text
stringlengths 184
4.48M
|
---|
"""
URL configuration for groceryshop project.
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/4.2/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: path('', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: path('', Home.as_view(), name='home')
Including another URLconf
1. Import the include() function: from django.urls import include, path
2. Add a URL to urlpatterns: path('blog/', include('blog.urls'))
"""
from django.conf.urls.static import static
from django.contrib import admin
from django.urls import path, include
from django.shortcuts import redirect
from . import settings
urlpatterns = [
path('', lambda req: redirect('/accounts/login/')),
path('admin/', admin.site.urls),
path('accounts/', include('allauth.urls')),
path('dashboard/', include('dashboard.urls', namespace='dashboard')),
path('products/', include('product.urls', namespace='products')),
path('checkout/', include('checkout.urls', namespace='checkout')),
path('order/', include('order.urls', namespace='order')),
path('coupons/', include('coupons.urls', namespace='coupons'))
]
if settings.DEBUG:
urlpatterns += static(settings.STATIC_URL, document_root=settings.STATIC_ROOT)
urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT) |
<template>
<div class="send d-flex justify-content-between align-items-center px-2">
<div class="d-flex comment flex-row align-items-center chatbox m-3 mr-2 ">
<span
@click.exact="pickEmoji($event)"
ref="emojiPicker"
class=" text-grey-500 "
>
<icon class="" size="lg" name="smile" />
</span>
<textarea
:value="value"
ref="chatArea"
@change="emitValue($event)"
@input="
emitValue($event);
resize();
"
@keydown.enter.exact="emitEnter"
placeholder="Type your comment"
class="text-bold width-100 text-grey-500 p-1"
type="text"
>
</textarea>
<div class="comment">
<button
@click="$emit('send', $event)"
class="d-flex justify-content-center send position-sticky"
>
<icon size="sm" class="mt-2 ml-1 " name="send" />
</button>
</div>
</div>
</div>
</template>
<script>
import EmojiButton from "@joeattardi/emoji-button";
export default {
props: {
value: {
required: false
}
},
data() {
return {
picker: ""
};
},
watch: {
value: function(newVal, oldVal) {
if (newVal == "") {
this.$refs.chatArea.style.height = `55px`;
}
}
},
components: {
Icon: () => import("@/components/SlaIcon")
},
methods: {
emitEnter(e) {
e.preventDefault();
this.$emit("keyup");
},
pickEmoji(e) {
this.picker.togglePicker(this.$refs.emojiPicker);
},
emitValue(e) {
this.$emit("input", e.target.value);
},
resize() {
if (this.$refs.chatArea.value == "") {
this.$refs.chatArea.style.height = `55px`;
}
let h = parseInt(this.$refs.chatArea.scrollHeight, 10);
if (h < 150) {
this.$refs.chatArea.style.height = `auto`;
this.$refs.chatArea.style.height = `${this.$refs.chatArea.scrollHeight}px`;
return;
} else if (h > 150) {
this.$refs.chatArea.style.height = `150px`;
}
}
},
mounted() {
this.picker.on("emoji", emoji => {
let chatArea = this.$refs.chatArea;
let cursorPosition = chatArea.selectionEnd;
let currentChat = chatArea.value;
let start = currentChat.substring(0, chatArea.selectionStart);
let end = currentChat.substring(chatArea.selectionStart);
chatArea.value = `${start}${emoji}${end}`;
chatArea.focus();
this.$nextTick(() => {
chatArea.selectionEnd = cursorPosition + emoji.length;
});
this.$emit("input", chatArea.value);
});
},
created() {
this.picker = new EmojiButton({
autoHide: false,
position: "top-start",
showVariants: false,
rootElement: this.$refs.emojiPicker
});
}
};
</script>
<style lang="scss" scoped>
.send {
.chatbox {
width: 80%;
border-radius: 30px;
background-color: #f4f4f4;
textarea {
max-height: 28px;
border-radius: 30px;
resize: none;
font-size: 14px;
background-color: #f4f4f4;
border: none;
padding-top: 1rem;
font-family: "Open sans";
margin-left: 1.8rem;
&:focus {
outline: none;
}
}
}
button {
background-color: color(bv-primary);
border-radius: 50%;
height: 48px;
width: 48px;
&:focus {
outline: none;
}
}
}
.comment {
button {
background-color: #0087db;
border-radius: 50%;
border: none;
height: 43px;
width: 43px;
&:focus {
outline: none;
}
}
}
.width- {
&25 {
width: 25% !important;
}
&50 {
width: 50% !important;
}
&100 {
width: 100% !important;
}
&auto {
width: auto !important;
}
&fit-content {
width: fit-content !important;
}
&fill-screen {
width: 100vh !important;
}
}
</style> |
<app-product-detail-skeleton *ngIf="!isLoaded"></app-product-detail-skeleton>
<div
*ngIf="isLoaded"
class="w-full mx-auto max-w-[70rem] gap-16 flex lg:flex-row flex-col p-6"
>
<div class="lg:w-[60%] w-full flex flex-col gap-6">
<img
[src]="item?.imageUrl || '../../../assets/images/lazy-image.svg'"
alt="Cover-Image"
id="cover-img"
class="rounded-[10px]"
/>
</div>
<div class="lg:w-[40%] min-w-[15rem] w-full flex flex-col gap-3">
<span class="font-medium text-3xl">{{ item?.name }}</span>
<span class="font-medium">{{ item?.shortDes }}</span>
<span class="font-medium text-lg" *ngIf="!item?.discountPrice"
>{{ item?.price | number : "1.2-2" }} โฌ</span
>
<div *ngIf="item?.discountPrice" class="flex gap-2">
<span class="font-medium text-lg"
>{{ item?.discountPrice | number : "1.2-2" }} โฌ</span
>
<span class="font-medium text-lg text-[#505050] line-through"
>{{ item?.price | number : "1.2-2" }} โฌ</span
>
</div>
<span
class="font-medium text-lg"
[ngClass]="{ 'text-[#ff5050]': showError }"
>Select size</span
>
<div
class="grid sm:grid-cols-3 grid-cols-2 gap-3"
[ngClass]="{ 'error-border': showError }"
>
<button
*ngFor="let size of item?.sizes"
class="border-2 border-[#ececec] hover:border-black py-3 px-6 rounded-[10px] font-medium"
(click)="onSelectSize(size)"
[ngClass]="{ 'border-black': selectedSize === size }"
>
{{ size }}
</button>
</div>
<button
class="bg-black hover:bg-[#404040] text-white rounded-full py-5 leading-[1.125rem]"
(click)="onAddToCart()"
>
<span class="font-medium text-lg">Add to cart</span>
</button>
<button
class="flex gap-3 justify-center items-center rounded-full py-5 border-2 border-[#dedede] hover:border-black"
(click)="onAddToFavorites()"
>
<span class="font-medium text-lg leading-[1.125rem]"
>Add to Favorites</span
>
<svg
class="w-5 h-5"
fill="none"
stroke="currentColor"
stroke-width="1.5"
viewBox="0 0 24 24"
xmlns="http://www.w3.org/2000/svg"
aria-hidden="true"
>
<path
stroke-linecap="round"
stroke-linejoin="round"
d="M21 8.25c0-2.485-2.099-4.5-4.688-4.5-1.935 0-3.597 1.126-4.312 2.733-.715-1.607-2.377-2.733-4.313-2.733C5.1 3.75 3 5.765 3 8.25c0 7.22 9 12 9 12s9-4.78 9-12z"
></path>
</svg>
</button>
</div>
</div> |
import {
AppBar,
Toolbar,
IconButton,
List,
ListItem,
ListItemButton,
ListItemIcon,
ListItemText,
Typography,
Box
} from "@mui/material";
import {
Dehaze,
AccountCircle,
Inbox,
Settings,
ExitToApp,
} from "@mui/icons-material";
import { useNavigate } from "react-router-dom";
import { useDispatch, useSelector } from "react-redux";
import { setDrawer } from "./../../store/aside";
import HoverPopover from "material-ui-popup-state/HoverPopover";
import PopupState, { bindHover, bindPopover } from "material-ui-popup-state";
import { useTheme } from "@mui/material";
const TopBar = () => {
const dispatch = useDispatch();
const navigate = useNavigate();
const { drawerStatus } = useSelector((state: any) => state.aside);
const theme = useTheme();
const onToggleAside = () => {
dispatch(setDrawer({ drawerStatus: !drawerStatus }));
};
return (
<AppBar
position="static"
elevation={0}
color="secondary"
sx={{
flexShrink: 0,
width: drawerStatus ? "calc(100% - 260px)" : "100%",
position: "fixed",
top: "0px",
left: "auto",
right: "0px",
zIndex: 1000,
transition: "width 195ms cubic-bezier(0.4, 0, 0.6, 1) 0ms",
borderBottom: `${theme.palette.divider} 1px solid`
}}
>
<Toolbar
sx={{
flexWrap: "wrap",
justifyContent: "space-between",
px: 1,
}}
disableGutters={true}
>
<IconButton onClick={onToggleAside}>
<Dehaze fontSize="small" />
</IconButton>
<PopupState variant="popover" popupId="demo-popup-popover">
{(popupState) => (
<>
<Box {...bindHover(popupState)} sx={{ display: "flex", justifyContent: 'center', alignItems: 'center' }}>
<Typography sx={{ fontSize: ".875rem" }} variant="body1">admin</Typography>
<IconButton>
<AccountCircle fontSize="small" />
</IconButton>
</Box>
<HoverPopover
{...bindPopover(popupState)}
anchorOrigin={{
vertical: "bottom",
horizontal: "center",
}}
transformOrigin={{
vertical: "top",
horizontal: "center",
}}
>
<nav aria-label="main mailbox folders">
<List>
<ListItem disablePadding>
<ListItemButton>
<ListItemIcon sx={{ marginRight: 1, minWidth: 0 }}>
<Inbox fontSize="small" />
</ListItemIcon>
<ListItemText primaryTypographyProps={{fontSize: '.875rem'}} primary="Inbox" />
</ListItemButton>
</ListItem>
<ListItem
disablePadding
onClick={() => {
navigate("/configuration");
}}
>
<ListItemButton>
<ListItemIcon sx={{ marginRight: 1, minWidth: 0 }}>
<Settings fontSize="small" />
</ListItemIcon>
<ListItemText primaryTypographyProps={{fontSize: '.875rem'}} primary="Configuration" />
</ListItemButton>
</ListItem>
<ListItem
disablePadding
onClick={() => {
navigate("/login");
}} >
<ListItemButton>
<ListItemIcon sx={{ marginRight: 1, minWidth: 0 }}>
<ExitToApp fontSize="small" />
</ListItemIcon>
<ListItemText primaryTypographyProps={{fontSize: '.875rem'}} primary="Logout" />
</ListItemButton>
</ListItem>
</List>
</nav>
</HoverPopover>
</>
)}
</PopupState>
</Toolbar>
</AppBar>
);
};
export default TopBar; |
package org.broadinstitute.ddp.environment;
import java.io.IOException;
import java.net.InetAddress;
import java.net.UnknownHostException;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.fluent.Request;
import org.apache.http.util.EntityUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class HostUtil {
private static final Logger LOG = LoggerFactory.getLogger(HostUtil.class);
// required header for accessing google's VM metadata
public static final String METADATA_FLAVOR_HEADER_VALUE = "Google";
public static final String METADATA_FLAVOR_HEADER = "Metadata-Flavor";
private static String hostName;
public static final String GOOGLE_VM_NAME_METADATA_URL = "http://metadata.google"
+ ".internal/computeMetadata/v1/instance/name";
private static String lookupGoogleVMName() {
String vmName = null;
try {
HttpResponse httpResponse = Request.Get(GOOGLE_VM_NAME_METADATA_URL)
.addHeader(METADATA_FLAVOR_HEADER, METADATA_FLAVOR_HEADER_VALUE)
.execute().returnResponse();
int statusCode = httpResponse.getStatusLine().getStatusCode();
String metadataTextResponse = EntityUtils.toString(httpResponse.getEntity());
if (statusCode == HttpStatus.SC_OK) {
vmName = metadataTextResponse;
} else {
LOG.warn("Attempt to lookup google VM returned " + statusCode + ": " + metadataTextResponse);
}
} catch (IOException e) {
LOG.warn("Could not lookup google VM name; hopefully this isn't running in a google VM.", e);
}
return vmName;
}
/**
* If running in google, returns the google VM name. Otherwise, returns
* the host's name.
*/
public static synchronized String getHostName() {
if (hostName == null) {
hostName = lookupGoogleVMName();
if (hostName == null) {
try {
hostName = InetAddress.getLocalHost().getHostName();
} catch (UnknownHostException hostNameException) {
LOG.warn("Could not resolve hostname", hostNameException);
hostName = "unknown";
}
}
}
return hostName;
}
} |
---
title: revalidatePath
description: API Reference for the revalidatePath function.
---
`revalidatePath` allows you to purge [cached data](/docs/app/building-your-application/caching) on-demand for a specific path.
> **Good to know**:
>
> - `revalidatePath` is available in both [Node.js and Edge runtimes](/docs/app/building-your-application/rendering/edge-and-nodejs-runtimes).
> - `revalidatePath` only invalidates the cache when the included path is next visited. This means calling `revalidatePath` with a dynamic route segment will not immediately trigger many revalidations at once. The invalidation only happens when the path is next visited.
> - Currently, `revalidatePath` invalidates all the routes in the [client-side Router Cache](/docs/app/building-your-application/caching#router-cache). This behavior is temporary and will be updated in the future to apply only to the specific path.
> - Using `revalidatePath` invalidates **only the specific path** in the [server-side Route Cache](/docs/app/building-your-application/caching#full-route-cache).
## Parameters
```tsx
revalidatePath(path: string, type?: 'page' | 'layout'): void;
```
- `path`: Either a string representing the filesystem path associated with the data you want to revalidate (for example, `/product/[slug]/page`), or the literal route segment (for example, `/product/123`). Must be less than 1024 characters. This value is case-sensitive.
- `type`: (optional) `'page'` or `'layout'` string to change the type of path to revalidate. If `path` contains a dynamic segment (for example, `/product/[slug]/page`), this parameter is required.
## Returns
`revalidatePath` does not return any value.
## Examples
### Revalidating A Specific URL
```ts
import { revalidatePath } from 'next/cache'
revalidatePath('/blog/post-1')
```
This will revalidate one specific URL on the next page visit.
### Revalidating A Page Path
```ts
import { revalidatePath } from 'next/cache'
revalidatePath('/blog/[slug]', 'page')
// or with route groups
revalidatePath('/(main)/post/[slug]', 'page')
```
This will revalidate any URL that matches the provided `page` file on the next page visit. This will _not_ invalidate pages beneath the specific page. For example, `/blog/[slug]` won't invalidate `/blog/[slug]/[author]`.
### Revalidating A Layout Path
```ts
import { revalidatePath } from 'next/cache'
revalidatePath('/blog/[slug]', 'layout')
// or with route groups
revalidatePath('/(main)/post/[slug]', 'layout')
```
This will revalidate any URL that matches the provided `layout` file on the next page visit. This will cause pages beneath with the same layout to revalidate on the next visit. For example, in the above case, `/blog/[slug]/[another]` would also revalidate on the next visit.
### Revalidating All Data
```ts
import { revalidatePath } from 'next/cache'
revalidatePath('/', 'layout')
```
This will purge the Client-side Router Cache, and revalidate the Data Cache on the next page visit.
### Server Action
```ts filename="app/actions.ts" switcher
'use server'
import { revalidatePath } from 'next/cache'
export default async function submit() {
await submitForm()
revalidatePath('/')
}
```
### Route Handler
```ts filename="app/api/revalidate/route.ts" switcher
import { revalidatePath } from 'next/cache'
import { NextRequest } from 'next/server'
export async function GET(request: NextRequest) {
const path = request.nextUrl.searchParams.get('path')
if (path) {
revalidatePath(path)
return Response.json({ revalidated: true, now: Date.now() })
}
return Response.json({
revalidated: false,
now: Date.now(),
message: 'Missing path to revalidate',
})
}
```
```js filename="app/api/revalidate/route.js" switcher
import { revalidatePath } from 'next/cache'
export async function GET(request) {
const path = request.nextUrl.searchParams.get('path')
if (path) {
revalidatePath(path)
return Response.json({ revalidated: true, now: Date.now() })
}
return Response.json({
revalidated: false,
now: Date.now(),
message: 'Missing path to revalidate',
})
}
``` |
import pandas as pd
def output_text_console(text):
"""
Function to output text to the console.
Parameters:
text (str): The text to be output.
Returns:
None
"""
print("Output text: ", text)
def write_file_builtin(text, file_path):
"""
Function to write to a file using Python's built-in capabilities.
Parameters:
text (str or pandas.DataFrame): The text or DataFrame to be written to the file.
file_path (str): The path to the file to be written.
Returns:
None
"""
if isinstance(text, str):
with open(file_path, 'w', newline='') as file:
file.write(text)
elif isinstance(text, pd.DataFrame):
with open(file_path, 'w', newline='') as file:
column_names = text.columns.tolist()
file.write(','.join(column_names) + '\n')
for index, row in text.iterrows():
file.write(','.join(map(str, row)) + '\n')
else:
raise ValueError("Unsupported type for text. Supported types are str and pandas.DataFrame.") |
import React from 'react';
import { useWallets } from '@rango-dev/wallets-react';
function Wallets() {
const { connect, providers, state, disconnect } = useWallets();
const list = Object.keys(providers());
return (
<div>
<h3>Available Wallets</h3>
<div className="wallets">
{list.map((type) => {
const wallet_type = type;
const wallet_state = state(wallet_type);
return (
<div className="wallet">
<h5>{wallet_type}</h5>
<p>Address: {wallet_state.accounts?.join(',')}</p>
<button
onClick={() => {
if (wallet_state.connected) {
disconnect(wallet_type);
} else {
connect(wallet_type);
}
}}>
{wallet_state.connected ? 'Disconnect' : 'Connect'}
</button>
</div>
);
})}
</div>
</div>
);
}
export { Wallets }; |
import {
Button,
Flex,
Stack
} from "@mantine/core"
import React from "react"
import ImageContext from "./ImgCtx"
export type PixelateImgProps = {
src: string
width?: number
height?: number
pixelSize?: number
centered?: boolean
fillTransparencyColor?: string
scrl: () => void
clbck?: (pixelArray: number[])=>void
}
const PixelateImg = ({
src,
width,
height,
pixelSize = 5,
centered,
fillTransparencyColor,
scrl,
clbck
}: PixelateImgProps) => {
const [pixelArray, setPixelArray] = React.useState([] as number[]);
const canvasRef = React.useRef<HTMLCanvasElement>()
const { setImg, applyImg } = React.useContext(ImageContext);
React.useEffect(() => {
const paintPixels = (
ctx: CanvasRenderingContext2D,
img: HTMLImageElement,
pixelSize?: number,
centered?: boolean,
fillTransparencyColor?: string
) => {
let pixelArray: Array<Array<number>> = [];
if (pixelSize !== undefined && Number.isFinite(pixelSize) && pixelSize > 0) {
for (let x = 0; x < img.width + pixelSize; x += pixelSize) {
for (let y = 0; y < img.height + pixelSize; y += pixelSize) {
let xColorPick = x
let yColorPick = y
if (x >= img.width) {
xColorPick = x - (pixelSize - (img.width % pixelSize) / 2) + 1
}
if (y >= img.height) {
yColorPick = y - (pixelSize - (img.height % pixelSize) / 2) + 1
}
const rgba = ctx.getImageData(xColorPick, yColorPick, 1, 1).data
const clr = rgba[3] === 0 ? fillTransparencyColor || "#ffffff"
: `rgba(${rgba[0]},${rgba[1]},${rgba[2]},${rgba[3]})`
ctx.fillStyle = clr;
if (x !== img.width && y !== img.height)
{
if (x / pixelSize >= pixelArray.length)
pixelArray.push([]);
pixelArray[x / pixelSize].push((rgba[0] << 16) + (rgba[1] << 8) + (rgba[2]));
}
if (centered) {
ctx.fillRect(
Math.floor(x - (pixelSize - (img.width % pixelSize) / 2)),
Math.floor(y - (pixelSize - (img.height % pixelSize) / 2)),
pixelSize,
pixelSize
)
} else {
ctx.fillRect(x, y, pixelSize, pixelSize)
}
let resArray: number[] = [];
for (let col in pixelArray[0]) {
for (let row of pixelArray) {
resArray.push(row[col]);
}
}
setPixelArray([...resArray])
}
}
}
}
const pixelate = ({
src,
width,
height,
pixelSize,
centered,
scrl,
fillTransparencyColor
}: PixelateImgProps) => {
let img: HTMLImageElement | undefined = new Image()
img.crossOrigin = "anonymous"
img.src = src
img.onload = () => {
const canvas: HTMLCanvasElement | undefined = canvasRef?.current
if (canvas && img) {
const ctx = canvas.getContext("2d") as CanvasRenderingContext2D
img.width = width ? width : img.width
img.height = height ? height : img.height
canvas.width = img.width
canvas.height = img.height
ctx.drawImage(img, 0, 0, img.width, img.height)
paintPixels(ctx, img, pixelSize, centered, fillTransparencyColor)
img = undefined
}
}
};
pixelate({
src,
width,
height,
pixelSize,
centered,
scrl,
fillTransparencyColor
})
}, [src, width, height, pixelSize, centered, fillTransparencyColor, scrl])
return (
<Stack>
<canvas ref={canvasRef as React.MutableRefObject<HTMLCanvasElement>} />
<Flex gap={20}>
<Button
fullWidth
ml={10}
onClick={()=>{
if (clbck) {
clbck(pixelArray);
}
}}
>
Send
</Button>
<Button
mr={10}
onClick={()=>{
setImg([...pixelArray.map(
val=>"#" + ("00000" + val.toString(16)).slice(-6)
)]);
applyImg();
scrl();
}}
fullWidth
>
Open in editor
</Button>
</Flex>
</Stack>
)
}
export default PixelateImg; |
๏ปฟusing System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Text.Json.Nodes;
using System.Threading.Tasks;
using Utilities.Communication;
namespace DoctorWPFApp.Networking
{
/// <summary>
/// Can format messages to send to the server. This hides the implementation of
/// for example the command and data field.
/// </summary>
class DoctorFormat
{
public static JsonObject BaseMessage(string command)
{
return BaseMessage(command, new JsonObject());
}
public static JsonObject BaseMessage(string command, JsonObject data)
{
return new JsonObject
{
{ "command", command },
{ "data", data }
};
}
public static JsonObject LoginMessage(string username, string password)
{
return BaseMessage("login", new JsonObject
{
{ "username", username },
{ "password", password }
});
}
public static JsonObject GetPatientsMessage()
{
return BaseMessage("session/getUsers", new JsonObject());
}
public static JsonObject SessionStartMessage(string patientName)
{
return BaseMessage("session/start", new JsonObject() { { "username", patientName } });
}
public static JsonObject SessionStopMessage(string patientName)
{
return BaseMessage("session/stop", new JsonObject() { { "username", patientName } });
}
//public static JsonObject SessionPauseMessage()
//{
// return BaseMessage("session/pause");
//}
//public static JsonObject SessionResumeMessage()
//{
// return BaseMessage("session/resume");
//}
public static JsonObject StatsSummaryMessage(string patientName)
{
return BaseMessage("stats/summary", new JsonObject() { { "username", patientName } });
}
public static JsonObject ChatsSendMessage(string chatMessage, string patientName)
{
return BaseMessage("chats/send", new JsonObject
{
{ "message", chatMessage },
{ "username", patientName }
});
}
public static JsonObject SetResistanceMessage(int value, string patientName)
{
return BaseMessage("resistance/set", new JsonObject() { { "value", value }, { "username", patientName } });
}
/// <summary>
/// Gets a key in a JsonObject (cannot retrieve nested keys).
/// </summary>
/// <param name="key">The name of the key to look for.</param>
/// <returns>The value of the given key, otherwise a CommunicationException.</returns>
public static JsonNode GetKey(JsonObject dataObject, string key)
{
if (dataObject.ContainsKey(key))
return dataObject[key];
else
throw new CommunicationException($"The message did not contain the JSON key '{key}'.");
}
/// <summary>
/// Checks if the correct command is received and returns the value of the given key in a JsonObject (cannot retrieve nested keys).
/// </summary>
/// <param name="expectedCommand">The command expected to be contained by the received message.</param>
/// <param name="keys">The name of the key to look for.</param>
/// <returns>The value of the given key, otherwise a CommunicationException.</returns>
public static JsonNode[] GetKeys(JsonObject fullMessage, string expectedCommand, params string[] keys)
{
JsonObject dataObject = GetDataObject(fullMessage, expectedCommand);
List<JsonNode> nodes = new List<JsonNode>();
foreach (var key in keys)
{
if (dataObject.ContainsKey(key))
nodes.Add(dataObject[key]);
else
throw new CommunicationException($"The message did not contain the JSON key '{key}'.");
}
return nodes.ToArray();
}
/// <summary>
/// Checks if the correct command is received and returns the data field as a JsonObject.
/// </summary>
/// <param name="expectedCommand">The command expected to be contained by the received message.</param>
public static JsonObject GetDataObject(JsonObject receivedMessage, string expectedCommand)
{
// Check if the message has a command field
if (!receivedMessage.ContainsKey("command"))
throw new CommunicationException("The message did not contain the JSON key 'command'.");
// Check if the message has a data field
if (!receivedMessage.ContainsKey("data"))
throw new CommunicationException("The message did not contain the JSON key 'data'.");
// Validate command field
string receivedCommand = receivedMessage["command"].ToString();
if (!expectedCommand.Equals("") && !receivedCommand.Equals(expectedCommand))
throw new CommunicationException($"Expected command '{expectedCommand}' but received '{receivedCommand}'.");
JsonObject dataObject = receivedMessage["data"].AsObject();
return dataObject;
}
}
} |
import time
import pandas as pd
from scipy.sparse import csr_matrix
from utils import get_prime_map_from_rel, get_sparsity, load_data, set_all_seeds
set_all_seeds(42)
# Path to projects
project_to_path = {
"codex-s": "./data/codex-s/",
"WN18RR": "./data/WN18RR/",
"FB15k-237": "./data/FB15k-237/",
"YAGO3-10-DR": "./data/YAGO3-10-DR/",
"hetionet": "./data/Hetionet/hetionet-v1.0-edges.tsv",
}
res = []
max_order = 5
for project_name, path in project_to_path.items():
print(project_name)
# speciic loaders for hetionet
if project_name == "hetionet":
df_train = pd.read_csv(path, sep="\t")
df_train.dropna(inplace=True)
df_train.columns = ["head", "rel", "tail"]
else:
_, df_train, _, _, _ = load_data(path, project_name, add_inverse_edges="NO")
# Statistics
unique_rels = sorted(list(df_train["rel"].unique()))
unique_nodes = sorted(
set(df_train["head"].values.tolist() + df_train["tail"].values.tolist())
)
print(
f"# of unique rels: {len(unique_rels)} \t | # of unique nodes: {len(unique_nodes)}"
)
node2id = {}
id2node = {}
for i, node in enumerate(unique_nodes):
node2id[node] = i
id2node[i] = node
time_s = time.time()
# Map the relations to primes
rel2id, id2rel = get_prime_map_from_rel(
unique_rels, starting_value=2, spacing_strategy="step_1"
)
# Create the adjacency matrix
df_train["rel_mapped"] = df_train["rel"].map(rel2id)
df_train["head_mapped"] = df_train["head"].map(node2id)
df_train["tail_mapped"] = df_train["tail"].map(node2id)
A_big = csr_matrix(
(df_train["rel_mapped"], (df_train["head_mapped"], df_train["tail_mapped"])),
shape=(len(unique_nodes), len(unique_nodes)),
)
# Calculate sparsity
sparsity = get_sparsity(A_big)
print(A_big.shape, f"Sparsity: {sparsity:.2f} %")
time_prev = time.time()
time_setup = time_prev - time_s
print(f"Total setup: {time_setup:.5f} secs ({time_setup/60:.2f} mins)")
# Generate the PAM^k matrices
power_A = [A_big]
for ii in range(1, max_order):
updated_power = power_A[-1] * A_big
updated_power.sort_indices()
updated_power.eliminate_zeros()
power_A.append(updated_power)
print(
f"Sparsity {ii + 1}-hop: {100 * (1 - updated_power.nnz/(updated_power.shape[0]**2)):.2f} %"
)
time_stop = time.time()
time_calc = time_stop - time_prev
print(f"A^k calc time: {time_calc:.5f} secs ({time_calc/60:.2f} mins)")
time_all = time_stop - time_s
print(f"All time: {time_all:.5f} secs ({time_all/60:.2f} mins)")
res.append(
{
"dataset": project_name,
"nodes": len(unique_nodes),
"rels": len(unique_rels),
"edges": df_train.shape[0],
"sparsity_start": sparsity,
"sparsity_end": get_sparsity(power_A[-1]),
"setup_time": time_setup,
"cacl_time": time_calc,
"total_time": time_all,
# 'total_time_plus': time_setup + time_calc
}
)
print(res[-1])
# break
print("\n\n")
res = pd.DataFrame(res)
print(res.to_string()) |
package model;
import gui.*;
import org.mindrot.jbcrypt.BCrypt;
import javax.xml.parsers.ParserConfigurationException;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class App {
private final String filename = "usuarios.bin";
private Users users;
private Session session;
private FileHandler fhandler;
public App() throws IOException {
session = new Session();
fhandler = new FileHandler(filename);
users = fhandler.load();
showLoginWindow();
}
public boolean login(String userName, String passwd) {
if (users.userExists(userName)) {
User usuario = users.getUserByName(userName);
//String hash = usuario.getPasswordHash();
System.out.println(usuario);
if(usuario.checkLogin(passwd)) {
System.out.println("Logged in");
session.setCurrentUser(users.getUserByName(userName));
session.logSessionEvent("LOGIN");
return true;
}
}
System.out.println("Log In Failed");
return false;
}
/*public void openUserWindow() {
gui.User userWindow = new gui.User(this, currUser.getName());
userWindow.setVisible(true);
}*/
public void showUserDetailsWindow() {
String name = session.getCurrentUser().getName();
String age = session.getCurrentUser().getAge();
String mail = session.getCurrentUser().getEmail();
UserDetails userDetailsWindow = new UserDetails(this, name, age, mail);
userDetailsWindow.setVisible(true);
}
public void exportXmlCurrUser(File file) throws ParserConfigurationException {
XML.exportXmlUser(session.getCurrentUser(), file);
}
public void exportToJson(File file) {
JSON.exportToJson(session.getCurrentUser(), file);
}
public void showChangePasswordWindow() {
UserChangePassword userChangePasswordWindow = new UserChangePassword(this, session.getCurrentUser().getName());
userChangePasswordWindow.setVisible(true);
}
public void changePassword(String newPassword) {
String hashedPassword = BCrypt.hashpw(newPassword, BCrypt.gensalt(10));
session.getCurrentUser().setPasswordHash(hashedPassword);
fhandler.saveUsers(users);
}
public void closeSession() {
session.logSessionEvent("LOGOUT");
session.setCurrentUser(null);
Login loginWindow = new Login(this);
loginWindow.setVisible(true);
}
public Session getSession() {
return session;
}
public void createUserWindow() {
UserCreate createUserWindow = new UserCreate(this);
createUserWindow.setVisible(true);
}
public void createUser(String name, String passwd, String age, String mail) {
User newUser = new User(name, passwd, age, mail);
users.addUser(newUser);
fhandler.saveUsers(users);
}
public void deleteUserWindow() {
UserDelete deleteUserWindow = new UserDelete(this, session.getCurrentUser().getName());
deleteUserWindow.setVisible(true);
}
public void delUser() {
users.deleteUser(session.getCurrentUser().getName());
fhandler.saveUsers(users);
}
public void showUserWindow() {
gui.User userWindow = new gui.User(this, session.getCurrentUser().getName());
userWindow.setVisible(true);
}
public void showLoginWindow() {
Login loginWindow = new Login(this);
loginWindow.setVisible(true);
}
public void exportAllUsersToXML(File file) throws ParserConfigurationException {
XML.exportAllUsersXML(users, file);
}
public void exportAllUsersToJSON(File file) {
JSON.exportAllUsersJSON(users, file);
}
public void zipExport (File file) throws ParserConfigurationException, IOException {
ZIP.exportAllUsersZip(this, file);
}
} |
# Templating
This section describes the platform reference implementation templating process.
All the readme files prefixed with `tpl_` will be parametrized and added to the user's GitOps repository.
All the values in files templated with - `<key>` under
`/platform` folder will be replaced with corresponding values generated by the system during the setup process.
In terraform files (`*.tf`) sections to be templated are also prefixed with comment symbol (`#`),
e.g. `# <key>` for integrity.
## Input values placeholder reference
Templating variables reference to input parameters
- `CLOUD_PROVIDER` - Cloud provider type
- `CLOUD_REGION` - Cloud region, replaced by cloud provider default when not specified
- `PRIMARY_CLUSTER_NAME` - Primary K8s cluster name
- `DOMAIN_NAME` - Domain name used for the deployment
- `OWNER_EMAIL` - Deployment owner URL, used for alerting
- `GIT_ORGANIZATION_NAME` - Git Organisation name
- `GIT_PROVIDER` - Git provider type
- `GITOPS_REPOSITORY_NAME` - Platform GitOps repository name
## Generated
Templating variables generated during setup process
### IAM roles
IAM roles for core components created during the setup process
- `CERT_MANAGER_IAM_ROLE_RN` - Certificate Manager IAM role for a K8s service account
- `CI_IAM_ROLE_RN` - Continuous Integration (Argo Workflow / Git* runners) IAM role for K8s service account
- `EXTERNAL_DNS_IAM_ROLE_RN` - External DNS IAM role for a K8s service account
- `IAC_PR_AUTOMATION_IAM_ROLE_RN` - IaC PR automation IAM role for a K8s service account
- `SECRET_MANAGER_IAM_ROLE_RN` - Secrets Manager (Vault) IAM role for a K8s service account
### Ingress
Ingress URLs for core components. Note!: URL does not contain protocol prefix
- `CC_CLUSTER_FQDN` - Primary K8s cluster FQDN
- `CD_INGRESS_URL` - Continuous Delivery (ArgoCD) ingress URL
- `CI_INGRESS_URL` - Continuous Integration (Argo Workflow) ingress URL
- `GRAFANA_INGRESS_URL` - Metrics / Logs visualization system (Grafana) ingress URL
- `IAC_PR_AUTOMATION_INGRESS_URL` - IaC PR automation (Atlantis) ingress URL
- `REGISTRY_INGRESS_URL` - Registry (Harbor) ingress URL
- `REGISTRY_REGISTRY_URL` - Registry (Harbor) entrypoint
- `SECRET_MANAGER_INGRESS_URL` - Secrets Manager (Vault) ingress URL
- `CODE_QUALITY_INGRESS_URL` - Code Quality (SonarQube) ingress URL
### Git
- `GIT_REPOSITORY_GIT_URL` - Platform GitOps repository Git URL
- `GIT_REPOSITORY_ROOT` - Git organisation root
- `GIT_REPOSITORY_URL` - Platform GitOps repository Http URL
- `GIT_USER_NAME` - Git machine user name used by Platform
- `GIT_USER_LOGIN` - Git machine user login used by Platform
- `IAC_PR_AUTOMATION_WEBHOOK_SECRET` - IaC PR automation (Atlantis) webhook secret
- `IAC_PR_AUTOMATION_WEBHOOK_URL` - IaC PR automation (Atlantis) webhook
- `VCS_BOT_SSH_PUBLIC_KEY` - Git machine user SSH public key
### OIDC
OIDC provider configuration Note!: URL does not contain protocol prefix
- `OIDC_PROVIDER_AUTHORIZE_URL` OIDC provider (Vault) authorise URL
- `OIDC_PROVIDER_TOKEN_URL` - OIDC provider (Vault) token URL
- `OIDC_PROVIDER_URL` - OIDC provider (Vault) URL
- `OIDC_PROVIDER_USERINFO_URL` - OIDC provider (Vault) user info URL
- `CD_OAUTH_CALLBACK_URL` - Continuous Delivery (ArgoCD) OAuth callback URL
- `CI_OAUTH_CALLBACK_URL` - Continuous Integration (Argo Workflow) OAuth callback URL
### K8s
- `CC_CLUSTER_SSH_PUBLIC_KEY` Primary K8s cluster SSH public key
- `K8S_ROLE_MAPPING` - K8s service account IAM role mapping attribute, cloud provider specific
- `KUBECTL_VERSION` - kubectl version
### Terraform snippets
- `GIT_PROVIDER_MODULE` - Terraform Git provider definition
- `TF_HOSTING_PROVIDER` - Terraform Cloud provider definition, eg AWS, Azure, GCP
- `TF_HOSTING_REMOTE_BACKEND` - Terraform state storage backend definition for cloud infrastructure, cloud provider
specific
- `TF_SECRETS_REMOTE_BACKEND`- Terraform state storage backend definition for secrets, cloud provider specific
- `TF_USERS_REMOTE_BACKEND` - Terraform state storage backend definition for users, cloud provider specific
- `TF_VCS_REMOTE_BACKEND`- Terraform state storage backend definition for version control (Git), cloud provider specific
### Manifest snippets
- `SECRET_MANAGER_SEAL` - Secret Manager (Vault) seal configuration
### Cloud
- `CLOUD_ACCOUNT` - Cloud account number, e.g., AWS account number, cloud provider specific
- `CLOUD_BINARY_ARTIFACTS_STORE` - Continuous Integration (Argo Workflow) Artifact Repository, cloud provider specific
- `NETWORK_ID` - Platform primary K8s cluster network ID
- `SECRET_MANAGER_SEAL_RN` - Secrets Manager (Vault) seal key ID
## Internal params
- `ARGOCD_PASSWORD` - Continuous Delivery (ArgoCD) admin password
- `ARGOCD_TOKEN` - Continuous Delivery (ArgoCD) admin token
- `ARGOCD_USER` - Continuous Delivery (ArgoCD) admin username
- `CC_CLUSTER_CA_CERT_DATA` - K8s cluster Certificate Authority certificate data
- `CC_CLUSTER_CA_CERT_PATH` - K8s cluster Certificate Authority certificate path
- `CC_CLUSTER_ENDPOINT` - Primary K8s cluster admin API endpoint
- `CLUSTER_SSH_PRIVATE_KEY` - K8s cluster SSH private key
- `CLUSTER_SSH_PRIVATE_KEY_PATH` - K8s cluster SSH private key path
- `CLUSTER_SSH_PUBLIC_KEY_PATH` - K8s cluster SSH public key path
- `DEFAULT_SSH_PRIVATE_KEY` - Default platform SSH private key
- `DEFAULT_SSH_PRIVATE_KEY_PATH` - Default platform SSH private key path
- `DEFAULT_SSH_PUBLIC_KEY` - Default platform SSH public key
- `DEFAULT_SSH_PUBLIC_KEY_PATH` - Default platform SSH public key path
- `GIT_ACCESS_TOKEN` - Git access token
- `GIT_USER_EMAIL` - Git machine user email
- `GIT_USER_LOGIN` - Git machine user login
- `GIT_USER_NAME` - Git machine user name
- `KCTL_CONFIG_PATH` - Primary K8s cluster kubectl config path
- `REGISTRY_ROBO_USER` - Registry (Harbor) machine username
- `REGISTRY_ROBO_USER_AUTH` - Registry (Harbor) auth string
- `REGISTRY_ROBO_USER_PASSWORD` - Registry (Harbor) machine user password
- `TF_BACKEND_STORAGE_NAME` - Terraform state storage backend location
- `VAULT_ROOT_TOKEN` - Secrets Manager (Vault) root access token |
import axios from "axios";
import React, { useEffect, useState } from "react";
import Card from "@mui/material/Card";
import CardActions from "@mui/material/CardActions";
import CardContent from "@mui/material/CardContent";
import CardMedia from "@mui/material/CardMedia";
import Button from "@mui/material/Button";
import Typography from "@mui/material/Typography";
import { Navigate, json, useNavigate } from "react-router-dom";
import Appbar from "./Appbar";
function ShowCourses() {
const [courses, setCourses] = useState([]);
const headers = {
Authorization: "Bearer " + localStorage.getItem("token"),
};
useEffect(() => {
axios
.get("http://localhost:3000/admin/courses", { headers })
.then((resp) => {
setCourses(resp.data.courses);
});
}, []);
return (
<>
<div
style={{
width: "100vw",
height: "100vh",
backgroundColor: "#eeeeee",
}}
>
<div>
<Appbar />
</div>
<div
style={{
display: "flex",
flexWrap: "wrap",
justifyContent: "center",
}}
>
{courses.map((course) => (
<Course
image={course.image}
title={course.title}
description={course.description}
key={course.id}
id={course.id}
/>
))}
</div>
</div>
</>
);
}
function Course({ image, title, description, id }) {
const navigate = useNavigate();
return (
<div
style={{
margin: 10,
}}
>
<Card sx={{ maxWidth: 400 }} variant="outlined">
<CardMedia
sx={{
height: 200,
width: 250,
margin: 0.5,
}}
image={image}
title={title}
onClick={() => {
navigate(`/course/${id}`);
}}
/>
<CardContent>
<Typography gutterBottom variant="h5" component="div">
{title}
</Typography>
<Typography variant="body2" color="text.secondary">
{description}
</Typography>
</CardContent>
<CardActions>
<Button variant="text" size="medium">
Delete
</Button>
</CardActions>
</Card>
</div>
);
}
export default ShowCourses; |
<!doctype html>
<html>
<head>
<title>simple plage</title>
<style>
:root{
--rotate: -26deg;
/* to make it responsive */
--unit: 1vmin;
--zoom: 160;
--workspace-min: 768; /* device screen height = 768px */
--upx: calc(var(--zoom) * (var(--unit) / var(--workspace-min))); /* upx = units per pixel */
/* DNA Helix loop */
--animation-speed: 1;
--helix-duration: 0.5s;
--helix-iteration-factor: 0.05s;
/* DNA model */
--dna-max-height: calc(140 * var(--upx));
--dna-nitrogenous-base-width: calc(6 * var(--upx));
--dna-nitrogenous-base-margin: calc(8 * var(--upx));
--dna-sugar-diameter: calc(15 * var(--upx));
--dna-sugar-border-size: calc(6 * var(--upx));
/* colors */
--color-background: #151515;
--color-sugar: #f2f1d5;
--color-A: #ffba36;
--color-T: #26f826;
--color-C: #439AD9;
--color-G: #E96451;
}
/* rotate the dna container for smart mobile devices */
@media (orientation: portrait){
:root{
--rotate: -58deg;
}
}
/* to make things look pretty */
html, body{
padding: 0;
margin: 0;
width: 100%;
height: 100%;
display: block;
}
body{
background: var(--color-background);
overflow: auto;
}
html{
scrollbar-width: thin;
scrollbar-color: rgba(255,255,255,0.4) rgba(0,0,0,0);
}
/* align the DNA to middle */
.wrapper{
position: absolute;
left: 50%;
top: 50%;
transform: translate(-50%, -50%) rotate(var(--rotate));
transform-origin: 50% 50%;
}
/* DNA is a flex container with n-double helix */
.dna{
display: flex;
align-items: center;
position: relative;
height: var(--dna-max-height);
}
/* helix (nitrogenous base) */
.dna > div{
font-size: 0;
position: relative;
width: var(--dna-nitrogenous-base-width);
height: 0;
background: linear-gradient(0deg, var(--color-G) 50%, var(--color-C) 50%);
display: inline-block;
margin: 0 var(--dna-nitrogenous-base-margin);
animation:
double-helix
ease
calc(var(--helix-duration) / var(--animation-speed))
calc((var(--i) * var(--helix-iteration-factor)) / var(--animation-speed))
alternate
infinite;
}
.dna > div:nth-child(2n){
background: linear-gradient(0deg, var(--color-A) 50%, var(--color-T) 50%);
}
@keyframes double-helix{
to{
height: var(--dna-max-height);
}
}
/* sugar */
.dna > div::before,
.dna > div::after{
content: "";
position: absolute;
left: 50%;
width: var(--dna-sugar-diameter);
height: var(--dna-sugar-diameter);
display: block;
border-radius: 50%;
background: var(--color-sugar);
border: var(--dna-sugar-border-size) solid var(--color-background);
box-sizing: content-box;
}
.dna > div::before{
top: 0;
transform: translate(-50%, -50%);
}
.dna > div::after{
bottom: 0;
transform: translate(-50%, 50%);
}
</style>
</head>
<body>
<div class="wrapper">
<div class="dna">
<div style="--i: 0">0</div>
<div style="--i: 1">1</div>
<div style="--i: 2">2</div>
<div style="--i: 3">3</div>
<div style="--i: 4">4</div>
<div style="--i: 5">5</div>
<div style="--i: 6">6</div>
<div style="--i: 7">7</div>
<div style="--i: 8">8</div>
<div style="--i: 9">9</div>
<div style="--i: 10">10</div>
<div style="--i: 11">11</div>
<div style="--i: 12">12</div>
<div style="--i: 13">13</div>
<div style="--i: 14">14</div>
<div style="--i: 15">15</div>
<div style="--i: 16">16</div>
<div style="--i: 17">17</div>
<div style="--i: 18">18</div>
<div style="--i: 19">19</div>
<div style="--i: 20">20</div>
<div style="--i: 21">21</div>
<div style="--i: 22">22</div>
<div style="--i: 23">23</div>
</div>
</div>
</body>
</html> |
// ้ฎ้ข:
// ๅฆๆ 1 ๅฏนๅ
ๅญๆฏๆ่ฝ็นๆฎ 1 ๅฏนๅฐๅ
ๅญ๏ผ่ๆฏๅฏนๅ
ๅญๅจๅฎๅบ็ๅ็็ฌฌ 3 ไธชๆ๏ผๅ่ฝๅผๅง็นๆฎ 1 ๅฏนๅฐๅ
ๅญใ
// ๅๅฎๅจไธๅ็ๆญปไบก็ๆ
ๅตไธ๏ผ็ฑ 1 ๅฏนๅ
ๅญๅผๅง๏ผ1 ๅนดๅ่ฝ็นๆฎๆๅคๅฐๅฏนๅ
ๅญ๏ผ
// ไบ่งฃ็้ฝ็ฅ้ๅ
ๅญๅจๅๆ็็นๆฎๆฐ็ฌฆๅ fibonacci ๆฐๅ๏ผไธ่ฟ่ฟ้ๅนถไธ็ดๆฅไป fibonacci ๆฐๅๅบๅ๏ผ่ๆฏ้็จ้ๆจๆ่ทฏ
// ่ฟฝ่ธชๆฏไธๅฏนๅ
ๅญๅจๅฝๆ็็นๆฎๆฐๆฏๆฏ่พๅฐ้พใๅฏไปฅๅฐๅ
ๅญๅไธบไธ็ฑป๏ผๅๆๆฏ็ฑปๅ
ๅญๅจๅฝๆ็็นๆฎๆฐใ
// ๅ
ๅญๅฏนๅฏไปฅๅไธบไธ็ฑป๏ผ1 ไธชๆๅคง็ๅ
ๅญ๏ผ2 ไธชๆๅคง็ๅ
ๅญ๏ผๅคงๅ
ๅญใ
// ๅ่ฎพ 1 ไธชๆๅคง็ๅ
ๅญๅฏนๆฐ้ไธบ x1, 2 ไธชๆๅคง็ๅ
ๅญๅฏนๆฐ้ไธบ x2, ๅคงๅ
ๅญๅฏนๆฐ้ไธบ x3
// x3 = x3 + x2
// x2 = x1
// x1 = x3
#include <stdio.h>
// n ไปฃ่กจๆๆฐ
int rabbit_breeding(int n)
{
int x1 = 1;
int x2 = 0;
int x3 = 0;
for (int i = 1; i <= n; i++) {
x3 = x3 + x2;
x2 = x1;
x1 = x3;
}
return x1 + x2 + x3;
}
int main()
{
for (int i = 1; i <= 12; i++) {
printf("็ฌฌ %d ไธชๆ็ๅ
ๅญๆฐ: %d\n", i, rabbit_breeding(i));
}
return 0;
} |
import * as service from '../services/productService.js';
export const getAll = async (req, res, next) => {
try{
const { limit, page, sort, query } = req.query;
const response = await service.getAll(limit, page, sort, query);
const next = response.hasNextPage ? `http://localhost:8080/users/all?page=${response.nextPage}` : null;
const prev = response.hasPrevPage ? `http://localhost:8080/users/all?page=${response.prevPage}` : null;
res.json({
payload: response.docs,
info: {
totalDocs: response.totalDocs,
totalPages: response.totalPages,
page: response.page,
prevPage: response.prevPage,
nextPage: response.nextPage,
prev,
next
}
});
}catch(error){
next(error.message);
}
}
export const getById = async (req, res, next) => {
try{
const { id } = req.params;
const response = await service.getById(id);
if(!response){
res.status(404).json({ msg: "Product not found!"});
}else{
res.status(200).json(response);
}
}catch(error){
next(error.message);
}
}
export const create = async (req, res, next) => {
try{
const newProd = await service.create(req.body);
if(!newProd){
throw new Error("Product creation error!");
}else{
res.json({data: newProd});
}
}catch(error){
next(error.message);
}
}
export const update = async (req, res, next) => {
try{
const { id } = req.params;
const prodUpd = await service.update(id, req.body);
if(!prodUpd){
res.status(404).json({ msg: 'Error with product update!'});
}else{
res.status(200).json(prodUpd);
}
}catch(error){
next(error.message);
}
}
export const remove = async (req, res, next) => {
try{
const { id } = req.params;
const prodDel = await service.remove(id);
if(!prodDel){
res.status(404).json({ msg: 'Error with product deletion!'});
}else{
res.status(200).json({ msg: `Product with id: ${id} deleted!`});
}
}catch(error){
next(error.message);
}
} |
*{
margin: 0;
padding: 0;
box-sizing: border-box;
}
body{
background: #f2f2f2;
font-family: Arial, Helvetica, sans-serif;
}
.contenedor{
width: 80%;
max-width: 700px;
height: 500px;
background: #212d40;
/* Los elementos hijos se hacen flexibles, similar a float:left */
display: flex;
/* FLEX-DIRECTION: define la direccion de las cajas dentro de un contenedor */
/* row, es por defecto */
/* flex-direction: row; */
/* flex-direction: row-reverse; */
/* flex-direction: column; */
/* flex-direction: column-reverse; */
flex-direction: row;
/* FLEX-WRAP: sirve para que las cajas se envuelvan en la siguiente linea
Utilizado para disenos flexibles y responsivos */
flex-wrap: wrap;
/* FLEX-FLOW: es la union de flex-direction y flex-wrap */
/* flex-flow: row wrap; */
/* JUSTIFY-CONTENT: Se usa para alinear horizontalmente las cajas dentro del contenedor */
/* Se usa con varias lineas de cajas */
/* justify-content: flex-start; */
/* justify-content: flex-end; */
/* justify-content: center; */
justify-content: space-between;
/* ALIGN-ITEMS: Se usa para alinear verticalmente las cajas dentro del contenedor */
/* para usar align-items, quitar el alto de la caja (alineacion vertical) */
/* align-items: flex-end; */
/* align-items: center; */
/* align-items: stretch; */
/* align-items: baseline; */
/* ALIGN-CONTENT: Se usa para alinear verticalmente las cajas dentro del contenedor */
/* align-content es lo mismo que align-items, ajusta las cajas al contenido */
/* align-content: flex-end; */
/* align-content: center; */
}
.caja{
width: 100px;
height: 100px;
background: #f79256;
text-align: center;
margin: 20px;
padding: 20px;
font-size: 40px;
color: #fff;
/* ORDER: Cambia el orden de las cajas o elementos */
/* order: 1; */
/* FLEX-GROW:Controla la capacidad de crecer de una caja o elemento mas alla de su tamano pred.
