rinput()
#n, m, k = rinput()
#k, n, m = rinput()
#k, m, n = rinput()
#m, k, n = rinput()
#m, n, k = rinput()
q = list(map(int, sys.stdin.readline().split()))
#q = linput()
clovar, p, x = {}, [], 1e9
for i in range(n):
if q[i] in clovar:
clovar[q[i]].append(i)
else:
clovar[q[i]] = [i]
for o in clovar:
t = clovar[o]
ma = max(t[0] + 1, n - t[-1])
dlinat = len(t) - 1
for i in range(dlinat):
ma = max(t[i + 1] - t[i], ma)
p.append([ma, o])
p.sort()
ans = [p[0]]
dlinap = len(p)
for i in range(1, dlinap):
if ans[-1][0] != p[i][0]:
ans.append(p[i])
ans.append([n + 1, 1e9])
dlina_1 = ans[0][0] - 1
print(*[-1 for i in range(dlina_1)], end=" ")
dlinaans = len(ans) - 1
for i in range(dlinaans):
x = min(x, ans[i][1])
dlinax = ans[i + 1][0] - ans[i][0]
print(*[x for o in range(dlinax)], end=" ")
print()
for i in range(int(sys.stdin.readline().strip()) ):
main() | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/problems/POTATOES:
Farmer Feb has three fields with potatoes planted in them. He harvested x potatoes from the first field, y potatoes from the second field and is yet to harvest potatoes from the third field. Feb is very superstitious and believes that if the sum of potatoes he harvests from the three fields is a prime number (http://en.wikipedia.org/wiki/Prime_number), he'll make a huge profit. Please help him by calculating for him the minimum number of potatoes that if harvested from the third field will make the sum of potatoes prime. At least one potato should be harvested from the third field.
-----Input-----
The first line of the input contains an integer T denoting the number of test cases. Each of the next T lines contain 2 integers separated by single space: x and y.
-----Output-----
For each test case, output a single line containing the answer.
-----Constraints-----
- 1 ≤ T ≤ 1000
- 1 ≤ x ≤ 1000
- 1 ≤ y ≤ 1000
-----Example-----
Input:
2
1 3
4 3
Output:
1
4
-----Explanation-----
In example case 1: the farmer harvested a potato from the first field and 3 potatoes from the second field. The sum is 4. If he is able to harvest a potato from the third field, that will make the sum 5, which is prime. Hence the answer is 1(he needs one more potato to make the sum of harvested potatoes prime.)
I tried it in Python, but could not do it. Can you solve it? | def factors(n):
c=0
for i in range(1,n+1):
if n%i==0:
c+=1
return c
t=int(input())
for _ in range(t):
z=1
x,y=map(int,input().split(" "))
k=x+y
while(True):
t=k+z
if factors(t)==2:
break
else:
z+=1
print(z)
t-=1 | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/sum-root-to-leaf-numbers/:
Given a binary tree containing digits from 0-9 only, each root-to-leaf path could represent a number.
An example is the root-to-leaf path 1->2->3 which represents the number 123.
Find the total sum of all root-to-leaf numbers.
Note: A leaf is a node with no children.
Example:
Input: [1,2,3]
1
/ \
2 3
Output: 25
Explanation:
The root-to-leaf path 1->2 represents the number 12.
The root-to-leaf path 1->3 represents the number 13.
Therefore, sum = 12 + 13 = 25.
Example 2:
Input: [4,9,0,5,1]
4
/ \
9 0
/ \
5 1
Output: 1026
Explanation:
The root-to-leaf path 4->9->5 represents the number 495.
The root-to-leaf path 4->9->1 represents the number 491.
The root-to-leaf path 4->0 represents the number 40.
Therefore, sum = 495 + 491 + 40 = 1026.
I tried it in Python, but could not do it. Can you solve it? | # Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
from copy import deepcopy
class Solution:
def sumNumbers(self, root):
"""
:type root: TreeNode
:rtype: int
"""
self.treeSum = 0
self.tree(root, [])
return self.treeSum
def tree(self, node, path):
if not node:
return
path.append(str(node.val))
self.tree(node.left, copy.deepcopy(path))
self.tree(node.right, copy.deepcopy(path))
if not node.left and not node.right:
self.treeSum += int(''.join(path))
'''
class Solution:
def sumNumbers(self, root):
self.res = 0
self.dfs(root, 0)
return self.res
def dfs(self, root, value):
if root:
self.dfs(root.left, value*10+root.val)
self.dfs(root.right, value*10+root.val)
if not root.left and not root.right:
self.res += value*10 + root.val
''' | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
My friend wants a new band name for her band. She like bands that use the formula: "The" + a noun with the first letter capitalized, for example:
`"dolphin" -> "The Dolphin"`
However, when a noun STARTS and ENDS with the same letter, she likes to repeat the noun twice and connect them together with the first and last letter, combined into one word (WITHOUT "The" in front), like this:
`"alaska" -> "Alaskalaska"`
Complete the function that takes a noun as a string, and returns her preferred band name written as a string. | def band_name_generator(name):
return name.capitalize()+name[1:] if name[0]==name[-1] else 'The '+ name.capitalize() | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/643/B:
Bearland has n cities, numbered 1 through n. Cities are connected via bidirectional roads. Each road connects two distinct cities. No two roads connect the same pair of cities.
Bear Limak was once in a city a and he wanted to go to a city b. There was no direct connection so he decided to take a long walk, visiting each city exactly once. Formally: There is no road between a and b. There exists a sequence (path) of n distinct cities v_1, v_2, ..., v_{n} that v_1 = a, v_{n} = b and there is a road between v_{i} and v_{i} + 1 for $i \in \{1,2, \ldots, n - 1 \}$.
On the other day, the similar thing happened. Limak wanted to travel between a city c and a city d. There is no road between them but there exists a sequence of n distinct cities u_1, u_2, ..., u_{n} that u_1 = c, u_{n} = d and there is a road between u_{i} and u_{i} + 1 for $i \in \{1,2, \ldots, n - 1 \}$.
Also, Limak thinks that there are at most k roads in Bearland. He wonders whether he remembers everything correctly.
Given n, k and four distinct cities a, b, c, d, can you find possible paths (v_1, ..., v_{n}) and (u_1, ..., u_{n}) to satisfy all the given conditions? Find any solution or print -1 if it's impossible.
-----Input-----
The first line of the input contains two integers n and k (4 ≤ n ≤ 1000, n - 1 ≤ k ≤ 2n - 2) — the number of cities and the maximum allowed number of roads, respectively.
The second line contains four distinct integers a, b, c and d (1 ≤ a, b, c, d ≤ n).
-----Output-----
Print -1 if it's impossible to satisfy all the given conditions. Otherwise, print two lines with paths descriptions. The first of these two lines should contain n distinct integers v_1, v_2, ..., v_{n} where v_1 = a and v_{n} = b. The second line should contain n distinct integers u_1, u_2, ..., u_{n} where u_1 = c and u_{n} = d.
Two paths generate at most 2n - 2 roads: (v_1, v_2), (v_2, v_3), ..., (v_{n} - 1, v_{n}), (u_1, u_2), (u_2, u_3), ..., (u_{n} - 1, u_{n}). Your answer will be considered wrong if contains more than k distinct...
I tried it in Python, but could not do it. Can you solve it? | n, k = list(map(int, input().split()))
a, b, c, d = list(map(int, input().split()))
if n == 4 or k < n + 1:
print(-1)
return
extra = 1
while extra == a or extra == b or extra == c or extra == d:
extra += 1
v = [a, c, extra, d]
k = extra + 1
while k <= n:
if k != a and k != b and k != c and k != d and k != extra:
v.append(k)
k += 1
v.append(b)
print(*v)
u = [c, a, extra] + list(reversed(v[3:]))
print(*u) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
There is a row of m houses in a small city, each house must be painted with one of the n colors (labeled from 1 to n), some houses that has been painted last summer should not be painted again.
A neighborhood is a maximal group of continuous houses that are painted with the same color. (For example: houses = [1,2,2,3,3,2,1,1] contains 5 neighborhoods [{1}, {2,2}, {3,3}, {2}, {1,1}]).
Given an array houses, an m * n matrix cost and an integer target where:
houses[i]: is the color of the house i, 0 if the house is not painted yet.
cost[i][j]: is the cost of paint the house i with the color j+1.
Return the minimum cost of painting all the remaining houses in such a way that there are exactly target neighborhoods, if not possible return -1.
Example 1:
Input: houses = [0,0,0,0,0], cost = [[1,10],[10,1],[10,1],[1,10],[5,1]], m = 5, n = 2, target = 3
Output: 9
Explanation: Paint houses of this way [1,2,2,1,1]
This array contains target = 3 neighborhoods, [{1}, {2,2}, {1,1}].
Cost of paint all houses (1 + 1 + 1 + 1 + 5) = 9.
Example 2:
Input: houses = [0,2,1,2,0], cost = [[1,10],[10,1],[10,1],[1,10],[5,1]], m = 5, n = 2, target = 3
Output: 11
Explanation: Some houses are already painted, Paint the houses of this way [2,2,1,2,2]
This array contains target = 3 neighborhoods, [{2,2}, {1}, {2,2}].
Cost of paint the first and last house (10 + 1) = 11.
Example 3:
Input: houses = [0,0,0,0,0], cost = [[1,10],[10,1],[1,10],[10,1],[1,10]], m = 5, n = 2, target = 5
Output: 5
Example 4:
Input: houses = [3,1,2,3], cost = [[1,1,1],[1,1,1],[1,1,1],[1,1,1]], m = 4, n = 3, target = 3
Output: -1
Explanation: Houses are already painted with a total of 4 neighborhoods [{3},{1},{2},{3}] different of target = 3.
Constraints:
m == houses.length == cost.length
n == cost[i].length
1 <= m <= 100
1 <= n <= 20
1 <= target <= m
0 <= houses[i] <= n
1 <= cost[i][j] <= 10^4 | from functools import lru_cache
class Solution:
def minCost(self, houses: List[int], cost: List[List[int]], m: int, n: int, target: int) -> int:
@lru_cache(None)
def dfs(i,prev,k):
if i>=m:
return 0 - int(k!=0)
if k<0:
return -1
if houses[i]!=0:
return dfs(i+1, houses[i], k - int(prev!=houses[i]))
else:
temp = float('inf')
for c in range(1,n+1):
c_cost = cost[i][c-1]
other = dfs(i+1,c, k-int(prev!=c))
if other>=0:
temp = min(temp, c_cost+other)
if temp == float('inf'):
return -1
return temp
return dfs(0,0,target) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/59476f9d7325addc860000b9:
Mr. Khalkhoul, an amazing teacher, likes to answer questions sent by his students via e-mail, but he often doesn't have the time to answer all of them. In this kata you will help him by making a program that finds
some of the answers.
You are given a `question` which is a string containing the question and some `information` which is a list of strings representing potential answers.
Your task is to find among `information` the UNIQUE string that has the highest number of words in common with `question`. We shall consider words to be separated by a single space.
You can assume that:
* all strings given contain at least one word and have no leading or trailing spaces,
* words are composed with alphanumeric characters only
You must also consider the case in which you get no matching words (again, ignoring the case): in such a case return `None/nil/null`.
Mr. Khalkhoul is countin' on you :)
I tried it in Python, but could not do it. Can you solve it? | def answer(question,information):
info=[ i.split(" ") for i in information]
ques=question.lower().split(" ")
high=0 ; iHigh=-1
for i in range(len(info)):
score=0
for j in info[i]:
if j.lower() in ques:
score+=1
if score>high:
iHigh=i
high=score
if iHigh==-1:return None
a="".join(j+" " for j in info[iHigh])[:-1]
return a | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/255/C:
Gena loves sequences of numbers. Recently, he has discovered a new type of sequences which he called an almost arithmetical progression. A sequence is an almost arithmetical progression, if its elements can be represented as: a_1 = p, where p is some integer; a_{i} = a_{i} - 1 + ( - 1)^{i} + 1·q (i > 1), where q is some integer.
