text
stringlengths 184
4.48M
|
---|
// SPDX-License-Identifier: MIT;
pragma solidity ^0.8.7;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
import "hardhat/console.sol";
error NotOwner();
contract AfterLife is ERC20, Ownable {
/**
* Create a token contract with name and symbol
*
* Sets the owner of the contract
* Mint a total supply of 1000000000000000(1 Quadrillion) AfterLife tokens to contract creator
*/
constructor() ERC20("AfterLife", "AL") {
_mint(msg.sender, 1000000000000000 * 10**18);
}
/**
* Changes the owner of the contract.
* @notice Can only be called by the current contract owner
*/
function _beforeTokenTransfer(
address from,
address to,
uint256 amount
) internal view override onlyOwner {}
} |
/* eslint-disable react/no-string-refs */
import React, {Component} from 'react';
import {
TouchableOpacity,
StyleSheet,
TextInput,
View,
Text,
Alert,
} from 'react-native';
function RegisterFetch(username, password) {
return fetch(
'https://backend-vegeteam.app.secoder.net/api/mobile/admin/regis/',
{
method: 'POST',
body: JSON.stringify({
user_name: username,
user_pwd: password,
}),
},
)
.then((response) => response.json())
.then((data) => {
if (data.code === 200) {
return 1;
} else {
return 0;
}
})
.catch((error) => {
console.warn(error);
});
}
export default class RegisterScene extends Component {
username = ''; //保存用户名
password = ''; //保存密码
confirmPassword = ''; //保存确认密码
/**
* 当用户名输入框值改变时,保存改变的值
* @param {[type]} newUsername [输入的用户名]
*/
onUsernameChanged = (newUsername) => {
//console.log(newUsername);//运行后可以在输入框随意输入内容并且查看log验证!
this.username = newUsername;
};
/**
* 当密码输入框值改变时,保存改变的值
* @param {[type]} newUsername [输入的密码]
*/
onPasswordChanged = (newPassword) => {
//console.log(newPassword);//运行后可以在输入框随意输入内容并且查看log验证!
this.password = newPassword;
};
/**
* 当确认密码输入框值改变时,保存改变的值
* @param {[type]} newUsername [输入的确认密码]
*/
onConfirmPasswordChanged = (newConfirmPassword) => {
//console.log(newConfirmPassword); //运行后可以在输入框随意输入内容并且查看log验证!
this.confirmPassword = newConfirmPassword;
};
/**
* 注册按钮,根据输入的内容判断注册是否成功
*/
register = () => {
if (
this.username !== '' &&
this.password !== '' &&
this.password.length >= 6
) {
if (this.password === this.confirmPassword) {
RegisterFetch(this.username, this.password).then((val) => {
if (val === 1) {
const {goBack} = this.props.navigation; //获取navigation的goBack方法
Alert.alert('注册成功', '返回登陆', [
{
text: '确定',
onPress: () => {
goBack();
},
},
]); //给弹出的提示框添加事件
} else {
Alert.alert('注册失败', '用户名重复!');
}
});
} else {
Alert.alert('注册失败', '密码与确认密码不同');
}
} else {
Alert.alert('注册失败', '用户名或密码不能为空,且密码长度不少于6位');
}
};
back = () => {
const {goBack} = this.props.navigation; //获取navigation的goBack方法
goBack();
};
render() {
return (
<TouchableOpacity
activeOpacity={1.0} //设置背景被点击时,透明度不变
style={styles.container}>
<View style={styles.inputBox}>
<TextInput
ref="username"
onChangeText={this.onUsernameChanged} //添加值改变事件
style={styles.input}
autoCapitalize="none" //设置首字母不自动大写
underlineColorAndroid={'transparent'} //将下划线颜色改为透明
placeholderTextColor={'#ccc'} //设置占位符颜色
placeholder={'username'} //设置占位符
/>
</View>
<View style={styles.inputBox}>
<TextInput
ref="password"
onChangeText={this.onPasswordChanged} //添加值改变事件
style={styles.input}
secureTextEntry={true} //设置为密码输入框
autoCapitalize="none" //设置首字母不自动大写
underlineColorAndroid={'transparent'} //将下划线颜色改为透明
placeholderTextColor={'#ccc'} //设置占位符颜色
placeholder={'password'} //设置占位符
/>
</View>
<View style={styles.inputBox}>
<TextInput
ref="confirmPassword"
onChangeText={this.onConfirmPasswordChanged} //添加值改变事件
style={styles.input}
secureTextEntry={true} //设置为密码输入框
autoCapitalize="none" //设置首字母不自动大写
underlineColorAndroid={'transparent'} //将下划线颜色改为透明
placeholderTextColor={'#ccc'} //设置占位符颜色
placeholder={'confirm password'} //设置占位符
/>
</View>
<TouchableOpacity onPress={this.register} style={styles.button}>
<Text style={styles.btText}>注册</Text>
</TouchableOpacity>
<TouchableOpacity onPress={this.back} style={styles.button}>
<Text style={styles.btText}>返回登陆</Text>
</TouchableOpacity>
</TouchableOpacity>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
input: {
width: 200,
height: 40,
fontSize: 20,
color: '#fff', //输入框输入的文本为白色
},
inputBox: {
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'center',
width: 280,
height: 50,
borderRadius: 8,
backgroundColor: '#66f',
marginBottom: 8,
padding: 0,
},
button: {
height: 50,
width: 280,
justifyContent: 'center',
alignItems: 'center',
borderRadius: 8,
backgroundColor: '#66f',
marginTop: 20,
},
btText: {
color: '#fff',
fontSize: 20,
},
}); |
{% extends 'base.html' %}
{% block additional_styles %}
<style>
table {
border-collapse: collapse;
width: 100%;
margin-bottom: 20px; /* Add margin between tables */
}
th, td {
border: 1px solid black;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
.table-heading {
font-weight: bold;
}
</style>
{% endblock %}
{% block content %}
<form action="{% url 'led' %}" method="GET">
<label for="date">Select Date:</label>
<input type="date" id="date" name="date">
<input type="submit" value="Filter">
</form>
<h2 class="table-heading">누적시간</h2>
<table>
<tr>
<th>Date</th>
<th>On Time (mins)</th>
<th>Off Time (mins)</th>
</tr>
{% for on_off_time in on_off_times %}
<tr>
<td>{{ on_off_time.date }}</td>
<td>{{ on_off_time.on_time }}</td>
<td>{{ on_off_time.off_time }}</td>
</tr>
{% endfor %}
</table>
<h2 class="table-heading">실 데이터</h2>
<table>
<tr>
<th>Start Time</th>
<th>End Time</th>
<th>LED</th>
</tr>
{% for daily_time in daily_times %}
<tr>
<td>{{ daily_time.start_time }}</td>
<td>{{ daily_time.end_time }}</td>
<td>{{ daily_time.led }}</td>
</tr>
{% endfor %}
</table>
{% if selected_date %}
{% block chart %}
<div id="chart"></div>
<script>
// Extract the required data for the chart
const chartData = [];
{% for daily_time in daily_times %}
{
const startTime = new Date('{{ daily_time.start_time }}');
const endTime = new Date('{{ daily_time.end_time }}');
const led = {% if daily_time.led == 'ON' %}1{% else %}0{% endif %};
chartData.push({
date: new Date(startTime),
led: led
});
chartData.push({
date: new Date(endTime),
led: led
});
}
{% endfor %}
// Generate the chart using C3.js and D3.js
const chart = c3.generate({
bindto: '#chart',
data: {
json: chartData,
keys: {
x: 'date',
value: ['led'],
},
// type: 'line'
type: 'area', // Use area-spline type for a filled line graph
colors: {
led: '#3366ff' // Set the color for the line and area fill
}
},
axis: {
x: {
type: 'timeseries',
tick: {
format: '%Y-%m-%d %H:%M' // Adjust the date format as per your needs
},
},
},
grid: {
x: {
show: true // Show y-axis grid lines
}
}
});
</script>
{% endblock %}
{% endif %}
{% endblock %} |
# 一、准备
## 1. 安装
安装webpack 和webpack命令行
```bash
pnpm install webpack webpack-cli -D
```
安装自动生成html文件的插件
```bash
pnpm install html-webpack-plugin -D
```
安装webpack服务器
```bash
pnpm install webpack-dev-server -D
```
## 2. 配置
### 2.1 在 `package.json` 中加入快捷命令
```json
"scripts": {
"dev": "webpack-dev-server",
"build": "webpack --mode development" // --mode [mode] 可以指定 development或production模式
},
```
### 2.2 在 `webpack.config.js` 中进行配置
```javascript
import path from "node:path";
import HtmlWebpackPlugin from "html-webpack-plugin";
// esm规范下没有__dirname和__filename,可以通过 import.meta.url 获取当前文件的绝对路径
import { fileURLToPath } from "node:url";
const __filename = fileURLToPath(import.meta.url);
const __dirname = path.dirname(__filename);
// 提供类型提示
/**
* @type {import("webpack").Configuration}
*/
export default {
mode: "development",
entry: "./src/index.ts", // 入口文件
output: {
filename: "index.js", // 打包后的文件名,[fullhash]是webpack内置的变量,表示每次打包后的hash值
path: path.resolve(__dirname, "dist"), // 打包后的目录
clean: true, // 打包前清空dist目录
},
plugins: [
new HtmlWebpackPlugin({
template: "./index.html", // 指定模板文件
}),
],
};
```
### 2.3 配置 `tsconfig.json`
```json
{
"compilerOptions": {
"module": "ESNext", // ts 文件中使用的模块化方案
"target": "ESNext", // 编译后生成的js文件应该使用哪个版本
"esModuleInterop": true, // 对接不同的模块化方案,使得在es6模块中能够像导入es6模块一样导入commonjs模块
"lib": ["ESNext", "DOM", "DOM.Iterable"], // 指定编译过程中需要引入的库文件或类型声明文件,用于提供类型检查等功能
"skipLibCheck": true, // 编译时跳过对声明文件(.d.ts)的检查
"sourceMap": false, // 是否生成sourceMap文件,用于调试ts文件
"importHelpers": true, // 减少因ts自动生成的辅助代码导致的重复
// 如果不配置moduleResolution,那么在使用import导入文件时,会报错:无法找到模块
"moduleResolution": "Bundler", // 模块解析策略,配合打包工具时用Bundler
"isolatedModules": true, // 将每个ts文件作为单独的模块,不会在全局作用域添加任何内容
"strict": true,
"baseUrl": "./", // ts文件中使用的路径别名的基础路径
"paths": {
// ts文件中使用的路径别名
"@/*": ["src/*"]
}
},
"include": ["src/**/*.ts", "src/**/*.d.ts", "src/**/*.vue"]
}
```
### 2.4 支持vue
安装 vue
```bash
pnpm install vue
```
为webpack安装解析vue文件的插件,https://vue-loader.vuejs.org/zh/guide/#vue-cli
```bash
pnpm install -D vue-loader vue-template-compiler
```
配置插件
```javascript
// webpack.config.js
import { VueLoaderPlugin } from "vue-loader";
// ...
export default {
// ...
module: {
rules: [
{
test: /\.vue$/, // 所有 .vue 文件
// 一个loader用loader,多个用use
loader: "vue-loader", // 使用 vue-loader 进行解析
},
],
},
plugins: [
new VueLoaderPlugin(),
// ...
],
};
```
配置路径别名和省略后缀名
```javascript
export default {
// ...
resolve: {
alias: {
"@": path.resolve(__dirname, "src"),
},
// extensions: [".js", ".ts", ".vue"], // import时尝试自动补全文件后缀
},
};
```
设置 [Feature Flags](https://github.com/vuejs/core/tree/main/packages/vue#bundler-build-feature-flags)
```javascript
// webpack.config.js
// webpack是CommonJS规范,直接使用 import { DefinePlugin } from "webpack"; 会报错
import webpack from "webpack";
const { DefinePlugin } = webpack;
// 在 export default 中添加
plugins: [
// ...
new DefinePlugin({
__VUE_OPTIONS_API__: false, // 是否启用选项式api(vue2)
__VUE_PROD_DEVTOOLS__: false, // 是否在生产环境下支持devtools
}),
],
```
### 2.5 支持css
安装用于解析css的 `css-loader` 和用于将 css 插入到 DOM 中的 `style-loader`
```bash
pnpm install -D css-loader style-loader
```
配置
```javascript
// webpack.config.js
export default {
// ...
module: {
// rules 从下到上执行
rules: [
// ...
{
test: /\.css$/, // 所有css文件
use: ["style-loader", "css-loader"], // 从右向左执行
},
],
}
};
```
### 2.6 支持sass
安装 sass 和 sass 解析器
```bash
pnpm install -D sass sass-loader
```
配置
```javascript
rules: [
{
test: /\.scss$/, // 所有scss文件
use: ["style-loader", "css-loader", "sass-loader"],
},
],
```
### 2.7 支持 typescript
安装 typescript 和 typescript 解析器
```bash
pnpm install -D typescript ts-loader
```
配置
```javascript
{
test: /\.ts/, // 所有 ts 文件
loader: "ts-loader",
options: {
configFile: path.resolve(process.cwd(), "tsconfig.json"), // 指定ts配置文件
appendTsSuffixTo: [/\.vue$/], // 给vue文件添加ts后缀,让vue文件也能使用ts
},
},
```
为了让ts识别 vue 后缀,需要在 index.ts同级目录下创建声明文件 xxx.d.ts
<span style="color:red">注意:不要和其他 ts 文件同名,否则会报错(如不要同时存在 index.ts 和 index.d.tsf)</span>
```typescript
// shims.d.ts
declare module "*.vue" {
import { DefineComponent } from "vue";
const component: DefineComponent<{}, {}, any>;
export default component;
}
```
### 2.8 美化 webpack-server 的输出
```bash
pnpm install -D friendly-errors-webpack-plugin
```
```javascript
// webpack.config.js
plugins: [
// ...
new FriendlyErrorsWebpackPlugin({
compilationSuccessInfo: {
messages: ["You application is running here http://localhost:8080"],
},
}),
],
stats: "errors-only", // 终端仅打印error
devServer: {
port: 8080, // 启动端口
open: true, // 自动打开浏览器
hot: true, // 开启热更新
client: {
logging: "warn", // 关闭热更新时控制台的打印信息
},
},
```
### 2.9 使用 CDN 引入以减小打包后的文件体积
```javascript
// webpack.config.js
export default {
// ...
externals: {
// 配置不打包的模块(可以转而使用cdn引入)
vue: "Vue",
},
};
```
```html
<!-- index.html -->
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
``` |
import React from 'react';
interface FilterComponentProps {
filter: string;
onFilterChange: (event: React.ChangeEvent<HTMLSelectElement>) => void;
}
const Filter: React.FC<FilterComponentProps> = ({ filter, onFilterChange }) => {
return (
<div className="mb-5">
<label htmlFor="filter" className="mr-2">Filter:</label>
<select id="filter" value={filter} onChange={onFilterChange} className="p-2 border rounded">
<option value="all">All</option>
<option value="price_above_1000">Price above 1000</option>
<option value="discount_percentage">Discount</option>
<option value="sort_by_rating">Sort by Rating (High to Low)</option>
<option value="sort_by_price">Sort by Price (Low to High)</option>
</select>
</div>
);
};
export default Filter; |
<template>
<aside class="bg-white flex-shrink-0 p-3 shadow"
style="width: 250px;min-height: 100vh;">
<ul class="list-unstyled ps-0">
<li class="mb-1">
<router-link :to="{name:'adminhome'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='adminhome'}">
<i class="bi bi-house-fill text-blue me-2"></i>Trang chủ
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'statistics_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='statistics_manager'}">
<i class="bi bi-speedometer2 text-red me-2"></i> Thống kê
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'user_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='user_manager'}">
<i class="bi bi-person text-pink me-2"></i> Tài khoản
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'orders_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='orders_manager'}">
<i class="bi bi-shop-window text-blue me-2"></i> Đơn đặt hàng
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'vouchers_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='vouchers_manager'}">
<i class="bi bi-gift-fill text-pink me-2"></i> Voucher
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'products_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='products_manager'}">
<i class="bi bi-laptop-fill text-blue me-2"></i> Sản phẩm
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'categories_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='categories_manager'}">
<i class="bi bi-list-ul text-blue me-2"></i>Danh mục
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'payments_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='payments_manager'}">
<i class="bi bi-currency-bitcoin text-yellow me-2"></i>Thanh toán
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'chats_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='chats_manager'}">
<i class="bi bi-messenger text-blue me-2"></i>Chăm sóc khách hàng
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'news_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='news_manager'}">
<i class="bi bi-newspaper text-blue me-2"></i>Tin đăng
</router-link>
</li>
<li class="mb-1">
<router-link :to="{name:'comments_manager'}" :class="{'w-100 text-start btn align-items-center rounded':true,'active':page_name=='comments_manager'}">
<i class="bi bi-star-fill text-yellow me-2"></i>Đánh giá
</router-link>
</li>
<li class="mb-1" @click="onLogout">
<a role="button" class="w-100 text-start btn align-items-center rounded">
<i class="bi bi-box-arrow-left text-red me-2"></i>Đăng xuất
</a>
</li>
</ul>
</aside>
</template>
<script>
import { ref,computed } from 'vue';
import router from '../../router';
import { useStore } from 'vuex';
export default {
setup(){
const store=useStore()
const onLogout=()=>{
store.dispatch("Logout")
router.push('/admin/dang-nhap')
}
const page_name =computed(()=>router.currentRoute.value.name)
return {page_name,onLogout}
}
}
</script>
<style scoped>
.active{
background-color: blue;
color:white;
}
</style> |
import {TreeItem, treeItemClasses, TreeItemProps} from "@mui/lab"
import * as React from "react"
import {MouseEventHandler} from "react"
import {Menu, MenuItem, SvgIconProps} from "@mui/material"
import {styled} from "@mui/material/styles"
import Box from "@mui/material/Box"
import Typography from "@mui/material/Typography"
import {useContextMenu} from "./useContextMenu"
import ListItemIcon from "@mui/material/ListItemIcon"
import ListItemText from "@mui/material/ListItemText"
import ArrowRightIcon from "@mui/icons-material/ArrowRight"
import {NestedMenuItem} from "mui-nested-menu"
declare module 'react' {
interface CSSProperties {
'--tree-view-color'?: string
'--tree-view-bg-color'?: string
}
}
/**
* Props for the StyledTreeItem component.
*
* @property bgColor background color of the tree item
* @property color color of the tree item
* @property labelIcon icon of the tree item
* @property rightContent content to display on the right side of the tree item
* @property labelText text of the tree item
* @property contextMenuItems items to display in the context menu
*/
type StyledTreeItemProps = TreeItemProps & {
bgColor?: string
color?: string
labelIcon?: React.ElementType<SvgIconProps>
rightContent?: React.ReactNode
labelText: string
contextMenuItems?: ContextMenuItem[]
}
/**
* An item in the context menu.
*
* @property label label of the item
* @property icon icon of the item
* @property onClick click handler of the item
* @property nestedItems nested items of the item
*/
interface ContextMenuItem {
label: string
icon: React.ElementType<SvgIconProps>
onClick?: MouseEventHandler<HTMLLIElement>
nestedItems?: ContextMenuItem[]
}
const StyledTreeItemRoot = styled(TreeItem)(({theme}) => ({
color: theme.palette.text.secondary,
[`& .${treeItemClasses.content}`]: {
color: theme.palette.text.secondary,
borderTopRightRadius: theme.spacing(1),
borderBottomRightRadius: theme.spacing(1),
paddingRight: theme.spacing(1),
fontWeight: theme.typography.fontWeightMedium,
'&.Mui-expanded': {
fontWeight: theme.typography.fontWeightRegular,
},
'&:hover': {
backgroundColor: theme.palette.action.hover,
},
'&.Mui-focused, &.Mui-selected, &.Mui-selected.Mui-focused': {
backgroundColor: `var(--tree-view-bg-color, ${theme.palette.action.selected})`,
color: 'var(--tree-view-color)',
},
[`& .${treeItemClasses.label}`]: {
fontWeight: 'inherit',
color: 'inherit',
},
}
}))
/**
* Styled tree item for the files tree.
*/
export function StyledTreeItem(
{bgColor, color, labelIcon: LabelIcon, rightContent, labelText, contextMenuItems, ...other}: StyledTreeItemProps
) {
const {
contextMenu,
handleContextMenu,
handleClose
} = useContextMenu()
return (
<>
<StyledTreeItemRoot
label={
<div
style={{'cursor': contextMenuItems ? 'context-menu' : 'default'}}
onContextMenu={contextMenuItems ? handleContextMenu : undefined}
onDoubleClick={contextMenuItems ? handleContextMenu : undefined}
>
<Box sx={{
display: 'flex', alignItems: 'center', p: 0.5, pr: 0,
height: '100%',
textOverflow: 'ellipsis',
whiteSpace: 'nowrap',
}}>
{LabelIcon && <LabelIcon color="inherit" sx={{mr: 1}}/>}
<Typography variant="body2" sx={{fontWeight: 'inherit'}}>
{labelText}
</Typography>
<Box>{rightContent}</Box>
</Box>
</div>
}
style={{
'--tree-view-color': color,
'--tree-view-bg-color': bgColor
}}
{...other}
/>
{
contextMenuItems &&
<Menu
open={contextMenu !== null}
onClose={handleClose}
anchorReference="anchorPosition"
anchorPosition={
contextMenu !== null
? {top: contextMenu.mouseY, left: contextMenu.mouseX}
: undefined
}
>
<ContextMenuItems items={contextMenuItems} handleClose={handleClose}/>
</Menu>
}
</>
)
}
/**
* Context menu items.
*
* @param items items to display in the context menu
* @param handleClose close handler of the context menu
*/
function ContextMenuItems({items, handleClose}: { items: ContextMenuItem[], handleClose: () => void }) {
return (
<>
{
items.map((item) => (
item.nestedItems
? <NestedMenuItem
leftIcon={<item.icon color={"primary"}/>}
rightIcon={<ArrowRightIcon/>}
label={item.label}
key={item.label}
parentMenuOpen={true}
sx={{pl: 2}}
>
<ContextMenuItems items={item.nestedItems} handleClose={handleClose}/>
</NestedMenuItem>
: <MenuItem key={item.label} onClick={(event) => {
handleClose()
if (item.onClick)
item.onClick(event)
}}>
<ListItemIcon>
{item.icon && <item.icon color={"primary"}/>}
</ListItemIcon>
<ListItemText>{item.label}</ListItemText>
</MenuItem>
))
}
</>
)
} |
import '/components/create_photo_string/create_photo_string_widget.dart';
import '/flutter_flow/flutter_flow_theme.dart';
import '/flutter_flow/flutter_flow_util.dart';
import '/flutter_flow/flutter_flow_widgets.dart';
import 'package:flutter/material.dart';
import 'package:google_fonts/google_fonts.dart';
import 'package:provider/provider.dart';
import 'binding_done_model.dart';
export 'binding_done_model.dart';
class BindingDoneWidget extends StatefulWidget {
const BindingDoneWidget({Key? key}) : super(key: key);
@override
_BindingDoneWidgetState createState() => _BindingDoneWidgetState();
}
class _BindingDoneWidgetState extends State<BindingDoneWidget> {
late BindingDoneModel _model;
@override
void setState(VoidCallback callback) {
super.setState(callback);
_model.onUpdate();
}
@override
void initState() {
super.initState();
_model = createModel(context, () => BindingDoneModel());
WidgetsBinding.instance.addPostFrameCallback((_) => setState(() {}));
}
@override
void dispose() {
_model.maybeDispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
context.watch<FFAppState>();
return Column(
mainAxisSize: MainAxisSize.max,
children: [
Align(
alignment: AlignmentDirectional(0.00, 0.00),
child: Padding(
padding: EdgeInsetsDirectional.fromSTEB(16.0, 16.0, 16.0, 16.0),
child: InkWell(
splashColor: Colors.transparent,
focusColor: Colors.transparent,
hoverColor: Colors.transparent,
highlightColor: Colors.transparent,
onTap: () async {
logFirebaseEvent('BINDING_DONE_Container_uf8v421e_ON_TAP');
logFirebaseEvent('Container_bottom_sheet');
await showModalBottomSheet(
isScrollControlled: true,
backgroundColor: Colors.transparent,
barrierColor: Color(0x00000000),
context: context,
builder: (context) {
return Padding(
padding: MediaQuery.viewInsetsOf(context),
child: CreatePhotoStringWidget(),
);
},
).then((value) => safeSetState(() {}));
},
child: Container(
width: 352.0,
height: 181.0,
constraints: BoxConstraints(
maxWidth: 400.0,
maxHeight: 900.0,
),
decoration: BoxDecoration(
color: FlutterFlowTheme.of(context).secondaryBackground,
borderRadius: BorderRadius.circular(13.0),
),
child: Column(
mainAxisSize: MainAxisSize.max,
children: [
Padding(
padding:
EdgeInsetsDirectional.fromSTEB(0.0, 25.0, 0.0, 0.0),
child: Text(
'Карта привязана и выдана',
style: FlutterFlowTheme.of(context).bodyMedium.override(
fontFamily: 'Montserrat',
fontSize: 18.0,
letterSpacing: 0.18,
lineHeight: 1.1,
),
),
),
Padding(
padding:
EdgeInsetsDirectional.fromSTEB(10.0, 10.0, 10.0, 0.0),
child: Text(
'Вы можете выбрать дополнительные продукты.',
textAlign: TextAlign.center,
style: FlutterFlowTheme.of(context).bodyMedium.override(
fontFamily: 'Montserrat',
fontSize: 12.0,
letterSpacing: 0.18,
lineHeight: 1.1,
),
),
),
Padding(
padding:
EdgeInsetsDirectional.fromSTEB(15.0, 34.0, 15.0, 0.0),
child: Row(
mainAxisSize: MainAxisSize.max,
children: [
Expanded(
child: Padding(
padding: EdgeInsetsDirectional.fromSTEB(
5.0, 0.0, 0.0, 0.0),
child: FFButtonWidget(
onPressed: () async {
logFirebaseEvent(
'BINDING_DONE_COMP_ГОТОВО_BTN_ON_TAP');
logFirebaseEvent(
'Button_close_dialog,_drawer,_etc');
Navigator.pop(context);
},
text: 'ГОТОВО',
options: FFButtonOptions(
width: double.infinity,
height: 40.0,
padding: EdgeInsetsDirectional.fromSTEB(
0.0, 0.0, 0.0, 0.0),
iconPadding: EdgeInsetsDirectional.fromSTEB(
0.0, 0.0, 0.0, 0.0),
color: Color(0xFF4460F0),
textStyle: FlutterFlowTheme.of(context)
.titleSmall
.override(
fontFamily: 'Montserrat',
color: FlutterFlowTheme.of(context)
.primaryBtnText,
fontSize: 12.0,
fontWeight: FontWeight.bold,
),
elevation: 0.0,
borderSide: BorderSide(
color: Colors.transparent,
width: 1.0,
),
borderRadius: BorderRadius.circular(50.0),
),
),
),
),
],
),
),
],
),
),
),
),
),
],
);
}
} |
//
// DO NOT REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
//
// @Authors:
// timop
//
// Copyright 2004-2012 by OM International
//
// This file is part of OpenPetra.org.
//
// OpenPetra.org is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// OpenPetra.org is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with OpenPetra.org. If not, see <http://www.gnu.org/licenses/>.
//
using System;
using System.IO;
using System.Xml;
using GNU.Gettext;
using Ict.Common;
using System.Windows.Forms;
namespace Ict.Common.IO
{
/// <summary>
/// support data liberation;
/// see also http://sourceforge.net/apps/mediawiki/openpetraorg/index.php?title=Data_liberation
///
/// </summary>
public class TImportExportDialogs
{
/// <summary>
/// Select a path and filename for file export
/// </summary>
/// <param name="ADialogTitle"></param>
/// <returns>Local path, or empty sctring if no path selected.</returns>
public static String GetExportFilename(string ADialogTitle)
{
SaveFileDialog DialogSave = new SaveFileDialog();
DialogSave.DefaultExt = "yml";
DialogSave.Filter = Catalog.GetString(
"Text file (*.yml)|*.yml|XML file (*.xml)|*.xml|Petra export (*.ext)|*.ext|Spreadsheet file (*.csv)|*.csv");
DialogSave.AddExtension = true;
DialogSave.RestoreDirectory = true;
DialogSave.Title = ADialogTitle;
if (DialogSave.ShowDialog() == DialogResult.OK)
{
return DialogSave.FileName.ToLower();
}
else
{
return String.Empty;
}
}
/// <summary>
/// Put this (ext formatted) string onto a file
/// </summary>
/// <param name="doc"></param>
/// <param name="FileName"></param>
/// <returns></returns>
public static bool ExportTofile(string doc, string FileName)
{
if (FileName.EndsWith("ext"))
{
StreamWriter outfile = new StreamWriter(FileName);
outfile.Write(doc);
outfile.Close();
return true;
}
return false;
}
/// <summary>
/// Put this (XML formatted) data on a local file
/// </summary>
/// <param name="doc">XML data to be exported</param>
/// <param name="FileName">Filename from GetExportFilename, above</param>
/// <returns>true if successful</returns>
public static bool ExportTofile(XmlDocument doc, string FileName)
{
if (FileName.EndsWith("xml"))
{
doc.Save(FileName);
return true;
}
else if (FileName.EndsWith("csv"))
{
return TCsv2Xml.Xml2Csv(doc, FileName);
}
else if (FileName.EndsWith("yml"))
{
return TYml2Xml.Xml2Yml(doc, FileName);
}
return false;
}
/// <summary>
/// Export data to a range of different file formats;
/// ask the user for filename and file format
///
/// NOTE this has been replaced by the two-part scheme above:
/// first get the filename, then the caller loads the data
/// from the server, then it calls ExportToFile.
/// </summary>
/// <param name="doc"></param>
/// <param name="ADialogTitle"></param>
/// <returns></returns>
public static bool ExportWithDialog(XmlDocument doc, string ADialogTitle)
{
// TODO: TExcel excel = new TExcel();
// TODO: openoffice
// See also http://sourceforge.net/apps/mediawiki/openpetraorg/index.php?title=Data_liberation
SaveFileDialog DialogSave = new SaveFileDialog();
DialogSave.DefaultExt = "yml";
DialogSave.Filter = Catalog.GetString(
"Text file (*.yml)|*.yml|XML file (*.xml)|*.xml|Petra export (*.ext)|*.ext|Spreadsheet file (*.csv)|*.csv");
DialogSave.AddExtension = true;
DialogSave.RestoreDirectory = true;
DialogSave.Title = ADialogTitle;
if (DialogSave.ShowDialog() == DialogResult.OK)
{
if (DialogSave.FileName.ToLower().EndsWith("xml"))
{
doc.Save(DialogSave.FileName);
return true;
}
else if (DialogSave.FileName.ToLower().EndsWith("ext"))
{
return false;
}
else if (DialogSave.FileName.ToLower().EndsWith("csv"))
{
return TCsv2Xml.Xml2Csv(doc, DialogSave.FileName);
}
else if (DialogSave.FileName.ToLower().EndsWith("yml"))
{
return TYml2Xml.Xml2Yml(doc, DialogSave.FileName);
}
}
return false;
}
/// <summary>
/// export zipped yml string to file.
/// ask the user for filename
/// </summary>
/// <param name="AZippedYML"></param>
/// <param name="ADialogTitle"></param>
/// <returns></returns>
public static bool ExportWithDialogYMLGz(string AZippedYML, string ADialogTitle)
{
SaveFileDialog DialogSave = new SaveFileDialog();
DialogSave.DefaultExt = "yml.gz";
DialogSave.Filter = Catalog.GetString("Zipped Text file (*.yml.gz)|*.yml.gz");
DialogSave.AddExtension = true;
DialogSave.RestoreDirectory = true;
DialogSave.Title = ADialogTitle;
if (DialogSave.ShowDialog() == DialogResult.OK)
{
string filename = DialogSave.FileName;
// it seems there was a bug that only .gz was added if user did not type the extension
if (!filename.EndsWith(".yml.gz"))
{
filename = Path.GetFileNameWithoutExtension(filename) + ".yml.gz";
}
FileStream fs = new FileStream(filename, FileMode.Create);
byte[] buffer = Convert.FromBase64String(AZippedYML);
fs.Write(buffer, 0, buffer.Length);
fs.Close();
return true;
}
return false;
}
/// <summary>
/// import a zipped yml file.
/// if the file is a plain yml file, the content will be zipped.
/// shows a dialog to the user to select the file to import
/// </summary>
/// <returns></returns>
public static string ImportWithDialogYMLGz(string ADialogTitle)
{
OpenFileDialog DialogOpen = new OpenFileDialog();
DialogOpen.Filter = Catalog.GetString(
"Zipped Text file (*.yml.gz)|*.yml.gz|Text file (*.yml)|*.yml");
DialogOpen.FilterIndex = 0;
DialogOpen.RestoreDirectory = true;
DialogOpen.Title = ADialogTitle;
if (DialogOpen.ShowDialog() == DialogResult.OK)
{
if (DialogOpen.FileName.ToLower().EndsWith("gz"))
{
FileStream fs = new FileStream(DialogOpen.FileName, FileMode.Open);
byte[] buffer = new byte[fs.Length];
fs.Read(buffer, 0, buffer.Length);
fs.Close();
return Convert.ToBase64String(buffer);
}
else if (DialogOpen.FileName.ToLower().EndsWith("yml"))
{
TextReader reader = new StreamReader(DialogOpen.FileName, true);
string ymlData = reader.ReadToEnd();
reader.Close();
return PackTools.ZipString(ymlData);
}
}
return null;
}
/// <summary>
/// convert from all sorts of formats into xml document;
/// shows a dialog to the user to select the file to import
/// </summary>
/// <returns></returns>
public static XmlDocument ImportWithDialog(string ADialogTitle)
{
string temp;
return ImportWithDialog(ADialogTitle, out temp);
}
/// <summary>
/// convert from all sorts of formats into xml document;
/// shows a dialog to the user to select the file to import
/// </summary>
/// <returns></returns>
public static XmlDocument ImportWithDialog(string ADialogTitle, out string AFilename)
{
// TODO support import from Excel and OpenOffice files
// See also http://sourceforge.net/apps/mediawiki/openpetraorg/index.php?title=Data_liberation
OpenFileDialog DialogOpen = new OpenFileDialog();
AFilename = "";
DialogOpen.Filter = Catalog.GetString(
"Text file (*.yml)|*.yml|XML file (*.xml)|*.xml|Spreadsheet file (*.csv)|All supported file formats (*.yml, *.xml, *.csv)|*.csv;*.yml;*.xml|");
DialogOpen.FilterIndex = 4;
DialogOpen.RestoreDirectory = true;
DialogOpen.Title = ADialogTitle;
if (DialogOpen.ShowDialog() == DialogResult.OK)
{
AFilename = DialogOpen.FileName;
if (DialogOpen.FileName.ToLower().EndsWith("csv"))
{
// select separator, make sure there is a header line with the column captions/names
TDlgSelectCSVSeparator dlgSeparator = new TDlgSelectCSVSeparator(true);
Boolean fileCanOpen = dlgSeparator.OpenCsvFile(DialogOpen.FileName);
if (!fileCanOpen)
{
throw new Exception(String.Format(Catalog.GetString("File {0} Cannot be opened."), DialogOpen.FileName));
}
if (dlgSeparator.ShowDialog() == DialogResult.OK)
{
XmlDocument doc = TCsv2Xml.ParseCSV2Xml(DialogOpen.FileName, dlgSeparator.SelectedSeparator);
return doc;
}
}
else if (DialogOpen.FileName.ToLower().EndsWith("xml"))
{
XmlDocument doc = new XmlDocument();
doc.Load(DialogOpen.FileName);
return doc;
}
else if (DialogOpen.FileName.ToLower().EndsWith("yml"))
{
TYml2Xml yml = new TYml2Xml(DialogOpen.FileName);
return yml.ParseYML2XML();
}
}
return null;
}
}
} |
import React from 'react'
import { TextInputStyled } from './TextInput.Styles'
const TextInput = ({
label,
type,
name,
register,
errors,
value,
required,
pattern,
maxLength,
subname,
placeholder,
}) => {
const Regex = new RegExp(pattern)
const propForm = subname ? `${subname}.${name}` : name
return (
<>
<TextInputStyled>
{label}
<input
value={value}
placeholder={placeholder}
type={type}
{...register(propForm, {
required: required,
maxLength: maxLength || false,
pattern: Regex,
})}
/>
{errors[name]?.type === `required` && type !== 'radio' && (
<span>{`* Este campo es requerido`}</span>
)}
{errors[name]?.type === `maxLength` && (
<span>{`Este campo acepta como maximo 15 digitos`}</span>
)}
{errors[name]?.type === `pattern` && (
<span>{`El formato de este campo es incorrecto`}</span>
)}
</TextInputStyled>
</>
)
}
export default TextInput |
import sets.algebra categories.subobj categories.subcat categories.examples
categories.strict_cat categories.diagrams
universes v v' u u' w
hott_theory
namespace hott
open hott.eq hott.is_trunc hott.trunc hott.is_equiv hott.set hott.subset
hott.precategories hott.categories hott.categories.strict
/- We introduce limits of diagrams mapped to categories, by using cones to
pick the universal object and encode the universal property.
As far as possible we copy the mathlib-code in [category_theory.limits]. -/
namespace categories.limits
set_option pp.universes true
@[hott]
structure cone {J : Type _} [is_strict_cat J] {C : Type _}
[is_precat C] (F : J ⥤ C) :=
(X : C)
(π : (@constant_functor J _ C _ X) ⟹ F)
@[hott, reducible, hsimp]
def cone.leg {J : Type _} [is_strict_cat J] {C : Type _}
[is_precat C] {F : J ⥤ C} (cF : cone F) :
Π j : J, cF.X ⟶ F.obj j :=
begin intro j, exact cF.π.app j end
@[hott]
def cone.fac {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] {F : J ⥤ C} (s : cone F) :
∀ {j k : J} (f : j ⟶ k), s.π.app j ≫ F.map f = s.π.app k :=
begin intros j k f, rwr <- s.π.naturality f, hsimp end
@[hott]
def cone_eq {J : Type _} [is_strict_cat J] {C : Type _}
[is_precat C] {F : J ⥤ C} (cF₁ cF₂ : cone F) :
Π (p : cF₁.X = cF₂.X), (Π j : J, cone.leg cF₁ j =[p; λ c, c ⟶ F.obj j]
cone.leg cF₂ j) -> cF₁ = cF₂ :=
begin
hinduction cF₁ with X₁ π₁, hinduction cF₂ with X₂ π₂,
intro vertex_eq, change X₁ = X₂ at vertex_eq, hinduction vertex_eq,
intro legs_eq,
fapply apd011 cone.mk, exact idp, apply pathover_idp_of_eq,
hinduction π₁ with app₁ nat₁, hinduction π₂ with app₂ nat₂,
fapply apd011 nat_trans.mk,
{ apply eq_of_homotopy, intro j, exact eq_of_pathover_idp (legs_eq j) },
{ apply pathover_of_tr_eq, exact is_prop.elim _ _ }
end
@[hott]
structure cone_map {J : Type _} [is_strict_cat J] {C : Type _}
[is_precat C] {F : J ⥤ C} (cF₁ cF₂ : cone F) :=
(v_lift : cF₁.X ⟶ cF₂.X)
(fac : Π (j : J), v_lift ≫ cF₂.π.app j = cF₁.π.app j)
@[hott]
def cone_map_eq {J : Type _} [is_strict_cat J] {C : Type _}
[is_precat C] {F : J ⥤ C} {cF₁ cF₂ : cone F} (m₁ m₂ : cone_map cF₁ cF₂) :
m₁.v_lift = m₂.v_lift -> m₁ = m₂ :=
begin
hinduction m₁ with vl₁ fac₁, hinduction m₂ with vl₂ fac₂, intro v_lift_eq,
fapply apd011, exact v_lift_eq,
apply pathover_of_tr_eq, apply eq_of_homotopy, intro j, exact is_prop.elim _ _
end
@[hott]
structure is_limit {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] {F : J ⥤ C} (t : cone F) :=
(lift : Π (s : cone F), cone_map s t)
(uniq : ∀ (s : cone F) (m : cone_map s t), m.v_lift = (lift s).v_lift)
@[hott, instance]
def is_limit_is_prop {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] {F : J ⥤ C} (t : cone F) : is_prop (is_limit t) :=
begin
apply is_prop.mk, intros l₁ l₂,
hinduction l₁ with lift₁ uniq₁, hinduction l₂ with lift₂ uniq₂,
fapply apd011,
{ apply eq_of_homotopy, intro s, apply cone_map_eq, exact uniq₂ s (lift₁ s) },
{ apply pathover_of_tr_eq, apply eq_of_homotopy2, intros s m, exact is_prop.elim _ _ }
end
@[hott]
def lift_itself_id {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] {F : J ⥤ C} {t : cone F} (l : is_limit t) :
(l.lift t).v_lift = 𝟙 t.X :=
have t_fac : ∀ j : J, 𝟙 t.X ≫ t.π.app j = t.π.app j, by intro j; hsimp,
(l.uniq _ (cone_map.mk (𝟙 t.X) t_fac))⁻¹
@[hott]
def limit_cone_point_iso {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] {F : J ⥤ C} {s t : cone F} (lₛ : is_limit s)
(lₜ : is_limit t) : Σ i : s.X ≅ t.X, i.hom = (lₜ.lift s).v_lift :=
let st := (lₜ.lift s).v_lift, ts := (lₛ.lift t).v_lift in
have s_fac : ∀ j : J, (st ≫ ts) ≫ s.π.app j = s.π.app j, from assume j,
calc (st ≫ ts) ≫ s.π.app j = st ≫ (ts ≫ s.π.app j) : is_precat.assoc _ _ _
... = st ≫ t.π.app j : by rwr (lₛ.lift t).fac j
... = s.π.app j : by rwr (lₜ.lift s).fac j,
have t_fac : ∀ j : J, (ts ≫ st) ≫ t.π.app j = t.π.app j, from assume j,
calc (ts ≫ st) ≫ t.π.app j = ts ≫ (st ≫ t.π.app j) : is_precat.assoc _ _ _
... = ts ≫ s.π.app j : by rwr (lₜ.lift s).fac j
... = t.π.app j : by rwr (lₛ.lift t).fac j,
have comp_s : st ≫ ts = 𝟙 s.X, from (lₛ.uniq _ (cone_map.mk _ s_fac)) ⬝ lift_itself_id lₛ,
have comp_t : ts ≫ st = 𝟙 t.X, from (lₜ.uniq _ (cone_map.mk _ t_fac)) ⬝ lift_itself_id lₜ,
⟨iso.mk st (is_iso.mk ts comp_t comp_s), rfl⟩
/- `limit_cone F` contains a cone over `F` together with the information that
it is a limit. -/
@[hott]
structure limit_cone {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] (F : J ⥤ C) :=
(cone : cone F)
(is_limit : is_limit cone)
@[hott]
def limit_cone_eq {J : Type _} [is_strict_cat J]
{C : Type u} [is_precat.{v} C] (F : J ⥤ C) (lc₁ lc₂ : limit_cone F) :
lc₁.cone = lc₂.cone -> lc₁ = lc₂ :=
begin
hinduction lc₁ with cone₁ is_limit₁, hinduction lc₂ with cone₂ is_limit₂,
intro cone_eq, fapply apd011, exact cone_eq,
apply pathover_of_tr_eq, exact is_prop.elim _ _
end
/- `has_limit F` represents the mere existence of a limit for `F`. This allows
to define it as a class with instances. -/
@[hott]
class has_limit {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] (F : J ⥤ C) :=
mk' :: (exists_limit : ∥limit_cone F∥)
@[hott]
def has_limit.mk {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] {F : J ⥤ C} (d : limit_cone F) :=
has_limit.mk' (tr d)
@[hott]
def has_limit_eq {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] {F : J ⥤ C} (hlF₁ hlF₂ : has_limit F) : hlF₁ = hlF₂ :=
begin
hinduction hlF₁ with el₁, hinduction hlF₂ with el₂,
apply ap has_limit.mk', exact is_prop.elim _ _
end
@[hott, instance]
def has_limit_is_prop {J : Type _} [is_strict_cat J] {C : Type u}
[is_precat.{v} C] {F : J ⥤ C} : is_prop (has_limit F) :=
is_prop.mk has_limit_eq
/- If `C` is a category, the limit cone vertices of two instances of
`limit_cone F` are equal since they are determined up to isomorphism. Then
the "legs" of the cones and the lifts of the universal property are
determined up to composition with the automorphism associated to this
equality of limit cone points, and limit cones are equal.
Thus, we can produce a `limit_cone F` from `has_limit F`. -/
@[hott]
def limit_cone_is_unique {J : Type _} [is_strict_cat J]
{C : Type u} [is_cat.{v} C] (F : J ⥤ C) :
∀ lc₁ lc₂ : limit_cone F, lc₁ = lc₂ :=
begin
intros lc₁ lc₂,
hinduction lc₁ with cone₁ is_limit₁, hinduction lc₂ with cone₂ is_limit₂,
let lcp_iso := limit_cone_point_iso is_limit₁ is_limit₂,
apply limit_cone_eq, fapply cone_eq,
{ exact idtoiso⁻¹ᶠ lcp_iso.1 },
{ intro j, apply pathover_of_tr_eq,
change idtoiso⁻¹ᶠ lcp_iso.1 ▸[λ c : C, c ⟶ F.obj j] (cone.leg cone₁ j) = _,
apply eq.concat (@iso_hom_tr_comp (Category.mk C _) _ _ _ lcp_iso.1 (cone.leg cone₁ j)),
apply inverse, apply iso_move_lr,
have p : lcp_iso.fst.hom = (is_limit₂.lift cone₁).v_lift, from lcp_iso.2,
rwr p, exact (is_limit₂.lift _).fac _ }
end
@[hott, instance]
def limit_cone_is_prop {J : Type _} [is_strict_cat J] {C : Type u}
[is_cat.{v} C] (F : J ⥤ C) : is_trunc -1 (limit_cone F) :=
is_prop.mk (limit_cone_is_unique F)
@[hott]
def get_limit_cone {J : Type _} [is_strict_cat J] {C : Type u}
[is_cat.{v} C] (F : J ⥤ C) [has_limit F] : limit_cone F :=
untrunc_of_is_trunc (has_limit.exists_limit F)
@[hott]
def limit.cone {J : Type _} [is_strict_cat J] {C : Type u}
[is_cat.{v} C] (F : J ⥤ C) [has_limit F] : cone F :=
(get_limit_cone F).cone
@[hott]
def limitcone_is_limit {J : Type _} [is_strict_cat J] {C : Type u}
[is_cat.{v} C] (F : J ⥤ C) [has_limit F] : is_limit (limit.cone F) :=
(get_limit_cone F).is_limit
@[hott]
def limit {J : Type _} [is_strict_cat J] {C : Type u} [is_cat.{v} C]
(F : J ⥤ C) [has_limit F] : C := (limit.cone F).X
@[hott]
def limit_leg {J : Type _} [is_strict_cat J] {C : Type u}
[is_cat.{v} C] (F : J ⥤ C) [has_limit F] (j : J) :
limit F ⟶ F.obj j := (limit.cone F).π.app j
@[hott]
def diag_eq_lim_eq_lim {J : strict_Category} {C : Type u} [is_cat.{v} C]
{F F' : J.obj ⥤ C} (p : F = F') [hlF : has_limit F] [hlF' : has_limit F'] :
@limit _ _ _ _ F hlF = @limit _ _ _ _ F' hlF' :=
apd011 (@limit _ _ _ _) p (pathover_of_tr_eq (has_limit_eq _ _))
@[hott]
def diag_inv_eq_lim_eq {J : strict_Category} {C : Type u} [is_cat.{v} C]
{F F' : J.obj ⥤ C} (p : F = F') [hlF : has_limit F] [hlF' : has_limit F'] :
(diag_eq_lim_eq_lim p⁻¹) = (diag_eq_lim_eq_lim p)⁻¹ :=
begin
change apd011 _ _ _ = eq.inverse (apd011 _ _ _),
rwr apd011_inv, apply ap (apd011 limit p⁻¹), rwr pathover_of_tr_eq_inv,
apply ap pathover_of_tr_eq, exact is_prop.elim _ _
end
@[hott]
def diag_eq_leg_eq {J : strict_Category} {C : Type _} [is_cat C]
{F F' : J.obj ⥤ C} (p : F = F') (j : J) [hlF : has_limit F] [hlF' : has_limit F'] :
limit_leg F' j = ((ap (λ F : J.obj ⥤ C, F.obj j) p) ▸[λ d : C, limit F' ⟶ d]
((diag_eq_lim_eq_lim p) ▸[λ c : C, c ⟶ F.obj j] limit_leg F j)) :=
@tr_tr_fn2_fn2_fn _ C C _ _ p _ _ _ (pathover_of_tr_eq (has_limit_eq (p ▸ hlF) hlF'))
(λ d e : C, d ⟶ e) _ _ (λ (F : J.obj ⥤ C) (hlF : has_limit F),
@limit_leg _ _ _ _ F hlF j)
/- Limits of diagrams of shapes with a functor between them are not necessarily naturally
mapped to each other. But limits of diagrams of isomorphic shapes are naturally
isomorphic and hence equal. -/
@[hott]
def cone_to_diag_iso_cone {J₁ J₂ : Strict_Categories} {C : Type u} [is_cat.{v} C]
(H : J₁ ≅ J₂) {F : J₂.obj ⥤ C} (cF : cone F) : cone (H.hom ⋙ F) :=
begin
revert J₁ J₂ H F cF,
apply @iso_ind _ (λ {J₁ J₂ : Strict_Categories} H, Π {F : J₂.obj ⥤ C},
cone F → cone (H.hom ⋙ F)),
intros J₁ F cF, fapply cone.mk, exact cF.X,
fapply nat_trans.mk, exact cF.π.app, exact cF.π.naturality
end
@[hott]
def diag_id_iso_cone {J : Strict_Categories} {C : Type u} [is_cat.{v} C]
{F : J.obj ⥤ C} (cF : cone F) :
cone_to_diag_iso_cone (id_iso J) cF =
cone.mk cF.X (nat_trans.mk cF.π.app cF.π.naturality) :=
begin
change @iso_ind _ (λ {J₁ J₂ : Strict_Categories} H, Π {F : J₂.obj ⥤ C},
cone F → cone (H.hom ⋙ F)) _ _ _ (id_iso J) F cF = _,
rwr iso_ind_id
end
@[hott]
def diag_id_iso_cone' {J : Strict_Categories} {C : Type u} [is_cat.{v} C]
{F : J.obj ⥤ C} (cF : cone F) :
cone_to_diag_iso_cone (id_iso J) cF = (funct_id_comp F)⁻¹ ▸ cF :=
begin
rwr diag_id_iso_cone,
hinduction cF with X π, hinduction π with app naturality,
change @cone.mk _ _ _ _ ((id_iso J).hom ⋙ F) X (nat_trans.mk app @naturality) =
_ ▸ @cone.mk _ _ _ _ F X (nat_trans.mk app @naturality),
rwr tr_fn3_ev_fn3_tr_tr_ev (λ (F : J.obj ⥤ C) (app : Π j : J.obj, X ⟶ F.obj j)
(naturality : Π (j j' : J.obj) (f : j ⟶ j'), (constant_functor X).map f ≫ app j' = app j ≫ F.map f),
@cone.mk _ _ _ _ F X (nat_trans.mk app naturality)), hsimp,
fapply apd011, exact idp, apply pathover_idp_of_eq,
fapply apd011,
{ apply eq_of_homotopy, intro j, rwr tr_fn_tr_eval,
rwr tr_compose (λ f : J.obj -> C, X ⟶ f j) functor.obj, rwr ap_inv,
have r : funct_id_comp F = functor_eq _ _, from rfl,
rwr r, rwr functor_eq_obj },
{ apply pathover_of_tr_eq, exact is_prop.elim _ _ }
end
@[hott]
def diag_iso_cone_vertex {J₁ J₂ : Strict_Categories} {C : Type u} [is_cat.{v} C]
(H : J₁ ≅ J₂) {F : J₂.obj ⥤ C} (cF : cone F) :
(cone_to_diag_iso_cone H cF).X = cF.X :=
begin
revert J₁ J₂ H F cF,
apply @iso_ind _ (λ {J₁ J₂ : Strict_Categories} H, Π {F : J₂.obj ⥤ C} {cF : cone F},
(cone_to_diag_iso_cone H cF).X = cF.X),
intros J F cF, exact ap cone.X (diag_id_iso_cone cF)
end
@[hott]
def diag_iso_cone_legs {J₁ J₂ : Strict_Categories} {C : Type u} [is_cat.{v} C]
(H : J₁ ≅ J₂) {F : J₂.obj ⥤ C} (cF : cone F) : Π (j₁ : J₁.obj),
(cone.leg (cone_to_diag_iso_cone H cF) j₁) =
((diag_iso_cone_vertex H cF)⁻¹ ▸[λ c : C, c ⟶ F.obj (H.hom.obj j₁)]
cone.leg cF (H.hom.obj j₁)) :=
begin
revert J₁ J₂ H F cF,
apply @iso_ind _ (λ {J₁ J₂ : Strict_Categories} H, Π {F : J₂.obj ⥤ C} {cF : cone F},
Π (j₁ : J₁.obj), (cone.leg (cone_to_diag_iso_cone H cF) j₁) =
((diag_iso_cone_vertex H cF)⁻¹ ▸[λ c : C, c ⟶ F.obj (H.hom.obj j₁)]
cone.leg cF (H.hom.obj j₁))),
intros J₁ F cF j₁,
apply @eq_inv_tr_of_tr_eq _ (λ c : C, c ⟶ ((id_iso J₁).hom ⋙ F).obj j₁) _ _
((diag_iso_cone_vertex (id_iso J₁) cF))
(cone.leg (cone_to_diag_iso_cone (id_iso J₁) cF) j₁)
(cone.leg cF (functor.obj (id_iso J₁).hom j₁)),
change _ = cone.leg cF j₁,
change (@iso_ind _ (λ {J₁ J₂ : Strict_Categories} H, Π {F : J₂.obj ⥤ C}
{cF : cone F}, (cone_to_diag_iso_cone H cF).X = cF.X) _ _ _ (id_iso J₁) F cF) ▸ _ = _,
rwr iso_ind_id, rwr tr_ap, apply tr_eq_of_pathover,
exact apd (λ cHF : cone ((id_iso J₁).hom ⋙ F),
@cone.leg _ _ _ _ ((id_iso J₁).hom ⋙ F) cHF j₁) (diag_id_iso_cone cF)
end
@[hott]
def diag_iso_cone_legs_fac {J₁ J₂ : Strict_Categories} {C : Type u} [is_cat.{v} C]
(H : J₁ ≅ J₂) {F : J₂.obj ⥤ C} (cF : cone F) : Π (j₁ : J₁.obj),
((idtoiso (diag_iso_cone_vertex H cF)).hom ≫ cone.leg cF (H.hom.obj j₁)) =
cone.leg (cone_to_diag_iso_cone H cF) j₁ :=
begin intro j₁, rwr diag_iso_cone_legs, rwr id_hom_tr_comp, rwr id_inv_iso_inv end
@[hott]
def diag_iso_cone_is_lim {J₁ J₂ : strict_Category} {C : Type u} [is_cat.{v} C]
(H : J₁ ≅ J₂) {F : J₂.obj ⥤ C} {cF : cone F} (is_lim : is_limit cF) :
is_limit (cone_to_diag_iso_cone H cF) :=
begin
revert J₁ J₂ H F cF,
apply @iso_ind _ (λ {J₁ J₂ : Strict_Categories} H, Π {F : J₂.obj ⥤ C} {cF : cone F},
is_limit cF → is_limit (cone_to_diag_iso_cone H cF)),
intros J₁ F cF lcF, rwr diag_id_iso_cone', apply transportD, exact lcF,
end
@[hott, instance]
def diag_iso_has_lim_to_has_lim {J₁ J₂ : strict_Category} {C : Type u} [is_cat.{v} C]
(H : J₁ ≅ J₂) {F : J₂.obj ⥤ C} [hlF : has_limit F] : has_limit (H.hom ⋙ F) :=
begin
apply has_limit.mk, fapply limit_cone.mk,
exact cone_to_diag_iso_cone H (limit.cone F),
exact diag_iso_cone_is_lim H (limitcone_is_limit _)
end
@[hott]
def diag_iso_lim_eq_lim {J₁ J₂ : strict_Category} {C : Type u} [is_cat.{v} C]
(H : J₁ ≅ J₂) (F : J₂.obj ⥤ C) [hlF : has_limit F] :
@limit _ _ _ _ (H.hom ⋙ F) (diag_iso_has_lim_to_has_lim H) = limit F :=
begin
change (cone_to_diag_iso_cone H (limit.cone F)).X = (limit.cone F).X,
exact (diag_iso_cone_vertex H (limit.cone F))
end
@[hott]
def diag_iso_lim_legs_eq {J₁ J₂ : strict_Category} {C : Type u} [is_cat.{v} C]
(H : J₁ ≅ J₂) (F : J₂.obj ⥤ C) [hlF : has_limit F] :
Π (j₁ : J₁), (idtoiso (diag_iso_lim_eq_lim H F)).hom ≫ limit_leg F (H.hom.obj j₁) =
limit_leg (H.hom ⋙ F) j₁ :=
assume j₁, diag_iso_cone_legs_fac H (limit.cone F) j₁
/- More general classes of existence of limits -/
@[hott]
class has_limits_of_shape (J : Type _) [is_strict_cat J]
(C : Type u) [is_cat.{v} C] :=
(has_limit : Π F : J ⥤ C, has_limit F)
@[hott, instance]
def has_lims_shape_is_prop (J : Type _) [is_strict_cat J] (C : Type u) [is_cat.{v} C] :
is_prop (has_limits_of_shape J C) :=
begin
apply is_prop.mk, intros hls₁ hls₂, hinduction hls₁, hinduction hls₂,
apply ap has_limits_of_shape.mk, exact is_prop.elim _ _
end
@[hott, priority 100]
instance has_limit_of_has_limits_of_shape
{J : Type _} [is_strict_cat J] (C : Type u) [is_cat.{v} C]
[has_limits_of_shape J C] (F : J ⥤ C) : has_limit F :=
has_limits_of_shape.has_limit F
@[hott]
class has_limits (C : Type u) [is_cat.{v} C] :=
(has_limit_of_shape : Π {J : Type _} [is_strict_cat J],
has_limits_of_shape J C )
@[hott]
class has_product {C : Type u} [is_cat.{v} C] {J : Set.{u'}}
(f : J -> C) := (has_limit : has_limit (discrete.functor f))
@[hott, priority 100]
instance has_limit_of_has_product {C : Type u} [is_cat.{v} C]
{J : Set.{u'}} (f : J -> C) [has_product f] : has_limit (discrete.functor f) :=
has_product.has_limit f
@[hott]
abbreviation pi_obj {C : Type u} [is_cat.{v} C] {J : Set.{u'}} (f : J → C)
[has_product f] := limit (discrete.functor f)
notation `∏ ` f:20 := pi_obj f
@[hott]
class has_products (C : Type u) [is_cat.{v} C] :=
(has_limit_of_shape : Π J : Set.{u'}, has_limits_of_shape (discrete J) C)
@[hott, instance, priority 100]
def has_limits_of_shape_of_has_products
(J : Set.{u'}) (C : Type u) [is_cat.{v} C] [has_products.{v u u'} C] :
has_limits_of_shape (discrete J) C :=
has_products.has_limit_of_shape C J
@[hott]
instance has_product_of_has_products {C : Type u} [is_cat.{v} C]
[has_products C] {J : Set.{u'}} (f : J -> C) : has_product f :=
⟨@has_limits_of_shape.has_limit _ _ _ _
(has_products.has_limit_of_shape C J) (discrete.functor f)⟩
@[hott, instance]
def has_product_of_has_limits_of_shape {C : Type u} [is_cat.{v} C]
{J : Set.{u'}} [has_limits_of_shape (discrete J) C] (f : J -> C) :
has_product f :=
⟨has_limits_of_shape.has_limit (discrete.functor f)⟩
@[hott, instance]
def has_products_of_has_limits (C : Type u) [is_cat.{v} C] [c : has_limits C] :
has_products C :=
has_products.mk (λ J, @has_limits.has_limit_of_shape C _ c (discrete J) _)
/-- A fan over `f : J → C` consists of a collection of maps from an object `P`
to every `f j`. This is enough to determine a cone which factorizes through
the product. -/
@[hott]
abbreviation fan {J : Set.{u'}} {C : Type u} [is_cat.{v} C] (f : J → C) :=
cone (discrete.functor f)
@[hott, hsimp]
def fan.mk {J : Set.{u'}} (C : Type u) [is_cat.{v} C] {f : J → C} {P : C}
(p : Π j, P ⟶ f j) : fan f :=
cone.mk P (discrete.nat_trans p)
@[hott, hsimp]
def pi.lift {J : Set.{u'}} {C : Type u} [is_cat.{v} C] {f : J → C} [has_product f]
{P : C} (p : Π j, P ⟶ f j) : P ⟶ ∏ f :=
((get_limit_cone (discrete.functor f)).is_limit.lift (fan.mk _ p)).v_lift
@[hott, hsimp]
def pi.π {J : Set.{u'}} {C : Type u} [is_cat.{v} C] (f : J → C) [has_product f]
(j : J) : ∏ f ⟶ f j :=
(limit.cone (discrete.functor f)).π.app j
@[hott]
def pi.hom_is_lift {J : Set.{u'}} {C : Type u} [is_cat.{v} C] {f : J → C}
[has_product f] {P : C} (h : P ⟶ ∏ f) :
h = pi.lift (λ j : J, h ≫ (pi.π _ j)) :=
let p := λ j : J, h ≫ (pi.π f j),
c := fan.mk _ p,
lc := get_limit_cone (discrete.functor f) in
begin
change h = (lc.is_limit.lift c).v_lift,
apply is_limit.uniq lc.is_limit c (cone_map.mk h (λ j, rfl))
end
@[hott]
def pi.lift_π_eq {J : Set.{u'}} (C : Type u) [is_cat.{v} C] {f : J → C}
[has_product f] {P : C} (p : Π j : J, P ⟶ f j) :
∀ j : J, pi.lift p ≫ pi.π _ j = p j :=
((get_limit_cone (discrete.functor f)).is_limit.lift (fan.mk _ p)).fac
@[hott]
def pi.lift_uniq {J : Set.{u'}} (C : Type u) [is_cat.{v} C] {f : J → C}
[has_product f] {P : C} {p : Π j : J, P ⟶ f j} :=
((get_limit_cone (discrete.functor f)).is_limit.lift (fan.mk _ p)).fac
@[hott]
def pi.lift_fac {J : Set.{u'}} {C : Type u} [is_cat.{v} C] {f : J → C}
[has_product f] {P Q : C} (g : Q ⟶ P) (h : Π j : J, P ⟶ f j) :
pi.lift (λ j, g ≫ h j) = g ≫ pi.lift h :=
let ch := fan.mk _ h, p := λ j : J, g ≫ h j, cp := fan.mk _ p,
lc := get_limit_cone (discrete.functor f) in
begin
have p_fac : Π j : J, (g ≫ pi.lift h) ≫ pi.π _ j = g ≫ h j, from
begin intro j, rwr is_precat.assoc, rwr pi.lift_π_eq end,
exact (is_limit.uniq lc.is_limit cp (cone_map.mk (g ≫ pi.lift h) p_fac))⁻¹
end
@[hott]
def pi_hom {J : Set.{u'}} {C : Type u} [is_cat.{v} C] {f g : J -> C}
[has_product f] [has_product g] (h : Π j : J, f j ⟶ g j) : ∏ f ⟶ ∏ g :=
pi.lift (λ j : J, pi.π f j ≫ h j)
notation `∏h ` h:20 := pi_hom h
@[hott]
def pi_hom_id {J : Set.{u'}} {C : Type u} [is_cat.{v} C] (f : J -> C) [has_product f] :
pi_hom (λ j, 𝟙 (f j)) = 𝟙 (∏ f) :=
have H : (λ j, pi.π f j ≫ 𝟙 (f j)) = λ j, 𝟙 (∏ f) ≫ pi.π f j, from
begin apply eq_of_homotopy, intro j, hsimp end,
begin change pi.lift (λ j, pi.π f j ≫ 𝟙 (f j)) = _, rwr H, rwr <- pi.hom_is_lift end
@[hott]
def pi_hom_comp {J : Set.{u'}} {C : Type u} [is_cat.{v} C] {f g h : J -> C}
[has_product f] [has_product g] [has_product h] (i₁ : Π j : J, f j ⟶ g j)
(i₂ : Π j : J, g j ⟶ h j) : (∏h i₁) ≫ (∏h i₂) = ∏h (λ j, i₁ j ≫ i₂ j) :=
have H : (λ j, pi.lift (λ j, pi.π f j ≫ i₁ j) ≫ pi.π g j ≫ i₂ j) =
λ j, pi.π f j ≫ i₁ j ≫ i₂ j, from
begin
apply eq_of_homotopy, intro j,
change pi.lift (λ j, pi.π f j ≫ i₁ j) ≫ pi.π g j ≫ i₂ j = _,
rwr <- is_precat.assoc, rwr pi.lift_π_eq,
change (pi.π f j ≫ i₁ j) ≫ i₂ j = pi.π f j ≫ i₁ j ≫ i₂ j, rwr is_precat.assoc
end,
calc pi.lift (λ j, pi.π f j ≫ i₁ j) ≫ pi.lift (λ j, pi.π g j ≫ i₂ j) =
pi.lift (λ j, pi.lift (λ j, pi.π f j ≫ i₁ j) ≫ pi.π g j ≫ i₂ j) :
by rwr <- pi.lift_fac
... = pi.lift (λ j, pi.π f j ≫ i₁ j ≫ i₂ j) : by rwr H
@[hott]
def pi_proj_sset {J : Set.{u'}} {A B : Subset J} (inc : A ⊆ B) {C : Type u}
[is_cat.{v} C] (f : pred_Set B -> C) [has_product f]
[has_product (f ∘ pred_Set_inc inc)] : ∏ f ⟶ ∏ (f ∘ pred_Set_inc inc) :=
pi.lift (λ j : pred_Set A, pi.π f (pred_Set_inc inc j))
/- `parallel_pair f g` is the diagram in `C` consisting of the two morphisms `f` and `g`
with common domain and codomain. -/
@[hott, hsimp]
def parallel_pair_obj {C : Type _} [is_cat C] {a b : C}
(f g : a ⟶ b) : walking_parallel_pair -> C :=
λ s, match s with
| wp_pair.up := a
| wp_pair.down := b
end
@[hott, hsimp]
def parallel_pair_map {C : Type _} [is_cat C] {a b : C}
(f g : a ⟶ b) : Π {s t : walking_parallel_pair},
(s ⟶ t) -> (parallel_pair_obj f g s ⟶ parallel_pair_obj f g t) :=
assume s t h,
begin
hinduction s,
{ hinduction t,
{ exact 𝟙 a },
{ hinduction h,
{ exact f },
{ exact g } } },
{ hinduction t,
{ hinduction h },
{ exact 𝟙 b } }
end
@[hott, hsimp]
def parallel_pair_map_id {C : Type _} [is_cat C] {a b : C}
(f g : a ⟶ b) : ∀ s : walking_parallel_pair,
parallel_pair_map f g (𝟙 s) = 𝟙 (parallel_pair_obj f g s) :=
by intro s; hinduction s; hsimp; hsimp
@[hott, hsimp]
def parallel_pair_map_comp {C : Type _} [is_cat C]
{a b : C} (f g : a ⟶ b) : ∀ {s t u : walking_parallel_pair}
(h : s ⟶ t) (i : t ⟶ u), parallel_pair_map f g (h ≫ i) =
(parallel_pair_map f g h) ≫ (parallel_pair_map f g i) :=
assume s t u h i,
begin
hinduction s,
{ hinduction t,
{ hsimp },
{ hinduction u,
{ hinduction i },
{ rwr wpp_ci, hsimp } } },
{ hinduction t,
{ induction h },
{ hsimp } }
end
@[hott]
def parallel_pair {C : Type _} [is_cat C] {a b : C}
(f g : a ⟶ b) : walking_parallel_pair ⥤ C :=
precategories.functor.mk (parallel_pair_obj f g)
(@parallel_pair_map _ _ _ _ f g)
(parallel_pair_map_id f g)
(@parallel_pair_map_comp _ _ _ _ f g)
/- A cone over a parallel pair is called a `fork`. -/
@[hott]
abbreviation fork {C : Type _} [is_cat C] {a b : C} (f g : a ⟶ b) :=
@cone walking_parallel_pair _ _ _ (parallel_pair f g)
set_option trace.class_instances false
@[hott]
def fork_map {C : Type u} [is_cat.{v} C] {a b : C} {f g : a ⟶ b} (fk : fork f g) :
↥(fk.X ⟶ a) := fk.π.app wp_up
@[hott]
def fork_eq {C : Type u} [is_cat.{v} C] {a b : C} {f g : a ⟶ b} (fk : fork f g) :
(fork_map fk) ≫ f = (fork_map fk) ≫ g :=
cone.fac fk wp_left ⬝ (cone.fac fk wp_right)⁻¹
@[hott]
def fork.of_i {C : Type u} [is_cat.{v} C] {a b c : C}
{f g : a ⟶ b} (i : c ⟶ a) (w : i ≫ f = i ≫ g) : fork f g :=
have π : @constant_functor ↥walking_parallel_pair _ C _ c ⟹ parallel_pair f g, from
let app := @wp_pair.rec (λ x, c ⟶ (parallel_pair f g).obj x) i (i ≫ f) in
have naturality : ∀ (x x' : walking_parallel_pair) (h : x ⟶ x'),
((@constant_functor ↥walking_parallel_pair _ C _ c).map h) ≫ (app x') =
(app x) ≫ ((parallel_pair f g).map h), from
begin
intros x x' h,
hinduction x,
{ hinduction x',
{ hinduction h, hsimp },
{ hinduction h,
{ hsimp, refl },
{ hsimp, exact w } } },
{ hinduction x',
{ hinduction h },
{ hinduction h, hsimp } }
end,
nat_trans.mk app naturality,
cone.mk c π
/- Limits of parallel pairs are `equalizers`. -/
@[hott]
class has_equalizer {C : Type u} [is_cat.{v} C] {a b : C} (f g : a ⟶ b) :=
(has_limit : has_limit (parallel_pair f g))
@[hott, priority 100]
instance has_limit_of_has_equalizer {C : Type u} [is_cat.{v} C] {a b : C} (f g : a ⟶ b)
[has_equalizer f g] : has_limit (parallel_pair f g) :=
has_equalizer.has_limit f g
@[hott]
def equalizer {C : Type u} [is_cat.{v} C] {a b : C} (f g : a ⟶ b) [has_equalizer f g] :=
limit (parallel_pair f g)
@[hott, reducible]
def equalizer_map {C : Type u} [is_cat.{v} C] {a b : C} (f g : a ⟶ b) [has_equalizer f g] :
equalizer f g ⟶ a := fork_map (limit.cone (parallel_pair f g))
@[hott]
def equalizer_eq {C : Type u} [is_cat.{v} C] {a b : C} (f g : a ⟶ b) [has_equalizer f g] :
equalizer_map f g ≫ f = equalizer_map f g ≫ g := fork_eq (limit.cone (parallel_pair f g))
@[hott]
def fork_lift {C : Type u} [is_cat.{v} C] {a b : C} {f g : a ⟶ b} [has_equalizer f g]
(fk : fork f g) : fk.X ⟶ equalizer f g :=
((get_limit_cone (parallel_pair f g)).is_limit.lift fk).v_lift
@[hott]
def fork_lift_uniq {C : Type u} [is_cat.{v} C] {a b : C} {f g : a ⟶ b} [has_equalizer f g]
(fk : fork f g) (m : fk.X ⟶ equalizer f g) :
m ≫ (equalizer_map f g) = fk.π.app wp_up -> m = fork_lift fk :=
begin
let equ := limit.cone (parallel_pair f g),
intro H,
have m_fac : Π j : walking_parallel_pair, m ≫ equ.π.app j = fk.π.app j, from
begin
intro j, hinduction j,
{ exact H },
{ change m ≫ (limit.cone (parallel_pair f g)).π.app wp_down = fk.π.app wp_down,
rwr <- cone.fac (limit.cone (parallel_pair f g)) wp_left,
rwr <- cone.fac fk wp_left, rwr <- is_precat.assoc m _ _,
change (m ≫ equalizer_map f g) ≫ _ = _, rwr H }
end,
exact (get_limit_cone (parallel_pair f g)).is_limit.uniq fk (cone_map.mk m m_fac),
end
@[hott]
class has_equalizers (C : Type u) [is_cat.{v} C] :=
(has_limit_of_shape : has_limits_of_shape walking_parallel_pair C)
@[hott, instance]
def has_equalizer_of_has_equalizers {C : Type u} [is_cat.{v} C]
[has_equalizers C] {a b : C} (f g : a ⟶ b) : has_equalizer f g :=
⟨@has_limits_of_shape.has_limit _ _ _ _
(has_equalizers.has_limit_of_shape C) (parallel_pair f g)⟩
@[hott, instance]
def has_equalizer_of_has_limits_of_shape {C : Type u} [is_cat.{v} C]
[H : has_limits_of_shape walking_parallel_pair C] {a b : C} (f g : a ⟶ b) :
has_equalizer f g :=
⟨@has_limits_of_shape.has_limit _ _ _ _ H (parallel_pair f g)⟩
@[hott, instance]
def has_equalizers_of_has_limits (C : Type u) [is_cat.{v} C] [H : has_limits C] :
has_equalizers C :=
has_equalizers.mk (@has_limits.has_limit_of_shape C _ H walking_parallel_pair _)
/- An equalizer is a subobject of the domain of the parallel pair. -/
@[hott]
def equalizer_as_subobject {C : Category.{u v}} {a b : C} (f g : a ⟶ b)
[H : has_equalizer f g] : @subobject C a :=
begin
let e := equalizer_map f g, let He : e ≫ f = e ≫ g := equalizer_eq f g,
fapply subobject.mk,
{ exact @equalizer _ _ _ _ f g H},
{ exact e },
{ intros d h h' Hm,
have Hhe : (h ≫ e) ≫ f = (h ≫ e) ≫ g, from
(is_precat.assoc h e f) ⬝ ap (category_struct.comp h) He ⬝ (is_precat.assoc h e g)⁻¹,
have Hhf : h = fork_lift (fork.of_i (h ≫ e) Hhe), from
fork_lift_uniq (fork.of_i (h ≫ e) Hhe) h rfl,
have Hh'e : (h' ≫ e) ≫ f = (h' ≫ e) ≫ g, from
(is_precat.assoc h' e f) ⬝ ap (category_struct.comp h') He ⬝ (is_precat.assoc h' e g)⁻¹,
have Hh'f : h' = fork_lift (fork.of_i (h' ≫ e) Hh'e), from
fork_lift_uniq (fork.of_i (h' ≫ e) Hh'e) h' rfl,
rwr Hhf, rwr Hh'f,
let F : Π (h'': d ⟶ a), (h'' ≫ f = h'' ≫ g) -> (d ⟶ equalizer f g) :=
(λ h'' p, fork_lift (fork.of_i h'' p)),
change F (h ≫ e) Hhe = F (h' ≫ e) Hh'e, fapply apd011 F,
exact Hm, apply pathover_of_tr_eq, exact is_set.elim _ _ }
end
/- The full subcategory on a subtype of a category with limits has limits if the limit
of a diagram of objects of the subtype is also in the subtype. -/
@[hott]
def limit_closed_subtype {J : Type _} [H : is_strict_cat J] {C : Type u} [is_cat.{v} C]
(P : C -> trunctype.{0} -1) (F : J ⥤ (sigma.subtype (λ c : C, ↥(P c)))) :=
∀ (lc : limit_cone (embed F)), (P lc.cone.X).carrier
@[hott]
def emb_cone {J : Type _} [H : is_strict_cat J] {C : Type u} [is_cat.{v} C]
{P : C -> trunctype.{0} -1} {F : J ⥤ (sigma.subtype (λ c : C, ↥(P c)))}
(s : cone F) : cone (embed F) :=
begin
fapply cone.mk,
{ exact s.X.1 },
{ fapply nat_trans.mk,
{ intro j, exact s.π.app j },
{ intros j k f, exact s.π.naturality f } }
end
@[hott]
def subcat_limit_cone {J : Type _} [H : is_strict_cat J] {C : Type u} [is_cat.{v} C]
{P : C -> trunctype.{0} -1} {F : J ⥤ (sigma.subtype (λ c : C, ↥(P c)))}
(lc : limit_cone (embed F)) (lim_clos : (P lc.cone.X).carrier) :
limit_cone F :=
begin
fapply limit_cone.mk,
{ fapply cone.mk,
{ exact ⟨lc.cone.X, lim_clos⟩ },
{ fapply nat_trans.mk,
{ intro j, exact lc.cone.π.app j },
{ intros j k f, exact lc.cone.π.naturality f } } },
{ fapply is_limit.mk,
{ intro s, fapply cone_map.mk,
{ exact (lc.is_limit.lift (emb_cone s)).v_lift },
{ intro j, exact (lc.is_limit.lift (emb_cone s)).fac j } },
{ intros s m,
exact lc.is_limit.uniq (emb_cone s) (cone_map.mk m.v_lift (λ j, m.fac j)) } }
end
@[hott, instance]
def subcat_has_limit {J : Type _} [H : is_strict_cat J] {C : Type u} [is_cat.{v} C]
{P : C -> trunctype.{0} -1} {F : J ⥤ (sigma.subtype (λ c : C, ↥(P c)))}
[has_limit (embed F)] (lim_clos : limit_closed_subtype P F) : has_limit F :=
has_limit.mk (subcat_limit_cone (get_limit_cone (embed F))
(lim_clos (get_limit_cone (embed F))))
@[hott, instance]
def subcat_has_limits_of_shape {J : Type _} [H : is_strict_cat J] {C : Type u} [is_cat.{v} C]
{P : C -> trunctype.{0} -1} [has_limits_of_shape J C]
(lim_clos : ∀ F : J ⥤ (sigma.subtype (λ c : C, ↥(P c))),
@limit_closed_subtype J _ _ _ P F) :
has_limits_of_shape J (sigma.subtype (λ c : C, ↥(P c))) :=
has_limits_of_shape.mk (λ F, subcat_has_limit (lim_clos F))
@[hott, instance]
def subcat_has_products {C : Type u} [is_cat.{v} C] {P : C -> trunctype.{0} -1}
[has_products C]
(lim_clos : ∀ (J : Set) (F : (discrete J) ⥤ (sigma.subtype (λ c : C, ↥(P c)))),
limit_closed_subtype P F) :
has_products (sigma.subtype (λ c : C, ↥(P c))) :=
⟨λ J : Set, @subcat_has_limits_of_shape (discrete J) _ _ _ _
(has_limits_of_shape_of_has_products J C) (lim_clos J)⟩
/- We introduce the terminal object in a category together with some of its properties;
it exists if the category has limits. -/
@[hott]
structure terminal (C : Type u) [is_cat.{v} C] :=
(star : C)
(map : Π (c : C), c ⟶ star)
(uniq : Π {c : C} (f g : c ⟶ star), f = g)
@[hott]
class has_terminal (C : Type u) [is_cat.{v} C] :=
(str : terminal C)
@[hott]
def terminal_obj (C : Type u) [is_cat.{v} C] [H : has_terminal C] : C :=
H.str.star
@[hott]
def terminal_map {C : Type u} [is_cat.{v} C] [H : has_terminal C] (c : C) :=
H.str.map c
@[hott, instance]
def has_terminal_of_has_product (C : Type u) [is_cat.{v} C]
[H : has_product (empty_map.{u u} C)] : has_terminal C :=
begin
apply has_terminal.mk, fapply terminal.mk,
{ exact @pi_obj _ _ _ (empty_map C) H },
{ intro c, apply pi.lift, intro j, hinduction j },
{ intros c f g,
let cc := @fan.mk _ C _ (empty_map.{u u} C) c (λ j : false, false.rec _ j),
let mcf : cone_map cc (get_limit_cone (discrete.functor (empty_map C))).cone :=
begin fapply cone_map.mk, exact f, exact (λ j : false, false.rec _ j) end,
let mcg : cone_map cc (get_limit_cone (discrete.functor (empty_map C))).cone :=
begin fapply cone_map.mk, exact g, exact (λ j : false, false.rec _ j) end,
change mcf.v_lift = mcg.v_lift,
let p := (get_limit_cone (discrete.functor (empty_map.{u u} C))).is_limit.uniq,
rwr p cc mcf, rwr p cc mcg }
end
@[hott]
def terminal_map_is_mono {C : Category} [H : has_terminal C] {c : C} :
Π (f : terminal_obj C ⟶ c), is_mono f :=
begin intros f d g₁ g₂ p, exact H.str.uniq g₁ g₂ end
@[hott]
def term_subobj {C : Category} [H : has_terminal C] {c : C} (f : terminal_obj C ⟶ c) :
subobject c := (subobject.mk (terminal_obj C) f (terminal_map_is_mono f))
end categories.limits
end hott |
/**
* 图片base64转blob
* @param image
* @returns {Blob}
*/
export function base64toBlob(base64Buf: string): Blob {
const arr = base64Buf.split(',');
const typeItem = arr[0];
const mime = typeItem.match(/:(.*?);/)![1];
const bstr = window.atob(arr[1]);
let n = bstr.length;
const u8arr = new Uint8Array(n);
while (n--) {
u8arr[n] = bstr.charCodeAt(n);
}
return new Blob([u8arr], { type: mime });
}
/**
* 图片url转base64
* @param url
* @param mimeType
*/
export function urlToBase64(url: string, mimeType?: string): Promise<string> {
return new Promise((resolve, reject) => {
let canvas = document.createElement('CANVAS') as Nullable<HTMLCanvasElement>;
const ctx = canvas!.getContext('2d');
const img = new Image();
img.crossOrigin = '';
// eslint-disable-next-line consistent-return
img.onload = function () {
if (!canvas || !ctx) {
return reject();
}
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0);
const dataURL = canvas.toDataURL(mimeType || 'image/png');
canvas = null;
resolve(dataURL);
};
img.src = url;
});
}
/**
* 获取图片宽高
* @param url
* @returns {Promise<{width: number; height: number}>}
*/
export function getImageSize(url: string): Promise<{ width: number; height: number }> {
return new Promise((resolve, reject) => {
const img = new Image();
img.onload = function () {
resolve({
width: img.width,
height: img.height,
});
};
img.onerror = function () {
reject();
};
img.src = url;
});
}
/**
* 压缩图片
* @param url
* @param options
* @returns {Promise<string>}
*/
export function compressImage(
url: string,
options: { width: number; height: number; quality: number; mimeType: string },
): Promise<string> {
return new Promise((resolve, reject) => {
const { width, height, quality, mimeType } = options;
let canvas = document.createElement('CANVAS') as Nullable<HTMLCanvasElement>;
const ctx = canvas!.getContext('2d');
const img = new Image();
img.crossOrigin = '';
// eslint-disable-next-line consistent-return
img.onload = function () {
if (!canvas || !ctx) {
return reject();
}
canvas.width = width;
canvas.height = height;
ctx.drawImage(img, 0, 0, width, height);
const dataURL = canvas.toDataURL(mimeType || 'image/png', quality);
canvas = null;
resolve(dataURL);
};
img.src = url;
});
}
/**
* 计算图片宽高及比率
* @param imageTrueW 图片实际宽
* @param imageTrueH 图片实际高
* @param showAreaW 展示区宽度
* @param showAreaH 展示区高度
* @returns {{width: number; height: number; ratio: number}}
*/
export function calcImageSize(
imageTrueW: number,
imageTrueH: number,
showAreaW: number,
showAreaH: number,
): { width: number; height: number; ratio: number } {
let [width, height, ratio] = [0, 0, 0];
// 图片真实宽大于真实高
if (imageTrueW > imageTrueH) {
if (imageTrueW >= showAreaW) {
// 真实宽大于或等于展示区最大宽
const imageRatioH = imageTrueH * (showAreaW / imageTrueW);
// 按展示区最大宽与实际宽比率换算后,高度大于显示高度时
if (imageRatioH >= showAreaW) {
width = imageTrueW * (showAreaH / imageTrueH);
height = showAreaH;
ratio = imageTrueH / showAreaH;
} else {
width = showAreaW;
height = imageRatioH;
ratio = imageTrueW / showAreaW;
}
} else {
width = imageTrueW;
height = imageTrueH;
ratio = 1;
}
} else {
// 图片真实宽小于或等于真实高
// eslint-disable-next-line no-lonely-if
if (imageTrueH >= showAreaH) {
// 真实高大于或等于展示区最大高
width = imageTrueW * (showAreaH / imageTrueH);
height = showAreaH;
ratio = imageTrueH / showAreaH;
} else {
width = imageTrueW;
height = imageTrueH;
ratio = 1;
}
}
return {
width,
height,
ratio,
};
} |
##DESCRIPTION
## Algebra problem: true or false for inequality
##ENDDESCRIPTION
##KEYWORDS('algebra', 'inequality', 'fraction')
## DBsubject('History')
## DBchapter('')
## DBsection('')
## Date('2/4/2010')
## Author('Darwyn Cook')
## Institution('Alfred University')
## TitleText1('A History of Mathematics: An Introduction')
## EditionText1('3')
## AuthorText1('Katz')
## Section1('4')
########################################################################
DOCUMENT();
loadMacros(
"PGstandard.pl",
"MathObjects.pl",
"PGcourse.pl"
);
# Print problem number and point value (weight) for the problem
TEXT(beginproblem());
# Show which answers are correct and which ones are incorrect
$showPartialCorrectAnswers = 1;
##############################################################
#
# Setup
#
#
Context()->strings->add("Arabic");
$answer = String("Arabic");
Context()->texStrings;
BEGIN_TEXT
Interestingly, John of Palermo was not only a mathematician but also an \{$answer->ans_rule(20)\} scholar, who may well have been familiar with this problem from the work of al-Karaji.