Es similar a establecer un porcentaje relativo. Util para disenos responsivos */
/* flex-grow: 1; */
/* FLEX-SHRINK: Controla la capacidad de reducirse de una caja o elemento dentro de un contenedor;
es lo contrario que flex-grow */
/* flex-shrink: 1 */
/* FLEX-BASIS: Estable el tamano base de las cajas o elementos antes de que se aplique cualquier ajuste de crecimiento o reduccion (flex-grow o flex-shrink) */
/* flex-basis: 200px */
/* FLEX: flex-grow + flex-shrink + flex-basis */
/* Permite que los elementos puedan ser flexibles, crecer o reducir segun sea necesario */
/* Util donde se desea que los elementos sean flexibles y se adapten a diferentes tamanos de pantallas y dispositivos */
flex: 1 1 auto;
}
/* .uno{
order:3;
}
.dos{
order: 2;
}
.tres{
order:1;
} */
/* .dos{
flex-shrink: 1;
flex-grow: 2; */
/* width: 200px; */
/* flex-basis, similar a width */
/* flex-basis: 200px;
flex:1 2 auto;
} */
.dos{
/* align-self: flex-end; */
/* align-self: center; */
/* strech, funciona junto con el height=auto */
align-self: stretch;
height: auto;
} |
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta http-equiv="Access-Control-Allow-Origin" content="*">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.4.0/css/font-awesome.min.css">
<title>
Velkommen til chatbot
</title>
<style class="cp-pen-styles">@import url(https://fonts.googleapis.com/css?family=Lato:400,700);
*, *:before, *:after {
box-sizing: border-box;
}
body {
background: #C5DDEB;
font: 14px/20px "Lato", Arial, sans-serif;
padding: 40px 0;
color: white;
}
.container {
margin: 0 auto;
width: 750px;
background: #444753;
border-radius: 5px;
}
.chat {
width: 100%;
float: left;
background: #F2F5F8;
border-top-right-radius: 5px;
border-bottom-right-radius: 5px;
color: #434651;
}
.chat .chat-header {
padding: 20px;
border-bottom: 2px solid white;
}
.chat .chat-header img {
float: left;
}
.chat .chat-header .chat-about {
float: left;
padding-left: 10px;
margin-top: 6px;
}
.chat .chat-header .chat-with {
font-weight: bold;
font-size: 16px;
}
.chat .chat-header .chat-num-messages {
color: #92959E;
}
.chat .chat-header .fa-star {
float: right;
color: #D8DADF;
font-size: 20px;
margin-top: 12px;
}
.chat .chat-history {
padding: 30px 30px 20px;
border-bottom: 2px solid white;
overflow-y: scroll;
height: 60%;
}
.chat .chat-history .message-data {
margin-bottom: 15px;
}
.chat .chat-history .message-data-time {
color: #a8aab1;
padding-left: 6px;
}
.chat .chat-history .message {
color: white;
padding: 18px 20px;
line-height: 26px;
font-size: 16px;
border-radius: 7px;
margin-bottom: 30px;
width: 90%;
position: relative;
}
.chat .chat-history .message:after {
bottom: 100%;
left: 7%;
border: solid transparent;
content: " ";
height: 0;
width: 0;
position: absolute;
pointer-events: none;
border-bottom-color: #86BB71;
border-width: 10px;
margin-left: -10px;
}
.chat .chat-history .my-message {
background: #86BB71;
}
.chat .chat-history .other-message {
background: #94C2ED;
}
.chat .chat-history .other-message:after {
border-bottom-color: #94C2ED;
left: 93%;
}
.chat .chat-message {
padding: 30px;
}
.chat .chat-message textarea {
width: 100%;
border: none;
padding: 10px 20px;
font: 14px/22px "Lato", Arial, sans-serif;
margin-bottom: 10px;
border-radius: 5px;
resize: none;
}
.chat .chat-message .fa-file-o, .chat .chat-message .fa-file-image-o {
font-size: 16px;
color: gray;
cursor: pointer;
}
.chat .chat-message button {
float: right;
color: #94C2ED;
font-size: 16px;
text-transform: uppercase;
border: none;
cursor: pointer;
font-weight: bold;
background: #F2F5F8;
}
.chat .chat-message button:hover {
color: #75b1e8;
}
.online, .offline, .me {
margin-right: 3px;
font-size: 10px;
}
.online {
color: #86BB71;
}
.offline {
color: #E38968;
}
.me {
color: #94C2ED;
}
.align-left {
text-align: left;
}
.align-right {
text-align: right;
}
.float-right {
float: right;
}
.clearfix:after {
visibility: hidden;
display: block;
font-size: 0;
content: " ";
clear: both;
height: 0;
}
.bot-image{
height:55px;
width:55px;
}
.message.my-message ul li {
background: #fff;
color: black;
margin: 8px 12px;
padding: 4px 15px;
border-radius: 10px;
text-align: center;
display: inline-block;
cursor: pointer;
}
img.response-image {
width: 100%;
}
</style>
</head>
<body>
<div class="container clearfix">
<div class="chat">
<div class="chat-header clearfix">
<img src="https://www.rebotify.com/style/img/bot.png" class="bot-image" alt="avatar">
<div class="chat-about">
<div class="chat-with">Chat med bot</div>
<div class="chat-num-messages"></div>
</div>
<i class="fa fa-star"></i>
</div>
<!-- end chat-header -->
<div class="chat-history">
<ul class="all">
<li>
<div class="message-data">
<span class="message-data-name"><i class="fa fa-circle online"></i> Bot</span>
<span class="message-data-time"><span id="current-time"></span>, I dag</span>
</div>
<div class="message my-message">
Hei der venner! Jeg er chatbot. Spรธr meg om at jeg skal prรธve รฅ svare.
</div>
</li>
</ul>
</div>
<!-- end chat-history -->
<div class="chat-message clearfix">
<textarea name="message-to-send" id="message-to-send" placeholder="Skriv inn meldingen din" rows="3"></textarea>
<i class="fa fa-file-o"></i>
<i class="fa fa-file-image-o"></i>
<button>Sende</button>
</div>
<!-- end chat-message -->
</div>
<!-- end chat -->
</div>
<!-- end container -->
<script id="message-template" type="text/x-handlebars-template">
<li class="clearfix">
<div class="message-data align-right">
<span class="message-data-time" >{{time}}, I dag</span>
<span class="message-data-name" >Du</span> <i class="fa fa-circle me"></i>
</div>
<div class="message other-message float-right">
{{messageOutput}}
</div>
</li>
</script>
<script id="message-response-template" type="text/x-handlebars-template">
<li>
<div class="message-data">
<span class="message-data-name"><i class="fa fa-circle online"></i> Bot</span>
<span class="message-data-time">{{time}}, I dag</span>
</div>
<div class="message my-message">
{{response}}
</div>
</li>
</script>
<script src="//static.codepen.io/assets/common/stopExecutionOnTimeout-41c52890748cd7143004e05d3c5f786c66b19939c4500ce446314d1748483e13.js"></script><script src="//cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script><script src="//cdnjs.cloudflare.com/ajax/libs/handlebars.js/3.0.0/handlebars.min.js"></script><script src="//cdnjs.cloudflare.com/ajax/libs/list.js/1.1.1/list.min.js"></script>
<script>(function () {
$(window).load(function(){
var currentdate = new Date();
var datetime = new Date().toLocaleString('en-US', { hour: 'numeric', minute: 'numeric', hour12: true });
$('#current-time').html(datetime);
});
var chat = {
messageToSend: '',
init: function () {
this.cacheDOM();
this.bindEvents();
this.render();
},
cacheDOM: function () {
this.$chatHistory = $('.chat-history');
this.$button = $('button');
this.$textarea = $('#message-to-send');
this.$chatHistoryList = this.$chatHistory.find('ul.all');
},
bindEvents: function () {
this.$button.on('click', this.addMessage.bind(this));
this.$textarea.on('keyup', this.addMessageEnter.bind(this));
},
render: function () {
this.scrollToBottom();
if (this.messageToSend.trim() !== '') {
var template = Handlebars.compile($("#message-template").html());
var context = {
messageOutput: this.messageToSend,
time: this.getCurrentTime()
};
this.$chatHistoryList.append(template(context));
this.scrollToBottom();
this.$textarea.val('');
// responses
var contextResponse = {};
var templateResponse = Handlebars.compile($("#message-response-template").html());
var responseMessage =
$.ajax({
type: 'GET',
url: 'http://18.191.166.225:8080/send',
data: { msg: this.messageToSend },
success: function(resultData) {
contextResponse = {
response: resultData,
time: new Date().toLocaleString('en-US', { hour: 'numeric', minute: 'numeric', hour12: true })
};
}
});
setTimeout(function () {
new_li = '<li><div class="message-data"><span class="message-data-name"><i class="fa fa-circle online"></i> Bot</span><span class="message-data-time">'+contextResponse.time+' I dag</span></div><div class="message my-message">'+contextResponse.response+'</div></li>';
this.$chatHistoryList.append(new_li);
this.scrollToBottom();
}.bind(this), 1500);
}
},
addMessage: function () {
this.messageToSend = this.$textarea.val();
this.render();
},
addMessageEnter: function (event) {
// enter was pressed
if (event.keyCode === 13) {
this.addMessage();
}
},
scrollToBottom: function () {
this.$chatHistory.scrollTop(this.$chatHistory[0].scrollHeight);
},
getCurrentTime: function () {
return new Date().toLocaleTimeString().
replace(/([\d]+:[\d]{2})(:[\d]{2})(.*)/, "$1$3");
},
getRandomItem: function (arr) {
return arr[Math.floor(Math.random() * arr.length)];
}
}
chat.init();
})();
function select_file(e){
get_file(e.type);
}
function get_file(file){
var template = Handlebars.compile($("#message-template").html());
var context = {
messageOutput: file,
time: new Date().toLocaleString('en-US', { hour: 'numeric', minute: 'numeric', hour12: true })
};
$('.chat-history').find('ul.all').append(template(context));
$('.chat-history').animate({scrollTop: $('.chat-history')[0].scrollHeight}, 500);
$.ajax({
type: 'GET',
url: 'http://18.191.166.225:8080/getfile',
data: { type: file },
success: function(resultData) {
new_response_li = '<li><div class="message-data"><span class="message-data-name"><i class="fa fa-circle online"></i> Bot</span><span class="message-data-time">'+new Date().toLocaleString('en-US', { hour: 'numeric', minute: 'numeric', hour12: true })+' I dag</span></div><div class="message my-message">'+resultData+'</div></li>';
$('.chat-history').find('ul.all').append(new_response_li);
$('.chat-history').animate({scrollTop: $('.chat-history')[0].scrollHeight}, 500);
}
});
}
</script>
</body>
</html> |
from django.test import TestCase, Client
from django.contrib.auth import get_user_model
from django.urls import reverse
class AdminTests(TestCase):
def setUp(self) -> None:
self.client = Client()
self.admin_user = get_user_model().objects.create_superuser(
email='admin@company.com', password='admin@1234')
self.client.force_login(self.admin_user)
self.user = get_user_model().objects.create_user(
email='user@company.com',
password='user@1234',
name='Test user full name')
def test_user_listed(self) -> None:
""" Test that users are listed on user page """
url = reverse('admin:core_user_changelist')
res = self.client.get(url)
self.assertContains(res, self.user.name)
self.assertContains(res, self.user.email)
def test_user_change_page(self) -> None:
""" Test that the user edit page works """
url = reverse('admin:core_user_change', args=[self.user.id])
# /admin/core/user/id
res = self.client.get(url)
self.assertEqual(res.status_code, 200)
def test_create_user_page(self):
""" Test that the create user page works """
url = reverse('admin:core_user_add')
res = self.client.get(url)
self.assertEqual(res.status_code, 200) |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Panda Commerce</title>
<!-- bootstrap stylesheet -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous">
<!-- custom stylesheet -->
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<!-- header starts -->
<header>
<!-- navigation starts -->
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<div class="container">
<a class="navbar-brand" href="#">
<img src="images/logo.png">
</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav"
aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link active" aria-current="page" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#shoe">Shoes</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#backpacks">Backpack</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#subscribe">Subscribe</a>
</li>
</ul>
</div>
</div>
</nav>
<!-- slider starts -->
<section class="container my-5">
<div id="carouselExampleControls" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner rounded-3">
<!-- slide 1 -->
<div class="carousel-item active">
<div class="row d-flex align-items-center panda-bg p-5">
<div class="col-6">
<h1>Cool Dude Headphone</h1>
<p>This is the best headphone in the world for people who just want to waste time in
front of funky world.</p>
<h1>$420</h1>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
<div class="col-6">
<img src="images/banner-images/headphone.png" class="d-block w-100" alt="...">
</div>
</div>
</div>
<!-- slide 2 -->
<div class="carousel-item">
<div class="row d-flex align-items-center panda-bg p-5">
<div class="col-6">
<h1>Cool Dude Headphone</h1>
<p>This is the best headphone in the world for people who just want to waste time in
front
of funky world.</p>
<h1>$420</h1>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
<div class="col-6">
<img src="images/banner-images/tv.png" class="d-block w-100" alt="...">
</div>
</div>
</div>
<!-- slide 3 -->
<div class="carousel-item">
<div class="row d-flex align-items-center panda-bg p-5">
<div class="col-6">
<h1>Cool Dude Headphone</h1>
<p>This is the best headphone in the world for people who just want to waste time in
front
of funky world.</p>
<h1>$420</h1>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
<div class="col-6">
<img src="images/banner-images/xbox.png" class="d-block w-100" alt="...">
</div>
</div>
</div>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleControls"
data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#carouselExampleControls"
data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
</section>
</header>
<main>
<!-- categories -->
<section class="container overflow-hidden categories">
<div class="row gy-4">
<!-- watch -->
<div class="col-lg-4 col-md-6">
<div
class="p-4 border cat1 text-white d-flex align-items-center justify-content-between rounded-3">
<h1>Watch</h1>
<img src="images/categories/watch.png" alt="">
</div>
</div>
<!-- bags -->
<div class="col-lg-4 col-md-6">
<div
class="p-4 border cat2 text-white d-flex align-items-center justify-content-between rounded-3">
<h1>Bags</h1>
<img src="images/categories/bag.png" alt="">
</div>
</div>
<!-- shoes -->
<div class="col-lg-4 col-md-6">
<div
class="p-4 border cat3 text-white d-flex align-items-center justify-content-between rounded-3">
<h1>Shoes</h1>
<img src="images/categories/shoes.png" alt="">
</div>
</div>
</div>
</section>
<!-- shoes section -->
<section class="container my-5" id="shoe">
<h1>Shoes</h1>
<div class="row row-cols-1 row-cols-md-2 row-cols-lg-3 g-4">
<!-- shoe 1 -->
<div class="col">
<div class="card border-0 shadow-lg h-100">
<img src="images/shoes/shoe-1.png" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Supply 350</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to
additional content. This content is a little bit longer.</p>
</div>
<div class="m-3">
<h4>$120</h4>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
</div>
</div>
<!-- shoe 2 -->
<div class="col">
<div class="card border-0 shadow-lg h-100">
<img src="images/shoes/shoe-2.png" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Nike 360</h5>
<p class="card-text">This card has supporting text below as a natural lead-in to additional
content.</p>
</div>
<div class="m-3">
<h4>$320</h4>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
</div>
</div>
<!-- shoe 3 -->
<div class="col">
<div class="card border-0 shadow-lg h-100">
<img src="images/shoes/shoe-3.png" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Red Airmax</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to
additional content. This card has even longer content than the first to show that equal
height action.</p>
</div>
<div class="m-3">
<h4>$350</h4>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
</div>
</div>
</div>
</section>
<!-- bags section -->
<section class="container my-5" id="backpacks">
<h1>Backpacks</h1>
<div class="row row-cols-1 row-cols-md-2 row-cols-lg-3 g-4">
<!-- bag 1 -->
<div class="col">
<div class="card border-0 shadow-lg h-100">
<img src="images/bags/bag-1.png" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Supply 350</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to
additional content. This content is a little bit longer.</p>
</div>
<div class="m-3">
<h4>$120</h4>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
</div>
</div>
<!-- bag 2 -->
<div class="col">
<div class="card border-0 shadow-lg h-100">
<img src="images/bags/bag-2.png" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Nike 360</h5>
<p class="card-text">This card has supporting text below as a natural lead-in to additional
content.</p>
</div>
<div class="m-3">
<h4>$320</h4>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
</div>
</div>
<!-- bag 3 -->
<div class="col">
<div class="card border-0 shadow-lg h-100">
<img src="images/bags/bag-3.png" class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Red Airmax</h5>
<p class="card-text">This is a wider card with supporting text below as a natural lead-in to
additional content. This card has even longer content than the first to show that equal
height action.</p>
</div>
<div class="m-3">
<h4>$350</h4>
<button class="btn panda-button text-white">BUY NOW</button>
</div>
</div>
</div>
</div>
</section>
<!-- subscribe section -->
<section style="height: 300px" class="container panda-bg d-flex align-items-center justify-content-center my-5 rounded-3">
<div>
<h1>LET'S STAY IN TOUCH</h1>
<h6>Get updates on sales, specials and more</h6>
<input class="form-control" style="margin-bottom: 5px;" type="text" placeholder="Email">
<br>
<div class="btn panda-button text-white">SUBMIT</div>
</div>
</section>
</main>
<footer>
<p id="subscribe" class="text-secondary text-center">ยฉ2020. Panda Commerce. All rights reserved. Sofia, Bulgaria.</p>
</footer>
<!-- bootstrap javascript -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js"
integrity="sha384-ka7Sk0Gln4gmtz2MlQnikT1wXgYsOg+OMhuP+IlRH9sENBO0LRn5q+8nbTov4+1p"
crossorigin="anonymous"></script>
</body>
</html> |
abstract class Animal {
String nome;
String onomatopeia; // representacao sonora emitida pelo animal
String cor;
Integer idade;
String raca;
Animal(String nome, String cor, String raca, Integer idade, String onomatopeia) {
this.nome = nome;
this.cor = cor;
this.raca = raca;
this.idade = idade;
this.onomatopeia = onomatopeia;
}
public void fazBarulho() {
System.out.println(this.onomatopeia);
}
public String toString() { // representa a classe atual em forma de string
return "Nome: " + this.nome + "\n"+
"Raรงa: " + this.raca + "\n"+
"Cor: " + this.cor + "\n"+
"Idade: " + this.idade + "\n"+
"Som: " + this.onomatopeia+"\n";
}
} |
import React, { useState } from 'react';
import { Bloglist, SearchBar, NotFound } from '../../components';
import { bloglist } from '../../demoData';
const Home = () => {
const [blogs, setBlogs] = useState(bloglist);
const [searchKey, setSearchKey] = useState('');
const formSubmit = (e) => {
e.preventDefault();
handleSearchResults();
};
//search for blogs by category
const handleSearchResults = (e) => {
const allBlogs = bloglist;
const filterBlogs = allBlogs.filter((blog) =>
blog.category.toLowerCase().includes(searchKey.toLowerCase().trim())
);
setBlogs(filterBlogs);
};
const clearSearch = () => {
setBlogs(bloglist);
setSearchKey('');
};
return (
<>
<h1 className="head-text">
All <span> New </span>Blogs
</h1>
<p className="p-text" style={{ textAlign: 'center' }}>
awesome place to make onself <br />
productive and entertained through daily updates
</p>
<SearchBar
value={searchKey}
formSubmit={formSubmit}
handleSearchKey={(e) => setSearchKey(e.target.value)}
clearSearch={clearSearch}
/>
{!blogs.length ? <NotFound /> : <Bloglist blogs={blogs} />}
</>
);
};
export default Home; |
from fairseq.criterions.sentence_prediction import SentencePredictionCriterion
from fairseq.criterions import register_criterion
from dataclasses import dataclass, field
from fairseq.dataclass import FairseqDataclass
import torch
import torch.nn as nn
import torch.nn.functional as F
@register_criterion("distillation_multitask")
class DistillationMultitaskCriterion(SentencePredictionCriterion):
def __init__(self, task, classification_head_name, regression_target):
super().__init__(task, classification_head_name, regression_target)
self.temperature = 1.0
def forward(self, model, sample, mode="supervised", reduce=True):
"""Compute the loss for the given sample.
Returns a tuple with three elements:
1) the loss
2) the sample size, which is used as the denominator for the gradient
3) logging outputs to display while training
"""
assert (
hasattr(model, "classification_heads")
and self.classification_head_name in model.classification_heads
), "model must provide sentence classification head for --criterion=distillation_multitask"
if mode == "supervised":
logits, _ = model(
**sample["net_input"],
features_only=True,
classification_head_name=self.classification_head_name,
)
targets = model.get_targets(sample, [logits]).view(-1)
sample_size = targets.numel()
lprobs = F.log_softmax(logits, dim=-1)
supervised_loss = F.nll_loss(lprobs, targets, reduction="sum")
logging_output = {
"supervised_loss": supervised_loss.data,
"ntokens": sample["ntokens"],
"nsentences": sample_size,
"sample_size": sample_size,
}
if not self.regression_target:
preds = logits.argmax(dim=1)
logging_output["ncorrect"] = (preds == targets).sum()
loss = supervised_loss
elif mode == "distillation":
logits, _ = model(
**sample["net_input"],
features_only=True,
classification_head_name=self.classification_head_name,
)
# according to https://github.com/peterliht/knowledge-distillation-pytorch/blob/master/model/net.py#L100
# teacher output gets F.softmax; student output gets F.log_softmax
lprobs = F.log_softmax(logits, dim=-1)
# distill_lprobs = F.log_softmax(logits / self.temperature, dim=-1)
distill_target = F.softmax(sample["distill_target"] / self.temperature, dim=-1)
distill_loss = F.kl_div(lprobs, distill_target, reduction="sum") * self.temperature * self.temperature
sample_size = logits.size()[-1]
logging_output = {
"distill_loss": distill_loss.data,
}
loss = distill_loss
else:
raise NotImplementedError
return loss, sample_size, logging_output |
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateQuizSoalTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('quiz_soal', function (Blueprint $table) {
$table->id();
$table->foreignId('quiz_id');
$table->text("soal");
$table->string('a');
$table->string('b');
$table->string('c');
$table->string('d');
$table->enum('kunci_jawaban', ['A', 'B', 'C', 'D']);
$table->string('pembahasan')->nullable();
$table->string('file')->nullable();
$table->enum('aktif', ['Y', 'N']);
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('quiz_soal');
}
} |
"use client";
import { PatientSortOrder } from "@bonfhir/core/r4b";
import { useFhirForm } from "@bonfhir/mantine/r4b";
import { useFhirSearchControllerNext } from "@bonfhir/next/r4b/client";
import { useFhirSearch } from "@bonfhir/query/r4b";
import {
FhirInput,
FhirPagination,
FhirQueryLoader,
FhirTable,
FhirValue,
} from "@bonfhir/react/r4b";
import { Box, Button, Flex, Paper, Stack } from "@mantine/core";
import { IconSearch } from "@tabler/icons-react";
export default function Patients() {
const searchController = useFhirSearchControllerNext<
PatientSortOrder,
{
patientName: string | undefined;
practitioner: string | undefined;
managingOrganization: string | undefined;
}
>("patients", {
pageSize: 15,
defaultSearch: {
patientName: undefined,
practitioner: undefined,
managingOrganization: undefined,
},
});
const form = useFhirForm({
initialValues: {
patientName: "",
practitioner: "",
managingOrganization: "",
},
});
const patientsQuery = useFhirSearch(
"Patient",
(search) =>
search
.name(searchController.search?.patientName)
.generalPractitioner(searchController.search?.practitioner)
.organization(searchController.search?.managingOrganization)
._total("accurate")
._count(searchController.pageSize)
._sort(searchController.sort),
searchController.pageUrl,
);
const handleReset = () => {
form.reset();
searchController.onSearch({
patientName: "",
practitioner: "",
managingOrganization: "",
});
};
const getAge = (birthDate: string) => {
// Thx copilot for this function
const today = new Date();
const birthDateObj = new Date(birthDate);
let age = today.getFullYear() - birthDateObj.getFullYear();
const m = today.getMonth() - birthDateObj.getMonth();
if (m < 0 || (m === 0 && today.getDate() < birthDateObj.getDate())) {
age--;
}
return age;
};
return (
<Paper>
<Stack>
<Box>
<form onSubmit={form.onSubmit(searchController.onSearch)}>
<Flex gap="md" align="flex-end" direction="row">
<FhirInput
type="string"
label="Patient name"
{...form.getInputProps("patientName")}
/>
<FhirInput
type="Reference"
resourceType="Practitioner"
label="Provider"
style={{ width: "100%" }}
search={(query) => `name=${query}`}
{...form.getInputProps("practitioner")}
/>
<FhirInput
type="Reference"
resourceType="Organization"
label="Clinic"
style={{ width: "100%" }}
{...form.getInputProps("managingOrganization")}
/>
<Button type="submit" leftSection={<IconSearch />}>
Search
</Button>
<Button onClick={handleReset}>Reset</Button>
</Flex>
</form>
</Box>
<Box>
<FhirQueryLoader query={patientsQuery}>
<FhirTable
{...patientsQuery}
{...searchController}
onRowNavigate={(patient) => `/patients/${patient.id}`}
columns={[
{
key: "name",
title: "Patient name",
sortable: true,
render: (patient) => (
<FhirValue type="HumanName" value={patient.name} />
),
},
{
key: "birthdate",
title: "Date of birth",
sortable: true,
render: (patient) => (
<>
<FhirValue
type="date"
value={patient.birthDate}
options={{
dateStyle: "medium",
}}
/>
{` (${getAge(patient?.birthDate ?? "")}yo)`}
</>
),
},
{
key: "ssn",
title: "SSN",
render: (patient) => (
<FhirValue
type="Identifier"
value={patient.identifier}
options={{
max: 1,
systemFilterOrder: ["http://hl7.org/fhir/sid/us-ssn"],
style: "value",
default: "Unknown",
}}
/>
),
},
{
key: "practitioner",
title: "Provider",
render: (patient) => (
<FhirValue
type="string"
value={patient.generalPractitioner?.[0]?.display}
/>
),
},
{
key: "clinic",
title: "Clinic",
render: (patient) => (
<FhirValue
type="string"
value={patient.managingOrganization?.display}
/>
),
},
]}
/>
<FhirPagination {...patientsQuery} {...searchController} />
</FhirQueryLoader>
</Box>
</Stack>
</Paper>
);
} |
<?php
namespace App\Http\Controllers\Api\v1\Devs;
use App\Http\Controllers\Controller;
use App\Http\Resources\DevsResource;
use App\Models\DevsPost;
use Illuminate\Database\Eloquent\ModelNotFoundException;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use Illuminate\Support\Facades\Validator;
use function PHPUnit\Framework\isEmpty;
class DevsController extends Controller
{
/**
* Display a listing of the resource.
*/
public function index()
{
$post = DevsPost::where('is_active', 1)->orderBy('updated_at', 'desc')->paginate(5);
return new DevsResource(true, 'List data devs post', $post);
}
/**
* Show the form for creating a new resource.
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*/
public function store(Request $request)
{
// Make validation
$validator = Validator::make($request->all(), [
'title' => 'required',
'content' => 'required',
]);
// Check validation
if ( $validator->fails() ) {
return response()->json($validator->errors(), 442);
}
$slug = str_replace(' ', '-', strtolower($request->title));
// return response(Auth::user()->id);
$post = DevsPost::create([
'title' => $request->title,
'content' => $request->content,
'slug' => $slug,
'is_active' => 1,
'category_id' => $request->category_id ?? 1,
'user_id' => 1,
// 'user_id' => Auth::user()->id,
]);
return new DevsResource(true, 'Data post berhasil ditambahkan!', $post);
}
/**
* Display the specified resource.
*/
public function show(DevsPost $devspost)
{
return new DevsResource(true, 'Data post ditemukan!', $devspost);
}
/**
* Show the form for editing the specified resource.
*/
public function edit(string $id)
{
//
}
/**
* Update the specified resource in storage.
*/
public function update(Request $request, $id)
// public function update(Request $request, Devspost $devspost)
{
// Make validation
$validator = Validator::make($request->all(), [
'title' => 'required',
'content' => 'required',
]);
// Check validation
if ( $validator->fails() ) {
return response()->json($validator->errors(), 442);
}
$devspost = DevsPost::find($id);
if (isEmpty($devspost)) {
return response()->json('Post data not found or incorrect', 404);
}
$slug = str_replace(' ', '-', strtolower($request->title));
$devspost->update([
'title' => $request->title,
'content' => $request->content,
'slug' => $slug,
'is_active' => 1,
// 'category_id' => $request->category_id,
// 'user_id' => Auth::user()->id,
]);
return new DevsResource(true, 'Data Post berhasil diubah!', $devspost);
}
/**
* Remove the specified resource from storage.
*/
public function destroy($id)
{
// $devspost = DevsPost::findOrFail($id);
$devspost = DevsPost::find($id);
if (isEmpty($devspost)) {
return response()->json('Post data not found or incorrect', 404);
}
$devspost->delete();
return new DevsResource(true, 'Data post berhasil dihapus', null);
}
} |
import React, { useCallback, useRef } from "react";
import styled from "@emotion/styled";
export interface OverlayProps {
children: React.ReactNode;
isOpen: boolean;
onClose?: () => void;
}
function Overlay({ children, isOpen, onClose }: OverlayProps) {
const overlayRef = useRef<HTMLDivElement | null>(null);
const mouseDownRef = useRef<HTMLElement | null>(null);
const handleClose = useCallback(
(e: React.MouseEvent | React.KeyboardEvent) => {
e.stopPropagation();
e.preventDefault();
onClose && onClose();
},
[onClose]
);
const handleMouseDown = useCallback((e: React.MouseEvent<HTMLElement>) => {
mouseDownRef.current = e.target as HTMLElement;
}, []);
const handleMouseUp = useCallback(
(e: React.MouseEvent<HTMLElement>) => {
if (
e.target === overlayRef.current &&
mouseDownRef.current === overlayRef.current
)
handleClose(e);
},
[handleClose]
);
const handleKeyPress = useCallback(
(e: React.KeyboardEvent) => {
console.log(e.key);
if (e.key === "Escape") handleClose(e);
},
[handleClose]
);
return (
<Wrapper
isOpen={isOpen}
ref={overlayRef}
onKeyPress={handleKeyPress}
onMouseDown={handleMouseDown}
onMouseUp={handleMouseUp}
>
{children}
</Wrapper>
);
}
export default Overlay;
const Wrapper = styled.div<{ isOpen: boolean }>`
position: absolute;
display: ${({ isOpen }) => (isOpen ? "flex" : "none")};
align-items: center;
justify-content: center;
top: 0;
left: 0;
height: 100%;
width: 100%;
background-color: rgba(0, 0, 0, 0.3);
`; |
// Copyright 2023 Cisco Systems, Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import last from 'lodash/last.js';
import {default as jsonata, ExprNode} from "jsonata";
/*
There are cases where we need to generate some AST nodes the JSONata does not generate. Instead of referring to
their ExprNode 'type' we say the generated node has a pseudoType
*/
interface GeneratedExprNode extends jsonata.ExprNode {
pseudoType?: string;
}
/*
Used to record a Step like 'b' in 'a.b.c'
*/
interface StepRecord{
type:string;
value: string;
emit:boolean; //not every step gets emitted as a dependency
}
export default class DependencyFinder {
compiledExpression: jsonata.Expression;
private ast: jsonata.ExprNode;
private readonly currentSteps: StepRecord[][]; //logically, [[a,b,c],[d,e,f]]
private nodeStack: GeneratedExprNode[];
private readonly dependencies: string[][]; //during tree walking we collect these dependencies like [["a", "b", "c"], ["foo", "bar"]] which means the dependencies are a.b.c and foo.bar
/**
* program can be either a string to be compiled, or an already-compiled AST
* @param program
*/
constructor(program: string | ExprNode) {
if (typeof program === 'string') {
// Handle the case where program is a string
this.compiledExpression = jsonata(program);
this.ast = this.compiledExpression.ast();
} else {
this.ast = program;
}
this.currentSteps = [];
this.dependencies = [];
this.nodeStack = [];
}
/**
* If we are looking to analyze only a portion of the jsonata program we can provide another jsonata expression
* such as '**[procedure.value='serial']' which will filter the AST down to what is defined. In the case of
* '**[procedure.value='serial']' the expression will extract the AST for $serial(...) as it may exist in the
* original program.
* @param jsonatExpr
*/
async withAstFilterExpression(jsonatExpr:string):Promise<DependencyFinder>{
const filter = jsonata(jsonatExpr);
this.ast = await filter.evaluate(this.ast);
return this;
}
/*
Walk the AST of the JSONata program
*/
findDependencies(node:GeneratedExprNode = this.ast):string[][] {
if (this.currentSteps.length === 0) {
this.currentSteps.push([]); //initialize a container for steps
}
if (node === undefined) {
return;
}
if (Array.isArray(node)) {
node.forEach(c => {
this.findDependencies(c);
});
return;
}
if (typeof node === 'object' && node !== null) {
const {
type,
} = node;
this.capturePathExpressions(node);
this.captureArrayIndexes(node);
this.nodeStack.push(node);
switch (type) {
case "bind": // :=
case "path": //a.b.c
//dependencies can happen inside dependencies. a.b[something].[zz] contains dependencies within dependencies
//so each time the path is broken we push the current dependencies and reset it.
const {pseudoType} = node;
if(pseudoType !== "procedure") { //this means we are entering a function call, and we want to collect the function name as part of the path we are currently traversing, not as a new path
this.currentSteps.push([]);
}
break;
}
//the children above require the special processing above. But then there are all the other
//children which we deal with, and we don't need to write any special code for them.
const children = this.collectChildren(node);
children.forEach(c => {
this.findDependencies(c);
});
//now we are coming out of the recursion, so the switch below is over the just-finished subtree
switch (type) {
case "variable":
if (!this.hasAncestor(n => n.type === "path")) {
this.emitPaths(); //every independent variable should be separately emitted. But if it is under a path then don't emit it since it should be glimmered onto the path
}
break;
case "path":
this.emitPaths(); //every independent path should be separately emitted
break;
}
this.nodeStack.pop();
}
return this.dependencies;
}
collectChildren(node: GeneratedExprNode):GeneratedExprNode[] {
function ensureProcedureBeforeArguments(arr):string[] {
const procedureIndex = arr.indexOf("procedure");
const argumentsIndex = arr.indexOf("arguments");
// If both strings are in the array and "arguments" comes before "procedure"
if (procedureIndex !== -1 && argumentsIndex !== -1 && argumentsIndex < procedureIndex) {
// Swap the strings
[arr[procedureIndex], arr[argumentsIndex]] = [arr[argumentsIndex], arr[procedureIndex]];
}
return arr;
}
function introducePseudoTypes(node, key):GeneratedExprNode {
const value:jsonata.ExprNode = node[key];
if (!value) return null;
if (Array.isArray(value)) {
if (key === "arguments") {
return { ...value, "pseudoType": key };
}
return value;
} else if (typeof value === 'object') {
return { ...value, "pseudoType": key };
}
return null;
}
//any property of node that is not type, value, or position is a child of the node
const keysToIgnore: string[] = ["type", "value", "position", "pseudoType"];
const filteredKeys: string[] = Object.keys(node).filter(key => !keysToIgnore.includes(key));
const orderedKeys: string[] = ensureProcedureBeforeArguments(filteredKeys);
const result = [];
for (const key of orderedKeys) {
const processedAttribute = introducePseudoTypes(node, key);
if (processedAttribute) {
result.push(processedAttribute);
}
}
return result;
}
captureArrayIndexes(node):void {
const {type, expr} = node;
if (type === "filter" && expr?.type === "number") {
last(this.currentSteps).push({type, "value": expr.value, "emit": expr?.type === "number"});
}
}
//this is where we capture the path and name expressions like a.b.c or $.a
capturePathExpressions(node) {
const {type, value} = node;
if (type !== "name" && type !== "variable") {
return;
}
if (this.isRootedIn$$(value)) { //if the root of the expression is $$ then we will always accept the navigation downwards
last(this.currentSteps).push({type, value, "emit": true});
return;
}
if (this.isInsideAScopeWhere$IsLocal(node)) { //path expressions inside a transform are ignored modulo the $$ case just checked for above
last(this.currentSteps).push({type, value, "emit": false});
return;
}
if (this.isSingle$Var(type, value)) { //accept the "" variable which comes from single-dollar like $.a.b when we are not inside a transform. We won't accept $foo.a.b
last(this.currentSteps).push({type, value, "emit": true});
return;
}
if (type === "variable") {
//if we are here then the variable must be an ordinary locally named variable since it is neither $$ or $.
//variables local to a closure cannot cause/imply a dependency for this expression
if (!this.hasParent("function")) { //the function name is actually a variable, we want to skip such variables
last(this.currentSteps).push({type, value, "emit": false});
}
return;
}
//if we are here then we are dealing with names, which are identifiers like 'a' which can occur in a.b.c or
// just plain a
//if the name is an argument to a function, then it should be emitted as a dependency
if(this.hasAncestor(n=>n.pseudoType === "arguments")){
last(this.currentSteps).push({type, value, "emit": true});
return;
}
/*
if (!this.isUnderTreeShape(["path", "function"])) {
last(this.currentSteps).push({type, value, "emit": true}); //tree shape like a.$sum(x,y) we cannot count x and y as dependencies because a can be an array that is mapped over
}
*/
const stepRecord: StepRecord = {type, value, "emit": true};
last(this.currentSteps).push(stepRecord);
}
hasParent(parentType) {
return this.nodeStack.length !==0 &&
( last(this.nodeStack).type === parentType || last(this.nodeStack).pseudoType === parentType);
}
hasAncestor(matcher) {
return this.nodeStack.some(matcher);
}
ancestors(matcher) {
return this.nodeStack.filter(matcher);
}
isSingle$Var(type, value) {
return type === "variable" && value === ""; // $ or $.<whatever> means the variable whose name is empty/"".
}
isRootedIn$$(value) {
const _last = last(this.currentSteps);
return _last && (_last.length === 0 && value === "$"
|| _last.length > 0 && _last[0].value === "$");
}
isInsideAScopeWhere$IsLocal(node): boolean {
let matches = this.ancestors(n => {
const {type:t, pseudoType:p, value:v} = n;
return (t === "unary" && ["(", "{", "[", "^"].includes(v)) //(map, {reduce, [filter, ^sort
|| t === "filter"
|| p === "predicate";
});
//the local scope is equal to the input scope to the expression the first time we enter one of these blocks
//However the second time the scope is the output from a prior expression, not the input. Therefore, the scope
//is local to the expression when there is more than one match in the ancestors
if (matches.length > 1){
return true;
}
//if we are following a consarray like [1..count].{"foo"+$}} then $ is a local scope. So we have to make
//sure we are not IN a consarray as count is in and then if we are not in a consarray, are we following
//a consarray (immediately or later)
matches = !this.hasAncestor(n=>n.consarray !== undefined) && this.ancestors(n => {
const {type:t, steps} = n;
//iterate the steps array and determine if there is a consarray that comes before this node's position in the steps
return t==="path" && steps.find(nn=>nn.consarray && nn.position < node.position);
});
if (matches.length > 0){
return true;
}
//If we are in a transform, scope is local
matches = this.ancestors(n => {
const {type:t} = n;
return t === "transform";
});
return matches.length > 0;
}
emitPaths() {
const emitted: string[] = [];
const steps: StepRecord[] = this.currentSteps.flat(); //[[],["a","b"], ["c"]] -> ["a","b","c"]
const lastStepsArray = last(this.currentSteps);
if (lastStepsArray.length > 0 && lastStepsArray.every(s => s.emit)) {
if (lastStepsArray[0].value === "$") { //corresponding to '$$' variable
//in this case the chain of steps must be broken as '$$' is an absolute reference to root document
lastStepsArray.forEach(s => emitted.push(s.value));
} else {
steps.forEach(s => s.emit && emitted.push(s.value));
}
}
this.currentSteps.pop();
if(emitted[emitted.length-1]===""){
emitted.pop(); //foo.{$} would result in [foo, ""] as dependency. Trailing "" must be removed.