Right now Gena has a piece of paper with sequence b, consisting of n integers. Help Gena, find there the longest subsequence of integers that is an almost arithmetical progression.
Sequence s_1, s_2, ..., s_{k} is a subsequence of sequence b_1, b_2, ..., b_{n}, if there is such increasing sequence of indexes i_1, i_2, ..., i_{k} (1 ≤ i_1 < i_2 < ... < i_{k} ≤ n), that b_{i}_{j} = s_{j}. In other words, sequence s can be obtained from b by crossing out some elements.
-----Input-----
The first line contains integer n (1 ≤ n ≤ 4000). The next line contains n integers b_1, b_2, ..., b_{n} (1 ≤ b_{i} ≤ 10^6).
-----Output-----
Print a single integer — the length of the required longest subsequence.
-----Examples-----
Input
2
3 5
Output
2
Input
4
10 20 10 30
Output
3
-----Note-----
In the first test the sequence actually is the suitable subsequence.
In the second test the following subsequence fits: 10, 20, 10.
I tried it in Python, but could not do it. Can you solve it? | import sys
from math import log2,floor,ceil,sqrt
# import bisect
# from collections import deque
Ri = lambda : [int(x) for x in sys.stdin.readline().split()]
ri = lambda : sys.stdin.readline().strip()
def input(): return sys.stdin.readline().strip()
def list2d(a, b, c): return [[c] * b for i in range(a)]
def list3d(a, b, c, d): return [[[d] * c for j in range(b)] for i in range(a)]
def list4d(a, b, c, d, e): return [[[[e] * d for j in range(c)] for j in range(b)] for i in range(a)]
def ceil(x, y=1): return int(-(-x // y))
def INT(): return int(input())
def MAP(): return list(map(int, input().split()))
def LIST(N=None): return list(MAP()) if N is None else [INT() for i in range(N)]
def Yes(): print('Yes')
def No(): print('No')
def YES(): print('YES')
def NO(): print('NO')
INF = 10 ** 18
MOD = 10**9+7
n = int(ri())
arr = Ri()
a = sorted(arr)
dic = {}
ite = 1
for i in range(n):
if a[i] not in dic:
dic[a[i]] = ite
ite+=1
for i in range(n):
arr[i] = dic[arr[i]]
dp = list2d(n,n+1,0)
for i in range(n):
for j in range(n+1):
dp[i][j] = 1
maxx = 1
for i in range(1,n):
for j in range(i-1,-1,-1):
dp[i][arr[j]] = max(dp[i][arr[j]], dp[j][arr[i]]+1)
maxx = max(maxx,dp[i][arr[j]])
print(maxx) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Pied Piper is a startup company trying to build a new Internet called Pipernet. Currently, they have $A$ users and they gain $X$ users everyday. There is also another company called Hooli, which has currently $B$ users and gains $Y$ users everyday.
Whichever company reaches $Z$ users first takes over Pipernet. In case both companies reach $Z$ users on the same day, Hooli takes over.
Hooli is a very evil company (like E-Corp in Mr. Robot or Innovative Online Industries in Ready Player One). Therefore, many people are trying to help Pied Piper gain some users.
Pied Piper has $N$ supporters with contribution values $C_1, C_2, \ldots, C_N$. For each valid $i$, when the $i$-th supporter contributes, Pied Piper gains $C_i$ users instantly. After contributing, the contribution value of the supporter is halved, i.e. $C_i$ changes to $\left\lfloor C_i / 2 \right\rfloor$. Each supporter may contribute any number of times, including zero. Supporters may contribute at any time until one of the companies takes over Pipernet, even during the current day.
Find the minimum number of times supporters must contribute (the minimum total number of contributions) so that Pied Piper gains control of Pipernet.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains six space-separated integers $N$, $A$, $B$, $X$, $Y$ and $Z$.
- The second line contains $N$ space-separated integers $C_1, C_2, \ldots, C_N$ — the initial contribution values.
-----Output-----
For each test case, if Hooli will always gain control of Pipernet, print a single line containing the string "RIP" (without quotes). Otherwise, print a single line containing one integer — the minimum number of times supporters must contribute.
-----Constraints-----
- $1 \le T \le 10$
- $1 \le N \le 10^5$
- $1 \le A, B, X, Y, Z \le 10^9$
- $A, B < Z$
- $0 \le C_i \le 10^9$ for each valid $i$
-----Example Input-----
3
3 10 15 5 10 100
12... | # cook your dish here
import heapq as hq
from math import floor
for _ in range(int(input())):
n,a,b,x,y,z=list(map(int,input().split()))
arr=[-int(i) for i in input().split()]
days=floor((z-b)/y)
if b+y*days==z:
z+=1
ans=0
hq.heapify(arr)
curr=a+days*x
while curr<z :
u=hq.heappop(arr)
u=-u
if u==0 :
break
else:
curr+=u
ans+=1
hq.heappush(arr,-(u//2))
if curr>=z:
print(ans)
else:
print("RIP") | python | train | qsol | codeparrot/apps | all |
Solve in Python:
You have a multiset containing several integers. Initially, it contains $a_1$ elements equal to $1$, $a_2$ elements equal to $2$, ..., $a_n$ elements equal to $n$.
You may apply two types of operations: choose two integers $l$ and $r$ ($l \le r$), then remove one occurrence of $l$, one occurrence of $l + 1$, ..., one occurrence of $r$ from the multiset. This operation can be applied only if each number from $l$ to $r$ occurs at least once in the multiset; choose two integers $i$ and $x$ ($x \ge 1$), then remove $x$ occurrences of $i$ from the multiset. This operation can be applied only if the multiset contains at least $x$ occurrences of $i$.
What is the minimum number of operations required to delete all elements from the multiset?
-----Input-----
The first line contains one integer $n$ ($1 \le n \le 5000$).
The second line contains $n$ integers $a_1$, $a_2$, ..., $a_n$ ($0 \le a_i \le 10^9$).
-----Output-----
Print one integer — the minimum number of operations required to delete all elements from the multiset.
-----Examples-----
Input
4
1 4 1 1
Output
2
Input
5
1 0 1 0 1
Output
3 | n = int(input()) + 1
l = list(map(int, input().split())) + [0]
out = 0
q = []
for v in l:
if v == 0:
dp = []
n = len(q)
for i in range(n):
curr = q[i] + i
smol = q[i]
for j in range(i - 1, -1, -1):
smol = min(q[j], smol)
diff = q[i] - smol
curr = min(curr, diff + dp[j] + i - j - 1)
dp.append(curr)
real = [n - i + dp[i] - 1 for i in range(n)] + [n]
out += min(real)
q = []
else:
q.append(v)
print(out) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/799/C:
Arkady plays Gardenscapes a lot. Arkady wants to build two new fountains. There are n available fountains, for each fountain its beauty and cost are known. There are two types of money in the game: coins and diamonds, so each fountain cost can be either in coins or diamonds. No money changes between the types are allowed.
Help Arkady to find two fountains with maximum total beauty so that he can buy both at the same time.
-----Input-----
The first line contains three integers n, c and d (2 ≤ n ≤ 100 000, 0 ≤ c, d ≤ 100 000) — the number of fountains, the number of coins and diamonds Arkady has.
The next n lines describe fountains. Each of these lines contain two integers b_{i} and p_{i} (1 ≤ b_{i}, p_{i} ≤ 100 000) — the beauty and the cost of the i-th fountain, and then a letter "C" or "D", describing in which type of money is the cost of fountain i: in coins or in diamonds, respectively.
-----Output-----
Print the maximum total beauty of exactly two fountains Arkady can build. If he can't build two fountains, print 0.
-----Examples-----
Input
3 7 6
10 8 C
4 3 C
5 6 D
Output
9
Input
2 4 5
2 5 C
2 1 D
Output
0
Input
3 10 10
5 5 C
5 5 C
10 11 D
Output
10
-----Note-----
In the first example Arkady should build the second fountain with beauty 4, which costs 3 coins. The first fountain he can't build because he don't have enough coins. Also Arkady should build the third fountain with beauty 5 which costs 6 diamonds. Thus the total beauty of built fountains is 9.
In the second example there are two fountains, but Arkady can't build both of them, because he needs 5 coins for the first fountain, and Arkady has only 4 coins.
I tried it in Python, but could not do it. Can you solve it? | from operator import itemgetter
def get_one_max(arr, resource):
res = 0
for beauty, _ in [x for x in arr if x[1] <= resource]:
res = max(res, beauty)
return res
def get_two_max(arr, resource):
arr.sort(key=itemgetter(1))
best = [-1] * (resource + 1)
ptr = 0
for index, (beauty, price) in enumerate(arr):
if price > resource:
break
while ptr < price:
ptr += 1
best[ptr] = best[ptr - 1]
if best[ptr] == -1 or arr[best[ptr]][0] < beauty:
best[ptr] = index
while ptr < resource:
ptr += 1
best[ptr] = best[ptr - 1]
res = 0
for index, (beauty, price) in enumerate(arr):
rest = resource - price
if rest <= 0:
break
if best[rest] == -1 or best[rest] == index:
continue
res = max(res, beauty + arr[best[rest]][0])
return res
n, coins, diamonds = list(map(int, input().split()))
with_coins = []
with_diamonds = []
for i in range(n):
beauty, price, tp = input().split()
if tp == 'C':
with_coins.append((int(beauty), int(price)))
else:
with_diamonds.append((int(beauty), int(price)))
with_coins_max = get_one_max(with_coins, coins)
with_diamonds_max = get_one_max(with_diamonds, diamonds)
ans = 0 if with_coins_max == 0 or with_diamonds_max == 0 \
else with_coins_max + with_diamonds_max
ans = max(ans, get_two_max(with_coins, coins))
ans = max(ans, get_two_max(with_diamonds, diamonds))
print(ans) | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5736378e3f3dfd5a820000cb:
It's been a tough week at work and you are stuggling to get out of bed in the morning.
While waiting at the bus stop you realise that if you could time your arrival to the nearest minute you could get valuable extra minutes in bed.
There is a bus that goes to your office every 15 minute, the first bus is at `06:00`, and the last bus is at `00:00`.
Given that it takes 5 minutes to walk from your front door to the bus stop, implement a function that when given the curent time will tell you much time is left, before you must leave to catch the next bus.