END_TEXT
Context()->normalStrings;
ANS($answer->cmp());
ENDDOCUMENT(); |
:
#
# E-Mail address directory lookup script to read $HOME/.addresses
#
# The format of the $HOME/.addresses file is simple:
# Place one E-Mail address on each line.
# Descriptive comments that should be displayed but NOT included in
# the address headers may follow the address, enclosed in [ ].
#
# Samples:
# "Fred Jones" <fjones@jones.com> [President of Jones Inc.]
# mary@ucla.edu (Mary Johnson)
# herbert (Herb Smith) [Herb, down in Accounting]
#
# The environment variable ADDRESSES can be used to reference another file
# instead of $HOME/.addresses, in the same format.
#
if test -z "$ADDRESSES"
then
ADDRESSES=$HOME/.addresses
fi
if test $# -ne 2
then
echo "`basename $0`: Expected 2 args, got $#"
exit 3 # Incorrect arguments
fi
matches=${TMPDIR-/tmp}/admatch$$
# make SP = space + tab
SP=`echo x | tr x '\011'``echo x | tr x '\040'`
# extract address part if this doesn't look like it's already a regex
# this won't work absolutely 100% but it should get it right on
# non-perverse cases
addressonly=$2
if echo "$addressonly" | grep -v '[]\*]' >/dev/null
then
# the following sed expressions do this, in order:
# - assume that anything in double quotes is a comment and drop it
# - pull out the address from any remaining comments
# - squeeze adjacent whitespace, strip leading/trailing whitespace
# - drop anything that looks like a relay/host name; in particular
# user@host, host!user@relay, user%host@relay, host1!host2!...!user
addressonly=`echo " $addressonly " \
| sed -e 's/\([^!]\)"[^"]*"\([^@]\)/\1\2/g' \
-e 's/([^)]*)//g' -e 's/^.*<\([^>]*\)>.*$/\1/' \
-e "s/[$SP][$SP]*/ /g" -e "s/^[$SP]//" -e "s/[$SP]$//" \
-e 's/@.*$//' -e 's/%.*$//' -e 's/^.*!\([^!]*\)$/\1/'`
# if we somehow nuked the entire string, fall back on the original
# string, stripping one level of quoting if it exists
test -z "$addressonly" && addressonly=`echo "$2" \
| sed -e 's/^"\(.*\)"$/\1/'`
fi
(egrep -i "$addressonly" $ADDRESSES || fgrep -i "$addressonly" $ADDRESSES) > $matches 2>/dev/null
count=`wc -l < $matches`
EXIT=1 # Execution failure
if test $count -eq 0
then
echo "$2"
EXIT=4 # No matches found
elif test $count -gt $1 -a $1 -gt 0
then
echo "Matched $count names (max $1)"
echo "Use a more specific pattern please."
EXIT=2 # Too many matches
else
cat $matches
if test $count -eq 1
then
EXIT=5 # Exactly one match
else
EXIT=0 # At least one match
fi
fi
rm -f $matches
exit $EXIT |
class Programa :
def __init__(self, nome, ano) -> None:
self._nome = nome.title()
self.ano = ano
self._likes = 0
@property
def likes(self) :
return self._likes
def dar_like(self) :
self._likes += 1
@property
def nome(self) :
return self._nome
@nome.setter
def nome(self, novo_nome) :
self._nome = novo_nome.title()
def __str__(self) :
return f'{self._nome} - {self.ano} - {self._likes} Likes'
class Filme(Programa) :
def __init__(self, nome, ano, duracao) -> None:
super().__init__(nome, ano)
self.duracao = duracao
def __str__(self) :
return f'{self._nome} - {self.ano} - {self.duracao} min - {self._likes} Likes'
class Serie(Programa) :
def __init__(self, nome, ano, temporadas) -> None:
super().__init__(nome, ano)
self.temporadas = temporadas
def __str__(self) :
return f'{self._nome} - {self.ano} - {self.temporadas} temporadas - {self._likes} Likes'
class Playlist :
def __init__(self, nome, programas) -> None:
self.nome = nome
self._programas = programas
@property
def listagem(self) :
return self._programas
@property
def tamanho(self) :
return len(self._programas)
vingadores = Filme("vingadores - guerra infinita", 2018, 160)
atlanta = Serie("atlanta", 2018, 2)
tmep = Filme("Todo mundo em panico", 199, 100)
demolidor = Serie("demolidor", 2016, 2)
vingadores.dar_like()
tmep.dar_like()
demolidor.dar_like()
demolidor.dar_like()
atlanta.dar_like()
atlanta.nome = "atlanta - a terra esquecida"
filmes_e_series = [vingadores, atlanta, demolidor, tmep]
playlist_fim_de_semana = Playlist("fim de semana", filmes_e_series)
print(f"Tamanho da Playlist: {len(playlist_fim_de_semana.listagem)}")
for programa in playlist_fim_de_semana.listagem :
print(programa) |
# Given a string s, find the longest palindromic subsequence's length in s.
# A subsequence is a sequence that can be derived from another sequence by deleting some or no elements without changing the order of the remaining elements.
# @param {String} s
# @return {Integer}
def longest_palindrome_subseq(s)
@string = s
@result = []
max_len(0, s.length-1)
end
def max_len(i, j)
return 0 if i > j
return 1 if i == j
return @result[i][j] if @result[i] && @result[i][j]
@result[i] ||= []
@result[i][j] = ((@string[i] == @string[j]) ? max_len(i+1, j-1)+2 : [max_len(i+1, j), max_len(i, j-1)].max)
end
pp longest_palindrome_subseq("bbbab")
pp longest_palindrome_subseq("cbbd")
# Example 1:
# Input: s = "bbbab"
# Output: 4
# Explanation: One possible longest palindromic subsequence is "bbbb".
# Example 2:
# Input: s = "cbbd"
# Output: 2
# Explanation: One possible longest palindromic subsequence is "bb". |
/**
* Grazie
* @copyright Copyright (c) 2024 David Dyess II
* @license MIT see LICENSE
*/
// Import styles of packages that you've installed.
// All packages except `@mantine/hooks` require styles imports
import '@mantine/core/styles.css';
import '@mantine/dates/styles.css';
import '@mantine/notifications/styles.css';
import '@mantine/tiptap/styles.css';
import {
Button,
ColorSchemeScript,
Container,
Group,
MantineProvider,
Title,
Text
} from '@mantine/core';
import { Notifications } from '@mantine/notifications';
import { cssBundleHref } from '@remix-run/css-bundle';
import {
json,
type LinksFunction,
type LoaderFunctionArgs
} from '@remix-run/node';
import {
isRouteErrorResponse,
Links,
LiveReload,
Meta,
Outlet,
Scripts,
ScrollRestoration,
useLoaderData,
useRouteError
} from '@remix-run/react';
import { getToast } from 'remix-toast';
import { AbilityProvider } from '~/components/AbilityProvider';
import { ThemeProvider } from '~/components/ThemeProvider';
import { setting } from '~/lib/setting.server';
import classes from '~/styles/NotFound.module.css';
import { getUser } from '~/utils/session.server';
import { site, theme } from '@/grazie';
export const links: LinksFunction = () => [
...(cssBundleHref ? [{ rel: 'stylesheet', href: cssBundleHref }] : [])
];
export const loader = async ({ request }: LoaderFunctionArgs) => {
const user = await getUser(request);
const { toast, headers } = await getToast(request);
return json(
{
user,
theme: {
footer: {
links: await setting({ name: 'footer.links', defaultValue: [] })
},
navbar: {
links: await setting({ name: 'navbar.links', defaultValue: [] })
}
},
site: await setting({ name: 'site', group: true, defaultValue: site }),
toast
},
{ headers }
);
};
export default function App() {
const data = useLoaderData();
return (
<html lang="en">
<head>
<meta charSet="utf-8" />
<meta name="viewport" content="width=device-width,initial-scale=1" />
<meta property="og:site_name" content={data?.site?.name} />
<Meta />
<Links />
<ColorSchemeScript defaultColorScheme="auto" />
</head>
<body>
<AbilityProvider>
<MantineProvider defaultColorScheme="auto" theme={theme}>
<ThemeProvider>
<Outlet />
<ScrollRestoration />
<Scripts />
<LiveReload />
</ThemeProvider>
<Notifications />
</MantineProvider>
</AbilityProvider>
</body>
</html>
);
}
function ErrorPage({ children }) {
return (
<html lang="en">
<head>
<meta charSet="utf-8" />
<meta name="viewport" content="width=device-width,initial-scale=1" />
<meta title="Error" />
<Meta />
<Links />
<ColorSchemeScript />
</head>
<body>
<MantineProvider defaultColorScheme="auto">
<ThemeProvider>
{children}
<ScrollRestoration />
<Scripts />
<LiveReload />
</ThemeProvider>
</MantineProvider>
</body>
</html>
);
}
export function ErrorBoundary() {
const error = useRouteError();
// when true, this is what used to go to `CatchBoundary`
if (isRouteErrorResponse(error)) {
switch (error.status) {
case 404:
return (
<ErrorPage>
<Container className={classes.root}>
<div className={classes.label}>404</div>
<Title className={classes.title}>
Unable to find the page you requested.
</Title>
<Text
c="dimmed"
size="lg"
ta="center"
className={classes.description}
>
Please check the URL, and failing that, report the issue to us.
</Text>
<Group justify="center">
<Button variant="subtle" size="md">
Take me back to home page
</Button>
</Group>
</Container>
</ErrorPage>
);
default:
return (
<ErrorPage>
<Container className={classes.root}>
<div className={classes.label}>{error.status}</div>
<Text
c="dimmed"
size="lg"
ta="center"
className={classes.description}
>
{error.data.message}
</Text>
</Container>
</ErrorPage>
);
}
}
// Don't forget to typecheck with your own logic.
// Any value can be thrown, not just errors!
let errorMessage = 'Unknown error';
if (error?.message) {
errorMessage = error.message;
}
return (
<ErrorPage>
<Container className={classes.root}>
<div className={classes.label}>Error</div>
<Title className={classes.title}>Something went wrong.</Title>
<Text c="dimmed" size="lg" ta="center" className={classes.description}>
{errorMessage}
</Text>
<Group justify="center">
<Button variant="subtle" size="md">
Take me back to home page
</Button>
</Group>
</Container>
</ErrorPage>
);
} |
<%@ page contentType="text/html;charset=UTF-8" %>
<%@ include file="/WEB-INF/views/include/taglib.jsp"%>
<html>
<head>
<title>老师管理</title>
<meta name="decorator" content="default"/>
<script type="text/javascript">
$(document).ready(function() {
});
function page(n,s){
$("#pageNo").val(n);
$("#pageSize").val(s);
$("#searchForm").submit();
return false;
}
</script>
</head>
<body>
<ul class="nav nav-tabs">
<li class="active"><a href="${ctx}/zxy/teachers/">老师列表</a></li>
<shiro:hasPermission name="zxy:teachers:edit"><li><a href="${ctx}/zxy/teachers/form">老师添加</a></li></shiro:hasPermission>
</ul>
<form:form id="searchForm" modelAttribute="teachers" action="${ctx}/zxy/teachers/" method="post" class="breadcrumb form-search">
<input id="pageNo" name="pageNo" type="hidden" value="${page.pageNo}"/>
<input id="pageSize" name="pageSize" type="hidden" value="${page.pageSize}"/>
<ul class="ul-form">
<li><label>登录账号:</label>
<form:input path="user.mobile" htmlEscape="false" maxlength="64" class="input-medium"/>
</li>
<li><label>姓名:</label>
<form:input path="name" htmlEscape="false" maxlength="16" class="input-medium"/>
</li>
<li><label>类型:</label>
<form:select path="type" class="input-medium">
<form:option value="" label=""/>
<form:options items="${fns:getDictList('teacher_type')}" itemLabel="label" itemValue="value" htmlEscape="false"/>
</form:select>
</li>
<li class="btns"><input id="btnSubmit" class="btn btn-primary" type="submit" value="查询"/></li>
<li class="clearfix"></li>
</ul>
</form:form>
<sys:message content="${message}"/>
<table id="contentTable" class="table table-striped table-bordered table-condensed">
<thead>
<tr>
<th>登录账号</th>
<th>姓名</th>
<th>头衔</th>
<th>类型</th>
<th>更新时间</th>
<shiro:hasPermission name="zxy:teachers:edit"><th>操作</th></shiro:hasPermission>
</tr>
</thead>
<tbody>
<c:forEach items="${page.list}" var="teachers">
<tr>
<td>
${teachers.user.mobile}
</td>
<td><a href="${ctx}/zxy/teachers/form?id=${teachers.id}">
${teachers.name}
</a></td>
<td>
${teachers.title}
</td>
<td>
${fns:getDictLabel(teachers.type, 'teacher_type', '')}
</td>
<td>
<fmt:formatDate value="${teachers.updateDate}" pattern="yyyy-MM-dd HH:mm:ss"/>
</td>
<shiro:hasPermission name="zxy:teachers:edit"><td>
<a href="${ctx}/zxy/teachers/form?id=${teachers.id}">修改</a>
<a href="${ctx}/zxy/teachers/delete?id=${teachers.id}" onclick="return confirmx('确认要删除该老师吗?', this.href)">删除</a>
</td></shiro:hasPermission>
</tr>
</c:forEach>
</tbody>
</table>
<div class="pagination">${page}</div>
</body>
</html> |
/* Copyright 1993-2003 The MathWorks, Inc. */
/*
* EROBW Binary image erosion (MEX-file)
*
* BW2 = EROBW(BW1, SE) erodes the binary image BW1 by the
* structuring element SE. BW1 must be a 2-D real uint8 array.
* SE must be a 2-D real double array. BW2 is a logical uint8 array.
*
*/
#include <string.h>
#include "mex.h"
static char rcsid[] = "$Revision: 1.11.4.2 $";
/*
* ValidateInputs checks to make sure the user has supplied valid
* input and output arguments.
*/
void ValidateInputs(int nlhs, mxArray *plhs[],
int nrhs, const mxArray *prhs[])
{
if (nrhs < 2) mexErrMsgIdAndTxt("Images:erobw:tooFewInputs",
"%s","Too few input arguments");
if (nrhs > 2) mexErrMsgIdAndTxt("Images:erobw:tooManyInputs",
"%s","Too many input arguments");
if (nlhs > 1) mexErrMsgIdAndTxt("Images:erobw:tooManyOutputs",
"%s","Too many output arguments");
if ((mxGetNumberOfDimensions(prhs[0]) != 2) ||
(!mxIsLogical(prhs[0])))
{
mexErrMsgIdAndTxt("Images:erobw:firstInputMustBe2DLogicalArray","%s",
"First input argument must be a 2-D logical array.");
}
if ((mxGetNumberOfDimensions(prhs[1]) != 2) ||
(mxGetClassID(prhs[1]) != mxDOUBLE_CLASS) ||
(mxIsComplex(prhs[1])))
{
mexErrMsgIdAndTxt("Images:erobw:secondInputMustBeRealDoubleVector",
"%s", "Second input argument must be a real"
" double vector.");
}
}
int NumberOfNonzeroElements(const mxArray *SE)
{
int counter = 0;
double *pr;
int MN;
int k;
MN = mxGetNumberOfElements(SE);
pr = (double *) mxGetData(SE);
for (k = 0; k < MN; k++)
{
if (*pr++)
{
counter++;
}
}
return(counter);
}
void ComputeStructuringElementOffsets(const mxArray *SE, int **rowOffsets,
int **colOffsets, int *numOffsets)
{
double *pr;
int r;
int c;
int counter;
int rowCenter;
int colCenter;
int M;
int N;
*numOffsets = NumberOfNonzeroElements(SE);
*rowOffsets = (int *) mxCalloc(*numOffsets, sizeof(**rowOffsets));
*colOffsets = (int *) mxCalloc(*numOffsets, sizeof(**colOffsets));
M = mxGetM(SE);
N = mxGetN(SE);
rowCenter = (int) (((double) M - 1.0) / 2.0);
colCenter = (int) (((double) N - 1.0) / 2.0);
counter = 0;
pr = (double *) mxGetData(SE);
for (c = 0; c < N; c++)
{
for (r = 0; r < M; r++)
{
if (*pr++)
{
(*rowOffsets)[counter] = r - rowCenter;
(*colOffsets)[counter] = c - colCenter;
counter++;
}
}
}
}
void erode(mxLogical *pout, mxLogical *pin, int M, int N,
int numOffsets, int *rowOffsets, int *colOffsets)
{
int col;
int row;
int k;
int c;
int r;
for (k = 0; k < M*N; k++)
{
pout[k] = 1;
}
for (col = 0; col < N; col++)
{
for (row = 0; row < M; row++)
{
for (k = 0; k < numOffsets; k++)
{
c = col + colOffsets[k];
r = row + rowOffsets[k];
if ((r >= 0) && (r < M) && (c >= 0) && (c < N))
{
if (*(pin + c*M + r) == 0)
{
*pout = 0;
break;
}
}
else
{
*pout = 0;
break;
}
}
pout++;
}
}
}
void mexFunction(int nlhs, mxArray *plhs[],
int nrhs, const mxArray *prhs[])
{
const mxArray *BWin;
mxArray *BWout;
const mxArray *SE;
int numOffsets;
int *rowOffsets;
int *colOffsets;
int M;
int N;
int size[2];
/*
* Check input argument validity
*/
ValidateInputs(nlhs, plhs, nrhs, prhs);
BWin = prhs[0];
SE = prhs[1];
/*
* Create the output array
*/
M = mxGetM(BWin);
N = mxGetN(BWin);
size[0] = M;
size[1] = N;
BWout = mxCreateLogicalArray(2, size);
ComputeStructuringElementOffsets(SE, &rowOffsets, &colOffsets, &numOffsets);
erode(mxGetLogicals(BWout), mxGetLogicals(BWin),
M, N, numOffsets, rowOffsets, colOffsets);
plhs[0] = BWout;
} |
// SPDX-License-Identifier: BUSL-1.1
pragma solidity ^0.8.23;
/// @title IBroadcastRegistry
/// @dev Interface for BroadcastRegistry
/// @author ZeroPoint Labs
interface IBroadcastRegistry {
// EXTERNAL WRITE FUNCTIONS //
/// @dev emitted when a payload is broadcasted
event PayloadSent(address indexed sender);
/// @dev emitted when a broadcast payload is received
event PayloadReceived(uint256 indexed payloadId, uint64 indexed srcChainId);
// EXTERNAL WRITE FUNCTIONS //
/// @dev allows core contracts to send payload to all configured destination chain.
/// @param srcSender_ is the caller of the function (used for gas refunds).
/// @param ambId_ is the identifier of the arbitrary message bridge to be used
/// @param gasFee_ is the gas fee to be used for broadcasting
/// @param message_ is the crosschain payload to be broadcasted
/// @param extraData_ defines all the message bridge related overrides
function broadcastPayload(
address srcSender_,
uint8 ambId_,
uint256 gasFee_,
bytes memory message_,
bytes memory extraData_
)
external
payable;
/// @dev allows ambs to write broadcasted payloads
function receiveBroadcastPayload(uint64 srcChainId_, bytes memory message_) external;
/// @dev allows privileged actors to process broadcasted payloads
/// @param payloadId_ is the identifier of the cross-chain payload
function processPayload(uint256 payloadId_) external;
} |
<template>
<section>
<base-card>
<h2>Submitted Experiences</h2>
<div>
<base-button @click="loadExperiences"
>Load Submitted Experiences</base-button
>
</div>
<p v-if="isLoading">Loading...</p>
<p v-else-if="!isLoading && error">{{ error }}</p>
<p v-else-if="!isLoading && (!results || results.length === 0)">
No stored experiences found.
</p>
<!-- v-else-if="!isLoading && results && results.length > 0" -->
<ul v-else>
<survey-result
v-for="result in results"
:key="result.id"
:name="result.name"
:rating="result.rating"
></survey-result>
</ul>
</base-card>
</section>
</template>
<script>
import SurveyResult from "./SurveyResult.vue";
export default {
// props: ["results"],
components: {
SurveyResult,
},
data() {
return {
results: [],
isLoading: false,
error: null,
};
},
methods: {
loadExperiences() {
this.isLoading = true;
this.error = null;
fetch(
"https://learing-vue-js-backend-default-rtdb.firebaseio.com/surveys.json"
)
.then(function (response) {
if (response.ok) {
return response.json(); //parsing data
}
})
.then((data) => {
this.isLoading = false;
console.log(data);
const results = [];
for (const id in data) {
results.push({
id: id,
name: data[id].name,
rating: data[id].rating,
});
}
this.results = results;
})
.catch(() => {
console.log(this.error);
this.isLoading = false;
this.error = "failed to fetch data-please try again later.";
});
//// {
//?The DEFAULT method is GET so we don't need the headers,body or method for carrying data from the database
//*get,post,delete,update, available options
//* method: "GET",
//* headers: {
//* "Content-Type": "application/json",
//* },
//chosen from the emit method
//* body: JSON.stringify({
//* name: this.userName,
//* rating: this.chosenRating,
//* }),
// }
},
},
mounted() {
//automatically load in the data without pressing the button
this.loadExperiences();
},
};
</script>
<style scoped>
ul {
list-style: none;
margin: 0;
padding: 0;
}
</style> |
Team Omega Tiger Woods OS Lab Project 3
Andrew Baker
Russell White
The purpose of this project is to create a program to simulate the synchronization
problem of the classic Producer-Consumer problem. This program does this by
creating a thread for each producer and consumer, and creating a single buffer
that each producer and consumer can access. This buffer is limited to a size of
10. Each producer and consumer can access the buffer only when the buffer is not
being accessed by another producer or consumer. Producers can add numbers to the
buffer, and consumers can remove numbers from it. Each thread waits a random
time from 0 to 1000 milliseconds before trying to access the buffer. Tjreads are
created using <pthreads.h>.
To compile this program, a Makefile has been included. Just run make in the same
directory the source code is included in.
To run this program, 3 arguments are required. The first is an integer for how
many producers are to be created. The second is an integer for how many consumers
are to be created. The third determines if the buffer is "First In, First Out"
or "First In, Last Out", which is a 0 or 1 respectively. This program is supposed
to handle up to 9 threads and 9 consumers; however, any integer greater than 0
will work. 0 or smaller integers create no threads.
A script has been included to run through all 200 possibilities to be tested.
This script will take over 16 hours to fully execute. If desired, change the
sleep timer in p3.c to be shorter (such as 5 seconds instead of 5 minutes).
The script may also need permissions to execute. Change appropriately.
The order this program works is it will read in command line arguments. Then it
will initialize the buffer to -1 in all 10 spots. Then it will create the
specified producer threads, followed by the specified consumer threads. After
that, the program will sleep for 300 seconds, while the producer and consumer
threads interact with the buffer. After the 300 seconds, the threads and buffer
will be cleared, and the program will exit.
To operate correctly, we created several functions:
producer_func(): Creates producers, and attempts to add integers to the buffer.
consumer_func(): Creates consumers, and attmpts to remove integers from the
buffer.
add_to(): Adds integers to the buffer.
pop(): Removes integers from the front of the buffer.
is_empty(): Checks to see if the buffer is empty.
is_full(): Checks to see if the buffer is full.
print_buffer(): Prints the buffer, including underscore characters where the
buffer has a -1.
To test this, we ran the program multiple times using different configurations.
Everything required for this project is included, implemented, and working
correctly. |
# Rumbleverse Download API
This repository contains the source code for the Rumbleverse Download API, an Express.js application designed to serve downloadable builds of Rumbleverse. The API provides an easy-to-use interface for listing and downloading different versions of the game.
## Features
- **Static File Serving:** The API serves zip files containing different builds of Rumbleverse.
- **Version Listing:** It dynamically lists available versions based on the files present in the `versions` directory.
- **Cross-Origin Resource Sharing (CORS):** Enabled CORS to allow requests from different domains.
- **Docker Support:** Includes a Dockerfile for easy deployment and containerization.
## Setup
1. **Prerequisites:**
- Node.js
- NPM (Node Package Manager)
- Docker (optional for containerized deployment)
2. **Installation:**
- Clone the repository.
- Run `npm install` to install the required Node.js packages.
3. **Adding Builds:**
- Place your Rumbleverse build files in the `versions` directory. For downloading builds join into our Discord Server. https://discord.gg/HU3YUaFcAG
- Ensure each build is compressed as a `.zip` file.
4. **Configuration:**
- Modify the domain in the `getVersionsData` function to point to your own domain.
- Example: Replace `https://rvapi.ferivoq.me/versions/${encodeURIComponent(file)}` with your domain.
5. **Docker Setup (Optional):**
- Build the Docker image using `docker build -t rumbleverse-api .`
- Run the container using `docker run -p 4000:4000 rumbleverse-api`
6. **Running the API:**
- Start the server using `npm run dev` or with your preferred process manager.
- Access the API at `http://localhost:4000`.
## API Endpoints
- `GET /api`: Returns a JSON object listing all available Rumbleverse versions with download links.
- `GET /`: A simple endpoint that returns the text 'Rumbleverse Download API'.
- Static files are served under `/versions`.
## Dockerfile
A Dockerfile is included for building a Docker image of the API. This allows for easy deployment and scaling.
## Contribution
Contributions to the project are welcome. Please ensure you follow the existing code style and include proper documentation for your changes.
---
Note: This documentation assumes basic familiarity with Node.js, Express.js, and Docker. Ensure you update the domain in the API as per your deployment needs. |
import personCSS from "./Person.module.css";
import { useSelector, useDispatch } from "react-redux";
import { deletePerson } from "../../redux/store/index";
// import { v4 as uuidv4 } from "uuid";
const Person = () => {
const dispatch = useDispatch();
//今持ってるStoreの情報をゲットできる
const peopleData = useSelector((state) => state.person);
console.log(peopleData);
return (
<>
{peopleData.length > 0 && (
<>
{peopleData.map((person) => (
<div key={person.id} className={personCSS.Person}>
<h1>{person.name}</h1>
<p>Age: {person.age}</p>
<button onClick={() => dispatch(deletePerson(person.id))}>
Delete
</button>
</div>
))}
</>
)}
</>
);
};
export default Person; |
package com.lefarmico.navigation.params
import android.os.Parcelable
import kotlinx.parcelize.Parcelize
sealed class LibraryParams : Parcelable {
@Parcelize
data class CategoryList(
val isFromWorkoutScreen: Boolean
) : LibraryParams()
@Parcelize
data class SubcategoryList(
val categoryId: Int,
val isFromWorkoutScreen: Boolean
) : LibraryParams()
@Parcelize
data class ExerciseList(
val categoryId: Int,
val subCategoryId: Int,
val isFromWorkoutScreen: Boolean
) : LibraryParams()
@Parcelize
data class Exercise(
val exerciseId: Int
) : LibraryParams()
@Parcelize
data class NewExercise(
val subCategoryId: Int,
val isFromWorkoutScreen: Boolean
) : LibraryParams()
} |
/*
* Copyright (C) 2016 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License
*/
package com.android.systemui.statusbar;
import android.animation.Animator;
import android.view.View;
import android.view.ViewPropertyAnimator;
import androidx.annotation.Nullable;
import com.android.app.animation.Interpolators;
import com.android.systemui.res.R;
import com.android.systemui.statusbar.notification.stack.StackStateAnimator;
/**
* A helper to fade views in and out.
*/
public class CrossFadeHelper {
public static final long ANIMATION_DURATION_LENGTH = 210;
public static void fadeOut(final View view) {
fadeOut(view, (Runnable) null);
}
public static void fadeOut(final View view, final Runnable endRunnable) {
fadeOut(view, ANIMATION_DURATION_LENGTH, 0, endRunnable);
}
public static void fadeOut(final View view, final Animator.AnimatorListener listener) {
fadeOut(view, ANIMATION_DURATION_LENGTH, 0, listener);
}
public static void fadeOut(final View view, long duration, int delay) {
fadeOut(view, duration, delay, (Runnable) null);
}
/**
* Perform a fade-out animation, invoking {@code endRunnable} when the animation ends. It will
* not be invoked if the animation is cancelled.
*
* @deprecated Use {@link #fadeOut(View, long, int, Animator.AnimatorListener)} instead.
*/
@Deprecated
public static void fadeOut(final View view, long duration, int delay,
@Nullable final Runnable endRunnable) {
view.animate().cancel();
view.animate()
.alpha(0f)
.setDuration(duration)
.setInterpolator(Interpolators.ALPHA_OUT)
.setStartDelay(delay)
.withEndAction(() -> {
if (endRunnable != null) {
endRunnable.run();
}
if (view.getVisibility() != View.GONE) {
view.setVisibility(View.INVISIBLE);
}
});
if (view.hasOverlappingRendering()) {
view.animate().withLayer();
}
}
public static void fadeOut(final View view, long duration, int delay,
@Nullable final Animator.AnimatorListener listener) {
view.animate().cancel();
ViewPropertyAnimator animator = view.animate()
.alpha(0f)
.setDuration(duration)
.setInterpolator(Interpolators.ALPHA_OUT)
.setStartDelay(delay)
.withEndAction(() -> {
if (view.getVisibility() != View.GONE) {
view.setVisibility(View.INVISIBLE);
}
});
if (listener != null) {
animator.setListener(listener);
}
if (view.hasOverlappingRendering()) {
view.animate().withLayer();
}
}
public static void fadeOut(View view, float fadeOutAmount) {
fadeOut(view, fadeOutAmount, true /* remap */);
}
/**
* Fade out a view by a given progress amount
* @param view the view to fade out
* @param fadeOutAmount how much the view is faded out. 0 means not at all and 1 means fully
* faded out
* @param remap whether the fade amount should be remapped to the shorter duration
* {@link #ANIMATION_DURATION_LENGTH} from the normal fade duration
* {@link StackStateAnimator#ANIMATION_DURATION_STANDARD} in order to have a faster fading.
*
* @see #fadeIn(View, float, boolean)
*/
public static void fadeOut(View view, float fadeOutAmount, boolean remap) {
view.animate().cancel();
if (fadeOutAmount == 1.0f && view.getVisibility() != View.GONE) {
view.setVisibility(View.INVISIBLE);
} else if (view.getVisibility() == View.INVISIBLE) {
view.setVisibility(View.VISIBLE);
}
if (remap) {
fadeOutAmount = mapToFadeDuration(fadeOutAmount);
}
float alpha = Interpolators.ALPHA_OUT.getInterpolation(1.0f - fadeOutAmount);
view.setAlpha(alpha);
updateLayerType(view, alpha);
}
private static float mapToFadeDuration(float fadeOutAmount) {
// Assuming a linear interpolator, we can easily map it to our new duration
float endPoint = (float) ANIMATION_DURATION_LENGTH
/ (float) StackStateAnimator.ANIMATION_DURATION_STANDARD;
return Math.min(fadeOutAmount / endPoint, 1.0f);
}
private static void updateLayerType(View view, float alpha) {
if (view.hasOverlappingRendering() && alpha > 0.0f && alpha < 1.0f) {
if (view.getLayerType() != View.LAYER_TYPE_HARDWARE) {
view.setLayerType(View.LAYER_TYPE_HARDWARE, null);
view.setTag(R.id.cross_fade_layer_type_changed_tag, true);
}
} else if (view.getLayerType() == View.LAYER_TYPE_HARDWARE
&& view.getTag(R.id.cross_fade_layer_type_changed_tag) != null) {
view.setLayerType(View.LAYER_TYPE_NONE, null);
}
}
public static void fadeIn(final View view) {
fadeIn(view, ANIMATION_DURATION_LENGTH, 0);
}
public static void fadeIn(final View view, Runnable endRunnable) {
fadeIn(view, ANIMATION_DURATION_LENGTH, /* delay= */ 0, endRunnable);
}
public static void fadeIn(final View view, Animator.AnimatorListener listener) {
fadeIn(view, ANIMATION_DURATION_LENGTH, /* delay= */ 0, listener);
}
public static void fadeIn(final View view, long duration, int delay) {
fadeIn(view, duration, delay, /* endRunnable= */ (Runnable) null);
}
/**
* Perform a fade-in animation, invoking {@code endRunnable} when the animation ends. It will
* not be invoked if the animation is cancelled.
*
* @deprecated Use {@link #fadeIn(View, long, int, Animator.AnimatorListener)} instead.
*/
@Deprecated
public static void fadeIn(final View view, long duration, int delay,
@Nullable Runnable endRunnable) {
view.animate().cancel();
if (view.getVisibility() == View.INVISIBLE) {
view.setAlpha(0.0f);
view.setVisibility(View.VISIBLE);
}
view.animate()
.alpha(1f)
.setDuration(duration)
.setStartDelay(delay)
.setInterpolator(Interpolators.ALPHA_IN)
.withEndAction(endRunnable);
if (view.hasOverlappingRendering() && view.getLayerType() != View.LAYER_TYPE_HARDWARE) {
view.animate().withLayer();
}
}
public static void fadeIn(final View view, long duration, int delay,
@Nullable Animator.AnimatorListener listener) {
view.animate().cancel();
if (view.getVisibility() == View.INVISIBLE) {
view.setAlpha(0.0f);
view.setVisibility(View.VISIBLE);
}
ViewPropertyAnimator animator = view.animate()
.alpha(1f)
.setDuration(duration)
.setStartDelay(delay)
.setInterpolator(Interpolators.ALPHA_IN);
if (listener != null) {
animator.setListener(listener);
}
if (view.hasOverlappingRendering() && view.getLayerType() != View.LAYER_TYPE_HARDWARE) {
view.animate().withLayer();
}
}
public static void fadeIn(View view, float fadeInAmount) {
fadeIn(view, fadeInAmount, false /* remap */);
}
/**
* Fade in a view by a given progress amount
* @param view the view to fade in
* @param fadeInAmount how much the view is faded in. 0 means not at all and 1 means fully
* faded in.
* @param remap whether the fade amount should be remapped to the shorter duration
* {@link #ANIMATION_DURATION_LENGTH} from the normal fade duration
* {@link StackStateAnimator#ANIMATION_DURATION_STANDARD} in order to have a faster fading.
*
* @see #fadeOut(View, float, boolean)
*/
public static void fadeIn(View view, float fadeInAmount, boolean remap) {
view.animate().cancel();
if (view.getVisibility() == View.INVISIBLE) {
view.setVisibility(View.VISIBLE);
}
if (remap) {
fadeInAmount = mapToFadeDuration(fadeInAmount);
}
float alpha = Interpolators.ALPHA_IN.getInterpolation(fadeInAmount);
view.setAlpha(alpha);
updateLayerType(view, alpha);
}
} |
/*
* Copyright (c) 2006-2016 Marvisan Pty. Ltd. All rights reserved.
* Use is subject to license terms.
*/
/*
* BidResponseMsg44TestData.java
*
* $Id: BidResponseMsg44TestData.java,v 1.1 2011-04-14 11:44:44 vrotaru Exp $
*/
package net.hades.fix.message.impl.v44.data;
import java.io.UnsupportedEncodingException;
import static org.junit.Assert.*;
import net.hades.fix.TestUtils;
import net.hades.fix.message.BidResponseMsg;
import net.hades.fix.message.MsgTest;
import net.hades.fix.message.group.impl.v44.BidRespComponentGroup44TestData;
/**
* Test utility for BidResponseMsg44 message class.
*
* @author <a href="mailto:support@marvisan.com">Support Team</a>
* @version $Revision: 1.1 $
* @created 11/05/2009, 12:08:30 PM
*/
public class BidResponseMsg44TestData extends MsgTest {
private static final BidResponseMsg44TestData INSTANCE;
static {
INSTANCE = new BidResponseMsg44TestData();
}
public static BidResponseMsg44TestData getInstance() {
return INSTANCE;
}
public void populate( BidResponseMsg msg) throws UnsupportedEncodingException {
TestUtils.populate44HeaderAll(msg);
msg.setBidID("BIDID5555722");
msg.setClientBidID("CLIBID7364844");
msg.setNoBidComponents(2);
BidRespComponentGroup44TestData.getInstance().populate1(msg.getBidComponentGroups()[0]);
BidRespComponentGroup44TestData.getInstance().populate2(msg.getBidComponentGroups()[1]);
}
public void check( BidResponseMsg expected, BidResponseMsg actual) throws Exception {
assertEquals(expected.getBidID(), actual.getBidID());
assertEquals(expected.getClientBidID(), actual.getClientBidID());
assertEquals(expected.getNoBidComponents(), actual.getNoBidComponents());
BidRespComponentGroup44TestData.getInstance().check(expected.getBidComponentGroups()[0], actual.getBidComponentGroups()[0]);
BidRespComponentGroup44TestData.getInstance().check(expected.getBidComponentGroups()[1], actual.getBidComponentGroups()[1]);
}
} |
// Copyright Epic Games, Inc. All Rights Reserved.
#pragma once
#include "FractureToolCutter.h"
#include "ModelingOperators.h"
#include "FractureToolPlaneCut.generated.h"
class FFractureToolContext;
class UMeshOpPreviewWithBackgroundCompute;
struct FNoiseOffsets;
struct FNoiseSettings;
UCLASS(config = EditorPerProjectUserSettings)
class UFracturePlaneCutSettings : public UFractureToolSettings
{
public:
GENERATED_BODY()
UFracturePlaneCutSettings(const FObjectInitializer& ObjInit)
: Super(ObjInit)
, NumberPlanarCuts(1) {}
/** Number of cutting planes. Only used when "Use Gizmo" is disabled */
UPROPERTY(EditAnywhere, Category = PlaneCut, meta = (DisplayName = "Number of Cuts", UIMin = "1", UIMax = "20", ClampMin = "1", EditCondition = "bCanCutWithMultiplePlanes", HideEditConditionToggle))
int32 NumberPlanarCuts;
UPROPERTY()
bool bCanCutWithMultiplePlanes = false;
};
UCLASS(DisplayName = "Plane Cut Tool", Category = "FractureTools")
class UFractureToolPlaneCut : public UFractureToolCutterBase, public UE::Geometry::IDynamicMeshOperatorFactory
{
public:
GENERATED_BODY()
UFractureToolPlaneCut(const FObjectInitializer& ObjInit);
// UFractureTool Interface
virtual FText GetDisplayText() const override;
virtual FText GetTooltipText() const override;
virtual FSlateIcon GetToolIcon() const override;
virtual void SelectedBonesChanged() override;
virtual void OnTick(float DeltaTime) override;
virtual void Render(const FSceneView* View, FViewport* Viewport, FPrimitiveDrawInterface* PDI) override;
virtual void RegisterUICommand(FFractureEditorCommands* BindingContext) override;
virtual TArray<UObject*> GetSettingsObjects() const override;
virtual void FractureContextChanged() override;
virtual int32 ExecuteFracture(const FFractureToolContext& FractureContext) override;
virtual void Setup() override;
virtual void Shutdown() override;
// IDynamicMeshOperatorFactory API, for generating noise preview meshes
virtual TUniquePtr<UE::Geometry::FDynamicMeshOperator> MakeNewOperator() override;
protected:
virtual void ClearVisualizations() override;
virtual void PostEditChangeChainProperty(struct FPropertyChangedChainEvent& PropertyChangedEvent) override;
private:
// Slicing
UPROPERTY(EditAnywhere, Category = Slicing)
TObjectPtr<UFracturePlaneCutSettings> PlaneCutSettings;
UPROPERTY(EditAnywhere, Category = Uniform)
TObjectPtr<UFractureTransformGizmoSettings> GizmoSettings;
UPROPERTY()
TObjectPtr<UMeshOpPreviewWithBackgroundCompute> NoisePreview;
void GenerateSliceTransforms(const FFractureToolContext& Context, TArray<FTransform>& CuttingPlaneTransforms);
float RenderCuttingPlaneSize;
const float GizmoPlaneSize = 100.f;
TArray<FTransform> RenderCuttingPlanesTransforms;
TArray<FNoiseOffsets> NoiseOffsets;
TArray<FVector> NoisePivots;
FVisualizationMappings PlanesMappings;
float NoisePreviewExplodeAmount = 0;
}; |
import * as express from "express";
import * as bodyParser from "body-parser";
import * as mongoose from "mongoose";
import * as session from "express-session";
import * as connectMongo from "connect-mongo";
const MongoStore = connectMongo(session);
const mongoDB = "mongodb://127.0.0.1:27017";
const db = mongoose.connection;
// require('./database');
// require('./seed');
mongoose.connect(mongoDB);
db.on("error", console.error.bind(console, "MongoDB connection error"));
const app = express();
import songsRouter from "./routes/song";
import artistsRouter from "./routes/artist";
import usersRouter from "./routes/user";
const PORT = 8000;
// currently this places the SPA on the homepage
// var DIST_DIR = path.join(__dirname, '/../../dist');
// app.use('/', express.static(DIST_DIR));
// app.use - mounts the specified middleware function or functions at the specified path
app.use((req, res, next) => {
res.header("Access-Control-Allow-Origin", "http://localhost:3000");
res.header("Access-Control-Allow-Credentials", "true");
res.header(
"Access-Control-Allow-Methods",
"GET, HEAD, OPTIONS, POST, PUT, DELETE"
);
res.header(
"Access-Control-Allow-Headers",
"Origin, X-Requested-With, Content-Type, Accept"
);
next();
});
app.use(
session({
secret: "giddy up",
resave: false,
saveUninitialized: false,
cookie: {
httpOnly: false // NOTE: look into changing this
},
store: new MongoStore({ mongooseConnection: db })
})
);
app.use(bodyParser.json());
app.use("/api", songsRouter);
app.use("/api", artistsRouter);
app.use("/api", usersRouter);
// app.listen - Binds and listens for connections on the specified host and port
app.listen(PORT, () => {
console.log("The server is running on port 8000!");
}); |
/* (c) Magnus Auvinen. See licence.txt in the root of the distribution for more information. */
/* If you are missing that file, acquire a complete release at teeworlds.com. */
#ifndef GAME_LOCALIZATION_H
#define GAME_LOCALIZATION_H
#include <base/system.h> // GNUC_ATTRIBUTE
#include <engine/shared/memheap.h>
#include <string>
#include <vector>
class CLanguage
{
public:
CLanguage() = default;
CLanguage(const char *pName, const char *pFileName, int Code, const std::vector<std::string> &vLanguageCodes) :
m_Name(pName), m_FileName(pFileName), m_CountryCode(Code), m_vLanguageCodes(vLanguageCodes) {}
std::string m_Name;
std::string m_FileName;
int m_CountryCode;
std::vector<std::string> m_vLanguageCodes;
bool operator<(const CLanguage &Other) const { return m_Name < Other.m_Name; }
};
class CLocalizationDatabase
{
class CString
{
public:
unsigned m_Hash;
unsigned m_ContextHash;
const char *m_pReplacement;
CString() {}
CString(unsigned Hash, unsigned ContextHash, const char *pReplacement) :
m_Hash(Hash), m_ContextHash(ContextHash), m_pReplacement(pReplacement)
{
}
bool operator<(const CString &Other) const { return m_Hash < Other.m_Hash || (m_Hash == Other.m_Hash && m_ContextHash < Other.m_ContextHash); }
bool operator<=(const CString &Other) const { return m_Hash < Other.m_Hash || (m_Hash == Other.m_Hash && m_ContextHash <= Other.m_ContextHash); }
bool operator==(const CString &Other) const { return m_Hash == Other.m_Hash && m_ContextHash == Other.m_ContextHash; }
};
std::vector<CLanguage> m_vLanguages;
std::vector<CString> m_vStrings;
CHeap m_StringsHeap;
int m_VersionCounter;
int m_CurrentVersion;
public:
CLocalizationDatabase();
void LoadIndexfile(class IStorage *pStorage, class IConsole *pConsole);
const std::vector<CLanguage> &Languages() const { return m_vLanguages; }
void SelectDefaultLanguage(class IConsole *pConsole, char *pFilename, size_t Length) const;
bool Load(const char *pFilename, class IStorage *pStorage, class IConsole *pConsole);
int Version() const { return m_CurrentVersion; }
void AddString(const char *pOrgStr, const char *pNewStr, const char *pContext);
const char *FindString(unsigned Hash, unsigned ContextHash) const;
};
extern CLocalizationDatabase g_Localization;
class CLocConstString
{
const char *m_pDefaultStr;
const char *m_pCurrentStr;
unsigned m_Hash;
unsigned m_ContextHash;
int m_Version;
public:
CLocConstString(const char *pStr, const char *pContext = "");
void Reload();
inline operator const char *()
{
if(m_Version != g_Localization.Version())
Reload();
return m_pCurrentStr;
}
};
extern const char *Localize(const char *pStr, const char *pContext = "")
GNUC_ATTRIBUTE((format_arg(1)));
#endif |
//===- ScopDetectionDiagnostic.cpp - Error diagnostics --------------------===//
//
// Part of the LLVM Project, under the Apache License v2.0 with LLVM Exceptions.
// See https://llvm.org/LICENSE.txt for license information.
// SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
//
//===----------------------------------------------------------------------===//
//
// Small set of diagnostic helper classes to encapsulate any errors occurred
// during the detection of Scops.
//
// The ScopDetection defines a set of error classes (via Statistic variables)
// that groups a number of individual errors into a group, e.g. non-affinity
// related errors.
// On error we generate an object that carries enough additional information
// to diagnose the error and generate a helpful error message.
//
//===----------------------------------------------------------------------===//
#include "polly/ScopDetectionDiagnostic.h"
#include "llvm/ADT/SmallPtrSet.h"
#include "llvm/ADT/SmallVector.h"
#include "llvm/ADT/Statistic.h"
#include "llvm/ADT/StringRef.h"
#include "llvm/ADT/Twine.h"
#include "llvm/Analysis/AliasSetTracker.h"
#include "llvm/Analysis/LoopInfo.h"
#include "llvm/Analysis/OptimizationRemarkEmitter.h"
#include "llvm/Analysis/RegionInfo.h"
#include "llvm/Analysis/ScalarEvolution.h"
#include "llvm/IR/BasicBlock.h"
#include "llvm/IR/CFG.h"
#include "llvm/IR/DebugLoc.h"
#include "llvm/IR/DiagnosticInfo.h"
#include "llvm/IR/Instruction.h"
#include "llvm/IR/Value.h"
#include "llvm/Support/raw_ostream.h"
#include <algorithm>
#include <cassert>
#include <string>
#include <utility>
using namespace llvm;
#define DEBUG_TYPE "polly-detect"
#define SCOP_STAT(NAME, DESC) \
{ \
"polly-detect", "NAME", "Number of rejected regions: " DESC, {0}, { \
false \
} \
}
Statistic RejectStatistics[] = {
SCOP_STAT(CFG, ""),
SCOP_STAT(InvalidTerminator, "Unsupported terminator instruction"),
SCOP_STAT(UnreachableInExit, "Unreachable in exit block"),
SCOP_STAT(IrreducibleRegion, "Irreducible loops"),
SCOP_STAT(LastCFG, ""),
SCOP_STAT(AffFunc, ""),
SCOP_STAT(UndefCond, "Undefined branch condition"),
SCOP_STAT(InvalidCond, "Non-integer branch condition"),
SCOP_STAT(UndefOperand, "Undefined operands in comparison"),
SCOP_STAT(NonAffBranch, "Non-affine branch condition"),
SCOP_STAT(NoBasePtr, "No base pointer"),
SCOP_STAT(UndefBasePtr, "Undefined base pointer"),
SCOP_STAT(VariantBasePtr, "Variant base pointer"),
SCOP_STAT(NonAffineAccess, "Non-affine memory accesses"),
SCOP_STAT(DifferentElementSize, "Accesses with differing sizes"),
SCOP_STAT(LastAffFunc, ""),
SCOP_STAT(LoopBound, "Uncomputable loop bounds"),
SCOP_STAT(LoopHasNoExit, "Loop without exit"),
SCOP_STAT(LoopHasMultipleExits, "Loop with multiple exits"),
SCOP_STAT(LoopOnlySomeLatches, "Not all loop latches in scop"),
SCOP_STAT(FuncCall, "Function call with side effects"),
SCOP_STAT(NonSimpleMemoryAccess,
"Compilated access semantics (volatile or atomic)"),
SCOP_STAT(Alias, "Base address aliasing"),
SCOP_STAT(Other, ""),
SCOP_STAT(IntToPtr, "Integer to pointer conversions"),
SCOP_STAT(Alloca, "Stack allocations"),
SCOP_STAT(UnknownInst, "Unknown Instructions"),
SCOP_STAT(Entry, "Contains entry block"),
SCOP_STAT(Unprofitable, "Assumed to be unprofitable"),
SCOP_STAT(LastOther, ""),
};
namespace polly {
/// Small string conversion via raw_string_stream.
template <typename T> std::string operator+(Twine LHS, const T &RHS) {
std::string Buf;
raw_string_ostream fmt(Buf);
fmt << RHS;
fmt.flush();
return LHS.concat(Buf).str();
}
} // namespace polly
namespace llvm {
// Lexicographic order on (line, col) of our debug locations.
static bool operator<(const DebugLoc &LHS, const DebugLoc &RHS) {
return LHS.getLine() < RHS.getLine() ||
(LHS.getLine() == RHS.getLine() && LHS.getCol() < RHS.getCol());
}
} // namespace llvm
namespace polly {
BBPair getBBPairForRegion(const Region *R) {
return std::make_pair(R->getEntry(), R->getExit());
}
void getDebugLocations(const BBPair &P, DebugLoc &Begin, DebugLoc &End) {
SmallPtrSet<BasicBlock *, 32> Seen;
SmallVector<BasicBlock *, 32> Todo;
Todo.push_back(P.first);
while (!Todo.empty()) {
auto *BB = Todo.pop_back_val();
if (BB == P.second)
continue;
if (!Seen.insert(BB).second)
continue;
Todo.append(succ_begin(BB), succ_end(BB));
for (const Instruction &Inst : *BB) {
DebugLoc DL = Inst.getDebugLoc();
if (!DL)
continue;
Begin = Begin ? std::min(Begin, DL) : DL;
End = End ? std::max(End, DL) : DL;
}
}
}
void emitRejectionRemarks(const BBPair &P, const RejectLog &Log,
OptimizationRemarkEmitter &ORE) {
DebugLoc Begin, End;
getDebugLocations(P, Begin, End);
ORE.emit(
OptimizationRemarkMissed(DEBUG_TYPE, "RejectionErrors", Begin, P.first)
<< "The following errors keep this region from being a Scop.");
for (RejectReasonPtr RR : Log) {
if (const DebugLoc &Loc = RR->getDebugLoc())
ORE.emit(OptimizationRemarkMissed(DEBUG_TYPE, RR->getRemarkName(), Loc,
RR->getRemarkBB())
<< RR->getEndUserMessage());
else
ORE.emit(OptimizationRemarkMissed(DEBUG_TYPE, RR->getRemarkName(), Begin,
RR->getRemarkBB())
<< RR->getEndUserMessage());
}
/* Check to see if Region is a top level region, getExit = NULL*/
if (P.second)
ORE.emit(
OptimizationRemarkMissed(DEBUG_TYPE, "InvalidScopEnd", End, P.second)
<< "Invalid Scop candidate ends here.");
else
ORE.emit(
OptimizationRemarkMissed(DEBUG_TYPE, "InvalidScopEnd", End, P.first)
<< "Invalid Scop candidate ends here.");
}
//===----------------------------------------------------------------------===//
// RejectReason.
RejectReason::RejectReason(RejectReasonKind K) : Kind(K) {
RejectStatistics[static_cast<int>(K)]++;
}
const DebugLoc RejectReason::Unknown = DebugLoc();
const DebugLoc &RejectReason::getDebugLoc() const {
// Allocate an empty DebugLoc and return it a reference to it.
return Unknown;
}
// RejectLog.
void RejectLog::print(raw_ostream &OS, int level) const {
int j = 0;
for (auto Reason : ErrorReports)
OS.indent(level) << "[" << j++ << "] " << Reason->getMessage() << "\n";
}
//===----------------------------------------------------------------------===//
// ReportCFG.
ReportCFG::ReportCFG(const RejectReasonKind K) : RejectReason(K) {}
bool ReportCFG::classof(const RejectReason *RR) {
return RR->getKind() >= RejectReasonKind::CFG &&
RR->getKind() <= RejectReasonKind::LastCFG;
}
//===----------------------------------------------------------------------===//
// ReportInvalidTerminator.
std::string ReportInvalidTerminator::getRemarkName() const {
return "InvalidTerminator";
}
const Value *ReportInvalidTerminator::getRemarkBB() const { return BB; }
std::string ReportInvalidTerminator::getMessage() const {
return ("Invalid instruction terminates BB: " + BB->getName()).str();
}
const DebugLoc &ReportInvalidTerminator::getDebugLoc() const {
return BB->getTerminator()->getDebugLoc();
}
bool ReportInvalidTerminator::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::InvalidTerminator;
}
//===----------------------------------------------------------------------===//
// UnreachableInExit.
std::string ReportUnreachableInExit::getRemarkName() const {
return "UnreachableInExit";
}
const Value *ReportUnreachableInExit::getRemarkBB() const { return BB; }
std::string ReportUnreachableInExit::getMessage() const {
std::string BBName = BB->getName();
return "Unreachable in exit block" + BBName;
}
const DebugLoc &ReportUnreachableInExit::getDebugLoc() const { return DbgLoc; }
std::string ReportUnreachableInExit::getEndUserMessage() const {
return "Unreachable in exit block.";
}
bool ReportUnreachableInExit::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::UnreachableInExit;
}
//===----------------------------------------------------------------------===//
// ReportIrreducibleRegion.
std::string ReportIrreducibleRegion::getRemarkName() const {
return "IrreducibleRegion";
}
const Value *ReportIrreducibleRegion::getRemarkBB() const {
return R->getEntry();
}
std::string ReportIrreducibleRegion::getMessage() const {
return "Irreducible region encountered: " + R->getNameStr();
}
const DebugLoc &ReportIrreducibleRegion::getDebugLoc() const { return DbgLoc; }
std::string ReportIrreducibleRegion::getEndUserMessage() const {
return "Irreducible region encountered in control flow.";
}
bool ReportIrreducibleRegion::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::IrreducibleRegion;
}
//===----------------------------------------------------------------------===//
// ReportAffFunc.