}
if(emitted.length === 1 && emitted[0]==="$"){
return; // a solo dependency of "$" gets scrapped. We don't track dependencies on the root of the template itself
}
if (emitted.length > 0) {
this.dependencies.push(emitted);
}
}
} |
<template>
<div class="z-carousel" ref="rootRef" @mouseover="showArrow" @mouseleave="hideArrow">
<div ref="carouselContainerRef" class="z-carousel-container" :style="containerStyle">
<!-- ๅทฆๅณไธค่พน็ๆ้ฎ-->
<transition name="leftArrow">
<button v-show="(isArrowShow&&direction!=='vertical'&&props.arrow!=='never')||props.arrow==='always'"
class="z-carousel-arrow z-carousel-arrow-left"
@click="prev">
<svg class="icon">
<use xlink:href="#icon-arrow-left"></use>
</svg>
</button>
</transition>
<transition name="rightArrow">
<button v-show="(isArrowShow&&direction!=='vertical'&&props.arrow!=='never')||props.arrow==='always'"
class="z-carousel-arrow z-carousel-arrow-right"
@click="next">
<svg class="icon">
<use xlink:href="#icon-arrow-right"></use>
</svg>
</button>
</transition>
<!-- ๆปๅจๆก-->
<slot></slot>
</div>
<!-- ไธๆนๅฎไฝๆก-->
<ul ref="carouselIndicatorRef" class="z-carousel-indicators" :class="indicatorClass">
<li class="z-carousel-indicator" v-for="(item,index) in slots"
@click="handleIndicator(index+1)" @mouseover="hoverIndicator(index+1)">
<button :class="{'is-active':activeKey===index+1}"></button>
</li>
</ul>
</div>
</template>
<script lang='ts' setup>
import {computed, onMounted, provide, ref, useSlots} from 'vue';
import CarouselItem from './CarouselItem.vue';
const rootRef = ref<HTMLDivElement>();
const activeKey = ref(1);
const carouselItem = ref({});
const carouselContainerRef = ref();
const carouselIndicatorRef = ref();
let intervalId;
let slots;
const props = defineProps({
'indicatorPosition': {type: String, values: ['outside', 'inside', 'none']},
'arrow': {type: String, values: ['always', 'never', 'default'], default: 'default'},
'direction': {type: String, values: ['horizontal', 'vertical'], 'default': 'horizontal'},
'trigger': {type: String, values: ['hover', 'click'], 'default': 'hover'},
'height': {type: [String, Number]}
});
const indicatorClass = computed(() => {
return {
[`z-carousel-indicators-${props.direction}`]: props.direction,
[`z-carousel-indicators-pos-${props.indicatorPosition}`]: props.indicatorPosition
};
});
const containerStyle = computed(() => {
return {
height: Number(props.height) + 'px'
};
});
function useArrowDisplay() {
const isArrowShow = ref(false);
function showArrow() {
isArrowShow.value = true;
clearTimer();
}
function hideArrow() {
isArrowShow.value = false;
startTimer();
}
function prev() {
activeKey.value -= 1;
if (activeKey.value <= 0) {
activeKey.value = slots.value.length;
}
}
function next() {
activeKey.value += 1;
if (activeKey.value > slots.value.length) {
activeKey.value = 1;
}
}
return {isArrowShow, showArrow, hideArrow, prev, next};
}
function useDefaultSlots() {
slots = ref<Array<CarouselItem>>((useSlots().default?.().filter(compo => compo.type === CarouselItem) ?? []));
if (slots.value.length === 0) {
useSlots().default?.().forEach(item => {
const res = (item['children'] as Array<CarouselItem>).filter(compo => compo.type === CarouselItem);
slots.value = slots.value.concat(res);
});
}
}
useDefaultSlots();
function clearTimer() {
clearInterval(intervalId);
}
function handleIndicator(index) {
if (props.trigger === 'hover') return;
activeKey.value = index;
}
function hoverIndicator(index) {
if (props.trigger === 'click') return;
setTimeout(() => {
activeKey.value = index;
}, 200);
}
provide('carouselBox', {
rootRef,
isVertical: props.direction === 'vertical',
activeKey,
carouselItem,
slotLength: slots.value.length
});
function startTimer() {
intervalId = setInterval(() => {
if (activeKey.value < slots.value.length) {
activeKey.value += 1;
} else {
activeKey.value = 1;
}
// eslint-disable-next-line no-magic-numbers
}, 2000);
}
const {isArrowShow, showArrow, hideArrow, prev, next} = useArrowDisplay();
onMounted(() => {
startTimer();
});
</script>
<style lang='scss' scoped>
.z-carousel {
overflow: hidden;
position: relative;
.z-carousel-container {
height: 300px;
position: relative;
.z-carousel-arrow {
position: absolute;
top: 50%;
transform: translateY(-50%);
width: 36px;
height: 36px;
border-radius: 50%;
border: none;
outline: none;
background-color: rgba(31, 45, 61, .11);
cursor: pointer;
text-align: center;
z-index: 2;
font-size: 16px;
display: inline-flex;
justify-content: center;
align-items: center;
color: #fff;
&:hover {
background-color: #a9b3bf;
}
svg {
fill: currentColor;
color: inherit;
}
&.z-carousel-arrow-left {
left: 16px;
}
&.z-carousel-arrow-right {
right: 16px;
}
&.leftArrow-enter-from {
left: 0;
}
&.leftArrow-enter-to {
left: 16px;
}
&.leftArrow-leave-from {
left: 16px;
opacity: 1;
}
&.leftArrow-leave-to {
left: 0;
opacity: 0;
}
&.rightArrow-enter-from {
right: 0;
}
&.rightArrow-enter-to {
right: 16px;
}
&.rightArrow-leave-from {
right: 16px;
opacity: 1;
}
&.rightArrow-leave-to {
right: 0;
opacity: 0;
}
}
.leftArrow-enter-active,
.leftArrow-leave-active, .rightArrow-enter-active,
.rightArrow-leave-active {
transition: all 0.25s linear;
}
}
.z-carousel-indicators {
position: absolute;
z-index: 2;
display: flex;
list-style: none;
cursor: pointer;
&.z-carousel-indicators-pos-none {
display: none;
}
&.z-carousel-indicators-pos-outside {
position: relative;
display: flex;
justify-content: center;
align-items: center;
&.z-carousel-indicators-vertical {
top: 0;
left: 2px;
transform: none;
justify-content: flex-start;
align-items: flex-start;
.z-carousel-indicator {
width: 100%;
}
}
}
.z-carousel-indicator {
display: inline-block;
list-style: none;
padding: 10px 4px;
margin-right: 2px;
cursor: pointer;
button {
height: 2px;
width: 30px;
outline: none;
border: none;
margin: 0;
padding: 0;
border-radius: 10px;
background-color: #cad2de;
cursor: pointer;
&:hover {
background-color: #f3f5f8;
}
&.is-active {
background-color: #1890ff;
}
}
}
&.z-carousel-indicators-horizontal {
left: 50%;
bottom: 0;
transform: translateX(-50%);
}
&.z-carousel-indicators-vertical {
top: 50%;
transform: translateY(-50%);
right: 14px;
flex-direction: column;
& .z-carousel-indicator {
padding: 0;
button {
width: 2px;
height: 20px;
}
}
}
}
}
</style> |
import Image from "next/image";
import EditClipboardIcon from "../Icons/EditClipboardIcon";
import BookmarkIcon from "../Icons/BookmarkIcon";
import { Bookmark } from "../types";
import { useRef, useState } from "react";
interface ArticleButtonMobileProps {
imageURL: string;
headline: string;
summary: string;
url: string;
bookmarked: boolean;
addTopicsCallback: (value: { title: string, topics: string[]; }) => void;
toggleBookmarkCallback: (value: Bookmark) => void;
byline: string;
topics: (string[])[];
bookmarkInfo: Bookmark;
uid: string | null | undefined;
}
export default function ArticleButtonNonMobile({
imageURL,
headline,
summary,
url,
bookmarked,
addTopicsCallback,
toggleBookmarkCallback,
topics,
byline,
bookmarkInfo,
uid
}: ArticleButtonMobileProps) {
const imageRef = useRef<HTMLDivElement>(null);
const regexPattern = /(?:By|,|and)\s+/gi;
const allTopics = byline.split(regexPattern).filter(Boolean);
allTopics.push(...topics.flat());
function handleShowTopics() {
const articleTopics = {
title: headline,
topics: allTopics,
};
// Calling back on button click
addTopicsCallback(articleTopics);
}
function handleBookmark() {
toggleBookmarkCallback(bookmarkInfo);
}
return (
<article
className="article_tablet_base"
onClick={(e) => {
e.stopPropagation();
window.open(url, "_blank", "noopener noreferrer");
}}>
<div className="article_tablet_content--top">
<div className="image_headline">
<div key={imageURL+'div'} ref={imageRef} className='image_wrap loading'>
<Image
key={imageURL}
src={imageURL}
alt={headline}
unoptimized
fill
priority
placeholder="empty"
blurDataURL="data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 1 1'%3E%3C/svg%3E"
onLoad={() => {
// Add a small delay to ensure the image is fully loaded
setTimeout(() => {
const imageWrap = imageRef.current;
if (imageWrap) {
imageWrap.classList.add('resolved');
}
}, 100);
}}
/>
</div>
<h2>{headline}</h2>
</div>
<summary>{summary}</summary>
</div>
<div className="article_tablet_content--bottom">
{uid && <div className="icon_wrap">
<BookmarkIcon key="bookmark-icon" callback={handleBookmark} selected={bookmarked} />
{/* Occasionally there are no topics - so we don't render a button */}
{!!allTopics.length && <EditClipboardIcon key="clipboard-icon" callback={handleShowTopics} />}
</div>}
{!uid && <div className="icon_wrap">
<EditClipboardIcon key="clipboard-icon" callback={handleShowTopics} />
</div>}
</div>
</article>
);
} |
---
description: "Cara Membuat Kepiting Kuah Pedas yang praktis"
title: "Cara Membuat Kepiting Kuah Pedas yang praktis"
slug: 2114-cara-membuat-kepiting-kuah-pedas-yang-praktis
date: 2020-07-07T07:01:35.213Z
image: https://img-global.cpcdn.com/recipes/e522afa92ff0d3c2/751x532cq70/kepiting-kuah-pedas-foto-resep-utama.jpg
thumbnail: https://img-global.cpcdn.com/recipes/e522afa92ff0d3c2/751x532cq70/kepiting-kuah-pedas-foto-resep-utama.jpg
cover: https://img-global.cpcdn.com/recipes/e522afa92ff0d3c2/751x532cq70/kepiting-kuah-pedas-foto-resep-utama.jpg
author: Christian Price
ratingvalue: 3.6
reviewcount: 8
recipeingredient:
- "1/2 kg kepiting"
- "4 bawang merah"
- "3 bawang putih"
- "10 cabe besar"
- "5 cabe rawit"
- "1/2 ruas kunyit"
- "1/2 ruas jahe"
- " Daun jeruk"
- " Daun salam"
- "Sejumput merica"
- "Sejumput ketumbar"
- " Gulgar"
- " Penyedap"
- "2 sendok santan bubuk"
- "400 ml air"
recipeinstructions:
- "Bersihkan kepiting. Cuci bersih. Siram dg air mendidih agar amis nya hilang"
- "Haluskan semua bumbu, kecuali daun salam"
- "Tumis bumbu halus + daun salam sampai harum"
- "Tambahkan air. Masak hingga mendidih"
- "Setelah mendidih, masukkan bubuk santan. Aduk agar kuah tidak pecah"
- "Masukkan kepiting. Aduk sebentar. Tambahkan gulgar + penyedap. Diamkan hingga matang"
- "Koreksi rasa, siap sajikan"
categories:
- Resep
tags:
- kepiting
- kuah
- pedas
katakunci: kepiting kuah pedas
nutrition: 133 calories
recipecuisine: Indonesian
preptime: "PT21M"
cooktime: "PT38M"
recipeyield: "1"
recipecategory: Lunch
---
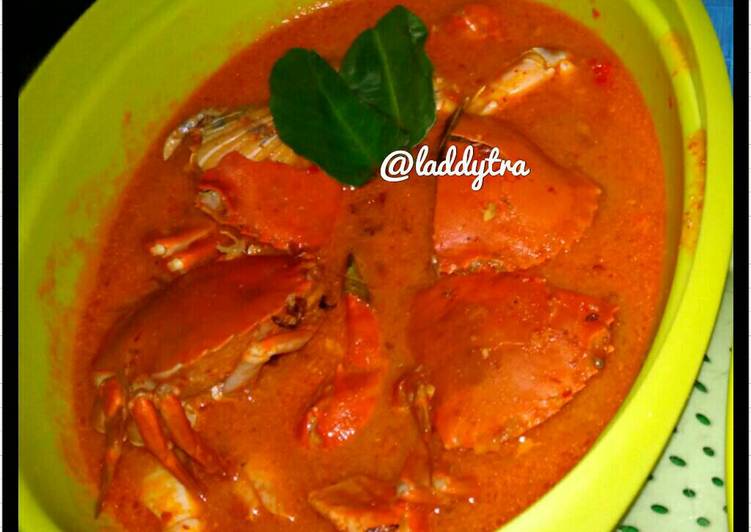
<b><i>kepiting kuah pedas</i></b>, Memasak bisa menjadi bentuk kegiatan yang membahagiakan dilakukan oleh banyak orang. tidaklah hanya para perempuan, sebagian laki laki juga banyak juga yang senang dengan kegemaran ini. walaupun hanya untuk sekedar bersenang senang dengan sahabat atau memang sudah menjadi kegemaran dalam dirinya. tak heran dalam dunia chef sekarang banyak ditemukan cowok dengan skill memasak yang sempurna, dan banyak juga kita lihat di banyak depot dan stand makanan mall yang menggunakan chef laki laki sebagai koki terbaik nya.
Baik, kita mulai ke pembahasan bumbu bumbu menu <i>kepiting kuah pedas</i>. di sela sela kegiatan kita, kemungkinan akan terasa menyenangkan jika sejenak kita menyediakan sedikit waktu untuk membuat kepiting kuah pedas ini. dengan kesuksesan anda dalam mengolah masakan tersebut, dapat menjadikan diri kita bangga dengan hasil hidangan kalian sendiri. dan juga disini dengan situs ini kita akan mempunyai rujukan untuk membuat masakan <u>kepiting kuah pedas</u> tersebut menjadi hidangan yang enak dan sempurna, oleh sebab itu ingat ingat alamat laman ini di gadget anda sebagai sebagian rujukan anda dalam meracik menu baru yang lezat.
Hay semua,, nah kali ini di dapur mbak mita aku mau bagi resep kepiting kuah pedas yang nampol abis pedasnya lhoooo cara bikinnya gampang banget bahan bahan. Kepiting adalah salah satu hidangan seafood paling populer. Anda dapat menyajikannya langsung dari dapur dengan sensasi pedas yang lezat dan membangkitkan selera.
Mari langsung saja kita start untuk membeli bahan baku yang dibutuhkan dalam membuat masakan <u><i>kepiting kuah pedas</i></u> ini. seenggaknya dibutuhkan <b>15</b> bahan yang diperlukan untuk olahan ini. agar nanti dapat menghasilkan rasa yang yummy dan nikmat. dan juga sediakan waktu anda sedikit, karena kita akan memprosesnya antara lain dengan <b>7</b> tahap. saya harap berbagai hal yang diperlukan sudah anda sediakan disini, Baiklah mari kita awali dengan mencatat dulu bahan bahan dibawah ini.
<!--inarticleads1-->
##### Komposisi dan bumbu yang digunakan untuk menyiapkan Kepiting Kuah Pedas:
1. Sediakan 1/2 kg kepiting
1. Ambil 4 bawang merah
1. Gunakan 3 bawang putih
1. Ambil 10 cabe besar
1. Ambil 5 cabe rawit
1. Siapkan 1/2 ruas kunyit
1. Siapkan 1/2 ruas jahe
1. Siapkan Daun jeruk
1. Sediakan Daun salam
1. Ambil Sejumput merica
1. Gunakan Sejumput ketumbar
1. Siapkan Gulgar
1. Siapkan Penyedap
1. Siapkan 2 sendok santan bubuk
1. Gunakan 400 ml air
Resep Hari Ini - Kali ini saya akan membagikan resep cara membuat kepiting saus pedas untuk anda. Yuk simak resep kepiting saus padang yang pedas dan nikmat di sini. Kepiting saus padang menawarkan sensasi rasa kenikmatan yang berbeda dari kuliner seafood lainnya. Ambil kepiting yang sudah bersih, kemudian masukkan ke dalam penggorengan bersama dengan semua bahan sisa termasuk telur.
<!--inarticleads2-->
##### Langkah-langkah membuat Kepiting Kuah Pedas:
1. Bersihkan kepiting. Cuci bersih. Siram dg air mendidih agar amis nya hilang
1. Haluskan semua bumbu, kecuali daun salam
1. Tumis bumbu halus + daun salam sampai harum
1. Tambahkan air. Masak hingga mendidih
1. Setelah mendidih, masukkan bubuk santan. Aduk agar kuah tidak pecah
1. Masukkan kepiting. Aduk sebentar. Tambahkan gulgar + penyedap. Diamkan hingga matang
1. Koreksi rasa, siap sajikan
Tongkol Kuah Pedas, masakan khas dari propinsi Riau Daratan. Penduduk Riau sepertinya suka masakan Sekilas masakan ini mirip Asam Pedas Ikan, hanya bedanya kuahnya memakai santan. Kepiting adalah binatang anggota krustasea berkaki sepuluh dari upabangsa (infraordo) Brachyura, yang dikenal mempunyai "ekor" yang sangat pendek (bahasa Yunani: brachy = pendek, ura = ekor), atau yang perutnya (abdomen) sama sekali tersembunyi di bawah dada (thorax). Bintang kita malam ini adalah Kepiting Saus Tiram Pedas, cocok untuk dipadukan dengan resep-resep seafood lainnya. Meski dimasak sederhana, olahan kepiting segar sudah akan memberikan citarasa maksimal.
Berikut sedikit ulasan masakan perihal resep resep <u>kepiting kuah pedas</u> yang endess. saya harapkan anda sudah memahami dengan tulisan diatas, dan kamu dapat memasak lagi di lain waktu untuk di sajikan dalam berbagai even even keluarga atau kolega anda. kita bs mengulik bumbu bumbu yang ditampilkan diatas selaras dengan harapan anda, sehingga hidangan <b>kepiting kuah pedas</b> ini bs menjadi lebih endess dan nikmat lagi. demikianlah penjelasan singkat ini, sampai bertemu lagi di lain hal. kami harap hari kalian menyenangkan. |
import 'server-only';
import { StaticImageData } from 'next/image';
import applylink_dashboard_helpboard_bookmarks from './gallery/applylink_dashboard_helpboard_bookmarks.png';
import applylink_dashboard_helpboard_overview from './gallery/applylink_dashboard_helpboard_overview.png';
import applylink_helpboard from './gallery/applylink_helpboard.png';
import applylink_helpboard_2 from './gallery/applylink_helpboard_2.png';
import applylink_home_en_dark from './gallery/applylink_home_en_dark.png';
import applylink_home_en_light from './gallery/applylink_home_en_light.png';
import applylink_home_fa_dark from './gallery/applylink_home_fa_dark.png';
import applylink_profile_hejazizo from './gallery/applylink_profile_hejazizo.png';
import applylink_event from './gallery/applylink_event.png';
import applylink_profile_hejazizo_experiences from './gallery/applylink_profile_hejazizo_experiences.png';
import applylink_roadmap from './gallery/applylink_roadmap.png';
import applylink_signup from './gallery/applylink_signup.png';
import oya_home from './gallery/oya_home.png';
import oya_report from './gallery/oya_report.png';
import oya_calendar from './gallery/oya_calendar.png';
import oya_clocks from './gallery/oya_clocks.png';
import oya_charts from './gallery/oya_charts.png';
import filmnet_home from './gallery/filmnet_home.jpg';
import filmnet_kids from './gallery/filmnet_kids.jpg';
import filmnet_single from './gallery/filmnet_single.jpg';
import filmnet_videos from './gallery/filmnet_videos.jpg';
import filmnet_profile from './gallery/filmnet_profile.jpg';
import tv_filmnet_home from './gallery/tv_filmnet_home.jpg';
import tv_filmnet_search from './gallery/tv_filmnet_search.jpg';
import tv_filmnet_single from './gallery/tv_filmnet_single.jpg';
import tv_filmnet_details from './gallery/tv_filmnet_details.jpg';
import tv_filmnet_login from './gallery/tv_filmnet_login.jpg';
export type Project = {
slug: string;
title: string;
description?: string;
points?: string[];
tools?: string[];
links?: string[];
gallery?: (StaticImageData)[];
date?: string;
};
export const getProjects: () => Project[] = () => [
{
slug: 'applylink',
title: 'ApplyLink',
description: 'A platform for linking students with each other to facilitate the application process for universities.',
points: [
'Blazingly fast with a 100% score on gtmatrix and lighthouse',
'Using server components to reduce the bundle size and improve the performance',
'All client-side interactions are handled by React-Query queries and mutations',
'Dark and light themes',
'Multiple languages support with Next-INTL',
],
tools: ['NextJS', 'Tailwind-CSS', 'React-Query', 'Next-INTL'],
date: '2024',
links: ['https://applylink.ai/'],
gallery: [
applylink_home_en_dark,
applylink_home_en_light,
applylink_home_fa_dark,
applylink_helpboard_2,
applylink_event,
applylink_profile_hejazizo,
applylink_helpboard,
// applylink_profile_hejazizo_experiences,
applylink_roadmap,
applylink_signup,
applylink_dashboard_helpboard_bookmarks,
// applylink_dashboard_helpboard_overview,
],
},
{
slug: 'gp',
title: 'NEZAJA Recruitment Platform',
description: 'Recruitment and evaluation platform for NEZAJA',
points: [
'Multiple micro frontend applications with a shared layout and authentication system',
'Handling lots of large data tables by virtualizing them with react-window',
],
tools: ['ReactJS', 'Material-UI', 'React-Router', 'SWR', 'FastAPI', 'SQLAlchemy', 'SQL Server'],
date: '2023',
},
{
slug: 'oya',
title: 'OYA',
description: 'A personal time tracking platform for logging and visualizing the time spent on different activities.',
points: [
'Data visualization with all kinds of charts and graphs',
'Client side data caching with SWR',
'Dark and light themes',
'Support both Jalaali and Gregorian calendars'
],
tools: ['ReactJS', 'Material-UI', 'React-Router', 'SWR', 'Nivo-Charts', 'FastAPI', 'SQLAlchemy', 'PostgreSQL'],
date: '2023',
links: ['https://github.com/doiali/oya/'],
gallery: [
oya_home,
oya_report,
oya_calendar,
oya_clocks,
oya_charts,
],
},
{
slug: 'filmnet',
title: 'Film Net',
description: 'A platform for watching movies and TV shows.',
points: [
'Using ISR to improve the performance of the website',
'Complete test coverage with jest and React-Testing-Library',
],
tools: ['NextJS', 'Emotion', 'swr', 'jest', 'React-Testing-Library'],
date: '2022',
links: ['https://filmnet.ir/'],
gallery: [
filmnet_home,
filmnet_kids,
filmnet_single,
filmnet_videos,
filmnet_profile,
],
},
{
slug: 'tv-filmnet',
title: 'Film Net for TV',
description: 'A platform for watching movies and TV shows on TV.',
points: [
'Featuring spatial navigation using arrow keys',
'Support a wide range of old browsers',
],
tools: ['NextJS', 'Emotion', 'swr', 'jest', 'React-Testing-Library'],
date: '2022',
links: ['https://tv.filmnet.ir/'],
gallery: [
tv_filmnet_home,
tv_filmnet_search,
tv_filmnet_single,
tv_filmnet_details,
tv_filmnet_login,
],
},
{
slug: 'project-manager',
title: 'Project Manager App',
description: 'A project manager application for managing projects, contracts and tasks.',
tools: ['ReactJS', 'Material-UI', 'React-Router', 'Redux', 'React-Redux'],
date: '2021',
},
{
slug: 'petus',
title: 'LDM Panel',
description: 'A panel for managing the LDM system for PETUS company.',
tools: ['ReactJS', 'Material-UI', 'React-Router', 'Redux', 'React-Redux'],
date: '2020',
},
{
slug: 'italian-dream',
title: 'Italian Dream',
description: 'A website for guiding students to study in Italy.',
tools: ['HTML5', 'CSS3', 'JavaScript', 'BootStrap', 'JQuery', 'PHP'],
date: '2020',
}
]; |
<template>
<button
id="button-compo"
:disabled="disabled"
:width="width"
type="button"
style="outline: none !important"
class="inline-flex items-center transition focus:outline-none"
:class="classAtt"
>
<icon-component
:data-svg="type"
class="icon-comp"
v-if="icon"
:name="icon"
:type="positionIcon"
:color="iconCol"
/>
<div
v-if="type === 'count'"
:class="disabled ? 'bg-primary-100' : 'bg-primary-500'"
class="h-20 w-20 mr-8p rounded-full flex items-center justify-center"
>
<span class="text-white text-14">{{ count }}</span>
</div>
<!-- <div :class="icon && !isEmptySlot ? 'mx-4p' : ''" class="flex items-center"> -->
<div
:class="{
'mx-4p': icon && !isEmptySlot,
'opacity-80': disabled,
}"
class="flex items-center"
>
<slot></slot>
</div>
</button>
</template>
<script lang="ts">
import { defineComponent, computed } from 'vue';
import IconComponent from './IconComponent.vue';
export default defineComponent({
components: {
IconComponent,
// IconComponent: () => import('./IconComponent.vue')
},
props: {
size: {
type: String,
default: 'small',
},
type: {
type: String,
default: 'primary',
},
disabled: {
type: Boolean,
default: false,
},
icon: {
type: String,
},
positionIcon: {
type: String,
default: null,
},
width: {
type: String,
default: '',
},
disclosure: {
type: Boolean,
default: false,
},
count: {
type: String,
default: '0',
},
active: {
type: Boolean,
default: false,
},
colorIcon: {
type: String,
default: '',
},
isFocus: {
type: Boolean,
default: false,
},
},
setup(props, { slots }) {
const isEmptySlot = computed(() => !slots.default);
const iconCol = computed(() => {
if (props.type === 'destructive-sublte') return 'error-3';
else if (props.colorIcon) return props.colorIcon;
else
return ['subtle', 'outline', 'secondary', 'link', 'destructive-link'].includes(props.type)
? 'black'
: 'white';
});
const classAtt = computed(() => {
const cursorIcon = {
'cursor-not-allowed': props.disabled,
};
const classSize = {
'text-16': ['link', 'destructive-link'].includes(props.type) && props.size === 'large',
'py-10p px-20p font-medium h-40':
props.size === 'large' && !['link', 'destructive-link'].includes(props.type) && !isEmptySlot.value,
'py-8p px-16p font-medium h-36':
props.size === 'medium' && !['link', 'destructive-link'].includes(props.type) && !isEmptySlot.value,
'py-6p px-12p font-medium h-32':
props.size === 'small' && !['link', 'destructive-link'].includes(props.type) && !isEmptySlot.value,
'py-0p px-4p font-normal': ['link', 'destructive-link'].includes(props.type),
'flex justify-center': isEmptySlot.value,
'w-32 h-32': isEmptySlot.value && props.size === 'small',
'w-36 h-36': isEmptySlot.value && props.size === 'medium',
'w-40 h-40': isEmptySlot.value && props.size === 'large',
'w-16 h-20': isEmptySlot.value && props.size === 'tiny',
};
const classIcon = {
'flex-row-reverse': props.positionIcon === 'right',
'pr-6p': props.icon && props.positionIcon === 'right',
'pl-6p': props.icon && props.positionIcon === 'left',
};
const classTypeDefault = {
'bg-primary-500 border-primary-500 hover:bg-primary-700 active:shadow-inner-primary active:bg-primary-500 active:bg-primary-700':
props.type === 'primary' && !props.disabled,
'bg-primary-100': props.type === 'primary' && props.disabled,
'bg-error-3 border hover:bg-error-2 active:bg-error-2 active:shadow-inner-destructive active:bg-error-3':
props.type === 'destructive' && !props.disabled,
'bg-error-6': props.type === 'destructive' && props.disabled,
'text-error-3 border hover:bg-error-5 active:bg-white active:shadow-inner-destructive active:bg-white ':
props.type === 'destructive-sublte' && !props.disabled,
'': props.type === 'destructive-sublte' && props.disabled,
'bg-neutrals-100 border hover:bg-neutrals-200 active:bg-neutrals-200 active:border-neutrals-200 active:outline-none active:border-primary-500 active:bg-neutrals-50 ':
props.type === 'secondary' && !props.disabled,
'bg-neutrals-50 text-neutrals-300': props.type === 'secondary' && props.disabled,
'bg-transparent border border-neutrals-100 hover:bg-neutrals-100 active:bg-neutrals-50 active:border-neutrals-100 active:outline-none active:border-primary-500 active:bg-white outline-none':
props.type === 'outline' && !props.disabled,
'bg-white border border-neutrals-100 text-neutrals-300': props.type === 'outline' && props.disabled,
'hover:bg-neutrals-50 hover:border-neutrals-50 border active:bg-neutrals-200 active:border-neutrals-100 active:border-primary-500 outline-none':
props.type === 'subtle' && !props.disabled,
'text-neutrals-300': props.type === 'subtle' && props.disabled,
'text-primary-500 border active:bg-neutrals-50 active:text-primary-700 active:underline hover:underline active:outline-none active:border-primary-500 hover:text-primary-700':
props.type === 'link' && !props.disabled,
'text-primary-100 border': props.type === 'link' && props.disabled,
'text-error-3 border active:bg-neutrals-50 hover:underline hover:text-error-2 active:outline-none active:border-primary-500 active:text-error-2 active:underline':
props.type === 'destructive-link' && !props.disabled,
'text-error-6 border': props.type === 'destructive-link' && props.disabled,
'bg-primary-50 hover:bg-primary-100 active:bg-primary-200 active:bg-primary-50 border active:outline-none active:border-primary-500 text-primary-700':
props.type === 'count' && !props.disabled,
'bg-primary-50 text-primary-100': props.type === 'count' && props.disabled,
'shadow-sm': !['secondary', 'subtle', 'outline', 'link', 'destructive-link', 'destructive-sublte'].includes(props.type),
};
const classTypeFocus = {
'bg-primary-500 border-primary-500 hover:bg-primary-700 focus:shadow-inner-primary focus:bg-primary-500 active:bg-primary-700':
props.type === 'primary' && !props.disabled,
'bg-primary-100': props.type === 'primary' && props.disabled,
'bg-error-3 border hover:bg-error-2 active:bg-error-2 focus:shadow-inner-destructive focus:bg-error-3':
props.type === 'destructive' && !props.disabled,
'bg-error-6': props.type === 'destructive' && props.disabled,
'border hover:bg-error-5 active:bg-white focus:shadow-inner-destructive focus:bg-white ':
props.type === 'destructive-sublte' && !props.disabled,
'': props.type === 'destructive-sublte' && props.disabled,
'bg-neutrals-100 border hover:bg-neutrals-200 active:bg-neutrals-200 active:border-neutrals-200 focus:outline-none focus:border-primary-500 focus:bg-neutrals-50 ':
props.type === 'secondary' && !props.disabled,
'bg-neutrals-50 text-neutrals-300': props.type === 'secondary' && props.disabled,
'bg-transparent border border-neutrals-100 hover:bg-neutrals-100 active:bg-neutrals-50 active:border-neutrals-100 focus:outline-none focus:border-primary-500 focus:bg-white outline-none':
props.type === 'outline' && !props.disabled,
'bg-white border border-neutrals-100 text-neutrals-300': props.type === 'outline' && props.disabled,
'hover:bg-neutrals-50 hover:border-neutrals-50 border active:bg-neutrals-200 active:border-neutrals-100 focus:border-primary-500 outline-none':
props.type === 'subtle' && !props.disabled,
'text-neutrals-300': props.type === 'subtle' && props.disabled,
'text-primary-500 border active:bg-neutrals-50 active:text-primary-700 active:underline hover:underline focus:outline-none focus:border-primary-500 hover:text-primary-700':
props.type === 'link' && !props.disabled,
'text-primary-100 border': props.type === 'link' && props.disabled,
'text-error-3 border active:bg-neutrals-50 hover:underline hover:text-error-2 focus:outline-none focus:border-primary-500 active:text-error-2 active:underline':
props.type === 'destructive-link' && !props.disabled,
'text-error-6 border': props.type === 'destructive-link' && props.disabled,
'bg-primary-50 hover:bg-primary-100 active:bg-primary-200 focus:bg-primary-50 border focus:outline-none focus:border-primary-500 text-primary-700':
props.type === 'count' && !props.disabled,
'bg-primary-50 text-primary-100': props.type === 'count' && props.disabled,
'shadow-sm': !['secondary', 'subtle', 'outline', 'link', 'destructive-link'].includes(props.type),
};
const classType = props.isFocus ? classTypeFocus : classTypeDefault;
const classTextCol = {
'text-error-3': ['destructive-subtle'].includes(props.type),
'text-white': ['primary', 'destructive'].includes(props.type),
'text-neutrals-900': ['secondary', 'outline', 'subtle'].includes(props.type) && !props.disabled,
};
const active = {
'active-btn': props.active,
};
return {
...active,
...classSize,
...classType,
...classTextCol,
...classIcon,
...cursorIcon,
'inline-flex items-center border-transparent text-14 rounded-4p focus:outline-none focus:ring-2 focus:ring-offset-2 focus:ring-indigo-500':
true,
};
});
return { classAtt, isEmptySlot, iconCol };
},
});
</script>
<style lang="scss" scoped>
button.active-btn {
border-color: #3e6dda;
border-color: rgba(62, 109, 218, var(--border-opacity));
}
button {
transition: 0.5s all;
// > div {
// transition: 0.5s all;
// }
.icon-comp[data-svg='primary'] {
:deep(svg) {
fill: white;
}
}
.icon-comp[data-svg='secondary'] {
:deep(svg) {
fill: black;
}
}
.icon-comp[data-svg='outline'] {
:deep(svg) {
fill: currentColor;
}
}
.icon-comp[data-svg='subtle'] {
:deep(svg) {
fill: black;
}
}
.icon-comp[data-svg='link'] {
:deep(svg) {
fill: #3e6dda;
}
}
.icon-comp[data-svg='destructive'] {
:deep(svg) {
fill: white;
}
}
.icon-comp[data-svg='destructive-link'] {
:deep(svg) {
fill: #ec2b08;
}
}
&:hover {
.icon-comp[data-svg='link'] {
:deep(svg) {
fill: #1b3d8d;
}
}
.icon-comp[data-svg='destructive-link'] {
:deep(svg) {
fill: #b12006;
}
}
}
&:focus {
.icon-comp[data-svg='link'] {
:deep(svg) {
fill: #3e6dda;
}
}
.icon-comp[data-svg='destructive-link'] {
:deep(svg) {
fill: #ec2b08;
}
}
}
&:active {
.icon-comp[data-svg='link'] {
:deep(svg) {
fill: #1b3d8d;
}
}
.icon-comp[data-svg='destructive-link'] {
:deep(svg) {
fill: #b12006;
}
}
}
&[disabled],
&:disabled {
.icon-comp[data-svg='subtle'],
.icon-comp[data-svg='outline'],
.icon-comp[data-svg='secondary'] {
color: white;
:deep(svg) {
fill: #9b9ba8;
}
}
.icon-comp[data-svg='link'] {
:deep(svg) {
fill: #bfcef3;
}
}
.icon-comp[data-svg='destructive-link'] {
:deep(svg) {
fill: #f29f8f;
}
}
}
}
</style> |
<template>
<field-set-component legend="Listado">
<header-search-detail-component
v-if="stateArticle._id"
id="header-search-children-feature"
:columns-filter="columnsFilterArticleChildrenFeatureDetail"
:column-filter-selected-default="'a.nombrecaracteristica'"
@on-change-field="onChangeField"
@on-search-for-value="onSearchForValue"
/>
<table-good-component
:title-notification="titleNotificationArticleChildrenFeatureDetail"
:columns="columnsArticleChildrenFeatureDetail"
:server-query="serverQueryArticleChildrenFeatureDetail"
:data-table="dataTableArticleChildrenFeatureDetail"
:load-items="loadItemsArticleChildrenFeatureDetail"
:manage-row="sendArticleChildrenFeatureDetail"
:pagination-enabled="!!stateArticle._id"
:option-show="false"
:option-edit="false"
:option-delete="false"
:option-status="false"
:slot-options="true"
@open-modal-for-edit="row=>openModalFor(row, 'edit')"
>
<template
#options="{ props }"
>
<template
v-if="props.row.tipo === 'H'"
>
<b-dropdown
variant="link"
toggle-class="text-decoration-none"
no-caret
size="sm"
>
<template v-slot:button-content>
<feather-icon
icon="MoreHorizontalIcon"
size="16"
class="text-body align-middle mr-25"
/>
</template>
<!-- INICIAR CAMBIAR ESTADO -->
<template v-if="optionsPermissions.includes(CAMBIAR_ESTADO)">
<b-dropdown-item
@click.stop="changeStatus(props.row.idt)"
>
<div class="d-flex align-items-center">
<feather-icon
:icon="props.row.activo ? 'SlashIcon' : 'CheckCircleIcon'"
class="mr-50"
/>
<span class="d-inline-block">
{{ props.row.activo ? 'Desactivar' : 'Activar' }}
</span>
</div>
</b-dropdown-item>
</template>
<!-- FINAL CAMBIAR ESTADO -->
<!-- INICIO EDITAR -->
<template v-if="optionsPermissions.includes(EDITAR)">
<b-dropdown-item
@click.stop="openModalFor(props.row)"
>
<div class="d-flex align-items-center">
<feather-icon
icon="Edit2Icon"
class="mr-50"
/>
<span class="d-inline-block">Editar</span>
</div>
</b-dropdown-item>
</template>
<!-- FINAL EDITAR -->
<!-- INICIO ELIMINAR -->
<template v-if="optionsPermissions.includes(ELIMINAR)">
<b-dropdown-item
@click.stop="deleteRow(props.row.idt)"
>
<div class="d-flex align-items-center">
<feather-icon
icon="TrashIcon"
class="mr-50"
/>
<span class="d-inline-block">Eliminar</span>
</div>
</b-dropdown-item>
</template>
<!-- FINAL ELIMINAR -->
</b-dropdown>
</template>
<template
v-else-if="props.row.tipo === 'P'"
>
<feather-icon
icon="LockIcon"
/>
</template>
</template>
</table-good-component>
</field-set-component>
</template>
<script>
import {
BDropdown,
BDropdownItem,
} from 'bootstrap-vue'
import HeaderSearchDetailComponent from '@/components/HeaderSearchDetailComponent/HeaderSearchDetailComponent.vue'
import FieldSetComponent from '@/components/FieldSetComponent/FieldSetComponent.vue'
import TableGoodComponent from '@/components/TableComponent/TableGoodComponent.vue'
import { confirmSwal } from '@/helpers/messageExtensions'
import { ACTION_DELETE, ACTION_STATUS } from '@/helpers/actionsApi'
import { endPointsCombo, loadCombos } from '@/helpers/combos'
import {
CAMBIAR_ESTADO,
EDITAR,
ELIMINAR,
} from '@/options'
import { stateArticle } from '../ServicesArticle/useVariablesArticle'
import {
columnsArticleChildrenFeatureDetail, serverQueryArticleChildrenFeatureDetail, dataTableArticleChildrenFeatureDetail, columnsFilterArticleChildrenFeatureDetail, titleNotificationArticleChildrenFeatureDetail, stateArticleChildrenFeatureDetail, combosArticleChildrenFeatureDetail,
} from '../ServicesArticleChildrenFeatureDetail/useVariablesArticleChildrenFeatureDetail'
import { loadItemsArticleChildrenFeatureDetail, sendArticleChildrenFeatureDetail } from '../ServicesArticleChildrenFeatureDetail/useServicesArticleChildrenFeatureDetail'
export default {
name: 'DetailTableChildrenFeature',
components: {
BDropdown,
BDropdownItem,
FieldSetComponent,
TableGoodComponent,
HeaderSearchDetailComponent,
},
computed: {
optionsPermissions() {
if (this.$store.state.rolesAndPermissions.options[this.$route.name]) {
return this.$store.state.rolesAndPermissions.options[this.$route.name]
}
return []
},
},
setup() {
let timer = null
const timeForLoad = 500
const changeStatus = async _id => {
const confirm = await confirmSwal(titleNotificationArticleChildrenFeatureDetail, ACTION_STATUS)
if (confirm) {
dataTableArticleChildrenFeatureDetail.value.loading = true
const { status } = await sendArticleChildrenFeatureDetail(ACTION_STATUS, _id)
dataTableArticleChildrenFeatureDetail.value.loading = false
if (status) loadItemsArticleChildrenFeatureDetail(1)
}
}
const openModalFor = async ({
idt, idTArticulo, idCaracteristica, idDtlCaracteristica, orden,
}) => {
dataTableArticleChildrenFeatureDetail.value.loading = true
await loadCombos(combosArticleChildrenFeatureDetail, ['featureDetail'], `${endPointsCombo.detalleCaracteristica}/1/${idCaracteristica}/0`, 'Valores Caracterรญstica')
stateArticleChildrenFeatureDetail.value._id = idt
stateArticleChildrenFeatureDetail.value.idTArticulo = idTArticulo
stateArticleChildrenFeatureDetail.value.idCaracteristica = idCaracteristica
stateArticleChildrenFeatureDetail.value.idDtlCaracteristica = idDtlCaracteristica
stateArticleChildrenFeatureDetail.value.orden = orden
dataTableArticleChildrenFeatureDetail.value.loading = false
return true
}
const deleteRow = async _id => {
const confirm = await confirmSwal(titleNotificationArticleChildrenFeatureDetail, ACTION_DELETE)
if (confirm) {
dataTableArticleChildrenFeatureDetail.value.loading = true
const { status } = await sendArticleChildrenFeatureDetail(ACTION_DELETE, _id)
dataTableArticleChildrenFeatureDetail.value.loading = false
if (status) loadItemsArticleChildrenFeatureDetail(1)
}
}
const onChangeField = (field, value) => {
serverQueryArticleChildrenFeatureDetail.value.campofiltro = field
serverQueryArticleChildrenFeatureDetail.value.filtro = value
loadItemsArticleChildrenFeatureDetail(1)
}
const onSearchForValue = (field, value) => {
dataTableArticleChildrenFeatureDetail.value.loading = true
clearTimeout(timer)
timer = setTimeout(() => {
serverQueryArticleChildrenFeatureDetail.value.campofiltro = field
serverQueryArticleChildrenFeatureDetail.value.filtro = value
loadItemsArticleChildrenFeatureDetail(1)
}, timeForLoad)
}
return {
stateArticle,
columnsArticleChildrenFeatureDetail,
serverQueryArticleChildrenFeatureDetail,
dataTableArticleChildrenFeatureDetail,
loadItemsArticleChildrenFeatureDetail,
sendArticleChildrenFeatureDetail,
columnsFilterArticleChildrenFeatureDetail,
titleNotificationArticleChildrenFeatureDetail,
changeStatus,
openModalFor,
deleteRow,
onChangeField,
onSearchForValue,
CAMBIAR_ESTADO,
EDITAR,
ELIMINAR,
}
},
}
</script> |
context("get_regulators")
test_that("Functions to retrieve gene regulation work as expected", {
## Connect to the RegulonDB database if necessary
if (!exists("regulondb_conn")) {
regulondb_conn <- connect_database()
}
## Build a regulondb object
regdb <-
regulondb(
database_conn = regulondb_conn,
organism = "prueba",
genome_version = "prueba",
database_version = "prueba"
)
rs1 <- get_gene_regulators(regdb, c("araC", "fis", "crp"))
expect_s4_class(rs1, "regulondb_result")
expect_true(metadata(rs1)$format == "multirow")
rs2 <-
get_gene_regulators(regdb, c("araC", "fis", "crp"), format = "onerow")
expect_s4_class(rs2, "regulondb_result")
expect_true(metadata(rs2)$format == "onerow")
rs3 <-
get_gene_regulators(regdb, c("araC", "fis", "crp"), format = "table")
expect_s4_class(rs3, "regulondb_result")
expect_true(metadata(rs3)$format == "table")
expect_s4_class(get_regulatory_network(regdb), "regulondb_result")
expect_s4_class(
get_regulatory_summary(regdb, gene_regulators = c("araC", "modB")),
"regulondb_result"
)
expect_error(get_regulatory_summary(regdb, gene_regulators = c(1, 2, 3)))
regulation_table <-
get_gene_regulators(regdb,
genes = c("araC", "modB"),
format = "table"
)
expect_s4_class(
get_regulatory_summary(regdb, regulation_table),
"regulondb_result"
)
regulation_onerow <-
get_gene_regulators(regdb,
genes = c("araC", "modB"),
format = "onerow"
)
expect_s4_class(
get_regulatory_summary(regdb, regulation_onerow),
"regulondb_result"
)
expect_error(
get_gene_regulators(regdb, genes = NULL),
"Parameter 'genes' must be a character vector or list."
)
expect_error(
get_gene_regulators(regdb, genes = c("araC"), output.type = "TFS"),
"Parameter 'output.type' must be either 'TF' or 'GENE'"
)
expect_error(
get_regulatory_network(regdb, type = "GEN-GENE"),
"Parameter 'type' must be TF-GENE, TF-TF, or GENE-GENE."