## Examples
```
"05:00" => 55
"10:00" => 10
"12:10" => 0
"12:11" => 14
```
### Notes
1. Return the number of minutes till the next bus
2. Input will be formatted as `HH:MM` (24-hour clock)
3. The input time might be after the buses have stopped running, i.e. after `00:00`
I tried it in Python, but could not do it. Can you solve it? | def bus_timer(time):
c = time.split(":")
z = int(c[1]) + 5
t = 0
if int(c[0]) == 23 and int(c[1]) >= 55:
c[0] = 0
c[1] = (int(c[1]) + 5) - 60
if int(c[1]) == 0:
t = 0
elif int(c[1]) > 0:
t = (((5 - int(c[0]))*60) + (60 - int(c[1])))
elif int(c[0]) == 5 and int(c[1]) >= 55:
c[0] = 6
c[1] = (int(c[1]) + 5) - 60
if int(c[1]) == 0:
t = 0
elif int(c[1]) > 0:
t = 15 - int(c[1])
elif int(c[0]) < 6 :
if int(c[1]) == 0:
t = ((6 - int(c[0]))*60) - 5
elif int(c[1]) > 0:
t = (((5 - int(c[0]))*60) + (60 - int(c[1]))) - 5
else:
if z > 60:
z = z - 60
if z < 15:
t = 15 - z
elif z > 15 and z < 30:
t = 30 - z
elif z > 30 and z < 45:
t = 45 - z
elif z > 45:
t = 60 - z
return t | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Lеt's create function to play cards. Our rules:
We have the preloaded `deck`:
```
deck = ['joker','2♣','3♣','4♣','5♣','6♣','7♣','8♣','9♣','10♣','J♣','Q♣','K♣','A♣',
'2♦','3♦','4♦','5♦','6♦','7♦','8♦','9♦','10♦','J♦','Q♦','K♦','A♦',
'2♥','3♥','4♥','5♥','6♥','7♥','8♥','9♥','10♥','J♥','Q♥','K♥','A♥',
'2♠','3♠','4♠','5♠','6♠','7♠','8♠','9♠','10♠','J♠','Q♠','K♠','A♠']
```
We have 3 arguments:
`card1` and `card2` - any card of our deck.
`trump` - the main suit of four ('♣', '♦', '♥', '♠').
If both cards have the same suit, the big one wins.
If the cards have different suits (and no one has trump) return 'Let's play again.'
If one card has `trump` unlike another, wins the first one.
If both cards have `trump`, the big one wins.
If `card1` wins, return 'The first card won.' and vice versa.
If the cards are equal, return 'Someone cheats.'
A few games:
```
('3♣', 'Q♣', '♦') -> 'The second card won.'
('5♥', 'A♣', '♦') -> 'Let us play again.'
('8♠', '8♠', '♣') -> 'Someone cheats.'
('2♦', 'A♠', '♦') -> 'The first card won.'
('joker', 'joker', '♦') -> 'Someone cheats.'
```
P.S. As a card you can also get the string 'joker' - it means this card always wins. | deck = ['joker','2♣','3♣','4♣','5♣','6♣','7♣','8♣','9♣','10♣','J♣','Q♣','K♣','A♣',
'2♦','3♦','4♦','5♦','6♦','7♦','8♦','9♦','10♦','J♦','Q♦','K♦','A♦',
'2♥','3♥','4♥','5♥','6♥','7♥','8♥','9♥','10♥','J♥','Q♥','K♥','A♥',
'2♠','3♠','4♠','5♠','6♠','7♠','8♠','9♠','10♠','J♠','Q♠','K♠','A♠']
def card_game(card_1, card_2, trump):
if card_1 == card_2:
return "Someone cheats."
ordinal, trumps = ["first", "second"], f"{card_1}{card_2}".count(trump)
if "joker" in (card_1, card_2):
winner = ordinal[card_2 == "joker"]
elif trumps == 1:
winner = ordinal[trump in card_2]
elif card_1[-1] == card_2[-1]:
winner = ordinal[deck.index(card_2) > deck.index(card_1)]
elif trumps == 0:
return "Let us play again."
return f"The {winner} card won." | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/272/D:
Little Dima has two sequences of points with integer coordinates: sequence (a_1, 1), (a_2, 2), ..., (a_{n}, n) and sequence (b_1, 1), (b_2, 2), ..., (b_{n}, n).
Now Dima wants to count the number of distinct sequences of points of length 2·n that can be assembled from these sequences, such that the x-coordinates of points in the assembled sequence will not decrease. Help him with that. Note that each element of the initial sequences should be used exactly once in the assembled sequence.
Dima considers two assembled sequences (p_1, q_1), (p_2, q_2), ..., (p_{2·}n, q_{2·}n) and (x_1, y_1), (x_2, y_2), ..., (x_{2·}n, y_{2·}n) distinct, if there is such i (1 ≤ i ≤ 2·n), that (p_{i}, q_{i}) ≠ (x_{i}, y_{i}).
As the answer can be rather large, print the remainder from dividing the answer by number m.
-----Input-----
The first line contains integer n (1 ≤ n ≤ 10^5). The second line contains n integers a_1, a_2, ..., a_{n} (1 ≤ a_{i} ≤ 10^9). The third line contains n integers b_1, b_2, ..., b_{n} (1 ≤ b_{i} ≤ 10^9). The numbers in the lines are separated by spaces.
The last line contains integer m (2 ≤ m ≤ 10^9 + 7).
-----Output-----
In the single line print the remainder after dividing the answer to the problem by number m.
-----Examples-----
Input
1
1
2
7
Output
1
Input
2
1 2
2 3
11
Output
2
-----Note-----
In the first sample you can get only one sequence: (1, 1), (2, 1).
In the second sample you can get such sequences : (1, 1), (2, 2), (2, 1), (3, 2); (1, 1), (2, 1), (2, 2), (3, 2). Thus, the answer is 2.
I tried it in Python, but could not do it. Can you solve it? | from math import sqrt,ceil,gcd
from collections import defaultdict
def modInverse(b,m):
g = gcd(b, m)
if (g != 1):
# print("Inverse doesn't exist")
return -1
else:
# If b and m are relatively prime,
# then modulo inverse is b^(m-2) mode m
return pow(b, m - 2, m)
def sol(n,m,rep):
r = 1
for i in range(2,n+1):
j = i
while j%2 == 0 and rep>0:
j//=2
rep-=1
r*=j
r%=m
return r
def solve():
n = int(input())
a = list(map(int,input().split()))
b = list(map(int,input().split()))
m = int(input())
hash = defaultdict(int)
e = defaultdict(int)
for i in range(n):
hash[a[i]]+=1
hash[b[i]]+=1
if a[i] == b[i]:
e[a[i]]+=1
ans = 1
for i in hash:
z1 = hash[i]
z2 = e[i]
ans*=sol(z1,m,z2)
ans%=m
print(ans)
# t = int(input())
# for _ in range(t):
solve() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
The chef is trying to solve some pattern problems, Chef wants your help to code it. Chef has one number K to form a new pattern. Help the chef to code this pattern problem.
-----Input:-----
- First-line will contain $T$, the number of test cases. Then the test cases follow.
- Each test case contains a single line of input, one integer $K$.
-----Output:-----
For each test case, output as the pattern.
-----Constraints-----
- $1 \leq T \leq 100$
- $1 \leq K \leq 100$
-----Sample Input:-----
3
2
3
4
-----Sample Output:-----
1121
1222
112131
122232
132333
11213141
12223242
13233343
14243444
-----EXPLANATION:-----
No need, else pattern can be decode easily. | # cook your dish here
t=int(input(""))
while(t!=0):
s=''
k=int(input(""))
#print(k)
for i in range(k):
for j in range(k):
s=s+str(j+1)+str(i+1)
print(s)
s=''
t-=1 | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Takahashi loves takoyaki - a ball-shaped snack.
With a takoyaki machine, he can make at most X pieces of takoyaki at a time, taking T minutes regardless of the number of pieces to make.
How long does it take to make N takoyaki?
-----Constraints-----
- 1 \leq N,X,T \leq 1000
- All values in input are integers.
-----Input-----
Input is given from Standard Input in the following format:
N X T
-----Output-----
Print an integer representing the minimum number of minutes needed to make N pieces of takoyaki.
-----Sample Input-----
20 12 6
-----Sample Output-----
12
He can make 12 pieces of takoyaki in the first 6 minutes and 8 more in the next 6 minutes, so he can make 20 in a total of 12 minutes.
Note that being able to make 12 in 6 minutes does not mean he can make 2 in 1 minute. | a=list(map(int,input().split()))
if a[0]%a[1]==0:
b=(a[0]//a[1])
else:
b=(a[0]//a[1])+1
c=b*a[2]
print(c) | python | test | qsol | codeparrot/apps | all |
Solve in Python:
You are given an empty grid with $N$ rows (numbered $1$ through $N$) and $M$ columns (numbered $1$ through $M$). You should fill this grid with integers in a way that satisfies the following rules:
- For any three cells $c_1$, $c_2$ and $c_3$ such that $c_1$ shares a side with $c_2$ and another side with $c_3$, the integers written in cells $c_2$ and $c_3$ are distinct.
- Let's denote the number of different integers in the grid by $K$; then, each of these integers should lie between $1$ and $K$ inclusive.
- $K$ should be minimum possible.
Find the minimum value of $K$ and a resulting (filled) grid. If there are multiple solutions, you may find any one.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains two space-separated integers $N$ and $M$.
-----Output-----
- For each test case, print $N+1$ lines.
- The first line should contain a single integer — the minimum $K$.
- Each of the following $N$ lines should contain $M$ space-separated integers between $1$ and $K$ inclusive. For each valid $i, j$, the $j$-th integer on the $i$-th line should denote the number in the $i$-th row and $j$-th column of the grid.
-----Constraints-----
- $1 \le T \le 500$
- $1 \le N, M \le 50$
- the sum of $N \cdot M$ over all test cases does not exceed $7 \cdot 10^5$
-----Subtasks-----
Subtask #1 (100 points): original constraints
-----Example Input-----
2
1 1
2 3
-----Example Output-----
1
1
3
1 1 2
2 3 3
-----Explanation-----
Example case 1: There is only one cell in the grid, so the only valid way to fill it is to write $1$ in this cell. Note that we cannot use any other integer than $1$.
Example case 2: For example, the integers written in the neighbours of cell $(2, 2)$ are $1$, $2$ and $3$; all these numbers are pairwise distinct and the integer written inside the cell $(2, 2)$ does not matter. Note that there are pairs of neighbouring cells with the same integer... | for _ in range(int(input())):
n, m = list(map(int, input().split()))
ans = [[0 for i in range(m)] for i in range(n)]
k = 0
if n == 1:
for i in range(m):
if i%4 == 0 or i%4 == 1:
t = 1
else:
t = 2
ans[0][i] = t
k = max(k, ans[0][i])
elif m == 1:
for i in range(n):
if i%4 == 0 or i%4 == 1:
t = 1
else:
t = 2
ans[i][0] = t
k = max(k, ans[i][0])
elif n == 2:
t = 1
for i in range(m):
ans[0][i] = t
ans[1][i] = t
t = (t)%3 + 1
k = max(k, ans[0][i])
elif m == 2:
t = 1
for i in range(n):
ans[i][0] = t
ans[i][1] = t
t = t%3 + 1
k = max(k, ans[i][0])
else:
for i in range(n):
if i%4 == 0 or i%4 == 1:
t = 0
else:
t = 2
for j in range(m):
ans[i][j] = (t%4) + 1
t += 1
k = max(k, ans[i][j])
print(k)
for i in range(n):
print(*ans[i]) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/57aaaada72292d3b8f0001b4:
This the second part part of the kata:
https://www.codewars.com/kata/the-sum-and-the-rest-of-certain-pairs-of-numbers-have-to-be-perfect-squares
In this part we will have to create a faster algorithm because the tests will be more challenging.
The function ```n_closestPairs_tonum()```, (Javascript: ```nClosestPairsToNum()```), will accept two arguments, ```upper_limit``` and ```k```.
The function should return the ```k``` largest pair of numbers [m, n] and the closest to ```upper_limit```. Expressing it in code:
```python
n_closestPairs_tonum(upper_limit, k) #-> [[m1, n1], [m2, n2], ...., [mk, nk]]
```
Such that:
```
upper_limit >= m1 >= m2 >= ....>= mk > 0
```
Examples:
```python
upper_limit = 1000; k = 4
n_closestPairs_tonum(upper_limit, k) == [[997, 372], [986, 950], [986, 310], [985, 864]]
upper_limit = 10000; k = 8
n_closestPairs_tonum(upper_limit, k) == [[9997, 8772], [9997, 2772], [9992, 6392], [9986, 5890], [9985, 7176], [9985, 4656], [9981, 9900], [9973, 9348]]
```
Features of the tests:
```
1000 ≤ upper_limit ≤ 200000
2 ≤ k ≤ 20
```
Enjoy it!