ReportAffFunc::ReportAffFunc(const RejectReasonKind K, const Instruction *Inst)
: RejectReason(K), Inst(Inst) {}
bool ReportAffFunc::classof(const RejectReason *RR) {
return RR->getKind() >= RejectReasonKind::AffFunc &&
RR->getKind() <= RejectReasonKind::LastAffFunc;
}
//===----------------------------------------------------------------------===//
// ReportUndefCond.
std::string ReportUndefCond::getRemarkName() const { return "UndefCond"; }
const Value *ReportUndefCond::getRemarkBB() const { return BB; }
std::string ReportUndefCond::getMessage() const {
return ("Condition based on 'undef' value in BB: " + BB->getName()).str();
}
bool ReportUndefCond::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::UndefCond;
}
//===----------------------------------------------------------------------===//
// ReportInvalidCond.
std::string ReportInvalidCond::getRemarkName() const { return "InvalidCond"; }
const Value *ReportInvalidCond::getRemarkBB() const { return BB; }
std::string ReportInvalidCond::getMessage() const {
return ("Condition in BB '" + BB->getName()).str() +
"' neither constant nor an icmp instruction";
}
bool ReportInvalidCond::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::InvalidCond;
}
//===----------------------------------------------------------------------===//
// ReportUndefOperand.
std::string ReportUndefOperand::getRemarkName() const { return "UndefOperand"; }
const Value *ReportUndefOperand::getRemarkBB() const { return BB; }
std::string ReportUndefOperand::getMessage() const {
return ("undef operand in branch at BB: " + BB->getName()).str();
}
bool ReportUndefOperand::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::UndefOperand;
}
//===----------------------------------------------------------------------===//
// ReportNonAffBranch.
std::string ReportNonAffBranch::getRemarkName() const { return "NonAffBranch"; }
const Value *ReportNonAffBranch::getRemarkBB() const { return BB; }
std::string ReportNonAffBranch::getMessage() const {
return ("Non affine branch in BB '" + BB->getName()).str() +
"' with LHS: " + *LHS + " and RHS: " + *RHS;
}
bool ReportNonAffBranch::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::NonAffBranch;
}
//===----------------------------------------------------------------------===//
// ReportNoBasePtr.
std::string ReportNoBasePtr::getRemarkName() const { return "NoBasePtr"; }
const Value *ReportNoBasePtr::getRemarkBB() const { return Inst->getParent(); }
std::string ReportNoBasePtr::getMessage() const { return "No base pointer"; }
bool ReportNoBasePtr::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::NoBasePtr;
}
//===----------------------------------------------------------------------===//
// ReportUndefBasePtr.
std::string ReportUndefBasePtr::getRemarkName() const { return "UndefBasePtr"; }
const Value *ReportUndefBasePtr::getRemarkBB() const {
return Inst->getParent();
}
std::string ReportUndefBasePtr::getMessage() const {
return "Undefined base pointer";
}
bool ReportUndefBasePtr::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::UndefBasePtr;
}
//===----------------------------------------------------------------------===//
// ReportVariantBasePtr.
std::string ReportVariantBasePtr::getRemarkName() const {
return "VariantBasePtr";
}
const Value *ReportVariantBasePtr::getRemarkBB() const {
return Inst->getParent();
}
std::string ReportVariantBasePtr::getMessage() const {
return "Base address not invariant in current region:" + *BaseValue;
}
std::string ReportVariantBasePtr::getEndUserMessage() const {
return "The base address of this array is not invariant inside the loop";
}
bool ReportVariantBasePtr::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::VariantBasePtr;
}
//===----------------------------------------------------------------------===//
// ReportDifferentArrayElementSize
std::string ReportDifferentArrayElementSize::getRemarkName() const {
return "DifferentArrayElementSize";
}
const Value *ReportDifferentArrayElementSize::getRemarkBB() const {
return Inst->getParent();
}
std::string ReportDifferentArrayElementSize::getMessage() const {
return "Access to one array through data types of different size";
}
bool ReportDifferentArrayElementSize::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::DifferentElementSize;
}
std::string ReportDifferentArrayElementSize::getEndUserMessage() const {
StringRef BaseName = BaseValue->getName();
std::string Name = BaseName.empty() ? "UNKNOWN" : BaseName;
return "The array \"" + Name +
"\" is accessed through elements that differ "
"in size";
}
//===----------------------------------------------------------------------===//
// ReportNonAffineAccess.
std::string ReportNonAffineAccess::getRemarkName() const {
return "NonAffineAccess";
}
const Value *ReportNonAffineAccess::getRemarkBB() const {
return Inst->getParent();
}
std::string ReportNonAffineAccess::getMessage() const {
return "Non affine access function: " + *AccessFunction;
}
bool ReportNonAffineAccess::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::NonAffineAccess;
}
std::string ReportNonAffineAccess::getEndUserMessage() const {
StringRef BaseName = BaseValue->getName();
std::string Name = BaseName.empty() ? "UNKNOWN" : BaseName;
return "The array subscript of \"" + Name + "\" is not affine";
}
//===----------------------------------------------------------------------===//
// ReportLoopBound.
ReportLoopBound::ReportLoopBound(Loop *L, const SCEV *LoopCount)
: RejectReason(RejectReasonKind::LoopBound), L(L), LoopCount(LoopCount),
Loc(L->getStartLoc()) {}
std::string ReportLoopBound::getRemarkName() const { return "LoopBound"; }
const Value *ReportLoopBound::getRemarkBB() const { return L->getHeader(); }
std::string ReportLoopBound::getMessage() const {
return "Non affine loop bound '" + *LoopCount +
"' in loop: " + L->getHeader()->getName();
}
const DebugLoc &ReportLoopBound::getDebugLoc() const { return Loc; }
bool ReportLoopBound::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::LoopBound;
}
std::string ReportLoopBound::getEndUserMessage() const {
return "Failed to derive an affine function from the loop bounds.";
}
//===----------------------------------------------------------------------===//
// ReportLoopHasNoExit.
std::string ReportLoopHasNoExit::getRemarkName() const {
return "LoopHasNoExit";
}
const Value *ReportLoopHasNoExit::getRemarkBB() const { return L->getHeader(); }
std::string ReportLoopHasNoExit::getMessage() const {
return "Loop " + L->getHeader()->getName() + " has no exit.";
}
bool ReportLoopHasNoExit::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::LoopHasNoExit;
}
const DebugLoc &ReportLoopHasNoExit::getDebugLoc() const { return Loc; }
std::string ReportLoopHasNoExit::getEndUserMessage() const {
return "Loop cannot be handled because it has no exit.";
}
//===----------------------------------------------------------------------===//
// ReportLoopHasMultipleExits.
std::string ReportLoopHasMultipleExits::getRemarkName() const {
return "ReportLoopHasMultipleExits";
}
const Value *ReportLoopHasMultipleExits::getRemarkBB() const {
return L->getHeader();
}
std::string ReportLoopHasMultipleExits::getMessage() const {
return "Loop " + L->getHeader()->getName() + " has multiple exits.";
}
bool ReportLoopHasMultipleExits::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::LoopHasMultipleExits;
}
const DebugLoc &ReportLoopHasMultipleExits::getDebugLoc() const { return Loc; }
std::string ReportLoopHasMultipleExits::getEndUserMessage() const {
return "Loop cannot be handled because it has multiple exits.";
}
//===----------------------------------------------------------------------===//
// ReportLoopOnlySomeLatches
std::string ReportLoopOnlySomeLatches::getRemarkName() const {
return "LoopHasNoExit";
}
const Value *ReportLoopOnlySomeLatches::getRemarkBB() const {
return L->getHeader();
}
std::string ReportLoopOnlySomeLatches::getMessage() const {
return "Not all latches of loop " + L->getHeader()->getName() +
" part of scop.";
}
bool ReportLoopOnlySomeLatches::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::LoopHasNoExit;
}
const DebugLoc &ReportLoopOnlySomeLatches::getDebugLoc() const { return Loc; }
std::string ReportLoopOnlySomeLatches::getEndUserMessage() const {
return "Loop cannot be handled because not all latches are part of loop "
"region.";
}
//===----------------------------------------------------------------------===//
// ReportFuncCall.
ReportFuncCall::ReportFuncCall(Instruction *Inst)
: RejectReason(RejectReasonKind::FuncCall), Inst(Inst) {}
std::string ReportFuncCall::getRemarkName() const { return "FuncCall"; }
const Value *ReportFuncCall::getRemarkBB() const { return Inst->getParent(); }
std::string ReportFuncCall::getMessage() const {
return "Call instruction: " + *Inst;
}
const DebugLoc &ReportFuncCall::getDebugLoc() const {
return Inst->getDebugLoc();
}
std::string ReportFuncCall::getEndUserMessage() const {
return "This function call cannot be handled. "
"Try to inline it.";
}
bool ReportFuncCall::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::FuncCall;
}
//===----------------------------------------------------------------------===//
// ReportNonSimpleMemoryAccess
ReportNonSimpleMemoryAccess::ReportNonSimpleMemoryAccess(Instruction *Inst)
: ReportOther(RejectReasonKind::NonSimpleMemoryAccess), Inst(Inst) {}
std::string ReportNonSimpleMemoryAccess::getRemarkName() const {
return "NonSimpleMemoryAccess";
}
const Value *ReportNonSimpleMemoryAccess::getRemarkBB() const {
return Inst->getParent();
}
std::string ReportNonSimpleMemoryAccess::getMessage() const {
return "Non-simple memory access: " + *Inst;
}
const DebugLoc &ReportNonSimpleMemoryAccess::getDebugLoc() const {
return Inst->getDebugLoc();
}
std::string ReportNonSimpleMemoryAccess::getEndUserMessage() const {
return "Volatile memory accesses or memory accesses for atomic types "
"are not supported.";
}
bool ReportNonSimpleMemoryAccess::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::NonSimpleMemoryAccess;
}
//===----------------------------------------------------------------------===//
// ReportAlias.
ReportAlias::ReportAlias(Instruction *Inst, AliasSet &AS)
: RejectReason(RejectReasonKind::Alias), Inst(Inst) {
for (const auto &I : AS)
Pointers.push_back(I.getValue());
}
std::string ReportAlias::formatInvalidAlias(std::string Prefix,
std::string Suffix) const {
std::string Message;
raw_string_ostream OS(Message);
OS << Prefix;
for (PointerSnapshotTy::const_iterator PI = Pointers.begin(),
PE = Pointers.end();
;) {
const Value *V = *PI;
assert(V && "Diagnostic info does not match found LLVM-IR anymore.");
if (V->getName().empty())
OS << "\" <unknown> \"";
else
OS << "\"" << V->getName() << "\"";
++PI;
if (PI != PE)
OS << ", ";
else
break;
}
OS << Suffix;
return OS.str();
}
std::string ReportAlias::getRemarkName() const { return "Alias"; }
const Value *ReportAlias::getRemarkBB() const { return Inst->getParent(); }
std::string ReportAlias::getMessage() const {
return formatInvalidAlias("Possible aliasing: ");
}
std::string ReportAlias::getEndUserMessage() const {
return formatInvalidAlias("Accesses to the arrays ",
" may access the same memory.");
}
const DebugLoc &ReportAlias::getDebugLoc() const { return Inst->getDebugLoc(); }
bool ReportAlias::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::Alias;
}
//===----------------------------------------------------------------------===//
// ReportOther.
std::string ReportOther::getRemarkName() const { return "UnknownRejectReason"; }
std::string ReportOther::getMessage() const { return "Unknown reject reason"; }
ReportOther::ReportOther(const RejectReasonKind K) : RejectReason(K) {}
bool ReportOther::classof(const RejectReason *RR) {
return RR->getKind() >= RejectReasonKind::Other &&
RR->getKind() <= RejectReasonKind::LastOther;
}
//===----------------------------------------------------------------------===//
// ReportIntToPtr.
ReportIntToPtr::ReportIntToPtr(Instruction *BaseValue)
: ReportOther(RejectReasonKind::IntToPtr), BaseValue(BaseValue) {}
std::string ReportIntToPtr::getRemarkName() const { return "IntToPtr"; }
const Value *ReportIntToPtr::getRemarkBB() const {
return BaseValue->getParent();
}
std::string ReportIntToPtr::getMessage() const {
return "Find bad intToptr prt: " + *BaseValue;
}
const DebugLoc &ReportIntToPtr::getDebugLoc() const {
return BaseValue->getDebugLoc();
}
bool ReportIntToPtr::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::IntToPtr;
}
//===----------------------------------------------------------------------===//
// ReportAlloca.
ReportAlloca::ReportAlloca(Instruction *Inst)
: ReportOther(RejectReasonKind::Alloca), Inst(Inst) {}
std::string ReportAlloca::getRemarkName() const { return "Alloca"; }
const Value *ReportAlloca::getRemarkBB() const { return Inst->getParent(); }
std::string ReportAlloca::getMessage() const {
return "Alloca instruction: " + *Inst;
}
const DebugLoc &ReportAlloca::getDebugLoc() const {
return Inst->getDebugLoc();
}
bool ReportAlloca::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::Alloca;
}
//===----------------------------------------------------------------------===//
// ReportUnknownInst.
ReportUnknownInst::ReportUnknownInst(Instruction *Inst)
: ReportOther(RejectReasonKind::UnknownInst), Inst(Inst) {}
std::string ReportUnknownInst::getRemarkName() const { return "UnknownInst"; }
const Value *ReportUnknownInst::getRemarkBB() const {
return Inst->getParent();
}
std::string ReportUnknownInst::getMessage() const {
return "Unknown instruction: " + *Inst;
}
const DebugLoc &ReportUnknownInst::getDebugLoc() const {
return Inst->getDebugLoc();
}
bool ReportUnknownInst::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::UnknownInst;
}
//===----------------------------------------------------------------------===//
// ReportEntry.
ReportEntry::ReportEntry(BasicBlock *BB)
: ReportOther(RejectReasonKind::Entry), BB(BB) {}
std::string ReportEntry::getRemarkName() const { return "Entry"; }
const Value *ReportEntry::getRemarkBB() const { return BB; }
std::string ReportEntry::getMessage() const {
return "Region containing entry block of function is invalid!";
}
std::string ReportEntry::getEndUserMessage() const {
return "Scop contains function entry (not yet supported).";
}
const DebugLoc &ReportEntry::getDebugLoc() const {
return BB->getTerminator()->getDebugLoc();
}
bool ReportEntry::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::Entry;
}
//===----------------------------------------------------------------------===//
// ReportUnprofitable.
ReportUnprofitable::ReportUnprofitable(Region *R)
: ReportOther(RejectReasonKind::Unprofitable), R(R) {}
std::string ReportUnprofitable::getRemarkName() const { return "Unprofitable"; }
const Value *ReportUnprofitable::getRemarkBB() const { return R->getEntry(); }
std::string ReportUnprofitable::getMessage() const {
return "Region can not profitably be optimized!";
}
std::string ReportUnprofitable::getEndUserMessage() const {
return "No profitable polyhedral optimization found";
}
const DebugLoc &ReportUnprofitable::getDebugLoc() const {
for (const BasicBlock *BB : R->blocks())
for (const Instruction &Inst : *BB)
if (const DebugLoc &DL = Inst.getDebugLoc())
return DL;
return R->getEntry()->getTerminator()->getDebugLoc();
}
bool ReportUnprofitable::classof(const RejectReason *RR) {
return RR->getKind() == RejectReasonKind::Unprofitable;
}
} // namespace polly |
import React, { useContext, useState } from 'react'
import './Navbar.css'
import logo from '../Assets/logo.png'
import cart_icon from '../Assets/cart_icon.png'
import { Link } from 'react-router-dom'
import { ShopContext } from '../../Context/ShopContext'
import burger_menu from '../Assets/burger-menu.svg'
import close_menu from '../Assets/close.svg'
const Navbar = () => {
let { getTotalCartItems } = useContext(ShopContext)
const [menu, setMenu] = useState('shop')
const [active, setActive] = useState(false)
const menuHandler = (activeName) => {
setMenu(activeName)
setActive(!active)
}
return (
<div className="navbar">
<div className="nav-logo">
<img src={logo} alt="" />
<p>SHOPPER</p>
</div>
<img onClick={() => setActive(!active)} src={burger_menu} className='menu' alt="" />
<ul className={active ? 'nav-menu active' : 'nav-menu'}>
<li onClick={() => menuHandler('shop')}> <Link to='/'> Shop </Link> {menu === 'shop' && <hr />} </li>
<li onClick={() => menuHandler('Mens')}> <Link to='/mens'> Men </Link> {menu === 'Mens' && <hr />} </li>
<li onClick={() => menuHandler('Womens')}> <Link to='/womens'> Women </Link> {menu === 'Womens' && <hr />} </li>
<li onClick={() => menuHandler('Kids')}> <Link to='/kids'> Kids </Link> {menu === 'Kids' && <hr />} </li>
<Link to='/login' ><button onClick={() => setActive(!active)}>Login</button></Link>
<img src={close_menu} onClick={() => setActive(!active)} className='close-menu' alt="" />
</ul>
<div className="nav-login-cart">
<Link to='/cart'><img src={cart_icon} alt="" /></Link>
<div className="nav-cart-count">{getTotalCartItems()}</div>
</div>
</div>
)
}
export default Navbar |
---
title: In 2024, How To Change Your Apple ID on iPhone 12 Pro With or Without Password | Dr.fone
date: 2024-05-19T07:28:02.894Z
updated: 2024-05-20T07:28:02.894Z
tags:
- unlock
- remove screen lock
categories:
- ios
- iphone
description: This article describes How To Change Your Apple ID on iPhone 12 Pro With or Without Password
excerpt: This article describes How To Change Your Apple ID on iPhone 12 Pro With or Without Password
keywords: unlock iphone with apple watch,fix iphone backup password never set but still asking,unlock iphone screen passcode,change country on iphone app store,unlock disabled iphone without itunes,iphone auto lock greyed out,how to unlock iphone 12,iphone x iphone xr lock screen
thumbnail: https://www.lifewire.com/thmb/x0mFXdBc-1Q_2JlWzJG0rvyCFFg=/400x300/filters:no_upscale():max_bytes(150000):strip_icc():format(webp)/dormroomentfeatured-5b5e9dd3c9e77c004f28632e.jpg
---
## How To Change Your Apple ID on Apple iPhone 12 Pro With or Without Password
The Apple ID is a unique identifier used to log in to all Apple services, including iCloud, the App Store, Apple Music, and more. You can track purchases and manage your account settings through your Apple ID. But what if you need a new one for security or moving to a new location?
Whatever the reason, learning **how to change your Apple ID on your iPad** is straightforward. And this guide will show the steps and provide some tips at the end.
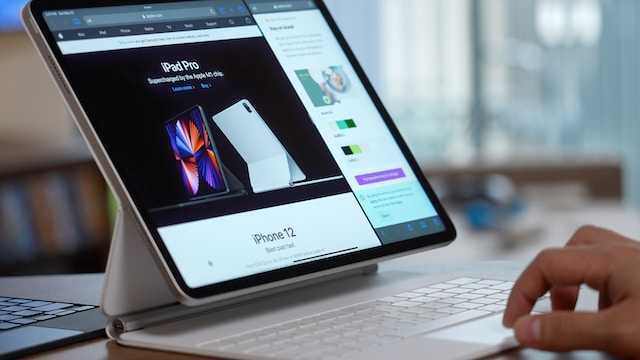
## Part 1: Reasons Why You Might Need To Change Your Apple ID
Your iOS devices contain most of your digital data. That includes your contact list, email, financial information, and social media passwords. All this data is valuable - others can use it to do identity theft, fraud, and other crimes.
If you feel the existing Apple ID isn't secure enough, it's a good idea to **change your Apple ID on your iPad** or other devices. Aside from this, there are a few scenarios where you might find yourself needing to change your details:
You No Longer Use the Email Address or Phone Number Associated With Your Apple ID
Life moves fast, and sometimes contact info changes. If the email or phone number linked to your Apple ID becomes outdated, it's a good idea to update it. That way, you won't miss out on vital notifications or have trouble recovering your account if needed.
You Want To Simplify Your Online Life
If you have multiple email addresses, you may want to consolidate them into one email address. It can make it easier to manage and remember your online accounts, including your Apple ID.
You Are Relocating to a Different Region or Country
Moving abroad? Your Apple ID may need an update to match your new location. It ensures access to region-specific apps and services. You need to have a few things in place before you can do that: add a payment method for your new location, cancel your subscriptions, and spend your store credit. Aside from that, you will also need to change your Apple ID country or region.
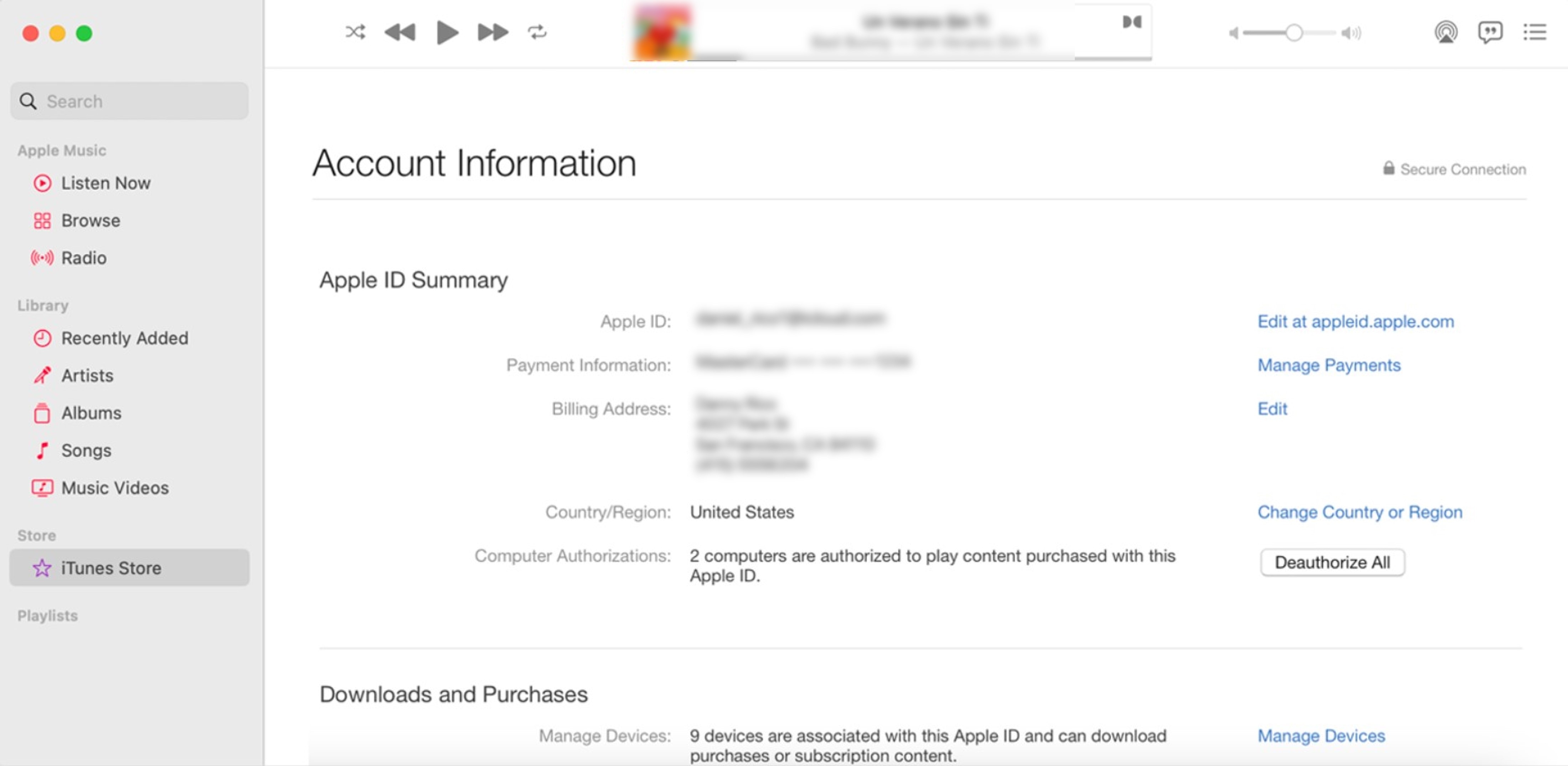
Changing your Apple ID can be smart, ensuring your digital life stays up-to-date, secure, and tailored to your needs. It's not complicated, and it's all about making your Apple experience better suited to you. So, go ahead and learn **how to change your Apple ID on your iPad** in the following sections.
## Part 2: How To Change Apple ID on iPad Without Losing Data?
If you receive a notification from Apple about suspicious account activity, it's time to act. Before you learn **how to change the Apple ID on an iPad** – for yourself or others, here are some common signs of a compromised Apple ID:
- A login attempt from a device or location that you don't recognize
- A password change that you didn't know about
- Unauthorized messages or purchases
- A password that no longer works
- Unfamiliar account details
If you suspect unauthorized access to your Apple ID, below is what you can do.
Steps To Change Your Apple ID to a Different Email Address
If you are worried about losing data such as purchases and contacts, don't worry. You can update your Apple ID account anytime without disrupting your data. Here's a step-by-step guide on **how to change your Apple ID** email address. Just sign out of all Apple services and devices that use your account first.
- **Step 1:** Visit the [<u>Apple ID</u>](https://appleid.apple.com/) official website and log in to your account credentials.
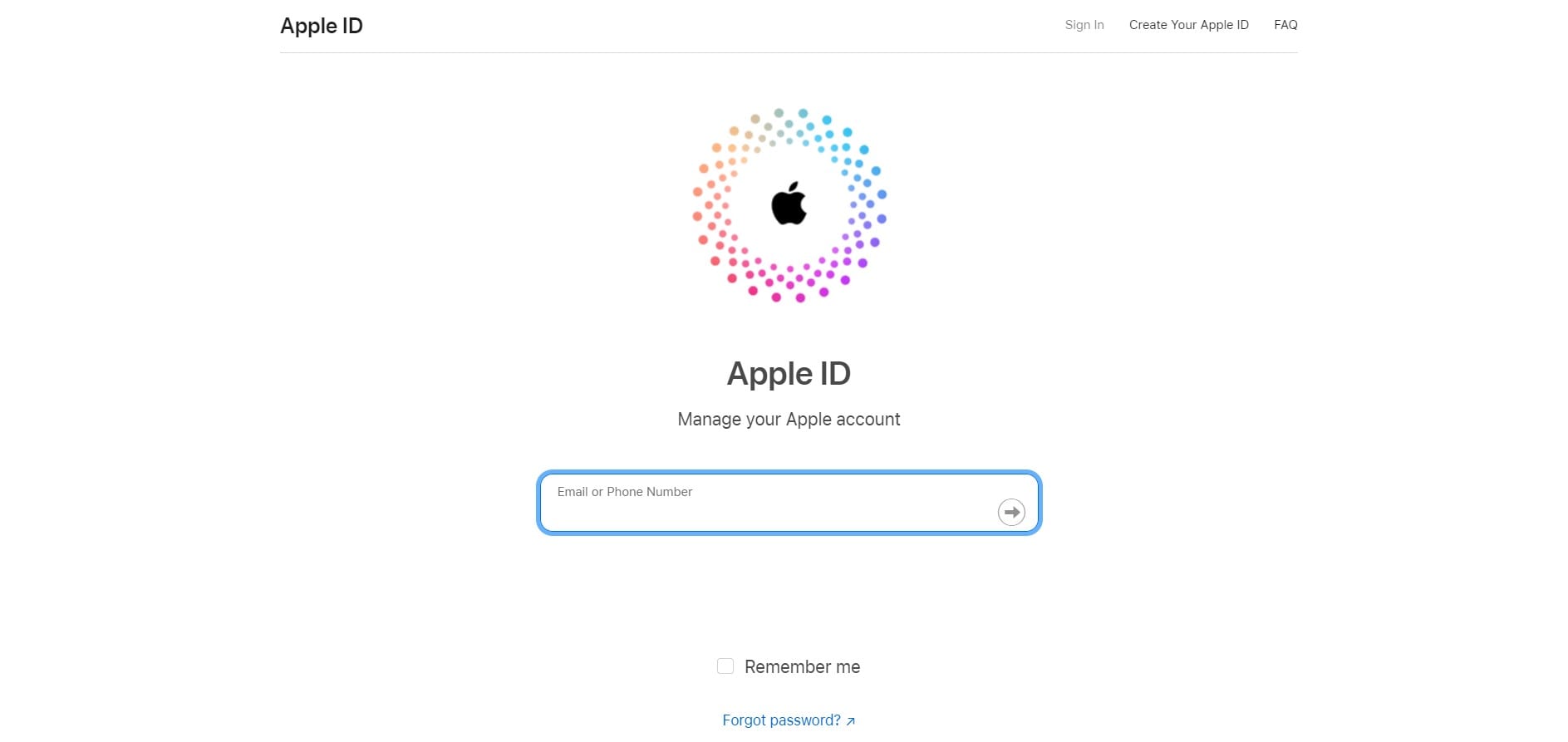
- **Step 2:** Once logged in, select **Apple ID** in the **Sign-In and Security** section.
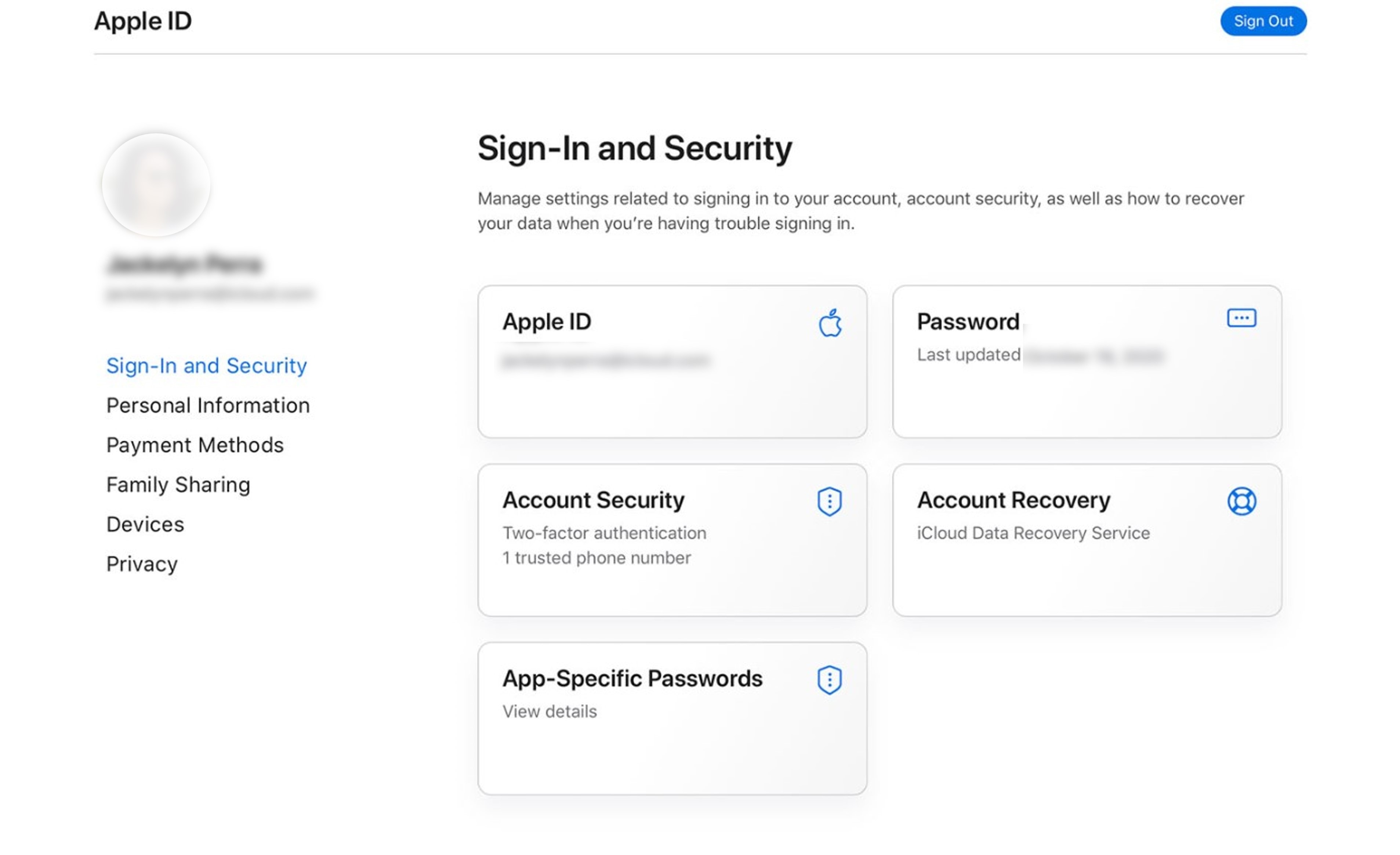
- **Step 3:** From the **Apple ID** pop-up window, enter your new Apple ID in the **Change your Apple ID** text field.
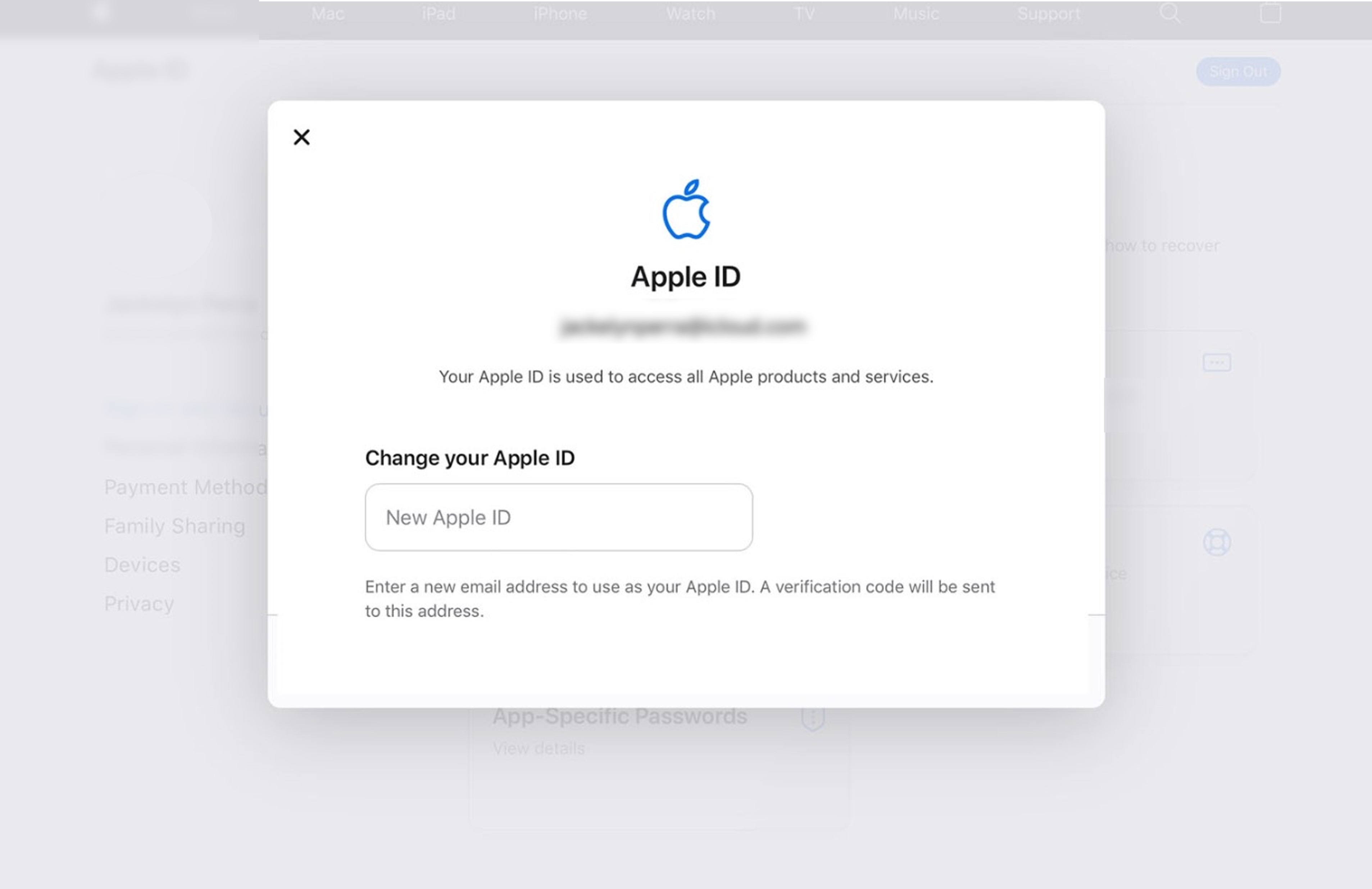
- **Step 4:** Select **Change Apple ID** to proceed.
- **Step 5:** Check your email to get a verification code sent by Apple to ensure that only you can change it to a third-party email address. Enter the code to complete the update.
**Step 6:** Sign in to Apple services such as iCloud and Messages, using your new Apple ID to continue sharing with others.
Steps To Change Your Apple ID to a Different Mobile Number
Do you want to use Your Apple iPhone 12 Pro number as your Apple ID username? It's possible, but it depends on your location, how you created your account, and what version of iOS you're using.
If you're in China mainland or India, and you're using iOS 11 or later, you can create a new Apple ID with Your Apple iPhone 12 Pro number as the username. However, Your Apple iPhone 12 Pro number must be (+86) or (+91). So, if you're thinking of, "**How can I change my Apple ID on my iPad** if it's a mobile phone number?" then this guide is for you:
- **Step 1:** Log out of all Apple services and devices currently signed in with your Apple ID, except the one you're using to change your Apple ID.
- **Step 2:** On your iPad, go to **Settings** > **\[your account name\]** > **Sign-in & Security**.
- **Step 3:** Tap **Edit** next to **Email & Phone Numbers**.
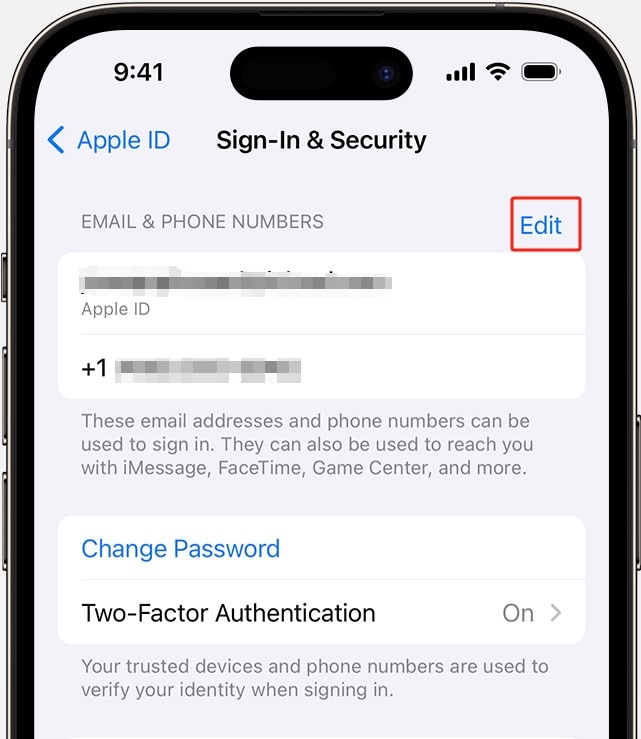
- **Step 4:** Go to the **Delete** icon next to your mobile number and follow the directions given.
- **Step 5:** Enter the code sent to the mobile number you added as your new Apple ID to verify it.
- **Step 6:** Log into all Apple services using your new Apple ID.
Even if you can't **change your Apple ID on your iPad** to a mobile phone number, you can still associate it with a phone number on your account page. It means you can [<u>use a different email address or mobile phone number to log in to your Apple ID</u>](https://drfone.wondershare.com/apple-account/how-to-remove-phone-number-from-apple-id.html). See the next sections for more information on the workarounds you can try.
## Part 3: What To Do if You Cannot Change Your Apple ID on iPad?
**Changing your Apple ID on an iPad** is usually easy. However, there can be setbacks during the process. Now, here's what to do if you find yourself unable to do it and why it might be happening:
Try Again Later
If you encounter errors during the process, give it a little time and try again. Mayne it is because you **changed your Apple ID on your iPad** to an iCloud email address within 30 days.
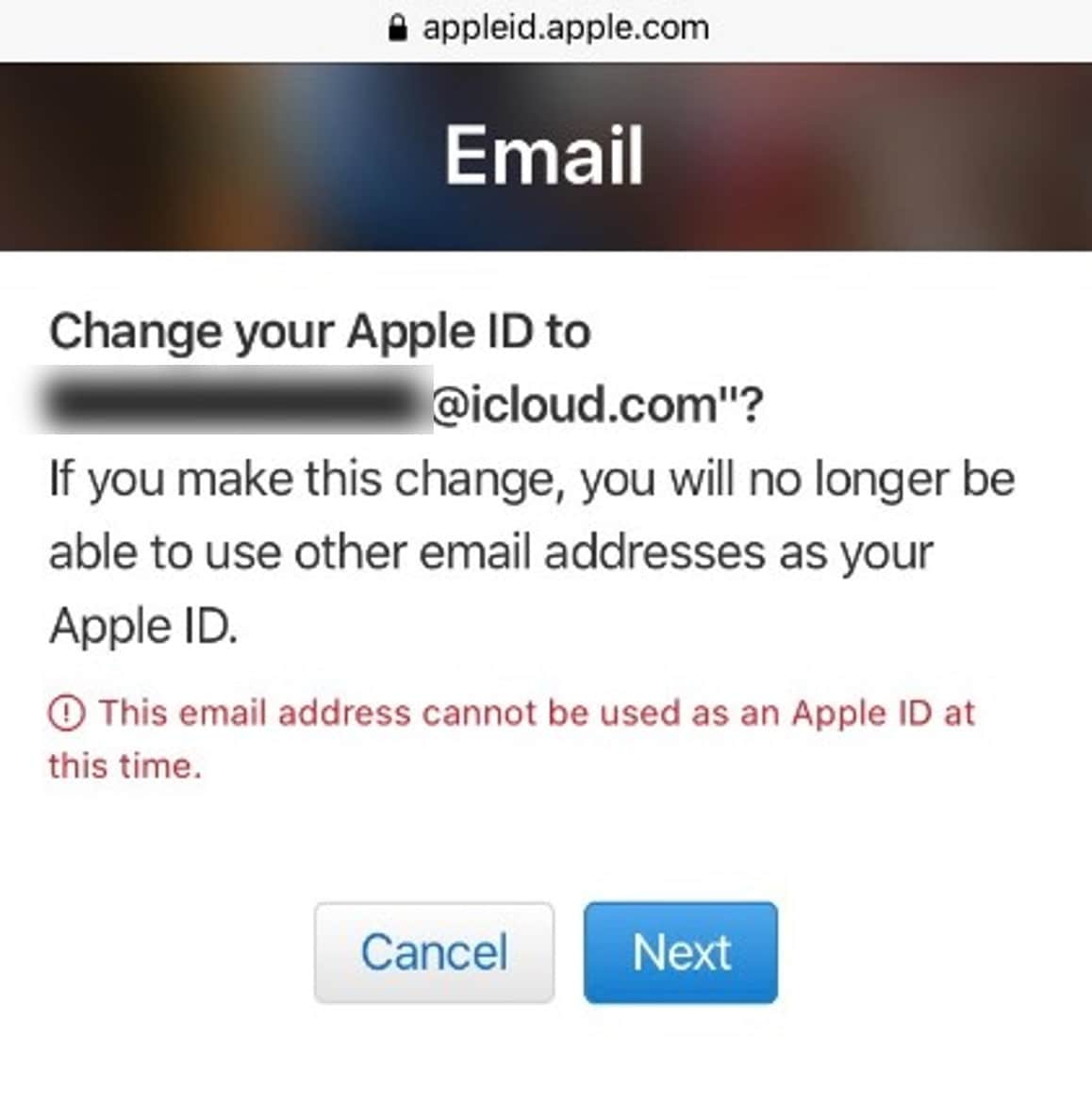
Check if Apple ID Is Already in Use
Apple IDs are unique, and you can't have two identical ones. If you're trying to change your Apple ID to one that's already in use, you'll hit a roadblock. Ensure that the new Apple ID you want isn't already taken. Remove it from the family member's account if they are using it.
Your Apple ID Is an Email Address
If your current Apple ID is an email address, you won't be able to change it to a mobile phone number directly. Instead, you can add an email or phone number to your account for contact purposes.
If you've addressed these issues and still can't change your Apple ID, don't worry. Apple customer support is available to assist you with account-related challenges. Contact them for guidance and solutions tailored to your situation.
However, if you can't **change your Apple ID on your iPad** because you forgot or don't have access to the password, you can use a third-party tool such as Dr.Fone. This tool is easy to use and can help you unlock your iPad and remove the Apple ID in a few clicks.
## Part 4: Using [<u>Wondershare Dr.Fone</u>](https://tools.techidaily.com/wondershare/drfone/drfone-toolkit/) To Remove Apple ID Without Password
Dr.Fone Screen Unlock (iOS) tool is your go-to solution for unlocking iOS devices without a passcode. The best part? It is easy to use for users of all technical backgrounds. With Dr.Fone, you can unlock iOS screens protected by 4 to 6-digit passcodes, Face ID, Touch ID, and more.
But it doesn't stop there; Dr.Fone can also help [<u>remove the iCloud activation lock</u>](https://drfone.wondershare.com/icloud/unlock-icloud-activation.html), unlock Apple ID without a password, and much more. This powerful tool is a lifesaver for iOS users, supporting the latest iPadOS 17, iOS 17, and iPhone 14.
Step-by-Step Guide to Removing Apple ID on iPad Using Dr.Fone
Dr.Fone can bypass the Find My/Find My iPhone feature to remove your Apple ID from your iPad, iPhone, or iPod Touch. Follow the steps below to learn how to use this feature on your iOS device.
- **Step 1:** Get [<u>Wondershare Dr.Fone</u>](https://tools.techidaily.com/wondershare/drfone/drfone-toolkit/) from their website and install it on your computer.
- **Step 2:** From the app's **Toolbox** homepage, click **Screen Unlock**, and select **iOS**.
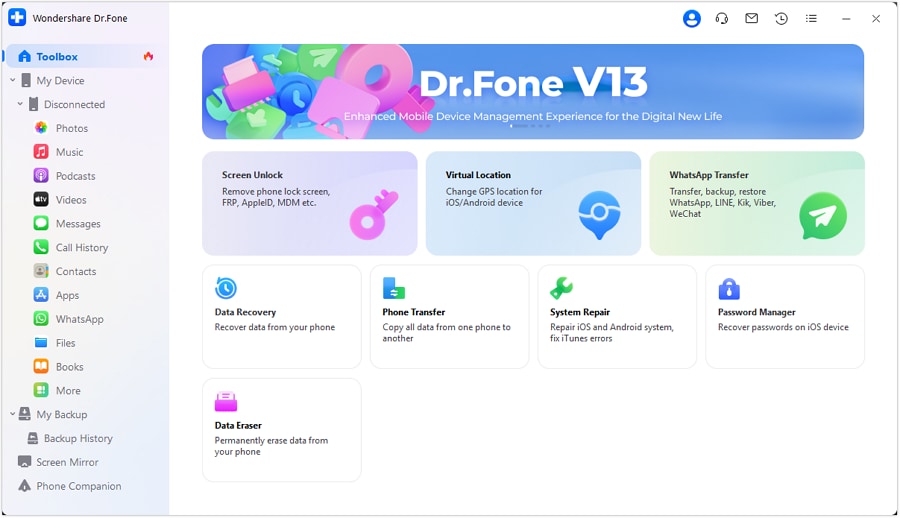
- **Step 3:** Select **Remove AppleID** from the available **Screen Unlock** tools.
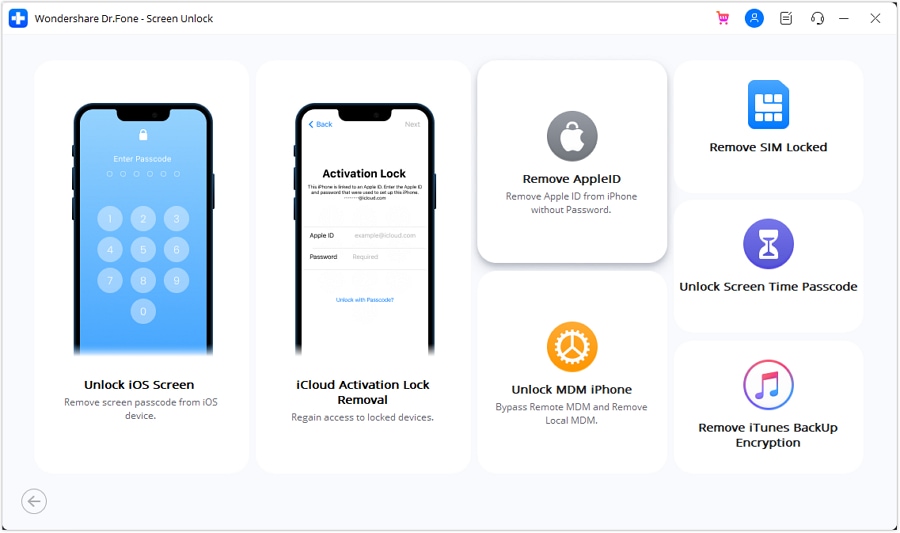
- **Step 4:** Connect your iPad or other iOS device to your computer, then click **Unlock Now** to continue.
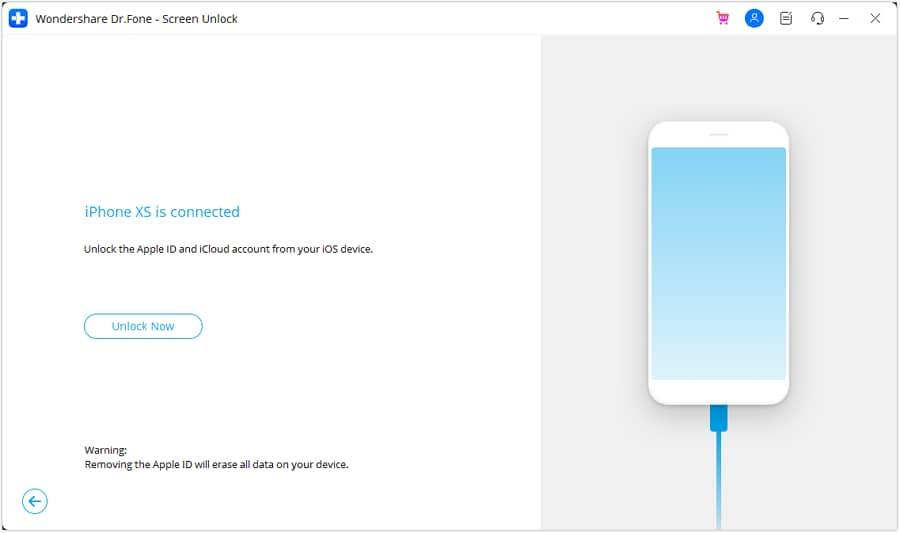
- **Step 5:** Click **Yes** to confirm your device has a screen lock. Otherwise, it won't work.
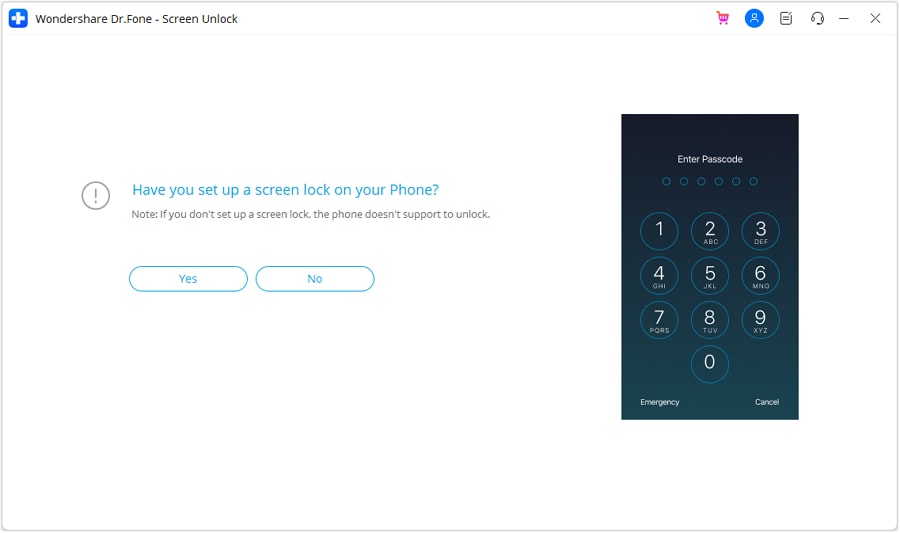
- **Step 6:** Enable the Two-Factor Authentication on your iPad so you can unlock your Apple ID. Then, click **Yes** to proceed.
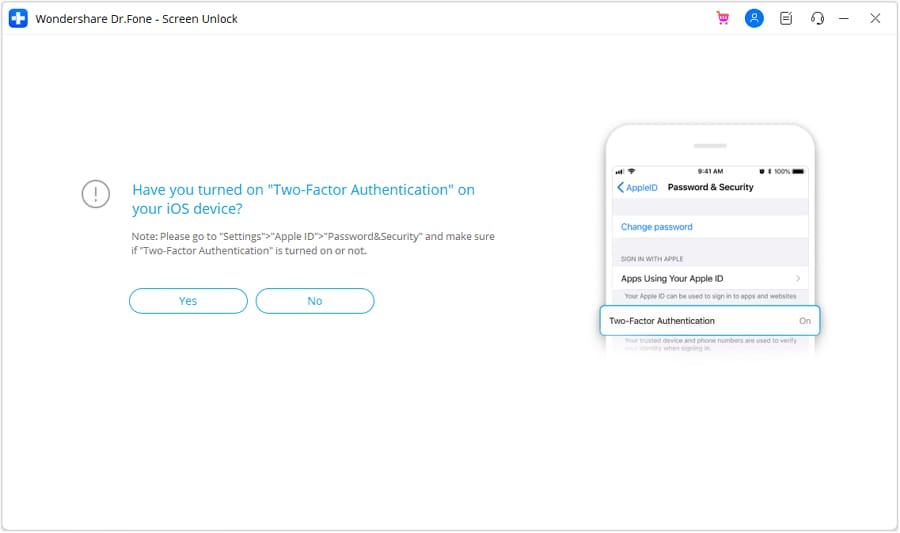
- **Step 7:** Follow the on-screen instructions to put your iPad in [<u>Recovery Mode</u>](https://drfone.wondershare.com/recovery-mode/iphone-stuck-in-recovery-mode.html).
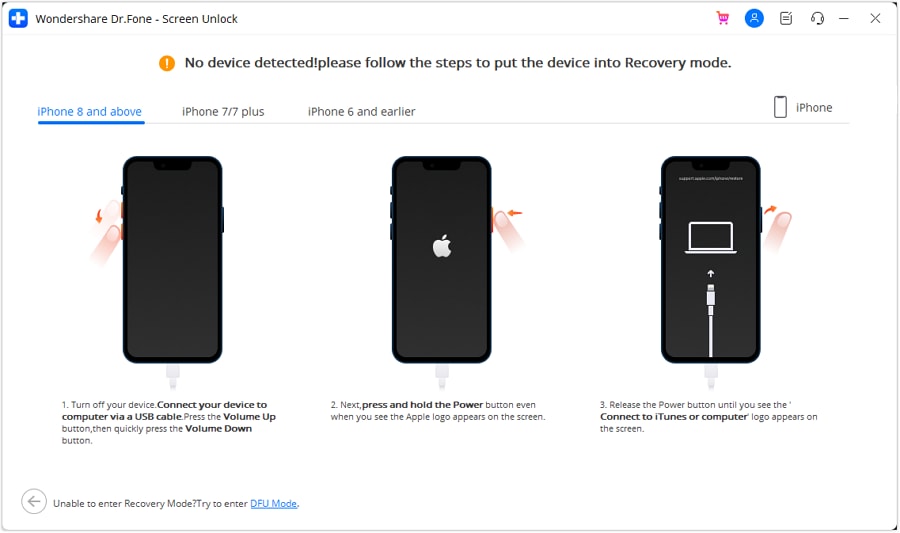
- **Step 8:** The next screen will demonstrate the **Device Model** information. Select an option from the **System Version** dropdown list and click **Start**.
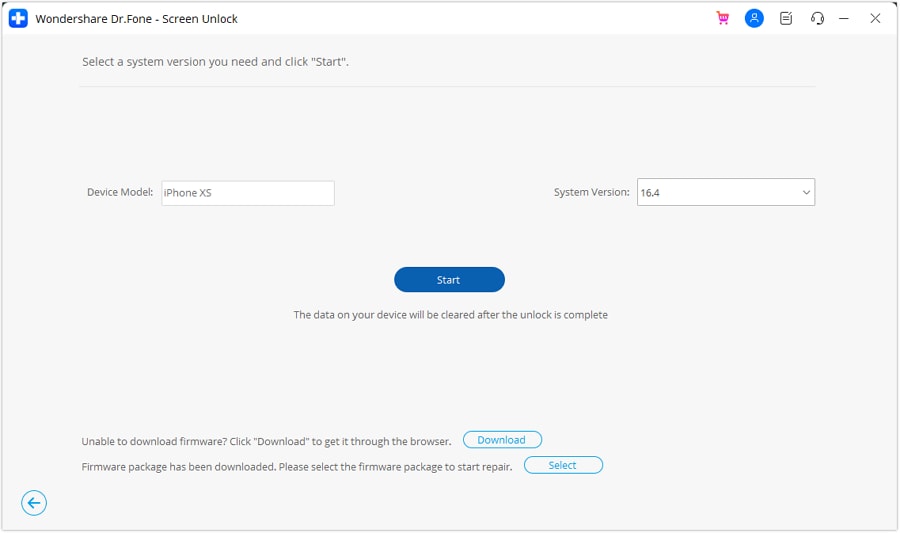
- **Step 9:** The [<u>iOS firmware</u>](https://drfone.wondershare.com/ios-upgrade/how-to-upgrade-with-firmware-files.html) will start downloading, and you can see the progress on the next screen. If you're experiencing slow download speeds, click **Copy** to get the link and download it.
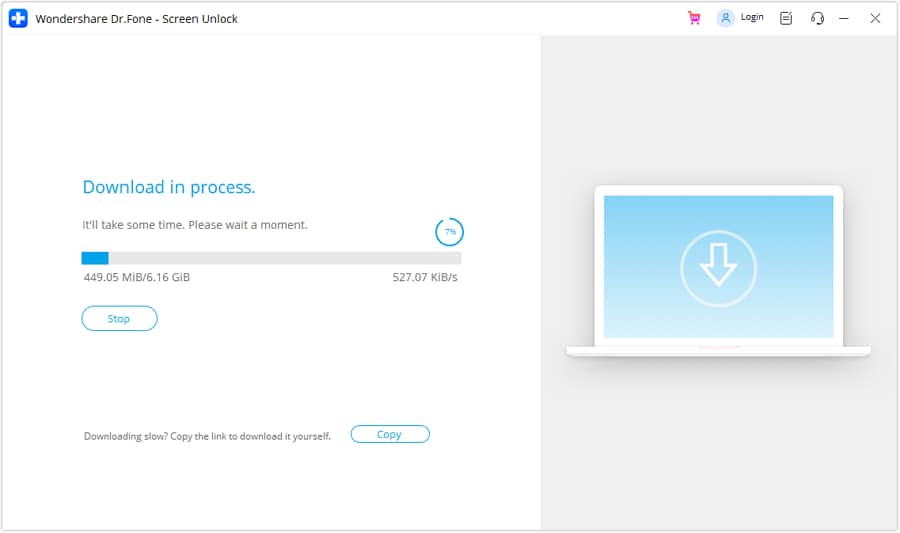
- **Step 10:** Review the **Device Model** and **System Version**, then click **Unlock Now** to proceed.
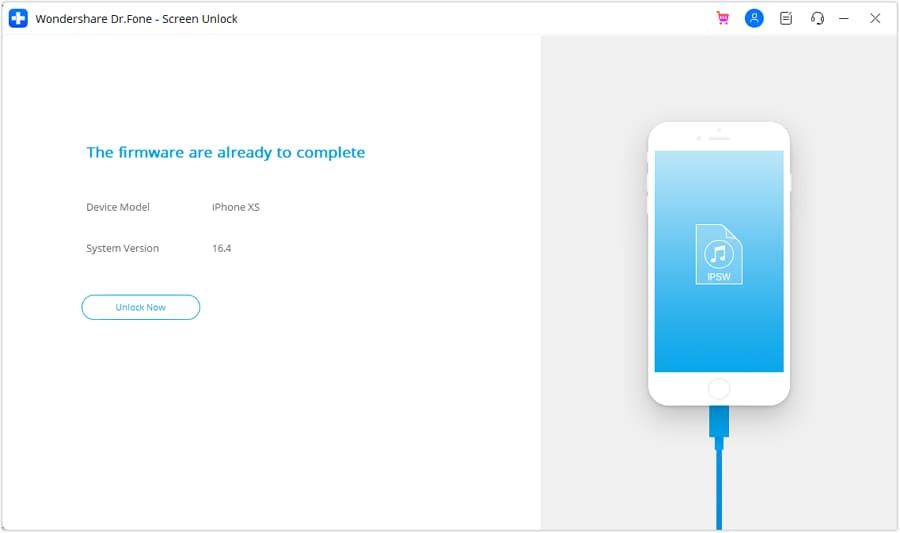
- **Step 11:** From the **Warning** dialogue box, enter the code shown in the text field, then click **Unlock**.
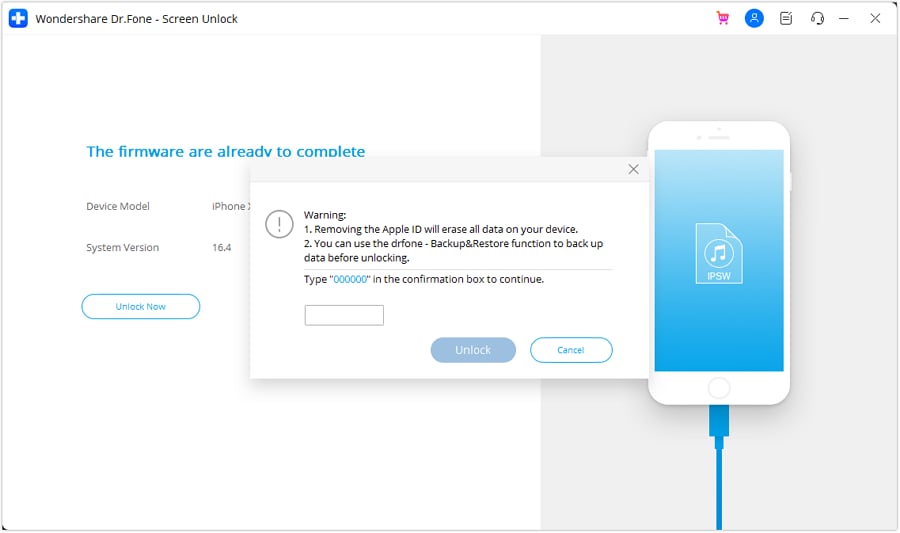
- **Step 12:** Wait and refrain from using your device while it's connected to avoid a [<u>bricked device</u>](https://drfone.wondershare.com/iphone-problems/how-to-fix-bricked-iphone.html).
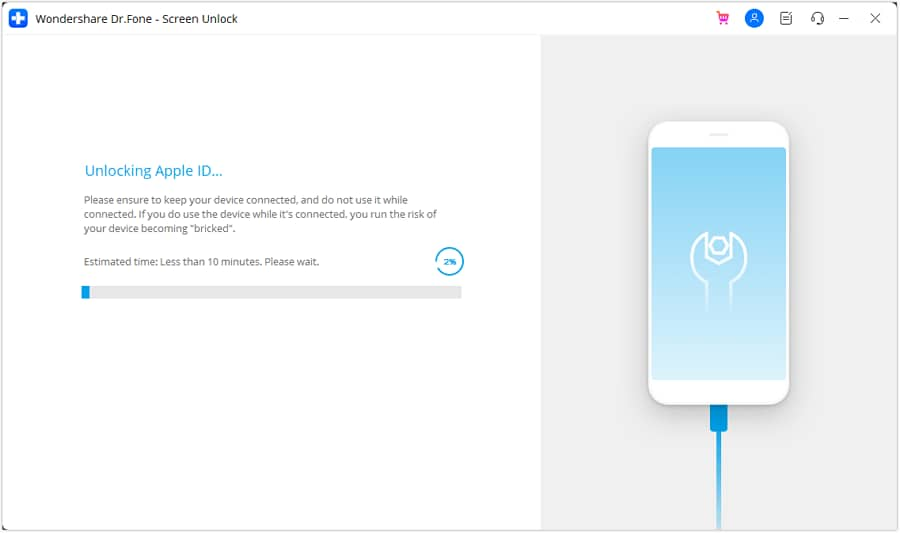
- **Step 13:** Once finished, click **Done**, then check your iPad. If your Apple ID is not unlocked, you may need to click **Try again** to restart.
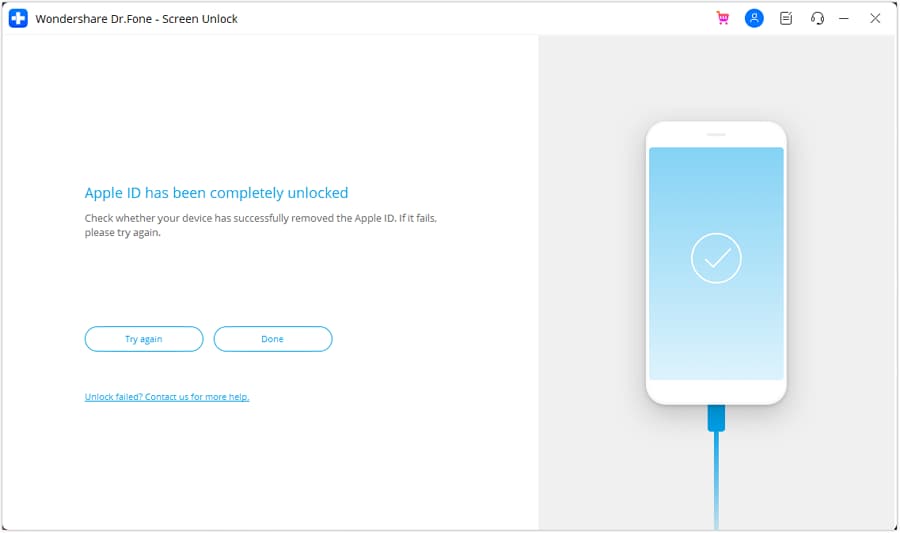
Best Practices To Secure Your iOS Data
**Changing your Apple ID on your iPad** can be a great way to protect your privacy and security. However, it's important to take steps to ensure that your iOS data remains safe during the process. Here are some tips to help you do just that:
- **Backup Your Files**
Before you **change your Apple ID on your iPad**, create a [<u>backup of your data</u>](https://drfone.wondershare.com/iphone-backup/backup-iphone-with-broken-screen.html) so you don't lose important files. You can create a backup using iCloud or Dr.Fone.
Dr.Fone also allows you to view the contents of your backup before you restore it, so you can ensure that you're getting back exactly what you want. This way, you can restore your data to your new Apple ID if needed.
- **Password Check**
Review your current passwords. Change any weak or compromised ones, including your Apple ID password, and never share it with anyone.
- **Avoid Sharing an Apple ID**
It's best practice for each family member to have their own Apple ID. Sharing one can lead to problems with app purchases, data syncing, and privacy.
- **Enable Two-Factor Authentication (2FA)**
If not already enabled, turn on 2FA for an extra layer of security. It's simple and highly effective.
## Conclusion
**Changing your Apple ID on your iPad** or other iOS devices is a simple process that can help you protect your privacy and information. With the tips above, you can ensure your iOS data is safe and secure during the switch.
And if you need to remove your Apple ID without a password, Dr.Fone is a good option. Dr.Fone is a powerful iOS toolkit that can help you with various phone solutions. It's easy to use and can help you solve mobile problems in just a few minutes.
## How to Unlock Apple iPhone 12 Pro Passcode without iTunes without Knowing Passcode?
If you have been locked out of your iOS device and would like to know how to unlock Apple iPhone 12 Pro passcode without iTunes, then you have come to the right place. Unlike Android, iOS is quite particular when it comes to passcode security and doesn’t provide too many ways to reset the passcode. Therefore, users have to take added measures to unlock their screens. Even though this article focuses on the Apple iPhone 12 Pro screen lock, you can follow the same instructions for other iOS devices. Read on and learn how to unlock Apple iPhone 12 Pro passcode without iTunes.
<iframe width="560" height="315" src="https://www.youtube.com/embed/wXbqfC7JTKc" title="YouTube video player" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen="allowfullscreen"></iframe>
safe & secure
## Part 1: How to unlock Apple iPhone 12 Pro passcode with Dr.Fone - Screen Unlock (iOS)?
Most of the users find it hard to unlock their devices by taking the assistance of iTunes. After all, it is a more complicated and time-consuming process. Ideally, you can take the assistance of a third-party tool like [Dr.Fone - Screen Unlock (iOS)](https://tools.techidaily.com/wondershare/drfone/iphone-unlock/) to [bypass the Apple iPhone 12 Pro passcode.](https://drfone.wondershare.com/unlock/bypass-iphone-passcode.html) This tool will wipe out all the data after unlocking iPhone. It provides extremely reliable and easy solutions regarding the removal of the Apple iPhone 12 Pro screen lock. Besides that, the tool can also be used to recover any kind of problem related to your iOS device.
It is compatible with all the leading iOS versions and devices. All you need to do is access its user-friendly interface and follow simple click-through steps to unlock your device. To learn how to unlock Apple iPhone 12 Pro passcode without iTunes (using Dr.Fone toolkit), follow these steps:
### [Dr.Fone - Screen Unlock (iOS)](https://tools.techidaily.com/wondershare/drfone/iphone-unlock/)
Unlock iPhone Screen Without Password
- Unlock screen passwords from all iPhone, iPad, and iPod Touch.
- Bypass iCloud activation lock and Apple ID without password.
- No tech knowledge is required; everybody can handle it.
- Fully compatible with the latest iOS/iPadOS.
**3981454** people have downloaded it
1\. To start with, download Dr.Fone - Screen Unlock (iOS) and install it on your computer. Launch it and select the option of "Screen Unlock" from the home screen.
2\. Now, connect your Apple iPhone 12 Pro to your system and wait for a while as Dr.Fone will detect it automatically. Click on the “Unlock iOS Screen” button to initiate the process.
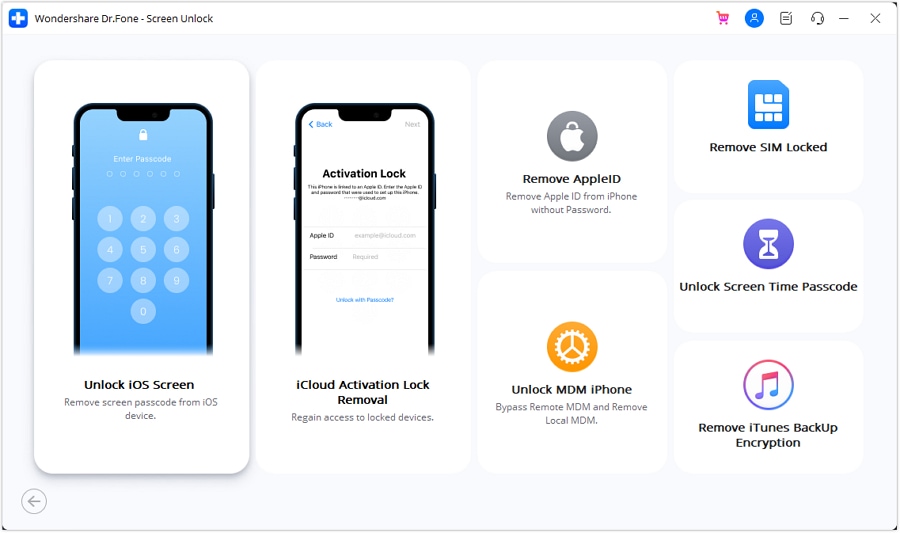
3\. As you would connect your Apple iPhone 12 Pro to your system, you will get a “Trust this Computer” prompt. Make sure that you close this window by tapping on the “x” button. Once connected, Dr.Fone will ask you to follow some steps to set your device in Recovery mode, allowing it to be detected.