)
expect_s4_class(
get_regulatory_network(regdb, regulator = "Fis"),
"regulondb_result"
)
expect_error(
get_regulatory_network(regdb, cytograph = TRUE),
"To use integration with Cytoscape, please launch Cytoscape before running get_regulatory_network()"
)
}) |
// @ts-nocheck
import type { NextPage } from "next";
import Head from "next/head";
import Image from "next/image";
import styles from "../styles/Home.module.css";
import React from "react";
const Home: NextPage = ({ comments }) => {
return (
<div className={styles.container}>
<h1>Homepage</h1>
{comments.map((comment) => (
<p key={comment.id}>{comment.name}</p>
))}
</div>
);
};
export async function getStaticProps(_context) {
let comments;
const result = await fetch("https://jsonplaceholder.cypress.io/comments");
if (result.ok) {
const json = await result.json();
comments = json;
}
return {
props: {
comments: comments ?? [],
},
};
}
export default Home; |
import React, { useContext, useEffect, useState } from 'react';
import { Link, useHistory } from 'react-router-dom';
import { ApiContext } from '../../contexts/apiContext'; // Import the ApiContext for accessing product data
import api from '../../services/api'; // Import the API service for making requests
import { toast } from 'react-toastify'; // Import toast notifications for user feedback
import 'react-toastify/dist/ReactToastify.css'; // Import toast styles
import { FiShoppingCart } from 'react-icons/fi'; // Import the shopping cart icon
import Loading from '../../components/Loading'; // Import the Loading component
import * as S from "./styles"; // Import styled components from './styles'
export default function Inspect(props) {
const history = useHistory(); // Initialize useHistory for navigation
const { allProducts } = useContext(ApiContext); // Access product data from ApiContext
const id = props.match.params.id; // Get the product ID from the URL parameter
const index = parseInt(id); // Convert the ID to an integer
const [product, setProduct] = useState({}); // Initialize state for the selected product
const [loading, setLoading] = useState(false); // Initialize loading state
const [quantity, setQuantity] = useState(1); // Initialize state for the quantity of the product
useEffect(() => {
async function loadProduct() {
setLoading(true);
const response = await api.get(`products/${index}`); // Fetch the product data from the API
if (response.data === null) {
history.replace('/'); // Redirect to the home page if the product doesn't exist
return;
}
let p = {
category: response.data.category,
description: response.data.description,
id: response.data.id,
image: response.data.image,
price: response.data.price,
rating: response.data.rating,
title: response.data.title,
quantity: 1 // Set the initial quantity to 1
};
setProduct(p); // Set the fetched product data
setLoading(false); // Finish loading
}
loadProduct(); // Call the function to load the product data
}, []);
// Function to add the product to the cart
function addItemOnCart() {
const myList = localStorage.getItem('products');
let savedProducts = JSON.parse(myList) || [];
const hasProduct = savedProducts.some(
(savedProduct) => savedProduct.id === product.id
);
if (hasProduct) {
// Show a toast notification if the product is already in the cart
toast.info('This product is already in the cart.', {
position: "top-left",
autoClose: 2000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined,
});
return;
}
savedProducts.push(product); // Add the product to the cart
localStorage.setItem('products', JSON.stringify(savedProducts));
// Show a toast notification for adding the product to the cart
toast.success('Product added to the cart!', {
position: "top-left",
autoClose: 1500,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined,
});
}
// Function to decrease the quantity of the product
function removeQuantity() {
if (product.quantity === 0) {
return; // Prevent negative quantity
}
product.quantity -= 1; // Decrease the quantity
setQuantity(quantity - 1); // Update the state
}
// Function to increase the quantity of the product
function addQuantity() {
product.quantity += 1; // Increase the quantity
setQuantity(quantity + 1); // Update the state
}
// Render the component elements
return (
<>
<S.Breadcrumb>
<Link to='/'>Home</Link>
<span>/</span>
<Link to="/products">Products</Link>
<span>/</span>
<p>{product.title}</p>
</S.Breadcrumb>
{loading && <Loading />} {/* Display loading spinner if loading is true */}
{!loading && (
<S.InspectItem>
<S.ImageProduct>
<img src={product.image} alt={product.title} />
</S.ImageProduct>
<S.InfoProduct>
<S.TitleCompany>E-Shop</S.TitleCompany>
<h1>{product.title}</h1>
<p>{product.description}</p>
<span>${product.price}</span>
<S.Purchase>
<div className="quantity">
<button type="button" onClick={removeQuantity}>-</button>
<input type="text" value={quantity} readOnly />
<button type="button" onClick={addQuantity}>+</button>
</div>
<button className="buttonPurchase" onClick={addItemOnCart}>
<FiShoppingCart /> Add to Cart
</button>
</S.Purchase>
</S.InfoProduct>
</S.InspectItem>
)}
</>
);
} |
<div class="container" style="margin-top:90px">
<div class="row">
<div class="col-md-12">
<form [formGroup]="signupForm">
<mat-form-field>
<input matInput placeholder="First Name" formControlName="firstName" required>
</mat-form-field>
<!-- <mat-error>
<span *ngIf="!signupForm.get('firstName').valid &&
signupForm.get('firstName').touched">First Name can't be empty.</span>
</mat-error> -->
<mat-form-field>
<input matInput placeholder="Last Name" formControlName="lastName" required>
</mat-form-field>
<mat-form-field>
<input matInput placeholder="Email ID" formControlName="email" required>
</mat-form-field>
<!-- <mat-error>
<span *ngIf="!signupForm.get('lastName').valid &&
signupForm.get('lastName').touched">Last Name can't be empty.</span>
</mat-error> -->
<mat-form-field>
<input matInput placeholder="Password" formControlName="password" type="password" required>
</mat-form-field>
<!-- <mat-error>
<span *ngIf="!signupForm.get('password').valid &&
signupForm.get('password').touched">Password can't be empty.</span>
</mat-error> -->
<mat-form-field>
<input matInput placeholder="Confirm Password" formControlName="confirmPassword" type="password" required>
</mat-form-field>
<!-- <mat-error>
<span *ngIf="!signupForm.get('confirmPassword').valid &&
signupForm.get('confirmPassword').touched">Confirm Password can't be empty.</span>
</mat-error> -->
<mat-form-field>
<input matInput placeholder="Display Name" formControlName="displayName" required>
</mat-form-field>
<!-- <mat-error>
<span *ngIf="!signupForm.get('displayName').valid &&
signupForm.get('displayName').touched">Display Name can't be empty.</span>
</mat-error> -->
<div>
<button mat-button [disabled]="!signupForm.valid" class="btn btn-primary" (click)="addUser()" >Submit</button>
</div>
<div>
<div>
<span *ngIf="isSuccess">User has been added successfully.</span>
</div>
<mat-error>
<span *ngIf="!isSuccess">{{responseMessage}}</span>
</mat-error>
</div>
</form>
</div>
</div>
</div> |
/* Variables */
// Colors:
// Should be assigned to "roles", never used directly.
$jade : #00C1A1;
$jade-dark : #455E57;
$coral : #F46969;
$rouge : #FF3B00;
$grey-dark : #444444;
$grey : #7E7E7E;
$grey-med : #E0E6E9;
$grey-light : #F2F2F2;
$dark-slate : #171B1E;
$dim-slate : #23282B;
$dank-slate : #2A3235;
$dead-slate : #1E2428;
$slate : #48565C;
$dark-label : white;
$dim-label : #A4AAAE;
$dank-label : #62656B;
// Color Roles:
// Roles can change more easily than named colors.
$accent : $jade;
$emphasis : $coral;
$alert : $rouge;
$background-color : $grey-med;
$foreground-color : white;
$base-font-color : $grey;
$base-heading-color : $jade-dark;
$base-link-color : $accent;
$base-link-hover : $emphasis;
$base-border-color : $grey-med;
$base-icon-color : $grey;
$alt-icon-color : $dim-label;
$progress-track : $slate;
$progress-status : $accent;
// Fonts:
$base-font-family : "Lato", "Helvetica Neue", Helvetica, Arial, sans-serif;
$base-font-size : em( 16 );
$base-line-height : 1.6;
// Measurements:
$toolbar-height : 60px;
$menu-width : 200px;
$menu-compact-width : 60px;
$session-width : 200px;
// Depths:
$background : 1000;
$middleground : 2000;
$foreground : 3000;
// Animation Styles:
$layout-shift: 333ms ease-out;
$overlay-shift: 456ms ease-out;
// Grid & Layout Values:
@import 'neat-helpers';
// Neat Overrides
// $column : em(90);
// $gutter : em(18);
// $grid-columns : 12;
$max-width : em(1200);
// Neat Utilities
// $visual-grid: true; // Un-comment for visual grid.
$visual-grid-color : black;
$visual-grid-opacity : 0.1;
$visual-grid-index : front;
// Neat Breakpoints
$screen-xs : em(480);
$screen-sm : em(768);
$screen-md : em(970);
$screen-lg : em(1200);
$screen-short : em(900);
// Media Queries:
// For min-width queries, above the breakpoint
$min-xs : new-breakpoint(min-width $screen-xs);
$min-sm : new-breakpoint(min-width $screen-sm);
$min-md : new-breakpoint(min-width $screen-md);
$min-lg : new-breakpoint(min-width $screen-lg);
$min-short : new-breakpoint(min-height $screen-short);
// For max-width queries, below the breakpoint
$max-xs : new-breakpoint(max-width $screen-xs - 1);
$max-sm : new-breakpoint(max-width $screen-sm - 1);
$max-md : new-breakpoint(max-width $screen-md - 1);
$max-lg : new-breakpoint(max-width $screen-lg - 1);
$max-short : new-breakpoint(max-height $screen-short - 1);
// Combos: Between the min and max breakpoints
$btw-xs-sm : new-breakpoint( min-width $screen-xs max-width $screen-sm - 1 );
$btw-sm-md : new-breakpoint( min-width $screen-sm max-width $screen-md - 1 );
$btw-md-lg : new-breakpoint( min-width $screen-md max-width $screen-lg - 1 );
// Usage: @include media($min-sm) { ... } |
export enum Role {
visitor = "visitor",
manager = "manager",
supermanager = "supermanager",
contentManager = "contentManager",
operator = "operator",
admin = "admin",
}
const rules = {
visitor: {
static: [
"auth:login",
"faq:pretty",
"fund:description-pretty",
"news:public",
],
},
contentManager: {
static: [
"applications:show",
"settings:index",
"users:show",
"fund:index",
"fund:faq-index",
"fund:description",
"fund:description-edit",
"faq:edit",
"news:edit",
"news:create",
"news:index",
"notifications:index",
],
},
operator: {
static: [
"chats:show",
"chats:index",
"settings:index",
"notifications:index",
],
},
manager: {
static: [
"applications:index",
"settings:index",
"application:edit",
"applications:show",
"applications:create",
"users:show",
"user:view-applications",
"notifications:index",
],
},
supermanager: {
static: [
"applications:index",
"applications:show",
"application:edit",
"application:can-vote",
"applications:create",
"settings:index",
"categories:index",
"users:show",
"user:view-applications",
"fund:index",
"fund:description",
"transactions:show",
"transactions:index",
"transactions:create",
"managers:index",
"managers:show",
"fund:faq-index",
"notifications:index",
"transactions:distribute",
],
},
admin: {
static: [
"users:index",
"users:show",
"user:edit",
"users:create",
"user:view-sessions",
"user:show-admin",
"settings:index",
"logs:index",
"notifications:index",
],
},
};
export function check(role: Role, action: string): boolean {
const permissions = rules[role];
if (!permissions) {
// role is not present in the rules
return false;
}
const staticPermissions = permissions.static;
if (staticPermissions && staticPermissions.includes(action)) {
// static rule not provided for action
return true;
}
return false;
}
export default rules; |
import './index.css';
// Fibonacci logic
function validateFibInput(inp) {
const i = Number(inp.trim());
return [i, Number.isInteger(i) && i >= 0 && i <= 1000];
}
function fibonacci(num) {
const result = [];
const memo = {};
const fib = (n) => {
if (!Number.isInteger(memo[n])) {
memo[n] = n < 2 ? n : fib(n - 1) + fib(n - 2);
}
return memo[n];
};
for (let i = 0; i < num; i++) {
result[i] = fib(i);
}
return result;
}
// Fibonacci UI
const fibResult = document.querySelector('#fibonacci .result');
const fibForm = document.querySelector('#fibonacci form');
fibForm['fib-btn'].disabled = true;
fibForm.addEventListener('input', () => {
fibResult.textContent = '';
fibForm['fib-btn'].disabled = !fibForm.checkValidity();
});
fibForm.addEventListener('submit', (event) => {
event.preventDefault();
const fibNum = event.target['fib-number'].value;
const [num, isValid] = validateFibInput(fibNum);
if (isValid) {
fibResult.textContent = fibonacci(num).join(',');
}
});
// Merge Sort logic
function validateNumList(numbers) {
const numArr = numbers
.trim()
.split(' ')
.filter((num) => num !== '')
.map((num) => Number(num));
return [numArr, numArr.every((num) => Number.isInteger(num))];
}
function mergeSort(numArr) {
if (numArr.length < 2) return numArr;
const arrCenter = Math.floor(numArr.length / 2);
const firstSortedHalf = mergeSort(numArr.slice(0, arrCenter));
const secondSortedHalf = mergeSort(numArr.slice(arrCenter));
const newArr = [];
while (firstSortedHalf.length > 0 && secondSortedHalf.length > 0) {
newArr.push(
firstSortedHalf[0] < secondSortedHalf[0]
? firstSortedHalf.shift()
: secondSortedHalf.shift(),
);
}
if (firstSortedHalf.length > 0) {
newArr.push(...firstSortedHalf);
} else {
newArr.push(...secondSortedHalf);
}
return newArr;
}
// Merge Sort UI
const sortResult = document.querySelector('#merge-sort .result');
const sortForm = document.querySelector('#merge-sort form');
sortForm['sort-btn'].disabled = true;
sortForm.addEventListener('input', () => {
sortResult.textContent = '';
sortForm['sort-btn'].disabled = !sortForm.checkValidity();
});
sortForm.addEventListener('submit', (event) => {
event.preventDefault();
const numList = event.target['number-list'].value;
const [numArr, isValid] = validateNumList(numList);
if (isValid) {
sortResult.textContent = mergeSort(numArr).join(' ');
}
}); |
๏ปฟusing System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
using System.Windows;
using sysWindows = System.Windows;
namespace AppDevTools.Extensions
{
/// <summary>
/// Provides a collection of methods that extend the functionality of the System.Windows.Window class
/// </summary>
public class Window : sysWindows.Window
{
#region Enums
#region Public
public enum WindowStateWin32
{
SW_HIDE = 0,
SW_SHOWNORMAL = 1,
SW_SHOWMINIMIZED = 2,
SW_SHOWMAXIMIZED = 3,
SW_SHOWNOACTIVATE = 4,
SW_SHOW = 5,
SW_MINIMIZE = 6,
SW_SHOWMINNOACTIVE = 7,
SW_SHOWNA = 8,
SW_RESTORE = 9,
SW_SHOWDEFAULT = 10,
SW_FORCEMINIMIZE = 11
}
#endregion Public
#endregion Enums
#region Methods
#region Private
[DllImport("user32.dll")]
private static extern bool ShowWindow(IntPtr handle, int cmdShow);
[DllImport("user32.dll")]
private static extern int SetForegroundWindow(IntPtr handle);
#endregion Private
#region Public
#region Method to check the window is open or not
/// <summary>
/// Checks window is open or not
/// </summary>
/// <param name="app">
/// The application that the window belongs to
/// </param>
/// <param name="titleWindow">
/// Window title
/// </param>
/// <returns>
/// true - if is opened, otherwise false
/// </returns>
/// <exception cref="ArgumentNullException"></exception>
public static bool IsOpened(Application app, string titleWindow)
{
if (app == null)
{
throw new ArgumentNullException(nameof(app));
}
if (titleWindow == null)
{
throw new ArgumentNullException(nameof(titleWindow));
}
List<sysWindows.Window> windows = app.Windows.Cast<sysWindows.Window>().Where(x => x.Title == titleWindow).ToList();
if (windows.Count >= 1)
{
windows.First().Activate();
return true;
}
return false;
}
#endregion Method to check the window is open or not
#region Method for getting window
/// <summary>
/// Gets window of application
/// </summary>
/// <param name="app">
/// The application that the window belongs to
/// </param>
/// <param name="titleWindow">
/// Window title
/// </param>
/// <returns>
/// Window
/// </returns>
/// <exception cref="ArgumentNullException"></exception>
public static sysWindows.Window? Get(Application app, string titleWindow)
{
if (app == null)
{
throw new ArgumentNullException(nameof(app));
}
if (titleWindow == null)
{
throw new ArgumentNullException(nameof(titleWindow));
}
return app.Windows.Cast<sysWindows.Window>().Where(x => x.Title == titleWindow).ToList().FirstOrDefault();
}
#endregion Method for getting window
#region Methods for activating window
/// <summary>
/// Activates window of application
/// </summary>
/// <param name="app">
/// The application that the window belongs to
/// </param>
/// <param name="titleWindow">
/// Window title
/// </param>
public static void Activate(Application app, string titleWindow)
{
sysWindows.Window? window = Get(app, titleWindow);
if (window != null)
{
window.Activate();
}
}
/// <summary>
/// Activates window by the specified handle
/// </summary>
/// <param name="handleWindow">
/// The specified handle for window
/// </param>
public static void Activate(IntPtr handleWindow)
{
SetForegroundWindow(handleWindow);
}
#endregion Methods for activating window
#region Methods for showing window
/// <summary>
/// Shows window of application
/// </summary>
/// <param name="app">
/// The application that the window belongs to
/// </param>
/// <param name="titleWindow">
/// Window title
/// </param>
public static void Show(Application app, string titleWindow)
{
sysWindows.Window? window = Get(app, titleWindow);
if (window != null)
{
window.Activate();
window.Show();
}
}
/// <summary>
/// Shows window by the specified handle
/// </summary>
/// <param name="handleWindow">
/// The specified handle for window
/// </param>
/// <param name="windowState">
/// The state of the window in which it will be shown
/// </param>
public static void Show(IntPtr handleWindow, WindowStateWin32 windowState)
{
ShowWindow(handleWindow, (int)windowState);
}
#endregion Methods for showing window
#region Method for hiding window
/// <summary>
/// Hides window of application
/// </summary>
/// <param name="app">
/// The application that the window belongs to
/// </param>
/// <param name="titleWindow">
/// Window title
/// </param>
public static void Hide(Application app, string titleWindow)
{
sysWindows.Window? window = Get(app, titleWindow);
if (window != null)
{
window.Hide();
}
}
#endregion Method for hiding window
#region Method for closing window
/// <summary>
/// Closes window of application
/// </summary>
/// <param name="app">
/// The application that the window belongs to
/// </param>
/// <param name="titleWindow">
/// Window title
/// </param>
public static void Close(Application app, string titleWindow)
{
sysWindows.Window? window = Get(app, titleWindow);
if (window != null)
{
window.Close();
}
}
#endregion Method for closing window
#endregion Public
#endregion Methods
}
} |
import {
View,
Text,
ScrollView,
Image,
TouchableOpacity,
TextInput,
PermissionsAndroid,
Modal,
Pressable,
Alert,
} from 'react-native';
import React, {useState} from 'react';
import styles from './styles';
import {useDispatch, useSelector} from 'react-redux';
import {
getDataUserById,
updateDataUser,
updateImageUser,
} from '../../stores/actions/user';
import {launchCamera, launchImageLibrary} from 'react-native-image-picker';
import {Picker} from '@react-native-picker/picker';
import {RadioButton} from 'react-native-paper';
export default function EditProfile(props) {
const dispatch = useDispatch();
const [modalVisible, setModalVisible] = useState(false);
const [editableUsername, setEditableUsername] = useState(true);
const [editableEmail, setEditableEmail] = useState(true);
const [editablePhone, setEditablePhone] = useState(true);
const [editableBirthday, setEditableBirthday] = useState(true);
const [checked, setChecked] = useState('male');
const userData = useSelector(state => state.user.userData[0]);
const [form, setForm] = useState({});
const [formImage, setFormImage] = useState({});
const handleChange = (name, value) => {
setForm({...form, [name]: value});
};
const handleSubmit = () => {
try {
dispatch(updateDataUser(userData.userId, form)).then(
response => alert('Update Data Successfull!'),
dispatch(getDataUserById(userData.userId)),
// props.navigation.navigate('Profile'),
);
} catch (error) {
console.log(error.value);
}
};
const handleUpdatePhoto = () => {
// console.log(formImage);
try {
const formDataImage = new FormData();
formDataImage.append('image', formImage.image);
dispatch(updateImageUser(userData.userId, formDataImage))
.then(
response => alert(response.value.data.message),
dispatch(getDataUserById(userData.userId)),
)
.catch(error => {
// console.log(error);
alert(error);
});
} catch (error) {
console.log(error);
}
};
const cameraLaunched = async () => {
try {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.CAMERA,
{
title: 'Cool Photo App Camera Permission',
message: 'Cool Photo App needs access to your camera ',
buttonNeutral: 'Ask Me Later',
buttonNegative: 'Cancel',
buttonPositive: 'OK',
},
);
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
launchCamera(
{
mediaType: 'photo',
includeBase64: false,
maxHeight: 200,
maxWidth: 200,
},
response => {
if (response.didCancel) {
console.log('User cancelled image picker');
}
if (response.errorCode) {
console.log('ImagePicker Error: ', response.errorMessage);
}
if (response.assets) {
const source = {
uri: response.assets[0].uri,
type: response.assets[0].type,
name: response.assets[0].fileName,
};
setFormImage({...formImage, image: source});
}
},
);
} else {
console.log('Camera permission denied');
}
} catch (error) {
console.log(error);
}
};
const galleryLaunched = async () => {
try {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.READ_EXTERNAL_STORAGE,
{
title: 'Cool Photo App Camera Permission',
message: 'Cool Photo App needs access to your camera ',
buttonNeutral: 'Ask Me Later',
buttonNegative: 'Cancel',
buttonPositive: 'OK',
},
);
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
launchImageLibrary(
{
mediaType: 'photo',
includeBase64: false,
maxHeight: 200,
maxWidth: 200,
},
response => {
if (response.didCancel) {
console.log('User cancelled image picker');
}
if (response.errorCode) {
console.log('ImagePicker Error: ', response.errorMessage);
}
if (response.assets) {
const source = {
uri: response.assets[0].uri,
type: response.assets[0].type,
name: response.assets[0].fileName,
};
setFormImage({...formImage, image: source});
}
},
);
} else {
console.log('Camera permission denied');
}
} catch (error) {
console.log(error);
}
};
const handleAppNavigation = path => {
props.navigation.navigate('AppScreen', {screen: path});
};
const userImage = {
uri: `https://res.cloudinary.com/drkoj1bvv/image/upload/v1663649636/${userData.image}`,
};
const randomImage = {
uri: `https://ui-avatars.com/api/?size=512&background=random&name=${userData.username}`,
};
return (
<ScrollView style={styles.backgroundTop}>
<View style={styles.container}>
<View style={styles.profileContainer}>
<TouchableOpacity
style={styles.profileBorder}
onPress={() => setModalVisible(true)}>
<Image
source={userData.image !== null ? userImage : randomImage}
style={styles.profilePicture}
/>
</TouchableOpacity>
<Text style={styles.profileName}>
{userData.name ? userData.name : 'Not set'}
</Text>
<Text style={styles.profileJob}>
{userData.profession ? userData.profession : 'Not set'}
</Text>
</View>
{/* Form Edit */}
<Text style={styles.labelForm}>Name</Text>
<TextInput
placeholder={userData.name ? userData.name : 'Not set'}
placeholderTextColor="gray"
style={styles.nameForm}
onChangeText={text => handleChange('name', text)}
/>
<Text style={styles.labelForm}>Username</Text>
<View style={styles.flexForm}>
<TextInput
placeholder={
userData.username ? `@${userData.username}` : 'Not set'
}
placeholderTextColor="gray"
style={
editableUsername ? styles.uneditableForm : styles.editableForm
}
editable={editableUsername ? false : true}
onChangeText={text => handleChange('username', text)}
/>
<TouchableOpacity>
<Text
style={styles.editForm}
onPress={() => setEditableUsername(!editableUsername)}>
Edit
</Text>
</TouchableOpacity>
</View>
<Text style={styles.labelForm}>Email</Text>
<View style={styles.flexForm}>
<TextInput
placeholder={userData.email ? userData.email : 'Not set'}
placeholderTextColor="gray"
style={editableEmail ? styles.uneditableForm : styles.editableForm}
editable={editableEmail ? false : true}
onChangeText={text => handleChange('email', text)}
/>
</View>
<Text style={styles.labelForm}>Phone</Text>
<View style={styles.flexForm}>
<TextInput
placeholder={userData.phone ? userData.phone : 'Not Set'}
placeholderTextColor="gray"
keyboardType="numeric"
// {userData.phone ? userData.phone : 'Not set'}
style={editablePhone ? styles.uneditableForm : styles.editableForm}
editable={editablePhone ? false : true}
onChangeText={text => handleChange('phone', text)}
/>
<TouchableOpacity>
<Text
style={styles.editForm}
onPress={() => setEditablePhone(!editablePhone)}>
Edit
</Text>
</TouchableOpacity>
</View>
<Text style={styles.labelForm}>Gender</Text>
<View style={styles.flexForm}>
<RadioButton.Group
onValueChange={text => handleChange('gender', text)}>
<View style={styles.radioButtons}>
<RadioButton value="Male" />
<Text style={{marginRight: 30}}>Male</Text>
<RadioButton value="Female" />
<Text>Female</Text>
</View>
</RadioButton.Group>
</View>
<Text style={styles.labelForm}>Profession</Text>
<View style={styles.editableFormPicker}>
<Picker
selectedValue={form.profession}
onValueChange={itemValue => handleChange('profession', itemValue)}>
<Picker.Item
label={
userData.profession
? userData.profession
: 'Choose your profession'
}
value=""
/>
<Picker.Item label="Student" value="Student" />
<Picker.Item label="Teacher" value="Teacher" />
<Picker.Item label="Doctor" value="Doctor" />
<Picker.Item label="Engineer" value="Engineer" />
<Picker.Item label="Other" value="Other" />
</Picker>
</View>
<Text style={styles.labelForm}>Nationality</Text>
<View style={styles.editableFormPicker}>
<Picker
selectedValue={form.nationality}
onValueChange={itemValue => handleChange('nationality', itemValue)}>
<Picker.Item
label={
userData.nationality
? userData.nationality
: 'Choose your nationality'
}
value=""
/>
<Picker.Item label="Indonesia" value="Indonesia" />
<Picker.Item label="Malaysia" value="Malaysia" />
<Picker.Item label="Singapore" value="Singapore" />
<Picker.Item label="India" value="India" />
<Picker.Item label="Japan" value="Japan" />
<Picker.Item label="Other" value="Other" />
</Picker>
</View>
<Text style={styles.labelForm}>Birthday Date</Text>
<View style={styles.flexForm}>
<TextInput
placeholder={
userData.dateOfBirth ? userData.dateOfBirth : 'Not Set'
}
placeholderTextColor="gray"
style={
editableBirthday ? styles.uneditableForm : styles.editableForm
}
editable={editableBirthday ? false : true}
onChangeText={text => handleChange('dateOfBirth', text)}
/>
<TouchableOpacity>
<Text
style={styles.editForm}
onPress={() => setEditableBirthday(!editableBirthday)}>
Edit
</Text>
</TouchableOpacity>
</View>
<TouchableOpacity style={styles.buyButton} onPress={handleUpdatePhoto}>
<Text style={styles.textButton}>Save</Text>
</TouchableOpacity>
<View style={styles.centeredView}>
<Modal
animationType="fade"
transparent={true}
visible={modalVisible}
onRequestClose={() => {
Alert.alert('Modal has been closed.');
setModalVisible(!modalVisible);
}}>
<View style={styles.centeredView}>
<View style={styles.modalView}>
<Text style={styles.modalText}>Update Image</Text>
<TouchableOpacity
style={styles.buttonUpdateImage}
onPress={cameraLaunched}>
<Text style={styles.textButtonUpdateCamera}>Camera</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.buttonUpdateImage}
onPress={galleryLaunched}>
<Text style={styles.textButtonUpdateGalery}>Gallery</Text>
</TouchableOpacity>
<Pressable
style={[styles.button, styles.buttonClose]}
onPress={() => setModalVisible(!modalVisible)}>
<Text style={styles.textStyle}>Cancel</Text>
</Pressable>
</View>
</View>
</Modal>
</View>
</View>
</ScrollView>
);
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Rainy Days Information about us</title>
<link rel="stylesheet" href="style.css">
<link href="https://fonts.googleapis.com/css2?family=Bebas+Neue&display=swap" rel="stylesheet">
<link href="https://fonts.googleapis.com/css2?family=Oswald:wght@200;400&display=swap" rel="stylesheet">
<meta name="description" content="All information about our company Rainy Days.">
</head>
<body>
<header>
<div class="container">
<div class="navbar">
<div class="logo">
<a href="index.html"><img src="images/image 1.png" width="125px"></a>
</div>
<nav>
<ul id="MenuItems">
<li><a href="index.html">Home</a></li>
<li><a href="products.html">Products</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
</ul>
</nav>
<a href="cart.html"><img src="images/cart.png" width="30px" height="30px"></a>
<img src="images/menu.png" onclick="menutoggle()" class="menu-icon">
</div>
</div>
</header>
<main>
<div class="container">
<div class="row">
<div class="col-2">
<h1>ABOUT RAINYDAYS</h1>
<br>
<p>We are here to develop the best rain jackets which will meet your requirements in any weather conditions. Our company is proud of our relationship with customers thru providing the best possible option, in terms of quality and sustainability. Rain jackets that will always be with you, everytime and everywhere.</p>
<a class="btn" href="#">HOME</a>
</div>
<div class="col-4">
<img src="images/about_img.png" alt="">
</div>
</div>
</div>
</main>
<footer>
<div class="footer">
<div class="container">
<div class="row">
<div class="footer-col1">
<h3>Download Our App</h3>
<p>Download App for Android and ios mobile phone.</p>
<div class="app-logo">
<img src="images/play-store.png">
<img src="images/app-store.png">
</div>
</div>
<div class="footer-col2">
<img src="images/image 1.png">
<p>Our Purpose Is To Sustainably Make the Pleasure and Benefits of Sports Accessible to the Many.</p>
</div>
<div class="footer-col3">
<h3>Useful Links</h3>
<ul>
<li>Coupons</li>
<li>Blog Post</li>
<li>Return Policy</li>
<li>Join Affiliate</li>
</ul>
</div>
</div>
</div>
</div>
</footer>
<!-------------js for toggle menu-------------->
<script>
var MenuItems = document.getElementById("MenuItems");
MenuItems.style.maxHeight = "0px";
function menutoggle()
{
if(MenuItems.style.maxHeight == "0px")
{
MenuItems.style.maxHeight = "200px";
}else
{
MenuItems.style.maxHeight = "0px"
}
}
</script>
</body>
</html> |
function [ combineddataout ] = streams_combinedata_iscontent( combineddatain )
%streams_combinedata_iscontent() adds .iscontent field to the combineddata
% structure indicating whether or not the word is content or function word
% based on the Corpus Gesproken Dutch (CGN) POS tag system
%
% CGN tag system reference:
% https://pdfs.semanticscholar.org/f3b4/676b6ce2f16883c3b8253b8b8cb312576db9.pdf
combineddataout = combineddatain;
% add content word field
% CGN tag system here: https://pdfs.semanticscholar.org/f3b4/676b6ce2f16883c3b8253b8b8cb312576db9.pdf
% WW - werkworden - verbs
% N - substantieven - nouns
% ADJ - adjectieven - adjectives
% BW - bijworden - adverbs
content = {'N', 'WW', 'ADJ', 'BW'};
% LET() - leestekens - punctuation
% VG() - voegworden - conjunctions
% LID() - lidworden - articles
% TSW() - tussenverpsels - interjections
% VZ() - voorzetsels - prepositions
% TW() - teelworden - numerals
% VNW() - voornaamwoorden - pronouns
closed = {'LET', 'VZ', 'LID', 'VG', 'TSW', 'TW', 'VNW', 'SPEC'};
fulltags = {combineddatain(:).POS}; % make a cell array from struct fields
wordPOS = cellfun(@(x) strtok(x, '('), fulltags); % retain only the string before the parenthesis
classvec = num2cell(ismember(wordPOS, content)); % create a logical cell array
[combineddataout(1:end).iscontent] = classvec{:};
end |
// JavaScript Document
"use strict";
$(document).ready( () => {
/*The following interactions are based on scrolling*/
$(window).scroll(function() {
/*The keyword this looks at the 'current element'. We decide the current element with the following selectors.*/
/*scrollTop looks at the position of the vertical scrollbar. If the position is above 100px run the next statements.*/
if ($(this).scrollTop()> 100) {
if (!$('.square').is('.run')) {
$('.evanescere').fadeOut(1000); /*In one second anything with the evanescere class will fade out.*/
$('.square').animate({width: "100%"}, 1000).addClass('run'); /*In one second everything with the class square will animate the width wider.*/
}
}
else { /*If the scroll bar is above 100px run these statements*/
if ($('.square').is('.run')) {
$('.evanescere').fadeIn(1000); /*In one second anything with the evanescere class will fade in.*/
$('.square').animate({width: "0px"}, 1000).removeClass('run'); /*In one second everything with the class square will animate the width smaller.*/
}
}
if ($(this).scrollTop()> 200) {
$('.content').fadeIn({queue: false, duration: 500}).animate({top: "0px"}, 1000); /*In 1/2 a second the content will fade in and in one second the content will move up.*/
}
}); /*END Scroll*/
// $('#peeka').mouseenter(() => {
// $('#peeka').animate({right: "90px"}, 500);
// });
// $('#peeka').mouseleave(() => {
// $('#peeka').animate({right: "0px"}, 500);
// });
$('#peeka').click(() => {
if ($('#peeka').hasClass('open')) {
$('#peeka').animate({right: "0px"}, 500).removeClass('open'); /*This will close the footer in 1/2 a second.*/
$('#peeka').html('<-- © Max Reitmayer')
} else {
$('#peeka').animate({right: "90px"}, 500).addClass('open'); /*This will open the footer in 1/2 a second.*/
$('#peeka').html('© Max Reitmayer -->')
}
});
}); |
const React = require('react');
const ReactDOM = require('react-dom');
const IMAGE_ROOT_URL = "/front-page-assets/";
const icons = {
github: <i className="fab fa-github"></i>,
html: <i className="fab fa-html5"></i>,
css: <i className="fab fa-css3-alt"></i>,
js: <i className="fab fa-js"></i>,
node: <i className="fab fa-node-js"></i>,
react: <i className="fab fa-react"></i>,
codepen: <i className="fab fa-codepen"></i>,
unity: <i className="fab fa-unity"></i>,
python: <i className="fab fa-python"></i>,
java: <i className="fab fa-java"></i>,
};
const IconTag = ({icon, href}) => {
const iconContent = icons[icon] ?? icon;
if (href) return (
<a className='icon-tag' href={href}>{iconContent}</a>
);
return (<a className='icon-tag' href={href}>{iconContent}</a>);
};
const Project = (props) => {
const makeTags = (tags) => {
return tags.map(tag =>
<li key={tag}><IconTag icon={tag}/></li>
);
}
return (
<section className="project-container">
<figure>
{props.image ? <img src={`${IMAGE_ROOT_URL}${props.image}`} alt={props.alt}/> : ''}
<figcaption>
<a href={`blogs${props.href}`}>
<h3>{props.title}</h3>
</a>
<IconTag icon="github" href={props.repository}></IconTag>
<ul className="tag-list">
{makeTags(props.tags)}
</ul>
<p>
{props.description}
</p>
</figcaption>
</figure>
</section>
);
};
module.exports = async () => {
const makeProjectList = (projects) => {
return projects.map(project =>
<li key={project.title}><Project
title={project.title}
description={project.description}
image={project.image}
alt={project.alt}
tags={project.tags}
repository={project.repository}
href={project.blog}
/></li>
);
}
const projectDataResponse = await fetch('/front-page-assets/projects.json', {
method: "GET",
headers: {
"Accept": "application/json",
},
});
const projectData = await projectDataResponse.json();
projectData.forEach(category => {
ReactDOM.render(
<ul>
{makeProjectList(category.projects)}
</ul>,
document.querySelector(`#${category.container} .project-list-container`)
);
});
}; |
public with sharing class EMS_Sidebar_Controller {
public String pageName { get; set; }
public List<Note> notes {
get {
if (notes == null) {
notes = new List<Note>();
List<EMS_Sidebar_Configuration__c> sidebarNotes = [
SELECT Id, Page_Name__c, Section_Title__c, Section_Body__c, Start_Date__c, End_Date__c, Active__c, Position__c, (SELECT Datetime_gne__c, User_gne__c, Viewed_gne__c FROM EMS_Sidebar_Viewers__r WHERE User_gne__c = :UserInfo.getUserId() ORDER BY LastModifiedDate ASC)
FROM EMS_Sidebar_Configuration__c
WHERE (Page_Name__c = :pageName OR Page_Name__c = NULL)
AND Active__c = true
AND ((Start_Date__c <= TODAY OR Start_Date__c = NULL) AND (End_Date__c >= TODAY OR End_Date__c = NULL))
ORDER BY Position__c ASC
];
for (EMS_Sidebar_Configuration__c sidebarNote :sidebarNotes) {
notes.add(new Note(sidebarNote));
}
}
return notes;
}
set;
}
public EMS_Sidebar_Controller() {
}
public String getNotesJSON() {
return JSON.serialize(notes);
}
public class Note {
private List<EMS_Sidebar_Viewer__c> views {
get {
if (views == null) views = new List<EMS_Sidebar_Viewer__c>();
return views;
}
set;
}
private Date startDate { get; set; }
private Date endDate { get; set; }
public String pageName { get; set; }
public String title { get; set; }
public String body { get; set; }
public String noteID { get; set; }
public Boolean active { get; set; }
public Boolean viewed { get; set; }
public Note(EMS_Sidebar_Configuration__c noteData) {
this.pageName = noteData.Page_Name__c;
this.title = noteData.Section_Title__c;
this.body = noteData.Section_Body__c;
this.noteID = noteData.Id;
this.startDate = noteData.Start_Date__c;
this.endDate = noteData.End_date__c;
this.active = noteData.Active__c;
this.viewed = false;
for (EMS_Sidebar_Viewer__c view :noteData.EMS_Sidebar_Viewers__r) {
views.add(view);
if (view.Viewed_gne__c == true) {
this.viewed = true;
}
}
}
}
@RemoteAction
public static EMS_Remote_Response readNotes(String noteID) {
EMS_Remote_Response response = new EMS_Remote_Response();
EMS_Sidebar_Viewer__c view = new EMS_Sidebar_Viewer__c(
Datetime_gne__c = DateTime.now(),
User_gne__c = UserInfo.getUserId(),
Viewed_gne__c = true,
EMS_Sidebar_Configuration_gne__c = noteID
);
try {
insert view;
response.isSuccess = true;
} catch (Exception e) {
response.addError(e.getMessage());
response.isSuccess = false;
}
return response;
}
} |
import 'package:blog_models/blog_models.dart';
import 'package:blog_repository/blog_repository.dart';
import 'package:blog_ui/blog_ui.dart';
import 'package:flutter/material.dart';
import 'package:flutter_bloc/flutter_bloc.dart';
import 'package:go_router/go_router.dart';
import 'package:personal_blog_flutter/blog_overview/bloc/blog_overview_bloc.dart';
import 'package:personal_blog_flutter/blog_overview/widgets/widgets.dart';
class BlogOverviewPage extends StatelessWidget {
const BlogOverviewPage({super.key});
factory BlogOverviewPage.routeBuilder(
_,
__,
) =>
const BlogOverviewPage(
key: Key('blog_overview_page'),
);
@override
Widget build(BuildContext context) {
return BlocProvider(
create: (context) =>
BlogOverviewBloc(blogRepository: context.read<BlogRepository>())
..add(const BlogOverviewPostsRequested()),
child: const BlogOverview(),
);
}
}
class BlogOverview extends StatelessWidget {
const BlogOverview({super.key});
@override
Widget build(BuildContext context) {
return BlocBuilder<BlogOverviewBloc, BlogOverviewState>(
builder: (context, state) => switch (state) {
BlogOverviewInitial() || BlogOverviewLoading() => const Center(
child: CircularProgressIndicator(),
),
BlogOverviewFailure(error: final error) => Center(
child: Container(
color: Theme.of(context).colorScheme.error,
padding: BlogSpacing.allPadding,
child: Text(
error.toString(),
style: BlogTextStyles.errorTextStyle.copyWith(
color: Theme.of(context).colorScheme.onError,
),
),
),
),
BlogOverviewLoaded(previews: final previews) => _BlogOverviewContent(
previews: previews,
)
},
);
}
}
class _BlogOverviewContent extends StatelessWidget {
const _BlogOverviewContent({
required this.previews,
});
final List<BlogPreview> previews;
@override
Widget build(BuildContext context) {
return Center(
child: Container(
margin: BlogSpacing.topMargin,
child: Column(
children: [
const BlogOverviewHeader(),
Expanded(
child: Padding(
padding: BlogSpacing.horizontalPadding,
child: ConstrainedBox(
constraints: const BoxConstraints(maxWidth: 1200),
child: ListView.builder(
itemCount: previews.length,
itemBuilder: (context, index) {
final preview = previews[index];
return BlogCard(
title: preview.title,
subtitle: preview.description,
published: preview.published,
imageUrl: preview.image,
onTap: () {
context.go(
'/${preview.slug}',
);
},
);
},
),
),
),
),
],
),
),
);
}
} |
import pyttsx3
import re
import os
from pydub import AudioSegment
from pydub.silence import split_on_silence
from pydub import AudioSegment
from pydub.utils import mediainfo
# Set the path to ffprobe executable
os.environ["PATH"] += os.pathsep + r"\ffmpeg-master-latest-win64-gpl-shared\ffmpeg-master-latest-win64-gpl-shared\bin"
def merge_audio_files(audio_files, output_file):
# Load each audio file
segments = [AudioSegment.from_file(file) for file in audio_files]
# Add a brief silence between segments (adjust duration as needed)
silence = AudioSegment.silent(duration=1000) # 1 second silence
combined = silence
# Concatenate audio segments with silence in between
for segment in segments:
combined += segment + silence
# Export the combined audio to the output file
combined.export(output_file, format="mp3")
def text_to_speech(text, file_path, voice_gender):
engine = pyttsx3.init()
# Set voice properties based on gender
if voice_gender == 'female':
engine.setProperty('voice', engine.getProperty('voices')[1].id) # Index 1 corresponds to a female voice
else:
engine.setProperty('voice', engine.getProperty('voices')[0].id) # Use the default (male) voice
engine.setProperty('rate', 190) # You can experiment with different values
engine.setProperty('volume', 0.9) # Adjust volume (0.0 to 1.0)
engine.save_to_file(text, file_path)
engine.runAndWait()
def process_conversation(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
conversation = []
current_speaker = None
current_message = ""
for line in lines:
#match = re.match(r'\(\s*\d+h?\s*\d+m\s*\d+s\s*\)\s*([\w\s]+):\s*(.*)', line)
match = re.match(r'\(\s*(?:(\d+h?)\s*)?(?:(\d+m)\s*)?(\d+s)\s*\)\s*([\w\s]+):\s*(.*)', line)
# print("match------",match)
if match:
groups = match.groups()
speaker = groups[-2]
message = groups[-1]
if current_speaker:
conversation.append((current_speaker, current_message))
current_speaker = speaker
current_message = message
else:
current_message += line.strip()
if current_speaker:
conversation.append((current_speaker, current_message))
return conversation
def main(uploaded_file):
conversation_file = uploaded_file
output_folder = 'audio_files'
final_audio_folder = 'final_audio'
merged_output_file = 'merged_audio.mp3'
# Ensure the output folder exists
os.makedirs(output_folder, exist_ok=True)
os.makedirs(final_audio_folder,exist_ok=True)
conversation = process_conversation(conversation_file)
for index, (speaker, message) in enumerate(conversation, start=1):
# Determine voice gender based on the speaker
voice_gender = 'female' if speaker.lower() == 'robin j' else 'male'
# Generate output file path
output_file = os.path.join(output_folder, f'{index}_{speaker.lower()}.mp3')
# Convert text to speech
text_to_speech(message, output_file, voice_gender)
# Get a list of all generated audio files
audio_files = [os.path.join(output_folder, f'{index}_{speaker.lower()}.mp3') for index, (speaker, _) in enumerate(conversation, start=1)]
# Merge the audio files
merge_audio_files(audio_files, os.path.join(final_audio_folder, merged_output_file))
if __name__ == "__main__":
main() |
import 'dart:math';
import 'package:borough_king/main.dart';
import 'package:flutter/cupertino.dart';
import "package:flutter/material.dart";
import "package:borough_king/widgets/preferiti.dart";
import "package:borough_king/widgets/occasioni_fotografiche.dart";
import 'package:folding_cell/folding_cell.dart';
import 'package:borough_king/widgets/search.dart';
import 'package:borough_king/widgets/Preferiti/preferitiSubiaco.dart';
class OccSubiaco extends StatelessWidget {
final _foldingCellKey1 = GlobalKey<SimpleFoldingCellState>();
final _foldingCellKey2 = GlobalKey<SimpleFoldingCellState>();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(
"Occasioni Fotografiche", style: TextStyle(color: Colors.black)),
backgroundColor: Color(0xff90EE90),
iconTheme: IconThemeData(color: Colors.black),
actions: <Widget>[
IconButton(
icon: Icon(
Icons.search,
color: Colors.black,
size: 30,
),
onPressed: () {
List<String> borghi = [
"Tolfa",
"Anagni",
"Subiaco",
"Bracciano",
"Calcata"
];
showSearch(context: context, delegate: Search(borghi));
},
)
],
),
body: Container(
child: ListView(
scrollDirection: Axis.vertical,
children: <Widget>[
GestureDetector(
onTap: () => _foldingCellKey1?.currentState?.toggleFold(),
child: Container(
child: SimpleFoldingCell.create(
key: _foldingCellKey1,
frontWidget: FrontWidget(_foldingCellKey1),
innerWidget: InnerWidget(_foldingCellKey1),
cellSize: Size(MediaQuery
.of(context)
.size
.width, 175),
padding: EdgeInsets.all(10.0)
),
),),
GestureDetector(
onTap: () => _foldingCellKey2?.currentState?.toggleFold(),
child: Container(
child: SimpleFoldingCell.create(
key: _foldingCellKey2,
frontWidget: FrontWidget(_foldingCellKey2),
innerWidget: InnerWidget(_foldingCellKey2),
cellSize: Size(MediaQuery
.of(context)
.size
.width, 175),
padding: EdgeInsets.all(10.0)
),
),),
]
)
),
bottomNavigationBar: BottomNavigationBar(
// Server per inserire la barra inferiore
currentIndex: 2,
type: BottomNavigationBarType.fixed,
backgroundColor: Color(0xff90EE90),
selectedItemColor: Colors.black.withOpacity(.60),
unselectedItemColor: Colors.black.withOpacity(.60),
selectedFontSize: 13.5,
unselectedFontSize: 13.5,
onTap: (value) {
// Respond to item press.