I tried it in Python, but could not do it. Can you solve it? | def n_closestPairs_tonum(num, k):
r=[]
m=2
while(m*m<num):
for n in range(1,m):
if (m*m+n*n>=num):
break
r.append([m*m+n*n,2*m*n])
m+=1
return sorted(r,reverse=True)[:k] | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5888145122fe8620950000f0:
# Task
`N` candles are placed in a row, some of them are initially lit. For each candle from the 1st to the Nth the following algorithm is applied: if the observed candle is lit then states of this candle and all candles before it are changed to the opposite. Which candles will remain lit after applying the algorithm to all candles in the order they are placed in the line?
# Example
For `a = [1, 1, 1, 1, 1]`, the output should be `[0, 1, 0, 1, 0].`
Check out the image below for better understanding:
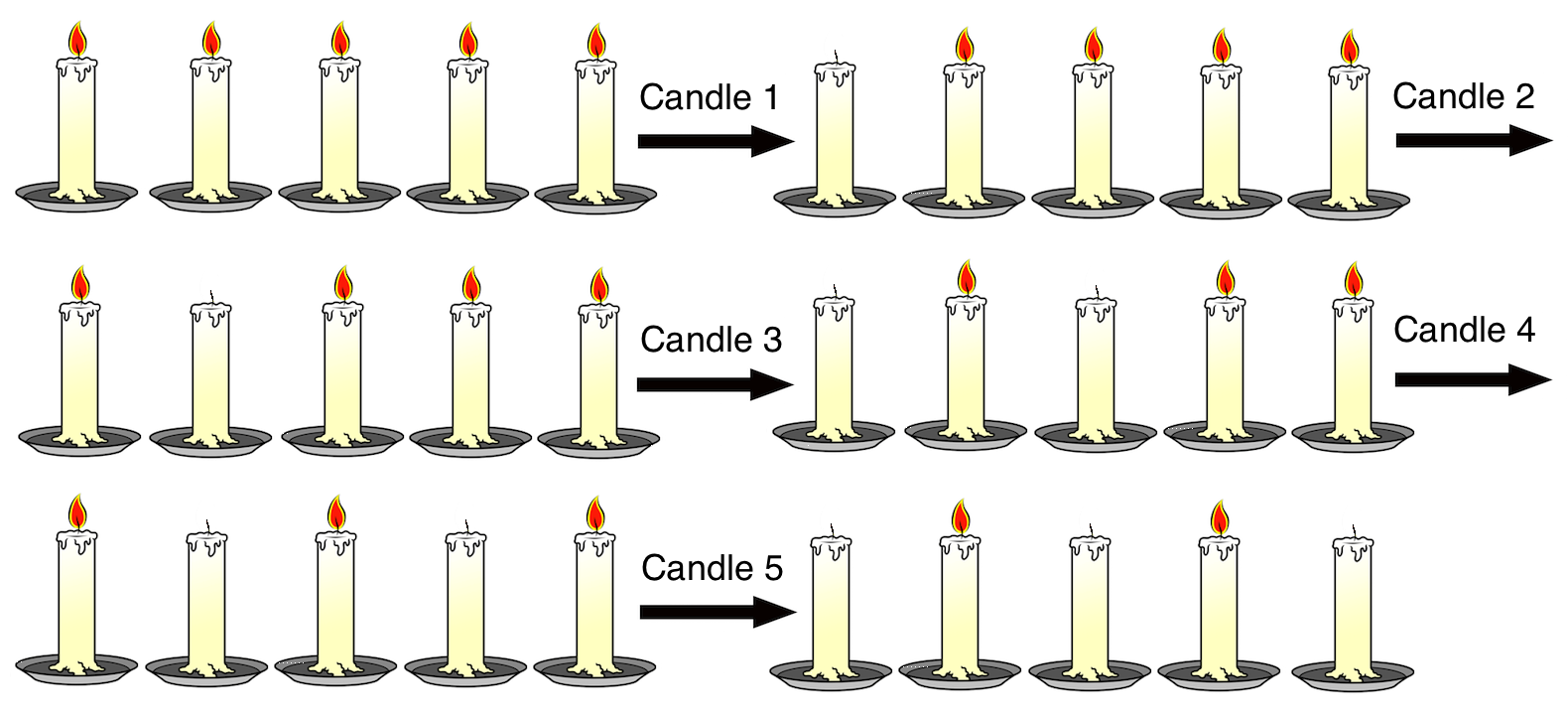
For `a = [0, 0]`, the output should be `[0, 0].`
The candles are not initially lit, so their states are not altered by the algorithm.
# Input/Output
- `[input]` integer array `a`
Initial situation - array of zeros and ones of length N, 1 means that the corresponding candle is lit.
Constraints: `2 ≤ a.length ≤ 5000.`
- `[output]` an integer array
Situation after applying the algorithm - array in the same format as input with the same length.
I tried it in Python, but could not do it. Can you solve it? | def switch_lights(initial_states):
states = list(initial_states)
parity = 0
for i in reversed(range(len(states))):
parity ^= initial_states[i]
states[i] ^= parity
return states | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
Santa Claus has Robot which lives on the infinite grid and can move along its lines. He can also, having a sequence of m points p_1, p_2, ..., p_{m} with integer coordinates, do the following: denote its initial location by p_0. First, the robot will move from p_0 to p_1 along one of the shortest paths between them (please notice that since the robot moves only along the grid lines, there can be several shortest paths). Then, after it reaches p_1, it'll move to p_2, again, choosing one of the shortest ways, then to p_3, and so on, until he has visited all points in the given order. Some of the points in the sequence may coincide, in that case Robot will visit that point several times according to the sequence order.
While Santa was away, someone gave a sequence of points to Robot. This sequence is now lost, but Robot saved the protocol of its unit movements. Please, find the minimum possible length of the sequence.
-----Input-----
The first line of input contains the only positive integer n (1 ≤ n ≤ 2·10^5) which equals the number of unit segments the robot traveled. The second line contains the movements protocol, which consists of n letters, each being equal either L, or R, or U, or D. k-th letter stands for the direction which Robot traveled the k-th unit segment in: L means that it moved to the left, R — to the right, U — to the top and D — to the bottom. Have a look at the illustrations for better explanation.
-----Output-----
The only line of input should contain the minimum possible length of the sequence.
-----Examples-----
Input
4
RURD
Output
2
Input
6
RRULDD
Output
2
Input
26
RRRULURURUULULLLDLDDRDRDLD
Output
7
Input
3
RLL
Output
2
Input
4
LRLR
Output
4
-----Note-----
The illustrations to the first three tests are given below.
[Image] [Image] [Image]
The last example illustrates that each point in the sequence should be counted as many times as it is presented in the sequence. | n = int(input())
a = input()
s = set()
ans = 0
for j in range(n):
if a[j] == 'R':
if 'L' in s:
ans += 1
s = set()
s.add(a[j])
elif a[j] == 'L':
if 'R' in s:
ans += 1
s = set()
s.add(a[j])
elif a[j] == 'U':
if 'D' in s:
ans += 1
s = set()
s.add(a[j])
elif a[j] == 'D':
if 'U' in s:
ans += 1
s = set()
s.add(a[j])
print(ans+1) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/727/D:
The organizers of a programming contest have decided to present t-shirts to participants. There are six different t-shirts sizes in this problem: S, M, L, XL, XXL, XXXL (sizes are listed in increasing order). The t-shirts are already prepared. For each size from S to XXXL you are given the number of t-shirts of this size.
During the registration, the organizers asked each of the n participants about the t-shirt size he wants. If a participant hesitated between two sizes, he could specify two neighboring sizes — this means that any of these two sizes suits him.
Write a program that will determine whether it is possible to present a t-shirt to each participant of the competition, or not. Of course, each participant should get a t-shirt of proper size: the size he wanted, if he specified one size; any of the two neibouring sizes, if he specified two sizes.
If it is possible, the program should find any valid distribution of the t-shirts.
-----Input-----
The first line of the input contains six non-negative integers — the number of t-shirts of each size. The numbers are given for the sizes S, M, L, XL, XXL, XXXL, respectively. The total number of t-shirts doesn't exceed 100 000.
The second line contains positive integer n (1 ≤ n ≤ 100 000) — the number of participants.
The following n lines contain the sizes specified by the participants, one line per participant. The i-th line contains information provided by the i-th participant: single size or two sizes separated by comma (without any spaces). If there are two sizes, the sizes are written in increasing order. It is guaranteed that two sizes separated by comma are neighboring.
-----Output-----
If it is not possible to present a t-shirt to each participant, print «NO» (without quotes).
Otherwise, print n + 1 lines. In the first line print «YES» (without quotes). In the following n lines print the t-shirt sizes the orginizers should give to participants, one per line. The order of the participants should be the same as in the input.
If there are...
I tried it in Python, but could not do it. Can you solve it? | shorts = [int(t) for t in input().split()]
n = int(input())
d = dict()
d["S"] = 0
d["M"] = 1
d["L"] = 2
d["XL"] = 3
d["XXL"] = 4
d["XXXL"] = 5
people = []
order = [t for t in range(n)]
for _ in range(n):
people.append(input().split(","))
order.sort(key=lambda x: [d[people[x][0]], len(people[x])])
ans = dict()
for i in order:
p = people[i]
if shorts[d[p[0]]] != 0:
ans[i] = p[0]
shorts[d[p[0]]] -= 1
elif len(p) == 2 and shorts[d[p[1]]] != 0:
ans[i] = p[1]
shorts[d[p[1]]] -= 1
else:
print("NO")
return
print("YES")
for i in ans.keys():
print(ans[i]) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc079/tasks/abc079_a:
We call a 4-digit integer with three or more consecutive same digits, such as 1118, good.
You are given a 4-digit integer N. Answer the question: Is N good?
-----Constraints-----
- 1000 ≤ N ≤ 9999
- N is an integer.
-----Input-----
Input is given from Standard Input in the following format:
N
-----Output-----
If N is good, print Yes; otherwise, print No.
-----Sample Input-----
1118
-----Sample Output-----
Yes
N is good, since it contains three consecutive 1.
I tried it in Python, but could not do it. Can you solve it? | n = input()
arr = []
for i in range(10):
s = ""
for j in range(3):
s += str(i)
arr.append(s)
if n[0:3] in arr or n[1:4] in arr:
print("Yes")
else:
print("No") | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/412/A:
The R1 company has recently bought a high rise building in the centre of Moscow for its main office. It's time to decorate the new office, and the first thing to do is to write the company's slogan above the main entrance to the building.
The slogan of the company consists of n characters, so the decorators hung a large banner, n meters wide and 1 meter high, divided into n equal squares. The first character of the slogan must be in the first square (the leftmost) of the poster, the second character must be in the second square, and so on.
Of course, the R1 programmers want to write the slogan on the poster themselves. To do this, they have a large (and a very heavy) ladder which was put exactly opposite the k-th square of the poster. To draw the i-th character of the slogan on the poster, you need to climb the ladder, standing in front of the i-th square of the poster. This action (along with climbing up and down the ladder) takes one hour for a painter. The painter is not allowed to draw characters in the adjacent squares when the ladder is in front of the i-th square because the uncomfortable position of the ladder may make the characters untidy. Besides, the programmers can move the ladder. In one hour, they can move the ladder either a meter to the right or a meter to the left.