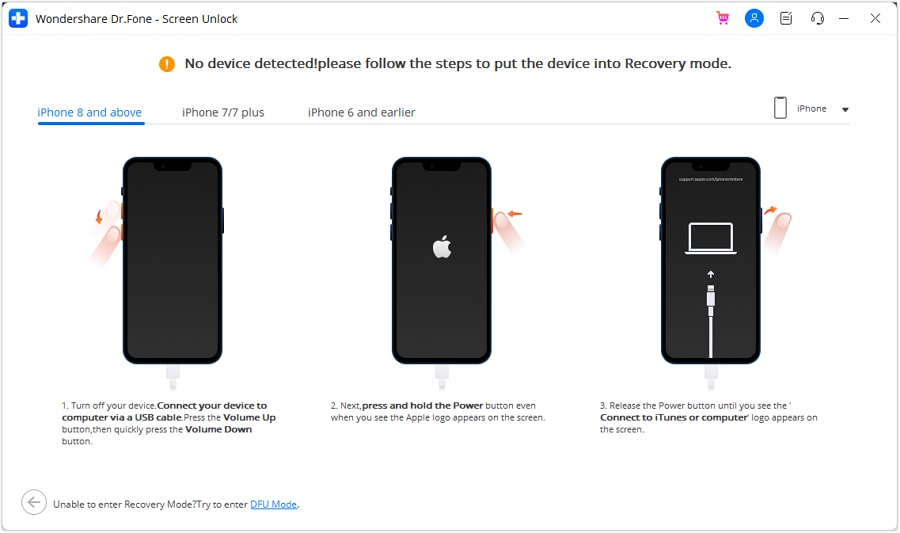
4\. Meanwhile, the Dr.Fone interface will provide the following screen, asking for various details related to your device. Provide crucial information related to your device (model, iOS version, and more) and click on the “Download” button.
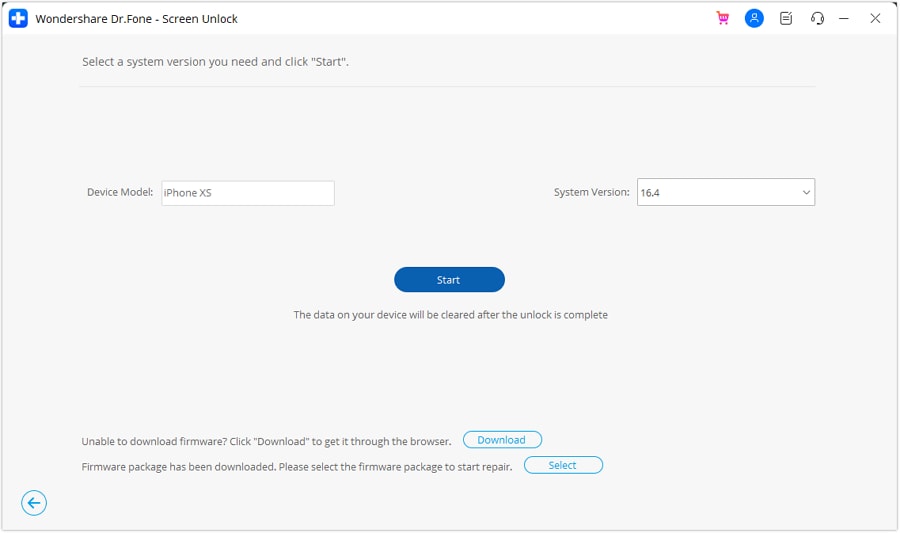
5\. Wait for a while, as the application will download the related firmware for your device and make it ready. It might take a while for the firmware to be downloaded completely.
6\. Once it is done, you will get the following prompt. To unlock your device, you need to uncheck the “Retain native data” feature, since the passcode can’t be removed without your Apple iPhone 12 Pro’s data loss. Click on the “Unlock Now” button.
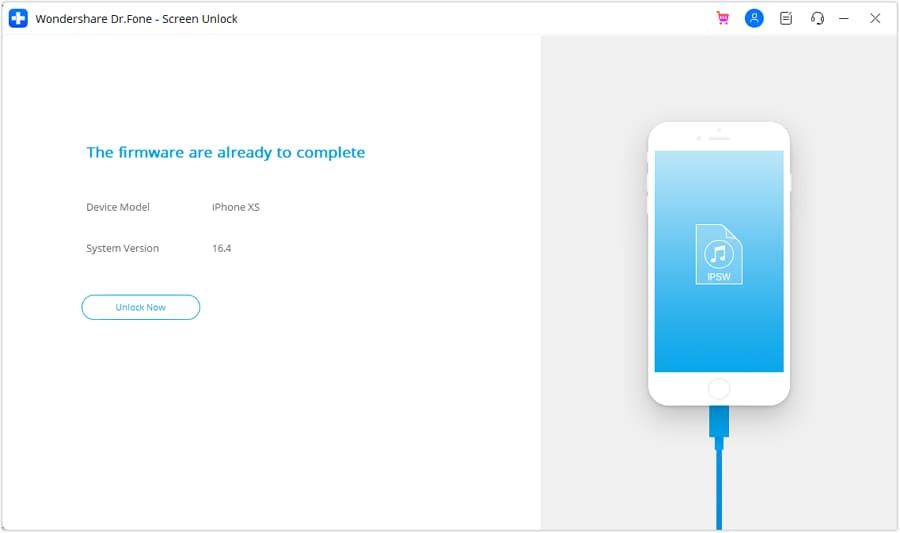
7\. You would be asked to confirm your choice, as the process will reset your device. After providing the on-screen confirmation code, click on the “Unlock” button and let the application unlock your device.
8\. In a matter of a few seconds, your device will be reset, and its passcode would also be removed. You will get the following message once the process is completed.
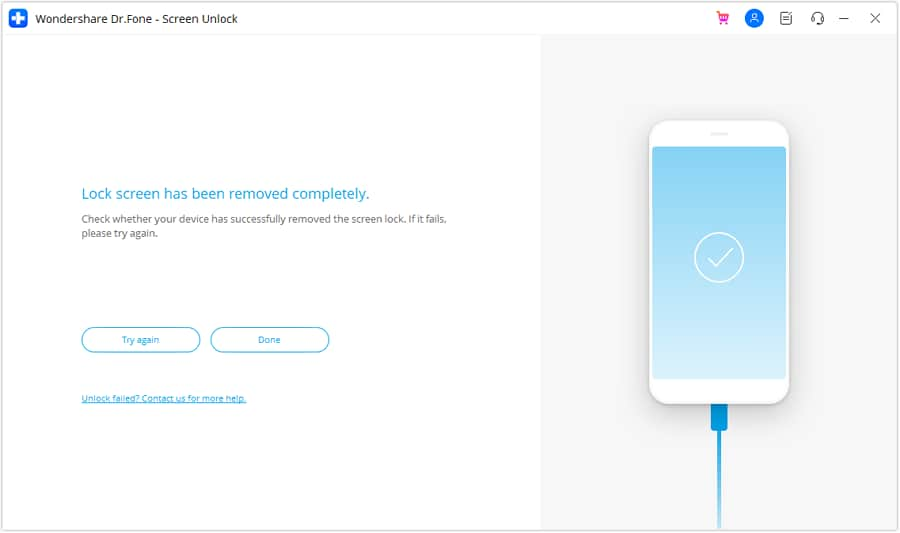
In the end, you can simply disconnect your Apple iPhone 12 Pro safely from the system and restart it. It would be restarted without any passcode, letting you access it in a trouble-free manner.
safe & secure
#### **You may also be interested in:**
- [4 Ways to Unlock iPhone without Passcode](https://drfone.wondershare.com/unlock/unlock-iphone-without-passcode.html)
- [3 Ways to Unlock A Disabled iPhone Without iTunes](https://drfone.wondershare.com/unlock/unlock-disabled-iphone-without-itunes.html)
- [4 Ways to Bypass iPhone Passcode Easily](https://drfone.wondershare.com/unlock/bypass-iphone-passcode.html)
- [How to Fix It If We've Are Locked Out of iPad?](https://drfone.wondershare.com/unlock/locked-out-of-ipad.html)
## Part 2: How to unlock Apple iPhone 12 Pro passcode with Find My iPhone?
Apple also allows its users to remotely locate, lock, and erase their devices. Though, this feature can also be used to reset a device and remove its passcode. Needless to say, while doing so, you will reset your device. To learn how to unlock Apple iPhone 12 Pro passcode without iTunes (with the Find My iPhone feature), follow these steps:
1\. To start with, open the iCloud website on your system and login using your Apple ID and password.
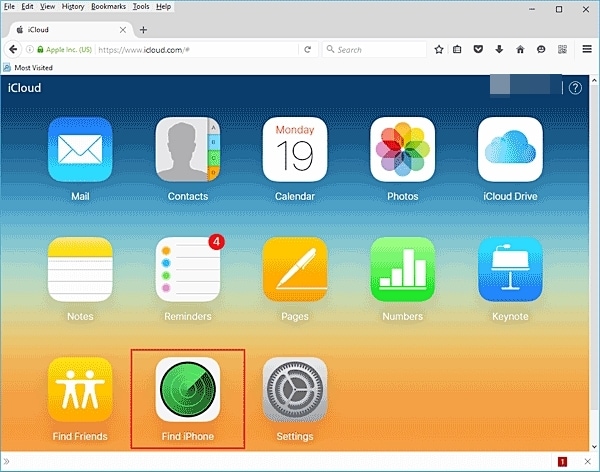
2\. From the home screen, you can access several features. Select “Find my iPhone” to proceed.
3\. Now, click on the “All Device” dropdown button to select the Apple iPhone 12 Pro device that you want to unlock.
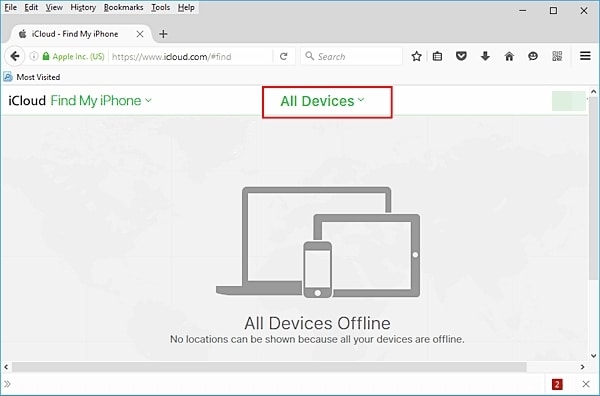
4\. After selecting your device, you will get an option to ring it, lock it, or erase it. Click on the “Erase iPhone” option.
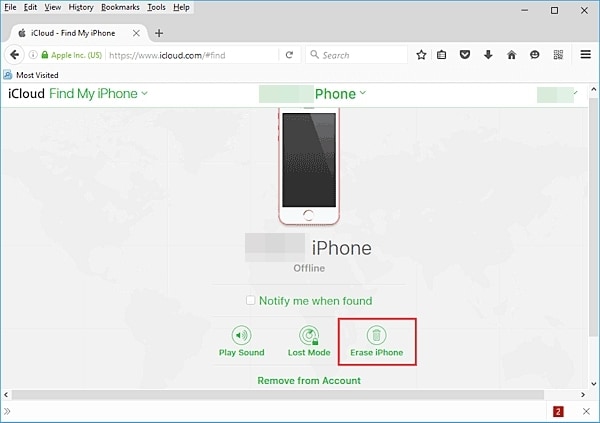
5\. Agree with the pop-up message and choose to restore your device. Once it is done, your phone will be restarted without any lock.
## Part 3: How to unlock Apple iPhone 12 Pro passcode in Recovery Mode?
If none of the above-mentioned solutions would work, then you can always choose to put your Apple iPhone 12 Pro in recovery mode and restore it. After when your Apple iPhone 12 Pro would be restored, you can access it without any lock. It can be done by following these steps:
1\. Firstly, you need to put your device in recovery mode. Beforehand, you need to make sure that your device is turned off. If not, press the Power button and slide the screen to turn your Apple iPhone 12 Pro off.
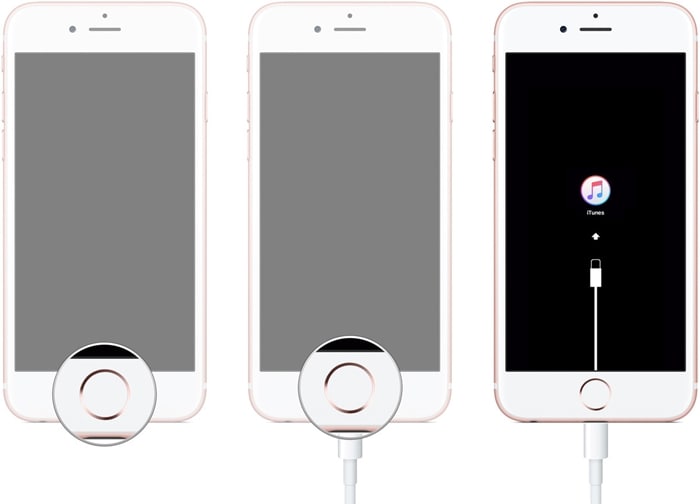
2\. Now, launch iTunes on your Mac or Windows system. Afterward, press and hold the Home button on your Apple iPhone 12 Pro. While holding the Home button, connect it to your system.
3\. You will get an iTunes symbol on the screen. In no time, iTunes will also detect your device.
4\. As iTunes will detect your device in recovery mode, it will display a prompt similar to this.
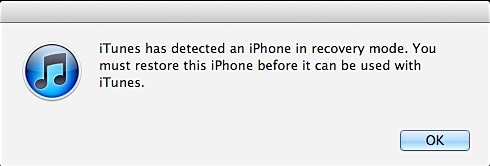
5\. Simply agree to it and let iTunes restore your device.
Once your device has been restored, you can access it without any screen lock.
## Part 4: About data loss after unlocking Apple iPhone 12 Pro passcode
As you can see, in all the above-mentioned solutions, your Apple iPhone 12 Pro data would be lost while unlocking its passcode. This is because, as of now, there is no way to unlock an iPhone without restoring it. Needless to say, while restoring a device, its data is automatically lost. Since Apple is quite concerned about the security of the Apple iPhone 12 Pro and the sensitivity of its data, it doesn’t let users unlock the Apple iPhone 12 Pro device without losing their data.
Even though lots of users have complained about this issue, Apple hasn’t come up with a solution yet. The best way to avoid this scenario is by taking a regular backup of your data. You can either backup your data on iCloud, via iTunes, or by using any third-party tool as well. In this way, you won’t be able to lose your important files while unlocking your device’s passcode.
## Conclusion
Now when you know how to unlock Apple iPhone 12 Pro passcode without iTunes, you can easily access your device. Ideally, you can simply take the assistance of [Dr.Fone - Screen Unlock (iOS)](https://tools.techidaily.com/wondershare/drfone/iphone-unlock/) to unlock your device. It can also be used to resolve any other problem related to your Apple iPhone 12 Pro/iPad as well. Feel free to give it a try and let us know if you face any problems while using it.
safe & secure
## Different Methods To Unlock Your Apple iPhone 12 Pro
The Apple iPhone 12 Pro 14 and iPhone 14 Pro are the latest flagship smartphones from Apple. They come equipped with advanced security features such as passcodes and Face ID. However, there may be instances where you find yourself locked out of your device. It can happen due to a forgotten passcode or Face ID not matching.
This article will explore different methods to unlock your Apple iPhone 12 Pro in such scenarios. Thus, providing you with the necessary steps to get your **iPhone 14 unlocked**.
## Part 1: About the Apple iPhone 12 Pro Passcode and Face ID
Before talking about the **unlocked iPhone 14 Pro**, it is crucial to understand its security features. The Apple iPhone 12 Pro 14 and iPhone 14 Pro offer robust security features. It is to protect your data and ensure your device's privacy. The primary methods of unlocking these devices include the passcode and Face ID. Both of which provide different levels of security and convenience.
### Passcode
The passcode is a numeric or alphanumeric code that you set up to secure your Apple iPhone 12 Pro. It acts as a barrier between unauthorized users and your device's contents. When you set up your Apple iPhone 12 Pro for the first time, you are prompted to create a passcode. You can choose a 6-digit or 4-digit passcode or a custom alphanumeric or numeric code. It all depends on your preference and the level of security you desire.
After setting up a passcode, it will be required every time you unlock your Apple iPhone 12 Pro. It is also required when accessing sensitive information or making changes to security settings. Moreover, it serves as the first line of defense in protecting your device from unauthorized access. If an incorrect passcode is entered many times, your Apple iPhone 12 Pro will be disabled for a specific time. It increases your device's security.
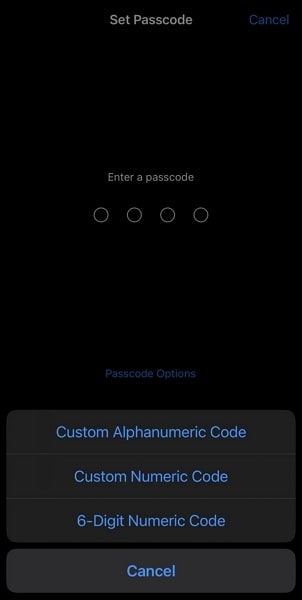
### Face ID
Face ID is an advanced biometric authentication system on Apple iPhone 12 Pro. It utilizes the Apple iPhone 12 Pro device's TrueDepth camera system. This includes sensors and an infrared camera to create a detailed map of your face. This facial recognition technology allows you to unlock your Apple iPhone 12 Pro by looking at it. During the initial setup, you are prompted to enroll your face by positioning it within the frame.
You also need to move your head in a circular motion. Face ID captures and analyzes various facial features. These include the unique patterns of your eyes, nose, and mouth. You can set up two types of Face IDs on iPhone 14 and iPhone 14 Pro. These include one with a mask and one without a mask.
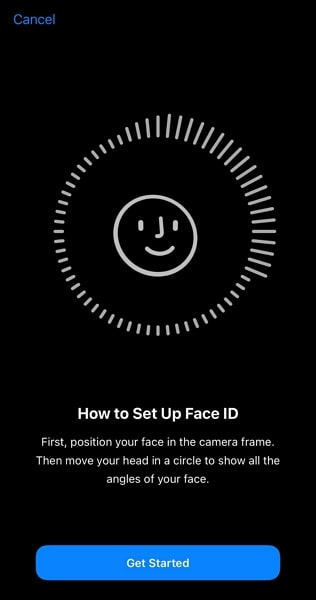
## Part 2: Methods To Unlock Your Apple iPhone 12 Pro
Losing access to your Apple iPhone 12 Pro 14 after forgetting its passcode can be frustrating. When looking for **how to unlock iPhone 14**, you will find that various methods are available. Below we have discussed the three most common methods to unlock your Apple iPhone 12 Pro 14:
In this method, you will be erasing the iPhone, meaning all your data on the Apple iPhone 12 Pro will be lost. Use the following steps to unlock your Apple iPhone 12 Pro via iCloud:
### Method 1: Using iCloud via Find My
This method lets you unlock your Apple iPhone 12 Pro using the Find My iPhone feature on iCloud. To use this method, ensure you have previously enabled Find My iPhone on your device. Moreover, you will also need Apple ID credentials to use your iCloud account.
In this method, you will be erasing the iPhone, meaning all your data on the Apple iPhone 12 Pro will be lost. Use the following steps to unlock your Apple iPhone 12 Pro via iCloud:
- **Step 1.** Open a web browser and go to the [iCloud website](https://www.icloud.com/) on a computer or another device. Sign in using your Apple ID, which should be the same credentials linked to your locked iPhone.
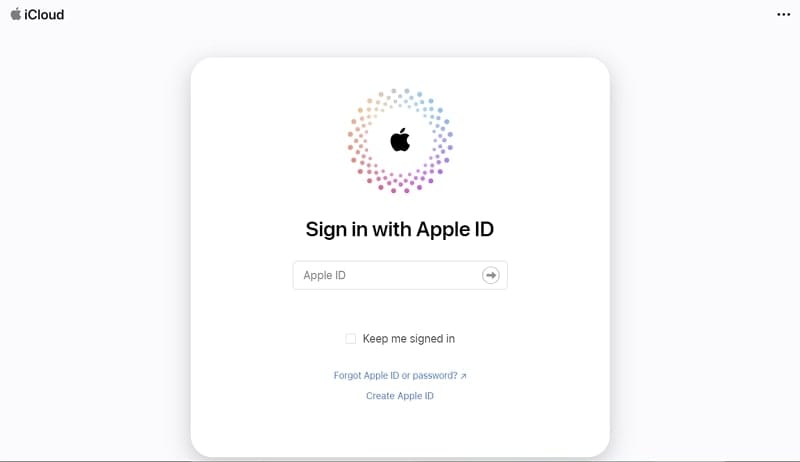
- **Step 2.** After signing in, click the "App Grid" icon on the top right corner and choose "Find My" from the options. On the Find iPhone page, you will see a list of devices associated with your iCloud account. Select your Apple iPhone 12 Pro from the list.
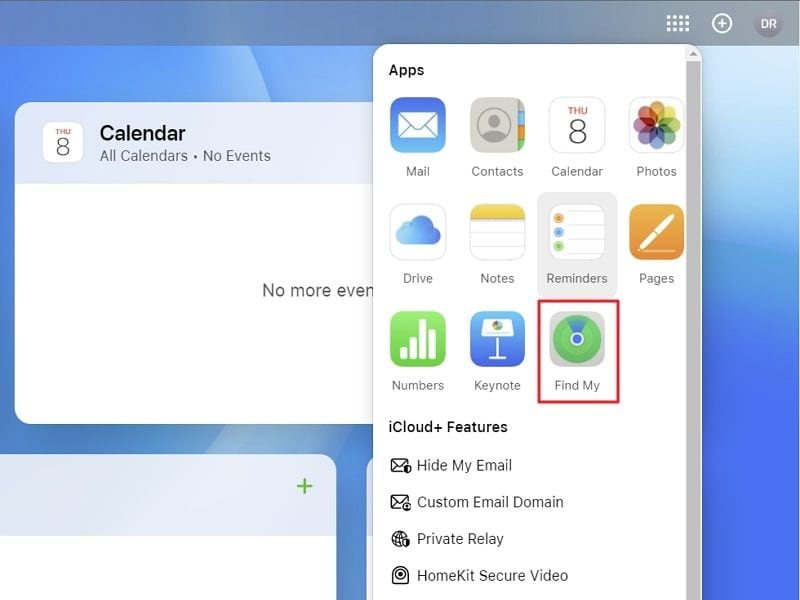
- **Step 3.** Look for the "Erase iPhone" option from the Apple iPhone 12 Pro device details and tap on it. This will start the erasure process on your Apple iPhone 12 Pro. Afterward, follow the on-screen instructions to erase your Apple iPhone 12 Pro. Once it is done, your device will be unlocked, and you will be able to set it up.
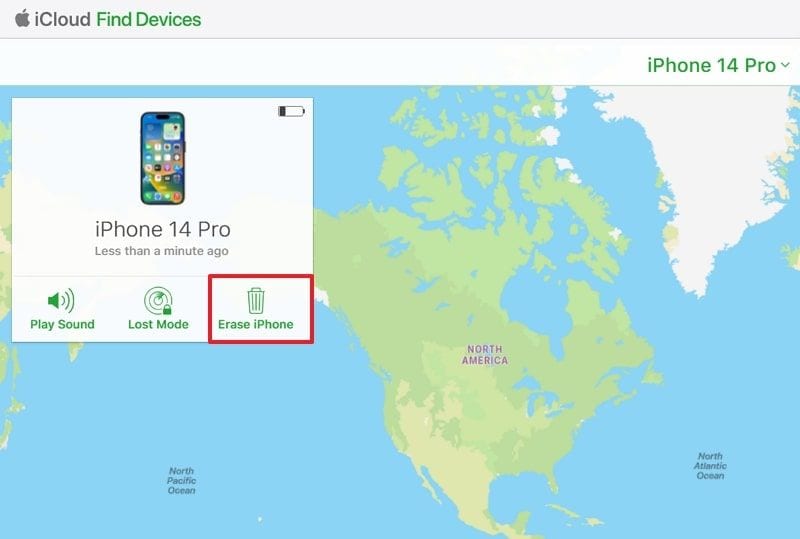
#### Pros
- With internet access, you can unlock your Apple iPhone 12 Pro remotely from any computer or device.
- It allows you to locate your Apple iPhone 12 Pro 14 or iPhone 14 Pro in case of you misplacing your device.
#### Cons
- You must have previously enabled Find My iPhone on your locked device to use this method.
### Method 2: Using iTunes on Your Computer
Restoring your Apple iPhone 12 Pro with iTunes is another method to unlock your device when you have forgotten the passcode. This method involves using iTunes on a computer to erase your Apple iPhone 12 Pro and install the latest iOS version.
For it, ensure you have the latest version of iTunes installed on your computer. Using this method will also result in losing all your data on the iPhone. Follow these steps to unlock **iPhone 14 Pro Max** with iTunes:
- **Step 1.** Launch iTunes on your computer and connect your Apple iPhone 12 Pro to your computer using a USB cable. Now first press and release the "Volume Up" button. Then repeat the same process with the "Volume Down" button.
- **Step 2.** Finally, press and hold the "Side" button until your Apple iPhone 12 Pro transitions to Recovery Mode. Now iTunes will detect your Apple iPhone 12 Pro in Recovery Mode. It will display a pop-up message asking whether you want to restore or update your device.
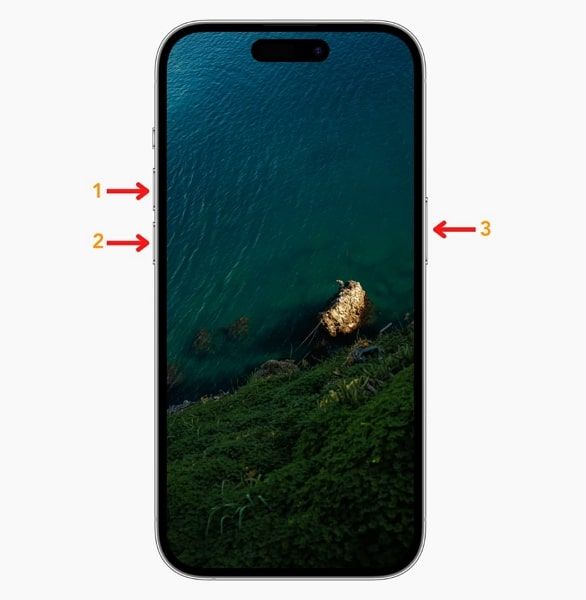
- **Step 3.** Here, choose the "Restore" option. It will erase all data on your device and install the latest iOS version. Once it is complete, you will see the initial setup screen on your Apple iPhone 12 Pro.
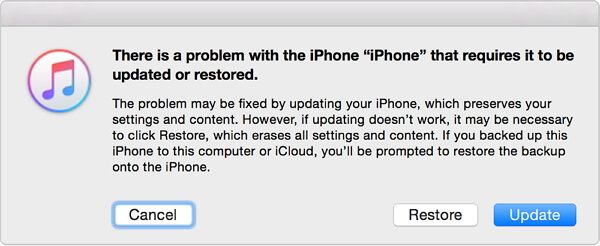
#### Pros
- Recovery Mode is supported by iTunes on Windows or macOS versions prior to Catalina and by Finder on macOS Catalina and later.
- If the passcode or Face ID issue is related to a software glitch or corruption, Recovery Mode can help restore your device to a stable state.
#### Cons
- Users unfamiliar with Recovery Mode can get their devices stuck in it if they make a mistake.
### Method 3: Using the Screen Unlock Feature of Wondershare Dr.Fone
You will come across many methods when looking for how to unlock iPhone 14. However, no way is simpler or safer than using [Wondershare Dr.Fone - Screen Unlock (iOS)](https://tools.techidaily.com/wondershare/drfone/iphone-unlock/). It can remove all screen locks from iPhone, iPad, and iPad Touch. These types include 4-digit passcode, 6-digit passcode, Touch ID, and Face IDs.
### [Dr.Fone - Screen Unlock (iOS)](https://tools.techidaily.com/wondershare/drfone/iphone-unlock/)
Fix iPad, iPod Touch, and iPhone Lock Screen Password Forgot Issue
- Remove Apple ID/iCloud Account efficiently.
- Save your Apple iPhone 12 Pro quickly from the disabled state.
- Free your sim out of any carrier worldwide.
- Bypass iCloud activation lock and enjoy all iCloud services.
- Works for all models of iPhone, iPad, and iPod touch.
- Fully compatible with the latest iOS.
**4,008,670** people have downloaded it
#### Steps to Use Wondershare Dr.Fone to Unlock Apple iPhone 12 Pro
Learning **how do you unlock an iPhone** becomes a lot easier with Wondershare Dr.Fone. Here is how you can unlock your Apple iPhone 12 Pro 14 with this iPhone screen unlock tool:
- **Step 1. Navigate to Unlock iOS Screen in Dr.Fone**
Launch Wondershare Dr.Fone on your computer and select "Screen Unlock" from the available options in the "Toolbox" menu. On the next screen, choose "iOS" and then click on "Unlock iOS Screen".
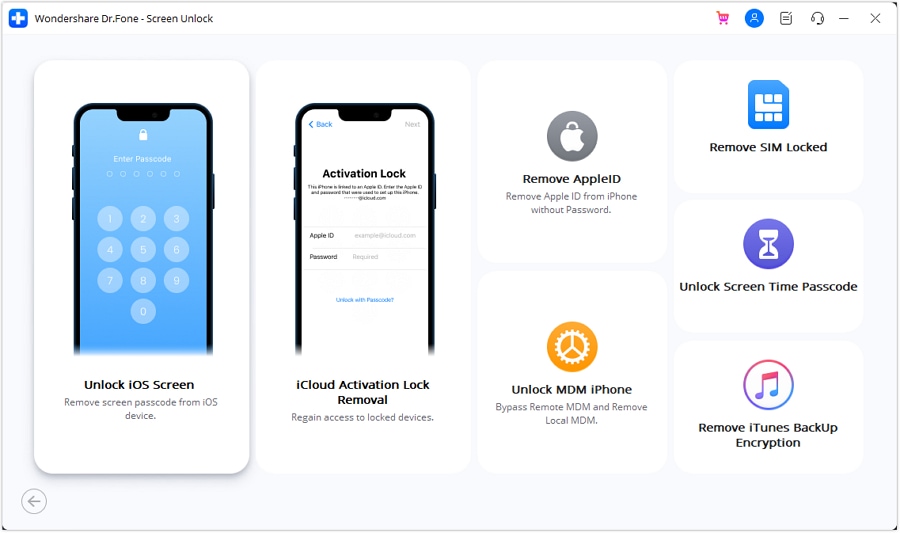
safe & secure
- **Step 2. Enable Recovery Mode on Your Apple iPhone 12 Pro**
Connect your Apple iPhone 12 Pro to the computer. Once Dr.Fone detects your Apple iPhone 12 Pro, click on "Start". Follow the on-screen instructions to put your device into Recovery Mode. Wondershare Dr.Fone will automatically detect the mode and iOS version of your Apple iPhone 12 Pro.
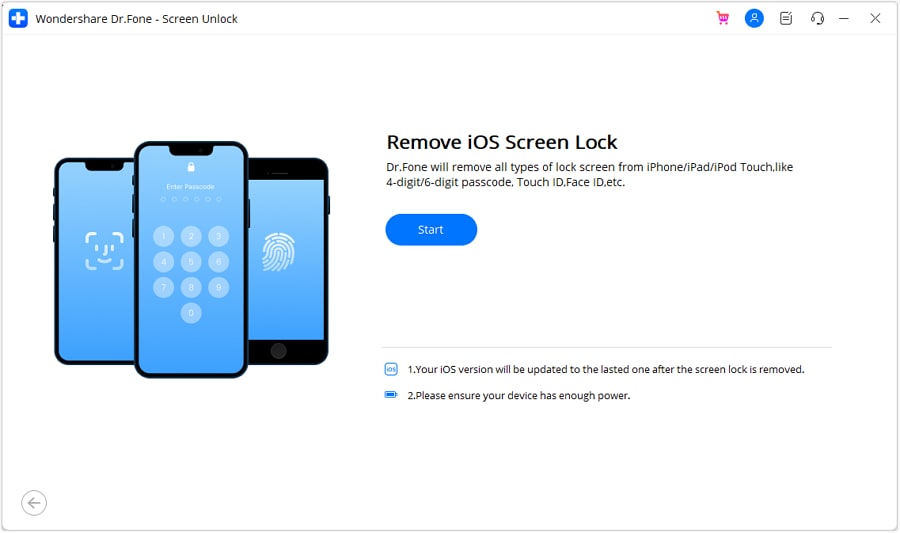
- **Step 3. Unlock Your Apple iPhone 12 Pro**
If the detected details are correct, click on "Start" to initiate the firmware downloading process. Once the firmware is downloaded, click on "Unlock Now." A prompt window will appear asking for confirmation. Enter the code "000000" to confirm. The firmware will then be installed, which will unlock your Apple iPhone 12 Pro.
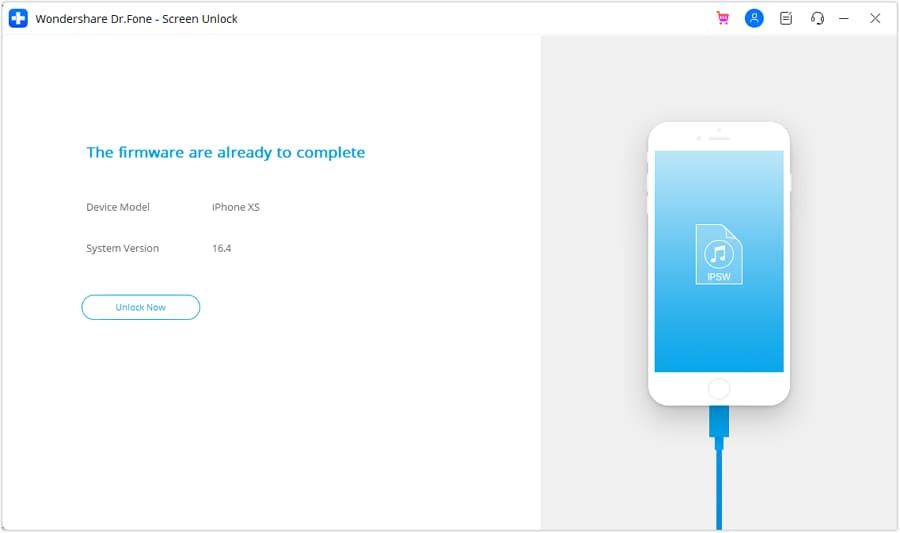
#### Pros
- With Wondershare Dr.Fone's help, you can also remove screen time passcodes.
- It also lets you remove the SIM lock on your Apple iPhone 12 Pro with ease.
#### Cons
- When using Wondershare Dr.Fone to unlock your Apple iPhone 12 Pro, you will lose your data.
safe & secure
## Part 3: Tips for Successful Apple iPhone 12 Pro Unlocking
Unlocking your Apple iPhone 12 Pro can be a delicate process. To increase your chances of success and avoid potential pitfalls, consider the following tips:
### 1\. Check Device Eligibility
Make sure your Apple iPhone 12 Pro is eligible for unlocking using the methods mentioned in this article. Different ways may have specific requirements or limitations, so verify compatibility beforehand. For example, if you want to use iCloud to unlock your Apple iPhone 12 Pro 14, you need to have Find My enabled on the targeted device. For iTunes, you need to have the latest version of iTunes installed.
### 2\. Backup Your Data
Using all the methods mentioned in this article will result in data loss. It is an important reminder that you should always create backups of your important data. If you are unsatisfied with native backup methods on your Apple iPhone 12 Pro, [Wondershare Dr.Fone](https://drfone.wondershare.com/iphone-backup-and-restore.html) is an excellent alternative. With it, you can create backups on your computer whenever you want using a one-click procedure.
If you find the manual backing up hectic, Wondershare Dr.Fone permits you to create an automatic incremental backup on your computer. These backups are done wirelessly at your specified time when both devices are connected to the same network.
### 3\. Understand Terms and Conditions
Familiarize yourself with the terms and conditions associated with the unlocking methods you plan to use. This includes any potential data loss, warranty implications, or risks involved. Be informed before proceeding. All unlocking methods, such as using iCloud's Find My iPhone or restoring with iTunes, involve erasing your Apple iPhone 12 Pro's data.
Understand the potential data loss implications and ensure you have a recent backup to restore your data if needed. Moreover, you also need to ensure that you have the legal right to unlock the iPhone. Unlocking methods should only be used on devices you own or have explicit permission to unlock.
### 4\. Follow Instructions Carefully
Following the instructions provided for each unlocking method is crucial to increase your chances of successful unlocking. Read the instructions thoroughly before starting the unlocking process. Familiarize yourself with each step and ensure you understand them. Take note of any prerequisites, such as having the latest version of iTunes installed, enabling Find My iPhone, or establishing a stable internet connection.
Avoid skipping or rushing through any steps, and carefully perform each action as instructed. Pay attention to details, such as button combinations, specific software settings, or prompts that may appear on your device or computer screen.
### 5\. Troubleshooting Common Issues
Unlocking an iPhone may encounter certain obstacles or issues along the way. First, you need to ensure that your Apple iPhone 12 Pro 14 actually needs unlocking. For example, in the case of Face ID, you are required to enter the passcode after a particular time. In the case of a simple Face ID, you will need to enter a password every 48 hours if it hasn't been unlocked during this time.
When both Face IDs, such as simple and mask, are enabled, every successful attempt adds 6.5 hours to the time before you are required to enter a passcode. Moreover, always use original or MFi-Certified cables to connect your Apple iPhone 12 Pro to the computer.
## Conclusion
To summarize, unlocking your Apple iPhone 12 Pro can be daunting when you've forgotten your passcode or encounter Face ID issues. Thankfully, there are different methods available to regain access to your device. This article explored three methods: using iCloud's Find My iPhone, restoring with iTunes, and utilizing Dr.Fone. Each way offers its advantages and considerations.
After careful evaluation, we recommend Wondershare Dr.Fone as the best choice to [unlock your Apple iPhone 12 Pro](https://drfone.wondershare.com/solutions/iphone-lock-screen-password-forgot.html). It provides comprehensive features to unlock your device efficiently. Moreover, it offers a local backup option, ensuring that your data is safeguarded.
safe & secure
<ins class="adsbygoogle"
style="display:block"
data-ad-format="autorelaxed"
data-ad-client="ca-pub-7571918770474297"
data-ad-slot="1223367746"></ins>
<ins class="adsbygoogle"
style="display:block"
data-ad-client="ca-pub-7571918770474297"
data-ad-slot="8358498916"
data-ad-format="auto"
data-full-width-responsive="true"></ins>
<span class="atpl-alsoreadstyle">Also read:</span>
<div><ul>
<li><a href="https://iphone-unlock.techidaily.com/how-to-unlock-iphone-14-passcode-screen-drfone-by-drfone-ios/"><u>How to Unlock iPhone 14 Passcode Screen? | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/how-to-fix-auto-lock-greyed-out-on-apple-iphone-8-drfone-by-drfone-ios/"><u>How To Fix Auto Lock Greyed Out on Apple iPhone 8 | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-how-to-change-country-on-app-store-for-iphone-6s-plus-with-7-methods-drfone-by-drfone-ios/"><u>In 2024, How To Change Country on App Store for iPhone 6s Plus With 7 Methods | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/complete-guide-on-unlocking-apple-iphone-11-with-a-broken-screen-drfone-by-drfone-ios/"><u>Complete Guide on Unlocking Apple iPhone 11 with a Broken Screen? | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-full-guide-to-unlock-iphone-8-plus-with-itunes-drfone-by-drfone-ios/"><u>In 2024, Full Guide to Unlock iPhone 8 Plus with iTunes | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-iphone-is-disabled-here-is-the-way-to-unlock-disabled-apple-iphone-15-drfone-by-drfone-ios/"><u>In 2024, iPhone Is Disabled? Here Is The Way To Unlock Disabled Apple iPhone 15 | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-did-your-iphone-6s-plus-passcode-change-itself-unlock-it-now-drfone-by-drfone-ios/"><u>In 2024, Did Your iPhone 6s Plus Passcode Change Itself? Unlock It Now | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-how-to-fix-iphone-7-plus-unavailable-issue-with-ease-drfone-by-drfone-ios/"><u>In 2024, How To Fix iPhone 7 Plus Unavailable Issue With Ease | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-how-to-turn-off-find-my-apple-iphone-13-mini-when-phone-is-broken-drfone-by-drfone-ios/"><u>In 2024, How to Turn Off Find My Apple iPhone 13 mini when Phone is Broken? | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-how-to-unlock-disabled-apple-iphone-xs-maxipad-without-computer-drfone-by-drfone-ios/"><u>In 2024, How to Unlock Disabled Apple iPhone XS Max/iPad Without Computer | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/disabled-apple-iphone-14-how-to-unlock-a-disabled-apple-iphone-14-drfone-by-drfone-ios/"><u>Disabled Apple iPhone 14 How to Unlock a Disabled Apple iPhone 14? | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-how-to-bypass-the-required-apple-store-verification-for-iphone-11-pro-drfone-by-drfone-ios/"><u>In 2024, How To Bypass the Required Apple Store Verification For iPhone 11 Pro | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/did-your-iphone-15-plus-passcode-change-itself-unlock-it-now-drfone-by-drfone-ios/"><u>Did Your iPhone 15 Plus Passcode Change Itself? Unlock It Now | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-how-many-attempts-to-unlock-apple-iphone-14-pro-max-drfone-by-drfone-ios/"><u>In 2024, How Many Attempts To Unlock Apple iPhone 14 Pro Max | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-the-best-methods-to-unlock-the-iphone-locked-to-owner-for-apple-iphone-xr-drfone-by-drfone-ios/"><u>In 2024, The Best Methods to Unlock the iPhone Locked to Owner for Apple iPhone XR | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/reset-itunes-backup-password-of-iphone-6s-plus-prevention-and-solution-drfone-by-drfone-ios/"><u>Reset iTunes Backup Password Of iPhone 6s Plus Prevention & Solution | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-unlock-apple-iphone-se-2022-with-forgotten-passcode-different-methods-you-can-try-drfone-by-drfone-ios/"><u>In 2024, Unlock Apple iPhone SE (2022) With Forgotten Passcode Different Methods You Can Try | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/how-can-i-unlock-my-iphone-12-pro-max-after-forgetting-my-pin-code-drfone-by-drfone-ios/"><u>How Can I Unlock My iPhone 12 Pro Max After Forgetting my PIN Code? | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/how-to-unlock-stolen-iphone-13-pro-max-in-different-conditionsin-drfone-by-drfone-ios/"><u>How To Unlock Stolen iPhone 13 Pro Max In Different Conditionsin | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/how-to-unlock-stolen-apple-iphone-8-in-different-conditionsin-drfone-by-drfone-ios/"><u>How To Unlock Stolen Apple iPhone 8 In Different Conditionsin | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-how-do-you-unlock-your-apple-iphone-8-plus-learn-all-4-methods-drfone-by-drfone-ios/"><u>In 2024, How Do You Unlock your Apple iPhone 8 Plus? Learn All 4 Methods | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/in-2024-how-to-unlock-apple-iphone-12-mini-with-an-apple-watch-and-what-to-do-if-it-doesnt-work-drfone-by-drfone-ios/"><u>In 2024, How to Unlock Apple iPhone 12 mini With an Apple Watch & What to Do if It Doesnt Work | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/iphone-6-asking-for-passcode-after-ios-1714-update-what-to-do-drfone-by-drfone-ios/"><u>iPhone 6 Asking for Passcode after iOS 17/14 Update, What to Do? | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/unlock-your-disabled-iphone-13-pro-without-itunes-in-5-ways-drfone-by-drfone-ios/"><u>Unlock Your Disabled iPhone 13 Pro Without iTunes in 5 Ways | Dr.fone</u></a></li>
<li><a href="https://iphone-unlock.techidaily.com/unlock-apple-iphone-xs-max-with-forgotten-passcode-different-methods-you-can-try-drfone-by-drfone-ios/"><u>Unlock Apple iPhone XS Max With Forgotten Passcode Different Methods You Can Try | Dr.fone</u></a></li>
<li><a href="https://change-location.techidaily.com/in-2024-what-is-the-best-pokemon-for-pokemon-pvp-ranking-on-samsung-galaxy-m54-5g-drfone-by-drfone-virtual-android/"><u>In 2024, What is the best Pokemon for pokemon pvp ranking On Samsung Galaxy M54 5G? | Dr.fone</u></a></li>
<li><a href="https://bypass-frp.techidaily.com/in-2024-how-to-bypass-google-frp-lock-from-vivo-s18-pro-devices-by-drfone-android/"><u>In 2024, How to Bypass Google FRP Lock from Vivo S18 Pro Devices</u></a></li>
<li><a href="https://review-topics.techidaily.com/how-to-upgrade-iphone-6-without-itunes-drfone-by-drfone-ios-system-repair-ios-system-repair/"><u>How to Upgrade iPhone 6 without iTunes? | Dr.fone</u></a></li>
<li><a href="https://android-transfer.techidaily.com/in-2024-how-to-migrate-android-data-from-honor-x9a-to-new-android-phone-drfone-by-drfone-transfer-from-android-transfer-from-android/"><u>In 2024, How to Migrate Android Data From Honor X9a to New Android Phone? | Dr.fone</u></a></li>
<li><a href="https://ai-video-apps.techidaily.com/new-2024-approved-best-movie-makers-for-windows-10-free-and-paid/"><u>New 2024 Approved Best Movie Makers for Windows 10 Free and Paid</u></a></li>
<li><a href="https://iphone-location.techidaily.com/in-2024-5-ways-change-your-home-address-in-googleapple-map-on-apple-iphone-12-miniipad-drfone-by-drfone-virtual-ios/"><u>In 2024, 5 Ways Change Your Home Address in Google/Apple Map on Apple iPhone 12 mini/iPad | Dr.fone</u></a></li>
<li><a href="https://blog-min.techidaily.com/how-to-recover-deleted-photos-from-android-gallery-app-on-xiaomi-mix-fold-3-by-stellar-photo-recovery-android-mobile-photo-recover/"><u>How to Recover Deleted Photos from Android Gallery App on Xiaomi Mix Fold 3</u></a></li>
<li><a href="https://phone-solutions.techidaily.com/in-2024-how-to-watch-hulu-outside-us-on-vivo-s17t-drfone-by-drfone-virtual-android/"><u>In 2024, How to Watch Hulu Outside US On Vivo S17t | Dr.fone</u></a></li>
<li><a href="https://activate-lock.techidaily.com/in-2024-how-to-unlock-icloud-lock-from-your-apple-iphone-6s-and-ipad-by-drfone-ios/"><u>In 2024, How to Unlock iCloud lock from your Apple iPhone 6s and iPad?</u></a></li>
<li><a href="https://android-frp.techidaily.com/in-2024-how-to-bypass-google-frp-lock-from-huawei-nova-y71-devices-by-drfone-android/"><u>In 2024, How to Bypass Google FRP Lock from Huawei Nova Y71 Devices</u></a></li>
<li><a href="https://location-social.techidaily.com/in-2024-how-to-sharefake-location-on-whatsapp-for-oppo-a78-drfone-by-drfone-virtual-android/"><u>In 2024, How to Share/Fake Location on WhatsApp for Oppo A78 | Dr.fone</u></a></li>
<li><a href="https://techidaily.com/how-to-repair-apple-iphone-x-system-issues-drfone-by-drfone-ios-system-repair-ios-system-repair/"><u>How To Repair Apple iPhone X System Issues? | Dr.fone</u></a></li>
<li><a href="https://unlock-android.techidaily.com/in-2024-how-to-show-wi-fi-password-on-xiaomi-redmi-12-5g-by-drfone-android/"><u>In 2024, How to Show Wi-Fi Password on Xiaomi Redmi 12 5G</u></a></li>
<li><a href="https://unlock-android.techidaily.com/universal-unlock-pattern-for-xiaomi-redmi-k70-by-drfone-android/"><u>Universal Unlock Pattern for Xiaomi Redmi K70</u></a></li>
</ul></div> |
import React, { useEffect, useState } from "react";
import Sidebar from "../Sidebar/Sidebar";
import DashboardHeader from "../Dashboard/DashboardHeader";
import Table from "react-bootstrap/Table";
import EditIcon from "@mui/icons-material/Edit";
import DeleteIcon from "@mui/icons-material/Delete";
import { useDispatch, useSelector } from "react-redux";
import "../Posts/post.css"
import axios from "axios";
import { toast } from "react-toastify"
import Button from 'react-bootstrap/Button';
import Modal from 'react-bootstrap/Modal';
import { allUsers, deleteUser } from "../../action/userAction";
import UpdateUser from "./UpdateUser";
const UsersList = () => {
const dispatch = useDispatch();
const { isDeleted } = useSelector((state) => state.profile)
const { loading, users } = useSelector((state) => state.allUsers);
// const { post } = useSelector((state) => state.postDetails);
// console.log(post)
const [userId, setUserId] = useState()
const [show, setShow] = useState(false);
const handleClose = () => setShow(false);
const handleShow = (id) => {
// console.log(id)
setUserId(id)
setShow(true)
};
const delUser = (id) => {
// console.log("delete")
dispatch(deleteUser(id))
}
useEffect(() => {
if (isDeleted) {
toast.success("User deleted successfully")
}
dispatch(allUsers());
}, [dispatch,isDeleted]);
let id = 1
return (
<>
<div className="dasboard_home">
<div className="dasboard_home_sidebar">
<Sidebar />
</div>
<div className="dashboard_post">
<Table striped bordered hover>
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Email</th>
<th>Role</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
{users &&
users.map((user) => {
return (
<tr>
<td>{id++}</td>
<td>{user.name}</td>
<td>{user.email}</td>
<td>{user.role}</td>
<td colSpan={2}>
<span id="edit" onClick={()=>handleShow(user._id)}>
<EditIcon />
</span>
<span id="delete" onClick={() => delUser(user._id)}>
<DeleteIcon />
</span>
</td>
</tr>
);
})}
</tbody>
</Table>
</div>
</div>
{/* updating post */}
<Modal show={show} onHide={handleClose}>
<Modal.Header closeButton>
<Modal.Title>Update User</Modal.Title>
</Modal.Header>
<Modal.Body>
<UpdateUser userId={userId}/>
</Modal.Body>
<Modal.Footer>
<Button variant="secondary" onClick={handleClose}>
Close
</Button>
<Button variant="primary" onClick={handleClose}>
Save Changes
</Button>
</Modal.Footer>
</Modal>
</>
);
};
export default UsersList; |
import joi from "joi";
export const register = joi
.object({
userName: joi.string().alphanum().min(3).max(20).required(),
email: joi
.string()
.email({
minDomainSegments: 2,
maxDomainSegments: 4,
tlds: {
allow: ["com", "net", "org", "edue", "gov", "mil", "edu", "eg"],
},
})
.required(),
password: joi.string().pattern(RegExp("^[a-zA-Z0-9]{3,30}$")).required(),
confirmPassword: joi.string().valid(joi.ref("password")).required(),
gender: joi.string().valid("male", "female", "not specified").required(),
phone: joi.string().min(11).max(11).required(),
})
.required();
export const activationCode = joi
.object({
activationCode: joi.string().required(),
})
.required();
export const login = joi
.object({
email: joi
.string()
.email({
minDomainSegments: 2,
maxDomainSegments: 4,
tlds: {
allow: ["com", "net", "org", "edue", "gov", "mil", "edu", "eg"],
},
})
.required(),
password: joi.string().pattern(RegExp("^[a-zA-Z0-9]{3,30}$")).required(),
})
.required();
export const forgetPass = joi
.object({
email: joi
.string()
.email({
minDomainSegments: 2,
maxDomainSegments: 4,
tlds: {
allow: ["com", "net", "org", "edue", "gov", "mil", "edu", "eg"],
},
})
.required(),
})
.required();
export const resetPass = joi
.object({
forgetCode: joi.string().required(),
password: joi.string().pattern(RegExp("^[a-zA-Z0-9]{3,30}$")).required(),
confirmPassword: joi.string().valid(joi.ref("password")).required(),
})
.required(); |
// 49) Faça um programa em C que leia um vetor de 30 inteiros e implemente as funções a seguir, utilizando,
// OBRIGATORIAMENTE, ponteiros e passagem de parâmetros por referência:
// a) void zeraPares (int *vet): recebe um vetor e troca todos os números pares contidos nele pelo zero.
// b) void dobra (int *vet): recebe um vetor e multiplica todos os seus elementos por dois.
// c) void soma (int *vet1, int * vet2): recebe dois vetores de inteiros e soma cada par de elementos
// de mesmo índice, colocando o resultado no primeiro vetor.
#include <stdio.h>
// a) void zeraPares (int *vet): recebe um vetor e troca todos os números pares contidos nele pelo zero.
void zeraPares(int *vet) {
for (int i = 0; i < 30 ; i++) {
if (*(vet+i) % 2 == 0) {
*(vet+i) = 0;
} // Final do if
} // Final do for
} // Final da função zeraPares
// b) void dobra (int *vet): recebe um vetor e multiplica todos os seus elementos por dois.
void dobra(int *vet) {
for (int i = 0; i < 30; i++) {
*(vet+i) *= 2; // Esse código é o mesmo que vet[i] = vet[i] * 2;
} // Final do for
} // Final da função dobra
// c) void soma (int *vet1, int *vet2): recebe dois vetores de inteiros e soma cada par de elementos
// de mesmo índice, colocando o resultado no primeiro vetor.
void soma(int *vet1, int *vet2) {
for (int i = 0; i < 30; i++) {
*(vet1+i) += *(vet2+i); // Esse código é o mesmo que vet1[i] = vet1[i] + vet2[i];
} // Final do for
} // Final da função soma
void main() {
int i, vetor1[30], vetor1Copia1[30], vetor1Copia2[30], vetor2[30];
printf("\nPreencha os valores do primeiro vetor:\n");
for (i = 0; i < 30; i++) {
printf("Digite o %dº valor do vetor: ", i+1);
scanf("%d", &vetor1[i]);
vetor1Copia1[i] = vetor1[i]; // vetor1Copia1[30] terá seus elementos multiplicados
vetor1Copia2[i] = vetor1[i]; // vetor1Copia2[30] terá seus elementos somados com o segundo vetor
} // Final do for
// a) void zeraPares (int *vet): recebe um vetor e troca todos os números pares contidos nele pelo zero.
zeraPares(vetor1);
printf("\nOs números pares do primeiro vetor:\n");
for (i = 0; i < 30; i++) {
printf("%dº valor: %d\n", i+1, vetor1[i]);
} // Final do for
// b) void dobra (int *vet): recebe um vetor e multiplica todos os seus elementos por dois.
printf("\nOs valores do primeiro vetor sendo multiplicados por 2:\n");
dobra(vetor1Copia1);
for (i = 0; i < 30; i++) {
printf("%dº valor: %d\n", i+1, vetor1Copia1[i]);
} // Final do for
// c) void soma (int *vet1, int * vet2): recebe dois vetores de inteiros e soma cada par de elementos
// de mesmo índice, colocando o resultado no primeiro vetor.
printf("\nPreencha os valores do segundo vetor:\n");
for (i = 0; i < 30; i++) {
printf("Digite o %dº valor do vetor: ", i+1);
scanf("%d", &vetor2[i]);
} // Final do for
soma(vetor1Copia2, vetor2);
printf("\nA soma do primeiro e segundo vetor que estão armazenados no primeiro vetor:\n");
for (i = 0; i < 30; i++) {
printf("%dº valor: %d\n", i+1, vetor1Copia2[i]);
} // Final do for
} |
import copy
import random
class RHC:
def __init__(self, filename):
self.__obj = []
self.__weight = 0
self.__nr_objects = 0
self.__best_solution = 0
self.__solutions = []
self.__file_configuration(filename)
def __file_configuration(self, filename):
with open(filename, 'r') as file:
lines = file.readlines()
self.__nr_objects = int(lines[0])
lines = [line.strip() for line in lines if line.strip()]
for line in lines[1:-1]:
obj = line.split()
obj_nr = int(obj[0])
weight = int(obj[1])
value = int(obj[2])
self.__obj.append({"nr": obj_nr, "weight": weight, "value": value})
self.__max_weight = int(lines[-1])
# this method evaluates the value of a solution for a knapsack problem , each item has a weight and a value
# and there is a constraint of the maximum weight that can be carried
def __eval(self, solution):
total_weight = 0
total_value = 0
for i in range(len(solution)):
if solution[i] == 1:
total_weight += self.__obj[i]["weight"]
total_value += self.__obj[i]["value"]
if total_weight <= self.__max_weight:
return total_value
return 0
# this method generates a random solution, where each item is represented either by '0' or by '1'.
# 0 is not included, 1 is included. The decision the include is made randomly.
def __generate(self):
solution = []
for _ in range(len(self.__obj)):
choice = random.choice([0,1])
solution.append(choice)
return solution
#this method generates the neighbours of a solution. Each neighbour si obtained by flipping the value.
@staticmethod
def __generate_neighbours(solution):
neighbours = []
for i in range(len(solution)):
sol_copy = copy.deepcopy(solution)
sol_copy[i] = 1 - sol_copy[i]
neighbours.append(sol_copy)
return neighbours
#chooses a random neighbour from the list
def __random_neighbours(self, neighbours):
return random.choice(neighbours)
#this method implements a local search(random hill climbing). Generates a solution and than a random neighbour for that solution.
#It eavluates the quality of the random neighbour and it updates the solution if the neighbour is better.
#after a specified number of iterations and if a better neighbour is not found , it breaks the loop.
def search(self, iteration, max_iteration):
solution = self.__generate()
best_solution = self.__eval(solution)
nr = 0
for i in range(iteration):
neighbours = self.__generate_neighbours(solution)
random_neighbour = self.__random_neighbours(neighbours)
best_neighbour = self.__eval(random_neighbour)
if best_neighbour > best_solution:
nr = 1
solution = random_neighbour
best_solution = best_neighbour
break
if i == max_iteration - 1 and nr == 0:
solution = self.__generate()
best_solution = self.__eval(solution)
self.__best_solution = best_solution
self.__solutions.append(self.__best_solution)
return self.__best_solution |
interface user_registration {
firstName: string
middleName: string
lastName: string
status: boolean
emailVerified: boolean
password: string
mobile: string
userInfo?: object
isStaff?: boolean
}
interface add_employees {
email: string
roleId?: bigint
userPositionId?: bigint
departmentId?: bigint
manager?: bigint
clientId?: bigint
isStaff?: boolean
}
interface update_employees {
firstName: string
middleName: string
lastName: string
isSubAdmin: boolean
status: boolean
mobile: string
userInfo: object
roleId?: bigint | null
}
interface update_self {
firstName: string
middleName: string
lastName: string
mobile: string
userInfo: object
bankInfo: object
}
interface set_permissions {
roleID: bigint
componentName: string
add: boolean
update: boolean
delete: boolean
view: boolean
}
interface add_roles {
role: string
code: string
}
interface update_roles {
role: string
code?: string
status: boolean
}
interface view_employee_where_clause {
isStaff?: boolean
isAdmin?: boolean
isSubAdmin?: boolean
status?: boolean
email?: string
emailVerified?: boolean
softDelete: boolean
AND?: Array<object>
}
interface bank_info {
employeeID: string
bankName: string
acNumber: string
ifscCode: string
panNumber: string
pfNo: string
pfAUN: string
}
interface role_permission {
roleId: bigint
permissionId: bigint
add: boolean
update: boolean
delete: boolean
view: boolean
}
interface view_roles {
softDelete: boolean
status?: boolean
AND?: Array<object>
}
interface view_brand_where_clause {
softDelete: boolean
status?: boolean
prioritize?: boolean
AND?: Array<object>
}
interface update_customers {
firstName: string
middleName: string
lastName: string
isSubAdmin: boolean
status: boolean
mobile: string
userInfo: object
roleId?: bigint | null
}
export {
add_roles,
bank_info,
view_roles,
update_self,
update_roles,
add_employees,
set_permissions,
role_permission,
update_customers,
update_employees,
user_registration,
view_brand_where_clause,
view_employee_where_clause
}; |
//
// AboutViewTests.swift
// MuseuZapTests
//
// Created by Ignácio Espinoso Ribeiro on 20/04/20.
// Copyright © 2020 Bernardo. All rights reserved.
//
import XCTest
@testable import Blin
class MockAboutViewModel: AboutViewModel {
init() {
// swiftlint:disable line_length
let aboutDescription1 = "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nisi elementum nunc, sollicitudin non pellentesque. In egestas adipiscing vestibulum varius "
let aboutDescription2 = "urna sed ornare consectetur. Convallis in volutpat fermentum ipsum in condimentum ut. Odio ornare id ornare augue. Aliquam sit cras arcu amet erat maecenas mi, amet."
// swiftlint:enable line_length
super.init(email: "mock@email.com", description: aboutDescription1 + aboutDescription2)
}
required init(email: String, description: String) {
fatalError("init(email:description:) has not been implemented")
}
}
class MockAboutViewModelDelegate: AboutViewModel {
override func sendEmail() throws {
return
}
}
class AboutViewTests: XCTestCase {
var aboutView: AboutView!
var aboutViewModel: MockAboutViewModel!
override func setUpWithError() throws {
aboutView = AboutView(frame: CGRect.zero)
aboutViewModel = MockAboutViewModel()
aboutView.viewModel = aboutViewModel
}
override func tearDownWithError() throws {
aboutView = nil
aboutViewModel = nil
UIPasteboard.general.string = ""
}
func testBackgroundColor() throws {
XCTAssertEqual(self.aboutView.contentView.backgroundColor, UIColor.Default.background)
}
func testLabelColor() throws {
XCTAssertEqual(self.aboutView.mailLabel.tintColor, UIColor.Default.label)
}
func testHandleTap() throws {
XCTAssertNotNil(aboutView)
XCTAssertNoThrow(try aboutView.handleTap())
}
func testHandleTapFail() throws {
aboutView.viewModel = nil
XCTAssertThrowsError(try aboutView.handleTap())
}
func testCopy() throws {
XCTAssertNoThrow(try aboutView.addToClipboard())
XCTAssertEqual(aboutViewModel.email, UIPasteboard.general.string)
}
func testCopyFail() throws {
aboutView.viewModel = nil
XCTAssertThrowsError(try aboutView.addToClipboard())
}
} |
import { DebugElement, NO_ERRORS_SCHEMA } from "@angular/core";
import { ComponentFixture, TestBed, waitForAsync } from "@angular/core/testing";
import { ControlContainer, FormsModule, NgForm } from "@angular/forms";
import { FormControlService } from "src/app-shared/services/form-control.service";
import { FormAttributeMapper } from "src/app-shared/services/mappers/form-attribute.mapper";
import { LoggedInUserService } from "src/app-shared/services/user/logged-in-user.service";
import { SharedModule } from "src/app-shared/shared.module";
import { FPSelectList } from "src/app-shared/types/support-data";
import { LookupModel } from "src/app-shared/views/lookup-editor/lookup/models/lookup.model";
import { HolidayRecordStore } from "src/app/business-admin/holiday-record/services/holiday-record.store";
import { LookupGroupModel } from "src/app/system-admin/lookup-group/models/lookup-group.model";
import { TestExtensions } from "src/testing/test-extensions";
import { LookupComponent } from "./lookup.component";
describe("LookupComponent", () => {
HolidayRecordStore;
let debugElement: DebugElement;
let component: LookupComponent;
let fixture: ComponentFixture<LookupComponent>;
let group: LookupGroupModel;
// let store: any = ;
const store: any = TestExtensions.GetFakeStore();
let lookupModelarray: LookupModel[];
beforeAll(() => {
const lookup1: LookupModel = {
displayOrder: 0,
groupId: 10143,
id: 21507,
isActive: true,
itemCanBeRemoved: false,
name: "PersonalLeave",
text: "Personal",
value: null,
valueIsEditable: false,
colorLID: 2,
};
const lookup2: LookupModel = {
displayOrder: 0,
groupId: 10143,
id: 21508,
isActive: true,
itemCanBeRemoved: false,
name: "SickLeave",
text: "Sick",
value: null,
valueIsEditable: false,
colorLID: 2,
};
const lookup3: LookupModel = {
displayOrder: 0,
groupId: 10143,
id: 21509,
isActive: true,
itemCanBeRemoved: false,
name: "MaternityLeave",
text: "Maternity",
value: null,
valueIsEditable: true,
colorLID: 2,
};
lookupModelarray = [lookup1, lookup2, lookup3];
group = {
id: 10143,
groupName: "LookDemo",
itemsCanBeAddedOrRemoved: false,
lookups: lookupModelarray,
};
});
beforeEach(
waitForAsync(() => {
TestBed.configureTestingModule({
imports: [FormsModule, SharedModule],
declarations: [LookupComponent],
schemas: [NO_ERRORS_SCHEMA],
providers: [
TestExtensions.BaseProviders,
ControlContainer,
FormAttributeMapper,
FormControlService,
NgForm,
// ExpandedViewExpectations.providersFunc(HolidayRecordStore),
],
})
.compileComponents()
.then(() => {
TestExtensions.InitiateAppInjector();
});
})
);
beforeEach(() => {
fixture = TestBed.createComponent(LookupComponent);
component = fixture.componentInstance;
component.store = store;
debugElement = fixture.debugElement;
TestBed.inject(LoggedInUserService).getUser = () =>
TestExtensions.getFakeUser();
store.getFormChanges = () => {};
fixture.detectChanges();
});
it("Should Create", () => {
component.group = group;
component.options = group.lookups;
fixture.detectChanges();
expect(component).toBeTruthy();
});
it("Shoud give error when store is not provided", () => {
expect(component.afterBaseInit()).toBeFalsy();
});
it("Shoud run After base Init if group not provided", () => {
component.store = store;
component.group = group;
component.options = group.lookups;
component.isNullable = true;
component.afterBaseInit();
expect(component).toBeTruthy();
});
it("Shoud run After base Init and change this.default when control.value is grater then 0 ", () => {
component.store = store;
component.group = group;
component.options = group.lookups;
component.isNullable = true;
component.control.value = 2;
component.afterBaseInit();
expect(component).toBeTruthy();
expect(component.default).toEqual(2);
});
// it("should open when clicked", () => {
// component.group = group;
// component.options = lookupModelarray;
// fixture.detectChanges();
// const container = fixture.debugElement.query(
// By.css(".p-dropdown")
// ).nativeElement;
// container.click();
// fixture.detectChanges();
// const dropdownPanel = fixture.debugElement.query(
// By.css(".p-dropdown-panel")
// );
// expect(container.className).toContain("p-dropdown-open");
// expect(dropdownPanel).toBeTruthy();
// });
it("Should handle hide event", () => {
spyOn(component.select, "emit").and.callThrough();
fixture.detectChanges();
component.emitOnClose = true;
component.handleHide();
expect(component.select.emit).toHaveBeenCalledTimes(1);
expect(component.handleHide).toBeTruthy();
spyOn(component.enter, "emit").and.callThrough();
fixture.detectChanges();
component.emitOnClose = false;
component.handleHide();
expect(component.enter.emit).toHaveBeenCalledTimes(1);
expect(component.handleHide).toBeTruthy();
});
it("Should open Edit Lookup Popup", () => {
const event = new MouseEvent("click");
TestExtensions.getFakeUser();
component.store = store;
component.group = group;
component.options = group.lookups;
component.loggedInUser.rolesHasCustomRightClickMenu = true;
component.openEditLookupPopup(event);
expect(component.openEditLookupPopup).toBeTruthy();
});
it("Shoud run handle Value change Method", () => {
spyOn(component.select, "emit").and.callThrough();
spyOn(component, "updateFormChanges").and.callThrough();
const value = 2;
component.group = group;
component.options = group.lookups;
component.emitOnClose = false;
component.alwaysPristine = true;
component.store = store;
component.handleChange(value);
expect(component.updateFormChanges).toHaveBeenCalledTimes(0);
expect(component.handleChange).toBeTruthy();
});
it("should set server control required to true when it is undefined", () => {
component.group = group;
component.control.required = undefined;
component.control.isEditFormControl = true;
component.afterBaseInit();
expect(component.control.required).toEqual(true);
});
it("should have run mapLookupGrouptoList ", () => {
spyOn(component.selectItemMapper, "mapLookupGrouptoList").and.returnValue(
new FPSelectList()
);
component.group = group;
component.control.required = false;
component.afterBaseInit();
expect(component.selectItemMapper.mapLookupGrouptoList).toHaveBeenCalled();
});
}); |
from pygame import Surface
from src.auxiliary_modules import *
from src.auxiliary_modules import graphics as grp
# Manages the User's information in the Game Menu
class User:
def __init__(self, name=""):
self.name = name
self.password = ""
self.best_speed = 0
self.best_time = 0
self.level = 1
self.parts = 0
self.image = grp.load_image(f"menu/interfaces/User/user_info/level1.png")
self.parts_text, self.coo_p_t = None, (0, 0)
self.best_speed_text, self.coo_bs_t = None, (0, 0)
self.best_time_text, self.coo_bt_t = None, (0, 0)
self.name_text, self.coo_n_t = None, (0, 0)
self.music_volume = 8
self.sound_volume = 8
def get_info(self) -> None:
data = files.read_file_content(f"saves/{self.name}/data.txt", 1)[0].split(" ")
self.best_speed, self.best_time, self.level, self.parts, self.password, self.music_volume, self.sound_volume = \
int(data[0]), int(data[1]), int(data[2]), int(data[3]), data[4], int(data[5]), int(data[6])
self.image = grp.load_image(f"menu/interfaces/User/user_info/level{self.level}.png")
def get_texts(self) -> None:
self.best_time_text, self.coo_bt_t = grp.writable_best_time(self.best_time)
self.best_speed_text, self.coo_bs_t = grp.writable_best_speed(self.best_speed)
self.parts_text, self.coo_p_t = grp.writable_parts_number(self.parts)
self.name_text, self.coo_n_t = grp.writable_user_name(self.name)
def draw_text(self, screen: Surface) -> None:
screen.blit(self.best_time_text, self.coo_bt_t)
screen.blit(self.best_speed_text, self.coo_bs_t)
screen.blit(self.parts_text, self.coo_p_t)
screen.blit(self.name_text, self.coo_n_t)
def get_active_user(self) -> None:
data = files.read_file_content("saves/active_user.txt", 1)[0].split(" ")
self.name, self.best_speed, self.best_time = data[0], int(data[1]), int(data[2])
self.level, self.parts, self.password = int(data[3]), int(data[4]), data[5]
self.music_volume, self.sound_volume = data[6], data[7]
self.image = grp.load_image(f"menu/interfaces/User/user_info/level{self.level}.png")
def get_string_attributes(self):
return f"{self.best_speed} {self.best_time} {self.level} {self.parts} {self.password} {self.music_volume}" \
f" {self.sound_volume}"
def turn_active(self) -> None:
files.write_file_content("saves/active_user.txt", f"{self.name} {self.get_string_attributes()}")
def save_info(self) -> None:
files.write_file_content(f"saves/{self.name}/data.txt", self.get_string_attributes()) |
/*
Copyright (c) Jon Newman (jpnewman ~at~ mit <dot> edu)
All right reserved.