//Ogni bottone ha un indice e gli indici partono da 0 quindi il primo รจ la home e gli altri a seguire
if (value == 0) {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => HomePage()),
);
} else if (value == 1) {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => preferiti()),
);
} else if (value == 2) {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => occasioniFotografiche()),
);
}
},
items: [
BottomNavigationBarItem(
label: 'Home',
icon: Icon(Icons.home),
),
BottomNavigationBarItem(
label: 'Preferiti',
icon: Icon(Icons.favorite),
),
BottomNavigationBarItem(
label: ' Occasioni\n Fotografiche',
icon: Icon(Icons.panorama),
),
],
),);
}
Widget FrontWidget(GlobalKey<SimpleFoldingCellState> key) {
if(key == _foldingCellKey1){
return Container(
color: Color(0xFFecf2f9),
alignment: Alignment.center,
child: Row(children: <Widget>[
Expanded(
flex: 1,
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(15.0),
image: DecorationImage(
image: AssetImage('assets/images/Subiaco/Monastero di Santa Scolastica.jpg'),
fit: BoxFit.cover),
),
),
),
Expanded(
flex: 2,
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(15.0),
color: Color(0xFFecf2f9),
),
child: Column(children: <Widget>[
Padding(
padding: EdgeInsets.all(10.0),
child: Center(child: Text(
"Monastero\ndi Santa Scolastica", style: TextStyle(fontSize: 23,fontFamily: "Times New Roman"),))
),
Padding(
padding: EdgeInsets.all(10.0),
child: Row(mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Padding(padding: EdgeInsets.only(right: 10),
child: Icon(Icons.room)),
Center(child: Text(
"Subiaco", style: TextStyle(
fontSize: 18),))
])
)
]),
),
),
])
);
} else if (key == _foldingCellKey2){
return Container(
color: Color(0xFFecf2f9),
alignment: Alignment.center,
child: Row(children: <Widget>[
Expanded(
flex: 1,
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(15.0),
image: DecorationImage(
image: AssetImage('assets/images/Subiaco/Aniene.jpg'),
fit: BoxFit.cover),
),
),
),
Expanded(
flex: 2,
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(15.0),
color: Color(0xFFecf2f9),
),
child: Column(children: <Widget>[
Padding(
padding: EdgeInsets.all(12.0),
child: Center(child: Text(
"Aniene", style: TextStyle(fontSize: 23,fontFamily: "Times New Roman"),))
),
Padding(
padding: EdgeInsets.all(10.0),
child: Row(mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Padding(padding: EdgeInsets.only(right: 10),
child: Icon(Icons.room)),
Center(child: Text(
"Subiaco", style: TextStyle(
fontSize: 18),))
])
)
]),
),
),
])
);
}
}
Widget InnerWidget(GlobalKey<SimpleFoldingCellState> key) {
if (key == _foldingCellKey2){ return Post1();}
else if( key == _foldingCellKey1){
return Post();
}
}
}
class Post extends StatefulWidget{
@override
PostState createState() => new PostState();
}
bool liked = false;
class PostState extends State<Post> {
_pressed() {
setState(() {
liked = !liked;
cards.contains('Monastero di Santa Scolastica') ? cards.remove('Monastero di Santa Scolastica') : cards.add('Monastero di Santa Scolastica');
!borghi.contains('Subiaco') ? borghi.add('Subiaco') : null;
});
}
@override
Widget build(BuildContext context) {
return Container(
color: Color(0xFFecf2f9),
padding: EdgeInsets.only(top: 10),
child: Column(
mainAxisAlignment: MainAxisAlignment.start, children: <Widget>[
Padding(
padding: EdgeInsets.all(15.0),
child: Column(
children: <Widget>[
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Monastero di \nSanta Scolastica",
style: TextStyle(fontSize: 25),),
Padding(padding: EdgeInsets.only(left: 100),
child: IconButton( icon: Icon(liked ? Icons.favorite : Icons.favorite_border_outlined,
color: liked ? Colors.redAccent : Colors.black),
iconSize: 30,
onPressed: () => _pressed(),
),),
])),
SizedBox(height: 10,),
Row(children: <Widget>[
Padding(padding: EdgeInsets.only(right: 10),
child: Icon(Icons.room)),
Center(child: Text("Subiaco",
style: TextStyle(fontSize: 20),))
]),
SizedBox(height: 10,),
Align(alignment: Alignment.topLeft,
child: Text(
"Il Monastero di Santa Scolastica รจ uno dei luoghi piรน suggestivi del Lazio.",
style: TextStyle(fontSize: 18),)),
SizedBox(height: 10,),
Align(alignment: Alignment.topLeft,
child: Text("Orario", style: TextStyle(fontSize: 16),)),
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Lun-Dom", style: TextStyle(fontSize: 16),),
Padding(padding: EdgeInsets.only(left: 50),
child: Text(
"09:30-12:15 15:30-18:15",
style: TextStyle(fontSize: 16),)),
])),
SizedBox(height: 20,),
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Costo", style: TextStyle(fontSize: 16),),
Padding(padding: EdgeInsets.only(left: 150),
child: Text("โฌโฌ", style: TextStyle(fontSize: 16),))
])),
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Tempo", style: TextStyle(fontSize: 16),),
Padding(padding: EdgeInsets.only(left: 125),
child: Text(
"1h-1.5h", style: TextStyle(fontSize: 16),)),
])),
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Fatica", style: TextStyle(fontSize: 16),),
Padding(padding: EdgeInsets.only(left: 140),
child: Text("๐ง๐ง"),),
])),
]),
),
],)
);
}
}
class Post1 extends StatefulWidget{
@override
Post1State createState() => new Post1State();
}
bool like = false;
class Post1State extends State<Post1> {
_pressed() {
setState(() {
like = !like;
cards.contains('Aniene') ? cards.remove('Aniene') : cards.add('Aniene');
!borghi.contains('Subiaco') ? borghi.add('Subiaco') : null;
});
}
@override
Widget build(BuildContext context) {
return Container(
color: Color(0xFFecf2f9),
padding: EdgeInsets.only(top: 10),
child: Column(
mainAxisAlignment: MainAxisAlignment.start, children: <Widget>[
Padding(
padding: EdgeInsets.all(15.0),
child: Column(
children: <Widget>[
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Aniene", style: TextStyle(fontSize: 25),),
Padding(padding: EdgeInsets.only(left: 200),
child: IconButton(icon: Icon(like ? Icons.favorite : Icons.favorite_border_outlined,
color: like ? Colors.redAccent : Colors.black),
iconSize: 30,
onPressed: () => _pressed(),
),),
])),
Row(children: <Widget>[
Padding(padding: EdgeInsets.only(right: 10),
child: Icon(Icons.room)),
Center(child: Text("Subiaco",
style: TextStyle(fontSize: 18),))
]),
SizedBox(height: 10,),
Align(alignment: Alignment.topLeft,
child: Text(
"Da Subiaco si possono ammirare le bellissime acque del fiume Aniene, non "
"contaminate dallo smog della cittร . E' anche possibile fare rafting!",
style: TextStyle(fontSize: 18),)),
SizedBox(height: 20,),
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Costo", style: TextStyle(fontSize: 18),),
Padding(padding: EdgeInsets.only(left: 150),
child: Text("โฌ", style: TextStyle(fontSize: 18),))
])),
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Tempo", style: TextStyle(fontSize: 18),),
Padding(padding: EdgeInsets.only(left: 125),
child: Text(
"45m-1h", style: TextStyle(fontSize: 18),)),
])),
Align(alignment: Alignment.topLeft,
child: Row(children: <Widget>[
Text("Fatica", style: TextStyle(fontSize: 18),),
Padding(padding: EdgeInsets.only(left: 150),
child: Text("๐ง๐ง")),
])),
]),
),
],)
);
}
} |
import csv
import random
from faker import Faker
# Initialize Faker to generate fake data
fake = Faker()
# Specify the number of users you want to generate
num_users = random.randint(50000, 99000)
# Create a list to store user data
user_data = []
# Generate data for each user
for _ in range(num_users):
first_name = fake.first_name()
last_name = fake.last_name()
age = random.randint(18, 65)
street_address = fake.street_address()
zip_code = fake.zipcode()
account_number = "".join([str(random.randint(0, 9)) for _ in range(12)])
user_data.append(
[first_name, last_name, age, street_address, zip_code, account_number]
)
# Write the data to a CSV file
csv_filename = "fake_user_data.csv"
with open(csv_filename, "w", newline="") as csv_file:
csv_writer = csv.writer(csv_file)
# Write header row
csv_writer.writerow(
[
"First Name",
"Last Name",
"Age",
"Street Address",
"Zip Code",
"Account Number",
]
)
# Write user data
csv_writer.writerows(user_data)
print(f"Generated {num_users} fake user records in '{csv_filename}'") |
import { useCallback, useEffect } from 'react';
import debounce from 'lodash/debounce';
import { useAppDispatch } from '../../redux-store/redux-store';
import { updateScreenDimensions } from '../../redux-store/layout/layout-slice';
export default function LayoutChangeDetector(): JSX.Element | null {
const appDispatch = useAppDispatch();
const debounceCallback = useCallback(debounce(() => {
appDispatch(updateScreenDimensions());
}, 300), []);
const handleWindowSizeChange = (): void => {
debounceCallback();
};
useEffect(() => {
appDispatch(updateScreenDimensions());
}, [])
useEffect(() => {
window.addEventListener('resize', handleWindowSizeChange);
return () => {
window.removeEventListener('resize', handleWindowSizeChange);
}
}, []);
return null;
} |
package com.cht.admin.catalogo.application.video.create;
import com.cht.admin.catalogo.application.Fixture;
import com.cht.admin.catalogo.application.UseCaseTest;
import com.cht.admin.catalogo.domain.Genre.GenreGateway;
import com.cht.admin.catalogo.domain.castmember.CastMemberGateway;
import com.cht.admin.catalogo.domain.category.CategoryGateway;
import com.cht.admin.catalogo.domain.video.Resource;
import com.cht.admin.catalogo.domain.video.*;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import java.time.Year;
import java.util.*;
import static org.mockito.AdditionalAnswers.returnsFirstArg;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.ArgumentMatchers.argThat;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
public class CreateVideoUseCaseTest extends UseCaseTest {
@InjectMocks
private DefaultCreateVideoUseCase useCase;
@Mock
private VideoGateway videoGateway;
@Mock
private CategoryGateway categoryGateway;
@Mock
private CastMemberGateway castMemberGateway;
@Mock
private GenreGateway genreGateway;
@Mock
private MediaResourceGateway mediaResourceGateway;
@Override
protected List<Object> getMocks() {
return List.of(videoGateway, categoryGateway, genreGateway, castMemberGateway, mediaResourceGateway);
}
@Test
public void givenAValidCommand_whenCallsCreateVideo_shouldReturnVideoId() {
// given
final var expectedTitle = Fixture.title();
final var expectedDescription = Fixture.Videos.description();
final var expectedLaunchYear = Year.of(Fixture.year());
final var expectedDuration = Fixture.duration();
final var expectedOpened = Fixture.bool();
final var expectedPublished = Fixture.bool();
final var expectedRating = Fixture.Videos.rating();
final var expectedCategories = Set.of(Fixture.Categories.aulas().getId());
final var expectedGenres = Set.of(Fixture.Genres.tech().getId());
final var expectedMembers = Set.of(
Fixture.CastMembers.wesley().getId(),
Fixture.CastMembers.gabriel().getId()
);
final Resource expectedVideo = Fixture.Videos.resource(VideoMediaType.VIDEO);
final Resource expectedTrailer = Fixture.Videos.resource(VideoMediaType.TRAILER);
final Resource expectedBanner = Fixture.Videos.resource(VideoMediaType.BANNER);
final Resource expectedThumb = Fixture.Videos.resource(VideoMediaType.THUMBNAIL);
final Resource expectedThumbHalf = Fixture.Videos.resource(VideoMediaType.THUMBNAIL_HALF);
final var aCommand = CreateVideoCommand.with(
expectedTitle,
expectedDescription,
expectedLaunchYear.getValue(),
expectedDuration,
expectedOpened,
expectedPublished,
expectedRating.getName(),
asString(expectedCategories),
asString(expectedGenres),
asString(expectedMembers),
expectedVideo,
expectedTrailer,
expectedBanner,
expectedThumb,
expectedThumbHalf
);
when(categoryGateway.existsByIds(any()))
.thenReturn(new ArrayList<>(expectedCategories));
when(castMemberGateway.existsByIds(any()))
.thenReturn(new ArrayList<>(expectedMembers));
when(genreGateway.existsByIds(any()))
.thenReturn(new ArrayList<>(expectedGenres));
mockImageMedia();
mockAudioVideoMedia();
when(videoGateway.create(any()))
.thenAnswer(returnsFirstArg());
// when
final var actualResult = useCase.execute(aCommand);
// then
Assertions.assertNotNull(actualResult);
Assertions.assertNotNull(actualResult.id());
verify(videoGateway).create(argThat(actualVideo ->
Objects.equals(expectedTitle, actualVideo.getTitle())
&& Objects.equals(expectedDescription, actualVideo.getDescription())
&& Objects.equals(expectedLaunchYear, actualVideo.getLaunchedAt())
&& Objects.equals(expectedDuration, actualVideo.getDuration())
&& Objects.equals(expectedOpened, actualVideo.getOpened())
&& Objects.equals(expectedPublished, actualVideo.getPublished())
&& Objects.equals(expectedRating, actualVideo.getRating())
&& Objects.equals(expectedCategories, actualVideo.getCategories())
&& Objects.equals(expectedGenres, actualVideo.getGenres())
&& Objects.equals(expectedMembers, actualVideo.getCastMembers())
&& Objects.equals(expectedVideo.name(), actualVideo.getVideo().get().name())
&& Objects.equals(expectedTrailer.name(), actualVideo.getTrailer().get().name())
&& Objects.equals(expectedBanner.name(), actualVideo.getBanner().get().name())
&& Objects.equals(expectedThumb.name(), actualVideo.getThumbnail().get().name())
&& Objects.equals(expectedThumbHalf.name(), actualVideo.getThumbnailHalf().get().name())
));
}
private void mockImageMedia() {
when(mediaResourceGateway.storeImage(any(), any())).thenAnswer(t -> {
final var resource = t.getArgument(1, Resource.class);
return ImageMedia.with(UUID.randomUUID().toString(), resource.name(), "/img");
});
}
private void mockAudioVideoMedia() {
when(mediaResourceGateway.storeAudioVideo(any(), any())).thenAnswer(t -> {
final var videoResource = t.getArgument(1, VideoResource.class);
final var resource = videoResource.resource();
return AudioVideoMedia.with(
resource.checksum(),
resource.name(),
"/img"
);
});
}
} |
package me.matsumo.klms.core.model.lms
import kotlinx.datetime.Instant
import kotlinx.serialization.Serializable
import me.matsumo.klms.core.model.lms.entity.OutcomeImportEntity
@Serializable
data class OutcomeImport(
val id: Int,
val learningOutcomeGroupId: Int?,
val createdAt: Instant,
val endedAt: Instant?,
val updatedAt: Instant,
val workflowState: String,
val data: OutcomeImportData?,
val progress: Int,
val user: LmsUser?,
val processingErrors: List<List<Map<Int, String>>>,
)
fun OutcomeImportEntity.translate(): OutcomeImport {
return OutcomeImport(
id = id,
learningOutcomeGroupId = learningOutcomeGroupId,
createdAt = Instant.parse(createdAt),
endedAt = endedAt?.let { Instant.parse(it) },
updatedAt = Instant.parse(updatedAt),
workflowState = workflowState,
data = data?.translate(),
progress = progress,
user = user?.translate(),
processingErrors = processingErrors,
)
} |
import Dashboard from "@/shared/layout/Dashboard";
import { attendenceService } from "@/shared/services/attendence.service";
import { IAttendence } from "@/shared/typeDef/attendence.type";
import { DeleteOutlined, EditOutlined } from "@ant-design/icons";
import { Button, Col, message, Popconfirm, Row, Space, Table, Tag } from "antd";
import Search from "antd/es/input/Search";
import { ColumnType } from "antd/es/table";
import { useState } from "react";
import { useMutation, useQuery } from "react-query";
import FormAttendence from "./form";
type Props = {};
const AttendenceManagement = ({}: Props) => {
const [open, setOpen] = useState(false);
const [action, setAtion] = useState<string>("");
const [rowId, setRowId] = useState<number>();
const { data: dataAttendence, refetch } = useQuery(
['listAttendence'],
() => attendenceService.getAllAttendence()
);
const deleteMutation = useMutation({
mutationKey: ["deleteAttendenceMutation"],
mutationFn: (id: number) => attendenceService.deleteAttendence(id),
onSuccess: () => {
message.success("Xoรก thร nh cรดng");
refetch();
},
onError() {
message.error("Xoรก khรดng thร nh cรดng");
},
});
const columns: ColumnType<IAttendence>[] = [
{
title: "#",
key: "id",
render: (value, record, index) => (
<div>
<p>{index}</p>
</div>
),
},
{
title: "Tรชn khoa",
key: "department_id",
render: (_, record) => (
<p>{record && record.department.name}</p>
)
},
{
title: "Tรชn lแปp",
key: "class_id",
render: (_, record) => (
<p>{record && record.class.name}</p>
)
},
{
title: "Mรฃ sinh viรชn",
key: "ma_sinh_vien",
render: (_, record) => (
<p>{record && record.student.ma_sinh_vien}</p>
)
},
{
title: "Tรชn sinh viรชn",
key: "student_id",
render: (_, record) => (
<p>{record && record.student.name}</p>
)
},
{
title: "Tรชn hแปc phแบงn",
key: "subject_id",
render: (_, record) => (
<p>{record && record.subject.name}</p>
)
},
{
title: "Sแป buแปi",
key: "number",
render: (value, record, index) => (
<Tag color={"blue"}>
{record.number}
</Tag>
),
},
{
title: "Hร nh ฤแปng",
key: "action",
render: (_, record) => (
<Space size="middle">
<div
className="cursor-pointer"
onClick={() => {
setAtion("edit");
setOpen(true);
setRowId(record.id);
}}
>
<EditOutlined />
</div>
<Popconfirm
okButtonProps={{ style: { background: 'red', color: 'white' }, loading: deleteMutation.isLoading }}
onConfirm={() => {
deleteMutation.mutate(record.id);
}}
title={"Xoรก"}
>
<DeleteOutlined className="cursor-pointer"></DeleteOutlined>
</Popconfirm>
</Space>
),
},
];
return (
<>
{dataAttendence && (
<Dashboard>
<Row justify={"space-between"} align="middle" gutter={16}>
<Col span={12}>
<h1 className="font-bold text-2xl">Quแบฃn lรฝ ฤiแปm danh</h1>
</Col>
<Col span={12}>
<div className="flex py-2 justify-between items-center gap-3">
<Search
className="bg-blue-300 rounded-lg"
placeholder="Tรฌm kiแบฟm"
onSearch={() => {}}
enterButton
/>
<Button
onClick={() => {
setAtion("create");
setRowId(NaN);
setOpen(true);
}}
>
Tแบกo mแปi
</Button>
</div>
</Col>
</Row>
<Table dataSource={dataAttendence.data} columns={columns} />
{action === "create" && !rowId ? (
<FormAttendence refetch={refetch} open={open} setOpen={setOpen} />
) : (
<FormAttendence refetch={refetch} editId={rowId} open={open} setOpen={setOpen} />
)}
</Dashboard>
)}
</>
);
};
export default AttendenceManagement; |
import Image from "next/image";
import { useEffect, useState } from "react";
import { useAccount, useConnect, useSignMessage } from "wagmi";
import { defaultAvatar, snapshotSpace } from "../../../config";
import { useVote } from "../../../hooks/snapshot";
import { postComment } from "../../../utils/firebase/post";
import { useGetUserProfile } from "../../../hooks/users/useGetUserProfile";
import { useListenUserTags } from "../../../hooks/database/useListenUserTags";
import { useSignIn } from "@/hooks/sign-in/useSignIn";
interface Homie {
name: String;
src: String;
}
interface Comment {
body: String;
author: Homie;
}
export default function CommentView({ proposal, back, choice }: any) {
const vote = useVote(snapshotSpace, proposal.id, choice);
const { data: account } = useAccount();
const user = useGetUserProfile();
const authorTags = useListenUserTags(user?.address);
const { data: signature, isSuccess, signMessage } = useSignMessage();
const [message, setMessage] = useState("");
const { signedIn } = useSignIn();
const canPost = signedIn;
useEffect(() => {
if (isSuccess && signature && signedIn) {
postComment(proposal.id, comment, signature).then((res) => {
console.log("Posted Comment: ", res);
setMessage("");
back();
});
}
}, [isSuccess]);
const comment = {
body: message,
author: account?.address,
choice: choice + 1,
};
return (
<div className="flex flex-col space-y-0 rounded-lg px-8 py-5">
<p className="text-base-100 pb-4 text-2xl font-semibold">Vote</p>
<div className="flex w-full flex-col space-y-6 overflow-clip">
<textarea
className="border-base-content/50 text-md w-full rounded-lg border bg-transparent p-4 font-light outline-0"
rows={2}
value={canPost ? message : "Please sign in to comment."}
disabled={!canPost}
onChange={(e) => setMessage(e.target.value)}
/>
{/* <div className="flex flex-row items-end justify-between border-gray-900 px-6">
<p className="text-4xl font-bold text-gray-900">
{proposal.choices[choice]}
</p>
<p
className="text-neutral w-full cursor-pointer font-normal hover:text-gray-700"
onClick={back}
>
Back
</p>
</div> */}
<div className="flex flex-row items-center justify-between">
<div className="flex flex-row items-center space-x-2">
<div className="min-w-fit">
<Image
src={user?.avatarSrc || defaultAvatar}
width={50}
height={50}
className="rounded-full"
/>
</div>
<div className="space-between flex flex-col items-baseline space-y-0">
<p className="text-xl font-semibold">{user?.name || "You"}</p>
<div className="flex flex-row space-x-2">
<p>
{authorTags
.slice(0, 3)
.map(({ tag }) => tag)
.join(", ")}
</p>
</div>
</div>
</div>
{canPost && (
<button
className="border-base-content group rounded-md border px-3 py-1"
onClick={() => {
signMessage({
message: JSON.stringify(comment),
});
// vote(message)
}}
>
<p className="">Submit</p>
</button>
)}
</div>
</div>
</div>
);
} |
/* $Id$ */
/*
* This file is part of FreeRCT.
* FreeRCT is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, version 2.
* FreeRCT is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU General Public License for more details. You should have received a copy of the GNU General Public License along with FreeRCT. If not, see <http://www.gnu.org/licenses/>.
*/
/** @file shop_placement.h Definition of coordination code for shop placement. */
#ifndef SHOP_PLACEMENT_H
#define SHOP_PLACEMENT_H
#include "viewport.h"
class ShopType;
/** States in the shop placement. */
enum ShopPlacementState {
SPS_OFF, ///< Shop placement is off.
SPS_OPENED, ///< A shop placement window is open.
SPS_BAD_POS, ///< A shop has been selected, but the mouse is at a bad spot for building a shop.
SPS_GOOD_POS, ///< A shop has been selected, and the mouse if at a good spot (is displayed in the world additions).
};
/** Result codes in trying to place a shop in the world. */
enum RidePlacementResult {
RPR_FAIL, ///< Ride could not be placed in the world.
RPR_SAMEPOS, ///< Ride got placed at the same spot as previously.
RPR_CHANGED, ///< Ride got placed at a different spot in the world.
};
/**
* Class interacting between #RideSelectGui, and the #Viewport mouse mode #MM_SHOP_PLACEMENT.
* @todo [low] Other rides will handle construction from their own instance window, perhaps the shop should do that too?
*/
class ShopPlacementManager : public MouseMode {
public:
ShopPlacementManager();
bool MayActivateMode() override;
void ActivateMode(const Point16 &pos) override;
void LeaveMode() override;
bool EnableCursors() override;
void OnMouseMoveEvent(Viewport *vp, const Point16 &old_pos, const Point16 &pos) override;
void OnMouseButtonEvent(Viewport *vp, uint8 state) override;
void OpenWindow();
void CloseWindow();
bool SetSelection(int ride_type);
void Rotated();
ShopPlacementState state; ///< Current state of the shop placement manager.
TileEdge orientation; ///< Orientation of the shop that will be placed.
int selected_ride; ///< Selected type of ride (negative means nothing selected).
uint16 instance; ///< Allocated ride instance, is #INVALID_RIDE_INSTANCE if not active.
Point16 mouse_pos; ///< Stored mouse position.
private:
bool CanPlaceShop(const ShopType *selected_shop, const XYZPoint16 &pos);
RidePlacementResult ComputeShopVoxel(XYZPoint32 world_pos);
void PlaceShop(const Point16 &pos);
};
extern ShopPlacementManager _shop_placer;
#endif |
import TreeModelHelper from './TreeModelHelper';
import TaskFactory from '../modules/tasks/TaskFactory';
import { IJsonTaskModel } from '../modules/tasks/models/TaskModel';
import { TasksByProject } from '../modules/tasks/models/TasksByProject';
describe('TreeModelHelper', () => {
let testTasks: TasksByProject | undefined;
beforeEach(() => {
const factory = new TaskFactory();
/* Structure:
-task1
--task1-1
---task-1-1-1
-task2
--task21
--task22
*/
const task111: Partial<IJsonTaskModel> = {
key: '111',
title: 'task1-1-1',
parent: undefined,
children: [],
};
const task11: Partial<IJsonTaskModel> = {
key: '11',
title: 'task1-1',
parent: undefined,
children: [task111 as IJsonTaskModel],
};
const task1: Partial<IJsonTaskModel> = {
key: '1',
title: 'task1',
parent: undefined,
children: [task11 as IJsonTaskModel],
};
task111.parent = task11 as IJsonTaskModel;
task11.parent = task1 as IJsonTaskModel;
const task21: Partial<IJsonTaskModel> = {
key: '21',
title: 'task21',
parent: undefined,
children: [],
};
const task22: Partial<IJsonTaskModel> = {
key: '22',
title: 'task22',
parent: undefined,
children: [],
};
const task2: Partial<IJsonTaskModel> = {
key: '2',
title: 'task2',
parent: undefined,
children: [task21, task22] as IJsonTaskModel[],
};
task21.parent = task2 as IJsonTaskModel;
task22.parent = task2 as IJsonTaskModel;
testTasks = factory.createTasks(({
proj: [task1, task2],
} as unknown) as TasksByProject);
});
test('getPathToNode #1', () => {
if (!testTasks) {
throw new Error();
}
const task111 = testTasks?.proj?.[0]?.children?.[0]?.children?.[0];
const result = task111 ? TreeModelHelper.getPathToNode(task111) : undefined;
expect(result).toStrictEqual(['1', '11', '111']);
});
test('getPathToNode #2', () => {
if (!testTasks) {
throw new Error();
}
const task22 = testTasks?.proj?.[1]?.children?.[0];
const result = task22 ? TreeModelHelper.getPathToNode(task22) : undefined;
expect(result).toStrictEqual(['2', '21']);
});
}); |
import React from 'react';
// @ts-ignore
import { action } from '@storybook/addon-actions';
import { skipVisualTest } from 'z-frontend-app-bootstrap';
import { Box } from 'zbase';
import { storiesOf } from '../../.storybook/storyHelpers';
import Checkbox from './Checkbox';
storiesOf('forms|Checkbox', module)
.addDecorator((getStory: Function) => (
<Box p={20} w={[1, 1 / 2]} bg="grayscale.white">
{getStory()}
</Box>
))
.add('default', () => <Checkbox label="Label" />)
.add('hover', () => <Checkbox label="Label" className="simulate-hover" />)
.add('default value (active)', () => <Checkbox defaultChecked name="name" label="Label" />)
.add('error', () => <Checkbox label="Label" className="error" />)
.add('disabled', () => (
<Box>
<Checkbox disabled label="Label" />
<Checkbox checked disabled label="Label" />
</Box>
))
.add('util props', () => <Checkbox label="Label" my={50} />)
.add(
'fires events',
() => (
<Checkbox
label="Try interacting"
onFocus={action('checkbox-onfocus')}
onBlur={action('checkbox-onblur')}
onKeyUp={action('checkbox-onkeyup')}
onChange={action('checkbox-onchange')}
/>
),
skipVisualTest,
); |
const mongoose = require('mongoose');
const Schema = mongoose.Schema; // Asegรบrate de que esta lรญnea estรฉ presente
/**
* @swagger
* components:
* schemas:
* Post:
* type: object
* required:
* - title
* - content
* - date_created
* - date_created_gmt
* - taxonomies
* properties:
* title:
* type: string
* description: The title of the post.
* resumedContent:
* type: string
* description: The resumed content of the post.
* content:
* type: string
* description: The content of the post.
* date_created:
* type: string
* description: The date the post was created.
* date_created_gmt:
* type: string
* description: The date the post was created in GMT.
* taxonomies:
* type: array
* items:
* type: string
* description: The taxonomies of the post.
* category:
* type: string
* description: The category of the post.
* createdBy:
* type: string
* description: The ID of the user who created the post.
* image:
* type: string
* description: The URL of the image.
* imageDescription:
* type: string
* description: The description of the image.
* example:
* title: Post Title
* resumedContent: Post Resumed Content
* content: Post Content
* date_created: 2022-01-01
* date_created_gmt: 2022-01-01
* taxonomies: ["taxonomy1", "taxonomy2"]
* category: Category
* createdBy: 60d0fe4b6469270015c2e9c1
* image: https://example.com/image.jpg
* imageDescription: Image description
*/
const PostSchema = new mongoose.Schema({
title: { type: String, required: true },
resumedContent: { type: String, required: true},
content: { type: String, required: true },
date_created: { type: String, required: true },
date_created_gmt: { type: String, required: true },
taxonomies: { type: [String], required: true },
category: { type: String ,required : true},
createdBy: { type: Schema.Types.ObjectId, ref: 'Usuario'},
createdByName: { type: String, required: false },
image: { type: String ,required : true},//solo va a ser una url
imageDescription: { type: String ,required : true},
});
PostSchema.pre('save', function(next) {
for (let i = 0; i < this.taxonomies.length; i++) {
if (this.taxonomies[i] === this.taxonomies[i].toUpperCase()) {
this.category = this.taxonomies[i];
break;
}
}
next();
});
const Post = mongoose.model('Post', PostSchema);
module.exports = Post; |
//
// CurrencyNetwork.swift
// AllYouNeed
//
// Created by Shubham Kumar on 07/02/22.
//
import Foundation
//MARK: CURRECY NETWORK LAYER
class CurrencyNetworkLayer {
static let shared = CurrencyNetworkLayer()
//MARK: FETCH CURRENCY
//Using @escaping for completion handler and error handler
func fetchCurrencies(completionHandler: @escaping ([Currency]) -> Void, errorHandler: @escaping (Error) -> Void) {
URLSession.shared.dataTask(with: getComponentsForCurrencyList().url!) { (data, response, error) in
do {
if let data = data {
let response = try JSONSerialization.jsonObject(with: data)
if let dictionary = response as? [String: Any], let currenciesJSON = dictionary["currencies"] as? [String: String] {
DispatchQueue.main.async {
completionHandler(currenciesJSON.map { Currency(code: $0.key, name: $0.value) })
}
}
}
} catch let error {
errorHandler(error)
}
}.resume()
}
//MARK: FETCH RATES
func fetchRates(completionHandler: @escaping ([Rate]) -> Void, errorHandler: @escaping (Error) -> Void) {
URLSession.shared.dataTask(with: getComponentsForCurrencyLive().url!) { (data, response, error) in
do {
if let data = data {
let response = try JSONSerialization.jsonObject(with: data)
if let dictionary = response as? [String: Any], let ratesJSON = dictionary["quotes"] as? [String: Double] {
DispatchQueue.main.async {
completionHandler(ratesJSON.map { Rate(code: $0.key, value: $0.value) })
}
}
}
} catch let error {
errorHandler(error)
}
}.resume()
}
} |
import { z } from "zod";
import { NEW_REPLY_ERROR } from "../../../commons/constants/domains/replies/new-reply-error";
const NewReplyPayloadSchema = z.object(
{
content: z.string({
required_error: NEW_REPLY_ERROR.MISSING_PROPERTY,
invalid_type_error: NEW_REPLY_ERROR.INVALID_DATA_TYPE,
}),
owner: z.string({
required_error: NEW_REPLY_ERROR.MISSING_PROPERTY,
invalid_type_error: NEW_REPLY_ERROR.INVALID_DATA_TYPE,
}),
threadId: z.string({
required_error: NEW_REPLY_ERROR.MISSING_PROPERTY,
invalid_type_error: NEW_REPLY_ERROR.INVALID_DATA_TYPE,
}),
commentId: z.string({
required_error: NEW_REPLY_ERROR.MISSING_PROPERTY,
invalid_type_error: NEW_REPLY_ERROR.INVALID_DATA_TYPE,
}),
},
{
required_error: NEW_REPLY_ERROR.MISSING_PROPERTY,
invalid_type_error: NEW_REPLY_ERROR.INVALID_DATA_TYPE,
}
);
export class NewReply {
readonly content: string;
readonly owner: string;
readonly threadId: string;
readonly commentId: string;
constructor(payload: unknown) {
const result = NewReplyPayloadSchema.safeParse(payload);
if (!result.success) {
const errorMessage = result.error.issues[0].message;
throw new Error(errorMessage);
}
const { content, owner, threadId, commentId } = result.data;
this.content = content;
this.owner = owner;
this.threadId = threadId;
this.commentId = commentId;
}
} |
import { NextResponse } from 'next/server';
import { print } from 'graphql';
import {
GetSessionDocument,
GetSessionQuery,
LoginDocument,
LoginMutation,
getClient,
} from '@woographql/graphql';
type RequestBody = ({
auth: string;
username: undefined;
password: undefined;
} | {
auth: undefined;
username: string;
password: string;
})
export async function POST(request: Request) {
try {
const { username, password } = await request.json() as RequestBody;
const graphQLClient = getClient();
if (!username || !password) {
return NextResponse.json({
errors: {
message: 'Proper credential must be provided for authentication',
},
}, { status: 500 });
}
const data = await graphQLClient.request<LoginMutation>(LoginDocument, { username, password });
if (!data?.login) {
return NextResponse.json({ errors: { message: 'Login failed.' } }, { status: 500 });
}
const { authToken, refreshToken } = data?.login;
if (!authToken || !refreshToken) {
return NextResponse.json({ errors: { message: 'Failed to retrieve credentials.' } }, { status: 500 });
}
graphQLClient.setHeader('Authorization', `Bearer ${data.login.authToken}`);
const { data:_, headers } = await graphQLClient.rawRequest<GetSessionQuery>(print(GetSessionDocument));
const sessionToken = headers.get('woocommerce-session');
if (!sessionToken) {
return NextResponse.json({ errors: { message: 'Failed to retrieve session token.' } }, { status: 500 });
}
return NextResponse.json({ authToken, refreshToken, sessionToken });
} catch (err) {
console.log(err);
return NextResponse.json({ errors: { message: 'Login credentials invalid.' } }, { status: 500 });
}
} |
#include <stdio.h>
#define TILE_SIZE 16
__global__ void mysgemm(int m, int n, int k, const float *A, const float *B, float* C) {
/********************************************************************
*
* Compute C = A x B
* where A is a (m x k) matrix
* where B is a (k x n) matrix
* where C is a (m x n) matrix
*
* Use shared memory for tiling
*
********************************************************************/
__shared__ float A_tile[TILE_SIZE][TILE_SIZE];
__shared__ float B_tile[TILE_SIZE][TILE_SIZE];
int bx = blockIdx.x;
int by = blockIdx.y;
int tx = threadIdx.x;
int ty = threadIdx.y;
int row = by * blockDim.y + ty;
int col = bx * blockDim.x + tx;
float Cvalue = 0;
// Loop over tiles in A and B
for (int p = 0; p < (k-1)/TILE_SIZE+1; p++)
{
// Load tile into shared memory
if (row < m && p*TILE_SIZE+tx < k)
A_tile[ty][tx] = A[row*k + p*TILE_SIZE+tx];
else
A_tile[ty][tx] = 0.0;
if (p*TILE_SIZE+ty < k && col < n)
B_tile[ty][tx] = B[(p*TILE_SIZE+ty)*n + col];
else
B_tile[ty][tx] = 0.0;
__syncthreads();
if (row < m && col < n)
{
for (int i = 0; i < TILE_SIZE; i++)
Cvalue += A_tile[ty][i] * B_tile[i][tx];
}
__syncthreads();
}
if (row < m && col < n)
C[row*n + col] = Cvalue;
/*************************************************************************/
// INSERT KERNEL CODE HERE
/*************************************************************************/
}
void basicSgemm(int m, int n, int k, const float *A, const float *B, float *C)
{
// Initialize thread block and kernel grid dimensions ---------------------
const unsigned int BLOCK_SIZE = TILE_SIZE;
//INSERT CODE HERE
dim3 dim_grid, dim_block;
dim_block.x = dim_block.y = BLOCK_SIZE; dim_block.z = 1;
dim_grid.x = (n - 1) / BLOCK_SIZE + 1;
dim_grid.y = (m - 1) / BLOCK_SIZE + 1;
dim_grid.z = 1;
// Invoke CUDA kernel -----------------------------------------------------
//INSERT CODE HERE
mysgemm<<<dim_grid, dim_block>>>(m, n, k, A, B, C);
} |
<div class="card">
<span
class="card__situation"
[ngStyle]="{
'color': SituationColor[getSituation(task.deadline)]
}"
>
{{getSituation(task.deadline)}}
</span>
<div class="card__divider"></div>
<div class="card__info">
<div
class="card__info__content"
[ngStyle]="{
'border-color': SituationColor[getSituation(task.deadline)]
}"
>
<span class="card__info__title">{{task.description}}</span>
</div>
<div class="card__info__actions">
<button [matMenuTriggerFor]="menu" aria-label="Example icon-button with a menu"
class="card__info__actions__btn-arrow">
<svg-dots width="15" height="15"/>
</button>
<mat-menu #menu="matMenu">
<button mat-menu-item (click)="onConclude()">
<svg-checked/>
<span>Concluir</span>
</button>
<button mat-menu-item (click)="onEdit()">
<svg-pencil color="#25294C" height="12" width="12"/>
<span>Editar</span>
</button>
<button mat-menu-item (click)="onRemove()">
<svg-trash color="#25294C" height="12" width="12"/>
<span>Remover</span>
</button>
</mat-menu>
</div>
</div>
<div class="card__add-info">
<span class="card__add-info__type">
<svg-list color="rgba(51, 51, 51, 0.3)" width="15" height="15"></svg-list>
Tasks
</span>
<span class="card__add-info__date"><svg-clock></svg-clock>
{{formatDate(task.deadline)}}</span>
</div>
</div> |
/*
* Copyright (C) 2019 Richard Hughes <richard@hughsie.com>
*
* SPDX-License-Identifier: LGPL-2.1+
*/
#pragma once
#include <fwupd.h>
#include <xmlb.h>
#include "fu-chunk.h"
#include "fu-firmware.h"
#define FU_TYPE_FIRMWARE (fu_firmware_get_type())
G_DECLARE_DERIVABLE_TYPE(FuFirmware, fu_firmware, FU, FIRMWARE, GObject)
/**
* FU_FIRMWARE_EXPORT_FLAG_NONE:
*
* No flags set.
*
* Since: 1.6.0
**/
#define FU_FIRMWARE_EXPORT_FLAG_NONE (0u)
/**
* FU_FIRMWARE_EXPORT_FLAG_INCLUDE_DEBUG:
*
* Include debug information when exporting.
*
* Since: 1.6.0
**/
#define FU_FIRMWARE_EXPORT_FLAG_INCLUDE_DEBUG (1u << 0)
/**
* FU_FIRMWARE_EXPORT_FLAG_ASCII_DATA:
*
* Write the data as UTF-8 strings.
*
* Since: 1.6.0
**/
#define FU_FIRMWARE_EXPORT_FLAG_ASCII_DATA (1u << 1)
/**
* FuFirmwareExportFlags:
*
* The firmware export flags.
**/
typedef guint64 FuFirmwareExportFlags;
struct _FuFirmwareClass {
GObjectClass parent_class;
gboolean (*parse)(FuFirmware *self,
GBytes *fw,
gsize offset,
FwupdInstallFlags flags,
GError **error) G_GNUC_WARN_UNUSED_RESULT;
GByteArray *(*write)(FuFirmware *self, GError **error)G_GNUC_WARN_UNUSED_RESULT;
void (*export)(FuFirmware *self, FuFirmwareExportFlags flags, XbBuilderNode *bn);
gboolean (*tokenize)(FuFirmware *self, GBytes *fw, FwupdInstallFlags flags, GError **error)
G_GNUC_WARN_UNUSED_RESULT;
gboolean (*build)(FuFirmware *self, XbNode *n, GError **error) G_GNUC_WARN_UNUSED_RESULT;
gchar *(*get_checksum)(FuFirmware *self,
GChecksumType csum_kind,
GError **error)G_GNUC_WARN_UNUSED_RESULT;
gboolean (*check_magic)(FuFirmware *self, GBytes *fw, gsize offset, GError **error);
gboolean (*check_compatible)(FuFirmware *self,
FuFirmware *other,
FwupdInstallFlags flags,
GError **error);
};
/**
* FU_FIRMWARE_FLAG_NONE:
*
* No flags set.
*
* Since: 1.5.0
**/
#define FU_FIRMWARE_FLAG_NONE (0u)
/**
* FU_FIRMWARE_FLAG_DEDUPE_ID:
*
* Dedupe images by ID.
*
* Since: 1.5.0
**/
#define FU_FIRMWARE_FLAG_DEDUPE_ID (1u << 0)
/**
* FU_FIRMWARE_FLAG_DEDUPE_IDX:
*
* Dedupe images by IDX.
*
* Since: 1.5.0
**/
#define FU_FIRMWARE_FLAG_DEDUPE_IDX (1u << 1)
/**
* FU_FIRMWARE_FLAG_HAS_CHECKSUM:
*
* Has a CRC or checksum to test internal consistency.
*
* Since: 1.5.6
**/
#define FU_FIRMWARE_FLAG_HAS_CHECKSUM (1u << 2)
/**
* FU_FIRMWARE_FLAG_HAS_VID_PID:
*
* Has a vendor or product ID in the firmware.
*
* Since: 1.5.6
**/
#define FU_FIRMWARE_FLAG_HAS_VID_PID (1u << 3)
/**
* FU_FIRMWARE_FLAG_DONE_PARSE:
*
* The firmware object has been used by fu_firmware_parse_full().
*
* Since: 1.7.3
**/
#define FU_FIRMWARE_FLAG_DONE_PARSE (1u << 4)
/**
* FU_FIRMWARE_FLAG_HAS_STORED_SIZE:
*
* Encodes the image size in the firmware.
*
* Since: 1.8.2
**/
#define FU_FIRMWARE_FLAG_HAS_STORED_SIZE (1u << 5)
/**
* FU_FIRMWARE_FLAG_ALWAYS_SEARCH:
*
* Always searches for magic regardless of the install flags.
* This is useful for firmware that always has an *unparsed* variable-length
* header.
*
* Since: 1.8.6
**/
#define FU_FIRMWARE_FLAG_ALWAYS_SEARCH (1u << 6)
/**
* FU_FIRMWARE_FLAG_NO_AUTO_DETECTION:
*
* Do not use this firmware type when auto-detecting firmware.
* This should be used when there is no valid signature or CRC to check validity when parsing.
*
* Since: 1.9.3
**/
#define FU_FIRMWARE_FLAG_NO_AUTO_DETECTION (1u << 7)
/**
* FuFirmwareFlags:
*
* The firmware flags.
**/
typedef guint64 FuFirmwareFlags;
/**
* FU_FIRMWARE_ID_PAYLOAD:
*
* The usual firmware ID string for the payload.
*
* Since: 1.6.0
**/
#define FU_FIRMWARE_ID_PAYLOAD "payload"
/**
* FU_FIRMWARE_ID_SIGNATURE:
*
* The usual firmware ID string for the signature.
*
* Since: 1.6.0
**/
#define FU_FIRMWARE_ID_SIGNATURE "signature"
/**
* FU_FIRMWARE_ID_HEADER:
*
* The usual firmware ID string for the header.