Drawing characters and moving the ladder is very tiring, so the programmers want to finish the job in as little time as possible. Develop for them an optimal poster painting plan!
-----Input-----
The first line contains two integers, n and k (1 ≤ k ≤ n ≤ 100) — the number of characters in the slogan and the initial position of the ladder, correspondingly. The next line contains the slogan as n characters written without spaces. Each character of the slogan is either a large English letter, or digit, or one of the characters: '.', '!', ',', '?'.
-----Output-----
In t lines, print the actions the programmers need to make. In the i-th line print: "LEFT" (without the quotes), if the i-th action was "move the ladder to the...
I tried it in Python, but could not do it. Can you solve it? | def main():
n, k = map(int, input().split())
s = input()
if k - 1 <= n - k:
for i in range(k - 1):
print("LEFT")
for i in range(n - 1):
print("PRINT", s[i])
print("RIGHT")
print("PRINT", s[n - 1])
else:
for i in range(n - k):
print("RIGHT")
for i in range(n - 1, 0, -1):
print("PRINT", s[i])
print("LEFT")
print("PRINT", s[0])
main() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Create a function hollow_triangle(height) that returns a hollow triangle of the correct height. The height is passed through to the function and the function should return a list containing each line of the hollow triangle.
```
hollow_triangle(6) should return : ['_____#_____', '____#_#____', '___#___#___', '__#_____#__', '_#_______#_', '###########']
hollow_triangle(9) should return : ['________#________', '_______#_#_______', '______#___#______', '_____#_____#_____', '____#_______#____', '___#_________#___', '__#___________#__', '_#_____________#_', '#################']
```
The final idea is for the hollow triangle is to look like this if you decide to print each element of the list:
```
hollow_triangle(6) will result in:
_____#_____ 1
____#_#____ 2
___#___#___ 3
__#_____#__ 4
_#_______#_ 5
########### 6 ---- Final Height
hollow_triangle(9) will result in:
________#________ 1
_______#_#_______ 2
______#___#______ 3
_____#_____#_____ 4
____#_______#____ 5
___#_________#___ 6
__#___________#__ 7
_#_____________#_ 8
################# 9 ---- Final Height
```
Pad spaces with underscores i.e _ so each line is the same length.Goodluck and have fun coding ! | hollow_triangle=lambda n:["".join([['_','#'][k in[n-1-i,n-1+i]]for k in range(n*2-1)])for i in range(n-1)]+[f"{'#'*(n*2-1)}"] | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Takahashi is at an all-you-can-eat restaurant.
The restaurant offers N kinds of dishes. It takes A_i minutes to eat the i-th dish, whose deliciousness is B_i.
The restaurant has the following rules:
- You can only order one dish at a time. The dish ordered will be immediately served and ready to eat.
- You cannot order the same kind of dish more than once.
- Until you finish eating the dish already served, you cannot order a new dish.
- After T-0.5 minutes from the first order, you can no longer place a new order, but you can continue eating the dish already served.
Let Takahashi's happiness be the sum of the deliciousness of the dishes he eats in this restaurant.
What is the maximum possible happiness achieved by making optimal choices?
-----Constraints-----
- 2 \leq N \leq 3000
- 1 \leq T \leq 3000
- 1 \leq A_i \leq 3000
- 1 \leq B_i \leq 3000
- All values in input are integers.
-----Input-----
Input is given from Standard Input in the following format:
N T
A_1 B_1
:
A_N B_N
-----Output-----
Print the maximum possible happiness Takahashi can achieve.
-----Sample Input-----
2 60
10 10
100 100
-----Sample Output-----
110
By ordering the first and second dishes in this order, Takahashi's happiness will be 110.
Note that, if we manage to order a dish in time, we can spend any amount of time to eat it. | import numpy as np
N, T = map(int, input().split())
AB = []
for i in range(N):
A, B = map(int, input().split())
AB.append([A, B])
AB.sort()
dp = np.zeros(T, dtype=int)
ans = 0
for a, b in AB:
ans = max(ans, dp[-1] + b)
dp[a:] = np.maximum(dp[a:], dp[:-a] + b)
print(ans) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/990/C:
A bracket sequence is a string containing only characters "(" and ")".
A regular bracket sequence is a bracket sequence that can be transformed into a correct arithmetic expression by inserting characters "1" and "+" between the original characters of the sequence. For example, bracket sequences "()()", "(())" are regular (the resulting expressions are: "(1)+(1)", "((1+1)+1)"), and ")(" and "(" are not.
You are given $n$ bracket sequences $s_1, s_2, \dots , s_n$. Calculate the number of pairs $i, j \, (1 \le i, j \le n)$ such that the bracket sequence $s_i + s_j$ is a regular bracket sequence. Operation $+$ means concatenation i.e. "()(" + ")()" = "()()()".
If $s_i + s_j$ and $s_j + s_i$ are regular bracket sequences and $i \ne j$, then both pairs $(i, j)$ and $(j, i)$ must be counted in the answer. Also, if $s_i + s_i$ is a regular bracket sequence, the pair $(i, i)$ must be counted in the answer.
-----Input-----
The first line contains one integer $n \, (1 \le n \le 3 \cdot 10^5)$ — the number of bracket sequences. The following $n$ lines contain bracket sequences — non-empty strings consisting only of characters "(" and ")". The sum of lengths of all bracket sequences does not exceed $3 \cdot 10^5$.
-----Output-----
In the single line print a single integer — the number of pairs $i, j \, (1 \le i, j \le n)$ such that the bracket sequence $s_i + s_j$ is a regular bracket sequence.
-----Examples-----
Input
3
)
()
(
Output
2
Input
2
()
()
Output
4
-----Note-----
In the first example, suitable pairs are $(3, 1)$ and $(2, 2)$.
In the second example, any pair is suitable, namely $(1, 1), (1, 2), (2, 1), (2, 2)$.
I tried it in Python, but could not do it. Can you solve it? | import math,string,itertools,fractions,heapq,collections,re,array,bisect,sys,random,time,copy,functools
sys.setrecursionlimit(10**7)
inf = 10**20
eps = 1.0 / 10**10
mod = 998244353
def LI(): return [int(x) for x in sys.stdin.readline().split()]
def LI_(): return [int(x)-1 for x in sys.stdin.readline().split()]
def LF(): return [float(x) for x in sys.stdin.readline().split()]
def LS(): return sys.stdin.readline().split()
def I(): return int(sys.stdin.readline())
def F(): return float(sys.stdin.readline())
def S(): return input()
def pf(s): return print(s, flush=True)
def main():
n = I()
a = [S() for _ in range(n)]
d = collections.defaultdict(int)
for c in a:
k = 0
lk = 0
for t in c:
if t == ')':
k += 1
if lk < k:
lk = k
else:
k -= 1
k = 0
rk = 0
for t in c[::-1]:
if t == '(':
k += 1
if rk < k:
rk = k
else:
k -= 1
if lk > 0 and rk > 0:
continue
if lk == 0 and rk == 0:
d[0] += 1
if lk > 0:
d[-lk] += 1
if rk > 0:
d[rk] += 1
r = d[0] ** 2
for k in list(d.keys()):
if k <= 0:
continue
r += d[k] * d[-k]
return r
print(main()) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/641/E:
Little Artem has invented a time machine! He could go anywhere in time, but all his thoughts of course are with computer science. He wants to apply this time machine to a well-known data structure: multiset.
Artem wants to create a basic multiset of integers. He wants these structure to support operations of three types:
Add integer to the multiset. Note that the difference between set and multiset is that multiset may store several instances of one integer. Remove integer from the multiset. Only one instance of this integer is removed. Artem doesn't want to handle any exceptions, so he assumes that every time remove operation is called, that integer is presented in the multiset. Count the number of instances of the given integer that are stored in the multiset.
But what about time machine? Artem doesn't simply apply operations to the multiset one by one, he now travels to different moments of time and apply his operation there. Consider the following example.
First Artem adds integer 5 to the multiset at the 1-st moment of time. Then Artem adds integer 3 to the multiset at the moment 5. Then Artem asks how many 5 are there in the multiset at moment 6. The answer is 1. Then Artem returns back in time and asks how many integers 3 are there in the set at moment 4. Since 3 was added only at moment 5, the number of integers 3 at moment 4 equals to 0. Then Artem goes back in time again and removes 5 from the multiset at moment 3. Finally Artyom asks at moment 7 how many integers 5 are there in the set. The result is 0, since we have removed 5 at the moment 3.
Note that Artem dislikes exceptions so much that he assures that after each change he makes all delete operations are applied only to element that is present in the multiset. The answer to the query of the third type is computed at the moment Artem makes the corresponding query and are not affected in any way by future changes he makes.
Help Artem implement time travellers multiset.
-----Input-----
The first line of the input contains a...
I tried it in Python, but could not do it. Can you solve it? | from bisect import *
d = [{}, {}]
i = [0, 0]
for q in range(int(input())):
a, t, x = map(int, input().split())
for k in [0, 1]:
d[k][x] = d[k].get(x, [])
i[k] = bisect(d[k][x], t)
if a < 3: d[-a][x].insert(i[-a], t)
else: print(i[1] - i[0]) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Mike and some bears are playing a game just for fun. Mike is the judge. All bears except Mike are standing in an n × m grid, there's exactly one bear in each cell. We denote the bear standing in column number j of row number i by (i, j). Mike's hands are on his ears (since he's the judge) and each bear standing in the grid has hands either on his mouth or his eyes. [Image]
They play for q rounds. In each round, Mike chooses a bear (i, j) and tells him to change his state i. e. if his hands are on his mouth, then he'll put his hands on his eyes or he'll put his hands on his mouth otherwise. After that, Mike wants to know the score of the bears.
Score of the bears is the maximum over all rows of number of consecutive bears with hands on their eyes in that row.
Since bears are lazy, Mike asked you for help. For each round, tell him the score of these bears after changing the state of a bear selected in that round.
-----Input-----
The first line of input contains three integers n, m and q (1 ≤ n, m ≤ 500 and 1 ≤ q ≤ 5000).
The next n lines contain the grid description. There are m integers separated by spaces in each line. Each of these numbers is either 0 (for mouth) or 1 (for eyes).
The next q lines contain the information about the rounds. Each of them contains two integers i and j (1 ≤ i ≤ n and 1 ≤ j ≤ m), the row number and the column number of the bear changing his state.
-----Output-----
After each round, print the current score of the bears.
-----Examples-----
Input
5 4 5
0 1 1 0
1 0 0 1
0 1 1 0
1 0 0 1
0 0 0 0
1 1
1 4
1 1
4 2
4 3
Output
3
4
3
3
4 | n, m, q = list(map(int, input().split()))
def csum(row):
return max(list(map(len, "".join(map(str, row)).split("0"))))
grid = ["".join(input().split()) for i in range(n)]
score = [max(list(map(len, row.split("0")))) for row in grid]
for i in range(q):
i, j = list(map(int, input().split()))
row = grid[i-1]
row = row[:j-1] + ("1" if row[j-1] == "0" else "0") + row[j:]
grid[i-1] = row
score[i-1] = csum(row)
print(max(score)) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/263/A:
You've got a 5 × 5 matrix, consisting of 24 zeroes and a single number one. Let's index the matrix rows by numbers from 1 to 5 from top to bottom, let's index the matrix columns by numbers from 1 to 5 from left to right. In one move, you are allowed to apply one of the two following transformations to the matrix: Swap two neighboring matrix rows, that is, rows with indexes i and i + 1 for some integer i (1 ≤ i < 5). Swap two neighboring matrix columns, that is, columns with indexes j and j + 1 for some integer j (1 ≤ j < 5).