This file is part of the Cyclops Library (CL) for Arduino.
CL is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
CL is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with CL. If not, see <http://www.gnu.org/licenses/>.
*/
#include <Cyclops.h>
#define PI_CONST 3.14159265358979323846
#define FREQ 0.5f // Frequency in Hz of sine wave
const float freq_rad = 2 * PI_CONST * FREQ;
// Create two cyclops devices, each with its own current limit. e.g. CH0
// corresponds to a physical board with jumper pads soldered so that OC0, CS0,
// TRIG0, and A0 are used. Set the current limit to 500 mA on CH0 and 200 mA
// on CH1.
Cyclops cyclops0(CH0, 500);
Cyclops cyclops1(CH1, 200);
void setup()
{
// Start both cyclops devices
Cyclops::begin();
// Zero out the DACs
cyclops0.dac_load_voltage(0);
cyclops1.dac_load_voltage(0);
}
// NOTE: you do not need to use the onboard DAC to use overcurrent protection.
// If you want to enable overcurrent protection using the EXT input, simply
// provide an empty loop() function here. The overcurrent protection loop will
// run in the background and take over if it detects an overcurrent condition
// in the analog portion of the drive circuitry.
void loop()
{
// Each board includes an onboard 12-bit (4095 position) DAC spanning 0-5
// volts. Use the DAC to generate a sine wave and a randomly stepping
// random voltage from 0 to 5V. This voltage is always scaled by the front
// dial before being used as a feedback reference.
float now = ((float)millis()) / 1000.0;
unsigned int sine_volt
= (unsigned int)(4095.0 * (0.5 * sin(freq_rad * now) + 0.5));
// Cyclops0 produces slow sine wave
cyclops0.dac_prog_voltage(sine_volt);
// Cyclops1 produces random output
cyclops1.dac_prog_voltage(random(0, 4095));
// Update the outputs simulateously.
Cyclops::dac_load();
} |
<template>
<nav class="navbar navbar-default">
<div class="container-fluid">
<div class="navbar-header">
<router-link class="navbar-brand" to="/">Stock Trader</router-link>
</div>
<div class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<router-link to="/portfolio" activeClass="active" tag="li">
<a>Portfolio</a>
</router-link>
<router-link to="/stocks" activeClass="active" tag="li">
<a>Stocks</a>
</router-link>
</ul>
<strong class="navbar-text navbar-right">Funds: {{funds |currency}}</strong>
<ul class="nav navbar-nav navbar-right">
<li>
<a href="#" @click="endDay">End Day</a>
</li>
<li
class="dropdown"
:class="{open: isDropdownOpen}"
@click="isDropdownOpen = !isDropdownOpen"
>
<a
href="#"
class="dropdown-toggle"
data-toggle="dropdown"
role="button"
aria-hashpopup="true"
aria-expanded="false"
>
Save & Load
<span class="caret"></span>
</a>
<ul class="dropdown-menu">
<li>
<a href="#">Save</a>
</li>
<li>
<a href="#">Load</a>
</li>
</ul>
</li>
</ul>
</div>
</div>
</nav>
</template>
<script>
import { mapActions } from "vuex";
export default {
data() {
return {
isDropdownOpen: false
};
},
computed: {
funds() {
return this.$store.getters.funds;
}
},
methods: {
...mapActions(["randomizeStocks"]),
endDay() {
this.randomizeStocks();
}
}
};
</script> |
package main
import (
"bufio"
"flag"
"fmt"
"io"
"net"
"os"
"os/signal"
"syscall"
"time"
)
/*
=== Утилита telnet ===
Реализовать примитивный telnet клиент:
Примеры вызовов:
go-telnet --timeout=10s host port go-telnet mysite.ru 8080 go-telnet --timeout=3s 1.1.1.1 123
Программа должна подключаться к указанному хосту (ip или доменное имя) и порту по протоколу TCP.
После подключения STDIN программы должен записываться в сокет, а данные полученные и сокета должны выводиться в STDOUT
Опционально в программу можно передать таймаут на подключение к серверу (через аргумент --timeout, по умолчанию 10s).
При нажатии Ctrl+D программа должна закрывать сокет и завершаться. Если сокет закрывается со стороны сервера, программа должна также завершаться.
При подключении к несуществующему сервер, программа должна завершаться через timeout.
*/
func main() {
timeout := flag.Duration("timeout", 10*time.Second, "таймаут на подключение к серверу")
flag.Parse()
// Получаем адрес хоста и порт
args := flag.Args()
if len(args) != 2 {
fmt.Println("Usage: [--timeout=10s] host port")
os.Exit(1)
}
host := args[0]
port := args[1]
var conn net.Conn
var err error
// Подключаемся к серверу
start := time.Now()
for time.Since(start) < *timeout {
conn, err = net.DialTimeout("tcp", net.JoinHostPort(host, port), *timeout)
if err != nil {
continue
}
}
if err != nil {
fmt.Println("Error connecting to server:", err)
os.Exit(1)
}
defer conn.Close()
// Горутина для чтения данных из сокета и вывода их в STDOUT
go func() {
reader := bufio.NewReader(conn)
for {
msg, err := reader.ReadString('\n')
if err != io.EOF {
os.Exit(1)
}
if err != nil {
fmt.Println("Error reading from server:", err)
continue
}
fmt.Println(msg)
}
}()
// Горутина для чтения данных из STDIN и записи их в сокет
go func() {
scanner := bufio.NewScanner(os.Stdin)
for scanner.Scan() {
msg := scanner.Text()
_, err := fmt.Fprintf(conn, "%s\n", msg)
if err != nil {
fmt.Println("Error writing to server:", err)
os.Exit(1)
}
}
}()
quit := make(chan os.Signal, 1)
signal.Notify(quit, syscall.SIGTERM, syscall.SIGINT)
<-quit
} |
import React, { useState, useEffect, useRef } from 'react'
export const useHover = () => {
const [hovering, setHovering] = React.useState(false)
const onHoverProps = {
onMouseEnter: () => setHovering(true),
onMouseLeave: () => setHovering(false),
}
return [hovering, onHoverProps]
}
type CopiedValue = string | null
type CopyFn = (text: string) => Promise<boolean> // Return success
export function useCopyToClipboard(): [CopiedValue, CopyFn] {
const [copiedText, setCopiedText] = useState<CopiedValue>(null)
const copy: CopyFn = async (text) => {
if (!navigator?.clipboard) {
console.warn('Clipboard not supported')
return false
}
// Try to save to clipboard then save it in the state if worked
try {
await navigator.clipboard.writeText(text)
setCopiedText(text)
return true
} catch (error) {
console.warn('Copy failed', error)
setCopiedText(null)
return false
}
}
return [copiedText, copy]
}
export const useTimeRemaining = (endTime: string): { timeRemaining: string } => {
const now = new Date()
const end = new Date(endTime)
const diff = end.getTime() - now.getTime()
const timeLeft = useRef({
days: Math.floor(diff / (1000 * 60 * 60 * 24)),
hours: Math.floor((diff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)),
minutes: Math.floor((diff % (1000 * 60 * 60)) / (1000 * 60)),
seconds: Math.floor((diff % (1000 * 60)) / 1000),
})
const { days, hours, minutes, seconds } = timeLeft.current
let timeRemaining = `Ends in ${days} ${days === 1 ? 'day' : 'days'}`
useEffect(() => {
const updateCountdown = setInterval(() => {
timeLeft.current = {
days: Math.floor(diff / (1000 * 60 * 60 * 24)),
hours: Math.floor((diff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)),
minutes: Math.floor((diff % (1000 * 60 * 60)) / (1000 * 60)),
seconds: Math.floor((diff % (1000 * 60)) / 1000),
}
}, 1000)
return () => {
clearInterval(updateCountdown)
}
})
if (days === 0 && hours !== 0) {
timeRemaining = `Ends in ${hours} ${hours === 1 ? 'hour' : 'hours'}`
}
if (days === 0 && hours === 0 && minutes !== 0) {
timeRemaining = `Ends in ${minutes} ${minutes === 1 ? 'minute' : 'minutes'}`
}
if (days === 0 && hours === 0 && minutes === 0) {
timeRemaining = `Ends in ${seconds} ${seconds === 1 ? 'second' : 'seconds'}`
}
if (diff <= 0) {
timeRemaining = 'Ended'
}
return { timeRemaining }
} |
import { Component, OnInit, Inject } from '@angular/core';
import { NavController, AlertController } from '@ionic/angular';
import { APP_CONFIG, AppConfig } from '../app.config';
import { User } from 'src/models/user.models';
import { Helper } from 'src/models/helper.models';
import { UiElementsService } from '../services/common/ui-elements.service';
import { TranslateService } from '@ngx-translate/core';
import { MyEventsService } from '../services/events/my-events.service';
import { ECommerceService } from '../services/common/ecommerce.service';
import { ApiService } from '../services/network/api.service';
import { InAppBrowser } from '@ionic-native/in-app-browser/ngx';
@Component({
selector: 'app-account',
templateUrl: './account.page.html',
styleUrls: ['./account.page.scss']
})
export class AccountPage implements OnInit {
constructor(@Inject(APP_CONFIG) public config: AppConfig, private navCtrl: NavController, private myEvent: MyEventsService, public apiService: ApiService,
private uiElementService: UiElementsService, private translate: TranslateService, private alertCtrl: AlertController, private eComService: ECommerceService,
private inAppBrowser: InAppBrowser) { }
ngOnInit() {
}
viewProfile() {
if (this.apiService.getUserMe() != null) {
this.navCtrl.navigateForward(['./my-profile']);
} else {
this.alertLogin();
}
}
orderTracking() {
if (this.apiService.getUserMe() != null) {
this.navCtrl.navigateForward(['./order-tracking']);
} else {
this.alertLogin();
}
}
myAddress() {
if (this.apiService.getUserMe() != null) {
this.navCtrl.navigateForward(['./addresses']);
} else {
this.alertLogin();
}
}
pillReminders() {
if (this.apiService.getUserMe() != null) {
this.navCtrl.navigateForward(['./pill-reminders']);
} else {
this.alertLogin();
}
}
orders() {
if (this.apiService.getUserMe() != null) {
this.navCtrl.navigateForward(['./orders']);
} else {
this.alertLogin();
}
}
contactUs() {
if (this.apiService.getUserMe() != null) {
this.navCtrl.navigateForward(['./contact-us']);
} else {
this.alertLogin();
}
}
savedItems() {
if (this.apiService.getUserMe() != null) {
this.navCtrl.navigateForward(['./saved-items']);
} else {
this.alertLogin();
}
}
termsConditions() {
this.navCtrl.navigateForward(['./tnc']);
}
faqs() {
this.navCtrl.navigateForward(['./faqs']);
}
wallet() {
this.navCtrl.navigateForward(['./wallet']);
}
logout() {
this.translate.get(["logout_title", "logout_message", "no", "yes"]).subscribe(values => {
this.alertCtrl.create({
header: values["logout_title"],
message: values["logout_message"],
buttons: [{
text: values["no"],
handler: () => { }
}, {
text: values["yes"],
handler: () => {
this.eComService.clearCart();
Helper.setLoggedInUserResponse(null);
this.myEvent.setUserMeData(null);
this.myEvent.setAddressData(null);
}
}]
}).then(alert => alert.present());
});
}
changeLanguage() {
this.navCtrl.navigateForward(['./change-language']);
}
alertLogin() {
this.translate.get("alert_login_short").subscribe(value => this.uiElementService.presentToast(value));
this.navCtrl.navigateForward(['./sign-in']);
}
buyAppAction() {
this.translate.get("just_moment").subscribe(value => {
this.uiElementService.presentLoading(value);
this.apiService.getContactLink().subscribe(res => {
this.uiElementService.dismissLoading();
this.inAppBrowser.create((res.link ? res.link : "https://bit.ly/cc_DoctoWorld"), "_system");
}, err => {
console.log("getContactLink", err);
this.uiElementService.dismissLoading();
this.inAppBrowser.create("https://bit.ly/cc_DoctoWorld", "_system");
});
});
}
developed_by() {
this.inAppBrowser.create("https://verbosetechlabs.com/", "_system");
}
} |
import datetime
import logging
import os
from collections import Counter
from functools import wraps
from urllib.parse import quote_plus, urlencode
from authlib.integrations.flask_client import OAuth
from flask import (Blueprint, abort, current_app, jsonify, redirect,
render_template, render_template_string, request, session,
url_for)
from report_app.main.report_database import ReportDatabase
from report_app.main.repository_report import RepositoryReport
main = Blueprint("main", __name__)
logger = logging.getLogger(__name__)
AUTHLIB_CLIENT = "authlib.integrations.flask_client"
@main.record
def setup_auth0(setup_state):
"""This is a Blueprint function that is called during app.register_blueprint(main)
Use this function to set up Auth0
Args:
setup_state (Flask): The Flask app itself
"""
app = setup_state.app
OAuth(app)
auth0 = app.extensions.get(AUTHLIB_CLIENT)
auth0.register(
"auth0",
client_id=os.getenv("AUTH0_CLIENT_ID"),
client_secret=os.getenv("AUTH0_CLIENT_SECRET"),
client_kwargs={
"scope": "openid profile email",
},
server_metadata_url=f'https://{os.getenv("AUTH0_DOMAIN")}/'
+ ".well-known/openid-configuration",
)
def requires_auth(function_f):
"""Redirects the web page to /index if user is not logged in
Args:
function_f: The calling function
Returns:
A redirect to /index or continue with the function that was called
"""
@wraps(function_f)
def decorated(*args, **kwargs):
logger.debug("requires_auth()")
if "user" not in session:
return redirect("/index")
return function_f(*args, **kwargs)
return decorated
@main.route("/home")
@main.route("/index")
@main.route("/")
def index():
'''Entrypoint into the application'''
all_reports = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_repository_reports()
compliant_reports = [report for report in all_reports if report['data']['status']]
non_compliant_reports = [report for report in all_reports if not report['data']['status']]
all_infractions = [infraction for report in non_compliant_reports for infraction in report['data']['infractions']]
human_readable_infractions = []
for infraction in all_infractions:
match infraction:
case "administrators_require_review equalling False is not compliant":
human_readable_infractions.append("Administrators require review")
case "default_branch_main equalling False is not compliant":
human_readable_infractions.append("Default branch is not main")
case "has_default_branch_protection equalling False is not compliant":
human_readable_infractions.append("Default branch is not protected")
case "has_description equalling False is not compliant":
human_readable_infractions.append("Repository has no description")
case "has_license equalling False is not compliant":
human_readable_infractions.append("Repository has no license")
case "has_require_approvals_enabled equalling False is not compliant":
human_readable_infractions.append("Require approvals is not enabled")
case "issues_section_enabled equalling False is not compliant":
human_readable_infractions.append("Issues section is not enabled")
case "requires_approving_reviews equalling False is not compliant":
human_readable_infractions.append("Requires approving reviews is not enabled")
case _:
human_readable_infractions.append("Unknown infraction")
common_infractions = Counter(human_readable_infractions).most_common(3)
return render_template("home.html",
total=len(all_reports),
compliant=len(compliant_reports),
last_updated=all_reports[0]["stored_at"],
common_infractions=common_infractions,
non_compliant=len(non_compliant_reports))
@main.route("/login")
def login():
"""When click on the login button connect to Auth0
Returns:
Creates a redirect to /callback if succesful
"""
logger.debug("login()")
auth0 = current_app.extensions.get(AUTHLIB_CLIENT)
return auth0.auth0.authorize_redirect(
redirect_uri=url_for("main.callback", _external=True)
)
@main.route("/callback", methods=["GET", "POST"])
def callback():
"""If login succesful redirect to /index
Returns:
Redirects to /home if user has correct email domain else redirects to /logout
"""
try:
auth0 = current_app.extensions.get(AUTHLIB_CLIENT)
token = auth0.auth0.authorize_access_token()
session["user"] = token
except (KeyError, AttributeError):
return render_template("500.html"), 500
try:
user_email = session["user"]["userinfo"]["email"]
except KeyError:
logger.warning("Unauthed User does not have an email address")
return render_template("500.html"), 500
if user_email is None:
logger.warning("User %s does not have an email address", user_email)
return redirect("/logout")
if __is_allowed_email(user_email):
logger.info("User %s has approved email domain", user_email)
return redirect("/home")
logger.warning("User %s does not have an approved email domain", user_email)
return redirect("/logout")
def __is_allowed_email(email_address):
allowed_domains = (
"@digital.justice.gov.uk",
"@justice.gov.uk",
"@cica.gov.uk",
"@hmcts.net",
)
return any(email_address.endswith(domain) for domain in allowed_domains)
@main.route("/logout", methods=["GET", "POST"])
def logout():
"""When click on the logout button, clear the session, and log out of Auth0
Returns:
Redirects to /index
"""
logger.debug("logout()")
session.clear()
return redirect(
"https://"
+ os.getenv("AUTH0_DOMAIN")
+ "/v2/logout?"
+ urlencode(
{
"returnTo": url_for("main.index", _external=True),
"client_id": os.getenv("AUTH0_CLIENT_ID"),
},
quote_via=quote_plus,
)
)
@main.errorhandler(404)
def page_not_found(err):
"""Load 404.html when page not found error occurs
Args:
err : N/A
Returns:
Load the templates/404.html page
"""
logger.debug("A request was made to a page that doesn't exist %s", err)
return render_template("404.html"), 404
@main.errorhandler(403)
def server_forbidden(err):
"""Load 403.html when server forbids error occurs
Args:
err : N/A
Returns:
Load the templates/403.html page
"""
logger.debug("server_forbidden()")
return render_template("403.html"), 403
@main.errorhandler(500)
def unknown_server_error(err):
"""Load 500.html when unknown server error occurs
Args:
err : N/A
Returns:
Load the templates/500.html page
"""
logger.error("An unknown server error occurred: %s", err)
return render_template("500.html"), 500
@main.errorhandler(504)
def gateway_timeout(err):
"""Load 504.html when gateway timeout error occurs
Args:
err : N/A
Returns:
Load the templates/504.html page
"""
logger.error("A gateway timeout error occurred: %s", err)
return render_template("504.html"), 504
def _is_request_correct(the_request):
"""Check request is a POST and has the correct API key
Args:
the_request: the incoming data request object
"""
correct = False
if (
the_request.method == "POST"
and "X-API-KEY" in the_request.headers
and the_request.headers.get("X-API-KEY") == os.getenv("API_KEY")
):
logger.debug("is_request_correct(): api key correct")
correct = True
else:
logger.warning("is_request_correct(): incorrect api key")
return correct
@main.route("/api/v2/update-github-reports", methods=["POST"])
def update_github_reports():
"""Update all GitHub repository reports we hold
This will overwrite any existing reports storing each report
in the database as a new record.
"""
logger.info("update_github_reports(): received request from %s", request.remote_addr)
if _is_request_correct(request) is False:
logger.error("update_github_reports(): incorrect api key, from %s", request.remote_addr)
abort(400)
logging.info("update_github_reports(): updating all GitHub reports")
RepositoryReport(request.json).update_all_github_reports()
return jsonify({"message": "GitHub reports updated"}), 200
@main.route("/api/v2/compliant-repository/<repository_name>", methods=["GET"])
# Deprecated API endpoints: These will be removed in a future release
@main.route("/api/v1/compliant_public_repositories/endpoint/<repository_name>", methods=["GET"])
@main.route("/api/v1/compliant_public_repositories/<repository_name>", methods=["GET"])
def display_badge_if_compliant(repository_name: str) -> dict:
"""Display a badge if a repository is considered compliant.
Compliance is determined by the status field in the database.
Private repositories are not supported and will return a 403 error.
Args:
repository_name: the name of the repository to check
"""
dynamo_db = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
)
try:
repository = dynamo_db.get_repository_report(repository_name)
except KeyError:
abort(404)
if repository['data']['is_private']:
abort(403, "Private repositories are not supported")
if repository["data"]["status"]:
return {
"schemaVersion": 1,
"label": "MoJ Compliant",
"message": "PASS",
"color": "005ea5",
"labelColor": "231f20",
"style": "for-the-badge",
}
return {
"schemaVersion": 1,
"label": "MoJ Compliant",
"message": "FAIL",
"color": "d4351c",
"style": "for-the-badge",
"isError": "true",
}
@main.route("/public-github-repositories.html", methods=["GET"])
def public_github_repositories():
public_repositories = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_public_repositories()
compliant_repos = [repo for repo in public_repositories if repo['data']['status']]
non_compliant_repos = [repo for repo in public_repositories if not repo['data']['status']]
return render_template("public-github-repositories.html",
last_updated=public_repositories[0]["stored_at"],
total=len(public_repositories),
compliant=len(compliant_repos),
non_compliant=len(non_compliant_repos))
@main.route("/private-github-repositories.html")
@requires_auth
def private_github_repositories():
private_repositories = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_private_repositories()
compliant_repos = [repo for repo in private_repositories if repo['data']['status']]
non_compliant_repos = [repo for repo in private_repositories if not repo['data']['status']]
return render_template("private-github-repositories.html",
last_updated=private_repositories[0]["stored_at"],
total=len(private_repositories),
compliant=len(compliant_repos),
non_compliant=len(non_compliant_repos))
@main.route('/search-public-repositories', methods=['GET'])
def search_public_repositories():
query = request.args.get('q')
search_results = []
public_reports = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_public_repositories()
for repo in public_reports:
if query.lower() in repo['name'].lower():
search_results.append(repo)
# Render the search results to a string and return it
return render_template_string(
"""
<p class="govuk-heading-s">{{ search_results|length }} results found</p>
<ul class="govuk-list">
{% for repo in search_results %}
<p><a href="/public-report/{{ repo.name }}">{{ repo.name }}</a></p>
{% else %}
<p>No results found</p>
{% endfor %}
</ul>
""",
search_results=search_results
)
@main.route("/public-report/<repository_name>", methods=["GET"])
def display_individual_public_report(repository_name: str):
"""View the GitHub standards report for a repository"""
try:
report = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_repository_report(repository_name)
except KeyError:
logger.warning("display_individual_public_report(): repository not found")
abort(404)
return render_template("/public-report.html", report=report)
@main.route("/compliant-public-repositories.html", methods=["GET"])
def display_compliant_public_repositories():
"""View all repositories that adhere to the MoJ GitHub standards"""
compliant_repositories = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_compliant_repository_reports()
public_compliant_repositories = [repo for repo in compliant_repositories if not repo['data']['is_private']]
return render_template("/compliant-public-repositories.html", compliant_repos=public_compliant_repositories)
@main.route("/non-compliant-public-repositories.html", methods=["GET"])
def display_noncompliant_public_repositories():
"""View all repositories that do not adhere to the MoJ GitHub standards"""
non_compliant = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_non_compliant_repository_reports()
non_compliant_repositories = [repo for repo in non_compliant if not repo['data']['is_private']]
return render_template("/non-compliant-public-repositories.html", non_compliant_repos=non_compliant_repositories)
@main.route("/all-public-repositories.html", methods=["GET"])
def display_all_public_repositories():
"""View all repositories that do not adhere to the MoJ GitHub standards"""
all_reports = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_repository_reports()
public_reports = [repo for repo in all_reports if not repo['data']['is_private']]
return render_template("/all-public-repositories.html", public_reports=public_reports)
@main.route('/search-private-repositories', methods=['GET'])
@requires_auth
def search_private_repositories():
query = request.args.get('q')
search_results = []
private_repos = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_private_repositories()
for repo in private_repos:
if query.lower() in repo['name'].lower():
search_results.append(repo)
# Render the search results to a string and return it
return render_template_string(
"""
<p class="govuk-heading-s">{{ search_results|length }} results found</p>
<ul class="govuk-list">
{% for repo in search_results %}
<p><a href="/private-report/{{ repo.name }}">{{ repo.name }}</a></p>
{% else %}
<p>No results found</p>
{% endfor %}
</ul>
""",
search_results=search_results
)
@main.route("/private-report/<repository_name>", methods=["GET"])
@requires_auth
def display_individual_private_report(repository_name: str):
"""View the GitHub standards report for a private repository"""
try:
report = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_repository_report(repository_name)
except KeyError:
logger.warning("display_individual_private_report(): repository not found")
abort(404)
return render_template("/private-report.html", report=report)
@main.route("/compliant-private-repositories.html", methods=["GET"])
@requires_auth
def display_compliant_private_repositories():
"""View all private repositories that adhere to the MoJ GitHub standards"""
compliant_repositories = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_compliant_repository_reports()
private_compliant_repositories = [repo for repo in compliant_repositories if repo['data']['is_private']]
return render_template("/compliant-private-repositories.html", compliant_repos=private_compliant_repositories)
@main.route("/non-compliant-private-repositories.html", methods=["GET"])
@requires_auth
def display_noncompliant_private_repositories():
"""View all repositories that do not adhere to the MoJ GitHub standards"""
non_compliant = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_non_compliant_repository_reports()
non_compliant_repositories = [repo for repo in non_compliant if repo['data']['is_private']]
return render_template("/non-compliant-private-repositories.html", non_compliant_repos=non_compliant_repositories)
@main.route("/all-private-repositories.html", methods=["GET"])
@requires_auth
def display_all_private_repositories():
"""View all repositories that do not adhere to the MoJ GitHub standards"""
all_reports = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_repository_reports()
private_reports = [repo for repo in all_reports if repo['data']['is_private']]
return render_template("/all-private-repositories.html", private_reports=private_reports)
@main.route('/search-results-public', methods=['GET'])
def search_public_repositories_and_display_results():
"""Similar to search_public_repositories() but returns a results page instead of a string"""
template = "public-results.html"
query = request.args.get('q')
search_results = []
public_repos = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_public_repositories()
if query is None:
return render_template(template, results=search_results)
for repo in public_repos:
if query.lower() in repo['name'].lower():
search_results.append(repo)
return render_template(template, results=search_results)
@main.route('/search-results-private', methods=['GET'])
@requires_auth
def search_private_repositories_and_display_results():
"""Similar to search_private_repositories() but returns a results page instead of a string"""
template = "private-results.html"
query = request.args.get('q')
search_results = []
private_repos = ReportDatabase(
os.getenv("DYNAMODB_TABLE_NAME")
).get_all_private_repositories()
if query is None:
return render_template(template, results=search_results)
for repo in private_repos:
if query.lower() in repo['name'].lower():
search_results.append(repo)
return render_template(template, results=search_results) |
package main
import "testing"
func Test_Cakes(t *testing.T) {
type args struct {
recipe map[string]int
available map[string]int
}
type test struct {
name string
args args
want int
}
tests := []test{
{
name: "#1",
args: args{
recipe: map[string]int{"flour": 500, "sugar": 200, "eggs": 1},
available: map[string]int{"flour": 1200, "sugar": 1200, "eggs": 5, "milk": 200},
},
want: 2,
},
{
name: "#2",
args: args{
recipe: map[string]int{"flour": 500, "sugar": 200, "eggs": 1},
available: map[string]int{"flour": 200, "sugar": 1200, "eggs": 5, "milk": 200},
},
want: 0,
},
{
name: "#3",
args: args{
recipe: map[string]int{"flour": 500, "sugar": 200, "eggs": 1},
available: map[string]int{"flour": 2500, "sugar": 1200, "eggs": 1, "milk": 200},
},
want: 1,
},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := Cakes(tt.args.recipe, tt.args.available); got != tt.want {
t.Errorf("Cakes() %s = %v, want %v", tt.name, got, tt.want)
}
})
}
} |
import { Component, HostListener, OnInit } from '@angular/core';
import { PokemonService } from './services/pokemon.service';
import { PokemonResult, Result } from '../interfaces/pokemon-result';
import { LightPokemon } from '../interfaces/pokemon';
@Component({
selector: 'app-pokemon-list',
templateUrl: './pokemon-list.component.html',
styleUrls: ['./pokemon-list.component.scss'],
})
export class PokemonListComponent implements OnInit {
private _sortBy = 'number';
private _filterBy = 'all';
showFilterBox = false;
_searchText = '';
loading = true;
pokemonsCompleteList: Array<Result> = [];
pokemonsFiltered: Array<Result> = [];
pokemonsCurrentPage: Array<Result> = [];
pokemonDetails: LightPokemon | null = null;
// Pagination
pokemonsPerPage = 27;
currentPage = 1;
lastPage = 0;
totalPokemons = 0;
middlePages = [2, 3, 4];
constructor(private pokemonService: PokemonService) {}
ngOnInit(): void {
this.getPokemonResult(10000, 0);
this.setPokemonsPerPage(window.innerWidth);
}
/**
* Récupère les résultats des pokémons
* @param limit Nombre de résultats à récupérer
* @param offset Nombre de résultats à sauter
* @returns void
*/
getPokemonResult(limit: number, offset: number) {
this.loading = true;
this.pokemonService.getPokemonResult(limit, offset).subscribe({
next: (response: PokemonResult) => {
response.results.forEach(pokemon => {
// On récupère le numéro du pokémon à partir de l'url
pokemon.number = this.pokemonService.getPokemonId(pokemon.url);
// On set l'état de capture du pokémon
pokemon.catched = this.pokemonService.isCatched(pokemon.number);
// On ajoute le pokémon à la liste complète
this.pokemonsCompleteList.push(pokemon as Result);
});
this.pokemonsFiltered = this.pokemonsCompleteList;
},
complete: () => {
this.loading = false;
this.totalPokemons = this.pokemonsFiltered.length;
this.initPagination();
},
});
}
/**
* Ouvre la modal des détails du pokémon
* @param pokemon Pokémon à afficher
* @returns void
*/
openPokemonDetails(pokemon: LightPokemon) {
this.pokemonDetails = pokemon;
}
/**
* Ferme la modal des détails du pokémon
* @returns void
*/
closePokemonDetails() {
this.pokemonDetails = null;
}
/**
* Change l'état de capture du pokémon
* @param LightPokemon Pokémon à modifier
* @returns void
*/
togleCatchPokemon(pokemon: LightPokemon) {
const pokemonIndex = this.pokemonsCompleteList.findIndex(
p => p.number === pokemon.id
);
if (pokemonIndex) {
this.pokemonsCompleteList[pokemonIndex].catched = pokemon.catched;
}
}
//#region Pagination
/**
* Quand une page est changée
* @param pokemons Liste des pokémons à afficher
* @returns void
*/
onPageChanged(newPage: number) {
this.pokemonsCurrentPage = this.pokemonsFiltered.slice(
(newPage - 1) * this.pokemonsPerPage,
newPage * this.pokemonsPerPage
);
this.pagesUpdate(newPage);
}
/**
* Initialise la pagination
* @param nbResults Nombre de résultats
*/
initPagination() {
this.currentPage = 1;
this.lastPage = Math.ceil(this.totalPokemons / this.pokemonsPerPage);
this.onPageChanged(this.currentPage);
}
/**
* Update les pages à afficher au milieu de la pagination
* @param page Numéro de la page à afficher
* @returns void
*/
pagesUpdate(page: number) {
// Si la page demandée est entre 3 et l'avant dernière page on set les trois pages du milieu à afficher
if (page >= 4 && this.lastPage > 5) this.updatePagesArray(page);
// Si la page 1 est demandée on set les pages à afficher à 2 et 3
if (page === 1) {
this.middlePages = [2, 3, 4];
}
// Si la dernière page est demandée on set les pages à afficher à l'avant dernière et à l'avant avant dernière
if (page === this.lastPage && this.lastPage > 5) {
this.middlePages = [this.lastPage - 2, this.lastPage - 1];
}
this.currentPage = page;
}
/**
* Set l'array des pages à afficher au milieu de la pagination
* @param page Page courante (numéro de la page qui va être affichée au milieu)
*/
updatePagesArray(page: number) {
this.middlePages = [page - 1, page, page + 1];
}
@HostListener('window:resize', ['$event'])
onResize(event: Event) {
if (event.target && 'innerWidth' in event.target) {
this.setPokemonsPerPage(event.target.innerWidth as number);
}
this.initPagination();
}
/**
* Set le nombre de résultats par page suivant la taille de l'écran
* @param windowWidth Largeur de l'écran
* @returns void
*/
setPokemonsPerPage(windowWidth: number) {
if (windowWidth > 1200) {
this.pokemonsPerPage = 27;
} else if (windowWidth < 1200 && windowWidth > 992) {
this.pokemonsPerPage = 24;
} else if (windowWidth < 992 && windowWidth > 768) {
this.pokemonsPerPage = 18;
} else if (windowWidth < 768 && windowWidth > 376) {
this.pokemonsPerPage = 12;
} else {
this.pokemonsPerPage = 9;
}
}
//#endregion Pagination
// #region Filter
/**
* Filtrer les pokémons
* @param filterValue Valeur du filtre
* @returns void
*/
filterPokemon(filterValue: string) {
// Filtre les pokémons suivant le nom ou le numéro à partir de la list complète
this.pokemonsFiltered = this.pokemonsCompleteList.filter(pokemon => {
return (
pokemon.name
.toLocaleLowerCase()
.includes(filterValue.toLocaleLowerCase()) ||
pokemon.number?.toString().includes(filterValue)
);
});
}
get searchText(): string {
return this._searchText;
}
set searchText(value: string) {
this._searchText = value;
this.filterPokemon(value);
// On set le nombre de pokémons total et on initialise la pagination
this.totalPokemons = this.pokemonsFiltered.length;
this.initPagination();
}
get sortByValue(): string {
return this._sortBy;
}
set sortByValue(value: string) {
if (value === 'name') {
this.pokemonsFiltered.sort((a, b) => {
return a.name.localeCompare(b.name);
});
}
if (value === 'number') {
this.pokemonsFiltered.sort((a, b) => {
if (a.number === undefined || b.number === undefined) return -1;
return a.number - b.number;
});
}
this._sortBy = value;
this.initPagination();
this.togleFilterBox();
}
get filterByValue(): string {
return this._filterBy;
}
set filterByValue(value: string) {
// Permet de récupérer les pokémons filtrés par le nom ou le numéro et ensuite de filtrer par catched ou not-catched
this.filterPokemon(this.searchText);
if (value === 'catched') {
this.pokemonsFiltered = this.pokemonsFiltered.filter(pokemon => {
return pokemon.catched;
});
} else if (value === 'not-catched') {
this.pokemonsFiltered = this.pokemonsFiltered.filter(pokemon => {
return !pokemon.catched;
});
}
this._filterBy = value;
// On set le nombre de pokémons total et on initialise la pagination
this.totalPokemons = this.pokemonsFiltered.length;
this.initPagination();
this.togleFilterBox();
}
togleFilterBox() {
this.showFilterBox = !this.showFilterBox;
}
//#endregion Filter
} |
# Projeto de API Restful
# Sobre o projeto
Esse projeto foi proposto no curso "Programação Orientada a Objetos com Java" da plataforma Udemy, ministrado pelo professor Nélio Alves. O projeto foi estruturado conforme as camadas lógicas e o modelo de domínio. Com esse projeto, foi possível compreender as principais diferenças entre o paradigma orientado a documentos e o relacional. Uma implementação das operações de CRUD foi feita no projeto com as entidades do modelo de domínio.
## Funcionalidades
Na camada de serviço, é possível realizar as seguintes operações relacionadas ao usuário:
- Buscar todos os usuários
- Buscar usuário por id
- Inserir um novo usuário
- Remover um usuário
- Atualizar dados de um usuário
Já com a entidade Post, é possível realizar as seguintes operações na camada de serviço:
- Buscar um post por id
- Buscar post por título
- Buscar post por texto, data mínima ou data máxima
## Modelo do domínio

## Instância do domínio

## Camadas lógicas

# Tecnologias utilizadas
[](https://skillicons.dev)
# Ferramentas utilizadas
[](https://skillicons.dev)
# Como executar o projeto
Pré-requisitos: Java
```bash
# clonar repositório
git clone https://github.com/mariamourie/springboot-mongodb-project.git
# entrar na pasta da aplicação
cd src/main/java/com/mariamourie/workshopmongo
# executar o projeto
./mvnw spring-boot:run
```
# Autor
Maria Eduarda Leitão da Cruz
https://www.linkedin.com/in/maria-eduarda-cruz |
;;;; -*- Mode: Lisp; Syntax: ANSI-Common-Lisp; Base: 10; Package: modlisp -*-
;;;; *************************************************************************
;;;; FILE IDENTIFICATION
;;;;
;;;; Name: sockets.lisp
;;;; Purpose: Socket functions
;;;; Programmer: Kevin M. Rosenberg with excerpts from portableaserve
;;;; Date Started: Jun 2003
;;;;
;;;; $Id$
;;;; *************************************************************************
(in-package #:kmrcl)
(eval-when (:compile-toplevel :load-toplevel :execute)
#+sbcl (require :sb-bsd-sockets)
#+lispworks (require "comm")
#+allegro (require :socket))
#+sbcl
(defun listen-to-inet-port (&key (port 0) (kind :stream) (reuse nil))
"Create, bind and listen to an inet socket on *:PORT.
setsockopt SO_REUSEADDR if :reuse is not nil"
(let ((socket (make-instance 'sb-bsd-sockets:inet-socket
:type :stream
:protocol :tcp)))
(if reuse
(setf (sb-bsd-sockets:sockopt-reuse-address socket) t))
(sb-bsd-sockets:socket-bind
socket (sb-bsd-sockets:make-inet-address "0.0.0.0") port)
(sb-bsd-sockets:socket-listen socket 15)
socket))
(defun create-inet-listener (port &key (format :text) (reuse-address t))
#+cmu (declare (ignore format reuse-address))
#+cmu (ext:create-inet-listener port)
#+allegro
(socket:make-socket :connect :passive :local-port port :format format
:address-family
(if (stringp port)
:file
(if (or (null port) (integerp port))
:internet
(error "illegal value for port: ~s" port)))
:reuse-address reuse-address)
#+sbcl (declare (ignore format))
#+sbcl (listen-to-inet-port :port port :reuse reuse-address)
#+clisp (declare (ignore format reuse-address))
#+clisp (ext:socket-server port)
#+openmcl
(declare (ignore format))
#+openmcl
(ccl:make-socket :connect :passive :local-port port
:reuse-address reuse-address)
#-(or allegro clisp cmu sbcl openmcl)
(warn "create-inet-listener not supported on this implementation")
)
(defun make-fd-stream (socket &key input output element-type)
#+cmu
(sys:make-fd-stream socket :input input :output output
:element-type element-type)
#+sbcl
(sb-bsd-sockets:socket-make-stream socket :input input :output output
:element-type element-type)
#-(or cmu sbcl) (declare (ignore input output element-type))
#-(or cmu sbcl) socket
)
(defun accept-tcp-connection (listener)
"Returns (VALUES stream socket)"
#+allegro
(let ((sock (socket:accept-connection listener)))
(values sock sock))
#+clisp
(let ((sock (ext:socket-accept listener)))
(values sock sock))
#+cmu
(progn
(mp:process-wait-until-fd-usable listener :input)
(let ((sock (nth-value 0 (ext:accept-tcp-connection listener))))
(values (sys:make-fd-stream sock :input t :output t) sock)))
#+sbcl
(when (sb-sys:wait-until-fd-usable
(sb-bsd-sockets:socket-file-descriptor listener) :input)
(let ((sock (sb-bsd-sockets:socket-accept listener)))
(values
(sb-bsd-sockets:socket-make-stream
sock :element-type :default :input t :output t)
sock)))
#+openmcl
(let ((sock (ccl:accept-connection listener :wait t)))
(values sock sock))
#-(or allegro clisp cmu sbcl openmcl)
(warn "accept-tcp-connection not supported on this implementation")
)
(defmacro errorset (form display)
`(handler-case
,form
(error (e)
(declare (ignorable e))
(when ,display
(format t "~&Error: ~A~%" e)))))
(defun close-passive-socket (socket)
#+allegro (close socket)
#+clisp (ext:socket-server-close socket)
#+cmu (unix:unix-close socket)
#+sbcl (sb-unix:unix-close
(sb-bsd-sockets:socket-file-descriptor socket))
#+openmcl (close socket)
#-(or allegro clisp cmu sbcl openmcl)
(warn "close-passive-socket not supported on this implementation")
)
(defun close-active-socket (socket)
#+sbcl (sb-bsd-sockets:socket-close socket)
#-sbcl (close socket))
(defun ipaddr-to-dotted (ipaddr &key values)
"Convert from 32-bit integer to dotted string."
(declare (type (unsigned-byte 32) ipaddr))
(let ((a (logand #xff (ash ipaddr -24)))
(b (logand #xff (ash ipaddr -16)))
(c (logand #xff (ash ipaddr -8)))
(d (logand #xff ipaddr)))
(if values
(values a b c d)
(format nil "~d.~d.~d.~d" a b c d))))
(defun dotted-to-ipaddr (dotted &key (errorp t))
"Convert from dotted string to 32-bit integer."
(declare (string dotted))
(if errorp
(let ((ll (delimited-string-to-list dotted #\.)))
(+ (ash (parse-integer (first ll)) 24)
(ash (parse-integer (second ll)) 16)
(ash (parse-integer (third ll)) 8)
(parse-integer (fourth ll))))
(ignore-errors
(let ((ll (delimited-string-to-list dotted #\.)))
(+ (ash (parse-integer (first ll)) 24)
(ash (parse-integer (second ll)) 16)
(ash (parse-integer (third ll)) 8)
(parse-integer (fourth ll)))))))
#+sbcl
(defun ipaddr-to-hostname (ipaddr &key ignore-cache)
(when ignore-cache
(warn ":IGNORE-CACHE keyword in IPADDR-TO-HOSTNAME not supported."))
(sb-bsd-sockets:host-ent-name
(sb-bsd-sockets:get-host-by-address
(sb-bsd-sockets:make-inet-address ipaddr))))
#+sbcl
(defun lookup-hostname (host &key ignore-cache)
(when ignore-cache
(warn ":IGNORE-CACHE keyword in LOOKUP-HOSTNAME not supported."))
(if (stringp host)
(sb-bsd-sockets:host-ent-address
(sb-bsd-sockets:get-host-by-name host))
(dotted-to-ipaddr (ipaddr-to-dotted host))))
(defun make-active-socket (server port)
"Returns (VALUES STREAM SOCKET)"
#+allegro
(let ((sock (socket:make-socket :remote-host server
:remote-port port)))
(values sock sock))
#+lispworks
(let ((sock (comm:open-tcp-stream server port)))
(values sock sock))
#+sbcl
(let ((sock (make-instance 'sb-bsd-sockets:inet-socket
:type :stream
:protocol :tcp)))
(sb-bsd-sockets:socket-connect sock (lookup-hostname server) port)
(values
(sb-bsd-sockets:socket-make-stream
sock :input t :output t :element-type :default)
sock))
#+cmu
(let ((sock (ext:connect-to-inet-socket server port)))
(values
(sys:make-fd-stream sock :input t :output t :element-type 'base-char)
sock))
#+clisp
(let ((sock (ext:socket-connect port server)))
(values sock sock))
#+openmcl
(let ((sock (ccl:make-socket :remote-host server :remote-port port )))
(values sock sock))
)
(defun ipaddr-array-to-dotted (array)
(format nil "~{~D~^.~}" (coerce array 'list))
#+ignore
(format nil "~D.~D.~D.~D"
(aref 0 array) (aref 1 array) (aref 2 array) (array 3 array)))
(defun remote-host (socket)
#+allegro (socket:ipaddr-to-dotted (socket:remote-host socket))
#+lispworks (nth-value 0 (comm:get-socket-peer-address socket))
#+sbcl (ipaddr-array-to-dotted
(nth-value 0 (sb-bsd-sockets:socket-peername socket)))
#+cmu (nth-value 0 (ext:get-peer-host-and-port socket))
#+clisp (let* ((peer (ext:socket-stream-peer socket t))
(stop (position #\Space peer)))
;; 2.37-2.39 had do-not-resolve-p backwards
(if stop (subseq peer 0 stop) peer))
#+openmcl (ccl:remote-host socket)
) |
package com.gbdecastro.library.domain.author;
import com.gbdecastro.library.application.rest.controller.author.AuthorMapper;
import com.gbdecastro.library.domain.shared.DomainException;
import com.gbdecastro.library.domain.shared.annotation.BaseService;
import com.gbdecastro.library.domain.shared.message.MessageContext;
import com.gbdecastro.library.domain.shared.utils.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import javax.persistence.EntityNotFoundException;
import javax.transaction.Transactional;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
import static com.gbdecastro.library.domain.shared.utils.StringUtils.COMMA;
@BaseService
public class AuthorServiceImpl implements AuthorService {
@Autowired
private AuthorRepository repository;
@Autowired
private AuthorMapper mapper;
@Autowired
private MessageContext messageContext;
@Override
public Author create(Author author) {
this.validate(author);
return this.repository.save(author);
}
@Override
public List<Author> findAll() {
return this.repository.findAll();
}
@Override
public Set<Author> findAllOrCreate(Set<Author> authors) {
List<Author> entities = repository.findAllById(authors.stream().map(Author::getId).collect(Collectors.toSet()));
Set<Long> entityIds = entities.stream().map(Author::getId).collect(Collectors.toSet());
Set<Author> entitiesToCreate = authors.stream().filter(entity -> !entityIds.contains(entity.getId())).collect(Collectors.toSet());
if (!entitiesToCreate.isEmpty()) {
repository.saveAll(entitiesToCreate);
entities.addAll(entitiesToCreate);
}
return new HashSet<>(entities);
}
@Override
public Author findById(Long id) {
return repository.findById(id).orElseThrow(() -> new EntityNotFoundException(messageContext.getMessage("author_not_found")));
}
@Override
public Author update(Long id, Author toUpdate) {
Author author = findById(id);
author = mapper.update(author, toUpdate);
this.validate(author);
repository.saveAndFlush(author);
return author;
}
@Override
@Transactional
public void delete(Long id) {
Author author = findById(id);
if (!author.getBooks().isEmpty()) {
throw new DomainException(HttpStatus.BAD_REQUEST.value(), messageContext.getMessage("author_already_linked_a_book"));
}
repository.delete(author);
}
private void validate(Author author) {
List<String> errors = new ArrayList<>();
if (StringUtils.isEmpty(author.getName())) {
errors.add(messageContext.getMessage("name_required"));
}
if (StringUtils.isGreaterThan(author.getName(), 40)) {
errors.add(messageContext.getMessage("name_max_length"));
}
if (!errors.isEmpty()) {
throw new DomainException(HttpStatus.BAD_REQUEST.value(), String.join(COMMA, errors));
}
}
} |
package main;
import java.util.Random;
import itumulator.executable.DisplayInformation;
import itumulator.executable.DynamicDisplayInformationProvider;
import itumulator.simulator.Actor;
import itumulator.world.Location;
import itumulator.world.World;
/**
* Carcass is an object that represents a dead animal in the simulation world.
* It can be eaten by other animals and can spawn {@link Fungus}.
*
* @param mass - The mass of the carcass.
* @param myFungus - The fungus that is growing inside the carcass.
* @param hasFungus - True if the carcass has a fungus, false otherwise.
* @param shouldHaveFungus - If the carcass should have fungus.
* @implNote Implements {@link Actor} and {@link DynamicDisplayInformationProvider}
*/
public class Carcass extends Eatable implements Actor, DynamicDisplayInformationProvider {
private int mass;
private Fungus myFungus = null;
private boolean hasFungus;
private boolean shouldHaveFungus = false;
/**
* Constructs a Carcass object with the specified animal type and whether it should have a fungus.
* The mass of the carcass is determined based on the animal type and whether it is an adult or not.
* @param type the animal type of the carcass
* @param shouldHaveFungus true if the carcass should have a fungus, false otherwise
*/
public Carcass(Animal type, boolean shouldHaveFungus) {
this.shouldHaveFungus = shouldHaveFungus;
//
// Determine the mass of the carcass based on the animal type
//
if (type instanceof Rabbit) {
if (type.isAdult) {
mass = 5;
} else {
mass = 3;
}
}
if (type instanceof Wolf) {
if (type.isAdult) {
mass = 10;
} else {
mass = 5;
}
}
if (type instanceof Bear) {
if (type.isAdult) {
mass = 15;
} else {
mass = 8;
}
}
if (type instanceof Snake) {
if (type.isAdult) {
mass = 6;
} else {
mass = 4;
}
}
}
/**
* Performs the actions of the carcass in the simulation world.
* This includes decaying, spawning fungus, and handling the carcass's death.
* @param world the simulation world
*/
public void act(World world) {
if (shouldHaveFungus) {
addFungus(world.getLocation(this), world);
shouldHaveFungus = false;
}
// There's a 20% chance the carcass decays
if (new Random().nextInt(5) == 0) {
mass--;
}
// If no mass, decay totally
if (mass <= 0) {
Location deadLocation = world.getLocation(this);
world.delete(this);
if (myFungus != null) {
world.setTile(deadLocation, myFungus);
}
return;
}
// Chance for a fungus to spawn inside the carcass
if (!hasFungus && new Random().nextInt(5) == 0) {
addFungus(world.getLocation(this), world);
}
}
/**
* Checks if the carcass has a fungus growing inside it.
* @return true if the carcass has a fungus, false otherwise
*/
public boolean hasFungus() {
return hasFungus;
}
public int getMass() {
return mass;
}
/**
* Gets the amount of the carcass that is eaten by another animal.
* Updates the mass of the carcass accordingly.
* @param biteSize the size of the bite taken by the animal
* @param world the simulation world
* @return the actual amount of the carcass that is eaten
*/
public int getEaten(int biteSize, World world) {
int tempMass = mass;
mass -= biteSize;
if (tempMass - biteSize <= 0) {
return tempMass;
} else {
return biteSize;
}
}
/**
* Adds a fungus to the carcass at the specified location in the simulation world.
* @param location the location where the fungus should be added
* @param world the simulation world
*/
public void addFungus(Location location, World world) {
myFungus = new Fungus(this, location);
hasFungus = true;
world.add(myFungus);
}
public Fungus getFungus() {
return myFungus;
}
@Override
public DisplayInformation getInformation() {
if (mass <= 5) {
return new DisplayInformation(java.awt.Color.black, "carcass-small");
}
return new DisplayInformation(java.awt.Color.black, "carcass");
}
} |
import { Injectable } from '@nestjs/common';
import { User } from '@prisma/client';
import { UserInputError } from 'apollo-server-express';
import { CreateUserInputData } from '@/modules/users/dto/input/createUser.input';
import { UpdateUserInputData } from '@/modules/users/dto/input/updateUser.input';
import { UsersRepository } from '@/modules/users/users.repository';
@Injectable()
export class UsersService {
constructor(private readonly usersRepository: UsersRepository) {}
async getUserById(id: number): Promise<User> {
const user = await this.usersRepository.findUserById(id);
if (!user) {
throw new UserInputError(`User with id: ${id} does not exist`);
}
return user;
}
async createUser(values: CreateUserInputData): Promise<User> {
return this.usersRepository.createUser(values);
}
async updateUserById(id: number, values: UpdateUserInputData): Promise<User> {
return this.usersRepository.updateUserById(id, values);
}
} |
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { HttpResponse } from '@angular/common/http';
import { HttpClientTestingModule } from '@angular/common/http/testing';
import { FormBuilder } from '@angular/forms';
import { ActivatedRoute } from '@angular/router';
import { RouterTestingModule } from '@angular/router/testing';
import { of, Subject, from } from 'rxjs';
import { DesignationService } from '../service/designation.service';
import { IDesignation, Designation } from '../designation.model';
import { DesignationUpdateComponent } from './designation-update.component';
describe('Designation Management Update Component', () => {
let comp: DesignationUpdateComponent;
let fixture: ComponentFixture<DesignationUpdateComponent>;
let activatedRoute: ActivatedRoute;
let designationService: DesignationService;
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule, RouterTestingModule.withRoutes([])],
declarations: [DesignationUpdateComponent],
providers: [
FormBuilder,
{
provide: ActivatedRoute,
useValue: {
params: from([{}]),
},
},
],
})
.overrideTemplate(DesignationUpdateComponent, '')
.compileComponents();
fixture = TestBed.createComponent(DesignationUpdateComponent);
activatedRoute = TestBed.inject(ActivatedRoute);
designationService = TestBed.inject(DesignationService);
comp = fixture.componentInstance;
});
describe('ngOnInit', () => {
it('Should update editForm', () => {
const designation: IDesignation = { id: 'CBA' };
activatedRoute.data = of({ designation });
comp.ngOnInit();
expect(comp.editForm.value).toEqual(expect.objectContaining(designation));
});
});
describe('save', () => {
it('Should call update service on save for existing entity', () => {
// GIVEN
const saveSubject = new Subject<HttpResponse<Designation>>();
const designation = { id: 'ABC' };
jest.spyOn(designationService, 'update').mockReturnValue(saveSubject);
jest.spyOn(comp, 'previousState');
activatedRoute.data = of({ designation });
comp.ngOnInit();
// WHEN
comp.save();
expect(comp.isSaving).toEqual(true);
saveSubject.next(new HttpResponse({ body: designation }));
saveSubject.complete();
// THEN
expect(comp.previousState).toHaveBeenCalled();
expect(designationService.update).toHaveBeenCalledWith(designation);
expect(comp.isSaving).toEqual(false);
});
it('Should call create service on save for new entity', () => {
// GIVEN
const saveSubject = new Subject<HttpResponse<Designation>>();
const designation = new Designation();
jest.spyOn(designationService, 'create').mockReturnValue(saveSubject);
jest.spyOn(comp, 'previousState');
activatedRoute.data = of({ designation });
comp.ngOnInit();
// WHEN
comp.save();
expect(comp.isSaving).toEqual(true);
saveSubject.next(new HttpResponse({ body: designation }));
saveSubject.complete();
// THEN
expect(designationService.create).toHaveBeenCalledWith(designation);
expect(comp.isSaving).toEqual(false);
expect(comp.previousState).toHaveBeenCalled();
});
it('Should set isSaving to false on error', () => {
// GIVEN
const saveSubject = new Subject<HttpResponse<Designation>>();
const designation = { id: 'ABC' };
jest.spyOn(designationService, 'update').mockReturnValue(saveSubject);
jest.spyOn(comp, 'previousState');
activatedRoute.data = of({ designation });
comp.ngOnInit();
// WHEN
comp.save();
expect(comp.isSaving).toEqual(true);
saveSubject.error('This is an error!');
// THEN
expect(designationService.update).toHaveBeenCalledWith(designation);
expect(comp.isSaving).toEqual(false);
expect(comp.previousState).not.toHaveBeenCalled();
});
});
}); |
import React, { FC, HTMLAttributes } from 'react'
const Button: FC<IProps> = ({ children, size, inverted = false, ...rest }) => (
<button
style={{width: '76px', height: '76px'}}
className={`px-1 py-2 text-sm font-normal border rounded shadow-sm focus:none mb-3 flex flex-col justify-between items-center ${
inverted
? 'text-secondary bg-gray-300 border-secondary hover:border-2 hover:border-gray-500'
: 'text-secondary bg-white border-secondary hover:border-2 hover:border-gray-500'
}
${size === 'big' ? 'text-sm px-8' : ''}
`}
{...rest}
>
{children}
</button>
)
interface IProps extends HTMLAttributes<HTMLButtonElement> {
inverted?: boolean
size?: 'big'
}
export default Button |
import { ApolloDriver, ApolloDriverConfig } from "@nestjs/apollo"
import { Module } from "@nestjs/common"
import { ConfigModule } from "@nestjs/config"
import { GraphQLModule } from "@nestjs/graphql"
import { MongooseModule } from "@nestjs/mongoose"
import { ApolloServerPluginLandingPageLocalDefault } from "@apollo/server/plugin/landingPage/default"
import { ScheduleModule } from "@nestjs/schedule"
import * as redisStore from "cache-manager-redis-store"
import { CacheModule } from "@nestjs/cache-manager"
import { AnimeModule } from "../anime/anime.module"
import { AnimeCommentModule } from "../animeComment/animeComment.module"
import { FactModule } from "../fact/fact.module"
import { FranchiseModule } from "../franchise/franchise.module"
import { GenreModule } from "../genre/genre.module"
import { PlatformModule } from "../platform/platform.module"
import { StudioModule } from "../studio/studio.module"
import { UserModule } from "../user/user.module"
import { AuthModule } from "../auth/auth.module"
import { ParseAnimeCronModule } from "../parseAnimeCron/parseAnimeCron.module"
import { UploadModule } from "../upload/upload.module"
import { AppController } from "./controllers/app.controller"
@Module({
imports: [
ScheduleModule.forRoot(),
ConfigModule.forRoot({ isGlobal: true }),
MongooseModule.forRoot(process.env.MONGODB_URI),
GraphQLModule.forRoot<ApolloDriverConfig>({
driver: ApolloDriver,
autoSchemaFile: true,
playground: false,
plugins: [ApolloServerPluginLandingPageLocalDefault()],
sortSchema: true,
introspection: true,
context: ({ req }) => ({ req }),
apollo: {
graphRef: process.env.APOLLO_GRAPH_REF,
key: process.env.APOLLO_KEY,
},
}),
CacheModule.register({
isGlobal: true,
useFactory: () => ({ store: redisStore }),
url: process.env.REDIS_URL,
ttl: 7200000,
}),
FactModule,
AnimeModule,
FranchiseModule,
GenreModule,
PlatformModule,
StudioModule,
AnimeCommentModule,
UserModule,
AuthModule,
ParseAnimeCronModule,
UploadModule,
],
controllers: [AppController],
})
class AppModule {}
export { AppModule } |
import { GoogleLogin } from 'react-google-login';
import { useMutation } from '@apollo/client';
import { AUTH_MUTATION } from '../../mutations/authMutations';
import { GOOGLE_CLIENT_ID } from '../../config';
import { setLocalStorage } from '../../utils/localStorage';
function Auth({ setUser }) {
const [authGoogle, { loading, error }] = useMutation(AUTH_MUTATION);
const googleResponse = (response) => {
authGoogle({
variables: {
input: {
accessToken: response.accessToken,
},
},
}).then((res) => {
setLocalStorage('user', JSON.stringify(res.data.authGoogle));
setUser(res.data.authGoogle);
});
};
const onFailure = (error) => {
console.log(error);
};
if (loading) return 'Submitting...';
if (error) return `Submission error! ${error.message}`;
return (
<div className="App">
<GoogleLogin
clientId={GOOGLE_CLIENT_ID}
buttonText="Login to Social media"
onSuccess={googleResponse}
onFailure={onFailure}
/>
</div>
);
}
export default Auth; |
# Specification
This project provides an implementation using Java of a Recipe book program that can be used and added to by Users.
Users of the program have the following access and capabilities when using the recipe book.
* Manually adding a new *Dish* to the *Recipe Book* from within the program. For example, adding 'Pepperoni Pizza' to the *Recipe Book* during runtime.
* View *Dishes* inside the recipe book with search functionality
* Reading *Dish* information from a specifically formatted file and adding to the *Recipe Book*
* Searching for a *Dish* or Dishes in the *Recipe Book* by name, favourites, cook time, user preferences, certain attributes (Vegetarian, Non-Vegetarian, Vegan)
and whether it contains certain *Ingredient*(s) (eg: Searching for dishes that contain *Potato*)
* Create favourites and preferences for the *User* within the program which can then be used with search functionality to sort through recipes
* Serialize the recipe book via a database
Each *Dish* in the *Recipe Book* must store information such as name, *Ingredient*(s), cook time, attributes (Veg, Non-Veg, etc.) and
cooking instructions.
These can either be input manually during runtime by the user or uploaded through a file. The structure splits each dish by the @ symbol in the file.
Each *User* will be able to add dishes to the communal *Recipe Book* (If allowed access) and have their own implementation of *favourites* and *Preferences*
When a new *User* is created, the program will ask for and store *Preferences* but these can be edited at anytime in the program
These can be saved as well as the current status of the dishes and ingredients known to the recipe book.\
*IMPORTANT*: Since the program will be tested without our assistance, we have decided to allow the User to give themselves editorial access, since otherwise the TA would have to email us in order to gain access to the functionality
Commands:
* "end" terminates the program
* "create" creates a new user
* Entering a username that exist prompts a password entry
* UserConsole Commands:
* "favourites" lists out the names of the dish the user has added to their favourites
* "preferences" lists out the user's preferences
* "add to preferences" adds a preference to the user's preferences
* "remove from preferences" removes a preference from the user's preferences
* BookConsole Commands:
* Typing in a name of a dish or ingredient will show its description. If there's a dish and an ingredient sharing
* the same name, the dish is displayed. The user can use "view ingredient" to view the ingredient
* "search" starts a search function with difference search filters
* -n dishname : returns a list of dishes containing dishname in their names
* -p : returns a list of dishes matching the user's preferences
* -t time : returns a list of dishes with cook time less than or equal to time
* -i ing1/ing2/... : returns a list of dishes with ing1, ing2, ... as their ingredients
* -a att1/att2/... : returns a list of dishes with att1, att2, ... as their attributes
* EXAMPLE: search -n burger -t 15 -i lettuce/pickles -a vegan
* this will return a list of dishes that has "burger" in its name, cook time no more than 15 minutes,
* lettuce, pickles as ingredients, and the vegan attribute.
* "view dishes" lists out the dishes in the dish manager
* "view ingredients" lists out the ingredients in the ingredient manager
* "view ingredient" allows user to input the ingredient name to view attributes of the ingredient
* "favourites" lists out the favourite dishes of the user
* "add to favourites" adds a specified dish to the user's favourites list
* "remove from favourites" removes a specified dish from the user's favourites list
* "preferences" shows the dishes matching the users preferences
* "create dish" starts the dish creator
* "create ingredient" starts the ingredient creator |
/*
* Copyright 1991-1996, Silicon Graphics, Inc.
* ALL RIGHTS RESERVED
*
* UNPUBLISHED -- Rights reserved under the copyright laws of the United
* States. Use of a copyright notice is precautionary only and does not
* imply publication or disclosure.
*
* U.S. GOVERNMENT RESTRICTED RIGHTS LEGEND:
* Use, duplication or disclosure by the Government is subject to restrictions
* as set forth in FAR 52.227.19(c)(2) or subparagraph (c)(1)(ii) of the Rights
* in Technical Data and Computer Software clause at DFARS 252.227-7013 and/or
* in similar or successor clauses in the FAR, or the DOD or NASA FAR
* Supplement. Contractor/manufacturer is Silicon Graphics, Inc.,
* 2011 N. Shoreline Blvd. Mountain View, CA 94039-7311.
*
* THE CONTENT OF THIS WORK CONTAINS CONFIDENTIAL AND PROPRIETARY
* INFORMATION OF SILICON GRAPHICS, INC. ANY DUPLICATION, MODIFICATION,
* DISTRIBUTION, OR DISCLOSURE IN ANY FORM, IN WHOLE, OR IN PART, IS STRICTLY
* PROHIBITED WITHOUT THE PRIOR EXPRESS WRITTEN PERMISSION OF SILICON
* GRAPHICS, INC.
*/
// -*- C++ -*-
/*
*
_______________________________________________________________________
______________ S I L I C O N G R A P H I C S I N C . ____________
|
| $Revision: 2.2 $
|
| Description:
| This file defines the SoFaceDetail class.
|
| Author(s) : Thaddeus Beier, Dave Immel, Howard Look
|
______________ S I L I C O N G R A P H I C S I N C . ____________
_______________________________________________________________________
*/
#ifndef _SO_FACE_DETAIL_
#define _SO_FACE_DETAIL_
#include <Inventor/details/SoPointDetail.h>
//
// Class: SoFaceDetail
//
// Detail information about vertex-based shapes made of faces. It
// adds indices of various items that vary among faces and vertices.
//
class SoFaceDetail : public SoDetail {
SO_DETAIL_HEADER(SoFaceDetail);
public:
// Constructor and destructor.
SoFaceDetail();
virtual ~SoFaceDetail();
// Returns the number of points in the face
int32_t getNumPoints() const { return numPoints; }
// Returns the point detail for the indexed point of the face
const SoPointDetail * getPoint(int i) const { return &point[i]; }
// Returns the index of the face within a shape
int32_t getFaceIndex() const { return faceIndex; }
// Returns the index of the part within a shape
int32_t getPartIndex() const { return partIndex; }
// Returns an instance that is a copy of this instance. The caller
// is responsible for deleting the copy when done.
virtual SoDetail * copy() const;
SoEXTENDER public:
// Sets the number of points in the face and allocates room for the points
void setNumPoints(int32_t num);
// Copies a point detail from the given detail
void setPoint(int32_t index, const SoPointDetail *pd);
// Sets the face index and part index
void setFaceIndex(int32_t i) { faceIndex = i; }
void setPartIndex(int32_t i) { partIndex = i; }
// Return a pointer to the point details.
SoPointDetail * getPoints() { return &point[0]; }
#ifndef IV_STRICT
void setNumPoints(long num) // System long
{ setNumPoints ((int32_t) num); }
void setPoint(long index, const SoPointDetail *pd) // System long
{ setPoint ((int32_t) index, pd); }
void setFaceIndex(long i) // System long
{ setFaceIndex ((int32_t) i); }
void setPartIndex(long i) // System long
{ setPartIndex ((int32_t) i); }
#endif
SoINTERNAL public:
static void initClass();
private:
SoPointDetail *point;
int32_t numPoints;
int32_t faceIndex, partIndex;
};
#endif /* _SO_FACE_DETAIL_ */ |
package com.example.shopstop;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.AppCompatButton;
import android.app.AlertDialog;
import android.content.Intent;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import android.widget.Toolbar;
import com.example.shopstop.model.Product;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import com.razorpay.Checkout;
import com.razorpay.PaymentResultListener;
import org.jetbrains.annotations.NotNull;
import org.json.JSONException;
import org.json.JSONObject;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.HashMap;
public class PlaceOrderActivity extends AppCompatActivity implements PaymentResultListener {
EditText shipName, shipPhone, shipAddress, shipCity;
AppCompatButton confirmOrder;
FirebaseAuth auth;
Intent intent;
String totalAmount;
TextView cartpricetotal;
Toolbar cartToolbar;
int amount;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_place_order);
shipName=findViewById(R.id.shipName);
shipPhone=findViewById(R.id.shipPhone);
shipAddress=findViewById(R.id.shipAddress);
shipCity=findViewById(R.id.shipCity);
confirmOrder=findViewById(R.id.confirmOrder);
cartpricetotal=findViewById(R.id.cartpricetotal);
cartToolbar=findViewById(R.id.cart_toolbar);
auth=FirebaseAuth.getInstance();
cartToolbar.setBackgroundResource(R.drawable.bg_color);
confirmOrder.setBackgroundResource(R.drawable.bg_color);
intent=getIntent();
totalAmount = intent.getStringExtra("totalAmount");
cartpricetotal.setText(totalAmount);
String sAmount="100";
//convert and round off
amount=Math.round(Float.parseFloat(sAmount)*100);
confirmOrder.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
check();
}
});
}
private void check() {
if(TextUtils.isEmpty(shipName.getText().toString())){
shipName.setError("Enter name");
Toast.makeText(this, "Please enter your full name", Toast.LENGTH_SHORT).show();
}else if(TextUtils.isEmpty(shipPhone.getText().toString())){
shipPhone.setError("Enter phone no.");
Toast.makeText(this, "Please enter your phone no.", Toast.LENGTH_SHORT).show();
}else if(TextUtils.isEmpty(shipAddress.getText().toString())){
shipAddress.setError("Enter address");
Toast.makeText(this, "Please enter your address", Toast.LENGTH_SHORT).show();
}else if(TextUtils.isEmpty(shipCity.getText().toString())){
shipCity.setError("Enter phone no.");
Toast.makeText(this, "Please enter your city", Toast.LENGTH_SHORT).show();
}else{
paymentFunc();
}
}
private void paymentFunc(){
Checkout checkout= new Checkout();
//set key id
checkout.setKeyID("rzp_test_k2xvsKoKqhTaa0");
//set image
checkout.setImage(R.drawable.rzp_logo);
//initialize JSON object
JSONObject object= new JSONObject();
try {
//put name
object.put("name","Android User");
//put description
object.put("description","Test Payment");
//put currency unit
object.put("currency","INR");
//put amount
object.put("amount",amount);
//put mobile number
object.put("prefill.contact","9876543210");
//put email
object.put("prefill.email","androiduser@rzp.com");
//open razorpay checkout activity
checkout.open(PlaceOrderActivity.this,object);
} catch (JSONException e) {
e.printStackTrace();
}
}
private void confirmOrderFunc() {
final String saveCurrentDate, saveCurrentTime;
Calendar calendar=Calendar.getInstance();
SimpleDateFormat currentDate= new SimpleDateFormat("dd/MM/yyyy");
saveCurrentDate=currentDate.format(calendar.getTime());
SimpleDateFormat currentTime= new SimpleDateFormat("HH:mm:ss a");
saveCurrentTime=currentTime.format(calendar.getTime());
final DatabaseReference ordersRef= FirebaseDatabase.getInstance().getReference()
.child("Orders").child(auth.getCurrentUser().getUid()).child("History")
.child(saveCurrentDate.replaceAll("/","-")+" "+saveCurrentTime);
HashMap<String, Object> ordersMap= new HashMap<>();
ordersMap.put("totalAmount",totalAmount);
ordersMap.put("name",shipName.getText().toString());
ordersMap.put("phone",shipPhone.getText().toString());
ordersMap.put("address",shipAddress.getText().toString());
ordersMap.put("city",shipCity.getText().toString());
ordersMap.put("date",saveCurrentDate);
ordersMap.put("time",saveCurrentTime);
ordersRef.updateChildren(ordersMap).addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull @NotNull Task<Void> task) {
//empty user's cart after confirming order
if(task.isSuccessful()) {
FirebaseDatabase.getInstance().getReference().child("Cart List")
.child("User View").child(auth.getCurrentUser().getUid())
.removeValue()
.addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull @NotNull Task<Void> task) {
if(task.isSuccessful()){
Toast.makeText(PlaceOrderActivity.this, "Your order has been placed successfully", Toast.LENGTH_SHORT).show();
Intent intentcart= new Intent(PlaceOrderActivity.this, HomeActivity.class);
intentcart.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intentcart);
finish();
}
}
});
}
}
});
}
@Override
public void onPaymentSuccess(String s) {
confirmOrderFunc();
AlertDialog.Builder builder= new AlertDialog.Builder(this);
builder.setTitle("Payment ID");
builder.setMessage(s);
builder.show();
}
@Override
public void onPaymentError(int i, String s) {
Toast.makeText(this, s, Toast.LENGTH_SHORT).show();
}
} |
/*
* Copyright (C) 2018 evove.tech
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <https://www.gnu.org/licenses/>.