*
* Since: 1.6.0
**/
#define FU_FIRMWARE_ID_HEADER "header"
#define FU_FIRMWARE_ALIGNMENT_1 0x00
#define FU_FIRMWARE_ALIGNMENT_2 0x01
#define FU_FIRMWARE_ALIGNMENT_4 0x02
#define FU_FIRMWARE_ALIGNMENT_8 0x03
#define FU_FIRMWARE_ALIGNMENT_16 0x04
#define FU_FIRMWARE_ALIGNMENT_32 0x05
#define FU_FIRMWARE_ALIGNMENT_64 0x06
#define FU_FIRMWARE_ALIGNMENT_128 0x07
#define FU_FIRMWARE_ALIGNMENT_256 0x08
#define FU_FIRMWARE_ALIGNMENT_512 0x09
#define FU_FIRMWARE_ALIGNMENT_1K 0x0A
#define FU_FIRMWARE_ALIGNMENT_2K 0x0B
#define FU_FIRMWARE_ALIGNMENT_4K 0x0C
#define FU_FIRMWARE_ALIGNMENT_8K 0x0D
#define FU_FIRMWARE_ALIGNMENT_16K 0x0E
#define FU_FIRMWARE_ALIGNMENT_32K 0x0F
#define FU_FIRMWARE_ALIGNMENT_64K 0x10
#define FU_FIRMWARE_ALIGNMENT_128K 0x11
#define FU_FIRMWARE_ALIGNMENT_256K 0x12
#define FU_FIRMWARE_ALIGNMENT_512K 0x13
#define FU_FIRMWARE_ALIGNMENT_1M 0x14
#define FU_FIRMWARE_ALIGNMENT_2M 0x15
#define FU_FIRMWARE_ALIGNMENT_4M 0x16
#define FU_FIRMWARE_ALIGNMENT_8M 0x17
#define FU_FIRMWARE_ALIGNMENT_16M 0x18
#define FU_FIRMWARE_ALIGNMENT_32M 0x19
#define FU_FIRMWARE_ALIGNMENT_64M 0x1A
#define FU_FIRMWARE_ALIGNMENT_128M 0x1B
#define FU_FIRMWARE_ALIGNMENT_256M 0x1C
#define FU_FIRMWARE_ALIGNMENT_512M 0x1D
#define FU_FIRMWARE_ALIGNMENT_1G 0x1E
#define FU_FIRMWARE_ALIGNMENT_2G 0x1F
#define FU_FIRMWARE_ALIGNMENT_4G 0x20
#define FU_FIRMWARE_SEARCH_MAGIC_BUFSZ_MAX (32 * 1024 * 1024)
const gchar *
fu_firmware_flag_to_string(FuFirmwareFlags flag);
FuFirmwareFlags
fu_firmware_flag_from_string(const gchar *flag);
FuFirmware *
fu_firmware_new(void);
FuFirmware *
fu_firmware_new_from_bytes(GBytes *fw);
FuFirmware *
fu_firmware_new_from_gtypes(GBytes *fw, gsize offset, FwupdInstallFlags flags, GError **error, ...);
gchar *
fu_firmware_to_string(FuFirmware *self);
void
fu_firmware_export(FuFirmware *self, FuFirmwareExportFlags flags, XbBuilderNode *bn);
gchar *
fu_firmware_export_to_xml(FuFirmware *self, FuFirmwareExportFlags flags, GError **error);
const gchar *
fu_firmware_get_version(FuFirmware *self);
void
fu_firmware_set_version(FuFirmware *self, const gchar *version);
guint64
fu_firmware_get_version_raw(FuFirmware *self);
void
fu_firmware_set_version_raw(FuFirmware *self, guint64 version_raw);
void
fu_firmware_add_flag(FuFirmware *firmware, FuFirmwareFlags flag);
gboolean
fu_firmware_has_flag(FuFirmware *firmware, FuFirmwareFlags flag);
const gchar *
fu_firmware_get_filename(FuFirmware *self);
void
fu_firmware_set_filename(FuFirmware *self, const gchar *filename);
const gchar *
fu_firmware_get_id(FuFirmware *self);
void
fu_firmware_set_id(FuFirmware *self, const gchar *id);
guint64
fu_firmware_get_addr(FuFirmware *self);
void
fu_firmware_set_addr(FuFirmware *self, guint64 addr);
guint64
fu_firmware_get_offset(FuFirmware *self);
void
fu_firmware_set_offset(FuFirmware *self, guint64 offset);
gsize
fu_firmware_get_size(FuFirmware *self);
void
fu_firmware_set_size(FuFirmware *self, gsize size);
void
fu_firmware_set_size_max(FuFirmware *self, gsize size_max);
gsize
fu_firmware_get_size_max(FuFirmware *self);
void
fu_firmware_set_images_max(FuFirmware *self, guint images_max);
guint
fu_firmware_get_images_max(FuFirmware *self);
guint64
fu_firmware_get_idx(FuFirmware *self);
void
fu_firmware_set_idx(FuFirmware *self, guint64 idx);
GBytes *
fu_firmware_get_bytes(FuFirmware *self, GError **error);
GBytes *
fu_firmware_get_bytes_with_patches(FuFirmware *self, GError **error);
void
fu_firmware_set_bytes(FuFirmware *self, GBytes *bytes);
guint8
fu_firmware_get_alignment(FuFirmware *self);
void
fu_firmware_set_alignment(FuFirmware *self, guint8 alignment);
void
fu_firmware_add_chunk(FuFirmware *self, FuChunk *chk);
GPtrArray *
fu_firmware_get_chunks(FuFirmware *self, GError **error);
FuFirmware *
fu_firmware_get_parent(FuFirmware *self);
void
fu_firmware_set_parent(FuFirmware *self, FuFirmware *parent);
gboolean
fu_firmware_tokenize(FuFirmware *self, GBytes *fw, FwupdInstallFlags flags, GError **error)
G_GNUC_WARN_UNUSED_RESULT;
gboolean
fu_firmware_build(FuFirmware *self, XbNode *n, GError **error) G_GNUC_WARN_UNUSED_RESULT;
gboolean
fu_firmware_build_from_xml(FuFirmware *self,
const gchar *xml,
GError **error) G_GNUC_WARN_UNUSED_RESULT;
gboolean
fu_firmware_parse(FuFirmware *self, GBytes *fw, FwupdInstallFlags flags, GError **error)
G_GNUC_WARN_UNUSED_RESULT;
gboolean
fu_firmware_parse_file(FuFirmware *self, GFile *file, FwupdInstallFlags flags, GError **error)
G_GNUC_WARN_UNUSED_RESULT;
gboolean
fu_firmware_parse_full(FuFirmware *self,
GBytes *fw,
gsize offset,
FwupdInstallFlags flags,
GError **error) G_GNUC_WARN_UNUSED_RESULT;
GBytes *
fu_firmware_write(FuFirmware *self, GError **error) G_GNUC_WARN_UNUSED_RESULT;
GBytes *
fu_firmware_write_chunk(FuFirmware *self, guint64 address, guint64 chunk_sz_max, GError **error)
G_GNUC_WARN_UNUSED_RESULT;
gboolean
fu_firmware_write_file(FuFirmware *self, GFile *file, GError **error) G_GNUC_WARN_UNUSED_RESULT;
gchar *
fu_firmware_get_checksum(FuFirmware *self, GChecksumType csum_kind, GError **error);
gboolean
fu_firmware_check_compatible(FuFirmware *self,
FuFirmware *other,
FwupdInstallFlags flags,
GError **error);
void
fu_firmware_add_image(FuFirmware *self, FuFirmware *img);
gboolean
fu_firmware_add_image_full(FuFirmware *self, FuFirmware *img, GError **error);
gboolean
fu_firmware_remove_image(FuFirmware *self, FuFirmware *img, GError **error);
gboolean
fu_firmware_remove_image_by_idx(FuFirmware *self, guint64 idx, GError **error);
gboolean
fu_firmware_remove_image_by_id(FuFirmware *self, const gchar *id, GError **error);
GPtrArray *
fu_firmware_get_images(FuFirmware *self);
FuFirmware *
fu_firmware_get_image_by_id(FuFirmware *self, const gchar *id, GError **error);
GBytes *
fu_firmware_get_image_by_id_bytes(FuFirmware *self, const gchar *id, GError **error);
FuFirmware *
fu_firmware_get_image_by_idx(FuFirmware *self, guint64 idx, GError **error);
GBytes *
fu_firmware_get_image_by_idx_bytes(FuFirmware *self, guint64 idx, GError **error);
FuFirmware *
fu_firmware_get_image_by_gtype(FuFirmware *self, GType gtype, GError **error);
GBytes *
fu_firmware_get_image_by_gtype_bytes(FuFirmware *self, GType gtype, GError **error);
FuFirmware *
fu_firmware_get_image_by_checksum(FuFirmware *self, const gchar *checksum, GError **error);
void
fu_firmware_add_patch(FuFirmware *self, gsize offset, GBytes *blob); |
document.addEventListener('DOMContentLoaded', () => {
const apiKey = '4da392491f9a4cc485873437240806'; // Your WeatherAPI key
const weatherInfoDiv = document.getElementById('weather-info');
const forecastInfoDiv = document.getElementById('forecast-info');
const locationInputDiv = document.getElementById('location-input');
const manualLocationInput = document.getElementById('manual-location');
const getWeatherButton = document.getElementById('get-weather');
const changeLocationButton = document.getElementById('change-location');
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition, showError);
} else {
console.error('Geolocation is not supported by this browser.');
askForLocation();
}
// Event listener for changing location
changeLocationButton.addEventListener('click', () => {
showLocationInput();
});
// Event listener for getting weather by manual location input
getWeatherButton.addEventListener('click', () => {
const newLocation = manualLocationInput.value.trim();
if (newLocation !== '') {
getWeatherByLocation(newLocation);
}
});
function showPosition(position) {
const lat = position.coords.latitude;
const lon = position.coords.longitude;
console.log(`Current Position: lat=${lat}, lon=${lon}`);
getWeatherByCoords(lat, lon);
}
function showError(error) {
switch (error.code) {
case error.PERMISSION_DENIED:
console.error("User denied the request for Geolocation.");
break;
case error.POSITION_UNAVAILABLE:
console.error("Location information is unavailable.");
break;
case error.TIMEOUT:
console.error("The request to get user location timed out.");
break;
case error.UNKNOWN_ERROR:
console.error("An unknown error occurred.");
break;
}
askForLocation();
}
function askForLocation() {
const savedLocation = localStorage.getItem('location');
if (savedLocation) {
getWeatherByLocation(savedLocation);
} else {
locationInputDiv.style.display = 'block';
}
}
function getWeatherByCoords(lat, lon) {
showLoadingIndicator(); // Show loading indicator
fetch(`https://api.weatherapi.com/v1/forecast.json?key=${apiKey}&q=${lat},${lon}&days=5`)
.then(response => response.json())
.then(data => {
console.log(data);
displayWeather(data);
hideLoadingIndicator(); // Hide loading indicator after data is loaded
})
.catch(error => {
console.error('Error fetching weather data:', error);
hideLoadingIndicator(); // Hide loading indicator if there's an error
});
}
function getWeatherByLocation(location) {
showLoadingIndicator(); // Show loading indicator
const loc = location || manualLocationInput.value;
if (loc) {
localStorage.setItem('location', loc);
fetch(`https://api.weatherapi.com/v1/forecast.json?key=${apiKey}&q=${loc}&days=5`)
.then(response => response.json())
.then(data => {
forecastInfoDiv.innerHTML = '';
displayWeather(data);
hideLoadingIndicator(); // Hide loading indicator after data is loaded
})
.catch(error => {
console.error('Error fetching weather data:', error);
hideLoadingIndicator(); // Hide loading indicator if there's an error
});
}
}
// to convert date
function getDayFromDate(dateString) {
const daysOfWeek = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'];
const monthsOfYear = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
const date = new Date(dateString);
const dayOfWeek = daysOfWeek[date.getDay()];
const dayOfMonth = date.getDate();
const month = monthsOfYear[date.getMonth()];
const year = date.getFullYear();
return `${dayOfWeek}, ${month} ${dayOfMonth}, ${year}`;
}
function displayWeather(data) {
if (data.error) {
weatherInfoDiv.innerHTML = `<p>Error: ${data.error.message}</p>`;
} else {
const { temp_c, condition, wind_kph, humidity, feelslike_c, vis_km, gust_kph, precip_mm, pressure_mb } = data.current;
const { name, country } = data.location;
document.getElementById('location').textContent = `${name}, ${country}`;
document.getElementById('temperature').textContent = `${temp_c}ยฐC`;
document.getElementById('condition').innerHTML = `
<img src="https:${condition.icon}" alt="${condition.text}">
<p class="badge ${getConditionBadgeClass(condition.code)}">${condition.text}</p>
`;
document.getElementById('additional-info').innerHTML = `
<p>Feels Like: ${feelslike_c}ยฐC</p>
<p>Wind: ${wind_kph} km/h</p>
<p>Humidity: ${humidity}%</p>
<p>Visibility: ${vis_km} km</p>
<p>Gust: ${gust_kph} km/h</p>
<p>Precip: ${precip_mm} mm</p>
<p>Pressure: ${pressure_mb} mb</p>
`;
// forecastInfoDiv.innerHTML = `<h1>5-Day Forecast</h1>`;
forecastInfoDiv.innerHTML += `<div class="forecast-days">`; // Start a container for forecast days
data.forecast.forecastday.forEach(day => {
const { date, day: { maxtemp_c, mintemp_c, condition, maxwind_kph, avghumidity } } = day;
const formattedDate = getDayFromDate(date); // Convert date to "Day, Month Date, Year" format
forecastInfoDiv.innerHTML += `
<div class="forecast-day">
<h4>${formattedDate}</h4>
<img src="https:${condition.icon}" alt="${condition.text}">
<p class="badge ${getConditionBadgeClass(condition.code)}">${condition.text}</p>
<p>Max Temp: ${maxtemp_c}ยฐC</p>
<p>Min Temp: ${mintemp_c}ยฐC</p>
<p>Max Wind: ${maxwind_kph} kph</p>
<p>Avg Humidity: ${avghumidity}%</p>
</div>
`;
});
forecastInfoDiv.innerHTML += `</div>`; // End the container for forecast days
}
locationInputDiv.style.display = 'none';
hideLoadingIndicator(); // Hide loading indicator once weather data is displayed
}
function showLocationInput() {
locationInputDiv.style.display = 'block';
}
function showLoadingIndicator() {
weatherInfoDiv.classList.add('loading');
}
function hideLoadingIndicator() {
weatherInfoDiv.classList.remove('loading');
}
function getConditionBadgeClass(conditionCode) {
// badge classes based on weather
switch (conditionCode) {
case 1000: // Sunny
return 'sunny';
case 1003: // Partly Cloudy
case 1006: // Cloudy
case 1009: // Overcast
return 'cloudy';
case 1063: // Patchy rain possible
case 1180: // Patchy rain nearby
case 1183: // Patchy light rain
case 1186: // Light rain
case 1189: // Moderate rain at times
case 1192: // Moderate rain
case 1195: // Heavy rain at times
case 1198: // Heavy rain
case 1201: // Light freezing rain
case 1204: // Moderate or heavy freezing rain
case 1207: // Light sleet
case 1210: // Moderate or heavy sleet
case 1213: // Patchy light snow
case 1216: // Light snow
case 1219: // Patchy moderate snow
case 1222: // Moderate snow
case 1225: // Patchy heavy snow
case 1228: // Heavy snow
case 1237: // Ice pellets
case 1240: // Light rain shower
case 1243: // Moderate or heavy rain shower
case 1246: // Torrential rain shower
case 1249: // Light sleet showers
case 1252: // Moderate or heavy sleet showers
case 1255: // Light snow showers
case 1258: // Moderate or heavy snow showers
return 'rainy';
case 1066: // Patchy snow possible
case 1069: // Patchy sleet possible
case 1072: // Patchy freezing drizzle possible
case 1135: // Fog
case 1147: // Freezing fog
return 'snowy';
default:
return '';
}
}
}); |
package com.ssafy.tab.service;
import com.ssafy.tab.domain.Survey;
import com.ssafy.tab.domain.User;
import com.ssafy.tab.dto.SurveyDto;
import com.ssafy.tab.dto.SurveyResponseDto;
import com.ssafy.tab.repository.SurveyRepository;
import lombok.RequiredArgsConstructor;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.beans.factory.annotation.Value;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
@Service
@Transactional
@RequiredArgsConstructor
public class SurveyService {
private final UserService userService;
private final SurveyRepository surveyRepository;
@Value("${naver.client.id.key}")
private String clientId;
@Value("${naver.client.secret.key}")
private String clientSecret;
//์ฌ์ฉ์์ ์์ ๋ฑ๋ก
public void createSurvey(SurveyDto surveyDto, String userId) {
User user = userService.findByUserId(userId);
Survey survey = new Survey(user, surveyDto.getStartLatitude(), surveyDto.getStartLongtitude(), surveyDto.getDestinationLatitude(), surveyDto.getDestinationLongtitude());
surveyRepository.save(survey);
}
//๋ด ์์์กฐ์ฌ ์ญ์ ํ๊ธฐ
public void deleteSurvey(String userId) {
surveyRepository.delete(surveyRepository.findByUser(userService.findByUserId(userId)).get());
}
//๋ด๊ฐ ์์ฑํ ์์์กฐ์ฌ๋ง ๊ฐ์ ธ์ค๊ธฐ.
@Transactional(readOnly = true)
public SurveyDto selectMySurvey(String userId) throws Exception {
Survey survey = surveyRepository.findByUser(userService.findByUserId(userId)).orElse(null);
if (survey != null) {
SurveyDto surveyDto = new SurveyDto(survey.getStartLatitude(), survey.getStartLontitude(), survey.getDestinationLatitude(), survey.getDestinationLongtitude());
return surveyDto;
} else {
throw new Exception();
}
}
//๋ชจ๋ ์์์กฐ์ฌ๋ฅผ ๊ฐ์ ธ์ค๊ธฐ
@Transactional(readOnly = true)
public List<SurveyDto> selectAllSurvey() {
List<Survey> SurveyList = surveyRepository.findAll();
List<SurveyDto> result = new ArrayList<>();
for (Survey survey : SurveyList) {
result.add(SurveyDto.toDto(survey));
}
return result;
}
//๋ค์ด๋ฒ api๋ฅผ ์ฌ์ฉํด์ ๊ฒฝ๋์ ์๋ ๋ฟ๋ ค์ฃผ๊ธฐ.
public List<SurveyResponseDto> findBestRoute() throws Exception {
List<Survey> listSurvey = surveyRepository.findAll();
List<SurveyResponseDto> result = new ArrayList<>();
for (Survey survey : listSurvey) {
String apiUrl = "https://naveropenapi.apigw.ntruss.com/map-direction/v1/driving?start=" + survey.getStartLontitude() + "," + survey.getStartLatitude() + "&goal=" + survey.getDestinationLongtitude() + "," + survey.getDestinationLatitude() + "&option=trafast";
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("X-NCP-APIGW-API-KEY-ID", clientId);
conn.setRequestProperty("X-NCP-APIGW-API-KEY", clientSecret);
int responseCode = conn.getResponseCode();
//API ํธ์ถ์ด ์ ์ ์ผ ๋
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonResponse = objectMapper.readTree(response.toString());
JsonNode pathArray = jsonResponse.get("route").get("trafast").get(0).get("path");
// ์๋์ ๊ฒฝ๋ ์ ๋ณด ์ถ์ถ
for (JsonNode locationNode : pathArray) {
double longitude = locationNode.get(0).asDouble();
double latitude = locationNode.get(1).asDouble();
result.add(SurveyResponseDto.builder().longtitude(longitude).latitude(latitude).build());
}
}
else {
throw new Exception("APIํธ์ถ ํ๋๋ฐ ์ค๋ฅ๊ฐ ๋ฐ์ํ์ต๋๋ค.");
}
}
return result;
}
} |
#include "../inc/Fixed.hpp"
const int Fixed::bits = 8;
Fixed::Fixed(void) : val(0){}
Fixed::Fixed(int num)
{
this->val = num * (1 << bits);
}
Fixed::Fixed(float num)
{
this->val = roundf(num * (1 << bits));
}
Fixed::~Fixed(void)
{
}
Fixed::Fixed(const Fixed &cp)
{
*this = cp;
}
Fixed &Fixed::operator=(const Fixed &cp)
{
this->val = cp.val;
return (*this);
}
bool Fixed::operator>(const Fixed &cp)
{
return(this->val > cp.val);
}
bool Fixed::operator<(const Fixed &cp)
{
return (this->val < cp.val);
}
bool Fixed::operator>=(const Fixed &cp)
{
return (this->val >= cp.val);
}
bool Fixed::operator<=(const Fixed &cp)
{
return (this->val <= cp.val);
}
bool Fixed::operator==(const Fixed &cp)
{
return (this->val == cp.val);
}
bool Fixed::operator!=(const Fixed &cp)
{
return (this->val != cp.val);
}
Fixed Fixed::operator+(const Fixed &cp)
{
Fixed ans;
ans.val = (this->val + cp.val);
return (ans);
}
Fixed Fixed::operator-(const Fixed &cp)
{
Fixed ans;
ans.val = (this->val - cp.val);
return (ans);
}
Fixed Fixed::operator*(const Fixed &cp)
{
Fixed ans;
ans.val = (this->val * cp.val);
return (ans);
}
Fixed Fixed::operator/(const Fixed &cp)
{
Fixed ans;
ans.val = (this->val * cp.val);
return (ans);
}
Fixed &Fixed::operator++(void)
{
this->val++;
return (*this);
}
Fixed Fixed::operator++(int)
{
Fixed ans(*this);
this->val++;
return (ans);
}
Fixed &Fixed::operator--(void)
{
this->val--;
return (*this);
}
Fixed Fixed::operator--(int)
{
Fixed ans(*this);
this->val--;
return(ans);
}
Fixed &Fixed::min(Fixed &a, Fixed &b)
{
if (a > b)
return b;
else
return a;
}
const Fixed &Fixed::min(const Fixed &a, const Fixed &b)
{
if ((Fixed &)a > (Fixed &)b)
return (b);
else
return (a);
}
Fixed &Fixed::max(Fixed &a, Fixed &b)
{
if (a < b)
return (b);
else
return (a);
}
const Fixed &Fixed::max(const Fixed &a, const Fixed &b)
{
if ((Fixed &)a < (Fixed &)b)
return (b);
else
return (a);
}
float Fixed::toFloat(void) const
{
return (float)val / (1 << bits);
}
int Fixed::toInt(void) const
{
return (int)val / (1 << bits);
}
int Fixed::getRawBits(void) const
{
return (this->val);
}
void Fixed::setRawBits(int const raw)
{
this->val = raw;
}
std::ostream &operator<<(std::ostream &stream, const Fixed &fixed_class)
{
stream << fixed_class.toFloat();
return stream;
} |
package org.Learning.MyBag;
import java.util.List;
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserType;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
import com.microsoft.playwright.options.SelectOption;
public class Handling_Dropdown {
public static void main(String[] args) {
// TODO Auto-generated method stub
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(new BrowserType.LaunchOptions().setHeadless(false).setChannel("chrome"));
Page page = browser.newPage();
page.navigate("https://letcode.in/dropdowns");
//When Using selectOptions is one method to use Dropdown
page.selectOption("//select[@id='fruits']", "4");
//When Using Locator is another options to use Dropdown
//Locator locator = page.locator("//select[@id='fruits']");
//locator.selectOption("1");//select by value
//locator.selectOption(new SelectOption().setValue("0"));// By value
//locator.selectOption(new SelectOption().setLabel("Banana"));//By visible text
//locator.selectOption(new SelectOption().setIndex(3)); //By index
String text = page.locator("//div[@class='notification is-success']").textContent();
//textContent is like getText method
System.out.println(text);
/*
* Locator superHero = page.locator("//div[@class='select is-multiple']");
* superHero.selectOption(new String[] {"aq", "bt", "xm"});
*/
Locator prgln = page.locator("//select[@id='lang']");
Locator options = prgln.locator("option");
int values= options.count();
System.out.println("Length :" + values);
prgln.selectOption(new SelectOption().setIndex(values-1));
//Print all the dropdown values in the web page
List<String> all = options.allInnerTexts();
all.forEach(i->System.out.println(i));
}
} |
function [wrappedValues, multiplier] = wraparound(values,interval)
%WRAPAROUND wraps values onto an interval [a, b)
%
% SYNOPSIS [wrappedValues, multiplier] = wraparound(values,interval)
%
% INPUT values : n-by-d array of values to be wrapped
% interval : 2-by-1 o4 2-by-d array of intervals. The first row is
% the lower, the second row the upper limit of the
% interval. If a 2-by-1 interval is given with a
% n-by-d array of values, the same interval is
% applied for all dimensions
%
% OUTPUT wrappedValues : n-by-d values as projected onto the interval
% multiplier : multiplier of interval to reconstitute original
% value
%
% REMARKS The final value will lie within the interval [lower, upper).
% It will never have th value of upper. To find out how
% far away from the lower limit the value lies, you have to
% subtract that lower limit yourself
% Remember: If the allowed values range from 1 to 10, lower=1 and
% upper = 11, because you want to allow a value of 10, but you
% want to turn 11 into 1.
%
% EXAMPLES With an interval [0;10], 19 becomes [[9], [1]]
% With an interval [2;4], [9.2;-2.6] becomes [[3.2;3.4], [3;-3]]
% With an interval [-180,180], [270;360] becomes [[-90;0], [1;1]]
%
% c: 7/05 jonas
%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
% TEST INPUT
if nargin ~= 2 || isempty(values) || isempty(interval)
error('You have to specify two non-empty input arguments!')
end
% get number of dimensions
sizeValues = size(values);
if length(sizeValues) > 2
error('only 2D arrays allowed for values!')
end
% make sure interval is a 2-by-nDims array
interval = returnRightVector(interval, 2, 'r');
% fill up interval if necessary
if size(interval,2) == 1 && sizeValues(2) > 1
interval = repmat(interval, [1, sizeValues(2)]);
end
if ~all(interval(1,:) < interval(2,:))
error('lower limits have to be strictly smaller than upper limits!')
end
% test whether we have all "integers"
if all(all(isApproxEqual(values,round(values),1e-15))) && ...
all(all(isApproxEqual(interval,round(interval),1e-15)))
roundValues = 1;
else
roundValues = 0;
end
% make arrays filled with lower and upper limits
lower = repmat(interval(1,:),[sizeValues(1),1]);
upper = repmat(interval(2,:),[sizeValues(1),1]);
% WRAP AROUND
% Strategy: Transform interval and data to [0,1], then separate integer
% part as multiplier and transform the rest back onto the original interval
% Since we round towards minus infinity when calculating the multiplier, we
% can just subtract the multiplier from everything and get the corect
% wrappedValue: For example, -0.9 will be rounded down to -1, -0.9+1=0.1,
% which is at the right position on the interval.
wrappedValues = values - lower;
upper = upper - lower;
% divide by upper limit
wrappedValues = wrappedValues ./ upper;
% make multiplier. Floor rounds toward -inf, which works well with negative
% values
multiplier = floor(wrappedValues);
% now get remainder.
wrappedValues = wrappedValues - multiplier;
% transform wrappedValues back onto the original interval
wrappedValues = (wrappedValues .* upper) + lower;
% if the input was "integer", we want to return "integers"
if roundValues
wrappedValues = round(wrappedValues);
end |
//
// AppState.swift
// MLCChat
//
// Created by Yaxing Cai on 5/13/23.
//
import Foundation
final class AppState: ObservableObject {
@Published var models = [ModelState]()
@Published var exampleModels = [ExampleModelConfig]()
@Published var chatState = ChatState()
@Published var alertMessage = "" // TODO: Should move out
@Published var alertDisplayed = false // TODO: Should move out
private var appConfig: AppConfig?
private var localModelIDs = Set<String>()
private let fileManager: FileManager = FileManager.default
private lazy var cacheDirectoryURL: URL = {
fileManager.urls(for: .cachesDirectory, in: .userDomainMask)[0]
}()
private let jsonDecoder = JSONDecoder()
private let jsonEncoder = JSONEncoder()
func loadAppConfigAndModels() {
appConfig = loadAppConfig()
// Can't do anything without a valid app config
guard let appConfig else {
return
}
loadPrebuiltModels()
loadModelsConfig(modelList: appConfig.modelList)
loadExampleModelsConfig(exampleModels: appConfig.exampleModels)
}
func requestAddModel(url: String, localID: String?) {
if let localID, localModelIDs.contains(localID) {
showAlert(message: "Local ID: \(localID) has been occupied")
} else {
if let modelURL = URL(string: url) {
downloadConfig(modelURL: modelURL, localID: localID, isBuiltin: false)
} else {
showAlert(message: "Failed to resolve the given url")
}
}
}
func requestDeleteModel(localId: String) {
// model dir should have been deleted in ModelState
assert(!fileManager.fileExists(atPath: cacheDirectoryURL.appending(path: localId).path()))
localModelIDs.remove(localId)
models.removeAll(where: {$0.modelConfig.localID == localId})
updateAppConfig {
appConfig?.modelList.removeAll(where: {$0.localID == localId})
}
}
}
private extension AppState {
func loadAppConfig() -> AppConfig? {
// models in cache to download
var appConfigFileURL = cacheDirectoryURL.appending(path: Constants.appConfigFileName)
if !fileManager.fileExists(atPath: appConfigFileURL.path()) {
appConfigFileURL = Bundle.main.bundleURL.appending(path: Constants.appConfigFileName)
}
assert(fileManager.fileExists(atPath: appConfigFileURL.path()))
do {
let fileHandle = try FileHandle(forReadingFrom: appConfigFileURL)
let data = fileHandle.readDataToEndOfFile()
let appConfig = try jsonDecoder.decode(AppConfig.self, from: data)
return appConfig
} catch {
showAlert(message: "Failed to load app config: \(error.localizedDescription)")
return nil
}
}
func loadModelsConfig(modelList: [AppConfig.ModelRecord]) {
for model in modelList {
let modelConfigFileURL = cacheDirectoryURL
.appending(path: model.localID)
.appending(path: Constants.modelConfigFileName)
if fileManager.fileExists(atPath: modelConfigFileURL.path()) {
if let modelConfig = loadModelConfig(modelConfigURL: modelConfigFileURL) {
addModelConfig(
modelConfig: modelConfig,
modelURL: URL(string: model.modelURL),
isBuiltin: true
)
}
} else {
downloadConfig(
modelURL: URL(string: model.modelURL),
localID: model.localID,
isBuiltin: true)
}
}
}
func loadExampleModelsConfig(exampleModels: [AppConfig.ModelRecord]) {
self.exampleModels = exampleModels.map{
ExampleModelConfig(modelURL: $0.modelURL, localID: $0.localID)
}
}
func loadPrebuiltModels() {
// models in dist
do {
let distDirURL = Bundle.main.bundleURL.appending(path: Constants.prebuiltModelDir)
let contents = try fileManager.contentsOfDirectory(at: distDirURL, includingPropertiesForKeys: nil)
let modelDirs = contents.filter{ $0.hasDirectoryPath }
for modelDir in modelDirs {
let modelConfigURL = modelDir.appending(path: Constants.modelConfigFileName)
if fileManager.fileExists(atPath: modelConfigURL.path()) {
if let modelConfig = loadModelConfig(modelConfigURL: modelConfigURL) {
assert(modelDir.lastPathComponent == modelConfig.localID)
addModelConfig(modelConfig: modelConfig, modelURL: nil, isBuiltin: true)
}
}
}
} catch {
showAlert(message: "Failed to load prebuilt models: \(error.localizedDescription)")
}
}
func loadModelConfig(modelConfigURL: URL) -> ModelConfig? {
do {
assert(fileManager.fileExists(atPath: modelConfigURL.path()))
let fileHandle = try FileHandle(forReadingFrom: modelConfigURL)
let data = fileHandle.readDataToEndOfFile()
let modelConfig = try jsonDecoder.decode(ModelConfig.self, from: data)
if !isModelConfigAllowed(modelConfig: modelConfig) {
return nil
}
return modelConfig
} catch {
showAlert(message: "Failed to resolve model config: \(error.localizedDescription)")
}
return nil
}
func showAlert(message: String) {
DispatchQueue.main.async { [weak self] in
guard let self = self else { return }
if !self.alertDisplayed {
self.alertMessage = message
self.alertDisplayed = true
} else {
self.alertMessage.append("\n" + message)
}
}
}
func isModelConfigAllowed(modelConfig: ModelConfig) -> Bool {
if appConfig?.modelLibs.contains(modelConfig.modelLib) ?? true {
return true
}
showAlert(message: "Model lib \(modelConfig.modelLib) is not supported")
return false
}
func downloadConfig(modelURL: URL?, localID: String?, isBuiltin: Bool) {
guard let modelConfigURL = modelURL?.appending(path: "resolve").appending(path: "main").appending(path: Constants.modelConfigFileName) else {
return
}
let downloadTask = URLSession.shared.downloadTask(with: modelConfigURL) {
[weak self] urlOrNil, responseOrNil, errorOrNil in
guard let self else {
return
}
if let error = errorOrNil {
self.showAlert(message: "Failed to download model config: \(error.localizedDescription)")
return
}
guard let fileUrl = urlOrNil else {
self.showAlert(message: "Failed to download model config")
return
}
// cache temp file to avoid being deleted by system automatically
let tempName = UUID().uuidString
let tempFileURL = self.cacheDirectoryURL.appending(path: tempName)
do {
try self.fileManager.moveItem(at: fileUrl, to: tempFileURL)
} catch {
self.showAlert(message: "Failed to cache downloaded file: \(error.localizedDescription)")
return
}
do {
guard let modelConfig = loadModelConfig(modelConfigURL: tempFileURL) else {
try fileManager.removeItem(at: tempFileURL)
return
}
if localID != nil {
assert(localID == modelConfig.localID)
}
if localModelIDs.contains(modelConfig.localID) {
try fileManager.removeItem(at: tempFileURL)
return
}
let modelBaseUrl = cacheDirectoryURL.appending(path: modelConfig.localID)
try fileManager.createDirectory(at: modelBaseUrl, withIntermediateDirectories: true)
let modelConfigUrl = modelBaseUrl.appending(path: Constants.modelConfigFileName)
try fileManager.moveItem(at: tempFileURL, to: modelConfigUrl)
assert(fileManager.fileExists(atPath: modelConfigUrl.path()))
assert(!fileManager.fileExists(atPath: tempFileURL.path()))
addModelConfig(modelConfig: modelConfig, modelURL: modelURL, isBuiltin: isBuiltin)
} catch {
showAlert(message: "Failed to import model: \(error.localizedDescription)")
}
}
downloadTask.resume()
}
func addModelConfig(modelConfig: ModelConfig, modelURL: URL?, isBuiltin: Bool) {
assert(!localModelIDs.contains(modelConfig.localID))
localModelIDs.insert(modelConfig.localID)
let modelBaseURL: URL
// local-id dir should exist
if modelURL == nil {
// prebuilt model in dist
modelBaseURL = Bundle.main.bundleURL.appending(path: Constants.prebuiltModelDir).appending(path: modelConfig.localID)
} else {
// download model in cache
modelBaseURL = cacheDirectoryURL.appending(path: modelConfig.localID)
}
assert(fileManager.fileExists(atPath: modelBaseURL.path()))
// mlc-chat-config.json should exist
let modelConfigURL = modelBaseURL.appending(path: Constants.modelConfigFileName)
assert(fileManager.fileExists(atPath: modelConfigURL.path()))
let model = ModelState(modelConfig: modelConfig, modelLocalBaseURL: modelBaseURL, startState: self, chatState: chatState)
model.checkModelDownloadState(modelURL: modelURL)
models.append(model)
if modelURL != nil && !isBuiltin {
updateAppConfig {
appConfig?.modelList.append(AppConfig.ModelRecord(modelURL: modelURL!.absoluteString, localID: modelConfig.localID))
}
}
}
func updateAppConfig(action: () -> Void) {
action()
let appConfigURL = cacheDirectoryURL.appending(path: Constants.appConfigFileName)
do {
let data = try jsonEncoder.encode(appConfig)
try data.write(to: appConfigURL, options: Data.WritingOptions.atomic)
} catch {
print(error.localizedDescription)
}
}
} |
<?php
defined('BASEPATH') or exit('No se permite acceso directo'); //si la constante esta definida se ejecuta el resto del codigo, sino se detiene la ejecuciรณn y tira un mensaje
require_once ROOT . '/mvc/modelos/tareas_enviar/tareas_enviarmodel.php'; //carga el archivo con el modelo
require_once ROOT . '/mvc/system/session.php'; //carga el archivo de la clase 'session'
class tareas_enviarcontroller extends controller //Crea la clase "tareas_enviarcontroller" con herencia de la clase "controller"
{
private $session; //Crea la variable 'session'
private $model; //Crea la variable 'model'
public function __construct() //funciรณn que se ejecuta al asignarle la clase a un objeto
{
$this->model = new tareas_enviarmodel(); //creamos el objeto 'model' con la clase 'tareas_enviarmodel' para poder comunicarse con la base de datos a traves del modelo
$this->session = new session(); //creamos el objeto 'session' con la clase 'session' para poder obtener informacion de la sesiรณn
$this->session->iniciar(); //iniciamos la sesiรณn
if (empty($this->session->getAll())) { //pedimos la variable completa de la sesiรณn, si existe se sigue con el cรณdigo, sino nos manda al login
header('location: /mvc/login'); //usamos la funciรณn header(propia de php) para ir al login
}
if ($this->session->get('rol') == 3) { //Todos los roles diferentes a los alumnos pueden ver esto
header('location: /mvc/errorpage'); //Sino te manda a la pantalla de error
}
}
public function salir() //Funciรณn para cerrar la sesion
{
$this->session->close(); //Cerramos la sesion
$this->model->close(); //le decimos al modelo que finalice la conexiรณn con la base de datos por seguridad
header('location: /mvc/login'); //Nos manda al login
}
public function eliminar_tarea($idtarea) //funciรณn para eliminar una tarea
{
$this->model->eliminarTarea($idtarea); //le pedimos al modelo eliminar la tarea que tenga la id que recibimos como parametro
$this->model->close(); //le decimos al modelo que finalice la conexiรณn con la base de datos por seguridad
header('location: /mvc/tareas_enviar'); //te recarga la pรกgina para ver el cambio
}
public function crear_tarea($parametros) //funciรณn para crear una tarea
{
date_default_timezone_set('America/Argentina/Buenos_Aires'); //Establecemos la zona horaria en Buenos Aires, Argentina
$fecha = date('Y-m-d H:i:s'); //La fecha se compone del aรฑo, mes, dรญa, hora, minutos y segundos, en ese orden
if (isset($_FILES) && $_FILES['archivo']['name'] != '') { //Si existe la variable $_FILES(es decir, que se haya subido un archivo) y si el archivo tiene un nombre diferente a vacio sucede lo siguiente
$archivo = $_FILES['archivo']; //Crea un array con los datos del archivo
$nom_arch = $archivo['name']; //Nombre del archivo
$tipo_arch = $archivo['type']; //Extensiรณn del archivo
$tmp_arch = $archivo['tmp_name']; //Ubicacion temporal
$err_arch = $archivo['error']; //Errores del archivo
$tam_arch = $archivo['size']; //El tamaรฑo del archivo
$ext_arch = explode('.', $nom_arch); //Utilizamos explode para sacar el '.' de la extensiรณn del archivo
$ext_arch2 = strtolower(end($ext_arch)); //Utilizamos strtolower(funciรณn de php) para poner en minuscula la extensiรณn del archivo, la funciรณn 'end' solo sirve para seleccionar el ultimo elemento de un array, que en este caso seria la extensiรณn
$ext_permitidas = array('jpg', 'jpeg', 'png', 'pdf', 'doc', 'docx', 'xls', 'xlsx', 'ppt', 'pptx', 'rar', 'zip'); //Las extensiones permitidas
if (in_array($ext_arch2, $ext_permitidas)) { //Si el tipo de extension es una de las permitidas se procede a continuar
if ($err_arch === 0) { //Si no hay errores se procede a continuar
if ($tam_arch < 10000000) { //El peso del archivo sera el limite de tamaรฑo, en este caso son 10mb
$ubicacion_arch = 'archivos_subidos/'.$nom_arch; //Declaramos la ruta a la que va a ir el archivo, a la carpeta de 'archivos_subidos'
move_uploaded_file($tmp_arch, $ubicacion_arch); //Movemos el archivo de su ubicacion temporal a la ruta anteriormente definida con la funciรณn de php 'move_uploaded_file'
} else {
exit("El archivo es muy pesado, el maximo es 10mb"); //mensaje que aparece si el archivo es demasiado grande
}
} else {
exit("Hubo un error al subir el archivo"); //mensaje que aparece si el archivo produjo errores
}
} else {
exit("No se pueden enviar archivos con esa extension"); //mensaje que aparece si tiene una extensiรณn no permitida
}
}
if (!empty($parametros['nom_tarea']) and !empty($parametros['desc_tarea'])) { //verifica que no haya datos vacรญos
$this->model->crearTarea($parametros, $fecha, $nom_arch); //le pedimos al modelo que envie los datos de la tarea a la base de datos
$this->model->close(); //le decimos al modelo que finalice la conexiรณn con la base de datos por seguridad
header('location: /mvc/tareas_enviar'); //nos manda a la misma pรกgina para simular una recarga de pรกgina y ver los datos nuevos
} else {
header('location: /mvc/tareas_enviar'); //nos recarga la pรกgina
}
}
public function exec() //funciรณn que se encarga de obtener los datos para la vista y mostrarla
{
$query_cursos = $this->model->cursos(); //le pedimos al modelo informaciรณn de los cursos
$query_materias = $this->model->materias(); //le pedimos al modelo informaciรณn de las materias
$query_tareas = $this->model->tareas($this->session->get('idUser')); //le pedimos al modelo informaciรณn de las tareas segรบn la id del usuario
$params = array('nombre' => $this->session->get('nombre'), 'rol' => $this->session->get('rol'), 'tema' => $this->session->get('temavis'), 'id_user' => $this->session->get('idUser'), 'query_cursos' => $query_cursos, 'query_materias' => $query_materias, 'query_tareas' => $query_tareas); //asignamos los parametros que vamos a enviar a la vista
$this->render(__CLASS__, $params); //funciรณn heredada que permite mostrar la vista y enviarle los datos que queramos, dentro de 'params'
$this->model->close(); //le decimos al modelo que finalice la conexiรณn con la base de datos por seguridad
}
}
?> |
#include "bmp.h" // Simple .bmp library
#include <iostream>
#include <fstream>
#include <string>
#include <math.h>
#include <vector>
using namespace std;
#define PI 3.14159265358979
int QuantizationMatrix[8][8] = {
{3, 5, 7, 9, 11, 13, 15, 17},
{5, 7, 9, 11, 13, 15, 17, 19},
{7, 9, 11, 13, 15, 17, 19, 21},
{9, 11, 13, 15, 17, 19, 21, 23},
{11, 13, 15, 17, 19, 21, 23, 25},
{13, 15, 17, 19, 21, 23, 25, 27},
{15, 17, 19, 21, 23, 25, 27, 29},
{17, 19, 21, 23, 25, 27, 29, 31}};
double c(int k)
{
return k != 0 ? 1 : 1 / sqrt(2);
}
double **createDoubleArray(int m, int n)
{
double *values = (double *)calloc(m * n, sizeof(double));
double **rows = (double **)malloc(m * sizeof(double *));
for (int i = 0; i < m; ++i)
{
rows[i] = values + i * n;
}
return rows;
}
double **resizedImage(double **inputArry, int inputWidth, int inputHeight, int width, int height)
{
double **resizedArr = createDoubleArray(height + 1, width + 1);
double x_ratio = (inputWidth - 1) * 1.0f / (width - 1);
double y_ratio = (inputHeight - 1) * 1.0f / (height - 1);
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
int x_l = floor(x_ratio * j);
int y_l = floor(y_ratio * i);
int x_h = ceil(x_ratio * j);
int y_h = ceil(y_ratio * i);
double x_weight = x_ratio * j - x_l;
double y_weight = y_ratio * i - y_l;
double a = inputArry[y_l][x_l];
double b = inputArry[y_l][x_h];
double c = inputArry[y_h][x_l];
double d = inputArry[y_h][x_h];
double pixel = a * (1 - x_weight) * (1 - y_weight) + b * x_weight * (1 - y_weight) +
c * y_weight * (1 - x_weight) +
d * x_weight * y_weight;
resizedArr[i][j] = pixel;
}
}
return resizedArr;
}
int main(int argc, char **argv)
{
if (argc <= 2 || argc >= 5)
{
cout << "Arguments prompt: dct.exe <img_path> <apply_idct> <scale>" << endl;
return 0;
}
string imgPath = argv[1];
bool need_idct = stoi(argv[2]);
float scale = 1;
if (argc == 4)
{
scale = stof(argv[3]);
if (scale <= 0)
scale = 1;
}
//! read input image
Bitmap s_img(imgPath.c_str());
int rows = s_img.getHeight(), cols = s_img.getWidth();
cout << "Apply DCT on image (" << rows << ", " << cols << ")." << endl;
//! preprocess by shifting pixel values by 128
//! 2D DCT for every 8x8 block (assume that the input image resolution is fixed to 256)
// The quantized coefficients stored into 'coeffArray'
double coeffArray[256][256] = {0};
int blockRow = rows / 8, blockCol = cols / 8;
for (int i = 0; i < blockRow; i++)
{
for (int j = 0; j < blockCol; j++)
{
int xpos = j * 8, ypos = i * 8;
double F_r[8][8];
for (int u = 0; u < 8; u++)
{
for (int v = 0; v < 8; v++)
{
double rowSum = 0;
for (int x = 0; x < 8; x++)
{
unsigned char val;
s_img.getPixel(xpos + x, ypos + v, val);
rowSum += cos((2 * x + 1) * u * PI / 16) * (val - 128);
}
F_r[u][v] = rowSum * c(u) / 2;
}
}
for (int u = 0; u < 8; u++)
{
for (int v = 0; v < 8; v++)
{
double colSum = 0;
for (int y = 0; y < 8; y++)
{
colSum += cos((2 * y + 1) * v * PI / 16) * F_r[u][y];
}
coeffArray[xpos + u][ypos + v] = c(v) * colSum / 2;
}
}
// quantize the frequency coefficient of this block
for (int u = 0; u < 8; u++)
{
for (int v = 0; v < 8; v++)
{
coeffArray[u + xpos][v + ypos] = round(coeffArray[u + xpos][v + ypos] / QuantizationMatrix[u][v]);
}
}
}
}
//! output the computed coefficient array
FILE *fp = fopen("coeffs.txt", "w");
for (int r = 0; r < rows; r++)
{
for (int c = 0; c < cols; c++)
{
fprintf(fp, "%3.3lf ", coeffArray[c][r]);
}
fprintf(fp, "\n");
}
cout << "Quantized coefficients saved!" << endl;
if (need_idct)
{
Bitmap reconstructedImg(cols, rows);
double **ImageArr = createDoubleArray(rows, cols);
for (int i = 0; i < blockRow; i++)
{
for (int j = 0; j < blockCol; j++)
{
int xpos = j * 8, ypos = i * 8;
// apply de-quantization on block_ij
double F_1[8][8];
for (int u = 0; u < 8; u++)
{
for (int v = 0; v < 8; v++)
{
F_1[u][v] = coeffArray[xpos + u][ypos + v] * QuantizationMatrix[u][v];
}
}
// apply IDCT on this block
double F_2[8][8];
for (int u = 0; u < 8; u++)
{
for (int v = 0; v < 8; v++)
{
double colSum = 0;
for (int y = 0; y < 8; y++)
{
colSum += cos((2 * v + 1) * y * PI / 16) * F_1[u][y] * c(y);
}
F_2[u][v] = colSum / 2;
}
}
double F_3[8][8];
for (int u = 0; u < 8; u++)
{
for (int v = 0; v < 8; v++)
{
double rowSum = 0;
for (int x = 0; x < 8; x++)
{
rowSum += cos((2 * u + 1) * x * PI / 16) * F_2[x][v] * c(x);
}
F_3[u][v] = rowSum / 2;
}
}
// shiftting back the pixel value range to 0~255
for (int u = 0; u < 8; u++)
{
for (int v = 0; v < 8; v++)
{
ImageArr[xpos + u][ypos + v] = round(F_3[u][v] + 128);
}
}
}
}
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
if (ImageArr[i][j] > 255)
ImageArr[i][j] = 255;
if (ImageArr[i][j] < 0)
ImageArr[i][j] = 0;
reconstructedImg.setPixel(i, j, ImageArr[i][j]);
}
}
if (scale != 1)
{
int size = (int)(scale * rows);
double **newArr = resizedImage(ImageArr, cols - 1, rows - 1, size, size);
Bitmap newImg = Bitmap(size, size);
for (int i = 0; i < size; i++)
for (int j = 0; j < size; j++)
{
if (newArr[i][j] > 255)
newArr[i][j] = 255;
if (newArr[i][j] < 0)
newArr[i][j] = 0;
newImg.setPixel(i, j, (unsigned char)newArr[i][j]);
}
newImg.save("reconstructedImg.bmp");
return 0;
}
string savePath = "reconstructedImg.bmp";
reconstructedImg.save(savePath.c_str());
cout << "reconstructed image saved!" << endl;
}
return 0;
} |
/**
* Loads country markers, and highlights a polygon when the user hovers over it.
*
* This example starts in Southeast Asia to demonstrate hovering multi-marker polygons such as Indonesia, Phillipines,
* etc.
*/
import de.fhpotsdam.unfolding.*;
import de.fhpotsdam.unfolding.data.*;
import de.fhpotsdam.unfolding.geo.*;
import de.fhpotsdam.unfolding.marker.*;
import de.fhpotsdam.unfolding.utils.*;
import processing.opengl.*;
import codeanticode.glgraphics.*;
UnfoldingMap map;
List<Marker> countryMarkers = new ArrayList<Marker>();
Location indonesiaLocation = new Location(-6.175, 106.82);
void setup() {
size(800, 600, GLConstants.GLGRAPHICS);
map = new UnfoldingMap(this);
map.zoomAndPanTo(indonesiaLocation, 3);
MapUtils.createDefaultEventDispatcher(this, map);
List<Feature> countries = GeoJSONReader.loadData(this, "countries.geo.json");
List<Marker> countryMarkers = MapUtils.createSimpleMarkers(countries);
map.addMarkers(countryMarkers);
}
void draw() {
background(240);
map.draw();
}
void mouseMoved() {
// Deselect all marker
for (Marker marker : map.getMarkers()) {
marker.setSelected(false);
}
// Select hit marker
// Note: Use getHitMarkers(x, y) if you want to allow multiple selection.
Marker marker = map.getFirstHitMarker(mouseX, mouseY);
if (marker != null) {
marker.setSelected(true);
}
} |
---
id: index-gettingstarted
title: Getting Started with Node and Redis
sidebar_label: Getting Started
slug: /develop/node/gettingstarted
authors: [ajeet, simon]
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
import Authors from '@theme/Authors';
<Authors frontMatter={frontMatter} />
Find tutorials, examples and technical articles that will help you to develop with Redis and Node.js/JavaScript:
## Introduction
Redis is an open source, in-memory, key-value data store most commonly used as a primary database, cache, message broker, and queue. Redis cache delivers sub-millisecond response times, enabling fast and powerful real-time applications in industries such as gaming, fintech, ad-tech, social media, healthcare, and IoT.
Redis is a great database for use with Node. Both Redis and Node share similar type conventions and threading models, which makes for a very predictable development experience. By pairing Node and Redis together you can achieve a scalable and productive development platform.
Redis has two primary Node clients which are [node-redis](https://github.com/redis/node-redis) and [ioredis](https://github.com/luin/ioredis). Both are available through npm. We generally suggest using node-redis, as it has wide support for Redis modules, is easily extended, and is widely used.
Check out a list of Redis clients that the community has built for Node [here](https://redis.io/clients#nodejs).
This article shows how to get started with the recommended libraries: [node-redis](https://github.com/redis/node-redis) and [ioredis](https://github.com/luin/ioredis).