You think that a matrix looks beautiful, if the single number one of the matrix is located in its middle (in the cell that is on the intersection of the third row and the third column). Count the minimum number of moves needed to make the matrix beautiful.
-----Input-----
The input consists of five lines, each line contains five integers: the j-th integer in the i-th line of the input represents the element of the matrix that is located on the intersection of the i-th row and the j-th column. It is guaranteed that the matrix consists of 24 zeroes and a single number one.
-----Output-----
Print a single integer — the minimum number of moves needed to make the matrix beautiful.
-----Examples-----
Input
0 0 0 0 0
0 0 0 0 1
0 0 0 0 0
0 0 0 0 0
0 0 0 0 0
Output
3
Input
0 0 0 0 0
0 0 0 0 0
0 1 0 0 0
0 0 0 0 0
0 0 0 0 0
Output
1
I tried it in Python, but could not do it. Can you solve it? | a = []
for i in range(5):
a.extend(map(int, input().split()))
a = a.index(1)
print(abs(a // 5 - 2) + abs(a % 5 - 2)) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codewars.com/kata/5c80b55e95eba7650dc671ea:
__Definition:__ According to Wikipedia, a [complete binary tree](https://en.wikipedia.org/wiki/Binary_tree#Types_of_binary_trees) is a binary tree _"where every level, except possibly the last, is completely filled, and all nodes in the last level are as far left as possible."_
The Wikipedia page referenced above also mentions that _"Binary trees can also be stored in breadth-first order as an implicit data structure in arrays, and if the tree is a complete binary tree, this method wastes no space."_
Your task is to write a method (or function) that takes an array (or list, depending on language) of integers and, assuming that the array is ordered according to an _in-order_ traversal of a complete binary tree, returns an array that contains the values of the tree in breadth-first order.
__Example 1:__
Let the input array be `[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]`. This array contains the values of the following complete binary tree.
```
_ 7_
/ \
4 9
/ \ / \
2 6 8 10
/ \ /
1 3 5
```
In this example, the input array happens to be sorted, but that is _not_ a requirement.
__Output 1:__ The output of the function shall be an array containing the values of the nodes of the binary tree read top-to-bottom, left-to-right. In this example, the returned array should be:
```[7, 4, 9, 2, 6, 8, 10, 1, 3, 5]```
__Example 2:__
Let the input array be `[1, 2, 2, 6, 7, 5]`. This array contains the values of the following complete binary tree.
```
6
/ \
2 5
/ \ /
1 2 7
```
Note that an in-order traversal of this tree produces the input array.
__Output 2:__ The output of the function shall be an array containing the values of the nodes of the binary tree read top-to-bottom, left-to-right. In this example, the returned array should be:
```[6, 2, 5, 1, 2, 7]```
I tried it in Python, but could not do it. Can you solve it? | def mid(n):
x = 1 << (n.bit_length() - 1)
return x-1 if x//2 - 1 <= n-x else n-x//2
def complete_binary_tree(a):
res, queue = [], [a]
while queue:
L = queue.pop(0)
m = mid(len(L))
res.append(L[m])
if m: queue.append(L[:m])
if m < len(L)-1: queue.append(L[m+1:])
return res | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://www.codechef.com/problems/WATMELON:
Let's call a sequence good if the sum of all its elements is $0$.
You have a sequence of integers $A_1, A_2, \ldots, A_N$. You may perform any number of operations on this sequence (including zero). In one operation, you should choose a valid index $i$ and decrease $A_i$ by $i$. Can you make the sequence good using these operations?
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains a single integer $N$.
- The second line contains $N$ space-separated integers $A_1, A_2, \ldots, A_N$.
-----Output-----
For each test case, print a single line containing the string "YES" if it is possible to make the given sequence good or "NO" if it is impossible.
-----Constraints-----
- $1 \le T \le 1,000$
- $1 \le N \le 10$
- $|A_i| \le 100$ for each valid $i$
-----Subtasks-----
Subtask #1 (10 points): $N = 1$
Subtask #2 (30 points): $N \le 2$
Subtask #3 (60 points): original constraints
-----Example Input-----
2
1
-1
2
1 2
-----Example Output-----
NO
YES
-----Explanation-----
Example case 2: We can perform two operations ― subtract $1$ from $A_1$ and $2$ from $A_2$.
I tried it in Python, but could not do it. Can you solve it? | n=int(input())
for i in range(n):
t=int(input())
m=list(map(int,input().split()))
p,q=0,0
if t==1:
if m[0]>=0:
print('YES')
else:
print('NO')
else:
for i in m:
if i<0:
q+=1
else:
p+=1
if p>=abs(q):
print('YES')
else:
print('NO') | python | train | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1129/C:
In Morse code, an letter of English alphabet is represented as a string of some length from $1$ to $4$. Moreover, each Morse code representation of an English letter contains only dots and dashes. In this task, we will represent a dot with a "0" and a dash with a "1".
Because there are $2^1+2^2+2^3+2^4 = 30$ strings with length $1$ to $4$ containing only "0" and/or "1", not all of them correspond to one of the $26$ English letters. In particular, each string of "0" and/or "1" of length at most $4$ translates into a distinct English letter, except the following four strings that do not correspond to any English alphabet: "0011", "0101", "1110", and "1111".
You will work with a string $S$, which is initially empty. For $m$ times, either a dot or a dash will be appended to $S$, one at a time. Your task is to find and report, after each of these modifications to string $S$, the number of non-empty sequences of English letters that are represented with some substring of $S$ in Morse code.
Since the answers can be incredibly tremendous, print them modulo $10^9 + 7$.
-----Input-----
The first line contains an integer $m$ ($1 \leq m \leq 3\,000$) — the number of modifications to $S$.
Each of the next $m$ lines contains either a "0" (representing a dot) or a "1" (representing a dash), specifying which character should be appended to $S$.
-----Output-----
Print $m$ lines, the $i$-th of which being the answer after the $i$-th modification to $S$.
-----Examples-----
Input
3
1
1
1
Output
1
3
7
Input
5
1
0
1
0
1
Output
1
4
10
22
43
Input
9
1
1
0
0
0
1
1
0
1
Output
1
3
10
24
51
109
213
421
833
-----Note-----
Let us consider the first sample after all characters have been appended to $S$, so S is "111".
As you can see, "1", "11", and "111" all correspond to some distinct English letter. In fact, they are translated into a 'T', an 'M', and an 'O', respectively. All non-empty sequences of English letters that are represented with some substring of $S$ in Morse code, therefore, are as follows. "T"...
I tried it in Python, but could not do it. Can you solve it? | MOD = 10 ** 9 + 7
BAD = ([0, 0, 1, 1], [0, 1, 0, 1], [1, 1, 1, 0], [1, 1, 1, 1])
def zfunc(s):
z = [0] * len(s)
l = r = 0
for i in range(1, len(s)):
if i <= r:
z[i] = min(r - i + 1, z[i - l])
while i + z[i] < len(s) and s[z[i]] == s[i + z[i]]:
z[i] += 1
if i + z[i] - 1 > r:
l, r = i, i + z[i] - 1
return z
n = int(input())
s = []
sm = 0
for i in range(1, n + 1):
s.append(int(input()))
cur = 0
f = [0] * (i + 1)
sum4 = f[i] = 1
for j in range(i - 1, -1, -1):
if j + 4 < i:
sum4 -= f[j + 5]
if j + 4 <= i and s[j : j + 4] in BAD:
f[j] -= f[j + 4]
f[j] = (f[j] + sum4) % MOD
sum4 += f[j]
z = zfunc(s[::-1])
new = i - max(z)
sm = (sm + sum(f[:new])) % MOD
print(sm) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Boboniu likes bit operations. He wants to play a game with you.
Boboniu gives you two sequences of non-negative integers $a_1,a_2,\ldots,a_n$ and $b_1,b_2,\ldots,b_m$.
For each $i$ ($1\le i\le n$), you're asked to choose a $j$ ($1\le j\le m$) and let $c_i=a_i\& b_j$, where $\&$ denotes the bitwise AND operation. Note that you can pick the same $j$ for different $i$'s.
Find the minimum possible $c_1 | c_2 | \ldots | c_n$, where $|$ denotes the bitwise OR operation.
-----Input-----
The first line contains two integers $n$ and $m$ ($1\le n,m\le 200$).
The next line contains $n$ integers $a_1,a_2,\ldots,a_n$ ($0\le a_i < 2^9$).
The next line contains $m$ integers $b_1,b_2,\ldots,b_m$ ($0\le b_i < 2^9$).
-----Output-----
Print one integer: the minimum possible $c_1 | c_2 | \ldots | c_n$.
-----Examples-----
Input
4 2
2 6 4 0
2 4
Output
2
Input
7 6
1 9 1 9 8 1 0
1 1 4 5 1 4
Output
0
Input
8 5
179 261 432 162 82 43 10 38
379 357 202 184 197
Output
147
-----Note-----
For the first example, we have $c_1=a_1\& b_2=0$, $c_2=a_2\& b_1=2$, $c_3=a_3\& b_1=0$, $c_4 = a_4\& b_1=0$.Thus $c_1 | c_2 | c_3 |c_4 =2$, and this is the minimal answer we can get. | n,m = [int(i) for i in input().split()]
a = [int(i) for i in input().split()]
b = [int(i) for i in input().split()]
c=0
for i in range(0,513):
c=1
for j in a:
u = 0
for k in b:
if(i|(j&k)==i):
u = 1
break
if(u==0):
c=0
break
if(c==1):
print(i)
break | python | test | qsol | codeparrot/apps | all |
Solve in Python:
The famous joke programming language HQ9+ has only 4 commands. In this problem we will explore its subset — a language called HQ...
-----Input-----
The only line of the input is a string between 1 and 10^6 characters long.
-----Output-----
Output "Yes" or "No".
-----Examples-----
Input
HHHH
Output
Yes
Input
HQHQH
Output
No
Input
HHQHHQH
Output
No
Input
HHQQHHQQHH
Output
Yes
-----Note-----
The rest of the problem statement was destroyed by a stray raccoon. We are terribly sorry for the inconvenience. | import sys
s = input()
qc = s.count('Q')
qs = int(qc ** 0.5)
hc = s.count('H')
if qs == 0:
print('Yes')
return
if not qc == qs ** 2:
print('No')
return
if not hc % (qs + 1) == 0:
print('No')
return
t = s.split('Q')
pre = len(t[0]) // 2
suf = 0 if len(t) == 1 else len(t[-1]) // 2
a = ['H' * pre] + t[1 : qs] + ['H' * suf]
o = [c for c in 'Q'.join(a)]
g = []
for c in o:
if c == 'H':
g += ['H']
else:
g += o
print('Yes' if ''.join(g) == s else 'No') | python | test | qsol | codeparrot/apps | all |
Solve in Python:
You have unweighted tree of $n$ vertices. You have to assign a positive weight to each edge so that the following condition would hold:
For every two different leaves $v_{1}$ and $v_{2}$ of this tree, bitwise XOR of weights of all edges on the simple path between $v_{1}$ and $v_{2}$ has to be equal to $0$.
Note that you can put very large positive integers (like $10^{(10^{10})}$).
It's guaranteed that such assignment always exists under given constraints. Now let's define $f$ as the number of distinct weights in assignment.
[Image] In this example, assignment is valid, because bitwise XOR of all edge weights between every pair of leaves is $0$. $f$ value is $2$ here, because there are $2$ distinct edge weights($4$ and $5$).
[Image] In this example, assignment is invalid, because bitwise XOR of all edge weights between vertex $1$ and vertex $6$ ($3, 4, 5, 4$) is not $0$.
What are the minimum and the maximum possible values of $f$ for the given tree? Find and print both.