*/
package sourcerer
import com.google.common.collect.ImmutableList
import com.google.common.collect.ImmutableSet
import javax.annotation.processing.RoundEnvironment
import javax.lang.model.element.Element
import javax.lang.model.element.TypeElement
data
class ParentRound(
val number: Int = 0,
val prev: ParentRound? = null,
val elements: ImmutableSet<TypeElement> = ImmutableSet.of(),
val rootElements: ImmutableSet<Element> = ImmutableSet.of(),
val rounds: ImmutableList<Round> = ImmutableList.of(),
val parentOutputs: ImmutableList<Output> = ImmutableList.of()
) {
val outputs: ImmutableList<Output> by lazy {
rounds.flatMap(Round::outputs)
.toImmutableList()
}
val deferredElements: ImmutableSet<Element> by lazy {
rounds.flatMap(Round::deferredElements)
.toImmutableSet()
}
fun process(
elements: Set<TypeElement>,
roundEnv: RoundEnvironment,
steps: RoundSteps,
processFunc: () -> Boolean
): ParentRound {
val parentRound = ParentRound(
number + 1,
this,
elements.toImmutableSet(),
roundEnv.rootElements.toImmutableSet()
)
steps.preRound(parentRound)
processFunc()
val rounds = steps.postRound(roundEnv)
.toImmutableList()
return parentRound.copy(rounds = rounds)
}
} |
using System.Numerics;
using System.Runtime.CompilerServices;
namespace Common;
public enum Base4Direction2d : byte
{
U,
L,
D,
R
}
public enum Base8Direction2d : byte
{
U,
LU,
L,
LD,
D,
RD,
R,
RU
}
public enum Base6Direction3d : byte
{
L = 0,
R = 1,
U = 2,
D = 3,
F = 4,
B = 5
}
[Flags]
public enum Base6Direction3dMask : byte
{
None = 0,
L = 1 << 0,
R = 1 << 1,
U = 1 << 2,
D = 1 << 3,
F = 1 << 4,
B = 1 << 5,
}
public readonly struct Vector2d
{
public static readonly Vector2d Zero = new(0, 0);
public static readonly Vector2d One = new(1, 1);
public static readonly Vector2d UnitX = new(1, 0);
public static readonly Vector2d UnitY = new(0, 1);
public double X { get; }
public double Y { get; }
public Vector2d(double x, double y)
{
X = x;
Y = y;
}
public Vector2d(double value) : this(value, value)
{
}
// We may consider using functions here. Normalized() is already using it.
public double NormSqr => X * X + Y * Y;
public double Norm => Math.Sqrt(NormSqr);
public Vector2d Normalized() => this / Norm;
public override bool Equals(object? obj)
{
if (obj is not Vector2d other)
{
return false;
}
return Equals(other);
}
public bool Equals(Vector2d other) => X.Equals(other.X) && Y.Equals(other.Y);
public bool ApproxEq(Vector2d other, double eps = 1e-7) => X.ApproxEq(other.X, eps) && Y.ApproxEq(other.Y, eps);
public override int GetHashCode() => HashCode.Combine(X, Y);
public override string ToString() => $"X={X}, Y={Y}";
public Vector2ds Floor() => new((int)Math.Floor(X), (int)Math.Floor(Y));
public Vector2ds Round() => new((int)Math.Round(X), (int)Math.Round(Y));
public Vector2ds Ceiling() => new((int)Math.Ceiling(X), (int)Math.Ceiling(Y));
public static bool operator ==(Vector2d a, Vector2d b) => a.Equals(b);
public static bool operator !=(Vector2d a, Vector2d b) => !a.Equals(b);
public static Vector2d operator +(Vector2d v) => new(+v.X, +v.Y);
public static Vector2d operator -(Vector2d v) => new(-v.X, -v.Y);
public static Vector2d operator +(Vector2d a, Vector2d b) => new(a.X + b.X, a.Y + b.Y);
public static Vector2d operator -(Vector2d a, Vector2d b) => new(a.X - b.X, a.Y - b.Y);
public static Vector2d operator *(Vector2d a, Vector2d b) => new(a.X * b.X, a.Y * b.Y);
public static Vector2d operator /(Vector2d a, Vector2d b) => new(a.X / b.X, a.Y / b.Y);
public static Vector2d operator *(Vector2d v, double scalar) => new(v.X * scalar, v.Y * scalar);
public static Vector2d operator /(Vector2d v, double scalar) => new(v.X / scalar, v.Y / scalar);
public static implicit operator Vector2d(Vector2 v) => new(v.X, v.Y);
public static implicit operator Vector2(Vector2d v) => new((float)v.X, (float)v.Y);
}
public readonly struct Vector2ds : IComparable<Vector2ds>
{
public static readonly Vector2ds Zero = new(0, 0);
public static readonly Vector2ds UnitX = new(1, 0);
public static readonly Vector2ds UnitY = new(0, 1);
public static readonly Vector2ds One = new(1, 1);
public short X { get; init; }
public short Y { get; init; }
public int NormSqr => X * X + Y * Y;
public double Norm => Math.Sqrt(NormSqr);
public float NormF => MathF.Sqrt(NormSqr);
private static readonly Base4Direction2d[] Directions4 = Enum.GetValues<Base4Direction2d>();
private static readonly Base8Direction2d[] Directions8 = Enum.GetValues<Base8Direction2d>();
public Vector2ds(short x, short y)
{
X = x;
Y = y;
}
public Vector2ds(int x, int y)
{
X = (short)x;
Y = (short)y;
}
public override string ToString() => $"X={X}, Y={Y}";
public override bool Equals(object? obj) => obj is Vector2ds v && this.Equals(v);
public bool Equals(Vector2ds other) => X == other.X && Y == other.Y;
public override int GetHashCode() => HashCode.Combine(X, Y);
public Base4Direction2d Base4DirectionTo(Vector2ds b)
{
if (this == b)
{
throw new ArgumentException("Cannot get direction to same point", nameof(b));
}
var a = this;
return Directions4.MinBy(n => DistanceSqr(a + n, b));
}
public Base8Direction2d Base8DirectionTo(Vector2ds b)
{
if (this == b)
{
throw new ArgumentException("Cannot get direction to same point", nameof(b));
}
var a = this;
return Directions8.MinBy(n => DistanceSqr(a + n, b));
}
public static Vector2ds operator +(Vector2ds a, Vector2ds b) => new(a.X + b.X, a.Y + b.Y);
public static Vector2ds operator +(Vector2ds a, Base4Direction2d d) => a + d.Step();
public static Vector2ds operator +(Vector2ds a, Base8Direction2d d) => a + d.Step();
public static Vector2ds operator -(Vector2ds a, Vector2ds b) => new(a.X - b.X, a.Y - b.Y);
public static Vector2ds operator -(Vector2ds a, Base4Direction2d d) => a - d.Step();
public static Vector2ds operator -(Vector2ds a, Base8Direction2d d) => a - d.Step();
public static Vector2ds operator /(Vector2ds a, Vector2ds b) => new(a.X / b.X, a.Y / b.Y);
public static Vector2ds operator /(Vector2ds a, int s) => new(a.X / s, a.Y / s);
public static Vector2ds operator *(Vector2ds a, Vector2ds b) => new(a.X * b.X, a.Y * b.Y);
public static Vector2ds operator *(Vector2ds a, int s) => new(a.X * s, a.Y * s);
public static bool operator ==(Vector2ds a, Vector2ds b) => a.Equals(b);
public static bool operator !=(Vector2ds a, Vector2ds b) => !a.Equals(b);
public static implicit operator Vector2(Vector2ds v) => new(v.X, v.Y);
public static implicit operator Vector2d(Vector2ds v) => new(v.X, v.Y);
public static int DistanceSqr(Vector2ds a, Vector2ds b) => (a - b).NormSqr;
public static double Distance(Vector2ds a, Vector2ds b) => (a - b).Norm;
public static float DistanceF(Vector2ds a, Vector2ds b) => (a - b).NormF;
public static int Manhattan(Vector2ds a, Vector2ds b) => Math.Abs(a.X - b.X) + Math.Abs(a.Y - b.Y);
public int CompareTo(Vector2ds other) => this.NormSqr.CompareTo(other.NormSqr);
public static Vector2d BarycenterMany(IEnumerable<Vector2ds> points)
{
var x = 0d;
var y = 0d;
var i = 0;
foreach (var p in points)
{
x += (p.X - x) / (i + 1);
y += (p.Y - y) / (i + 1);
i++;
}
return new Vector2d(x, y);
}
public static Vector2ds Clamp(Vector2ds v, Vector2ds min, Vector2ds max) => new(
Math.Clamp(v.X, min.X, max.X),
Math.Clamp(v.Y, min.Y, max.Y)
);
public static Vector2ds Clamp(Vector2ds v, int min, int max) => new(
Math.Clamp(v.X, min, max),
Math.Clamp(v.Y, min, max)
);
}
public readonly struct Vector3di : IComparable<Vector3di>
{
public static readonly Vector3di Zero = new(0, 0, 0);
public static readonly Vector3di UnitX = new(1, 0, 0);
public static readonly Vector3di UnitY = new(0, 1, 0);
public static readonly Vector3di UnitZ = new(0, 0, 1);
public static readonly Vector3di One = new(1, 1, 1);
public int X { get; init; }
public int Y { get; init; }
public int Z { get; init; }
public int NormSqr => X * X + Y * Y + Z * Z;
public double Norm => Math.Sqrt(NormSqr);
public float NormF => MathF.Sqrt(NormSqr);
public Vector3di(int x, int y, int z)
{
X = x;
Y = y;
Z = z;
}
public Vector3di(int value)
{
X = value;
Y = value;
Z = value;
}
public override string ToString() => $"X={X}, Y={Y}, Z={Z}";
public override bool Equals(object? obj) => obj is Vector3di v && this.Equals(v);
public bool Equals(Vector3di other) => X == other.X && Y == other.Y && Z == other.Z;
public override int GetHashCode() => HashCode.Combine(X, Y, Z);
public int CompareTo(Vector3di other) => NormSqr.CompareTo(other.NormSqr);
public static Vector3di operator +(Vector3di a) => a;
public static Vector3di operator -(Vector3di a) => new(-a.X, -a.Y, -a.Z);
public static Vector3di operator ~(Vector3di a) => new(~a.X, ~a.Y, ~a.Z);
public static Vector3di operator +(Vector3di a, Vector3di b) => new(a.X + b.X, a.Y + b.Y, a.Z + b.Z);
public static Vector3di operator +(Vector3di a, int b) => new(a.X + b, a.Y + b, a.Z + b);
public static Vector3di operator -(Vector3di a, Vector3di b) => new(a.X - b.X, a.Y - b.Y, a.Z - b.Z);
public static Vector3di operator -(Vector3di a, int b) => new(a.X - b, a.Y - b, a.Z - b);
public static Vector3di operator *(Vector3di a, Vector3di b) => new(a.X * b.X, a.Y * b.Y, a.Z * b.Z);
public static Vector3di operator *(Vector3di a, int scalar) => new(a.X * scalar, a.Y * scalar, a.Z * scalar);
public static Vector3di operator /(Vector3di a, Vector3di b) => new(a.X / b.X, a.Y / b.Y, a.Z / b.Z);
public static Vector3di operator /(Vector3di a, int scalar) => new(a.X / scalar, a.Y / scalar, a.Z / scalar);
public static Vector3di operator <<(Vector3di a, int shl) => new(a.X << shl, a.Y << shl, a.Z << shl);
public static Vector3di operator >>(Vector3di a, int shr) => new(a.X >> shr, a.Y >> shr, a.Z >> shr);
public static Vector3di operator <<(Vector3di a, Vector3di b) => new(a.X << b.X, a.Y << b.Y, a.Z << b.Z);
public static Vector3di operator >>(Vector3di a, Vector3di b) => new(a.X >> b.X, a.Y >> b.Y, a.Z >> b.Z);
public static Vector3di operator &(Vector3di a, int b) => new(a.X & b, a.Y & b, a.Z & b);
public static Vector3di operator &(Vector3di a, Vector3di b) => new(a.X & b.X, a.Y & b.Y, a.Z & b.Z);
public static Vector3di operator |(Vector3di a, int b) => new(a.X | b, a.Y | b, a.Z | b);
public static Vector3di operator |(Vector3di a, Vector3di b) => new(a.X | b.X, a.Y | b.Y, a.Z | b.Z);
public static Vector3di operator ^(Vector3di a, int b) => new(a.X ^ b, a.Y ^ b, a.Z ^ b);
public static Vector3di operator ^(Vector3di a, Vector3di b) => new(a.X ^ b.X, a.Y ^ b.Y, a.Z ^ b.Z);
public static Vector3di operator %(Vector3di a, int b) => new(a.X % b, a.Y % b, a.Z % b);
public static Vector3di operator %(Vector3di a, Vector3di b) => new(a.X % b.X, a.Y % b.Y, a.Z % b.Z);
public static bool operator <(Vector3di a, int b) => a.X < b && a.Y < b && a.Z < b;
public static bool operator >(Vector3di a, int b) => a.X > b && a.Y > b && a.Z > b;
public static bool operator <=(Vector3di a, int b) => a.X <= b && a.Y <= b && a.Z <= b;
public static bool operator >=(Vector3di a, int b) => a.X >= b && a.Y >= b && a.Z >= b;
public static bool operator ==(Vector3di a, Vector3di b) => a.Equals(b);
public static bool operator !=(Vector3di a, Vector3di b) => !a.Equals(b);
public static implicit operator Vector3(Vector3di v) => new(v.X, v.Y, v.Z);
public static implicit operator Vector3d(Vector3di v) => new(v.X, v.Y, v.Z);
public static explicit operator Vector3di(Vector3 v) => new((int)v.X, (int)v.Y, (int)v.Z);
public static int DistanceSqr(Vector3di a, Vector3di b) => (a - b).NormSqr;
public static double Distance(Vector3di a, Vector3di b) => (a - b).Norm;
public static float DistanceF(Vector3di a, Vector3di b) => (a - b).NormF;
public static int Manhattan(Vector3di a, Vector3di b) => Math.Abs(a.X - b.X) + Math.Abs(a.Y - b.Y) + Math.Abs(a.Z - b.Z);
public static Vector3di Clamp(Vector3di v, Vector3di min, Vector3di max) => new(
Math.Clamp(v.X, min.X, max.X),
Math.Clamp(v.Y, min.Y, max.Y),
Math.Clamp(v.Z, min.Z, max.Z)
);
public static Vector3di Clamp(Vector3di v, int min, int max) => new(
Math.Clamp(v.X, min, max),
Math.Clamp(v.Y, min, max),
Math.Clamp(v.Z, min, max)
);
public static Vector3di Min(Vector3di a, Vector3di b) => new(
Math.Min(a.X, b.X),
Math.Min(a.Y, b.Y),
Math.Min(a.Z, b.Z)
);
public static Vector3di Max(Vector3di a, Vector3di b) => new(
Math.Max(a.X, b.X),
Math.Max(a.Y, b.Y),
Math.Max(a.Z, b.Z)
);
}
public readonly struct Vector3d
{
public static readonly Vector3d Zero = new(0, 0, 0);
public static readonly Vector3d One = new(1, 1, 1);
public static readonly Vector3d UnitX = new(1, 0, 0);
public static readonly Vector3d UnitY = new(0, 1, 0);
public static readonly Vector3d UnitZ = new(0, 0, 1);
public double X { get; }
public double Y { get; }
public double Z { get; }
public Vector3d(double x, double y, double z)
{
X = x;
Y = y;
Z = z;
}
public Vector3d(double value)
{
X = value;
Y = value;
Z = value;
}
public double NormSqr => Dot(this, this);
public double Norm => Math.Sqrt(NormSqr);
public Vector3d Normalized() => this / Norm;
public static double Dot(Vector3d a, Vector3d b) => a.X * b.X + a.Y * b.Y + a.Z * b.Z;
public static Vector3d Cross(Vector3d a, Vector3d b) => new(a.Y * b.Z - a.Z * b.Y, a.Z * b.X - a.X * b.Z, a.X * b.Y - a.Y * b.X);
public static double DistanceSqr(Vector3d a, Vector3d b) => (a - b).NormSqr;
public static double Distance(Vector3d a, Vector3d b) => (a - b).Norm;
public static Vector3d Lerp(Vector3d a, Vector3d b, double t) => new(Mathx.Lerp(a.X, b.X, t), Mathx.Lerp(a.Y, b.Y, t), Mathx.Lerp(a.Z, b.Z, t));
public static Vector3d operator +(Vector3d v) => v;
public static Vector3d operator -(Vector3d v) => new(-v.X, -v.Y, -v.Z);
public static Vector3d operator +(Vector3d a, Vector3d b) => new(a.X + b.X, a.Y + b.Y, a.Z + b.Z);
public static Vector3d operator -(Vector3d a, Vector3d b) => new(a.X - b.X, a.Y - b.Y, a.Z - b.Z);
public static Vector3d operator *(Vector3d a, Vector3d b) => new(a.X * b.X, a.Y * b.Y, a.Z * b.Z);
public static Vector3d operator /(Vector3d a, Vector3d b) => new(a.X / b.X, a.Y / b.Y, a.Z / b.Z);
public static Vector3d operator *(Vector3d a, double scalar) => new(a.X * scalar, a.Y * scalar, a.Z * scalar);
public static Vector3d operator /(Vector3d a, double scalar) => new(a.X / scalar, a.Y / scalar, a.Z / scalar);
public static implicit operator Vector3d(Vector3 v) => new(v.X, v.Y, v.Z);
public static implicit operator Vector3(Vector3d v) => new((float)v.X, (float)v.Y, (float)v.Z);
public static Vector3 Max(Vector3 a, Vector3 b) => new(
a.X > b.X ? a.X : b.X,
a.Y > b.Y ? a.Y : b.Y,
a.Z > b.Z ? a.Z : b.Z
);
public static Vector3d Min(Vector3d a, Vector3d b) => new(
a.X < b.X ? a.X : b.X,
a.Y < b.Y ? a.Y : b.Y,
a.Z < b.Z ? a.Z : b.Z
);
public static Vector3d Clamp(Vector3d value, Vector3d min, Vector3d max) =>
Min(Max(value, min), max);
}
public readonly struct BoundingBox3di
{
public Vector3di Min { get; init; }
public Vector3di Max { get; init; }
public BoundingBox3di(Vector3di min, Vector3di max)
{
Min = min;
Max = max;
}
public BoundingBox3di(int minX, int minY, int minZ, int maxX, int maxY, int maxZ)
{
Min = new Vector3di(minX, minY, minZ);
Max = new Vector3di(maxX, maxY, maxZ);
}
public bool IsValid => Min.X <= Max.X && Min.Y <= Max.Y && Min.Z <= Max.Z;
public int Volume => (Max.X - Min.X) * (Max.Y - Min.Y) * (Max.Z - Min.Z);
public Vector3d Center => new((Min.X + Max.X) / 2d, (Min.Y + Max.Y) / 2d, (Min.Z + Max.Z) / 2d);
public bool Contains(Vector3di point) =>
point.X >= Min.X && point.X <= Max.X &&
point.Y >= Min.Y && point.Y <= Max.Y &&
point.Z >= Min.Z && point.Z <= Max.Z;
public bool Contains(int x, int y, int z) =>
x >= Min.X && x <= Max.X &&
y >= Min.Y && y <= Max.Y &&
z >= Min.Z && z <= Max.Z;
public bool Contains(Vector3 point) =>
point.X >= Min.X && point.X <= Max.X &&
point.Y >= Min.Y && point.Y <= Max.Y &&
point.Z >= Min.Z && point.Z <= Max.Z;
public bool ContainsExclusive(Vector3di point) =>
point.X >= Min.X && point.X < Max.X &&
point.Y >= Min.Y && point.Y < Max.Y &&
point.Z >= Min.Z && point.Z < Max.Z;
public bool ContainsExclusive(int x, int y, int z) =>
x >= Min.X && x < Max.X &&
y >= Min.Y && y < Max.Y &&
z >= Min.Z && z < Max.Z;
public bool Intersects(BoundingBox3di box) =>
Max.X >= box.Min.X && Min.X <= box.Max.X &&
Max.Y >= box.Min.Y && Min.Y <= box.Max.Y &&
Max.Z >= box.Min.Z && Min.Z <= box.Max.Z;
public int DistanceToSqr(Vector3di point) => Contains(point) ? 0 : Vector3di.DistanceSqr(point, Vector3di.Clamp(point, Min, Max));
public float DistanceToSqr(Vector3 point) => Contains(point) ? 0 : Vector3.DistanceSquared(point, Vector3.Clamp(point, Min, Max));
public double DistanceTo(Vector3di point) => Math.Sqrt(DistanceToSqr(point));
public float DistanceFTo(Vector3di point) => MathF.Sqrt(DistanceToSqr(point));
public static BoundingBox3di Intersect(BoundingBox3di a, BoundingBox3di b) => new(
Math.Max(a.Min.X, b.Min.X),
Math.Max(a.Min.Y, b.Min.Y),
Math.Max(a.Min.Z, b.Min.Z),
Math.Min(a.Max.X, b.Max.X),
Math.Min(a.Max.Y, b.Max.Y),
Math.Min(a.Max.Z, b.Max.Z)
);
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="apple-touch-icon" sizes="180x180" href="/apple-touch-icon.png">
<link rel="icon" type="image/png" sizes="32x32" href="/favicon-32x32.png">
<link rel="icon" type="image/png" sizes="16x16" href="/favicon-16x16.png">
<link rel="manifest" href="/site.webmanifest">
<link rel="mask-icon" href="/safari-pinned-tab.svg" color="#5bbad5">
<meta name="msapplication-TileColor" content="#da532c">
<meta name="theme-color" content="#ffffff">
<meta property="og:title" content="Entries">
<!-- <meta property="og:description" content="Your website description goes here."> -->
<meta property="og:image" content="https://golf.contact/logo.png">
<meta property="og:url" content="https://golf.contact/">
<link rel="shortcut icon" href="logo.png" type="image/x-icon">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.1/dist/css/bootstrap.min.css" rel="stylesheet" />
<link href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.10.0/font/bootstrap-icons.css" rel="stylesheet">
<!-- ? root styles -->
<link rel="stylesheet" href="./styles/main.css">
<!-- Add Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.1/dist/css/bootstrap.min.css" rel="stylesheet" />
<link href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.10.0/font/bootstrap-icons.css" rel="stylesheet">
<title>Entries</title>
<script src="https://code.jquery.com/jquery-3.7.0.min.js"></script>
<style>
html,
body {
height: 100%;
margin: 0;
overflow: hidden;
/* Prevent scrolling */
}
/* The outer container */
.table-container {
height: 80%;
/* or a suitable value for your design */
overflow-x: auto;
overflow-y: auto;
/* Allow vertical scrolling if the table content exceeds the container height */
}
.table thead th:first-child {
position: sticky;
top: 0;
left: 0;
z-index: 10;
/* Highest z-index */
/* background-color: white; */
}
/* Adjusted z-index for the other headers */
.table thead th {
position: sticky;
top: 0;
z-index: 5;
/* Lesser z-index than the first column header */
/* background-color: white; */
}
/* Adjusted z-index for the sticky first column cells (non-header) */
.table td:first-child {
position: sticky;
left: 0;
z-index: 3;
/* Lesser z-index than the headers */
/* background-color: white; */
}
/* This ensures the table takes up its full width and doesn't wrap */
.table-responsive>.table {
margin-bottom: 0;
max-width: none;
}
/* Center text in the header */
.table thead th {
text-align: center;
white-space: nowrap;
/* Prevent text wrapping */
}
/* If you need to increase header height for aesthetic reasons, adjust the padding */
.table thead th {
padding: 1rem 0.5rem;
/* You can adjust this value to your preference */
}
/* Ensure that text in headers and cells doesn't wrap and is centered */
.table th,
.table td {
text-align: center;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
/* Use this to indicate that the text has been clipped if too long */
}
/* If you need to increase cell and header height for aesthetic reasons, adjust the padding */
.table th,
.table td {
padding: 1rem 0.5rem;
/* You can adjust this value to your preference */
}
#tablecard {
margin-bottom: 20px;
/* Adjusted margin to make sure it's not hidden behind bottom nav */
border-collapse: separate;
border-radius: 15px;
overflow: hidden;
background-color: #f9f9f9;
border: 2px solid #ccc;
background: linear-gradient(to bottom, #f9f9f9, #e5e5e5);
max-height: 75vh;
}
#tablecard th {
text-align: center;
background: linear-gradient(to top, #204870, #003e7e);
color: #fff;
border-right: 2px solid #ccc;
}
#tablecard th:last-child {
border-right: none;
}
#tablecard tbody tr:hover {
background-color: #f2f2f2;
}
#tablecard td,
#tablecard th {
padding: 15px;
}
</style>
</head>
<body id="entries">
<div style="height: 50px;"></div>
<script src="js/header-footer.js"></script>
<div class="card card-body bg-light rounded container mt-3" id="tablecard">
<div class="table-container">
<table class="table table-bordered table-striped table-responsive">
<thead id="table-head"></thead>
<tbody id="table-body"></tbody>
</table>
</div>
</div>
<script>
// Replace with your API key and spreadsheet ID
const apiKey = 'AIzaSyCTIOtXB0RDa5Y5gubbRn328WIrqHwemrc';
const spreadsheetId = '1_bP0NUG6XqrF0XQvKXNm3b07QuHABfvWotsemGToyYg';
// Function to fetch Google Sheets data
async function fetchGoogleSheetsData() {
try {
const response = await fetch(
`https://sheets.googleapis.com/v4/spreadsheets/${spreadsheetId}/values/Sheet1!A:K?key=${apiKey}`
);
const data = await response.json();
// Check if there's an error in the response
if (data.error) {
throw new Error(data.error.message);
}
displayTable(data);
} catch (error) {
console.error('Error fetching Google Sheets data:', error);
}
}
function displayTable(data) {
const {
values
} = data;
if (!values || values.length === 0) {
console.error("No data available.");
return;
}
// Extract headers
const headers = values[0];
let theadContent = '<tr>';
for (let header of headers) {
theadContent += `<th>${header}</th>`;
}
theadContent += '</tr>';
document.getElementById('table-head').innerHTML = theadContent;
// Extract rows
let tbodyContent = '';
for (let i = 1; i < values.length; i++) { // Starting from 1 because 0 is headers
tbodyContent += '<tr>';
for (let cell of values[i]) {
tbodyContent += `<td>${cell}</td>`;
}
tbodyContent += '</tr>';
}
document.getElementById('table-body').innerHTML = tbodyContent;
}
// Automatically fetch and display data when the page loads
window.onload = fetchGoogleSheetsData;
</script>
</body>
</html> |
from flask import Flask, request
import tkinter as tk
from tkinter import ttk
import threading
import params
app = Flask(__name__)
class BagStatus:
ON_BELT = "On belt"
OFF_BELT = "Off belt"
LOADED = "Loaded"
off_belt = []
closed = []
error_bag = None
# Create a Tkinter window
window = tk.Tk()
window.title("BagInfo v0.0.1")
screen_width = window.winfo_screenwidth()
screen_height = window.winfo_screenheight()
window.geometry(f"{screen_width // 2}x{screen_height // 4}+{screen_width // 2}+0")
COLUMNS = ("BagId", "Class", "Status", "ULD")
table = ttk.Treeview(window, columns=COLUMNS)
uld_closing = {"one": 0, "two": 0}
@app.after_request
def log(response):
print(response.get_data())
return response
@app.route("/scan", methods=["POST"])
def scan():
content = request.get_json()
cartid = content["cart"]
if cartid in closed:
return "ULD closed", 200
global uld_closing
uld_closing = {id: 0 if id != cartid else uld_closing[cartid] for id in uld_closing}
global error_bag
if not error_bag and not off_belt:
return "Bag not found", 400
bagId = error_bag or off_belt.pop()
row, values = get_row(bagId)
table.item(row, values=(values[0], values[1], BagStatus.LOADED, cartid))
if values[1] not in params.SEGREGATION[cartid]:
table.item(row, tags=("error"))
error_bag = bagId
return "INCORRECT CART", 200
table.item(row, tags=("success"))
error_bag = None
return "SUCCESS", 200
@app.route("/ULD", methods=["POST"])
def uld():
content = request.get_json()
cartid = content["cart"]
if cartid in closed:
return "ULD closed", 200
global uld_closing
uld_closing = {id: 0 if id != cartid else uld_closing[cartid] for id in uld_closing}
uld_closing[cartid] += 1
if uld_closing[cartid] < 2:
return f"ULD closing {uld_closing[cartid]}/2", 200
update_uld_status(cartid, "ULD closed")
closed.append(cartid)
return "ULD closed", 200
@app.route("/bag", methods=["POST"])
def bag():
content = request.get_json()
bagId = content["bagId"]
action = content["action"]
if action == "on-belt":
bag_type = content["bag_type"]
table.insert("", "end", values=(bagId, bag_type, BagStatus.ON_BELT, ""))
return "OK", 200
elif action == "off-belt":
global error_bag
error_bag = None
row, values = get_row(bagId)
if row is None:
return "Bag not found", 400
table.item(row, values=(values[0], values[1], BagStatus.OFF_BELT, values[3]))
off_belt.append(bagId)
return "OK", 200
return "Invalid action", 400
def get_row(bagId=None, uld=None):
for row in table.get_children():
values = table.item(row, "values")
if bagId is not None and int(values[0]) != bagId:
continue
if uld is not None and values[3] != uld:
continue
return row, values
return None, None
def update_uld_status(uld, status):
for row in table.get_children():
values = table.item(row, "values")
if values[3] == uld:
table.item(row, values=(values[0], values[1], status, uld))
def run_server():
app.run(host="0.0.0.0", port=params.SERVER_PORT, threaded=True)
server_thread = threading.Thread(target=run_server)
server_thread.start()
for i, col in enumerate(COLUMNS):
table.heading("#" + str(i + 1), text=col)
table.column("#" + str(i + 1), anchor="center")
table.tag_configure("error", background="orange")
table.tag_configure("success", background="green")
# table.insert("", "end", values=(1, "ECO", BagStatus.OFF_BELT, ""))
# off_belt.append(1)
def reset():
table.delete(*table.get_children())
off_belt.clear()
closed.clear()
global error_bag
error_bag = None
global uld_closing
uld_closing = {id: 0 for id in uld_closing}
reset_button = tk.Button(window, text="Reset", command=reset)
table.pack()
reset_button.pack()
window.mainloop()
server_thread.join() |
from fastapi import FastAPI, File, UploadFile,Form
from fastapi.responses import JSONResponse
import whisper
from fastapi.middleware.cors import CORSMiddleware
import tempfile
from transformers import AutoTokenizer, AutoModelWithLMHead
import torch
import nltk
nltk.download('punkt')
from nltk.tokenize import sent_tokenize
app = FastAPI()
app.add_middleware(
CORSMiddleware,
allow_origins=["*"], # Allows all origins
allow_credentials=True,
allow_methods=["*"], # Allows all methods
allow_headers=["*"], # Allows all headers
)
# Function to transcribe audio using Whisper
async def transcribe_audio(audio_path):
model = whisper.load_model("large") # Adjust model size as needed
result = model.transcribe(audio_path,fp16=False)
return result["text"]
@app.post("/transcribe/")
async def transcribe_audio_file(file: UploadFile = File(...)):
try:
# Save uploaded audio to a temporary file
with tempfile.NamedTemporaryFile(delete=False, suffix=".mp3") as tmp_audio:
tmp_audio.write(await file.read())
audio_path = tmp_audio.name
# Transcribe audio to text
text = await transcribe_audio(audio_path)
return JSONResponse(status_code=200, content={"transcription": text})
except Exception as e:
return JSONResponse(status_code=500, content={"message": str(e)})
@app.post("/summarize/")
async def summarzie_audio_file(text: str = Form(...)):
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
m_name = "marefa-nlp/summarization-arabic-english-news"
tokenizer = AutoTokenizer.from_pretrained(m_name)
model = AutoModelWithLMHead.from_pretrained(m_name).to(device)
def get_summary(text, tokenizer, model, device, num_beams=8, max_length=2048, length_penalty=5.0):
if len(text.strip()) < 50:
return ["Please provide a longer text."]
text = "summarize in detail: <paragraph> " + " <paragraph> ".join([s.strip() for s in sent_tokenize(text) if s.strip() != ""]) + " </s>"
text = text.strip().replace("\n","")
tokenized_text = tokenizer.encode(text, return_tensors="pt").to(device)
summary_ids = model.generate(
tokenized_text,
max_length=max_length,
num_beams=num_beams,
repetition_penalty=1.5,
length_penalty=length_penalty,
early_stopping=True
)
output = tokenizer.decode(summary_ids[0], skip_special_tokens=True)
return [s.strip() for s in output.split("<hl>") if s.strip() != ""]
hls = get_summary(text, tokenizer, model, device)
summary_text = " ".join(hls)
return JSONResponse(status_code=200, content={"summary": summary_text}) |
---
title: Analyse des accidents de la route pendant l'année 2021 en fonction du lieu,
de la période, de l'age et du sexe
author: "Groupe FLAL"
output:
pdf_document:
toc: yes
toc_depth: 2
html_document:
toc: yes
toc_depth: 2
---
Sources de nos jeux de données : https://www.data.gouv.fr/fr/datasets/bases-de-donnees-annuelles-des-accidents-corporels-de-la-circulation-routiere-annees-de-2005-a-2021/
##### Alex Delagrange, Léo Bouvier, Lucas Giry, Farah Seifeddine
```{r setup, include=FALSE}
library(xfun)
library(dplyr)
library(readxl)
library(tidyverse)
library(ggplot2)
library(leaflet)
library(maps)
library(sf)
library(lubridate)
```
\newpage !!!!! A faire !!!!! :
\newline - Télécharger le dossier "data" dans le même répertoire que les fichiers FLAL_stats.rmd et data.rmd
\newline - Run le fichier data.rmd
\newline - Knit le fichier FLAL_stats.rmd en PDF
Contexte : En France en 2021, le début d'année est accompagné d'un confinement, il est interéssant de voir quels sont les chiffres à propos des accidents mais aussi, pour une étude plus globale de prévention, savoir quels sont les facteurs qui influencent la gravité des accidents.
Ainsi, pour progresser de manière structurée dans notre étude, nous nous sommes posés la question suivante :
Quels sont les facteurs importants impactant la gravité des accidents en France en 2021 ?
Après avoir établi les différents facteurs nous avons effectué plusieurs croisements pour expliquer la gravité : Les usagers (age et sexe), les vehicules (catégorie), le lieu (régions et départements), l'environnement (équipement de sécurité et état de la route)
### Quelques éléments nécessaires à la compréhension :
Dans notre étude, un accident corporel est défini comme un accident survenu sur une voie ouverte à la circulation publique, impliquant au moins un véhicule et ayant fait au moins une victime ayant nécessité des soins. Cette définition prend en compte tous les accidents qui ont des conséquences physiques pour les personnes impliquées, qu'il s'agisse de blessures mineures ou de décès, ce qui nous permettra d'analyser les facteurs de risque des accidents de la route.
Nous étudions les accidents de la route en France en 2021 et un individu de notre jeu de données est une personne touchée par un accidents.
## Nettoyage et représentation du jeu de données
En ce qui concerne la représentation des données nous avons choisi de représenter toutes les données dans une grande dataframe dont on récupérera les colonnes necessaires pour les différents facteurs.
Pour nettoyer notre jeu de données, nous avons d'abord examiné les valeurs manquantes, les doublons et les valeurs aberrantes. Par la suite nous avons enlevé les lignes de la dataframe sans données dans lesquelles les colonnes étaient remplies de -1. Nous avons aussi créer une nouvelle variable "region" en fonction de la valeur de la variable "dep", qui correspond à un code départemental. Après ce processus, nous avons constaté qu'environ 0,04 % des lignes ont été éliminées en raison de valeurs manquantes ou de doublons.
En plus, 50 % des colonnes ont été éliminées car elles ne contenaient pas des informations pertinentes ou utiles pour notre analyse. Par exemple, la variable occutc qui indique le nombre d’occupants dans le transport en commun s'est avérée inutile, ainsi que le type de moteur du véhicule. Pour finir, il fallait enlever les caractères spéciaux des régions.
Ce nettoyage nous a permis d'avoir un jeu de données plus cohérent, propre et exploitable pour notre analyse ultérieure dont voici un aperçu :
```{r echo=FALSE}
df <- read_delim("data/data.csv", delim = ";",
escape_double = FALSE, col_types = cols(Num_Acc = col_character(),
id_vehicule = col_character(), hrmn = col_time(format = "%H:%M"),
lat = col_character()), trim_ws = TRUE)
# ajustement sur les données
# long et lat as numeric
df$lat <- sub(",", ".", df$lat)
df$long <- sub(",", ".", df$long)
df$lat <- as.numeric(df$lat)
df$long <- as.numeric(df$long)
# magouille pour éliminer les caractères spéciaux de région
# df$region <- iconv(df$region, from = "ISO-8859-1", to = "UTF-8")
# df$region <- sub("�î", "î", df$region)
# df$region <- sub("Ã*", "î", df$region)
# charcter bizarre dans id_vehicule
df$id_vehicule <- iconv(df$id_vehicule, from = "ISO-8859-1", to = "UTF-8")
df$id_vehicule <- as.character(df$id_vehicule)
head(df)
```
## Etude globale sur les accidents en 2021 en France
On regarde d'abord juste une étude globale sur le nombre d'accidents en France en fonction du mois de l'année.
```{r pressure, echo=FALSE, warning=FALSE}
# accidents par mois en 2021
plot_acc <- df %>% group_by(mois) %>% summarize("count" = n())
# mois nom
noms_mois <- c("janv", "févr", "mars", "avr", "mai", "juin", "juil", "août", "sept", "oct", "nov", "déc")
mois_ordre <- factor(noms_mois, levels = noms_mois)
# fonction pour associer le numéro de mois à son nom abrégé
nom_mois <- function(num_mois) {
return(noms_mois[num_mois])
}
plot_acc$nom_mois <- factor(sapply(plot_acc$mois, nom_mois), levels = mois_ordre)
# plot
ggplot(data = plot_acc, aes(x=nom_mois, y=count)) +
geom_col(stat="identity", fill="darkred") +
ylab("nombre d'accidents") +
xlab("") +
ggtitle("Nombre d'accidents par mois en 2021")
rm(plot_acc, noms_mois)
```
Ici on voit un gros pique en juin qui pourrait s'expliquer par la fin du confinement de 2021 ce qui a poussé les gens à sortir et donc à plus prendre la voiture ce qui implique forcément il y plus d'accidents.
On peut aussi regarder le nombre d'accidents en fonction de la période de la journée : nuit, matin, après-midi ou soir
```{r echo=FALSE, warning=FALSE}
# créer la colonne "heure"
# df$heure <- hms(sprintf("%02d:%02d:00", df$hrmn %/% 100, df$hrmn %% 100))
# Créer une colonne "heure_de_la_journee" contenant la tranche horaire de l'accident
df$heure_de_la_journee <- cut(as.integer(substr(df$hrmn, 1, 2)), breaks = c(-Inf, 6, 12, 18, Inf), labels = c("Nuit", "Matin", "Après-midi", "Soir"))
# Compter le nombre d'accidents par heure de la journée
accidents_par_heure <- df %>%
group_by(heure_de_la_journee) %>%
summarise(nombre_accidents = n()) %>%
arrange(heure_de_la_journee)
# Visualiser le nombre d'accidents par heure de la journée
ggplot(accidents_par_heure, aes(x = heure_de_la_journee, y = nombre_accidents)) +
geom_bar(stat = "identity", fill = "darkred") +
xlab("") +
ylab("Nombre d'accidents") +
ggtitle("Nombre d'accidents par période de la journée en 2021")
```
On s'attend à voir ce graphique comme résultat, avec plus d'accidents en après-midi et le matin puisque c'est dans ces moments là de la journée que l'activité française est au plus haut avec les départs au travail et les enfants à amener à l'école.
## Est ce que les usagers influencent la gravité des accidents ?
### Commençons par étudier si l'âge du conducteur est en corrélation avec la gravité de l'accident
```{r echo=FALSE, warning=FALSE}
# Sous-ensemble de données contenant la gravité, l'âge et le nombre d'accidents pour chaque âge
sub_df <- df %>%
filter(place == 1, catu == 1, age > 13) %>%
group_by(grav, age) %>%
summarise(nombre_accidents = n()) %>%
filter(!is.na(age))
sub_df$grav <- factor(sub_df$grav, levels = 1:4,
labels = c("Indemne", "Mort", "Blessé hospitalisé", "Blessé léger"))
# Créer un boxplot avec le nombre d'accidents sur l'axe x et l'âge sur l'axe y, en facet_wrap selon la gravité
ggplot(sub_df, aes(x = nombre_accidents, y = age)) +
geom_boxplot() +
ylab("Âge du conducteur") +
xlab("Nombre d'accidents") +
coord_flip() +
ggtitle("Boxplot sur l'âge des conducteurs/conductrices en fonction de la gravité") +
facet_wrap(~grav, scales = "free")
# Afficher les statistiques du boxplot pour chaque niveau de gravité
print(by(sub_df$age, sub_df$grav, boxplot.stats))
```
- Le nombre d'observations varie de 83 à 86.
- La médiane de l'âge est d'environ 35 ans pour tous les groupes de gravité.
- Le groupe "Indemne" a la valeur minimale la plus élevée et le groupe "Blessé léger" a la valeur maximale la plus élevée. - Cela indique que les conducteurs indemnes ont tendance à être plus âgés que les autres groupes de gravité, tandis que les conducteurs blessés légèrement ont tendance à être plus jeunes.
- Il n'y a pas de valeurs aberrantes pour chaque groupe de gravité.
Voici ce que nous pouvons observer sur l'ensemble du jeu de données :
```{r echo=FALSE, warning=FALSE}
# Sous-ensemble de données contenant l'âge et le nombre d'accidents pour chaque âge
sub_df <- df %>% filter(place == 1, catu == 1, age > 13) %>%
group_by(age) %>%
summarise(nombre_accidents = n()) %>%
filter(!is.na(age))
# Créer un boxplot avec le nombre d'accidents sur l'axe x et l'âge sur l'axe y
ggplot(sub_df, aes(x = nombre_accidents, y = age)) +
geom_boxplot() +
ylab("Âge du conducteur") +
xlab("Nombre d'accidents") +
coord_flip() + ggtitle("Boxplot sur l'âge des conducteurs/conductrices")
# Afficher les statistiques du boxplot
print(boxplot.stats(sub_df$age))
```
Sur 126086 accidents de la route en 2021, 50 % des conducteurs ont moins de 57 ans. 50 % d'entre eux ont entre 35.5 et 78.5 ans.
Dans ce cas, l'intervalle de confiance est [49.7,64.3], ce qui signifie que l'on peut être raisonnablement sûr que la moyenne de l'âge dans la population dont l'échantillon a été prélevé se trouve dans cette plage avec une probabilité de 95%.
Pour un autre point de vue sur le nombre d'accidents par age (conducteur ou non conducteur) :
```{r echo=FALSE, message=FALSE, warning=FALSE}
## remettre dans l'ordre les chiffres de grav
# accidents par année de naissance en 2021
plot_usagers <- df %>% filter(catu == 1, age > 13) %>% group_by(age, grav) %>% summarize("count" = n()) %>% filter(grav == 2)
ggplot(data = plot_usagers, aes(x=age, y=count, fill=grav)) +
geom_bar(stat="identity", fill = "#339966") + geom_smooth(se=FALSE, color = "orange") + ggtitle("Effectif des accidents mortels par âge du conducteurs")+ theme(legend.position='none') + xlab("Âge du conducteur") + ylab("Effectif")
plot_usagers <- df %>% group_by(age, grav) %>% summarize("count" = n()) %>% filter(grav == 2)
ggplot(data = plot_usagers, aes(x=age, y=count, fill=grav)) +
geom_bar(stat="identity", fill = "#339966") + geom_smooth(se=FALSE, color = "orange") + ggtitle("Effectif des accidents mortels par âge des personnes touchées")+ theme(legend.position='none') + xlab("Âge") + ylab("Effectif")
rm(plot_usagers, plot_sexe)
```
On constate la même croissance du nombre d'accidents mortels (conducteur ou non) de 0 à 23 ans, les personnes touchées sont plus nombreuses, ce qui est normal il y a les passagers ajoutés. La tendance décroît fortement de 23 à 40 ans avant de rester plus ou moins au même niveau jusqu'à environ 70 ans.
Pour les conducteurs, on constate une décroissance nette plus tôt que chez les personnes touchées, une hypothèse serait que plus l'âge augmente, moins il y a de conducteurs.
Le fort pique autour de 20 ans pourrait s'expliquer par l'âge de l'obtention du permis de conduire qui se traduit par un manque d'expérience en tant que conducteur. Cela pourrait aussi s'expliquer, dans le cas des personnes touchées, par le fait que pas 100% des jeunes d'environ 20 ans ont une voiture, donc une personne de 20 ans avec une voiture va plus avoir tendance à amener avec lui ses amis ainsi un accident d'une voiture d'une personne de 20 ans compte possiblement 2, 3 voire 4 personnes en plus.
De ces données nous pouvons en déduire le graphique de densité suivant :
```{r echo=FALSE, warning=FALSE}
# Sous-ensemble de données contenant la gravité de l'accident et l'âge du conducteur
sub_df <- df[, c("grav", "age")]
# Supprimer les lignes où l'âge est manquant
sub_df <- na.omit(sub_df)
# Créer un graphique de densité pour la gravité de l'accident par tranche d'âge
ggplot(sub_df, aes(x = age, fill = factor(grav))) +
geom_density(alpha = 0.5) +
xlab("Âge") +
ylab("Densité") +
scale_fill_discrete(name = "Gravité de l'accident",
labels = c("Indemne", "Blessé léger", "Blessé hospitalisé", "Mort")) + ggtitle("Densité des accidents par âge des personnes touchées")
```
De manière générale, le graphique de densité montre comment les distributions de l'âge des conducteurs diffèrent selon la gravité de l'accident. On remarque un fort pic de densité autour de 20 ans ce qui laisse penser à une forte corrélation entre l'age et la gravité de l'accident.
Pour déterminer si il y a une corrélation ou non entre l'âge et la gravité de l'accident, nous avons établi une régression logistique que voici :
```{r echo=FALSE, warning=FALSE}
## concept de regression logistique : il s'agit d'expliquer au mieux une variable binaire (la présence ou l'absence d'une caractéristique donnée) par des observations réelles nombreuses
# Créer une variable binaire pour la gravité de l'accident (1 si l'accident est grave, 0 sinon)
sub_df$grav_bin <- ifelse(sub_df$grav > 1, 1, 0)
# Supprimer les lignes où l'âge est manquant
sub_df <- na.omit(sub_df)
# Effectuer la régression logistique
model <- glm(grav_bin ~ age, data = sub_df, family = binomial())
# Résumé de la régression logistique
summary(model)
##############################################################################################
######################### Notes sur les significations des résultats #########################
##############################################################################################
# Call : indique la formule utilisée pour ajuster le modèle, les options et les données.
#
# Deviance Residuals : affiche les résidus de deviance pour chaque observation. La deviance est un critère qui mesure l'ajustement du modèle aux données. Les résidus de deviance indiquent si le modèle prédit correctement la gravité de l'accident.
#
# Coefficients : fournit les coefficients de régression estimés pour chaque variable d'entrée. Dans ce cas, il y a une seule variable d'entrée, l'âge. Pour chaque année d'augmentation de l'âge, la probabilité de subir un accident mortel diminue de 0,6 % (coefficient négatif). Le coefficient de l'interception indique la probabilité de subir un accident mortel pour une personne de 0 an (en pratique cela n'a pas de sens, il s'agit d'une propriété mathématique du modèle).
#
# Signif. codes : indique le niveau de signification de chaque coefficient estimé. Les étoiles servent à représenter différents niveaux de significativité : *** pour un niveau très significatif, ** pour un niveau significatif, * pour un niveau de signification modéré, . pour un niveau de signification faible.
#
# Dispersion parameter : fournit l'estimation de la variance de la distribution des résidus.
#
# Null deviance : indique la deviance du modèle nul, c'est-à-dire un modèle qui ne contient aucune variable explicative.
#
# Residual deviance : indique la deviance résiduelle du modèle, c'est-à-dire la deviance restante après avoir ajusté le modèle aux données.
#
# AIC : fournit le critère d'information d'Akaike, un critère d'ajustement qui tient compte de la complexité du modèle et de l'ajustement aux données.
#
# Number of Fisher Scoring iterations : indique le nombre d'itérations nécessaires pour estimer les paramètres du modèle.
```
Les résultats de la régression logistique indiquent que l'âge est significativement associé à la gravité des accidents. Plus précisément, pour une unité d'augmentation de l'âge, la log-odds d'avoir une gravité plus élevée diminue de 0,00667. Cela peut être interprété comme une diminution de la probabilité d'avoir une gravité plus élevée pour chaque année supplémentaire.
Le rapport des deviances (null deviance et residual deviance) montre que le modèle ajuste bien les données, car il y a une réduction significative de la deviance résiduelle par rapport à la deviance nulle. En outre, l'AIC est relativement faible, ce qui indique que le modèle est un ajustement approprié pour les données.
Le test de significativité indique que la relation entre l'âge et la gravité de l'accident est très significative (p-value < 2e-16), ce qui renforce l'idée que l'âge est un facteur important à prendre en compte dans l'évaluation de la gravité des accidents de la route.
En résumé, les résultats de la régression logistique soutiennent l'idée que l'âge est significativement associé à la gravité des accidents de la route et que le modèle est un ajustement approprié pour les données.
### Est-ce que le sexe influence la gravité des accidents ?
Ici, à l'aide de représentations graphique et de test du Chi2 nous allons observer si le sexe et la gravité ont une corrélation ou non en distinguant les personnes touchées et les conducteurs/trices.
```{r echo=FALSE, warning=FALSE}
sub_df <- df[, c("grav", "sexe")]
# enleve les na
sub_df <- na.omit(sub_df) %>% filter(sexe != -1)
tab <- table(sub_df$sexe, sub_df$grav)
# Conversion de la table croisée en un data frame
df_s <- as.data.frame.matrix(tab)
df_s$sexe <- rownames(df_s)
# Mise en forme des données pour un diagramme en barres empilées
df_long <- tidyr::gather(df_s, key = "grav", value = "count", -sexe)
df_long$grav <- factor(df_long$grav, levels = c("1", "2", "3", "4"))
df_long$sexe <- factor(df_long$sexe, levels = c("1", "2"))
# Convertir la variable grav en factor avec les niveaux correspondants
df_long$grav <- factor(df_long$grav, levels = 1:4,
labels = c("Indemne", "Mort", "Blessé hospitalisé", "Blessé léger"))
df_long$sexe <- factor(df_long$sexe, levels = 1:2,
labels = c("Homme", "Femme"))
# Définir les couleurs personnalisées
colors <- c("Homme" = "pink",
"Femme" = "lightblue")
# Réorganiser les niveaux de la variable "grav" en fonction de la fréquence
df_long$grav <- reorder(df_long$grav, desc(df_long$count))
# Créer le graphique
ggplot(df_long, aes(x = grav, fill = sexe)) +
geom_col(position = "dodge", aes(y = count)) +
scale_fill_manual(values = colors, name = "Sexe",
labels = c("Homme", "Femme")) +
labs(title = "Gravité des accidents par sexe", x = "", y = "Nombre d'accidents") + theme_gray()
```
```{r echo=FALSE, warning=FALSE}
d <- df %>% filter(age > 13, catu == 1)
sub_df <- d[, c("grav", "sexe")]
# enleve les na
sub_df <- na.omit(sub_df) %>% filter(sexe != -1)
tab <- table(sub_df$sexe, sub_df$grav)
# Conversion de la table croisée en un data frame
df_s <- as.data.frame.matrix(tab)
df_s$sexe <- rownames(df_s)
# Mise en forme des données pour un diagramme en barres empilées
df_long <- tidyr::gather(df_s, key = "grav", value = "count", -sexe)
df_long$grav <- factor(df_long$grav, levels = c("1", "2", "3", "4"))
df_long$sexe <- factor(df_long$sexe, levels = c("1", "2"))
# Convertir la variable grav en factor avec les niveaux correspondants
df_long$grav <- factor(df_long$grav, levels = 1:4,
labels = c("Indemne", "Mort", "Blessé hospitalisé", "Blessé léger"))
df_long$sexe <- factor(df_long$sexe, levels = 1:2,
labels = c("Homme", "Femme"))
# Définir les couleurs personnalisées
colors <- c("Homme" = "pink",
"Femme" = "lightblue")
# Réorganiser les niveaux de la variable "grav" en fonction de la fréquence
df_long$grav <- reorder(df_long$grav, desc(df_long$count))
# Créer le graphique
ggplot(df_long, aes(x = grav, fill = sexe)) +
geom_col(position = "dodge", aes(y = count)) +
scale_fill_manual(values = colors, name = "Sexe",
labels = c("Homme", "Femme")) +
labs(title = "Gravité des accidents par sexe du conducteur", x = "", y = "Nombre d'accidents") + theme_gray()
```
On remarque que, conducteur.trice ou non, la tendance reste la même au niveau de la gravité. La quantité d'accidents reste plus importante chez les hommes que chez les femmes. Cependant, nous n'avons pas la quantité totale de conducteur.trice en France donc on ne peut pas avoir une proportion du nombre d'accidents.
```{r echo=FALSE, warning=FALSE}
#test du X²
df_conducteurs <- df %>% filter(catu == 1, age > 13)
chisq.test(table(df_conducteurs$sexe, df_conducteurs$grav))
```
Puisque la p-value est inférieure au seuil de significativité communément utilisé de 0,05, on peut rejeter l'hypothèse nulle selon laquelle il n'y a pas d'association entre le sexe et la gravité des accidents de la route. On peut donc conclure qu'il y a une association significative entre le sexe et la gravité des accidents de la route en France en 2021.
Cela signifie que le sexe des conducteurs est statistiquement significatif pour prédire la gravité des accidents de la route en France en 2021. Cependant, il est important de noter que les résultats de ce test ne permettent pas de déterminer la direction de l'association (c'est-à-dire, si les accidents graves sont plus fréquents chez les hommes ou chez les femmes).
## Est ce que le lieu influence la gravité des accidents ?
Un autre facteur de gravité pourrait être le lieu de l'accidents, voyons si il y a des lieux où la gravité des accidents est plus forte.
```{r echo=FALSE, warning=FALSE}
################### carte du rapport accident_mortel / nb accidents * 100 en France en 2021
# Importer les données géographiques
regions_geojson <- st_read("https://raw.githubusercontent.com/gregoiredavid/france-geojson/master/regions-version-simplifiee.geojson")
# Calculer le nombre d'accidents par région
df_region <- df %>%
group_by(region) %>%
summarise(n_accidents = n())
# Fusionner les données géographiques et les données de nombre d'accidents
map_df <- left_join(regions_geojson, df_region, by = c("nom" = "region"))
# Créer la carte
ggplot() +
geom_sf(data = map_df, aes(fill = n_accidents)) +
scale_fill_gradient(low = "lightyellow", high = "darkred") +
ggtitle("Nombre d'accidents par région en France en 2021") +
labs(fill="Nombre d'accidents") +
theme_void()
########### carte du nombre d'accidents par région en France en 2021
# Calculer le nombre d'accidents par région
df_region <- df %>%
group_by(region) %>%
summarise(n_accidents = n(),
n_accidents_mortels = sum(grav == 2),
taux_accidents_mortels = round(sum(grav == 2)/n_accidents, 4)*100)
# Fusionner les données géographiques et les données de nombre d'accidents
map_df <- left_join(regions_geojson, df_region, by = c("nom" = "region"))
# Créer la carte
ggplot() +
geom_sf(data = map_df, aes(fill = taux_accidents_mortels)) +
scale_fill_gradient(low = "lightyellow", high = "darkred") +
ggtitle("Rapport du nombre d'accidents mortels sur le nombre d'accidents par région") +
labs(fill = "Taux d'accidents graves") +
theme_void()
# Créer une table de contingence croisant la région et la gravité de l'accident
cont_table <- table(df$region, df$grav)
# Effectuer le test du chi2
chi2 <- chisq.test(cont_table)
chi2
```
Le résultat du test montre une statistique de test de 6065.5 et un degré de liberté de 39, ce qui donne une p-value inférieure à 2.2e-16.
La p-value est très faible, ce qui suggère qu'il y a une forte association entre la région et la gravité de l'accident. Autrement dit, la gravité des accidents semble varier significativement selon la région où ils se produisent.
La région Parisienne a le plus de nombre d'accidents, mais c'est aussi la plus peuplée, on constate à la suite que cette région a le plus d'accidents mais les moins graves. On peut émettre l'hypothèse que la limitation de vitesse peut peut-être influer la gravité des accidents.
Seulement, notre variable sur la limitation de vitesse n'est pas utilisable en vue des valeurs au-delà de 130 km/h.
```{r echo=FALSE, warning=FALSE}
# Importer les données géographiques
deps_geojson <- st_read("https://france-geojson.gregoiredavid.fr/repo/departements.geojson")
# Calculer le nombre d'accidents par région
df_dep <- df %>%
group_by(dep) %>%
summarise(n_accidents = n())
# Fusionner les données géographiques et les données de nombre d'accidents
map_df <- left_join(deps_geojson, df_dep, by = c("code" = "dep"))
# Créer la carte
ggplot() +
geom_sf(data = map_df, aes(fill = n_accidents)) +
scale_fill_gradient2(low = "lightyellow", mid = "orange",high = "darkred", midpoint = 6000) +
ggtitle("Nombre d'accidents par département en France en 2021") +
labs(fill="Nombre d'accidents") +
theme_void()
########### carte du nombre d'accidents par région en France en 2021
# Calculer le nombre d'accidents par région
df_dep <- df %>%
group_by(dep) %>%
summarise(n_accidents = n(),
n_accidents_mortels = sum(grav == 2),
taux_accidents_mortels = round(sum(grav == 2)/n_accidents, 4)*100)
# Fusionner les données géographiques et les données de nombre d'accidents
map_df <- left_join(deps_geojson, df_dep, by = c("code" = "dep"))
# Créer la carte
ggplot() +
geom_sf(data = map_df, aes(fill = taux_accidents_mortels)) +
scale_fill_gradient(low = "lightyellow", high = "darkred") +
ggtitle("Rapport du nombre d'accidents mortels sur le nombre d'accidents par département") +
labs(fill = "Taux d'accidents graves") +
theme_void()
# Créer une table de contingence croisant la région et la gravité de l'accident
cont_table <- table(df$dep, df$grav)
# Effectuer le test du chi2
chi2 <- chisq.test(cont_table)
chi2
```
Dans les deux résultats, la statistique de test est très élevée (6065.5 pour les régions et 10960 pour les départements), ce qui suggère qu'il existe un lien significatif entre la région/departement et la gravité de l'accident. On remarque que les deux cartes précédentes sont quasiment inverse c'est-à-dire que dans les zones où le nombre d'accidents est élévé, le taux d'accidents mortels l'est moins. On remarque ceci notamment pour la région d'île de france et pour le département du rhône. Cela pourrait s'expliquer par le fait que dans les grandes villes, les accidents se font à des vitesses réduites.
## Est ce que l'environnement influence la gravité des accidents ?
### Est ce que la catégorie de véhicule influence la gravité ?
```{r echo=FALSE, warning=FALSE}
#Créer une nouvelle variable "categorie" à partir de la variable "catv"
df$categorie <- ifelse(df$catv %in% c("01", "02", "03", "05", "06", "30", "31", "32", "33", "34", "41", "42", "43"), "Deux-roues",
ifelse(df$catv %in% c("04", "07", "10", "11", "12"), "Voiture",
ifelse(df$catv %in% c("13", "14", "15", "16", "17"), "Camion",
ifelse(df$catv %in% c("18", "19", "37", "38", "40"), "Transport en commun",
ifelse(df$catv %in% c("20", "21"), "Engin spécial", "Autre")))))
# Créer un tableau croisé entre catv et grav
ctable <- table(df$categorie, df$grav)
# Convertir en data frame et renommer les colonnes
df_ctable <- as.data.frame.matrix(ctable)
colnames(df_ctable) <- c("Indemne", "Mort", "Blessé hospitalisé", "Blessé léger")
# Ajouter une colonne avec le total de chaque catégorie de véhicule
# Ajouter la variable "catv" et convertir en format long pour pouvoir utiliser ggplot
df_ctable_long <- df_ctable %>%
mutate(catv = rownames(df_ctable)) %>%
gather(key = "grav", value = "count", -catv) %>%
group_by(catv) %>%
mutate(percent = count/sum(count)*100)
# Créer le graphique
ggplot(df_ctable_long, aes(x = percent, y = catv, fill = grav)) +
geom_bar(stat = "identity") +
labs(title = "Répartition des catégories de véhicules par niveau de gravité",
x = "Catégorie de véhicule", y = "Pourcentage") +
scale_fill_manual(name = "Gravité de l'accident",
labels = c("Indemne", "Blessé léger", "Blessé hospitalisé", "Mort"),
values = c("green", "yellow", "orange", "red")) +
theme_classic()
df$grav_binary <- ifelse(df$grav %in% c(2,3), 1, 0)
model <- glm(grav_binary ~ categorie, data = df, family = binomial)
summary(model)
```
La première partie de la sortie donne quelques informations sur la qualité de l'ajustement du modèle, notamment les résidus de deviance. Dans l'ensemble, les résidus semblent assez faibles, ce qui suggère que le modèle s'adapte bien aux données.
La deuxième partie de la sortie présente les coefficients estimés pour chaque niveau de la variable explicative (les différentes catégories de véhicules). Les coefficients indiquent l'effet de chaque niveau de la variable explicative sur la probabilité de gravité de l'accident. Par exemple, la variable categorieTransport en commun a un coefficient négatif (-1.050340), ce qui signifie qu'être impliqué dans un accident avec un transport en commun diminue la probabilité de gravité de l'accident par rapport aux autres catégories de véhicules.
En somme, ces résultats indiquent que la catégorie de véhicule est un facteur significatif pour prédire la gravité de l'accident, et que certains types de véhicules ont une probabilité de gravité plus élevée ou plus faible que d'autres.
### Est ce que l'équipement de sécurité influence la gravité ?
```{r echo=FALSE, warning=FALSE}
df_secu = data.frame(df$grav)
df_secu$grav = df$grav
df_secu$grav <- factor(df$grav, levels = c(1, 2, 3, 4),
labels = c("Indemne", "Mort","Blessé hospitalisé", "Blessé léger"))
df_secu$secu1 <- factor(df$secu1, levels = c(0, 1, 2, 3, 4, 5, 6, 7, 8, 9, -1),
labels = c("Aucun équipement", "Ceinture", "Casque", "Dispositif enfants",
"Gilet réfléchissant", "Airbag", "Gants", "Gants + Airbag",
"Non déterminable", "Autre", "Non renseigné"))
df_secu$secu2 <- factor(df$secu2, levels = c(0, 1, 2, 3, 4, 5, 6, 7, 8, 9),
labels = c("Aucun équipement", "Ceinture", "Casque", "Dispositif enfants",
"Gilet réfléchissant", "Airbag", "Gants", "Gants + Airbag",
"Non déterminable", "Autre"))
df_secu$secu3 <- factor(df$secu3, levels = c(0, 1, 2, 3, 4, 5, 6, 7, 8, 9),
labels = c("Aucun équipement", "Ceinture", "Casque", "Dispositif enfants",
"Gilet réfléchissant", "Airbag", "Gants", "Gants + Airbag",
"Non déterminable", "Autre"))
# ggplot(df_secu, aes(x = grav, fill = secu1)) +
# geom_bar(position = "fill") +
# scale_fill_manual(values = c("#E69F00", "#56B4E9", "#009E73", "#F0E442", "#0072B2",
# "#D55E00", "#CC79A7", "#999999", "#000000" ,"#570B66", "#382B53")) +
# labs(title = "Lien entre l'équipement de sécurité et la gravité de l'accident",
# x = "Gravité de l'accident", y = "Proportion") +
# theme_classic() +
# scale_y_continuous(trans = "log")
# Créer un dataframe avec les données de secu1
df_secu1 <- data.frame(table(df_secu$secu1))
ggplot(df_secu, aes(x = grav, fill = secu1)) +
labs(title = "Gravité et nombre d'accidents par rapport à l'équipement de sécurité porté par les passagers",x ="Gravité", y="Nombre d'accidents", fill = "Sécurité") +
geom_bar()
# Création de la table de contingence
tab <- table(df_secu$grav, df_secu$secu1)
# Ajout des marges
tab_marg <- addmargins(tab)
# Arrondi des valeurs de la table à deux décimales
tab_marg <- round(tab_marg, 2)
# Formatage de la table sous forme de tableau
# tab_marg_format <- format(tab_marg, nsmall = 2, justify = "right")
# colnames(tab_marg_format) <- c("Secu 1", "Total")
# rownames(tab_marg_format) <- c("Indemne", "Tué", "Blessé hospitalisé", "Blessé léger", "Total")
```
On constate que, parmis les accidents de la route de notre jeu de données, une grande majorité a un équipement de sécurité équipé tel que la ceinture ou un casque pour les 2 roues.
```{r echo=FALSE, warning=FALSE}
# Créer le tableau de fréquences conditionnelles entre grav et secu1
freq_table <- prop.table(table(df_secu$grav, df_secu$secu1), margin = 1) * 100
# Ajouter des totaux de colonne et de ligne
# freq_table <- addmargins(freq_table, margin = 1)
# freq_table <- addmargins(freq_table, margin = 2)
# Afficher le tableau de fréquences conditionnelles avec les totaux
freq_table
```
Dans les faits, 79.8% des accidents où les passagers ont porté une ceinture de sécurité, ils sont sortis indemnes.
On peut tirer plusieurs conclusions de ce tableau, par exemple :
- Dans l'ensemble des accidents, la majorité des passagers portent une ceinture de sécurité.
- Les passagers qui ne portent aucun équipement de sécurité ont un risque accru d'être gravement blessés ou tués dans un accident.
- Le port du casque est associé à une gravité plus élevée des accidents, probablement en raison du fait que les accidents où le casque est porté sont principalement des accidents de moto.
- Les passagers qui portent un gilet réfléchissant ou un dispositif pour enfants ont des taux de mortalité et de blessures graves relativement faibles.
- Les valeurs "Non déterminable" et "Non renseigné" pour l'équipement de sécurité sont relativement élevées, ce qui peut indiquer des lacunes dans la collecte de données ou des erreurs dans la déclaration des équipements de sécurité portés.
### Est ce que la luminosité influence la gravité des accidents ?
```{r echo=FALSE, warning=FALSE}
d <- df %>% filter(age > 13, catu == 1)
sub_df <- d[, c("grav", "lum")]
# enleve les na
sub_df <- na.omit(sub_df) %>% filter(lum != -1)
tab <- table(sub_df$lum, sub_df$grav)
# Conversion de la table croisée en un data frame
df_s <- as.data.frame.matrix(tab)
df_s$lum <- rownames(df_s)
# Mise en forme des données pour un diagramme en barres empilées
df_long <- tidyr::gather(df_s, key = "grav", value = "count", -lum)
df_long$grav <- factor(df_long$grav, levels = c("1", "2", "3", "4"))
df_long$sexe <- factor(df_long$lum, levels = c("1", "2", "3", "4", "5"))
# Convertir la variable grav en factor avec les niveaux correspondants
df_long$grav <- factor(df_long$grav, levels = 1:4,
labels = c("Indemne", "Mort", "Blessé hospitalisé", "Blessé léger"))
df_long$lum <- factor(df_long$sexe, levels = 1:2:3:4:5,
labels = c("Plein jour",
"Crépuscule ou aube",
"Nuit sans éclairage public",
"Nuit avec éclairage public non allumé",
"Nuit avec éclairage public allumé"))
# Définir les couleurs personnalisées
colors <- c("Plein jour"="yellow",
"Crépuscule ou aube"="orange",
"Nuit sans éclairage public"="darkblue",
"Nuit avec éclairage public non allumé"="purple",
"Nuit avec éclairage public allumé"="lightblue")
# Réorganiser les niveaux de la variable "grav" en fonction de la fréquence
df_long$grav <- reorder(df_long$grav, desc(df_long$count))
# Créer le graphique
ggplot(df_long, aes(x = grav, fill = lum)) +
geom_col(position = "dodge", aes(y = count), alpha = 0.8) +
scale_fill_manual(values = colors, name = "Conditions d'eclairage",
labels = c("Plein jour",
"Crépuscule ou aube",
"Nuit sans éclairage public",
"Nuit avec éclairage public non allumé",
"Nuit avec éclairage public allumé")) +
labs(title = "Gravité des accidents par condition d'eclairage", x = "", y = "Nombre d'accidents") + theme_gray()+
coord_flip()
```
#### On effectue un test du Chi² pour tester la corrélation entre la gravité et l'état de la route
```{r echo=FALSE, warning=FALSE}
chisq.test(df$grav, df$surf)
```
La p-value élevée de 1 indique que l'hypothèse nulle, selon laquelle il n'y a pas de corrélation entre les deux variables, ne peut être rejetée. Autrement dit, il n'y a pas de preuve statistique pour affirmer qu'il y a une corrélation significative entre la gravité des blessures (variable "grav") et l'état de la surface (variable "surf") dans les accidents de la route en France en 2021.
## Conclusion
Les conclusions que l'on peut tirer de cette étude sont les suivantes :
- La fin du confinement en juin 2021 a entraîné une augmentation du nombre d'accidents de la route en France, probablement en raison de l'augmentation du nombre de voitures sur les routes.
- Les accidents sont plus fréquents l'après-midi et le matin, pendant les périodes de pointe de la circulation, lorsque de nombreuses personnes vont au travail ou emmènent leurs enfants à l'école.
- L'âge des conducteurs est significativement associé à la gravité des accidents, avec une diminution de la probabilité d'avoir une gravité plus élevée pour chaque année supplémentaire. Les conducteurs indemnes ont tendance à être plus âgés que les autres groupes de gravité, tandis que les conducteurs blessés légèrement ont tendance à être plus jeunes.
- Le sexe ne semble pas influencer significativement la gravité des accidents, bien que le nombre total de conducteurs soit plus élevé chez les hommes que chez les femmes.
Ces conclusions peuvent être utilisées pour élaborer des politiques visant à améliorer la sécurité routière, telles que la sensibilisation aux dangers de la conduite pendant les périodes de pointe de la circulation, la formation des conducteurs plus jeunes et l'amélioration de la sécurité des routes pour les conducteurs plus âgés. |
///
//
//M2TWEOP structures and functions. There are not many examples and descriptions here. Also note that the examples do not include many of the checks that would be required when creating modifications.