<Tabs
defaultValue="node-redis"
values={[
{label: 'node-redis', value: 'node-redis'},
{label: 'ioredis', value: 'ioredis'},
]}>
<TabItem value="node-redis">
#### Step 1. Run a Redis server
You can either run Redis in a Docker container or directly on your Mac OS.
Use the following commands to setup a Redis server locally:
```
brew tap redis-stack/redis-stack
brew install --cask redis-stack
```
:::info INFO
Redis Stack unifies and simplifies the developer experience of the leading Redis data store, modules and the capabilities they provide. Redis Stack supports the folliwng in additon to Redis: JSON, Search, Time Series, Triggers and Functions, and Probilistic data structures.
:::
Ensure that you are able to use the following Redis command to connect to the Redis instance.
```bash
redis-cli
localhost>
```
Now you should be able to perform CRUD operations with Redis keys.
The above Redis client command might require password if you have setup authentication in your Redis configuration file. Refer [Redis Command Reference](https://redis.io/commands/)
#### Step 2. Install node redis using `NPM` (or `YARN`)
Run the following NPM command to install the Redis client.
```bash
npm install redis
```
#### Step 2. Write your Application Code
Use the following sample code for our Node.js application:
```javascript
import { createClient } from 'redis';
async function nodeRedisDemo() {
try {
const client = createClient();
await client.connect();
await client.set('mykey', 'Hello from node redis');
const myKeyValue = await client.get('mykey');
console.log(myKeyValue);
const numAdded = await client.zAdd('vehicles', [
{
score: 4,
value: 'car',
},
{
score: 2,
value: 'bike',
},
]);
console.log(`Added ${numAdded} items.`);
for await (const { score, value } of client.zScanIterator('vehicles')) {
console.log(`${value} -> ${score}`);
}
await client.quit();
} catch (e) {
console.error(e);
}
}
nodeRedisDemo();
```
</TabItem>
<TabItem value="ioredis">
#### Step 1. Install ioredis using `npm` (or `yarn`)
```bash
npm install ioredis
```
#### Step 2. Write your Application Code
```javascript
const Redis = require('ioredis');
async function ioredisDemo() {
try {
const client = new Redis();
await client.set('mykey', 'Hello from io-redis!');
const myKeyValue = await client.get('mykey');
console.log(myKeyValue);
const numAdded = await client.zadd('vehicles', 4, 'car', 2, 'bike');
console.log(`Added ${numAdded} items.`);
const stream = client.zscanStream('vehicles');
stream.on('data', (items) => {
// items = array of value, score, value, score...
for (let n = 0; n < items.length; n += 2) {
console.log(`${items[n]} -> ${items[n + 1]}`);
}
});
stream.on('end', async () => {
await client.quit();
});
} catch (e) {
console.error(e);
}
}
ioredisDemo();
```
</TabItem>
</Tabs>
---
### Redis Launchpad
Redis Launchpad is like an โApp Storeโ for Redis sample apps. You can easily find apps for your preferred frameworks and languages.
Check out a few of these apps below, or [click here to access the complete list](https://launchpad.redis.com).
<div class="row text--center">
<div class="col ">
<div className="ri-container">
#### Hacker News Clone in NodeJS

[A Hacker News Clone project](https://launchpad.redis.com/?id=project%3Aredis-hacker-news-demo) built in NextJS, NodeJS and Express based on Search and JSON
</div>
</div>
<div class="col">
<div className="ri-container">
#### Shopping Cart application in NodeJS

[Shopping Cart app in NodeJS](https://launchpad.redis.com/?id=project%3Abasic-redis-shopping-chart-nodejs) module functionalities
</div>
</div>
</div>
---
### More Developer Resources
<div class="row">
<div class="col">
#### Sample Code
**[Basic Redis Caching](https://developer.redis.com/howtos/caching/)**
This application calls the GitHub API and caches the results into Redis.
**[Redis Rate-Limiting](https://developer.redis.com/howtos/ratelimiting/)**
This is a very simple app that demonstrates rate-limiting feature using Redis.
**[Notifications with WebSocket, Vue & Redis](https://github.com/redis-developer/redis-websockets-vue-notifications)**
This project allows you to push notifications in a Vue application from a Redis `PUBLISH` using WebSockets.
</div>
<div class="col">
#### Technical Articles & Videos
**[Redis Rapid Tips: ioredis](https://www.youtube.com/watch?v=H6rikGCYPUk)** (YouTube)
**[Mapping Objects between Node and Redis](https://www.youtube.com/watch?v=dukkMLbzPfA)** (YouTube)
</div>
</div>
---
### Redis University
### [Redis for JavaScript Developers](https://university.redis.com/courses/ru102js/)
Build full-fledged Redis applications with Node.js and Express.
<div class="text--center">
<iframe
width="560"
height="315"
src="https://www.youtube.com/embed/Ik1WXPX3WNU"
frameborder="0"
allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture"
allowfullscreen></iframe>
</div> |
//
// NetworkingServiceTests.swift
// Currency ConverterTests
//
// Created by Aleksei Kudriashov on 4/1/24.
//
import XCTest
@testable import Currency_Converter
final class NetworkingServiceTests: XCTestCase {
func testLoadCurrencies() async throws {
// Given
let networking: Networking = HTTPClient()
// Action
let response = try await networking.loadCurrencies()
// Result
XCTAssertTrue(!response.currenciesInfo.isEmpty, "Currencies should be available")
}
func testLoadExchangeRate() async throws {
// Given
let networking: Networking = HTTPClient()
let currencyCode = "USD"
// Action
let curencyList = try await networking.loadExchangeRate(for: currencyCode).data
// Result
XCTAssertNotNil(curencyList[currencyCode], "Currency should be available")
XCTAssertTrue(curencyList[currencyCode] == 1, "Currency rate should be 1")
}
func testLoadUnavailableExchangeRate() async throws {
// Given
let networking: Networking = HTTPClient()
let currencyCode = "Converter"
// Action
let curencyList = try await networking.loadExchangeRate(for: currencyCode).data
// Result
XCTAssertNil(curencyList[currencyCode], "Searching currency shouldn't be available")
}
} |
package org.example.utils;
import io.github.bonigarcia.wdm.WebDriverManager;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class BrowserFactory {
public static WebDriver createInstance(BrowserTypeEnum browserTypeEnum) {
WebDriver driver;
switch (browserTypeEnum) {
case CHROME:
ChromeOptions options = new ChromeOptions();
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver(options);
break;
case FIREFOX:
System.setProperty("webdriver.gecko.driver", "src/test/resources/geckodriver.exe");
driver = new FirefoxDriver();
break;
case EDGE:
driver = new EdgeDriver();
break;
case MOBILE:
ChromeOptions mobileOptions = new ChromeOptions();
mobileOptions.addArguments("--window-size=375,812"); // ะัะธะผะตั ะผะพะฑะธะปัะฝะพะณะพ ัะฐะทัะตัะตะฝะธั iPhone X
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
driver = new ChromeDriver(mobileOptions);
break;
default:
throw new IllegalArgumentException(STR."Unsupported browser type: \{browserTypeEnum}");
}
return driver;
}
} |
import 'package:flutter/material.dart';
import 'package:wordum/models/dictionary_word.dart';
/// Page about details of a word.
///
/// The page for adding and displaying all word data as in [DictionaryWord] model.
class WordPage extends StatelessWidget {
final DictionaryWord dictionaryWord;
final String translation;
final TextStyle headerStyle = const TextStyle(fontWeight: FontWeight.bold, fontSize: 20.0);
final TextStyle labelStyle = const TextStyle(fontWeight: FontWeight.bold, fontSize: 16.0);
final TextStyle bodyStyle = const TextStyle(fontSize: 16.0);
const WordPage({
super.key,
required this.dictionaryWord,
required this.translation,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Word details: ${dictionaryWord.word}'),
),
body: Center(
child: Column(
children: [
Container(
padding: const EdgeInsets.all(40),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(dictionaryWord.word, style: headerStyle),
const SizedBox(height: 20),
Row(
mainAxisAlignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
children: [
Text('translation: ', style: labelStyle),
Text(translation, style: bodyStyle),
],
),
const SizedBox(height: 15),
const TextField(
decoration: InputDecoration(
labelText: 'Definition',
border: OutlineInputBorder(),
),
),
const SizedBox(height: 30),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('Save')
),
const SizedBox(width: 30),
ElevatedButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('Back')
),
],
),
],
),
),
],
),
),
);
}
} |
import { jsx as _jsx, Fragment as _Fragment, jsxs as _jsxs } from "react/jsx-runtime";
/**
* External dependencies
*/
import { PlainText } from '@wordpress/block-editor';
import { Button, ButtonGroup } from '@wordpress/components';
import { useKeyboardShortcut } from '@wordpress/compose';
import { forwardRef, useImperativeHandle, useRef, useEffect, useCallback, } from '@wordpress/element';
import { __ } from '@wordpress/i18n';
import { Icon, closeSmall, check, arrowUp, trash, reusableBlock } from '@wordpress/icons';
import classNames from 'classnames';
import React from 'react';
/**
* Internal dependencies
*/
import './style.scss';
import AiStatusIndicator from '../ai-status-indicator';
import { GuidelineMessage } from './message';
// eslint-disable-next-line @typescript-eslint/no-empty-function
const noop = () => { };
/**
* AI Control component.
*
* @param {AiControlProps} props - Component props.
* @param {React.MutableRefObject} ref - Ref to the component.
* @returns {React.ReactElement} Rendered component.
*/
export function AIControl({ disabled = false, value = '', placeholder = '', showAccept = false, acceptLabel = __('Accept', 'jetpack-ai-client'), showButtonLabels = true, isTransparent = false, state = 'init', showGuideLine = false, customFooter = null, onChange = noop, onSend = noop, onStop = noop, onAccept = noop, onDiscard = null, showRemove = false, }, ref // eslint-disable-line @typescript-eslint/ban-types
) {
const promptUserInputRef = useRef(null);
const loading = state === 'requesting' || state === 'suggesting';
const [editRequest, setEditRequest] = React.useState(false);
const [lastValue, setLastValue] = React.useState(value || null);
useEffect(() => {
if (editRequest) {
promptUserInputRef?.current?.focus();
}
if (!editRequest && lastValue !== null && value !== lastValue) {
onChange?.(lastValue);
}
}, [editRequest, lastValue, value]);
const sendRequest = useCallback(() => {
setLastValue(value);
setEditRequest(false);
onSend?.(value);
}, [value]);
const changeHandler = useCallback((newValue) => {
onChange?.(newValue);
if (state === 'init') {
return;
}
if (!lastValue) {
// here we're coming from a one-click action
setEditRequest(newValue.length > 0);
}
else {
// here we're coming from an edit action
setEditRequest(newValue !== lastValue);
}
}, [lastValue, state]);
const discardHandler = useCallback(() => {
onDiscard?.();
onAccept?.();
}, []);
const cancelEdit = useCallback(() => {
onChange(lastValue || '');
setEditRequest(false);
}, [lastValue]);
// Pass the ref to forwardRef.
useImperativeHandle(ref, () => promptUserInputRef.current);
useKeyboardShortcut('mod+enter', () => {
if (showAccept) {
onAccept?.();
}
}, {
target: promptUserInputRef,
});
useKeyboardShortcut('enter', e => {
e.preventDefault();
sendRequest();
}, {
target: promptUserInputRef,
});
return (_jsx("div", { className: "jetpack-components-ai-control__container-wrapper", children: _jsxs("div", { className: "jetpack-components-ai-control__container", children: [_jsxs("div", { className: classNames('jetpack-components-ai-control__wrapper', {
'is-transparent': isTransparent,
}), children: [_jsx(AiStatusIndicator, { state: state }), _jsx("div", { className: "jetpack-components-ai-control__input-wrapper", children: _jsx(PlainText, { value: value, onChange: changeHandler, placeholder: placeholder, className: "jetpack-components-ai-control__input", disabled: loading || disabled, ref: promptUserInputRef }) }), (!showAccept || editRequest) && (_jsx("div", { className: "jetpack-components-ai-control__controls-prompt_button_wrapper", children: !loading ? (_jsxs(_Fragment, { children: [editRequest && (_jsx(Button, { className: "jetpack-components-ai-control__controls-prompt_button", onClick: cancelEdit, variant: "secondary", label: __('Cancel', 'jetpack-ai-client'), children: showButtonLabels ? (__('Cancel', 'jetpack-ai-client')) : (_jsx(Icon, { icon: closeSmall })) })), showRemove && !editRequest && !value?.length && onDiscard && (_jsx(Button, { className: "jetpack-components-ai-control__controls-prompt_button", onClick: discardHandler, variant: "secondary", label: __('Cancel', 'jetpack-ai-client'), children: showButtonLabels ? (__('Cancel', 'jetpack-ai-client')) : (_jsx(Icon, { icon: closeSmall })) })), value?.length > 0 && (_jsx(Button, { className: "jetpack-components-ai-control__controls-prompt_button", onClick: sendRequest, variant: "primary", disabled: !value?.length || disabled, label: __('Send request', 'jetpack-ai-client'), children: showButtonLabels ? (__('Generate', 'jetpack-ai-client')) : (_jsx(Icon, { icon: arrowUp })) }))] })) : (_jsx(Button, { className: "jetpack-components-ai-control__controls-prompt_button", onClick: onStop, variant: "secondary", label: __('Stop request', 'jetpack-ai-client'), children: showButtonLabels ? (__('Stop', 'jetpack-ai-client')) : (_jsx(Icon, { icon: closeSmall })) })) })), showAccept && !editRequest && (_jsxs("div", { className: "jetpack-components-ai-control__controls-prompt_button_wrapper", children: [(value?.length > 0 || lastValue === null) && (_jsxs(ButtonGroup, { children: [_jsx(Button, { className: "jetpack-components-ai-control__controls-prompt_button", label: __('Discard', 'jetpack-ai-client'), onClick: discardHandler, tooltipPosition: "top", children: _jsx(Icon, { icon: trash }) }), _jsx(Button, { className: "jetpack-components-ai-control__controls-prompt_button", label: __('Regenerate', 'jetpack-ai-client'), onClick: () => onSend?.(value), tooltipPosition: "top", disabled: !value?.length || value === null || disabled, children: _jsx(Icon, { icon: reusableBlock }) })] })), _jsx(Button, { className: "jetpack-components-ai-control__controls-prompt_button", onClick: onAccept, variant: "primary", label: acceptLabel, children: showButtonLabels ? acceptLabel : _jsx(Icon, { icon: check }) })] }))] }), showGuideLine && !loading && !editRequest && (customFooter || _jsx(GuidelineMessage, {}))] }) }));
}
export default forwardRef(AIControl); |
<?php
namespace Tests\Feature;
use App\Models\Contact;
use Database\Seeders\ContactSeeder;
use Database\Seeders\SearchSeeder;
use Database\Seeders\UserSeeder;
use Illuminate\Foundation\Testing\RefreshDatabase;
use Illuminate\Foundation\Testing\WithFaker;
use Illuminate\Support\Facades\DB;
use Illuminate\Support\Facades\Log;
use Tests\TestCase;
class ContactTest extends TestCase
{
protected function setUp(): void
{
parent::setUp();
DB::delete('DELETE FROM addresses');
DB::delete('DELETE FROM contacts');
DB::delete('DELETE FROM users');
}
public function testCreateSuccess()
{
$this->seed(UserSeeder::class);
$this->post('/api/contacts',
[
'first_name' => 'Raka',
'last_name' => 'Febrian',
'email' => 'raka@gmail.com',
'phone' => '081242526'
],
[
'Authorization' => 'test'
])->assertStatus(201)->assertJson([
'data' => [
'first_name' => 'Raka',
'last_name' => 'Febrian',
'email' => 'raka@gmail.com',
'phone' => '081242526'
]
]);
}
public function testCreateFailed()
{
$this->seed(UserSeeder::class);
$this->post('/api/contacts',
[
'first_name' => '',
'last_name' => 'Febrian',
'email' => 'raka',
'phone' => '081242526'
],
[
'Authorization' => 'test'
])->assertStatus(400)->assertJson([
'errors' => [
'first_name' => ['The first name field is required.'],
'email' => ['The email field must be a valid email address.'],
]
]);
}
public function testCreateUnauthorized()
{
$this->seed(UserSeeder::class);
$this->post('/api/contacts',
[
'first_name' => '',
'last_name' => 'Febrian',
'email' => 'raka',
'phone' => '081242526'
],
[
'Authorization' => 'wrong'
])->assertStatus(401)->assertJson([
'errors' => [
'message' => ['unauthorized'],
]
]);
}
public function testGetSuccess()
{
$this->seed([UserSeeder::class,ContactSeeder::class]);
$contact = Contact::limit(1)->first();
$this->get('/api/contacts/' . $contact->id,[
'Authorization' => 'test'
])->assertStatus(200)->assertJson([
'data' => [
'first_name' => 'test',
'last_name' => 'test',
'email' => 'test@example.com',
'phone' => '111111'
]
]);
}
public function testGetNotFound()
{
$this->seed([UserSeeder::class,ContactSeeder::class]);
$contact = Contact::limit(1)->first();
$this->get('/api/contacts/' . $contact->id + 1,[
'Authorization' => 'test'
])->assertStatus(404)->assertJson([
'errors' => [
'message' => ['not found']
]
]);
}
public function testGetOtherUserContact()
{
$this->seed([UserSeeder::class,ContactSeeder::class]);
$contact = Contact::limit(1)->first();
$this->get('/api/contacts/' . $contact->id,[
'Authorization' => 'test2'
])->assertStatus(404)->assertJson([
'errors' => [
'message' => ['not found']
]
]);
}
public function testUpdateSuccess()
{
$this->seed([UserSeeder::class,ContactSeeder::class]);
$contact = Contact::limit(1)->first();
$this->put('/api/contacts/' . $contact->id,
[
'first_name' => 'test2',
'last_name' => 'test2',
'email' => 'test2@example.com',
'phone' => '111112'
],
[
'Authorization' => 'test'
])->assertStatus(200)->assertJson([
'data' => [
'first_name' => 'test2',
'last_name' => 'test2',
'email' => 'test2@example.com',
'phone' => '111112'
]
]);
}
public function testUpdateValidationError()
{
$this->seed([UserSeeder::class,ContactSeeder::class]);
$contact = Contact::limit(1)->first();
$this->put('/api/contacts/' . $contact->id,
[
'first_name' => '',
'last_name' => 'test2',
'email' => 'test2@example.com',
'phone' => '111112'
],
[
'Authorization' => 'test'
])->assertStatus(400)->assertJson([
'errors' => [
'first_name' => ['The first name field is required.'],
]
]);
}
public function testDeleteSuccess()
{
$this->seed([UserSeeder::class,ContactSeeder::class]);
$contact = Contact::limit(1)->first();
$this->delete('/api/contacts/' . $contact->id,[],[
'Authorization' => 'test'
])->assertStatus(200)->assertJson([
'data' => true
]);
}
public function testDeleteNotFound()
{
$this->seed([UserSeeder::class,ContactSeeder::class]);
$contact = Contact::limit(1)->first();
$this->delete('/api/contacts/' . $contact->id + 1,[],[
'Authorization' => 'test'
])->assertStatus(404)->assertJson([
'errors' => [
'message' => ['not found']
]
]);
}
public function testSearchByFirstName()
{
$this->seed([UserSeeder::class,SearchSeeder::class]);
$response = $this->get('/api/contacts?name=first', [
'Authorization' => 'test'
])->assertStatus(200)->json();
// Log::channel('stderr')->info(json_encode($response,JSON_PRETTY_PRINT));
self::assertEquals(10,count($response['data']));
self::assertEquals(20,$response['meta']['total']);
}
public function testSearchByLastName()
{
$this->seed([UserSeeder::class,SearchSeeder::class]);
$response = $this->get('/api/contacts?name=last', [
'Authorization' => 'test'
])->assertStatus(200)->json();
// Log::channel('stderr')->info(json_encode($response,JSON_PRETTY_PRINT));
self::assertEquals(10,count($response['data']));
self::assertEquals(20,$response['meta']['total']);
}
public function testSearchByEmail()
{
$this->seed([UserSeeder::class,SearchSeeder::class]);
$response = $this->get('/api/contacts?email=test', [
'Authorization' => 'test'
])->assertStatus(200)->json();
// Log::channel('stderr')->info(json_encode($response,JSON_PRETTY_PRINT));
self::assertEquals(10,count($response['data']));
self::assertEquals(20,$response['meta']['total']);
}
public function testSearchByPhone()
{
$this->seed([UserSeeder::class,SearchSeeder::class]);
$response = $this->get('/api/contacts?phone=11111', [
'Authorization' => 'test'
])->assertStatus(200)->json();
// Log::channel('stderr')->info(json_encode($response,JSON_PRETTY_PRINT));
self::assertEquals(10,count($response['data']));
self::assertEquals(20,$response['meta']['total']);
}
public function testSearchNotFound()
{
$this->seed([UserSeeder::class,SearchSeeder::class]);
$response = $this->get('/api/contacts?name=wrong', [
'Authorization' => 'test'
])->assertStatus(200)->json();
// Log::channel('stderr')->info(json_encode($response,JSON_PRETTY_PRINT));
self::assertEquals(0,count($response['data']));
self::assertEquals(0,$response['meta']['total']);
}
public function testSearchWithPage()
{
$this->seed([UserSeeder::class,SearchSeeder::class]);
$response = $this->get('/api/contacts?size=5&page=2', [
'Authorization' => 'test'
])->assertStatus(200)->json();
// Log::channel('stderr')->info(json_encode($response,JSON_PRETTY_PRINT));
self::assertEquals(5,count($response['data']));
self::assertEquals(20,$response['meta']['total']);
self::assertEquals(2,$response['meta']['current_page']);
}
} |
#header
<<
#include <string>
#include <iostream>
using namespace std;
// struct to store information about tokens
typedef struct {
string kind;
string text;
} Attrib;
// function to fill token information (predeclaration)
void zzcr_attr(Attrib *attr, int type, char *text);
// fields for AST nodes
#define AST_FIELDS string kind; string text;
#include "ast.h"
// macro to create a new AST node (and function predeclaration)
#define zzcr_ast(as,attr,ttype,textt) as=createASTnode(attr,ttype,textt)
AST* createASTnode(Attrib* attr, int ttype, char *textt);
>>
<<
#include <cstdlib>
#include <cmath>
#include <map>
map<string,int> keysMap;
// function to fill token information
void zzcr_attr(Attrib *attr, int type, char *text) {
if (type == NUM) {
attr->kind = "intconst";
attr->text = text;
}
else if (type == TBOOL or type == FBOOL) {
attr->kind = "boolean";
attr->text = text;
}
else if (type == PLUS or type == TIMES) {
attr->kind = "intoperator";
attr->text = text;
}
else if (type == COMP) {
attr->kind = "booleanoperator";
attr->kind = text;
}
else if (type == ID) {
attr->kind = "id";
attr->text = text;
}
else {
attr->kind = text;
attr->text = "";
}
}
// function to create a new AST node
AST* createASTnode(Attrib* attr, int type, char* text) {
AST* as = new AST;
as->kind = attr->kind;
as->text = attr->text;
as->right = NULL;
as->down = NULL;
return as;
}
/// get nth child of a tree. Count starts at 0.
/// if no such child, returns NULL
AST* child(AST *a,int n) {
AST *c=a->down;
for (int i=0; c!=NULL && i<n; i++) c=c->right;
return c;
}
/// print AST, recursively, with indentation
void ASTPrintIndent(AST *a,string s)
{
if (a==NULL) return;
//TODO: modificar per a que no pinti el tipus (o que ho faci nomes en alguns casos).
cout<<a->kind;
if (a->text!="") cout<<"("<<a->text<<")";
cout<<endl;
AST *i = a->down;
while (i!=NULL && i->right!=NULL) {
cout<<s+" \\__";
ASTPrintIndent(i,s+" |"+string(i->kind.size()+i->text.size(),' '));
i=i->right;
}
if (i!=NULL) {
cout<<s+" \\__";
ASTPrintIndent(i,s+" "+string(i->kind.size()+i->text.size(),' '));
i=i->right;
}
}
/// print AST
void ASTPrint(AST *a)
{
while (a!=NULL) {
cout<<" ";
ASTPrintIndent(a,"");
a=a->right;
}
}
//PLUS "[\+\-]"
//TIMES "[\*\/]"
int operate(AST *a) {
if (a->text == "+") return operate(child(a,0)) + operate(child(a,1));
else if (a->text == "-") return operate(child(a,0)) - operate(child(a,1));
else if (a->text == "*") return operate(child(a,0)) * operate(child(a,1));
else if (a->text == "/") return operate(child(a,0)) / operate(child(a,1));
return atoi(a->text.c_str());
}
//COMP "[<>] | [!<>=][=]"
bool compare(AST *a) {
if (a->text == "<") return evaluate(child(a,0)) < evaluate(child(a,1));
else if (a->text == ">") return evaluate(child(a,0)) > evaluate(child(a,1));
else if (a->text == "==") return evaluate(child(a,0)) == evaluate(child(a,1));
else if (a->text == "!=") return evaluate(child(a,0)) != evaluate(child(a,1));
else if (a->text == "<=") return evaluate(child(a,0)) <= evaluate(child(a,1));
else if (a->text == ">=") return evaluate(child(a,0)) >= evaluate(child(a,1));
else if (a->kind == "boolean" and a->text == "TRUE") return true;
else return false;
}
int evaluate(AST *a){
if (a == NULL) return 0;
else if (a->kind == "intconst") return atoi(a->text.c_str());
else if (a->kind == "intoperator") return operate(a);
else if (a->kind == "booleanoperator") return compare(a);
else return 0;
}
int main() {
AST *root = NULL;
ANTLR(expr(&root), stdin);
ASTPrint(root);
cout << evaluate(root) << endl;
}
>>
#lexclass START
//Keywords
#token WRITE "write"
#token IF "if"
#token THEN "then"
#token ELSE "else"
#token ENDIF "endif"
#token WHILE "while"
#token ENDWHILE "endwhile"
#token DO "do"
//Operators
#token PLUS "[\+\-]"
#token TIMES "[\*\/]"
#token LP "\("
#token RP "\)"
#token ASIG ":="
#token COMP "[<>] | [!<>=][=]"
#token TBOOL "TRUE"
#token FBOOL "FALSE"
#token NUM "[0-9]+"
#token ID "[a-zA-Z] [a-zA-Z0-9]*"
//WhiteSpace
#token SPACE "[\ \n\t]" << zzskip();>>
//expr: NUM (PLUS^ NUM)* ; //non recursive version - only PLUS
//expr: NUM (PLUS^ expr | ); //recursive version - only PLUS
program: (instruction)* "@";
instruction: expr ;//(WRITE^ expr) | (ID ASIG^ expr);
expr: term (PLUS^ term)*;
term: subexpr (TIMES^ subexpr)*;
subexpr: NUM | LP! expr RP! | ID; |
from datetime import datetime, timedelta
from rest_framework import status
# models
from clientboards.api.models import Sessions, Users
# serializers
from clientboards.api.serializers.accounts.login_serializer import LoginSerializer
from clientboards.api.serializers.sessions.sessions_serializer import SessionsSerializer
# errors
from clientboards.api.services.ServicesError import ServicesError
class LoginServices():
@staticmethod
def login(email: str, password: str, request=None):
"""
Creates a session model and return it.
"""
loginSerializer = LoginSerializer(
data={'email': email, 'password': password})
if not loginSerializer.is_valid() or not loginSerializer.save():
print('logger: login is not valid')
raise ServicesError(
message='Login is not valid', details=loginSerializer.errors, status_code=status.HTTP_400_BAD_REQUEST)
# create the session
try:
user = Users.objects.get(email=email)
except Exception:
print('logger: user does not exist')
raise ServicesError(
message="User does not exist", status_code=status.HTTP_400_BAD_REQUEST)
# check if the user has a session or not, given their email. If so, then return that session.
sessionsQuerySet = Sessions.objects.filter(
user_id__exact=user.id)
# save the last login
user.last_login = datetime.now()
user.save()
existingSession = sessionsQuerySet.first()
if existingSession:
print('logger: user already has a session')
return (user, existingSession)
# TODO: differentiate it by ip address in the future.
currentTime = datetime.now()
sessionExpiry = currentTime + timedelta(days=60)
sessionData = {'user_id': user.id,
'start_date': currentTime, 'end_date': sessionExpiry}
sessionSerializer = SessionsSerializer(data=sessionData)
if not sessionSerializer.is_valid():
print('logger: session is not valid')
raise ServicesError(
message="Session is not valid", details=sessionSerializer.errors, status_code=status.HTTP_400_BAD_REQUEST)
savedSession = sessionSerializer.save()
if not isinstance(savedSession, Sessions):
raise ServicesError(
message="Not a Session", status_code=status.HTTP_500_INTERNAL_SERVER_ERROR)
return (user, savedSession)
@staticmethod
def logout():
pass |
<div [@routerTransition]>
<page-header [autoBreadcrumb]="false" title="{{l('TenantsHeaderInfo')}}" [action]="action">
<ng-template #action>
<button nz-button ng-if="isGranted('Pages.Tenants.Create')" [nzType]="'primary'" (click)="createTenant()">
<i class="anticon anticon-plus"></i>{{l("CreateNewTenant")}}
</button>
</ng-template>
</page-header>
<form nz-form (submit)="refresh()" class="search-form" autocomplete="off">
<div nz-row [nzGutter]="24">
<nz-col nzLg="12" nzMd="12" nzSm="24">
<nz-form-item>
<nz-form-label>{{l('TenantNameOrTenancyCode')}}</nz-form-label>
<nz-form-control>
<nz-input-group nzSearch [nzSuffix]="suffix" style="width: 100%">
<input type="text" nz-input name="filterText" [(ngModel)]="filters.filterText"
placeholder="{{l('SearchWithThreeDot')}}">
<ng-template #suffix>
<button nz-button nzType="primary" nzSearch (click)="refresh()" type="submit">
{{l('Search')}}
</button>
</ng-template>
</nz-input-group>
</nz-form-control>
</nz-form-item>
</nz-col>
<nz-col nzLg="12" [nzMd]="12" [nzSm]="24">
<nz-form-item>
<nz-form-label>{{l('Edition')}}</nz-form-label>
<nz-form-control>
<edition-combo [(selectedEdition)]="filters.selectedEditionId"></edition-combo>
</nz-form-control>
</nz-form-item>
</nz-col>
</div>
<div nz-row class="text-right mb-md">
<button nz-button [nzType]="'primary'" type="button" (click)="refresh()">
<i class="anticon anticon-reload"> </i> {{l('Refresh')}}
</button>
</div>
</form>
<nz-table #nzTable [nzFrontPagination]="false" [nzData]="dataItems" [nzTotal]="totalItems"
[(nzPageIndex)]="pageNumber"
[(nzPageSize)]="pageSize" [nzLoading]="isTableLoading" [nzBordered]="true"
[nzShowSizeChanger]="true" (nzPageIndexChange)="refresh()" (nzPageSizeChange)="refresh()">
<thead (nzSortChange)="sort($event)" nzSingleSort>
<tr>
<th nzShowSort nzSortKey="tenancyName">{{l('TenancyCodeName')}}</th>
<th nzShowSort nzSortKey="name">{{l('Name')}}</th>
<th nzShowSort nzSortKey="editionDisplayName">{{l('Edition')}}</th>
<th nzShowSort nzSortKey="subscriptionEndDateUtc">{{l('SubscriptionEndDateUtc')}}</th>
<th nzShowSort nzSortKey="isActive">{{l('Active')}}</th>
<th nzShowSort nzSortKey="creationTime">{{l('CreationTime')}}</th>
<th nzWidth="130px" nzRight="0px" style="text-align: center">
<span>{{l('Actions')}}</span>
</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let item of nzTable.data">
<td>
<i *ngIf="item.connectionString" class="anticon anticon-database"
title="{{l('HasOwnDatabase')}}"></i>
{{item.tenancyName}}
</td>
<td>{{item.name}}</td>
<td>{{item.editionDisplayName}}</td>
<td>
{{ item.subscriptionEndDateUtc ? (item.subscriptionEndDateUtc | momentFormat:'L') : '-'}}
</td>
<td>
<span *ngIf="item.isActive" class="label label-success">{{l('Yes')}}</span>
<span *ngIf="!item.isActive" class="label label-default">{{l('No')}}</span>
</td>
<td>
{{item.creationTime | momentFormat:'L'}}
</td>
<td nzRight="0px">
<nz-dropdown [nzTrigger]="'click'">
<a nz-dropdown>
<button nz-button [nzType]="'primary'">
<i class="anticon anticon-setting"></i>
{{l("Actions")}}
<i class="anticon anticon-down"></i>
</button>
</a>
<ul nz-menu>
<li [class.disabled]="!item.isActive" nz-menu-item>
<a *ngIf="permission.isGranted('Pages.Tenants.Impersonation')"
(click)="item.isActive && showUserImpersonateLookUpModal(item)">{{l('LoginAsThisTenant')}}</a>
</li>
<li nz-menu-item>
<a *ngIf="permission.isGranted('Pages.Tenants.Edit')"
(click)="editTenant(item.id, item.name)">{{l('Edit')}}</a>
</li>
<li nz-menu-item>
<a *ngIf="permission.isGranted('Pages.Tenants.ChangeFeatures')"
(click)="showTenantFeature(item.id, item.name)">{{l('Features')}}</a>
</li>
<li nz-menu-item>
<a *ngIf="permission.isGranted('Pages.Tenants.Delete')"
(click)="delete (item)">{{l('Delete')}}</a>
</li>
<li nz-menu-item>
<a (click)="unlockUser(item)">{{l('Unlock')}}</a>
</li>
</ul>
</nz-dropdown>
</td>
</tr>
</tbody>
</nz-table>
</div> |
import { useEffect, useState } from "react"
import { useDispatch } from "react-redux"
import { useNavigate, useParams } from "react-router-dom"
import axios from "axios"
import Swal from "sweetalert2"
import Cookies from "universal-cookie"
import { updateBlog } from "./../redux/api/BlogAPI"
import { Form } from "../redux/api/submitFormApi"
import { fetchPostURL } from "../constants/apis"
export default function EditPostForm() {
const editBlogApi = async () => {
try {
const cookies = new Cookies()
const token = cookies.get("user_jwt")
const BEARER = "bearer"
const posts = await axios.get(`${fetchPostURL}/${id}`, {
headers: { Authorization: `${BEARER} ${token}` }
})
if (posts["status"] == 200) {
setLoading(false)
setPostForm({
title: posts["data"]["data"]["attributes"]["title"],
post: posts["data"]["data"]["attributes"]["post"]
})
}
} catch (error) {
setLoading(false)
console.log(error)
}
}
const dispatch = useDispatch()
const navigate = useNavigate()
const { id } = useParams()
useEffect(() => {
editBlogApi()
}, [])
const [postForm, setPostForm] = useState({ title: "", post: "" })
const [loading, setLoading] = useState(true)
const formChangeFunc = (e) => {
const name = e.target.name
const value = e.target.value
setPostForm({ ...postForm, [name]: value })
}
const submitFunc = (e) => {
e.preventDefault()
if (postForm.title == "" || postForm.post == "") {
Swal.fire({
icon: "error",
title: "Error",
text: "Field(s) cannot be empty"
})
return
}
updateBlog(dispatch, navigate, postForm, id)
}
if (loading) {
return <p> Loading </p>
}
return (
<div className="container w-4/6 mx-auto mt-8">
<form onSubmit={submitFunc}>
<div className="max-w-2xl mx-auto lg:mx-0">
<h2 className="text-3xl font-bold tracking-tight text-gray-900 sm:text-4xl">
Edit Post
</h2>
</div>
<div className="space-y-12">
<div className="pb-12 border-b border-gray-900/10">
<div className="grid grid-cols-1 mt-10 gap-x-6 gap-y-8 sm:grid-cols-6">
<div className="sm:col-span-4">
<label
htmlFor="username"
className="block text-sm font-medium leading-6 text-gray-900"
>
Title
</label>
{postForm && (
<div className="mt-2">
<input
type="text"
name="title"
value={postForm["title"]}
onChange={formChangeFunc}
autoComplete="given-name"
className="block p-2 w-full rounded-md border-0 py-1.5 text-gray-900 shadow-sm ring-1 ring-inset ring-gray-300 placeholder:text-gray-400 focus:ring-2 focus:ring-inset focus:ring-indigo-600 sm:text-sm sm:leading-6"
/>
</div>
)}
</div>
<div className="col-span-full">
<label
htmlFor="about"
className="block text-sm font-medium leading-6 text-gray-900"
>
Post
</label>
<div className="mt-2">
<textarea
name="post"
value={postForm["post"]}
onChange={formChangeFunc}
rows={3}
className="p-3 block w-full rounded-md border-0 py-1.5 text-gray-900 shadow-sm ring-1 ring-inset ring-gray-300 placeholder:text-gray-400 focus:ring-2 focus:ring-inset focus:ring-indigo-600 sm:text-sm sm:leading-6"
/>
</div>
</div>
</div>
<button className="px-4 py-2 mt-5 font-bold text-white bg-blue-500 border border-blue-700 rounded hover:bg-blue-700">
Update
</button>
</div>
</div>
</form>
</div>
)
} |
// ROS functions for publishing messages
void publishIMU(unsigned long time) { // require getGyroReadings() and getAccReadings()
current_time = nh.now();
dt = double(time) / 1000; //Time in s
// integrate angular velocity by timestep to get yaw
dth_gyro = gyroZ * dt;
theta_gyro += dth_gyro;
// account for rad overflow
if (theta_gyro >= two_pi) theta_gyro -= two_pi;
if (theta_gyro <= -two_pi) theta_gyro += two_pi;
imu_msg.header.stamp = current_time;
imu_msg.header.frame_id = imu_link;
imu_msg.linear_acceleration.x = accelX;
imu_msg.linear_acceleration.y = 0; // accelY = 0, nonholonomic robot
imu_msg.linear_acceleration.z = 0; // accelZ = 0, planar robot
imu_msg.linear_acceleration_covariance[0] = 0.04;
imu_msg.linear_acceleration_covariance[1] = 0;
imu_msg.linear_acceleration_covariance[2] = 0;
imu_msg.linear_acceleration_covariance[3] = 0;
imu_msg.linear_acceleration_covariance[4] = 0.04;
imu_msg.linear_acceleration_covariance[5] = 0;
imu_msg.linear_acceleration_covariance[6] = 0;
imu_msg.linear_acceleration_covariance[7] = 0;
imu_msg.linear_acceleration_covariance[8] = 0.04;
imu_msg.angular_velocity.x = 0; // gyroX = 0, planar robot
imu_msg.angular_velocity.y = 0; // gyroY = 0, planar robot
imu_msg.angular_velocity.z = gyroZ;
imu_msg.angular_velocity_covariance[0] = 0.02;
imu_msg.angular_velocity_covariance[1] = 0;
imu_msg.angular_velocity_covariance[2] = 0;
imu_msg.angular_velocity_covariance[3] = 0;
imu_msg.angular_velocity_covariance[4] = 0.02;
imu_msg.angular_velocity_covariance[5] = 0;
imu_msg.angular_velocity_covariance[6] = 0;
imu_msg.angular_velocity_covariance[7] = 0;
imu_msg.angular_velocity_covariance[8] = 0.02;
geometry_msgs::Quaternion gyro_quat = tf::createQuaternionFromYaw(theta_gyro);
imu_msg.orientation = gyro_quat;
imu_msg.orientation_covariance[0] = 0.0025;
imu_msg.orientation_covariance[1] = 0;
imu_msg.orientation_covariance[2] = 0;
imu_msg.orientation_covariance[3] = 0;
imu_msg.orientation_covariance[4] = 0.0025;
imu_msg.orientation_covariance[5] = 0;
imu_msg.orientation_covariance[6] = 0;
imu_msg.orientation_covariance[7] = 0;
imu_msg.orientation_covariance[8] = 0.0025;
imu.publish(&imu_msg);
}
void publishLIDAR(unsigned long time) {
current_time = nh.now();
lidar_msg.header.stamp = current_time;
lidar_msg.header.frame_id = laser;
lidar_msg.angle_min = 0.0;
lidar_msg.angle_max = two_pi;
lidar_msg.angle_increment = (two_pi / 360);
lidar_msg.time_increment = (double(time) / 1000) / 360; // scan time / points
lidar_msg.scan_time = double(time) / 1000; // time since last call, ms to s
lidar_msg.range_min = 0.06;
lidar_msg.range_max = 5.00;
lidar_msg.ranges_length = 360;
lidar_msg.ranges = range;
lidar_msg.intensities = intensity;
lidar.publish(&lidar_msg);
lastAngle = currentAngle; // register index of latest range data for validation purposes
memset(range, 0, sizeof(range)); //clear array in the event errors preserve old data
memset(intensity, 0, sizeof(intensity));
}
void publishODOM(unsigned long time) { // require getMotorDataPub()
current_time = nh.now();
// register time step duration
dt = double(time) / 1000; // Change in time, time step, in s
// calculate velocities
vx = (dt == 0) ? 0 : (speed_act1Pub + speed_act2Pub) / 2; // linear velocity, in m
vth = (dt == 0) ? 0 : (speed_act2Pub - speed_act1Pub) / base_diameter; // angular velocity, in m
// scale velocities by set factor
if (vx > 0) vx *= linear_scale_positive;
if (vx < 0) vx *= linear_scale_negative;
if (vth > 0) vth *= angular_scale_positive;
if (vth < 0) vth *= angular_scale_negative;
// differentiate velocities into displacement
dxy = vx * dt; // Change in position, displacement, in m
dth_odom = vth * dt; // Change in yaw, angular displacement, in m
// extract change in x and change in y
dx = cos(dth_odom) * dxy; // Change in x position
dy = sin(dth_odom) * dxy; // Change in y position
// add change in x and change in y to position variable
x_pos += dx;
y_pos += dy;
// add change in yaw to yaw variable
theta_odom += dth_odom;
// account for rad overflow
if (theta_odom >= two_pi) theta_odom -= two_pi;
if (theta_odom <= -two_pi) theta_odom += two_pi;
geometry_msgs::Quaternion odom_quat = tf::createQuaternionFromYaw(theta_odom);
geometry_msgs::Quaternion empty_quat = tf::createQuaternionFromYaw(0);
odom_msg.header.stamp = current_time;
odom_msg.header.frame_id = odom;
odom_msg.pose.pose.position.x = x_pos;
odom_msg.pose.pose.position.y = y_pos;
odom_msg.pose.pose.position.z = 0.0;
odom_msg.pose.pose.orientation = odom_quat;
odom_msg.twist.twist.linear.x = vx;
odom_msg.twist.twist.linear.y = 0.0;
odom_msg.twist.twist.angular.z = vth;
// writing covariances
if (speed_act1Pub == 0 && speed_act2Pub == 0) {
odom_msg.pose.covariance[0] = 1e-9;
odom_msg.pose.covariance[7] = 1e-3;
odom_msg.pose.covariance[8] = 1e-9;
odom_msg.pose.covariance[14] = 1e6;
odom_msg.pose.covariance[21] = 1e6;
odom_msg.pose.covariance[28] = 1e6;
odom_msg.pose.covariance[35] = 1e-9;
odom_msg.twist.covariance[0] = 1e-9;
odom_msg.twist.covariance[7] = 1e-3;
odom_msg.twist.covariance[8] = 1e-9;
odom_msg.twist.covariance[14] = 1e6;
odom_msg.twist.covariance[21] = 1e6;
odom_msg.twist.covariance[28] = 1e6;
odom_msg.twist.covariance[35] = 1e-9;
} else {
odom_msg.pose.covariance[0] = 1e-3;
odom_msg.pose.covariance[7] = 1e-3;
odom_msg.pose.covariance[8] = 0.0;
odom_msg.pose.covariance[14] = 1e6;
odom_msg.pose.covariance[21] = 1e6;
odom_msg.pose.covariance[28] = 1e6;
odom_msg.pose.covariance[35] = 1e3;
odom_msg.twist.covariance[0] = 1e-3;
odom_msg.twist.covariance[7] = 1e-3;
odom_msg.twist.covariance[8] = 0.0;
odom_msg.twist.covariance[14] = 1e6;
odom_msg.twist.covariance[21] = 1e6;
odom_msg.twist.covariance[28] = 1e6;
odom_msg.twist.covariance[35] = 1e3;
}
odom_pub.publish(&odom_msg);
} |
"use client";
import { ChevronsLeftRight } from "lucide-react";
import { useUser, SignOutButton } from "@clerk/clerk-react";
import {
Avatar,
AvatarImage
} from "@/components/ui/avatar";
import {
DropdownMenu,
DropdownMenuContent,
DropdownMenuItem,
DropdownMenuLabel,
DropdownMenuSeparator,
DropdownMenuTrigger,
} from "@/components/ui/dropdown-menu";
export const UserItem = () => {
const { user } = useUser();
return (
<DropdownMenu >
<DropdownMenuTrigger asChild>
<div role="button" className="flex items-center justify-center text-sm p-3 w-full hover:bg-primary/5 pb-3">
<div className=" flex items-center justify-center ">
<Avatar className="h-12 w-12 rounded-xl object-contain">
<AvatarImage src={user?.imageUrl} />
</Avatar>
{/** <span className="text-start font-medium line-clamp-1">
{user?.fullName}'s
</span> */}
</div>
{/** <ChevronsLeftRight className="rotate-90 ml-2 text-muted-foreground h-4 w-4" /> */}
</div>
</DropdownMenuTrigger>
<DropdownMenuContent
className="w-80"
align="start"
alignOffset={11}
forceMount
>
<div className="flex flex-col space-y-4 p-2">
<p className="text-xs font-medium leading-none text-muted-foreground">
{user?.emailAddresses[0].emailAddress}
</p>
<div className="flex items-center gap-x-2">
<div className="rounded-md bg-secondary p-1">
<Avatar className="h-10 w-10 rounded-xl">
<AvatarImage src={user?.imageUrl} />
</Avatar>
</div>
<div className="space-y-1">
<p className="text-sm line-clamp-1">
{user?.fullName}
</p>
</div>
</div>
</div>
<DropdownMenuSeparator />
<DropdownMenuItem asChild className="w-full cursor-pointer text-muted-foreground">
<SignOutButton>
Log out
</SignOutButton>
</DropdownMenuItem>
</DropdownMenuContent>
</DropdownMenu>
)
} |
const arrayNumbers= [10, 3, 4, 50, 6];
arrayNumbers.forEach(function(currentValue, index, array){
console.log(currentValue, index, array);
});
arrayNumbers.forEach(function(currentValue){
console.log(currentValue);
});
arrayNumbers.forEach((currentValue)=>{
console.log(currentValue);
});
arrayNumbers.forEach(currentValue=>console.log(currentValue));
console.log("======== Find even numbers==========");
const array = [2, 3, 4, 5, 6, 7, 0, 34, 57];
console.log(array);
array.forEach( (currentValue) => {
if(currentValue%2==0) {
console.log(currentValue);
}
})
const arrayn = [2, 3, 4, 5, 6, 7, 0, 34, 57];
const arrayOfEven = [];
console.log(arrayn);
array.forEach( (currentValue) => {
if(currentValue%2==0) {
arrayOfEven.push(currentValue);
console.log(currentValue);
}
});
console.log("Array of even numbers");
console.log(arrayOfEven);
console.log("Callback with arg functions");
let add = function (n1, n2){
console.log(n1+n2);
}
let multiply = function (n1, n2){
console.log(n1*n2);
}
function operation(callbackAdd, callbackMultiply) {
console.log('operation');
callbackAdd(5, 5);
callbackMultiply(2, 2);
}
operation(add, multiply);
class Vehicle {
constructor(company,model,fuel,drive,price){
this.company=company;
this.model=model;
this.fuel=fuel;
this.drive=drive;
this.price=price;
}
}
let audiA3 = new Vehicle("Audi","A3","Petrol","Four Wheel Drive",8000000);
let audiQ3= new Vehicle("Audi","Q3","Diseal","Two Wheel Drive",4900000);
let mahindra = new Vehicle("Mahindra","Thar","Petrol","Four Wheel Drive",2000000);
let honda =new Vehicle("Honda","Unicorn","Petrol","Two Wheeler",125000);
let hero =new Vehicle("Hero","Splender","Petrol","Two Wheeler",85000);
const arrayOfVehicles = [ audiA3, audiQ3, mahindra, honda, hero];
arrayOfVehicles.forEach( (vehicle) => {
if (vehicle.price<150000) {
console.log(vehicle.model,vehicle.company, vehicle.drive, vehicle.fuel, vehicle.price);
}
} );
console.log("====== Set of Vehicles =======");
const setOfVehicles = new Set();
setOfVehicles.add(audiA3);
setOfVehicles.add(audiQ3);
setOfVehicles.add(mahindra);
setOfVehicles.add(honda);
setOfVehicles.add(hero);
setOfVehicles.forEach( (itemVehicle)=> {
console.log(itemVehicle.model);
} );
console.log("====== Map of Vehicles =======");
let mapOfVehicles = new Map();
mapOfVehicles.set("REG_11", audiA3);
mapOfVehicles.set("REG_22", audiQ3);
mapOfVehicles.set("REG_33", mahindra);
mapOfVehicles.set("REG_44", hero);
mapOfVehicles.set("REG_55", honda);
mapOfVehicles.forEach((vehicleObject, regNo) => {
console.log(vehicleObject.model, regNo);
} ); |
// Game constants
let inputDir = { x: 0, y: 0 }
const foodSound = new Audio('food.mp3');
const gameOverSound = new Audio('gameover.wav');
const moveSound = new Audio('snaketurns.wav');
const musicSound = new Audio('bgmusic.mp3');
let speed = 4;
let score = 0;
let lastpainttime = 0;
let snakeArr = [{ x: 13, y: 15 }]
food = { x: 9, y: 10 }
let dummyfood = { x: 0, y: 0 }
var up = true
var down = true
var left = true
var right = true
// Game Functions
function main(ctime) {
// musicSound.play()
window.requestAnimationFrame(main); //it will call main function again and again , a game loop is formed
// console.log(ctime)
if ((ctime - lastpainttime) / 1000 < 1 / speed) {
return;
}
lastpainttime = ctime
gameEngine();
}
function gameEngine() {
// part 1 : updating the snake array & Food
function isCollide(snake) {
// return false;
// if you bump into youself
for (let i = 1; i < snakeArr.length; i++) {
if (snake[0].x === snake[i].x && snake[0].y === snake[i].y) {
return true;
}
}
// if you bump into wall
if (snake[0].x >= 18 || snake[0].x <= 0 || snake[0].y <= 0 || snake[0].y >= 18) {
return true;
}
}
if (isCollide(snakeArr)) {
gameOverSound.play()
musicSound.pause()
inputDir = { x: 0, y: 0 }
alert('Game Over, press any key to play again!')