-----Input-----
The first line contains integer $n$ ($3 \le n \le 10^{5}$) — the number of vertices in given tree.
The $i$-th of the next $n-1$ lines contains two integers $a_{i}$ and $b_{i}$ ($1 \le a_{i} \lt b_{i} \le n$) — it means there is an edge between $a_{i}$ and $b_{i}$. It is guaranteed that given graph forms tree of $n$ vertices.
-----Output-----
Print two integers — the minimum and maximum possible value of $f$ can be made from valid assignment of given tree. Note that it's always possible to make an assignment under given constraints.
-----Examples-----
Input
6
1 3
2 3
3 4
4 5
5 6
Output
1 4
Input
6
1 3
2 3
3 4
4 5
4 6
Output
3 3
Input
7
1 2
2 7
3 4
4 7
5 6
6 7
Output
1 6
-----Note-----
In the first example, possible assignments for each minimum and maximum are described in picture below. Of course, there are multiple possible assignments for each minimum and maximum. [Image]
In the second example, possible assignments for each minimum and maximum are described in picture below. The $f$ value of... | import sys
input = sys.stdin.readline
n = int(input())
neigh = [[] for i in range(n)]
l = []
for i in range(n - 1):
a, b = list(map(int, input().split()))
a -= 1
b -= 1
neigh[a].append(b)
neigh[b].append(a)
l.append((a,b))
#Max
edges = set()
for a, b in l:
if len(neigh[a]) == 1:
a = -1
if len(neigh[b]) == 1:
b = -1
if a > b:
a, b = b, a
edges.add((a,b))
MAX = len(edges)
#Min
leafDepth = []
visited = [False] * n
from collections import deque
q = deque()
q.append((0,0))
while q:
nex, d = q.popleft()
if not visited[nex]:
visited[nex] = True
if len(neigh[nex]) == 1:
leafDepth.append(d)
for v in neigh[nex]:
q.append((v,d+1))
MIN = 1
corr = leafDepth.pop() % 2
for d in leafDepth:
if d % 2 != corr:
MIN = 3
#Out
print(MIN, MAX) | python | train | qsol | codeparrot/apps | all |
Solve in Python:
Uppal-balu is busy in extraction of gold from ores. He got an assignment from his favorite professor MH sir, but he got stuck in a problem. Can you help Uppal-balu to solve the problem.
You are given an array $a_1$, $a_2$, $\dots$, $a_n$ of length $n$. You can perform the following operations on it:
- Choose an index $i$ ($1 \leq i \leq n$), and replace $a_i$ by $a_{i} + 1$ or $a_{i} - 1$, which means add or subtract one to the element at index $i$.
Beauty of the array is defined as maximum length of a subarray containing numbers which give same remainder upon dividing it by $k$ (that is, ($a_l$ mod $k$) = ($a_{l+1}$ mod $k$) = $\dots$ = ($a_r$ mod $k$) for some $1 \leq l \leq r \leq n$).
You need to calculate the beauty of the array $a_1$, $a_2$, $\dots$, $a_n$ after applying at most $m$ operations.
NOTE:
- The subarray of a is a contiguous part of the array $a$, i. e. the array $a_l$, $a_{l+1}$, $\dots$, $a_r$ for some $1 \leq l \leq r \leq n$.
- $x$ mod $y$ means the remainder of $x$ after dividing it by $y$.
-----Input:-----
- First line of input contains 3 integers $n$, $m$ and $k$.
- The second line contains $n$ integers $a_1$, $a_2$, $\dots$, $a_n$.
-----Output:-----
Output in a single line the beauty of the array after applying at most m operations.
-----Constraints-----
- $1 \leq n,m \leq 2\cdot10^{5}$
- $1 \leq k \leq 7$
- $1 \leq a_i \leq 10^9$
-----Sample Input 1:-----
7 3 4
8 2 3 7 8 1 1
-----Sample Output 1:-----
5
-----Sample Input 2:-----
8 3 5
7 2 1 3 6 5 6 2
-----Sample Output 2:-----
5 | t=1
for _ in range(t):
n,m,k=(list(map(int,input().split())))
a=list(map(int,input().split()))
for i in range(n):
a[i]=a[i]%k
s=1
for j in range(k):
ss=0
v=0
for i in range(n):
v+=min(abs(a[i]-j),k-abs(a[i]-j))
while ss<i and v>m:
v-=min(abs(a[ss]-j),k-abs(a[ss]-j))
ss+=1
s=max(s,i-ss+1)
print(s) | python | test | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/895/A:
Students Vasya and Petya are studying at the BSU (Byteland State University). At one of the breaks they decided to order a pizza. In this problem pizza is a circle of some radius. The pizza was delivered already cut into n pieces. The i-th piece is a sector of angle equal to a_{i}. Vasya and Petya want to divide all pieces of pizza into two continuous sectors in such way that the difference between angles of these sectors is minimal. Sector angle is sum of angles of all pieces in it. Pay attention, that one of sectors can be empty.
-----Input-----
The first line contains one integer n (1 ≤ n ≤ 360) — the number of pieces into which the delivered pizza was cut.
The second line contains n integers a_{i} (1 ≤ a_{i} ≤ 360) — the angles of the sectors into which the pizza was cut. The sum of all a_{i} is 360.
-----Output-----
Print one integer — the minimal difference between angles of sectors that will go to Vasya and Petya.
-----Examples-----
Input
4
90 90 90 90
Output
0
Input
3
100 100 160
Output
40
Input
1
360
Output
360
Input
4
170 30 150 10
Output
0
-----Note-----
In first sample Vasya can take 1 and 2 pieces, Petya can take 3 and 4 pieces. Then the answer is |(90 + 90) - (90 + 90)| = 0.
In third sample there is only one piece of pizza that can be taken by only one from Vasya and Petya. So the answer is |360 - 0| = 360.
In fourth sample Vasya can take 1 and 4 pieces, then Petya will take 2 and 3 pieces. So the answer is |(170 + 10) - (30 + 150)| = 0.
Picture explaning fourth sample:
[Image]
Both red and green sectors consist of two adjacent pieces of pizza. So Vasya can take green sector, then Petya will take red sector.
I tried it in Python, but could not do it. Can you solve it? | n = int( input() )
a = list( map( int, input().split() ) )
a = a + a
#print( a )
mid = n//2
best = 99999999999999999999999
for i in range( n ):
sub = a[i:i+n]
#print( sub )
d = sum(sub)
best = min( best, d )
s = 0
#print( d, s )
for j in range( n ):
s += sub[j]
d -= sub[j]
diff = abs( s - d)
best = min( best, diff )
print( best ) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/801/A:
Tonio has a keyboard with only two letters, "V" and "K".
One day, he has typed out a string s with only these two letters. He really likes it when the string "VK" appears, so he wishes to change at most one letter in the string (or do no changes) to maximize the number of occurrences of that string. Compute the maximum number of times "VK" can appear as a substring (i. e. a letter "K" right after a letter "V") in the resulting string.
-----Input-----
The first line will contain a string s consisting only of uppercase English letters "V" and "K" with length not less than 1 and not greater than 100.
-----Output-----
Output a single integer, the maximum number of times "VK" can appear as a substring of the given string after changing at most one character.
-----Examples-----
Input
VK
Output
1
Input
VV
Output
1
Input
V
Output
0
Input
VKKKKKKKKKVVVVVVVVVK
Output
3
Input
KVKV
Output
1
-----Note-----
For the first case, we do not change any letters. "VK" appears once, which is the maximum number of times it could appear.
For the second case, we can change the second character from a "V" to a "K". This will give us the string "VK". This has one occurrence of the string "VK" as a substring.
For the fourth case, we can change the fourth character from a "K" to a "V". This will give us the string "VKKVKKKKKKVVVVVVVVVK". This has three occurrences of the string "VK" as a substring. We can check no other moves can give us strictly more occurrences.
I tried it in Python, but could not do it. Can you solve it? | def alternate(c):
if c == "V":
return "K"
return "V"
s = input()
res = s.count("VK")
for i in range(len(s)):
res = max(res, (s[:i]+alternate(s[i])+s[i+1:]).count("VK"))
print(res) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Petr likes to come up with problems about randomly generated data. This time problem is about random permutation. He decided to generate a random permutation this way: he takes identity permutation of numbers from $1$ to $n$ and then $3n$ times takes a random pair of different elements and swaps them. Alex envies Petr and tries to imitate him in all kind of things. Alex has also come up with a problem about random permutation. He generates a random permutation just like Petr but swaps elements $7n+1$ times instead of $3n$ times. Because it is more random, OK?!
You somehow get a test from one of these problems and now you want to know from which one.
-----Input-----
In the first line of input there is one integer $n$ ($10^{3} \le n \le 10^{6}$).
In the second line there are $n$ distinct integers between $1$ and $n$ — the permutation of size $n$ from the test.
It is guaranteed that all tests except for sample are generated this way: First we choose $n$ — the size of the permutation. Then we randomly choose a method to generate a permutation — the one of Petr or the one of Alex. Then we generate a permutation using chosen method.
-----Output-----
If the test is generated via Petr's method print "Petr" (without quotes). If the test is generated via Alex's method print "Um_nik" (without quotes).
-----Example-----
Input
5
2 4 5 1 3
Output
Petr
-----Note-----
Please note that the sample is not a valid test (because of limitations for $n$) and is given only to illustrate input/output format. Your program still has to print correct answer to this test to get AC.
Due to randomness of input hacks in this problem are forbidden. | n = int(input())
l = list(map(int, input().split()))
c = 1
ans = 0
vis = [0] * 1000005
for i in range(n):
p = i
vis[i + 1] = 1
while vis[l[p]] != 1:
ans -= -1
vis[l[p]] = 1
p = l[p] - 1
if 3 * n % 2 == ans % 2: print("Petr")
else: print("Um_nik") | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://leetcode.com/problems/largest-rectangle-in-histogram/:
Given n non-negative integers representing the histogram's bar height where the width of each bar is 1, find the area of largest rectangle in the histogram.
Above is a histogram where width of each bar is 1, given height = [2,1,5,6,2,3].
The largest rectangle is shown in the shaded area, which has area = 10 unit.
Example:
Input: [2,1,5,6,2,3]
Output: 10
I tried it in Python, but could not do it. Can you solve it? | class Solution:
def largestRectangleArea(self, heights):
n = len(heights)
if n == 0:
return 0
left = [i for i in range(n)]
right = [i+1 for i in range(n)]
print(heights)
for i in range(1, n):
# indicates the next value to compare
cur = i - 1
# import pdb
# pdb.set_trace()
while cur >= 0 and heights[cur] >= heights[i]:
left[i] = left[cur]
cur = left[cur] - 1
for j in range(n-1, -1, -1):
cur = j + 1
while cur < n and heights[cur] >= heights[j]:
# import pdb
# pdb.set_trace()
right[j] = right[cur]
cur = right[cur]
print(left)
print(right)
max_val = 0
for i in range(n):
tmp = heights[i] * (right[i] - left[i])
if max_val < tmp:
max_val = tmp
return max_val | python | train | abovesol | codeparrot/apps | all |
Solve in Python:
A triangle is called an equable triangle if its area equals its perimeter. Return `true`, if it is an equable triangle, else return `false`. You will be provided with the length of sides of the triangle. Happy Coding! | def equable_triangle(a,b,c):
return (a + b + c) * 16 == (a + b - c) * (b + c - a) * (c + a - b) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/1203/E:
There are $n$ boxers, the weight of the $i$-th boxer is $a_i$. Each of them can change the weight by no more than $1$ before the competition (the weight cannot become equal to zero, that is, it must remain positive). Weight is always an integer number.