//@module LuaPlugin
//@author youneuoy
//@license GPL-3.0
#include "luaP.h"
#include "plugData.h"
#include "eopEduHelpers.h"
#include "buildingStructHelpers.h"
void luaP::initEopEdu()
{
struct
{
sol::table M2TWEOPEDUTable;
sol::table EDB;
}tables;
struct
{
sol::usertype<edbEntry>edbEntry;
sol::usertype<BuildingLvlCapability>capability;
sol::usertype<recruitPool>recruitpool;
}types;
using namespace UnitEnums;
//@section attackAttr
/***
Enum with a list of attack attributes.
@tfield int nothing
@tfield int spear
@tfield int light_spear
@tfield int prec
@tfield int ap
@tfield int bp
@tfield int area
@tfield int fire
@tfield int launching
@tfield int thrown
@tfield int short_pike
@tfield int long_pike
@tfield int spear_bonus_12
@tfield int spear_bonus_10
@tfield int spear_bonus_8
@tfield int spear_bonus_6
@tfield int spear_bonus_4
@table attackAttr
*/
luaState.new_enum(
"attackAttr",
"nothing", attackAttr::nothing,
"spear", attackAttr::spear,
"light_spear", attackAttr::light_spear,
"prec", attackAttr::prec,
"ap", attackAttr::ap,
"bp", attackAttr::bp,
"area", attackAttr::area,
"fire", attackAttr::fire,
"launching", attackAttr::launching,
"thrown", attackAttr::thrown,
"short_pike", attackAttr::short_pike,
"long_pike", attackAttr::long_pike,
"spear_bonus_12", attackAttr::spear_bonus_12,
"spear_bonus_10", attackAttr::spear_bonus_10,
"spear_bonus_8", attackAttr::spear_bonus_8,
"spear_bonus_6", attackAttr::spear_bonus_6,
"spear_bonus_4", attackAttr::spear_bonus_4
);
//@section eduStat
/***
Enum with a list of stats.
@tfield int none
@tfield int armour
@tfield int defense
@tfield int shield
@tfield int attack
@tfield int charge
@table eduStat
*/
luaState.new_enum(
"eduStat",
"none", eduStat::none,
"armour", eduStat::armour,
"defense", eduStat::defense,
"shield", eduStat::shield,
"attack", eduStat::attack,
"charge", eduStat::charge
);
///M2TWEOPDU
//@section M2TWEOPDUTable
/***
Basic M2TWEOPDU table. Contains descriptions of M2TWEOP unit types.
@tfield addEopEduEntryFromFile addEopEduEntryFromFile
@tfield addEopEduEntryFromEDUID addEopEduEntryFromEDUID
@tfield getEopEduEntryByID getEopEduEntryByID Needed for change many parameters of entry.
@tfield getDataEopDu getDataEopDu
@tfield getEduEntry getEduEntry
@tfield getEduEntryByType getEduEntryByType
@tfield getEduIndexByType getEduIndexByType
@tfield setEntryUnitCardTga setEntryUnitCardTga (only for eopdu units added by file!)
@tfield setEntryInfoCardTga setEntryInfoCardTga (only for eopdu units added by file!)
@tfield setEntrySoldierModel setEntrySoldierModel
@tfield getArmourUpgradeLevelsNum getArmourUpgradeLevelsNum
@tfield setArmourUpgradeLevelsNum setArmourUpgradeLevelsNum (only for eopdu units added by file!)
@tfield getArmourUpgradeLevel getArmourUpgradeLevel
@tfield setArmourUpgradeLevel setArmourUpgradeLevel (only for eopdu units added by file!)
@tfield getArmourUpgradeModelsNum getArmourUpgradeModelsNum
@tfield setArmourUpgradeModelsNum setArmourUpgradeModelsNum (only for eopdu units added by file!)
@tfield getArmourUpgradeModel getArmourUpgradeModel
@tfield setArmourUpgradeModel setArmourUpgradeModel (only for eopdu units added by file!)
@tfield setEntryAttackAttribute setEntryAttackAttribute
@tfield getEntryAttackAttribute getEntryAttackAttribute
@tfield setEntryStat setEntryStat
@tfield getEntryStat getEntryStat
@tfield setEntryLocalizedName setEntryLocalizedName
@tfield setEntryLocalizedDescr setEntryLocalizedDescr
@tfield setEntryLocalizedShortDescr setEntryLocalizedShortDescr
@table M2TWEOPDU
*/
tables.M2TWEOPEDUTable = luaState.create_table("M2TWEOPDU");
/***
Create new M2TWEOPDU entry from a file describing it.
@function M2TWEOPDU.addEopEduEntryFromFile
@tparam string filepath path to file with unit type description(like in export\_descr\_unit.txt, but only with one record and without comments)
@tparam int eopEnryIndex Entry index, which will be assigned to a new record in DU (recommend starting from 1000, so that there is no confusion with records from EDU).
@treturn eduEntry retEntry Usually you shouldn't use this value.
@usage
M2TWEOPDU.addEopEduEntryFromFile(M2TWEOP.getModPath().."/youneuoy_Data/unitTypes/myTestType.txt",1000);
*/
tables.M2TWEOPEDUTable.set_function("addEopEduEntryFromFile", &eopEduHelpers::addEopEduEntryFromFile);
/***
Create new M2TWEOPDU entry.
@function M2TWEOPDU.addEopEduEntryFromEDUID
@tparam int baseEnryIndex Entry index number, which will be taken as the base for this DU record.
@tparam int eopEnryIndex Entry index, which will be assigned to a new record in DU (recommend starting from 1000, so that there is no confusion with records from EDU).
@treturn eduEntry retEntry Usually you shouldn't use this value.
@usage
M2TWEOPDU.addEopEduEntryFromEDUID(1,1000);
*/
tables.M2TWEOPEDUTable.set_function("addEopEduEntryFromEDUID", &eopEduHelpers::addEopEduEntry);
/***
Get eduEntry of a M2TWEOPDU entry. Needed to change many parameters of the entry.
@function M2TWEOPDU.getEopEduEntryByID
@tparam int eopEnryIndex Entry index in M2TWEOPDU.
@treturn eduEntry retEntry
@usage
local eduEntryOfEOPDU=M2TWEOPDU.getEopEduEntryByID(1000);
eduEntryOfEOPDU.SoldierCount=20;
eduEntryOfEOPDU.Width=1.5;
*/
tables.M2TWEOPEDUTable.set_function("getEopEduEntryByID", &eopEduHelpers::getEopEduEntry);
/***
Get eduEntry by index. Needed to change many parameters of the entry.
@function M2TWEOPDU.getEduEntry
@tparam int EnryIndex Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@treturn eduEntry retEntry
@usage
local eduEntry=M2TWEOPDU.getEduEntry(5);
eduEntry.SoldierCount=20;
eduEntry.Width=1.5;
*/
tables.M2TWEOPEDUTable.set_function("getEduEntry", &eopEduHelpers::getEduEntry);
/***
Get eduEntry by edu type name. Needed to change many parameters of the entry.
@function M2TWEOPDU.getEduEntryByType
@tparam string type Unit type as in export_descr_unit.
@treturn eduEntry retEntry
@usage
local eduEntry=M2TWEOPDU.getEduEntryByType("Peasants");
eduEntry.SoldierCount=20;
eduEntry.Width=1.5;
*/
tables.M2TWEOPEDUTable.set_function("getEduEntryByType", &eopEduHelpers::getEduEntryByType);
/***
Get edu index by edu type name. Needed to use many edu functions.
@function M2TWEOPDU.getEduIndexByType
@tparam string type Unit type as in export_descr_unit.
@treturn int eduindex
@usage
local eduindex=M2TWEOPDU.getEduIndexByType("Peasants");
M2TWEOPDU.setEntryStat(eduindex, eduStat.armour, 5, 1);
*/
tables.M2TWEOPEDUTable.set_function("getEduIndexByType", &eopEduHelpers::getEduIndexByType);
/***
Get data of a M2TWEOPDU entry. You usually won't need this.
@function M2TWEOPDU.getDataEopDu
@tparam int eopEnryIndex Entry index in M2TWEOPDU.
@treturn int retEntry Usually you shouldn't use this value.
@usage
local eopEntry=M2TWEOPDU.getDataEopDu(1000);
*/
tables.M2TWEOPEDUTable.set_function("getDataEopDu", &eopEduHelpers::getDataEopDu);
/***
Set unit card for a M2TWEOPDU entry. Requirements for the location and parameters of the image are unchanged in relation to the game (only for eopdu units added by file!).
@function M2TWEOPDU.setEntryUnitCardTga
@tparam int eopEnryIndex Entry index in M2TWEOPDU.
@tparam string newCardTga
@usage
M2TWEOPDU.setEntryUnitCardTga(1000,"#akavir_swordsmen.tga");
*/
tables.M2TWEOPEDUTable.set_function("setEntryUnitCardTga", &eopEduHelpers::setEntryUnitCardTga);
/***
Set unit info card for M2TWEOPDU entry. Requirements for the location and parameters of the image are unchanged in relation to the game (only for eopdu units added by file!).
@function M2TWEOPDU.setEntryInfoCardTga
@tparam int eopEnryIndex Entry index in M2TWEOPDU.
@tparam string newInfoCardTga
@usage
M2TWEOPDU.setEntryInfoCardTga(1000,"akavir_swordsmen_info.tga");
*/
tables.M2TWEOPEDUTable.set_function("setEntryInfoCardTga", &eopEduHelpers::setEntryInfoCardTga);
/***
Set unit info card for a M2TWEOPDU entry. Requirements for the location and parameters of the image are unchanged in relation to the game.
@function M2TWEOPDU.setEntrySoldierModel
@tparam int eopEnryIndex Entry index in M2TWEOPDU.
@tparam string newSoldierModel
@usage
M2TWEOPDU.setEntrySoldierModel(1000,"Sword_and_Buckler_Men");
*/
tables.M2TWEOPEDUTable.set_function("setEntrySoldierModel", &eopEduHelpers::setEntrySoldierModel);
/***
Get the amount of numbers in the armour_upg_levels line in export_descr_unit.
@function M2TWEOPDU.getArmourUpgradeLevelsNum
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@treturn int ArmourUpgradeLevelsNum
@usage
M2TWEOPDU.getArmourUpgradeLevelsNum(1000);
*/
tables.M2TWEOPEDUTable.set_function("getArmourUpgradeLevelsNum", &eopEduHelpers::getArmourUpgradeLevelsNum);
/***
Set the amount of armour_upg_levels, if you increase the amount of levels the last number entry will be repeated (only for eopdu units added by file!).
@function M2TWEOPDU.setArmourUpgradeLevelsNum
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int amount
@usage
M2TWEOPDU.setArmourUpgradeLevelsNum(1000, 3);
*/
tables.M2TWEOPEDUTable.set_function("setArmourUpgradeLevelsNum", &eopEduHelpers::setArmourUpgradeLevelsNum);
/***
Get armour upgrade level number at specified index.
@function M2TWEOPDU.getArmourUpgradeLevel
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int levelidx
@treturn int level
@usage
M2TWEOPDU.getArmourUpgradeLevel(1000, 0);
*/
tables.M2TWEOPEDUTable.set_function("getArmourUpgradeLevel", &eopEduHelpers::getArmourUpgradeLevel);
/***
Set armour upgrade level number at specified index (only for eopdu units added by file!).
@function M2TWEOPDU.setArmourUpgradeLevel
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int levelidx
@tparam int newlevel
@usage
M2TWEOPDU.setArmourUpgradeLevel(1000, 1, 4);
*/
tables.M2TWEOPEDUTable.set_function("setArmourUpgradeLevel", &eopEduHelpers::setArmourUpgradeLevel);
/***
Get the amount of models in the armour_upg_models line in export_descr_unit.
@function M2TWEOPDU.getArmourUpgradeModelsNum
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@treturn int ArmourUpgradeLevelsNum
@usage
M2TWEOPDU.getArmourUpgradeModelsNum(1000);
*/
tables.M2TWEOPEDUTable.set_function("getArmourUpgradeModelsNum", &eopEduHelpers::getArmourUpgradeModelsNum);
/***
Set the amount of armour_upg_levels, if you increase the amount of models the last model entry will be repeated (only for eopdu units added by file!).
@function M2TWEOPDU.setArmourUpgradeModelsNum
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int amount Maximum: 4
@usage
M2TWEOPDU.setArmourUpgradeModelsNum(1000, 3);
*/
tables.M2TWEOPEDUTable.set_function("setArmourUpgradeModelsNum", &eopEduHelpers::setArmourUpgradeModelsNum);
/***
Get armour upgrade level number at specified index.
@function M2TWEOPDU.getArmourUpgradeModel
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int levelidx
@treturn string modelName
@usage
M2TWEOPDU.getArmourUpgradeModel(1000, 0);
*/
tables.M2TWEOPEDUTable.set_function("getArmourUpgradeModel", &eopEduHelpers::getArmourUpgradeModel);
/***
Set the unit model at specified index (only for eopdu units added by file!).
@function M2TWEOPDU.setArmourUpgradeModel
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int levelidx
@tparam string modelName
@usage
M2TWEOPDU.setArmourUpgradeModel(1000, 1, 4);
*/
tables.M2TWEOPEDUTable.set_function("setArmourUpgradeModel", &eopEduHelpers::setArmourUpgradeModel);
/***
Set a primary or secondary attack attribute of an edu entry.
@function M2TWEOPDU.setEntryAttackAttribute
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int attribute Use the attackAttr enum: attackAttr.spear, attackAttr.light\_spear, attackAttr.prec, attackAttr.ap, attackAttr.bp, attackAttr.area, attackAttr.fire, attackAttr.launching, attackAttr.thrown, attackAttr.short\_pike, attackAttr.long\_pike, attackAttr.spear\_bonus\_12, attackAttr.spear\_bonus\_10, attackAttr.spear\_bonus\_8, attackAttr.spear\_bonus\_6, attackAttr.spear\_bonus\_4.
@tparam boolean enable
@tparam int sec 1 = primary, 2 = secondary.
@usage
M2TWEOPDU.setEntryAttackAttribute(1000, attackAttr.ap, true, 1);
*/
tables.M2TWEOPEDUTable.set_function("setEntryAttackAttribute", &eopEduHelpers::setEntryAttackAttribute);
/***
Get a primary or secondary attack attribute from an edu entry.
@function M2TWEOPDU.getEntryAttackAttribute
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int attribute Use the attackAttr enum: attackAttr.spear, attackAttr.light\_spear, attackAttr.prec, attackAttr.ap, attackAttr.bp, attackAttr.area, attackAttr.fire, attackAttr.launching, attackAttr.thrown, attackAttr.short\_pike, attackAttr.long\_pike, attackAttr.spear\_bonus\_12, attackAttr.spear\_bonus\_10, attackAttr.spear\_bonus\_8, attackAttr.spear\_bonus\_6, attackAttr.spear\_bonus\_4.
@tparam int sec 1 = primary, 2 = secondary.
@treturn boolean hasAttackAttribute
@usage
M2TWEOPDU.getEntryAttackAttribute(1000, attackAttr.ap, 1);
*/
tables.M2TWEOPEDUTable.set_function("getEntryAttackAttribute", &eopEduHelpers::getEntryAttackAttribute);
/***
Set any of the basic unit stats of an edu entry.
@function M2TWEOPDU.setEntryStat
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int eduStat Use the eduStat enum: eduStat.armour, eduStat.defense, eduStat.shield, eduStat.attack, eduStat.charge.
@tparam int value
@tparam int sec 1 = primary, 2 = secondary.
@usage
M2TWEOPDU.setEntryStat(1000, attackAttr.attack, 1);
*/
tables.M2TWEOPEDUTable.set_function("setEntryStat", &eopEduHelpers::setEntryStat);
/***
Get any of the basic unit stats of an edu entry.
@function M2TWEOPDU.getEntryStat
@tparam int index Entry index (Values lower then 500 look for edu entry, values over 500 look for EOP edu entry).
@tparam int eduStat Use the eduStat enum: eduStat.armour, eduStat.defense, eduStat.shield, eduStat.attack, eduStat.charge.
@tparam int sec 1 = primary, 2 = secondary.
@treturn int unitStat
@usage
M2TWEOPDU.getEntryStat(1000, attackAttr.attack, 1);
*/
tables.M2TWEOPEDUTable.set_function("getEntryStat", &eopEduHelpers::getEntryStat);
/***
Set localized name for a M2TWEOPDU entry. This does not require any entries in the text folder.
@function M2TWEOPDU.setEntryLocalizedName
@tparam int eopEnryIndex Entry index in M2TWEOPDU.
@tparam string newLocalizedName
@usage
M2TWEOPDU.setEntryLocalizedName(1000,"Test unit");
*/
tables.M2TWEOPEDUTable.set_function("setEntryLocalizedName", &eopEduHelpers::setEntryLocalizedName);
//tables.M2TWEOPEDUTable.set_function("addUnitToRQ", &eopEduHelpers::addUnitToRQ); comment out not good implementation
/***
Set localized description for M2TWEOPDU entry. This does not require any entries in the text folder.
@function M2TWEOPDU.setEntryLocalizedDescr
@tparam int eopEnryIndex Entry index in M2TWEOPDU.
@tparam string newLocalizedDescr
@usage
M2TWEOPDU.setEntryLocalizedDescr(1000,"This is test unit description\n123321\nCreated with m2tweop");
*/
tables.M2TWEOPEDUTable.set_function("setEntryLocalizedDescr", &eopEduHelpers::setEntryLocalizedDescr);
/***
Set localized short description for M2TWEOPDU entry. This does not require any entries in the text folder.
@function M2TWEOPDU.setEntryLocalizedShortDescr
@tparam int eopEnryIndex Entry index in M2TWEOPDU.
@tparam string newLocalizedShortDescr
@usage
M2TWEOPDU.setEntryLocalizedShortDescr(1000,"This is test unit short description\n123321\nCreated with m2tweop");
*/
tables.M2TWEOPEDUTable.set_function("setEntryLocalizedShortDescr", &eopEduHelpers::setEntryLocalizedShortDescr);
///EdbEntry
//@section edbEntry
/***
Basic edbEntry table.
@tfield int buildingID
@tfield int classification
@tfield int isCoreBuilding
@tfield int isPort
@tfield int isCoreBuilding2
@tfield int hasReligion
@tfield int religionID
@tfield int isHinterland
@tfield int isFarm
@tfield int buildingLevelCount
@table edbEntry
*/
types.edbEntry = luaState.new_usertype<edbEntry>("edbEntry");
types.edbEntry.set("buildingID", &edbEntry::buildingID);
types.edbEntry.set("classification", &edbEntry::classification);
types.edbEntry.set("isCoreBuilding", &edbEntry::isCoreBuilding);
types.edbEntry.set("isPort", &edbEntry::isPort);
types.edbEntry.set("isCoreBuilding2", &edbEntry::isCoreBuilding2);
types.edbEntry.set("hasReligion", &edbEntry::hasReligion);
types.edbEntry.set("religionID", &edbEntry::religionID);
types.edbEntry.set("isHinterland", &edbEntry::isHinterland);
types.edbEntry.set("isFarm", &edbEntry::isFarm);
types.edbEntry.set("buildingLevelCount", &edbEntry::buildingLevelCount);
///Capability
//@section capability
/***
Basic capability table.
@tfield int capabilityType
@tfield int capabilityLvl
@tfield int bonus
@tfield int capabilityID
@table capability
*/
types.capability = luaState.new_usertype<BuildingLvlCapability>("capability");
types.capability.set("capabilityType", &BuildingLvlCapability::capabilityType);
types.capability.set("capabilityLvl", &BuildingLvlCapability::capabilityLvl);
types.capability.set("bonus", &BuildingLvlCapability::bonus);
types.capability.set("capabilityID", &BuildingLvlCapability::capabilityID);
///RecruitPool
//@section recruitpool
/***
Basic recruitpool table.
@tfield int capabilityType
@tfield int capabilityLvlorExp Difference is for agents
@tfield int unitID
@tfield float initialSize
@tfield float gainPerTurn
@tfield float maxSize
@table recruitpool
*/
types.recruitpool = luaState.new_usertype<recruitPool>("recruitpool");
types.recruitpool.set("capabilityType", &recruitPool::capabilityType);
types.recruitpool.set("capabilityLvlorExp", &recruitPool::capabilityLvlorExp);
types.recruitpool.set("unitID", &recruitPool::unitID);
types.recruitpool.set("initialSize", &recruitPool::initialSize);
types.recruitpool.set("gainPerTurn", &recruitPool::gainPerTurn);
types.recruitpool.set("maxSize", &recruitPool::maxSize);
///EDB
//@section EDB
/***
Basic EDB table.
@tfield addEopBuildEntry addEopBuildEntry
@tfield getEopBuildEntry getEopBuildEntry
@tfield setBuildingPic setBuildingPic
@tfield setBuildingPicConstructed setBuildingPicConstructed
@tfield setBuildingPicConstruction setBuildingPicConstruction
@tfield setBuildingLocalizedName setBuildingLocalizedName
@tfield setBuildingLocalizedDescr setBuildingLocalizedDescr
@tfield setBuildingLocalizedDescrShort setBuildingLocalizedDescrShort
@tfield addBuildingCapability addBuildingCapability
@tfield removeBuildingCapability removeBuildingCapability
@tfield getBuildingCapability getBuildingCapability
@tfield getBuildingCapabilityNum getBuildingCapabilityNum
@tfield addBuildingPool addBuildingPool
@tfield removeBuildingPool removeBuildingPool
@tfield getBuildingPool getBuildingPool
@tfield getBuildingPoolNum getBuildingPoolNum
@tfield getBuildingByName getBuildingByName
@table EDB
*/
tables.EDB = luaState.create_table("EDB");
/***
Create new EOP Building entry
@function EDB.addEopBuildEntry
@tparam edbEntry edbEntry Old entry.
@tparam int newIndex New index of new entry. Use index > 127!
@treturn edbEntry eopentry.
@usage
-- Basic Example
oldBuilding = EDB.getBuildingByName("market")
newBuilding = EDB.addEopBuildEntry(oldBuilding,150);
-- Full example
local oldBuilding = EDB.getBuildingByName("market");
local eopBuilding = EDB.addEopBuildEntry(oldBuilding, 150);
-- Set pictures, names and descriptions by culture and faction
for c = 0, 6 do --every culture
EDB.setBuildingPic(eopBuilding, 'some path to pic', 0, c)
EDB.setBuildingPicConstructed(eopBuilding,'some path to pic', 0, c)
end
for f = 0, 30 do --every faction
EDB.setBuildingLocalizedName(eopBuilding, 'some name', 0, f)
EDB.setBuildingLocalizedDescr(eopBuilding, 'some description', 0, f)
end
-- Add in an income bonus of 500
EDB.addBuildingCapability(eopBuilding, 0, buildingCapability.income_bonus, 500, true)
-- Add a recruit pool
EDB.addBuildingPool(eopBuilding, 0, M2TWEOPDU.getEduIndexByType("Peasants"), 1, 0.1, 2, 0, "");
-- Create a dummy building and get it
sett:createBuilding("market");; --just assuming you have got a sett with some loop or function
-- Set the existing building in the settlement to be the EOP building we just created
local dummyBuilding = sett:getBuilding(5)
dummyBuilding.edbEntry = eopBuilding
*/
tables.EDB.set_function("addEopBuildEntry", &buildingStructHelpers::addEopBuildEntry);
/***
Get EOP Building entry. Returns vanilla build entry if you use a vanilla building index (< 128).
@function EDB.getEopBuildEntry
@tparam int index Index of eop entry.
@treturn edbEntry eopentry.
@usage
building = EDB.getEopBuildEntry(150);
*/
tables.EDB.set_function("getEopBuildEntry", &buildingStructHelpers::getEopBuildEntry);
/***
Set picture of building.
@function EDB.setBuildingPic
@tparam edbEntry edbEntry Entry to set.
@tparam string newPic Path to new pic.
@tparam int level Building level to set pic for.
@tparam int culture ID of the culture to set the pic for.
@usage
building = EDB.getBuildingByName("market")
EDB.setBuildingPic(building, modPath .. mp_path_mods .. "data/ui/northern_european/buildings/#northern_european_vintner.tga", 0, 4);
*/
tables.EDB.set_function("setBuildingPic", &buildingStructHelpers::setBuildingPic);
/***
Set constructed picture of building.
@function EDB.setBuildingPicConstructed
@tparam edbEntry edbEntry Entry to set.
@tparam string newPic Path to new pic.
@tparam int level Building level to set pic for.
@tparam int culture ID of the culture to set the pic for.
@usage
building = EDB.getBuildingByName("market")
EDB.setBuildingPicConstructed(building, modPath .. mp_path_mods .. "data/ui/northern_european/buildings/#northern_european_vintner.tga", 0, 4);
*/
tables.EDB.set_function("setBuildingPicConstructed", &buildingStructHelpers::setBuildingPicConstructed);
/***
Set construction picture of building.
@function EDB.setBuildingPicConstruction
@tparam edbEntry edbEntry Entry to set.
@tparam string newPic Path to new pic.
@tparam int level Building level to set pic for.
@tparam int culture ID of the culture to set the pic for.
@usage
building = EDB.getBuildingByName("market")
EDB.setBuildingPicConstruction(building, modPath .. mp_path_mods .. "data/ui/northern_european/buildings/#northern_european_vintner.tga", 0, 4);
*/
tables.EDB.set_function("setBuildingPicConstruction", &buildingStructHelpers::setBuildingPicConstruction);
/***
Set name of a building.
@function EDB.setBuildingLocalizedName
@tparam edbEntry edbEntry Entry to set.
@tparam string newName New name.
@tparam int level Building level.
@tparam int facnum Faction ID of the faction to set it for (dipNum).
@usage
building = EDB.getBuildingByName("market")
EDB.setBuildingLocalizedName(building, modPath .. mp_path_mods .. "data/ui/northern_european/buildings/#northern_european_vintner.tga", 0, 4);
*/
tables.EDB.set_function("setBuildingLocalizedName", &buildingStructHelpers::setBuildingLocalizedName);
/***
Set description of a building.
@function EDB.setBuildingLocalizedDescr
@tparam edbEntry edbEntry Entry to set.
@tparam string newName New description.
@tparam int level Building level.
@tparam int facnum Faction ID of the faction to set it for (dipNum).
@usage
building = EDB.getBuildingByName("market")
EDB.setBuildingLocalizedDescr(building, modPath .. mp_path_mods .. "data/ui/northern_european/buildings/#northern_european_vintner.tga", 0, 4);
*/
tables.EDB.set_function("setBuildingLocalizedDescr", &buildingStructHelpers::setBuildingLocalizedDescr);
/***
Set short description of a building.
@function EDB.setBuildingLocalizedDescrShort
@tparam edbEntry edbEntry Entry to set.
@tparam string newName New short description.
@tparam int level Building level.
@tparam int facnum Faction ID of the faction to set it for (dipNum).
@usage
building = EDB.getBuildingByName("market")
EDB.setBuildingLocalizedDescrShort(building, modPath .. mp_path_mods .. "data/ui/northern_european/buildings/#northern_european_vintner.tga", 0, 4);
*/
tables.EDB.set_function("setBuildingLocalizedDescrShort", &buildingStructHelpers::setBuildingLocalizedDescrShort);
/***
Add a capability to a building.
@function EDB.addBuildingCapability
@tparam edbEntry edbEntry Entry to set.
@tparam int level Building level.
@tparam int capability ID of capability to set. Use buildingCapability enum.
@tparam int value Value to set.
@tparam bool bonus Is it bonus or not.
@usage
building = EDB.getBuildingByName("market")
-- Add a population growth bonus to the market building
EDB.addBuildingCapability(building, 0, 0, 5, true);
-- Add a 500 income bonus to the market building
EDB.addBuildingCapability(building, 0, buildingCapability.income_bonus, 500, true)
*/
tables.EDB.set_function("addBuildingCapability", &buildingStructHelpers::addBuildingCapability);
/***
Remove a capability from a building.
@function EDB.removeBuildingCapability
@tparam edbEntry edbEntry Entry to set.
@tparam int level Building level.
@tparam int index Which capability to remove (In order of iterating).
@usage
building = EDB.getBuildingByName("market")
EDB.removeBuildingCapability(building, 0, 3);
*/
tables.EDB.set_function("removeBuildingCapability", &buildingStructHelpers::removeBuildingCapability);
/***
Get capability from a building at an index.
@function EDB.getBuildingCapability
@tparam edbEntry edbEntry Entry to set.
@tparam int level Building level.
@tparam int index
@treturn capability capability.
@usage
building = EDB.getBuildingByName("market")
cap = EDB.getBuildingCapability(building, 0, 3);
*/
tables.EDB.set_function("getBuildingCapability", &buildingStructHelpers::getBuildingCapability);
/***
Get capability amount from a building.
@function EDB.getBuildingCapabilityNum
@tparam edbEntry edbEntry Entry to set.
@tparam int level Building level.
@treturn int capabilityNum.
@usage
building = EDB.getBuildingByName("market")
EDB.getBuildingCapabilityNum(building, 0);
*/
tables.EDB.set_function("getBuildingCapabilityNum", &buildingStructHelpers::getBuildingCapabilityNum);
/***
Add a recruitment pool to a building.
@function EDB.addBuildingPool
@tparam edbEntry edbEntry Entry to set.
@tparam int level Building level.
@tparam int eduIndex edu index of unit to add.
@tparam float initialSize Initial pool.
@tparam float gainPerTurn Replenishment per turn.
@tparam float maxSize Maximum size.
@tparam int exp Initial experience.
@tparam string condition Like in export_descr_buildings but without "requires".
@usage
building = EDB.getBuildingByName("market")
EDB.addBuildingPool(building, 0, 55, 1, 0.1, 2, 0, "region_religion catholic 34");
*/
tables.EDB.set_function("addBuildingPool", &buildingStructHelpers::addBuildingPool);
/***
Remove a recruitment pool from a building.
@function EDB.removeBuildingPool
@tparam edbEntry edbEntry Entry to set.
@tparam int level Building level.
@tparam int index Which pool to remove (In order of iterating).
@usage
building = EDB.getBuildingByName("market")
EDB.removeBuildingPool(building, 0, 3);
*/
tables.EDB.set_function("removeBuildingPool", &buildingStructHelpers::removeBuildingPool);
/***
Get a recruitment pool from a building by index.
@function EDB.getBuildingPool
@tparam edbEntry edbEntry Entry to set.
@tparam int level Building level.
@tparam int index Which pool to get (In order of iterating).
@treturn recruitpool pool.
@usage
building = EDB.getBuildingByName("market")
EDB.getBuildingPool(building, 0, 3);
*/
tables.EDB.set_function("getBuildingPool", &buildingStructHelpers::getBuildingPool);
/***
Get a recruitment pool count.
@function EDB.getBuildingPoolNum
@tparam edbEntry edbEntry Entry to set.
@tparam int level Building level.
@treturn int poolNum.
@usage
building = EDB.getBuildingByName("market")
EDB.getBuildingPoolNum(building, 0);
*/
tables.EDB.set_function("getBuildingPoolNum", &buildingStructHelpers::getBuildingPoolNum);
//tables.EDB.set_function("createEOPBuilding", &buildingStructHelpers::createEOPBuilding);
/***
Get a building edb entry by name.
@function EDB.getBuildingByName
@tparam string buildingname
@treturn edbEntry entry
@usage
building = EDB.getBuildingByName("market")
*/
tables.EDB.set_function("getBuildingByName", &buildingStructHelpers::getBuildingByName);
} |
import React from "react";
import { fetchTodos } from "../api/todo";
import { revalidatePath } from "next/cache";
import { fetchPaginatedTodos } from "../api/actions";
//import { getTokenCookie } from "../utils/auth";
interface Pagination {
currentPage: number;
totalPages: number;
hasNextPage: boolean;
hasPrevPage: boolean;
}
const Pagination = ({ pagination }: { pagination: any }) => {
const { currentPage, totalPages, hasNextPage, hasPrevPage }: Pagination =
pagination;
let newPage = currentPage;
const limit = 10; // default from api
const handlePrevious = () => {
if (currentPage > 1) {
//const skip = limit * currentPage;
const skip = limit * currentPage - 2;
// await fetchTodos({ skip });
revalidatePath("/todos");
}
};
const handleNext = async () => {
if (currentPage < totalPages) {
const skip = limit * currentPage;
await fetchPaginatedTodos(skip);
}
};
return (
<nav className="flex justify-center my-4">
<ul className="flex pagination space-x-2">
<li className={`page-item ${hasPrevPage ? "" : "disabled"}`}>
<button
className="page-link px-3 py-1 rounded-md bg-gray-200 hover:bg-gray-300 text-gray-700 hover:text-gray-800"
onClick={handlePrevious}
disabled={!hasPrevPage}
>
Previous
</button>
</li>
<li className="page-item">
<span className="page-link px-3 py-1 bg-gray-200 text-gray-700 rounded-md">
Page {currentPage} of {totalPages}
</span>
</li>
<li className={`page-item ${hasNextPage ? "" : "disabled"}`}>
<button
className="page-link px-3 py-1 rounded-md bg-gray-200 hover:bg-gray-300 text-gray-700 hover:text-gray-800"
onClick={handleNext}
disabled={!hasNextPage}
>
Next
</button>
</li>
</ul>
</nav>
);
};
export default Pagination; |
import type { Meta, StoryObj } from '@storybook/react';
// import { fn } from '@storybook/test';
import IconButton from '../components/IconButton.tsx';
// More on how to set up stories at: https://storybook.js.org/docs/writing-stories#default-export
const meta = {
title: 'Example/Button/IconButton',
component: IconButton,
parameters: {
// Optional parameter to center the component in the Canvas. More info: https://storybook.js.org/docs/configure/story-layout
layout: 'centered',
},
// This component will have an automatically generated Autodocs entry: https://storybook.js.org/docs/writing-docs/autodocs
tags: ['autodocs'],
// More on argTypes: https://storybook.js.org/docs/api/argtypes
argTypes: {
alt: { control: 'text', description: 'alt text' },
iconPath: { control: 'text', description: 'icon path' },
onClick: { action: 'onClick', description: 'onClick' },
width: { control: 'number', description: 'width' },
className: { control: 'text', description: 'className' },
},
// Use `fn` to spy on the onClick arg, which will appear in the actions panel once invoked: https://storybook.js.org/docs/essentials/actions#action-args
// args: { onClick: fn() },
} satisfies Meta<typeof IconButton>;
export default meta;
type Story = StoryObj<typeof meta>;
// import cancelIcon from '../assets/icons/cancel.svg';
// More on writing stories with args: https://storybook.js.org/docs/writing-stories/args
export const Primary: Story = {
args: {
alt: 'icon',
iconPath: '/icons/cancel.svg',
onClick: () => {
console.log('clicked');
},
width: 14,
className: '',
},
}; |
import java.io.*;
import java.util.*;
public class DBManager {
private static final String METADATA_FILE = "metadata.txt";
private static final String TABLE_DIR = "tables";
public static void createTable(String query) {
try {
File metadataFile = new File(METADATA_FILE);
FileWriter metadataWriter = new FileWriter(metadataFile, true);
BufferedWriter metadataBufferedWriter = new BufferedWriter(metadataWriter);
metadataBufferedWriter.write(query);
metadataBufferedWriter.newLine();
metadataBufferedWriter.close();
String[] parts = query.split("\\(");
String tableName = parts[0].split("CREATE TABLE ")[1].trim();
File tableFile = new File(TABLE_DIR + File.separator + tableName + ".txt");
tableFile.createNewFile();
System.out.println("Table '" + tableName + "' created successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
public static void insertIntoTable(String query) {
try {
String[] splitQuery = query.split("VALUES");
String tableName = splitQuery[0].split("INSERT INTO ")[1].trim();
File tableFile = new File(TABLE_DIR + File.separator + tableName + ".txt");
FileWriter tableWriter = new FileWriter(tableFile, true);
BufferedWriter tableBufferedWriter = new BufferedWriter(tableWriter);
String values = splitQuery[1].replaceAll("[(),']", "").trim();
tableBufferedWriter.write(values);
tableBufferedWriter.newLine();
tableBufferedWriter.close();
System.out.println("Values inserted into table '" + tableName + "'.");
} catch (IOException e) {
e.printStackTrace();
}
}
public static void showTableContents(String tableName) {
try {
File tableFile = new File(TABLE_DIR + File.separator + tableName + ".txt");
BufferedReader br = new BufferedReader(new FileReader(tableFile));
String line;
System.out.println("Table Contents for '" + tableName + "':");
while ((line = br.readLine()) != null) {
System.out.println(line);
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Choose an option:");
System.out.println("1. Create Table");
System.out.println("2. Insert Into Table");
System.out.println("3. Show Table Contents");
System.out.println("4. Exit");
int option = scanner.nextInt();
scanner.nextLine(); // Consume newline character
switch (option) {
case 1:
System.out.println("Enter CREATE TABLE query:");
String createQuery = scanner.nextLine();
createTable(createQuery);
break;
case 2:
System.out.println("Enter INSERT INTO query:");
String insertQuery = scanner.nextLine();
insertIntoTable(insertQuery);
break;
case 3:
System.out.println("Enter table name:");
String tableName = scanner.nextLine();
showTableContents(tableName);
break;
case 4:
System.out.println("Exiting...");
scanner.close();
System.exit(0);
default:
System.out.println("Invalid option.");
break;
}
}
}
} |
# coding: utf8
import clinica.pipelines.engine as cpe
__author__ = "Junhao Wen"
__copyright__ = "Copyright 2016-2018, The Aramis Lab Team"
__credits__ = ["Junhao Wen"]
__license__ = "See LICENSE.txt file"
__version__ = "0.1.0"
__maintainer__ = "Junhao Wen"
__email__ = "Junhao.Wen@inria.fr"
__status__ = "Development"
class StatisticsSurface(cpe.Pipeline):
"""
Based on the Matlab toolbox [SurfStat](http://www.math.mcgill.ca/keith/surfstat/), which performs statistical
analyses of univariate and multivariate surface and volumetric data using the generalized linear model (GLM),
this pipelines performs analyses including group comparison and correlation with the surface-based features.
Currently, this pipelines fits the normalised cortical thickness on FsAverage from `t1-freesurfer` pipelines.
New features will be added in the future.
Args:
caps_directory: str, the output folder of recon-all which will contain the result files: ?h.thickness.fwhm**.mgh.
tsv_file: str, Path to the tsv containing the information for GLM.
design_matrix: str, the linear model that fits into the GLM, for example '1+group'.
contrast: string, the contrast matrix for GLM, if the factor you choose is categorized variable, clinica_surfstat will create two contrasts,
for example, contrast = 'Label', this will create contrastpos = Label.AD - Label.CN, contrastneg = Label.CN - Label.AD; if the fac-
tory that you choose is a continuous factor, clinica_surfstat will just create one contrast, for example, contrast = 'Age', but note,
the string name that you choose should be exactly the same with the columns names in your subjects_visits_tsv.
str_format: string, the str_format which uses to read your tsv file, the typy of the string should corresponds exactly with the columns in the tsv file.
Defaut parameters, we set these parameters to be some default values, but you can also set it by yourself:
group_label: current group name for this analysis
glm_type: based on the hypothesis, you should define one of the glm types, "group_comparison", "correlation"
full_width_at_half_maximum: fwhm for the surface smoothing, default is 20, integer.
threshold_uncorrected_pvalue: threshold to display the uncorrected Pvalue, float, default is 0.001.
threshold_corrected_pvalue: the threshold to display the corrected cluster, default is 0.05, float.
cluster_threshold: threshold to define a cluster in the process of cluster-wise correction, default is 0.001, float.
working_directory: define where to put the infomartion of the nipype workflow.
n_procs: define how many cores to run this workflow.
Returns:
A clinica pipelines object containing the StatisticsSurface pipelines.
"""
def check_custom_dependencies(self):
"""Check dependencies that can not be listed in the `info.json` file.
"""
pass
def get_input_fields(self):
"""Specify the list of possible inputs of this pipelines.
Returns:
A list of (string) input fields name.
"""
return ['design_matrix',
'contrast',
'str_format',
'group_label',
'glm_type',
'surface_file',
'full_width_at_half_maximum',
'threshold_uncorrected_pvalue',
'threshold_corrected_pvalue',
'cluster_threshold',
'feature_label']
def get_output_fields(self):
"""Specify the list of possible outputs of this pipelines.
Returns:
A list of (string) output fields name.
"""
return []
def build_input_node(self):
"""Build and connect an input node to the pipelines.
"""
import nipype.interfaces.utility as nutil
import nipype.pipeline.engine as npe
read_parameters_node = npe.Node(name="LoadingCLIArguments",
interface=nutil.IdentityInterface(
fields=self.get_input_fields(),
mandatory_inputs=True))
read_parameters_node.inputs.design_matrix = self.parameters['design_matrix']
read_parameters_node.inputs.contrast = self.parameters['contrast']
read_parameters_node.inputs.str_format = self.parameters['str_format']
read_parameters_node.inputs.group_label = self.parameters['group_label']
read_parameters_node.inputs.glm_type = self.parameters['glm_type']
read_parameters_node.inputs.surface_file = self.parameters['custom_file']
read_parameters_node.inputs.full_width_at_half_maximum = self.parameters['full_width_at_half_maximum']
read_parameters_node.inputs.threshold_uncorrected_pvalue = self.parameters['threshold_uncorrected_pvalue']
read_parameters_node.inputs.threshold_corrected_pvalue = self.parameters['threshold_corrected_pvalue']
read_parameters_node.inputs.cluster_threshold = self.parameters['cluster_threshold']
read_parameters_node.inputs.feature_label = self.parameters['feature_label']
self.connect([
(read_parameters_node, self.input_node, [('design_matrix', 'design_matrix')]), # noqa
(read_parameters_node, self.input_node, [('surface_file', 'surface_file')]), # noqa
(read_parameters_node, self.input_node, [('contrast', 'contrast')]), # noqa
(read_parameters_node, self.input_node, [('str_format', 'str_format')]), # noqa
(read_parameters_node, self.input_node, [('group_label', 'group_label')]), # noqa
(read_parameters_node, self.input_node, [('glm_type', 'glm_type')]), # noqa
(read_parameters_node, self.input_node, [('full_width_at_half_maximum', 'full_width_at_half_maximum')]), # noqa
(read_parameters_node, self.input_node, [('threshold_uncorrected_pvalue', 'threshold_uncorrected_pvalue')]), # noqa
(read_parameters_node, self.input_node, [('threshold_corrected_pvalue', 'threshold_corrected_pvalue')]), # noqa
(read_parameters_node, self.input_node, [('cluster_threshold', 'cluster_threshold')]), # noqa
(read_parameters_node, self.input_node, [('feature_label', 'feature_label')])
])
def build_output_node(self):
"""Build and connect an output node to the pipelines.
"""
pass
def build_core_nodes(self):
"""Build and connect the core nodes of the pipelines.
"""
import statistics_surface_utils as utils
import nipype.interfaces.utility as nutil
import nipype.pipeline.engine as npe
from nipype.interfaces.io import JSONFileSink
# Node to fetch the input variables
data_prep = npe.Node(name='inputnode',
interface=nutil.Function(
input_names=['input_directory', 'subjects_visits_tsv', 'group_label', 'glm_type'],
output_names=['path_to_matscript', 'surfstat_input_dir', 'output_directory',
'freesurfer_home', 'out_json'],
function=utils.prepare_data))
data_prep.inputs.input_directory = self.caps_directory
data_prep.inputs.subjects_visits_tsv = self.tsv_file
# Node to wrap the SurfStat matlab script
surfstat = npe.Node(name='surfstat',
interface=nutil.Function(
input_names=['input_directory',
'output_directory',
'subjects_visits_tsv',
'design_matrix',
'contrast',
'str_format',
'glm_type',
'group_label',
'freesurfer_home',
'surface_file',
'path_to_matscript',
'full_width_at_half_maximum',
'threshold_uncorrected_pvalue',
'threshold_corrected_pvalue',
'cluster_threshold',
'feature_label'],
output_names=['out_images'],
function=utils.runmatlab))
surfstat.inputs.subjects_visits_tsv = self.tsv_file
# Node to create the dictionary for JSONFileSink
jsondict = npe.Node(name='Jsondict',
interface=nutil.Function(
input_names=['glm_type', 'design_matrix', 'str_format', 'contrast', 'group_label', 'full_width_at_half_maximum',
'threshold_uncorrected_pvalue', 'threshold_corrected_pvalue', 'cluster_threshold'],
output_names=['json_dict'],
function=utils.json_dict_create))
# Node to write the GLM information into a JSON file
jsonsink = npe.Node(JSONFileSink(input_names=['out_file']), name='jsonsinker')
# Connection
# ==========
self.connect([
(self.input_node, data_prep, [('group_label', 'group_label')]), # noqa
(self.input_node, data_prep, [('glm_type', 'glm_type')]), # noqa
(self.input_node, surfstat, [('design_matrix', 'design_matrix')]), # noqa
(self.input_node, surfstat, [('contrast', 'contrast')]), # noqa
(self.input_node, surfstat, [('str_format', 'str_format')]), # noqa
(self.input_node, surfstat, [('glm_type', 'glm_type')]), # noqa
(self.input_node, surfstat, [('group_label', 'group_label')]), # noqa
(self.input_node, surfstat, [('full_width_at_half_maximum', 'full_width_at_half_maximum')]), # noqa
(self.input_node, surfstat, [('threshold_uncorrected_pvalue', 'threshold_uncorrected_pvalue')]), # noqa
(self.input_node, surfstat, [('threshold_corrected_pvalue', 'threshold_corrected_pvalue')]), # noqa
(self.input_node, surfstat, [('cluster_threshold', 'cluster_threshold')]), # noqa
(self.input_node, surfstat, [('surface_file', 'surface_file')]), # noqa
(self.input_node, surfstat, [('feature_label', 'feature_label')]),
(data_prep, surfstat, [('surfstat_input_dir', 'input_directory')]), # noqa
(data_prep, surfstat, [('path_to_matscript', 'path_to_matscript')]), # noqa
(data_prep, surfstat, [('output_directory', 'output_directory')]), # noqa
(data_prep, surfstat, [('freesurfer_home', 'freesurfer_home')]), # noqa
(self.input_node, jsondict, [('glm_type', 'glm_type')]), # noqa
(self.input_node, jsondict, [('design_matrix', 'design_matrix')]), # noqa
(self.input_node, jsondict, [('str_format', 'str_format')]), # noqa
(self.input_node, jsondict, [('contrast', 'contrast')]), # noqa
(self.input_node, jsondict, [('group_label', 'group_label')]), # noqa
(self.input_node, jsondict, [('full_width_at_half_maximum', 'full_width_at_half_maximum')]), # noqa
(self.input_node, jsondict, [('threshold_uncorrected_pvalue', 'threshold_uncorrected_pvalue')]), # noqa
(self.input_node, jsondict, [('threshold_corrected_pvalue', 'threshold_corrected_pvalue')]), # noqa
(self.input_node, jsondict, [('cluster_threshold', 'cluster_threshold')]), # noqa
(data_prep, jsonsink, [('out_json', 'out_file')]), # noqa
(jsondict, jsonsink, [('json_dict', 'in_dict')]), # noqa
]) |
import { NotFoundException } from '@nestjs/common';
import { Test } from '@nestjs/testing';
import { TaskRepository } from 'src/entities/task.repository';
import { GetTasksFilterDto } from './dto/get-task.dto';
import { TaskStatus } from './task.enum';
import { TasksService } from './tasks.service';
const mockUser = {
id: 'b7eca235-9847-4ea3-ba75-796f79bd9b8f',
username: 'Test User',
};
const mockTaskRepository = () => ({
getTasks: jest.fn(),
findOne: jest.fn(),
});
describe('TasksService', () => {
let taskService: any;
let taskRepository: any;
beforeEach(async () => {
const module = await Test.createTestingModule({
providers: [
TasksService,
{ provide: TaskRepository, useFactory: mockTaskRepository },
],
}).compile();
taskService = await module.get<TasksService>(TasksService);
taskRepository = await module.get<TaskRepository>(TaskRepository);
});
describe('getTasks', () => {
it('get all task from repository', async () => {
taskRepository.getTasks.mockResolvedValue('someVal');
expect(taskRepository.getTasks).not.toHaveBeenCalled();
const filters: GetTasksFilterDto = {
search: 'Some search',
status: TaskStatus.IN_PROGRESS,
};
const result = await taskService.getTasks(filters, mockUser);
taskService.getTasks(filters, mockUser);
expect(taskRepository.getTasks).toHaveBeenCalled();
expect(result).toEqual('someVal');
});
});
describe('getTaskById', () => {
it('calls taskRepository.findOne() and succesffuly retrieve and return the task', async () => {
const mockTask = { title: 'Test task', description: 'Test desc' };
taskRepository.findOne.mockResolvedValue(mockTask);
const result = await taskService.getTaskById(
'b7eca235-9847-4ea3-ba75-796f79bd9b8f',
mockUser,
);
expect(result).toEqual(mockTask);
expect(taskRepository.findOne).toHaveBeenCalledWith({
where: {
id: 'b7eca235-9847-4ea3-ba75-796f79bd9b8f',
userId: mockUser.id,
},
});
});
it('throws an error as task is not found', () => {
taskRepository.findOne.mockResolvedValue(null);
expect(
taskService.getTaskById(
'b7eca235-9847-4ea3-ba75-796f79bd9b8f',
mockUser,
),
).rejects.toThrow(NotFoundException);
});
});
}); |
<?php
namespace App\Console\Commands;
use App\Interfaces\CategoryInterface;
use App\Interfaces\ProductInterface;
use App\Repositories\CategoryRepository;
use App\Repositories\ProductRepository;
use Exception;
use Illuminate\Console\Command;
class Product extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'product:create';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Execute the console command.
*/
public function handle(CategoryRepository $categoryRepository, ProductRepository $productRepository)
{
$categories = $categoryRepository->getForConsole();
$names = [];
$selectedCategories = [];
$names[0] = 'Select this when you done choosing categories';
foreach ($categories as $value) {
$names[$value->id] = $value->name;
}
$name = $this->ask('enter product name: ');
$price = $this->ask('enter product price: ');
$description = $this->ask('enter product description: ');
do {
$category_name = $this->choice('Choose Category: ', $names);
$category_id = array_search($category_name, $names);
if ($category_id != 0 && !in_array($category_id, $selectedCategories)) {
array_push($selectedCategories, $category_id);
}
} while ($category_id);
$data = ['name' => $name ];
$data['price'] = $price;
$data['description'] = $description;
$data['categories'] = $selectedCategories;
$data['image'] = 'default.png';
try {
$productRepository->create($data);
$this->info('Product has been created successfully');
} catch (Exception $e) {
$this->error("Error: error occured when trying to create product, please verify you inputs and try again");
}
}
} |
# dashboard7.py
import streamlit as st
import pandas as pd
import plotly.express as px
import plotly.graph_objects as go
def main():
# Assuming df_startup is already defined and contains the necessary data
# If not, load your data and perform the required processing
# Load data
file_path = r"startup_funding_all_years.csv"
df_startup = pd.read_csv(file_path)
# Group the DataFrame by 'HeadQuarter' and calculate the total funding amount received by startups in each region
funding_by_region = df_startup.groupby('HeadQuarter')['Amount($)'].sum()
# Sort the regions by total funding amount and take only the top 20
top20 = funding_by_region.sort_values(ascending=False)[:20]
# Create an interactive scatter plot using Plotly Express with a different color scale (Viridis)
fig = px.scatter(
x=top20.index,
y=[1] * len(top20),
size=top20.values / 5000000,
size_max=50,
color=top20.values,
labels={'size': 'Total Funding Amount ($ Billions)'},
title='Total Funding Received by the Top 20 Startups in Each Region',
template='plotly_dark',
color_continuous_scale='teal', # Change color scale here
hover_name=top20.index, # Show region names on hover
)
# # Customize layout for the plot
# fig.update_layout(
# xaxis_title='Region',
# yaxis_title='Size of bubble represents total funding amount ($ Billions)',
# xaxis=dict(tickangle=45),
# )
fig.update_layout(
height=600, # Adjust the height
width=800, # Adjust the width
)
# Display the interactive plot
st.plotly_chart(fig)
# Additional interactivity
st.markdown("## Additional Options")
# Option for selected region details
selected_region = st.selectbox('Select a Region:', top20.index)
st.write(f"Total funding received by {selected_region}: ${funding_by_region[selected_region] / 1e9:.2f} Billion")
# Highlight the selected region on the plot
selected_city_mask = df_startup['HeadQuarter'] == selected_region
fig.add_trace(
go.Scatter(
x=[selected_region],
y=[1],
mode='markers',
marker=dict(size=funding_by_region[selected_region] / 5000000, color='red'),
name=f'Selected: {selected_region}',
showlegend=False,
)
)
# Option for summary statistics
st.markdown("## Summary Statistics")
st.write(f"Total funding received by the top 20 startups: ${top20.sum() / 1e9:.2f} Billion")
if __name__ == "__main__":
main() |
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 100
struct Node
{
int data;
struct Node *next;
};
struct Graph
{
int numVertices;
struct Node *adjList[MAX_VERTICES];
int visited[MAX_VERTICES];
};
struct QueueNode
{
int data;
struct QueueNode *next;
};
struct Queue
{
struct QueueNode *front, *rear;
};
struct Node *createNode(int data)
{
struct Node *newNode = (struct Node *)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
struct Graph *createGraph(int vertices)
{
struct Graph *graph = (struct Graph *)malloc(sizeof(struct Graph));
graph->numVertices = vertices;
for (int i = 0; i < vertices; ++i)
{
graph->adjList[i] = NULL;
graph->visited[i] = 0;
}
return graph;
}
void addEdge(struct Graph *graph, int src, int dest)
{
struct Node *newNode = createNode(dest);
newNode->next = graph->adjList[src];
graph->adjList[src] = newNode;
newNode = createNode(src);
newNode->next = graph->adjList[dest];
graph->adjList[dest] = newNode;
}
struct QueueNode *createQueueNode(int data)
{
struct QueueNode *newNode = (struct QueueNode *)malloc(sizeof(struct QueueNode));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
struct Queue *createQueue()
{
struct Queue *queue = (struct Queue *)malloc(sizeof(struct Queue));
queue->front = queue->rear = NULL;
return queue;
}
void enqueue(struct Queue *queue, int data)
{
struct QueueNode *newNode = createQueueNode(data);
if (queue->rear == NULL)
{
queue->front = queue->rear = newNode;
return;
}
queue->rear->next = newNode;
queue->rear = newNode;
}
int dequeue(struct Queue *queue)
{
if (queue->front == NULL)
return -1; // Queue is empty
struct QueueNode *temp = queue->front;
int data = temp->data;
queue->front = temp->next;
if (queue->front == NULL)
queue->rear = NULL;
free(temp);
return data;
}
void BFS(struct Graph *graph, int startVertex)
{
struct Queue *queue = createQueue();
graph->visited[startVertex] = 1;
enqueue(queue, startVertex);
while (queue->front != NULL)
{
int currentVertex = dequeue(queue);
printf("%d ", currentVertex);
struct Node *temp = graph->adjList[currentVertex];
while (temp != NULL)
{
int adjVertex = temp->data;
if (!graph->visited[adjVertex])
{
graph->visited[adjVertex] = 1;
enqueue(queue, adjVertex);
}
temp = temp->next;
}
}
free(queue);
}
int main()
{
int vertices = 6;
struct Graph *graph = createGraph(vertices);
addEdge(graph, 0, 1);
addEdge(graph, 0, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 4);
addEdge(graph, 3, 5);
printf("Breadth-First Traversal starting from vertex 0:\n");
BFS(graph, 0);
return 0;
} |
package apps
import (
"e_wallet/domain/entities"
"e_wallet/domain/payload/request"
"e_wallet/infrastructure/persistences"
"sync"
)
type userApp struct {
repo persistences.Repositories
}
// AddUser implements UserAppInterface.
func (app *userApp) AddUser(request *request.UserRequest) (*entities.UserDetail, error) {
user := entities.UserFromUserRequest(request)
user, err := app.repo.User.Save(user)
if err != nil {
return nil, err
}
var (
profile *entities.UserProfile
wallet *entities.Wallet
)
var profileErr, walletErr error
var wg sync.WaitGroup
wg.Add(2)
go func() {
defer wg.Done()
profile = entities.NewUserProfile(user.ID, request)
profile, profileErr = app.repo.UserProfile.Save(profile)
}()
go func() {
defer wg.Done()
wallet = entities.NewWallet(user.ID)
wallet, walletErr = app.repo.Wallet.Save(wallet)
}()
wg.Wait()
if profileErr != nil {
return nil, profileErr
}
if walletErr != nil {
return nil, walletErr
}
userDetail := entities.NewUserDetail(user, profile, wallet)
return userDetail, nil
}
// GetAllUsers implements UserAppInterface.
func (app *userApp) GetAllUsers() ([]entities.User, error) {
return app.repo.User.FindAll()
}
// GetUserById implements UserAppInterface.
func (app *userApp) GetUserById(id string) (*entities.UserDetail, error) {
return app.repo.User.GetUserById(id)
}
func NewUserApp(repo persistences.Repositories) *userApp {
return &userApp{repo}
}
var _ UserAppInterface = &userApp{}
type UserAppInterface interface {
AddUser(request *request.UserRequest) (*entities.UserDetail, error)
GetAllUsers() ([]entities.User, error)
GetUserById(id string) (*entities.UserDetail, error)
} |
/*
* Copyright (C) 2020 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.build.api.component.analytics
import com.android.build.api.variant.AndroidResources
import com.android.build.api.variant.ApkPackaging
import com.android.build.api.variant.JniLibsApkPackaging
import com.android.build.api.variant.Renderscript
import com.android.build.api.variant.ResourcesPackaging
import com.android.build.api.variant.TestVariant
import com.android.build.gradle.internal.fixtures.FakeGradleProperty
import com.android.build.gradle.internal.fixtures.FakeGradleProvider
import com.android.build.gradle.internal.fixtures.FakeObjectFactory
import com.android.tools.build.gradle.internal.profile.VariantPropertiesMethodType
import com.google.common.truth.Truth
import com.google.wireless.android.sdk.stats.GradleBuildVariant
import org.junit.Before
import org.junit.Test
import org.mockito.Mock
import org.mockito.Mockito
import org.mockito.MockitoAnnotations
class AnalyticsEnabledTestVariantTest {
@Mock
lateinit var delegate: TestVariant
private val stats = GradleBuildVariant.newBuilder()
private lateinit var proxy: AnalyticsEnabledTestVariant
@Before
fun setup() {
MockitoAnnotations.initMocks(this)
proxy = AnalyticsEnabledTestVariant(delegate, stats, FakeObjectFactory.factory)
}
@Test
fun getApplicationId() {
Mockito.`when`(delegate.applicationId).thenReturn(FakeGradleProperty("myApp"))
Truth.assertThat(proxy.applicationId.get()).isEqualTo("myApp")
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(1)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.first().type
).isEqualTo(VariantPropertiesMethodType.APPLICATION_ID_VALUE)
Mockito.verify(delegate, Mockito.times(1))
.applicationId
}
@Test
fun getAndroidResources() {
val androidResources = Mockito.mock(AndroidResources::class.java)
Mockito.`when`(delegate.androidResources).thenReturn(androidResources)
Truth.assertThat(proxy.androidResources).isEqualTo(androidResources)
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(1)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.first().type
).isEqualTo(VariantPropertiesMethodType.AAPT_OPTIONS_VALUE)
Mockito.verify(delegate, Mockito.times(1))
.androidResources
}
@Test
fun testedApplicationId() {
Mockito.`when`(delegate.testedApplicationId).thenReturn(FakeGradleProvider("myApp"))
Truth.assertThat(proxy.testedApplicationId.get()).isEqualTo("myApp")
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(1)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.first().type
).isEqualTo(VariantPropertiesMethodType.TESTED_APPLICATION_ID_VALUE)
Mockito.verify(delegate, Mockito.times(1))
.testedApplicationId
}
@Test
fun instrumentationRunner() {
Mockito.`when`(delegate.instrumentationRunner).thenReturn(FakeGradleProperty("my_runner"))
Truth.assertThat(proxy.instrumentationRunner.get()).isEqualTo("my_runner")
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(1)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.first().type
).isEqualTo(VariantPropertiesMethodType.INSTRUMENTATION_RUNNER_VALUE)
Mockito.verify(delegate, Mockito.times(1))
.instrumentationRunner
}
@Test
fun handleProfiling() {
Mockito.`when`(delegate.handleProfiling).thenReturn(FakeGradleProperty(true))
Truth.assertThat(proxy.handleProfiling.get()).isEqualTo(true)
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(1)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.first().type
).isEqualTo(VariantPropertiesMethodType.HANDLE_PROFILING_VALUE)
Mockito.verify(delegate, Mockito.times(1))
.handleProfiling
}
@Test
fun functionalTest() {
Mockito.`when`(delegate.functionalTest).thenReturn(FakeGradleProperty(true))
Truth.assertThat(proxy.functionalTest.get()).isEqualTo(true)
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(1)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.first().type
).isEqualTo(VariantPropertiesMethodType.FUNCTIONAL_TEST_VALUE)
Mockito.verify(delegate, Mockito.times(1))
.functionalTest
}
@Test
fun testLabel() {
Mockito.`when`(delegate.testLabel).thenReturn(FakeGradleProperty("some_label"))
Truth.assertThat(proxy.testLabel.get()).isEqualTo("some_label")
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(1)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.first().type
).isEqualTo(VariantPropertiesMethodType.TEST_LABEL_VALUE)
Mockito.verify(delegate, Mockito.times(1))
.testLabel
}
@Test
fun getRenderscript() {
val renderscript = Mockito.mock(Renderscript::class.java)
Mockito.`when`(delegate.renderscript).thenReturn(renderscript)
// simulate a user configuring packaging options for jniLibs and resources
proxy.renderscript
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(1)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.first().type
).isEqualTo(VariantPropertiesMethodType.RENDERSCRIPT_VALUE)
Mockito.verify(delegate, Mockito.times(1)).renderscript
}
@Test
fun getApkPackaging() {
val apkPackaging = Mockito.mock(ApkPackaging::class.java)
val jniLibsApkPackagingOptions = Mockito.mock(JniLibsApkPackaging::class.java)
val resourcesPackagingOptions = Mockito.mock(ResourcesPackaging::class.java)
Mockito.`when`(apkPackaging.jniLibs).thenReturn(jniLibsApkPackagingOptions)
Mockito.`when`(apkPackaging.resources).thenReturn(resourcesPackagingOptions)
Mockito.`when`(delegate.packaging).thenReturn(apkPackaging)
// simulate a user configuring packaging options for jniLibs and resources
proxy.packaging.jniLibs
proxy.packaging.resources
Truth.assertThat(stats.variantApiAccess.variantPropertiesAccessCount).isEqualTo(4)
Truth.assertThat(
stats.variantApiAccess.variantPropertiesAccessList.map { it.type }
).containsExactlyElementsIn(
listOf(
VariantPropertiesMethodType.PACKAGING_OPTIONS_VALUE,
VariantPropertiesMethodType.JNI_LIBS_PACKAGING_OPTIONS_VALUE,
VariantPropertiesMethodType.PACKAGING_OPTIONS_VALUE,
VariantPropertiesMethodType.RESOURCES_PACKAGING_OPTIONS_VALUE
)
)
Mockito.verify(delegate, Mockito.times(1)).packaging
}
} |
module SessionsHelper
def log_in(person, person_type)
session["#{person_type}_id".to_sym] = person.id
end
def remember(person, person_type)
person.remember
cookies.permanent.encrypted["#{person_type}_id".to_sym] = person.id
cookies.permanent[:remember_token] = { value: person.remember_token, expires: 1.day.from_now.utc }
end
def forget(person, person_type)
person.forget
cookies.delete("#{person_type}_id".to_sym)
cookies.delete(:remember_token)
end
def current_person(person_type)
person_id = "#{person_type}_id".to_sym
if session[person_id]
@current_person ||= person_type.capitalize.constantize.find_by(id: session[person_id])
elsif cookies.encrypted[person_id]
person = person_type.capitalize.constantize.find_by(id: cookies.encrypted[person_id])
if person && person.authenticated?(:remember, cookies[:remember_token])
log_in(person, person_type)
@current_person = person
end
end
end
def logged_in?(person_type)
!current_person(person_type).nil?
end
def log_out(person_type)
forget(current_person(person_type), person_type)
person_id = "#{person_type}_id".to_sym
session.delete(person_id)
@current_person = nil
end
def current_person?(person, person_type)
person && person == current_person(person_type)
end
def redirect_back_or(default)
redirect_to(session[:forwarding_url] || default)
session.delete(:forwarding_url)
end
def store_location
# if request.get?
session[:forwarding_url] = request.original_url
end
end |
<mat-card>
<mat-toolbar color="primary">Cursos Disponíveis</mat-toolbar>
<div *ngIf="courses$ | async as courses; else loading">
<table mat-table [dataSource]="courses" class="mat-elevation-z8">
<!-- Position Column -->
<ng-container matColumnDef="position">
<th mat-header-cell *matHeaderCellDef>No.</th>
<td mat-cell *matCellDef="let course">{{ course.position }}</td>
</ng-container>
<!-- Name Column -->
<ng-container matColumnDef="name">
<th mat-header-cell *matHeaderCellDef>Curso</th>
<td mat-cell *matCellDef="let course">
{{ course.name }}
<mat-icon aria-hidden="false" arial-label="Categoria do Curso">{{
course.category | category
}}</mat-icon>
</td>
</ng-container>
<!-- Weight Column -->
<ng-container matColumnDef="weight">
<th mat-header-cell *matHeaderCellDef>Weight</th>
<td mat-cell *matCellDef="let element">{{ element.weight }}</td>
</ng-container>
<!-- Symbol Column -->
<ng-container matColumnDef="symbol">
<th mat-header-cell *matHeaderCellDef>Symbol</th>
<td mat-cell *matCellDef="let element">{{ element.symbol }}</td>
</ng-container>
<tr mat-header-row *matHeaderRowDef="displayedColums"></tr>
<tr mat-row *matRowDef="let row; columns: displayedColums"></tr>
</table>
</div>
<ng-template #loading>
<div class="loading-spinner">
<mat-spinner></mat-spinner>
</div>
</ng-template>
</mat-card> |
package com.fhzc.app.android.android.ui.view.adapter;
import android.content.Context;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import com.fhzc.app.android.android.ui.view.widget.im.AudioRenderView;
import com.fhzc.app.android.android.ui.view.widget.im.ImageRenderView;
import com.fhzc.app.android.android.ui.view.widget.im.ShareRenderView;
import com.fhzc.app.android.android.ui.view.widget.im.TextRenderView;
import com.fhzc.app.android.android.ui.view.widget.im.TimeRenderView;
import com.fhzc.app.android.configer.constants.Constant;
import com.fhzc.app.android.configer.enums.MessageType;
import com.fhzc.app.android.db.UserPreference;
import com.fhzc.app.android.models.AudioMessage;
import com.fhzc.app.android.models.ContactModel;
import com.fhzc.app.android.models.ImageMessage;
import com.fhzc.app.android.models.MessageEntity;
import com.fhzc.app.android.models.TextMessage;
import com.fhzc.app.android.utils.DateUtil;
import com.fhzc.app.android.utils.im.CommonUtil;
import java.util.ArrayList;
/**
* @author : yingmu on 15-1-8.
* @email : yingmu@mogujie.com.