snakeArr = [{ x: 13, y: 15 }]
musicSound.play()
score = 0;
scoreVal.innerHTML = "Score : " + score
}
// if you have eaten the food, regenrate the food and also incrase the score
if (snakeArr[0].x === food.x && snakeArr[0].y === food.y) {
foodSound.play()
score += 1
if ((localStorage.getItem("hiscore")) < score) {
hiscoreval = score
localStorage.setItem("hiscore", JSON.stringify(hiscoreval))
highScore.innerHTML = "HiScore : " + hiscoreval
}
scoreVal.innerHTML = "Score : " + score
snakeArr.unshift({ x: snakeArr[0].x + inputDir.x, y: snakeArr[0].y + inputDir.y })
let a = 2;
let b = 16;
food = { x: Math.round(a + (b - a) * Math.random()), y: Math.round(a + (b - a) * Math.random()) }
console.log('1', food)
for (let i = snakeArr.length - 2; i >= 0; i--) {
while ((snakeArr[i].x == food.x) && (snakeArr[i].y == food.y)) {
food = { x: Math.round(a + (b - a) * Math.random()), y: Math.round(a + (b - a) * Math.random()) }
}
}
}
for (let i = snakeArr.length - 2; i >= 0; i--) {
// const element = array[i]
snakeArr[i + 1] = { ...snakeArr[i] }
}
snakeArr[0].x += inputDir.x
snakeArr[0].y += inputDir.y
// part 2 : Display the snake and food
// Display the snake
board.innerHTML = ""
snakeArr.forEach((e, index) => {
snakeElement = document.createElement('div')
snakeElement.style.gridRowStart = e.y;
snakeElement.style.gridColumnStart = e.x;
if (index == 0) {
snakeElement.classList.add('head')
}
else {
snakeElement.classList.add('snake')
}
board.appendChild(snakeElement)
})
//Display the food
foodElement = document.createElement('div')
foodElement.style.gridRowStart = food.y;
foodElement.style.gridColumnStart = food.x;
foodElement.classList.add('food')
board.appendChild(foodElement)
}
// Main logic starts here
musicSound.play()
let hiscore = localStorage.getItem("hiscore")
if (hiscore === null) {
hiscoreval = 0
localStorage.setItem("hiscore", JSON.stringify(hiscoreval))
}
else {
highScore.innerHTML = "HiScore : " + hiscore
}
window.requestAnimationFrame(main) //it will call main function
window.addEventListener('keydown', e => {
inputDir = { x: 0, y: 1 }; // start the game
moveSound.play();
console.log('event triggered')
switch (e.key) {
case "ArrowUp":
if (up) {
inputDir.x = 0;
inputDir.y = -1;
break;
}
case "ArrowDown":
if (down) {
inputDir.x = 0;
inputDir.y = 1;
break;
}
case "ArrowRight":
if (right) {
inputDir.x = 1;
inputDir.y = 0;
break;
}
case "ArrowLeft":
if (left) {
inputDir.x = -1;
inputDir.y = 0;
break;
}
default:
break;
}
}); |
/*
Insecure Web App (IWA)
Copyright (C) 2020 Micro Focus or one of its affiliates
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
package com.microfocus.example.payload.request;
import org.springframework.context.annotation.Bean;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import javax.persistence.Column;
import javax.validation.constraints.Email;
import javax.validation.constraints.NotEmpty;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Size;
/**
* Subscribe User Request DTO
*
* @author Kevin A. Lee
*/
public class SubscribeUserRequest {
private Integer id;
private String firstName;
private String lastName;
private String email;
public SubscribeUserRequest() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "SubscribeUserRequest{" +
"id='" + id + '\'' +
", firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
", email=" + email +
'}';
}
} |
<div fxLayout="column" fxLayoutAlign="center center" fxFlexFill class="item-container">
<h1> Showing all your items </h1>
<div fxLayout="row" fxLayoutGap="80px" fxLayoutAlign="center center" class="action-buttons">
<button mat-button (click)="goBack()"><mat-icon>arrow_back</mat-icon>Go Back</button>
<button mat-raised-button color="primary" (click)="exportCollection()">Export collection</button>
</div>
<div class="table-container">
<ng-container *ngIf="(dataSource?.data ?? []).length > 0; else noDataTemplate">
<mat-paginator [pageSizeOptions]="[5, 10, 25, 100]" showFirstLastButtons></mat-paginator>
<mat-table mat-table [dataSource]="dataSource" matSort class="mat-elevation-z8">
<ng-container matColumnDef="name">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Name </th>
<td mat-cell *matCellDef="let element"> {{element.name}} </td>
</ng-container>
<ng-container matColumnDef="price">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Price </th>
<td mat-cell *matCellDef="let element"> {{ element.price ? element.price : 'No value set' }} </td>
</ng-container>
<ng-container matColumnDef="type">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Type </th>
<td mat-cell *matCellDef="let element"> {{element.type}} </td>
</ng-container>
<ng-container matColumnDef="dimensions">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Dimensions (WxH) </th>
<td mat-cell *matCellDef="let element">
{{ (element.width && element.height) ? (element.width + ' x ' + element.height) : 'No dimensions set' }}
</td>
</ng-container>
<ng-container matColumnDef="color">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Color </th>
<td mat-cell *matCellDef="let element"> {{element.color ? element.color: 'No color set'}} </td>
</ng-container>
<ng-container matColumnDef="amount">
<th mat-header-cell *matHeaderCellDef mat-sort-header> Amount </th>
<td mat-cell *matCellDef="let element"> {{element.amount}} </td>
</ng-container>
<ng-container matColumnDef="actions">
<mat-header-cell *matHeaderCellDef> Actions </mat-header-cell>
<mat-cell *matCellDef="let element">
<button mat-icon-button color="warn" (click)="deleteItem(element)" aria-label="Delete">
<mat-icon>close</mat-icon>
</button>
</mat-cell>
</ng-container>
<tr mat-header-row *matHeaderRowDef="displayedColumns"></tr>
<tr mat-row *matRowDef="let row; columns: displayedColumns;"></tr>
</mat-table>
</ng-container>
<ng-template #noDataTemplate>
<div style="text-align: center;">There are no items to show yet</div>
</ng-template>
</div>
</div> |
from flux_query_builder.functions.base import FluxQueryFunction
class Measurements(FluxQueryFunction):
"""schema.measurements() returns a list of measurements in a specific bucket.Results include a single table with a single column, _value.(Required)
Bucket to retrieve measurements from.Oldest time to include in results. Default is -30d.Newest time include in results.
The stop time is exclusive, meaning values with a time equal to stop time are excluded from the results.
Default is now().
Params:
bucket
- (Required)
Bucket to retrieve measurements from.
start
- Oldest time to include in results. Default is -30d.
stop
- Newest time include in results.
The stop time is exclusive, meaning values with a time equal to stop time are excluded from the results.
Default is now().
"""
name = "measurements"
package="schema"
def __init__(self, bucket, start=None, stop=None, ):
super().__init__(bucket=bucket, start=start, stop=stop, ) |
@extends('layouts.kampus')
@section('nama-kampus', $kampus->nama_kampus)
@section('page-title', 'Tambah Prodi')
@section('content')
<form action="{{ route('detail-kampus.prodi.store', ['kampus' => $kampus->id]) }}" method="POST">
<div class="card">
<div class="card-header">Form Prodi</div>
<div class="card-body">
@csrf
<div class="mb-3">
<label for="kode_prodi" class="form-label">Kode Prodi</label>
<input type="text" name="kode_prodi" value="{{ old('kode_prodi') }}" class="form-control @error('kode_prodi') is-invalid @enderror" id="kode_prodi" aria-describedby="kode_prodi" />
@error('kode_prodi')
<div class="invalid-feedback">{{ $message }}</div>
@enderror
</div>
<div class="mb-3">
<label for="nama" class="form-label">Nama Prodi</label>
<input type="text" name="nama" value="{{ old('nama') }}" class="form-control @error('nama') is-invalid @enderror" id="nama" aria-describedby="nama" />
@error('nama')
<div class="invalid-feedback">{{ $message }}</div>
@enderror
</div>
<div class="mb-3">
<label for="jenjang" class="form-label">Jenjang</label>
<input type="text" name="jenjang" value="{{ old('jenjang') }}" class="form-control @error('jenjang') is-invalid @enderror" id="jenjang" aria-describedby="jenjang" />
@error('jenjang')
<div class="invalid-feedback">{{ $message }}</div>
@enderror
</div>
<div class="mb-3">
<label for="masa_studi" class="form-label">Jenjang</label>
<small>(Tahun)</small>
<input type="text" name="masa_studi" value="{{ old('masa_studi') }}" class="form-control @error('masa_studi') is-invalid @enderror" id="masa_studi" aria-describedby="masa_studi" />
@error('masa_studi')
<div class="invalid-feedback">{{ $message }}</div>
@enderror
</div>
</div>
<div class="card-footer text-muted">
<button type="submit" class="btn btn-primary">Simpan</button>
</div>
</div>
</form>
@endsection |
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<%--
# ๋ฐฐ์ด ๋ฐ์ดํฐ ์ฒ๋ฆฌ
1. ๋ฐฐ์ด์ ์ด์ฉํ๋ฉด for๋ฌธ๊ณผ if๋ฌธ์ ํตํด์ ๋ค์ํ ํ์์ ์นํ๋ฉด์ ์ฒ๋ฆฌํ ์ ์๋ค.
2. ์ค๋ฌด ํ๋ก๊ทธ๋
1) combo
2) radio
3) checkbox
ex) ๊ฒ์:
์ฌ์๋ช
[ ]
๋ถ์๋ช
[select]
์ ์ฒด
10 ์ธ์ฌ
20 ์ฌ๋ฌด
DB์์ dao๋ก ๋ถ๋ฌ์จ ๋ฐ์ดํฐ๋ฅผ ์ ํํด์ ๊ฒ์ํ ์ ์๊ฒ ์ฒ๋ฆฌํด์ผ ํ๋ค.
==> ๋ฑ๋ก/์์ /์์ ์์๋ ์ dao์์ ๋ถ๋ฌ์จ ๋ฐ์ดํฐ ๊ธฐ์ค์ผ๋ก ์ ํํด์ ์ฒ๋ฆฌํจ์ผ๋ก ๋ฐ์ดํฐ์ ๋ฌด๊ฒฐ์ฑ/์ผ๊ด์ฑ์ ๋ฌธ์ ๊ฐ ์์ด ์ฒ๋ฆฌ๋๋ค.
--%>
<ol>
<%
String []fruits = {"์ฌ๊ณผ","๋ฐ๋๋","๋ธ๊ธฐ"};
for(String fruit:fruits){
%>
<li><%=fruit%></li>
<%} %>
</ol>
<%--
ex) select์ option ๋ด์ฉ์ ๋ง๋ ๋ถ์ ์ ๋ณด๋ฅผ ๋ฐฐ์ด๋ก ์ ์ธํ๊ณ ์ถ๋ ฅํ์ธ์ --%>
<%
String [] dNames = {"์ธ์ฌ","์ด๋ฌด","๊ฒฝ์์ง์","์์
"};
int pcodes[] = {10,20,30,40};
%>
๋ถ์:
<select onchange="alert('์ ํ๊ฐ'+this.value)">
<option value='00'>๋ถ์ ์ ํํ์ธ์</option>
<%for(int idx=0;idx<dNames.length;idx++){
%>
<option value="<%=pcodes[idx]%>"><%=dNames[idx]%></option>
<%}%>
</select>
</body>
</html> |
import logging
from typing import Any, Dict, List
# The latest migration version of the database.
#
# Database migrations are applied starting from the number specified in the database's
# `migration_version` table + 1 (or from 0 if this table does not yet exist) up until
# the version specified here.
#
# When a migration is performed, the `migration_version` table should be incremented.
latest_migration_version = 2
logger = logging.getLogger(__name__)
class Storage:
def __init__(self, database_config: Dict[str, str]):
"""Setup the database.
Runs an initial setup or migrations depending on whether a database file has already
been created.
Args:
database_config: a dictionary containing the following keys:
* type: A string, one of "sqlite" or "postgres".
* connection_string: A string, featuring a connection string that
be fed to each respective db library's `connect` method.
"""
self.conn = self._get_database_connection(
database_config["type"], database_config["connection_string"]
)
self.cursor = self.conn.cursor()
self.db_type = database_config["type"]
# Try to check the current migration version
migration_level = 0
try:
self._execute("SELECT version FROM migration_version")
row = self.cursor.fetchone()
migration_level = row[0]
except Exception:
self._initial_setup()
finally:
if migration_level < latest_migration_version:
self._run_migrations(migration_level)
logger.info(f"Database initialization of type '{self.db_type}' complete")
def _get_database_connection(
self, database_type: str, connection_string: str
) -> Any:
"""Creates and returns a connection to the database"""
if database_type == "sqlite":
import sqlite3
# Initialize a connection to the database, with autocommit on
return sqlite3.connect(connection_string, isolation_level=None)
elif database_type == "postgres":
import psycopg2
conn = psycopg2.connect(connection_string)
# Autocommit on
conn.set_isolation_level(0)
return conn
def _initial_setup(self) -> None:
"""Initial setup of the database"""
logger.info("Performing initial database setup...")
# Set up the migration_version table
self._execute(
"""
CREATE TABLE migration_version (
version INTEGER PRIMARY KEY
)
"""
)
# Initially set the migration version to 0
self._execute(
"""
INSERT INTO migration_version (
version
) VALUES (?)
""",
(0,),
)
# Set up any other necessary database tables here
logger.info("Database setup complete")
def _run_migrations(self, current_migration_version: int) -> None:
"""Execute database migrations. Migrates the database to the
`latest_migration_version`.
Args:
current_migration_version: The migration version that the database is
currently at.
"""
logger.debug("Checking for necessary database migrations...")
if current_migration_version < 1:
logger.info("Migrating the database from v0 to v1...")
# Add new table, delete old ones, etc.
# Add table for storing uploaded media file uris, so we don't have to reupload them to the server each time
self._execute(
"""
CREATE TABLE static_media_uris (
filename TEXT UNIQUE NOT NULL,
uri TEXT NOT NULL
)
"""
)
# Update the stored migration version
self._execute("UPDATE migration_version SET version = 1")
logger.info("Database migrated to v1")
if current_migration_version < 2:
logger.info("Migrating the database from v1 to v2...")
# Add new table, delete old ones, etc.
# Add table for storing last to be destroyed events and their timestamps for rooms the bot is in.
self._execute(
"""
CREATE TABLE last_room_events (
room_id VARCHAR(80) PRIMARY KEY,
event_id VARCHAR(80),
timestamp TEXT,
delete_after TEXT,
deletion_turned_on VARCHAR(1),
batch_token_start VARCHAR(80),
batch_token_end VARCHAR(80)
)
"""
)
# Update the stored migration version
self._execute("UPDATE migration_version SET version = 2")
logger.info("Database migrated to v2")
def _execute(self, *args) -> None:
"""A wrapper around cursor.execute that transforms placeholder ?'s to %s for postgres.
This allows for the support of queries that are compatible with both postgres and sqlite.
Args:
args: Arguments passed to cursor.execute.
"""
if self.db_type == "postgres":
self.cursor.execute(args[0].replace("?", "%s"), *args[1:])
else:
self.cursor.execute(*args)
def delete_uri(self, filename: str):
"""Delete a uri entry via its filename"""
self._execute(
"""
DELETE FROM static_media_uris WHERE filename = ?
""",
((filename,)),
)
def get_uri(self, filename):
"""Get the uri of a file by the filename"""
self._execute(
"""
SELECT uri FROM static_media_uris
WHERE filename = ?
""",
((filename,)),
)
row = self.cursor.fetchone()
if row is not None:
return row[0]
return None
def set_uri(self, filename, uri):
"""Create a new URI for a file with filename"""
self._execute(
"""
INSERT INTO static_media_uris (
filename,
uri
) VALUES(
?, ?
)
""",
(
filename,
uri,
),
)
def create_room(self, room_id:str):
self._execute(
"""
INSERT INTO last_room_events (room_id) VALUES(?)
""",
(
room_id,
),
)
def get_room(self, room_id:str) -> str:
self._execute("SELECT room_id FROM last_room_events WHERE room_id= ?;", (room_id,))
id = self.cursor.fetchone()
if id:
return id[0]
return id
def get_all_rooms(self) -> List[str]:
self._execute("SELECT room_id FROM last_room_events;", ())
rows = self.cursor.fetchall()
return [row[0] for row in rows]
def set_room_event(self, room_id:str, event_id:str, timestamp:str, batch_token_start:str, batch_token_end:str):
self._execute(
"""
UPDATE last_room_events SET event_id= ?, timestamp=?, batch_token_start=?, batch_token_end=? WHERE room_id =?
""",
(
event_id,
timestamp,
batch_token_start,
batch_token_end,
room_id,
),
)
def set_delete_after(self, room_id:str, delete_after: str):
self._execute(
"""
UPDATE last_room_events SET delete_after= ? WHERE room_id =?
""",
(
delete_after,
room_id,
),
)
def set_deletion_turned_on(self, room_id:str, deletion_turned_on: bool):
self._execute(
"""
UPDATE last_room_events SET deletion_turned_on= ? WHERE room_id =?
""",
(
deletion_turned_on,
room_id,
),
)
def get_room_event(self, room_id:str):
self._execute(
"""
SELECT event_id, timestamp, batch_token_start, batch_token_end FROM last_room_events WHERE room_id =?
""",
(
room_id,
),
)
row = self.cursor.fetchone()
if row:
return {
"room_id": room_id,
"event_id": row[0],
"timestamp": row[1],
"batch_token_start": row[2],
"batch_token_end": row[3]
}
return None
def get_room_all(self, room_id:str):
self._execute(
"""
SELECT room_id, event_id, timestamp, delete_after, deletion_turned_on, batch_token_start, batch_token_end FROM last_room_events WHERE room_id =?
""",
(
room_id,
),
)
row = self.cursor.fetchone()
if row:
return {
"room_id": row[0],
"event_id": row[1],
"timestamp": row[2],
"delete_after": row[3],
"deletion_turned_on": row[4],
"batch_token_start": row[5],
"batch_token_end": row[6]
}
return None
def get_room_deletion(self, room_id:str):
self._execute(
"""
SELECT deletion_turned_on, delete_after FROM last_room_events WHERE room_id =?
""",
(
room_id,
),
)
row = self.cursor.fetchone()
if row:
return {
"room_id": room_id,
"deletion_turned_on": row[0],
"delete_after": row[1]
}
return None |
/*
Copyright (C) 2013, 2015, 2018, 2021 Bengt Martensson.
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 3 of the License, or (at
your option) any later version.
This program is distributed in the hope that it will be useful, but
WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
General Public License for more details.
You should have received a copy of the GNU General Public License along with
this program. If not, see http://www.gnu.org/licenses/.
*/
package org.harctoolbox.girr;
import java.io.IOException;
import java.io.Reader;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import java.util.logging.Level;
import java.util.logging.Logger;
import static org.harctoolbox.girr.AdminData.printIfNonempty;
import static org.harctoolbox.girr.Command.INITIAL_HASHMAP_CAPACITY;
import static org.harctoolbox.girr.XmlStatic.ADMINDATA_ELEMENT_NAME;
import static org.harctoolbox.girr.XmlStatic.APPLICATIONDATA_ELEMENT_NAME;
import static org.harctoolbox.girr.XmlStatic.APPLICATION_ATTRIBUTE_NAME;
import static org.harctoolbox.girr.XmlStatic.APPPARAMETER_ELEMENT_NAME;
import static org.harctoolbox.girr.XmlStatic.COMMANDSET_ELEMENT_NAME;
import static org.harctoolbox.girr.XmlStatic.COMMENT_ATTRIBUTE_NAME;
import static org.harctoolbox.girr.XmlStatic.DEVICECLASS_ATTRIBUTE_NAME;
import static org.harctoolbox.girr.XmlStatic.DISPLAYNAME_ATTRIBUTE_NAME;
import static org.harctoolbox.girr.XmlStatic.GIRR_NAMESPACE;
import static org.harctoolbox.girr.XmlStatic.MANUFACTURER_ATTRIBUTE_NAME;
import static org.harctoolbox.girr.XmlStatic.MODEL_ATTRIBUTE_NAME;
import static org.harctoolbox.girr.XmlStatic.NAME_ATTRIBUTE_NAME;
import static org.harctoolbox.girr.XmlStatic.NOTES_ELEMENT_NAME;
import static org.harctoolbox.girr.XmlStatic.REMOTENAME_ATTRIBUTE_NAME;
import static org.harctoolbox.girr.XmlStatic.REMOTE_ELEMENT_NAME;
import static org.harctoolbox.girr.XmlStatic.VALUE_ATTRIBUTE_NAME;
import org.harctoolbox.ircore.IrCoreException;
import org.harctoolbox.ircore.IrSignal;
import org.harctoolbox.irp.IrpException;
import org.harctoolbox.xml.XmlUtils;
import static org.harctoolbox.xml.XmlUtils.ENGLISH;
import static org.harctoolbox.xml.XmlUtils.XML_LANG_ATTRIBUTE_NAME;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
/**
* This class describes a remote in Girr.
* A Remote is essentially an abstraction of a hand-held "clicker" for controlling one device.
* It has a name for identification, and a number of comment-like text fields. Most importantly,
* it has a dictionary of CommandSets, indexed by their names.
* The CommandSets contain Commands, having names that are unique within their CommandSet.
* Note that Commands with the same name can be present in different CommandSets.
* For example, some TVs have both an RC5 and an RC6 Command set, both containing a command "power",
* but these are or course different.
*/
public final class Remote extends XmlExporter implements Named, Iterable<CommandSet> {
private final static Logger logger = Logger.getLogger(Remote.class.getName());
private final MetaData metaData;
private final AdminData adminData;
private String comment;
private final Map<String, String> notes;
private final Map<String, CommandSet> commandSets;
private final Map<String, Map<String, String>> applicationParameters;
/**
* This constructor is used to read a Girr file into a Remote.
* @param file Girr file; must have "remote" as the root element.
* @throws org.harctoolbox.girr.GirrException
* @throws java.io.IOException
* @throws org.xml.sax.SAXException
*/
public Remote(String file) throws GirrException, IOException, SAXException {
this(XmlUtils.openXmlThing(file));
}
/**
* This constructor is used to read a Reader into a Remote.
* @param reader Reader producing a Document with a top level element "remote".
* @throws org.harctoolbox.girr.GirrException
* @throws java.io.IOException
* @throws org.xml.sax.SAXException
*/
public Remote(Reader reader) throws IOException, SAXException, GirrException {
this(XmlUtils.openXmlReader(reader, null, true, true));
}
/**
* This constructor is used to import a Document.
* @param doc W3C Document with root element of type "remote".
* @throws org.harctoolbox.girr.GirrException
*/
public Remote(Document doc) throws GirrException {
this(doc.getDocumentElement(), null);
}
/**
* XML import function.
*
* @param element Element to read from. Must be tag name "remote".
* @param source Textual representation of the origin of the information.
* @throws org.harctoolbox.girr.GirrException
*/
public Remote(Element element, String source) throws GirrException {
if (!element.getTagName().equals(REMOTE_ELEMENT_NAME))
throw new GirrException("Element name is not " + REMOTE_ELEMENT_NAME);
metaData = new MetaData(element.getAttribute(NAME_ATTRIBUTE_NAME),
element.getAttribute(DISPLAYNAME_ATTRIBUTE_NAME),
element.getAttribute(MANUFACTURER_ATTRIBUTE_NAME),
element.getAttribute(MODEL_ATTRIBUTE_NAME),
element.getAttribute(DEVICECLASS_ATTRIBUTE_NAME),
element.getAttribute(REMOTENAME_ATTRIBUTE_NAME));
NodeList nl = element.getElementsByTagName(ADMINDATA_ELEMENT_NAME);
adminData = nl.getLength() > 0 ? new AdminData((Element) nl.item(0)) : new AdminData();
if (source != null && !source.isEmpty())
adminData.setSourceIfEmpty(source);
applicationParameters = new LinkedHashMap<>(INITIAL_HASHMAP_CAPACITY);
comment = element.getAttribute(COMMENT_ATTRIBUTE_NAME);
notes = XmlStatic.parseElementsByLanguage(element.getElementsByTagName(NOTES_ELEMENT_NAME));
nl = element.getElementsByTagName(APPLICATIONDATA_ELEMENT_NAME);
for (int i = 0; i < nl.getLength(); i++) {
Element el = (Element) nl.item(i);
NodeList nodeList = el.getElementsByTagName(APPPARAMETER_ELEMENT_NAME);
Map<String, String> map = new HashMap<>(32);
for (int index = 0; index < nodeList.getLength(); index++) {
Element par = (Element) nodeList.item(index);
map.put(par.getAttribute(NAME_ATTRIBUTE_NAME), par.getAttribute(VALUE_ATTRIBUTE_NAME));
}
applicationParameters.put(el.getAttribute(APPLICATION_ATTRIBUTE_NAME), map);
}
nl = element.getElementsByTagName(COMMANDSET_ELEMENT_NAME);
commandSets = new LinkedHashMap<>(nl.getLength());
for (int i = 0; i < nl.getLength(); i++) {
CommandSet commandSet = new CommandSet((Element) nl.item(i));
commandSets.put(commandSet.getName(), commandSet);
}
}
/**
* Construct a Remote from its arguments, general case.
*
* @param metaData Remote.MetaData meta data
* @param source Textual representation of the origin of the information
* @param comment Textual comment
* @param notes Textual notes
* @param commandSetsCollection Collection<CommandSet>
* @param applicationParameters
*/
// The silly type of the commandSetsCollection is to be avoid name clashes with another constructor.
// Sorry for that.
public Remote(MetaData metaData, String source, String comment, Map<String, String> notes,
Collection<CommandSet> commandSetsCollection, Map<String, Map<String, String>> applicationParameters) {
this.adminData = new AdminData(source);
this.metaData = metaData;
this.comment = comment;
this.notes = notes != null ? notes : new HashMap<>(2);
this.commandSets = new LinkedHashMap<>(INITIAL_HASHMAP_CAPACITY);
if (commandSetsCollection != null)
commandSetsCollection.forEach(cmdSet -> {
commandSets.put(cmdSet.getName(), cmdSet);
});
this.applicationParameters = applicationParameters;
}
public Remote(MetaData metaData, String comment, Map<String, String> notes,
Collection<CommandSet> commandSetsCollection, Map<String, Map<String, String>> applicationParameters) {
this(metaData, null, comment, notes, commandSetsCollection, applicationParameters);
}
public Remote(MetaData metaData, String comment, Map<String, String> notes,
CommandSet commandSet, Map<String, Map<String, String>> applicationParameters) {
this(metaData, comment, notes, Named.toList(commandSet), applicationParameters);
}
public Remote(MetaData metaData, String comment, Map<String, String> notes,
Map<String, Command> commands, Map<String, Map<String, String>> applicationParameters, String protocolName, Map<String, Long> parameters) {
this(metaData, comment, notes, new CommandSet("commandSet", null, commands, protocolName, parameters), applicationParameters);
}
public Remote(MetaData metaData, String source, String comment, Map<String, String> notes,
Map<String, Command> commands, Map<String, Map<String, String>> applicationParameters, String protocolName, Map<String, Long> parameters) {
this(metaData, source, comment, notes, Named.toList(new CommandSet("commandSet", null, commands, protocolName, parameters)), applicationParameters);
}
public Remote(MetaData metaData, String comment, Map<String, String> notes,
Map<String, Command> commands, Map<String, Map<String, String>> applicationParameters) {
this(metaData, comment, notes, commands, applicationParameters, null, null);
}
/**
* This constructor constructs a Remote from one single IrSignal.
*
* @param irSignal
* @param name Name of command to be constructed.
* @param comment Comment for command to be constructed.
* @param deviceName
*/
public Remote(IrSignal irSignal, String name, String comment, String deviceName) {
this(new MetaData(deviceName),
null, // comment
null, // notes
new CommandSet(new Command(name, comment, irSignal)),
null /* applicationParameters */);
}
public Remote(CommandSet commandSet) {
this(new MetaData("unnamed"), null, null, commandSet, null);
}
@Override
public Element toElement(Document doc, boolean fatRaw, boolean generateParameters, boolean generateProntoHex, boolean generateRaw) {
Element element = doc.createElementNS(GIRR_NAMESPACE, REMOTE_ELEMENT_NAME);
Element adminDataEl = adminData.toElement(doc);
if (adminDataEl.hasChildNodes() || adminDataEl.hasAttributes())
element.appendChild(adminDataEl);
element.setAttribute(NAME_ATTRIBUTE_NAME, metaData.name);
if (metaData.displayName != null && !metaData.displayName.isEmpty())
element.setAttribute(DISPLAYNAME_ATTRIBUTE_NAME, metaData.displayName);
if (metaData.manufacturer != null && !metaData.manufacturer.isEmpty())
element.setAttribute(MANUFACTURER_ATTRIBUTE_NAME, metaData.manufacturer);
if (metaData.model != null && !metaData.model.isEmpty())
element.setAttribute(MODEL_ATTRIBUTE_NAME, metaData.model);
if (metaData.deviceClass != null && !metaData.deviceClass.isEmpty())
element.setAttribute(DEVICECLASS_ATTRIBUTE_NAME, metaData.deviceClass);
if (metaData.remoteName != null && !metaData.remoteName.isEmpty())
element.setAttribute(REMOTENAME_ATTRIBUTE_NAME, metaData.remoteName);
if (comment != null && !comment.isEmpty())
element.setAttribute(COMMENT_ATTRIBUTE_NAME, comment);
if (notes != null) {
notes.entrySet().stream().map((note) -> {
Element notesEl = doc.createElementNS(GIRR_NAMESPACE, NOTES_ELEMENT_NAME);
notesEl.setAttribute(XML_LANG_ATTRIBUTE_NAME, note.getKey());
notesEl.setTextContent(note.getValue());
return notesEl;
}).forEachOrdered((notesEl) -> {
element.appendChild(notesEl);
});
}
if (applicationParameters != null) {
applicationParameters.entrySet().forEach((kvp) -> {
if (kvp.getValue() != null) {
Element appEl = doc.createElementNS(GIRR_NAMESPACE, APPLICATIONDATA_ELEMENT_NAME);
appEl.setAttribute(APPLICATION_ATTRIBUTE_NAME, kvp.getKey());
element.appendChild(appEl);
kvp.getValue().entrySet().stream().map((param) -> {
Element paramEl = doc.createElementNS(GIRR_NAMESPACE, APPPARAMETER_ELEMENT_NAME);
paramEl.setAttribute(NAME_ATTRIBUTE_NAME, param.getKey());
paramEl.setAttribute(VALUE_ATTRIBUTE_NAME, param.getValue());
return paramEl;
}).forEachOrdered((paramEl) -> {
appEl.appendChild(paramEl);
});
}
});
}
for (CommandSet commandSet : this)
element.appendChild(commandSet.toElement(doc, fatRaw, generateParameters, generateProntoHex, generateRaw));
return element;
}
/**
* Apply the sort function to all contained CommandSets.
* @param comparator
*/
public void sort(Comparator<? super Named> comparator) {
List<CommandSet> list = new ArrayList<>(commandSets.values());
Collections.sort(list, comparator);
Named.populateMap(commandSets, list);
}
public void sortCommands(Comparator<? super Named> comparator) {
for (CommandSet commandSet : this)
commandSet.sort(comparator);
}
public void sortCommands() {
sortCommands(new Named.CompareNameCaseSensitive());
}
public void sortCommandsIgnoringCase() {
sort(new Named.CompareNameCaseInsensitive());
}
public void sort() {
sort(new Named.CompareNameCaseSensitive());
}
public void sortIgnoringCase() {
sort(new Named.CompareNameCaseInsensitive());
}
/**
* Replaces all CommandSets with a single one,
* containing all the commands of the original CommandSets.
* Note that only one commend with a particular name is present after this operation,
* so information may be lost.
*/
public void normalize() {
if (commandSets.size() < 2)
return;
int numberOfOriginalCommands = getNumberOfCommands();
Map<String, Command> commands = new LinkedHashMap<>(numberOfOriginalCommands);
StringJoiner stringJoiner = new StringJoiner(", ", "Merge of CommandSets ", "");
for (CommandSet cmdSet : this) {
for (Command cmd : cmdSet)
commands.put(cmd.getName(), cmd);
stringJoiner.add(cmdSet.getName());
}
Map<String, String> notesCmdSet = new HashMap<>(1);
String noteString = stringJoiner.toString();
CommandSet commandSet = new CommandSet("MergedCommandSet", notesCmdSet, commands, null, null);
commandSets.clear();
commandSets.put(commandSet.getName(), commandSet);
int missing = numberOfOriginalCommands - commandSet.size();
if (missing > 0)
noteString += "\n" + missing + " commands lost in merge";
notesCmdSet.put(XmlUtils.ENGLISH, noteString);
}
/**
* Applies the format argument to all Command's in the Remote.
*
* @param format
* @param repeatCount
*/
public void addFormat(Command.CommandTextFormat format, int repeatCount) {
for (CommandSet commandSet : this) {
for (Command command : commandSet) {
try {
command.addFormat(format, repeatCount);
} catch (IrCoreException | IrpException ex) {
logger.log(Level.WARNING, null, ex);
}
}
}
}
/**
* Returns true if and only if all contained commands has the protocol in the argument.
* @param protocolName
* @return
* @throws org.harctoolbox.irp.IrpException
* @throws org.harctoolbox.ircore.IrCoreException
*/
public boolean hasThisProtocol(String protocolName) throws IrpException, IrCoreException {
for (CommandSet commandSet : this) {
for (Command command : commandSet) {
String prtcl = command.getProtocolName();
if (prtcl == null || !prtcl.equalsIgnoreCase(protocolName))
return false;
}
}
return true;
}
/**
* Returns true if and only if at least one contained commands has the protocol in the argument.
* @param protocolName
* @return
* @throws org.harctoolbox.irp.IrpException
* @throws org.harctoolbox.ircore.IrCoreException
*/
public boolean containsThisProtocol(String protocolName) throws IrpException, IrCoreException {
for (CommandSet commandSet : this) {
for (Command command : commandSet) {
String prtcl = command.getProtocolName();
if (prtcl != null && prtcl.equalsIgnoreCase(protocolName))
return true;
}
}
return false;
}
/**
*
* @return the metaData
*/
public MetaData getMetaData() {
return metaData;
}
/**
*
* @return Name of the Remote.
*/
@Override
public String getName() {
return metaData.name;
}
public void setName(String name) {
metaData.name = name;
}
/**
*
* @return displayName of the Remote.
*/
public String getDisplayName() {
return metaData.displayName;
}
/**
* @return the comment
*/
public String getComment() {
return comment;
}
/**
* @deprecated Loop the CommandSets instead.
* @return
*/
public int getNumberOfCommands() {
int sum = 0;
for (CommandSet cmdSet : this)
sum += cmdSet.size();
return sum;
}
/**
* Returns all commands contained.
* If there are several CommandSets, the index names will be two part: commandSet : command.
* @return the commands
* @deprecated Loop the CommandSets instead.
*/
public Collection<Command> getCommands() {
if (commandSets.isEmpty())
return new ArrayList<>(0);
if (commandSets.size() == 1)
return iterator().next().getCommands();
List<Command> allCommands = new ArrayList<>(getNumberOfCommands());
for (CommandSet cmdSet : this) {
//String prefix = cmdSet.getName() + ':';
for (Command cmd : cmdSet) {
//String newName = prefix + cmd.getName();
allCommands.add(cmd);
}
}
return allCommands;
}
/**
* @deprecated Loop the CommandSets instead.
* @param name
* @return
*/
public List<Command> getCommand(String name) {
List<Command> commands = new ArrayList<>(commandSets.size());
for (CommandSet cmdSet : this) {
Command cmd = cmdSet.getCommand(name);
if (cmd != null)
commands.add(cmd);
}
return commands;
}
/**
* @return the applicationParameters
*/
public Map<String, Map<String, String>> getApplicationParameters() {
return Collections.unmodifiableMap(applicationParameters);
}
/**
* @return the manufacturer
*/
public String getManufacturer() {
return metaData.manufacturer;
}
/**
* @return the model
*/
public String getModel() {
return metaData.model;
}
/**
* @return the deviceClass
*/
public String getDeviceClass() {
return metaData.deviceClass;
}
/**
* @return the remoteName
*/
public String getRemoteName() {
return metaData.remoteName;
}
AdminData getAdminData() {
return adminData;
}
public String getFormattedAdminData() {
return adminData.toFormattedString();
}
/**
* @return the notes
*/
Map<String, String> getAllNotes() {
return Collections.unmodifiableMap(notes);
}
/**
* @return the notes
*/
public String getNotes() {
return notes.get(ENGLISH);
}
/**
* @param lang
* @return the notes
*/
public String getNotes(String lang) {
return notes.get(lang);
}
public void setNotes(String lang, String string) {
this.notes.put(lang, string);
}
public void setNotes(String string) {
this.notes.put(ENGLISH, string);
}
void setComment(String comment) {
this.comment = comment;
}
public void checkForParameters() throws IrpException, IrCoreException {
for (CommandSet commandSet : this)
for (Command command : commandSet)
command.checkForParameters();
}
@Override
public Iterator<CommandSet> iterator() {
return commandSets.values().iterator();
}
public Map<String, CommandSet> getCommandSets() {
return Collections.unmodifiableMap(commandSets);
}
public CommandSet getCommandSet(String name) {
return commandSets.get(name);
}
public Command getCommand(String commandSetName, String name) {
return getCommandSet(commandSetName).getCommand(name);
}
/**
* Apply the strip function to all the CommandSets.
*/
public void strip() {
for (CommandSet commandSet : this)
commandSet.strip();
}
/**
* This class bundles different meta data for a remote together.
*/
public static final class MetaData implements Serializable {
public static final String DEFAULT_REMOTENAME = "remote";
private static boolean isVoid(String s) {
return s == null || s.isEmpty();
}
private String name;
private String displayName;
private String manufacturer;
private String model;
private String deviceClass;
private String remoteName;
/**
* Constructor for empty MetaData.
*/
public MetaData() {
this.name = DEFAULT_REMOTENAME;
this.displayName = null;
this.manufacturer = null;
this.model = null;
this.deviceClass = null;
this.remoteName = null;
}
/**
* Constructor with name.
* @param name
*/
public MetaData(String name) {
this();
this.name = name;
}
/**
* Generic constructor.
* @param name Name of the remote, as how users and other program is referring to it. Should be in ENglish and not containing special characters.
* @param displayName A "nicely looking" displayable name. May contain spaces and special characters.
* @param manufacturer Manufacturer of the device.
* @param model Model name, to be identified by humans-
* @param deviceClass Device class, for example "TV".
* @param remoteName Manufacturers name of original remote.
*/
public MetaData(String name, String displayName, String manufacturer, String model,
String deviceClass, String remoteName) {
this.name = name;
this.displayName = displayName;
this.manufacturer = manufacturer;
this.model = model;
this.deviceClass = deviceClass;
this.remoteName = remoteName;
}
public String toFormattedString() {
StringBuilder sb = new StringBuilder(256);
printIfNonempty(sb, NAME_ATTRIBUTE_NAME, name);
printIfNonempty(sb, DISPLAYNAME_ATTRIBUTE_NAME, displayName);
printIfNonempty(sb, MANUFACTURER_ATTRIBUTE_NAME, manufacturer);
printIfNonempty(sb, MODEL_ATTRIBUTE_NAME, model);
printIfNonempty(sb, DEVICECLASS_ATTRIBUTE_NAME, deviceClass);
printIfNonempty(sb, REMOTENAME_ATTRIBUTE_NAME, remoteName);
int len = sb.length();
if (len > 1)
sb.deleteCharAt(sb.length() - 1);
return sb.toString();
}
/**
* Returns true if there is no non-trivial content.
* @return
*/
public boolean isEmpty() {
return isVoid(name)
&& isVoid(displayName)
&& isVoid(manufacturer)
&& isVoid(model)
&& isVoid(deviceClass)
&& isVoid(remoteName);
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @return the displayName
*/
public String getDisplayName() {
return displayName;
}
/**
* @return the manufacturer
*/
public String getManufacturer() {
return manufacturer;
}
/**
* @return the model
*/
public String getModel() {
return model;
}
/**
* @return the deviceClass
*/
public String getDeviceClass() {
return deviceClass;
}
/**
* @return the remoteName
*/
public String getRemoteName() {
return remoteName;
}
}
} |
/*
* @lc app=leetcode.cn id=46 lang=cpp
*
* [46] ๅ
จๆๅ
*/
#include <iostream>
#include <vector>
#include <algorithm>
#include <unordered_set>
using namespace std;// @lc code=start
class Solution {
public:
vector<vector<int>> result;
vector<int> path;
void backtracking (vector<int>& nums, vector<bool>& used) {
// ๆญคๆถ่ฏดๆๆพๅฐไบไธ็ป
if (path.size() == nums.size()) {
result.push_back(path);
return;
}
for (int i = 0; i < nums.size(); i++) {
if (used[i] == true) continue; // path้ๅทฒ็ปๆถๅฝ็ๅ
็ด ๏ผ็ดๆฅ่ทณ่ฟ
used[i] = true;
path.push_back(nums[i]);
backtracking(nums, used);
path.pop_back();
used[i] = false;
}
}
vector<vector<int>> permute(vector<int>& nums) {
result.clear();
path.clear();
vector<bool> used(nums.size(), false);
backtracking(nums, used);
return result;
}
};
// @lc code=end |
import { Command } from '../lib/Command';
import { CommandResult } from '../lib/CommandResult';
import { CommandMessage } from '../lib/CommandMessage';
import { Bot } from '../Bot';
import { MessageEmbed, MessageReaction, ClientUser } from 'discord.js';
import { emojis } from '../lib/Emojis';
import { Question } from '../../Questions/Question';
export class Next implements Command {
private question: Question;
public readonly config = {
name: 'Next',
description: 'Get the next question.',
command: 'next'
};
public constructor() {
}
public async handle(commandMessage: CommandMessage, bot: Bot): Promise<CommandResult> {
const questions = await bot.botService.questionsService.getAll();
this.question = questions[ 0 ];
const embed = new MessageEmbed();
embed.setTitle('Next question:');
embed.setDescription(`${ this.question.question }\n \n:regional_indicator_t: **true** or :regional_indicator_f: **false**? \n \n`);
embed.addFields([
{
name: 'Correct Answers',
value: '3 / 123',
inline: true
}, {
name: 'Tags',
value: this.question.tags.map(tag => tag.name).join(', '),
inline: true
}
]);
return {
message: embed,
reactions: [
{
emoji: emojis.t,
handler: this.reacted
}, {
emoji: emojis.f,
handler: this.reacted
}
]
};
}
public reacted(reaction: MessageReaction, user: ClientUser) {
if (reaction.emoji.name === emojis.f && Boolean(this.question.options.find(option => option.isCorrect).option)) {
const e = new MessageEmbed();
e.setTitle('Correct answer guessed!');
e.setDescription(`<@${ user.id }> guessed right :clap:`);
reaction.message.reply(e);
}
}
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.