It is necessary to choose the largest boxing team in terms of the number of people, that all the boxers' weights in the team are different (i.e. unique).
Write a program that for given current values $a_i$ will find the maximum possible number of boxers in a team.
It is possible that after some change the weight of some boxer is $150001$ (but no more).
-----Input-----
The first line contains an integer $n$ ($1 \le n \le 150000$) — the number of boxers. The next line contains $n$ integers $a_1, a_2, \dots, a_n$, where $a_i$ ($1 \le a_i \le 150000$) is the weight of the $i$-th boxer.
-----Output-----
Print a single integer — the maximum possible number of people in a team.
-----Examples-----
Input
4
3 2 4 1
Output
4
Input
6
1 1 1 4 4 4
Output
5
-----Note-----
In the first example, boxers should not change their weights — you can just make a team out of all of them.
In the second example, one boxer with a weight of $1$ can be increased by one (get the weight of $2$), one boxer with a weight of $4$ can be reduced by one, and the other can be increased by one (resulting the boxers with a weight of $3$ and $5$, respectively). Thus, you can get a team consisting of boxers with weights of $5, 4, 3, 2, 1$.
I tried it in Python, but could not do it. Can you solve it? | def main():
n = int(input())
arr = list(map(int,input().split()))
arr.sort()
nums = set()
for i in arr:
if i-1 > 0 and ((i-1) not in nums):
nums.add(i-1)
elif i not in nums:
nums.add(i)
elif (i+1) not in nums:
nums.add(i+1)
print(len(nums))
main() | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Given an integer array nums, find the contiguous subarray within an array (containing at least one number) which has the largest product.
Example 1:
Input: [2,3,-2,4]
Output: 6
Explanation: [2,3] has the largest product 6.
Example 2:
Input: [-2,0,-1]
Output: 0
Explanation: The result cannot be 2, because [-2,-1] is not a subarray. | class Solution:
def maxProduct(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
n = len(nums)
dp = [[nums[0], nums[0]] for _ in range(n)]
ans = nums[0]
for i in range(1, n):
temp = [dp[i-1][0] * nums[i], dp[i-1][1] * nums[i], nums[i]]
dp[i][0], dp[i][1] = max(temp), min(temp)
if dp[i][0] > ans:
ans = dp[i][0]
return ans
# n = len(nums)
# dp = [[nums[0], nums[0]]] * n 尽量不要使用乘法
# ans = nums[0]
# for i in range(1, n):
# temp = [dp[i-1][0] * nums[i], dp[i-1][1] * nums[i], nums[i]]
# dp[i][0], dp[i][1] = max(temp), min(temp) 这样赋值会改变所有值
# if dp[i][0] > ans:
# ans = dp[i][0]
# return dp[-1][0] | python | train | qsol | codeparrot/apps | all |
Solve in Python:
You are given Name of chef's friend and using chef's new method of calculating value of string , chef have to find the value of all the names. Since chef is busy , he asked you to do the work from him .
The method is a function $f(x)$ as follows -
-
$f(x)$ = $1$ , if $x$ is a consonent
-
$f(x)$ = $0$ , if $x$ is a vowel
Your task is to apply the above function on all the characters in the string $S$ and convert the obtained binary string in decimal number N. Since the number N can be very large, compute it modulo $10^9+7$ .
Input:
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each test line contains one String $S$ composed of lowercase English alphabet letters.
-----Output:-----
For each case, print a single line containing one integer $N$ modulo $10^9+7$ .
-----Constraints-----
- $1 \leq T \leq 50$
- $1 \leq |S| \leq 10^5$
-----Sample Input:-----
1
codechef
-----Sample Output:-----
173
-----EXPLANATION:-----
The string "codechef" will be converted to 10101101 ,using the chef's method function . Which is equal to 173. | for _ in range(int(input().strip())):
s = list(input().strip())
n = len(s)
MOD = 1000000007
vow = ['a' , 'e' , 'i' , 'o' , 'u']
K = ""
for i in range(n):
if s[i] not in vow:
K = K + "1"
else:
K = K + "0"
co = int(K,2)
print(str(co%MOD)) | python | train | qsol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/610/C:
The semester is already ending, so Danil made an effort and decided to visit a lesson on harmony analysis to know how does the professor look like, at least. Danil was very bored on this lesson until the teacher gave the group a simple task: find 4 vectors in 4-dimensional space, such that every coordinate of every vector is 1 or - 1 and any two vectors are orthogonal. Just as a reminder, two vectors in n-dimensional space are considered to be orthogonal if and only if their scalar product is equal to zero, that is: $\sum_{i = 1}^{n} a_{i} \cdot b_{i} = 0$.
Danil quickly managed to come up with the solution for this problem and the teacher noticed that the problem can be solved in a more general case for 2^{k} vectors in 2^{k}-dimensinoal space. When Danil came home, he quickly came up with the solution for this problem. Can you cope with it?
-----Input-----
The only line of the input contains a single integer k (0 ≤ k ≤ 9).
-----Output-----
Print 2^{k} lines consisting of 2^{k} characters each. The j-th character of the i-th line must be equal to ' * ' if the j-th coordinate of the i-th vector is equal to - 1, and must be equal to ' + ' if it's equal to + 1. It's guaranteed that the answer always exists.
If there are many correct answers, print any.
-----Examples-----
Input
2
Output
++**
+*+*
++++
+**+
-----Note-----
Consider all scalar products in example: Vectors 1 and 2: ( + 1)·( + 1) + ( + 1)·( - 1) + ( - 1)·( + 1) + ( - 1)·( - 1) = 0 Vectors 1 and 3: ( + 1)·( + 1) + ( + 1)·( + 1) + ( - 1)·( + 1) + ( - 1)·( + 1) = 0 Vectors 1 and 4: ( + 1)·( + 1) + ( + 1)·( - 1) + ( - 1)·( - 1) + ( - 1)·( + 1) = 0 Vectors 2 and 3: ( + 1)·( + 1) + ( - 1)·( + 1) + ( + 1)·( + 1) + ( - 1)·( + 1) = 0 Vectors 2 and 4: ( + 1)·( + 1) + ( - 1)·( - 1) + ( + 1)·( - 1) + ( - 1)·( + 1) = 0 Vectors 3 and 4: ( + 1)·( + 1) + ( + 1)·( - 1) + ( + 1)·( - 1) + ( + 1)·( + 1) = 0
I tried it in Python, but could not do it. Can you solve it? | k = int(input())
if (k == 0):
print('+')
return
answer = [['+', '+'], ['+', '*']]
length = 2
for i in range(k - 1):
new = []
for i in answer:
temp = []
for j in range(length):
if i[j] == '+':
temp += ['+', '+']
else:
temp += ['*', '*']
new.append(temp)
for i in answer:
temp = []
for j in range(length):
if i[j] == '+':
temp += ['+', '*']
else:
temp += ['*', '+']
new.append(temp)
answer = new
length *= 2
print('\n'.join([''.join(i) for i in answer])) | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://codeforces.com/problemset/problem/998/A:
There are quite a lot of ways to have fun with inflatable balloons. For example, you can fill them with water and see what happens.
Grigory and Andrew have the same opinion. So, once upon a time, they went to the shop and bought $n$ packets with inflatable balloons, where $i$-th of them has exactly $a_i$ balloons inside.
They want to divide the balloons among themselves. In addition, there are several conditions to hold:
Do not rip the packets (both Grigory and Andrew should get unbroken packets); Distribute all packets (every packet should be given to someone); Give both Grigory and Andrew at least one packet; To provide more fun, the total number of balloons in Grigory's packets should not be equal to the total number of balloons in Andrew's packets.
Help them to divide the balloons or determine that it's impossible under these conditions.
-----Input-----
The first line of input contains a single integer $n$ ($1 \le n \le 10$) — the number of packets with balloons.
The second line contains $n$ integers: $a_1$, $a_2$, $\ldots$, $a_n$ ($1 \le a_i \le 1000$) — the number of balloons inside the corresponding packet.
-----Output-----
If it's impossible to divide the balloons satisfying the conditions above, print $-1$.
Otherwise, print an integer $k$ — the number of packets to give to Grigory followed by $k$ distinct integers from $1$ to $n$ — the indices of those. The order of packets doesn't matter.
If there are multiple ways to divide balloons, output any of them.
-----Examples-----
Input
3
1 2 1
Output
2
1 2
Input
2
5 5
Output
-1
Input
1
10
Output
-1
-----Note-----
In the first test Grigory gets $3$ balloons in total while Andrey gets $1$.
In the second test there's only one way to divide the packets which leads to equal numbers of balloons.
In the third test one of the boys won't get a packet at all.
I tried it in Python, but could not do it. Can you solve it? | # python3
def readline(): return list(map(int, input().split()))
def main():
n, = readline()
a = tuple(readline())
if n == 1 or n == 2 and a[0] == a[1]:
print(-1)
else:
print(1)
print(a.index(min(a)) + 1)
main() | python | test | abovesol | codeparrot/apps | all |
I found an interesting problem on https://atcoder.jp/contests/abc122/tasks/abc122_d:
You are given an integer N. Find the number of strings of length N that satisfy the following conditions, modulo 10^9+7:
- The string does not contain characters other than A, C, G and T.
- The string does not contain AGC as a substring.
- The condition above cannot be violated by swapping two adjacent characters once.
-----Notes-----
A substring of a string T is a string obtained by removing zero or more characters from the beginning and the end of T.
For example, the substrings of ATCODER include TCO, AT, CODER, ATCODER and (the empty string), but not AC.
-----Constraints-----
- 3 \leq N \leq 100
-----Input-----
Input is given from Standard Input in the following format:
N
-----Output-----
Print the number of strings of length N that satisfy the following conditions, modulo 10^9+7.
-----Sample Input-----
3
-----Sample Output-----
61
There are 4^3 = 64 strings of length 3 that do not contain characters other than A, C, G and T. Among them, only AGC, ACG and GAC violate the condition, so the answer is 64 - 3 = 61.
I tried it in Python, but could not do it. Can you solve it? | import numpy as np
N = int(input())
mod = 10**9+7
dp = np.zeros([N+1,4,4,4],dtype=int)
dp[0,-1,-1,-1] = 1
for i in range(N):
for c1 in range(4):
for c2 in range(4):
for c3 in range(4):
if not dp[i,c1,c2,c3]:continue
for a in range(4):
if c1 == 0 and c3 == 1 and a == 2:continue
if c1 == 0 and c2 == 1 and a == 2:continue
if c2 == 0 and c3 == 1 and a == 2:continue
if c2 == 0 and c3 == 2 and a == 1:continue
if c2 == 1 and c3 == 0 and a == 2:continue
dp[i+1,c2,c3,a] += dp[i,c1,c2,c3]
dp[i+1,c2,c3,a] %= mod
print(dp[-1].sum()%mod) | python | test | abovesol | codeparrot/apps | all |
Solve in Python:
Ms. E.T. came from planet Hex. She has 8 fingers in each hand which makes her count in hexadecimal way. When she meets you, she tells you that she came from 7E light years from the planet Earth. You see she means that it is 126 light years far away and she is telling you the numbers in hexadecimal. Now, you are in trouble to understand what those numbers really mean. Therefore, you have to convert the hexadecimal numbers to decimals.
Input:
First line of code contain T test cases.
every line of text case contain a Hex-value
Output:
Every line of output contain a decimal conversion of given nunmber
Sample Input:
3
A
1A23
2C2A
Sample Output:
10
6691
11306 | # cook your dish here
try:
n=int(input())
for i in range(n):
a=input()
print(int(a, 16))
except EOFError as e:
pass | python | train | qsol | codeparrot/apps | all |