*/
public class MessageAdapter extends BaseAdapter {
private ArrayList<Object> msgObjectList = new ArrayList<>();
private Context ctx;
/**
* 依赖整体session状态的
*/
public MessageAdapter(Context ctx) {
this.ctx = ctx;
}
/**
* ----------------------添加历史消息-----------------
*/
public void addItem(final MessageEntity msg) {
int nextTime = msg.getCreated();
if (getCount() > 0) {
Object object = msgObjectList.get(getCount() - 1);
if (object instanceof MessageEntity) {
long preTime = ((MessageEntity) object).getCreated();
boolean needTime = DateUtil.needDisplayTime(preTime, nextTime);
if (needTime) {
int in = nextTime;
msgObjectList.add(in);
}
}
} else {
int in = msg.getCreated();
msgObjectList.add(in);
}
if (msg.getDisplayType().equals(Constant.SHOW_ORIGIN_TEXT_TYPE)) {
msgObjectList.add(new TextMessage(msg));
}
if (msg.getDisplayType().equals(Constant.SHOW_AUDIO_TYPE)) {
msgObjectList.add(AudioMessage.parse(msg));
}
if (msg.getDisplayType().equals(Constant.SHOW_IMAGE_TYPE)) {
msgObjectList.add(new ImageMessage(msg));
}
notifyDataSetChanged();
}
@Override
public int getCount() {
if (null == msgObjectList) {
return 0;
} else {
return msgObjectList.size();
}
}
@Override
public int getViewTypeCount() {
return MessageType.values().length;
}
@Override
public int getItemViewType(int position) {
try {
/**默认是失败类型*/
MessageType type = MessageType.MESSAGE_TYPE_INVALID;
Object obj = msgObjectList.get(position);
if (obj instanceof Integer) {
type = MessageType.MESSAGE_TYPE_TIME_TITLE;
} else if (obj instanceof MessageEntity) {
MessageEntity info = (MessageEntity) obj;
boolean isMine = info.getFromId() == UserPreference.getUid();
switch (info.getDisplayType()) {
case Constant.SHOW_AUDIO_TYPE:
type = isMine ? MessageType.MESSAGE_TYPE_MINE_AUDIO
: MessageType.MESSAGE_TYPE_OTHER_AUDIO;
break;
case Constant.SHOW_IMAGE_TYPE:
type = isMine ? MessageType.MESSAGE_TYPE_MINE_IMAGE
: MessageType.MESSAGE_TYPE_OTHER_IMAGE;
break;
case Constant.SHOW_ORIGIN_TEXT_TYPE:
if (CommonUtil.isShareText(info.getContent())) {
type = isMine ? MessageType.MESSAGE_TYPE_MINE_SHARE
: MessageType.MESSAGE_TYPE_OTHER_SHARE;
} else {
type = isMine ? MessageType.MESSAGE_TYPE_MINE_TEXT
: MessageType.MESSAGE_TYPE_OTHER_TEXT;
}
break;
default:
break;
}
}
return type.ordinal();
} catch (Exception e) {
return MessageType.MESSAGE_TYPE_INVALID.ordinal();
}
}
@Override
public Object getItem(int position) {
if (position >= getCount() || position < 0) {
return null;
}
return msgObjectList.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
/**
* 时间气泡的渲染展示
*/
private View timeBubbleRender(int position, View convertView, ViewGroup parent) {
TimeRenderView timeRenderView;
Integer timeBubble = (Integer) msgObjectList.get(position);
if (null == convertView) {
timeRenderView = TimeRenderView.inflater(ctx, parent);
} else {
// 不用再使用tag 标签了
timeRenderView = (TimeRenderView) convertView;
}
timeRenderView.setTime(timeBubble);
return timeRenderView;
}
public void updateItemState(MessageEntity messageEntity, boolean success) {
for (Object o : msgObjectList) {
if (!(o instanceof Integer)) {
if (((MessageEntity) o).getLocId() == messageEntity.getLocId()) {
((MessageEntity) o).setStatus(success ? Constant.MSG_SUCCESS : Constant.MSG_FAILURE);
((MessageEntity) o).setId(success ? messageEntity.getId() : 0);
notifyDataSetChanged();
return;
}
}
}
}
/**
* 1.头像事件
* mine:事件 other事件
* 图片的状态 消息收到,没收到,图片展示成功,没有成功
* 触发图片的事件 【长按】
* <p/>
* 图片消息类型的render
*
* @param position
* @param convertView
* @param parent
* @param isMine
* @return
*/
private View imageMsgRender(final int position, View convertView, final ViewGroup parent, final boolean isMine) {
ImageRenderView imageRenderView;
final ImageMessage imageMessage = (ImageMessage) msgObjectList.get(position);
ContactModel userEntity = new ContactModel();
if (null == convertView) {
imageRenderView = ImageRenderView.inflater(ctx, parent, isMine);
} else {
imageRenderView = (ImageRenderView) convertView;
}
imageRenderView.render(imageMessage, userEntity, ctx);
return imageRenderView;
}
/**
* 语音的路径,判断收发的状态
* 展现的状态
* 播放动画相关
* 获取语音的读取状态/
* 语音长按事件
*
* @param position
* @param convertView
* @param parent
* @param isMine
* @return
*/
private View audioMsgRender(final int position, View convertView, final ViewGroup parent, final boolean isMine) {
AudioRenderView audioRenderView;
final AudioMessage audioMessage = (AudioMessage) msgObjectList.get(position);
ContactModel entity = new ContactModel();
if (null == convertView) {
audioRenderView = AudioRenderView.inflater(ctx, parent, isMine);
} else {
audioRenderView = (AudioRenderView) convertView;
}
audioRenderView.setBtnImageListener(new AudioRenderView.BtnImageListener() {
@Override
public void onClickUnread() {
}
@Override
public void onClickReaded() {
}
});
audioRenderView.render(audioMessage, entity, ctx);
return audioRenderView;
}
/**
* text类型的: 1. 设定内容Emoparser
* 2. 点击事件 单击跳转、 双击方法、长按pop menu
* 点击头像的事件 跳转
*
* @param position
* @param convertView
* @param viewGroup
* @param isMine
* @return
*/
private View textMsgRender(final int position, View convertView, final ViewGroup viewGroup, final boolean isMine) {
TextRenderView textRenderView;
final TextMessage textMessage = (TextMessage) msgObjectList.get(position);
ContactModel userEntity = new ContactModel();
if (null == convertView) {
textRenderView = TextRenderView.inflater(ctx, viewGroup, isMine); //new TextRenderView(ctx,viewGroup,isMine);
} else {
textRenderView = (TextRenderView) convertView;
}
textRenderView.render(textMessage, userEntity, ctx);
return textRenderView;
}
/**
* text类型的: 1. 设定内容Emoparser
* 2. 点击事件 单击跳转、 双击方法、长按pop menu
* 点击头像的事件 跳转
*
* @param position
* @param convertView
* @param viewGroup
* @param isMine
* @return
*/
private View shareMsgRender(final int position, View convertView, final ViewGroup viewGroup, final boolean isMine) {
ShareRenderView textRenderView;
final TextMessage textMessage = (TextMessage) msgObjectList.get(position);
if (null == convertView) {
textRenderView = ShareRenderView.inflater(ctx, viewGroup, isMine); //new TextRenderView(ctx,viewGroup,isMine);
} else {
textRenderView = (ShareRenderView) convertView;
}
textRenderView.render(textMessage, ctx);
return textRenderView;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
final int typeIndex = getItemViewType(position);
MessageType renderType = MessageType.values()[typeIndex];
// 改用map的形式
switch (renderType) {
case MESSAGE_TYPE_INVALID:
// 直接返回
break;
case MESSAGE_TYPE_TIME_TITLE:
convertView = timeBubbleRender(position, convertView, parent);
break;
case MESSAGE_TYPE_MINE_AUDIO:
convertView = audioMsgRender(position, convertView, parent, true);
break;
case MESSAGE_TYPE_OTHER_AUDIO:
convertView = audioMsgRender(position, convertView, parent, false);
break;
case MESSAGE_TYPE_MINE_IMAGE:
convertView = imageMsgRender(position, convertView, parent, true);
break;
case MESSAGE_TYPE_OTHER_IMAGE:
convertView = imageMsgRender(position, convertView, parent, false);
break;
case MESSAGE_TYPE_MINE_TEXT:
convertView = textMsgRender(position, convertView, parent, true);
break;
case MESSAGE_TYPE_OTHER_TEXT:
convertView = textMsgRender(position, convertView, parent, false);
break;
case MESSAGE_TYPE_MINE_SHARE:
convertView = shareMsgRender(position, convertView, parent, true);
break;
case MESSAGE_TYPE_OTHER_SHARE:
convertView = shareMsgRender(position, convertView, parent, false);
break;
}
return convertView;
}
} |
extends RichTextLabel
class_name LiveCaption
export(float) var duration = 4.0
export(float, 0, 0.5) var inter_duration = 0.1
export(String, "center", "left", "right", "fill") var alignment = "center"
export(Color) var default_color = Color.white
export(int) var shake_rate = 40
export(int) var shake_level = 7
enum CAPTION_MOOD {
DEFAULT,
UPPERCASE,
QUOTE,
ITALIC,
BOLD,
SHAKE
}
var _timers = []
var _characters = {}
var _last_character: String
func _enter_tree():
bbcode_enabled = true
func _ready():
bbcode_enabled = true
scroll_active = true
scroll_following = true
func caption(text: String, force_clear: bool = false, timeout: float = duration):
if force_clear:
bbcode_text = ""
for timer in _timers:
timer.disconnect("timeout", self, "on_timeout")
_timers.clear()
yield(get_tree().create_timer(inter_duration), "timeout")
var caption = align_text("%s" % text)
append_bbcode(caption)
newline()
var timer = get_tree().create_timer(timeout)
timer.connect("timeout", self, "on_timeout", [timer])
_timers.append(timer)
func dialog(character: String, text: String, mood: int = CAPTION_MOOD.DEFAULT, force_clear: bool = false, timeout: float = duration):
var config = _get_character(character)
var template = mood_text(text, mood)
if _last_character != character:
template = "[%s] %s" % [character, mood_text(text, mood)]
template = color_text(template, config.color)
_last_character = character
caption(template, force_clear, timeout)
func descriptive(text: String, force_clear: bool = false, timeout: float = duration):
_last_character = ""
var template = color_text("[%s]" % text, default_color)
caption(template, force_clear, timeout)
func set_character_color(character: String, color: Color):
var config = _get_character(character)
config['color'] = color
_characters[character] = config
func _get_character(character: String):
if _characters.has(character):
return _characters[character]
else:
return {
'color': default_color
}
func color_text(caption: String, color: Color):
return "[color=#%s]%s[/color]" % [color.to_html(), caption]
func align_text(caption: String):
match alignment:
"left":
return caption
"center":
return "[center]%s[/center]" % caption
"right":
return "[right]%s[/right]" % caption
"fill":
return "[fill]%s[/fill]" % caption
func mood_text(caption: String, mood: int):
match mood:
CAPTION_MOOD.DEFAULT:
return caption
CAPTION_MOOD.UPPERCASE:
return caption.to_upper()
CAPTION_MOOD.QUOTE:
return '[i]"%s"[/i]' % caption
CAPTION_MOOD.ITALIC:
return '[i]%s[/i]' % caption
CAPTION_MOOD.BOLD:
return '[b]%s[/b]' % caption
CAPTION_MOOD.SHAKE:
return '[shake rate=%s level=%s]%s[/shake]' % [shake_rate, shake_level, caption]
func on_timeout(timer: SceneTreeTimer):
_timers.erase(timer)
if get_line_count() > 2:
remove_line(0)
else:
_last_character = ""
remove_line(0)
update() |
using System.Security;
using Core.Entities;
using Core.Interfaces;
using Core.Specifications;
using Microsoft.EntityFrameworkCore;
namespace Infrastructure.Data
{
public class GenericRepository<T> : IGenericRepository<T> where T : BaseEntity // We need to match the constraints here
{
private readonly StoreContext _context;
public GenericRepository(StoreContext context)
{
_context = context;
}
public async Task<T> GetByIdAsync(int id)
{
// Set creates a .DbSet around the T type which we can use to query
// We cant use .Include() here as we don't know what type T is
return await _context.Set<T>().FindAsync(id);
}
public async Task<T> GetEntityWithSpec(ISpecification<T> spec)
{
return await ApplySpecification(spec).FirstOrDefaultAsync();
}
public async Task<IReadOnlyList<T>> ListAllAsync()
{
return await _context.Set<T>().ToListAsync();
}
public async Task<IReadOnlyList<T>> ListAsync(ISpecification<T> spec)
{
return await ApplySpecification(spec).ToListAsync();
}
public async Task<int> CountAsync(ISpecification<T> spec)
{
return await ApplySpecification(spec).CountAsync();
}
private IQueryable<T> ApplySpecification(ISpecification<T> spec)
{
// Note that .AsQueryable is not really needed here, as .Set<T> returns a DbSet<T> which already derives from IQueryable<T>
return SpecificationEvaluator<T>.GetQuery(_context.Set<T>().AsQueryable(), spec);
}
public void Add(T entity)
{
// DbSet.Add tracks an adding change
_context.Set<T>().Add(entity);
}
public void Update(T entity)
{
// Marking the entity as modified to be changed when .SaveChangesAsync is called
_context.Set<T>().Attach(entity);
_context.Entry(entity).State = EntityState.Modified;
}
public void Delete(T entity)
{
// Track the remove
_context.Set<T>().Remove(entity);
}
}
} |
/**
* File: ParagraphSeparator.ts
*
* Author: Herbert Baier (herbert.baier@uni-wuerzburg.de)
* Date: 06.03.2023
*/
import {LineEntity} from '../LineEntity';
import {XmlElementNode, xmlElementNode} from 'simple_xml';
/**
* Defines paragraph separators.
*
* @author <a href="mailto:herbert.baier@uni-wuerzburg.de">Herbert Baier</a>
* @version 1.0
*/
export class ParagraphSeparator implements LineEntity {
static readonly xmlTag_single: string = 'parsep';
static readonly xmlTag_double: string = 'parsep_dbl';
/**
* The pattern for single paragraph separators.
*/
public static readonly patternSingles = ['§', '¬¬¬'];
/**
* The pattern for double paragraph separators.
*/
public static readonly patternDoubles = ['§§', '==='];
/**
* True if the paragraph separator is single. Otherwise it is double.
*/
private readonly isSingle: boolean;
/**
* Creates a paragraph separator.
*
* @param isSingle True if the paragraph separator is simple.
*/
public constructor(isSingle: boolean) {
this.isSingle = isSingle;
}
public exportXml(): XmlElementNode {
return xmlElementNode(this.isSingle ? ParagraphSeparator.xmlTag_single : ParagraphSeparator.xmlTag_double, {}, []);
}
} |
//
// Created by tu on 02/12/2023.
//
#include "heap_sort.h"
// To heapify a subtree rooted with node i
// which is an index in arr[].
// n is size of heap
void heapify(void *arr[], int n, int i, CompareFunction compare)
{
// Find largest among root,
// left child and right child
// Initialize largest as root
int largestIndex = i;
// left = 2*i + 1
int leftIndex = 2 * i + 1;
// right = 2*i + 2
int rightIndex = 2 * i + 2;
// If left child is larger than root
if (leftIndex < n && compare(arr[leftIndex], arr[largestIndex]) > 0)
largestIndex = leftIndex;
// If right child is larger than largest
// so far
if (rightIndex < n && compare(arr[rightIndex], arr[largestIndex]) > 0)
largestIndex = rightIndex;
// Swap and continue heapify
// if root is not the largest
// If largest is not root
if (largestIndex != i) {
void *tmpV = arr[i];
arr[i] = arr[largestIndex];
arr[largestIndex] = tmpV;
// Recursively heapify the affected
// subtree
heapify(arr, n, largestIndex, compare);
}
}
void heapSort(void *arr[], size_t arrSize, CompareFunction compareFunction) {
// build max heap
for (int i = (arrSize / 2) - 1; i >= 0; i-- ) {
heapify(arr, arrSize, i, compareFunction);
}
// sort
for (int i = arrSize - 1; i >= 0; i--) {
void *tmpV = arr[0];
arr[0] = arr[i];
arr[i] = tmpV;
// Heapify root element
// to get highest element at
// root again
heapify(arr, i, 0, compareFunction);
}
} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - checking email</title>
<link rel="stylesheet" href="bootstrap.min.css" type="text/css" />
<style>
.gradient-custom {
display: block;
width: 100vw;
height: 100vh;
position: fixed;
top: 0;
left: 0;
/* fallback for old browsers */
background: #6a11cb;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right, rgba(106, 17, 203, 1), rgba(37, 117, 252, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right, rgba(106, 17, 203, 1), rgba(37, 117, 252, 1));
}
</style>
</head>
<body>
<div class="gradient-custom"></div>
<div class="container">
<section class="vh-100">
<div class="row d-flex justify-content-center align-items-center h-100">
<div class="col-12 col-md-8 col-lg-6 col-xl-5">
<div class="card bg-dark text-white" style="border-radius: 1rem;">
<div class="card-body p-5 text-center">
<form name="form1" action="/auth" method="post">
<div class="mb-md-5">
<h2 class="fw-bold mb-2 text-uppercase">Login</h2>
<p class="text-white-50 mb-5 pb-lg-2">Please enter your login and password!</p>
<div class="form-floating form-white mb-3">
<input type="email" id="typeEmailX" class="form-control form-control-lg"
placeholder="name@example.com" name="username"/>
<label class="form-label" for="typeEmailX">Email</label>
</div>
<div class="form-floating form-white mb-3">
<input type="password" id="typePasswordX" class="form-control form-control-lg"
placeholder="password" name="password"/>
<label class="form-label" for="typePasswordX">Password</label>
</div>
<p class="small mb-5 pb-lg-2"><a class="text-white-50" href="#">Forgot
password?</a></p>
<button class="btn btn-outline-light btn-lg px-5" type="submit">Login</button>
</div>
</form>
<div>
<span class="mb-0">Don't have an account? <a href="./register.html"
class="text-white-50 fw-bold">Sign Up</a>
</span>
</div>
</div>
</div>
</div>
</div>
</section>
</div>
<script>
function validateEmail(e) {
e.preventDefault();
const user_email = document.form1.typeEmailX;
const user_pass = document.form1.typePasswordX;
var mailformat = /^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/;
if (!user_email.value.match(mailformat)) {
alert("Invalid email address!");
user_email.focus();
return false;
}
else{
alert("Valid email");
}
}
</script>
</body>
</html> |
<?php
/**
* @package Lastfm Connector
*/
namespace Inc\Api;
class SettingsApi {
public $admin_pages = array();
public $settings = array();
public $sections = array();
public $fields = array();
public function register() {
if ( ! empty( $this->admin_pages ) ) {
add_action( 'admin_menu', array( $this, 'addAdminMenu' ) );
}
if ( ! empty( $this->settings ) ) {
add_action( 'admin_init', array( $this, 'registerCustomFields' ) );
}
}
public function addPages( array $pages ) {
$this->admin_pages = $pages;
return $this;
}
public function addAdminMenu() {
foreach ( $this->admin_pages as $page ) {
add_menu_page( $page['page_title'], $page['menu_title'], $page['capability'], $page['menu_slug'], $page['callback'], $page['icon_url'], $page['position'] );
}
}
public function setSettings( array $settings ) {
$this->settings = $settings;
return $this;
}
public function setSections( array $sections ) {
$this->sections = $sections;
return $this;
}
public function setFields( array $fields ) {
$this->fields = $fields;
return $this;
}
public function registerCustomFields() {
// register the setting
foreach ( $this->settings as $setting ) {
register_setting( $setting['option_group'], $setting['option_name'], ( isset( $setting['callback'] ) ? $setting['callback'] : '' ) );
}
// add settings section
foreach ( $this->sections as $section ) {
add_settings_section( $section['id'], $section['title'], ( isset( $section['callback'] ) ? $section['callback'] : '' ), $section['page'] );
}
foreach ( $this->fields as $field ) {
add_settings_field( $field['id'], $field['title'], ( isset( $field['callback'] ) ? $field['callback'] : '' ), $field['page'], $field['section'], ( isset( $field['args'] ) ? $field['args'] : '' ) );
}
// add settings field
}
} |
'use client'
import Image from "next/image";
import Link from 'next/link'
import Header from "@/components/Header";
import Modal from "@/components/Modal";
import { useState } from "react";
type SearchParamProps = {
searchParams: Record<string, string> | null | undefined;
};
export default function Home({ searchParams }: SearchParamProps) {
const show = searchParams?.show;
const [bookmark,setBookmark] = useState(false);
return (
<div className={ show ? "overflow-y-hidden relative flex flex-col bg-mobile md:bg-desktop bg-contain bg-top bg-no-repeat w-full h-screen" : " overflow-y-auto relative flex flex-col bg-mobile md:bg-desktop bg-contain bg-top bg-no-repeat w-full h-screen"}>
{show && (
<Modal />)}
<Header/>
<div className=" relative flex flex-col mt-20 md:mt-28 lg:mt-40 xl:mt-60 items-center space-y-10 pb-40">
<div className=" w-full absolute top-2 left-0 flex justify-center">
<Image
src={"/images/logo-mastercraft.svg"}
width={56}
height={56}
alt="mastercraft"/>
</div>
<div className=" w-11/12 md:w-3/4 lg:w-3/5 xl:w-1/2 bg-white rounded-xl shadow space-y-7 pt-16 pb-10 px-5 md:px-10">
<div className=" text-center font-bold text-3xl ">
Mastercraft Bamboo Monitor Riser
</div>
<div className=" text-gray-500 text-center">
A beautiful & handcrafted monitor stand to reduce neck and eye strain.
</div>
<div className=" w-full flex flex-row space-x-3 min-[410px]:space-x-5 justify-between">
<Link href="/?show=true" className=" text-center w-full min-[410px]:text-base text-xs md:w-auto bg-teal-500 hover:bg-teal-700 px-10 py-5 font-medium text-white rounded-full">
Back This Project
</Link>
<button onClick={()=>setBookmark(!bookmark)} className={ !bookmark ?"bg-gray-100 relative w-16 md:w-52 px-8 md:px-10 py-5 font-medium text-end rounded-full" : " bg-teal-50 relative w-16 md:w-52 px-8 md:px-10 py-5 font-medium text-end rounded-full"}>
<div className=" h-full absolute top-0 left-0">
<Image
src={!bookmark ? "/images/icon-bookmark.svg" : "/images/icon-bookmark2.svg"}
width={56}
height={56}
className=" bg-cover h-full w-16 md:w-full"
alt="bookmark"/>
</div>
<div className={!bookmark ? " hidden md:flex text-neutral-500 absolute top-0 right-0 h-full w-4/5 items-center justify-center " : "hidden md:flex text-teal-500 absolute top-0 right-0 h-full w-4/5 items-center justify-center "}>
{ !bookmark ? "Bookmark" : "Bookmarked"}
</div>
</button>
</div>
</div>
<div className=" w-11/12 md:w-3/4 lg:w-3/5 xl:w-1/2 bg-white flex flex-col p-10 space-y-10 rounded-xl shadow">
<div className=" flex flex-col md:flex-row space-y-7 md:space-y-0 justify-around items-center">
<div className=" flex flex-col space-y-2 md:pr-20 pb-10 md:pb-0 border-b md:border-b-0 border-gray-300 md:border-r text-center md:text-start">
<div className=" text-3xl font-bold ">
$89,914
</div>
<div className=" text-gray-500">
of $100,000 backed
</div>
</div>
<div className=" flex flex-col space-y-2 md:pr-20 pb-10 md:pb-0 border-b md:border-b-0 border-gray-300 md:border-r text-center md:text-start">
<div className=" text-3xl font-bold">
5,007
</div>
<div className=" text-gray-500">
total backers
</div>
</div>
<div className=" flex flex-col space-y-2 text-center md:text-start">
<div className=" text-3xl font-bold">
56
</div>
<div className=" text-gray-500">
days left
</div>
</div>
</div>
<div className=" w-full h-3 bg-gray-50 rounded-full relative">
<div className=" absolute top-0 left-0 h-3 w-[89%] bg-teal-500 rounded-full">
</div>
</div>
</div>
<div className=" w-11/12 md:w-3/4 lg:w-3/5 xl:w-1/2 bg-white flex flex-col p-8 md:p-10 space-y-10 rounded-xl shadow">
<div className=" font-semibold text-lg">
About this project
</div>
<div className=" text-gray-500 text-xs min-[425px]:text-base">
The Mastercraft Bamboo Monitor Riser is a sturdy and stylish platform that elevates your screen to a more comfortable viewing height. Placing your monitor at eye level has the potential to improve your posture and make you more comfortable while at work, helping you stay focused on the task at hand.
</div>
<div className=" text-gray-500 text-xs min-[425px]:text-base">
Featuring artisan craftsmanship, the simplicity of design creates extra desk space below your computer to allow notepads, pens, and USB sticks to be stored under the stand.
</div>
<div className=" flex flex-col space-y-5">
<div className=" flex flex-col px-7 md:px-10 py-7 space-y-7 border border-gray-300 rounded-md">
<div className=" flex flex-col md:flex-row justify-between">
<div className=" font-semibold text-lg">
Bamboo Stand
</div>
<div className=" text-teal-500 font-semibold">
Pledge $25 or more
</div>
</div>
<div className=" text-gray-500 text-xs min-[425px]:text-base">
You get an ergonomic stand made of natural bamboo. You've helped us launch our promotional campaign, and you’ll be added to a special Backer member list.
</div>
<div className=" flex flex-col space-y-3 md:space-y-0 md:flex-row justify-between">
<div className="flex flex-row items-center">
<div className=" text-3xl font-bold text-black">101</div>
<div className=" text-gray-500 ml-2">left</div>
</div>
<button className=" w-fit md:w-auto px-5 rounded-full font-medium py-3 bg-teal-500 hover:bg-teal-700 text-white">
Select Reward
</button>
</div>
</div>
<div className=" flex flex-col px-7 md:px-10 py-7 space-y-7 border border-gray-300 rounded-md">
<div className=" flex flex-col md:flex-row justify-between">
<div className=" font-semibold text-lg">
Black Edition Stand
</div>
<div className=" text-teal-500 font-semibold">
Pledge $75 or more
</div>
</div>
<div className=" text-gray-500 text-xs min-[425px]:text-base">
You get a Black Special Edition computer stand and a personal thank you. You’ll be added to our Backer member list. Shipping is included.
</div>
<div className=" flex flex-col space-y-3 md:space-y-0 md:flex-row justify-between">
<div className="flex flex-row items-center">
<div className=" text-3xl font-bold text-black">64</div>
<div className=" text-gray-500 ml-2">left</div>
</div>
<button className=" w-fit md:w-auto px-5 rounded-full font-medium py-3 bg-teal-500 hover:bg-teal-700 text-white">
Select Reward
</button>
</div>
</div>
<div className=" flex flex-col px-7 md:px-10 py-7 space-y-7 border border-gray-300 rounded-md">
<div className=" flex flex-col md:flex-row justify-between">
<div className=" font-semibold text-lg text-zinc-500">
Mahogany Special Stand
</div>
<div className=" text-[#90d5cd] font-semibold">
Pledge $200 or more
</div>
</div>
<div className=" text-gray-400 text-xs min-[425px]:text-base">
You get two Special Edition Mahogany stands, a Backer T-Shirt, and a personal thank you. You’ll be added to our Backer member list. Shipping is included.
</div>
<div className=" flex flex-col space-y-3 md:space-y-0 md:flex-row justify-between">
<div className="flex flex-row items-center">
<div className=" text-3xl font-bold text-zinc-500">0</div>
<div className=" text-gray-400 ml-2">left</div>
</div>
<button disabled className=" w-fit md:w-auto px-5 rounded-full font-medium py-3 bg-zinc-300 text-white">
Out of stock
</button>
</div>
</div>
</div>
</div>
</div>
</div>
);
} |
package dao
import (
"context"
"time"
"restapi-golang-gin-gen/model"
"github.com/guregu/null"
"github.com/satori/go.uuid"
)
var (
_ = time.Second
_ = null.Bool{}
_ = uuid.UUID{}
)
// GetAllMaps_ is a function to get a slice of record(s) from maps table in the rocket_development database
// params - page - page requested (defaults to 0)
// params - pagesize - number of records in a page (defaults to 20)
// params - order - db sort order column
// error - ErrNotFound, db Find error
func GetAllMaps_(ctx context.Context, page, pagesize int64, order string) (results []*model.Maps_, totalRows int, err error) {
resultOrm := DB.Model(&model.Maps_{})
resultOrm.Count(&totalRows)
if page > 0 {
offset := (page - 1) * pagesize
resultOrm = resultOrm.Offset(offset).Limit(pagesize)
} else {
resultOrm = resultOrm.Limit(pagesize)
}
if order != "" {
resultOrm = resultOrm.Order(order)
}
if err = resultOrm.Find(&results).Error; err != nil {
err = ErrNotFound
return nil, -1, err
}
return results, totalRows, nil
}
// GetMaps_ is a function to get a single record from the maps table in the rocket_development database
// error - ErrNotFound, db Find error
func GetMaps_(ctx context.Context, argID int64) (record *model.Maps_, err error) {
record = &model.Maps_{}
if err = DB.First(record, argID).Error; err != nil {
err = ErrNotFound
return record, err
}
return record, nil
}
// AddMaps_ is a function to add a single record to maps table in the rocket_development database
// error - ErrInsertFailed, db save call failed
func AddMaps_(ctx context.Context, record *model.Maps_) (result *model.Maps_, RowsAffected int64, err error) {
db := DB.Save(record)
if err = db.Error; err != nil {
return nil, -1, ErrInsertFailed
}
return record, db.RowsAffected, nil
}
// UpdateMaps_ is a function to update a single record from maps table in the rocket_development database
// error - ErrNotFound, db record for id not found
// error - ErrUpdateFailed, db meta data copy failed or db.Save call failed
func UpdateMaps_(ctx context.Context, argID int64, updated *model.Maps_) (result *model.Maps_, RowsAffected int64, err error) {
result = &model.Maps_{}
db := DB.First(result, argID)
if err = db.Error; err != nil {
return nil, -1, ErrNotFound
}
if err = Copy(result, updated); err != nil {
return nil, -1, ErrUpdateFailed
}
db = db.Save(result)
if err = db.Error; err != nil {
return nil, -1, ErrUpdateFailed
}
return result, db.RowsAffected, nil
}
// DeleteMaps_ is a function to delete a single record from maps table in the rocket_development database
// error - ErrNotFound, db Find error
// error - ErrDeleteFailed, db Delete failed error
func DeleteMaps_(ctx context.Context, argID int64) (rowsAffected int64, err error) {
record := &model.Maps_{}
db := DB.First(record, argID)
if db.Error != nil {
return -1, ErrNotFound
}
db = db.Delete(record)
if err = db.Error; err != nil {
return -1, ErrDeleteFailed
}
return db.RowsAffected, nil
} |
package service
import (
"context"
"fmt"
"sync"
"time"
"github.com/google/uuid"
coapSync "github.com/plgd-dev/go-coap/v3/pkg/sync"
"github.com/plgd-dev/hub/v2/pkg/log"
kitNetGrpc "github.com/plgd-dev/hub/v2/pkg/net/grpc"
pkgTime "github.com/plgd-dev/hub/v2/pkg/time"
"github.com/plgd-dev/hub/v2/resource-aggregate/commands"
cqrsAggregate "github.com/plgd-dev/hub/v2/resource-aggregate/cqrs/aggregate"
"github.com/plgd-dev/hub/v2/resource-aggregate/cqrs/eventbus"
"github.com/plgd-dev/hub/v2/resource-aggregate/cqrs/eventstore"
"github.com/plgd-dev/hub/v2/resource-aggregate/cqrs/eventstore/mongodb"
"github.com/plgd-dev/hub/v2/resource-aggregate/events"
"go.uber.org/atomic"
"google.golang.org/grpc/codes"
"google.golang.org/grpc/status"
)
var ServiceUserID = uuid.NullUUID{Valid: true}.UUID.String()
const errFmtUpdateServiceMetadata = "cannot update service metadata: %w"
func validateUpdateServiceMetadata(request *commands.UpdateServiceMetadataRequest) error {
if request.GetUpdate() == nil {
return status.Errorf(codes.InvalidArgument, "invalid update")
}
if request.GetHeartbeat() == nil {
return status.Errorf(codes.InvalidArgument, "unexpected update %T", request.GetUpdate())
}
if request.GetHeartbeat().GetServiceId() == "" {
return status.Errorf(codes.InvalidArgument, "invalid heartbeat.serviceId")
}
if request.GetHeartbeat().GetTimeToLive() < int64(time.Second) {
return status.Errorf(codes.InvalidArgument, "invalid heartbeat.timeToLive(%v): is less than 1s", time.Duration(request.GetHeartbeat().GetTimeToLive()))
}
return nil
}
func (a *Aggregate) UpdateServiceMetadata(ctx context.Context, request *commands.UpdateServiceMetadataRequest) (events []eventstore.Event, err error) {
events, err = a.HandleCommand(ctx, request)
if err != nil {
err = fmt.Errorf("unable to process update service metadata command command: %w", err)
return
}
cleanUpToSnapshot(ctx, a, events)
return
}
func (a *Aggregate) ConfirmExpiredServices(ctx context.Context, request *events.ConfirmExpiredServicesRequest) (events []eventstore.Event, err error) {
events, err = a.HandleCommand(ctx, request)
if err != nil {
err = fmt.Errorf("unable to process update service metadata command command: %w", err)
return
}
cleanUpToSnapshot(ctx, a, events)
return
}
func (r RequestHandler) UpdateServiceMetadata(ctx context.Context, request *commands.UpdateServiceMetadataRequest) (*commands.UpdateServiceMetadataResponse, error) {
if err := validateUpdateServiceMetadata(request); err != nil {
return nil, log.LogAndReturnError(kitNetGrpc.ForwardErrorf(codes.InvalidArgument, errFmtUpdateServiceMetadata, err))
}
respChan := make(chan UpdateServiceMetadataResponseChanData, 1)
if err := r.serviceHeartbeat.ProcessRequest(UpdateServiceMetadataReqResp{
Request: request,
ResponseChan: respChan,
}); err != nil {
return nil, log.LogAndReturnError(kitNetGrpc.ForwardErrorf(codes.Internal, errFmtUpdateServiceMetadata, err))
}
select {
case <-ctx.Done():
return nil, log.LogAndReturnError(kitNetGrpc.ForwardErrorf(codes.Canceled, errFmtUpdateServiceMetadata, ctx.Err()))
case resp := <-respChan:
if resp.Err != nil {
return nil, resp.Err
}
return resp.Response, nil
}
}
type UpdateServiceMetadataReqResp struct {
Request *commands.UpdateServiceMetadataRequest
ResponseChan chan UpdateServiceMetadataResponseChanData
}
type ServiceHeartbeat struct {
logger log.Logger
config Config
eventstore *mongodb.EventStore
publisher eventbus.Publisher
wake chan struct{}
wakeExpired chan struct{}
closed atomic.Bool
wg sync.WaitGroup
offlineServices *coapSync.Map[string, *events.ServicesHeartbeat_Heartbeat]
private struct {
mutex sync.Mutex
requestQueue []UpdateServiceMetadataReqResp
}
}
func NewServiceHeartbeat(config Config, eventstore *mongodb.EventStore, publisher eventbus.Publisher, logger log.Logger) *ServiceHeartbeat {
s := &ServiceHeartbeat{
logger: logger,
config: config,
eventstore: eventstore,
publisher: publisher,
wake: make(chan struct{}, 1),
wakeExpired: make(chan struct{}, 1),
offlineServices: coapSync.NewMap[string, *events.ServicesHeartbeat_Heartbeat](),
private: struct {
mutex sync.Mutex
requestQueue []UpdateServiceMetadataReqResp
}{
requestQueue: make([]UpdateServiceMetadataReqResp, 0, 8),
},
}
s.wg.Add(2)
go func() {
defer s.wg.Done()
s.run()
}()
go func() {
defer s.wg.Done()
s.runProcessExpiredServices()
}()
return s
}
func (s *ServiceHeartbeat) handleExpiredService(ctx context.Context, aggregate *Aggregate, service *events.ServicesHeartbeat_Heartbeat) error {
const limitNumDevices = 256
for {
devices, err := s.eventstore.LoadDeviceMetadataByServiceIDs(ctx, []string{service.GetServiceId()}, limitNumDevices)
if err != nil {
return fmt.Errorf("cannot load devices for expired services %v: %w", service.GetServiceId(), err)
}
if len(devices) == 0 {
_, err := aggregate.ConfirmExpiredServices(ctx, &events.ConfirmExpiredServicesRequest{
Heartbeat: []*events.ServicesHeartbeat_Heartbeat{service},
})
if err != nil {
return fmt.Errorf("cannot confirm expired services metadata: %w", err)
}
return nil
}
for _, d := range devices {
err := s.updateDeviceToExpired(ctx, d.ServiceID, d.DeviceID, ServiceUserID)
if err != nil {
return fmt.Errorf("cannot update device %v to expired because service %v is expired: %w", d.DeviceID, d.ServiceID, err)
}
}
}
}
type UpdateServiceMetadataResponseChanData struct {
Response *commands.UpdateServiceMetadataResponse
Err error
}
func (s *ServiceHeartbeat) updateServiceMetadata(aggregate *Aggregate, r UpdateServiceMetadataReqResp) {
publishEvents, err := aggregate.UpdateServiceMetadata(context.Background(), r.Request)
if err != nil {
select {
case r.ResponseChan <- UpdateServiceMetadataResponseChanData{
Err: log.LogAndReturnError(kitNetGrpc.ForwardErrorf(codes.InvalidArgument, errFmtUpdateServiceMetadata, err)),
}: // sent
default:
}
}
var heartbeatValidUntil int64
for _, e := range publishEvents {
if ev, ok := e.(*events.ServiceMetadataUpdated); ok {
for _, s := range ev.GetServicesHeartbeat().GetValid() {
if s.GetServiceId() == r.Request.GetHeartbeat().GetServiceId() {
heartbeatValidUntil = s.GetValidUntil()
break
}
}
}
}
select {
case r.ResponseChan <- UpdateServiceMetadataResponseChanData{
Response: &commands.UpdateServiceMetadataResponse{
HeartbeatValidUntil: heartbeatValidUntil,
},
}: // sent
default:
}
}
func (s *ServiceHeartbeat) processRequest(r UpdateServiceMetadataReqResp) time.Time {
resID := commands.NewResourceID(s.config.HubID, commands.ServicesResourceHref)
var snapshot *events.ServiceMetadataSnapshotTakenForCommand
newServicesMetadataFactoryModelFunc := func(ctx context.Context) (cqrsAggregate.AggregateModel, error) {
snapshot = events.NewServiceMetadataSnapshotTakenForCommand(ServiceUserID, ServiceUserID, s.config.HubID)
return snapshot, nil
}
nextWakeUp := pkgTime.MaxTime
aggregate, err := NewAggregate(resID, s.config.Clients.Eventstore.SnapshotThreshold, s.eventstore, newServicesMetadataFactoryModelFunc, cqrsAggregate.NewDefaultRetryFunc(s.config.Clients.Eventstore.ConcurrencyExceptionMaxRetry))
if err != nil {
s.logger.Errorf(errFmtUpdateServiceMetadata, err)
return nextWakeUp
}
s.updateServiceMetadata(aggregate, r)
for _, expired := range snapshot.GetServiceMetadataUpdated().GetServicesHeartbeat().GetExpired() {
s.offlineServices.Store(expired.GetServiceId(), expired)
}
for _, valid := range snapshot.GetServiceMetadataUpdated().GetServicesHeartbeat().GetValid() {
validUntil := pkgTime.Unix(0, valid.GetValidUntil())
if validUntil.Before(nextWakeUp) {
nextWakeUp = time.Unix(0, valid.GetValidUntil())
}
}
if s.offlineServices.Length() > 0 {
s.wakeUpProcessingExpired()
}
return nextWakeUp
}
func (s *ServiceHeartbeat) processRequests() time.Time {
nextWakeUp := pkgTime.MaxTime
for {
reqs := s.pop()
if len(reqs) == 0 {
return nextWakeUp
}
for _, r := range reqs {
if t := s.processRequest(r); t.Before(nextWakeUp) {
nextWakeUp = t
}
}
}
}
func (s *ServiceHeartbeat) processExpiredServices() {
offlineServices := s.offlineServices.CopyData()
ctx := context.Background()
resID := commands.NewResourceID(s.config.HubID, commands.ServicesResourceHref)
aggregate, err := NewAggregate(resID, s.config.Clients.Eventstore.SnapshotThreshold, s.eventstore, NewServicesMetadataFactoryModel(ServiceUserID, ServiceUserID, s.config.HubID), cqrsAggregate.NewDefaultRetryFunc(s.config.Clients.Eventstore.ConcurrencyExceptionMaxRetry))
if err != nil {
s.logger.Errorf(errFmtUpdateServiceMetadata, err)
return
}
for _, off := range offlineServices {
err := s.handleExpiredService(ctx, aggregate, off)
if err != nil {
s.logger.Errorf("cannot handle expired service %v: %w", off.GetServiceId(), err)
} else {
s.logger.Infof("all devices associated with expired service %v have been marked as offline", off.GetServiceId())
s.offlineServices.Delete(off.GetServiceId())
}
}
}
func (s *ServiceHeartbeat) runProcessExpiredServices() {
for {
if s.closed.Load() {
return
}
<-s.wakeExpired
s.processExpiredServices()
}
}
func (s *ServiceHeartbeat) pushCheckExpiredServices() {
s.private.mutex.Lock()
defer s.private.mutex.Unlock()
for _, req := range s.private.requestQueue {
// check if request is already in queue
if req.Request.GetHeartbeat() != nil && req.Request.GetHeartbeat().GetServiceId() == "" && req.Request.GetHeartbeat().GetTimeToLive() == 0 {
return
}
}
s.private.requestQueue = append(s.private.requestQueue, UpdateServiceMetadataReqResp{
Request: &commands.UpdateServiceMetadataRequest{
Update: &commands.UpdateServiceMetadataRequest_Heartbeat{
Heartbeat: &commands.ServiceHeartbeat{},
},
},
ResponseChan: make(chan UpdateServiceMetadataResponseChanData, 1),
})
}
func (s *ServiceHeartbeat) run() {
// wake up immediately to check expired services
timer := time.NewTimer(0)
defer timer.Stop()
for {
if s.closed.Load() {
return
}
select {
case <-s.wake:
case <-timer.C:
// push heartbeat to check expired services
s.pushCheckExpiredServices()
}
nextWakeUp := s.processRequests()
d := time.Until(nextWakeUp)
if d <= 0 {
d = time.Minute * 10
}
// stop timer and drain if it was not already expired
if !timer.Stop() {
select {
case <-timer.C:
default:
}
}
timer.Reset(d)
}
}
func (s *ServiceHeartbeat) push(req UpdateServiceMetadataReqResp) {
s.private.mutex.Lock()
defer s.private.mutex.Unlock()
s.private.requestQueue = append(s.private.requestQueue, req)
}
func (s *ServiceHeartbeat) pop() []UpdateServiceMetadataReqResp {
s.private.mutex.Lock()
defer s.private.mutex.Unlock()
reqs := s.private.requestQueue
s.private.requestQueue = make([]UpdateServiceMetadataReqResp, 0, 8)
return reqs
}
func (s *ServiceHeartbeat) ProcessRequest(r UpdateServiceMetadataReqResp) error {
if r.Request == nil {
return fmt.Errorf("invalid request")
}
if r.ResponseChan == nil {
return fmt.Errorf("invalid response channel")
}
s.push(r)
s.wakeUp()
return nil
}
func (s *ServiceHeartbeat) wakeUpProcessingExpired() {
select {
case s.wakeExpired <- struct{}{}:
default:
}
}
func (s *ServiceHeartbeat) wakeUp() {
select {
case s.wake <- struct{}{}:
default:
}
}
func (s *ServiceHeartbeat) Close() {
if s.closed.CompareAndSwap(false, true) {
s.wakeUp()
s.wakeUpProcessingExpired()
s.wg.Wait()
}
}
func (s *ServiceHeartbeat) updateDeviceToExpired(ctx context.Context, serviceID, deviceID, userID string) error {
resID := commands.NewResourceID(deviceID, commands.StatusHref)
var latestSnapshot *events.DeviceMetadataSnapshotTakenForCommand
deviceMetadataFactoryModel := func(ctx context.Context) (cqrsAggregate.AggregateModel, error) {
latestSnapshot = events.NewDeviceMetadataSnapshotTakenForCommand(userID, "", s.config.HubID)
return latestSnapshot, nil
}
aggregate, err := NewAggregate(resID, s.config.Clients.Eventstore.SnapshotThreshold, s.eventstore, deviceMetadataFactoryModel, cqrsAggregate.NewDefaultRetryFunc(s.config.Clients.Eventstore.ConcurrencyExceptionMaxRetry))
if err != nil {
return log.LogAndReturnError(kitNetGrpc.ForwardErrorf(codes.InvalidArgument, "cannot set device('%v') to offline state: %v", deviceID, err))
}
publishEvents, err := aggregate.UpdateDeviceToOffline(ctx, &events.UpdateDeviceToOfflineRequest{
DeviceID: deviceID,
ServiceID: serviceID,
})
if err != nil {
return log.LogAndReturnError(kitNetGrpc.ForwardErrorf(codes.Internal, "cannot set device('%v') to offline state: %v", deviceID, err))
}
// TODO: In the future, we need to retrieve the owner from the identity-store service.
PublishEvents(s.publisher, latestSnapshot.GetDeviceMetadataUpdated().GetAuditContext().GetOwner(), aggregate.DeviceID(), aggregate.ResourceID(), publishEvents, s.logger)
return nil
} |
# MemoTeca
This project is the result of learning from [Alura's Angular course](https://cursos.alura.com.br/course/angular-explorando-framework) and was generated with [Angular CLI](https://github.com/angular/angular-cli) version 15.2.1.
Briefly, MemoTeca is a platform to post, edit and delete (CRUD) memories, thoughts, quotes and reflections about your life.
## Technologies used





## Development server
Run `ng serve` for a dev server, or `ng serve --open` to automatically open in your web browser. Navigate to `http://localhost:4200/`. The application will automatically reload if you change any of the source files.
## Code scaffolding
Run `ng generate component component-name` to generate a new component. You can also use `ng generate directive|pipe|service|class|guard|interface|enum|module`.
## Build
Run `ng build` to build the project. The build artifacts will be stored in the `dist/` directory.
## Running unit tests
Run `ng test` to execute the unit tests via [Karma](https://karma-runner.github.io).
## Running end-to-end tests
Run `ng e2e` to execute the end-to-end tests via a platform of your choice. To use this command, you need to first add a package that implements end-to-end testing capabilities.
## Further help
To get more help on the Angular CLI use `ng help` or go check out the [Angular CLI Overview and Command Reference](https://angular.io/cli) page. |
import { useState } from 'react'
import {v4 as uuidv4} from 'uuid'
import './App.css'
import Header from './components/header/header'
import Formulario from './components/Formulario/Formulario'
import MiOrg from './components/MiOrg'
import Equipo from './components/Equipo'
import Footer from './components/Footer'
function App() {
const [mostarFormulario, actualizarMostrar] = useState(false)
const [colaboradores, actualizarColaboradores] = useState([
{
id: uuidv4(),
equipo: "Front End",
foto: "https://github.com/daveoval.png",
nombre: "Carlos Daivd",
puesto: "Programador",
fav: true,
},
{
id: uuidv4(),
equipo: "Front End",
foto: "https://images.pexels.com/photos/2896853/pexels-photo-2896853.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Lucio Sandalio",
puesto: "Programador",
fav: true,
},
{
id: uuidv4(),
equipo: "Front End",
foto: "https://images.pexels.com/photos/774909/pexels-photo-774909.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Corina Yanira",
puesto: "Programador",
fav: true,
},
{
id: uuidv4(),
equipo: "Front End",
foto: "https://images.pexels.com/photos/3992656/pexels-photo-3992656.png?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Maria Carmen Sara",
puesto: "Programador",
fav: false,
},
{
id: uuidv4(),
equipo: "Programacion",
foto: "https://github.com/daveoval.png",
nombre: "Albino Azahar",
puesto: "Programador",
fav: false,
},
{
id: uuidv4(),
equipo: "Programacion",
foto: "https://images.pexels.com/photos/2896853/pexels-photo-2896853.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Ylenia Fanny",
puesto: "Programador",
fav: true,
},
{
id: uuidv4(),
equipo: "Programacion",
foto: "https://images.pexels.com/photos/774909/pexels-photo-774909.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Ovidio Yasmin",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "Programacion",
foto: "https://images.pexels.com/photos/3992656/pexels-photo-3992656.png?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Crisóstomo Eva María",
puesto: "Programador"
},
//
{
id: uuidv4(),
equipo: "Data Science",
foto: "https://github.com/daveoval.png",
nombre: "Ximena Yairr",
puesto: "Data Science"
},
{
id: uuidv4(),
equipo: "Data Science",
foto: "https://images.pexels.com/photos/2896853/pexels-photo-2896853.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Plinio Héctor",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "Data Science",
foto: "https://images.pexels.com/photos/774909/pexels-photo-774909.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Dionisia Javi",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "Data Science",
foto: "https://images.pexels.com/photos/3992656/pexels-photo-3992656.png?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "María Josefa Ildefonso",
puesto: "Programador"
},
//
{
id: uuidv4(),
equipo: "Devops",
foto: "https://github.com/daveoval.png",
nombre: "José María Mía",
puesto: "Data Science"
},
{
id: uuidv4(),
equipo: "Devops",
foto: "https://images.pexels.com/photos/2896853/pexels-photo-2896853.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Ciriaco Florindar",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "Devops",
foto: "https://images.pexels.com/photos/774909/pexels-photo-774909.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Leopoldo Consuelo",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "Devops",
foto: "https://images.pexels.com/photos/3992656/pexels-photo-3992656.png?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Jéssica Amancio",
puesto: "Programador"
},
//
{
id: uuidv4(),
equipo: "UX y Diseño",
foto: "https://github.com/daveoval.png",
nombre: "Alexandra Yago",
puesto: "Data Science"
},
{
id: uuidv4(),
equipo: "UX y Diseño",
foto: "https://images.pexels.com/photos/2896853/pexels-photo-2896853.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Janeth Catalina",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "UX y Diseño",
foto: "https://images.pexels.com/photos/774909/pexels-photo-774909.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Ana Belén Verónica",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "UX y Diseño",
foto: "https://images.pexels.com/photos/3992656/pexels-photo-3992656.png?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Abundio Encarnación",
puesto: "Programador"
},
//
{
id: uuidv4(),
equipo: "Movil",
foto: "https://github.com/daveoval.png",
nombre: "Alexandra Yago",
puesto: "Data Science"
},
{
id: uuidv4(),
equipo: "Movil",
foto: "https://images.pexels.com/photos/2896853/pexels-photo-2896853.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Janeth Catalina",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "Movil",
foto: "https://images.pexels.com/photos/774909/pexels-photo-774909.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Ana Belén Verónica",
puesto: "Programador"
},
{
id: uuidv4(),
equipo: "Movil",
foto: "https://images.pexels.com/photos/3992656/pexels-photo-3992656.png?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1",
nombre: "Abundio Encarnación",
puesto: "Programador"
},
])
const [equipos, actualizarEquipos] = useState([
{
id: uuidv4(),
titulo: 'Programacion',
colorPrimario: '#57C278 ',
colorSecundario: '#D9F7E9'
},
{
id: uuidv4(),
titulo: 'Front End',
colorPrimario: '#82CFFA',
colorSecundario: '#E8F8FF'
},
{
id: uuidv4(),
titulo: 'Data Science',
colorPrimario: '#A6D157',
colorSecundario: '#F0F8E2'
},
{
id: uuidv4(),
titulo: 'Devops',
colorPrimario: '#E06B69',
colorSecundario: '#FDE7E8'
},
{
id: uuidv4(),
titulo: 'UX y Diseño',
colorPrimario: '#DB6EBF',
colorSecundario: '#FAE9F5'
},
{
id: uuidv4(),
titulo: 'Movil',
colorPrimario: '#FFBA05',
colorSecundario: '#FFF5D9'
},
{
id: uuidv4(),
titulo: 'Innovacion y Gestion',
colorPrimario: '#FF8A29',
colorSecundario: '#FFEEDF'
},
])
//Ternario -> condicion ? seMuestra : noSeMuestra
const cambiarMostrar = () =>{
actualizarMostrar(!mostarFormulario)
}
//Registrar colaborador
const registrarColaborador = (colaborador) => {
console.log("Nuevo colaborador", colaborador)
//Spread operator
actualizarColaboradores([...colaboradores, colaborador])
}
//Eliminar colaborador
const eliminarColaborador = (id) =>{
console.log("Eliminar colaborador", id)
const nuevosColaboradores = colaboradores.filter((colaborador) => colaborador.id !== id)
actualizarColaboradores(nuevosColaboradores)
}
//Actualizar color de equipo
const actualizarColor = (color, id) =>{
const equiposActualizados = equipos.map((equipo) =>{
if(equipo.id === id){
equipo.colorPrimario = color
}
return equipo
})
actualizarEquipos(equiposActualizados)
}
//Crear equipo
const crearEquipo = (nuevoEquipo) =>{
actualizarEquipos([...equipos, {...nuevoEquipo, id: uuidv4()}])
}
//
const like = (id) =>{
console.log("Like", id)
const colaboradoresAcualizados = colaboradores.map((colaborador) =>{
if(colaborador.id === id){
colaborador.fav = !colaborador.fav
}
return colaborador
})
actualizarColaboradores(colaboradoresAcualizados)
}
return (
<div>
<Header/>
{/* { mostarFormulario === true ? <Formulario/> : <></>} */}
{
mostarFormulario && <Formulario
equipos={equipos.map((equipo) => equipo.titulo)}
registrarColaborador={registrarColaborador}
crearEquipo={crearEquipo}
/>
}
<MiOrg cambiarMostrar={cambiarMostrar}/>
{
equipos.map((equipo, ) => <Equipo
datos={equipo}
key={equipo.titulo}
colaboradores={colaboradores.filter(colaborador => colaborador.equipo === equipo.titulo)}
eliminarColaborador={eliminarColaborador}
actualizarColor={actualizarColor}
like={like}
/>
)
}
<Footer/>
</div>
)
}
export default App |
import React, {useState, useEffect} from 'react'
import { Link, useLocation, useNavigate } from 'react-router-dom'
import {useDispatch, useSelector} from 'react-redux'
import {Form, Button, Row, Col} from 'react-bootstrap'
import Loader from '../components/Loader'
import Message from '../components/Message'
import { register } from '../actions/userActions'
import FormContainer from '../components/FormContainer'
function RegisterScreen() {
const [name, setName ] = useState('')
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const [confirmPassword, setConfirmPassword] = useState('')
const [message, setMessage ] = useState('')
const navigate = useNavigate()
const dispatch = useDispatch()
const location = useLocation()
const redirect = location.search
? location.search.split("=")[1]
: "/";
const userRegister = useSelector(state => state.userRegister)
const{error, loading, userInfo} = userRegister
useEffect(() => {
if (userInfo) {
navigate(redirect)
}
},[navigate,userInfo, redirect])
const submitHandler = (e) => {
e.preventDefault()
if (password !== confirmPassword) {
setMessage('Passwords do not match!')
}
else{
dispatch(register (name,email,password))
}
}
return (
<FormContainer>
<h1>Sign In</h1>
{message && <Message variant='primary'>{message}</Message>}
{loading && <Loader />}
{error && <Message variant='primary'>{error}</Message>}
<Form onSubmit={submitHandler}>
<Form.Group controlId='name'>
<Form.Label>Name:</Form.Label>
<Form.Control
required
type='name'
placeholder='Enter Name'
value={name}
onChange= {(e) => setName(e.target.value)}
>
</Form.Control>
</Form.Group>
<Form.Group controlId='email'>
<Form.Label>Email Address:</Form.Label>
<Form.Control
required
type='email'
placeholder='Enter Email'
value={email}
onChange= {(e) => setEmail(e.target.value)}
>
</Form.Control>
</Form.Group>
<Form.Group controlId='password'>
<Form.Label>Password:</Form.Label>
<Form.Control
required
type='password'
placeholder='Enter Password'
value={password}
onChange= {(e) => setPassword(e.target.value)}
>
</Form.Control>
</Form.Group>
<Form.Group controlId='confirmPassword'>
<Form.Label>Confirm Password:</Form.Label>
<Form.Control
required
type='password'
placeholder='Confirm Password'
value={confirmPassword}
onChange= {(e) =>setConfirmPassword(e.target.value)}
>
</Form.Control>
</Form.Group>
<Button type='submit' variant='primary' id="signup"> Sign In</Button>
<Row className='py-3'>
<Col>
Have an account? <Link to={ redirect ? `/login?redirect=${redirect}` : `/login`}>Sign In!</Link>
</Col>
</Row>
</Form>
</FormContainer>
)
}
export default RegisterScreen |
// Structurally identical
// Send Feedback
// Given two Generic trees, return true if they are structurally identical i.e. they are made of nodes with the same values arranged in the same way.
// Input format :
// Line 1 : Tree 1 elements in level order form separated by space (as per done in class). Order is -
// Root_data, n (No_Of_Child_Of_Root), n children, and so on for every element
// Line 2 : Tree 2 elements in level order form separated by space (as per done in class). Order is -
// Root_data, n (No_Of_Child_Of_Root), n children, and so on for every element
// Output format : true or false
// Sample Input 1 :
// 10 3 20 30 40 2 40 50 0 0 0 0
// 10 3 20 30 40 2 40 50 0 0 0 0
// Sample Output 1 :
// true
// Sample Input 2 :
// 10 3 20 30 40 2 40 50 0 0 0 0
// 10 3 2 30 40 2 40 50 0 0 0 0
// Sample Output 2:
// false
package Assignment;
public class CheckStructurallyIdentical {
public static boolean checkIdentical(TreeNode<Integer> root1, TreeNode<Integer> root2){
if (root1 == null && root2 == null)
return true;
if (root1.children.size() != root2.children.size())
return false;
if (root1.data != root2.data)
return false;
for (int i = 0; i < root1.children.size(); i++){
if (!checkIdentical(root1.children.get(i), root2.children.get(i)))
return false;
}
return true;
}
} |
package com.algotic.controllers;
import com.algotic.base.LogHandlerConfiguration;
import com.algotic.config.JwtHelper;
import com.algotic.data.repositories.TradesRepo;
import com.algotic.exception.AlgoticException;
import com.algotic.exception.CommonErrorCode;
import com.algotic.model.request.TradeRequest;
import com.algotic.model.response.*;
import com.algotic.services.TradeService;
import com.algotic.utils.AlgoticUtils;
import jakarta.validation.Valid;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.*;
@CrossOrigin
@RestController
@Slf4j
@RequestMapping("/api")
public class TradeController {
@Autowired
private TradeService tradeService;
@Autowired
private TradesRepo tradesRepo;
@Autowired
private LogHandlerConfiguration logConfig;
@Autowired
JwtHelper jwtHelper;
@CrossOrigin
@GetMapping("/trades")
public ResponseEntity<TradeSetupResponse> getTrades(int limit, int offset) {
log.info(logConfig
.getLogHandler()
.getInfoLog("Get All Trades", "All the trades will be shown here ", jwtHelper.getUserId(), null));
return tradeService.getTrades(limit, offset);
}
@CrossOrigin
@PostMapping("/trade")
public ResponseEntity<TradeWebhookResponse> saveTrade(
@Valid @RequestBody TradeRequest tradeRequest, BindingResult errors) {
if (errors.hasErrors()) {
AlgoticException exception = new AlgoticException(CommonErrorCode.BAD_REQUEST);
exception
.getErrorCode()
.setErrorMessage(errors.getAllErrors().stream()
.sorted((error1, error2) ->
error1.getDefaultMessage().compareTo(error2.getDefaultMessage()))
.toList()
.get(0)
.getDefaultMessage());
throw exception;
}
log.info(logConfig
.getLogHandler()
.getInfoLog("Save Trade", AlgoticUtils.objectToJsonString(tradeRequest), jwtHelper.getUserId(), null));
return tradeService.saveTrade(tradeRequest);
}
@CrossOrigin
@PostMapping("/trade/{tradeId}/{type}")
public ResponseEntity<GlobalMessageResponse> deleteTrade(@PathVariable String tradeId, @PathVariable String type) {
log.info(logConfig
.getLogHandler()
.getInfoLog("delete trade", "Delete the trade by the particular id", jwtHelper.getUserId(), null));
return tradeService.tradeActiveInactive(tradeId, type);
}
@CrossOrigin
@DeleteMapping("/trade/{id}")
public ResponseEntity<GlobalMessageResponse> deleteUser(@PathVariable String id) {
log.info(logConfig
.getLogHandler()
.getInfoLog("Delete trade ", "Delete trade by id ", jwtHelper.getUserId(), null));
return tradeService.deleteTrade(id);
}
} |
/* -*- Mode: C++; tab-width: 2; indent-tabs-mode: nil; c-basic-offset: 2; -*- */
/* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
#ifndef mozilla_ExtensionPolicyService_h
#define mozilla_ExtensionPolicyService_h
#include "mozilla/MemoryReporting.h"
#include "mozilla/extensions/WebExtensionPolicy.h"
#include "mozIExtensionProcessScript.h"
#include "nsCOMPtr.h"
#include "nsCycleCollectionParticipant.h"
#include "nsHashKeys.h"
#include "nsIAddonPolicyService.h"
#include "nsAtom.h"
#include "nsIMemoryReporter.h"
#include "nsIObserver.h"
#include "nsIObserverService.h"
#include "nsISupports.h"
#include "nsPointerHashKeys.h"
#include "nsRefPtrHashtable.h"
#include "nsTHashSet.h"
class nsIChannel;
class nsIObserverService;
class nsIPIDOMWindowInner;
class nsIPIDOMWindowOuter;
namespace mozilla {
namespace dom {
class Promise;
} // namespace dom
namespace extensions {
class DocInfo;
class DocumentObserver;
class WebExtensionContentScript;
} // namespace extensions
using extensions::DocInfo;
using extensions::WebExtensionPolicy;
class ExtensionPolicyService final : public nsIAddonPolicyService,
public nsIObserver,
public nsIMemoryReporter {
public:
NS_DECL_CYCLE_COLLECTION_CLASS_AMBIGUOUS(ExtensionPolicyService,
nsIAddonPolicyService)
NS_DECL_CYCLE_COLLECTING_ISUPPORTS
NS_DECL_NSIADDONPOLICYSERVICE
NS_DECL_NSIOBSERVER
NS_DECL_NSIMEMORYREPORTER
static mozIExtensionProcessScript& ProcessScript();
static ExtensionPolicyService& GetSingleton();
// Helper for fetching an AtomSet of restricted domains as configured by the
// extensions.webextensions.restrictedDomains pref. Safe to call from any
// thread.
static RefPtr<extensions::AtomSet> RestrictedDomains();
// Thread-safe AtomSet from extensions.quarantinedDomains.list.
static RefPtr<extensions::AtomSet> QuarantinedDomains();
static already_AddRefed<ExtensionPolicyService> GetInstance() {
return do_AddRef(&GetSingleton());
}
// Unlike the other methods on the ExtensionPolicyService, this method is
// threadsafe, and can look up a WebExtensionPolicyCore by hostname on any
// thread.
static RefPtr<extensions::WebExtensionPolicyCore> GetCoreByHost(
const nsACString& aHost);
WebExtensionPolicy* GetByID(const nsAtom* aAddonId) {
return mExtensions.GetWeak(aAddonId);
}
WebExtensionPolicy* GetByID(const nsAString& aAddonId) {
RefPtr<nsAtom> atom = NS_AtomizeMainThread(aAddonId);
return GetByID(atom);
}
WebExtensionPolicy* GetByURL(const extensions::URLInfo& aURL);
WebExtensionPolicy* GetByHost(const nsACString& aHost) const;
void GetAll(nsTArray<RefPtr<WebExtensionPolicy>>& aResult);
bool RegisterExtension(WebExtensionPolicy& aPolicy);
bool UnregisterExtension(WebExtensionPolicy& aPolicy);
bool RegisterObserver(extensions::DocumentObserver& aPolicy);
bool UnregisterObserver(extensions::DocumentObserver& aPolicy);
bool UseRemoteExtensions() const;
bool IsExtensionProcess() const;
bool GetQuarantinedDomainsEnabled() const;
nsresult InjectContentScripts(WebExtensionPolicy* aExtension);
protected:
virtual ~ExtensionPolicyService();
private:
ExtensionPolicyService();
void RegisterObservers();
void UnregisterObservers();
void CheckRequest(nsIChannel* aChannel);
void CheckDocument(dom::Document* aDocument);
void CheckContentScripts(const DocInfo& aDocInfo, bool aIsPreload);
already_AddRefed<dom::Promise> ExecuteContentScript(
nsPIDOMWindowInner* aWindow,
extensions::WebExtensionContentScript& aScript);
RefPtr<dom::Promise> ExecuteContentScripts(
JSContext* aCx, nsPIDOMWindowInner* aWindow,
const nsTArray<RefPtr<extensions::WebExtensionContentScript>>& aScripts);
void UpdateRestrictedDomains();
void UpdateQuarantinedDomains();
nsRefPtrHashtable<nsPtrHashKey<const nsAtom>, WebExtensionPolicy> mExtensions;
nsRefPtrHashtable<nsPtrHashKey<const extensions::DocumentObserver>,
extensions::DocumentObserver>
mObservers;
nsCOMPtr<nsIObserverService> mObs;
nsString mDefaultCSP;
nsString mDefaultCSPV3;
};
} // namespace mozilla
#endif // mozilla_ExtensionPolicyService_h |
# Wi-Fi CSI Human Activity Recognition Model
This project implements a human activity recognition (HAR) model using Wi-Fi Channel State Information (CSI) signals. CSI data, obtained from Wi-Fi devices, provides fine-grained information about the wireless channel, including signal amplitude, phase, and frequency. Leveraging this rich data source, our model accurately classifies human activities in a given environment.
## Key Features:
- **CSI Signal Processing:** Preprocessing involves filtering the CSI data using a Butterworth filter to enhance signal quality and remove noise.
- **Deep Learning Architecture:** The model architecture incorporates Bidirectional Long Short-Term Memory (BiLSTM) networks, known for their ability to capture temporal dependencies in sequential data, making them ideal for HAR tasks. The BiLSTM layers consist of 200 units each.
- **Attention Mechanism:** An attention mechanism with 400 hidden units is employed to focus on relevant parts of the input sequence during model training.
- **Real-time Inference:** Efficient algorithms for real-time inference on streaming CSI data enable quick and accurate activity recognition.
- **Confusion Matrix for Accuracy:** Model accuracy can be obtained using the provided confusion matrix, allowing users to assess the performance across different activity classes.
- **Optimizer and Learning Rate:** The model is trained using RMSprop optimizer with a learning rate of 1e-4.
- **Training Epochs:** The model is trained for 50 epochs to ensure convergence and optimal performance.
- **Robustness:** Designed to handle variations in Wi-Fi environment and user movements.
## How to Use:
1. **Data Collection:** Gather Wi-Fi CSI data using compatible hardware and software tools.
2. **Data Preprocessing:** Apply a Butterworth filter to clean and enhance the collected CSI data before feeding it into the model.
3. **Model Training:** Utilize the provided scripts to train the HAR model, employing BiLSTM networks with attention mechanisms and specified parameters.
4. **Evaluation:** Evaluate model performance using standard metrics, including the confusion matrix, and test datasets to ensure accuracy and robustness.
5. **Deployment:** Integrate the trained model into your application for real-world activity recognition tasks.
## Requirements:
- Python
- TensorFlow
- NumPy, Pandas, Scikit-learn |
package com.example.api.factories;
import com.example.api.dto.OwnerCarDto;
import com.example.store.model.OwnerCarEntity;
import org.springframework.stereotype.Component;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
@Component
public class OwnerCarDtoFactory {
public OwnerCarDto makeOwnerCarDto(OwnerCarEntity ownerCar) {
return OwnerCarDto.builder()
.id(ownerCar.getId())
.address(ownerCar.getAddress())
.firstName(ownerCar.getFirstName())
.middleName(ownerCar.getMiddleName())
.secondName(ownerCar.getSecondName())
.build();
}
public List<OwnerCarDto> makeOwnersCarDto(List<OwnerCarEntity> ownersCar){
return ownersCar.stream().map(this::makeOwnerCarDto).collect(Collectors.toList());
}
} |
package com.pollub.lab_6.controllers;
import com.pollub.lab_6.configuration.UserAuthenticationDetails;
import com.pollub.lab_6.dao.UserDao;
import com.pollub.lab_6.entities.User;
import jakarta.validation.Valid;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import java.security.Principal;
@Controller
public class UserController {
@Autowired
private PasswordEncoder passwordEncoder;
@Autowired
private UserAuthenticationDetails userAuthenticationDetails;
@Autowired
private UserDao dao;
@GetMapping("/login")
public String loginPage() {
// zwrócenie nazwy widoku logowania — login.html
return "login";
}
@GetMapping("/register")
public String registerPage(Model m) {
// dodanie do modelu nowego użytkownika
m.addAttribute("user", new User());
// zwrócenie nazwy widoku rejestracji — register.html
return "register";
}
@PostMapping("/register")
public String registerPagePOST(@Valid User user, BindingResult binding) {
if (binding.hasErrors()) {
return "register"; // powrót do rejestracji
}
User existingUser = dao.findByLogin(user.getLogin());
if (existingUser != null) {
binding.rejectValue("login", "error.user", "User with that login already exists");
return "register";
}
user.setPassword(passwordEncoder.encode(user.getPassword()));
dao.save(user);
return "redirect:/login";
}
@GetMapping("/profile")
public String profilePage(Model m, Principal principal) {
// dodanie do modelu aktualnie zalogowanego użytkownika:
m.addAttribute("user", dao.findByLogin(principal.getName()));
// zwrócenie nazwy widoku profilu użytkownika — profile.html
return "profile";
}
@GetMapping("/users")
public String usersPage(Model m) {
// dodanie do modelu listy użytkowników
m.addAttribute("users", dao.findAll());
// zwrócenie nazwy widoku users.html
return "users";
}
@GetMapping("/users/current/edit")
public String editCurrentUser(Model m, Principal principal) {
User user = dao.findByLogin(principal.getName());
m.addAttribute("user", user);
return "user_edit";
}
@PostMapping("/users/current/edit")
public String updateCurrentUser(@Valid User user, BindingResult bindingResult, Principal principal) {
if (bindingResult.hasErrors()) {
return "user_edit"; // Return the view if there are validation errors
}
User currentUser = dao.findByLogin(principal.getName());
User existingUser = dao.findByLogin(user.getLogin());
if (existingUser != null && !existingUser.getId().equals(currentUser.getId()) && existingUser.getLogin().equals(user.getLogin())) {
bindingResult.rejectValue("login", "error.user", "Login already exists");
return "user_edit";
}
currentUser.setName(user.getName());
currentUser.setSurname(user.getSurname());
currentUser.setLogin(user.getLogin());
currentUser.setPassword(passwordEncoder.encode(user.getPassword()));
dao.save(currentUser);
var userDetails = userAuthenticationDetails.loadUserByUsername(currentUser.getLogin());
Authentication authentication = new UsernamePasswordAuthenticationToken(
userDetails,
userDetails.getPassword(),
userDetails.getAuthorities()
);
SecurityContextHolder.getContext().setAuthentication(authentication);
return "redirect:/profile";
}
@GetMapping("/users/current/delete")
public String deleteCurrentUser(Principal principal) {
User user = dao.findByLogin(principal.getName());
dao.delete(user);
return "redirect:/logout";
}
} |
{% extends 'landing/base.html' %}
{% load crispy_forms_tags %}
{% block content %}
{% if user.is_authenticated %}
<div class="container">
<div class="row justify-content-center mt3">
<div class="col-md-5 col-sm-12 border-bottom">
<a href="{% url 'templates' %}" class="btn btn-dark">Draw</a>
</div>
</div>
<div class="row justify-content-center mt-3 mb-5">
<div class="col-md-5 col-sm-12">
<form method="POST" enctype="multipart/form-data">
{% csrf_token %}
{{ form | crispy }}
<div class="d-grid gap-2">
<button class="btn btn-success mt-3">Submit</button></div>
</form>
{% endif %}
</div>
</div>
</div>
{% for post in post_list %}
<div class="row justify-content-center mt-3">
<div class="col-md-5 col-sm-12 border-bottom <position-relative>">
<div>
<a href="{% url 'profile' post.author.profile.pk %}">
<img class="round-circle post-img" height="30" width="30" src="{{ post.author.profile.picture.url }}"/>
</a>
<p class="post-text">
<a class="text-primary post-link" href="{% url 'profile' post.author.profile.pk %}">
</p>
</div>
</div>
</div>
</div>
<div class="row justify-content-center mt-3">
<p><a style="text-decoration: none;" class="text-primary"
href="{% url 'profile' post.author.profile.pk %}">@{{ post.author }}</a>
{{ post.created_on }}</p>
<div class="position-relative">
<p{{post.comic}}></p>
<img src = "{{ post.comic.url }}" class = "post-comic"/>
<p>{{ post.body }}</p>
<a class="stretched-link" href="{% url 'post-detail' post.pk %}"></a>
</div>
<div class="position-relative">
<div class = "d-flex flex-row"><form method = "POST" action = "{% url 'like' post.pk %}">
{% csrf_token %}
<input type = "hidden" name = "next" value = "{{ request.path }}">
<button class="remove-default-btn" type="submit"><i class = "far fa-thumbs-up"> <span>{{ post.likes.all.count }}</span></i></button>
</form>
<form method="POST" action="{% url 'dislike' post.pk %}">
{% csrf_token %}
<input type="hidden" name="next" value="{{ request.path }}">
<button class="remove-default-btn" type="submit"><i class="far fa-thumbs-down"> <span>{{ post.dislikes.all.count }}</span></i></button>
</form>
</div>
</div>
</div>
</div>
<div>
</div>
{% endfor %}
<p></p><p></p><p></p>
{% endblock content %} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.